branch_name
stringclasses 22
values | content
stringlengths 18
81.8M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
36
| num_files
int64 1
7.38k
| repo_language
stringclasses 151
values | repo_name
stringlengths 7
101
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
|
---|---|---|---|---|---|---|---|---|
refs/heads/master | <file_sep>module Objecthash.JsonTest exposing (..)
import Dict
import Expect exposing (Expectation)
import Objecthash.Json exposing (..)
import Objecthash.Value exposing (Value(..))
import Test exposing (..)
suite : Test
suite =
describe "Json decoder"
[ test "null" <| \() -> testNull
, test "bool" <| \() -> testBool
, test "int" <| \() -> testInt
, test "float" <| \() -> testFloat
, test "string" <| \() -> testString
, test "list" <| \() -> testList
, test "set" <| \() -> testSet
, test "dict" <| \() -> testDict
]
testNull : Expectation
testNull =
Expect.equal (decodeWith null "null") (Ok VNull)
testBool : Expectation
testBool =
Expect.equal
[ decodeWith bool "true", decodeWith bool "false" ]
[ Ok (VBool True), Ok (VBool False) ]
testInt : Expectation
testInt =
Expect.equal
[ decodeWith int "1"
, decodeWith int "42"
, decodeWith int "0"
]
[ Ok (VInteger 1)
, Ok (VInteger 42)
, Ok (VInteger 0)
]
testFloat : Expectation
testFloat =
Expect.equal
[ decodeWith float "1.0"
, decodeWith float "42.0"
, decodeWith float "0.0"
, decodeWith float "-0.0"
]
[ Ok (VFloat 1.0)
, Ok (VFloat 42.0)
, Ok (VFloat 0.0)
, Ok (VFloat 0.0)
]
testString : Expectation
testString =
Expect.equal
[ decodeWith string "\"foo\""
, decodeWith string "\"42\""
]
[ Ok (VString "foo")
, Ok (VString "42")
]
testList : Expectation
testList =
Expect.equal
[ decodeWith list """["foo"]"""
, decodeWith list """[42.1, "foo"]"""
]
[ Ok (VList [ VString "foo" ])
, Ok (VList [ VFloat 42.1, VString "foo" ])
]
testSet : Expectation
testSet =
Expect.equal
[ decodeWith set """["foo"]"""
, decodeWith set """[42.0, "foo"]"""
, decodeWith set """["foo", "foo"]"""
]
[ Ok (VSet [ VString "foo" ])
, Ok (VSet [ VFloat 42.0, VString "foo" ])
, Ok (VSet [ VString "foo" ])
]
testDict : Expectation
testDict =
Expect.equal
[ decodeWith dict """{"foo": "bar"}"""
, decodeWith dict """{"x": 42.0}"""
]
[ Ok (VDict <| Dict.fromList [ ( "foo", VString "bar" ) ])
, Ok (VDict <| Dict.fromList [ ( "x", VFloat 42.0 ) ])
]
<file_sep>module Crypto.SHA.Constants exposing (initialHashValues, roundConstants)
import Crypto.SHA.Alg exposing (Alg(..))
import Crypto.SHA.Types exposing (RoundConstants, WorkingVars)
import Word exposing (Word(..))
initialHashValues : Alg -> WorkingVars
initialHashValues alg =
case alg of
SHA224 ->
WorkingVars
(W 0xC1059ED8)
(W 0x367CD507)
(W 0x3070DD17)
(W 0xF70E5939)
(W 0xFFC00B31)
(W 0x68581511)
(W 0x64F98FA7)
(W 0xBEFA4FA4)
SHA256 ->
WorkingVars
(W 0x6A09E667)
(W 0xBB67AE85)
(W 0x3C6EF372)
(W 0xA54FF53A)
(W 0x510E527F)
(W 0x9B05688C)
(W 0x1F83D9AB)
(W 0x5BE0CD19)
SHA384 ->
WorkingVars
(D 0xCBBB9D5D 0xC1059ED8)
(D 0x629A292A 0x367CD507)
(D 0x9159015A 0x3070DD17)
(D 0x152FECD8 0xF70E5939)
(D 0x67332667 0xFFC00B31)
(D 0x8EB44A87 0x68581511)
(D 0xDB0C2E0D 0x64F98FA7)
(D 0x47B5481D 0xBEFA4FA4)
SHA512 ->
WorkingVars
(D 0x6A09E667 0xF3BCC908)
(D 0xBB67AE85 0x84CAA73B)
(D 0x3C6EF372 0xFE94F82B)
(D 0xA54FF53A 0x5F1D36F1)
(D 0x510E527F 0xADE682D1)
(D 0x9B05688C 0x2B3E6C1F)
(D 0x1F83D9AB 0xFB41BD6B)
(D 0x5BE0CD19 0x137E2179)
SHA512_224 ->
WorkingVars
-- http://nvlpubs.nist.gov/nistpubs/FIPS/NIST.FIPS.180-4.pdf
-- Section 5.3.6.1
(D 0x8C3D37C8 0x19544DA2)
(D 0x73E19966 0x89DCD4D6)
(D 0x1DFAB7AE 0x32FF9C82)
(D 0x679DD514 0x582F9FCF)
(D 0x0F6D2B69 0x7BD44DA8)
(D 0x77E36F73 0x04C48942)
(D 0x3F9D85A8 0x6A1D36C8)
(D 0x1112E6AD 0x91D692A1)
SHA512_256 ->
WorkingVars
-- http://nvlpubs.nist.gov/nistpubs/FIPS/NIST.FIPS.180-4.pdf
-- Section 5.3.6.2
(D 0x22312194 0xFC2BF72C)
(D 0x9F555FA3 0xC84C64C2)
(D 0x2393B86B 0x6F53B151)
(D 0x96387719 0x5940EABD)
(D 0x96283EE2 0xA88EFFE3)
(D 0xBE5E1E25 0x53863992)
(D 0x2B0199FC 0x2C85B8AA)
(D 0x0EB72DDC 0x81C52CA2)
roundConstants : Alg -> RoundConstants
roundConstants alg =
case alg of
SHA224 ->
roundConstants SHA256
SHA256 ->
[ W 0x428A2F98
, W 0x71374491
, W 0xB5C0FBCF
, W 0xE9B5DBA5
, W 0x3956C25B
, W 0x59F111F1
, W 0x923F82A4
, W 0xAB1C5ED5
, W 0xD807AA98
, W 0x12835B01
, W 0x243185BE
, W 0x550C7DC3
, W 0x72BE5D74
, W 0x80DEB1FE
, W 0x9BDC06A7
, W 0xC19BF174
, W 0xE49B69C1
, W 0xEFBE4786
, W 0x0FC19DC6
, W 0x240CA1CC
, W 0x2DE92C6F
, W 0x4A7484AA
, W 0x5CB0A9DC
, W 0x76F988DA
, W 0x983E5152
, W 0xA831C66D
, W 0xB00327C8
, W 0xBF597FC7
, W 0xC6E00BF3
, W 0xD5A79147
, W 0x06CA6351
, W 0x14292967
, W 0x27B70A85
, W 0x2E1B2138
, W 0x4D2C6DFC
, W 0x53380D13
, W 0x650A7354
, W 0x766A0ABB
, W 0x81C2C92E
, W 0x92722C85
, W 0xA2BFE8A1
, W 0xA81A664B
, W 0xC24B8B70
, W 0xC76C51A3
, W 0xD192E819
, W 0xD6990624
, W 0xF40E3585
, W 0x106AA070
, W 0x19A4C116
, W 0x1E376C08
, W 0x2748774C
, W 0x34B0BCB5
, W 0x391C0CB3
, W 0x4ED8AA4A
, W 0x5B9CCA4F
, W 0x682E6FF3
, W 0x748F82EE
, W 0x78A5636F
, W 0x84C87814
, W 0x8CC70208
, W 0x90BEFFFA
, W 0xA4506CEB
, W 0xBEF9A3F7
, W 0xC67178F2
]
SHA384 ->
roundConstants SHA512
SHA512 ->
[ D 0x428A2F98 0xD728AE22
, D 0x71374491 0x23EF65CD
, D 0xB5C0FBCF 0xEC4D3B2F
, D 0xE9B5DBA5 0x8189DBBC
, D 0x3956C25B 0xF348B538
, D 0x59F111F1 0xB605D019
, D 0x923F82A4 0xAF194F9B
, D 0xAB1C5ED5 0xDA6D8118
, D 0xD807AA98 0xA3030242
, D 0x12835B01 0x45706FBE
, D 0x243185BE 0x4EE4B28C
, D 0x550C7DC3 0xD5FFB4E2
, D 0x72BE5D74 0xF27B896F
, D 0x80DEB1FE 0x3B1696B1
, D 0x9BDC06A7 0x25C71235
, D 0xC19BF174 0xCF692694
, D 0xE49B69C1 0x9EF14AD2
, D 0xEFBE4786 0x384F25E3
, D 0x0FC19DC6 0x8B8CD5B5
, D 0x240CA1CC 0x77AC9C65
, D 0x2DE92C6F 0x592B0275
, D 0x4A7484AA 0x6EA6E483
, D 0x5CB0A9DC 0xBD41FBD4
, D 0x76F988DA 0x831153B5
, D 0x983E5152 0xEE66DFAB
, D 0xA831C66D 0x2DB43210
, D 0xB00327C8 0x98FB213F
, D 0xBF597FC7 0xBEEF0EE4
, D 0xC6E00BF3 0x3DA88FC2
, D 0xD5A79147 0x930AA725
, D 0x06CA6351 0xE003826F
, D 0x14292967 0x0A0E6E70
, D 0x27B70A85 0x46D22FFC
, D 0x2E1B2138 0x5C26C926
, D 0x4D2C6DFC 0x5AC42AED
, D 0x53380D13 0x9D95B3DF
, D 0x650A7354 0x8BAF63DE
, D 0x766A0ABB 0x3C77B2A8
, D 0x81C2C92E 0x47EDAEE6
, D 0x92722C85 0x1482353B
, D 0xA2BFE8A1 0x4CF10364
, D 0xA81A664B 0xBC423001
, D 0xC24B8B70 0xD0F89791
, D 0xC76C51A3 0x0654BE30
, D 0xD192E819 0xD6EF5218
, D 0xD6990624 0x5565A910
, D 0xF40E3585 0x5771202A
, D 0x106AA070 0x32BBD1B8
, D 0x19A4C116 0xB8D2D0C8
, D 0x1E376C08 0x5141AB53
, D 0x2748774C 0xDF8EEB99
, D 0x34B0BCB5 0xE19B48A8
, D 0x391C0CB3 0xC5C95A63
, D 0x4ED8AA4A 0xE3418ACB
, D 0x5B9CCA4F 0x7763E373
, D 0x682E6FF3 0xD6B2B8A3
, D 0x748F82EE 0x5DEFB2FC
, D 0x78A5636F 0x43172F60
, D 0x84C87814 0xA1F0AB72
, D 0x8CC70208 0x1A6439EC
, D 0x90BEFFFA 0x23631E28
, D 0xA4506CEB 0xDE82BDE9
, D 0xBEF9A3F7 0xB2C67915
, D 0xC67178F2 0xE372532B
, D 0xCA273ECE 0xEA26619C
, D 0xD186B8C7 0x21C0C207
, D 0xEADA7DD6 0xCDE0EB1E
, D 0xF57D4F7F 0xEE6ED178
, D 0x06F067AA 0x72176FBA
, D 0x0A637DC5 0xA2C898A6
, D 0x113F9804 0xBEF90DAE
, D 0x1B710B35 0x131C471B
, D 0x28DB77F5 0x23047D84
, D 0x32CAAB7B 0x40C72493
, D 0x3C9EBE0A 0x15C9BEBC
, D 0x431D67C4 0x9C100D4C
, D 0x4CC5D4BE 0xCB3E42B6
, D 0x597F299C 0xFC657E2A
, D 0x5FCB6FAB 0x3AD6FAEC
, D 0x6C44198C 0x4A475817
]
SHA512_224 ->
roundConstants SHA512
SHA512_256 ->
roundConstants SHA512
<file_sep>module Crypto.SHA exposing (digest)
import Array exposing (Array)
import Crypto.SHA.Alg as Alg exposing (Alg(..))
import Crypto.SHA.Preprocess
import Crypto.SHA.Process
import Word exposing (Word)
digest : Alg -> List Int -> Array Word
digest alg =
Crypto.SHA.Preprocess.preprocess alg
>> Word.fromBytes (Alg.wordSize alg)
>> Crypto.SHA.Process.chunks alg
<file_sep>module Crypto.SHA.Types exposing
( RoundConstants
, WorkingVars
, addWorkingVars
, workingVarsToWords
)
import Array exposing (Array)
import Crypto.SHA.Alg exposing (Alg(..))
import Word exposing (Word(..))
type alias RoundConstants =
List Word
type alias WorkingVars =
{ a : Word
, b : Word
, c : Word
, d : Word
, e : Word
, f : Word
, g : Word
, h : Word
}
addWorkingVars : WorkingVars -> WorkingVars -> WorkingVars
addWorkingVars x y =
WorkingVars
(Word.add x.a y.a)
(Word.add x.b y.b)
(Word.add x.c y.c)
(Word.add x.d y.d)
(Word.add x.e y.e)
(Word.add x.f y.f)
(Word.add x.g y.g)
(Word.add x.h y.h)
workingVarsToWords : Alg -> WorkingVars -> Array Word
workingVarsToWords alg { a, b, c, d, e, f, g, h } =
case alg of
SHA224 ->
Array.fromList [ a, b, c, d, e, f, g ]
SHA256 ->
Array.fromList [ a, b, c, d, e, f, g, h ]
SHA384 ->
Array.fromList [ a, b, c, d, e, f ]
SHA512 ->
Array.fromList [ a, b, c, d, e, f, g, h ]
SHA512_224 ->
[ a, b, c, d ]
|> List.concatMap toSingleWord
|> List.take 7
|> Array.fromList
SHA512_256 ->
Array.fromList [ a, b, c, d ]
toSingleWord : Word -> List Word
toSingleWord word =
case word of
D xh xl ->
[ W xh, W xl ]
_ ->
[ word ]
<file_sep>module Objecthash exposing (objecthash, fromJson)
{-| Objecthash implementation.
Based on the implementation from <NAME> <https://github.com/benlaurie/objecthash>
@docs objecthash, fromJson
-}
import Json.Decode as Decode
import Objecthash.Hash exposing (bytes, toHex)
import Objecthash.Json as Json
import Objecthash.Value exposing (Value(..))
{-| Hash an `Objecthash.Value` and return it as a hexadecimal string
representation.
If you need more control over the hash, `Objecthash.Hash` offers a range of
functions to operate on elm values and lists of bytes.
-}
objecthash : Value -> String
objecthash value =
(toHex << bytes) value
{-| Hash a Common JSON blob and return it as a hexadecimal string
representation.
If you need more control over the JSON decoder, check the `Objecthash.Json`
module.
-}
fromJson : String -> Result Decode.Error String
fromJson input =
input
|> Json.decode
|> Result.map objecthash
<file_sep>module Objecthash.Value exposing
( Value(..)
, toJsonValue
, null, list, int, float, string, dict, bool
)
{-| Value operations.
@docs Value
@docs toJsonValue
@docs null, list, int, float, string, dict, bool
-}
import Dict exposing (Dict)
import Json.Encode as Json
{-| Objecthash Value.
-}
type Value
= VBool Bool
| VDict (Dict String Value)
| VFloat Float
| VInteger Int
| VList (List Value)
| VNull
| VSet (List Value)
| VString String
-- {-| -}
-- toString : Value -> String
-- toString value =
-- case value of
-- VBool inner ->
-- case inner of
-- True ->
-- "true"
-- False ->
-- "false"
-- VDict inner ->
-- inner
-- |> Dict.toList
-- |> List.map (\( key, val ) -> ( key, toString val ))
-- |> Debug.toString
-- VFloat inner ->
-- String.fromFloat inner
-- VInteger inner ->
-- String.fromInt inner
-- VList inner ->
-- Debug.toString (List.map toString inner)
-- VNull ->
-- ""
-- VSet inner ->
-- Debug.toString (List.map toString inner)
-- VString inner ->
-- inner
{-| -}
toJsonValue : Value -> Json.Value
toJsonValue value =
case value of
VBool inner ->
Json.bool inner
VDict inner ->
inner
|> Dict.toList
|> List.map (\( key, val ) -> ( key, toJsonValue val ))
|> Json.object
VFloat inner ->
Json.float inner
VInteger inner ->
Json.int inner
VList inner ->
Json.list toJsonValue inner
VNull ->
Json.null
VSet inner ->
Json.list toJsonValue inner
VString inner ->
Json.string inner
{-| -}
bool : Bool -> Value
bool value =
VBool value
{-| -}
dict : Dict String Value -> Value
dict value =
VDict value
{-| -}
float : Float -> Value
float value =
VFloat value
{-| -}
int : Int -> Value
int value =
VInteger value
{-| -}
list : List Value -> Value
list value =
VList value
{-| -}
null : Value
null =
VNull
{-| -}
set : List Value -> Value
set value =
VSet value
{-| -}
string : String -> Value
string value =
VString value
<file_sep># Objecthash in Elm
Implementation of [<NAME>'s
objecthash](https://github.com/benlaurie/objecthash) in Elm.
## Usage
There are a few interfaces available. The main module offers two functions
`objecthash` and `fromJson`. The former expects an `Objecthash.Value` and the
latter a JSON string.
```elm
import Objecthash exposing (fromJson, objecthash)
import Objecthash.Value exposing (Value(..))
fromJson """{"k1":"v1","k2":"v2","k3":"v3"}"""
-- Ok "ddd65f1f7568269a30df7cafc26044537dc2f02a1a0d830da61762fc3e687057"
objecthash <| VSet [VInteger 1, VInteger 2]
-- "ee104c03e5465735a9fb3fa5d0f19199297a135486fa76930c69cec825f8dac8"
```
The `Objecthash.Json` module exposes a set of functions to decode JSON built
on top of the `Json.Decode` from core. For example, the `Objecthash.fromJson`
mentioned above is implemented as:
```elm
import Objecthash.Json exposing (decode)
fromJson : String -> Result String String
fromJson input =
input
|> decode
|> Result.map objecthash
```
The function `Json.decode` is a combined decoder that assumes all arrays in
the JSON blob are lists and all numbers floats. You can use your own decoder
with the `Json.decodeWith` function.
Note that `Json.decode` aligns with other objecthash “Common JSON”
implementations. This is the reason for having numbers as floats.
Finally, the `Objecthash.Hash` module exposes a set of functions to operate on
Elm values and return the hashed bytes.
```elm
import Dict
import Objecthash.Hash exposing (dict, unicode, toHex)
toHex <| dict (Dict.fromList [ ( "foo", unicode "bar" ) ])
-- "7ef5237c3027d6c58100afadf37796b3d351025cf28038280147d42fdc53b960"
```
## Redaction
This implementation offers a similar mechanism for redaction as the one
offered by [<NAME>'s Python implementation](https://github.com/benlaurie/objecthash/blob/master/objecthash.py).
In short, objecthash lets you redact parts of a data structure and keep the
final hash intact. To do that, is represents redacted parts as tagged strings
of the form `**REDACTED***{hexadecimal representation of the hashed value}`.
For example, given the JSON blob:
```elm
fromJson """{"foo": {"x": 1}, "bar": {"x": 2}}"""
Ok "56b425f5e640238f9481dbf227d3b3aa023905b91e9941e6c987e56bd37ec6a3"
```
And the fact that `{"x": 1}` has the hash `480499ec4efe0e177793c217c8227d4096d2352beee2d6816ba8f4e8a421a138`:
```elm
fromJson """{"x": 1}"""
Ok "480499ec4efe0e177793c217c8227d4096d2352beee2d6816ba8f4e8a421a138"
```
You can redact the original value for the `foo` key as follows:
```elm
fromJson """{"foo": "**REDACTED**480499ec4efe0e177793c217c8227d4096d2352beee2d6816ba8f4e8a421a138", "bar": {"x
": 2}}"""
Ok "56b425f5e640238f9481dbf227d3b3aa023905b91e9941e6c987e56bd37ec6a3"
```
And still get the same result.
## Contribute
To run a clone of this project locally, you need
[Yarn](https://yarnpkg.com/) (and nodejs):
```sh
yarn install
```
To run the test suite:
```sh
yarn test
```
Note that the elm tooling is installed local to the project so as long as you
use commands via `yarn` you should be fine.
## Notice
This library inlines [elm-crypto](https://github.com/ktonon/elm-crypto) to
address the need for composing a sha256 hash from a list of words rather than
from a string. This is the corresponding license:
Copyright (c) 2017 <NAME> <<EMAIL>>
Licensed under the MIT license <LICENSE or http://opensource.org/licenses/MIT>,
at your option. This file may not be copied, modified, or distributed except
according to those terms.
## License
Copyright (c) 2018-2019 elm-objecthash contributors licensed under [BSD-3-clause](LICENSE).
<file_sep>module Objecthash.Json exposing
( decode, decodeWith
, dict, list, bool, float, int, null, set, string
)
{-| Helper functions to transform JSON to the Objecthash values.
@docs decode, decodeWith
# Decoders
@docs dict, list, bool, float, int, null, set, string
-}
import Json.Decode as Decode exposing (Decoder, decodeString)
import Objecthash.Value exposing (Value(..))
{-| Expects a JSON string and returns the result of attempting to decode it
into an AST of `Objecthash.Value`.
-}
decode : String -> Result Decode.Error Value
decode input =
decodeString decoder input
{-| Decodes a JSON string with the given decoder.
-}
decodeWith : Decoder Value -> String -> Result Decode.Error Value
decodeWith decoder_ input =
decodeString decoder_ input
{-| Default decoder. Common JSON.
TODO: Handle raw values (e.g. `VRaw`)
-}
decoder : Decoder Value
decoder =
Decode.oneOf
[ Decode.lazy (\() -> dict)
, Decode.lazy (\() -> list)
, bool
, null
, string
-- , int
, float
]
{-| TODO: Parametrise internal decoder
-}
dict : Decoder Value
dict =
Decode.lazy (\() -> decoder)
|> Decode.dict
|> Decode.map VDict
{-| TODO: Parametrise internal decoder
-}
list : Decoder Value
list =
Decode.lazy (\() -> decoder)
|> Decode.list
|> Decode.map VList
{-| TODO: Parametrise internal decoder
-}
set : Decoder Value
set =
Decode.lazy (\() -> decoder)
|> Decode.list
|> Decode.map collectSet
collectSet : List Value -> Value
collectSet xs =
VSet
(xs
|> List.foldr
(\x xs_ ->
if List.member x xs_ then
xs_
else
x :: xs_
)
[]
)
{-| -}
string : Decoder Value
string =
Decode.map VString Decode.string
{-| -}
float : Decoder Value
float =
Decode.map VFloat Decode.float
{-| -}
int : Decoder Value
int =
Decode.map VInteger Decode.int
{-| -}
bool : Decoder Value
bool =
Decode.map VBool Decode.bool
{-| -}
null : Decoder Value
null =
Decode.null VNull
<file_sep>module Objecthash.ObjecthashTest exposing (..)
import Dict
import Expect exposing (Expectation)
import Objecthash exposing (fromJson, objecthash)
import Objecthash.Value exposing (Value(..))
import Test exposing (..)
suite : Test
suite =
describe "Objecthash"
[ test "null" <| \() -> testNull
, test "dict of lists" <| \() -> testListDict
, test "dict ordering" <| \() -> testDictOrder
, test "mix" <| \() -> testMix
, describe "redaction"
[ test "redacted list" <| \() -> testRedactedList
, test "redacted mix" <| \() -> testRedactedMix
]
, describe "sets"
[ test "set" <| \() -> testSet
, test "complex set" <| \() -> testComplexSet
, test "complex set repeated" <| \() -> testComplexSetRepeated
]
, describe "fromJson"
[ test "string list" <| \() -> testJsonStringList
, test "float and int" <| \() -> testJsonFloatAndInt
, test "key change" <| \() -> testJsonKeyChange
, test "key order" <| \() -> testJsonKeyOrderIndependence
, test "redaction" <| \() -> testRedaction
-- , test "unicode normalisation" <| \() -> testJsonUnicodeNormalisation
]
]
testNull : Expectation
testNull =
Expect.equal (objecthash VNull) "1b16b1df538ba12dc3f97edbb85caa7050d46c148134290feba80f8236c83db9"
testListDict : Expectation
testListDict =
let
dict =
Dict.fromList
[ ( "foo", VList [ VString "bar", VString "baz" ] )
, ( "qux", VList [ VString "norf" ] )
]
in
Expect.equal (objecthash (VDict dict)) "f1a9389f27558538a064f3cc250f8686a0cebb85f1cab7f4d4dcc416ceda3c92"
testDictOrder : Expectation
testDictOrder =
let
dict1 =
Dict.fromList
[ ( "k1", VString "v1" )
, ( "k2", VString "v2" )
, ( "k3", VString "v3" )
]
dict2 =
Dict.fromList
[ ( "k2", VString "v2" )
, ( "k1", VString "v1" )
, ( "k3", VString "v3" )
]
in
Expect.equal (objecthash (VDict dict1)) (objecthash (VDict dict2))
testMix : Expectation
testMix =
let
raw =
VList
[ VString "foo"
, VDict
(Dict.fromList
[ ( "bar"
, VList
[ VString "baz"
, VNull
, VFloat 1.0
, VFloat 1.5
, VFloat 0.0001
, VFloat 1000.0
, VFloat 2.0
, VFloat -23.1234
, VFloat 2.0
]
)
]
)
]
in
Expect.equal (objecthash raw) "783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213"
testRedactedList : Expectation
testRedactedList =
Expect.equal
(objecthash <|
VList
[ VString "foo"
, VString "**REDACTED**96e2aab962831956c80b542f056454be411f870055d37805feb3007c855bd823"
]
)
"783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213"
testRedactedMix : Expectation
testRedactedMix =
Expect.equal
(objecthash <|
VList
[ VString "foo"
, VDict <|
Dict.fromList
[ ( "bar"
, VList
[ VString "**REDACTED**82f70430fa7b78951b3c4634d228756a165634df977aa1fada051d6828e78f30"
, VNull
, VFloat 1.0
, VFloat 1.5
, VString "**REDACTED**1195afc7f0b70bb9d7960c3615668e072a1cbfbbb001f84871fd2e222a87be1d"
, VFloat 1000.0
, VFloat 2.0
, VFloat -23.1234
, VFloat 2.0
]
)
]
]
)
"783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213"
testSet : Expectation
testSet =
Expect.equal
(objecthash <|
VDict <|
Dict.fromList
[ ( "thing1"
, VDict <|
Dict.fromList
[ ( "thing2"
, VSet
[ VInteger 1
, VInteger 2
, VString "s"
]
)
]
)
, ( "thing3", VFloat 1234.567 )
]
)
"618cf0582d2e716a70e99c2f3079d74892fec335e3982eb926835967cb0c246c"
testComplexSet : Expectation
testComplexSet =
Expect.equal
(objecthash <|
VSet
[ VString "foo"
, VFloat 23.6
, VSet [ VSet [] ]
, VSet [ VSet [ VInteger 1 ] ]
]
)
"3773b0a5283f91243a304d2bb0adb653564573bc5301aa8bb63156266ea5d398"
testComplexSetRepeated : Expectation
testComplexSetRepeated =
Expect.equal
(objecthash <|
VSet
[ VString "foo"
, VFloat 23.6
, VSet [ VSet [] ]
, VSet [ VSet [ VInteger 1 ] ]
, VSet [ VSet [] ]
]
)
"3773b0a5283f91243a304d2bb0adb653564573bc5301aa8bb63156266ea5d398"
-- fromJson
testJsonStringList : Expectation
testJsonStringList =
Expect.equal
(fromJson """["foo", "bar"]""")
(Ok "32ae896c413cfdc79eec68be9139c86ded8b279238467c216cf2bec4d5f1e4a2")
testJsonFloatAndInt : Expectation
testJsonFloatAndInt =
Expect.equal
[ fromJson """["foo", {"bar":["baz", null, 1.0, 1.5, 0.0001, 1000.0, 2.0, -23.1234, 2.0]}]"""
, fromJson """["foo", {"bar":["baz", null, 1, 1.5, 0.0001, 1000, 2, -23.1234, 2]}]"""
]
[ Ok "783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213"
, Ok "783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213"
]
testJsonKeyChange : Expectation
testJsonKeyChange =
Expect.equal
(fromJson """["foo", {"b4r":["baz", null, 1, 1.5, 0.0001, 1000, 2, -23.1234, 2]}]""")
(Ok "7e01f8b45da35386e4f9531ff1678147a215b8d2b1d047e690fd9ade6151e431")
testJsonKeyOrderIndependence : Expectation
testJsonKeyOrderIndependence =
Expect.equal
[ fromJson """{"k1":"v1","k2":"v2","k3":"v3"}"""
, fromJson """{"k2":"v2","k1":"v1","k3":"v3"}"""
]
[ Ok "ddd65f1f7568269a30df7cafc26044537dc2f02a1a0d830da61762fc3e687057"
, Ok "ddd65f1f7568269a30df7cafc26044537dc2f02a1a0d830da61762fc3e687057"
]
{-| TODO: Review
-}
testJsonUnicodeNormalisation : Expectation
testJsonUnicodeNormalisation =
Expect.equal
[ fromJson "\"\\u03d3\""
, fromJson "\"\\u03d2\\u0301\""
]
[ Ok "f72826713a01881404f34975447bd6edcb8de40b191dc57097ebf4f5417a554d"
, Ok "f72826713a01881404f34975447bd6edcb8de40b191dc57097ebf4f5417a554d"
]
-- Redaction
testRedaction : Expectation
testRedaction =
Expect.equal
[ fromJson """["foo", "bar"]"""
, fromJson "\"bar\""
, fromJson """["foo", "**REDACTED**e303ce0bd0f4c1fdfe4cc1e837d7391241e2e047df10fa6101733dc120675dfe"]"""
]
[ Ok "32ae896c413cfdc79eec68be9139c86ded8b279238467c216cf2bec4d5f1e4a2"
, Ok "e303ce0bd0f4c1fdfe4cc1e837d7391241e2e047df10fa6101733dc120675dfe"
, Ok "32ae896c413cfdc79eec68be9139c86ded8b279238467c216cf2bec4d5f1e4a2"
]
-- def test_float_and_int(self):
-- self.verify_json('{"bar":["baz", null, 1.0, 1.5, 0.0001, 1000.0, 2.0, -23.1234, 2.0]}',
-- '96e2aab962831956c80b542f056454be411f870055d37805feb3007c855bd823')
-- self.verify_json('["foo", "**REDACTED**96e2aab962831956c80b542f056454be411f870055d37805feb3007c855bd823"]',
-- '783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213')
-- self.verify_json('["foo", {"bar":["baz", null, 1.0, 1.5, 0.0001, 1000.0, 2.0, -23.1234, 2.0]}]',
-- '783a423b094307bcb28d005bc2f026ff44204442ef3513585e7e73b66e3c2213')
-- self.verify_json('"baz"', '82f70430fa7b78951b3c4634d228756a165634df977aa1fada051d6828e78f30')
-- self.verify_json('0.0001', '1195afc7f0b70bb9d7960c3615668e072a1cbfbbb001f84871fd2e222a87be1d')
-- self.verify_json('["foo", {"bar": ["**REDACTED**82f70430fa7b78951b3c4634d228756a165634df977aa1fada051d6828e78f30", null, 1.0, 1.5, "**REDACTED**1195afc7f0b70bb9d7960c3615668e072a1cbfbbb001f84871fd2e222a87be1d", 1000.0, 2.0, -23.1234, 2.0]}]',
-- '<KEY>')
-- self.verify_json('"bar"', 'e303ce0bd0f4c1fdfe4cc1e837d7391241e2e047df10fa6101733dc120675dfe')
-- self.verify_json('["foo", {"**REDACTED**e303ce0bd0f4c1fdfe4cc1e837d7391241e2e047df10fa6101733dc120675dfe": ["baz", null, 1.0, 1.5, 0.0001, 1000.0, 2.0, -23.1234, 2.0]}]',
-- '<KEY>')
<file_sep>module Crypto.SHA.Process exposing (chunks)
{-| Chunk processing.
import Array
import Crypto.SHA.Types exposing (Alg(..))
-}
import Array exposing (Array)
import Crypto.SHA.Alg exposing (Alg(..))
import Crypto.SHA.Chunk as Chunk exposing (Chunk)
import Crypto.SHA.Constants exposing (initialHashValues, roundConstants)
import Crypto.SHA.MessageSchedule as MessageSchedule exposing (MessageSchedule)
import Crypto.SHA.Types exposing (..)
import Word exposing (Word)
{-| Process chunks producing an array of hashed words.
-}
chunks : Alg -> Array Word -> Array Word
chunks alg words =
chunks_ alg (Array.toList words) (initialHashValues alg)
|> workingVarsToWords alg
chunks_ : Alg -> List Word -> WorkingVars -> WorkingVars
chunks_ alg words currentHash =
case Chunk.next alg words of
( Nothing, _ ) ->
currentHash
( Just chunk, rest ) ->
let
vars =
chunk
|> MessageSchedule.fromChunk alg
|> compressLoop alg currentHash
|> addWorkingVars currentHash
in
chunks_ alg rest vars
compressLoop : Alg -> WorkingVars -> MessageSchedule -> WorkingVars
compressLoop alg workingVars messageSchedule =
List.foldl
(compress alg)
workingVars
(List.map2 (\a b -> ( a, b )) (roundConstants alg) (Array.toList messageSchedule))
{-| for i from 0 to 63
S1 := (e rightrotate 6) xor (e rightrotate 11) xor (e rightrotate 25)
ch := (e and f) xor ((not e) and g)
temp1 := h + S1 + ch + k[i] + w[i]
S0 := (a rightrotate 2) xor (a rightrotate 13) xor (a rightrotate 22)
maj := (a and b) xor (a and c) xor (b and c)
temp2 := S0 + maj
h := g
g := f
f := e
e := d + temp1
d := c
c := b
b := a
a := temp1 + temp2
-}
compress : Alg -> ( Word, Word ) -> WorkingVars -> WorkingVars
compress alg ( k, w ) { a, b, c, d, e, f, g, h } =
let
s1 =
-- S1 := (e rightrotate 6) xor (e rightrotate 11) xor (e rightrotate 25)
sum1 alg e
ch =
-- ch := (e and f) xor ((not e) and g)
Word.and e f
|> Word.xor (Word.and g <| Word.complement e)
temp1 =
-- temp1 := h + S1 + ch + k[i] + w[i]
h
|> Word.add s1
|> Word.add ch
|> Word.add k
|> Word.add w
s0 =
-- S0 := (a rightrotate 2) xor (a rightrotate 13) xor (a rightrotate 22)
sum0 alg a
maj =
-- maj := (a and b) xor (a and c) xor (b and c)
Word.and a b
|> Word.xor (Word.and a c)
|> Word.xor (Word.and b c)
temp2 =
-- temp2 := S0 + maj
Word.add s0 maj
in
-- h := g
-- g := f
-- f := e
-- e := d + temp1
-- d := c
-- c := b
-- b := a
-- a := temp1 + temp2
WorkingVars
(Word.add temp1 temp2)
a
b
c
(Word.add d temp1)
e
f
g
sum1 : Alg -> Word -> Word
sum1 alg word =
case alg of
SHA224 ->
sum1 SHA256 word
SHA384 ->
sum1 SHA512 word
SHA256 ->
Word.rotateRightBy 6 word
|> Word.xor (Word.rotateRightBy 11 word)
|> Word.xor (Word.rotateRightBy 25 word)
SHA512 ->
Word.rotateRightBy 14 word
|> Word.xor (Word.rotateRightBy 18 word)
|> Word.xor (Word.rotateRightBy 41 word)
SHA512_224 ->
sum1 SHA512 word
SHA512_256 ->
sum1 SHA512 word
sum0 : Alg -> Word -> Word
sum0 alg word =
case alg of
SHA224 ->
sum0 SHA256 word
SHA384 ->
sum0 SHA512 word
SHA256 ->
Word.rotateRightBy 2 word
|> Word.xor (Word.rotateRightBy 13 word)
|> Word.xor (Word.rotateRightBy 22 word)
SHA512 ->
Word.rotateRightBy 28 word
|> Word.xor (Word.rotateRightBy 34 word)
|> Word.xor (Word.rotateRightBy 39 word)
SHA512_224 ->
sum0 SHA512 word
SHA512_256 ->
sum0 SHA512 word
<file_sep>module Crypto.HMAC exposing
( digest, Key, Message
, digestBytes, KeyBytes, MessageBytes
, Hash, sha224, sha256, sha384, sha512, sha512_224, sha512_256
)
{-| Compute HMAC SHA message digests.
@docs digest, Key, Message
## Input and Output as Bytes
@docs digestBytes, KeyBytes, MessageBytes
## Hash Algorithms
@docs Hash, sha224, sha256, sha384, sha512, sha512_224, sha512_256
-}
import Array exposing (Array)
import Crypto.HMAC.Digest
import Crypto.SHA
import Crypto.SHA.Alg exposing (Alg(..))
import Word exposing (Word)
import Word.Bytes as Bytes
import Word.Hex as Hex
-- EXPOSED API
{-| Secret key
-}
type alias Key =
String
{-| Secret key, as a list of bytes.
You must ensure each `Int` is an 8-bit value.
-}
type alias KeyBytes =
List Int
{-| Message to be hashed
-}
type alias Message =
String
{-| Message to be hashed, as a list of bytes.
You must ensure each `Int` is an 8-bit value.
-}
type alias MessageBytes =
List Int
{-| Type of hash algorithm.
-}
type Hash
= SHA Crypto.SHA.Alg.Alg
{-| Use SHA224 as the hash algorithm.
-}
sha224 : Hash
sha224 =
SHA Crypto.SHA.Alg.SHA224
{-| Use SHA256 as the hash algorithm.
-}
sha256 : Hash
sha256 =
SHA Crypto.SHA.Alg.SHA256
{-| Use SHA384 as the hash algorithm.
-}
sha384 : Hash
sha384 =
SHA Crypto.SHA.Alg.SHA384
{-| Use SHA512 as the hash algorithm.
-}
sha512 : Hash
sha512 =
SHA Crypto.SHA.Alg.SHA512
{-| Use SHA512/224 as the hash algorithm.
-}
sha512_224 : Hash
sha512_224 =
SHA Crypto.SHA.Alg.SHA512_224
{-| Use SHA512/256 as the hash algorithm.
-}
sha512_256 : Hash
sha512_256 =
SHA Crypto.SHA.Alg.SHA512_256
{-| HMAC digest using UTF-8 strings as input.
Outputs bytes encoded as a hexadecimal string. Prefer this function when your
key and message are UTF-8 encoded strings.
Crypto.HMAC.digest sha256 "key" "The quick brown fox jumps over the lazy dog"
--> "f7bc83f430538424b13298e6aa6fb143ef4d59a14946175997479dbc2d1a3cd8"
Crypto.HMAC.digest sha512 "key" "I ❤ cheese"
--> "a885c96140f95cb0b326306edfba49afbb5d38d3a7ed6ccfd67153429cbd3c56d0c514fcaa53b710bb7ba6cc0dfedfdb4d53795acbeb48eb23aa93e5ce9760dd"
-}
digest : Hash -> Key -> Message -> String
digest type_ key message =
Crypto.HMAC.Digest.digestBytes
(hash type_)
(blockSize type_)
(Bytes.fromUTF8 key)
(Bytes.fromUTF8 message)
|> Hex.fromWordArray
{-| HMAC digest using raw bytes as input and output.
Prefer `digest` when your key and message are UTF-8 strings. This function
(`digestBytes`) is unsafe, in that it does not ensure each `Int` fits into an
8-bit value.
Prefer `digestBytes` when you need to chain digests. That is, to use the output
of a digest as the input (either key or message) to another digest.
See the [AWS Signature V4 Example](http://docs.aws.amazon.com/general/latest/gr/signature-v4-test-suite.html#signature-v4-test-suite-example)
for an explanation of the following algorithm:
import Word.Bytes as Bytes
import Word.Hex as Hex
let
digest =
\message key ->
Crypto.HMAC.digestBytes sha256
key
(Bytes.fromUTF8 message)
in
("AWS4" ++ "w<KEY>EMI/K7MDENG+bPxRfiCYEXAMPLEKEY")
|> Bytes.fromUTF8
|> digest "20150830"
|> digest "us-east-1"
|> digest "service"
|> digest "aws4_request"
|> digest "AWS4-HMAC-SHA256\n20150830T123600Z\n20150830/us-east-1/service/aws4_request\n816cd5b414d056048ba4f7c5386d6e0533120fb1fcfa93762cf0fc39e2cf19e0"
|> Hex.fromByteList
--> "b97d918cfa904a5beff61c982a1b6f458b799221646efd99d3219ec94cdf2500"
-}
digestBytes : Hash -> KeyBytes -> MessageBytes -> List Int
digestBytes type_ key message =
Crypto.HMAC.Digest.digestBytes
(hash type_)
(blockSize type_)
key
message
|> Word.toBytes
blockSize : Hash -> Int
blockSize (SHA alg) =
case alg of
SHA224 ->
blockSize (SHA SHA256)
SHA256 ->
64
SHA384 ->
blockSize (SHA SHA512)
SHA512 ->
128
SHA512_224 ->
blockSize (SHA SHA512)
SHA512_256 ->
blockSize (SHA SHA512)
hash : Hash -> List Int -> Array Word
hash (SHA alg) =
Crypto.SHA.digest alg
<file_sep>module Objecthash.ValueTest exposing (suite, testNull)
import Dict
import Expect exposing (Expectation)
import Objecthash.Value as Value exposing (..)
import Test exposing (..)
suite : Test
suite =
describe "Value"
[ test "null" <| \() -> testNull
-- , describe "toString"
-- [ test "null" <| \() -> testNullToString
-- ]
]
testNull : Expectation
testNull =
Expect.equal null VNull
-- testNullToString : Expectation
-- testNullToString =
-- Expect.equal (Value.toString VNull) ""
<file_sep>module Crypto.SHA.MessageSchedule exposing (MessageSchedule, fromChunk)
import Array exposing (Array)
import Crypto.SHA.Alg exposing (Alg(..))
import Crypto.SHA.Chunk exposing (Chunk)
import Crypto.SHA.Constants exposing (roundConstants)
import Word exposing (Word)
type alias MessageSchedule =
Array Word
{-| Copy chunk into first 16 words w[0..15] of the message schedule array.
Extend the first 16 words into the remaining 48 words w[16..63] of the message schedule array:
for i from 16 to 63
-}
fromChunk : Alg -> Chunk -> MessageSchedule
fromChunk alg chunk =
let
n =
List.length (roundConstants alg)
in
List.foldl (nextPart alg) (Array.fromList chunk) (List.range 16 (n - 1))
{-| Get the next value in the message schedule.
s0 := (w[i-15] rightrotate 7) xor (w[i-15] rightrotate 18) xor (w[i-15] rightshift 3)
s1 := (w[i-2] rightrotate 17) xor (w[i-2] rightrotate 19) xor (w[i-2] rightshift 10)
w[i] := w[i-16] + s0 + w[i-7] + s1
-}
nextPart : Alg -> Int -> MessageSchedule -> MessageSchedule
nextPart alg i w =
let
i15 =
at (i - 15) w
i2 =
at (i - 2) w
s0 =
sigma0 alg i15
s1 =
sigma1 alg i2
in
Array.append
w
(Array.fromList
[ at (i - 16) w
|> Word.add s0
|> Word.add (at (i - 7) w)
|> Word.add s1
]
)
sigma0 : Alg -> Word -> Word
sigma0 alg word =
case alg of
SHA224 ->
sigma0 SHA256 word
SHA384 ->
sigma0 SHA512 word
SHA256 ->
Word.rotateRightBy 7 word
|> Word.xor (Word.rotateRightBy 18 word)
|> Word.xor (Word.shiftRightZfBy 3 word)
SHA512 ->
Word.rotateRightBy 1 word
|> Word.xor (Word.rotateRightBy 8 word)
|> Word.xor (Word.shiftRightZfBy 7 word)
SHA512_224 ->
sigma0 SHA512 word
SHA512_256 ->
sigma0 SHA512 word
sigma1 : Alg -> Word -> Word
sigma1 alg word =
case alg of
SHA224 ->
sigma1 SHA256 word
SHA384 ->
sigma1 SHA512 word
SHA256 ->
Word.rotateRightBy 17 word
|> Word.xor (Word.rotateRightBy 19 word)
|> Word.xor (Word.shiftRightZfBy 10 word)
SHA512 ->
Word.rotateRightBy 19 word
|> Word.xor (Word.rotateRightBy 61 word)
|> Word.xor (Word.shiftRightZfBy 6 word)
SHA512_224 ->
sigma1 SHA512 word
SHA512_256 ->
sigma1 SHA512 word
at : Int -> MessageSchedule -> Word
at i =
Array.get i
>> Maybe.withDefault Word.Mismatch
<file_sep>module Objecthash.HashTest exposing (..)
import Dict
import Expect exposing (Expectation)
import Objecthash.Hash exposing (..)
import Test exposing (..)
suite : Test
suite =
describe "Hash"
[ test "null" <| \() -> testNull
, test "raw" <| \() -> testRaw
, describe "bool"
[ test "true" <| \() -> testBoolTrue
, test "false" <| \() -> testBoolFalse
]
, describe "integer"
[ test "0" <| \() -> testInteger0
, test "42" <| \() -> testInteger42
, test "dangerous" <| \() -> testIntDangerous
]
, describe "string"
[ test "unicode" <| \() -> testUnicode ]
, describe "redacted"
[ test "string" <| \() -> testRedacted
]
, describe "list"
[ test "empty" <| \() -> testEmptyList
, test "string" <| \() -> testStringLists
]
, describe "set"
[ test "empty" <| \() -> testEmptySet
, test "mixed" <| \() -> testMixedSet
]
, describe "dict"
[ test "empty" <| \() -> testEmptyDict
]
, describe "float"
[ test "0.0" <| \() -> testFloat0
, test "other" <| \() -> testFloats
, test "dangerous" <| \() -> testFloatDangerous
, test "special" <| \() -> testFloatSpecialValues
]
]
testNull : Expectation
testNull =
Expect.equal (toHex null) "1b16b1df538ba12dc3f97edbb85caa7050d46c148134290feba80f8236c83db9"
testRaw : Expectation
testRaw =
Expect.equal
(toHex <| raw "6b18693874513ba13da54d61aafa7cad0c8f5573f3431d6f1c04b07ddb27d6bb")
"e318859db4d2acc89c0d503ddbcf8331625125a79018d19cf8f8d1336b7eb39e"
-- Unicode
testUnicode : Expectation
testUnicode =
Expect.equal
[ toHex (unicode "ԱԲաբ")
, toHex (unicode "ϓ")
, toHex (unicode "foo")
]
[ "<KEY>"
, "<KEY>"
, "<KEY>"
]
-- Redaction
testRedacted : Expectation
testRedacted =
Expect.equal
(toHex <| redacted "**REDACTED**<KEY>")
"<KEY>"
-- Bool
testBoolTrue : Expectation
testBoolTrue =
Expect.equal (toHex <| bool True) "7dc96f776c8423e57a2785489a3f9c43fb6e756876d6ad9a9cac4aa4e72ec193"
testBoolFalse : Expectation
testBoolFalse =
Expect.equal (toHex <| bool False) "c02c0b965e023abee808f2b548d8d5193a8b5229be6f3121a6f16e2d41a449b3"
-- Integer
testInteger0 : Expectation
testInteger0 =
Expect.equal (toHex (int 0)) "a4e167a76a05add8a8654c169b07b0447a916035aef602df103e8ae0fe2ff390"
testInteger42 : Expectation
testInteger42 =
Expect.equal (toHex (int 42)) "ebc35dc1b8e2602b72beb8d8e5bcdb2babe90f57bcb54ad7282ec798659d2196"
testIntDangerous : Expectation
testIntDangerous =
Expect.equal
[ toHex <| int 9007199254740992
, toHex <| int (9007199254740992 + 1)
]
[ "<KEY>"
, "<KEY>"
]
-- Float
testFloat0 : Expectation
testFloat0 =
Expect.equal
[ toHex <| float 0.0
, toHex <| float -0.0
]
[ "60101d8c9cb988411468e38909571f357daa67bff5a7b0a3f9ae295cd4aba33d"
, "60101d8c9cb988411468e38909571f357daa67bff5a7b0a3f9ae295cd4aba33d"
]
testFloats : Expectation
testFloats =
Expect.equal
[ toHex <| float -0.1
, toHex <| float 1.2345
, toHex <| float -10.1234
]
[ "55ab03db6fbb5e6de473a612d7e462ca8fd2387266080980e87f021a5c7bde9f"
, "844e08b1195a93563db4e5d4faa59759ba0e0397caf065f3b6bc0825499754e0"
, "59b49ae24998519925833e3ff56727e5d4868aba4ecf4c53653638ebff53c366"
]
testFloatDangerous : Expectation
testFloatDangerous =
Expect.equal
[ toHex <| float 9007199254740992
, toHex <| float (9007199254740992 + 1)
]
[ "9e7d7d02dacab24905c2dc23391bd61d4081a9d541dfafd2469c881cc6c748e4"
, "9e7d7d02dacab24905c2dc23391bd61d4081a9d541dfafd2469c881cc6c748e4"
]
testFloatSpecialValues : Expectation
testFloatSpecialValues =
Expect.equal
[ toHex <| float (0 / 0) -- NaN
, toHex <| float (1 / 0) -- Infinity
, toHex <| float (-1 / 0) -- -Infinity
]
[ "5d6c301a98d835732d459d7018a8d546872f7ba3c39a45ba481746d2c6d566d9"
, "<KEY>"
, "1167518d5554ba86d9b176af0a57f29d425bedaa9847c245cc397b37533228f7"
]
-- List
testEmptyList : Expectation
testEmptyList =
Expect.equal
(toHex <| list [])
"acac86c0e609ca906f632b0e2dacccb2b77d22b0621f20ebece1a4835b93f6f0"
testStringLists : Expectation
testStringLists =
Expect.equal
[ toHex (list [ unicode "foo" ])
, toHex (list [ unicode "foo", unicode "bar" ])
]
[ "268bc27d4974d9d576222e4cdbb8f7c6bd<KEY>"
, "32ae896c413cfdc79eec68be9139c86ded8b279238467c216cf2bec4d5f1e4a2"
]
-- Set
testEmptySet : Expectation
testEmptySet =
Expect.equal
(toHex <| set [])
"043a718774c572bd8a25adbeb1bfcd5c0256ae11cecf9f9c3f925d0e52beaf89"
testMixedSet : Expectation
testMixedSet =
Expect.equal
(toHex <| set [ int 1, int 2, unicode "foo" ])
"7b0bde44f8fa6fb6e1f56f5290b1815766c16a06d70258dc3ad501b27f59741d"
-- Dict
testEmptyDict : Expectation
testEmptyDict =
Expect.equal
(toHex <| dict (Dict.fromList []))
"18ac3e7343f016890c510e93f935261169d9e3f565436429830faf0934f4f8e4"
testStringDict : Expectation
testStringDict =
Expect.equal
(toHex <| dict (Dict.fromList [ ( "foo", unicode "bar" ) ]))
"7ef5237c3027d6c58100afadf37796b3d351025cf28038280147d42fdc53b960"
<file_sep>module Objecthash.Tag exposing
( Tag(..)
, toChar, toByte
)
{-| Functions to operate Tags.
# Types
@docs Tag
# Transformers
@docs toChar, toByte
-}
import Char exposing (fromCode)
{-| -}
type Tag
= Bool
| Dict
| Float
| Integer
| List
| Null
| Set
| Unicode
| Raw
| Custom Int
{-| -}
toChar : Tag -> Char
toChar tag =
fromCode (toByte tag)
{-| -}
toByte : Tag -> Int
toByte tag =
case tag of
Bool ->
0x62
Dict ->
0x64
Float ->
0x66
Integer ->
0x69
List ->
0x6C
Null ->
0x6E
Set ->
0x73
Unicode ->
0x75
Raw ->
0x72
Custom int ->
int
<file_sep>module Crypto.SHA.Alg exposing (Alg(..), toString, wordSize)
import Word exposing (Size(..))
type Alg
= SHA224
| SHA256
| SHA384
| SHA512
| SHA512_224
| SHA512_256
toString : Alg -> String
toString alg =
case alg of
SHA224 ->
"SHA224"
SHA256 ->
"SHA256"
SHA384 ->
"SHA384"
SHA512 ->
"SHA512"
SHA512_224 ->
"SHA512_224"
SHA512_256 ->
"SHA512_256"
wordSize : Alg -> Size
wordSize alg =
case alg of
SHA224 ->
wordSize SHA256
SHA256 ->
Bit32
SHA384 ->
wordSize SHA512
SHA512 ->
Bit64
SHA512_224 ->
wordSize SHA512
SHA512_256 ->
wordSize SHA512
<file_sep>module Objecthash.Hash exposing
( ByteList
, bytes, toHex
, unicode, redacted, null, int, float, bool, raw, pair
, list, set, dict, bag, untagged
)
{-| Functions to hash values.
# Types
@docs ByteList
# Convert from and to lists of bytes.
@docs bytes, toHex
# Primitives
@docs unicode, redacted, null, int, float, bool, raw, pair
# Collection primitives
@docs list, set, dict, bag, untagged
-}
import Crypto.SHA as SHA
import Crypto.SHA.Alg exposing (Alg(..))
import Dict exposing (Dict)
import Objecthash.Tag as Tag exposing (Tag)
import Objecthash.Value exposing (Value(..))
import Word.Bytes
import Word.Hex as Hex
{-| A list of bytes.
-}
type alias ByteList =
List Int
sha256 : List Int -> String
sha256 bytes_ =
bytes_
|> SHA.digest SHA256
|> Hex.fromWordArray
{-| Transform a list of bytes into its hexadecimal string representation.
toHex [ 27, 22, 177, 223, 83, 139, 161, 45, 195, 249, 126, 219, 184, 92, 170, 112, 80, 212, 108, 20, 129, 52, 41, 15, 235, 168, 15, 130, 54, 200, 61, 185 ] == "1b16b1df538ba12dc3f97edbb85caa7050d46c148134290feba80f8236c83db9"
-}
toHex : ByteList -> String
toHex bytes_ =
Hex.fromByteList bytes_
{-| Hash an Objecthash Value.
bytes VNull == [ 27, 22, 177, 223, 83, 139, 161, 45, 195, 249, 126, 219, 184, 92, 170, 112, 80, 212, 108, 20, 129, 52, 41, 15, 235, 168, 15, 130, 54, 200, 61, 185 ]
-}
bytes : Value -> ByteList
bytes value =
case value of
VBool inner ->
bool inner
VDict inner ->
inner
|> Dict.map (\k v -> bytes v)
|> dict
VFloat inner ->
float inner
VInteger inner ->
int inner
VList inner ->
list (List.map bytes inner)
VNull ->
null
VSet inner ->
set (List.map bytes inner)
VString inner ->
if String.startsWith "**REDACTED**" inner then
redacted inner
else
unicode inner
-- Primitives
{-| Hashes strings with the given tag
-}
primitive : Tag -> String -> ByteList
primitive tag input =
input
|> String.cons (Tag.toChar tag)
|> Word.Bytes.fromUTF8
|> sha256
|> Hex.toByteList
{-| Hashes collections of bytes
-}
bag : Tag -> List ByteList -> ByteList
bag tag input =
([ Tag.toByte tag ] :: input)
|> List.concat
|> sha256
|> Hex.toByteList
{-| Hashes a raw list of bytes, untagged
-}
untagged : List ByteList -> ByteList
untagged input =
input
|> List.concat
|> sha256
|> Hex.toByteList
{-| Hashes a raw hash.
toHex (raw "7dc96f776c8423e57a2785489a3f9c43fb6e756876d6ad9a9cac4aa4e72ec193") == "72e68c48e6e01b3d898bf9d907938459cb80d6abec2078df7f19271ff9eb19e4"
-}
raw : String -> ByteList
raw input =
Tag.toByte Tag.Raw
:: Hex.toByteList input
|> sha256
|> Hex.toByteList
{-| Hashes a boolean.
toHex (bool True) == "7dc96f776c8423e57a2785489a3f9c43fb6e756876d6ad9a9cac4aa4e72ec193"
-}
bool : Bool -> ByteList
bool input =
let
input_ =
case input of
True ->
"1"
False ->
"0"
in
primitive Tag.Bool input_
{-| Hashes a unicode string.
toHex (unicode "foo") == "a6a6e5e783c363cd95693ec189c2682315d956869397738679b56305f2095038"
-}
unicode : String -> ByteList
unicode input =
primitive Tag.Unicode input
{-| Hashes a redacted stringified list of bytes.
toHex (redacted "**REDACTED**480499ec4efe0e177793c217c8227d4096d2352beee2d6816ba8f4e8a421a138") == "480499ec4efe0e177793c217c8227d4096d2352beee2d6816ba8f4e8a421a138"
-}
redacted : String -> ByteList
redacted input =
input
|> String.dropLeft 12
|> Hex.toByteList
{-| Hashes a null value.
toHex null == "1b16b1df538ba12dc3f97edbb85caa7050d46c148134290feba80f8236c83db9"
-}
null : ByteList
null =
primitive Tag.Null ""
{-| Hashes an integer.
toHex (int 6) == "396ee89382efc154e95d7875976cce373a797fe93687ca8a27589116644c4bcd"
-}
int : Int -> ByteList
int input =
primitive Tag.Integer (String.fromInt input)
{-| Hashes a list of ByteList.
[ unicode "foo", int 6 ]
|> list
|> toHex
== "28dbb78890fb7b0462c62de04bcf165c69bd65b9f992f2edd89ae7369afa7005"
-}
list : List ByteList -> ByteList
list input =
bag Tag.List input
{-| Hashes a set of ByteList. Note that this function receives a `List` but
treats it as a Set (i.e. removes duplicates).
[ unicode "foo", int 6 ]
|> set
|> toHex
== "cf38664185ed5377fee384d0a37bdb36681a16d72480f21336e38a493a8851b9"
-}
set : List ByteList -> ByteList
set input =
input
|> List.foldr collectUnique []
|> List.sort
|> bag Tag.Set
collectUnique : ByteList -> List ByteList -> List ByteList
collectUnique bytes_ acc =
if List.member bytes_ acc then
acc
else
bytes_ :: acc
{-| Hahes a dictionary of ByteList.
Dict.fromList [ ( "foo", int 1 ) ]
|> dict
|> toHex
== "bf4c58f5e308e31e2cd64bdbf7a01b9b595a13602438be5e912c7d94f6d8177a"
-}
dict : Dict String ByteList -> ByteList
dict input =
input
|> Dict.toList
|> List.map pair
|> List.sort
|> bag Tag.Dict
{-| -}
pair : ( String, ByteList ) -> ByteList
pair ( key, value ) =
unicode key ++ value
{-| Hashes a float number. Note this function normalises values following the
same algorithm implemented in the original Objecthash implementation.
toHex (float 6.1) == "43f5ebd1617989a69b819ed3a246c9e59468d6db90c29abdd3c8c1f17ffc365a"
-}
float : Float -> ByteList
float input =
if isNaN input || isInfinite input then
primitive Tag.Float (String.fromFloat input)
else
primitive Tag.Float (normaliseFloat input)
normaliseFloat : Float -> String
normaliseFloat n =
if n == 0 then
"+0:"
else
let
( f, e ) =
( abs n, 0 )
|> exponent
|> floatReduce
( _, s ) =
mantissa ( f, sign n ++ String.fromInt e ++ ":" )
in
s
sign : Float -> String
sign f =
if f < 0 then
"-"
else
"+"
exponent : ( Float, Int ) -> ( Float, Int )
exponent ( f, e ) =
if f > 1 then
exponent ( f / 2, e + 1 )
else
( f, e )
floatReduce : ( Float, Int ) -> ( Float, Int )
floatReduce ( f, e ) =
if f <= 0.5 then
floatReduce ( f * 2, e - 1 )
else
( f, e )
{-| TODO: Handle errors
<https://github.com/benlaurie/objecthash/blob/master/go/objecthash/objecthash.go#L133>
-}
mantissa : ( Float, String ) -> ( Float, String )
mantissa ( f, s ) =
if String.length s >= 1000 then
( 0, "ERROR" )
else if f /= 0 then
if f >= 1 then
mantissa ( (f - 1) * 2, s ++ "1" )
else
mantissa ( f * 2, s ++ "0" )
else
( f, s )
<file_sep>module Crypto.Hash exposing (sha224, sha256, sha384, sha512, sha512_224, sha512_256)
{-| Secure Hash Algorithms.
@docs sha224, sha256, sha384, sha512, sha512_224, sha512_256
-}
import Crypto.SHA as SHA
import Crypto.SHA.Alg exposing (Alg(..))
import Word.Bytes as Bytes
import Word.Hex as Hex
{-| Secure Hash Algorithm using 32-bit words and 64 rounds (truncated).
Crypto.Hash.sha224 ""
--> "d14a028c2a3a2bc9476102bb288234c415a2b01f828ea62ac5b3e42f"
Crypto.Hash.sha224 "The quick brown fox jumps over the lazy dog"
--> "730e109bd7a8a32b1cb9d9a09aa2325d2430587ddbc0c38bad911525"
-}
sha224 : String -> String
sha224 message =
message
|> Bytes.fromUTF8
|> SHA.digest SHA224
|> Hex.fromWordArray
{-| Secure Hash Algorithm using 32-bit words and 64 rounds.
Crypto.Hash.sha256 ""
--> "e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855"
-}
sha256 : String -> String
sha256 message =
message
|> Bytes.fromUTF8
|> SHA.digest SHA256
|> Hex.fromWordArray
{-| Secure Hash Algorithm using 64-bit words and 80 rounds (truncated).
Crypto.Hash.sha384 ""
--> "38b060a751ac96384cd9327eb1b1e36a21fdb71114be07434c0cc7bf63f6e1da274edebfe76f65fbd51ad2f14898b95b"
-}
sha384 : String -> String
sha384 message =
message
|> Bytes.fromUTF8
|> SHA.digest SHA384
|> Hex.fromWordArray
{-| Secure Hash Algorithm using 64-bit words and 80 rounds.
Crypto.Hash.sha512 ""
--> "cf83e1357eefb8bdf1542850d66d8007d620e4050b5715dc83f4a921d36ce9ce47d0d13c5d85f2b0ff8318d2877eec2f63b931bd47417a81a538327af927da3e"
-}
sha512 : String -> String
sha512 message =
message
|> Bytes.fromUTF8
|> SHA.digest SHA512
|> Hex.fromWordArray
{-| Secure Hash Algorithm using 64-bit words and 80 rounds (truncated to 224).
Crypto.Hash.sha512_224 ""
--> "6ed0dd02806fa89e25de060c19d3ac86cabb87d6a0ddd05c333b84f4"
-}
sha512_224 : String -> String
sha512_224 message =
message
|> Bytes.fromUTF8
|> SHA.digest SHA512_224
|> Hex.fromWordArray
{-| Secure Hash Algorithm using 64-bit words and 80 rounds (truncated to 256).
Crypto.Hash.sha512_256 ""
--> "c672b8d1ef56ed28ab87c3622c5114069bdd3ad7b8f9737498d0c01ecef0967a"
-}
sha512_256 : String -> String
sha512_256 message =
message
|> Bytes.fromUTF8
|> SHA.digest SHA512_256
|> Hex.fromWordArray
<file_sep>module Crypto.HMAC.Digest exposing (digestBytes)
import Array exposing (Array)
import Bitwise
import Word exposing (Word)
type alias Hash =
List Int -> Array Word
digestBytes : Hash -> Int -> List Int -> List Int -> Array Word
digestBytes hash blockSize key message =
key
|> normalizeKey hash blockSize
|> hmac_ hash message
hmac_ : Hash -> List Int -> List Int -> Array Word
hmac_ hash message key =
let
oKeyPad =
key
|> List.map (Bitwise.xor 0x5C)
iKeyPad =
key
|> List.map (Bitwise.xor 0x36)
in
List.append iKeyPad message
|> hash
|> Word.toBytes
|> List.append oKeyPad
|> hash
normalizeKey : Hash -> Int -> List Int -> List Int
normalizeKey hash blockSize key =
case compare blockSize <| List.length key of
EQ ->
key
GT ->
key
|> padEnd blockSize
LT ->
key
|> hash
|> Word.toBytes
|> padEnd blockSize
padEnd : Int -> List Int -> List Int
padEnd blockSize bytes =
List.append bytes <|
List.repeat
(blockSize - List.length bytes)
0
| fba16fcaf837ea2d225d7ba9c3218c7ac5303513 | [
"Markdown",
"Elm"
] | 19 | Markdown | arnau/elm-objecthash | 86121954bff9e1ea59e9d04f62fa940089a8dd7d | 8f1af92785c7a52287b5ed66f45e543fd92e3299 |
refs/heads/master | <repo_name>RuslanSayko/cityCountryList<file_sep>/counries.sql
INSERT INTO countries VALUES (30, "Afghanistan");
INSERT INTO countries VALUES (21, "Albania");
INSERT INTO countries VALUES (22, "Algeria");
INSERT INTO countries VALUES (23, "American Samoa");
INSERT INTO countries VALUES (26, "Andorra");
INSERT INTO countries VALUES (25, "Angola");
INSERT INTO countries VALUES (24, "Anguilla");
INSERT INTO countries VALUES (27, "Antigua and Barbuda");
INSERT INTO countries VALUES (28, "Argentina");
INSERT INTO countries VALUES (6, "Armenia");
INSERT INTO countries VALUES (29, "Aruba");
INSERT INTO countries VALUES (19, "Australia");
INSERT INTO countries VALUES (20, "Austria");
INSERT INTO countries VALUES (5, "Azerbaijan");
INSERT INTO countries VALUES (31, "Bahamas");
INSERT INTO countries VALUES (34, "Bahrain");
INSERT INTO countries VALUES (32, "Bangladesh");
INSERT INTO countries VALUES (33, "Barbados");
INSERT INTO countries VALUES (3, "Belarus");
INSERT INTO countries VALUES (36, "Belgium");
INSERT INTO countries VALUES (35, "Belize");
INSERT INTO countries VALUES (37, "Benin");
INSERT INTO countries VALUES (38, "Bermuda");
INSERT INTO countries VALUES (47, "Bhutan");
INSERT INTO countries VALUES (40, "Bolivia");
INSERT INTO countries VALUES (235, "Bonaire, Sint Eustatius and Saba");
INSERT INTO countries VALUES (41, "Bosnia and Herzegovina");
INSERT INTO countries VALUES (42, "Botswana");
INSERT INTO countries VALUES (43, "Brazil");
INSERT INTO countries VALUES (52, "British Virgin Islands");
INSERT INTO countries VALUES (44, "Brunei Darussalam");
INSERT INTO countries VALUES (39, "Bulgaria");
INSERT INTO countries VALUES (45, "Burkina Faso");
INSERT INTO countries VALUES (46, "Burundi");
INSERT INTO countries VALUES (103, "Côte d`Ivoire");
INSERT INTO countries VALUES (91, "Cambodia");
INSERT INTO countries VALUES (92, "Cameroon");
INSERT INTO countries VALUES (10, "Canada");
INSERT INTO countries VALUES (90, "Cape Verde");
INSERT INTO countries VALUES (149, "Cayman Islands");
INSERT INTO countries VALUES (213, "Central African Republic");
INSERT INTO countries VALUES (214, "Chad");
INSERT INTO countries VALUES (216, "Chile");
INSERT INTO countries VALUES (97, "China");
INSERT INTO countries VALUES (98, "Colombia");
INSERT INTO countries VALUES (99, "Comoros");
INSERT INTO countries VALUES (100, "Congo");
INSERT INTO countries VALUES (101, "Congo, Democratic Republic");
INSERT INTO countries VALUES (150, "Cook Islands");
INSERT INTO countries VALUES (102, "Costa Rica");
INSERT INTO countries VALUES (212, "Croatia");
INSERT INTO countries VALUES (104, "Cuba");
INSERT INTO countries VALUES (138, "Curaçao");
INSERT INTO countries VALUES (95, "Cyprus");
INSERT INTO countries VALUES (215, "Czech Republic");
INSERT INTO countries VALUES (73, "Denmark");
INSERT INTO countries VALUES (231, "Djibouti");
INSERT INTO countries VALUES (74, "Dominica");
INSERT INTO countries VALUES (75, "Dominican Republic");
INSERT INTO countries VALUES (54, "East Timor");
INSERT INTO countries VALUES (221, "Ecuador");
INSERT INTO countries VALUES (76, "Egypt");
INSERT INTO countries VALUES (166, "El Salvador");
INSERT INTO countries VALUES (222, "Equatorial Guinea");
INSERT INTO countries VALUES (223, "Eritrea");
INSERT INTO countries VALUES (14, "Estonia");
INSERT INTO countries VALUES (224, "Ethiopia");
INSERT INTO countries VALUES (208, "Falkland Islands");
INSERT INTO countries VALUES (204, "Faroe Islands");
INSERT INTO countries VALUES (205, "Fiji");
INSERT INTO countries VALUES (207, "Finland");
INSERT INTO countries VALUES (209, "France");
INSERT INTO countries VALUES (210, "French Guiana");
INSERT INTO countries VALUES (211, "French Polynesia");
INSERT INTO countries VALUES (56, "Gabon");
INSERT INTO countries VALUES (59, "Gambia");
INSERT INTO countries VALUES (7, "Georgia");
INSERT INTO countries VALUES (65, "Germany");
INSERT INTO countries VALUES (60, "Ghana");
INSERT INTO countries VALUES (66, "Gibraltar");
INSERT INTO countries VALUES (71, "Greece");
INSERT INTO countries VALUES (70, "Greenland");
INSERT INTO countries VALUES (69, "Grenada");
INSERT INTO countries VALUES (61, "Guadeloupe");
INSERT INTO countries VALUES (72, "Guam");
INSERT INTO countries VALUES (62, "Guatemala");
INSERT INTO countries VALUES (63, "Guinea");
INSERT INTO countries VALUES (64, "Guinea-Bissau");
INSERT INTO countries VALUES (58, "Guyana");
INSERT INTO countries VALUES (57, "Haiti");
INSERT INTO countries VALUES (67, "Honduras");
INSERT INTO countries VALUES (68, "Hong Kong");
INSERT INTO countries VALUES (50, "Hungary");
INSERT INTO countries VALUES (86, "Iceland");
INSERT INTO countries VALUES (80, "India");
INSERT INTO countries VALUES (81, "Indonesia");
INSERT INTO countries VALUES (84, "Iran");
INSERT INTO countries VALUES (83, "Iraq");
INSERT INTO countries VALUES (85, "Ireland");
INSERT INTO countries VALUES (147, "Isle of Man");
INSERT INTO countries VALUES (8, "Israel");
INSERT INTO countries VALUES (88, "Italy");
INSERT INTO countries VALUES (228, "Jamaica");
INSERT INTO countries VALUES (229, "Japan");
INSERT INTO countries VALUES (82, "Jordan");
INSERT INTO countries VALUES (4, "Kazakhstan");
INSERT INTO countries VALUES (94, "Kenya");
INSERT INTO countries VALUES (96, "Kiribati");
INSERT INTO countries VALUES (105, "Kuwait");
INSERT INTO countries VALUES (11, "Kyrgyzstan");
INSERT INTO countries VALUES (106, "Laos");
INSERT INTO countries VALUES (12, "Latvia");
INSERT INTO countries VALUES (109, "Lebanon");
INSERT INTO countries VALUES (107, "Lesotho");
INSERT INTO countries VALUES (108, "Liberia");
INSERT INTO countries VALUES (110, "Libya");
INSERT INTO countries VALUES (111, "Liechtenstein");
INSERT INTO countries VALUES (13, "Lithuania");
INSERT INTO countries VALUES (112, "Luxembourg");
INSERT INTO countries VALUES (116, "Macau");
INSERT INTO countries VALUES (117, "Macedonia");
INSERT INTO countries VALUES (115, "Madagascar");
INSERT INTO countries VALUES (118, "Malawi");
INSERT INTO countries VALUES (119, "Malaysia");
INSERT INTO countries VALUES (121, "Maldives");
INSERT INTO countries VALUES (120, "Mali");
INSERT INTO countries VALUES (122, "Malta");
INSERT INTO countries VALUES (125, "Marshall Islands");
INSERT INTO countries VALUES (124, "Martinique");
INSERT INTO countries VALUES (114, "Mauritania");
INSERT INTO countries VALUES (113, "Mauritius");
INSERT INTO countries VALUES (126, "Mexico");
INSERT INTO countries VALUES (127, "Micronesia");
INSERT INTO countries VALUES (15, "Moldova");
INSERT INTO countries VALUES (129, "Monaco");
INSERT INTO countries VALUES (130, "Mongolia");
INSERT INTO countries VALUES (230, "Montenegro");
INSERT INTO countries VALUES (131, "Montserrat");
INSERT INTO countries VALUES (123, "Morocco");
INSERT INTO countries VALUES (128, "Mozambique");
INSERT INTO countries VALUES (132, "Myanmar");
INSERT INTO countries VALUES (133, "Namibia");
INSERT INTO countries VALUES (134, "Nauru");
INSERT INTO countries VALUES (135, "Nepal");
INSERT INTO countries VALUES (139, "Netherlands");
INSERT INTO countries VALUES (143, "New Caledonia");
INSERT INTO countries VALUES (142, "New Zealand");
INSERT INTO countries VALUES (140, "Nicaragua");
INSERT INTO countries VALUES (136, "Niger");
INSERT INTO countries VALUES (137, "Nigeria");
INSERT INTO countries VALUES (141, "Niue");
INSERT INTO countries VALUES (148, "Norfolk Island");
INSERT INTO countries VALUES (173, "North Korea");
INSERT INTO countries VALUES (174, "Northern Mariana Islands");
INSERT INTO countries VALUES (144, "Norway");
INSERT INTO countries VALUES (146, "Oman");
INSERT INTO countries VALUES (152, "Pakistan");
INSERT INTO countries VALUES (153, "Palau");
INSERT INTO countries VALUES (154, "Palestine");
INSERT INTO countries VALUES (155, "Panama");
INSERT INTO countries VALUES (156, "Papua New Guinea");
INSERT INTO countries VALUES (157, "Paraguay");
INSERT INTO countries VALUES (158, "Peru");
INSERT INTO countries VALUES (206, "Philippines");
INSERT INTO countries VALUES (159, "Pitcairn Islands");
INSERT INTO countries VALUES (160, "Poland");
INSERT INTO countries VALUES (161, "Portugal");
INSERT INTO countries VALUES (162, "Puerto Rico");
INSERT INTO countries VALUES (93, "Qatar");
INSERT INTO countries VALUES (163, "Réunion");
INSERT INTO countries VALUES (165, "Romania");
INSERT INTO countries VALUES (1, "Russia");
INSERT INTO countries VALUES (164, "Rwanda");
INSERT INTO countries VALUES (169, "São Tomé and Príncipe");
INSERT INTO countries VALUES (172, "Saint Helena");
INSERT INTO countries VALUES (178, "Saint Kitts and Nevis");
INSERT INTO countries VALUES (179, "Saint Lucia");
INSERT INTO countries VALUES (180, "Saint Pierre and Miquelon");
INSERT INTO countries VALUES (177, "Saint Vincent and the Grenadines");
INSERT INTO countries VALUES (167, "Samoa");
INSERT INTO countries VALUES (168, "San Marino");
INSERT INTO countries VALUES (170, "Saudi Arabia");
INSERT INTO countries VALUES (176, "Senegal");
INSERT INTO countries VALUES (181, "Serbia");
INSERT INTO countries VALUES (175, "Seychelles");
INSERT INTO countries VALUES (190, "Sierra Leone");
INSERT INTO countries VALUES (182, "Singapore");
INSERT INTO countries VALUES (234, "Sint Maarten");
INSERT INTO countries VALUES (184, "Slovakia");
INSERT INTO countries VALUES (185, "Slovenia");
INSERT INTO countries VALUES (186, "Solomon Islands");
INSERT INTO countries VALUES (187, "Somalia");
INSERT INTO countries VALUES (227, "South Africa");
INSERT INTO countries VALUES (226, "South Korea");
INSERT INTO countries VALUES (232, "South Sudan");
INSERT INTO countries VALUES (87, "Spain");
INSERT INTO countries VALUES (220, "Sri Lanka");
INSERT INTO countries VALUES (188, "Sudan");
INSERT INTO countries VALUES (189, "Suriname");
INSERT INTO countries VALUES (219, "Svalbard and Jan Mayen");
INSERT INTO countries VALUES (171, "Swaziland");
INSERT INTO countries VALUES (218, "Sweden");
INSERT INTO countries VALUES (217, "Switzerland");
INSERT INTO countries VALUES (183, "Syria");
INSERT INTO countries VALUES (192, "Taiwan");
INSERT INTO countries VALUES (16, "Tajikistan");
INSERT INTO countries VALUES (193, "Tanzania");
INSERT INTO countries VALUES (191, "Thailand");
INSERT INTO countries VALUES (194, "Togo");
INSERT INTO countries VALUES (195, "Tokelau");
INSERT INTO countries VALUES (196, "Tonga");
INSERT INTO countries VALUES (197, "Trinidad and Tobago");
INSERT INTO countries VALUES (199, "Tunisia");
INSERT INTO countries VALUES (200, "Turkey");
INSERT INTO countries VALUES (17, "Turkmenistan");
INSERT INTO countries VALUES (151, "Turks and Caicos Islands");
INSERT INTO countries VALUES (198, "Tuvalu");
INSERT INTO countries VALUES (53, "US Virgin Islands");
INSERT INTO countries VALUES (9, "USA");
INSERT INTO countries VALUES (201, "Uganda");
INSERT INTO countries VALUES (2, "Ukraine");
INSERT INTO countries VALUES (145, "United Arab Emirates");
INSERT INTO countries VALUES (49, "United Kingdom");
INSERT INTO countries VALUES (203, "Uruguay");
INSERT INTO countries VALUES (18, "Uzbekistan");
INSERT INTO countries VALUES (48, "Vanuatu");
INSERT INTO countries VALUES (233, "Vatican");
INSERT INTO countries VALUES (51, "Venezuela");
INSERT INTO countries VALUES (55, "Vietnam");
INSERT INTO countries VALUES (202, "Wallis and Futuna");
INSERT INTO countries VALUES (78, "Western Sahara");
INSERT INTO countries VALUES (89, "Yemen");
INSERT INTO countries VALUES (77, "Zambia");
INSERT INTO countries VALUES (79, "Zimbabwe");
<file_sep>/regions.sql
INSERT INTO regions VALUES (3513674, 80, "State of Andhra Pradesh");
INSERT INTO regions VALUES (3513455, 80, "State of Arunāchal Pradesh");
INSERT INTO regions VALUES (3513388, 80, "State of Assam");
INSERT INTO regions VALUES (3511329, 80, "State of Bihār");
INSERT INTO regions VALUES (3621886, 80, "State of Chhattīsgarh");
INSERT INTO regions VALUES (3507837, 80, "State of Goa");
INSERT INTO regions VALUES (3507542, 80, "State of Gujarāt");
INSERT INTO regions VALUES (3507140, 80, "State of Haryāna");
INSERT INTO regions VALUES (3507018, 80, "State of Himāchal Pradesh");
INSERT INTO regions VALUES (3506398, 80, "State of Jammu and Kashmīr");
INSERT INTO regions VALUES (3621887, 80, "State of Jharkhand");
INSERT INTO regions VALUES (3505138, 80, "State of Karnātaka");
INSERT INTO regions VALUES (3504796, 80, "State of Kerala");
INSERT INTO regions VALUES (3502648, 80, "State of Madhya Pradesh");
INSERT INTO regions VALUES (3502551, 80, "State of Mahārāshtra");
INSERT INTO regions VALUES (3501997, 80, "State of Manipur");
INSERT INTO regions VALUES (3501610, 80, "State of Meghālaya");
INSERT INTO regions VALUES (3501433, 80, "State of Mizoram");
INSERT INTO regions VALUES (3500882, 80, "State of Nāgāland");
INSERT INTO regions VALUES (3499903, 80, "State of Odisha");
INSERT INTO regions VALUES (3498477, 80, "State of Punjab");
INSERT INTO regions VALUES (3498202, 80, "State of Rājasthān");
INSERT INTO regions VALUES (3496138, 80, "State of Sikkim");
INSERT INTO regions VALUES (3495172, 80, "State of Tamil Nādu");
INSERT INTO regions VALUES (5469479, 80, "State of Telangana");
INSERT INTO regions VALUES (3494505, 80, "State of Tripura");
INSERT INTO regions VALUES (3494100, 80, "State of Uttar Pradesh");
INSERT INTO regions VALUES (3621888, 80, "State of Uttarakhand");
INSERT INTO regions VALUES (3493524, 80, "State of West Bengal");
INSERT INTO regions VALUES (3513685, 80, "Union Territory of Andaman and Nicobar Islands");
INSERT INTO regions VALUES (3510605, 80, "Union Territory of Chandīgarh");
INSERT INTO regions VALUES (3509820, 80, "Union Territory of Dādra and Nagar Haveli");
INSERT INTO regions VALUES (3507835, 80, "Union Territory of Damān and Diu");
INSERT INTO regions VALUES (3509476, 80, "Union Territory of Delhi");
INSERT INTO regions VALUES (3503152, 80, "Union Territory of Lakshadweep");
INSERT INTO regions VALUES (3498620, 80, "Union Territory of Puducherry");
INSERT INTO regions VALUES (3476618, 81, "Daerah Istimewa Aceh");
INSERT INTO regions VALUES (3694419, 81, "Daerah Istimewa Yogyakarta");
INSERT INTO regions VALUES (3707008, 81, "Daerah Khusus Ibukota Jakarta Raya");
INSERT INTO regions VALUES (3711404, 81, "Propinsi Bali");
INSERT INTO regions VALUES (3886039, 81, "Propinsi Banten");
INSERT INTO regions VALUES (3710638, 81, "Propinsi Bengkulu");
INSERT INTO regions VALUES (3706977, 81, "Propinsi Jambi");
INSERT INTO regions VALUES (3706850, 81, "Propinsi Jawa Barat");
INSERT INTO regions VALUES (3706847, 81, "Propinsi Jawa Tengah");
INSERT INTO regions VALUES (3706846, 81, "Propinsi Jawa Timur");
INSERT INTO regions VALUES (3706396, 81, "Propinsi Kalimantan Barat");
INSERT INTO regions VALUES (3706395, 81, "Propinsi Kalimantan Selatan");
INSERT INTO regions VALUES (3706394, 81, "Propinsi Kalimantan Tengah");
INSERT INTO regions VALUES (3706393, 81, "Propinsi Kalimantan Timur");
INSERT INTO regions VALUES (3886041, 81, "Propinsi Kepulauan Bangka Belitung");
INSERT INTO regions VALUES (3704375, 81, "Propinsi Lampung");
INSERT INTO regions VALUES (3703319, 81, "Propinsi Maluku");
INSERT INTO regions VALUES (3911098, 81, "Propinsi Maluku Utara");
INSERT INTO regions VALUES (3701727, 81, "Propinsi Nusa Tenggara Barat");
INSERT INTO regions VALUES (3701726, 81, "Propinsi Nusa Tenggara Timur");
INSERT INTO regions VALUES (3697417, 81, "Propinsi Sulawesi Tengah");
INSERT INTO regions VALUES (3697416, 81, "Propinsi Sulawesi Tenggara");
INSERT INTO regions VALUES (3697415, 81, "Propinsi Sulawesi Utara");
INSERT INTO regions VALUES (3697401, 81, "Propinsi Sumatera Barat");
INSERT INTO regions VALUES (3697400, 81, "Propinsi Sumatera Selatan");
INSERT INTO regions VALUES (3475297, 81, "Propinsi Sumatera Utara");
INSERT INTO regions VALUES (3886040, 81, "Provinsi Gorontalo");
INSERT INTO regions VALUES (3944018, 81, "Provinsi Irian Jaya Barat");
INSERT INTO regions VALUES (3944020, 81, "Provinsi Kepulauan Riau");
INSERT INTO regions VALUES (3707056, 81, "Provinsi Papua");
INSERT INTO regions VALUES (3699190, 81, "Provinsi Riau");
INSERT INTO regions VALUES (3944019, 81, "Provinsi Sulawesi Barat");
INSERT INTO regions VALUES (3697418, 81, "Provinsi Sulawesi Selatan");
INSERT INTO regions VALUES (3050544, 83, "Muḩāfaz̧at al Anbār");
INSERT INTO regions VALUES (3043655, 83, "Muḩāfaz̧at al Başrah");
INSERT INTO regions VALUES (3050136, 83, "Muḩāfaz̧at al Muthanná");
INSERT INTO regions VALUES (3050128, 83, "Muḩāfaz̧at al Qādisīyah");
INSERT INTO regions VALUES (3050025, 83, "Muḩāfaz̧at an Najaf");
INSERT INTO regions VALUES (3047734, 83, "Muḩāfaz̧at Arbīl");
INSERT INTO regions VALUES (3049746, 83, "Muḩāfaz̧at as Sulaymānīyah");
INSERT INTO regions VALUES (3049713, 83, "Muḩāfaz̧at at Ta'mīm");
INSERT INTO regions VALUES (3049593, 83, "Muḩāfaz̧at Bābil");
INSERT INTO regions VALUES (3049556, 83, "Muḩāfaz̧at Baghdād");
INSERT INTO regions VALUES (3048909, 83, "Muḩāfaz̧at Dahūk");
INSERT INTO regions VALUES (3048727, 83, "Muḩāfaz̧at Dhī Qār");
INSERT INTO regions VALUES (3048696, 83, "Muḩāfaz̧at Diyālá");
INSERT INTO regions VALUES (3047311, 83, "Muḩāfaz̧at Karbalā'");
INSERT INTO regions VALUES (3046455, 83, "Muḩāfaz̧at Maysān");
INSERT INTO regions VALUES (3046024, 83, "Muḩāfaz̧at Nīnawá");
INSERT INTO regions VALUES (3045219, 83, "Muḩāfaz̧at Şalāḩ ad Dīn");
INSERT INTO regions VALUES (3043897, 83, "Muḩāfaz̧at Wāsiţ");
INSERT INTO regions VALUES (5324588, 83, "Ostān-e Dīāleh");
INSERT INTO regions VALUES (5324574, 83, "Ostān-e Soleymānīyeh");
INSERT INTO regions VALUES (5324643, 83, "Ostān-e Soleymānīyeh");
INSERT INTO regions VALUES (3064799, 84, "Khorasan Ostan-e");
INSERT INTO regions VALUES (3198247, 84, "Ostān-e Ardabīl");
INSERT INTO regions VALUES (3076360, 84, "Ostān-e Āz̄arbāyjān-e Gharbī");
INSERT INTO regions VALUES (3076359, 84, "Ostān-e Āz̄arbāyjān-e Sharqī");
INSERT INTO regions VALUES (3074290, 84, "Ostān-e Būshehr");
INSERT INTO regions VALUES (3074179, 84, "Ostān-e Chahār Maḩāll va Bakhtīārī");
INSERT INTO regions VALUES (3200562, 84, "Ostān-e Eşfahān");
INSERT INTO regions VALUES (3070430, 84, "Ostān-e Fārs");
INSERT INTO regions VALUES (3069390, 84, "Ostān-e Gīlān");
INSERT INTO regions VALUES (3207619, 84, "Ostān-e Golestān");
INSERT INTO regions VALUES (3068501, 84, "Ostān-e Hamadān");
INSERT INTO regions VALUES (3067779, 84, "Ostān-e Hormozgān");
INSERT INTO regions VALUES (3067431, 84, "Ostān-e Īlām");
INSERT INTO regions VALUES (3065449, 84, "Ostān-e Kermān");
INSERT INTO regions VALUES (3065443, 84, "Ostān-e Kermānshāh");
INSERT INTO regions VALUES (5133581, 84, "Ostān-e Khorāsān-e Jonūbī");
INSERT INTO regions VALUES (5133582, 84, "Ostān-e Khorāsān-e Raẕavī");
INSERT INTO regions VALUES (5133583, 84, "Ostān-e Khorāsān-e Shomālī");
INSERT INTO regions VALUES (3064573, 84, "Ostān-e Khūzestān");
INSERT INTO regions VALUES (3064413, 84, "Ostān-e Kohgīlūyeh va Būyer Aḩmad");
INSERT INTO regions VALUES (3064218, 84, "Ostān-e Kordestān");
INSERT INTO regions VALUES (3063555, 84, "Ostān-e Lorestān");
INSERT INTO regions VALUES (3062905, 84, "Ostān-e Markazī");
INSERT INTO regions VALUES (3062748, 84, "Ostān-e Māzandarān");
INSERT INTO regions VALUES (3207620, 84, "Ostān-e Qazvīn");
INSERT INTO regions VALUES (3207621, 84, "Ostān-e Qom");
INSERT INTO regions VALUES (3056644, 84, "Ostān-e Semnān");
INSERT INTO regions VALUES (3429447, 84, "Ostān-e Sīstān va Balūchestān");
INSERT INTO regions VALUES (3052652, 84, "Ostān-e Tehrān");
INSERT INTO regions VALUES (3053415, 84, "<NAME>");
INSERT INTO regions VALUES (3053117, 84, "Ostān-<NAME>");
INSERT INTO regions VALUES (4396993, 85, "<NAME>");
INSERT INTO regions VALUES (4396956, 85, "<NAME>");
INSERT INTO regions VALUES (4394890, 85, "An Iarmhí");
INSERT INTO regions VALUES (4395726, 85, "An Longfort");
INSERT INTO regions VALUES (4395649, 85, "An Mhí");
INSERT INTO regions VALUES (4396119, 85, "Ciarraí");
INSERT INTO regions VALUES (4396038, 85, "Cill Chainnigh");
INSERT INTO regions VALUES (4396063, 85, "<NAME>");
INSERT INTO regions VALUES (4394871, 85, "<NAME>");
INSERT INTO regions VALUES (4397106, 85, "County Carlow");
INSERT INTO regions VALUES (4396782, 85, "County Cork");
INSERT INTO regions VALUES (4396614, 85, "County Donegal");
INSERT INTO regions VALUES (4396524, 85, "County Dublin");
INSERT INTO regions VALUES (4396369, 85, "County Galway");
INSERT INTO regions VALUES (4395795, 85, "County Leitrim");
INSERT INTO regions VALUES (4395780, 85, "County Limerick");
INSERT INTO regions VALUES (4395604, 85, "County Monaghan");
INSERT INTO regions VALUES (4395003, 85, "County Tipperary");
INSERT INTO regions VALUES (4395827, 85, "Laois");
INSERT INTO regions VALUES (4394885, 85, "<NAME>");
INSERT INTO regions VALUES (4395710, 85, "Lú");
INSERT INTO regions VALUES (4395651, 85, "<NAME>");
INSERT INTO regions VALUES (4394898, 85, "Port Láirge");
INSERT INTO regions VALUES (4395233, 85, "<NAME>");
INSERT INTO regions VALUES (4395111, 85, "Sligeach");
INSERT INTO regions VALUES (4395437, 85, "Uíbh Fhailí");
INSERT INTO regions VALUES (4607072, 86, "Austurland");
INSERT INTO regions VALUES (4636480, 86, "Höfuðborgarsvæði");
INSERT INTO regions VALUES (4607071, 86, "Norðurland Eystra");
INSERT INTO regions VALUES (4607070, 86, "Norðurland Vestra");
INSERT INTO regions VALUES (4607073, 86, "Suðurland");
INSERT INTO regions VALUES (4636481, 86, "Suðurnes");
INSERT INTO regions VALUES (4636483, 86, "Vestfirðir");
INSERT INTO regions VALUES (4636482, 86, "Vesturland");
INSERT INTO regions VALUES (4215483, 87, "Andalucía");
INSERT INTO regions VALUES (4607020, 87, "Aragón");
INSERT INTO regions VALUES (4215484, 87, "Canarias");
INSERT INTO regions VALUES (4607019, 87, "Cantabria");
INSERT INTO regions VALUES (4607021, 87, "Castilla y León");
INSERT INTO regions VALUES (4215485, 87, "Castilla-La Mancha");
INSERT INTO regions VALUES (4607022, 87, "Catalunya");
INSERT INTO regions VALUES (5464793, 87, "Ceuta");
INSERT INTO regions VALUES (4518423, 87, "Comunidad de Madrid");
INSERT INTO regions VALUES (4215487, 87, "Comunitat Valenciana");
INSERT INTO regions VALUES (4607024, 87, "Euskal Autonomia Erkidegoa");
INSERT INTO regions VALUES (4215486, 87, "Extremadura");
INSERT INTO regions VALUES (4607023, 87, "Galicia");
INSERT INTO regions VALUES (4189525, 87, "Illes Balears");
INSERT INTO regions VALUES (4607018, 87, "La Rioja");
INSERT INTO regions VALUES (5173454, 87, "Melilla");
INSERT INTO regions VALUES (4516818, 87, "Navarra");
INSERT INTO regions VALUES (4516092, 87, "Principado de Asturias");
INSERT INTO regions VALUES (4185354, 87, "Región de Murcia");
INSERT INTO regions VALUES (4551683, 88, "Regione Abruzzo");
INSERT INTO regions VALUES (4190429, 88, "Regione Autonoma della Sardegna");
INSERT INTO regions VALUES (4546485, 88, "Regione Autonoma <NAME>");
INSERT INTO regions VALUES (4190346, 88, "Regione Autonoma Siciliana");
INSERT INTO regions VALUES (4538511, 88, "Regione Autonoma Trentino-Alto Adige");
INSERT INTO regions VALUES (4538251, 88, "Regione Autonoma Valle d'Aosta");
INSERT INTO regions VALUES (4550824, 88, "Regione Basilicata");
INSERT INTO regions VALUES (4192131, 88, "Regione Calabria");
INSERT INTO regions VALUES (4549883, 88, "Regione Campania");
INSERT INTO regions VALUES (4538067, 88, "Regione del Veneto");
INSERT INTO regions VALUES (4547069, 88, "Regione Emilia-Romagna");
INSERT INTO regions VALUES (4545429, 88, "Regione Lazio");
INSERT INTO regions VALUES (4545274, 88, "Regione Liguria");
INSERT INTO regions VALUES (4545201, 88, "Regione Lombardia");
INSERT INTO regions VALUES (4544797, 88, "Regione Marche");
INSERT INTO regions VALUES (4544264, 88, "Regione Molise");
INSERT INTO regions VALUES (4542561, 88, "Regione Piemonte");
INSERT INTO regions VALUES (4541747, 88, "Regione Puglia");
INSERT INTO regions VALUES (4538589, 88, "Regione Toscana");
INSERT INTO regions VALUES (4538387, 88, "Regione Umbria");
INSERT INTO regions VALUES (3178917, 76, "Al Sharqia Governorate");
INSERT INTO regions VALUES (3179541, 76, "Alexandria Governorate");
INSERT INTO regions VALUES (3178754, 76, "Aswan Governorate");
INSERT INTO regions VALUES (3178750, 76, "Asyut Governorate");
INSERT INTO regions VALUES (3179770, 76, "Beheira Governorate");
INSERT INTO regions VALUES (3178483, 76, "Beni Suef Governorate");
INSERT INTO regions VALUES (3179218, 76, "Cairo Governorate");
INSERT INTO regions VALUES (3180022, 76, "Dakahlia Governorate");
INSERT INTO regions VALUES (3178144, 76, "Damietta Governorate");
INSERT INTO regions VALUES (3179736, 76, "Faiyum Governorate");
INSERT INTO regions VALUES (3179714, 76, "Gharbia Governorate");
INSERT INTO regions VALUES (3179494, 76, "Giza Governorate");
INSERT INTO regions VALUES (3179538, 76, "Ismailia Governorate");
INSERT INTO regions VALUES (3176694, 76, "Kafr el-Sheikh Governorate");
INSERT INTO regions VALUES (5456064, 76, "Luxor Governorate");
INSERT INTO regions VALUES (3175918, 76, "Matrouh Governorate");
INSERT INTO regions VALUES (3179258, 76, "Minya Governorate");
INSERT INTO regions VALUES (3179259, 76, "Monufia Governorate");
INSERT INTO regions VALUES (3179114, 76, "New Valley Governorate");
INSERT INTO regions VALUES (3174813, 76, "North Sinai Governorate");
INSERT INTO regions VALUES (3178321, 76, "Port Said Governorate");
INSERT INTO regions VALUES (3179210, 76, "Qalyubia Governorate");
INSERT INTO regions VALUES (3175012, 76, "Qena Governorate");
INSERT INTO regions VALUES (3179847, 76, "Red Sea Governorate");
INSERT INTO regions VALUES (3174638, 76, "Sohag Governorate");
INSERT INTO regions VALUES (3177122, 76, "South Sinai Governorate");
INSERT INTO regions VALUES (3178758, 76, "Suez Governorate");
INSERT INTO regions VALUES (4299973, 20, "Bundesland Burgenland");
INSERT INTO regions VALUES (4296437, 20, "Bundesland Kärnten");
INSERT INTO regions VALUES (4294004, 20, "Bundesland Niederösterreich");
INSERT INTO regions VALUES (4293504, 20, "Bundesland Oberösterreich");
INSERT INTO regions VALUES (4291659, 20, "Bundesland Salzburg");
INSERT INTO regions VALUES (4290422, 20, "Bundesland Steiermark");
INSERT INTO regions VALUES (4289881, 20, "Bundesland Tirol");
INSERT INTO regions VALUES (4289074, 20, "Bundesland Vorarlberg");
INSERT INTO regions VALUES (4288533, 20, "Bundesland Wien");
INSERT INTO regions VALUES (4007061, 19, "Australian Capital Territory");
INSERT INTO regions VALUES (5308503, 19, "Heard Island and McDonald Islands (general)");
INSERT INTO regions VALUES (3977860, 19, "Northern Territory");
INSERT INTO regions VALUES (4002131, 19, "State of New South Wales");
INSERT INTO regions VALUES (4001529, 19, "State of Queensland");
INSERT INTO regions VALUES (3977604, 19, "State of South Australia");
INSERT INTO regions VALUES (4000719, 19, "State of Tasmania");
INSERT INTO regions VALUES (4000210, 19, "State of Victoria");
INSERT INTO regions VALUES (3977392, 19, "State of Western Australia");
INSERT INTO regions VALUES (3314789, 21, "Qarku i Beratit");
INSERT INTO regions VALUES (3314790, 21, "Qarku i Dibrës");
INSERT INTO regions VALUES (4609715, 21, "Qarku i Durrësit");
INSERT INTO regions VALUES (3314791, 21, "Qarku i Elbasanit");
INSERT INTO regions VALUES (4609716, 21, "Qarku i Fierit");
INSERT INTO regions VALUES (3314792, 21, "Qarku i Gjirokastrës");
INSERT INTO regions VALUES (3314793, 21, "Qarku i Korçës");
INSERT INTO regions VALUES (3314794, 21, "Qarku i Kukësit");
INSERT INTO regions VALUES (4609717, 21, "Qarku i Lezhës");
INSERT INTO regions VALUES (4609718, 21, "Qarku i Shkodrës");
INSERT INTO regions VALUES (4609719, 21, "Qarku i Tiranës");
INSERT INTO regions VALUES (4609720, 21, "Qarku i Vlorës");
INSERT INTO regions VALUES (4183555, 22, "Wilaya d'Adrar");
INSERT INTO regions VALUES (4183146, 22, "Wilaya d'Alger");
INSERT INTO regions VALUES (4183101, 22, "Wilaya d'Annaba");
INSERT INTO regions VALUES (4181560, 22, "Wilaya d'El Bayadh");
INSERT INTO regions VALUES (4181240, 22, "Wilaya d'El Oued");
INSERT INTO regions VALUES (4181218, 22, "<NAME>'<NAME>");
INSERT INTO regions VALUES (4180818, 22, "Wilaya d'Illizi");
INSERT INTO regions VALUES (4179241, 22, "Wilaya d'Oran");
INSERT INTO regions VALUES (4178702, 22, "Wilaya d'Oum el Bouaghi");
INSERT INTO regions VALUES (4183455, 22, "<NAME>");
INSERT INTO regions VALUES (4183280, 22, "<NAME>");
INSERT INTO regions VALUES (4182959, 22, "W<NAME>");
INSERT INTO regions VALUES (4182945, 22, "<NAME>");
INSERT INTO regions VALUES (4182930, 22, "Wilaya de Bejaïa");
INSERT INTO regions VALUES (4182667, 22, "Wilaya de Biskra");
INSERT INTO regions VALUES (4182653, 22, "Wilaya de Blida");
INSERT INTO regions VALUES (4182619, 22, "Wilaya de B<NAME>");
INSERT INTO regions VALUES (4182445, 22, "Wilaya de Bouira");
INSERT INTO regions VALUES (4182405, 22, "Wilaya de Boumerdes");
INSERT INTO regions VALUES (4182206, 22, "Wilaya de Chlef");
INSERT INTO regions VALUES (4182174, 22, "Wilaya de Constantine");
INSERT INTO regions VALUES (4182039, 22, "Wilaya de Djelfa");
INSERT INTO regions VALUES (4181083, 22, "Wilaya de Ghardaïa");
INSERT INTO regions VALUES (4181046, 22, "Wilaya de Guelma");
INSERT INTO regions VALUES (4180739, 22, "Wilaya de Jijel");
INSERT INTO regions VALUES (4180649, 22, "Wilaya de Khenchela");
INSERT INTO regions VALUES (4180578, 22, "Wilaya de Laghouat");
INSERT INTO regions VALUES (4179303, 22, "Wilaya de M'Sila");
INSERT INTO regions VALUES (4180409, 22, "Wilaya de Mascara");
INSERT INTO regions VALUES (4179510, 22, "Wilaya de Médéa");
INSERT INTO regions VALUES (4179382, 22, "Wilaya de Mila");
INSERT INTO regions VALUES (4179337, 22, "Wilaya de Mostaganem");
INSERT INTO regions VALUES (4179287, 22, "Wilaya de Naama");
INSERT INTO regions VALUES (4179223, 22, "Wilaya de Ouargla");
INSERT INTO regions VALUES (4178592, 22, "Wilaya de Relizane");
INSERT INTO regions VALUES (4178513, 22, "Wilaya de Saïda");
INSERT INTO regions VALUES (4178428, 22, "Wilaya de Sétif");
INSERT INTO regions VALUES (4178368, 22, "Wilaya de Sidi Bel Abbès");
INSERT INTO regions VALUES (4178219, 22, "Wilaya de Skikda");
INSERT INTO regions VALUES (4178185, 22, "Wilaya de Souk Ahras");
INSERT INTO regions VALUES (4177986, 22, "Wilaya de Tamanghasset");
INSERT INTO regions VALUES (4177843, 22, "Wilaya de Tébessa");
INSERT INTO regions VALUES (4177777, 22, "Wilaya de Tiaret");
INSERT INTO regions VALUES (4177706, 22, "Wilaya de Tindouf");
INSERT INTO regions VALUES (4177686, 22, "Wilaya de Tipaza");
INSERT INTO regions VALUES (4177667, 22, "Wilaya de Tissemsilt");
INSERT INTO regions VALUES (4177630, 22, "Wilaya de T<NAME>");
INSERT INTO regions VALUES (4177611, 22, "Wilaya de Tlemcen");
INSERT INTO regions VALUES (4611697, 25, "Província da Huíla");
INSERT INTO regions VALUES (3078804, 25, "Província da Lunda Norte");
INSERT INTO regions VALUES (3078803, 25, "Província da Lunda Sul");
INSERT INTO regions VALUES (4613698, 25, "Província de Benguela");
INSERT INTO regions VALUES (4024321, 25, "Província de Cabinda");
INSERT INTO regions VALUES (4022918, 25, "Província de Luanda");
INSERT INTO regions VALUES (4022745, 25, "Província de Malanje");
INSERT INTO regions VALUES (4610997, 25, "Província de Namibe");
INSERT INTO regions VALUES (4024471, 25, "Província do Bengo");
INSERT INTO regions VALUES (4613687, 25, "Província do Bié");
INSERT INTO regions VALUES (3315334, 25, "Província do Cuando Cubango");
INSERT INTO regions VALUES (4023576, 25, "Província do Cuanza Norte");
INSERT INTO regions VALUES (4612237, 25, "Província do Cuanza Sul");
INSERT INTO regions VALUES (4612183, 25, "Província do Cunene");
INSERT INTO regions VALUES (4611700, 25, "Província do Huambo");
INSERT INTO regions VALUES (3315145, 25, "Província do Moxico");
INSERT INTO regions VALUES (4020823, 25, "Província do Uíge");
INSERT INTO regions VALUES (4020724, 25, "Província do Zaire");
INSERT INTO regions VALUES (4455977, 26, "Parròquia d'Andorra la Vella");
INSERT INTO regions VALUES (4455914, 26, "Parròquia d'Encamp");
INSERT INTO regions VALUES (4607437, 26, "Parròquia d'Escaldes-Engordany");
INSERT INTO regions VALUES (4455825, 26, "Parròquia d'Ordino");
INSERT INTO regions VALUES (4455960, 26, "Parròquia de Canillo");
INSERT INTO regions VALUES (4455878, 26, "Parròquia de la Massana");
INSERT INTO regions VALUES (4455799, 26, "Parròquia de Sant Julià de Lòria");
INSERT INTO regions VALUES (4696394, 27, "Barbuda");
INSERT INTO regions VALUES (4696303, 27, "Parish of Saint George");
INSERT INTO regions VALUES (4696302, 27, "Parish of Saint John");
INSERT INTO regions VALUES (4696298, 27, "Parish of Saint Mary");
INSERT INTO regions VALUES (4696297, 27, "Parish of Saint Paul");
INSERT INTO regions VALUES (4696296, 27, "Parish of Saint Peter");
INSERT INTO regions VALUES (4696295, 27, "Parish of Saint Philip");
INSERT INTO regions VALUES (4637590, 28, "Distrito Federal");
INSERT INTO regions VALUES (4637893, 28, "Provincia de Buenos Aires");
INSERT INTO regions VALUES (4829490, 28, "Provincia de Catamarca");
INSERT INTO regions VALUES (4829080, 28, "Provincia de Córdoba");
INSERT INTO regions VALUES (4637721, 28, "Provincia de Corrientes");
INSERT INTO regions VALUES (4637622, 28, "Provincia de Entre Ríos");
INSERT INTO regions VALUES (4637574, 28, "Provincia de Formosa");
INSERT INTO regions VALUES (4827898, 28, "Provincia de Jujuy");
INSERT INTO regions VALUES (4827592, 28, "Provincia de La Pampa");
INSERT INTO regions VALUES (4827516, 28, "Provincia de La Rioja");
INSERT INTO regions VALUES (4826833, 28, "Provincia de Mendoza");
INSERT INTO regions VALUES (4637176, 28, "Provincia de Misiones");
INSERT INTO regions VALUES (1955670, 28, "Provincia de Río Negro");
INSERT INTO regions VALUES (4825789, 28, "Provincia de Salta");
INSERT INTO regions VALUES (4825592, 28, "Provincia de San Juan");
INSERT INTO regions VALUES (4825565, 28, "Provincia de San Luis");
INSERT INTO regions VALUES (4825431, 28, "Provincia de Santa Cruz");
INSERT INTO regions VALUES (4825424, 28, "Provincia de Santa Fe");
INSERT INTO regions VALUES (4825348, 28, "Provincia de Santiago del Estero");
INSERT INTO regions VALUES (4824930, 28, "Provincia de Tucumán");
INSERT INTO regions VALUES (4829422, 28, "Provincia del Chaco");
INSERT INTO regions VALUES (4829315, 28, "Provincia del Chubut");
INSERT INTO regions VALUES (4826658, 28, "Provincia del Neuquén");
INSERT INTO regions VALUES (4825064, 28, "Tierra del Fuego, Antártida e Islas del Atlántico Sur");
INSERT INTO regions VALUES (5308500, 29, "Aruba (general)");
INSERT INTO regions VALUES (3423229, 30, "Velāyat-e Badakhshān");
INSERT INTO regions VALUES (3423200, 30, "Velāyat-e Bādghīs");
INSERT INTO regions VALUES (3423063, 30, "Velāyat-e Baghlān");
INSERT INTO regions VALUES (3422888, 30, "Velāyat-e Balkh");
INSERT INTO regions VALUES (3422852, 30, "Velāyat-e Bāmīān");
INSERT INTO regions VALUES (3419449, 30, "Velāyat-e Farāh");
INSERT INTO regions VALUES (3419426, 30, "Velāyat-e Fāryāb");
INSERT INTO regions VALUES (3418822, 30, "Velāyat-e Ghaznī");
INSERT INTO regions VALUES (3418714, 30, "Velāyat-e Ghowr");
INSERT INTO regions VALUES (3417994, 30, "Velāyat-e Helmand");
INSERT INTO regions VALUES (3417982, 30, "Velāyat-e Herāt");
INSERT INTO regions VALUES (3417311, 30, "Velāyat-e Jowzjān");
INSERT INTO regions VALUES (3417236, 30, "Velāyat-e Kābol");
INSERT INTO regions VALUES (3416871, 30, "Velāyat-e Kandahār");
INSERT INTO regions VALUES (3416823, 30, "Velāyat-e Kāpīsā");
INSERT INTO regions VALUES (3415154, 30, "Velāyat-e Konar");
INSERT INTO regions VALUES (3415145, 30, "Velāyat-e Kondoz");
INSERT INTO regions VALUES (3414685, 30, "Velāyat-e Laghmān");
INSERT INTO regions VALUES (3414381, 30, "Velāyat-e Lowgar");
INSERT INTO regions VALUES (3412944, 30, "Velāyat-e Nangarhār");
INSERT INTO regions VALUES (3412568, 30, "Velāyat-e Nīmrūz");
INSERT INTO regions VALUES (3412304, 30, "Velāyat-e Orūzgān");
INSERT INTO regions VALUES (3412178, 30, "Velāyat-e Paktīā");
INSERT INTO regions VALUES (3412177, 30, "Velāyat-e Paktīkā");
INSERT INTO regions VALUES (3412053, 30, "Velāyat-e Parvān");
INSERT INTO regions VALUES (3409696, 30, "Velāyat-e Samangān");
INSERT INTO regions VALUES (3409242, 30, "Velāyat-e Sar-e Pol");
INSERT INTO regions VALUES (3406864, 30, "Velāyat-e Takhār");
INSERT INTO regions VALUES (3405996, 30, "Velāyat-e Vardak");
INSERT INTO regions VALUES (3405542, 30, "Velāyat-e Zābol");
INSERT INTO regions VALUES (3621884, 30, "Velāyat-e [provisional] Khowst");
INSERT INTO regions VALUES (3621885, 30, "Velāyat-e [provisional] Nūrestān");
INSERT INTO regions VALUES (1600001, 3, "Брестская область");
INSERT INTO regions VALUES (1600563, 3, "Витебская область");
INSERT INTO regions VALUES (1601201, 3, "Гомельская область");
INSERT INTO regions VALUES (1601806, 3, "Гродненская область");
INSERT INTO regions VALUES (1602269, 3, "Минская область");
INSERT INTO regions VALUES (1602923, 3, "Могилевская область");
INSERT INTO regions VALUES (4695480, 31, "Acklins and Crooked Islands District");
INSERT INTO regions VALUES (4695447, 31, "Bimini District");
INSERT INTO regions VALUES (4695419, 31, "Cat Island District");
INSERT INTO regions VALUES (4695375, 31, "Exuma District");
INSERT INTO regions VALUES (1902549, 31, "Freeport District");
INSERT INTO regions VALUES (4695359, 31, "Fresh Creek District");
INSERT INTO regions VALUES (4695346, 31, "Governor's Harbour District");
INSERT INTO regions VALUES (4695341, 31, "Green Turtle Cay District");
INSERT INTO regions VALUES (4695336, 31, "Harbour Island District");
INSERT INTO regions VALUES (4695322, 31, "High Rock District");
INSERT INTO regions VALUES (4695315, 31, "Inagua District");
INSERT INTO regions VALUES (4695309, 31, "Kemps Bay District");
INSERT INTO regions VALUES (4695299, 31, "Long Island District");
INSERT INTO regions VALUES (4695282, 31, "Marsh Harbour District");
INSERT INTO regions VALUES (4695277, 31, "Mayaguana District");
INSERT INTO regions VALUES (4695248, 31, "New Providence District");
INSERT INTO regions VALUES (4695246, 31, "Nichollstown and Berry Islands District");
INSERT INTO regions VALUES (4695221, 31, "Ragged Island District");
INSERT INTO regions VALUES (4695213, 31, "Rock Sound District");
INSERT INTO regions VALUES (4695200, 31, "San Salvador and Rum Cay District");
INSERT INTO regions VALUES (4695201, 31, "Sandy Point District");
INSERT INTO regions VALUES (3559040, 32, "Borishal");
INSERT INTO regions VALUES (3559019, 32, "Chittagong");
INSERT INTO regions VALUES (3558999, 32, "Dhaka Division");
INSERT INTO regions VALUES (3559029, 32, "Khulna Division");
INSERT INTO regions VALUES (3558986, 32, "Rājshāhi Division");
INSERT INTO regions VALUES (3634625, 32, "Sylhet Division");
INSERT INTO regions VALUES (4617570, 33, "<NAME>");
INSERT INTO regions VALUES (4617270, 33, "Saint Andrew");
INSERT INTO regions VALUES (4617266, 33, "Saint George");
INSERT INTO regions VALUES (4617265, 33, "Saint James");
INSERT INTO regions VALUES (4617264, 33, "Saint John");
INSERT INTO regions VALUES (4617263, 33, "Saint Joseph");
INSERT INTO regions VALUES (4617260, 33, "Saint Lucy");
INSERT INTO regions VALUES (4617255, 33, "Saint Michael");
INSERT INTO regions VALUES (4617253, 33, "Saint Peter");
INSERT INTO regions VALUES (4617252, 33, "Saint Philip");
INSERT INTO regions VALUES (4617251, 33, "Saint Thomas");
INSERT INTO regions VALUES (3138262, 34, "<NAME>ḩarraq");
INSERT INTO regions VALUES (5447246, 34, "Capital");
INSERT INTO regions VALUES (5447245, 34, "Central");
INSERT INTO regions VALUES (5447243, 34, "Northern");
INSERT INTO regions VALUES (5447244, 34, "Southern");
INSERT INTO regions VALUES (4698052, 35, "Belize District");
INSERT INTO regions VALUES (4698005, 35, "Cayo District");
INSERT INTO regions VALUES (4697973, 35, "Corozal District");
INSERT INTO regions VALUES (4697825, 35, "Orange Walk District");
INSERT INTO regions VALUES (4697691, 35, "Stann Creek District");
INSERT INTO regions VALUES (4697685, 35, "Toledo District");
INSERT INTO regions VALUES (4308109, 36, "Province de Liège");
INSERT INTO regions VALUES (4306644, 36, "Province de Namur");
INSERT INTO regions VALUES (4606525, 36, "Province du Brabant Wallon");
INSERT INTO regions VALUES (4311509, 36, "Province du Hainaut");
INSERT INTO regions VALUES (4307789, 36, "Province du Luxembourg");
INSERT INTO regions VALUES (4316213, 36, "Provincie Antwerpen");
INSERT INTO regions VALUES (4308061, 36, "Provincie Limburg");
INSERT INTO regions VALUES (4306059, 36, "Provincie Oost-Vlaanderen");
INSERT INTO regions VALUES (4606524, 36, "Provincie Vlaams-Brabant");
INSERT INTO regions VALUES (4301623, 36, "Provincie West-Vlaanderen");
INSERT INTO regions VALUES (4314504, 36, "Région de Bruxelles-Capitale");
INSERT INTO regions VALUES (4218590, 37, "Département de l' Alibori");
INSERT INTO regions VALUES (4131930, 37, "Département de l' Atakora");
INSERT INTO regions VALUES (4131918, 37, "Département de l' Atlantique");
INSERT INTO regions VALUES (4129711, 37, "Département de l' Ouémé");
INSERT INTO regions VALUES (4218593, 37, "Département de la Donga");
INSERT INTO regions VALUES (4131552, 37, "Département du Borgou");
INSERT INTO regions VALUES (4218591, 37, "Département du Collines");
INSERT INTO regions VALUES (4218592, 37, "Département du Kouffo");
INSERT INTO regions VALUES (4218594, 37, "Département du Littoral");
INSERT INTO regions VALUES (4129940, 37, "Département du Mono");
INSERT INTO regions VALUES (4218595, 37, "Département du Plateau");
INSERT INTO regions VALUES (4128614, 37, "Département du Zou");
INSERT INTO regions VALUES (4695507, 38, "Devonshire Parish");
INSERT INTO regions VALUES (4695506, 38, "Hamilton");
INSERT INTO regions VALUES (4695504, 38, "Hamilton Parish");
INSERT INTO regions VALUES (4695497, 38, "Paget Parish");
INSERT INTO regions VALUES (4695496, 38, "Pembroke Parish");
INSERT INTO regions VALUES (4695495, 38, "Saint George");
INSERT INTO regions VALUES (4695493, 38, "Saint George's Parish");
INSERT INTO regions VALUES (4695492, 38, "Sandys Parish");
INSERT INTO regions VALUES (4695490, 38, "Smith's Parish");
INSERT INTO regions VALUES (4695488, 38, "Southampton Parish");
INSERT INTO regions VALUES (4695483, 38, "Warwick Parish");
INSERT INTO regions VALUES (4845148, 40, "Departamento de Chuquisaca");
INSERT INTO regions VALUES (4845050, 40, "Departamento de Cochabamba");
INSERT INTO regions VALUES (4840003, 40, "Departamento de La Paz");
INSERT INTO regions VALUES (4838666, 40, "Departamento de Oruro");
INSERT INTO regions VALUES (4838347, 40, "Departamento de Pando");
INSERT INTO regions VALUES (4837875, 40, "Departamento de Potosí");
INSERT INTO regions VALUES (4836317, 40, "Departamento de Santa Cruz");
INSERT INTO regions VALUES (4835492, 40, "Departamento de Tarija");
INSERT INTO regions VALUES (4846673, 40, "Departamento del Beni");
INSERT INTO regions VALUES (3260328, 39, "Oblast Blagoevgrad");
INSERT INTO regions VALUES (3259977, 39, "<NAME>");
INSERT INTO regions VALUES (3254978, 39, "Oblast Dobrich");
INSERT INTO regions VALUES (3314320, 39, "<NAME>");
INSERT INTO regions VALUES (3258182, 39, "Oblast Khaskovo");
INSERT INTO regions VALUES (3314321, 39, "Oblast Kŭrdzhali");
INSERT INTO regions VALUES (3314322, 39, "Oblast Kyustendil");
INSERT INTO regions VALUES (3257491, 39, "<NAME>");
INSERT INTO regions VALUES (3210935, 39, "Oblast Montana");
INSERT INTO regions VALUES (3256538, 39, "Oblast Pazardzhik");
INSERT INTO regions VALUES (3256497, 39, "<NAME>");
INSERT INTO regions VALUES (3256391, 39, "Oblast Pleven");
INSERT INTO regions VALUES (3256383, 39, "Oblast Plovdiv");
INSERT INTO regions VALUES (3210934, 39, "Oblast Razgrad");
INSERT INTO regions VALUES (3255838, 39, "Oblast Ruse");
INSERT INTO regions VALUES (3314323, 39, "Oblast Shumen");
INSERT INTO regions VALUES (3314324, 39, "Oblast Silistra");
INSERT INTO regions VALUES (3314325, 39, "Oblast Sliven");
INSERT INTO regions VALUES (3314326, 39, "Oblast Smolyan");
INSERT INTO regions VALUES (3255429, 39, "Oblast Sofiya");
INSERT INTO regions VALUES (3258626, 39, "Oblast Sofiya-Grad");
INSERT INTO regions VALUES (3314327, 39, "Oblast Stara Zagora");
INSERT INTO regions VALUES (3314328, 39, "Oblast Tŭrgovishte");
INSERT INTO regions VALUES (3254701, 39, "Oblast Varna");
INSERT INTO regions VALUES (3314329, 39, "Oblast Veliko Tŭrnovo");
INSERT INTO regions VALUES (3314330, 39, "Oblast Vidin");
INSERT INTO regions VALUES (3254442, 39, "Oblast Vratsa");
INSERT INTO regions VALUES (3314331, 39, "Oblast Yambol");
INSERT INTO regions VALUES (4595537, 41, "Brčko District");
INSERT INTO regions VALUES (4574979, 41, "Federation of Bosnia and Herzegovina");
INSERT INTO regions VALUES (4574980, 41, "Republika Srpska");
INSERT INTO regions VALUES (3336334, 42, "Central District");
INSERT INTO regions VALUES (3336331, 42, "Chobe District");
INSERT INTO regions VALUES (3336301, 42, "Ghanzi District");
INSERT INTO regions VALUES (3336260, 42, "Kgalagadi District");
INSERT INTO regions VALUES (3336258, 42, "Kgatleng District");
INSERT INTO regions VALUES (3336222, 42, "Kweneng District");
INSERT INTO regions VALUES (3336057, 42, "North East District");
INSERT INTO regions VALUES (3336063, 42, "North West District");
INSERT INTO regions VALUES (3335972, 42, "South East District");
INSERT INTO regions VALUES (3335971, 42, "Southern District");
INSERT INTO regions VALUES (4651311, 43, "Distrito Federal");
INSERT INTO regions VALUES (4655525, 43, "Estado da Bahia");
INSERT INTO regions VALUES (4624629, 43, "Estado da Paraíba");
INSERT INTO regions VALUES (4633761, 43, "Estado de Alagoas");
INSERT INTO regions VALUES (4651547, 43, "Estado de Espírito Santo");
INSERT INTO regions VALUES (4650779, 43, "Estado de Goiás");
INSERT INTO regions VALUES (4648051, 43, "Estado de Mato Grosso");
INSERT INTO regions VALUES (4648050, 43, "Estado de Mato Grosso do Sul");
INSERT INTO regions VALUES (4647935, 43, "Estado de Minas Gerais");
INSERT INTO regions VALUES (4624131, 43, "Estado de Pernambuco");
INSERT INTO regions VALUES (4847534, 43, "Estado de Rondônia");
INSERT INTO regions VALUES (4743088, 43, "Estado de Roraima");
INSERT INTO regions VALUES (4644136, 43, "Estado de Santa Catarina");
INSERT INTO regions VALUES (4642892, 43, "Estado de São Paulo");
INSERT INTO regions VALUES (4642514, 43, "Estado de Sergipe");
INSERT INTO regions VALUES (4656777, 43, "Estado de Tocantins");
INSERT INTO regions VALUES (4744080, 43, "Estado do Acre");
INSERT INTO regions VALUES (4633530, 43, "Estado do Amapá");
INSERT INTO regions VALUES (4744050, 43, "Estado do Amazonas");
INSERT INTO regions VALUES (4630226, 43, "Estado do Ceará");
INSERT INTO regions VALUES (4625997, 43, "Estado do Maranhão");
INSERT INTO regions VALUES (4624645, 43, "Estado do Pará");
INSERT INTO regions VALUES (4646774, 43, "Estado do Parana");
INSERT INTO regions VALUES (4624095, 43, "Estado do Piauí");
INSERT INTO regions VALUES (4644590, 43, "Estado do Rio de Janeiro");
INSERT INTO regions VALUES (4622903, 43, "Estado do Rio Grande do Norte");
INSERT INTO regions VALUES (4644541, 43, "Estado do Rio Grande do Sul");
INSERT INTO regions VALUES (3812868, 44, "Belait District");
INSERT INTO regions VALUES (3812867, 44, "Brunei and Muara District");
INSERT INTO regions VALUES (3812650, 44, "Temburong District");
INSERT INTO regions VALUES (3812647, 44, "Tutong District");
INSERT INTO regions VALUES (4111062, 45, "Province d' Oubritenga");
INSERT INTO regions VALUES (4111061, 45, "Province de l' Oudalan");
INSERT INTO regions VALUES (4115446, 45, "Province de la Bougouriba");
INSERT INTO regions VALUES (4113025, 45, "Province de la Comoé");
INSERT INTO regions VALUES (4114145, 45, "Province de la Gnagna");
INSERT INTO regions VALUES (4218578, 45, "Province de la Komondjari");
INSERT INTO regions VALUES (4218579, 45, "Province de la Kompienga");
INSERT INTO regions VALUES (4112865, 45, "Province de la Kossi");
INSERT INTO regions VALUES (4218582, 45, "Province de la Léraba");
INSERT INTO regions VALUES (4109735, 45, "Province de la Sissili");
INSERT INTO regions VALUES (4109113, 45, "Province de la Tapoa");
INSERT INTO regions VALUES (4218571, 45, "Province des Balé");
INSERT INTO regions VALUES (4218572, 45, "Province des Banwa");
INSERT INTO regions VALUES (4116246, 45, "Province du Bam");
INSERT INTO regions VALUES (4115961, 45, "Province du Bazèga");
INSERT INTO regions VALUES (4115413, 45, "Province du Boulgou");
INSERT INTO regions VALUES (4115399, 45, "Province du Boulkiemdé");
INSERT INTO regions VALUES (4114223, 45, "Province du Ganzourgou");
INSERT INTO regions VALUES (4113882, 45, "Province du Gourma");
INSERT INTO regions VALUES (4113749, 45, "Province du Houet");
INSERT INTO regions VALUES (4218573, 45, "Province du Ioba");
INSERT INTO regions VALUES (4113622, 45, "Province du Kadiogo");
INSERT INTO regions VALUES (4113321, 45, "Province du Kénédougou");
INSERT INTO regions VALUES (4218580, 45, "Province du Koulpélogo");
INSERT INTO regions VALUES (4112579, 45, "Province du Kouritenga");
INSERT INTO regions VALUES (4218581, 45, "Province du Kourwéogo");
INSERT INTO regions VALUES (4218583, 45, "Province du Loroum");
INSERT INTO regions VALUES (4111849, 45, "Province du Mouhoun");
INSERT INTO regions VALUES (4111569, 45, "Province du Nahouri");
INSERT INTO regions VALUES (4111623, 45, "Province du Namentenga");
INSERT INTO regions VALUES (4218584, 45, "Province du Nayala");
INSERT INTO regions VALUES (4218585, 45, "Province du Noumbiel");
INSERT INTO regions VALUES (4110783, 45, "Province du Passore");
INSERT INTO regions VALUES (4110557, 45, "Province du Poni");
INSERT INTO regions VALUES (4110134, 45, "Province du Sanguié");
INSERT INTO regions VALUES (4110123, 45, "Province du Sanmatenga");
INSERT INTO regions VALUES (4109963, 45, "Province du Séno");
INSERT INTO regions VALUES (4109542, 45, "Province du Soum");
INSERT INTO regions VALUES (4109508, 45, "Province du Sourou");
INSERT INTO regions VALUES (4218586, 45, "Province du Tuy");
INSERT INTO regions VALUES (4218587, 45, "Province du Yagha");
INSERT INTO regions VALUES (4108098, 45, "Province du Yatenga");
INSERT INTO regions VALUES (4218588, 45, "Province du Ziro");
INSERT INTO regions VALUES (4218589, 45, "Province du Zondoma");
INSERT INTO regions VALUES (4107728, 45, "Province du Zoundwéogo");
INSERT INTO regions VALUES (3203107, 46, "iProvense ya Bubanza");
INSERT INTO regions VALUES (3202488, 46, "iProvense ya Bujumbura");
INSERT INTO regions VALUES (3202043, 46, "iProvense ya Bururi");
INSERT INTO regions VALUES (3203393, 46, "iProvense ya Cibitoke");
INSERT INTO regions VALUES (3202622, 46, "iProvense ya Gitega");
INSERT INTO regions VALUES (3203053, 46, "iProvense ya Karuzi");
INSERT INTO regions VALUES (3203508, 46, "iProvense ya Kayanza");
INSERT INTO regions VALUES (3203773, 46, "iProvense ya Kirundo");
INSERT INTO regions VALUES (3202501, 46, "iProvense ya Muramvya");
INSERT INTO regions VALUES (3203641, 46, "iProvense ya Muyinga");
INSERT INTO regions VALUES (3204623, 46, "iProvense ya Mwaro");
INSERT INTO regions VALUES (3204436, 46, "iProvense ya Rutana");
INSERT INTO regions VALUES (3202948, 46, "Province de Cankuzo");
INSERT INTO regions VALUES (3201796, 46, "Province de Makamba");
INSERT INTO regions VALUES (3203473, 46, "Province de Ngozi");
INSERT INTO regions VALUES (3202754, 46, "Province de Ruyigi");
INSERT INTO regions VALUES (3676731, 55, "An Giang");
INSERT INTO regions VALUES (3668470, 55, "Bà Rịa-Vũng Tàu");
INSERT INTO regions VALUES (3870277, 55, "Bắc Giang");
INSERT INTO regions VALUES (3870527, 55, "Bắc Kạn");
INSERT INTO regions VALUES (3870533, 55, "Bạc Liêu");
INSERT INTO regions VALUES (3870270, 55, "Bắc Ninh");
INSERT INTO regions VALUES (3670730, 55, "Bến Tre");
INSERT INTO regions VALUES (3870333, 55, "Bình Dương");
INSERT INTO regions VALUES (3870338, 55, "Bình Phước");
INSERT INTO regions VALUES (3666584, 55, "Bình Thuận");
INSERT INTO regions VALUES (3670664, 55, "Bình Định");
INSERT INTO regions VALUES (3870536, 55, "Cà Mau");
INSERT INTO regions VALUES (3666161, 55, "Can Tho Municipality");
INSERT INTO regions VALUES (3669461, 55, "Cao Bằng");
INSERT INTO regions VALUES (3870326, 55, "Da Nang Municipality");
INSERT INTO regions VALUES (3666091, 55, "Gia Lai");
INSERT INTO regions VALUES (3666039, 55, "Hà Giang");
INSERT INTO regions VALUES (3870495, 55, "Hà Nam");
INSERT INTO regions VALUES (3665818, 55, "Hà Tĩnh");
INSERT INTO regions VALUES (3870544, 55, "Hải Dương");
INSERT INTO regions VALUES (3666231, 55, "Haiphong Municipality");
INSERT INTO regions VALUES (3666121, 55, "Hanoi Municipality");
INSERT INTO regions VALUES (5420747, 55, "<NAME>");
INSERT INTO regions VALUES (1712200, 55, "Ho Chi Minh City");
INSERT INTO regions VALUES (3659788, 55, "<NAME>");
INSERT INTO regions VALUES (3870557, 55, "Hưng Yên");
INSERT INTO regions VALUES (3665163, 55, "<NAME>");
INSERT INTO regions VALUES (5470719, 55, "<NAME>");
INSERT INTO regions VALUES (3654237, 55, "<NAME>");
INSERT INTO regions VALUES (3663962, 55, "Lai Châu");
INSERT INTO regions VALUES (3663910, 55, "Lâm Đồng");
INSERT INTO regions VALUES (3662726, 55, "Lạng Sơn");
INSERT INTO regions VALUES (3652274, 55, "Lào Cai");
INSERT INTO regions VALUES (3662101, 55, "Long An");
INSERT INTO regions VALUES (3870484, 55, "Nam Định");
INSERT INTO regions VALUES (3650049, 55, "Nghệ An");
INSERT INTO regions VALUES (3650050, 55, "Ninh Bình");
INSERT INTO regions VALUES (3650051, 55, "Ninh Thuận");
INSERT INTO regions VALUES (3870435, 55, "Phú Thọ");
INSERT INTO regions VALUES (3657673, 55, "Phú Yên");
INSERT INTO regions VALUES (3656896, 55, "Quảng Bình");
INSERT INTO regions VALUES (3870374, 55, "Quảng Nam");
INSERT INTO regions VALUES (3656829, 55, "Quảng Ngãi");
INSERT INTO regions VALUES (3656819, 55, "Quảng Ninh");
INSERT INTO regions VALUES (3656796, 55, "Quảng Trị");
INSERT INTO regions VALUES (3650052, 55, "Sóc Trăng");
INSERT INTO regions VALUES (3656232, 55, "Sơn La");
INSERT INTO regions VALUES (3655479, 55, "Tây Ninh");
INSERT INTO regions VALUES (3655318, 55, "Thái Bình");
INSERT INTO regions VALUES (3870355, 55, "Thái Nguyên");
INSERT INTO regions VALUES (3655182, 55, "Thanh Hóa");
INSERT INTO regions VALUES (3654187, 55, "Thừa Thiên-Huế");
INSERT INTO regions VALUES (3653901, 55, "Tiền Giang");
INSERT INTO regions VALUES (3650053, 55, "Trà Vinh");
INSERT INTO regions VALUES (3650054, 55, "Tuyên Quang");
INSERT INTO regions VALUES (3650055, 55, "Vĩnh Long");
INSERT INTO regions VALUES (3652448, 55, "Vinh Phúc");
INSERT INTO regions VALUES (3650056, 55, "Yên Bái");
INSERT INTO regions VALUES (3668200, 55, "Đắk Lắk");
INSERT INTO regions VALUES (5420745, 55, "Đăk Nông");
INSERT INTO regions VALUES (5420744, 55, "Điện Biên");
INSERT INTO regions VALUES (3667166, 55, "Đồng Nai");
INSERT INTO regions VALUES (3667021, 55, "Đồng Tháp");
INSERT INTO regions VALUES (2200001, 6, "Арагацотнская");
INSERT INTO regions VALUES (2200123, 6, "Араратская");
INSERT INTO regions VALUES (2200222, 6, "Армавирская");
INSERT INTO regions VALUES (2200321, 6, "Вайоцдзорская");
INSERT INTO regions VALUES (2200367, 6, "Гехаркуникская");
INSERT INTO regions VALUES (2200465, 6, "Котайкская");
INSERT INTO regions VALUES (2200535, 6, "Лорийская");
INSERT INTO regions VALUES (2200663, 6, "Сюникская");
INSERT INTO regions VALUES (2200791, 6, "Тавушская");
INSERT INTO regions VALUES (2200857, 6, "Ширакская");
INSERT INTO regions VALUES (3998727, 48, "Ambrym");
INSERT INTO regions VALUES (3998710, 48, "Aoba/Maéwo");
INSERT INTO regions VALUES (3998602, 48, "Éfaté");
INSERT INTO regions VALUES (3998584, 48, "Épi");
INSERT INTO regions VALUES (3998169, 48, "Malakula");
INSERT INTO regions VALUES (4011633, 48, "Malampa Province");
INSERT INTO regions VALUES (3997950, 48, "Paama");
INSERT INTO regions VALUES (4011634, 48, "Penama Province");
INSERT INTO regions VALUES (3997915, 48, "Pentecôte");
INSERT INTO regions VALUES (3997805, 48, "Sanma Province");
INSERT INTO regions VALUES (4011635, 48, "Shefa Province");
INSERT INTO regions VALUES (3997790, 48, "Shepherd");
INSERT INTO regions VALUES (3997765, 48, "Tafea Province");
INSERT INTO regions VALUES (3998678, 48, "Torba Province");
INSERT INTO regions VALUES (5145349, 49, "England");
INSERT INTO regions VALUES (4233181, 49, "Northern Ireland");
INSERT INTO regions VALUES (4231395, 49, "Scotland");
INSERT INTO regions VALUES (4229320, 49, "Wales");
INSERT INTO regions VALUES (4731494, 51, "Dependencias Federales");
INSERT INTO regions VALUES (4731495, 51, "Distrito Federal");
INSERT INTO regions VALUES (4735768, 51, "Estado Amazonas");
INSERT INTO regions VALUES (4735728, 51, "Estado Anzoátegui");
INSERT INTO regions VALUES (4735711, 51, "Estado Apure");
INSERT INTO regions VALUES (4735702, 51, "Estado Aragua");
INSERT INTO regions VALUES (4735497, 51, "Estado Barinas");
INSERT INTO regions VALUES (4735261, 51, "Estado Bolívar");
INSERT INTO regions VALUES (4734600, 51, "Estado Carabobo");
INSERT INTO regions VALUES (4734037, 51, "Estado Cojedes");
INSERT INTO regions VALUES (4731508, 51, "Estado Falcón");
INSERT INTO regions VALUES (4731198, 51, "Estado Guárico");
INSERT INTO regions VALUES (4729237, 51, "Estado Lara");
INSERT INTO regions VALUES (4726926, 51, "Estado Mérida");
INSERT INTO regions VALUES (4726866, 51, "Estado Miranda");
INSERT INTO regions VALUES (4726828, 51, "Estado Monagas");
INSERT INTO regions VALUES (4726603, 51, "Estado Nueva Esparta");
INSERT INTO regions VALUES (4725892, 51, "Estado Portuguesa");
INSERT INTO regions VALUES (4723969, 51, "Estado Sucre");
INSERT INTO regions VALUES (4723928, 51, "Estado Táchira");
INSERT INTO regions VALUES (4723688, 51, "Estado Trujillo");
INSERT INTO regions VALUES (4824219, 51, "Estado Vargas");
INSERT INTO regions VALUES (4723377, 51, "Estado Yaracuy");
INSERT INTO regions VALUES (4723322, 51, "Estado Zulia");
INSERT INTO regions VALUES (4733684, 51, "Territorio Federal Delta Amacuro");
INSERT INTO regions VALUES (4782025, 57, "Département de l' Artibonite");
INSERT INTO regions VALUES (4773611, 57, "Département de l' Ouest");
INSERT INTO regions VALUES (4777400, 57, "Département de la Grand'Anse");
INSERT INTO regions VALUES (4779862, 57, "Département du Centre");
INSERT INTO regions VALUES (4773678, 57, "Département du Nord");
INSERT INTO regions VALUES (4773677, 57, "Département du Nord-Est");
INSERT INTO regions VALUES (4773674, 57, "Département du Nord-Ouest");
INSERT INTO regions VALUES (4771983, 57, "Département du Sud");
INSERT INTO regions VALUES (4771982, 57, "Département du Sud-Est");
INSERT INTO regions VALUES (4134943, 56, "Province de l' Estuaire");
INSERT INTO regions VALUES (4132786, 56, "Province de l' Ogooué-Ivindo");
INSERT INTO regions VALUES (4132785, 56, "Province de l' Ogooué-Lolo");
INSERT INTO regions VALUES (4132784, 56, "Province de l' Ogooué-Maritime");
INSERT INTO regions VALUES (4133109, 56, "Province de la Ngounié");
INSERT INTO regions VALUES (4132927, 56, "Province de la Nyanga");
INSERT INTO regions VALUES (4134818, 56, "Province du Haut-Ogooué");
INSERT INTO regions VALUES (4133308, 56, "Province du Moyen-Ogooué");
INSERT INTO regions VALUES (4132330, 56, "Province du Woleu-Ntem");
INSERT INTO regions VALUES (4618338, 58, "Barima-Waini Region");
INSERT INTO regions VALUES (4618286, 58, "Cuyuni-Mazaruni Region");
INSERT INTO regions VALUES (4618278, 58, "Demerara-Mahaica Region");
INSERT INTO regions VALUES (4618273, 58, "East Berbice-Corentyne Region");
INSERT INTO regions VALUES (4618262, 58, "Essequibo Islands-West Demerara Region");
INSERT INTO regions VALUES (4618143, 58, "Mahaica-Berbice Region");
INSERT INTO regions VALUES (4618060, 58, "Pomeroon-Supenaam Region");
INSERT INTO regions VALUES (4618056, 58, "Potaro-Siparuni Region");
INSERT INTO regions VALUES (4617971, 58, "Upper Demerara-Berbice Region");
INSERT INTO regions VALUES (4617969, 58, "Upper Takutu-Upper Essequibo Region");
INSERT INTO regions VALUES (4144527, 59, "Central River Division");
INSERT INTO regions VALUES (4145540, 59, "City of Banjul");
INSERT INTO regions VALUES (4144535, 59, "Lower River Division");
INSERT INTO regions VALUES (4144205, 59, "North Bank Division");
INSERT INTO regions VALUES (4143638, 59, "Upper River Division");
INSERT INTO regions VALUES (4143611, 59, "Western Division");
INSERT INTO regions VALUES (4066457, 60, "Ashanti Region");
INSERT INTO regions VALUES (4065296, 60, "Brong-Ahafo Region");
INSERT INTO regions VALUES (4065164, 60, "Central Region");
INSERT INTO regions VALUES (4064388, 60, "Eastern Region");
INSERT INTO regions VALUES (4063773, 60, "Greater Accra Region");
INSERT INTO regions VALUES (4061099, 60, "Northern Region");
INSERT INTO regions VALUES (4058918, 60, "Upper East Region");
INSERT INTO regions VALUES (4058915, 60, "Upper West Region");
INSERT INTO regions VALUES (4058875, 60, "Volta Region");
INSERT INTO regions VALUES (4058744, 60, "Western Region");
INSERT INTO regions VALUES (4151164, 63, "Capitale d'État-Zone Spéciale de Conakry");
INSERT INTO regions VALUES (4151553, 63, "Préfecture de Beyla");
INSERT INTO regions VALUES (4151456, 63, "Préfecture de Boffa");
INSERT INTO regions VALUES (4151427, 63, "Préfecture de Boké");
INSERT INTO regions VALUES (4217455, 63, "Préfecture de Coyah");
INSERT INTO regions VALUES (4151149, 63, "Préfecture de Dabola");
INSERT INTO regions VALUES (4151107, 63, "Préfecture de Dalaba");
INSERT INTO regions VALUES (4150758, 63, "Préfecture de Dinguiraye");
INSERT INTO regions VALUES (4150525, 63, "Préfecture de Dubréka");
INSERT INTO regions VALUES (4150346, 63, "Préfecture de Faranah");
INSERT INTO regions VALUES (4150118, 63, "Préfecture de Forécariah");
INSERT INTO regions VALUES (4150046, 63, "Préfecture de Fria");
INSERT INTO regions VALUES (4150004, 63, "Préfecture de Gaoual");
INSERT INTO regions VALUES (4149854, 63, "Préfecture de Guékédou");
INSERT INTO regions VALUES (4149485, 63, "Préfecture de Kankan");
INSERT INTO regions VALUES (4149228, 63, "Préfecture de Kérouané");
INSERT INTO regions VALUES (4149181, 63, "Préfecture de Kindia");
INSERT INTO regions VALUES (4149136, 63, "Préfecture de Kissidougou");
INSERT INTO regions VALUES (4217458, 63, "Préfecture de Koubia");
INSERT INTO regions VALUES (4148582, 63, "Préfecture de Koundara");
INSERT INTO regions VALUES (4148483, 63, "Préfecture de Kouroussa");
INSERT INTO regions VALUES (4148439, 63, "Préfecture de Labé");
INSERT INTO regions VALUES (4217459, 63, "Préfecture de Lélouma");
INSERT INTO regions VALUES (4217460, 63, "Préfecture de Lola");
INSERT INTO regions VALUES (4148216, 63, "Préfecture de Macenta");
INSERT INTO regions VALUES (4148141, 63, "Préfecture de Mali");
INSERT INTO regions VALUES (4148105, 63, "Préfecture de Mamou");
INSERT INTO regions VALUES (4217461, 63, "Préfecture de Mandiana");
INSERT INTO regions VALUES (4147531, 63, "Préfecture de Nzérékoré");
INSERT INTO regions VALUES (4147233, 63, "Préfecture de Pita");
INSERT INTO regions VALUES (4146735, 63, "Préfecture de Siguiri");
INSERT INTO regions VALUES (4146229, 63, "Préfecture de Télimélé");
INSERT INTO regions VALUES (4146001, 63, "Préfecture de Tougué");
INSERT INTO regions VALUES (4145696, 63, "Préfecture de Yomou");
INSERT INTO regions VALUES (4124399, 64, "Bafatá");
INSERT INTO regions VALUES (4124114, 64, "Biombo");
INSERT INTO regions VALUES (4124087, 64, "Bissau");
INSERT INTO regions VALUES (4124021, 64, "Bolama");
INSERT INTO regions VALUES (4123811, 64, "Cacheu");
INSERT INTO regions VALUES (4122830, 64, "Gabú");
INSERT INTO regions VALUES (4121968, 64, "Oio");
INSERT INTO regions VALUES (4121539, 64, "Quinara");
INSERT INTO regions VALUES (4120427, 64, "Tombali");
INSERT INTO regions VALUES (5325900, 61, "Région Guadeloupe");
INSERT INTO regions VALUES (4707583, 62, "Departamento de Alta Verapaz");
INSERT INTO regions VALUES (4707511, 62, "Departamento de Baja Verapaz");
INSERT INTO regions VALUES (4706984, 62, "Departamento de Chimaltenango");
INSERT INTO regions VALUES (4706919, 62, "Departamento de Chiquimula");
INSERT INTO regions VALUES (4705881, 62, "Departamento de El Progreso");
INSERT INTO regions VALUES (4705589, 62, "Departamento de Escuintla");
INSERT INTO regions VALUES (4705474, 62, "Departamento de Guatemala");
INSERT INTO regions VALUES (4705422, 62, "Departamento de Huehuetenango");
INSERT INTO regions VALUES (4705359, 62, "Departamento de Izabal");
INSERT INTO regions VALUES (4705348, 62, "Departamento de Jalapa");
INSERT INTO regions VALUES (4705257, 62, "Departamento de Jutiapa");
INSERT INTO regions VALUES (4703322, 62, "Departamento de Quetzaltenango");
INSERT INTO regions VALUES (4703264, 62, "Departamento de Retalhuleu");
INSERT INTO regions VALUES (4703158, 62, "Departamento de Sacatepéquez");
INSERT INTO regions VALUES (4702734, 62, "Departamento de San Marcos");
INSERT INTO regions VALUES (4702419, 62, "Departamento de Santa Rosa");
INSERT INTO regions VALUES (4702179, 62, "Departamento de Sololá");
INSERT INTO regions VALUES (4702159, 62, "Departamento de Suchitepéquez");
INSERT INTO regions VALUES (4701936, 62, "Departamento de Totonicapán");
INSERT INTO regions VALUES (4701588, 62, "Departamento de Zacapa");
INSERT INTO regions VALUES (4703578, 62, "Departamento del Petén");
INSERT INTO regions VALUES (4703314, 62, "Departamento del Quiché");
INSERT INTO regions VALUES (2722591, 67, "Choluteca");
INSERT INTO regions VALUES (4717970, 67, "Departamento de Atlántida");
INSERT INTO regions VALUES (4717140, 67, "Departamento de Choluteca");
INSERT INTO regions VALUES (4717033, 67, "Departamento de Colón");
INSERT INTO regions VALUES (4717021, 67, "Departamento de Comayagua");
INSERT INTO regions VALUES (4716964, 67, "Departamento de Copán");
INSERT INTO regions VALUES (4716904, 67, "Departamento de Cortés");
INSERT INTO regions VALUES (4715185, 67, "Departamento de El Paraíso");
INSERT INTO regions VALUES (4714125, 67, "Departamento de Gracias a Dios");
INSERT INTO regions VALUES (4713790, 67, "Departamento de Intibucá");
INSERT INTO regions VALUES (4713776, 67, "Departamento de Islas de la Bahía");
INSERT INTO regions VALUES (4711693, 67, "Departamento de Lempira");
INSERT INTO regions VALUES (4710522, 67, "Departamento de Ocotepeque");
INSERT INTO regions VALUES (4710470, 67, "Departamento de Olancho");
INSERT INTO regions VALUES (4708704, 67, "Departamento de Santa Bárbara");
INSERT INTO regions VALUES (4707925, 67, "Departamento de Valle");
INSERT INTO regions VALUES (4707763, 67, "Departamento de Yoro");
INSERT INTO regions VALUES (4714159, 67, "Departmento de Francisco Morazán");
INSERT INTO regions VALUES (4712624, 67, "Departmento de La Paz");
INSERT INTO regions VALUES (4697365, 69, "Saint Andrew");
INSERT INTO regions VALUES (4697361, 69, "Saint David");
INSERT INTO regions VALUES (4697359, 69, "Saint George");
INSERT INTO regions VALUES (4697356, 69, "Saint John");
INSERT INTO regions VALUES (4697352, 69, "Saint Mark");
INSERT INTO regions VALUES (4697349, 69, "Saint Patrick");
INSERT INTO regions VALUES (5470147, 71, "Attica Region");
INSERT INTO regions VALUES (5470153, 71, "Central Greece Region");
INSERT INTO regions VALUES (5470149, 71, "Central Macedonia Region");
INSERT INTO regions VALUES (5470159, 71, "Crete Region");
INSERT INTO regions VALUES (5470148, 71, "East Macedonia and Thrace Region");
INSERT INTO regions VALUES (5470150, 71, "Epirus Region");
INSERT INTO regions VALUES (5470156, 71, "Ionian Islands Region");
INSERT INTO regions VALUES (3132967, 71, "<NAME>");
INSERT INTO regions VALUES (3132976, 71, "Nomós <NAME> Akarnanías");
INSERT INTO regions VALUES (3132391, 71, "Nomós Argolídos");
INSERT INTO regions VALUES (3132370, 71, "Nomós Arkadías");
INSERT INTO regions VALUES (3132314, 71, "<NAME>");
INSERT INTO regions VALUES (3262186, 71, "Nomós Chalkidikís");
INSERT INTO regions VALUES (3129367, 71, "Nomós Chíou");
INSERT INTO regions VALUES (3131337, 71, "<NAME>");
INSERT INTO regions VALUES (3262582, 71, "Nomós Drámas");
INSERT INTO regions VALUES (3262526, 71, "<NAME>");
INSERT INTO regions VALUES (3131025, 71, "<NAME>");
INSERT INTO regions VALUES (3131022, 71, "<NAME>");
INSERT INTO regions VALUES (3262485, 71, "Nomós Florínis");
INSERT INTO regions VALUES (3130871, 71, "Nomós Fokídos");
INSERT INTO regions VALUES (3130793, 71, "Nomós Fthiótidos");
INSERT INTO regions VALUES (3262437, 71, "Nomós Grevenón");
INSERT INTO regions VALUES (3130583, 71, "Nomós Ileías");
INSERT INTO regions VALUES (3262414, 71, "Nomós Imathías");
INSERT INTO regions VALUES (3130571, 71, "Nomós Ioannínon");
INSERT INTO regions VALUES (3130075, 71, "Nomós Kardhítsas");
INSERT INTO regions VALUES (3262275, 71, "<NAME>");
INSERT INTO regions VALUES (3262220, 71, "<NAME>");
INSERT INTO regions VALUES (3129591, 71, "<NAME>");
INSERT INTO regions VALUES (4176187, 71, "<NAME>");
INSERT INTO regions VALUES (3262130, 71, "<NAME>");
INSERT INTO regions VALUES (3128974, 71, "<NAME>");
INSERT INTO regions VALUES (3262014, 71, "<NAME>");
INSERT INTO regions VALUES (3129269, 71, "<NAME>");
INSERT INTO regions VALUES (3128609, 71, "<NAME>");
INSERT INTO regions VALUES (3128553, 71, "<NAME>");
INSERT INTO regions VALUES (3128470, 71, "<NAME>");
INSERT INTO regions VALUES (3128484, 71, "<NAME>");
INSERT INTO regions VALUES (3128201, 71, "<NAME>");
INSERT INTO regions VALUES (3127716, 71, "Nomós Messinías");
INSERT INTO regions VALUES (3261368, 71, "<NAME>");
INSERT INTO regions VALUES (3261309, 71, "<NAME>");
INSERT INTO regions VALUES (3126151, 71, "<NAME>");
INSERT INTO regions VALUES (3261116, 71, "Nomós Rodópis");
INSERT INTO regions VALUES (3125821, 71, "<NAME>");
INSERT INTO regions VALUES (3261088, 71, "<NAME>");
INSERT INTO regions VALUES (3125114, 71, "<NAME>");
INSERT INTO regions VALUES (3260904, 71, "Nomós Thessaloníkis");
INSERT INTO regions VALUES (3125001, 71, "<NAME>");
INSERT INTO regions VALUES (3260763, 71, "<NAME>");
INSERT INTO regions VALUES (3124198, 71, "<NAME>");
INSERT INTO regions VALUES (5470157, 71, "North Aegean Region");
INSERT INTO regions VALUES (5470154, 71, "Peloponnese Region");
INSERT INTO regions VALUES (5470158, 71, "Southern Aegean Region");
INSERT INTO regions VALUES (5470152, 71, "Thessaly Region");
INSERT INTO regions VALUES (5470155, 71, "West Greece Region");
INSERT INTO regions VALUES (5470151, 71, "West Macedonia Region");
INSERT INTO regions VALUES (2400001, 7, "Абхазия");
INSERT INTO regions VALUES (2400037, 7, "Аджария");
INSERT INTO regions VALUES (2400074, 7, "Гурийский");
INSERT INTO regions VALUES (2400154, 7, "Имеретинский");
INSERT INTO regions VALUES (1927460, 7, "Кахетинский");
INSERT INTO regions VALUES (2400337, 7, "Квемо-Картлийский");
INSERT INTO regions VALUES (2400407, 7, "Мцхета-Мтианетский");
INSERT INTO regions VALUES (2400483, 7, "Рача-Лечхумский и Квемо-Сванетский");
INSERT INTO regions VALUES (2400542, 7, "Самегрело и Земо-Сванетский");
INSERT INTO regions VALUES (2400621, 7, "Самцхе-Джавахетский");
INSERT INTO regions VALUES (2400681, 7, "Шида-Картлийский");
INSERT INTO regions VALUES (5208152, 73, "Region Hovedstaden");
INSERT INTO regions VALUES (5208153, 73, "Region Midtjylland");
INSERT INTO regions VALUES (5208154, 73, "Region Nordjylland");
INSERT INTO regions VALUES (5208155, 73, "Region Sjæland");
INSERT INTO regions VALUES (5208156, 73, "Region Syddanmark");
INSERT INTO regions VALUES (3113861, 231, "Région d' Ali Sabieh");
INSERT INTO regions VALUES (3210378, 231, "Région d' Arta");
INSERT INTO regions VALUES (3113838, 231, "Région d' Obock");
INSERT INTO regions VALUES (3113852, 231, "Région de Dikhil");
INSERT INTO regions VALUES (3113834, 231, "Région de Tadjourah");
INSERT INTO regions VALUES (3113851, 231, "Ville de Djibouti");
INSERT INTO regions VALUES (4696191, 74, "Saint Andrew");
INSERT INTO regions VALUES (4696189, 74, "Saint David");
INSERT INTO regions VALUES (4696188, 74, "Saint George");
INSERT INTO regions VALUES (4696187, 74, "Saint John");
INSERT INTO regions VALUES (4696186, 74, "<NAME>");
INSERT INTO regions VALUES (4696184, 74, "<NAME>");
INSERT INTO regions VALUES (4696183, 74, "Saint Mark");
INSERT INTO regions VALUES (4696182, 74, "Saint Patrick");
INSERT INTO regions VALUES (4696181, 74, "<NAME>");
INSERT INTO regions VALUES (4696180, 74, "<NAME>");
INSERT INTO regions VALUES (4663639, 75, "Distrito Nacional");
INSERT INTO regions VALUES (4669940, 75, "Provincia de Azua");
INSERT INTO regions VALUES (4669896, 75, "Provincia de Baoruco");
INSERT INTO regions VALUES (4669894, 75, "Provincia de Barahona");
INSERT INTO regions VALUES (4668786, 75, "Provincia de Dajabón");
INSERT INTO regions VALUES (4667590, 75, "Provincia de El Seíbo");
INSERT INTO regions VALUES (4668075, 75, "Provincia de Elías Piña");
INSERT INTO regions VALUES (4667188, 75, "Provincia de Hato Mayor");
INSERT INTO regions VALUES (4667041, 75, "Provincia de Independencia");
INSERT INTO regions VALUES (4666813, 75, "Provincia de La Altagracia");
INSERT INTO regions VALUES (4665585, 75, "Provincia de La Romana");
INSERT INTO regions VALUES (4665150, 75, "Provincia de La Vega");
INSERT INTO regions VALUES (4663734, 75, "Provincia de Monseñor Nouel");
INSERT INTO regions VALUES (4663698, 75, "Provincia de Monte Cristi");
INSERT INTO regions VALUES (4663659, 75, "Provincia de Monte Plata");
INSERT INTO regions VALUES (4663348, 75, "Provincia de Pedernales");
INSERT INTO regions VALUES (4663312, 75, "Provincia de Peravia");
INSERT INTO regions VALUES (4663131, 75, "Provincia de Puerto Plata");
INSERT INTO regions VALUES (4662726, 75, "Provincia de Salcedo");
INSERT INTO regions VALUES (4662716, 75, "Provincia de Samaná");
INSERT INTO regions VALUES (4662694, 75, "Provincia de San Cristóbal");
INSERT INTO regions VALUES (5133579, 75, "Provincia de San José de Ocoa");
INSERT INTO regions VALUES (4662640, 75, "Provincia de San Juan");
INSERT INTO regions VALUES (4662615, 75, "Provincia de San Pedro de Macorís");
INSERT INTO regions VALUES (4662556, 75, "Provincia de Santiago");
INSERT INTO regions VALUES (4662552, 75, "Provincia de Santiago Rodríguez");
INSERT INTO regions VALUES (5133580, 75, "Provincia de Santo Domingo");
INSERT INTO regions VALUES (4662367, 75, "Provincia de Valverde");
INSERT INTO regions VALUES (4668717, 75, "Provincia Duarte");
INSERT INTO regions VALUES (4667463, 75, "Provincia Espaillat");
INSERT INTO regions VALUES (4663885, 75, "Provincia María Trinidad Sánchez");
INSERT INTO regions VALUES (4662696, 75, "Provincia <NAME>");
INSERT INTO regions VALUES (4742175, 221, "Provincia de Bolívar");
INSERT INTO regions VALUES (4741576, 221, "Provincia de Cotopaxi");
INSERT INTO regions VALUES (4741282, 221, "Provincia de El Oro");
INSERT INTO regions VALUES (4741137, 221, "Provincia de Esmeraldas");
INSERT INTO regions VALUES (4824216, 221, "Provincia de Francisco de Orellana");
INSERT INTO regions VALUES (4741069, 221, "Provincia de Galápagos");
INSERT INTO regions VALUES (4739172, 221, "Provincia de Imbabura");
INSERT INTO regions VALUES (4738548, 221, "Provincia de Loja");
INSERT INTO regions VALUES (4738495, 221, "Provincia de Los Ríos");
INSERT INTO regions VALUES (4738430, 221, "Provincia de Manabí");
INSERT INTO regions VALUES (4738209, 221, "Provincia de Morona Santiago");
INSERT INTO regions VALUES (4738167, 221, "Provincia de Napo");
INSERT INTO regions VALUES (4737868, 221, "Provincia de Pichincha");
INSERT INTO regions VALUES (4824215, 221, "Provincia de Sucumbíos");
INSERT INTO regions VALUES (4736069, 221, "Provincia de Zamora Chinchipe");
INSERT INTO regions VALUES (4742330, 221, "Provincia del Azuay");
INSERT INTO regions VALUES (4742044, 221, "Provincia del Cañar");
INSERT INTO regions VALUES (4741986, 221, "Provincia del Carchi");
INSERT INTO regions VALUES (4741773, 221, "Provincia del Chimborazo");
INSERT INTO regions VALUES (4740882, 221, "Provincia del Guayas");
INSERT INTO regions VALUES (4737935, 221, "Provincia del Pastaza");
INSERT INTO regions VALUES (4736288, 221, "Provincia del Tungurahua");
INSERT INTO regions VALUES (4070131, 222, "Provincia de Annobón");
INSERT INTO regions VALUES (4208752, 222, "Provincia de Bioko Norte");
INSERT INTO regions VALUES (4208753, 222, "Provincia de Bioko Sur");
INSERT INTO regions VALUES (4208754, 222, "Provincia de Centro Sur");
INSERT INTO regions VALUES (4208755, 222, "Provincia de Kié-Ntem");
INSERT INTO regions VALUES (4208756, 222, "Provincia de Litoral");
INSERT INTO regions VALUES (4208757, 222, "Provincia de Wele-Nzas");
INSERT INTO regions VALUES (3209834, 223, "Zoba Ānseba");
INSERT INTO regions VALUES (3209835, 223, "Zoba Debub");
INSERT INTO regions VALUES (3209836, 223, "Zoba Debubawī K'eyih Bahrī");
INSERT INTO regions VALUES (3209837, 223, "Zoba Gash Barka");
INSERT INTO regions VALUES (3209838, 223, "Zoba Ma'ākel");
INSERT INTO regions VALUES (3209839, 223, "Zoba Semēnawī K'eyih Bahrī");
INSERT INTO regions VALUES (1803175, 14, "Hiiu");
INSERT INTO regions VALUES (1803181, 14, "Ida-Viru");
INSERT INTO regions VALUES (1803215, 14, "Järva");
INSERT INTO regions VALUES (1803201, 14, "Jõgeva");
INSERT INTO regions VALUES (1803228, 14, "Lääne");
INSERT INTO regions VALUES (1803241, 14, "Lääne-Viru");
INSERT INTO regions VALUES (1803272, 14, "Pärnu");
INSERT INTO regions VALUES (1803257, 14, "Põlva");
INSERT INTO regions VALUES (1803293, 14, "Rapla");
INSERT INTO regions VALUES (1803304, 14, "Saare");
INSERT INTO regions VALUES (1803321, 14, "Tartu");
INSERT INTO regions VALUES (1803343, 14, "Valga");
INSERT INTO regions VALUES (1803357, 14, "Viljandi");
INSERT INTO regions VALUES (1803373, 14, "Võru");
INSERT INTO regions VALUES (1803151, 14, "Harju");
INSERT INTO regions VALUES (3207885, 224, "Ādīs Ābeba Āstedader");
INSERT INTO regions VALUES (3207886, 224, "Āfar Kilil");
INSERT INTO regions VALUES (3207887, 224, "Āmara Kilil");
INSERT INTO regions VALUES (3207888, 224, "Bīnshangul Gumuz Kilil");
INSERT INTO regions VALUES (3207889, 224, "Dirē Dawa Āstedader");
INSERT INTO regions VALUES (3207890, 224, "Gambēla Hizboch Kilil");
INSERT INTO regions VALUES (3207891, 224, "Hārerī Hizb Kilil");
INSERT INTO regions VALUES (3207892, 224, "Oromīya Kilil");
INSERT INTO regions VALUES (3207893, 224, "Sumalē Kilil");
INSERT INTO regions VALUES (3207894, 224, "Tigray Kilil");
INSERT INTO regions VALUES (3163784, 224, "Welega Kifle Hāger");
INSERT INTO regions VALUES (3207895, 224, "YeDebub Bihēroch Bihēreseboch na Hizboch Kilil");
INSERT INTO regions VALUES (3395905, 79, "Bulawayo Province");
INSERT INTO regions VALUES (3395906, 79, "Harare Province");
INSERT INTO regions VALUES (3317072, 79, "Manicaland Province");
INSERT INTO regions VALUES (3317049, 79, "Mashonaland Central Province");
INSERT INTO regions VALUES (3317048, 79, "Mashonaland East Province");
INSERT INTO regions VALUES (3317047, 79, "Mashonaland West Province");
INSERT INTO regions VALUES (3317042, 79, "Masvingo Province");
INSERT INTO regions VALUES (3317040, 79, "Matabeleland North Province");
INSERT INTO regions VALUES (3317039, 79, "Matabeleland South Province");
INSERT INTO regions VALUES (3316993, 79, "Midlands Province");
INSERT INTO regions VALUES (3329373, 77, "Central Province");
INSERT INTO regions VALUES (3327843, 77, "Copperbelt Province");
INSERT INTO regions VALUES (3327787, 77, "Eastern Province");
INSERT INTO regions VALUES (3324668, 77, "Luapula Province");
INSERT INTO regions VALUES (3324448, 77, "Lusaka Province");
INSERT INTO regions VALUES (3320289, 77, "North-Western Province");
INSERT INTO regions VALUES (3320290, 77, "Northern Province");
INSERT INTO regions VALUES (3318020, 77, "Southern Province");
INSERT INTO regions VALUES (3317673, 77, "Western Province");
INSERT INTO regions VALUES (5256014, 78, "Oued Ed-Dahab-Lagouira");
INSERT INTO regions VALUES (5458515, 89, "Amanat Al-Asemah");
INSERT INTO regions VALUES (3043443, 89, "Muḩāfaz̧at Abyan");
INSERT INTO regions VALUES (5133405, 89, "Muḩāfaz̧at aḑ Ḑāli`");
INSERT INTO regions VALUES (3043121, 89, "Muḩāfaz̧at al Bayḑā'");
INSERT INTO regions VALUES (3042776, 89, "Muḩāfaz̧at al Ḩudaydah");
INSERT INTO regions VALUES (3040009, 89, "Muḩāfaz̧at al Jawf");
INSERT INTO regions VALUES (3042443, 89, "Muḩāfaz̧at al Mahrah");
INSERT INTO regions VALUES (3039590, 89, "Muḩāfaz̧at al Maḩwīt");
INSERT INTO regions VALUES (5458511, 89, "Muḩāfaz̧at Dhamar");
INSERT INTO regions VALUES (3040486, 89, "Muḩāfaz̧at Ḩaḑramawt");
INSERT INTO regions VALUES (5133407, 89, "Muḩāfaz̧at Ḩajjah");
INSERT INTO regions VALUES (5133408, 89, "Muḩāfaz̧at Ibb");
INSERT INTO regions VALUES (5133409, 89, "Muḩāfaz̧at Laḩij");
INSERT INTO regions VALUES (5458507, 89, "Muḩāfaz̧at Ma'rib");
INSERT INTO regions VALUES (5458513, 89, "Muḩāfaz̧at Raymah");
INSERT INTO regions VALUES (5458509, 89, "Muḩāfaz̧at Sa'dah");
INSERT INTO regions VALUES (5458505, 89, "Muḩāfaz̧at Sana'a");
INSERT INTO regions VALUES (3038749, 89, "Muḩāfaz̧at Shabwah");
INSERT INTO regions VALUES (3038457, 89, "Muḩāfaz̧at Ta`izz");
INSERT INTO regions VALUES (3043435, 89, "Muḩāfaz̧at `Adan");
INSERT INTO regions VALUES (5133406, 89, "Muḩāfaz̧at `Amrān");
INSERT INTO regions VALUES (3207391, 82, "Jerash");
INSERT INTO regions VALUES (3124075, 82, "Muḩāfaz̧at al Balqā'");
INSERT INTO regions VALUES (3124025, 82, "Muḩāfaz̧at al Karak");
INSERT INTO regions VALUES (3124006, 82, "Muḩāfaz̧at al Mafraq");
INSERT INTO regions VALUES (3207392, 82, "Muḩāfaz̧at al `Aqabah");
INSERT INTO regions VALUES (3123881, 82, "Muḩāfaz̧at aţ Ţafīlah");
INSERT INTO regions VALUES (3123855, 82, "Muḩāfaz̧at az Zarqā'");
INSERT INTO regions VALUES (3123762, 82, "Muḩāfaz̧at Irbid");
INSERT INTO regions VALUES (3207393, 82, "Muḩāfaz̧at Mādabā");
INSERT INTO regions VALUES (3123625, 82, "Muḩāfaz̧at Ma`ān");
INSERT INTO regions VALUES (3207390, 82, "Muḩāfaz̧at `Ajlūn");
INSERT INTO regions VALUES (3123948, 82, "Muḩāfaz̧at `Ammān");
INSERT INTO regions VALUES (3079466, 95, "Famagusta District");
INSERT INTO regions VALUES (3079324, 95, "Kyrenia District");
INSERT INTO regions VALUES (3079315, 95, "Larnaca District");
INSERT INTO regions VALUES (3079301, 95, "Limassol District");
INSERT INTO regions VALUES (3079212, 95, "Nicosia District");
INSERT INTO regions VALUES (3079170, 95, "Paphos District");
INSERT INTO regions VALUES (3993104, 96, "Gilbert Islands District");
INSERT INTO regions VALUES (4904805, 96, "Line Islands District");
INSERT INTO regions VALUES (4617901, 90, "Concelho da Boa Vista");
INSERT INTO regions VALUES (4617896, 90, "Concelho da Brava");
INSERT INTO regions VALUES (4617732, 90, "Concelho da Praia");
INSERT INTO regions VALUES (4617713, 90, "Concelho da Ribeira Grande");
INSERT INTO regions VALUES (4617700, 90, "Concelho de Santa Catarina");
INSERT INTO regions VALUES (4635784, 90, "Concelho de Santa Cruz");
INSERT INTO regions VALUES (4635785, 90, "Concelho de São Domingos");
INSERT INTO regions VALUES (4635786, 90, "Concelho de São Filipe");
INSERT INTO regions VALUES (4635787, 90, "Concelho de São Miguel");
INSERT INTO regions VALUES (4617689, 90, "Concelho de São Nicolau");
INSERT INTO regions VALUES (4617687, 90, "Concelho de São Vicente");
INSERT INTO regions VALUES (4617793, 90, "Concelho do Maio");
INSERT INTO regions VALUES (4617754, 90, "Concelho do Paul");
INSERT INTO regions VALUES (4617705, 90, "Concelho do Sal");
INSERT INTO regions VALUES (4617676, 90, "Concelho do Tarrafal");
INSERT INTO regions VALUES (4635783, 90, "Concelho dos Mosteiros");
INSERT INTO regions VALUES (4617827, 90, "Fogo Concelho do");
INSERT INTO regions VALUES (1700002, 4, "Акмолинская область");
INSERT INTO regions VALUES (1700309, 4, "Актюбинская область");
INSERT INTO regions VALUES (1700503, 4, "Алма-Атинская область");
INSERT INTO regions VALUES (1700945, 4, "Атырауская область");
INSERT INTO regions VALUES (1701029, 4, "Восточно-Казахстанская область");
INSERT INTO regions VALUES (1701315, 4, "Жамбылская область");
INSERT INTO regions VALUES (1701514, 4, "Западно-Казахстанская область");
INSERT INTO regions VALUES (1701716, 4, "Карагандинская область");
INSERT INTO regions VALUES (1702235, 4, "Кзыл-Ординская область");
INSERT INTO regions VALUES (1701955, 4, "Кустанайская область");
INSERT INTO regions VALUES (1702346, 4, "Мангистауская область");
INSERT INTO regions VALUES (1702392, 4, "Павлодарская область");
INSERT INTO regions VALUES (1702577, 4, "Северо-Казахстанская область");
INSERT INTO regions VALUES (1702873, 4, "Южно-Казахстанская область");
INSERT INTO regions VALUES (3813049, 91, "<NAME>-Ŏ<NAME>");
INSERT INTO regions VALUES (3867312, 91, "<NAME>");
INSERT INTO regions VALUES (3812888, 91, "Khétt Bătdâmbâng");
INSERT INTO regions VALUES (3820672, 91, "<NAME>mpó<NAME>");
INSERT INTO regions VALUES (3820669, 91, "Khétt Kâmpóng Chhnăng");
INSERT INTO regions VALUES (3820654, 91, "Khétt Kâmpóng Spœ");
INSERT INTO regions VALUES (3820651, 91, "Khétt Kâmpóng Thum");
INSERT INTO regions VALUES (3820643, 91, "Khétt Kâmpôt");
INSERT INTO regions VALUES (3820638, 91, "Khétt Kândal");
INSERT INTO regions VALUES (3820631, 91, "Khétt Kaôh Kŏng");
INSERT INTO regions VALUES (3820474, 91, "<NAME>");
INSERT INTO regions VALUES (3820442, 91, "<NAME>");
INSERT INTO regions VALUES (3812881, 91, "<NAME>ŭthĭsăt");
INSERT INTO regions VALUES (3813127, 91, "Khétt Pre<NAME>ĭhéar");
INSERT INTO regions VALUES (3813102, 91, "Khé<NAME>");
INSERT INTO regions VALUES (3813090, 91, "<NAME>");
INSERT INTO regions VALUES (3813051, 91, "Khétt Siĕ<NAME>");
INSERT INTO regions VALUES (3813012, 91, "Khétt Stœ̆ng Trêng");
INSERT INTO regions VALUES (3813003, 91, "<NAME>ng");
INSERT INTO regions VALUES (3812994, 91, "Khétt Takêv");
INSERT INTO regions VALUES (3820409, 91, "Krŏng Phnum Pénh");
INSERT INTO regions VALUES (3867308, 91, "Krŏng Preăh Seihânŭ");
INSERT INTO regions VALUES (4020532, 92, "Adamaoua Province");
INSERT INTO regions VALUES (4018989, 92, "Centre Province");
INSERT INTO regions VALUES (4018047, 92, "East Province");
INSERT INTO regions VALUES (4017993, 92, "Far North Province");
INSERT INTO regions VALUES (4016675, 92, "Littoral Province");
INSERT INTO regions VALUES (4013810, 92, "Province du Nord");
INSERT INTO regions VALUES (4013809, 92, "Province du Nord-Ouest");
INSERT INTO regions VALUES (4012899, 92, "Province du Sud");
INSERT INTO regions VALUES (4012898, 92, "Province du Sud-Ouest");
INSERT INTO regions VALUES (4013472, 92, "West Province");
INSERT INTO regions VALUES (1953862, 10, "Alberta");
INSERT INTO regions VALUES (5122981, 10, "British Columbia");
INSERT INTO regions VALUES (5126967, 10, "Manitoba");
INSERT INTO regions VALUES (1910632, 10, "New Brunswick");
INSERT INTO regions VALUES (5165608, 10, "Newfoundland and Labrador");
INSERT INTO regions VALUES (5127822, 10, "Northwest Territories");
INSERT INTO regions VALUES (5127854, 10, "Nova Scotia");
INSERT INTO regions VALUES (5127862, 10, "Nunavut");
INSERT INTO regions VALUES (1711248, 10, "Ontario");
INSERT INTO regions VALUES (1932549, 10, "Prince Edward Island");
INSERT INTO regions VALUES (5164658, 10, "Québec");
INSERT INTO regions VALUES (5129212, 10, "Saskatchewan");
INSERT INTO regions VALUES (5130807, 10, "Yukon");
INSERT INTO regions VALUES (3190858, 93, "Baladīyat ad Dawḩah");
INSERT INTO regions VALUES (3190853, 93, "Baladīyat al Ghuwayrīyah");
INSERT INTO regions VALUES (3190856, 93, "Baladīyat al Jumaylīyah");
INSERT INTO regions VALUES (3190854, 93, "Baladīyat al Khawr");
INSERT INTO regions VALUES (3190859, 93, "Baladīyat al Wakrah");
INSERT INTO regions VALUES (3190857, 93, "Baladīyat ar Rayyān");
INSERT INTO regions VALUES (3190860, 93, "Baladīyat Jarayān al Bāţinah");
INSERT INTO regions VALUES (3190852, 93, "Baladīyat Madīnat ash Shamāl");
INSERT INTO regions VALUES (3190855, 93, "Baladīyat Umm Şalāl");
INSERT INTO regions VALUES (5133403, 93, "Baladīyat Umm Sa`īd");
INSERT INTO regions VALUES (3192688, 94, "Central Province");
INSERT INTO regions VALUES (3192686, 94, "Coast Province");
INSERT INTO regions VALUES (3192687, 94, "Eastern Province");
INSERT INTO regions VALUES (3095066, 94, "Nairobi Area");
INSERT INTO regions VALUES (3192691, 94, "North Eastern Province");
INSERT INTO regions VALUES (3094746, 94, "Nyanza Province");
INSERT INTO regions VALUES (3192690, 94, "Rift Valley Province");
INSERT INTO regions VALUES (3192689, 94, "Western Province");
INSERT INTO regions VALUES (2300001, 11, "Баткенская");
INSERT INTO regions VALUES (2300050, 11, "Джалал-Абадская");
INSERT INTO regions VALUES (1703239, 11, "Иссык-Кульская");
INSERT INTO regions VALUES (2300227, 11, "Нарынская");
INSERT INTO regions VALUES (2300290, 11, "Ошская");
INSERT INTO regions VALUES (2300445, 11, "Таласская");
INSERT INTO regions VALUES (2300474, 11, "Чуйская");
INSERT INTO regions VALUES (3811422, 97, "Anhui Province");
INSERT INTO regions VALUES (3965211, 97, "Beijing Municipality");
INSERT INTO regions VALUES (2763, 97, "Chongqing Municipality");
INSERT INTO regions VALUES (3805562, 97, "Fujian Province");
INSERT INTO regions VALUES (3805271, 97, "Gansu Province");
INSERT INTO regions VALUES (3804600, 97, "Guangdong Province");
INSERT INTO regions VALUES (3804541, 97, "Guangxi Zhuang Autonomous Region");
INSERT INTO regions VALUES (3804172, 97, "Guizhou Province");
INSERT INTO regions VALUES (3803831, 97, "Hainan Province");
INSERT INTO regions VALUES (3803590, 97, "Hebei Province");
INSERT INTO regions VALUES (3963941, 97, "Heilongjiang Province");
INSERT INTO regions VALUES (3803370, 97, "Henan Province");
INSERT INTO regions VALUES (3802020, 97, "Hubei Province");
INSERT INTO regions VALUES (3801809, 97, "Hunan Province");
INSERT INTO regions VALUES (3962719, 97, "Inner Mongolia Autonomous Region");
INSERT INTO regions VALUES (3801439, 97, "Jiangsu Province");
INSERT INTO regions VALUES (3801404, 97, "Jiangxi Province");
INSERT INTO regions VALUES (3963518, 97, "Jilin Province");
INSERT INTO regions VALUES (1704222, 97, "Liaoning Province");
INSERT INTO regions VALUES (3795499, 97, "Ningxia Huizu Autonomous Region");
INSERT INTO regions VALUES (3514972, 97, "Qinghai Province");
INSERT INTO regions VALUES (3793064, 97, "Shaanxi Province");
INSERT INTO regions VALUES (3792937, 97, "Shandong Province");
INSERT INTO regions VALUES (3792848, 97, "Shanghai Municipality");
INSERT INTO regions VALUES (3792558, 97, "Shanxi Province");
INSERT INTO regions VALUES (3791125, 97, "Sichuan Province");
INSERT INTO regions VALUES (3789910, 97, "Tianjin Municipality");
INSERT INTO regions VALUES (3514517, 97, "Tibet Autonomous Region");
INSERT INTO regions VALUES (3638211, 97, "Xinjiang Uygur Autonomous Region");
INSERT INTO regions VALUES (3783629, 97, "Yunnan Province");
INSERT INTO regions VALUES (3782793, 97, "Zhejiang Province");
INSERT INTO regions VALUES (1969571, 98, "Chita");
INSERT INTO regions VALUES (4747536, 98, "Departamemnto del Putumayo");
INSERT INTO regions VALUES (4759073, 98, "Departamento de Antioquia");
INSERT INTO regions VALUES (4759013, 98, "Departamento de Arauca");
INSERT INTO regions VALUES (4758352, 98, "Departamento de Bolívar");
INSERT INTO regions VALUES (4758290, 98, "Departamento de Boyacá");
INSERT INTO regions VALUES (4757937, 98, "Departamento de Caldas");
INSERT INTO regions VALUES (4757461, 98, "Departamento de Casanare");
INSERT INTO regions VALUES (4756773, 98, "Departamento de Córdoba");
INSERT INTO regions VALUES (4756526, 98, "Departamento de Cundinamarca");
INSERT INTO regions VALUES (4752225, 98, "Departamento de La Guajira");
INSERT INTO regions VALUES (4749231, 98, "Departamento de Nariño");
INSERT INTO regions VALUES (4749148, 98, "Departamento de Norte de Santander");
INSERT INTO regions VALUES (4747271, 98, "Departamento de Risaralda");
INSERT INTO regions VALUES (4745812, 98, "Departamento de Santander");
INSERT INTO regions VALUES (4745297, 98, "Departamento de Sucre");
INSERT INTO regions VALUES (4744896, 98, "Departamento de Tolima");
INSERT INTO regions VALUES (4759156, 98, "Departamento del Amazonas");
INSERT INTO regions VALUES (4758844, 98, "Departamento del Atlántico");
INSERT INTO regions VALUES (4757648, 98, "Departamento del Caquetá");
INSERT INTO regions VALUES (4757371, 98, "Departamento del Cauca");
INSERT INTO regions VALUES (4757286, 98, "Departamento del Cesar");
INSERT INTO regions VALUES (4757059, 98, "Departamento del Chocó");
INSERT INTO regions VALUES (4754017, 98, "Departamento del Guainía");
INSERT INTO regions VALUES (4753873, 98, "Departamento del Guaviare");
INSERT INTO regions VALUES (4753492, 98, "Departamento del Huila");
INSERT INTO regions VALUES (4750103, 98, "Departamento del Magdalena");
INSERT INTO regions VALUES (4749642, 98, "Departamento del Meta");
INSERT INTO regions VALUES (4747489, 98, "Departamento del Quindío");
INSERT INTO regions VALUES (4744569, 98, "Departamento del Valle del Cauca");
INSERT INTO regions VALUES (4744535, 98, "Departamento del Vaupés");
INSERT INTO regions VALUES (4744436, 98, "Departamento del Vichada");
INSERT INTO regions VALUES (4758380, 98, "Distrito Capital de Bogotá");
INSERT INTO regions VALUES (4748543, 98, "Pasto");
INSERT INTO regions VALUES (4746998, 98, "Providencia y Santa Catalina, Departamento de Archipiélago de Sa");
INSERT INTO regions VALUES (3329820, 99, "Anjouan");
INSERT INTO regions VALUES (3329763, 99, "Grande Comore");
INSERT INTO regions VALUES (3329715, 99, "Mayotte");
INSERT INTO regions VALUES (3329694, 99, "Mohéli");
INSERT INTO regions VALUES (4211427, 100, "Commune de Brazzaville");
INSERT INTO regions VALUES (4037172, 100, "Région de la Bouenza");
INSERT INTO regions VALUES (4037067, 100, "Région de la Cuvette");
INSERT INTO regions VALUES (4215488, 100, "Région de la Cuvette-Ouest");
INSERT INTO regions VALUES (4035809, 100, "Région de la Lékoumou");
INSERT INTO regions VALUES (4035768, 100, "Région de la Likouala");
INSERT INTO regions VALUES (4033964, 100, "Région de la Sangha");
INSERT INTO regions VALUES (4034027, 100, "Région des Plateaux");
INSERT INTO regions VALUES (4035914, 100, "Région du Kouilou");
INSERT INTO regions VALUES (4034548, 100, "Région du Niari");
INSERT INTO regions VALUES (4034020, 100, "Région du Pool");
INSERT INTO regions VALUES (3106760, 101, "Kivu Région du");
INSERT INTO regions VALUES (4076196, 101, "Province de Bandundu");
INSERT INTO regions VALUES (3110439, 101, "Province de l' Équateur");
INSERT INTO regions VALUES (4076081, 101, "Province du Bas-Congo");
INSERT INTO regions VALUES (3108231, 101, "Province du Kasaï-Occidental");
INSERT INTO regions VALUES (3108230, 101, "Province du Kasaï-Oriental");
INSERT INTO regions VALUES (3101542, 101, "Province du Katanga");
INSERT INTO regions VALUES (3104812, 101, "Province du Maniema");
INSERT INTO regions VALUES (3102568, 101, "Province du Nord-Kivu");
INSERT INTO regions VALUES (3101280, 101, "Province du Sud-Kivu");
INSERT INTO regions VALUES (3110014, 101, "Province Orientale");
INSERT INTO regions VALUES (4073380, 101, "Ville de Kinshasa");
INSERT INTO regions VALUES (4723287, 102, "Provincia de Alajuela");
INSERT INTO regions VALUES (4722944, 102, "Provincia de Cartago");
INSERT INTO regions VALUES (4722524, 102, "Provincia de Guanacaste");
INSERT INTO regions VALUES (4722457, 102, "Provincia de Heredia");
INSERT INTO regions VALUES (4722252, 102, "Provincia de Limón");
INSERT INTO regions VALUES (4721742, 102, "Provincia de Puntarenas");
INSERT INTO regions VALUES (4721464, 102, "Provincia de San José");
INSERT INTO regions VALUES (4218628, 103, "Région de l' Agnéby");
INSERT INTO regions VALUES (4218642, 103, "Région de la Marahoué");
INSERT INTO regions VALUES (4218631, 103, "Région de la Vallée du Bandama");
INSERT INTO regions VALUES (4218633, 103, "Région des Dix-Huit Montagnes");
INSERT INTO regions VALUES (4218634, 103, "Région des Lacs");
INSERT INTO regions VALUES (4218626, 103, "Région des Lagunes");
INSERT INTO regions VALUES (4218630, 103, "Région des Savanes");
INSERT INTO regions VALUES (4218644, 103, "Région du Bafing");
INSERT INTO regions VALUES (4218636, 103, "Région du Bas-Sassandra");
INSERT INTO regions VALUES (4218638, 103, "Région du Denguélé");
INSERT INTO regions VALUES (4218640, 103, "Région du Fromager");
INSERT INTO regions VALUES (4218629, 103, "Région du Haut-Sassandra");
INSERT INTO regions VALUES (4218643, 103, "Région du Moyen-Cavally");
INSERT INTO regions VALUES (4218632, 103, "Région du Moyen-Comoé");
INSERT INTO regions VALUES (4218641, 103, "Région du N'zi-Comoé");
INSERT INTO regions VALUES (4218639, 103, "Région du Sud-Bandama");
INSERT INTO regions VALUES (4218627, 103, "Région du Sud-Comoé");
INSERT INTO regions VALUES (4218637, 103, "Région du Worodougou");
INSERT INTO regions VALUES (4218635, 103, "Région du Zanzan");
INSERT INTO regions VALUES (4691615, 104, "Municipio Especial Isla de la Juventud");
INSERT INTO regions VALUES (4693775, 104, "Provincia de Camagüey");
INSERT INTO regions VALUES (4693400, 104, "Provincia de Ciego de Ávila");
INSERT INTO regions VALUES (4693386, 104, "Provincia de Cienfuegos");
INSERT INTO regions VALUES (4693369, 104, "Provincia de Ciudad de La Habana");
INSERT INTO regions VALUES (4691844, 104, "Provincia de Guantánamo");
INSERT INTO regions VALUES (4691683, 104, "Provincia de Holguín");
INSERT INTO regions VALUES (4690848, 104, "Provincia de La Habana");
INSERT INTO regions VALUES (4690101, 104, "Provincia de Las Tunas");
INSERT INTO regions VALUES (4689352, 104, "Provincia de Matanzas");
INSERT INTO regions VALUES (4688635, 104, "Provincia de Pinar del Río");
INSERT INTO regions VALUES (4687765, 104, "Provincia de Sancti Spíritus");
INSERT INTO regions VALUES (4686793, 104, "Provincia de Santiago de Cuba");
INSERT INTO regions VALUES (4686244, 104, "Provincia de Villa Clara");
INSERT INTO regions VALUES (4691925, 104, "Provincia Granma");
INSERT INTO regions VALUES (3136802, 105, "Muḩāfaz̧at al Aḩmadī");
INSERT INTO regions VALUES (3136797, 105, "Muḩāfaz̧at al Farwānīyah");
INSERT INTO regions VALUES (3136786, 105, "Muḩāfaz̧at al `Āşimah");
INSERT INTO regions VALUES (3136756, 105, "Muḩāfaz̧at Ḩawallī");
INSERT INTO regions VALUES (3136788, 105, "Muḩāfaz̧at Jahrā'");
INSERT INTO regions VALUES (3136777, 105, "Muḩāfaz̧atalWafrah");
INSERT INTO regions VALUES (4049058, 108, "Bomi County");
INSERT INTO regions VALUES (4049028, 108, "Bong County");
INSERT INTO regions VALUES (4215489, 108, "Gbarpolu County");
INSERT INTO regions VALUES (4047749, 108, "Grand Bassa County");
INSERT INTO regions VALUES (4047748, 108, "Grand Cape Mount County");
INSERT INTO regions VALUES (4047745, 108, "Grand Gedeh County");
INSERT INTO regions VALUES (4213213, 108, "Grand Kru County");
INSERT INTO regions VALUES (4046689, 108, "Lofa County");
INSERT INTO regions VALUES (4213214, 108, "Margibi County");
INSERT INTO regions VALUES (4046526, 108, "Maryland County");
INSERT INTO regions VALUES (4046369, 108, "Montserrado County");
INSERT INTO regions VALUES (4046210, 108, "Nimba County");
INSERT INTO regions VALUES (4213215, 108, "River Cess County");
INSERT INTO regions VALUES (4215490, 108, "River Gee County");
INSERT INTO regions VALUES (4045584, 108, "Sinoe County");
INSERT INTO regions VALUES (5466163, 110, "Baladiyah Al Boutnan البطنان");
INSERT INTO regions VALUES (5466164, 110, "Baladiyah Al Djabal al Akhdar الجبل الاخضر");
INSERT INTO regions VALUES (5466177, 110, "Baladiyah Al Djabal al Gharbi الجبل الغربي");
INSERT INTO regions VALUES (5466174, 110, "Baladiyah Al Djfara الجفارة");
INSERT INTO regions VALUES (5466178, 110, "Baladiyah Al Djoufrah الجفرة");
INSERT INTO regions VALUES (5466168, 110, "Baladiyah Al Koufrah الكفرة");
INSERT INTO regions VALUES (5466165, 110, "Baladiyah Al Marj المرج");
INSERT INTO regions VALUES (5466169, 110, "Baladiyah Al Mourqoub المرقب");
INSERT INTO regions VALUES (5466167, 110, "Baladiyah Al Wahat الواحات");
INSERT INTO regions VALUES (5466176, 110, "Baladiyah An Nouqat al Khams النقاط الخمس");
INSERT INTO regions VALUES (5466175, 110, "Baladiyah Az Zaouiyah الزاوية");
INSERT INTO regions VALUES (5466166, 110, "Baladiyah Benghazi بنغازي");
INSERT INTO regions VALUES (5466162, 110, "Baladiyah Darnah درنة");
INSERT INTO regions VALUES (5466170, 110, "Baladiyah Ghat غات");
INSERT INTO regions VALUES (5466171, 110, "Baladiyah Misratah مصراتة");
INSERT INTO regions VALUES (5466183, 110, "<NAME> مرزق");
INSERT INTO regions VALUES (5466172, 110, "<NAME> نالوت");
INSERT INTO regions VALUES (5466179, 110, "<NAME> سبها");
INSERT INTO regions VALUES (5466180, 110, "<NAME> سرت");
INSERT INTO regions VALUES (5466173, 110, "<NAME>i طرابلس");
INSERT INTO regions VALUES (5466182, 110, "<NAME> ach Chatii وادي الشاطئ");
INSERT INTO regions VALUES (5466181, 110, "<NAME> al Hayaat وادي الحياة");
INSERT INTO regions VALUES (5133577, 109, "Mohafazat Aakâr");
INSERT INTO regions VALUES (5133578, 109, "Mohafazat Baalbek-Hermel");
INSERT INTO regions VALUES (3135850, 109, "Mohafazat Béqaa");
INSERT INTO regions VALUES (3134870, 109, "Mohafazat Beyrouth");
INSERT INTO regions VALUES (3135210, 109, "Mohafazat Liban-Nord");
INSERT INTO regions VALUES (3135699, 109, "Mohafazat Liban-Sud");
INSERT INTO regions VALUES (3134180, 109, "Mohafazat Mont-Liban");
INSERT INTO regions VALUES (3207896, 109, "Mohafazat Nabatîyé");
INSERT INTO regions VALUES (4456089, 111, "Gemeinde Balzers");
INSERT INTO regions VALUES (4456086, 111, "<NAME>");
INSERT INTO regions VALUES (4456084, 111, "<NAME>");
INSERT INTO regions VALUES (4456080, 111, "<NAME>");
INSERT INTO regions VALUES (4456076, 111, "<NAME>");
INSERT INTO regions VALUES (4456074, 111, "<NAME>");
INSERT INTO regions VALUES (4456072, 111, "<NAME>");
INSERT INTO regions VALUES (4456069, 111, "<NAME>");
INSERT INTO regions VALUES (4456067, 111, "<NAME>");
INSERT INTO regions VALUES (4456065, 111, "<NAME>");
INSERT INTO regions VALUES (4456063, 111, "<NAME>");
INSERT INTO regions VALUES (3869765, 106, "<NAME>");
INSERT INTO regions VALUES (3869766, 106, "<NAME>");
INSERT INTO regions VALUES (3720411, 106, "<NAME>");
INSERT INTO regions VALUES (3869763, 106, "Khouè<NAME>");
INSERT INTO regions VALUES (3869764, 106, "Khouèng Bolikhamxai");
INSERT INTO regions VALUES (3713355, 106, "Khouèng Champasak");
INSERT INTO regions VALUES (3713254, 106, "Khouèng Houaphan");
INSERT INTO regions VALUES (3713168, 106, "Khouèng Khammouan");
INSERT INTO regions VALUES (3713015, 106, "Khouèng Louangnamtha");
INSERT INTO regions VALUES (3713012, 106, "Khouèng Louangphrabang");
INSERT INTO regions VALUES (3712688, 106, "Khouèng Oudômxai");
INSERT INTO regions VALUES (3712593, 106, "Khouèng Phôngsali");
INSERT INTO regions VALUES (3712480, 106, "Khouèng Salavan");
INSERT INTO regions VALUES (3712476, 106, "Khouèng Savannakhét");
INSERT INTO regions VALUES (3712203, 106, "Khouèng Viangchan");
INSERT INTO regions VALUES (3712196, 106, "Khouèng Xaignabouli");
INSERT INTO regions VALUES (3869762, 106, "Khouèng Xékong");
INSERT INTO regions VALUES (3712179, 106, "Khouèng Xiangkhoang");
INSERT INTO regions VALUES (2607558, 12, "Ādažu novads");
INSERT INTO regions VALUES (5422548, 12, "Aglonas novads");
INSERT INTO regions VALUES (5422601, 12, "Aizkraukles novads");
INSERT INTO regions VALUES (5422602, 12, "Aizputes novads");
INSERT INTO regions VALUES (5422603, 12, "Aknīstes novads");
INSERT INTO regions VALUES (5422604, 12, "Alojas novads");
INSERT INTO regions VALUES (5422605, 12, "Alsungas novads");
INSERT INTO regions VALUES (5422606, 12, "Alūksnes novads");
INSERT INTO regions VALUES (2601206, 12, "Amatas novads");
INSERT INTO regions VALUES (5422607, 12, "Apes novads");
INSERT INTO regions VALUES (5422608, 12, "Auces novads");
INSERT INTO regions VALUES (5422609, 12, "Babītes novads");
INSERT INTO regions VALUES (5422610, 12, "Baldones novads");
INSERT INTO regions VALUES (5422611, 12, "Baltinavas novads");
INSERT INTO regions VALUES (5422612, 12, "Balvu novads");
INSERT INTO regions VALUES (5422613, 12, "Bauskas novads");
INSERT INTO regions VALUES (5422614, 12, "Beverīnas novads");
INSERT INTO regions VALUES (2607772, 12, "Brocēnu novads");
INSERT INTO regions VALUES (5422615, 12, "Burtnieku novads");
INSERT INTO regions VALUES (2607602, 12, "Carnikavas novads");
INSERT INTO regions VALUES (5422616, 12, "Cēsu novads");
INSERT INTO regions VALUES (5422617, 12, "Cesvaines novads");
INSERT INTO regions VALUES (2604269, 12, "Ciblas novads");
INSERT INTO regions VALUES (5422618, 12, "Dagdas novads");
INSERT INTO regions VALUES (5422619, 12, "Daugavpils novads");
INSERT INTO regions VALUES (5422620, 12, "Dobeles novads");
INSERT INTO regions VALUES (5422621, 12, "Dundagas novads");
INSERT INTO regions VALUES (2603934, 12, "Durbes novads");
INSERT INTO regions VALUES (5422622, 12, "Engures novads");
INSERT INTO regions VALUES (5422623, 12, "Ērgļu novads");
INSERT INTO regions VALUES (2607616, 12, "Garkalnes novads");
INSERT INTO regions VALUES (5422624, 12, "Grobiņas novads");
INSERT INTO regions VALUES (5422625, 12, "Gulbenes novads");
INSERT INTO regions VALUES (2601050, 12, "Iecavas novads");
INSERT INTO regions VALUES (2605461, 12, "Ikšķiles novads");
INSERT INTO regions VALUES (2601459, 12, "Ilūkstes novads");
INSERT INTO regions VALUES (5422626, 12, "Inčukalna novads");
INSERT INTO regions VALUES (5422627, 12, "Jaunjelgavas novads");
INSERT INTO regions VALUES (5422628, 12, "Jaunpiebalgas novads");
INSERT INTO regions VALUES (5422629, 12, "Jaunpils novads");
INSERT INTO regions VALUES (5422630, 12, "Jēkabpils novads");
INSERT INTO regions VALUES (5422631, 12, "Jelgavas novads");
INSERT INTO regions VALUES (2608154, 12, "Kandavas novads");
INSERT INTO regions VALUES (5422632, 12, "Kārsavas novads");
INSERT INTO regions VALUES (2605476, 12, "Ķeguma novads");
INSERT INTO regions VALUES (5422638, 12, "Ķekavas novads");
INSERT INTO regions VALUES (5422633, 12, "Kocēnu novads");
INSERT INTO regions VALUES (5422634, 12, "Kokneses novads");
INSERT INTO regions VALUES (2602867, 12, "Krāslavas novads");
INSERT INTO regions VALUES (5422635, 12, "Krimuldas novads");
INSERT INTO regions VALUES (5422636, 12, "Krustpils novads");
INSERT INTO regions VALUES (5422637, 12, "Kuldīgas novads");
INSERT INTO regions VALUES (2605512, 12, "Lielvārdes novads");
INSERT INTO regions VALUES (5422639, 12, "Līgatnes novads");
INSERT INTO regions VALUES (5422640, 12, "Limbažu novads");
INSERT INTO regions VALUES (2605649, 12, "Līvānu novads");
INSERT INTO regions VALUES (5422641, 12, "Lubānas novads");
INSERT INTO regions VALUES (5422642, 12, "Ludzas novads");
INSERT INTO regions VALUES (5422643, 12, "Madonas novads");
INSERT INTO regions VALUES (5422644, 12, "Mālpils novads");
INSERT INTO regions VALUES (5422645, 12, "Mārupes novads");
INSERT INTO regions VALUES (5422646, 12, "Mazsalacas novads");
INSERT INTO regions VALUES (5422647, 12, "Mērsraga novads");
INSERT INTO regions VALUES (5422648, 12, "Naukšēnu novads");
INSERT INTO regions VALUES (5422565, 12, "Neretas novads");
INSERT INTO regions VALUES (5422649, 12, "Nīcas novads");
INSERT INTO regions VALUES (2605524, 12, "Ogres novads");
INSERT INTO regions VALUES (5422650, 12, "Olaines novads");
INSERT INTO regions VALUES (2602727, 12, "Ozolnieku novads");
INSERT INTO regions VALUES (5422651, 12, "Pārgaujas novads");
INSERT INTO regions VALUES (2604057, 12, "Pāvilostas novads");
INSERT INTO regions VALUES (5422652, 12, "Pļaviņu novads");
INSERT INTO regions VALUES (2605775, 12, "Preiļu novads");
INSERT INTO regions VALUES (5422653, 12, "Priekules novads");
INSERT INTO regions VALUES (5422654, 12, "Priekuļu novads");
INSERT INTO regions VALUES (5422655, 12, "Raunas novads");
INSERT INTO regions VALUES (5422656, 12, "Rēzeknes novads");
INSERT INTO regions VALUES (2605882, 12, "Riebiņu novads");
INSERT INTO regions VALUES (5422657, 12, "Rojas novads");
INSERT INTO regions VALUES (2607704, 12, "Ropažu novads");
INSERT INTO regions VALUES (5422658, 12, "Rucavas novads");
INSERT INTO regions VALUES (5422659, 12, "Rugāju novads");
INSERT INTO regions VALUES (5422661, 12, "Rūjienas novads");
INSERT INTO regions VALUES (5422660, 12, "Rundāles novads");
INSERT INTO regions VALUES (5422662, 12, "Salacgrīvas novads");
INSERT INTO regions VALUES (5422663, 12, "Salas novads");
INSERT INTO regions VALUES (2607722, 12, "Salaspils novads");
INSERT INTO regions VALUES (5422664, 12, "Saldus novads");
INSERT INTO regions VALUES (5422665, 12, "Saulkrastu novads");
INSERT INTO regions VALUES (5422666, 12, "Sējas novads");
INSERT INTO regions VALUES (2607752, 12, "Siguldas novads");
INSERT INTO regions VALUES (5422667, 12, "Skrīveru novads");
INSERT INTO regions VALUES (5422668, 12, "Skrundas novads");
INSERT INTO regions VALUES (5422669, 12, "Smiltenes novads");
INSERT INTO regions VALUES (5422670, 12, "Stopiņu novads");
INSERT INTO regions VALUES (5422671, 12, "Strenču novads");
INSERT INTO regions VALUES (5422672, 12, "Talsu novads");
INSERT INTO regions VALUES (2602114, 12, "Tērvetes novads");
INSERT INTO regions VALUES (5422673, 12, "Tukuma novads");
INSERT INTO regions VALUES (5422674, 12, "Vaiņodes novads");
INSERT INTO regions VALUES (5422675, 12, "Valkas novads");
INSERT INTO regions VALUES (5422676, 12, "Varakļānu novads");
INSERT INTO regions VALUES (2606274, 12, "Vārkavas novads");
INSERT INTO regions VALUES (5422677, 12, "Vecpiebalgas novads");
INSERT INTO regions VALUES (5422678, 12, "Vecumnieku novads");
INSERT INTO regions VALUES (5422679, 12, "Ventspils novads");
INSERT INTO regions VALUES (5422680, 12, "Viesītes novads");
INSERT INTO regions VALUES (5422681, 12, "Viļakas novads");
INSERT INTO regions VALUES (5422682, 12, "Viļānu novads");
INSERT INTO regions VALUES (2605030, 12, "Zilupes novads");
INSERT INTO regions VALUES (3335922, 107, "Berea District");
INSERT INTO regions VALUES (3335913, 107, "Butha-Buthe District");
INSERT INTO regions VALUES (3335859, 107, "Leribe District");
INSERT INTO regions VALUES (3335839, 107, "Mafeteng District");
INSERT INTO regions VALUES (3335812, 107, "Maseru District");
INSERT INTO regions VALUES (3335795, 107, "Mohale's Hoek District");
INSERT INTO regions VALUES (3335784, 107, "Mokhotlong District");
INSERT INTO regions VALUES (3335731, 107, "Qacha's Nek District");
INSERT INTO regions VALUES (3335725, 107, "Quthing District");
INSERT INTO regions VALUES (3335676, 107, "Thaba-Tseka District");
INSERT INTO regions VALUES (2709502, 13, "Akmenės rajonas");
INSERT INTO regions VALUES (5423850, 13, "Alytaus miesto savivaldybė");
INSERT INTO regions VALUES (2700002, 13, "Alytaus rajonas");
INSERT INTO regions VALUES (2715083, 13, "Anykščių rajonas");
INSERT INTO regions VALUES (2701217, 13, "Birštono savivaldybė");
INSERT INTO regions VALUES (2706630, 13, "Biržų rajonas");
INSERT INTO regions VALUES (2700445, 13, "Druskininkų savivaldybė");
INSERT INTO regions VALUES (2718341, 13, "Elektrėnų savivaldybė");
INSERT INTO regions VALUES (2715863, 13, "Ignalinos rajonas");
INSERT INTO regions VALUES (2701269, 13, "Jonavos rajonas");
INSERT INTO regions VALUES (2709678, 13, "Joniškio rajonas");
INSERT INTO regions VALUES (2712524, 13, "Jurbarko rajonas");
INSERT INTO regions VALUES (2701559, 13, "Kaišiadorių rajonas");
INSERT INTO regions VALUES (2705300, 13, "Kalvarijos savivaldybė");
INSERT INTO regions VALUES (5423810, 13, "Kauno miesto savivaldybė");
INSERT INTO regions VALUES (2701985, 13, "Kauno rajonas");
INSERT INTO regions VALUES (2705478, 13, "Kazlų Rūdos savivaldybė");
INSERT INTO regions VALUES (2702387, 13, "Kėdainių rajonas");
INSERT INTO regions VALUES (2709983, 13, "Kelmės rajonas");
INSERT INTO regions VALUES (5423822, 13, "Klaipėdos miesto savivaldybė");
INSERT INTO regions VALUES (2703966, 13, "Klaipėdos rajonas");
INSERT INTO regions VALUES (2704289, 13, "Kretingos rajonas");
INSERT INTO regions VALUES (2707182, 13, "Kupiškio rajonas");
INSERT INTO regions VALUES (2700513, 13, "Lazdijų rajonas");
INSERT INTO regions VALUES (2705010, 13, "Marijampolės savivaldybė");
INSERT INTO regions VALUES (2713930, 13, "Mažeikių rajonas");
INSERT INTO regions VALUES (2716587, 13, "Molėtų rajonas");
INSERT INTO regions VALUES (5423782, 13, "Neringos savivaldybė");
INSERT INTO regions VALUES (2712927, 13, "Pagėgių savivaldybė");
INSERT INTO regions VALUES (2710818, 13, "Pakruojo rajonas");
INSERT INTO regions VALUES (5423859, 13, "Palangos miesto savivaldybė");
INSERT INTO regions VALUES (5423829, 13, "Panevėžio miesto savivaldybė");
INSERT INTO regions VALUES (2707598, 13, "Panevėžio rajonas");
INSERT INTO regions VALUES (2708372, 13, "Pasvalio rajonas");
INSERT INTO regions VALUES (2714140, 13, "Plungės rajonas");
INSERT INTO regions VALUES (2702941, 13, "Prienų rajonas");
INSERT INTO regions VALUES (2711208, 13, "Radviliškio rajonas");
INSERT INTO regions VALUES (2703357, 13, "Raseinių rajonas");
INSERT INTO regions VALUES (2714362, 13, "Rietavo savivaldybė");
INSERT INTO regions VALUES (1948799, 13, "Rokiškio rajonas");
INSERT INTO regions VALUES (2705667, 13, "Šakių rajonas");
INSERT INTO regions VALUES (2718615, 13, "Šalčininkų rajonas");
INSERT INTO regions VALUES (5423839, 13, "Šiaulių miesto savivaldybė");
INSERT INTO regions VALUES (2711652, 13, "Šiaulių rajonas");
INSERT INTO regions VALUES (2713034, 13, "Šilalės rajonas");
INSERT INTO regions VALUES (2704685, 13, "Šilutės rajonas");
INSERT INTO regions VALUES (2719084, 13, "Širvintų rajonas");
INSERT INTO regions VALUES (2704500, 13, "Skuodo rajonas");
INSERT INTO regions VALUES (2719582, 13, "Švenčionių rajonas");
INSERT INTO regions VALUES (2712193, 13, "Tauragės rajonas");
INSERT INTO regions VALUES (1942670, 13, "Telšių rajonas");
INSERT INTO regions VALUES (2720201, 13, "Trakų rajonas");
INSERT INTO regions VALUES (2720654, 13, "Ukmergės rajonas");
INSERT INTO regions VALUES (2714473, 13, "Utenos rajonas");
INSERT INTO regions VALUES (2700884, 13, "Varėnos rajonas");
INSERT INTO regions VALUES (2706225, 13, "Vilkaviškio rajonas");
INSERT INTO regions VALUES (5423861, 13, "Vilniaus miesto savivaldybė");
INSERT INTO regions VALUES (2721289, 13, "Vilniaus rajonas");
INSERT INTO regions VALUES (5423794, 13, "Visagino savivaldybė");
INSERT INTO regions VALUES (2717533, 13, "Zarasų rajonas");
INSERT INTO regions VALUES (4394712, 112, "District de Diekirch");
INSERT INTO regions VALUES (4394600, 112, "District de Grevenmacher");
INSERT INTO regions VALUES (4394467, 112, "District de Luxembourg");
INSERT INTO regions VALUES (3547947, 132, "Ayeyarwady Division");
INSERT INTO regions VALUES (3530667, 132, "Bago Division");
INSERT INTO regions VALUES (3551925, 132, "Chin State");
INSERT INTO regions VALUES (3547844, 132, "Kachin State");
INSERT INTO regions VALUES (3546078, 132, "Kayah State");
INSERT INTO regions VALUES (3546654, 132, "Kayin State");
INSERT INTO regions VALUES (3540459, 132, "Magway Division");
INSERT INTO regions VALUES (3539875, 132, "Mandalay Division");
INSERT INTO regions VALUES (3537114, 132, "Mon State");
INSERT INTO regions VALUES (3529485, 132, "Rakhine State");
INSERT INTO regions VALUES (3529241, 132, "Sagaing Division");
INSERT INTO regions VALUES (3528228, 132, "Shan State");
INSERT INTO regions VALUES (3525163, 132, "Tanintharyi Division");
INSERT INTO regions VALUES (3529466, 132, "Yangon Division");
INSERT INTO regions VALUES (3979269, 127, "State of Chuuk");
INSERT INTO regions VALUES (3979238, 127, "State of Kosrae");
INSERT INTO regions VALUES (3979171, 127, "State of Pohnpei");
INSERT INTO regions VALUES (3979113, 127, "State of Yap");
INSERT INTO regions VALUES (3209656, 113, "Agalega Islands");
INSERT INTO regions VALUES (3336618, 113, "Black River District");
INSERT INTO regions VALUES (3396279, 113, "Cargados Carajos");
INSERT INTO regions VALUES (3336563, 113, "Flacq District");
INSERT INTO regions VALUES (3336549, 113, "Grand Port District");
INSERT INTO regions VALUES (3336478, 113, "Moka District");
INSERT INTO regions VALUES (3336454, 113, "Pamplemousses District");
INSERT INTO regions VALUES (3336443, 113, "Plaines Wilhems District");
INSERT INTO regions VALUES (3336436, 113, "Port Louis District");
INSERT INTO regions VALUES (3336413, 113, "Rivière du Rempart District");
INSERT INTO regions VALUES (3638996, 113, "Rodrigues");
INSERT INTO regions VALUES (3336386, 113, "Savanne District");
INSERT INTO regions VALUES (4124804, 114, "Nouakchott District de");
INSERT INTO regions VALUES (4125224, 114, "Région de Dakhlet Nouadhibou");
INSERT INTO regions VALUES (4125413, 114, "Région de l' Adrar");
INSERT INTO regions VALUES (4125362, 114, "Région de l' Assaba");
INSERT INTO regions VALUES (4125002, 114, "Région de l' Inchiri");
INSERT INTO regions VALUES (4125265, 114, "Région du Brakna");
INSERT INTO regions VALUES (4125079, 114, "Région du Gorgol");
INSERT INTO regions VALUES (4125035, 114, "Région du Guidimaka");
INSERT INTO regions VALUES (4125008, 114, "Région du Hod<NAME>");
INSERT INTO regions VALUES (4125007, 114, "Région du Hodh El Gharbi");
INSERT INTO regions VALUES (4124679, 114, "Région du Tagant");
INSERT INTO regions VALUES (4124624, 114, "Région du Tiris Zemmour");
INSERT INTO regions VALUES (4124601, 114, "Région du Trarza");
INSERT INTO regions VALUES (3376196, 115, "Faritanin' Antananarivo");
INSERT INTO regions VALUES (3371483, 115, "Faritanin' i Fianarantsoa");
INSERT INTO regions VALUES (3370119, 115, "Faritanin' i Mahajanga");
INSERT INTO regions VALUES (3364853, 115, "Faritanin' i Toamasina");
INSERT INTO regions VALUES (3364822, 115, "Faritanin' i Toliara");
INSERT INTO regions VALUES (3374693, 115, "Faritany d' Antsiranana");
INSERT INTO regions VALUES (5424359, 117, "Општина Јегуновце");
INSERT INTO regions VALUES (5424450, 117, "Општина Аеродром");
INSERT INTO regions VALUES (5424330, 117, "Општина Арачиново");
INSERT INTO regions VALUES (5424331, 117, "Општина Берово");
INSERT INTO regions VALUES (5424332, 117, "Општина Битола");
INSERT INTO regions VALUES (5424333, 117, "Општина Богданци");
INSERT INTO regions VALUES (5424334, 117, "Општина Боговиње");
INSERT INTO regions VALUES (5424335, 117, "Општина Босилово");
INSERT INTO regions VALUES (5424336, 117, "Општина Брвеница");
INSERT INTO regions VALUES (5424451, 117, "Општина Бутел");
INSERT INTO regions VALUES (5424337, 117, "Општина Валандово");
INSERT INTO regions VALUES (5424338, 117, "Општина Василево");
INSERT INTO regions VALUES (5424339, 117, "Општина Вевчани");
INSERT INTO regions VALUES (5424340, 117, "Општина Велес");
INSERT INTO regions VALUES (5424341, 117, "Општина Виница");
INSERT INTO regions VALUES (5424342, 117, "Општина Вранештица");
INSERT INTO regions VALUES (5424343, 117, "Општина Врапчиште");
INSERT INTO regions VALUES (5424452, 117, "Општина Гази Баба");
INSERT INTO regions VALUES (5424344, 117, "Општина Гевгелија");
INSERT INTO regions VALUES (5424453, 117, "Општина Ѓорче Петров");
INSERT INTO regions VALUES (5424345, 117, "Општина Гостивар");
INSERT INTO regions VALUES (5424346, 117, "Општина Градско");
INSERT INTO regions VALUES (5424348, 117, "Општина Дебaрца");
INSERT INTO regions VALUES (5424347, 117, "Општина Дебар");
INSERT INTO regions VALUES (5424349, 117, "Општина Делчево");
INSERT INTO regions VALUES (5424350, 117, "Општина Демир Капија");
INSERT INTO regions VALUES (5424351, 117, "Општина Демир Хисар");
INSERT INTO regions VALUES (5424352, 117, "Општина Дојран");
INSERT INTO regions VALUES (5424353, 117, "Општина Долнени");
INSERT INTO regions VALUES (5424354, 117, "Општина Другово");
INSERT INTO regions VALUES (5424355, 117, "Општина Желино");
INSERT INTO regions VALUES (5424356, 117, "Општина Зајас");
INSERT INTO regions VALUES (5424357, 117, "Општина Зелениково");
INSERT INTO regions VALUES (5424358, 117, "Општина Зрновци");
INSERT INTO regions VALUES (5424605, 117, "Општина Илинден");
INSERT INTO regions VALUES (5424360, 117, "Општина Кавадарци");
INSERT INTO regions VALUES (5424361, 117, "Општина Карбинци");
INSERT INTO regions VALUES (5424454, 117, "Општина Карпош");
INSERT INTO regions VALUES (5424455, 117, "Општина Кисела Вода");
INSERT INTO regions VALUES (5424362, 117, "Општина Кичево");
INSERT INTO regions VALUES (5424363, 117, "Општина Конче");
INSERT INTO regions VALUES (5424364, 117, "Општина Кочани");
INSERT INTO regions VALUES (5424365, 117, "Општина Кратово");
INSERT INTO regions VALUES (5424366, 117, "Општина К<NAME>");
INSERT INTO regions VALUES (5424367, 117, "Општина Кривогаштани");
INSERT INTO regions VALUES (5424368, 117, "Општина Крушево");
INSERT INTO regions VALUES (5424369, 117, "Општина Куманово");
INSERT INTO regions VALUES (5424370, 117, "Општина Липково");
INSERT INTO regions VALUES (5424371, 117, "Општина Лозово");
INSERT INTO regions VALUES (5424372, 117, "Општина Маврово и Ростуша");
INSERT INTO regions VALUES (5424373, 117, "Општина Македонска Каменица");
INSERT INTO regions VALUES (5424374, 117, "Општина Македонски Брод");
INSERT INTO regions VALUES (5424375, 117, "Општина Могила");
INSERT INTO regions VALUES (5424376, 117, "Општина Неготино");
INSERT INTO regions VALUES (5424377, 117, "Општина Новаци");
INSERT INTO regions VALUES (5424378, 117, "Општина Ново Село");
INSERT INTO regions VALUES (5424379, 117, "Општина Осломеј");
INSERT INTO regions VALUES (5424380, 117, "Општина Охрид");
INSERT INTO regions VALUES (5424381, 117, "Општина Петровец");
INSERT INTO regions VALUES (5424382, 117, "Општина Пехчево");
INSERT INTO regions VALUES (5424383, 117, "Општина Пласница");
INSERT INTO regions VALUES (5424384, 117, "Општина Прилеп");
INSERT INTO regions VALUES (5424385, 117, "Општина Пробиштип");
INSERT INTO regions VALUES (5424386, 117, "Општина Радовиш");
INSERT INTO regions VALUES (5424387, 117, "Општина Ранковце");
INSERT INTO regions VALUES (5424388, 117, "Општина Ресен");
INSERT INTO regions VALUES (5424389, 117, "Општина Росоман");
INSERT INTO regions VALUES (5424456, 117, "Општина Сарај");
INSERT INTO regions VALUES (5424390, 117, "Општина Свети Николе");
INSERT INTO regions VALUES (5424391, 117, "Општина Сопиште");
INSERT INTO regions VALUES (5424392, 117, "Општина Старо Нагоричане");
INSERT INTO regions VALUES (5424393, 117, "Општина Струга");
INSERT INTO regions VALUES (5424394, 117, "Општина Струмица");
INSERT INTO regions VALUES (5424395, 117, "Општина Студеничани");
INSERT INTO regions VALUES (5424396, 117, "Општина Теарце");
INSERT INTO regions VALUES (5424397, 117, "Општина Тетово");
INSERT INTO regions VALUES (5424457, 117, "Општина Центар");
INSERT INTO regions VALUES (5424398, 117, "Општина Центар Жупа");
INSERT INTO regions VALUES (5424458, 117, "Општина Чаир");
INSERT INTO regions VALUES (5424399, 117, "Општина Чашка");
INSERT INTO regions VALUES (5424400, 117, "Општина Чешиново-Облешево");
INSERT INTO regions VALUES (5424401, 117, "Општина Чучер Сандево");
INSERT INTO regions VALUES (5424402, 117, "Општина Штип");
INSERT INTO regions VALUES (5424459, 117, "Општина Шуто Оризари");
INSERT INTO regions VALUES (4175702, 120, "District de Bamako");
INSERT INTO regions VALUES (4172926, 120, "Région de Gao");
INSERT INTO regions VALUES (4172021, 120, "Région de Kayes");
INSERT INTO regions VALUES (4218695, 120, "Région de Kidal");
INSERT INTO regions VALUES (4171193, 120, "Région de Koulikoro");
INSERT INTO regions VALUES (4170194, 120, "Région de Mopti");
INSERT INTO regions VALUES (4168644, 120, "Région de Ségou");
INSERT INTO regions VALUES (4168394, 120, "Région de Sikasso");
INSERT INTO regions VALUES (4167228, 120, "Région de Tombouctou");
INSERT INTO regions VALUES (3395902, 118, "Balaka District");
INSERT INTO regions VALUES (3335543, 118, "Blantyre District");
INSERT INTO regions VALUES (3335137, 118, "Chikwawa District");
INSERT INTO regions VALUES (3334801, 118, "Chiradzulu District");
INSERT INTO regions VALUES (3119464, 118, "Chitipa District");
INSERT INTO regions VALUES (3334484, 118, "Dedza District");
INSERT INTO regions VALUES (3334460, 118, "Dowa District");
INSERT INTO regions VALUES (3333604, 118, "Karonga District");
INSERT INTO regions VALUES (3333542, 118, "Kasungu District");
INSERT INTO regions VALUES (3395903, 118, "Likoma District");
INSERT INTO regions VALUES (3333230, 118, "Lilongwe District");
INSERT INTO regions VALUES (3333142, 118, "Machinga District");
INSERT INTO regions VALUES (3332912, 118, "Mangochi District");
INSERT INTO regions VALUES (3332646, 118, "Mchinji District");
INSERT INTO regions VALUES (3332067, 118, "Mulanje District");
INSERT INTO regions VALUES (3331965, 118, "Mwanza District");
INSERT INTO regions VALUES (3331906, 118, "Mzimba District");
INSERT INTO regions VALUES (3331605, 118, "Nkhata Bay District");
INSERT INTO regions VALUES (3331590, 118, "Nkhotakota District");
INSERT INTO regions VALUES (3331522, 118, "Nsanje District");
INSERT INTO regions VALUES (3331784, 118, "Ntcheu District");
INSERT INTO regions VALUES (3331487, 118, "Ntchisi District");
INSERT INTO regions VALUES (3395904, 118, "Phalombe District");
INSERT INTO regions VALUES (3331298, 118, "Rumphi District");
INSERT INTO regions VALUES (3331275, 118, "Salima District");
INSERT INTO regions VALUES (3334643, 118, "Thyolo District");
INSERT INTO regions VALUES (3330829, 118, "Zomba District");
INSERT INTO regions VALUES (3767484, 119, "Johor Darul Ta'zim");
INSERT INTO regions VALUES (3767483, 119, "K<NAME>");
INSERT INTO regions VALUES (3767479, 119, "<NAME>");
INSERT INTO regions VALUES (3767470, 119, "Melaka");
INSERT INTO regions VALUES (1923106, 119, "<NAME>");
INSERT INTO regions VALUES (3767477, 119, "<NAME>");
INSERT INTO regions VALUES (3767476, 119, "<NAME>");
INSERT INTO regions VALUES (3767475, 119, "<NAME>");
INSERT INTO regions VALUES (3767482, 119, "<NAME>");
INSERT INTO regions VALUES (3767474, 119, "S<NAME> Di Bawah Bayu");
INSERT INTO regions VALUES (3767473, 119, "<NAME>");
INSERT INTO regions VALUES (3767472, 119, "<NAME>");
INSERT INTO regions VALUES (3767471, 119, "<NAME>");
INSERT INTO regions VALUES (3767481, 119, "Wilayah Persekutuan Kuala Lumpur");
INSERT INTO regions VALUES (3767915, 119, "Wilayah Persekutuan Labuan");
INSERT INTO regions VALUES (3944021, 119, "Wilayah Persekutuan Putrajaya");
INSERT INTO regions VALUES (5425956, 122, "Attard");
INSERT INTO regions VALUES (5425958, 122, "Balzan");
INSERT INTO regions VALUES (5425959, 122, "Birgu");
INSERT INTO regions VALUES (5425961, 122, "Birkirkara");
INSERT INTO regions VALUES (5425960, 122, "Birżebbuġa");
INSERT INTO regions VALUES (5425962, 122, "Bormla");
INSERT INTO regions VALUES (5425967, 122, "Dingli");
INSERT INTO regions VALUES (5426006, 122, "Fgura");
INSERT INTO regions VALUES (5426007, 122, "Floriana");
INSERT INTO regions VALUES (5426021, 122, "Fontana");
INSERT INTO regions VALUES (5425966, 122, "Gudja");
INSERT INTO regions VALUES (5425965, 122, "Gżira");
INSERT INTO regions VALUES (5426010, 122, "Għajnsielem");
INSERT INTO regions VALUES (5426011, 122, "Għarb");
INSERT INTO regions VALUES (5425964, 122, "Għargħur");
INSERT INTO regions VALUES (5426012, 122, "Għasri");
INSERT INTO regions VALUES (5425957, 122, "Għaxaq");
INSERT INTO regions VALUES (5425972, 122, "Iklin");
INSERT INTO regions VALUES (5425973, 122, "Kalkara");
INSERT INTO regions VALUES (5426016, 122, "Kerċem");
INSERT INTO regions VALUES (5425974, 122, "Kirkop");
INSERT INTO regions VALUES (5425977, 122, "Lija");
INSERT INTO regions VALUES (5425978, 122, "Luqa");
INSERT INTO regions VALUES (5425979, 122, "Marsa");
INSERT INTO regions VALUES (5425980, 122, "Marsaskala");
INSERT INTO regions VALUES (5425981, 122, "Marsaxlokk");
INSERT INTO regions VALUES (5425983, 122, "Mdina");
INSERT INTO regions VALUES (5425984, 122, "Mellieħa");
INSERT INTO regions VALUES (5425982, 122, "Mġarr");
INSERT INTO regions VALUES (5425986, 122, "Mosta");
INSERT INTO regions VALUES (5425985, 122, "Mqabba");
INSERT INTO regions VALUES (5425987, 122, "Msida");
INSERT INTO regions VALUES (5425988, 122, "Mtarfa");
INSERT INTO regions VALUES (5426017, 122, "Munxar");
INSERT INTO regions VALUES (5426018, 122, "Nadur");
INSERT INTO regions VALUES (5425989, 122, "Naxxar");
INSERT INTO regions VALUES (5425990, 122, "Paola");
INSERT INTO regions VALUES (5425991, 122, "Pembroke");
INSERT INTO regions VALUES (5425992, 122, "Pietà");
INSERT INTO regions VALUES (5426015, 122, "Qala");
INSERT INTO regions VALUES (5425975, 122, "Qormi");
INSERT INTO regions VALUES (5425976, 122, "Qrendi");
INSERT INTO regions VALUES (5425993, 122, "Rabat");
INSERT INTO regions VALUES (5425999, 122, "Safi");
INSERT INTO regions VALUES (5425994, 122, "<NAME>");
INSERT INTO regions VALUES (5425995, 122, "<NAME>");
INSERT INTO regions VALUES (5426019, 122, "<NAME>");
INSERT INTO regions VALUES (5425996, 122, "San Pawl il-Baħar");
INSERT INTO regions VALUES (5426020, 122, "Sannat");
INSERT INTO regions VALUES (5425998, 122, "Santa Luċija");
INSERT INTO regions VALUES (5425997, 122, "Santa Venera");
INSERT INTO regions VALUES (5426000, 122, "Senglea");
INSERT INTO regions VALUES (5426001, 122, "Siġġiewi");
INSERT INTO regions VALUES (5426002, 122, "Sliema");
INSERT INTO regions VALUES (5426003, 122, "Swieqi");
INSERT INTO regions VALUES (5426005, 122, "Ta' Xbiex");
INSERT INTO regions VALUES (5426004, 122, "Tarxien");
INSERT INTO regions VALUES (5425963, 122, "Valletta");
INSERT INTO regions VALUES (5426013, 122, "Victoria");
INSERT INTO regions VALUES (5426022, 122, "Xagħra");
INSERT INTO regions VALUES (5426023, 122, "Xewkija");
INSERT INTO regions VALUES (5426009, 122, "Xgħajra");
INSERT INTO regions VALUES (5425968, 122, "Żabbar");
INSERT INTO regions VALUES (5425969, 122, "Żebbuġ");
INSERT INTO regions VALUES (5425970, 122, "Żejtun");
INSERT INTO regions VALUES (5425971, 122, "Żurrieq");
INSERT INTO regions VALUES (5426008, 122, "Ħamrun");
INSERT INTO regions VALUES (5426024, 122, "Ħaż-Żebbuġ");
INSERT INTO regions VALUES (5254777, 123, "Ait n'Yoult");
INSERT INTO regions VALUES (5255080, 123, "Chouihia");
INSERT INTO regions VALUES (5254138, 123, "Laâyoune-Boujdour-Sakia El Hamra");
INSERT INTO regions VALUES (5255089, 123, "Mestegmer");
INSERT INTO regions VALUES (5457895, 123, "Région d'Oued Ed-Dahab-Lagouira");
INSERT INTO regions VALUES (4218696, 123, "Région de Chaouia-Ouardigha");
INSERT INTO regions VALUES (4218697, 123, "Région de Doukkala-Abda");
INSERT INTO regions VALUES (4200531, 123, "Région de Fès-Boulemane");
INSERT INTO regions VALUES (4218698, 123, "Région de Gharb-Chrarda-Beni Hssen");
INSERT INTO regions VALUES (4218699, 123, "Région de Guelmim-Es Smara");
INSERT INTO regions VALUES (4218700, 123, "Région de L'Oriental");
INSERT INTO regions VALUES (4198057, 123, "Région de Marrakech-Tensift-Al Haouz");
INSERT INTO regions VALUES (4197973, 123, "Région de Meknès-Tafilalet");
INSERT INTO regions VALUES (4196243, 123, "Région de Rabat-Salé-Zemmour-Zaër");
INSERT INTO regions VALUES (4218701, 123, "Région de Souss-Massa-Drâa");
INSERT INTO regions VALUES (4218702, 123, "Région de Tadla-Azilal");
INSERT INTO regions VALUES (4218703, 123, "Région de Tanger-Tétouan");
INSERT INTO regions VALUES (4218704, 123, "Région de Taza-Al Hoceima-Taounate");
INSERT INTO regions VALUES (4203411, 123, "Région du Grand Casablanca");
INSERT INTO regions VALUES (5255535, 123, "Zaouiat Lakouacem");
INSERT INTO regions VALUES (5255786, 123, "Zeghanghane");
INSERT INTO regions VALUES (5325996, 124, "Région Martinique");
INSERT INTO regions VALUES (4681526, 126, "Distrito Federal");
INSERT INTO regions VALUES (4897077, 126, "Estado de Aguascalientes");
INSERT INTO regions VALUES (4896292, 126, "Estado de Baja California");
INSERT INTO regions VALUES (4896291, 126, "Estado de Baja California Sur");
INSERT INTO regions VALUES (4684764, 126, "Estado de Campeche");
INSERT INTO regions VALUES (4684226, 126, "Estado de Chiapas");
INSERT INTO regions VALUES (4894474, 126, "Estado de Chihuahua");
INSERT INTO regions VALUES (4894124, 126, "Estado de Coahuila de Zaragoza");
INSERT INTO regions VALUES (4894029, 126, "Estado de Colima");
INSERT INTO regions VALUES (4893056, 126, "Estado de Durango");
INSERT INTO regions VALUES (4889165, 126, "Estado de Guanajuato");
INSERT INTO regions VALUES (4681204, 126, "Estado de Guerrero");
INSERT INTO regions VALUES (4681125, 126, "Estado de Hidalgo");
INSERT INTO regions VALUES (4888504, 126, "Estado de Jalisco");
INSERT INTO regions VALUES (4678050, 126, "Estado de México");
INSERT INTO regions VALUES (4883525, 126, "Estado de Michoacán de Ocampo");
INSERT INTO regions VALUES (4677822, 126, "Estado de Morelos");
INSERT INTO regions VALUES (4883046, 126, "Estado de Nayarit");
INSERT INTO regions VALUES (4677517, 126, "Estado de Nuevo León");
INSERT INTO regions VALUES (4677486, 126, "Estado de Oaxaca");
INSERT INTO regions VALUES (4676418, 126, "Estado de Puebla");
INSERT INTO regions VALUES (4676282, 126, "Estado de Querétaro de Arteaga");
INSERT INTO regions VALUES (4676261, 126, "Estado de Quintana Roo");
INSERT INTO regions VALUES (4876896, 126, "Estado de San Luis Potosí");
INSERT INTO regions VALUES (4875276, 126, "Estado de Sinaloa");
INSERT INTO regions VALUES (4875185, 126, "Estado de Sonora");
INSERT INTO regions VALUES (4672390, 126, "Estado de Tabasco");
INSERT INTO regions VALUES (4672337, 126, "Estado de Tamaulipas");
INSERT INTO regions VALUES (4671506, 126, "Estado de Tlaxcala");
INSERT INTO regions VALUES (4671101, 126, "Estado de Veracruz-Llave");
INSERT INTO regions VALUES (4670648, 126, "Estado de Yucatán");
INSERT INTO regions VALUES (4873463, 126, "Estado de Zacatecas");
INSERT INTO regions VALUES (3395907, 128, "Cidade de Maputo");
INSERT INTO regions VALUES (3362337, 128, "Província de Cabo Delgado");
INSERT INTO regions VALUES (3359218, 128, "Província de Gaza");
INSERT INTO regions VALUES (3358687, 128, "Província de Inhambane");
INSERT INTO regions VALUES (3356333, 128, "Província de Manica");
INSERT INTO regions VALUES (3356135, 128, "Província de Maputo");
INSERT INTO regions VALUES (3352911, 128, "Província de Nampula");
INSERT INTO regions VALUES (3352158, 128, "Província de Niassa");
INSERT INTO regions VALUES (3350346, 128, "Província de Sofala");
INSERT INTO regions VALUES (3349836, 128, "Província de Tete");
INSERT INTO regions VALUES (3348820, 128, "Província de Zambézia");
INSERT INTO regions VALUES (5423497, 15, "АТО Гагаузия");
INSERT INTO regions VALUES (5423652, 15, "АТО Приднестровье");
INSERT INTO regions VALUES (5423445, 15, "Бессарабский район");
INSERT INTO regions VALUES (5423457, 15, "Бричанский район");
INSERT INTO regions VALUES (5423480, 15, "Глодянский район");
INSERT INTO regions VALUES (5423474, 15, "Дондюшанский район");
INSERT INTO regions VALUES (5423475, 15, "Дрокиевский район");
INSERT INTO regions VALUES (5423476, 15, "Дубоссарский район");
INSERT INTO regions VALUES (5423477, 15, "Единецкий район");
INSERT INTO regions VALUES (5423461, 15, "Кагульский район");
INSERT INTO regions VALUES (5423467, 15, "Каларашский район");
INSERT INTO regions VALUES (5423462, 15, "Кантемирский район");
INSERT INTO regions VALUES (5423468, 15, "Каушанский район");
INSERT INTO regions VALUES (5423473, 15, "Криулянский район");
INSERT INTO regions VALUES (5423483, 15, "Леовский район");
INSERT INTO regions VALUES (5423484, 15, "Ниспоренский район");
INSERT INTO regions VALUES (5423444, 15, "Новоаненский район");
INSERT INTO regions VALUES (5423485, 15, "Окницкий район");
INSERT INTO regions VALUES (5423486, 15, "Оргеевский район");
INSERT INTO regions VALUES (5423487, 15, "Резинский район");
INSERT INTO regions VALUES (5423488, 15, "Рышканский район");
INSERT INTO regions VALUES (5423490, 15, "Сорокский район");
INSERT INTO regions VALUES (5423491, 15, "Страшенский район");
INSERT INTO regions VALUES (5423489, 15, "Сынджерейский район");
INSERT INTO regions VALUES (5423494, 15, "Тараклийский район");
INSERT INTO regions VALUES (5423495, 15, "Теленештский район");
INSERT INTO regions VALUES (5423496, 15, "Унгенский район");
INSERT INTO regions VALUES (5423478, 15, "Фалештский район");
INSERT INTO regions VALUES (5423479, 15, "Флорештский район");
INSERT INTO regions VALUES (5423481, 15, "Хынчештский район");
INSERT INTO regions VALUES (5423472, 15, "Чимишлийский район");
INSERT INTO regions VALUES (5423492, 15, "Шолданештский район");
INSERT INTO regions VALUES (5423493, 15, "Штефан-Водский район");
INSERT INTO regions VALUES (5423482, 15, "Яловенский район");
INSERT INTO regions VALUES (4604078, 129, "Commune de Monaco");
INSERT INTO regions VALUES (3960316, 130, "Arhangay Aymag");
INSERT INTO regions VALUES (3638117, 130, "Bayan-Ölgiy Aymag");
INSERT INTO regions VALUES (3638122, 130, "Bayanhongor Aymag");
INSERT INTO regions VALUES (3960129, 130, "Bulgan Aymag");
INSERT INTO regions VALUES (3960042, 130, "Darhan Hot (historical)");
INSERT INTO regions VALUES (5421176, 130, "Darhan Uul Aymag");
INSERT INTO regions VALUES (20573, 130, "Dornod Aymag");
INSERT INTO regions VALUES (3959994, 130, "Dornogovĭ Aymag");
INSERT INTO regions VALUES (3959979, 130, "Dundgovĭ Aymag");
INSERT INTO regions VALUES (3638035, 130, "Dzavhan Aymag");
INSERT INTO regions VALUES (3973855, 130, "Erdenet Hot (historical)");
INSERT INTO regions VALUES (3638018, 130, "Govĭ-Altay Aymag");
INSERT INTO regions VALUES (3976737, 130, "Govĭ-Sumber");
INSERT INTO regions VALUES (3959788, 130, "Hentiy Aymag");
INSERT INTO regions VALUES (3637982, 130, "Hovd Aymag");
INSERT INTO regions VALUES (3959715, 130, "Hövsgöl Aymag");
INSERT INTO regions VALUES (3959486, 130, "Ömnögovĭ Aymag");
INSERT INTO regions VALUES (3976738, 130, "Orhon Aymag");
INSERT INTO regions VALUES (3959459, 130, "Övörhangay Aymag");
INSERT INTO regions VALUES (3959430, 130, "Selenge Aymag");
INSERT INTO regions VALUES (3959370, 130, "Sühbaatar Aymag");
INSERT INTO regions VALUES (3959308, 130, "Töv Aymag");
INSERT INTO regions VALUES (5421178, 130, "Ulaanbaatar");
INSERT INTO regions VALUES (3637826, 130, "Uvs Aymag");
INSERT INTO regions VALUES (4696823, 131, "Parish of Saint Anthony");
INSERT INTO regions VALUES (4696822, 131, "Parish of Saint Georges");
INSERT INTO regions VALUES (4696818, 131, "Parish of Saint Peter");
INSERT INTO regions VALUES (4166469, 136, "Département d' Agadez");
INSERT INTO regions VALUES (4164933, 136, "Département de Diffa");
INSERT INTO regions VALUES (4164775, 136, "Département de Dosso");
INSERT INTO regions VALUES (4161902, 136, "Département de Maradi");
INSERT INTO regions VALUES (4160791, 136, "Département de Tahoua");
INSERT INTO regions VALUES (4217449, 136, "Département de Tillabéri");
INSERT INTO regions VALUES (4159909, 136, "Département de Zinder");
INSERT INTO regions VALUES (4217450, 136, "Niamey");
INSERT INTO regions VALUES (4161477, 136, "Niamey Département de");
INSERT INTO regions VALUES (4207796, 137, "Abia State");
INSERT INTO regions VALUES (4107354, 137, "Abuja Federal Capital Territory");
INSERT INTO regions VALUES (4207798, 137, "Adamawa State");
INSERT INTO regions VALUES (4105508, 137, "Akwa Ibom State");
INSERT INTO regions VALUES (4104711, 137, "Anambra State");
INSERT INTO regions VALUES (4102486, 137, "Bauchi State");
INSERT INTO regions VALUES (4217490, 137, "Bayelsa State");
INSERT INTO regions VALUES (4218659, 137, "Bendel State");
INSERT INTO regions VALUES (4102319, 137, "Benue State");
INSERT INTO regions VALUES (4102320, 137, "Benue-Plateau State");
INSERT INTO regions VALUES (4101911, 137, "Borno State");
INSERT INTO regions VALUES (4101154, 137, "Cross River State");
INSERT INTO regions VALUES (4207797, 137, "Delta State");
INSERT INTO regions VALUES (4099685, 137, "East-Central State");
INSERT INTO regions VALUES (4217491, 137, "Ebonyi State");
INSERT INTO regions VALUES (4217492, 137, "Ekiti State");
INSERT INTO regions VALUES (4207799, 137, "Ekpoma");
INSERT INTO regions VALUES (4207800, 137, "Enugu State");
INSERT INTO regions VALUES (4217493, 137, "Gombe State");
INSERT INTO regions VALUES (4096344, 137, "Gongola State");
INSERT INTO regions VALUES (875, 137, "Imo State");
INSERT INTO regions VALUES (4207801, 137, "Jigawa State");
INSERT INTO regions VALUES (4092178, 137, "Kaduna State");
INSERT INTO regions VALUES (4091743, 137, "Kano State");
INSERT INTO regions VALUES (4091404, 137, "Katsina State");
INSERT INTO regions VALUES (4218660, 137, "Kebbi State");
INSERT INTO regions VALUES (4218661, 137, "Kogi State");
INSERT INTO regions VALUES (4089731, 137, "Kwara State");
INSERT INTO regions VALUES (4089455, 137, "Lagos State");
INSERT INTO regions VALUES (4217494, 137, "Nassarawa State");
INSERT INTO regions VALUES (4086426, 137, "Niger State");
INSERT INTO regions VALUES (4086242, 137, "North-Eastern State");
INSERT INTO regions VALUES (4086241, 137, "North-Western State");
INSERT INTO regions VALUES (13330, 137, "Ogun State");
INSERT INTO regions VALUES (4083918, 137, "Ondo State");
INSERT INTO regions VALUES (4218662, 137, "Osun State");
INSERT INTO regions VALUES (4083066, 137, "Oyo State");
INSERT INTO regions VALUES (1706162, 137, "Plateau State");
INSERT INTO regions VALUES (4082438, 137, "Rivers State");
INSERT INTO regions VALUES (4081108, 137, "Sokoto State");
INSERT INTO regions VALUES (4218663, 137, "Taraba State");
INSERT INTO regions VALUES (4077613, 137, "Western State");
INSERT INTO regions VALUES (4218664, 137, "Yobe State");
INSERT INTO regions VALUES (4217495, 137, "Zamfara State");
INSERT INTO regions VALUES (4286352, 139, "Provincie Drenthe");
INSERT INTO regions VALUES (4604079, 139, "Provincie Flevoland");
INSERT INTO regions VALUES (4285944, 139, "Provincie Friesland");
INSERT INTO regions VALUES (4285868, 139, "Provincie Gelderland");
INSERT INTO regions VALUES (4285680, 139, "Provincie Groningen");
INSERT INTO regions VALUES (4284022, 139, "Provincie Limburg");
INSERT INTO regions VALUES (4283263, 139, "Provincie Noord-Brabant");
INSERT INTO regions VALUES (4283231, 139, "Provincie Noord-Holland");
INSERT INTO regions VALUES (4282750, 139, "Provincie Overijssel");
INSERT INTO regions VALUES (4281368, 139, "Provincie Utrecht");
INSERT INTO regions VALUES (4280496, 139, "Provincie Zeeland");
INSERT INTO regions VALUES (4280381, 139, "Provincie Zuid-Holland");
INSERT INTO regions VALUES (4720798, 140, "Departamento de Boaco");
INSERT INTO regions VALUES (4720726, 140, "Departamento de Carazo");
INSERT INTO regions VALUES (4720684, 140, "Departamento de Chinandega");
INSERT INTO regions VALUES (4720681, 140, "Departamento de Chontales");
INSERT INTO regions VALUES (4720091, 140, "Departamento de Estelí");
INSERT INTO regions VALUES (4720075, 140, "Departamento de Granada");
INSERT INTO regions VALUES (4720010, 140, "Departamento de Jinotega");
INSERT INTO regions VALUES (4719527, 140, "Departamento de León");
INSERT INTO regions VALUES (4719393, 140, "Departamento de Madriz");
INSERT INTO regions VALUES (4719384, 140, "Departamento de Managua");
INSERT INTO regions VALUES (4719371, 140, "Departamento de Masaya");
INSERT INTO regions VALUES (4719365, 140, "Departamento de Matagalpa");
INSERT INTO regions VALUES (4719268, 140, "Departamento de Nueva Segovia");
INSERT INTO regions VALUES (4719104, 140, "Departamento de Río San Juan");
INSERT INTO regions VALUES (4719100, 140, "Departamento de Rivas");
INSERT INTO regions VALUES (4824217, 140, "Región Autónoma Atlántico Norte");
INSERT INTO regions VALUES (4824218, 140, "Región Autónoma Atlántico Sur");
INSERT INTO regions VALUES (4369922, 65, "Freie und Hansestadt Hamburg");
INSERT INTO regions VALUES (4389991, 65, "Freistaat Bayern");
INSERT INTO regions VALUES (4335567, 65, "Freistaat Sachsen");
INSERT INTO regions VALUES (4326698, 65, "Freistaat Thüringen");
INSERT INTO regions VALUES (4390844, 65, "Land Baden-Württemberg");
INSERT INTO regions VALUES (4389002, 65, "Land Berlin");
INSERT INTO regions VALUES (4386474, 65, "Land Brandenburg");
INSERT INTO regions VALUES (4386042, 65, "Land Bremen");
INSERT INTO regions VALUES (4367194, 65, "Land Hessen");
INSERT INTO regions VALUES (4352094, 65, "Land Mecklenburg-Vorpommern");
INSERT INTO regions VALUES (4346621, 65, "Land Niedersachsen");
INSERT INTO regions VALUES (4345997, 65, "Land Nordrhein-Westfalen");
INSERT INTO regions VALUES (4335566, 65, "Land Sachsen-Anhalt");
INSERT INTO regions VALUES (4333897, 65, "Land Schleswig-Holstein");
INSERT INTO regions VALUES (4337956, 65, "Rheinland-Pfalz");
INSERT INTO regions VALUES (4335604, 65, "Saarland");
INSERT INTO regions VALUES (4615484, 133, "Bethanien");
INSERT INTO regions VALUES (3315997, 133, "Boesmanland");
INSERT INTO regions VALUES (3389807, 133, "Caprivi");
INSERT INTO regions VALUES (3315992, 133, "<NAME>");
INSERT INTO regions VALUES (4615446, 133, "Damaraland");
INSERT INTO regions VALUES (4616148, 133, "Erongo");
INSERT INTO regions VALUES (4615274, 133, "Gobabis");
INSERT INTO regions VALUES (4615259, 133, "Grootfontein");
INSERT INTO regions VALUES (4616149, 133, "Hardap");
INSERT INTO regions VALUES (4615219, 133, "<NAME>");
INSERT INTO regions VALUES (4615218, 133, "<NAME>");
INSERT INTO regions VALUES (4615151, 133, "Kaokoland");
INSERT INTO regions VALUES (4616150, 133, "Karas");
INSERT INTO regions VALUES (4615141, 133, "Karasburg");
INSERT INTO regions VALUES (4615137, 133, "Karibib");
INSERT INTO regions VALUES (4615119, 133, "Kavango");
INSERT INTO regions VALUES (4615116, 133, "Keetmanshoop");
INSERT INTO regions VALUES (4616159, 133, "Khomas");
INSERT INTO regions VALUES (4616151, 133, "Kunene");
INSERT INTO regions VALUES (4615040, 133, "Lüderitz");
INSERT INTO regions VALUES (4615029, 133, "Maltahöhe");
INSERT INTO regions VALUES (4615023, 133, "Mariental");
INSERT INTO regions VALUES (4614963, 133, "Namaland");
INSERT INTO regions VALUES (4616152, 133, "Ohangwena");
INSERT INTO regions VALUES (4614838, 133, "Okahandja");
INSERT INTO regions VALUES (4616153, 133, "Okavango");
INSERT INTO regions VALUES (4616154, 133, "Omaheke");
INSERT INTO regions VALUES (4614627, 133, "Omaruru");
INSERT INTO regions VALUES (4616155, 133, "Omusati");
INSERT INTO regions VALUES (4616156, 133, "Oshana");
INSERT INTO regions VALUES (4616157, 133, "Oshikoto");
INSERT INTO regions VALUES (4614188, 133, "Otjiwarongo");
INSERT INTO regions VALUES (4616158, 133, "Otjozondjupa");
INSERT INTO regions VALUES (4614143, 133, "Outjo");
INSERT INTO regions VALUES (4614136, 133, "Owambo");
INSERT INTO regions VALUES (4614106, 133, "Rehoboth");
INSERT INTO regions VALUES (4614004, 133, "Swakopmund");
INSERT INTO regions VALUES (4613972, 133, "Tsumeb");
INSERT INTO regions VALUES (3517061, 135, "Bāgmatī Zone");
INSERT INTO regions VALUES (3516974, 135, "Bherī Zone");
INSERT INTO regions VALUES (3516850, 135, "Dhawalāgiri Zone");
INSERT INTO regions VALUES (3516813, 135, "Gandakī Zone");
INSERT INTO regions VALUES (3516738, 135, "Janakpur Zone");
INSERT INTO regions VALUES (3516686, 135, "Karnālī Zone");
INSERT INTO regions VALUES (3516642, 135, "Kosī Zone");
INSERT INTO regions VALUES (1708606, 135, "Lumbinī Zone");
INSERT INTO regions VALUES (3516590, 135, "Mahākālī Zone");
INSERT INTO regions VALUES (3516556, 135, "Mechī Zone");
INSERT INTO regions VALUES (3516521, 135, "Nārāyanī Zone");
INSERT INTO regions VALUES (3516404, 135, "Rāptī Zone");
INSERT INTO regions VALUES (3516381, 135, "Sagarmāthā Zone");
INSERT INTO regions VALUES (3516354, 135, "Setī Zone");
INSERT INTO regions VALUES (4010056, 142, "Auckland");
INSERT INTO regions VALUES (4008215, 142, "Bay of Plenty");
INSERT INTO regions VALUES (4009929, 142, "Canterbury");
INSERT INTO regions VALUES (4904941, 142, "Chatham Islands");
INSERT INTO regions VALUES (4009706, 142, "Gisborne");
INSERT INTO regions VALUES (4009593, 142, "Hawkes Bay");
INSERT INTO regions VALUES (4009094, 142, "Marlborough");
INSERT INTO regions VALUES (5301622, 142, "Nelson");
INSERT INTO regions VALUES (5301623, 142, "Otago");
INSERT INTO regions VALUES (4008209, 142, "Southland");
INSERT INTO regions VALUES (4008109, 142, "Taranaki");
INSERT INTO regions VALUES (4007798, 142, "Waikato");
INSERT INTO regions VALUES (4007653, 142, "Wanganui");
INSERT INTO regions VALUES (4007628, 142, "Wellington");
INSERT INTO regions VALUES (5301627, 142, "West Coast");
INSERT INTO regions VALUES (4007573, 142, "Whangarei");
INSERT INTO regions VALUES (4537400, 144, "Akershus Fylke");
INSERT INTO regions VALUES (4537045, 144, "Aust-Agder Fylke");
INSERT INTO regions VALUES (5308502, 144, "Bouvetøya (general)");
INSERT INTO regions VALUES (4536346, 144, "Buskerud Fylke");
INSERT INTO regions VALUES (3299023, 144, "Finnmark Fylke");
INSERT INTO regions VALUES (4534636, 144, "Hedmark Fylke");
INSERT INTO regions VALUES (4534229, 144, "Hordaland Fylke");
INSERT INTO regions VALUES (4532542, 144, "Møre og Romsdal Fylke");
INSERT INTO regions VALUES (4532186, 144, "Nord-Trøndelag Fylke");
INSERT INTO regions VALUES (4532216, 144, "Nordland Fylke");
INSERT INTO regions VALUES (4532010, 144, "Oppland Fylke");
INSERT INTO regions VALUES (4531943, 144, "Oslo Fylke");
INSERT INTO regions VALUES (4531522, 144, "Rogaland Fylke");
INSERT INTO regions VALUES (4530621, 144, "Sogn og Fjordane Fylke");
INSERT INTO regions VALUES (4530465, 144, "Sør-Trøndelag Fylke");
INSERT INTO regions VALUES (4529901, 144, "Telemark Fylke");
INSERT INTO regions VALUES (4529693, 144, "Troms Fylke");
INSERT INTO regions VALUES (4529225, 144, "Vest-Agder Fylke");
INSERT INTO regions VALUES (4529213, 144, "Vestfold Fylke");
INSERT INTO regions VALUES (4531923, 144, "Østfold Fylke");
INSERT INTO regions VALUES (3138594, 145, "Abū Z̧aby");
INSERT INTO regions VALUES (3138576, 145, "Al Fujayrah");
INSERT INTO regions VALUES (3138524, 145, "Ash Shāriqah");
INSERT INTO regions VALUES (3138487, 145, "Dubayy");
INSERT INTO regions VALUES (3138367, 145, "Ra's al Khaymah");
INSERT INTO regions VALUES (1932288, 145, "Umm al Qaywayn");
INSERT INTO regions VALUES (3138590, 145, "`Ajmān");
INSERT INTO regions VALUES (3197517, 146, "Muḩāfaz̧at ad Dākhilīyah");
INSERT INTO regions VALUES (3197518, 146, "Muḩāfaz̧at al Bāţinah");
INSERT INTO regions VALUES (5457908, 146, "Muḩāfaz̧at Al Buraimi");
INSERT INTO regions VALUES (3197519, 146, "Muḩāfaz̧at al Wusţá");
INSERT INTO regions VALUES (3197520, 146, "Muḩāfaz̧at ash Sharqīyah");
INSERT INTO regions VALUES (3197521, 146, "Muḩāfaz̧at az̧ Z̧āhirah");
INSERT INTO regions VALUES (3197522, 146, "Muḩāfaz̧at Masqaţ");
INSERT INTO regions VALUES (3197523, 146, "Muḩāfaz̧at Musandam");
INSERT INTO regions VALUES (3197524, 146, "Muḩāfaz̧at Z̧ufār");
INSERT INTO regions VALUES (5426446, 147, "Andreas");
INSERT INTO regions VALUES (5426447, 147, "Arbory");
INSERT INTO regions VALUES (5426448, 147, "Ballaugh");
INSERT INTO regions VALUES (5426449, 147, "Braddan");
INSERT INTO regions VALUES (5426450, 147, "Bride");
INSERT INTO regions VALUES (5426438, 147, "Castletown");
INSERT INTO regions VALUES (5426437, 147, "Douglas");
INSERT INTO regions VALUES (5426451, 147, "German");
INSERT INTO regions VALUES (5426452, 147, "Jurby");
INSERT INTO regions VALUES (5426443, 147, "Laxey");
INSERT INTO regions VALUES (5426453, 147, "Lezayre");
INSERT INTO regions VALUES (5426454, 147, "Lonan");
INSERT INTO regions VALUES (5426455, 147, "Malew");
INSERT INTO regions VALUES (5426456, 147, "Marown");
INSERT INTO regions VALUES (5426457, 147, "Maughold");
INSERT INTO regions VALUES (5426442, 147, "Michael");
INSERT INTO regions VALUES (5426441, 147, "Onchan");
INSERT INTO regions VALUES (5426458, 147, "Patrick");
INSERT INTO regions VALUES (5426439, 147, "Peel");
INSERT INTO regions VALUES (5426444, 147, "<NAME>");
INSERT INTO regions VALUES (5426445, 147, "Port St Mary");
INSERT INTO regions VALUES (5426440, 147, "Ramsey");
INSERT INTO regions VALUES (5426459, 147, "Rushen");
INSERT INTO regions VALUES (5426460, 147, "Santon");
INSERT INTO regions VALUES (3829545, 226, "Chungcheong North Province");
INSERT INTO regions VALUES (3829544, 226, "Chungcheong South Province");
INSERT INTO regions VALUES (3823048, 226, "Daegu Metropolitan City");
INSERT INTO regions VALUES (3822994, 226, "Daejeon Metropolitan City");
INSERT INTO regions VALUES (3828232, 226, "Gangwon Province");
INSERT INTO regions VALUES (3827369, 226, "Gwangju Metropolitan City");
INSERT INTO regions VALUES (3827232, 226, "Gyeonggi Province");
INSERT INTO regions VALUES (3827224, 226, "Gyeongsang North Province");
INSERT INTO regions VALUES (3868558, 226, "Gyeongsang South Province");
INSERT INTO regions VALUES (3828501, 226, "Incheon Metropolitan City");
INSERT INTO regions VALUES (3830282, 226, "Jeju Province");
INSERT INTO regions VALUES (3829978, 226, "Jeolla North Province");
INSERT INTO regions VALUES (3829977, 226, "Jeolla South Province");
INSERT INTO regions VALUES (3823380, 226, "Seoul Special City");
INSERT INTO regions VALUES (3822009, 226, "Ulsan Metropolitan City");
INSERT INTO regions VALUES (3340370, 227, "Free State");
INSERT INTO regions VALUES (3387881, 227, "Gauteng");
INSERT INTO regions VALUES (3387884, 227, "Limpopo Province");
INSERT INTO regions VALUES (3387882, 227, "Mpumalanga");
INSERT INTO regions VALUES (3387880, 227, "Province of Eastern Cape");
INSERT INTO regions VALUES (3341201, 227, "Province of KwaZulu-Natal");
INSERT INTO regions VALUES (3387885, 227, "Province of North-West");
INSERT INTO regions VALUES (3387883, 227, "Province of Northern Cape");
INSERT INTO regions VALUES (3387886, 227, "Province of the Western Cape");
INSERT INTO regions VALUES (3970389, 173, "Chagang Province");
INSERT INTO regions VALUES (3969600, 173, "Hamgyong North Province");
INSERT INTO regions VALUES (3851076, 173, "Hamgyong South Province");
INSERT INTO regions VALUES (3850602, 173, "Hwanghae North Province");
INSERT INTO regions VALUES (3850599, 173, "Hwanghae South Province");
INSERT INTO regions VALUES (3850191, 173, "Kaeseong");
INSERT INTO regions VALUES (3849968, 173, "Kangwon Province");
INSERT INTO regions VALUES (3976593, 173, "Najin Sŏnbong-si");
INSERT INTO regions VALUES (3848141, 173, "Nampo");
INSERT INTO regions VALUES (3846685, 173, "Pyongan North Province");
INSERT INTO regions VALUES (3846684, 173, "Pyongan South Province");
INSERT INTO regions VALUES (3846605, 173, "Pyongyang");
INSERT INTO regions VALUES (3965971, 173, "Ryanggang Province");
INSERT INTO regions VALUES (3447179, 152, "Azad Kashmir");
INSERT INTO regions VALUES (3446719, 152, "Balochistān");
INSERT INTO regions VALUES (3443358, 152, "Federally Administered Tribal Areas");
INSERT INTO regions VALUES (3431125, 152, "Islāmābād Capital Territory");
INSERT INTO regions VALUES (3435767, 152, "North-West Frontier Province");
INSERT INTO regions VALUES (3434952, 152, "Punjab");
INSERT INTO regions VALUES (3432894, 152, "Sind");
INSERT INTO regions VALUES (3136735, 154, "West Bank");
INSERT INTO regions VALUES (4765067, 155, "Comarca de San Blas");
INSERT INTO regions VALUES (4770642, 155, "Provincia de Bocas del Toro");
INSERT INTO regions VALUES (4769995, 155, "Provincia de Chiriquí");
INSERT INTO regions VALUES (4769904, 155, "Provincia de Coclé");
INSERT INTO regions VALUES (4769874, 155, "Provincia de Colón");
INSERT INTO regions VALUES (4768313, 155, "Provincia de Herrera");
INSERT INTO regions VALUES (4766442, 155, "Provincia de Los Santos");
INSERT INTO regions VALUES (4765910, 155, "Provincia de Panamá");
INSERT INTO regions VALUES (4764543, 155, "Provincia de Veraguas");
INSERT INTO regions VALUES (4769772, 155, "Provincia del Darién");
INSERT INTO regions VALUES (3982051, 156, "Sandaun Province");
INSERT INTO regions VALUES (4656775, 157, "Asunción");
INSERT INTO regions VALUES (4639624, 157, "Departamento Central");
INSERT INTO regions VALUES (4639764, 157, "Departamento de Alto Paraguay");
INSERT INTO regions VALUES (4830600, 157, "Departamento de Boquerón");
INSERT INTO regions VALUES (4639703, 157, "Departamento de Caaguazú");
INSERT INTO regions VALUES (4639695, 157, "Departamento de Caazapá");
INSERT INTO regions VALUES (4639657, 157, "Departamento de Canindeyú");
INSERT INTO regions VALUES (4639396, 157, "Departamento de Concepción");
INSERT INTO regions VALUES (4638713, 157, "Departamento de Itapúa");
INSERT INTO regions VALUES (4639392, 157, "Departamento de la Cordillera");
INSERT INTO regions VALUES (4638618, 157, "Departamento de Misiones");
INSERT INTO regions VALUES (4638595, 157, "Departamento de Ñeembucú");
INSERT INTO regions VALUES (4638570, 157, "Departamento de Paraguarí");
INSERT INTO regions VALUES (4638524, 157, "Departamento de Presidente Hayes");
INSERT INTO regions VALUES (4638224, 157, "Departamento de San Pedro");
INSERT INTO regions VALUES (4639763, 157, "Departamento del Alto Paraná");
INSERT INTO regions VALUES (4639758, 157, "Departamento del Amambay");
INSERT INTO regions VALUES (4638752, 157, "Departamento del Guairá");
INSERT INTO regions VALUES (4764375, 158, "Departamento de Amazonas");
INSERT INTO regions VALUES (4764362, 158, "Departamento de Ancash");
INSERT INTO regions VALUES (4859054, 158, "Departamento de Apurímac");
INSERT INTO regions VALUES (4859010, 158, "Departamento de Arequipa");
INSERT INTO regions VALUES (4858872, 158, "Departamento de Ayacucho");
INSERT INTO regions VALUES (4764071, 158, "Departamento de Cajamarca");
INSERT INTO regions VALUES (4856149, 158, "Departamento de Cusco");
INSERT INTO regions VALUES (4854998, 158, "Departamento de Huancavelica");
INSERT INTO regions VALUES (4762704, 158, "Departamento de Huánuco");
INSERT INTO regions VALUES (4854483, 158, "Departamento de Ica");
INSERT INTO regions VALUES (4853960, 158, "Departamento de Junín");
INSERT INTO regions VALUES (4762349, 158, "Departamento de La Libertad");
INSERT INTO regions VALUES (4762330, 158, "Departamento de Lambayeque");
INSERT INTO regions VALUES (4853473, 158, "Departamento de Lima");
INSERT INTO regions VALUES (4762056, 158, "Departamento de Loreto");
INSERT INTO regions VALUES (4853084, 158, "Departamento de Madre de Dios");
INSERT INTO regions VALUES (4852583, 158, "Departamento de Moquegua");
INSERT INTO regions VALUES (4851611, 158, "Departamento de Pasco");
INSERT INTO regions VALUES (4761246, 158, "Departamento de Piura");
INSERT INTO regions VALUES (4850846, 158, "Departamento de Puno");
INSERT INTO regions VALUES (4760527, 158, "Departamento de San Martín");
INSERT INTO regions VALUES (4849168, 158, "Departamento de Tacna");
INSERT INTO regions VALUES (4759770, 158, "Departamento de Tumbes");
INSERT INTO regions VALUES (4759750, 158, "Departamento de Ucayali");
INSERT INTO regions VALUES (4858433, 158, "Provincia Constitucional del Callao");
INSERT INTO regions VALUES (4607120, 160, "Województwo Dolnośląskie");
INSERT INTO regions VALUES (4607128, 160, "Województwo Kujawsko-Pomorskie");
INSERT INTO regions VALUES (3312575, 160, "Województwo Lubelskie");
INSERT INTO regions VALUES (4607122, 160, "Województwo Lubuskie");
INSERT INTO regions VALUES (3312577, 160, "Województwo Mazowieckie");
INSERT INTO regions VALUES (3312576, 160, "Województwo Małopolskie");
INSERT INTO regions VALUES (4607123, 160, "Województwo Opolskie");
INSERT INTO regions VALUES (3312578, 160, "Województwo Podkarpackie");
INSERT INTO regions VALUES (3312579, 160, "Województwo Podlaskie");
INSERT INTO regions VALUES (4607124, 160, "Województwo Pomorskie");
INSERT INTO regions VALUES (4607125, 160, "Województwo Śląskie");
INSERT INTO regions VALUES (3312580, 160, "Województwo Świętokrzyskie");
INSERT INTO regions VALUES (3312581, 160, "Województwo Warmińsko-Mazurskie");
INSERT INTO regions VALUES (4607126, 160, "<NAME>");
INSERT INTO regions VALUES (4607127, 160, "<NAME>");
INSERT INTO regions VALUES (4607121, 160, "<NAME>");
INSERT INTO regions VALUES (4276474, 161, "Distrito da Guarda");
INSERT INTO regions VALUES (4279570, 161, "Distrito de Aveiro");
INSERT INTO regions VALUES (4043564, 161, "Distrito de Beja");
INSERT INTO regions VALUES (4279098, 161, "Distrito de Braga");
INSERT INTO regions VALUES (4279093, 161, "Distrito de Bragança");
INSERT INTO regions VALUES (4042591, 161, "Distrito de Castelo Branco");
INSERT INTO regions VALUES (4277961, 161, "Distrito de Coimbra");
INSERT INTO regions VALUES (4041919, 161, "Distrito de Évora");
INSERT INTO regions VALUES (4041880, 161, "Distrito de Faro");
INSERT INTO regions VALUES (4041237, 161, "Distrito de Leiria");
INSERT INTO regions VALUES (4041220, 161, "Distrito de Lisboa");
INSERT INTO regions VALUES (4039608, 161, "Distrito de Portalegre");
INSERT INTO regions VALUES (4038883, 161, "Distrito de Santarém");
INSERT INTO regions VALUES (4038561, 161, "Distrito de Setúbal");
INSERT INTO regions VALUES (4271665, 161, "Distrito de Viana do Castelo");
INSERT INTO regions VALUES (4271356, 161, "Distrito de Vila Real");
INSERT INTO regions VALUES (4271195, 161, "Distrito de Viseu");
INSERT INTO regions VALUES (4274232, 161, "Distrito do Porto");
INSERT INTO regions VALUES (4215482, 161, "Região Autónoma da Madeira");
INSERT INTO regions VALUES (4635732, 161, "Região Autónoma dos Açores");
INSERT INTO regions VALUES (5325867, 163, "Région Réunion");
INSERT INTO regions VALUES (1000001, 1, "Адыгея");
INSERT INTO regions VALUES (1121540, 1, "Алтай");
INSERT INTO regions VALUES (1121829, 1, "Алтайский край");
INSERT INTO regions VALUES (1123488, 1, "Амурская область");
INSERT INTO regions VALUES (1000236, 1, "Архангельская область");
INSERT INTO regions VALUES (1004118, 1, "Астраханская область");
INSERT INTO regions VALUES (1004565, 1, "Башкортостан");
INSERT INTO regions VALUES (1009404, 1, "Белгородская область");
INSERT INTO regions VALUES (1011109, 1, "Брянская область");
INSERT INTO regions VALUES (1124157, 1, "Бурятия");
INSERT INTO regions VALUES (1124833, 1, "Владимирская область");
INSERT INTO regions VALUES (1014032, 1, "Волгоградская область");
INSERT INTO regions VALUES (1015702, 1, "Вологодская область");
INSERT INTO regions VALUES (1023816, 1, "Воронежская область");
INSERT INTO regions VALUES (1025654, 1, "Дагестан");
INSERT INTO regions VALUES (1127400, 1, "Еврейская АОбл");
INSERT INTO regions VALUES (1159987, 1, "Забайкальский край");
INSERT INTO regions VALUES (1027297, 1, "Ивановская область");
INSERT INTO regions VALUES (1030371, 1, "Ингушетия");
INSERT INTO regions VALUES (1127513, 1, "Иркутская область");
INSERT INTO regions VALUES (1030428, 1, "Кабардино-Балкарская");
INSERT INTO regions VALUES (1030632, 1, "Калининградская область");
INSERT INTO regions VALUES (1031793, 1, "Калмыкия");
INSERT INTO regions VALUES (1032084, 1, "Калужская область");
INSERT INTO regions VALUES (1128991, 1, "Камчатский край");
INSERT INTO regions VALUES (1035359, 1, "Карачаево-Черкесская");
INSERT INTO regions VALUES (1035522, 1, "Карелия");
INSERT INTO regions VALUES (1129059, 1, "Кемеровская область");
INSERT INTO regions VALUES (1130218, 1, "Кировская область");
INSERT INTO regions VALUES (1036606, 1, "Коми");
INSERT INTO regions VALUES (1134737, 1, "Корякский АО");
INSERT INTO regions VALUES (1037344, 1, "Костромская область");
INSERT INTO regions VALUES (1040652, 1, "Краснодарский край");
INSERT INTO regions VALUES (1134771, 1, "Красноярский край");
INSERT INTO regions VALUES (1137144, 1, "Курганская область");
INSERT INTO regions VALUES (1042388, 1, "Курская область");
INSERT INTO regions VALUES (1045244, 1, "Ленинградская область");
INSERT INTO regions VALUES (1048584, 1, "Липецкая область");
INSERT INTO regions VALUES (1138434, 1, "Магаданская область");
INSERT INTO regions VALUES (1050307, 1, "<NAME>");
INSERT INTO regions VALUES (1052052, 1, "Мордовия");
INSERT INTO regions VALUES (1053480, 1, "Московская область");
INSERT INTO regions VALUES (1060316, 1, "Мурманская область");
INSERT INTO regions VALUES (5331184, 1, "Ненецкий АО");
INSERT INTO regions VALUES (1138534, 1, "Нижегородская область");
INSERT INTO regions VALUES (1060458, 1, "Новгородская область");
INSERT INTO regions VALUES (1143518, 1, "Новосибирская область");
INSERT INTO regions VALUES (1145150, 1, "Омская область");
INSERT INTO regions VALUES (1146712, 1, "Оренбургская область");
INSERT INTO regions VALUES (1064424, 1, "Орловская область");
INSERT INTO regions VALUES (1067455, 1, "Пензенская область");
INSERT INTO regions VALUES (1148549, 1, "Пермский край");
INSERT INTO regions VALUES (1152714, 1, "Приморский край");
INSERT INTO regions VALUES (1069004, 1, "Псковская область");
INSERT INTO regions VALUES (1077676, 1, "Ростовская область");
INSERT INTO regions VALUES (1080077, 1, "Рязанская область");
INSERT INTO regions VALUES (1082931, 1, "Самарская область");
INSERT INTO regions VALUES (1084332, 1, "Саратовская область");
INSERT INTO regions VALUES (1153366, 1, "Саха /Якутия/");
INSERT INTO regions VALUES (1153840, 1, "Сахалинская область");
INSERT INTO regions VALUES (1154131, 1, "Свердловская область");
INSERT INTO regions VALUES (1086244, 1, "Северная Осетия - Алания");
INSERT INTO regions VALUES (1086468, 1, "Смоленская область");
INSERT INTO regions VALUES (1091406, 1, "Ставропольский край");
INSERT INTO regions VALUES (1156333, 1, "Таймырский (Долгано-Ненецкий) АО");
INSERT INTO regions VALUES (1092174, 1, "Тамбовская область");
INSERT INTO regions VALUES (1094197, 1, "Татарстан");
INSERT INTO regions VALUES (1097508, 1, "Тверская область");
INSERT INTO regions VALUES (1156388, 1, "Томская область");
INSERT INTO regions VALUES (1105465, 1, "Тульская область");
INSERT INTO regions VALUES (1157049, 1, "Тыва");
INSERT INTO regions VALUES (1157218, 1, "Тюменская область");
INSERT INTO regions VALUES (1109098, 1, "Удмуртская");
INSERT INTO regions VALUES (1111137, 1, "Ульяновская область");
INSERT INTO regions VALUES (1158917, 1, "Хабаровский край");
INSERT INTO regions VALUES (1159424, 1, "Хакасия");
INSERT INTO regions VALUES (1159710, 1, "Ханты-Мансийский Автономный округ - Югра АО");
INSERT INTO regions VALUES (1112201, 1, "Челябинская область");
INSERT INTO regions VALUES (1113642, 1, "Чеченская");
INSERT INTO regions VALUES (1113937, 1, "Чувашская");
INSERT INTO regions VALUES (1160844, 1, "Чукотский АО");
INSERT INTO regions VALUES (1160930, 1, "Ямало-Ненецкий АО");
INSERT INTO regions VALUES (1115658, 1, "Ярославская область");
INSERT INTO regions VALUES (5205441, 164, "Eastern Province");
INSERT INTO regions VALUES (5205445, 164, "Intara y' Amajyepfo");
INSERT INTO regions VALUES (3098690, 164, "Préfecture de Kibuye");
INSERT INTO regions VALUES (3098688, 164, "Préfecture de Kigali");
INSERT INTO regions VALUES (3098631, 164, "Préfecture de Ruhengeri");
INSERT INTO regions VALUES (3099331, 164, "Prefegitura ya Butare");
INSERT INTO regions VALUES (3099334, 164, "Prefegitura ya Byumba");
INSERT INTO regions VALUES (3098706, 164, "Prefegitura ya Cyangugu");
INSERT INTO regions VALUES (3098701, 164, "Prefegitura ya Gikongoro");
INSERT INTO regions VALUES (3098700, 164, "Prefegitura ya Gisenyi");
INSERT INTO regions VALUES (3098698, 164, "Prefegitura ya Gitarama");
INSERT INTO regions VALUES (3098692, 164, "Prefegitura ya Kibungo");
INSERT INTO regions VALUES (5205444, 164, "Province de l' Ouest");
INSERT INTO regions VALUES (5205443, 164, "Province du Nord");
INSERT INTO regions VALUES (5205442, 164, "Umujyi wa Kigali");
INSERT INTO regions VALUES (3246003, 165, "Judeţ<NAME>");
INSERT INTO regions VALUES (3245707, 165, "Judeţ<NAME>");
INSERT INTO regions VALUES (3245653, 165, "Judeţul Argeş");
INSERT INTO regions VALUES (3245431, 165, "Judeţul Bacău");
INSERT INTO regions VALUES (3244474, 165, "Judeţul Bihor");
INSERT INTO regions VALUES (3244278, 165, "Judeţul Bistriţa-Năsăud");
INSERT INTO regions VALUES (3243727, 165, "Judeţul Botoşani");
INSERT INTO regions VALUES (3243605, 165, "Judeţul Brăila");
INSERT INTO regions VALUES (3243553, 165, "Judeţul Braşov");
INSERT INTO regions VALUES (3242895, 165, "Judeţul Buzău");
INSERT INTO regions VALUES (3242802, 165, "Judeţul Călăraşi");
INSERT INTO regions VALUES (3242544, 165, "Judeţul Caraş-Severin");
INSERT INTO regions VALUES (3241249, 165, "Judeţul Cluj");
INSERT INTO regions VALUES (3240946, 165, "Judeţul Constanţa");
INSERT INTO regions VALUES (3240457, 165, "Judeţul Covasna");
INSERT INTO regions VALUES (3239507, 165, "Judeţul Dâmboviţa");
INSERT INTO regions VALUES (3239288, 165, "Judeţul Dolj");
INSERT INTO regions VALUES (3237971, 165, "Judeţul Galaţi");
INSERT INTO regions VALUES (3237435, 165, "Judeţul Giurgiu");
INSERT INTO regions VALUES (3237252, 165, "Judeţul Gorj");
INSERT INTO regions VALUES (3236708, 165, "Judeţ<NAME>");
INSERT INTO regions VALUES (3236360, 165, "Judeţul Hunedoara");
INSERT INTO regions VALUES (3236301, 165, "Judeţul Ialomiţa");
INSERT INTO regions VALUES (3236267, 165, "Judeţul Iaşi");
INSERT INTO regions VALUES (3314752, 165, "Judeţul Ilfov");
INSERT INTO regions VALUES (3234506, 165, "Judeţul Maramureş");
INSERT INTO regions VALUES (3234262, 165, "Judeţul Mehedinţi");
INSERT INTO regions VALUES (3233372, 165, "Judeţul Mureş");
INSERT INTO regions VALUES (3233232, 165, "Judeţul Neamţ");
INSERT INTO regions VALUES (3232689, 165, "Judeţul Olt");
INSERT INTO regions VALUES (3230737, 165, "Judeţul Prahova");
INSERT INTO regions VALUES (3229400, 165, "Judeţul Sălaj");
INSERT INTO regions VALUES (3229052, 165, "Judeţul Satu Mare");
INSERT INTO regions VALUES (3228505, 165, "Judeţul Sibiu");
INSERT INTO regions VALUES (3227213, 165, "Judeţul Suceava");
INSERT INTO regions VALUES (3226724, 165, "Judeţul Teleorman");
INSERT INTO regions VALUES (3226552, 165, "Judeţul Timiş");
INSERT INTO regions VALUES (3226035, 165, "Judeţul Tulcea");
INSERT INTO regions VALUES (3224506, 165, "Judeţul Vâlcea");
INSERT INTO regions VALUES (3224713, 165, "Judeţul Vaslui");
INSERT INTO regions VALUES (3224105, 165, "Judeţul Vrancea");
INSERT INTO regions VALUES (3243238, 165, "Municipiul Bucureşti");
INSERT INTO regions VALUES (3092515, 183, "Muḩāfaz̧at al Ḩasakah");
INSERT INTO regions VALUES (3092361, 183, "Muḩāfaz̧at al Lādhiqīyah");
INSERT INTO regions VALUES (3092196, 183, "Muḩāfaz̧at al Qunayţirah");
INSERT INTO regions VALUES (3092002, 183, "Muḩāfaz̧at ar Raqqah");
INSERT INTO regions VALUES (3091783, 183, "Muḩāfaz̧at as Suwaydā'");
INSERT INTO regions VALUES (3090845, 183, "Muḩāfaz̧at Dar`ā");
INSERT INTO regions VALUES (3090785, 183, "Muḩāfaz̧at Dayr az Zawr");
INSERT INTO regions VALUES (3089013, 183, "Muḩāfaz̧at Dimashq");
INSERT INTO regions VALUES (3090468, 183, "Muḩāfaz̧at Ḩalab");
INSERT INTO regions VALUES (3090443, 183, "Muḩāfaz̧at Ḩamāh");
INSERT INTO regions VALUES (3090217, 183, "Muḩāfaz̧at Ḩimş");
INSERT INTO regions VALUES (3090121, 183, "Muḩāfaz̧at Idlib");
INSERT INTO regions VALUES (3090707, 183, "Muḩāfaz̧at Rīf Dimashq");
INSERT INTO regions VALUES (3086915, 183, "Muḩāfaz̧at Ţarţūs");
INSERT INTO regions VALUES (5022370, 9, "Alabama");
INSERT INTO regions VALUES (5121904, 9, "Alaska");
INSERT INTO regions VALUES (5102686, 9, "Arizona");
INSERT INTO regions VALUES (4913862, 9, "Arkansas");
INSERT INTO regions VALUES (5090053, 9, "California");
INSERT INTO regions VALUES (5096212, 9, "Colorado");
INSERT INTO regions VALUES (5022640, 9, "Connecticut");
INSERT INTO regions VALUES (4920357, 9, "Delaware");
INSERT INTO regions VALUES (4919775, 9, "District of Columbia");
INSERT INTO regions VALUES (4922758, 9, "Florida");
INSERT INTO regions VALUES (1948650, 9, "Georgia");
INSERT INTO regions VALUES (5121081, 9, "Hawaii");
INSERT INTO regions VALUES (5104769, 9, "Idaho");
INSERT INTO regions VALUES (5029761, 9, "Illinois");
INSERT INTO regions VALUES (5032525, 9, "Indiana");
INSERT INTO regions VALUES (5025808, 9, "Iowa");
INSERT INTO regions VALUES (4939607, 9, "Kansas");
INSERT INTO regions VALUES (5135819, 9, "Kentucky");
INSERT INTO regions VALUES (4948591, 9, "Louisiana");
INSERT INTO regions VALUES (5037706, 9, "Maine");
INSERT INTO regions VALUES (4956003, 9, "Maryland");
INSERT INTO regions VALUES (5135820, 9, "Massachusetts");
INSERT INTO regions VALUES (5041735, 9, "Michigan");
INSERT INTO regions VALUES (5046564, 9, "Minnesota");
INSERT INTO regions VALUES (4967628, 9, "Mississippi");
INSERT INTO regions VALUES (4963022, 9, "Missouri");
INSERT INTO regions VALUES (5106727, 9, "Montana");
INSERT INTO regions VALUES (5052130, 9, "Nebraska");
INSERT INTO regions VALUES (5100181, 9, "Nevada");
INSERT INTO regions VALUES (5053846, 9, "New Hampshire");
INSERT INTO regions VALUES (5055537, 9, "New Jersey");
INSERT INTO regions VALUES (5098753, 9, "New Mexico");
INSERT INTO regions VALUES (5060716, 9, "New York");
INSERT INTO regions VALUES (4973955, 9, "North Carolina");
INSERT INTO regions VALUES (5108325, 9, "North Dakota");
INSERT INTO regions VALUES (5066570, 9, "Ohio");
INSERT INTO regions VALUES (4983519, 9, "Oklahoma");
INSERT INTO regions VALUES (5110997, 9, "Oregon");
INSERT INTO regions VALUES (5135821, 9, "Pennsylvania");
INSERT INTO regions VALUES (5079226, 9, "Rhode Island");
INSERT INTO regions VALUES (4992815, 9, "South Carolina");
INSERT INTO regions VALUES (5112275, 9, "South Dakota");
INSERT INTO regions VALUES (5000392, 9, "Tennessee");
INSERT INTO regions VALUES (5007584, 9, "Texas");
INSERT INTO regions VALUES (5102480, 9, "Utah");
INSERT INTO regions VALUES (5082321, 9, "Vermont");
INSERT INTO regions VALUES (2204128, 9, "Virginia");
INSERT INTO regions VALUES (5118746, 9, "Washington");
INSERT INTO regions VALUES (5021644, 9, "West Virginia");
INSERT INTO regions VALUES (5086755, 9, "Wisconsin");
INSERT INTO regions VALUES (5119491, 9, "Wyoming");
INSERT INTO regions VALUES (4701508, 166, "Departamento de Ahuachapán");
INSERT INTO regions VALUES (4701373, 166, "Departamento de Cabañas");
INSERT INTO regions VALUES (4701280, 166, "Departamento de Chalatenango");
INSERT INTO regions VALUES (4701110, 166, "Departamento de Cuscatlán");
INSERT INTO regions VALUES (4699894, 166, "Departamento de La Libertad");
INSERT INTO regions VALUES (4699851, 166, "Departamento de La Paz");
INSERT INTO regions VALUES (4699627, 166, "Departamento de La Unión");
INSERT INTO regions VALUES (4699304, 166, "Departamento de Morazán");
INSERT INTO regions VALUES (4698606, 166, "Departamento de San Miguel");
INSERT INTO regions VALUES (4698514, 166, "Departamento de San Salvador");
INSERT INTO regions VALUES (4698350, 166, "Departamento de San Vicente");
INSERT INTO regions VALUES (4698489, 166, "Departamento de Santa Ana");
INSERT INTO regions VALUES (4698299, 166, "Departamento de Sonsonate");
INSERT INTO regions VALUES (4698158, 166, "Departamento de Usulután");
INSERT INTO regions VALUES (4609903, 168, "Castello di Acquaviva");
INSERT INTO regions VALUES (4609904, 168, "Castello di B<NAME>");
INSERT INTO regions VALUES (4548093, 168, "Cast<NAME>");
INSERT INTO regions VALUES (4609905, 168, "Cast<NAME>");
INSERT INTO regions VALUES (4609906, 168, "Castello di Faetano");
INSERT INTO regions VALUES (4609907, 168, "Castello di Fiorentino");
INSERT INTO regions VALUES (4609908, 168, "Castello di Montegiardino");
INSERT INTO regions VALUES (4609902, 168, "Castello di San Marino Città");
INSERT INTO regions VALUES (4539463, 168, "Castello di Serravalle");
INSERT INTO regions VALUES (4143442, 169, "Príncipe");
INSERT INTO regions VALUES (4143374, 169, "São Tomé");
INSERT INTO regions VALUES (3051954, 170, "Al Minţaqah ash Sharqīyah");
INSERT INTO regions VALUES (3052486, 170, "Minţaqat al Bāḩah");
INSERT INTO regions VALUES (3052361, 170, "Minţaqat al Ḩudūd ash Shamālīyah");
INSERT INTO regions VALUES (3052328, 170, "Minţaqat al Jawf");
INSERT INTO regions VALUES (3052247, 170, "Minţaqat al Madīnah");
INSERT INTO regions VALUES (3052132, 170, "Minţaqat al Qaşīm");
INSERT INTO regions VALUES (3051993, 170, "Minţaqat ar Riyāḑ");
INSERT INTO regions VALUES (3051662, 170, "Minţaqat Ḩā'il");
INSERT INTO regions VALUES (3051545, 170, "Minţaqat Jīzān");
INSERT INTO regions VALUES (3051440, 170, "Minţaqat Makkah");
INSERT INTO regions VALUES (3051330, 170, "Minţaqat Najrān");
INSERT INTO regions VALUES (3051055, 170, "Minţaqat Tabūk");
INSERT INTO regions VALUES (3051927, 170, "Minţaqat `Asīr");
INSERT INTO regions VALUES (5421651, 171, "Hhohho District");
INSERT INTO regions VALUES (5421652, 171, "Lubombo District");
INSERT INTO regions VALUES (5421650, 171, "Manzini District");
INSERT INTO regions VALUES (5421653, 171, "Shiselweni District");
INSERT INTO regions VALUES (3336728, 172, "Hhohho District");
INSERT INTO regions VALUES (3336713, 172, "Lubombo District");
INSERT INTO regions VALUES (3336701, 172, "Manzini District");
INSERT INTO regions VALUES (3336677, 172, "Shiselweni District");
INSERT INTO regions VALUES (3121458, 175, "Anse aux Pins");
INSERT INTO regions VALUES (3121457, 175, "Anse Boileau");
INSERT INTO regions VALUES (3121455, 175, "Anse Etoile");
INSERT INTO regions VALUES (3121454, 175, "Anse Louis");
INSERT INTO regions VALUES (3121453, 175, "Anse Royale");
INSERT INTO regions VALUES (3209774, 175, "Au Cap");
INSERT INTO regions VALUES (3121450, 175, "Baie Lazare");
INSERT INTO regions VALUES (3121449, 175, "Baie Sainte Anne");
INSERT INTO regions VALUES (3121448, 175, "Beau Vallon");
INSERT INTO regions VALUES (3121446, 175, "Bel Air");
INSERT INTO regions VALUES (3121445, 175, "Bel Ombre");
INSERT INTO regions VALUES (3121443, 175, "Cascade");
INSERT INTO regions VALUES (3121435, 175, "English River");
INSERT INTO regions VALUES (3121440, 175, "Glacis");
INSERT INTO regions VALUES (3121438, 175, "Grand Anse Mahe");
INSERT INTO regions VALUES (3121439, 175, "Grand Anse Praslin");
INSERT INTO regions VALUES (3121437, 175, "Inner Islands");
INSERT INTO regions VALUES (3209772, 175, "Les Mamelles");
INSERT INTO regions VALUES (3121431, 175, "Mont Buxton");
INSERT INTO regions VALUES (3121430, 175, "<NAME>");
INSERT INTO regions VALUES (3121429, 175, "Plaisance");
INSERT INTO regions VALUES (3121428, 175, "Pointe Larue");
INSERT INTO regions VALUES (3121427, 175, "Port Glaud");
INSERT INTO regions VALUES (3209773, 175, "Roche Caiman");
INSERT INTO regions VALUES (3121425, 175, "Saint Louis");
INSERT INTO regions VALUES (3121423, 175, "Takamaka");
INSERT INTO regions VALUES (4032544, 176, "Région de Dakar");
INSERT INTO regions VALUES (4031656, 176, "Région de Diourbel");
INSERT INTO regions VALUES (4031347, 176, "Région de Fatick");
INSERT INTO regions VALUES (4030490, 176, "Région de Kaolack");
INSERT INTO regions VALUES (4029542, 176, "Région de Kolda");
INSERT INTO regions VALUES (4029128, 176, "Région de Louga");
INSERT INTO regions VALUES (4028772, 176, "Région de Matam");
INSERT INTO regions VALUES (4026836, 176, "Région de Saint-Louis");
INSERT INTO regions VALUES (4025540, 176, "Région de Tambacounda");
INSERT INTO regions VALUES (4025386, 176, "Région de Thiès");
INSERT INTO regions VALUES (4024696, 176, "Région de Ziguinchor");
INSERT INTO regions VALUES (4696771, 177, "Grenadines");
INSERT INTO regions VALUES (4696787, 177, "Parish of Charlotte");
INSERT INTO regions VALUES (4696747, 177, "Parish of Saint Andrew");
INSERT INTO regions VALUES (4696746, 177, "Parish of Saint David");
INSERT INTO regions VALUES (4696745, 177, "Parish of Saint George");
INSERT INTO regions VALUES (4696744, 177, "Parish of Saint Patrick");
INSERT INTO regions VALUES (4696133, 178, "Christ Church Nichola Town");
INSERT INTO regions VALUES (4696060, 178, "Saint Anne Sandy Point");
INSERT INTO regions VALUES (4696059, 178, "Saint George Basseterre");
INSERT INTO regions VALUES (4696058, 178, "Saint George Gingerland");
INSERT INTO regions VALUES (4696057, 178, "Saint <NAME>ward");
INSERT INTO regions VALUES (4696056, 178, "Saint John Capesterre");
INSERT INTO regions VALUES (4696055, 178, "Saint <NAME>");
INSERT INTO regions VALUES (4696053, 178, "Saint Mary Cayon");
INSERT INTO regions VALUES (4696052, 178, "Saint Paul Capesterre");
INSERT INTO regions VALUES (4696051, 178, "Saint Paul Charlestown");
INSERT INTO regions VALUES (4696049, 178, "Saint Peter Basseterre");
INSERT INTO regions VALUES (4696047, 178, "Saint Thomas Lowland");
INSERT INTO regions VALUES (4696046, 178, "Saint Thomas Middle Island");
INSERT INTO regions VALUES (4696036, 178, "Trinity Palmetto Point");
INSERT INTO regions VALUES (4696582, 179, "Quarter of Anse-la-Raye");
INSERT INTO regions VALUES (4696545, 179, "Quarter of Castries");
INSERT INTO regions VALUES (4696539, 179, "Quarter of Choiseul");
INSERT INTO regions VALUES (4696531, 179, "Quarter of Dauphin");
INSERT INTO regions VALUES (4696525, 179, "Quarter of Dennery");
INSERT INTO regions VALUES (4696495, 179, "Quarter of Gros-Islet");
INSERT INTO regions VALUES (4696486, 179, "Quarter of Laborie");
INSERT INTO regions VALUES (4696454, 179, "Quarter of Micoud");
INSERT INTO regions VALUES (4696426, 179, "Quarter of Praslin");
INSERT INTO regions VALUES (4696408, 179, "Quarter of Soufrière");
INSERT INTO regions VALUES (4696401, 179, "Quarter of Vieux-Fort");
INSERT INTO regions VALUES (5326638, 180, "Collectivité territoriale de Saint-Pierre-et-Miquelon");
INSERT INTO regions VALUES (5423553, 181, "Autonomna Pokrajina Kosovo i Metohija");
INSERT INTO regions VALUES (5423550, 181, "Autonomna Pokrajina Vojvodina");
INSERT INTO regions VALUES (5423551, 181, "Grad Beograd");
INSERT INTO regions VALUES (5423552, 181, "Južna i istočna Srbija");
INSERT INTO regions VALUES (5439506, 181, "Šumadija i zapadna Srbija");
INSERT INTO regions VALUES (4609300, 184, "Banskobystrický Kraj");
INSERT INTO regions VALUES (4609301, 184, "Bratislavský Kraj");
INSERT INTO regions VALUES (3314544, 184, "Košický Kraj");
INSERT INTO regions VALUES (4609302, 184, "Nitriansky Kraj");
INSERT INTO regions VALUES (3314545, 184, "Prešovský Kraj");
INSERT INTO regions VALUES (4609303, 184, "Trenčiansky Kraj");
INSERT INTO regions VALUES (4609304, 184, "Trnavský Kraj");
INSERT INTO regions VALUES (4465797, 184, "Žilinský Kraj");
INSERT INTO regions VALUES (4565054, 185, "Mestna Občina Celje");
INSERT INTO regions VALUES (4561404, 185, "Mestna Občina Koper-Capodistria");
INSERT INTO regions VALUES (4561145, 185, "Mestna Občina Kranj");
INSERT INTO regions VALUES (4576795, 185, "Mestna Občina Ljubljana");
INSERT INTO regions VALUES (4559910, 185, "Mestna Občina Maribor");
INSERT INTO regions VALUES (4559343, 185, "Mestna Občina Murska Sobota");
INSERT INTO regions VALUES (4559198, 185, "Mestna Občina Nova Gorica");
INSERT INTO regions VALUES (4559108, 185, "Mestna Občina Novo Mesto");
INSERT INTO regions VALUES (4557622, 185, "Mestna Občina Ptuj");
INSERT INTO regions VALUES (4556419, 185, "Mestna Občina Slovenj Gradec");
INSERT INTO regions VALUES (4555456, 185, "Mestna Občina Velenje");
INSERT INTO regions VALUES (4566549, 185, "Občina Ajdovščina");
INSERT INTO regions VALUES (5470258, 185, "Občina Apače");
INSERT INTO regions VALUES (4576787, 185, "Občina Beltinci");
INSERT INTO regions VALUES (4609699, 185, "Občina Benedikt");
INSERT INTO regions VALUES (4609681, 185, "Občina Bistrica ob Sotli");
INSERT INTO regions VALUES (4576694, 185, "Občina Bled");
INSERT INTO regions VALUES (4609668, 185, "Občina Bloke");
INSERT INTO regions VALUES (4576693, 185, "Občina Bohinj");
INSERT INTO regions VALUES (4576674, 185, "Občina Borovnica");
INSERT INTO regions VALUES (4576692, 185, "Občina Bovec");
INSERT INTO regions VALUES (4609674, 185, "Občina Braslovče");
INSERT INTO regions VALUES (4576681, 185, "Občina Brda");
INSERT INTO regions VALUES (4565531, 185, "Občina Brežice");
INSERT INTO regions VALUES (4576672, 185, "Občina Brezovica");
INSERT INTO regions VALUES (4609707, 185, "Občina Cankova");
INSERT INTO regions VALUES (4576698, 185, "Občina Cerklje na Gorenjskem");
INSERT INTO regions VALUES (4565001, 185, "Občina Cerknica");
INSERT INTO regions VALUES (4576685, 185, "Občina Cerkno");
INSERT INTO regions VALUES (4609698, 185, "Občina Cerkvenjak");
INSERT INTO regions VALUES (5470260, 185, "Občina Cirkulane");
INSERT INTO regions VALUES (4576790, 185, "Občina Črenšovci");
INSERT INTO regions VALUES (4576722, 185, "Občina Črna na Koroškem");
INSERT INTO regions VALUES (4564788, 185, "Občina Črnomelj");
INSERT INTO regions VALUES (4609695, 185, "Občina Destrnik");
INSERT INTO regions VALUES (4576661, 185, "Občina Divača");
INSERT INTO regions VALUES (4609682, 185, "Občina Dobje");
INSERT INTO regions VALUES (4576733, 185, "Občina Dobrepolje");
INSERT INTO regions VALUES (4609683, 185, "Občina Dobrna");
INSERT INTO regions VALUES (4576689, 185, "Občina Dobrova-Horjul-Polhov Gradec");
INSERT INTO regions VALUES (4609705, 185, "Občina Dobrovnik-Dobronak");
INSERT INTO regions VALUES (4576703, 185, "Občina Dol pri Ljubljani");
INSERT INTO regions VALUES (4609679, 185, "Občina Dolenjske Toplice");
INSERT INTO regions VALUES (4564332, 185, "Občina Domžale");
INSERT INTO regions VALUES (4576771, 185, "Obč<NAME>");
INSERT INTO regions VALUES (4563879, 185, "Občina Dravograd");
INSERT INTO regions VALUES (4576750, 185, "Občina Duplek");
INSERT INTO regions VALUES (4576688, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4576769, 185, "Občina Gorišnica");
INSERT INTO regions VALUES (4563109, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4576709, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4576782, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4609708, 185, "Občina Grad");
INSERT INTO regions VALUES (4562555, 185, "Občina Grosuplje");
INSERT INTO regions VALUES (4609691, 185, "Občina Hajdina");
INSERT INTO regions VALUES (4609689, 185, "Občina Hoče-Slivnica");
INSERT INTO regions VALUES (4609709, 185, "Občina Hodoš-Hodos");
INSERT INTO regions VALUES (4609666, 185, "Občina Horjul");
INSERT INTO regions VALUES (4562393, 185, "Občina Hrastnik");
INSERT INTO regions VALUES (4576660, 185, "Občina Hrpelje-Kozina");
INSERT INTO regions VALUES (4562297, 185, "Občina Idrija");
INSERT INTO regions VALUES (4576670, 185, "Občina Ig");
INSERT INTO regions VALUES (4562275, 185, "Občina I<NAME>");
INSERT INTO regions VALUES (4576732, 185, "Občina I<NAME>");
INSERT INTO regions VALUES (4562185, 185, "Občina Izola-Isola");
INSERT INTO regions VALUES (4561968, 185, "Občina Jesenice");
INSERT INTO regions VALUES (4609663, 185, "Občina Jezersko");
INSERT INTO regions VALUES (4576772, 185, "Občina Juršinci");
INSERT INTO regions VALUES (4561787, 185, "Občina Kamnik");
INSERT INTO regions VALUES (4576683, 185, "Občina Kanal");
INSERT INTO regions VALUES (4576757, 185, "Občina Kidričevo");
INSERT INTO regions VALUES (4576690, 185, "Občina Kobarid");
INSERT INTO regions VALUES (4576786, 185, "Občina Kobilje");
INSERT INTO regions VALUES (4561516, 185, "Občina Kočevje");
INSERT INTO regions VALUES (4576678, 185, "Občina Komen");
INSERT INTO regions VALUES (4609665, 185, "Občina Komenda");
INSERT INTO regions VALUES (5470264, 185, "Občina Kostanjevica na Krki");
INSERT INTO regions VALUES (4609677, 185, "Občina Kostel");
INSERT INTO regions VALUES (4576767, 185, "Občina Kozje");
INSERT INTO regions VALUES (4576696, 185, "Občina Kranjska Gora");
INSERT INTO regions VALUES (4609701, 185, "Obč<NAME>");
INSERT INTO regions VALUES (4560993, 185, "Občina Krško");
INSERT INTO regions VALUES (4576747, 185, "Občina Kungota");
INSERT INTO regions VALUES (4576781, 185, "Občina Kuzma");
INSERT INTO regions VALUES (4560718, 185, "Občina Laško");
INSERT INTO regions VALUES (4560658, 185, "Občina Lenart");
INSERT INTO regions VALUES (4609704, 185, "Občina Lendava-Lendva");
INSERT INTO regions VALUES (4560493, 185, "Obč<NAME>");
INSERT INTO regions VALUES (4576725, 185, "Občina Ljubno");
INSERT INTO regions VALUES (4560416, 185, "Občina Ljutomer");
INSERT INTO regions VALUES (4560405, 185, "Občina Logatec");
INSERT INTO regions VALUES (4576664, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4576666, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4609687, 185, "Občina <NAME> Pohorju");
INSERT INTO regions VALUES (4576710, 185, "Občina Luče");
INSERT INTO regions VALUES (4576729, 185, "Občina Lukovica");
INSERT INTO regions VALUES (4576758, 185, "Občina Majšperk");
INSERT INTO regions VALUES (4609694, 185, "Občina Markovci");
INSERT INTO regions VALUES (4576701, 185, "Občina Medvode");
INSERT INTO regions VALUES (4576702, 185, "Občina Mengeš");
INSERT INTO regions VALUES (4559726, 185, "Občina Metlika");
INSERT INTO regions VALUES (4576718, 185, "Občina Mežica");
INSERT INTO regions VALUES (4609690, 185, "Občina Miklavž na Dravskem Polju");
INSERT INTO regions VALUES (4576679, 185, "Občina Miren-Kostanjevica");
INSERT INTO regions VALUES (4609680, 185, "Občina <NAME>");
INSERT INTO regions VALUES (4576744, 185, "Občina Mislinja");
INSERT INTO regions VALUES (5470279, 185, "Občina Mokronog-Trebelno");
INSERT INTO regions VALUES (4576708, 185, "Občina Moravče");
INSERT INTO regions VALUES (4576785, 185, "Občina Moravske Toplice");
INSERT INTO regions VALUES (4559433, 185, "Občina Mozirje");
INSERT INTO regions VALUES (4576720, 185, "Občina Muta");
INSERT INTO regions VALUES (4576695, 185, "Občina Naklo");
INSERT INTO regions VALUES (4576791, 185, "Občina Nazarje");
INSERT INTO regions VALUES (4576789, 185, "Občina Odranci");
INSERT INTO regions VALUES (4609684, 185, "Občina Oplotnica");
INSERT INTO regions VALUES (4558846, 185, "Občina Ormož");
INSERT INTO regions VALUES (4576667, 185, "Občina Osilnica");
INSERT INTO regions VALUES (4576749, 185, "Občina Pesnica");
INSERT INTO regions VALUES (4558451, 185, "Občina Piran-Pirano");
INSERT INTO regions VALUES (4576663, 185, "Občina Pivka");
INSERT INTO regions VALUES (4576766, 185, "Občina Podčetrtek");
INSERT INTO regions VALUES (4609693, 185, "Občina Podlehnik");
INSERT INTO regions VALUES (4609685, 185, "Občina Podvelka");
INSERT INTO regions VALUES (5470261, 185, "Občina Poljčane");
INSERT INTO regions VALUES (4609675, 185, "Občina Polzela");
INSERT INTO regions VALUES (4557956, 185, "Občina Postojna");
INSERT INTO regions VALUES (4609676, 185, "Občina Prebold");
INSERT INTO regions VALUES (4576697, 185, "Občina Preddvor");
INSERT INTO regions VALUES (4609671, 185, "Občina Prevalje");
INSERT INTO regions VALUES (4576779, 185, "Občina Puconci");
INSERT INTO regions VALUES (4576755, 185, "Občina Rače-Fram");
INSERT INTO regions VALUES (4576730, 185, "Občina Radeče");
INSERT INTO regions VALUES (4576776, 185, "Občina Radenci");
INSERT INTO regions VALUES (4557534, 185, "Občina Radlje ob Dravi");
INSERT INTO regions VALUES (4557496, 185, "Občina Radovljica");
INSERT INTO regions VALUES (4576717, 185, "Občina Ravne na Koroškem");
INSERT INTO regions VALUES (5443121, 185, "Obcina Ravne-Prevalje");
INSERT INTO regions VALUES (4609710, 185, "Občina Razkrižje");
INSERT INTO regions VALUES (5470276, 185, "Občina Rečica ob Savinji");
INSERT INTO regions VALUES (5470262, 185, "Občina Renče - Vogrsko");
INSERT INTO regions VALUES (4557227, 185, "Občina Ribnica");
INSERT INTO regions VALUES (4609686, 185, "Občina Ribnica na Pohorju");
INSERT INTO regions VALUES (4576763, 185, "Občina Rogaška Slatina");
INSERT INTO regions VALUES (4576780, 185, "Občina Rogašovci");
INSERT INTO regions VALUES (4576765, 185, "Občina Rogatec");
INSERT INTO regions VALUES (4576746, 185, "Občina Ruše");
INSERT INTO regions VALUES (4609712, 185, "Občina Šalovci");
INSERT INTO regions VALUES (4609688, 185, "Občina Selnica ob Dravi");
INSERT INTO regions VALUES (4576735, 185, "Občina Semič");
INSERT INTO regions VALUES (4609667, 185, "Občina Šempeter-Vrtojba");
INSERT INTO regions VALUES (4576699, 185, "Občina Šenčur");
INSERT INTO regions VALUES (4576748, 185, "Občina Šentilj");
INSERT INTO regions VALUES (4576737, 185, "Občina Šentjernej");
INSERT INTO regions VALUES (4556774, 185, "Občina Šentjur pri Celju");
INSERT INTO regions VALUES (5470259, 185, "Občina Šentrupert");
INSERT INTO regions VALUES (4556712, 185, "Občina Sevnica");
INSERT INTO regions VALUES (4556707, 185, "Občina Sežana");
INSERT INTO regions VALUES (4576739, 185, "Občina Škocjan");
INSERT INTO regions VALUES (4556554, 185, "Občina Škofja Loka");
INSERT INTO regions VALUES (4576669, 185, "Občina Škofljica");
INSERT INTO regions VALUES (4556417, 185, "Občina Slovenska Bistrica");
INSERT INTO regions VALUES (4556414, 185, "Občina Slovenske Konjice");
INSERT INTO regions VALUES (4556399, 185, "Občina Šmarje pri Jelšah");
INSERT INTO regions VALUES (5470257, 185, "Občina Šmarješke Toplice");
INSERT INTO regions VALUES (4576728, 185, "Občina Šmartno ob Paki");
INSERT INTO regions VALUES (4609711, 185, "Občina Šmartno pri Litiji");
INSERT INTO regions VALUES (4609669, 185, "Občina Sodražica");
INSERT INTO regions VALUES (4609664, 185, "Občina Solčava");
INSERT INTO regions VALUES (4576726, 185, "Občina Šoštanj");
INSERT INTO regions VALUES (4576756, 185, "Občina Starše");
INSERT INTO regions VALUES (4576740, 185, "Občina Štore");
INSERT INTO regions VALUES (4609700, 185, "Občina Sveta Ana");
INSERT INTO regions VALUES (5470255, 185, "Občina Sveta Trojica v Slovenskih goricah");
INSERT INTO regions VALUES (4609697, 185, "Občina Sveti Andraž v Slovenskih Goricah");
INSERT INTO regions VALUES (4576774, 185, "Občina Sveti Jurij ob Ščavnici");
INSERT INTO regions VALUES (5470256, 185, "Občina <NAME> v Slovenskih goricah");
INSERT INTO regions VALUES (5443065, 185, "Občina Sveti Tomaž");
INSERT INTO regions VALUES (4609673, 185, "Občina Tabor");
INSERT INTO regions VALUES (4609706, 185, "Občina Tišina");
INSERT INTO regions VALUES (4555432, 185, "Občina Tolmin");
INSERT INTO regions VALUES (4555344, 185, "Občina Trbovlje");
INSERT INTO regions VALUES (4555323, 185, "Občina Trebnje");
INSERT INTO regions VALUES (4609696, 185, "Občina Trnovska Vas");
INSERT INTO regions VALUES (4555190, 185, "Občina Tržič");
INSERT INTO regions VALUES (4609670, 185, "Občina Trzin");
INSERT INTO regions VALUES (4576788, 185, "Občina Turnišče");
INSERT INTO regions VALUES (4609703, 185, "Občina V<NAME>");
INSERT INTO regions VALUES (4576668, 185, "Občina V<NAME>");
INSERT INTO regions VALUES (4609702, 185, "Občina Veržej");
INSERT INTO regions VALUES (4576760, 185, "Občina Videm");
INSERT INTO regions VALUES (4576675, 185, "Občina Vipava");
INSERT INTO regions VALUES (4576742, 185, "Občina Vitanje");
INSERT INTO regions VALUES (4576700, 185, "Občina Vodice");
INSERT INTO regions VALUES (4576741, 185, "Občina Vojnik");
INSERT INTO regions VALUES (4609672, 185, "Občina Vransko");
INSERT INTO regions VALUES (4554290, 185, "Občina Vrhnika");
INSERT INTO regions VALUES (4576721, 185, "Občina Vuzenica");
INSERT INTO regions VALUES (4554052, 185, "Občina Zagorje ob Savi");
INSERT INTO regions VALUES (4553998, 185, "Občina Žalec");
INSERT INTO regions VALUES (4576770, 185, "Občina Zavrč");
INSERT INTO regions VALUES (4576686, 185, "Občina Železniki");
INSERT INTO regions VALUES (4609692, 185, "Občina Žetale");
INSERT INTO regions VALUES (4576684, 185, "Občina Žiri");
INSERT INTO regions VALUES (4609662, 185, "Občina Žirovnica");
INSERT INTO regions VALUES (4576743, 185, "<NAME>");
INSERT INTO regions VALUES (4609678, 185, "<NAME>");
INSERT INTO regions VALUES (3992828, 186, "Central Province");
INSERT INTO regions VALUES (3992621, 186, "Guadalcanal Province");
INSERT INTO regions VALUES (3992411, 186, "Isabel Province");
INSERT INTO regions VALUES (4007429, 186, "Makira Province");
INSERT INTO regions VALUES (3991886, 186, "Malaita Province");
INSERT INTO regions VALUES (4007305, 186, "Temotu Province");
INSERT INTO regions VALUES (3990219, 186, "Western Province");
INSERT INTO regions VALUES (5145346, 187, "Gobolka Awdal");
INSERT INTO regions VALUES (3036451, 187, "Gobolka Bakool");
INSERT INTO regions VALUES (3036391, 187, "Gobolka Banaadir");
INSERT INTO regions VALUES (3036326, 187, "Gobolka Bari");
INSERT INTO regions VALUES (3036288, 187, "Gobolka Bay");
INSERT INTO regions VALUES (3034839, 187, "Gobolka Galguduud");
INSERT INTO regions VALUES (3034682, 187, "Gobolka Gedo");
INSERT INTO regions VALUES (3034267, 187, "Gobolka Hiiraan");
INSERT INTO regions VALUES (3034001, 187, "Gobolka Jubbada Dhexe");
INSERT INTO regions VALUES (3034000, 187, "Gobolka Jubbada Hoose");
INSERT INTO regions VALUES (3033368, 187, "Gobolka Mudug");
INSERT INTO regions VALUES (3033293, 187, "Gobolka Nugaal");
INSERT INTO regions VALUES (3032992, 187, "Gobolka Sanaag");
INSERT INTO regions VALUES (3032928, 187, "Gobolka Shabeellaha Dhexe");
INSERT INTO regions VALUES (3032927, 187, "Gobolka Shabeellaha Hoose");
INSERT INTO regions VALUES (5145347, 187, "Gobolka Sool");
INSERT INTO regions VALUES (3032742, 187, "Gobolka Togdheer");
INSERT INTO regions VALUES (3032510, 187, "Gobolka Woqooyi Galbeed");
INSERT INTO regions VALUES (3186806, 188, "Al Wilāyah al Istiwā'īyah");
INSERT INTO regions VALUES (3186965, 188, "Al Wilāyah al Wusţá");
INSERT INTO regions VALUES (3186371, 188, "Al Wilāyah ash Shamālīyah");
INSERT INTO regions VALUES (3186367, 188, "Al Wilāyah ash Sharqīyah");
INSERT INTO regions VALUES (3186127, 188, "Bahr al Ghazal Wilayat");
INSERT INTO regions VALUES (3183715, 188, "Kurdufan Wilayat");
INSERT INTO regions VALUES (3196590, 188, "Wilāyat al Baḩr al Aḩmar");
INSERT INTO regions VALUES (3196591, 188, "Wilāyat al Buḩayrāt");
INSERT INTO regions VALUES (3196592, 188, "Wilāyat al Jazīrah");
INSERT INTO regions VALUES (3186759, 188, "Wilāyat al Kharţūm");
INSERT INTO regions VALUES (3196593, 188, "Wilāyat al Qaḑārif");
INSERT INTO regions VALUES (3196595, 188, "Wilāyat an Nīl al Abyaḑ");
INSERT INTO regions VALUES (3196596, 188, "Wilāyat an Nīl al Azraq");
INSERT INTO regions VALUES (3187572, 188, "Wilāyat A`ālī an Nīl");
INSERT INTO regions VALUES (3196597, 188, "Wilāyat Baḩr al Jabal");
INSERT INTO regions VALUES (3196598, 188, "Wilāyat Gharb al Istiwā'īyah");
INSERT INTO regions VALUES (3196599, 188, "Wilāyat Gharb Baḩr al Ghazāl");
INSERT INTO regions VALUES (5468664, 188, "Wilāyat Gharb Dārfūr");
INSERT INTO regions VALUES (5468663, 188, "Wilāyat Gharb Kurdufān");
INSERT INTO regions VALUES (3196602, 188, "Wilāyat Janūb Dārfūr");
INSERT INTO regions VALUES (3196603, 188, "Wilāyat Janūb Kurdufān");
INSERT INTO regions VALUES (3196604, 188, "Wilāyat Junqalī");
INSERT INTO regions VALUES (3196605, 188, "Wilāyat Kassalā");
INSERT INTO regions VALUES (3196606, 188, "Wilāyat Nahr an Nīl");
INSERT INTO regions VALUES (5468684, 188, "Wilayat Sannār");
INSERT INTO regions VALUES (3196607, 188, "Wilāyat Shamāl Baḩr al Ghazāl");
INSERT INTO regions VALUES (3196608, 188, "Wilāyat Shamāl Dārfūr");
INSERT INTO regions VALUES (3196609, 188, "Wilāyat Shamāl Kurdufān");
INSERT INTO regions VALUES (4619369, 189, "Distrikt Brokopondo");
INSERT INTO regions VALUES (4619351, 189, "Distrikt Commewijne");
INSERT INTO regions VALUES (4619344, 189, "Distrikt Coronie");
INSERT INTO regions VALUES (4619183, 189, "Distrikt Marowijne");
INSERT INTO regions VALUES (4619161, 189, "Distrikt Nickerie");
INSERT INTO regions VALUES (4619137, 189, "Distrikt Para");
INSERT INTO regions VALUES (4619133, 189, "Distrikt Paramaribo");
INSERT INTO regions VALUES (4619076, 189, "Distrikt Saramacca");
INSERT INTO regions VALUES (4619066, 189, "Distrikt Sipaliwini");
INSERT INTO regions VALUES (4619016, 189, "Distrikt Wanica");
INSERT INTO regions VALUES (5421094, 54, "Aileu");
INSERT INTO regions VALUES (5421098, 54, "Ainaro");
INSERT INTO regions VALUES (5421090, 54, "Baucau");
INSERT INTO regions VALUES (5421099, 54, "Bobonaro");
INSERT INTO regions VALUES (5421100, 54, "Cova Lima");
INSERT INTO regions VALUES (5421093, 54, "Dili");
INSERT INTO regions VALUES (5421097, 54, "Ermera");
INSERT INTO regions VALUES (5421089, 54, "Lautém");
INSERT INTO regions VALUES (5421096, 54, "Liquiçá");
INSERT INTO regions VALUES (5421092, 54, "Manatuto");
INSERT INTO regions VALUES (5421095, 54, "Manufahi");
INSERT INTO regions VALUES (5421101, 54, "Oecussi-Ambeno");
INSERT INTO regions VALUES (3695172, 54, "Timor-Leste");
INSERT INTO regions VALUES (5421091, 54, "Viqueque");
INSERT INTO regions VALUES (4142296, 190, "Eastern Province");
INSERT INTO regions VALUES (4138012, 190, "Northern Province");
INSERT INTO regions VALUES (4137067, 190, "Southern Province");
INSERT INTO regions VALUES (4136507, 190, "Western Area");
INSERT INTO regions VALUES (3685509, 191, "Changawat Chanthaburi");
INSERT INTO regions VALUES (3871376, 191, "Changwat Amnat Charoen");
INSERT INTO regions VALUES (3694396, 191, "Changwat Ang Thong");
INSERT INTO regions VALUES (3685528, 191, "Changwat Buriram");
INSERT INTO regions VALUES (3685526, 191, "Changwat Chachoengsao");
INSERT INTO regions VALUES (3685521, 191, "Changwat Chai Nat");
INSERT INTO regions VALUES (3685518, 191, "Changwat Chaiyaphum");
INSERT INTO regions VALUES (3424841, 191, "Changwat Chiang Mai");
INSERT INTO regions VALUES (3424839, 191, "Changwat Chiang Rai");
INSERT INTO regions VALUES (3685481, 191, "Changwat Chon Buri");
INSERT INTO regions VALUES (3424833, 191, "Changwat Chumphon");
INSERT INTO regions VALUES (3685418, 191, "Changwat Kalasin");
INSERT INTO regions VALUES (3424808, 191, "Changwat Kamphaeng Phet");
INSERT INTO regions VALUES (3424806, 191, "Changwat Kanchanaburi");
INSERT INTO regions VALUES (3685154, 191, "Changwat Khon Kaen");
INSERT INTO regions VALUES (3424760, 191, "Changwat Krabi");
INSERT INTO regions VALUES (3424753, 191, "Changwat Lampang");
INSERT INTO regions VALUES (3424751, 191, "Changwat Lamphun");
INSERT INTO regions VALUES (3685081, 191, "Changwat Loei");
INSERT INTO regions VALUES (3685073, 191, "Changwat Lop Buri");
INSERT INTO regions VALUES (3424726, 191, "Changwat Mae Hong Son");
INSERT INTO regions VALUES (3685066, 191, "Changwat Maha Sarakham");
INSERT INTO regions VALUES (3684994, 191, "Changwat Mukdahan");
INSERT INTO regions VALUES (3684979, 191, "Changwat Nakhon Nayok");
INSERT INTO regions VALUES (3684977, 191, "Changwat Nakhon Pathom");
INSERT INTO regions VALUES (3684975, 191, "Changwat Nakhon Phanom");
INSERT INTO regions VALUES (3684973, 191, "Changwat Nakhon Ratchasima");
INSERT INTO regions VALUES (3684971, 191, "Changwat Nakhon Sawan");
INSERT INTO regions VALUES (3684970, 191, "Changwat Nakhon Si Thammarat");
INSERT INTO regions VALUES (3684954, 191, "Changwat Nan");
INSERT INTO regions VALUES (3684947, 191, "Changwat Narathiwat");
INSERT INTO regions VALUES (3871377, 191, "Changwat Nong Bua Lamphu");
INSERT INTO regions VALUES (3684920, 191, "Changwat Nong Khai");
INSERT INTO regions VALUES (3684897, 191, "Changwat Nonthaburi");
INSERT INTO regions VALUES (3684871, 191, "Changwat Pathum Thani");
INSERT INTO regions VALUES (3684867, 191, "Changwat Pattani");
INSERT INTO regions VALUES (3424654, 191, "Changwat Phangnga");
INSERT INTO regions VALUES (3684840, 191, "Changwat Phatthalung");
INSERT INTO regions VALUES (3684836, 191, "Changwat Phayao");
INSERT INTO regions VALUES (3684829, 191, "Changwat Phetchabun");
INSERT INTO regions VALUES (3424645, 191, "Changwat Phetchaburi");
INSERT INTO regions VALUES (3684823, 191, "<NAME>");
INSERT INTO regions VALUES (3684819, 191, "Changwat Phitsanulok");
INSERT INTO regions VALUES (3684794, 191, "Changwat Phra Nakhon Si Ayutthaya");
INSERT INTO regions VALUES (3684802, 191, "Changwat Phrae");
INSERT INTO regions VALUES (3424630, 191, "Changwat Phuket");
INSERT INTO regions VALUES (3684758, 191, "Changwat Pr<NAME>");
INSERT INTO regions VALUES (3424625, 191, "Changwat Pr<NAME>");
INSERT INTO regions VALUES (3424621, 191, "<NAME>");
INSERT INTO regions VALUES (3424619, 191, "Changwat Ratchaburi");
INSERT INTO regions VALUES (3684728, 191, "<NAME>");
INSERT INTO regions VALUES (3684725, 191, "Changwat R<NAME>");
INSERT INTO regions VALUES (3871378, 191, "Changwat Sa Kaeo");
INSERT INTO regions VALUES (3684704, 191, "Changwat S<NAME>akhon");
INSERT INTO regions VALUES (3684685, 191, "Changwat Sam<NAME>");
INSERT INTO regions VALUES (3684683, 191, "Changwat Samut Sakhon");
INSERT INTO regions VALUES (3684681, 191, "Changwat Samut Songkhram");
INSERT INTO regions VALUES (3684664, 191, "Changwat S<NAME>");
INSERT INTO regions VALUES (3684654, 191, "Changwat Satun");
INSERT INTO regions VALUES (3684628, 191, "<NAME>");
INSERT INTO regions VALUES (3684621, 191, "Changwat Sisaket");
INSERT INTO regions VALUES (3684607, 191, "Changwat Songkhla");
INSERT INTO regions VALUES (3424572, 191, "Changwat Sukhothai");
INSERT INTO regions VALUES (3684592, 191, "Changwat Sup<NAME>");
INSERT INTO regions VALUES (3424570, 191, "Changwat Surat Thani");
INSERT INTO regions VALUES (3684590, 191, "Changwat Surin");
INSERT INTO regions VALUES (3424568, 191, "Changwat Tak");
INSERT INTO regions VALUES (3424523, 191, "Changwat Trang");
INSERT INTO regions VALUES (3684521, 191, "Changwat Trat");
INSERT INTO regions VALUES (3684515, 191, "Changwat Ubon Ratchathani");
INSERT INTO regions VALUES (3684513, 191, "Changwat Udon Thani");
INSERT INTO regions VALUES (3424520, 191, "Changwat Uthai Thani");
INSERT INTO regions VALUES (3684506, 191, "Changwat Uttaradit");
INSERT INTO regions VALUES (3684465, 191, "Changwat Yala");
INSERT INTO regions VALUES (3684454, 191, "Changwat Yasothon");
INSERT INTO regions VALUES (3685116, 191, "Krung Thep Mahanakhon");
INSERT INTO regions VALUES (3729089, 192, "Fujian Province");
INSERT INTO regions VALUES (3726885, 192, "Kaohsiung City");
INSERT INTO regions VALUES (5451154, 192, "New Taipei City");
INSERT INTO regions VALUES (5451153, 192, "Taichung City");
INSERT INTO regions VALUES (5451152, 192, "Tainan City");
INSERT INTO regions VALUES (3722832, 192, "Taipei City");
INSERT INTO regions VALUES (3720463, 192, "Taiwan Province");
INSERT INTO regions VALUES (5470178, 192, "Taoyuan City");
INSERT INTO regions VALUES (3086381, 193, "Arusha Region");
INSERT INTO regions VALUES (3085800, 193, "Dar es Salaam Region");
INSERT INTO regions VALUES (3085765, 193, "Dodoma Region");
INSERT INTO regions VALUES (3085186, 193, "Iringa Region");
INSERT INTO regions VALUES (3079667, 193, "Kagera Region");
INSERT INTO regions VALUES (3084410, 193, "Kigoma Region");
INSERT INTO regions VALUES (3084241, 193, "Kilimanjaro Region");
INSERT INTO regions VALUES (3083383, 193, "Lindi Region");
INSERT INTO regions VALUES (3204990, 193, "Manyara Region");
INSERT INTO regions VALUES (3082812, 193, "Mara Region");
INSERT INTO regions VALUES (3082593, 193, "Mbeya Region");
INSERT INTO regions VALUES (3081915, 193, "Morogoro Region");
INSERT INTO regions VALUES (3316245, 193, "Mtwara Region");
INSERT INTO regions VALUES (3081387, 193, "Mwanza Region");
INSERT INTO regions VALUES (3080668, 193, "Pemba North Region");
INSERT INTO regions VALUES (3080667, 193, "Pemba South Region");
INSERT INTO regions VALUES (3080591, 193, "Pwani Region");
INSERT INTO regions VALUES (3080524, 193, "Rukwa Region");
INSERT INTO regions VALUES (3316041, 193, "Ruvuma Region");
INSERT INTO regions VALUES (3080326, 193, "Shinyanga Region");
INSERT INTO regions VALUES (3080270, 193, "Singida Region");
INSERT INTO regions VALUES (3080175, 193, "Tabora Region");
INSERT INTO regions VALUES (3080151, 193, "Tanga Region");
INSERT INTO regions VALUES (3079694, 193, "Zanzibar Central/South Region");
INSERT INTO regions VALUES (3079693, 193, "Zanzibar North Region");
INSERT INTO regions VALUES (3079692, 193, "Zanzibar Urban/West Region");
INSERT INTO regions VALUES (4119222, 194, "Région Centrale");
INSERT INTO regions VALUES (4218685, 194, "Région de la Kara");
INSERT INTO regions VALUES (4117270, 194, "Région des Plateaux");
INSERT INTO regions VALUES (4117151, 194, "Région des Savanes");
INSERT INTO regions VALUES (4117784, 194, "Région Maritime");
INSERT INTO regions VALUES (4696021, 197, "Arima");
INSERT INTO regions VALUES (4695959, 197, "County of Caroni");
INSERT INTO regions VALUES (4695769, 197, "County of Mayaro");
INSERT INTO regions VALUES (4695732, 197, "County of Nariva");
INSERT INTO regions VALUES (4695657, 197, "County of Saint Andrew");
INSERT INTO regions VALUES (4695651, 197, "County of Saint David");
INSERT INTO regions VALUES (4695646, 197, "County of Saint George");
INSERT INTO regions VALUES (4695631, 197, "County of Saint Patrick");
INSERT INTO regions VALUES (4695561, 197, "County of Victoria");
INSERT INTO regions VALUES (4695685, 197, "Port-of-Spain");
INSERT INTO regions VALUES (4695627, 197, "<NAME>");
INSERT INTO regions VALUES (4695588, 197, "Ward of Tobago");
INSERT INTO regions VALUES (4177225, 199, "Gouvernorat de Ariana");
INSERT INTO regions VALUES (4177159, 199, "Gouvernorat de Béja");
INSERT INTO regions VALUES (4177121, 199, "Gouvernorat de <NAME>");
INSERT INTO regions VALUES (4177137, 199, "Gouvernorat de Bizerte");
INSERT INTO regions VALUES (4176628, 199, "Gouvernorat de Gabès");
INSERT INTO regions VALUES (4176625, 199, "Gouvernorat de Gafsa");
INSERT INTO regions VALUES (4176754, 199, "Gouvernorat de Jendouba");
INSERT INTO regions VALUES (4177263, 199, "Gouvernorat de Kairouan");
INSERT INTO regions VALUES (4177267, 199, "Gouvernorat de Kasserine");
INSERT INTO regions VALUES (4176544, 199, "Gouvernorat de Kebili");
INSERT INTO regions VALUES (4177350, 199, "Gouvernorat de Kef");
INSERT INTO regions VALUES (5133404, 199, "Gouvernorat de la Manouba");
INSERT INTO regions VALUES (4177326, 199, "Gouvernorat de Mahdia");
INSERT INTO regions VALUES (4176719, 199, "Gouvernorat de Médenine");
INSERT INTO regions VALUES (4177283, 199, "Gouvernorat de Monastir");
INSERT INTO regions VALUES (4176645, 199, "Gouvernorat de Nabeul");
INSERT INTO regions VALUES (4176503, 199, "Gouvernorat de Sfax");
INSERT INTO regions VALUES (4176419, 199, "Gouvernorat de Sidi Bou Zid");
INSERT INTO regions VALUES (4176395, 199, "Gouvernorat de Siliana");
INSERT INTO regions VALUES (4176378, 199, "Gouvernorat de Sousse");
INSERT INTO regions VALUES (4176347, 199, "Gouvernorat de Tataouine");
INSERT INTO regions VALUES (4176343, 199, "Gouvernorat de Tozeur");
INSERT INTO regions VALUES (4176322, 199, "Gouvernorat de Tunis");
INSERT INTO regions VALUES (4176299, 199, "Gouvernorat de Zaghouan");
INSERT INTO regions VALUES (4176321, 199, "Tunis al Janubiyah Wilayat");
INSERT INTO regions VALUES (3163134, 200, "<NAME>");
INSERT INTO regions VALUES (3163110, 200, "Adıyaman İli");
INSERT INTO regions VALUES (3163089, 200, "Afyonkarahisar İli");
INSERT INTO regions VALUES (3162960, 200, "Ağrı İli");
INSERT INTO regions VALUES (3207412, 200, "Aksaray İli");
INSERT INTO regions VALUES (3275557, 200, "Amasya İli");
INSERT INTO regions VALUES (3161839, 200, "Ankara İli");
INSERT INTO regions VALUES (3161832, 200, "Antalya İli");
INSERT INTO regions VALUES (3313738, 200, "Ardahan İli");
INSERT INTO regions VALUES (3275390, 200, "Artvin İli");
INSERT INTO regions VALUES (3160978, 200, "Aydın İli");
INSERT INTO regions VALUES (3160446, 200, "Balıkesir İli");
INSERT INTO regions VALUES (3313735, 200, "Bartın İli");
INSERT INTO regions VALUES (3207413, 200, "Batman İli");
INSERT INTO regions VALUES (3313739, 200, "Bayburt İli");
INSERT INTO regions VALUES (3159569, 200, "Bilecik İli");
INSERT INTO regions VALUES (3159535, 200, "Bingöl İli");
INSERT INTO regions VALUES (3159487, 200, "Bitlis İli");
INSERT INTO regions VALUES (3274260, 200, "Bolu İli");
INSERT INTO regions VALUES (3158973, 200, "Burdur İli");
INSERT INTO regions VALUES (3274056, 200, "Bursa İli");
INSERT INTO regions VALUES (3273666, 200, "Çanakkale İli");
INSERT INTO regions VALUES (3273643, 200, "Çankırı İli");
INSERT INTO regions VALUES (3272885, 200, "Çorum İli");
INSERT INTO regions VALUES (3156239, 200, "Denizli İli");
INSERT INTO regions VALUES (3155759, 200, "Diyarbakır İli");
INSERT INTO regions VALUES (3314753, 200, "Düzce İli");
INSERT INTO regions VALUES (3271902, 200, "Edirne İli");
INSERT INTO regions VALUES (3155144, 200, "Elazığ İli");
INSERT INTO regions VALUES (3154800, 200, "Erzincan İli");
INSERT INTO regions VALUES (3154797, 200, "Erzurum İli");
INSERT INTO regions VALUES (3154656, 200, "Eskişehir İli");
INSERT INTO regions VALUES (3154351, 200, "Gaziantep İli");
INSERT INTO regions VALUES (3271222, 200, "Giresun İli");
INSERT INTO regions VALUES (3270857, 200, "Gümüşhane İli");
INSERT INTO regions VALUES (3152728, 200, "Hakkari İli");
INSERT INTO regions VALUES (3152314, 200, "Hatay İli");
INSERT INTO regions VALUES (3207411, 200, "Iğdır İli");
INSERT INTO regions VALUES (3151209, 200, "Isparta İli");
INSERT INTO regions VALUES (3269689, 200, "İstanbul İli");
INSERT INTO regions VALUES (3151189, 200, "İzmir İli");
INSERT INTO regions VALUES (3151029, 200, "Kahramanmaraş İli");
INSERT INTO regions VALUES (3313736, 200, "Karabük İli");
INSERT INTO regions VALUES (3207414, 200, "Karaman İli");
INSERT INTO regions VALUES (3268817, 200, "<NAME>");
INSERT INTO regions VALUES (3268769, 200, "Kastamonu İli");
INSERT INTO regions VALUES (3149136, 200, "Kayseri İli");
INSERT INTO regions VALUES (3207433, 200, "Kilis İli");
INSERT INTO regions VALUES (3207415, 200, "Kırıkkale İli");
INSERT INTO regions VALUES (3268190, 200, "Kırklareli İli");
INSERT INTO regions VALUES (3148392, 200, "Kırşehir İli");
INSERT INTO regions VALUES (1951468, 200, "Kocaeli İli");
INSERT INTO regions VALUES (3147672, 200, "Konya İli");
INSERT INTO regions VALUES (3146626, 200, "Kütahya İli");
INSERT INTO regions VALUES (3146334, 200, "Malatya İli");
INSERT INTO regions VALUES (3146264, 200, "Manisa İli");
INSERT INTO regions VALUES (3146242, 200, "<NAME>li");
INSERT INTO regions VALUES (3151742, 200, "Mersin İli");
INSERT INTO regions VALUES (3145739, 200, "Muğla İli");
INSERT INTO regions VALUES (3145623, 200, "<NAME>li");
INSERT INTO regions VALUES (3145454, 200, "Nevşehir İli");
INSERT INTO regions VALUES (3145451, 200, "Niğde İli");
INSERT INTO regions VALUES (3266494, 200, "Ordu İli");
INSERT INTO regions VALUES (3207410, 200, "Osmaniye İli");
INSERT INTO regions VALUES (3265957, 200, "Rize İli");
INSERT INTO regions VALUES (3265848, 200, "Sakarya İli");
INSERT INTO regions VALUES (3265776, 200, "Samsun İli");
INSERT INTO regions VALUES (3140854, 200, "Şanlıurfa İli");
INSERT INTO regions VALUES (3142908, 200, "Siirt İli");
INSERT INTO regions VALUES (3265200, 200, "Sinop İli");
INSERT INTO regions VALUES (3207416, 200, "Şırnak İli");
INSERT INTO regions VALUES (3142738, 200, "Sivas İli");
INSERT INTO regions VALUES (3264645, 200, "Tekirdağ İli");
INSERT INTO regions VALUES (3264483, 200, "Tokat İli");
INSERT INTO regions VALUES (3264411, 200, "Trabzon İli");
INSERT INTO regions VALUES (3141279, 200, "Tunceli İli");
INSERT INTO regions VALUES (3140825, 200, "Uşak İli");
INSERT INTO regions VALUES (3140669, 200, "Van İli");
INSERT INTO regions VALUES (3313737, 200, "Yalova İli");
INSERT INTO regions VALUES (3139287, 200, "Yozgat İli");
INSERT INTO regions VALUES (3263018, 200, "Zonguldak İli");
INSERT INTO regions VALUES (3117373, 201, "(( Moroto");
INSERT INTO regions VALUES (3207462, 201, "Apac District");
INSERT INTO regions VALUES (3207463, 201, "Arua District");
INSERT INTO regions VALUES (3207611, 201, "Bugiri District");
INSERT INTO regions VALUES (3207464, 201, "Bundibugyo District");
INSERT INTO regions VALUES (3207465, 201, "Bushenyi District");
INSERT INTO regions VALUES (3207612, 201, "Busia District");
INSERT INTO regions VALUES (3118387, 201, "Busoga Province");
INSERT INTO regions VALUES (3118076, 201, "Eastern Province");
INSERT INTO regions VALUES (3207466, 201, "Gulu District");
INSERT INTO regions VALUES (3207467, 201, "Hoima District");
INSERT INTO regions VALUES (3207469, 201, "Iganga District");
INSERT INTO regions VALUES (3207470, 201, "Jinja District");
INSERT INTO regions VALUES (3207471, 201, "Kabale District");
INSERT INTO regions VALUES (3207472, 201, "Kabarole District");
INSERT INTO regions VALUES (3209625, 201, "Kaberamaido District");
INSERT INTO regions VALUES (3207473, 201, "Kalangala District");
INSERT INTO regions VALUES (3207474, 201, "Kampala District");
INSERT INTO regions VALUES (3207475, 201, "Kamuli District");
INSERT INTO regions VALUES (3209626, 201, "Kamwenge District");
INSERT INTO regions VALUES (3209627, 201, "Kanungu District");
INSERT INTO regions VALUES (3207476, 201, "Kapchorwa District");
INSERT INTO regions VALUES (3207477, 201, "Kasese District");
INSERT INTO regions VALUES (3207613, 201, "Katakwi District");
INSERT INTO regions VALUES (3209628, 201, "Kayunga District");
INSERT INTO regions VALUES (3207478, 201, "Kibale District");
INSERT INTO regions VALUES (3207479, 201, "Kiboga District");
INSERT INTO regions VALUES (3207480, 201, "Kisoro District");
INSERT INTO regions VALUES (3207481, 201, "Kitgum District");
INSERT INTO regions VALUES (3207482, 201, "Kotido District");
INSERT INTO regions VALUES (3207483, 201, "Kumi District");
INSERT INTO regions VALUES (3209629, 201, "Kyenjojo District");
INSERT INTO regions VALUES (3207484, 201, "Lira District");
INSERT INTO regions VALUES (3207485, 201, "Luwero District");
INSERT INTO regions VALUES (3114071, 201, "Masaka District");
INSERT INTO regions VALUES (3207486, 201, "Masindi District");
INSERT INTO regions VALUES (3209630, 201, "Mayuge District");
INSERT INTO regions VALUES (3207488, 201, "Mbale District");
INSERT INTO regions VALUES (3207490, 201, "Mbarara District");
INSERT INTO regions VALUES (3207491, 201, "Moroto District");
INSERT INTO regions VALUES (3207492, 201, "Moyo District");
INSERT INTO regions VALUES (3118138, 201, "Mpigi District");
INSERT INTO regions VALUES (3207493, 201, "Mubende District");
INSERT INTO regions VALUES (3207494, 201, "Mukono District");
INSERT INTO regions VALUES (3209631, 201, "Nakapiripirit District");
INSERT INTO regions VALUES (3207614, 201, "Nakasongola District");
INSERT INTO regions VALUES (3207495, 201, "Nebbi District");
INSERT INTO regions VALUES (3114927, 201, "Nile Province");
INSERT INTO regions VALUES (3114866, 201, "North Buganda Province");
INSERT INTO regions VALUES (3114864, 201, "Northern Province");
INSERT INTO regions VALUES (3207496, 201, "Ntungamo District");
INSERT INTO regions VALUES (3209632, 201, "Pader District");
INSERT INTO regions VALUES (3207497, 201, "Pallisa District");
INSERT INTO regions VALUES (3207498, 201, "Rakai District");
INSERT INTO regions VALUES (3207499, 201, "Rukungiri District");
INSERT INTO regions VALUES (3207615, 201, "Sembabule District");
INSERT INTO regions VALUES (3209633, 201, "Sironko District");
INSERT INTO regions VALUES (3207500, 201, "Soroti District");
INSERT INTO regions VALUES (3114069, 201, "Southern Province");
INSERT INTO regions VALUES (3207501, 201, "Tororo District");
INSERT INTO regions VALUES (3209634, 201, "Wakiso District");
INSERT INTO regions VALUES (3113896, 201, "Western Province");
INSERT INTO regions VALUES (3209635, 201, "Yumbe District");
INSERT INTO regions VALUES (4465214, 50, "Bács-Kiskun megye");
INSERT INTO regions VALUES (4464994, 50, "Baranya megye");
INSERT INTO regions VALUES (3251923, 50, "Békés megye");
INSERT INTO regions VALUES (3251667, 50, "Borsod-Abaúj-Zemplén megye");
INSERT INTO regions VALUES (4464449, 50, "Budapest főváros");
INSERT INTO regions VALUES (3251340, 50, "Csongrád megye");
INSERT INTO regions VALUES (4463335, 50, "Fejér megye");
INSERT INTO regions VALUES (4462568, 50, "Győr-Moson-Sopron megye");
INSERT INTO regions VALUES (1907379, 50, "Hajdú-Bihar megye");
INSERT INTO regions VALUES (3250248, 50, "Heves megye");
INSERT INTO regions VALUES (3250009, 50, "Jász-Nagykun-Szolnok megye");
INSERT INTO regions VALUES (4460879, 50, "Komárom-Esztergom megye");
INSERT INTO regions VALUES (4459447, 50, "Nógrád megye");
INSERT INTO regions VALUES (4458856, 50, "Pest megye");
INSERT INTO regions VALUES (4458067, 50, "Somogy megye");
INSERT INTO regions VALUES (3247327, 50, "Szabolcs-Szatmár-Bereg megye");
INSERT INTO regions VALUES (4457158, 50, "Tolna megye");
INSERT INTO regions VALUES (4456568, 50, "Vas megye");
INSERT INTO regions VALUES (4456488, 50, "Veszprém megye");
INSERT INTO regions VALUES (4456258, 50, "Zala megye");
INSERT INTO regions VALUES (2100001, 18, "Андижанская");
INSERT INTO regions VALUES (2100224, 18, "Бухарская");
INSERT INTO regions VALUES (2100367, 18, "Джизакская");
INSERT INTO regions VALUES (2102522, 18, "Каракалпакия");
INSERT INTO regions VALUES (2100516, 18, "Кашкадарьинская");
INSERT INTO regions VALUES (2100772, 18, "Навоийская");
INSERT INTO regions VALUES (2100875, 18, "Наманганская");
INSERT INTO regions VALUES (2101236, 18, "Самаркандская");
INSERT INTO regions VALUES (2101551, 18, "Сурхандарьинская");
INSERT INTO regions VALUES (2101749, 18, "Сырдарьинская");
INSERT INTO regions VALUES (2101844, 18, "Ташкентская");
INSERT INTO regions VALUES (2102099, 18, "Ферганская");
INSERT INTO regions VALUES (2102342, 18, "Хорезмская");
INSERT INTO regions VALUES (1500595, 2, "Вінницька область");
INSERT INTO regions VALUES (1501549, 2, "Волинська область");
INSERT INTO regions VALUES (1502117, 2, "Дніпропетровська область");
INSERT INTO regions VALUES (1502709, 2, "Донецька область");
INSERT INTO regions VALUES (1503296, 2, "Житомирська область");
INSERT INTO regions VALUES (1504102, 2, "Закарпатська область");
INSERT INTO regions VALUES (1504503, 2, "Запорізька область");
INSERT INTO regions VALUES (1504975, 2, "Івано-Франківська область");
INSERT INTO regions VALUES (1505578, 2, "Київська область");
INSERT INTO regions VALUES (1506310, 2, "Кіровоградська область");
INSERT INTO regions VALUES (1500001, 2, "Крим");
INSERT INTO regions VALUES (1506831, 2, "Луганська область");
INSERT INTO regions VALUES (1507378, 2, "Львівська область");
INSERT INTO regions VALUES (1508347, 2, "Миколаївська область");
INSERT INTO regions VALUES (1508808, 2, "Одеська область");
INSERT INTO regions VALUES (1509477, 2, "Полтавська область");
INSERT INTO regions VALUES (1510213, 2, "Рівненська область");
INSERT INTO regions VALUES (1510799, 2, "Сумська область");
INSERT INTO regions VALUES (1511345, 2, "Тернопільська область");
INSERT INTO regions VALUES (1512004, 2, "Харківська область");
INSERT INTO regions VALUES (1512710, 2, "Херсонська область");
INSERT INTO regions VALUES (1513133, 2, "Хмельницька область");
INSERT INTO regions VALUES (1513930, 2, "Черкаська область");
INSERT INTO regions VALUES (1514896, 2, "Чернігівська область");
INSERT INTO regions VALUES (1514541, 2, "Чернівецька область");
INSERT INTO regions VALUES (4640489, 203, "Departamento de Artigas");
INSERT INTO regions VALUES (4640439, 203, "Departamento de Canelones");
INSERT INTO regions VALUES (4640402, 203, "Departamento de Cerro Largo");
INSERT INTO regions VALUES (4640386, 203, "Departamento de Colonia");
INSERT INTO regions VALUES (4640335, 203, "Departamento de Durazno");
INSERT INTO regions VALUES (4640295, 203, "Departamento de Flores");
INSERT INTO regions VALUES (4640293, 203, "Departamento de Florida");
INSERT INTO regions VALUES (4640192, 203, "Departamento de Lavalleja");
INSERT INTO regions VALUES (4640165, 203, "Departamento de Maldonado");
INSERT INTO regions VALUES (4640130, 203, "Departamento de Montevideo");
INSERT INTO regions VALUES (4640069, 203, "Departamento de Paysandú");
INSERT INTO regions VALUES (4639929, 203, "Departamento de Río Negro");
INSERT INTO regions VALUES (4639926, 203, "Departamento de Rivera");
INSERT INTO regions VALUES (4639924, 203, "Departamento de Rocha");
INSERT INTO regions VALUES (4639914, 203, "Departamento de Salto");
INSERT INTO regions VALUES (4639897, 203, "Departamento de San José");
INSERT INTO regions VALUES (4639852, 203, "Departamento de Soriano");
INSERT INTO regions VALUES (4639847, 203, "Departamento de Tacuarembó");
INSERT INTO regions VALUES (4639827, 203, "Departamento de Treinta y Tres");
INSERT INTO regions VALUES (4011323, 205, "Central Division");
INSERT INTO regions VALUES (4905737, 205, "Eastern Division");
INSERT INTO regions VALUES (4010569, 205, "Northern Division");
INSERT INTO regions VALUES (5163974, 205, "Rotuma");
INSERT INTO regions VALUES (4010168, 205, "Western Division");
INSERT INTO regions VALUES (3765957, 206, "Angeles City");
INSERT INTO regions VALUES (3765164, 206, "Bacolod City");
INSERT INTO regions VALUES (3764838, 206, "Bago City");
INSERT INTO regions VALUES (3764714, 206, "Baguio City");
INSERT INTO regions VALUES (3764600, 206, "Bais City");
INSERT INTO regions VALUES (3764533, 206, "Balabagan");
INSERT INTO regions VALUES (3762860, 206, "Batangas City");
INSERT INTO regions VALUES (3760063, 206, "Butuan City");
INSERT INTO regions VALUES (3759874, 206, "Cabanatuan City");
INSERT INTO regions VALUES (3759334, 206, "Cadiz City");
INSERT INTO regions VALUES (3759263, 206, "Cagayan de Oro City");
INSERT INTO regions VALUES (3758817, 206, "Calbayog City");
INSERT INTO regions VALUES (3758633, 206, "Caloocan City");
INSERT INTO regions VALUES (3757860, 206, "Canlaon City");
INSERT INTO regions VALUES (3756898, 206, "Cavite City");
INSERT INTO regions VALUES (3756796, 206, "Cebu City");
INSERT INTO regions VALUES (5275987, 206, "Chartered City of Cagayan de Oro");
INSERT INTO regions VALUES (5275864, 206, "Chartered City of Iligan");
INSERT INTO regions VALUES (5275873, 206, "Chartered City of Marawi");
INSERT INTO regions VALUES (3746295, 206, "City of Manila");
INSERT INTO regions VALUES (3756357, 206, "Cotabato City");
INSERT INTO regions VALUES (3755976, 206, "Dagupan City");
INSERT INTO regions VALUES (3755691, 206, "Danao City");
INSERT INTO regions VALUES (3755524, 206, "Dapitan City");
INSERT INTO regions VALUES (3755397, 206, "Davao City");
INSERT INTO regions VALUES (3755018, 206, "Dipolog City");
INSERT INTO regions VALUES (3754714, 206, "Dumaguete City");
INSERT INTO regions VALUES (3753927, 206, "General Santos City");
INSERT INTO regions VALUES (3753831, 206, "Gingoog City");
INSERT INTO regions VALUES (3752656, 206, "Iligan City");
INSERT INTO regions VALUES (3752617, 206, "Iloilo City");
INSERT INTO regions VALUES (3752330, 206, "Iriga City");
INSERT INTO regions VALUES (3750761, 206, "La Carlota City");
INSERT INTO regions VALUES (3750205, 206, "Laoag City");
INSERT INTO regions VALUES (3750109, 206, "Lapu-Lapu City");
INSERT INTO regions VALUES (3749857, 206, "Legaspi City");
INSERT INTO regions VALUES (3749285, 206, "Lipa City");
INSERT INTO regions VALUES (3748784, 206, "Lucena City");
INSERT INTO regions VALUES (3746477, 206, "Mandaue City");
INSERT INTO regions VALUES (3745919, 206, "Marawi City");
INSERT INTO regions VALUES (3744719, 206, "Mountain Province");
INSERT INTO regions VALUES (3744506, 206, "Naga City");
INSERT INTO regions VALUES (3743433, 206, "Olongapo City");
INSERT INTO regions VALUES (3743336, 206, "Ormoc City");
INSERT INTO regions VALUES (3743331, 206, "Oroquieta City");
INSERT INTO regions VALUES (3743251, 206, "Ozamis City");
INSERT INTO regions VALUES (3743111, 206, "Pagadian City");
INSERT INTO regions VALUES (3742736, 206, "Palayan City");
INSERT INTO regions VALUES (3741756, 206, "Pasay City");
INSERT INTO regions VALUES (3731223, 206, "Province of Zamboanga del Sur");
INSERT INTO regions VALUES (3767065, 206, "Province of Abra");
INSERT INTO regions VALUES (3766683, 206, "Province of Agusan del Norte");
INSERT INTO regions VALUES (3766682, 206, "Province of Agusan del Sur");
INSERT INTO regions VALUES (3766651, 206, "Province of Aklan");
INSERT INTO regions VALUES (3766553, 206, "Province of Albay");
INSERT INTO regions VALUES (3765770, 206, "Province of Antique");
INSERT INTO regions VALUES (3765415, 206, "Province of Aurora");
INSERT INTO regions VALUES (3762942, 206, "Province of Basilan");
INSERT INTO regions VALUES (3762912, 206, "Province of Bataan");
INSERT INTO regions VALUES (3762881, 206, "Province of Batanes");
INSERT INTO regions VALUES (3762861, 206, "Province of Batangas");
INSERT INTO regions VALUES (3762388, 206, "Province of Benguet");
INSERT INTO regions VALUES (3761627, 206, "Province of Bohol");
INSERT INTO regions VALUES (3760707, 206, "Province of Bukidnon");
INSERT INTO regions VALUES (3760674, 206, "Province of Bulacan");
INSERT INTO regions VALUES (3759267, 206, "Province of Cagayan");
INSERT INTO regions VALUES (3758421, 206, "Province of Camarines Norte");
INSERT INTO regions VALUES (3758420, 206, "Province of Camarines Sur");
INSERT INTO regions VALUES (3758322, 206, "Province of Camiguin");
INSERT INTO regions VALUES (3757555, 206, "Province of Capiz");
INSERT INTO regions VALUES (3757084, 206, "Province of Catanduanes");
INSERT INTO regions VALUES (3756899, 206, "Province of Cavite");
INSERT INTO regions VALUES (3756800, 206, "Province of Cebu");
INSERT INTO regions VALUES (5420937, 206, "Province of Compostela Valley");
INSERT INTO regions VALUES (3755398, 206, "Province of Davao del Norte");
INSERT INTO regions VALUES (3755396, 206, "Province of Davao del Sur");
INSERT INTO regions VALUES (3755395, 206, "Province of Davao Oriental");
INSERT INTO regions VALUES (3754582, 206, "Province of Eastern Samar");
INSERT INTO regions VALUES (3752831, 206, "Province of Ifugao");
INSERT INTO regions VALUES (3752631, 206, "Province of Ilocos Norte");
INSERT INTO regions VALUES (3752630, 206, "Province of Ilocos Sur");
INSERT INTO regions VALUES (3752618, 206, "Province of Iloilo");
INSERT INTO regions VALUES (3752312, 206, "Province of Isabela");
INSERT INTO regions VALUES (3751712, 206, "Province of Kalinga-Apayao");
INSERT INTO regions VALUES (3749968, 206, "Province of La Union");
INSERT INTO regions VALUES (3750625, 206, "Province of Laguna");
INSERT INTO regions VALUES (3750389, 206, "Province of Lanao del Norte");
INSERT INTO regions VALUES (3750388, 206, "Province of Lanao del Sur");
INSERT INTO regions VALUES (3749805, 206, "Province of Leyte");
INSERT INTO regions VALUES (3747623, 206, "Province of Maguindanao");
INSERT INTO regions VALUES (3745823, 206, "Province of Marinduque");
INSERT INTO regions VALUES (3745716, 206, "Province of Masbate");
INSERT INTO regions VALUES (3745009, 206, "Province of Mindoro Occidental");
INSERT INTO regions VALUES (3745008, 206, "Province of Mindoro Oriental");
INSERT INTO regions VALUES (3744950, 206, "Province of Misamis Occidental");
INSERT INTO regions VALUES (3744949, 206, "Province of Misamis Oriental");
INSERT INTO regions VALUES (3743836, 206, "Province of Negros Occidental");
INSERT INTO regions VALUES (3743835, 206, "Province of Negros Oriental");
INSERT INTO regions VALUES (3743666, 206, "Province of North Cotabato");
INSERT INTO regions VALUES (3743665, 206, "Province of Northern Samar");
INSERT INTO regions VALUES (3743640, 206, "Province of Nueva Ecija");
INSERT INTO regions VALUES (3743623, 206, "Province of Nueva Vizcaya");
INSERT INTO regions VALUES (3742744, 206, "Province of Palawan");
INSERT INTO regions VALUES (3742538, 206, "Province of Pampanga");
INSERT INTO regions VALUES (3742268, 206, "Province of Pangasinan");
INSERT INTO regions VALUES (3740139, 206, "Province of Quezon");
INSERT INTO regions VALUES (3740004, 206, "Province of Quirino");
INSERT INTO regions VALUES (3739747, 206, "Province of Rizal");
INSERT INTO regions VALUES (3739726, 206, "Province of Romblon");
INSERT INTO regions VALUES (3739139, 206, "Province of Samar");
INSERT INTO regions VALUES (5420938, 206, "Province of Sarangani");
INSERT INTO regions VALUES (3735467, 206, "Province of Siquijor");
INSERT INTO regions VALUES (3735290, 206, "Province of Sorsogon");
INSERT INTO regions VALUES (3735283, 206, "Province of South Cotabato");
INSERT INTO regions VALUES (3735280, 206, "Province of Southern Leyte");
INSERT INTO regions VALUES (3735087, 206, "Province of Sultan Kudarat");
INSERT INTO regions VALUES (3735082, 206, "Province of Sulu");
INSERT INTO regions VALUES (3734971, 206, "Province of Surigao del Norte");
INSERT INTO regions VALUES (3734970, 206, "Province of Surigao del Sur");
INSERT INTO regions VALUES (3733439, 206, "Province of Tarlac");
INSERT INTO regions VALUES (3733352, 206, "Province of Tawi-Tawi");
INSERT INTO regions VALUES (3731228, 206, "Province of Zambales");
INSERT INTO regions VALUES (3731224, 206, "Province of Zamboanga del Norte");
INSERT INTO regions VALUES (3740460, 206, "Puerto Princesa City");
INSERT INTO regions VALUES (3740138, 206, "Quezon City");
INSERT INTO regions VALUES (3739663, 206, "Roxas City");
INSERT INTO regions VALUES (3738755, 206, "San Carlos City");
INSERT INTO regions VALUES (3738042, 206, "San Jose City");
INSERT INTO regions VALUES (3737513, 206, "San Pablo City");
INSERT INTO regions VALUES (3735731, 206, "Silay City");
INSERT INTO regions VALUES (3734972, 206, "Surigao City");
INSERT INTO regions VALUES (3734639, 206, "Tacloban City");
INSERT INTO regions VALUES (3734543, 206, "Tagaytay City");
INSERT INTO regions VALUES (3734511, 206, "Tagbilaran City");
INSERT INTO regions VALUES (3733622, 206, "Tangub City");
INSERT INTO regions VALUES (3732682, 206, "Toledo City");
INSERT INTO regions VALUES (3732504, 206, "Trece Martires City");
INSERT INTO regions VALUES (3731225, 206, "Zamboanga City");
INSERT INTO regions VALUES (1967330, 207, "Ahvenanmaa");
INSERT INTO regions VALUES (5423530, 207, "Etelä-Karjala");
INSERT INTO regions VALUES (5423523, 207, "Etelä-Pohjanmaa");
INSERT INTO regions VALUES (5423522, 207, "Etelä-Savo");
INSERT INTO regions VALUES (5423519, 207, "Kainuu");
INSERT INTO regions VALUES (5423532, 207, "Kanta-Häme");
INSERT INTO regions VALUES (5423524, 207, "Keski-Pohjanmaa");
INSERT INTO regions VALUES (5423528, 207, "Keski-Suomi");
INSERT INTO regions VALUES (5423534, 207, "Kymenlaakso");
INSERT INTO regions VALUES (5423517, 207, "Lappi");
INSERT INTO regions VALUES (5423531, 207, "Päijät-Häme");
INSERT INTO regions VALUES (5423526, 207, "Pirkanmaa");
INSERT INTO regions VALUES (5423525, 207, "Pohjanmaa");
INSERT INTO regions VALUES (5423520, 207, "Pohjois-Karjala");
INSERT INTO regions VALUES (5423518, 207, "Pohjois-Pohjanmaa");
INSERT INTO regions VALUES (5423521, 207, "Pohjois-Savo");
INSERT INTO regions VALUES (5423527, 207, "Satakunta");
INSERT INTO regions VALUES (5423533, 207, "Uusimaa");
INSERT INTO regions VALUES (5423529, 207, "Varsinais-Suomi");
INSERT INTO regions VALUES (4226493, 204, "Faroe Islands");
INSERT INTO regions VALUES (4455222, 209, "Région Alsace");
INSERT INTO regions VALUES (4454681, 209, "Région Aquitaine");
INSERT INTO regions VALUES (4453582, 209, "Région Auvergne");
INSERT INTO regions VALUES (4452683, 209, "Région Basse-Normandie");
INSERT INTO regions VALUES (4449778, 209, "Région Bourgogne");
INSERT INTO regions VALUES (4449226, 209, "Région Bretagne");
INSERT INTO regions VALUES (4447410, 209, "Région Centre");
INSERT INTO regions VALUES (4446863, 209, "Région Champagne-Ardenne");
INSERT INTO regions VALUES (4443892, 209, "Région Corse");
INSERT INTO regions VALUES (4439125, 209, "Région Franche-Comté");
INSERT INTO regions VALUES (4436536, 209, "Région Haute-Normandie");
INSERT INTO regions VALUES (4435840, 209, "Région Île-de-France");
INSERT INTO regions VALUES (4431261, 209, "Région Languedoc-Roussillon");
INSERT INTO regions VALUES (4422857, 209, "Région Limousin");
INSERT INTO regions VALUES (4422300, 209, "Région Lorraine");
INSERT INTO regions VALUES (4419467, 209, "Région Midi-Pyrénées");
INSERT INTO regions VALUES (4416408, 209, "Région Nord-Pas-de-Calais");
INSERT INTO regions VALUES (4415039, 209, "Région Pays de la Loire");
INSERT INTO regions VALUES (4414421, 209, "Région Picardie");
INSERT INTO regions VALUES (4413754, 209, "Région Poitou-Charentes");
INSERT INTO regions VALUES (4412758, 209, "Région Provence-Alpes-Côte d'Azur");
INSERT INTO regions VALUES (4411607, 209, "Région Rhône-Alpes");
INSERT INTO regions VALUES (5325998, 210, "Région Guyane");
INSERT INTO regions VALUES (4607134, 212, "Bjelovarsko-Bilogorska Županija");
INSERT INTO regions VALUES (4607135, 212, "Brodsko-Posavska Županija");
INSERT INTO regions VALUES (4607136, 212, "Dubrovačko-Neretvanska Županija");
INSERT INTO regions VALUES (4607153, 212, "Grad Zagreb");
INSERT INTO regions VALUES (4607137, 212, "Istarska Županija");
INSERT INTO regions VALUES (4607138, 212, "Karlovačka Županija");
INSERT INTO regions VALUES (4607139, 212, "Koprivničko-Križevačka Županija");
INSERT INTO regions VALUES (4607140, 212, "Krapinsko-Zagorska Županija");
INSERT INTO regions VALUES (4607141, 212, "Ličko-Senjska Županija");
INSERT INTO regions VALUES (4607142, 212, "Međimurska Županija");
INSERT INTO regions VALUES (4607143, 212, "Osječko-Baranjska Županija");
INSERT INTO regions VALUES (4607144, 212, "Požeško-Slavonska Županija");
INSERT INTO regions VALUES (4607145, 212, "Primorsko-Goranska Županija");
INSERT INTO regions VALUES (4607146, 212, "Šibensko-Kninska Županija");
INSERT INTO regions VALUES (4607147, 212, "Sisačko-Moslavačka Županija");
INSERT INTO regions VALUES (4607148, 212, "Splitsko-Dalmatinska Županija");
INSERT INTO regions VALUES (4607149, 212, "Varaždinska Županija");
INSERT INTO regions VALUES (4607154, 212, "Virovitičko-Podravska Županija");
INSERT INTO regions VALUES (4607150, 212, "Vukovarsko-Srijemska Županija");
INSERT INTO regions VALUES (4607151, 212, "Zadarska Županija");
INSERT INTO regions VALUES (4607152, 212, "Zagrebačka Županija");
INSERT INTO regions VALUES (4218311, 213, "Commune de Bangui");
INSERT INTO regions VALUES (4125942, 213, "Préfecture de l' Ombella-Mpoko");
INSERT INTO regions VALUES (4125910, 213, "Préfecture de l' Ouham");
INSERT INTO regions VALUES (4125909, 213, "Préfecture de l' Ouham-Pendé");
INSERT INTO regions VALUES (3121219, 213, "Préfecture de la Bamingui-Bangoran");
INSERT INTO regions VALUES (3121120, 213, "Préfecture de la Basse-Kotto");
INSERT INTO regions VALUES (3120562, 213, "Préfecture de la Haute-Kotto");
INSERT INTO regions VALUES (4126495, 213, "Préfecture de la Kémo");
INSERT INTO regions VALUES (4126298, 213, "Préfecture de la Lobaye");
INSERT INTO regions VALUES (4126611, 213, "Préfecture de la Mambéré-Kadéï");
INSERT INTO regions VALUES (4126631, 213, "Préfecture de la Nana-Grébizi");
INSERT INTO regions VALUES (4126065, 213, "Préfecture de la Nana-Mambéré");
INSERT INTO regions VALUES (3119921, 213, "Préfecture de la Ouaka");
INSERT INTO regions VALUES (4125780, 213, "Préfecture de la Sangha-Mbaéré");
INSERT INTO regions VALUES (3119625, 213, "Préfecture de la Vakaga");
INSERT INTO regions VALUES (3120561, 213, "Préfecture du Haut-Mbomou");
INSERT INTO regions VALUES (3120129, 213, "Préfecture du Mbomou");
INSERT INTO regions VALUES (4158868, 214, "Préfecture du Batha");
INSERT INTO regions VALUES (3122969, 214, "Préfecture du Biltine");
INSERT INTO regions VALUES (4158096, 214, "Préfecture du Borkou-Ennedi-Tibesti");
INSERT INTO regions VALUES (4157877, 214, "Préfecture du Chari-Baguirmi");
INSERT INTO regions VALUES (4156219, 214, "Préfecture du Guéra");
INSERT INTO regions VALUES (4155819, 214, "Préfecture du Kanem");
INSERT INTO regions VALUES (4154944, 214, "Préfecture du Lac");
INSERT INTO regions VALUES (4154800, 214, "Préfecture du Logone Occidental");
INSERT INTO regions VALUES (4154799, 214, "Préfecture du Logone Oriental");
INSERT INTO regions VALUES (4154185, 214, "Préfecture du Mayo-Kébbi");
INSERT INTO regions VALUES (4153686, 214, "Préfecture du Moyen-Chari");
INSERT INTO regions VALUES (3121803, 214, "Préfecture du Ouaddaï");
INSERT INTO regions VALUES (3121724, 214, "Préfecture du Salamat");
INSERT INTO regions VALUES (4152651, 214, "Préfecture du Tandjilé");
INSERT INTO regions VALUES (4474808, 215, "Hlavní město Praha");
INSERT INTO regions VALUES (4607897, 215, "Jihočeský Kraj");
INSERT INTO regions VALUES (4607896, 215, "Jihomoravský Kraj");
INSERT INTO regions VALUES (4607899, 215, "Karlovarský Kraj");
INSERT INTO regions VALUES (4607900, 215, "Královéhradecký Kraj");
INSERT INTO regions VALUES (2209031, 215, "Liberecký Kraj");
INSERT INTO regions VALUES (4607909, 215, "Moravskoslezský Kraj");
INSERT INTO regions VALUES (4607902, 215, "Olomoucký Kraj");
INSERT INTO regions VALUES (4607910, 215, "Pardubický kraj");
INSERT INTO regions VALUES (4607911, 215, "Plzeňský Kraj");
INSERT INTO regions VALUES (4607912, 215, "Středočeský Kraj");
INSERT INTO regions VALUES (4607913, 215, "Ústecký Kraj");
INSERT INTO regions VALUES (5425867, 215, "Vysočina");
INSERT INTO regions VALUES (4607914, 215, "Zlínský Kraj");
INSERT INTO regions VALUES (4834170, 216, "Región Aisén del General Carlos Ibáñez del Campo");
INSERT INTO regions VALUES (4834081, 216, "Región de Antofagasta");
INSERT INTO regions VALUES (4834053, 216, "Región de Atacama");
INSERT INTO regions VALUES (4833465, 216, "Región de Coquimbo");
INSERT INTO regions VALUES (4834074, 216, "Región de la Araucanía");
INSERT INTO regions VALUES (4832241, 216, "Región de Los Lagos");
INSERT INTO regions VALUES (4905738, 216, "Región de Magallanes y de la Antártica Chilena");
INSERT INTO regions VALUES (4830892, 216, "Región de Tarapacá");
INSERT INTO regions VALUES (4830714, 216, "Región de Valparaíso");
INSERT INTO regions VALUES (4833996, 216, "Región del Biobío");
INSERT INTO regions VALUES (4832414, 216, "Región del Libertador General Bernardo O'Higgins");
INSERT INTO regions VALUES (4832069, 216, "Región del Maule");
INSERT INTO regions VALUES (4831294, 216, "Región Metropolitana de Santiago");
INSERT INTO regions VALUES (5466395, 230, "Opština Andrijevica");
INSERT INTO regions VALUES (5466396, 230, "Opština Bar");
INSERT INTO regions VALUES (5466397, 230, "Opština Berane");
INSERT INTO regions VALUES (5466398, 230, "Opština Bi<NAME>");
INSERT INTO regions VALUES (5466399, 230, "Opština Budva");
INSERT INTO regions VALUES (5466400, 230, "Opština Cetinje");
INSERT INTO regions VALUES (5466401, 230, "Opšt<NAME>");
INSERT INTO regions VALUES (5466402, 230, "Opština Herceg-Novi");
INSERT INTO regions VALUES (5466403, 230, "Opština Kolašin");
INSERT INTO regions VALUES (5466404, 230, "Opština Kotor");
INSERT INTO regions VALUES (5466405, 230, "Opština Mojkovac");
INSERT INTO regions VALUES (5466406, 230, "Opština Nikšić");
INSERT INTO regions VALUES (5466407, 230, "Opština Plav");
INSERT INTO regions VALUES (5466409, 230, "Opština Pljevlja");
INSERT INTO regions VALUES (5466408, 230, "Opština Plužine");
INSERT INTO regions VALUES (5466410, 230, "Opština Podgorica");
INSERT INTO regions VALUES (5466411, 230, "Opština Rožaje");
INSERT INTO regions VALUES (5466412, 230, "Opšt<NAME>");
INSERT INTO regions VALUES (5466413, 230, "<NAME>");
INSERT INTO regions VALUES (5466414, 230, "<NAME>");
INSERT INTO regions VALUES (4554127, 230, "<NAME>");
INSERT INTO regions VALUES (4245320, 217, "<NAME>");
INSERT INTO regions VALUES (4245271, 217, "<NAME>");
INSERT INTO regions VALUES (4244392, 217, "<NAME>");
INSERT INTO regions VALUES (4243352, 217, "<NAME>");
INSERT INTO regions VALUES (4244949, 217, "<NAME>");
INSERT INTO regions VALUES (4243371, 217, "<NAME>");
INSERT INTO regions VALUES (4243492, 217, "<NAME>");
INSERT INTO regions VALUES (4246242, 217, "<NAME>");
INSERT INTO regions VALUES (4246133, 217, "<NAME>");
INSERT INTO regions VALUES (4246135, 217, "<NAME>");
INSERT INTO regions VALUES (4246029, 217, "Kanton Basel-Landschaft");
INSERT INTO regions VALUES (4246028, 217, "<NAME>l-Stadt");
INSERT INTO regions VALUES (4245983, 217, "<NAME>");
INSERT INTO regions VALUES (4245232, 217, "<NAME>");
INSERT INTO regions VALUES (4245180, 217, "<NAME>");
INSERT INTO regions VALUES (4244640, 217, "<NAME>");
INSERT INTO regions VALUES (4244373, 217, "<NAME>");
INSERT INTO regions VALUES (4244236, 217, "<NAME>");
INSERT INTO regions VALUES (4243840, 217, "<NAME>");
INSERT INTO regions VALUES (4243790, 217, "<NAME>");
INSERT INTO regions VALUES (4243715, 217, "<NAME>");
INSERT INTO regions VALUES (4243646, 217, "<NAME>");
INSERT INTO regions VALUES (4243494, 217, "<NAME>");
INSERT INTO regions VALUES (4243385, 217, "<NAME>");
INSERT INTO regions VALUES (4243121, 217, "<NAME>");
INSERT INTO regions VALUES (4243111, 217, "<NAME>");
INSERT INTO regions VALUES (1708737, 218, "Blekinge Län");
INSERT INTO regions VALUES (4260063, 218, "Dalarnas Län");
INSERT INTO regions VALUES (4264991, 218, "Gävleborgs Län");
INSERT INTO regions VALUES (1906635, 218, "Gotlands Län");
INSERT INTO regions VALUES (4263577, 218, "Hallands Län");
INSERT INTO regions VALUES (4261395, 218, "Jämtlands Län");
INSERT INTO regions VALUES (4261278, 218, "Jönköpings Län");
INSERT INTO regions VALUES (4261002, 218, "Kalmar Län");
INSERT INTO regions VALUES (4259800, 218, "Kronobergs Län");
INSERT INTO regions VALUES (3211540, 218, "Norrbottens Län");
INSERT INTO regions VALUES (4255371, 218, "Örebro Län");
INSERT INTO regions VALUES (4255018, 218, "Östergötlands Län");
INSERT INTO regions VALUES (4607063, 218, "Skåne Län");
INSERT INTO regions VALUES (4251422, 218, "Södermanlands Län");
INSERT INTO regions VALUES (4250390, 218, "Stockholms Län");
INSERT INTO regions VALUES (4248022, 218, "Uppsala Län");
INSERT INTO regions VALUES (1705481, 218, "Värmlands Län");
INSERT INTO regions VALUES (1904536, 218, "Västerbottens Län");
INSERT INTO regions VALUES (1973862, 218, "Västernorrlands Län");
INSERT INTO regions VALUES (4247176, 218, "Västmanlands Län");
INSERT INTO regions VALUES (2211169, 218, "Västra Götalands Län");
INSERT INTO regions VALUES (3491453, 220, "Central Province");
INSERT INTO regions VALUES (3482233, 220, "North Central Province");
INSERT INTO regions VALUES (3482232, 220, "North Eastern Province");
INSERT INTO regions VALUES (3482231, 220, "North Western Province");
INSERT INTO regions VALUES (3479773, 220, "Province of Sabaragamuwa");
INSERT INTO regions VALUES (3478099, 220, "Province of Uva");
INSERT INTO regions VALUES (3479443, 220, "Southern Province");
INSERT INTO regions VALUES (3477043, 220, "Western Province");
INSERT INTO regions VALUES (4661797, 228, "Parish of Clarendon");
INSERT INTO regions VALUES (4661335, 228, "Parish of Hanover");
INSERT INTO regions VALUES (4661159, 228, "Parish of Kingston");
INSERT INTO regions VALUES (4661027, 228, "Parish of Manchester");
INSERT INTO regions VALUES (4660694, 228, "Parish of Portland");
INSERT INTO regions VALUES (4660523, 228, "Parish of Saint Andrew");
INSERT INTO regions VALUES (4660522, 228, "Parish of Saint Ann");
INSERT INTO regions VALUES (4660520, 228, "Parish of Saint Catherine");
INSERT INTO regions VALUES (4660517, 228, "Parish of Saint Elizabeth");
INSERT INTO regions VALUES (4660511, 228, "Parish of Saint James");
INSERT INTO regions VALUES (4660507, 228, "Parish of Saint Mary");
INSERT INTO regions VALUES (4660502, 228, "Parish of Saint Thomas");
INSERT INTO regions VALUES (4660245, 228, "Parish of Trelawny");
INSERT INTO regions VALUES (4660163, 228, "Parish of Westmoreland");
INSERT INTO regions VALUES (3841417, 229, "Aichi-ken");
INSERT INTO regions VALUES (3994823, 229, "Akita-ken");
INSERT INTO regions VALUES (3996922, 229, "Aomori-ken");
INSERT INTO regions VALUES (3994768, 229, "Chiba-ken");
INSERT INTO regions VALUES (2201038, 229, "Ehime-ken");
INSERT INTO regions VALUES (3840532, 229, "Fukui-ken");
INSERT INTO regions VALUES (3840511, 229, "Fukuoka-ken");
INSERT INTO regions VALUES (3994717, 229, "Fukushima-ken");
INSERT INTO regions VALUES (3840331, 229, "Gifu-ken");
INSERT INTO regions VALUES (3840266, 229, "Gunma-ken");
INSERT INTO regions VALUES (3839634, 229, "Hiroshima-ken");
INSERT INTO regions VALUES (3996587, 229, "Hokkaidō");
INSERT INTO regions VALUES (3839433, 229, "Hyōgo-ken");
INSERT INTO regions VALUES (3994568, 229, "Ibaraki-ken");
INSERT INTO regions VALUES (3839022, 229, "Ishikawa-ken");
INSERT INTO regions VALUES (3994463, 229, "Iwate-ken");
INSERT INTO regions VALUES (3838724, 229, "Kagawa-ken");
INSERT INTO regions VALUES (3838720, 229, "Kagoshima-ken");
INSERT INTO regions VALUES (3838382, 229, "Kanagawa-ken");
INSERT INTO regions VALUES (3837713, 229, "Kōchi-ken");
INSERT INTO regions VALUES (3837338, 229, "Kumamoto-ken");
INSERT INTO regions VALUES (3837114, 229, "Kyōto-fu");
INSERT INTO regions VALUES (3836834, 229, "Mie-ken");
INSERT INTO regions VALUES (3994072, 229, "Miyagi-ken");
INSERT INTO regions VALUES (3836454, 229, "Miyazaki-ken");
INSERT INTO regions VALUES (3836170, 229, "Nagano-ken");
INSERT INTO regions VALUES (3836152, 229, "Nagasaki-ken");
INSERT INTO regions VALUES (3835817, 229, "Nara-ken");
INSERT INTO regions VALUES (3835717, 229, "Niigata-ken");
INSERT INTO regions VALUES (3835130, 229, "Ōita-ken");
INSERT INTO regions VALUES (3835066, 229, "Okayama-ken");
INSERT INTO regions VALUES (3835046, 229, "Okinawa-ken");
INSERT INTO regions VALUES (1517590, 229, "Ōsaka-fu");
INSERT INTO regions VALUES (3834589, 229, "Saga-ken");
INSERT INTO regions VALUES (1703286, 229, "Saitama-ken");
INSERT INTO regions VALUES (3834168, 229, "Shiga-ken");
INSERT INTO regions VALUES (3834102, 229, "Shimane-ken");
INSERT INTO regions VALUES (3833653, 229, "Shizuoka-ken");
INSERT INTO regions VALUES (3832872, 229, "Tochigi-ken");
INSERT INTO regions VALUES (3832780, 229, "Tokushima-ken");
INSERT INTO regions VALUES (3832772, 229, "Tokyo Metropolis");
INSERT INTO regions VALUES (3832639, 229, "Tottori-ken");
INSERT INTO regions VALUES (3832630, 229, "Toyama-ken");
INSERT INTO regions VALUES (3832112, 229, "Wakayama-ken");
INSERT INTO regions VALUES (3993231, 229, "Yamagata-ken");
INSERT INTO regions VALUES (3831963, 229, "Yamaguchi-ken");
INSERT INTO regions VALUES (3831938, 229, "Yamanashi-ken");
<file_sep>/CountryRegionCity.sql
CREATE TABLE `countries` (
`id` INT(10) UNSIGNED NOT NULL,
`title` VARCHAR(100) NOT NULL,
PRIMARY KEY (`id`)
)
COLLATE='utf8_general_ci'
ENGINE=InnoDB
;
CREATE TABLE `regions` (
`id` INT(10) UNSIGNED NOT NULL,
`country_id` INT(10) UNSIGNED NOT NULL,
`title` VARCHAR(100) NOT NULL,
PRIMARY KEY (`id`),
INDEX `countries_fk` (`country_id`),
CONSTRAINT `countries_fk` FOREIGN KEY (`country_id`) REFERENCES `countries` (`id`) ON UPDATE CASCADE ON DELETE CASCADE
)
COLLATE='utf8_general_ci'
ENGINE=InnoDB
;
CREATE TABLE `cities` (
`id` INT(10) UNSIGNED NOT NULL,
`country_id` INT(10) UNSIGNED NOT NULL,
`region_id` INT(10) UNSIGNED NOT NULL,
`title` VARCHAR(80) NOT NULL,
PRIMARY KEY (`id`),
INDEX `country_fk` (`country_id`),
INDEX `region_fk` (`region_id`),
CONSTRAINT `country_fk` FOREIGN KEY (`country_id`) REFERENCES `countries` (`id`) ON UPDATE CASCADE ON DELETE CASCADE,
CONSTRAINT `region_fk` FOREIGN KEY (`region_id`) REFERENCES `regions` (`id`) ON UPDATE CASCADE ON DELETE CASCADE
)
COLLATE='utf8_general_ci'
ENGINE=InnoDB
;
<file_sep>/Scripts/GenerateStatusCodeClass.py
import os; # os.linesep
import requests; # requests
import codecs; # work with file
import re; #regex
status_codes_request = requests.get('https://en.wikipedia.org/wiki/List_of_HTTP_status_codes');
if status_codes_request.status_code == 200:
with codecs.open('StatusCode.py', 'w', encoding='utf8') as file:
file.writelines(['from enum import Enum;' + os.linesep, 'class StatusCode(Enum):' + os.linesep])
matchObj = re.finditer(u'<(dt)>.*?(\\d+) ([^()<>\\d]+).*?</(\\1)>', status_codes_request.text , re.M | re.I);
for x in matchObj:
file.write((' {0} = {1}' + os.linesep).format(re.sub('[\s\/\'\"`-]', '', x.group(3)), x.group(2).strip()));<file_sep>/classes/StatusCode.py
from enum import Enum
class StatusCode(Enum):
"""doc string for ClassName"""
# 1xxInformational
Continue = 100
SwitchingProtocols = 101
Processing = 102
NameNotResolved = 105
# 2xxSuccess
Ok = 200
Created = 201
Accepted = 202
NonAuthoritativeInformation = 203
NoContent = 204
ResetContent = 205
PartialContent = 206
MultiStatus = 207
AlreadyReported = 208
IMUsed = 226
# 3xxRedirection
MultipleChoices = 300
MovedPermanently = 301
Found = 302
SeeOther = 303
NotModified = 304
UseProxy = 305
SwitchProxy= 306
TemporaryRedirect = 307
PermanentRedirect = 308
ResumeIncomplete = 308
# 4xxClientError
BadRequest = 400
Unauthorized = 401
PaymentRequired = 402
Forbidden = 403
NotFound = 404
MethodNotAllowed = 405
NotAcceptable = 406
ProxyAuthenticationRequired = 407
RequestTimeout = 408
Conflict = 409
Gone = 410
LengthRequired = 411
PreconditionFailed = 412
PayloadTooLarge = 413
RequestURITooLong = 414
UnsupportedMediaType = 415
<file_sep>/StatusCode.py
from enum import Enum;
class StatusCode(Enum):
Continue = 100
SwitchingProtocols = 101
Processing = 102
OK = 200
Created = 201
Accepted = 202
NonAuthoritativeInformation = 203
NoContent = 204
ResetContent = 205
PartialContent = 206
MultiStatus = 207
AlreadyReported = 208
IMUsed = 226
MultipleChoices = 300
MovedPermanently = 301
Found = 302
SeeOther = 303
NotModified = 304
UseProxy = 305
SwitchProxy = 306
TemporaryRedirect = 307
PermanentRedirect = 308
ResumeIncomplete = 308
BadRequest = 400
Unauthorized = 401
PaymentRequired = 402
Forbidden = 403
NotFound = 404
MethodNotAllowed = 405
NotAcceptable = 406
ProxyAuthenticationRequired = 407
RequestTimeout = 408
Conflict = 409
Gone = 410
LengthRequired = 411
PreconditionFailed = 412
PayloadTooLarge = 413
RequestURITooLong = 414
UnsupportedMediaType = 415
RequestedRangeNotSatisfiable = 416
ExpectationFailed = 417
Imateapot = 418
AuthenticationTimeout = 419
MethodFailure = 420
EnhanceYourCalm = 420
MisdirectedRequest = 421
UnprocessableEntity = 422
Locked = 423
FailedDependency = 424
UpgradeRequired = 426
PreconditionRequired = 428
TooManyRequests = 429
RequestHeaderFieldsTooLarge = 431
LoginTimeout = 440
NoResponse = 444
RetryWith = 449
BlockedbyWindowsParentalControls = 450
UnavailableForLegalReasons = 451
Redirect = 451
RequestHeaderTooLarge = 494
CertError = 495
NoCert = 496
HTTPtoHTTPS = 497
Tokenexpiredinvalid = 498
ClientClosedRequest = 499
Tokenrequired = 499
InternalServerError = 500
NotImplemented = 501
BadGateway = 502
ServiceUnavailable = 503
GatewayTimeout = 504
HTTPVersionNotSupported = 505
VariantAlsoNegotiates = 506
InsufficientStorage = 507
LoopDetected = 508
BandwidthLimitExceeded = 509
NotExtended = 510
NetworkAuthenticationRequired = 511
UnknownError = 520
OriginConnectionTimeout = 522
Networkreadtimeouterror = 598
Networkconnecttimeouterror = 599
<file_sep>/classes/User.py
class User:
"""First class"""
def firstFunction(self, arg):
print(arg);<file_sep>/Scripts/InsertRegions.py
import os;
import requests;
import codecs;
import re;
import time;
import pymysql;
import json;
cookies = dict(remixlang='1');
countries = requests.get('http://api.vk.com/method/database.getCountries?v=5.5&need_all=1&count=1000', cookies=cookies);
countriesJson = json.loads(countries.text);
query = '';
for country in countriesJson['response']['items']:
regions = requests.get('http://api.vk.com/method/database.getRegions?v=5.5&need_all=1&offset=0&count=1000&country_id='+str(country['id']), cookies=cookies);
regionsJson = json.loads(regions.text);
for region in regionsJson['response']['items']:
query += ('INSERT INTO regions VALUES ({0}, {1}, "{2}");' + os.linesep).format(region['id'], country['id'], region['title']);
time.sleep(0.3);
with codecs.open('regions.json', 'r', encoding='utf8') as f:
query = f.read();
# conn = pymysql.connect(host='127.0.0.1', user='root', passwd=None, db='skoal.com', charset='utf8');
# cur = conn.cursor();
# cur.execute(query.encode('utf-8'));
# conn.commit();
# cur.close();
# conn.close();<file_sep>/Scripts/InsertCoutris.py
import os;
import requests;
import codecs;
import re;
import time;
import pymysql;
import json;
cookies = dict(remixlang='3');
countries = requests.get('http://api.vk.com/method/database.getCountries?v=5.5&need_all=1&count=1000', cookies=cookies);
countriesJson = json.loads(countries.text);
query = '';
for x in range(0, countriesJson['response']['count']):
query += ('INSERT INTO countries VALUES ({0}, "{1}");' + os.linesep).format(countriesJson['response']['items'][x]['id'], countriesJson['response']['items'][x]['title']);
# print(res);
with codecs.open('counries.sql', 'w', encoding='utf8') as f:
f.write(query);
# conn = pymysql.connect(host='127.0.0.1', user='root', passwd=<PASSWORD>, db='skoal.com');
# cur = conn.cursor();
# ree = cur.execute(query);
# print(ree);
# # for r in cur:
# # print(r);
# conn.commit()
# cur.close();
# conn.close();<file_sep>/Scripts/InsertCity.py
import os;
import requests;
import codecs;
import re;
import time;
import pymysql;
import json;
cookies = dict(remixlang='1');
countries = requests.get('http://api.vk.com/method/database.getCountries?v=5.5&need_all=1&count=1000', cookies=cookies);
countriesJson = json.loads(countries.text);
query = '';
index = 0;
url = 'http://api.vk.com/method/database.getCities?v=5.5&country_id={0}®ion_id={1}&offset=0&need_all=1&count=1000';
for country in countriesJson['response']['items']:
regions = requests.get('http://api.vk.com/method/database.getRegions?v=5.5&need_all=1&offset=0&count=1000&country_id='+str(country['id']), cookies=cookies);
regionsJson = json.loads(regions.text);
# http://api.vk.com/method/database.getCities?v=5.5&country_id=1®ion_id=1045244&offset=0&need_all=1&count=1000
for region in regionsJson['response']['items']:
cities = requests.get(url.format(country['id'], region['id']), cookies=cookies);
citiesJson = json.loads(cities.text);
for city in citiesJson['response']['items']:
query += ('INSERT INTO cities VALUES ({0}, {1}, {2}, "{3}");' + os.linesep).format(city['id'], country['id'], region['id'], city['title']);
time.sleep(0.4);
index += 1;
print(index);
with codecs.open('cities.sql', 'w', encoding='utf8') as f:
f.write(query);
# conn = pymysql.connect(host='127.0.0.1', user='root', passwd=None, db='skoal.com', charset='utf8');
# cur = conn.cursor();
# cur.execute(query.encode('utf-8'));
# conn.commit();
# cur.close();
# conn.close();
<file_sep>/Scripts/test.py
from classes.User import User;
test = User();
test.firstFunction('test'); | 33d7c939815feb12e72c7bd4f3c72eb892b279f3 | [
"SQL",
"Python"
] | 11 | SQL | RuslanSayko/cityCountryList | a925416d4786bfe63c0874727522097224e98459 | a47202d2d549ea7adfae0f9a57f5f33b8c6858c8 |
refs/heads/master | <repo_name>egorpushkin/docker-cluster<file_sep>/client/src/app/controls/modal.json.controller.js
(function() {
'use strict';
angular
.module('app.controls')
.controller('JsonController', JsonController);
function JsonController($scope, $modalInstance, title, content) {
$scope.title = title;
$scope.aceLoaded = function(editor) {
$scope.editor = editor;
$scope.editor.$blockScrolling = Infinity;
// $scope.editor.setReadOnly(true);
$scope.editor.setValue(content);
$scope.editor.session.selection.clearSelection();
};
$scope.ok = function() {
$modalInstance.close($scope.editor.getValue());
};
$scope.cancel = function() {
$modalInstance.dismiss('cancel');
};
}
})();
<file_sep>/client/src/app/controls/controls.service.js
(function() {
'use strict';
angular.module('app.controls').
service('controlsService', function ($modal) {
return {
showModal: function(config) {
var editorConfig = {
templateUrl: config.templateUrl ? config.templateUrl : '/app/controls/modal.json.html',
controller: config.controller ? config.controller : 'JsonController',
size: config.size ? config.size : "m",
resolve: {
title: function() {
return config.title ? config.title : "Config";
},
content: function () {
if ( ( typeof config.template ) == "string" ) {
return config.template;
} else {
return JSON.stringify(config.template, null, " ");
}
}
}
};
var editor = $modal.open(editorConfig);
editor.result.then(function(data) {
if ( config.ok ) {
var blob = JSON.parse(data);
config.ok(blob);
}
}, function () {});
}
};
});
})();
<file_sep>/client/src/app/api/sync/api.sync.fs.js
(function() {
'use strict';
angular.module('app.api.sync').
service('syncService', function ($http) {
return {
getList: function(base) {
return $http.get(base + '/api/1/list');
},
getItem: function(base, name) {
return $http.get(base + '/api/1/get?name=' + name);
},
updateItem: function(base, name, data) {
return $http.post(base + '/api/1/update?name=' + name, data);
}
};
});
})();
<file_sep>/docs/installation.md
# Installation
Docker Cluster can be installed via image available at Docker Hub.
## Host Discovery
Docker Cluster detects hosts via EC2 instance lookup. Instances with specified tag are added to the list.
## Environment Variables
The following environment variables are currently supported:
| Variable | Presence | Description
| --------------------- | -------- | -----------------------------
| AWS_REGION | required | AWS Region (e.g. "us-east-1")
| AWS_ACCESS_KEY_ID | optional | AWS Access Key ID
| AWS_SECRET_ACCESS_KEY | optional | AWS Secret Access Key
| CLUSTER_TAG_NAME | required | Cluster tag name
| CLUSTER_TAG_VALUE | required | Cluster tag value
Either AWS_ACCESS_KEY_ID, AWS_SECRET_ACCESS_KEY or instance IAM role is required in order for the backend service
to be able to list EC2 instances.
CLUSTER_TAG_NAME amd CLUSTER_TAG_VALUE are used to filter instances based on a tag.
## Command Line
Docker Cluster can be launched locally or on remote machine using this command:
```bash
docker run -d \
-p 8080:8080 \
-e AWS_REGION=us-east-1 \
-e AWS_ACCESS_KEY_ID=... \
-e AWS_SECRET_ACCESS_KEY=... \
-e CLUSTER_TAG_NAME=... \
-e CLUSTER_TAG_VALUE=... \
--name docker-cluster \
glympse/docker-cluster:latest
```
## Docker Remote API
This installation method assumes that you already have a host with Docker API exposed:
```
curl \
--header "Content-Type: application/json" \
--data '
{
"Image": "glympse/docker-cluster:latest",
"Env": [
"AWS_REGION=us-east-1",
"AWS_ACCESS_KEY_ID=...",
"AWS_SECRET_ACCESS_KEY=...",
"CLUSTER_TAG_NAME=...",
"CLUSTER_TAG_VALUE=..."
],
"HostConfig": {
"PortBindings": {
"8080/tcp": [
{
"HostPort": "8080"
}
]
},
"RestartPolicy": {
"Name": "always"
}
}
}' http://$DOCKER_HOST:$DOCKER_PORT/containers/create?name=cluster-demo
```
## Worker Node Setup
Docker Remote API endpoint must be exposed by the daemon in order for Docker Cluster to be able to interact with the host.
### Amazon Linux
The following script can be used to install and configure Docker daemon:
```bash
#!/bin/bash -ex
# Install updates
yum -y clean all
yum -y update
# Install docker
yum install docker -y
yum update docker -y
chkconfig docker on
# Configure docker deamon
echo OPTIONS=\"-H tcp://0.0.0.0:2375 -H unix:///var/run/docker.sock --api-cors-header=*\" > /etc/sysconfig/docker
# Start docker deamon
service docker start
```
<file_sep>/server/core/manager.py
import utilities
import cloud.aws
class Manager:
def __init__(self):
# Read app config
self.env = utilities.Env()
# Instantiate cloud provider
self.provider = cloud.aws.Provider(self.env.get("AWS_REGION"))
<file_sep>/server/api/rest.py
import json
import tornado
import tornado.web
"""
Implements JSON encoding and JSONP on top of tornado.web.RequestHandler.
"""
class JsonRequestHandler(tornado.web.RequestHandler):
def initialize(self, manager):
self.manager = manager
@tornado.web.asynchronous
def get(self, **args):
self.process(args)
@tornado.web.asynchronous
def post(self, **args):
self.process(args)
@tornado.web.asynchronous
def options(self, **args):
self.set_header("Access-Control-Allow-Methods",
self.request.headers.get("Access-Control-Request-Methods", default="*"))
self.set_header("Access-Control-Allow-Headers",
self.request.headers.get("Access-Control-Request-Headers", default="*"))
self.set_header("Content-Type", "text/html; charset=utf-8")
self.finish()
def set_default_headers(self):
self.set_header("Access-Control-Allow-Origin", "*")
def process(self, args):
# Handle the call
try:
# Handle authentication
self.handle_auth()
# Propagate to business logic
response = { "result": "ok", "body": self.handle(args) }
except NameError as e:
response = { "result": "failure", "error": str(e) }
except:
response = { "result": "failure" }
# Serialize response to JSON.
try:
output = json.dumps(response)
except:
output = '{ "result": "failure", "error": "serialization" }'
# See if JSONP response is expected by the caller.
callback = self.get_argument("callback", None)
if callback:
self.set_header("Content-Type", "application/javascript")
output = callback + "(" + output + ")"
else:
self.set_header("Content-Type", "application/json")
# Send the response.
self.write(output)
self.finish()
def handle_auth(self):
pass
def handle(self, args):
pass
"""
Provides skeleton of authenticated endpoint.
"""
class ApiHandler(JsonRequestHandler):
def initialize(self, manager):
self.manager = manager
def handle_auth(self):
try:
if self.manager.env.get("API_IMPORT_SECRET") != self.get_argument("secret"):
raise()
except:
raise NameError("invalid_token")
"""
Provided to prevent dyno from sleeping.
"""
class AwakeEndpoint(JsonRequestHandler):
def handle(self, args):
return {}
<file_sep>/server/api/hosts/list.py
import api.rest
class Endpoint(api.rest.JsonRequestHandler):
def handle(self, args):
try:
tag_name = self.manager.env.get("CLUSTER_TAG_NAME")
tag_value = self.manager.env.get("CLUSTER_TAG_VALUE")
compute_instances = self.manager.provider.find_instances_by_tag(tag_name, tag_value)
instances = []
for compute_instance in compute_instances:
if "running" != compute_instance.state:
continue
instance = {
"_id": compute_instance.id,
"name": compute_instance.tags["Name"],
"network": {
"public_ip": compute_instance.ip_address,
"private_ip": compute_instance.private_ip_address
},
"cloud": {
"provider": "aws",
"region": compute_instance.region.name,
"zone": compute_instance.placement,
"instance_type": compute_instance.instance_type
},
"docker": {
"protocol": "http",
"port": 2375
},
"tags": {
"cloud": "aws",
"region": compute_instance.region.name,
"zone": compute_instance.placement
}
}
instances.append(instance)
return instances
except Exception as e:
raise NameError("failed_to_find")
<file_sep>/README.md
# Docker Cluster
Docker Cluster tool is aimed to provide convenient way of experimenting with a cluster of Docker machines.
It uses [Docker Remote API](https://docs.docker.com/reference/api/docker_remote_api/)
to perform routine Docker tasks on those instances.
<div align="center">
<img width="60%" src="https://drive.google.com/uc?id=0B9NxURKU5b4SZEx6MzR6dm9SSUk&export=view">
</div>
## Features
- Ability to pull images from Docker Hub and private registries.
- Authenticated access to Docker Registries is supported.
- Access to all container properties exposed by Remote API (port bindings, volume mappings, environment variables, etc.).
- Complete control over container lifecycle.
## Documentation
- [Installation](docs/installation.md)
- [Tutorial](docs/tutorial.md)
## Disclaimer
Docker Cluster interacts with Docker daemon via Remote API. Exposing Remote API on production machines is considered
being security vulnerability. It is highly recommended to use Docker Cluster for educational and experimental purposes
only.
From [Docker documentation](https://docs.docker.com/articles/basics/#bind-docker-to-another-hostport-or-a-unix-socket):
> Warning: Changing the default docker daemon binding to a TCP port or Unix docker user group will increase your
> security risks by allowing non-root users to gain root access on the host. Make sure you control access to docker.
> If you are binding to a TCP port, anyone with access to that port has full Docker access; so it is not advisable
> on an open network.
## License
Code is licensed under the [The MIT License](http://opensource.org/licenses/MIT). <br>
Documentation is licensed under [Creative Commons Attribution 4.0 International License](https://creativecommons.org/licenses/by/4.0/).
## Author
Docker Cluster is developed by <NAME>. My recent efforts are targeted towards designing connected systems (mostly in mobile space) with focus on cross-platform development methodologies, modern communication paradigms and highly automated workflows.
LinkedIn - [https://www.linkedin.com/in/egorpushkin](https://www.linkedin.com/in/egorpushkin) <br>
Twitter - [https://twitter.com/egorpushkin](https://twitter.com/egorpushkin)
<file_sep>/client/src/app/hosts/hosts.controller.js
(function() {
'use strict';
angular
.module('app.hosts')
.controller('HostsController', HostsController);
function HostsController($scope, hostsService) {
$scope.layout_horizontal = true;
hostsService.getList().success(function(data) {
$scope.hosts = data.body;
});
}
})();
<file_sep>/client/src/app/sync/sync.module.js
(function() {
'use strict';
angular.module('app.sync', [
'ui.tree',
'app.api.sync'
]).
config(['$routeProvider', function($routeProvider) {
$routeProvider.when('/sync', {
controller: 'SyncController',
templateUrl: '/app/sync/sync.html'
});
}]);
})();
<file_sep>/client/src/app/hosts/hosts.module.js
(function() {
'use strict';
angular.module('app.hosts', [
'app.api.cluster',
'app.api.docker',
'app.controls'
]).
config(['$routeProvider', function($routeProvider) {
$routeProvider.when('/hosts', {
controller: 'HostsController',
templateUrl: '/app/hosts/hosts.html'
});
}]);
})();
<file_sep>/server/app.py
import os
import tornado.web
import utilities
import core.manager
import api.hosts.list
root = os.path.dirname(os.path.realpath(__file__))
manager = core.manager.Manager()
class ConsoleIndexHandler(tornado.web.RequestHandler):
def get(self):
self.render(root + "/../client/src/index.html")
app = tornado.web.Application([
(r'/api/1/hosts/list', api.hosts.list.Endpoint, dict(manager=manager)),
(r'/', ConsoleIndexHandler),
(r'/(.*)', tornado.web.StaticFileHandler, {'path': root + "/../client/src/"})
])
if __name__ == '__main__':
server = utilities.TornadoServer(app, 8080)
server.start()
<file_sep>/server/requirements.txt
tornado==4.2
boto==2.38.0
<file_sep>/Dockerfile
FROM python:latest
# Ass and install requirements
RUN mkdir -p /src/server
COPY ./server/requirements.txt /src/server/
RUN pip install -r /src/server/requirements.txt
# Set working directory
WORKDIR /src/server
# Container port
EXPOSE 8080
# Set default container command
ENTRYPOINT ["python3"]
# Bundle app source
COPY . /src
# Run the app
CMD ["/src/server/app.py"]
<file_sep>/client/src/app/api/docker/api.docker.module.js
(function() {
'use strict';
angular.module('app.api.docker', []);
})();
<file_sep>/build.sh
#!/bin/sh
# App name/home registry
REGISTRY_URL=registry.sandbox.glympse.com
IMAGE_NAME=tools/docker-cluster
# Helpers
IMAGE_TAG=$(git rev-parse --short HEAD)
TAGGED_IMAGE=$REGISTRY_URL/$IMAGE_NAME:$IMAGE_TAG
LATEST_IMAGE=$REGISTRY_URL/$IMAGE_NAME:latest
# Build the image
docker build -t $IMAGE_NAME .
# Mark image with registry URL and tag
docker tag -f $IMAGE_NAME $TAGGED_IMAGE
docker tag -f $IMAGE_NAME $LATEST_IMAGE
# Push image to repote registry
docker push $TAGGED_IMAGE
docker push $LATEST_IMAGE
<file_sep>/client/src/app/sync/sync.controller.js
(function() {
'use strict';
angular.module('app.sync').
controller('SyncController', function($rootScope, $scope, $modal, syncService, controlsService) {
function clear() {
var template = [{
"id": 1,
"title": "/",
"nodes": [],
}];
$scope.data = angular.copy(template);
$scope.current = angular.copy(template);
if ( $scope.editor ) {
$scope.editor.setValue("");
}
}
function init() {
$scope.base = "";
clear();
if ( $rootScope.currentTool["sync"] ) {
$scope.base
= "http://"
+ $rootScope.currentTool.sync.host.network.private_ip + ":"
+ $rootScope.currentTool.sync.port.PublicPort;
$scope.connect();
}
}
$scope.aceLoaded = function(editor) {
$scope.editor = editor;
$scope.editor.$blockScrolling = Infinity;
}
$scope.connect = function() {
clear();
syncService.getList($scope.base).success(function(data) {
$scope.data[0].nodes = [];
angular.forEach(data.body, function(value, key) {
$scope.data[0].nodes.push({
"id": value.name,
"title": value.name
});
});
});
};
$scope.selected = function(node) {
$scope.current = [ node ];
syncService.getItem($scope.base, node.id).success(function(data) {
$scope.editor.setValue(data);
$scope.editor.session.selection.clearSelection();
});
};
$scope.update = function() {
var node = $scope.current[0];
var data = $scope.editor.getValue(data);
syncService.updateItem($scope.base, node.id, data).success(function(data) {
});
};
$scope.create = function() {
var template = {
"name": "",
};
controlsService.showModal({
title: "Create New File",
template: template,
size: "lg",
ok: function(params) {
var name = params["name"];
syncService.updateItem($scope.base, name, "").success(function(data) {
$scope.connect();
});
}
});
};
init();
});
})();
<file_sep>/docs/tutorial.md
# Tutorial
The tutorial walks you through the process of setting up Wordpress application using Docker Cluster.
Corresponding video tutorial ia available here:
[](http://www.youtube.com/watch?v=28yTgcNzwjk)
Here is an accompanying step-by-step guide with required JSON snippets.
- Install MySQL on one of your boxes using the following template.
```json
{
"Name": "mysql-wordpress",
"Image": "mysql:latest",
"Env": [
"MYSQL_ROOT_PASSWORD=root",
"MYSQL_DATABASE=wp_db",
"MYSQL_USER=wp_user",
"MYSQL_PASSWORD=<PASSWORD>"
],
"HostConfig": {
"PortBindings": {
"3306/tcp": [
{
"HostPort": "3306"
}
]
},
"RestartPolicy": {
"Name": "always"
}
}
}
```
- Install phpMyAdmin.
Replace ${HOST_IP} with IP address of MySQL host.
```json
{
"Name": "phpmyadmin",
"Image": "silintl/phpmyadmin:latest",
"Env": [
"MYSQL_HOST=${HOST_IP}"
],
"HostConfig": {
"PortBindings": {
"80/tcp": [
{
"HostPort": "80"
}
]
},
"RestartPolicy": {
"Name": "always"
}
}
}
```
- Install Wordpress itself.
Replace ${HOST_IP} with IP address of MySQL host.
```json
{
"Name": "wordpress",
"Image": "wordpress:latest",
"Env": [
"WORDPRESS_DB_HOST=${HOST_IP}",
"WORDPRESS_DB_NAME=wp_db",
"WORDPRESS_DB_USER=wp_user",
"WORDPRESS_DB_PASSWORD=<PASSWORD>"
],
"HostConfig": {
"PortBindings": {
"80/tcp": [
{
"HostPort": "80"
}
]
},
"RestartPolicy": {
"Name": "always"
}
}
}
```
- Select "launch (http)" from dropdown menu on a port exposed by Wordpress container to launch
Wordpress installation wizard.
<file_sep>/client/src/app/hosts/host.controller.js
(function() {
'use strict';
angular
.module('app.hosts')
.controller('HostController', HostController);
function HostController($scope, $modal, containersService, controlsService) {
function init() {
$scope.containers = [];
$scope.refresh();
}
$scope.createImage = function() {
var template = {
"fromImage": "",
"X-Registry-Auth": ""
};
controlsService.showModal({
title: "Create an Image",
template: template,
size: "lg",
ok: function(params) {
// Extract arguments
var fromImage = params["fromImage"];
var xRegistryAuth = params["X-Registry-Auth"];
// Make API call to create an image.
containersService.createImage($scope.host, fromImage, xRegistryAuth).success(function(data) {
controlsService.showModal({
title: "Image Created",
template: data,
size: "lg"
});
$scope.refresh();
});
}
});
}
$scope.createContainer = function() {
var template = {
"Name": "",
"Image": "",
"HostConfig": {
"RestartPolicy": {
"Name": "always"
}
}
};
controlsService.showModal({
title: "Create a Container",
template: template,
size: "lg",
ok: function(params) {
// Extract the name.
var name = params["Name"];
// Make API call to create a container.
containersService.createContainer($scope.host, name, params).success(function(data) {
$scope.refresh();
});
}
});
};
$scope.refresh = function() {
containersService.getList($scope.host).success(function(data) {
$scope.containers = data;
});
};
$scope.showInfo = function() {
// Retrieve host info.
containersService.getHostInfo($scope.host).success(function(data) {
controlsService.showModal({
title: "Host Info",
template: data,
size: "lg"
});
});
};
$scope.showImages = function() {
// Retrieve the list of images.
containersService.getImagesList($scope.host).success(function(data) {
controlsService.showModal({
title: "Images List",
template: data,
size: "lg"
});
});
};
init();
}
})();
<file_sep>/client/src/app/layout/layout.module.js
(function() {
'use strict';
angular.module('app.layout', [
'app.hosts',
'app.sync'
]).
config(['$routeProvider', function($routeProvider) {
$routeProvider.when('/', {
controller: 'HostsController',
templateUrl: '/app/hosts/hosts.html'
})
.otherwise({redirectTo: '/'});
}]);
})();
<file_sep>/server/cloud/aws.py
import boto.ec2
class Provider:
def __init__(self, region):
self.region = region
self.conn = boto.ec2.connect_to_region(region)
def find_instances_by_tag(self, key, value):
reservations = self.conn.get_all_instances( filters={ "tag:" + key: value } )
instances = [index for reservation in reservations for index in reservation.instances]
return instances
| 6407d067db1c37be4a866698dedb68df207003ed | [
"Shell",
"Markdown",
"Dockerfile",
"JavaScript",
"Python",
"Text"
] | 21 | Shell | egorpushkin/docker-cluster | e06e3658bb72eec9bfb7ed8e28142f467a56fd3b | 2c472783448ea22a79a62a9e6fa3578390708963 |
refs/heads/master | <file_sep>from api import API
app = API()
@app.route("/home")
def home(request, response):
response.text = "This is HOME."
@app.route("/about")
def about(request, response):
response.text = "This is ABOUT."
@app.route("/hello/{name}")
def home(request, response, name):
response.text = f"Bonjour {name}!"
@app.route("/class")
class MyClass:
def get(self, request, response):
response.text = f"GET method"
def post(self, request, response):
response.text = f"POST method"
<file_sep># Web framework
My take on http://rahmonov.me/posts/write-python-framework-part-one/
<file_sep>from inspect import isclass
from webob import Request, Response
from parse import parse
class API:
def __init__(self):
self.routes = {}
def route(self, path):
"""Decorator to extract the routes ala Flask"""
if path in self.routes.keys():
raise AssertionError("The route already exists.")
def wrapper(handler):
self.routes[path] = handler
return handler
return wrapper
def default_response(self, request, response):
response.status = 404
response.text = "Page not found"
def find_handler(self, request_path):
"""Returns the handler corresponding to the requested path
aswell as the keywords arguments. If the requested path does
not exist, return the default 404 handler."""
selected_handler = self.default_response
kwargs = {}
for path, handler in self.routes.items():
parse_result = parse(path, request_path)
if parse_result is not None:
selected_handler = handler
kwargs = parse_result.named
return selected_handler, kwargs
def handle_request(self, request):
response = Response()
handler, kwargs = self.find_handler(request_path=request.path)
if isclass(handler):
# handler is now the method request.method.lower from
# the initial handler class (if the method exists)
handler = getattr(handler(), request.method.lower(), None)
if handler is None:
raise AttributeError(f"The method {request.method} is not allowed.")
handler(request, response, **kwargs)
return response
def __call__(self, environ, start_response):
request = Request(environ)
response = self.handle_request(request)
return response(environ, start_response)
<file_sep>import pytest
from api import API
@pytest.fixture
def api():
return API()
def test_add_route(api):
@api.route("/home")
def home(request, response):
response.text = "Test for adding a route"
with pytest.raises(AssertionError):
@api.route("/home")
def home(request, response):
response.text = "Test for adding a route"
| 28e36b6c31ca3bc4b95763002733a25088513bfc | [
"Markdown",
"Python"
] | 4 | Markdown | PetitLepton/web-framework | bf94de2d2918a53982071846b6aa9eba8f4ada00 | 45f32648ae96c882997b5d1354ce93b7b7a694f7 |
refs/heads/main | <repo_name>JayKhan4Real/ios-apps-projects<file_sep>/venpro/README.md
# VenPro-iOS-<file_sep>/Lab 3.8 Order Of Events/Order of Events/Order of Events/FirstVC.swift
//
// FirstVC.swift
// Order of Events
//
// Created by <NAME> on 6/6/20.
// Copyright © 2020 PretendCo. All rights reserved.
//
import UIKit
class FirstVC: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
}
<file_sep>/Lab 3.8 Order Of Events/Order of Events/Order of Events/MiddleVC.swift
//
// MiddleVC.swift
// Order of Events
//
// Created by <NAME> on 6/6/20.
// Copyright © 2020 PretendCo. All rights reserved.
//
import UIKit
class MiddleVC: UIViewController {
@IBOutlet weak var middleVCLabel: UILabel!
var eventNumber: Int = 1
override func viewDidLoad() {
super.viewDidLoad()
if let actualText = middleVCLabel.text {
middleVCLabel.text = "\(actualText)\nEvent number \(eventNumber) is viewDidLoad"
eventNumber += 1
}
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
if let actualText = middleVCLabel.text {
middleVCLabel.text = "\(actualText)\nEvent number \(eventNumber) is viewWillAppear"
eventNumber += 1
}
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
if let actualText = middleVCLabel.text {
middleVCLabel.text = "\(actualText)\nEvent number \(eventNumber) is viewDidAppear"
eventNumber += 1
}
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
if let actualText = middleVCLabel.text {
middleVCLabel.text = "\(actualText)\nEvent number \(eventNumber) is viewWillDisappear"
eventNumber += 1
}
}
override func viewDidDisappear(_ animated: Bool) {
super.viewDidDisappear(animated)
if let actualText = middleVCLabel.text {
middleVCLabel.text = "\(actualText)\nEvent number \(eventNumber) is viewDidDisappear"
eventNumber += 1
}
}
}
<file_sep>/Fav athlete/FavoriteAthlete/FavoriteAthlete/Controller/AthleteFormViewController.swift
//
// AthleteFormViewController.swift
// FavoriteAthlete
//
// Created by <NAME> on 6/6/20.
//
import UIKit
class AthleteFormViewController: UIViewController {
var athlete: Athlete?
@IBOutlet weak var nameText: UITextField!
@IBOutlet weak var ageText: UITextField!
@IBOutlet weak var leagueText: UITextField!
@IBOutlet weak var teamText: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
updateFormView()
}
func updateFormView() {
if let confirmedAthlete = athlete {
print("confirmed \(confirmedAthlete)!")
nameText.text = confirmedAthlete.name
teamText.text = confirmedAthlete.team
leagueText.text = confirmedAthlete.league
ageText.text = confirmedAthlete.age
}
}
@IBAction func persist(_ sender: Any) {
guard let name = nameText.text,
let age = ageText.text,
let league = leagueText.text,
let team = teamText.text else {return}
athlete = Athlete(name: name, age: age, league: league, team: team)
performSegue(withIdentifier: "UnwindToAthleteTable", sender: self)
}
}
<file_sep>/Lab 1.8/InterfaceBuilderBasics/InterfaceBuilderBasics/ViewController.swift
//
// ViewController.swift
// InterfaceBuilderBasics
//
// Created by <NAME> on 12/8/19.
// Copyright © 2019 PretendCo. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var mainLabel: UILabel!
@IBAction func changeTitle(_ sender: UIButton) {
mainLabel.text = "This app rocks!"
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
}
| f0781586895efa7ff54eb4c2e4b9cfe4f71cedb2 | [
"Swift",
"Markdown"
] | 5 | Swift | JayKhan4Real/ios-apps-projects | e82cb8ac78ae91d15abc00793ba1f6316735feff | ba1ea683b1036337a95883ed58ea9d53b907c2cb |
refs/heads/master | <repo_name>rhwy/NFluent<file_sep>/code/tests/NFluent.Tests/BatchChecksShould.cs
using NUnit.Framework;
using System;
using System.Collections.Generic;
using System.Text;
namespace NFluent.Tests
{
using NFluent.Helpers;
[TestFixture]
public class BatchChecksShould
{
[Test]
public void
WorkForSingleCheck()
{
var sut = Check.StartBatch();
Check.That(2).IsEqualTo(3);
Check.ThatCode(() => sut.Dispose()).IsAFailingCheckWithMessage("",
"The checked value is different from the expected one.",
"The checked value:",
"\t[2]",
"The expected value:",
"\t[3]");
}
[Test]
public void
WorkForTwoChecks()
{
var sut = Check.StartBatch();
Check.That(2).IsPositiveOrZero();
Check.That(2).IsEqualTo(3);
Check.ThatCode(() => sut.Dispose()).IsAFailingCheckWithMessage("",
"The checked value is different from the expected one.",
"The checked value:",
"\t[2]",
"The expected value:",
"\t[3]");
}
[Test]
public void
WorkForTwoFailingChecks()
{
var sut = Check.StartBatch();
Check.That(2).IsNegativeOrZero();
Check.That(2).IsEqualTo(3);
Check.ThatCode(() => sut.Dispose()).IsAFailingCheckWithMessage("",
"The checked value is not negative or equal to zero.",
"The checked value:",
"\t[2]",
"** And **",
"The checked value is different from the expected one.",
"The checked value:",
"\t[2]",
"The expected value:",
"\t[3]");
}
[Test]
public void
WorkWhenExceptionRaised()
{
Check.ThatCode(() =>
{
using (Check.StartBatch())
{
Check.That(2).IsNegativeOrZero();
throw new ApplicationException("Random exception");
}
}).IsAFailingCheckWithMessage("",
"The checked value is not negative or equal to zero.",
"The checked value:",
"\t[2]");
}
}
}
| e8ea9a9ca97262799343af0dac4819b07ed57341 | [
"C#"
] | 1 | C# | rhwy/NFluent | a4335d5968fb56fb6b92389fa87e111b920b6231 | bb404bd53966571dd574288603abab14bb10c1ea |
refs/heads/master | <repo_name>jgrantbrown/travel-backend<file_sep>/app/models/user.rb
class User < ApplicationRecord
has_secure_password
belongs_to :hometown, class_name:"Location"
has_many :trips
has_many :attractions, through: :trips
has_many :visits, through: :trips
has_many :locations, through: :trips
has_many :comments
end
<file_sep>/db/migrate/20191122150607_change_description_to_be_descritpion_in_attractions.rb
class ChangeDescriptionToBeDescritpionInAttractions < ActiveRecord::Migration[5.2]
def change
rename_column :attractions, :desctription, :description
end
end
<file_sep>/app/models/comment.rb
class Comment < ApplicationRecord
belongs_to :user
belongs_to :attraction
end
<file_sep>/dev-log.md
11-22-19
-add some seed data double-check models rails c
-add a serializer fast json Api
12-3-19
-Watched globetrotter-4
-Begin watching globetrotter-5
<file_sep>/db/seeds.rb
# This file should contain all the record creation needed to seed the database with its default values.
# The data can then be loaded with the rails db:seed command (or created alongside the database with db:setup).
#
# Examples:
#
# movies = Movie.create([{ name: 'Star Wars' }, { name: 'Lord of the Rings' }])
# Character.create(name: 'Luke', movie: movies.first)
# Commited by seed
User.create(name: "Jay", username: "jayb", password: "<PASSWORD>", hometown_id: 1)
anaheim = Location.create(city:'Anaheim' , state: "California", country: "USA")
disney = anaheim.attractions.create(name: "Disneyland", description: "Happiest place on Earth")
jay = User.first
jason_disney = jay.trips.create(start_date: "2019-06-01", end_date: "2019-06-07", location_id: 1)
jason_disney.visits.create(attraction: disney, date: "2019-06-01")
jay.comments.create(content: "Fun for all", attraction: disney)
<file_sep>/app/serializers/user_serializer.rb
class UserSerializer
include FastJsonapi::ObjectSerializer
attributes :username, :name
# Created serliazer
# belongs_to :hometown, seralizer: HometownSerializer
attribute :hometown_json do |user|
{
city: user.hometown.city,
state: user.hometown.state,
country: user.hometown.country
}
end
end
<file_sep>/app/controllers/api/v1/trips_controller.rb
class Api::V1::TripsController < ApplicationController
def index
if logged_in?
@trips = current_user.trips
render json: @trips
else
render json: {
error: "You must be logged in to see trips"
}
end
end
end
| 75ce78b28fbe9ae7500cd3c58d61191d22d0a5ca | [
"Markdown",
"Ruby"
] | 7 | Markdown | jgrantbrown/travel-backend | 0e5890ab8ee91c668f21dd189c555e68237b5493 | b8019109ecace61e66e0377ff86ba1ab9eaff32e |
refs/heads/master | <file_sep>package tests.threads;
import service.StoreImpl;
public class QCClientThread extends Thread {
private StoreImpl quebecStore;
private StoreImpl ontarioStore;
private StoreImpl britishColumbiaStore;
public QCClientThread(StoreImpl quebecStore, StoreImpl ontarioStore, StoreImpl britishColumbiaStore){
this.quebecStore = quebecStore;
this.ontarioStore = ontarioStore;
this.britishColumbiaStore = britishColumbiaStore;
}
@Override
public void run() {
pause(1000);
String response = "";
response = quebecStore.purchaseItem("qcc1111", "qc1234", "25/10/2020 20:00")+"\n";
System.out.println(response);
pause(2000);
response = quebecStore.exchange("qcc1111", "qc1111", "qc1234","25/10/2020 20:00")+"\n";
System.out.println(response);
}
private void pause(long time) {
try {
Thread.sleep(time);
} catch (InterruptedException e) {
// e.printStackTrace();
}
}
}
<file_sep>package tests.threads;
import service.StoreImpl;
public class ManagerThread extends Thread {
private StoreImpl quebecStore;
private StoreImpl ontarioStore;
private StoreImpl britishColumbiaStore;
public ManagerThread(StoreImpl quebecStore, StoreImpl britishColumbiaStore, StoreImpl ontarioStore) {
this.quebecStore = quebecStore;
this.ontarioStore = ontarioStore;
this.britishColumbiaStore = britishColumbiaStore;
}
@Override
public void run() {
StringBuilder response = new StringBuilder();
response.append(britishColumbiaStore.addItem("bcm1111", "bc1111", "TEA", 2, 980.00));
//System.out.println(response.toString());
response.append(britishColumbiaStore.addItem("bcm1111", "bc1234", "LAPTOP", 2, 30.00));
//System.out.println(response.toString());
response.append(quebecStore.addItem("qcm1111", "qc1234", "TEA", 2, 30.00));
//System.out.println(response.toString());
response.append(quebecStore.addItem("qcm1111", "qc1111", "XYZ", 2, 40.00)+"\n");
response.append(quebecStore.listItemAvailability("qcm1111"));
//System.out.println(response.toString());
System.out.println(response.toString());
}
}
<file_sep>package tests.main;
import org.omg.CORBA.ORB;
import org.omg.CosNaming.NamingContextExt;
import org.omg.CosNaming.NamingContextExtHelper;
import service.StoreImpl;
import tests.threads.BCClientThread;
import tests.threads.ManagerThread;
import tests.threads.ONClientThread;
import tests.threads.QCClientThread;
public class ConcurrencyTest {
private static QCClientThread qcClientThread;
private static ONClientThread onClientThread;
private static BCClientThread bcClientThread;
private static ManagerThread genericManager;
private static StoreImpl quebecStore;
private static StoreImpl britishColumbiaStore;
private static StoreImpl ontarioStore;
public static void main(String[] args) {
initializeStores(args);
runTest();
}
private static void runTest() {
genericManager.run();
onClientThread.start();
qcClientThread.start();
bcClientThread.start();
}
private static void initializeStores(String[] args) {
try {
// quebecStore = StoreHelper.narrow(ncRef.resolve_str("quebecStore"));
// britishColumbiaStore = StoreHelper.narrow(ncRef.resolve_str("britishColumbiaStore"));
// ontarioStore = StoreHelper.narrow(ncRef.resolve_str("ontarioStore"));
//
// initializeClientThreads(quebecStore, britishColumbiaStore, ontarioStore);
}
catch(Exception e) {
System.out.println("Hello Client exception: " + e);
}
}
private static void initializeClientThreads(StoreImpl quebecStore, StoreImpl britishColumbiaStore, StoreImpl ontarioStore) {
genericManager = new ManagerThread(quebecStore, britishColumbiaStore, ontarioStore);
qcClientThread = new QCClientThread(quebecStore, ontarioStore, britishColumbiaStore);
onClientThread = new ONClientThread(quebecStore, ontarioStore, britishColumbiaStore);
bcClientThread = new BCClientThread(quebecStore, ontarioStore, britishColumbiaStore);
}
private static void pause(long time) {
try {
Thread.sleep(time);
} catch (InterruptedException e) {
// e.printStackTrace();
}
}
}
| ddcd9e4ef15342c8c0955c87b490cddbb9f1bb1a | [
"Java"
] | 3 | Java | mcalixte/SOEN423-A3 | 90f9c48ee1ea45c438da615a39d73c04e8fccbdc | 979293767c83a9ee1fdbea7d4b51f8f8069fb724 |
refs/heads/master | <repo_name>rofrol/data-canvas<file_sep>/flowtype/types.js
// These are type declarations for use with Flow
// http://flowtype.org/
declare module "data-canvas" {
declare class DataCanvasRenderingContext2D extends CanvasRenderingContext2D {
pushObject(o: any): void;
popObject(): void;
reset(): void;
}
declare function getDataContext(ctx: CanvasRenderingContext2D): DataCanvasRenderingContext2D;
declare function getDataContext(canvas: HTMLCanvasElement): DataCanvasRenderingContext2D;
declare class DataContext extends DataCanvasRenderingContext2D {
constructor(ctx: CanvasRenderingContext2D): void;
}
declare class RecordingContext extends DataCanvasRenderingContext2D {
constructor(ctx: CanvasRenderingContext2D): void;
calls: Object[];
drawnObjectsWith(predicate: (o: Object)=>boolean): Object[];
callsOf(type: string): Array<any>[];
static recordAll(): void;
static reset(): void;
static drawnObjectsWith(div: HTMLElement, selector: string, predicate:(o: Object)=>boolean): Object[];
static drawnObjectsWith(predicate:(o: Object)=>boolean): Object[];
static drawnObjects(div: HTMLElement, selector: string): Object[];
static drawnObjects(): Object[];
static callsOf(div: HTMLElement, selector: string, type: string): Array<any>[];
static callsOf(type: string): Array<any>[];
}
declare class ClickTrackingContext extends DataCanvasRenderingContext2D {
constructor(ctx: CanvasRenderingContext2D, x: number, y: number): void;
hit: ?any[];
hits: any[][];
}
}
<file_sep>/generate-coverage.sh
#!/bin/bash
# Generate code coverage data for posting to Coveralls.
# Output is coverage/lcov.info
set -o errexit
set -x
# Instrument the source code with Istanbul's __coverage__ variable.
rm -rf coverage/* # Clear out everything to ensure a hermetic run.
istanbul instrument --output coverage src
# Run the tests using mocha-phantomjs & mocha-phantomjs-istanbul
# This produces coverage/coverage.json
phantomjs \
./node_modules/mocha-phantomjs/lib/mocha-phantomjs.coffee \
test/coverage.html \
spec '{"hooks": "mocha-phantomjs-istanbul", "coverageFile": "coverage/coverage.json"}'
if [ $CI ]; then
# Convert the JSON coverage to LCOV for coveralls.
istanbul report --include coverage/*.json lcovonly
# Post the results to coveralls.io
set +o errexit
cat coverage/lcov.info | coveralls
echo '' # reset exit code -- failure to post coverage shouldn't be an error.
else
# Convert the JSON coverage to HTML for viewing
istanbul report --include coverage/*.json html
set +x
echo 'To browse coverage, run:'
echo
echo ' open coverage/index.html'
echo
fi
<file_sep>/index.js
module.exports = require('./src/data-canvas');
<file_sep>/README.md
[](https://travis-ci.org/hammerlab/data-canvas) [](https://coveralls.io/github/hammerlab/data-canvas?branch=master)[](https://gitter.im/hammerlab/data-canvas?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)
data-canvas
===========
data-canvas allows you to add event tracking and tests to existing [canvas][1]
code without sacrificing performance and without forcing you to refactor.
It does this by introducing a new abstraction to canvas: the data stack.
Background
----------
The [HTML5 canvas][1] has several advantages over [SVG][], its main rival for
graphics on the web:
- Depending on the benchmark, it's anywhere from 10x to 300x faster than SVG.
- It allows for a simpler coding style. Rather than setting up [elaborate data
binding][2] to track updates, you just redraw the scene from scratch every
time.
That being said, canvas also has some major drawbacks:
- It can only be used through JavaScript. Rather than styling elements
declaratively using CSS, you have to style them in code.
- It's harder to work with events. For example, when the user clicks on the
canvas, it's difficult to determine exactly which element they clicked on.
- It's harder to test. Assertions about individual pixels are hard to
understand and can break easily. With SVG, you can find complete shapes using
selectors and make assertions about them.
data-canvas aims to overcome some of these drawbacks without compromising
canvas's speed and simplicity.
A canvas example
----------------
Here's a bit of data representing a car:
```javascript
var car = {
name: '<NAME>',
x: 100,
y: 100,
width: 200,
wheels: [
{name: 'back wheel', x: 20, radius: 15},
{name: 'front wheel', x: 180, radius: 10}
]
};
```
You might render it using canvas like so:
```javascript
function renderScene(ctx, car) {
ctx.fillStyle = 'red';
ctx.fillRect(car.x, car.y, car.width, -25);
ctx.fillRect(car.x + 50, car.y - 25, car.width - 100, -25);
ctx.fillStyle = 'black';
ctx.strokeStyle = 'gray';
car.wheels.forEach(function(wheel) {
ctx.beginPath();
ctx.arc(car.x + wheel.x, car.y, wheel.radius, 0, Math.PI*2, true);
ctx.fill();
ctx.stroke();
});
}
function renderBoxyCar() {
var ctx = canvas.getContext('2d');
renderScene(ctx, car);
}
```

_(see [full demo][4])_
This is a beautiful car and a faithful rendering of the data. But what if you
wanted to add a click handler to it? What if you wanted to write a test which
asserted that there were two wheels?
data-canvas can help you do both of these.
The data stack
--------------
[_"All problems in computer science can be solved by another level of indirection."_][3]
data-canvas wraps the browser's canvas rendering context with a `DataContext`,
which adds two new primitives:
```
declare class DataCanvasRenderingContext2D extends CanvasRenderingContext2D {
pushObject(o: any): void;
popObject(): void;
}
```
These primitives associate a _data stack_ with the canvas rendering context.
Whenever you render a bit of data, you should push it onto the data stack. When
you're done with it, you pop it off.
Here's what the car example looks like using a `DataContext`:
```javascript
function renderScene(ctx) {
ctx.pushObject(car); // <---
ctx.fillStyle = 'red';
ctx.fillRect(car.x, car.y, car.width, -25);
ctx.fillRect(car.x + 50, car.y - 25, car.width - 100, -25);
ctx.fillStyle = 'black';
ctx.strokeStyle = 'gray';
car.wheels.forEach(function(wheel) {
ctx.pushObject(wheel); // <---
ctx.beginPath();
ctx.arc(car.x + wheel.x, car.y, wheel.radius, 0, Math.PI*2, true);
ctx.fill();
ctx.stroke();
ctx.popObject(); // <---
});
ctx.popObject(); // <---
}
function renderBoxyCar() {
var ctx = dataCanvas.getDataContext(canvas.getContext('2d')); // <---
renderScene(ctx);
}
```
The new code is marked by comments. The `pushObject`/`popObject` calls fit
nicely into the existing code without changing its style.
Here's what the data stack looks like while the rendering happens:
<!--
Code for generating this image is here: http://jsfiddle.net/7nkbfbkb/6/
convert -background white -alpha remove -layers OptimizePlus -delay 75 -dispose Background -loop 0 frame*.png data-canvas-stack.gif
-->
<img src="data-canvas-stack.gif" width=400 height=125>
Testing
-------
Using this modified code, we can write a test:
```javascript
describe('boxy car', function() {
it('should have two wheels', function() {
var RecordingContext = dataCanvas.RecordingContext;
RecordingContext.recordAll(); // stub in a recording data context
renderBoxyCar();
var wheels = RecordingContext.drawnObjectsWith(x => x.radius);
expect(wheels).to.have.length(2);
expect(wheels[0].name).to.equal('back wheel');
expect(wheels[1].name).to.equal('front wheel');
RecordingContext.reset(); // restore the usual data context
});
});
```
The `RecordingContext.recordAll()` call swaps in an alternate implementation of
`DataContext` which records every method called on it. This includes the
`pushObject` calls. After the drawing is done, we can access the drawn objects
using its helper methods, such as `drawnObjectsWith`.
It's typically easiest to make assertions about the objects pushed onto the
data stack, but you can make assertions about the underlying drawing commands, too:
```javascript
describe('boxy car', function() {
it('should draw two wheels', function() {
var RecordingContext = dataCanvas.RecordingContext;
RecordingContext.recordAll(); // stub in a recording data context
renderBoxyCar();
RecordingContext.reset(); // restore the usual data context
var wheels = RecordingContext.callsOf('arc');
expect(wheels).to.have.length(2);
expect(wheels[0].slice(0, 3)).to.deep.equal(['arc', 120, 100, 15]);
expect(wheels[1].slice(0, 3)).to.deep.equal(['arc', 280, 100, 10]);
});
});
```
Writing the test required no modifications to the rendering code beyond the
`pushObject`/`popObject` calls.
Click tracking
--------------
data-canvas also facilitates mapping (x, y) coordinates to objects in the scene.
Suppose you wanted to add click handlers to the wheels and the car itself.
Here's how you might do that:
```javascript
canvas.onclick = function(e) {
var ctx = canvas.getContext('2d');
var trackingContext = new dataCanvas.ClickTrackingContext(ctx, e.offsetX, e.offsetY);
renderScene(trackingContext);
if (trackingContext.hit) {
alert(trackingContext.hit[0].name);
}
};
```
_(Try it with [this fiddle][5])_
Again, no modifications to the scene rendering code were required. To determine
which object (if any) was clicked on, we swapped in an alternate data context
(`ClickTrackingContext`) and redrew the scene.
While redrawing the scene may feel inefficient, it rarely is.
`ClickTrackingContext` doesn't need to draw any shapes, only check whether they
contain the relevant point.
After rendering the scene, `ClickTrackingContext` will have both a `hit` and a
`hits` property. `hit` records the contents of the data stack for the last
(top-most) shape which contains the point. `hits` records the contents for all
shapes which contained the point, ordered from top to bottom.
For example, if you click the top part of the back wheel, then we'll have:
`hit = [back wheel, car]`
Because both the car and the back wheel were on the data stack when the car was drawn.
We'll also have:
`hits = [[back wheel, car], [car]]`
This is because only `car` was on the stack when the car rectangle was drawn,
and another shape (the wheel) was drawn on top of it.
Usage
-----
To install data-canvas, use NPM:
npm install data-canvas
Then include it either in your page:
<script src="node_modules/data-canvas/data-canvas.js"></script>
or require it:
var dataCanvas = require('data-canvas');
data-canvas comes with type bindings for [Flow][]. To use these, add the
following to your `.flowconfig`:
```
[ignore]
\.*node_modules[/\\]data-canvas\.*
[libs]
node_modules[/\\]data-canvas[/\\]flowtype
```
[Windows binaries for flow](http://www.ocamlpro.com/pub/ocpwin/flow-builds/)
[1]: https://developer.mozilla.org/en-US/docs/Web/API/Canvas_API
[svg]: https://developer.mozilla.org/en-US/docs/Web/SVG
[2]: http://alignedleft.com/tutorials/d3/binding-data/
[3]: http://www.dmst.aueb.gr/dds/pubs/inbook/beautiful_code/html/Spi07g.html
[4]: http://jsfiddle.net/7nkbfbkb/1/
[5]: http://jsfiddle.net/7nkbfbkb/3/
[flow]: http://flowtype.org
<file_sep>/test/data-canvas-test.js
(function() {
'use strict';
var expect = chai.expect;
describe('data-canvas', function() {
var testDiv = document.getElementById('testdiv');
var canvas;
before(function() {
canvas = document.createElement('canvas');
canvas.width = 600;
canvas.height = 200;
testDiv.appendChild(canvas);
});
after(function() {
// testDiv.innerHTML = ''; // avoid pollution between tests.
});
function rgbAtPos(im, x, y) {
var index = y * (im.width * 4) + x * 4;
return [
im.data[index],
im.data[index + 1],
im.data[index + 2]
];
}
describe('DataContext', function() {
it('should put pixels on the canvas', function() {
if (!canvas) throw 'bad'; // for flow
var ctx = canvas.getContext('2d');
var dtx = dataCanvas.getDataContext(ctx);
dtx.fillStyle = 'red';
dtx.fillRect(100, 50, 200, 25);
dtx.pushObject({something: 'or other'});
dtx.popObject();
var im = ctx.getImageData(0, 0, 600, 400);
expect(rgbAtPos(im, 50, 50)).to.deep.equal([0, 0, 0]);
expect(rgbAtPos(im, 200, 60)).to.deep.equal([255, 0, 0]);
});
it('should cache calls', function() {
if (!canvas) throw 'bad'; // for flow
var ctx = canvas.getContext('2d');
var dtx = dataCanvas.getDataContext(canvas);
var dtx2 = dataCanvas.getDataContext(ctx);
expect(dtx2).to.equal(dtx);
});
it('should support read/write to properties', function() {
var dtx = dataCanvas.getDataContext(canvas);
dtx.lineWidth = 10;
expect(dtx.lineWidth).to.equal(10);
});
});
describe('ClickTrackingContext', function() {
var ctx;
before(function() {
if (!canvas) throw 'bad'; // for flow
ctx = canvas.getContext('2d');
ctx.clearRect(0, 0, ctx.canvas.width, ctx.canvas.height);
});
function getObjectsAt(draw, x, y) {
var dtx = new dataCanvas.ClickTrackingContext(ctx, x, y);
draw(dtx);
return dtx.hits;
}
// To draw any of these:
// draw(dataCanvas.getDataContext(ctx));
it('should track clicks on rects', function() {
function draw(dtx) {
dtx.pushObject('r');
dtx.fillStyle = 'red';
dtx.fillRect(100, 50, 100, 25);
dtx.popObject();
dtx.pushObject('b');
dtx.fillStyle = 'blue';
dtx.fillRect(300, 100, 200, 25);
dtx.popObject();
}
expect(getObjectsAt(draw, 150, 60)).to.deep.equal([['r']]);
expect(getObjectsAt(draw, 350, 110)).to.deep.equal([['b']]);
expect(getObjectsAt(draw, 250, 110)).to.deep.equal([]);
});
it('should track clicks on complex shapes', function() {
function draw(dtx) {
// This is the upper right half of a rectangle, i.e. a triangle.
dtx.pushObject('triangle');
dtx.beginPath();
dtx.moveTo(100, 100);
dtx.lineTo(400, 100);
dtx.lineTo(400, 200);
dtx.closePath();
dtx.fill();
dtx.popObject();
}
// This point is in the top right (and hence in the triangle)
expect(getObjectsAt(draw, 300, 110)).to.deep.equal([['triangle']]);
// This poitn is in the bottom left (and hence not in the triangle)
expect(getObjectsAt(draw, 200, 180)).to.deep.equal([]);
});
it('should track clicks on stacked shapes', function() {
function draw(dtx) {
dtx.pushObject('bottom');
dtx.fillStyle = 'red';
dtx.fillRect(100, 50, 400, 100);
dtx.pushObject('top');
dtx.fillStyle = 'blue';
dtx.fillRect(200, 75, 100, 50);
dtx.popObject();
dtx.popObject();
dtx.pushObject('side');
dtx.fillStyle = 'green';
dtx.fillRect(450, 75, 100, 50);
dtx.popObject();
}
draw(dataCanvas.getDataContext(ctx));
expect(getObjectsAt(draw, 110, 60)).to.deep.equal([['bottom']]);
expect(getObjectsAt(draw, 250, 100)).to.deep.equal([['top', 'bottom'], ['bottom']]);
expect(getObjectsAt(draw, 475, 100)).to.deep.equal([['side'], ['bottom']]);
});
it('should reset hit tracker', function() {
function draw(dtx) {
dtx.reset();
dtx.clearRect(0, 0, dtx.canvas.width, dtx.canvas.height);
dtx.pushObject('rect');
dtx.fillRect(100, 10, 200, 30);
dtx.popObject();
}
function doubledraw(dtx) {
draw(dtx);
draw(dtx);
}
// Despite the double-drawing, only one object matches, not two.
// This is because of the reset() call.
doubledraw(dataCanvas.getDataContext(ctx));
expect(getObjectsAt(doubledraw, 110, 30)).to.deep.equal([['rect']]);
});
// PhantomJS 1.9.x does not support isStrokeInPath
// When Travis-CI updates to Phantom2, this can be re-enabled.
// See https://github.com/ariya/phantomjs/issues/12948
if (!navigator.userAgent.match(/PhantomJS\/1.9/)) {
it('should detect clicks in strokes', function() {
function draw(dtx) {
dtx.save();
dtx.pushObject('shape');
dtx.lineWidth = 5;
dtx.beginPath();
dtx.moveTo(100, 10);
dtx.lineTo(200, 10);
dtx.lineTo(200, 30);
dtx.lineTo(100, 30);
dtx.closePath();
dtx.stroke();
dtx.popObject();
dtx.restore();
}
draw(dataCanvas.getDataContext(ctx));
// a click on the stroke is a hit...
expect(getObjectsAt(draw, 100, 10)).to.deep.equal([['shape']]);
// ... while a click in the interior is not.
expect(getObjectsAt(draw, 150, 20)).to.deep.equal([]);
});
}
});
describe('RecordingContext', function() {
var RecordingContext = dataCanvas.RecordingContext;
var ctx;
before(function() {
if (!canvas) throw 'bad'; // for flow
ctx = canvas.getContext('2d');
ctx.clearRect(0, 0, ctx.canvas.width, ctx.canvas.height);
});
describe('single canvas', function() {
it('should record calls', function() {
var dtx = new RecordingContext(ctx);
dtx.fillStyle = 'red';
dtx.pushObject('a');
dtx.fillRect(100, 50, 200, 25);
dtx.popObject();
expect(dtx.calls).to.have.length(3); // push, fill, pop
expect(dtx.drawnObjectsWith(function(x) { return x == 'a' })).to.have.length(1);
expect(dtx.drawnObjectsWith(function(x) { return x == 'b' })).to.have.length(0);
// TODO: check drawing styles
});
it('should return values from proxied functions', function() {
var dtx = new RecordingContext(ctx);
var metrics = dtx.measureText('Hello');
expect(dtx.calls).to.deep.equal([['measureText', 'Hello']]);
expect(metrics.width).to.be.greaterThan(0);
});
it('should provid static testing methods', function() {
RecordingContext.recordAll();
var dtx = dataCanvas.getDataContext(ctx);
dtx.pushObject('hello');
dtx.fillText('hello', 100, 10);
dtx.popObject();
expect(RecordingContext.drawnObjects()).to.deep.equal(['hello']);
expect(RecordingContext.drawnObjectsWith(function(x) { return x == 'hello' })).to.deep.equal(['hello']);
expect(RecordingContext.callsOf('fillText')).to.deep.equal(
[['fillText', 'hello', 100, 10]]);
RecordingContext.reset();
});
it('should reset the list of calls', function() {
function render(dtx) {
dtx.reset(); // this clears the list of calls
dtx.pushObject('hello');
dtx.fillText('hello', 100, 10);
dtx.popObject();
}
RecordingContext.recordAll();
var dtx = dataCanvas.getDataContext(ctx);
render(dtx);
render(dtx);
// Only one object, not two (even though there are two render calls).
expect(RecordingContext.drawnObjects()).to.have.length(1);
RecordingContext.reset();
});
});
describe('multiple canvases', function() {
var canvas2;
before(function() {
canvas2 = document.createElement('canvas');
canvas2.width = 400;
canvas2.height = 100;
canvas2.setAttribute('class', 'canvas2');
canvas.setAttribute('class', 'canvas1');
testDiv.appendChild(canvas2);
});
it('should record calls to both canvases', function() {
function render(dtx, text) {
dtx.pushObject(text);
dtx.fillText(text, 100, 10);
dtx.popObject();
}
RecordingContext.recordAll();
var dtx1 = dataCanvas.getDataContext(canvas),
dtx2 = dataCanvas.getDataContext(canvas2);
render(dtx1, 'Hello #1');
render(dtx2, 'Hello #2');
expect(function() {
RecordingContext.drawnObjects();
}).to.throw(/multiple canvases/);
expect(RecordingContext.drawnObjects(testdiv, '.canvas1'))
.to.deep.equal(['Hello #1']);
expect(RecordingContext.drawnObjects(testdiv, '.canvas2'))
.to.deep.equal(['Hello #2']);
expect(RecordingContext.callsOf(testdiv, '.canvas1', 'fillText'))
.to.deep.equal([['fillText', 'Hello #1', 100, 10]]);
expect(RecordingContext.callsOf(testdiv, '.canvas2', 'fillText'))
.to.deep.equal([['fillText', 'Hello #2', 100, 10]]);
expect(function() {
RecordingContext.drawnObjects(testdiv, '.canvas3');
}).to.throw(/Unable to find.*\.canvas3/);
RecordingContext.reset();
});
it('should throw on matching non-canvas', function() {
testDiv.innerHTML += '<div class=foo>Foo</div>';
RecordingContext.recordAll();
expect(function() {
RecordingContext.drawnObjects(testdiv, '.foo');
}).to.throw(/.foo neither matches nor contains/);
RecordingContext.reset();
});
it('should throw before recording', function() {
// TODO: this error message doesn't make much sense for a user.
expect(function() {
RecordingContext.drawnObjects(testdiv, '.canvas1');
}).to.throw(/must call .*recordAll.*other.*static methods/);
});
});
describe('error cases', function() {
it('should throw on reset before record', function() {
expect(function() {
RecordingContext.reset();
}).to.throw(/reset.*before.*recordAll/);
});
it('should throw on double record', function() {
expect(function() {
RecordingContext.recordAll();
RecordingContext.recordAll();
}).to.throw(/forgot.*reset/);
RecordingContext.reset();
});
it('should throw on access without recording', function() {
expect(function() {
RecordingContext.drawnObjects();
}).to.throw(/You must call .*recordAll/);
});
it('should throw on access with nothing recorded', function() {
expect(function() {
RecordingContext.recordAll();
RecordingContext.drawnObjects();
}).to.throw(/no canvases are being recorded/);
RecordingContext.reset();
});
});
describe('drawImage', function() {
beforeEach(function() {
RecordingContext.recordAll();
});
afterEach(function() {
RecordingContext.reset();
});
function makeOffscreenImage() {
var image = document.createElement('canvas');
image.width = 100;
image.height = 100;
var dtx = dataCanvas.getDataContext(image);
dtx.pushObject('A');
dtx.fillRect(0, 0, 50, 50);
dtx.popObject();
return image;
}
it('should transfer recorded calls', function() {
var image = makeOffscreenImage();
var dtx = dataCanvas.getDataContext(canvas);
dtx.drawImage(image, 0, 0);
expect(dtx.calls).to.have.length(3);
expect(dtx.drawnObjects()).to.deep.equal(['A']);
expect(dtx.callsOf('fillRect')).to.deep.equal([['fillRect', 0, 0, 50, 50]]);
// The drawImage call is elided.
// This could be changed -- either way would be reasonable.
expect(dtx.callsOf('drawImage')).to.deep.equal([]);
});
it('should translate recorded calls', function() {
var image = makeOffscreenImage();
var dtx = dataCanvas.getDataContext(canvas);
dtx.drawImage(image, 50, 0); // dx=50
expect(dtx.calls).to.have.length(3);
expect(dtx.callsOf('fillRect')).to.deep.equal([['fillRect', 50, 0, 50, 50]]);
});
it('should transform recorded calls', function() {
var image = makeOffscreenImage();
var dtx = dataCanvas.getDataContext(canvas);
dtx.drawImage(image, 50, 0, 75, 50); // dx=50, dWidth=75, dHeight=50
expect(dtx.calls).to.have.length(3);
expect(dtx.callsOf('fillRect')).to.deep.equal([['fillRect', 50, 0, 37.5, 25]]);
});
it('should support a source rectangle', function() {
var image = makeOffscreenImage();
var dtx = dataCanvas.getDataContext(canvas);
// This copies x=75-100 and y=50-100 from source to dest
dtx.drawImage(image, 25, 50, 75, 50, 0, 0, 75, 50);
expect(dtx.calls).to.have.length(3);
expect(dtx.callsOf('fillRect')).to.deep.equal([['fillRect', -25, -50, 50, 50]]);
});
it('should reject invalid drawImage calls', function() {
var image = makeOffscreenImage();
var dtx = dataCanvas.getDataContext(canvas);
expect(function() {
dtx.drawImage(image, 50, 0, 75); // four params, should be 3, 5 or 9
}).to.throw(); // exact error depends on browser
});
it('should transform paths', function() {
var image = makeOffscreenImage();
var ctx = dataCanvas.getDataContext(image);
ctx.beginPath();
ctx.moveTo(20, 10);
ctx.lineTo(30, 20);
ctx.quadraticCurveTo(50, 20, 40, 30);
ctx.closePath();
var dtx = dataCanvas.getDataContext(canvas);
dtx.drawImage(image, 0, 10, 50, 25); // dx=0, dy=10, dWidth=50, dHeight=25
expect(dtx.callsOf('moveTo')).to.deep.equal([['moveTo', 10, 12.5]]);
expect(dtx.callsOf('lineTo')).to.deep.equal([['lineTo', 15, 15]]);
expect(dtx.callsOf('quadraticCurveTo')).to.deep.equal(
[['quadraticCurveTo', 25, 15, 20, 17.5]]);
});
it('should not transfer calls from unrecorded canvases', function() {
var image = document.createElement('canvas');
image.width = 100;
image.height = 100;
image.getContext('2d').fillRect(0, 0, 100, 100);
var dtx = dataCanvas.getDataContext(canvas);
dtx.drawImage(image, 0, 0);
// The fillRect call should not be transferred over.
expect(dtx.callsOf('drawImage')).to.deep.equal(
[['drawImage', image, 0, 0]]);
expect(dtx.callsOf('fillRect')).to.deep.equal([]);
});
// Regression test for #13
it('should record calls after drawImage', function() {
var image = makeOffscreenImage();
var dtx = dataCanvas.getDataContext(canvas);
dtx.clearRect(0, 0, 200, 50);
dtx.drawImage(image, 0, 0);
dtx.fillRect(20, 10, 100, 40);
expect(dtx.calls).to.have.length(5);
expect(dtx.drawnObjects()).to.deep.equal(['A']);
expect(dtx.callsOf('clearRect')).to.deep.equal([['clearRect', 0, 0, 200, 50]]);
expect(dtx.callsOf('fillRect')).to.deep.equal([
['fillRect', 0, 0, 50, 50],
['fillRect', 20, 10, 100, 40]
]);
});
});
});
});
})();
<file_sep>/src/data-canvas.js
/**
* Wrappers around CanvasRenderingContext2D to facilitate testing and click-tracking.
*
* This adds the concept of a "data stack" to the canvas. When shapes are
* drawn, they represent the objects currently on the stack. This stack can be
* manipulated using context.pushObject() and context.popObject().
*
* See test file for sample usage.
*/
(function() {
'use strict';
// Turn obj into a proxy for target. This forwards both function calls and
// property setters/getters.
function forward(obj, target, onlyAccessors) {
onlyAccessors = onlyAccessors || false;
for (var k in target) {
(function(k) {
if (typeof(target[k]) == 'function') {
if (!onlyAccessors) {
obj[k] = target[k].bind(target);
}
} else {
Object.defineProperty(obj, k, {
get: function() { return target[k]; },
set: function(x) { target[k] = x; }
});
}
})(k);
}
}
// The most basic data-aware canvas. This throws away all data information.
// Use this for basic drawing
function DataContext(ctx) {
forward(this, ctx);
this.pushObject = this.popObject = this.reset = function() {};
}
var stubGetDataContext = null;
/**
* Get a DataContext for the built-in CanvasRenderingContext2D.
*
* This caches DataContexts and facilitates stubbing in tests.
*
* As a convenience, you may pass in a Canvas element instead of a
* CanvasRenderingContext2D. data-canvas will call getContext('2d') for you.
*/
function getDataContext(ctxOrCanvas) {
if (ctxOrCanvas instanceof HTMLCanvasElement) {
return getDataContext(ctxOrCanvas.getContext('2d'));
}
var ctx = ctxOrCanvas;
if (stubGetDataContext) {
return stubGetDataContext(ctx);
} else {
for (var i = 0; i < getDataContext.cache.length; i++) {
var pair = getDataContext.cache[i];
if (pair[0] == ctx) return pair[1];
}
var dtx = new DataContext(ctx);
getDataContext.cache.push([ctx, dtx]);
return dtx;
}
}
getDataContext.cache = []; // (CanvasRenderingContext2D, DataContext) pairs
/**
* A context which records what it does (for testing).
*
* This proxies all calls to the underlying canvas, so they do produce visible
* drawing. Use `drawnObjectsWith` or `calls` to test what was drawn.
*/
function RecordingContext(ctx) {
forward(this, ctx, true /* only foward accessors */);
var calls = [];
this.calls = calls;
for (var k in ctx) {
(function(k) {
if (typeof(ctx[k]) != 'function') return;
this[k] = function() {
// TODO: record current drawing style
var args = Array.prototype.slice.call(arguments);
calls.push([k].concat(args));
return ctx[k].apply(ctx, arguments);
};
}).bind(this)(k);
}
this.pushObject = function(o) {
calls.push(['pushObject', o]);
};
this.popObject = function() {
calls.push(['popObject']);
};
this.reset = function() {
this.calls = calls = [];
};
var recordingDrawImage = this.drawImage; // plain recording drawImage()
this.drawImage = function(image) {
// If the drawn image has recorded calls, then they need to be transferred over.
var recorder = RecordingContext.recorderForCanvas(image);
if (!recorder) {
recordingDrawImage.apply(ctx, arguments);
} else {
ctx.drawImage.apply(ctx, arguments);
calls = calls.concat(transformedCalls(recorder.calls, arguments));
this.calls = calls;
}
}
}
// Transform the calls to a new coordinate system.
// The arguments are those to drawImage().
function transformedCalls(calls, args) {
var image = args[0],
sx = 0,
sy = 0,
sWidth = image.width,
sHeight = image.height,
dx,
dy,
dWidth = image.width,
dHeight = image.height;
if (args.length == 3) {
// void ctx.drawImage(image, dx, dy);
dx = args[1];
dy = args[2];
} else if (args.length == 5) {
// void ctx.drawImage(image, dx, dy, dWidth, dHeight);
dx = args[1];
dy = args[2];
dWidth = args[3];
dHeight = args[4];
} else if (args.length == 9) {
// void ctx.drawImage(image, sx, sy, sWidth, sHeight, dx, dy, dWidth, dHeight);
sx = args[1];
sy = args[2];
sWidth = args[3];
sHeight = args[4];
dx = args[5];
dy = args[6];
dWidth = args[7];
dHeight = args[8];
}
// Other arities will make the browser throw an error on ctx.drawImage.apply
var xScaling = getScaleFactor(sx, sx + sWidth, dx, dx + dWidth),
xScale = makeScale( sx, sx + sWidth, dx, dx + dWidth),
yScaling = getScaleFactor(sy, sy + sHeight, dy, dy + dHeight),
yScale = makeScale( sy, sy + sHeight, dy, dy + dHeight);
// These calls are more complex:
// arc
// arcTo
// ellipse
// TODO: clip calls outside of the source rectangle.
var transformCall = function(originalCall) {
var call = originalCall.slice(), // make a copy
type = call[0];
if (type in CALLS_XY) {
var xys = CALLS_XY[type];
if (typeof(xys) == 'number') xys = [xys];
xys.forEach(function(pos) {
call[1 + pos] = xScale(call[1 + pos]);
call[2 + pos] = yScale(call[2 + pos]);
});
}
if (type in CALLS_WH) {
var whs = CALLS_WH[type];
if (typeof(whs) == 'number') whs = [whs];
whs.forEach(function(pos) {
call[1 + pos] *= xScaling;
call[2 + pos] *= yScaling;
});
}
return call;
};
return calls.map(transformCall);
}
// Helpers for transformedCalls
// Map (x1, x2) --> (y1, y2)
function getScaleFactor(x1, x2, y1, y2) {
return (y2 - y1) / (x2 - x1);
};
function makeScale(x1, x2, y1, y2) {
var scale = getScaleFactor(x1, x2, y1, y2);
return function(x) {
return y1 + scale * (x - x1);
};
};
// These calls all have (x, y) as args at the specified positions.
var CALLS_XY = {
clearRect: 0,
fillRect: 0,
strokeRect: 0,
fillText: 1,
strokeText: 1,
moveTo: 0,
lineTo: 0,
bezierCurveTo: [0, 2, 4],
quadraticCurveTo: [0, 2],
rect: 0
};
// These calls have (width, height) as args at the specified positions.
var CALLS_WH = {
clearRect: 2,
fillRect: 2,
strokeRect: 2,
// fillText has an optional `max_width` param
rect: 2,
};
/**
* Get a list of objects which have been pushed to the data canvas that match
* the particular predicate.
* If no predicate is specified, all objects are returned.
*/
RecordingContext.prototype.drawnObjectsWith = function(predicate) {
if (!predicate) predicate = function() { return true; };
return this.callsOf('pushObject')
.filter(function(x) { return predicate(x[1]) })
.map(function(x) { return x[1]; });
};
// This version reads better if there's no predicate.
RecordingContext.prototype.drawnObjects = RecordingContext.prototype.drawnObjectsWith;
/**
* Find calls of a particular type, e.g. `fillText` or `pushObject`.
*
* Returns an array of the calls and their parameters, e.g.
* [ ['fillText', 'Hello!', 20, 10] ]
*/
RecordingContext.prototype.callsOf = function(type) {
return this.calls.filter(function(call) { return call[0] == type });
};
/**
* Static method to begin swapping in RecordingContext in place of DataContext.
* Don't forget to call RecordingContext.reset() after the test completes!
*/
RecordingContext.recordAll = function() {
if (stubGetDataContext != null) {
throw 'You forgot to call RecordingContext.reset()';
}
RecordingContext.recorders = [];
stubGetDataContext = function(ctx) {
var recorder = RecordingContext.recorderForCanvas(ctx.canvas);
if (recorder) return recorder;
recorder = new RecordingContext(ctx);
RecordingContext.recorders.push([ctx.canvas, recorder]);
return recorder;
};
};
/**
* Revert the stubbing performed by RecordingContext.recordAll.
*/
RecordingContext.reset = function() {
if (!stubGetDataContext) {
throw 'Called RecordingContext.reset() before RecordingContext.recordAll()';
}
stubGetDataContext = null;
RecordingContext.recorders = null;
};
// Get the recording context for a canvas.
RecordingContext.recorderForCanvas = function(canvas) {
var recorders = RecordingContext.recorders;
if (recorders == null) {
throw 'You must call RecordingContext.recordAll() before using other RecordingContext static methods';
}
for (var i = 0; i < recorders.length; i++) {
var r = recorders[i];
if (r[0] == canvas) return r[1];
}
return null;
};
/**
* Get the recording context for a canvas inside of div.querySelector(selector).
*
* This is useful when you have a test div and several canvases.
*/
RecordingContext.recorderForSelector = function(div, selector) {
var canvas = div.querySelector(selector + ' canvas') || div.querySelector(selector);
if (!canvas) {
throw 'Unable to find a canvas matching ' + selector;
} else if (!(canvas instanceof HTMLCanvasElement)) {
throw 'Selector ' + selector + ' neither matches nor contains a canvas';
}
return RecordingContext.recorderForCanvas(canvas);
};
// Resolves arguments for RecordingContext helpers.
// You can either specify a div & selector to find the canvas, or omit this if
// there's only one canvas being recorded.
function findRecorder(div, selector) {
if (!div) {
if (!RecordingContext.recorders) {
throw 'You must call RecordingContext.recordAll() before using other RecordingContext static methods';
} else if (RecordingContext.recorders.length == 0) {
throw 'Called a RecordingContext method, but no canvases are being recorded.';
} else if (RecordingContext.recorders.length > 1) {
throw 'Called a RecordingContext method while multiple canvases were being recorded. Specify one using a div and selector.';
} else {
return RecordingContext.recorders[0][1];
}
} else {
return RecordingContext.recorderForSelector(div, selector);
}
}
// Find objects pushed onto a particular recorded canvas.
RecordingContext.drawnObjectsWith = function(div, selector, predicate) {
// Check for the zero-argument or one-argument version.
if (typeof(div) == 'function' || arguments.length == 0) {
predicate = div;
div = null;
}
var recorder = findRecorder(div, selector);
predicate = predicate || function() { return true; };
return recorder ? recorder.drawnObjectsWith(predicate) : [];
};
// This version reads better if there's no predicate.
RecordingContext.drawnObjects = RecordingContext.drawnObjectsWith;
// Find calls of particular drawing functions (e.g. fillText)
RecordingContext.callsOf = function (div, selector, type) {
// Check for the one-argument version.
if (typeof(div) == 'string') {
type = div;
div = null;
}
var recorder = findRecorder(div, selector);
return recorder ? recorder.callsOf(type) : [];
};
/**
* A context which determines the data at a particular location.
*
* When drawing methods are called on this class, nothing is rendered. Instead,
* each shape is checked to see if it includes the point of interest. If it
* does, the current data stack is saved as a "hit".
*
* The `hits` property records all such hits.
* The `hit` property records only the last (top) hit.
*/
function ClickTrackingContext(ctx, px, py) {
forward(this, ctx);
var stack = [];
this.hits = [];
this.hit = null;
var that = this;
function recordHit() {
that.hits.unshift(Array.prototype.slice.call(stack));
that.hit = that.hits[0];
}
this.pushObject = function(o) {
stack.unshift(o);
};
this.popObject = function() {
stack.shift();
};
this.reset = function() {
this.hits = [];
this.hit = null;
};
// These are (most of) the canvas methods which draw something.
// TODO: would it make sense to purge existing hits covered by this?
this.clearRect = function(x, y, w, h) { };
this.fillRect = function(x, y, w, h) {
if (px >= x && px <= x + w && py >= y && py <= y + h) recordHit();
};
this.strokeRect = function(x, y, w, h) {
// ...
};
this.fill = function(fillRule) {
// TODO: implement fillRule
if (ctx.isPointInPath(px, py)) recordHit();
};
this.stroke = function() {
if (ctx.isPointInStroke(px, py)) recordHit();
};
this.fillText = function(text, x, y, maxWidth) {
// ...
};
this.strokeText = function(text, x, y, maxWidth) {
// ...
};
}
var exports = {
DataContext: DataContext,
RecordingContext: RecordingContext,
ClickTrackingContext: ClickTrackingContext,
getDataContext: getDataContext
};
if (typeof(module) !== 'undefined') {
/* istanbul ignore next */
module.exports = exports;
} else {
window.dataCanvas = exports;
}
})();
<file_sep>/.travis.yml
sudo: false # Use container-based infrastructure
language: node_js
node_js:
- "0.12"
script:
- npm run test
- ./generate-coverage.sh
| f23eb5975826159538ab81505680a246c51e2d95 | [
"Markdown",
"Shell",
"JavaScript",
"YAML"
] | 7 | Markdown | rofrol/data-canvas | f63d16e4291739e463fd9f1cb0d8ab481381cc60 | ca24d63bbfa2bcb37b530a3f9c45b71876eda9fa |
refs/heads/master | <file_sep># hopur58
prufa
| ac4886b2b3952359b31e58c12de80c009be59784 | [
"Markdown"
] | 1 | Markdown | steinijons/hopur58 | 39285665da909aaaeb992e22a4d319ecbfa2d453 | 2b14c3b028784923bbd91c67fe7fddbcbeffb853 |
refs/heads/master | <repo_name>vseledkin/LifelongVAE<file_sep>/decoders.py
import math
import tensorflow as tf
import tensorflow.contrib.slim as slim
from encoders import _get_normalizer
class CNNDecoder(object):
def __init__(self, sess, input_size, is_training,
gf_dim=64, double_channels=False,
activation=tf.nn.elu, use_bn=False,
use_ln=False, scope="cnn_decoder"):
self.sess = sess
self.layer_type = "cnn"
self.input_size = input_size
self.gf_dim = gf_dim
self.activation = activation
self.double_channels = double_channels
self.use_bn = use_bn
self.use_ln = use_ln
self.scope = scope
self.is_training = is_training
# compute the sizing automatically
self.s_h, self.s_w, self.s_h2, self.s_w2, self.s_h4, \
self.s_w4, self.s_h8, self.s_w8, self.s_h16, self.s_w16 \
= self._compute_sizing()
def get_info(self):
return {'activation': self.activation.__name__,
'sizes': self.get_sizing(),
'use_bn': str(self.use_bn),
'use_ln': str(self.use_ln)}
def get_sizing(self):
return 'fc%d_4_5x5xN_s2' % (self.gf_dim*8*self.s_h16*self.s_w16)
def get_detailed_sizing(self):
return 'fc%d_' % (self.gf_dim*8*self.s_h16*self.s_w16) \
+ 's2_5x5x%d_' % (self.gf_dim*4) \
+ 's2_5x5x%d_' % (self.gf_dim*2) \
+ 's2_5x5x%d_' % (self.gf_dim*1) \
+ 's2_5x5x%d_' % (self.gf_dim*1)
# return str(self.gf_dim*8*self.s_h16*self.s_w16) \
# + str([self.s_h8, self.s_w8, self.gf_dim*4]) \
# + str([self.s_h4, self.s_w4, self.gf_dim*2]) \
# + str([self.s_h2, self.s_w2, self.gf_dim]) \
# + str([self.s_h, self.s_w, self.input_size[-1]])
@staticmethod
def conv_out_size_same(size, stride):
'''From DCGAN (carpedm20)'''
return int(math.ceil(float(size) / float(stride)))
def _compute_sizing(self):
s_h, s_w = self.input_size[0], self.input_size[1]
s_h2, s_w2 = [self.conv_out_size_same(s_h, 2),
self.conv_out_size_same(s_w, 2)]
s_h4, s_w4 = [self.conv_out_size_same(s_h2, 2),
self.conv_out_size_same(s_w2, 2)]
s_h8, s_w8 = [self.conv_out_size_same(s_h4, 2),
self.conv_out_size_same(s_w4, 2)]
s_h16, s_w16 = [self.conv_out_size_same(s_h8, 2),
self.conv_out_size_same(s_w8, 2)]
return [s_h, s_w, s_h2, s_w2, s_h4,
s_w4, s_h8, s_w8, s_h16, s_w16]
def get_model(self, z):
# get the normalizer function and parameters
normalizer_fn, normalizer_params = _get_normalizer(self.is_training,
self.use_bn,
self.use_ln)
winit = tf.contrib.layers.xavier_initializer_conv2d()
# winit = tf.truncated_normal_initializer(stddev=0.01)
with tf.variable_scope(self.scope):
with slim.arg_scope([slim.conv2d_transpose],
activation_fn=self.activation,
weights_initializer=winit,
biases_initializer=tf.constant_initializer(0),
normalizer_fn=normalizer_fn,
normalizer_params=normalizer_params):
proj_z = slim.fully_connected(z, self.gf_dim*8*self.s_h16*self.s_w16,
activation_fn=None,
weights_initializer=tf.contrib.layers.xavier_initializer(),
biases_initializer=tf.constant_initializer(0),
scope='proj_z')
z_flat = self.activation(tf.reshape(proj_z, [-1, self.s_h16,
self.s_w16,
self.gf_dim*8]))
h0 = slim.conv2d_transpose(z_flat, num_outputs=self.gf_dim*4,
kernel_size=[5, 5],
stride=[2, 2],
padding='SAME')
h1 = slim.conv2d_transpose(h0, num_outputs=self.gf_dim*2,
kernel_size=[5, 5],
stride=[2, 2],
padding='SAME')
h2 = slim.conv2d_transpose(h1, num_outputs=self.gf_dim,
kernel_size=[5, 5],
stride=[2, 2],
padding='SAME')
channels = self.input_size[-1] if len(self.input_size) > 2 else 1
if self.double_channels:
channels *= 2
h3 = slim.conv2d_transpose(h2, num_outputs=channels,
kernel_size=[5, 5],
stride=[2, 2],
padding='SAME',
normalizer_fn=None,
normalizer_params=None,
activation_fn=None)
print 'h3 = ', h3.get_shape().as_list()
return slim.flatten(h3) if channels == 1 else h3
| d74218a1d5e7e614e6f3c6d97acd8990265e9205 | [
"Python"
] | 1 | Python | vseledkin/LifelongVAE | 2391edc63b77def5b63a1836cf1b7512734b9192 | ba02e054f88016602e34c7ca9cc0394db8525ec9 |
refs/heads/master | <file_sep>* description
a simple interactive program written in C to draw the Mandelbrot set https://en.wikipedia.org/wiki/Mandelbrot_set
it provides keys to zoom and move around the Mandelbrot drawing
#+CAPTION: image of drawn Mandelbrot
#+NAME: fig:SED-HR4049
[[./image.png]]
* how to run
#+BEGIN_SRC bash
gcc main.c -lSDL2
./a.out
#+END_SRC
* keys to interact with Mandelbrot
l -> go to right
h -> go to left
k -> go up
j -> go down
d -> go to right (big step)
a -> go to left (big step)
w -> go up (big step)
s -> go down (big step)
i -> zoom in
o -> zoom out
<file_sep>#include <SDL2/SDL.h>
#include <stdio.h>
/* read about mandelbrot set here: https://en.wikipedia.org/wiki/Mandelbrot_set */
int iterations_per_pixel = 1;
int mandelbrot_scale(double real_component, double imaginary_component) {
double z_a = real_component;
double z_b = imaginary_component;
int i;
for (i = 0; i < iterations_per_pixel; ++i) {
double tmp_z_a = z_a;
z_a = z_a * z_a - z_b * z_b + real_component;
z_b = 2 * tmp_z_a * z_b + imaginary_component;
if (abs(z_a + z_b) >= 2)
break;
}
return i;
}
double scale(double num, double src_min, double src_max, double dest_min, double dest_max) {
return ((num - src_min) * (dest_max - dest_min) + (dest_min * src_max) -
(dest_min * src_min)) / (src_max - src_min);
}
void draw_mandelbrot(SDL_Renderer* renderer, int width, int height, double x_mov, double y_mov, double zoom) {
for (int a = 0; a < width; ++a) {
for (int b = 0; b < height; ++b) {
double imaginary_component = scale(b, 0, height - 1, -2/zoom, 2/zoom);
double real_component = scale(a, 0, width - 1, -2/zoom, 2/zoom);
imaginary_component += y_mov;
real_component += x_mov;
double color_scale = scale(mandelbrot_scale(real_component, imaginary_component), 0,
iterations_per_pixel - 1, 0, 255);
SDL_SetRenderDrawColor(renderer, color_scale, color_scale, color_scale, 0x00);
SDL_RenderDrawPoint(renderer, a, b);
}
}
}
int main(int argc, char *argv[])
{
SDL_Window *window;
SDL_Renderer *renderer;
SDL_Event event;
if (SDL_Init(SDL_INIT_VIDEO) < 0) {
SDL_LogError(SDL_LOG_CATEGORY_APPLICATION, "Couldn't initialize SDL: %s", SDL_GetError());
return 3;
}
if (SDL_CreateWindowAndRenderer(320, 240, SDL_WINDOW_RESIZABLE, &window, &renderer)) {
SDL_LogError(SDL_LOG_CATEGORY_APPLICATION, "Couldn't create window and renderer: %s", SDL_GetError());
return 3;
}
SDL_PollEvent(&event);
/* control variables */
double zoom = 1;
double y_mov = 0;
double x_mov = -0.1;
/* stop the loop */
int quit = 0;
int zoom_cnt = 0;
double zoom_value = 0.1;
/* used for zooming in because variables cant be declared in case statements */
double to_zoom = zoom_value;
while (!quit) {
while (SDL_PollEvent(&event)) {
switch (event.type) {
case SDL_KEYDOWN:
switch (event.key.keysym.sym)
{
case SDLK_LEFT:
case SDLK_h:
x_mov -= 0.001;
printf("x_mov: %f\n", x_mov);
break;
case SDLK_RIGHT:
case SDLK_l:
x_mov += 0.001;
printf("x_mov: %f\n", x_mov);
break;
case SDLK_UP:
case SDLK_k:
y_mov -= 0.001;
printf("y_mov: %f\n", y_mov);
break;
case SDLK_DOWN:
case SDLK_j:
y_mov += 0.001;
printf("y_mov: %f\n", y_mov);
break;
case SDLK_i:
zoom *= 2;
printf("zoom: %f\n", zoom);
break;
case SDLK_o:
zoom /= 2;
printf("zoom: %f\n", zoom);
break;
case SDLK_w:
y_mov -= 0.01;
printf("y_mov: %f\n", y_mov);
break;
case SDLK_s:
y_mov += 0.01;
printf("y_mov: %f\n", y_mov);
break;
case SDLK_d:
x_mov += 0.01;
printf("x_mov: %f\n", x_mov);
break;
case SDLK_a:
x_mov -= 0.01;
printf("x_mov: %f\n", x_mov);
break;
}
break;
case SDL_QUIT:
quit = 1;
break;
default:
break;
}
fflush(stdout);
}
SDL_SetRenderDrawColor(renderer, 0x00, 0x00, 0xff, 0x00);
SDL_RenderClear(renderer);
int width;
int height;
SDL_GetWindowSize(window, &width, &height);
draw_mandelbrot(renderer, width, height, x_mov, y_mov, zoom);
SDL_RenderPresent(renderer);
iterations_per_pixel += 1;
}
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
| 95c217c33a58d572a175d295477b1280e384d26b | [
"C",
"Org"
] | 2 | C | mahmoodsheikh36/mandelbrot | ea212153f29d92332f3c70854d0a2b8ee6f9160a | 98644d035cdf304936ee86bd4ba1a7347ffc6da8 |
refs/heads/master | <file_sep>/* 4b – Week 4: Setting up your own database */
/* Modifying tables 1 */
/* Modifying data in existing tables
The previous activity covered a broad range of standard SQL syntax for querying data in existing tables. Now we're going to have a look at how we can set these tables up from scratch and how we can manipulate them in more advanced ways.
As a starting point, let's have a look at how we can modify data in an existing table.
There are three basic commands to manipulate a table's content:
INSERT: inserts a new row into a table;
DELETE: deletes a specified row;
UPDATE: changes attributes within a row.
We're going to look at each of them in turn using a small version of our Star table from the previous activity.
*/
/* The INSERT statement */
/* The INSERT statement adds a row to an existing table using the following syntax:
INSERT INTO <tablename> (<attributes>) VALUES (...);
You can optionally pass a list of attributes that define the order. The data goes into the VALUES (...) clause. For example, let's add a new star to our Star table:
INSERT INTO Star (kepler_id, t_eff, radius)
VALUES (2713050, 5000, 0.956);
Have a look at the table before and after the INSERT command using SELECT * FROM Star.
You can insert multiple rows at once using multiple tuples of values:
INSERT INTO Star (kepler_id, t_eff, radius) VALUES
(2713050, 5000, 0.956),
(2713051, 3100, 1.321);
It is not always necessary to include a list of the attributes, it is still good practice. If the order of the columns in the table change, you don't have to change the INSERT command if you've set the order of attributes in there. For example, the following command works just as well as the one above:
INSERT INTO Star (kepler_id, radius, t_eff)
VALUES (2713050, 0.956, 5000);
*/
<file_sep>Week 3 Notes, Practice Exercises and Programming Assignments.
<file_sep>Week 1 exercises:
Hello World of NN
Housing Prices
<file_sep>/* 3b – Week 3: Writing your own SQL queries */
/* Write an SQL query to find the radius and temperature of the stars in the Star table that are larger than our sun. */
SELECT radius, t_eff
FROM Star
WHERE radius > 1;
/* Write a range query which returns the kepler_id and the t_eff attributes of all those stars in the Star table whose temperature lies between 5000 and 6000 Kelvin (inclusive). */
SELECT kepler_id, t_eff
FROM Star
WHERE t_eff BETWEEN 5000 AND 6000;
/* Write a query to find the kepler_name and radius of each planet in the Planet table which is a confirmed exoplanet, meaning that their kepler_name is not NULL, or, equivalently, whose status is 'CONFIRMED'.
Restrict your results to those planets whose radius lies between one and three earth radii, and remember that the radius of the planets is relative to the earth radius. */
SELECT kepler_name, radius
FROM Planet
WHERE (kepler_name IS NOT NULL OR status = 'CONFIRMED') AND radius BETWEEN 1 AND 3;
/* Write a query that calculates the:
-minimum radius;
-maximum radius;
-average radius; and
-standard deviation of the radii
of unconfirmed planets (with a NULL value in kepler_name) in the Planet table. */
SELECT MIN(radius), MAX(radius), AVG(radius), STDDEV(radius)
FROM Planet
WHERE kepler_name IS NULL;
/* How many planets in the Planet database are in a multi-planet system. Planets sharing the same star will have the same kepler_id, but different koi_name values.
Your query should return a table in which each row contains the kepler_id of the star and the number of planets orbiting that star (i.e. that share this kepler_id).
Limit your results to counts above one and order the rows in descending order based on the number of planets. */
SELECT kepler_id, COUNT(*)
FROM Planet
GROUP BY 1
HAVING COUNT(*) > 1
ORDER BY COUNT(*) DESC;
/* 4a – Week 3: Joining tables with SQL */
/* Write a query that returns the radius of each star and planet pair whose radii have a ratio greater than the Sun-to-Earth radius ratio. Order the results in descending order based on the stellar radii. Use sun_radius and planet_radius as attribute aliases for the star and planet radii.
For this problem you will have to join the two tables to find all planets belonging to a given star and use a condition to select those results which fulfill the size requirement above. */
SELECT s.radius AS sun_radius, p.radius AS planet_radius
FROM Star s INNER JOIN Planet p ON s.kepler_id = p.kepler_id
WHERE (s.radius/p.radius) > 1
ORDER BY sun_radius DESC;
/* Write a query which counts the number of planets in each solar system where the corresponding stars are larger than our sun (i.e. their radius is larger than 1).
Your query should return the star's radius and its number of planets, showing only rows where the number of planets is more than one. Sort the rows in descending order based on the star radii. */
SELECT s.radius, COUNT(*)
FROM Star s INNER JOIN Planet p ON s.kepler_id = p.kepler_id
WHERE s.radius > 1
GROUP BY s.kepler_id
HAVING COUNT(*) > 1
ORDER BY s.radius DESC;
/* Write a query which returns the kepler_id, t_eff and radius for all stars in the Star table which haven't got a planet as join partner. Order the resulting table based on the t_eff attribute in descending order. */
SELECT s.kepler_id, s.t_eff, s.radius
FROM Star s LEFT OUTER JOIN Planet p ON s.kepler_id = p.kepler_id
WHERE p.koi_name IS NULL
ORDER BY t_eff DESC;
/* Write a query which queries both the Star and the Planet table and calculates the following quantities:
-the average value of the planets' equilibrium temperature t_eq, rounded to one decimal place;
-the minimum effective temperature t_eff of the stars;
-the maximum value of t_eff;
Your query should only use those star-planet pairs whose stars have a higher temperature (t_eff) than the average star temperature in the table. Try to use a subquery to solve this problem! */
SELECT ROUND(AVG(p.t_eq), 1), MIN(s.t_eff), MAX(s.t_eff)
FROM Star s JOIN Planet p USING(kepler_id)
WHERE s.t_eff > (SELECT AVG(t_eff) AS t_eff FROM Star);
/* Write a query which finds the radii of those planets in the Planet table which orbit the five largest stars in the Star table. Your query should return the planet's koi_name and radius as well as the corresponding star radius. */
SELECT koi_name, p.radius, s.radius
FROM Planet p JOIN Star s USING (kepler_id)
WHERE s.radius IN (SELECT radius FROM Star ORDER BY radius DESC LIMIT 5);
<file_sep>A quick intro notes on derivatives.
<file_sep>Summary:
We started this module with a question about pulsars. How many pulsars were emitting at the radio frequencies detected by the MWA. Initially, our investigation showed that most were not detected. But we decided to use a clever technique called stacking to improve our signal to noise ratio and make a statistical detection of the invisible population.
Setting out on this mission, we implemented a really simple mean stacking program and then decided to extend it to a median stack, as the median can be a more robust statistic. Implementing the median stack on a small amount of data was fine, but when we increased the data size, things immediately became problematic.
This is a really typical situation in modern science. Even a scientist who does not consider themselves a computational scientist or programmer has to work out how to analyze data efficiently.
There are many different technologies that are important and it's important that you have a good toolkit available to help you solve the problems. But, in this course, we want to focus on the underlying approach to problem solving, and this is often called computational thinking.
Computational thinking allows you to identify the problem, think about ways of framing problems computationally, think about possible solutions, and also be aware of the pitfalls of different solutions. In this case, running out of memory on your computer.
You can apply this approach to any data problems you encounter in your studies or your research. Being able to think about data and algorithms gives you a huge advantage in whatever you choose to do next.
<file_sep>Week 2 exercises:
-
<file_sep>Summary:
We started this module with a question about exoplanets when we asked 'How many of the earth sized exoplanets discovered so far lie in the habitable zone of their host star?'
These the planets with the potential to harbor life and finding them is a very active area of scientific research.
Questions like ours lend themselves to filtering data, and as we have seen, there can be an abundance of data about each object. And to answer questions you need easy access to the data relevant to your science.
This is true not only in exoplanetary science, but across a broad array of disciplines. Sometimes information is missing, so we have to deal with NULL values. And sometimes data is duplicated, leaving multiple entries of the same information.
You should now possess the skills to handle these cases and be able to extract only the data that you want. With these skills, we have explored the NASA Exoplanet Archive and seen the extreme diversity of exoplanet properties. With space missions like TESS and the James Webb Space Telescope, exoplanetary data will continue to pour in, so efficient organization of those data will only increase in importance.
We've seen how databases provide that organizational framework. You'll come across databases in all aspects of life, including in most major scientific projects. Most of the time you'll have a user friendly interface, such as a webpage that you can use to query the database.
However, sometimes you want more flexibility and control. Understanding how you can query databases directly using SQL opens up a whole new way of working with data, without the limitations of other peoples' thinking. You can now explore huge archives of data, both in astronomy and beyond in the rapidly expanding data science industry.
<file_sep>Hello World!
Here is the Week 2 Python Programming section from Data Driven Astronomy course on Coursera.
<file_sep>Week 4 Notes, Practice Exercises and Programming Assignments.
<file_sep>Week 2 Notes, Practice Exercises and Programming Assignments.
| d648b508bf187bd0d9ce4a6fbd20ebf9d2507c92 | [
"Markdown",
"SQL"
] | 11 | Markdown | manojmukkamala/Coursera | 965b2d794fd6113661b0af3f76f04b61094450d2 | f9d0c10bd32ad3b32c3f9aaf08d3534804a33591 |
refs/heads/main | <repo_name>rafaelgauderio/java-academia<file_sep>/src/academia/Equipment.java
package academia;
public class Equipment {
private String name;
public Equipment(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "[" + name + "]";
}
}
<file_sep>/src/academia/Session.java
package academia;
public class Session {
private String duration;
private People instructor;
private People client;
private People client2;
private People cliente3;
public Session(String duration, People instructor, People client, People client2, People cliente3) {
super();
this.duration = duration;
this.instructor = instructor;
this.client = client;
this.client2 = client2;
this.cliente3 = cliente3;
}
public String getDuration() {
return duration;
}
public void setDuration(String duration) {
this.duration = duration;
}
public People getInstructor() {
return instructor;
}
public void setInstructor(People instructor) {
this.instructor = instructor;
}
public People getClient() {
return client;
}
public void setClient(People client) {
this.client = client;
}
public People getClient2() {
return client2;
}
public void setClient2(People client2) {
this.client2 = client2;
}
public People getCliente3() {
return cliente3;
}
public void setCliente3(People cliente3) {
this.cliente3 = cliente3;
}
@Override
public String toString() {
return "\nSession \n[duration=" + duration + ", instructor=" + instructor + client + client2 + cliente3 ;
}
// I desire 1 instructor and three clients
}
<file_sep>/src/academia/Client.java
package academia;
public class Client extends People {
private Equipment equipment;
public Client(String name, int age, Equipment equipment) {
super(name, age);
this.equipment = equipment;
}
public Equipment getEquipment() {
return equipment;
}
public void setEquipment(Equipment equipment) {
this.equipment = equipment;
}
@Override
public String toString() {
return "\nClient: "+ super.toString()+ "[Equipment used= " + equipment;
}
}
| eeeb5f052abfb12177a8793293fb920276b5f4ec | [
"Java"
] | 3 | Java | rafaelgauderio/java-academia | f6d5e24300211834cab55a3e17702456fe08905c | 4c7167706d7906ba3c0b2e48d884e349dceee6b9 |
refs/heads/master | <file_sep>#include "Player.h"
GLuint Player::p1UP = 0;
GLuint Player::p1DOWN = 0;
GLuint Player::p1RIGHT = 0;
GLuint Player::p1LEFT = 0;
GLuint Player::p2UP = 0;
GLuint Player::p2DOWN = 0;
GLuint Player::p2RIGHT = 0;
GLuint Player::p2LEFT = 0;
Player::Player(float _x,float _y,float _w,float _h,int _playerNumber)
{
w = _w;
h = _h;
x = _x;
y = _y;
lastTime = 0.0f;
lives = 3;
points = 0;
playerNumber = _playerNumber;
direction = DOWN;
if (playerNumber == 1)
{
p1UP = TextureLoader::getTexture("resources/textures/p1up.png");
p1DOWN = TextureLoader::getTexture("resources/textures/p1down.png");
p1RIGHT = TextureLoader::getTexture("resources/textures/p1right.png");
p1LEFT = TextureLoader::getTexture("resources/textures/p1left.png");
}
else if(playerNumber == 2){
p2UP = TextureLoader::getTexture("resources/textures/p2up.png");
p2DOWN = TextureLoader::getTexture("resources/textures/p2down.png");
p2RIGHT = TextureLoader::getTexture("resources/textures/p2right.png");
p2LEFT = TextureLoader::getTexture("resources/textures/p2left.png");
}
sprite = new Sprite(_w, _h, _x, _y, DYNAMIC);
sprite->setTextureMode(STATIC_TEXTURE);
if (playerNumber == 1) sprite->setTexture(p1DOWN);
else if (playerNumber == 2) sprite->setTexture(p2DOWN);
}
Player::~Player()
{
delete sprite;
for (short i = 0; i < shells.size(); i++)
{
delete shells[i];
}
}
void Player::setTexture(const char *pathTexture)
{
sprite->setTexture(pathTexture);
}
void Player::setTexture(GLuint texture)
{
sprite->setTexture(texture);
}
void Player::draw()
{
sprite->x = this->x;
sprite->y = this->y;
sprite->draw();
drawShells();
}
void Player::drawShells()
{
for (short i = 0; i < shells.size(); i++)
{
shells[i]->draw();
}
}
void Player::move(KEY_TYPE key)
{
if (key == KEY_W)
{
this->y -= PLAYER_SPEED;
if (direction != UP)
{
this->direction = UP;
if (playerNumber == 1) this->setTexture(p1UP);
else if (playerNumber == 2) this->setTexture(p2UP);
}
}
else if (key == KEY_S)
{
this->y += PLAYER_SPEED;
if (this->direction != DOWN)
{
this->direction = DOWN;
if (playerNumber == 1) this->setTexture(p1DOWN);
else if (playerNumber == 2) this->setTexture(p2DOWN);
}
}
else if (key == KEY_D)
{
this->x += PLAYER_SPEED;
if (this->direction != RIGHT)
{
this->direction = RIGHT;
if (playerNumber == 1) this->setTexture(p1RIGHT);
else if (playerNumber == 2) this->setTexture(p2RIGHT);
}
}
else if (key == KEY_A)
{
this->x -= PLAYER_SPEED;
if (this->direction != LEFT)
{
this->direction = LEFT;
if (playerNumber == 1) this->setTexture(p1LEFT);
else if (playerNumber == 2) this->setTexture(p2LEFT);
}
}
}
void Player::instantiateShell(float currTime)
{
float deltaTime = currTime - lastTime;
std::cout << deltaTime << std::endl;
if (deltaTime < 1) return;
lastTime = glfwGetTime();
float shell_size = 10;
if (direction == UP)
{
Shell *shell = new Shell(x + shell_size, y, shell_size, shell_size, SHELL_UP);
this->shells.push_back(shell);
}
else if (direction == DOWN)
{
Shell *shell = new Shell(x + shell_size, y + w, shell_size, shell_size, SHELL_DOWN);
this->shells.push_back(shell);
}
else if (direction == RIGHT)
{
Shell *shell = new Shell(x + w, y + (h / 2), shell_size, shell_size, SHELL_RIGHT);
this->shells.push_back(shell);
}
else if (direction == LEFT)
{
Shell *shell = new Shell(x, y + (w / 2), shell_size, shell_size, SHELL_LEFT);
this->shells.push_back(shell);
}
}
int& Player::getLives()
{
return lives;
}
int& Player::getPoints()
{
return points;
}<file_sep>#pragma once
#include <GL\glew.h>
#include <SOIL.h>
#include "Log.h"
class TextureLoader
{
public:
static GLuint getTexture(const char *filepath);
static void freeTexture(GLuint texture);
};<file_sep>#include "TextureLoader.h"
GLuint TextureLoader::getTexture(const char *pathTexture)
{
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
int w, h;
unsigned char *image = SOIL_load_image(pathTexture, &w, &h, 0, SOIL_LOAD_RGB);
if (!image)
{
Log::error("image error : ");
return 0;
}
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, w, h, 0, GL_RGB, GL_UNSIGNED_BYTE, image);
SOIL_free_image_data(image);
glBindTexture(GL_TEXTURE_2D, 0);
return texture;
}
void TextureLoader::freeTexture(GLuint texture)
{
glDeleteTextures(1, &texture);
}<file_sep>#include "Map.h"
Map::~Map()
{
short size_sections = sections.size();
for (short i = 0; i < size_sections; i++)
{
delete sections[i];
}
}
void Map::draw()
{
short size_sections = sections.size();
for (short i = 0; i < size_sections; i++)
{
sections[i]->draw();
}
}<file_sep>#include "InputManager.h"
bool InputManager::key_pressed(KEY_TYPE key)
{
if (glfwGetKey(window, key) == GLFW_PRESS) return true;
else return false;
}<file_sep>#pragma once
#include "Map.h"
#include "Player.h"
enum COLLISION_TYPE
{
RIGHT_COLLISION,
LEFT_COLLISION,
UP_COLLISION,
DOWN_COLLISION,
NO_COLLISION
};
class Collision
{
public:
static COLLISION_TYPE player_collision(Player *player,Map *map);
static void shell_collision(Player *player1,Player *player2,Map *map);
};<file_sep>#include "Application.h"
Application::Application(const char *title)
{
if (glfwInit() != GLFW_TRUE)
{
window = nullptr;
return;
}
w = MAP_SIZE * MAP_SIZE;
h = MAP_SIZE * MAP_SIZE;
window = new Window(w, h, title);
if (!initGL())
{
window->deleteWindow();
window = nullptr;
}
initAppObjects();
initOrtho();
}
Application::~Application()
{
delete window;
delete map;
delete shader;
delete player1;
delete soundEngine;
}
void Application::start()
{
soundEngine->play2D("resources/music/breakout.mp3",GL_TRUE);
if (window) {
while (window->is_open())
{
clear();
key();
collision();
checkGame();
shader->use();
shader->SetMatrix4fv("Ortho",Ortho);
map->draw();
player1->draw();
player2->draw();
gui->draw();
}
}
else {
Sleep(50000);
}
}
void Application::restartGame()
{
if (player1->getLives() > player2->getLives()) player1->getPoints()++;
else if(player2->getLives() > player1->getLives()) player2->getPoints()++;
else player1->getLives() = player2->getLives()++;
if (player1->getPoints() >= 9) player1->getPoints() = 0;
if (player2->getPoints() >= 9) player2->getPoints() = 0;
player1->getLives() = 3;
player2->getLives() = 3;
MapLoader::setPlayersPosition(player1, player2, "resources/maps/map1.txt", SECTION_SIZE);
gui->restart();
}
void Application::checkGame()
{
if (player1->getLives() <= 0) restartGame();
else if (player2->getLives() <= 0) restartGame();
}
void Application::key()
{
COLLISION_TYPE player1_collision = Collision::player_collision(player1, map);
COLLISION_TYPE player2_collision = Collision::player_collision(player2, map);
if (inputManager->key_pressed(KEY_W)) { if (player1_collision != UP_COLLISION && player1_collision != LEFT_COLLISION && player1_collision != RIGHT_COLLISION) player1->move(KEY_W); }
else if (inputManager->key_pressed(KEY_S)) { if (player1_collision != DOWN_COLLISION && player1_collision != LEFT_COLLISION && player1_collision != RIGHT_COLLISION) player1->move(KEY_S); }
else if (inputManager->key_pressed(KEY_D)) { if (player1_collision != RIGHT_COLLISION && player1_collision != UP_COLLISION && player1_collision != DOWN_COLLISION) player1->move(KEY_D); }
else if (inputManager->key_pressed(KEY_A)) { if (player1_collision != LEFT_COLLISION && player1_collision != DOWN_COLLISION && player1_collision != UP_COLLISION) player1->move(KEY_A); }
else if (inputManager->key_pressed(KEY_LEFT_ALT)) { player1->instantiateShell((float)glfwGetTime()); }
if (inputManager->key_pressed(KEY_UP)) { if (player2_collision != UP_COLLISION && player2_collision != LEFT_COLLISION && player2_collision != RIGHT_COLLISION) player2->move(KEY_W); }
else if (inputManager->key_pressed(KEY_DOWN)) { if (player2_collision != DOWN_COLLISION && player2_collision != LEFT_COLLISION && player2_collision != RIGHT_COLLISION) player2->move(KEY_S); }
else if (inputManager->key_pressed(KEY_RIGHT)){ if (player2_collision != RIGHT_COLLISION && player2_collision != UP_COLLISION && player2_collision != DOWN_COLLISION) player2->move(KEY_D); }
else if (inputManager->key_pressed(KEY_LEFT)) { if (player2_collision != LEFT_COLLISION && player2_collision != DOWN_COLLISION && player2_collision != UP_COLLISION) player2->move(KEY_A); }
else if (inputManager->key_pressed(KEY_RIGHT_ALT)) { player2->instantiateShell((float)glfwGetTime()); }
}
void Application::collision()
{
Collision::shell_collision(player1, player2, map);
}
void Application::initAppObjects()
{
shader = new Shader();
shader->loadShaders("VertexShader.txt", "FragmentShader.txt", LOAD_FROM_FILE);
player1 = new Player(100, 100, SECTION_SIZE +15, SECTION_SIZE +15,1);
player2 = new Player(200, 200, SECTION_SIZE +15, SECTION_SIZE +15,2);
map = new Map();
MapLoader::loadMap(map, "resources/maps/map1.txt", MAP_SIZE, SECTION_SIZE);
MapLoader::setPlayersPosition(player1, player2, "resources/maps/map1.txt",SECTION_SIZE);
inputManager = new InputManager(window->getWindow());
gui = new GUI(player1, player2,SECTION_SIZE * SECTION_SIZE, SECTION_SIZE * SECTION_SIZE);
gui->setLivesSize(SECTION_SIZE, SECTION_SIZE);
gui->setPointsSize(SECTION_SIZE*2, SECTION_SIZE*2);
gui->initGUI();
soundEngine = createIrrKlangDevice();
}
void Application::initOrtho()
{
glm::mat4 projection = glm::ortho(0.0f,(float)w,(float)h,0.0f,-1.0f,1.0f);
glm::mat4 model = glm::mat4(1.0f);
Ortho = projection * model;
}
void Application::clear()
{
glClearColor(0.0, 0.0, 0.0, 1.0);
glClear(GL_COLOR_BUFFER_BIT);
}
bool Application::initGL()
{
if (glewInit() != GLEW_OK)
{
Log::error("glew error");
return false;
}
return true;
}<file_sep>#include "Sprite.h"
Sprite::Sprite(float _w,float _h,float _x,float _y,RECT_TYPE type)
{
w = _w;
h = _h;
x = _x;
y = _y;
rect = new Rect(_w, _h, _x, _y,WITH_TEXTURE,type);
}
Sprite::~Sprite()
{
rect->freeRes();
glDeleteTextures(1, &texture);
}
void Sprite::freeRes()
{
this->freeTexture();
rect->freeRes();
}
void Sprite::draw()
{
rect->x = this->x;
rect->y = this->y;
glBindTexture(GL_TEXTURE_2D, texture);
rect->draw();
glBindTexture(GL_TEXTURE_2D, 0);
}
void Sprite::setTexture(const char *pathTexture)
{
this->freeTexture();
texture = TextureLoader::getTexture(pathTexture);
}
void Sprite::setTexture(GLuint _texture)
{
this->freeTexture();
texture = _texture;
}
void Sprite::freeTexture()
{
if(texture_mode != STATIC_TEXTURE) glDeleteTextures(1, &texture);
}
void Sprite::setTextureMode(TEXTURE_MODE mode)
{
texture_mode = mode;
}
GLuint Sprite::getTexture()
{
return texture;
}
<file_sep>#pragma once
#include "Player.h"
#include "Sprite.h"
#include "TextureLoader.h"
class GUI
{
private:
GLuint live_texture;
GLuint empty_live_texture;
GLuint p1_textures_points[10];
GLuint p2_textures_points[10];
Sprite *p1_lives[3];
Sprite *p2_lives[3];
Sprite *p1_points;
Sprite *p2_points;
Player *p1;
Player *p2;
float size_livesW;
float size_livesH;
float size_pointsW;
float size_pointsH;
float w, h;
void initTextures();
void initLives();
void initPoints();
void drawLives();
void drawPoints();
public:
GUI(Player *p1, Player *p2,float w,float h);
~GUI();
void setLivesSize(float w, float h);
void setPointsSize(float w, float h);
void initGUI();
void restart();
void draw();
};<file_sep>#pragma once
#include "Sprite.h"
enum SECTION_TYPE
{
GRASS_SECTION,
GROUND_SECTION,
WALL_SECTION,
WATER_SECTION,
FLAG_SECTION,
OLD_WALL_SECTION
};
class Section
{
private:
Sprite *sprite;
public:
SECTION_TYPE type;
float w, h, x, y;
Section(float _w,float _h,float _x,float _y,SECTION_TYPE _type);
~Section();
void setTexture(const char *setTexture);
void draw();
};<file_sep>#pragma once
#include <GL\glew.h>
#include <GLFW\glfw3.h>
#include <irrKlang.h>
#include <Windows.h>
#include <iostream>
#include <thread>
#include "Player.h"
#include "Math.h"
#include "Log.h"
#include "Window.h"
#include "Shader.h"
#include "Sprite.h"
#include "Map.h"
#include "MapLoader.h"
#include "InputManager.h"
#include "Collision.h"
#include "GUI.h"
#define MAP_SIZE 30
#define SECTION_SIZE 30
#define PLAYER_SPEED 5
using namespace irrklang;
class Application
{
private:
int w, h;
ISoundEngine *soundEngine;
Player *player1;
Player *player2;
Map *map;
GUI *gui;
InputManager *inputManager;
Window *window;
Shader *shader;
glm::mat4 Ortho;
void clear();
void initAppObjects();
void initOrtho();
void key();
void collision();
void restartGame();
void checkGame();
bool initGL();
public:
Application(const char *title);
~Application();
void start();
};<file_sep>#include "Collision.h"
COLLISION_TYPE Collision::player_collision(Player *player, Map *map)
{
float pX = player->x;
float pY = player->y;
float pW = player->w;
float pH = player->h;
short size_map = map->sections.size();
for (short i = 0; i < size_map; i++)
{
float sX = map->sections[i]->x;
float sY = map->sections[i]->y;
float sW = map->sections[i]->w;
float sH = map->sections[i]->h;
//Up collision
if (((pY <= (sY + sH) && (pY+pH) >= sY)) && ((pX <= (sX + sW) && (pX + pW) >= sX)))
{
if (player->direction == UP) return UP_COLLISION;
else if (player->direction == DOWN) return DOWN_COLLISION;
else if (player->direction == RIGHT) return RIGHT_COLLISION;
else if (player->direction == LEFT) return LEFT_COLLISION;
}
}
return NO_COLLISION;
}
void Collision::shell_collision(Player *player1,Player *player2,Map *map)
{
short shell_size1 = player1->shells.size();
short shell_size2 = player2->shells.size();
short sections_size = map->sections.size();
//chek player1 shells
for (short i = 0; i < shell_size1; i++)
{
float shellX = player1->shells[i]->x;
float shellY = player1->shells[i]->y;
float shellW = player1->shells[i]->w;
float shellH = player1->shells[i]->h;
//first chek player and shell collision
float pX = player2->x;
float pY = player2->y;
float pW = player2->w;
float pH = player2->h;
if (((shellY <= (pY + pH) && (shellY + shellH) >= pY)) && ((shellX <= (pX + pW) && (shellX + shellW) >= pX)))
{
player2->getLives()--;
delete player1->shells[i];
player1->shells.erase(player1->shells.begin() + i);
break;
}
for (short j = 0; j < sections_size; j++)
{
float sX = map->sections[j]->x;
float sY = map->sections[j]->y;
float sW = map->sections[j]->w;
float sH = map->sections[j]->h;
if (((shellY <= (sY + sH) && (shellY + shellH) >= sY)) && ((shellX <= (sX + sW) && (shellX + shellW) >= sX)))
{
delete player1->shells[i];
player1->shells.erase(player1->shells.begin() + i);
break;
}
}
}
for (short i = 0; i < shell_size2; i++)
{
float shellX = player2->shells[i]->x;
float shellY = player2->shells[i]->y;
float shellW = player2->shells[i]->w;
float shellH = player2->shells[i]->h;
float pX = player1->x;
float pY = player1->y;
float pW = player1->w;
float pH = player1->h;
if (((shellY <= (pY + pH) && (shellY + shellH) >= pY)) && ((shellX <= (pX + pW) && (shellX + shellW) >= pX)))
{
player1->getLives()--;
delete player2->shells[i];
player2->shells.erase(player2->shells.begin() + i);
continue;
}
for (short j = 0; j < sections_size; j++)
{
float sX = map->sections[j]->x;
float sY = map->sections[j]->y;
float sW = map->sections[j]->w;
float sH = map->sections[j]->h;
if (((shellY <= (sY + sH) && (shellY + shellH) >= sY)) && ((shellX <= (sX + sW) && (shellX + shellW) >= sX)))
{
delete player2->shells[i];
player2->shells.erase(player2->shells.begin() + i);
break;
}
}
}
}<file_sep>#pragma once
#include <glm\glm.hpp>
#include <glm\vec3.hpp>
#include <glm\mat4x4.hpp>
#include <glm\gtc\matrix_transform.hpp>
struct vertices
{
float x, y, z;
vertices(float _x, float _y, float _z) : x(_x), y(_y), z(_z) {};
vertices(const vertices &v)
{
x = v.x;
y = v.y;
z = v.z;
}
};<file_sep>#pragma once
#include <iostream>
#include <vector>
#include "Sprite.h"
#include "InputManager.h"
#include "Shell.h"
#include "TextureLoader.h"
enum DIRECTION
{
UP = 0,
DOWN = 1,
RIGHT = 2,
LEFT = 3
};
#define PLAYER_SPEED 5
class Player
{
private:
static GLuint p1UP;
static GLuint p1DOWN;
static GLuint p1RIGHT;
static GLuint p1LEFT;
static GLuint p2UP;
static GLuint p2DOWN;
static GLuint p2RIGHT;
static GLuint p2LEFT;
Sprite *sprite;
float lastTime;
int lives;
int points;
int playerNumber;
public:
std::vector<Shell*> shells;
DIRECTION direction;
float x,y,w,h;
Player(float _x,float _y,float _w,float _h,int _playerNumber);
~Player();
void setTexture(const char *pathTexture);
void setTexture(GLuint texture);
void draw();
void drawShells();
void instantiateShell(float currTime);
void move(KEY_TYPE key);
int& getLives();
int& getPoints();
};<file_sep>#include "Rect.h"
Rect::Rect(float _w, float _h, float _x, float _y,RECT_DRAW_TYPE _type_draw,RECT_TYPE _type_rect)
{
rect_type = _type_rect;
draw_type = _type_draw;
w = _w;
h = _h;
this->x = _x;
this->y = _y;
VAO = 0;
init();
}
Rect::~Rect()
{
freeRes();
}
void Rect::init()
{
GLuint VBO, IBO;
float z = 0.0f;
if (draw_type == NON_TEXTURE)
{
float vertices[] =
{
this->x,this->y,z,
this->x,this->y + h,z,
this->x + w,this->y + h,z,
this->x + w,this->y,z
};
unsigned int indices[] =
{
0,1,2,
0,2,3
};
glGenVertexArrays(1, &VAO);
glGenBuffers(1, &VBO);
glGenBuffers(1, &IBO);
glBindVertexArray(VAO);
glBindBuffer(GL_ARRAY_BUFFER, VBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, IBO);
glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), indices, GL_STATIC_DRAW);
glEnableVertexAttribArray(0);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(float), 0);
glBindVertexArray(0);
}
else if (draw_type == WITH_TEXTURE) {
float vertices[] =
{
this->x,this->y,z, 0.0,0.0,
this->x,this->y + h,z, 0.0,1.0,
this->x + w,this->y + h,z, 1.0,1.0,
this->x + w,this->y,z, 1.0,0.0
};
unsigned int indices[] =
{
0,1,2,
0,2,3
};
glGenVertexArrays(1, &VAO);
glGenBuffers(1, &VBO);
glGenBuffers(1, &IBO);
glBindVertexArray(VAO);
glBindBuffer(GL_ARRAY_BUFFER, VBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, IBO);
glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), indices, GL_STATIC_DRAW);
glEnableVertexAttribArray(0);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 5 * sizeof(float), 0);
glEnableVertexAttribArray(1);
glVertexAttribPointer(1, 2, GL_FLOAT, GL_FALSE, 5 * sizeof(float), (void*)(3*sizeof(float)));
glBindVertexArray(0);
}
}
void Rect::freeRes()
{
glDeleteVertexArrays(1, &VAO);
}
void Rect::draw()
{
if (rect_type == DYNAMIC)
{
freeRes();
init();
}
glBindVertexArray(VAO);
glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_INT, 0);
glBindVertexArray(0);
}<file_sep>#pragma once
#include <fstream>
#include <string>
#include <iostream>
#include "TextureLoader.h"
#include "Section.h"
#include "Map.h"
#include "Log.h"
#include "Player.h"
enum
{
WALL = '#',
OLD_WALL = '|',
WATER = '&',
GRASS = '$',
WINN_1 = 'g',
WINN_2 = 'z',
PLAYER_1 = '1',
PLAYER_2 = '2'
};
class MapLoader
{
public:
static void loadMap(Map *map,const char *mapPath,int mapSize,int sectionSize);
static void setPlayersPosition(Player *p1, Player *p2,const char *mapFile,int sectionSize);
};<file_sep>#include "Shell.h"
GLuint Shell::shell_texture = 0;
Shell::Shell(float _x, float _y, float _w, float _h, SHELL_DIRECTION _direction)
{
w = _w;
h = _h;
x = _x;
y = _y;
shell_texture = TextureLoader::getTexture("resources/textures/shell3.png");
direction = _direction;
sprite = new Sprite(w, h, x, y, DYNAMIC);
sprite->setTexture(shell_texture);
sprite->setTextureMode(STATIC_TEXTURE);
}
Shell::~Shell()
{
sprite->freeRes();
delete sprite;
}
void Shell::move()
{
if (this->direction == SHELL_UP) y -= SHELL_SPEED;
else if (this->direction == SHELL_DOWN) y += SHELL_SPEED;
else if (this->direction == SHELL_RIGHT) x += SHELL_SPEED;
else if (this->direction == SHELL_LEFT) x -= SHELL_SPEED;
}
void Shell::draw()
{
move();
sprite->x = this->x;
sprite->y = this->y;
sprite->draw();
}<file_sep>#include "Section.h"
Section::Section(float _w, float _h, float _x, float _y, SECTION_TYPE _type)
{
this->w = _w;
this->h = _h;
this->x = _x;
this->y = _y;
this->type = _type;
sprite = new Sprite(w, h, x, y, STATIC);
}
Section::~Section()
{
sprite->freeRes();
delete sprite;
}
void Section::setTexture(const char *pathTexture)
{
sprite->setTexture(pathTexture);
}
void Section::draw()
{
sprite->x = this->x;
sprite->y = this->y;
sprite->draw();
}<file_sep>#pragma once
#include "Sprite.h"
#include "TextureLoader.h"
#define SHELL_SPEED 10
enum SHELL_DIRECTION
{
SHELL_UP,
SHELL_DOWN,
SHELL_RIGHT,
SHELL_LEFT
};
class Shell
{
private:
static GLuint shell_texture;
Sprite *sprite;
void move();
public:
SHELL_DIRECTION direction;
float x, y, w, h;
Shell(float x,float y,float w,float h,SHELL_DIRECTION direction);
~Shell();
void draw();
};
<file_sep>#include "Log.h"
void Log::error(const char *str)
{
std::cout << "\nError : " << str << "\n" << std::endl;
}
void Log::info(const char *str)
{
std::cout << "\nInfo : " << str << "\n" << std::endl;
}<file_sep>#include "Shader.h"
Shader::Shader()
{
vsShader = fsShader = program = 0;
}
Shader::~Shader()
{
this->deleteProgram();
}
void Shader::loadShaders(const char *vs, const char *fs, LOAD_TYPE load_type)
{
if (load_type == LOAD_FROM_FILE) loadFromFile(vs, fs);
else if (load_type == LOAD_FROM_STRING) loadFromString(vs, fs);
}
void Shader::loadFromFile(const char *vsPath, const char *fsPath)
{
std::ifstream vsFile, fsFile;
vsFile.open(vsPath);
fsFile.open(fsPath);
if (!vsFile.is_open()){
Log::error("Vertex shader file did not find");
return;
}
else if (!vsFile.is_open()){
Log::error("Fragment shader file did not find");
return;
}
std::stringstream vs_streamStr, fs_streamStr;
std::string vsStr, fsStr;
vs_streamStr << vsFile.rdbuf();
fs_streamStr << fsFile.rdbuf();
vsStr = vs_streamStr.str();
fsStr = fs_streamStr.str();
const char *sourceVs = vsStr.c_str();
const char *sourceFs = fsStr.c_str();
initProgram(sourceVs, sourceFs);
}
void Shader::loadFromString(const char *vsStr, const char *fsStr)
{
initProgram(vsStr, fsStr);
}
void Shader::initProgram(const char *vsSource, const char *fsSource)
{
createShaders(vsSource, fsSource);
createProgram();
}
void Shader::createShaders(const char *vsSource, const char *fsSource)
{
vsShader = glCreateShader(GL_VERTEX_SHADER);
glShaderSource(vsShader, 1, &vsSource, NULL);
glCompileShader(vsShader);
fsShader = glCreateShader(GL_FRAGMENT_SHADER);
glShaderSource(fsShader, 1, &fsSource, NULL);
glCompileShader(fsShader);
error(vsShader, SHADER_ERROR);
error(fsShader, SHADER_ERROR);
}
void Shader::createProgram()
{
program = glCreateProgram();
glAttachShader(program, vsShader);
glAttachShader(program, fsShader);
glLinkProgram(program);
error(program, PROGRAM_ERROR);
}
void Shader::error(GLuint object, ERROR_TYPE error_type)
{
if (error_type == SHADER_ERROR) errorShader(object);
else if (error_type = PROGRAM_ERROR) errorProgram(object);
}
void Shader::errorShader(GLuint shader)
{
int compile_status = 0;
glGetShaderiv(shader, GL_COMPILE_STATUS, &compile_status);
if (!compile_status)
{
char buffer[512];
glGetShaderInfoLog(shader, 512, NULL, buffer);
Log::error(buffer);
}
}
void Shader::errorProgram(GLuint program)
{
int link_status = 0;
glGetProgramiv(program, GL_LINK_STATUS, &link_status);
if (!link_status)
{
char buffer[512];
glGetProgramInfoLog(program, 512, NULL, buffer);
Log::error(buffer);
}
}
void Shader::deleteProgram()
{
deleteShader(vsShader);
deleteShader(fsShader);
this->stop();
glDeleteProgram(program);
}
void Shader::deleteShader(GLuint shader)
{
glDeleteShader(shader);
}
void Shader::use()
{
glUseProgram(program);
}
void Shader::stop()
{
glUseProgram(0);
}
GLuint Shader::getProgramId()
{
return program;
}
//SetUniforms
void Shader::SetMatrix4fv(const char *uniName, glm::mat4 &Matrix)
{
glUniformMatrix4fv(glGetUniformLocation(program, uniName), 1, GL_FALSE, &Matrix[0][0]);
}<file_sep>#include "GUI.h"
GUI::GUI(Player *_p1, Player *_p2,float _w,float _h)
{
w = _w;
h = _h;
p1 = _p1;
p2 = _p2;
size_livesH = 30;
size_livesW = 30;
size_pointsH = 30;
size_pointsW = 30;
initTextures();
p1_points = nullptr;
p2_points = nullptr;
for (short i = 0; i < 3; i++)
{
p1_lives[i] = p2_lives[i] = nullptr;
}
}
GUI::~GUI()
{
for (short i = 0; i < 3; i++)
{
delete p1_lives[i];
delete p2_lives[i];
}
TextureLoader::freeTexture(live_texture);
TextureLoader::freeTexture(empty_live_texture);
for (short i = 0; i < 9; i++)
{
TextureLoader::freeTexture(p1_textures_points[i]);
TextureLoader::freeTexture(p2_textures_points[i]);
}
}
void GUI::initTextures()
{
live_texture = TextureLoader::getTexture("resources/textures/live1.png");
empty_live_texture = TextureLoader::getTexture("resources/textures/emptyLive.png");
p1_textures_points[0] = TextureLoader::getTexture("resources/textures/numbers/p2_0.png");
p1_textures_points[1] = TextureLoader::getTexture("resources/textures/numbers/p2_1.png");
p1_textures_points[2] = TextureLoader::getTexture("resources/textures/numbers/p2_2.png");
p1_textures_points[3] = TextureLoader::getTexture("resources/textures/numbers/p2_3.png");
p1_textures_points[4] = TextureLoader::getTexture("resources/textures/numbers/p2_4.png");
p1_textures_points[5] = TextureLoader::getTexture("resources/textures/numbers/p2_5.png");
p1_textures_points[6] = TextureLoader::getTexture("resources/textures/numbers/p2_6.png");
p1_textures_points[7] = TextureLoader::getTexture("resources/textures/numbers/p2_7.png");
p1_textures_points[8] = TextureLoader::getTexture("resources/textures/numbers/p2_8.png");
p1_textures_points[9] = TextureLoader::getTexture("resources/textures/numbers/p2_9.png");
p2_textures_points[0] = TextureLoader::getTexture("resources/textures/numbers/p1_0.png");
p2_textures_points[1] = TextureLoader::getTexture("resources/textures/numbers/p1_1.png");
p2_textures_points[2] = TextureLoader::getTexture("resources/textures/numbers/p1_2.png");
p2_textures_points[3] = TextureLoader::getTexture("resources/textures/numbers/p1_3.png");
p2_textures_points[4] = TextureLoader::getTexture("resources/textures/numbers/p1_4.png");
p2_textures_points[5] = TextureLoader::getTexture("resources/textures/numbers/p1_5.png");
p2_textures_points[6] = TextureLoader::getTexture("resources/textures/numbers/p1_6.png");
p2_textures_points[7] = TextureLoader::getTexture("resources/textures/numbers/p1_7.png");
p2_textures_points[8] = TextureLoader::getTexture("resources/textures/numbers/p1_8.png");
p2_textures_points[9] = TextureLoader::getTexture("resources/textures/numbers/p1_9.png");
}
void GUI::setLivesSize(float _w, float _h)
{
size_livesH = _h;
size_livesW = _w;
}
void GUI::setPointsSize(float _w, float _h)
{
size_pointsW = _w;
size_pointsH = _h;
}
void GUI::initPoints()
{
p1_points = new Sprite(size_pointsW, size_pointsH, (w / 2) - size_pointsW, 0, STATIC);
p1_points->setTextureMode(STATIC_TEXTURE);
p1_points->setTexture(p1_textures_points[0]);
p2_points = new Sprite(size_pointsW, size_pointsH, (w / 2), 0, STATIC);
p2_points->setTextureMode(STATIC_TEXTURE);
p2_points->setTexture(p1_textures_points[0]);
}
void GUI::initLives()
{
float y = 0;
float _w = w - size_livesW;
for (short x = 0,i = 0; x < p1->getLives() * size_livesW; x+=size_livesW,i++)
{
p1_lives[i] = new Sprite(size_livesW, size_livesH, x, y, STATIC);
p1_lives[i]->setTextureMode(STATIC_TEXTURE);
p1_lives[i]->setTexture(live_texture);
p2_lives[i] = new Sprite(size_livesW, size_livesH, _w - x, y, STATIC);
p2_lives[i]->setTextureMode(STATIC_TEXTURE);
p2_lives[i]->setTexture(live_texture);
}
}
void GUI::restart()
{
p1_points->setTexture(p1_textures_points[0]);
p2_points->setTexture(p2_textures_points[0]);
for (short i = 0; i < p1->getLives(); i++)
{
p1_lives[i]->setTexture(live_texture);
p2_lives[i]->setTexture(live_texture);
}
}
void GUI::initGUI()
{
initLives();
initPoints();
}
void GUI::drawPoints()
{
if (p1_points == nullptr) return;
p1_points->setTexture(p1_textures_points[p1->getPoints()]);
p2_points->setTexture(p2_textures_points[p2->getPoints()]);
p1_points->draw();
p2_points->draw();
}
void GUI::drawLives()
{
//draw player 1 lives
if (p1_lives[0] == nullptr) return;
for (short i = 0; i < p1->getLives(); i++)
{
p1_lives[i]->draw();
}
for (short i = p1->getLives(); i < 3; i++)
{
p1_lives[i]->setTexture(empty_live_texture);
p1_lives[i]->draw();
}
//draw player 2 lives
for (short i = 0; i < p2->getLives(); i++)
{
p2_lives[i]->draw();
}
for (short i = p2->getLives(); i < 3; i++)
{
p2_lives[i]->setTexture(empty_live_texture);
p2_lives[i]->draw();
}
}
void GUI::draw()
{
drawLives();
drawPoints();
}<file_sep>#pragma once
#include <GL/glew.h>
#include <GLFW\glfw3.h>
#include "Log.h"
class Window
{
private:
GLFWwindow *window;
int w, h;
public:
Window(int w, int h, const char *title);
~Window();
void deleteWindow();
bool is_open();
GLFWwindow* getWindow() const;
};<file_sep>#pragma once
#include "TextureLoader.h"
#include "Rect.h"
enum TEXTURE_MODE
{
STATIC_TEXTURE, //ALL GAME
DYNAMIC_TEXTURE //FREE TEXTURE
};
class Sprite
{
private:
GLuint texture;
Rect *rect;
TEXTURE_MODE texture_mode;
public:
float w, h, x, y;
Sprite(float _w,float _h,float x,float y,RECT_TYPE type);
~Sprite();
void draw();
void freeTexture();
void setTexture(const char *filepath);
void setTexture(GLuint _texture);
void setTextureMode(TEXTURE_MODE mode);
void freeRes();
GLuint getTexture();
};<file_sep>#pragma once
#include <iostream>
#include <vector>
#include "Section.h"
class Map
{
public:
~Map();
std::vector<Section*> sections;
void draw();
};<file_sep>#pragma once
#include <GL\glew.h>
#include <fstream>
#include <sstream>
#include "Math.h"
#include "Log.h"
enum ERROR_TYPE{
SHADER_ERROR,
PROGRAM_ERROR,
};
enum LOAD_TYPE{
LOAD_FROM_FILE,
LOAD_FROM_STRING,
};
class Shader
{
private:
GLuint vsShader, fsShader, program;
void error(GLuint object, ERROR_TYPE type);
void errorProgram(GLuint program);
void errorShader(GLuint shader);
void loadFromFile(const char *vsFilepath, const char *fsFilepath);
void loadFromString(const char *vsStr, const char *fsStr);
void initProgram(const char *vsSource, const char *fsSource);
void createShaders(const char *vs, const char *fs);
void createProgram();
void deleteShader(GLuint shader);
public:
Shader();
~Shader();
void loadShaders(const char *vs,const char *fs,LOAD_TYPE type);
void use();
void stop();
void deleteProgram();
GLuint getProgramId();
//SetUniform
void SetMatrix4fv(const char *uniName, glm::mat4 &Matrix);
};<file_sep>#pragma once
#include <iostream>
class Log
{
public:
static void error(const char *errorStrig);
static void info(const char *errorStrig);
};<file_sep>#pragma once
#include <GLFW\glfw3.h>
enum KEY_TYPE
{
KEY_W = GLFW_KEY_W,
KEY_D = GLFW_KEY_D,
KEY_A = GLFW_KEY_A,
KEY_S = GLFW_KEY_S,
KEY_UP = GLFW_KEY_UP,
KEY_DOWN = GLFW_KEY_DOWN,
KEY_LEFT = GLFW_KEY_LEFT,
KEY_RIGHT = GLFW_KEY_RIGHT,
KEY_ESCAPE = GLFW_KEY_ESCAPE,
KEY_LEFT_ALT = GLFW_KEY_LEFT_ALT,
KEY_RIGHT_ALT = GLFW_KEY_RIGHT_ALT
};
class InputManager
{
private:
GLFWwindow *window;
public:
InputManager(GLFWwindow *_window) : window(_window) {};
bool key_pressed(KEY_TYPE key);
};<file_sep>#include "MapLoader.h"
void MapLoader::loadMap(Map *map,const char *mapPath,int mapSize,int sectionSize)
{
std::ifstream file(mapPath);
if (!file.is_open())
{
Log::error("error map file");
return;
}
std::string str;
float x = 0.0f;
float y = 0.0f;
short m = 0;
while (std::getline(file,str) && m < mapSize)
{
x = 0.0f;
for (short i = 0;i < str.size(); i++)
{
if (str[i] == WALL)
{
Section *section = new Section(sectionSize,sectionSize, x, y,WALL_SECTION);
section->setTexture("resources/textures/wall.png");
map->sections.push_back(section);
}
else if (str[i] == WATER)
{
Section *section = new Section(sectionSize, sectionSize, x, y, WATER_SECTION);
section->setTexture("resources/textures/water.jpg");
map->sections.push_back(section);
}
else if (str[i] == GRASS)
{
Section *section = new Section(sectionSize, sectionSize, x, y, GRASS_SECTION);
section->setTexture("resources/textures/grass.png");
map->sections.push_back(section);
}
else if (str[i] == OLD_WALL)
{
Section *section = new Section(sectionSize, sectionSize, x, y, OLD_WALL_SECTION);
section->setTexture("resources/textures/oldWall.png");
map->sections.push_back(section);
}
else if (str[i] == WINN_1)
{
Section *section = new Section(sectionSize, sectionSize, x, y, OLD_WALL_SECTION);
section->setTexture("resources/textures/winn1.png");
map->sections.push_back(section);
}
else if (str[i] == WINN_2)
{
Section *section = new Section(sectionSize, sectionSize, x, y, OLD_WALL_SECTION);
section->setTexture("resources/textures/winn2.png");
map->sections.push_back(section);
}
x += sectionSize;
}
m++;
y += sectionSize;
}
}
void MapLoader::setPlayersPosition(Player *p1, Player *p2,const char *mapFile,int sectionSize)
{
std::ifstream file(mapFile);
if (!file.is_open())
{
Log::error("bad map filepath");
return;
}
std::string str;
float x = 0.0f;
float y = 0.0f;
int i = 0;
while (getline(file, str))
{
for (short i = 0; i < str.size(); i++)
{
if (str[i] == PLAYER_1)
{
std::cout << "юсссс" << std::endl;
p1->x = x;
p1->y = y;
i++;
}
else if (str[i] == PLAYER_2)
{
p2->x = x;
p2->y = y;
i++;
}
if (i >= 2) break;
x += sectionSize;
}
y += sectionSize;
}
}<file_sep>#pragma once
#include <GL\glew.h>
enum RECT_DRAW_TYPE{
NON_TEXTURE,
WITH_TEXTURE
};
enum RECT_TYPE
{
DYNAMIC,
STATIC
};
class Rect
{
private:
GLuint VAO;
float w, h;
void init();
RECT_DRAW_TYPE draw_type;
RECT_TYPE rect_type;
public:
float x, y;
Rect(float _w, float _h, float _x, float _y,RECT_DRAW_TYPE _type,RECT_TYPE type);
~Rect();
void freeRes();
void draw();
};<file_sep>#include "Application.h"
int main()
{
Application game("Packman");
game.start();
return 0;
}<file_sep>#include "Window.h"
Window::Window(int _w, int _h, const char *title)
{
w = _w;
h = _h;
window = glfwCreateWindow(w,h,title,NULL,NULL);
if (!window)
{
Log::error("Window did not create");
return;
}
glfwMakeContextCurrent(window);
glewExperimental = true;
}
Window::~Window()
{
this->deleteWindow();
}
void Window::deleteWindow()
{
if (window) {
glfwTerminate();
glfwDestroyWindow(window);
}
}
bool Window::is_open()
{
if (!window) return false;
glfwPollEvents();
glfwSwapBuffers(window);
return !glfwWindowShouldClose(window);
}
GLFWwindow* Window::getWindow() const
{
return window;
} | bda076afe661fa90f4a6c567345f78a88c6713da | [
"C",
"C++"
] | 32 | C | lamiral/TanksGame | 63000b66d035729caef9442ee45707fd4723d938 | 280f151363ef2531c2ba85bb8d4cbfdff1283af8 |
refs/heads/master | <repo_name>Vikkas18/Ecamp-AWS<file_sep>/UpdateDBUtility.java
package com.ipru.tcs.cloud.ecamp.Constants;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.ipru.tcs.cloud.db.utils.GenericDAO;
import com.ipru.tcs.cloud.rdbms.entity.campaignrecord.CampaignRecordStatusEntity;
import com.ipru.tcs.cloud.utils.UtilsException;
@Component
public class UpdateDBUtility {
@Autowired
GenericDAO genericDao;
static final String DATE_PATTERN = "yyyy-MM-dd";
public CampaignRecordStatusEntity updateCampaignRecordStatusToRetry(Long campaignRecordId, String serviceName, String remark, int substatus) throws UtilsException {
CampaignRecordStatusEntity[] campaignRecordStatusEntityArray =
genericDao.findByField(Constants.CAMPAIGN_RECORD_ENTITY, "recordid",
Long.toString(campaignRecordId), CampaignRecordStatusEntity.class);
CampaignRecordStatusEntity campaignRecordStatusEntity = campaignRecordStatusEntityArray[0];
campaignRecordStatusEntity.setStatus(Constants.RETRY);
// Not sure till now
if(remark != null) {
campaignRecordStatusEntity.setRemarks(remark);
campaignRecordStatusEntity.setSubstatus(substatus);
}
else {
campaignRecordStatusEntity.setRemarks("Technical Error");
campaignRecordStatusEntity.setSubstatus(0);
}
campaignRecordStatusEntity.setUpdateddate(Constants.getDate("yyyy-MM-dd"));
campaignRecordStatusEntity.setUpdatedby(serviceName);
// GenericDao call to update DB
CampaignRecordStatusEntity campaignRecordStatusEntityResponse =
genericDao.save(Constants.CAMPAIGN_RECORD_ENTITY, campaignRecordStatusEntity);
return campaignRecordStatusEntityResponse;
}
}
<file_sep>/Constants.java
package com.ipru.tcs.cloud.ecamp.Constants;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class Constants {
//Statuses and Substatuses
public static final int NEW = 000;
public static final int TRANSIT = 500;
public static final int SENT = 200;
public static final int DELIVERED = 300;
public static final int RETRY = 800;
public static final int NOT_TO_SEND = 900;
public static final int READ = 310;
public static final int NAVIGATE = 320;
public static final int SYSTEM_ISSUE = 801;
public static final int GATEWAY_ISSUE = 802;
public static final int TIME_WINDOW_LAPSE = 803;
public static final int DND = 901;
public static final int MAX_ATTEMPT_REACHED = 902;
public static final int BOUNCE = 903;
public static final int FAILED = 700;
public static final int PROCESSING = 500;
public static final int ENQUEUE_FAILD = 112;
public static final int EVALUATED = 101;
public static final int ENQUEUEING = 101;
//Netcore Pepipost events
public static final String NETCORE_PEPIPOST_STATUS_SENT = "sent";
public static final String NETCORE_PEPIPOST_STATUS_OPENED = "opened";
public static final String NETCORE_PEPIPOST_STATUS_CLICKED = "clicked";
public static final String NETCORE_PEPIPOST_STATUS_BOUNCED = "bounced";
public static final String NETCORE_PEPIPOST_STATUS_DROPPED = "dropped";
public static final String NETCORE_PEPIPOST_STATUS_INVALID = "invalid";
public static final String NETCORE_PEPIPOST_STATUS_UNSUBSCRIBED = "unsubscribed";
public static final String NETCORE_PEPIPOST_STATUS_SPAM = "spam";
//IBank SMTP events
public static final String IBANK_SMTP_STATUS_READ = "read";
public static final String IBANK_SMTP_STATUS_NAVIGATE = "navigate";
//Vendors
public static final String IBANK_SMTP = "ibank_smtp";
public static final String NETCORE_PEPIPOST = "netcore_pepipost";
//Entities
public static final String CAMPAIGN_RECORD_ENTITY = "campaignrecord";
public static final String CAMPAIGN_DEFINITION_ENTITY = "campaigndefinition";
public static final String CAMPAIGN_TEMPLATE_ENTITY = "campaigntemplate";
public static final String RETRY__ENTITY = "retry";
public static final String RETRYSCHEDULERSERVICE = "RetrySchedulerService";
//Services
public static final String EMAIL_SERVICE = "emailservice";
public static final String IBANK_STATUS_SERVICE = "ibankstatusservice";
public static final String NETCORE_PEPIPOST_STATUS_SERVICE = "netcorepepipoststatusservice";
//LoadDistibutionService Statuses
public static final String LOADDISTRIBUTION_SUCCESS_STATUS ="Processing";
public static final String LOADDISTRIBUTION_SUCCESS_SUBSTATUS ="QueueAssigned";
public static final String LOADDISTRIBUTION_FAILURE_STATUS ="Failed";
public static final String LOADDISTRIBUTION_FALIURE_SUBSTATUS ="Invalid Input paramters";
public static final String LOADDISTRIBUTION_FALIURE1_SUBSTATUS ="InvalidProcessingCategory";
public static final String LOADDISTRIBUTION_FALIURE2_SUBSTATUS ="No_Recordswith_preference1";
public static final String LOADDISTRIBUTION_RETRY_SUBSTATUS ="TechError";
public static final String LOADDISTRIBUTION_RETRY_STATUS ="Retry";
public static final String LOADDISTRIBUTION_REMARK ="loadbalancer";
public static final String CONFIGURATIONTAG ="TAG";
public static final String VERY_HIGH_VOLUME ="VERYHIGHVOLUME";
public static final String HIGH_VOLUME ="HIGHVOLUME";
public static final String MODARATE_VOLUME ="MODARATEVOLUME";
public static final String LOW_VOLUME ="LOWVOLUME";
// Get Current Date
public static String getDate(String pattern) {
DateFormat dateFormat = new SimpleDateFormat(pattern);
Calendar cal = Calendar.getInstance();
Date date=cal.getTime();
String currentDate=dateFormat.format(date);
return currentDate;
}
// Get Current Time
public static String getTime(String pattern) {
DateFormat timeFormat = new SimpleDateFormat(pattern);
Calendar cal = Calendar.getInstance();
Date date=cal.getTime();
String currentTime=timeFormat.format(date);
return currentTime;
}
}
| 7b69b2b442578f729e30d4757f0f40be5248b6a0 | [
"Java"
] | 2 | Java | Vikkas18/Ecamp-AWS | fbea715c15aef374d7e027c4f2687a54b36cab06 | b2edb9688a637a720a344136302def8ee78bb06e |
refs/heads/main | <file_sep><!DOCTYPE HTML>
<html lang="zh-CN">
<head>
<meta charset="utf-8">
<meta name="keywords" content="Nginx, Yuimm">
<meta name="description" content="Nginx。-------------------------------------------------">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=no">
<meta name="renderer" content="webkit|ie-stand|ie-comp">
<meta name="mobile-web-app-capable" content="yes">
<meta name="format-detection" content="telephone=no">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black-translucent">
<title>Nginx | Yuimm</title>
<link rel="icon" type="image/png" href="/favicon.png">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/awesome/css/all.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/materialize/materialize.min.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/aos/aos.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/animate/animate.min.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/lightGallery/css/lightgallery.min.css">
<link rel="stylesheet" type="text/css" href="/css/matery.css">
<link rel="stylesheet" type="text/css" href="/css/my.css">
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/jquery/jquery.min.js"></script>
<meta name="generator" content="Hexo 4.2.1"><link rel="stylesheet" href="/css/prism-tomorrow.css" type="text/css"></head>
<body>
<header class="navbar-fixed">
<nav id="headNav" class="bg-color nav-transparent">
<div id="navContainer" class="nav-wrapper head-container">
<div class="brand-logo">
<a href="/" class="waves-effect waves-light">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/logo.png" class="logo-img" alt="LOGO">
<span class="logo-span">Yuimm</span>
</a>
</div>
<a href="#" data-target="mobile-nav" class="sidenav-trigger button-collapse"><i class="fas fa-bars"></i></a>
<ul class="right nav-menu">
<li class="hide-on-med-and-down nav-item">
<a href="/" class="waves-effect waves-light">
<i class="fas fa-home" style="zoom: 0.6;"></i>
<span>首页</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/tags" class="waves-effect waves-light">
<i class="fas fa-tags" style="zoom: 0.6;"></i>
<span>标签</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/categories" class="waves-effect waves-light">
<i class="fas fa-bookmark" style="zoom: 0.6;"></i>
<span>分类</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/archives" class="waves-effect waves-light">
<i class="fas fa-archive" style="zoom: 0.6;"></i>
<span>归档</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/about" class="waves-effect waves-light">
<i class="fas fa-user-circle" style="zoom: 0.6;"></i>
<span>关于</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/contact" class="waves-effect waves-light">
<i class="fas fa-comments" style="zoom: 0.6;"></i>
<span>留言板</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/friends" class="waves-effect waves-light">
<i class="fas fa-address-book" style="zoom: 0.6;"></i>
<span>友情链接</span>
</a>
</li>
<li>
<a href="#searchModal" class="modal-trigger waves-effect waves-light">
<i id="searchIcon" class="fas fa-search" title="搜索" style="zoom: 0.85;"></i>
</a>
</li>
</ul>
<div id="mobile-nav" class="side-nav sidenav">
<div class="mobile-head bg-color">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/logo.png" class="logo-img circle responsive-img">
<div class="logo-name">Yuimm</div>
<div class="logo-desc">
Never really desperate, only the lost of the soul.
</div>
</div>
<ul class="menu-list mobile-menu-list">
<li class="m-nav-item">
<a href="/" class="waves-effect waves-light">
<i class="fa-fw fas fa-home"></i>
首页
</a>
</li>
<li class="m-nav-item">
<a href="/tags" class="waves-effect waves-light">
<i class="fa-fw fas fa-tags"></i>
标签
</a>
</li>
<li class="m-nav-item">
<a href="/categories" class="waves-effect waves-light">
<i class="fa-fw fas fa-bookmark"></i>
分类
</a>
</li>
<li class="m-nav-item">
<a href="/archives" class="waves-effect waves-light">
<i class="fa-fw fas fa-archive"></i>
归档
</a>
</li>
<li class="m-nav-item">
<a href="/about" class="waves-effect waves-light">
<i class="fa-fw fas fa-user-circle"></i>
关于
</a>
</li>
<li class="m-nav-item">
<a href="/contact" class="waves-effect waves-light">
<i class="fa-fw fas fa-comments"></i>
Contact
</a>
</li>
<li class="m-nav-item">
<a href="/friends" class="waves-effect waves-light">
<i class="fa-fw fas fa-address-book"></i>
友情链接
</a>
</li>
<li><div class="divider"></div></li>
<li>
<a href="https://github.com/Yuimm" class="waves-effect waves-light" target="_blank">
<i class="fab fa-github-square fa-fw"></i>Fork Me
</a>
</li>
</ul>
</div>
</div>
<style>
.nav-transparent .github-corner {
display: none !important;
}
.github-corner {
position: absolute;
z-index: 10;
top: 0;
right: 0;
border: 0;
transform: scale(1.1);
}
.github-corner svg {
color: #0f9d58;
fill: #fff;
height: 64px;
width: 64px;
}
.github-corner:hover .octo-arm {
animation: a 0.56s ease-in-out;
}
.github-corner .octo-arm {
animation: none;
}
@keyframes a {
0%,
to {
transform: rotate(0);
}
20%,
60% {
transform: rotate(-25deg);
}
40%,
80% {
transform: rotate(10deg);
}
}
</style>
<a href="https://github.com/Yuimm" class="github-corner tooltipped hide-on-med-and-down" target="_blank"
data-tooltip="Fork Me" data-position="left" data-delay="50">
<svg viewBox="0 0 250 250" aria-hidden="true">
<path d="M0,0 L115,115 L130,115 L142,142 L250,250 L250,0 Z"></path>
<path d="M128.3,109.0 C113.8,99.7 119.0,89.6 119.0,89.6 C122.0,82.7 120.5,78.6 120.5,78.6 C119.2,72.0 123.4,76.3 123.4,76.3 C127.3,80.9 125.5,87.3 125.5,87.3 C122.9,97.6 130.6,101.9 134.4,103.2"
fill="currentColor" style="transform-origin: 130px 106px;" class="octo-arm"></path>
<path d="M115.0,115.0 C114.9,115.1 118.7,116.5 119.8,115.4 L133.7,101.6 C136.9,99.2 139.9,98.4 142.2,98.6 C133.8,88.0 127.5,74.4 143.8,58.0 C148.5,53.4 154.0,51.2 159.7,51.0 C160.3,49.4 163.2,43.6 171.4,40.1 C171.4,40.1 176.1,42.5 178.8,56.2 C183.1,58.6 187.2,61.8 190.9,65.4 C194.5,69.0 197.7,73.2 200.1,77.6 C213.8,80.2 216.3,84.9 216.3,84.9 C212.7,93.1 206.9,96.0 205.4,96.6 C205.1,102.4 203.0,107.8 198.3,112.5 C181.9,128.9 168.3,122.5 157.7,114.1 C157.9,116.9 156.7,120.9 152.7,124.9 L141.0,136.5 C139.8,137.7 141.6,141.9 141.8,141.8 Z"
fill="currentColor" class="octo-body"></path>
</svg>
</a>
</nav>
</header>
<div class="bg-cover pd-header post-cover" style="background-image: url('https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/java.jpg')">
<div class="container" style="right: 0px;left: 0px;">
<div class="row">
<div class="col s12 m12 l12">
<div class="brand">
<h1 class="description center-align post-title">Nginx</h1>
</div>
</div>
</div>
</div>
</div>
<main class="post-container content">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/tocbot/tocbot.css">
<style>
#articleContent h1::before,
#articleContent h2::before,
#articleContent h3::before,
#articleContent h4::before,
#articleContent h5::before,
#articleContent h6::before {
display: block;
content: " ";
height: 100px;
margin-top: -100px;
visibility: hidden;
}
#articleContent :focus {
outline: none;
}
.toc-fixed {
position: fixed;
top: 64px;
}
.toc-widget {
width: 345px;
padding-left: 20px;
}
.toc-widget .toc-title {
margin: 35px 0 15px 0;
padding-left: 17px;
font-size: 1.5rem;
font-weight: bold;
line-height: 1.5rem;
}
.toc-widget ol {
padding: 0;
list-style: none;
}
#toc-content {
height: calc(100vh - 250px);
overflow: auto;
}
#toc-content ol {
padding-left: 10px;
}
#toc-content ol li {
padding-left: 10px;
}
#toc-content .toc-link:hover {
color: #42b983;
font-weight: 700;
text-decoration: underline;
}
#toc-content .toc-link::before {
background-color: transparent;
max-height: 25px;
}
#toc-content .is-active-link {
color: #42b983;
}
#toc-content .is-active-link::before {
background-color: #42b983;
}
#floating-toc-btn {
position: fixed;
right: 15px;
bottom: 76px;
padding-top: 15px;
margin-bottom: 0;
z-index: 998;
}
#floating-toc-btn .btn-floating {
width: 48px;
height: 48px;
}
#floating-toc-btn .btn-floating i {
line-height: 48px;
font-size: 1.4rem;
}
</style>
<div class="row">
<div id="main-content" class="col s12 m12 l9">
<!-- 文章内容详情 -->
<div id="artDetail">
<div class="card">
<div class="card-content article-info">
<div class="row tag-cate">
<div class="col s7">
<div class="article-tag">
<a href="/tags/%E8%B4%9F%E8%BD%BD%E5%9D%87%E8%A1%A1%E4%B8%AD%E9%97%B4%E4%BB%B6/">
<span class="chip bg-color">负载均衡中间件</span>
</a>
</div>
</div>
<div class="col s5 right-align">
<div class="post-cate">
<i class="fas fa-bookmark fa-fw icon-category"></i>
<a href="/categories/%E5%88%86%E5%B8%83%E5%BC%8F%E4%B8%AD%E9%97%B4%E4%BB%B6/" class="post-category">
分布式中间件
</a>
</div>
</div>
</div>
<div class="post-info">
<div class="post-date info-break-policy">
<i class="far fa-calendar-minus fa-fw"></i>发布日期:
2021-04-03
</div>
<div class="post-date info-break-policy">
<i class="far fa-calendar-check fa-fw"></i>更新日期:
2021-11-22
</div>
<div class="info-break-policy">
<i class="far fa-file-word fa-fw"></i>文章字数:
10.5k
</div>
<div class="info-break-policy">
<i class="far fa-clock fa-fw"></i>阅读时长:
43 分
</div>
<div id="busuanzi_container_page_pv" class="info-break-policy">
<i class="far fa-eye fa-fw"></i>阅读次数:
<span id="busuanzi_value_page_pv"></span>
</div>
</div>
</div>
<hr class="clearfix">
<div class="card-content article-card-content">
<div id="articleContent">
<h1 id="1-什么是nginx"><a href="#1-什么是nginx" class="headerlink" title="1. 什么是nginx"></a>1. 什么是nginx</h1><p>Nginx (“engine x”) 是一个高性能的 HTTP 和反向代理服务器,特点是占有内存少,并发能力强。</p>
<p>Nginx 可以作为静态页面的 web 服务器,同时还支持 CGI 协议的动态语言,比如 perl、php等,但是不支持 java。Java 程序只能通过与 tomcat 配合完成。Nginx 专为性能优化而开发,性能是其最重要的考量,实现上非常注重效率 ,能经受高负载的考验,有报告表明能支持高达 50,000 个并发连接数。</p>
<h1 id="2-正向代理与反向代理"><a href="#2-正向代理与反向代理" class="headerlink" title="2. 正向代理与反向代理"></a>2. 正向代理与反向代理</h1><h2 id="2-1-概念"><a href="#2-1-概念" class="headerlink" title="2.1 概念"></a>2.1 概念</h2><blockquote>
<p>在客户端配置代理服务器,通过代理服务器进行互联网访问</p>
</blockquote>
<p><strong>正向代理</strong>是一个位于客户端和目标服务器之间的代理服务器(中间服务器)。为了从原始服务器取得内容,客户端向代理服务器发送一个请求,并且指定目标服务器,之后代理向目标服务器转交并且将获得的内容返回给客户端。正向代理的情况下客户端必须要进行一些特别的设置才能使用。</p>
<blockquote>
<p>客户端是无感知的</p>
</blockquote>
<p><strong>反向代理</strong>正好相反。对于客户端来说,反向代理就好像目标服务器。并且客户端不需要进行任何设置。客户端向反向代理发送请求,接着反向代理服务器去选择目标服务器获取数据,再返回给客户端,此时反向代理服务器和目标服务器对外就是一个服务器。暴露的是代理服务器的地址,隐藏了真实服务器的IP地址。客户端并不会感知到反向代理后面的服务,也因此不需要客户端做任何设置,只需要把反向代理服务器当成真正的服务器就好了。</p>
<h2 id="2-2-区别"><a href="#2-2-区别" class="headerlink" title="2.2 区别"></a>2.2 区别</h2><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200519095355.png" alt=""></p>
<p>正向代理中,proxy和client同属一个LAN,对server透明; 反向代理中,proxy和server同属一个LAN,对client透明。 实际上proxy在两种代理中做的事都是代为收发请求和响应,不过从结构上来看正好左右互换了下,所以把前者那种代理方式叫做正向代理,后者叫做反向代理。</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200519095419.png" alt="img"></p>
<h3 id="正向代理-客户端-lt-一-gt-代理-一-gt-服务端"><a href="#正向代理-客户端-lt-一-gt-代理-一-gt-服务端" class="headerlink" title="正向代理:客户端 <一> 代理 一>服务端"></a>正向代理:客户端 <一> 代理 一>服务端</h3><p>正向代理简单地打个租房的比方:</p>
<p>A(客户端)想租C(服务端)的房子,但是A(客户端)并不认识C(服务端)租不到。<br> B(代理)认识C(服务端)能租这个房子所以你找了B(代理)帮忙租到了这个房子。</p>
<p>这个过程中C(服务端)不认识A(客户端)只认识B(代理)<br> C(服务端)并不知道A(客户端)租了房子,只知道房子租给了B(代理)。</p>
<h3 id="反向代理-客户端-一-gt-代理-lt-一-gt-服务端"><a href="#反向代理-客户端-一-gt-代理-lt-一-gt-服务端" class="headerlink" title="反向代理:客户端 一>代理 <一> 服务端"></a>反向代理:客户端 一>代理 <一> 服务端</h3><p>反向代理也用一个租房的例子:</p>
<p>A(客户端)想租一个房子,B(代理)就把这个房子租给了他。<br> 这时候实际上C(服务端)才是房东。<br> B(代理)是中介把这个房子租给了A(客户端)。</p>
<p>这个过程中A(客户端)并不知道这个房子到底谁才是房东<br> 他都有可能认为这个房子就是B(代理)的</p>
<p><strong>由上的例子和图,我们可以知道正向代理和反向代理的区别在于代理的对象不一样,正向代理的代理对象是客户端,反向代理的代理对象是服务端。</strong></p>
<h1 id="3-负载均衡"><a href="#3-负载均衡" class="headerlink" title="3. 负载均衡"></a>3. 负载均衡</h1><p><strong><em>增加服务器的数量,然后将请求分发到各个服务器上,将原先请求集中到单个服务器上的情况改为将请求分发到多个服务器上,将负载分发到不同的服务器,也就是我们所说的负载均衡</em></strong></p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200519100327.png" alt="img"></p>
<h1 id="4-动静分离"><a href="#4-动静分离" class="headerlink" title="4. 动静分离"></a>4. 动静分离</h1><p>为了加快网站的解析速度,可以把动态页面和静态页面由不同的服务器来解析,加快解析速度。降低原来单个服务器的压力。</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200519100459.png" alt="img"></p>
<h1 id="5-基于apt源安装"><a href="#5-基于apt源安装" class="headerlink" title="5. 基于apt源安装"></a>5. 基于apt源安装</h1><h2 id="5-1-安装"><a href="#5-1-安装" class="headerlink" title="5.1 安装"></a>5.1 安装</h2><pre class=" language-shell"><code class="language-shell">// 更新包
sudo apt-get update
// 下载安装nginx
sudo apt-get install nginx</code></pre>
<p>Ubuntu安装之后的文件结构大致为:</p>
<ul>
<li>所有的配置文件都在/etc/nginx下,并且每个虚拟主机已经安排在了/etc/nginx/sites-available下</li>
<li>程序文件在/usr/sbin/nginx</li>
<li>日志放在了/var/log/nginx中</li>
<li>并已经在/etc/init.d/下创建了启动脚本nginx</li>
<li>默认的虚拟主机的目录设置在了/var/www/nginx-default (有的版本 默认的虚拟主机的目录设置在了/var/www, 请参考/etc/nginx/sites-available里的配置)</li>
</ul>
<p>其实从上面的根目录文件夹可以知道,Linux系统的配置文件一般放在/etc,日志一般放在/var/log,运行的程序一般放在/usr/sbin或者/usr/bin。</p>
<p>当然,如果要更清楚Nginx的配置项放在什么地方,可以打开/etc/nginx/nginx.conf</p>
<p>然后通过这种方式安装的,会自动创建服务,会自动在/etc/init.d/nginx新建服务脚本,然后就可以使用一下命令来启动</p>
<pre class=" language-shell"><code class="language-shell">sudo service nginx {start|stop|restart|reload|force-reload|status|configtest|rotate|upgrade}</code></pre>
<p>脚本如下:</p>
<pre class=" language-shell"><code class="language-shell">#!/bin/sh
### BEGIN INIT INFO
# Provides: nginx
# Required-Start: $local_fs $remote_fs $network $syslog $named
# Required-Stop: $local_fs $remote_fs $network $syslog $named
# Default-Start: 2 3 4 5
# Default-Stop: 0 1 6
# Short-Description: starts the nginx web server
# Description: starts nginx using start-stop-daemon
### END INIT INFO
PATH=/usr/local/sbin:/usr/local/bin:/sbin:/bin:/usr/sbin:/usr/bin
DAEMON=/usr/sbin/nginx
NAME=nginx
DESC=nginx
# Include nginx defaults if available
if [ -r /etc/default/nginx ]; then
. /etc/default/nginx
fi
STOP_SCHEDULE="${STOP_SCHEDULE:-QUIT/5/TERM/5/KILL/5}"
test -x $DAEMON || exit 0
. /lib/init/vars.sh
. /lib/lsb/init-functions
# Try to extract nginx pidfile
PID=$(cat /etc/nginx/nginx.conf | grep -Ev '^\s*#' | awk 'BEGIN { RS="[;{}]" } { if ($1 == "pid") print $2 }' | head -n1)
if [ -z "$PID" ]; then
PID=/run/nginx.pid
fi
if [ -n "$ULIMIT" ]; then
# Set ulimit if it is set in /etc/default/nginx
ulimit $ULIMIT
fi
start_nginx() {
# Start the daemon/service
#
# Returns:
# 0 if daemon has been started
# 1 if daemon was already running
# 2 if daemon could not be started
start-stop-daemon --start --quiet --pidfile $PID --exec $DAEMON --test > /dev/null \
|| return 1
start-stop-daemon --start --quiet --pidfile $PID --exec $DAEMON -- \
$DAEMON_OPTS 2>/dev/null \
|| return 2
}
test_config() {
# Test the nginx configuration
$DAEMON -t $DAEMON_OPTS >/dev/null 2>&1
}
stop_nginx() {
# Stops the daemon/service
#
# Return
# 0 if daemon has been stopped
# 1 if daemon was already stopped
# 2 if daemon could not be stopped
# other if a failure occurred
start-stop-daemon --stop --quiet --retry=$STOP_SCHEDULE --pidfile $PID --name $NAME
RETVAL="$?"
sleep 1
return "$RETVAL"
}
reload_nginx() {
# Function that sends a SIGHUP to the daemon/service
start-stop-daemon --stop --signal HUP --quiet --pidfile $PID --name $NAME
return 0
}
rotate_logs() {
# Rotate log files
start-stop-daemon --stop --signal USR1 --quiet --pidfile $PID --name $NAME
return 0
}
upgrade_nginx() {
# Online upgrade nginx executable
# http://nginx.org/en/docs/control.html
#
# Return
# 0 if nginx has been successfully upgraded
# 1 if nginx is not running
# 2 if the pid files were not created on time
# 3 if the old master could not be killed
if start-stop-daemon --stop --signal USR2 --quiet --pidfile $PID --name $NAME; then
# Wait for both old and new master to write their pid file
while [ ! -s "${PID}.oldbin" ] || [ ! -s "${PID}" ]; do
cnt=`expr $cnt + 1`
if [ $cnt -gt 10 ]; then
return 2
fi
sleep 1
done
# Everything is ready, gracefully stop the old master
if start-stop-daemon --stop --signal QUIT --quiet --pidfile "${PID}.oldbin" --name $NAME; then
return 0
else
return 3
fi
else
return 1
fi
}
case "$1" in
start)
log_daemon_msg "Starting $DESC" "$NAME"
start_nginx
case "$?" in
0|1) log_end_msg 0 ;;
2) log_end_msg 1 ;;
esac
;;
stop)
log_daemon_msg "Stopping $DESC" "$NAME"
stop_nginx
case "$?" in
0|1) log_end_msg 0 ;;
2) log_end_msg 1 ;;
esac
;;
restart)
log_daemon_msg "Restarting $DESC" "$NAME"
# Check configuration before stopping nginx
if ! test_config; then
log_end_msg 1 # Configuration error
exit $?
fi
stop_nginx
case "$?" in
0|1)
start_nginx
case "$?" in
0) log_end_msg 0 ;;
1) log_end_msg 1 ;; # Old process is still running
*) log_end_msg 1 ;; # Failed to start
esac
;;
*)
# Failed to stop
log_end_msg 1
;;
esac
;;
reload|force-reload)
log_daemon_msg "Reloading $DESC configuration" "$NAME"
# Check configuration before stopping nginx
#
# This is not entirely correct since the on-disk nginx binary
# may differ from the in-memory one, but that's not common.
# We prefer to check the configuration and return an error
# to the administrator.
if ! test_config; then
log_end_msg 1 # Configuration error
exit $?
fi
reload_nginx
log_end_msg $?
;;
configtest|testconfig)
log_daemon_msg "Testing $DESC configuration"
test_config
log_end_msg $?
;;
status)
status_of_proc -p $PID "$DAEMON" "$NAME" && exit 0 || exit $?
;;
upgrade)
log_daemon_msg "Upgrading binary" "$NAME"
upgrade_nginx
log_end_msg $?
;;
rotate)
log_daemon_msg "Re-opening $DESC log files" "$NAME"
rotate_logs
log_end_msg $?
;;
*)
echo "Usage: $NAME {start|stop|restart|reload|force-reload|status|configtest|rotate|upgrade}" >&2
exit 3
;;
esac
</code></pre>
<p>还有一个好处,创建好的文件由于放在/usr/sbin目录下,所以能直接在终端中使用nginx命令而无需指定路径。</p>
<h2 id="5-2-测试安装是否成功"><a href="#5-2-测试安装是否成功" class="headerlink" title="5.2 测试安装是否成功"></a>5.2 测试安装是否成功</h2><p>在命令行中输入:</p>
<pre class=" language-shell"><code class="language-shell">sudo nginx -t</code></pre>
<p>窗口显示:</p>
<pre class=" language-shell"><code class="language-shell">nginx: the configuration file /etc/nginx/nginx.conf syntax is ok
nginx: configuration file /etc/nginx/nginx.conf test is successful</code></pre>
<p>在浏览器中输入ip地址:</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200519123550.png" alt=""></p>
<h2 id="5-3-卸载"><a href="#5-3-卸载" class="headerlink" title="5.3 卸载"></a>5.3 卸载</h2><h3 id="5-3-1-停止nginx服务"><a href="#5-3-1-停止nginx服务" class="headerlink" title="5.3.1. 停止nginx服务"></a>5.3.1. 停止nginx服务</h3><pre class=" language-shell"><code class="language-shell">sudo service nginx stop</code></pre>
<h3 id="5-3-2-删除nginx,-purge包括配置文件"><a href="#5-3-2-删除nginx,-purge包括配置文件" class="headerlink" title="5.3.2. 删除nginx,-purge包括配置文件"></a>5.3.2. 删除nginx,-purge包括配置文件</h3><pre class=" language-shell"><code class="language-shell">sudo apt-get --purge remove nginx</code></pre>
<h3 id="5-3-3-移除全部不使用的软件包"><a href="#5-3-3-移除全部不使用的软件包" class="headerlink" title="5.3.3. 移除全部不使用的软件包"></a>5.3.3. 移除全部不使用的软件包</h3><pre class=" language-shell"><code class="language-shell">sudo apt-get autoremove</code></pre>
<h3 id="5-3-4-罗列出与nginx相关的软件并删除"><a href="#5-3-4-罗列出与nginx相关的软件并删除" class="headerlink" title="5.3.4. 罗列出与nginx相关的软件并删除"></a>5.3.4. 罗列出与nginx相关的软件并删除</h3><pre class=" language-shell"><code class="language-shell">dpkg --get-selections|grep nginx
sudo apt-get --purge remove nginx
sudo apt-get --purge remove nginx-common
sudo apt-get --purge remove nginx-core</code></pre>
<h3 id="5-3-5-查看nginx正在运行的进程,如果有就kill掉"><a href="#5-3-5-查看nginx正在运行的进程,如果有就kill掉" class="headerlink" title="5.3.5. 查看nginx正在运行的进程,如果有就kill掉"></a>5.3.5. 查看nginx正在运行的进程,如果有就kill掉</h3><pre class=" language-shell"><code class="language-shell">ps -ef |grep nginx
sudo kill -9 XXX</code></pre>
<h1 id="6-通过源码包编译安装"><a href="#6-通过源码包编译安装" class="headerlink" title="6. 通过源码包编译安装"></a>6. 通过源码包编译安装</h1><p>这种方式可以自定安装指定的模块以及最新的版本。方式更灵活。</p>
<p>官方下载页面:<a href="http://nginx.org/en/download.html" target="_blank" rel="noopener">http://nginx.org/en/download.html</a></p>
<p>configure配置文件详解:<a href="http://nginx.org/en/docs/configure.html" target="_blank" rel="noopener">http://nginx.org/en/docs/configure.html</a></p>
<h2 id="6-1-安装各种依赖库"><a href="#6-1-安装各种依赖库" class="headerlink" title="6.1. 安装各种依赖库"></a>6.1. 安装各种依赖库</h2><p> 安装gcc g++的依赖库</p>
<pre class=" language-shell"><code class="language-shell">sudo apt-get install build-essential
sudo apt-get install libtool</code></pre>
<p>安装pcre依赖库(<a href="http://www.pcre.org/)" target="_blank" rel="noopener">http://www.pcre.org/)</a></p>
<pre class=" language-shell"><code class="language-shell">sudo apt-get update
sudo apt-get install libpcre3 libpcre3-dev</code></pre>
<p>安装zlib依赖库(<a href="http://www.zlib.net/" target="_blank" rel="noopener">http://www.zlib.net</a>)</p>
<pre class=" language-shell"><code class="language-shell">sudo apt-get install zlib1g-dev</code></pre>
<p>安装SSL依赖库(18.04默认已经安装了)</p>
<pre class=" language-shell"><code class="language-shell">sudo apt-get install openssl</code></pre>
<h2 id="6-2-安装Nginx"><a href="#6-2-安装Nginx" class="headerlink" title="6.2. 安装Nginx"></a>6.2. 安装Nginx</h2><pre class=" language-shell"><code class="language-shell">#下载最新版本:
wget http://nginx.org/download/nginx-1.13.6.tar.gz
#解压:
tar -zxvf nginx-1.13.6.tar.gz
#进入解压目录:
cd nginx-1.13.6
#配置:
./configure --prefix=/usr/local/nginx
#编译:
make
#安装:
sudo make install
#启动:
sudo /usr/local/nginx/sbin/nginx -c /usr/local/nginx/conf/nginx.conf
注意:-c 指定配置文件的路径,不加的话,nginx会自动加载默认路径的配置文件,可以通过-h查看帮助命令。
#查看进程:
ps -ef | grep nginx</code></pre>
<h2 id="6-3-配置软链接"><a href="#6-3-配置软链接" class="headerlink" title="6.3. 配置软链接"></a>6.3. 配置软链接</h2><pre class=" language-shell"><code class="language-shell">sudo ln -s /usr/local/nginx/sbin/nginx /usr/bin/nginx</code></pre>
<p>现在就可以不用路径直接输入nginx启动。</p>
<h2 id="6-4-配置开机启动服务"><a href="#6-4-配置开机启动服务" class="headerlink" title="6.4. 配置开机启动服务"></a>6.4. 配置开机启动服务</h2><p>在/etc/init.d/下创建nginx文件,sudo vim /etc/init.d/nginx,内容如下:</p>
<pre class=" language-shell"><code class="language-shell">#!/bin/sh
### BEGIN INIT INFO
# Provides: nginx
# Required-Start: $local_fs $remote_fs $network $syslog $named
# Required-Stop: $local_fs $remote_fs $network $syslog $named
# Default-Start: 2 3 4 5
# Default-Stop: 0 1 6
# Short-Description: starts the nginx web server
# Description: starts nginx using start-stop-daemon
### END INIT INFO
PATH=/usr/local/sbin:/usr/local/bin:/sbin:/bin:/usr/sbin:/usr/bin
DAEMON=/usr/sbin/nginx
NAME=nginx
DESC=nginx
# Include nginx defaults if available
if [ -r /etc/default/nginx ]; then
. /etc/default/nginx
fi
STOP_SCHEDULE="${STOP_SCHEDULE:-QUIT/5/TERM/5/KILL/5}"
test -x $DAEMON || exit 0
. /lib/init/vars.sh
. /lib/lsb/init-functions
# Try to extract nginx pidfile
PID=$(cat /etc/nginx/nginx.conf | grep -Ev '^\s*#' | awk 'BEGIN { RS="[;{}]" } { if ($1 == "pid") print $2 }' | head -n1)
if [ -z "$PID" ]; then
PID=/run/nginx.pid
fi
if [ -n "$ULIMIT" ]; then
# Set ulimit if it is set in /etc/default/nginx
ulimit $ULIMIT
fi
start_nginx() {
# Start the daemon/service
#
# Returns:
# 0 if daemon has been started
# 1 if daemon was already running
# 2 if daemon could not be started
start-stop-daemon --start --quiet --pidfile $PID --exec $DAEMON --test > /dev/null \
|| return 1
start-stop-daemon --start --quiet --pidfile $PID --exec $DAEMON -- \
$DAEMON_OPTS 2>/dev/null \
|| return 2
}
test_config() {
# Test the nginx configuration
$DAEMON -t $DAEMON_OPTS >/dev/null 2>&1
}
stop_nginx() {
# Stops the daemon/service
#
# Return
# 0 if daemon has been stopped
# 1 if daemon was already stopped
# 2 if daemon could not be stopped
# other if a failure occurred
start-stop-daemon --stop --quiet --retry=$STOP_SCHEDULE --pidfile $PID --name $NAME
RETVAL="$?"
sleep 1
return "$RETVAL"
}
reload_nginx() {
# Function that sends a SIGHUP to the daemon/service
start-stop-daemon --stop --signal HUP --quiet --pidfile $PID --name $NAME
return 0
}
rotate_logs() {
# Rotate log files
start-stop-daemon --stop --signal USR1 --quiet --pidfile $PID --name $NAME
return 0
}
upgrade_nginx() {
# Online upgrade nginx executable
# http://nginx.org/en/docs/control.html
#
# Return
# 0 if nginx has been successfully upgraded
# 1 if nginx is not running
# 2 if the pid files were not created on time
# 3 if the old master could not be killed
if start-stop-daemon --stop --signal USR2 --quiet --pidfile $PID --name $NAME; then
# Wait for both old and new master to write their pid file
while [ ! -s "${PID}.oldbin" ] || [ ! -s "${PID}" ]; do
cnt=`expr $cnt + 1`
if [ $cnt -gt 10 ]; then
return 2
fi
sleep 1
done
# Everything is ready, gracefully stop the old master
if start-stop-daemon --stop --signal QUIT --quiet --pidfile "${PID}.oldbin" --name $NAME; then
return 0
else
return 3
fi
else
return 1
fi
}
case "$1" in
start)
log_daemon_msg "Starting $DESC" "$NAME"
start_nginx
case "$?" in
0|1) log_end_msg 0 ;;
2) log_end_msg 1 ;;
esac
;;
stop)
log_daemon_msg "Stopping $DESC" "$NAME"
stop_nginx
case "$?" in
0|1) log_end_msg 0 ;;
2) log_end_msg 1 ;;
esac
;;
restart)
log_daemon_msg "Restarting $DESC" "$NAME"
# Check configuration before stopping nginx
if ! test_config; then
log_end_msg 1 # Configuration error
exit $?
fi
stop_nginx
case "$?" in
0|1)
start_nginx
case "$?" in
0) log_end_msg 0 ;;
1) log_end_msg 1 ;; # Old process is still running
*) log_end_msg 1 ;; # Failed to start
esac
;;
*)
# Failed to stop
log_end_msg 1
;;
esac
;;
reload|force-reload)
log_daemon_msg "Reloading $DESC configuration" "$NAME"
# Check configuration before stopping nginx
#
# This is not entirely correct since the on-disk nginx binary
# may differ from the in-memory one, but that's not common.
# We prefer to check the configuration and return an error
# to the administrator.
if ! test_config; then
log_end_msg 1 # Configuration error
exit $?
fi
reload_nginx
log_end_msg $?
;;
configtest|testconfig)
log_daemon_msg "Testing $DESC configuration"
test_config
log_end_msg $?
;;
status)
status_of_proc -p $PID "$DAEMON" "$NAME" && exit 0 || exit $?
;;
upgrade)
log_daemon_msg "Upgrading binary" "$NAME"
upgrade_nginx
log_end_msg $?
;;
rotate)
log_daemon_msg "Re-opening $DESC log files" "$NAME"
rotate_logs
log_end_msg $?
;;
*)
echo "Usage: $NAME {start|stop|restart|reload|force-reload|status|configtest|rotate|upgrade}" >&2
exit 3
;;
esac</code></pre>
<pre class=" language-shell"><code class="language-shell">#设置服务脚本有执行权限
sudo chmod +x /etc/init.d/nginx
#注册服务cd /etc/init.d/
sudo update-rc.d nginx defaults</code></pre>
<p>现在基本上就可以开机启动了,常用的命令如下:</p>
<pre><code>sudo service nginx {start|stop|restart|reload|force-reload|status|configtest|rotate|upgrade}</code></pre><h1 id="7-常用命令"><a href="#7-常用命令" class="headerlink" title="7. 常用命令"></a>7. 常用命令</h1><p>以下命令nginx已经通过apt安装好了,有nginx命令。</p>
<h2 id="7-1-查看版本"><a href="#7-1-查看版本" class="headerlink" title="7.1. 查看版本"></a>7.1. 查看版本</h2><pre class=" language-shell"><code class="language-shell">nginx -v</code></pre>
<p>如果你想同时看到更详细的配置项,使用 -V</p>
<pre class=" language-shell"><code class="language-shell">nginx -V</code></pre>
<h2 id="7-2-查看-Nginx-配置语法的正确性"><a href="#7-2-查看-Nginx-配置语法的正确性" class="headerlink" title="7.2. 查看 Nginx 配置语法的正确性"></a>7.2. 查看 Nginx 配置语法的正确性</h2><pre class=" language-shell"><code class="language-shell">sudo nginx -t</code></pre>
<p>这里如果用大写 T,效果就是 测试、丢弃并退出</p>
<pre class=" language-shell"><code class="language-shell">sudo nginx -T</code></pre>
<h2 id="7-3-为Nginx指定一个配置文件"><a href="#7-3-为Nginx指定一个配置文件" class="headerlink" title="7.3. 为Nginx指定一个配置文件"></a>7.3. 为Nginx指定一个配置文件</h2><pre class=" language-shell"><code class="language-shell">nginx -c </path/config> #为Nginx指定一个配置文件,来代替缺省值conf/nginx.conf</code></pre>
<h2 id="7-4-启动-Nginx-服务"><a href="#7-4-启动-Nginx-服务" class="headerlink" title="7.4. 启动 Nginx 服务"></a>7.4. 启动 Nginx 服务</h2><pre class=" language-shell"><code class="language-shell">sudo systemctl start nginx #systemd
OR
sudo service nginx start #sysvinit</code></pre>
<h2 id="7-5-开机自启动"><a href="#7-5-开机自启动" class="headerlink" title="7.5. 开机自启动"></a>7.5. 开机自启动</h2><pre class=" language-shell"><code class="language-shell">sudo systemctl enable nginx #systemd
或
sudo service nginx enable #sysv init</code></pre>
<h2 id="7-6-重启-Nginx-服务"><a href="#7-6-重启-Nginx-服务" class="headerlink" title="7.6. 重启 Nginx 服务"></a>7.6. 重启 Nginx 服务</h2><p>重启的意思是,停止 然后 启动</p>
<pre class=" language-shell"><code class="language-shell">sudo systemctl restart nginx #systemd
或
sudo service nginx restart #sysv init</code></pre>
<h2 id="7-7-查看-Nginx-服务状态"><a href="#7-7-查看-Nginx-服务状态" class="headerlink" title="7.7. 查看 Nginx 服务状态"></a>7.7. 查看 Nginx 服务状态</h2><p>这条是看运行时的服务状态信息的。</p>
<pre class=" language-shell"><code class="language-shell">sudo systemctl status nginx #systemd
或
sudo service nginx status #sysvinit</code></pre>
<h2 id="7-8-重载-Nginx-服务"><a href="#7-8-重载-Nginx-服务" class="headerlink" title="7.8. 重载 Nginx 服务"></a>7.8. 重载 Nginx 服务</h2><p>重载是重新加载 配置文件,看命令:</p>
<pre class=" language-shell"><code class="language-shell">sudo systemctl reload nginx #systemd
或
sudo service nginx reload #sysvinit</code></pre>
<h2 id="7-9-停止-Nginx-服务"><a href="#7-9-停止-Nginx-服务" class="headerlink" title="7.9. 停止 Nginx 服务"></a>7.9. 停止 Nginx 服务</h2><p>不管你是什么原因想停了它,都可以用:</p>
<pre class=" language-shell"><code class="language-shell">sudo systemctl stop nginx #systemd
OR
sudo service nginx stop #sysvinit</code></pre>
<h2 id="7-10-查看命令帮助"><a href="#7-10-查看命令帮助" class="headerlink" title="7.10. 查看命令帮助"></a>7.10. 查看命令帮助</h2><p>如果还有什么不懂,或者想知道其他命令,就用这个</p>
<pre class=" language-shell"><code class="language-shell">systemctl -h nginx</code></pre>
<h1 id="8-配置文件"><a href="#8-配置文件" class="headerlink" title="8. 配置文件"></a>8. 配置文件</h1><p>nginx 安装目录下,其默认的配置文件都放在这个目录的 conf 目录下,而主配置文件 nginx.conf 也在其中,后续对 nginx 的使用基本上都是对此配置文件进行相应的修改</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200519132450.png" alt="img"></p>
<h2 id="组成"><a href="#组成" class="headerlink" title="组成"></a>组成</h2><blockquote>
<p>第一部分 全局块</p>
</blockquote>
<p>从配置文件开始到 events 块之间的内容,主要会设置一些影响 nginx 服务器整体运行的配置指令,主要包括配置运行 Nginx 服务器的用户(组)、允许生成的 worker process 数,进程 PID 存放路径、日志存放路径和类型以及配置文件的引入等。</p>
<p><strong>worker_processes 1;</strong> 这是 Nginx 服务器并发处理服务的关键配置,worker_processes 值越大,可以支持的并发处理量也越多,但是会受到硬件、软件等设备的制约</p>
<blockquote>
<p>第二部分 events块</p>
</blockquote>
<p>events 块涉及的指令主要影响 Nginx 服务器与用户的网络连接,常用的设置包括是否开启对多 work process 下的网络连接进行序列化,是否允许同时接收多个网络连接,选取哪种事件驱动模型来处理连接请求,每个 word process 可以同时支持的最大连接数等。</p>
<p> <strong>worker_connections 1024;</strong>表示每个 work process 支持的最大连接数为 1024. 这部分的配置对 Nginx 的性能影响较大,在实际中应该灵活配置。</p>
<blockquote>
<p>第三部分 http块</p>
</blockquote>
<p>这算是 Nginx 服务器配置中最频繁的部分,代理、缓存和日志定义等绝大多数功能和第三方模块的配置都在这里。</p>
<p>需要注意的是:http 块也可以包括 <strong>http</strong> <strong>全局块</strong>、<strong>server</strong> <strong>块</strong>。</p>
<h3 id="1-http全局块"><a href="#1-http全局块" class="headerlink" title="1. http全局块"></a>1. http全局块</h3><p>http 全局块配置的指令包括文件引入、MIME-TYPE 定义、日志自定义、连接超时时间、单链接请求数上限等。</p>
<p><strong>upstream</strong>(上游服务器设置,主要为反向代理、负载均衡相关配置,upstream 的指令用于设置一系列的后端服务器,设置反向代理及后端服务器的负载均衡</p>
<h3 id="2-server块"><a href="#2-server块" class="headerlink" title="2. server块"></a>2. server块</h3><p>这块和虚拟主机有密切关系,虚拟主机从用户角度看,和一台独立的硬件主机是完全一样的,该技术的产生是为了节省互联网服务器硬件成本。</p>
<p>每个 http 块可以包括多个 server 块,而每个 server 块就相当于一个虚拟主机。</p>
<p>而每个 server 块也分为全局 server 块,以及可以同时包含多个 locaton 块。</p>
<h4 id="1-全局-server-块"><a href="#1-全局-server-块" class="headerlink" title="1.全局 server 块"></a>1.全局 server 块</h4><p>最常见的配置是本虚拟机主机的监听配置和本虚拟主机的名称或 IP 配置。</p>
<h4 id="2-location块"><a href="#2-location块" class="headerlink" title="2. location块"></a>2. location块</h4><p>一个 server 块可以配置多个 location 块。</p>
<p>这块的主要作用是基于 Nginx 服务器接收到的请求字符串(例如 server_name/uri-string),对虚拟主机名称(也可以是 IP 别名)之外的字符串(例如 前面的 /uri-string)进行匹配,对特定的请求进行处理。地址定向、数据缓存和应答控制等功能,还有许多第三方模块的配置也在这里进行。</p>
<h2 id="nginx-conf-详细的配置文件介绍"><a href="#nginx-conf-详细的配置文件介绍" class="headerlink" title="nginx.conf 详细的配置文件介绍"></a>nginx.conf 详细的配置文件介绍</h2><pre class=" language-properties"><code class="language-properties"><span class="token comment" spellcheck="true">#定义Nginx运行的用户和用户组</span>
<span class="token attr-name">user</span> <span class="token attr-value">www www; </span>
<span class="token comment" spellcheck="true">#nginx进程数,通常设置成和cpu的数量相等</span>
<span class="token attr-name">worker_processes</span> <span class="token attr-value">4; </span>
<span class="token comment" spellcheck="true">#全局错误日志定义类型,[debug | info | notice | warn | error | crit]</span>
<span class="token comment" spellcheck="true">#error_log logs/error.log;</span>
<span class="token comment" spellcheck="true">#error_log logs/error.log notice;</span>
<span class="token comment" spellcheck="true">#error_log logs/error.log info;</span>
<span class="token comment" spellcheck="true">#进程pid文件</span>
<span class="token comment" spellcheck="true">#pid logs/nginx.pid;</span>
<span class="token comment" spellcheck="true">#指定进程可以打开的最大描述符:数目</span>
<span class="token comment" spellcheck="true">#工作模式与连接数上限</span>
<span class="token comment" spellcheck="true">##这个指令是指当一个nginx进程打开的最多文件描述符数目,理论值应该是最多打开文件数(ulimit -n)与nginx进程数相除,但是nginx分配请求并不是那么均匀,所以最好与ulimit -n 的值保持一致。</span>
<span class="token comment" spellcheck="true">#这是因为nginx调度时分配请求到进程并不是那么的均衡,所以假如填写10240,总并发量达到3-4万时就有进程可能超过10240了,这时会返回502错误。</span>
<span class="token attr-name">worker_rlimit_nofile</span> <span class="token attr-value">65535;</span>
<span class="token attr-name">events</span> <span class="token attr-value">{</span>
<span class="token comment" spellcheck="true"> #参考事件模型,use [ kqueue | rtsig | epoll | /dev/poll | select | poll ]; epoll模型</span>
<span class="token comment" spellcheck="true"> #是Linux 2.6以上版本内核中的高性能网络I/O模型,linux建议epoll,如果跑在FreeBSD上面,就用kqueue模型。</span>
<span class="token comment" spellcheck="true"> #补充说明:</span>
<span class="token comment" spellcheck="true"> #与apache相类,nginx针对不同的操作系统,有不同的事件模型</span>
<span class="token comment" spellcheck="true"> #A)标准事件模型</span>
<span class="token comment" spellcheck="true"> #Select、poll属于标准事件模型,如果当前系统不存在更有效的方法,nginx会选择select或poll</span>
<span class="token comment" spellcheck="true"> #B)高效事件模型</span>
<span class="token comment" spellcheck="true"> #Kqueue:使用于FreeBSD 4.1+, OpenBSD 2.9+, NetBSD 2.0 和 MacOS X.使用双处理器的MacOS X系统使用kqueue可能会造成内核崩溃。</span>
<span class="token comment" spellcheck="true"> #Epoll:使用于Linux内核2.6版本及以后的系统。</span>
<span class="token comment" spellcheck="true"> #/dev/poll:使用于Solaris 7 11/99+,HP/UX 11.22+ (eventport),IRIX 6.5.15+ 和 Tru64 UNIX 5.1A+。</span>
<span class="token comment" spellcheck="true"> #Eventport:使用于Solaris 10。 为了防止出现内核崩溃的问题, 有必要安装安全补丁。</span>
<span class="token attr-name"> use</span> <span class="token attr-value">epoll</span>
<span class="token comment" spellcheck="true"> #单个进程最大连接数(最大连接数=连接数+进程数)</span>
<span class="token comment" spellcheck="true"> #根据硬件调整,和前面工作进程配合起来用,尽量大,但是别把cup跑到100%就行。</span>
<span class="token attr-name"> worker_connections</span> <span class="token attr-value"> 1024;</span>
<span class="token comment" spellcheck="true"> #keepalive 超时时间</span>
<span class="token attr-name"> keepalive_timeout</span> <span class="token attr-value">60;</span>
<span class="token comment" spellcheck="true"> #客户端请求头部的缓冲区大小。这个可以根据你的系统分页大小来设置,一般一个请求头的大小不会超过1k,不过由于一般系统分页都要大于1k,所以这里设置为分页大小。</span>
<span class="token comment" spellcheck="true"> #分页大小可以用命令getconf PAGESIZE 取得。</span>
<span class="token comment" spellcheck="true"> #[root@web001 ~]# getconf PAGESIZE</span>
<span class="token comment" spellcheck="true"> #但也有client_header_buffer_size超过4k的情况,但是client_header_buffer_size该值必须设置为“系统分页大小”的整倍数。</span>
<span class="token attr-name"> client_header_buffer_size</span> <span class="token attr-value">4k;</span>
<span class="token comment" spellcheck="true"> #这个将为打开文件指定缓存,默认是没有启用的,max指定缓存数量,建议和打开文件数一致,inactive是指经过多长时间文件没被请求后删除缓存。</span>
<span class="token attr-name"> open_file_cache</span> <span class="token attr-value">max=65535 inactive=60s;</span>
<span class="token comment" spellcheck="true"> #这个是指多长时间检查一次缓存的有效信息。</span>
<span class="token comment" spellcheck="true"> #语法:open_file_cache_valid time 默认值:open_file_cache_valid 60 使用字段:http, server, location 这个指令指定了何时需要检查open_file_cache中缓存项目的有效信息.</span>
<span class="token attr-name"> open_file_cache_valid</span> <span class="token attr-value">80s;</span>
<span class="token comment" spellcheck="true"> #open_file_cache指令中的inactive参数时间内文件的最少使用次数,如果超过这个数字,文件描述符一直是在缓存中打开的,如上例,如果有一个文件在inactive时间内一次没被使用,它将被移除。</span>
<span class="token comment" spellcheck="true"> #语法:open_file_cache_min_uses number 默认值:open_file_cache_min_uses 1 使用字段:http, server, location 这个指令指定了在open_file_cache指令无效的参数中一定的时间范围内可以使用的最小文件数,如果使用更大的值,文件描述符在cache中总是打开状态.</span>
<span class="token attr-name"> open_file_cache_min_uses</span> <span class="token attr-value">1;</span>
<span class="token comment" spellcheck="true"> #语法:open_file_cache_errors on | off 默认值:open_file_cache_errors off 使用字段:http, server, location 这个指令指定是否在搜索一个文件是记录cache错误.</span>
<span class="token attr-name"> open_file_cache_errors</span> <span class="token attr-value">on;</span>
}
<span class="token comment" spellcheck="true">#设定http服务器,利用它的反向代理功能提供负载均衡支持</span>
http{
<span class="token comment" spellcheck="true"> #文件扩展名与文件类型映射表</span>
<span class="token attr-name"> include</span> <span class="token attr-value">mime.types;</span>
<span class="token comment" spellcheck="true"> #默认文件类型</span>
<span class="token attr-name"> default_type</span> <span class="token attr-value">application/octet-stream;</span>
<span class="token comment" spellcheck="true"> #默认编码</span>
<span class="token attr-name"> charset</span> <span class="token attr-value">utf-8;</span>
<span class="token comment" spellcheck="true"> #服务器名字的hash表大小</span>
<span class="token comment" spellcheck="true"> #保存服务器名字的hash表是由指令server_names_hash_max_size 和server_names_hash_bucket_size所控制的。参数hash bucket size总是等于hash表的大小,并且是一路处理器缓存大小的倍数。在减少了在内存中的存取次数后,使在处理器中加速查找hash表键值成为可能。如果hash bucket size等于一路处理器缓存的大小,那么在查找键的时候,最坏的情况下在内存中查找的次数为2。第一次是确定存储单元的地址,第二次是在存储单元中查找键 值。因此,如果Nginx给出需要增大hash max size 或 hash bucket size的提示,那么首要的是增大前一个参数的大小.</span>
<span class="token attr-name"> server_names_hash_bucket_size</span> <span class="token attr-value">128;</span>
<span class="token comment" spellcheck="true"> #客户端请求头部的缓冲区大小。这个可以根据你的系统分页大小来设置,一般一个请求的头部大小不会超过1k,不过由于一般系统分页都要大于1k,所以这里设置为分页大小。分页大小可以用命令getconf PAGESIZE取得。</span>
<span class="token attr-name"> client_header_buffer_size</span> <span class="token attr-value">32k;</span>
<span class="token comment" spellcheck="true"> #客户请求头缓冲大小。nginx默认会用client_header_buffer_size这个buffer来读取header值,如果header过大,它会使用large_client_header_buffers来读取。</span>
<span class="token attr-name"> large_client_header_buffers</span> <span class="token attr-value">4 64k;</span>
<span class="token comment" spellcheck="true"> #设定通过nginx上传文件的大小</span>
<span class="token attr-name"> client_max_body_size</span> <span class="token attr-value">8m;</span>
<span class="token comment" spellcheck="true"> #开启高效文件传输模式,sendfile指令指定nginx是否调用sendfile函数来输出文件,对于普通应用设为 on,如果用来进行下载等应用磁盘IO重负载应用,可设置为off,以平衡磁盘与网络I/O处理速度,降低系统的负载。注意:如果图片显示不正常把这个改成off。</span>
<span class="token comment" spellcheck="true"> #sendfile指令指定 nginx 是否调用sendfile 函数(zero copy 方式)来输出文件,对于普通应用,必须设为on。如果用来进行下载等应用磁盘IO重负载应用,可设置为off,以平衡磁盘与网络IO处理速度,降低系统uptime。</span>
<span class="token attr-name"> sendfile</span> <span class="token attr-value">on;</span>
<span class="token comment" spellcheck="true"> #开启目录列表访问,合适下载服务器,默认关闭。</span>
<span class="token attr-name"> autoindex</span> <span class="token attr-value">on;</span>
<span class="token comment" spellcheck="true"> #此选项允许或禁止使用socke的TCP_CORK的选项,此选项仅在使用sendfile的时候使用</span>
<span class="token attr-name"> tcp_nopush</span> <span class="token attr-value">on;</span>
<span class="token attr-name"> tcp_nodelay</span> <span class="token attr-value">on;</span>
<span class="token comment" spellcheck="true"> #长连接超时时间,单位是秒</span>
<span class="token attr-name"> keepalive_timeout</span> <span class="token attr-value">120;</span>
<span class="token comment" spellcheck="true"> #FastCGI相关参数是为了改善网站的性能:减少资源占用,提高访问速度。下面参数看字面意思都能理解。</span>
<span class="token attr-name"> fastcgi_connect_timeout</span> <span class="token attr-value">300;</span>
<span class="token attr-name"> fastcgi_send_timeout</span> <span class="token attr-value">300;</span>
<span class="token attr-name"> fastcgi_read_timeout</span> <span class="token attr-value">300;</span>
<span class="token attr-name"> fastcgi_buffer_size</span> <span class="token attr-value">64k;</span>
<span class="token attr-name"> fastcgi_buffers</span> <span class="token attr-value">4 64k;</span>
<span class="token attr-name"> fastcgi_busy_buffers_size</span> <span class="token attr-value">128k;</span>
<span class="token attr-name"> fastcgi_temp_file_write_size</span> <span class="token attr-value">128k;</span>
<span class="token comment" spellcheck="true"> #gzip模块设置</span>
<span class="token attr-name"> gzip</span> <span class="token attr-value">on; #开启gzip压缩输出</span>
<span class="token attr-name"> gzip_min_length</span> <span class="token attr-value">1k; #最小压缩文件大小</span>
<span class="token attr-name"> gzip_buffers</span> <span class="token attr-value">4 16k; #压缩缓冲区</span>
<span class="token attr-name"> gzip_http_version</span> <span class="token attr-value">1.0; #压缩版本(默认1.1,前端如果是squid2.5请使用1.0)</span>
<span class="token attr-name"> gzip_comp_level</span> <span class="token attr-value">2; #压缩等级</span>
<span class="token attr-name"> gzip_types</span> <span class="token attr-value">text/plain application/x-javascript text/css application/xml; #压缩类型,默认就已经包含textml,所以下面就不用再写了,写上去也不会有问题,但是会有一个warn。</span>
<span class="token attr-name"> gzip_vary</span> <span class="token attr-value">on;</span>
<span class="token comment" spellcheck="true"> #开启限制IP连接数的时候需要使用</span>
<span class="token comment" spellcheck="true"> #limit_zone crawler $binary_remote_addr 10m;</span>
<span class="token comment" spellcheck="true"> #负载均衡配置</span>
<span class="token attr-name"> upstream</span> <span class="token attr-value">piao.jd.com {</span>
<span class="token comment" spellcheck="true"> #upstream的负载均衡,weight是权重,可以根据机器配置定义权重。weigth参数表示权值,权值越高被分配到的几率越大。</span>
<span class="token attr-name"> server</span> <span class="token attr-value">192.168.80.121:80 weight=3;</span>
<span class="token attr-name"> server</span> <span class="token attr-value">192.168.80.122:80 weight=2;</span>
<span class="token attr-name"> server</span> <span class="token attr-value">192.168.80.123:80 weight=3;</span>
<span class="token comment" spellcheck="true"> #nginx的upstream目前支持4种方式的分配</span>
<span class="token comment" spellcheck="true"> #1、轮询(默认)</span>
<span class="token comment" spellcheck="true"> #每个请求按时间顺序逐一分配到不同的后端服务器,如果后端服务器down掉,能自动剔除。</span>
<span class="token comment" spellcheck="true"> #2、weight</span>
<span class="token comment" spellcheck="true"> #指定轮询几率,weight和访问比率成正比,用于后端服务器性能不均的情况。</span>
<span class="token comment" spellcheck="true"> #例如:</span>
<span class="token comment" spellcheck="true"> #upstream bakend {</span>
<span class="token comment" spellcheck="true"> # server 192.168.0.14 weight=10;</span>
<span class="token comment" spellcheck="true"> # server 192.168.0.15 weight=10;</span>
<span class="token comment" spellcheck="true"> #}</span>
<span class="token comment" spellcheck="true"> #2、ip_hash</span>
<span class="token comment" spellcheck="true"> #每个请求按访问ip的hash结果分配,这样每个访客固定访问一个后端服务器,可以解决session的问题。</span>
<span class="token comment" spellcheck="true"> #例如:</span>
<span class="token comment" spellcheck="true"> #upstream bakend {</span>
<span class="token comment" spellcheck="true"> # ip_hash;</span>
<span class="token comment" spellcheck="true"> # server 192.168.0.14:88;</span>
<span class="token comment" spellcheck="true"> # server 192.168.0.15:80;</span>
<span class="token comment" spellcheck="true"> #}</span>
<span class="token comment" spellcheck="true"> #3、fair(第三方)</span>
<span class="token comment" spellcheck="true"> #按后端服务器的响应时间来分配请求,响应时间短的优先分配。</span>
<span class="token comment" spellcheck="true"> #upstream backend {</span>
<span class="token comment" spellcheck="true"> # server server1;</span>
<span class="token comment" spellcheck="true"> # server server2;</span>
<span class="token comment" spellcheck="true"> # fair;</span>
<span class="token comment" spellcheck="true"> #}</span>
<span class="token comment" spellcheck="true"> #4、url_hash(第三方)</span>
<span class="token comment" spellcheck="true"> #按访问url的hash结果来分配请求,使每个url定向到同一个后端服务器,后端服务器为缓存时比较有效。</span>
<span class="token comment" spellcheck="true"> #例:在upstream中加入hash语句,server语句中不能写入weight等其他的参数,hash_method是使用的hash算法</span>
<span class="token comment" spellcheck="true"> #upstream backend {</span>
<span class="token comment" spellcheck="true"> # server squid1:3128;</span>
<span class="token comment" spellcheck="true"> # server squid2:3128;</span>
<span class="token comment" spellcheck="true"> # hash $request_uri;</span>
<span class="token comment" spellcheck="true"> # hash_method crc32;</span>
<span class="token comment" spellcheck="true"> #}</span>
<span class="token comment" spellcheck="true"> #tips:</span>
<span class="token comment" spellcheck="true"> #upstream bakend</span>
<span class="token comment" spellcheck="true"> #在需要使用负载均衡的server中增加 proxy_pass http://bakend/;</span>
<span class="token comment" spellcheck="true"> #每个设备的状态设置为:</span>
<span class="token comment" spellcheck="true"> #1.down表示单前的server暂时不参与负载</span>
<span class="token comment" spellcheck="true"> #2.weight为weight越大,负载的权重就越大。</span>
<span class="token comment" spellcheck="true"> #3.max_fails:允许请求失败的次数默认为1.当超过最大次数时,返回proxy_next_upstream模块定义的错误</span>
<span class="token comment" spellcheck="true"> #4.fail_timeout:max_fails次失败后,暂停的时间。</span>
<span class="token comment" spellcheck="true"> #5.backup: 其它所有的非backup机器down或者忙的时候,请求backup机器。所以这台机器压力会最轻。</span>
<span class="token comment" spellcheck="true"> #nginx支持同时设置多组的负载均衡,用来给不用的server来使用。</span>
<span class="token comment" spellcheck="true"> #client_body_in_file_only设置为On 可以讲client post过来的数据记录到文件中用来做debug</span>
<span class="token comment" spellcheck="true"> #client_body_temp_path设置记录文件的目录 可以设置最多3层目录</span>
<span class="token comment" spellcheck="true"> #location对URL进行匹配.可以进行重定向或者进行新的代理 负载均衡</span>
}
<span class="token comment" spellcheck="true"> #虚拟主机的配置</span>
<span class="token attr-name"> server</span> <span class="token attr-value">{</span>
<span class="token comment" spellcheck="true"> #监听端口</span>
<span class="token attr-name"> listen</span> <span class="token attr-value">80;</span>
<span class="token comment" spellcheck="true"> #域名可以有多个,用空格隔开</span>
<span class="token attr-name"> server_name</span> <span class="token attr-value">www.jd.com jd.com;</span>
<span class="token comment" spellcheck="true"> #默认入口文件名称</span>
<span class="token attr-name"> index</span> <span class="token attr-value">index.html index.htm index.php;</span>
<span class="token attr-name"> root</span> <span class="token attr-value">/data/www/jd;</span>
<span class="token comment" spellcheck="true"> #对******进行负载均衡</span>
<span class="token attr-name"> location</span> <span class="token attr-value">~ .*.(php|php5)?$</span>
{
<span class="token attr-name"> fastcgi_pass</span> <span class="token attr-value">127.0.0.1:9000;</span>
<span class="token attr-name"> fastcgi_index</span> <span class="token attr-value">index.php;</span>
<span class="token attr-name"> include</span> <span class="token attr-value">fastcgi.conf;</span>
}
<span class="token comment" spellcheck="true"> #图片缓存时间设置</span>
<span class="token attr-name"> location</span> <span class="token attr-value">~ .*.(gif|jpg|jpeg|png|bmp|swf)$</span>
{
<span class="token attr-name"> expires</span> <span class="token attr-value">10d;</span>
}
<span class="token comment" spellcheck="true"> #JS和CSS缓存时间设置</span>
<span class="token attr-name"> location</span> <span class="token attr-value">~ .*.(js|css)?$</span>
{
<span class="token attr-name"> expires</span> <span class="token attr-value">1h;</span>
}
<span class="token comment" spellcheck="true"> #日志格式设定</span>
<span class="token comment" spellcheck="true"> #$remote_addr与$http_x_forwarded_for用以记录客户端的ip地址;</span>
<span class="token comment" spellcheck="true"> #$remote_user:用来记录客户端用户名称;</span>
<span class="token comment" spellcheck="true"> #$time_local: 用来记录访问时间与时区;</span>
<span class="token comment" spellcheck="true"> #$request: 用来记录请求的url与http协议;</span>
<span class="token comment" spellcheck="true"> #$status: 用来记录请求状态;成功是200,</span>
<span class="token comment" spellcheck="true"> #$body_bytes_sent :记录发送给客户端文件主体内容大小;</span>
<span class="token comment" spellcheck="true"> #$http_referer:用来记录从那个页面链接访问过来的;</span>
<span class="token comment" spellcheck="true"> #$http_user_agent:记录客户浏览器的相关信息;</span>
<span class="token comment" spellcheck="true"> #通常web服务器放在反向代理的后面,这样就不能获取到客户的IP地址了,通过$remote_add拿到的IP地址是反向代理服务器的iP地址。反向代理服务器在转发请求的http头信息中,可以增加x_forwarded_for信息,用以记录原有客户端的IP地址和原来客户端的请求的服务器地址。</span>
<span class="token attr-name"> log_format</span> <span class="token attr-value">access '$remote_addr - $remote_user [$time_local] "$request" '</span>
<span class="token attr-name"> '$status</span> <span class="token attr-value">$body_bytes_sent "$http_referer" '</span>
<span class="token attr-name"> '"$http_user_agent"</span> <span class="token attr-value">$http_x_forwarded_for';</span>
<span class="token comment" spellcheck="true"> #定义本虚拟主机的访问日志</span>
<span class="token attr-name"> access_log</span> <span class="token attr-value"> /usr/local/nginx/logs/host.access.log main;</span>
<span class="token attr-name"> access_log</span> <span class="token attr-value"> /usr/local/nginx/logs/host.access.404.log log404;</span>
<span class="token comment" spellcheck="true"> #对 "/connect-controller" 启用反向代理</span>
<span class="token attr-name"> location</span> <span class="token attr-value">/connect-controller {</span>
<span class="token attr-name"> proxy_pass</span> <span class="token attr-value">http://127.0.0.1:88; #请注意此处端口号不能与虚拟主机监听的端口号一样(也就是server监听的端口)</span>
<span class="token attr-name"> proxy_redirect</span> <span class="token attr-value">off;</span>
<span class="token attr-name"> proxy_set_header</span> <span class="token attr-value">X-Real-IP $remote_addr;</span>
<span class="token comment" spellcheck="true"> #后端的Web服务器可以通过X-Forwarded-For获取用户真实IP</span>
<span class="token attr-name"> proxy_set_header</span> <span class="token attr-value">X-Forwarded-For $proxy_add_x_forwarded_for;</span>
<span class="token comment" spellcheck="true"> #以下是一些反向代理的配置,可选。</span>
<span class="token attr-name"> proxy_set_header</span> <span class="token attr-value">Host $host;</span>
<span class="token comment" spellcheck="true"> #允许客户端请求的最大单文件字节数</span>
<span class="token attr-name"> client_max_body_size</span> <span class="token attr-value">10m;</span>
<span class="token comment" spellcheck="true"> #缓冲区代理缓冲用户端请求的最大字节数,</span>
<span class="token comment" spellcheck="true"> #如果把它设置为比较大的数值,例如256k,那么,无论使用firefox还是IE浏览器,来提交任意小于256k的图片,都很正常。如果注释该指令,使用默认的client_body_buffer_size设置,也就是操作系统页面大小的两倍,8k或者16k,问题就出现了。</span>
<span class="token comment" spellcheck="true"> #无论使用firefox4.0还是IE8.0,提交一个比较大,200k左右的图片,都返回500 Internal Server Error错误</span>
<span class="token attr-name"> client_body_buffer_size</span> <span class="token attr-value">128k;</span>
<span class="token comment" spellcheck="true"> #表示使nginx阻止HTTP应答代码为400或者更高的应答。</span>
<span class="token attr-name"> proxy_intercept_errors</span> <span class="token attr-value">on;</span>
<span class="token comment" spellcheck="true"> #后端服务器连接的超时时间_发起握手等候响应超时时间</span>
<span class="token comment" spellcheck="true"> #nginx跟后端服务器连接超时时间(代理连接超时)</span>
<span class="token attr-name"> proxy_connect_timeout</span> <span class="token attr-value">90;</span>
<span class="token comment" spellcheck="true"> #后端服务器数据回传时间(代理发送超时)</span>
<span class="token comment" spellcheck="true"> #后端服务器数据回传时间_就是在规定时间之内后端服务器必须传完所有的数据</span>
<span class="token attr-name"> proxy_send_timeout</span> <span class="token attr-value">90;</span>
<span class="token comment" spellcheck="true"> #连接成功后,后端服务器响应时间(代理接收超时)</span>
<span class="token comment" spellcheck="true"> #连接成功后_等候后端服务器响应时间_其实已经进入后端的排队之中等候处理(也可以说是后端服务器处理请求的时间)</span>
<span class="token attr-name"> proxy_read_timeout</span> <span class="token attr-value">90;</span>
<span class="token comment" spellcheck="true"> #设置代理服务器(nginx)保存用户头信息的缓冲区大小</span>
<span class="token comment" spellcheck="true"> #设置从被代理服务器读取的第一部分应答的缓冲区大小,通常情况下这部分应答中包含一个小的应答头,默认情况下这个值的大小为指令proxy_buffers中指定的一个缓冲区的大小,不过可以将其设置为更小</span>
<span class="token attr-name"> proxy_buffer_size</span> <span class="token attr-value">4k;</span>
<span class="token comment" spellcheck="true"> #proxy_buffers缓冲区,网页平均在32k以下的设置</span>
<span class="token comment" spellcheck="true"> #设置用于读取应答(来自被代理服务器)的缓冲区数目和大小,默认情况也为分页大小,根据操作系统的不同可能是4k或者8k</span>
<span class="token attr-name"> proxy_buffers</span> <span class="token attr-value">4 32k;</span>
<span class="token comment" spellcheck="true"> #高负荷下缓冲大小(proxy_buffers*2)</span>
<span class="token attr-name"> proxy_busy_buffers_size</span> <span class="token attr-value">64k;</span>
<span class="token comment" spellcheck="true"> #设置在写入proxy_temp_path时数据的大小,预防一个工作进程在传递文件时阻塞太长</span>
<span class="token comment" spellcheck="true"> #设定缓存文件夹大小,大于这个值,将从upstream服务器传</span>
<span class="token attr-name"> proxy_temp_file_write_size</span> <span class="token attr-value">64k;</span>
}
<span class="token comment" spellcheck="true"> #本地动静分离反向代理配置</span>
<span class="token comment" spellcheck="true"> #所有jsp的页面均交由tomcat或resin处理</span>
<span class="token attr-name"> location</span> <span class="token attr-value">~ .(jsp|jspx|do)?$ {</span>
<span class="token attr-name"> proxy_set_header</span> <span class="token attr-value">Host $host;</span>
<span class="token attr-name"> proxy_set_header</span> <span class="token attr-value">X-Real-IP $remote_addr;</span>
<span class="token attr-name"> proxy_set_header</span> <span class="token attr-value">X-Forwarded-For $proxy_add_x_forwarded_for;</span>
<span class="token attr-name"> proxy_pass</span> <span class="token attr-value">http://127.0.0.1:8080;</span>
}
}
}</code></pre>
<h2 id="总结"><a href="#总结" class="headerlink" title="总结"></a>总结</h2><p>Nginx 配置文件主要分成四部分:main(全局设置)、server(主机设置)、upstream(上游服务器设置,主要为反向代理、负载均衡相关配置)和 location(URL匹配特定位置后的设置)。</p>
<p>main 部分设置的指令影响其他所有部分的设置;</p>
<p>server 部分的指令主要用于制定虚拟主机域名、IP 和端口号;</p>
<p>upstream 的指令用于设置一系列的后端服务器,设置反向代理及后端服务器的负载均衡;</p>
<p>location 部分用于匹配网页位置(比如,根目录“/”,“/images”,等等)。</p>
<p>他们之间的关系:server 继承 main,location 继承 server;upstream 既不会继承指令也不会被继承。</p>
<h1 id="9-反向代理实例一"><a href="#9-反向代理实例一" class="headerlink" title="9. 反向代理实例一"></a>9. 反向代理实例一</h1><p>实现效果:使用 nginx 反向代理,访问 <a href="http://www.123.com" target="_blank" rel="noopener">www.123.com</a> 直接跳转到 127.0.0.1:8080</p>
<h2 id="实现过程"><a href="#实现过程" class="headerlink" title="实现过程"></a>实现过程</h2><h3 id="9-1-启动一个-tomcat,浏览器地址栏输入-127-0-0-1-8080,出现如下界面"><a href="#9-1-启动一个-tomcat,浏览器地址栏输入-127-0-0-1-8080,出现如下界面" class="headerlink" title="9.1. 启动一个 tomcat,浏览器地址栏输入 127.0.0.1:8080,出现如下界面"></a>9.1. 启动一个 tomcat,浏览器地址栏输入 127.0.0.1:8080,出现如下界面</h3><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521173446.png" alt=""></p>
<h3 id="9-2-通过修改本地-host-文件,将-www-123-com-映射到-127-0-0-1"><a href="#9-2-通过修改本地-host-文件,将-www-123-com-映射到-127-0-0-1" class="headerlink" title="9.2. 通过修改本地 host 文件,将 www.123.com 映射到 127.0.0.1"></a>9.2. 通过修改本地 host 文件,将 <a href="http://www.123.com" target="_blank" rel="noopener">www.123.com</a> 映射到 127.0.0.1</h3><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521173537.png" alt=""></p>
<p>配置完成之后,我们便可以通过 <a href="http://www.123.com:8080" target="_blank" rel="noopener">www.123.com:8080</a> 访问到第一步出现的 Tomcat 初始界面。那么如何只需要输入 <a href="http://www.123.com" target="_blank" rel="noopener">www.123.com</a> 便可以跳转到 Tomcat 初始界面呢?便用到 nginx 的反向代理。</p>
<h3 id="9-3-在-nginx-conf-配置文件中增加如下配置"><a href="#9-3-在-nginx-conf-配置文件中增加如下配置" class="headerlink" title="9.3. 在 nginx.conf 配置文件中增加如下配置"></a>9.3. <strong>在 nginx.conf 配置文件中增加如下配置</strong></h3><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521173630.png" alt=""></p>
<p>如上配置,我们监听 80 端口,访问域名为 <a href="http://www.123.com,不加端口号时默认为" target="_blank" rel="noopener">www.123.com,不加端口号时默认为</a> 80 端口,故访问该域名时会跳转到 127.0.0.1:8080 路径上。在浏览器端输入 <a href="http://www.123.com" target="_blank" rel="noopener">www.123.com</a> 结果如下:</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521173703.png" alt=""></p>
<h1 id="10-反向代理实例二"><a href="#10-反向代理实例二" class="headerlink" title="10. 反向代理实例二"></a>10. 反向代理实例二</h1><p>实现效果:使用 nginx 反向代理,根据访问的路径跳转到不同端口的服务中 nginx 监听端口为 9001,</p>
<blockquote>
<p>访问 <a href="http://127.0.0.1:9001/edu/" target="_blank" rel="noopener">http://127.0.0.1:9001/edu/</a> 直接跳转到 127.0.0.1:8081 </p>
<p>访问 <a href="http://127.0.0.1:9001/vod/" target="_blank" rel="noopener">http://127.0.0.1:9001/vod/</a> 直接跳转到 127.0.0.1:8082</p>
</blockquote>
<h2 id="实现过程-1"><a href="#实现过程-1" class="headerlink" title="实现过程"></a>实现过程</h2><h3 id="10-1-准备两个-tomcat,一个-8001-端口,一个-8002-端口,并准备好测试的页面"><a href="#10-1-准备两个-tomcat,一个-8001-端口,一个-8002-端口,并准备好测试的页面" class="headerlink" title="10.1.准备两个 tomcat,一个 8001 端口,一个 8002 端口,并准备好测试的页面"></a>10.1.准备两个 tomcat,一个 8001 端口,一个 8002 端口,并准备好测试的页面</h3><h3 id="10-2-修改-nginx-的配置文件在-http-块中添加-server"><a href="#10-2-修改-nginx-的配置文件在-http-块中添加-server" class="headerlink" title="10.2. 修改 nginx 的配置文件在 http 块中添加 server{}"></a>10.2. 修改 nginx 的配置文件在 http 块中添加 server{}</h3><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521173939.png" alt=""></p>
<p><strong>location</strong> <strong>指令说明</strong> 该指令用于匹配 URL。</p>
<p>语法如下:</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521174037.png" alt=""></p>
<ol>
<li>= :用于不含正则表达式的 uri 前,要求请求字符串与 uri 严格匹配,如果匹配成功,就停止继续向下搜索并立即处理该请求。</li>
<li>~:用于表示 uri 包含正则表达式,并且区分大小写。</li>
<li>~*:用于表示 uri 包含正则表达式,并且不区分大小写。</li>
<li>^~:用于不含正则表达式的 uri 前,要求 Nginx 服务器找到标识 uri 和请求字符串匹配度最高的 location 后,立即使用此 location 处理请求,而不再使用 location 块中的正则 uri 和请求字符串做匹配。</li>
</ol>
<p><strong>注意:如果 uri 包含正则表达式,则必须要有 ~ 或者 ~* 标识。</strong></p>
<h1 id="11负载均衡"><a href="#11负载均衡" class="headerlink" title="11负载均衡"></a>11负载均衡</h1><h2 id="11-1首先准备两个同时启动的-Tomcat"><a href="#11-1首先准备两个同时启动的-Tomcat" class="headerlink" title="11.1首先准备两个同时启动的 Tomcat"></a>11.1<strong>首先准备两个同时启动的</strong> <strong>Tomcat</strong></h2><h2 id="11-2-在-nginx-conf-中进行配置"><a href="#11-2-在-nginx-conf-中进行配置" class="headerlink" title="11.2.在 nginx.conf 中进行配置"></a>11.2.<strong>在 nginx.conf 中进行配置</strong></h2><p><strong>在 nginx.conf 中进行配置</strong></p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521183833.png" alt=""></p>
<p>随着互联网信息的爆炸性增长,负载均衡(load balance)已经不再是一个很陌生的话题,顾名思义,负载均衡即是将负载分摊到不同的服务单元,既保证服务的可用性,又保证响应足够快,给用户很好的体验。快速增长的访问量和数据流量催生了各式各样的负载均衡产品,很多专业的负载均衡硬件提供了很好的功能,但却价格不菲,这使得负载均衡软件大受欢迎, nginx 就是其中的一个,在 linux 下有 Nginx、LVS、Haproxy 等等服务可以提供负载均衡服务,而且 Nginx 提供了几种分配方式(策略):</p>
<p> <strong>1、轮询(默认)</strong></p>
<p>每个请求按时间顺序逐一分配到不同的后端服务器,如果后端服务器 down 掉,能自动剔除。</p>
<p><strong>2、weight</strong></p>
<p>weight 代表权,重默认为 1,权重越高被分配的客户端越多</p>
<p> 指定轮询几率,weight 和访问比率成正比,用于后端服务器性能不均的情况。 例如:</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521184047.png" alt=""></p>
<p><strong>3、ip_hash</strong></p>
<p>每个请求按访问 ip 的 hash 结果分配,这样每个访客固定访问一个后端服务器,可以解决 session 的问题。例如:</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521184132.png" alt=""></p>
<p><strong>4、fair(第三方)</strong></p>
<p>按后端服务器的响应时间来分配请求,响应时间短的优先分配。</p>
<p> <img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521184224.png" alt=""></p>
<h1 id="12-动静分离"><a href="#12-动静分离" class="headerlink" title="12.动静分离"></a>12.动静分离</h1><h2 id="12-1-什么是动静分离"><a href="#12-1-什么是动静分离" class="headerlink" title="12.1 什么是动静分离"></a>12.1 什么是动静分离</h2><p>Nginx 动静分离简单来说就是把动态跟静态请求分开,不能理解成只是单纯的把动态页面和静态页面物理分离。严格意义上说应该是动态请求跟静态请求分开,可以理解成使用 Nginx 处理静态页面,Tomcat 处理动态页面。动静分离从目前实现角度来讲大致分为两种,</p>
<p><strong>一种是纯粹把静态文件独立成单独的域名,放在独立的服务器上,也是目前主流推崇的方案</strong>;</p>
<p><strong>另外一种方法就是动态跟静态文件混合在一起发布,通过 nginx 来分开</strong>。</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521204548.png" alt=""></p>
<p>通过 location 指定不同的后缀名实现不同的请求转发。通过 expires 参数设置,可以使浏览器缓存过期时间,减少与服务器之前的请求和流量。具体 Expires 定义:是给一个资源设定一个过期时间,也就是说无需去服务端验证,直接通过浏览器自身确认是否过期即可,所以不会产生额外的流量。此种方法非常适合不经常变动的资源。(如果经常更新的文件,不建议使用 Expires 来缓存),我这里设置 3d,表示在这 3 天之内访问这个 URL,发送一个请求,比对服务器该文件最后更新时间没有变化,则不会从服务器抓取,返回状态码304,如果有修改,则直接从服务器重新下载,返回状态码 200。</p>
<h2 id="12-2准备工作"><a href="#12-2准备工作" class="headerlink" title="12.2准备工作"></a>12.2准备工作</h2><p><strong>在 liunx 系统中准备静态资源,用于进行访问</strong></p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521204736.png" alt=""></p>
<h2 id="12-3-具体配置"><a href="#12-3-具体配置" class="headerlink" title="12.3 具体配置"></a>12.3 具体配置</h2><p><strong>在</strong> <strong>nginx</strong> <strong>配置文件中进行配置</strong></p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521204831.png" alt=""></p>
<h2 id="12-4-最终测试"><a href="#12-4-最终测试" class="headerlink" title="12.4 最终测试"></a>12.4 最终测试</h2><p><strong>浏览器中输入地址</strong> </p>
<p>http://服务器ip/image/01.jpg</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521205251.png" alt=""></p>
<p><strong>因为配置文件</strong> *<em>autoindex on *</em> 列出文件夹中的文件</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521205456.png" alt=""></p>
<p><strong>在浏览器地址栏输入地址</strong></p>
<p>http://服务器ip/www/a.html</p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521205633.png" alt=""></p>
<h1 id="13-高可用集群"><a href="#13-高可用集群" class="headerlink" title="13.高可用集群"></a>13.高可用集群</h1><h2 id="13-1-Keepalived-Nginx-高可用集群(主从模式)"><a href="#13-1-Keepalived-Nginx-高可用集群(主从模式)" class="headerlink" title="13.1. Keepalived+Nginx 高可用集群(主从模式)"></a>13.1. Keepalived+Nginx 高可用集群(主从模式)</h2><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521211609.png" alt=""></p>
<h1 id=""><a href="#" class="headerlink" title=""></a></h1><h2 id="13-2-配置高可用的准备工作"><a href="#13-2-配置高可用的准备工作" class="headerlink" title="13.2. 配置高可用的准备工作"></a>13.2. 配置高可用的准备工作</h2><ol>
<li>需要两台服务器</li>
<li>需要keepalived</li>
<li>需要虚拟ip</li>
</ol>
<h2 id="13-3-在两台服务器上安装keepalived"><a href="#13-3-在两台服务器上安装keepalived" class="headerlink" title="13.3. 在两台服务器上安装keepalived"></a>13.3. 在两台服务器上安装keepalived</h2><ol>
<li><p>使用yum命令安装</p>
<pre class=" language-shell"><code class="language-shell">yum install keepalived-v</code></pre>
</li>
<li><p>安装完成之后,在etc里面生成keepalived,有文件keepalived.conf</p>
</li>
</ol>
<h2 id="13-4-完成高可用配置-主从配置"><a href="#13-4-完成高可用配置-主从配置" class="headerlink" title="13.4. 完成高可用配置(主从配置)"></a>13.4. 完成高可用配置(主从配置)</h2><ol>
<li>修改<code>/etc/keepalived/keepalived.conf</code>配置文件</li>
</ol>
<pre class=" language-properties"><code class="language-properties"><span class="token attr-name">global_defs</span> <span class="token attr-value">{</span>
<span class="token attr-name">notification_email</span> <span class="token attr-value">{</span>
<EMAIL>
<EMAIL>
<EMAIL>
}
<span class="token attr-name">notification_email_from</span> <span class="token attr-value"><EMAIL></span>
<span class="token attr-name">smtp_server</span> <span class="token attr-value">192.168.17.129</span>
<span class="token attr-name">smtp_connect_timeout</span> <span class="token attr-value">30</span>
<span class="token attr-name">router_id</span> <span class="token attr-value">LVS_DEVEL</span>
}
<span class="token attr-name">vrrp_script</span> <span class="token attr-value">chk_http_port {</span>
<span class="token attr-name">script</span> <span class="token attr-value">"/usr/local/src/nginx_check.sh"</span>
<span class="token attr-name">interval</span> <span class="token attr-value">2 #(检测脚本执行的间隔)</span>
<span class="token attr-name">weight</span> <span class="token attr-value">2</span>
}
<span class="token attr-name">vrrp_instance</span> <span class="token attr-value">VI_1 {</span>
<span class="token attr-name">state</span> <span class="token attr-value">BACKUP # 备份服务器上将 MASTER 改为 BACKUP</span>
<span class="token attr-name">interface</span> <span class="token attr-value">ens33 //网卡</span>
<span class="token attr-name">virtual_router_id</span> <span class="token attr-value">51 # 主、备机的 virtual_router_id 必须相同</span>
<span class="token attr-name">priority</span> <span class="token attr-value">100 # 主、备机取不同的优先级,主机值较大,备份机值较小</span>
<span class="token attr-name">advert_int</span> <span class="token attr-value">1</span>
<span class="token attr-name">authentication</span> <span class="token attr-value">{</span>
<span class="token attr-name">auth_type</span> <span class="token attr-value">PASS</span>
<span class="token attr-name">auth_pass</span> <span class="token attr-value"><PASSWORD></span>
}
<span class="token attr-name">virtual_ipaddress</span> <span class="token attr-value">{</span>
<span class="token attr-name">192.168.17.50</span> <span class="token attr-value">// VRRP H 虚拟地址</span>
}
}
</code></pre>
<ol start="2">
<li>在<code>/usr/local/src</code>添加检测脚本</li>
</ol>
<pre class=" language-bash"><code class="language-bash"><span class="token shebang important">#!/bin/bash</span>
A<span class="token operator">=</span><span class="token variable"><span class="token variable">`</span><span class="token function">ps</span> -C nginx –no-header <span class="token operator">|</span><span class="token function">wc</span> -l<span class="token variable">`</span></span>
<span class="token keyword">if</span> <span class="token punctuation">[</span> <span class="token variable">$A</span> -eq 0 <span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token keyword">then</span> /usr/local/nginx/sbin/nginx
<span class="token function">sleep</span> 2
<span class="token keyword">if</span> <span class="token punctuation">[</span> <span class="token variable"><span class="token variable">`</span><span class="token function">ps</span> -C nginx --no-header <span class="token operator">|</span><span class="token function">wc</span> -l<span class="token variable">`</span></span> -eq 0 <span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token keyword">then</span> <span class="token function">killall</span> keepalived
<span class="token keyword">fi</span>
<span class="token keyword">fi</span></code></pre>
<ol start="3">
<li>把两台服务器上nginx和keepalived启动</li>
</ol>
<p>启动nginx </p>
<pre class=" language-shell"><code class="language-shell">systemctl start nginx</code></pre>
<p>启动keepalived</p>
<pre class=" language-shell"><code class="language-shell">systemctl start keepalived.service</code></pre>
<h2 id="13-5-最终测试"><a href="#13-5-最终测试" class="headerlink" title="13.5. 最终测试"></a>13.5. 最终测试</h2><ol>
<li>在浏览器地址栏输入虚拟地址ip 192.168.17.50</li>
</ol>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521213142.png" alt=""></p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521213213.png" alt=""></p>
<ol start="2">
<li>把主服务器(192.168.17.129) nginx和keepalived停止,在输入192.168.17.50</li>
</ol>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521213341.png" alt=""></p>
<p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521213419.png" alt=""></p>
<h1 id="14-Nginx原理及优化参数配置"><a href="#14-Nginx原理及优化参数配置" class="headerlink" title="14.Nginx原理及优化参数配置"></a>14.Nginx原理及优化参数配置</h1><h2 id="14-1master和worker"><a href="#14-1master和worker" class="headerlink" title="14.1master和worker"></a>14.1master和worker</h2><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521205820.png" alt=""></p>
<h2 id="14-2worker如何进行工作"><a href="#14-2worker如何进行工作" class="headerlink" title="14.2worker如何进行工作"></a>14.2worker如何进行工作</h2><p><img src="https://gitee.com/krislin_zhao/IMGcloud/raw/master/img/20200521205949.png" alt=""></p>
<h2 id="14-3-一个master和多个woker有好处"><a href="#14-3-一个master和多个woker有好处" class="headerlink" title="14.3 一个master和多个woker有好处"></a>14.3 一个master和多个woker有好处</h2><p>首先,对于每个 worker 进程来说,独立的进程,不需要加锁,所以省掉了锁带来的开销,同时在编程以及问题查找时,也会方便很多。其次,采用独立的进程,可以让互相之间不会影响,一个进程退出后,其它进程还在工作,服务不会中断,master 进程则很快启动新的 worker 进程。当然,worker 进程的异常退出,肯定是程序有 bug 了,异常退出,会导致当前 worker 上的所有请求失败,不过不会影响到所有请求,所以降低了风险。</p>
<ol>
<li>可以使用nginx –s reload热部署,利用nginx进行热部署操作</li>
<li>每个woker是独立的进程,如果有其中的一个woker出现问题,其他woker独立的,继续进行争抢,实现请求过程,不会造成服务中断</li>
</ol>
<h2 id="14-4设置多少个worker合适"><a href="#14-4设置多少个worker合适" class="headerlink" title="14.4设置多少个worker合适"></a>14.4设置多少个worker合适</h2><p>Nginx 同 redis 类似都采用了 io 多路复用机制,每个 worker 都是一个独立的进程,但每个进程里只有一个主线程,通过异步非阻塞的方式来处理请求, 即使是千上万个请求也不在话下。每个 worker 的线程可以把一个 cpu 的性能发挥到极致。所以 worker 数和服务器的 cpu 数相等是最为适宜的。设少了会浪费 cpu,设多了会造成 cpu 频繁切换上下文带来的损耗。</p>
<p>设置worker 数量: worker_processes 4 work 绑定 cpu(4 work 绑定 4cpu)。</p>
<h2 id="14-5-连接数worker-connection"><a href="#14-5-连接数worker-connection" class="headerlink" title="14.5 连接数worker_connection"></a>14.5 连接数worker_connection</h2><p>这个值是表示每个 worker 进程所能建立连接的最大值,所以,一个 nginx 能建立的最大连接数,应该是 worker_connections * worker_processes。当然,这里说的是最大连接数,对于 HTTP 请 求 本 地 资 源来 说 , 能 够 支 持 的 最大 并 发 数 量 是 worker_connections * worker_processes,如果是支持 http1.1 的浏览器每次访问要占两个连接,所以普通的静态访问最大并发数是: worker_connections * worker_processes /2,而如果是 HTTP 作 为反向代理来说,最大并发数量应该是 worker_connections *worker_processes/4。因为作为反向代理服务器,每个并发会建立与客户端的连接和与后端服务的连接,会占用两个连接。</p>
<p>第一个:发送请求,占用了woker 的几个连接数?</p>
<p> 答案:2或者4个</p>
<p>第二个:nginx有一个master,有四个woker,每个woker支持最大的连接数1024,支持的最大并发数是多少?</p>
<p>普通的静态访问最大并发数是: worker_connections * worker_processes /2,</p>
<p>而如果是HTTP作为反向代理来说,最大并发数量应该是worker_connections * worker_processes/4。</p>
<script>
document.querySelectorAll('.github-emoji')
.forEach(el => {
if (!el.dataset.src) { return; }
const img = document.createElement('img');
img.style = 'display:none !important;';
img.src = el.dataset.src;
img.addEventListener('error', () => {
img.remove();
el.style.color = 'inherit';
el.style.backgroundImage = 'none';
el.style.background = 'none';
});
img.addEventListener('load', () => {
img.remove();
});
document.body.appendChild(img);
});
</script>
</div>
<hr/>
<div class="reprint" id="reprint-statement">
<div class="reprint__author">
<span class="reprint-meta" style="font-weight: bold;">
<i class="fas fa-user">
文章作者:
</i>
</span>
<span class="reprint-info">
<a href="http://yuimm.github.io" rel="external nofollow noreferrer">Yuimm</a>
</span>
</div>
<div class="reprint__type">
<span class="reprint-meta" style="font-weight: bold;">
<i class="fas fa-link">
文章链接:
</i>
</span>
<span class="reprint-info">
<a href="http://yuimm.github.io/posts/nginx.html">http://yuimm.github.io/posts/nginx.html</a>
</span>
</div>
<div class="reprint__notice">
<span class="reprint-meta" style="font-weight: bold;">
<i class="fas fa-copyright">
版权声明:
</i>
</span>
<span class="reprint-info">
本博客所有文章除特別声明外,均采用
<a href="https://creativecommons.org/licenses/by/4.0/deed.zh" rel="external nofollow noreferrer" target="_blank">CC BY 4.0</a>
许可协议。转载请注明来源
<a href="http://yuimm.github.io" target="_blank">Yuimm</a>
!
</span>
</div>
</div>
<script async defer>
document.addEventListener("copy", function (e) {
let toastHTML = '<span>复制成功,请遵循本文的转载规则</span><button class="btn-flat toast-action" onclick="navToReprintStatement()" style="font-size: smaller">查看</a>';
M.toast({html: toastHTML})
});
function navToReprintStatement() {
$("html, body").animate({scrollTop: $("#reprint-statement").offset().top - 80}, 800);
}
</script>
<div class="tag_share" style="display: block;">
<div class="post-meta__tag-list" style="display: inline-block;">
<div class="article-tag">
<a href="/tags/%E8%B4%9F%E8%BD%BD%E5%9D%87%E8%A1%A1%E4%B8%AD%E9%97%B4%E4%BB%B6/">
<span class="chip bg-color">负载均衡中间件</span>
</a>
</div>
</div>
<div class="post_share" style="zoom: 80%; width: fit-content; display: inline-block; float: right; margin: -0.15rem 0;">
<link rel="stylesheet" type="text/css" href="/libs/share/css/share.min.css">
<div id="article-share">
<div class="social-share" data-sites="twitter,facebook,google,qq,qzone,wechat,weibo,douban,linkedin" data-wechat-qrcode-helper="<p>微信扫一扫即可分享!</p>"></div>
<script src="/libs/share/js/social-share.min.js"></script>
</div>
</div>
</div>
<style>
#reward {
margin: 40px 0;
text-align: center;
}
#reward .reward-link {
font-size: 1.4rem;
line-height: 38px;
}
#reward .btn-floating:hover {
box-shadow: 0 6px 12px rgba(0, 0, 0, 0.2), 0 5px 15px rgba(0, 0, 0, 0.2);
}
#rewardModal {
width: 320px;
height: 350px;
}
#rewardModal .reward-title {
margin: 15px auto;
padding-bottom: 5px;
}
#rewardModal .modal-content {
padding: 10px;
}
#rewardModal .close {
position: absolute;
right: 15px;
top: 15px;
color: rgba(0, 0, 0, 0.5);
font-size: 1.3rem;
line-height: 20px;
cursor: pointer;
}
#rewardModal .close:hover {
color: #ef5350;
transform: scale(1.3);
-moz-transform:scale(1.3);
-webkit-transform:scale(1.3);
-o-transform:scale(1.3);
}
#rewardModal .reward-tabs {
margin: 0 auto;
width: 210px;
}
.reward-tabs .tabs {
height: 38px;
margin: 10px auto;
padding-left: 0;
}
.reward-content ul {
padding-left: 0 !important;
}
.reward-tabs .tabs .tab {
height: 38px;
line-height: 38px;
}
.reward-tabs .tab a {
color: #fff;
background-color: #ccc;
}
.reward-tabs .tab a:hover {
background-color: #ccc;
color: #fff;
}
.reward-tabs .wechat-tab .active {
color: #fff !important;
background-color: #22AB38 !important;
}
.reward-tabs .alipay-tab .active {
color: #fff !important;
background-color: #019FE8 !important;
}
.reward-tabs .reward-img {
width: 210px;
height: 210px;
}
</style>
<div id="reward">
<a href="#rewardModal" class="reward-link modal-trigger btn-floating btn-medium waves-effect waves-light red">赏</a>
<!-- Modal Structure -->
<div id="rewardModal" class="modal">
<div class="modal-content">
<a class="close modal-close"><i class="fas fa-times"></i></a>
<h4 class="reward-title">你的赏识是我前进的动力</h4>
<div class="reward-content">
<div class="reward-tabs">
<ul class="tabs row">
<li class="tab col s6 alipay-tab waves-effect waves-light"><a href="#alipay">支付宝</a></li>
<li class="tab col s6 wechat-tab waves-effect waves-light"><a href="#wechat">微 信</a></li>
</ul>
<div id="alipay">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/alipay.jpg" class="reward-img" alt="支付宝打赏二维码">
</div>
<div id="wechat">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/wechat.png" class="reward-img" alt="微信打赏二维码">
</div>
</div>
</div>
</div>
</div>
</div>
<script>
$(function () {
$('.tabs').tabs();
});
</script>
</div>
</div>
<style>
.valine-card {
margin: 1.5rem auto;
}
.valine-card .card-content {
padding: 20px 20px 5px 20px;
}
#vcomments textarea {
box-sizing: border-box;
background: url("https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/comment_bg.png") 100% 100% no-repeat;
}
#vcomments p {
margin: 2px 2px 10px;
font-size: 1.05rem;
line-height: 1.78rem;
}
#vcomments blockquote p {
text-indent: 0.2rem;
}
#vcomments a {
padding: 0 2px;
color: #4cbf30;
font-weight: 500;
text-decoration: none;
}
#vcomments img {
max-width: 100%;
height: auto;
cursor: pointer;
}
#vcomments ol li {
list-style-type: decimal;
}
#vcomments ol,
ul {
display: block;
padding-left: 2em;
word-spacing: 0.05rem;
}
#vcomments ul li,
ol li {
display: list-item;
line-height: 1.8rem;
font-size: 1rem;
}
#vcomments ul li {
list-style-type: disc;
}
#vcomments ul ul li {
list-style-type: circle;
}
#vcomments table, th, td {
padding: 12px 13px;
border: 1px solid #dfe2e5;
}
#vcomments table, th, td {
border: 0;
}
table tr:nth-child(2n), thead {
background-color: #fafafa;
}
#vcomments table th {
background-color: #f2f2f2;
min-width: 80px;
}
#vcomments table td {
min-width: 80px;
}
#vcomments h1 {
font-size: 1.85rem;
font-weight: bold;
line-height: 2.2rem;
}
#vcomments h2 {
font-size: 1.65rem;
font-weight: bold;
line-height: 1.9rem;
}
#vcomments h3 {
font-size: 1.45rem;
font-weight: bold;
line-height: 1.7rem;
}
#vcomments h4 {
font-size: 1.25rem;
font-weight: bold;
line-height: 1.5rem;
}
#vcomments h5 {
font-size: 1.1rem;
font-weight: bold;
line-height: 1.4rem;
}
#vcomments h6 {
font-size: 1rem;
line-height: 1.3rem;
}
#vcomments p {
font-size: 1rem;
line-height: 1.5rem;
}
#vcomments hr {
margin: 12px 0;
border: 0;
border-top: 1px solid #ccc;
}
#vcomments blockquote {
margin: 15px 0;
border-left: 5px solid #42b983;
padding: 1rem 0.8rem 0.3rem 0.8rem;
color: #666;
background-color: rgba(66, 185, 131, .1);
}
#vcomments pre {
font-family: monospace, monospace;
padding: 1.2em;
margin: .5em 0;
background: #272822;
overflow: auto;
border-radius: 0.3em;
tab-size: 4;
}
#vcomments code {
font-family: monospace, monospace;
padding: 1px 3px;
font-size: 0.92rem;
color: #e96900;
background-color: #f8f8f8;
border-radius: 2px;
}
#vcomments pre code {
font-family: monospace, monospace;
padding: 0;
color: #e8eaf6;
background-color: #272822;
}
#vcomments pre[class*="language-"] {
padding: 1.2em;
margin: .5em 0;
}
#vcomments code[class*="language-"],
pre[class*="language-"] {
color: #e8eaf6;
}
#vcomments [type="checkbox"]:not(:checked), [type="checkbox"]:checked {
position: inherit;
margin-left: -1.3rem;
margin-right: 0.4rem;
margin-top: -1px;
vertical-align: middle;
left: unset;
visibility: visible;
}
#vcomments b,
strong {
font-weight: bold;
}
#vcomments dfn {
font-style: italic;
}
#vcomments small {
font-size: 85%;
}
#vcomments cite {
font-style: normal;
}
#vcomments mark {
background-color: #fcf8e3;
padding: .2em;
}
#vcomments table, th, td {
padding: 12px 13px;
border: 1px solid #dfe2e5;
}
table tr:nth-child(2n), thead {
background-color: #fafafa;
}
#vcomments table th {
background-color: #f2f2f2;
min-width: 80px;
}
#vcomments table td {
min-width: 80px;
}
#vcomments [type="checkbox"]:not(:checked), [type="checkbox"]:checked {
position: inherit;
margin-left: -1.3rem;
margin-right: 0.4rem;
margin-top: -1px;
vertical-align: middle;
left: unset;
visibility: visible;
}
</style>
<div class="card valine-card" data-aos="fade-up">
<div class="comment_headling" style="font-size: 20px; font-weight: 700; position: relative; left: 20px; top: 15px; padding-bottom: 5px;">
<i class="fas fa-comments fa-fw" aria-hidden="true"></i>
<span>评论</span>
</div>
<div id="vcomments" class="card-content" style="display: grid">
</div>
</div>
<script src="/libs/valine/av-min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/valine/Valine.min.js"></script>
<script>
new Valine({
el: '#vcomments',
appId: '<KEY>',
appKey: '<KEY>IQ',
notify: 'false' === 'true',
verify: 'false' === 'true',
visitor: 'true' === 'true',
avatar: 'mm',
pageSize: '10',
lang: 'zh-cn',
placeholder: 'ヾノ≧∀≦)o来啊,快活啊!'
});
</script>
<article id="prenext-posts" class="prev-next articles">
<div class="row article-row">
<div class="article col s12 m6" data-aos="fade-up">
<div class="article-badge left-badge text-color">
<i class="fas fa-chevron-left"></i> 上一篇</div>
<div class="card">
<a href="/posts/java-bing-fa-bian-cheng-xia-pian.html">
<div class="card-image">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/java.jpg" class="responsive-img" alt="Java并发编程--下篇">
<span class="card-title">Java并发编程--下篇</span>
</div>
</a>
<div class="card-content article-content">
<div class="summary block-with-text">
多线程并发。--------------------------------------------
</div>
<div class="publish-info">
<span class="publish-date">
<i class="far fa-clock fa-fw icon-date"></i>2021-04-03
</span>
<span class="publish-author">
<i class="fas fa-bookmark fa-fw icon-category"></i>
<a href="/categories/%E5%A4%9A%E7%BA%BF%E7%A8%8B%E5%B9%B6%E5%8F%91/" class="post-category">
多线程并发
</a>
</span>
</div>
</div>
<div class="card-action article-tags">
<a href="/tags/%E5%A4%9A%E7%BA%BF%E7%A8%8B%E5%B9%B6%E5%8F%91/">
<span class="chip bg-color">多线程并发</span>
</a>
</div>
</div>
</div>
<div class="article col s12 m6" data-aos="fade-up">
<div class="article-badge right-badge text-color">
下一篇 <i class="fas fa-chevron-right"></i>
</div>
<div class="card">
<a href="/posts/rabbitmq.html">
<div class="card-image">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/java.jpg" class="responsive-img" alt="RabbitMQ">
<span class="card-title">RabbitMQ</span>
</div>
</a>
<div class="card-content article-content">
<div class="summary block-with-text">
RabbitMQ。-------------------------------------------------
</div>
<div class="publish-info">
<span class="publish-date">
<i class="far fa-clock fa-fw icon-date"></i>2021-04-02
</span>
<span class="publish-author">
<i class="fas fa-bookmark fa-fw icon-category"></i>
<a href="/categories/%E5%88%86%E5%B8%83%E5%BC%8F%E4%B8%AD%E9%97%B4%E4%BB%B6/" class="post-category">
分布式中间件
</a>
</span>
</div>
</div>
<div class="card-action article-tags">
<a href="/tags/%E6%B6%88%E6%81%AF%E4%B8%AD%E9%97%B4%E4%BB%B6/">
<span class="chip bg-color">消息中间件</span>
</a>
</div>
</div>
</div>
</div>
</article>
</div>
<script>
$('#articleContent').on('copy', function (e) {
// IE8 or earlier browser is 'undefined'
if (typeof window.getSelection === 'undefined') return;
var selection = window.getSelection();
// if the selection is short let's not annoy our users.
if (('' + selection).length < Number.parseInt('100')) {
return;
}
// create a div outside of the visible area and fill it with the selected text.
var bodyElement = document.getElementsByTagName('body')[0];
var newdiv = document.createElement('div');
newdiv.style.position = 'absolute';
newdiv.style.left = '-99999px';
bodyElement.appendChild(newdiv);
newdiv.appendChild(selection.getRangeAt(0).cloneContents());
// we need a <pre> tag workaround.
// otherwise the text inside "pre" loses all the line breaks!
if (selection.getRangeAt(0).commonAncestorContainer.nodeName === 'PRE') {
newdiv.innerHTML = "<pre>" + newdiv.innerHTML + "</pre>";
}
var url = document.location.href;
newdiv.innerHTML += '<br />'
+ '来源: Yuimm<br />'
+ '文章作者: Yuimm<br />'
+ '文章链接: <a href="' + url + '">' + url + '</a><br />'
+ '本文章著作权归作者所有,任何形式的转载都请注明出处。';
selection.selectAllChildren(newdiv);
window.setTimeout(function () {bodyElement.removeChild(newdiv);}, 200);
});
</script>
<!-- 代码块功能依赖 -->
<script type="text/javascript" src="/libs/codeBlock/codeBlockFuction.js"></script>
<!-- 代码语言 -->
<script type="text/javascript" src="/libs/codeBlock/codeLang.js"></script>
<!-- 代码块复制 -->
<script type="text/javascript" src="/libs/codeBlock/codeCopy.js"></script>
<!-- 代码块收缩 -->
<script type="text/javascript" src="/libs/codeBlock/codeShrink.js"></script>
<!-- 代码块折行 -->
<style type="text/css">
code[class*="language-"], pre[class*="language-"] { white-space: pre !important; }
</style>
</div>
<div id="toc-aside" class="expanded col l3 hide-on-med-and-down">
<div class="toc-widget">
<div class="toc-title"><i class="far fa-list-alt"></i> 目录</div>
<div id="toc-content"></div>
</div>
</div>
</div>
<!-- TOC 悬浮按钮. -->
<div id="floating-toc-btn" class="hide-on-med-and-down">
<a class="btn-floating btn-large bg-color">
<i class="fas fa-list-ul"></i>
</a>
</div>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/tocbot/tocbot.min.js"></script>
<script>
$(function () {
tocbot.init({
tocSelector: '#toc-content',
contentSelector: '#articleContent',
headingsOffset: -($(window).height() * 0.4 - 45),
collapseDepth: Number('0'),
headingSelector: 'h2, h3, h4'
});
// modify the toc link href to support Chinese.
let i = 0;
let tocHeading = 'toc-heading-';
$('#toc-content a').each(function () {
$(this).attr('href', '#' + tocHeading + (++i));
});
// modify the heading title id to support Chinese.
i = 0;
$('#articleContent').children('h2, h3, h4').each(function () {
$(this).attr('id', tocHeading + (++i));
});
// Set scroll toc fixed.
let tocHeight = parseInt($(window).height() * 0.4 - 64);
let $tocWidget = $('.toc-widget');
$(window).scroll(function () {
let scroll = $(window).scrollTop();
/* add post toc fixed. */
if (scroll > tocHeight) {
$tocWidget.addClass('toc-fixed');
} else {
$tocWidget.removeClass('toc-fixed');
}
});
/* 修复文章卡片 div 的宽度. */
let fixPostCardWidth = function (srcId, targetId) {
let srcDiv = $('#' + srcId);
if (srcDiv.length === 0) {
return;
}
let w = srcDiv.width();
if (w >= 450) {
w = w + 21;
} else if (w >= 350 && w < 450) {
w = w + 18;
} else if (w >= 300 && w < 350) {
w = w + 16;
} else {
w = w + 14;
}
$('#' + targetId).width(w);
};
// 切换TOC目录展开收缩的相关操作.
const expandedClass = 'expanded';
let $tocAside = $('#toc-aside');
let $mainContent = $('#main-content');
$('#floating-toc-btn .btn-floating').click(function () {
if ($tocAside.hasClass(expandedClass)) {
$tocAside.removeClass(expandedClass).hide();
$mainContent.removeClass('l9');
} else {
$tocAside.addClass(expandedClass).show();
$mainContent.addClass('l9');
}
fixPostCardWidth('artDetail', 'prenext-posts');
});
});
</script>
</main>
<footer class="page-footer bg-color">
<div class="container row center-align" style="margin-bottom: 15px !important;">
<div class="col s12 m8 l8 copy-right">
Copyright ©
<span id="year">2020</span>
<a href="http://yuimm.github.io" target="_blank">Yuimm</a>
| Powered by <a href="https://hexo.io/" target="_blank">Hexo</a>
| Theme <a href="https://github.com/blinkfox/hexo-theme-matery" target="_blank">Matery</a>
<br>
<i class="fas fa-chart-area"></i> 站点总字数: <span
class="white-color">544.9k</span> 字
<span id="busuanzi_container_site_pv">
| <i class="far fa-eye"></i> 总访问量: <span id="busuanzi_value_site_pv"
class="white-color"></span> 次
</span>
<span id="busuanzi_container_site_uv">
| <i class="fas fa-users"></i> 总访问人数: <span id="busuanzi_value_site_uv"
class="white-color"></span> 人
</span>
<br>
<span id="sitetime">载入运行时间...</span>
<script>
function siteTime() {
var seconds = 1000;
var minutes = seconds * 60;
var hours = minutes * 60;
var days = hours * 24;
var years = days * 365;
var today = new Date();
var startYear = "2020";
var startMonth = "3";
var startDate = "30";
var startHour = "0";
var startMinute = "0";
var startSecond = "0";
var todayYear = today.getFullYear();
var todayMonth = today.getMonth() + 1;
var todayDate = today.getDate();
var todayHour = today.getHours();
var todayMinute = today.getMinutes();
var todaySecond = today.getSeconds();
var t1 = Date.UTC(startYear, startMonth, startDate, startHour, startMinute, startSecond);
var t2 = Date.UTC(todayYear, todayMonth, todayDate, todayHour, todayMinute, todaySecond);
var diff = t2 - t1;
var diffYears = Math.floor(diff / years);
var diffDays = Math.floor((diff / days) - diffYears * 365);
var diffHours = Math.floor((diff - (diffYears * 365 + diffDays) * days) / hours);
var diffMinutes = Math.floor((diff - (diffYears * 365 + diffDays) * days - diffHours * hours) /
minutes);
var diffSeconds = Math.floor((diff - (diffYears * 365 + diffDays) * days - diffHours * hours -
diffMinutes * minutes) / seconds);
if (startYear == todayYear) {
document.getElementById("year").innerHTML = todayYear;
document.getElementById("sitetime").innerHTML = "本站已安全运行 " + diffDays + " 天 " + diffHours +
" 小时 " + diffMinutes + " 分钟 " + diffSeconds + " 秒";
} else {
document.getElementById("year").innerHTML = startYear + " - " + todayYear;
document.getElementById("sitetime").innerHTML = "本站已安全运行 " + diffYears + " 年 " + diffDays +
" 天 " + diffHours + " 小时 " + diffMinutes + " 分钟 " + diffSeconds + " 秒";
}
}
setInterval(siteTime, 1000);
</script>
<br>
</div>
<div class="col s12 m4 l4 social-link social-statis">
<a href="https://github.com/Yuimm" class="tooltipped" target="_blank" data-tooltip="访问我的GitHub" data-position="top" data-delay="50">
<i class="fab fa-github"></i>
</a>
<a href="https://gitee.com/Yuimm" class="tooltipped" target="_blank" data-tooltip="Gitee: https://gitee.com/Yuimm" data-position="top" data-delay="50">
<i class="fab fa-git"></i>
</a>
<a href="mailto:<EMAIL>" class="tooltipped" target="_blank" data-tooltip="邮件联系我" data-position="top" data-delay="50">
<i class="fas fa-envelope-open"></i>
</a>
<a href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/qq.jpg" class="tooltipped" target="_blank" data-tooltip="QQ联系我: https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/qq.jpg" data-position="top" data-delay="50">
<i class="fab fa-qq"></i>
</a>
<a href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/wechat.jpg" class="tooltipped" target="_blank" data-tooltip="微信联系我: " data-position="top" data-delay="50">
<i class="fab fa-weixin"></i>
</a>
</div>
</div>
</footer>
<div class="progress-bar"></div>
<!-- 搜索遮罩框 -->
<div id="searchModal" class="modal">
<div class="modal-content">
<div class="search-header">
<span class="title"><i class="fas fa-search"></i> 搜索</span>
<input type="search" id="searchInput" name="s" placeholder="请输入搜索的关键字"
class="search-input">
</div>
<div id="searchResult"></div>
</div>
</div>
<script src="/js/search.js"></script>
<script type="text/javascript">
$(function () {
searchFunc("/" + "search.xml", 'searchInput', 'searchResult');
});
</script>
<!-- 回到顶部按钮 -->
<div id="backTop" class="top-scroll">
<a class="btn-floating btn-large waves-effect waves-light" href="#!">
<i class="fas fa-arrow-up"></i>
</a>
</div>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/materialize/materialize.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/masonry/masonry.pkgd.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/aos/aos.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/scrollprogress/scrollProgress.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/lightGallery/js/lightgallery-all.min.js"></script>
<script src="/js/matery.js"></script>
<!--文字点击-->
<!-- <script src="/js/click_show_text.js"></script> -->
<!--鼠标点击烟花特效-->
<!-- <canvas class="fireworks" style="position: fixed;left: 0;top: 0;z-index: 1; pointer-events: none;" ></canvas>
<script type="text/javascript" src="//cdn.bootcss.com/animejs/2.2.0/anime.min.js"></script>
<script type="text/javascript" src="/js/firework.js"></script> -->
<!-- Global site tag (gtag.js) - Google Analytics -->
<!-- Baidu Analytics -->
<!-- Baidu Push -->
<script>
(function () {
var bp = document.createElement('script');
var curProtocol = window.location.protocol.split(':')[0];
if (curProtocol === 'https') {
bp.src = 'https://zz.bdstatic.com/linksubmit/push.js';
} else {
bp.src = 'http://push.zhanzhang.baidu.com/push.js';
}
var s = document.getElementsByTagName("script")[0];
s.parentNode.insertBefore(bp, s);
})();
</script>
<script async src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/others/busuanzi.pure.mini.js"></script>
<script type="text/javascript" color="0,0,255"
pointColor="0,0,255" opacity='0.7'
zIndex="-1" count="99"
src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/background/canvas-nest.js"></script>
<script type="text/javascript" size="150" alpha='0.6'
zIndex="-1" src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/background/ribbon.min.js" async="async"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/background/ribbon-dynamic.js" async="async"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/instantpage/instantpage.js" type="module"></script>
</body>
</html>
<file_sep><!DOCTYPE HTML>
<html lang="zh-CN">
<head>
<meta charset="utf-8">
<meta name="keywords" content="JDBC, Yuimm">
<meta name="description" content="------------------------------------------------------------------------------------">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=no">
<meta name="renderer" content="webkit|ie-stand|ie-comp">
<meta name="mobile-web-app-capable" content="yes">
<meta name="format-detection" content="telephone=no">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black-translucent">
<title>JDBC | Yuimm</title>
<link rel="icon" type="image/png" href="/favicon.png">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/awesome/css/all.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/materialize/materialize.min.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/aos/aos.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/animate/animate.min.css">
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/lightGallery/css/lightgallery.min.css">
<link rel="stylesheet" type="text/css" href="/css/matery.css">
<link rel="stylesheet" type="text/css" href="/css/my.css">
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/jquery/jquery.min.js"></script>
<meta name="generator" content="Hexo 4.2.1"><link rel="stylesheet" href="/css/prism-tomorrow.css" type="text/css"></head>
<body>
<header class="navbar-fixed">
<nav id="headNav" class="bg-color nav-transparent">
<div id="navContainer" class="nav-wrapper head-container">
<div class="brand-logo">
<a href="/" class="waves-effect waves-light">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/logo.png" class="logo-img" alt="LOGO">
<span class="logo-span">Yuimm</span>
</a>
</div>
<a href="#" data-target="mobile-nav" class="sidenav-trigger button-collapse"><i class="fas fa-bars"></i></a>
<ul class="right nav-menu">
<li class="hide-on-med-and-down nav-item">
<a href="/" class="waves-effect waves-light">
<i class="fas fa-home" style="zoom: 0.6;"></i>
<span>首页</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/tags" class="waves-effect waves-light">
<i class="fas fa-tags" style="zoom: 0.6;"></i>
<span>标签</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/categories" class="waves-effect waves-light">
<i class="fas fa-bookmark" style="zoom: 0.6;"></i>
<span>分类</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/archives" class="waves-effect waves-light">
<i class="fas fa-archive" style="zoom: 0.6;"></i>
<span>归档</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/about" class="waves-effect waves-light">
<i class="fas fa-user-circle" style="zoom: 0.6;"></i>
<span>关于</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/contact" class="waves-effect waves-light">
<i class="fas fa-comments" style="zoom: 0.6;"></i>
<span>留言板</span>
</a>
</li>
<li class="hide-on-med-and-down nav-item">
<a href="/friends" class="waves-effect waves-light">
<i class="fas fa-address-book" style="zoom: 0.6;"></i>
<span>友情链接</span>
</a>
</li>
<li>
<a href="#searchModal" class="modal-trigger waves-effect waves-light">
<i id="searchIcon" class="fas fa-search" title="搜索" style="zoom: 0.85;"></i>
</a>
</li>
</ul>
<div id="mobile-nav" class="side-nav sidenav">
<div class="mobile-head bg-color">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/logo.png" class="logo-img circle responsive-img">
<div class="logo-name">Yuimm</div>
<div class="logo-desc">
Never really desperate, only the lost of the soul.
</div>
</div>
<ul class="menu-list mobile-menu-list">
<li class="m-nav-item">
<a href="/" class="waves-effect waves-light">
<i class="fa-fw fas fa-home"></i>
首页
</a>
</li>
<li class="m-nav-item">
<a href="/tags" class="waves-effect waves-light">
<i class="fa-fw fas fa-tags"></i>
标签
</a>
</li>
<li class="m-nav-item">
<a href="/categories" class="waves-effect waves-light">
<i class="fa-fw fas fa-bookmark"></i>
分类
</a>
</li>
<li class="m-nav-item">
<a href="/archives" class="waves-effect waves-light">
<i class="fa-fw fas fa-archive"></i>
归档
</a>
</li>
<li class="m-nav-item">
<a href="/about" class="waves-effect waves-light">
<i class="fa-fw fas fa-user-circle"></i>
关于
</a>
</li>
<li class="m-nav-item">
<a href="/contact" class="waves-effect waves-light">
<i class="fa-fw fas fa-comments"></i>
Contact
</a>
</li>
<li class="m-nav-item">
<a href="/friends" class="waves-effect waves-light">
<i class="fa-fw fas fa-address-book"></i>
友情链接
</a>
</li>
<li><div class="divider"></div></li>
<li>
<a href="https://github.com/Yuimm" class="waves-effect waves-light" target="_blank">
<i class="fab fa-github-square fa-fw"></i>Fork Me
</a>
</li>
</ul>
</div>
</div>
<style>
.nav-transparent .github-corner {
display: none !important;
}
.github-corner {
position: absolute;
z-index: 10;
top: 0;
right: 0;
border: 0;
transform: scale(1.1);
}
.github-corner svg {
color: #0f9d58;
fill: #fff;
height: 64px;
width: 64px;
}
.github-corner:hover .octo-arm {
animation: a 0.56s ease-in-out;
}
.github-corner .octo-arm {
animation: none;
}
@keyframes a {
0%,
to {
transform: rotate(0);
}
20%,
60% {
transform: rotate(-25deg);
}
40%,
80% {
transform: rotate(10deg);
}
}
</style>
<a href="https://github.com/Yuimm" class="github-corner tooltipped hide-on-med-and-down" target="_blank"
data-tooltip="Fork Me" data-position="left" data-delay="50">
<svg viewBox="0 0 250 250" aria-hidden="true">
<path d="M0,0 L115,115 L130,115 L142,142 L250,250 L250,0 Z"></path>
<path d="M128.3,109.0 C113.8,99.7 119.0,89.6 119.0,89.6 C122.0,82.7 120.5,78.6 120.5,78.6 C119.2,72.0 123.4,76.3 123.4,76.3 C127.3,80.9 125.5,87.3 125.5,87.3 C122.9,97.6 130.6,101.9 134.4,103.2"
fill="currentColor" style="transform-origin: 130px 106px;" class="octo-arm"></path>
<path d="M115.0,115.0 C114.9,115.1 118.7,116.5 119.8,115.4 L133.7,101.6 C136.9,99.2 139.9,98.4 142.2,98.6 C133.8,88.0 127.5,74.4 143.8,58.0 C148.5,53.4 154.0,51.2 159.7,51.0 C160.3,49.4 163.2,43.6 171.4,40.1 C171.4,40.1 176.1,42.5 178.8,56.2 C183.1,58.6 187.2,61.8 190.9,65.4 C194.5,69.0 197.7,73.2 200.1,77.6 C213.8,80.2 216.3,84.9 216.3,84.9 C212.7,93.1 206.9,96.0 205.4,96.6 C205.1,102.4 203.0,107.8 198.3,112.5 C181.9,128.9 168.3,122.5 157.7,114.1 C157.9,116.9 156.7,120.9 152.7,124.9 L141.0,136.5 C139.8,137.7 141.6,141.9 141.8,141.8 Z"
fill="currentColor" class="octo-body"></path>
</svg>
</a>
</nav>
</header>
<div class="bg-cover pd-header post-cover" style="background-image: url('https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/java.jpg')">
<div class="container" style="right: 0px;left: 0px;">
<div class="row">
<div class="col s12 m12 l12">
<div class="brand">
<h1 class="description center-align post-title">JDBC</h1>
</div>
</div>
</div>
</div>
</div>
<main class="post-container content">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/tocbot/tocbot.css">
<style>
#articleContent h1::before,
#articleContent h2::before,
#articleContent h3::before,
#articleContent h4::before,
#articleContent h5::before,
#articleContent h6::before {
display: block;
content: " ";
height: 100px;
margin-top: -100px;
visibility: hidden;
}
#articleContent :focus {
outline: none;
}
.toc-fixed {
position: fixed;
top: 64px;
}
.toc-widget {
width: 345px;
padding-left: 20px;
}
.toc-widget .toc-title {
margin: 35px 0 15px 0;
padding-left: 17px;
font-size: 1.5rem;
font-weight: bold;
line-height: 1.5rem;
}
.toc-widget ol {
padding: 0;
list-style: none;
}
#toc-content {
height: calc(100vh - 250px);
overflow: auto;
}
#toc-content ol {
padding-left: 10px;
}
#toc-content ol li {
padding-left: 10px;
}
#toc-content .toc-link:hover {
color: #42b983;
font-weight: 700;
text-decoration: underline;
}
#toc-content .toc-link::before {
background-color: transparent;
max-height: 25px;
}
#toc-content .is-active-link {
color: #42b983;
}
#toc-content .is-active-link::before {
background-color: #42b983;
}
#floating-toc-btn {
position: fixed;
right: 15px;
bottom: 76px;
padding-top: 15px;
margin-bottom: 0;
z-index: 998;
}
#floating-toc-btn .btn-floating {
width: 48px;
height: 48px;
}
#floating-toc-btn .btn-floating i {
line-height: 48px;
font-size: 1.4rem;
}
</style>
<div class="row">
<div id="main-content" class="col s12 m12 l9">
<!-- 文章内容详情 -->
<div id="artDetail">
<div class="card">
<div class="card-content article-info">
<div class="row tag-cate">
<div class="col s7">
<div class="article-tag">
<a href="/tags/JDBC/">
<span class="chip bg-color">JDBC</span>
</a>
</div>
</div>
<div class="col s5 right-align">
<div class="post-cate">
<i class="fas fa-bookmark fa-fw icon-category"></i>
<a href="/categories/java/" class="post-category">
java
</a>
</div>
</div>
</div>
<div class="post-info">
<div class="post-date info-break-policy">
<i class="far fa-calendar-minus fa-fw"></i>发布日期:
2021-01-06
</div>
<div class="post-date info-break-policy">
<i class="far fa-calendar-check fa-fw"></i>更新日期:
2021-11-22
</div>
<div class="info-break-policy">
<i class="far fa-file-word fa-fw"></i>文章字数:
12.5k
</div>
<div class="info-break-policy">
<i class="far fa-clock fa-fw"></i>阅读时长:
53 分
</div>
<div id="busuanzi_container_page_pv" class="info-break-policy">
<i class="far fa-eye fa-fw"></i>阅读次数:
<span id="busuanzi_value_page_pv"></span>
</div>
</div>
</div>
<hr class="clearfix">
<div class="card-content article-card-content">
<div id="articleContent">
<h2 id="第1章:JDBC概述"><a href="#第1章:JDBC概述" class="headerlink" title="第1章:JDBC概述"></a>第1章:JDBC概述</h2><h3 id="1-1-数据的持久化"><a href="#1-1-数据的持久化" class="headerlink" title="1.1 数据的持久化"></a>1.1 数据的持久化</h3><ul>
<li><p>持久化(persistence):<strong>把数据保存到可掉电式存储设备中以供之后使用</strong>。大多数情况下,特别是企业级应用,<strong>数据持久化意味着将内存中的数据保存到硬盘</strong>上加以”固化”<strong>,而持久化的实现过程大多通过各种关系数据库来完成</strong>。</p>
</li>
<li><p>持久化的主要应用是将内存中的数据存储在关系型数据库中,当然也可以存储在磁盘文件、XML数据文件中。</p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_01.png" alt="jdbc_01"></p>
</li>
</ul>
<h3 id="1-2-Java中的数据存储技术"><a href="#1-2-Java中的数据存储技术" class="headerlink" title="1.2 Java中的数据存储技术"></a>1.2 Java中的数据存储技术</h3><ul>
<li>在Java中,数据库存取技术可分为如下几类:<ul>
<li><strong>DBC</strong>直接访问数据库</li>
<li>JDO (Java Data Object )技术</li>
<li><strong>第三方O/R工具</strong>,如Hibernate, Mybatis 等</li>
</ul>
</li>
<li>JDBC是java访问数据库的基石,JDO、Hibernate、MyBatis等只是更好的封装了JDBC。</li>
</ul>
<h3 id="1-3-JDBC介绍"><a href="#1-3-JDBC介绍" class="headerlink" title="1.3 JDBC介绍"></a>1.3 JDBC介绍</h3><ul>
<li><p>JDBC(Java Database Connectivity)是一个<strong>独立于特定数据库管理系统、通用的SQL数据库存取和操作的公共接口</strong>(一组API),定义了用来访问数据库的标准Java类库,(<strong>java.sql,javax.sql</strong>)使用这些类库可以以一种<strong>标准</strong>的方法、方便地访问数据库资源。</p>
</li>
<li><p>JDBC为访问不同的数据库提供了一种<strong>统一的途径</strong>,为开发者屏蔽了一些细节问题。</p>
</li>
<li><p>JDBC的目标是使Java程序员使用JDBC可以连接任何<strong>提供了JDBC驱动程序</strong>的数据库系统,这样就使得程序员无需对特定的数据库系统的特点有过多的了解,从而大大简化和加快了开发过程。</p>
</li>
<li><p>如果没有JDBC,那么Java程序访问数据库时是这样的:</p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_02.png" alt="jdbc_02"></p>
</li>
<li><p>有了JDBC,Java程序访问数据库时是这样的:</p>
</li>
</ul>
<p> <img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_03.png" alt="jdbc_03"></p>
<ul>
<li><p>总结如下:</p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_04.png" alt="jdbc_04"></p>
</li>
</ul>
<h3 id="1-4-JDBC体系结构"><a href="#1-4-JDBC体系结构" class="headerlink" title="1.4 JDBC体系结构"></a>1.4 JDBC体系结构</h3><ul>
<li>DBC接口(API)包括两个层次:<ul>
<li><strong>面向应用的API</strong>:Java API,抽象接口,供应用程序开发人员使用(连接数据库,执行SQL语句,获得结果)。</li>
<li><strong>面向数据库的API</strong>:Java Driver API,供开发商开发数据库驱动程序用.</li>
</ul>
</li>
</ul>
<blockquote>
<p><strong>JDBC是sun公司提供一套用于数据库操作的接口,java程序员只需要面向这套接口编程即可。</strong></p>
<p><strong>不同的数据库厂商,需要针对这套接口,提供不同实现。不同的实现的集合,即为不同数据库的驱动。 ————面向接口编程</strong></p>
</blockquote>
<h3 id="1-5-JDBC程序编写步骤"><a href="#1-5-JDBC程序编写步骤" class="headerlink" title="1.5 JDBC程序编写步骤"></a>1.5 JDBC程序编写步骤</h3><p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_05.png" alt="jdbc_05"></p>
<blockquote>
<p><strong>补充:ODBC(Open Database Connectivity,开放式数据库连接),是微软在Windows平台下推出的。使用者在程序中只需要调用ODBC API,由 ODBC 驱动程序将调用转换成为对特定的数据库的调用请求。</strong></p>
</blockquote>
<h2 id="第2章:获取数据库连接"><a href="#第2章:获取数据库连接" class="headerlink" title="第2章:获取数据库连接"></a>第2章:获取数据库连接</h2><h3 id="2-1-要素一:Driver接口实现类"><a href="#2-1-要素一:Driver接口实现类" class="headerlink" title="2.1 要素一:Driver接口实现类"></a>2.1 要素一:Driver接口实现类</h3><h4 id="2-1-1-Driver接口介绍"><a href="#2-1-1-Driver接口介绍" class="headerlink" title="2.1.1 Driver接口介绍"></a>2.1.1 Driver接口介绍</h4><ul>
<li>java.sql.Driver 接口是所有 JDBC 驱动程序需要实现的接口。这个接口是提供给数据库厂商使用的,不同数据库厂商提供不同的实现。</li>
<li>在程序中不需要直接去访问实现了 Driver 接口的类,而是由驱动程序管理器类(java.sql.DriverManager)去调用这些Driver实现。<ul>
<li>Oracle的驱动:<strong>oracle.jdbc.driver.OracleDriver</strong></li>
<li>MySql的驱动: <strong>com.mysql.jdbc.Driver</strong></li>
</ul>
</li>
</ul>
<h4 id="2-1-2-加载与注册JDBC驱动"><a href="#2-1-2-加载与注册JDBC驱动" class="headerlink" title="2.1.2 加载与注册JDBC驱动"></a>2.1.2 加载与注册JDBC驱动</h4><ul>
<li><p>加载驱动:加载 JDBC 驱动需调用 Class 类的静态方法 forName(),向其传递要加载的 JDBC 驱动的类名</p>
<ul>
<li><strong>Class.forName(“com.mysql.cj.jdbc.Driver”);</strong></li>
</ul>
</li>
<li><p>注册驱动:DriverManager 类是驱动程序管理器类,负责管理驱动程序</p>
<ul>
<li><p><strong>使用DriverManager.registerDriver(com.mysql.jdbc.Driver)来注册驱动</strong></p>
</li>
<li><p>通常不用显式调用 DriverManager 类的 registerDriver() 方法来注册驱动程序类的实例,因为 Driver 接口的驱动程序类<strong>都</strong>包含了静态代码块,在这个静态代码块中,会调用 DriverManager.registerDriver() 方法来注册自身的一个实例。下图是MySQL的Driver实现类的源码:</p>
<pre class=" language-java"><code class="language-java"> <span class="token keyword">static</span> <span class="token punctuation">{</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
DriverManager<span class="token punctuation">.</span><span class="token function">registerDriver</span><span class="token punctuation">(</span><span class="token keyword">new</span> <span class="token class-name">Driver</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> var1<span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token keyword">throw</span> <span class="token keyword">new</span> <span class="token class-name">RuntimeException</span><span class="token punctuation">(</span><span class="token string">"Can't register driver!"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
</li>
</ul>
</li>
</ul>
<h3 id="2-2-要素二:URL"><a href="#2-2-要素二:URL" class="headerlink" title="2.2 要素二:URL"></a>2.2 要素二:URL</h3><ul>
<li><p>JDBC URL 用于标识一个被注册的驱动程序,驱动程序管理器通过这个 URL 选择正确的驱动程序,从而建立到数据库的连接。</p>
</li>
<li><p>JDBC URL的标准由三部分组成,各部分间用冒号分隔。</p>
<ul>
<li><p><strong>jdbc:子协议:子名称</strong></p>
</li>
<li><p><strong>协议</strong>:JDBC URL中的协议总是jdbc</p>
</li>
<li><p><strong>子协议</strong>:子协议用于标识一个数据库驱动程序</p>
</li>
<li><p><strong>子名称</strong>:一种标识数据库的方法。子名称可以依不同的子协议而变化,用子名称的目的是为了<strong>定位数据库</strong>提供足够的信息。包含<strong>主机名</strong>(对应服务端的ip地址)<strong>,端口号,数据库名</strong></p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_06.png" alt="jdbc_06"></p>
</li>
</ul>
</li>
<li><p><strong>几种常用数据库的 JDBC URL</strong></p>
<ul>
<li><p>MySQL的连接URL编写方式:</p>
<ul>
<li><pre><code>jdbc:mysql://主机名称:mysql服务端口号/数据库名称?参数=值&参数=值
jdbc:mysql://IP地址:3306/数据库名?rewriteBatchedStatements=true&useSSL=false&serverTimezone=GMT</code></pre></li>
</ul>
</li>
<li><p>Oracle 9i的连接URL编写方式:</p>
<ul>
<li><pre><code>jdbc:oracle:thin:@主机名称:oracle服务端口号:数据库名称</code></pre></li>
</ul>
</li>
<li><p>SQLServer的连接URL编写方式:</p>
<ul>
<li><pre><code>jdbc:sqlserver://主机名称:sqlserver服务端口号:DatabaseName=数据库名称</code></pre></li>
</ul>
</li>
</ul>
</li>
</ul>
<h3 id="2-3-要素三:用户名和密码"><a href="#2-3-要素三:用户名和密码" class="headerlink" title="2.3 要素三:用户名和密码"></a>2.3 要素三:用户名和密码</h3><ul>
<li>user,password可以用“属性名=属性值”方式告诉数据库</li>
<li>可以调用 DriverManager 类的 getConnection() 方法建立到数据库的连接</li>
</ul>
<pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>connection<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>io<span class="token punctuation">.</span>InputStream<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Connection<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Driver<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>DriverManager<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>SQLException<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>Properties<span class="token punctuation">;</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">ConnectionTest</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//方式一</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> SQLException <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取Driver的实现类对象</span>
Driver driver <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">com<span class="token punctuation">.</span>mysql<span class="token punctuation">.</span>cj<span class="token punctuation">.</span>jdbc<span class="token punctuation">.</span>Driver</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String url <span class="token operator">=</span> <span class="token string">"jdbc:mysql://IP地址:3306/test?rewriteBatchedStatements=true&useSSL=false&serverTimezone=GMT"</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//将用户名和密码封装在Properties中</span>
Properties info <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Properties</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
info<span class="token punctuation">.</span><span class="token function">setProperty</span><span class="token punctuation">(</span><span class="token string">"user"</span><span class="token punctuation">,</span><span class="token string">"root"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
info<span class="token punctuation">.</span><span class="token function">setProperty</span><span class="token punctuation">(</span><span class="token string">"password"</span><span class="token punctuation">,</span><span class="token string">"hct"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Connection conn <span class="token operator">=</span> driver<span class="token punctuation">.</span><span class="token function">connect</span><span class="token punctuation">(</span>url<span class="token punctuation">,</span>info<span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>conn<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//方式二,不出现第三方的API,使得程序有更好的可移植性</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testConnection2</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> SQLException<span class="token punctuation">,</span> ClassNotFoundException<span class="token punctuation">,</span> IllegalAccessException<span class="token punctuation">,</span> InstantiationException <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取Driver的实现类对象,使用反射</span>
Class <span class="token class-name">aClass</span> <span class="token operator">=</span> Class<span class="token punctuation">.</span><span class="token function">forName</span><span class="token punctuation">(</span><span class="token string">"com.mysql.cj.jdbc.Driver"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Driver driver <span class="token operator">=</span> <span class="token punctuation">(</span>Driver<span class="token punctuation">)</span> aClass<span class="token punctuation">.</span><span class="token function">newInstance</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//提供要连接的数据库</span>
String url <span class="token operator">=</span> <span class="token string">"jdbc:mysql://IP地址:3306/test?rewriteBatchedStatements=true&useSSL=false&serverTimezone=GMT"</span><span class="token punctuation">;</span>
Properties info <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Properties</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
info<span class="token punctuation">.</span><span class="token function">setProperty</span><span class="token punctuation">(</span><span class="token string">"user"</span><span class="token punctuation">,</span><span class="token string">"root"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
info<span class="token punctuation">.</span><span class="token function">setProperty</span><span class="token punctuation">(</span><span class="token string">"password"</span><span class="token punctuation">,</span><span class="token string">"hct"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Connection conn <span class="token operator">=</span> driver<span class="token punctuation">.</span><span class="token function">connect</span><span class="token punctuation">(</span>url<span class="token punctuation">,</span>info<span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>conn<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//方式三,使用DriverManager替换Driver</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testConnection3</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> ClassNotFoundException<span class="token punctuation">,</span> IllegalAccessException<span class="token punctuation">,</span> InstantiationException<span class="token punctuation">,</span> SQLException <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//1.提供连接信息</span>
String url <span class="token operator">=</span> <span class="token string">"jdbc:mysql://IP地址:3306/test?rewriteBatchedStatements=true&useSSL=false&serverTimezone=GMT"</span><span class="token punctuation">;</span>
String user <span class="token operator">=</span> <span class="token string">"root"</span><span class="token punctuation">;</span>
String password <span class="token operator">=</span> <span class="token string">"hct"</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//2.获取Driver实现类对象</span>
Class <span class="token class-name">aClass</span> <span class="token operator">=</span> Class<span class="token punctuation">.</span><span class="token function">forName</span><span class="token punctuation">(</span><span class="token string">"com.mysql.cj.jdbc.Driver"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Driver driver <span class="token operator">=</span> <span class="token punctuation">(</span>Driver<span class="token punctuation">)</span> aClass<span class="token punctuation">.</span><span class="token function">newInstance</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//注册驱动</span>
DriverManager<span class="token punctuation">.</span><span class="token function">registerDriver</span><span class="token punctuation">(</span>driver<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取连接</span>
Connection connection <span class="token operator">=</span> DriverManager<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span>url<span class="token punctuation">,</span> user<span class="token punctuation">,</span> password<span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>connection<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//方式四</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testConnection4</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> ClassNotFoundException<span class="token punctuation">,</span> IllegalAccessException<span class="token punctuation">,</span> InstantiationException<span class="token punctuation">,</span> SQLException <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//1.提供连接信息</span>
String url <span class="token operator">=</span> <span class="token string">"jdbc:mysql://IP地址:3306/test?rewriteBatchedStatements=true&useSSL=false&serverTimezone=GMT"</span><span class="token punctuation">;</span>
String user <span class="token operator">=</span> <span class="token string">"root"</span><span class="token punctuation">;</span>
String password <span class="token operator">=</span> <span class="token string">"hct"</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//2.获取Driver实现类对象</span>
Class<span class="token punctuation">.</span><span class="token function">forName</span><span class="token punctuation">(</span><span class="token string">"com.mysql.cj.jdbc.Driver"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//下面的两行代码可以省</span>
<span class="token comment" spellcheck="true">/*
Driver driver = (Driver) aClass.newInstance();
//注册驱动
DriverManager.registerDriver(driver);
*/</span>
<span class="token comment" spellcheck="true">//因为mysql的Driver实现类中,声明了如下操作</span>
<span class="token comment" spellcheck="true">/*
static {
try {
DriverManager.registerDriver(new Driver());
} catch (SQLException var1) {
throw new RuntimeException("Can't register driver!");
}
}
* */</span>
<span class="token comment" spellcheck="true">//获取连接</span>
Connection connection <span class="token operator">=</span> DriverManager<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span>url<span class="token punctuation">,</span> user<span class="token punctuation">,</span> password<span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>connection<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//最终版,将连接信息声明在配置文件中</span>
<span class="token comment" spellcheck="true">/*
* 优点:
* 1.实现了数据与代码的分离,解耦合
* 2.只需要修改配置信息,避免重新打包
* */</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testConnection5</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> Exception <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//1.读取配置文件中的4个基本信息,通过类加载器</span>
InputStream in <span class="token operator">=</span> ConnectionTest<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">.</span><span class="token function">getClassLoader</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">.</span><span class="token function">getResourceAsStream</span><span class="token punctuation">(</span><span class="token string">"jdbc.properties"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Properties pros <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Properties</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
pros<span class="token punctuation">.</span><span class="token function">load</span><span class="token punctuation">(</span>in<span class="token punctuation">)</span><span class="token punctuation">;</span>
String url <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"url"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String username <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"username"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String password <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"password"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String driverClassName <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"driverClassName"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//2.获取Driver实现类对象</span>
Class<span class="token punctuation">.</span><span class="token function">forName</span><span class="token punctuation">(</span>driverClassName<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取连接</span>
Connection connection <span class="token operator">=</span> DriverManager<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span>url<span class="token punctuation">,</span> username<span class="token punctuation">,</span> password<span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>connection<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<h2 id="第3章:使用PreparedStatement实现CRUD操作"><a href="#第3章:使用PreparedStatement实现CRUD操作" class="headerlink" title="第3章:使用PreparedStatement实现CRUD操作"></a>第3章:使用PreparedStatement实现CRUD操作</h2><h3 id="3-1-操作和访问数据库"><a href="#3-1-操作和访问数据库" class="headerlink" title="3.1 操作和访问数据库"></a>3.1 操作和访问数据库</h3><ul>
<li><p>数据库连接被用于向数据库服务器发送命令和 SQL 语句,并接受数据库服务器返回的结果。其实一个数据库连接就是一个Socket连接。</p>
</li>
<li><p>在 java.sql 包中有 3 个接口分别定义了对数据库的调用的不同方式:</p>
<ul>
<li><p>Statement:用于执行静态 SQL 语句并返回它所生成结果的对象。</p>
</li>
<li><p>PrepatedStatement:SQL 语句被预编译并存储在此对象中,可以使用此对象多次高效地执行该语句。</p>
</li>
<li><p>CallableStatement:用于执行 SQL 存储过程</p>
</li>
</ul>
</li>
</ul>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_07.png" alt="jdbc_07"></p>
<h3 id="3-2-使用Statement操作数据表的弊端"><a href="#3-2-使用Statement操作数据表的弊端" class="headerlink" title="3.2 使用Statement操作数据表的弊端"></a>3.2 使用Statement操作数据表的弊端</h3><ul>
<li><p>通过调用 Connection 对象的 createStatement() 方法创建该对象。该对象用于执行静态的 SQL 语句,并且返回执行结果。</p>
</li>
<li><p>Statement 接口中定义了下列方法用于执行 SQL 语句:</p>
<pre><code>int excuteUpdate(String sql):执行更新操作INSERT、UPDATE、DELETE
ResultSet executeQuery(String sql):执行查询操作SELECT</code></pre></li>
<li><p>但是使用Statement操作数据表存在弊端:</p>
<ul>
<li><strong>问题一:存在拼串操作,繁琐</strong></li>
<li><strong>问题二:存在SQL注入问题</strong></li>
</ul>
</li>
<li><p>SQL 注入是利用某些系统没有对用户输入的数据进行充分的检查,而在用户输入数据中注入非法的 SQL 语句段或命令(如:SELECT user, password FROM user_table WHERE user=‘a’ OR 1 = ’ AND password = ’ OR ‘1’ = ‘1’) ,从而利用系统的 SQL 引擎完成恶意行为的做法。</p>
</li>
<li><p>对于 Java 而言,要防范 SQL 注入,只要用 PreparedStatement(从Statement扩展而来) 取代 Statement 就可以了。</p>
</li>
</ul>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_08.png" alt="jdbc_08"></p>
<h3 id="3-3-PreparedStatement的使用"><a href="#3-3-PreparedStatement的使用" class="headerlink" title="3.3 PreparedStatement的使用"></a>3.3 PreparedStatement的使用</h3><h4 id="3-3-1-PreparedStatement介绍"><a href="#3-3-1-PreparedStatement介绍" class="headerlink" title="3.3.1 PreparedStatement介绍"></a>3.3.1 PreparedStatement介绍</h4><ul>
<li>可以通过调用 Connection 对象的 <strong>preparedStatement(String sql)</strong> 方法获取 PreparedStatement 对象</li>
<li><strong>PreparedStatement 接口是 Statement 的子接口,它表示一条预编译过的 SQL 语句</strong></li>
<li>PreparedStatement 对象所代表的 SQL 语句中的参数用问号(?)来表示,调用 PreparedStatement 对象的 setXxx() 方法来设置这些参数. setXxx() 方法有两个参数,第一个参数是要设置的 SQL 语句中的参数的索引(从 1 开始),第二个是设置的 SQL 语句中的参数的值</li>
</ul>
<h4 id="3-3-2-PreparedStatement-vs-Statement"><a href="#3-3-2-PreparedStatement-vs-Statement" class="headerlink" title="3.3.2 PreparedStatement vs Statement"></a>3.3.2 PreparedStatement vs Statement</h4><ul>
<li>代码的可读性和可维护性。</li>
<li><strong>PreparedStatement 能最大可能提高性能:</strong><ul>
<li>DBServer会对<strong>预编译</strong>语句提供性能优化。因为预编译语句有可能被重复调用,所以语句在被DBServer的编译器编译后的执行代码被缓存下来,那么下次调用时只要是相同的预编译语句就不需要编译,只要将参数直接传入编译过的语句执行代码中就会得到执行。</li>
<li>在statement语句中,即使是相同操作但因为数据内容不一样,所以整个语句本身不能匹配,没有缓存语句的意义.事实是没有数据库会对普通语句编译后的执行代码缓存。这样每执行一次都要对传入的语句编译一次。</li>
<li>(语法检查,语义检查,翻译成二进制命令,缓存)</li>
</ul>
</li>
<li>PreparedStatement 可以防止 SQL 注入</li>
</ul>
<h4 id="3-3-3-Java与SQL对应数据类型转换表"><a href="#3-3-3-Java与SQL对应数据类型转换表" class="headerlink" title="3.3.3 Java与SQL对应数据类型转换表"></a>3.3.3 Java与SQL对应数据类型转换表</h4><table>
<thead>
<tr>
<th align="center">Java类型</th>
<th align="center">SQL类型</th>
</tr>
</thead>
<tbody><tr>
<td align="center">boolean</td>
<td align="center">BIT</td>
</tr>
<tr>
<td align="center">byte</td>
<td align="center">TINYINT</td>
</tr>
<tr>
<td align="center">short</td>
<td align="center">SMALLINT</td>
</tr>
<tr>
<td align="center">int</td>
<td align="center">INTEGER</td>
</tr>
<tr>
<td align="center">long</td>
<td align="center">BIGINT</td>
</tr>
<tr>
<td align="center">String</td>
<td align="center">CHAR,VARCHAR,LONGVARCHAR</td>
</tr>
<tr>
<td align="center">byte array</td>
<td align="center">BINARY , VAR BINARY</td>
</tr>
<tr>
<td align="center">java.sql.Date</td>
<td align="center">DATE</td>
</tr>
<tr>
<td align="center">java.sql.Time</td>
<td align="center">TIME</td>
</tr>
<tr>
<td align="center">java.sql.Timestamp</td>
<td align="center">TIMESTAMP</td>
</tr>
</tbody></table>
<h4 id="3-3-4-使用PreparedStatement实现增、删、改操作"><a href="#3-3-4-使用PreparedStatement实现增、删、改操作" class="headerlink" title="3.3.4 使用PreparedStatement实现增、删、改操作"></a>3.3.4 使用PreparedStatement实现增、删、改操作</h4><pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>preparedstatement_3<span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">/*
* 使用PreparedStatement替换statement,实现对数据表的增删改查
*
*
* */</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>connection_1<span class="token punctuation">.</span>ConnectionTest<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>util_4<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>io<span class="token punctuation">.</span>IOException<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>io<span class="token punctuation">.</span>InputStream<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Connection<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>DriverManager<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>PreparedStatement<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>SQLException<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>text<span class="token punctuation">.</span>ParseException<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>text<span class="token punctuation">.</span>SimpleDateFormat<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Date<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>Properties<span class="token punctuation">;</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">PreparedStatementUpdateTest</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//向customers表中添加一条记录</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testInsert</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
Connection connection <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//1.读取配置文件中的4个基本信息,通过类加载器</span>
InputStream in <span class="token operator">=</span> ConnectionTest<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">.</span><span class="token function">getClassLoader</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">.</span><span class="token function">getResourceAsStream</span><span class="token punctuation">(</span><span class="token string">"jdbc.properties"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Properties pros <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Properties</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
pros<span class="token punctuation">.</span><span class="token function">load</span><span class="token punctuation">(</span>in<span class="token punctuation">)</span><span class="token punctuation">;</span>
String url <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"url"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String username <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"username"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String password <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"password"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String driverClassName <span class="token operator">=</span> pros<span class="token punctuation">.</span><span class="token function">getProperty</span><span class="token punctuation">(</span><span class="token string">"driverClassName"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//2.获取Driver实现类对象</span>
Class<span class="token punctuation">.</span><span class="token function">forName</span><span class="token punctuation">(</span>driverClassName<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//3.获取连接</span>
connection <span class="token operator">=</span> DriverManager<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span>url<span class="token punctuation">,</span> username<span class="token punctuation">,</span> password<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//4.预编译sql语句,返回PreparedStatement实例。?为占位符,解决sql注入</span>
String sql <span class="token operator">=</span> <span class="token string">" insert into customers (name,email,birth) values (?,?,?) "</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> connection<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//5.填充占位符</span>
ps<span class="token punctuation">.</span><span class="token function">setString</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">,</span><span class="token string">"哪吒"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
ps<span class="token punctuation">.</span><span class="token function">setString</span><span class="token punctuation">(</span><span class="token number">2</span><span class="token punctuation">,</span><span class="token string">"<EMAIL>"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
SimpleDateFormat simpleDateFormat <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">SimpleDateFormat</span><span class="token punctuation">(</span><span class="token string">"yy-MM-dd"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>Date date <span class="token operator">=</span> simpleDateFormat<span class="token punctuation">.</span><span class="token function">parse</span><span class="token punctuation">(</span><span class="token string">"1000-10-23"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
ps<span class="token punctuation">.</span><span class="token function">setDate</span><span class="token punctuation">(</span><span class="token number">3</span><span class="token punctuation">,</span><span class="token keyword">new</span> <span class="token class-name">Date</span><span class="token punctuation">(</span>date<span class="token punctuation">.</span><span class="token function">getTime</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//6.执行操作</span>
ps<span class="token punctuation">.</span><span class="token function">execute</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">IOException</span> <span class="token operator">|</span> ClassNotFoundException <span class="token operator">|</span> SQLException <span class="token operator">|</span> ParseException e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//7.关闭资源</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>ps <span class="token operator">!=</span> null<span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">close</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>connection <span class="token operator">!=</span> null<span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
connection<span class="token punctuation">.</span><span class="token function">close</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//修改customers表的一条记录</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testUpdate</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//1.获取数据库连接</span>
Connection connection <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
connection <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//2.预编译sql语句,返回Preparedstatement实例</span>
String sql <span class="token operator">=</span> <span class="token string">"update customers set name = ? where id = ?"</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> connection<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//3.填充占位符</span>
ps<span class="token punctuation">.</span><span class="token function">setString</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">,</span><span class="token string">"张三三三"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
ps<span class="token punctuation">.</span><span class="token function">setInt</span><span class="token punctuation">(</span><span class="token number">2</span><span class="token punctuation">,</span><span class="token number">18</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//4.执行操作</span>
ps<span class="token punctuation">.</span><span class="token function">execute</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//5.关闭资源</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>connection<span class="token punctuation">,</span>ps <span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">test</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//String sql = "delete from customers where id = ?";</span>
<span class="token comment" spellcheck="true">//update(sql,3);</span>
String sql <span class="token operator">=</span> <span class="token string">"update `order` set order_name = ? where order_id = ?"</span><span class="token punctuation">;</span>
<span class="token function">update</span><span class="token punctuation">(</span>sql<span class="token punctuation">,</span><span class="token string">"DD"</span><span class="token punctuation">,</span><span class="token number">2</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<p><strong>通用的增删改</strong></p>
<pre><code>//通用的增删改操作方法
public void update(String sql,Object ...args){
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
//1.建立连接
connection = JDBCUtils.getConnection();
//2.预编译sql语句,返回Preparedstatement实例
preparedStatement = connection.prepareStatement(sql);
//3.填充占位符
for (int i = 0; i < args.length;i++){
preparedStatement.setObject(i+1,args[i]);
}
//4.执行操作
preparedStatement.execute();
} catch (Exception e) {
e.printStackTrace();
}
//5.关闭资源
JDBCUtils.closeResource(connection,preparedStatement);
}
</code></pre><h4 id="3-3-5-使用PreparedStatement实现查询操作"><a href="#3-3-5-使用PreparedStatement实现查询操作" class="headerlink" title="3.3.5 使用PreparedStatement实现查询操作"></a>3.3.5 使用PreparedStatement实现查询操作</h4><pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>preparedstatement_3<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>bean<span class="token punctuation">.</span>Order<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>util_4<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>lang<span class="token punctuation">.</span>reflect<span class="token punctuation">.</span>Field<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>*<span class="token punctuation">;</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">OrderQuery</span> <span class="token punctuation">{</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">tesstQuery1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select order_id,order_name,order_date from `order` where order_id = ?"</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">,</span><span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
rs <span class="token operator">=</span> ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token keyword">int</span> id <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getInt</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String name <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getString</span><span class="token punctuation">(</span><span class="token number">2</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Date date <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getDate</span><span class="token punctuation">(</span><span class="token number">3</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Order order <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Order</span><span class="token punctuation">(</span>id<span class="token punctuation">,</span> name<span class="token punctuation">,</span> date<span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>order<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//针对ORder表的通用查询操作</span>
<span class="token keyword">public</span> Order <span class="token function">orderForQuery</span><span class="token punctuation">(</span>String sql<span class="token punctuation">,</span>Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span>null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span>null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//获取结果集</span>
rs <span class="token operator">=</span>ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取结果集的元数据</span>
ResultSetMetaData rsmd <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getMetaData</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取列(字段)的个数</span>
<span class="token keyword">int</span> columnCount <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnCount</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Order order <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Order</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span><span class="token punctuation">(</span><span class="token keyword">int</span> i<span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> columnCount<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的列值:通过ResultSet</span>
Object columnValue <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getObject</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的名子:通过ResultSetMetaData 不推荐使用</span>
<span class="token comment" spellcheck="true">//String columnName = rsmd.getColumnName(i + 1);</span>
<span class="token comment" spellcheck="true">//获取列的别名</span>
String columnLabel <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnLabel</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//通过反射,将对象指定名columnName的属性赋值为指定的值cliumnValue</span>
Field declaredField <span class="token operator">=</span> Order<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">.</span><span class="token function">getDeclaredField</span><span class="token punctuation">(</span>columnLabel<span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">setAccessible</span><span class="token punctuation">(</span><span class="token boolean">true</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">set</span><span class="token punctuation">(</span>order<span class="token punctuation">,</span>columnValue<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> order<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">test</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
String sql <span class="token operator">=</span> <span class="token string">"select order_id orderId,order_name orderName,order_date orderDate from `order` where order_id = ?"</span><span class="token punctuation">;</span>
Order order <span class="token operator">=</span> <span class="token function">orderForQuery</span><span class="token punctuation">(</span>sql<span class="token punctuation">,</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>order<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<p><strong>针对不同表的通用的查询操作:</strong></p>
<pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>preparedstatement_3<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>bean<span class="token punctuation">.</span>Customer<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>bean<span class="token punctuation">.</span>Order<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>util_4<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>lang<span class="token punctuation">.</span>reflect<span class="token punctuation">.</span>Field<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Connection<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>PreparedStatement<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>ResultSet<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>ResultSetMetaData<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>ArrayList<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>List<span class="token punctuation">;</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">PreparedStatementQueryTest</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">/**
* 针对不同表的查询,返回一条数据
* @param clazz
* @param sql
* @param args
* @param <T>
* @return
*/</span>
<span class="token keyword">public</span> <span class="token operator"><</span>T<span class="token operator">></span> T <span class="token function">getInstance</span><span class="token punctuation">(</span>Class<span class="token operator"><</span>T<span class="token operator">></span> clazz <span class="token punctuation">,</span>String sql<span class="token punctuation">,</span> Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span>null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span>null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//获取结果集</span>
rs <span class="token operator">=</span>ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取结果集的元数据</span>
ResultSetMetaData rsmd <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getMetaData</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取列(字段)的个数</span>
<span class="token keyword">int</span> columnCount <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnCount</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
T t <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">newInstance</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span><span class="token punctuation">(</span><span class="token keyword">int</span> i<span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> columnCount<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的列值:通过ResultSet</span>
Object columnValue <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getObject</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的名子:通过ResultSetMetaData 不推荐使用</span>
<span class="token comment" spellcheck="true">//String columnName = rsmd.getColumnName(i + 1);</span>
<span class="token comment" spellcheck="true">//获取列的别名</span>
String columnLabel <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnLabel</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//通过反射,将对象指定名columnName的属性赋值为指定的值cliumnValue</span>
Field declaredField <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">getDeclaredField</span><span class="token punctuation">(</span>columnLabel<span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">setAccessible</span><span class="token punctuation">(</span><span class="token boolean">true</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">set</span><span class="token punctuation">(</span>t<span class="token punctuation">,</span>columnValue<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> t<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">/**
* 针对不同表的查询,返回多条数据
* @param clazz
* @param sql
* @param args
* @param <T>
* @return
*/</span>
<span class="token keyword">public</span> <span class="token operator"><</span>T<span class="token operator">></span> List<span class="token operator"><</span>T<span class="token operator">></span> <span class="token function">getForList</span><span class="token punctuation">(</span>Class<span class="token operator"><</span>T<span class="token operator">></span> clazz <span class="token punctuation">,</span>String sql<span class="token punctuation">,</span> Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span>null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span>null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//获取结果集</span>
rs <span class="token operator">=</span>ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取结果集的元数据</span>
ResultSetMetaData rsmd <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getMetaData</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取列(字段)的个数</span>
<span class="token keyword">int</span> columnCount <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnCount</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//创建集合对象</span>
ArrayList<span class="token operator"><</span>T<span class="token operator">></span> list <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">ArrayList</span><span class="token operator"><</span><span class="token operator">></span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">while</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
T t <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">newInstance</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//处理结果集的一行数据中的每一列:给t对象指定的属性赋值</span>
<span class="token keyword">for</span><span class="token punctuation">(</span><span class="token keyword">int</span> i<span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> columnCount<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的列值:通过ResultSet</span>
Object columnValue <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getObject</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的名子:通过ResultSetMetaData 不推荐使用</span>
<span class="token comment" spellcheck="true">//String columnName = rsmd.getColumnName(i + 1);</span>
<span class="token comment" spellcheck="true">//获取列的别名</span>
String columnLabel <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnLabel</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//通过反射,将对象指定名columnName的属性赋值为指定的值cliumnValue</span>
Field declaredField <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">getDeclaredField</span><span class="token punctuation">(</span>columnLabel<span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">setAccessible</span><span class="token punctuation">(</span><span class="token boolean">true</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">set</span><span class="token punctuation">(</span>t<span class="token punctuation">,</span>columnValue<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
list<span class="token punctuation">.</span><span class="token function">add</span><span class="token punctuation">(</span>t<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> list<span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">test</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">/* String sql = "select id,name,email,birth from customers where id = ?";
Customer instance = getInstance(Customer.class, sql, 12);
System.out.println(instance);*/</span>
String sql <span class="token operator">=</span> <span class="token string">"select order_id orderId,order_name orderName,order_date orderDate from `order` where order_id = ?"</span><span class="token punctuation">;</span>
Order order <span class="token operator">=</span> <span class="token function">getInstance</span><span class="token punctuation">(</span>Order<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">,</span>sql<span class="token punctuation">,</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>order<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">test1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">/*String sql = "select id,name,email,birth from customers where id < ?";
List<Customer> list = getForList(Customer.class, sql, 12);
list.forEach(System.out::println);*/</span>
String sql <span class="token operator">=</span> <span class="token string">"select order_id orderId,order_name orderName,order_date orderDate from `order` where order_id < ?"</span><span class="token punctuation">;</span>
List<span class="token operator"><</span>Order<span class="token operator">></span> list <span class="token operator">=</span> <span class="token function">getForList</span><span class="token punctuation">(</span>Order<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">,</span> sql<span class="token punctuation">,</span> <span class="token number">4</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
list<span class="token punctuation">.</span><span class="token function">forEach</span><span class="token punctuation">(</span>System<span class="token punctuation">.</span>out<span class="token operator">:</span><span class="token operator">:</span>println<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<h3 id="3-4-ResultSet与ResultSetMetaData"><a href="#3-4-ResultSet与ResultSetMetaData" class="headerlink" title="3.4 ResultSet与ResultSetMetaData"></a>3.4 ResultSet与ResultSetMetaData</h3><h4 id="3-4-1-ResultSet"><a href="#3-4-1-ResultSet" class="headerlink" title="3.4.1 ResultSet"></a>3.4.1 ResultSet</h4><ul>
<li><p>查询需要调用PreparedStatement 的 executeQuery() 方法,查询结果是一个ResultSet 对象</p>
</li>
<li><p>ResultSet 对象以逻辑表格的形式封装了执行数据库操作的结果集,ResultSet 接口由数据库厂商提供实现</p>
</li>
<li><p>ResultSet 返回的实际上就是一张数据表。有一个指针指向数据表的第一条记录的前面。</p>
</li>
<li><p>ResultSet 对象维护了一个指向当前数据行的<strong>游标</strong>,初始的时候,游标在第一行之前,可以通过 ResultSet 对象的 next() 方法移动到下一行。调用 next()方法检测下一行是否有效。若有效,该方法返回 true,且指针下移。相当于Iterator对象的 hasNext() 和 next() 方法的结合体。</p>
</li>
<li><p>当指针指向一行时, 可以通过调用 getXxx(int index) 或 getXxx(int columnName) 获取每一列的值。</p>
<ul>
<li>例如: getInt(1), getString(“name”)</li>
<li><strong>注意:Java与数据库交互涉及到的相关Java API中的索引都从1开始。</strong></li>
</ul>
</li>
<li><p>ResultSet 接口的常用方法:</p>
<ul>
<li>boolean next()</li>
<li>getString()</li>
<li>…</li>
</ul>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_09.png" alt="jdbc_09"></p>
</li>
</ul>
<h4 id="3-4-2-ResultSetMetaData"><a href="#3-4-2-ResultSetMetaData" class="headerlink" title="3.4.2 ResultSetMetaData"></a>3.4.2 ResultSetMetaData</h4><ul>
<li><p>可用于获取关于 ResultSet 对象中列的类型和属性信息的对象</p>
</li>
<li><p>ResultSetMetaData meta = rs.getMetaData();</p>
<ul>
<li><strong>getColumnName</strong>(int column):获取指定列的名称</li>
<li><strong>getColumnLabel</strong>(int column):获取指定列的别名</li>
<li><strong>getColumnCount</strong>():返回当前 ResultSet 对象中的列数。</li>
<li>getColumnTypeName(int column):检索指定列的数据库特定的类型名称。</li>
<li>getColumnDisplaySize(int column):指示指定列的最大标准宽度,以字符为单位。</li>
<li><strong>isNullable</strong>(int column):指示指定列中的值是否可以为 null。</li>
<li>isAutoIncrement(int column):指示是否自动为指定列进行编号,这样这些列仍然是只读的。</li>
</ul>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_10.png" alt="jdbc_10"></p>
</li>
</ul>
<p><strong>问题1:得到结果集后, 如何知道该结果集中有哪些列 ? 列名是什么?</strong></p>
<p> 需要使用一个描述 ResultSet 的对象, 即 ResultSetMetaData</p>
<p><strong>问题2:关于ResultSetMetaData</strong></p>
<ol>
<li><strong>如何获取 ResultSetMetaData</strong>: 调用 ResultSet 的 getMetaData() 方法即可</li>
<li><strong>获取 ResultSet 中有多少列</strong>:调用 ResultSetMetaData 的 getColumnCount() 方法</li>
<li><strong>获取 ResultSet 每一列的列的别名是什么</strong>:调用 ResultSetMetaData 的getColumnLabel() 方法</li>
</ol>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_11.png" alt="jdbc_11"></p>
<h3 id="3-5-资源的释放"><a href="#3-5-资源的释放" class="headerlink" title="3.5 资源的释放"></a>3.5 资源的释放</h3><ul>
<li>放ResultSet, Statement,Connection。</li>
<li>数据库连接(Connection)是非常稀有的资源,用完后必须马上释放,如果Connection不能及时正确的关闭将导致系统宕机。Connection的使用原则是<strong>尽量晚创建,尽量早的释放。</strong></li>
<li>可以在finally中关闭,保证及时其他代码出现异常,资源也一定能被关闭。</li>
</ul>
<h3 id="3-6-JDBC-API小结"><a href="#3-6-JDBC-API小结" class="headerlink" title="3.6 JDBC API小结"></a>3.6 JDBC API小结</h3><ul>
<li><p>两种思想</p>
<ul>
<li>面向接口编程的思想</li>
<li>ORM思想(object relational mapping)<ul>
<li>一个数据表对应一个java类</li>
<li>表中的一条记录对应java类的一个对象</li>
<li>表中的一个字段对应java类的一个属性</li>
</ul>
</li>
</ul>
<blockquote>
<p>sql是需要结合列名和表的属性名来写。注意起别名。</p>
</blockquote>
</li>
<li><p>两种技术</p>
<ul>
<li>JDBC结果集的元数据:ResultSetMetaData<ul>
<li>获取列数:getColumnCount()</li>
<li>获取列的别名:getColumnLabel()</li>
</ul>
</li>
<li>通过反射,创建指定类的对象,获取指定的属性并赋值</li>
</ul>
</li>
</ul>
<h2 id="第4章-操作BLOB类型字段"><a href="#第4章-操作BLOB类型字段" class="headerlink" title="第4章 操作BLOB类型字段"></a>第4章 操作BLOB类型字段</h2><h3 id="4-1-MySQL-BLOB类型"><a href="#4-1-MySQL-BLOB类型" class="headerlink" title="4.1 MySQL BLOB类型"></a>4.1 MySQL BLOB类型</h3><ul>
<li><p>MySQL中,BLOB是一个二进制大型对象,是一个可以存储大量数据的容器,它能容纳不同大小的数据。</p>
</li>
<li><p>插入BLOB类型的数据必须使用PreparedStatement,因为BLOB类型的数据无法使用字符串拼接写的。</p>
</li>
<li><p>MySQL的四种BLOB类型(除了在存储的最大信息量上不同外,他们是等同的)</p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_12.png" alt="jdbc_12"></p>
</li>
<li><p>实际使用中根据需要存入的数据大小定义不同的BLOB类型。</p>
</li>
<li><p>需要注意的是:如果存储的文件过大,数据库的性能会下降。</p>
</li>
<li><p>如果在指定了相关的Blob类型以后,还报错:xxx too large,那么在mysql的安装目录下,找my.ini文件加上如下的配置参数: <strong>max_allowed_packet=16M</strong>。同时注意:修改了my.ini文件之后,需要重新启动mysql服务。</p>
<pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>blob_6<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>bean<span class="token punctuation">.</span>Customer<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>util_4<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>io<span class="token punctuation">.</span>*<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>*<span class="token punctuation">;</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">BlobTest</span> <span class="token punctuation">{</span>
</code></pre>
</li>
</ul>
<pre><code> //插入Blob数据
@Test
public void testInsert(){
Connection conn = null;
PreparedStatement ps = null;
try {
conn = JDBCUtils.getConnection();
String sql = "insert into customers(name,email,birth,photo) values(?,?,?,?)";
ps = conn.prepareStatement(sql);
ps.setObject(1,"asda");
ps.setObject(2,"<EMAIL>");
ps.setObject(3,"1234-02-21");
FileInputStream in = new FileInputStream(new File("I:\\IDEA_Projects\\JDBC\\src\\12.jpg"));
ps.setBlob(4,in);
ps.execute();
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.closeResource(conn,ps);
}
}
//查询Blob数据
@Test
public void testQuery() {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
InputStream binaryStream = null;
FileOutputStream out = null;
try {
conn = JDBCUtils.getConnection();
String sql = "select id,name,email,birth,photo from customers where id = ?";
ps = conn.prepareStatement(sql);
ps.setObject(1,21);
rs = ps.executeQuery();
if (rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
String email = rs.getString("email");
Date birth = rs.getDate("birth");
Customer customer = new Customer(id,name,email,birth);
System.out.println(customer);
Blob ptoto = rs.getBlob("photo");
binaryStream = ptoto.getBinaryStream();
out = new FileOutputStream("aaa.jpg");
byte[] buffer = new byte[1024*1024];
int len;
while ((len = binaryStream.read()) != -1){
out.write(buffer,0,len);
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.closeResource(conn,ps,rs);
if (binaryStream != null){
try {
binaryStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (out != null){
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}</code></pre><p> }</p>
<pre><code>
## 第5章 批量插入
### 5.1 批量执行SQL语句
当需要成批插入或者更新记录时,可以采用Java的批量**更新**机制,这一机制允许多条语句一次性提交给数据库批量处理。通常情况下比单独提交处理更有效率
JDBC的批量处理语句包括下面三个方法:
- **addBatch(String):添加需要批量处理的SQL语句或是参数;**
- **executeBatch():执行批量处理语句;**
- **clearBatch():清空缓存的数据**
通常我们会遇到两种批量执行SQL语句的情况:
- 多条SQL语句的批量处理;
- 一个SQL语句的批量传参;
### 5.2 高效的批量插入
#### 5.2.1 实现层次一:使用Statement
```java
Connection conn = JDBCUtils.getConnection();
Statement statement = conn.createStatement();
for (int i = 0;i < 20000;i++){
String sql = "insert into goods(name) values('name_" + i + "')";
statement.execute(sql);
}</code></pre><h4 id="5-2-2-实现层次二:使用PreparedStatement"><a href="#5-2-2-实现层次二:使用PreparedStatement" class="headerlink" title="5.2.2 实现层次二:使用PreparedStatement"></a>5.2.2 实现层次二:使用PreparedStatement</h4><pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>preparedstatement_3<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>util_4<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Connection<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>ParameterMetaData<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>PreparedStatement<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Statement<span class="token punctuation">;</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">BatchOperation</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//批量插入方式二:使用PreparedStatement</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
<span class="token keyword">long</span> start <span class="token operator">=</span> System<span class="token punctuation">.</span><span class="token function">currentTimeMillis</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"insert into goods(name) values(?)"</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span> <span class="token number">1</span><span class="token punctuation">;</span>i <span class="token operator"><</span> <span class="token number">20000</span><span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">,</span><span class="token string">"name_"</span> <span class="token operator">+</span> i<span class="token punctuation">)</span><span class="token punctuation">;</span>
ps<span class="token punctuation">.</span><span class="token function">execute</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">long</span> end <span class="token operator">=</span> System<span class="token punctuation">.</span><span class="token function">currentTimeMillis</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span><span class="token string">"总用时:"</span> <span class="token operator">+</span> <span class="token punctuation">(</span>end<span class="token operator">-</span>start<span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">/**
* 批量插入方式三
* 1.addBatch()、executeBatch()、clearBatch()
* 3.mysql服务器默认是关闭批处理的,需要一个参数,让mysql开启批处理的支持
* 把rewriteBatchedStatements=true写在配置文件url的后面
* 3.使用更新mysql驱动:mysql-connection-java-5.1.37-bin.jar
*/</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testBatch1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
<span class="token keyword">long</span> start <span class="token operator">=</span> System<span class="token punctuation">.</span><span class="token function">currentTimeMillis</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"insert into goods(name) values(?)"</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span> <span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> <span class="token number">20000</span><span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">,</span><span class="token string">"name_"</span> <span class="token operator">+</span> i<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//1.攒 sql</span>
ps<span class="token punctuation">.</span><span class="token function">addBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span> <span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">)</span> <span class="token operator">%</span> <span class="token number">500</span> <span class="token operator">==</span> <span class="token number">0</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//2.执行batch</span>
ps<span class="token punctuation">.</span><span class="token function">executeBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//3.清空batch</span>
ps<span class="token punctuation">.</span><span class="token function">clearBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token keyword">long</span> end <span class="token operator">=</span> System<span class="token punctuation">.</span><span class="token function">currentTimeMillis</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span><span class="token string">"总用时:"</span> <span class="token operator">+</span> <span class="token punctuation">(</span>end<span class="token operator">-</span>start<span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">/**
* 批量插入方式四:设置连接不允许自动提交数据
*/</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testBatch2</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
<span class="token keyword">long</span> start <span class="token operator">=</span> System<span class="token punctuation">.</span><span class="token function">currentTimeMillis</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//设置不允许自动提交</span>
conn<span class="token punctuation">.</span><span class="token function">setAutoCommit</span><span class="token punctuation">(</span><span class="token boolean">false</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"insert into goods(name) values(?)"</span><span class="token punctuation">;</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span> <span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> <span class="token number">1000000</span><span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">,</span><span class="token string">"name_"</span> <span class="token operator">+</span> i<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//1.攒 sql</span>
ps<span class="token punctuation">.</span><span class="token function">addBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span> <span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">)</span> <span class="token operator">%</span> <span class="token number">500</span> <span class="token operator">==</span> <span class="token number">0</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//2.执行batch</span>
ps<span class="token punctuation">.</span><span class="token function">executeBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//3.清空batch</span>
ps<span class="token punctuation">.</span><span class="token function">clearBatch</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//提交数据</span>
conn<span class="token punctuation">.</span><span class="token function">commit</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">long</span> end <span class="token operator">=</span> System<span class="token punctuation">.</span><span class="token function">currentTimeMillis</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span><span class="token string">"总用时:"</span> <span class="token operator">+</span> <span class="token punctuation">(</span>end<span class="token operator">-</span>start<span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>ps<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<h2 id="第6章:-数据库事务"><a href="#第6章:-数据库事务" class="headerlink" title="第6章: 数据库事务"></a>第6章: 数据库事务</h2><h3 id="6-1-数据库事务介绍"><a href="#6-1-数据库事务介绍" class="headerlink" title="6.1 数据库事务介绍"></a>6.1 数据库事务介绍</h3><ul>
<li><strong>事务:一组逻辑操作单元,使数据从一种状态变换到另一种状态。</strong></li>
<li><strong>事务处理(事务操作):\</strong>保证所有事务都作为一个工作单元来执行,即使出现了故障,都不能改变这种执行方式。当在一个事务中执行多个操作时,要么所有的事务都*<em>被提交(commit)*</em>,那么这些修改就永久地保存下来;要么数据库管理系统将放弃所作的所有修改,整个事务<strong>回滚(rollback)</strong>到最初状态。</li>
<li>为确保数据库中数据的<strong>一致性</strong>,数据的操纵应当是离散的成组的逻辑单元:当它全部完成时,数据的一致性可以保持,而当这个单元中的一部分操作失败,整个事务应全部视为错误,所有从起始点以后的操作应全部回退到开始状态。</li>
</ul>
<h3 id="6-2-JDBC事务处理"><a href="#6-2-JDBC事务处理" class="headerlink" title="6.2 JDBC事务处理"></a>6.2 JDBC事务处理</h3><ul>
<li><p>数据一旦提交,就不可回滚。</p>
</li>
<li><p>数据什么时候意味着提交?</p>
<ul>
<li><strong>当一个连接对象被创建时,默认情况下是自动提交事务</strong>:每次执行一个 SQL 语句时,如果执行成功,就会向数据库自动提交,而不能回滚。</li>
<li><strong>关闭数据库连接,数据就会自动的提交。</strong>如果多个操作,每个操作使用的是自己单独的连接,则无法保证事务。即同一个事务的多个操作必须在同一个连接下。</li>
</ul>
</li>
<li><p><strong>JDBC程序中为了让多个 SQL 语句作为一个事务执行:</strong></p>
<ul>
<li>调用 Connection 对象的 <strong>setAutoCommit(false);</strong> 以取消自动提交事务</li>
<li>在所有的 SQL 语句都成功执行后,调用 <strong>commit();</strong> 方法提交事务</li>
<li>在出现异常时,调用 <strong>rollback();</strong> 方法回滚事务</li>
</ul>
<blockquote>
<p>若此时 Connection 没有被关闭,还可能被重复使用,则需要恢复其自动提交状态 setAutoCommit(true)。尤其是在使用数据库连接池技术时,执行close()方法前,建议恢复自动提交状态。</p>
</blockquote>
</li>
</ul>
<h3 id="6-3-事务的ACID属性"><a href="#6-3-事务的ACID属性" class="headerlink" title="6.3 事务的ACID属性"></a>6.3 事务的ACID属性</h3><ol>
<li><strong>原子性(Atomicity)</strong><br>原子性是指事务是一个不可分割的工作单位,事务中的操作要么都发生,要么都不发生。</li>
<li><strong>一致性(Consistency)</strong><br>事务必须使数据库从一个一致性状态变换到另外一个一致性状态。</li>
<li><strong>隔离性(Isolation)</strong><br>事务的隔离性是指一个事务的执行不能被其他事务干扰,即一个事务内部的操作及使用的数据对并发的其他事务是隔离的,并发执行的各个事务之间不能互相干扰。</li>
<li><strong>持久性(Durability)</strong><br>持久性是指一个事务一旦被提交,它对数据库中数据的改变就是永久性的,接下来的其他操作和数据库故障不应该对其有任何影响。</li>
</ol>
<h4 id="6-3-1-数据库的并发问题"><a href="#6-3-1-数据库的并发问题" class="headerlink" title="6.3.1 数据库的并发问题"></a>6.3.1 数据库的并发问题</h4><ul>
<li>对于同时运行的多个事务, 当这些事务访问数据库中相同的数据时, 如果没有采取必要的隔离机制, 就会导致各种并发问题:<ul>
<li><strong>脏读</strong>: 对于两个事务 T1, T2, T1 读取了已经被 T2 更新但还<strong>没有被提交</strong>的字段。之后, 若 T2 回滚, T1读取的内容就是临时且无效的。</li>
<li><strong>不可重复读</strong>: 对于两个事务T1, T2, T1 读取了一个字段, 然后 T2 <strong>更新</strong>了该字段。之后, T1再次读取同一个字段, 值就不同了。</li>
<li><strong>幻读</strong>: 对于两个事务T1, T2, T1 从一个表中读取了一个字段, 然后 T2 在该表中<strong>插入</strong>了一些新的行。之后, 如果 T1 再次读取同一个表, 就会多出几行。</li>
</ul>
</li>
<li><strong>数据库事务的隔离性</strong>: 数据库系统必须具有隔离并发运行各个事务的能力, 使它们不会相互影响, 避免各种并发问题。</li>
<li>一个事务与其他事务隔离的程度称为隔离级别。数据库规定了多种事务隔离级别, 不同隔离级别对应不同的干扰程度, <strong>隔离级别越高, 数据一致性就越好, 但并发性越弱。</strong></li>
</ul>
<h4 id="6-3-2-四种隔离级别"><a href="#6-3-2-四种隔离级别" class="headerlink" title="6.3.2 四种隔离级别"></a>6.3.2 四种隔离级别</h4><ul>
<li><p>数据库提供的4种事务隔离级别:</p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_13.png" alt="jdbc_13"></p>
</li>
<li><p>Oracle 支持的 2 种事务隔离级别:<strong>READ COMMITED</strong>, SERIALIZABLE。 Oracle 默认的事务隔离级别为: <strong>READ COMMITED</strong> 。</p>
</li>
<li><p>Mysql 支持 4 种事务隔离级别。Mysql 默认的事务隔离级别为: <strong>REPEATABLE READ。</strong></p>
</li>
</ul>
<h4 id="6-3-3-在MySql中设置隔离级别"><a href="#6-3-3-在MySql中设置隔离级别" class="headerlink" title="6.3.3 在MySql中设置隔离级别"></a>6.3.3 在MySql中设置隔离级别</h4><ul>
<li><p>每启动一个 mysql 程序, 就会获得一个单独的数据库连接. 每个数据库连接都有一个全局变量 @@tx_isolation, 表示当前的事务隔离级别。</p>
</li>
<li><p>查看当前的隔离级别:</p>
<pre><code>SELECT @@tx_isolation;</code></pre></li>
<li><p>设置当前 mySQL 连接的隔离级别:</p>
<pre><code>set transaction isolation level read committed;</code></pre></li>
<li><p>设置数据库系统的全局的隔离级别:</p>
<pre><code>set global transaction isolation level read committed;</code></pre></li>
<li><p>补充操作:</p>
<ul>
<li><p>创建mysql数据库用户:</p>
<pre><code>create user tom identified by 'abc123';</code></pre><p>授予权限</p>
<pre><code>#授予通过网络方式登录的tom用户,对所有库所有表的全部权限,密码设为<PASSWORD>.
grant all privileges on *.* to tom@'%' identified by 'abc123';
#给tom用户使用本地命令行方式,授予atguigudb这个库下的所有表的插删改查的权限。
grant select,insert,delete,update on atguigudb.* to tom@localhost identified by 'abc123'; </code></pre></li>
</ul>
</li>
</ul>
<h2 id="第7章:DAO及相关实现类"><a href="#第7章:DAO及相关实现类" class="headerlink" title="第7章:DAO及相关实现类"></a>第7章:DAO及相关实现类</h2><ul>
<li><p>DAO:Data Access Object访问数据信息的类和接口,包括了对数据的CRUD(Create、Retrival、Update、Delete),而不包含任何业务相关的信息。有时也称作:BaseDAO</p>
</li>
<li><p>作用:为了实现功能的模块化,更有利于代码的维护和升级。</p>
</li>
<li><p>层次结构:</p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_14.png" alt="jdbc_14"></p>
</li>
</ul>
<h3 id="【BaseDAO-java】"><a href="#【BaseDAO-java】" class="headerlink" title="【BaseDAO.java】"></a>【BaseDAO.java】</h3><pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>Dao<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>utils<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>lang<span class="token punctuation">.</span>reflect<span class="token punctuation">.</span>Field<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>*<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>ArrayList<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>List<span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">/**
* 封装了数据表的通用操作
*/</span>
<span class="token keyword">public</span> <span class="token keyword">abstract</span> <span class="token keyword">class</span> <span class="token class-name">BaseDao</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">/**
* 通用的增删改操作方法
* @param conn
* @param sql
* @param args
*/</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">update</span><span class="token punctuation">(</span>Connection conn<span class="token punctuation">,</span>String sql<span class="token punctuation">,</span>Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
PreparedStatement preparedStatement <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//2.预编译sql语句,返回Preparedstatement实例</span>
preparedStatement <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//3.填充占位符</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span> <span class="token number">0</span><span class="token punctuation">;</span> i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span> i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
preparedStatement<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//4.执行操作</span>
preparedStatement<span class="token punctuation">.</span><span class="token function">execute</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//5.关闭资源</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>null<span class="token punctuation">,</span>preparedStatement<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">/**
* 针对不同表的查询,返回一条数据
* @param conn
* @param clazz
* @param sql
* @param args
* @param <T>
* @return
*/</span>
<span class="token keyword">public</span> <span class="token operator"><</span>T<span class="token operator">></span> T <span class="token function">queryForOne</span><span class="token punctuation">(</span>Connection conn<span class="token punctuation">,</span>Class<span class="token operator"><</span>T<span class="token operator">></span> clazz <span class="token punctuation">,</span>String sql<span class="token punctuation">,</span> Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
PreparedStatement ps <span class="token operator">=</span>null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span>null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//获取结果集</span>
rs <span class="token operator">=</span>ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取结果集的元数据</span>
ResultSetMetaData rsmd <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getMetaData</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取列(字段)的个数</span>
<span class="token keyword">int</span> columnCount <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnCount</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
T t <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">newInstance</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span><span class="token punctuation">(</span><span class="token keyword">int</span> i<span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> columnCount<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的列值:通过ResultSet</span>
Object columnValue <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getObject</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的名子:通过ResultSetMetaData 不推荐使用</span>
<span class="token comment" spellcheck="true">//String columnName = rsmd.getColumnName(i + 1);</span>
<span class="token comment" spellcheck="true">//获取列的别名</span>
String columnLabel <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnLabel</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//通过反射,将对象指定名columnName的属性赋值为指定的值cliumnValue</span>
Field declaredField <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">getDeclaredField</span><span class="token punctuation">(</span>columnLabel<span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">setAccessible</span><span class="token punctuation">(</span><span class="token boolean">true</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">set</span><span class="token punctuation">(</span>t<span class="token punctuation">,</span>columnValue<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> t<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>null<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">/**
* 针对不同表的查询,返回多条数据
* @param conn
* @param clazz
* @param sql
* @param args
* @param <T>
* @return
*/</span>
<span class="token keyword">public</span> <span class="token operator"><</span>T<span class="token operator">></span> List<span class="token operator"><</span>T<span class="token operator">></span> <span class="token function">queryForList</span><span class="token punctuation">(</span>Connection conn<span class="token punctuation">,</span>Class<span class="token operator"><</span>T<span class="token operator">></span> clazz <span class="token punctuation">,</span> String sql<span class="token punctuation">,</span> Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
PreparedStatement ps <span class="token operator">=</span>null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span>null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
ps <span class="token operator">=</span> conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//获取结果集</span>
rs <span class="token operator">=</span>ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取结果集的元数据</span>
ResultSetMetaData rsmd <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getMetaData</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取列(字段)的个数</span>
<span class="token keyword">int</span> columnCount <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnCount</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//创建集合对象</span>
ArrayList<span class="token operator"><</span>T<span class="token operator">></span> list <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">ArrayList</span><span class="token operator"><</span><span class="token operator">></span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">while</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
T t <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">newInstance</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//处理结果集的一行数据中的每一列:给t对象指定的属性赋值</span>
<span class="token keyword">for</span><span class="token punctuation">(</span><span class="token keyword">int</span> i<span class="token operator">=</span><span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> columnCount<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的列值:通过ResultSet</span>
Object columnValue <span class="token operator">=</span> rs<span class="token punctuation">.</span><span class="token function">getObject</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//获取每个列(字段)的名子:通过ResultSetMetaData 不推荐使用</span>
<span class="token comment" spellcheck="true">//String columnName = rsmd.getColumnName(i + 1);</span>
<span class="token comment" spellcheck="true">//获取列的别名</span>
String columnLabel <span class="token operator">=</span> rsmd<span class="token punctuation">.</span><span class="token function">getColumnLabel</span><span class="token punctuation">(</span>i <span class="token operator">+</span> <span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//通过反射,将对象指定名columnName的属性赋值为指定的值cliumnValue</span>
Field declaredField <span class="token operator">=</span> clazz<span class="token punctuation">.</span><span class="token function">getDeclaredField</span><span class="token punctuation">(</span>columnLabel<span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">setAccessible</span><span class="token punctuation">(</span><span class="token boolean">true</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
declaredField<span class="token punctuation">.</span><span class="token function">set</span><span class="token punctuation">(</span>t<span class="token punctuation">,</span>columnValue<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
list<span class="token punctuation">.</span><span class="token function">add</span><span class="token punctuation">(</span>t<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> list<span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>null<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">/**
* 用于其他查询
* @param conn
* @param sql
* @param args
* @param <T>
* @return
*/</span>
<span class="token keyword">public</span> <span class="token operator"><</span>T<span class="token operator">></span> T <span class="token function">getValue</span><span class="token punctuation">(</span>Connection conn<span class="token punctuation">,</span>String sql<span class="token punctuation">,</span>Object <span class="token punctuation">.</span><span class="token punctuation">.</span><span class="token punctuation">.</span>args<span class="token punctuation">)</span><span class="token punctuation">{</span>
PreparedStatement ps <span class="token operator">=</span> null<span class="token punctuation">;</span>
ResultSet rs <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
conn<span class="token punctuation">.</span><span class="token function">prepareStatement</span><span class="token punctuation">(</span>sql<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">for</span> <span class="token punctuation">(</span><span class="token keyword">int</span> i <span class="token operator">=</span> <span class="token number">0</span><span class="token punctuation">;</span>i <span class="token operator"><</span> args<span class="token punctuation">.</span>length<span class="token punctuation">;</span>i<span class="token operator">++</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
ps<span class="token punctuation">.</span><span class="token function">setObject</span><span class="token punctuation">(</span>i<span class="token operator">+</span><span class="token number">1</span><span class="token punctuation">,</span>args<span class="token punctuation">[</span>i<span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
rs <span class="token operator">=</span> ps<span class="token punctuation">.</span><span class="token function">executeQuery</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>rs<span class="token punctuation">.</span><span class="token function">next</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
<span class="token keyword">return</span> <span class="token punctuation">(</span>T<span class="token punctuation">)</span> rs<span class="token punctuation">.</span><span class="token function">getObject</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>null<span class="token punctuation">,</span>ps<span class="token punctuation">,</span>rs<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<h2 id="第8章:数据库连接池"><a href="#第8章:数据库连接池" class="headerlink" title="第8章:数据库连接池"></a>第8章:数据库连接池</h2><h3 id="8-1-JDBC数据库连接池的必要性"><a href="#8-1-JDBC数据库连接池的必要性" class="headerlink" title="8.1 JDBC数据库连接池的必要性"></a>8.1 JDBC数据库连接池的必要性</h3><ul>
<li>在使用开发基于数据库的web程序时,传统的模式基本是按以下步骤:<ul>
<li><strong>在主程序(如servlet、beans)中建立数据库连接</strong></li>
<li><strong>进行sql操作</strong></li>
<li><strong>断开数据库连接</strong></li>
</ul>
</li>
<li>这种模式开发,存在的问题:<ul>
<li>普通的JDBC数据库连接使用 DriverManager 来获取,每次向数据库建立连接的时候都要将 Connection 加载到内存中,再验证用户名和密码(得花费0.05s~1s的时间)。需要数据库连接的时候,就向数据库要求一个,执行完成后再断开连接。这样的方式将会消耗大量的资源和时间。<strong>数据库的连接资源并没有得到很好的重复利用。</strong>若同时有几百人甚至几千人在线,频繁的进行数据库连接操作将占用很多的系统资源,严重的甚至会造成服务器的崩溃。</li>
<li><strong>对于每一次数据库连接,使用完后都得断开。</strong>否则,如果程序出现异常而未能关闭,将会导致数据库系统中的内存泄漏,最终将导致重启数据库。(回忆:何为Java的内存泄漏?)</li>
<li><strong>这种开发不能控制被创建的连接对象数</strong>,系统资源会被毫无顾及的分配出去,如连接过多,也可能导致内存泄漏,服务器崩溃。</li>
</ul>
</li>
</ul>
<h3 id="8-2-数据库连接池技术"><a href="#8-2-数据库连接池技术" class="headerlink" title="8.2 数据库连接池技术"></a>8.2 数据库连接池技术</h3><ul>
<li><p>为解决传统开发中的数据库连接问题,可以采用数据库连接池技术。</p>
</li>
<li><p><strong>数据库连接池的基本思想</strong>:就是为数据库连接建立一个“缓冲池”。预先在缓冲池中放入一定数量的连接,当需要建立数据库连接时,只需从“缓冲池”中取出一个,使用完毕之后再放回去。</p>
</li>
<li><p><strong>数据库连接池</strong>负责分配、管理和释放数据库连接,它<strong>允许应用程序重复使用一个现有的数据库连接,而不是重新建立一个</strong>。</p>
</li>
<li><p>数据库连接池在初始化时将创建一定数量的数据库连接放到连接池中,这些数据库连接的数量是由<strong>最小数据库连接数来设定</strong>的。无论这些数据库连接是否被使用,连接池都将一直保证至少拥有这么多的连接数量。连接池的<strong>最大数据库连接数量</strong>限定了这个连接池能占有的最大连接数,当应用程序向连接池请求的连接数超过最大连接数量时,这些请求将被加入到等待队列中。</p>
</li>
<li><p><strong>工作原理:</strong></p>
<p><img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/jdbc_15.png" alt="jdbc_15"></p>
</li>
<li><p><strong>数据库连接池技术的优点</strong></p>
<p><strong>1. 资源重用</strong></p>
<p>由于数据库连接得以重用,避免了频繁创建,释放连接引起的大量性能开销。在减少系统消耗的基础上,另一方面也增加了系统运行环境的平稳性。</p>
<p><strong>2. 更快的系统反应速度</strong></p>
<p>数据库连接池在初始化过程中,往往已经创建了若干数据库连接置于连接池中备用。此时连接的初始化工作均已完成。对于业务请求处理而言,直接利用现有可用连接,避免了数据库连接初始化和释放过程的时间开销,从而减少了系统的响应时间</p>
<p><strong>3. 新的资源分配手段</strong></p>
<p>对于多应用共享同一数据库的系统而言,可在应用层通过数据库连接池的配置,实现某一应用最大可用数据库连接数的限制,避免某一应用独占所有的数据库资源</p>
<p><strong>4. 统一的连接管理,避免数据库连接泄漏</strong></p>
<p>在较为完善的数据库连接池实现中,可根据预先的占用超时设定,强制回收被占用连接,从而避免了常规数据库连接操作中可能出现的资源泄露</p>
</li>
</ul>
<h3 id="8-3-多种开源的数据库连接池"><a href="#8-3-多种开源的数据库连接池" class="headerlink" title="8.3 多种开源的数据库连接池"></a>8.3 多种开源的数据库连接池</h3><ul>
<li>JDBC 的数据库连接池使用 javax.sql.DataSource 来表示,DataSource 只是一个接口,该接口通常由服务器(Weblogic, WebSphere, Tomcat)提供实现,也有一些开源组织提供实现:<ul>
<li><strong>DBCP</strong> 是Apache提供的数据库连接池。tomcat 服务器自带dbcp数据库连接池。<strong>速度相对c3p0较快</strong>,但因自身存在BUG,Hibernate3已不再提供支持。</li>
<li><strong>C3P0</strong> 是一个开源组织提供的一个数据库连接池,<strong>速度相对较慢,稳定性还可以。</strong>hibernate官方推荐使用</li>
<li><strong>Proxool</strong> 是sourceforge下的一个开源项目数据库连接池,有监控连接池状态的功能,<strong>稳定性较c3p0差一点</strong></li>
<li><strong>BoneCP</strong> 是一个开源组织提供的数据库连接池,速度快</li>
<li><strong>Druid</strong> 是阿里提供的数据库连接池,据说是集DBCP 、C3P0 、Proxool 优点于一身的数据库连接池,但是速度不确定是否有BoneCP快</li>
</ul>
</li>
<li>DataSource 通常被称为数据源,它包含连接池和连接池管理两个部分,习惯上也经常把 DataSource 称为连接池</li>
<li><strong>DataSource用来取代DriverManager来获取Connection,获取速度快,同时可以大幅度提高数据库访问速度。</strong></li>
<li>特别注意:<ul>
<li>数据源和数据库连接不同,数据源无需创建多个,它是产生数据库连接的工厂,因此<strong>整个应用只需要一个数据源即可。</strong></li>
<li>当数据库访问结束后,程序还是像以前一样关闭数据库连接:conn.close(); 但conn.close()并没有关闭数据库的物理连接,它仅仅把数据库连接释放,归还给了数据库连接池。</li>
</ul>
</li>
</ul>
<h4 id="8-3-1-C3P0数据库连接池"><a href="#8-3-1-C3P0数据库连接池" class="headerlink" title="8.3.1 C3P0数据库连接池"></a>8.3.1 C3P0数据库连接池</h4><ul>
<li><p>获取连接方式一</p>
<pre class=" language-java"><code class="language-java"><span class="token comment" spellcheck="true">//使用C3P0数据库连接池的方式,获取数据库的连接:不推荐</span>
<span class="token comment" spellcheck="true">//方式一</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testGetConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> PropertyVetoException<span class="token punctuation">,</span> SQLException <span class="token punctuation">{</span>
<span class="token comment" spellcheck="true">//获取C3P0数据库连接池</span>
ComboPooledDataSource cpds <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">ComboPooledDataSource</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
cpds<span class="token punctuation">.</span><span class="token function">setDriverClass</span><span class="token punctuation">(</span> <span class="token string">"com.mysql.cj.jdbc.Driver"</span> <span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token comment" spellcheck="true">//loads the jdbc driver</span>
cpds<span class="token punctuation">.</span><span class="token function">setJdbcUrl</span><span class="token punctuation">(</span> <span class="token string">"jdbc:mysql://IP:3306/test?rewriteBatchedStatements=true&useSSL=false&serverTimezone=GMT"</span> <span class="token punctuation">)</span><span class="token punctuation">;</span>
cpds<span class="token punctuation">.</span><span class="token function">setUser</span><span class="token punctuation">(</span><span class="token string">"root"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
cpds<span class="token punctuation">.</span><span class="token function">setPassword</span><span class="token punctuation">(</span><span class="token string">"hct11"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//设置初始的连接池的连接数</span>
cpds<span class="token punctuation">.</span><span class="token function">setInitialPoolSize</span><span class="token punctuation">(</span><span class="token number">10</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Connection conn <span class="token operator">=</span> cpds<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>conn<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//销毁C3P0连接池</span>
DataSources<span class="token punctuation">.</span><span class="token function">destroy</span><span class="token punctuation">(</span>cpds<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span></code></pre>
</li>
<li><p>获取连接方式二:</p>
<pre class=" language-java"><code class="language-java"><span class="token comment" spellcheck="true">//方式二,这里使用的时配置xml文件(测试未通过,或许与jar和mysql版本有关,未再验证),还可以使用Properties</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testGetConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> PropertyVetoException<span class="token punctuation">,</span> SQLException <span class="token punctuation">{</span>
ComboPooledDataSource cpds <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">ComboPooledDataSource</span><span class="token punctuation">(</span><span class="token string">"helloc3p0"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
Connection conn <span class="token operator">=</span> cpds<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>conn<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span></code></pre>
</li>
<li><p>其中,src下的配置文件为:【c3p0-config.xml】</p>
<pre class=" language-xml"><code class="language-xml"><span class="token prolog"><?xml version="1.0" encoding="UTF-8"?></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>c3p0-config</span><span class="token punctuation">></span></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>named-config</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>helloc3p0<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- 获取连接的4个基本信息 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>user<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>root<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>password<span class="token punctuation">"</span></span><span class="token punctuation">></span></span><PASSWORD><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>jdbcUrl<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>jdbc:mysql:///test<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>driverClass<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>com.mysql.jdbc.Driver<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- 涉及到数据库连接池的管理的相关属性的设置 --></span>
<span class="token comment" spellcheck="true"><!-- 若数据库中连接数不足时, 一次向数据库服务器申请多少个连接 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>acquireIncrement<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>5<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- 初始化数据库连接池时连接的数量 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>initialPoolSize<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>5<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- 数据库连接池中的最小的数据库连接数 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>minPoolSize<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>5<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- 数据库连接池中的最大的数据库连接数 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>maxPoolSize<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>10<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- C3P0 数据库连接池可以维护的 Statement 的个数 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>maxStatements<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>20<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token comment" spellcheck="true"><!-- 每个连接同时可以使用的 Statement 对象的个数 --></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>property</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation">=</span><span class="token punctuation">"</span>maxStatementsPerConnection<span class="token punctuation">"</span></span><span class="token punctuation">></span></span>5<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>property</span><span class="token punctuation">></span></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>named-config</span><span class="token punctuation">></span></span>
<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>c3p0-config</span><span class="token punctuation">></span></span>
</code></pre>
</li>
</ul>
<h4 id="8-3-2-DBCP数据库连接池"><a href="#8-3-2-DBCP数据库连接池" class="headerlink" title="8.3.2 DBCP数据库连接池"></a>8.3.2 DBCP数据库连接池</h4><ul>
<li>DBCP 是 Apache 软件基金组织下的开源连接池实现,该连接池依赖该组织下的另一个开源系统:Common-pool。如需使用该连接池实现,应在系统中增加如下两个 jar 文件:<ul>
<li>Commons-dbcp.jar:连接池的实现</li>
<li>Commons-pool.jar:连接池实现的依赖库</li>
</ul>
</li>
<li><strong>Tomcat 的连接池正是采用该连接池来实现的。</strong>该数据库连接池既可以与应用服务器整合使用,也可由应用程序独立使用。</li>
<li>数据源和数据库连接不同,数据源无需创建多个,它是产生数据库连接的工厂,因此整个应用只需要一个数据源即可。</li>
<li>当数据库访问结束后,程序还是像以前一样关闭数据库连接:conn.close(); 但上面的代码并没有关闭数据库的物理连接,它仅仅把数据库连接释放,归还给了数据库连接池。</li>
<li>配置属性说明</li>
</ul>
<table>
<thead>
<tr>
<th align="center">属性</th>
<th align="center">默认值</th>
<th align="center">说明</th>
</tr>
</thead>
<tbody><tr>
<td align="center">initialSize</td>
<td align="center">0</td>
<td align="center">连接池启动时创建的初始化连接数量</td>
</tr>
<tr>
<td align="center">maxActive</td>
<td align="center">8</td>
<td align="center">连接池中可同时连接的最大的连接数</td>
</tr>
<tr>
<td align="center">maxIdle</td>
<td align="center">8</td>
<td align="center">连接池中最大的空闲的连接数,超过的空闲连接将被释放,如果设置为负数表示不限制</td>
</tr>
<tr>
<td align="center">minIdle</td>
<td align="center">0</td>
<td align="center">连接池中最小的空闲的连接数,低于这个数量会被创建新的连接。该参数越接近maxIdle,性能越好,因为连接的创建和销毁,都是需要消耗资源的;但是不能太大。</td>
</tr>
<tr>
<td align="center">maxWait</td>
<td align="center">无限制</td>
<td align="center">最大等待时间,当没有可用连接时,连接池等待连接释放的最大时间,超过该时间限制会抛出异常,如果设置-1表示无限等待</td>
</tr>
<tr>
<td align="center">poolPreparedStatements</td>
<td align="center">false</td>
<td align="center">开启池的Statement是否prepared</td>
</tr>
<tr>
<td align="center">maxOpenPreparedStatements</td>
<td align="center">无限制</td>
<td align="center">开启池的prepared 后的同时最大连接数</td>
</tr>
<tr>
<td align="center">minEvictableIdleTimeMillis</td>
<td align="center"></td>
<td align="center">连接池中连接,在时间段内一直空闲, 被逐出连接池的时间</td>
</tr>
<tr>
<td align="center">removeAbandonedTimeout</td>
<td align="center">300</td>
<td align="center">超过时间限制,回收没有用(废弃)的连接</td>
</tr>
<tr>
<td align="center">removeAbandoned</td>
<td align="center">false</td>
<td align="center">超过removeAbandonedTimeout时间后,是否进 行没用连接(废弃)的回收</td>
</tr>
</tbody></table>
<ul>
<li><p>获取连接方式</p>
<pre class=" language-java"><code class="language-java"> <span class="token comment" spellcheck="true">/**
* DBCP连接池
* 使用静态代码块,连接池只需要创建一个就可以了,
* 防止每次调用getConnection()方法都要创建一个
*/</span>
<span class="token keyword">private</span> <span class="token keyword">static</span> DataSource source <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">static</span><span class="token punctuation">{</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
Properties pros <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Properties</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
InputStream is <span class="token operator">=</span> DBCPTest<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">.</span><span class="token function">getClassLoader</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">.</span><span class="token function">getResourceAsStream</span><span class="token punctuation">(</span><span class="token string">"dbcp.properties"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
pros<span class="token punctuation">.</span><span class="token function">load</span><span class="token punctuation">(</span>is<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">//根据提供的BasicDataSourceFactory创建对应的DataSource对象</span>
source <span class="token operator">=</span> BasicDataSourceFactory<span class="token punctuation">.</span><span class="token function">createDataSource</span><span class="token punctuation">(</span>pros<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token keyword">public</span> <span class="token keyword">static</span> Connection <span class="token function">getConnection4</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> Exception <span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> source<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">return</span> conn<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
</code></pre>
</li>
</ul>
<h4 id="8-3-3-Druid(德鲁伊)数据库连接池"><a href="#8-3-3-Druid(德鲁伊)数据库连接池" class="headerlink" title="8.3.3 Druid(德鲁伊)数据库连接池"></a>8.3.3 Druid(德鲁伊)数据库连接池</h4><p>Druid是阿里巴巴开源平台上一个数据库连接池实现,它结合了C3P0、DBCP、Proxool等DB池的优点,同时加入了日志监控,可以很好的监控DB池连接和SQL的执行情况,可以说是针对监控而生的DB连接池,<strong>可以说是目前最好的连接池之一。</strong></p>
<pre class=" language-java"><code class="language-java"><span class="token comment" spellcheck="true">/**
* Druid连接池
* 使用静态代码块,连接池只需要创建一个就可以了,
* 防止每次调用getConnection()方法都要创建一个
*/</span>
<span class="token keyword">private</span> <span class="token keyword">static</span> DataSource dataSource1<span class="token punctuation">;</span>
<span class="token keyword">static</span> <span class="token punctuation">{</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
Properties pro <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Properties</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
InputStream is <span class="token operator">=</span> ClassLoader<span class="token punctuation">.</span><span class="token function">getSystemClassLoader</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">.</span><span class="token function">getResourceAsStream</span><span class="token punctuation">(</span><span class="token string">"jdbc.properties"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
pro<span class="token punctuation">.</span><span class="token function">load</span><span class="token punctuation">(</span>is<span class="token punctuation">)</span><span class="token punctuation">;</span>
dataSource <span class="token operator">=</span> DruidDataSourceFactory<span class="token punctuation">.</span><span class="token function">createDataSource</span><span class="token punctuation">(</span>pro<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">IOException</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">Exception</span> e<span class="token punctuation">)</span> <span class="token punctuation">{</span>
e<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token keyword">public</span> <span class="token keyword">static</span> Connection <span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">throws</span> SQLException <span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> dataSource<span class="token punctuation">.</span><span class="token function">getConnection</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token keyword">return</span> conn<span class="token punctuation">;</span>
<span class="token punctuation">}</span></code></pre>
<ul>
<li>详细配置参数:(略)</li>
</ul>
<h2 id="第9章:Apache-DBUtils实现CRUD操作"><a href="#第9章:Apache-DBUtils实现CRUD操作" class="headerlink" title="第9章:Apache-DBUtils实现CRUD操作"></a>第9章:Apache-DBUtils实现CRUD操作</h2><h3 id="9-1-Apache-DBUtils简介"><a href="#9-1-Apache-DBUtils简介" class="headerlink" title="9.1 Apache-DBUtils简介"></a>9.1 Apache-DBUtils简介</h3><ul>
<li>commons-dbutils 是 Apache 组织提供的一个开源 JDBC工具类库,它是对JDBC的简单封装,学习成本极低,并且使用dbutils能极大简化jdbc编码的工作量,同时也不会影响程序的性能。</li>
<li>API介绍:<ul>
<li>org.apache.commons.dbutils.QueryRunner</li>
<li>org.apache.commons.dbutils.ResultSetHandler</li>
<li>工具类:org.apache.commons.dbutils.DbUtils</li>
</ul>
</li>
</ul>
<h3 id="9-2-主要API的使用"><a href="#9-2-主要API的使用" class="headerlink" title="9.2 主要API的使用"></a>9.2 主要API的使用</h3><h4 id="9-2-1-DbUtils"><a href="#9-2-1-DbUtils" class="headerlink" title="9.2.1 DbUtils"></a>9.2.1 DbUtils</h4><ul>
<li>DbUtils :提供如关闭连接、装载JDBC驱动程序等常规工作的工具类,里面的所有方法都是静态的。主要方法如下:<ul>
<li><strong>public static void close(…) throws java.sql.SQLException</strong>: DbUtils类提供了三个重载的关闭方法。这些方法检查所提供的参数是不是NULL,如果不是的话,它们就关闭Connection、Statement和ResultSet。</li>
<li>public static void closeQuietly(…): 这一类方法不仅能在Connection、Statement和ResultSet为NULL情况下避免关闭,还能隐藏一些在程序中抛出的SQLEeception。</li>
<li>public static void commitAndClose(Connection conn)throws SQLException: 用来提交连接的事务,然后关闭连接</li>
<li>public static void commitAndCloseQuietly(Connection conn): 用来提交连接,然后关闭连接,并且在关闭连接时不抛出SQL异常。</li>
<li>public static void rollback(Connection conn)throws SQLException:允许conn为null,因为方法内部做了判断</li>
<li>public static void rollbackAndClose(Connection conn)throws SQLException</li>
<li>rollbackAndCloseQuietly(Connection)</li>
<li>public static boolean loadDriver(java.lang.String driverClassName):这一方装载并注册JDBC驱动程序,如果成功就返回true。使用该方法,你不需要捕捉这个异常ClassNotFoundException。</li>
</ul>
</li>
</ul>
<h4 id="9-2-2-QueryRunner类"><a href="#9-2-2-QueryRunner类" class="headerlink" title="9.2.2 QueryRunner类"></a>9.2.2 QueryRunner类</h4><ul>
<li><strong>该类简单化了SQL查询,它与ResultSetHandler组合在一起使用可以完成大部分的数据库操作,能够大大减少编码量。</strong></li>
<li>QueryRunner类提供了两个构造器:<ul>
<li>默认的构造器</li>
<li>需要一个 javax.sql.DataSource 来作参数的构造器</li>
</ul>
</li>
<li>QueryRunner类的主要方法:<ul>
<li>更新<ul>
<li>public int update(Connection conn, String sql, Object… params) throws SQLException:用来执行一个更新(插入、更新或删除)操作。</li>
<li>…</li>
</ul>
</li>
<li>插入<ul>
<li>public T insert(Connection conn,String sql,ResultSetHandler rsh, Object… params) throws SQLException:只支持INSERT语句,其中 rsh - The handler used to create the result object from the ResultSet of auto-generated keys. 返回值: An object generated by the handler.即自动生成的键值</li>
<li>…</li>
</ul>
</li>
<li>批处理<ul>
<li>public int[] batch(Connection conn,String sql,Object[][] params)throws SQLException: INSERT, UPDATE, or DELETE语句</li>
<li>public T insertBatch(Connection conn,String sql,ResultSetHandler rsh,Object[][] params)throws SQLException:只支持INSERT语句</li>
<li>…</li>
</ul>
</li>
<li>查询<ul>
<li>public Object query(Connection conn, String sql, ResultSetHandler rsh,Object… params) throws SQLException:执行一个查询操作,在这个查询中,对象数组中的每个元素值被用来作为查询语句的置换参数。该方法会自行处理 PreparedStatement 和 ResultSet 的创建和关闭。</li>
</ul>
</li>
</ul>
</li>
</ul>
<h4 id="9-2-3-ResultSetHandler接口及实现类"><a href="#9-2-3-ResultSetHandler接口及实现类" class="headerlink" title="9.2.3 ResultSetHandler接口及实现类"></a>9.2.3 ResultSetHandler接口及实现类</h4><ul>
<li>该接口用于处理 java.sql.ResultSet,将数据按要求转换为另一种形式。</li>
<li>ResultSetHandler 接口提供了一个单独的方法:Object handle (java.sql.ResultSet .rs)。</li>
<li>接口的主要实现类:<ul>
<li>ArrayHandler:把结果集中的第一行数据转成对象数组。</li>
<li>ArrayListHandler:把结果集中的每一行数据都转成一个数组,再存放到List中。</li>
<li><strong>BeanHandler:</strong>将结果集中的第一行数据封装到一个对应的JavaBean实例中。</li>
<li><strong>BeanListHandler:</strong>将结果集中的每一行数据都封装到一个对应的JavaBean实例中,存放到List里。</li>
<li>ColumnListHandler:将结果集中某一列的数据存放到List中。</li>
<li>KeyedHandler(name):将结果集中的每一行数据都封装到一个Map里,再把这些map再存到一个map里,其key为指定的key。</li>
<li><strong>MapHandler:</strong>将结果集中的第一行数据封装到一个Map里,key是列名,value就是对应的值。</li>
<li><strong>MapListHandler:</strong>将结果集中的每一行数据都封装到一个Map里,然后再存放到List</li>
<li><strong>ScalarHandler:</strong>查询单个值对象</li>
</ul>
</li>
</ul>
<pre class=" language-java"><code class="language-java"><span class="token keyword">package</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>dbUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>bean<span class="token punctuation">.</span>Customer<span class="token punctuation">;</span>
<span class="token keyword">import</span> com<span class="token punctuation">.</span>hct<span class="token punctuation">.</span>utils_2<span class="token punctuation">.</span>JDBCUtils<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>apache<span class="token punctuation">.</span>commons<span class="token punctuation">.</span>dbutils<span class="token punctuation">.</span>QueryRunner<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>apache<span class="token punctuation">.</span>commons<span class="token punctuation">.</span>dbutils<span class="token punctuation">.</span>ResultSetHandler<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>apache<span class="token punctuation">.</span>commons<span class="token punctuation">.</span>dbutils<span class="token punctuation">.</span>handlers<span class="token punctuation">.</span>*<span class="token punctuation">;</span>
<span class="token keyword">import</span> org<span class="token punctuation">.</span>junit<span class="token punctuation">.</span>Test<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>Connection<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>ResultSet<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>sql<span class="token punctuation">.</span>SQLException<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>List<span class="token punctuation">;</span>
<span class="token keyword">import</span> java<span class="token punctuation">.</span>util<span class="token punctuation">.</span>Map<span class="token punctuation">;</span>
<span class="token comment" spellcheck="true">/**
* @Author HCT
* @Date 2021/1/7 23:19
* @Version 1.0
*/</span>
<span class="token keyword">public</span> <span class="token keyword">class</span> <span class="token class-name">QueryRunnerTest</span> <span class="token punctuation">{</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testInsert</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"insert into customers(name,email,birth) values(?,?,?)"</span><span class="token punctuation">;</span>
runner<span class="token punctuation">.</span><span class="token function">update</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>sql<span class="token punctuation">,</span><span class="token string">"12ii"</span><span class="token punctuation">,</span><span class="token string">"<EMAIL>"</span><span class="token punctuation">,</span><span class="token string">"1988-12-30"</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//查询一条数据</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testQuery1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select id,name,email,birth from customers where id = ?"</span><span class="token punctuation">;</span>
Customer customer <span class="token operator">=</span> runner<span class="token punctuation">.</span><span class="token function">query</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span> sql<span class="token punctuation">,</span> <span class="token keyword">new</span> <span class="token class-name">BeanHandler</span><span class="token operator"><</span>Customer<span class="token operator">></span><span class="token punctuation">(</span>Customer<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">)</span><span class="token punctuation">,</span> <span class="token number">16</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>customer<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//查询多条数据</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testQuery2</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select id,name,email,birth from customers "</span><span class="token punctuation">;</span>
List<span class="token operator"><</span>Customer<span class="token operator">></span> customers <span class="token operator">=</span> runner<span class="token punctuation">.</span><span class="token function">query</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span> sql<span class="token punctuation">,</span> <span class="token keyword">new</span> <span class="token class-name">BeanListHandler</span><span class="token operator"><</span>Customer<span class="token operator">></span><span class="token punctuation">(</span>Customer<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
customers<span class="token punctuation">.</span><span class="token function">forEach</span><span class="token punctuation">(</span>System<span class="token punctuation">.</span>out <span class="token operator">:</span><span class="token operator">:</span> println<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//MapHandler,对应表中的一条数据</span>
<span class="token comment" spellcheck="true">//将字段及相应字段的值作为map的key和value</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testQuery3</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select id,name,email,birth from customers where id = ?"</span><span class="token punctuation">;</span>
Map<span class="token operator"><</span>String<span class="token punctuation">,</span> Object<span class="token operator">></span> map <span class="token operator">=</span> runner<span class="token punctuation">.</span><span class="token function">query</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span> sql<span class="token punctuation">,</span> <span class="token keyword">new</span> <span class="token class-name">MapHandler</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">,</span> <span class="token number">16</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>map<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//MapListHandler,对应表中的多条数据</span>
<span class="token comment" spellcheck="true">//将字段及相应字段的值作为map的key和value,将map封装到list</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testQuery4</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select id,name,email,birth from customers"</span><span class="token punctuation">;</span>
List<span class="token operator"><</span>Map<span class="token operator"><</span>String<span class="token punctuation">,</span> Object<span class="token operator">>></span> query <span class="token operator">=</span> runner<span class="token punctuation">.</span><span class="token function">query</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span> sql<span class="token punctuation">,</span> <span class="token keyword">new</span> <span class="token class-name">MapListHandler</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
query<span class="token punctuation">.</span><span class="token function">forEach</span><span class="token punctuation">(</span>System<span class="token punctuation">.</span>out <span class="token operator">:</span><span class="token operator">:</span> println<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//自定义</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testQuery5</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select id,name,email,birth from customers where id = ?"</span><span class="token punctuation">;</span>
ResultSetHandler<span class="token operator"><</span>Customer<span class="token operator">></span> handler <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">ResultSetHandler</span><span class="token operator"><</span>Customer<span class="token operator">></span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token annotation punctuation">@Override</span>
<span class="token keyword">public</span> Customer <span class="token function">handle</span><span class="token punctuation">(</span>ResultSet resultSet<span class="token punctuation">)</span> <span class="token keyword">throws</span> SQLException <span class="token punctuation">{</span>
<span class="token keyword">return</span> null<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span><span class="token punctuation">;</span>
Customer query <span class="token operator">=</span> runner<span class="token punctuation">.</span><span class="token function">query</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span> sql<span class="token punctuation">,</span> handler<span class="token punctuation">,</span><span class="token number">23</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>query<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token comment" spellcheck="true">//自定义ResultSetHandle的实现类</span>
<span class="token annotation punctuation">@Test</span>
<span class="token keyword">public</span> <span class="token keyword">void</span> <span class="token function">testQuery6</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">{</span>
Connection conn <span class="token operator">=</span> null<span class="token punctuation">;</span>
<span class="token keyword">try</span> <span class="token punctuation">{</span>
QueryRunner runner <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">QueryRunner</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
conn <span class="token operator">=</span> JDBCUtils<span class="token punctuation">.</span><span class="token function">getConnection1</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
String sql <span class="token operator">=</span> <span class="token string">"select count(*) from customers"</span><span class="token punctuation">;</span>
Long count <span class="token operator">=</span> <span class="token punctuation">(</span>Long<span class="token punctuation">)</span>runner<span class="token punctuation">.</span><span class="token function">query</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span> sql<span class="token punctuation">,</span> <span class="token keyword">new</span> <span class="token class-name">ScalarHandler</span><span class="token operator"><</span><span class="token operator">></span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
System<span class="token punctuation">.</span>out<span class="token punctuation">.</span><span class="token function">println</span><span class="token punctuation">(</span>count<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">catch</span> <span class="token punctuation">(</span><span class="token class-name">SQLException</span> throwables<span class="token punctuation">)</span> <span class="token punctuation">{</span>
throwables<span class="token punctuation">.</span><span class="token function">printStackTrace</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">finally</span> <span class="token punctuation">{</span>
JDBCUtils<span class="token punctuation">.</span><span class="token function">closeResource</span><span class="token punctuation">(</span>conn<span class="token punctuation">,</span>null<span class="token punctuation">)</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre>
<script>
document.querySelectorAll('.github-emoji')
.forEach(el => {
if (!el.dataset.src) { return; }
const img = document.createElement('img');
img.style = 'display:none !important;';
img.src = el.dataset.src;
img.addEventListener('error', () => {
img.remove();
el.style.color = 'inherit';
el.style.backgroundImage = 'none';
el.style.background = 'none';
});
img.addEventListener('load', () => {
img.remove();
});
document.body.appendChild(img);
});
</script>
</div>
<hr/>
<div class="reprint" id="reprint-statement">
<div class="reprint__author">
<span class="reprint-meta" style="font-weight: bold;">
<i class="fas fa-user">
文章作者:
</i>
</span>
<span class="reprint-info">
<a href="http://yuimm.github.io" rel="external nofollow noreferrer">Yuimm</a>
</span>
</div>
<div class="reprint__type">
<span class="reprint-meta" style="font-weight: bold;">
<i class="fas fa-link">
文章链接:
</i>
</span>
<span class="reprint-info">
<a href="http://yuimm.github.io/posts/jdbc.html">http://yuimm.github.io/posts/jdbc.html</a>
</span>
</div>
<div class="reprint__notice">
<span class="reprint-meta" style="font-weight: bold;">
<i class="fas fa-copyright">
版权声明:
</i>
</span>
<span class="reprint-info">
本博客所有文章除特別声明外,均采用
<a href="https://creativecommons.org/licenses/by/4.0/deed.zh" rel="external nofollow noreferrer" target="_blank">CC BY 4.0</a>
许可协议。转载请注明来源
<a href="http://yuimm.github.io" target="_blank">Yuimm</a>
!
</span>
</div>
</div>
<script async defer>
document.addEventListener("copy", function (e) {
let toastHTML = '<span>复制成功,请遵循本文的转载规则</span><button class="btn-flat toast-action" onclick="navToReprintStatement()" style="font-size: smaller">查看</a>';
M.toast({html: toastHTML})
});
function navToReprintStatement() {
$("html, body").animate({scrollTop: $("#reprint-statement").offset().top - 80}, 800);
}
</script>
<div class="tag_share" style="display: block;">
<div class="post-meta__tag-list" style="display: inline-block;">
<div class="article-tag">
<a href="/tags/JDBC/">
<span class="chip bg-color">JDBC</span>
</a>
</div>
</div>
<div class="post_share" style="zoom: 80%; width: fit-content; display: inline-block; float: right; margin: -0.15rem 0;">
<link rel="stylesheet" type="text/css" href="/libs/share/css/share.min.css">
<div id="article-share">
<div class="social-share" data-sites="twitter,facebook,google,qq,qzone,wechat,weibo,douban,linkedin" data-wechat-qrcode-helper="<p>微信扫一扫即可分享!</p>"></div>
<script src="/libs/share/js/social-share.min.js"></script>
</div>
</div>
</div>
<style>
#reward {
margin: 40px 0;
text-align: center;
}
#reward .reward-link {
font-size: 1.4rem;
line-height: 38px;
}
#reward .btn-floating:hover {
box-shadow: 0 6px 12px rgba(0, 0, 0, 0.2), 0 5px 15px rgba(0, 0, 0, 0.2);
}
#rewardModal {
width: 320px;
height: 350px;
}
#rewardModal .reward-title {
margin: 15px auto;
padding-bottom: 5px;
}
#rewardModal .modal-content {
padding: 10px;
}
#rewardModal .close {
position: absolute;
right: 15px;
top: 15px;
color: rgba(0, 0, 0, 0.5);
font-size: 1.3rem;
line-height: 20px;
cursor: pointer;
}
#rewardModal .close:hover {
color: #ef5350;
transform: scale(1.3);
-moz-transform:scale(1.3);
-webkit-transform:scale(1.3);
-o-transform:scale(1.3);
}
#rewardModal .reward-tabs {
margin: 0 auto;
width: 210px;
}
.reward-tabs .tabs {
height: 38px;
margin: 10px auto;
padding-left: 0;
}
.reward-content ul {
padding-left: 0 !important;
}
.reward-tabs .tabs .tab {
height: 38px;
line-height: 38px;
}
.reward-tabs .tab a {
color: #fff;
background-color: #ccc;
}
.reward-tabs .tab a:hover {
background-color: #ccc;
color: #fff;
}
.reward-tabs .wechat-tab .active {
color: #fff !important;
background-color: #22AB38 !important;
}
.reward-tabs .alipay-tab .active {
color: #fff !important;
background-color: #019FE8 !important;
}
.reward-tabs .reward-img {
width: 210px;
height: 210px;
}
</style>
<div id="reward">
<a href="#rewardModal" class="reward-link modal-trigger btn-floating btn-medium waves-effect waves-light red">赏</a>
<!-- Modal Structure -->
<div id="rewardModal" class="modal">
<div class="modal-content">
<a class="close modal-close"><i class="fas fa-times"></i></a>
<h4 class="reward-title">你的赏识是我前进的动力</h4>
<div class="reward-content">
<div class="reward-tabs">
<ul class="tabs row">
<li class="tab col s6 alipay-tab waves-effect waves-light"><a href="#alipay">支付宝</a></li>
<li class="tab col s6 wechat-tab waves-effect waves-light"><a href="#wechat">微 信</a></li>
</ul>
<div id="alipay">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/alipay.jpg" class="reward-img" alt="支付宝打赏二维码">
</div>
<div id="wechat">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/wechat.png" class="reward-img" alt="微信打赏二维码">
</div>
</div>
</div>
</div>
</div>
</div>
<script>
$(function () {
$('.tabs').tabs();
});
</script>
</div>
</div>
<style>
.valine-card {
margin: 1.5rem auto;
}
.valine-card .card-content {
padding: 20px 20px 5px 20px;
}
#vcomments textarea {
box-sizing: border-box;
background: url("https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/images/comment_bg.png") 100% 100% no-repeat;
}
#vcomments p {
margin: 2px 2px 10px;
font-size: 1.05rem;
line-height: 1.78rem;
}
#vcomments blockquote p {
text-indent: 0.2rem;
}
#vcomments a {
padding: 0 2px;
color: #4cbf30;
font-weight: 500;
text-decoration: none;
}
#vcomments img {
max-width: 100%;
height: auto;
cursor: pointer;
}
#vcomments ol li {
list-style-type: decimal;
}
#vcomments ol,
ul {
display: block;
padding-left: 2em;
word-spacing: 0.05rem;
}
#vcomments ul li,
ol li {
display: list-item;
line-height: 1.8rem;
font-size: 1rem;
}
#vcomments ul li {
list-style-type: disc;
}
#vcomments ul ul li {
list-style-type: circle;
}
#vcomments table, th, td {
padding: 12px 13px;
border: 1px solid #dfe2e5;
}
#vcomments table, th, td {
border: 0;
}
table tr:nth-child(2n), thead {
background-color: #fafafa;
}
#vcomments table th {
background-color: #f2f2f2;
min-width: 80px;
}
#vcomments table td {
min-width: 80px;
}
#vcomments h1 {
font-size: 1.85rem;
font-weight: bold;
line-height: 2.2rem;
}
#vcomments h2 {
font-size: 1.65rem;
font-weight: bold;
line-height: 1.9rem;
}
#vcomments h3 {
font-size: 1.45rem;
font-weight: bold;
line-height: 1.7rem;
}
#vcomments h4 {
font-size: 1.25rem;
font-weight: bold;
line-height: 1.5rem;
}
#vcomments h5 {
font-size: 1.1rem;
font-weight: bold;
line-height: 1.4rem;
}
#vcomments h6 {
font-size: 1rem;
line-height: 1.3rem;
}
#vcomments p {
font-size: 1rem;
line-height: 1.5rem;
}
#vcomments hr {
margin: 12px 0;
border: 0;
border-top: 1px solid #ccc;
}
#vcomments blockquote {
margin: 15px 0;
border-left: 5px solid #42b983;
padding: 1rem 0.8rem 0.3rem 0.8rem;
color: #666;
background-color: rgba(66, 185, 131, .1);
}
#vcomments pre {
font-family: monospace, monospace;
padding: 1.2em;
margin: .5em 0;
background: #272822;
overflow: auto;
border-radius: 0.3em;
tab-size: 4;
}
#vcomments code {
font-family: monospace, monospace;
padding: 1px 3px;
font-size: 0.92rem;
color: #e96900;
background-color: #f8f8f8;
border-radius: 2px;
}
#vcomments pre code {
font-family: monospace, monospace;
padding: 0;
color: #e8eaf6;
background-color: #272822;
}
#vcomments pre[class*="language-"] {
padding: 1.2em;
margin: .5em 0;
}
#vcomments code[class*="language-"],
pre[class*="language-"] {
color: #e8eaf6;
}
#vcomments [type="checkbox"]:not(:checked), [type="checkbox"]:checked {
position: inherit;
margin-left: -1.3rem;
margin-right: 0.4rem;
margin-top: -1px;
vertical-align: middle;
left: unset;
visibility: visible;
}
#vcomments b,
strong {
font-weight: bold;
}
#vcomments dfn {
font-style: italic;
}
#vcomments small {
font-size: 85%;
}
#vcomments cite {
font-style: normal;
}
#vcomments mark {
background-color: #fcf8e3;
padding: .2em;
}
#vcomments table, th, td {
padding: 12px 13px;
border: 1px solid #dfe2e5;
}
table tr:nth-child(2n), thead {
background-color: #fafafa;
}
#vcomments table th {
background-color: #f2f2f2;
min-width: 80px;
}
#vcomments table td {
min-width: 80px;
}
#vcomments [type="checkbox"]:not(:checked), [type="checkbox"]:checked {
position: inherit;
margin-left: -1.3rem;
margin-right: 0.4rem;
margin-top: -1px;
vertical-align: middle;
left: unset;
visibility: visible;
}
</style>
<div class="card valine-card" data-aos="fade-up">
<div class="comment_headling" style="font-size: 20px; font-weight: 700; position: relative; left: 20px; top: 15px; padding-bottom: 5px;">
<i class="fas fa-comments fa-fw" aria-hidden="true"></i>
<span>评论</span>
</div>
<div id="vcomments" class="card-content" style="display: grid">
</div>
</div>
<script src="/libs/valine/av-min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/valine/Valine.min.js"></script>
<script>
new Valine({
el: '#vcomments',
appId: '<KEY>',
appKey: '<KEY>',
notify: 'false' === 'true',
verify: 'false' === 'true',
visitor: 'true' === 'true',
avatar: 'mm',
pageSize: '10',
lang: 'zh-cn',
placeholder: 'ヾノ≧∀≦)o来啊,快活啊!'
});
</script>
<article id="prenext-posts" class="prev-next articles">
<div class="row article-row">
<div class="article col s12 m6" data-aos="fade-up">
<div class="article-badge left-badge text-color">
<i class="fas fa-chevron-left"></i> 上一篇</div>
<div class="card">
<a href="/posts/servlet.html">
<div class="card-image">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/java.jpg" class="responsive-img" alt="Servlet">
<span class="card-title">Servlet</span>
</div>
</a>
<div class="card-content article-content">
<div class="summary block-with-text">
------------------------------------------------------------------------------------
</div>
<div class="publish-info">
<span class="publish-date">
<i class="far fa-clock fa-fw icon-date"></i>2021-01-07
</span>
<span class="publish-author">
<i class="fas fa-bookmark fa-fw icon-category"></i>
<a href="/categories/javaWeb/" class="post-category">
javaWeb
</a>
</span>
</div>
</div>
<div class="card-action article-tags">
<a href="/tags/javaWeb/">
<span class="chip bg-color">javaWeb</span>
</a>
</div>
</div>
</div>
<div class="article col s12 m6" data-aos="fade-up">
<div class="article-badge right-badge text-color">
下一篇 <i class="fas fa-chevron-right"></i>
</div>
<div class="card">
<a href="/posts/javase-ji-chu.html">
<div class="card-image">
<img src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/java.jpg" class="responsive-img" alt="javaSE基础">
<span class="card-title">javaSE基础</span>
</div>
</a>
<div class="card-content article-content">
<div class="summary block-with-text">
javaSE是java的基础内容。------------------------------------
</div>
<div class="publish-info">
<span class="publish-date">
<i class="far fa-clock fa-fw icon-date"></i>2020-05-02
</span>
<span class="publish-author">
<i class="fas fa-bookmark fa-fw icon-category"></i>
<a href="/categories/%E7%BC%96%E7%A8%8B%E8%AF%AD%E8%A8%80/" class="post-category">
编程语言
</a>
</span>
</div>
</div>
<div class="card-action article-tags">
<a href="/tags/JAVA/">
<span class="chip bg-color">JAVA</span>
</a>
<a href="/tags/javaSE/">
<span class="chip bg-color">javaSE</span>
</a>
</div>
</div>
</div>
</div>
</article>
</div>
<script>
$('#articleContent').on('copy', function (e) {
// IE8 or earlier browser is 'undefined'
if (typeof window.getSelection === 'undefined') return;
var selection = window.getSelection();
// if the selection is short let's not annoy our users.
if (('' + selection).length < Number.parseInt('100')) {
return;
}
// create a div outside of the visible area and fill it with the selected text.
var bodyElement = document.getElementsByTagName('body')[0];
var newdiv = document.createElement('div');
newdiv.style.position = 'absolute';
newdiv.style.left = '-99999px';
bodyElement.appendChild(newdiv);
newdiv.appendChild(selection.getRangeAt(0).cloneContents());
// we need a <pre> tag workaround.
// otherwise the text inside "pre" loses all the line breaks!
if (selection.getRangeAt(0).commonAncestorContainer.nodeName === 'PRE') {
newdiv.innerHTML = "<pre>" + newdiv.innerHTML + "</pre>";
}
var url = document.location.href;
newdiv.innerHTML += '<br />'
+ '来源: Yuimm<br />'
+ '文章作者: Yuimm<br />'
+ '文章链接: <a href="' + url + '">' + url + '</a><br />'
+ '本文章著作权归作者所有,任何形式的转载都请注明出处。';
selection.selectAllChildren(newdiv);
window.setTimeout(function () {bodyElement.removeChild(newdiv);}, 200);
});
</script>
<!-- 代码块功能依赖 -->
<script type="text/javascript" src="/libs/codeBlock/codeBlockFuction.js"></script>
<!-- 代码语言 -->
<script type="text/javascript" src="/libs/codeBlock/codeLang.js"></script>
<!-- 代码块复制 -->
<script type="text/javascript" src="/libs/codeBlock/codeCopy.js"></script>
<!-- 代码块收缩 -->
<script type="text/javascript" src="/libs/codeBlock/codeShrink.js"></script>
<!-- 代码块折行 -->
<style type="text/css">
code[class*="language-"], pre[class*="language-"] { white-space: pre !important; }
</style>
</div>
<div id="toc-aside" class="expanded col l3 hide-on-med-and-down">
<div class="toc-widget">
<div class="toc-title"><i class="far fa-list-alt"></i> 目录</div>
<div id="toc-content"></div>
</div>
</div>
</div>
<!-- TOC 悬浮按钮. -->
<div id="floating-toc-btn" class="hide-on-med-and-down">
<a class="btn-floating btn-large bg-color">
<i class="fas fa-list-ul"></i>
</a>
</div>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/tocbot/tocbot.min.js"></script>
<script>
$(function () {
tocbot.init({
tocSelector: '#toc-content',
contentSelector: '#articleContent',
headingsOffset: -($(window).height() * 0.4 - 45),
collapseDepth: Number('0'),
headingSelector: 'h2, h3, h4'
});
// modify the toc link href to support Chinese.
let i = 0;
let tocHeading = 'toc-heading-';
$('#toc-content a').each(function () {
$(this).attr('href', '#' + tocHeading + (++i));
});
// modify the heading title id to support Chinese.
i = 0;
$('#articleContent').children('h2, h3, h4').each(function () {
$(this).attr('id', tocHeading + (++i));
});
// Set scroll toc fixed.
let tocHeight = parseInt($(window).height() * 0.4 - 64);
let $tocWidget = $('.toc-widget');
$(window).scroll(function () {
let scroll = $(window).scrollTop();
/* add post toc fixed. */
if (scroll > tocHeight) {
$tocWidget.addClass('toc-fixed');
} else {
$tocWidget.removeClass('toc-fixed');
}
});
/* 修复文章卡片 div 的宽度. */
let fixPostCardWidth = function (srcId, targetId) {
let srcDiv = $('#' + srcId);
if (srcDiv.length === 0) {
return;
}
let w = srcDiv.width();
if (w >= 450) {
w = w + 21;
} else if (w >= 350 && w < 450) {
w = w + 18;
} else if (w >= 300 && w < 350) {
w = w + 16;
} else {
w = w + 14;
}
$('#' + targetId).width(w);
};
// 切换TOC目录展开收缩的相关操作.
const expandedClass = 'expanded';
let $tocAside = $('#toc-aside');
let $mainContent = $('#main-content');
$('#floating-toc-btn .btn-floating').click(function () {
if ($tocAside.hasClass(expandedClass)) {
$tocAside.removeClass(expandedClass).hide();
$mainContent.removeClass('l9');
} else {
$tocAside.addClass(expandedClass).show();
$mainContent.addClass('l9');
}
fixPostCardWidth('artDetail', 'prenext-posts');
});
});
</script>
</main>
<footer class="page-footer bg-color">
<div class="container row center-align" style="margin-bottom: 15px !important;">
<div class="col s12 m8 l8 copy-right">
Copyright ©
<span id="year">2020</span>
<a href="http://yuimm.github.io" target="_blank">Yuimm</a>
| Powered by <a href="https://hexo.io/" target="_blank">Hexo</a>
| Theme <a href="https://github.com/blinkfox/hexo-theme-matery" target="_blank">Matery</a>
<br>
<i class="fas fa-chart-area"></i> 站点总字数: <span
class="white-color">544.9k</span> 字
<span id="busuanzi_container_site_pv">
| <i class="far fa-eye"></i> 总访问量: <span id="busuanzi_value_site_pv"
class="white-color"></span> 次
</span>
<span id="busuanzi_container_site_uv">
| <i class="fas fa-users"></i> 总访问人数: <span id="busuanzi_value_site_uv"
class="white-color"></span> 人
</span>
<br>
<span id="sitetime">载入运行时间...</span>
<script>
function siteTime() {
var seconds = 1000;
var minutes = seconds * 60;
var hours = minutes * 60;
var days = hours * 24;
var years = days * 365;
var today = new Date();
var startYear = "2020";
var startMonth = "3";
var startDate = "30";
var startHour = "0";
var startMinute = "0";
var startSecond = "0";
var todayYear = today.getFullYear();
var todayMonth = today.getMonth() + 1;
var todayDate = today.getDate();
var todayHour = today.getHours();
var todayMinute = today.getMinutes();
var todaySecond = today.getSeconds();
var t1 = Date.UTC(startYear, startMonth, startDate, startHour, startMinute, startSecond);
var t2 = Date.UTC(todayYear, todayMonth, todayDate, todayHour, todayMinute, todaySecond);
var diff = t2 - t1;
var diffYears = Math.floor(diff / years);
var diffDays = Math.floor((diff / days) - diffYears * 365);
var diffHours = Math.floor((diff - (diffYears * 365 + diffDays) * days) / hours);
var diffMinutes = Math.floor((diff - (diffYears * 365 + diffDays) * days - diffHours * hours) /
minutes);
var diffSeconds = Math.floor((diff - (diffYears * 365 + diffDays) * days - diffHours * hours -
diffMinutes * minutes) / seconds);
if (startYear == todayYear) {
document.getElementById("year").innerHTML = todayYear;
document.getElementById("sitetime").innerHTML = "本站已安全运行 " + diffDays + " 天 " + diffHours +
" 小时 " + diffMinutes + " 分钟 " + diffSeconds + " 秒";
} else {
document.getElementById("year").innerHTML = startYear + " - " + todayYear;
document.getElementById("sitetime").innerHTML = "本站已安全运行 " + diffYears + " 年 " + diffDays +
" 天 " + diffHours + " 小时 " + diffMinutes + " 分钟 " + diffSeconds + " 秒";
}
}
setInterval(siteTime, 1000);
</script>
<br>
</div>
<div class="col s12 m4 l4 social-link social-statis">
<a href="https://github.com/Yuimm" class="tooltipped" target="_blank" data-tooltip="访问我的GitHub" data-position="top" data-delay="50">
<i class="fab fa-github"></i>
</a>
<a href="https://gitee.com/Yuimm" class="tooltipped" target="_blank" data-tooltip="Gitee: https://gitee.com/Yuimm" data-position="top" data-delay="50">
<i class="fab fa-git"></i>
</a>
<a href="mailto:<EMAIL>" class="tooltipped" target="_blank" data-tooltip="邮件联系我" data-position="top" data-delay="50">
<i class="fas fa-envelope-open"></i>
</a>
<a href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/qq.jpg" class="tooltipped" target="_blank" data-tooltip="QQ联系我: https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/qq.jpg" data-position="top" data-delay="50">
<i class="fab fa-qq"></i>
</a>
<a href="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_blog_picture/posts/wechat.jpg" class="tooltipped" target="_blank" data-tooltip="微信联系我: " data-position="top" data-delay="50">
<i class="fab fa-weixin"></i>
</a>
</div>
</div>
</footer>
<div class="progress-bar"></div>
<!-- 搜索遮罩框 -->
<div id="searchModal" class="modal">
<div class="modal-content">
<div class="search-header">
<span class="title"><i class="fas fa-search"></i> 搜索</span>
<input type="search" id="searchInput" name="s" placeholder="请输入搜索的关键字"
class="search-input">
</div>
<div id="searchResult"></div>
</div>
</div>
<script src="/js/search.js"></script>
<script type="text/javascript">
$(function () {
searchFunc("/" + "search.xml", 'searchInput', 'searchResult');
});
</script>
<!-- 回到顶部按钮 -->
<div id="backTop" class="top-scroll">
<a class="btn-floating btn-large waves-effect waves-light" href="#!">
<i class="fas fa-arrow-up"></i>
</a>
</div>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/materialize/materialize.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/masonry/masonry.pkgd.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/aos/aos.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/scrollprogress/scrollProgress.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/lightGallery/js/lightgallery-all.min.js"></script>
<script src="/js/matery.js"></script>
<!--文字点击-->
<!-- <script src="/js/click_show_text.js"></script> -->
<!--鼠标点击烟花特效-->
<!-- <canvas class="fireworks" style="position: fixed;left: 0;top: 0;z-index: 1; pointer-events: none;" ></canvas>
<script type="text/javascript" src="//cdn.bootcss.com/animejs/2.2.0/anime.min.js"></script>
<script type="text/javascript" src="/js/firework.js"></script> -->
<!-- Global site tag (gtag.js) - Google Analytics -->
<!-- Baidu Analytics -->
<!-- Baidu Push -->
<script>
(function () {
var bp = document.createElement('script');
var curProtocol = window.location.protocol.split(':')[0];
if (curProtocol === 'https') {
bp.src = 'https://zz.bdstatic.com/linksubmit/push.js';
} else {
bp.src = 'http://push.zhanzhang.baidu.com/push.js';
}
var s = document.getElementsByTagName("script")[0];
s.parentNode.insertBefore(bp, s);
})();
</script>
<script async src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/others/busuanzi.pure.mini.js"></script>
<script type="text/javascript" color="0,0,255"
pointColor="0,0,255" opacity='0.7'
zIndex="-1" count="99"
src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/background/canvas-nest.js"></script>
<script type="text/javascript" size="150" alpha='0.6'
zIndex="-1" src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/background/ribbon.min.js" async="async"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/background/ribbon-dynamic.js" async="async"></script>
<script src="https://cdn.jsdelivr.net/gh/Yuimm/yuimm_CDN@V1.0.1/source/libs/instantpage/instantpage.js" type="module"></script>
</body>
</html>
| 1746deb0c3576f232f4d1d4365ad462d99e33371 | [
"HTML"
] | 2 | HTML | Yuimm/yuimm.github.io | 64a4685c7290790736bd951595e81c835d3c5b53 | 23c640181978887404622d764738b3b819721857 |
refs/heads/master | <file_sep>Typescript version of BramboraSK's string-edit package.
<!-- BADGES/ -->
<span class="badge-npmversion"><a href="https://npmjs.org/package/string-edit" title="View this project on NPM"><img src="https://img.shields.io/npm/v/string-edit.svg" alt="NPM version" /></a></span>
<span class="badge-npmdownloads"><a href="https://npmjs.org/package/string-edit" title="View this project on NPM"><img src="https://img.shields.io/npm/dm/string-edit.svg" alt="NPM downloads" /></a></span>
<!-- /BADGES -->
# String Edit
- String Edit and String Tools
- Easy-to-Use
## Installation
```
npm install --save string-edit
```
## Features
- Latinization of text
- Removal of letters, number or special characters
- Random case, alternating case
- And many more...
## Usage
``` js
const stringEdit = require("string-edit");
//Alternate case
stringEdit.alternateCase("my name is john"); // => "My NaMe Is JoHn"
stringEdit.alternateCase("my name is john", false, false); // => "mY NaMe iS JoHn"
//Reverse case
stringEdit.reverseCase("hEllO, WhaT'S Up?"); // => "HeLLo, wHAt's uP?"
//Find character's indexes
stringEdit.findCharIndexes("potato potatoes", "o"); // => [ 1, 5, 8, 12 ]
```
## Functions
### latinize(string)
This function latinizes the string.
For example: "héľĺô woŕlď" -> "hello world"
##### string
Type: `string`
The string to be latinized.
### removeNumbers(string)
This function removes numbers.
For example: "1orange 2apples 3strawberries" -> "orange apples strawberries"
##### string
Type: `string`
The string where the numbers will be removed.
### removeLetters(string)
This function removes letters.
For example: "1orange 2apples 3strawberries 4úäňô" -> "1 2 3 4"
##### string
Type: `string`
The string where the letters will be removed.
### removeSpecialCharacters(string)
This function removes special characters except whitespace.
For example: "hel-lo. wor,ld!" -> "hello world"
##### string
Type: `string`
The string where the special characters will be removed.
### randomCase(string)
This function randomizes the case of characters.
For example: "apple" -> "aPPlE"
##### string
Type: `string`
The string where the case will be randomized.
### alternateCase(string, start_with_uppercase, ignore_whitespace)
This function alternates the case of characters.
For example: "strawberry" -> "StRaWbErRy"
##### string
Type: `string`
The string where the case will be alternated.
##### start_with_uppercase
Type: `boolean`
Default value: `true`
The alternating case will start with uppercase if true.
##### ignore_whitespace
Type: `boolean`
Default value: `true`
Whitespaces will be ignored (they won't count as character) if true.
### reverseContent(string)
This function reverses the string.
For example: "orange" -> "egnaro"
##### string
Type: `string`
The string to be reversed.
### reverseCase(string)
This function reverses case of every character in the string.
For example: "HelLo" -> "hELlO"
##### string
Type: `string`
The string where the case will be reversed.
### findCharIndexes(string, to_find)
This function finds every specific character's index in the string.
For example: "potato", "o" -> [ 1, 5 ]
##### string
Type: `string`
The string that you want to search through.
##### to_find
Type: `string`
The character to be found.
### extractCharacters(string, to_array, repeat_characters)
This function extracts every character in the string to array or string.
For example: "feeling!" -> [ "f", "e", "l", "i", "n", "g", "!" ] or "fe.el" -> "fe.l"
##### string
Type: `string`
The string with characters that will extracted.
##### to_array
Type: `boolean`
Default value: `true`
Characters will be extracted to array if true (otherwise to string).
##### repeat_characters
Type: `boolean`
Default value: `false`
Characters won't repeat if false.
### shuffle(string)
This function shuffles the string.
For example: "entity" -> "tyetin"
##### string
Type: `string`
The string to be shuffled.
### randomJoin(array, join_specific_amount)
This function joins strings from array randomly.
For example: [ "ab", "cd", "ef" ] -> "cdefab" or [ "ab", "cd", "ef" ] -> "efcd"
##### array
Type: `array`
The array from which the strings will be joined.
##### join_specific_amount
Type: `number`
Default value: `0`
The amount of strings to be joined (needs to be greater than 0).<file_sep>//Some very important things :o
import { latins_map, letters } from "./utils";
/**
* This function latinizes the string.
* For example: "héľĺô woŕlď" -> "hello world"
* @param {String} str
* @returns {String} Latinized string.
*/
export function latinize(str: string): string {
return str.replace(/[^A-Za-z0-9\[\] ]/g, (substring: string) => {
return latins_map.get(substring) ?? substring;
});
}
/**
* Alias for latinize().
* This function latinizes the string.
* For example: "héľĺô woŕlď" -> "hello world"
* @param {String} str
* @returns {String} Latinized string.
*/
export const latinise = latinize;
/**
* This function removes numbers.
* For example: "1orange 2apples 3strawberries" -> "orange apples strawberries"
* @param {String} str
* @returns {String} String without numbers.
*/
export function removeNumbers(str: string): string {
return str.replace(/[0-9]/g, "");
}
/**
* This function removes letters.
* For example: "1orange 2apples 3strawberries 4úäňô" -> "1 2 3 4"
* @param {String} str
* @returns {String} String without letters.
*/
export function removeLetters(str: string): string {
return str.replace(new RegExp("["+decodeURI(letters)+"]","g"), "");
}
/**
* This function removes special characters except whitespace.
* For example: "hel-lo. wor,ld!" -> "hello world"
* @param {String} str
* @returns {String} String without special characters except whitespace.
*/
export function removeSpecialCharacters(str: string): string {
return str.replace(new RegExp("[^0-9"+decodeURI(letters)+" ]","g"), "");
}
/**
* This function randomizes the case of characters.
* For example: "apple" -> "aPPlE"
* @param {String} str
* @returns {String} String with randomized the case of characters.
*/
export function randomCase(str: string): string {
var edited = "";
for(let i=0; i < str.length; i++) {
//Generate letter with random case
var random = Math.round(Math.random());
var new_letter = random === 0 ? str[i].toLowerCase() : str[i].toUpperCase();
edited = edited + new_letter;
}
return edited;
}
/**
* This function alternates the case of characters.
* For example: "strawberry" -> "StRaWbErRy"
* @param {String} str
* @param {Boolean} start_with_uppercase Defaultly true
* @param {Boolean} ignore_whitespace Defaultly true
* @returns {String} String with alternated case.
*/
export function alternateCase(str: string, start_with_uppercase: boolean, ignore_whitespace: boolean): string {
if(!start_with_uppercase && start_with_uppercase !== false) start_with_uppercase = true;
if(!ignore_whitespace && ignore_whitespace !== false) ignore_whitespace = true;
var edit = ignore_whitespace ? str.replace(/ /g, "") : str;
var edited = "";
//Alternate case based on given params
for(let i=0,y=0; i < edit.length, y < str.length; i++, y++) {
if(edit[i] === undefined) continue;
//Check if the current character is whitespace and ignore it
if(ignore_whitespace && str[y] == " ") {
edited = edited + " ";
i--;
continue;
}
//Alternate
if(i % 2 == 0) {
edited += start_with_uppercase ? edit[i].toUpperCase() : edit[i].toLowerCase();
} else {
edited += !start_with_uppercase ? edit[i].toUpperCase() : edit[i].toLowerCase();
}
}
return edited;
}
/**
* This function reverses the string.
* For example: "orange" -> "egnaro"
* @param {String} str
* @returns {String} String with reversed content.
*/
export function reverseContent(str: string): string {
return str.split("").reverse().join("");
}
/**
* This function reverses case of every character in the string.
* For example: "HelLo" -> "hELlO"
* @param {String} str
* @returns {String} String with reversed case.
*/
export function reverseCase(str: string): string {
var edited = "";
//Reverse case of every letter
for(let i=0; i < str.length; i++) {
edited += str[i] === str[i].toLowerCase() ? str[i].toUpperCase() : str[i].toLowerCase();
}
return edited;
}
/**
* This function finds every specific character's index in the string.
* For example: "potato", "o" -> [ 1, 5 ]
* @param {String} str
* @param {String} to_find
* @returns {Array} Array with found indexes.
*/
export function findCharIndexes(str: string, to_find: string): Array<any> {
var found = [];
for(let i=0; i < str.length; i++) {
if(str[i] === to_find) found.push(i);
}
return found;
}
/**
* This function extracts every character in the string to array or string.
* For example: "feeling!" -> [ "f", "e", "l", "i", "n", "g", "!" ] or "fe.el" -> "fe.l"
* @param {String} str
* @param {Boolean} to_array Defaulty true
* @param {Boolean} repeat_characters Defaulty false
* @returns {any} Array or string with extracted characters.
*/
export function extractCharacters(str: string, to_array: boolean = true, repeat_characters: boolean = false): Array<String> | string {
var res: String[] = str.split("");
if (!repeat_characters) {
res = Array.from(new Set<String>(res));
}
return to_array ? res : res.join("");
}
/**
* This function shuffles the string.
* For example: "entity" -> "tyetin"
* @param {String} str
* @returns {String} Shuffled string.
*/
export function shuffle(str: string): string {
var array = str.split("");
//Shuffle
for(let i=0; i < array.length; i++) {
const y = Math.floor(Math.random() * (i + 1));
[array[i], array[y]] = [array[y], array[i]];
}
return array.join("");
}
/**
* This function joins strings from array randomly.
* For example: [ "ab", "cd", "ef" ] -> "cdefab" or [ "ab", "cd", "ef" ] -> "efcd"
* @param {Array} array
* @param {Number} join_specific_amount Defaultly 0
* @returns {String} String, that's made from randomly joined strings.
*/
export function randomJoin(array: Array<string>, join_specific_amount: number): string {
if(!join_specific_amount) { //Join everything together
//Shuffle
for(let i=0; i < array.length; i++) {
const y = Math.floor(Math.random() * (i + 1));
[array[i], array[y]] = [array[y], array[i]];
}
return array.join("");
} else { //Join only two strings together
if(array.length < join_specific_amount) throw new Error(`Error: Array length (${array.length}) is shorter than given amount ${join_specific_amount}.`);
if(join_specific_amount < 0) throw new Error(`Error: Given amount ${join_specific_amount} is invalid. Choose number that's greater than 0.`);
var new_array = [];
for(let i=0; i < join_specific_amount; i++) {
let random_index = Math.floor(Math.random() * array.length);
new_array.push(array[random_index]);
array.splice(random_index, 1);
}
return new_array.join("");
}
}<file_sep>on:
push:
branches:
- master
jobs:
publish:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v1
- uses: actions/setup-node@v1
with:
node-version: 10
- run: npm install
- run: npx tsc
- run: rm -r src && rm -r .github && mv dist/* .
- run: ls -l
- uses: JS-DevTools/npm-publish@v1
with:
token: ${{ secrets.NPM_AUTH_TOKEN }}
registry: https://npm.pkg.github.com | 7a9428c47650921abbbfaaac218ccf99fffc41ae | [
"Markdown",
"TypeScript",
"YAML"
] | 3 | Markdown | 0xWryth/string-edit | 6d1f1a746f54eb4f67018ba8fd5317b2a33a6829 | 042686ef0f436582dcf221db1aaea24111df7dce |
refs/heads/master | <repo_name>sheelkhanna/groovy-standalone<file_sep>/src/main/groovy/test.groovy
println 'hi' + new Date() | 51aac6f7c6d6dede3dd1954dec4df051cce01d2b | [
"Groovy"
] | 1 | Groovy | sheelkhanna/groovy-standalone | 2b0106d617b7544b9b876d50502c99c2e311f183 | 0df08ca530f3cc67bf5f8c8264351b0d752d229a |
refs/heads/master | <repo_name>mitchellmui17/UnityGameProj1<file_sep>/README.md
# UnityGameProj1
A repository used for personal interest in game app development using Unity
| 596e48bafdb569cda12abdc9a83ce3270988c5b5 | [
"Markdown"
] | 1 | Markdown | mitchellmui17/UnityGameProj1 | 91ed7de6fe44c8f2799dababd8478bdd19173cd7 | b9e7173fa4860eddaf07cae40b5e326d8d086c93 |
refs/heads/master | <file_sep>import React from 'react';
import {
ChakraProvider,
Box,
Text,
Link,
VStack,
Code,
Grid,
Flex,
Heading,
} from '@chakra-ui/react';
import ARButton from '../ARButton/ARButton.js'
function Feature({ title, desc, ...rest }) {
return (
<Flex bgGradient="linear(to-l, #7928CA, #FF0080)" minH="100vh" minW="100vw" justify="center" fontSize="xl">
<Box p={5} mt="30vh" bg="white" maxW="40rem" maxH="25rem" borderRadius="xl" shadow="md" borderWidth="1px" {...rest}>
<Heading color="black" fontSize="4xl">{title}</Heading>
<Text color="black" mt={4}>Visit this link on the laptop to view our dashboard!</Text>
<Text color="black" mt={4}>{desc}</Text>
<Box
as="button"
p={2}
mt={5}
color="white"
fontWeight="bold"
borderRadius="md"
bgGradient="linear(to-r, teal.500,green.500)"
_hover={{
bgGradient: "linear(to-r, red.500, yellow.500)",
}}
>
<a target="_blank" href="https://ecocrib-ar.netlify.app/">Go View</a>
</Box>
</Box>
</Flex>
)
}
function MobileView() {
return (
<>
<VStack spacing={8}>
<Feature
title="Looks like you're on a phone..."
desc="You can test our AR feature from your phone right now! Just tap the button below!"
/>
</VStack>
</>
);
}
export default MobileView;
| 8d508f2d33f1789a6de001c2862dba34c3ec4bb0 | [
"JavaScript"
] | 1 | JavaScript | Adub17030/HackUconn2021 | 58327cc9206fabb316e9ae96a077c320488ca4f2 | 863b0da9e50f9446ec600f8e460334374fbda6dd |
refs/heads/main | <repo_name>Xavier577/Covid-19-tracker<file_sep>/README.md
# App Overview
This is a react web app that displays the stats on covid-19. It connects to an api namelly disease.sh fetches the data from it and displays it in structured manner. It is made with react and using other libraries such as material-ui, chartjs and leaflets. To view this app i have setup a github page here https://xavier577.github.io/Covid-19-tracker/
permalink: /index.html
<file_sep>/src/App.js
import React, { Component } from "react";
import "./App.css";
import InfoBox from "./component/InfoBox/InfoBox";
import numeral from "numeral";
import Map from "./component/Map/Map";
import LineGraph from "./component/LineGraph/lineGraph";
import Table from "./component/Table/table";
import { prettyShowStat, sortData } from "./util";
import "leaflet/dist/leaflet.css";
import {
Card,
CardContent,
FormControl,
Select,
MenuItem,
} from "@material-ui/core";
export default class App extends Component {
constructor(props) {
super(props);
this.state = {
countries: [],
country: "worldwide",
casesType: "cases",
countryInfo: {},
tableData: [],
mapCenter: { lat: 34.80746, lng: -40.4796 },
mapZoom: 3,
mapCountries: [],
};
}
componentDidMount() {
const allInfo = async () => {
fetch("https://disease.sh/v3/covid-19/all")
.then((response) => response.json())
.then((data) => {
this.setState({
countryInfo: data,
});
});
};
allInfo();
const getCountriesData = async () => {
try {
const fetchData = await fetch(
"https://disease.sh/v3/covid-19/countries"
);
const data = await fetchData.json();
const countries = data.map((country) => ({
name: country.country,
value: country.countryInfo.iso2,
}));
let sortedData = sortData(data);
this.setState({
countries: countries,
tableData: sortedData,
mapCountries: data,
});
console.log(data);
} catch (err) {
console.log(err.message);
}
};
getCountriesData();
}
onCountryChange = (e) => {
const countryCode = e.target.value;
const url =
countryCode === "worldwide"
? "https://disease.sh/v3/covid-19/all"
: `https://disease.sh/v3/covid-19/countries/${countryCode}`;
fetch(url)
.then((response) => response.json())
.then((data) => {
this.setState({
mapCenter: [data.countryInfo.lat, data.countryInfo.long],
mapZoom: 4,
country: countryCode,
countryInfo: data,
});
});
};
render() {
return (
<div className="app">
<div className="app-left">
<div className="app-header">
<h1> COVID-19 Tracker</h1>
<FormControl className="app-dropdowm">
<Select
variant="outlined"
value={this.state.country}
onChange={this.onCountryChange}>
<MenuItem value="worldwide">Worldwide</MenuItem>
{this.state.countries.map((country) => (
<MenuItem key={country.name} value={country.value}>
{country.name}
</MenuItem>
))}
{/* <MenuItem value = 'Nigeria'>Nigeria</MenuItem>
<MenuItem value = 'China'>China</MenuItem> */}
</Select>
</FormControl>
</div>
<div className="app-stats">
<InfoBox
onClick={(e) => {
this.setState({
casesType: "cases",
});
}}
title="Coronavirus Cases"
cases={prettyShowStat(this.state.countryInfo.todayCases)}
total={numeral(this.state.countryInfo.cases).format("0.0a")}
active={this.state.casesType === "cases"}
isRed
/>
<InfoBox
onClick={(e) => {
this.setState({
casesType: "recovered",
});
}}
title="Recovered"
active={this.state.casesType === "recovered"}
cases={prettyShowStat(this.state.countryInfo.todayRecovered)}
total={numeral(this.state.countryInfo.recovered).format("0.0a")}
/>
<InfoBox
onClick={(e) => {
this.setState({
casesType: "deaths",
});
}}
title="Death"
isRed
active={this.state.casesType === "deaths"}
cases={prettyShowStat(this.state.countryInfo.todayDeaths)}
total={numeral(this.state.countryInfo.deaths).format("0.0a")}
/>
</div>
<Map
countries={this.state.mapCountries}
casesType={this.state.casesType}
center={this.state.mapCenter}
zoom={this.state.mapZoom}
/>
</div>
<Card className="app-right">
<CardContent>
<div className="app-information">
<h3>Live Cases by Country</h3>
<Table countries={this.state.tableData} />
<h3>Worldwide new {this.state.casesType}</h3>
<LineGraph casesType={this.state.casesType} />
</div>
</CardContent>
</Card>
</div>
);
}
}
| 13938ad6116ed9428710365f373b55fdb84b03fd | [
"Markdown",
"JavaScript"
] | 2 | Markdown | Xavier577/Covid-19-tracker | 0d79eb0bdc8f8abe72b4520b86587d27eefcdb0d | cea7f866070987bfac477bf0ffb95ee084e4e553 |
refs/heads/master | <repo_name>jadar/BackgroundView<file_sep>/BackgroundView/ViewController.m
//
// ViewController.m
// BackgroundView
//
// Created by <NAME> on 12/18/14.
// Copyright (c) 2014 <NAME>. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
- (instancetype)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil {
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
}
return self;
}
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
UIView *view = [[UIView alloc] initWithFrame:self.tableView.frame];
view.backgroundColor = [UIColor redColor];
UITapGestureRecognizer *tgr = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(tgrHit:)];
[view addGestureRecognizer:tgr];
self.tableView.backgroundView = view;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)tgrHit:(id)view {
NSLog(@"hit");
}
@end
| 1d10b977b2e82444de6bd55b1022166deb78de0c | [
"Objective-C"
] | 1 | Objective-C | jadar/BackgroundView | 207da62f0383f8d3c3065a4fe0cbec465a88442b | 22191fbb94d4a6d28101cc6771ff0951b8ca394f |
refs/heads/master | <repo_name>sadeghmohebbi/porsan-api-for-hmp-docs<file_sep>/apis/single-question.md
[Back To Home](/)
**Single Question**
----
Returns json data of question with its answers
* **URL**
/Questions/{question_id}
* **Method:**
`GET`
* **URL Params**
**Required:**
`question_id=[integer] valid question id`
* **Data Params**
None
* **Success Response:**
* **Code:** 200 OK
* **Sample Response:**
```json
{
"question": {
"id": "4184",
"title": "در توسعه ی سایت جوابینا، چرا phalcon و چرا node js نه؟",
"question": "در توسعه ی سایت جوابینا، چرا phalcon و چرا node js نه؟",
"numUpvotes": 0,
"numFollows": 0,
"isUpvoted": true,
"isFollowed": false,
"user": {
"id": "45",
"fullName": "sadeghmohebbi",
"profileImageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
}
},
"answers": [
{
"id": "520",
"answer": "سرعت بسیار بالای فالکون و البته سلیقه ی توسعه دهنده و تمایل به استفاده از php منجر شده",
"user": {
"id": "46",
"fullName": "<NAME>",
"profileImageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
},
"numUpvotes": "1",
"numDownvotes": "0",
"isUpvoted": true,
"isDownvoted": false
},
{
"id": "524",
"answer": "اینم یه عکس افقی",
"user": {
"id": "53",
"fullName": "<NAME>",
"profileImageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
},
"numUpvotes": "0",
"numDownvotes": "2",
"isUpvoted": false,
"isDownvoted": true
}
]
}
```
* **Error Response:**
* **Code:** 404 Not Found <br />
**Content:** `{ title: string, description: string }`
[Back To Home](/)<file_sep>/README.md
# Porsan API for Hamdam Docs
developed by [<NAME>](https://github.com/sadeghmohebbi) | powered by [docsify](https://docsify.js.org)<file_sep>/homepage.md
# Porsan API for Hamdam (HMP)
## Base URL and Version
Version1 (v1) <br>
https://porsan.com/api/v1
### Notes
1. Pagination System Structure is the same as [hamdam-core-api](http://hamdam-core-api.tebyansmart.com) specification
## APIs
* [GET /VerifiedUsers](/apis/verified-users)
* [GET /Topics](/apis/topics)
* [GET /TopicContent/{topic_id}](/apis/topic-content)
* [GET /Questions/{question_id}](/apis/single-question)
* [POST /Questions/{question_id}/Upvote](/apis/question-upvote)
* [POST /Questions/{question_id}/Follow](/apis/question-follow)
* [POST /Questions](/apis/create-question)
* [DELETE /Questions/{question_id}](/apis/remove-question)
* [GET /Accounts/Me](/apis/accounts-me)
* [POST /Answers/{answer_id}/Upvote](/apis/answer-upvote)
* [POST /Answers/{answer_id}/Downvote](/apis/answer-downvote)
* [GET /search/questions](/apis/search-questions)
___
_developed by [sadegh mohebbi](https://github.com/sadeghmohebbi) | powered by [docsify](https://docsify.js.org)_
<file_sep>/apis/answer-upvote.md
[Back To Home](/)
**Upvote Answer**
----
set upvote or undo upvote answer
* **URL**
/Answers/{answer_id}/Upvote
* **Method:**
`POST`
* **URL Params**
**Required:**
`answer_id=[integer] valid answer id`
* **Data Params**
None
* **Success Response:**
* **Code:** 202 Accepted
OR
* **Code:** 205 Reset Content
* **Error Response:**
* **Code:** 404 Not Found <br />
**Content:** `{ title: string, description: string }`
[Back To Home](/)<file_sep>/apis/search-questions.md
[Back To Home](/)
**Search Questions**
----
Search in questions
* **URL**
/search/questions
* **Method:**
`GET`
* **URL Params**
None
* **Data Params**
**Required:**
`q=[string] text to search` <br>
`scope=[string] should be "hmp"`
**Optional:**
`PageNumber=[integer]` <br>
`PageSize=[integer]` <br>
no need total counts is true by default and client can't change it
* **Success Response:**
* **Code:** 200 OK
* **Sample Response:**
```json
[
{
"id":3852,
"userId":18,
"slug":"سلام-من-و-همسرم-یک-سال-و-نیم-هست-اعتیاد-داریم-و-متادون-مصرف-میکنیم-خیلی-سعی-کردی",
"question":"سلام من و همسرم یک سال و نیم هست اعتیاد داریم و متادون مصرف میکنیم. خیلی سعی کردیم شده ۲ هفته خوابیدیم دوباره وسوسه شدیم. به کمک شدید نیاز دارم چون برای بارداری هم میخوام اقدام کنم. چکار باید بکنیم؟",
"description":null,
"isCommentable":1,
"isAnonymous":0,
"numTags":0,
"numFollows":0,
"numAnswers":0,
"numRequests":0,
"numUpvotes":0,
"numDownvotes":0,
"numViews":32,
"createdAt":"2019-08-30T14:33:04.000Z",
"updatedAt":"2020-06-28T22:48:36.000Z",
"numComments":0,
"user_id":18,
"user":{
"id":18,
"email":null,
"mobile":null,
"username":"mostafa",
"fullname":"<NAME>",
"brief":null,
"biography":null,
"avatar":"AVATAR_8601586978342MOSTAFA_USER.png",
"numAnswers":0,
"numQuestions":0,
"numFollowers":3,
"createdAt":"2019-07-17T20:03:39.000Z",
"updatedAt":"2020-05-30T08:03:00.000Z"
}
}
]
```
[Back To Home](/)<file_sep>/apis/verified-users.md
[Back To Home](/)
**Verified Users**
----
Returns json data list of verified users.
* **URL**
/VerifiedUsers
* **Method:**
`GET`
* **URL Params**
**Required:**
`scope=[string] should equal to "hmp"`
**Optional:**
`PageNumber=[integer]` <br>
`PageSize=[integer]` <br>
`job=[string] example: "consultant"`
* **Success Response:**
* **Code:** 200 OK
* **Sample Response:**
```json
[
{
"id": "1",
"fullName": "<NAME>",
"profileImageUrl": "http:\/\/localhost:8000\/contents\/users\/avatars\/AVATAR_1561585563737FARVISUN_USER.png",
"tags": [
"استارتاپ",
"پزشکی",
"اینترنت (Internet)",
"طراحی سایت",
"سرمایهگذاری",
"کتاب",
"زیبایی",
"موسیقی",
"زبان انگلیسی",
"پوست"
]
}
]
```
[Back To Home](/)<file_sep>/apis/topic-content.md
[Back To Home](/)
**Topic Content**
----
Returns json data list of topic contents <br>
Topic content is contain question and answer properties (qa)
* **URL**
/TopicContent/{topic_id}
* **Method:**
`GET`
* **URL Params**
**Required:**
`topic_id=[integer] valid topic id`
* **Data Params**
**Optional:**
`PageNumber=[integer]` <br>
`PageSize=[integer]`
* **Success Response:**
* **Code:** 200 OK
* **Sample Response:**
when isAnonymous is 1 for question user or answer user, each questionUserOwner or answerUserOwner are null<br>
```json
{
"topic": {
"id": "58",
"name": "اختلالات شخصیتی",
"imageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
},
"qas": [
{
"questionId": "4184",
"question": "در توسعه ی سایت جوابینا، چرا phalcon و چرا node js نه؟",
"questionTitle": "در توسعه ی سایت جوابینا، چرا phalcon و چرا node js نه؟",
"answer": "اینم یه عکس افقی",
"numAnswerUpvotes": "1",
"numAnswerDownvotes": "0",
"numQuestionUpvotes": "4",
"numQuestionFollows": "2",
"meIsAnswerUpvoted": true,
"meIsAnswerDownvoted": false,
"meIsQuestionFollowed": false,
"questionUserOwner": {
"id": "45",
"fullName": "sadeghmohebbi",
"profileImageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
},
"answerUserOwner": {
"id": "53",
"fullName": "<NAME>",
"profileImageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
},
"dateQuestionCreatedAt": "1 ماه پیش",
"dateAnswerCreatedAt": "1 هفته پیش"
}
]
}
```
* **Error Response:**
* **Code:** 404 Not Found <br />
**Content:** `{ title: string, description: string }`
[Back To Home](/)<file_sep>/apis/topics.md
[Back To Home](/)
**Topics**
----
Returns json data list of topics
* **URL**
/Topics
* **Method:**
`GET`
* **URL Params**
None
* **Data Params**
**Required:**
`scope=[string] should be "hmp"`
**Optional:**
`PageNumber=[integer]` <br>
`PageSize=[integer]`
* **Success Response:**
* **Code:** 200 OK
* **Sample Response:**
```json
[
{
"id": "58",
"name": "<NAME>",
"imageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
}
]
```
[Back To Home](/)<file_sep>/apis/create-question.md
[Back To Home](/)
**Create Question**
----
create new question
* **URL**
/Questions
* **Method:**
`POST`
* **URL Params**
None
* **Data Params**
**Required:**
`isAnonymous=[integer] 1 mean true and 0 mean false` <br>
`scope=[string] enum: "hmp", "public"` <br>
`question=[string] question entered text (max-length: 280)`
* **Success Response:**
* **Code:** 201 Created
* **Sample Response:**
user is null because isAnonymous is 1 <br>
```json
{
"id": "4214",
"title": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم",
"question": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم",
"user": null
}
```
user is not null when isAnonymous is 0 <br>
```json
{
"id": "9632",
"title": "عنوان تستس",
"question": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم",
"user": {
"id": "109",
"fullName": "porsan_bceecf1a-bd8f-463a-870b-77999da6112a",
"profileImageUrl": "http:\/\/localhost:8000\/assets\/imgs\/defaults\/avatar.png"
}
}
```
* **Error Response:**
* **Code:** 500 Internal Server Error <br />
**Content:** `{ title: string, description: string }`
[Back To Home](/)<file_sep>/Dockerfile
FROM node:10.13-alpine
ENV NODE_ENV production
WORKDIR /usr/src/app
RUN npm i docsify-cli -g
COPY . .
EXPOSE 3000
CMD docsify serve<file_sep>/apis/remove-question.md
[Back To Home](/)
**Remove Question**
----
Returns json data of question with its answers
* **URL**
/Questions/{question_id}
* **Method:**
`DELETE`
* **URL Params**
**Required:**
`question_id=[integer] valid question id`
* **Data Params**
None
* **Success Response:**
* **Code:** 204 No Content
* **Error Response:**
* **Code:** 404 Not Found <br />
**Content:** `{ title: string, description: string }`
OR
* **Code:** 500 Internal Server Error <br />
**Content:** `{ title: string, description: string }`
[Back To Home](/)<file_sep>/apis/accounts-me.md
[Back To Home](/)
**My Accounts**
----
Returns json data list of user questions
* **URL**
/Accounts/Me
* **Method:**
`GET`
* **URL Params**
None
* **Data Params**
**Optional:**
`PageNumber=[integer]` <br>
`PageSize=[integer]`
* **Success Response:**
* **Code:** 200 OK
* **Sample Response:**
```json
{
"questions": [
{
"id": "4214",
"title": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم",
"question": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم"
},
{
"id": "4213",
"title": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم",
"question": "یه سوال تستی پرسیدم الآن از طریق وب سرویس پرسان برای همدم"
}
]
}
```
[Back To Home](/) | dce0b40e6577755d1dd8f5fe32b1c969e424e876 | [
"Markdown",
"Dockerfile"
] | 12 | Markdown | sadeghmohebbi/porsan-api-for-hmp-docs | 428e6f450c96b17597cdfe01b847e98026428b3e | a61d926c8e725fb16c75c6070f037b73e226da3d |
refs/heads/master | <repo_name>angelahpark/ios-interview<file_sep>/Questions.md
## Questions
###1. Swift is a work-in-progress language; how do you stay informed and connected to the community?
I started off learning Swift with the book 'Swift Programming: The Big Nerd Ranch Guide' and I use it to review from time to time. I try to stay up to date by subscribing to blogs like NSHipster. And I've joined the Swift iOS Development group on facebook to stay connected to the community.
###3. What is your favorite open source Swift library?
I've used Alamofire in all the iOS projects I've worked on thus far. It simplifies making requests and handling responses. I also use the AlamofireImage component library to deal with asynchronous network downloading of images.
###4. Do you usually build interfaces in code or with interface builder?
My coding style in Xcode has been a hybrid. I stick with the interface builder for basic styles. I find it to be cleaner because there's less code and the components are reusable. It's also nice that it catches and gives more insight to runtime errors and doesn't break when I'm updating to the newest version of Xcode. When necessary, for more dynamic content or interactive UIs, I build out the components in code. Because the NBC application is the base of other brands under the NBC Universal umbrella, we've created a themable UI styles and copy in code. In all cases, I make it a point to confer and determine what works best for the project at hand with the team to build the project out adhering to the same coding styles.
<file_sep>/ios-interview/ViewController.swift
//
// ViewController.swift
// ios-interview
//
// Copyright © 2016 Headspace. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
// MARK: Variables
// *leaving these vars here but best to move out of vc
var userID = "HSUSER_234234"
var sessionID = "HSSESSION_123111"
// MARK: Actions
@IBAction func login(_ sender: UIButton) {
// Send login event to Google Analytics and Snowplow.
AnalyticsTracker.sharedInstance.trackLogin(userID: userID, data: ["buttonColor": "blue"])
}
@IBAction func completeMeditation(_ sender: UIButton) {
// Send meditation completion event to Snowplow
AnalyticsTracker.sharedInstance.trackMeditationComplete(userID: userID, sessionID: sessionID)
}
}
<file_sep>/ios-interview/Analytics/AnalyticsTracker.swift
//
// AnalyticsTracker.swift
// ios-interview
//
// Created by Apple on 12/2/16.
// Copyright © 2016 Headspace. All rights reserved.
//
import Foundation
class AnalyticsTracker: NSObject {
static let sharedInstance = AnalyticsTracker()
func trackLogin(userID: String, data: [String : Any]) {
if let data = data as? [String : String] {
GoogleAnalytics.sharedInstance.login(userID: userID, withData: data)
}
SnowPlow.sharedInstance.login(userID: userID)
}
func trackMeditationComplete(userID: String, sessionID: String) {
SnowPlow.sharedInstance.meditationComplete(userID: userID, sessionID: sessionID)
}
}
<file_sep>/ios-interview/Analytics/GoogleAnalytics.swift
//
// GoogleAnalytics.swift
// ios-interview
//
// Created by Apple on 12/2/16.
// Copyright © 2016 Headspace. All rights reserved.
//
import Foundation
// Properties
// * simplifying event renaming process
enum GoogleAnalyticsProperty: String {
case Login = "login"
}
class GoogleAnalytics {
static let sharedInstance = GoogleAnalytics()
func sendEvent(_ identifier: String, forUser userID: String, withData data: [String : String]) {
GoogleAnalyticsSDK.sharedInstance.sendEvent(identifier, forUser: userID, withData: data)
}
// convenience methods
func login(userID: String, withData data: [String : String]) {
sendEvent(GoogleAnalyticsProperty.Login.rawValue, forUser: userID, withData: data)
}
}
<file_sep>/ios-interview/Analytics/Snowplow.swift
//
// Snowplow.swift
// ios-interview
//
// Created by Apple on 12/2/16.
// Copyright © 2016 Headspace. All rights reserved.
//
import Foundation
// Properties
// * simplifying event renaming process
enum SnowPlowAnalyticsProperty: String {
case Login = "log_in"
case CompleteMeditation = "complete_meditation"
}
class SnowPlow {
static let sharedInstance = SnowPlow()
func sendEvent(_ eventID: String, withPayload payload: [String : Any]) {
SnowplowSDK.sharedInstance.dispatchEvent(eventID, withPayload: payload)
}
// convenience methods
func login(userID: String) {
sendEvent(SnowPlowAnalyticsProperty.Login.rawValue, withPayload: [
"userID": userID
])
}
func meditationComplete(userID: String, sessionID: String) {
sendEvent(SnowPlowAnalyticsProperty.CompleteMeditation.rawValue, withPayload: [
"userID": userID,
"sessionID": sessionID
])
}
}
| d8959e87326f497d281f390b55708f81b7665063 | [
"Swift",
"Markdown"
] | 5 | Swift | angelahpark/ios-interview | 0ba514f5953e1ed3db64ccc769a33b54585ad89b | 8267689106d392e2dbecd1ed3c926a35bc5a3419 |
refs/heads/master | <repo_name>yaohaif/github-service-35fc73d4-46db-4bcf-b7b5-6c62ee1062a9<file_sep>/README.md
# github-service-35fc73d4-46db-4bcf-b7b5-6c62ee1062a9 | 5caa418389546ec72f7e35ae5c6452254fc06770 | [
"Markdown"
] | 1 | Markdown | yaohaif/github-service-35fc73d4-46db-4bcf-b7b5-6c62ee1062a9 | 2ba9351eaf841df20ce311afea028e378995230f | 8a2f0dc68c6598d55d3a753511f2b6887c82c78a |
refs/heads/master | <repo_name>AL-WebApps/DevHub-Web-Admin<file_sep>/app/partials/courses/coursesController.js
var coursesController = angular.module('coursesController',[]);
coursesController.controller('CoursesController', ['$scope','$http','ngTableParams','$filter',
function($scope, $http, ngTableParams, $filter)
{
// Import
var Course = Parse.Object.extend("Course");
// Variables
$scope.allCourses = [];
// Get all courses and fill table
$http({
method: 'GET',
url: url_courses + "?include=category,owner",
headers: {'X-Parse-Application-Id': appId, 'X-Parse-REST-API-Key': restId}
}).success(function(data, status) {
$scope.allCourses = data.results;
setOwnerNamesFirstLevel();
setCategoryNamesFirstLevel();
fillTable();
$('table').show();
}).error(function(data, status) {
swal("Error!", "An unexpected error have ocurred", "error");
$('table').hide();
});
/** Document Ready! **/
angular.element(document).ready(function(){
});
// Functions
var fillTable = function(){
var data = $scope.allCourses;
$scope.tableParams = new ngTableParams({ page: 1,count: 10,sorting: {createdAt: 'desc'}},
{
total: data.length,
getData: function($defer, params) {
var orderedData = params.sorting() ? $filter('orderBy')(data, params.orderBy()) : data;
orderedData = params.filter ? $filter('filter')(orderedData, params.filter()) :orderedData;
courses = orderedData.slice((params.page() - 1) * params.count(), params.page() * params.count());
params.total(orderedData.length); // set total for recalc pagination
$defer.resolve(courses);
}
});
}
var setOwnerNamesFirstLevel = function(){
for (var i = 0; i < $scope.allCourses.length; i++) {
$scope.allCourses[i]['ownername'] = $scope.allCourses[i].owner.name;
}
}
var setCategoryNamesFirstLevel = function(){
for (var i = 0; i < $scope.allCourses.length; i++) {
$scope.allCourses[i]['categoryname'] = $scope.allCourses[i].category.name;
}
}
var addCourseOnTable = function(course){
$scope.allCourses.push(course);
$scope.tableParams.reload();
}
var deleteCourseOnTable = function(id){
var index = _.indexOf(_.pluck($scope.allCourses, 'objectId'), id);
$scope.allCourses.splice(index,1);
$scope.tableParams.reload();
}
// CRUD
$scope.addCourse = function(){
}
$scope.editCourse = function(){
}
$scope.verification = function(id, check){
var query = new Parse.Query(Course);
query.get(id, {
success: function(course) {
course.set("isValid", check);
course.save();
swal("Edited!", "Course was edited successfully!", "success");
},
error: function(course, error) {
swal("Error!", "Course wasn't edited, please, try again!", "error");
}
});
}
$scope.deleteCourse = function(id){
swal({
title: "Are you sure?",
text: "You are going to delete this course",
type: "warning",
showCancelButton: true,
confirmButtonColor: "#DD6B55",
confirmButtonText: "Yes, delete it!",
closeOnConfirm: false
},
function(){
$http({
method: "DELETE",
url: url_courses + id,
headers: {'X-Parse-Application-Id': appId,
'X-Parse-REST-API-Key': restId}
}).success(function(data){
swal("Deleted!", "This course has been deleted", "success");
deleteCourseOnTable(id);
}).error(function(data){
swal("Error!", "An unexpected error ocurred, try again!", "error");
});
});
}
}]);
<file_sep>/README.md
## DevHub
Project Final to Salesianos Triana. DevHub is an app developed by <NAME>, to administration data of DevHub Android App

<file_sep>/config.js
var appId = '<KEY>';
var restId = '<KEY>';
var jsId = '<KEY>';
var url_categories = 'https://api.parse.com/1/classes/Category/';
var url_courses = 'https://api.parse.com/1/classes/Course/';
var url_videos = 'https://api.parse.com/1/classes/Video/';
var url_comments = 'https://api.parse.com/1/classes/Comment/';
var url_users = 'https://api.parse.com/1/classes/_User/';
var url_batch = 'https://api.parse.com/1/batch';
| 87e417d30dba0856ca2b9807ea965ed10fb89679 | [
"Markdown",
"JavaScript"
] | 3 | Markdown | AL-WebApps/DevHub-Web-Admin | 605cd842357d25ed13a7bfdb31958fff4f66ee39 | 37d95dea6b7df22e06e61c7d040cf6060629bb80 |
refs/heads/master | <repo_name>xuemoz2012/Exploitme1_ret_eip_overwrite<file_sep>/Exploitme1_ret_eip_overwrite/Exploitme1_ret_eip_overwrite.cpp
// Exploitme1_ret_eip_overwrite.cpp : 定义控制台应用程序的入口点。
//
#include "stdafx.h"
int main()
{
char name[32];
printf("Enter your name and press ENTER\n");
scanf("%s", name);
printf("Hi, %s!\n", name);
return 0;
}
| 2f718f74a06c1dcf7537946efee16ccf2e1be0e4 | [
"C++"
] | 1 | C++ | xuemoz2012/Exploitme1_ret_eip_overwrite | 7a400448475303deeea1fac7934e3bcf37524fc1 | 7a6ad1786d7eee791915abfe9811996b97127d6d |
refs/heads/master | <file_sep>package br.com.evegermano.semafaro;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JLabel;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class Semafarotela extends JFrame {
private JPanel contentPane;
private static JLabel label;
private ThreadSemaforo semaforo = new ThreadSemaforo();
/**
* Launch the application.
*/
// public static void main(String[] args) {
// EventQueue.invokeLater(new Runnable() {
// public void run() {
// try {
// Semafarotela frame = new Semafarotela();
// frame.setVisible(true);
// } catch (Exception e) {
// e.printStackTrace();
// }
// }
// });
// }
/**
* Create the frame.
*/
public static void main(String[] args) {
ThreadSemaforo semaforo = new ThreadSemaforo();
Semafarotela sem = new Semafarotela();
sem.setVisible(true);
for(int i=1; i<=12; i++) {
// Semafarotela sem = new Semafarotela(semaforo.getCor());
// sem.setVisible(true);
switch (semaforo.getCor()){
case VERDE:
label.setIcon(new ImageIcon("C:\\Users\\evelin.santos\\Pictures\\traffic-light-149580_960_720.png"));
break;
case AMARELO:
label.setIcon(new ImageIcon("C:\\Users\\evelin.santos\\Pictures\\Yellow-Stoplight.png"));
break;
case VERMELHO:
label.setIcon(new ImageIcon("C:\\Users\\evelin.santos\\Pictures\\traffic-light-149581_960_720.png"));
break;
default:;
}
semaforo.esperaCorMudar();
}
semaforo.desligarSemaforo();
}
public Semafarotela() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
label = new JLabel("");
label.setBounds(10, 32, 299, 199);
contentPane.add(label);
}
}
| 8bc98643712a83c59f65d2d41e8b4ef73db0c3c8 | [
"Java"
] | 1 | Java | evelingermano/evelingermano | 692ad4d451d5308ccbeeeddd18b053675389108b | 02bc7af16a1d3633a1dfd40b9b2d6835e0007e3f |
refs/heads/master | <repo_name>kobleistvan/PinewoodPC<file_sep>/Pinewood PC project files/Splash.vb
Public Class Form1
Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
Timer1.Stop()
Form2.Show()
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Timer1.Start()
End Sub
End Class<file_sep>/Pinewood PC project files/PC Derby.vb
Public Class Form2
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
MaskedTextBox1.Text = ""
MaskedTextBox2.Text = ""
MaskedTextBox3.Text = ""
MaskedTextBox4.Text = ""
MaskedTextBox1.Select()
Timer1.Stop()
Label14.Text = "0"
Label13.Text = "0"
Label10.Text = "0"
Label11.Text = "0"
Label12.Text = "0"
Label15.Text = "0"
Label16.Text = "0"
Label17.Text = "0"
Label18.Text = "0"
Label9.Text = "0"
Label9.Hide()
Label10.Hide()
Label11.Hide()
Label12.Hide()
Label15.Hide()
Label16.Hide()
Label17.Hide()
Label18.Hide()
Label19.Hide()
Label20.Hide()
Label22.Hide()
Label23.Hide()
Button2.Enabled = True
End Sub
Private Sub ExitToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ExitToolStripMenuItem.Click
Form1.Close()
Me.Close()
Dialog1.Close()
AboutBox1.Close()
End Sub
Private Sub PinewoodPCToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles PinewoodPCToolStripMenuItem.Click
AboutBox1.Show()
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
keybd_event(VK_SCROLL, 0, 0, 0) 'Press Scroll Lock
keybd_event(VK_SCROLL, 0, KEYEVENTF_KEYUP, 0) 'Release Scroll Lock
Sleep(200) 'Wait a bit
keybd_event(VK_SCROLL, 0, 0, 0) 'Press Scroll Lock
keybd_event(VK_SCROLL, 0, KEYEVENTF_KEYUP, 0) 'Release Scroll Lock
MaskedTextBox1.Select()
Timer1.Start()
Button2.Enabled = False
End Sub
Private Declare Sub keybd_event Lib "user32.dll" _
(ByVal bVk As Byte, ByVal bScan As Byte, _
ByVal dwFlags As Long, ByVal dwExtraInfo As Long)
Private Declare Sub Sleep Lib "kernel32" _
(ByVal dwMilliseconds As Long)
Const VK_SCROLL = &H91
Const KEYEVENTF_KEYUP = &H2
Private Sub MaskedTextBox1_MaskInputRejected(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MaskInputRejectedEventArgs)
Activate()
End Sub
Private Sub Form2_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Form1.Close()
Label9.Hide()
Label10.Hide()
Label11.Hide()
Label12.Hide()
Label15.Hide()
Label16.Hide()
Label17.Hide()
Label18.Hide()
Label19.Hide()
Label20.Hide()
Label22.Hide()
Label23.Hide()
MaskedTextBox1.Select()
End Sub
Private Sub MaskedTextBox1_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MaskedTextBox1.TextChanged
If MaskedTextBox1.MaxLength Then
Label9.Text = Label14.Text
Label10.Text = Label13.Text
Label9.Show()
Label10.Show()
Label23.Show()
MaskedTextBox2.Select()
End If
End Sub
Private Sub MaskedTextBox2_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MaskedTextBox2.TextChanged
If MaskedTextBox2.MaxLength Then
Label11.Text = Label14.Text
Label12.Text = Label13.Text
Label11.Show()
Label12.Show()
Label22.Show()
MaskedTextBox3.Select()
End If
End Sub
Private Sub MaskedTextBox3_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MaskedTextBox3.TextChanged
If MaskedTextBox3.MaxLength Then
Label15.Text = Label14.Text
Label16.Text = Label13.Text
Label15.Show()
Label16.Show()
Label20.Show()
MaskedTextBox4.Select()
End If
End Sub
Private Sub MaskedTextBox4_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MaskedTextBox4.TextChanged
If MaskedTextBox4.MaxLength Then
Label17.Text = Label14.Text
Label18.Text = Label13.Text
Label17.Show()
Label18.Show()
Label19.Show()
Timer1.Stop()
End If
End Sub
Dim tmr1 As Integer
Dim tmr2 As Integer
Dim tmr3 As Integer
Dim tmr4 As Integer
Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
tmr1 = tmr1 + 1
Label13.Text = tmr1
If tmr1 = 9 Then
Label14.Text = Label14.Text + 1
tmr1 = 0
End If
If Label14.Text = 9 Then
Timer1.Stop()
If MaskedTextBox1.Text = "" Then
MaskedTextBox1.Text = "0"
End If
If MaskedTextBox2.Text = "" Then
MaskedTextBox2.Text = "0"
End If
If MaskedTextBox3.Text = "" Then
MaskedTextBox3.Text = "0"
End If
If MaskedTextBox4.Text = "" Then
MaskedTextBox4.Text = "0"
End If
End If
End Sub
Private Sub ÚtmutatásToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ÚtmutatásToolStripMenuItem.Click
Dialog1.Show()
End Sub
Private Sub MagyarToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MagyarToolStripMenuItem.Click
Me.MagyarToolStripMenuItem.Checked = True
Me.AngolToolStripMenuItem.Checked = False
Me.NyelvToolStripMenuItem.Text = "Nyelv"
Me.FileToolStripMenuItem.Text = "Fájl"
Me.AboutToolStripMenuItem.Text = "Segítség"
Me.ExitToolStripMenuItem.Text = "Kilépés"
Me.ÚtmutatásToolStripMenuItem.Text = "Útmutatás"
Me.PinewoodPCToolStripMenuItem.Text = "Pinewood PC-ről"
Me.MagyarToolStripMenuItem.Text = "Magyar"
Me.AngolToolStripMenuItem.Text = "Angol"
Me.Button1.Text = "Következő futam"
Me.Label5.Text = "Sáv"
Me.Label6.Text = "Sáv"
Me.Label7.Text = "Sáv"
Me.Label8.Text = "Sáv"
Me.Label1.Text = "l. Hely"
Me.Label2.Text = "ll. Hely"
Me.Label3.Text = "lll. Hely"
Me.Label4.Text = "lV. Hely"
Dialog1.TextBox1.Text = "A START gomb elindítja a stopperórát és a kocsikat."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "A KÖVETKEZŐ FUTAM gomb lenullázza a stopperórát és kitőrli az elöző futamban nyert adatokat."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "A kocsi beérkezésénél a hozzá illő stopper leáll. A programhoz illő műszer automatikusan betáplálja a sávok számát a kocsik beérkezésénél."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "A műszernek szükséges egy működő 2.0 USB port."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "A program műkődéséért a NUM LOCK gomb illetve billentyüzeten lévő LED kötelező, hogy aktív legyen!"
Dialog1.Text = "Útmutatás"
Dialog1.Button1.Text = "Értettem"
AboutBox1.Label1.Text = "A Pinewood PC egy számitógép program, amely a cserkészetbeli Pinewood Derby kisautó versenynek van szánva. A program optimális műkődéséért ajánolva van a hozzá illő hardware használata. Készitette Köble István <NAME> Minden jog fenntartva"
End Sub
Private Sub AngolToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles AngolToolStripMenuItem.Click
Me.MagyarToolStripMenuItem.Checked = False
Me.AngolToolStripMenuItem.Checked = True
Me.NyelvToolStripMenuItem.Text = "Language"
Me.FileToolStripMenuItem.Text = "File"
Me.AboutToolStripMenuItem.Text = "Help"
Me.ExitToolStripMenuItem.Text = "Exit"
Me.ÚtmutatásToolStripMenuItem.Text = "Instructions"
Me.PinewoodPCToolStripMenuItem.Text = "About Pinewood PC"
Me.MagyarToolStripMenuItem.Text = "Hungarian"
Me.AngolToolStripMenuItem.Text = "English"
Me.Button1.Text = "Next race"
Me.Label5.Text = "Lane"
Me.Label6.Text = "Lane"
Me.Label7.Text = "Lane"
Me.Label8.Text = "Lane"
Me.Label1.Text = "1st Place"
Me.Label2.Text = "2nd Place"
Me.Label3.Text = "3rd Place"
Me.Label4.Text = "4th Place"
Dialog1.TextBox1.Text = "The START button starts the timers and the cars."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "The NEXT RACE button resets the timers and the previous results."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "When a car crosses the finish line, it's timer stops automatically. The module of this software automatically inputs the number of the lanes at the arriving of the cars."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "The module requires a functional 2.0 USB port."
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += Environment.NewLine
Dialog1.TextBox1.Text += "For the proper operation of this program, the NUM LOCK key must be turned on!"
Dialog1.Text = "Instructions"
Dialog1.Button1.Text = "Got it"
AboutBox1.Label1.Text = "Pinewood PC is a computer software specially developed for Pinewood Derby racing in scouting. For the optimal behaviour of the software, the use of a hardware, specially developed for it is recommended. Created,and developed by <NAME> A.K.A. PityX All rights reserved"
End Sub
End Class
| 38681c73bf01dca558c48af98cc2695b1098aab4 | [
"Visual Basic .NET"
] | 2 | Visual Basic .NET | kobleistvan/PinewoodPC | 248280a4fc6462b8b38331e05f943235bdaa1f8a | 6f85b8144d55596959b51301753fc5f4e4f3e966 |
refs/heads/master | <file_sep>package de.rixtrick.demo.dao.impl;
import org.springframework.stereotype.Repository;
import de.rixtrick.demo.dao.iface.MatchDayDao;
import de.rixtrick.demo.model.MatchDay;
/**
* @author buehner
*
*/
@Repository
public class MatchDayDaoImpl extends GenericHibernateDaoImpl<MatchDay, Integer>
implements MatchDayDao {
protected MatchDayDaoImpl() {
super(MatchDay.class);
}
}
<file_sep>package de.rixtrick.demo.service.iface;
import de.rixtrick.demo.model.GoalGetter;
/**
* @author buehner
*
*/
public interface GoalGetterService {
void saveGoalGetter(GoalGetter goalGetter);
}
<file_sep>package de.rixtrick.demo.model;
import java.util.HashSet;
import java.util.Set;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.ManyToMany;
import javax.persistence.OneToMany;
import javax.persistence.Table;
/**
* @author buehner
*
*/
@Entity
@Table
public class Team extends PersistentObject {
private static final long serialVersionUID = -3347746010176522188L;
@Column(nullable = false, unique = true, length = 64)
private String name;
@Column
private String iconUrl;
@OneToMany(mappedBy = "currentTeam", cascade = { CascadeType.ALL })
private Set<GoalGetter> squad = new HashSet<GoalGetter>();
@ManyToMany(mappedBy = "teams")
private Set<Competition> competitions = new HashSet<Competition>();
public Team() {
}
public Team(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getIconUrl() {
return iconUrl;
}
public void setIconUrl(String iconUrl) {
this.iconUrl = iconUrl;
}
public Set<GoalGetter> getSquad() {
return squad;
}
public void setSquad(Set<GoalGetter> squad) {
this.squad = squad;
}
@Override
public String toString() {
return name + ", id : " + getId() + ", created at " + getCreated()
+ ", updated at " + getModified();
}
}
<file_sep>Basic Java Web Project for Eclipse, created with Maven and used as a Sandbox.
Using
- Spring 3
- Hibernate 4
- PostgreSQL 9.1
- c3p0 Connection Pooling
- Jodatime
Inspired by
- http://www.mkyong.com/maven/how-to-create-a-web-application-project-with-maven/
- http://ocpsoft.org/java/hibernate-use-a-base-class-to-map-common-fields/
- https://community.jboss.org/wiki/GenericDataAccessObjects<file_sep>package de.rixtrick.demo.service.impl;
import java.util.List;
import org.hibernate.criterion.Criterion;
import org.hibernate.criterion.Restrictions;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import de.rixtrick.demo.dao.iface.TeamDao;
import de.rixtrick.demo.model.Team;
import de.rixtrick.demo.service.iface.TeamService;
/**
* @author buehner
*
*/
@Service("teamService")
@Transactional(readOnly = true)
public class TeamServiceImpl implements TeamService {
@Autowired
private TeamDao teamDao;
public Team findByTeamName(String name) {
Criterion criterion = Restrictions.eq("name", name);
List<Team> teamList = teamDao.findByCriteria(criterion);
if (teamList.size() > 0) {
return teamList.get(0);
}
return null;
}
@Transactional(readOnly = false)
public void saveTeam(Team team) {
teamDao.saveOrUpdate(team);
}
@Transactional(readOnly = false)
public void deleteTeam(String name) {
Team team = findByTeamName(name);
teamDao.delete(team);
}
public List<Team> findTeamsLike(String name) {
Criterion criterion = Restrictions.ilike("name", "%" + name + "%");
return teamDao.findByCriteria(criterion);
}
}
<file_sep>package de.rixtrick.demo.service.iface;
import java.util.Set;
import de.rixtrick.demo.model.Competition;
/**
* @author buehner
*
*/
public interface CompetitionService {
void saveCompetition(Competition competition);
Set<Competition> findAllCompetitions();
}
<file_sep>/**
*
*/
package de.rixtrick.demo.service.iface;
import de.rixtrick.demo.model.Match;
/**
* @author buehner
*
*/
public interface MatchService {
void saveMatch(Match match);
}
<file_sep>package de.rixtrick.demo.dao.iface;
import java.util.List;
import de.rixtrick.demo.model.Team;
public interface TeamDao extends GenericHibernateDao<Team, String> {
public List<Team> findByExample(Team example);
}
<file_sep>package de.rixtrick.demo.controller;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import de.rixtrick.demo.model.Team;
import de.rixtrick.demo.service.iface.TeamService;
@Controller
@RequestMapping("/")
public class BaseController {
private static final Logger LOGGER = Logger.getLogger(BaseController.class);
/**
* The Service to manipulate the Teams
*/
@Autowired
private TeamService teamService;
@RequestMapping(value = "/welcome", method = RequestMethod.GET)
public String welcome(ModelMap model) {
LOGGER.info("starting welcome() method");
model.addAttribute("message",
"Maven Web Project + Spring 3 MVC - welcome()");
LOGGER.debug("executed default welcome");
// Spring uses InternalResourceViewResolver and return back index.jsp
return "index";
}
@RequestMapping(value = "/welcome/{name}", method = RequestMethod.GET)
public String welcomeName(@PathVariable String name, ModelMap model) {
LOGGER.info("starting welcomeName() method with name '" + name + "'");
model.addAttribute("message", "Maven Web Project + Spring 3 MVC - "
+ name);
Team team = new Team(name);
// team.
// team.setLastName(name);
// team.setPassword(name);
teamService.saveTeam(team);
LOGGER.debug("executed enhanced welcome");
return "index";
}
}<file_sep>package de.rixtrick.demo.model;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.ManyToMany;
import javax.persistence.OneToMany;
import javax.persistence.OrderBy;
import javax.persistence.Table;
/**
* @author buehner
*
*/
@Entity
@Table
public class Competition extends PersistentObject {
private static final long serialVersionUID = 1867737649008505171L;
@Column(length = 64)
private String name;
@Column(length = 64)
private String sport;
@Column(length = 32)
private String code;
private Integer level;
private Integer startYear;
private Integer endYear;
@OneToMany(mappedBy = "competition", cascade = { CascadeType.ALL }, fetch = FetchType.EAGER)
@OrderBy("orderIndex")
private List<MatchDay> matchDays = new ArrayList<MatchDay>();
@ManyToMany(cascade = { CascadeType.ALL })
private Set<Team> teams = new HashSet<Team>();
public Competition() {
}
public Competition(String name, String sport, Integer level,
Integer startYear, Integer endYear) {
this.name = name;
this.sport = sport;
this.level = level;
this.startYear = startYear;
this.endYear = endYear;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSport() {
return sport;
}
public void setSport(String sport) {
this.sport = sport;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public Integer getLevel() {
return level;
}
public void setLevel(Integer level) {
this.level = level;
}
public Integer getStartYear() {
return startYear;
}
public void setStartYear(Integer startYear) {
this.startYear = startYear;
}
public Integer getEndYear() {
return endYear;
}
public void setEndYear(Integer endYear) {
this.endYear = endYear;
}
public List<MatchDay> getMatchDays() {
return matchDays;
}
public void setMatchDays(List<MatchDay> matchDays) {
this.matchDays = matchDays;
}
public Set<Team> getTeams() {
return teams;
}
public void setTeams(Set<Team> teams) {
this.teams = teams;
}
}
<file_sep>package de.rixtrick.demo.dao.iface;
import de.rixtrick.demo.model.Goal;
/**
* @author buehner
*
*/
public interface GoalDao extends GenericHibernateDao<Goal, Integer> {
}
<file_sep>jdbc.driverClassName=org.postgresql.Driver
jdbc.url=jdbc:postgresql://localhost:5432/mydatabase
jdbc.username=myuser
jdbc.password=<PASSWORD><file_sep><project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>de.rixtrick</groupId>
<artifactId>SimpleJavaWebApp</artifactId>
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version>
<name>Simple Java, Maven and Eclipse Webapp</name>
<url>http://maven.apache.org</url>
<properties>
<spring.version>3.2.3.RELEASE</spring.version>
<junit.version>4.11</junit.version>
<jdk.version>1.6</jdk.version>
<log4j.version>1.2.17</log4j.version>
<jackson.version>1.9.12</jackson.version>
<hibernate.version>4.2.3.Final</hibernate.version>
<postgresql.version>9.1-901-1.jdbc4</postgresql.version>
<c3p0.version>0.9.2.1</c3p0.version>
<joda-time.version>2.2</joda-time.version>
<jadira-usertype-jodatime.version>2.0.1</jadira-usertype-jodatime.version>
<maven-compiler-plugin.version>3.1</maven-compiler-plugin.version>
<downloadSources>true</downloadSources>
<downloadJavadocs>false</downloadJavadocs>
</properties>
<dependencies>
<!-- Spring 3 dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- JUnit dependencies -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<!-- Logging dependencies -->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>${log4j.version}</version>
</dependency>
<!-- Jackson JSON Mapper -->
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>${jackson.version}</version>
</dependency>
<!-- Hibernate -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>${hibernate.version}</version>
</dependency>
<!-- PostgreSQL -->
<dependency>
<groupId>postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>${postgresql.version}</version>
</dependency>
<!-- Data Source -->
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>${c3p0.version}</version>
</dependency>
<!-- Joda Time Library -->
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
<version>${joda-time.version}</version>
</dependency>
<!-- Jadira Usertype (Joda Mapping Classes for Hibernate) -->
<dependency>
<groupId>org.jadira.usertype</groupId>
<artifactId>usertype.jodatime</artifactId>
<version>${jadira-usertype-jodatime.version}</version>
</dependency>
</dependencies>
<build>
<finalName>SimpleJavaWebApp</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>${maven-compiler-plugin.version}</version>
<configuration>
<source>${jdk.version}</source>
<target>${jdk.version}</target>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-eclipse-plugin</artifactId>
<configuration>
<downloadSources>${downloadSources}</downloadSources>
<downloadJavadocs>${downloadJavadocs}</downloadJavadocs>
</configuration>
</plugin>
</plugins>
</build>
</project>
<file_sep>package de.rixtrick.demo.dao.impl;
import org.springframework.stereotype.Repository;
import de.rixtrick.demo.dao.iface.GoalDao;
import de.rixtrick.demo.model.Goal;
/**
* @author buehner
*
*/
@Repository
public class GoalDaoImpl extends GenericHibernateDaoImpl<Goal, Integer> implements
GoalDao {
protected GoalDaoImpl() {
super(Goal.class);
}
}
<file_sep>package de.rixtrick.demo.dao.impl;
import java.util.List;
import org.springframework.stereotype.Repository;
import de.rixtrick.demo.dao.iface.TeamDao;
import de.rixtrick.demo.model.Team;
/**
* @author buehner
*
*/
@Repository
public class TeamDaoImpl extends GenericHibernateDaoImpl<Team, String>
implements TeamDao {
protected TeamDaoImpl() {
super(Team.class);
}
@Override
public List<Team> findByExample(Team example) {
// TODO some logic
return null;
}
}
<file_sep>package de.rixtrick.demo.dao.iface;
import de.rixtrick.demo.model.Match;
/**
* @author buehner
*
*/
public interface MatchDao extends GenericHibernateDao<Match, Integer> {
}
<file_sep>/**
*
*/
package de.rixtrick.demo.service.impl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import de.rixtrick.demo.dao.iface.GoalGetterDao;
import de.rixtrick.demo.model.GoalGetter;
import de.rixtrick.demo.service.iface.GoalGetterService;
/**
* @author buehner
*
*/
@Service("goalGetterService")
@Transactional(readOnly = true)
public class GoalGetterServiceImpl implements GoalGetterService {
@Autowired
private GoalGetterDao goalGetterDao;
@Transactional(readOnly = false)
public void saveGoalGetter(GoalGetter goalGetter) {
goalGetterDao.saveOrUpdate(goalGetter);
}
}
<file_sep>package de.rixtrick.demo.model;
/**
* @author buehner
*
*/
public enum Position {
GOALKEEPER, DEFENSE, MIDFIELD, FORWARD
}
| 2aa0e30840904e8c07722f89f449ba954e7cdde3 | [
"Java",
"Markdown",
"INI",
"Maven POM"
] | 18 | Java | buehner/SimpleJavaWebApp | 1ae427b385aac4e00847969336a881f7615a3d9b | ba1dbf1700aa0489ecda21faaa4148212b67b221 |
refs/heads/master | <file_sep># Week 8 Application Layer
## Domain Name System (DNS)
* Distributed database implemented in a hierarchy of many name servers
* Application Layer protocol that allows a host to query the database in order to resolve names
* Used by application layer protocols (http, ftp, smtp)
### DNS Defined
* Number of RFSs are directly related to DNS:
* RFC 1034: Domain Names: Concepts and Facilities
* RFC 1035: Domain Names: Implementation and Specification
* RFC 1519: Domain Name System Structure and Delegation
* RFC 2219: Use of DNS Aliases for Network Services
* RFC 2606: Reserved Top Level DNS Names
* RFC 3647: Role of the Domain Name System
### Conceptual Divisions of DNS Namespace
* A hierarchical naming convention
* The top of the hierarchy is managed by ICANN (The Internet Corporation for Assigned Names and Numbers)
> Example of DNS Namespace
>> <img src='DNS_Namespace.png' width=50%>
### Why note centralize DNS
* Single point of failure
* Traffic volume
* Distant centralized database
* Maintenance
* Doe not scale well
### DNS Services
* Hostname to IP address translation
* Host aliasing: Alias names for canonical names
* E.g. Canonical relay1.westcoast.enterprise.com -> www.enterprise.com
* Mail server aliasing
* E.g. <EMAIL> -> <EMAIL>
* Load distribution
* Busy sites are replicated over multiple servers
* A set of IP addresses is associated with one canonical name
* DNS server rotates the order of the addresses to distribute the load
## Domain Name Characteristics
* Domain Name:
* Are case insensitive
* Can have up to 63 characters per constitute
* Can have up to 255 chars per path
* Can be internationalized
* Naming conventions usually follow either organizational or physical boundaries
* Absolute domain names ends in a .
* Relative domain names partially specify the location and can be used only within the context of an absolute domain name
## Zone Name Servers
* DNS namespace divided non-overlapping zones
* Each zone contains a part of the DNS tree and also name servers authoritative for that zone
* Usually 2 name servers for a zone
* Sometimes secondary is actually outside the zone
* Name servers are arranged in a hierarchical manner extending from a set of root servers
## Root Name Servers
* The root servers form the authoritative cluster for enquiry in the event of locally unresolvable name queries
* There are 13 root servers globally
## Resource Records
* The resources records are the key objects in the Domain Name System
* A RR consists of a domain name, TTL, class, type, value
* Domain name: Which domain this record applies to
* TTL: Indicates stability or temporal extent of the record
* Class: IN for internet
* Type: a closed vocabulary of the following:
* A: The Internet address of the host
* CNAME: The canonical name of an alias
* MX: The mail exchanger
* NS: The name server
* PTR: The host name if the query is in the form of an Internet address; Otherwise the pointer to other information
* SOA: The domain's start-of-authority information
* Value: data
### Example of asking for domain name
1. User machine runs the client side of the DNS software
2. Browser extracts the hostname from the URL, and passes it to the client-side of the DNS application
3. DNS client send a query containing the hostname to a DNS server
4. DNS client eventually receives a reply containing the IP address for the hostname
5. Browser initiates a TCP connection to the process located at port 80 at the IP address
## DNS in Action
* Finding the IP address for a given hostname is called name resolution and is done with the DNS protocol
* Resolution:
* Computer requests local name server to resolve
* Local name server asks the root name server
* Root returns the name server for a lower zone
* Continue down zones until name server can answer
* DNS protocol:
* Runs on UDP port 53, retransmits when lost messages
* Caches name server answers for better performance
> Example of resolution
>> <img src='Resolution.png' width=50%>
* Iterated query: Contacted server replies with name of server to contact
* Recursive query: Server obtains mapping on client's behalf
## DNS Caching and Updating Records
* Once name server learns a mapping, it caches the mapping
* IP addresses of TLD servers typically cached in local name servers
* Cache entries timeout after some time
## World Wide Web (WWW)
* Client and server software:
* Firefox is the client software for web access where Apache is on the server side
* Web markup languages:
* HTML is how webpages are coded
* Web scripting languages:
* Javascript adds more dynamicity to web webpages
* HTTp is about how to transfer
## Web Access
* A web page consists of objects
* An object can be HTML file but also JPEG image, Java applet, audio file
* A web page consists of a base HTML file which includes several referenced objects
* Each object is addressable by a URL (Uniform Resource Locator)
> Example of a URL:
>> <img src='URL.png' width=50%>
## Hypertext Transfer Protocol (HTTP)
* HTTP is at the application layer
* Client/Server Model
* Client: Browser that requests, receives and displays Web objects
* Server: Web server sends object in response to requests
> Illustration of HTTP
>> <img src='HTTP.png' width=50%>
### HTTP Connections
* Non-persistent HTTP: at most one object sent over a TCP connection
* Persistent HTTP: Multiple objects can be sent over a single TCP connection between client and server
### Non-persistent HTTP
* Example of visiting www.someSchool.edu/someDepartment/home.index:
1. a) HTTP clients initiates TCP connection to HTTP server process at www.someSchool.edu on port 80 \
b) HTTP server at host www.someSchool.edu waiting for TCP connection at port 80. Accepts connection and notify client
2. HTTP client sends a HTTP request message, containing URL, into TCO connection socket. Message indicates that client wants object someDepartment/home.index
3. HTTP server receives request message, form response message containing requested object, and sends message into its socket
4. HTTP client receives response message containing HTML file
5. HTTP server closes TCP connection
6. Parses HTML file and finds 10 referenced jpeg objects
7. Steps 1-6 repeated for each pf the 10 jpeg objects
### Response Time
* Round Trip Time (RTT): Time for a small packet to travel from client to server and back
* Response time:
* One RTT to initiate TCP connection
* One RTT for HTTP request and first few bytes of HTTP response to return
* File transmission time
* Total Response time = 2 RTT + file transmission time
> Illustration of HTTP response
>> <img src='Response_Time.png' width=50%>
### Non-persistent HTTP issues
* Requires new connection per requested object
* OS overhead for each TCP connection
* Delivery delay of 2 RTTs per requested object
### Persistant HTTP
* Server leaves connection open after sending response
* Subsequent HTTP messages between same client/server sent over open connection
* Pipelining: Client sends request as soon as it encounters a referenced object
* As little as one RTT for all the referenced objects
* Server closes a connection if it hasn't been used for some time
### HTTP Request Methods
|Method|Description|
|----|----|
|GET|Request to read a web page|
|HEAD|Request to read a web page's header|
|PUT|Request to store a web page|
|POST|Append to a named resource|
|DELETE|Remove the web page|
|TRACE|Echo the incoming request|
|CONNECT|Reserved for future use|
|OPTIONS|Query certain options|
### HTTP Error Codes
|Code|Meaning|Examples|
|----|----|----|
|1xx|Information|100 = server agrees to handle client's request|
|2xx|Success|200 = request succeeded; 204 = no content present|
|3xx|Redirection|301 = page moved; 304 = cached page still valid|
|4xx|Client error|403 = forbidden page; 404 = page not found|
|5xx|Server error|500 = internal server error; 503 = try again later|
## Cookies
* The http servers are stateless
* Cookies to place small amount of info on users computer and reuse deterministically
* Questionable mechanism for tracking users
* Advantages:
* Authorization
* Shopping carts
* Recommendations
* User session state
### Cookies vs. Sessions
|Sessions|Cookies|
|----|----|
|Sessions information regarding visitor's interaction stored at the server side: upto some hours|Cookies are transferred between server and client|
|When user closes the website, the session ends|Cookie information stored at both client and server|
|Sessions information size can be large|Maintain client information until deleted|
||Cookies information size limited|
## Web Caches (Proxy Server)
* Goal is to satisfy client request without involving origin server
* User sets browser to access web via cache
* Browser sends all HTTP requests to cache
* If object in cache, cache returns object, else cache requests object from origin server, then returns object to client
* Cache acts as both client and server
* Typically cache in installed by ISP
* Causes problems for frequently changing data
* Advantages:
* Reduce response time for client request
* Reduce traffic on an institution's access link
## Dealing with Multimedia Data
* Higher bandwidth requirements
* Higher QoS requirement
* Separate providers
* Not all communication is one-to-one. Many is multicast/broadcast which is different to most traffic
* Specialized infrastructure also needs special attention
## A basic model for multimedia on the web
> Illustration of the multimedia basic model
>> <img src='Basic_Model.png' width=50%>
* Problems:
* The entire media file must be transmitted over the network before playback starts
* Basic model assumes mainly point-to-point data distribution rather than a point-to-multipoint distribution model
* Basic model relies on simple browser/plugin/helper integration and traditional service types
## Streaming Media Protocols
* HTTP
* RTP: Real-time Protocol
* MPEG-4
* Microsoft's Windows Media
## Specialized Multimedia Software
* 4 main tasks of the multimedia playback software:
* To deal with the user interface side:
* Functions such as volume control, playback, next
* Handle transmission errors in conjunction with transport protocols
* Using RTP/UDP errors will likely occur, playback software must manage/mask them gracefully
* Eliminate jitter
* Small buffer, quick playback but susceptible to jitter/delay
* Large buffer, delay at start of playback while buffer fills, but less susceptible to delay/jitter
* Sometimes compress and almost always decompress the multimedia files to reduce size
> Specialized Model
>> <img src='Specialized_Model.png' width=50%>
## Handling Errors
* Forward Error Correction: The error-correcting encoding of data. For every X data packets Y new packets are added similar to methods. These contains redundant bits that are used to deal with errors
* Methods used parity or exclusive-OR sums of the bits in each of the data packets but are more complex
* Error Resilience: Remarking for re-sync so that a packet loss does not create a total loss, mainly on sender side
* Error Concealment: Done by the receiver
* Retransmission: Less meaningful for streaming data but for watching a movie. This can be deployed for lost packets of the movie.
## Jitter management
* Jitter happens because of variable bandwidth and loss/retransmissions. Therefore, we use buffers.
> Illustration of buffers
>> <img src='Buffer.png' width=50%>
## Compression
* ADC (Analog-to-Digital Converter) produces digital data
> Example of ADC
>> <img src='ADC.png' width=50%>
### Audio compression
* Use Nyquist and Shannon theorems to convert analog data to digital. Then apply techniques to eliminate some data
* E.g.
* Perceptual coding is that some data can mask other data
* Frequency masking: Some sounds mask/hide others so there is no point encoding them
* Temporal masking: Human ears can miss soft sounds immediately after loud sounds, takes time for ear to adjust, no need to store them.
### MP3
* MP3 is MPEG Audio Player 3
* MP3's compression is based on perceptual coding
* MP3 audio compression results in significant file size savings without a perceived loss of audio quality
* Typical MP3 audio compression rates for CS quality audio reduce the need for bandwidth for 1.4 Mbps for stereo down to 96-128 Kbps
### For digital video
* Video is digitized as pixels
* Video is sent compressed due to its large bandwidth requirements
* Lossy compression exploits human perception
* Large compression ratios achieved
### JPEG
* JPEG is lossy compression
* JPEG often provides compression ratios of 20:1
* JPEG compression is symmetric, decoding takes as long as encoding
### MPEG
* MPEG is Motion Picture Experts Group
* MPEG can compress both audio and video together
* The evolution of MPEG:
* MPEG-1: VCR quality at 1.2 Mbps (40:1)
* MPEG-2: Broadcast quality at 4-6Mbps (200:1)
* MPEG-4: DVD quality at 10Mbps (1200:1)
<file_sep># Week 1 Introduction to Networking
## Terminologies
* A <font color=red>network device</font>: E.g. <font color=pink>PC, Router, Switch</font>
* <font color=red>Server</font>: Provider of a service. Accept requests from clients.
* <font color=red>Client</font>: A network device connecting to a server and requesting a service.
* <font color=red>Computer Network</font>: A collection of autonomous computers interconnected by a single technology
* <font color=red>Packet</font>: A message send between two network devices.
* <font color=red>IP Address</font>: A unique number identifying a network device.
## Network vs Computer Network
* Network (Noun):
* An intricately connected system of things or people
* An interconnected or intersecting configuration or system of components.
* Computer Network:
* A data network with computers at one or more of nodes
* A collection of autonomous computers interconnected by a single technology
### Internet and World Wide Web
* Neither the Internet nor the World Wide Web is a computer network
* The Internet is not a single network but a network of networks
* The World Wide Web is a distributed system that runs on top of the Internet
## Computer networks
* Usage
* Business Applications:
* Resources sharing
* Home Applications:
* Access to remote information
* Interactive entertainment
* E-commerce
* Mobile Users
* Mobility
* Internet-of-things <font color=pink>(E.g. parking, smart-center, vending machines)</font>
* Social Interactions
* Origins: Simple Client-Server Networking
* A network with two clients and one server
> Example of Client and Server Model
>> <img src='Client_and_Server.png' width=50%>
## Differentiating Factors of Networks
* Types of transmission technology
* <font color=red>Broadcast link</font>
* Broadcast networks have a single communication channel shared bu all machines on a network. Packets sent by any machine are received by all others, an address field in the packet specifies the intended recipient. Intended recipients process the packet contents others simply ignore it.
* Broadcasting is a mode of operation which allows a packet to be transmitted that every machines in the network must process
* <font color=red>Point-to-point Links</font>
* Data from sender machine is not seen and process by other machines
* Point to point networks consist of connections between individual pairs of machines. Packets travelling from source to destination must visit intermediate machines to determine a route or multiple routes of variant efficiencies are available and optimization is an important principle
* Unicasting is the term where point-to-point networks with a single sender and receiver pair can exchange data
* <font color=red>Multicasting</font>
* Transmission to a subset of the machines
### Differentiating by Scale
> |Interprocessor Distance|Processors located in same|Example|
>|----|----|----|----|
>|1m|Square Meter|Personal Area Network|
>|10m|Room|Local Area Network|
>|100m|Building|Local Area Network|
>|1km|Campus|Local Area Network|
>|10km|City|Metropolitan Area Network|
>|100km|Country|Wide Area Network|
>|1000km|Continent|Wide Area Network|
>|10000km|Planet|The Internet|
### Mixing taxonomies
* Local Area Networks can further be distinguished by three factors:
* Size
* Transmission Technology: <font color=pink>Physically wired network</font>
* Topology:
* <font color=red>Bus</font>: Only a single machine on the network can transmit at any point in time requires a negotiation mechanism to resolve transmission conflicts: <font color=pink>Ethernet is the most common bus network</font>
> Example of Bus network structure:
>> <img src='Bus.png' width=50%>
* <font color=red>Ring</font>: Each transmission bit is propagated individually and requires access control to resolve propagation queuing. <font color=pink>E.g. Token Ring</font>
> Example of Ring network structure:
>> <img src='Ring.png' width=50%>
## Components of the Internet
* <font color=red>Protocols, Layers, and Services</font>
* Protocol Hierarchies
* Design of Layer Models
* Connection-Oriented and Connectionless Service Types
* Service Primitives
* Service and Protocols
* <font color=red>Network Reference Models</font>
* Open Systems Interconnect
* TCP/IP
* <font color=red>Network Standards</font>
## Protocol Hierarchies
* Consider the network as a stack of layers
* Each layer offers services to layer above it
* Inter-layer exchanges are conducted according to a protocol
> Example of a protocol hierarchies
>> <img src='Protocol_Hierarchies.png' width=50%>
> Example of information flow supporting virtual communication in Layer 5
>> <img src='Layer5.png' width=50%>
## Design Issues for the Layers
* <font color=red>Connection Oriented</font>: Connect -> Use -> Disconnect <font color=pink>(similar to telephone service)</font>
* Negotiation inherent in connection setup
* <font color=red>Connectionless</font>: Just sent <font color=pink>(similar to postal service)</font>
* Choice of service type has corresponding impact on the reliability and quality of the service itself.
## Connection-Oriented and Connectionless services
* Six different types of services:
>|Type|Service|Example|
>|----|----|----|
>|Connection-Oriented|Reliable Message Stream|Sequence of pages|
>|Connection-Oriented|Reliable byte stream|Remote login|
>|Connection-Oriented|Unreliable connection|Digitized voice|
>|Connectionless|Unreliable datagram|Electronic junk mail|
>|Connectionless|Acknowledge datagram|Registered mail|
>|Connectionless|Request-reply|Database query|
## Service Primitives
* Primitives are formal set of operations for services
* The number and type of primitives in any particular context is dependent on nature of service itself - in general more complex services require more primitive service
* Six service primitive for implementing a simple connection-oriented service:
>|Primitive|Meaning|
>|----|----|
>|<font color=pink>LISTEN</font>|Block waiting for an incoming connection|
>|<font color=pink>CONNECT</font>|Establish a connection with a waiting peer|
>|<font color=pink>ACCEPT</font>|Accept an incoming connection from a peer|
>|<font color=pink>RECEIVE</font>|Block waiting for an incoming message|
>|<font color=pink>SEND</font>|Send a message to the peer|
>|<font color=pink>DISCONNECT</font>|Terminate a connection|
## Relationship of Services and Protocols
* Service is a set of primitives that a layer provides to a layer above it
* Defines what operations the layer is prepared to perform on behalf of its users
* It says nothing about how these operations are implemented
* Interfaces between layers
* Protocol is a set of rules governing the format and meaning of packets that are exchanged by peers within a layer
* Packets sent between peer entities
> The relationship between Services and Protocol:
>> <img src='Service_Protocols.png' width=50%>
## Reference Models
* Example of reference model
* The <font color=red> OSI</font> Reference Model
* The <font color=red> TCP/IP</font> Reference Model
* A reference model provides a common baseline for the development of many services and protocols by independent parties
* Since networks are very complex systems, a reference model can serve to simplify the design process
* It's engineering best practice to have an abstract reference model, and corresponding implementations are always required for validation purposes. <file_sep># Week 3 Data Link Layer
## The Data Link Layer in OSI and TCP/IP
* Reliable, efficient communication of frames between two adjacent machines
* Handles transmission errors and flow control
## Functions and Methods of the Data Link Layer
* Function of the data link layer:
* Provide a well-defined service interface to network layer
* Handling transmission errors
* Data flow regulation
* Primary method: Take packets from network layer, and encapsulate them into frames. A frame contains a header, a payload and a trailer.
### Relationship between Packets and Frames
* Data Link layer accepts packets from the network layer, and encapsulates them into frames that it sends using the physical layer, reception is the opposite process
> Implementation of a Data Link Layer:
>> <img src='Implementation.png' width=50%>
## Type of Services
* Connection-Oriented vs. Connectionless: Whether a connection is setup before sending a message
* Acknowledged vs Unacknowledged: Whether the service provider give the service user an acknowledgement upon delivering the message
## Service Provided to Network Layer
* Principal concern is transferring data from network layer on source host to network layer on destination host
* Services provided:
* Unacknowledged connectionless service
* Source host transmits independent frames to recipient host with no acknowledgement
* No logical connection establishment or release
* No lost frame recovery mechanism
* E.g. Ethernet LANs
* Real-time traffic
* Acknowledged connectionless service
* Source host transmits independent frames to recipient host with acknowledgement
* No logical connection establishment or release
* Each frame individually acknowledged and retransmission if lost or errors
* E.g. Wireless(IEEE 802.11 WiFi)
* Acknowledged connection-oriented service
* Source host transmits independent frames to recipient host after connection establishment and with acknowledgement
* Connection established and released. Communicate rate and details of message
* Frames are numbered, counted, acknowledged with logical order enforced
* Unreliable links such as statellite channel or long distance telephone circuit
## Framing
* Physical layer provides no guarantee a raw stream of bits is error free
* Framing is the method used by data link layer to break raw bit stream into discrete unites and generate a checksum for the unit
* Checksums can be computed and embedded at the source, then computed and compared at the destination. Checksum = f(payload)
* The primary purpose of framing is to provide some level of reliability over the unreliable physical layer
### Framing Methods
* Framing methods:
* Character count
* Use a field in the frame header to specify the number of characters in a frame
> Example of character counts
>> <img src='Character_Counts.png' width=50%>
* Flag bytes with byte stuffing
* Each frame starts and ends with a special byte: flag byte
> Example of flag bytes with byte stuffing
>> <img src='Flag_Byte.png' width=50%>
* Start and end flags with bit stuffing
* Frames contain an arbitrary number of bits and allow character codes
* With an arbitrary number of bits per character
* Each frame begins and ends with a special bit pattern
> Start and end flags with bit stuffing
>> <img src='Start_End.png' width=50%>
* Most data link protocols use a combination of character count and one other method
## Error Control
* Ensuring that a garbled message by the physical layer is not considered as the original message by the receiver by adding check bits
* Error control deals with:
* Detecting the error
* Correcting the error
* Re-transmitting lost frames
* Data link layer deals with bit errors
### Error Detection and Correction
* Physical media may be subject to errors
* Errors may occur randomly or in bursts
* Bursts of errors are easier to detect but harder to resolve
* Resolution needs to occur before handing data to network layer
* Key issues:
* Fast mechanism and low computational overhead
* Detection of different kinds of error
* Minimum amount of extra bits send with the data
### Error Bounds: Hamming distance
* Code turns data of n bits into codewords of n+k
* Hamming distance is the minimum bit flips to turn one valid codeword into any other valid one.
> Example with 4 codewords ob 10 bits (n=2, k=8)
> * 0000000000
> * 0000011111
> * 1111100000
> * 1111111111
> The Hamming distance is 5
* Bounds for a code with distance:
* 2d+1 can correct d errors
* d+1 can detect d errors
### Hamming Codes
* n = 2*k - k - 1 (n is the number of data, k is the check bits)
* Put check bits in positions p that are power of 2, starting with position 1.
> Example of Hamming Codes
> Data: 0101, Requires 3 check bits: n = 4, therefore:
> 4 = (2^3) - 3 - 1
> Example of Hamming Code
>> <img src='Hamming_Code.png' width=50%><file_sep># Week 6 Network Layer
## Flooding
* A non-adaptive algorithm
* Every incoming packet is sent out on every outgoing line except the one on which it arrived
* Generates a large number of duplicate packets, so it is inefficient
* Selective flooding is routers send packets only on links which are in approximately the right direction. This is an improved variation.
> Example of Flooding
>> <img src='Flooding.png' width=50%>
## Distance Vector Routing
* A dynamic algorithm
* Each router maintains a table which includes the best known distance to each destination and which line to use to get there
* Tables are exchanged with neighboring routers
* Global information shared locally
* Algorithm:
* Each node knows distance of links to its neighbors
* Each node advertises vector of lowest known distances to all neighbors
* Each node uses received vectors to update its own
* Repeat periodically
> Example of Distance Vector Routing
>> <img src='Distance_Vector_Routing.png' width=50%>
## Link State Routing
* A dynamic algorithm
* An alternative to distance vector
* DV: primary problem that caused its demise was that the algorithm often took too long to converge after the network topology changed
* Widely used in the Internet
* More computation but simpler dynamics
* Each router has to do 5 steps:
1. Discover neighbors and learn network addresses
2. Measure delay or cost to each neighbors
3. Construct packet resulting from previous steps
4. Send this packet to all other routers
5. Compute the shortest path to every other router
### Building link state packets
* LSP for a node lists neighbors and weights of links to reach them
* The hard part is determining when to build LSP
* Periodically at regular intervals
* Build them when some significant event occurs, such as a line or neighbor going down or coming back up again or changing its properties appreciably
> Example of Link State Packet
>> <img src='LSP.png' width=50%>
## Hierarchical Routing
* As networks grow in size, routing tables expand but this impacts CPU and memory requirements
* Dividing all routers into regions allows efficiencies
* Each router knows everything about other routers in its region but nothing about routers in other regions
* Router which connect to two regions act as exchange points for routing decisions
* Hierarchical routing reduces the work of route computation but may result in slightly longer paths than flat routing
> Example of Hierarchical Routing
>> <img src='Hierarchical_Routing.png' width=50%>
## Broadcast Routing
* Broadcast routing allows hosts to send messages to many or all other hosts
* Single distinct packet
* Inefficient, sources, needs all destination addresses
* Flooding
* Multi-destination routing
* Efficient but source needs to know all the destinations
* A router receives a single packet which encapsulates the list of destinations, and then constructs a specific packet for each one
* Acts as a relay
* Reverse path forwarding
* When a broadcast packet arrives at a router, the router checks to see if the packet arrived on the line normally used for sending packets to use broadcast. If so there is a high probability that the route used to transmit the received packet is the best route. The router then to forwards the packet onto all other lines.
* If the broadcast packet arrived on a link other than the preferred one for reaching the source, the packet is discarded as a likely duplicate.
## Multicast Routing
* A routing algorithm used to send a message oto a well-defined group within the whole network
* Each router computes a spanning tree covering all other routers: The first router to receive the packet prunes the spanning tree to eliminate all lines which do not lead to members of the group
## Addressing
* Routing tables needs addressing to work/route
* IP addresses are used for this purpose
* They are hierarchical
* There is a network portion to an address
* And also a host portion
* The network portion is same for all hosts on a network
* The host portion grabs a continuous block of addresses and is called the prefix
## IP Addresses
* Addresses are allocated in prefixes
* Prefix is determined by a LAN
* IP addresses are written in dotted decimals
* 4 bytes * 4 = 32 bit addresses
* Written as lowest address + length
* E.g. 192.168.127.12/24
* /24 states the length of the network part in bits, so here 8 bits are left for hosts
* Overall IP allocation responsibility of Internet Corporation for Assigned Names and Numbers (ICANN) by delegation to Internet Assigned Numbers Authority (IANA) and Regional Internet Registries (RIR's)
> Example of IP Address
>> <img src='IP_Address.png' width=50%>
## Subnets
* Subnetting allows networks to be split into several parts for internal used whilst acting like a single network for external use
> Example of Subnets
>> <img src='Subnets.png' width=50%>
## IP Addressing and Routing Tables
* Routing tables are typically based around a triplet:
* IP Address
* Subnet Mask
* Outgoing Line (Physical or virtual)
> Example of a routing table
>> <img src='Routing_Table.png' width=50%>
## Aggregation of IP addresses
* Backbone router connection networks around the world
* Aggregation: Process of joining multiple IP prefixes into a single larger prefix to reduce size of routing table
> Example of Aggregation of IP Addresses
>> <img src='Aggregation.png' width=50%>
### Longest Matching Prefix
* Packets are forwarded to the entry with the longest matching prefix or smallest address block
* Complicates forwarding but adds flexibility
* Processes:
1. Check address whether matches the longest prefix
2. If not then see if it matches others
> Example of Longest Matching Prefix
>> <img src='Longest_Matching_Prefix.png' width=50%>
## Classful Addressing
* Part of history now-old addresses came in blocks of fixed size (A, B, C)
* Carries size as part of address, but lacks flexibility
* Called classful addressing
> Class of Addresses
>> <img src='Class_Of_Addressing.png' width=50%>
## Private IP Ranges
* Range of IP addresses that cannot appear in the Internet
* Only for private networks
* 10.0.0.0/8
* 172.16.0.0/12
* 192.168.0.0/17
## Network Address Translation (NAT)
* NAT box maps one external IP address to many internal IP addresses
* Uses TCP/UDP port to tell connections apart
* Violates layering; Very common in homes
> Example of NAT
>> <img src='NAT.png' width=50%>
## Internet Control Protocols
* IP works with the help of several control protocols:
* ICMP is a companion to IP that returns error info
* Required and used in many ways
* ARP finds MAC address of a local IP address
* Glue that is needed to send any IP packets
* Host queries an address and the owner replies
* DHCP assigns a local IP address to a host
* Gets host started by automatically configuring it
* Host sends request to server, which grants a lease
## Mobile IP
* Handle it at data link layer
* For IPv4 the solution is through ICMP
## ICMP
* Internet Control Message Protocol
* Used for testing and monitoring ambient conditions between hosts and routers
>|Message Type|Description|
>|----|----|
>|Destination unreachable|Packet could not be delivered|
>|Time exceeded|Time to live field hit 0|
>|Parameter problem|Invalid header field|
>|Source quench|Choke packet|
>|Redirect|Teach a router about geography|
>|Echo and Echo reply|Check if a machine is alive|
>|Timestamp request/reply|Same as Echo, but with timestamp|
>|Router advertisement/solicitation|Find a nearby router|
## Other Considerations
* Congestion Control Algorithms
* Quality of Service
# Transport Layer
## Transport Layer Function
* Main function: Provide efficient, reliable and cost-effective data transmission service to the processes in the application layer. Independent of physical and data networks
# Transport Layer services
* Transport layer services provide interfaces between the application lay and the network layer
* Transport entities is the hardware or softeware which actually does the work. It can exist in multiple locations:
* OS kernel
* System Library
* But not so much in:
* User process
* Network interface card
* Transport layer adds reliability to the network layer
* Offers connectionless in addition to connection-oriented services to applications
> Relationship between network, transport and application layes:
>> <img src='Relationship_Transport.png' width=50%>
## Transport Layer and Network Layer Service Comparison
* Transport layer code runs entirely on hosts. Network layer code runs almost entirely on routers
* Users have no real control over the network layer. But can improve QoS in transport layer.
* Transport layer fixes reliability problems caused by the network layer
## Position of the Transport Layer
* The transport layer occupies a key position in the layer hierarchy because it clearly delineates
* Providers of data transmissions services are at the network, data link, and physical layers
* Users of reliable data transmission services are at the application layer
* In particular, users commonly access connection-oriented transport services for a reliable service on top of an unreliable network
## Features of a simple transport layer
* Abstraction and primitives provide a simpler API for application developers independent of network layer
> |Primitive|Meaning|
> |----|----|
> |LISTEN|Block waiting for an incoming connection|
> |CONNECT|Establish a connection with a waiting peer|
> |RECEIVE|Block waiting for an incoming message|
> |SEND|Send a message to the peer|
> |DISCONNECT|Terminate a connection|
## Transport Layer Encapsulation
* Abstract representation of messages sent to and from transport entities: Transport Protocol Data Unit (TPDU)
* Encapsulation of TPDUs transport layer unites to network layer units to frames in data layer units
> Example of Transport Layer Encapsulation
>> <img src='Transport_Encapsulation.png' width=50%>
## Transport Services Primitives/Segments
* Primitives that applications might call to transport data for a simple connection-oriented service:
* Server executes LISTEN
* Client executes CONNECT
* Sends CONNECTION REQUEST TPDU to server
* Receives CONNECTION ACCEPTED TPDU to client
* Data exchanged using SEND and RECEIVE
* Either party executes DISCONNECT
> |Primitive|Segment sent|Meaning|
> |----|----|----|
> |LISTEN|(none)|Block until some process tries to connect|
> |CONNECT|CONNECTION REQ|Actively attempt to establish a connection|
> |SEND|DATA|Send Information|
> |RECEIVE|(none)|Block until a DATA packet arrives|
> |DISCONNECT|DISCONNECTION REQ|This side wants to release the connection|
> Illustration of a Simple Connection
>> <img src='Simple_Connection.png' width=50%>
> * Solid lines show client state sequence
> * Dashed lines show server state sequence
> * Transitions in italics are due to segments arrivals
## Elements of Transport Protocols
* Connection establishment
* When networks can lose, store and duplicate packets, connection establishment can be complicated:
* Congested networks may delay acknowledgements
* Incurring repeated multiple transmissions
* Any of which may not arrive at all or out of sequence: Delayed duplicates
* Application degenerate with such congestion
* Reliable Connection Establishment
* Key challenge is to ensure reliability even though packets may be lost, corrupted, delayed and duplicated
* Don't treat an old or duplicate packet as new
* Approach:
* Don't reuse sequence numbers within maximum segment lifetime
* Use a sequence number space large enough that it will nor wrap, even when sending at full rate
* Three-way handshake for establishing connection
### Three Way Handshake
* Three-way handshake used for initial packet
* Since no state from previous connection
* Both hosts contribute fresh sequence numbers
* CR = Connect Request
> Example of Three Way Handshake
>> <img src='Three_Way_Handshake.png' width=50%>
* Connection Release
* Asymmetric Disconnection
* Either party can issue a DISCONNECT, which results in DISCONNECT TPDU and transmission ends in both directions
* Symmetric Disconnection
* Both parties issue DISCONNECT, closing only one direction at a time. Allows flexibility to remain in receive mode.
* Asymmetric release may result in data loss hence symmetric release is more attractive
* Symmetric release works well where each process has a set amount of data to transmit and knows when it has been sent.
<file_sep># Week 10 Security
## Public Key Algorithms
* Fundamentally different to symmetric key ones
* Difff & Hellman proposed the new mode: Asymmetric Keys:
* Two keys are used
* Not easily derivable from each other
* Hence addressing a fundamental issue of key sharing
## Asymmetric Keys
* Key 1, public key: Usable by anyone to encrypt messages to the owner of the key, this key known to all
* Key 2, private key: Required to decrypt the message and know only by the owner of this key
## The process of asymmetric keys
* C = ciphertext, P = plaintext, E = Encryption, D = Decryption, K1, K2 = keys
### RSA Algorithm
* Key generation:
* Choose two large primes, p and q
* Compute n = p * q and z = (p-1) * (q-1)
* Choose d to be relatively prime to z which means no common factors
* Find e such that: (d * e) mod z = 1
* Public key is (e, n) and private key is (d, n)
* Encryption: C = $P^e \ mod \ n$
* Decryption: P = $C^d \ mod \ n$
### RSA Security
* RSA's security is based on the difficulty involved in factoring large numbers in math theory: Approximately $10^25$ years to factor a 500 digit number and RSA uses 1024 bits
* Disadvantage: Too slow for encrypting/decrypting large volumes of data
* RSA is widely used for other things such as secure key distribution
## Digital Signatures
* Cryptographic approaches can also be used to ensure authenticity and allow for non-repudiation
* Requirements:
* Receiver can verify the claimed identity of the sender
* Sender cannot deny the created contents of the message
* Receiver cannot have derived the message themselves
* Approaches:
* Using symmetric keys via an intermediary
* Using public keys as individuals
## Signatures with Message Digests
* Basic concept of a message digest is to use a one-way hash function for an arbitrary length of plaintext, so that it becomes a unique small fixed-length bit string
* Thus no need to deal with huge message text and encryption just for authentication purposes
* A message digest (MD) has four important properties:
1. Given P, it is easy to compute MD(P)
2. Given MD(P), it is effectively impossible to find P
3. Given P, no one can find P' such that MD(P') = MD(P)
4. A change in even a single bit of input produces a very different output
* Famous Message Digest Algorithms:
* MD5
* SHA-1
## Public Key Management
* There is specific PK infrastructure to avoid compromising the security of PK's during the initial distribution process
* Certification Authority (CA): A trust intermediary who uses non-electronic identification to identify users prior to certifying keys and certificates
* X.509: An international standard for certificate expression
* PKI (Public Key Infrastructure): Hierarchically structured certificate authorities allow for the establishment of a chain of trust or certification path
## Authentication
* Authentication is a primary tenet of network security
* Authentication process itself needs to be secure also.
* A fundamental principle: Minimize the use of permanent keys in establishment of secure connections. The less packets are exchanged using such keys, the less exposure to potential attackers
* Four methods in common use:
* Shared keys
* Key distribution
* Kerberos
* Public keys
## Shared Secret Key
> Illustration of Shared Secret Key
>> <img src='Secret_Key.png' width=50%>
## Key Distribution Center
* A trusted intermediary is used to facilitate
* Users each share a key with a central key distribution center, and authenticate to the KDC directly
* The KDC acts as a relay between the two parties
* Issues: Replay-attack
* Solution: Timestamps
## Kerberos
* Similar to KDC, Kerberos is a popluar emerged and in frequent use
* A multi-component system is required:
* Authentication Server
* Ticket Granting Server (TGS)
* Recipient
* Authentication is managed centrally, and then party to party communication is facilitated by single use tickets
* Disadvantages: Does not scale to large numbers. Different businesses need to trust each other's TGSs
## Public Keys Cryptography
> Illustration of public key
>> <img src='Public_Key.png' width=50%>
## IPSec
* Position of security: Should be in multiple layers
* One can put security at application level but also other layers
* IPSec (RFC 2401) puts at the network level as well
* In the IPSec model, encryption is compulsory, but a null encryption algorithm can be used between points
* The main IPSec framework features are secrecy, data integrity, and replay attack protection
* the IPSec framework allows multiple algorithms and multiple levels of granularity, connection-oriented
### Implementation
* IPSec has two main implementation components:
* Things being added to packets in transit
* ISAKMP key management: Internet Security Association and Key Management Protocol for establishing keys
* IPSec has 2 modes
* Transport mode: Uses header insertion after IP header
* Tunnel mode: Uses packet encapsulation
## Virtual Private Networks
* Unlike a physical network based on leased lines between locations for which secure transit is required
* A virtual private network (VPN) is a virtual layer on top of an IP network which provides a secure end-to-end connection over public infrastructure
* A common VPN implementation model:
* Use a firewall at each end of a connection
* Setup a SA to create an IPSec tunnel between the two end points
* Communication on this infrastructure is transparent to end users
> Illustration of VPN
>> <img src='VPN.png' width=50%>
## Firewalls
* While IPSec ensures security in transit, a firewall ensures security at the network perimeter
* Firewalls are positioned at the network boundary, and provide a controlled series of routes between the internal and external networks
* Three characteristics of firewalls:
* All inbound and outbound traffic must transit the firewall
* Only authorized traffic must pass through the firewall
* Firewalls should be immune to penetration themselves
### Firewall scope
* Check packets for bad packets
* Administrators can write rules for this
* Not everything is inside the wall
* Web servers and email servers need to be exposed to allow more open communication
* Best firewall is not disconnection everything from the internet
* Through further rules packets go in-between this gray area and the LAN
* Firewalls do not provide protection against inhouse threats
* Applications can still distribute viruses via bad attachments
## Wireless Security Context
* Wired networks are relatively easy to secure because they require physical access to intercept traffic
* Wireless networks are more difficult to secure because of omnidirectional signal propagation
* Additionally by default most wireless network equipment operates in an insecure and promiscuous manner
* 802.11 has a native secure protocol, Wired Equivalency Protocol, which is a 40-bit encryption based on RC4 algorithm
* Two inherent insecurities:
* 40 bit encryption is breakable with low-moderate computational resources
* RC4 re-uses keys, so capturing a small volume of encrypted traffic will guarantee key identification
* Additional encryption (128 bit WEP)
* Increased security through longer key lengths
* MAC Address Filtering
* Only allow specified MAC interfaces to establish connection
* WPA2
* Multilayered security<file_sep># Week 7 Transport Layer
## Strategies for Connection Release
* Three way handshake
* Finite retry
* Timeouts
* Normal release sequence initiated by transport user on sender host
* DR = Disconnect Request
* Both DRs are ACKed by the other side
> Examples of error cases for Connection Releases
>> <img src='Error_Case.png' width=50%>
## Addressing
* Specification of remote process to connect to is required at application and transport layers
* Addressing in transport layer is typically done using Transport Service Access Points (TSAPs)
* On the Internet, a TSAP in commonly referred to as a port
* Addressing in the network layer is typically done using Network Service Access Points (NSAPs)
* On the Internet, the concept of an NSAP is commonly interpreted as simply an IP address
> Illustration of TSAP and NSAP
>> <img src='TSAP_NSAP.png' width=50%>
### Type of TSAP Allocation
* Static: Well known services have standard allocated TSAPs/ports, which are embedded in OS
* Directory Assistance - Port-mapper:
* A new service must register itself with the portmapper, giving both its services name and TSAP
* Mediated:
* A process server intercepts inbound connections and spawns requested server and attaches inbound connection
## Sockets
* Sockets widely used for interconnections
* Berkeley sockets are predominant in internet applications
* Notion of sockets as transport endpoints
* Like the simple set plus SOCKET, BIND, ACCEPT
>|Primitive|Meaning|
>|----|----|
>|SOCKET|Create a new communication end point|
>|BIND|Associate a local address with a socket|
>|LISTEN|Announce willingness to accept connections; give queue size|
>|ACCEPT|Passively establish an incoming connection|
>|CONNECT|Actively attempt to establish a connection|
>|SEND|Send some data over the connection|
>|RECEIVE|Receive some data from the connection|
>|CLOSE|Release the connection|
## UDP
* User Datagram Protocol
* Does not add much to the Network Layer functionality
* TCP just does the realiability for this layer
* UDP remove connection primitives to use it in a program
* UDP is good for apps like video streaming and gaming regularly
* The reliability issue is left to the application layer. Retransmission decision as well as congestion control
* Provides a protocol whereby applications can transmit encapsulated IP datagrams without a connection establishment
* UDP transmits in segments consisting of an 8-byte header followed by the payload
* UDP headers contain source and destination ports
* Payload is handed to the process which is attached to the particular port at destination
* The main advantage of using UDP over raw IP is the ability to specify ports for source and destination pairs
* Both source and destination ports are required. Destination allows for incoming segments, sources allows reply for outgoing segments
> Example of a UDP header
>> <img src='UDP_Header.png' width=50%>
### Strengths and Weakness of UDP
* Strength: Provides an IP interface with multiplexing/de-multiplexing capabilities and related transmission efficiencies
* Weakness: UDP does not include support for flow control, error control/retransmission of bad segments
* Where applications require a precise level of control over packet flow/error/timing, UDP is good choice as application layer can make choices.
## Remote Procedure Call
* Sending a message and getting a reply back in analogous to making a function all in programming languages
* To call a remote procedure, the client is bound to a small library call the client stub that represents the server procedure in the client's address space
* Similarly the server is bound with a procedure call the server stub
* These stubs hide the fact that the procedure itself is not local
> Illustration of RPC
>> <img src='RPC.png' width=50%>
## Transmission Control Protocol (TCP)
* Provides a protocol by which application can transmit IP datagrams within a connection-oriented framework, thus increasing reliability
* TCP transport entity manages TCP streams and interfaces to the IP layer - can exist in numerous locations (kernel, library, user process)
* TCP entity accepts user data streams, and segments them into pieces < 64KB (Often at a size in order so that the IP and TCP headers can fit into a single Ethernet frame), and sends each piece as a separate IP datagram.
* Recipient TCP entities reconstruct the original byte streams from the encapsulation
## The TCP service Model
* Sender and receiver both create sockets, consisting of the IP address of the host and a port number.
* For TCP Service to be activated, connections must be explicitly established between a socket at a sending host and a socket at a receiving host
* Special one-way server sockets may be used for multiple connections simultaneously
> Example of TCP Service model
>> <img src='TCP.png' width=50%>
### Port Allocations
* Port numbers can range from 0 to 65535
* Port numbers are regulated by IANA
* Ports are classified into segments
* Well Known Ports (0-1023)
* Registered Ports (1024-49151)
* Dynamic Ports (49152-65535)
>|Port|Protocol|Use|
>|----|----|----|
>|20, 21|FTP|File transfer|
>|22|SSH|Remote login, replacement for Telnet|
>|25|SMTP|Email|
>|80|HTTP|World Wide Web|
>|110|POP-3|Remote email access|
>|143|IMAP|Remote email access|
>|443|HTTPS|Secure Web|
>|543|RTSP|Media Player Control|
>|631|IPP|Printer|
### Socket Library: Multiplexing
* Socket library provides a multiplexing tool on top of TSAPs to allow servers to service multiple clients
* It simulate the server using a different port to connect back to the client
> Example of Socket Library
>> <img src='Socket_Library.png' width=50%>
### Features of TCP Connections
* Full duplex: Data in both directions simultaneously
* Point to point: Exact pairs of senders and receivers
* Byte streams, not message streams: Message boundaries are not preserved
* Buffer options: TCP entity can choose to buffer prior to sending or not depending on the context
### TCP entities
* Data sent between TCP entities in segments: a 20 byte header plus zero or more data bytes
* TCP entities decide how large segments should be mainly with 2 constraints:
* 65515 byte IP payload
* Ethernet unit size, generally 1500 bytes
* Sliding window:
* Sender transmits and starts a timer
* Receiver sends back an acknowledgement which is the next sequence number expected. If sender's timer expires before acknowledgement, then the sender transmits the original segment again
### TCP Segment Header
* TCP header includes addressing (ports), sliding window (seq/ack), flow control (window), error control (checksum) and more
> Example of TCP header
>> <img src='TCP_Header.png' width=50%>
> * Source port and destination port: Identify the local end points of the connection
> * Sequence number and Acknowledgement number: Perform their usual functions
> * TCP header length: Tells how many 32-bit words are contained in the TCP header
> * Window size: Tells how many bytes may be sent starting at the byte acknowledged
> * Checksum: Provided for extra reliability. It checksums the header, the data
> * Options: Provides a way to add extra facilities not covered by the regular header
> * URG: Set to 1 if the Urgent pointer is in use. The Urgent pointer is used to indicate a byte offset from the current sequence number at which urgent data are to be found
> * CWR and ECE: Used to signal congestion when ECN (Explicit Congestion Notification) is used
> * ECE: Set to signal an ECN-Echo to a TCP sender to tell it to slow down when the TCP receiver gets a congestion indication from the network
> * CWR: Set to signal Congestion Window Reduced from the TCP sender to the TCP receiver so that it knows the sender has slowed down can stop sending the ECN-Echo
> * ACK: Set to 1 to indicate that the Acknowledgment number is valid. This is the case for nearly all packets. 0 means ignore ACK number field
> * PSH: Indicates PUSHed data. The receiver is hereby kindly requested to deliver the data to application upon arrival and not buffer it until a full buffer has been received
> * RST: Used to abruptly reset a connection that has become confused due to a host crash or some other reason. It is also used to reject an invalid segment or refuse an attempt to open a connection
> * SYN: Used to establish connections. The connection request has SYN=1 and ACK=0. The connection reply does bear an acknowledgement, so it has SYN=1 and ACK=1. The SYn bit is used to denote both CONNECTION REQUEST and CONNECTION ACCEPTED, with the ACK bit used to distinguish between these two possibilities
> * FIN: Used to release a connection. It specifies that the sender has no more data to transmit. However, after closing a connection, the closing process may continue to receive data.
### TCP Connection Establishment and Release
* Connections established using three-way handshake
* Two simultaneous connection attempts reults in only one connection uniquely identified by end points
* Connections released with symmetric release
* Timers used for lost connection release
### TCP Connection Management
* The full TCP connection finite state machine has more states than the simple example from earlier
>|State|Description|
>|----|----|
>|CLOSED|No connection is active or pending|
>|LISTEN|The server is waiting for an incoming call|
>|SYN RCVD|A connection request has arrived; Wait for ACK|
>|SYN SENT|The application has started to open a connection|
>|ESTABLISHED|The normal data transfer state|
>|FIN WAIT 1|The application has said it is finished|
>|FIN WAIT 2|The other side has agreed to release|
>|TIME WAIT|Wait for all packets to die off|
>|CLOSING|Both side have tried to close simultaneously|
>|CLOSE WAIT|The other side has initiated a release|
>|LAST ACK|Wait for all packets to die off|
### TCP Transmission Policy
* TCP acknowledges bytes
* Receiver advertises window based on available buffer space
> Example of TCP Transmission
>> <img src='TCP_Transmission_Policy.png' width=50%>
## Congestion
* Congestion results when too much traffic is offered; Performance degrades due to loss/retransmissions
> Illustration of Congestion
>> <img src='Congestion.png' width=50%>
### Congestion Control vs. Flow Control
* Flow control is an issue for point to point traffic, primarily concerned with preventing sender transmitting data faster than receiver can receive it
* Congestion control is an issue affecting the ability of the subnet to actually carry the available traffic in a global context
## Load Shedding
* When congestion control mechanisms fail, load shedding is the key remaining possibility.
* In order to ameliorate impact, applications can mark certain packets as priority to avoid discard policy
## Key problem if network is not delivering properly
* Quality of Service becomes low
* Expected network performance is an important criterion for a wide range of network applications
* Some engineering techniques are available to guarantee QoS
* Four things to watch out for: Bandwidth, reliability, delay, jitter
## Jitter
* Jitter is the variation in packet arrival times
> Example of High Jitter
>> <img src='High_Jitter.png' width=50%>
> Example of Low Jitter
>> <img src='Low_Jitter.png' width=50%>
* Jitter is an issue for some applications
* Jitter can be contained by determined the expected transit time of a packet
* Packets can be shuffled at each hop in order to minimize jitter: Slower packets sent first, faster packets wait in a queue.
* For certain applications jitter control is extremely important as it mainly directly affects the quality perceived by the application user
## QoS Requirements
* Different applications care about different properties
>|Application|Bandwidth|Delay|Jitter|Loss|
>|----|----|----|----|----|----|
>|Email|Low|Low|Low|Medium|
>|File Sharing|High|Low|Low|Medium|
>|Web Access|Medium|Medium|Low|Medium|
>|Remote Login|Low|Medium|Medium|Medium|
>|Audio on demand|Low|Low|High|Low|
>|Video on demand|High|Low|High|Low|
>|Telephony|Low|High|High|Low|
>|Videoconferencing|High|High|High|Low|
## Techniques for Achieving QoS
* Over-provisioning: More tha adequate buffer, router CPU, and bandwidth
* Buffering: Buffer received flows before delivery: Increase delay, but smoothes out jitter, no effect in reliability or bandwidth
* Traffic Shaping: Regulate the average rate of transmission and burstiness of transmission
* Leaky Bucket
* Token Bucket
* Resource reservation: Reserve bandwidth, buffer space, CPU in advance
* Admission Control: Routers can decide based on traffic patterns whether to accept new flows, or reject/reroute them
* Proportional routing: Traffic for same destination split across multiple routes
* Packet scheduling: Create queues based on priority.
* Fair queuing, weighted fair queuing
### Leaky Bucket
* Large bursts of traffic is buffer and smoothed while sending
> Illustration of Leaky Bucket
>> <img src='Leaky_Bucket.png' width=50%>
## TCP Congestion Control
* When networks are overloaded, congestion occurs, potentially affecting all layers. Although lower layers attempt to ameliorate congestion, in reality TCP impacts congestion most significantly because TCP offers best methods to reduce the data rate, and hence reduce congestion
* TCP adopts a defensive stance:
* At connection establishment, a suitable window size is chosen by the receiver based on its buffer size
* If the sender is constrained to this size, then congestion problems will not occur due to overflow at the receiver itself, but may still occur due to congestion within the network
### Design of Congestion Control
* Two different problems exist
* Network capacity and receiver capacity
* These should be dealt with separately, but compatibly
* Sender maintains two windows:
* Window described by the receiver
* Congestion window
* Each regulates the number of bytes the sender can transmit. The maximum transmission rate is the minimum of the two windows
## Slow Start
* On connection establishment, the sender initializes the congestion window to a size, and transmits one segment
* If this segment is acknowledged before the timer expires, the sender adds another segment's worth of bytes to the congestion window, and transmits two segments
* As each new segment is acknowledged, the congestion window is increased by one more segment
* In effect, each set of acknowledgements doubles the congestion window which grows until either a timeout occurs or the receiver's specified window is reached.
> Illustration of Slow Start
>> <img src='Slow_Start.png' width=50%>
### Additive Increase
> Illustration of Additive Increase
>> <img src='Additive_Increase.png' width=50%>
### TCP Tahoe
* Slow start followed by additive increase.
* Threshold is half of previous
> Illustration of TCP Tahoe
>> <img src='TCP_Tahoe.png' width=50%>
### TCP Reno
> Illustration of TCP Reno
>> <img src='TCP_Reno.png' width=50%>
## Congestion Control and Wireless
* Much harder to deal with:
* Things are increasingly wireless
* Not everything is wireless, but parts of a path
* More variety on wireless links as well
* SNR varies when people more
* Delay is different if it is WiFi
## TCP Timer
* A key worry is when timers go out
* Too early means too many resends
* Too late means reliability comes with more additional cost
* Solutions rely on dynamicity as network conditions change
* One needs to measure network performance and adapt timers<file_sep># Week 9 Application Layer
## Email
* User Agent: Thunderbird
* Message Transfer Agent: Exchange
* Message Transfer Protocol
## Email Services
* Standards for Internet-enabled email are based on 2 RFC's
* RFC 821 (transmission)
* RFC 822 (message format)
* RFC 2821 and RFC 2822 (revised versions of earlier RFCs)
## Architecture and Services of Email
* User agents: Allow user to read and send mail
* Message transfer agents: Transport messages from source to destination
* Simple Mail Transfer Protocol (SMTP): Used to send messages from the sender
* Mail server to the receiver's mail server
* User agent to the sender's mail server
> Architecture of Email Service
>> <img src='Email_Architecture.png' width=50%>
## User agent
* Basic functions: compose, report, display and dispose
* Envelope and contents: encapsulation of transport related information
* Envelope: Destination address, priority, and security level, all of which are distinct from the message itself
* Mail servers use the envelope for routing
* Header and body:
* Header: User agent control info
* Body: For human recipient
* Contains contains control information for the user agents
* User must provide message, destination, optional other parameters
## RFC 822: Message
* RFC 822 doesn't distinguish header and envelope fields
* RFC 822 allows users to invent new headers for private use but they must start with X-
## Multipurpose Internet Mail Extensions (MIME)
* RFC822 was enough for these simple constraints
* In time the inadequacy of RFC822 became apparent
* Languages with accents
* Non-Latin alphabets
* Non-alphabetic language
* Messages with content other than text
* As a result, MIME (RFC 1341) was written
* MIME retains RFC822 format but adds structural elements to the message body and defines encoding rules for non-ASCII messages
* MIME has 5 additional message headers:
* MIME-Version: Identifies the MIME version
* Content-Description: Human readable describing contents
* Content-Id: Unique Identifier
* Content-Transfer-Encoding: How body is wrapped for transmission
* Content-Type: Type and format of content
### MIME Types and Subtypes
|Type|Example subtypes|Description|
|----|----|----|
|text|plain, html, xml, css|Text in various formats|
|image|gif, jpeg, tiff|Pictures|
|audio|basic, mpeg, mp4|Sounds|
|video|mpeg, mp4, quicktime|Movies|
|model|vrml|3D model|
|application|octet-stream, pdf, javascript, zip|Data produced by applications|
|message|http, rfc822|Encapsulated message|
|multipart|mixed, alternative, parallel, digest|Combination of multiple types|
## Message Transfer
* Transfer: SMTP (Simple Message Transfer Protocol)
* Delivery:
* POP3 (Post Office Protocol 3): Download to a single device
* IMAP (Internet Message Access Protocol): Designed with multiple devices in mind
## SMTP
* Simple Message Transfer Protocol
* Simple ASCII protocol, operating on TCP port 25
* RFC 821: Simple Mail Transfer Protocol
* RFC 2821: Extended Simple Mail Transfer Protocol
* Basic steps:
* User agent submits to mail transfer agent on port 587
* One mail transfer agent to the next one on port 25
* Other protocols used for final delivery (IMAP, POP3)
## IMAP
* Used for final delivery
* Internet Message Access Protocol
* RFC 3501 defines version 4
* User agent runs an IMAP client for this
* Protocol has command like: Login, List, Copy, Create, Delete
* Main difference with POP3 is mail remains on server
* Complex protocol but makes mail machine independent
## POP3
* Email is taken into the user computer
* Simpler protocol
* Ties emails to one's machine
## Webmail
* Gmail and alike
* A service run by a company server
* An interface to managing email over the Web
* Mainly it is an user interface
## Spam
* Unwanted email
* Main countermeasures are:
* Filters based on email content
* Blacklisting known spam addresses
* Parking email from unknown sources
* Collection spam and creating a knowledge-base
* Detecting mass emails
# Other Layers
## Two more layers from the OSI model
* Presentation layer
* Session layer
* They did not see a distinct use and labeling
## Presentation Layer
* Formatting related issues
* Encryption and Decryption
* Should be done in presentation layer as well although it is commonly done at application layer
* Compression
* It should be done at this layer rather than in application layer
### Why not have an explicit presentation layer
* Internet does not have this clear distinction
* A key reason is many presentation layer list of things to do that is considered to be application specific. Thus, application layer and presentation layer is not explicitly separated for internet
## Session layer
* Common service of this layer:
* Authentication
* Session management
* Monitoring connections
* Disconnect if not used
* Reconnect if needed
* These are also seen as a part of the application layer duties today depending on different requirements of applications of today's Internet
* A few are done at Transport layer
* Especially session management in a simple client server architecture is trivial
## Existence of these layers
* If you have need to do compression, session management, then think before implementing
* You should probably create a better software design by creating your own little session layer as a separate layer in your software architecture
* This is what most software architects do: Create a middleware layer in their software
## P2P systems
* Client server systems dominated the Internet for a while because they are simple to implement
* Peer-to-Peer systems (P2P) are more complex:
* A P2P system:
* Does not have client and server but just peers
* Does not have a central point of control
* Advantages:
* No central point of control or failure
* Potential to scale without a bottleneck
* Disadvantages:
* Harder to develop applications on a dynamic platform where PCs come and go
* Not all peers can know about all others in a large system.
> Example P2P system
>> <img src='P2P.png' width=50%>
> * The black dots depict live nodes. The blue ones are files. The address space is considered as circular: node 0 is adjacent to node $(2^{128}-1)$. The diagram illustrates the routing from node 65A1FC to D46A1C. This happens at application layer at each PC
# Network Security
## What is network security
* Network security is a combo of 4 related areas:
* Secrecy: Keep information hidden from a general audience
* Authentication: Ensuring the user you are giving content to has valid credentials
* Non-repudiation: Prove a content was created by a named user
* Integrity control: Ensure that a content has not been tampered with
* All of those are equally valid and has been around for all systems for some time, but have different and sometimes more challenging implications in a networked environment
* Aspects of security can be found at all layers of a protocol stack, there is no way to secure a network by building security into one layer only
* Most security implementations are based on common cryptographic principles and appear on almost all layers
## Cryptography
* A key area/set of algorithms for creating secrets, authenticating users, making sure messages are not tampered with, and edits are not denied by the original author
## Encryption
> Encryption Model
>> <img src='Encryption_Model.png' width=50%>
### Key Cryptography Concepts
* Three foundations:
* Plaintext: Messages to be encrypted can be transformed by a function.
* Key: A function that parameterized the transformation
* Ciphertext: The output of the transformation process
* Kerckhoff's principle: Cryptographic algorithms and related functions (E, D) are public; Only the keys (K) are secret
### The notations
* C = Ciphertext
* P = plaintext
* E = Encryption
* D = Decryption
* K = Key
### Keys
* A key is a string that allows the selection of one of many potential encryptions
* The key can be changed as often as required
* Algorithms are more likely to be at the hands of attackers anyhow, not changed as frequently
* Cipher is a term commonly used as the term for algorithm
* The size of the overall key space is determined by the number of bits in the key string
* The longer the key, the more effort is required to break a given encryption
### XOR
* An XOR is an exclusive or function used regularly
* A XOR B means A or B, but not both
* XOR is commonly used in cryptography
> Exclusive ORs
>> <img src='XOR.png' width=50%>
## Main Types of Ciphers
* Substitution cipher: Each letter of group of letters is replaced systematically by other letters or groups of letters
* Transposition cipher: All letters are re-ordered without disguising them
* One-time pad:
* Uses a random bit string as the key: convert the plaintext into a bit string, then XOR the two strings bit by bit
* Harder to break
# Key-based Algorithms
* Symmetric key algorithms: Use the same key for both encryption and decryption. Symmetric key algorithms can use permutation, substitution and a combination of both to encrypt and decrypt
* 2 Symmetric Key Algorithms:
* Data Encryption Standard (DES)
* Uses 64 bit blocks and 56 bit keys
* $2^{56}$ key space
* Triple DES has a $3*2^{56}$ key space
* Advance Encryption Standard(AES)
* Uses 128 bit blocks and 128 bit keys
* $2^{128}$ key space
* Still substitution and permutation based with multiple rounds
## Cipher Block Chaining Mode
* Same text leads to same ciphertext unless something else is done
* In block chaining mode, each plaintext block is XOR with the previous ciphertext block being encrypted
> Example of CBC
>> <img src='CBC.png' width=50%>
## Cipher Feedback Mode
* In cipher feedback mode, byte-by-byte encryption is used rather than block-by-block encryption
* Good for things like encryption someones key strokes on a keyboard where a lot of data is not immediately available
## Stream Cipher Mode
* In stream cipher mode, recursive sequential block encryption is sued has one-time pad, and XOR with plaintext to generate ciphertext
> Illustration of Stream Cipher Mode
>> <img src='Stream_Cipher.png' width=50%>
## Counter mode
* In counter mode, plaintext is not directly encrypted, but an initialization parameter plus an arbitrary constant is encrypted and the resulting ciphertext is XOR with plaintext
> Illustration of Counter Mode
>> <img src='Counter_Mode.png' width=50%>
## Symmetric Key Algorithms
|Cipher|Author|Key Length|Comments|
|----|----|----|----|
|Blowfish|B<NAME>|1-448 bits|Old and Slow|
|DES|IBM|56 bits|Too weak to use now|
|IDEA|Massey and Xuejia|128 bits|Good, but patented|
|RC4|<NAME>|1-2048 bits|Some keys are weak|
|RC5|<NAME>|128-256 bits|Good, but patented|
|Rijndael|Daemen and Rijmen|128-256 bits|Best choice|
|Serpent|Anderson, Biham, Knudsem|128-256 bits|Very strong|
|Triple DES|IBM|168 bits|Second best choice|
|Twofish|<NAME>|128-256 bits|Very strongly; Widely used|<file_sep># Week 5 MAC Sub-Layer
## CSMA with Collision Detection
* Principle that transmission aborted when collision detected:
* After collision detected, abort, wait random period, try again
* Channel must be continually monitored
* Used in half-duplex system
## Classic Ethernet Minimum Packet Size
* Collisions can occur and take as long as 2 $\tau$ to detect
* $\tau$ is the time it takes to propagate over the ethernet
* Leads to minimum packet size for reliable detection
> Illustration of the collision detecting time
>> <img src='Collision_Time.png' width=50%>
## Collision Free Protocols
### Bit Map Protocol
* Reservation-based protocol
* 1 bit per station overhead
* Division of transmission right, and transmission event. No collisions as this is a reservation based protocol
> Example of Bit Map Protocol
>> <img src='Bit_Map_Protocol.png' width=50%>
### Binary Countdown Protocol
* Avoid the 1 bit per station scalability problem by using binary station addressing
* No collisions as higher-order bit positions are used to arbitrate between stations wanting to transmit
* Higher numbered stations have a higher priority
* Process:
* Stations send their address in contention slot (log N bits instead of N bits)
* Channel medium ORs bits, stations give up when they send a 0 but see a 1
* Station that ses its full address in next to send
> Example of Binary Countdown Protocol
>> <img src='Binary_Countdown.png' width=50%>
## Limited Contention Protocols
* Both contention and collision free become inefficient at different points
* Under low loads, collision free is less attractive because of a higher delay between transmissions
* Under higher loads, contention is less attractive because overhead associated with channel arbitration becomes greater
* Limited content protocols increase the probability of stations acquiring transmission rights by arbitrarily dividing stations and using a binary algorithm to determine rights allocation
### Adaptive Tree Walk Protocol
* All stations compete for right to transmit, if a collision occurs, binary division is used to resolve contention
* Tree divides stations into groups of nodes to pull
* Depth First Search under nodes will poll collisions
* Start search at lower levels if more than one station expected
> Example of Adaptive Tree Walk Protocol
>> <img src='Adaptive_Tree_Walk.png' width=50%>
### Wireless LAN Protocols
* Wireless Complications: When a station is in the range of two transmitters or relays, interference affects signal reception
* This leads to hidden and exposed terminal problems
* Require detection of transmissions to receiver, not just carrier sensing
* Transmission Protocols for Wireless LANs (802.11)
* Multiple Access with Collision Avoidance for Wireless (MACAW)
### Hidden and Exposed Terminals
* Hidden terminals are senders that cannot sense each other but nonetheless collide at intended receiver
* Want to prevent but will leads to loss efficiency
> Illustration of Hidden Terminals
>> <img src='Hidden_Terminals.png' width=50%>
> A and C are hidden terminals when sending to B
* Exposed terminals are senders who can sense each other but still transmit safely to different receivers
> Illustration of Exposed Terminals
>> <img src='Exposed_Terminals.png' width=50%>
> B -> A and C -> D are exposed terminals
### Multiple Access with Collision Avoidance (MACA)
* Sender asks receiver to transmit short control frame
* Stations near receiver hear control frame
* Sender can then transmit data to receiver
> Illustration of MACA
>> <img src='MACA.png' width=50%>
> MACA protocol grants access for A to send to B
> * A sends RTS to B; B replies with CTS
> * A can send with exposed but no hiddent terminals
## Classic Ethernet
* Each type of Ethernet has a maximum cable length per segment
* Multiple cable lengths can be connected by repeaters - a physical device which receives, amplifies and retransmits signals in both directions
> Construction of a classic Ethernet
>> <img src='Classic_Ethernet.png' width=50%>
## Ethernet Frame Format
* MAC protocol is 1-persistent CSMA/CD
* Random delay after collision is computed with BEB (Binary Exponential Backoff)
* Frame format is still used in modern Ethernet
> Example of Ethernet Frame Format
>> <img src='Ethernet_Frame_Format.png' width=50%>
> * Preamble (7B): Synchronization between sender and receiver
> * Start of Frame (1B): FLAG bytes
> * Destinations and Source Addresses: Identify who send, who receive
> * Type and Length (2B): Specifies which process to give the frame to
> * Pad (0-46B): Minimum size of the message of the Ethernet
> * CRC (4B): 32 bits checksum
## MAC Addressing
* Sources and destination addressing can be done at a local or global levels
* The MAC Address provides the unique identifier for a physical interface
* MAC Address is a 48-bit number encoded in the frame
* E.g. 00:02:2D:66:7C:2C
## Ethernet Performance
$$ Channel \ Efficiency = \frac{1}{1+\frac{2BLe}{cF}}$$
* F: Frame Length
* B: Band Width
* L: Cable Length
* c: Speed of Light
* Optimal case of e contention slow per frame
* When cF is large, the channel efficiency will be high
* Increasing network bandwidth or distance reduces the efficiency for a given frame size
## Switched Ethernet
* Hubs wire all lines into a single CSMA/CD domain
* Switches isolate each port to a separate domain
* Much greater throughput for multiple ports
* No need for CSMA/CD with full-duplex lines
> Illustration of Hubs and Switches
>> <img src='Hubs_and_Switches.png' width=50%>
# Network Layer
## Principles of Internet Design
1. Make sure it works
2. Keep it simple
3. Make clear choices
4. Exploit modularity
5. Expect heterogeneity
6. Avoid static options and parameters
7. Choose a good, but not necessarily perfect design
8. Be strict in sending and tolerant in receiving
9. Consider scalability
10. Consider performance vs costs
## Network Layer in the Internet
* Internet is an interconnected collection of many networks of Autonomous systems that is held together by the IP protocol
## Internet Protocol (IP)
* The glue that holds the whole Internet together in the Network layer protocol, IP
* Provides a best-effort service to route datagrams from source host to destination host
* These hosts may be on the same network or different networks
* Each network is called an Autonomous System(AS)
## Service Provided to the Transport Layer
* Design goals:
* Services should be independent of router technologies
* Transport layer should be shielded from number, type, and topology of routers
* Network addressing should use a uniform numbering plan
## Store and Forward Packet Switching
* Hosts generate packets and injects into the network
* Routers treat packets as messages, receiving them and then forwarding them based on how the message is addressed
* Router routes packets through the network
> Example of packets routing with datagram subnet
>> <img src='Packet_Routing.png' width=50%>
## Routing within a datagram subnet
* Post office model: Packets are routed individually based on destination addresses in them. Packets can take different paths
* Routing table can be fixed or change over time
* Routing algorithm manages the routing table
> Example of P1 sends a long message to P2
>> <img src='Routing_Datagram.png' width=50%>
## Routing with virtual-circuit subnet
* Model is like a telephone network
* Packets are routed through virtual circuits based on tag number (not full address but unique at a given link) in them.
* Packets take the same path to avoid having to choose a new route for every packet sent
* E.g. Multi-protocol Label Switching Network
> Example of routing with virtual-circuit subnet
>> <img src='Routing_Virtual_Circuit.png' width=50%>
## Difference in Virtual Circuit and Datagram Subnets
|Issue|Datagram Network|Virtual-circuit Network|
|----|----|----|
|Circuit Setup|Not needed|Required|
|Addressing|Each packet contains the full source and destination address|Each packet contains a short VC number|
|State Information|Routers do not hold state information about connections|Each VC requires router table space per connection|
|Effect of router failures|Each packet is routed independently|Route chosen when VC is set up; All packets follow it|
|Quality of Service|Difficult|Easy if enough resources can be allocated in advance for each VC|
|Congestion control|Difficult|Easy if enough resources can be allocated in advance for each VC|
## Compromises of VC and Datagram Subnets
* Memories vs Bandwidth
* VC require more space in router memory but save potential overhead in full addressing of each packet and computation of path
* Setup time vs Address Parsing Time
* VC require setup time and resources, but packet transmission is very fast
* Amount of memory
* Datagram subnets require large tables of every possible destination routes, whereas VC requires entry per link
* QoS and Congestion avoidance
* VC can use tighter QoS to be able to reserve CPU, bandwidth, and buffer in advance
* Longevity
* VC can exist for a long time
* Vulnerability
* VC particularly vulnerable to hardware/software crashes - all VC aborted and no traffic until they are rebuilt. Datagram uses an alternative route
## Issue of Internetworking
* Internetworking joins multiple, different networks into a single larger network
* Issues when connecting networks:
* Different network types and protocols
* Different motivations for network choices
* Different technologies at both hardware and software levels
## Different Dealt at the Network Layer
|Item|Some Possibilities|
|----|----|
|Service Offered|Connectionless vs connection oriented|
|Addressing|Different sizes, flat or hierarchical|
|Broadcasting|Present or absent|
|Packet size|Every network has its own maximum|
|Ordering|Ordered and unordered delivery|
|Quality of service|Present or absent|
|Reliability|Different levels of loss|
|Security|Privacy rules, encryption|
|Parameters|Different timeouts, flow specifications|
|Accounting|By connect time, packet, byte or not at all|
## Tunneling
* Tunneling is a special case used when the source and destination are on the same network, but there is a different network in between
* Source packets are encapsulated over the packets in the connecting network
> Example of Tunneling IPv6 packets through IPv4
>> <img src='Tunneling.png' width=50% >
## Fragmentation
* All networks have a maximum size for packets, could be motivated by:
* Hardware
* Operating system
* Protocols
* Standards compliance
* Desire to reduce transmissions due to errors
* Desire for efficiency in communication channel
* Fragmentation is the division of packets into fragments. It allows network gateways to meet size constraints
## Types of Fragmentation
* Transparent: Packets fragmented and reassembled in each network.
* Route constrained, need more work
> Example of transparent fragmentation
>> <img src='Transparent.png' width=50%>
* Non-transparent: Fragments are reassembled at destination
* Less work: packet number, byte offset, end of packet flag
> Example of non-transparent fragmentation
>> <img src='Non-transparent.png' width=50%>
> Example of IP style fragmentation
>> <img src='IP_Style.png' width=50%>
## Path MTU Discovery
* An alternative to fragmentation
* Advantage: The source now knows what length packet to send but if the routes and path MTU change, new error packets will be triggered and the source will adapt to the new path.
> Example of Path MTU Discovery
>> <img src='MTU.png' width=50%>
## IP Datagram
* IPv4 header is carried on all packets and has fields for the key parts of the protocol
> Example of IPv4 Datagram header
>> <img src='IPv4_Datagram.png' width=50%>
* IPv4 datagram consists of a header and some text
* Header is 20 byte fixed part + variable length optional part
* Version: IPv4 or IPv6
* IHL: Header Length, in 32bits units, min 5 and max 15
* Type: Differentiates different classes of service
* Total Length: Header and payload, maximum length 65535 bytes
* Identification: Allows host to determine which datagram the new fragment belongs to - all fragments of same datagram have same ID
* DF: Don't Fragment Byte
* MF: More Fragment Byte, determine whether it is the last one or not
* Fragment offset: Where in the datagram the current fragment belongs
* TTL: Limits packet lifetimes, hops or seconds
* Protocol: TCP, UDP, or others
* Header Checksum: verifies the header only
* Source Address: IP, host/network
* Destination Address: IP, host/network
* Options: Security, strict vs loose source routing, record route, timestamp
## Routing Algorithms
* Consider the network as a graph of nodes and links:
* Decide what to optimize
* Update routes for changes in topology
* Routing is the process of discovering network path
* The routing algorithm is responsible for deciding on which output line an incoming packet should be transmitted
* Non-adaptive Algorithms
* Static decision making process
* Adaptive Algorithms
* Dynamic decision making process
* Changes in network topology, traffic
## Sink Tree/Spanning Tree
* Sink Tree/Spanning Tree: The set of optimal routes from all sources to a given destination form a tree rooted at the destination
* The goal of a routing algorithm is to discover and utilize the spanning trees for all routers
> Example of a spanning for a graph of routers
>> <img src='Spanning_Tree.png' width=50%>
## Shortest Path Routing
* A non-adaptive algorithm
* Shortest path can be determined by building a graph with each node representing a router, and each edge representing a communication link
* To choose a path between 2 routers, the algorithm finds the shortest path between them on the graph
## Dijkstra Algorithm
* Dijkstra algorithm computes a spanning tree on the graph:
* Each link is assigned a non-negative weight/distance
* Shortest path is the one with lowest total weight
* Using weights of 1 gives paths with fewest hops
* Algorithm:
* Start with sink, set distance at other nodes to infinity
* Relax distance to other nodes
* Pick the lowest distance node, add it to the spanning tree
* Repeat until all nodes are in the spanning tree
> Example to Dijkstra Algorithm
>> <img src='Dijkstra.png' width=50%><file_sep>* [Introduction to Networking](#Intro)
* [Physical Layer](#Physical)
* [Data Link Layer](#Data)
* [MAC Sub Layer](#MAC)
* [Network Layer](#Network)
* [Transport Layer](#Transport)
* [Application Layer](#Application)
* [Security](#Security)
<h1 id='Intro'>Introduction to Networking</h1>
## Terminologies
* A <font color=red>network device</font>: E.g. <font color=pink>PC, Router, Switch</font>
* <font color=red>Server</font>: Provider of a service. Accept requests from clients.
* <font color=red>Client</font>: A network device connecting to a server and requesting a service.
* <font color=red>Computer Network</font>: A collection of autonomous computers interconnected by a single technology
* <font color=red>Packet</font>: A message send between two network devices.
* <font color=red>IP Address</font>: A unique number identifying a network device.
## Network vs Computer Network
* Network (Noun):
* An intricately connected system of things or people
* An interconnected or intersecting configuration or system of components.
* Computer Network:
* A data network with computers at one or more of nodes
* A collection of autonomous computers interconnected by a single technology
### Internet and World Wide Web
* Neither the Internet nor the World Wide Web is a computer network
* The Internet is not a single network but a network of networks
* The World Wide Web is a distributed system that runs on top of the Internet
## Computer networks
* Usage
* Business Applications:
* Resources sharing
* Home Applications:
* Access to remote information
* Interactive entertainment
* E-commerce
* Mobile Users
* Mobility
* Internet-of-things <font color=pink>(E.g. parking, smart-center, vending machines)</font>
* Social Interactions
* Origins: Simple Client-Server Networking
* A network with two clients and one server
> Example of Client and Server Model
>> <img src='images/Client_and_Server.png' width=50%>
## Differentiating Factors of Networks
* Types of transmission technology
* <font color=red>Broadcast link</font>
* Broadcast networks have a single communication channel shared bu all machines on a network. Packets sent by any machine are received by all others, an address field in the packet specifies the intended recipient. Intended recipients process the packet contents others simply ignore it.
* Broadcasting is a mode of operation which allows a packet to be transmitted that every machines in the network must process
* <font color=red>Point-to-point Links</font>
* Data from sender machine is not seen and process by other machines
* Point to point networks consist of connections between individual pairs of machines. Packets travelling from source to destination must visit intermediate machines to determine a route or multiple routes of variant efficiencies are available and optimization is an important principle
* Unicasting is the term where point-to-point networks with a single sender and receiver pair can exchange data
* <font color=red>Multicasting</font>
* Transmission to a subset of the machines
### Differentiating by Scale
> |Interprocessor Distance|Processors located in same|Example|
>|----|----|----|----|
>|1m|Square Meter|Personal Area Network|
>|10m|Room|Local Area Network|
>|100m|Building|Local Area Network|
>|1km|Campus|Local Area Network|
>|10km|City|Metropolitan Area Network|
>|100km|Country|Wide Area Network|
>|1000km|Continent|Wide Area Network|
>|10000km|Planet|The Internet|
### Mixing taxonomies
* Local Area Networks can further be distinguished by three factors:
* Size
* Transmission Technology: <font color=pink>Physically wired network</font>
* Topology:
* <font color=red>Bus</font>: Only a single machine on the network can transmit at any point in time requires a negotiation mechanism to resolve transmission conflicts: <font color=pink>Ethernet is the most common bus network</font>
> Example of Bus network structure:
>> <img src='images/Bus.png' width=50%>
* <font color=red>Ring</font>: Each transmission bit is propagated individually and requires access control to resolve propagation queuing. <font color=pink>E.g. Token Ring</font>
> Example of Ring network structure:
>> <img src='images/Ring.png' width=50%>
## Components of the Internet
* <font color=red>Protocols, Layers, and Services</font>
* Protocol Hierarchies
* Design of Layer Models
* Connection-Oriented and Connectionless Service Types
* Service Primitives
* Service and Protocols
* <font color=red>Network Reference Models</font>
* Open Systems Interconnect
* TCP/IP
* <font color=red>Network Standards</font>
## Protocol Hierarchies
* Consider the network as a stack of layers
* Each layer offers services to layer above it
* Inter-layer exchanges are conducted according to a protocol
> Example of a protocol hierarchies
>> <img src='images/Protocol_Hierarchies.png' width=50%>
> Example of information flow supporting virtual communication in Layer 5
>> <img src='images/Layer5.png' width=50%>
## Design Issues for the Layers
* <font color=red>Connection Oriented</font>: Connect -> Use -> Disconnect <font color=pink>(similar to telephone service)</font>
* Negotiation inherent in connection setup
* <font color=red>Connectionless</font>: Just sent <font color=pink>(similar to postal service)</font>
* Choice of service type has corresponding impact on the reliability and quality of the service itself.
## Connection-Oriented and Connectionless services
* Six different types of services:
>|Type|Service|Example|
>|----|----|----|
>|Connection-Oriented|Reliable Message Stream|Sequence of pages|
>|Connection-Oriented|Reliable byte stream|Remote login|
>|Connection-Oriented|Unreliable connection|Digitized voice|
>|Connectionless|Unreliable datagram|Electronic junk mail|
>|Connectionless|Acknowledge datagram|Registered mail|
>|Connectionless|Request-reply|Database query|
## Service Primitives
* Primitives are formal set of operations for services
* The number and type of primitives in any particular context is dependent on nature of service itself - in general more complex services require more primitive service
* Six service primitive for implementing a simple connection-oriented service:
>|Primitive|Meaning|
>|----|----|
>|<font color=pink>LISTEN</font>|Block waiting for an incoming connection|
>|<font color=pink>CONNECT</font>|Establish a connection with a waiting peer|
>|<font color=pink>ACCEPT</font>|Accept an incoming connection from a peer|
>|<font color=pink>RECEIVE</font>|Block waiting for an incoming message|
>|<font color=pink>SEND</font>|Send a message to the peer|
>|<font color=pink>DISCONNECT</font>|Terminate a connection|
## Relationship of Services and Protocols
* Service is a set of primitives that a layer provides to a layer above it
* Defines what operations the layer is prepared to perform on behalf of its users
* It says nothing about how these operations are implemented
* Interfaces between layers
* Protocol is a set of rules governing the format and meaning of packets that are exchanged by peers within a layer
* Packets sent between peer entities
> The relationship between Services and Protocol:
>> <img src='images/Service_Protocols.png' width=50%>
## Reference Models
* Example of reference model
* The <font color=red> OSI</font> Reference Model
* The <font color=red> TCP/IP</font> Reference Model
* A reference model provides a common baseline for the development of many services and protocols by independent parties
* Since networks are very complex systems, a reference model can serve to simplify the design process
* It's engineering best practice to have an abstract reference model, and corresponding implementations are always required for validation purposes.
## OSI Reference Model
* Open Systems Interconnection
* Has 7 layers
* Layer divisions based on principled decisions
### OSI Layer Division Principles
1. A layer should be created where a different abstraction is needed
2. Each layer should perform a well defined function
3. The function of each layer should be chosen with a view toward defining internationally standardized protocols
4. The layer boundaries should be chosen to minimize the information flow across the interfaces
5. The number of layers should be large enough that distinct functions need not to be thrown together in the same layer out of necessity, and small enough that the architecture does not become unwieldy
> Structure of OSI Model
>> <img src='images/OSI_Model.png' width=50%>
## TCP/IP Model
* Transmission Control Protocol/Internet Protocol
* Has 4 layers
> Structure of TCP/IP Model
>> <img src='images/TCP/IP_Model.png' width=50%>
## Comparison of OSI and TCP/IP Models
### Critique of OSI Model
* Bad Timing
* Bad Technology
* Bad implementations
* Bad politics
### Critique of TCP/IP Model
* Service, interface and protocol not distinguished
* Not a general model
* Host-to-Network layer not really a lay but an interface between network and data link layers
* No mention of physical and data link layers
* Minor protocols deeply entrenched, hard to replace
## Hybrid model
* An hybrid model of OSI model and TCP/IP model
> Structure of the Hybrid Model
>> <img src='images/Hybrid_Model.png' width=50%>
## Network Standardization
|Body|Area|Examples|
|----|----|----|
|<font color=pink>ITU</font> (International Telecommunication Union)|Telecommunications|ADSL, PON, MPEG4|
|<font color=pink>IEEE</font> (Institute of Electrical and Electronics Engineers)|Communications|Ethernet, WiFi|
|<font color=pink>IETF</font> (Internet Engineering Task Force)|Internet|HTTP/1.1, DNS|
|<font color=pink>W3C</font> (The World Wide Web Consortium)|Web|HTML5 standard|
<h1 id='Physical'>Physical Layer</h1>
## What is the Physical Layer
* The physical layer is the lowest layer in OSI model
* The physical layer's properties in TCP/IP model are in the host-to-network division
* The physical layer is concerned with the mechanical, electrical and timing interfaces of the network
* Various physical media can be sued to transmit data, but all of them are affected by a range of physical properties and hence have distinct differences
### Link Model
* <font color=red>Simplified Link Model</font>: Consider the network as a connected link between computers.
> Example of Link Model
>> <img src='images/Link_Model.png' width=50%>
* <font color=pink>Bandwidth</font> is usually treated as rate of transmission in bits/second
* <font color=pink>Delay (in seconds)</font> is the time required for the first bit to travel from computer A to computer B.
## Message Latency
* <font color=pink>Latency</font> is the delay associated with sending a message over a link
* This is made up of two parts related to the link model:
* Transmission delay:
* T-delay = Message in bits / Rate of transmission = M/R (seconds)
* Propagation delay:
* P-delay = Length of the channel / speed of signals
* Latency = M/R + P-delay
## Twisted Pair
* Construction:
* Two insulated copper wires, twisted in helical form
* Twisting reduces radiance of waves from effectively parallel antennae
* Distance up to < 5km, repeaters can extend this distance
* Twisting reduces interference
* Properties and Types of Twisted Pair:
* Bandwidth dependent on distance, wire quality/density
* <font color=pink>Cat 3</font>: 2 wires, 4 pairs in sheath, 16 Mhz
* <font color=pink>Cat 5</font>: 2 wires, 4 pair in sheath, more twists, 100 Mhz
* <font color=pink>Cat 6</font>: 250 Mhz
## Coaxial Cable ("Co-ax")
* Better shielding than twisted pair = higher speeds
* Copper core with insulation, mesh, and sheath
* Bandwidth approaches 1 Ghz
* Still widely used for cable TV/Internet
## Fiber Optics
* Optical transmission has three components:
* Light source
* Transmission medium
* Detector
* Semantics: light = 1, no light = 0
* Data transmission over a fiber of glass
* A detector generates electrical pulse when light hits it
* Common for high rates and long distances
* Fiber has enormous bandwidth and tiny signal loss - hence high rates over long distances
* Types of Fiber Optics:
* Single-mode:
* Core so narrow light can't even bounce around
* Used with laser for long distances
* Multi-mode:
* Other main type of fiber
* Light can bounce
* Used with LEDs for cheaper, shorter distance links
* Fiber optic connections:
* Connector and Fiber sockets (10-20% loss, but easy to configure)
* Mechanical Splice (10% loss, labor intensive)
* Fusion (<1% loss, but very specialized)
* Fiber optic cable is a scalable network media - LAN, WAN
* Fiber optic cable can be considered either as a ring or as a bus network type
## Comparison of Wires and Fiber
|Property|Wires|Fiber|
|----|----|----|
|Distance|Short (100s of m)| Long (10s of km)|
|Bandwidth|Moderate|Very high|
|Cost|Inexpensive|More Expensive|
|Convenience|Easy to use|Harder to use|
|Security|Easy to tap|Hard to tap|
## Wireless Transmission
* Mobile users requires a mobility enabled network
* Wireless networks can provide advantages even in fixed location environments
* There are many types of wireless data transmission networks, but they all have a common basis: radio wave propagation
* Wireless signals are broadcasted over a region
* Potential signal collisions
### Electromagnetic Spectrum
* Number of oscillations per second of a wave is called frequency , measured in Hz
* Distance between two consecutive minima or maxima is called wavelength
* All electromagnetic waves travel at same speed
* Fundamental relationship:
* Wavelength * Frequency = Speed of Light
## Wireless vs. Wires/Fiber
* Wireless:
* Easy and inexpensive to deploy
* Naturally supports mobility
* Naturally supports broadcast
* Transmissions interfere and must be managed
* Signal strengths hence data rates vary greatly
* Wires/Fiber
* Easy to engineer a fixed data rate over point-to-point links
* Can be expensive to deploy
* Does not readily support mobility or broadcast
## Communication Satellites
* Satellites are effective for broadcast distribution and anywhere/anytime communications
### Kinds of Satellites
* Geostationary Satellite (GEO):
* 35000 km above a fixed location
* VSAT computers can communicate with the help of a hub
* Different bands in the GHz are in use but may be crowded or susceptible to rain
* Low-Earth Orbit Satellites (LEO)
* Systems such as Iridium use many low-latency satellites for coverage and route communications via them
> Different Satellites' high distribution
>> <img src='images/Satellites.png' width=50%>
## Satellite vs. Fiber
* Satellite:
* Can rapidly set up anywhere/anytime communications after satellites have been launched
* Can broadcast to large regions
* Limited bandwidth and interference to manage
* Fiber:
* Enormous bandwidth over long distances
* Installation can be more expensive/difficult
## Bits to a Link
* Model types:
* Full-duplex Link: Used for transmission in both directions at once. <font color=pink>E.g. Use different twisted pairs for each direction</font>
* Half-duplex Link: Both directions, but not at the same time. <font color=pink>Senders take turns on a wireless channel</font>
* Simplex Link: Only one fixed directions at all times; Not very common
### Multiplexing
* When multiple sources want to put things onto a medium
* Time Division Multiplexing:
* Users can send according to a fixed schedule
* Slotted access to the full speed of the media
> Example of Time Division Multiplexing:
>> <img src='images/Time_Division.png' width=50%>
* Frequency Division Multiplexing
* Users can only use specific frequencies to send their data
* Continuous access with lower speed
> Example of Frequency Division Multiplexing:
>> <img src='images/Frequency_Division.png' width=50%>
* Statistical Multiplexing
* Code Division Multiple Access
## How bits look as a signal
* Information on wire transmitted by variance of a physical property
* Generating a periodic function
* Three things can change the behaviour of the function: Amplitude, Frequency, and Phase
> Change in Amplitude, Frequency, and Phase of a function:
>> <img src='images/Change_Amplitude.png' width=50%>
### Modulation Types
> Modulating the amplitude, frequency or phase of a carrier signal sends bits in a frequency range:
>> <img src='images/Modulation.png' width=50%>
## Maximum Data Rate of a Channel
### Nyquist's theorem
* If a function contains no frequencies higher than B Hz then it is completely determined by looking at a set of points spaced 1/2B apart
* Nyquist's theorem relates the data rate to the bandwidth (B) in Hz of a signal and the number of signal levels V used:
> <img src='images/Nyquist.png' width=50%>
### Shannon's Theorem
* Shannon's theorem relates the data rate to the bandwidth (B) and signal strength (S) relative to the noise (N):
> <img src='images/Shannon.png' width=50%>
<h1 id='Data'>Data Link Layer</h1>
## The Data Link Layer in OSI and TCP/IP
* Reliable, efficient communication of frames between two adjacent machines
* Handles transmission errors and flow control
## Functions and Methods of the Data Link Layer
* Function of the data link layer:
* Provide a well-defined service interface to network layer
* Handling transmission errors
* Data flow regulation
* Primary method: Take packets from network layer, and encapsulate them into frames. A frame contains a header, a payload and a trailer.
### Relationship between Packets and Frames
* Data Link layer accepts packets from the network layer, and encapsulates them into frames that it sends using the physical layer, reception is the opposite process
> Implementation of a Data Link Layer:
>> <img src='images/Implementation.png' width=50%>
## Type of Services
* Connection-Oriented vs. Connectionless: Whether a connection is setup before sending a message
* Acknowledged vs Unacknowledged: Whether the service provider give the service user an acknowledgement upon delivering the message
## Service Provided to Network Layer
* Principal concern is transferring data from network layer on source host to network layer on destination host
* Services provided:
* Unacknowledged connectionless service
* Source host transmits independent frames to recipient host with no acknowledgement
* No logical connection establishment or release
* No lost frame recovery mechanism
* E.g. Ethernet LANs
* Real-time traffic
* Acknowledged connectionless service
* Source host transmits independent frames to recipient host with acknowledgement
* No logical connection establishment or release
* Each frame individually acknowledged and retransmission if lost or errors
* E.g. Wireless(IEEE 802.11 WiFi)
* Acknowledged connection-oriented service
* Source host transmits independent frames to recipient host after connection establishment and with acknowledgement
* Connection established and released. Communicate rate and details of message
* Frames are numbered, counted, acknowledged with logical order enforced
* Unreliable links such as statellite channel or long distance telephone circuit
## Framing
* Physical layer provides no guarantee a raw stream of bits is error free
* Framing is the method used by data link layer to break raw bit stream into discrete unites and generate a checksum for the unit
* Checksums can be computed and embedded at the source, then computed and compared at the destination. Checksum = f(payload)
* The primary purpose of framing is to provide some level of reliability over the unreliable physical layer
### Framing Methods
* Framing methods:
* Character count
* Use a field in the frame header to specify the number of characters in a frame
> Example of character counts
>> <img src='images/Character_Counts.png' width=50%>
* Flag bytes with byte stuffing
* Each frame starts and ends with a special byte: flag byte
> Example of flag bytes with byte stuffing
>> <img src='images/Flag_Byte.png' width=50%>
* Start and end flags with bit stuffing
* Frames contain an arbitrary number of bits and allow character codes
* With an arbitrary number of bits per character
* Each frame begins and ends with a special bit pattern
> Start and end flags with bit stuffing
>> <img src='images/Start_End.png' width=50%>
* Most data link protocols use a combination of character count and one other method
## Error Control
* Ensuring that a garbled message by the physical layer is not considered as the original message by the receiver by adding check bits
* Error control deals with:
* Detecting the error
* Correcting the error
* Re-transmitting lost frames
* Data link layer deals with bit errors
### Error Detection and Correction
* Physical media may be subject to errors
* Errors may occur randomly or in bursts
* Bursts of errors are easier to detect but harder to resolve
* Resolution needs to occur before handing data to network layer
* Key issues:
* Fast mechanism and low computational overhead
* Detection of different kinds of error
* Minimum amount of extra bits send with the data
### Error Bounds: Hamming distance
* Code turns data of n bits into codewords of n+k
* Hamming distance is the minimum bit flips to turn one valid codeword into any other valid one.
> Example with 4 codewords ob 10 bits (n=2, k=8)
> * 0000000000
> * 0000011111
> * 1111100000
> * 1111111111
> The Hamming distance is 5
* Bounds for a code with distance:
* 2d+1 can correct d errors
* d+1 can detect d errors
### Hamming Codes
* n = 2*k - k - 1 (n is the number of data, k is the check bits)
* Put check bits in positions p that are power of 2, starting with position 1.
> Example of Hamming Codes
> Data: 0101, Requires 3 check bits: n = 4, therefore:
> 4 = (2^3) - 3 - 1
> Example of Hamming Code
>> <img src='images/Hamming_Code.png' width=50%>
## Error Correcting Codes Key Points
* More efficient in noisy transmission media
* Challenge is that the error can be in the check bits
* Assumption on a specific number of errors occurring in transmission
## Error Detecting Codes
* More efficient in some transmission media
* Types of error detecting codes:
* Parity (1 bit): Hamming distance = 2
* Checksum (16 bits): Hamming distance = 2
* Cyclical Redundancy Check (Standard 32 bit CRC): Hamming distance = 4
### How does error detecting codes works
* Sender calculates R check bits using a function of data bits
* Receiver receive the codeword and calculates the same function on the data and match the results with received data check bits
### Parity Bit
* E.g. 10001110 is data and count the number of 1s
> Received: 100011100(For even parity)/100011101(For odd parity) and then transfer
> Check the transferred data for errors on arrival and can catch:
>> 2 - 1 = 1 error bit can be detected
>> and
>> (2 - 1)/2 = 0.5 which not even 1 bit error can be corrected
## Checksum
* There are different variation of checksum
* Internet checksum (16-bit word): Sum modulo 2^16 and adding any overflow of high order bits back into low-order bits
> Example of Checksum for data 00110 10001 11001 01011
>> <img src='images/Checksum.png' width=50%>
## Cyclic Redundancy Check
* Based on a generator polynomial G(x)
> Example: G(x) = x^4 + x + 1(10011)
> * Let r be the degree of G(x) therefore r = 4. Append r zero bits to the low-order end of the frame so it now contains m + r bits and corresponds to the polynomial x^r * M(x)
> * Divide the bit string corresponding to G(x) into the bit string corresponding to x^r * M(x), using modulo 2 division
> * Subtract the remainder from the bit string corresponding to x^r * M(x) using modulo 2 subtraction. The result is the checksum frame to be transmitted. Call its polynomial T(x)
>> <img src='images/CRC.png' width=50%>
## Data Transmission
* A service to send message should have:
* Reliability
* Each layer need to make sure the service provided to other layers is reliable
* Retransmission with error detection is a way of ensuring reliability
* Error correction is another way but has its own shortcomings
* Flow Control
* The fast sender vs. slow receivers requires a solution
* Principles to control when sender can send next frame
* Feedback based flow control is usually used in Data Link Layer
* Rate based flow control
## Acknowledged transmission
* Data transmitted in one direction
* Time is relatively important, buffer space constrained
> Example of Acknowledged Transmission
>> <img src='images/Acknowledged_Transmission.png' width=50%>
## Noisy Channel Protocol
* Frames can be lost either entirely or partially
* Requires distinction between frames already sent/received and those being re-transmitted
* Requires timeout function to determine arrival or non-arrival of complete frames
## Stop and Wait Protocol
* Concept of ARQ (Automatic Repeat reQuest)
* Ack and Timeout
* Stop and Wait
* One bit Ack
> Example of Stop and Wait Protocol
>> <img src='images/Stop_and_Wait.png' width=50%>
### Link Utilization
* Principle of efficiency in communication is measured by Link Utilization (U)
> Let B be the bit-rate of the link and L be the length of the frame.
> Tf = Time needed to transmit a frame of length L
> Tp = Propagation delay of the channel
> Ta = Time for transmitting an Ack
> So Tf = L/B. Assume that Ta = 0, Tt = Tf + 2 * Tp
> U = (Time of transmitting a frame)/(Total time for the transfer) = Tf/Tt = Tf / (Tf+2Tp) = (L/B)/(L/B + 2Tp) = L/(L + 2 * Tp * B)
## Sliding Window Protocols
* Data is commonly transmitted in both direction simultaneously
* Sender maintains a set of sequence numbers corresponding to frames it is allowed to send
* Receiver maintains a set of sequence numbers corresponding to frames it is allowed to accept
* Stop and Wait can be seen as a special case with window size 1
### Protocol Using Go-Back-N
* Long transmission times need to be taken into account when programming timeouts
* Senders don't need to wait for acknowledgement for each frame before sending next frame
> Example of Go-Back-N protocol
>> <img src='images/Go_Back_N.png' width=50%>
### Selective Repeat
* Receiver accepts frames anywhere in receive window
* Cumulative ack indicate highest in-order frame
* NAK (negative ack) causes sender retransmission of missing frame before a timeout resend window
> Example of Selective Repeat
>> <img src='images/Selective_Repeat.png' width=50%>
### Go-Back-N vs. Selective Repeat
* Go-Back-N: Receiver discards all subsequent frames from error point, sending no acknowledgement, until the next frame in sequence
* Selective Repeat: Receiver buffers good frames after an error point, and relies on sender to resend oldest unacknowledged frames
* Trade-off between efficient use of bandwidth and data link layer buffer space
## Packet over SONET
* Packer over SONET is the method used to carry IP packets over SONET optical fiber links
* Uses PPP (Point-to-Point Protocol) for framing
> Structure of Packet over SONET
>> <img src='images/SONET.png' width=50%>
### PPP
* PPP (Point-to-Point Protocol) is a general method for delivering packets across links
* Framing uses a flag (0x7E) and byte stuffing
* Unnumbered mode (connectionless unacknowledged service) is used
* Errors are detected with checksum
> Example of a PPP packet
>> <img src='images/PPP.png' width=50%>
## ADSL
* Widely used for broadband Internet over local loops
* ADSL runs from modem to DSLAM
* IP packets are sent over PPP and AAL5/ATM
> Example structure of ADSL
>> <img src='images/ADSL.png' width=50%>
* PPP data is sent in AAL5 frames over ATM cells:
* ATM is a data link layer that uses short, fixed-size cells and each cell has a virtual circuit identifier
* ALL5 is a format to send packets over ATM
* PPP frame is converted to a AAL5 frame (PPPoA)
> Example of AAL5 Frame
>> <img src='images/AAL5_Frame.png' width=50%>
<h1 id='MAC'>MAC Sub-layer</h1>
## MAC Sub-layer
* On point to point networks, there are only singular sender and receiver pairs, eliminating transmission contention
* On broadcast networks, determining right to transmit is a complex problem
* Medium Access Control (MAC) sub-layer is used to assist in resolving transmission conflicts
## Channel Allocation Mechanisms
* Various methods exist for allocation a single broadcast channel amongst competing users
* Static Channel Allocation
* Dynamic Channel Allocation
### Static Channel Allocation
* Arbitrary division of a channel into segments and each user allocated allocated a dedicated segment for transmission
* Frequency Division Multiplexing (FDM) is typically used
* Significant inefficiencies arise when:
* Number of senders is greater than allocated segments
* Number of senders is not static
* Traffic is bursty
* Downfalls:
* Usually good for fixed number of users
* Network traffic is busty
* TDM and FDM try to give consistent access to the network leading to inefficiency in the use of network resources
## Dynamic Channel Allocation
* Channel segmentation is dynamic, segment allocation is dynamic
* Assumption for dynamic channel allocation:
* Independent transmission stations
* Single channel for all communication
* Simultaneous transmission results in damaged frames
* Time
* Transmission can begin at any time
* Transmission can begin only within discrete intervals
* Carrier Sense
* Detection of channel use prior to transmission
* No detection of channel use prior to transmission
## Multiple Access Protocols
### ALOHA
* Users transmit frames whenever they have data. Users retry after a random time if there are collision
* Requires no central control mechanism
* Efficient under low load but inefficient under high traffic loads
* Slotted ALOHA: Allows the users to start sending only at the beginning of defined slots. Increase efficiency of pure ALOHA by reducing possibility of collisions
### Carrier Sense Multiple Access Protocols (CSMA)
* In networks which require transmission state detection to determine transmission rights dynamically, there are specific protocols which are used:
* Persistent and Non-persistent CSMA
* CSMA with Collision Detection
#### Persistent and Non-Persistent CSMA
* When a sender has data to transmit, first check channel to detect other active transmission
* 1-persistent CSMA: Wait until channel idle. Transmit one frame and check collisions. If collision, wait for a random time and repeat.
* Non-persistent CSMA: If channel busy, wait random period and check again. If not, start transmitting.
* P-persistent CSMA: If channel idle, transmit with probability p, or wait with probability (1-p), and check again
> Example binary tree of 1-persistent, non-persistent and p-persistent
>> <img src='images/CSMA.png' width=50%>
## CSMA with Collision Detection
* Principle that transmission aborted when collision detected:
* After collision detected, abort, wait random period, try again
* Channel must be continually monitored
* Used in half-duplex system
## Classic Ethernet Minimum Packet Size
* Collisions can occur and take as long as 2 $\tau$ to detect
* $\tau$ is the time it takes to propagate over the ethernet
* Leads to minimum packet size for reliable detection
> Illustration of the collision detecting time
>> <img src='images/Collision_Time.png' width=50%>
## Collision Free Protocols
### Bit Map Protocol
* Reservation-based protocol
* 1 bit per station overhead
* Division of transmission right, and transmission event. No collisions as this is a reservation based protocol
> Example of Bit Map Protocol
>> <img src='images/Bit_Map_Protocol.png' width=50%>
### Binary Countdown Protocol
* Avoid the 1 bit per station scalability problem by using binary station addressing
* No collisions as higher-order bit positions are used to arbitrate between stations wanting to transmit
* Higher numbered stations have a higher priority
* Process:
* Stations send their address in contention slot (log N bits instead of N bits)
* Channel medium ORs bits, stations give up when they send a 0 but see a 1
* Station that ses its full address in next to send
> Example of Binary Countdown Protocol
>> <img src='images/Binary_Countdown.png' width=50%>
## Limited Contention Protocols
* Both contention and collision free become inefficient at different points
* Under low loads, collision free is less attractive because of a higher delay between transmissions
* Under higher loads, contention is less attractive because overhead associated with channel arbitration becomes greater
* Limited content protocols increase the probability of stations acquiring transmission rights by arbitrarily dividing stations and using a binary algorithm to determine rights allocation
### Adaptive Tree Walk Protocol
* All stations compete for right to transmit, if a collision occurs, binary division is used to resolve contention
* Tree divides stations into groups of nodes to pull
* Depth First Search under nodes will poll collisions
* Start search at lower levels if more than one station expected
> Example of Adaptive Tree Walk Protocol
>> <img src='images/Adaptive_Tree_Walk.png' width=50%>
### Wireless LAN Protocols
* Wireless Complications: When a station is in the range of two transmitters or relays, interference affects signal reception
* This leads to hidden and exposed terminal problems
* Require detection of transmissions to receiver, not just carrier sensing
* Transmission Protocols for Wireless LANs (802.11)
* Multiple Access with Collision Avoidance for Wireless (MACAW)
### Hidden and Exposed Terminals
* Hidden terminals are senders that cannot sense each other but nonetheless collide at intended receiver
* Want to prevent but will leads to loss efficiency
> Illustration of Hidden Terminals
>> <img src='images/Hidden_Terminals.png' width=50%>
> A and C are hidden terminals when sending to B
* Exposed terminals are senders who can sense each other but still transmit safely to different receivers
> Illustration of Exposed Terminals
>> <img src='images/Exposed_Terminals.png' width=50%>
> B -> A and C -> D are exposed terminals
### Multiple Access with Collision Avoidance (MACA)
* Sender asks receiver to transmit short control frame
* Stations near receiver hear control frame
* Sender can then transmit data to receiver
> Illustration of MACA
>> <img src='images/MACA.png' width=50%>
> MACA protocol grants access for A to send to B
> * A sends RTS to B; B replies with CTS
> * A can send with exposed but no hiddent terminals
## Classic Ethernet
* Each type of Ethernet has a maximum cable length per segment
* Multiple cable lengths can be connected by repeaters - a physical device which receives, amplifies and retransmits signals in both directions
> Construction of a classic Ethernet
>> <img src='images/Classic_Ethernet.png' width=50%>
## Ethernet Frame Format
* MAC protocol is 1-persistent CSMA/CD
* Random delay after collision is computed with BEB (Binary Exponential Backoff)
* Frame format is still used in modern Ethernet
> Example of Ethernet Frame Format
>> <img src='images/Ethernet_Frame_Format.png' width=50%>
> * Preamble (7B): Synchronization between sender and receiver
> * Start of Frame (1B): FLAG bytes
> * Destinations and Source Addresses: Identify who send, who receive
> * Type and Length (2B): Specifies which process to give the frame to
> * Pad (0-46B): Minimum size of the message of the Ethernet
> * CRC (4B): 32 bits checksum
## MAC Addressing
* Sources and destination addressing can be done at a local or global levels
* The MAC Address provides the unique identifier for a physical interface
* MAC Address is a 48-bit number encoded in the frame
* E.g. 00:02:2D:66:7C:2C
## Ethernet Performance
$$ Channel \ Efficiency = \frac{1}{1+\frac{2BLe}{cF}}$$
* F: Frame Length
* B: Band Width
* L: Cable Length
* c: Speed of Light
* Optimal case of e contention slow per frame
* When cF is large, the channel efficiency will be high
* Increasing network bandwidth or distance reduces the efficiency for a given frame size
## Switched Ethernet
* Hubs wire all lines into a single CSMA/CD domain
* Switches isolate each port to a separate domain
* Much greater throughput for multiple ports
* No need for CSMA/CD with full-duplex lines
> Illustration of Hubs and Switches
>> <img src='images/Hubs_and_Switches.png' width=50%>
<h1 id='Network'>Network Layer</h1>
## Principles of Internet Design
1. Make sure it works
2. Keep it simple
3. Make clear choices
4. Exploit modularity
5. Expect heterogeneity
6. Avoid static options and parameters
7. Choose a good, but not necessarily perfect design
8. Be strict in sending and tolerant in receiving
9. Consider scalability
10. Consider performance vs costs
## Network Layer in the Internet
* Internet is an interconnected collection of many networks of Autonomous systems that is held together by the IP protocol
## Internet Protocol (IP)
* The glue that holds the whole Internet together in the Network layer protocol, IP
* Provides a best-effort service to route datagrams from source host to destination host
* These hosts may be on the same network or different networks
* Each network is called an Autonomous System(AS)
## Service Provided to the Transport Layer
* Design goals:
* Services should be independent of router technologies
* Transport layer should be shielded from number, type, and topology of routers
* Network addressing should use a uniform numbering plan
## Store and Forward Packet Switching
* Hosts generate packets and injects into the network
* Routers treat packets as messages, receiving them and then forwarding them based on how the message is addressed
* Router routes packets through the network
> Example of packets routing with datagram subnet
>> <img src='images/Packet_Routing.png' width=50%>
## Routing within a datagram subnet
* Post office model: Packets are routed individually based on destination addresses in them. Packets can take different paths
* Routing table can be fixed or change over time
* Routing algorithm manages the routing table
> Example of P1 sends a long message to P2
>> <img src='images/Routing_Datagram.png' width=50%>
## Routing with virtual-circuit subnet
* Model is like a telephone network
* Packets are routed through virtual circuits based on tag number (not full address but unique at a given link) in them.
* Packets take the same path to avoid having to choose a new route for every packet sent
* E.g. Multi-protocol Label Switching Network
> Example of routing with virtual-circuit subnet
>> <img src='images/Routing_Virtual_Circuit.png' width=50%>
## Difference in Virtual Circuit and Datagram Subnets
|Issue|Datagram Network|Virtual-circuit Network|
|----|----|----|
|Circuit Setup|Not needed|Required|
|Addressing|Each packet contains the full source and destination address|Each packet contains a short VC number|
|State Information|Routers do not hold state information about connections|Each VC requires router table space per connection|
|Effect of router failures|Each packet is routed independently|Route chosen when VC is set up; All packets follow it|
|Quality of Service|Difficult|Easy if enough resources can be allocated in advance for each VC|
|Congestion control|Difficult|Easy if enough resources can be allocated in advance for each VC|
## Compromises of VC and Datagram Subnets
* Memories vs Bandwidth
* VC require more space in router memory but save potential overhead in full addressing of each packet and computation of path
* Setup time vs Address Parsing Time
* VC require setup time and resources, but packet transmission is very fast
* Amount of memory
* Datagram subnets require large tables of every possible destination routes, whereas VC requires entry per link
* QoS and Congestion avoidance
* VC can use tighter QoS to be able to reserve CPU, bandwidth, and buffer in advance
* Longevity
* VC can exist for a long time
* Vulnerability
* VC particularly vulnerable to hardware/software crashes - all VC aborted and no traffic until they are rebuilt. Datagram uses an alternative route
## Issue of Internetworking
* Internetworking joins multiple, different networks into a single larger network
* Issues when connecting networks:
* Different network types and protocols
* Different motivations for network choices
* Different technologies at both hardware and software levels
## Different Dealt at the Network Layer
|Item|Some Possibilities|
|----|----|
|Service Offered|Connectionless vs connection oriented|
|Addressing|Different sizes, flat or hierarchical|
|Broadcasting|Present or absent|
|Packet size|Every network has its own maximum|
|Ordering|Ordered and unordered delivery|
|Quality of service|Present or absent|
|Reliability|Different levels of loss|
|Security|Privacy rules, encryption|
|Parameters|Different timeouts, flow specifications|
|Accounting|By connect time, packet, byte or not at all|
## Tunneling
* Tunneling is a special case used when the source and destination are on the same network, but there is a different network in between
* Source packets are encapsulated over the packets in the connecting network
> Example of Tunneling IPv6 packets through IPv4
>> <img src='images/Tunneling.png' width=50% >
## Fragmentation
* All networks have a maximum size for packets, could be motivated by:
* Hardware
* Operating system
* Protocols
* Standards compliance
* Desire to reduce transmissions due to errors
* Desire for efficiency in communication channel
* Fragmentation is the division of packets into fragments. It allows network gateways to meet size constraints
## Types of Fragmentation
* Transparent: Packets fragmented and reassembled in each network.
* Route constrained, need more work
> Example of transparent fragmentation
>> <img src='images/Transparent.png' width=50%>
* Non-transparent: Fragments are reassembled at destination
* Less work: packet number, byte offset, end of packet flag
> Example of non-transparent fragmentation
>> <img src='images/Non-transparent.png' width=50%>
> Example of IP style fragmentation
>> <img src='images/IP_Style.png' width=50%>
## Path MTU Discovery
* An alternative to fragmentation
* Advantage: The source now knows what length packet to send but if the routes and path MTU change, new error packets will be triggered and the source will adapt to the new path.
> Example of Path MTU Discovery
>> <img src='images/MTU.png' width=50%>
## IP Datagram
* IPv4 header is carried on all packets and has fields for the key parts of the protocol
> Example of IPv4 Datagram header
>> <img src='images/IPv4_Datagram.png' width=50%>
* IPv4 datagram consists of a header and some text
* Header is 20 byte fixed part + variable length optional part
* Version: IPv4 or IPv6
* IHL: Header Length, in 32bits units, min 5 and max 15
* Type: Differentiates different classes of service
* Total Length: Header and payload, maximum length 65535 bytes
* Identification: Allows host to determine which datagram the new fragment belongs to - all fragments of same datagram have same ID
* DF: Don't Fragment Byte
* MF: More Fragment Byte, determine whether it is the last one or not
* Fragment offset: Where in the datagram the current fragment belongs
* TTL: Limits packet lifetimes, hops or seconds
* Protocol: TCP, UDP, or others
* Header Checksum: verifies the header only
* Source Address: IP, host/network
* Destination Address: IP, host/network
* Options: Security, strict vs loose source routing, record route, timestamp
## Routing Algorithms
* Consider the network as a graph of nodes and links:
* Decide what to optimize
* Update routes for changes in topology
* Routing is the process of discovering network path
* The routing algorithm is responsible for deciding on which output line an incoming packet should be transmitted
* Non-adaptive Algorithms
* Static decision making process
* Adaptive Algorithms
* Dynamic decision making process
* Changes in network topology, traffic
## Sink Tree/Spanning Tree
* Sink Tree/Spanning Tree: The set of optimal routes from all sources to a given destination form a tree rooted at the destination
* The goal of a routing algorithm is to discover and utilize the spanning trees for all routers
> Example of a spanning for a graph of routers
>> <img src='images/Spanning_Tree.png' width=50%>
## Shortest Path Routing
* A non-adaptive algorithm
* Shortest path can be determined by building a graph with each node representing a router, and each edge representing a communication link
* To choose a path between 2 routers, the algorithm finds the shortest path between them on the graph
## Dijkstra Algorithm
* Dijkstra algorithm computes a spanning tree on the graph:
* Each link is assigned a non-negative weight/distance
* Shortest path is the one with lowest total weight
* Using weights of 1 gives paths with fewest hops
* Algorithm:
* Start with sink, set distance at other nodes to infinity
* Relax distance to other nodes
* Pick the lowest distance node, add it to the spanning tree
* Repeat until all nodes are in the spanning tree
> Example to Dijkstra Algorithm
>> <img src='images/Dijkstra.png' width=50%>
## Flooding
* A non-adaptive algorithm
* Every incoming packet is sent out on every outgoing line except the one on which it arrived
* Generates a large number of duplicate packets, so it is inefficient
* Selective flooding is routers send packets only on links which are in approximately the right direction. This is an improved variation.
> Example of Flooding
>> <img src='images/Flooding.png' width=50%>
## Distance Vector Routing
* A dynamic algorithm
* Each router maintains a table which includes the best known distance to each destination and which line to use to get there
* Tables are exchanged with neighboring routers
* Global information shared locally
* Algorithm:
* Each node knows distance of links to its neighbors
* Each node advertises vector of lowest known distances to all neighbors
* Each node uses received vectors to update its own
* Repeat periodically
> Example of Distance Vector Routing
>> <img src='images/Distance_Vector_Routing.png' width=50%>
## Link State Routing
* A dynamic algorithm
* An alternative to distance vector
* DV: primary problem that caused its demise was that the algorithm often took too long to converge after the network topology changed
* Widely used in the Internet
* More computation but simpler dynamics
* Each router has to do 5 steps:
1. Discover neighbors and learn network addresses
2. Measure delay or cost to each neighbors
3. Construct packet resulting from previous steps
4. Send this packet to all other routers
5. Compute the shortest path to every other router
### Building link state packets
* LSP for a node lists neighbors and weights of links to reach them
* The hard part is determining when to build LSP
* Periodically at regular intervals
* Build them when some significant event occurs, such as a line or neighbor going down or coming back up again or changing its properties appreciably
> Example of Link State Packet
>> <img src='images/LSP.png' width=50%>
## Hierarchical Routing
* As networks grow in size, routing tables expand but this impacts CPU and memory requirements
* Dividing all routers into regions allows efficiencies
* Each router knows everything about other routers in its region but nothing about routers in other regions
* Router which connect to two regions act as exchange points for routing decisions
* Hierarchical routing reduces the work of route computation but may result in slightly longer paths than flat routing
> Example of Hierarchical Routing
>> <img src='images/Hierarchical_Routing.png' width=50%>
## Broadcast Routing
* Broadcast routing allows hosts to send messages to many or all other hosts
* Single distinct packet
* Inefficient, sources, needs all destination addresses
* Flooding
* Multi-destination routing
* Efficient but source needs to know all the destinations
* A router receives a single packet which encapsulates the list of destinations, and then constructs a specific packet for each one
* Acts as a relay
* Reverse path forwarding
* When a broadcast packet arrives at a router, the router checks to see if the packet arrived on the line normally used for sending packets to use broadcast. If so there is a high probability that the route used to transmit the received packet is the best route. The router then to forwards the packet onto all other lines.
* If the broadcast packet arrived on a link other than the preferred one for reaching the source, the packet is discarded as a likely duplicate.
## Multicast Routing
* A routing algorithm used to send a message oto a well-defined group within the whole network
* Each router computes a spanning tree covering all other routers: The first router to receive the packet prunes the spanning tree to eliminate all lines which do not lead to members of the group
## Addressing
* Routing tables needs addressing to work/route
* IP addresses are used for this purpose
* They are hierarchical
* There is a network portion to an address
* And also a host portion
* The network portion is same for all hosts on a network
* The host portion grabs a continuous block of addresses and is called the prefix
## IP Addresses
* Addresses are allocated in prefixes
* Prefix is determined by a LAN
* IP addresses are written in dotted decimals
* 4 bytes * 4 = 32 bit addresses
* Written as lowest address + length
* E.g. 172.16.17.32/24
* /24 states the length of the network part in bits, so here 8 bits are left for hosts
* Overall IP allocation responsibility of Internet Corporation for Assigned Names and Numbers (ICANN) by delegation to Internet Assigned Numbers Authority (IANA) and Regional Internet Registries (RIR's)
> Example of IP Address
>> <img src='images/IP_Address.png' width=50%>
## Subnets
* Subnetting allows networks to be split into several parts for internal used whilst acting like a single network for external use
> Example of Subnets
>> <img src='images/Subnets.png' width=50%>
## IP Addressing and Routing Tables
* Routing tables are typically based around a triplet:
* IP Address
* Subnet Mask
* Outgoing Line (Physical or virtual)
> Example of a routing table
>> <img src='images/Routing_Table.png' width=50%>
## Aggregation of IP addresses
* Backbone router connection networks around the world
* Aggregation: Process of joining multiple IP prefixes into a single larger prefix to reduce size of routing table
> Example of Aggregation of IP Addresses
>> <img src='images/Aggregation.png' width=50%>
### Longest Matching Prefix
* Packets are forwarded to the entry with the longest matching prefix or smallest address block
* Complicates forwarding but adds flexibility
* Processes:
1. Check address whether matches the longest prefix
2. If not then see if it matches others
> Example of Longest Matching Prefix
>> <img src='images/Longest_Matching_Prefix.png' width=50%>
## Classful Addressing
* Part of history now-old addresses came in blocks of fixed size (A, B, C)
* Carries size as part of address, but lacks flexibility
* Called classful addressing
> Class of Addresses
>> <img src='images/Class_Of_Addressing.png' width=50%>
## Private IP Ranges
* Range of IP addresses that cannot appear in the Internet
* Only for private networks
* 10.0.0.0/8
* 172.16.0.0/12
* 192.168.0.0/17
## Network Address Translation (NAT)
* NAT box maps one external IP address to many internal IP addresses
* Uses TCP/UDP port to tell connections apart
* Violates layering; Very common in homes
> Example of NAT
>> <img src='images/NAT.png' width=50%>
## Internet Control Protocols
* IP works with the help of several control protocols:
* ICMP is a companion to IP that returns error info
* Required and used in many ways
* ARP finds MAC address of a local IP address
* Glue that is needed to send any IP packets
* Host queries an address and the owner replies
* DHCP assigns a local IP address to a host
* Gets host started by automatically configuring it
* Host sends request to server, which grants a lease
## Mobile IP
* Handle it at data link layer
* For IPv4 the solution is through ICMP
## ICMP
* Internet Control Message Protocol
* Used for testing and monitoring ambient conditions between hosts and routers
>|Message Type|Description|
>|----|----|
>|Destination unreachable|Packet could not be delivered|
>|Time exceeded|Time to live field hit 0|
>|Parameter problem|Invalid header field|
>|Source quench|Choke packet|
>|Redirect|Teach a router about geography|
>|Echo and Echo reply|Check if a machine is alive|
>|Timestamp request/reply|Same as Echo, but with timestamp|
>|Router advertisement/solicitation|Find a nearby router|
## Other Considerations
* Congestion Control Algorithms
* Quality of Service
<h1 id='Transport'>Transport Layer</h1>
## Transport Layer Function
* Main function: Provide efficient, reliable and cost-effective data transmission service to the processes in the application layer. Independent of physical and data networks
# Transport Layer services
* Transport layer services provide interfaces between the application lay and the network layer
* Transport entities is the hardware or softeware which actually does the work. It can exist in multiple locations:
* OS kernel
* System Library
* But not so much in:
* User process
* Network interface card
* Transport layer adds reliability to the network layer
* Offers connectionless in addition to connection-oriented services to applications
> Relationship between network, transport and application layes:
>> <img src='images/Relationship_Transport.png' width=50%>
## Transport Layer and Network Layer Service Comparison
* Transport layer code runs entirely on hosts. Network layer code runs almost entirely on routers
* Users have no real control over the network layer. But can improve QoS in transport layer.
* Transport layer fixes reliability problems caused by the network layer
## Position of the Transport Layer
* The transport layer occupies a key position in the layer hierarchy because it clearly delineates
* Providers of data transmissions services are at the network, data link, and physical layers
* Users of reliable data transmission services are at the application layer
* In particular, users commonly access connection-oriented transport services for a reliable service on top of an unreliable network
## Features of a simple transport layer
* Abstraction and primitives provide a simpler API for application developers independent of network layer
> |Primitive|Meaning|
> |----|----|
> |LISTEN|Block waiting for an incoming connection|
> |CONNECT|Establish a connection with a waiting peer|
> |RECEIVE|Block waiting for an incoming message|
> |SEND|Send a message to the peer|
> |DISCONNECT|Terminate a connection|
## Transport Layer Encapsulation
* Abstract representation of messages sent to and from transport entities: Transport Protocol Data Unit (TPDU)
* Encapsulation of TPDUs transport layer unites to network layer units to frames in data layer units
> Example of Transport Layer Encapsulation
>> <img src='images/Transport_Encapsulation.png' width=50%>
## Transport Services Primitives/Segments
* Primitives that applications might call to transport data for a simple connection-oriented service:
* Server executes LISTEN
* Client executes CONNECT
* Sends CONNECTION REQUEST TPDU to server
* Receives CONNECTION ACCEPTED TPDU to client
* Data exchanged using SEND and RECEIVE
* Either party executes DISCONNECT
> |Primitive|Segment sent|Meaning|
> |----|----|----|
> |LISTEN|(none)|Block until some process tries to connect|
> |CONNECT|CONNECTION REQ|Actively attempt to establish a connection|
> |SEND|DATA|Send Information|
> |RECEIVE|(none)|Block until a DATA packet arrives|
> |DISCONNECT|DISCONNECTION REQ|This side wants to release the connection|
> Illustration of a Simple Connection
>> <img src='images/Simple_Connection.png' width=50%>
> * Solid lines show client state sequence
> * Dashed lines show server state sequence
> * Transitions in italics are due to segments arrivals
## Elements of Transport Protocols
* Connection establishment
* When networks can lose, store and duplicate packets, connection establishment can be complicated:
* Congested networks may delay acknowledgements
* Incurring repeated multiple transmissions
* Any of which may not arrive at all or out of sequence: Delayed duplicates
* Application degenerate with such congestion
* Reliable Connection Establishment
* Key challenge is to ensure reliability even though packets may be lost, corrupted, delayed and duplicated
* Don't treat an old or duplicate packet as new
* Approach:
* Don't reuse sequence numbers within maximum segment lifetime
* Use a sequence number space large enough that it will nor wrap, even when sending at full rate
* Three-way handshake for establishing connection
### Three Way Handshake
* Three-way handshake used for initial packet
* Since no state from previous connection
* Both hosts contribute fresh sequence numbers
* CR = Connect Request
> Example of Three Way Handshake
>> <img src='images/Three_Way_Handshake.png' width=50%>
* Connection Release
* Asymmetric Disconnection
* Either party can issue a DISCONNECT, which results in DISCONNECT TPDU and transmission ends in both directions
* Symmetric Disconnection
* Both parties issue DISCONNECT, closing only one direction at a time. Allows flexibility to remain in receive mode.
* Asymmetric release may result in data loss hence symmetric release is more attractive
* Symmetric release works well where each process has a set amount of data to transmit and knows when it has been sent.
## Strategies for Connection Release
* Three way handshake
* Finite retry
* Timeouts
* Normal release sequence initiated by transport user on sender host
* DR = Disconnect Request
* Both DRs are ACKed by the other side
> Examples of error cases for Connection Releases
>> <img src='images/Error_Case.png' width=50%>
## Addressing
* Specification of remote process to connect to is required at application and transport layers
* Addressing in transport layer is typically done using Transport Service Access Points (TSAPs)
* On the Internet, a TSAP in commonly referred to as a port
* Addressing in the network layer is typically done using Network Service Access Points (NSAPs)
* On the Internet, the concept of an NSAP is commonly interpreted as simply an IP address
> Illustration of TSAP and NSAP
>> <img src='images/TSAP_NSAP.png' width=50%>
### Type of TSAP Allocation
* Static: Well known services have standard allocated TSAPs/ports, which are embedded in OS
* Directory Assistance - Port-mapper:
* A new service must register itself with the portmapper, giving both its services name and TSAP
* Mediated:
* A process server intercepts inbound connections and spawns requested server and attaches inbound connection
## Sockets
* Sockets widely used for interconnections
* Berkeley sockets are predominant in internet applications
* Notion of sockets as transport endpoints
* Like the simple set plus SOCKET, BIND, ACCEPT
>|Primitive|Meaning|
>|----|----|
>|SOCKET|Create a new communication end point|
>|BIND|Associate a local address with a socket|
>|LISTEN|Announce willingness to accept connections; give queue size|
>|ACCEPT|Passively establish an incoming connection|
>|CONNECT|Actively attempt to establish a connection|
>|SEND|Send some data over the connection|
>|RECEIVE|Receive some data from the connection|
>|CLOSE|Release the connection|
## UDP
* User Datagram Protocol
* Does not add much to the Network Layer functionality
* TCP just does the realiability for this layer
* UDP remove connection primitives to use it in a program
* UDP is good for apps like video streaming and gaming regularly
* The reliability issue is left to the application layer. Retransmission decision as well as congestion control
* Provides a protocol whereby applications can transmit encapsulated IP datagrams without a connection establishment
* UDP transmits in segments consisting of an 8-byte header followed by the payload
* UDP headers contain source and destination ports
* Payload is handed to the process which is attached to the particular port at destination
* The main advantage of using UDP over raw IP is the ability to specify ports for source and destination pairs
* Both source and destination ports are required. Destination allows for incoming segments, sources allows reply for outgoing segments
> Example of a UDP header
>> <img src='images/UDP_Header.png' width=50%>
### Strengths and Weakness of UDP
* Strength: Provides an IP interface with multiplexing/de-multiplexing capabilities and related transmission efficiencies
* Weakness: UDP does not include support for flow control, error control/retransmission of bad segments
* Where applications require a precise level of control over packet flow/error/timing, UDP is good choice as application layer can make choices.
## Remote Procedure Call
* Sending a message and getting a reply back in analogous to making a function all in programming languages
* To call a remote procedure, the client is bound to a small library call the client stub that represents the server procedure in the client's address space
* Similarly the server is bound with a procedure call the server stub
* These stubs hide the fact that the procedure itself is not local
> Illustration of RPC
>> <img src='images/RPC.png' width=50%>
## Transmission Control Protocol (TCP)
* Provides a protocol by which application can transmit IP datagrams within a connection-oriented framework, thus increasing reliability
* TCP transport entity manages TCP streams and interfaces to the IP layer - can exist in numerous locations (kernel, library, user process)
* TCP entity accepts user data streams, and segments them into pieces < 64KB (Often at a size in order so that the IP and TCP headers can fit into a single Ethernet frame), and sends each piece as a separate IP datagram.
* Recipient TCP entities reconstruct the original byte streams from the encapsulation
## The TCP service Model
* Sender and receiver both create sockets, consisting of the IP address of the host and a port number.
* For TCP Service to be activated, connections must be explicitly established between a socket at a sending host and a socket at a receiving host
* Special one-way server sockets may be used for multiple connections simultaneously
> Example of TCP Service model
>> <img src='images/TCP.png' width=50%>
### Port Allocations
* Port numbers can range from 0 to 65535
* Port numbers are regulated by IANA
* Ports are classified into segments
* Well Known Ports (0-1023)
* Registered Ports (1024-49151)
* Dynamic Ports (49152-65535)
>|Port|Protocol|Use|
>|----|----|----|
>|20, 21|FTP|File transfer|
>|22|SSH|Remote login, replacement for Telnet|
>|25|SMTP|Email|
>|80|HTTP|World Wide Web|
>|110|POP-3|Remote email access|
>|143|IMAP|Remote email access|
>|443|HTTPS|Secure Web|
>|543|RTSP|Media Player Control|
>|631|IPP|Printer|
### Socket Library: Multiplexing
* Socket library provides a multiplexing tool on top of TSAPs to allow servers to service multiple clients
* It simulate the server using a different port to connect back to the client
> Example of Socket Library
>> <img src='images/Socket_Library.png' width=50%>
### Features of TCP Connections
* Full duplex: Data in both directions simultaneously
* Point to point: Exact pairs of senders and receivers
* Byte streams, not message streams: Message boundaries are not preserved
* Buffer options: TCP entity can choose to buffer prior to sending or not depending on the context
### TCP entities
* Data sent between TCP entities in segments: a 20 byte header plus zero or more data bytes
* TCP entities decide how large segments should be mainly with 2 constraints:
* 65515 byte IP payload
* Ethernet unit size, generally 1500 bytes
* Sliding window:
* Sender transmits and starts a timer
* Receiver sends back an acknowledgement which is the next sequence number expected. If sender's timer expires before acknowledgement, then the sender transmits the original segment again
### TCP Segment Header
* TCP header includes addressing (ports), sliding window (seq/ack), flow control (window), error control (checksum) and more
> Example of TCP header
>> <img src='images/TCP_Header.png' width=50%>
> * Source port and destination port: Identify the local end points of the connection
> * Sequence number and Acknowledgement number: Perform their usual functions
> * TCP header length: Tells how many 32-bit words are contained in the TCP header
> * Window size: Tells how many bytes may be sent starting at the byte acknowledged
> * Checksum: Provided for extra reliability. It checksums the header, the data
> * Options: Provides a way to add extra facilities not covered by the regular header
> * URG: Set to 1 if the Urgent pointer is in use. The Urgent pointer is used to indicate a byte offset from the current sequence number at which urgent data are to be found
> * CWR and ECE: Used to signal congestion when ECN (Explicit Congestion Notification) is used
> * ECE: Set to signal an ECN-Echo to a TCP sender to tell it to slow down when the TCP receiver gets a congestion indication from the network
> * CWR: Set to signal Congestion Window Reduced from the TCP sender to the TCP receiver so that it knows the sender has slowed down can stop sending the ECN-Echo
> * ACK: Set to 1 to indicate that the Acknowledgment number is valid. This is the case for nearly all packets. 0 means ignore ACK number field
> * PSH: Indicates PUSHed data. The receiver is hereby kindly requested to deliver the data to application upon arrival and not buffer it until a full buffer has been received
> * RST: Used to abruptly reset a connection that has become confused due to a host crash or some other reason. It is also used to reject an invalid segment or refuse an attempt to open a connection
> * SYN: Used to establish connections. The connection request has SYN=1 and ACK=0. The connection reply does bear an acknowledgement, so it has SYN=1 and ACK=1. The SYn bit is used to denote both CONNECTION REQUEST and CONNECTION ACCEPTED, with the ACK bit used to distinguish between these two possibilities
> * FIN: Used to release a connection. It specifies that the sender has no more data to transmit. However, after closing a connection, the closing process may continue to receive data.
### TCP Connection Establishment and Release
* Connections established using three-way handshake
* Two simultaneous connection attempts reults in only one connection uniquely identified by end points
* Connections released with symmetric release
* Timers used for lost connection release
### TCP Connection Management
* The full TCP connection finite state machine has more states than the simple example from earlier
>|State|Description|
>|----|----|
>|CLOSED|No connection is active or pending|
>|LISTEN|The server is waiting for an incoming call|
>|SYN RCVD|A connection request has arrived; Wait for ACK|
>|SYN SENT|The application has started to open a connection|
>|ESTABLISHED|The normal data transfer state|
>|FIN WAIT 1|The application has said it is finished|
>|FIN WAIT 2|The other side has agreed to release|
>|TIME WAIT|Wait for all packets to die off|
>|CLOSING|Both side have tried to close simultaneously|
>|CLOSE WAIT|The other side has initiated a release|
>|LAST ACK|Wait for all packets to die off|
### TCP Transmission Policy
* TCP acknowledges bytes
* Receiver advertises window based on available buffer space
> Example of TCP Transmission
>> <img src='images/TCP_Transmission_Policy.png' width=50%>
## Congestion
* Congestion results when too much traffic is offered; Performance degrades due to loss/retransmissions
> Illustration of Congestion
>> <img src='images/Congestion.png' width=50%>
### Congestion Control vs. Flow Control
* Flow control is an issue for point to point traffic, primarily concerned with preventing sender transmitting data faster than receiver can receive it
* Congestion control is an issue affecting the ability of the subnet to actually carry the available traffic in a global context
## Load Shedding
* When congestion control mechanisms fail, load shedding is the key remaining possibility.
* In order to ameliorate impact, applications can mark certain packets as priority to avoid discard policy
## Key problem if network is not delivering properly
* Quality of Service becomes low
* Expected network performance is an important criterion for a wide range of network applications
* Some engineering techniques are available to guarantee QoS
* Four things to watch out for: Bandwidth, reliability, delay, jitter
## Jitter
* Jitter is the variation in packet arrival times
> Example of High Jitter
>> <img src='images/High_Jitter.png' width=50%>
> Example of Low Jitter
>> <img src='images/Low_Jitter.png' width=50%>
* Jitter is an issue for some applications
* Jitter can be contained by determined the expected transit time of a packet
* Packets can be shuffled at each hop in order to minimize jitter: Slower packets sent first, faster packets wait in a queue.
* For certain applications jitter control is extremely important as it mainly directly affects the quality perceived by the application user
## QoS Requirements
* Different applications care about different properties
>|Application|Bandwidth|Delay|Jitter|Loss|
>|----|----|----|----|----|----|
>|Email|Low|Low|Low|Medium|
>|File Sharing|High|Low|Low|Medium|
>|Web Access|Medium|Medium|Low|Medium|
>|Remote Login|Low|Medium|Medium|Medium|
>|Audio on demand|Low|Low|High|Low|
>|Video on demand|High|Low|High|Low|
>|Telephony|Low|High|High|Low|
>|Videoconferencing|High|High|High|Low|
## Techniques for Achieving QoS
* Over-provisioning: More tha adequate buffer, router CPU, and bandwidth
* Buffering: Buffer received flows before delivery: Increase delay, but smoothes out jitter, no effect in reliability or bandwidth
* Traffic Shaping: Regulate the average rate of transmission and burstiness of transmission
* Leaky Bucket
* Token Bucket
* Resource reservation: Reserve bandwidth, buffer space, CPU in advance
* Admission Control: Routers can decide based on traffic patterns whether to accept new flows, or reject/reroute them
* Proportional routing: Traffic for same destination split across multiple routes
* Packet scheduling: Create queues based on priority.
* Fair queuing, weighted fair queuing
### Leaky Bucket
* Large bursts of traffic is buffer and smoothed while sending
> Illustration of Leaky Bucket
>> <img src='images/Leaky_Bucket.png' width=50%>
## TCP Congestion Control
* When networks are overloaded, congestion occurs, potentially affecting all layers. Although lower layers attempt to ameliorate congestion, in reality TCP impacts congestion most significantly because TCP offers best methods to reduce the data rate, and hence reduce congestion
* TCP adopts a defensive stance:
* At connection establishment, a suitable window size is chosen by the receiver based on its buffer size
* If the sender is constrained to this size, then congestion problems will not occur due to overflow at the receiver itself, but may still occur due to congestion within the network
### Design of Congestion Control
* Two different problems exist
* Network capacity and receiver capacity
* These should be dealt with separately, but compatibly
* Sender maintains two windows:
* Window described by the receiver
* Congestion window
* Each regulates the number of bytes the sender can transmit. The maximum transmission rate is the minimum of the two windows
## Slow Start
* On connection establishment, the sender initializes the congestion window to a size, and transmits one segment
* If this segment is acknowledged before the timer expires, the sender adds another segment's worth of bytes to the congestion window, and transmits two segments
* As each new segment is acknowledged, the congestion window is increased by one more segment
* In effect, each set of acknowledgements doubles the congestion window which grows until either a timeout occurs or the receiver's specified window is reached.
> Illustration of Slow Start
>> <img src='images/Slow_Start.png' width=50%>
### Additive Increase
> Illustration of Additive Increase
>> <img src='images/Additive_Increase.png' width=50%>
### TCP Tahoe
* Slow start followed by additive increase.
* Threshold is half of previous
> Illustration of TCP Tahoe
>> <img src='images/TCP_Tahoe.png' width=50%>
### TCP Reno
> Illustration of TCP Reno
>> <img src='images/TCP_Reno.png' width=50%>
## Congestion Control and Wireless
* Much harder to deal with:
* Things are increasingly wireless
* Not everything is wireless, but parts of a path
* More variety on wireless links as well
* SNR varies when people more
* Delay is different if it is WiFi
## TCP Timer
* A key worry is when timers go out
* Too early means too many resends
* Too late means reliability comes with more additional cost
* Solutions rely on dynamicity as network conditions change
* One needs to measure network performance and adapt timers
<h1 id='Application'>Application Layer</h1>
## Domain Name System (DNS)
* Distributed database implemented in a hierarchy of many name servers
* Application Layer protocol that allows a host to query the database in order to resolve names
* Used by application layer protocols (http, ftp, smtp)
### DNS Defined
* Number of RFSs are directly related to DNS:
* RFC 1034: Domain Names: Concepts and Facilities
* RFC 1035: Domain Names: Implementation and Specification
* RFC 1519: Domain Name System Structure and Delegation
* RFC 2219: Use of DNS Aliases for Network Services
* RFC 2606: Reserved Top Level DNS Names
* RFC 3647: Role of the Domain Name System
### Conceptual Divisions of DNS Namespace
* A hierarchical naming convention
* The top of the hierarchy is managed by ICANN (The Internet Corporation for Assigned Names and Numbers)
> Example of DNS Namespace
>> <img src='images/DNS_Namespace.png' width=50%>
### Why note centralize DNS
* Single point of failure
* Traffic volume
* Distant centralized database
* Maintenance
* Doe not scale well
### DNS Services
* Hostname to IP address translation
* Host aliasing: Alias names for canonical names
* E.g. Canonical relay1.westcoast.enterprise.com -> www.enterprise.com
* Mail server aliasing
* E.g. <EMAIL> -> <EMAIL>
* Load distribution
* Busy sites are replicated over multiple servers
* A set of IP addresses is associated with one canonical name
* DNS server rotates the order of the addresses to distribute the load
## Domain Name Characteristics
* Domain Name:
* Are case insensitive
* Can have up to 63 characters per constitute
* Can have up to 255 chars per path
* Can be internationalized
* Naming conventions usually follow either organizational or physical boundaries
* Absolute domain names ends in a .
* Relative domain names partially specify the location and can be used only within the context of an absolute domain name
## Zone Name Servers
* DNS namespace divided non-overlapping zones
* Each zone contains a part of the DNS tree and also name servers authoritative for that zone
* Usually 2 name servers for a zone
* Sometimes secondary is actually outside the zone
* Name servers are arranged in a hierarchical manner extending from a set of root servers
## Root Name Servers
* The root servers form the authoritative cluster for enquiry in the event of locally unresolvable name queries
* There are 13 root servers globally
## Resource Records
* The resources records are the key objects in the Domain Name System
* A RR consists of a domain name, TTL, class, type, value
* Domain name: Which domain this record applies to
* TTL: Indicates stability or temporal extent of the record
* Class: IN for internet
* Type: a closed vocabulary of the following:
* A: The Internet address of the host
* CNAME: The canonical name of an alias
* MX: The mail exchanger
* NS: The name server
* PTR: The host name if the query is in the form of an Internet address; Otherwise the pointer to other information
* SOA: The domain's start-of-authority information
* Value: data
### Example of asking for domain name
1. User machine runs the client side of the DNS software
2. Browser extracts the hostname from the URL, and passes it to the client-side of the DNS application
3. DNS client send a query containing the hostname to a DNS server
4. DNS client eventually receives a reply containing the IP address for the hostname
5. Browser initiates a TCP connection to the process located at port 80 at the IP address
## DNS in Action
* Finding the IP address for a given hostname is called name resolution and is done with the DNS protocol
* Resolution:
* Computer requests local name server to resolve
* Local name server asks the root name server
* Root returns the name server for a lower zone
* Continue down zones until name server can answer
* DNS protocol:
* Runs on UDP port 53, retransmits when lost messages
* Caches name server answers for better performance
> Example of resolution
>> <img src='images/Resolution.png' width=50%>
* Iterated query: Contacted server replies with name of server to contact
* Recursive query: Server obtains mapping on client's behalf
## DNS Caching and Updating Records
* Once name server learns a mapping, it caches the mapping
* IP addresses of TLD servers typically cached in local name servers
* Cache entries timeout after some time
## World Wide Web (WWW)
* Client and server software:
* Firefox is the client software for web access where Apache is on the server side
* Web markup languages:
* HTML is how webpages are coded
* Web scripting languages:
* Javascript adds more dynamicity to web webpages
* HTTp is about how to transfer
## Web Access
* A web page consists of objects
* An object can be HTML file but also JPEG image, Java applet, audio file
* A web page consists of a base HTML file which includes several referenced objects
* Each object is addressable by a URL (Uniform Resource Locator)
> Example of a URL:
>> <img src='images/URL.png' width=50%>
## Hypertext Transfer Protocol (HTTP)
* HTTP is at the application layer
* Client/Server Model
* Client: Browser that requests, receives and displays Web objects
* Server: Web server sends object in response to requests
> Illustration of HTTP
>> <img src='images/HTTP.png' width=50%>
### HTTP Connections
* Non-persistent HTTP: at most one object sent over a TCP connection
* Persistent HTTP: Multiple objects can be sent over a single TCP connection between client and server
### Non-persistent HTTP
* Example of visiting www.someSchool.edu/someDepartment/home.index:
1. a) HTTP clients initiates TCP connection to HTTP server process at www.someSchool.edu on port 80 \
b) HTTP server at host www.someSchool.edu waiting for TCP connection at port 80. Accepts connection and notify client
2. HTTP client sends a HTTP request message, containing URL, into TCO connection socket. Message indicates that client wants object someDepartment/home.index
3. HTTP server receives request message, form response message containing requested object, and sends message into its socket
4. HTTP client receives response message containing HTML file
5. HTTP server closes TCP connection
6. Parses HTML file and finds 10 referenced jpeg objects
7. Steps 1-6 repeated for each pf the 10 jpeg objects
### Response Time
* Round Trip Time (RTT): Time for a small packet to travel from client to server and back
* Response time:
* One RTT to initiate TCP connection
* One RTT for HTTP request and first few bytes of HTTP response to return
* File transmission time
* Total Response time = 2 RTT + file transmission time
> Illustration of HTTP response
>> <img src='images/Response_Time.png' width=50%>
### Non-persistent HTTP issues
* Requires new connection per requested object
* OS overhead for each TCP connection
* Delivery delay of 2 RTTs per requested object
### Persistant HTTP
* Server leaves connection open after sending response
* Subsequent HTTP messages between same client/server sent over open connection
* Pipelining: Client sends request as soon as it encounters a referenced object
* As little as one RTT for all the referenced objects
* Server closes a connection if it hasn't been used for some time
### HTTP Request Methods
|Method|Description|
|----|----|
|GET|Request to read a web page|
|HEAD|Request to read a web page's header|
|PUT|Request to store a web page|
|POST|Append to a named resource|
|DELETE|Remove the web page|
|TRACE|Echo the incoming request|
|CONNECT|Reserved for future use|
|OPTIONS|Query certain options|
### HTTP Error Codes
|Code|Meaning|Examples|
|----|----|----|
|1xx|Information|100 = server agrees to handle client's request|
|2xx|Success|200 = request succeeded; 204 = no content present|
|3xx|Redirection|301 = page moved; 304 = cached page still valid|
|4xx|Client error|403 = forbidden page; 404 = page not found|
|5xx|Server error|500 = internal server error; 503 = try again later|
## Cookies
* The http servers are stateless
* Cookies to place small amount of info on users computer and reuse deterministically
* Questionable mechanism for tracking users
* Advantages:
* Authorization
* Shopping carts
* Recommendations
* User session state
### Cookies vs. Sessions
|Sessions|Cookies|
|----|----|
|Sessions information regarding visitor's interaction stored at the server side: upto some hours|Cookies are transferred between server and client|
|When user closes the website, the session ends|Cookie information stored at both client and server|
|Sessions information size can be large|Maintain client information until deleted|
||Cookies information size limited|
## Web Caches (Proxy Server)
* Goal is to satisfy client request without involving origin server
* User sets browser to access web via cache
* Browser sends all HTTP requests to cache
* If object in cache, cache returns object, else cache requests object from origin server, then returns object to client
* Cache acts as both client and server
* Typically cache in installed by ISP
* Causes problems for frequently changing data
* Advantages:
* Reduce response time for client request
* Reduce traffic on an institution's access link
## Dealing with Multimedia Data
* Higher bandwidth requirements
* Higher QoS requirement
* Separate providers
* Not all communication is one-to-one. Many is multicast/broadcast which is different to most traffic
* Specialized infrastructure also needs special attention
## A basic model for multimedia on the web
> Illustration of the multimedia basic model
>> <img src='images/Basic_Model.png' width=50%>
* Problems:
* The entire media file must be transmitted over the network before playback starts
* Basic model assumes mainly point-to-point data distribution rather than a point-to-multipoint distribution model
* Basic model relies on simple browser/plugin/helper integration and traditional service types
## Streaming Media Protocols
* HTTP
* RTP: Real-time Protocol
* MPEG-4
* Microsoft's Windows Media
## Specialized Multimedia Software
* 4 main tasks of the multimedia playback software:
* To deal with the user interface side:
* Functions such as volume control, playback, next
* Handle transmission errors in conjunction with transport protocols
* Using RTP/UDP errors will likely occur, playback software must manage/mask them gracefully
* Eliminate jitter
* Small buffer, quick playback but susceptible to jitter/delay
* Large buffer, delay at start of playback while buffer fills, but less susceptible to delay/jitter
* Sometimes compress and almost always decompress the multimedia files to reduce size
> Specialized Model
>> <img src='images/Specialized_Model.png' width=50%>
## Handling Errors
* Forward Error Correction: The error-correcting encoding of data. For every X data packets Y new packets are added similar to methods. These contains redundant bits that are used to deal with errors
* Methods used parity or exclusive-OR sums of the bits in each of the data packets but are more complex
* Error Resilience: Remarking for re-sync so that a packet loss does not create a total loss, mainly on sender side
* Error Concealment: Done by the receiver
* Retransmission: Less meaningful for streaming data but for watching a movie. This can be deployed for lost packets of the movie.
## Jitter management
* Jitter happens because of variable bandwidth and loss/retransmissions. Therefore, we use buffers.
> Illustration of buffers
>> <img src='images/Buffer.png' width=50%>
## Compression
* ADC (Analog-to-Digital Converter) produces digital data
> Example of ADC
>> <img src='images/ADC.png' width=50%>
### Audio compression
* Use Nyquist and Shannon theorems to convert analog data to digital. Then apply techniques to eliminate some data
* E.g.
* Perceptual coding is that some data can mask other data
* Frequency masking: Some sounds mask/hide others so there is no point encoding them
* Temporal masking: Human ears can miss soft sounds immediately after loud sounds, takes time for ear to adjust, no need to store them.
### MP3
* MP3 is MPEG Audio Player 3
* MP3's compression is based on perceptual coding
* MP3 audio compression results in significant file size savings without a perceived loss of audio quality
* Typical MP3 audio compression rates for CS quality audio reduce the need for bandwidth for 1.4 Mbps for stereo down to 96-128 Kbps
### For digital video
* Video is digitized as pixels
* Video is sent compressed due to its large bandwidth requirements
* Lossy compression exploits human perception
* Large compression ratios achieved
### JPEG
* JPEG is lossy compression
* JPEG often provides compression ratios of 20:1
* JPEG compression is symmetric, decoding takes as long as encoding
### MPEG
* MPEG is Motion Picture Experts Group
* MPEG can compress both audio and video together
* The evolution of MPEG:
* MPEG-1: VCR quality at 1.2 Mbps (40:1)
* MPEG-2: Broadcast quality at 4-6Mbps (200:1)
* MPEG-4: DVD quality at 10Mbps (1200:1)
## Email
* User Agent: Thunderbird
* Message Transfer Agent: Exchange
* Message Transfer Protocol
## Email Services
* Standards for Internet-enabled email are based on 2 RFC's
* RFC 821 (transmission)
* RFC 822 (message format)
* RFC 2821 and RFC 2822 (revised versions of earlier RFCs)
## Architecture and Services of Email
* User agents: Allow user to read and send mail
* Message transfer agents: Transport messages from source to destination
* Simple Mail Transfer Protocol (SMTP): Used to send messages from the sender
* Mail server to the receiver's mail server
* User agent to the sender's mail server
> Architecture of Email Service
>> <img src='images/Email_Architecture.png' width=50%>
## User agent
* Basic functions: compose, report, display and dispose
* Envelope and contents: encapsulation of transport related information
* Envelope: Destination address, priority, and security level, all of which are distinct from the message itself
* Mail servers use the envelope for routing
* Header and body:
* Header: User agent control info
* Body: For human recipient
* Contains contains control information for the user agents
* User must provide message, destination, optional other parameters
## RFC 822: Message
* RFC 822 doesn't distinguish header and envelope fields
* RFC 822 allows users to invent new headers for private use but they must start with X-
## Multipurpose Internet Mail Extensions (MIME)
* RFC822 was enough for these simple constraints
* In time the inadequacy of RFC822 became apparent
* Languages with accents
* Non-Latin alphabets
* Non-alphabetic language
* Messages with content other than text
* As a result, MIME (RFC 1341) was written
* MIME retains RFC822 format but adds structural elements to the message body and defines encoding rules for non-ASCII messages
* MIME has 5 additional message headers:
* MIME-Version: Identifies the MIME version
* Content-Description: Human readable describing contents
* Content-Id: Unique Identifier
* Content-Transfer-Encoding: How body is wrapped for transmission
* Content-Type: Type and format of content
### MIME Types and Subtypes
|Type|Example subtypes|Description|
|----|----|----|
|text|plain, html, xml, css|Text in various formats|
|image|gif, jpeg, tiff|Pictures|
|audio|basic, mpeg, mp4|Sounds|
|video|mpeg, mp4, quicktime|Movies|
|model|vrml|3D model|
|application|octet-stream, pdf, javascript, zip|Data produced by applications|
|message|http, rfc822|Encapsulated message|
|multipart|mixed, alternative, parallel, digest|Combination of multiple types|
## Message Transfer
* Transfer: SMTP (Simple Message Transfer Protocol)
* Delivery:
* POP3 (Post Office Protocol 3): Download to a single device
* IMAP (Internet Message Access Protocol): Designed with multiple devices in mind
## SMTP
* Simple Message Transfer Protocol
* Simple ASCII protocol, operating on TCP port 25
* RFC 821: Simple Mail Transfer Protocol
* RFC 2821: Extended Simple Mail Transfer Protocol
* Basic steps:
* User agent submits to mail transfer agent on port 587
* One mail transfer agent to the next one on port 25
* Other protocols used for final delivery (IMAP, POP3)
## IMAP
* Used for final delivery
* Internet Message Access Protocol
* RFC 3501 defines version 4
* User agent runs an IMAP client for this
* Protocol has command like: Login, List, Copy, Create, Delete
* Main difference with POP3 is mail remains on server
* Complex protocol but makes mail machine independent
## POP3
* Email is taken into the user computer
* Simpler protocol
* Ties emails to one's machine
## Webmail
* Gmail and alike
* A service run by a company server
* An interface to managing email over the Web
* Mainly it is an user interface
## Spam
* Unwanted email
* Main countermeasures are:
* Filters based on email content
* Blacklisting known spam addresses
* Parking email from unknown sources
* Collection spam and creating a knowledge-base
* Detecting mass emails
# Other Layers
## Two more layers from the OSI model
* Presentation layer
* Session layer
* They did not see a distinct use and labeling
## Presentation Layer
* Formatting related issues
* Encryption and Decryption
* Should be done in presentation layer as well although it is commonly done at application layer
* Compression
* It should be done at this layer rather than in application layer
### Why not have an explicit presentation layer
* Internet does not have this clear distinction
* A key reason is many presentation layer list of things to do that is considered to be application specific. Thus, application layer and presentation layer is not explicitly separated for internet
## Session layer
* Common service of this layer:
* Authentication
* Session management
* Monitoring connections
* Disconnect if not used
* Reconnect if needed
* These are also seen as a part of the application layer duties today depending on different requirements of applications of today's Internet
* A few are done at Transport layer
* Especially session management in a simple client server architecture is trivial
## Existence of these layers
* If you have need to do compression, session management, then think before implementing
* You should probably create a better software design by creating your own little session layer as a separate layer in your software architecture
* This is what most software architects do: Create a middleware layer in their software
## P2P systems
* Client server systems dominated the Internet for a while because they are simple to implement
* Peer-to-Peer systems (P2P) are more complex:
* A P2P system:
* Does not have client and server but just peers
* Does not have a central point of control
* Advantages:
* No central point of control or failure
* Potential to scale without a bottleneck
* Disadvantages:
* Harder to develop applications on a dynamic platform where PCs come and go
* Not all peers can know about all others in a large system.
> Example P2P system
>> <img src='images/P2P.png' width=50%>
> * The black dots depict live nodes. The blue ones are files. The address space is considered as circular: node 0 is adjacent to node $(2^{128}-1)$. The diagram illustrates the routing from node 65A1FC to D46A1C. This happens at application layer at each PC
<h1 id='Security'>Security</h1>
## What is network security
* Network security is a combo of 4 related areas:
* Secrecy: Keep information hidden from a general audience
* Authentication: Ensuring the user you are giving content to has valid credentials
* Non-repudiation: Prove a content was created by a named user
* Integrity control: Ensure that a content has not been tampered with
* All of those are equally valid and has been around for all systems for some time, but have different and sometimes more challenging implications in a networked environment
* Aspects of security can be found at all layers of a protocol stack, there is no way to secure a network by building security into one layer only
* Most security implementations are based on common cryptographic principles and appear on almost all layers
## Cryptography
* A key area/set of algorithms for creating secrets, authenticating users, making sure messages are not tampered with, and edits are not denied by the original author
## Encryption
> Encryption Model
>> <img src='images/Encryption_Model.png' width=50%>
### Key Cryptography Concepts
* Three foundations:
* Plaintext: Messages to be encrypted can be transformed by a function.
* Key: A function that parameterized the transformation
* Ciphertext: The output of the transformation process
* Kerckhoff's principle: Cryptographic algorithms and related functions (E, D) are public; Only the keys (K) are secret
### The notations
* C = Ciphertext
* P = plaintext
* E = Encryption
* D = Decryption
* K = Key
### Keys
* A key is a string that allows the selection of one of many potential encryptions
* The key can be changed as often as required
* Algorithms are more likely to be at the hands of attackers anyhow, not changed as frequently
* Cipher is a term commonly used as the term for algorithm
* The size of the overall key space is determined by the number of bits in the key string
* The longer the key, the more effort is required to break a given encryption
### XOR
* An XOR is an exclusive or function used regularly
* A XOR B means A or B, but not both
* XOR is commonly used in cryptography
> Exclusive ORs
>> <img src='images/XOR.png' width=50%>
## Main Types of Ciphers
* Substitution cipher: Each letter of group of letters is replaced systematically by other letters or groups of letters
* Transposition cipher: All letters are re-ordered without disguising them
* One-time pad:
* Uses a random bit string as the key: convert the plaintext into a bit string, then XOR the two strings bit by bit
* Harder to break
# Key-based Algorithms
* Symmetric key algorithms: Use the same key for both encryption and decryption. Symmetric key algorithms can use permutation, substitution and a combination of both to encrypt and decrypt
* 2 Symmetric Key Algorithms:
* Data Encryption Standard (DES)
* Uses 64 bit blocks and 56 bit keys
* $2^{56}$ key space
* Triple DES has a $3*2^{56}$ key space
* Advance Encryption Standard(AES)
* Uses 128 bit blocks and 128 bit keys
* $2^{128}$ key space
* Still substitution and permutation based with multiple rounds
## Cipher Block Chaining Mode
* Same text leads to same ciphertext unless something else is done
* In block chaining mode, each plaintext block is XOR with the previous ciphertext block being encrypted
> Example of CBC
>> <img src='images/CBC.png' width=50%>
## Cipher Feedback Mode
* In cipher feedback mode, byte-by-byte encryption is used rather than block-by-block encryption
* Good for things like encryption someones key strokes on a keyboard where a lot of data is not immediately available
## Stream Cipher Mode
* In stream cipher mode, recursive sequential block encryption is sued has one-time pad, and XOR with plaintext to generate ciphertext
> Illustration of Stream Cipher Mode
>> <img src='images/Stream_Cipher.png' width=50%>
## Counter mode
* In counter mode, plaintext is not directly encrypted, but an initialization parameter plus an arbitrary constant is encrypted and the resulting ciphertext is XOR with plaintext
> Illustration of Counter Mode
>> <img src='images/Counter_Mode.png' width=50%>
## Symmetric Key Algorithms
|Cipher|Author|Key Length|Comments|
|----|----|----|----|
|Blowfish|<NAME>|1-448 bits|Old and Slow|
|DES|IBM|56 bits|Too weak to use now|
|IDEA|Massey and Xuejia|128 bits|Good, but patented|
|RC4|<NAME>|1-2048 bits|Some keys are weak|
|RC5|Ronald Rivest|128-256 bits|Good, but patented|
|Rijndael|Daemen and Rijmen|128-256 bits|Best choice|
|Serpent|Anderson, Biham, Knudsem|128-256 bits|Very strong|
|Triple DES|IBM|168 bits|Second best choice|
|Twofish|<NAME>|128-256 bits|Very strongly; Widely used|
## Public Key Algorithms
* Fundamentally different to symmetric key ones
* Difff & Hellman proposed the new mode: Asymmetric Keys:
* Two keys are used
* Not easily derivable from each other
* Hence addressing a fundamental issue of key sharing
## Asymmetric Keys
* Key 1, public key: Usable by anyone to encrypt messages to the owner of the key, this key known to all
* Key 2, private key: Required to decrypt the message and know only by the owner of this key
## The process of asymmetric keys
* C = ciphertext, P = plaintext, E = Encryption, D = Decryption, K1, K2 = keys
### RSA Algorithm
* Key generation:
* Choose two large primes, p and q
* Compute n = p * q and z = (p-1) * (q-1)
* Choose d to be relatively prime to z which means no common factors
* Find e such that: (d * e) mod z = 1
* Public key is (e, n) and private key is (d, n)
* Encryption: C = $P^e \ mod \ n$
* Decryption: P = $C^d \ mod \ n$
### RSA Security
* RSA's security is based on the difficulty involved in factoring large numbers in math theory: Approximately $10^25$ years to factor a 500 digit number and RSA uses 1024 bits
* Disadvantage: Too slow for encrypting/decrypting large volumes of data
* RSA is widely used for other things such as secure key distribution
## Digital Signatures
* Cryptographic approaches can also be used to ensure authenticity and allow for non-repudiation
* Requirements:
* Receiver can verify the claimed identity of the sender
* Sender cannot deny the created contents of the message
* Receiver cannot have derived the message themselves
* Approaches:
* Using symmetric keys via an intermediary
* Using public keys as individuals
## Signatures with Message Digests
* Basic concept of a message digest is to use a one-way hash function for an arbitrary length of plaintext, so that it becomes a unique small fixed-length bit string
* Thus no need to deal with huge message text and encryption just for authentication purposes
* A message digest (MD) has four important properties:
1. Given P, it is easy to compute MD(P)
2. Given MD(P), it is effectively impossible to find P
3. Given P, no one can find P' such that MD(P') = MD(P)
4. A change in even a single bit of input produces a very different output
* Famous Message Digest Algorithms:
* MD5
* SHA-1
## Public Key Management
* There is specific PK infrastructure to avoid compromising the security of PK's during the initial distribution process
* Certification Authority (CA): A trust intermediary who uses non-electronic identification to identify users prior to certifying keys and certificates
* X.509: An international standard for certificate expression
* PKI (Public Key Infrastructure): Hierarchically structured certificate authorities allow for the establishment of a chain of trust or certification path
## Authentication
* Authentication is a primary tenet of network security
* Authentication process itself needs to be secure also.
* A fundamental principle: Minimize the use of permanent keys in establishment of secure connections. The less packets are exchanged using such keys, the less exposure to potential attackers
* Four methods in common use:
* Shared keys
* Key distribution
* Kerberos
* Public keys
## Shared Secret Key
> Illustration of Shared Secret Key
>> <img src='images/Secret_Key.png' width=50%>
## Key Distribution Center
* A trusted intermediary is used to facilitate
* Users each share a key with a central key distribution center, and authenticate to the KDC directly
* The KDC acts as a relay between the two parties
* Issues: Replay-attack
* Solution: Timestamps
## Kerberos
* Similar to KDC, Kerberos is a popluar emerged and in frequent use
* A multi-component system is required:
* Authentication Server
* Ticket Granting Server (TGS)
* Recipient
* Authentication is managed centrally, and then party to party communication is facilitated by single use tickets
* Disadvantages: Does not scale to large numbers. Different businesses need to trust each other's TGSs
## Public Keys Cryptography
> Illustration of public key
>> <img src='images/Public_Key.png' width=50%>
## IPSec
* Position of security: Should be in multiple layers
* One can put security at application level but also other layers
* IPSec (RFC 2401) puts at the network level as well
* In the IPSec model, encryption is compulsory, but a null encryption algorithm can be used between points
* The main IPSec framework features are secrecy, data integrity, and replay attack protection
* the IPSec framework allows multiple algorithms and multiple levels of granularity, connection-oriented
### Implementation
* IPSec has two main implementation components:
* Things being added to packets in transit
* ISAKMP key management: Internet Security Association and Key Management Protocol for establishing keys
* IPSec has 2 modes
* Transport mode: Uses header insertion after IP header
* Tunnel mode: Uses packet encapsulation
## Virtual Private Networks
* Unlike a physical network based on leased lines between locations for which secure transit is required
* A virtual private network (VPN) is a virtual layer on top of an IP network which provides a secure end-to-end connection over public infrastructure
* A common VPN implementation model:
* Use a firewall at each end of a connection
* Setup a SA to create an IPSec tunnel between the two end points
* Communication on this infrastructure is transparent to end users
> Illustration of VPN
>> <img src='images/VPN.png' width=50%>
## Firewalls
* While IPSec ensures security in transit, a firewall ensures security at the network perimeter
* Firewalls are positioned at the network boundary, and provide a controlled series of routes between the internal and external networks
* Three characteristics of firewalls:
* All inbound and outbound traffic must transit the firewall
* Only authorized traffic must pass through the firewall
* Firewalls should be immune to penetration themselves
### Firewall scope
* Check packets for bad packets
* Administrators can write rules for this
* Not everything is inside the wall
* Web servers and email servers need to be exposed to allow more open communication
* Best firewall is not disconnection everything from the internet
* Through further rules packets go in-between this gray area and the LAN
* Firewalls do not provide protection against inhouse threats
* Applications can still distribute viruses via bad attachments
## Wireless Security Context
* Wired networks are relatively easy to secure because they require physical access to intercept traffic
* Wireless networks are more difficult to secure because of omnidirectional signal propagation
* Additionally by default most wireless network equipment operates in an insecure and promiscuous manner
* 802.11 has a native secure protocol, Wired Equivalency Protocol, which is a 40-bit encryption based on RC4 algorithm
* Two inherent insecurities:
* 40 bit encryption is breakable with low-moderate computational resources
* RC4 re-uses keys, so capturing a small volume of encrypted traffic will guarantee key identification
* Additional encryption (128 bit WEP)
* Increased security through longer key lengths
* MAC Address Filtering
* Only allow specified MAC interfaces to establish connection
* WPA2
* Multilayered security<file_sep># Week 2 Introduction to Networking
## OSI Reference Model
* Open Systems Interconnection
* Has 7 layers
* Layer divisions based on principled decisions
### OSI Layer Division Principles
1. A layer should be created where a different abstraction is needed
2. Each layer should perform a well defined function
3. The function of each layer should be chosen with a view toward defining internationally standardized protocols
4. The layer boundaries should be chosen to minimize the information flow across the interfaces
5. The number of layers should be large enough that distinct functions need not to be thrown together in the same layer out of necessity, and small enough that the architecture does not become unwieldy
> Structure of OSI Model
>> <img src='OSI_Model.png' width=50%>
## TCP/IP Model
* Transmission Control Protocol/Internet Protocol
* Has 4 layers
> Structure of TCP/IP Model
>> <img src='TCP/IP_Model.png' width=50%>
## Comparison of OSI and TCP/IP Models
### Critique of OSI Model
* Bad Timing
* Bad Technology
* Bad implementations
* Bad politics
### Critique of TCP/IP Model
* Service, interface and protocol not distinguished
* Not a general model
* Host-to-Network layer not really a lay but an interface between network and data link layers
* No mention of physical and data link layers
* Minor protocols deeply entrenched, hard to replace
## Hybrid model
* An hybrid model of OSI model and TCP/IP model
> Structure of the Hybrid Model
>> <img src='Hybrid_Model.png' width=50%>
## Network Standardization
|Body|Area|Examples|
|----|----|----|
|<font color=pink>ITU</font> (International Telecommunication Union)|Telecommunications|ADSL, PON, MPEG4|
|<font color=pink>IEEE</font> (Institute of Electrical and Electronics Engineers)|Communications|Ethernet, WiFi|
|<font color=pink>IETF</font> (Internet Engineering Task Force)|Internet|HTTP/1.1, DNS|
|<font color=pink>W3C</font> (The World Wide Web Consortium)|Web|HTML5 standard|
# Physical Layer
## What is the Physical Layer
* The physical layer is the lowest layer in OSI model
* The physical layer's properties in TCP/IP model are in the host-to-network division
* The physical layer is concerned with the mechanical, electrical and timing interfaces of the network
* Various physical media can be sued to transmit data, but all of them are affected by a range of physical properties and hence have distinct differences
### Link Model
* <font color=red>Simplified Link Model</font>: Consider the network as a connected link between computers.
> Example of Link Model
>> <img src='Link_Model.png' width=50%>
* <font color=pink>Bandwidth</font> is usually treated as rate of transmission in bits/second
* <font color=pink>Delay (in seconds)</font> is the time required for the first bit to travel from computer A to computer B.
## Message Latency
* <font color=pink>Latency</font> is the delay associated with sending a message over a link
* This is made up of two parts related to the link model:
* Transmission delay:
* T-delay = Message in bits / Rate of transmission = M/R (seconds)
* Propagation delay:
* P-delay = Length of the channel / speed of signals
* Latency = M/R + P-delay
## Twisted Pair
* Construction:
* Two insulated copper wires, twisted in helical form
* Twisting reduces radiance of waves from effectively parallel antennae
* Distance up to < 5km, repeaters can extend this distance
* Twisting reduces interference
* Properties and Types of Twisted Pair:
* Bandwidth dependent on distance, wire quality/density
* <font color=pink>Cat 3</font>: 2 wires, 4 pairs in sheath, 16 Mhz
* <font color=pink>Cat 5</font>: 2 wires, 4 pair in sheath, more twists, 100 Mhz
* <font color=pink>Cat 6</font>: 250 Mhz
## Coaxial Cable ("Co-ax")
* Better shielding than twisted pair = higher speeds
* Copper core with insulation, mesh, and sheath
* Bandwidth approaches 1 Ghz
* Still widely used for cable TV/Internet
## Fiber Optics
* Optical transmission has three components:
* Light source
* Transmission medium
* Detector
* Semantics: light = 1, no light = 0
* Data transmission over a fiber of glass
* A detector generates electrical pulse when light hits it
* Common for high rates and long distances
* Fiber has enormous bandwidth and tiny signal loss - hence high rates over long distances
* Types of Fiber Optics:
* Single-mode:
* Core so narrow light can't even bounce around
* Used with laser for long distances
* Multi-mode:
* Other main type of fiber
* Light can bounce
* Used with LEDs for cheaper, shorter distance links
* Fiber optic connections:
* Connector and Fiber sockets (10-20% loss, but easy to configure)
* Mechanical Splice (10% loss, labor intensive)
* Fusion (<1% loss, but very specialized)
* Fiber optic cable is a scalable network media - LAN, WAN
* Fiber optic cable can be considered either as a ring or as a bus network type
## Comparison of Wires and Fiber
|Property|Wires|Fiber|
|----|----|----|
|Distance|Short (100s of m)| Long (10s of km)|
|Bandwidth|Moderate|Very high|
|Cost|Inexpensive|More Expensive|
|Convenience|Easy to use|Harder to use|
|Security|Easy to tap|Hard to tap|
## Wireless Transmission
* Mobile users requires a mobility enabled network
* Wireless networks can provide advantages even in fixed location environments
* There are many types of wireless data transmission networks, but they all have a common basis: radio wave propagation
* Wireless signals are broadcasted over a region
* Potential signal collisions
### Electromagnetic Spectrum
* Number of oscillations per second of a wave is called frequency , measured in Hz
* Distance between two consecutive minima or maxima is called wavelength
* All electromagnetic waves travel at same speed
* Fundamental relationship:
* Wavelength * Frequency = Speed of Light
## Wireless vs. Wires/Fiber
* Wireless:
* Easy and inexpensive to deploy
* Naturally supports mobility
* Naturally supports broadcast
* Transmissions interfere and must be managed
* Signal strengths hence data rates vary greatly
* Wires/Fiber
* Easy to engineer a fixed data rate over point-to-point links
* Can be expensive to deploy
* Does not readily support mobility or broadcast
## Communication Satellites
* Satellites are effective for broadcast distribution and anywhere/anytime communications
### Kinds of Satellites
* Geostationary Satellite (GEO):
* 35000 km above a fixed location
* VSAT computers can communicate with the help of a hub
* Different bands in the GHz are in use but may be crowded or susceptible to rain
* Low-Earth Orbit Satellites (LEO)
* Systems such as Iridium use many low-latency satellites for coverage and route communications via them
> Different Satellites' high distribution
>> <img src='Satellites.png' width=50%>
## Satellite vs. Fiber
* Satellite:
* Can rapidly set up anywhere/anytime communications after satellites have been launched
* Can broadcast to large regions
* Limited bandwidth and interference to manage
* Fiber:
* Enormous bandwidth over long distances
* Installation can be more expensive/difficult
## Bits to a Link
* Model types:
* Full-duplex Link: Used for transmission in both directions at once. <font color=pink>E.g. Use different twisted pairs for each direction</font>
* Half-duplex Link: Both directions, but not at the same time. <font color=pink>Senders take turns on a wireless channel</font>
* Simplex Link: Only one fixed directions at all times; Not very common
### Multiplexing
* When multiple sources want to put things onto a medium
* Time Division Multiplexing:
* Users can send according to a fixed schedule
* Slotted access to the full speed of the media
> Example of Time Division Multiplexing:
>> <img src='Time_Division.png' width=50%>
* Frequency Division Multiplexing
* Users can only use specific frequencies to send their data
* Continuous access with lower speed
> Example of Frequency Division Multiplexing:
>> <img src='Frequency_Division.png' width=50%>
* Statistical Multiplexing
* Code Division Multiple Access
## How bits look as a signal
* Information on wire transmitted by variance of a physical property
* Generating a periodic function
* Three things can change the behaviour of the function: Amplitude, Frequency, and Phase
> Change in Amplitude, Frequency, and Phase of a function:
>> <img src='Change_Amplitude.png' width=50%>
### Modulation Types
> Modulating the amplitude, frequency or phase of a carrier signal sends bits in a frequency range:
>> <img src='Modulation.png' width=50%>
## Maximum Data Rate of a Channel
### Nyquist's theorem
* If a function contains no frequencies higher than B Hz then it is completely determined by looking at a set of points spaced 1/2B apart
* Nyquist's theorem relates the data rate to the bandwidth (B) in Hz of a signal and the number of signal levels V used:
> <img src='Nyquist.png' width=50%>
### Shannon's Theorem
* Shannon's theorem relates the data rate to the bandwidth (B) and signal strength (S) relative to the noise (N):
> <img src='Shannon.png' width=50%>
<file_sep># Week 4 Data Link Layer
## Error Correcting Codes Key Points
* More efficient in noisy transmission media
* Challenge is that the error can be in the check bits
* Assumption on a specific number of errors occurring in transmission
## Error Detecting Codes
* More efficient in some transmission media
* Types of error detecting codes:
* Parity (1 bit): Hamming distance = 2
* Checksum (16 bits): Hamming distance = 2
* Cyclical Redundancy Check (Standard 32 bit CRC): Hamming distance = 4
### How does error detecting codes works
* Sender calculates R check bits using a function of data bits
* Receiver receive the codeword and calculates the same function on the data and match the results with received data check bits
### Parity Bit
* E.g. 10001110 is data and count the number of 1s
> Received: 100011100(For even parity)/100011101(For odd parity) and then transfer
> Check the transferred data for errors on arrival and can catch:
>> 2 - 1 = 1 error bit can be detected
>> and
>> (2 - 1)/2 = 0.5 which not even 1 bit error can be corrected
## Checksum
* There are different variation of checksum
* Internet checksum (16-bit word): Sum modulo 2^16 and adding any overflow of high order bits back into low-order bits
> Example of Checksum for data 00110 10001 11001 01011
>> <img src='Checksum.png' width=50%>
## Cyclic Redundancy Check
* Based on a generator polynomial G(x)
> Example: G(x) = x^4 + x + 1(10011)
> * Let r be the degree of G(x) therefore r = 4. Append r zero bits to the low-order end of the frame so it now contains m + r bits and corresponds to the polynomial x^r * M(x)
> * Divide the bit string corresponding to G(x) into the bit string corresponding to x^r * M(x), using modulo 2 division
> * Subtract the remainder from the bit string corresponding to x^r * M(x) using modulo 2 subtraction. The result is the checksum frame to be transmitted. Call its polynomial T(x)
>> <img src='CRC.png' width=50%>
## Data Transmission
* A service to send message should have:
* Reliability
* Each layer need to make sure the service provided to other layers is reliable
* Retransmission with error detection is a way of ensuring reliability
* Error correction is another way but has its own shortcomings
* Flow Control
* The fast sender vs. slow receivers requires a solution
* Principles to control when sender can send next frame
* Feedback based flow control is usually used in Data Link Layer
* Rate based flow control
## Acknowledged transmission
* Data transmitted in one direction
* Time is relatively important, buffer space constrained
> Example of Acknowledged Transmission
>> <img src='Acknowledged_Transmission.png' width=50%>
## Noisy Channel Protocol
* Frames can be lost either entirely or partially
* Requires distinction between frames already sent/received and those being re-transmitted
* Requires timeout function to determine arrival or non-arrival of complete frames
## Stop and Wait Protocol
* Concept of ARQ (Automatic Repeat reQuest)
* Ack and Timeout
* Stop and Wait
* One bit Ack
> Example of Stop and Wait Protocol
>> <img src='Stop_and_Wait.png' width=50%>
### Link Utilization
* Principle of efficiency in communication is measured by Link Utilization (U)
> Let B be the bit-rate of the link and L be the length of the frame.
> Tf = Time needed to transmit a frame of length L
> Tp = Propagation delay of the channel
> Ta = Time for transmitting an Ack
> So Tf = L/B. Assume that Ta = 0, Tt = Tf + 2 * Tp
> U = (Time of transmitting a frame)/(Total time for the transfer) = Tf/Tt = Tf / (Tf+2Tp) = (L/B)/(L/B + 2Tp) = L/(L + 2 * Tp * B)
## Sliding Window Protocols
* Data is commonly transmitted in both direction simultaneously
* Sender maintains a set of sequence numbers corresponding to frames it is allowed to send
* Receiver maintains a set of sequence numbers corresponding to frames it is allowed to accept
* Stop and Wait can be seen as a special case with window size 1
### Protocol Using Go-Back-N
* Long transmission times need to be taken into account when programming timeouts
* Senders don't need to wait for acknowledgement for each frame before sending next frame
> Example of Go-Back-N protocol
>> <img src='Go_Back_N.png' width=50%>
### Selective Repeat
* Receiver accepts frames anywhere in receive window
* Cumulative ack indicate highest in-order frame
* NAK (negative ack) causes sender retransmission of missing frame before a timeout resend window
> Example of Selective Repeat
>> <img src='Selective_Repeat.png' width=50%>
### Go-Back-N vs. Selective Repeat
* Go-Back-N: Receiver discards all subsequent frames from error point, sending no acknowledgement, until the next frame in sequence
* Selective Repeat: Receiver buffers good frames after an error point, and relies on sender to resend oldest unacknowledged frames
* Trade-off between efficient use of bandwidth and data link layer buffer space
## Packet over SONET
* Packer over SONET is the method used to carry IP packets over SONET optical fiber links
* Uses PPP (Point-to-Point Protocol) for framing
> Structure of Packet over SONET
>> <img src='SONET.png' width=50%>
### PPP
* PPP (Point-to-Point Protocol) is a general method for delivering packets across links
* Framing uses a flag (0x7E) and byte stuffing
* Unnumbered mode (connectionless unacknowledged service) is used
* Errors are detected with checksum
> Example of a PPP packet
>> <img src='PPP.png' width=50%>
## ADSL
* Widely used for broadband Internet over local loops
* ADSL runs from modem to DSLAM
* IP packets are sent over PPP and AAL5/ATM
> Example structure of ADSL
>> <img src='ADSL.png' width=50%>
* PPP data is sent in AAL5 frames over ATM cells:
* ATM is a data link layer that uses short, fixed-size cells and each cell has a virtual circuit identifier
* ALL5 is a format to send packets over ATM
* PPP frame is converted to a AAL5 frame (PPPoA)
> Example of AAL5 Frame
>> <img src='AAL5_Frame.png' width=50%>
# MAC Sub-layer
## MAC Sub-layer
* On point to point networks, there are only singular sender and receiver pairs, eliminating transmission contention
* On broadcast networks, determining right to transmit is a complex problem
* Medium Access Control (MAC) sub-layer is used to assist in resolving transmission conflicts
## Channel Allocation Mechanisms
* Various methods exist for allocation a single broadcast channel amongst competing users
* Static Channel Allocation
* Dynamic Channel Allocation
### Static Channel Allocation
* Arbitrary division of a channel into segments and each user allocated allocated a dedicated segment for transmission
* Frequency Division Multiplexing (FDM) is typically used
* Significant inefficiencies arise when:
* Number of senders is greater than allocated segments
* Number of senders is not static
* Traffic is bursty
* Downfalls:
* Usually good for fixed number of users
* Network traffic is busty
* TDM and FDM try to give consistent access to the network leading to inefficiency in the use of network resources
## Dynamic Channel Allocation
* Channel segmentation is dynamic, segment allocation is dynamic
* Assumption for dynamic channel allocation:
* Independent transmission stations
* Single channel for all communication
* Simultaneous transmission results in damaged frames
* Time
* Transmission can begin at any time
* Transmission can begin only within discrete intervals
* Carrier Sense
* Detection of channel use prior to transmission
* No detection of channel use prior to transmission
## Multiple Access Protocols
### ALOHA
* Users transmit frames whenever they have data. Users retry after a random time if there are collision
* Requires no central control mechanism
* Efficient under low load but inefficient under high traffic loads
* Slotted ALOHA: Allows the users to start sending only at the beginning of defined slots. Increase efficiency of pure ALOHA by reducing possibility of collisions
### Carrier Sense Multiple Access Protocols (CSMA)
* In networks which require transmission state detection to determine transmission rights dynamically, there are specific protocols which are used:
* Persistent and Non-persistent CSMA
* CSMA with Collision Detection
#### Persistent and Non-Persistent CSMA
* When a sender has data to transmit, first check channel to detect other active transmission
* 1-persistent CSMA: Wait until channel idle. Transmit one frame and check collisions. If collision, wait for a random time and repeat.
* Non-persistent CSMA: If channel busy, wait random period and check again. If not, start transmitting.
* P-persistent CSMA: If channel idle, transmit with probability p, or wait with probability (1-p), and check again
> Example binary tree of 1-persistent, non-persistent and p-persistent
>> <img src='CSMA.png' width=50%> | 7ab551c604a55cd615d7491676195c3c70beacc3 | [
"Markdown"
] | 11 | Markdown | Stevey0714/COMP90007 | 7680362e94f2cf4fe2631a908096a4204ac451e6 | 1232b333439d0978f669bdbe4bd0c0de214b271e |
refs/heads/master | <repo_name>stevehan12138/3-Heart-Plugin<file_sep>/README.md
# 3-Heart-Plugin
A Plugin for stoneserver
Set player health depend on player's xp
<file_sep>/src/main.ts
const sys = server.registerSystem(0, 0);
sys.initialize = function (){
server.log("3 heart mod by Fjun loaded");
sys.listenForEvent<IBlockDestructionStartedEventData>("minecraft:block_destruction_started", eventData =>{
let player = eventData.data.player;
let playerName = sys.getComponent<INameableComponent>(player, MinecraftComponent.Nameable).data.name;
setHeart(player, playerName, 0, 4, 6);
setHeart(player, playerName, 5, 9, 8);
setHeart(player, playerName, 10, 14, 10);
setHeart(player, playerName, 15, 19, 12);
setHeart(player, playerName, 20, 24, 14);
setHeart(player, playerName, 25, 29, 16);
setHeart(player, playerName, 30, 34, 18);
setHeart(player, playerName, 35, 39, 20);
setHeart(player, playerName, 40, 44, 22);
setHeart(player, playerName, 45, 49, 24);
setHeart(player, playerName, 50, 54, 26);
setHeart(player, playerName, 55, 59, 28);
setHeart(player, playerName, 60, 64, 30);
setHeart(player, playerName, 65, 69, 32);
setHeart(player, playerName, 70, 74, 34);
setHeart(player, playerName, 75, 79, 36);
setHeart(player, playerName, 80, 84, 38);
setHeart(player, playerName, 85, 89, 40);
setHeart(player, playerName, 90, 94, 42);
setHeart(player, playerName, 95, 99, 44);
setHeart(player, playerName, 100, 104, 46);
setHeart(player, playerName, 105, 109, 48);
setHeart(player, playerName, 110, 114, 50);
setHeart(player, playerName, 115, 119, 52);
setHeart(player, playerName, 120, 124, 54);
setHeart(player, playerName, 125, 129, 56);
setHeart(player, playerName, 130, 134, 58);
setHeart(player, playerName, 135, 23333, 60);
});
}
function setHeart(player: IEntity, playerName: string, min: number, max: number, maxhp: number){
sys.executeCommand("testfor @a[name=" + playerName + ",lm=" + min + ",l=" + max + "]", data =>{
let jsonData: ITestFor = JSON.parse(JSON.stringify(data));
//当有玩家时statusCode为0
if(jsonData.statusCode == 0){
if(jsonData.victim[0] == playerName){
let health = sys.getComponent<IHealthComponent>(player, MinecraftComponent.Health);
health.data.max = maxhp;
if(health.data.value > maxhp){health.data.value = maxhp}
sys.applyComponentChanges(player, health);
}
}
})
}
interface ITestFor{
statusCode: number;
statusMessage : string;
victim : any;
} | d1629e92ed74d4578a000c62ed99c785e11072be | [
"Markdown",
"TypeScript"
] | 2 | Markdown | stevehan12138/3-Heart-Plugin | d609b8762010d6e095f4025bdb194451e3f544f1 | bd1d760d0ad958d59f493965d8b4d562b1a3faf6 |
refs/heads/main | <repo_name>digitallyamar/Flexbox-Examples<file_sep>/README.md
# Flexbox-Examples
All about Flexbox
| 63eb3241579ccfaea519e57232d08b1bb696024f | [
"Markdown"
] | 1 | Markdown | digitallyamar/Flexbox-Examples | 44eed98ae58b9e621a2ab6d0f17185b1a02247a9 | 8ed9a3fe110583021f8f099548502bd2c239ebdc |
refs/heads/master | <repo_name>carlosenciso/IVRHH-PRESENTATION<file_sep>/config.tex
\usepackage[Peru]{babel}
\usepackage[utf8]{inputenc}
\usepackage{graphicx}
\usepackage{booktabs}
\institute[UNALM]
{
%================= logos no meio =====================
\vspace*{-0.35cm}
\includegraphics[width=1.8cm]{img/UNALM.jpg}
\vspace*{0.35cm}\\
Universidad Nacional Agraria La Molina -- UNALM \\
Maestría en Ingeniería de Recursos Hídricos -- RRHH\\
Investigación en la Variabilidad Climática en Recursos Hídricos -- IVRRHH\\
%\medskip
%\texttt{\{<EMAIL>,<EMAIL>} % emails
}
\date{\today}
\AtBeginSection[]
{
\begin{frame}
\frametitle{Conteúdo}
\tableofcontents[currentsection]
\end{frame}
}<file_sep>/main.tex
\documentclass[11pt]{beamer}
%%%%%%%% tema e cor %%%%%%%%
\mode<presentation> {
\usetheme{Madrid}
%\usecolortheme{albatross}
}
\input{config.tex}
%%%%%%%% titulo e subtitulo %%%%%%%%
\title[IVRRHH Exposición]{Does extreme precipitation scaling depend on emissions scenario ?}
%%%%%%%% nome dos autores %%%%%%%%
\author[<NAME>.]{
<NAME>}
\begin{document}
\input{files/pre-slides.tex}
%%%%%%%% slides %%%%%%%%
\section{Introducción}
\input{files/introducao.tex}
\section{Justificación}
\input{files/justificativa.tex}
\section{Objetivos}
\input{files/objetivos.tex}
\section{Fundamento Teórico}
\input{files/fundamentacao.tex}
\section{Metodología}
\input{files/metodologia.tex}
\section{Resultados}
\input{files/resultados.tex}
\section{Conclusión}
\input{files/conclusao.tex}
%%%%%%%% agradecimentos %%%%%%%%
\begin{frame}{Agradecimentos}
\large{Agradeço a fulano, ciclano e beltrano que apoiaram o desenvolvimento dessa pesquisa.}
\end{frame}
%------------------------------------------------
%------------------------------------------------
%------------------------------------------------
%%%%%%%% referencias %%%%%%%%
\nocite{*}
\begin{frame}[allowframebreaks]{Referências}
\bibliographystyle{unsrt}
\bibliography{ref.bib}
\end{frame}
%%%%%%%% repete primeiro slide %%%%%%%%
\begin{frame}
\titlepage
\end{frame}
\end{document} | 95e5bb632951e8a7151050525e614ea7b12fb51b | [
"TeX"
] | 2 | TeX | carlosenciso/IVRHH-PRESENTATION | 13774d38df553e237a09aad65114edc87e2b2fb2 | 4342f5389f0502c1a1f2454f2c2d9f59edb7bdf5 |
refs/heads/master | <repo_name>SumitDahiya0/FlashChat<file_sep>/FlashChat/Configurations.swift
//
// Configurations.swift
// FlashChat
//
// Created by <NAME> on 20/04/21.
//
import Foundation
let apiKey = "1234567890"
let secretKey = "myNameIsSumitDahiya"
| 89b20fa1b65aba545752b67e0c5efcbacea371bd | [
"Swift"
] | 1 | Swift | SumitDahiya0/FlashChat | a785c52a4524b9b0a8d0411f008ae3ccc4ed3dfb | 46948394ac1977fbb6a47a00464939e5c1ca4a88 |
refs/heads/master | <repo_name>krloxz/pluralsight-spring-cloudconfig-wa-tolls-perf<file_sep>/README.md
# pluralsight-spring-cloudconfig-wa-tolls-perf
Another sample Spring Cloud Config Repository
| afa8fcbac24947902c36d494811411ff36672680 | [
"Markdown"
] | 1 | Markdown | krloxz/pluralsight-spring-cloudconfig-wa-tolls-perf | c752c4c4368880b5e71598605d315d6d31bce905 | 636004b7d694c041b04f4661441fc6281442ab9a |
refs/heads/main | <file_sep># Hello-World2
2020/08/24
Learning how to use github for the first time!
| 1c97ca6363d44be4f967c129d53e939c957830a0 | [
"Markdown"
] | 1 | Markdown | Y-coder725/Hello-World2 | cd9ddcfd4a68fc1fa8e2e965d355419158be45fa | b2f001c4aaf2346f497d75569e5f1e59b4f5246c |
refs/heads/master | <repo_name>helsingborg-stad/student-council-protocols<file_sep>/source/php/Helper/RenderBlade.php
<?php
namespace StudentCouncilProtocols\Helper;
use Philo\Blade\Blade;
class RenderBlade
{
/**
* Return markup from a Blade template
* @param string $view View name
* @param array $data View data
* @param string $viewPath Path to the blade views
* @return string The markup
*/
public static function blade($view, $data = array(), $viewPath = STUDENTCOUNCILPROTOCOLS_VIEW_PATH)
{
if (!file_exists(STUDENTCOUNCILPROTOCOLS_CACHE_DIR)) {
mkdir(STUDENTCOUNCILPROTOCOLS_CACHE_DIR, 0777, true);
}
$blade = new Blade($viewPath, STUDENTCOUNCILPROTOCOLS_CACHE_DIR);
return $blade->view()->make($view, $data)->render();
}
}
<file_sep>/views/archive-protocol.blade.php
@extends('templates.master')
@section('content')
<div class="react-root"></div>
@stop
<file_sep>/source/js/Module/Protocol/Components/Card.js
import React from 'react';
const editBtn = (protocol) => {
if (protocol.metadata.data.currentUserId === protocol.author) {
return (
<a href={`${protocol.link}#modal-edit-post`} class="card-edit-btn">
<i class="pricon pricon-pen pricon-pen-white"></i>
</a>
)
}
}
const commentLink = (protocol, authenticated, comments) => {
if (authenticated) {
return (
<a href={`${protocol.link}#comments`} className="card-small-text card-comments-link">{comments} ({protocol.metadata.data.commentsCount})</a>
);
}
}
const truncate = (text, size) => {
return text.length > size ? text.slice(0, size - 1) + '...' : text;
}
const Card = ({ protocols, translations, state }) => {
const {
noProtocolsFound,
comments
} = translations;
const {
showAs,
protocolTruncateSize,
authenticated
} = state;
const gridClass = (showAs == 'cards') ? 'grid-sm-6 grid-md-4 grid-lg-3' : '';
const cardClass = (showAs == 'cards') ? '' : 'card-as-list';
if (protocols.length > 0) {
return (
<div className="grid grid--columns">
{protocols.map((protocol, i) => {
return (
<div className={`u-flex grid-xs-12 ${gridClass}`} key={i}>
<a href={protocol.link} className={`card ${cardClass}`} data-id={protocol.id}>
<div className="card-body">
<h4 className="card-title">{truncate(protocol.metadata.data.title, 75)}</h4>
{editBtn(protocol)}
<div className="card-info">
<span className="card-small-text">{protocol.metadata.data.name_of_council_or_politician}</span>
<br></br>
<time className="card-small-text">{protocol.date.split('T')[0]}</time>
{commentLink(protocol, authenticated, comments)}
</div>
<p class="card-paragraph">
{truncate(protocol.metadata.data.content, protocolTruncateSize)}
</p>
</div>
</a>
</div>
);
})}
</div>
);
} else {
return (
<div className="grid grid--columns">
<div className="u-flex grid-xs-12 grid-md-4">
<h4>{noProtocolsFound}</h4>
</div>
</div>
);
}
};
export default Card;
<file_sep>/source/js/Module/Protocol/Components/Filters.js
import React from 'react';
const renderAuthors = (authors, filterHandler, targetAuthorValue) => {
return (
<select className="input-select" name="yummie" data-option="authors" onChange={filterHandler} value={targetAuthorValue}>
{authors.map((author, i) => {
return (
<option value={author} key={i}>{author}</option>
);
})}
</select>
);
};
const renderSubjects = (allSubjects, filterHandler, targetSubjectValue) => {
return (
<select className="input-select" name="yummie" data-option="subjects" onChange={filterHandler} value={targetSubjectValue} selected={targetSubjectValue}>
{allSubjects.map((subject, i) => {
return (
<option value={subject} key={i}>{subject}</option>
);
})}
</select>
);
};
const Filters = ({ filterHandler, state, translations }) => {
const authorClass = (state.targetGroupValue == 'all') ? 'hidden' : '';
return (
<div className="filter-content">
<h3 className="filter-content-title">{translations.filterTitle}</h3>
<div className="filter-radios">
<label class="radio-container">
<input
className="radio-input"
type="radio"
name="Council"
value="council"
data-option="radio"
onChange={filterHandler}
checked={state.targetGroupValue == "council"}
></input>
<span class="radio-btn"></span>
<span className="radio-title">{translations.council}</span>
</label>
<label class="radio-container">
<input
className="radio-input"
type="radio"
name="Politician"
value="politician"
data-option="radio"
onChange={filterHandler}
checked={state.targetGroupValue == "politician"}
></input>
<span class="radio-btn"></span>
<span className="radio-title">{translations.politician}</span>
</label>
<label class="radio-container">
<input
className="radio-input"
type="radio"
name="All"
value="all"
data-option="radio"
onChange={filterHandler}
checked={state.targetGroupValue == "all"}
></input>
<span class="radio-btn"></span>
<span className="radio-title">{translations.all}</span>
</label>
</div>
<div className="filter-selects">
<div className={`author-select ${authorClass}`}>
<span className="authors-label">{translations.writtenBy}</span>
{state.authors.length > 0 ? renderAuthors(state.authors, filterHandler, state.targetAuthorValue) : ''}
</div>
<div className="subject-select">
<span className="subjects-label">{translations.subject}</span>
{state.allSubjects.length > 0 ? renderSubjects(state.allSubjects, filterHandler, state.targetSubjectValue) : ''}
</div>
</div>
</div>
);
};
export default Filters;
<file_sep>/source/js/Module/Protocol/Components/ShowAsList.js
import React from 'react';
const ShowAsList = ({ showAsHandler, state, translations }) => (
<div className="show-list-wrap">
<span>{`${translations.found} ${state.totalProtocols} ${translations.protocols}`}</span>
<a href="" className="show-list" data-showAs={state.showAs} onClick={showAsHandler}>{state.showAsText}</a>
</div>
);
export default ShowAsList;
<file_sep>/source/js/Module/Protocol/Components/SearchBar.js
const SearchBar = ({ updateSearchString, handleKeyUp, searchSubmitHandler, searchPlaceholderString, searchAllPostsString, searchError }) => (
<div className="search-field">
<div className="input-group">
<input
type="text"
id="filter-keyword"
className={`form-control search-input ${searchError ? 'search-error' : ''}`}
onChange={updateSearchString}
onKeyUp={handleKeyUp}
placeholder={searchPlaceholderString}
/>
<a onClick={searchSubmitHandler} class="input-search-submit-icon">
<i class="fa fa-search"></i>
</a>
</div>
<a class="btn-oval search-submit-btn" onClick={searchSubmitHandler}>
<span className="input-group-addon">
<i className="fa fa-search fa-search-white" />
{searchAllPostsString}
</span>
</a>
</div>
);
export default SearchBar;
<file_sep>/README.md
# protocol manager
Plugin to gather and manage protocols
## Constants
#### Google Maps Geocoding API key
Used server side to gather the protocols locations.
```
define('G_GEOCODE_SERVER_KEY', 'YOUR_API_KEY');
```
<file_sep>/source/js/Module/Protocol/Components/SearchingOverlay.js
import React from 'react';
const SearchingOverlay = ({ searching }) => {
const searchingClass = !searching ? 'hidden' : '';
return (
<div className={`searching ${searchingClass}`}>
<div className={`overlay ${searchingClass}`}></div>
<div className={`loading loading-blue ${searchingClass}`}>
<div></div>
<div></div>
<div></div>
<div></div>
</div>
</div>
);
};
export default SearchingOverlay;
<file_sep>/source/php/Helper/File.php
<?php
namespace StudentCouncilProtocols\Helper;
class File
{
/**
* Return readable filenames
* @param string $string Filename string
* @return string Cleaned filename
*/
public static function cleanFileName($string)
{
$pathParts = pathinfo($string);
$name = preg_replace('/([A-Za-z0-9]+-)/i', '', $pathParts['filename'], 1);
$name = str_replace('_', ' ', $name);
$name = str_replace('-', ' ', $name);
return $name;
}
}
<file_sep>/source/js/Module/Protocol/Components/ProtocolsButtons.js
import React from 'react';
const ProtocolsButtons = ({ translations, orderButtonClick, state }) => {
const viewedClass = (state.targetOrderValue == 'viewed') ? 'btn-active' : '';
const latestClass = (state.targetOrderValue == 'latest') ? 'btn-active' : '';
return (
<div className="protocols-btn-field">
<a href={`${window.location.origin}/create-protocol`} className="btn-oval new-protocol-btn"><i class="pricon pricon-plus"></i><span>{translations.writeNewPost}</span></a>
<div className="order-btns">
<a href="" className={`btn-square ${latestClass}`} onClick={orderButtonClick} data-order="latest">{translations.latest}</a>
<a href="" className={`btn-square ${viewedClass}`} onClick={orderButtonClick} data-order="viewed">{translations.mostViewed}</a>
</div>
</div>
);
};
export default ProtocolsButtons;
<file_sep>/student-council-protocols.php
<?php
/**
* Plugin Name: Student Council Protocols
* Plugin URI: https://github.com/helsingborg-stad/student-council-protocols
* Description: Plugin to post and view protocols.
* Version: 1.0.0
* Author: <NAME>
* Author URI: https://github.com/helsingborg-stad
* License: MIT
* License URI: https://opensource.org/licenses/MIT
* Text Domain: student-council-protocols
* Domain Path: /languages
*/
// Protect agains direct file access
if (! defined('WPINC')) {
die;
}
define('STUDENTCOUNCILPROTOCOLS_PATH', plugin_dir_path(__FILE__));
define('STUDENTCOUNCILPROTOCOLS_URL', plugins_url('', __FILE__));
define('STUDENTCOUNCILPROTOCOLS_VIEW_PATH', STUDENTCOUNCILPROTOCOLS_PATH . 'views/');
define('STUDENTCOUNCILPROTOCOLS_CACHE_DIR', trailingslashit(wp_upload_dir()['basedir']) . 'cache/blade-cache/');
load_plugin_textdomain('student-council-protocols', false, plugin_basename(dirname(__FILE__)) . '/languages');
require_once STUDENTCOUNCILPROTOCOLS_PATH . 'source/php/Vendor/Psr4ClassLoader.php';
require_once STUDENTCOUNCILPROTOCOLS_PATH . 'Public.php';
if (file_exists(STUDENTCOUNCILPROTOCOLS_PATH . 'vendor/autoload.php')) {
require_once STUDENTCOUNCILPROTOCOLS_PATH . 'vendor/autoload.php';
}
// Acf auto import and export
add_action('plugins_loaded', function () {
$acfExportManager = new \AcfExportManager\AcfExportManager();
$acfExportManager->setTextdomain('student-council-protocols');
$acfExportManager->setExportFolder(STUDENTCOUNCILPROTOCOLS_PATH . 'acf-fields/');
$acfExportManager->autoExport(array(
'protocol_status' => 'group_5a134a48de846',
'administration_unit' => 'group_5a134bb83af1a',
'tax_color' => 'group_5ab3a45759ba5'
));
$acfExportManager->import();
});
// Instantiate and register the autoloader
$loader = new StudentCouncilProtocols\Vendor\Psr4ClassLoader();
$loader->addPrefix('StudentCouncilProtocols', STUDENTCOUNCILPROTOCOLS_PATH);
$loader->addPrefix('StudentCouncilProtocols', STUDENTCOUNCILPROTOCOLS_PATH . 'source/php/');
$loader->register();
// Start application
new StudentCouncilProtocols\App();
<file_sep>/source/sass/student-council-protocols.scss
$color-blue: #0069b4;
$color-orange: #f9a301;
$color-gray: #ededed;
$color-text: #252525;
$color-danger: #dc3545;
$shadow: 0px 3px 3px 1px rgba(0,0,0,0.15);
a {
cursor: pointer;
}
html, body, #main-content, #react-root, #react-root>div {
height: 100%
}
.hidden {
display: none;
}
.protocols-title {
margin: 20px 0;
color: $color-text;
}
input[type="radio"] {
top:0 !important;
}
.btn-oval {
border-radius: 20px !important;
width: 200px;
height: 40px;
text-align: center;
cursor: pointer;
display: flex;
align-items:center;
justify-content: center;
padding: 5px 10px;
border: 0px solid transparent;
font-size: 15px;
transform: translateY(0px);
transition:.2s;
}
.btn-oval:hover {
color: $color-blue;
box-shadow: $shadow;
transform: translateY(-1px);
}
.btn-oval:hover > span,
.btn-oval:hover > i {
color: $color-blue;
}
.input-group > .form-control.search-input {
border-radius: 20px !important;
width:100%;
box-shadow: none;
border: 1px solid black;
z-index: 1;
}
.input-search-submit-icon {
display: none;
position: absolute;
right: 0;
padding-top:8px;
width: 40px;
height: 100%;
z-index: 1;
}
.input-group {
min-width: 275px;
max-width: 400px;
}
.input-select {
font-size: 15px;
background-image: url('data:image/svg+xml;base64,<KEY>ZnPg==') !important;
border-radius: 4px;
border: 1px solid $color-text;
}
.input-select:hover {
background-image: url('data:image/svg+xml;base64,<KEY>ZnPg==') !important;
background-color: $color-blue;
border-color: $color-blue;
color: white;
cursor: pointer;
}
.input-select:hover ~ .pricon-caret-right {
color: white;
}
.pricon-caret-right {
font-size: 12px;
transform: rotate(90deg);
position: absolute;
right: 12px;
bottom: 15px;
color:$color-text;
}
.search-field {
margin: 20px 0;
flex-flow: row nowrap;
display: flex !important;
justify-content: flex-start !important;
}
.search-field > .input-group {
width: 100%;
}
.search-submit-btn {
font-size: 15px;
margin-left: 15px;
background-color: $color-blue !important;
color: white;
transition: .1s;
}
.search-submit-btn:hover > span {
color: white !important;
}
::placeholder {
opacity: 1;
}
:-ms-input-placeholder { /* Internet Explorer 10-11 */
opacity: 1;
}
::-ms-input-placeholder { /* Microsoft Edge */
opacity: 1;
}
.search-error {
color: $color-danger !important;
font-weight: 500;
opacity: 1;
-webkit-text-fill-color: $color-danger !important;
}
.input-group-addon {
width: auto !important;
padding: 0 !important;
height: auto !important;
display: inline-block !important;
background-color: transparent !important;
border: 0px solid transparent !important;
color: white;
}
.fa.fa-search {
font-size:17px;
margin-right: 10px;
}
.fa-search-white {
color: white;
}
.filter-content {
background-color: $color-gray;
padding: 20px;
}
.filter-content-title {
margin-bottom: 10px;
}
.filter-selects {
margin-top: 20px;
max-width:500px;
display: flex;
flex-flow: row nowrap;
justify-content: flex-start;
align-items: center;
}
.filter-radios {
display: block;
}
.author-select {
margin-right: 15px;
}
.author-select,
.subject-select {
position: relative;
flex-basis: 50%;
}
.subjects-label,
.authors-label {
font-weight: 500;
}
.radio-container:not(:first-child) {
margin-left: 12px;
}
.show-list-wrap {
margin: 20px 0;
}
.show-list {
margin-left: 15px;
text-decoration: underline !important;
}
.protocols {
position: relative;
background-color: $color-orange;
padding: 20px 0;
}
.searching {
padding-top: 100px;
position: absolute;
width: 100%;
height: 100%;
top: 0;
z-index: 1;
display: flex;
justify-content: center;
align-items: flex-start;
}
.overlay {
width:100%;
height:100%;
top: 0;
position: absolute;
z-index: 2;
background-color: white;
opacity: .7;
}
.loading {
min-width:300px;
z-index: 2 !important;
}
.protocols-btn-field {
display: flex;
flex-flow: row wrap;
justify-content: space-between;
align-items: center;
margin-bottom: 30px;
}
.pricon-pen-white {
color: white;
}
.new-protocol-btn {
background-color:white !important;
color: $color-text !important;
margin-top: 15px;
}
.order-btns {
display: flex;
flex-flow:row nowrap;
margin-top: 15px;
}
.order-btns > .btn-square:last-child {
margin-left:10px;
}
.new-protocol-btn > .pricon-plus {
margin-right: 15px;
}
.btn-square {
display: inline-block;
font-size: 15px;
width: 180px;
height:40px;
display: flex;
align-items: center;
justify-content: center;
border: 0px solid transparent;
background-color: white;
opacity: .6;
color: $color-text !important;
border-radius: 5px;
cursor: pointer;
transition: .3s;
}
.btn-square:not(:last-child) {
margin-right: 10px;
}
.btn-square:hover {
color: $color-blue;
opacity: 1;
}
.btn-active {
opacity: 1;
}
.btn-active:hover {
opacity:1;
color: $color-text;
}
.card {
position: relative;
width: 100%;
min-height: 304px;
background-color: white;
border-radius: 20px;
padding: 20px;
margin: 0 auto;
overflow:hidden;
}
.card-title {
display: inline-block;
max-width: 80%;
}
.card-body {
color: $color-text;
}
.card-comments-link {
text-decoration: underline !important;
margin-left: 10px;
}
.card-info {
margin: 10px 0;
}
.card-info,
.card-paragraph {
font-size: 15px;
font-weight: 300;
}
.grid-xs-12 {
margin-bottom: 16px !important;
margin-top: 16px !important;
}
.pagination {
display: flex;
flex-flow: row nowrap;
justify-content: center;
align-items: center;
max-width: 300px;
margin: 20px auto;
}
.pagination > li {
width: auto;
margin: 0 15px;
}
.pagination > li > a:focus {
background-color: white;
}
.pagination > li > a:active {
outline: 0 solid transparent;
}
.pagination > .next > a,
.pagination > .previous > a {
width: 100% !important;
padding: 0 5px !important;
}
.pagination a {
border: 1px solid $color-blue !important;
box-shadow: none !important;
}
.pagination a:hover {
background-color: $color-blue !important;
color: white !important;
}
.card-edit-btn {
background-color: $color-blue;
position: absolute;
top: 0;
right: 0;
width: 40px;
height:40px;
text-align:center;
padding-top: 5px;
border-top-right-radius: 20px;
transition: .3s;
}
.pagination > li > a[aria-current="page"] {
background-color: $color-blue !important;
color: white !important;
}
.card-edit-btn:hover {
opacity: .8;
}
.container {
padding: 0 15px !important;
}
.card-as-list {
min-height: 200px;
}
/* CUSTOM RADIO BUTTON */
/* Customize the label (the container) */
.radio-container {
display: inline-block;
position: relative;
cursor: pointer;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.radio-title {
font-size:15px;
margin-left: 30px;
}
/* Hide the browser's default radio button */
.radio-container .radio-input {
position: absolute;
opacity: 0;
cursor: pointer;
height: 0;
width: 0;
}
/* Create a custom radio button */
.radio-btn {
border: 1px solid $color-text;
position: absolute;
top: 0;
left: 0;
height: 22px;
width: 22px;
background-color: white;
border-radius: 50%;
}
/* Create the indicator (the dot/circle - hidden when not checked) */
.radio-btn:after {
content: "";
position: absolute;
display: none;
}
/* Show the indicator (dot/circle) when checked */
.radio-container .radio-input:checked ~ .radio-btn:after {
display: block;
}
/* Style the indicator (dot/circle) */
.radio-container .radio-btn:after {
top: 4px;
left:4px;
width:12px;
height: 12px;
border-radius: 50%;
background: $color-text;
}
/* MEDIA QUERIES */
@media screen and (max-width: 672px) {
.card {
min-height: 200px;
}
.search-submit-btn {
display: none;
}
.input-search-submit-icon {
display: block;
}
.btn-square {
width:125px;
}
.protocols-btn-field {
flex-flow: column nowrap;
}
.filter-content {
padding: 10px;
}
}
@media screen and (max-width: 450px) {
.radio-container,
.radio-container:not(:first-child) {
display: block;
margin: 10px 0;
}
.container {
padding: 0 5px !important;
}
.show-list-wrap {
text-align:center;
margin: 15px 0;
}
.show-list {
display: none;
}
}
<file_sep>/source/js/front/student-council-protocols.js
var studentCouncilProtocols = {};
studentCouncilProtocols = studentCouncilProtocols || {};
studentCouncilProtocols.student = studentCouncilProtocols.student || {};
studentCouncilProtocols.student.userVisit = (function ($) {
function userVisit() {
$(function() {
this.init();
}.bind(this));
}
userVisit.prototype.init = function() {
var posts_visited = JSON.parse(localStorage.getItem('posts_visited'));
var is_visited = posts_visited ? posts_visited.indexOf(userVisitData.post_id) : null;
var increment = false;
if (!is_visited || is_visited === -1) {
// if a post has never been visited before
if (!posts_visited) {
localStorage.setItem('posts_visited', JSON.stringify([userVisitData.post_id]));
} else {
posts_visited.push(userVisitData.post_id)
localStorage.setItem('posts_visited', JSON.stringify(posts_visited));
}
increment = true;
}
$.ajax({
url: userVisitData.ajax_url,
type: 'post',
data: {
action: 'userVisitAjax',
increment: increment,
postId: userVisitData.post_id,
nonce: userVisitData.nonce
},
beforeSend: function (xhr) {
xhr.setRequestHeader('X-WP-Nonce', userVisitData.nonce );
},
success: function(response) {
console.log(response);
},
error: function(xhr, error){
console.debug(xhr);
console.debug(error);
},
});
}
return new userVisit();
})(jQuery);
<file_sep>/source/php/App.php
<?php
namespace StudentCouncilProtocols;
use Philo\Blade\Blade as Blade;
class App
{
public function __construct()
{
new PostTypes\Protocol();
add_action('wp_ajax_nopriv_userVisitAjax', array($this, 'userVisitAjax'));
add_action('wp_ajax_userVisitAjax', array($this, 'userVisitAjax'));
add_action('wp_enqueue_scripts', array($this, 'script'), 5);
add_filter('Municipio/blade/view_paths', array($this, 'viewPaths'), 10, 1);
add_action('rest_api_init', array($this, 'registerRestApiMeta'));
}
public function registerRestApiMeta()
{
register_rest_field('protocol', 'metadata', array(
'get_callback' => function ($data) {
$auth = is_user_logged_in();
$userId = get_current_user_id();
$postMeta = get_post_meta($data['id']);
$content = $data['content']['raw'];
$title = $data['title']['raw'];
$postFormData = unserialize($postMeta['form-data'][0]);
$allSubjects = get_fields($postFormData['modularity-form-id'])['form_fields'][0]['values'];
$subjects = $postFormData['valj-amnen-kategorier'];
$postComments = get_comments(array('post_id' => $data['id']));
$name = $postMeta['name_of_council_or_politician'];
$targetGroup = $postMeta['target_group'];
$response = array(
'authenticated' => $auth,
'currentUserId' => $userId,
'postMeta' => $postMeta,
'data' => $data,
'postFormData' => $postFormData,
'content' => $content,
'title' => $title,
'target_group' => $targetGroup,
'name_of_council_or_politician' => $name,
'subjects' => $subjects,
'allSubjects' => $allSubjects,
'commentsCount' => count($postComments)
);
return rest_ensure_response($response);
}));
register_rest_route(
'wp/v2',
'/protocols/users',
array(
'methods' => \WP_REST_Server::READABLE,
'callback' => array($this, 'getUsers'),
)
);
}
public function getSchoolsAndPoliticians($users)
{
$response = array(
'politicians' => array(),
'schools' => array()
);
foreach ($users as $user_ids) {
$userMeta = get_user_meta($user_ids->ID);
$targetGroup = $userMeta["target_group"][0];
if ($targetGroup == "council") {
array_push($response["schools"], $userMeta["name_of_council_or_politician"][0]);
} elseif ($targetGroup == "politician") {
array_push($response["politicians"], $userMeta["name_of_council_or_politician"][0]);
}
}
return $response;
}
public function getUsers()
{
$users = get_users(array('fields' => array( 'ID' )));
$schoolsAndPoliticians = $this->getSchoolsAndPoliticians($users);
return rest_ensure_response($schoolsAndPoliticians);
}
public function userVisitAjax() {
$postId = $_POST['postId'];
$increment = $_POST['increment'];
$numberOfVisits = get_post_meta($postId, 'number_of_visits');
if ($increment === 'true') {
if (empty($numberOfVisits)) {
update_post_meta($postId, 'number_of_visits', 1);
} else {
update_post_meta($postId, 'number_of_visits', ++$numberOfVisits[0]);
}
}
return true;
}
public function viewPaths($paths)
{
$paths[] = STUDENTCOUNCILPROTOCOLS_PATH . 'views';
return $paths;
}
/**
* Enqueue scripts and styles for front ui
* @return void
*/
public function script()
{
global $post;
wp_register_script(
'student-council-protocols',
STUDENTCOUNCILPROTOCOLS_URL.'/dist/'.\StudentCouncilProtocols\Helper\CacheBust::name(
'js/student-council-protocols.js',
false
),
array('jQuery'),
'',
true
);
wp_register_style('student-council-protocols', STUDENTCOUNCILPROTOCOLS_URL.'/dist/'.\StudentCouncilProtocols\Helper\CacheBust::name(
'css/student-styles.css',
false
));
if (is_object($post) && $post->post_type == 'protocol') {
wp_enqueue_script('student-council-protocols');
wp_enqueue_style('student-council-protocols');
}
if (is_single()) {
wp_localize_script('student-council-protocols', 'userVisitData', array('ajax_url' => admin_url('admin-ajax.php'),'nonce' => wp_create_nonce('userVisitNonce'),'post_id' => $post->ID));
}
$translations = array(
'pageTitle' => __('All Protocols', 'student-council-protocols'),
'searchPlaceholder' => __('Search all posts...', 'student-council-protocols'),
'searchPlaceholderError' => __('You have to fill in this field.', 'student-council-protocols'),
'searchAllPosts' => __('Search all posts', 'student-council-protocols'),
'filterTitle' => __('Filter', 'student-council-protocols'),
'council' => __('Council', 'student-council-protocols'),
'politician' => __('Politician', 'student-council-protocols'),
'all' => __('All', 'student-council-protocols'),
'allCouncils' => __('All councils', 'student-council-protocols'),
'allPoliticians' => __('All politicians', 'student-council-protocols'),
'allSubjectsText' => __('All subjects', 'student-council-protocols'),
'writtenBy' => __('Written By', 'student-council-protocols'),
'subject' => __('Subject', 'student-council-protocols'),
'found' => __('Found', 'student-council-protocols'),
'protocols' => __('protocols', 'student-council-protocols'),
'showAsList' => __('Show as list', 'student-council-protocols'),
'showAsCards' => __('Show as cards', 'student-council-protocols'),
'writeNewPost' => __('Write a new post', 'student-council-protocols'),
'latest' => __('Latest', 'student-council-protocols'),
'mostViewed' => __('Most viewed', 'student-council-protocols'),
'comments' => __('Comments', 'student-council-protocols'),
'previous' => __('Previous', 'student-council-protocols'),
'next' => __('Next', 'student-council-protocols'),
'noProtocolsFound' => __('No protocols found', 'student-council-protocols'),
);
wp_enqueue_script(
'protocol-react-index',
STUDENTCOUNCILPROTOCOLS_URL.'/dist/'.\StudentCouncilProtocols\Helper\CacheBust::name(
'js/student-react.js',
false
),
array('jquery', 'react', 'react-dom'),
false,
true
);
wp_localize_script('protocol-react-index', 'reactData', array(
'nonce' => wp_create_nonce('wp_rest'),
'rest_url' => rest_url(),
'translations' => $translations
));
}
/**
* Return markup from a Blade template
* @param string $view View name
* @param array $data View data
* @return string The markup
*/
public static function blade($view, $data = array())
{
if (!file_exists(STUDENTCOUNCILPROTOCOLS_CACHE_DIR)) {
mkdir(STUDENTCOUNCILPROTOCOLS_CACHE_DIR, 0777, true);
}
$paths = array(
STUDENTCOUNCILPROTOCOLS_VIEW_PATH,
get_template_directory() . '/views',
);
$blade = new Blade($paths, STUDENTCOUNCILPROTOCOLS_CACHE_DIR);
return $blade->view()->make($view, $data)->render();
}
}
<file_sep>/source/php/PostTypes/Protocol.php
<?php
namespace StudentCouncilProtocols\PostTypes;
class Protocol
{
public $postTypeSlug;
public function __construct()
{
$this->postTypeSlug = 'protocol';
$this->slug = 'protocol_subjects';
$this->namePlural = 'Subjects';
$this->nameSingular = 'Subject';
$this->args = array(
'hierarchical' => true,
'public' => true,
'show_ui' => true,
'show_in_nav_menus' => true,
'_builtin' => false,
);
$this->taxonomyStatus();
$this->taxonomyTargetGroup();
add_action('init', array($this, 'register'), 9);
add_action('save_post_' . $this->postTypeSlug, array($this, 'setDefaultData'), 10, 3);
add_action('Municipio/blog/post_info', array($this, 'addProtocolStatusPost'), 9, 1);
add_filter('wp_insert_post_data', array($this, 'allowComments'), 99, 2);
add_filter('ModularityFormBuilder/excluded_fields/front', array($this, 'excludedFields'), 10, 3);
add_filter('Municipio/taxonomy/tag_style', array($this, 'setStatusColor'), 10, 3);
add_filter('manage_edit-' . $this->postTypeSlug . '_columns', array($this, 'tableColumns'), 11);
add_filter('ModularityFormBuilder/options/post_types', array($this, 'addSelectablePostType'), 10);
}
public function addProtocolStatusPost($post)
{
if (is_object($post) && is_singular() && $post->post_type == $this->postTypeSlug) {
$statuses = !is_wp_error(wp_get_post_terms($post->ID, 'protocol_statuses')) ? wp_get_post_terms($post->ID, 'protocol_statuses') : null;
if (!empty($statuses[0])) {
echo '<li><i class="pricon pricon-info-o"></i> ' . $statuses[0]->name . '</li>';
}
}
}
public function isProtocolPage()
{
global $post;
if (is_object($post) && $post->post_type == $this->postTypeSlug && !is_archive() && !is_admin()) {
return true;
}
return false;
}
/**
* Registers protocol post type
* @return void
*/
public function register()
{
$args = array(
'hierarchical' => false,
'description' => 'Post type for managing protocols',
'public' => true,
'show_ui' => true,
'show_in_menu' => true,
'show_in_admin_bar' => true,
'menu_position' => 50,
'menu_icon' => 'dashicons-lightbulb',
'show_in_nav_menus' => true,
'publicly_queryable' => true,
'exclude_from_search' => false,
'has_archive' => true,
'query_var' => true,
'can_export' => true,
'rewrite' => array(
'with_front' => false,
'slug' => $this->postTypeSlug
),
'supports' => array('title', 'author', 'editor', 'comments', 'thumbnail'),
'show_in_rest' => true,
'rest_base' => $this->postTypeSlug,
'capabilities' => array(
'create_posts' => 'edit_posts',
),
);
new \ModularityFormBuilder\Entity\PostType(
$this->postTypeSlug,
__('protocol', 'student-council-protocols'),
__('Protocols', 'student-council-protocols'),
$args
);
$this->registerTaxonomyCategory();
}
/**
* Create status taxonomy
* @return string
*/
public function taxonomyStatus()
{
// Register new taxonomy
$taxonomyStatus = new \ModularityFormBuilder\Entity\Taxonomy(
__('Status', 'student-council-protocols'),
__('Statuses', 'student-council-protocols'),
'protocol_statuses',
array($this->postTypeSlug),
array(
'hierarchical' => false,
'public' => true,
'show_ui' => true,
'show_in_nav_menus' => true,
'_builtin' => false,
)
);
// Remove default UI
add_action('admin_menu', function () {
remove_meta_box('tagsdiv-protocol_statuses', $this->postTypeSlug, 'side');
});
//Add filter
new \ModularityFormBuilder\Entity\Filter(
$taxonomyStatus->slug,
$this->postTypeSlug
);
}
/**
* Create administration unit taxonomy
* @return string
*/
public function taxonomyTargetGroup()
{
// Register new taxonomy
$taxonomyTargetGroup = new \ModularityFormBuilder\Entity\Taxonomy(
__('Target group', 'student-council-protocols'),
__('Target groups', 'student-council-protocols'),
'protocol_target_groups',
array($this->postTypeSlug),
array(
'hierarchical' => false,
'public' => true,
'show_ui' => false,
'show_in_nav_menus' => false,
'_builtin' => false,
'show_in_rest' => true
)
);
// Remove default UI
add_action('admin_menu', function () {
remove_meta_box('tagsdiv-protocol_administration_units', $this->postTypeSlug, 'side');
});
//Add filter
new \ModularityFormBuilder\Entity\Filter(
$taxonomyTargetGroup->slug,
$this->postTypeSlug
);
}
/**
* Create status taxonomy
* @return string
*/
public function registerTaxonomyCategory()
{
$labels = array(
'name' => $this->namePlural,
'singular_name' => $this->nameSingular,
'search_items' => sprintf(__('Search %s', 'modularity-form-builder'), $this->namePlural),
'all_items' => sprintf(__('All %s', 'modularity-form-builder'), $this->namePlural),
'parent_item' => sprintf(__('Parent %s:', 'modularity-form-builder'), $this->nameSingular),
'parent_item_colon' => sprintf(__('Parent %s:', 'modularity-form-builder'), $this->nameSingular) . ':',
'edit_item' => sprintf(__('Edit %s', 'modularity-form-builder'), $this->nameSingular),
'update_item' => sprintf(__('Update %s', 'modularity-form-builder'), $this->nameSingular),
'add_new_item' => sprintf(__('Add New %s', 'modularity-form-builder'), $this->nameSingular),
'new_item_name' => sprintf(__('New %s Name', 'modularity-form-builder'), $this->nameSingular),
'menu_name' => $this->namePlural,
);
$this->args['labels'] = $labels;
register_taxonomy($this->slug, $this->postTypeSlug, $this->args);
}
public function setDefaultData($postId, $post, $update)
{
$userMeta = get_user_meta(get_current_user_id());
$postFormData = $_POST;
if (!empty($userMeta['name_of_council_or_politician']) && !empty($userMeta['target_group'])) {
$name = $userMeta['name_of_council_or_politician'][0];
$targetGroup = $userMeta['target_group'][0];
$term = term_exists($targetGroup, 'protocol_target_groups');
if ($term !== 0 && $term !== null) {
wp_set_object_terms($postId, (int)$term['term_id'], 'protocol_target_groups');
} else {
$newTerm = wp_insert_term($targetGroup, 'protocol_target_groups');
if (!is_wp_error($newTerm)) {
wp_set_object_terms($postId, (int)$newTerm['term_id'], 'protocol_target_groups');
}
}
if (!empty($postFormData) && !empty($postFormData['valj-amnen-kategorier'])) {
$subjects = $postFormData['valj-amnen-kategorier'];
}
update_post_meta($postId, 'subjects', $subjects);
update_post_meta($postId, 'name_of_council_or_politician', $name);
update_post_meta($postId, 'target_group', $targetGroup);
update_post_meta($postId, 'number_of_visits', 0);
}
if (!$update) {
// Set default status
wp_set_object_terms($postId, __('Incoming', 'student-council-protocols'), 'protocol_statuses');
// Hide Share icons
update_field('field_56c33d008efe3', false, $postId);
// Hide Author
update_field('field_56cadc4e0480b', false, $postId);
// Hide Author image
update_field('field_56cadc7b0480c', false, $postId);
// Save administration unit
if (class_exists('\\Intranet\\User\\AdministrationUnits')) {
$unit = \Intranet\User\AdministrationUnits::getUsersAdministrationUnitIntranet();
if ($unit) {
$term = term_exists($unit->name, 'protocol_administration_units');
if ($term !== 0 && $term !== null) {
wp_set_object_terms($postId, (int)$term['term_id'], 'protocol_administration_units');
} else {
$newTerm = wp_insert_term($unit->name, 'protocol_administration_units');
if (!is_wp_error($newTerm)) {
wp_set_object_terms($postId, (int)$newTerm['term_id'], 'protocol_administration_units');
}
}
}
}
}
}
/**
* Allow comments by default for this post type
* @param array $data [description]
* @param array $postarr [description]
* @return array Modified data list
*/
public function allowComments($data, $postarr) {
if ($data['post_type'] == $this->postTypeSlug) {
$data['comment_status'] = 'open';
}
return $data;
}
/**
* Table columns
* @param array $columns
* @return array
*/
public function tableColumns($columns)
{
return array(
'cb' => '',
'title' => __('Title'),
'date' => __('Date')
);
}
public function excludedFields($exclude, $postType, $postId)
{
if ($postType === $this->postTypeSlug) {
$exclude[] = 'sender-firstname';
$exclude[] = 'sender-lastname';
$exclude[] = 'sender-email';
$exclude[] = 'sender-phone';
$exclude[] = 'sender-address';
$exclude[] = 'file_upload';
$exclude[] = 'input';
$exclude[] = 'checkbox';
}
return $exclude;
}
/**
* Adds custom style to taxonomy tags
* @param string $attr Default style string
* @param string $term The term
* @param string $taxonomy Taxnomy name
* @param obj $post Post object
* @return string Modified style string
*/
public function setStatusColor($style, $term, $taxonomy)
{
if ($taxonomy == 'protocol_statuses') {
$color = get_field('taxonomy_color', $term);
if (!empty($color)) {
$style .= sprintf('background:%s;color:#fff;', $color);
}
}
return $style;
}
/**
* Add protocol post type to selectable post types option
* @param $postTypes
* @return array
*/
public function addSelectablePostType($postTypes)
{
$postTypes[$this->postTypeSlug] = __('Protocols', 'student-council-protocols');
return $postTypes;
}
}
<file_sep>/source/js/Module/Protocol/Index.js
// Polyfills
import 'es6-promise';
import 'isomorphic-fetch';
// Components
import Protocols from './Components/Protocols';
const element = document.getElementsByClassName('react-root')[0];
ReactDOM.render(
<Protocols/>,
element
);<file_sep>/gulpfile.js
// Include gulp
var gulp = require('gulp');
// Include Our Plugins
var sass = require('gulp-sass');
var concat = require('gulp-concat');
var uglify = require('gulp-uglify');
var cssnano = require('gulp-cssnano');
var rename = require('gulp-rename');
var autoprefixer = require('gulp-autoprefixer');
var plumber = require('gulp-plumber');
var sourcemaps = require('gulp-sourcemaps');
var terser = require('gulp-terser');
var notifier = require('node-notifier');
//Dependecies required to compile ES6 Scripts
var browserify = require('browserify');
var reactify = require('reactify');
var source = require('vinyl-source-stream');
var buffer = require('vinyl-buffer');
var babelify = require('babelify');
var es = require('event-stream');
// Compile Our Sass
gulp.task('sass-dist', function() {
gulp.src('source/sass/student-council-protocols.scss')
.pipe(plumber())
.pipe(sass())
.pipe(autoprefixer('last 2 version', 'safari 5', 'ie 8', 'ie 9', 'opera 12.1'))
.pipe(rename({suffix: '.min'}))
.pipe(cssnano())
.pipe(gulp.dest('dist/css'));
});
gulp.task('sass-dev', function() {
gulp.src('source/sass/student-council-protocols.scss')
.pipe(plumber())
.pipe(sass())
.pipe(autoprefixer('last 2 version', 'safari 5', 'ie 8', 'ie 9', 'opera 12.1'))
.pipe(rename({suffix: '.dev'}))
.pipe(gulp.dest('dist/css'));
});
gulp.task('sass', function() {
var filePath = 'source/sass/';
var files = ['archive-filtering.scss'];
var tasks = files.map(function(entry) {
return gulp
.src(filePath + entry)
.pipe(plumber())
.pipe(sourcemaps.init())
.pipe(
sass().on('error', function(err) {
console.log(err.message);
notifier.notify({
title: 'SASS Compile Error',
message: err.message,
});
})
)
.pipe(autoprefixer('last 2 version', 'safari 5', 'ie 8', 'ie 9', 'opera 12.1'))
.pipe(sourcemaps.write())
.pipe(gulp.dest('dist/css'))
.pipe(cleanCSS({ debug: true }))
.pipe(gulp.dest('dist/.tmp/css'));
});
return es.merge.apply(null, tasks);
});
// Concatenate & Minify JS
gulp.task('scripts-dist', function() {
gulp.src([
'source/js/front/**/*.js',
])
.pipe(concat('student-council-protocols.dev.js'))
.pipe(gulp.dest('dist/js'))
.pipe(rename('student-council-protocols.min.js'))
.pipe(uglify())
.pipe(gulp.dest('dist/js'));
var filePath = 'source/js/';
var files = ['Module/Event/Index.js'];
var tasks = files.map(function(entry) {
return (
browserify({
entries: [filePath + entry],
debug: true,
})
.transform([babelify])
.bundle()
.on('error', function(err) {
console.log(err.message);
notifier.notify({
title: 'Compile Error',
message: err.message,
});
this.emit('end');
})
.pipe(source(entry)) // Converts To Vinyl Stream
.pipe(buffer()) // Converts Vinyl Stream To Vinyl Buffer
// Gulp Plugins Here!
.pipe(sourcemaps.init())
.pipe(sourcemaps.write())
.pipe(gulp.dest('dist/js'))
.pipe(terser())
.pipe(gulp.dest('dist/.tmp/js'))
);
});
return es.merge.apply(null, tasks);
});
// Watch Files For Changes
gulp.task('watch', function() {
gulp.watch('source/js/**/*.js', ['scripts-dist']);
gulp.watch('source/sass/**/*.scss', ['sass-dist', 'sass-dev']);
});
// Default Task
gulp.task('default', ['sass-dist', 'sass-dev', 'scripts-dist', 'watch']);
| 2c743ed1935abc4942481bdc279336f344980861 | [
"SCSS",
"Markdown",
"JavaScript",
"PHP",
"Blade"
] | 17 | SCSS | helsingborg-stad/student-council-protocols | c79192835adadf5a5b41d3e2706559b06b0b9fc8 | c981865158b81d15e610fe873b3f3341a9d595be |
refs/heads/master | <repo_name>ombelinedej/JavaScript<file_sep>/script_3.js
let answer = "";
answer = prompt("Salut, bienvenue dans ma super pyramide ! Combien d'étages veux-tu ?");
function repeatStringNumTimes(string, times) {
var repeatedString = "";
while (times > 0) {
repeatedString += string;
times++;
}
return repeatedString;
}
for(let count = 0; count <=answer; count++){
console.log(repeatStringNumTimes("#" , count));
}<file_sep>/script_2.js
let answer = "";
answer = prompt("De quel nombre veux-tu calculer la factorielle ?");
function factorialize(num) {
let result = num;
/*--cas ou answer = 0 ou answer = 1 -------*/
if (num === 0 || num === 1)
return 1;
/*--boucle while pour multiplier à chaque fois par le num - 1-------*/
while (num > 1) {
num--; /*. = num - 1. */
result = result * num;
}
return result;
}
console.log( "Le résultat est" + " " + ":" + " " + factorialize(answer));<file_sep>/README.md
[FR] (ENGLISH BELLOW, because I am bilingue)
Hello mes petits correcteurs, pour me corriger facilement :
$ git clone https://github.com/ombelinedej/JavaScript
$ Sublime Javascript (ou autre commande si vous n'utilisez pas Sublime Text).
Dans Index.html, j'ai fait appel à chaque fichier avec la commande <script src="script_1.js"></script> ; <script src="script_2.js"></script> etc.
Vous pouvez simplement afficher la ligne du ficher JS que vous souhaitez ouvrir et cacher les autre ( "cmd + maj + /" sur mac pour mettre la ligne en commentaire et mêmes commandes pour décommenter).
Puis dans votre navigateur : ouvrez une nouvelle fenêtre et cmd + O pour ouvrir le fichier index.html puis cmd + alt + i pour ouvrir la console.
Soyez gentils <3 <3
---------------------------------------------------------------
[EN]
*add file to your computer
$ git clone https://github.com/ombelinedej/JavaScript
*open file
$ Sublime Javascript (or other command if you are not using Sublime Text)
*open index.html
Here you can use "cmd + maj + /" (mac) to comment the JS files you don't want to show and only keep the file you want
*open browser
*open index.html
"cmd + o" (mac)
*open console
"cmd + alt + i"
See the magic.
Be nice, I did my best.
If you don't have a mac, I'm very sorry, I don't know the commands on other computers.
| 5d13c76fdb0e82ae7672a5886542183a553b7684 | [
"Markdown",
"JavaScript"
] | 3 | Markdown | ombelinedej/JavaScript | b21fa1d30a6f12cb53d07edc952085cbf9885c68 | 5ad2fa45859a4716f7c2073a0c14cff488b14071 |
refs/heads/master | <file_sep>package main
import (
"fmt"
"flag"
"github.com/ardeshir/simple"
)
var debug bool = false
func main() {
fmt.Println(simple.Add(1,2))
minusO := flag.Bool("o", false, "o")
minusC := flag.Bool("c", false, "c")
minusK := flag.Int("k", 0, "an int")
flag.Parse()
fmt.Println("-o:", *minusO)
fmt.Println("-c:", *minusC)
fmt.Println("-k:", *minusK)
for index, val := range flag.Args() {
fmt.Println(index, ":" , val)
}
if debug == true {
simple.Version()
}
}
<file_sep>#!/bin/bash
MAIN=""
if [[ -z $1 ]]; then
MAIN=main.go
else
MAIN=$1
fi
go run $MAIN
<file_sep># scratch
## PATH=$PATH:.
## run
<file_sep>package main
// go build -ldflags "-X main.version=0.0.1"
import (
v "github.com/ardeshir/version"
)
var version string
func main() {
v.V(version)
}
| 6a0764ec968140fa3303418fafe1ee5fdaeed7d5 | [
"Markdown",
"Shell",
"Go"
] | 4 | Markdown | ardeshir/scratch | a0f934851465342e296c8eb705873cd91b6a770d | 52ee47fd9e7ffe909d1d1ddc7659785bb02b1373 |
refs/heads/main | <file_sep>use ipnetwork::IpNetwork;
use log::info;
use rand::{
prelude::{IteratorRandom, SliceRandom},
thread_rng,
};
use trust_dns_resolver::{
config::{NameServerConfig, ResolverConfig, ResolverOpts},
proto::rr::RecordType,
IntoName, Name, Resolver,
};
use crate::{
authority::{find_members, init_trust_dns_authority, new_ptr_authority},
hosts::parse_hosts,
integration_tests::{init_test_logger, init_test_runtime, TestContext, TestNetwork},
tests::HOSTS_DIR,
utils::{
authtoken_path, domain_or_default, get_listen_ips, init_authority, parse_ip_from_cidr,
},
};
use std::{
collections::HashMap,
net::{IpAddr, Ipv4Addr, SocketAddr},
str::FromStr,
sync::{mpsc::sync_channel, Arc, Mutex},
thread::{self, sleep},
time::Duration,
};
use tokio::runtime::Runtime;
#[derive(Clone)]
struct Service {
runtime: Arc<Mutex<Runtime>>,
tn: Arc<TestNetwork>,
resolvers: Arc<Vec<Arc<Resolver>>>,
update_interval: Option<Duration>,
pub listen_ips: Vec<String>,
pub listen_cidrs: Vec<String>,
}
pub(crate) trait Lookup {
fn lookup_a(&self, record: String) -> Vec<Ipv4Addr>;
fn lookup_ptr(&self, record: String) -> Vec<String>;
}
impl Lookup for Resolver {
fn lookup_a(&self, record: String) -> Vec<Ipv4Addr> {
self.lookup(record, RecordType::A)
.unwrap()
.record_iter()
.map(|r| r.rdata().clone().into_a().unwrap())
.collect()
}
fn lookup_ptr(&self, record: String) -> Vec<String> {
self.lookup(record, RecordType::PTR)
.unwrap()
.record_iter()
.map(|r| r.rdata().clone().into_ptr().unwrap().to_string())
.collect()
}
}
enum HostsType {
Path(&'static str),
Fixture(&'static str),
None,
}
fn create_listeners(
runtime: Arc<Mutex<Runtime>>,
tn: &TestNetwork,
hosts: HostsType,
update_interval: Option<Duration>,
wildcard_everything: bool,
) -> (Vec<String>, Vec<String>) {
let listen_cidrs = runtime
.lock()
.unwrap()
.block_on(get_listen_ips(
&authtoken_path(None),
&tn.network.clone().id.unwrap(),
))
.unwrap();
let mut listen_ips = Vec::new();
let (s, r) = sync_channel(listen_cidrs.len());
let mut ipmap = HashMap::new();
let mut authority_map = HashMap::new();
let authority = init_trust_dns_authority(domain_or_default(None).unwrap());
for cidr in listen_cidrs.clone() {
let listen_ip = parse_ip_from_cidr(cidr.clone());
listen_ips.push(listen_ip.clone());
let cidr = IpNetwork::from_str(&cidr.clone()).unwrap();
if !ipmap.contains_key(&listen_ip) {
ipmap.insert(listen_ip, cidr.network());
}
if !authority_map.contains_key(&cidr.network()) {
let ptr_authority = new_ptr_authority(cidr).unwrap();
let mut ztauthority = init_authority(
ptr_authority,
tn.central(),
tn.network.clone().id.unwrap(),
domain_or_default(None).unwrap(),
match hosts {
HostsType::Fixture(hosts) => Some(format!("{}/{}", HOSTS_DIR, hosts)),
HostsType::Path(hosts) => Some(hosts.to_string()),
HostsType::None => None,
},
update_interval.unwrap_or(Duration::new(30, 0)),
authority.clone(),
);
if wildcard_everything {
ztauthority.wildcard_everything();
}
let arc_authority = Arc::new(tokio::sync::RwLock::new(ztauthority));
authority_map.insert(cidr.network(), arc_authority.to_owned());
let lock = runtime.lock().unwrap();
lock.spawn(find_members(arc_authority));
drop(lock);
}
}
for ip in listen_ips.clone() {
let cidr = ipmap.get(&ip).unwrap();
let authority = authority_map.get(cidr).unwrap();
let sync = s.clone();
let rt = &mut runtime.lock().unwrap();
let server = crate::server::Server::new(authority.to_owned());
rt.spawn({
sync.send(()).unwrap();
drop(sync);
info!("Serving {}", ip.clone());
server.listen(format!("{}:53", ip.clone()), Duration::new(0, 1000))
});
}
drop(s);
loop {
if r.recv().is_err() {
break;
}
}
sleep(Duration::new(2, 0)); // FIXME this sleep should not be necessary
(listen_cidrs, listen_ips.clone())
}
fn create_resolvers(ips: Vec<String>) -> Vec<Arc<Resolver>> {
let mut resolvers = Vec::new();
for ip in ips {
let mut resolver_config = ResolverConfig::new();
resolver_config.add_search(domain_or_default(None).unwrap());
resolver_config.add_name_server(NameServerConfig {
socket_addr: SocketAddr::new(IpAddr::from_str(&ip).unwrap(), 53),
protocol: trust_dns_resolver::config::Protocol::Udp,
tls_dns_name: None,
trust_nx_responses: true,
});
let mut opts = ResolverOpts::default();
opts.cache_size = 0;
opts.rotate = true;
opts.use_hosts_file = false;
opts.positive_min_ttl = Some(Duration::new(0, 0));
opts.positive_max_ttl = Some(Duration::new(0, 0));
opts.negative_min_ttl = Some(Duration::new(0, 0));
opts.negative_max_ttl = Some(Duration::new(0, 0));
resolvers.push(Arc::new(
trust_dns_resolver::Resolver::new(resolver_config, opts).unwrap(),
));
}
resolvers
}
pub(crate) struct ServiceConfig {
hosts: HostsType,
update_interval: Option<Duration>,
ips: Option<Vec<&'static str>>,
wildcard_everything: bool,
}
impl Default for ServiceConfig {
fn default() -> Self {
Self {
hosts: HostsType::None,
update_interval: None,
ips: None,
wildcard_everything: false,
}
}
}
impl ServiceConfig {
fn hosts(mut self, h: HostsType) -> Self {
self.hosts = h;
self
}
fn update_interval(mut self, u: Option<Duration>) -> Self {
self.update_interval = u;
self
}
fn ips(mut self, ips: Option<Vec<&'static str>>) -> Self {
self.ips = ips;
self
}
fn wildcard_everything(mut self, w: bool) -> Self {
self.wildcard_everything = w;
self
}
}
impl Service {
fn new(sc: ServiceConfig) -> Self {
let runtime = init_test_runtime();
let tn = if let Some(ips) = sc.ips {
TestNetwork::new_multi_ip(
runtime.clone(),
"basic-ipv4",
&mut TestContext::default(),
ips,
)
.unwrap()
} else {
TestNetwork::new(runtime.clone(), "basic-ipv4", &mut TestContext::default()).unwrap()
};
let (listen_cidrs, listen_ips) = create_listeners(
runtime.clone(),
&tn,
sc.hosts,
sc.update_interval,
sc.wildcard_everything,
);
Self {
runtime,
tn: Arc::new(tn),
listen_ips: listen_ips.clone(),
listen_cidrs,
resolvers: Arc::new(create_resolvers(listen_ips)),
update_interval: sc.update_interval,
}
}
pub fn any_listen_ip(&self) -> Ipv4Addr {
Ipv4Addr::from_str(
&self
.listen_ips
.clone()
.into_iter()
.choose(&mut rand::thread_rng())
.unwrap()
.clone(),
)
.unwrap()
}
pub fn runtime(&self) -> Arc<Mutex<Runtime>> {
self.runtime.clone()
}
pub fn network(&self) -> Arc<TestNetwork> {
self.tn.clone()
}
pub fn resolvers(&self) -> Arc<Vec<Arc<Resolver>>> {
self.resolvers.clone()
}
pub fn any_resolver(&self) -> Arc<Resolver> {
self.resolvers()
.choose(&mut rand::thread_rng())
.to_owned()
.unwrap()
.clone()
}
pub fn lookup_a(&self, record: String) -> Vec<Ipv4Addr> {
self.any_resolver().lookup_a(record)
}
pub fn lookup_ptr(self, record: String) -> Vec<String> {
self.any_resolver().lookup_ptr(record)
}
pub fn member_record(&self) -> String {
format!("zt-{}.domain.", self.network().identity().clone())
}
pub fn change_name(&self, name: &'static str) {
let mut member = self
.runtime()
.lock()
.unwrap()
.block_on(
zerotier_central_api::apis::network_member_api::get_network_member(
&self.network().central(),
&self.network().network.clone().id.unwrap(),
&self.network().identity(),
),
)
.unwrap();
member.name = Some(name.to_string());
self.runtime()
.lock()
.unwrap()
.block_on(
zerotier_central_api::apis::network_member_api::update_network_member(
&self.network().central(),
&self.network().network.clone().id.unwrap(),
&self.network().identity(),
member,
),
)
.unwrap();
if self.update_interval.is_some() {
thread::sleep(self.update_interval.unwrap()); // wait for it to update
}
}
}
#[test]
#[ignore]
fn test_wildcard_ipv4_central() {
init_test_logger();
let service = Service::new(
ServiceConfig::default()
.update_interval(Some(Duration::new(1, 0)))
.wildcard_everything(true),
);
let member_record = service.member_record();
let named_record = Name::from_str("islay.domain.").unwrap();
service.change_name("islay");
assert_eq!(
service.lookup_a(named_record.to_string()).first().unwrap(),
&service.any_listen_ip(),
);
assert_eq!(
service.lookup_a(member_record.to_string()).first().unwrap(),
&service.any_listen_ip(),
);
for host in vec!["one", "ten", "zt-foo", "another-record"] {
for rec in vec![named_record.to_string(), member_record.clone()] {
let lookup = Name::from_str(&host)
.unwrap()
.append_domain(&Name::from_str(&rec).unwrap())
.to_string();
assert_eq!(
service.lookup_a(lookup).first().unwrap(),
&service.any_listen_ip()
);
}
}
}
#[test]
#[ignore]
fn test_hosts_file_reloading() {
init_test_logger();
let hosts_path = "/tmp/zeronsd-test-hosts";
std::fs::write(hosts_path, "127.0.0.2 islay\n").unwrap();
let service = Service::new(
ServiceConfig::default()
.hosts(HostsType::Path(hosts_path))
.update_interval(Some(Duration::new(1, 0))),
);
assert_eq!(
service
.lookup_a("islay.domain.".to_string())
.first()
.unwrap(),
&Ipv4Addr::from_str("127.0.0.2").unwrap()
);
std::fs::write(hosts_path, "127.0.0.3 islay\n").unwrap();
sleep(Duration::new(3, 0)); // wait for bg update
assert_eq!(
service
.lookup_a("islay.domain.".to_string())
.first()
.unwrap(),
&Ipv4Addr::from_str("127.0.0.3").unwrap()
);
}
#[test]
#[ignore]
fn test_battery_single_domain() {
init_test_logger();
let service = Service::new(ServiceConfig::default().ips(Some(vec![
"172.16.240.2",
"172.16.240.3",
"172.16.240.4",
])));
let record = service.member_record();
info!("Looking up {}", record);
let mut listen_ips = service.listen_ips.clone();
listen_ips.sort();
for _ in 0..10000 {
let mut ips = service
.lookup_a(record.clone())
.into_iter()
.map(|i| i.to_string())
.collect::<Vec<String>>();
ips.sort();
assert_eq!(ips, listen_ips);
}
let ptr_records: Vec<Name> = service
.listen_ips
.clone()
.into_iter()
.map(|ip| IpAddr::from_str(&ip).unwrap().into_name().unwrap())
.collect();
for ptr_record in ptr_records.clone() {
info!("Looking up {}", ptr_record);
for _ in 0..10000 {
let service = service.clone();
assert_eq!(
service.lookup_ptr(ptr_record.to_string()).first().unwrap(),
&record.to_string()
);
}
}
info!("Interleaved lookups of PTR and A records");
for _ in 0..10000 {
// randomly switch order
if rand::random::<bool>() {
assert_eq!(
service
.lookup_a(record.clone())
.into_iter()
.map(|i| i.to_string())
.collect::<Vec<String>>(),
service.listen_ips,
);
assert_eq!(
service
.clone()
.lookup_ptr(ptr_records.choose(&mut thread_rng()).unwrap().to_string())
.first()
.unwrap(),
&record.to_string()
);
} else {
assert_eq!(
service
.clone()
.lookup_ptr(ptr_records.choose(&mut thread_rng()).unwrap().to_string())
.first()
.unwrap(),
&record.to_string()
);
assert_eq!(
service
.lookup_a(record.clone())
.into_iter()
.map(|i| i.to_string())
.collect::<Vec<String>>(),
service.listen_ips,
);
}
}
}
#[test]
#[ignore]
fn test_battery_multi_domain_hosts_file() {
init_test_logger();
let ips = vec!["172.16.240.2", "172.16.240.3", "172.16.240.4"];
let service = Service::new(
ServiceConfig::default()
.hosts(HostsType::Fixture("basic"))
.ips(Some(ips.clone())),
);
let record = service.member_record();
info!("Looking up random domains");
let mut hosts_map = parse_hosts(
Some(format!("{}/basic", HOSTS_DIR)),
"domain.".into_name().unwrap(),
)
.unwrap();
for ip in ips {
hosts_map.insert(
IpAddr::from_str(&ip).unwrap(),
vec![record.clone().into_name().unwrap()],
);
}
let mut hosts = hosts_map.values().flatten().collect::<Vec<&Name>>();
for _ in 0..10000 {
hosts.shuffle(&mut rand::thread_rng());
let host = *hosts.first().unwrap();
let ips = service.lookup_a(host.to_string());
assert!(hosts_map
.get(&IpAddr::from(*ips.first().unwrap()))
.unwrap()
.contains(host));
}
}
#[test]
#[ignore]
fn test_battery_single_domain_named() {
init_test_logger();
let update_interval = Duration::new(1, 0);
let service = Service::new(
ServiceConfig::default()
.update_interval(Some(update_interval))
.ips(Some(vec!["172.16.240.2", "172.16.240.3", "172.16.240.4"])),
);
let member_record = service.member_record();
service.change_name("islay");
let named_record = "islay.domain.".to_string();
for record in vec![member_record, named_record.clone()] {
info!("Looking up {}", record);
let mut listen_ips = service.listen_ips.clone();
listen_ips.sort();
for _ in 0..10000 {
let mut ips = service
.lookup_a(record.clone())
.into_iter()
.map(|i| i.to_string())
.collect::<Vec<String>>();
ips.sort();
assert_eq!(ips, listen_ips.clone(),);
}
}
let ptr_records: Vec<Name> = service
.listen_ips
.clone()
.into_iter()
.map(|ip| IpAddr::from_str(&ip).unwrap().into_name().unwrap())
.collect();
for ptr_record in ptr_records {
info!("Looking up {}", ptr_record);
for _ in 0..10000 {
let service = service.clone();
assert_eq!(
service.lookup_ptr(ptr_record.to_string()).first().unwrap(),
&named_record.to_string()
);
}
}
}
<file_sep>use std::{
collections::HashMap,
net::IpAddr,
str::FromStr,
sync::{Arc, RwLock, RwLockWriteGuard},
time::Duration,
};
use ipnetwork::IpNetwork;
use log::{error, info, warn};
use tokio::sync::RwLockReadGuard;
use trust_dns_resolver::{
config::{NameServerConfigGroup, ResolverOpts},
proto::rr::{dnssec::SupportedAlgorithms, rdata::SOA, RecordSet},
IntoName,
};
use trust_dns_server::{
authority::{AuthorityObject, Catalog},
client::rr::{Name, RData, Record, RecordType, RrKey},
store::forwarder::ForwardAuthority,
store::{forwarder::ForwardConfig, in_memory::InMemoryAuthority},
};
use zerotier_central_api::{apis::configuration::Configuration, models::Member};
use crate::{
hosts::{parse_hosts, HostsFile},
utils::{parse_member_name, ToHostname},
};
pub(crate) type TokioZTAuthority = Arc<tokio::sync::RwLock<ZTAuthority>>;
pub(crate) type Authority = Box<Arc<RwLock<InMemoryAuthority>>>;
pub(crate) type PtrAuthority = Option<Authority>;
pub(crate) fn new_ptr_authority(ip: IpNetwork) -> Result<PtrAuthority, anyhow::Error> {
Ok(match ip {
IpNetwork::V4(ip) => {
let domain_name = ip
.network()
.into_name()?
// round off the subnet, account for in-addr.arpa.
.trim_to(8 - (ip.prefix() as usize / 8) * 8 / 8);
let mut authority = InMemoryAuthority::empty(
domain_name.clone(),
trust_dns_server::authority::ZoneType::Primary,
false,
);
set_soa(&mut authority, domain_name);
Some(Box::new(Arc::new(RwLock::new(authority))))
}
IpNetwork::V6(_) => None,
})
}
pub(crate) async fn find_members(zt: TokioZTAuthority) {
let read = zt.read().await;
let mut interval = tokio::time::interval(read.update_interval.clone());
drop(read);
loop {
interval.tick().await;
zt.write()
.await
.configure_hosts()
.expect("Could not configure authority from hosts file");
match get_members(zt.read().await).await {
Ok(members) => match zt.write().await.configure_members(members) {
Ok(_) => {}
Err(e) => {
error!("error configuring authority: {}", e)
}
},
Err(e) => {
error!("error syncing members: {}", e)
}
}
}
}
async fn get_members(zt: RwLockReadGuard<'_, ZTAuthority>) -> Result<Vec<Member>, anyhow::Error> {
let config = zt.config.clone();
let network = zt.network.clone();
Ok(
zerotier_central_api::apis::network_member_api::get_network_member_list(&config, &network)
.await?,
)
}
fn prune_records(authority: Authority, written: Vec<Name>) -> Result<(), anyhow::Error> {
let mut rrkey_list = Vec::new();
let mut lock = authority
.write()
.expect("Could not acquire write lock on authority");
let rr = lock.records_mut();
for (rrkey, _) in rr.clone() {
let key = &rrkey.name().into_name()?;
if !written.contains(key) {
rrkey_list.push(rrkey);
}
}
for rrkey in rrkey_list {
warn!("Removing expired record {}", rrkey.name());
rr.remove(&rrkey);
}
Ok(())
}
fn upsert_address(
authority: &mut RwLockWriteGuard<InMemoryAuthority>,
fqdn: Name,
rt: RecordType,
rdatas: Vec<RData>,
) {
let serial = authority.serial() + 1;
let records = authority.records_mut();
let key = records
.into_iter()
.map(|(key, _)| key)
.find(|key| key.name().into_name().unwrap().eq(&fqdn));
if let Some(key) = key {
let key = key.clone();
records
.get_mut(&key)
.replace(&mut Arc::new(RecordSet::new(&fqdn.clone(), rt, serial)));
}
for rdata in rdatas {
if match rt {
RecordType::A => match rdata {
RData::A(_) => Some(()),
_ => None,
},
RecordType::AAAA => match rdata {
RData::AAAA(_) => Some(()),
_ => None,
},
RecordType::PTR => match rdata {
RData::PTR(_) => Some(()),
_ => None,
},
_ => None,
}
.is_some()
{
let mut address = Record::with(fqdn.clone(), rt, 60);
address.set_rdata(rdata.clone());
info!("Adding new record {}: ({})", fqdn.clone(), rdata.clone());
authority.upsert(address, serial);
}
}
}
fn set_ptr_record(
authority: &mut RwLockWriteGuard<InMemoryAuthority>,
ip_name: Name,
canonical_name: Name,
) {
warn!(
"Replacing PTR record {}: ({})",
ip_name.clone(),
canonical_name
);
authority.records_mut().remove(&RrKey::new(
ip_name
.clone()
.into_name()
.expect("Could not coerce IP address into DNS name")
.into(),
RecordType::PTR,
));
upsert_address(
authority,
ip_name.clone(),
RecordType::PTR,
vec![RData::PTR(canonical_name)],
);
}
fn set_ip_record(
authority: &mut RwLockWriteGuard<InMemoryAuthority>,
name: Name,
rt: RecordType,
newips: Vec<IpAddr>,
) {
upsert_address(
authority,
name.clone(),
rt,
newips
.into_iter()
.map(|i| match i {
IpAddr::V4(i) => RData::A(i),
IpAddr::V6(i) => RData::AAAA(i),
})
.collect(),
);
}
fn configure_ptr(
authority: Authority,
ip: IpAddr,
canonical_name: Name,
) -> Result<(), anyhow::Error> {
let lock = &mut authority
.write()
.expect("Could not acquire authority write lock");
match lock
.records()
.get(&RrKey::new(ip.into_name()?.into(), RecordType::PTR))
{
Some(records) => {
if !records
.records(false, SupportedAlgorithms::all())
.any(|rec| rec.rdata().eq(&RData::PTR(canonical_name.clone())))
{
set_ptr_record(lock, ip.into_name()?, canonical_name.clone());
}
}
None => set_ptr_record(lock, ip.into_name()?, canonical_name.clone()),
}
Ok(())
}
pub(crate) fn set_soa(authority: &mut InMemoryAuthority, domain_name: Name) {
let mut soa = Record::new();
soa.set_name(domain_name.clone());
soa.set_rr_type(RecordType::SOA);
soa.set_rdata(RData::SOA(SOA::new(
domain_name.clone(),
Name::from_str("administrator")
.unwrap()
.append_domain(&domain_name.clone()),
authority.serial() + 1,
30,
0,
-1,
0,
)));
authority.upsert(soa, authority.serial() + 1);
}
pub(crate) fn init_trust_dns_authority(domain_name: Name) -> Authority {
let mut authority = InMemoryAuthority::empty(
domain_name.clone(),
trust_dns_server::authority::ZoneType::Primary,
false,
);
set_soa(&mut authority, domain_name.clone());
Box::new(Arc::new(RwLock::new(authority)))
}
pub(crate) async fn init_catalog(zt: TokioZTAuthority) -> Result<Catalog, std::io::Error> {
let read = zt.read().await;
let mut catalog = Catalog::default();
catalog.upsert(read.domain_name.clone().into(), read.authority.box_clone());
if read.ptr_authority.is_some() {
let unwrapped = read.ptr_authority.clone().unwrap();
catalog.upsert(unwrapped.origin(), unwrapped.box_clone());
} else {
warn!("PTR records are not supported on IPv6 networks (yet!)");
}
drop(read);
let resolvconf = trust_dns_resolver::config::ResolverConfig::default();
let mut nsconfig = NameServerConfigGroup::new();
for server in resolvconf.name_servers() {
nsconfig.push(server.clone());
}
let options = Some(ResolverOpts::default());
let config = &ForwardConfig {
name_servers: nsconfig.clone(),
options,
};
let forwarder = ForwardAuthority::try_from_config(
Name::root(),
trust_dns_server::authority::ZoneType::Primary,
config,
)
.await
.expect("Could not initialize forwarder");
catalog.upsert(
Name::root().into(),
Box::new(Arc::new(RwLock::new(forwarder))),
);
Ok(catalog)
}
pub(crate) struct ZTRecord {
fqdn: Name,
canonical_name: Option<Name>,
ptr_name: Name,
ips: Vec<IpAddr>,
wildcard_everything: bool,
}
impl ZTRecord {
pub(crate) fn new(
member: &Member,
domain_name: Name,
wildcard_everything: bool,
) -> Result<Self, anyhow::Error> {
let member_name = format!(
"zt-{}",
member
.clone()
.node_id
.expect("Node ID for member does not exist")
);
let fqdn = member_name.clone().to_fqdn(domain_name.clone())?;
// this is default the zt-<member id> but can switch to a named name if
// tweaked in central. see below.
let mut canonical_name = None;
let mut ptr_name = fqdn.clone();
if let Some(name) = parse_member_name(member.name.clone(), domain_name.clone()) {
canonical_name = Some(name.clone());
ptr_name = name;
}
let ips: Vec<IpAddr> = member
.clone()
.config
.expect("Member config does not exist")
.ip_assignments
.expect("IP assignments for member do not exist")
.into_iter()
.map(|s| IpAddr::from_str(&s).expect("Could not parse IP address"))
.collect();
Ok(Self {
wildcard_everything,
fqdn,
canonical_name,
ptr_name,
ips,
})
}
pub(crate) fn insert(&self, records: &mut Vec<Name>) {
records.push(self.fqdn.clone());
for ip in self.ips.clone() {
records.push(ip.into_name().expect("Could not coerce IP into name"));
}
if self.canonical_name.is_some() {
records.push(self.canonical_name.clone().unwrap());
if self.wildcard_everything {
records.push(self.get_canonical_wildcard().unwrap())
}
}
if self.wildcard_everything {
records.push(self.get_wildcard())
}
}
pub(crate) fn get_wildcard(&self) -> Name {
Name::from_str("*")
.unwrap()
.append_name(&self.fqdn)
.into_wildcard()
}
pub(crate) fn get_canonical_wildcard(&self) -> Option<Name> {
if self.canonical_name.is_none() {
return None;
}
Some(
Name::from_str("*")
.unwrap()
.append_name(&self.canonical_name.clone().unwrap())
.into_wildcard(),
)
}
pub(crate) fn insert_member(&self, authority: &ZTAuthority) -> Result<(), anyhow::Error> {
authority.match_or_insert(self.fqdn.clone(), self.ips.clone());
if self.wildcard_everything {
authority.match_or_insert(self.get_wildcard(), self.ips.clone());
}
if self.canonical_name.is_some() {
authority.match_or_insert(self.canonical_name.clone().unwrap(), self.ips.clone());
if self.wildcard_everything {
authority.match_or_insert(self.get_canonical_wildcard().unwrap(), self.ips.clone())
}
}
Ok(())
}
pub(crate) fn insert_member_ptr(
&self,
authority: &PtrAuthority,
records: &mut Vec<Name>,
) -> Result<(), anyhow::Error> {
if authority.is_some() {
for ip in self.ips.clone() {
records.push(ip.into_name().expect("Could not coerce IP into name"));
configure_ptr(authority.clone().unwrap(), ip, self.ptr_name.clone())?;
}
}
Ok(())
}
}
#[derive(Clone)]
pub(crate) struct ZTAuthority {
ptr_authority: PtrAuthority,
authority: Authority,
domain_name: Name,
network: String,
config: Configuration,
hosts_file: Option<String>,
hosts: Option<Box<HostsFile>>,
update_interval: Duration,
wildcard_everything: bool,
}
impl ZTAuthority {
pub(crate) fn new(
domain_name: Name,
network: String,
config: Configuration,
hosts_file: Option<String>,
ptr_authority: PtrAuthority,
update_interval: Duration,
authority: Authority,
) -> Self {
Self {
update_interval,
domain_name: domain_name.clone(),
network,
config,
authority,
ptr_authority,
hosts_file,
hosts: None,
wildcard_everything: false,
}
}
pub(crate) fn wildcard_everything(&mut self) {
self.wildcard_everything = true;
}
fn match_or_insert(&self, name: Name, newips: Vec<IpAddr>) {
let rdatas: Vec<RData> = newips
.clone()
.into_iter()
.map(|ip| match ip {
IpAddr::V4(ip) => RData::A(ip),
IpAddr::V6(ip) => RData::AAAA(ip),
})
.collect();
for rt in vec![RecordType::A, RecordType::AAAA] {
let lock = self
.authority
.read()
.expect("Could not get authority read lock");
let records = lock
.records()
.get(&RrKey::new(name.clone().into(), rt))
.clone();
match records {
Some(records) => {
let records = records.records(false, SupportedAlgorithms::all());
if records.is_empty()
|| !records.into_iter().all(|r| rdatas.contains(r.rdata()))
{
drop(lock);
set_ip_record(
&mut self.authority.write().expect("write lock"),
name.clone(),
rt,
newips.clone(),
);
}
}
None => {
drop(lock);
set_ip_record(
&mut self.authority.write().expect("write lock"),
name.clone(),
rt,
newips.clone(),
);
}
}
}
}
fn configure_members(&mut self, members: Vec<Member>) -> Result<(), anyhow::Error> {
let mut records = vec![self.domain_name.clone()];
if let Some(hosts) = self.hosts.to_owned() {
self.prune_hosts();
records.append(&mut hosts.values().flatten().map(|v| v.clone()).collect());
}
for member in members {
let record =
ZTRecord::new(&member, self.domain_name.clone(), self.wildcard_everything)?;
record.insert_member(self)?;
record.insert_member_ptr(&self.ptr_authority, &mut records)?;
record.insert(&mut records);
}
prune_records(self.authority.to_owned(), records.clone())?;
if let Some(ptr_authority) = self.ptr_authority.to_owned() {
prune_records(ptr_authority, records.clone())?;
}
Ok(())
}
fn configure_hosts(&mut self) -> Result<(), anyhow::Error> {
self.hosts = Some(Box::new(parse_hosts(
self.hosts_file.clone(),
self.domain_name.clone(),
)?));
for (ip, hostnames) in self.hosts.clone().unwrap().iter() {
for hostname in hostnames {
self.match_or_insert(hostname.clone(), vec![ip.clone()]);
}
}
Ok(())
}
fn prune_hosts(&mut self) {
if self.hosts.is_none() {
return;
}
let mut lock = self.authority.write().expect("Pruning hosts write lock");
let serial = lock.serial();
let rr = lock.records_mut();
let mut hosts_map = HashMap::new();
for (ip, hosts) in self.hosts.to_owned().unwrap().into_iter() {
for host in hosts {
if !hosts_map.contains_key(&host) {
hosts_map.insert(host.clone(), vec![]);
}
hosts_map.get_mut(&host).unwrap().push(ip);
}
}
for (host, ips) in hosts_map.into_iter() {
for (rrkey, rset) in rr.clone() {
let key = &rrkey.name().into_name().expect("could not parse name");
let records = rset.records(false, SupportedAlgorithms::all());
let rt = rset.record_type();
let rdatas: Vec<RData> = ips
.clone()
.into_iter()
.filter_map(|i| match i {
IpAddr::V4(ip) => {
if rt == RecordType::A {
Some(RData::A(ip))
} else {
None
}
}
IpAddr::V6(ip) => {
if rt == RecordType::AAAA {
Some(RData::AAAA(ip))
} else {
None
}
}
})
.collect();
if key.eq(&host)
&& (records.is_empty()
|| !records.map(|r| r.rdata()).all(|rd| rdatas.contains(rd)))
{
let mut new_rset = RecordSet::new(key, rt, serial + 1);
for rdata in rdatas.clone() {
new_rset.add_rdata(rdata);
}
warn!("Replacing host record for {} with {:?}", key, ips);
rr.remove(&rrkey);
rr.insert(rrkey, Arc::new(new_rset));
}
}
}
}
}
#[cfg(test)]
mod tests;
| f4639194bf38a37fcb122440ae57490cf7e3b043 | [
"Rust"
] | 2 | Rust | DavidAlphaFox/zeronsd | bdc9c80ca71b5265a561f67fa3da229d4e10c7fc | 2e6a5e7e70c6f05b0617609c0ab48739c610258b |
refs/heads/master | <file_sep><!DOCTYPE html>
<html>
<head>
<title>Meme Calendar - October</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href="main.css" rel="stylesheet">
<!--
<link href="https://fonts.googleapis.com/css?family=Limelight" rel="stylesheet">
-->
</head>
<body>
<h1>Memes from October</h1>
<img class="center" src="images/moth.jpg">
<p>
First there was the moth meme <br>
<a href=https://oschre7741.github.io/website1/>a website about moth memes</a><br>
<img class="center" src="images/printer.jpg">
In a similar format, the printer memes <br>
<img class="center" src="images/sans.jpg">
<a href="https://www.youtube.com/watch?v=jAHfD-xA0qo">Then came the rise of Sans memes </a>
</p>
</body>
</html>
| 303423bbf40530daa074f75cb739545cd55108e2 | [
"HTML"
] | 1 | HTML | oschre7741/meme-site | 95ac421bdc0c28d66712155a0870f66a5d471661 | 767e9a0e80af6cf74fe2ad510d73281bd6145e65 |
refs/heads/master | <repo_name>digideskio/Lifa-Barber-android<file_sep>/app/src/main/java/porterapptest/rmk/com/lifabarberapp/DashBoard.java
package porterapptest.rmk.com.lifabarberapp;
import android.content.res.Configuration;
import android.os.Bundle;
import android.os.PersistableBundle;
import android.support.design.widget.NavigationView;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.view.GravityCompat;
import android.support.v4.widget.DrawerLayout;
import android.support.v7.app.ActionBar;
import android.support.v7.app.ActionBarDrawerToggle;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.Menu;
import android.view.MenuItem;
public class DashBoard extends AppCompatActivity {
private DrawerLayout mDrawerLayout;
private Toolbar mToolbar;
private NavigationView navDrawerView;
private ActionBarDrawerToggle actionBarDrawerToggle;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_dash_board);
mDrawerLayout = (DrawerLayout) findViewById(R.id.drawer_layout);
mToolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(mToolbar);
navDrawerView = (NavigationView) findViewById(R.id.nvView);
//Disabling icon tint in side drawer
navDrawerView.setItemIconTintList(null);
// Set the menu icon instead of the launcher icon.
final ActionBar ab = getSupportActionBar();
if (ab != null) {
ab.setDisplayHomeAsUpEnabled(true);
ab.setHomeAsUpIndicator(R.drawable.ic_action);
}
//Setting up content based on Drawer Item.
setupDrawerContent(navDrawerView);
//Connecting DrawerLayout and Toolbar
actionBarDrawerToggle = setupDrawerToggle();
mDrawerLayout.setDrawerListener(actionBarDrawerToggle);
}
private void setupDrawerContent(NavigationView navDrawerView) {
navDrawerView.setNavigationItemSelectedListener(new NavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(MenuItem menuItem) {
selectedNavDrawerItem(menuItem);
return false;
}
});
}
private void selectedNavDrawerItem(MenuItem menuItem) {
Fragment selectedFragment = null;
Class fragmentClass;
switch (menuItem.getItemId()) {
case R.id.parcelList:
fragmentClass = BlankFragment.class;
break;
case R.id.liveLocation:
fragmentClass = BlankFragment2.class;
break;
case R.id.apiData:
fragmentClass = BlankFragment3.class;
break;
default:
fragmentClass = BlankFragment3.class;
}
try {
selectedFragment = (Fragment) fragmentClass.newInstance();
} catch (Exception e) {
e.printStackTrace();
}
FragmentManager fragmentManager = getSupportFragmentManager();
fragmentManager.beginTransaction().replace(R.id.flContent, selectedFragment);
menuItem.setChecked(true);
setTitle(menuItem.getTitle());
mDrawerLayout.closeDrawers();
}
private ActionBarDrawerToggle setupDrawerToggle() {
return new ActionBarDrawerToggle(this, mDrawerLayout, mToolbar, R.string.drawer_open, R.string.drawer_close);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_dash_board, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == android.R.id.home) {
mDrawerLayout.openDrawer(GravityCompat.START);
return true;
}
if (actionBarDrawerToggle.onOptionsItemSelected(item)) {
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onPostCreate(Bundle savedInstanceState, PersistableBundle persistentState) {
super.onPostCreate(savedInstanceState, persistentState);
actionBarDrawerToggle.syncState();
}
@Override
public void onConfigurationChanged(Configuration newConfig) {
super.onConfigurationChanged(newConfig);
actionBarDrawerToggle.onConfigurationChanged(newConfig);
}
}
| 6fdd827d593ce2793074ee80cdceadfe5ba46c34 | [
"Java"
] | 1 | Java | digideskio/Lifa-Barber-android | 35a97b0b29062c79dfb67a60c9dc332994c1f3ce | c63e90ce3b2aaedbd4080961d7eed220cb75db80 |
refs/heads/main | <file_sep>#/bin/sh
PYSPARK_DRIVER_PYTHON=/gext/jean-marc.gratien/home/hduser/local/anaconda3/bin/python
PYSPARK_PYTHON=/gext/jean-marc.gratien/home/hduser/local/anaconda3/bin/python
spark-submit \
--conf spark.yarn.appMasterEnv.PYSPARK_PYTHON=/gext/jean-marc.gratien/home/hduser/local/anaconda3/bin/python \
--master local[*] \
--deploy-mode client \
$1
<file_sep># BigData_exemples
## A/ Spark ML : Iris classification
**_Data : iris.csv_**
The notebook **iris_ml_spark.ipynb** script, present a Spark Pipeline that transform and process the data before applying differnet classification model:
- Decision tree Classifier;
- The Random Forest Classifier ;
- LogisticRegression Classifier.
Then Compare their performance
## B/ Spark : parallelisation of the image processing algorithm « MedianFilter »
The median filter consists in replace the value of a pixel _p[i,j]_ by the median value of the list :
_[p[i-1,j-1],p[i-1,j],p[i-1,j+1],p[i,j-1],p[i,j],p[i,j+1],p[i+1,j-1],p[i+1,j],p[i+1,j+1]]_
The notebook **median_filter_spark.ipynb** present a parallisation of this filter using **spark**.
The script is executed on the **lena_noizy.jpg** image and generate the new file **lena_filter.jpg**
## C/ Dask ML : flower classification
**_Data : flower_images.zip_**
the notebook **iris_ml_spark.ipynb** use Dask to apply to apply a pipeline on different images before segmenting them.
The following pipeline is:
- convert to gray scale
- apply segmentation
- get num of labels
Then plot hystogram of numbers of labels.
## D/ Dask : parallelisation of the image processing algorithm « MedianFilter »
The notebook **median_filter_dask.ipynb** present a parallisation of the Median filter presented above using **dask**.
The script is executed on the **lena_noizy.jpg** image and generate the new file **lena_filter.jpg**
| ca2023910efd4b1513a44919e121b361e7c5168e | [
"Markdown",
"Shell"
] | 2 | Markdown | NezLheimeur/BigData_examples | 41637b1bd8f4e47c939ae03eafe808e305273308 | 89f58d8d6b16e37d0a6e2d45c5209ab100745156 |
refs/heads/master | <repo_name>samarco123/samra<file_sep>/README.md
# samra
front end web developer
| 343fb43612e27c2b1e224c9578978f21abde525f | [
"Markdown"
] | 1 | Markdown | samarco123/samra | d00be31ef738d16b84021dd695a8dc870eba5c6a | f1db2f6a8ec0549d5b66302604fad779b3a8d649 |
refs/heads/master | <file_sep>## Image Recognition ARDrone Program
This project uses my other project SiegDrone.py for a backbone.
The added features for this repository is a fully trained CNN using the CIFAR 10 imageset, and trained with CAFFE.
Standard connection to the drone, with controls setup for a PS4 controller.
New feature is the aiming square on the video feed. Aim at your desired object and press the "O" circle button to
see if the image is recognized as a deer. Code can be chaged to focus on the other 9 images that CIFAR was trained
with, so it can be adapted to the user. Modifiable code resides in the SiegDrone.py file.
| 675140084f6a68c8810a76ae4343d9c9a8a0b9cd | [
"Markdown"
] | 1 | Markdown | siegfriedgreg/MLDrone | 8313e2f90cc7b0382abedbaefd5fbfa81a0b6b9f | 553b3ffd4ad2dd3c242408fc6240fb530d3e5614 |
refs/heads/master | <file_sep>import { Request, Response } from 'express'
/**
* GET /api/welcome
* Hello, World! 👋
*/
export const welcome = (req: Request, res: Response) => {
const { name } = req.params
name ? res.send(`Hello, ${name}!`) : res.send('Hello, World!')
}
<file_sep>import express from 'express'
import expressValidator from 'express-validator'
import helmet from 'helmet'
import { handleError } from '../utils/errors'
interface App {
use: (fn: any, cb?: any) => void
}
export = (app: App): void => {
app.use(express.json())
app.use(helmet())
app.use(expressValidator())
app.use('/api', require('./routes'))
app.use(handleError)
}
<file_sep>import { Request, Response } from 'express'
import { Budget } from '../models'
/**
* POST /api/budget
* Create a new budget.
*/
export const post = async (req: Request, res: Response) => {
req.assert('budgetAmount', 'Budget amount is not valid').isNumeric()
req
.assert('title', 'Title must be valid word characters')
.isLength({ min: 1 })
.matches(/\w/g)
const errors = req.validationErrors()
if (errors) return res.status(422).json({ errors })
const budget = new Budget(req.body)
const promise = await budget.save()
res.status(201).json(promise)
}
/**
* GET /api/budget
* Fetch all budgets.
*/
export const get = async (req: Request, res: Response) => {
const promise = await Budget.find().select('-__v').exec()
promise && promise.length
? res.json(promise)
: res.status(404).json({ error: 'No budgets found' })
}
<file_sep>import { Document, model, Schema } from 'mongoose'
export type CategoryModel = Document & {
title: string
}
const categorySchema = new Schema({
title: {
required: true,
type: String,
unique: true
}
})
const Category = model('Category', categorySchema)
export default Category
<file_sep>import { NextFunction, Request, Response } from 'express'
export const catchError = (fn?: any) =>
(req?: Request, res?: Response, next?: NextFunction) =>
fn(req, res, next).catch(next)
interface IError extends Error {
status: number
}
export const handleError = (err: IError, req: Request, res: Response, next?: NextFunction) => {
if (err && err.message.includes('E11000 duplicate key error')) {
return res
.status(422)
.json({ message: `${err.message.split('\"')[1]} is a duplicate key`, err })
}
const errorDetails = {
message: err.message,
stack: err.stack || '',
status: err.status
}
res.status(err.status || 500).json(errorDetails)
}
<file_sep>export { get as getBudget, post as postBudget } from './budget'
export { get as getCategory, post as postCategory } from './category'
export { aggregate, get as getExpense, post as postExpense } from './expense'
export * from './welcome'
<file_sep>import { Router } from 'express'
import {
aggregate,
getBudget,
getCategory,
getExpense,
postBudget,
postCategory,
postExpense,
welcome
} from '../controllers'
import { catchError } from '../utils/errors'
const router: Router = Router()
router.get('/welcome', welcome)
/* Budget Endpoints */
router.get('/budget', catchError(getBudget))
router.post('/budget', catchError(postBudget))
/* Category Endpoints */
router.get('/category', catchError(getCategory))
router.post('/category', catchError(postCategory))
/* Expense Endpoints */
router.get('/aggregate', catchError(aggregate))
router.get('/expense', catchError(getExpense))
router.post('/expense', catchError(postExpense))
export = router
<file_sep>import { Document, model, Schema } from 'mongoose'
export type BudgetModel = Document & {
budgetAmount: number,
title: string
}
const budgetSchema = new Schema({
budgetAmount: Number,
title: {
required: true,
type: String,
unique: true
}
})
const Budget = model('Budget', budgetSchema)
export default Budget
<file_sep>export = {
db: process.env.DATABASE || 'mongodb://localhost:27017/budgettracker',
port: process.env.PORT || 8080
}
<file_sep>import mongoose from 'mongoose'
interface Config {
db: string
}
export = (config: Config): void => {
mongoose
.connect(config.db)
.then(() => console.log('📙 connected to MongoDB'))
.catch((err: Error) => console.log(err.message))
mongoose.Promise = global.Promise
}
<file_sep>import dotenv from 'dotenv'
import express from 'express'
import config from './config/config'
dotenv.config({ path: '.env' })
const app: express.Application = express()
require('./config/express')(app)
require('./config/mongoose')(config)
app.listen(config.port, () =>
console.log(`🤖 listening: http://localhost:${config.port}/ in ${app.get('env')} mode`))
<file_sep>export { default as Budget } from './Budget'
export { CategoryModel, default as Category } from './Category'
export { ExpenseModel, default as Expense } from './Expense'
<file_sep>import { Document, model, Schema, Types } from 'mongoose'
export type ExpenseModel = Document & {
amount: number,
budget: Types.ObjectId | null,
category: Types.ObjectId | null,
description: string
}
const expenseSchema = new Schema({
amount: {
required: true,
type: Number
},
budget: {
ref: 'Budget',
type: Schema.Types.ObjectId
},
category: {
ref: 'Category',
type: Schema.Types.ObjectId
},
description: {
required: true,
type: String
}
})
const Expense = model('Expense', expenseSchema)
export default Expense
<file_sep>import { Request, Response } from 'express'
import { Budget, Category, Expense } from '../models'
/**
* POST /api/expense
* Create a new expense.
*/
export const post = async (req: Request, res: Response) => {
const { amount, description } = req.body
let { budget, category } = req.body
req.assert('amount', 'Invalid amount').isNumeric()
req
.assert('description', 'Description must be valid word characters')
.matches(/\w/g)
const errors = req.validationErrors()
if (errors) return res.status(422).json({ errors })
if (budget) {
budget = await Budget.findOne({ title: budget }).exec()
if (!budget) {
return res
.status(404)
.json({ error: `Budget ${budget} could not be found` })
}
}
if (category) {
category = await Category.findOne({ title: category }).exec()
if (!category) {
return res
.status(404)
.json({ error: `Category ${category} could not be found` })
}
}
const expense = new Expense({
amount,
budget: budget ? budget._id : undefined,
category: category ? category._id : undefined,
description
})
expense.save()
const promise = await expense.populate('budget category').execPopulate()
res.status(201).json(promise)
}
/**
* GET /api/expense
* Fetch all expenses.
*/
export const get = async (req: Request, res: Response) => {
const promise = await Expense.find()
.select('-__v')
.populate('budget category', '-_id title')
.exec()
promise && promise.length
? res.json(promise)
: res.status(404).json({ error: 'No budgets found' })
}
/**
* GET /api/aggregate
* Aggregates all expenses and subtracts them from their respective budgets
*/
export const aggregate = async (req: Request, res: Response) => {
const budgets = await Expense
.aggregate([{
$group: {
_id: '$budget',
count: { $sum: 1 },
totalExpenses: { $sum: '$amount' },
}
}])
.exec()
const expenses = await Expense
.find()
.select('-_id -__v')
.populate('budget category', '-__v')
res.json({ budgets, expenses })
}
<file_sep>import { Request, Response } from 'express'
import { Category } from '../models'
/**
* POST /api/category
* Create a new category.
*/
export const post = async (req: Request, res: Response) => {
req.assert('title', 'Title cannot be blank').isLength({ min: 1 }).matches(/\w/g)
const errors = req.validationErrors()
if (errors) return res.status(422).json({ errors })
const category = new Category(req.body)
const response = await category.save()
res.status(201).json(response)
}
/**
* GET /api/category
* Fetch all categories.
*/
export const get = async (req: Request, res: Response) => {
const promise = await Category.find().select('-__v').exec()
promise && promise.length
? res.json(promise)
: res.status(404).json({ error: 'No categories found' })
}
| c4176449180c5012d00ca478ebd655e1ebb9332d | [
"TypeScript"
] | 15 | TypeScript | frankfaustino/Sprint-Challenge-Mongo | de17288bb60c189dd6d5cc7897d5d9d424e9154c | 720ef0c91c5430e8c5f71559da9c13e133c5962f |
refs/heads/master | <repo_name>cronJ/EagleLibrary<file_sep>/README.md
# EagleLibrary
My librarys for Cadsoft Eagle
| 1edfca12377e4a647185bf5af4fdec20f4953192 | [
"Markdown"
] | 1 | Markdown | cronJ/EagleLibrary | 9c3b7467722e1973d90e474cefc45e0bb32c861e | f3414ce92ebdb175b814467bc36fccf906b714ed |
refs/heads/master | <file_sep>#!/usr/bin/env python
#-*- coding: utf-8 -*-
from collections import namedtuple
import json
import sys
"""
Ce fichier convertit les données brutes de bureaux provenant de
data.nantes.fr en format lisible par le scrit Leaflet dans index.hmtl
"""
candidates = ['AMADOU',
'EGRON',
'NICOLAS',
'MERAND',
'KORIAT',
'SCALES',
'RINEAU',
'AVELLO',
'PERNOT',
'SEMSAR_BEHAGUE',
'CRAM',
'MAGAUD',
'REBORA',
'RAIMBOURG',
'CALONNE',
'RAYNAUD',
'BARRELY',
'LEGEAY']
OfficeResults = namedtuple('OfficeResult', ' ,'.join(candidates))
if __name__ == '__main__':
if len(sys.argv) != 4:
msg = ('Usage : {command} <input.json>'
' <outputd.json> <outputg.json>').format(
command=sys.argv[0])
print(msg)
exit()
offices = {}
general = {'votants': 0,
'exprimes': 0,
'inscrits': 0}
gresults = {}
with open(sys.argv[1], 'r') as json_file:
reader = json.load(json_file, encoding='utf8')
for row in reader['data']:
# Get results for each political list
results = sorted(
[{candidate.lower():
float(row[candidate]) * 100. / float(row['VOTANTS'])}
for candidate in candidates],
key=lambda k: k.values(),
reverse=True)
parts = row['BUREAU_DE_VOTE'].split()
if parts[0] == "1":
bureau = " ".join(parts[2:])
else:
bureau = "Nantes-"+parts[0]
offices[bureau] = results
for i in row:
try:
vval = row[i]
if not vval:
vval = 0
val = int(vval)
if i.lower() in general:
general[i.lower()] += val
else:
general[i.lower()] = val
except ValueError:
continue
# Get results for each political list
gresults = sorted(
[{candidate.lower():
float(general[candidate.lower()]) * 100. / float(general['votants'])}
for candidate in candidates],
key=lambda k: k.values(),
reverse=True)
with open(sys.argv[2], 'w') as outfile:
response_json = json.dump(offices, outfile, indent=2)
with open(sys.argv[3], 'w') as outfile:
response_json = json.dump(gresults, outfile, indent=2)
<file_sep>#!/usr/bin/env python
#-*- coding: utf-8 -*-
from collections import namedtuple
from collections import OrderedDict
import re
import json
import six
import sys
import csv
candidates = ['AMADOU',
'EGRON',
'NICOLAS',
'MERAND',
'KORIAT',
'SCALES',
'RINEAU',
'AVELLO',
'PERNOT',
'SEMSAR_BEHAGUE',
'CRAM',
'MAGAUD',
'REBORA',
'RAIMBOURG',
'CALONNE',
'RAYNAUD',
'BARRELY',
'LEGEAY']
data = OrderedDict()
if __name__ == '__main__':
if len(sys.argv) != 3:
msg = ('Usage : {command} <input.json>'
' <outputd.json> <outputg.json>').format(
command=sys.argv[0])
print(msg)
exit()
with open(sys.argv[1], 'rb') as csvfile:
spamreader = csv.DictReader(csvfile, delimiter=',', quotechar='"')
for row in spamreader:
candidat = row.get('candidat')
if not candidat:
continue
for item, val in six.iteritems(row):
if re.search('Boug|Rez|saintseb', item):
recorder = data.setdefault(item, [])
recorder.append({candidat: val.replace(',', '.').replace('%', '') })
with open(sys.argv[2], 'w') as outfile:
response_json = json.dump(data, outfile, indent=2)
<file_sep>NantesFI44
============
## Technologies
* Leaflet
## Crédits
### Données
### Code
<file_sep>// get color depending on the candidate
var colors = {
'amadou': '#FB7102'
, 'nicolas': '#FB7132'
, 'avello': '#1B2638'
, 'calonne': '#1B2640'
, 'scales': '#7AB42D'
, 'egron': '#E50000'
, 'pernot': '#FF0011'
, 'raimbourg': '#8297BE'
, 'rebora': '#8297CE'
, 'barrely': '#8297DE'
, 'rineau': '#8297EE'
, 'koriat': '#8298EE'
, 'raynaud': '#4299EE'
, 'legeay': '#8299EE'
, 'semsar_behague': '#8291EE'
, 'magaud': '#8292EE'
, 'merand': '#8293EE'
, 'cram': '#8294EE'
};
var fullNames = {
'amadou': '<NAME>'
, 'avello': 'avellot'
, 'nicolas': 'nicolas'
, 'calonne': 'calonne'
, 'scales': 'scales'
, 'egron': '<NAME>'
, 'pernot': 'pernot'
, 'raimbourg': 'raimbourg'
, 'raynaud': 'raynaud'
, 'rebora': 'rebora'
, 'barrely': 'barrely'
, 'rineau': 'rineau'
, 'koriat': 'koriat'
, 'legeay': 'legeay'
, 'magaud': 'magaud'
, 'semsar_behague': 'semsar_behague'
, 'merand': 'merand'
, 'cram': 'cram'
};
var partis = {
'amadou': 'LREM'
, 'avello': 'FN'
, 'cram': 'UPR'
, 'raynaud': 'LO'
, 'nicolas': 'EELV'
, 'calonne': 'DLF'
, 'scales': 'LCPHAP'
, 'egron': 'FI'
, 'pernot': 'PCF'
, 'raimbourg': 'PS'
, 'rebora': 'PFE'
, 'koriat': 'blancs'
, 'barrely': 'REG'
, 'rineau': 'Pchrétien'
, 'legeay': 'animalistes'
, 'magaud': 'PCR'
, 'semsar_behague': 'RDG'
, 'merand': 'UDI'
};
//
var resultsd = {};
var resultsdreze = {};
var resultsdbouguenais = {};
var resultsdsaintseb = {};
var resultsg = {};
var geojson;
var map;
var legend;
var osmAttrib = '<a href="http://data.nantes.fr/donnees/detail/decoupage-geographique-des-bureaux-de-vote-de-la-ville-de-nantes/" target="_blank">Découpage</a>';
var osm;
//
$.ajax({
url: "results_1detail.json",
dataType: 'json',
async: false,
success: function(data) {resultsd = data;}
});
$.ajax({
url: "bouguenais.json",
dataType: 'json',
async: false,
success: function(data) {resultsdbouguenais = data;}
});
$.ajax({
url: "saintseb.json",
dataType: 'json',
async: false,
success: function(data) {resultsdsaintseb = data;}
});
$.ajax({
url: "reze.json",
dataType: 'json',
async: false,
success: function(data) {resultsdreze = data;}
});
$.ajax({
url: "results_1general.json",
dataType: 'json',
async: false,
success: function(data) {resultsg = data;}
})
$.extend(resultsd, resultsdreze);
$.extend(resultsd, resultsdbouguenais);
$.extend(resultsd, resultsdsaintseb);
function resetHighlight(e) {
geojson.setStyle(bureauxStyle);
legend.update();
}
function onEachFeature(feature, layer) {
layer.on({mouseover: highlightFeature, mouseout: resetHighlight});
}
function highlightFeature(e) {
var layer = e.target;
legend.update(layer.feature.properties);
layer.setStyle({weight: 4});
}
function launchmap() {
map = L.map('map', {maxZoom: 17, minZoom: 11}).setView([47.22, -1.55], 12);
map.attributionControl.setPrefix(osmAttrib);
map.setMaxBounds(new L.LatLngBounds(new L.LatLng(47.230752, -1.694697),new L.LatLng(47.128512, -1.413313)));
L.tileLayer("https://tilestream.makina-corpus.net/v2/nantes-desaturate/{z}/{x}/{y}.png",{attribution:'© Contributeurs <a href="http://osm.org/copyright">OpenStreetMap</a>'}).addTo(map)
if (L.Browser.touch) { L.control.touchHover().addTo(map); }
geojson = L.geoJson(
bureaux,
{style: bureauxStyle, onEachFeature: onEachFeature}).addTo(map);
legend = L.control({position: 'topright'});
legend.onAdd = function (map) {
this._div = L.DomUtil.create('div', 'legend info');
this.update();
return this._div;
};
};
| 6743fa12b00fb9422686a24aaf859a4d9badbf91 | [
"Markdown",
"JavaScript",
"Python"
] | 4 | Markdown | kiorky/fi44maps | 77ace924ae2c39551494f47eef887dac2f36e92c | 531e8a8751dd78eb425a6c16e3eb80a5a85fd99e |
refs/heads/master | <file_sep># ReduxSimpleStarter
此程式碼為 Udemy
<NAME> 影片 教學程式碼
This code only for my pratice
| c908c87b67b053c186ceef8f9421efb3f7de45df | [
"Markdown"
] | 1 | Markdown | jim25522532/reactPratice | a83330559358b17bf920315764db15ef81a01296 | cf976e6eab20399fc30de7956496fa2e60a41d00 |
refs/heads/master | <file_sep>"""
Class that represents a single linked
list node that holds a single value
and a reference to the next node in the list
"""
import math
class Node:
def __init__(self, value=None, next_node=None):
self.value = value
self.next_node = next_node
def get_value(self):
return self.value
def get_next(self):
return self.next_node
def set_next(self, new_next):
self.next_node = new_next
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def add_to_tail(self, value):
# wrap it in a node instance
new_node = Node(value)
# check if there's no head
if self.head is None:
self.head = new_node
self.tail = new_node
else:
# set the current tail's next reference to the new node
self.tail.set_next(new_node)
# update the List's tail reference
self.tail = new_node
def remove_head(self):
node_to_remove = self.head
# check to see if there is a head
if node_to_remove is None:
return None
# check if the head node has a next node
elif node_to_remove == self.tail:
self.head = None
self.tail = None
return node_to_remove.get_value()
else:
self.head = self.head.get_next()
return node_to_remove.get_value()
def contains(self, value):
# get reference to current node
current_node = self.head
# walk along the list so long as curent node is a node
while current_node is not None:
# return true if the current value we're looking for matches our target
if current_node.get_value() == value:
return True
# update our current reference
current_node = current_node.get_next()
return False
def get_max(self):
if self.head:
current_node = self.head
max_value = math.inf * -1
while current_node is not None:
if current_node.get_value() > max_value:
max_value = current_node.get_value()
current_node = current_node.get_next()
return max_value
| 560cbe8ea2b0d6c1f8b9ab6725d57c604898d61e | [
"Python"
] | 1 | Python | meltzerkyle/Data-Structures | 964b7b956eee89fa5fd9f8f195588dfab9b01be8 | edbac80f1a7482fe736089c9218f50aa4367d61f |
refs/heads/master | <repo_name>JacobBennett/StorageSpy<file_sep>/README.md
# StorageSpy :floppy_disk::squirrel:
| 7262f516e546fbec6c7926fef42d55b553fb8a7f | [
"Markdown"
] | 1 | Markdown | JacobBennett/StorageSpy | 707c3d94eeae17d097fee493bd8105abd9f419c0 | 169e90e2ddaf6f0d03cca6369bcca811187d9508 |
refs/heads/master | <file_sep>body{
background-color: #250352;
color:white;
}
input{
color:black;
}
#header{
ul{
a{
color:black;
}
}
a{
color:white;
cursor:pointer;
}
}
.content{
-webkit-transition: 0.3s linear;
-moz-transition: 0.3s linear;
-o-transition: 0.3s linear;
transition: 0.3s linear;
margin-top:10%;
}
.title, .subtitle{
-webkit-transition: 0.1s linear;
-moz-transition: 0.1s linear;
-o-transition: 0.1s linear;
transition: 0.1s linear;
opacity:1;
}
.afterSubmitSearch{
margin-top:-13%;
}
.afterSumitInfo{
opacity:0;
}
.search{
margin-top:100px;
}
.dropdown-menu{
width:100%;
}
.empty, .notFound{
margin-top: -20px;
}
.people{
opacity:0;
margin-left:-70px;
-webkit-transition: 0.3s linear all;
-moz-transition: 0.3s linear all;
-o-transition: 0.3s linear all;
transition: 0.3s linear all;
}
.person{
min-height:100px;
}
.poepleEntering{
margin-left: 0px;
opacity:1;
}<file_sep>extends layout
block content
if edit
form(method="post" novalidate action="")
label(for="email") Email
input(type="text" value="#{details.email}" disabled)
label(for="city") Miasto
input(type="text" name="city" value="#{details.city}")
label(for="photo") Avatar:
input(type="file" name="avatar")
input(type="submit" value="Zapisz")
else
p Email:
p=details.email
p Miasto
p=details.city
a.btn.btn-default(href='profile/edit') Edytuj<file_sep>var mongoose = require('mongoose')
// Schema
var ActivitiesSchema = new mongoose.Schema({
name: String,
})
ActivitiesSchema.statics = {
searchForActivities: function(activity,callback){
var model = mongoose.model('Activities', ActivitiesSchema);
model.find({'name': {'$regex': activity}},{'name':1}).exec(function(err,data){
if(err) console.log(err);
var activities = [];
for(var i=0;i<data.length;i++){
activities.push(data[i].name);
}
callback(activities);
})
}
}
module.exports = mongoose.model('Activities', ActivitiesSchema);<file_sep>doctype html
html(ng-app="NoFriends")
head
meta(charset='utf-8')
meta(http-equiv='X-UA-Compatible', content='IE=edge')
meta(name='viewport', content='width=device-width, initial-scale=1.0')
meta(name='csrf-token', content=_csrf)
link(href='//maxcdn.bootstrapcdn.com/bootstrap/3.3.4/css/bootstrap.min.css' rel="stylesheet")
link(href='/css/main.css' rel="stylesheet")
script(src='https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.js')
script(src='//maxcdn.bootstrapcdn.com/bootstrap/3.3.4/js/bootstrap.min.js')
script(src='https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.14/angular.min.js')
script(src='/js/angular-bootstrap.js')
script(src='/js/main-page.js')
title #{title} - NpFriendsApp
body
include header
.container
block content<file_sep>extends layout
block content
.content(ng-controller="MainPageController" ng-class="{'afterSubmitSearch': afterSubmit}")
h1.title.text-center(ng-class="{'afterSubmitInfo':afterSubmit}") Are you bored?
.subtitle.text-center(ng-class="{'afterSubmitInfo':afterSubmit}") Lets find someting interesting to do
form(ng-submit="submitAndSearchActivities()")
.input-group.text-center.search
.empty(ng-show="inputIsEmpty") Musisz cos wpisac. Pole jest puste
.notFound(ng-show="notFound") Nie znaleziono aktywnosci
input.form-control(type="text", ng-model="asyncSelected", placeholder="Locations loaded via $http", typeahead="activity for activity in getActivities($viewValue)",typeahead-on-select='submitAndSearchActivities()', typeahead-loading="loadingLocations", class="form-control")
span.input-group-btn
button.btn.btn-default(type='button') Do It!
div(ng-controller="PeopleController")
{{found}}
.people(ng-class="{'poepleEntering': startEntering}")
h2 Ludzie w twojej okolicy
.col-xs-2.person(ng-repeat="person in UsersService.people")
img.img-responsive(src="img/photo.jpg")
div `
div
span.name {{person.name}}
span.surname {{person.surname}}
div
span.dbo {{person.dbo}}
span.city {{person.city}}
div.text-centet {{person.activity}}
<file_sep>extends layout
block content
.col-md-offset-4.col-md-4.text-center
form(method="post" novalidate action="")
div
label(for="email") Login:
input(type="email" name="email")
div
label(for="password") Hasło:
input(type="<PASSWORD>" name="<PASSWORD>")
div
input(type="submit")
<file_sep>// Dependeciens
var express = require('express');
var mongoose = require('mongoose');
var bodyParser = require('body-parser');
var expressValidator = require('express-validator');
var path = require('path');
var passport = require('passport');
var expressSession = require('express-session');
var flash = require('connect-flash');
var cookieParser = require('cookie-parser')
var morgan = require('morgan');
var MongoStore = require('connect-mongo')(expressSession);
//Mongodb
mongoose.connect('mongodb://localhost/rest_test');
//Express
var app = express();
app.use(bodyParser.urlencoded({extended: true}));
app.use(bodyParser.json());
app.use(expressValidator());
app.set('port', process.env.PORT || 3000);
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'jade');
app.set('view options', {
layout: 'layout'
});
app.use(morgan('dev'));
app.use(express.static(path.join(__dirname, 'public'), { maxAge: 31557600000 }));
app.use(flash());
// Passport - obsluga sesji
app.use(expressSession({
resave: true,
saveUninitialized: true,
secret: 'ilovenodejsverymuch',
store: new MongoStore({ url: 'mongodb://localhost/rest_test', autoReconnect: true })
}));
app.use(passport.initialize());
app.use(passport.session());
app.use(cookieParser())
app.use(function(req, res, next) {
res.locals.user = req.user;
next();
});
require('./config/passport.js');
// Plik z autentykacja
// Routers
var index = require('./routes/index');
app.get('/', function(req,res){
console.log('user');
console.log(req.user)
res.render('index');
});
app.get('/api', require('./routes/api'));
app.get('/get/activities/:activity',index.searchForActivities);
app.get('/get/UsersWithActivity/:activity',index.getUsersWithActivity);
app.get('/register',index.register);
app.post('/register', index.postRegister);
app.get('/login',index.login)
app.post('/login',index.postLogin);
app.get('/logout',index.logout);
app.get('/profile', index.profile);
app.get('/profile/edit',index.profileEdit)
app.post('/profile/edit',index.postProfileEdit);
// Start server
app.listen('3000');
console.log('Server is runing on port 3000');
<file_sep>var activities_model = require('../models/activities.js');
var user_model = require('../models/user.js');
var passport = require('passport');
exports.searchForActivities = function(req,res){
var activity = req.param('activity');
activities_model.searchForActivities(activity, function(activities){
res.send(activities);
})
}
exports.getUsersWithActivity = function(req,res){
var activity = req.param('activity');
user_model.getUsersWithActivity(activity,function(users){
res.send(users)
});
}
exports.login = function(req,res){
console.log(req);
res.render('login');
}
exports.postLogin = function(req, res, next) {
req.assert('email', 'Email is not valid').isEmail();
req.assert('password', 'Password cannot be blank').notEmpty();
var errors = req.validationErrors();
if (errors) {
console.log('error');
req.flash('errors', errors);
return res.redirect('/login');
}
passport.authenticate('login', function(err, user, info) {
if (err) return next(err);
if (!user) {
req.flash('errors', { msg: info.message });
return res.redirect('/login');
}
req.logIn(user, function(err) {
if (err) return next(err);
req.flash('success', { msg: 'Success! You are logged in.' });
res.redirect(req.session.returnTo || '/');
});
})(req, res, next);
};
exports.register = function(req,res){
res.render('register');
}
exports.postRegister = function(req,res){
req.assert('email', 'Email is not valid').isEmail();
req.assert('password', 'Password must be at least 4 characters long').len(4);
req.assert('confirmPassword', 'Passwords do not match').equals(req.body.password);
var errors = req.validationErrors();
if (errors) {
req.flash('errors', errors);
return res.redirect('/signup');
}
var user = new user_model({
email: req.body.email,
password: req.body.<PASSWORD>
});
user_model.findOne({ email: req.body.email }, function(err, existingUser) {
if (existingUser) {
console.log(existingUser)
req.flash('errors', { msg: 'Account with that email address already exists.' });
return res.redirect('/register');
}
user.save(function(err) {
if (err) return next(err);
req.logIn(user, function(err) {
if (err) return next(err);
res.redirect('/');
});
});
});
}
exports.logout = function(req, res) {
req.logout();
res.redirect('/');
};
exports.profile = function(req,res){
if(req.user){
user_model.findOne({'email': req.user.email},function(err,user){
user.getDetails(function(details){
res.render('profile', {'details': details});
});
});
}else{
res.redirect('/login')
}
}
exports.profileEdit = function(req,res){
if(req.user){
user_model.findOne({'email': req.user.email},function(err,user){
user.getDetails(function(details){
res.render('profile', {'details': details, 'edit':true});
});
});
}else{
res.redirect('/login')
}
}
exports.postProfileEdit = function(req,res){
if(req.user){
user_model.findOne({'email': req.user.email},function(err,user){
console.log(req.body.city);
user.city = req.body.city
user.save(function(err){
if(err){
console.log('Nie udalo sie zapisac');
res.redirect('/profile/edit');
}
console.log("Zapisalem");
console.log(user)
res.redirect('/profile');
})
});
}else{
res.redirect('/login')
}
}
<file_sep>#header.text-right
if user
.dropdown.col-md-offset-10.col-md-2.text-right
a#dropdownMenu1.dropdown-toggle(data-toggle='dropdown' aria-expanded='true')=user.email
ul.dropdown-menu(role='menu' aria-labelledby='dropdownMenu1')
li(role='presentation')
a(role='menuitem' tabindex='-1' href='/profile') Profil
a(role='menuitem' tabindex='-1' href='/logout') Logout
else
.col-xs-12
a(href="/login") Zaloguj
a(href="/register") Zarejestruj<file_sep>// Dependeciens
var express = require('express');
var router = express.Router();
// Models
var Product = require('../models/product.js');
// Routes
Product.before('post',function(req, res, next){
console.log('xxx');
next();
}).methods(['post','put','delete',{
method: 'get',
before: function(req,res,next){
}
}]);
Product.register(router,'/products');
// Retrun router
module.exports = router; | dd9f87a36a5d6521ba3503a696f037f6bdcd98b4 | [
"JavaScript",
"Less",
"Pug"
] | 10 | JavaScript | Tarvald/NoFriends | 1f1f3f8c06c4036778931dd53a69186e104b8a5b | 89f0f5a38d4d916d296fac7c684320decc616d30 |
refs/heads/master | <repo_name>kgx/prefect_logger_repro<file_sep>/research/flow.py
from prefect import task, Flow
from prefect.engine.executors import LocalDaskExecutor
from prefect.environments import LocalEnvironment
from prefect.environments.storage import Docker
from research.example_pkg import things
@task(log_stdout=True)
def run_thing1():
if things.thing1():
return True
@task
def run_thing2(log_stdout=True):
if things.thing2():
return True
def main():
with Flow("test_flow") as flow:
run_thing1()
run_thing2()
flow.environment = LocalEnvironment(executor=LocalDaskExecutor(scheduler="processes"))
flow.storage = Docker(
base_image="prefect_logger_repro",
local_image=True,
ignore_healthchecks=True,
registry_url="artifactory.aq.tc/prefect/",
)
flow.register(project_name="default")
if __name__ == "__main__":
main()
| 4fb11b6facd2de217b3f3a53c44cf0d506567cda | [
"Python"
] | 1 | Python | kgx/prefect_logger_repro | 4fd61a863c2bbeeb9f313278430c38f52a42a26a | 86bb2b41f8fc6cb3e12670b6d4193195d1eb01db |
refs/heads/main | <file_sep>using System.Collections.Generic;
namespace AlyaDiscord
{
public class TicketRemoveMainQuestion : DatBase
{
public TicketRemoveMainQuestion(List<DialogData> rootList) : base(rootList){}
protected override string processingInternalAsync(string input)
{
if(input == "1")
{
var WebshareID = new DialogData();
var Reason = new DialogData();
WebshareID.ChatQuestion = "Jaké **Webshare streamy** chcete smazat?\nMůžete napsat **ID** nebo **URL odkazy**\nvedle sebe oddělené mezerou nebo na nový řadek.\nNapřiklad: **wsid1 wsid2 wsid3**";
WebshareID.StatusReportDescription = "Webshare Streamy";
WebshareID.AnswerTypeObject = new DatWebshareUrlID(rootList);
WebshareID.InternalDescription = "Webshare_Streams";
Reason.ChatQuestion = "Napiš důvod smazání";
Reason.StatusReportDescription = "Důvod";
rootList.Insert(index+1,Reason);
rootList.Insert(index+1,WebshareID);
return "Mazat Streamy";
}
if(input == "2")
{
var Service = new DialogData();
var Reason = new DialogData();
var ServiceID = new DialogData();
Service.ChatQuestion = "Jakou službu? [**csfd** | **tmdb** | **imdb** | **trakt** | **tvdb**]\nMůžete vybrat **jen jednu**!";
Service.StatusReportDescription = "Default services";
Service.AnswerTypeObject = null;
Service.InternalDescription = "default_services_flag";
Reason.ChatQuestion = "Napiš důvod smazání";
Reason.StatusReportDescription = "Důvod";
ServiceID.ChatQuestion = $"Zadej **ID** pro **servisní službu**";
ServiceID.StatusReportDescription = $"{Service.Answer} ID";
ServiceID.InternalDescription = $"{Service.Answer}_id";
ServiceID.AnswerTypeObject = null;
rootList.Insert(index+1,Reason);
rootList.Insert(index+1,ServiceID);
rootList.Insert(index+1,Service);
return "Maz<NAME>";
}
if(input == "3")
{
var Service = new DialogData();
var Reason = new DialogData();
var ServiceID = new DialogData();
Service.ChatQuestion = "Jakou službu? [**csfd** | **tmdb** | **imdb** | **trakt**]\nMůžete vybrat **jen jednu**!";
Service.StatusReportDescription = "Default services";
Service.AnswerTypeObject = null;
Service.InternalDescription = "default_services_flag";
Reason.ChatQuestion = "Napiš důvod smazaní";
Reason.StatusReportDescription = "Důvod";
ServiceID.ChatQuestion = $"Zadej **ID** pro **servisní službu**";
ServiceID.StatusReportDescription = $"{Service.Answer} ID";
ServiceID.InternalDescription = $"{Service.Answer}_id";
ServiceID.AnswerTypeObject = null;
rootList.Insert(index+1,Reason);
rootList.Insert(index+1,ServiceID);
rootList.Insert(index+1,Service);
return "Mazat seriály";
}
if(input == "4")
{
return null;
}
if(input == "5")
{
return null;
}
return null;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace AlyaDiscord
{
public class DatWebshareUrlID : DatBase
{
public DatWebshareUrlID(List<DialogData> rootList) : base(rootList){}
protected override string processingInternalAsync(string input)
{
string[] lines = input.Split(
new[] { "\r\n", "\r", "\n", " " },
StringSplitOptions.None
);
lines.ToList();
outputvar = new List<string>();
Regex r = new Regex(@"(?<=file/)[a-zA-Z0-9]+", RegexOptions.Compiled);
foreach (var item in lines)
{
if (item.Contains("/"))
{
Match match = r.Match(item);
outputvar.Add(match.Value);
}
else
{
outputvar.Add(item);
}
}
// maybe return none
return input;
}
}
}
<file_sep>using RestSharp;
namespace AlyaDiscord
{
public class CideFCApis
{
static public dynamic getAllFleetCarrier()
{
var client = new RestClient("http://0.0.0.0:5000/api/FleetCarrierItem");
client.RemoteCertificateValidationCallback = (sender, certificate, chain, sslPolicyErrors) => true;
client.Timeout = -1;
var request = new RestRequest(Method.GET);
IRestResponse response = client.Execute(request);
var cideFcObject = CideFcObject.FromJson(response.Content);
return cideFcObject;
}
}
}
<file_sep>namespace AlyaDiscord
{
using System;
using System.Collections.Generic;
using System.Globalization;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
public partial class CideFcObject
{
[JsonProperty("id", NullValueHandling = NullValueHandling.Ignore)]
public string Id { get; set; }
[JsonProperty("callsign", NullValueHandling = NullValueHandling.Ignore)]
public string Callsign { get; set; }
[JsonProperty("name", NullValueHandling = NullValueHandling.Ignore)]
public string Name { get; set; }
[JsonProperty("ownerCmdr", NullValueHandling = NullValueHandling.Ignore)]
public string OwnerCmdr { get; set; }
[JsonProperty("location", NullValueHandling = NullValueHandling.Ignore)]
public string Location { get; set; }
[JsonProperty("nextJump", NullValueHandling = NullValueHandling.Ignore)]
public string NextJump { get; set; }
[JsonProperty("nextJumpDateTime", NullValueHandling = NullValueHandling.Ignore)]
public string NextJumpDateTime { get; set; }
[JsonProperty("destination", NullValueHandling = NullValueHandling.Ignore)]
public string Destination { get; set; }
[JsonProperty("dockingAccess", NullValueHandling = NullValueHandling.Ignore)]
public string DockingAccess { get; set; }
[JsonProperty("allowNotorious", NullValueHandling = NullValueHandling.Ignore)]
public bool? AllowNotorious { get; set; }
[JsonProperty("fuelLevel", NullValueHandling = NullValueHandling.Ignore)]
public long? FuelLevel { get; set; }
[JsonProperty("jumpRangeCurr", NullValueHandling = NullValueHandling.Ignore)]
public long? JumpRangeCurr { get; set; }
[JsonProperty("jumpRangeMax", NullValueHandling = NullValueHandling.Ignore)]
public long? JumpRangeMax { get; set; }
[JsonProperty("jumpLeft", NullValueHandling = NullValueHandling.Ignore)]
public long? JumpLeft { get; set; }
[JsonProperty("jumpMax", NullValueHandling = NullValueHandling.Ignore)]
public long? JumpMax { get; set; }
[JsonProperty("statusMode", NullValueHandling = NullValueHandling.Ignore)]
public long? StatusMode { get; set; }
[JsonProperty("jumpDateTime", NullValueHandling = NullValueHandling.Ignore)]
public string JumpDateTime { get; set; }
[JsonProperty("fuelInMarket", NullValueHandling = NullValueHandling.Ignore)]
public long? FuelInMarket { get; set; }
[JsonProperty("fuelNeeded", NullValueHandling = NullValueHandling.Ignore)]
public long? FuelNeeded { get; set; }
}
public partial class CideFcObject
{
public static List<CideFcObject> FromJson(string json) => JsonConvert.DeserializeObject<List<CideFcObject>>(json, AlyaDiscord.Converter.Settings);
}
public static class Serialize
{
public static string ToJson(this List<CideFcObject> self) => JsonConvert.SerializeObject(self, AlyaDiscord.Converter.Settings);
}
internal static class Converter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
Converters =
{
new IsoDateTimeConverter { DateTimeStyles = DateTimeStyles.AssumeUniversal }
},
};
}
}
<file_sep>using System.Collections.Generic;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.Entities;
namespace AlyaDiscord
{
abstract public class DatBase : IDatBase
{
public List<DialogData> rootList { get; set; }
public string input { get; set; }
public int index { get; set; }
public List<string> outputvar { get; set; }
public DiscordMessage StatusReportMessageID { get; set; }
public DiscordMessage DialogMessageID { get; set; }
public CommandContext ctx { get; set; }
public DatBase(List<DialogData> rootList)
{
this.rootList = rootList;
}
protected abstract string processingInternalAsync(string input);
internal string processing()
{
if (input != null)
{
return processingInternalAsync(input);
}
return null;
}
public virtual async Task<dynamic> output()
{
await Task.FromResult(0);
return processing();
}
}
}
<file_sep>using System.Collections.Generic;
using System.IO;
using System.Text.Json;
using System.Text.Json.Serialization;
using DSharpPlus;
namespace AlyaDiscord
{
public static class CideStoreTimer
{
private static List<CideTimerData> timerList = new List<CideTimerData>();
[JsonInclude]
internal static List<CideTimerData> TimerList { get => timerList; set => timerList = value; }
}
public class CideTimerData
{
[JsonIgnore]
public dynamic timer { get; set; }
[JsonInclude]
public ulong Guild { get; set; }
[JsonInclude]
public ulong Channel { get; set; }
}
public static class CideTimerOnStartup
{
internal static async System.Threading.Tasks.Task LoadAsync(DiscordClient client)
{
string jsonString = null;
try
{
jsonString = File.ReadAllText("CideData/ActiveChannel.json");
if (jsonString != null)
{
await System.Threading.Tasks.Task.Delay(System.TimeSpan.FromSeconds(10));
CideStoreTimer.TimerList = new List<CideTimerData>();
var LoadedTimerData = JsonSerializer.Deserialize<List<CideTimerData>>(jsonString);
foreach (var item in LoadedTimerData)
{
CideTimerData data = new CideTimerData();
data.timer = await CideFCTimer.runAsync(client, (ulong)item.Guild, (ulong)item.Channel);
data.Guild = item.Guild;
data.Channel = item.Channel;
CideStoreTimer.TimerList.Add(data);
await CideCommands.SaveToJsonAsync(CideStoreTimer.TimerList);
}
}
}
catch (System.Exception)
{}
}
}
}<file_sep>using System;
using System.Threading.Tasks;
class SCC
{
private string DiscordToken;
public SCC(string discordtoken) => DiscordToken = discordtoken;
public async Task<dynamic> deleteStreamAsync(string wsident)
{
// the method contains sensitive information.
throw new NotImplementedException();
}
public async Task<dynamic> deleteMediaAsync(string type,string service, string serviceID)
{
// the method contains sensitive information.
throw new NotImplementedException();
}
public static async Task<string> SearchStreamAsync(string find)
{
// the method contains sensitive information.
throw new NotImplementedException();
}
public static async Task<dynamic> GetInfoParentAsync(string parentID)
{
// the method contains sensitive information.
throw new NotImplementedException();
}
public static async Task<dynamic> GetInfoStreamAsync(string parentID)
{
// the method contains sensitive information.
throw new NotImplementedException();
}
}<file_sep>using System;
using System.Threading.Tasks;
using DSharpPlus.Entities;
namespace AlyaDiscord.Cide
{
public class CideMessages
{
public static async Task MakeAndSendMessagesAsync(CideFcObject FC, DiscordMessage msg)
{
switch (FC.StatusMode)
{
case 1:
await RespondMethodOneAsync(FC, msg, RespondMessageBaseBuilder(FC));
break;
case 2:
await RespondMethodTwoAsync(FC, msg, RespondMessageBaseBuilder(FC));
break;
case 3:
await RespondMethodThreeAsync(FC, msg, RespondMessageBaseBuilder(FC));
break;
case 4:
await RespondMethodFourAsync(FC, msg, RespondMessageBaseBuilder(FC));
break;
default:
await RespondMethodOneAsync(FC, msg, RespondMessageBaseBuilder(FC));
break;
}
}
public static DiscordEmbedBuilder RespondMessageBaseBuilder(CideFcObject FC)
{
string CzechTitle = $"Fleet Carrier - {FC.Name}";
string CzechFCName = "Název";
string CzechCallSign = "Označení";
string CzechID = "Identifikátor";
string CzechDescription = "Base message";
string CzechCurrentFuel = "Aktuální počet paliva";
string CzechLocationNow = "Aktuální poloha";
// if status 2 >> we know + - how many fuel needed for trip
string CzechNeededFuel = "Palivo potřebné na cestu";
var BaseRespond = new DiscordEmbedBuilder
{
Title = CzechTitle,
Description = CzechDescription,
Footer = new DiscordEmbedBuilder.EmbedFooter
{
Text = "Poslední aktualizace"
},
Color = new DiscordColor(25, 118, 210),
ThumbnailUrl = "https://android.arwwarr.eu/cide_full_white.png"
};
// if not null >> add new field and use it!
if (FC.Name != null)
{
BaseRespond.AddField(CzechFCName, FC.Name, true);
}
if (FC.Callsign != null)
{
BaseRespond.AddField(CzechCallSign, FC.Callsign, true);
}
if (FC.Id != null)
{
BaseRespond.AddField(CzechID, FC.Id, true);
}
if (FC.OwnerCmdr != null)
{
BaseRespond.AddField("Majitel",FC.OwnerCmdr);
}
if (FC.FuelLevel != null)
{
BaseRespond.AddField(CzechCurrentFuel,FC.FuelLevel.ToString(),true);
}
if (FC.StatusMode == 2 | FC.StatusMode == 3 && FC.FuelLevel != null && FC.FuelNeeded != null)
{
BaseRespond.AddField(CzechNeededFuel,FC.FuelNeeded.ToString(),true);
}
if (FC.Location != null)
{
BaseRespond.AddField(CzechLocationNow,FC.Location);
}
BaseRespond.WithTimestamp(DateTime.Now);
return BaseRespond;
}
public static async Task RespondMethodOneAsync(CideFcObject FC, DiscordMessage msg, DiscordEmbedBuilder BaseEmbed)
{
string CzechDescription = "Fáze konstantního monitorování bez určitého cílového systému.";
string CzechNextJump = "Plánování skoku do systému";
string CzechNextJumpDateTime = "Skok se uskuteční v";
BaseEmbed.Description = CzechDescription;
if (FC.NextJump != null && FC.NextJumpDateTime != null)
{
BaseEmbed.AddField(CzechNextJump, FC.NextJump, true);
BaseEmbed.AddField(CzechNextJumpDateTime, FC.NextJumpDateTime, true);
}
await SendToDiscordAsync(msg, BaseEmbed);
}
public static async Task RespondMethodTwoAsync(CideFcObject FC, DiscordMessage msg, DiscordEmbedBuilder BaseEmbed)
{
BaseEmbed.Description = "Fáze přípravy na dlouhý let, Fleet Carrier zůstane určitý čas v tomto systému pro zadockovaní všech CMDR a nabrání paliva na let.";
string CzechNextJump = "Destinace plánované cesty";
string CzechDepartDate = "Datum začátku cesty";
string CzechDepartTime = "Čas začátku cesty";
DateTime DateDepart = DateTime.ParseExact(FC.NextJumpDateTime, "HH:mm-dd-MM-yyyy",System.Globalization.CultureInfo.InvariantCulture);
string DateDeparting = DateDepart.ToString("dd.MM.yyyy");
string TimeDeparting = DateDepart.ToString("HH:mm");
string CzechJumps = "Počet skoků do destinace";
string CzechdockingAccess = "Docking přístup";
string CzechAllowNotorious = "Přístup s Notorious";
string CzechAllowNotoriousAnswer = "";
BaseEmbed.Color = new DiscordColor(124, 77, 255);
// bool AllowNotorious to string ANO < > NE
if(FC.AllowNotorious == true){CzechAllowNotoriousAnswer = "Ano";}else{CzechAllowNotoriousAnswer = "Ne";}
if (FC.NextJump != null)
{
BaseEmbed.AddField(CzechNextJump, FC.NextJump, true);
}
if (DateDeparting != null)
{
BaseEmbed.AddField(CzechDepartDate, DateDeparting, true);
}
if (TimeDeparting != null)
{
BaseEmbed.AddField(CzechDepartTime, TimeDeparting, true);
}
if (FC.JumpMax != null)
{
BaseEmbed.AddField(CzechJumps, FC.JumpMax.ToString(), true);
}
if (FC.DockingAccess != null)
{
BaseEmbed.AddField(CzechdockingAccess, char.ToUpper(FC.DockingAccess[0]) + FC.DockingAccess.Substring(1), true);
}
if (CzechAllowNotoriousAnswer != null)
{
BaseEmbed.AddField(CzechAllowNotorious, CzechAllowNotoriousAnswer, true);
}
await SendToDiscordAsync(msg, BaseEmbed);
}
public static async Task RespondMethodThreeAsync(CideFcObject FC, DiscordMessage msg, DiscordEmbedBuilder BaseEmbed)
{
BaseEmbed.Color = new DiscordColor(199, 44, 65);
BaseEmbed.Description = "Fleet Carrier je na cestě do specifické destinace.";
string CzechNextJump = "Cíl dalšího skoku";
string CzechDepartTime = "Čas skoku";
string TimeDeparting = null;
if (FC.NextJumpDateTime != null)
{
DateTime DateDepart = DateTime.ParseExact(FC.NextJumpDateTime, "HH:mm-dd-MM-yyyy",System.Globalization.CultureInfo.InvariantCulture);
TimeDeparting = DateDepart.ToString("HH:mm");
}
else
{
System.TimeSpan cdjump = new System.TimeSpan(0, 1, 15, 0);
DateTime localDate = DateTime.Now;
System.DateTime result = localDate + cdjump;
TimeDeparting = result.ToString("HH:mm");
}
string CzechJumpLeft = "Skoků zbývá";
string CzechJumpMax = "Skoků celkem";
string CzechFinalDestination = "Finální destinace";
string CzechTimeDepartTimeFinal = "Čas příletu";
string CzechTimeDepartDateFinal = "Datum příletu";
string DepartTimeFinal = null;
string DepartDateFinal = null;
if (FC.JumpLeft != null && FC.JumpMax != null)
{
BaseEmbed.Color = CalculateColorFromJumps(FC.JumpLeft, FC.JumpMax);
}
if (FC.JumpDateTime != null && FC.JumpLeft != null)
{
DateTime DateDepartFinal = FinalDateTime(FC.JumpDateTime, FC.JumpLeft);
DepartTimeFinal = DateDepartFinal.ToString("HH:mm");
DepartDateFinal = DateDepartFinal.ToString("dd.MM.yyyy");
}
if (FC.NextJump != null)
{
// next jump
BaseEmbed.AddField(CzechNextJump, FC.NextJump, true);
}
if (TimeDeparting!= null)
{
// when will the next jump be time
BaseEmbed.AddField(CzechDepartTime, TimeDeparting, true);
}
if (FC.JumpLeft != null)
{
// how many jump remains
BaseEmbed.AddField(CzechJumpLeft, FC.JumpLeft.ToString(),true);
}
if (FC.JumpMax != null)
{
// how many jumps remain maximum
BaseEmbed.AddField(CzechJumpMax, FC.JumpMax.ToString(), false);
}
if (FC.Destination != null)
{
// the name of the final destination system
BaseEmbed.AddField(CzechFinalDestination, FC.Destination, true);
}
if (DepartTimeFinal != null)
{
// estimated date of arrival at the final destination
BaseEmbed.AddField(CzechTimeDepartTimeFinal, DepartTimeFinal, true);
}
if (DepartDateFinal != null)
{
// estimated time of arrival at the final destination
BaseEmbed.AddField(CzechTimeDepartDateFinal, DepartDateFinal, true);
}
await SendToDiscordAsync(msg, BaseEmbed);
}
private static async Task RespondMethodFourAsync(CideFcObject FC, DiscordMessage msg, DiscordEmbedBuilder BaseEmbed)
{
BaseEmbed.Color = new DiscordColor(53, 255, 105);
BaseEmbed.Description = "Fleet Carrier dorazil do cíle.";
await SendToDiscordAsync(msg, BaseEmbed);
}
private static DateTime FinalDateTime(string jumpDateTime, long? jumpLeft)
{
DateTime Time = DateTime.ParseExact(jumpDateTime, "HH:mm-dd-MM-yyyy",System.Globalization.CultureInfo.InvariantCulture);
for (int i = 0; i < jumpLeft; i++)
{
System.TimeSpan cdjump = new System.TimeSpan(0, 0, 21, 0);
Time = Time + cdjump;
}
return Time;
}
public static async Task SendToDiscordAsync(DiscordMessage msg,DiscordEmbedBuilder emb)
{
await msg.ModifyAsync(embed: emb, content: "");
}
private static DiscordColor CalculateColorFromJumps(long? jumpLeft, long? jumpMax)
{
float r = ((float)jumpMax-(float)jumpLeft)/(float)jumpMax * ((float)180/(float)255);
float g = ((float)1 - (float)r);
float b = (float)0;
return new DiscordColor(g,r,b);
}
}
}
<file_sep>namespace AlyaBackend
{
using System;
using System.Collections.Generic;
using System.Globalization;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
using J = Newtonsoft.Json.JsonPropertyAttribute;
using R = Newtonsoft.Json.Required;
using N = Newtonsoft.Json.NullValueHandling;
public partial class SccParentObject
{
[J("totalCount", NullValueHandling = N.Ignore)] public long? TotalCount { get; set; }
[J("data", NullValueHandling = N.Ignore)] public SccParentObjectDatum[] Data { get; set; }
}
public partial class SccParentObjectDatum
{
[J("_id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("_score")] public dynamic Score { get; set; }
[J("_source", NullValueHandling = N.Ignore)] public PurpleSource Source { get; set; }
}
public partial class PurpleSource
{
[J("info_labels", NullValueHandling = N.Ignore)] public PurpleInfoLabels InfoLabels { get; set; }
[J("cast", NullValueHandling = N.Ignore)] public PurpleCast[] Cast { get; set; }
[J("services", NullValueHandling = N.Ignore)] public PurpleServices Services { get; set; }
[J("i18n_info_labels", NullValueHandling = N.Ignore)] public PurpleI18NInfoLabel[] I18NInfoLabels { get; set; }
[J("play_count", NullValueHandling = N.Ignore)] public long? PlayCount { get; set; }
[J("available_streams", NullValueHandling = N.Ignore)] public AvailableStreams AvailableStreams { get; set; }
[J("root_parent", NullValueHandling = N.Ignore)] public string RootParent { get; set; }
[J("children_count", NullValueHandling = N.Ignore)] public long? ChildrenCount { get; set; }
[J("stream_info", NullValueHandling = N.Ignore)] public StreamInfo StreamInfo { get; set; }
}
public partial class AvailableStreams
{
[J("count", NullValueHandling = N.Ignore)] public long? Count { get; set; }
[J("audio_languages", NullValueHandling = N.Ignore)] public AudioLanguage[] AudioLanguages { get; set; }
[J("subtitles_languages", NullValueHandling = N.Ignore)] public string[] SubtitlesLanguages { get; set; }
}
public partial class AudioLanguage
{
[J("lang", NullValueHandling = N.Ignore)] public string Lang { get; set; }
[J("date_added", NullValueHandling = N.Ignore)] public string DateAdded { get; set; }
}
public partial class PurpleCast
{
[J("name", NullValueHandling = N.Ignore)] public string Name { get; set; }
[J("role", NullValueHandling = N.Ignore)] public string Role { get; set; }
[J("thumbnail", NullValueHandling = N.Ignore)] public Uri Thumbnail { get; set; }
}
public partial class PurpleI18NInfoLabel
{
[J("lang", NullValueHandling = N.Ignore)] public string Lang { get; set; }
[J("title", NullValueHandling = N.Ignore)] public string Title { get; set; }
[J("plot", NullValueHandling = N.Ignore)] public string Plot { get; set; }
[J("rating", NullValueHandling = N.Ignore)] public double? Rating { get; set; }
[J("votes", NullValueHandling = N.Ignore)] public string Votes { get; set; }
[J("art", NullValueHandling = N.Ignore)] public PurpleArt Art { get; set; }
[J("parent_titles", NullValueHandling = N.Ignore)] public string[] ParentTitles { get; set; }
[J("trailer", NullValueHandling = N.Ignore)] public string Trailer { get; set; }
}
public partial class PurpleArt
{
[J("poster")] public Uri Poster { get; set; }
}
public partial class PurpleInfoLabels
{
[J("originaltitle", NullValueHandling = N.Ignore)] public string Originaltitle { get; set; }
[J("genre", NullValueHandling = N.Ignore)] public string[] Genre { get; set; }
[J("year", NullValueHandling = N.Ignore)] public long? Year { get; set; }
[J("duration", NullValueHandling = N.Ignore)] public long? Duration { get; set; }
[J("director", NullValueHandling = N.Ignore)] public string[] Director { get; set; }
[J("studio", NullValueHandling = N.Ignore)] public dynamic[] Studio { get; set; }
[J("writer", NullValueHandling = N.Ignore)] public string[] Writer { get; set; }
[J("aired", NullValueHandling = N.Ignore)] public string Aired { get; set; }
[J("dateadded", NullValueHandling = N.Ignore)] public string Dateadded { get; set; }
[J("mediatype", NullValueHandling = N.Ignore)] public string Mediatype { get; set; }
[J("season", NullValueHandling = N.Ignore)] public long? Season { get; set; }
[J("episode", NullValueHandling = N.Ignore)] public long? Episode { get; set; }
[J("premiered", NullValueHandling = N.Ignore)] public string Premiered { get; set; }
}
public partial class PurpleServices
{
[J("csfd", NullValueHandling = N.Ignore)] public string Csfd { get; set; }
[J("trakt", NullValueHandling = N.Ignore)] public string Trakt { get; set; }
[J("tmdb", NullValueHandling = N.Ignore)] public string Tmdb { get; set; }
[J("tvdb", NullValueHandling = N.Ignore)] public string Tvdb { get; set; }
[J("trakt_with_type", NullValueHandling = N.Ignore)] public string TraktWithType { get; set; }
[J("imdb", NullValueHandling = N.Ignore)] public string Imdb { get; set; }
}
public partial class StreamInfo
{
[J("audio", NullValueHandling = N.Ignore)] public StreamInfoAudio Audio { get; set; }
[J("video", NullValueHandling = N.Ignore)] public StreamInfoVideo Video { get; set; }
[J("subtitles")] public Subtitles Subtitles { get; set; }
}
public partial class StreamInfoAudio
{
[J("language", NullValueHandling = N.Ignore)] public string Language { get; set; }
[J("codec", NullValueHandling = N.Ignore)] public string Codec { get; set; }
[J("channels", NullValueHandling = N.Ignore)] public long? Channels { get; set; }
}
public partial class Subtitles
{
[J("language", NullValueHandling = N.Ignore)] public string Language { get; set; }
}
public partial class StreamInfoVideo
{
[J("width", NullValueHandling = N.Ignore)] public long? Width { get; set; }
[J("height", NullValueHandling = N.Ignore)] public long? Height { get; set; }
[J("codec", NullValueHandling = N.Ignore)] public string Codec { get; set; }
[J("aspect", NullValueHandling = N.Ignore)] public double? Aspect { get; set; }
[J("duration", NullValueHandling = N.Ignore)] public double? Duration { get; set; }
}
public partial class SccSearchObject
{
[J("totalCount", NullValueHandling = N.Ignore)] public long? TotalCount { get; set; }
[J("data", NullValueHandling = N.Ignore)] public SccSearchObjectDatum[] Data { get; set; }
[J("pagination", NullValueHandling = N.Ignore)] public Pagination Pagination { get; set; }
}
public partial class SccSearchObjectDatum
{
[J("_id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("_score", NullValueHandling = N.Ignore)] public double? Score { get; set; }
[J("_source", NullValueHandling = N.Ignore)] public FluffySource Source { get; set; }
}
public partial class FluffySource
{
[J("info_labels", NullValueHandling = N.Ignore)] public FluffyInfoLabels InfoLabels { get; set; }
[J("cast", NullValueHandling = N.Ignore)] public FluffyCast[] Cast { get; set; }
[J("services", NullValueHandling = N.Ignore)] public FluffyServices Services { get; set; }
[J("i18n_info_labels", NullValueHandling = N.Ignore)] public FluffyI18NInfoLabel[] I18NInfoLabels { get; set; }
[J("play_count", NullValueHandling = N.Ignore)] public long? PlayCount { get; set; }
[J("budget", NullValueHandling = N.Ignore)] public long? Budget { get; set; }
[J("revenue", NullValueHandling = N.Ignore)] public long? Revenue { get; set; }
[J("language", NullValueHandling = N.Ignore)] public string Language { get; set; }
[J("country", NullValueHandling = N.Ignore)] public string Country { get; set; }
[J("adult", NullValueHandling = N.Ignore)] public bool? Adult { get; set; }
[J("available_streams", NullValueHandling = N.Ignore)] public AvailableStreams AvailableStreams { get; set; }
[J("children_count", NullValueHandling = N.Ignore)] public long? ChildrenCount { get; set; }
[J("stream_info", NullValueHandling = N.Ignore)] public StreamInfo StreamInfo { get; set; }
[J("root_parent", NullValueHandling = N.Ignore)] public string RootParent { get; set; }
}
public partial class FluffyCast
{
[J("name", NullValueHandling = N.Ignore)] public string Name { get; set; }
[J("role", NullValueHandling = N.Ignore)] public string Role { get; set; }
[J("thumbnail", NullValueHandling = N.Ignore)] public string Thumbnail { get; set; }
}
public partial class FluffyI18NInfoLabel
{
[J("lang", NullValueHandling = N.Ignore)] public string Lang { get; set; }
[J("title", NullValueHandling = N.Ignore)] public string Title { get; set; }
[J("plot", NullValueHandling = N.Ignore)] public string Plot { get; set; }
[J("plotoutline", NullValueHandling = N.Ignore)] public string Plotoutline { get; set; }
[J("trailer", NullValueHandling = N.Ignore)] public string Trailer { get; set; }
[J("rating", NullValueHandling = N.Ignore)] public double? Rating { get; set; }
[J("votes", NullValueHandling = N.Ignore)] public string Votes { get; set; }
[J("art", NullValueHandling = N.Ignore)] public FluffyArt Art { get; set; }
[J("parent_titles", NullValueHandling = N.Ignore)] public dynamic[] ParentTitles { get; set; }
}
public partial class FluffyArt
{
[J("poster")] public string Poster { get; set; }
[J("fanart")] public Uri Fanart { get; set; }
[J("thumb")] public Uri Thumb { get; set; }
[J("banner")] public Uri Banner { get; set; }
[J("clearart")] public Uri Clearart { get; set; }
[J("clearlogo")] public Uri Clearlogo { get; set; }
[J("landscape")] public dynamic Landscape { get; set; }
[J("icon")] public dynamic Icon { get; set; }
}
public partial class FluffyInfoLabels
{
[J("originaltitle", NullValueHandling = N.Ignore)] public string Originaltitle { get; set; }
[J("genre", NullValueHandling = N.Ignore)] public string[] Genre { get; set; }
[J("year", NullValueHandling = N.Ignore)] public long? Year { get; set; }
[J("duration", NullValueHandling = N.Ignore)] public long? Duration { get; set; }
[J("director", NullValueHandling = N.Ignore)] public string[] Director { get; set; }
[J("mpaa", NullValueHandling = N.Ignore)] public string Mpaa { get; set; }
[J("studio", NullValueHandling = N.Ignore)] public string[] Studio { get; set; }
[J("writer", NullValueHandling = N.Ignore)] public string[] Writer { get; set; }
[J("premiered", NullValueHandling = N.Ignore)] public string Premiered { get; set; }
[J("trailer", NullValueHandling = N.Ignore)] public string Trailer { get; set; }
[J("dateadded", NullValueHandling = N.Ignore)] public string Dateadded { get; set; }
[J("mediatype", NullValueHandling = N.Ignore)] public string Mediatype { get; set; }
[J("season", NullValueHandling = N.Ignore)] public long? Season { get; set; }
[J("episode", NullValueHandling = N.Ignore)] public long? Episode { get; set; }
[J("aired", NullValueHandling = N.Ignore)] public string Aired { get; set; }
}
public partial class FluffyServices
{
[J("csfd", NullValueHandling = N.Ignore)] public string Csfd { get; set; }
[J("trakt", NullValueHandling = N.Ignore)] public string Trakt { get; set; }
[J("slug", NullValueHandling = N.Ignore)] public string Slug { get; set; }
[J("tmdb", NullValueHandling = N.Ignore)] public string Tmdb { get; set; }
[J("imdb", NullValueHandling = N.Ignore)] public string Imdb { get; set; }
[J("trakt_with_type", NullValueHandling = N.Ignore)] public string TraktWithType { get; set; }
[J("tvdb", NullValueHandling = N.Ignore)] public string Tvdb { get; set; }
[J("fanart", NullValueHandling = N.Ignore)] public string Fanart { get; set; }
}
public partial class Pagination
{
[J("next", NullValueHandling = N.Ignore)] public string Next { get; set; }
[J("prev", NullValueHandling = N.Ignore)] public string Prev { get; set; }
[J("page", NullValueHandling = N.Ignore)] public long? Page { get; set; }
[J("pageCount", NullValueHandling = N.Ignore)] public long? PageCount { get; set; }
[J("from", NullValueHandling = N.Ignore)] public long? From { get; set; }
[J("limit", NullValueHandling = N.Ignore)] public long? Limit { get; set; }
}
public partial class SccStreamObject
{
[J("_id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("name", NullValueHandling = N.Ignore)] public string Name { get; set; }
[J("provider", NullValueHandling = N.Ignore)] public string Provider { get; set; }
[J("ident", NullValueHandling = N.Ignore)] public string Ident { get; set; }
[J("size", NullValueHandling = N.Ignore)] public long? Size { get; set; }
[J("validated", NullValueHandling = N.Ignore)] public bool? Validated { get; set; }
[J("audio", NullValueHandling = N.Ignore)] public AudioElement[] Audio { get; set; }
[J("video", NullValueHandling = N.Ignore)] public VideoElement[] Video { get; set; }
[J("subtitles", NullValueHandling = N.Ignore)] public Subtitle[] Subtitles { get; set; }
}
public partial class AudioElement
{
[J("_id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("language", NullValueHandling = N.Ignore)] public string Language { get; set; }
[J("codec", NullValueHandling = N.Ignore)] public string Codec { get; set; }
[J("channels", NullValueHandling = N.Ignore)] public long? Channels { get; set; }
}
public partial class Subtitle
{
[J("_id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("language", NullValueHandling = N.Ignore)] public string Language { get; set; }
[J("forced", NullValueHandling = N.Ignore)] public bool? Forced { get; set; }
}
public partial class VideoElement
{
[J("_id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("width", NullValueHandling = N.Ignore)] public long? Width { get; set; }
[J("height", NullValueHandling = N.Ignore)] public long? Height { get; set; }
[J("codec", NullValueHandling = N.Ignore)] public string Codec { get; set; }
[J("aspect", NullValueHandling = N.Ignore)] public double? Aspect { get; set; }
[J("hdr", NullValueHandling = N.Ignore)] public bool? Hdr { get; set; }
[J("3d", NullValueHandling = N.Ignore)] public bool? The3D { get; set; }
[J("duration", NullValueHandling = N.Ignore)] public double? Duration { get; set; }
}
public partial class SccParentObject
{
public static SccParentObject FromJson(string json) => JsonConvert.DeserializeObject<SccParentObject>(json, AlyaBackend.Converter.Settings);
}
public partial class SccSearchObject
{
public static SccSearchObject FromJson(string json) => JsonConvert.DeserializeObject<SccSearchObject>(json, AlyaBackend.Converter.Settings);
}
public partial class SccStreamObject
{
public static SccStreamObject[] FromJson(string json) => JsonConvert.DeserializeObject<SccStreamObject[]>(json, AlyaBackend.Converter.Settings);
}
public static class Serialize
{
public static string ToJson(this SccParentObject self) => JsonConvert.SerializeObject(self, AlyaBackend.Converter.Settings);
public static string ToJson(this SccSearchObject self) => JsonConvert.SerializeObject(self, AlyaBackend.Converter.Settings);
public static string ToJson(this SccStreamObject[] self) => JsonConvert.SerializeObject(self, AlyaBackend.Converter.Settings);
}
internal static class Converter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
Converters =
{
new IsoDateTimeConverter { DateTimeStyles = DateTimeStyles.AssumeUniversal }
},
};
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.CommandsNext.Attributes;
using DSharpPlus.Interactivity;
using Newtonsoft.Json;
namespace AlyaDiscord
{
public class SCCTicketCommands
{
[Command("scc_remove_ticket"), Description("Inicializovat konverzaci pro vyvolání mazacího ticketu."),Hidden]
public async Task scc_remove_ticket(CommandContext ctx)
{
await ctx.Message.DeleteAsync();
var interactivity = ctx.Client.GetInteractivityModule();
List<DialogData> Questions = new List<DialogData>();
Questions.Add(new DialogData());
Questions[0].ChatQuestion = @"Typ operace: vyberte si, napište číslo:
[
1. **Smazat WS ID**
2. **Smazat celý film**
3. **Smazat celý seriál**
]";
Questions[0].StatusReportDescription = "Typ operace";
Questions[0].InternalDescription = "";
Questions[0].AnswerTypeObject = new TicketRemoveMainQuestion(Questions);
var DialogThread = await dialog.Create(ctx,Questions);
var Result = await DialogThread.FinalOutputAsync(true);
if (Result[0].AnswerTypeObject.input == 1.ToString())
{
var TicketRemoveWsID = new AlyaDiscord.TicketRemove.API.WsId.Remove();
TicketRemoveWsID.DiscordUuid = ctx.User.Id.ToString();
TicketRemoveWsID.DiscordUserName = ctx.User.Username;
TicketRemoveWsID.DiscordMention = ctx.User.Mention;
TicketRemoveWsID.TimeStampCreation = DateTime.Now.ToString("HH:mm-dd-MM-yyyy");
TicketRemoveWsID.WebshareIdString = Result[1].AnswerTypeObject.input;
TicketRemoveWsID.Reason = Result[2].Answer;
var json = JsonConvert.SerializeObject(TicketRemoveWsID);
AlyaDiscord.TicketRemove.API.Call.requestwsid.post(json);
}
else if(Result[0].AnswerTypeObject.input == 2.ToString())
{
var TicketRemoveRoot = new AlyaDiscord.TicketRemove.API.Root.Remove();
TicketRemoveRoot.DiscordUuid = ctx.User.Id.ToString();
TicketRemoveRoot.DiscordUserName = ctx.User.Username;
TicketRemoveRoot.DiscordMention = ctx.User.Mention;
TicketRemoveRoot.TimeStampCreation = DateTime.Now.ToString("HH:mm-dd-MM-yyyy");
if (Result[1].Answer == "csfd")
{
TicketRemoveRoot.ServiceType = 1;
}
else if (Result[1].Answer == "tmdb")
{
TicketRemoveRoot.ServiceType = 2;
}
else if (Result[1].Answer == "trakt")
{
TicketRemoveRoot.ServiceType = 3;
}
else if (Result[1].Answer == "imdb")
{
TicketRemoveRoot.ServiceType = 4;
}
else if (Result[1].Answer == "tvdb")
{
TicketRemoveRoot.ServiceType = 5;
}
TicketRemoveRoot.ServiceId = Result[2].Answer;
TicketRemoveRoot.WhatIsTheGoal = "movie";
TicketRemoveRoot.Reason = Result[3].Answer;
var json = JsonConvert.SerializeObject(TicketRemoveRoot);
AlyaDiscord.TicketRemoveAPICall.requestRoot.post(json);
}
else if(Result[0].AnswerTypeObject.input == 3.ToString())
{
var TicketRemoveRoot = new AlyaDiscord.TicketRemove.API.Root.Remove();
TicketRemoveRoot.DiscordUuid = ctx.User.Id.ToString();
TicketRemoveRoot.DiscordUserName = ctx.User.Username;
TicketRemoveRoot.DiscordMention = ctx.User.Mention;
TicketRemoveRoot.TimeStampCreation = DateTime.Now.ToString("HH:mm-dd-MM-yyyy");
if (Result[1].Answer == "csfd")
{
TicketRemoveRoot.ServiceType = 1;
}
else if (Result[1].Answer == "tmdb")
{
TicketRemoveRoot.ServiceType = 2;
}
else if (Result[1].Answer == "trakt")
{
TicketRemoveRoot.ServiceType = 3;
}
else if (Result[1].Answer == "imdb")
{
TicketRemoveRoot.ServiceType = 4;
}
else if (Result[1].Answer == "tvdb")
{
TicketRemoveRoot.ServiceType = 5;
}
TicketRemoveRoot.ServiceId = Result[2].Answer;
TicketRemoveRoot.WhatIsTheGoal = "tvshow";
TicketRemoveRoot.Reason = Result[3].Answer;
var json = JsonConvert.SerializeObject(TicketRemoveRoot);
AlyaDiscord.TicketRemoveAPICall.requestRoot.post(json);
}
}
[Command("scc_remove_ticket_ticket"), Description("Vše smazat."),Hidden,RequireOwner]
public async Task scc_remove_ticket_ticket(CommandContext ctx, string token)
{
var stringObjectRoot = AlyaDiscord.TicketRemoveAPICall.requestRoot.getall();
var stringObjectWsid = AlyaDiscord.TicketRemove.API.Call.requestwsid.getall();
var objectRoot = AlyaDiscord.TicketRemoveAdminRoot.Object.FromJson(stringObjectRoot);
var objectWsid = AlyaDiscord.TicketRemoveAdminwsid.Object.FromJson(stringObjectWsid);
await Task.Run(async () =>
{
foreach (var item in objectRoot)
{
await item.proceedAsync(ctx,token);
await Task.Delay(TimeSpan.FromSeconds(5));
}
});
await Task.Run(async () =>
{
foreach (var item in objectWsid)
{
await item.proceedAsync(ctx,token);
await Task.Delay(TimeSpan.FromSeconds(5));
}
});
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.IO;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace AlyaDiscord
{
public class DATSccSearchnext : DatBaseAsync
{
public List<DATSccSearchData> searchList;
private DATSccSearchData selected;
public DATSccSearchnext(List<DialogData> rootList) : base(rootList){}
protected override async Task<string> processingInternalAsync(string input)
{
if (input != null)
{
foreach (var item in searchList)
{
if (item.index == Convert.ToInt32(input))
{
selected = item;
var ParentInfo = await SCC.GetInfoParentAsync(item.sccid);
AlyaBackend.SccParentObject sccParentObject = AlyaBackend.SccParentObject.FromJson(ParentInfo);
var SeasonCheck = new List<bool>();
var EpisodeCheck = new List<bool>();
foreach (var objekt in sccParentObject.Data)
{
if (objekt.Source.InfoLabels.Mediatype == "season")
{
SeasonCheck.Add(true);
}
else
{
SeasonCheck.Add(false);
}
if (objekt.Source.InfoLabels.Mediatype == "episode")
{
EpisodeCheck.Add(true);
}
else
{
EpisodeCheck.Add(false);
}
}
if (SeasonCheck.Contains(true))
{
List<DATSccSearchData> EpisodesInternalProcessing = new List<DATSccSearchData>();
foreach (var obsahSezon in sccParentObject.Data)
{
var SeasonInfo = await SCC.GetInfoParentAsync(obsahSezon.Id);
AlyaBackend.SccParentObject SeasonInfoSeri = AlyaBackend.SccParentObject.FromJson(SeasonInfo);
foreach (var Episodes in SeasonInfoSeri.Data)
{
if (Episodes.Source.InfoLabels.Mediatype == "episode")
{
var newdata = new DATSccSearchData();
if (Episodes.Source.InfoLabels.Episode != null)
{
newdata.IndexEpisode = Convert.ToInt32(Episodes.Source.InfoLabels.Episode);
}
if (Episodes.Source.InfoLabels.Season != null)
{
newdata.IndexSeason = Convert.ToInt32(Episodes.Source.InfoLabels.Season);
}
newdata.name = Episodes.Source.InfoLabels.Originaltitle;
newdata.type = Episodes.Source.InfoLabels.Mediatype;
newdata.year = Episodes.Source.InfoLabels.Year.ToString();
newdata.sccid = Episodes.Id;
EpisodesInternalProcessing.Add(newdata);
}
}
}
foreach (var jednotlivedily in EpisodesInternalProcessing)
{
await jednotlivedily.getStreamsAsync();
}
var fullObj = new SccObjectMovie();
var servicedb = new SccObjectServices();
List<SccObjectItem> EpisodesObj = new List<SccObjectItem>();
servicedb.csfd = selected.csfd;
servicedb.imdb = selected.imdb;
servicedb.tmdb = selected.tmdb;
servicedb.trakt = selected.trakt;
servicedb.tvdb = selected.tvdb;
fullObj.services = servicedb;
foreach (var item5 in EpisodesInternalProcessing)
{
SccObjectItem OneEpisodesObj = new SccObjectItem();
OneEpisodesObj.episode = item5.IndexEpisode;
OneEpisodesObj.season = item5.IndexSeason;
OneEpisodesObj.streams = item5.streams;
EpisodesObj.Add(OneEpisodesObj);
}
fullObj.items = EpisodesObj;
var output = JsonConvert.SerializeObject(fullObj);
byte[] byteArray = Encoding.ASCII.GetBytes( output );
MemoryStream stream = new MemoryStream( byteArray );
await StatusReportMessageID.RespondWithFileAsync(stream,"result_tvshow.json",content:ctx.User.Mention);
}
if (EpisodeCheck.Contains(true))
{
List<DATSccSearchData> EpisodesInternalProcessing = new List<DATSccSearchData>();
foreach (var Item6 in sccParentObject.Data)
{
if (Item6.Source.InfoLabels.Mediatype == "episode")
{
var newdata = new DATSccSearchData();
if (Item6.Source.InfoLabels.Episode != null)
{
newdata.IndexEpisode = Convert.ToInt32(Item6.Source.InfoLabels.Episode);
}
if (Item6.Source.InfoLabels.Season != null)
{
newdata.IndexSeason = Convert.ToInt32(Item6.Source.InfoLabels.Season);
}
newdata.name = Item6.Source.InfoLabels.Originaltitle;
newdata.type = Item6.Source.InfoLabels.Mediatype;
newdata.year = Item6.Source.InfoLabels.Year.ToString();
newdata.sccid = Item6.Id;
EpisodesInternalProcessing.Add(newdata);
}
}
foreach (var jednotlivedily in EpisodesInternalProcessing)
{
await jednotlivedily.getStreamsAsync();
}
var fullObj = new SccObjectMovie();
var servicedb = new SccObjectServices();
List<SccObjectItem> EpisodesObj = new List<SccObjectItem>();
servicedb.csfd = selected.csfd;
servicedb.imdb = selected.imdb;
servicedb.tmdb = selected.tmdb;
servicedb.trakt = selected.trakt;
servicedb.tvdb = selected.tvdb;
fullObj.services = servicedb;
foreach (var item5 in EpisodesInternalProcessing)
{
SccObjectItem OneEpisodesObj = new SccObjectItem();
OneEpisodesObj.episode = item5.IndexEpisode;
OneEpisodesObj.streams = item5.streams;
EpisodesObj.Add(OneEpisodesObj);
}
fullObj.items = EpisodesObj;
var output = JsonConvert.SerializeObject(fullObj);
byte[] byteArray = Encoding.ASCII.GetBytes( output );
MemoryStream stream = new MemoryStream( byteArray );
await StatusReportMessageID.RespondWithFileAsync(stream,"result_tvshow_episode_in_root.json",content:ctx.User.Mention);
}
if (selected.type == "movie")
{
await selected.getStreamsAsync();
var fullObj = new SccObjectMovie();
var servicedb = new SccObjectServices();
List<SccObjectItem> EpisodesObj = new List<SccObjectItem>();
servicedb.csfd = selected.csfd;
servicedb.imdb = selected.imdb;
servicedb.tmdb = selected.tmdb;
servicedb.trakt = selected.trakt;
servicedb.tvdb = selected.tvdb;
fullObj.services = servicedb;
SccObjectItem OneEpisodesObj = new SccObjectItem();
OneEpisodesObj.streams = selected.streams;
EpisodesObj.Add(OneEpisodesObj);
fullObj.items = EpisodesObj;
var output = JsonConvert.SerializeObject(fullObj);
byte[] byteArray = Encoding.ASCII.GetBytes( output );
MemoryStream stream = new MemoryStream( byteArray );
await StatusReportMessageID.RespondWithFileAsync(stream,"result_movie.json",content:ctx.User.Mention);
}
}
}
return selected.name;
}
return null;
}
}
}
<file_sep>using System.Collections.Generic;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.Entities;
namespace AlyaDiscord
{
abstract public class DatBaseAsync : IDatBase
{
public List<DialogData> rootList { get; set; }
public string input { get; set; }
public int index { get; set; }
public List<string> outputvar { get; set; }
public DiscordMessage StatusReportMessageID { get; set; }
public DiscordMessage DialogMessageID { get; set; }
public CommandContext ctx { get; set; }
public DatBaseAsync(List<DialogData> rootList)
{
this.rootList = rootList;
}
protected abstract Task<string> processingInternalAsync(string input);
internal async Task<string> processingAsync()
{
if (input != null)
{
return await processingInternalAsync(input);
}
return null;
}
public virtual async Task<dynamic> output()
{
return await processingAsync();
}
}
}
<file_sep>using System.Collections.Generic;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.CommandsNext.Attributes;
using System.Text.Json;
using System.IO;
namespace AlyaDiscord
{
public class CideCommands
{
List<CideTimerData> TimerList = new List<CideTimerData>();
[Command("cide_fc_status_start"), Hidden]
public async Task cide_fleetcarrier_status_start(CommandContext ctx)
{
CideTimerData data = new CideTimerData();
data.timer = await CideFCTimer.runAsync(ctx.Client, ctx.Guild.Id, ctx.Channel.Id);
data.Guild = ctx.Guild.Id;
data.Channel = ctx.Channel.Id;
CideStoreTimer.TimerList.Add(data);
await SaveToJsonAsync(CideStoreTimer.TimerList);
}
[Command("cide_fc_status_stop"), Hidden]
public async Task cide_fleetcarrier_status_stop(CommandContext ctx)
{
if (CideStoreTimer.TimerList != null)
{
foreach (var item in CideStoreTimer.TimerList)
{
if (item.Channel == ctx.Channel.Id)
{
await item.timer.DisposeAsync();
}
}
}
await SaveToJsonAsync(CideStoreTimer.TimerList);
}
public static async Task SaveToJsonAsync(List<CideTimerData> timerList)
{
using FileStream createStream = File.Create("CideData/ActiveChannel.json");
await JsonSerializer.SerializeAsync(createStream, timerList);
}
}
}
<file_sep>namespace AlyaDiscord.TicketRemoveAdminRoot
{
using System;
using System.Collections.Generic;
using System.Globalization;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
using J = Newtonsoft.Json.JsonPropertyAttribute;
using R = Newtonsoft.Json.Required;
using N = Newtonsoft.Json.NullValueHandling;
using DSharpPlus.Interactivity;
using DSharpPlus.Entities;
using DSharpPlus.CommandsNext;
public partial class Object
{
[J("id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("serviceType", NullValueHandling = N.Ignore)] public long? ServiceType { get; set; }
[J("serviceID", NullValueHandling = N.Ignore)] public string ServiceId { get; set; }
[J("reason", NullValueHandling = N.Ignore)] public string Reason { get; set; }
[J("whatIsTheGoal", NullValueHandling = N.Ignore)] public string WhatIsTheGoal { get; set; }
[J("discordUUID", NullValueHandling = N.Ignore)] public string DiscordUuid { get; set; }
[J("discordUserName", NullValueHandling = N.Ignore)] public string DiscordUserName { get; set; }
[J("discordMention", NullValueHandling = N.Ignore)] public string DiscordMention { get; set; }
[J("timeStampCreation", NullValueHandling = N.Ignore)] public string TimeStampCreation { get; set; }
public async System.Threading.Tasks.Task<dynamic> TryRemoveAsync(string token)
{
var scc = new SCC(token);
var ResultFromSccAPIS = await scc.deleteMediaAsync(WhatIsTheGoal,TheServiceType(),ServiceId);
return ResultFromSccAPIS;
}
public void RemoveFromDB()
{
AlyaDiscord.TicketRemoveAPICall.requestRoot.delete(Id);
}
public string TheServiceType()
{
if (ServiceType == 1)
{
return "csfd";
}
else if (ServiceType == 2)
{
return "tmdb";
}
else if (ServiceType == 3)
{
return "trakt";
}
else if (ServiceType == 4)
{
return "imdb";
}
else if (ServiceType == 5)
{
return "tvdb";
}
return "error";
}
public async System.Threading.Tasks.Task FeedbackToAdminAsync(DSharpPlus.CommandsNext.CommandContext ctx, DiscordEmbedBuilder emb)
{
emb.AddField("Žadatel",DiscordUserName);
var guild = await ctx.Client.GetGuildAsync(12345678912345);
var personArwwarr = await guild.GetMemberAsync(12345678912345);
await personArwwarr.SendMessageAsync(embed: emb);
}
private DiscordEmbedBuilder CreateBaseDiscordEmbed()
{
var BaseRespond = new DiscordEmbedBuilder
{
Title = "Ticket Remove - User Report",
Description = "Oznamení pro správce db SCC, informace ohledně ticketu.",
Footer = new DiscordEmbedBuilder.EmbedFooter
{
Text = "Report vytvořen"
},
Color = new DiscordColor(25, 118, 210),
};
BaseRespond.AddField(TheServiceType(), ServiceId, true);
BaseRespond.AddField("Důvod vymazání", Reason, true);
BaseRespond.WithTimestamp(DateTime.Now);
return BaseRespond;
}
public async System.Threading.Tasks.Task FeedbackToUserAsync(DSharpPlus.CommandsNext.CommandContext ctx, DiscordEmbedBuilder emb)
{
var guild = await ctx.Client.GetGuildAsync(706602726565609563);
var person = await guild.GetMemberAsync(Convert.ToUInt64(DiscordUuid));
await person.SendMessageAsync(embed: emb);
}
public async System.Threading.Tasks.Task proceedAsync(DSharpPlus.CommandsNext.CommandContext ctx, string token)
{
var UserMessage = CreateBaseDiscordEmbed();
var AdminMessage = CreateBaseDiscordEmbed();
AdminMessage.Title = "Ticket Remove - Admin Report";
AdminMessage.Description = "Oznámení pro adminy SCC, informace ohledně ticketu.";
var Result = await TryRemoveAsync(token);
if (Result[0] == "1")
{
UserMessage.AddField("Status", "Smazáno :white_check_mark:", false);
AdminMessage.AddField("Status", "Smazáno :white_check_mark:", false);
UserMessage.Color = new DiscordColor(84, 227, 70);
AdminMessage.Color = new DiscordColor(84, 227, 70);
}
else
{
UserMessage.AddField("Status", "Error :red_square:", false);
AdminMessage.AddField("Status", "Error :red_square:", false);
UserMessage.Color = new DiscordColor(255, 0, 0);
AdminMessage.Color = new DiscordColor(255, 0, 0);
}
await FeedbackToUserAsync(ctx,UserMessage);
await FeedbackToAdminAsync(ctx,UserMessage);
AlyaDiscord.TicketRemoveAPICall.requestRoot.delete(Id);
}
}
public partial class Object
{
public static List<Object> FromJson(string json) => JsonConvert.DeserializeObject<List<Object>>(json, AlyaDiscord.TicketRemoveAdminRoot.Converter.Settings);
}
public static class Serialize
{
public static string ToJson(this List<Object> self) => JsonConvert.SerializeObject(self, AlyaDiscord.TicketRemoveAdminRoot.Converter.Settings);
}
internal static class Converter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
Converters =
{
new IsoDateTimeConverter { DateTimeStyles = DateTimeStyles.AssumeUniversal }
},
};
}
}
<file_sep>using System;
using System.Linq;
using System.Threading.Tasks;
using DSharpPlus;
using DSharpPlus.Entities;
using System.Collections.Generic;
using AlyaDiscord.Cide;
namespace AlyaDiscord
{
public class CideFCTimer
{
static private async Task DeleteAllInChannel(DiscordClient client, ulong channel)
{
var channelContext = await client.GetChannelAsync(channel);
var messageList = await channelContext.GetMessagesAsync();
if (messageList.Count != 0)
{
await channelContext.DeleteMessagesAsync(messageList);
}
}
static private async Task<List<DiscordMessage>> DiscordCreateBlankMessages(DiscordClient client, ulong id)
{
var FClist = CideFCApis.getAllFleetCarrier();
var MessageIDList = new List<DiscordMessage>();
if (FClist != null)
{
foreach (var item in FClist)
{
if (item != null)
{
DiscordChannel channel = await client.GetChannelAsync(id);
MessageIDList.Add(await channel.SendMessageAsync("Alya: Starting Preworking"));
}
}
return MessageIDList;
}
return MessageIDList;
}
static public async Task processingAsync(DiscordClient client, ulong channel, ulong guild)
{
var guildContext = await client.GetGuildAsync(guild);
var channelContext = guildContext.GetChannel(channel);
// ziska list z počtu id ze instanci db
//var MessageIDList = await DiscordCreateBlankMessages(client, channel);
IReadOnlyList<DiscordMessage> MessageInChannel = await DiscordCountMessageInChannelAsync(client, channel);
List<CideFcObject> FClist = CideFCApis.getAllFleetCarrier();
if (FClist != null)
{
if (MessageInChannel.Count == FClist.Count)
{
var FCAndMSG = FClist.Zip(MessageInChannel, (n, w) => new { FC = n, MSG = w });
foreach (var item in FCAndMSG)
{
await CideMessages.MakeAndSendMessagesAsync(item.FC, item.MSG);
}
if (guildContext.Name != null)
{
Console.WriteLine($"CIDE: Update executed in {guildContext.Name} inside channel {channelContext.Name}");
}
else
{
Console.WriteLine($"CIDE: Update executed inside channel {channelContext.Name}");
}
}
else
{
await DeleteAllInChannel(client, channel);
await DiscordCreateBlankMessages(client, channel);
await processingAsync(client,channel,guild);
}
}
}
private static async Task<IReadOnlyList<DiscordMessage>> DiscordCountMessageInChannelAsync(DiscordClient client, ulong channel)
{
DiscordChannel DiscordChannel = await client.GetChannelAsync(channel);
return await DiscordChannel.GetMessagesAsync();
}
static public async Task<dynamic> runAsync(DiscordClient client, ulong guild, ulong channel)
{
await DeleteAllInChannel(client, channel);
var timer = new System.Threading.Timer(async e => await processingAsync(client, channel, guild), null, TimeSpan.Zero, TimeSpan.FromMinutes(1));
return timer;
}
}
}
<file_sep>namespace AlyaDiscord.TicketRemove.API.Root
{
using System.Globalization;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
using J = Newtonsoft.Json.JsonPropertyAttribute;
using R = Newtonsoft.Json.Required;
using N = Newtonsoft.Json.NullValueHandling;
public partial class Remove
{
[J("discordUUID", NullValueHandling = N.Ignore)] public string DiscordUuid { get; set; }
[J("discordUserName", NullValueHandling = N.Ignore)] public string DiscordUserName { get; set; }
[J("discordMention", NullValueHandling = N.Ignore)] public string DiscordMention { get; set; }
[J("timeStampCreation", NullValueHandling = N.Ignore)] public string TimeStampCreation { get; set; }
[J("serviceType", NullValueHandling = N.Ignore)] public long? ServiceType { get; set; }
[J("serviceID", NullValueHandling = N.Ignore)] public string ServiceId { get; set; }
[J("reason", NullValueHandling = N.Ignore)] public string Reason { get; set; }
[J("whatIsTheGoal", NullValueHandling = N.Ignore)] public string WhatIsTheGoal { get; set; }
}
public partial class Remove
{
public static Remove FromJson(string json) => JsonConvert.DeserializeObject<Remove>(json, AlyaDiscord.TicketRemove.API.Root.Converter.Settings);
}
public static class Serialize
{
public static string ToJson(this Remove self) => JsonConvert.SerializeObject(self, AlyaDiscord.TicketRemove.API.Root.Converter.Settings);
}
internal static class Converter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
Converters =
{
new IsoDateTimeConverter { DateTimeStyles = DateTimeStyles.AssumeUniversal }
},
};
}
}
<file_sep>FROM mcr.microsoft.com/dotnet/sdk:5.0 AS build
WORKDIR /source
COPY *.csproj .
RUN dotnet restore
COPY . .
RUN dotnet publish -c release -o /app
FROM mcr.microsoft.com/dotnet/runtime:5.0
WORKDIR /app
COPY config.json .
COPY Cide/ActiveChannel.json .
COPY config.example.json .
COPY --from=build /app .
ENTRYPOINT ["dotnet", "AlyaDiscord.dll"]<file_sep>namespace AlyaDiscord.TicketRemoveAdminwsid
{
using System;
using System.Collections.Generic;
using System.Globalization;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
using J = Newtonsoft.Json.JsonPropertyAttribute;
using R = Newtonsoft.Json.Required;
using N = Newtonsoft.Json.NullValueHandling;
using DSharpPlus.Entities;
using System.Text.RegularExpressions;
using System.Linq;
using System.Threading.Tasks;
public partial class Object
{
[J("id", NullValueHandling = N.Ignore)] public string Id { get; set; }
[J("webshareIDString", NullValueHandling = N.Ignore)] public string WebshareIdString { get; set; }
[J("reason", NullValueHandling = N.Ignore)] public string Reason { get; set; }
[J("whatIsTheGoal")] public dynamic WhatIsTheGoal { get; set; }
[J("discordUUID", NullValueHandling = N.Ignore)] public string DiscordUuid { get; set; }
[J("discordUserName", NullValueHandling = N.Ignore)] public string DiscordUserName { get; set; }
[J("discordMention", NullValueHandling = N.Ignore)] public string DiscordMention { get; set; }
[J("timeStampCreation", NullValueHandling = N.Ignore)] public string TimeStampCreation { get; set; }
public async Task<dynamic> TryRemoveAsync(string token,string wsid)
{
var scc = new SCC(token);
var ResultFromSccAPIS = await scc.deleteStreamAsync(wsid);
return ResultFromSccAPIS;
}
public void RemoveFromDB()
{
AlyaDiscord.TicketRemoveAPICall.requestRoot.delete(Id);
}
public List<string> GetList()
{
List<string> outputvar;
string[] lines = WebshareIdString.Split(new[] { "\r\n", "\r", "\n", " " },StringSplitOptions.None);
lines.ToList();
outputvar = new List<string>();
Regex r = new Regex(@"(?<=file/)[a-zA-Z0-9]+", RegexOptions.Compiled);
foreach (var item in lines)
{
if (item.Contains("/"))
{
Match match = r.Match(item);
outputvar.Add(match.Value);
}
else
{
outputvar.Add(item);
}
}
return outputvar;
}
public async System.Threading.Tasks.Task FeedbackToAdminAsync(DSharpPlus.CommandsNext.CommandContext ctx, DiscordEmbedBuilder emb)
{
emb.AddField("Žadatel",DiscordUserName);
var guild = await ctx.Client.GetGuildAsync(12345678912345);
var personArwwarr = await guild.GetMemberAsync(12345678912345);
await personArwwarr.SendMessageAsync(embed: emb);
}
private DiscordEmbedBuilder CreateBaseDiscordEmbed()
{
var BaseRespond = new DiscordEmbedBuilder
{
Title = "Ticket Remove - User Report",
Description = "Oznámení pro správce db SCC, informace ohledně ticketu.",
Footer = new DiscordEmbedBuilder.EmbedFooter
{
Text = "Report vytvořen"
},
Color = new DiscordColor(25, 118, 210),
};
BaseRespond.AddField("Důvod vymazání", Reason, false);
BaseRespond.WithTimestamp(DateTime.Now);
return BaseRespond;
}
public async System.Threading.Tasks.Task FeedbackToUserAsync(DSharpPlus.CommandsNext.CommandContext ctx, DiscordEmbedBuilder emb)
{
var guild = await ctx.Client.GetGuildAsync(706602726565609563);
var person = await guild.GetMemberAsync(Convert.ToUInt64(DiscordUuid));
await person.SendMessageAsync(embed: emb);
}
public async System.Threading.Tasks.Task proceedAsync(DSharpPlus.CommandsNext.CommandContext ctx, string token)
{
int DoneItems = 0;
var UserMessage = CreateBaseDiscordEmbed();
var AdminMessage = CreateBaseDiscordEmbed();
AdminMessage.Title = "Ticket Remove - Admin Report";
AdminMessage.Description = "Oznámení pro adminy SCC, informace ohledně ticketu.";
UserMessage.Color = new DiscordColor(84, 227, 70);
AdminMessage.Color = new DiscordColor(84, 227, 70);
foreach (var item in GetList())
{
var Result = await TryRemoveAsync(token,item);
if (Result[0] == "1")
{
UserMessage.AddField("WS ID", item, true);
AdminMessage.AddField("WS ID", item, true);
UserMessage.AddField("Status", "Smazáno :white_check_mark:", false);
AdminMessage.AddField("Status", "Smazáno :white_check_mark:", false);
DoneItems = DoneItems + 1;
}
else
{
UserMessage.AddField("WS ID", item, true);
AdminMessage.AddField("WS ID", item, true);
UserMessage.AddField("Status", "Error :red_square:", false);
AdminMessage.AddField("Status", "Error :red_square:", false);
UserMessage.Color = new DiscordColor(255, 0, 0);
AdminMessage.Color = new DiscordColor(255, 0, 0);
DoneItems = DoneItems + 1;
}
if (DoneItems == 5)
{
await FeedbackToUserAsync(ctx,UserMessage);
await FeedbackToAdminAsync(ctx,UserMessage);
UserMessage = CreateBaseDiscordEmbed();
AdminMessage = CreateBaseDiscordEmbed();
UserMessage.Color = new DiscordColor(84, 227, 70);
AdminMessage.Color = new DiscordColor(84, 227, 70);
DoneItems = 0;
}
await Task.Delay(TimeSpan.FromSeconds(1));
}
if (DoneItems > 0)
{
await FeedbackToUserAsync(ctx,UserMessage);
await FeedbackToAdminAsync(ctx,UserMessage);
}
AlyaDiscord.TicketRemove.API.Call.requestwsid.delete(Id);
}
}
public partial class Object
{
public static List<Object> FromJson(string json) => JsonConvert.DeserializeObject<List<Object>>(json, AlyaDiscord.TicketRemoveAdminwsid.Converter.Settings);
}
public static class Serialize
{
public static string ToJson(this List<Object> self) => JsonConvert.SerializeObject(self, AlyaDiscord.TicketRemoveAdminwsid.Converter.Settings);
}
internal static class Converter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
Converters =
{
new IsoDateTimeConverter { DateTimeStyles = DateTimeStyles.AssumeUniversal }
},
};
}
}
<file_sep>using RestSharp;
using System.Net;
namespace AlyaDiscord.TicketRemove.API.Call
{
public class requestwsid
{
static public dynamic post(string json)
{
var client = new RestClient("http://0.0.0.0:5005/api/SCCRemoveWSID");
client.RemoteCertificateValidationCallback = (sender, certificate, chain, sslPolicyErrors) => true;
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader("Content-Type", "application/json");
request.AddParameter("application/json", json, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
return response.Content;
}
else
{
return "error";
}
}
static public dynamic put(string json, string id)
{
var client = new RestClient($"http://0.0.0.0:5005/api/SCCRemoveWSID/{id}");
client.RemoteCertificateValidationCallback = (sender, certificate, chain, sslPolicyErrors) => true;
client.Timeout = -1;
var request = new RestRequest(Method.PUT);
request.AddHeader("Content-Type", "application/json");
request.AddParameter("application/json", json, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK | response.StatusCode == HttpStatusCode.NoContent)
{
return response.Content;
}
else
{
return "error";
}
}
static public dynamic delete(string id)
{
var client = new RestClient($"http://0.0.0.0:5005/api/SCCRemoveWSID/{id}");
client.RemoteCertificateValidationCallback = (sender, certificate, chain, sslPolicyErrors) => true;
client.Timeout = -1;
var request = new RestRequest(Method.DELETE);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK | response.StatusCode == HttpStatusCode.NoContent)
{
return response.Content;
}
else
{
return "error";
}
}
static public dynamic get(string id)
{
var client = new RestClient($"http://0.0.0.0:5005/api/SCCRemoveWSID/{id}");
client.RemoteCertificateValidationCallback = (sender, certificate, chain, sslPolicyErrors) => true;
client.Timeout = -1;
var request = new RestRequest(Method.GET);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
return response.Content;
}
else
{
return "error";
}
}
static public dynamic getall()
{
var client = new RestClient($"http://0.0.0.0:5005/api/SCCRemoveWSID/");
client.RemoteCertificateValidationCallback = (sender, certificate, chain, sslPolicyErrors) => true;
client.Timeout = -1;
var request = new RestRequest(Method.GET);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
return response.Content;
}
else
{
return "error";
}
}
}
}<file_sep># AlyaDiscord
Jedná se Discord bota, který vykonavá určité commandy, bot není celý dokončen.
po technické stránce tu není moc zajimavych věci, ale i přesto odkažu tady na dvě věci > root/dialog/dialog.cs, root/Cide
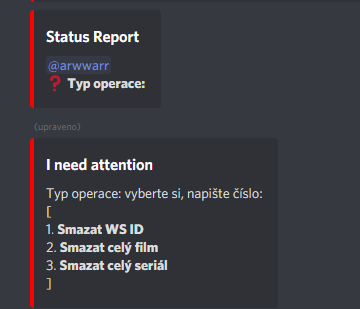
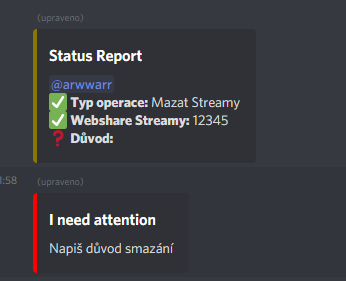
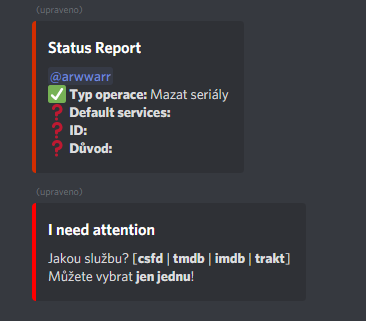
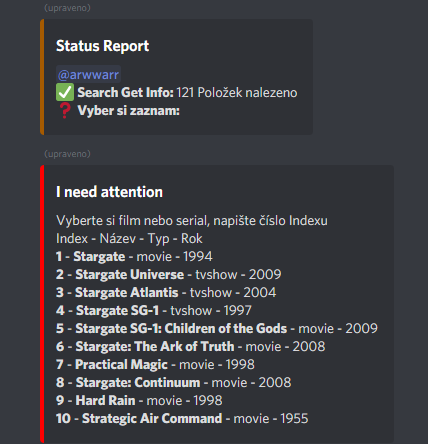
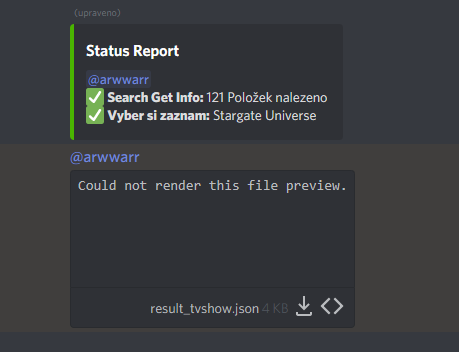
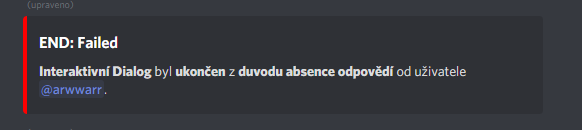
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.Entities;
using DSharpPlus.Interactivity;
namespace AlyaDiscord
{
public class dialog
{
private CommandContext ctx;
private List<DialogData> dialogData;
private DiscordMessage StatusReportMessageID;
private DiscordMessage DialogMessageID;
public dialog(CommandContext CTX, List<DialogData> DATA)
{
ctx = CTX;
dialogData = DATA;
}
public static async Task<dialog> Create(CommandContext ss, List<DialogData> sdsd)
{
var dialogo = new dialog(ss,sdsd);
await dialogo.Initialize();
return dialogo;
}
private async Task Initialize()
{
await StatusReportFirst();
await konverzace();
}
private async Task StatusReportFirst()
{
string DescriptionFunc = $"{ctx.User.Mention}\n";
foreach (var item in dialogData)
{
DescriptionFunc += String.Format($"{item.emojiidk} **{item.StatusReportDescription}:** {item.Answer}\n");
}
var TemplateChannelNoneStatus = new DiscordEmbedBuilder
{
Title = "Status Report",
Description = DescriptionFunc,
Color = new DiscordColor(255, 0, 0)
};
StatusReportMessageID = await ctx.RespondAsync(embed: TemplateChannelNoneStatus);
DialogMessageID = await ctx.RespondAsync(content: "Starting");
}
private async Task StatusReportApplyChange()
{
string DescriptionFunc = $"{ctx.User.Mention}\n";
int answerCounter = 0;
float r = 1;
float g = 1;
float b = 1;
foreach (var item in dialogData)
{
if (item.Answer != null)
{
item.emojiidk = item.emojigood;
answerCounter= answerCounter +1;
}
DescriptionFunc += String.Format($"{item.emojiidk} **{item.StatusReportDescription}:** {item.Answer}\n");
}
var TemplateChannelNoneStatus = new DiscordEmbedBuilder
{
Title = "Status Report",
Description = DescriptionFunc
};
r = (float)answerCounter/(float)dialogData.Count() * ((float)180/(float)255);
g = ((float)1 - (float)r);
b = (float)0;
TemplateChannelNoneStatus.Color = new DiscordColor(g,r,b);
await StatusReportMessageID.ModifyAsync(embed: TemplateChannelNoneStatus);
}
private bool AnswerGrapper(DiscordMessage msg)
{
if (msg.Author.Username == ctx.User.Username && msg.Channel == ctx.Channel)
{
return true;
}
else
{
return false;
}
}
private async Task konverzace()
{
var interactivity = ctx.Client.GetInteractivityModule();
for (int i = 0; i < dialogData.Count; i++)
{
var DialogQuestion = new DiscordEmbedBuilder
{
Title = "I need attention",
Color = new DiscordColor(255, 0, 0)
};
if (dialogData[i].ChatQuestion.Length < 1999)
{
DialogQuestion.Description = dialogData[i].ChatQuestion;
await DialogMessageID.ModifyAsync(embed:DialogQuestion,content: "");
}
else if(dialogData[i].ChatQuestion.Length > 1999)
{
var PageObj = interactivity.GeneratePagesInEmbeds(dialogData[i].ChatQuestion);
await interactivity.SendPaginatedMessage(ctx.Channel,ctx.User,PageObj);
Console.WriteLine("sdsd");
}
var userAnswer = await interactivity.WaitForMessageAsync(AnswerGrapper);
//null check if null == no reaction >> maybe user not respond, delete board, throw message about too much waiting and END
if (userAnswer == null)
{
DialogQuestion.Title = "END: Failed";
DialogQuestion.Description = $"**Interaktivní Dialog** byl **ukončen** z **duvodu absence odpovědí** od uživatele {ctx.User.Mention}.";
await StatusReportMessageID.DeleteAsync();
await DialogMessageID.ModifyAsync(embed: DialogQuestion);
throw new System.ArgumentException("None input from user", "userAnswer");
}
dialogData[i].Answer = userAnswer.Message.Content;
if (dialogData[i].AnswerTypeObject != null)
{
dialogData[i].AnswerTypeObject.ctx = ctx;
dialogData[i].AnswerTypeObject.DialogMessageID = DialogMessageID;
dialogData[i].AnswerTypeObject.StatusReportMessageID = StatusReportMessageID;
dialogData[i].AnswerTypeObject.index = i;
dialogData[i].AnswerTypeObject.input = userAnswer.Message.Content;
dialogData[i].Answer = await dialogData[i].AnswerTypeObject.output();
}
await userAnswer.Message.DeleteAsync();
await StatusReportApplyChange();
}
await StatusReportApplyChange();
}
public dynamic FinalGetDialogMessageID()
{
return DialogMessageID;
}
public dynamic FinalGetStatusReportMessageID()
{
return StatusReportMessageID;
}
public async Task<dynamic> FinalOutputAsync(bool del)
{
if (del == true)
{
await DialogMessageID.DeleteAsync();
}
return dialogData;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace AlyaDiscord
{
public class DATSccSearch : DatBaseAsync
{
public int Page = 1;
public DATSccSearch(List<DialogData> rootList) : base(rootList){}
protected override async Task<string> processingInternalAsync(string input)
{
var resultfromapi = await SCC.SearchStreamAsync(input);
var sccParentObject = AlyaBackend.SccParentObject.FromJson(resultfromapi);
List<DATSccSearchData> SearchData = new List<DATSccSearchData>();
int abc = 0;
foreach (var item in sccParentObject.Data)
{
abc = abc + 1;
var newdata = new DATSccSearchData();
newdata.index = abc;
newdata.name = item.Source.InfoLabels.Originaltitle;
newdata.type = item.Source.InfoLabels.Mediatype;
newdata.year = item.Source.InfoLabels.Year.ToString();
newdata.sccid = item.Id;
if(item.Source.Services.Csfd != null){newdata.csfd = item.Source.Services.Csfd;}
if(item.Source.Services.Imdb != null){newdata.imdb = item.Source.Services.Imdb;}
if(item.Source.Services.Tmdb != null){newdata.tmdb = item.Source.Services.Tmdb;}
if(item.Source.Services.Trakt != null){newdata.trakt = item.Source.Services.Trakt;}
if(item.Source.Services.Tvdb != null){newdata.tvdb = item.Source.Services.Tvdb;}
SearchData.Add(newdata);
if (abc > 9)
{
break;
}
}
string DescriptionFunc = String.Format("Vyberte si film nebo serial, napište číslo Indexu\n");
DescriptionFunc = DescriptionFunc + String.Format($"Index - Název - Typ - Rok\n");
foreach (var item in SearchData)
{
DescriptionFunc += String.Format($"**{item.index}** - **{item.name}** - {item.type} - {item.year}\n");
}
var ChoiceAndShow = new DialogData();
ChoiceAndShow.ChatQuestion = DescriptionFunc;
ChoiceAndShow.StatusReportDescription = "Vyber si zaznam";
ChoiceAndShow.AnswerTypeObject = new DATSccSearchnext(rootList);
ChoiceAndShow.AnswerTypeObject.searchList = SearchData;
ChoiceAndShow.InternalDescription = "";
rootList.Insert(index+1,ChoiceAndShow);
return String.Format($"{sccParentObject.TotalCount} Položek nalezeno");
}
}
}
<file_sep>namespace AlyaDiscord
{
public class DialogData
{
public string ChatQuestion {get;set;}
public string StatusReportDescription {get;set;}
public string InternalDescription {get;set;}
public dynamic AnswerTypeObject {get;set;}
public dynamic Answer {get;set;}
public string emojigood = ":white_check_mark:";
public string emojiidk = ":question:";
public string emojibad = ":red_square:";
}
}
<file_sep>using System.Collections.Generic;
using System.Threading.Tasks;
namespace AlyaDiscord
{
public class DATSccSearchData
{
public int index {get;set;}
public string name {get;set;}
public string type {get;set;}
public string year {get;set;}
public string sccid {get;set;}
public int IndexSeason {get;set;}
public int IndexEpisode {get;set;}
public List<string> streams { get; set; }
public string csfd {get;set;}
public string tmdb {get;set;}
public string imdb {get;set;}
public string trakt {get;set;}
public string tvdb {get;set;}
public async Task getStreamsAsync()
{
streams = new List<string>();
if (sccid != null)
{
var resultFromApi = await SCC.GetInfoStreamAsync(sccid);
var sccStreamObject = AlyaBackend.SccStreamObject.FromJson(resultFromApi);
if (sccStreamObject != null)
{
foreach (var item in sccStreamObject)
{
System.Threading.Thread.Sleep(1);
streams.Add(item.Ident);
}
}
}
}
}
}
<file_sep>using System.Collections.Generic;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.Entities;
namespace AlyaDiscord
{
interface IDatBase
{
// base list for dialogs
List<DialogData> rootList {get;set;}
// way how add input to this class
string input {get;set;}
// index of list
int index {get;set;}
// vystup
List<string> outputvar {get;set;}
// Status message id
DiscordMessage StatusReportMessageID {get;set;}
// dialog message id
DiscordMessage DialogMessageID {get;set;}
CommandContext ctx {get;set;}
Task<dynamic> output();
}
}
<file_sep>using System.Collections.Generic;
using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.CommandsNext.Attributes;
using DSharpPlus.Entities;
using DSharpPlus.Interactivity;
using Newtonsoft.Json;
namespace AlyaDiscord
{
public class dialogCommands
{
[Command("scc_dialog_alpha"), Description("ziskat ws id ze scc db")]
public async Task scc_dialog_alpha(CommandContext ctx)
{
await ctx.Message.DeleteAsync();
List<DialogData> Questions = new List<DialogData>();
Questions.Add(new DialogData());
Questions[0].ChatQuestion = @"Napište prosím Název **Filmu** nebo **Serialu**, stejně jak by jste to hledaly ve **SCC**";
Questions[0].StatusReportDescription = "Search Get Info";
Questions[0].InternalDescription = "SCC_SEARCH_ENTRY";
Questions[0].AnswerTypeObject = new DATSccSearch(Questions);
var testovani = await dialog.Create(ctx,Questions);
var Result = await testovani.FinalOutputAsync(true);
}
}
}
<file_sep>using System.Collections.Generic;
using Newtonsoft.Json;
namespace AlyaDiscord
{
public partial class SccObjectMovie
{
public SccObjectServices services { get; set; }
public List<SccObjectItem> items { get; set; }
public bool IsConcert { get; set; }
}
public partial class SccObjectItem
{
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public long? season { get; set; }
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public long? episode { get; set; }
public List<string> streams { get; set; }
}
public partial class SccObjectServices
{
#nullable enable
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public string? tmdb { get; set; }
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public string? trakt { get; set; }
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public string? csfd { get; set; }
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public string? imdb { get; set; }
[JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
public string? tvdb { get; set; }
}
}
<file_sep>using System.Threading.Tasks;
using DSharpPlus.CommandsNext;
using DSharpPlus.Entities;
using DSharpPlus.Interactivity;
class scc_getDiscordToken
{
private CommandContext ctx;
public scc_getDiscordToken(CommandContext ctx)
{
this.ctx = ctx;
}
internal bool validator(DiscordMessage a)
{
if (a.Author.Username == ctx.User.Username && a.Channel == ctx.Channel)
{
return true;
}
return false;
}
public async Task<dynamic> getDiscordTokenFromPMAsync()
{
var interactivity = ctx.Client.GetInteractivityModule();
var DialogQuestion = new DiscordEmbedBuilder
{
Title = "I need attention",
Description = "Zadej tvuj Discord Token.",
Color = new DiscordColor(255, 0, 0)
};
var message = await ctx.Member.SendMessageAsync(embed: DialogQuestion);
var userReaction = await interactivity.WaitForMessageAsync(validator);
if (userReaction != null)
{
return userReaction.Message.Content;
}
else
{
return null;
}
}
} | 85cc91546ad41d31922d0c6ff27e0c89643395bd | [
"C#",
"Markdown",
"Dockerfile"
] | 28 | C# | LukePavelka/AlyaDiscord-example | d66e3d2ccdefb9834ce20382c3fe780acbb9f458 | 7495d84a91b762f7edbaae89c6300a78d9d1c71a |
refs/heads/master | <repo_name>Garin151/DeepIn-NewsSystem<file_sep>/src/com/news/dao/CommentDao.java
package com.news.dao;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.List;
import com.news.po.Comment;
import com.news.util.DB_util;
public class CommentDao {
//新增一条评论
public void addComment(int fromId,String user,String comment,String creatTime) {
String sql = "insert into comment_db (fromId,user,comment,creatTime) values (?,?,?,?)";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setInt(1, fromId);
pstmt.setString(2, user);
pstmt.setString(3, comment);
pstmt.setString(4, creatTime);
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
}
//查找所有评论
public List<Comment> getAllComment() {
String sql = "select * from comment_db order by creatTime asc";
List<Comment> commentList = new ArrayList<Comment>();
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
ResultSet res = pstmt.executeQuery();
while(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
int id = res.getInt("id");
int fromId = res.getInt("fromId");
String user = res.getString("user");
String comment = res.getString("comment");
String creatTime = sdf.format(res.getDate("creatTime"));
Comment one = new Comment(id, fromId, user, comment, creatTime);
commentList.add(one);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return commentList;
}
//根据新闻id查找评论
public List<Comment> getFromId(int idd) {
String sql = "select * from comment_db where fromId = ? order by creatTime asc";
List<Comment> commentList = new ArrayList<Comment>();
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setInt(1,idd);
ResultSet res = pstmt.executeQuery();
while(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
int id = res.getInt("id");
int fromId = res.getInt("fromId");
String user = res.getString("user");
String comment = res.getString("comment");
String creatTime = sdf.format(res.getDate("creatTime"));
Comment one = new Comment(id, fromId, user, comment, creatTime);
commentList.add(one);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return commentList;
}
}
<file_sep>/src/com/news/po/User.java
package com.news.po;
public class User {
private String user;
private String password;
private String findBack;
private String answer;
private int isBan;
private String sex;
private String city;
private String birthday;
private String avatarUrl;
public User(String user,String password,String findBack,String answer) {
super();
this.user = user;
this.password = <PASSWORD>;
this.findBack = findBack;
this.answer = answer;
}
public User(String user,String sex,String city,String birthday,String avatarUrl) {
super();
this.user = user;
this.sex = sex;
this.city = city;
this.birthday = birthday;
this.avatarUrl = avatarUrl;
}
public User(String user,String password) {
super();
this.user = user;
this.password = <PASSWORD>;
}
public String getUser() {
return user;
}
public void setUser(String user) {
this.user = user;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = <PASSWORD>;
}
public String getFindBack() {
return findBack;
}
public void setFindBack(String findBack) {
this.findBack = findBack;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getAvatarUrl() {
return avatarUrl;
}
public void setAvatarUrl(String avatarUrl) {
this.avatarUrl = avatarUrl;
}
public String getAnswer() {
return answer;
}
public void setAnswer(String answer) {
this.answer = answer;
}
public int getIsBan() {
return isBan;
}
public void setIsBan(int isBan) {
this.isBan = isBan;
}
}
<file_sep>/WebContent/ban.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>账号被封</title>
<link rel="stylesheet" type="text/css" href="../iconfont/iconfont.css">
<link rel="stylesheet" href="https://cdn.staticfile.org/twitter-bootstrap/4.3.1/css/bootstrap.min.css">
<script src="https://cdn.staticfile.org/jquery/3.2.1/jquery.min.js"></script>
<script src="https://cdn.staticfile.org/popper.js/1.15.0/umd/popper.min.js"></script>
<script src="https://cdn.staticfile.org/twitter-bootstrap/4.3.1/js/bootstrap.min.js"></script>
<style type="text/css">
.wholeBody {width: 100%;height: 907px;display: flex;background-color: #F2F2F2;justify-content:center;align-items:center;}
.bbbox {position: relative;width: 600px;height: 600px;background-color: #FFFFFF;border-radius: 12px;display: flex;justify-content:center;align-items:center;}
.errorTip {position: absolute;top: 70px;font-size: 32px;font-weight: bold;color: #FF2727;letter-spacing: 8px;}
.tipss {position: absolute;top: 200px;font-size: 26px;font-weight: bold;letter-spacing: 8px;}
.btnnn {position: absolute;bottom: 80px;}
</style>
<script type="text/javascript">
function backLogin() {
window.location.href = "login.jsp"
}
</script>
</head>
<body>
<div class="wholeBody">
<div class="bbbox">
<p class="errorTip">您的账号已经被封</p>
<p class="tipss">不接受任何申诉!</p>
<button class="btn btn-success btnnn" onclick="backLogin()">返回登录</button>
</div>
</div>
</body>
</html><file_sep>/src/com/news/dao/NewsDao.java
package com.news.dao;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.List;
import com.news.po.News;
import com.news.util.DB_util;
public class NewsDao {
//根据id查找新闻
public News findID(int id) {
String sql = "select * from news_db where id = ?";
News news = new News("","","","","",0);
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setInt(1, id);
ResultSet res = pstmt.executeQuery();
if(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String author = res.getString("author");
String creatTime = sdf.format(res.getDate("creatTime"));
String title = res.getString("title");
String image = res.getString("image");
String content = res.getString("content");
int num_view = res.getInt("num_view");
News oneNew = new News(author, creatTime, title, content, image, num_view);
news = oneNew;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return news;
}
public List<News> searchNews(String data) {
List<News> newsList = new ArrayList<News>();
String sql = "select * from news_db where title like '%" + data + "%' order by creatTime desc";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
ResultSet res = pstmt.executeQuery();
while(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
int id = res.getInt("id");
String author = res.getString("author");
String creatTime = sdf.format(res.getDate("creatTime"));
String title = res.getString("title");
String image = res.getString("image");
String content = res.getString("content");
int num_view = res.getInt("num_view");
News oneNew = new News(id, author, creatTime, title, content, image, num_view);
newsList.add(oneNew);
}
res.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return newsList;
}
//根据id删除一条新闻
public void deleteNews(int id) {
String sql = "delete from news_db where id = ?";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setInt(1,id);
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
}
//新增新闻的阅读量
public void updateView(int id) {
String sql = "update news_db set num_view = num_view + 1 where id = ?";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setInt(1,id);
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
}
//新增一条新闻
public void addNews(String author,String creatTime,String title,String content,String image) {
String sql = "insert into news_db (author,creatTime,title,content,image,num_view) values (?,?,?,?,?,?)";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setString(1, author);
pstmt.setString(2, creatTime);
pstmt.setString(3, title);
pstmt.setString(4, content);
pstmt.setString(5, image);
pstmt.setInt(6, 0);
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
}
//查找同一作者的新闻
public List<News> authorNews(String username) {
List<News> newsList = new ArrayList<News>();
String sql = "select * from news_db where author = ? order by creatTime desc";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
pstmt.setString(1, username);
ResultSet res = pstmt.executeQuery();
while(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
int id = res.getInt("id");
String author = res.getString("author");
String creatTime = sdf.format(res.getDate("creatTime"));
String title = res.getString("title");
String image = res.getString("image");
String content = res.getString("content");
int num_view = res.getInt("num_view");
News oneNew = new News(id, author, creatTime, title, content, image, num_view);
newsList.add(oneNew);
}
res.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return newsList;
}
//查询所有新闻并按时间排序
public List<News> findAllNews() {
List<News> newsList = new ArrayList<News>();
String sql = "select * from news_db order by creatTime desc";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
ResultSet res = pstmt.executeQuery();
while(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
int id = res.getInt("id");
String author = res.getString("author");
String creatTime = sdf.format(res.getDate("creatTime"));
String title = res.getString("title");
String image = res.getString("image");
String content = res.getString("content");
int num_view = res.getInt("num_view");
News oneNew = new News(id, author, creatTime, title, content, image, num_view);
newsList.add(oneNew);
}
res.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return newsList;
}
//查询首页推荐新闻
public News findRecommend() {
News recommend = new News(0,"","","","","",0);
String sql = "select * from recommend_db";
PreparedStatement pstmt = DB_util.getPreparedStatement(sql);
try {
ResultSet res = pstmt.executeQuery();
while(res.next()) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
int id = res.getInt("id");
String author = res.getString("author");
String creatTime = sdf.format(res.getDate("creatTime"));
String title = res.getString("title");
String image = res.getString("image");
String content = res.getString("content");
int num_view = res.getInt("num_view");
News oneNew = new News(id, author, creatTime, title, content, image, num_view);
recommend = oneNew;
}
res.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DB_util.closeDB();
}
return recommend;
}
}
<file_sep>/src/com/news/util/DB_util.java
package com.news.util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Statement;
public class DB_util {
private static Connection conn;
private static Statement stmt;
private static PreparedStatement pstmt;
private static String driver = "com.mysql.cj.jdbc.Driver";
private static String url = "jdbc:mysql://localhost:3306/systemnews?serverTimezone=UTC&characterEncoding=utf-8";
private static String user = "root";
private static String password = "<PASSWORD>";
static {
//加载Mysql驱动
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
System.out.println("数据库驱动加载失败");
}
}
//连接数据库
public static Connection getConnection() {
try {
conn = DriverManager.getConnection(url,user,password);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
System.out.println("数据库连接失败");
}
return conn;
}
//创建执行环境
public static Statement getStatement() {
Connection conn = getConnection();
try {
if(conn != null){
stmt = conn.createStatement();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return stmt;
}
//实例化SQL执行语句柄pstmt
public static PreparedStatement getPreparedStatement(String sql) {
Connection conn = getConnection();
try {
if(conn != null){
pstmt = conn.prepareStatement(sql);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return pstmt;
}
//关闭数据库连接资源
public static void closeDB() {
try {
if(pstmt != null && !pstmt.isClosed()){
pstmt.close();
}
if(stmt != null && !stmt.isClosed()){
stmt.close();
}
if(conn != null && !conn.isClosed()){
conn.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
<file_sep>/src/com/news/servlet/commentServlet.java
package com.news.servlet;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.news.business.CommentService;
public class commentServlet extends HttpServlet{
private CommentService commentService = new CommentService();
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String param = request.getParameter("param");
if("add".equals(param)) {
String user = request.getParameter("username");
String idd = request.getParameter("newsID");
int newsID = Integer.parseInt(request.getParameter("newsID"));
String comment = request.getParameter("comment");
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date nowDay = new Date();
String creatTime = sdf.format(nowDay);
commentService.addComFX(newsID, user, comment, creatTime);
response.sendRedirect("newServlet?param=detail&id="+ idd);
}else if("delete".equals(param)){
}
}
}
<file_sep>/WebContent/login.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>登录页面</title>
<link rel="stylesheet" href="https://cdn.staticfile.org/twitter-bootstrap/4.3.1/css/bootstrap.min.css">
<script src="https://cdn.staticfile.org/jquery/3.2.1/jquery.min.js"></script>
<script src="https://cdn.staticfile.org/popper.js/1.15.0/umd/popper.min.js"></script>
<script src="https://cdn.staticfile.org/twitter-bootstrap/4.3.1/js/bootstrap.min.js"></script>
<style type="text/css">
a:link {color: #AAAAAA;text-decoration:none;} /* 未被访问的链接 */
a:visited {color: #AAAAAA;text-decoration:none;} /* 已被访问过的链接*/
a:hover {color: #DC3545;text-decoration:none;} /* 鼠标悬浮在上的链接 */
a:active {color: #DC3545;text-decoration:none;} /* 鼠标点中激活链接 */
.wholeBox {width: 100%;height: 937px;position: relative;display: flex;}
.leftBox {height: 100%;flex:35;background-color: #FFFFFF;position: relative;display: flex;justify-content:center;}
.rightBox {height: 100%;flex:65;background-color: #3D86EF;position: relative;}
.r_topBox {width: 100%;height:68%;background-color: #D3D3D3;position: relative;}
.r_bottomBox {width: 100%;height:32%;background-color: #0C0C0C;position: relative;display: flex;justify-content:center;align-items:center;}
.swiperMask {width: 100%;height: 100%;background-color: #1D1D1D;position: absolute;z-index: 99;opacity: 0.3;}
.carousel-inner img {width: 100%;height: 100%;object-fit: cover;}
.carousel-inner {width: 100%;height: 100%;}
.control {z-index: 9999}
.officialName {margin-left: 8px;font-family: "华文新魏";font-weight: bold;font-size: 74px;top: 200px;position: absolute;letter-spacing: 20px;}
.officialName_02 {font-family: "幼圆";font-size: 24px;top: 350px;position: absolute;letter-spacing: 8px;}
.carousel-item {width: 100%;height: 100%;}
.bottomtext {font-size: 18px;position: absolute;bottom: 30px;color: #FFFFFF;}
.s-title {position: absolute;font-size: 40px;top: -100px;line-height: 60px;white-space: pre-line;text-align: center;}
.btn_group {bottom: 300px;position: absolute;width: 60%;height: 100px;display: flex;justify-content: space-around;align-items:center;}
.Modalclose {width: 50px;height: 50px;position: absolute;z-index: 9999}
.loginForm {width: 100%;height: 200px;}
.registerForm {width: 100%;height: 500px;}
.findback {position: absolute;bottom: 10px;left: 20px;}
.adminPage {position: absolute;bottom: 10px;right: 20px;}
.loginContain {width: 100%;height: 60px;display: flex;justify-content:center;align-items:center;}
</style>
<script type="text/javascript">
$(document).ready(function() {
$("#loginForm").submit(function() {
let b = $("#inputPassword").val();
let a = $("#inputUser").val();
if(a == "" || b == "") {
alert("请填写正确的账号和密码")
return false;
}else {
return true;
}
});
$("#registerForm").submit(function() {
let a = $("#rinputUser").val();
let b = $("#rinputPassword").val();
let c = $("#rinputRepassword").val();
let d = $("#rinputAnswer").val();
if(a == "" || b == "" || c == "" || d == "") {
alert("请填写完整注册信息")
return false;
}else {
if(b != c) {
alert("两次密码不一致")
return false;
}else {
return true;
}
}
});
})
function ChangeSelect() {
let data = $("#ControlSelect option:selected").val()
console.log(data)
};
function addRegister() {
let f = document.getElementsByTagName("form")[1];
if(f.action == "http://localhost:8080/systemNews/login?param=register") {
return 0
}else {
f.action=f.action+"?param=register"
}
}
function addLogin() {
let f = document.getElementsByTagName("form")[0];
if(f.action == "http://localhost:8080/systemNews/login?param=login") {
return 0
}else {
f.action=f.action+"?param=login"
}
}
function ToadminPage() {
let f = document.getElementsByTagName("form")[0];
if(f.action == "http://localhost:8080/systemNews/login?param=admin") {
}else {
f.action=f.action+"?param=admin"
}
document.getElementById("loginForm").submit();
}
</script>
</head>
<body>
<!-- 登录模态框 -->
<div class="modal fade" style="z-index: 99999" id="loginModal" tabindex="-1" role="dialog" aria-labelledby="loginModalTitle" aria-hidden="true">
<div class="modal-dialog modal-dialog-centered" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="loginModalTitle">登录账户</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body" style="height: 200px;">
<form id="loginForm" action="login" autocomplete="off" method="post" class="loginForm">
<div class="form-group row">
<label for="inputEmail3" class="col-sm-2 col-form-label">用户:</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="inputUser" id="inputUser">
</div>
</div>
<div class="form-group row">
<label for="inputPassword3" class="col-sm-2 col-form-label">密码:</label>
<div class="col-sm-10">
<input type="<PASSWORD>" class="form-control" name="inputPassword" id="inputPassword">
</div>
</div>
<div>
<div class="loginContain">
<button type="submit" style="padding: 6px 20px;" class="btn btn-outline-dark" onclick="addLogin()">登录</button>
</div>
<div>
<a class="findback" href="findback.jsp">找回密码</a>
<a class="adminPage" onclick="ToadminPage()">管理员登录</a>
</div>
</div>
</form>
</div>
<div class="modal-footer"></div>
</div>
</div>
</div>
<!-- 模态框 -->
<!-- 注册模态框 -->
<div class="modal fade" style="z-index: 99999" id="registerModal" tabindex="-1" role="dialog" aria-labelledby="loginModalTitle" aria-hidden="true">
<div class="modal-dialog modal-dialog-centered" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="registerModalTitle">注册账户</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body" style="height: 360px;">
<form id="registerForm" action="login" autocomplete="off" method="post" class="registerForm">
<div class="form-group row">
<label for="inputEmail3" class="col-sm-2 col-form-label">用户:</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="inputUser" id="rinputUser">
</div>
</div>
<div class="form-group row">
<label for="inputPassword3" class="col-sm-2 col-form-label">密码:</label>
<div class="col-sm-10">
<input type="<PASSWORD>" class="form-control" name="inputPassword" id="rinputPassword">
</div>
</div>
<div class="form-group row">
<label for="inputPassword3" style="width: 100px!important;white-space: nowrap;" class="col-sm-2 col-form-label">确认密码:</label>
<div class="col-sm-10">
<input type="password" class="form-control" name="inputRepassword" id="rinputRepassword">
</div>
</div>
<div class="form-group row">
<label for="exampleFormControlSelect1" style="margin-left: 16px;">找回密码:</label>
<select onchange="ChangeSelect()" class="form-control" style="width: 383px!important;margin-left: 2px;" name="findBack" id="ControlSelect">
<option value="高中暗恋对象的名字">高中暗恋对象的名字 ?</option>
<option value="父亲的电话号码">父亲的电话号码 ?</option>
<option value="母亲的电话号码">母亲的电话号码 ?</option>
<option value="就读小学的名称">就读小学的名称 ?</option>
</select>
</div>
<div class="form-group row">
<label for="inputEmail3" class="col-sm-2 col-form-label">答案:</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="inputAnswer" id="rinputAnswer" placeholder="请务必牢记问题答案!">
</div>
</div>
<div>
<div class="loginContain">
<button type="submit" style="padding: 6px 20px;" class="btn btn-outline-danger" onclick="addRegister()">注册</button>
</div>
</div>
</form>
</div>
<div class="modal-footer"></div>
</div>
</div>
</div>
<!-- 模态框 -->
<div class="wholeBox">
<div class="leftBox">
<p class="officialName">深度新闻网</p>
<p class="officialName_02">有深度,有情怀,有热点</p>
<div class="btn_group">
<button type="button" id="login" data-toggle="modal" data-target="#loginModal" class="btn btn-outline-dark" style="padding: 10px 25px;">登录</button>
<button type="button" id="register" data-toggle="modal" data-target="#registerModal" class="btn btn-outline-danger" style="padding: 10px 25px;">注册</button>
</div>
</div>
<div class="rightBox">
<div id="loginSwiper" class="carousel slide r_topBox" data-pause=false data-ride="carousel" data-interval="3000">
<div class="swiperMask"></div>
<ul class="carousel-indicators control">
<li data-target="#loginSwiper" data-slide-to="0" class="active"></li>
<li data-target="#loginSwiper" data-slide-to="1"></li>
<li data-target="#loginSwiper" data-slide-to="2"></li>
</ul>
<div class="carousel-inner">
<div class="carousel-item active">;
<img src="static/picture/309736.jpg">
<div class="carousel-caption d-none d-md-block" style="z-index: 999;">
<p class="s-title">深圳最新规划透露:出门10分钟有轨道交通,新增住房170万套</p>
<p class="s-content">Shenzhen's latest planning revealed: 10 minutes out of the door rail transit, 1.7 million new housing</p>
</div>
</div>
<div class="carousel-item">
<img src="static/picture/2018593.jpg">
<div class="carousel-caption d-none d-md-block" style="z-index: 999;">
<p class="s-title">中国动漫文娱产业投资攀升,或将推动产业升级</p>
<p class="s-content">China's animation and entertainment industry investment is rising, or will promote industrial upgrading</p>
</div>
</div>
<div class="carousel-item">
<img src="static/picture/2009490.jpg">
<div class="carousel-caption d-none d-md-block" style="z-index: 999;">
<p class="s-title">迈凯伦亚洲官方体验中心,2024年将落户杭州</p>
<p class="s-content">The official Asia experience center of McLaren will be settled in Hangzhou in 2024</p>
</div>
</div>
</div>
</div>
<div class="r_bottomBox">
<p class="bottomtext">Copyright© 2020-2050 DeepIn News All Right Reserved | 中华人民共和国增值电信业务经营许可证: 粤SB-25041 粤ICP备14250SB号</p>
</div>
</div>
</div>
</body>
</html><file_sep>/src/com/news/business/userService.java
package com.news.business;
import com.news.dao.userDao;
import java.util.ArrayList;
import java.util.List;
import com.news.po.User;
import com.news.po.Show;
public class userService {
//ÅжϵǼÊÇ·ñºÏ·¨
private userDao userDao = new userDao();
public boolean legal(User user) {
return userDao.isExists(user.getUser(),user.getPassword());
}
public User getInfoFX(String username) {
return userDao.getInfo(username);
}
public boolean isBanFX(String user,String password) {
return userDao.isBan(user, password);
}
public void banorActUserFX(String name,String data) {
userDao.banorActUser(name, data);
}
public List<Show> getAllShowFX() {
return userDao.getAllShow();
}
}
<file_sep>/src/com/news/servlet/findbackServlet.java
package com.news.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.news.dao.FindBackDao;
import com.news.po.User;
public class findbackServlet extends HttpServlet{
private FindBackDao FindBackDao = new FindBackDao();
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String findBack = request.getParameter("findBack");
String inputAnswer = request.getParameter("inputAnswer");
User one = FindBackDao.findBack(findBack, inputAnswer);
String name = one.getUser();
if(name == "") {
response.sendRedirect("error.jsp");
}else {
request.setAttribute("user", one.getUser());
request.setAttribute("password", <PASSWORD>());
request.getRequestDispatcher("findBackOK.jsp").forward(request, response);
}
}
}
| 3bf0e6f502b7e86f3000fb6755a3124813c6b8bf | [
"Java",
"Java Server Pages"
] | 9 | Java | Garin151/DeepIn-NewsSystem | 08e0bd32710accc7d51ce9e664c429f025351f57 | 44d3cc9d3c98dbf5850f58bc78c87b846641ea64 |
refs/heads/master | <file_sep>#!/usr/bin/python
from ansible.module_utils.basic import AnsibleModule
from subprocess import Popen
from subprocess import PIPE
def check_guest_additions():
p1 = Popen(['ps', 'aux'], stdout=PIPE)
p2 = Popen(['grep', 'VBoxService'], stdin=p1.stdout, stdout=PIPE)
p1.stdout.close()
output = p2.communicate()[0]
if '/usr/sbin/VBoxService' in output:
return True
else:
return False
def main():
module = AnsibleModule(argument_spec = dict())
if check_guest_additions() == True:
chg = False
else:
chg = True
module.exit_json(
changed=chg,
ansible_facts=dict(additions_is_installed=check_guest_additions())
)
if __name__ == '__main__':
main()
| ebc0b199b8a8dc813ffa1bc1cf394cd530fe3ebd | [
"Python"
] | 1 | Python | tmolnar0831/ansible-guest_additions | 9af44db9a3c8f8be0bc001e0190b31a6eb11abe0 | a774effb2d83eb185fc2ee98ab7fcda85e1c6c5d |
refs/heads/master | <repo_name>iwabuchiken/WS_Others.Art<file_sep>/JVEMV6/46_art/2_image-prog/1_gimp/2_/plugin_libs.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
########################################################################
# version 1.0.0
#
# GimpPythonFuLayerAction-2-8.py
# Copyright (C) 2012 かんら・から http://www.pixiv.net/member.php?id=3098715
#
# GimpPythonFuLayerAction-2-8.py is Python-fu plugin for GIMP 2.8
#
# GimpPythonFuLayerAction-2-8.py is free software: you can redistribute it and/or modify it
# under the terms of the GNU General Public License as published by the
# Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# GimpPythonFuLayerAction-2-8.py is distributed in the hope that it will be useful, but
# WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
# See the GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License along
# with this program. If not, see <http://www.gnu.org/licenses/>.
#
# GPLv3 ライセンス
# かんら・から http://www.pixiv.net/member.php?id=3098715
# バグレポート・アイデアなどは pixiv メッセージでお願いします。
#
# ダウンロード
# http://www.magic-object.mydns.jp/
#
# このスクリプトを使用して発生した問題に関し、作成者は如何なる保証などを行う事はありません。
# 自己責任でのみの使用を許可します。
########################################################################
from gimpfu import *
# from time import time
########################################################################
########################################################################
# レイヤー操作クラス
#
# レイヤー転写機能群など
'''
@param string_type
serial "20160604_193404"
basic "2016/06/04 19:34:04"
'''
# def get_TimeLabel_Now(string_type="serial", mili=False):
# # def get_TimeLabel_Now(string_type="serial"):
#
# t = time()
#
# # str = strftime("%Y%m%d_%H%M%S", t)
# # str = strftime("%Y%m%d_%H%M%S", localtime())
#
# '''###################
# build string
# ###################'''
# if string_type == "serial" : #if string_type == "serial"
#
# str = strftime("%Y%m%d_%H%M%S", localtime(t))
#
# elif string_type == "basic" : #if string_type == "serial"
#
# str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
#
# else : #if string_type == "serial"
#
# str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
#
# #/if string_type == "serial"
#
#
# # str = strftime("%Y%m%d_%H%M%S", localtime(t))
#
# #ref https://stackoverflow.com/questions/5998245/get-current-time-in-milliseconds-in-python "answered May 13 '11 at 22:21"
# if mili == True :
#
# if string_type == "serial" : #if string_type == "serial"
#
# str = "%s_%03d" % (str, int(t % 1 * 1000))
#
# else : #if string_type == "serial"
#
# str = "%s.%03d" % (str, int(t % 1 * 1000))
#
# #ref decimal value https://stackoverflow.com/questions/30090072/get-decimal-part-of-a-float-number-in-python "answered May 7 '15 at 1:56"
# # str = "%s_%03d" % (str, int(t % 1 * 1000))
#
# return str
#
# #ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
# # return strftime("%Y%m%d_%H%M%S", localtime())
# # return strftime("%Y%m%d_%H%M%S", gmtime())
#
# #]]get_TimeLabel_Now():
#ref https://jacksonbates.wordpress.com/2015/09/14/python-fu-4-hello-warning/
def hello_warning(image, drawable):
# function code goes here...
pdb.gimp_message("Hello, world!")
#ref https://www.ibm.com/developerworks/jp/opensource/library/os-autogimp/
def plugin_main(timg, tdrawable):
dpath = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\2_"
fpath = "%s\\python_fu.txt" % (dpath)
# fpath = "%s\\python_fu.%s.txt" % (dpath, get_TimeLabel_Now())
# fpath = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\2_\\python_fu.txt"
f = open(fpath, "a")
f.write("リサイズするスクリプトを作成する")
# f.write("time is : %s" % get_TimeLabel_Now())
f.write("\n")
f.close()
# print "Hello, world!"
register(
"python_fu_resize",
"Saves the image at a maximum width and height",
"Saves the image at a maximum width and height",
"<NAME>",
"<NAME>",
"2010",
"<Image>/Image/Resize to max...",
"RGB*, GRAY*",
[],
[],
plugin_main)
register(
"hello_warning",
"display message",
"display message",
"https://jacksonbates.wordpress.com",
"https://jacksonbates.wordpress.com",
"September 14, 2015",
"<Image>/Image/Hello warning !",
"RGB*, GRAY*",
[],
[],
hello_warning)
# hello_warning, menu="/File/Hello warning!") # second item is menu location
# register(
# "python-fu-hello-warning",
# "Hello world warning",
# "Prints 'Hello, world!' to the error console",
# "<NAME>", "<NAME>", "2015",
# "Hello warning",
# "", # type of image it works on (*, RGB, RGB*, RGBA, GRAY etc...)
# [
# (PF_IMAGE, "image", "takes current image", None),
# (PF_DRAWABLE, "drawable", "Input layer", None)
# ],
# [],
# hello_warning, menu="/File") # second item is menu location
# # hello_warning, menu="/File/Hello warning!") # second item is menu location
main() # プラグインを駆動させるための関数
<file_sep>/JVEMV6/46_art/2_image-prog/1_gimp/4_/plugin_2_1_4 (2).py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\2_image-prog\1_gimp\4_\plugin_2_1_4.py
at : 2018/04/06 14:34:49
plugin at : C:\WORKS_2\Programs\GIMP 2\plugins
'''
from gimpfu import *
from time import time, localtime, strftime
import inspect, os
from fileinput import lineno
########################################################################
def write_Log(msg):
dpath = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\4_"
fname = "debug.log"
fpath = "%s\\%s" % (dpath, fname)
f = open(fpath, "a")
f.write("===============================\n")
f.write(msg)
f.write("\n\n")
f.close()
#/ def write_Log(msg):
def thisfile(depth=0):
# def _file(depth=0):
# print "line"
callerframerecord = inspect.stack()[1] # 0 represents this line
# 1 represents line at caller
frame = callerframerecord[0]
info = inspect.getframeinfo(frame)
return info.filename # __FILE__ -> Test.py
# print info.filename # __FILE__ -> Test.py
# print info.function # __FUNCTION__ -> Main
# print info.lineno # __LINE__ -> 13
'''
frame = inspect.currentframe(0)
# frame = inspect.currentframe(depth+1)
return os.path.basename(frame.f_code.co_filename)
# return frame.f_code.co_filename
'''
#/def thisfile(depth=0):
def linenum(depth=0):
# print "line"
#ref https://stackoverflow.com/questions/6810999/how-to-determine-file-function-and-line-number 'answered Jul 25 '11 at 1:31'
callerframerecord = inspect.stack()[1] # 0 represents this line
# 1 represents line at caller
frame = callerframerecord[0]
info = inspect.getframeinfo(frame)
# return info.filename # __FILE__ -> Test.py
# print info.filename # __FILE__ -> Test.py
# print info.function # __FUNCTION__ -> Main
# print info.lineno # __LINE__ -> 13
return info.lineno # __LINE__ -> 13
'''
frame = inspect.currentframe(depth+1)
return frame.f_lineno
'''
'''
@param string_type
serial "20160604_193404"
basic "2016/06/04 19:34:04"
'''
def get_TimeLabel_Now(string_type="serial", mili=False):
# def get_TimeLabel_Now(string_type="serial"):
t = time()
# str = strftime("%Y%m%d_%H%M%S", t)
# str = strftime("%Y%m%d_%H%M%S", localtime())
'''###################
build string
###################'''
if string_type == "serial" : #if string_type == "serial"
str = strftime("%Y%m%d_%H%M%S", localtime(t))
elif string_type == "basic" : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
else : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
#/if string_type == "serial"
# str = strftime("%Y%m%d_%H%M%S", localtime(t))
#ref https://stackoverflow.com/questions/5998245/get-current-time-in-milliseconds-in-python "answered May 13 '11 at 22:21"
if mili == True :
if string_type == "serial" : #if string_type == "serial"
str = "%s_%03d" % (str, int(t % 1 * 1000))
else : #if string_type == "serial"
str = "%s.%03d" % (str, int(t % 1 * 1000))
#ref decimal value https://stackoverflow.com/questions/30090072/get-decimal-part-of-a-float-number-in-python "answered May 7 '15 at 1:56"
# str = "%s_%03d" % (str, int(t % 1 * 1000))
return str
#ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
# return strftime("%Y%m%d_%H%M%S", localtime())
# return strftime("%Y%m%d_%H%M%S", gmtime())
#]]get_TimeLabel_Now():
class ImageObject :
image = None
layer = None
def __init__( self, image ):
self.image = image
return
def get_Image(self):
return self.image
def add_Layer(self, layer_Name):
#debug
msg = "[%s / %s:%d]\nadd_Layer => starting..." \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
width = self.image.width
height = self.image.height
type = RGB_IMAGE
opacity = 100
mode = NORMAL_MODE
#debug
msg = "[%s / %s:%d]\ngimp.Layer() ---> calling..." \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
self.layer = gimp.Layer(self.image, layer_Name, width, height, type, opacity, mode)
# self.layer = gimp.Layer(image, name, width, height, type, opacity, mode)
#debug
msg = "[%s / %s:%d]\ngimp.Layer() ---> done" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
self.layer.fill(1)
#
# 画像データの 0 番目の位置にレイヤーを挿入する
position = 0
self.image.add_layer(self.layer, position)
# self.image.add_layer(layer, position)
# return
return
#/def add_Layer(self, layer_Name):
def get_Layer(self):
if self.layer == None :
pdb.gimp_message("layer ==> None !!!")
return False
# return layer
return self.layer
def create_image(width, height):
# 画像データを生成
return gimp.Image(width, height, RGB)
def display_image(image):
gimp.Display(image)
def add_layer(image, name):
# レイヤーの作成に必要なパラメータ
width = image.width
height = image.height
type = RGB_IMAGE
opacity = 100
mode = NORMAL_MODE
#
# パラメータをもとにレイヤーを作成
layer = gimp.Layer(image, name, width, height, type, opacity, mode)
#
# レイヤーを背景色で塗りつぶす(GIMP のデフォルトの挙動に合わせています)
layer.fill(1)
#
# 画像データの 0 番目の位置にレイヤーを挿入する
position = 0
image.add_layer(layer, position)
#
return layer
def draw_pencil_lines(drawable, lines):
# ペンシルツールで線を描画する
pdb.gimp_pencil(drawable, len(lines), lines)
def draw_rect(drawable, x1, y1, x2, y2):
lines = [x1, y1, x2, y1, x2, y2, x1, y2, x1, y1]
draw_pencil_lines(drawable, lines)
def test_2(timg, tdrawable):
image = create_image(640, 400)
name_Layer = "L-1"
# image instance
imageObj = ImageObject( image )
im = imageObj.get_Image()
# #debug
# t = time()
# str_Time = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
# num_Line = linenum(0)
#
# lenOf_Stacks = len(inspect.stack())
#
# num_Line_EndStack = linenum(lenOf_Stacks - 1)
#
# # ref https://stackoverflow.com/questions/6810999/how-to-determine-file-function-and-line-number 'answered Jul 25 '11 at 1:31'
# callerframerecord = inspect.stack()[1] # 0 represents this line
# # 1 represents line at caller
#
# lenOf_Stacks = len(inspect.stack())
#
# frame = inspect.currentframe(1)
# num_Line_Current = frame.f_lineno
# msg = "[%s / %d] imageObj ==> instance made (lenOf_Stacks = %d)" % \
# msg = "[%s / %d] imageObj ==> instance made (num_Line_EndStack = %d / num_Line_Current = %d)" \
msg = "[%s / %s:%d]\nimageObj ==> instance made" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
msg = "[%s / %s:%d]\ncalling ---> im.add_Layer()" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
imageObj.add_Layer(name_Layer)
# im.add_Layer(name_Layer)
msg = "[%s / %s:%d]\nimageObj ==> Layer added" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
#
# #debug
# pdb.gimp_message("layer => added")
#
# layer = im.get_Layer()
#
# draw_rect(layer, 190, 210, 490, 310)
# # draw_rect(layer, 390, 210, 490, 310)
display_image(im)
# display_image(image)
def test_1(timg, tdrawable):
image = create_image(640, 400)
name_Layer = "L-1"
layer = add_layer(image, name_Layer)
# layer = add_layer(image, "背景")
draw_rect(layer, 390, 210, 490, 310)
display_image(image)
def plugin_main(timg, tdrawable):
test_2(timg, tdrawable)
########################################################################
register(
'plugin_main', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'new image', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
], # プロシジャの引数
[], # 戻り値の定義
plugin_main, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
main() # プラグインを駆動させるための関数
<file_sep>/JVEMV6/46_art/11_guitar/jvemv6_37_12_1_guitar-memo.bat
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
C:\WORKS_2\Programs\Python\Python_3.5.1\python.exe jvemv6_37_12_1_guitar-memo.py
pause
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgShader.js
/*---------- myGlsl.js -------------------------------
プログラムオブジェクト(シェーダプログラム)を生成する.
gl : GLコンテキスト
vs_source : 頂点シェーダのプログラム(文字列)
fs_source : フラグメントシェーダのプログラム(文字列)
プログラムオブジェクトprogramを生成し,成功したらtrueを返す
------------------------------------------------------*/
function initGlsl(gl, vs_source, fs_source)
{
var vertexShader = gl.createShader(gl.VERTEX_SHADER);
var fragmentShader = gl.createShader(gl.FRAGMENT_SHADER);
gl.shaderSource(vertexShader, vs_source);
gl.shaderSource(fragmentShader, fs_source);
// シェーダをコンパイルする
gl.compileShader(vertexShader);
gl.compileShader(fragmentShader);
//プログラムオブジェクトの作成
var program = gl.createProgram();
if (!program)
{
alert("プログラムオブジェクトの作成に失敗");
return false;
}
// シェーダオブジェクトを設定する
gl.attachShader(program, vertexShader);
gl.attachShader(program, fragmentShader);
// プログラムオブジェクトをリンクする
gl.linkProgram(program);
// リンク結果をチェックする
var linked = gl.getProgramParameter(program, gl.LINK_STATUS);
if (!linked)
{
var error = gl.getProgramInfoLog(program);
alert('プログラムオブジェクトのリンクに失敗 ' + error);
gl.deleteProgram(program);
gl.deleteShader(fragmentShader);
gl.deleteShader(vertexShader);
return false;
}
gl.useProgram(program);
gl.program = program;
return true;
}
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgSpring.js
//swgSpring.js
var MAX_SPRING = 360;
var numSpring = 1 ;
//enum SKind { S_SPRING, S_CYLINDER};
function Spring()
{
//プロパティ
this.kind = "SPRING";
this.vPos = new Vector3();//位置(m)
this.vEuler = new Vector3();//回転角度(オイラー角で指定,更新後)
this.vSize = new Vector3(1.0, 1.0, 1.0);//スケーリング
this.diffuse = [0.8, 0.8, 0.6, 1.0];
this.ambient = [0.4, 0.4, 0.3, 1.0];
this.specular = [0.8, 0.8, 0.8, 1.0];
this.shininess = 100.0;
this.constK = 10;
this.num1 = 10; //分割数(Nm:主円周分割点数)
this.num2 = 8; //分割数(Ns:断面円周分割点数)
this.num3 = 10; //バネの巻き数
this.radius = 0.03; //バネの主円周軸半径(実寸)
this.length0 = 1;//バネの自然長
this.length; //バネの長さ(自然長+変位量)
this.angle0; //springの角度(若い番号のspringとのなす角, rad)
//Vector vDir, vDir0;//vAngle0;
//this.disp0; //初期変位量
this.ratio = 0.5; //Torus,Spring(中心軸半径に対する断面半径比率
//this.row1; this.col1, row2, col2, stk1, stk2;
//this.n0, np1, np2, np = [];
//hinge(2次元)
this.num; //hingeに隣接する質点のペア個数
this.row = [];//hingeに隣接する質点の行番号
this.col = [];//h = ingeに隣接する質点の列番号
this stk = [];//hingeに隣接する質点の積番号
}
//-----------------------------------------------------------------
void CSpring::draw(bool flagShadow)
{
static float shadowDiffuse[] = {0.2f,0.2f,0.2f,0.3f};//影の拡散光
static float shadowSpecular[] = {0.0f,0.0f,0.0f,1.0f};//影の鏡面光
//環境光と反射光はR,G,B同じ係数で指定されている
static float diffuse[] = {0.9, 0.9, 0.5, 1.0};
static float ambient[] = {0.5, 0.5, 0.2, 1.0};
static float specular[] = {1.0, 1.0, 1.0, 1.0};
if(flagShadow == false){
glMaterialfv(GL_FRONT,GL_DIFFUSE, diffuse);
glMaterialfv(GL_FRONT,GL_AMBIENT, ambient);
glMaterialfv(GL_FRONT,GL_SPECULAR, specular);
glMaterialf(GL_FRONT,GL_SHININESS, 100.0);
}
else{//影
glMaterialfv(GL_FRONT,GL_AMBIENT_AND_DIFFUSE,shadowDiffuse);
glMaterialfv(GL_FRONT,GL_SPECULAR,shadowSpecular);
}
glPushMatrix();
if(kind == S_SPRING)
{
//現在位置
glTranslated(vPos.x, vPos.y, vPos.z);//平行移動
//回転
glRotated(vEuler.z, 0.0, 0.0, 1.0);//z軸回転
glRotated(vEuler.y, 0.0, 1.0, 0.0);//y軸回転
glRotated(vEuler.x, 1.0, 0.0, 0.0);//x軸回転
drawSpring(num1, num2, num3, radius, ratio, length) ;
}
else if(kind == S_CYLINDER)
{
//円柱
vSize.x = radius ;//円柱のサイズ
vSize.y = radius ;
vSize.z = length;
//現在位置
glTranslated(vPos.x, vPos.y, vPos.z);//平行移動
//回転
glRotated(vEuler.z, 0.0, 0.0, 1.0);//z軸回転
glRotated(vEuler.y, 0.0, 1.0, 0.0);//y軸回転
glRotated(vEuler.x, 1.0, 0.0, 0.0);//x軸回転
//スケーリング
glScaled(vSize.x, vSize.y, vSize.z);
glCallList(cylinder);
}
glPopMatrix();
}
<file_sep>/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/2_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\2_\2_1.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\1_1.py
at : 2018/05/13 12:06:10
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\2_\
python 2_1.py
'''
# from __future__ import print_function
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\2_image-prog') # libs_mm
# sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_image_prog import libs
# from libs_mm import libs
# from libs_VX7GLZ import libs_VX7GLZ
# from libs_VX7GLZ import wablibs
'''###################
import : specifics
###################'''
# import midi, pretty_midi
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, cv2, \
math as math, random as rnd, numpy as np, \
matplotlib.pyplot as plt, matplotlib.style as ms
###############################################
from matplotlib import pylab as plt
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def get_mean(lo_Data):
sumOf_Data = 0
for d in lo_Data:
sumOf_Data += d
#/for d in lo_Data:
# return
return sumOf_Data
#/ def get_mean(lo_Data):
def test_6():
print("[%s:%d] test_6()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
load : image
###################'''
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\images"
dpath_Img = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images"
fname_Img = "IMG_3171.JPG"
fpath_Img = "%s/%s" % (dpath_Img, fname_Img)
img = cv2.imread(fpath_Img)
# img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
'''###################
get : data
###################'''
# data
height, width, channels = img2.shape
off_set = 280
tlabel = libs.get_TimeLabel_Now()
plt_ = plt
# save image
'''###################
corner : LB
###################'''
titles = ["clp_LB", "clp_RB", "clp_LU", "clp_RU"]
clips = [
img2[(height - off_set) : height, 0 : off_set], # clp_LB
img2[(height - off_set) : height, width - off_set : width], # clp_RB
img2[0 : off_set, 0 : off_set], # clp_LU
img2[0 : off_set, width - off_set : width], # clp_RU
]
'''###################
max, min
###################'''
# idxOf_Clips = 1
idxOf_Clips = 0
clip_0 = clips[idxOf_Clips]
# clip_0 = clips[0]
max_R = -1; max_G = -1; max_B = -1
# counter
cntOf_Row = 0
cntOf_Cell = 0
# values
valsOf_R = [0] * 255
valsOf_G = [0] * 255
valsOf_B = [0] * 255
colNames = [str(x) for x in range(255)]
# colNames = [x for x in range(255)]
str_ColNames = "\t".join(colNames)
# str_ColNames = colNames.join("\t")
for row in clip_0:
for cell in row:
# get value
R = cell[0]; G = cell[1]; B = cell[2]
# addition
valsOf_R[R] += 1
valsOf_G[G] += 1
valsOf_B[B] += 1
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
# count
cntOf_Cell += 1
# reset count of cells
cntOf_Cell = 0
# count
cntOf_Row += 1
#/for row in clip_0:
'''###################
log
###################'''
dpath_Log = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6" \
+ "/46_art/2_image-prog/2_projects/1_sort-out/2_" \
+ "/data"
# validate dir
if not os.path.exists(dpath): os.makedirs(dpath)
fname_Log = "3_.%s.log" % libs.get_TimeLabel_Now()
fpath_Log = "%s/%s" % (dpath_Log, fname_Log)
f = open(fpath_Log, "a")
f.write("===================================")
f.write("\n")
msg = "[%s:%s:%d] result =>" % \
(libs.get_TimeLabel_Now(), os.path.basename(libs.thisfile()), libs.linenum() \
)
f.write(msg)
f.write("\n")
msg = "file : %s" % \
(fpath_Img)
f.write(msg)
f.write("\n")
msg = "rows, cells : %d x %d" % (cntOf_Row, cntOf_Cell)
f.write(msg)
f.write("\n")
# index
msg = "index of clips : %d (%s)" % (idxOf_Clips, titles[idxOf_Clips])
f.write(msg)
f.write("\n")
msg = "min, max : "
f.write(msg)
# f.write("\n")
msg = "max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B)
f.write(msg)
f.write("\n")
# f.write("\n")
# print("[%s:%d] min, max -----------" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# # print("max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B))
'''###################
vals
###################'''
msg = "color\t%s" % str_ColNames
f.write(msg)
f.write("\n")
lineOf_R = ""
lineOf_G = ""
lineOf_B = ""
for i in range(255):
lineOf_R += str(valsOf_R[i]) + "\t"
lineOf_G += str(valsOf_G[i]) + "\t"
lineOf_B += str(valsOf_B[i]) + "\t"
#/for i in range(255):
msg = "Rs\t%s" % lineOf_R
f.write(msg)
f.write("\n")
msg = "Gs\t%s" % lineOf_G
f.write(msg)
f.write("\n")
msg = "Bs\t%s" % lineOf_B
f.write(msg)
f.write("\n")
'''###################
stats
###################'''
sumOf_Rs = 0
# sum
for d in valsOf_R: sumOf_Rs += d
# mean
mean_R = sumOf_Rs * 1.0 / len(valsOf_R)
# summation
summation = 0
for d in valsOf_R:
summation += np.power(d - mean_R, 4)
#/for d in valsOf_R:
# summation divided by length
summation = summation / len(valsOf_R)
# standard dev
lo_tmp = [np.power(x, 2) for x in valsOf_R]
# sum of squares
sumOf_Squares_R = sum(lo_tmp)
# dev
deviation = sumOf_Squares_R * 1.0 / len(valsOf_R) - np.power(mean_R, 2)
# std dev
std_dev = np.sqrt(deviation)
# skew
#ref http://hs-www.hyogo-dai.ac.jp/~kawano/HStat/?plugin=cssj&page=2010%2F3rd%2FSkewness_Kurtosis
skew = summation * 1.0 / np.power(std_dev, 4)
# write to file
msg = "sumOf_Rs = %d, mean_R = %.03f summation = %.03f std_dev = %.03f skew = %.03f" % \
(sumOf_Rs, mean_R, summation, std_dev, skew)
# msg = "sumOf_Rs = %d, mean_R = %.03f" % (sumOf_Rs, mean_R)
f.write(msg)
f.write("\n")
# index of max
idxOf_Max_R = -1
maxOf_R = -1
idx = 0
for d in valsOf_R:
if d > maxOf_R : #if d > maxOf_R
# update : index of max
idxOf_Max_R = idx
# update : max of Rs
maxOf_R = d
#/if d > maxOf_R
# increment
idx += 1
#/for d in valsOf_R:
# write
msg = "idxOf_Max_R = %d" % \
(idxOf_Max_R)
# msg = "sumOf_Rs = %d, mean_R = %.03f" % (sumOf_Rs, mean_R)
f.write(msg)
f.write("\n")
#/for d in valsOf_R:
# sumOf_Rs = get_mean(valsOf_R)
#
# mean_R = sumOf_Rs * 1.0 / len(valsOf_R)
'''###################
dividing line
###################'''
f.write("\n")
'''###################
close
###################'''
f.close()
#/ def test_5():
def test_5():
print("[%s:%d] test_5()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
load : image
###################'''
dpath_Img = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images"
fname_Img = "IMG_3171.JPG"
fpath_Img = "%s/%s" % (dpath_Img, fname_Img)
img = cv2.imread(fpath_Img)
# img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
'''###################
get : data
###################'''
# data
height, width, channels = img2.shape
off_set = 280
tlabel = libs.get_TimeLabel_Now()
plt_ = plt
# save image
'''###################
corner : LB
###################'''
titles = ["clp_LB", "clp_RB", "clp_LU", "clp_RU"]
clips = [
img2[(height - off_set) : height, 0 : off_set], # clp_LB
img2[(height - off_set) : height, width - off_set : width], # clp_RB
img2[0 : off_set, 0 : off_set], # clp_LU
img2[0 : off_set, width - off_set : width], # clp_RU
]
'''###################
max, min
###################'''
idxOf_Clips = 1
# idxOf_Clips = 0
clip_0 = clips[idxOf_Clips]
# clip_0 = clips[0]
max_R = -1; max_G = -1; max_B = -1
# counter
cntOf_Row = 0
cntOf_Cell = 0
# values
valsOf_R = [0] * 255
valsOf_G = [0] * 255
valsOf_B = [0] * 255
colNames = [str(x) for x in range(255)]
# colNames = [x for x in range(255)]
str_ColNames = "\t".join(colNames)
# str_ColNames = colNames.join("\t")
for row in clip_0:
for cell in row:
# get value
R = cell[0]; G = cell[1]; B = cell[2]
# addition
valsOf_R[R] += 1
valsOf_G[G] += 1
valsOf_B[B] += 1
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
# count
cntOf_Cell += 1
# reset count of cells
cntOf_Cell = 0
# count
cntOf_Row += 1
#/for row in clip_0:
'''###################
log
###################'''
dpath_Log = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_"
fname_Log = "2_.log"
fpath_Log = "%s/%s" % (dpath_Log, fname_Log)
f = open(fpath_Log, "a")
f.write("===================================")
f.write("\n")
msg = "[%s:%s:%d] result =>" % \
(libs.get_TimeLabel_Now(), os.path.basename(libs.thisfile()), libs.linenum() \
)
f.write(msg)
f.write("\n")
msg = "file : %s" % \
(fpath_Img)
f.write(msg)
f.write("\n")
msg = "rows, cells : %d x %d" % (cntOf_Row, cntOf_Cell)
f.write(msg)
f.write("\n")
# index
msg = "index of clips : %d (%s)" % (idxOf_Clips, titles[idxOf_Clips])
f.write(msg)
f.write("\n")
msg = "min, max : "
f.write(msg)
# f.write("\n")
msg = "max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B)
f.write(msg)
f.write("\n")
# f.write("\n")
# print("[%s:%d] min, max -----------" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# # print("max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B))
'''###################
vals
###################'''
msg = "color\t%s" % str_ColNames
f.write(msg)
f.write("\n")
lineOf_R = ""
lineOf_G = ""
lineOf_B = ""
for i in range(255):
lineOf_R += str(valsOf_R[i]) + "\t"
lineOf_G += str(valsOf_G[i]) + "\t"
lineOf_B += str(valsOf_B[i]) + "\t"
#/for i in range(255):
msg = "Rs\t%s" % lineOf_R
f.write(msg)
f.write("\n")
msg = "Gs\t%s" % lineOf_G
f.write(msg)
f.write("\n")
msg = "Bs\t%s" % lineOf_B
f.write(msg)
f.write("\n")
f.write("\n")
'''###################
close
###################'''
f.close()
#/ def test_5():
def test_4():
print("[%s:%d] test_4()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
load : image
###################'''
dpath_Img = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images"
fname_Img = "IMG_3171.JPG"
fpath_Img = "%s/%s" % (dpath_Img, fname_Img)
img = cv2.imread(fpath_Img)
# img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
'''###################
get : data
###################'''
# data
height, width, channels = img2.shape
off_set = 280
tlabel = libs.get_TimeLabel_Now()
plt_ = plt
# save image
'''###################
corner : LB
###################'''
titles = ["clp_LB", "clp_RB", "clp_LU", "clp_RU"]
clips = [
img2[(height - off_set) : height, 0 : off_set], # clp_LB
img2[(height - off_set) : height, width - off_set : width], # clp_RB
img2[0 : off_set, 0 : off_set], # clp_LU
img2[0 : off_set, width - off_set : width], # clp_RU
]
'''###################
max, min
###################'''
clip_0 = clips[0]
max_R = -1; max_G = -1; max_B = -1
for item in clip_0[0]:
R = item[0]; G = item[1]; B = item[2]
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
#/for item in clip_0[0]:
'''###################
log
###################'''
dpath_Log = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_"
fname_Log = "2_.log"
fpath_Log = "%s/%s" % (dpath_Log, fname_Log)
f = open(fpath_Log, "a")
f.write("===================================")
f.write("\n")
msg = "[%s:%s:%d] file : %s" % \
(libs.get_TimeLabel_Now(), os.path.basename(libs.thisfile()), libs.linenum() \
, fpath_Img)
f.write(msg)
f.write("\n")
msg = "[%s:%s:%d] min, max -----------" % \
(libs.get_TimeLabel_Now(), os.path.basename(libs.thisfile()), libs.linenum())
f.write(msg)
f.write("\n")
msg = "max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B)
f.write(msg)
f.write("\n")
f.write("\n")
# print("[%s:%d] min, max -----------" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# # print("max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B))
f.close()
#/ def test_4():
def test_3():
print("[%s:%d] test_3()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
load : image
###################'''
img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# plt.imshow(img2)
#
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
#
# fname = "image.%s.png" % (libs.get_TimeLabel_Now())
#
# fpath = "%s/%s" % (dpath, fname)
#
# plt.savefig(fpath)
#ref display image https://github.com/PrinzEugen7/ImageProcessing/blob/master/Image/Python/Matplotlib/draw_opencv_img.py
# plt.show()
'''###################
get : data
###################'''
# data
height, width, channels = img2.shape
off_set = 280
tlabel = libs.get_TimeLabel_Now()
plt_ = plt
# save image
'''###################
corner : LB
###################'''
titles = ["clp_LB", "clp_RB", "clp_LU", "clp_RU"]
clips = [
img2[(height - off_set) : height, 0 : off_set], # clp_LB
img2[(height - off_set) : height, width - off_set : width], # clp_RB
img2[0 : off_set, 0 : off_set], # clp_LU
img2[0 : off_set, width - off_set : width], # clp_RU
]
# iterate
cnt = 0 # counter
for item in titles:
# file name
fname = "image.%s.%s.png" % (tlabel, item)
# save fig
test_2__SaveFig(item, dpath, fname, clips[cnt], plt_)
# clear plot
plt_.clf()
# count
cnt += 1
#/for item in titles:
# title = "clp_LB"
# fname = "image.%s.%s.png" % (tlabel, title)
# clp_LB = img2[(height - off_set) : height, 0 : off_set]
# h_Clp_LB, w_Clp_LB, ch_Clp_Lb = clp_LB.shape
# test_2__SaveFig(title, dpath, fname, clp_LB, plt_)
'''###################
corner : RB
###################'''
# title = "clp_RB"
# fname = "image.%s.%s.png" % (tlabel, title)
# clp_RB = img2[(height - off_set) : height, width - off_set : width]
# plt_.clf()
# plt_ = plt
# test_2__SaveFig(title, dpath, fname, clp_RB, plt_)
'''###################
corner : LU
###################'''
# title = "clp_LU"
# fname = "image.%s.%s.png" % (tlabel, title)
# clp_LU = img2[0 : off_set, 0 : off_set]
# plt_.clf()
# # plt_ = plt
#
# test_2__SaveFig(title, dpath, fname, clp_LU, plt_)
'''###################
corner : RU
###################'''
# title = "clp_RU"
# fname = "image.%s.%s.png" % (tlabel, title)
# clp_RU = img2[0 : off_set, width - off_set : width]
# plt_.clf()
# # plt_ = plt
#
# test_2__SaveFig(title, dpath, fname, clp_RU, plt_)
# title = "clp_LB"
#
# fname = "image.%s.%s.png" % (title, libs.get_TimeLabel_Now())
#
# fpath = "%s/%s" % (dpath, fname)
#
# xpixels = clp_LB.shape[1]
# ypixels = clp_LB.shape[0]
#
# dpi = 72
# scalefactor = 1
#
# xinch = xpixels * scalefactor / dpi
# yinch = ypixels * scalefactor / dpi
#
# fig = plt.figure(figsize=(xinch,yinch))
#
# plt.imshow(clp_LB)
#
# # plt.savefig(fpath)
# plt.savefig(fpath, dpi=dpi)
#/ def test_2():
def test_2__SaveFig(title, dpath, fname, clp_, plt_):
# save image
# title = "clp_LB"
# fname = "image.%s.%s.png" % (title, libs.get_TimeLabel_Now())
fpath = "%s/%s" % (dpath, fname)
xpixels = clp_.shape[1]
ypixels = clp_.shape[0]
dpi = 72
scalefactor = 1
xinch = xpixels * scalefactor / dpi
yinch = ypixels * scalefactor / dpi
fig = plt_.figure(figsize=(xinch,yinch))
plt_.imshow(clp_)
# plt.savefig(fpath)
plt_.savefig(fpath, dpi=dpi)
#/ def test_2():
def test_2():
print("[%s:%d] test_2()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
load : image
###################'''
img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# plt.imshow(img2)
#
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
#
# fname = "image.%s.png" % (libs.get_TimeLabel_Now())
#
# fpath = "%s/%s" % (dpath, fname)
#
# plt.savefig(fpath)
#ref display image https://github.com/PrinzEugen7/ImageProcessing/blob/master/Image/Python/Matplotlib/draw_opencv_img.py
# plt.show()
'''###################
clip
###################'''
# data
height, width, channels = img2.shape
off_set = 280
tlabel = libs.get_TimeLabel_Now()
plt_ = plt
# save image
'''###################
corner : LB
###################'''
title = "clp_LB"
fname = "image.%s.%s.png" % (tlabel, title)
clp_LB = img2[(height - off_set) : height, 0 : off_set]
# h_Clp_LB, w_Clp_LB, ch_Clp_Lb = clp_LB.shape
test_2__SaveFig(title, dpath, fname, clp_LB, plt_)
'''###################
corner : RB
###################'''
title = "clp_RB"
fname = "image.%s.%s.png" % (tlabel, title)
clp_RB = img2[(height - off_set) : height, width - off_set : width]
plt_.clf()
# plt_ = plt
test_2__SaveFig(title, dpath, fname, clp_RB, plt_)
'''###################
corner : LU
###################'''
title = "clp_LU"
fname = "image.%s.%s.png" % (tlabel, title)
clp_LU = img2[0 : off_set, 0 : off_set]
plt_.clf()
# plt_ = plt
test_2__SaveFig(title, dpath, fname, clp_LU, plt_)
'''###################
corner : RU
###################'''
title = "clp_RU"
fname = "image.%s.%s.png" % (tlabel, title)
clp_RU = img2[0 : off_set, width - off_set : width]
plt_.clf()
# plt_ = plt
test_2__SaveFig(title, dpath, fname, clp_RU, plt_)
# title = "clp_LB"
#
# fname = "image.%s.%s.png" % (title, libs.get_TimeLabel_Now())
#
# fpath = "%s/%s" % (dpath, fname)
#
# xpixels = clp_LB.shape[1]
# ypixels = clp_LB.shape[0]
#
#ref https://stackoverflow.com/questions/13623301/convert-contour-matplotlib-or-opencv-to-image-of-the-same-size-as-the-original#13623960
# dpi = 72
# scalefactor = 1
#
# xinch = xpixels * scalefactor / dpi
# yinch = ypixels * scalefactor / dpi
#
# fig = plt.figure(figsize=(xinch,yinch))
#
# plt.imshow(clp_LB)
#
# # plt.savefig(fpath)
# plt.savefig(fpath, dpi=dpi)
#/ def test_2():
def test_1():
print("[%s:%d] test_1()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
load : image
###################'''
img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# plt.imshow(img2)
#
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
#
# fname = "image.%s.png" % (libs.get_TimeLabel_Now())
#
# fpath = "%s/%s" % (dpath, fname)
#
# plt.savefig(fpath)
#ref display image https://github.com/PrinzEugen7/ImageProcessing/blob/master/Image/Python/Matplotlib/draw_opencv_img.py
# plt.show()
'''###################
clip
###################'''
height, width, channels = img2.shape
#debug
print()
print("[%s:%d] height = %d, width = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, height, width
), file=sys.stderr)
off_set = 280
clp_LB = img2[(height - off_set) : height, 0 : off_set]
h_Clp_LB, w_Clp_LB, ch_Clp_Lb = clp_LB.shape
#debug
print()
print("[%s:%d] h_Clp_LB = %d, w_Clp_LB = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, h_Clp_LB, w_Clp_LB
), file=sys.stderr)
# plt.imshow(clp_LB)
# save image
title = "clp_LB"
fname = "image.%s.%s.png" % (title, libs.get_TimeLabel_Now())
fpath = "%s/%s" % (dpath, fname)
xpixels = clp_LB.shape[1]
ypixels = clp_LB.shape[0]
dpi = 72
scalefactor = 1
xinch = xpixels * scalefactor / dpi
yinch = ypixels * scalefactor / dpi
fig = plt.figure(figsize=(xinch,yinch))
plt.imshow(clp_LB)
# plt.savefig(fpath)
plt.savefig(fpath, dpi=dpi)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_6()
# test_5()
# test_4()
# test_3()
# test_2()
# test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/libs_admin/cons_ip.py
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\libs_admin\cons_ip.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\libs_admin\lib_ip.py
at : 2018/06/08 10:10:11
'''
'''###################
import : built-in modules
###################'''
import sys, os
#!C:\WORKS_2\Programs\Python\Python_3.5.1\python.exe
from enum import Enum
from time import gmtime, strftime, localtime, time
'''###################
import : orig modules
###################'''
# sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects')
# from libs_admin import libs
'''###################
@param string_type
serial "20160604_193404"
basic "2016/06/04 19:34:04"
###################'''
def get_TimeLabel_Now(string_type="serial", mili=False):
# def get_TimeLabel_Now(string_type="serial"):
# t = time.time()
t = time()
# str = strftime("%Y%m%d_%H%M%S", t)
# str = strftime("%Y%m%d_%H%M%S", localtime())
'''###################
build string
###################'''
if string_type == "serial" : #if string_type == "serial"
str = strftime("%Y%m%d_%H%M%S", localtime(t))
elif string_type == "basic" : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
else : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
#/if string_type == "serial"
# str = strftime("%Y%m%d_%H%M%S", localtime(t))
#ref https://stackoverflow.com/questions/5998245/get-current-time-in-milliseconds-in-python "answered May 13 '11 at 22:21"
if mili == True :
if string_type == "serial" : #if string_type == "serial"
str = "%s_%03d" % (str, int(t % 1 * 1000))
else : #if string_type == "serial"
str = "%s.%03d" % (str, int(t % 1 * 1000))
#ref decimal value https://stackoverflow.com/questions/30090072/get-decimal-part-of-a-float-number-in-python "answered May 7 '15 at 1:56"
# str = "%s_%03d" % (str, int(t % 1 * 1000))
return str
#ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
# return strftime("%Y%m%d_%H%M%S", localtime())
# return strftime("%Y%m%d_%H%M%S", gmtime())
#]]get_TimeLabel_Now():
class DfltVals(Enum):
#get_4Corners__Corner_Width = 200
get_4Corners__Corner_Width = 100
# get_4Corners__Corner_Width = 280
#get_4Corners__Corner_Padding = 20
get_4Corners__Corner_Padding = 10
'''###################
dos attack
###################'''
numOf_DosAttack = 0
class ColorThresholds(Enum):
'''######################################
Red
######################################'''
'''###################
thresholds : max values
###################'''
# R
# isRed_Max_Val_R__Lower = 2.2 * 1000
# isRed_Max_Val_R__Lower = 2.0 * 1000
# isRed_Max_Val_R__Lower = 1.85 * 1000
#isRed_Max_Val_R__Lower = 1.70 * 1000
isRed_Max_Val_R__Lower = 1.60 * 1000
isRed_Max_Val_R__Upper = 6.0 * 1000
# isRed_Max_Val_R__Upper = 4.7 * 1000
# isRed_Max_Val_R__Upper = 3.9 * 1000
# G
# isRed_Max_Val_G__Lower = 2.3 * 1000
# isRed_Max_Val_G__Lower = 2.0 * 1000
# isRed_Max_Val_G__Lower = 1.85 * 1000
isRed_Max_Val_G__Lower = 1.70 * 1000
isRed_Max_Val_G__Upper = 5.5 * 1000 #=> 20180709_060511
# isRed_Max_Val_G__Upper = 4.7 * 1000
# isRed_Max_Val_G__Upper = 3.9 * 1000
# B
# isRed_Max_Val_B__Lower = 1.6 * 1000
# isRed_Max_Val_B__Lower = 1.35 * 1000
isRed_Max_Val_B__Lower = 1.00 * 1000
# isRed_Max_Val_B__Lower = 1.8 * 1000
# isRed_Max_Val_B__Upper = 2.5 * 1000
isRed_Max_Val_B__Upper = 4.25 * 1000
# isRed_Max_Val_B__Upper = 3.5 * 1000
# isRed_Max_Val_B__Upper = 3.1 * 1000
'''###################
thresholds : index of max values
###################'''
# R
# isRed_IdxOf_Max_R__Upper = 50
# isRed_IdxOf_Max_R__Upper = 70
isRed_IdxOf_Max_R__Upper = 91
isRed_IdxOf_Max_R__Lower = 0
# G
#isRed_IdxOf_Max_G__Upper = 60
#isRed_IdxOf_Max_G__Upper = 80
isRed_IdxOf_Max_G__Upper = 105
isRed_IdxOf_Max_G__Lower = 0
# B
# isRed_IdxOf_Max_B__Upper = 200
# isRed_IdxOf_Max_B__Upper = 210
# isRed_IdxOf_Max_B__Upper = 230
isRed_IdxOf_Max_B__Upper = 250
isRed_IdxOf_Max_B__Lower = 150
'''######################################
Green
######################################'''
'''###################
thresholds : max values
###################'''
# R
# isGreen_Max_Val_R__Lower = 3.0 * 1000
# isGreen_Max_Val_R__Lower = 2.6 * 1000
isGreen_Max_Val_R__Lower = 1.8 * 1000
isGreen_Max_Val_R__Upper = 5.4 * 1000
#isGreen_Max_Val_R__Upper = 5.3 * 1000
# isGreen_Max_Val_R__Upper = 4.1 * 1000
# G
isGreen_Max_Val_G__Lower = 1.4 * 1000
isGreen_Max_Val_G__Upper = 4.95 * 1000
# isGreen_Max_Val_G__Upper = 4.5 * 1000
# isGreen_Max_Val_G__Upper = 3.7 * 1000
# isGreen_Max_Val_G__Upper = 3.2 * 1000
# isGreen_Max_Val_G__Upper = 2.8 * 1000
# isGreen_Max_Val_G__Upper = 2.6 * 1000
# B
#isGreen_Max_Val_B__Lower = 2.0 * 1000
isGreen_Max_Val_B__Lower = 1.85 * 1000
isGreen_Max_Val_B__Upper = 4.85 * 1000
#isGreen_Max_Val_B__Upper = 4.3 * 1000
# isGreen_Max_Val_B__Upper = 3.2 * 1000
'''###################
thresholds : index of max values
###################'''
# R
# isGreen_IdxOf_Max_R__Upper = 50
# isGreen_IdxOf_Max_R__Upper = 65
#isGreen_IdxOf_Max_R__Upper = 120
isGreen_IdxOf_Max_R__Upper = 130
isGreen_IdxOf_Max_R__Lower = 0
# G
# isGreen_IdxOf_Max_G__Upper = 170
# isGreen_IdxOf_Max_G__Upper = 210
# isGreen_IdxOf_Max_G__Upper = 240
isGreen_IdxOf_Max_G__Upper = 255
isGreen_IdxOf_Max_G__Lower = 80
# B
# isGreen_IdxOf_Max_B__Upper = 200
# isGreen_IdxOf_Max_B__Upper = 120
# isGreen_IdxOf_Max_B__Upper = 145
#isGreen_IdxOf_Max_B__Upper = 160
isGreen_IdxOf_Max_B__Upper = 175
isGreen_IdxOf_Max_B__Lower = 50
'''######################################
Yellow
######################################'''
'''###################
thresholds : max values
###################'''
# max values
# R
# ts_Max_Val_R__Lower = 4000
# isYellow_Max_Val_R__Lower = 2500
# isYellow_Max_Val_R__Lower = 2.2 * 1000
isYellow_Max_Val_R__Lower = 1.6 * 1000
isYellow_Max_Val_R__Upper = 6.4 * 1000
# isYellow_Max_Val_R__Upper = 5.8 * 1000
# isYellow_Max_Val_R__Upper = 5000
# G
# isYellow_Max_Val_G__Lower = 3000
# isYellow_Max_Val_G__Lower = 1.6 * 1000
isYellow_Max_Val_G__Lower = 1.1 * 1000
isYellow_Max_Val_G__Upper = 6.3 * 1000
# isYellow_Max_Val_G__Upper = 4000
# isYellow_Max_Val_G__Upper = 5.4 * 1000
# isYellow_Max_Val_G__Upper = 4.8 * 1000
# B
# isYellow_Max_Val_B__Lower = 3500
# isYellow_Max_Val_B__Lower = 2000
# isYellow_Max_Val_B__Lower = 1.6 * 1000
# isYellow_Max_Val_B__Lower = 1.4 * 1000
isYellow_Max_Val_B__Lower = 1.2 * 1000
isYellow_Max_Val_B__Upper = 10.8 * 1000 #=> 20180709_064100
# isYellow_Max_Val_B__Upper = 6.2 * 1000
# isYellow_Max_Val_B__Upper = 5.8 * 1000
# isYellow_Max_Val_B__Upper = 4500
# isYellow_Max_Val_G = 2000
# isYellow_Max_Val_B = 2000
'''###################
thresholds : index of max values
###################'''
# index of max values
# R
isYellow_IdxOf_Max_R__Upper = -1 # -1 ==> not used
# isYellow_IdxOf_Max_R__Lower = 80
isYellow_IdxOf_Max_R__Lower = 115
# isYellow_IdxOf_Max_R__Lower = 95
# G
isYellow_IdxOf_Max_G__Upper = 250
isYellow_IdxOf_Max_G__Lower = 115
#isYellow_IdxOf_Max_G__Lower = 130
# isYellow_IdxOf_Max_G__Upper = 220
# isYellow_IdxOf_Max_G__Lower = 145
# isYellow_IdxOf_Max_G__Lower = 165
# isYellow_IdxOf_Max_G__Lower = 180
# B
# isYellow_IdxOf_Max_B__Upper = 200
# isYellow_IdxOf_Max_B__Upper = 180
# isYellow_IdxOf_Max_B__Upper = 165
# isYellow_IdxOf_Max_B__Upper = 240
isYellow_IdxOf_Max_B__Upper = 255
isYellow_IdxOf_Max_B__Lower = 140 # -1 ==> not used
'''######################################
White
######################################'''
'''###################
thresholds : index of max values
###################'''
isWhite_IdxOf_Max = 245
# isWhite_IdxOf_Max = 255
'''###################
thresholds : max values
###################'''
# R
isWhite_Max_Val__Lower = 14 * 1000
# isWhite_Max_Val__Lower = 26 * 1000
# isYellow_Max_Val_R__Upper = 5000
'''######################################
Black
######################################'''
'''###################
thresholds : index of max values
###################'''
isBlack_IdxOf_Max = 0
'''###################
thresholds : max values
###################'''
# R
# isBlack_Max_Val__Lower = 21 * 1000
#isBlack_Max_Val__Lower = 16 * 1000
isBlack_Max_Val__Lower = 15 * 1000
# isBlack_Max_Val__Lower = 26 * 1000
'''######################################
thresholds : HSV
######################################'''
'''###################
yellow
###################'''
#isYellow_HSV_Variance__Upper = 0.3
isYellow_HSV_Variance__Upper = 0.65
isYellow_HSV_Variance__Lower = 0.0
#isYellow_HSV_Variance__Lower = 0.002
# isYellow_HSV_Variance__Lower = 0.01
# isYellow_HSV_Average__Upper = 90.0
#isYellow_HSV_Average__Upper = 93.0
#isYellow_HSV_Average__Upper = 97.0
#isYellow_HSV_Average__Upper = 98.0
isYellow_HSV_Average__Upper = 99.2
isYellow_HSV_Average__Lower = 85.500 # 20181217_112606
#isYellow_HSV_Average__Lower = 88.0
'''###################
red
###################'''
# isRed_HSV_Variance__Upper = 0.3
#isRed_HSV_Variance__Upper = 0.40
isRed_HSV_Variance__Upper = 0.43
isRed_HSV_Variance__Lower = 0.0
#isRed_HSV_Variance__Lower = 0.002
# isRed_HSV_Variance__Lower = 0.01
#isRed_HSV_Average__Upper = 124.0 # 20190215_070409
isRed_HSV_Average__Upper = 124.6
isRed_HSV_Average__Lower = 115.0
#isRed_HSV_Average__Lower = 116.0
# isRed_HSV_Average__Lower = 120.0
'''###################
green
###################'''
# isGreen_HSV_Variance__Upper = 0.3
#isGreen_HSV_Variance__Upper = 0.38
#isGreen_HSV_Variance__Upper = 0.60
# isGreen_HSV_Variance__Upper = 0.75
isGreen_HSV_Variance__Upper = 0.76 # 20181103_085711
isGreen_HSV_Variance__Lower = 0.0
#isGreen_HSV_Variance__Lower = 0.002
# isGreen_HSV_Variance__Lower = 0.01
# isGreen_HSV_Average__Upper = 72.0
# isGreen_HSV_Average__Upper = 75.0
#isGreen_HSV_Average__Upper = 79.0
#isGreen_HSV_Average__Upper = 83.0
isGreen_HSV_Average__Upper = 85.0 # 20190127_075112
isGreen_HSV_Average__Lower = 58.0
#isGreen_HSV_Average__Lower = 67.0
# isGreen_HSV_Average__Lower = 70.0
'''###################
blue
ref : https://docs.google.com/spreadsheets/d/1K0hGC_FFcnUhNEgWi2wYSeDBk0F4dDpSh0y5Dg1DOjE/edit#gid=0
###################'''
#isBlue_HSV_Variance__Upper = 0.25
#isBlue_HSV_Variance__Upper = 0.270 # 20181208_094922
#isBlue_HSV_Variance__Upper = 0.7000 # 20181209_071939
isBlue_HSV_Variance__Upper = 0.930 # 20181214_063434
isBlue_HSV_Variance__Lower = 0.01
#isBlue_HSV_Average__Upper = 13.30
isBlue_HSV_Average__Upper = 17.00 # 20181208_094215
isBlue_HSV_Average__Lower = 8.850 # 20181214_063812
#isBlue_HSV_Average__Lower = 11.00
#isBlue_HSV_Average__Lower = 9.500 # 20181212_064030
'''###################
pink
ref : https://docs.google.com/spreadsheets/d/1K0hGC_FFcnUhNEgWi2wYSeDBk0F4dDpSh0y5Dg1DOjE/edit#gid=0
###################'''
#isPink_HSV_Variance__Upper = 0.40
#isPink_HSV_Variance__Upper = 0.75 # 20190205_153002
isPink_HSV_Variance__Upper = 0.77 # 20200722_085321
isPink_HSV_Variance__Lower = 0.03 # 20190206_100002
#isPink_HSV_Variance__Lower = 0.18
#isPink_HSV_Average__Upper = 134.70
#isPink_HSV_Average__Upper = 136.0 # 20190205_152345
#isPink_HSV_Average__Upper = 141.0 # 20190208_114613
#isPink_HSV_Average__Upper = 144.0 # 20200720_085213
#isPink_HSV_Average__Upper = 147.0 # 20200722_085618
isPink_HSV_Average__Upper = 150.0 # 2020年7月31日13:20:52
isPink_HSV_Average__Lower = 127.9 # 20190205_153437
#isPink_HSV_Average__Lower = 129.5 # 20190205_150749
#isPink_HSV_Average__Lower = 131.00
'''###################
black
###################'''
isBlack_HSV_Variance__Upper = 0.00
isBlack_HSV_Variance__Lower = 0.00
isBlack_HSV_Average__Upper = 0.0
isBlack_HSV_Average__Lower = 0.0
#2020年7月31日13:19:30
#isPink_HSV_Average__Upper = 147.0 # 20200722_085618
#isPink_HSV_Average__Lower = 127.9 # 20190205_153437
#isRed_HSV_Average__Upper = 124.6
#isRed_HSV_Average__Lower = 115.0
#isYellow_HSV_Average__Upper = 99.2
#isGreen_HSV_Average__Upper = 85.0 # 20190127_075112
#isGreen_HSV_Average__Lower = 58.0
#isBlue_HSV_Average__Upper = 17.00 # 20181208_094215 isBlue_HSV_Average__Lower = 8.850 # 20181214_063812
#/ class ColorThresholds(Enum):
class FilePaths(Enum):
dpath_LogFile = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\logs"
fname_LogFile = "get_4_corners.%s.log" % get_TimeLabel_Now()
# fname_LogFile = "get_4_corners.%s.log" % libs.get_TimeLabel_Now()
# fname_LogFile = "get_4_corners.log"
fname_LogFile__Gradation = "gradation.log"
'''###################
anims
###################'''
dpath_Anims_Image_Files = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\anims\\data\\data_images"
fname_Anims_Image_Files = "leaf-2.1.png"
#/ class ColorThresholds(Enum):
class ColorNameSet(Enum):
lo_Color_Sets = [
"oooo" # other
# '''######################################
# r,g,y ==> each only # 3 types
# ######################################'''
# '''###################
# r : red
# ###################'''
, "ooor" # kb
#, "oorr" # 朝永
, "oorr" # シュッツ 20200105_100303
# '''###################
# b : blue
# ###################'''
#, "booo" # :m X-X*X RES / 245 / 『相対性理論』 メラー 永田恒夫、伊藤大介、訳 / p.XX
, "booo" # :m X-X*X RES / 245 / 『古典場から量子場への道』 高橋康 / p.XX #20190806_084436
#, "bboo" # :m X-X*X RES / 245 / 『不完全性定理』 ゲーデル 林晋、八杉満利子 / p.XX
#, "bboo" # :m X-X*X RES / 245 / 『四元数』 今野紀雄 / p.XX #20190510_080306
, "bboo" # :m RES 1-2*2 / free# VX7GLZ 27#8 / 27. math / 8. miscs / id=68OZ / マクローリン級数、テイラー級数 #20190611_105855
# '''###################
# g : green
# ###################'''
#, "gooo" # ":m 食べた物 間食 / 砂糖菓子"
, "gooo" # ":m 食べた物 間食 / 味噌スープ" # 20191209_091533
#, "ggoo" # :m 食べた物 間食 / ヨーグルト / +=
, "ggoo" # :m 食べた物 間食 / お菓子 / += #20200424_175358
# '''###################
# y : yellow
# ###################'''
, "oooy" # :m 食べた物
, "ooyy" # delete
# '''###################
# p : pink
# ###################'''
, "ooop" # :m 記録 / お酒
, "oopp" # : ":m to-write / diary"
, "ooyy" # delete
# '''###################
# a : black
# ###################'''
, "aaaa" # 読みたい本
, "aaao" # 読みたい本
, "aaoo" # 読みたい本
# '''###################
# w : white
# ###################'''
, "wwww" # 読みたい本
, "owww" # 読みたい本
# '''######################################
# r,g,y ==> each, 2
# ######################################'''
# '''###################
# blue red
# ###################'''
, "boor" # :diary / diary / 20181206 / (K,C,P,R,)
# '''###################
# blue green
# ###################'''
, "bgoo" # :m RES 1-3*3 / free# JVEMV6 44#5.1_2X / 44. currency / 5. ea tools / 1. first / memo
# '''###################
# blue yellow
# ###################'''
, "booy" # :m art / dessin / D-17 8# / des.竹林、巻雲
# '''###################
# yellow white
# ###################'''
, "oowy" # :m 食べた物
# '''###################
# yellow black
# ###################'''
, "aooy" # :m 食べた物
, "aaoy" # :m 食べた物
# '''###################
# green red
# ###################'''
, "goor" # :m XXX
# '''###################
# green yellow
# ###################'''
, "gooy"
# '''###################
# green black
# ###################'''
, "aggo" # :m 食べた物 間食 / ヨーグルト / +=
# '''###################
# red yellow
# ###################'''
, "oory" # 読んだ本
# '''###################
# red black
# ###################'''
, "aoor" # kb
# '''######################################
# pink
# ######################################'''
# '''###################
# pink : yellow
# ###################'''
, "oopy" # :m X-X*X RES / 245 / 『カオスとフラクタル』 山口昌哉 / p.XX
# '''###################
# r,g,y ==> each, 3 # 3 types
# ###################'''
# '''###################
# r,g,y ==> each, 4 # 3 types
# ###################'''
# '''###################
# r,g,y ==> 1 or 2 each, 2 kinds # 3 types
# ###################'''
# '''###################
# white black
# ###################'''
# 1 a / 2 w /
, "aoww" # 読みたい本
# 2 a / 2 1 /
, "aaow" # 読みたい本
]#lo_Color_Sets = [
do_Color_Sets_Memo = {
"oooo" : ""
# '''#########################################################
# r,g,y ==> each only # 3 types
# #########################################################'''
# '''######################################
# 1 letter
# ######################################'''
# '''###################
# red
# ###################'''
# #, "ooor" : "UNDEFINED"
#, "ooor" : u':m music / log / XXX / '
#, "ooor" : u':m music / log / kb / XXX' # 20181231_072022
#, "ooor" : u':m music / log / kb / s.1:XXX s.2:XXX s.3:XXX s.4:XXX s.5:XXX' # 20190505_075300
#, "ooor" : u':m music / log / kb / s.1:XXX s.2:XXX s.3:XXX s.4:XXX s.5:XXX s.6:XXX s.7:XXX' # 20190529_105137
#, "ooor" : u':m music / log / gt / || s.1:XXX || s.2:XXX || s.3:XXX || s.4:XXX || s.5:XXX || s.6:XXX || s.7:XXX' # 20190816_085002
#, "ooor" : u':m music / log / fl / || s.1:XXX || s.2:XXX || s.3:XXX || s.4:XXX || s.5:XXX || s.6:XXX || s.7:XXX' # 20200422_080206
, "ooor" : u':m music / log / kb / || s.1:XXX || s.2:XXX || s.3:XXX || s.4:XXX || s.5:XXX || s.6:XXX || s.7:XXX' # 20200521_130007
#, "oorr" : ":m X-X*X RES / 245 / 『量子力学 I』 朝永 / p.XX" # 朝永 # 20190113_063411
, "oorr" : ":m X-X*X RES / 245 / 『相対論』 シュッツ / p.XX" # シュッツ # 2020年1月5日10:01:28
# '''###################
# green
# ###################'''
#, "gooo" : ":m 食べた物 間食 / 砂糖菓子or乾物菓子"
#, "gooo" : ":m 食べた物 間食 / 砂糖菓子" # 20190204_095122
#, "ggoo" : u':m 食べた物 間食 / 味噌スープ' # 20191209_091705
, "gooo" : u':m 食べた物 間食 / 味噌スープ' # 20191209_091705
# '''###################
# yeloow
# ###################'''
, "oooy" : u':m 食べた物'
# '''###################
# b : blue
# ###################'''
#, "booo" : ":m X-X*X RES / 245 / 『相対性理論』 メラー 永田恒夫、伊藤大介、訳 / p.XX"
, "booo" : ":m X-X*X RES / 245 / 『古典場から量子場への道』 高橋康 / p.XX" #20190806_084536
# '''###################
# p : pink
# ###################'''
, "ooop" : ":m 記録 / お酒" # 20200720_085323
#, "ooop" : ":m 記録 / art:sculpture" # 20200702_130851
, "oopp" : ":m to-write / diary"
# '''######################################
# 2 letters
# ######################################'''
#, "bboo" : ":m X-X*X RES / 245 / 『不完全性定理』 ゲーデル 林晋、八杉満利子 / p.XX"
#, "bboo" : ":m X-X*X RES / 245 / 『四元数』 今野紀雄 / p.XX" #20190510_080306
#, "bboo" : ":m RES 1-2*2 / free# VX7GLZ 27#15_1 / 27. math / 15. quaternion / XXX" #20190611_105855
#, "bboo" : ":m 1-X*X RES / free# VX7GLZ 28#3CVF XX / 28. physics / 3CVF spinors / XXX" #20190624_150437
, "bboo" : ":m 1*1 res VX7GLZ / 28#12_1.XX / 28. physics / 12. twistor / 1. lie groups" #20190707_110442
#, "ggoo" : u':m 食べた物 間食 / ヨーグルト / += '
#, "ggoo" : u':m 食べた物 間食 / 味噌スープ' # 20190219_085106
, "ggoo" : u':m 食べた物 間食 / お菓子' #20200425_113322
, "ooyy" : u'\'-*'
#, "oorr" : u'MEMO'
, "oowy" : u':m 食べた物'
, "aooy" : u':m 食べた物'
, "aaoy" : u':m 食べた物'
, "aaoo" : u':m 読みたい本 / 『XXX』 / 著者=XXX' # 読みたい本
# '''###################
# red : black
# ###################'''
, "aoor" : u':m music / log / kb / ' # 20190329_101815
# '''######################################
# pink
# ######################################'''
# '''###################
# pink : yellow
# ###################'''
, "oopy" : ":m X-X*X RES / 245 / 『カオスとフラクタル』 山口昌哉 / p.XX"
# '''###################
# r,g,y ==> each, 2 # 3 types
# ###################'''
# , "oorr"
#, "oorr" : uMEMO'UNDEFINED'
# '''######################################
# 3 letters
# ######################################'''
, "owww" : u':m 読みたい本 / 『』 / 著者=XXX' # 読みたい本
, "aaao" : u':m 読みたい本 / 『』 / 著者=XXX' # 読みたい本
# '''###################
# r,g,y ==> each, 3 # 3 types
# ###################'''
# '''###################
# r,g,y ==> each, 4 # 3 types
# ###################'''
, "aaaa" : u':m 読みたい本 / 『』 / 著者=XXX' # 読みたい本
, "wwww" : u':m 読みたい本 / 『』 / 著者=XXX' # 読みたい本
# '''###################
# r,g,y ==> 1 each, 2 kinds # 3 types
# ###################'''
#, "goor" : u'UNDEFINED'
, "goor" : u':m XXX'
, "gooy" : u'\'-*'
, "aggo" : u':m 食べた物 間食 / ヨーグルト / +=' # 20181215_070822
, "oory" : u':bookmemo / 読んだ本 / 『XXX』 / 著者=XXX'
# '''###################
# r,g,y ==> 1 or 2 each, 2 kinds # 3 types
# ###################'''
, "aoww" : u':m 読みたい本 / 『』 / 著者=XXX' # 読みたい本
, "aaow" : u':m 読みたい本 / 『』 / 著者=XXX' # 読みたい本
# '''#########################################################
# 2 kinds
# #########################################################'''
# '''######################################
# blue, red
# ######################################'''
#, "boor" : ":diary / diary / 20181206 / (K,C,P,R,)"
#, "boor" : ":diary / diary / 20181206 / (K;C;P;R;)"
#, "boor" : ":diary / diary / 20181206 / (K*C*P*R*)" # 20181229_085109
#, "boor" : ":diary / diary / 20190211 / (K*C*P*R*)" # 20190128_062550
#, "boor" : ":diary / diary / 20190314 / (K*C*P*R*)" # 20190314_082519
#, "boor" : ":diary / diary / 20190403 / (K*C*P*R*)" # 20190403_073227
, "boor" : ":diary / memo;diary / 201905XX / (K*C*P*R*J*)" # 20190530_090842
# '''###################
# blue green
# ###################'''
, "bgoo" : ":m RES 1-3*3 / free# JVEMV6 44#5.1_2X / 44. currency / 5. ea tools / 1. first / memo"
# '''######################################
# blue, yellow
# ######################################'''
, "booy" : ":m art / dessin / D-17 8# / des.XXX"
}#do_Color_Sets_Memo = {
memo_Unknown = "UNKNOWN"
colName_Red = "red"
colName_Yellow = "yellow"
colName_Green = "green"
colName_White = "white"
colName_Black = "black"
colName_Blue = "blue" # symbol : "b"
colName_Pink = "pink" # symbol : "p"
#/ class ColorNameSet(Enum):
class Anims_Params(Enum):
'''###################
main
###################'''
PARAM__1_MOVE_LEAVES = "1"
PARAM__2_MOVE_LEAVES__V2 = "2"
PARAM__3_CLUSTERS ="3"
#/ class Anims_Params(Enum):<file_sep>/JVEMV6/46_art/8_kb/start_write-out.apps.filezilla.bat
REM start "C:\WORKS_2\Programs\FileZilla FTP Client\filezilla.exe"
call "C:\WORKS_2\Programs\FileZilla FTP Client\filezilla.exe"
REM pause
exit
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/anims/utils/dos_attack.py
'''###################
created : 2018/06/17 10:59:46
pushd
###################'''
import os, sys
sys.path.append('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/VIRTUAL/Admin_Projects')
from libs_admin import libs
# import urllib
# from lxml import html
# import urllib2
import requests, time
import threading
# import urllib3, requests
# from html.parser import HTMLParser
from bs4 import BeautifulSoup as BS
'''######################################
funcs
######################################'''
#ref https://pymotw.com/2/threading/
def worker():
"""thread worker function"""
r = requests.get('http://localhost:8001/ip/dos_attack/')
# r = requests.get('http://localhost:8001/ip/dos_attack')
#ref https://qiita.com/itkr/items/513318a9b5b92bd56185
#ref r.text https://stackoverflow.com/questions/36709165/beautifulsoup-object-of-type-response-has-no-len#36709275 answered Apr 19 '16 at 5:25
soup = BS(r.text, "html.parser")
# soup = BS(r.text)
print()
print("[%s:%d] soup => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# soup = BS(r)
# print ('Worker')
return
def test_4__Thread__V2():
threads = []
# for i in range(500):
for i in range(120):
# for i in range(130):
# for i in range(150):
# for i in range(160):
# for i in range(200):
# for i in range(50):
# for i in range(5):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
def test_4__Thread():
# Create two threads as follows
try:
thread.start_new_thread( print_time, ("Thread-1", 2, ) )
thread.start_new_thread( print_time, ("Thread-2", 4, ) )
except:
print ("Error: unable to start thread")
# #ref https://qiita.com/Taillook/items/a0f2c59d8e17381fc835
# r = requests.get('http://localhost:8001/ip/dos_attack')
#
# #ref https://qiita.com/itkr/items/513318a9b5b92bd56185
# #ref r.text https://stackoverflow.com/questions/36709165/beautifulsoup-object-of-type-response-has-no-len#36709275 answered Apr 19 '16 at 5:25
# soup = BS(r.text)
# # soup = BS(r)
#
# print()
# print("[%s:%d] soup => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , soup
# ), file=sys.stderr)
# r = urllib3.urlopen("http://localhost:8001/ip/dos_attack")
# print()
# print("[%s:%d] r.text => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , r.text
# ), file=sys.stderr)
def test_3():
#ref https://qiita.com/Taillook/items/a0f2c59d8e17381fc835
r = requests.get('http://localhost:8001/ip/dos_attack')
#ref https://qiita.com/itkr/items/513318a9b5b92bd56185
#ref r.text https://stackoverflow.com/questions/36709165/beautifulsoup-object-of-type-response-has-no-len#36709275 answered Apr 19 '16 at 5:25
soup = BS(r.text)
# soup = BS(r)
print()
print("[%s:%d] soup => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, soup
), file=sys.stderr)
# r = urllib3.urlopen("http://localhost:8001/ip/dos_attack")
# print()
# print("[%s:%d] r.text => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , r.text
# ), file=sys.stderr)
def test_2():
#ref https://qiita.com/Taillook/items/a0f2c59d8e17381fc835
r = requests.get('http://localhost:8001/ip/dos_attack')
print()
print("[%s:%d] r.text => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, r.text
), file=sys.stderr)
parser = Parser()
parser.feed(r.text)
parser.close()
print()
print("[%s:%d] parser => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, parser
), file=sys.stderr)
print()
print("[%s:%d] parser.data => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, parser.data
), file=sys.stderr)
for i in parser.data:
print("Title: " + i["title"], "\nLink: " + i["link"] + "\n")
# print()
# print("[%s:%d] r => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , r
# ), file=sys.stderr)
#
# print()
# print("[%s:%d] r.text => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , r.text
# ), file=sys.stderr)
# response = urllib2.urlopen('http://python.org/')
# html = response.read()
# print()
# print("[%s:%d] html => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , html
# ), file=sys.stderr)
# #ref https://stackoverflow.com/questions/4489550/how-to-get-an-html-file-using-python#4492108
# # url = "http://www.infolanka.com/miyuru_gee/art/art.html"
# url = "http://localhost:8001/ip/dos_attack/"
# page = html.fromstring(urllib.urlopen(url).read())
#
# print()
# print("[%s:%d] page => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , page
# ), file=sys.stderr)
#/ def test_1():
def test_1():
#ref https://urllib3.readthedocs.io/en/latest/
http = urllib3.PoolManager()
# r = http.request('GET', 'http://httpbin.org/robots.txt')
r = http.request('GET', 'http://localhost:8001/ip/dos_attack')
print()
print("[%s:%d] r => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, r
), file=sys.stderr)
print()
print("[%s:%d] r.data => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, r.data
), file=sys.stderr)
print()
print("[%s:%d] type(r.data) => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, type(r.data)
), file=sys.stderr)
print()
print("[%s:%d] r.text => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, r.text
), file=sys.stderr)
# response = urllib2.urlopen('http://python.org/')
# html = response.read()
# print()
# print("[%s:%d] html => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , html
# ), file=sys.stderr)
# #ref https://stackoverflow.com/questions/4489550/how-to-get-an-html-file-using-python#4492108
# # url = "http://www.infolanka.com/miyuru_gee/art/art.html"
# url = "http://localhost:8001/ip/dos_attack/"
# page = html.fromstring(urllib.urlopen(url).read())
#
# print()
# print("[%s:%d] page => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , page
# ), file=sys.stderr)
#/ def test_1():
#ref https://qiita.com/Taillook/items/a0f2c59d8e17381fc835
# class Parser(HTMLParser):
# def __init__(self):
# HTMLParser.__init__(self)
# self.title = False
# self.link = False
# self.data = []
#
# def handle_starttag(self, tag, attrs):
# attrs = dict(attrs)
# if tag == "h2" and "class" in attrs and attrs['class'] == "esc-lead-article-title":
# self.data.append({})
# self.title = True
# self.link = True
#
# if tag == "a" and self.link == True:
# self.data[-1].update({"link": attrs["href"]})
#
# def handle_data(self, data):
# if self.title == True or self.link == True:
# self.data[-1].update({"title": data})
# self.title = False
# self.link = False
#ref https://www.tutorialspoint.com/python/python_multithreading.htm
# Define a function for the thread
def print_time( threadName, delay):
count = 0
while count < 5:
time.sleep(delay)
count += 1
# print "%s: %s" % ( threadName, time.ctime(time.time()) )
print ("%s: %s") % ( threadName, time.ctime(time.time()) )
def exec_prog(): # from :
test_4__Thread__V2()
# test_4__Thread()
# test_3()
# test_2()
# test_1()
'''###################
Report
###################'''
print ("[%s:%d] exec_prog => done" % (os.path.basename(libs.thisfile()), libs.linenum()))
#/def exec_prog(): # from : 20180116_103908
'''
<usage>
'''
if __name__ == "__main__" :
'''###################
evecute
###################'''
exec_prog()
print()
print ("[%s:%d] all done" % (os.path.basename(os.path.basename(libs.thisfile())), libs.linenum()))
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgDog.js
/*---------------------------------------------------------------
Dogクラス
----------------------------------------------------------------*/
var MAXFRAME = 5000;//許容最大コマ数
var MAXKEYFRAME = 5;//最大キーフレーム数
function Dog()
{
this.numParts = 19;
this.numJoints = 10;
this.flagDebug = false;
this.shadow = 0.0;
//Dogの位置・姿勢(root位置)
this.vPos = new Vector3(0.0, 0.0, 0.0);//仮の位置
this.vEuler = new Vector3(0.0, 0.0, 0.0);
this.vSize = new Vector3(1.0, 1.0, 1.0);//全体のスケール変換に使用
this.q = new Quaternion();//1.0, 1.0, 0.0, 0.0);
this.angle = 0;
this.vAxis = new Vector3(0.0, 0.0, 1.0);
var i;
this.parts = [];
for(i = 0; i < this.numParts; i++)
{
this.parts[i] = new Rigid_HS();
this.parts[i].kind = "SUPER";
this.parts[i].nSlice = 10;
this.parts[i].nStack = 10;
this.parts[i].eps1 = 0.8;
this.parts[i].eps2 = 0.5;
}
this.parts[0].diffuse = [0.8, 0.5, 0.2, 1.0];//前胴体
this.parts[0].ambient = [0.5, 0.3, 0.1, 1.0];
this.parts[1].diffuse = [0.8, 0.5, 0.2, 1.0];//後胴体
this.parts[1].ambient = [0.5, 0.3, 0.1, 1.0];
this.parts[2].diffuse = [0.8, 0.4, 0.2, 1.0];//頭部
this.parts[2].ambient = [0.5, 0.3, 0.1, 1.0];
this.parts[3].diffuse = [0.2, 0.2, 0.6, 1.0];//右目
this.parts[3].ambient = [0.1, 0.1, 0.3, 1.0];
this.parts[4].diffuse = [0.2, 0.2, 0.6, 1.0];//左目
this.parts[4].ambient = [0.1, 0.1, 0.3, 1.0];
this.parts[5].diffuse = [0.8, 0.5, 0.2, 1.0];//右耳
this.parts[5].ambient = [0.4, 0.3, 0.1, 1.0];
this.parts[6].diffuse = [0.8, 0.5, 0.2, 1.0];//左耳
this.parts[6].ambient = [0.4, 0.3, 0.1, 1.0];
this.parts[7].diffuse = [0.8, 0.4, 0.2, 1.0];//口鼻を付けるparts
this.parts[7].ambient = [0.5, 0.3, 0.1, 1.0];
this.parts[8].diffuse = [0.2, 0.2, 0.4, 1.0];//鼻
this.parts[8].ambient = [0.1, 0.1, 0.2, 1.0];
this.parts[9].diffuse = [0.6, 0.2, 0.2, 1.0];//口
this.parts[9].ambient = [0.3, 0.1, 0.1, 1.0];
for(i = 10; i < 18; i++)
{//脚
this.parts[i].diffuse = [0.7, 0.4, 0.3, 1.0];
this.parts[i].ambient = [0.4, 0.2, 0.2, 1.0];
}
this.parts[18].diffuse = [0.7, 0.6, 0.4, 1.0];//尾
this.parts[18].ambient = [0.4, 0.3, 0.2, 1.0];
//サイズ
this.vTrunk = new Vector3(1.0, 1.0, 1.0);//胴体(前後共通)
this.vHead = new Vector3(1.2, 1.2, 1.0);
this.vEye = new Vector3(0.2, 0.2, 0.2);
this.vEar = new Vector3( 0.5, 0.15, 0.4);
this.vMouthNose = new Vector3(0.6, 0.7, 0.6);//口鼻を付けるパーツのサイズ
this.vMouth = new Vector3(0.3, 0.3, 0.1);
this.vNose = new Vector3(0.15, 0.15, 0.2);
this.vLeg1 = new Vector3(0.3, 0.3, 0.5);//上脚(前脚,後脚)
this.vLeg2 = new Vector3(0.3, 0.3, 0.3);//下脚(前脚,後脚
this.vTail = new Vector3( 0.5, 0.3, 0.3);//尾
//関節
this.vJoints = [];
this.vJoints[0] = new Vector3(0.0, 0.0, 0.0);//首
this.vJoints[1] = new Vector3(0.0, 0.0, 0.0);//前右股関節
this.vJoints[2] = new Vector3(0.0, 0.0, 0.0);//前右膝関節
this.vJoints[3] = new Vector3(0.0, 0.0, 0.0);//前左股関節
this.vJoints[4] = new Vector3(0.0, 0.0, 0.0);//前左膝関節
this.vJoints[5] = new Vector3(0.0, 0.0, 0.0);//後右股関節
this.vJoints[6] = new Vector3(0.0, 0.0, 0.0);//後右膝関節
this.vJoints[7] = new Vector3(0.0, 0.0, 0.0);//後左股関節
this.vJoints[8] = new Vector3(0.0, 0.0, 0.0);//後左膝関節
this.vJoints[9] = new Vector3(0.0, 60.0, 0.0);//尾の付け根
this.legLen0 = (0.9 * this.vLeg1.z + this.vLeg2.z);
this.height0 = (0.3 * this.vTrunk.z + 0.9 * this.vLeg1.z + this.vLeg2.z);// -0.02;
this.legLen = (0.9 * this.vLeg1.z + this.vLeg2.z);
this.height = (0.3 * this.vTrunk.z + 0.9 * this.vLeg1.z + this.vLeg2.z);// -0.02;
//animation用
this.f_vPos = [];
for(i = 0; i < MAXFRAME; i++) this.f_vPos[i] = new Vector3();
this.f_vEuler = [];
for(i = 0; i < MAXFRAME; i++) this.f_vEuler[i] = new Vector3();
this.k_vPos = [];
for(i = 0; i < MAXKEYFRAME; i++) this.k_vPos[i] = new Vector3();
this.k_vEuler = [];
for(i = 0; i < MAXKEYFRAME; i++) this.k_vEuler[i] = new Vector3();
this.f_vJoints = [];
for(i = 0; i < MAXFRAME*this.numJoints; i++) this.f_vJoints[i] = new Vector3();
this.k_vJoints = [];
for(i = 0; i < MAXKEYFRAME*this.numJoints; i++) this.k_vJoints[i] = new Vector3();
this.actTime = [];//keyFrame間の動作時間
this.unitTime;//1フレーム当たりの描画時間
this.numKeyFrame = 0;//キーフレーム数
this.numFrame = 0;//1つのシーンの全表示フレーム数
}
Dog.prototype.draw = function(gl)
{
for(var i = 0; i < this.numParts; i++)
{
this.parts[i].flagDebug = this.flagDebug;
this.parts[i].shadow = this.shadow;
}
var n = this.parts[0].initVertexBuffers(gl);//1種類のプリミティブを使うので1回だけでよい
//スタック行列の確保
var stackMat = [];
for(var i = 0; i < 10; i++) stackMat[i] = new Matrix4();
// モデル行列の初期化
var modelMatrix = new Matrix4();
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
//Webページでスケーリングを行ったとき,脚の長さルートの高さも変更する
this.legLen = this.legLen0 * this.vSize.z;
this.height = this.height0 * this.vSize.z;
//root
//平行移動
//modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z + this.height);//vPos.zは足の高さ
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z );//2013.7.5変更
//回転
//this.q = q_getQFromEulerXYZ(dog.vEuler);
if(this.q.s > 1.0) this.q.s = 1.0;
if(this.q.s < -1.0) this.q.s = -1.0;
this.angle = 2.0 * Math.acos(this.q.s);//[rad]
this.vAxis = norm(getVector(this.q));
if(this.vAxis.x == 0 && this.vAxis.y == 0 && this.vAxis.z == 0) this.vAxis.x = 1;
modelMatrix.rotate(this.angle * RAD_TO_DEG, this.vAxis.x, this.vAxis.y, this.vAxis.z); //任意軸回転
//スケーリング
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
stackMat[0].copy(modelMatrix);//rootのモデル行列を保存
//---------前胴体----------------------
modelMatrix.translate(0.45 * this.vTrunk.x, 0.0, 0.0);
stackMat[1].copy(modelMatrix);//前胴体のモデル行列を保存
modelMatrix.scale(this.vTrunk.x, this.vTrunk.y, this.vTrunk.z);
this.parts[0].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//rootのモデル行列に戻す
//後胴体
modelMatrix.translate(-0.4 * this.vTrunk.x, 0.0, 0.0);
stackMat[2].copy(modelMatrix);//後胴体のモデル行列を保存
modelMatrix.scale(this.vTrunk.x, this.vTrunk.y, this.vTrunk.z);
this.parts[1].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[1]);//前胴体のモデル行列に戻す
//---------頭部・顔全体-----------------------
modelMatrix.translate(this.vTrunk.x * 0.4, 0.0, this.vTrunk.z * 0.5 - 0.2);//首関節まで平行移動
modelMatrix.rotate(this.vJoints[0].z, 0, 0, 1);
modelMatrix.rotate(this.vJoints[0].y - 90.0, 0, 1, 0);
modelMatrix.rotate(this.vJoints[0].x, 1, 0, 0);
//頭部
modelMatrix.scale(this.vHead.x, this.vHead.y, this.vHead.z);
modelMatrix.translate(0.5, 0.0, 0.0);
this.parts[2].draw(gl, n, modelMatrix);
stackMat[3].copy(modelMatrix);//頭部のモデル行列を保存
//右目
modelMatrix.translate(this.vHead.x * 0.21, -this.vHead.y * 0.2, - this.vHead.z * 0.45 );
modelMatrix.scale(this.vEye.x, this.vEye.y, this.vEye.z);
this.parts[3].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[3]);//頭部のモデル行列に戻す
//左目
modelMatrix.translate(this.vHead.x * 0.21, this.vHead.y * 0.2, - this.vHead.z * 0.45 );
modelMatrix.scale(this.vEye.x, this.vEye.y, this.vEye.z);
this.parts[4].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[3]);//頭部のモデル行列に戻る
//右耳
modelMatrix.translate(this.vHead.x * 0.1, -this.vHead.y * 0.45, this.vHead.z * 0.2 );
modelMatrix.scale(this.vEar.x, this.vEar.y, this.vEar.z);
modelMatrix.translate(0.0, 0.0, -0.5);
this.parts[5].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[3]);//頭部のモデル行列に戻る
//左耳
modelMatrix.translate(this.vHead.x * 0.1, this.vHead.y * 0.45, this.vHead.z * 0.2 );
modelMatrix.scale(this.vEar.x, this.vEar.y, this.vEar.z);
modelMatrix.translate(0.0, 0.0, -0.5);
this.parts[6].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[3]);//頭部のモデル行列に戻る
//口鼻全体
modelMatrix.translate( -0.1 * this.vHead.x, 0.0, -this.vHead.z / 2.0);
modelMatrix.scale(this.vMouthNose.x, this.vMouthNose.y, this.vMouthNose.z);
this.parts[7].draw(gl, n, modelMatrix);
stackMat[4].copy(modelMatrix);//口鼻全体のモデル行列を保存
//鼻
modelMatrix.translate(this.vMouthNose.x * 0.45, 0.0, -this.vMouthNose.z * 0.85);
modelMatrix.scale(this.vNose.x, this.vNose.y, this.vNose.z);
this.parts[8].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[4]);//口鼻全体のモデル行列に戻る
//口
modelMatrix.translate(-this.vMouthNose.x * 0.3, 0.0, -this.vMouthNose.z * 0.85);
modelMatrix.scale(this.vMouth.x, this.vMouth.y, this.vMouth.z);
this.parts[9].draw(gl, n, modelMatrix);
//-------脚---------------------------------------
//前脚
modelMatrix.copy(stackMat[1]);//前胴体に戻す
//右側上
modelMatrix.translate(0.0, -0.4 * this.vTrunk.y, -0.3 * this.vTrunk.z);//前胴体の中心からの股関節の位置
modelMatrix.rotate(this.vJoints[1].y, 0.0, 1.0, 0.0);//y軸回転
stackMat[3].copy(modelMatrix);//前右股関節のモデル行列を保存
modelMatrix.scale(this.vLeg1.x, this.vLeg1.y, this.vLeg1.z );
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[10].draw(gl, n, modelMatrix);
//右側下
modelMatrix.copy(stackMat[3]);//股関節のモデル行列
modelMatrix.translate(0.0, 0.0, -this.vLeg1.z * 0.9);//下脚の位置へ平行移動
//膝の回転
modelMatrix.rotate(this.vJoints[2].y, 0.0, 1.0, 0.0);//y軸回転
modelMatrix.scale(this.vLeg2.x, this.vLeg2.y, this.vLeg2.z);
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[11].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[1]);//前胴体に戻す
//左側上
modelMatrix.translate(0.0, 0.4 * this.vTrunk.y, -0.3 * this.vTrunk.z);
modelMatrix.rotate(this.vJoints[3].y, 0.0, 1.0, 0.0);//y軸回転
stackMat[3].copy(modelMatrix);//前右股関節のモデル行列を保存
modelMatrix.scale(this.vLeg1.x, this.vLeg1.y, this.vLeg1.z );
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[12].draw(gl, n, modelMatrix);
//左側下
modelMatrix.copy(stackMat[3]);
modelMatrix.translate(0.0, 0.0, -this.vLeg1.z * 0.9);//下脚の位置へ平行移動
//膝の回転
modelMatrix.rotate(this.vJoints[4].y, 0.0, 1.0, 0.0);//y軸回転
modelMatrix.scale(this.vLeg2.x, this.vLeg2.y, this.vLeg2.z);
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[13].draw(gl, n, modelMatrix);
//後脚
modelMatrix.copy(stackMat[2]);//後胴体に戻す
//右側上
modelMatrix.translate(0.0, -0.4 * this.vTrunk.y, -0.3 * this.vTrunk.z);
modelMatrix.rotate(this.vJoints[5].y, 0.0, 1.0, 0.0);//股関節y軸回転
stackMat[3].copy(modelMatrix);//後右股関節のモデル行列を保存
modelMatrix.scale(this.vLeg1.x, this.vLeg1.y, this.vLeg1.z );
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[14].draw(gl, n, modelMatrix);
//右側下
modelMatrix.copy(stackMat[3]);
modelMatrix.translate(0.0, 0.0, -this.vLeg1.z * 0.9);//下脚の位置へ平行移動
modelMatrix.rotate(this.vJoints[6].y, 0.0, 1.0, 0.0);//膝関節y軸回転
modelMatrix.scale(this.vLeg2.x, this.vLeg2.y, this.vLeg2.z);
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[15].draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[2]);//後胴体に戻す
//左側上
modelMatrix.translate(0.0, 0.4 * this.vTrunk.y, -0.3 * this.vTrunk.z);
modelMatrix.rotate(this.vJoints[7].y, 0.0, 1.0, 0.0);//股関節y軸回転
stackMat[3].copy(modelMatrix);//後右股関節のモデル行列を保存
modelMatrix.scale(this.vLeg1.x, this.vLeg1.y, this.vLeg1.z );
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[16].draw(gl, n, modelMatrix);
//左側下
modelMatrix.copy(stackMat[3]);//
modelMatrix.translate(0.0, 0.0, -this.vLeg1.z * 0.9);//下脚の位置へ平行移動
modelMatrix.rotate(this.vJoints[8].y, 0.0, 1.0, 0.0);//膝関節y軸回転
modelMatrix.scale(this.vLeg2.x, this.vLeg2.y, this.vLeg2.z);
modelMatrix.translate(0.0, 0.0, -0.5);//原点の位置へ下げる
this.parts[17].draw(gl, n, modelMatrix);
//尾
modelMatrix.copy(stackMat[2]);//後胴体に戻す
modelMatrix.translate(-0.3 * this.vTrunk.x, 0.0, 0.3 * this.vTrunk.z);
modelMatrix.rotate(this.vJoints[9].y, 0.0, 1.0, 0.0);//y軸回転
//stackMat[3].copy(modelMatrix);//後右股関節のモデル行列を保存
modelMatrix.scale(this.vTail.x, this.vTail.y, this.vTail.z );
modelMatrix.translate(-0.5, 0.0, 0.0);//原点の位置へ下げる
this.parts[18].draw(gl, n, modelMatrix);
}
//----------------------------------------------------------------------------
// animation
//----------------------------------------------------------------------------
//keyframeを作成するメンバ関数において最初にcallする
Dog.prototype.initKeyAnimation = function()
{
//最初のキーフレーム値を現在の値にセット
for(var i = 0; i < this.numKeyFrame; i++)
{
this.k_vPos[i].copy(this.vPos);//new Vector3();
this.k_vEuler[i].copy(this.vEuler);
for(var j = 0; j < this.numJoints; j++) this.k_vJoints[i * this.numJoints + j].copy(this.vJoints[j]);
}
}
//----------------------------------------------------------------------------
//フレームデータ作成
//keyframeを作成するメンバ関数で最後にcall
Dog.prototype.makeFrameData = function ()
{
var i, j, k, numHokan, ss;
for(k = 1; k < this.numKeyFrame; k++)
{
numHokan = Math.floor(this.actTime[k] / this.unitTime);//補間点数
if(numHokan == 0) continue;
if(this.numFrame + numHokan > MAXFRAME)
{
alert("フレーム数が制限を越えるおそれがあります ");
return;
}
var vpos = new Vector3();
vpos.copy( sub(this.k_vPos[k], this.k_vPos[k-1]) );
var vang = new Vector3();
vang.copy( sub(this.k_vEuler[k], this.k_vEuler[k-1]) );
for(i = 0; i <= numHokan; i++)
{
ss = i / numHokan;
this.f_vPos[this.numFrame + i].x = this.k_vPos[k-1].x + vpos.x * ss;
this.f_vPos[this.numFrame + i].y = this.k_vPos[k-1].y + vpos.y * ss;
this.f_vPos[this.numFrame + i].z = this.k_vPos[k-1].z + vpos.z * ss;
this.f_vEuler[this.numFrame + i].x = this.k_vEuler[k-1].x + vang.x * ss;;
this.f_vEuler[this.numFrame + i].y = this.k_vEuler[k-1].y + vang.y * ss;;
this.f_vEuler[this.numFrame + i].z = this.k_vEuler[k-1].z + vang.z * ss;;
for(j = 0; j < this.numJoints; j++)
{//補間データを作成(線形補間)
this.f_vJoints[(this.numFrame + i) * this.numJoints + j].x = this.k_vJoints[(k-1) * this.numJoints + j].x
+ (this.k_vJoints[k*this.numJoints + j].x - this.k_vJoints[(k-1)*this.numJoints + j].x) * ss;
this.f_vJoints[(this.numFrame + i) * this.numJoints + j].y = this.k_vJoints[(k-1) * this.numJoints + j].y
+ (this.k_vJoints[k*this.numJoints + j].y - this.k_vJoints[(k-1)*this.numJoints + j].y) * ss;
this.f_vJoints[(this.numFrame + i) * this.numJoints + j].z = this.k_vJoints[(k-1) * this.numJoints + j].z
+ (this.k_vJoints[k*this.numJoints + j].z - this.k_vJoints[(k-1)*this.numJoints + j].z) * ss;
}
}
this.numFrame += numHokan;
}
this.vPos.copy(this.f_vPos[this.numFrame]);
this.vEuler.copy(this.f_vEuler[this.numFrame]);
for(i = 0; i < this.numJoints; i++) this.vJoints[i].copy(this.f_vJoints[this.numFrame*this.numJoints + i]);
}
//----------------------------------------------------------
//与えられた距離歩く
Dog.prototype.walk = function(dist, step, time)
{ //距離, 歩幅 , 所要時間(s)
var i, numStep, numStep2, rest;
numStep = Math.floor(dist / step);
//右足,左足2歩を単位とした歩数
numStep2 = Math.floor(numStep / 2);
var time1 = time * step / dist;
var flag = "RIGHT";//左右フラグ
while(dist > 0)
{
if(dist > 2 * step)
{
this.walkRight(step, time1);//前右,後左
this.walkLeft(step, time1); //前左,後右
dist -= 2 * step;
}
else if(dist > step)
{
this.walkRight(step, time1);//前右,後左
flag = "LEFT";
dist -= step;//alert("bbb dist=" + dist);
}
else if(dist > 0)
{
if(flag == "RIGHT") this.walkRight(dist, time1 * dist / step);//前右,後左
else this.walkLeft(dist, time1 * dist / step); //前左,後右
dist -= step;
}
}
}
//---------------------------------------------------------------------
Dog.prototype.walkRight = function(step, time)
{
var step2 = step / 2.0;//半歩
var pp = Math.PI / 180.0;
//股関節角度
var stepA = 180.0 * Math.asin(step2 / this.legLen) / Math.PI;
var cc = step2 * Math.cos(pp * this.vEuler.z) ;//半歩のx成分(基本姿勢ではx方向が前)
var ss = step2 * Math.sin(pp * this.vEuler.z) ;//y成分
this.numKeyFrame = 3;
this.initKeyAnimation();
//action1(右前脚一歩前へ)
this.k_vJoints[1*this.numJoints + 1].y = -stepA;//前右
this.k_vJoints[1*this.numJoints + 3].y = stepA; //前左
this.k_vJoints[1*this.numJoints + 5].y = stepA;//後右
this.k_vJoints[1*this.numJoints + 7].y = -stepA; //後左
this.k_vPos[1].x = this.k_vPos[0].x + cc;
this.k_vPos[1].y = this.k_vPos[0].y + ss;
this.actTime[1] = time / 2.0;
//action2(基本姿勢に戻る)
this.k_vJoints[2*this.numJoints + 1].y = 0.0;//前右
this.k_vJoints[2*this.numJoints + 3].y = 0.0;//前左
this.k_vJoints[2*this.numJoints + 5].y = 0.0;//後右
this.k_vJoints[2*this.numJoints + 7].y = 0.0;//後左
this.k_vPos[2].x = this.k_vPos[1].x + cc;
this.k_vPos[2].y = this.k_vPos[1].y + ss;
this.actTime[2] = time / 2.0;
this.makeFrameData();
}
//---------------------------------------------------------------------
Dog.prototype.walkLeft = function(step, time)
{
var step2 = step / 2.0;
var pp = Math.PI / 180.0;
//股関節角度
var stepA = 180.0 * Math.asin(step2 / this.legLen) / Math.PI;
var cc = step2 * Math.cos(pp * this.vEuler.z);//半歩のx成分
var ss = step2 * Math.sin(pp * this.vEuler.z);//y成分
this.numKeyFrame = 3;
this.initKeyAnimation();
//action1(右前脚一歩前へ)
this.k_vJoints[1*this.numJoints + 1].y = stepA; //前右
this.k_vJoints[1*this.numJoints + 3].y = -stepA;//前左
this.k_vJoints[1*this.numJoints + 5].y = -stepA;//後右
this.k_vJoints[1*this.numJoints + 7].y = stepA; //後左
this.k_vPos[1].x = this.k_vPos[0].x + cc;
this.k_vPos[1].y = this.k_vPos[0].y + ss;
this.actTime[1] = time / 2.0;
//action2(基本姿勢に戻る)
this.k_vJoints[2*this.numJoints + 1].y = 0.0;//前右
this.k_vJoints[2*this.numJoints + 3].y = 0.0;//前左
this.k_vJoints[2*this.numJoints + 5].y = 0.0;//後右
this.k_vJoints[2*this.numJoints + 7].y = 0.0;//後左
this.k_vPos[2].x = this.k_vPos[1].x + cc;
this.k_vPos[2].y = this.k_vPos[1].y + ss;
this.actTime[2] = time / 2.0;
this.makeFrameData();
}
//--------------------------------------------------------------------------
//正面方向の回転(angle>0で上から見て左回転)
Dog.prototype.turn = function(angle, time)
{
this.numKeyFrame = 2;
this.initKeyAnimation();
this.k_vEuler[1].copy(this.k_vEuler[0]);
this.k_vEuler[1].z += angle;
this.actTime[1] = time;
this.makeFrameData();
}
//後脚で立ち上がる
Dog.prototype.stand = function(time)
{
this.numKeyFrame = 2;
this.initKeyAnimation();
this.k_vJoints[this.numJoints + 5].y = 90.0;//後右
this.k_vJoints[this.numJoints + 7].y = 90.0;//後左
this.k_vEuler[1].y = -90.0;
this.k_vJoints[this.numJoints + 0].y = 90.0;
this.actTime[1] = time ;
this.makeFrameData();
}
//基本姿勢に戻る
Dog.prototype.initPose = function(time)
{
var j;
this.numKeyFrame = 2;
this.initKeyAnimation();
for(j = 0; j < this.numJoints; j++) this.k_vJoints[this.numJoints + j] = new Vector3();
this.k_vEuler[1].y = 0.0;
this.k_vEuler[1].x = 0.0;
this.actTime[1] = time;
this.makeFrameData();
}
Dog.prototype.wait = function(time)
{
this.numKeyFrame = 2;
this.initKeyAnimation();
this.actTime[1] = time;
this.makeFrameData();
}
Dog.prototype.swingTail = function(n, time)
{
var i;
for(i = 0; i < n; i++)
{
this.upTail(time/(2.0*n));
this.downTail(time/(2.0*n));
}
}
Dog.prototype.upTail = function(time)
{
this.numKeyFrame = 2;
this.initKeyAnimation();
this.k_vJoints[this.numJoints + 9].y = this.k_vJoints[9].y + 30.0;
this.actTime[1] = time;
this.makeFrameData();
}
Dog.prototype.downTail = function(time)
{
this.numKeyFrame = 2;
this.initKeyAnimation();
this.k_vJoints[this.numJoints + 9].y = this.k_vJoints[9].y -30.0;
this.actTime[1] = time;
this.makeFrameData();
}
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgMatrix4.js
/*------------------------------------------------------------------------------------------------------
松田晃一著:「WebGL+HTML5 3DCGプログラミング入門」,カットシステム,2012年
この書籍に添付されているCD-ROMのcuon-matrix.jsの1部を著者の許可を得て変更あるいは削除して使用している.
--------------------------------------------------------------------------------------------------------*/
/*----------------------------------------------
4x4の行列を実装したクラス.
この行列は、OpenGL,GLSLとおなじように列優先である.
行列演算は右方向から実行する.
------------------------------------------------*/
//単位行列に初期化するコンストラクタ
function Matrix4()
{
this.elements = new Float32Array([
1,0,0,0,
0,1,0,0,
0,0,1,0,
0,0,0,1
]);
};
Matrix4.prototype.set = function(e0, e1, e2, e3, e4, e5, e6, e7, e8, e9, e10, e11, e12, e13, e14, e15)
{
this.elements[0] = e0;
this.elements[1] = e1;
this.elements[2] = e2;
this.elements[3] = e3;
this.elements[4] = e4;
this.elements[5] = e5;
this.elements[6] = e6;
this.elements[7] = e7;
this.elements[8] = e8;
this.elements[9] = e9;
this.elements[10] = e10;
this.elements[11] = e11;
this.elements[12] = e12;
this.elements[13] = e13;
this.elements[14] = e14;
this.elements[15] = e15;
}
/*---------------------------------------------------
渡された行列の要素をコピーする。
src 要素をコピーしてくる行列
----------------------------------------------------*/
Matrix4.prototype.copy = function(src)
{
var i, s, d;
s = src.elements;
d = this.elements;
if (s === d) {
return;
}
for (i = 0; i < 16; ++i) {
d[i] = s[i];
}
return this;
};
/*-------------------------------
行列どうしの積
other:右側からかける行列
this:掛けられる行列(その結果を返す)
---------------------------------*/
Matrix4.prototype.multiply = function(other)
{
var i, e, a, b, ai0, ai1, ai2, ai3;
e = this.elements;
a = this.elements;
b = other.elements;
for (i = 0; i < 4; i++) {
ai0=a[i]; ai1=a[i+4]; ai2=a[i+8]; ai3=a[i+12];
e[i] = ai0 * b[0] + ai1 * b[1] + ai2 * b[2] + ai3 * b[3];
e[i+4] = ai0 * b[4] + ai1 * b[5] + ai2 * b[6] + ai3 * b[7];
e[i+8] = ai0 * b[8] + ai1 * b[9] + ai2 * b[10] + ai3 * b[11];
e[i+12] = ai0 * b[12] + ai1 * b[13] + ai2 * b[14] + ai3 * b[15];
}
};
/*-------------------------
転置行列をつくる。
----------------------------*/
Matrix4.prototype.transpose = function()
{
var e, t;
e = this.elements;
t = e[ 1]; e[ 1] = e[ 4]; e[ 4] = t;
t = e[ 2]; e[ 2] = e[ 8]; e[ 8] = t;
t = e[ 3]; e[ 3] = e[12]; e[12] = t;
t = e[ 6]; e[ 6] = e[ 9]; e[ 9] = t;
t = e[ 7]; e[ 7] = e[13]; e[13] = t;
t = e[11]; e[11] = e[14]; e[14] = t;
};
/*-----------------------------
other: 逆行列を計算する行列
-------------------------------*/
Matrix4.prototype.setInverseOf = function(other)
{
var i, s, d, inv, det;
s = other.elements;
d = this.elements;
inv = new Float32Array(16);
inv[0] = s[5]*s[10]*s[15] - s[5] *s[11]*s[14] - s[9] *s[6]*s[15]
+ s[9]*s[7] *s[14] + s[13]*s[6] *s[11] - s[13]*s[7]*s[10];
inv[4] = - s[4]*s[10]*s[15] + s[4] *s[11]*s[14] + s[8] *s[6]*s[15]
- s[8]*s[7] *s[14] - s[12]*s[6] *s[11] + s[12]*s[7]*s[10];
inv[8] = s[4]*s[9] *s[15] - s[4] *s[11]*s[13] - s[8] *s[5]*s[15]
+ s[8]*s[7] *s[13] + s[12]*s[5] *s[11] - s[12]*s[7]*s[9];
inv[12] = - s[4]*s[9] *s[14] + s[4] *s[10]*s[13] + s[8] *s[5]*s[14]
- s[8]*s[6] *s[13] - s[12]*s[5] *s[10] + s[12]*s[6]*s[9];
inv[1] = - s[1]*s[10]*s[15] + s[1] *s[11]*s[14] + s[9] *s[2]*s[15]
- s[9]*s[3] *s[14] - s[13]*s[2] *s[11] + s[13]*s[3]*s[10];
inv[5] = s[0]*s[10]*s[15] - s[0] *s[11]*s[14] - s[8] *s[2]*s[15]
+ s[8]*s[3] *s[14] + s[12]*s[2] *s[11] - s[12]*s[3]*s[10];
inv[9] = - s[0]*s[9] *s[15] + s[0] *s[11]*s[13] + s[8] *s[1]*s[15]
- s[8]*s[3] *s[13] - s[12]*s[1] *s[11] + s[12]*s[3]*s[9];
inv[13] = s[0]*s[9] *s[14] - s[0] *s[10]*s[13] - s[8] *s[1]*s[14]
+ s[8]*s[2] *s[13] + s[12]*s[1] *s[10] - s[12]*s[2]*s[9];
inv[2] = s[1]*s[6]*s[15] - s[1] *s[7]*s[14] - s[5] *s[2]*s[15]
+ s[5]*s[3]*s[14] + s[13]*s[2]*s[7] - s[13]*s[3]*s[6];
inv[6] = - s[0]*s[6]*s[15] + s[0] *s[7]*s[14] + s[4] *s[2]*s[15]
- s[4]*s[3]*s[14] - s[12]*s[2]*s[7] + s[12]*s[3]*s[6];
inv[10] = s[0]*s[5]*s[15] - s[0] *s[7]*s[13] - s[4] *s[1]*s[15]
+ s[4]*s[3]*s[13] + s[12]*s[1]*s[7] - s[12]*s[3]*s[5];
inv[14] = - s[0]*s[5]*s[14] + s[0] *s[6]*s[13] + s[4] *s[1]*s[14]
- s[4]*s[2]*s[13] - s[12]*s[1]*s[6] + s[12]*s[2]*s[5];
inv[3] = - s[1]*s[6]*s[11] + s[1]*s[7]*s[10] + s[5]*s[2]*s[11]
- s[5]*s[3]*s[10] - s[9]*s[2]*s[7] + s[9]*s[3]*s[6];
inv[7] = s[0]*s[6]*s[11] - s[0]*s[7]*s[10] - s[4]*s[2]*s[11]
+ s[4]*s[3]*s[10] + s[8]*s[2]*s[7] - s[8]*s[3]*s[6];
inv[11] = - s[0]*s[5]*s[11] + s[0]*s[7]*s[9] + s[4]*s[1]*s[11]
- s[4]*s[3]*s[9] - s[8]*s[1]*s[7] + s[8]*s[3]*s[5];
inv[15] = s[0]*s[5]*s[10] - s[0]*s[6]*s[9] - s[4]*s[1]*s[10]
+ s[4]*s[2]*s[9] + s[8]*s[1]*s[6] - s[8]*s[2]*s[5];
det = s[0]*inv[0] + s[1]*inv[4] + s[2]*inv[8] + s[3]*inv[12];
if (det === 0) {
console.log("Matrix4の逆行列を求めることができません");
}
det = 1 / det;
for (i = 0; i < 16; i++) {
d[i] = inv[i] * det;
}
};
/*-----------------------------
自身の逆行列を求める
-------------------------------*/
Matrix4.prototype.invert = function() {
return this.setInverseOf(this);
};
/*----------------------------------
正射影行列に設定しthisにかけられる
left: 左クリップ平面のX座標
right: 右クリップ平面のX座標
bottom: 下クリップ平面のY座標
top: 上クリップ平面のY座標
near: 近クリップ平面までの距離
far: 遠クリップ平面までの距離
-------------------------------------*/
Matrix4.prototype.ortho = function(left, right, bottom, top, near, far)
{
var e, rw, rh, rd;
if (left === right || bottom === top || near === far) {
console.log( 'ortho error!');
}
rw = 1 / (right - left);
rh = 1 / (top - bottom);
rd = 1 / (far - near);
var e = new Float32Array(16);
e[0] = 2 * rw;
e[1] = 0;
e[2] = 0;
e[3] = 0;
e[4] = 0;
e[5] = 2 * rh;
e[6] = 0;
e[7] = 0;
e[8] = 0;
e[9] = 0;
e[10] = -2 * rd;
e[11] = 0;
e[12] = -(right + left) * rw;
e[13] = -(top + bottom) * rh;
e[14] = -(far + near) * rd;
e[15] = 1;
var m = new Matrix4();
m.elements = e;
this.multiply(m);
};
/*---------------------------------------------------
透視射影行列を作成し右からthisに掛ける
fov: 垂直視野角 [度]
aspect: 視野のアスペクト比(幅 / 高さ)
near: 近クリップ平面までの距離。正数でなくてはならない
far: 遠クリップ平面までの距離。正数でなくてはならない
-------------------------------------------------------*/
Matrix4.prototype.perspective = function(fov, aspect, near, far)
{
var e, rd, s, ct;
if (near === far || aspect === 0) {
console.log('perspective error! near = far or aspect = 0');
}
if (near <= 0) {
console.log( 'perspective error! near <= 0');
}
if (far <= 0) {
console.log( 'perspective error! far <= 0');
}
fov = Math.PI * fov / 180 / 2;
s = Math.sin(fov);
if (s === 0) {
console.log( 'perspective error! fovY = 0');
}
rd = 1 / (far - near);
ct = Math.cos(fov) / s;
var e = new Float32Array(16);
e[0] = ct / aspect;
e[1] = 0;
e[2] = 0;
e[3] = 0;
e[4] = 0;
e[5] = ct;
e[6] = 0;
e[7] = 0;
e[8] = 0;
e[9] = 0;
e[10] = -(far + near) * rd;
e[11] = -1;
e[12] = 0;
e[13] = 0;
e[14] = -2 * near * far * rd;
e[15] = 0;
var m = new Matrix4();
m.elements = e;
this.multiply(m);
};
/*----------------------------------------
スケーリング行列をつくりthisに右からかける
x: X方向の倍率
y: Y方向の倍率
z: Z方向の倍率
-------------------------------------------*/
Matrix4.prototype.scale = function(x, y, z)
{
var e = new Float32Array(16);
e[0] = x; e[4] = 0; e[8] = 0; e[12] = 0;
e[1] = 0; e[5] = y; e[9] = 0; e[13] = 0;
e[2] = 0; e[6] = 0; e[10] = z; e[14] = 0;
e[3] = 0; e[7] = 0; e[11] = 0; e[15] = 1;
var m = new Matrix4();
m.elements = e;
this.multiply(m);
};
/*-----------------------------------
平行移動行列をつくり右からかける
x: X方向の移動量
y: Y方向の移動量
z: Z方向の移動量
-------------------------------------*/
Matrix4.prototype.translate = function(x, y, z)
{
var e = new Float32Array(16);
e[0] = 1; e[4] = 0; e[8] = 0; e[12] = x;
e[1] = 0; e[5] = 1; e[9] = 0; e[13] = y;
e[2] = 0; e[6] = 0; e[10] = 1; e[14] = z;
e[3] = 0; e[7] = 0; e[11] = 0; e[15] = 1;
var m = new Matrix4();
m.elements = e;
this.multiply(m);
};
/*------------------------------
* 回転行列をつくり右からかける
angle: 回転角 [度]
x: 回転軸方向ベクトルのX成分
y: 回転軸方向ベクトルのY成分
z: 回転軸方向ベクトルのZ成分
--------------------------------*/
Matrix4.prototype.rotate = function(angle, x, y, z)
{
var e, s, c, len, rlen, nc, xy, yz, zx, xs, ys, zs;
angle = Math.PI * angle / 180;
var e = new Float32Array(16);
s = Math.sin(angle);
c = Math.cos(angle);
if (0 !== x && 0 === y && 0 === z) {
// X軸まわりの回転
if (x < 0) {
s = -s;
}
e[0] = 1; e[4] = 0; e[ 8] = 0; e[12] = 0;
e[1] = 0; e[5] = c; e[ 9] =-s; e[13] = 0;
e[2] = 0; e[6] = s; e[10] = c; e[14] = 0;
e[3] = 0; e[7] = 0; e[11] = 0; e[15] = 1;
} else if (0 === x && 0 !== y && 0 === z) {
// Y軸まわりの回転
if (y < 0) {
s = -s;
}
e[0] = c; e[4] = 0; e[ 8] = s; e[12] = 0;
e[1] = 0; e[5] = 1; e[ 9] = 0; e[13] = 0;
e[2] =-s; e[6] = 0; e[10] = c; e[14] = 0;
e[3] = 0; e[7] = 0; e[11] = 0; e[15] = 1;
} else if (0 === x && 0 === y && 0 !== z) {
// Z軸まわりの回転
if (z < 0) {
s = -s;
}
e[0] = c; e[4] =-s; e[ 8] = 0; e[12] = 0;
e[1] = s; e[5] = c; e[ 9] = 0; e[13] = 0;
e[2] = 0; e[6] = 0; e[10] = 1; e[14] = 0;
e[3] = 0; e[7] = 0; e[11] = 0; e[15] = 1;
} else {
// その他の任意軸まわりの回転
len = Math.sqrt(x*x + y*y + z*z);
if (len !== 1) {
rlen = 1 / len;
x *= rlen;
y *= rlen;
z *= rlen;
}
nc = 1 - c;
xy = x * y;
yz = y * z;
zx = z * x;
xs = x * s;
ys = y * s;
zs = z * s;
e[ 0] = x*x*nc + c;
e[ 1] = xy *nc + zs;
e[ 2] = zx *nc - ys;
e[ 3] = 0;
e[ 4] = xy *nc - zs;
e[ 5] = y*y*nc + c;
e[ 6] = yz *nc + xs;
e[ 7] = 0;
e[ 8] = zx *nc + ys;
e[ 9] = yz *nc - xs;
e[10] = z*z*nc + c;
e[11] = 0;
e[12] = 0;
e[13] = 0;
e[14] = 0;
e[15] = 1;
}
var m = new Matrix4();
m.elements = e;
this.multiply(m);
};
/*------------------------------------------------
視野変換行列を設定する。
eyeX, eyeY, eyeZ: 視点の位置
centerX, centerY, centerZ: 注視点の位置
upX, upY, upZ: カメラの上方向を表す方向ベクトル
--------------------------------------------------*/
Matrix4.prototype.lookAt = function(eyeX, eyeY, eyeZ, centerX, centerY, centerZ, upX, upY, upZ)
{
var fx, fy, fz, rlf, sx, sy, sz, rls, ux, uy, uz;
fx = centerX - eyeX;
fy = centerY - eyeY;
fz = centerZ - eyeZ;
// fを正規化する
rlf = 1 / Math.sqrt(fx*fx + fy*fy + fz*fz);
fx *= rlf;
fy *= rlf;
fz *= rlf;
// fとupの外積を求める
sx = fy * upZ - fz * upY;
sy = fz * upX - fx * upZ;
sz = fx * upY - fy * upX;
// sを正規化する
rls = 1 / Math.sqrt(sx*sx + sy*sy + sz*sz);
sx *= rls;
sy *= rls;
sz *= rls;
// sとfの外積を求める
ux = sy * fz - sz * fy;
uy = sz * fx - sx * fz;
uz = sx * fy - sy * fx;
var e = new Float32Array(16);
e[0] = sx;
e[1] = ux;
e[2] = -fx;
e[3] = 0;
e[4] = sy;
e[5] = uy;
e[6] = -fy;
e[7] = 0;
e[8] = sz;
e[9] = uz;
e[10] = -fz;
e[11] = 0;
e[12] = 0;
e[13] = 0;
e[14] = 0;
e[15] = 1;
var m = new Matrix4();
m.elements = e;
// 平行移動する
m.translate(-eyeX, -eyeY, -eyeZ);
this.multiply(m);
};
/*-----------------------------------------------------------------------
頂点を平面上に射影するような行列を右からかける。
plane: 平面方程式 ax + by + cz + d = 0 の係数[a, b, c, d]を格納した配列
light: 光源の同次座標を格納した配列。light[3]=0の場合、平行光源を表す
------------------------------------------------------------------------*/
Matrix4.prototype.dropShadow = function(plane, light)
{
var e = new Float32Array(16);
var dot = plane[0] * light[0] + plane[1] * light[1] + plane[2] * light[2] + plane[3] * light[3];
e[ 0] = dot - light[0] * plane[0];
e[ 1] = - light[1] * plane[0];
e[ 2] = - light[2] * plane[0];
e[ 3] = - light[3] * plane[0];
e[ 4] = - light[0] * plane[1];
e[ 5] = dot - light[1] * plane[1];
e[ 6] = - light[2] * plane[1];
e[ 7] = - light[3] * plane[1];
e[ 8] = - light[0] * plane[2];
e[ 9] = - light[1] * plane[2];
e[10] = dot - light[2] * plane[2];
e[11] = - light[3] * plane[2];
e[12] = - light[0] * plane[3];
e[13] = - light[1] * plane[3];
e[14] = - light[2] * plane[3];
e[15] = dot - light[3] * plane[3];
var m = new Matrix4();
m.elements = e;
this.multiply(m);
}
<file_sep>/JVEMV6/46_art/6_visual-arts/end_visual-arts.bat
REM ************************
REM doc
REM app : gimp
REM app : git
REM ************************
REM ************************
REM doc
REM ************************
REM taskkill /f /im soffice.bin
REM ************************
REM app : gimp
REM ************************
taskkill /f /im gimp-2.8.exe
REM ************************
REM app : git
REM ************************
taskkill /im wish.exe
pause
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/data/ops/3_opencv/1_motion-detection/1_/ops_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\3_opencv\1_motion-detection\1_\ops_1.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\2_image-programming\2_projects\3_handle-exif-data\ops_1.py
at : 2018/08/23 12:33:00
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\3_opencv\1_motion-detection\1_
python ops_1.py
'''
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects') # libs_mm
from libs_admin import libs, lib_ip
'''###################
import : built-in modules
###################'''
# import getopt
import os, glob, getopt, math as math, numpy as np
# import inspect
'''###################
import : built-in modules
###################'''
import cv2
# from pandas.compat import str_to_bytes
from numpy.distutils.from_template import item_re
#ref https://stackoverflow.com/questions/3129322/how-do-i-get-monitor-resolution-in-python
# from win32api import GetSystemMetrics
# from matplotlib import pylab as plt
#ref https://www.lifewithpython.com/2014/12/python-extract-exif-data-like-data-from-images.html
# from PIL import Image
# from PIL.ExifTags import TAGS
###############################################
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
#ref https://www.lifewithpython.com/2014/12/python-extract-exif-data-like-data-from-images.html
'''###################
at : 2018/08/24 15:38:23
<usage>
0. C/P video file
from : anywhere
to : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\3_opencv\1_motion-detection\data
1. set
1. dir path : dpath_Ops_Videos
2. file name : fname_Ops_Video
2. console
exec
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\3_opencv\1_motion-detection\1_
python ops_1.py
3. ==> 3 windows show up
###################'''
def test_1():
#debug
print("[%s:%d] test_1() --> stareting..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
prep
######################################'''
dpath_Ops_Videos = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\3_opencv\\1_motion-detection\\data"
# C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\3_opencv\1_motion-detection\data
fname_Ops_Video = "2018-08-24_06-20-53_000.mov.0-10-sec.avi"
# fname_Ops_Video = "vtest.avi"
fpath_Ops_Video = os.path.join(dpath_Ops_Videos, fname_Ops_Video)
'''###################
ops
###################'''
# 定数定義
ESC_KEY = 27 # Escキー
INTERVAL= 33 # インターバル
FRAME_RATE = 30 # fps
WINDOW_ORG = "org"
WINDOW_BACK = "back"
WINDOW_DIFF = "diff"
FILE_ORG = fpath_Ops_Video
# FILE_ORG = "org_768x576.avi"
# ウィンドウの準備
cv2.namedWindow(WINDOW_ORG)
cv2.namedWindow(WINDOW_BACK)
cv2.namedWindow(WINDOW_DIFF)
# 元ビデオファイル読み込み
mov_org = cv2.VideoCapture(FILE_ORG)
# 最初のフレーム読み込み
has_next, i_frame = mov_org.read()
# 背景フレーム
back_frame = np.zeros_like(i_frame, np.float32)
#debug
print("[%s:%d] prep done. starting while loop..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# 変換処理ループ
while has_next == True:
# 入力画像を浮動小数点型に変換
f_frame = i_frame.astype(np.float32)
# 差分計算
diff_frame = cv2.absdiff(f_frame, back_frame)
# 背景の更新
cv2.accumulateWeighted(f_frame, back_frame, 0.025)
# フレーム表示
cv2.imshow(WINDOW_ORG, i_frame)
cv2.imshow(WINDOW_BACK, back_frame.astype(np.uint8))
cv2.imshow(WINDOW_DIFF, diff_frame.astype(np.uint8))
# Escキーで終了
key = cv2.waitKey(INTERVAL)
if key == ESC_KEY:
break
# 次のフレーム読み込み
has_next, i_frame = mov_org.read()
#debug
print("[%s:%d] while loop done..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# 終了処理
cv2.destroyAllWindows()
mov_org.release()
'''###################
message
###################'''
print()
# print("[%s:%d] test_1 =======================" % \
print("[%s:%d] test_1 ======================= done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
'''
ApertureValue
BrightnessValue
ColorSpace
ComponentsConfiguration
CustomRendered
DateTime
DateTimeDigitized
DateTimeOriginal
ExifImageHeight
ExifImageWidth
ExifOffset
ExifVersion
ExposureBiasValue
ExposureMode
ExposureProgram
ExposureTime
FNumber
Flash
FlashPixVersion
FocalLength
FocalLengthIn35mmFilm
GPSInfo
ISOSpeedRatings
LensMake
LensModel
LensSpecification
Make
MakerNote
MeteringMode
Model
Orientation
ResolutionUnit
SceneCaptureType
SceneType
SensingMethod
ShutterSpeedValue
Software
SubjectLocation
SubsecTimeDigitized
SubsecTimeOriginal
WhiteBalance
XResolution
YCbCrPositioning
YResolution
'''<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgSpringMass3.js
/*----------------------------------------------
spring_mass3.js
3次元バネ質点モデル
3次元弾性体(立方体、球、円柱)を表現
-----------------------------------------------*/
//初期のサイズとバネ全体の自然長は同じとする
function SpringMass3()
{
this.kind = "CUBE";//3次元SMMを1個の物体とみなしたときの種類(ほかに"SPHERE","CYLINDER")
this.numPole = 1;
this.numRow = 10;//1行当たりのバネ個数
this.numCol = 10;//1列当たりのバネ個数
this.numStk = 10;//1積当たりのバネ個数
this.numStruct;
this.numShear ;
this.numPoint ;//(this.nnumRow+1) * (this.numCol+1);
this.numHinge ;
this.mass = 0.1;//質点1個当たりの質量(kg)
this.structK = 100;//バネ1個当たり
this.shearK = 100;
this.hingeK = 0;//;
this.damping = 0.1;//0.5;
this.drag = 0.1;//0.1;
this.muK = 0.2;
this.restitution = 0.2;
this.lengthX0; //springの1個当たり自然長
this.lengthY0; //springの1個当たり自然長
this.lengthZ0; //springの1個当たり自然長
this.vPos = new Vector3();//3次元SMM全体の中心座標
this.vVel = new Vector3();//3次元SMM全体の速度
this.vOmg = new Vector3();//3次元SMM全体の角速度
this.vSize = new Vector3(1, 1, 1);
this.vEuler = new Vector3();//3次元SMM全体の姿勢
this.radius = 0.02 ;//質点の半径
this.height0 = 2;//初期状態の高さ
this.vForce0 = new Vector3();//外力の初期値
this.structS = [];//構造バネ
this.shearS = []; //せん断バネ
this.hingeS = []; //蝶番バネ
this.point = [];
this.pole = [];
this.shadow = 0;
this.dispType = "SMM";//"TEX", "CHECK",
this.flagShearDisp = false;
this.object = new Rigid();//2,3次元バネ質点モデル全体を1個のオブジェクトとして描画するときに使用
this.rigid2;//衝突する剛体
}
SpringMass3.prototype.initialize = function()
{
if(this.kind == "CUBE") this.initializeCube();
else if(this.kind == "SPHERE") this.initializeSphere();
else this.initializeCylinder();
}
//-----------------------------------------------------------------
SpringMass3.prototype.draw = function(gl)
{
var i, r1, r2;
if(this.dispType == "SMM")//"SPRING_MASS_MODEL" (ばね質点表示)
{
//チェック表示後、剛体が表示されなくなることを防ぐためのダミー
var dummy = new Rigid();
dummy.kind ="CHECK_PLATE";//"SPHERE";//
dummy.flagCheck = true;
dummy.nSlice = 20;
dummy.nStack = 20;
var n = dummy.initVertexBuffers(gl);
//質点
n = this.point[0].initVertexBuffers(gl);
for(i = 0; i < this.numPoint; i++)
{
if(this.point[i].flagFixed == true){
this.point[i].diffuse = [0.8, 0.2, 0.2, 1.0];
this.point[i].ambient = [0.4, 0.1, 0.1, 1.0];
}
else {
this.point[i].diffuse = [0.2, 0.9, 0.9, 1.0];
this.point[i].ambient = [0.1, 0.5, 0.5, 1.0];
}
this.point[i].shadow = this.shadow;
this.point[i].draw(gl, n);
}
var np1, np2;
//構造バネ
n = this.structS[0].initVertexBuffers(gl);
for(i = 0; i < this.numStruct; i++)
{
np1 = this.structS[i].np1; np2 = this.structS[i].np2;
var len = distance(this.point[np1].vPos, this.point[np2].vPos);
this.structS[i].vSize = new Vector3(this.radius, this.radius, len);
this.structS[i].vEuler = getEulerZ(this.point[np1].vPos, this.point[np2].vPos);
this.structS[i].vPos = div(add(this.point[np1].vPos, this.point[np2].vPos), 2.0);
this.structS[i].shadow = this.shadow;
this.structS[i].draw(gl, n);
}
//せん断ばね
if(this.flagShearDisp)
{
n = this.shearS[0].initVertexBuffers(gl);
for(i = 0; i < this.numShear; i++)
{
np1 = this.shearS[i].np1; np2 = this.shearS[i].np2;
var len = distance(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[i].vSize = new Vector3(this.radius, this.radius, len);
this.shearS[i].vEuler = getEulerZ(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[i].vPos = div(add(this.point[np1].vPos, this.point[np2].vPos), 2.0);
this.shearS[i].shadow = this.shadow;
this.shearS[i].draw(gl, n);
}
}
}
else //this.dispType == "TEX", "CHECK")
{//3次元SMM全体を1つのオブジェクトとしてテクスチャ、チェック模様を貼り付ける
this.object.data= [];
this.object.shadow = this.shadow;
if(this.dispType == "TEX")
{
if(this.kind == "CUBE") this.object.kind = "GRID_SQUARE";
else if(this.kind == "SPHERE") this.object.kind = "GRID_SPHERE";
else if(this.kind == "CYLINDER") this.object.kind = "GRID_CYLINDER";
this.object.flagTexture = true;
this.object.flagCheck = false;
}
else
{//"CHECK"
if(this.kind == "CUBE") this.object.kind = "CHECK_SQUARE";
else if(this.kind == "SPHERE") this.object.kind = "CHECK_SPHERE";
else if(this.kind == "CYLINDER") this.object.kind = "CHECK_CYLINDER";
this.object.col1 = [1.0, 0.2, 0.1, 1.0];
this.object.col2 = [0.1, 0.8, 0.9, 1.0];
this.object.flagTexture = false;
this.object.flagCheck = true;
}
if(this.object.flagDebug) {
this.object.flagTexture = false;
this.object.flagCheck = false;
}
var m;
if(this.kind == "CUBE")
{
//Top(k=0、上部)
k = 0;
for(j = 0; j <= this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{
m = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
this.object.nSlice = this.numRow;
this.object.nStack = this.numCol;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];
//Front(i=numROW)
// this.object.data= [];
i = this.numRow;
for(j = 0; j <= this.numCol; j++)
for(k = 0; k <= this.numStk; k++)
{
m = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
this.object.nSlice = this.numStk;
this.object.nStack = this.numCol;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];
//Right(j=0,オブジェクト自身から見て右側)
j = 0;
for(k = 0; k <= this.numStk; k++)
for(i = this.numRow; i >= 0; i--)
{
m = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
this.object.nSlice = this.numRow;
this.object.nStack = this.numStk;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];
//Left(j=0,オブジェクト自身から見て左側)
j = this.numCol;
for(i = this.numRow; i >= 0; i--)
for(k = 0; k <= this.numStk; k++)
{
m = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
this.object.nSlice = this.numStk;
this.object.nStack = this.numRow;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];
//Rear(j=0,オブジェクト自身から見て後ろ側)
i = 0;
for(j = this.numCol; j >= 0; j--)
for(k = 0; k <= this.numStk; k++)
{
m = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
this.object.nSlice = this.numStk;
this.object.nStack = this.numCol;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];
//Bottpom(k=numStk,オブジェクト自身から見て下部)
k = this.numStk;
for(j = this.numCol; j >= 0; j--)
for(i = 0; i <= this.numRow; i++)
{
m = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
this.object.nSlice = this.numRow;
this.object.nStack = this.numCol;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];
}
else if(this.kind == "SPHERE")
{
// k = 0;Top
this.object.data.push([this.point[0].vPos.x, this.point[0].vPos.y, this.point[0].vPos.z]);
for(k = 1; k < this.numStk; k++)
for(j = 0; j < this.numCol; j++)
{
m = j + (k-1) * this.numCol + 1;
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
k = this.numPoint-2;//球のSMMデータは中心のデータを含む、TEXおよびCHECK表示には中心点は必要ない
this.object.data.push([this.point[k].vPos.x, this.point[k].vPos.y, this.point[k].vPos.z]);
this.object.nSlice = this.numCol;
this.object.nStack = this.numStk;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];//クリア
}
else if(this.kind == "CYLINDER")
{
// k = 0;Topの中心
this.object.data.push([this.point[0].vPos.x, this.point[0].vPos.y, this.point[0].vPos.z]);
//side
for(k = 0; k <= this.numStk; k++)
for(j = 0; j < this.numCol; j++)
{
m = j + k * (this.numCol + 1) + 1;
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
}
m = this.numStk * (this.numCol+1);//Bottomの中心
this.object.data.push([this.point[m].vPos.x, this.point[m].vPos.y, this.point[m].vPos.z]);
this.object.nSlice = this.numCol;
this.object.nStack = this.numStk;
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
this.object.data= [];//クリア
}
}
}
//-----------------------------------------------------------------------------
//3次元のバネマスモデル
SpringMass3.prototype.calcSpringMass3 = function(tt)
{
var i, j, I, J, k, r1, r2, m;
var vDir1 = new Vector3();//hinge中心から#1へ向かう単位方向ベクトル(他にも使用)
var vDir2 = new Vector3();//hinge中心から#2へ向かう単位方向ベクトル(他にも使用)
var vFF = new Vector3();
var vRelativeVel = new Vector3();
var vNormal = new Vector3();
var vG = new Vector3(0.0, 0.0, -gravity * this.mass);//重力ベクトル
var dampingF, len, len1, len2, angle;
// var angle0 = Math.PI;
// var lenShear0 = Math.sqrt(this.lengthX0*this.lengthX0 + this.lengthY0*this.lengthY0);
//console.log("lenS0 = " + lenShear0);
//力の総和
//初期設定値(風などの外力)
for(i = 0; i < this.numPoint; i++){
this.point[i].vForce = add(this.point[i].vForce0, vG);
}
//var nrp = this.numRow +1;
var np1, np2;
//バネによる力
for(i = 0; i < this.numStruct; i++)
{
//弾性力
np1 = this.structS[i].np1;
np2 = this.structS[i].np2;
vDir1 = direction(this.point[np1].vPos , this.point[np2].vPos);//#1から#2への単位ベクトル
len = distance(this.point[np1].vPos, this.point[np2].vPos);
//console.log("len = " + len + " lenX0 = " + this.lengthX0 + " lenY0 = " + this.lengthY0);
//if(len < this.structS[i].length0 * 0.8) len = this.structS[i].length0 * 0.8;
//if(len > this.structS[i].length0 * 1.2) len = this.structS[i].length0 * 1.2;
vFF = mul(this.structK * (len - this.structS[i].length0), vDir1) ;
this.point[np1].vForce.add(vFF) ;//vDirと同方向
this.point[np2].vForce.sub(vFF) ;//反対方向
//減衰力
vRelativeVel = sub(this.point[np1].vVel , this.point[np2].vVel);
dampingF = this.damping * dot(vRelativeVel, vDir1);
this.point[np1].vForce.sub(mul(dampingF , vDir1));//相対速度とは反対方向
this.point[np2].vForce.add(mul(dampingF , vDir1));//同方向
}
//せん断バネによる力
if(this.shearK > 0)
{
for(i = 0; i < this.numShear; i++)
{
np1 = this.shearS[i].np1;
np2 = this.shearS[i].np2;
vDir1 = direction(this.point[np1].vPos , this.point[np2].vPos);//#1から#2への単位ベクトル
len = distance(this.point[np1].vPos, this.point[np2].vPos);
//if(len < this.shearS[i].length0 * 0.8) len = this.shearS[i].length0 * 0.8;
//if(len > this.shearS[i].length0 * 1.2) len = this.shearS[i].length0 * 1.2;
vDir1 = direction(this.point[np1].vPos , this.point[np2].vPos);
vFF = mul(this.shearK * (len - this.shearS[i].length0), vDir1) ;
this.point[np1].vForce.add(vFF) ;//vDirと同方向
this.point[np2].vForce.sub(vFF) ;//反対方向
//減衰力
vRelativeVel = sub(this.point[np1].vVel, this.point[np2].vVel);
dampingF = this.damping * dot(vRelativeVel, vDir1);
vFF = mul(dampingF, vDir1);
this.point[np1].vForce.sub(vFF);//相対速度とは反対方向
this.point[np2].vForce.add(vFF);//同方向
}
}
//console.log("e = " + this.restitution + " muK = " + this.muK);
//console.log("damping = " + this.damping + " drag = " + this.drag);
//粘性抵抗と床面処理
for(i = 0; i < this.numPoint; i++)
{
//if(this.point[i].vPos.z > 0.6) console.log(" i = " + i + " pz = " + this.point[i].vPos.z + " vy = " + this.point[i].vVel.y );
//if(this.point[i].vPos.z < 0.1) console.log(" i = " + i + " pz = " + this.point[i].vPos.z + " vy = " + this.point[i].vVel.y );
if(this.point[i].flagFixed) continue; //固定
//空気粘性抵抗(全ての質点に一様と仮定)
this.point[i].vForce.sub(mul(this.drag, this.point[i].vVel));
//床面処理
if(this.point[i].vPos.z < this.radius + 0.01)
{
//床面にある質点に対してはすべり摩擦を与える
this.point[i].vForce.sub(mul(this.muK * this.mass * gravity, norm(this.point[i].vVel)));
//床面上に制限
this.point[i].vPos.z = this.radius;
//床との衝突
if(this.point[i].vVel.z < 0.0)
{ //質点と床面とは弾性衝突とする
this.point[i].vVel.z = - this.restitution * this.point[i].vVel.z ;
}
}
//Euler法
//加速度
this.point[i].vAcc = div(this.point[i].vForce , this.mass);
//速度
this.point[i].vVel.add(mul(this.point[i].vAcc, tt));
//位置
this.point[i].vPos.add(mul(this.point[i].vVel, tt));
}
//全体の中心位置
var vPos = new Vector3();
for(i = 0; i < this.numPoint; i++) vPos.add(this.point[i].vPos);
this.vPos = div(vPos, this.numPoint);
}
//-----------------------------------------------------------------
SpringMass3.prototype.initializeCube = function()
{
var i, j, k;
var cnt = 0;
//質点のサイズ,位置
for(k = 0; k <= this.numStk; k++)
for(j = 0; j <= this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{
//初期状態では立方体の中心をオブジェクト座標の原点
this.point[cnt] = new Rigid();
this.point[cnt].kind = "SPHERE";
this.point[cnt].vSize = new Vector3(2.0*this.radius, 2.0*this.radius, 2.0*this.radius);
this.point[cnt].vPos.x = i * this.vSize.x / this.numRow - this.vSize.x / 2;
this.point[cnt].vPos.y = j * this.vSize.y / this.numCol - this.vSize.y / 2;
this.point[cnt].vPos.z =-k * this.vSize.z / this.numStk + this.vSize.z / 2;
this.point[cnt].vPos = rotate(this.point[cnt].vPos, this.vEuler);//3次元弾性体自身の回転も考慮
this.point[cnt].vPos.add(this.vPos);//全体の平行移動(this.vPosはサンプル・プログラム側で指定)
this.point[cnt].vVel = new Vector3();
this.point[cnt].nSlice = 6;
this.point[cnt].nStack = 6;
cnt ++;
}
this.numPoint = cnt;
//バネに接続する質点番号(row,col,stkで指定)など
var np1, np2;
cnt = 0;
//x軸に平行なバネ
for(k = 0; k <= this.numStk; k++)
for(j = 0; j <= this.numCol; j++)
for(i = 0; i < this.numRow; i++)
{
this.structS[cnt] = new Rigid();
this.structS[cnt].kind = "CYLINDER";
this.structS[cnt].numSlice = 6;
this.structS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i+1 + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
}
//y軸に平行なバネ
for(k = 0; k <= this.numStk; k++)
for(j = 0; j < this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{
this.structS[cnt] = new Rigid();
this.structS[cnt].kind = "CYLINDER";
this.structS[cnt].numSlice = 6;
this.structS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i + (j+1)*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
}
//z軸に平行なバネ
for(k = 0; k < this.numStk; k++)
for(j = 0; j <= this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{
this.structS[cnt] = new Rigid();
this.structS[cnt].kind = "CYLINDER";
this.structS[cnt].numSlice = 6;
this.structS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i + j*(this.numRow+1) + (k+1)*(this.numRow+1)*(this.numCol+1);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
}
this.numStruct = cnt;
//せん断バネ
cnt = 0;
//x-y平面
for(k = 0; k <= this.numStk; k++)
for(j = 0; j < this.numCol; j++)
for(i = 0; i < this.numRow; i++)
{
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i+1 + (j+1)*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
np1 = i+1 + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i + (j+1)*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
//this.shearS[cnt].row1 = i+1; this.shearS[cnt].col1 = j; this.shearS[cnt].stk1 = k;
//this.shearS[cnt].row2 = i; this.shearS[cnt].col2 = j+1;this.shearS[cnt].stk2 = k;
cnt++;
}
//y-z平面
for(k = 0; k < this.numStk; k++)
for(j = 0; j < this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i + (j+1)*(this.numRow+1) + (k+1)*(this.numRow+1)*(this.numCol+1);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + (k+1)*(this.numRow+1)*(this.numCol+1);
np2 = i + (j+1)*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
}
//x-z平面
for(k = 0; k < this.numStk; k++)
for(j = 0; j <= this.numCol; j++)
for(i = 0; i < this.numRow; i++)
{
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
np2 = i+1 + j*(this.numRow+1) + (k+1)*(this.numRow+1)*(this.numCol+1);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
np1 = i + j*(this.numRow+1) + (k+1)*(this.numRow+1)*(this.numCol+1);
np2 = i+1 + j*(this.numRow+1) + k*(this.numRow+1)*(this.numCol+1);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
}
this.numShear = cnt;
}
//-----------------------------------------------------------------------------
SpringMass3.prototype.initializeSphere = function()
{
var j, jp, kp, k, np, np1, np2, cnt;
var phi, theta;
var rx, ry, rz;//弾性体球全体の半径
//半径
rx = this.vSize.x / 2.0;
ry = this.vSize.y / 2.0;
rz = this.vSize.z / 2.0;
//全質点個数
this.numPoint = (this.numStk-1) * this.numCol + 3;
//alert("numPoint = " + this.numPoint);
for(i = 0; i < this.numPoint; i++)
{
this.point[i] = new Rigid();
this.point[i].kind = "SPHERE";
this.point[i].vSize = new Vector3(2.0*this.radius, 2.0*this.radius, 2.0*this.radius);
}
//numStk---z軸方向のバネ個数
//numCol---x-y面(方位角,経度)のバネ個数
//中心にも質点を置く(numPoint-1番目)
//質点のサイズ,位置
//Top
np = 0;
this.point[0].vPos.x = 0.0; //x座標
this.point[0].vPos.y = 0.0; //y
this.point[0].vPos.z = rz;//z軸方向の半径
this.point[0].vPos = rotate(this.point[0].vPos, this.vEuler);
this.point[0].vPos.add(this.vPos);
//Bottom
np = this.numPoint - 2;
this.point[np].vPos.x = 0.0; //x座標
this.point[np].vPos.y = 0.0; //y
this.point[np].vPos.z = - rz;//z
this.point[np].vPos = rotate(this.point[np].vPos, this.vEuler);
this.point[np].vPos.add(this.vPos);
//console.log("np="+np + " x= "+this.point[np].vPos.x+" y= "+ this.point[np].vPos.y+" z = "+this.point[np].vPos.z);
for(k = 1; k < this.numStk; k++)
{
theta = Math.PI/2.0 - Math.PI*k/this.numStk;
for(j = 0; j < this.numCol; j++)
{ //真後ろをj=0
np = (k-1)*this.numCol + j + 1;
phi = 2.0*Math.PI*j/this.numCol;
this.point[np].vPos.x = -rx*Math.cos(phi)*Math.cos(theta); //x座標
this.point[np].vPos.y = -ry*Math.sin(phi)*Math.cos(theta); //y
this.point[np].vPos.z = rz*Math.sin(theta); //z
this.point[np].vPos = rotate(this.point[np].vPos, this.vEuler);
this.point[np].vPos.add(this.vPos);
//console.log("np="+np + " x= "+this.point[np].vPos.x+" y= "+ this.point[np].vPos.y+" z = "+this.point[np].vPos.z);
}
}
//中心(球だけ中心にも質点)
np = this.numPoint-1;
this.point[np].vPos = this.vPos;
//バネのx,y,z座標(原点に近い質点で定義)と回転角
//バネに接続する質点番号
//横方向
cnt = 0;
for(k = 1; k < this.numStk; k++)
for(j = 0; j < this.numCol; j++)
{
np1 = (k-1)*this.numCol + j + 1;
jp = j + 1;
if(jp == this.numCol) jp = 0;
np2 = (k-1)*this.numCol + jp + 1;
this.structS[cnt] = new Rigid();
this.structS[cnt].vPos = this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
//縦方向
k = 0; //Top
for(j = 0; j < this.numCol; j++)
{
np1 = 0;
np2 = j + 1;
this.structS[cnt] = new Rigid();
//this.structS[cnt].vPos =this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
//中間
for(k = 1; k < this.numStk-1; k++)
{
kp = k + 1;
for(j = 0; j < this.numCol; j++)
{
np1 = (k-1)*this.numCol + j + 1;
np2 = (kp-1)*this.numCol + j + 1;
this.structS[cnt] = new Rigid();
//this.structS[cnt].vPos = this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
}
k = this.numStk-1;//Botom
for(j = 0; j < this.numCol; j++)
{
np1 = (k-1)*this.numCol + j + 1;
np2 = this.numPoint - 2;
this.structS[cnt] = new Rigid();
//this.structS[cnt].vPos = this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
//中心と質点間(中心に質点を)
for(k = 0; k < this.numPoint-1; k++)
{
np1 = k;
np2 = this.numPoint-1;//中心
this.structS[cnt] = new Rigid();
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
//this.structS[cnt].vPos = this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
cnt++;
}
this.numStruct = cnt;
//alert("numStruct = " + this.numStruct);
for(i = 0; i < this.numStruct; i++)
{
this.structS[i].kind = "CYLINDER";
this.structS[i].numSlice = 6;
this.structS[i].radiusRatio = 1 ;
}
//せん断バネ
cnt = 0;
for(k = 1; k < this.numStk-1; k++)
for(j = 0; j < this.numCol; j++)
{
jp = j + 1;
if(jp == this.numCol) jp = 0;
np1 = (k-1) * this.numCol + j + 1;
np2 = k * this.numCol + jp + 1;
this.shearS[cnt] = new Rigid();
//this.shearS[cnt].vPos = this.point[np1].vPos;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
cnt++;
np1 = (k-1) * this.numCol + jp + 1;
np2 = k * this.numCol + j + 1;
this.shearS[cnt] = new Rigid();
//this.shearS[cnt].vPos = this.point[np1].vPos;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
cnt++;
}
this.numShear = cnt;
for(i = 0; i < this.numShear; i++)
{
this.shearS[i].kind = "CYLINDER";
this.shearS[i].numSlice = 6;
this.shearS[i].radiusRatio = 1 ;
}
}
//-----------------------------------------------------------------------------
SpringMass3.prototype.initializeCylinder = function()
{
var j, jp, k, n, np1, np2, cnt;
var phi, rx, ry, h;
rx = this.vSize.x / 2.0;
ry = this.vSize.y / 2.0;
h = this.vSize.z;
lengthZ0 = h / this.numStk;
this.numPoint = (this.numStk+1) * (this.numCol + 1);
//alert(" numPoint = " + this.numPoint);
for(i = 0; i < this.numPoint; i++)
{
this.point[i] = new Rigid();
this.point[i].kind = "SPHERE";
this.point[i].vSize = new Vector3(2.0*this.radius, 2.0*this.radius, 2.0*this.radius);
}
//numStk---z軸方向の個数
//numCol---x-y面(方位角,経度,格段の上底下底の中心にも質点を置く)
//円周の質点個数+1(0番目が中心)
//質点の位置
for(k = 0; k <= this.numStk; k++)
{
//中心軸上
n = k * (this.numCol+1);
this.point[n].vPos.x = 0.0; //x
this.point[n].vPos.y = 0.0; //y
this.point[n].vPos.z = -k * lengthZ0 + h / 2.0;//z
this.point[n].vPos = rotate(this.point[n].vPos, this.vEuler);
this.point[n].vPos.add(this.vPos);
//円周上
for(j = 0; j < this.numCol; j++)
{ //真後ろをj=0
n = k * (this.numCol+1) + j + 1;
phi = 2.0* Math.PI* j / this.numCol;
this.point[n].vPos.x = -rx * Math.cos(phi); //x座標
this.point[n].vPos.y = -ry * Math.sin(phi); //y
this.point[n].vPos.z = -k * lengthZ0 + h / 2.0;//z
this.point[n].vPos = rotate(this.point[n].vPos, this.vEuler);
this.point[n].vPos.add(this.vPos);
}
}
//構造バネのx,y,z座標
//バネに接続する質点番号
cnt = 0;
for(k = 0; k <= this.numStk; k++)
{
//中心と円周上の質点
for(j = 1; j <= this.numCol; j++)
{
np1 = k * (this.numCol + 1);//中心
np2 = k * (this.numCol + 1) + j;
this.structS[cnt] = new Rigid();
//this.structS[cnt].vPos = this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos,this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
//横方向(円周上の質点間)
for(j = 1; j <= this.numCol; j++)
{
np1 = k * (this.numCol+1) + j ;
jp = j + 1;
if(jp == this.numCol+1) jp = 1;
np2 = k * (this.numCol+1) + jp;
this.structS[cnt] = new Rigid();
//this.structS[cnt].vPos =this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos,this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
}
//縦方向(上下の中心間および円周間)
for(k = 0; k < this.numStk; k++)
{
for(j = 0; j <= this.numCol; j++)
{
np1 = k * (this.numCol+1) + j;
np2 = (k+1) * (this.numCol+1) + j;
this.structS[cnt] = new Rigid();
//this.structS[cnt].vPos = this.point[np1].vPos;
this.structS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.structS[cnt].np1 = np1;
this.structS[cnt].np2 = np2;
cnt++;
}
}
this.numStruct = cnt;
for(i = 0; i < this.numStruct; i++)
{
this.structS[i].kind = "CYLINDER";
this.structS[i].numSlice = 6;
this.structS[i].radiusRatio = 1 ;
}
//円周上のせん断バネ
cnt = 0;
for(k = 0; k < this.numStk; k++)
{
for(j = 0; j < this.numCol; j++)
{
jp = j + 1;
if(jp == this.numCol) jp = 0;
np1 = k * (this.numCol+1) + j + 1;
np2 = (k+1) * (this.numCol+1) + jp + 1;
this.shearS[cnt] = new Rigid();
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
cnt++;
np1 = k * (this.numCol+1) + jp + 1;
np2 = (k+1) * (this.numCol+1) + j + 1;
this.shearS[cnt] = new Rigid();
this.shearS[cnt].vPos = this.point[np1].vPos;
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
cnt++;
}
}
/*
//せん断バネ(各要素の中心の反対側と結ぶ)
for(k = 0; k < this.numStk; k++)
{
for(j = 1; j <= this.numCol; j++)
{
jp = this.numCol/2 + j;
if(jp > this.numCol) jp -= this.numCol;
np1 = k * (this.numCol+1) + j;
np2 = (k+1) * (this.numCol+1) + jp;
this.shearS[cnt] = new Rigid();
//this.shearS[cnt].vPos = this.point[np1].vPos;
console.log(" np1 = " + np1 + " np2 = " + np2);
this.shearS[cnt].length0 = distance(this.point[np1].vPos, this.point[np2].vPos);
this.shearS[cnt].np1 = np1;
this.shearS[cnt].np2 = np2;
cnt++;
}
}*/
this.numShear = cnt;
for(i = 0; i < this.numShear; i++)
{
this.shearS[i].kind = "CYLINDER";
this.shearS[i].numSlice = 6;
this.shearS[i].radiusRatio = 1 ;
}
}
<file_sep>/JVEMV6/46_art/VIRTUAL/test_gmail.py
'''###################
file : C:\WORKS_2\WS\WS_Others\prog\D-7\2_2\VIRTUAL\test_gmail.py
at : 2018/02/08 21:19:37
pushd C:\WORKS_2\WS\WS_Others\prog\D-7\2_2\VIRTUAL
python test_gmail.py
###################'''
import sys
import os
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:/WORKS_2/WS/WS_Others/free/fx/82_')
from libs import libs
from libs import libfx
from libs import cons
# from libs_D_7 import cons_D_7
# from libs_31 import libmt
import getopt
import os
import inspect
import math as math
import struct
from shutil import copyfile
import xml.etree.ElementTree as ET
from smtplib import SMTP
from poplib import POP3_SSL
import imaplib, re, email, six, dateutil.parser
def exec_prog():
user = 'iwabuchik2010'
passwd = '<PASSWORD>'
gmail = imaplib.IMAP4_SSL("imap.gmail.com")
gmail.login(user, passwd)
# gmail.login("user","password")
gmail.close()
gmail.logout()
# File "C:\WORKS_2\Programs\Python\Python_3.5.1\lib\imaplib.py", line 582, in lo
# gin
# raise self.error(dat[-1])
# imaplib.error: b'[AUTHENTICATIONFAILED] Invalid credentials (Failure)'
# host, port = 'pop.gmail.com', 995
#
# user = 'iwabuchik2010'
# passwd = '<PASSWORD>'
#
# popServ = POP3_SSL(host)
# popServ.user(user)
# popServ.pass_(passwd)
#
# print()
# print("[%s:%d] popServ.pass_(passwd) => done" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# msg_ct, mbox_siz = popServ.stat()
#
# rsp, msg, siz = popServ.retr(msg_ct)
#
# for line in msg:
#
# print(line)
#
# popServ.quit()
# File "C:\WORKS_2\Programs\Python\Python_3.5.1\lib\poplib.py", line 152, in _ge
# tresp
# raise error_proto(resp)
# poplib.error_proto: b'-ERR [AUTH] Username and password not accepted.'
return None
#/def exec_prog():
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print ("[%s:%d] all done" % (os.path.basename(os.path.basename(libs.thisfile())), libs.linenum()))
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgTexture.js
//--------------------------------------------------
//kwgTexture.js
//--------------------------------------------------
function makeCubeTex(vertices, texCoords, normals, indices, flagDebug, nRepeatS, nRepeatT)
{
// 6面にテクスチャをマッピング
// 1辺が1の立方体を生成する
// p2----- p1
// /| /|
// p3------p0|
// | | | |
// | |p6---|-|p5
// |/ |/
// p7------p4
// 頂点座標
var vv = [
0.5, 0.5, 0.5, -0.5, 0.5, 0.5, -0.5,-0.5, 0.5, 0.5,-0.5, 0.5, //p0-p1-p2-p3 上(0,1,2,3)
0.5, 0.5, 0.5, 0.5,-0.5, 0.5, 0.5,-0.5,-0.5, 0.5, 0.5,-0.5, //p0-p3-p7-p4 前(4,5,6,7)
0.5, 0.5, 0.5, 0.5, 0.5,-0.5, -0.5, 0.5,-0.5, -0.5, 0.5, 0.5, //p0-p4-p5-p1 右(8,9,10,11)
-0.5, 0.5, 0.5, -0.5, 0.5,-0.5, -0.5,-0.5,-0.5, -0.5,-0.5, 0.5, //p1-p5-p6-p2 奥(12,13,14,15)
-0.5,-0.5,-0.5, 0.5,-0.5,-0.5, 0.5,-0.5, 0.5, -0.5,-0.5, 0.5, //p6-p7-p3-p2 左(16,17,18,19)
0.5, 0.5,-0.5, 0.5,-0.5,-0.5, -0.5,-0.5,-0.5, -0.5, 0.5,-0.5 //p4-p7-p6-p5 下(20,21,22,23)
];
for(var i = 0; i < vv.length; i++) vertices[i] = vv[i];
//テクスチャ座標
var s = nRepeatS;
var t = nRepeatT;
var tt = [ s, 0, s, t, 0, t, 0, 0,
s, t, 0, t, 0, 0, s, 0,
0, t, 0, 0, s, 0, s, t,
0, t, 0, 0, s, 0, s, t,
0, 0, s, 0, s, t, 0, t,
s, t, 0, t, 0, 0, s, 0
];
for(var i = 0; i < tt.length; i++) texCoords[i] = tt[i];
// 法線
var nn = [
0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, //p0-p1-p2-p3 上
1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, //p0-p3-p7-p4 前
0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, //p0-p4-p5-p1 右
-1.0, 0.0, 0.0, -1.0, 0.0, 0.0, -1.0, 0.0, 0.0, -1.0, 0.0, 0.0, //p1-p5-p6-p2 奥
0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, //p6-p7-p3-p2 左
0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0 //p4-p7-p6-p5 下
];
for(var i = 0; i < nn.length; i++) normals[i] = nn[i];
// インデックス
if(flagDebug == false)
{//各三角形頂点に対するインデックス
var ii = [
0, 1, 2, 0, 2, 3, // 上
4, 5, 6, 4, 6, 7, // 前
8, 9,10, 8,10,11, // 右
12,13,14, 12,14,15, // 奥
16,17,18, 16,18,19, // 左
20,21,22, 20,22,23 // 下
];
for(var i = 0; i < ii.length; i++) indices[i] = ii[i];
}
else
{
var i2 = [
0, 1, 2, 3,
4, 5, 6, 7,
8, 9, 10, 11,
12, 13, 14, 15,
16, 17, 18, 19,
22, 23, 20, 21
];
for(var i = 0; i < i2.length; i++) indices[i] = i2[i];
}
return indices.length;
}
//---------------------------------------------------------------------------------------------
function makeCubeBump(vertices, texCoords, normals, indices, flagDebug, nRepeatS, nRepeatT)
{ //バンプマッピング用
// 側面4面にテクスチャをマッピング
// 1辺が1の立方体を生成する
// p2----- p1
// /| /|
// p3------p0|
// | | | |
// | |p6---|-|p5
// |/ |/
// p7------p4
// 頂点座標
var vv = [
0.5, 0.5, 0.5, -0.5, 0.5, 0.5, -0.5,-0.5, 0.5, 0.5,-0.5, 0.5, //p0-p1-p2-p3 上(0,1,2,3)
0.5, 0.5, 0.5, 0.5,-0.5, 0.5, 0.5,-0.5,-0.5, 0.5, 0.5,-0.5, //p0-p3-p7-p4 前(4,5,6,7)
0.5, 0.5, 0.5, 0.5, 0.5,-0.5, -0.5, 0.5,-0.5, -0.5, 0.5, 0.5, //p0-p4-p5-p1 右(8,9,10,11)
-0.5, 0.5, 0.5, -0.5, 0.5,-0.5, -0.5,-0.5,-0.5, -0.5,-0.5, 0.5, //p1-p5-p6-p2 奥(12,13,14,15)
-0.5,-0.5,-0.5, 0.5,-0.5,-0.5, 0.5,-0.5, 0.5, -0.5,-0.5, 0.5, //p6-p7-p3-p2 左(16,17,18,19)
0.5, 0.5,-0.5, 0.5,-0.5,-0.5, -0.5,-0.5,-0.5, -0.5, 0.5,-0.5 //p4-p7-p6-p5 下(20,21,22,23)
];
for(var i = 0; i < vv.length; i++) vertices[i] = vv[i];
//テクスチャ座標
var s = nRepeatS;
var t = nRepeatT;
var tt = [
0, 0, 0, 0, 0, 0, 0, 0,//上
s, t, 0, t, 0, 0, s, 0,
0, t, 0, 0, s, 0, s, t,
0, t, 0, 0, s, 0, s, t,
0, 0, s, 0, s, t, 0, t,
0, 0, 0, 0, 0, 0, 0, 0//下
];
for(var i = 0; i < tt.length; i++) texCoords[i] = tt[i];
// 法線
var nn = [
0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, //p0-p1-p2-p3 上
1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, //p0-p3-p7-p4 前
0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, //p0-p4-p5-p1 右
-1.0, 0.0, 0.0, -1.0, 0.0, 0.0, -1.0, 0.0, 0.0, -1.0, 0.0, 0.0, //p1-p5-p6-p2 奥
0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, //p6-p7-p3-p2 左
0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0, 0.0, 0.0,-1.0 //p4-p7-p6-p5 下
];
for(var i = 0; i < nn.length; i++) normals[i] = nn[i];
// インデックス
if(flagDebug == false)
{//各三角形頂点に対するインデックス
var ii = [
0, 1, 2, 0, 2, 3, // 上
4, 5, 6, 4, 6, 7, // 前
8, 9,10, 8,10,11, // 右
12,13,14, 12,14,15, // 奥
16,17,18, 16,18,19, // 左
20,21,22, 20,22,23 // 下
];
for(var i = 0; i < ii.length; i++) indices[i] = ii[i];
}
else
{
var i2 = [
0, 1, 2, 3,
4, 5, 6, 7,
8, 9, 10, 11,
12, 13, 14, 15,
16, 17, 18, 19,
22, 23, 20, 21
];
for(var i = 0; i < i2.length; i++) indices[i] = i2[i];
}
return indices.length;
}
//-----------------------------------------------
function makeSphereTex(vertices, texCoords, normals, indices, nSlice, nStack, nRepeatS, nRepeatT)
{
//直径が1の球(鉛直軸はz)に球投影
//nSlice:経度方向(i)分割数
//nStack:緯度方向(j)分割数
var i, phi, si, ci;
var j, theta, sj, cj;
var r = 0.5;
var s, t;//テクスチャ座標
// 頂点座標を生成する
for (j = 0; j <= nStack; j++)
{
theta = j * Math.PI / nStack;
sj = r * Math.sin(theta);
cj = r * Math.cos(theta);
//北極点を1とするt座標
t = (1.0 - j / nStack) * nRepeatT;
for (i = 0; i <= nSlice; i++)
{
phi = i * 2 * Math.PI / nSlice;
si = Math.sin(phi);
ci = Math.cos(phi);
vertices.push(sj * ci);//x
vertices.push(sj * si);//y
vertices.push(cj); //z
//テクスチャのs座標
s = i * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
}
}
var k1, k2;
// インデックスを生成する
for (j = 0; j < nStack; j++)
{
for (i = 0; i < nSlice; i++)
{
k1 = j * (nSlice+1) + i;
k2 = k1 + (nSlice+1);
indices.push(k1);
indices.push(k2);
indices.push(k1 + 1);
indices.push(k1 + 1);
indices.push(k2);
indices.push(k2 + 1);
}
}
// 頂点座標と法線は同じものが使える
for(var i = 0; i < vertices.length; i++) normals[i] = vertices[i];
return indices.length;
}
//----------------------------------------------------------------------
function makeCylinderTex(vertices, texCoords, normals, indices, radiusRatio, nSlice, flagDebug, nRepeatS, nRepeatT)
{
//側面に円筒投影
//半径0.5,高さ1.0の円柱
//円柱(rBottom=rTop))、円錐台、円錐(rTop = 0.0)
//nSlice--xy断面分割数
var rBottom = 0.5;//下底半径
var rTop = rBottom * radiusRatio;//上底半径
var height = 1.0;//高さ
//物体の中心は下底と上底の中間
var i, j;
var phi;
var phi0 = 2.0*Math.PI/nSlice;
var s, t;
//上底(Top)
vertices[0] = 0.0; vertices[1] = 0.0; vertices[2] = height/2.0; //中心点
normals[0] = 0.0; normals[1] = 0.0; normals[2] = 1.0;
//側面にだけテクスチャをマッピングしますが,上下の頂点にもテクスチャ座標を与えないときは
//gl.drawElementsでGL_INVALID_OPERATIONのエラーになる
texCoords.push(0);
texCoords.push(0);
for(i = 0; i <= nSlice; i++)
{
phi = i * phi0;
vertices.push(rTop * Math.cos(phi));//x
vertices.push(rTop * Math.sin(phi));//y
vertices.push(height/2.0); //z
normals.push(0.0); //x
normals.push(0.0); //y
normals.push(1.0); //z
//空のデータをテクスチャ座標に与える
texCoords.push(0);
texCoords.push(0);
}
//側面(Side)
var rr = rBottom - rTop;//半径差
var ss = Math.sqrt(rr*rr + height*height);//斜辺
var nz = rr / ss;
var nxy = height / ss;
var hh, r0;
for(j = 0; j <= 1; j++)
{
if(j == 0) { hh = height / 2.0; r0 = rTop; }
else { hh = - height / 2.0; r0 = rBottom; }
t = (1 - j) * nRepeatT;//テクスチャ t 座標
for(i = 0; i <= nSlice; i++)
{
phi = i * phi0;
vertices.push(r0 * Math.cos(phi));//x座標
vertices.push(r0 * Math.sin(phi));//y座標
vertices.push(hh); //z座標
s = i * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
//法線ベクトル
normals.push(nxy * Math.cos(phi));//x
normals.push(nxy * Math.sin(phi));//y
normals.push(nz); //z
}
}
var nd = vertices.length;//これまでの頂点データ個数
//下底(Bottom)
vertices[nd] = 0.0; vertices[nd+1] = 0.0; vertices[nd+2] = -height/2.0; //中心点
normals[nd] = 0.0; normals[nd+1] = 0.0; normals[nd+2] = -1.0;
//空のデータをテクスチャ座標に与える
texCoords.push(0);
texCoords.push(0);
for(i = 0; i <= nSlice; i++)
{
phi = -i * phi0;//時計回り
vertices.push(rBottom * Math.cos(phi));//x
vertices.push(rBottom * Math.sin(phi));//y
vertices.push(-height/2.0); //z
normals.push( 0.0); //x
normals.push( 0.0); //y
normals.push(-1.0); //z
//空のデータをテクスチャ座標に与える
texCoords.push(0);
texCoords.push(0);
}
//index
if(flagDebug == false)
{
//Top
for(var i = 0; i < nSlice; i++)
{
indices.push(0); indices.push(i+1); indices.push(i+2);
}
for(i = 0; i < nSlice; i++)
{//各面に三角形要素が2つ
indices.push(nSlice + 2 + i);
indices.push(2*nSlice + 3 + i);
indices.push(nSlice + 3 + i);
indices.push(2*nSlice + 3 + i);
indices.push(2*nSlice + 4 + i);
indices.push(nSlice + 3 + i);
}
//Bottom
var nv = nd / 3; //中心点の頂点番号
for(i = 0; i < nSlice; i++)
{
indices.push(nv); indices.push(nv+i+1); indices.push(nv+i+2);
}
}
else //wireframe
{//側面だけでよい
for(i = 0; i < nSlice; i++)
{
indices.push(nSlice + 2 + i);
indices.push(2*nSlice + 3 + i);
indices.push(2*nSlice + 4 + i);
indices.push(nSlice + 3 + i);
indices.push(nSlice + 2 + i);
}
}
return indices.length;
}
//----------------------------------------------------------------------
function makePrismTex(vertices, texCoords, normals, indices, radiusRatio, nSlice, flagDebug, nRepeatS, nRepeatT)
{
//円筒投影でテクスチャマッピング
//半径0.5,高さ1.0の円柱に内接する多角柱
//多角柱(rBottom=rTop))、多角錐台、多角錐(rTop = 0.0)
//nSlice--xy断面分割数
var rBottom = 0.5;//下底半径
var rTop = rBottom * radiusRatio;//上底半径
var height = 1.0;//高さ
var i, j;
var phi, phi2;
var phi0 = 2.0 * Math.PI/nSlice;
var s, t;
//上底(Top)
vertices[0] = 0.0; vertices[1] = 0.0; vertices[2] = height/2.0; //中心点
normals[0] = 0.0; normals[1] = 0.0; normals[2] = 1.0;
//側面にだけテクスチャをマッピングしますが,
//上下の頂点にもテクスチャ座標を与えないと
//gl.drawElementsでGL_INVALID_OPERATIONのエラーになる
texCoords.push(0);
texCoords.push(0);
for(i = 0; i <= nSlice; i++)
{
phi = i * phi0 + phi0/2;//平らな面が正面を向くようにphi0/2を追加
vertices.push(rTop * Math.cos(phi));//x
vertices.push(rTop * Math.sin(phi));//y
vertices.push(height/2.0); //z
normals.push(0.0); //x
normals.push(0.0); //y
normals.push(1.0); //z
//空のデータをテクスチャ座標に与える
texCoords.push(0);
texCoords.push(0);
}
//側面(Side)
var alpha = (nSlice - 2)*Math.PI / (2.0 * nSlice);
var rr = (rBottom - rTop) * Math.sin(alpha);//半径差
var ss = Math.sqrt(rr*rr + height*height);//斜辺
var nz = rr / ss;
var nxy = height / ss;
var hh, r0;
for(j = 0; j <= 1; j++)
{
//半径
if(j == 0){ r0 = rTop; hh = height/2.0; }
else {r0 = rBottom; hh = -height/2.0; }
t = (1 - j) * nRepeatT;
for(i = 0; i < nSlice; i++)
{
//1つの頂点に番号を2個必要
phi = i * phi0 + phi0/2;
phi2 = phi + phi0/2.0;
//座標
vertices.push(r0 * Math.cos(phi));//x座標(外部から見て左側)
vertices.push(r0 * Math.sin(phi));//y座標
vertices.push(hh); //z座標
s = (i+0.5) * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
vertices.push(r0 * Math.cos(phi+phi0));//x座標(右側)
vertices.push(r0 * Math.sin(phi+phi0));//y座標
vertices.push(hh); //z座標
s = (i+1.5) * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
//法線ベクトル(隣り合う頂点は同じ法線ベクトル)
normals.push(nxy * Math.cos(phi2));//x
normals.push(nxy * Math.sin(phi2));//y
normals.push(nz); //z
normals.push(nxy * Math.cos(phi2));//x
normals.push(nxy * Math.sin(phi2));//y
normals.push(nz); //z
}
}
var nd = vertices.length;//これまでの頂点データ個数
//下底(Bottom)
vertices[nd] = 0.0; vertices[nd+1] = 0.0; vertices[nd+2] = -height/2.0; //中心点
normals[nd] = 0.0; normals[nd+1] = 0.0; normals[nd+2] = -1.0;
texCoords.push(0);
texCoords.push(0);
for(i = 0; i <= nSlice; i++)
{
phi = -i * phi0 - phi0/2;//時計回り
vertices.push(rBottom * Math.cos(phi));//x
vertices.push(rBottom * Math.sin(phi));//y
vertices.push(-height/2.0); //z
normals.push( 0.0); //x
normals.push( 0.0); //y
normals.push(-1.0); //z
//空のデータをテクスチャ座標に与える
texCoords.push(0);
texCoords.push(0);
}
//index
if(flagDebug == false)
{
//Top
for(var i = 0; i < nSlice; i++)
{
indices.push(0); indices.push(i+1); indices.push(i+2);
}
//Side
for(i = 0; i < nSlice; i++)
{//各面に三角形要素が2つ
indices.push(nSlice + 2 + i*2);
indices.push(3 * nSlice + 2 + i*2);
indices.push(nSlice + 3 + i*2);
indices.push(3 * nSlice + 2 + i*2);
indices.push(3 * nSlice + 3 + i*2);
indices.push(nSlice + 3 + i*2);
}
//Bottom
var nv = nd / 3; //中心点の頂点番号
for(i = 0; i < nSlice; i++)
{
indices.push(nv); indices.push(nv+i+1); indices.push(nv+i+2);
}
}
else//wireframe
{
//Top
for(i = 0; i < nSlice; i++)
{
indices.push(nSlice + 2 + i*2);
indices.push(3 * nSlice + 2 + i*2);
indices.push(3 * nSlice + 3 + i*2);
indices.push(nSlice + 3 + i*2);
indices.push(nSlice + 2 + i*2);
}
}
return indices.length;
}
//---------------------------------------------
function makeTorusTex(vertices, texCoords, normals, indices, radiusRatio, nSlice, nStack, nRepeatS, nRepeatT)
{
var radius1 = 0.5;//円環の中心軸半径(主半径)
var radius2 = radiusRatio * radius1;//断面半径(副半径)
//nSlice:円環断面における表面分割点数
//nStack:円環の分割数
if(radiusRatio > 1.0) { printf("radiusRatio < 1 としてください"); return;}
var i, j;
var rr, zz;
var theta0, theta1, phi;
var s, t;
//頂点座標,法線ベクトル
for(j = 0; j <= nStack; j++)
{
//i=0は基本断面(x=radius1を中心とする円, y=0)
theta0 = 2.0 * Math.PI * j / nStack;
s = j * nRepeatS / nStack;//s座標
for(i = 0; i <= nSlice; i++)
{
phi = -Math.PI + 2.0 * Math.PI * i / nSlice;
rr = radius1 + radius2 * Math.cos(phi);//z軸からの距離
zz = radius2 * Math.sin(phi);
//頂点のxyz座標(i=0を内側xy平面)
vertices.push(rr * Math.cos(theta0));//x座標
vertices.push(rr * Math.sin(theta0));//y
vertices.push(zz); //z
t = i * nRepeatT / nSlice;
texCoords.push(s);
texCoords.push(t);
normals.push(Math.cos(phi)*Math.cos(theta0));//x
normals.push(Math.cos(phi)*Math.sin(theta0));//y
normals.push(Math.sin(phi)); //z
}
}
//インデックス
for(j = 0; j < nStack; j++)
{
for(i = 0; i < nSlice; i++)
{
indices.push((nSlice+1) * j + i);
indices.push((nSlice+1) * (j+1) + i);
indices.push((nSlice+1) * j + i+1);
indices.push((nSlice+1) * (j+1) + i);
indices.push((nSlice+1) * (j+1) + i+1);
indices.push((nSlice+1) * j + i+1);
}
}
return indices.length;
}
//-----------------------------------------------------------------
function makeSuperTex(vertices, texCoords, normals, indices, nSlice, nStack, eps1, eps2, nRepeatS, nRepeatT)
{
//上下の中心が原点
var i,j,ip,im,np,npL,npR,npU,npD,k;
var ct,theta,phi,z,cc, ss, gg;
var r = 0.5;//基本球の半径
var s, t;//テクスチャ座標
//頂点座標
//j == 0;//Top(nSlice+1個)
for (i = 0 ;i <= nSlice; i++)
{
vertices.push(0.0);//x
vertices.push(0.0);//y
vertices.push(r); //z
s = i * nRepeatS / nSlice; t = nRepeatT;
texCoords.push(s);
texCoords.push(t);
}
for(j = 1 ;j < nStack;j++)
{
//北極点を1とするt座標
t = (1.0 - j / nStack) * nRepeatT;
theta = (Math.PI/nStack) * (nStack / 2.0 - j);
//thetaはx-y平面からの偏角となっている
if(theta >= 0.0) //上半分
{
z = r * Math.pow(Math.sin(Math.abs(theta)),eps1);
}
else//下半分
{
z = - r * Math.pow(Math.sin(Math.abs(theta)), eps1);
}
for (i = 0;i <= nSlice; i++)
{
phi = 2.0 * Math.PI * i/nSlice;
ct = Math.cos(phi);
if (ct >= 0) { cc = Math.pow(ct, eps2);}
else { cc = -Math.pow(Math.abs(ct),eps2); }
//座標
vertices.push(r * Math.pow(Math.cos(theta),eps1)*cc);//x
if(i == 0 || i == nSlice/2 || i == nSlice) vertices.push(0.0);//y
else
{
ss = Math.sin(phi);
gg = Math.pow(Math.abs(ss), eps2);
if(i > nSlice/2) gg = -gg;
vertices.push(r * Math.pow(Math.cos(theta),eps1) * gg);//y
}
vertices.push(z);//z
s = i * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
}//i
}//j
//j = nStack:Bottom(nSlice+1個)
for(i = 0; i <= nSlice; i++)
{
vertices.push(0.0);//x
vertices.push(0.0);//y
vertices.push(-r); //z
s = i * nRepeatS / nSlice; t = 0;
texCoords.push(s);
texCoords.push(t);
}
var p1 = [], p2 = [], p3 = [];
var n1 = [], n2 = [], n3 = [], n4 = [];
//法線ベクトル
//Top
for(i = 0;i <= nSlice;i++)
{
normals.push(0.0);//x
normals.push(0.0);//y
normals.push(1.0);//z
}
//Side
for(j = 1;j < nStack;j++)//隣り合う4個の三角形の法線ベクトルを平均化
{
for(i = 0;i <= nSlice;i++)
{
ip = i+1;
if(ip == nSlice+1) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = j*(nSlice+1)+i;//注目点
npL = j*(nSlice+1)+im;//左側
npR = j*(nSlice+1)+ip;//右側
npU = np-nSlice-1;//上
npD = np+nSlice+1;//下
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npU]; p2[1]=vertices[3*npU+1]; p2[2]=vertices[3*npU+2];
p3[0]=vertices[3*npL]; p3[1]=vertices[3*npL+1]; p3[2]=vertices[3*npL+2];
calcNormal(p1,p2,p3,n1);//外から見て左上
p2[0]=vertices[3*npR]; p2[1]=vertices[3*npR+1]; p2[2]=vertices[3*npR+2];
p3[0]=vertices[3*npU]; p3[1]=vertices[3*npU+1]; p3[2]=vertices[3*npU+2];
calcNormal(p1,p2,p3,n2);//右上
//p1[0]=vertices[3*np]; p1[1]=vertices[3*np+1]; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npL]; p2[1]=vertices[3*npL+1]; p2[2]=vertices[3*npL+2];
p3[0]=vertices[3*npD]; p3[1]=vertices[3*npD+1]; p3[2]=vertices[3*npD+2];
calcNormal(p1,p2,p3,n3);//外から見て左下
p2[0]=vertices[3*npD]; p2[1]=vertices[3*npD+1]; p2[2]=vertices[3*npD+2];
p3[0]=vertices[3*npR]; p3[1]=vertices[3*npR+1]; p3[2]=vertices[3*npR+2];
calcNormal(p1,p2,p3,n4);//右下
normals.push((n1[0]+n2[0]+n3[0]+n4[0])/4.0);//x方向
normals.push((n1[1]+n2[1]+n3[1]+n4[1])/4.0);//y
normals.push((n1[2]+n2[2]+n3[2]+n4[2])/4.0);//z
}
}
//Bottom
for(i = 0;i <= nSlice;i++)
{
normals.push(0.0); //x
normals.push(0.0); //y
normals.push(-1.0);//z
}
//インデックス
var k1, k2;
for (j = 0; j < nStack; j++)
{
for (i = 0; i < nSlice; i++)
{
k1 = j * (nSlice+1) + i;
k2 = k1 + (nSlice+1);
indices.push(k1);
indices.push(k2);
indices.push(k1 + 1);
indices.push(k2);
indices.push(k2 + 1);
indices.push(k1 + 1);
}
}
return indices.length;
}
//----------------------------------------------------------------
//法線方向計算ルーチン
function calcNormal(p1, p2, p3, nn)
{
var A = new Vector3(p2[0] - p1[0], p2[1] - p1[1], p2[2] - p1[2]);
var B = new Vector3(p3[0] - p2[0], p3[1] - p2[1], p3[2] - p2[2]);
var n = cross(A , B);//外積
n.norm();
nn[0] = n.x; nn[1] = n.y; nn[2] = n.z;
}
//--------------------------------------------------------
function makePlateZTex(vertices, texCoords, normals, indices, flagDebug, nRepeatS, nRepeatT)
{
var i;
//1辺が1.0の正方形
//vertices
var vv = [ 0.5, 0.5, 0.0,
-0.5, 0.5, 0.0,
-0.5,-0.5, 0.0,
0.5,-0.5, 0.0,
0.5, 0.5, 0.0];
for(i = 0; i < vv.length; i++) vertices[i] = vv[i];
//texCoords
var s = nRepeatS;
var t = nRepeatT;
var tt = [ s, 0,
s, t,
0, t,
0, 0,
s, 0
];
for(i = 0; i < tt.length; i++) texCoords[i] = tt[i];
//normals
for(i = 0; i < vv.length; i++)
{
normals.push(0.0); normals.push(0.0); normals.push(1.0);
}
//indices
if(flagDebug == false)
{
var ii = [ 0, 1, 2,
0, 2, 3];
for(var i = 0; i < ii.length; i++) indices[i] = ii[i];
}
else
{
var i2 = [ 0, 1, 2, 3, 4];
for(i = 0; i < i2.length; i++) indices[i] = i2[i];
}
return indices.length;
}
//-------------------------------------------------------------------------------------------------------
function makeGridPlateTex(vertices, texCoords, normals, indices, nSliceX, nSliceY, flagDebug, nRepeatS, nRepeatT)
{//1辺が1,xy平面,中心は原点(y方向をs座標に、負のx軸方向をt座標にしている)
var i, j;
var pitchX = 1.0 / nSliceX;
var pitchY = 1.0 / nSliceY;
var s = nRepeatS * pitchY;//y方向をs
var t = nRepeatT * pitchX;//-x方向をt
//verdices, normals, texCoords
for(i = 0; i <= nSliceX; i++)
{
for(j = 0; j <= nSliceY; j++)
{
//頂点座標
vertices.push(-i * pitchX + 0.5);//x
vertices.push(j * pitchY - 0.5); //y
vertices.push(0.0); //z
//テクスチャ座標
texCoords.push(j * s);//s
texCoords.push(i * t);//t
//法線
normals.push(0.0);//x
normals.push(0.0);//y
normals.push(1.0);//z
}
}
//indices
if(!flagDebug)
{
for(j = 0; j < nSliceY; j++)
{
for(i = 0; i < nSliceX; i++)
{
indices.push((nSliceY+1) * i + j);
indices.push((nSliceY+1) * i + j+1);
indices.push((nSliceY+1) * (i+1) + j+1);
indices.push((nSliceY+1) * i + j);
indices.push((nSliceY+1) * (i+1) + j+1);
indices.push((nSliceY+1) * (i+1) + j);
}
}
}
else
{
for(j = 0; j < nSliceY; j++)
{
for(i = 0; i < nSliceX; i++)
{
indices.push((nSliceY+1) * i + j); indices.push((nSliceY+1) * i + j+1);
indices.push((nSliceY+1) * i + j+1);indices.push((nSliceY+1) * (i+1) + j+1);
indices.push((nSliceY+1) * (i+1) + j+1);indices.push((nSliceY+1) * i + j);
indices.push((nSliceY+1) * (i+1) + j+1);indices.push((nSliceY+1) * (i+1) + j);
indices.push((nSliceY+1) * (i+1) + j);indices.push((nSliceY+1) * i + j);
}
}
}
return indices.length;
}
//--------------------------------------------------------------------------------------------------
function makeGridSquareTex(data, vertices, texCoords, normals, indices, nSliceX, nSliceY, flagDebug, nRepeatS, nRepeatT)
{
//例えば2次元バネ-質点モデル全体を1つの四辺形で表現
//data[k][0]~data[k][2]で格子点iのx,y,z成分が与えられる。
var s = nRepeatS / nSliceY;//y方向をs
var i, j, k;
//頂点座標vertices
for(j = 0; j <= nSliceY; j++)
{
for(i = 0; i <= nSliceX; i++)
{
k = i + j * (nSliceX + 1);
//座標
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
//テクスチャ座標
texCoords.push(j * s);//s
texCoords.push((1 - i/nSliceX) * nRepeatT);//t
}
}
//法線normals
//角は1つの三角形、辺は2つの三角形、内部は4個の三角形の平均
var np, npL, npR, npU, npL;
var n1 = [], n2 = [], n3 = [], n4 = [];
for(j = 0;j <= nSliceY;j++)
{
if(j == 0)
{
//i == 0
np = 0; npD = 1; npR = nSliceX+1;
calcNormal(data[np], data[npD], data[npR], n1);
normals.push(n1[0]);//x
normals.push(n1[1]);//y
normals.push(n1[2]);//z
for(i = 1; i < nSliceX; i++)
{//2つの三角形の平均
np = i; npR = i+nSliceX+1; npU = i-1; npD = i+1;
calcNormal(data[np], data[npR], data[npU], n1);
calcNormal(data[np], data[npD], data[npR], n2);
normals.push((n1[0] + n2[0]) / 2);//x
normals.push((n1[1] + n2[1]) / 2);//y
normals.push((n1[2] + n2[2]) / 2);//z
}
//i == nSliceX
np = nSliceX; npR = 2*nSliceX+1; npU = nSliceX-1;
calcNormal(data[np], data[npD], data[npR], n1);
normals.push(n1[0]);//x
normals.push(n1[1]);//y
normals.push(n1[2]);//z
}
else if(j < nSliceY)
{
//i = 0
//2つの三角形の平均
np = j * (nSliceX + 1); npL = np-(nSliceX+1); npD = np+1; npR = np+(nSliceX+1);
calcNormal(data[np], data[npL], data[npD], n1);
calcNormal(data[np], data[npD], data[npR], n2);
normals.push((n1[0] + n2[0]) / 2);//x
normals.push((n1[1] + n2[1]) / 2);//y
normals.push((n1[2] + n2[2]) / 2);//z
for(i = 1; i < nSliceX; i++)//
{ //隣り合う4個の三角形の法線ベクトルを平均化
np = i+j*(nSliceX+1); npU = np-1; npD = np+1;
npL = i+(j-1)*(nSliceX+1); npR = i+(j+1)*(nSliceX+1);
calcNormal(data[np], data[npU], data[npL], n1);
calcNormal(data[np], data[npL], data[npD], n2);
calcNormal(data[np], data[npD], data[npR], n3);
calcNormal(data[np], data[npR], data[npU], n4);
normals.push((n1[0] + n2[0] + n3[0] + n4[0]) / 4);//x
normals.push((n1[1] + n2[1] + n3[1] + n4[1]) / 4);//y
normals.push((n1[2] + n2[2] + n3[2] + n4[2]) / 4);//z
}
//i = nSliceX;
//2つの三角形の平均
np = nSliceX+j*(nSliceX+1); npL = np-(nSliceX+1); npU = np-1; npR = np+(nSliceX+1);
calcNormal(data[np], data[npU], data[npL], n1);
calcNormal(data[np], data[npR], data[npU], n2);
normals.push((n1[0] + n2[0]) / 2);//x
normals.push((n1[1] + n2[1]) / 2);//y
normals.push((n1[2] + n2[2]) / 2);//z
}
else//j= nSliceY
{
//i == 0
np = nSliceY*(nSliceX+1); npL = (nSliceY-1)*(nSliceX+1); npD = np+1;
calcNormal(data[np], data[npL], data[npD], n1);
normals.push(n1[0]);//x
normals.push(n1[1]);//y
normals.push(n1[2]);//z
for(i = 1; i < nSliceX; i++)
{//2つの三角形の平均
np = i+nSliceY*(nSliceX+1); npL = np-(nSliceX+1); npU = np-1; npD = np+1;
calcNormal(data[np], data[npU], data[npL], n1);
calcNormal(data[np], data[npL], data[npD], n2);
normals.push((n1[0] + n2[0]) / 2);//x
normals.push((n1[1] + n2[1]) / 2);//y
normals.push((n1[2] + n2[2]) / 2);//z
}
//i == nSliceX
np = nSliceX+nSliceY*(nSliceX+1); npU = np-1; npL = np-(nSliceX+1);
calcNormal(data[np], data[npU], data[npL], n1);
normals.push(n1[0]);//x
normals.push(n1[1]);//y
normals.push(n1[2]);//z
}
}
//indices
var np0, np1, np2, np3;
//if(flagDebug)//solid model
for(j = 0; j < nSliceY; j++)
{
for(i = 0; i < nSliceX; i++)
{
np0 = i + j*(nSliceX+1); np1 = np0+1; np2 = np1+nSliceX+1; np3 = np2-1;
if(flagDebug == false)//solid model
{
indices.push(np0); indices.push(np1); indices.push(np3);
indices.push(np1); indices.push(np2); indices.push(np3);
}
else//wireframe model
{
indices.push(np0); indices.push(np1); indices.push(np1); indices.push(np3);
indices.push(np3); indices.push(np0); indices.push(np1); indices.push(np2);
indices.push(np2); indices.push(np3);
}
}
}
return indices.length;
}
//-----------------------------------------------------------------
function makeGridSphereTex(data, vertices, texCoords, normals, indices, nSlice, nStack, nRepeatS, nRepeatT)
{
//上下の中心が原点
var i,j;//,ip,im,np,npL,npR,npU,npD,k;
var s, t;//テクスチャ座標
//頂点座標
//j == 0;//Top(nSlice+1個)
for (i = 0 ;i <= nSlice; i++)
{
vertices.push(data[0][0]);//x
vertices.push(data[0][1]);//y
vertices.push(data[0][2]);//z
s = i * nRepeatS / nSlice; t = nRepeatT;
texCoords.push(s);
texCoords.push(t);
}
for(j = 1 ;j < nStack;j++)
{
//北極点を1とするt座標
t = (1.0 - j / nStack) * nRepeatT;
for(i = 0 ; i <= nSlice; i++)
{
var i0 = i;
if(i == nSlice) i0 = 0;
k = i0 + (j-1) * nSlice + 1;
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
s = i * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
}
}
k = data.length - 1;
for(i = 0; i <= nSlice; i++)
{
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
s = i * nRepeatS / nSlice; t = 0;
texCoords.push(s);
texCoords.push(t);
}
var p1 = [], p2 = [], p3 = [];
var n1 = [], n2 = [], n3 = [], n4 = [];
//法線ベクトル
//Top
for(i = 0;i <= nSlice;i++)
{
normals.push(0.0);//x
normals.push(0.0);//y
normals.push(0.0);//z
}
//Side
for(j = 1;j < nStack;j++)//隣り合う4個の三角形の法線ベクトルを平均化
{
for(i = 0;i <= nSlice;i++)
{
ip = i+1;
if(ip == nSlice+1) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = j*(nSlice+1)+i;//注目点
npL = j*(nSlice+1)+im;//左側
npR = j*(nSlice+1)+ip;//右側
npU = np-nSlice-1;//上
npD = np+nSlice+1;//下
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npU]; p2[1]=vertices[3*npU+1]; p2[2]=vertices[3*npU+2];
p3[0]=vertices[3*npL]; p3[1]=vertices[3*npL+1]; p3[2]=vertices[3*npL+2];
calcNormal(p1,p2,p3,n1);//外から見て左上
p2[0]=vertices[3*npR]; p2[1]=vertices[3*npR+1]; p2[2]=vertices[3*npR+2];
p3[0]=vertices[3*npU]; p3[1]=vertices[3*npU+1]; p3[2]=vertices[3*npU+2];
calcNormal(p1,p2,p3,n2);//右上
p2[0]=vertices[3*npL]; p2[1]=vertices[3*npL+1]; p2[2]=vertices[3*npL+2];
p3[0]=vertices[3*npD]; p3[1]=vertices[3*npD+1]; p3[2]=vertices[3*npD+2];
calcNormal(p1,p2,p3,n3);//外から見て左下
p2[0]=vertices[3*npD]; p2[1]=vertices[3*npD+1]; p2[2]=vertices[3*npD+2];
p3[0]=vertices[3*npR]; p3[1]=vertices[3*npR+1]; p3[2]=vertices[3*npR+2];
calcNormal(p1,p2,p3,n4);//右下
normals.push((n1[0]+n2[0]+n3[0]+n4[0])/4.0);//x方向
normals.push((n1[1]+n2[1]+n3[1]+n4[1])/4.0);//y
normals.push((n1[2]+n2[2]+n3[2]+n4[2])/4.0);//z
}
}
//Bottom
for(i = 0;i <= nSlice;i++)
{
normals.push(0.0);//x
normals.push(0.0);//y
normals.push(0.0);//z
}
//インデックス
var k1, k2;
for (j = 0; j < nStack; j++)
{
for (i = 0; i < nSlice; i++)
{
k1 = j * (nSlice+1) + i;
k2 = k1 + (nSlice+1);
indices.push(k1);
indices.push(k2);
indices.push(k1 + 1);
indices.push(k2);
indices.push(k2 + 1);
indices.push(k1 + 1);
}
}
return indices.length;
}
//------------------------------------------------------------------------
function makeGridCylinderTex(data, vertices, texCoords, normals, indices, nSlice, nStack, nRepeatS, nRepeatT)
{ //SpringMassModelのDebugモードで使用
//上下の中心が原点
var i, i0, j, ip, im, np, npL, npR, npU, npD, k;
//Topの中心頂点座標
//座標
vertices.push(data[0][0]);//x
vertices.push(data[0][1]);//y
vertices.push(data[0][2]);//z
s = 0;
t = 1;
texCoords.push(s);
texCoords.push(t);
//Topの円周
for(i = 0 ; i <= nSlice; i++)
{
var i0 = i;
if(i == nSlice) i0 = 0;
k = i0 + 1;
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
s = 0;
t = 1;
texCoords.push(s);
texCoords.push(t);
}
//側面(Textureは側面だけ)
for(j = 0 ; j <= nStack; j++)
{
//Topを1とするt座標
t = (1.0 - j / nStack) * nRepeatT;
for(i = 0 ; i <= nSlice; i++)
{
var i0 = i;
if(i == nSlice) i0 = 0;
k = i0 + j * nSlice + 1;
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
//if(i < nSlice) cnt++;
s = i * nRepeatS / nSlice;
texCoords.push(s);
texCoords.push(t);
}
}
//Bottomの円周
for(i = 0 ; i <= nSlice; i++)
{
var i0 = i;
if(i == nSlice) i0 = 0;
k = i0 + nStack*nSlice + 1
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
s = 0; t = 0;
texCoords.push(s);
texCoords.push(t);
}
//Bottomの中心座標(nSlice+1個)
k = data.length-1;
vertices.push(data[k][0]);//x
vertices.push(data[k][1]);//y
vertices.push(data[k][2]);//z
s = 0; t = 0;
texCoords.push(s);
texCoords.push(t);
var p1 = [], p2 = [], p3 = [];
var n1 = [], n2 = [], n3 = [], n4 = [];
//法線ベクトル
//Topの中心
normals.push(0.0);//x
normals.push(0.0);//y
normals.push(0.0);//z
//Topの円周
for(i = 0;i <= nSlice;i++)//隣り合う2個の三角形の法線ベクトルを平均化
{
ip = i+1;
if(ip == nSlice+1) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = i + 1;//注目点
npL = im + 1;//左側
npR = ip + 1;//右側
npU = 0;//上
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npU]; p2[1]=vertices[3*npU+1]; p2[2]=vertices[3*npU+2];
p3[0]=vertices[3*npL]; p3[1]=vertices[3*npL+1]; p3[2]=vertices[3*npL+2];
calcNormal(p1,p2,p3,n1);//外から見て左上
p2[0]=vertices[3*npR]; p2[1]=vertices[3*npR+1]; p2[2]=vertices[3*npR+2];
p3[0]=vertices[3*npU]; p3[1]=vertices[3*npU+1]; p3[2]=vertices[3*npU+2];
calcNormal(p1,p2,p3,n2);//右上
normals.push((n1[0]+n2[0])/2.0);//x方向
normals.push((n1[1]+n2[1])/2.0);//y
normals.push((n1[2]+n2[2])/2.0);//z
}
var nn = nSlice + 2;//ここまでの頂点数
//SideのTop
j = 0;
for(i = 0;i <= nSlice;i++)//側面のTop
{//隣り合う2個の三角形の法線ベクトルを平均化
ip = i+1;
if(ip == nSlice+1) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = j*(nSlice+1)+i + nn;//注目点
npL = j*(nSlice+1)+im + nn;//左側
npR = j*(nSlice+1)+ip + nn;//右側
npD = np+nSlice+1 + nn;//下
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npL]; p2[1]=vertices[3*npL+1]; p2[2]=vertices[3*npL+2];
p3[0]=vertices[3*npD]; p3[1]=vertices[3*npD+1]; p3[2]=vertices[3*npD+2];
calcNormal(p1,p2,p3,n3);//外から見て左下
p2[0]=vertices[3*npD]; p2[1]=vertices[3*npD+1]; p2[2]=vertices[3*npD+2];
p3[0]=vertices[3*npR]; p3[1]=vertices[3*npR+1]; p3[2]=vertices[3*npR+2];
calcNormal(p1,p2,p3,n4);//右下
normals.push((n3[0]+n4[0])/2.0);//x方向
normals.push((n3[1]+n4[1])/2.0);//y
normals.push((n3[2]+n4[2])/2.0);//z
}
//sideの中間
for(j = 1;j < nStack;j++)
{
for(i = 0;i <= nSlice;i++)//隣り合う4個の三角形の法線ベクトルを平均化
{
ip = i+1;
if(ip == nSlice+1) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = j*(nSlice+1)+i + nn;//注目点
npL = j*(nSlice+1)+im + nn;//左側
npR = j*(nSlice+1)+ip + nn;//右側
npU = np-nSlice-1;//上
npD = np+nSlice+1;//下
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npU]; p2[1]=vertices[3*npU+1]; p2[2]=vertices[3*npU+2];
p3[0]=vertices[3*npL]; p3[1]=vertices[3*npL+1]; p3[2]=vertices[3*npL+2];
calcNormal(p1,p2,p3,n1);//外から見て左上
p2[0]=vertices[3*npR]; p2[1]=vertices[3*npR+1]; p2[2]=vertices[3*npR+2];
p3[0]=vertices[3*npU]; p3[1]=vertices[3*npU+1]; p3[2]=vertices[3*npU+2];
calcNormal(p1,p2,p3,n2);//右上
p2[0]=vertices[3*npL]; p2[1]=vertices[3*npL+1]; p2[2]=vertices[3*npL+2];
p3[0]=vertices[3*npD]; p3[1]=vertices[3*npD+1]; p3[2]=vertices[3*npD+2];
calcNormal(p1,p2,p3,n3);//外から見て左下
p2[0]=vertices[3*npD]; p2[1]=vertices[3*npD+1]; p2[2]=vertices[3*npD+2];
p3[0]=vertices[3*npR]; p3[1]=vertices[3*npR+1]; p3[2]=vertices[3*npR+2];
calcNormal(p1,p2,p3,n4);//右下
normals.push((n1[0]+n2[0]+n3[0]+n4[0])/4.0);//x方向
normals.push((n1[1]+n2[1]+n3[1]+n4[1])/4.0);//y
normals.push((n1[2]+n2[2]+n3[2]+n4[2])/4.0);//z
}
}
//sideのbottom
j = nStack;
for(i = 0;i <= nSlice;i++)//隣り合う2個の三角形の法線ベクトルを平均化
{
ip = i+1;
if(ip == nSlice+1) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = j*(nSlice+1)+i + nn;//注目点
npL = j*(nSlice+1)+im + nn;//左側
npR = j*(nSlice+1)+ip + nn;//右側
npU = np-nSlice-1;//上
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npU]; p2[1]=vertices[3*npU+1]; p2[2]=vertices[3*npU+2];
p3[0]=vertices[3*npL]; p3[1]=vertices[3*npL+1]; p3[2]=vertices[3*npL+2];
calcNormal(p1,p2,p3,n1);//外から見て左上
p2[0]=vertices[3*npR]; p2[1]=vertices[3*npR+1]; p2[2]=vertices[3*npR+2];
p3[0]=vertices[3*npU]; p3[1]=vertices[3*npU+1]; p3[2]=vertices[3*npU+2];
calcNormal(p1,p2,p3,n2);//右上
normals.push((n1[0]+n2[0])/2.0);//x方向
normals.push((n1[1]+n2[1])/2.0);//y
normals.push((n1[2]+n2[2])/2.0);//z
}
//Bottomの円周
nn = (nSlice+1) * (nStack+2) + 1;
for(i = 0;i <= nSlice;i++)
{//隣り合う2個の三角形の法線ベクトルを平均化
ip = i+1;
if(i == nSlice) ip = 1;
im = i-1;
if(i == 0) im = nSlice-1;
np = i + nn;//注目点
npL = im + nn;//左側
npR = ip + nn;//右側
npD = nSlice + nn+1;//Bottomの中心
p1[0]=vertices[3*np] ; p1[1]=vertices[3*np+1] ; p1[2]=vertices[3*np+2];
p2[0]=vertices[3*npL]; p2[1]=vertices[3*npL+1]; p2[2]=vertices[3*npL+2];
p3[0]=vertices[3*npD]; p3[1]=vertices[3*npD+1]; p3[2]=vertices[3*npD+2];
calcNormal(p1,p2,p3,n3);//外から見て左下
p2[0]=vertices[3*npD]; p2[1]=vertices[3*npD+1]; p2[2]=vertices[3*npD+2];
p3[0]=vertices[3*npR]; p3[1]=vertices[3*npR+1]; p3[2]=vertices[3*npR+2];
calcNormal(p1,p2,p3,n4);//右下
normals.push((n3[0]+n4[0])/2.0);//x方向
normals.push((n3[1]+n4[1])/2.0);//y
normals.push((n3[2]+n4[2])/2.0);//z
}
//Bottomの中心
normals.push(0.0);//x
normals.push(0.0);//y
normals.push(0.0);//z
//インデックス
var k1, k2;
//Top
for(i = 0; i < nSlice; i++)
{
ip = i + 1;
if(i == nSlice) ip = 1
indices.push(0);
indices.push(i + 1);
indices.push(ip + 1);
}
nn = nSlice + 2;
for (j = 0; j < nStack; j++)
{
for (i = 0; i < nSlice; i++)
{
k1 = j * (nSlice+1) + i + nn ;
k2 = k1 + (nSlice+1);
indices.push(k1);
indices.push(k2);
indices.push(k1 + 1);
indices.push(k2);
indices.push(k2 + 1);
indices.push(k1 + 1);
}
}
nn = (nStack+2) * (nSlice + 1) + 1;
//Bottom
for(i = 0; i < nSlice; i++)
{
ip = i + 1;
indices.push(vertices.length/3-1);//中心点
indices.push(nn + ip);
indices.push(nn + i);
}
return indices.length;
}
<file_sep>/JVEMV6/46_art/8_kb/voicememos.20190315_132810/2019-03/manage_voicememos.20190315_133842.rb
require 'pathname'
require 'fileutils'
=begin
file: C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\voicememos.20190315_132810\2019-03\manage_voicememos.20190315_133842.rb
created at: 2019/03/15 13:39:24
<Usage>
C:\WORKS_2\WS\WS_Others\prog\D-5\1#\get_folder_list.rb
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\voicememos.20190315_132810\2019-03\
manage_voicememos.20190315_133842.rb
=end
################################
#
# vars (global)
#
################################
VERSION = "1.0"
FILE_PATH = "C:\\WORKS_2\\WS\\WS_Others\\prog\\D-5\\1#\\get_folder_list.rb"
################################
# @param
# serial 20161221_141900
# @orig: C:\WORKS_2\WS\WS_Others\res.245\115\r.245-115.5#1.elec-conductivity.rb
#
################################
def get_time_label(type = "serial")
if type == "serial"
#ref http://stackoverflow.com/questions/7415982/how-do-i-get-the-current-date-time-in-dd-mm-yyyy-hhmm-format
return Time.now.strftime("%Y%m%d_%H%M%S")
elsif type == "display"
#ref http://stackoverflow.com/questions/7415982/how-do-i-get-the-current-date-time-in-dd-mm-yyyy-hhmm-format
return Time.now.strftime("%Y/%m/%d %H:%M:%S")
else
return Time.now.strftime("%Y%m%d_%H%M%S")
end
end
def test_1
####################
# step : A : 1
# prep
####################
#ref https://stackoverflow.com/questions/6705982/escaping-single-and-double-quotes-in-a-string-in-ruby#6706040
dpath_Src = %q[C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\voicememos.20190315_132810\2019-03]
fname_Src = "data.dat"
fpath_Src = "#{dpath_Src}\\#{fname_Src}"
puts
puts "[#{__FILE__} : #{__LINE__}] fpath_Src --> #{fpath_Src}"
####################
# step : A : 1.1
# validate
####################
if File.exist?(fpath_Src)
puts
puts "[#{__FILE__} : #{__LINE__}] file --> exists"
else#if (File.exists?)
puts
puts "[#{__FILE__} : #{__LINE__}] file --> NOT exists"
# exit
return
end#if (File.exists?)
####################
# step : A : 1.2
# validate : open file
####################
#ref http://rubylearning.com/satishtalim/ruby_exceptions.html
begin
f_in = File.open(fpath_Src, "r")
# f_in = File.open(fpath_Src + ".new", "r")
rescue StandardError => e
puts
puts "[#{__FILE__} : #{__LINE__}] ERROR!"
p e
return
end
# f_in = File.open(fpath_Src + ".new", "r")
# f_in = File.open(fpath_Src, "r")
####################
# step : A : 1.3
# file : read lines
####################
# lines = f_in.readlines
#ref https://stackoverflow.com/questions/25168662/how-to-read-lines-from-file-into-array
lines = File.readlines(fpath_Src)
#debug
puts
puts "[#{__FILE__} : #{__LINE__}] lines --> #{lines.length.to_s}"
####################
# step : A : 2
# build : new lines
####################
lines_New = []
#ref https://stackoverflow.com/questions/20258086/difference-between-each-with-index-and-each-with-index-in-ruby#20258160
lines.each_with_index do |val, idx|
tokens = val.strip.split("\t")
# tokens = val.split("\t")
#debug
puts
puts "[#{__FILE__} : #{__LINE__}] iter (#{idx.to_s} : "
p tokens
puts
strOf_TimeLabel = (tokens[0].split(".")[0]).split(" ").join("_")
# puts "tokens"
# p tokens
# p strOf_TimeLabel
val_New = "m=#{strOf_TimeLabel}.#{tokens[1]}.m4a"
p val_New
# # append
# lines_New << val_New
####################
# step : A : 2.1
# copy file
####################
####################
# step : A : 2.1 : 1
# path : source
####################
_fpath_Src = "#{dpath_Src}\\#{tokens[0]}"
####################
# step : A : 2.1 : 2
# validate : exitsts
####################
judge = File.exist?(_fpath_Src)
if judge == false
#debug
puts
puts "[#{__FILE__} : #{__LINE__}] file --> NOT exist : #{_fpath_Src}"
else#if (judge == false)
#debug
puts
puts "[#{__FILE__} : #{__LINE__}] file --> exists : #{_fpath_Src}"
end#if (judge == false)
####################
# step : A : 2.1 : 3
# dest file
####################
_fpath_Dst = "#{dpath_Src}\\#{val_New}"
####################
# step : A : 2.1 : 4
# copy
####################
FileUtils.cp(_fpath_Src, _fpath_Dst)
end
puts
p lines_New
# ####################
# # step : A : 3
# # new file
# ####################
# lines.each_with_index do |val, idx|
#
# ####################
# # step : A : 3.1
# # build : fpath source
# ####################
# _fpath_Src = "#{dpath_Src}\\#{val}"
#
# ####################
# # step : A : 3.2
# # validate : exitsts
# ####################
# judge = File.exist?(_fpath_Src)
#
# if judge == false
#
# #debug
# puts
# puts "[#{__FILE__} : #{__LINE__}] file --> NOT exist : #{_fpath_Src}"
#
# else#if (judge == false)
#
# #debug
# puts
# puts "[#{__FILE__} : #{__LINE__}] file --> exists : #{_fpath_Src}"
#
#
# end#if (judge == false)
#
# end#lines.each_with_index do |val, idx|
#_20190315_134150
####################
# step : X : 1.1
# close : file
####################
f_in.close
end#def test_1
def exec
####################
# ops
####################
test_1
puts
puts "[#{__FILE__} : #{__LINE__}] exec --> done"
end#exec
exec
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/data/ops/2_image-programming/2_projects/3_handle-exif-data/ops_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\2_image-programming\2_projects\3_handle-exif-data\ops_1.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\1_1.py
at : 2018/08/23 12:33:00
r w && r d4
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\2_image-programming\2_projects\3_handle-exif-data
python ops_1.py
'''
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects') # libs_mm
from libs_admin import libs, lib_ip
'''###################
import : built-in modules
###################'''
# import getopt
import os, glob, getopt, math as math, numpy as np
# import inspect
'''###################
import : built-in modules
###################'''
import cv2
# from pandas.compat import str_to_bytes
from numpy.distutils.from_template import item_re
#ref https://stackoverflow.com/questions/3129322/how-do-i-get-monitor-resolution-in-python
from win32api import GetSystemMetrics
from matplotlib import pylab as plt
#ref https://www.lifewithpython.com/2014/12/python-extract-exif-data-like-data-from-images.html
from PIL import Image
from PIL.ExifTags import TAGS
###############################################
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
#ref https://www.lifewithpython.com/2014/12/python-extract-exif-data-like-data-from-images.html
def get_exif_of_image(file):
"""Get EXIF of an image if exists.
指定した画像のEXIFデータを取り出す関数
@return exif_table Exif データを格納した辞書
"""
im = Image.open(file)
# Exif データを取得
# 存在しなければそのまま終了 空の辞書を返す
try:
exif = im._getexif()
except AttributeError:
return {}
# タグIDそのままでは人が読めないのでデコードして
# テーブルに格納する
exif_table = {}
for tag_id, value in exif.items():
tag = TAGS.get(tag_id, tag_id)
exif_table[tag] = value
return exif_table
'''###################
get_GPS_Data(fpath_Image)
@return: tuple
=> (('N', 35, 35, 24.14), ('E', 139, 34, 48.01))
@param fpath_Image: file full path
@example file : C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload\2018-08-22_15-42-10_000.jpg
[ops_1.py:126] dicOf_Exif['GPSInfo'] =>
{1: 'N', 2: ((35, 1), (35, 1), (2414, 100)), 3: 'E', 4: ((139, 1), (34, 1), (480
1, 100)), 5: b'\x00', 6: (24268, 387), 7: ((6, 1), (42, 1), (900, 100)), 12: 'K'
, 13: (0, 1), 16: 'T', 17: (80093, 231), 23: 'T', 24: (80093, 231), 29: '2018:08
:22', 31: (10, 1)}
###################'''
def get_GPS_Data(fpath_Image):
dicOf_Exif = get_exif_of_image(fpath_Image)# def get_GPS_Data():
gps_Info = dicOf_Exif['GPSInfo']
'''###################
lat, longi
###################'''
txt_Lat = "%s=" % (gps_Info[1])
txt_Longi = "%s=" % (gps_Info[3])
'''###################
values
###################'''
vals_Lat = gps_Info[2]
txt_Lat += "%d-%d-%.02f" % \
(vals_Lat[0][0], vals_Lat[1][0], vals_Lat[2][0] * 1.0 / vals_Lat[2][1])
# (vals_Lat[0][0], vals_Lat[1][0])
vals_Longi = gps_Info[4]
txt_Longi += "%d-%d-%.02f" % \
(vals_Longi[0][0], vals_Longi[1][0], vals_Longi[2][0] * 1.0 / vals_Longi[2][1])
# txt_Longi += "%d-%d-" % \
# (vals_Longi[0][0], vals_Longi[1][0])
'''###################
tuples
###################'''
data_Lat = (gps_Info[1], vals_Lat[0][0], vals_Lat[1][0], vals_Lat[2][0] * 1.0 / vals_Lat[2][1])
data_Longi = (gps_Info[3], vals_Longi[0][0], vals_Longi[1][0], vals_Longi[2][0] * 1.0 / vals_Longi[2][1])
data_Final = (data_Lat, data_Longi)
'''###################
report
###################'''
print("[%s:%d] txt_Lat = %s, txt_Longi = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, txt_Lat, txt_Longi
), file=sys.stderr)
'''###################
return
###################'''
return data_Final
#/ def get_GPS_Data(fpath_Image):
def test_1():
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Cake_IFM11\\iphone_to_upload"
# C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
fname_Ops_Image = "2018-08-22_15-32-53_000.jpg"
# fname_Ops_Image = "2018-08-22_15-42-10_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : exif data
###################'''
# print(get_exif_of_image(fpath_Ops_Image))
# # print get_exif_of_image(fpath_Ops_Image)
# # print get_exif_of_image("sample.jpg")
#ref https://stackoverflow.com/questions/15785719/how-to-print-a-dictionary-line-by-line-in-python
# for item in dicOf_Exif:
#
# print(item)
#
# #/for item in dicOf_Exif:
# for key in sorted(dicOf_Exif.keys()):
#
# print(key)
#
# #/for key in :
# print("[%s:%d] dicOf_Exif['SubjectLocation'] => " % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(dicOf_Exif['SubjectLocation'])
'''###################
gps info
###################'''
result = get_GPS_Data(fpath_Ops_Image)
#(('N', 35, 35, 24.14), ('E', 139, 34, 48.01))
print()
print("[%s:%d] GPS data =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(result)
return
dicOf_Exif = get_exif_of_image(fpath_Ops_Image)
print()
print("[%s:%d] dicOf_Exif['GPSInfo'] => " % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(dicOf_Exif['GPSInfo'])
'''###################
gps info
###################'''
gps_Info = dicOf_Exif['GPSInfo']
for item in gps_Info.keys():
# for item in gps_Info:
print("key => %d" % (item))
print(gps_Info[item])
print()
# print("key => %d" % (item))
# print(gps_Info[item])
# print("%s => %s" % (item, gps_Info[item]))
# print(item, type(item))
# #1 <class 'int'>
#/for item in gps_Info:
'''###################
message
###################'''
print()
print("[%s:%d] test_1 =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
'''
ApertureValue
BrightnessValue
ColorSpace
ComponentsConfiguration
CustomRendered
DateTime
DateTimeDigitized
DateTimeOriginal
ExifImageHeight
ExifImageWidth
ExifOffset
ExifVersion
ExposureBiasValue
ExposureMode
ExposureProgram
ExposureTime
FNumber
Flash
FlashPixVersion
FocalLength
FocalLengthIn35mmFilm
GPSInfo
ISOSpeedRatings
LensMake
LensModel
LensSpecification
Make
MakerNote
MeteringMode
Model
Orientation
ResolutionUnit
SceneCaptureType
SceneType
SensingMethod
ShutterSpeedValue
Software
SubjectLocation
SubsecTimeDigitized
SubsecTimeOriginal
WhiteBalance
XResolution
YCbCrPositioning
YResolution
'''<file_sep>/JVEMV6/46_art/11_guitar/end_gt.bat
taskkill /im iTunes.exe
taskkill /im audacity.exe
REM taskkill /f /im soffice.bin
REM taskkill /im vlc.exe
taskkill /im sakura.exe
<file_sep>/README.md
"# WS_Others.Art"
<file_sep>/JVEMV6/46_art/6_visual-arts/start_visual-arts.bat
REM ************************
REM folders
REM doc
REM app : gimp
REM app : mspaint
REM ************************
REM goto testing
REM ************************
REM folders
REM ************************
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts
REM : C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
REM ************************
REM doc
REM ************************
REM : log
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\log_46#6.odt
REM : sheet
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\46#6_visual-arts.ods
REM ************************
REM app : gimp
REM ************************
REM :testing
"C:\WORKS_2\Programs\GIMP 2\bin\gimp-2.8.exe"
REM call "C:\WORKS_2\Programs\GIMP 2\bin\gimp-2.8.exe"
REM start "C:\WORKS_2\Programs\GIMP 2\bin\gimp-2.8.exe" ==> prog doesn't start; console window opens #20191104_135645
REM ************************
REM app : mspaint
REM 2019/11/04 14:10:34
REM ************************
start mspaint.exe
<file_sep>/JVEMV6/46_art/11_guitar/end_guitar_2.bat
taskkill /im soffice.bin
taskkill /im sakura.exe
<file_sep>/JVEMV6/46_art/8_kb/start_conv.bat
REM *******************************
REM open folders
REM *******************************
echo start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\domino\memos
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\domino\memos
REM *******************************
REM apps : itunes
REM *******************************
REM call C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.bat
echo start C:\WORKS_2\Programs\itunes_12.6.2\iTunes.exe
start C:\WORKS_2\Programs\itunes_12.6.2\iTunes.exe
REM *******************************
REM browser
REM *******************************
echo start chrome.exe "https://www.online-convert.com/"
pushd "C:\Program Files (x86)\Google\Chrome\Application"
REM start chrome.exe "https://www.online-convert.com/"
start chrome.exe "https://audio.online-convert.com/convert-to-mp3"
start chrome.exe "https://audio.online-convert.com/convert-to-mp3"
start chrome.exe "https://audio.online-convert.com/convert-to-mp3"
REM *******************************
REM git pull
REM *******************************
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_command_prompot.bat
echo git pull....
pushd C:\WORKS_2\WS\WS_Others.Art
e
REM pause
REM exit
<file_sep>/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/2-1.for-memo.py
#https://postd.cc/image-processing-101/
#ref ipython notebook how to : https://qiita.com/payashim/items/d4fe5227b21a5215e78b
'''
file : 2-1.for-memo.py
date : 2018/05/13 11:42:34
r 3
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\2_\
ipython notebook
'''
# coding: utf-8
# In[1]:
import cv2, matplotlib
# In[9]:
import numpy as np
# In[10]:
abc.name
# In[11]:
import matplotlib.pyplot as plt
# In[15]:
img = cv2.imread('../images/IMG_3171.JPG')
# In[16]:
print(img)
# In[17]:
plt.imshow(img)
# In[18]:
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
plt.imshow(img2)
#aa
# In[20]:
plt.imshow(img2)
# In[21]:
img3 = cv2.imread('images/IMG_3171.JPG')
# In[23]:
plt.imshow(img3)
# In[24]:
img4 = cv2.imread('images/IMG_3166.PNG')
# In[25]:
plt.imshow(img4)
# In[26]:
gray_img = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
plt.imshow(gray_img)
gray_img[0]
# In[28]:
_, threshold_img = cv2.threshold(gray_img, 60, 255, cv2.THRESH_BINARY)
plt.imshow(threshold_img)
_, threshold_img = cv2.threshold(gray_img, 60, 100, cv2.THRESH_BINARY)
plt.imshow(threshold_img)
_, threshold_img = cv2.threshold(gray_img, 20, 255, cv2.THRESH_BINARY)
plt.imshow(threshold_img)
# In[30]:
_, threshold_img = cv2.threshold(gray_img, 100, 255, cv2.THRESH_BINARY)
plt.imshow(threshold_img)
#閾値より低い値を探さなくても、 =========================================
piet = cv2.imread('../images/IMG_3171.JPG')
# piet = cv2.imread('images/piet.png')
piet_hsv = cv2.cvtColor(piet, cv2.COLOR_BGR2HSV)
len(piet_hsv)
# threshold for hue channel in blue range
blue_min = np.array([100, 100, 20], np.uint8)
blue_min[0]
len(blue_min)
# blue_min = np.array([100, 100, 100], np.uint8)
blue_max = np.array([140, 255, 255], np.uint8)
threshold_blue_img = cv2.inRange(piet_hsv, blue_min, blue_max)
plt.imshow(threshold_blue_img)
val_max = 80
blue_min = np.array([20, 20, 20], np.uint8)
blue_max = np.array([val_max, val_max, val_max], np.uint8)
threshold_blue_img = cv2.inRange(piet_hsv, blue_min, blue_max)
plt.imshow(threshold_blue_img)
threshold_blue_img_Cnvtd = cv2.cvtColor(threshold_blue_img, cv2.COLOR_GRAY2RGB)
plt.imshow(threshold_blue_img_Cnvtd)
#------------------------------------------
piet = cv2.imread('images/IMG_3171.JPG')
piet_RGB = cv2.cvtColor(piet, cv2.COLOR_BGR2RGB)
plt.imshow(piet_RGB)
piet_hsv = cv2.cvtColor(piet_RGB, cv2.COLOR_BGR2HSV)
plt.imshow(piet_hsv)
blue_min = np.array([100, 100, 20], np.uint8)
blue_max = np.array([140, 255, 255], np.uint8)
threshold_blue_img = cv2.inRange(piet_hsv, blue_min, blue_max)
plt.imshow(threshold_blue_img)
mask_RGB = cv2.inRange(piet_RGB, blue_min, blue_max)
plt.imshow(mask_RGB)
plt.imshow(piet_RGB)
blue_min = np.array([0, 10, 10], np.uint8)
blue_max = np.array([20, 100, 100], np.uint8)
mask_RGB = cv2.inRange(piet_RGB, blue_min, blue_max)
plt.imshow(mask_RGB)
#ref https://stackoverflow.com/questions/2462725/cv-saveimage-in-opencv
cv2.imwrite('01.png',mask_RGB)
# -----------------------------------------
mask_RGB_cvted = cv2.cvtColor(mask_RGB, cv2.COLOR_GRAY2RGB)
plt.imshow(mask_RGB)
plt.imshow(mask_RGB_cvted)
# -----------------------------------------
# clipping
#ref https://qiita.com/satoshicano/items/bba9594a1203e24e2a31
height, width, channels = mask_RGB_cvted.shape
off_set = 280
clp = mask_RGB_cvted[(height - off_set) : height, (width - off_set) : width]
plt.imshow(clp)
# cv2.imshow(clp)
# clp = img[0:height/2, 0:width/2]
# -----------------------------------------
cv2.imwrite('images/clp.png',clp)
clp_LeftBottom = mask_RGB_cvted[(height - off_set) : height, 0 : off_set]
plt.imshow(clp_LeftBottom)
# ----------------------------------------- 20180513_120247
plt.imshow(img2)
height, width, channels = img2.shape
off_set = 280
clp = img2[(height - off_set) : height, 0 : off_set]
plt.imshow(clp)
# ----------------------------------------- 20180513_130130
img = cv2.imread('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/images/IMG_3171.JPG')
# img = cv2.imread('../images/IMG_3171.JPG')
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# plt.imshow(img2)
#
dpath = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/2_/images"
#
# fname = "image.%s.png" % (libs.get_TimeLabel_Now())
#
# fpath = "%s/%s" % (dpath, fname)
#
# plt.savefig(fpath)
#ref display image https://github.com/PrinzEugen7/ImageProcessing/blob/master/Image/Python/Matplotlib/draw_opencv_img.py
# plt.show()
'''###################
get : data
###################'''
# data
height, width, channels = img2.shape
off_set = 280
tlabel = "20180513_130233"
# tlabel = libs.get_TimeLabel_Now()
plt_ = plt
# save image
'''###################
corner : LB
###################'''
titles = ["clp_LB", "clp_RB", "clp_LU", "clp_RU"]
clips = [
img2[(height - off_set) : height, 0 : off_set], # clp_LB
img2[(height - off_set) : height, width - off_set : width], # clp_RB
img2[0 : off_set, 0 : off_set], # clp_LU
img2[0 : off_set, width - off_set : width], # clp_RU
]
clips[0]
clip_0 = clips[0]
len(clip_0)
len(clip_0[0])
clip_0[0][0]
# ----------------------------------------- 20180513_130451
max_R = -1
for item in clip_0[0]:
R = item[0]
if R > max_R : #if R > max_R
max_R = R
#/if R > max_R
#/for item in clip_0[0]:
print("max_R => %d" % max_R)
# ----------------------------------------- 20180513_130745
max_R = -1; max_G = -1; max_B = -1
for item in clip_0[0]:
R = item[0]; G = item[1]; B = item[2]
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
#/if G > max_G
#/for item in clip_0[0]:
print("max_R = %d, max_G = %d, max_B = %d" % (max_R, max_G, max_B))
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/libs_admin/lib_ip.py
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\libs_admin\lib_ip.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\libs_admin\libs.py
at : 2018/05/26 13:29:43
'''
# from sympy.matrices.densetools import row
'''###################
import : built-in modules
###################'''
import inspect, os, sys
# import inspect, os, sys, cv2
from enum import Enum
#ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
from time import gmtime, strftime, localtime, time
'''###################
import : user-installed modules
###################'''
# from sympy.physics.vector.printing import params
from scipy.stats import skew
from sympy.matrices.densetools import row
import cv2, numpy as np, matplotlib.pyplot as plt
# import numpy as np, matplotlib.pyplot as plt
from PIL import Image
from PIL.ExifTags import TAGS
'''###################
import : orig modules
###################'''
# sys.path.append('.')
#C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\libs_admin\libs.py
from libs_admin import libs, cons_ip
# from libs_admin import libs #=> working
# import libs
'''#########################################################
enums
#########################################################'''
'''#########################################################
functions
#########################################################'''
'''###################
get_Std_Dev(lo_Data)
at :
@return: dictionary of skew values
{'R' : 1.1002928031008152,
'G' : 4.955577672343114,
'B' : 6.775475308994965 }
###################'''
def get_Std_Dev(lo_Data):
'''###################
get : mean value
###################'''
# values of each color composite
# valsOf_R = [x * 0.001 for x in lo_Data]
# valsOf_R = [x * np.power(10, -3) for x in lo_Data]
valsOf_R = lo_Data
# valsOf_R = do_Image_MetaData['valsOf_R']
# #debug
# print()
# print("[%s:%d] valsOf_R[0:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(valsOf_R[0:10])
# sum
sumOf_ValsOf_R = sum(valsOf_R)
# mean
meanOf_R = sumOf_ValsOf_R / len(valsOf_R)
# #debug
# print()
# print("[%s:%d] meanOf_R = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , meanOf_R
# ), file=sys.stderr)
# vals squared
valsSquared_R = [np.power(x, 2) for x in valsOf_R]
# #debug
# print()
# print("[%s:%d] valsSquared_R[0:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(valsSquared_R[0:10])
# summation of vals squared
summationOf_ValsSquared_R = sum(valsSquared_R)
# summationOf_ValsSquared_R = sum[valsSquared_R]
# #debug
# print()
# print("[%s:%d] summationOf_ValsSquared_R = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , summationOf_ValsSquared_R
# ), file=sys.stderr)
# length
lenOf_R = len(valsOf_R)
# standard dev
variance_R = 1.0 * summationOf_ValsSquared_R / lenOf_R - np.power(meanOf_R, 2)
# #debug
# print()
# print("[%s:%d] variance_R = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , variance_R
# ), file=sys.stderr)
# standard dev
stdDev_R = np.sqrt(variance_R)
'''###################
return
###################'''
return stdDev_R
#/ def get_Std_Dev(lo__Data):
'''###################
get_Moment(valsOf_ColorComposite, moment_Num)
@return: Nnd moment
e.g. 4.955577672343114,
###################'''
def get_Moment(valsOf_ColorComposite, moment_Num):
# length
lenOf_Vals = len(valsOf_ColorComposite)
# # stddev
# stddev = get_Std_Dev(valsOf_ColorComposite)
# average
avg = sum(valsOf_ColorComposite) / lenOf_Vals
lo_Power = [np.power(x - avg, moment_Num) for x in valsOf_ColorComposite]
moment = sum(lo_Power) / lenOf_Vals
# moment = sum(lo_Power) / lenOf_Vals / np.power(stddev, moment_Num)
'''###################
return
###################'''
return moment
#/ def get_Moment(valsOf_ColorComposite, 3):
'''###################
get_Skew_Value(valsOf_ColorComposite)
@return: skew value (float)
e.g. 4.955577672343114,
###################'''
# def get_Skew_Value(lo_Image_Data):
def get_Skew_Value(valsOf_ColorComposite):
'''###################
prep
###################'''
'''###################
get : std dev
###################'''
# values of each color composite
# valsOf_R = valsOf_ColorComposite
# std dev
stdDev = get_Std_Dev(valsOf_ColorComposite)
# stdDev_R = get_Std_Dev(valsOf_R)
# #debug
# print()
# print("[%s:%d] stdDev_R = %.05f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , stdDev_R
# ), file=sys.stderr)
'''###################
get : moment : 3
###################'''
moment_Number = 3
moment_3 = get_Moment(valsOf_ColorComposite, moment_Number)
'''###################
skew
###################'''
skew = 1.0 * moment_3 / stdDev
'''###################
return
###################'''
return skew
# return -999
# pass
#/ def get_Skew_Value(list):
'''###################
get_Skews(img_Data)
@return: dictionary of skew values
{'R' : 1.1002928031008152,
'G' : 4.955577672343114,
'B' : 6.775475308994965 }
###################'''
def get_Skews(img_Data):
'''###################
vars
###################'''
do_Skews = {"skew_R" : -1, "skew_G" : -1, "skew_B" : -1}
'''###################
skew : prep
###################'''
# meta data
do_Image_MetaData = get_Image_MetaData_Basic(img_Data)
# tuple of vals
valsOf_R = do_Image_MetaData['valsOf_R']
valsOf_G = do_Image_MetaData['valsOf_G']
valsOf_B = do_Image_MetaData['valsOf_B']
# to_Vals = (valsOf_R, valsOf_G, valsOf_B)
'''###################
skew
ref : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\2_\2_1.py
###################'''
# adjust values
valOf_Adjust = 0.01
valsOf_R__ = [x * valOf_Adjust for x in valsOf_R]
valsOf_G__ = [x * valOf_Adjust for x in valsOf_G]
valsOf_B__ = [x * valOf_Adjust for x in valsOf_B]
do_Skews['skew_R'] = get_Skew_Value(valsOf_R__)
do_Skews['skew_G'] = get_Skew_Value(valsOf_G__)
do_Skews['skew_B'] = get_Skew_Value(valsOf_B__)
# do_Skews['skew_R'] = get_Skew_Value(valsOf_R)
# do_Skews['skew_G'] = get_Skew_Value(valsOf_G)
# do_Skews['skew_B'] = get_Skew_Value(valsOf_B)
# do_Skews['skew_R'] = skew(valsOf_R)
# do_Skews['skew_G'] = skew(valsOf_G)
# do_Skews['skew_B'] = skew(valsOf_B)
# # sum
# sumOf_ValsOf_R = sum(valsOf_R)
# sumOf_ValsOf_G = sum(valsOf_G)
# sumOf_ValsOf_B = sum(valsOf_B)
#
# # print()
# # print("[%s:%d] sumOf_ValsOf_R = %d, sumOf_ValsOf_G = %d, sumOf_ValsOf_B = %d" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# # , sumOf_ValsOf_R, sumOf_ValsOf_G, sumOf_ValsOf_B
# # ), file=sys.stderr)
# # print("[%s:%d] sumOf_ValsOf_R => %d" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# # , sumOf_ValsOf_R
# # ), file=sys.stderr)
#
# # means
# meanOf_R = sumOf_ValsOf_R / len(valsOf_R)
# meanOf_G = sumOf_ValsOf_G / len(valsOf_G)
# meanOf_B = sumOf_ValsOf_B / len(valsOf_B)
#
# # #debug
# # print()
# # print("[%s:%d] meanOf_R = %.03f, meanOf_G = %.03f, meanOf_B = %.03f" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# # , meanOf_R, meanOf_G, meanOf_B
# # ), file=sys.stderr)
#
# # summation
# lo_PowerOf_R_3 = [np.power((x - meanOf_R), 3) for x in valsOf_R]
# # lo_PowerOf_3_R = [x * 3 for x in valsOf_R]
# # summationOf_R = [x * 3 for x in valsOf_R]
# # summationOf_R = sum[np.power((x - meanOf_R), 3) for x in valsOf_R]
#
# summationOf_R_3 = sum[lo_PowerOf_R_3]
#
# # squares of Rs
#
'''###################
return
###################'''
return do_Skews
# return -1
#/ def get_Skew(img_Data):
'''###################
get_Image_MetaData_Basic(img_Data)
at : 2018/05/28 07:59:22
@return:
do_MetaData['max_R'] = max_R
do_MetaData['max_G'] = max_G
do_MetaData['max_B'] = max_B
do_MetaData['min_R'] = min_R
do_MetaData['min_G'] = min_G
do_MetaData['min_B'] = min_B
do_MetaData['valsOf_R'] = valsOf_R
do_MetaData['valsOf_G'] = valsOf_G
do_MetaData['valsOf_B'] = valsOf_B
###################'''
def get_Image_MetaData_Basic(img_Data):
'''###################
vars
###################'''
do_MetaData = {}
max_R = -1; max_G = -1; max_B = -1
min_R = 256; min_G = 256; min_B = 256
# min_R = 255; min_G = 255; min_B = 255
# counter
cntOf_Row = 0
cntOf_Cell = 0
# values
valsOf_R = [0] * 256
valsOf_G = [0] * 256
valsOf_B = [0] * 256
for row in img_Data:
# for row in item:
for cell in row:
# get value
R = cell[0]; G = cell[1]; B = cell[2]
# histogram
valsOf_R[R] += 1
valsOf_G[G] += 1
valsOf_B[B] += 1
# max value
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
# min value
if R < min_R : min_R = R
if G < min_G : min_G = G
if B < min_B : min_B = B
# count
cntOf_Cell += 1
# reset count of cells
cntOf_Cell = 0
# count
cntOf_Row += 1
#/for row in item:
# set : data
do_MetaData['max_R'] = max_R
do_MetaData['max_G'] = max_G
do_MetaData['max_B'] = max_B
do_MetaData['min_R'] = min_R
do_MetaData['min_G'] = min_G
do_MetaData['min_B'] = min_B
do_MetaData['valsOf_R'] = valsOf_R
do_MetaData['valsOf_G'] = valsOf_G
do_MetaData['valsOf_B'] = valsOf_B
# lo_Image_MetaData.append(
# [
# # valsOf_R
# # , valsOf_G
# # , valsOf_B
#
# # , max_R
# max_R
# , max_G
# , max_B
#
# , min_R
# , min_G
# , min_B
#
# , valsOf_R
# , valsOf_G
# , valsOf_B
# ]
# )
'''###################
return
###################'''
return do_MetaData
#/ def get_Image_MetaData_Basic(img_Data):
'''###################
get_IdxOf_Maxes(img_Data)
@param img_Data: [
[
[103 101 93], [100 98 90],..., [ 72 80 10]
],
[
[103 101 93], [100 98 90],..., [ 72 80 10]
],
...
]
###################'''
def get_IdxOf_Maxes(img_Data):
'''###################
metadata
###################'''
# meta data
do_Image_MetaData = get_Image_MetaData_Basic(img_Data)
# tuple of vals
valsOf_R = do_Image_MetaData['valsOf_R']
valsOf_G = do_Image_MetaData['valsOf_G']
valsOf_B = do_Image_MetaData['valsOf_B']
'''###################
vars
###################'''
# index
idxOf_Max_R = -1
idxOf_Max_G = -1
idxOf_Max_B = -1
# vals
maxVal_R = -1
maxVal_G = -1
maxVal_B = -1
# counter
cntOf_R = 0
cntOf_G = 0
cntOf_B = 0
'''###################
iteration
###################'''
'''###################
red
###################'''
for val in valsOf_R:
# judge
if val > maxVal_R :
maxVal_R = val; idxOf_Max_R = cntOf_R
# count
cntOf_R += 1
#/for val in valsOf_R:
'''###################
G
###################'''
for val in valsOf_G:
# judge
if val > maxVal_G :
maxVal_G = val; idxOf_Max_G = cntOf_G
# count
cntOf_G += 1
#/for val in valsOf_G:
'''###################
B
###################'''
for val in valsOf_B:
# judge
if val > maxVal_B :
maxVal_B = val; idxOf_Max_B = cntOf_B
# count
cntOf_B += 1
#/for val in valsOf_B:
# print()
# print("[%s:%d] idxOf_Max_R = %d, len(valsOf_R) = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , idxOf_Max_R, len(valsOf_R)
# ), file=sys.stderr)
#
# iteration
# for xs in img_Data:
#
# for ys in xs:
#
# # #debug
# # print()
# # print("[%s:%d] vals =>" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# #
# # ), file=sys.stderr)
# # print(vals)
#
# # get : elem vals
# R = ys[0]
# G = ys[1]
# B = ys[2]
#
# # update max val, idx of max
# if R > maxVal_R :
# maxVal_R = R
# idxOf_Max_R = cntOf_R
#
# if G > maxVal_G : maxVal_G = G; idxOf_Max_G = cntOf_G
# if B > maxVal_B : maxVal_B = B; idxOf_Max_B = cntOf_B
#
# # counter
# cnt
#
#
# #/for vals in img_Data:
'''###################
return
###################'''
return idxOf_Max_R, idxOf_Max_G, idxOf_Max_B, \
maxVal_R, maxVal_G, maxVal_B
#/ def get_IdxOf_Maxes(img_Data):
'''###################
is_CornerOf_Green__PhotoOf_Sweets
@return: res, msg
res boolean
msg string ==> if res is 'False', gives info
###################'''
def is_CornerOf_Green__PhotoOf_Sweets(image_StatsData):
'''###################
vars
###################'''
idxOf_Maxes = image_StatsData['idxOf_Maxes']
# print()
# print("[%s:%d] idxOf_Maxes =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(idxOf_Maxes)
max_Vals = image_StatsData['max_Vals']
# print()
# print("[%s:%d] max_Vals =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(max_Vals)
'''###################
judge
###################'''
# prep vars
idxOf_Maxes_R = idxOf_Maxes[0]
idxOf_Maxes_G = idxOf_Maxes[1]
idxOf_Maxes_B = idxOf_Maxes[2]
max_Val_R = max_Vals[0]
max_Val_G = max_Vals[1]
max_Val_B = max_Vals[2]
# thresholds
ts_Max_Val_R = 5500
ts_Max_Val_G = 5500
ts_Max_Val_B = 500
# judge : index of max val
# 'B' ===> color element of R (data is obtained in BGR format)
if idxOf_Maxes_B > 20 :
msg = "False : idxOf_Max_B > 20 (%d)" % idxOf_Maxes_B
# print()
# print("[%s:%d] False : idxOf_Max_B > 20 (%d)" % \
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
if idxOf_Maxes_G < 30 or idxOf_Maxes_G > 80 :
# print()
# print("[%s:%d] False : idxOf_Max_G < 30 or idxOf_Maxes_G > 80" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# ), file=sys.stderr)
#
# return False
msg = "False : idxOf_Max_G < 30 or idxOf_Maxes_G > 80 (%d)" % idxOf_Maxes_G
# print()
# print("[%s:%d] False : idxOf_Max_B > 20 (%d)" % \
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
if idxOf_Maxes_R < 30 or idxOf_Maxes_R > 80 :
# print()
# print("[%s:%d] False : idxOf_Max_R < 30 or idxOf_Maxes_R > 80" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# ), file=sys.stderr)
#
# return False
msg = "False : idxOf_Max_R < 30 or idxOf_Maxes_R > 80 (%d)" % idxOf_Maxes_R
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
# judge : max vals
# if max_Val_R < 5000 :
# if max_Val_R > 5000 :
if max_Val_R > ts_Max_Val_R :
# print()
# # print("[%s:%d] False : max_Val_R < 5000" % \
# print("[%s:%d] False : max_Val_R > 5000 (%d)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , max_Val_R
# ), file=sys.stderr)
#
# return False
msg = "False : max_Val_R > 5000 (%d)" % max_Val_R
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
# if max_Val_G < 5000 :
# if max_Val_G > 5000 :
if max_Val_G > ts_Max_Val_G :
# print()
# print("[%s:%d] False : max_Val_G > 5000" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# ), file=sys.stderr)
#
# return False
msg = "False : max_Val_G > 5000 (%d)" % max_Val_G
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
if max_Val_B < 5000 or max_Val_B > 7500 :
# print()
# print("[%s:%d] False : max_Val_B < 5000 or max_Val_B > 7500" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# ), file=sys.stderr)
#
# return False
'''###################
judge : index of max val ==> 0?
###################'''
if max_Val_B > 7500 and not idxOf_Maxes_B == 0 : #if max_Val_B > 7500 and not idxOf_Maxes_B == 0
msg = "False : max_Val_B < 5000 or max_Val_B > 7500 (%d, idxOf_Max = %d)" \
% (max_Val_B, idxOf_Maxes_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
#/if max_Val_B > 7500 and not idxOf_Maxes_B == 0
# msg = "False : max_Val_B < 5000 or max_Val_B > 7500 (%d)" % max_Val_B
msg = "False : max_Val_B < 5000 or max_Val_B > 7500 (%d, idxOf_Max = %d)" \
% (max_Val_B, idxOf_Maxes_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
#
# return False, msg
'''###################
return
###################'''
return True, "True"
#/ def is_CornerOf_Green(image_StatsData):
'''###################
is_ColorName_Green
description :
1. copy of is_CornerOf_Green__PhotoOf_Sweets
2. using RGB values for judgement
at : 2018/06/05 07:39:31
@return: res, msg
res boolean
msg string ==> if res is 'False', gives info
###################'''
def is_ColorName_Green(image_StatsData):
'''###################
get vars : indices, max vals
###################'''
idxOf_Maxes = image_StatsData['idxOf_Maxes']
max_Vals = image_StatsData['max_Vals']
'''###################
get vars : each color element
R
==> in the sheet graph and genreted data --> 'r'
==> in reality --> 'b'
G
==> in the sheet graph and genreted data --> 'g'
==> in reality --> 'g'
B
==> in the sheet graph and genreted data --> 'b'
==> in reality --> 'r'
###################'''
# prep vars
idxOf_Maxes_R = idxOf_Maxes[0]
idxOf_Maxes_G = idxOf_Maxes[1]
idxOf_Maxes_B = idxOf_Maxes[2]
max_Val_R = max_Vals[0]
max_Val_G = max_Vals[1]
max_Val_B = max_Vals[2]
'''###################
judge : order of idxOf_Maxes
###################'''
condition_IdxOf_Maxes_1 = \
(idxOf_Maxes_G > idxOf_Maxes_B \
and idxOf_Maxes_B > idxOf_Maxes_R)
# if not (idxOf_Maxes_G > idxOf_Maxes_R and idxOf_Maxes_R > idxOf_Maxes_B):
if not (condition_IdxOf_Maxes_1):
# msg = "False : order of idxOf_Maxes ==> incomplicit (idxOf_Maxes_G = %d, idxOf_Maxes_R = %d, idxOf_Maxes_B = %d)" \
msg = "False : order of idxOf_Maxes ==> incomplicit (should be : G > R > B | idxOf_Maxes_R = %d, idxOf_Maxes_G = %d, idxOf_Maxes_B = %d)" \
% (idxOf_Maxes_R, idxOf_Maxes_G, idxOf_Maxes_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
prep vars : thresholds
###################'''
'''###################
thresholds : max values
###################'''
# R
ts_Max_Val_R__Lower = cons_ip.ColorThresholds.isGreen_Max_Val_R__Lower.value
ts_Max_Val_R__Upper = cons_ip.ColorThresholds.isGreen_Max_Val_R__Upper.value
# ts_Max_Val_R__Lower = 2.2 * 1000
# ts_Max_Val_R__Upper = 3.9 * 1000
# G
ts_Max_Val_G__Lower = cons_ip.ColorThresholds.isGreen_Max_Val_G__Lower.value
ts_Max_Val_G__Upper = cons_ip.ColorThresholds.isGreen_Max_Val_G__Upper.value
# ts_Max_Val_G__Lower = 2.3 * 1000
# ts_Max_Val_G__Upper = 3.9 * 1000
# B
ts_Max_Val_B__Lower = cons_ip.ColorThresholds.isGreen_Max_Val_B__Lower.value
ts_Max_Val_B__Upper = cons_ip.ColorThresholds.isGreen_Max_Val_B__Upper.value
# ts_Max_Val_B__Lower = 1.8 * 1000
# ts_Max_Val_B__Upper = 2.5 * 1000
'''###################
thresholds : index of max values
###################'''
# R
ts_IdxOf_Max_R__Upper = cons_ip.ColorThresholds.isGreen_IdxOf_Max_R__Upper.value
ts_IdxOf_Max_R__Lower = cons_ip.ColorThresholds.isGreen_IdxOf_Max_R__Lower.value
# ts_IdxOf_Max_R__Upper = 50
# ts_IdxOf_Max_R__Lower = 0
# G
# ts_IdxOf_Max_G__Upper = 60
# ts_IdxOf_Max_G__Lower = 0
ts_IdxOf_Max_G__Upper = cons_ip.ColorThresholds.isGreen_IdxOf_Max_G__Upper.value
ts_IdxOf_Max_G__Lower = cons_ip.ColorThresholds.isGreen_IdxOf_Max_G__Lower.value
# B
# ts_IdxOf_Max_B__Upper = 200
# ts_IdxOf_Max_B__Lower = 150
ts_IdxOf_Max_B__Upper = cons_ip.ColorThresholds.isGreen_IdxOf_Max_B__Upper.value
ts_IdxOf_Max_B__Lower = cons_ip.ColorThresholds.isGreen_IdxOf_Max_B__Lower.value
'''###################
judge : max value : blue
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_R > ts_Max_Val_R__Upper \
or max_Val_R < ts_Max_Val_R__Lower :
msg = "False : max_Val_R ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_R__Upper, ts_Max_Val_R__Lower, max_Val_R)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : max value : green
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_G > ts_Max_Val_G__Upper \
or max_Val_G < ts_Max_Val_G__Lower :
msg = "False : max_Val_G ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_G__Upper, ts_Max_Val_G__Lower, max_Val_G)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : max value : red
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_B > ts_Max_Val_B__Upper \
or max_Val_B < ts_Max_Val_B__Lower :
msg = "False : max_Val_B ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_B__Upper, ts_Max_Val_B__Lower, max_Val_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : blue
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
if not (idxOf_Maxes_R > ts_IdxOf_Max_R__Lower \
and idxOf_Maxes_R < ts_IdxOf_Max_R__Upper) :
msg = "False : idxOf_Max_R ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_IdxOf_Max_R__Upper, ts_IdxOf_Max_R__Lower, idxOf_Maxes_R)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : green
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
if not (idxOf_Maxes_G > ts_IdxOf_Max_G__Lower \
and idxOf_Maxes_G < ts_IdxOf_Max_G__Upper) :
msg = "False : idxOf_Maxe_G ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_IdxOf_Max_G__Upper, ts_IdxOf_Max_G__Lower, idxOf_Maxes_G)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : red
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
# if not (idxOf_Maxes_R > ts_IdxOf_Max_R__Upper) :
if not (idxOf_Maxes_B > ts_IdxOf_Max_B__Lower \
and idxOf_Maxes_B < ts_IdxOf_Max_B__Upper) :
msg = "False : idxOf_Max_B ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_IdxOf_Max_B__Upper, ts_IdxOf_Max_B__Lower, idxOf_Maxes_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
return
###################'''
return True, "True"
#/ def is_ColorName_Red(image_StatsData):
'''###################
is_ColorName_Yellow
description :
at : 2018/06/08 09:42:37
@return: res, msg
res boolean
msg string ==> if res is 'False', gives info
###################'''
def is_ColorName_Yellow(image_StatsData):
'''###################
get vars : indices, max vals
###################'''
idxOf_Maxes = image_StatsData['idxOf_Maxes']
# print()
# print("[%s:%d] idxOf_Maxes =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(idxOf_Maxes)
max_Vals = image_StatsData['max_Vals']
# print()
# print("[%s:%d] max_Vals =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(max_Vals)
'''###################
get vars : each color element
R
==> in the sheet graph and genreted data --> 'r'
==> in reality --> 'b'
G
==> in the sheet graph and genreted data --> 'g'
==> in reality --> 'g'
B
==> in the sheet graph and genreted data --> 'b'
==> in reality --> 'r'
###################'''
# prep vars
idxOf_Maxes_R = idxOf_Maxes[0]
idxOf_Maxes_G = idxOf_Maxes[1]
idxOf_Maxes_B = idxOf_Maxes[2]
max_Val_R = max_Vals[0]
max_Val_G = max_Vals[1]
max_Val_B = max_Vals[2]
'''###################
judge : order of idxOf_Maxes
###################'''
condition_IdxOf_Maxes_1 = \
(idxOf_Maxes_B > idxOf_Maxes_R \
and idxOf_Maxes_G > idxOf_Maxes_R)
condition_IdxOf_Maxes_2 = \
(idxOf_Maxes_B >= idxOf_Maxes_G \
or np.abs(idxOf_Maxes_B - idxOf_Maxes_G) < 20)
# if not (idxOf_Maxes_R > idxOf_Maxes_G and idxOf_Maxes_G > idxOf_Maxes_B):
if not (condition_IdxOf_Maxes_1):
msg = "False : order of idxOf_Maxes ==> incomplicit (should be : R,G > B | idxOf_Maxes_R = %d, idxOf_Maxes_G = %d, idxOf_Maxes_B = %d)" \
% (idxOf_Maxes_R, idxOf_Maxes_G, idxOf_Maxes_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
if not (condition_IdxOf_Maxes_2):
msg = "False : order of idxOf_Maxes ==> incomplicit (should be : R >= G or abs(R - G) < 20 | idxOf_Maxes_R = %d, idxOf_Maxes_G = %d, idxOf_Maxes_B = %d)" \
% (idxOf_Maxes_R, idxOf_Maxes_G, idxOf_Maxes_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
prep vars : thresholds
###################'''
# max values
# R
# ts_Max_Val_R__Lower = 4000
ts_Max_Val_R__Lower = cons_ip.ColorThresholds.isYellow_Max_Val_R__Lower.value
ts_Max_Val_R__Upper = cons_ip.ColorThresholds.isYellow_Max_Val_R__Upper.value
# ts_Max_Val_R__Lower = 2500
# ts_Max_Val_R__Upper = 5000
# G
# ts_Max_Val_G__Lower = 3000
# ts_Max_Val_G__Lower = 2000
# ts_Max_Val_G__Upper = 4000
ts_Max_Val_G__Lower = cons_ip.ColorThresholds.isYellow_Max_Val_G__Lower.value
ts_Max_Val_G__Upper = cons_ip.ColorThresholds.isYellow_Max_Val_G__Upper.value
# B
# ts_Max_Val_B__Lower = 3500
# ts_Max_Val_B__Lower = 2000
# ts_Max_Val_B__Upper = 4500
ts_Max_Val_B__Lower = cons_ip.ColorThresholds.isYellow_Max_Val_B__Lower.value
ts_Max_Val_B__Upper = cons_ip.ColorThresholds.isYellow_Max_Val_B__Upper.value
# ts_Max_Val_G = 2000
# ts_Max_Val_B = 2000
# index of max values
# R
ts_IdxOf_Max_R__Upper = cons_ip.ColorThresholds.isYellow_IdxOf_Max_R__Upper.value
ts_IdxOf_Max_R__Lower = cons_ip.ColorThresholds.isYellow_IdxOf_Max_R__Lower.value
# ts_IdxOf_Max_R__Upper = -1 # -1 ==> not used
# ts_IdxOf_Max_R__Lower = 80
# G
# ts_IdxOf_Max_G__Upper = 220
# ts_IdxOf_Max_G__Lower = 165
ts_IdxOf_Max_G__Upper = cons_ip.ColorThresholds.isYellow_IdxOf_Max_G__Upper.value
ts_IdxOf_Max_G__Lower = cons_ip.ColorThresholds.isYellow_IdxOf_Max_G__Lower.value
# B
# ts_IdxOf_Max_B__Upper = 200
# ts_IdxOf_Max_B__Upper = 180
# ts_IdxOf_Max_B__Lower = -1 # -1 ==> not used
ts_IdxOf_Max_B__Upper = cons_ip.ColorThresholds.isYellow_IdxOf_Max_B__Upper.value
ts_IdxOf_Max_B__Lower = cons_ip.ColorThresholds.isYellow_IdxOf_Max_B__Lower.value
# #debug
# print()
# print("[%s:%d] ts_IdxOf_Max_B__Upper = %d, ts_IdxOf_Max_B__Lower = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , ts_IdxOf_Max_B__Upper, ts_IdxOf_Max_B__Lower
# ), file=sys.stderr)
# ts_IdxOf_Max_G = 110
# ts_IdxOf_Max_B = 120
'''###################
judge : max value : blue
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_R > ts_Max_Val_R__Upper \
or max_Val_R < ts_Max_Val_R__Lower :
msg = "False : max_Val_R ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_R__Upper, ts_Max_Val_R__Lower, max_Val_R)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : max value : green
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_G > ts_Max_Val_G__Upper \
or max_Val_G < ts_Max_Val_G__Lower :
msg = "False : max_Val_G ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_G__Upper, ts_Max_Val_G__Lower, max_Val_G)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : max value : red
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_B > ts_Max_Val_B__Upper \
or max_Val_B < ts_Max_Val_B__Lower :
msg = "False : max_Val_B ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_B__Upper, ts_Max_Val_B__Lower, max_Val_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : blue
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
if not (idxOf_Maxes_R < ts_IdxOf_Max_R__Lower) :
msg = "False : idxOf_Max_R ==> out of range (idxOf_Maxes_R = %d, ts_IdxOf_Max_R__Lower = %d)" \
% (idxOf_Maxes_R, ts_IdxOf_Max_R__Lower)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : green
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
if not (idxOf_Maxes_G < ts_IdxOf_Max_G__Upper \
and idxOf_Maxes_G > ts_IdxOf_Max_G__Lower) :
msg = "False : idxOf_Maxe_G ==> out of range (max = %d, min = %d, value = %d)" \
% (ts_IdxOf_Max_G__Upper, ts_IdxOf_Max_G__Lower, idxOf_Maxes_G)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : red
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
# if not (idxOf_Maxes_R > ts_IdxOf_Max_R__Upper) :
if not (idxOf_Maxes_B > ts_IdxOf_Max_B__Lower \
and idxOf_Maxes_B < ts_IdxOf_Max_B__Upper) :
# if not (idxOf_Maxes_B > ts_IdxOf_Max_B__Upper) :
msg = "False : idxOf_Max_B ==> out of range (max = %d, min = %d, value = %d)" \
% (ts_IdxOf_Max_B__Upper, ts_IdxOf_Max_B__Lower, idxOf_Maxes_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : span between indices of max valuea : B - G
###################'''
# condition
spanOf_IdxOf_Maxes_BG = 50
condition_Span__BG = (idxOf_Maxes_B - idxOf_Maxes_G) < spanOf_IdxOf_Maxes_BG
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
# if not (idxOf_Maxes_R > ts_IdxOf_Max_R__Upper) :
if not (condition_Span__BG) :
# if not (idxOf_Maxes_B > ts_IdxOf_Max_B__Upper) :
msg = "False : span between idxOf_Max B and G ==> out of range (idxOf_Maxes_B = %d, idxOf_Maxes_G = %d, spanOf_IdxOf_Maxes_BG = %d)" \
% (idxOf_Maxes_B, idxOf_Maxes_G, spanOf_IdxOf_Maxes_BG)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
return
###################'''
return True, "True"
#/ def is_ColorName_Yellow(image_StatsData):
'''###################
is_ColorName_White
description :
at : 2018/06/21 07:41:17
@return: res, msg
res boolean
msg string ==> if res is 'False', gives info
###################'''
def is_ColorName_White(image_StatsData):
'''###################
get vars : indices, max vals
###################'''
idxOf_Maxes = image_StatsData['idxOf_Maxes']
# print()
# print("[%s:%d] idxOf_Maxes =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(idxOf_Maxes)
max_Vals = image_StatsData['max_Vals']
# print()
# print("[%s:%d] max_Vals =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(max_Vals)
'''###################
get vars : each color element
R
==> in the sheet graph and genreted data --> 'r'
==> in reality --> 'b'
G
==> in the sheet graph and genreted data --> 'g'
==> in reality --> 'g'
B
==> in the sheet graph and genreted data --> 'b'
==> in reality --> 'r'
###################'''
# prep vars
idxOf_Maxes_R = idxOf_Maxes[0]
idxOf_Maxes_G = idxOf_Maxes[1]
idxOf_Maxes_B = idxOf_Maxes[2]
max_Val_R = max_Vals[0]
max_Val_G = max_Vals[1]
max_Val_B = max_Vals[2]
'''###################
judge : order of idxOf_Maxes
###################'''
condition_IdxOf_Maxes_1 = \
(idxOf_Maxes_B > cons_ip.ColorThresholds.isWhite_IdxOf_Max.value \
and idxOf_Maxes_R > cons_ip.ColorThresholds.isWhite_IdxOf_Max.value \
and idxOf_Maxes_G > cons_ip.ColorThresholds.isWhite_IdxOf_Max.value)
# (idxOf_Maxes_B == cons_ip.ColorThresholds.isWhite_IdxOf_Max.value \
# and idxOf_Maxes_R == cons_ip.ColorThresholds.isWhite_IdxOf_Max.value \
# and idxOf_Maxes_G == cons_ip.ColorThresholds.isWhite_IdxOf_Max.value)
condition_Max_Val_1 = \
(max_Val_B > cons_ip.ColorThresholds.isWhite_Max_Val__Lower.value \
and max_Val_G > cons_ip.ColorThresholds.isWhite_Max_Val__Lower.value \
and max_Val_R > cons_ip.ColorThresholds.isWhite_Max_Val__Lower.value
)
# if not (idxOf_Maxes_R > idxOf_Maxes_G and idxOf_Maxes_G > idxOf_Maxes_B):
if not (condition_IdxOf_Maxes_1):
msg = "False : order of idxOf_Maxes ==> incomplicit (should be : R,G,B == %d | idxOf_Maxes_R = %d, idxOf_Maxes_G = %d, idxOf_Maxes_B = %d)" \
% (cons_ip.ColorThresholds.isWhite_IdxOf_Max.value \
, idxOf_Maxes_R, idxOf_Maxes_G, idxOf_Maxes_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
if not (condition_Max_Val_1):
msg = "False : max vals ==> incomplicit (should be : R,G,B > %d | max_Val_R = %d, max_Val_G = %d, max_Val_B = %d)" \
% (cons_ip.ColorThresholds.isWhite_Max_Val__Lower.value \
, max_Val_R, max_Val_G, max_Val_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
return
###################'''
return True, "True"
#/ def is_ColorName_Yellow(image_StatsData):
'''###################
is_ColorName_Black(image_StatsData)
description :
at : 2018/06/21 17:04:48
@return: res, msg
res boolean
msg string ==> if res is 'False', gives info
###################'''
def is_ColorName_Black(image_StatsData):
'''###################
get vars : indices, max vals
###################'''
idxOf_Maxes = image_StatsData['idxOf_Maxes']
# print()
# print("[%s:%d] idxOf_Maxes =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(idxOf_Maxes)
max_Vals = image_StatsData['max_Vals']
# print()
# print("[%s:%d] max_Vals =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(max_Vals)
'''###################
get vars : each color element
R
==> in the sheet graph and genreted data --> 'r'
==> in reality --> 'b'
G
==> in the sheet graph and genreted data --> 'g'
==> in reality --> 'g'
B
==> in the sheet graph and genreted data --> 'b'
==> in reality --> 'r'
###################'''
# prep vars
idxOf_Maxes_R = idxOf_Maxes[0]
idxOf_Maxes_G = idxOf_Maxes[1]
idxOf_Maxes_B = idxOf_Maxes[2]
max_Val_R = max_Vals[0]
max_Val_G = max_Vals[1]
max_Val_B = max_Vals[2]
'''###################
judge : order of idxOf_Maxes
###################'''
condition_IdxOf_Maxes_1 = \
(idxOf_Maxes_B == cons_ip.ColorThresholds.isBlack_IdxOf_Max.value \
and idxOf_Maxes_R == cons_ip.ColorThresholds.isBlack_IdxOf_Max.value \
and idxOf_Maxes_G == cons_ip.ColorThresholds.isBlack_IdxOf_Max.value)
condition_Max_Val_1 = \
(max_Val_B > cons_ip.ColorThresholds.isBlack_Max_Val__Lower.value \
and max_Val_G > cons_ip.ColorThresholds.isBlack_Max_Val__Lower.value \
and max_Val_R > cons_ip.ColorThresholds.isBlack_Max_Val__Lower.value
)
# if not (idxOf_Maxes_R > idxOf_Maxes_G and idxOf_Maxes_G > idxOf_Maxes_B):
if not (condition_IdxOf_Maxes_1):
msg = "False : order of idxOf_Maxes ==> incomplicit (should be : R,G,B == %d | idxOf_Maxes_R = %d, idxOf_Maxes_G = %d, idxOf_Maxes_B = %d)" \
% (cons_ip.ColorThresholds.isWhite_IdxOf_Max.value \
, idxOf_Maxes_R, idxOf_Maxes_G, idxOf_Maxes_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
if not (condition_Max_Val_1):
msg = "False : max vals ==> incomplicit (should be : R,G,B > %d | max_Val_R = %d, max_Val_G = %d, max_Val_B = %d)" \
% (cons_ip.ColorThresholds.isWhite_Max_Val__Lower.value \
, max_Val_R, max_Val_G, max_Val_B)
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
return
###################'''
return True, "True"
#/ def is_ColorName_Yellow(image_StatsData):
'''###################
is_ColorName_Red
description :
at : 2018/06/10 12:24:45
@return: res, msg
res boolean
msg string ==> if res is 'False', gives info
###################'''
def is_ColorName_Red(image_StatsData):
'''###################
get vars : indices, max vals
###################'''
idxOf_Maxes = image_StatsData['idxOf_Maxes']
# print()
# print("[%s:%d] idxOf_Maxes =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(idxOf_Maxes)
max_Vals = image_StatsData['max_Vals']
# print()
# print("[%s:%d] max_Vals =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(max_Vals)
'''###################
get vars : each color element
R
==> in the sheet graph and genreted data --> 'r'
==> in reality --> 'b'
G
==> in the sheet graph and genreted data --> 'g'
==> in reality --> 'g'
B
==> in the sheet graph and genreted data --> 'b'
==> in reality --> 'r'
###################'''
# prep vars
idxOf_Maxes_R = idxOf_Maxes[0]
idxOf_Maxes_G = idxOf_Maxes[1]
idxOf_Maxes_B = idxOf_Maxes[2]
max_Val_R = max_Vals[0]
max_Val_G = max_Vals[1]
max_Val_B = max_Vals[2]
'''###################
prep vars : thresholds
###################'''
'''###################
thresholds : max values
###################'''
# R
ts_Max_Val_R__Lower = cons_ip.ColorThresholds.isRed_Max_Val_R__Lower.value
ts_Max_Val_R__Upper = cons_ip.ColorThresholds.isRed_Max_Val_R__Upper.value
# ts_Max_Val_R__Lower = 2.2 * 1000
# ts_Max_Val_R__Upper = 3.9 * 1000
# G
ts_Max_Val_G__Lower = cons_ip.ColorThresholds.isRed_Max_Val_G__Lower.value
ts_Max_Val_G__Upper = cons_ip.ColorThresholds.isRed_Max_Val_G__Upper.value
# ts_Max_Val_G__Lower = 2.3 * 1000
# ts_Max_Val_G__Upper = 3.9 * 1000
# B
ts_Max_Val_B__Lower = cons_ip.ColorThresholds.isRed_Max_Val_B__Lower.value
ts_Max_Val_B__Upper = cons_ip.ColorThresholds.isRed_Max_Val_B__Upper.value
# ts_Max_Val_B__Lower = 1.8 * 1000
# ts_Max_Val_B__Upper = 2.5 * 1000
'''###################
thresholds : index of max values
###################'''
# R
ts_IdxOf_Max_R__Upper = cons_ip.ColorThresholds.isRed_IdxOf_Max_R__Upper.value
ts_IdxOf_Max_R__Lower = cons_ip.ColorThresholds.isRed_IdxOf_Max_R__Lower.value
# ts_IdxOf_Max_R__Upper = 50
# ts_IdxOf_Max_R__Lower = 0
# G
# ts_IdxOf_Max_G__Upper = 60
# ts_IdxOf_Max_G__Lower = 0
ts_IdxOf_Max_G__Upper = cons_ip.ColorThresholds.isRed_IdxOf_Max_G__Upper.value
ts_IdxOf_Max_G__Lower = cons_ip.ColorThresholds.isRed_IdxOf_Max_G__Lower.value
# B
# ts_IdxOf_Max_B__Upper = 200
# ts_IdxOf_Max_B__Lower = 150
ts_IdxOf_Max_B__Upper = cons_ip.ColorThresholds.isRed_IdxOf_Max_B__Upper.value
ts_IdxOf_Max_B__Lower = cons_ip.ColorThresholds.isRed_IdxOf_Max_B__Lower.value
'''###################
judge : max value : blue
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_R > ts_Max_Val_R__Upper \
or max_Val_R < ts_Max_Val_R__Lower :
msg = "False : max_Val_R ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_R__Upper, ts_Max_Val_R__Lower, max_Val_R)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : max value : green
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_G > ts_Max_Val_G__Upper \
or max_Val_G < ts_Max_Val_G__Lower :
msg = "False : max_Val_G ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_G__Upper, ts_Max_Val_G__Lower, max_Val_G)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : max value : red
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
if max_Val_B > ts_Max_Val_B__Upper \
or max_Val_B < ts_Max_Val_B__Lower :
msg = "False : max_Val_B ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_Max_Val_B__Upper, ts_Max_Val_B__Lower, max_Val_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : blue
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
if not (idxOf_Maxes_R > ts_IdxOf_Max_R__Lower \
and idxOf_Maxes_R < ts_IdxOf_Max_R__Upper) :
msg = "False : idxOf_Max_R ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_IdxOf_Max_R__Upper, ts_IdxOf_Max_R__Lower, idxOf_Maxes_R)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : green
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
if not (idxOf_Maxes_G > ts_IdxOf_Max_G__Lower \
and idxOf_Maxes_G < ts_IdxOf_Max_G__Upper) :
msg = "False : idxOf_Maxe_G ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_IdxOf_Max_G__Upper, ts_IdxOf_Max_G__Lower, idxOf_Maxes_G)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
judge : index of max value : red
###################'''
# judge : index of max val
# 'R' ===> color element of R (data is obtained in BGR format)
# if idxOf_Maxes_R > ts_IdxOf_Max_R__Lower :
# if not (idxOf_Maxes_R > ts_IdxOf_Max_R__Upper) :
if not (idxOf_Maxes_B > ts_IdxOf_Max_B__Lower \
and idxOf_Maxes_B < ts_IdxOf_Max_B__Upper) :
msg = "False : idxOf_Max_B ==> out of range (max = %d, min = %d, actual = %d)" \
% (ts_IdxOf_Max_B__Upper, ts_IdxOf_Max_B__Lower, idxOf_Maxes_B)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg
# ), file=sys.stderr)
return False, msg
'''###################
return
###################'''
return True, "True"
#/ def is_ColorName_Red(image_StatsData):
'''###################
get_Corner_Images(img_Src, corner_Length)
@return: [left bottom, right bottom, left up, right up]
|------------------------|
|(2) (3)|
| |
| |
| |
|(0) (1)|
|------------------------|
###################'''
# def get_Corner_Images(img_Src, corner_Length) :
def get_Corner_Images(img_Src, corner_Length, padding = 0) :
height, width, channels = img_Src.shape
clips = [
img_Src[(height - corner_Length) : height - padding, \
0 + padding : corner_Length], # clp_LB
img_Src[(height - corner_Length) : height - padding, \
width - corner_Length : width - padding], # clp_RB
img_Src[0 + padding : corner_Length, \
0 + padding : corner_Length], # clp_LU
img_Src[0 + padding : corner_Length, \
width - corner_Length : width - padding], # clp_RU
# img_Src[(height - corner_Length) : height, 0 : corner_Length], # clp_LB
# img_Src[(height - corner_Length) : height, width - corner_Length : width], # clp_RB
# img_Src[0 : corner_Length, 0 : corner_Length], # clp_LU
# img_Src[0 : corner_Length, width - corner_Length : width], # clp_RU
]
# return
return clips
#/ def get_Corner_Images(img_RGB, corner_Length) :
# def _exec_get_4_corners__Write_Log(lo_Names_Of_Corner_Images, lo_Image_MetaData):
# def is_PhotoOf__Sweets(dpath_Images, fname_Image) :
'''###################
is_PhotoOf__Sweets
###################'''
def is_PhotoOf__Sweets \
(dpath_Images, fname_Image, flg_SaveImage = False, \
corner_Width = 280, param_Corner_Padding = 0) :
'''###################
###################'''
'''###################
get : cv instance
###################'''
fpath_Image = "%s\\%s" % (dpath_Images, fname_Image)
# validate
res = os.path.isfile(fpath_Image)
if res == False : #if res == True
print("[%s:%d] file NOT exist! => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image
), file=sys.stderr)
# set dic
msg = "file NOT exist : %s" % fpath_Image
# return
return False, msg
# cv instance
img_Orig = cv2.imread(fpath_Image)
print()
print("[%s:%d] cv2 image ==> loaded" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# convert to RGB
img_RGB = img_Orig
# #TEST
# get_ColorName_From_CornerImage(img_RGB, dpath_Images, fname_Image)
# return
'''###################
get : meta data
###################'''
# data
height, width, channels = img_RGB.shape
# print()
# print("[%s:%d] height = %d, width = %d, channels = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , height, width, channels
# ), file=sys.stderr)
'''###################
get : 4 corners
###################'''
corner_Length = corner_Width
# corner_Length = 280
padding = param_Corner_Padding
# padding = 0
img_Corners = get_Corner_Images(img_RGB, corner_Length, padding)
'''###################
save : images of 4 corners
###################'''
save_Image = flg_SaveImage
# save_Image = True
# save_Image = False
lo_Names_Of_Corner_Images = \
get_4_corners__SaveImage_4Corners(img_Corners, fname_Image, save_Image)
'''###################
get : basic data
###################'''
lo_Image_MetaData = get_4_corners__Get_MetaData(img_Corners)
'''###################
get : stat data
###################'''
lo_Image_StatsData = get_4_corners__Get_StatsData(img_Corners)
'''###################
filtering : a corner of green ?
###################'''
res, comment = is_CornerOf_Green__PhotoOf_Sweets(lo_Image_StatsData[0])
'''###################
get : color name of the corner
###################'''
lo_Color_Names = []
lenOf_Lo_Names_Of_Corner_Images = len(lo_Names_Of_Corner_Images)
for i in range(lenOf_Lo_Names_Of_Corner_Images) :
nameOf_CornerImage = lo_Names_Of_Corner_Images[i]
#debug
print()
msg = "[%s / %s:%d] inspecting ===> %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, nameOf_CornerImage
)
print(msg, file=sys.stderr)
#debug
# libs.write_Log(msg, True)
stats_Data = lo_Image_StatsData[i]
color_Name = get_Color_Name_From_StatsData(stats_Data)
# append
lo_Color_Names.append([nameOf_CornerImage, color_Name])
#/for i in lenOf_Lo_Names_Of_Corner_Images:
msg = "[%s / %s:%d] lo_Color_Names =>" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
#debug
libs.write_Log(msg, True)
for item in lo_Color_Names:
# print(item)
#debug
msg = "%s => %s" % (item[0], item[1])
libs.write_Log(msg, True)
#/for item in lo_Color_Names:
'''###################
write log : file names
###################'''
# _exec_get_4_corners__Write_Log(
get_4_corners__Write_Log(
lo_Names_Of_Corner_Images,
lo_Image_MetaData,
lo_Image_StatsData
, dpath_Images
, fname_Image
, res
, comment
)
'''###################
get color names : HSV
###################'''
lo_Color_Names_2 = []
for i in range(lenOf_Lo_Names_Of_Corner_Images) :
# name of the image
nameOf_CornerImage = lo_Names_Of_Corner_Images[i]
# corner image
img = img_Corners[i]
# inspect
msg = get_ColorName_From_CornerImage(img, dpath_Images, fname_Image, i)
# append
lo_Color_Names_2.append([nameOf_CornerImage, msg])
# lo_Color_Names_2.append([nameOf_CornerImage, color_Name])
'''###################
report
###################'''
msg = "lo_Color_Names_2 =>"
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
for item in lo_Color_Names_2:
msg = "file = %s / color name = %s" % (item[0], item[1])
msg_Log = "%s" % \
(
msg)
libs.write_Log(msg_Log, True)
#/for item in lo_Color_Names_2:
'''###################
return
###################'''
return res, comment, (height, width, channels)
# return res, comment
# return False, msg
#/ def is_PhotoOf__Sweets(dir_path, file_name) :
'''###################
get_ColorName_Set_From_Image
@return: color name
e.g. "others", "yellow"
###################'''
def get_ColorName_Set_From_Image(\
dpath_Images, fpath, flg_SaveImage, \
# dpath_Images, fname, flg_SaveImage, \
param_Corner_Width, param_Corner_Padding) :
# '''###################
# vars
# ###################'''
# lo_Color_Names = []
'''###################
get : cv instance
###################'''
fpath_Image = fpath
# fpath_Image = "%s\\%s" % (dpath_Images, fname)
#debug
print()
print("[%s:%d] os.path.basename(fpath) => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, os.path.basename(fpath)
), file=sys.stderr)
# validate
res = os.path.isfile(fpath_Image)
if res == False : #if res == True
print("[%s:%d] file NOT exist! => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image
), file=sys.stderr)
# set dic
msg = "file NOT exist : %s" % fpath_Image
# return
return False, msg
# cv instance
img_Orig = cv2.imread(fpath_Image)
# '''###################
# validate : image obtained
# ###################'''
# #ref https://stackoverflow.com/questions/541390/extracting-extension-from-filename-in-python#541394
# filename, file_extension = os.path.splitext('/path/to/somefile.ext')
#
# if file_extension == ".mov" \
# or file_extension == ".MOV" : #if file_extension ==
#
# msg = "NOT an image file : '%s'\nreturning default 'others'" %\
# (fpath_Image)
#
# msg_Log = "[%s / %s:%d] %s" % \
# (
# libs.get_TimeLabel_Now()
# , os.path.basename(libs.thisfile()), libs.linenum()
# , msg)
#
# libs.write_Log(msg_Log, True)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg_Log
# ), file=sys.stderr)
#
# # return default
# return "others"
# #/if file_extension ==
# #ref https://stackoverflow.com/questions/17198466/none-python-error-bug#17198511
# if isinstance(img_Orig, type(None)) : #if img_Orig == None
#
# msg = "file NOT obtained (NoneType) : '%s'\nreturning default 'others'" %\
# (fpath_Image)
#
# msg_Log = "[%s / %s:%d] %s" % \
# (
# libs.get_TimeLabel_Now()
# , os.path.basename(libs.thisfile()), libs.linenum()
# , msg)
#
# libs.write_Log(msg_Log, True)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg_Log
# ), file=sys.stderr)
#
# # return default
# return "others"
#
# if not img_Orig.all() : #if img_Orig == None
# # if (img_Orig == None) : #if img_Orig == None
# # if img_Orig == None : #if img_Orig == None
#
# msg = "file NOT obtained (all() returns False) : '%s'\nreturning default 'others'" %\
# (fpath_Image)
#
# msg_Log = "[%s / %s:%d] %s" % \
# (
# libs.get_TimeLabel_Now()
# , os.path.basename(libs.thisfile()), libs.linenum()
# , msg)
#
# libs.write_Log(msg_Log, True)
#
# print()
# print("[%s:%d] %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , msg_Log
# ), file=sys.stderr)
#
# # return default
# return "others"
#
# #/if img_Orig == None
print()
print("[%s:%d] cv2 image ==> loaded" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
set : RGB
###################'''
# convert to RGB
img_RGB = img_Orig
'''###################
get : meta data
###################'''
# data
height, width, channels = img_RGB.shape
# print()
# print("[%s:%d] height = %d, width = %d, channels = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , height, width, channels
# ), file=sys.stderr)
'''###################
get : 4 corners
###################'''
corner_Length = param_Corner_Width
# corner_Length = corner_Width
# corner_Length = 280
padding = param_Corner_Padding
# padding = 0
img_Corners = get_Corner_Images(img_RGB, corner_Length, padding)
# print()
# print("[%s:%d] len(img_Corners) = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(img_Corners)
# ), file=sys.stderr)
'''###################
save : images of 4 corners
###################'''
save_Image = flg_SaveImage
# save_Image = True
# save_Image = False
fname_Image = os.path.basename(fpath)
lo_Names_Of_Corner_Images = \
get_4_corners__SaveImage_4Corners(img_Corners, fname_Image, save_Image)
# saveImage_4Corners(img_Corners, fname_Image, save_Image)
# _exec_get_4_corners__SaveImage_4Corners(img_Corners, fname_Image)
# print()
# print("[%s:%d] lo_Names_Of_Corner_Images =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Names_Of_Corner_Images)
'''###################
get : basic data
###################'''
lo_Image_MetaData = get_4_corners__Get_MetaData(img_Corners)
# lo_Image_MetaData = _exec_get_4_corners__Get_MetaData(img_Corners)
'''###################
get : stat data
###################'''
lo_Image_StatsData = get_4_corners__Get_StatsData(img_Corners)
# lo_Image_StatsData = _exec_get_4_corners__Get_StatsData(img_Corners)
'''###################
get : color name of the corner
###################'''
lo_Color_Names = []
lo_Color_Names_2 = []
lenOf_Lo_Names_Of_Corner_Images = len(lo_Names_Of_Corner_Images)
# for i in lenOf_Lo_Names_Of_Corner_Images:
for i in range(lenOf_Lo_Names_Of_Corner_Images) :
nameOf_CornerImage = lo_Names_Of_Corner_Images[i]
#debug
# print()
# msg = "[%s:%d] inspecting ===> %s" % \
msg = "[%s / %s:%d] inspecting ===> %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, nameOf_CornerImage
)
# print(msg, file=sys.stderr)
# print("[%s:%d] inspecting ===> %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , nameOf_CornerImage
# ), file=sys.stderr)
#debug
libs.write_Log(msg, True)
stats_Data = lo_Image_StatsData[i]
color_Name = get_Color_Name_From_StatsData(stats_Data)
# append
lo_Color_Names.append([nameOf_CornerImage, color_Name])
'''###################
lo_Color_Names_2
###################'''
img_Corner = img_Corners[i]
dpath_Images = dpath_Images
fname_Image = nameOf_CornerImage
ind = i
color_Name_2 = get_ColorName_From_CornerImage(
img_Corner, dpath_Images, fname_Image, ind)
# append
lo_Color_Names_2.append([nameOf_CornerImage, color_Name_2])
#/for i in lenOf_Lo_Names_Of_Corner_Images:
# #debug
# print()
# print("[%s:%d] lo_Color_Names_2 =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# for item in lo_Color_Names_2:
#
# print(item)
#
#/for item in lo_Color_Names_2:
#debug
libs.write_Log(msg, True)
for item in lo_Color_Names:
# print(item)
#debug
msg = "%s => %s" % (item[0], item[1])
libs.write_Log(msg, True)
#/for item in lo_Color_Names:
# print(lo_Color_Names)
'''###################
write log : file names
###################'''
# _exec_get_4_corners__Write_Log(
# get_4_corners__Write_Log(
get_4_corners__Write_Log__V2(
lo_Names_Of_Corner_Images,
lo_Image_MetaData,
lo_Image_StatsData
, dpath_Images
, fname_Image
, res
)
'''###################
return
###################'''
return lo_Color_Names_2
# return lo_Color_Names
#/ get_ColorName_Set_From_Image(dpath_Images, file_Name)
'''###################
func : get_NamesOf_CornerColors
at : 2018/06/12 13:07:10
color names : (3 colors)
yellow red green
@return: list of color names
[
['img...1.png', 'other']
,['img...1.png', 'yellow']
,['img...1.png', 'yellow']
,['img...1.png', 'other']
]
###################'''
def get_NamesOf_CornerColors \
(dpath_Images, fname_Image, flg_SaveImage = False, \
corner_Width = 280, param_Corner_Padding = 0) :
'''###################
###################'''
'''###################
get : cv instance
###################'''
fpath_Image = "%s\\%s" % (dpath_Images, fname_Image)
# validate
res = os.path.isfile(fpath_Image)
if res == False : #if res == True
print("[%s:%d] file NOT exist! => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image
), file=sys.stderr)
# set dic
msg = "file NOT exist : %s" % fpath_Image
# return
return False, msg
# cv instance
img_Orig = cv2.imread(fpath_Image)
print()
print("[%s:%d] cv2 image ==> loaded" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# convert to RGB
img_RGB = img_Orig
'''###################
get : meta data
###################'''
# data
height, width, channels = img_RGB.shape
print()
print("[%s:%d] height = %d, width = %d, channels = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, height, width, channels
), file=sys.stderr)
'''###################
get : 4 corners
###################'''
corner_Length = corner_Width
# corner_Length = 280
padding = param_Corner_Padding
# padding = 0
img_Corners = get_Corner_Images(img_RGB, corner_Length, padding)
print()
print("[%s:%d] len(img_Corners) = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(img_Corners)
), file=sys.stderr)
'''###################
save : images of 4 corners
###################'''
save_Image = flg_SaveImage
# save_Image = True
# save_Image = False
lo_Names_Of_Corner_Images = \
get_4_corners__SaveImage_4Corners(img_Corners, fname_Image, save_Image)
# saveImage_4Corners(img_Corners, fname_Image, save_Image)
# _exec_get_4_corners__SaveImage_4Corners(img_Corners, fname_Image)
print()
print("[%s:%d] lo_Names_Of_Corner_Images =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_Names_Of_Corner_Images)
'''###################
get : basic data
###################'''
lo_Image_MetaData = get_4_corners__Get_MetaData(img_Corners)
# lo_Image_MetaData = _exec_get_4_corners__Get_MetaData(img_Corners)
'''###################
get : stat data
###################'''
lo_Image_StatsData = get_4_corners__Get_StatsData(img_Corners)
# lo_Image_StatsData = _exec_get_4_corners__Get_StatsData(img_Corners)
'''###################
filtering : a corner of green ?
###################'''
# lo_Image_StatsData[0] => left bottom corner
# res = lib_ip.is_CornerOf_Green(lo_Image_StatsData[0])
# res, comment = lib_ip.is_CornerOf_Green__PhotoOf_Sweets(lo_Image_StatsData[0])
res, comment = is_CornerOf_Green__PhotoOf_Sweets(lo_Image_StatsData[0])
'''###################
get : color name of the corner
###################'''
lo_Color_Names = []
lenOf_Lo_Names_Of_Corner_Images = len(lo_Names_Of_Corner_Images)
# for i in lenOf_Lo_Names_Of_Corner_Images:
for i in range(lenOf_Lo_Names_Of_Corner_Images) :
nameOf_CornerImage = lo_Names_Of_Corner_Images[i]
#debug
print()
# msg = "[%s:%d] inspecting ===> %s" % \
msg = "[%s / %s:%d] inspecting ===> %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, nameOf_CornerImage
)
print(msg, file=sys.stderr)
# print("[%s:%d] inspecting ===> %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , nameOf_CornerImage
# ), file=sys.stderr)
#debug
libs.write_Log(msg, True)
stats_Data = lo_Image_StatsData[i]
color_Name = get_Color_Name_From_StatsData(stats_Data)
# append
lo_Color_Names.append([nameOf_CornerImage, color_Name])
#/for i in lenOf_Lo_Names_Of_Corner_Images:
print()
# msg = "[%s:%d] lo_Color_Names =>" % \
msg = "[%s / %s:%d] lo_Color_Names =>" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
print(msg, file=sys.stderr)
# print("[%s:%d] lo_Color_Names =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#debug
libs.write_Log(msg, True)
for item in lo_Color_Names:
# print(item)
#debug
msg = "%s => %s" % (item[0], item[1])
libs.write_Log(msg, True)
#/for item in lo_Color_Names:
# print(lo_Color_Names)
'''###################
write log : file names
###################'''
# _exec_get_4_corners__Write_Log(
get_4_corners__Write_Log(
lo_Names_Of_Corner_Images,
lo_Image_MetaData,
lo_Image_StatsData
, dpath_Images
, fname_Image
, res
, comment
)
'''###################
return
###################'''
# msg = "done"
return res, comment, (height, width, channels)
# return res, comment
# return False, msg
#/ def is_PhotoOf__Sweets(dir_path, file_name) :
'''###################
get_Color_Name_From_StatsData(stats_Data)
@return: nameOf_Color :: string
==> name of color
"green"
"yellow"
"red"
###################'''
def get_Color_Name_From_StatsData(stats_Data) :
'''###################
vars
###################'''
nameOf_Color = "other"
'''###################
judge : green
###################'''
#debug
# print()
msg = "[%s / %s:%d] inspecting : green ------------" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
# print(msg, file=sys.stderr)
# libs.write_Log(msg, True)
# print("[%s:%d] inspecting : green ------------" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
res, msg = is_ColorName_Green(stats_Data)
# green ?
if res == True : #if res == True
nameOf_Color = "green"
return nameOf_Color
else :
#debug
msg = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, msg
)
# libs.write_Log(msg, True)
'''###################
judge : yellow
###################'''
#debug
# print()
msg = "[%s / %s:%d] inspecting : yellow ------------" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
# print(msg, file=sys.stderr)
# libs.write_Log(msg, True)
# print("[%s:%d] inspecting : yellow ------------" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
res, msg = is_ColorName_Yellow(stats_Data)
# green ?
if res == True : #if res == True
nameOf_Color = "yellow"
return nameOf_Color
else :
#debug
msg = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, msg
)
# libs.write_Log(msg, True)
#/if res == True
'''###################
judge : red
###################'''
# print()
# msg = "[%s:%d] inspecting : red ------------" % \
msg = "[%s / %s:%d] inspecting : red ------------" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
#libs.write_Log(msg, True)
# libs.write_Log(msg, dpath_Log, fname_Log, True)
res, msg = is_ColorName_Red(stats_Data)
# green ?
if res == True : #if res == True
nameOf_Color = "red"
return nameOf_Color
else :
#debug
msg = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, msg
)
#libs.write_Log(msg, True)
#/if res == True
'''###################
judge : white
###################'''
# print()
msg = "[%s / %s:%d] inspecting : white ------------" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
#libs.write_Log(msg, True)
res, msg = is_ColorName_White(stats_Data)
# white ?
if res == True : #if res == True
nameOf_Color = "white"
return nameOf_Color
else :
#debug
msg = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, msg
)
#libs.write_Log(msg, True)
#/if res == True
'''###################
judge : black
###################'''
# print()
msg = "[%s / %s:%d] inspecting : black ------------" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
#libs.write_Log(msg, True)
res, msg = is_ColorName_Black(stats_Data)
# white ?
if res == True : #if res == True
nameOf_Color = "black"
return nameOf_Color
else :
#debug
msg = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, msg
)
#libs.write_Log(msg, True)
#/if res == True
'''###################
return
###################'''
# nameOf_Color = "other"
return nameOf_Color
#/ def get_Color_Name_From_StatsData(stats_Data) :
def get_4_corners__SaveImage_4Corners(img_Corners, fname_Image, save_Image = True) :
# count
cntOf_Corners = 1
# time label
tlabel = libs.get_TimeLabel_Now()
# paths
dpath_Plot= "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\img.corners"
lo_Names_Of_Corner_Images = []
for item in img_Corners:
# xpixels = item.shape[1]
# ypixels = item.shape[0]
#
# dpi = 72
# scalefactor = 1
#
# xinch = xpixels * scalefactor / dpi
# yinch = ypixels * scalefactor / dpi
#
# fig = plt.figure(figsize=(xinch,yinch))
#
# plt.imshow(item)
# dpath_Plot= "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\img.corners"
fname_Plot = "img.%s.%s.%d.png" % (tlabel, fname_Image, cntOf_Corners)
# fname_Plot = "%s.%s.%d.png" % (fname_Image, tlabel, cntOf_Corners)
fpath_Plot = "%s\\%s" % (dpath_Plot, fname_Plot)
# increment
cntOf_Corners += 1
# plt.savefig(fpath_Plot, dpi=dpi)
# cv2 : save image
#ref https://www.tutorialkart.com/opencv/python/opencv-python-save-image-example/
if save_Image == True : cv2.imwrite(fpath_Plot, item)
# cv2.imwrite(fpath_Plot, item)
# #debug
# print()
# print("[%s:%d] fpath_Plot => '%s'" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , fpath_Plot
# ), file=sys.stderr)
# append file name
lo_Names_Of_Corner_Images.append(fname_Plot)
# # reset plot
# plt.clf()
# plt.cla()
#/for item in im_Corners:
'''###################
return
###################'''
return lo_Names_Of_Corner_Images
#/ def saveImage_4Corners(img_Corners, fname_Image) :
def get_4_corners__Get_MetaData(img_Corners) :
# data
lo_Image_MetaData = []
for item in img_Corners:
'''###################
vars
###################'''
max_R = -1; max_G = -1; max_B = -1
min_R = 256; min_G = 256; min_B = 256
# min_R = 255; min_G = 255; min_B = 255
# counter
cntOf_Row = 0
cntOf_Cell = 0
# values
valsOf_R = [0] * 256
valsOf_G = [0] * 256
valsOf_B = [0] * 256
for row in item:
for cell in row:
# #debug
# print()
# print("[%s:%d] len(cell) => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(cell)
# ), file=sys.stderr)
# return
# get value
R = cell[0]; G = cell[1]; B = cell[2]
# histogram
valsOf_R[R] += 1
valsOf_G[G] += 1
valsOf_B[B] += 1
# max value
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
# min value
if R < min_R : min_R = R
if G < min_G : min_G = G
if B < min_B : min_B = B
# count
cntOf_Cell += 1
# reset count of cells
cntOf_Cell = 0
# count
cntOf_Row += 1
#/for row in item:
# append data
lo_Image_MetaData.append(
[
# valsOf_R
# , valsOf_G
# , valsOf_B
# , max_R
max_R
, max_G
, max_B
, min_R
, min_G
, min_B
, valsOf_R
, valsOf_G
, valsOf_B
]
)
#/for item in img_Corners:
'''###################
return
###################'''
#debug
# print()
# print("[%s:%d] len(lo_Image_MetaData) => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(lo_Image_MetaData)
# ), file=sys.stderr)
return lo_Image_MetaData
#/ def get_4_corners__Get_MetaData(img_Corners) :
def get_4_corners__Get_StatsData(img_Corners) :
# data
lo_Image_StatsData = []
for img_Data in img_Corners:
# var
do_StasData = {}
'''###################
skews
###################'''
# print("[%s:%d] getting skew values..." % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# ), file=sys.stderr)
skew_Values = get_Skews(img_Data)
# skew = lib_ip.get_Skew(img_Data)
do_StasData['skew_values'] = skew_Values
# do_StasData['skew'] = skew
# append
lo_Image_StatsData.append(do_StasData)
'''###################
index of max
###################'''
# idxOf_Max_R, idxOf_Max_G, idxOf_Max_B = lib_ip.get_IdxOf_Maxes(img_Data)
idxOf_Max_R, idxOf_Max_G, idxOf_Max_B, maxVal_R, maxVal_G, maxVal_B \
= get_IdxOf_Maxes(img_Data)
# print()
# print("[%s:%d] idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , idxOf_Max_R, idxOf_Max_G, idxOf_Max_B
# ), file=sys.stderr)
do_StasData['idxOf_Maxes'] = [idxOf_Max_R, idxOf_Max_G, idxOf_Max_B]
do_StasData['max_Vals'] = [maxVal_R, maxVal_G, maxVal_B]
#/for img in img_Corners:
'''###################
return
###################'''
return lo_Image_StatsData
#/ def get_4_corners__Get_StatsData(img_Corners) :
def get_4_corners__Write_Log(
lo_Names_Of_Corner_Images,
lo_Image_MetaData,
lo_Image_StatsData
, dpath_Images
, fname_Image
, res
, comment
) :
dpath_Log = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\logs"
fname_Log = "get_4_corners.log"
fpath_Log = "%s\\%s" % (dpath_Log, fname_Log)
fout_Log = open(fpath_Log, "a")
# header
fout_Log.write(
"[%s %s:%s] =============== Get 4 corners" % \
(libs.get_TimeLabel_Now(),
os.path.basename(libs.thisfile()),
libs.linenum()))
fout_Log.write("\n")
'''###################
write : meta info
###################'''
msg = "dpath_Images = %s" % (dpath_Images)
fout_Log.write(msg)
fout_Log.write('\n')
msg = "fname_Image = %s" % (fname_Image)
fout_Log.write(msg)
fout_Log.write('\n')
fout_Log.write('\n')
# iterate
idxOf_Images = 0
lenOf_LO_Names_Of_Corner_Images = len(lo_Names_Of_Corner_Images)
# for item in lo_Names_Of_Corner_Images:
for i in range(lenOf_LO_Names_Of_Corner_Images):
# items
name = lo_Names_Of_Corner_Images[i]
# meta data
metaData = lo_Image_MetaData[i]
# stats
# [{'skew_values': {'skew_B': 533.0519884872008, 'skew_R': 236.23069368923885, 'sk
# ew_G': 238.3791814689376}}, {'skew_values': {'skew_B': 56.682440339675104, 'skew
# _R': 149.4848026940312, 'skew_G': 78.97239727258551}}, {'skew_values': {'skew_B'
# : 494.3158542205711, 'skew_R': 312.1150017522547, 'skew_G': 465.10712589168577}}
# , {'skew_values': {'skew_B': 481.71441048360913, 'skew_R': 323.66383568902853, '
# skew_G': 475.05481070433}}]
do_Stats = lo_Image_StatsData[i]
do_Skews = do_Stats['skew_values']
# print()
# print("[%s:%d] lo_Image_StatsData =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Image_StatsData)
#
# print()
# print("[%s:%d] do_Stats =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(do_Stats)
skew_R = do_Skews['skew_R']
skew_G = do_Skews['skew_G']
skew_B = do_Skews['skew_B']
# skew_R = do_Stats['skew_R']
# skew_G = do_Stats['skew_G']
# skew_B = do_Stats['skew_B']
'''###################
file name
###################'''
# file name
fout_Log.write(name)
# fout_Log.write(item)
fout_Log.write('\n')
# meta data
# print()
# print("[%s:%d] type(metaData) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData)
# ), file=sys.stderr)
#
# print("[%s:%d] type(metaData[0]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData[0])
# ), file=sys.stderr)
#
# print("[%s:%d] type(metaData[1]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData[1])
# ), file=sys.stderr)
# max_R
# , max_G
# , max_B
#
# , min_R
# , min_G
# , min_B
# , valsOf_R
# , valsOf_G
# , valsOf_B
msg = "R=(%d,%d) G=(%d,%d) B=(%d,%d)" % \
(metaData[0], metaData[3], metaData[1]
, metaData[4], metaData[2], metaData[5]
)
# msg = "\t".join(metaData)
#fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
#fout_Log.write('\n')
'''###################
skews
###################'''
msg = "skew_R = %.04f, skew_G = %.04f, skew_B = %.04f" % \
(
skew_R, skew_G, skew_B
)
# msg = "\t".join(metaData)
#fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
#fout_Log.write('\n')
'''###################
index of max values,
max values
###################'''
idxOf_MaxVals = do_Stats['idxOf_Maxes']
max_Vals = do_Stats['max_Vals']
# msg = "idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
msg = "idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
(
idxOf_MaxVals[0], idxOf_MaxVals[1], idxOf_MaxVals[2]
)
# msg = "\t".join(metaData)
#fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
#fout_Log.write('\n')
msg = "max_Vals_R = %d, max_Vals_G = %d, max_Vals_B = %d" % \
(
max_Vals[0], max_Vals[1], max_Vals[2]
)
# msg = "\t".join(metaData)
#fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
#fout_Log.write('\n')
'''###################
raw data : histogram
###################'''
dat = [str(x) for x in metaData[6]]
msg = "\t".join(dat)
# msg = "\t".join(metaData[6])
#fout_Log.write(msg)
#fout_Log.write('\n')
dat = [str(x) for x in metaData[7]]
msg = "\t".join(dat)
#fout_Log.write(msg)
#fout_Log.write('\n')
dat = [str(x) for x in metaData[8]]
msg = "\t".join(dat)
#fout_Log.write(msg)
#fout_Log.write('\n')
#fout_Log.write('\n')
#/for item in lo_Names_Of_Corner_Images:
# separator line
# fout_Log.write('\n')
'''###################
write : judge
###################'''
# fout_Log.write('\n')
# msg = "dpath_Images = %s" % (dpath_Images)
#
# fout_Log.write(msg)
# fout_Log.write('\n')
#
# msg = "fname_Image = %s" % (fname_Image)
#
# fout_Log.write(msg)
# fout_Log.write('\n')
msg = "is_CornerOf_Green__PhotoOf_Sweets => %s (%s)" % (res, comment)
fout_Log.write(msg)
fout_Log.write('\n')
# close file
fout_Log.close()
#/ get_4_corners__Write_Log
def get_4_corners__Write_Log__V2(
lo_Names_Of_Corner_Images,
lo_Image_MetaData,
lo_Image_StatsData
, dpath_Images
, fname_Image
, res
) :
dpath_Log = cons_ip.FilePaths.dpath_LogFile.value
fname_Log = cons_ip.FilePaths.fname_LogFile.value
# fname_Log = cons_fx.FPath.fname_LogFile.value
# dpath_Log = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\logs"
#
# fname_Log = "get_4_corners.log"
fpath_Log = "%s\\%s" % (dpath_Log, fname_Log)
fout_Log = open(fpath_Log, "a")
# header
fout_Log.write(
"[%s %s:%s] =============== Get 4 corners" % \
(libs.get_TimeLabel_Now(),
os.path.basename(libs.thisfile()),
libs.linenum()))
fout_Log.write("\n")
'''###################
write : meta info
###################'''
msg = "dpath_Images = %s" % (dpath_Images)
fout_Log.write(msg)
fout_Log.write('\n')
msg = "fname_Image = %s" % (fname_Image)
fout_Log.write(msg)
fout_Log.write('\n')
fout_Log.write('\n')
'''###################
return (forced)
###################'''
return
# iterate
idxOf_Images = 0
lenOf_LO_Names_Of_Corner_Images = len(lo_Names_Of_Corner_Images)
# for item in lo_Names_Of_Corner_Images:
for i in range(lenOf_LO_Names_Of_Corner_Images):
# items
name = lo_Names_Of_Corner_Images[i]
# meta data
metaData = lo_Image_MetaData[i]
# stats
# [{'skew_values': {'skew_B': 533.0519884872008, 'skew_R': 236.23069368923885, 'sk
# ew_G': 238.3791814689376}}, {'skew_values': {'skew_B': 56.682440339675104, 'skew
# _R': 149.4848026940312, 'skew_G': 78.97239727258551}}, {'skew_values': {'skew_B'
# : 494.3158542205711, 'skew_R': 312.1150017522547, 'skew_G': 465.10712589168577}}
# , {'skew_values': {'skew_B': 481.71441048360913, 'skew_R': 323.66383568902853, '
# skew_G': 475.05481070433}}]
do_Stats = lo_Image_StatsData[i]
do_Skews = do_Stats['skew_values']
# print()
# print("[%s:%d] lo_Image_StatsData =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Image_StatsData)
#
# print()
# print("[%s:%d] do_Stats =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(do_Stats)
skew_R = do_Skews['skew_R']
skew_G = do_Skews['skew_G']
skew_B = do_Skews['skew_B']
# skew_R = do_Stats['skew_R']
# skew_G = do_Stats['skew_G']
# skew_B = do_Stats['skew_B']
'''###################
file name
###################'''
# file name
fout_Log.write(name)
# fout_Log.write(item)
fout_Log.write('\n')
# meta data
# print()
# print("[%s:%d] type(metaData) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData)
# ), file=sys.stderr)
#
# print("[%s:%d] type(metaData[0]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData[0])
# ), file=sys.stderr)
#
# print("[%s:%d] type(metaData[1]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData[1])
# ), file=sys.stderr)
# max_R
# , max_G
# , max_B
#
# , min_R
# , min_G
# , min_B
# , valsOf_R
# , valsOf_G
# , valsOf_B
msg = "R=(%d,%d) G=(%d,%d) B=(%d,%d)" % \
(metaData[0], metaData[3], metaData[1]
, metaData[4], metaData[2], metaData[5]
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
'''###################
skews
###################'''
msg = "skew_R = %.04f, skew_G = %.04f, skew_B = %.04f" % \
(
skew_R, skew_G, skew_B
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
'''###################
index of max values,
max values
###################'''
idxOf_MaxVals = do_Stats['idxOf_Maxes']
max_Vals = do_Stats['max_Vals']
# msg = "idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
msg = "idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
(
idxOf_MaxVals[0], idxOf_MaxVals[1], idxOf_MaxVals[2]
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
msg = "max_Vals_R = %d, max_Vals_G = %d, max_Vals_B = %d" % \
(
max_Vals[0], max_Vals[1], max_Vals[2]
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
'''###################
raw data : histogram
###################'''
dat = [str(x) for x in metaData[6]]
msg = "\t".join(dat)
# msg = "\t".join(metaData[6])
fout_Log.write(msg)
fout_Log.write('\n')
dat = [str(x) for x in metaData[7]]
msg = "\t".join(dat)
fout_Log.write(msg)
fout_Log.write('\n')
dat = [str(x) for x in metaData[8]]
msg = "\t".join(dat)
fout_Log.write(msg)
fout_Log.write('\n')
fout_Log.write('\n')
#/for item in lo_Names_Of_Corner_Images:
# separator line
# fout_Log.write('\n')
'''###################
write : judge
###################'''
# msg = "is_CornerOf_Green__PhotoOf_Sweets => %s (%s)" % (res, comment)
#
# fout_Log.write(msg)
# fout_Log.write('\n')
# close file
fout_Log.close()
#/ get_4_corners__Write_Log
'''###################
@param color_Names: ["o", "r", "o", "o"]
###################'''
def get_Memo_From_ColorNames_Set(color_Names) :
#ref https://stackoverflow.com/questions/15046242/how-to-sort-the-letters-in-a-string-alphabetically-in-python
str_ColorNames = ''.join(sorted(color_Names))
memo = ""
'''###################
judge
###################'''
if str_ColorNames in cons_ip.ColorNameSet.lo_Color_Sets.value : #if str_ColorNames in cons_ip.ColorNameSet.lo_Color_Sets.value
do_Color_Sets_Memo = cons_ip.ColorNameSet.do_Color_Sets_Memo.value
memo = do_Color_Sets_Memo[str_ColorNames]
else :
memo = cons_ip.ColorNameSet.memo_Unknown.value
#/if str_ColorNames in cons_ip.ColorNameSet.lo_Color_Sets.value
# if str_ColorNames == cons_ip.ColorNameSet.colSet_OOOO.value: #if str_ColorNames ==
#
# memo = cons_ip.ColorNameSet.memo_OOOO.value
#
# elif str_ColorNames == cons_ip.ColorNameSet.colSet_GGOO.value : #if str_ColorNames ==
#
# memo = cons_ip.ColorNameSet.memo_GGOO.value
#
# elif str_ColorNames == cons_ip.ColorNameSet.colSet_OOOY.value : #if str_ColorNames ==
#
# memo = cons_ip.ColorNameSet.memo_OOOY.value
#
# else : #if str_ColorNames ==
#
# memo = "%s (%s)" % (cons_ip.ColorNameSet.memo_Unknown.value, str_ColorNames)
# # memo = cons_ip.ColorNameSet.memo_Unknown.value
#
# #/if str_ColorNames ==
# return
return memo
#/ def get_Memo_From_ColorNames_Set(color_Names) :
def test__get_Opt_IP():
'''###################
vars
###################'''
lo_Args_Pairs = []
charOf_Option_Switch = "-"
'''###################
get : args
###################'''
args = sys.argv
print()
print("[%s:%d] args =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(args)
'''###################
validate : no option elements
###################'''
if len(args) < 2 : #if len(args) < 2
print()
print("[%s:%d] no args =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(args)
return lo_Args_Pairs
#/if len(args) < 2
'''###################
ops
###################'''
# length : omit program name element
args_Options = args[1:]
lenOf_Args_Options = len(args_Options)
# lenOf_Args = len(args)
# each element in the args
for i in range(lenOf_Args_Options):
# start with : "-"
elem = args_Options[i]
#ref https://stackoverflow.com/questions/8802860/checking-whether-a-string-starts-with-xxxx#8802889
judge = elem.startswith(charOf_Option_Switch)
if judge == True : #if judge == True
print()
print("[%s:%d] option swith => %s (char is '%s', index = %d)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, elem, elem[1:], i
), file=sys.stderr)
'''###################
validate : list length --> enough
###################'''
if (lenOf_Args_Options - 2) >= i : #if (lenOf_Args_Options - 2) >= i
pass
else : #if (lenOf_Args_Options - 2) >= i
print()
print("[%s:%d] option-related value => not given" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if (lenOf_Args_Options - 2) >= i
else : #if judge == True
pass
#/if judge == True
#/for i in range(lenOf_Args):
'''###################
returen
###################'''
return lo_Args_Pairs
#/ def get_Opt_IP():
def get_ColorName_Set_From_CornerImage(img_Corner):
height, width, channels = img_Corner.shape
msg = "height = %d, width = %d, channels = %d" %\
(height, width, channels)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log
, cons_fx.FPath.dpath_LogFile.value
, cons_fx.FPath.fname_LogFile.value
, 1)
print()
print("[%s:%d] %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, msg_Log
), file=sys.stderr)
#/ def get_ColorName_Set_From_CornerImage(img_Corner):
'''###################
@param img: RGB data
###################'''
def get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image):
# def get_StatsData_Of_Image__HSV(img):
'''###################
convert : to HSV
###################'''
img_HSV = cv2.cvtColor(img, cv2.COLOR_RGB2HSV)
'''###################
vars
###################'''
Hs = []
Ss = []
Vs = []
'''###################
ops
###################'''
# #debug
# print("[%s:%d] img_HSV[0][:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# print(img_HSV[0][:10])
for row in img_HSV:
for pix in row:
Hs.append(pix[0])
Ss.append(pix[1])
Vs.append(pix[2])
#/for pix in row:
#/for row in img_HSV:
# #debug
# print()
# print("[%s:%d] Hs[:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(Hs[:10])
# #debug : average
# print()
# print("[%s:%d] Hs ==> average = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , sum(Hs) * 1.0 / len(Hs)
# ), file=sys.stderr)
#debug
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
# val_Average, val_Variance, sd = get_StatsData(Hs)
# print()
# print("[%s:%d] val_Average = %.03f, val_Variance = %.03f, sd = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , val_Average, val_Variance, sd
# ), file=sys.stderr)
'''###################
save : stats data
###################'''
msg = "file = %s / val_Average = %.04f\tval_Variance = %.04f\tsd = %.04f\tmax = %d\tmin = %d" %\
(fname_Image, val_Average, val_Variance, sd, val_Max, val_Min)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
# #debug : plot
# img_Plot_Target = Hs
# # img_Plot_Target = Hs[:1000]
#
# rng = np.arange(len(img_Plot_Target))
#
# # reset plot
# plt.clf()
# plt.cla()
#
#
# plt.plot(rng, img_Plot_Target, 'r-', label='Hs')
# # plt.plot(rng, Hs[:100], 'r-', label='Hs')
# # plt.plot(rng, Hs[:100])
# # plt.plot(Hs)
#
# plt.legend(loc='best')
#
# ax = plt.gca()
#
# #ref grid https://stackoverflow.com/questions/16074392/getting-vertical-gridlines-to-appear-in-line-plot-in-matplotlib
# ax.grid(which='major', axis='both', linestyle='--')
# ax.grid(which='minor', axis='both', linestyle='--')
#
# # ax.set(aspect=1,
# ax.set(
# xlim=(0, len(img_Plot_Target)),
# # xlim=(0, len(Hs[:100])),
# # xlim=(0, len(lo_Rs)),
# ylim=(0, 250))
#
#
# dpath_Savefig = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\data_images"
#
# fname = "savefig.%s.%s.png" % (libs.get_TimeLabel_Now(), fname_Image)
#
# fpath_Savefig = os.path.join(dpath_Savefig, fname)
#
# plt.savefig(fpath_Savefig)
#
# print("[%s:%d] plot ==> saved (%s)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , fpath_Savefig
# ), file=sys.stderr)
#
# # reset plot
# plt.clf()
# plt.cla()
'''###################
return
###################'''
return Hs, Ss, Vs
#/ def get_StatsData_Of_Image__HSV(img):
'''###################
@param img: HSV data
@return: res, msg
###################'''
def is_ColorName_Yellow__2(img, dpath_Images, fname_Image):
# def is_ColorName_Yellow__2(img):
'''###################
get : stats
###################'''
Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image)
# Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img)
#debug
msg = "len(Hs) = %d, len(Ss) = %d, len(Vs) = %d" %\
(len(Hs), len(Ss), len(Vs))
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : Hue data
###################'''
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
'''###################
judge
###################'''
# default values
result = False
msg = "other"
# variance
# (val_Variance < cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value \
# and val_Variance > cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value) :
if not \
(val_Variance <= cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value \
and val_Variance >= cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value) :
# msg = "variance --> out of range (variance = %.03f / upper = %.03f / loweer = %.03f " %\
msg = "yellow : variance --> out of range (variance = %.03f / upper = %.03f / lower = %.03f)" %\
(val_Variance
, cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value
, cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
# average
if not \
(val_Average < cons_ip.ColorThresholds.isYellow_HSV_Average__Upper.value \
and val_Average > cons_ip.ColorThresholds.isYellow_HSV_Average__Lower.value): #if val_Variance > cons_ip.ColorThresholds
# msg = "average --> out of range (average = %.03f / upper = %.03f / lower = %.03f " %\
msg = "yellow : average --> out of range (average = %.03f / upper = %.03f / lower = %.03f)" %\
(val_Average
, cons_ip.ColorThresholds.isYellow_HSV_Average__Upper.value
, cons_ip.ColorThresholds.isYellow_HSV_Average__Lower.value)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
'''###################
set : is yellow
###################'''
result = True
msg = "yellow"
'''###################
return
###################'''
# result = False
#
# msg = "other"
return result, msg
#/ def is_ColorName_Yellow__2(img):
'''###################
@param img: HSV data
@return: res, msg
###################'''
def is_ColorName_Red__2(img, dpath_Images, fname_Image):
# def is_ColorName_Yellow__2(img):
'''###################
get : stats
###################'''
Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image)
# Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img)
#debug
msg = "len(Hs) = %d, len(Ss) = %d, len(Vs) = %d" %\
(len(Hs), len(Ss), len(Vs))
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : Hue data
###################'''
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
'''###################
judge
###################'''
# default values
result = False
msg = "other"
color_Name = cons_ip.ColorNameSet.colName_Red.value
variance_Upper = cons_ip.ColorThresholds.isRed_HSV_Variance__Upper.value
variance_Lower = cons_ip.ColorThresholds.isRed_HSV_Variance__Lower.value
average_Upper = cons_ip.ColorThresholds.isRed_HSV_Average__Upper.value
average_Lower = cons_ip.ColorThresholds.isRed_HSV_Average__Lower.value
# variance
if not \
(val_Variance <= variance_Upper \
and val_Variance >= variance_Lower) :
# (val_Variance < variance_Upper \
# and val_Variance > variance_Lower) :
# (val_Variance < cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value \
# and val_Variance > cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value) :
# msg = "variance --> out of range (variance = %.03f / upper = %.03f / loweer = %.03f " %\
msg = "%s : variance --> out of range (variance = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Variance
, variance_Upper
, variance_Lower)
# , cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value
# , cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
# average
if not \
(val_Average < average_Upper \
and val_Average > average_Lower) :
# (val_Average < cons_ip.ColorThresholds.isYellow_HSV_Average__Upper.value \
# and val_Average > cons_ip.ColorThresholds.isYellow_HSV_Average__Lower.value): #if val_Variance > cons_ip.ColorThresholds
# msg = "average --> out of range (average = %.03f / upper = %.03f / lower = %.03f " %\
msg = "%s : average --> out of range (average = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Average
, average_Upper
, average_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
'''###################
set : is red
###################'''
result = True
msg = color_Name
'''###################
return
###################'''
# result = False
#
# msg = "other"
return result, msg
#/ def is_ColorName_Yellow__2(img):
'''###################
<descriptions>
1. using RGB values for judgement
@param img: HSV data
@return: res, msg
###################'''
def is_ColorName_Green__2(img, dpath_Images, fname_Image):
'''###################
get : stats
###################'''
Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image)
#debug
msg = "len(Hs) = %d, len(Ss) = %d, len(Vs) = %d" %\
(len(Hs), len(Ss), len(Vs))
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : Hue data
###################'''
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
'''###################
judge
###################'''
# default values
result = False
msg = "other"
color_Name = cons_ip.ColorNameSet.colName_Green.value
variance_Upper = cons_ip.ColorThresholds.isGreen_HSV_Variance__Upper.value
variance_Lower = cons_ip.ColorThresholds.isGreen_HSV_Variance__Lower.value
average_Upper = cons_ip.ColorThresholds.isGreen_HSV_Average__Upper.value
average_Lower = cons_ip.ColorThresholds.isGreen_HSV_Average__Lower.value
# variance
if not \
(val_Variance <= variance_Upper \
and val_Variance >= variance_Lower) :
# (val_Variance < variance_Upper \
# and val_Variance > variance_Lower) :
# (val_Variance < cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value \
# and val_Variance > cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value) :
# msg = "variance --> out of range (variance = %.03f / upper = %.03f / loweer = %.03f " %\
msg = "%s : variance --> out of range (variance = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Variance
, variance_Upper
, variance_Lower)
# , cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value
# , cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
# average
if not \
(val_Average < average_Upper \
and val_Average > average_Lower) :
# (val_Average < cons_ip.ColorThresholds.isYellow_HSV_Average__Upper.value \
# and val_Average > cons_ip.ColorThresholds.isYellow_HSV_Average__Lower.value): #if val_Variance > cons_ip.ColorThresholds
# msg = "average --> out of range (average = %.03f / upper = %.03f / lower = %.03f " %\
msg = "%s : average --> out of range (average = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Average
, average_Upper
, average_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
'''###################
set : is red
###################'''
result = True
msg = color_Name
'''###################
return
###################'''
# result = False
#
# msg = "other"
return result, msg
#/ def is_ColorName_Yellow__2(img):
'''###################
<descriptions>
1. using RGB values for judgement
@param img: HSV data
@return: res, msg
###################'''
def is_ColorName_Blue__2(img, dpath_Images, fname_Image):
'''###################
get : stats
###################'''
Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image)
#debug
msg = "len(Hs) = %d, len(Ss) = %d, len(Vs) = %d" %\
(len(Hs), len(Ss), len(Vs))
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : Hue data
###################'''
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
'''###################
judge
###################'''
# default values
result = False
msg = "other"
color_Name = cons_ip.ColorNameSet.colName_Blue.value
variance_Upper = cons_ip.ColorThresholds.isBlue_HSV_Variance__Upper.value
variance_Lower = cons_ip.ColorThresholds.isBlue_HSV_Variance__Lower.value
average_Upper = cons_ip.ColorThresholds.isBlue_HSV_Average__Upper.value
average_Lower = cons_ip.ColorThresholds.isBlue_HSV_Average__Lower.value
# variance
if not \
(val_Variance <= variance_Upper \
and val_Variance >= variance_Lower) :
msg = "%s : variance --> out of range (variance = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Variance
, variance_Upper
, variance_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
# average
if not \
(val_Average < average_Upper \
and val_Average > average_Lower) :
msg = "%s : average --> out of range (average = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Average
, average_Upper
, average_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
'''###################
set : is red
###################'''
result = True
msg = color_Name
'''###################
return
###################'''
# result = False
#
# msg = "other"
return result, msg
#/ is_ColorName_Blue__2(img, dpath_Images, fname_Image)
'''###################
<descriptions>
1. using RGB values for judgement
@param img: HSV data
@return: res, msg
###################'''
def is_ColorName_Pink__2(img, dpath_Images, fname_Image):
'''###################
get : stats
###################'''
Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image)
#debug
msg = "len(Hs) = %d, len(Ss) = %d, len(Vs) = %d" %\
(len(Hs), len(Ss), len(Vs))
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : Hue data
###################'''
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
'''###################
judge
###################'''
# default values
result = False
msg = "other"
color_Name = cons_ip.ColorNameSet.colName_Pink.value
variance_Upper = cons_ip.ColorThresholds.isPink_HSV_Variance__Upper.value
variance_Lower = cons_ip.ColorThresholds.isPink_HSV_Variance__Lower.value
average_Upper = cons_ip.ColorThresholds.isPink_HSV_Average__Upper.value
average_Lower = cons_ip.ColorThresholds.isPink_HSV_Average__Lower.value
# variance
if not \
(val_Variance <= variance_Upper \
and val_Variance >= variance_Lower) :
msg = "%s : variance --> out of range (variance = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Variance
, variance_Upper
, variance_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
# average
if not \
(val_Average < average_Upper \
and val_Average > average_Lower) :
msg = "%s : average --> out of range (average = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Average
, average_Upper
, average_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
'''###################
set : is red
###################'''
result = True
msg = color_Name
'''###################
return
###################'''
# result = False
#
# msg = "other"
return result, msg
#/ is_ColorName_Pink__2(img, dpath_Images, fname_Image)
'''###################
@param img: HSV data
@return: res, msg
###################'''
def is_ColorName_Black__2(img, dpath_Images, fname_Image):
'''###################
get : stats
###################'''
Hs, Ss, Vs = get_StatsData_Of_Image__HSV(img, dpath_Images, fname_Image)
#debug
msg = "len(Hs) = %d, len(Ss) = %d, len(Vs) = %d" %\
(len(Hs), len(Ss), len(Vs))
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : Hue data
###################'''
val_Average, val_Variance, sd, val_Max, val_Min = get_StatsData(Hs)
'''###################
judge
###################'''
# default values
result = False
msg = "other"
color_Name = cons_ip.ColorNameSet.colName_Black.value
variance_Upper = cons_ip.ColorThresholds.isBlack_HSV_Variance__Upper.value
variance_Lower = cons_ip.ColorThresholds.isBlack_HSV_Variance__Lower.value
average_Upper = cons_ip.ColorThresholds.isBlack_HSV_Average__Upper.value
average_Lower = cons_ip.ColorThresholds.isBlack_HSV_Average__Lower.value
# variance
if not \
(val_Variance <= variance_Upper \
and val_Variance >= variance_Lower) :
# (val_Variance < cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value \
# and val_Variance > cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value) :
# msg = "variance --> out of range (variance = %.03f / upper = %.03f / loweer = %.03f " %\
msg = "%s : variance --> out of range (variance = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Variance
, variance_Upper
, variance_Lower)
# , cons_ip.ColorThresholds.isYellow_HSV_Variance__Upper.value
# , cons_ip.ColorThresholds.isYellow_HSV_Variance__Lower.value)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
# average
if not \
(val_Average <= average_Upper \
and val_Average >= average_Lower) :
# (val_Average < cons_ip.ColorThresholds.isYellow_HSV_Average__Upper.value \
# and val_Average > cons_ip.ColorThresholds.isYellow_HSV_Average__Lower.value): #if val_Variance > cons_ip.ColorThresholds
# msg = "average --> out of range (average = %.03f / upper = %.03f / lower = %.03f " %\
msg = "%s : average --> out of range (average = %.03f / upper = %.03f / lower = %.03f)" %\
( color_Name
, val_Average
, average_Upper
, average_Lower)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return result, msg
'''###################
set : is red
###################'''
result = True
msg = color_Name
'''###################
return
###################'''
# result = False
#
# msg = "other"
return result, msg
#/ def is_ColorName_Yellow__2(img):
'''###################
@param ind: index of the img_Corner
|------------------------|
|(2) (3)|
| |
| |
| |
|(0) (1)|
|------------------------|
@return: msg
"other"
"yellow"
"green"
"red"
###################'''
# def get_ColorName_From_CornerImage(img_Corner, dpath_Images, fname_Image):
def get_ColorName_From_CornerImage(img_Corner, dpath_Images, fname_Image, ind):
height, width, channels = img_Corner.shape
'''###################
meta data
###################'''
# msg = "%s %d" %\
#msg = "\n%s %d" %\
msg = "\n%s %d ---------------------" %\
(fname_Image, ind)
# (fname_Image)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
print()
print("[%s:%d] %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, msg_Log
), file=sys.stderr)
'''######################################
get : color name
###################'''
# # default
# msg = "other"
'''###################
yellow
###################'''
res, msg = is_ColorName_Yellow__2(img_Corner, dpath_Images, fname_Image)
# yellow
if res == True : #if res == True
return msg
'''###################
red
###################'''
res, msg = is_ColorName_Red__2(img_Corner, dpath_Images, fname_Image)
# red
if res == True : #if res == True
return msg
'''###################
green
###################'''
res, msg = is_ColorName_Green__2(img_Corner, dpath_Images, fname_Image)
# green
if res == True : #if res == True
return msg
'''###################
blue
###################'''
res, msg = is_ColorName_Blue__2(img_Corner, dpath_Images, fname_Image)
# green
if res == True : #if res == True
return msg
'''###################
pink
###################'''
res, msg = is_ColorName_Pink__2(img_Corner, dpath_Images, fname_Image)
# green
if res == True : #if res == True
return msg
'''###################
black
###################'''
res, msg = is_ColorName_Black__2(img_Corner, dpath_Images, fname_Image)
# black
if res == True : #if res == True
return msg
'''###################
other
###################'''
# debug
msg = "file : %s %d (%s)" %\
(fname_Image, ind, msg)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
return
###################'''
# default
msg = "other"
return msg
# return res, msg
#/ def get_ColorName_Set_From_CornerImage(img_Corner):
'''###################
@param lo_Data: [188, 187, 188, ...]
@return: val_Average, val_Variance, sd
###################'''
def get_StatsData(lo_Data):
lenOf_LO_Data = len(lo_Data)
# print()
# print("[%s:%d] lo_Data[:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Data[:10])
val_Average = sum(lo_Data) * 1.0 / lenOf_LO_Data
# print()
# print("[%s:%d] val_Average =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(val_Average)
# [lib_ip.py:3837] val_Average =>
# 94.75623456790123
'''###################
variance
###################'''
#ref http://www.geisya.or.jp/~mwm48961/kou3/prob_variance1.htm
# �シ剃ケ励�ョ蟷ウ蝮�
lo_Squares = [1.0 * x * x for x in lo_Data]
# lo_Squares = [x * x for x in lo_Data]
# lo_Squares = np.power(lo_Data, 2)
# lo_Squares = [np.power(lo_Data, 2)]
# print()
# print("[%s:%d] lo_Squares[:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Squares[:10])
# print()
# print("[%s:%d] lo_Data[0] = %.03f, lo_Data[0] * lo_Data[0] = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , lo_Data[0], lo_Data[0] * lo_Data[0]
# ), file=sys.stderr)
# # print(lo_Data[0] * lo_Data[0])
# print()
# print("[%s:%d] lo_Data[:10] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Data[:10])
avgOf_LO_Squares = sum(lo_Squares) / lenOf_LO_Data
# avgOf_LO_Squares = lo_Squares / lenOf_LO_Data
# print()
# print("[%s:%d] avgOf_LO_Squares =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(avgOf_LO_Squares)
# variance : �シ剃ケ励�ョ蟷ウ蝮� - 蟷ウ蝮�縺ョ莠御ケ�
#ref power https://docs.scipy.org/doc/numpy/reference/generated/numpy.power.html
val_Variance = avgOf_LO_Squares - np.power(val_Average, 2)
# print()
# print("[%s:%d] val_Variance =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(val_Variance)
'''###################
standard dev
###################'''
sd = np.sqrt(val_Variance)
'''###################
min, max
###################'''
val_Max = max(lo_Data)
val_Min = min(lo_Data)
'''###################
return
###################'''
return val_Average, val_Variance, sd, val_Max, val_Min
# return val_Average, val_Variance, sd
# , val_Variance, sd
#/ def get_StatsData(img):
'''###################
@param file: file pull path
@return: dictionary
ref https://www.lifewithpython.com/2014/12/python-extract-exif-data-like-data-from-images.html
###################'''
def get_exif_of_image(file):
"""Get EXIF of an image if exists.
謖�螳壹@縺溽判蜒上�ョEXIF繝�繝シ繧ソ繧貞叙繧雁�コ縺咎未謨ー
@return exif_table Exif 繝�繝シ繧ソ繧呈�シ邏阪@縺溯セ樊嶌
"""
im = Image.open(file)
# Exif 繝�繝シ繧ソ繧貞叙蠕�
# 蟄伜惠縺励↑縺代l縺ー縺昴�ョ縺セ縺セ邨ゆコ� 遨コ縺ョ霎樊嶌繧定ソ斐☆
try:
exif = im._getexif()
except AttributeError:
return {}
# 繧ソ繧ーID縺昴�ョ縺セ縺セ縺ァ縺ッ莠コ縺瑚ェュ繧√↑縺�縺ョ縺ァ繝�繧ウ繝シ繝峨@縺ヲ
# 繝�繝シ繝悶Ν縺ォ譬シ邏阪☆繧�
exif_table = {}
for tag_id, value in exif.items():
tag = TAGS.get(tag_id, tag_id)
exif_table[tag] = value
return exif_table
'''###################
get_GPS_Data(fpath_Image)
@return: tuple
=> (('N', 35, 35, 24.14), ('E', 139, 34, 48.01))
False
=> no key "GPSInfo"
@param fpath_Image: file full path
@example file : C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload\2018-08-22_15-42-10_000.jpg
[ops_1.py:126] dicOf_Exif['GPSInfo'] =>
{1: 'N', 2: ((35, 1), (35, 1), (2414, 100)), 3: 'E', 4: ((139, 1), (34, 1), (480
1, 100)), 5: b'\x00', 6: (24268, 387), 7: ((6, 1), (42, 1), (900, 100)), 12: 'K'
, 13: (0, 1), 16: 'T', 17: (80093, 231), 23: 'T', 24: (80093, 231), 29: '2018:08
:22', 31: (10, 1)}
###################'''
def get_GPS_Data(fpath_Image):
#debug
print()
print("[%s:%d] getting GPS data for ... : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image
), file=sys.stderr)
dicOf_Exif = get_exif_of_image(fpath_Image)# def get_GPS_Data():
'''###################
validate : GPSInfo
###################'''
if not "GPSInfo" in dicOf_Exif : #if not "GPSInfo" in dicOf_Exif
msg = "no key 'GPSInfo' for file : %s" %\
(fpath_Image)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
return False
#/if not "GPSInfo" in dicOf_Exif
# get data
gps_Info = dicOf_Exif['GPSInfo']
'''###################
lat, longi
###################'''
txt_Lat = "%s=" % (gps_Info[1])
txt_Longi = "%s=" % (gps_Info[3])
'''###################
values
###################'''
vals_Lat = gps_Info[2]
txt_Lat += "%d-%d-%.02f" % \
(vals_Lat[0][0], vals_Lat[1][0], vals_Lat[2][0] * 1.0 / vals_Lat[2][1])
# (vals_Lat[0][0], vals_Lat[1][0])
vals_Longi = gps_Info[4]
txt_Longi += "%d-%d-%.02f" % \
(vals_Longi[0][0], vals_Longi[1][0], vals_Longi[2][0] * 1.0 / vals_Longi[2][1])
# txt_Longi += "%d-%d-" % \
# (vals_Longi[0][0], vals_Longi[1][0])
'''###################
tuples
###################'''
data_Lat = (gps_Info[1], vals_Lat[0][0], vals_Lat[1][0], vals_Lat[2][0] * 1.0 / vals_Lat[2][1])
data_Longi = (gps_Info[3], vals_Longi[0][0], vals_Longi[1][0], vals_Longi[2][0] * 1.0 / vals_Longi[2][1])
data_Final = (data_Lat, data_Longi)
'''###################
report
###################'''
print("[%s:%d] txt_Lat = %s, txt_Longi = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, txt_Lat, txt_Longi
), file=sys.stderr)
'''###################
return
###################'''
return data_Final
#/ def get_GPS_Data(fpath_Image):
<file_sep>/JVEMV6/46_art/3_sound-prog/1_/1_1 (2).py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_\1_\1_1.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\1_\1_1.py
at : 2018/03/21 11:55:27
pushd C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_\1_
python 1_1.py
'''
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_mm import libs
from libs_VX7GLZ import libs_VX7GLZ
from libs_VX7GLZ import wablibs
'''###################
import : specifics
###################'''
import midi, pretty_midi
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, math as math, random as rnd
###############################################
import wave
import struct
import numpy as np
# from pylab import *
from matplotlib import pylab as plt
# import random as rnd
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def test_5():
print("[%s:%d] test_5()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
for i in range(128):
name_Inst = pretty_midi.program_to_instrument_name(i)
print("num = %d / %s" % (i, name_Inst))
#/for i in range(127):
#/ def test_1():
def test_4():
print("[%s:%d] test_4()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# Create a PrettyMIDI object
cello_c_chord = pretty_midi.PrettyMIDI()
# Create an Instrument instance for a cello instrument
cello_program = pretty_midi.instrument_name_to_program('Cello')
cello = pretty_midi.Instrument(program=cello_program)
# Iterate over note names, which will be converted to note number later
for note_name in ['C5', 'E5', 'G5']:
# Retrieve the MIDI note number for this note name
note_number = pretty_midi.note_name_to_number(note_name)
# Create a Note instance for this note, starting at 0s and ending at .5s
note = pretty_midi.Note(velocity=100, pitch=note_number, start=0, end=.5)
# Add it to our cello instrument
cello.notes.append(note)
# Add the cello instrument to the PrettyMIDI object
cello_c_chord.instruments.append(cello)
# Write out the MIDI data
cello_c_chord.write('cello-C-chord.mid')
#/ def test_1():
def test_3():
print("[%s:%d] test_3()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# fname = "test.%s.mid" % (libs.get_TimeLabel_Now())
pitch_1 = midi.A_4
pitch_2 = midi.G_4
pitch_3 = midi.F_4
pitch_4 = midi.E_4
pitch_5 = midi.D_4
pitch_6 = midi.C_4
pitch_7 = midi.B_4
ps = [
midi.A_4,
midi.G_4,
midi.F_4,
midi.E_4,
midi.D_4,
midi.C_4,
midi.B_4,
]
# ps = [pitch_1, pitch_2, pitch_3, pitch_4]
lenOf_PS = len(ps)
numOf_Notes = 32
# file name
fname = "test.%s.ps-%02d.notes-%02d.mid" %\
(libs.get_TimeLabel_Now(), len(ps), numOf_Notes)
pattern = midi.Pattern(resolution=960) #このパターンがmidiファイルに対応しています。
track = midi.Track() #トラックを作ります
pattern.append(track) #パターンに作ったトラックを追加します。
ev = midi.SetTempoEvent(tick=0, bpm=120) #テンポを設定するイベントを作ります
track.append(ev) #イベントをトラックに追加します。
'''###################
set : notes
###################'''
for i in range(numOf_Notes):
# for i in range(8):
num = rnd.randint(0, lenOf_PS - 1)
# print("[%s:%d] num => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , num
# ), file=sys.stderr)
# num = rnd.randint(1, lenOf_PS)
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= ps[num]) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= ps[num]) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
# e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_1) #ソの音を鳴らし始めるイベントを作ります。
# track.append(e)
#
# e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_1) #ソの音を鳴らし終えるイベントを作ります。
# track.append(e)
#/for i in range(8):
'''###################
track : end
###################'''
eot = midi.EndOfTrackEvent(tick=1) #トラックを終えるイベントを作ります
track.append(eot)
midi.write_midifile(fname, pattern) #パターンをファイルに書き込みます。
'''###################
read mid
###################'''
# pattern = midi.read_midifile(fname)
#
# print(pattern)
#/ def test_1():
def test_2():
print("[%s:%d] test_2()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
file = "test.%s.mid" % (libs.get_TimeLabel_Now())
pitch_1 = midi.A_4
pitch_2 = midi.G_4
pattern = midi.Pattern(resolution=960) #このパターンがmidiファイルに対応しています。
track = midi.Track() #トラックを作ります
pattern.append(track) #パターンに作ったトラックを追加します。
ev = midi.SetTempoEvent(tick=0, bpm=120) #テンポを設定するイベントを作ります
track.append(ev) #イベントをトラックに追加します。
'''###################
set : notes
###################'''
# e = midi.NoteOnEvent(tick=0, velocity=100, pitch=midi.G_4) #ソの音を鳴らし始めるイベントを作ります。
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_1) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_1) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_2) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_2) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_1) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_1) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_2) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_2) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_1) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_1) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
e = midi.NoteOnEvent(tick=0, velocity=100, pitch= pitch_2) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch= pitch_2) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
'''###################
track : end
###################'''
eot = midi.EndOfTrackEvent(tick=1) #トラックを終えるイベントを作ります
track.append(eot)
midi.write_midifile(file, pattern) #パターンをファイルに書き込みます。
#/ def test_1():
def test_1():
print("[%s:%d] test_1()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
file = "test.mid"
pattern = midi.Pattern(resolution=960) #このパターンがmidiファイルに対応しています。
track = midi.Track() #トラックを作ります
pattern.append(track) #パターンに作ったトラックを追加します。
ev = midi.SetTempoEvent(tick=0, bpm=120) #テンポを設定するイベントを作ります
track.append(ev) #イベントをトラックに追加します。
e = midi.NoteOnEvent(tick=0, velocity=100, pitch=midi.G_4) #ソの音を鳴らし始めるイベントを作ります。
track.append(e)
e = midi.NoteOffEvent(tick=960, velocity=100, pitch=midi.G_4) #ソの音を鳴らし終えるイベントを作ります。
track.append(e)
eot = midi.EndOfTrackEvent(tick=1) #トラックを終えるイベントを作ります
track.append(eot)
midi.write_midifile(file, pattern) #パターンをファイルに書き込みます。
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_5()
# test_4()
# test_3()
# test_2()
# test_1()
# test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgHierarchy.js
//kwgHierarchy.js
function Table()
{
//部品の大きさは異なるが同じ色,同じ種類なのでparts個数は1
this.flagSolid = true;
this.shadow = 0.0;
//位置・姿勢(root位置)
this.vPos = new Vector3(0.0, 0.0, 0.0);//Topの中心の(x,y),脚の下端
this.vEuler = new Vector3(0.0, 0.0, 0.0);
this.vSize = new Vector3(1.0, 1.0, 1.0);//全体のスケール変換に使用
var i;
// this.parts;
this.parts = new Rigid_HS();
this.parts.kind = "SUPER";
this.parts.diffuse = [0.8, 0.5, 0.2, 1.0];
this.parts.ambient = [0.5, 0.3, 0.1, 1.0];
this.parts.nSlice = 10;
this.parts.nStack = 10;
this.parts.eps1 = 0.2;
this.parts.eps2 = 0.2;
//サイズ
this.vTop = new Vector3(1.0, 2.0, 0.1);//天板
this.vLeg = new Vector3(0.15, 0.15, 1.0);
//------------------------------
Table.prototype.draw = function(gl)
{
this.parts.flagSolid = this.flagSolid;
this.parts.shadow = this.shadow;
var n = this.parts.initVertexBuffers(gl);//1種類のプリミティブを使うので1回だけでよい
//スタック行列の確保
var stackMat = new Matrix4();//テーブルのときは1個でよい
// モデル行列の初期化
var modelMatrix = new Matrix4();
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
//全体(root)
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z);
modelMatrix.rotate(this.vEuler.z, 0, 0, 1); // z軸周りに回転
modelMatrix.rotate(this.vEuler.y, 0, 1, 0); // y軸周りに回転
modelMatrix.rotate(this.vEuler.x, 1, 0, 0); // x軸周りに回転
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
stackMat.copy(modelMatrix);//rootのモデル行列を保存
//天板
modelMatrix.translate(0.0, 0.0, this.vLeg.z);
modelMatrix.scale(this.vTop.x, this.vTop.y, this.vTop.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//前右脚
modelMatrix.translate(0.4 * this.vTop.x, -0.4 * this.vTop.y, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//前左脚
modelMatrix.translate(-0.4 * this.vTop.x, -0.4 * this.vTop.y, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//後右脚
modelMatrix.translate(0.4 * this.vTop.x, 0.4 * this.vTop.y, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//後左脚
modelMatrix.translate(-0.4 * this.vTop.x, 0.4 * this.vTop.y, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
}
}
//-------------------------------------------------------------------
function Chair()
{
this.flagSolid = true;
this.shadow = 0.0;
//位置・姿勢(root位置)
this.vPos = new Vector3(0.0, 0.0, 0.0);//Topの中心の(x,y),脚の下端
this.vEuler = new Vector3(0.0, 0.0, 0.0);
this.vSize = new Vector3(1.0, 1.0, 1.0);//全体のスケール変換に使用
this.parts = new Rigid_HS();
this.parts.kind = "SUPER";
this.parts.diffuse = [0.8, 0.2, 0.2, 1.0];
this.parts.ambient = [0.4, 0.1, 0.1, 1.0];
this.parts.nSlice = 10;
this.parts.nStack = 10;
this.parts.eps1 = 0.2;
this.parts.eps2 = 1.0;
//サイズ
this.vTop = new Vector3(0.5, 0.5, 0.05);//天板
this.vLeg = new Vector3(0.1, 0.1, 0.5);
//------------------------------
Chair.prototype.draw = function(gl)
{
this.parts.flagSolid = this.flagSolid;
this.parts.shadow = this.shadow;
var n = this.parts.initVertexBuffers(gl);//1種類のプリミティブを使うので1回だけでよい
//スタック行列の確保
var stackMat = new Matrix4();//テーブルのときは1個でよい
// モデル行列の初期化
var modelMatrix = new Matrix4();
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
//全体(root)
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z);
modelMatrix.rotate(this.vEuler.z, 0, 0, 1); // z軸周りに回転
modelMatrix.rotate(this.vEuler.y, 0, 1, 0); // y軸周りに回転
modelMatrix.rotate(this.vEuler.x, 1, 0, 0); // x軸周りに回転
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
stackMat.copy(modelMatrix);//rootのモデル行列を保存
//天板
modelMatrix.translate(0.0, 0.0, this.vLeg.z);
modelMatrix.scale(this.vTop.x, this.vTop.y, this.vTop.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//前脚
modelMatrix.translate(0.4 * this.vTop.x, 0.0, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//後脚
modelMatrix.translate(-0.4 * this.vTop.x, 0.0, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//右脚
modelMatrix.translate(0.0, -0.4 * this.vTop.y, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat);//rootのモデル行列に戻す
//左脚
modelMatrix.translate(0.0, 0.4 * this.vTop.y, 0.5 * this.vLeg.z);
modelMatrix.scale(this.vLeg.x, this.vLeg.y, this.vLeg.z);
this.parts.draw(gl, n, modelMatrix);
}
}
<file_sep>/JVEMV6/46_art/4_dtm/start_apps.bat
REM @ECHO OFF
REM *************************************
REM browser
REM *************************************
pushd "C:\Program Files (x86)\Google\Chrome\Application"
start chrome.exe https://audio.online-convert.com/convert-to-mp3
start chrome.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=jve+46%%234&RBs_AND_OR_Memo=AND&sort=file_name&direction=desc"
start chrome.exe "https://writer.zoho.com/writer/open/evojoe2141dae20014586ba9b199e668206cc"
start chrome.exe "http://benfranklin.chips.jp/PHP_server/D-2/time_calc.php"
REM pushd "C:\Program Files (x86)\MT4 Gaitame Finest Company Limited"
REM start terminal.exe
REM *************************************
REM folders
REM *************************************
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\4_dtm
start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
REM *************************************
REM MuLab
REM *************************************
pushd "C:\WORKS_2\Programs\freeware\music,sound\MuLab (64 bit)"
start MuLab.exe
REM *************************************
REM domino
REM 2019/06/12 17:30:07
REM *************************************
pushd C:\WORKS_2\Programs\Domino143
start Domino.exe
REM *************************************
REM itunes
REM 2019/06/17 14:16:02
REM *************************************
pushd C:\WORKS_2\Programs\itunes_12.6.2
start iTunes.exe
REM *************************************
REM git
REM *************************************
pushd C:\WORKS_2\WS\WS_Others.Art
REM call C:\WORKS_2\batches\s.bat
C:\WORKS_2\batches\s.bat
REM start start_git.bat
REM *************************************
REM console
REM *************************************
REM pushd C:\WORKS_2\Utils\shortcuts\proj_FX
REM start start_command_prompot.(1).bat
REM start "start_command_prompot.(1).bat"
REM call "start_command_prompot.(1).bat"
REM call start_command_prompot.(2).bat
REM pause
REM exit
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第3章/diffusion2D2.js
/***********************************************
diffusion2D2.js
2次元拡散方程式
解析領域は正方形
領域によって拡散係数が異なる場合
中心導体が直線状
D2
----------------------
D1
----------------------
D2
************************************************/
var canvas;//canvas要素
var gl; //WebGL描画用コンテキスト
var camera;//カメラ
var light; //光源
var flagDebug = false;
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var flagStart = false;
var flagStep = false;
var flagReset = false;
//表示オブジェクト
var object;//計算結果表示用
var adjustH = 0.5;//表示上の高さ調整
var colorMode = 0;//連続/段階表示
//数値計算
var flagConst = false;
var size = 1;//x,y軸方向サイズ(正方形)
var nMesh = 50;//x,y軸方向分割数
var delta;//Δx=Δy(格子間隔)
var radius = 0.1;//初期分布の半径
var x0 = 0;//物理量初期分布の中心(ワールド座標)
var y0 = 0;
var diffCoef1 = 0.01;//拡散係数1
var diffCoef2 = 0.0001; //拡散係数2
var width0 = 0.2;//クロス状中心導体の幅(拡散係数1)
var deltaT0 = 0.01;//数値計算上の時間刻み(初期設定値)
var deltaT; //実際の数値計算上の時間刻み(delta0/thinningN)
var thinningN = 1; //間引き回数
var diffNumber1; //拡散数
var diffNumber2;
var profile = 0; //Cylinder
var boundary = 0;//Dirichlet
var f0 = [];
var D = [];//拡散係数配列
function webMain()
{
// Canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);//webgl-utils.js
if(!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSL初期化に失敗");
return;
}
//Canvasをクリアする色を設定し、隠面消去機能を有効にする
gl.clearColor(0.1, 0.1, 0.1, 1.0);
gl.enable(gl.DEPTH_TEST);
form2.deltaT0.value = deltaT0;
form2.thinningN.value = thinningN;
form2.nMesh.value = nMesh;
form2.radius.value = radius;
form2.diffCoef1.value = diffCoef1;
form2.diffCoef2.value = diffCoef2;
form2.adjustH.value = adjustH;
form2.x0.value = x0;
form2.y0.value = y0;
initCamera();
initData();
display();
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
var timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += deltaT;//frameTime;//数値計算上の経過時間(実経過時間ではない)
for(var i = 0; i < thinningN; i++) calculate();
elapseTime0 = elapseTime;//現在の経過時間を保存
form1.time.value = elapseTime.toString();
if(flagStep) { flagStart = false; }
}
display();
}
animate();
}
//--------------------------------------------
function initCamera()
{
//光源インスタンスを作成
light = new Light();
//カメラ・インスタンスを作成
camera = new Camera();
camera.cnt[2] = 0.2;
camera.dist = 4;
camera.fovy = 15;
camera.getPos();//カメラ位置を計算
mouseOperation(canvas, camera);//swgSupport.js
}
function initData()
{
deltaT0 = parseFloat(form2.deltaT0.value);
thinningN = parseInt(form2.thinningN.value);
radius = parseFloat(form2.radius.value);
diffCoef1 = parseFloat(form2.diffCoef1.value);
diffCoef2 = parseFloat(form2.diffCoef2.value);
nMesh = parseInt(form2.nMesh.value);//空間分割数
delta = size / nMesh;//格子間隔
x0 = parseFloat(form2.x0.value);
y0 = parseFloat(form2.y0.value);
//物理量を表現するオブジェクト
object = new Rigid();
object.kind = "ELEVATION";
object.sizeX = size;
object.sizeY = size;
object.vSize = new Vector3(1, 1, adjustH);
object.nSlice = object.nStack = nMesh;
object.flagDebug = flagDebug;
//淡色表示のときのmaterial
object.diffuse = [ 0.4, 0.6, 0.9, 1.0] ;
object.ambient = [ 0.1, 0.2, 0.3, 1.0];
object.specular = [ 0.8, 0.8, 0.8, 1.0];
object.shininess = 100.0;
//フロア
floor0 = new Rigid();
floor0.kind = "PLATE_Z";
floor0.vPos = new Vector3(0, 0, -0.001);//Zファイティングを防ぐため少し下げる
var i, j, k;
deltaT = deltaT0 / thinningN;//数値計算上のタイムステップ
form2.deltaT.value = deltaT;
diffNumber1 = diffCoef1 * deltaT / (delta*delta);//拡散数
diffNumber2 = diffCoef2 * deltaT / (delta*delta);//拡散数
form2.diffNumber.value = diffNumber1;//1だけ表示
if(diffNumber1 > 0.25) alert("拡散数が0.5以上です")
flagConst = form2.Const.checked;
var i, j, k;
var x, y, r;
for(j = 0; j <= nMesh; j++)
for(i = 0; i <= nMesh; i++)
{
k = i + j * (nMesh + 1);
//物理量分布中心からの距離
x = (i - nMesh / 2) * delta - x0;
y = (j - nMesh / 2) * delta - y0;
if(profile == 0)//Cylinder
{
r = Math.sqrt(x * x + y * y);
if(r < radius) object.data[k] = f0[k] = 1.0;//物理量
else object.data[k] = f0[k] = 0.0;
}
else//Cube
{
if(Math.abs(x) < radius && Math.abs(y) < radius) object.data[k] = f0[k] = 1.0;
else object.data[k] = f0[k] = 0.0;
}
}
var b = delta / 2;//フェルミ分布関数の滑らかさ
var g;
//拡散係数
for(j = 0; j <= nMesh; j++)
for(i = 0; i <= nMesh; i++)
{
k = i + j * (nMesh + 1);
//解析領域中心を原点とした座標
x = i * delta - 0.5*size;
y = j * delta - 0.5*size;
if(x >= x0 ) g = (x - (x0 + width0/2)) / b;
else g = -(x - (x0 - width0/2)) / b;
D[k] = (diffCoef1 - diffCoef2) / (1 + Math.exp(g)) + diffCoef2;
}
}
function display()
{
//光源
var lightPosLoc = gl.getUniformLocation(gl.program, 'u_lightPos');
gl.uniform4fv(lightPosLoc, light.pos);
var lightColLoc = gl.getUniformLocation(gl.program, 'u_lightColor');
gl.uniform4fv(lightColLoc, light.color);
var cameraLoc = gl.getUniformLocation(gl.program, 'u_cameraPos');
gl.uniform3fv(cameraLoc, camera.pos);
//ビュー投影行列を計算する
var vpMatrix = new Matrix4();// 初期化
vpMatrix.perspective(camera.fovy, canvas.width/canvas.height, camera.near, camera.far);
if(Math.cos(Math.PI * camera.theta /180.0) >= 0.0)//カメラ仰角90度でビューアップベクトル切替
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, 1.0);
else
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, -1.0);
var vpMatrixLoc = gl.getUniformLocation(gl.program, 'u_vpMatrix');
gl.uniformMatrix4fv(vpMatrixLoc, false, vpMatrix.elements);
var colorLoc = gl.getUniformLocation(gl.program, 'u_colorMode');
gl.uniform1i(colorLoc, colorMode);
// カラーバッファとデプスバッファをクリアする
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
object.vSize = new Vector3(1, 1, adjustH);
var n = object.initVertexBuffers(gl);
object.draw(gl, n);
//floor
gl.uniform1i(colorLoc, 2);
n = floor0.initVertexBuffers(gl);
floor0.draw(gl, n);
}
function calculate()
{
var i, j, k;
var x, y, r;
var fim, fip, fjm, fjp;
var kim, kip, kjm, kjp;
var cc = deltaT / (delta * delta);
if(flagConst)
{
for(j = 0; j <= nMesh; j++)
for(i = 0; i <= nMesh; i++)
{
k = i + j * (nMesh + 1);
//矩形中心からの距離
x = (i - nMesh / 2) * delta - x0;
y = (j - nMesh / 2) * delta - y0;
if(profile == 0)//Cylinder
{
r = Math.sqrt(x * x + y * y);
if(r < radius) object.data[k] = f0[k] = 1.0;//物理量
}
else//Cube
{
if(Math.abs(x) < radius && Math.abs(y) < radius) object.data[k] = f0[k] = 1.0;
}
}
}
//陽解法
if(boundary == 0) //Dirichlet
{
for(j = 1; j < nMesh; j++)
for(i = 1; i < nMesh; i++)
{
k = i + j * (nMesh + 1);
kim = i-1 + j*(nMesh+1); kip = i+1 + j*(nMesh+1);
kjm = i + (j-1)*(nMesh+1); kjp = i + (j+1)*(nMesh+1);
fim = f0[kim]; if(i == 1) fim = 0;
fip = f0[kip]; if(i == nMesh-1) fip = 0;
fjm = f0[kjm]; if(j == 1) fjm = 0;
fjp = f0[kjp]; if(j == nMesh-1) fjp = 0;
object.data[k] = f0[k]
+ cc * ( ( (D[kip]-D[kim]) * (fip-fim) + (D[kjp]-D[kjm]) * (fjp-fjm) ) / 4
+ D[k] * (fim + fip + fjm + fjp - 4 * f0[k]) );
}
}
else//Neumann
{
for(j = 1; j < nMesh; j++)
for(i = 1; i < nMesh; i++)
{
k = i + j * (nMesh + 1);
kim = i-1 + j*(nMesh+1); kip = i+1 + j*(nMesh+1);
kjm = i + (j-1)*(nMesh+1); kjp = i + (j+1)*(nMesh+1);
fim = f0[kim]; if(i == 1) fim = object.data[kim] = f0[i + j*(nMesh+1)];
fip = f0[kip]; if(i == nMesh-1) fip = object.data[kip] = f0[i + j*(nMesh+1)];
fjm = f0[kjm]; if(j == 1) fjm = object.data[kjm] = f0[i + j*(nMesh+1)];
fjp = f0[kjp]; if(j == nMesh-1) fjp = object.data[kjp] = f0[i + j*(nMesh+1)];
object.data[k] = f0[k]
+ cc * ( ( (D[kip]-D[kim]) * (fip-fim) + (D[kjp]-D[kjm]) * (fjp-fjm) ) / 4
+ D[k] * (fim + fip + fjm + fjp - 4 * f0[k]) );
}
//4隅
object.data[0] = f0[1 + (nMesh+1)];
object.data[nMesh] = f0[nMesh-1 + nMesh+1];
object.data[nMesh*(nMesh+1)] = f0[1 + (nMesh-1)*(nMesh+1)];
object.data[nMesh+nMesh*(nMesh+1)] = f0[nMesh-1 + (nMesh-1)*(nMesh+1)];
}
//計算結果をf0[]へ
for(j = 0; j <= nMesh; j++)
for(i = 0; i <= nMesh; i++)
{
k = i + j * (nMesh + 1);
f0[k] = object.data[k];
}
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
flagStart = false;
initData();
}
function onChangeAdjustH()
{
adjustH = parseFloat(form2.adjustH.value);
if(adjustH == 0) adjustH = 0.001;// 完全に0にするとmatrix4の逆行列ルーチンでエラーになる
display();
}
function onChangeProfile()
{
var nn;
var radioP = document.getElementsByName("radioP");
for(var i = 0; i < radioP.length; i++)
{
if(radioP[i].checked) profile = i;
}
flagStart = false;
initData();
}
function onChangeBoundary()
{
var nn;
var radioB = document.getElementsByName("radioB");
for(var i = 0; i < radioB.length; i++)
{
if(radioB[i].checked) boundary = i;
}
flagStart = false;
initData();
}
function onChangeColorMode()
{
var nn;
var radioC = document.getElementsByName("radioC");
for(var i = 0; i < radioC.length; i++)
{
if(radioC[i].checked) colorMode = i;
}
flagStart = false;
// initData();
}
function onClickDebug()
{
if(form2.debug.checked) object.flagDebug = flagDebug = true;
else object.flagDebug = flagDebug = false;
display();
}
function onClickLight()
{
light.pos[0] = parseFloat(form2.lightX.value);
light.pos[1] = parseFloat(form2.lightY.value);
light.pos[2] = parseFloat(form2.lightZ.value);
display();
}
function onClickStart()
{
fps = 0;
elapseTime = elapseTime0;
elapseTime0 = 0;
elapseTime1 = 0;
flagStart = true;
flagStep = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
if(flagStart) { flagStart = false; }
else { flagStart = true; elapseTime = elapseTime0; }
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.time.value = "0";
display();
}
function onClickCameraReset()
{
initCamera();
display();
}
function onClickCameraAbove()
{
initCamera();
camera.theta = 90.01;
camera.getPos();//カメラ位置を計算
display();
}
<file_sep>/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/tut-1.for-memo.py
#https://postd.cc/image-processing-101/
#ref ipython notebook how to : https://qiita.com/payashim/items/d4fe5227b21a5215e78b
'''
r 3
ipython notebook
'''
# coding: utf-8
# In[1]:
import cv2, matplotlib
# In[9]:
import numpy as np
# In[10]:
abc.name
# In[11]:
import matplotlib.pyplot as plt
# In[15]:
img = cv2.imread('images/IMG_3171.JPG')
# In[16]:
print(img)
# In[17]:
plt.imshow(img)
# In[18]:
img2 = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# In[19]:
plt.imshow(img2)
# In[20]:
plt.imshow(img2)
# In[21]:
img3 = cv2.imread('images/IMG_3171.JPG')
# In[23]:
plt.imshow(img3)
# In[24]:
img4 = cv2.imread('images/IMG_3166.PNG')
# In[25]:
plt.imshow(img4)
# In[26]:
gray_img = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
# In[27]:
print(gray_img)
# In[28]:
_, threshold_img = cv2.threshold(gray_img, 60, 255, cv2.THRESH_BINARY)
# In[29]:
plt.imshow(threshold_img)
# In[30]:
_, threshold_img = cv2.threshold(gray_img, 100, 255, cv2.THRESH_BINARY)
plt.imshow(threshold_img)
#閾値より低い値を探さなくても、 =========================================
piet = cv2.imread('images/IMG_3171.JPG')
# piet = cv2.imread('images/piet.png')
piet_hsv = cv2.cvtColor(piet, cv2.COLOR_BGR2HSV)
# threshold for hue channel in blue range
blue_min = np.array([100, 100, 20], np.uint8)
# blue_min = np.array([100, 100, 100], np.uint8)
blue_max = np.array([140, 255, 255], np.uint8)
threshold_blue_img = cv2.inRange(piet_hsv, blue_min, blue_max)
threshold_blue_img = cv2.cvtColor(threshold_blue_img, cv2.COLOR_GRAY2RGB)
plt.imshow(threshold_blue_img)
#------------------------------------------
piet = cv2.imread('images/IMG_3171.JPG')
piet_RGB = cv2.cvtColor(piet, cv2.COLOR_BGR2RGB)
plt.imshow(piet_RGB)
piet_hsv = cv2.cvtColor(piet_RGB, cv2.COLOR_BGR2HSV)
plt.imshow(piet_hsv)
blue_min = np.array([100, 100, 20], np.uint8)
blue_max = np.array([140, 255, 255], np.uint8)
threshold_blue_img = cv2.inRange(piet_hsv, blue_min, blue_max)
plt.imshow(threshold_blue_img)
mask_RGB = cv2.inRange(piet_RGB, blue_min, blue_max)
plt.imshow(mask_RGB)
plt.imshow(piet_RGB)
blue_min = np.array([0, 10, 10], np.uint8)
blue_max = np.array([20, 100, 100], np.uint8)
mask_RGB = cv2.inRange(piet_RGB, blue_min, blue_max)
plt.imshow(mask_RGB)
#ref https://stackoverflow.com/questions/2462725/cv-saveimage-in-opencv
cv2.imwrite('01.png',mask_RGB)
# -----------------------------------------
mask_RGB_cvted = cv2.cvtColor(mask_RGB, cv2.COLOR_GRAY2RGB)
plt.imshow(mask_RGB)
plt.imshow(mask_RGB_cvted)
# -----------------------------------------
# clipping
#ref https://qiita.com/satoshicano/items/bba9594a1203e24e2a31
height, width, channels = mask_RGB_cvted.shape
off_set = 280
clp = mask_RGB_cvted[(height - off_set) : height, (width - off_set) : width]
plt.imshow(clp)
# cv2.imshow(clp)
# clp = img[0:height/2, 0:width/2]
# -----------------------------------------
cv2.imwrite('images/clp.png',clp)
clp_LeftBottom = mask_RGB_cvted[(height - off_set) : height, 0 : off_set]
plt.imshow(clp_LeftBottom) <file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第7章/waterSim5.js
/*-----------------------------------------------
waterSim5.js
波動シミュレーションのみ
コースティックス
水面の透明化+屈折効果
水面をクリックすると単一波が発生
------------------------------------------------*/
var canvas;//canvas要素
var gl; //WebGL描画用コンテキスト
var camera;//カメラ
var light; //光源
var flagDebug = false;
//animation
var fps = 0.0; //フレームレート
var lastTime = 0.0;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var elapseTimeN = 0.0;
var flagStart = true;//false;
var flagStep = false;
var flagReset = false;
var deltaT = 0.025;
//表示オブジェクト
var w_object;//水面
var obstacle;//移動障害物
var floor0; //水槽の底
var wall = [];//水槽の壁面
//水面オブジェクト
var NX = 63;
var NY = 63;
var DX, DY;
var sizeX = 10; //[m]
var sizeY = 10; //[m]
//波動パラメータ
var period = 1;//[s]
var freq = 1; //[Hz]
var lambda = 1;//[m]
var amp0 = 1; //[m]
var waveVel = 1;//伝搬速度
var mu0 = 0.8; //粘性抵抗
var adjustC = 1.0;//集光模様の強さ調整
var mode = "CONTINUOUS";//連続
//波動による変位量計算
var vel = []; //z軸方向の速度
var pos = []; //変位量
var sourceI, sourceJ;//波源格子点(移動障害物の中心点)
//集光模様
var caus;//データ
var fovy_proj = 12;
var texWidth = 64;
var texHeight = 64;
var flagCaustics = false;
//その他
var plane ;//影の対象平面の方程式(a,b,c,d)
var heightW = 4;//フロア(水槽の底)から水面までの高さ
var shadow_value = 0.2;//影
var transparency = 0.85;//透明度
//屈折効果
var index = 1.33;//屈折率
var stencil; //ステンシルオブジェクト
var fish0, fish1, fish2;
var vSource;// = new Vector3();//マウスでクリックされた水面位置(swgSupportW.jsのmouseOperation())
//ダミー
var dummy = new Rigid();
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas, {stencil: true});//stencil bufferを使えるようにする
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(0.1, 0.1, 0.1, 1);
gl.enable(gl.DEPTH_TEST);
gl.enable(gl.STENCIL_TEST);
form2.deltaT.value = deltaT;
form2.adjustC.value = adjustC;
form2.amp0.value = amp0;
form2.waveVel.value = waveVel;
// form2.speed.value = speed;
form2.lambda.value = lambda;
form2.mu0.value = mu0;
form2.shadow.value = shadow_value;
form2.transparency.value = transparency;
initCamera();
initData();
setCausTexture();
onClickStart();
display();
var timestep = 0;
var time = 0;
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;
elapseTimeN += deltaT;//数値計算上の経過時間(無次元時間)
//障害物の中心を波源位置にする
if(vSource.x > -sizeX/2 && vSource.x < sizeX/2 && vSource.y > -sizeY/2 && vSource.y < sizeY/2)
{
time += deltaT;
var sourceI = Math.round(NX * (vSource.x + sizeX/2.0) / sizeX);
var sourceJ = Math.round(NY * (vSource.y + sizeY/2.0) / sizeY);
if(mode == "SINGLE")
{
if(time < period)
vel[sourceI][sourceJ] = amp0 * Math.sin(2.0 * Math.PI * freq * time);
else{
time = 0;
vSource.x = 1000; vSource.y = 1000;
}
}
else
{
vel[sourceI][sourceJ] = amp0 * Math.sin(2.0 * Math.PI * freq * time);
}
}
var nSkip = 10;
var dt = deltaT / nSkip;
for(var i = 0; i < nSkip; i++) calcDisp(dt);
fish0.motion(elapseTimeN);
fish1.motion(elapseTimeN);
fish2.motion(elapseTimeN);
display();
elapseTime0 = elapseTime;//現在の経過時間を保存
form1.e_time.value = elapseTime.toString();
form1.n_time.value = elapseTimeN.toString();
if(flagStep) { flagStart = false; }
}
}
animate();
}
//--------------------------------------------
function initCamera()
{
//光源インスタンスを作成
light = new Light();
light.pos = [0.0, 0.0, 10.0, 1.0];
form2.lightX.value = light.pos[0];
form2.lightY.value = light.pos[1];
form2.lightZ.value = light.pos[2];
//カメラ・インスタンスを作成
camera = new Camera();
camera.theta = 30;
camera.cnt[2] = -1.5;
camera.dist = 35;
camera.delta = 1;
camera.fovy = 20;
camera.getPos();//カメラ位置を計算
mouseOperation(canvas, camera);//swgSupportW.js
}
function initObject()
{
waveVel = parseFloat(form2.waveVel.value);
lambda = parseFloat(form2.lambda.value);
period = lambda / waveVel;
freq = 1 / period;
amp0 = parseFloat(form2.amp0.value);
mu0 = parseFloat(form2.mu0.value);
// speed = parseFloat(form2.speed.value);
deltaT = parseFloat(form2.deltaT.value);
sourceI = NX / 2;
sourceJ = NY / 2;
DX = sizeX / NX;//格子間隔
DY = sizeY / NY;
//解析結果表示オブジェクト
w_object = new Rigid();//Rigidクラスのオブジェクトを表示オブジェクト
w_object.kind = "ELEVATION";
w_object.sizeX = sizeX;
w_object.sizeY = sizeY;
//w_object.vSize = new Vector3(1, 1, adjustC);//scaling
w_object.nSlice = NX;
w_object.nStack = NY;
w_object.diffuse = [ 0.0, 0., 0.2, 1.0] ;
w_object.ambient = [ 0.0, 0.2, 0.9, 1.0];
w_object.specular = [ 1, 1, 1, 0.2];
w_object.shininess = 200.0;
w_object.flagDebug = flagDebug;
//水槽の壁面
var WN1 = 10;
var WN2 = 5;
for(var i = 0; i < 4; i++)
{
wall[i] = new Rigid();
wall[i].kind = "CHECK_PLATE";
wall[i].flagCheck = true;
if(i == 0 || i == 1)
{
wall[i].nSlice = WN1;//x方向分割数
wall[i].nStack = WN2;//y方向分割数
}
else
{
wall[i].nSlice = WN2;//x方向分割数
wall[i].nStack = WN1;//y方向分割数
}
wall[i].col1 = [0.6, 0.5, 0.5, 1.0];
wall[i].col2 = [0.4, 0.4, 0.55, 1.0];
}
var h = heightW + 0.8;//壁面全体の高さ(heightWは水面以下の高さ)
//左端
wall[0].vPos = new Vector3(0, -sizeY/2-0.01, h/2 - heightW);
wall[0].vSize = new Vector3(sizeX+0.2, h, 1);
wall[0].vEuler = new Vector3(-90, 0, 0);
wall[0].plane = [0.0, 1.0, 0.0, sizeY/2];//平面方程式のa,b,c,d
//右端
wall[1].vPos = new Vector3(0, sizeY/2+0.01, h/2 - heightW);
wall[1].vSize = new Vector3(sizeX+0.2, h, 1);
wall[1].vEuler = new Vector3(90, 0, 0);
wall[1].plane = [0.0, -1.0, 0.0, sizeY/2];
//奥
wall[2].vPos = new Vector3(-sizeX/2-0.01, 0, h/2 - heightW);
wall[2].vSize = new Vector3( h, sizeY+0.2, 1);
wall[2].vEuler = new Vector3(0, 90, 0);
wall[2].plane = [1.0, 0.0, 0.0, sizeX/2];
//手前
wall[3].vPos = new Vector3( sizeX/2+0.01, -0, h/2 - heightW);
wall[3].vSize = new Vector3( h, sizeY+0.2, 1);
wall[3].vEuler = new Vector3(0, -90, 0);
wall[3].plane = [-1.0, 0.0, 0.0, sizeX/2];
//フロアの初期設定
floor0 = new Rigid();
floor0.kind = "CHECK_PLATE";
floor0.plane = [0.0, 0.0, 1.0, heightW];
floor0.vPos = new Vector3(0.0, 0.0, -heightW-0.01);//水面の高さは0
floor0.vSize = new Vector3(sizeX, sizeY, 1);
floor0.nSlice = WN1;//x方向分割数
floor0.nStack = WN1;//y方向分割数
floor0.col1 = [0.6, 0.5, 0.5, 1.0];
floor0.col2 = [0.4, 0.4, 0.55, 1.0];
floor0.specular = [0.1, 0.1, 0.1, 1.0];
floor0.shininess = 50;
floor0.flagCheck = true;
//ステンシル
stencil = new Rigid();
stencil.kind = "PLATE_Z";
stencil.vSize = new Vector3(sizeX, sizeY, 1);
//fish0の初期値
fish0 = new Fish0();
fish0.vSize = new Vector3(2.0, 2.0, 2.0);
fish0.vEuler = new Vector3(90.0, fish0.angleZ, 0.0);
fish0.vPos0 = new Vector3(-fish0.radius, 0.0, -2.2);
fish0.vPos = new Vector3(-fish0.radius, 0.0, -2.2);
//fish1の初期値
fish1 = new Fish1();
fish1.vSize = new Vector3(2.0, 2.0, 2.0);
fish1.vEuler = new Vector3(90.0, fish1.angleZ, 90.0);
fish1.vPos0 = new Vector3(0.0, -fish1.radius, -3.5);
fish1.vPos = new Vector3(0.0, -fish1.radius, -3.5);
//fish1の初期値
fish2 = new Fish2();
fish2.vSize = new Vector3(2.0, 2.0, 2.0);
fish2.vEuler = new Vector3(90.0, fish2.angleZ, -90.0);
fish2.vPos0 = new Vector3(0.0, fish2.radius, -1.0);
fish2.vPos = new Vector3(0.0, fish2.radius, -1.0);
//円形波の波源位置
vSource = new Vector3(0, 0, 0);
//ダミー
dummy.kind = "CHECK_PLATE";
dummy.nSlice = NX;
dummy.nStack = NY;
dummy.flagCheck = true;
}
function initData()
{
initObject();
var i, j;
for(i = 0; i <= NX; i++)
{
//配列の2次元化
vel[i] = [];
pos[i] = [];
}
//初期値
for(j = 0; j <= NY; j++)
for (i = 0; i <= NX; i++)
{
//波動計算用の格子点速度・位置
vel[i][j] = 0.0;//変位のz軸方向速度
pos[i][j] = 0.0;//変位
}
//集合模様データ
caus = new Uint8Array(texWidth*texHeight);
}
function display()
{
//水面の変位データ
var i, j, k;
for(j = 0; j <= NY; j++)
for(i = 0; i <= NX; i++)
{
k = i + j * (NX+ 1);
w_object.data[k] = pos[i][j] ;
//変位データを集合模様データに変換
caus[k] = 128 + w_object.data[k] * 10 ;
}
//テクスチャを設定
setCausTexture();
//テクスチャを投影
projectTexture();
//光源
var lightPosLoc = gl.getUniformLocation(gl.program, 'u_lightPos');
gl.uniform4fv(lightPosLoc, light.pos);
var lightColLoc = gl.getUniformLocation(gl.program, 'u_lightColor');
gl.uniform4fv(lightColLoc, light.color);
var cameraLoc = gl.getUniformLocation(gl.program, 'u_cameraPos');
gl.uniform3fv(cameraLoc, camera.pos);
//ビュー投影行列を計算する
var vpMatrix = new Matrix4();// 初期化
vpMatrix.perspective(camera.fovy, canvas.width/canvas.height, camera.near, camera.far);
if(Math.cos(Math.PI * camera.theta /180.0) >= 0.0)//カメラ仰角90度でビューアップベクトル切替
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, 1.0);
else
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, -1.0);
var vpMatrixLoc = gl.getUniformLocation(gl.program, 'u_vpMatrix');
gl.uniformMatrix4fv(vpMatrixLoc, false, vpMatrix.elements);
var indexLoc = gl.getUniformLocation(gl.program, 'u_index');
gl.uniform1f(indexLoc, index);
var adjustLoc = gl.getUniformLocation(gl.program, 'u_adjustC');
gl.uniform1f(adjustLoc, adjustC);
// カラーバッファ,デプスバッファ,ステンシルバッファをクリアする
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT | gl.STENCIL_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
gl.bindTexture(gl.TEXTURE_2D, texObj);
gl.colorMask(false, false, false, false);
gl.depthMask(false);
//ステンシルバッファに値を書き込む
gl.clearStencil(0);
gl.stencilFunc(gl.ALWAYS, 1, ~0);
gl.stencilOp(gl.REPLACE, gl.REPLACE, gl.REPLACE);
var n = stencil.initVertexBuffers(gl);//水面と同じサイズの平面
stencil.draw(gl, n);
//ステンシルを有効にして屈折を考慮した結果をレンダリング
gl.stencilFunc(gl.EQUAL, 1, ~0);
gl.stencilOp(gl.KEEP, gl.KEEP, gl.KEEP);
var stencilLoc = gl.getUniformLocation(gl.program, 'u_flagStencil');
gl.uniform1f(stencilLoc, true);
gl.colorMask(true, true, true, true);
gl.depthMask(true);
//不透明物体を先に描画
var causLoc = gl.getUniformLocation(gl.program, 'u_flagCaustics');
gl.uniform1i(causLoc, true);
var n = dummy.initVertexBuffers(gl);//ダミー
fish0.draw(gl);
fish1.draw(gl);
fish2.draw(gl);
//水槽の底と壁面
drawTiles();
gl.uniform1i(causLoc, false);
//影の表示
drawShadow();
//透明物体(水面)
gl.depthMask(false);
gl.blendFunc(gl.SRC_ALPHA,gl.ONE_MINUS_SRC_ALPHA);
gl.enable(gl.BLEND);
w_object.diffuse[3] = 1 - transparency;
w_object.ambient[3] = 1 - transparency;
w_object.vSize = new Vector3(1, 1, 1);
n = dummy.initVertexBuffers(gl);//ダミー
n = w_object.initVertexBuffers(gl);
w_object.draw(gl, n);
gl.disable(gl.BLEND);
gl.depthMask(true);
//ステンシルを無効に
gl.stencilFunc(gl.NOTEQUAL, 1, ~0);
gl.stencilOp(gl.KEEP, gl.KEEP, gl.KEEP);
gl.uniform1f(stencilLoc, false);
//そしてもう一度描く
var causLoc = gl.getUniformLocation(gl.program, 'u_flagCaustics');
gl.uniform1i(causLoc, true);
n = dummy.initVertexBuffers(gl);//ダミー
fish0.draw(gl);
fish1.draw(gl);
fish2.draw(gl);
//水槽の底と壁面
drawTiles();
gl.uniform1i(causLoc, false);
//影の表示
drawShadow();
//透明物体(水面)
gl.depthMask(false);
gl.blendFunc(gl.SRC_ALPHA,gl.ONE_MINUS_SRC_ALPHA);
gl.enable(gl.BLEND);
w_object.diffuse[3] = 1 - transparency;
w_object.ambient[3] = 1 - transparency;
n = dummy.initVertexBuffers(gl);//ダミー
n = w_object.initVertexBuffers(gl);
w_object.draw(gl, n);
gl.disable(gl.BLEND);
gl.depthMask(true);
gl.bindTexture(gl.TEXTURE_2D, null);
}
function drawTiles()
{
//水槽の壁面(視点側の壁はカット)
gl.enable(gl.CULL_FACE);
gl.cullFace(gl.BACK);
//底(フロア)
n = floor0.initVertexBuffers(gl);
floor0.draw(gl, n);
for(i = 0; i < 4; i++)
{
plane0 = wall[i].plane;
n = wall[i].initVertexBuffers(gl);
wall[i].draw(gl, n);
}
gl.disable(gl.CULL_FACE);
}
function drawShadow()
{
gl.depthMask(false);
gl.blendFunc(gl.SRC_ALPHA_SATURATE, gl.ONE_MINUS_SRC_ALPHA);
gl.enable(gl.BLEND);
var n = dummy.initVertexBuffers(gl);//ダミー
var vCameraPos = new Vector3(camera.pos[0], camera.pos[1], camera.pos[2]);
var vDirCamera = direction(floor0.vPos, vCameraPos);//フロア中心からのカメラ方向
plane = floor0.plane;//影を落とす面
var vDirPlane = new Vector3(plane[0], plane[1], plane[2]);
if(dot(vDirCamera, vDirPlane) > 0)//裏から見たときは表示しない
{
//魚の影をフロアへ
fish0.shadow = shadow_value;
fish0.draw(gl);
fish0.shadow = 0;
fish1.shadow = shadow_value;
fish1.draw(gl);
fish1.shadow = 0;
fish2.shadow = shadow_value;
fish2.draw(gl);
fish2.shadow = 0;
}
//fishの影を各壁面へ
fish0.shadow = shadow_value;
fish1.shadow = shadow_value;
fish2.shadow = shadow_value;
//n = obstacle.initVertexBuffers(gl);
for(var i = 0; i < 4; i++)
{
plane = wall[i].plane;//影を落とす面
if(plane[0]*camera.pos[0]+plane[1]*camera.pos[1]+plane[2]*camera.pos[2] < 0) continue;
fish0.draw(gl);
fish1.draw(gl);
fish2.draw(gl);
}
fish0.shadow = 0;//描画後は元に戻す
fish1.shadow = 0;//描画後は元に戻す
fish2.shadow = 0;//描画後は元に戻す
var plane0;
//壁面の影をフロアへ
for(i = 0; i < 4; i++)
{
plane0 = wall[i].plane;
wall[i].shadow = shadow_value;
n = wall[i].initVertexBuffers(gl);
wall[i].draw(gl, n);
wall[i].shadow = 0;
}
n = dummy.initVertexBuffers(gl);//ダミー
//フロアに対する水面の影
w_object.shadow = shadow_value * (1 - transparency);
n = w_object.initVertexBuffers(gl);
w_object.draw(gl, n);
w_object.shadow = 0;
gl.disable(gl.BLEND);
gl.depthMask(true);
}
function setCausTexture()
{
texObj = gl.createTexture();//テクスチャオブジェクトを作成
gl.activeTexture(gl.TEXTURE0);
//位置座標をテクスチャとして設定
gl.bindTexture(gl.TEXTURE_2D, texObj);
gl.pixelStorei(gl.UNPACK_ALIGNMENT, 1);
//数値テクスチャの割り当て
gl.texImage2D(gl.TEXTURE_2D, 0, gl.LUMINANCE, texWidth, texHeight, 0, gl.LUMINANCE, gl.UNSIGNED_BYTE, caus);
//テクスチャを拡大・縮小する方法の指定
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MAG_FILTER, gl.LINEAR);
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MIN_FILTER, gl.LINEAR);
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_WRAP_S, gl.CLAMP_TO_EDGE);
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_WRAP_T, gl.CLAMP_TO_EDGE);
// u_samplerの格納場所を取得する
var samplerLoc = gl.getUniformLocation(gl.program, 'u_sampler');
// サンプラにテクスチャユニット0を設定する
gl.uniform1i(samplerLoc, 0);
//バインドを解除
gl.bindTexture(gl.TEXTURE_2D, null);
}
function projectTexture()
{
//投影マッピングの視野角を計算
var distLight = Math.sqrt(light.pos[0]*light.pos[0] + light.pos[1]*light.pos[1] + light.pos[2]*light.pos[2]);
fovy_proj = 2 * Math.atan(sizeX / distLight) * 180.0 / Math.PI;
//テクスチャのビュー投影行列
var texVpMatrix = new Matrix4();
texVpMatrix.translate(0.5, 0.5, 0.0);
texVpMatrix.perspective(fovy_proj, 1, 1, 50);
texVpMatrix.lookAt(light.pos[0], light.pos[1], light.pos[2], 0.0, 0.0, 0.0, 0.0, 1.0, 0.0);
//テクスチャのビュー投影行列をシェーダへ
var texVpMatrixLoc = gl.getUniformLocation(gl.program, 'u_texVpMatrix');
gl.uniformMatrix4fv(texVpMatrixLoc, false, texVpMatrix.elements);
}
//----------------------------------------------------------------
function calcDisp(dt)
{
//波動方程式
var i, j;
var D = sizeX / NX;//格子間隔(sizeX0=sizeY0,NX0=NY0であること)
var D2 = D * D;
var cc = waveVel * waveVel / D2;
//格子点のz方向速度と位置の更新(Euler法)
for(j = 0; j <= NY; j++)
for(i = 0; i <= NX; i++)
{
//解析領域境界は常に自由境界
{
if(i == 0) pos[0][j] = -pos[1][j] ;
if(i == NX) pos[NX][j] = -pos[NX-1][j] ;
if(j == 0) pos[i][0] = -pos[i][1] ;
if(j == NY) pos[i][NY] = -pos[i][NY-1] ;
}
if(i == 0 || i == NX || j == 0 || j == NY) continue;
var accel = cc * (pos[i-1][j] + pos[i+1][j] + pos[i][j-1] + pos[i][j+1] - 4 * pos[i][j]);
accel -= mu0 * vel[i][j];
//速度
vel[i][j] += accel * dt;
//位置
pos[i][j] += vel[i][j] * dt;
}
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
initData();
}
function onDisplay()
{
display();
}
function onClickObsStop()
{
flagObsStop = !flagObsStop;
}
function onClickMode()
{
var nn;
var radioM = document.getElementsByName("radioM");
for(var i = 0; i < radioM.length; i++)
{
if(radioM[i].checked) nn = i;
}
if(nn == 0) mode = "SINGLE";
else if(nn == 1) mode = "CONTINUOUS";
}
function onChangeData2()
{
adjustC = parseFloat(form2.adjustC.value);
amp0 = parseFloat(form2.amp0.value);
mu0 = parseFloat(form2.mu0.value);
shadow_value = parseFloat(form2.shadow.value);
transparency = parseFloat(form2.transparency.value);
index = parseFloat(form2.index.value);
display();
}
function onClickStart()
{
fps = 0;
time = 0;
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
elapseTimeN = 0;
elapseTimeN0 = 0;
flagStart = true;
flagStep = false;
flagFreeze = false;
flagObsStop = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
flagStart = !flagStart;
flagFreeze = !flagFreeze;
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTimeN0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.e_time.value = "0";
display();
}
function onClickCameraReset()
{
initCamera();
display();
}
function onClickCameraAbove()
{
initCamera();
camera.theta = 90.01;
camera.getPos();//カメラ位置を計算
//console.log(" ccx = " + camera.pos[0] + " ccy = " + camera.pos[1] + " ccz = " + camera.pos[2]);
display();
}
function onClickLight()
{
light.pos[0] = parseFloat(form2.lightX.value);
light.pos[1] = parseFloat(form2.lightY.value);
light.pos[2] = parseFloat(form2.lightZ.value);
display();
}
<file_sep>/JVEMV6/46_art/6_visual-arts/3_free-drawing/1_use-gimp/2_/2_2.py
# -*- coding: utf-8 -*-
'''
C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\3_free-drawing\1_use-gimp\2_
python 2_2.py
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\3_free-drawing\1_use-gimp\2_
python 2_2.py
'''
'''
Regex
print "[%s:%d] result => %s" % (libs.thisfile(), libs.linenum(), result_HighLowDiffs)
^ +(print )(".+" %.+\(.+\).*)$
^( +)(print )(".+" %.+\(.+\).*)$
$1$2($3)
print "[%s:%d] result => %s" % \
(libs.thisfile(), libs.linenum(), result_HighLowDiffs)
^( +)(print )(".+" %) \\\r\n(.+)$
$1$2($3)$4$5
$1$2($3 \\\r\n$4)
print ("[%s:%d] all done" % (libs.thisfile(), libs.linenum()))
^( +)(print )(.+)(libs.+file\(\))(.+)
$1$2$3os.path.basename($4)$5
'''
import sys
from sympy.solvers.tests.test_constantsimp import C2
import os
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art')
# sys.path.append('C:/WORKS_2/WS/WS_Others/free/fx/82_')
from libs_46_art import cons_art
# from libs_31 import libmt
sys.path.append('C:/WORKS_2/WS/WS_Others/prog/D-7/2_2/VIRTUAL/Admin_Projects/mm')
from libs_mm import libs
# from libs import libs
import getopt
import os
import inspect
import math as math, struct, numpy as np
from shutil import copyfile
import xml.etree.ElementTree as ET
from PIL import Image, ImageDraw, ImageFont
from random import randint
###############################################
def test_3():
'''###################
params
###################'''
w = 640
h = 640
center_X = w / 2
center_Y = h / 2
r = 200
# r = 50
col_R = randint(0, 255)
col_G = randint(0, 255)
col_B = randint(0, 255)
colsOf_ImageNew = (col_R, col_G, col_B)
sizeOf_Canvas = (w, h)
dataOf_Ellipse = (center_X - r, center_Y - r,
center_X + r, center_X + r)
im = Image.new("RGB", sizeOf_Canvas, colsOf_ImageNew)
# im = Image.new("RGB", sizeOf_Canvas, (128, 128, 128))
# im = Image.new("RGB", (512, 512), (128, 128, 128))
'''###################
ellipse
###################'''
draw = ImageDraw.Draw(im)
#ref https://stackoverflow.com/questions/4789894/python-pil-how-to-draw-an-ellipse-in-the-middle-of-an-image#4790962
#ref outline https://endoyuta.com/2015/09/27/python3-pillowの使い方/
draw.ellipse(dataOf_Ellipse, outline=(0,0,0))
# draw.ellipse(dataOf_Ellipse, fill=(0, 0, 255))
# draw.ellipse((250, 300, 450, 400), fill=(0, 0, 255))
'''###################
lines
###################'''
coords_L1 = (w/2 - r, h/2, w/2 + r, h/2)
draw.line(coords_L1, fill=(255, 0, 0), width=2)
# draw.line(coords_L1, fill=(255, 0, 0), width=8)
# draw.line((0, im.height, im.width, 0), fill=(255, 0, 0), width=8)
coords_L2 = (w/2, h/2 - r, w/2, h/2 + r)
draw.line(coords_L2, fill=(255, 0, 0), width=2)
# # 45 degrees
# th = np.pi / 4
#
# dx = r * np.cos(th)
# dy = r * np.sin(th)
#
# coords_L3 = (w/2 + dx, h/2 - dy, w/2 - dx, h/2 + dy)
# draw.line(coords_L3, fill=(255, 0, 0), width=2)
for i in (1,2, 4, 5) :
# for i in (1,2) :
th = np.pi * i / 6
dx = r * np.cos(th)
dy = r * np.sin(th)
coords_L4 = (w/2 + dx, h/2 - dy, w/2 - dx, h/2 + dy)
draw.line(coords_L4, fill=(255, 0, 0), width=2)
'''###################
file
###################'''
dpath_Out = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/6_visual-arts/3_free-drawing/1_use-gimp/2_/data2"
fname = "image_%s.jpg" % libs.get_TimeLabel_Now()
fpath_Out = "%s/%s" % (dpath_Out, fname)
#ref https://endoyuta.com/2015/09/27/python3-pillowの使い方/
res = im.save(fpath_Out)
print()
print("[%s:%d] save image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, res
), file=sys.stderr)
print()
print ("[%s:%d] test_3 => done")
#/def test_2():
def test_2():
w = 640
h = 640
center_X = w / 2
center_Y = h / 2
radius = 200
# radius = 50
col_R = randint(0, 255)
col_G = randint(0, 255)
col_B = randint(0, 255)
colsOf_ImageNew = (col_R, col_G, col_B)
sizeOf_Canvas = (w, h)
dataOf_Ellipse = (center_X - radius, center_Y - radius,
center_X + radius, center_X + radius)
im = Image.new("RGB", sizeOf_Canvas, colsOf_ImageNew)
# im = Image.new("RGB", sizeOf_Canvas, (128, 128, 128))
# im = Image.new("RGB", (512, 512), (128, 128, 128))
draw = ImageDraw.Draw(im)
#ref https://stackoverflow.com/questions/4789894/python-pil-how-to-draw-an-ellipse-in-the-middle-of-an-image#4790962
#ref outline https://endoyuta.com/2015/09/27/python3-pillowの使い方/
draw.ellipse(dataOf_Ellipse, outline=(0,0,0))
# draw.ellipse(dataOf_Ellipse, fill=(0, 0, 255))
# draw.ellipse((250, 300, 450, 400), fill=(0, 0, 255))
'''###################
file
###################'''
dpath_Out = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/6_visual-arts/3_free-drawing/1_use-gimp/2_/data2"
fname = "image_%s.jpg" % libs.get_TimeLabel_Now()
fpath_Out = "%s/%s" % (dpath_Out, fname)
#ref https://endoyuta.com/2015/09/27/python3-pillowの使い方/
res = im.save(fpath_Out)
print()
print("[%s:%d] save image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, res
), file=sys.stderr)
print()
print ("[%s:%d] test_1 => done")
#/def test_2():
def test_1():
im = Image.new("RGB", (512, 512), (128, 128, 128))
dpath_Out = "C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/6_visual-arts/3_free-drawing/1_use-gimp/2_/data2"
fname = "image_%s.jpg" % libs.get_TimeLabel_Now()
fpath_Out = "%s/%s" % (dpath_Out, fname)
#ref https://endoyuta.com/2015/09/27/python3-pillowの使い方/
res = im.save(fpath_Out)
print()
print("[%s:%d] save image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, res
), file=sys.stderr)
print()
print ("[%s:%d] test_1 => done")
#/def test_2():
def exec_prog(): # from :
'''###################
ops
###################'''
test_3()
# test_2()
# test_1()
'''###################
Report
###################'''
print ("[%s:%d] exec_prog => done" % (os.path.basename(libs.thisfile()), libs.linenum()))
#/def exec_prog(): # from : 20180116_103908
'''
<usage>
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print ("[%s:%d] all done" % (os.path.basename(os.path.basename(libs.thisfile())), libs.linenum()))
<file_sep>/JVEMV6/46_art/4_dtm/end_apps.bat
REM @ECHO OFF
REM ***************************
REM taskkill : MuLab
REM note : must be executed before git operations
REM at : 2019/04/05 10:14:36
REM ***************************
taskkill /im MuLab.exe
REM ***************************
REM taskkill : git
REM at : 2019/06/10 16:42:01
REM ***************************
taskkill /im wish.exe
REM : domino
taskkill /im domino.exe
REM : itunes
taskkill /im itunes.exe
REM ***************************
REM git : push
REM at : 2019/04/05 10:14:45
REM ***************************
r a && e && p
REM taskkill /im MuLab.exe
REM takskill /im MuLab.exe
REM takskkill /im MuLab.exe
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/sp/templatetags/video_tags.py
from django import template
register = template.Library()
@register.simple_tag
def get_rate(crit, rates):
return rates.get(crit=crit).rate
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgSpringMass2.js
/*----------------------------------------------
spring_mass2.js
2次元バネ質点モデル
布やゴム板など2次元弾性体を表現
-----------------------------------------------*/
var gravity = 9.8;
//剛体の衝突に必要なパラメータ
var restitution = 0.5;
var muK = 0.2;
//var dampRotation = 3;//水平面内の摩擦による減衰
function SpringMass2()
{
this.flagCollision = false;//衝突処理有効/無効
this.numPole = 1;
this.numRow = 10;//1行当たりのバネ個数
this.numCol = 10;//1列当たりのバネ個数
this.numStruct = 220;
this.numShear = 200;
this.numPoint = 121;//(this.nnumRow+1) * (this.numCol+1);
this.numHinge = 121;
this.mass = 0.1;//質点1個当たりの質量(kg)
this.structK = 100;//バネ1個当たり
this.shearK = 100;
this.hingeK = 0;//;
this.damping = 0.1;//0.5;
this.drag = 0.1;//0.1;
this.muK = 0.2;
this.restitution = 0.2;
this.totalLenX = 1.0;//x軸方向全体の長さ
this.totalLenY = 1.0;//y軸方向全体の長さ
this.lengthX0; //springの1個当たり自然長
this.lengthY0; //springの1個当たり自然長
this.vPos = new Vector3();
this.vEuler = new Vector3();//2次元SMM全体の姿勢
this.radius = 0.02 ;//質点の半径
this.height0 = 2;//初期状態の高さ
this.vForce0 = new Vector3();//外力の初期値
this.structS = [];//構造バネ
this.shearS = []; //せん断バネ
this.hingeS = []; //蝶番バネ
this.point = [];
this.pole = [];
this.shadow = 0;
this.dispType = "SMM";//"TEX", "CHECK",
this.flagShearDisp = false;
this.object = new Rigid();//2次元バネ質点モデル全体を1個のオブジェクトとして描画するときに使用
this.rigid2;//衝突する剛体
}
//-----------------------------------------------------------------
SpringMass2.prototype.initialize = function()
{
var i, j, cnt;
cnt = 0;
//質点のサイズ,位置
for(j = 0; j <= this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{//基本姿勢はxy平面
this.point[cnt] = new Rigid();
this.point[cnt].kind = "SPHERE";
this.point[cnt].vSize = new Vector3(2.0*this.radius, 2.0*this.radius, 2.0*this.radius);
this.point[cnt].vPos.x = i * this.totalLenX / this.numRow;
this.point[cnt].vPos.y = j * this.totalLenY / this.numCol;
this.point[cnt].vPos.z = 0.0;
this.point[cnt].vPos = rotate(this.point[cnt].vPos, this.vEuler);
this.point[cnt].vPos.add(this.vPos);//全体の平行移動(this.vPosはサンプル・プログラム側で指定)
this.point[cnt].vVel = new Vector3();
this.point[cnt].nSlice = 6;
this.point[cnt].nStack = 6;
//alert("i="+i+" j="+j+" n="+cnt+" x="+this.point[cnt].vPos.x + " y="+this.point[cnt].vPos.y + " z="+this.point[cnt].vPos.z);
cnt ++;
}
this.numPoint = cnt;
//バネに接続する質点番号(row,colで指定)など
//x軸に平行な構造バネ
cnt = 0;
for(j = 0; j <= this.numCol; j++)
for(i = 0; i < this.numRow; i++)
{
this.structS[cnt] = new Rigid();
this.structS[cnt].kind = "CYLINDER";
this.structS[cnt].numSlice = 6;
this.structS[cnt].radiusRatio = 1 ;
this.structS[cnt].row1 = i;
this.structS[cnt].col1 = j;
this.structS[cnt].row2 = i + 1;
this.structS[cnt].col2 = j;
cnt++;
}
//y軸に平行な構造バネ
for(j = 0; j < this.numCol; j++)
for(i = 0; i <= this.numRow; i++)
{
this.structS[cnt] = new Rigid();
this.structS[cnt].kind = "CYLINDER";
this.structS[cnt].numSlice = 6;
this.structS[cnt].radiusRatio = 1 ;
this.structS[cnt].row1 = i;
this.structS[cnt].col1 = j;
this.structS[cnt].row2 = i;
this.structS[cnt].col2 = j + 1;
cnt++;
}
this.numStruct = cnt;
//せん断バネ
cnt = 0;
for(j = 0; j < this.numCol; j++)
for(i = 0; i < this.numRow; i++)
{
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
this.shearS[cnt].row1 = i; this.shearS[cnt].col1 = j;
this.shearS[cnt].row2 = i+1; this.shearS[cnt].col2 = j+1;
cnt++;
this.shearS[cnt] = new Rigid();
this.shearS[cnt].kind = "CYLINDER";
this.shearS[cnt].numSlice = 6;
this.shearS[cnt].radiusRatio = 1 ;
this.shearS[cnt].row1 = i+1; this.shearS[cnt].col1 = j;
this.shearS[cnt].row2 = i; this.shearS[cnt].col2 = j+1;
cnt++;
}
this.numShear = cnt;
//ヒンジバネを考慮
var nr = this.numRow;
var nc = this.numCol;
this.numHinge = (nr+1) * (nc+1);
for(i = 0; i < this.numHinge; i++) this.hingeS[i] = new Rigid();
//角にはヒンジバネを置かない
//隣接する質点番号,ペア個数
//j = 0
for(i = 1; i < this.numRow; i++)
{
this.hingeS[i].num = 1;//ペア個数
this.hingeS[i].row[0] = i-1; this.hingeS[i].col[0] = 0;
this.hingeS[i].row[1] = i+1; this.hingeS[i].col[1] = 0;
}
//j = numCol
for(i = 1; i < this.numRow; i++)
{
this.hingeS[i+nc*(nr+1)].num = 1;
this.hingeS[i+nc*(nr+1)].row[0] = i-1; this.hingeS[i+nc*(nr+1)].col[0] = nc;
this.hingeS[i+nc*(nr+1)].row[1] = i+1; this.hingeS[i+nc*(nr+1)].col[1] = nc;
}
//i = 0
for(j = 1; j < this.numCol; j++)
{
this.hingeS[j*(nr+1)].num = 1;
this.hingeS[j*(nr+1)].row[0] = 0; this.hingeS[j*(nr+1)].col[0] = j-1;
this.hingeS[j*(nr+1)].row[1] = 0; this.hingeS[j*(nr+1)].col[1] = j+1;
}
//i = numRow
for(j = 1; j < this.numCol; j++)
{
this.hingeS[nr+j*(nr+1)].num = 1;
this.hingeS[nr+j*(nr+1)].row[0] = nr; this.hingeS[nr+j*(nr+1)].col[0] = j-1;
this.hingeS[nr+j*(nr+1)].row[1] = nr; this.hingeS[nr+j*(nr+1)].col[1] = j+1;
}
//inside
for(i = 1; i < this.numRow; i++)
for(j = 1; j < this.numCol; j++)
{
this.hingeS[i+j*(nr+1)].num = 2;
this.hingeS[i+j*(nr+1)].row[0] = i-1; this.hingeS[i+j*(nr+1)].col[0] = j;
this.hingeS[i+j*(nr+1)].row[1] = i+1; this.hingeS[i+j*(nr+1)].col[1] = j;
this.hingeS[i+j*(nr+1)].row[2] = i ; this.hingeS[i+j*(nr+1)].col[2] = j-1;
this.hingeS[i+j*(nr+1)].row[3] = i ; this.hingeS[i+j*(nr+1)].col[3] = j+1;
}
//pole
for(i = 0; i < this.numPole; i++)
{
this.pole[i] = new Rigid();
this.pole[i].kind = "CYLINDER";
this.pole[i].mass = 100;
this.pole[i].vVel = new Vector3();//固定
if(i < 4) this.pole[i].vSize = new Vector3(0.06, 0.06, this.height0);
this.pole[i].radiusRatio = 1 ;
this.pole[i].nSlice = 6;
this.pole[i].diffuse = [0.8, 0.4, 0.2, 1.0];
this.pole[i].ambient = [0.4, 0.2, 0.1, 1.0];
this.pole[i].specular = [0.6, 0.6, 0.6, 1.0];
}
if(this.numPole == 1)
this.pole[0].vPos = new Vector3(0, 0, this.height0/2);
else if(this.numPole >= 4)
{
this.pole[0].vPos = new Vector3(-this.totalLenX/2, -this.totalLenY/2, this.height0/2);
this.pole[1].vPos = new Vector3( this.totalLenX/2, -this.totalLenY/2, this.height0/2);
this.pole[2].vPos = new Vector3( this.totalLenX/2, this.totalLenY/2, this.height0/2);
this.pole[3].vPos = new Vector3(-this.totalLenX/2, this.totalLenY/2, this.height0/2);
if(this.numPole == 8)
{
this.pole[4].vPos = new Vector3(0, -this.totalLenY/2, this.height0);
this.pole[4].vSize = new Vector3(0.06, 0.06, this.totalLenX);
this.pole[4].vEuler = new Vector3(0, 90, 0);
this.pole[5].vPos = new Vector3(this.totalLenX/2, 0, this.height0);
this.pole[5].vSize = new Vector3(0.06, 0.06, this.totalLenY);
this.pole[5].vEuler = new Vector3(90, 0, 0);
this.pole[6].vPos = new Vector3(0, this.totalLenY/2, this.height0);
this.pole[6].vSize = new Vector3(0.06, 0.06, this.totalLenX);
this.pole[6].vEuler = new Vector3(0, 90, 0);
this.pole[7].vPos = new Vector3(-this.totalLenX/2, 0, this.height0);
this.pole[7].vSize = new Vector3(0.06, 0.06, this.totalLenY);
this.pole[7].vEuler = new Vector3(90, 0, 0);
}
}
}
//-----------------------------------------------------------------
SpringMass2.prototype.draw = function(gl)
{
var i, r1, r2;
if(this.dispType == "SMM")//"SPRING_MASS_MODEL"
{//ばね質点表示
//質点
var n = this.point[0].initVertexBuffers(gl);
for(i = 0; i < this.numPoint; i++)
{
if(this.point[i].flagFixed == true){
this.point[i].diffuse = [0.8, 0.2, 0.2, 1.0];
this.point[i].ambient = [0.4, 0.1, 0.1, 1.0];
}
else {
this.point[i].diffuse = [0.2, 0.9, 0.9, 1.0];
this.point[i].ambient = [0.1, 0.5, 0.5, 1.0];
}
this.point[i].shadow = this.shadow;
this.point[i].draw(gl, n);
}
var nrp = this.numRow+1;
//構造バネ
n = this.structS[0].initVertexBuffers(gl);
for(i = 0; i < this.numStruct; i++)
{
r1 = this.structS[i].row1; c1 = this.structS[i].col1;
r2 = this.structS[i].row2; c2 = this.structS[i].col2;
var len = distance(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos);
this.structS[i].vSize = new Vector3(this.radius, this.radius, len);
this.structS[i].vEuler = getEulerZ(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos);
this.structS[i].vPos = div(add(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos), 2.0);
this.structS[i].shadow = this.shadow;
this.structS[i].draw(gl, n);
}
//せん断ばね
if(this.flagShearDisp)
{
n = this.shearS[0].initVertexBuffers(gl);
for(i = 0; i < this.numShear; i++)
{
r1 = this.shearS[i].row1; c1 = this.shearS[i].col1;
r2 = this.shearS[i].row2; c2 = this.shearS[i].col2;
var len = distance(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos);
this.shearS[i].vSize = new Vector3(this.radius, this.radius, len);
this.shearS[i].vEuler = getEulerZ(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos);
this.shearS[i].vPos = div(add(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos), 2.0);
this.shearS[i].shadow = this.shadow;
this.shearS[i].draw(gl, n);
}
}
}
else if(this.dispType == "TEX")
{//旗全体を1つのオブジェクトとしてテクスチャを貼り付ける
this.object.data = [];
this.object.kind = "GRID_SQUARE";
this.object.nSlice = this.numRow;
this.object.nStack = this.numCol;
this.object.shadow = this.shadow;
this.object.flagTexture = true;
this.object.flagCheck = false;
if(this.object.flagDebug) this.object.flagTexture = false;
for(i = 0; i < this.numPoint; i++){
//console.log("AAA i = " + i + " x = " + this.point[i].vPos.x + " y = " + this.point[i].vPos.y + " z = " + this.point[i].vPos.z);
this.object.data.push([this.point[i].vPos.x, this.point[i].vPos.y, this.point[i].vPos.z]);
}
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
}
else if(this.dispType == "CHECK")
{
this.object.data = [];
this.object.kind = "CHECK_SQUARE";
this.object.nSlice = this.numRow;
this.object.nStack = this.numCol;
this.object.shadow = this.shadow;
this.object.col1 = [1.0, 0.2, 0.1, 1.0];
this.object.col2 = [0.1, 0.8, 0.9, 1.0];
this.object.flagCheck = true;
this.object.flagTexture = false;
if(this.object.flagDebug) this.object.flagCheck = false;
for(i = 0; i < this.numPoint; i++){
this.object.data.push([this.point[i].vPos.x, this.point[i].vPos.y, this.point[i].vPos.z]);
}
n = this.object.initVertexBuffers(gl);
this.object.draw(gl, n);
//格子数の少ないチェック模様を描画した後に剛体が表示されなくなることを防ぐため
var dummy = new Rigid();
dummy.kind = "CHECK_PLATE";
dummy.flagCheck = true;
dummy.nSlice = 20;
dummy.nStack = 20;
n = dummy.initVertexBuffers(gl);
}
//ポール
if(this.numPole >= 1) n = this.pole[0].initVertexBuffers(gl);
for(i = 0; i < this.numPole; i++)
{
this.pole[i].shadow = this.shadow;
this.pole[i].draw(gl, n);
}
}
//-----------------------------------------------------------------------------
//2次元のバネマスモデル(hinge springも考慮,3次元空間で運動)
SpringMass2.prototype.calcSpringMass2 = function(tt)
{
var i, j, k, r1, r2, m;
var vDir1 = new Vector3();//hinge中心から#1へ向かう単位方向ベクトル(他にも使用)
var vDir2 = new Vector3();//hinge中心から#2へ向かう単位方向ベクトル(他にも使用)
var vFF = new Vector3();
var vRelativeVel = new Vector3();
var vNormal = new Vector3();
var vG = new Vector3(0.0, 0.0, -gravity * this.mass);//重力ベクトル
var dampingF, len, len1, len2, angle;
var angle0 = Math.PI;
var lenShear0 = Math.sqrt(this.lengthX0*this.lengthX0 + this.lengthY0*this.lengthY0);
//力の総和
//初期設定値(風などの外力)
for(i = 0; i < this.numPoint; i++){
this.point[i].vForce = add(this.point[i].vForce0, vG);
}
var nrp = this.numRow +1;
//バネによる力
for(i = 0; i < this.numStruct; i++)
{
//弾性力
r1 = this.structS[i].row1; c1 = this.structS[i].col1;
r2 = this.structS[i].row2; c2 = this.structS[i].col2;
//console.log("i = " + i + " r1 = " + r1 + " r2 = " + r2 + " c1 = " + c1 + " c2 = " + c2);
vDir1 = direction(this.point[r1+c1*nrp].vPos , this.point[r2+c2*nrp].vPos);//#1から#2への単位ベクトル
//console.log("x = " + vDir1.x + " y = " + vDir1.y + " z = " + vDir1.z)
len = distance(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos);
//console.log("len = " + len + " lenX0 = " + this.lengthX0 + " lenY0 = " + this.lengthY0);
if(r1 == r2)//行のバネ
vFF = mul(this.structK * (len - this.lengthY0), vDir1) ;
else//列のバネ
vFF = mul(this.structK * (len - this.lengthX0), vDir1) ;
this.point[r1+c1*nrp].vForce.add(vFF) ;//vDirと同方向
this.point[r2+c2*nrp].vForce.sub(vFF) ;//反対方向
//減衰力
vRelativeVel = sub(this.point[r1+c1*nrp].vVel , this.point[r2+c2*nrp].vVel);
dampingF = this.damping * dot(vRelativeVel, vDir1);
this.point[r1+c1*nrp].vForce.sub(mul(dampingF , vDir1));//相対速度とは反対方向
this.point[r2+c2*nrp].vForce.add(mul(dampingF , vDir1));//同方向
}
//せん断バネによる力
if(this.shearK > 0)
{
for(i = 0; i < this.numShear; i++)
{
r1 = this.shearS[i].row1; c1 = this.shearS[i].col1;
r2 = this.shearS[i].row2; c2 = this.shearS[i].col2;
len = distance(this.point[r1+c1*nrp].vPos, this.point[r2+c2*nrp].vPos);
//vDir1 = direction(this.point[r2+c2*nrp].vPos , this.point[r1+c1*nrp].vPos);
vDir1 = direction(this.point[r1+c1*nrp].vPos , this.point[r2+c2*nrp].vPos);
vFF = mul(this.shearK * (len - lenShear0), vDir1) ;
this.point[r1+c1*nrp].vForce.add(vFF) ;//vDirと同方向
this.point[r2+c2*nrp].vForce.sub(vFF) ;//反対方向
//減衰力
vRelativeVel = sub(this.point[r1+c1*nrp].vVel, this.point[r2+c2*nrp].vVel);
dampingF = this.damping * dot(vRelativeVel, vDir1);
vFF = mul(dampingF, vDir1);
this.point[r1+c1*nrp].vForce.sub(vFF);//相対速度とは反対方向
this.point[r2+c2*nrp].vForce.add(vFF);//同方向
}
}
if(this.hingeK > 0)
{
//ヒンジ(蝶番)バネを考慮
for(i = 0; i <= this.numRow; i++)
{
for(j = 0; j <= this.numCol; j++)
{
if(i == 0 && (j == 0 || j == this.numCol)) angle0 = Math.PI / 2;
else if(i == this.numRow && (j == 0 || j == this.numCol)) angle0 = Math.PI / 2;
else angle0 = Math.PI;
for(m = 0; m < this.hingeS[i+j*nrp].num; m++)
{
if(i == 0 && j == 0) continue;
if(i == 0 && j == this.numCol-1) continue;
if(i == this.numRow && j == 0) continue;
if(i == this.numRow && j == this.numCol) continue;
r1 = this.hingeS[i+j*nrp].row[2*m]; c1 = this.hingeS[i+j*nrp].col[2*m];
r2 = this.hingeS[i+j*nrp].row[2*m+1]; c2 = this.hingeS[i+j*nrp].col[2*m+1];
len1 = distance(this.point[i+j*nrp].vPos, this.point[r1+c1*nrp].vPos);
len2 = distance(this.point[i+j*nrp].vPos, this.point[r2+c2*nrp].vPos);
//hingeの中心から隣接質点へ向くベクトル方向
vDir1 = direction(this.point[i+j*nrp].vPos , this.point[r1+c1*nrp].vPos);
vDir2 = direction(this.point[i+j*nrp].vPos , this.point[r2+c2*nrp].vPos);
//2つのベクトルのなす角度
angle = getAngle_rad(vDir1, vDir2);
//法線方向
vNormal = cross(vDir1, vDir2);
//質点1に作用する力
vDir1 = cross(vNormal, vDir1);
vFF = mul(this.hingeK * (angle - angle0) / len1 , vDir1);
this.point[r1+c1*nrp].vForce.add(vFF) ;//vDirと同方向
this.point[i+j*nrp].vForce.sub(vFF) ;//注目点では反対方向
//質点2に作用する力
vDir2 = cross(vNormal, vDir2);
vFF = mul(this.hingeK*(angle - angle0)/len2 , vDir2);
this.point[r2+c2*nrp].vForce.sub(vFF) ;//vDir2の反対方向
this.point[i+j*nrp].vForce.add(vFF) ;//注目点では反対方向
}
}
}
}
/*
if(this.flagCollision)
{
//質点どうしの衝突
var dia = 0.2;
for(j = 0; j <= this.numCol; j++)
{
for(i = 0; i <= this.numRow; i++)
{
for(J = 0; J <= this.numCol; J++)
{
for(I = 0; I <= this.numRow; I++)
{
//隣同士は除く
if(Math.abs(i - I) <= 1 && Math.abs(j - J) <= 1) continue;
vDir1 = direction(this.point[i+j*nrp].vPos , this.point[I+J*nrp].vPos);//#1から#2への単位ベクトル
len = distance(this.point[i+j*nrp].vPos, this.point[I+J*nrp].vPos);
if(len < dia) {
vFF = mul( 0.001 / (0.01+len*len), vDir1) ;
this.point[i+j*nrp].vForce.sub(vFF) ;//vDirと反対方向
this.point[I+J*nrp].vForce.add(vFF) ;//同方向
}
}
}
}
}
var vPolePos = new Vector3();//質点と同じ高さのpoleの位置
//poleと質点
for(k = 0; k < this.numPole; k++)
{
for(i = 0; i <= this.numRow; i++)
{
for(j = 0; j <= this.numCol; j++)
{
if(this.point[i+j*nrp].flagFixed == true) continue; //固定
vPolePos.copy(this.pole[k].vPos);
vPolePos.z = this.point[i+j*nrp].vPos.z;//質点と同じ高さのpole位置
if(vPolePos.z > this.pole[k].vPos.z + this.pole[k].vSize.z/2.0) continue;
if(vPolePos.z < this.pole[k].vPos.z - this.pole[k].vSize.z/2.0) continue;
vDir1 = direction(vPolePos , this.point[i+j*nrp].vPos);//単位ベクトル
len = distance(vPolePos, this.point[i+j*nrp].vPos);
if(len < dia + this.pole[k].vSize.x/2.0)
{
vFF = mul( 0.005 / (0.0001+len*len) , vDir1) ;
this.point[i+j*nrp].vForce.add(vFF) ;//vDir1の方向へ
}
}
}
}
}
*/
//粘性抵抗と床面処理
for(i = 0; i <= this.numRow; i++)
for(j = 0; j <= this.numCol; j++)
{
if(this.point[i+j*nrp].flagFixed) continue; //固定
//空気粘性抵抗(全ての質点に一様と仮定)
this.point[i+j*nrp].vForce.sub(mul(this.drag, this.point[i+j*nrp].vVel));
//床面処理
if(this.point[i+j*nrp].vPos.z < this.radius)
{
//床面にある質点に対してはすべり摩擦を与える
this.point[i+j*nrp].vForce.sub(mul(this.muK * this.mass * gravity, norm(this.point[i+j*nrp].vVel)));
//床面上に制限
this.point[i+j*nrp].vPos.z = this.radius;
//床との衝突
if(this.point[i+j*nrp].vVel.z < 0.0)
{ //質点と床面とは弾性衝突とする
this.point[i+j*nrp].vVel.z = - this.restitution * this.point[i+j*nrp].vVel.z ;
}
}
//Euler法
//加速度
this.point[i+j*nrp].vAcc = div(this.point[i+j*nrp].vForce , this.mass);
//速度
this.point[i+j*nrp].vVel.add(mul(this.point[i+j*nrp].vAcc, tt));
//位置
this.point[i+j*nrp].vPos.add(mul(this.point[i+j*nrp].vVel, tt));
}
}
<file_sep>/JVEMV6/46_art/1_/1_1 (2).py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\1_\82_1.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\1_\1_1.py
at : 2018/02/25 20:55:36
pushd C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\1_\
python 1_1.py
ref : http://aidiary.hatenablog.com/entry/20110607/1307449007
'''
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_mm import libs
from libs_VX7GLZ import libs_VX7GLZ
from libs_VX7GLZ import wablibs
# from libs.libs import * #=> C:\WORKS_2\WS\Eclipse_Luna\C_SoundProg\python\libs
# import libs.libs as lib
# from libs.libs import * #=> in 'libs' subdirectory --> see : https://stackoverflow.com/questions/1260792/import-a-file-from-a-subdirectory 'community wiki'
#=> ref : http://qiita.com/Usek/items/86edfa0835292c80fff5
# from libs import * #=> in 'libs' subdirectory --> see : https://stackoverflow.com/questions/1260792/import-a-file-from-a-subdirectory 'community wiki'
#=> libs.py : C:\WORKS_2\WS\WS_Others\free\K6H7DD_schroedinger\28_1\libs.py
# import libs.labs2 as labs
# # import libs.labs as labs
#
# import libs.wablibs as wl
# import wablibs
# import wablibs as wl
'''###################
import : built-in modules
###################'''
import getopt
import os
import inspect
import math as math
###############################################
import wave
import struct
import numpy as np
# from pylab import *
from matplotlib import pylab as plt
import random as rnd
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def test_2():
A = 1 #振幅
fs = 16000 #サンプリング周波数
# fs = 8000 #サンプリング周波数
f0 = 392 #周波数
# f0 = 262 #周波数
# f0 = 440 #周波数
sec = 5 #秒
phase_Param = 0
# phase_Param = 4
phase = np.pi * 0
# phase = f0 * (4 / 2)
# phase = f0 / 2
# bin data
phase = np.pi * ( 1 / 4 )
pow_Param = 1
scalar_Param = 2
binwave = wablibs.gen_WaveData(fs, f0, phase, sec, A, pow_Param, scalar_Param)
# binwave = gen_WaveData(fs, f0, phase, sec, A)
# #サイン波をwavファイルとして書き出し
wave_Params = (1, 2, fs, len(binwave), 'NONE', 'not compressed')
# wave_Params = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
'''###################
dirs, paths
###################'''
dname_Audios = "data.46_1\\audios"
graph_Type = "sin"
if not os.path.isdir(dname_Audios) : os.makedirs(dname_Audios)
# fname_Out = "audio/output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# fname_Out = "%s\\output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
fname_Out = "%s\\output_%s.%s.fs-%d_f0-%d.(phase-%s)(pow-%.1f)(scalar-%.1f)(sec-%d).wav" % \
(dname_Audios
, libs.get_TimeLabel_Now()
, graph_Type
, fs, f0
, "%.1fpi" % (phase / np.pi)
, pow_Param
, scalar_Param
# , "%1.2f" % (phase_Param / 4.0) + "pi"
, sec)
'''###################
save
###################'''
wablibs.save_Wave(fname_Out, wave_Params, binwave)
# save_Wave(fname_Out, wave_Params, binwave)
print()
print("[%s:%d] save wave => done : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Out
), file=sys.stderr)
#/ def test_1():
def test_1():
A = 1 #振幅
fs = 16000 #サンプリング周波数
# fs = 8000 #サンプリング周波数
f0 = 392 #周波数
# f0 = 262 #周波数
# f0 = 440 #周波数
sec = 5 #秒
phase_Param = 0
# phase_Param = 4
phase = f0 * (phase_Param / 2)
# phase = f0 * (4 / 2)
# phase = f0 / 2
# bin data
phase = np.pi * ( 1 / 4 )
binwave = wablibs.gen_WaveData(fs, f0, phase, sec, A)
# binwave = gen_WaveData(fs, f0, phase, sec, A)
# #サイン波をwavファイルとして書き出し
wave_Params = (1, 2, fs, len(binwave), 'NONE', 'not compressed')
# wave_Params = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
'''###################
dirs, paths
###################'''
dname_Audios = "data.46_1\\audios"
if not os.path.isdir(dname_Audios) : os.makedirs(dname_Audios)
# fname_Out = "audio/output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
fname_Out = "%s\\output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
(dname_Audios
, libs.get_TimeLabel_Now()
, fs, f0
, "%1.2f" % (phase_Param / 4.0) + "pi", sec)
'''###################
save
###################'''
wablibs.save_Wave(fname_Out, wave_Params, binwave)
# save_Wave(fname_Out, wave_Params, binwave)
print()
print("[%s:%d] save wave => done : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Out
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_2()
# test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print ("[%s:%d] exec_prog" % (thisfile(), linenum()))
# #debug
# return
# A = 1 #振幅
# fs = 16000 #サンプリング周波数
# # fs = 8000 #サンプリング周波数
# f0 = 392 #周波数
# # f0 = 262 #周波数
# # f0 = 440 #周波数
# sec = 5 #秒
#
# phase_Param = 4
#
# phase = f0 * (phase_Param / 2)
# # phase = f0 * (4 / 2)
# # phase = f0 / 2
#
# # bin data
# binwave = gen_WaveData(fs, f0, phase, sec, A)
#
# # #サイン波をwavファイルとして書き出し
# wave_Params = (1, 2, fs, len(binwave), 'NONE', 'not compressed')
# # wave_Params = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
#
# '''###################
# dirs
# ###################'''
# dname_Audios = "data.46_1\\audios"
#
# if not os.path.isdir(dname_Audios) : os.makedirs(dname_Audios)
#
# # fname_Out = "audio/output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# fname_Out = "%s\\output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# (dname_Audios
# , libs.get_TimeLabel_Now()
# , fs, f0
# , "%1.2f" % (phase_Param / 4.0) + "pi", sec)
# # fname_Out = "audios/output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# # (libs.get_TimeLabel_Now(), fs, f0, "%1.2f" % (phase_Param / 4.0) + "pi", sec)
# # (get_TimeLabel_Now(), fs, f0, "%1.2f" % (phase_Param / 4.0) + "pi", sec)
# # fname_Out = "audio/output_%s.sin.fs-%d_f0-%d_phase-%d_sec-%d.wav" % \
# # (get_TimeLabel_Now(), fs, f0, phase, sec)
#
# save_Wave(fname_Out, wave_Params, binwave)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/d2.bat
@echo off
echo killing MuseScore.exe
taskkill /im MuseScore.exe
taskkill /im MuseScore3.exe
echo killing wish.exe
taskkill /im wish.exe
echo killing Domino.exe
taskkill /im Domino.exe
echo killing javaw.exe
taskkill /im javaw.exe
echo killing thunderbird.exe
taskkill /im thunderbird.exe
echo killing chrome.exe
taskkill /im chrome.exe
taskkill /f /im filezilla.exe
taskkill /f /im sakura.exe
exit
REM pause
REM exit
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第6章/waveEquation2D2.js
/*-----------------------------------------------
waveEuation2D2.js
2次元波動方程式の解
カラーマップ表示を追加
固定障害物を追加
------------------------------------------------*/
var canvas; //canvas要素
var gl; //WebGL描画用コンテキスト
var camera; //カメラ
var light; //光源
var rigid;//表示オブジェクト
var flagDebug = false;
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var flagStart = false;
var flagStep = false;
var flagReset = false;
//波
var NX0 = 50;//dummyを含まない有効領域分割数
var NY0 = 50;
var NX = 80;//dummyを含む分割数
var NY = 80;
var DX, DY;
var nDummy = 30;
var sizeX0 = 10; //dummyを含まない有効領域サイズ[m]
var sizeY0 = 10; //dummyを含む[m]
var sizeX = 10; //[m]
var sizeY = 10; //[m]
var period = 1; //[s]
var freq = 1; //[Hz]
var lambda = 1; //[m]
var amp0 = 0.4; //[m]
var waveVel = 1;//伝搬速度
var mu0 = 0.0;//粘性抵抗
var nSource = 1; //波源数
var sourceI = [];//波源座標
var sourceJ = [];
var sourceY0 = -5.0;//波源のy座標
var vel = []; //z軸方向の速度
var pos = []; //変位量
var type = [];//障害物判定に利用
var mode = "CONTINUOUS";//連続
var boundary = "B_FIXED";
var w_object;//波全体を1個のオブジェクトで表示
var colorMode = 0;
//固定物体
var obstacle = [];
var obstacle0 = [];
var pattern = 0;//障害物の配置パターン
var numObstacle = 1;
var nGap = 4;//pattern=1,2のときの障害物間隔(DXの整数倍で指定する、整数部分を指定))
function webMain()
{
// Canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);//webgl-utils.js
if(!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSL初期化に失敗");
return;
}
//Canvasをクリアする色を設定し、隠面消去機能を有効にする
gl.clearColor(0.1, 0.1, 0.1, 1.0);
gl.enable(gl.DEPTH_TEST);
initCamera();
initData();
display();
var timestep = 0;
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;//全経過時間
if(mode == "SINGLE")
{
for(var k = 0; k < nSource; k++)
{
if(elapseTime < period)
pos[sourceI[k]][sourceJ[k]] = amp0 * Math.sin(2.0 * Math.PI * freq * elapseTime);
}
}
else
{
for(var k = 0; k < nSource; k++)
{
pos[sourceI[k]][sourceJ[k]] = amp0 * Math.sin(2.0 * Math.PI * freq * elapseTime);
}
}
var nSkip = 20;//時間刻みを小さくするため間引き表示
var tt = frameTime / nSkip;
for(var j = 0; j < nSkip; j++)
{
calcDisp(tt);
}
elapseTime0 = elapseTime;//現在の経過時間を保存
form1.time.value = elapseTime.toString();
if(flagStep) { flagStart = false; }
display();
}
}
animate();
}
//--------------------------------------------
function initCamera()
{
//光源インスタンスを作成
light = new Light();
light.pos[1] = 50;
//初期設定値をHTMLのフォームに表示
form2.lightX.value = light.pos[0];
form2.lightY.value = light.pos[1];
form2.lightZ.value = light.pos[2];
//カメラ・インスタンスを作成
camera = new Camera();
camera.dist = 15;
camera.theta = 30;
camera.cnt[2] = 0.0;
camera.getPos();//カメラ位置を計算
camera.delta = 1;
mouseOperation(canvas, camera);//swgSupport.js
}
function initObstacle()
{
nGap = parseInt(form2.nGap.value);
var gap = nGap * DX;
//固定物体
for(i = 0; i < 3; i++)
{
obstacle0[i] = new Rigid();
obstacle[i] = new Rigid();
}
if(pattern == 0)
{
numObstacle = 1;
obstacle0[0] = new Rigid();//表示用
obstacle0[0].kind = "CUBE";
obstacle0[0].vPos = new Vector3(sizeX0/4, 0.0, 0.0);
obstacle0[0].vSize = new Vector3(sizeX0/2, 2*DY, 0.5);
obstacle0[0].flagDebug = flagDebug;
obstacle[0] = new Rigid();//解析用
obstacle[0].kind = "CUBE";
obstacle[0].vPos = new Vector3(sizeX/4, 0.0, 0.0);
obstacle[0].vSize = new Vector3(sizeX/2, 2*DY, 0.5);
}
else if(pattern == 1)
{
numObstacle = 2;
obstacle0[0] = new Rigid();//表示用
obstacle0[0].kind = "CUBE";
obstacle0[0].vPos = new Vector3((sizeX0 -gap)/4+gap/2, 0.0, 0.0);
obstacle0[0].vSize = new Vector3((sizeX0-gap)/2, 2*DY, 0.5);
obstacle0[0].flagDebug = flagDebug;
obstacle[0] = new Rigid();//解析用
obstacle[0].kind = "CUBE";
obstacle[0].vPos = new Vector3((sizeX-gap)/4+gap/2, 0.0, 0.0);
obstacle[0].vSize = new Vector3((sizeX-gap)/2, 2*DY, 0.5);
obstacle0[1] = new Rigid();//表示用
obstacle0[1].kind = "CUBE";
obstacle0[1].vPos = new Vector3(-(sizeX0 -gap)/4-gap/2, 0.0, 0.0);
obstacle0[1].vSize = new Vector3((sizeX0-gap)/2, 2*DY, 0.5);
obstacle0[1].flagDebug = flagDebug;
obstacle[1] = new Rigid();//解析用
obstacle[1].kind = "CUBE";
obstacle[1].vPos = new Vector3(-(sizeX-gap)/4-gap/2, 0.0, 0.0);
obstacle[1].vSize = new Vector3((sizeX-gap)/2, 2*DY, 0.5);
}
else if(pattern == 2)
{
numObstacle = 3;
obstacle0[0] = new Rigid();//表示用
obstacle0[0].kind = "CUBE";
obstacle0[0].vPos = new Vector3((sizeX0 -3*gap)/4+3*gap/2, 0.0, 0.0);
obstacle0[0].vSize = new Vector3((sizeX0-3*gap)/2, 2*DY, 0.5);
obstacle0[0].flagDebug = flagDebug;
obstacle[0] = new Rigid();//解析用
obstacle[0].kind = "CUBE";
obstacle[0].vPos = new Vector3((sizeX-3*gap)/4+3*gap/2, 0.0, 0.0);
obstacle[0].vSize = new Vector3((sizeX-3*gap)/2, 2*DY, 0.5);
obstacle0[1] = new Rigid();//表示用
obstacle0[1].kind = "CUBE";
obstacle0[1].vPos = new Vector3(-(sizeX0 -3*gap)/4-3*gap/2, 0.0, 0.0);
obstacle0[1].vSize = new Vector3((sizeX0-3*gap)/2, 2*DY, 0.5);
obstacle0[1].flagDebug = flagDebug;
obstacle[1] = new Rigid();//解析用
obstacle[1].kind = "CUBE";
obstacle[1].vPos = new Vector3(-(sizeX-3*gap)/4-3*gap/2, 0.0, 0.0);
obstacle[1].vSize = new Vector3((sizeX-3*gap)/2, 2*DY, 0.5);
//中心の障害物(gapと同じ長さ)
obstacle0[2] = new Rigid();//表示用
obstacle0[2].kind = "CUBE";
obstacle0[2].vPos = new Vector3(0.0, 0.0, 0.0);
obstacle0[2].vSize = new Vector3(gap, 2*DY, 0.5);
obstacle0[2].flagDebug = flagDebug;
obstacle[2] = new Rigid();//解析用
obstacle[2].kind = "CUBE";
obstacle[2].vPos = new Vector3(0.0, 0.0, 0.0);
obstacle[2].vSize = new Vector3(gap, 2*DY, 0.5);
}
}
function initData()
{
waveVel = parseFloat(form2.waveVel.value);
lambda = parseFloat(form2.lambda.value);
period = lambda / waveVel;
freq = 1 / period;
amp0 = parseFloat(form2.amp0.value);
mu0 = parseFloat(form2.mu0.value);
NX0 = parseInt(form2.nMesh.value);
NY0 = NX0;
DX = sizeX0 / NX0;
DY = sizeY0 / NY0;
nSource = parseInt(form2.nSource.value);
interval0 = parseFloat(form2.interval.value);//波源間隔
sourceY0 = parseFloat(form2.Y0.value);//波源のy座標
pattern = parseInt(form2.pattern.value);
w_object = new Rigid();//波を表示するオブジェクト
w_object.kind = "ELEVATION";
w_object.nSlice = NX0;
w_object.nStack = NY0;
w_object.sizeX = sizeX0;//ダミーを含まない領域のサイズ
w_object.sizeY = sizeY0;
w_object.diffuse = [ 0.4, 0.6, 0.9, 1.0] ;
w_object.ambient = [ 0.1, 0.2, 0.3, 1.0];
w_object.specular = [ 0.8, 0.8, 0.8, 1.0];
w_object.shininess = 100.0;
w_object.flagDebug = flagDebug;
w_object.data = [];
var i, j, k;
//解析領域境界は常に無反射
nDummy = 30;//最大で30
NX = NX0 + 2 * nDummy;
NY = NY0 + 2 * nDummy;
sizeX = sizeX0 + 2.0 * DX * nDummy;
sizeY = sizeY0 + 2.0 * DY * nDummy;
initObstacle();
//配列の2次元化
for(i = 0; i <= NX; i++)
{
vel[i] = [];
pos[i] = [];
type[i] = [];
}
//データをクリア,typeの決定
for(var j = 0; j <= NY; j++)
for(var i = 0; i <= NX; i++)
{
//格子点速度・位置
vel[i][j] = 0.0;
pos[i][j] = 0.0;
type[i][j] = 0;//障害物なし
//下の障害物(すべてのパターン)
if(i*DX >= sizeX/2 + obstacle[0].vPos.x - obstacle[0].vSize.x/2 &&
i*DX <= sizeX/2 + obstacle[0].vPos.x + obstacle[0].vSize.x/2 &&
j*DY >= sizeY/2 + obstacle[0].vPos.y - obstacle[0].vSize.y/2 &&
j*DY <= sizeY/2 + obstacle[0].vPos.y + obstacle[0].vSize.y/2) type[i][j] = 1;//障害物内部(境界を含む)
if(pattern >= 1)
{
//pattern == 1,2の上の障害物
if(i*DX >= sizeX/2 + obstacle[1].vPos.x - obstacle[1].vSize.x/2 &&
i*DX <= sizeX/2 + obstacle[1].vPos.x + obstacle[1].vSize.x/2 &&
j*DY >= sizeY/2 + obstacle[1].vPos.y - obstacle[1].vSize.y/2 &&
j*DY <= sizeY/2 + obstacle[1].vPos.y + obstacle[1].vSize.y/2) type[i][j] = 1;//障害物内部(境界を含む)
}
if(pattern == 2)
{
//pattern=2の中心障害物
if(i*DX >= sizeX/2 + obstacle[2].vPos.x - obstacle[2].vSize.x/2 &&
i*DX <= sizeX/2 + obstacle[2].vPos.x + obstacle[2].vSize.x/2 &&
j*DY >= sizeY/2 + obstacle[2].vPos.y - obstacle[2].vSize.y/2 &&
j*DY <= sizeY/2 + obstacle[2].vPos.y + obstacle[2].vSize.y/2) type[i][j] = 1;//障害物内部(境界を含む)
}
}
//波源の格子番号
if(!form2.plane.checked)
{
var xs, ys;
for(k = 0; k < nSource; k++)
{//interval0:波源間隔
xs = interval0 * (-0.5 * (nSource-1) + k);
ys = sourceY0;
sourceI[k] = Math.round(NX * (xs + sizeX/2.0) / sizeX);
sourceJ[k] = Math.round(NY * (ys + sizeY/2.0) / sizeY);
//console.log("k = " + k + " sx = " + sourceI[k] + " sy = " + sourceJ[k]);
}
}
else
{
//平面波(x軸に平行な1列の格子点をすべて波源にする
nSource = NX;
for(k = 0; k < nSource; k++)
{
ys = sourceY0;
sourceI[k] = k;
sourceJ[k] = Math.round(NY * (ys + sizeY/2.0) / sizeY);
}
}
flagStart = false;
}
//---------------------------------------------
function display()
{
//光源
var lightPosLoc = gl.getUniformLocation(gl.program, 'u_lightPos');
gl.uniform4fv(lightPosLoc, light.pos);
var lightColLoc = gl.getUniformLocation(gl.program, 'u_lightColor');
gl.uniform4fv(lightColLoc, light.color);
var cameraLoc = gl.getUniformLocation(gl.program, 'u_cameraPos');
gl.uniform3fv(cameraLoc, camera.pos);
//ビュー投影行列を計算する
var vpMatrix = new Matrix4();// 初期化
vpMatrix.perspective(camera.fovy, canvas.width/canvas.height, camera.near, camera.far);
if(Math.cos(Math.PI * camera.theta /180.0) >= 0.0)//カメラ仰角90度でビューアップベクトル切替
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, 1.0);
else
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, -1.0);
var vpMatrixLoc = gl.getUniformLocation(gl.program, 'u_vpMatrix');
gl.uniformMatrix4fv(vpMatrixLoc, false, vpMatrix.elements);
var colorLoc = gl.getUniformLocation(gl.program, 'u_colorMode');
gl.uniform1i(colorLoc, colorMode);
// カラーバッファとデプスバッファをクリアする
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
//各頂点の座標
w_object.data = [];
var i, j;
for(j = nDummy; j <= NY0 + nDummy; j++){
for(i = nDummy; i <= NX0 + nDummy; i++){
w_object.data.push(pos[i][j]);
}
}
//水面
var obstacleLoc = gl.getUniformLocation(gl.program, 'u_obstacle');
gl.uniform1i(obstacleLoc, 0);//障害物でない
var n = w_object.initVertexBuffers(gl);
w_object.draw(gl, n);
//固定物体
gl.uniform1i(obstacleLoc, 1);//障害物あり
for(i = 0; i < numObstacle; i++)
{
obstacle0[i].flagDebug = flagDebug;
n = obstacle0[i].initVertexBuffers(gl);
obstacle0[i].draw(gl, n);
}
}
function calcDisp(dt)
{
var i, j;
var D = sizeX0 / NX0;//格子間隔(sizeX0=sizeY0,NX0=NY0であること)
var D2 = D * D;
var mu = mu0;
var nm = nDummy;//無反射のときの有効領域境界番号
var np = NX0 + nDummy;
var muMax = 5.0;
var cc = waveVel * waveVel / D2;
//格子点のz方向速度と位置の更新(Euler法)
for(j = 1; j < NY; j++)
{
for(i = 1; i < NX; i++)
{
//解析領域境界は常に無反射境界とする
if(j < nm) mu = mu0 + muMax * (nm - j) / nDummy;
if(j > np) mu = mu0 + muMax * (j - np) / nDummy;
if(i < nm) mu = mu0 + muMax * (nm - i) / nDummy;
if(i > np) mu = mu0 + muMax * (i - np) / nDummy;
//障害物
if(boundary == "B_FIXED")
{
if(type[i-1][j] == 0 && type[i][j] == 1) pos[i][j] = 0;
if(type[i+1][j] == 0 && type[i][j] == 1) pos[i][j] = 0;
if(type[i][j-1] == 0 && type[i][j] == 1) pos[i][j] = 0;
if(type[i][j+1] == 0 && type[i][j] == 1) pos[i][j] = 0;
}
else if(boundary == "B_FREE")
{
//障害物
if(type[i-1][j] == 0 && type[i][j] == 1) pos[i][j] = pos[i-1][j];
if(type[i+1][j] == 0 && type[i][j] == 1) pos[i][j] = pos[i+1][j];
if(type[i][j-1] == 0 && type[i][j] == 1) pos[i][j] = pos[i][j-1];
if(type[i][j+1] == 0 && type[i][j] == 1) pos[i][j] = pos[i][j+1];
}
if(type[i][j] == 1) continue;
var accel = cc * (pos[i-1][j] + pos[i+1][j] + pos[i][j-1] + pos[i][j+1] - 4 * pos[i][j]);
//粘性抵抗
accel -= mu * vel[i][j];
//速度
vel[i][j] += accel * dt;
//位置
pos[i][j] += vel[i][j] * dt;
}
}
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
// initData();
}
function onClickBoundary()
{
var nn;
var radioB = document.getElementsByName("radioB");
for(var i = 0; i < radioB.length; i++)
{
if(radioB[i].checked) nn = i;
}
if(nn == 0) boundary = "B_FIXED";
else if(nn == 1) boundary = "B_FREE";
else if(nn == 2) boundary = "B_NON";
onClickReset();
}
function onClickMode()
{
var nn;
var radioM = document.getElementsByName("radioM");
for(var i = 0; i < radioM.length; i++)
{
if(radioM[i].checked) nn = i;
}
if(nn == 0) mode = "SINGLE";
else if(nn == 1) mode = "CONTINUOUS";
onClickReset();
}
function onClickDebug()
{
if(form2.debug.checked) w_object.flagDebug = flagDebug = true;
else w_object.flagDebug = flagDebug = false;
display();
}
function onClickColor()
{
if(form2.color.checked) colorMode = 1;
else colorMode = 0;
display();
}
function onClickLight()
{
light.pos[0] = parseFloat(form2.lightX.value);
light.pos[1] = parseFloat(form2.lightY.value);
light.pos[2] = parseFloat(form2.lightZ.value);
display();
}
function onClickStart()
{
fps = 0;
elapseTime = elapseTime0;
elapseTime0 = 0;
elapseTime1 = 0;
flagStart = true;
flagStep = false;
lastTime = new Date().getTime();
//count = 0;
}
function onClickFreeze()
{
if(flagStart) { flagStart = false; }
else { flagStart = true; elapseTime = elapseTime0; }
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.time.value = "0";
display();
}
function onClickCameraReset()
{
initCamera();
display();
}
function onClickCameraAbove()
{
initCamera();
camera.theta = 90.01;
camera.getPos();//カメラ位置を計算
display();
}
<file_sep>/JVEMV6/46_art/8_kb/open-folders.bat
start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\domino\memos\2018-10\2018-10.ms
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第7章/vortexWave3.js
/*-----------------------------------------------
vortexWave3.js
レギュラー格子による速度-圧力法(フラクショナル・ステップ法)
移動障害物(円柱)。
渦と波が共存
正の渦度を負に反転表示
------------------------------------------------*/
var canvas;//canvas要素
var gl; //WebGL描画用コンテキスト
var camera;//カメラ
var light; //光源
var flagDebug = false;
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var flagStart = false;
var flagStep = false;
var flagReset = false;
//表示オブジェクト
var w_object;//水面
var obstacle = [];//移動、固定障害物
var adjustH = 0.1;//表示上の高さ調整
var displayMode = 0;//渦度/流れ関数
var colorMode = 0;//連続/段階表示
//数値計算
var type = [];//格子点のタイプ
var Prs = [];//圧力
var Omg = [];//渦度(x,y速度で計算)
var velX = [];//格子点のx速度
var velY = [];//格子点のy速度
var velXgx = [];//速度微分
var velXgy = [];//速度微分
var velYgx = [];//速度微分
var velYgy = [];//速度微分
var deltaT = 0.05;
var Re = 3000.0;//レイノルズ数
var maxDisp = 2.0;
//移動障害物
var speed = 1;//障害物速度
var obsRadius = 3;// 4;//円運動時の半径
var moveMode = 0;//直線
var flagObsStop = false;
var rad; //球表示のときの半径
//波
var NX = 100;
var NY = 100;
var DX, DY;
var sizeX = 10; //[m]
var sizeY = 10; //[m]
var period = 1; //[s]
var freq = 1; //[Hz]
var lambda = 1; //[m]
var amp0 = 20; //[m]
var waveVel = 1;//伝搬速度
var mu0 = 1.5; //粘性抵抗
//波動による変位量計算
var vel = []; //z軸方向の速度
var pos = []; //変位量
//移動障害物
var obs_nWX = 6;//障害物の厚さ(x方向,格子間隔の整数倍)
var obs_nWY = 6; //障害物の幅(y方向,格子間隔の整数倍)
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(0.1, 0.1, 0.1, 1);
gl.enable(gl.DEPTH_TEST);
form2.deltaT.value = deltaT;
form2.Re.value = Re;
form2.nMesh.value = NX;
form2.adjustH.value = adjustH;
form2.maxDisp.value = maxDisp;
form2.amp0.value = amp0;
form2.waveVel.value = waveVel;
form2.lambda.value = lambda;
form2.mu0.value = mu0;
initCamera();
initData();
display();
var timestep = 0;
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;
elapseTimeN += deltaT;//数値計算上の経過時間(無次元時間)
renewObsPos(deltaT);
//障害物の中心を波源位置にする
var sourceI = Math.round(NY * (obstacle[0].vPos.x + sizeX/2.0) / sizeX);
var sourceJ = Math.round(NY * (obstacle[0].vPos.y + sizeY/2.0) / sizeY);
if(!flagObsStop) pos[sourceI][sourceJ] = amp0 * Math.sin(2.0 * Math.PI * freq * elapseTimeN);
var nSkip = 10;
var dt = deltaT / nSkip;
for(var i = 0; i < nSkip; i++)
{
calcVortex(dt);//渦度計算
calcWave(dt);
}
display();
elapseTime0 = elapseTime;//現在の経過時間を保存
form1.e_time.value = elapseTime.toString();
form1.n_time.value = elapseTimeN.toString();
if(flagStep) { flagStart = false; }
}
}
animate();
}
//--------------------------------------------
function initCamera()
{
//光源インスタンスを作成
light = new Light();
light.pos = [-200.0, 0.0, 100.0, 1.0];
form2.lightX.value = light.pos[0];
form2.lightY.value = light.pos[1];
form2.lightZ.value = light.pos[2];
//カメラ・インスタンスを作成
camera = new Camera();
camera.theta = 40;
camera.cnt[2] = 0.2;
camera.dist = 15;
camera.delta = 1;
camera.getPos();//カメラ位置を計算
mouseOperation(canvas, camera);//swgSupport.js
}
function initObject()
{
waveVel = parseFloat(form2.waveVel.value);
lambda = parseFloat(form2.lambda.value);
period = lambda / waveVel;
freq = 1 / period;
amp0 = parseFloat(form2.amp0.value);
mu0 = parseFloat(form2.mu0.value);
deltaT = parseFloat(form2.deltaT.value);
Re = parseFloat(form2.Re.value);
NX = parseInt(form2.nMesh.value);
NY = NX;
DX = sizeX / NX;//格子間隔
DY = sizeY / NY;
//解析結果表示オブジェクト
w_object = new Rigid();//Rigidクラスのオブジェクトを表示オブジェクト
w_object.kind = "ELEVATION";
w_object.sizeX = sizeX;
w_object.sizeY = sizeY;
w_object.vSize = new Vector3(1, 1, adjustH);//scaling
w_object.nSlice = NX;
w_object.nStack = NY;
w_object.diffuse = [ 0.4, 0.6, 0.9, 1.0] ;
w_object.ambient = [ 0.1, 0.2, 0.3, 1.0];
w_object.specular = [ 0.8, 0.8, 0.8, 1.0];
w_object.shininess = 100.0;
w_object.flagDebug = flagDebug;
//障害物
for(var i = 0; i < 5; i++) {
obstacle[i] = new Rigid();
if(i == 0) obstacle[i].kind = "SPHERE";
else obstacle[i].kind = "CUBE";
}
rad = obs_nWX * DX *0.564;//断面積がほぼ等しく
obstacle[0].vSize = new Vector3(rad*2, rad*2, rad*2);
obstacle[0].vPos = new Vector3(0, -4, 0);
obstacle[0].vVel = new Vector3(0, speed, 0);
obstacle[0].flagDebug = flagDebug;
speed = parseFloat(form2.obs_speed.value);
//obsRadius = Math.abs(obstacle[0].vPos.y);//円運動時の半径
//固定障害物(境界)
var w = 0.1;//固定障害物の幅
obstacle[1].vPos = new Vector3(0,-sizeY/2 - w, 0);
obstacle[1].vSize = new Vector3(sizeX, w, 0.5);
obstacle[2].vPos = new Vector3(0, sizeY/2 - w, 0);
obstacle[2].vSize = new Vector3(sizeX, w, 0.5);
obstacle[3].vPos = new Vector3(-sizeX/2, - w, 0);
obstacle[3].vSize = new Vector3( w, sizeY,0.5);
obstacle[4].vPos = new Vector3( sizeX/2, - w, 0);
obstacle[4].vSize = new Vector3( w, sizeY,0.5);
}
function initData()
{
initObject();
//障害物の左端・右端・上端・下端の格子単位の位置
nX1 = Math.round(NX/2 + obstacle[0].vPos.x/DX - obs_nWX/2);//障害物左端
nX2 = Math.round(NX/2 + obstacle[0].vPos.x/DX + obs_nWX/2);//障害物右端
nY2 = Math.round(NY/2 + obstacle[0].vPos.y/DY + obs_nWY/2);//障害物上端
nY1 = Math.round(NY/2 + obstacle[0].vPos.y/DY - obs_nWY/2);//障害物下端
var i, j;
for(i = 0; i <= NX; i++)
{
type[i] = [];//格子点のタイプ
Prs[i] = []; //圧力
Omg[i] = []; //渦度
velX[i] = []; //表示用も同じ
velY[i] = [];
velXgx[i] = [];//x方向微分
velXgy[i] = [];//y方向微分
velYgx[i] = [];//x方向微分
velYgy[i] = [];//y方向微分
//波動用
vel[i] = [];
pos[i] = [];
}
//格子点のタイプ
for(j = 0; j <= NY; j++)
{
for(i = 0; i <= NX; i++)
{
type[i][j] = "INSIDE";//内点
if(j == 0) type[i][j] = "BOTTOM";//下側壁面
if(j == NY) type[i][j] = "TOP";//上側壁面
if(i == 0) type[i][j] = "INLET";//左側壁面
if(i == NX) type[i][j] = "OUTLET";//右側壁面
if(i == nX1 && j > nY1 && j < nY2) type[i][j] = "OBS_LEFT";//障害物左端
if(i == nX2 && j > nY1 && j < nY2) type[i][j] = "OBS_RIGHT";//障害物右端
if(i > nX1 && i < nX2 && j == nY2) type[i][j] = "OBS_TOP";//障害物上端
if(i > nX1 && i < nX2 && j == nY1) type[i][j] = "OBS_BOTTOM";//障害物上端
if(i > nX1 && i < nX2 && j > nY1 && j < nY2) type[i][j] = "OBSTACLE";//障害物内部
//コーナー
if(i == nX1 && j == nY1) type[i][j] = "OBS_LL";
if(i == nX1 && j == nY2) type[i][j] = "OBS_UL";
if(i == nX2 && j == nY1) type[i][j] = "OBS_LR";
if(i == nX2 && j == nY2) type[i][j] = "OBS_UR";
}
}
//初期値
//左端/右端もは流速0
for(j = 0; j <= NY; j++)
for (i = 0; i <= NX; i++)
{
//圧力
Prs[i][j] = 0.0;
//速度
velX[i][j] = 0.0;
velY[i][j] = 0.0;
velXgx[i][j] = 0.0;
velXgy[i][j] = 0.0;
velYgx[i][j] = 0.0;
velYgy[i][j] = 0.0;
Omg[i][j] = 0.0;//渦度
//波動計算用の格子点速度・位置
vel[i][j] = 0.0;//変位のz軸方向速度
pos[i][j] = 0.0;//変位
}
}
function renewObsPos(dt)
{
if(moveMode == 0)//直線モード
{
obstacle[0].vVel.x = 0;
if(obstacle[0].vVel.y > 0) obstacle[0].vVel.y = speed;
else obstacle[0].vVel.y = -speed;
if(obstacle[0].vPos.y >= 4) obstacle[0].vVel.y = -speed;
if(obstacle[0].vPos.y <= -4) obstacle[0].vVel.y = speed;
if(!flagObsStop)obstacle[0].vPos.add(mul(obstacle[0].vVel, dt));
}
else//円運動
{//初期位置は左側
var xx = -obstacle[0].vPos.x;//中心からの距離(左側で正)
var yy = obstacle[0].vPos.y;//中心からの距離(上側で正)
obstacle[0].vVel.x = speed * yy / obsRadius;
obstacle[0].vVel.y = speed * xx / obsRadius;
if(!flagObsStop){
obstacle[0].vPos.x = -obsRadius * Math.sin(speed * elapseTimeN / obsRadius);
obstacle[0].vPos.y = -obsRadius * Math.cos(speed * elapseTimeN / obsRadius);
}
}
nX1 = Math.round(NX/2 + obstacle[0].vPos.x/DX - obs_nWX/2);//障害物左端位置
nX2 = Math.round(NX/2 + obstacle[0].vPos.x/DX + obs_nWX/2);//障害物右端位置
nY2 = Math.round(NY/2 + obstacle[0].vPos.y/DY + obs_nWY/2);//障害物上端位置
nY1 = Math.round(NY/2 + obstacle[0].vPos.y/DY - obs_nWY/2);//障害物下端位置
//障害物の新しい格子点のタイプ
var i, j;
for(i = 1; i < NX; i++)
for(j = 1; j < NY; j++)
{
type[i][j] = "INSIDE";//内点
if(i == nX1 && j > nY1 && j < nY2) type[i][j] = "OBS_LEFT";//障害物左端
if(i == nX2 && j > nY1 && j < nY2) type[i][j] = "OBS_RIGHT";//障害物右端
if(i >= nX1 && i <= nX2 && j == nY2) type[i][j] = "OBS_TOP";//障害物上端
if(i >= nX1 && i <= nX2 && j == nY1) type[i][j] = "OBS_BOTTOM";//障害物下端
if(i > nX1 && i < nX2 && j > nY1 && j < nY2) type[i][j] = "OBSTACLE";//障害物内部
if(i == nX1 && j == nY1) type[i][j] = "OBS_LL";//左下
if(i == nX1 && j == nY2) type[i][j] = "OBS_UL";
if(i == nX2 && j == nY1) type[i][j] = "OBS_LR";
if(i == nX2 && j == nY2) type[i][j] = "OBS_UR";
}
}
function display()
{
//光源
var lightPosLoc = gl.getUniformLocation(gl.program, 'u_lightPos');
gl.uniform4fv(lightPosLoc, light.pos);
var lightColLoc = gl.getUniformLocation(gl.program, 'u_lightColor');
gl.uniform4fv(lightColLoc, light.color);
var cameraLoc = gl.getUniformLocation(gl.program, 'u_cameraPos');
gl.uniform3fv(cameraLoc, camera.pos);
//ビュー投影行列を計算する
var vpMatrix = new Matrix4();// 初期化
vpMatrix.perspective(camera.fovy, canvas.width/canvas.height, camera.near, camera.far);
if(Math.cos(Math.PI * camera.theta /180.0) >= 0.0)//カメラ仰角90度でビューアップベクトル切替
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, 1.0);
else
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, -1.0);
var vpMatrixLoc = gl.getUniformLocation(gl.program, 'u_vpMatrix');
gl.uniformMatrix4fv(vpMatrixLoc, false, vpMatrix.elements);
var colorLoc = gl.getUniformLocation(gl.program, 'u_colorMode');
gl.uniform1i(colorLoc, colorMode);
// カラーバッファとデプスバッファをクリアする
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
var i, j, k;
var rangeDisp = maxDisp * 2;
for(j = 0; j <= NY; j++)
for(i = 0; i <= NX; i++)
{
k = i + j * (NX+ 1);
var omega = Omg[i][j];
if(omega > 0) omega = -omega;//正を負に反転
var zz = omega + pos[i][j];
//障害物の上部に変位を表示させないように
var x, y;
x = (i-NX/2)*DX - obstacle[0].vPos.x;
y = (j-NY/2)*DY - obstacle[0].vPos.y;
if( Math.sqrt(x * x + y * y) < rad) zz = 0;
w_object.data[k] = (zz + maxDisp) / rangeDisp;
}
w_object.vSize = new Vector3(1, 1, adjustH);
var n = w_object.initVertexBuffers(gl);
w_object.draw(gl, n);
//障害物
gl.uniform1i(colorLoc, 2);
for(i = 0; i < 5; i++)
{
n = obstacle[i].initVertexBuffers(gl);
obstacle[i].draw(gl, n);
}
}
function calcVortex(dt)
{
var i, j;
var iteration = 1;//最大繰り返し回数(Poisson方程式)
var tolerance = 0.0001;//許容誤差
var error = 0.0;
var maxError = 0.0;
var pp;
var DX2 = DX * DX;
var DY2 = DY * DY;
var A1 = 0.5 * DY2 / (DX2 + DY2);
var A2 = 0.5 * DX2 / (DX2 + DY2);
var A3 = 0.25 * DX2*DY2 / (DX2 + DY2);
var a, b, pp;
//障害物
for (j = 1; j < NY; j++)
for (i = 1; i < NX; i++)
{
if(type[i][j] == "INSIDE") continue;
{
if(flagObsStop)
{ velX[i][j] = 0; velY[i][j] = 0; }
else
{
velX[i][j] = obstacle[0].vVel.x;
velY[i][j] = obstacle[0].vVel.y;
}
}
}
//NS方程式による速度更新
methodCIP(velX, velXgx, velXgy, velX, velY, dt);
methodCIP(velY, velYgx, velYgy, velX, velY, dt);
//Poisson方程式の右辺
var D = [];
for(i = 0; i <= NX; i++) D[i] = [];
for (j = 1; j < NY; j++)
for (i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;//INSIDE以外の速度は初期設定値
a = (velX[i+1][j] - velX[i-1][j]) / DX;
b = (velY[i][j+1] - velY[i][j-1]) / DY;
D[i][j] = A3 * (a + b) / dt;
}
//Poissonの方程式を解く
var cnt = 0;
while (cnt < iteration)
{
maxError = 0.0;
for (i = 0; i <= NX; i++)
for (j = 0; j <= NY; j++)
{
if(type[i][j] == "INSIDE") continue;
else if(type[i][j] == "INLET") Prs[i][j] = Prs[1][j];//Neumann(左端)
else if(type[i][j] == "OUTLET") Prs[i][j] = 0;//(右端)
else if(type[i][j] == "TOP") Prs[i][j] = Prs[i][NY-1];
else if(type[i][j] == "BOTTOM") Prs[i][j] = Prs[i][1];
else if(type[i][j] == "OBS_LEFT") Prs[i][j] = Prs[i-1][j];
else if(type[i][j] == "OBS_RIGHT") Prs[i][j] = Prs[i+1][j];
else if(type[i][j] == "OBS_TOP") Prs[i][j] = Prs[i][j+1];
else if(type[i][j] == "OBS_BOTTOM") Prs[i][j] = Prs[i][j-1];
else if(type[i][j] == "OBS_UL") Prs[i][j] = Prs[i-1][j+1];
else if(type[i][j] == "OBS_UR") Prs[i][j] = Prs[i+1][j+1];
else if(type[i][j] == "OBS_LL") Prs[i][j] = Prs[i-1][j-1];
else if(type[i][j] == "OBS_LR") Prs[i][j] = Prs[i+1][j-1];
}
//反復計算
for (j = 1; j < NY; j++)
for (i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
pp = A1 * (Prs[i+1][j] + Prs[i-1][j]) + A2 * (Prs[i][j+1] + Prs[i][j-1]) - D[i][j];
error = Math.abs(pp - Prs[i][j]);
if (error > maxError) maxError = error;
Prs[i][j] = pp;//更新
}
if (maxError < tolerance) break;
cnt++;
}
//速度ベクトルの更新
for (j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
velX[i][j] += - 0.5 * dt * (Prs[i+1][j] - Prs[i-1][j]) / DX;
velY[i][j] += - 0.5 * dt * (Prs[i][j+1] - Prs[i][j-1]) / DY;
}
//渦度を速度から求める
for(i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
Omg[i][j] = 0.5 * ((velY[i+1][j] - velY[i-1][j]) / DX - (velX[i][j+1] - velX[i][j-1]) / DY);
}
}
//------------------------------------------------------------------------
function methodCIP(f, gx, gy, vx, vy, dt)
{
var newF = [];//関数
var newGx = [];//x方向微分
var newGy = [];//y方向微分
var i, j, ip, jp;
for(i = 0; i <= NX; i++)
{//配列の2次元化
newF[i] = [];
newGx[i] = [];
newGy[i] = [];
}
var c11, c12, c21, c02, c30, c20, c03, a, b, sx, sy, x, y, dx, dy, dx2, dy2, dx3, dy3;
var i, j, ip, jp;
for(i = 1; i < NX; i++)
for(j = 1; j < NY; j++)
{
if(type[i][j] != "INSIDE") continue;
if(vx[i][j] >= 0.0) sx = 1.0; else sx = -1.0;
if(vy[i][j] >= 0.0) sy = 1.0; else sy = -1.0;
x = - vx[i][j] * dt;
y = - vy[i][j] * dt;
ip = i - sx;//上流点
jp = j - sy;
dx = sx * DX;
dy = sy * DY;
dx2 = dx * dx;
dy2 = dy * dy;
dx3 = dx2 * dx;
dy3 = dy2 * dy;
c30 = ((gx[ip][j] + gx[i][j]) * dx - 2.0 * (f[i][j] - f[ip][j])) / dx3;
c20 = (3.0 * (f[ip][j] - f[i][j]) + (gx[ip][j] + 2.0 * gx[i][j]) * dx) / dx2;
c03 = ((gy[i][jp] + gy[i][j]) * dy - 2.0 * (f[i][j] - f[i][jp])) / dy3;
c02 = (3.0 * (f[i][jp] - f[i][j]) + (gy[i][jp] + 2.0 * gy[i][j]) * dy) / dy2;
a = f[i][j] - f[i][jp] - f[ip][j] + f[ip][jp];
b = gy[ip][j] - gy[i][j];
c12 = (-a - b * dy) / (dx * dy2);
c21 = (-a - (gx[i][jp] - gx[i][j]) * dx) / (dx2*dy);
c11 = -b / dx + c21 * dx;
newF[i][j] = f[i][j] + ((c30 * x + c21 * y + c20) * x + c11 * y + gx[i][j]) * x
+ ((c03 * y + c12 * x + c02) * y + gy[i][j]) * y;
newGx[i][j] = gx[i][j] + (3.0 * c30 * x + 2.0 * (c21 * y + c20)) * x + (c12 * y + c11) * y;
newGy[i][j] = gy[i][j] + (3.0 * c03 * y + 2.0 * (c12 * x + c02)) * y + (c21 * x + c11) * x;
//粘性項に中央差分
newF[i][j] += dt * ( (f[i-1][j] + f[i+1][j] - 2.0 * f[i][j]) / dx2
+ (f[i][j-1] + f[i][j+1] - 2.0 * f[i][j]) / dy2 ) / Re;
}
//更新
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
f[i][j] = newF[i][j];
gx[i][j] = newGx[i][j];
gy[i][j] = newGy[i][j];
}
}
//----------------------------------------------------------------
function calcWave(dt)
{
//波動方程式
var i, j;
var D = sizeX / NX;//格子間隔(sizeX0=sizeY0,NX0=NY0であること)
var D2 = D * D;
var cc = waveVel * waveVel / D2;
//格子点のz方向速度と位置の更新(Euler法)
for(j = 0; j <= NY; j++)
for(i = 0; i <= NX; i++)
{
//解析領域境界は常に自由境界
{
if(i == 0) pos[0][j] = -pos[1][j] ;
if(i == NX) pos[NX][j] = -pos[NX-1][j] ;
if(j == 0) pos[i][0] = -pos[i][1] ;
if(j == NY) pos[i][NY] = -pos[i][NY-1] ;
}
if(i == 0 || i == NX || j == 0 || j == NY) continue;
var accel = cc * (pos[i-1][j] + pos[i+1][j] + pos[i][j-1] + pos[i][j+1] - 4 * pos[i][j]);
accel -= mu0 * vel[i][j];
//速度
vel[i][j] += accel * dt;
//位置
pos[i][j] += vel[i][j] * dt;
}
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
initData();
}
function onDisplay()
{
display();
}
function onClickObsStop()
{
flagObsStop = !flagObsStop;
}
function onChangeMoveMode()
{
var nn;
var radioMM = document.getElementsByName("radioMM");
for(var i = 0; i < radioMM.length; i++)
{
if(radioMM[i].checked) moveMode = i;
}
onClickReset();
}
function onChangeData2()
{
adjustH = parseFloat(form2.adjustH.value);
if(adjustH == 0) adjustH = 0.001;// 完全に0にするとmatrix4の逆行列ルーチンでエラーになる
maxDisp = parseFloat(form2.maxDisp.value);
amp0 = parseFloat(form2.amp0.value);
mu0 = parseFloat(form2.mu0.value);
speed = parseFloat(form2.obs_speed.value);
display();
}
function onChangeDisplayMode()
{
var nn;
var radioD = document.getElementsByName("radioD");
for(var i = 0; i < radioD.length; i++)
{
if(radioD[i].checked) displayMode = i;
}
display();
}
function onChangeColorMode()
{
var nn;
var radioC = document.getElementsByName("radioC");
for(var i = 0; i < radioC.length; i++)
{
if(radioC[i].checked) colorMode = i;
}
display();
}
function onClickDebug()
{
w_object.flagDebug = obstacle[0].flagDebug = flagDebug = !flagDebug ;
display();
}
function onClickStart()
{
fps = 0;
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
elapseTimeN = 0;
elapseTimeN0 = 0;
flagStart = true;
flagStep = false;
flagFreeze = false;
flagObsStop = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
flagStart = !flagStart;
flagFreeze = !flagFreeze;
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTimeN0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.e_time.value = "0";
form1.n_time.value = "0";
display();
}
function onClickCameraReset()
{
initCamera();
display();
}
function onClickCameraAbove()
{
initCamera();
camera.theta = 90.01;
camera.getPos();//カメラ位置を計算
display();
}
function onClickLight()
{
light.pos[0] = parseFloat(form2.lightX.value);
light.pos[1] = parseFloat(form2.lightY.value);
light.pos[2] = parseFloat(form2.lightZ.value);
display();
}
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgCollision.js
/*------------------------------------------------------------
swgCollision.js
剛体同士の衝突
--------------------------------------------------------------*/
var flagCollision = true;
var flagSeparate = false;
var awakeValue = 1;//覚醒させる運動量のしきい値
function collision(dt)
{
var i, j;
for(i = 0; i < numRigid; i++)
{
rigid[i].action3D(dt);//放物運動(swgRigid.js)
//console.log(" i = " + i + " state = " + rigid[i].state);
if(!flagCollision) continue;//衝突判定せず
for(j = 0; j < numRigid; j++)
{
if(i == j) continue;
//console.log(" i = " + i + " state = " + rigid[i].state + " j = " + j + " state = " + rigid[j].state);
//境界球で予備判定
var d = distance2(rigid[i].vPos, rigid[j].vPos);//2乗距離
var a = rigid[i].boundingR + rigid[j].boundingR;
if(d > a*a) continue;
collisionWithRigid(i, j, dt);
}
}
//強制引き離し
var distEnforce = 0.2;//引き離す距離
var vDir = new Vector3();
for(i = 0; i < numRigid; i++)
{
if(flagSeparate)
{
//強制引き離し(x,y,z方向のサイズが同程度でなければ利用不可)
for(j = 0; j < numRigid; j++)
{
var a = (rigid[i].vSize.x + rigid[j].vSize.x)/2;
var d = distance(rigid[i].vPos, rigid[j].vPos);//中心間距離
if(d < 0.2) vDir = new Vector3(1.0, 1.0, 0.0);//深くめり込んだ場合
else vDir = direction(rigid[i].vPos , rigid[j].vPos);
if(d < a)
{
rigid[i].vPos.sub(mul(distEnforce, vDir));
rigid[j].vPos.add(mul(distEnforce, vDir));
}
}
}
}
}
//--------------------------------------------------------------------------
function collisionWithRigid(i, j, dt)
{
var rikiseki;
var vn1, vn2;//法線成分の大きさ
var muc,B, m1, m2;
var vVelocityP1, vVelocityP2;//合成速度
var vTangential;//接線方向
var vDir = new Vector3();
var vA1 = new Vector3();
var vA2 = new Vector3();
var vPos1 = new Vector3();
var vPos2 = new Vector3();
var vRg1 = new Vector3();
var vRg2 = new Vector3();
var depth = checkCollision(i, j);//衝突判定
if(depth < 0.0) return;
rigid[i].vForce = new Vector3();
rigid[i].vTorque = new Vector3();
rigid[j].vForce = new Vector3();
rigid[j].vTorque = new Vector3();
vRg1.copy(rigid[i].vGravityToPoint);
vRg2.copy(rigid[j].vGravityToPoint);
m1 = rigid[i].mass;
m2 = rigid[j].mass;
vVelocityP1 = add(rigid[i].vVel , cross(rigid[i].vOmega, vRg1));
vn1 = dot(vVelocityP1, rigid[i].vNormal);
vVelocityP2 = add(rigid[j].vVel , cross(rigid[j].vOmega, vRg2));
vn2 = dot(vVelocityP2, rigid[i].vNormal);
//衝突応答
vTangential = cross( rigid[i].vNormal, cross(sub(vVelocityP1, vVelocityP2), rigid[i].vNormal));
vTangential.norm();
//力積
rikiseki = - (restitution + 1.0) * (vn1 - vn2) / (1.0/m1 + 1.0/m2
+ dot(rigid[i].vNormal , cross(mulMV(rigid[i].mInertiaInverse, cross(vRg1, rigid[i].vNormal)), vRg1))
+ dot(rigid[i].vNormal , cross(mulMV(rigid[j].mInertiaInverse, cross(vRg2, rigid[i].vNormal)), vRg2)));
//強い運動量を受けたとき覚醒
if(Math.abs(rikiseki) > awakeValue)
{
rigid[i].state = "FREE";
rigid[j].state = "FREE";
}
//力の総和
rigid[i].vForce.add(mul(rigid[i].vNormal , rikiseki / dt));
rigid[j].vForce.sub(mul(rigid[i].vNormal , rikiseki / dt));
//力のモーメント(トルク)の総和
rigid[i].vTorque.add(mul(cross(vRg1, rigid[i].vNormal) , rikiseki / dt));
rigid[j].vTorque.sub(mul(cross(vRg2, rigid[i].vNormal) , rikiseki / dt));
//摩擦を考慮
vA1 = cross(mulMV(rigid[i].mInertiaInverse , cross(vRg1, vTangential)) , vRg1);
vA2 = cross(mulMV(rigid[j].mInertiaInverse , cross(vRg2, vTangential)) , vRg2);
B = - dot(vTangential, sub(vVelocityP1, vVelocityP2)) / (1.0/m1+1.0/m2+ dot(vTangential,add(vA1, vA2)));
muc = Math.abs(B / rikiseki);
if(muK >= muc)
{
rigid[i].vForce.add(vTangential, B / dt);
rigid[i].vTorque.add(mul(cross(vRg1, vTangential) , B / dt));
rigid[j].vForce.sub(mul(vTangential, B / dt));
rigid[j].vTorque.sub(mul(cross(vRg2, vTangential) , B / dt));
}
else
{
rigid[i].vForce.add(mul( muK * rikiseki / dt , vTangential));
rigid[i].vTorque.add(mul(cross(vRg1, vTangential) , muK * rikiseki / dt));
rigid[j].vForce.sub(mul(muK * rikiseki / dt , vTangential));
rigid[j].vTorque.sub(mul(cross(vRg2, vTangential) , muK * rikiseki / dt));
}
//位置回転角度の更新
if(rigid[i].state== "FREE")// || rigid[i].state== "FREE_ON_FLOOR" )
{
// i番目の剛体
//衝突時にめり込んだ分引き離す
rigid[i].vPos.sub(mul(depth/2.0+0.01 , rigid[i].vNormal));//0.01は分離を確実にするため
rigid[i].vAcc = div(rigid[i].vForce , m1); //加速度
rigid[i].vVel.add(mul(rigid[i].vAcc , dt));//速度
rigid[i].vAlpha = mulMV(rigid[i].mInertiaInverse , rigid[i].vTorque);//角加速度の更新
rigid[i].vOmega.add(mul(rigid[i].vAlpha , dt)); //角速度
}
if(rigid[j].state== "FREE")// || rigid[j].state== "FREE_ON_FLOOR" )
{
// j番の剛体
//衝突時にめり込んだ分引き離す
rigid[j].vPos.add(mul(depth/2.0+0.01 , rigid[i].vNormal));
rigid[j].vAcc = div(rigid[j].vForce , m2);
rigid[j].vVel.add(mul(rigid[j].vAcc , dt));
rigid[j].vAlpha = mulMV(rigid[j].mInertiaInverse , rigid[j].vTorque);
rigid[j].vOmega.add(mul(rigid[j].vAlpha , dt));
}
}
//--------------------------------------------------------------------------------------------
//衝突があれば衝突時の深さdepthを返す。
//depthが負のときは非衝突である
function checkCollision(i, j)
{
var depth;
if(rigid[i].kind == "SPHERE")
{
switch(rigid[j].kind )
{
case "SPHERE"://球同士
depth = rigid[i].collisionSphereWithSphere(rigid[j]);
return depth;
break;
case "CUBE":
depth = rigid[i].collisionSphereWithCube(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionCubeWithSphere(rigid[i]);
if(depth >= 0.0) rigid[i].vNormal.reverse();
return depth;
break;
case "CYLINDER"://円柱
depth = rigid[i].collisionSphereWithCylinder(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionCylinderWithSphere(rigid[i]);
if(depth >= 0.0) rigid[i].vNormal.reverse();
return depth;
break;
}
}
else if(rigid[i].kind == "CUBE")
{
switch(rigid[j].kind)
{
case "SPHERE":
depth = rigid[i].collisionCubeWithSphere(rigid[j]);
//console.log("BBB depth = " + depth + " x=" + rigid[i].vNormal.x + " y=" + rigid[i].vNormal.y + " z=" + rigid[i].vNormal.z);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionSphereWithCube(rigid[i]);
if(depth >= 0.0) rigid[i].vNormal.reverse();
//console.log("CCC depth = " + depth + " x=" + rigid[i].vNormal.x + " y=" + rigid[i].vNormal.y + " z=" + rigid[i].vNormal.z);
return depth;
break;
case "CUBE":
depth = rigid[i].collisionCubeWithCube(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionCubeWithCube(rigid[i]);
if(depth >= 0.0) rigid[i].vNormal.reverse();
return depth;
break;
case "CYLINDER"://円柱
depth = rigid[i].collisionCubeWithCylinder(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionCylinderWithCube(rigid[i]);
if(depth >= 0.0) rigid[i].vNormal.reverse();
return depth;
break;
}
}
else if(rigid[i].kind == "CYLINDER")//円柱
{
switch(rigid[j].kind)
{
case "SPHERE":
depth = rigid[i].collisionCylinderWithSphere(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionSphereWithCylinder(rigid[i]);
rigid[i].vNormal.reverse();
return depth;
break;
case "CUBE":
depth = rigid[i].collisionCylinderWithCube(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionCubeWithCylinder(rigid[i]);
rigid[i].vNormal.reverse();
return depth;
break;
case "CYLINDER"://円柱同士
depth = rigid[i].collisionCylinderWithCylinder(rigid[j]);
if(depth >= 0.0) return depth;
depth = rigid[j].collisionCylinderWithCylinder(rigid[i]);
if(depth >= 0.0) rigid[i].vNormal.reverse();
return depth;
break;
}
}
return NON_COLLISION;
}
<file_sep>/JVEMV6/46_art/8_kb/start_write-out.bat
REM start C:\WORKS_2\Programs\musescore\musescore_2.2.1\bin\MuseScore.exe
REM *******************************
REM command line
REM *******************************
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_command_prompot.bat
REM *******************************
REM open folders
REM *******************************
start open-folders.bat
REM *******************************
REM apps
REM *******************************
REM call C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.bat
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.bat
REM pause
exit
<file_sep>/JVEMV6/46_art/11_guitar/start_guitar.new.bat
REM ******************************* list
REM open folders
REM vlc player
REM browser
REM doc
REM metronome
REM *******************************
REM *******************************
REM open folders
REM *******************************
REM echo start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
echo start C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\15_flute
start C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\15_flute
echo start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
echo start C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\5_guitar
start C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\5_guitar
REM *******************************
REM vlc player
REM 2019/09/26 18:16:41
REM *******************************
pushd C:\Users\iwabuchiken\VLC
start vlc.exe
REM *******************************
REM browser
REM 2019/09/16 14:18:11
REM *******************************
:web_page_keyboard_opera
REM : opera
pushd "C:\WORKS_2\Programs\opera"
start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=gt+log&RBs_AND_OR_Memo=AND&sort=file_name&direction=desc"
REM start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=gt+video+g-1008&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
REM start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=gt+compi&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
REM start launcher.exe "https://docs.zoho.com/sheet/open/ek7i4683eb5df87d04a23acef09182e7df623/sheets/free/ranges/A3"
REM start launcher.exe "https://www.youtube.com/playlist?list=PLZe1Q2NRG8YXa2FCHsE3Ji3VCOg2Ey8LS"
goto exit_web_page_keyboard
:exit_web_page_keyboard
REM *******************************
REM doc
REM 2019/09/16 14:18:11
REM *******************************
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\list_of_memos.ods
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\memos_guitar.odt
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\15_flute_japanese\JVE_46#15.[flute-japanese].odt
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\list_session-menues.ods
REM *******************************
REM apps : metronome
REM 2019/10/24 15:11:38
REM *******************************
pushd "C:\WORKS_2\Programs\opera"
REM : metronome
REM start launcher.exe "https://www.metronomeonline.com"
REM start launcher.exe "https://www.imusic-school.com/en/tools/online-metronome/"
start launcher.exe https://www.flutetunes.com/metronome/
REM : keyboard
start launcher.exe https://pianoplays.com
REM start launcher.exe https://www.onlinepianist.com/virtual-piano
REM pause
REM exit
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgRigid_HS.js
/*----------------------------------------------------------------------------
階層構造用Rigidクラス
2014.9.17 更新
----------------------------------------------------------------------------*/
var MAX_VERTEX = 30;//多面体近似のときの頂点数
var muK = 1.0;//0.5;//動摩擦係数
var muS = 1.0;//静止摩擦係数
var restitution = 0.5;//跳ね返り係数
var dampRotation = 3.0;//回転減速係数
var gravity = 9.8;//重力加速度[m/s^2]
var restValue = 0.2; //静止状態にするしきい値(速度,回転速度)
var flagDrag = false;//空気抵抗フラグ
var flagMagnus = false;
var flagTumbling = false;
var flagQuaternion = false;
function Rigid_HS()
{
//プロパティ
this.kind = "SPHERE";
this.diffuse = [0.6, 0.6, 0.6, 1.0];
this.ambient = [0.4, 0.4, 0.4, 1.0];
//this.specular = [0.8, 0.8, 0.8, 1.0];
this.specular = [0.5, 0.5, 0.5, 1.0];
this.shininess = 200.0;
this.vVel = new Vector3();//速度(m/s)
this.vVel0 = new Vector3();//更新前の速度
this.vPos = new Vector3();//位置(m)
this.vPos0 = new Vector3();//初期位置
this.vForce = new Vector3();//外力(Newton)
this.vForce0 = new Vector3();//外力(初期値)
this.vOmega = new Vector3();//角速度(rad/s)
this.vOmega0 = new Vector3();//角速度(rad/s),更新前
this.vAcc = new Vector3();//加速度
this.vTorque = new Vector3();//トルク
this.vEuler0 = new Vector3();//回転角度(オイラー角で指定,更新前)
this.vEuler = new Vector3();//回転角度(オイラー角で指定,更新後)
this.vSize = new Vector3(1.0, 1.0, 1.0);//スケーリング
this.vGravityToPoint = new Vector3();//重心から衝突点までのベクトル
this.mass = 1;//質量[kg]
this.mInertia = new Matrix3();//慣性モーメントのテンソル
this.mInertiaInverse = new Matrix3();//その逆行列
this.vInertialResistance = new Vector3();
this.vRotationalResistance = new Vector3();
this.q = new Quaternion();
this.vAxis = new Vector3(1.0, 0.0, 0.0);//回転軸
this.angle = 0.0;
this.nSlice = 25;
this.nStack = 25;
this.radiusRatio = 0.2;//上底半径/下底半径(トーラスとバネの時はradius1/radius2)
this.eps1 = 1.0;//"SUPER"のパラメータ
this.eps2 = 1.0;//"SUPER"のパラメータ
this.flagDebug = false;//trueのときワイヤーフレームモデル
this.shadow = 0.0;//影の濃さを表す(0.0なら実オブジェクト)
//フロア表示のチェック模様
this.flagCheck = false;
this.col1 = [0.6, 0.5, 0.5, 1.0];
this.col2 = [0.4, 0.4, 0.6, 1.0];
this.plane = [0, 0, 1, 0];//簡易シャドウを描くときの平面の平面方程式
//テクスチャ
this.flagTexture = false;
this.nRepeatS = 1;
this.nRepeatT = 1;
//衝突判定などに使われるプロパティ
this.vP = [];
this.vP0 = [];//球以外の頂点座標
this.vNormal = new Vector3();
this.vNormalFacet = [];//直方体の面の法線ベクトル
this.numVertex;//球以外の多面体の頂点数
this.boundingR;//境界球の半径
this.state = "FREE";
this.flagFixed = false;
//tumbling特有のプロパティ
this.coefLift = 1.0;//揚力係数
this.delta = 0.5;//シフト率
//Spring特有のプロパティ
this.nPitch = 5;//バネ
this.radius = 0.5;//バネはvSizeを使用しない
this.len0 = 1;//バネの自然長
this.len = 1;//length0+変位量
this.constant;//バネ定数
this.row = [];
this.col = [];
//水面の波などの表現
this.sizeX = 10;
this.sizeY = 10;
this.data = [];//各格子点のx,y,z座標(kind = "GRID_SQUARE"などのときに必要なデータ)
//kind = "ELEVATION"のときはz成分だけ
//SUPER2のパラメータ
this.size1 = [0.5, 0.2, 0.5];
this.size2 = [0.5, 0.2, 0.5];
this.middle = 0.5; //中間のサイズ
this.angle2 = 0;//曲げる角度(度)
this.jStart = 1;
this.type = 0;//0,1,2だけ
}
Rigid_HS.prototype.initVertexBuffers = function(gl)
{
//頂点データをシェーダへアップロードするメソッド
var n;
var vertices = [];//頂点座標
var normals = []; //法線ベクトル
var indices = []; //頂点番号
var colors = [];//check模様のときだけ
var texCoords = [];//テクスチャ座標
if(!this.flagTexture)
{//非テクスチャ用
if (this.kind == "CUBE") n = makeCube(vertices, normals, indices, this.flagDebug);
else if(this.kind == "SPHERE") n = makeSphere(vertices, normals, indices, this.nSlice, this.nStack);
else if(this.kind == "CYLINDER")n = makeCylinder(vertices, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug);
else if(this.kind == "PRISM") n = makePrism(vertices, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug);
else if(this.kind == "TORUS") n = makeTorus(vertices, normals, indices, this.radiusRatio, this.nSlice, this.nStack);
else if(this.kind == "SUPER") n = makeSuper(vertices, normals, indices, this.nSlice, this.nStack, this.eps1, this.eps2);
else if(this.kind == "SUPER2") n = makeSuper2(vertices, normals, indices, this.size1, this.size2, this.nSlice, this.nStack, this.eps1, this.eps2, this.middle, this.angle2, this.jStart, this.type);
else if(this.kind == "SPRING") n = makeSpring(vertices, normals, indices, this.radius, this.radiusRatio, this.nSlice, this.nStack, this.nPitch, this.len);
else if(this.kind == "CYLINDER_X") n = makeCylinderX(vertices, normals, indices, this.nSlice, this.flagDebug);
else if(this.kind == "CYLINDER_Y") n = makeCylinderY(vertices, normals, indices, this.nSlice, this.flagDebug);
else if(this.kind == "CYLINDER_Z") n = makeCylinderZ(vertices, normals, indices, this.nSlice, this.flagDebug);
else if(this.kind == "PLATE_Z") n = makePlateZ(vertices, normals, indices, this.flagDebug);
else if(this.kind == "GRID_PLATE") n = makeGridPlate(vertices, normals, indices, this.nSlice, this.nStack, this.flagDebug);
else if(this.kind == "GRID_SQUARE") n = makeGridSquare(this.data, vertices, normals, indices, this.nSlice, this.nStack, this.flagDebug);
else if(this.kind == "ELEVATION") n = makeElevation(this.data, vertices, normals, indices, this.nSlice, this.nStack, this.sizeX, this.sizeY, this.flagDebug)
else if(this.kind == "CHECK_PLATE") n = makeCheckedPlate(vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2) ;
else if(this.kind == "CHECK_SQUARE") n = makeCheckedSquare(this.data, vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2) ;
else if(this.kind == "GRID_SPHERE") n = makeGridSphere(this.data, vertices, normals, indices, this.nSlice, this.nStack);
else if(this.kind == "CHECK_SPHERE") n = makeCheckedSphere(this.data, vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2);
else if(this.kind == "GRID_CYLINDER") n = makeGridCylinder(this.data, vertices, normals, indices, this.nSlice, this.nStack);
else if(this.kind == "CHECK_CYLINDER") n = makeCheckedCylinder(this.data, vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2);
}
else
{//テクスチャ用
if (this.kind == "CUBE") n = makeCubeTex(vertices, texCoords, normals, indices, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "CUBE_BUMP") n = makeCubeBump(vertices, texCoords, normals, indices, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "SPHERE") n = makeSphereTex(vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
else if(this.kind == "CYLINDER")n = makeCylinderTex(vertices, texCoords, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "PRISM") n = makePrismTex(vertices, texCoords, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "TORUS") n = makeTorusTex(vertices, texCoords, normals, indices, this.radiusRatio, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
else if(this.kind == "SUPER") n = makeSuperTex(vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.eps1, this.eps2, this.nRepeatS, this.nRepeatT);
else if(this.kind == "PLATE_Z") n = makePlateZTex(vertices, texCoords, normals, indices, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_PLATE") n = makeGridPlateTex(vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_SQUARE") n = makeGridSquareTex(this.data, vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_SPHERE") n = makeGridSphereTex(this.data, vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_CYLINDER") n = makeGridCylinderTex(this.data, vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
}
gl.disableVertexAttribArray(colorLoc);//colorのバッファオブジェクトの割り当てを無効化(フロアを表示したとき,フロアのインデックス以上の球やトーラスを描画できなくなることを防ぐため)
// バッファオブジェクトを作成する
var vertexBuffer = gl.createBuffer();
if(this.flagTexture) {var texCoordBuffer = gl.createBuffer();}
var normalBuffer = gl.createBuffer();
if(this.flagCheck) var colorBuffer = gl.createBuffer();
var indexBuffer = gl.createBuffer();
if (!vertexBuffer || !normalBuffer || !indexBuffer) return -1;
// 頂点の座標をバッファ・オブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices), gl.STATIC_DRAW);
// vertexLocにバッファ・オブジェクトを割り当てる
var vertexLoc = gl.getAttribLocation(gl.program, 'a_vertex');
gl.vertexAttribPointer(vertexLoc, 3, gl.FLOAT, false, 0, 0);
// バッファ・オブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(vertexLoc);//有効化する
if(this.flagTexture)
{
// 頂点のテクスチャ座標をバッファオブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, texCoordBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(texCoords), gl.STATIC_DRAW);
// texLocにバッファオブジェクトを割り当てる
var texLoc = gl.getAttribLocation(gl.program, 'a_texCoord');
gl.vertexAttribPointer(texLoc, 2, gl.FLOAT, false, 0, 0);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(texLoc);//有効化する
}
if(this.flagCheck)
{
// 頂点の色をバッファ・オブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, colorBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(colors), gl.STATIC_DRAW);
// a_colorの格納場所を取得し、バッファオブジェクトを割り当てる
var colorLoc = gl.getAttribLocation(gl.program, 'a_color');
gl.vertexAttribPointer(colorLoc, 3, gl.FLOAT, false, 0, 0);
// バッファ・オブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(colorLoc);//有効化
}
// 法線データをバッファ・オブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, normalBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(normals), gl.STATIC_DRAW);
// normalLocにバッファ・オブジェクトを割り当てる
var normalLoc = gl.getAttribLocation(gl.program, 'a_normal');
gl.vertexAttribPointer(normalLoc, 3, gl.FLOAT, false, 0, 0);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(normalLoc);//有効化する
//バッファ・オブジェクトをターゲットにバインドする
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, indexBuffer);
// インデックスデータをバッファ・オブジェクトに書き込む
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(indices), gl.STATIC_DRAW);
gl.bindBuffer(gl.ARRAY_BUFFER, null);
return n;
}
Rigid_HS.prototype.draw = function(gl, n, modelMatrix)
{
//マテリアル特性のユニフォーム変数格納場所を取得し値を設定する
var diffLoc = gl.getUniformLocation(gl.program, 'u_diffuseColor');
gl.uniform4fv(diffLoc, new Float32Array(this.diffuse));
var ambiLoc = gl.getUniformLocation(gl.program, 'u_ambientColor');
gl.uniform4fv(ambiLoc, new Float32Array(this.ambient));
var specLoc = gl.getUniformLocation(gl.program, 'u_specularColor');
gl.uniform4fv(specLoc, new Float32Array(this.specular));
var shinLoc = gl.getUniformLocation(gl.program, 'u_shininess');
gl.uniform1f(shinLoc, this.shininess);
var checkLoc = gl.getUniformLocation(gl.program, 'u_flagCheck');
gl.uniform1i(checkLoc, this.flagCheck);
var shadowLoc = gl.getUniformLocation(gl.program, 'u_shadow');
gl.uniform1f(shadowLoc, this.shadow);
var flagTexLoc = gl.getUniformLocation(gl.program, 'u_flagTexture');
gl.uniform1i(flagTexLoc, this.flagTexture);
//法線変換行列を計算する
var normalMatrix = new Matrix4();// 初期化
if(this.shadow < 0.01)//影でないときだけ
{
normalMatrix.setInverseOf(modelMatrix);//モデルリング行列の逆行列を求め
normalMatrix.transpose(); //さらに転置する
}
//それぞれの行列のuniform変数の格納場所を取得し値を設定する
var modelMatrixLoc = gl.getUniformLocation(gl.program, 'u_modelMatrix');
gl.uniformMatrix4fv(modelMatrixLoc, false, modelMatrix.elements);
var normalMatrixLoc = gl.getUniformLocation(gl.program, 'u_normalMatrix');
gl.uniformMatrix4fv(normalMatrixLoc, false, normalMatrix.elements);
//物体を描画する
if(this.flagDebug == false)//solidモデルで描画
gl.drawElements(gl.TRIANGLES, n, gl.UNSIGNED_SHORT, 0);
else//wireframeモデルで描画
{
if(this.kind == "GRID_SQUARE" || this.kind == "CHECK_SQUARE" || this.kind == "ELEVATION")
gl.drawElements(gl.LINES, n, gl.UNSIGNED_SHORT, 0);
else
gl.drawElements(gl.LINE_STRIP, n, gl.UNSIGNED_SHORT, 0);
}
}
<file_sep>/JVEMV6/others/voice_recog.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\others\voice_recog.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\1_\82_1.py
at : 2018/10/08 15:30:46
r d4
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\others\
python voice_recog.py
ref : http://aidiary.hatenablog.com/entry/20110607/1307449007
'''
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_mm import libs
# from libs_VX7GLZ import libs_VX7GLZ
# from libs_VX7GLZ import wablibs
# from libs.libs import * #=> C:\WORKS_2\WS\Eclipse_Luna\C_SoundProg\python\libs
# import libs.libs as lib
# from libs.libs import * #=> in 'libs' subdirectory --> see : https://stackoverflow.com/questions/1260792/import-a-file-from-a-subdirectory 'community wiki'
#=> ref : http://qiita.com/Usek/items/86edfa0835292c80fff5
# from libs import * #=> in 'libs' subdirectory --> see : https://stackoverflow.com/questions/1260792/import-a-file-from-a-subdirectory 'community wiki'
#=> libs.py : C:\WORKS_2\WS\WS_Others\free\K6H7DD_schroedinger\28_1\libs.py
# import libs.labs2 as labs
# # import libs.labs as labs
#
# import libs.wablibs as wl
# import wablibs
# import wablibs as wl
'''###################
import : built-in modules
###################'''
import getopt
import os
import inspect
import math as math
import requests
###############################################
import wave
import struct
import numpy as np
# from pylab import *
from matplotlib import pylab as plt
import random as rnd
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def test_1():
#ref https://qiita.com/kinpira/items/75513eaab6eed19da9a3
# path = 'audio_sample_amivoice.raw'
path = '20181008 151334.16bit-16khz.aiff'
# path = '20181008 151334.16bit-16khz.wav'
# path = '20181008-151334.wav'
# path = '/home/pi/test.wav'
#APIKEY = "58362f467950765273456f4652485a516c6258465a75794e79794a4835386869412f33576876774c767631"
APIKEY = "71596d4e512e752e4e7a44507463445767694c70485254485251674174384c4a33576a7253457478495431"
#ref https://dev.smt.docomo.ne.jp/?p=docs.api.page&api_name=speech_recognition&p_name=api_amivoice_1#tag01
# https://api.apigw.smt.docomo.ne.jp/amiVoice/v1/recognize
url = "https://api.apigw.smt.docomo.ne.jp/amiVoice/v1/recognize?APIKEY={}".format(APIKEY)
print("[%s:%d] url = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, url
), file=sys.stderr)
print("[%s:%d] path = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, path
), file=sys.stderr)
# [voice_recog.py:148]
# url = https://api.apigw.smt.docomo.ne.jp/amiVoice/v1/recognize?APIKEY=58362f467950765273456f4652485a516c6258465a75794e79794a4835386869412f33576876774c767631
# validate
# f = open(path, "rb")
if not os.path.isfile(path) : #if のtos.path.isfile(path)
print("[%s:%d] file not exist : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, path
), file=sys.stderr)
return
#/if のtos.path.isfile(path)
files = {"a": open(path, 'rb'), "v":"on"}
r = requests.post(url, files=files)
print("r =>")
print(r)
# r =>
# <Response [401]>
# print (r.json())
# print (r.json()['text'])
# r =>
# <Response [200]>
# {'utteranceid': '20181008/docomo-dds-test@1841153612-LOHwlMQ', 'results': [{'end
# time': 6410, 'tokens': [{'endtime': 1970, 'confidence': 0.87, 'spoken': 'はー',
# 'starttime': 1430, 'written': 'はあ'}, {'endtime': 2240, 'confidence': 0.3, 'spo
# ken': '_', 'starttime': 1970, 'written': '。'}, {'endtime': 2710, 'confidence':
# 0.67, 'spoken': 'はー', 'starttime': 2240, 'written': 'はあ'}, {'endtime': 2900,
# 'confidence': 0.86, 'spoken': '_', 'starttime': 2710, 'written': '。'}, {'endti
# me': 4130, 'confidence': 0.94, 'spoken': 'か', 'starttime': 3600, 'written': '下
# '}, {'endtime': 4850, 'confidence': 1.0, 'spoken': 'か', 'starttime': 4340, 'wri
# tten': 'か'}, {'endtime': 5540, 'confidence': 0.99, 'spoken': 'か', 'starttime':
# 5080, 'written': '下'}, {'endtime': 6120, 'confidence': 0.99, 'spoken': 'か', '
# starttime': 5590, 'written': 'か'}, {'endtime': 6410, 'confidence': 0.22, 'spoke
# n': '_', 'starttime': 6120, 'written': '。'}], 'rulename': '', 'confidence': 0.6
# 39, 'tags': [], 'starttime': 0, 'text': 'はあ。はあ。下か下か。'}], 'message': '
# ', 'code': '', 'text': 'はあ。はあ。下か下か。'}
# get keys
#ref https://stackoverflow.com/questions/15789059/python-json-only-get-keys-in-first-level#15789236
json = r.json()
keys = json.keys()
for item in keys:
print(item)
#/for item in keys:
for item in keys:
print("%s =>" % item)
print(json[item])
#/for item in keys:
# results
print()
results = json['results']
# keys_results = results.keys()
# print("tokens => %d tokens" % len(results[0]['tokens']))
# print(results[0]['tokens'])
for item in results[0]['tokens']:
print(item)
#/for item in results[0]['tokens']:
# {'starttime': 1430, 'endtime': 1970, 'confidence': 0.87, 'spoken': 'はー', 'writ
# ten': 'はあ'}
# {'starttime': 1970, 'endtime': 2240, 'confidence': 0.3, 'spoken': '_', 'written'
# : '。'}
# {'starttime': 2240, 'endtime': 2710, 'confidence': 0.67, 'spoken': 'はー', 'writ
# ten': 'はあ'}
# {'starttime': 2710, 'endtime': 2900, 'confidence': 0.86, 'spoken': '_', 'written
# ': '。'}
# {'starttime': 3600, 'endtime': 4130, 'confidence': 0.94, 'spoken': 'か', 'writte
# n': '下'}
# {'starttime': 4340, 'endtime': 4850, 'confidence': 1.0, 'spoken': 'か', 'written
# ': 'か'}
# {'starttime': 5080, 'endtime': 5540, 'confidence': 0.99, 'spoken': 'か', 'writte
# n': '下'}
# {'starttime': 5590, 'endtime': 6120, 'confidence': 0.99, 'spoken': 'か', 'writte
# n': 'か'}
# {'starttime': 6120, 'endtime': 6410, 'confidence': 0.22, 'spoken': '_', 'written
# ': '。'}
# # for item in keys_results:
# for item in results:
#
# print(item)
#
# # print("[%s:%d] keys_results : %s" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# # , item
# # ), file=sys.stderr)
#/for item in keys_results:
# for item in json['results']:
#
# print(item)
#
# #/for item in json['results']:
print("[%s:%d] test_1 : done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/11_guitar/start_guitar.bat
REM *******************************
REM open folders
REM apps : itunes
REM browser
REM apps
REM git pull
REM *******************************
REM *******************************
REM open folders
REM *******************************
echo start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
REM *******************************
REM apps : itunes
REM *******************************
REM call C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.bat
REM echo start C:\WORKS_2\Programs\itunes_12.6.2\iTunes.exe
REM start C:\WORKS_2\Programs\itunes_12.6.2\iTunes.exe
REM *******************************
REM browser
REM *******************************
pushd "C:\Program Files (x86)\Google\Chrome\Application"
REM : doc
start chrome.exe "https://docs.google.com/document/d/1SjUWaAOO4C19dSpDT0j5AqGwlsXeYaUZdkMMsZaU_bI/edit"
REM : image memos
start chrome.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_file_name=2018-07-22&filter_memo=guitar&RBs_AND_OR_Memo=AND&RBs_AND_OR_File_Name=AND"
REM : keyboard
https://www.apronus.com/music/flashpiano.htm
https://www.onlinepianist.com/virtual-piano
REM *******************************
REM apps
REM *******************************
REM : musescore
start C:\WORKS_2\Programs\musescore\ms_3.0.2.5315\bin\MuseScore3.exe
REM : domino
start C:\WORKS_2\Programs\Domino143\Domino.exe
REM *******************************
REM git pull
REM *******************************
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_command_prompot.bat
echo git pull....
pushd C:\WORKS_2\WS\WS_Others.Art
e
REM pause
REM exit
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgFish.js
/*********************************************************************
swgFish.js
***********************************************************************/
function Fish0()
{
//基本姿勢は+z方向を向き、左が+x、右が-X、魚の上方向は+y
this.flagDebug = false;
this.shadow = 0.0;
//robotの位置・姿勢
this.vPos = new Vector3();
this.vEuler = new Vector3();
this.vSize = new Vector3(1.0, 1.0, 1.0);//全体のスケール変換
this.radius = 3;//円運動の半径
this.period = 10;//1周回転の周期
this.periodSwing = 2;//胸鰭の周期
this.maxAngSwing = 20;//胸鰭の最大角度
this.angleZ = 20;//z軸に対する魚体の傾斜角
//partsの種類や色・サイズなど
this.trunk = new Rigid_HS();
this.trunk.kind = "SUPER2";//胴体
this.trunk.diffuse = [0.8, 0.7, 0.2, 1.0];
this.trunk.ambient = [0.6, 0.6, 0.1, 1.0];
this.trunk.size1 = [0.5, 0.1, 0.5];
this.trunk.size2 = [0.5, 0.1, 0.5];
this.trunk.nSlice = 20;
this.trunk.nStack = 20;
this.trunk.eps1 = 2.0;
this.trunk.eps2 = 1.5;
this.trunk.middle = 0.8;
this.trunk.angle2 = 0;
this.trunk.type = 0;
this.eyeL = new Rigid_HS();
this.eyeL.kind = "SPHERE"//左目
this.eyeL.diffuse = [0.3, 0.3, 0.6, 1.0];
this.eyeL.ambient = [0.2, 0.2, 0.4, 1.0];
this.eyeL.nSlice = 6;
this.eyeL.nStack = 6;
this.eyeL.vSize = new Vector3(0.1, 0.1, 0.1);
this.eyeR = new Rigid_HS();
this.eyeR.kind = "SPHERE"//右目
this.eyeR.diffuse = [0.3, 0.3, 0.6, 1.0];
this.eyeR.ambient = [0.2, 0.2, 0.4, 1.0];
this.eyeR.nSlice = 6;
this.eyeR.nStack = 6;
this.eyeR.vSize = new Vector3(0.1, 0.1, 0.1);
this.mouth = new Rigid_HS();
this.mouth.kind = "SPHERE"//口
this.mouth.diffuse = [0.8, 0.5, 0.3, 1.0];
this.mouth.ambient = [0.6, 0.4, 0.2, 1.0];
this.mouth.nSlice = 6;
this.mouth.nStack = 6;
this.mouth.vSize = new Vector3(0.2, 0.1, 0.2);
this.tail = new Rigid_HS();
this.tail.kind = "CYLINDER"//尾
this.tail.diffuse = [0.8, 0.7, 0.2, 1.0];
this.tail.ambient = [0.6, 0.6, 0.1, 1.0];
this.tail.nSlice = 8;
this.tail.nStack = 8;
this.tail.radiusRatio = 5.0;
this.tail.vSize = new Vector3(0.01, 0.005, 0.8);
}
Fish0.prototype.draw = function(gl)
{
this.trunk.shadow = this.shadow;
this.eyeL.shadow = this.shadow;
this.eyeR.shadow = this.shadow;
this.mouth.shadow = this.shadow;
this.tail.shadow = this.shadow;
//スタック行列の確保
var stackMat = [];
for(var i = 0; i < 2; i++) stackMat[i] = new Matrix4();
var modelMatrix = new Matrix4(); //モデル行列の初期化
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
//全体(root)
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z);
modelMatrix.rotate(this.vEuler.z, 0, 0, 1); // z軸周りに回転
modelMatrix.rotate(this.vEuler.y, 0, 1, 0); // y軸周りに回転
modelMatrix.rotate(this.vEuler.x, 1, 0, 0); // x軸周りに回転
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
stackMat[0].copy(modelMatrix);//モデル行列を保存(ここまでのモデル行列は他のpaarts[]にも影響する)
var n = this.trunk.initVertexBuffers(gl);
this.trunk.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeLに影響するモデル行列をスタックからpop
//左目
modelMatrix.translate(this.trunk.size1[0]*0.4, 0.05, this.trunk.size1[2]*0.5);//に平行移動
modelMatrix.scale(this.eyeL.vSize.x, this.eyeL.vSize.y, this.eyeL.vSize.z);
n = this.eyeL.initVertexBuffers(gl);
this.eyeL.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//右目
modelMatrix.translate(-this.trunk.size1[0]*0.4, 0.05, this.trunk.size1[2]*0.5);
modelMatrix.scale(this.eyeR.vSize.x, this.eyeR.vSize.y, this.eyeR.vSize.z);
n = this.eyeR.initVertexBuffers(gl);
this.eyeR.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//口
modelMatrix.translate(0, 0.0, this.trunk.size1[2]*0.85);
modelMatrix.scale(this.mouth.vSize.x, this.mouth.vSize.y, this.mouth.vSize.z);
n = this.mouth.initVertexBuffers(gl);
this.mouth.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//尾
modelMatrix.translate(0, 0.0, -this.trunk.size2[2]);
modelMatrix.scale(this.tail.vSize.x, this.tail.vSize.y, this.tail.vSize.z);
modelMatrix.translate(0.0, 0.0, -this.tail.vSize.z/2);//中心位置を持ち上げる
n = this.tail.initVertexBuffers(gl);
this.tail.draw(gl, n, modelMatrix);
}
Fish0.prototype.motion = function(t)
{
//左回り回転
var theta0 = Math.atan2(this.vPos0.y , this.vPos0.x);
var theta = 2*Math.PI * t / this.period + theta0;//x軸からの角度
this.vPos.x = this.radius * Math.cos( theta );
this.vPos.y = this.radius * Math.sin( theta );
this.vEuler.x = 90;
this.vEuler.y = this.angleZ;
this.vEuler.z = 180 * theta / Math.PI + 180;//方向
this.trunk.angle2 = this.maxAngSwing * Math.sin(2.0 * Math.PI * t / this.periodSwing);
}
//-------------------------------------------------------------------------------
Fish1
//------------------------------------------------------------------------------
function Fish1()
{
//基本姿勢は+z方向を向き、左が+x、右が-X、魚の上方向は+y
this.flagDebug = false;
this.shadow = 0.0;
//robotの位置・姿勢
this.vPos = new Vector3();
this.vEuler = new Vector3();
this.vSize = new Vector3(1.0, 1.0, 1.0);//全体のスケール変換
this.radius = 3.8;//円運動の半径
this.period = 20;//1周回転の周期
this.periodSwing = 1;//後胴体の周期
this.maxAngSwing = 10;//その最大角度
this.angleZ = 2;//z軸に対する魚体の傾斜角
//partsの種類や色・サイズなど
this.trunk = new Rigid_HS();
this.trunk.kind = "SUPER2";//胴体
this.trunk.diffuse = [0.8, 0.3, 0.2, 1.0];
this.trunk.ambient = [0.6, 0.2, 0.1, 1.0];
this.trunk.size1 = [0.5, 0.1, 0.5];
this.trunk.size2 = [0.5, 0.1, 0.5];
this.trunk.nSlice = 20;
this.trunk.nStack = 20;
this.trunk.eps1 = 1.5;
this.trunk.eps2 = 1.5;
this.trunk.middle = 0.8;
this.trunk.angle2 = 0;
this.trunk.type = 1;
this.eyeL = new Rigid_HS();
this.eyeL.kind = "SPHERE"//左目
this.eyeL.diffuse = [0.3, 0.3, 0.4, 1.0];
this.eyeL.ambient = [0.2, 0.2, 0.3, 1.0];
this.eyeL.nSlice = 6;
this.eyeL.nStack = 6;
this.eyeL.vSize = new Vector3(0.1, 0.1, 0.1);
this.eyeR = new Rigid_HS();
this.eyeR.kind = "SPHERE"//右目
this.eyeR.diffuse = [0.3, 0.3, 0.4, 1.0];
this.eyeR.ambient = [0.2, 0.2, 0.3, 1.0];
this.eyeR.nSlice = 6;
this.eyeR.nStack = 6;
this.eyeR.vSize = new Vector3(0.1, 0.1, 0.1);
this.mouth = new Rigid_HS();
this.mouth.kind = "SPHERE"//口
this.mouth.diffuse = [0.5, 0.3, 0.3, 1.0];
this.mouth.ambient = [0.4, 0.2, 0.2, 1.0];
this.mouth.nSlice = 6;
this.mouth.nStack = 6;
this.mouth.vSize = new Vector3(0.2, 0.1, 0.2);
this.tail = new Rigid_HS();
this.tail.kind = "CYLINDER"//尾
this.tail.diffuse = [0.8, 0.3, 0.2, 1.0];
this.tail.ambient = [0.6, 0.2, 0.1, 1.0];
this.tail.nSlice = 8;
this.tail.nStack = 8;
this.tail.radiusRatio = 0.2
this.tail.vSize = new Vector3(0.4, 0.05, 0.3);
}
Fish1.prototype.draw = function(gl)
{
this.trunk.shadow = this.shadow;
this.eyeL.shadow = this.shadow;
this.eyeR.shadow = this.shadow;
this.mouth.shadow = this.shadow;
this.tail.shadow = this.shadow;
//スタック行列の確保
var stackMat = [];
for(var i = 0; i < 2; i++) stackMat[i] = new Matrix4();
var modelMatrix = new Matrix4(); //モデル行列の初期化
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
//全体(root)
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z);
modelMatrix.rotate(this.vEuler.z, 0, 0, 1); // z軸周りに回転
modelMatrix.rotate(this.vEuler.y, 0, 1, 0); // y軸周りに回転
modelMatrix.rotate(this.vEuler.x, 1, 0, 0); // x軸周りに回転
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
stackMat[0].copy(modelMatrix);//モデル行列を保存(ここまでのモデル行列は他のpaarts[]にも影響する)
var n = this.trunk.initVertexBuffers(gl);
this.trunk.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeLに影響するモデル行列をスタックからpop
//左目
modelMatrix.translate(this.trunk.size1[0]*0.4, 0.0, this.trunk.size1[2]*0.8);//に平行移動
modelMatrix.scale(this.eyeL.vSize.x, this.eyeL.vSize.y, this.eyeL.vSize.z);
n = this.eyeL.initVertexBuffers(gl);
this.eyeL.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//右目
modelMatrix.translate(-this.trunk.size1[0]*0.4, 0.0, this.trunk.size1[2]*0.8);
modelMatrix.scale(this.eyeR.vSize.x, this.eyeR.vSize.y, this.eyeR.vSize.z);
n = this.eyeR.initVertexBuffers(gl);
this.eyeR.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//口
modelMatrix.translate(0, 0.0, this.trunk.size1[2]*0.85);
modelMatrix.scale(this.mouth.vSize.x, this.mouth.vSize.y, this.mouth.vSize.z);
n = this.mouth.initVertexBuffers(gl);
this.mouth.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//尾
modelMatrix.rotate(this.trunk.angle2*0.5, 1, 0, 0);//尾びれを揺らす
modelMatrix.translate(0, 0.0, -this.trunk.size2[2]*0.8);
modelMatrix.scale(this.tail.vSize.x, this.tail.vSize.y, this.tail.vSize.z);
modelMatrix.translate(0.0, 0.0, -this.tail.vSize.z/2);//中心位置を持ち上げる
n = this.tail.initVertexBuffers(gl);
this.tail.draw(gl, n, modelMatrix);
}
Fish1.prototype.motion = function(t)
{
//左回り回転
var theta0 = Math.atan2(this.vPos0.y , this.vPos0.x);
var theta = 2*Math.PI * t / this.period + theta0;//x軸からの角度
this.vPos.x = this.radius * Math.cos( theta );
this.vPos.y = this.radius * Math.sin( theta );
this.vEuler.x = 90;
this.vEuler.y = this.angleZ;
this.vEuler.z = 180 * theta / Math.PI + 180;//方向
this.trunk.angle2 = this.maxAngSwing * Math.sin(2.0 * Math.PI * t / this.periodSwing);
}
//-----------------------------------------------------------------------------------------------
Fish2
//-----------------------------------------------------------------------------------------------
function Fish2()
{
//基本姿勢は+z方向を向き、左が+x、右が-X、魚の上方向は+y
this.flagDebug = false;
this.shadow = 0.0;
//robotの位置・姿勢
this.vPos = new Vector3();
this.vPos0 = new Vector3();//初期位置
this.vEuler = new Vector3();
this.vSize = new Vector3(1.0, 1.0, 1.0);//全体のスケール変換
this.radius = 3.5;//円運動の半径
this.period = 15;//1周回転の周期
this.periodSwing = 2;//周期
this.maxAngSwing = 30;//最大角度
this.angleZ = 5;//z軸に対する魚体の傾斜角
//partsの種類や色・サイズなど
this.trunk = new Rigid_HS();
this.trunk.kind = "SUPER2";//胴体
this.trunk.diffuse = [0.2, 0.7, 0.9, 1.0];
this.trunk.ambient = [0.1, 0.4, 0.6, 1.0];
this.trunk.size1 = [0.4, 0.6, 1];
this.trunk.size2 = [0.4, 0.6, 0.7];
this.trunk.nSlice = 20;
this.trunk.nStack = 20;
this.trunk.eps1 = 1.8;
this.trunk.eps2 = 1.5;
this.trunk.middle = 0.6;;
this.trunk.angle2 = 0;
this.trunk.jStart = 6;
this.trunk.type = 2;
this.eyeL = new Rigid_HS();
this.eyeL.kind = "SPHERE"//左目
this.eyeL.diffuse = [0.3, 0.3, 0.6, 1.0];
this.eyeL.ambient = [0.2, 0.2, 0.4, 1.0];
this.eyeL.nSlice = 6;
this.eyeL.nStack = 6;
this.eyeL.vSize = new Vector3(0.1, 0.1, 0.1);
this.eyeR = new Rigid_HS();
this.eyeR.kind = "SPHERE"//右目
this.eyeR.diffuse = [0.3, 0.3, 0.6, 1.0];
this.eyeR.ambient = [0.2, 0.2, 0.4, 1.0];
this.eyeR.nSlice = 6;
this.eyeR.nStack = 6;
this.eyeR.vSize = new Vector3(0.1, 0.1, 0.1);
this.mouth = new Rigid_HS();
this.mouth.kind = "SPHERE"//口
this.mouth.diffuse = [0.5, 0.3, 0.3, 1.0];
this.mouth.ambient = [0.4, 0.2, 0.2, 1.0];
this.mouth.nSlice = 6;
this.mouth.nStack = 6;
this.mouth.vSize = new Vector3(0.2, 0.1, 0.1);
this.tail = new Rigid_HS();
this.tail.kind = "CYLINDER"//尾
this.tail.diffuse = [0.2, 0.7, 0.9, 1.0];
this.tail.ambient = [0.2, 0.6, 0.8, 1.0];
this.tail.nSlice = 8;
this.tail.nStack = 8;
this.tail.radiusRatio = 0.2
this.tail.vSize = new Vector3(0.05, 0.5, 0.4);
}
Fish2.prototype.draw = function(gl)
{
this.trunk.shadow = this.shadow;
this.eyeL.shadow = this.shadow;
this.eyeR.shadow = this.shadow;
this.mouth.shadow = this.shadow;
this.tail.shadow = this.shadow;
//スタック行列の確保
var stackMat = [];
for(var i = 0; i < 2; i++) stackMat[i] = new Matrix4();
var modelMatrix = new Matrix4(); //モデル行列の初期化
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
//全体(root)
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z);
modelMatrix.rotate(this.vEuler.z, 0, 0, 1); // z軸周りに回転
modelMatrix.rotate(this.vEuler.y, 0, 1, 0); // y軸周りに回転
modelMatrix.rotate(this.vEuler.x, 1, 0, 0); // x軸周りに回転
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
stackMat[0].copy(modelMatrix);//モデル行列を保存(ここまでのモデル行列は他のpaarts[]にも影響する)
var n = this.trunk.initVertexBuffers(gl);
this.trunk.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeLに影響するモデル行列をスタックからpop
//左目
modelMatrix.translate(this.trunk.size1[0]*0.3, 0.0, this.trunk.size1[2]*0.8);//に平行移動
modelMatrix.scale(this.eyeL.vSize.x, this.eyeL.vSize.y, this.eyeL.vSize.z);
n = this.eyeL.initVertexBuffers(gl);
this.eyeL.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//右目
modelMatrix.translate(-this.trunk.size1[0]*0.3, 0.0, this.trunk.size1[2]*0.8);
modelMatrix.scale(this.eyeR.vSize.x, this.eyeR.vSize.y, this.eyeR.vSize.z);
n = this.eyeR.initVertexBuffers(gl);
this.eyeR.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//eyeRに影響するモデル行列をスタックからpop
//口
modelMatrix.translate(0, 0.0, this.trunk.size1[2]*0.95);
modelMatrix.scale(this.mouth.vSize.x, this.mouth.vSize.y, this.mouth.vSize.z);
n = this.mouth.initVertexBuffers(gl);
this.mouth.draw(gl, n, modelMatrix);
modelMatrix.copy(stackMat[0]);//tailに影響するモデル行列をスタックからpop
//尾
modelMatrix.rotate(this.trunk.angle2*0.5, 0, 1, 0);//尾びれを揺らす
modelMatrix.translate(0, 0.0, -this.trunk.size2[2]*0.8);
modelMatrix.scale(this.tail.vSize.x, this.tail.vSize.y, this.tail.vSize.z);
modelMatrix.translate(0.0, 0.0, -this.tail.vSize.z/2);//中心位置を持ち上げる
n = this.tail.initVertexBuffers(gl);
this.tail.draw(gl, n, modelMatrix);
}
Fish2.prototype.motion = function(t)
{
//左回り回転
var theta0 = Math.atan2(this.vPos0.y , this.vPos0.x);
var theta = 2*Math.PI * t / this.period + theta0;//x軸からの角度
this.vPos.x = this.radius * Math.cos( theta );
this.vPos.y = this.radius * Math.sin( theta );
this.vEuler.x = 90;
this.vEuler.y = this.angleZ;
this.vEuler.z = 180 * theta / Math.PI + 180;//方向
this.trunk.angle2 = this.maxAngSwing * Math.sin(2.0 * Math.PI * t / this.periodSwing);
}
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgRigid.js
/*----------------------------------------------------------
Rigidクラス(3Dオブジェクトの定義と描画)
------------------------------------------------------------*/
var MAX_VERTEX = 30;//多面体近似のときの頂点数
var muK = 1.0;//0.5;//動摩擦係数
var muS = 1.0;//静止摩擦係数
var restitution = 0.5;//跳ね返り係数
var dampRotation = 3.0;//回転減速係数
var gravity = 9.8;//重力加速度[m/s^2]
var restValue = 0.2; //静止状態にするしきい値(速度,回転速度)
var flagDrag = false;//空気抵抗フラグ
var flagMagnus = false;
var flagTumbling = false;
var flagQuaternion = false;
function Rigid()
{
//プロパティ
this.kind = "SPHERE";
this.diffuse = [0.6, 0.6, 0.6, 1.0];
this.ambient = [0.4, 0.4, 0.4, 1.0];
this.specular = [0.5, 0.5, 0.5, 1.0];
this.shininess = 200.0;
this.vVel = new Vector3();//速度(m/s)
this.vVel0 = new Vector3();//更新前の速度
this.vPos = new Vector3();//位置(m)
this.vPos0 = new Vector3();//初期位置
this.vForce = new Vector3();//外力(Newton)
this.vForce0 = new Vector3();//外力(初期値)
this.vOmega = new Vector3();//角速度(rad/s)
this.vOmega0 = new Vector3();//角速度(rad/s),更新前
this.vAcc = new Vector3();//加速度
this.vTorque = new Vector3();//トルク
this.vEuler0 = new Vector3();//回転角度(オイラー角で指定,更新前)
this.vEuler = new Vector3();//回転角度(オイラー角で指定,更新後)
this.vSize = new Vector3(1.0, 1.0, 1.0);//スケーリング
this.vGravityToPoint = new Vector3();//重心から衝突点までのベクトル
this.mass = 1;//質量[kg]
this.mInertia = new Matrix3();//慣性モーメントのテンソル
this.mInertiaInverse = new Matrix3();//その逆行列
this.vInertialResistance = new Vector3();
this.vRotationalResistance = new Vector3();
this.q = new Quaternion();
this.vAxis = new Vector3(1.0, 0.0, 0.0);//回転軸
this.angle = 0.0;
this.nSlice = 25;
this.nStack = 25;
this.radiusRatio = 0.2;//上底半径/下底半径(トーラスとバネの時はradius1/radius2)
this.eps1 = 1.0;//"SUPER"のパラメータ
this.eps2 = 1.0;//"SUPER"のパラメータ
this.flagDebug = false;//trueのときワイヤーフレームモデル
this.shadow = 0.0;//影の濃さを表す(0.0なら実オブジェクト)
//フロア表示のチェック模様
this.flagCheck = false;
this.col1 = [0.6, 0.5, 0.5, 1.0];
this.col2 = [0.4, 0.4, 0.6, 1.0];
this.plane = [0, 0, 1, 0];//簡易シャドウを描くときの平面の平面方程式
//テクスチャ
this.flagTexture = false;
this.nRepeatS = 1;
this.nRepeatT = 1;
//衝突判定などに使われるプロパティ
this.vP = [];
this.vP0 = [];//球以外の頂点座標
this.vNormal = new Vector3();
this.vNormalFacet = [];//直方体の面の法線ベクトル
this.numVertex;//球以外の多面体の頂点数
this.boundingR;//境界球の半径
this.state = "FREE";
this.flagFixed = false;
//tumbling特有のプロパティ
this.coefLift = 1.0;//揚力係数
this.delta = 0.5;//シフト率
//Spring特有のプロパティ
this.nPitch = 5;//バネ
this.radius = 0.5;//バネはvSizeを使用しない
this.len0 = 1;//バネの自然長
this.len = 1;//length0+変位量
this.constant;//バネ定数
this.row = [];
this.col = [];
//水面の波などの表現
this.sizeX = 10;
this.sizeY = 10;
this.data = [];//各格子点のx,y,z座標(kind = "GRID_SQUARE"などのときに必要なデータ)
//kind = "ELEVATION"のときはz成分だけ
//SUPER2のパラメータ
this.size1 = [0.5, 0.1, 0.5];
this.size2 = [0.5, 0.1, 0.5];
this.middle = 0.5; //中間のサイズ
this.angle2 = 0;//曲げる角度(度)
this.jStart = 5;
this.type = 1;//0,1,2だけ
}
Rigid.prototype.initVertexBuffers = function(gl)
{
//頂点データをシェーダへアップロードするメソッド
var n;
var vertices = [];//頂点座標
var normals = []; //法線ベクトル
var indices = []; //頂点番号
var colors = [];//check模様のときだけ
var texCoords = [];//テクスチャ座標
if(!this.flagTexture)
{//非テクスチャ用
if (this.kind == "CUBE") n = makeCube(vertices, normals, indices, this.flagDebug);
else if(this.kind == "SPHERE") n = makeSphere(vertices, normals, indices, this.nSlice, this.nStack);
else if(this.kind == "CYLINDER")n = makeCylinder(vertices, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug);
else if(this.kind == "PRISM") n = makePrism(vertices, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug);
else if(this.kind == "TORUS") n = makeTorus(vertices, normals, indices, this.radiusRatio, this.nSlice, this.nStack);
else if(this.kind == "SUPER") n = makeSuper(vertices, normals, indices, this.nSlice, this.nStack, this.eps1, this.eps2);
else if(this.kind == "SUPER2") n = makeSuper2(vertices, normals, indices, this.size1, this.size2, this.nSlice, this.nStack, this.eps1, this.eps2, this.middle, this.angle2, this.jStart, this.type);
else if(this.kind == "SPRING") n = makeSpring(vertices, normals, indices, this.radius, this.radiusRatio, this.nSlice, this.nStack, this.nPitch, this.len);
else if(this.kind == "CYLINDER_X") n = makeCylinderX(vertices, normals, indices, this.nSlice, this.flagDebug);
else if(this.kind == "CYLINDER_Y") n = makeCylinderY(vertices, normals, indices, this.nSlice, this.flagDebug);
else if(this.kind == "CYLINDER_Z") n = makeCylinderZ(vertices, normals, indices, this.nSlice, this.flagDebug);
else if(this.kind == "PLATE_Z") n = makePlateZ(vertices, normals, indices, this.flagDebug);
else if(this.kind == "GRID_PLATE") n = makeGridPlate(vertices, normals, indices, this.nSlice, this.nStack, this.flagDebug);
else if(this.kind == "GRID_SQUARE") n = makeGridSquare(this.data, vertices, normals, indices, this.nSlice, this.nStack, this.flagDebug);
else if(this.kind == "ELEVATION") n = makeElevation(this.data, vertices, normals, indices, this.nSlice, this.nStack, this.sizeX, this.sizeY, this.flagDebug)
else if(this.kind == "CHECK_PLATE") n = makeCheckedPlate(vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2) ;
else if(this.kind == "CHECK_SQUARE") n = makeCheckedSquare(this.data, vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2) ;
else if(this.kind == "GRID_SPHERE") n = makeGridSphere(this.data, vertices, normals, indices, this.nSlice, this.nStack);
else if(this.kind == "CHECK_SPHERE") n = makeCheckedSphere(this.data, vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2);
else if(this.kind == "GRID_CYLINDER") n = makeGridCylinder(this.data, vertices, normals, indices, this.nSlice, this.nStack);
else if(this.kind == "CHECK_CYLINDER") n = makeCheckedCylinder(this.data, vertices, colors, normals, indices, this.nSlice, this.nStack, this.col1, this.col2);
}
else
{//テクスチャ用
if (this.kind == "CUBE") n = makeCubeTex(vertices, texCoords, normals, indices, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "CUBE_BUMP") n = makeCubeBump(vertices, texCoords, normals, indices, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "SPHERE") n = makeSphereTex(vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
else if(this.kind == "CYLINDER")n = makeCylinderTex(vertices, texCoords, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "PRISM") n = makePrismTex(vertices, texCoords, normals, indices, this.radiusRatio, this.nSlice, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "TORUS") n = makeTorusTex(vertices, texCoords, normals, indices, this.radiusRatio, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
else if(this.kind == "SUPER") n = makeSuperTex(vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.eps1, this.eps2, this.nRepeatS, this.nRepeatT);
else if(this.kind == "PLATE_Z") n = makePlateZTex(vertices, texCoords, normals, indices, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_PLATE") n = makeGridPlateTex(vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_SQUARE") n = makeGridSquareTex(this.data, vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.flagDebug, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_SPHERE") n = makeGridSphereTex(this.data, vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
else if(this.kind == "GRID_CYLINDER") n = makeGridCylinderTex(this.data, vertices, texCoords, normals, indices, this.nSlice, this.nStack, this.nRepeatS, this.nRepeatT);
}
gl.disableVertexAttribArray(colorLoc);//colorのバッファオブジェクトの割り当てを無効化(フロアを表示したとき,フロアのインデックス以上の球やトーラスを描画できなくなることを防ぐため)
// バッファオブジェクトを作成する
var vertexBuffer = gl.createBuffer();
if(this.flagTexture) {var texCoordBuffer = gl.createBuffer();}
var normalBuffer = gl.createBuffer();
if(this.flagCheck) var colorBuffer = gl.createBuffer();
var indexBuffer = gl.createBuffer();
if (!vertexBuffer || !normalBuffer || !indexBuffer) return -1;
// 頂点の座標をバッファ・オブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices), gl.STATIC_DRAW);
// vertexLocにバッファ・オブジェクトを割り当てる
var vertexLoc = gl.getAttribLocation(gl.program, 'a_vertex');
gl.vertexAttribPointer(vertexLoc, 3, gl.FLOAT, false, 0, 0);
// バッファ・オブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(vertexLoc);//有効化する
if(this.flagTexture)
{
// 頂点のテクスチャ座標をバッファオブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, texCoordBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(texCoords), gl.STATIC_DRAW);
// texLocにバッファオブジェクトを割り当てる
var texLoc = gl.getAttribLocation(gl.program, 'a_texCoord');
gl.vertexAttribPointer(texLoc, 2, gl.FLOAT, false, 0, 0);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(texLoc);//有効化する
}
if(this.flagCheck)
{
// 頂点の色をバッファ・オブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, colorBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(colors), gl.STATIC_DRAW);
// a_colorの格納場所を取得し、バッファオブジェクトを割り当てる
var colorLoc = gl.getAttribLocation(gl.program, 'a_color');
gl.vertexAttribPointer(colorLoc, 3, gl.FLOAT, false, 0, 0);
// バッファ・オブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(colorLoc);//有効化
}
// 法線データをバッファ・オブジェクトに書き込む
gl.bindBuffer(gl.ARRAY_BUFFER, normalBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(normals), gl.STATIC_DRAW);
// normalLocにバッファ・オブジェクトを割り当てる
var normalLoc = gl.getAttribLocation(gl.program, 'a_normal');
gl.vertexAttribPointer(normalLoc, 3, gl.FLOAT, false, 0, 0);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.enableVertexAttribArray(normalLoc);//有効化する
//バッファ・オブジェクトをターゲットにバインドする
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, indexBuffer);
// インデックスデータをバッファ・オブジェクトに書き込む
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(indices), gl.STATIC_DRAW);
gl.bindBuffer(gl.ARRAY_BUFFER, null);
return n;
}
Rigid.prototype.draw = function(gl, n)
{
//マテリアル特性のユニフォーム変数格納場所を取得し値を設定する
var diffLoc = gl.getUniformLocation(gl.program, 'u_diffuseColor');
gl.uniform4fv(diffLoc, new Float32Array(this.diffuse));
var ambiLoc = gl.getUniformLocation(gl.program, 'u_ambientColor');
gl.uniform4fv(ambiLoc, new Float32Array(this.ambient));
var specLoc = gl.getUniformLocation(gl.program, 'u_specularColor');
gl.uniform4fv(specLoc, new Float32Array(this.specular));
var shinLoc = gl.getUniformLocation(gl.program, 'u_shininess');
gl.uniform1f(shinLoc, this.shininess);
var checkLoc = gl.getUniformLocation(gl.program, 'u_flagCheck');
gl.uniform1i(checkLoc, this.flagCheck);
var shadowLoc = gl.getUniformLocation(gl.program, 'u_shadow');
gl.uniform1f(shadowLoc, this.shadow);
var flagTexLoc = gl.getUniformLocation(gl.program, 'u_flagTexture');
gl.uniform1i(flagTexLoc, this.flagTexture);
// モデル行列を計算する
var modelMatrix = new Matrix4(); // モデル行列の初期化
if(this.shadow >= 0.01) modelMatrix.dropShadow(plane, light.pos);//簡易シャドウ
modelMatrix.translate(this.vPos.x, this.vPos.y, this.vPos.z);
//回転
if(flagQuaternion)
{
if(this.q.s > 1.0) this.q.s = 1.0;
if(this.q.s < -1.0) this.q.s = -1.0;
this.angle = 2.0 * Math.acos(this.q.s) * RAD_TO_DEG;//[rad->deg]
this.vAxis = norm(getVector(this.q));
if(this.vAxis.x == 0 && this.vAxis.y == 0 && this.vAxis.z == 0) {
this.vAxis.x = 1;
}
modelMatrix.rotate(this.angle, this.vAxis.x, this.vAxis.y, this.vAxis.z); //任意軸回転
}
else
{//xyz軸の順番でオイラー角回転
modelMatrix.rotate(this.vEuler.z, 0, 0, 1); // z軸周りに回転
modelMatrix.rotate(this.vEuler.y, 0, 1, 0); // y軸周りに回転
modelMatrix.rotate(this.vEuler.x, 1, 0, 0); // x軸周りに回転
}
//拡大縮小
modelMatrix.scale(this.vSize.x, this.vSize.y, this.vSize.z);
//法線変換行列を計算する
var normalMatrix = new Matrix4();// 初期化
if(this.shadow < 0.01)//影でないときだけ
{
normalMatrix.setInverseOf(modelMatrix);//モデルリング行列の逆行列を求め
normalMatrix.transpose(); //さらに転置する
}
//それぞれの行列のuniform変数の格納場所を取得し値を設定する
var modelMatrixLoc = gl.getUniformLocation(gl.program, 'u_modelMatrix');
gl.uniformMatrix4fv(modelMatrixLoc, false, modelMatrix.elements);
var normalMatrixLoc = gl.getUniformLocation(gl.program, 'u_normalMatrix');
gl.uniformMatrix4fv(normalMatrixLoc, false, normalMatrix.elements);
//物体を描画する
if(this.flagDebug == false)//solidモデルで描画
gl.drawElements(gl.TRIANGLES, n, gl.UNSIGNED_SHORT, 0);
else//wireframeモデルで描画
{
if(this.kind == "GRID_SQUARE" || this.kind == "CHECK_SQUARE" || this.kind == "ELEVATION")
gl.drawElements(gl.LINES, n, gl.UNSIGNED_SHORT, 0);
else
gl.drawElements(gl.LINE_STRIP, n, gl.UNSIGNED_SHORT, 0);
}
}
//---------------------------------------------------------
Rigid.prototype.ready = function()
{
this.state = "FREE";
for(var i = 0; i < MAX_VERTEX; i++){
this.vP0[i] = new Vector3();
this.vP[i] = new Vector3();
this.vNormalFacet[i] = new Vector3();
}
this.calcMomentOfInertia();
this.calcInertialResistance();
this.calcRotationalResistance();
this.calcBoundingRadius();
if(this.kind == "CUBE") this.setVertexOfCube();
else if(this.kind == "CYLINDER") this.setVertexOfCylinder();
}
//----------------------------------------------------------------------------
Rigid.prototype.sphere2D = function()
{
var R = this.vSize.x / 2.0;
//接触面の法線方向(rigid側から見た方向)
var vNormal = new Vector3(0.0, 0.0, -1.0);
//重心から接触点へのベクトル
this.vGravityToPoint = new Vector3(0.0, 0.0, -this.vPos.z);
//回転による接触面の速度(vVelとは逆方向)
var vVelRotate = cross(this.vOmega , this.vGravityToPoint);
//接地点の合成速度
var vp = add(this.vVel, vVelRotate); //接地点速度
var vAxisRotate = cross(vp, vNormal);//回転軸
vAxisRotate.norm();
var a = this.mass * muK * gravity;
//線形速度の減速/加速
this.vForce.sub(mul(a, norm(vp)));
//回転速度の加速/減速
this.vTorque.add(mul(a * R, vAxisRotate));
//滑らずに転がる場合でもある程度減速
this.vForce.sub(mul(0.1*a, norm(this.vVel)));
//鉛直軸回転に対する減速
this.vTorque.z -= 0.1 * dampRotation * this.mass * muK * (norm(this.vOmega)).z;
}
//-----------------------------------------------------------------------------
Rigid.prototype.cylinder2D = function()
{
var eps = 0.0174;//1度以内
//円柱の中心軸(オブジェクト座標のz軸)方向
var vCenter = new Vector3(0, 0, 1);
vCenter = qvRotate(this.q, vCenter);
//中心軸方向をrigidの速度方向に揃える
if(dot(this.vVel, vCenter) < 0.0) vCenter.reverse();
var e0 = Math.abs(dot(vCenter , new Vector3(0.0, 0.0, 1.0)));
if( e0 < eps && Math.abs(this.vOmega.z) < 0.5)
{//中心軸が床面に水平で慣性軸のz軸回転が小さいとき
var R = this.vSize.x / 2.0;//円柱の半径
//rigid側から見た接触面の法線方向
var vNormal = new Vector3(0.0, 0.0, -1.0);
this.vGravityToPoint = new Vector3(0.0, 0.0, -this.vPos.z);
var vDir = cross(vCenter, vNormal);//中心軸に直交する水平方向
//中心軸に直交する方向および平行な方向の速度成分
var vVelV = mul(dot(this.vVel, vDir), vDir);
var vVelH = mul(dot(this.vVel, vCenter), vCenter);
if(mag(vVelH) < 3.0)
{//中心軸に平行な速度がある値以下になったとき転がり運動に移行
this.vVel = vVelV;
//回転による接触面の速度
var vVelRotate = cross(this.vOmega, this.vGravityToPoint);
var vp = add(vVelV, vVelRotate);//接地点合成速度
var vAxisRotate = cross(vp, vNormal);//回転軸
vAxisRotate.norm();//正規化
var a = this.mass * muK * gravity;
//線形速度の減速/加速
this.vForce.sub(mul(a , norm(vp)));
//回転速度の加速/減速
this.vTorque.add(mul(a * R, vAxisRotate));
//滑らずに転がる場合でもある程度減速
this.vForce.sub(mul(0.1 * a, norm(this.vVel)));
}
else //滑り運動に対する減速だけ
this.vForce.add(mul(this.mass * muK * gravity , reverse(norm(this.vVel))));
}
else
{//中心軸が床面に水平でない場合.水平でも鉛直軸回転が大きい場合
this.vForce.add(mul(this.mass * muK * gravity, reverse(norm(this.vVel))));
}
//鉛直軸回転に対する減速
this.vTorque.z -= dampRotation * this.mass * muK * (norm(this.vOmega)).z;
}
//----------------------------------------------------------
Rigid.prototype.action3D = function(dt)
{
//フロア(地面)に落下するまでは放物運動をおこなう
this.vForce = add(this.vForce0, new Vector3(0.0, 0.0, -this.mass * gravity));
this.vTorque = new Vector3() ;
var bottom = this.getBottom();//最低点およびvGravityToPointも取得
if(bottom < 0.0) this.vPos.z -= bottom;//沈み込み防止
if(this.state != "FREE" ) return;
if(flagTumbling) this.tumbling();
if(flagDrag)
{
//並進運動に対する慣性抵抗
this.vForce.sub(mul(Math.abs(dot(qvRotate(this.q, this.vInertialResistance), this.vVel)) , this.vVel)) ;
//回転運動に対する慣性抵抗
this.vTorque.sub(mul(Math.abs(dot(qvRotate(this.q, this.vRotationalResistance), this.vOmega)) , this.vOmega));
}
if(flagMagnus)
{
var r = this.vSize.x / 2.0;
var k = Math.PI * 1.2 * r * r * r * 0.001;//rは見かけのサイズの1/10
this.vForce.add(mul(k, cross(this.vOmega, this.vVel)));
}
var flagCollisionF = false;
if(bottom <= 0 && this.vVel.z <= 0 ) //フロア上または衝突
{
if(this.vVel.z < -0.3)//vzが負の大きな値のとき衝突とする
{
this.collisionWithFloor(dt);
flagCollisionF = true;
}
if(!flagCollisionF)
{
this.state = "FREE";
//重力と抗力によるトルク
if(this.kind == "CUBE" || this.kind == "CYLINDER")
{
var torq = mul(cross(this.vGravityToPoint, new Vector3(0.0, 0.0, this.mass * gravity)), 0.5);
this.vTorque.add(torq);
}
//床平面上の2次元運動
if(this.kind == "SPHERE") this.sphere2D();
else if(this.kind == "CYLINDER") this.cylinder2D();
else
{//直方体
var vDir = normXY(this.vVel);//z成分を無視
this.vForce.sub(mul(this.mass * muK * gravity, vDir));
//床面におけるz軸回転に対し逆方向のトルクを与える
this.vTorque.z -= dampRotation * this.mass * muK * (norm(this.vOmega)).z;
}
if(this.isStabilized())
{
if(mag(this.vVel) + this.boundingR * mag(this.vOmega) < restValue)
{//静止状態にする
this.state = "RESTING";
}
}
}
}
if(this.state == "RESTING")
{//静止状態にする
this.vVel = new Vector3();
this.vTorque = new Vector3();
this.vOmega = new Vector3();
}
//並進運動の速度・位置の更新
var vAcc = div(this.vForce , this.mass);
this.vVel.add(mul(vAcc , dt));
this.vPos.add(mul(this.vVel , dt));
//回転運動の角速度・クォータニオンの更新
//オブジェクト座標系に変換しEulerの運動方程式を適用
var vOmegaObj = qvRotate(conjugate(this.q), this.vOmega);
var vTorqueObj = qvRotate(conjugate(this.q), this.vTorque);
var cs = cross(vOmegaObj, mulMV(this.mInertia, vOmegaObj));
//角加速度(オブジェクト座標系)
var vAlpha = mulMV(this.mInertiaInverse, sub(vTorqueObj, cs));
//角加速度を慣性座標系にもどし角速度を更新
this.vOmega.add(qvRotate(this.q, mul(vAlpha, dt)));
var qq = mulVQ(this.vOmega, this.q);
this.q.add(mulQS(qq, (0.5 * dt)));
this.q.norm();
}
//-------------------------------------------------------------------------
//Floorとの衝突を調べFloorから最低位置の高さを返す
Rigid.prototype.getBottom = function()
{
var bottom, i, cnt;
var eps = 0.0;//0.1;
var vCollision = [];//衝突点の頂点番号(多面体)
for(i = 0; i < 30; i++) vCollision[i] = new Vector3();
var vPointCollision = new Vector3();
bottom = 1000.0;
if( this.vPos.z <= this.boundingR + eps)
{//床と衝突の可能性あり
if(this.kind == "SPHERE")
{
bottom = this.vPos.z - this.boundingR;
this.vGravityToPoint = new Vector3(0, 0, -this.boundingR);
return bottom;
}
else
{
//World座標に変換
for(i = 0; i < this.numVertex; i++)
{
this.vP[i] = qvRotate(this.q, this.vP0[i]);//慣性空間へ変換
//中心座標だけ平行移動
this.vP[i].add(this.vPos);//World座標
}
cnt = 0;//衝突点個数
vPointCollision = new Vector3();
for(i = 0 ; i < this.numVertex; i++) {
if(this.vP[i].z <= 0) { vPointCollision.add(this.vP[i]); cnt++; }
if(this.vP[i].z < bottom ) bottom = this.vP[i].z;
}
if(bottom <= eps) //Floorと衝突
{
vPointCollision.div(cnt);
this.vGravityToPoint = sub(vPointCollision, this.vPos);
return bottom;
}
}
}
return bottom;
}
//--------------------------------------------------------------
Rigid.prototype.collisionWithFloor = function(dt)
{
var c = 0.2;
var vNormal = new Vector3(0.0, 0.0, -1.0);//衝突面の法線方向(rigid側からの法線)
//衝突点の合成速度
var vp = add(this.vVel, cross(this.vOmega, this.vGravityToPoint));//衝突点速度(ベクトル)
var vTangent = cross(vNormal, cross(vp, vNormal)); //接線ベクトル
vTangent.norm();
var a1 = cross(this.vGravityToPoint, vNormal);
var a2 = mulMV(this.mInertiaInverse, a1);
var a3 = cross(a2, this.vGravityToPoint);
//力積
var rikiseki = - (restitution + 1.0) * dot(vNormal, vp) / (1.0/this.mass + dot(vNormal, a3));
//摩擦なし
//力の総和
this.vForce.add(mul(vNormal, rikiseki / dt));
//力のモーメント(トルク)の総和
this.vTorque.add(mul(cross(this.vGravityToPoint, vNormal), c*rikiseki / dt));
//摩擦を考慮した分を追加
var c2 = dot(vTangent, cross(mulMV(this.mInertiaInverse, cross(this.vGravityToPoint, vTangent)), this.vGravityToPoint));
var B = -dot(vTangent, vp) / (1.0/this.mass + c2);
var muC = Math.abs(B / rikiseki);
if(muK >= muC){
this.vForce.add(mul(B/dt, vTangent));
this.vTorque.add(mul(cross(this.vGravityToPoint, vTangent), c* B/dt));
}
else
{
this.vForce.add( mul(muK * rikiseki / dt , vTangent));
this.vTorque.add(mul(cross(this.vGravityToPoint, vTangent) , c*muK * rikiseki / dt));
}
}
//--------------------------------------------------------------
Rigid.prototype.isStabilized = function()
{
//安定な姿勢とは,
//直方体:P0-P1とP1-P2の両方が水平であるか,どちらかが水平面に垂直
//円柱:中心軸が水平面に直角または平行
var e0, e1;
var eps0 = 0.0174;//cos89 0.08715;//cos8
var eps1 = 0.9998;//cos1 0.99619;//cos5
var vNormal = new Vector3(0, 0, 1);
if(this.kind == "SPHERE") return true;
else if(this.kind == "CUBE")
{
e0 = norm(qvRotate(this.q, direction(this.vP0[4], this.vP0[0])));//慣性座標に変換
e0 = Math.abs(dot(e0, vNormal));
e1 = norm(qvRotate(this.q, direction(this.vP0[0], this.vP0[1])));
e1 = Math.abs(dot(e1, vNormal));
//どちらかが鉛直なら安定
if( e0 > eps1 ) return true;
if( e1 > eps1 ) return true;
if( e0 < eps0 && e1 < eps0) return true;//どちらも水平
}
else if(this.kind == "CYLINDER")
{
e0 = norm(qvRotate(this.q, direction(this.vP0[2*this.nSlice], this.vP0[2*this.nSlice+1])));//中心軸(基本姿勢のz軸)
e0 = Math.abs(dot(e0, vNormal));
if( e0 > eps1) return true;//z軸に平行(垂直)
if( e0 < eps0) return true;//フロアに平行
}
return false;
}
//------------------------------------------------------------------------------
Rigid.prototype.calcMomentOfInertia = function()
{
if(this.kind != "SPHERE" && this.kind != "CUBE" && this.kind != "CYLINDER") {
console.log("この立体の慣性モーメントは求めていません"); return;
}
var sx = this.vSize.x; var sy = this.vSize.y; var sz = this.vSize.z;
var mass = this.mass;
var Ixx, Iyy, Izz;
if(this.kind == "SPHERE")
{
if(sx != sy || sy != sz || sz != sx) { console.log("完全な球にしてください"); return; }
Ixx = Iyy = Izz = sx * sx * mass / 10.0; //vSizeは直径
}
else if(this.kind == "CYLINDER")//円柱
{
if(sx != sy) { console.log("完全な円柱にしてください"); return; }
Ixx = mass * (sy * sy / 16.0 + sz * sz / 12.0);
Iyy = mass * (sx * sx / 16.0 + sz * sz / 12.0);
Izz = mass * (sx * sx + sy * sy) / 16.0;
}
else if(this.kind == "CUBE")//直方体(直方体)
{
Ixx = mass * (sy * sy + sz * sz) / 12.0;
Iyy = mass * (sx * sx + sz * sz) / 12.0;
Izz = mass * (sx * sx + sy * sy) / 12.0;
}
//慣性モーメント のテンソル
this.mInertia.set(Ixx, 0.0, 0.0,
0.0, Iyy, 0.0,
0.0, 0.0, Izz );
this.mInertiaInverse.set(1/Ixx, 0.0, 0.0,
0.0, 1/Iyy, 0.0,
0.0, 0.0, 1/Izz);
}
//-----------------------------------------------------------------------------
Rigid.prototype.calcInertialResistance = function()
{ //x,y,z方向の断面積
var vArea = new Vector3();
if(this.kind == "SPHERE")
{
vArea.x = Math.PI * this.vSize.y * this.vSize.z / 4.0;
vArea.y = Math.PI * this.vSize.z * this.vSize.x / 4.0;
vArea.z = Math.PI * this.vSize.x * this.vSize.y / 4.0;
}
else if(this.kind == "CUBE")
{
vArea.x = this.vSize.y * this.vSize.z ;
vArea.y = this.vSize.z * this.vSize.x ;
vArea.z = this.vSize.x * this.vSize.y ;
}
else if(this.kind == "CYLINDER")//円柱
{
vArea.x = this.vSize.y * this.vSize.z ;
vArea.y = this.vSize.x * this.vSize.z ;
vArea.z = Math.PI * this.vSize.x * this.vSize.y / 4.0;
}
//ci=0.5*CD*ρ*A(CD=1.0, ρ=1.2)
//見かけの大きさの1/10,面積は1/100倍
this.vInertialResistance = mul(0.5 * 1.2 * 0.01, vArea);
}
//---------------------------------------------------------------------
Rigid.prototype.calcRotationalResistance = function()
{
var gamma = 0.1;//回転抵抗係数の直交成分に対する平行成分の比
var k_shape;
var shortR, longR;
var a = this.vSize.x / 2.0, b = this.vSize.y / 2.0, c = this.vSize.z / 2.0;
var a2 = a * a, b2 = b * b, c2 = c * c;
if(this.kind == "SPHERE")
{
k_shape = 1.0;
var ci = (1.0 - (1.0 - gamma) * k_shape) * Math.PI * Math.pow(a, 5.0);
this.vRotationalResistance = new Vector3(ci, ci, ci);
}
else if(this.kind == "CUBE")
{
longR = Math.sqrt(b*b + c*c); shortR = Math.min(b, c);
k_shape = shortR / longR;
this.vRotationalResistance.x = (1.0 - (1.0 - gamma) * k_shape) * Math.pow(longR, 3.0) * b * c * 4.0;
longR = Math.sqrt(a*a + c*c); shortR = Math.min(a, c);
k_shape = shortR / longR;
this.vRotationalResistance.y = (1.0 - (1.0 - gamma) * k_shape) * Math.pow(longR, 3.0) * c * a * 4.0;
longR = Math.sqrt(a*a + b*b ); shortR = Math.min(a, b);
k_shape = shortR / longR;
this.vRotationalResistance.z = (1.0 - (1.0 - gamma) * k_shape) * Math.pow(longR, 3.0) * a * b * 4.0;
}
else if(this.kind == "CYLINDER")
{
longR = Math.max(a, b); shortR = Math.min(b, c);
k_shape = shortR / longR;
this.vRotationalResistance.z = (1.0 - (1.0 - gamma) * k_shape) * Math.pow(longR, 3.0) * a * b * Math.PI;
longR = Math.sqrt(b*b + c*c); shortR = Math.min(b, c);
k_shape = shortR / longR;
this.vRotationalResistance.x = (1.0 - (1.0 - gamma) * k_shape) * Math.pow(longR, 3.0) * b * c * 4.0;
longR = Math.max(a, c); shortR = Math.min(a, c);
k_shape = shortR / longR;
this.vRotationalResistance.y = (1.0 - (1.0 - gamma) * k_shape) * Math.pow(longR, 4.0) * a * c * 4.0;
}
//見かけのサイズの1/10,CD=1.0としてρCD/2を乗じる(CR=(1/8)*CD*ρ*R^3*A)
this.vRotationalResistance.mul(0.125 * 1.2 * 0.00001);
}
//-------------------------------------------------------------------------
Rigid.prototype.calcBoundingRadius = function()
{
var sx = this.vSize.x;
var sy = this.vSize.y;
var sz = this.vSize.z;
if(this.kind == "SPHERE"){ //球
this.boundingR = sx / 2.0;
}
else if(this.kind == "CUBE" ) {//直方体(球体とみなしたときの半径)
this.boundingR = Math.sqrt(sx * sx + sy *sy + sz * sz) / 2.0;
}
else if(this.kind == "CYLINDER"){//円柱
var a = Math.max(sx, sy);
this.boundingR = Math.sqrt(a * a + sz * sz) / 2.0;
}
}
//-----------------------------------------------------------------------------
Rigid.prototype.setVertexOfCube = function()
{
var sx = this.vSize.x;
var sy = this.vSize.y;
var sz = this.vSize.z;
this.vP0[0].x = sx / 2.0; this.vP0[0].y = sy / 2.0; this.vP0[0].z = sz / 2.0;
this.vP0[1].x =-sx / 2.0; this.vP0[1].y = sy / 2.0; this.vP0[1].z = sz / 2.0;
this.vP0[2].x =-sx / 2.0; this.vP0[2].y =-sy / 2.0; this.vP0[2].z = sz / 2.0;
this.vP0[3].x = sx / 2.0; this.vP0[3].y =-sy / 2.0; this.vP0[3].z = sz / 2.0;
this.vP0[4].x = sx / 2.0; this.vP0[4].y = sy / 2.0; this.vP0[4].z =-sz / 2.0;
this.vP0[5].x =-sx / 2.0; this.vP0[5].y = sy / 2.0; this.vP0[5].z =-sz / 2.0;
this.vP0[6].x =-sx / 2.0; this.vP0[6].y =-sy / 2.0; this.vP0[6].z =-sz / 2.0;
this.vP0[7].x = sx / 2.0; this.vP0[7].y =-sy / 2.0; this.vP0[7].z =-sz / 2.0;
this.numVertex = 8;
}
//-----------------------------------------------------------------------------
Rigid.prototype.setVertexOfCylinder = function()
{
var i, phi, phi0;
this.numVertex = 2 * this.nSlice + 2;
if(this.numVertex > MAX_VERTEX) {
console.log("nSliceを小さくしてください in setVertexOfCylinder");
return;
}
phi0 = 2.0 * Math.PI / this.nSlice;
for(i = 0;i < this.nSlice;i++)
{
phi = phi0 * i;
this.vP0[i].x = 0.5 * Math.cos(phi) * this.vSize.x; //上底のx成分
this.vP0[i].y = 0.5 * Math.sin(phi) * this.vSize.y; //y成分
this.vP0[i].z = 0.5 * this.vSize.z; //z成分(高さ)
this.vP0[i+this.nSlice].x = this.vP0[i].x; //下底のx成分
this.vP0[i+this.nSlice].y = this.vP0[i].y; //y成分
this.vP0[i+this.nSlice].z = - 0.5 * this.vSize.z; //z成分
}
//上底の中心
this.vP0[2*this.nSlice].x = 0.0;
this.vP0[2*this.nSlice].y = 0.0;
this.vP0[2*this.nSlice].z = 0.5 * this.vSize.z;
//下底の中心
this.vP0[2*this.nSlice+1].x = 0.0;
this.vP0[2*this.nSlice+1].y = 0.0;
this.vP0[2*this.nSlice+1].z = -0.5 * this.vSize.z;
}
//-----------------------------------------------------------------------
Rigid.prototype.tumbling = function()
{
var sx = this.vSize.x;
var sy = this.vSize.y;
//慣性空間における法線ベクトル
var vNorm = qvRotate(this.q, new Vector3(0.0, 0.0, 1.0));
if(vNorm.z < 0.0) vNorm.reverse();
var vVelocityN = norm(this.vVel);//正規化速度
//紙片の接線ベクトル
var vTang = cross(vNorm, cross(vVelocityN, vNorm));//接線ベクトル
vTang.norm();
//迎え角の計算
var dotTV = dot(vTang, vVelocityN);
if(dotTV > 1.0) dotTV = 1.0;
var phi = Math.acos(dotTV);//迎え角
var S = sx * sy * 0.01;//紙片の面積
var rho = 1.2;//空気密度
var cc = 0.5 * this.coefLift * rho * mag(this.vVel) * Math.sin(2*phi) * S;
var vForceLift = mul(cc, cross(this.vVel, cross(vNorm, vTang)));//揚力
this.vForce.add(vForceLift);
//進行方向の紙片の長さ
var Length = 0.1 * Math.abs(dot(qvRotate(this.q, new Vector3(sx, sy, 0.0)), vTang));
//回転トルクの追加
this.vTorque.add(mul(cross(vTang, vForceLift), this.delta * Length));
}
/*-----------------------------------------------------------------------------
剛体と剛体の衝突判定
----------------------------------------------------------------------------- */
var NON_COLLISION = -999;
//球同士
Rigid.prototype.collisionSphereWithSphere = function(rigid)
{
this.vNormal = direction(this.vPos, rigid.vPos);//球1(this)から球2(rig)へ向かう単位法線ベクトル
//重心から衝突点までのベクトル
this.vGravityToPoint = mul(this.vSize.x/2.0, this.vNormal);
rigid.vGravityToPoint = mul(rigid.vSize.x/2.0, this.vNormal);
rigid.vGravityToPoint.reverse();
var depth = (this.vSize.x + rigid.vSize.x) / 2.0 - distance(this.vPos, rigid.vPos);
return depth;//球同士の場合,必ず衝突
}
//------------------------------------------------------------------------------
//球と直方体の衝突判定
//球の中心から辺までの距離 <= 球の半径でかつ
//球の中心が直方体の辺に対して正領域が1つの場合
//直方体の辺と交差するときも衝突(正領域が2つ)
Rigid.prototype.collisionSphereWithCube = function(rigid)
{
//直方体の面を構成する頂点番号
var vs = [ [0,1,2,3], [0,3,7,4], [0,4,5,1],
[1,5,6,2], [2,6,7,3], [4,7,6,5] ];
//辺を構成する頂点番号(最初の2個)と面番号
var ve = [ [0,1,0,2], [1,2,0,3], [2,3,0,4], [3,0,0,1],
[0,4,1,2], [1,5,2,3], [2,6,3,4], [3,7,1,4],
[4,5,2,5], [5,6,3,5], [6,7,4,5], [7,4,1,5] ];
var i, j, k, no1, no2;
var dist = [];//球の中心から面までの距離
var f, d;//判定式
var faceNo = [];
var cnt;
var rr = this.vSize.x / 2.0;//球の半径
var depth;
var vCollision = new Vector3();//衝突点
var vDir = new Vector3();
//衝突対象(#2)の頂点の位置を取得
for(i = 0; i < rigid.numVertex; i++)
rigid.vP[i] = add(qvRotate(rigid.q, rigid.vP0[i]) , rigid.vPos);
//衝突対象(#2)の面の法線ベクトル
for(j = 0; j < 6; j++)//jは#2の面番号
{
rigid.vNormalFacet[j] = cross(sub(rigid.vP[vs[j][1]], rigid.vP[vs[j][0]]) , sub(rigid.vP[vs[j][2]], rigid.vP[vs[j][1]])) ;
rigid.vNormalFacet[j].norm();
}
//球の中心から対象直方体の面までの距離を調査
cnt = 0;//球の中心が直方体の面の正領域にある回数
for(j = 0; j < 6; j++) //対象直方体の面番号
{
f = dot(rigid.vNormalFacet[j] , sub(this.vPos, rigid.vP[vs[j][0]]));
if( f >= 0.0 )
{
faceNo[cnt] = j;
dist[faceNo[cnt]] = f;//面から球中心点までの距離
cnt++;
vCollision = sub(this.vPos , mul(rigid.vNormalFacet[j], rr)); //cnt=1のときの交点候補
}
}
if(cnt == 1)
{ //面と衝突の可能性あり
this.vNormal = rigid.vNormalFacet[faceNo[0]] ;
this.vNormal.reverse();
depth = dot(this.vNormal , sub(vCollision, rigid.vP[vs[faceNo[0]][0]]));
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos) ;//対象剛体側
return depth;
}
else if(cnt == 2)
{ //辺と交差の可能性あり
//面を共有する辺番号
for(k = 0; k < 12; k++)
{
if( faceNo[0] == ve[k][2] && faceNo[1] == ve[k][3] ){
no1 = ve[k][0]; no2 = ve[k][1]; //交差する辺の頂点番号
break;
}
}
//辺の方向ベクトル
vDir = direction(rigid.vP[no1] , rigid.vP[no2]); //
f = dot(vDir , sub(rigid.vP[no1], this.vPos)) ;
vCollision = sub(rigid.vP[no1], mul(vDir, f));//球の中心から辺へ垂線を下ろした時の交点
d = mag(sub(vCollision , this.vPos));//その距離
if(d > rr) return NON_COLLISION;
depth = rr - d;
this.vNormal = direction(this.vPos , vCollision) ;//球の中心から衝突点への単位ベクトル
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは球の中心座標)
rigid.vGravityToPoint =sub(vCollision, rigid.vPos) ;//対象剛体側
return depth;
}
else return NON_COLLISION;
}
//------------------------------------------------------------------------------
//直方体(直方体)と球の衝突判定
//直方体の頂点が球の内部に存在するとき衝突
Rigid.prototype.collisionCubeWithSphere = function(rigid)
{
var i, cnt, dist;
var vCollision = new Vector3();//衝突点
//直方体の頂点の位置を取得(vP[i]に格納)
for(i = 0; i <this.numVertex; i++)
{
this.vP[i] = qvRotate(this.q, this.vP0[i]);
this.vP[i].add(this.vPos);
}
cnt = 0;
for(i = 0; i < 8; i++)
{
dist = distance(rigid.vPos, this.vP[i]);//球中心から直方体の頂点までの距離
if(dist < rigid.boundingR) //球の半径以下なら
{//衝突
cnt++;
vCollision.add(this.vP[i]);
}
}
if(cnt == 0) return NON_COLLISION;
vCollision.div(cnt);
this.vNormal = sub(rigid.vPos, vCollision);//法線方向
var depth = rigid.boundingR - mag(this.vNormal);
this.vNormal.norm();
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos); //直方体(中心から衝突点へ向かうベクトル)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos);
return depth;
}
//------------------------------------------------------------------------------
//直方体と直方体の衝突判定
//注目剛体の頂点が対象剛体の内部(境界上を含む)にあるとき衝突
//辺と辺が交差するとき衝突
Rigid.prototype.collisionCubeWithCube = function(rigid)
{
//面を構成する頂点番号
var vs = [ [0,1,2,3], [0,3,7,4], [0,4,5,1],
[1,5,6,2], [2,6,7,3], [4,7,6,5] ];
var i, j, k;
var nfvP = [];//交点と辺で作る面の法線ベクトル
for(i = 0; i < 4; i++) nfvP[i] = new Vector3();
var f;//判定式
var min0, dd;
var minNo, kaisu, cnt, VertexNo = [];
var vCollision = new Vector3();//衝突点
var vPoint = [];//面との交点(参照渡しに使用するため配列宣言)
vPoint[0] = new Vector3();
//注目剛体の頂点の世界座標空間における位置を取得(vP[i]に格納)
for(i = 0; i < this.numVertex; i++)
{
this.vP[i] = qvRotate(this.q, this.vP0[i]);
this.vP[i].add(this.vPos);
}
//衝突対象(#2)の頂点の世界座標空間における位置を取得
for(i = 0; i < rigid.numVertex; i++)
{
rigid.vP[i] = qvRotate(rigid.q, rigid.vP0[i]);
rigid.vP[i].add(rigid.vPos);
}
//#2の面の法線ベクトル
for(j = 0; j < 6; j++)//jは#2(対象剛体)の面番号
{
rigid.vNormalFacet[j] = cross(sub(rigid.vP[vs[j][1]], rigid.vP[vs[j][0]]) , sub(rigid.vP[vs[j][2]], rigid.vP[vs[j][1]])) ;
rigid.vNormalFacet[j].norm();
}
//注目直方体の全ての頂点について対象剛体の内部にあるかどうかを調査
cnt = 0;//対象剛体内部にある注目剛体の頂点個数
for(i = 0; i < 8; i++) //#1の頂点
{
kaisu = 0;//判定式が負となる回数
for(j = 0; j < 6; j++) //#2の面番号
{
f = dot(rigid.vNormalFacet[j] , sub(this.vP[i], rigid.vP[vs[j][0]]));
if( f > 0.001 ) break;//fが全て負のとき衝突
kaisu ++;
}
if( kaisu == 6) //#1の頂点が#2の内部
{
VertexNo[cnt] = i;//#2に衝突している#1の頂点番号
cnt++;
}
}
if(cnt != 0)
{
//#2の面に衝突している#1の頂点をすべて求め平均値を衝突点とする
vCollision = new Vector3();//衝突点のクリア
for(k = 0; k < cnt; k++)
{
vCollision.add(this.vP[VertexNo[k]]);//衝突点を追加
}
//衝突点
vCollision.div(cnt);//平均値
//辺と辺が交差していればその部分の交点を追加し平均
if(this.getPointCubeWithCube(rigid, vPoint) == true)
{
vCollision.add(vPoint[0]);
vCollision.div(2.0);
}
//最も近い面番号minNoを決定
f = dot(rigid.vNormalFacet[0] , sub(vCollision, rigid.vP[vs[0][0]]));
min0 = Math.abs(f) / mag(rigid.vNormalFacet[0]) ;//面までの距離(めり込み量)
minNo = 0;
for(j = 1; j < 6; j++)//jは対象剛体の面番号
{
f = dot(rigid.vNormalFacet[j] , sub(vCollision, rigid.vP[vs[j][0]]));
dd = Math.abs(f) / mag(rigid.vNormalFacet[j]) ;//面までの距離
if( dd < min0 )
{
min0 = dd;
minNo = j;
}
}
//その面の法線ベクトルを反転
this.vNormal = reverse(rigid.vNormalFacet[minNo]);
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos) ;//対象剛体側
return min0;
}
else//辺と辺の交差
{
if(!this.getPointCubeWithCube(rigid, vPoint)) return NON_COLLISION;
//最も近い面番号minNoを決定
f = dot(rigid.vNormalFacet[0] , sub(vPoint[0], rigid.vP[vs[0][0]]));
min0 = Math.abs(f) / mag(rigid.vNormalFacet[0]) ;//j=0の面までの距離
minNo = 0;
for(j = 1; j < 6; j++)//jは対象剛体の面番号
{
f = dot(rigid.vNormalFacet[j] , sub(vPoint[0], rigid.vP[vs[j][0]]));
dd = Math.abs(f) / mag(rigid.vNormalFacet[j]) ;//面までの距離
if( dd < min0)
{
min0 = dd;
minNo = j;
}
}
if(this.vNormal.x == 0.0 && this.vNormal.y == 0.0 && this.vNormal.z == 0.0 ) this.vNormal = rigid.vNormalFacet[minNo];
this.vNormal.norm();//隣り合う面のときは和の正規化で近似
//その面の法線ベクトルを反転
this.vNormal.reverse();
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vPoint[0], this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vPoint[0], rigid.vPos) ;//対象剛体側
return min0;
}
}
//---------------------------------------------------------------------
//注目剛体の辺が対象剛体の面と交差している点を加え平均をとり衝突点とする
//辺の両端が1つの面の外側であればその辺は交差しない
//面と面が十字状にクロスしているときも判定
Rigid.prototype.getPointCubeWithCube = function(rigid, vPoint)
{
//直方体の面を構成する頂点番号
var vs = [ [0,1,2,3], [0,3,7,4], [0,4,5,1],
[1,5,6,2], [2,6,7,3], [4,7,6,5] ];
//辺を構成する頂点番号
var ve = [ [0,1], [1,2], [2,3], [3,0],
[0,4], [1,5], [2,6], [3,7],
[4,5], [5,6], [6,7], [7,4] ];
var i, j, k, kp, kaisu, cnt;
var fa, fb, tt;
var vNormal0 = [];//交点と辺で作る面の法線ベクトル
for(i = 0; i < 4; i++) vNormal0[i] = new Vector3();
var vPoint0 = new Vector3(); //交点
kaisu = 0; //注目剛体の辺が対象剛体の面と交差する回数
vPoint[0] = new Vector3(); //交差点
this.vNormal = new Vector3(); //面の法線方向の和
for(i = 0; i < 12; i++) //注目直方体の辺
{
for(j = 0; j < 6; j++)//対象直方体の面
{ //辺の頂点が対象剛体の面の正領域か負領域か
fa = dot(rigid.vNormalFacet[j] , sub(this.vP[ve[i][0]], rigid.vP[vs[j][0]]));
fb = dot(rigid.vNormalFacet[j] , sub(this.vP[ve[i][1]], rigid.vP[vs[j][0]]));
if(fa * fb >= 0.0) continue;//同じ領域にあれば交差しない
tt = fa / (fa - fb);
vPoint0 = add(mul(sub(this.vP[ve[i][1]], this.vP[ve[i][0]]) , tt), this.vP[ve[i][0]]);//平面との交点
cnt = 0;
for(k = 0; k < 4; k++)//交点を持つ面の辺
{
kp = k+1;
if(kp == 4) kp = 0;
vNormal0[k] = cross(sub(rigid.vP[vs[j][k]], vPoint0) , sub(rigid.vP[vs[j][kp]], rigid.vP[vs[j][k]])) ;
if(dot(rigid.vNormalFacet[j] , vNormal0[k]) < 0.0) break;//1つでも負ならばこの面とは交差しない
cnt++;
}
if(cnt == 4)
{//交差
kaisu++;
vPoint[0].add(vPoint0);
this.vNormal.add(rigid.vNormalFacet[j]);
}
}
}
if(kaisu != 2 && kaisu != 4 && kaisu != 8) return false;//交差なし
vPoint[0].div(kaisu);
return true;
}
//------------------------------------------------------------------
//球と円柱の衝突判定
//球の中心から円柱の中心軸までの距離 <= 球の半径+円柱の半径(側面衝突)
//上底または下底と衝突(球の中心は上底または下底の外部)
//どちらも衝突点が円柱の上底と下底の中間に存在
Rigid.prototype.collisionSphereWithCylinder = function(rigid)
{
var dist;//球の中心から円柱中心軸までの距離
var depth;//めり込み量
var h1, h2;
var rr = this.vSize.x / 2.0;//球の半径
var vCollision;//衝突点
var vKoten;//球の中心から円柱中心軸へ下ろした垂線の交点
//球の頂点
for(var i = 0; i < this.numVertex; i++)
{
this.vP[i] = qvRotate(this.q, this.vP0[i]);
this.vP[i].add(this.vPos);
}
//対象円柱の頂点
for(var i = 0; i < rigid.numVertex; i++)
{
rigid.vP[i] = qvRotate(rigid.q, rigid.vP0[i]);
rigid.vP[i].add(rigid.vPos);
}
//円柱中心軸ベクトル
var vCenter = direction(rigid.vP[2*rigid.nSlice + 1], rigid.vP[2*rigid.nSlice]);
//球の中心から円柱中心軸へ下ろした垂線の交点
vKoten = sub(rigid.vP[2*rigid.nSlice+1] , mul(vCenter, dot(vCenter, sub(rigid.vP[2*rigid.nSlice+1], this.vPos))));//vPosは球の中心
//球の中心から交点までの距離
dist = mag(sub(vKoten, this.vPos));
//衝突の最低条件
if(dist > rr + Math.max(rigid.vSize.x, rigid.vSize.y) / 2.0) return NON_COLLISION;
//球の中心から上底、下底までの距離
h1 = Math.abs(dot(vCenter , sub(this.vPos, rigid.vP[2*rigid.nSlice]))); //上底までの距離
h2 = Math.abs(dot(vCenter , sub(this.vPos, rigid.vP[2*rigid.nSlice+1])));//下底までの距離
//側面衝突
if(h1 < rigid.vSize.z && h2 < rigid.vSize.z)
{
this.vNormal = sub(this.vPos, vKoten);
vCollision = add(vKoten, mul(this.vNormal, rigid.vSize.x / 2.0)) ;
depth = rr + rigid.vSize.x/2.0 - mag(this.vNormal);
this.vNormal.norm();
}
else//上底または下底と衝突
{
if((h1 < rr || h2 < rr) && dist > Math.max(rigid.vSize.x, rigid.vSize.y) / 2.0) return NON_COLLISION;
//if(h1 > rr || h2 > rr || dist > Math.max(rigid.vSize.x, rigid.vSize.y) / 2.0) return NON_COLLISION;
if(h1 <= rr )//上底側で衝突
{
this.vNormal = vCenter;
vCollision = sub(this.vPos, mul(this.vNormal, dot(this.vNormal , sub(this.vPos, rigid.vP[2*rigid.nSlice]))));
depth = rr - h1;
}
else if(h2 <= rr )//下底側で衝突
{
this.vNormal = reverse(vCenter);
vCollision = sub(this.vPos, mul(this.vNormal, dot(this.vNormal , sub(this.vPos, rigid.vP[2*rigid.nSlice+1]))));
depth = rr - h2;
}
else return NON_COLLISION;
}
this.vNormal.reverse();//注目剛体側から見た法線方向
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは球の中心座標)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos) ;//対象剛体側
return depth;
}
//------------------------------------------------------------------------------
//円柱と球の衝突判定
//円柱の頂点が球の内部に存在するとき衝突
Rigid.prototype.collisionCylinderWithSphere = function(rigid)
{
var i, cnt, dist;
var vCollision = new Vector3();//衝突点
for(i = 0; i < this.numVertex; i++)
{
this.vP[i] = qvRotate(this.q, this.vP0[i]);
this.vP[i].add(this.vPos);
}
cnt = 0;
for(i = 0; i < 2*this.nSlice; i++)
{
dist = mag(sub(rigid.vPos, this.vP[i])); //球中心から円柱の頂点までの距離
if(dist < rigid.vSize.x / 2.0) //球の半径以下なら
{//衝突
cnt++;
vCollision.add(this.vP[i]);
}
}
if(cnt == 0) return NON_COLLISION;
vCollision.div(cnt);
this.vNormal = sub(rigid.vPos, vCollision);//法線方向
var depth = rigid.vSize.x / 2.0 - mag(this.vNormal);
this.vNormal.norm();
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos); //直方体(中心から衝突点へ向かうベクトル)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos);
return depth;
}
//------------------------------------------------------------------------------
//円柱同士の衝突判定
//円柱の頂点が他方の円柱の内部(境界上を含む)にあるとき衝突
//辺と辺の交差による衝突
Rigid.prototype.collisionCylinderWithCylinder = function(rigid)
{
var h1, h2, aveH1, aveH2, dist0, dist;
var vCollision = new Vector3() ;//衝突点
var vKoten = new Vector3();//衝突点から対象円柱の中心軸へ下ろした垂線の交点
vKotenTop = new Vector3();
vKotenBtm = new Vector3();
vDir = new Vector3();
var i, cnt;
var flagCollisionInfinite = false;
var a2 = rigid.vSize.x * rigid.vSize.x / 4.0;
var b2 = rigid.vSize.y * rigid.vSize.y / 4.0;
var depth;
//注目円柱(#1,円柱)の頂点の位置を取得
for(i = 0; i < this.numVertex; i++)
{
this.vP[i] = add(qvRotate(this.q, this.vP0[i]), this.vPos);
}
//衝突対象(#2,円柱)の頂点の位置を取得
for(i = 0; i < rigid.numVertex; i++)
{
rigid.vP[i] = add(qvRotate(rigid.q, rigid.vP0[i]), rigid.vPos);
}
var vCenter1 = direction(this.vP[2*this.nSlice+1], this.vP[2*this.nSlice]);//注目円柱の中心軸ベクトル(下底->上底)
var vCenter2 = direction(rigid.vP[2*rigid.nSlice+1], rigid.vP[2*rigid.nSlice]);//対象円柱の中心軸ベクトル
//中心軸間の最短距離
if( mag(sub(vCenter1, vCenter2)) == 0.0 || mag(add(vCenter1, vCenter2)) == 0.0){ //2直線が平行
//注目円柱の中心から対象中心軸へ下ろした垂線の交点
vKoten = sub(rigid.vPos, mul(vCenter2, dot(vCenter2 , sub(rigid.vPos, this.vPos))));
dist0 = distance(vKoten, this.vPos);
}
else
dist0 = Math.abs(dot(sub(this.vPos, rigid.vPos) , cross(vCenter1 , vCenter2)));
//最初の衝突判定
if(dist0 > this.vSize.x/2.0 + rigid.vSize.x/2.0) return NON_COLLISION;
if(this.vSize.x <= this.vSize.z)
{
if(rigid.vSize.x <= rigid.vSize.z)
{
//円柱を球とみなして衝突判定
depth = this.collisionSphereWithSphere(rigid);
if(depth > 0) return depth;
}
else
{
depth = this.collisionSphereWithCylinder(rigid);
if(depth > 0) return depth;
}
}
//注目円柱の全ての頂点について対象剛体の内部にあるかどうかを調査
cnt = 0;//対象剛体内部にある注目剛体の頂点個数
aveH1 = 0.0; aveH2 = 0.0;
var vAveKoten = new Vector3();
for(i = 0; i < 2*this.nSlice; i++) //#1の頂点
{
//#1の頂点から#2中心軸に落とした垂線の交点
vKoten = sub(rigid.vP[2*rigid.nSlice+1], mul(vCenter2, dot(vCenter2, sub(rigid.vP[2*rigid.nSlice+1], this.vP[i]))));
dist = distance(vKoten, this.vP[i]);//注目円柱の頂点から対象円柱中心軸までの距離
if(dist <= rigid.vSize.x / 2.0 + 0.001) //円柱の半径以下なら衝突の可能性あり
{
flagCollisionInfinite = true;
h1 = Math.abs(dot(vCenter2 , sub(vKoten, rigid.vP[2*rigid.nSlice]))); //上底までの距離
h2 = Math.abs(dot(vCenter2 , sub(vKoten, rigid.vP[2*rigid.nSlice+1])));//下底までの距離
if(h1 <= rigid.vSize.z+0.0001 && h2 <= rigid.vSize.z+0.0001)
{//衝突
cnt++;
aveH1 += h1;
aveH2 += h2;
vAveKoten.add(vKoten);
vCollision.add(this.vP[i]);
}
}
}
if(flagCollisionInfinite == true && cnt == 0) return NON_COLLISION;//無限円柱の内部にあるが,有限円柱の外部
if(cnt != 0)
{
//#2の面に衝突している#1の頂点の平均値を衝突点とする
vCollision.div(cnt);
aveH1 /= cnt;
aveH2 /= cnt;
vAveKoten.div(cnt);
depth = this.getDepthOfCollisionInCylinder(rigid, vCenter2, vCollision, vAveKoten, aveH1, aveH2);
return depth;
}
//辺と辺の交差を調査
//注目円柱のside edgeが対象円柱と交差している点を加え平均をとり衝突点とする
//side edgeが無限長の対象円柱と交差するか調べ,交差したならば
//その交点が両方の円柱の上底と下底の間に存在するとき交差
//注目剛体の中心軸方向
var vCenter = qvRotate(conjugate(rigid.q), vCenter1);
//対象物体座標系の基本姿勢で調査
cnt = 0;
vCollision = new Vector3(0.0, 0.0, 0.0);
var vCollision0 = new Vector3();
var vCollision1 = new Vector3();
for(i = 0; i < this.nSlice; i++)
{
//注目円柱の side edge 起点(下底):方向はvCenter
var vQ = qvRotate(conjugate(rigid.q), sub(this.vP[i+this.nSlice], rigid.vPos));
var a = vCenter.x * vCenter.x + vCenter.y * vCenter.y;
var b = (vQ.x * vCenter.x + vQ.y * vCenter.y);
var c = vQ.x * vQ.x + vQ.y * vQ.y - rigid.vSize.x * rigid.vSize.x / 4.0;
//判別式
var D = b * b - a * c;
if( D < 0.0) continue;//外部
else if(D == 0.0)//対象剛体の側面上
{
vCollision0 = vQ + (- b / a) * vCenter;
if(vCollision0.z > -rigid.vSize.z/2 && vCollision0.z < rigid.vSize.z/2)
{
vCollision.add(vCollision0);
cnt++;
}
continue;
}
else//内部
{
vCollision0 = vQ + (-(b + Math.sqrt(D)) / a) * vCenter;
vCollision1 = vQ + (-(b - Math.sqrt(D)) / a) * vCenter;
if(vCollision0.z > -rigid.vSize.z/2 && vCollision0.z < rigid.vSize.z/2)
{
if(vCollision1.z < -rigid.vSize.z/2) vCollision1.z = - rigid.vSize.z/2;
if(vCollision1.z > rigid.vSize.z/2) vCollision1.z = rigid.vSize.z/2;
vCollision.add( add(vCollision0, vCollision1) );
cnt += 2;
continue;
}
else if(vCollision0.z < -rigid.vSize.z/2)
{
if(vCollision1.z < -rigid.vSize.z/2) continue;
if(vCollision1.z > -rigid.vSize.z/2 && vCollision1.z < rigid.vSize.z/2)
{
vCollision0.z = - rigid.vSize.z/2;
}
else if(vCollision1.z > rigid.vSize.z/2)
{
vCollision0.z = - rigid.vSize.z/2;
vCollision1.z = rigid.vSize.z/2;
}
vCollision.add( add(vCollision0, vCollision1) );
cnt += 2;
continue;
}
else if(vCollision0.z > rigid.vSize.z/2)
{
if(vCollision1.z > rigid.vSize.z/2) continue;
if(vCollision1.z > -rigid.vSize.z/2 && vCollision1.z < rigid.vSize.z/2)
{
vCollision0.z = rigid.vSize.z/2;
}
else if(vCollision1.z < -rigid.vSize.z/2)
{
vCollision0.z = rigid.vSize.z/2;
vCollision1.z = - rigid.vSize.z/2;
}
vCollision.add( add(vCollision0, vCollision1) );
cnt += 2;
continue;
}
}
}
if(cnt == 0) return NON_COLLISION;
vCollision.div(cnt);
//めり込み量
depth = rigid.vSize.x/2.0 - Math.sqrt(vCollision.x*vCollision.x + vCollision.y*vCollision.y);//長半径からの距離で近似
//対象楕円柱の法線方向
this.vNormal = new Vector3(vCollision.x / a2, vCollision.y / b2, 0.0);
this.vNormal = qvRotate(rigid.q, this.vNormal);//世界座標系に戻す
if(mag(this.vNormal) <= 0.0001) return NON_COLLISION;//相殺されたとき
this.vNormal.norm();
this.vNormal.reverse();//物体#1から見た法線方向
//衝突点を世界座標に戻す
vCollision = add(qvRotate(rigid.q, vCollision), rigid.vPos);
//衝突点が注目円柱の内部か
h1 = Math.abs(dot(vCenter1 , sub(vCollision, this.vP[2*nSlice])));//注目円柱の上底までの距離
h2 = Math.abs(dot(reverse(vCenter1) , sub(vCollision, this.vP[2*nSlice+1])));//注目円柱の下底までの距離
if(h1 > vSize.z) return NON_COLLISION;
if(h2 > vSize.z) return NON_COLLISION;
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos) ;//対象剛体側
return depth;
}
//---------------------------------------------------------------------------------------------
Rigid.prototype.getDepthOfCollisionInCylinder = function(rigid, vCenter, vCollision, vKoten, aveH1, aveH2)
{
var depth;
//相対速度ベクトル
var vDir = sub(add(this.vVel, cross(this.vOmega , sub(vCollision, this.vPos))),
add(rigid.vVel, cross(rigid.vOmega , sub(vCollision, rigid.vPos))));//#2に対する#1の衝突点へ向かう衝突点の速度ベクトル
vDir.norm();
var ss;
//側面から衝突点までの距離
ss = rigid.vSize.x / 2.0 - distance(vCollision, vKoten);
var c = dot(vCenter , vDir);
if(Math.abs(c) > 0.01)//中心軸に直交せずに衝突
{
if(ss > aveH1)
{//衝突点がTopに近い
this.vNormal = reverse(vCenter);
depth = aveH1;
}
else if(ss > aveH2)
{//Bottomに近い
this.vNormal = vCenter;
depth = aveH2;
}
else
{//側面に衝突
this.vNormal = direction(vCollision, vKoten) ;
this.vNormal.norm();
depth = ss;
}
}
else//側面同士の衝突
{
this.vNormal = direction(vCollision, vKoten) ;
this.vNormal.norm();
depth = ss;
}
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos) ;//対象剛体側
return depth;
}
//------------------------------------------------------------------------------
//直方体と円柱の衝突判定
//直方体の頂点が円柱の内部に存在するとき衝突
//面と側面(辺)の衝突
Rigid.prototype.collisionCubeWithCylinder = function(rigid)
{
var i, j, cnt;
var depth;//めり込み量
var aveH1, aveH2;//平均値
var vCollision;//衝突点
var vCenter;//円柱中心軸方向ベクトル
var vKoten, vAveKoten ;//直方体の頂点から円柱の中心軸へ下ろした垂線の交点とその平均値
var a2 = rigid.vSize.x * rigid.vSize.x / 4.0;
var b2 = rigid.vSize.y * rigid.vSize.y / 4.0;
//直方体の頂点の世界座標位置を取得(this.vP[i]に格納)
for(i = 0; i < this.numVertex; i++)
{
this.vP[i] = add(qvRotate(this.q, this.vP0[i]) , this.vPos);
}
//対象円柱の頂点の世界座標位置を取得(rigid.vP[i]に格納)
for(i = 0; i < rigid.numVertex; i++)
{
rigid.vP[i] = add(qvRotate(rigid.q, rigid.vP0[i]) , rigid.vPos);
}
//円柱の中心軸
vCenter = direction(rigid.vP[2*rigid.nSlice+1] , rigid.vP[2*rigid.nSlice]) ;//中心軸単位ベクトル(下底->上底)
vCollision = new Vector3();
cnt = 0;
vAveKoten = new Vector3();
aveH1 = 0.0; aveH2 = 0.0;
for(i = 0; i < this.numVertex; i++)//注目剛体の各頂点
{
//対象円柱の物体座標(基本姿勢)における直方体の侵入頂点位置
var vQ = qvRotate(conjugate(rigid.q), sub(this.vP[i], rigid.vPos));
var f = vQ.x*vQ.x / a2 + vQ.y*vQ.y / b2 -1.0;
if(f > 0.0) continue;
if(vQ.z <= rigid.vSize.z / 2.0 && vQ.z >= -rigid.vSize.z / 2.0)
{//衝突
cnt++;
vKoten = sub(rigid.vPos , mul(vCenter, dot(vCenter, sub(rigid.vPos, this.vP[i]))));//注目剛体の各頂点から円柱中心軸へ降ろした垂線の交点
vCollision.add(this.vP[i]);//侵入した頂点を衝突点に近似
vAveKoten.add(vKoten);
aveH1 += rigid.vSize.z/2.0 - vQ.z;//h1;//上底までの平均距離
aveH2 += vQ.z + rigid.vSize.z/2.0;//h2;//下底までの平均距離
}
}
if(cnt == 0) return NON_COLLISION;
vCollision.div(cnt);
vAveKoten.div(cnt);
aveH1 /= cnt;
aveH2 /= cnt;
//側面から衝突点までの距離
ss = rigid.vSize.x / 2.0 - distance(vCollision, vAveKoten);
if(ss > aveH1)
{//衝突点がTopに近い
this.vNormal = reverse(vCenter);
depth = aveH1;
}
else if(ss > aveH2)
{//Bottomに近い
this.vNormal = vCenter;
depth = aveH2;
}
else
{//側面に衝突
this.vNormal = direction(vCollision, vAveKoten) ;
this.vNormal.norm();
depth = ss;
}
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision, this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vCollision, rigid.vPos) ;//対象剛体側
return depth;
}
//------------------------------------------------------------------------------
//円柱と直方体の衝突判定
//円柱の頂点が直方体の内部(境界上を含む)にあるとき衝突とする
//辺と辺の交差も考慮
Rigid.prototype.collisionCylinderWithCube = function(rigid)
{
//面を構成する頂点番号(対象直方体)
var vs = [ [0,1,2,3], [0,3,7,4], [0,4,5,1],
[1,5,6,2], [2,6,7,3], [4,7,6,5] ];
var i, j, k;
var dist = [];//頂点から面までの距離
var f;//判定式
var dd, depth;
var VertexNo = [];//[MAX_VERTEX];
var kaisu, cnt;
var vCollision;//衝突点
var vPoint;
var vCenter;//円柱の中心軸
//最初に円柱を球とみなして判定
if(this.vSize.x <= this.vSize.z)
{
depth = this.collisionSphereWithCube(rigid);
if(depth > 0) return depth;
}
//注目剛体(#1,円柱)の頂点の位置を取得
for(i = 0; i < this.numVertex; i++)
{
this.vP[i] = qvRotate(this.q, this.vP0[i]);
this.vP[i].add(this.vPos);
}
//衝突対象(#2,直方体)の頂点の位置を取得
for(i = 0; i < rigid.numVertex; i++)
{
rigid.vP[i] = qvRotate(rigid.q, rigid.vP0[i]);
rigid.vP[i].add(rigid.vPos);
}
//#2の面の法線ベクトル
for(j = 0; j < 6; j++)//jは#2の面番号
{
rigid.vNormalFacet[j] = cross(sub(rigid.vP[vs[j][1]], rigid.vP[vs[j][0]]) , sub(rigid.vP[vs[j][2]], rigid.vP[vs[j][1]])) ;
rigid.vNormalFacet[j].norm();
}
//注目円柱の全ての頂点について対象剛体の内部にあるかどうかを調査
cnt = 0;//対象剛体内部にある注目剛体の頂点個数
for(i = 0; i < this.numVertex; i++) //#1の頂点
{
kaisu = 0;//判定式が負となる回数(負のとき直方体の内側)
for(j = 0; j < 6; j++) //#2の面番号
{
f = dot(rigid.vNormalFacet[j] , sub(this.vP[i], rigid.vP[vs[j][0]]));
if( f > 0.001 ) break;
//fが全て負のとき衝突
dist[6 * i + j] = Math.abs(f) / mag(rigid.vNormalFacet[j]) ;//面までの距離
kaisu ++;
}
if( kaisu == 6) //#1の頂点が#2の内部
{
VertexNo[cnt] = i;//#2に衝突している#1の頂点番号
cnt++;
}
}
if(cnt == 0) //辺対辺を調査
{
var vPoint = []//1個であるが配列扱い(参照渡し)
vPoint[0] = new Vector3();//衝突点
depth = this.getPointCylinderInCube(rigid, vPoint) ;
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vPoint[0] , this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vPoint[0] , rigid.vPos) ;//対象剛体側
return depth;
}
else//点対面を調査
{//円柱の点対直方体の面
vCollision = new Vector3();//衝突点のクリア
for(k = 0; k < cnt; k++)
{
vCollision.add(this.vP[VertexNo[k]]);//衝突点を追加
}
//衝突点
vCollision.div(cnt);//平均値
//円柱の頂点が1つでも直方体の内部のとき面までの距離と面番号を求める
var cnt0 = 0;
depth = 1000;//大きな値に設定
var candidateNo;
for(j = 0; j < 6; j++)
{
dd = Math.abs(dot(rigid.vNormalFacet[j] , sub(vCollision, rigid.vP[vs[j][0]])))/mag(rigid.vNormalFacet[j]) ;//面までの距離
if(dd < rigid.vSize.x / 4)
{
//最も近い面番号とその距離を求める
if(dd < depth) { depth = dd; candidateNo = j;}// depth = dd;}
cnt0++;
}
}
if(cnt0 == 0) return NON_COLLISION;
this.vNormal = reverse(rigid.vNormalFacet[candidateNo]);
this.vNormal.norm();
//重心から衝突点までのベクトル
this.vGravityToPoint = sub(vCollision , this.vPos) ; //注目剛体側(vPosは中心座標)
rigid.vGravityToPoint = sub(vCollision , rigid.vPos) ;//対象剛体側
return depth;
}
}
//---------------------------------------------------------------------
Rigid.prototype.getPointCylinderInCube = function(rigid, vPoint)
{
//注目剛体の辺(edge)が対象剛体の面と交差しているときその交点を加え平均をとり衝突点とする
//注目剛体の線分の両端が対象剛体の1つの面の外側であればその線分は交差しない
//面を構成する頂点番号(対象直方体)
var vs = [ [0,1,2,3], [0,3,7,4], [0,4,5,1],
[1,5,6,2], [2,6,7,3], [4,7,6,5] ];
var i, j, k, kp, cnt, kosu;
var fa, fb, tt;
var fNo = [];
var vNormal0 = [];//[4];//交点と辺で作る面の法線ベクトル
var vPoint0; //交点
//辺を構成する頂点番号(注目円柱)
var ve = [];
for(i = 0; i < this.nSlice; i++){
ve[2*i] = i; ve[2*i+1] = i + this.nSlice;
}
kosu = 0;
vPoint[0] = new Vector3();
for(i = 0; i < this.nSlice; i++) //注目円柱の線分
{
for(j = 0; j < 6; j++)//対象直方体の面
{
fa = dot(rigid.vNormalFacet[j] , sub(this.vP[ve[i*2]], rigid.vP[vs[j][0]]));
fb = dot(rigid.vNormalFacet[j] , sub(this.vP[ve[i*2+1]], rigid.vP[vs[j][0]]));
if(fa * fb >= 0.0) continue;//この面とは交差しない
tt = fa / (fa - fb);
vPoint0 = add(mul(sub(this.vP[ve[i*2+1]], this.vP[ve[i*2]]), tt), this.vP[ve[i*2]]);//平面との交点
cnt = 0;
for(k = 0; k < 4; k++)//交点を持つ直方体面の辺
{
kp = k+1;
if(kp == 4) kp = 0;
vNormal0[k] = cross(sub(rigid.vP[vs[j][k]], vPoint0) , sub(rigid.vP[vs[j][kp]], rigid.vP[vs[j][k]])) ;
if(dot(rigid.vNormalFacet[j] , vNormal0[k]) < 0.0) break;//1つでも負ならばこの面とは交差しない
cnt++;
}
if(cnt == 4)
{//交差
vPoint[0].add(vPoint0);
fNo[kosu] = j;
kosu++; //直方体の面と交差している回数
}
}
}
if(kosu == 0) return NON_COLLISION;//2つの剛体は独立
vPoint[0].div(kosu);
var vCenter = direction(this.vP[2*this.nSlice+1] , this.vP[2*this.nSlice]);//下底から上底の中心軸方向
var vA = sub(vPoint[0], this.vP[2*this.nSlice+1]);//中心軸から交点へ向かうベクトル
this.vNormal = sub(vA , mul(vCenter, dot(vCenter, vA)));
this.vNormal.norm();
var depth = dot(rigid.vNormalFacet[fNo[0]] , sub(rigid.vP[vs[fNo[0]][0]], vPoint[0])) * 1.2;
return depth;
}
<file_sep>/JVEMV6/46_art/8_kb/start_keyboard.bat
REM ============================== list
REM open folders
REM vlc player
REM browser
REM doc
REM metronome
REM console
REM mind map
REM musescore3
REM ==============================
REM ==============================
REM open folders
REM ==============================
echo start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb
echo start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
start C:\WORKS_2\WS\WS_Cake_IFM11\iphone_to_upload
rem echo C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\6_kb
rem start C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\6_kb
rem 2020年9月10日18:16:28
echo C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\a_15_flute
start C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\a_15_flute
REM ==============================
REM vlc player
REM 2019/09/26 18:16:41
REM ==============================
pushd C:\Users\iwabuchiken\VLC
start vlc.exe
rem REM ==============================
rem REM browser
rem REM 2019/09/16 14:18:11
rem REM ==============================
rem : outsource to a file : 2021年2月10日14:56:38
rem call C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_keyboard_web-pages.bat
rem :web_page_keyboard_opera
rem REM : opera
rem pushd "C:\WORKS_2\Programs\opera"
rem rem : set urls 2020年11月17日18:27:33
rem rem : log files / 2020年11月17日18:27:39
rem rem set url_1="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=music+log&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem set url_1="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=session+shino&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem rem : video files : bamboo / 2020年11月17日18:27:39
rem set url_2="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=video+bamboo&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem rem : video files : flute / 2020年11月17日18:39:02
rem set url_2="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=video+jap.flute&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem start launcher.exe %url_1%
rem start launcher.exe %url_2%
rem REM : log
rem rem start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=kb+log&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem REM : video
rem rem start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=video+kb&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem REM start launcher.exe "https://docs.zoho.com/sheet/open/ek7i4683eb5df87d04a23acef09182e7df623/sheets/free/ranges/A3"
rem rem start launcher.exe "https://www.youtube.com/playlist?list=PLZe1Q2NRG8YXa2FCHsE3Ji3VCOg2Ey8LS"
rem goto exit_web_page_keyboard
rem :exit_web_page_keyboard
rem REM ==============================
rem REM apps : metronome
rem REM 2019/10/24 15:11:38
rem REM ==============================
rem REM : metronome
rem rem pushd "C:\WORKS_2\Programs\opera"
rem rem : comment out 2020年10月11日18:13:24
rem rem start launcher.exe https://www.flutetunes.com/metronome/
rem REM pushd "C:\WORKS_2\Programs\opera"
rem REM : metronome
rem REM start launcher.exe "https://www.metronomeonline.com"
rem REM start launcher.exe "https://www.imusic-school.com/en/tools/online-metronome/"
rem REM : keyboard
rem pushd "C:\WORKS_2\Programs\opera"
rem start launcher.exe https://pianoplays.com
rem start launcher.exe https://metronom.us/en/
rem start launcher.exe https://www.musicca.com/jp/metronome
rem REM start launcher.exe https://www.onlinepianist.com/virtual-piano
REM ==============================
REM console
REM 2020年7月22日19:08:10
REM ==============================
rem : comment out : 2022年2月26日19:04:47
rem pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb
rem start start_command_prompot.bat
REM ==============================
REM mind map
REM 2020年7月20日18:35:01
REM ==============================
rem : comment in 2020年10月25日18:13:20
rem : c/o : 2021年3月3日17:35:37
rem pushd "C:\WORKS_2\WS\FM_2_20171104_225946\Projects\JVEMV6\46 Art\8 kb"
rem start 3_music-types.mm
rem : comment in 2020年10月21日19:08:01
rem start "C:\WORKS_2\WS\FM_2_20171104_225946\Projects\JVEMV6\46 Art\8 kb\3_music-types.mm"
REM ==============================
REM musescore3
REM 2020年8月13日18:35:12
REM ==============================
rem commet out 2020年9月4日18:18:30
rem commet in 2020年9月24日18:28:09
rem commet out : 2021年2月3日17:55:18
rem start C:\WORKS_2\Programs\musescore\ms_3.2.3.7635\bin\MuseScore3.exe
REM ==============================
REM doc
REM 2019/09/16 14:18:11
REM ==============================
rem : 2022年5月1日18:06:55
start C:\WORKS_2\shortcuts_docs\data_shino.ods
start C:\WORKS_2\shortcuts_docs\data_shaku.ods
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\list_of_memos_keyboard.ods
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\memos_keyboard.odt
rem C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\log_keyboard.odt
rem "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\46#8.[kb].[admin].ods"
rem C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\6_kb\log_kb.txt
rem "C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019\6_kb\log_kb.txt"
rem C:\WORKS_2\shortcuts_docs\log_kb.txt
rem start C:\WORKS_2\shortcuts_docs\log_kb.txt
rem start "C:\WORKS_2\shortcuts_docs\log_[flute].txt"
rem : comment out : 2021年1月10日18:02:07
rem set text_1="C:\WORKS_2\shortcuts_docs\log_[flute].txt"
rem : comment out : 2021年1月10日18:02:07
rem C:\WORKS_2\Programs\sakura\sakura.exe %text_1%
rem 2021年11月1日18:11:43
start C:\WORKS_2\shortcuts_docs\log_jap-flute_shino.log
rem : 2022年4月13日15:41:09
C:\WORKS_2\WS\WS_Others.JVEMV6\JVEMV6\37_miscs\9_prog_lang\diary.ods
REM pause
REM exit
<file_sep>/JVEMV6/46_art/2_image-prog/1_gimp/2_/GimpPythonFuLayerAction-2-8.py (2).orig
#!/usr/bin/env python
# -*- coding: utf-8 -*-
########################################################################
# version 1.0.0
#
# GimpPythonFuLayerAction-2-8.py
# Copyright (C) 2012 かんら・から http://www.pixiv.net/member.php?id=3098715
#
# GimpPythonFuLayerAction-2-8.py is Python-fu plugin for GIMP 2.8
#
# GimpPythonFuLayerAction-2-8.py is free software: you can redistribute it and/or modify it
# under the terms of the GNU General Public License as published by the
# Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# GimpPythonFuLayerAction-2-8.py is distributed in the hope that it will be useful, but
# WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
# See the GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License along
# with this program. If not, see <http://www.gnu.org/licenses/>.
#
# GPLv3 ライセンス
# かんら・から http://www.pixiv.net/member.php?id=3098715
# バグレポート・アイデアなどは pixiv メッセージでお願いします。
#
# ダウンロード
# http://www.magic-object.mydns.jp/
#
# このスクリプトを使用して発生した問題に関し、作成者は如何なる保証などを行う事はありません。
# 自己責任でのみの使用を許可します。
########################################################################
from gimpfu import *
########################################################################
########################################################################
# レイヤー操作クラス
#
# レイヤー転写機能群など
#
class LayserActions:
########################################################################
image = None
doPutMessage = True
lastMessage = ''
usableVersion = ( 2, 8, 0 )
canUseLayerGroup = False
########################################################################
def __init__( self, image ):
self.image = image
versionLength = len( self.usableVersion )
for index in range( 0, versionLength ) :
if self.usableVersion[index] < gimp.version[index] :
self.canUseLayerGroup = True
break
elif self.usableVersion[index] == gimp.version[index] :
if index == ( versionLength - 1 ) :
self.canUseLayerGroup = True
break
continue
else :
self.canUseLayerGroup = False
break
return
########################################################################
# エラーメッセージ機能
#
def errorMessage( self, message=None ) :
if self.doPutMessage :
if message is None :
message = self.lastMessage
else :
self.lastMessage = message
# print message
pdb.gimp_message_set_handler( MESSAGE_BOX )
pdb.gimp_message( message )
# MESSAGE_BOX CONSOLE ERROR_CONSOLE
#gimp.message( message )
return
########################################################################
# 対象レイヤーがイメージの中にあるかどうか?
#
def isInImage( self, targetLayer=None, parent=None ) :
##############################################################
if targetLayer is None :
return False
##############################################################
if parent is None :
if targetLayer in self.image.layers :
return True
elif not self.canUseLayerGroup :
return False
for item in self.image.layers :
if pdb.gimp_item_is_group( item ) :
if self.isInImage( targetLayer, item ) :
return True
##############################################################
elif self.canUseLayerGroup :
( num_children, child_ids ) = pdb.gimp_item_get_children( parent )
if num_children < 1 :
return False
for itemId in child_ids :
item = gimp.Item.from_id( itemId )
if pdb.gimp_item_is_group( item ) :
if self.isInImage( targetLayer, item ) :
return True
else :
continue
elif item == targetLayer :
return True
##############################################################
return False
########################################################################
# 対象がレイヤーグループかどうか?
#
def isLayerGroup( self, target=None ) :
if target is None :
return False
elif not self.canUseLayerGroup :
return False
else :
return pdb.gimp_item_is_group( target )
########################################################################
# 対象が通常レイヤーかどうか?
#
def isNormalLayer( self, target=None ) :
if target is None :
return False
elif self.isLayerGroup( target ) :
return False
elif pdb.gimp_item_is_text_layer( target ) :
return False
else :
return pdb.gimp_item_is_layer( target )
########################################################################
# レイヤーを拾い上げる
#
def pickUpLayersLayer( self,
targetLayer=None,
searchRecursive=True,
needCheckVisible=True,
searchLayerGroupNumMax=1,
searchLayerNumMax=100,
parent=None
) :
##############################################################
if targetLayer is None :
return []
##############################################################
pickUppedList = []
##############################################################
if parent is None :
# 直接呼び出し
##############################################################
belongLayers = self.image.layers
##############################################################
if targetLayer not in belongLayers :
# 対象レイヤーがトップレベルに無い
parent = pdb.gimp_item_get_parent( targetLayer )
if parent is None :
return []
else :
# 対象がレイヤーグループ内に存在
( num_children, child_ids ) = pdb.gimp_item_get_children( parent )
if num_children < 1 :
return []
for itemId in child_ids :
belongLayers.append( gimp.Item.from_id( itemId ) )
if len( belongLayers ) < 1 :
return []
##############################################################
targetIndex = -1
try :
targetIndex = belongLayers.index( targetLayer )
except :
self.lastMessage = '不適切なレイヤーが指定されています。'
return []
##############################################################
for layer in belongLayers[ targetIndex + 1 : ] :
if searchLayerNumMax < 1 and searchLayerGroupNumMax < 1 :
return pickUppedList
elif needCheckVisible and not pdb.gimp_item_get_visible( layer ) :
continue
elif self.isLayerGroup( layer ) :
##############################################
if searchLayerGroupNumMax < 1 :
continue
##############################################
searchLayerGroupNumMax -= 1
resultsLayer = self.pickUpLayersLayer(
targetLayer,
searchRecursive,
needCheckVisible,
searchLayerGroupNumMax,
searchLayerNumMax,
layer
)
pickUppedList += resultsLayer
elif self.isNormalLayer( layer ) :
##############################################
if searchLayerNumMax < 1 :
continue
##############################################
searchLayerNumMax -= 1
pickUppedList.append( layer )
##############################################################
return pickUppedList
##############################################################
elif self.canUseLayerGroup :
# 再帰呼び出し
##############################################################
( num_children, child_ids ) = pdb.gimp_item_get_children( parent )
if num_children < 1 :
return []
for itemId in child_ids :
##############################################################
item = gimp.Item.from_id( itemId )
if needCheckVisible and not pdb.gimp_item_get_visible( item ) :
continue
elif self.isLayerGroup( item ) :
if not searchRecursive :
continue
resultsLayer = self.pickUpLayersLayer(
targetLayer,
searchRecursive,
needCheckVisible,
searchLayerGroupNumMax,
searchLayerNumMax,
item
)
pickUppedList += resultsLayer
elif self.isNormalLayer( item ) :
pickUppedList.append( item )
##############################################################
return pickUppedList
##############################################################
return pickUppedList
########################################################################
# レイヤーを検索する
#
def searchLayer( self,
targetLayer=None,
searchRecursive=True,
needCheckVisible=True,
searchLayerGroupNumMax=1,
searchLayerNumMax=0
) :
##############################################################
if targetLayer is None :
if self.image is None :
self.lastMessage = 'パラメータが不正です。'
return []
targetLayer = self.image.active_layer
##############################################################
if targetLayer is None :
self.lastMessage = 'レイヤーが選択されていません。'
return []
elif not self.isInImage( targetLayer ) :
self.lastMessage = '不適切なレイヤーが指定されています。'
return []
elif not self.isNormalLayer( targetLayer ) :
self.lastMessage = '対象がレイヤーではありません。'
return []
elif self.isLayerGroup( targetLayer ) :
self.lastMessage = 'レイヤーグループを対象にする事はできません。'
return []
elif searchLayerGroupNumMax < 1 and searchLayerNumMax < 1 :
self.lastMessage = '制限数が設定されていません。'
return []
##############################################################
pickUppedList = self.pickUpLayersLayer(
targetLayer,
searchRecursive,
needCheckVisible,
searchLayerGroupNumMax,
searchLayerNumMax,
None
)
if len( pickUppedList ) < 1 :
self.lastMessage = '対象が見つかりませんでした。'
return pickUppedList
##############################################################
return pickUppedList
########################################################################
# ターゲットのレイヤーに対し転写が可能かどうかチェックする
#
def canDoTranscribe( self, targetLayer=None ) :
##############################################################
if targetLayer is None :
if self.image is None :
self.lastMessage = 'パラメータが不正です。'
return False
targetLayer = self.image.active_layer
##############################################################
if targetLayer is None :
self.lastMessage = 'レイヤーが選択されていません。'
return False
elif not self.isInImage( targetLayer ) :
self.lastMessage = '不適切なレイヤーが指定されています。'
return False
elif not self.isNormalLayer( targetLayer ) :
self.lastMessage = '対象がレイヤーではありません。'
return False
elif self.isLayerGroup( targetLayer ) :
self.lastMessage = 'レイヤーグループに対して転写を行う事は出来ません。'
return False
##############################################################
# 対象レイヤーを含む、連続する通常レイヤーのリストを作成
layerList = []
parent = pdb.gimp_item_get_parent( targetLayer )
if parent is None :
for item in self.image.layers :
if self.isNormalLayer( item ) :
if targetLayer in layerList :
return True
else :
layerList.append( item )
else :
layerList = []
continue
else :
( num_children, child_ids ) = pdb.gimp_item_get_children( parent )
for itemId in child_ids :
item = gimp.Item.from_id( itemId )
if self.isNormalLayer( item ) :
if targetLayer in layerList :
return True
else :
layerList.append( item )
else :
layerList = []
continue
##############################################################
# 問題が無ければ通常、ここまで来ない
# エラー解析
if len( layerList ) < 2 :
self.lastMessage = '転写を行うには、転写元と転写先のレイヤーが必要です。'
return False
elif targetLayer not in layerList :
self.lastMessage = '下位に適切なレイヤーがありません。'
return False
else :
targetIndex = -1
try :
targetIndex = layerList.index( targetLayer )
except :
self.lastMessage = '不適切なレイヤーが指定されています。'
return False
if targetIndex == ( len( layerList ) - 1 ) :
self.lastMessage = '下位に適切なレイヤーがありません。'
return False
##############################################################
self.lastMessage = 'エラーが発生しましたが、解析に失敗しました。'
return False
########################################################################
# 転写を実行する
#
def transcribe( self, targetLayer=None ) :
##############################################################
# 対象のレイヤーチェック
if not self.canDoTranscribe() :
self.errorMessage()
return False
##############################################################
if targetLayer is None :
if self.image is None :
self.lastMessage = 'パラメータが不正です。'
self.errorMessage()
return False
targetLayer = self.image.active_layer
##############################################################
if targetLayer is None :
self.lastMessage = 'レイヤーが選択されていません。'
self.errorMessage()
return False
##############################################################
# 転写先のレイヤーを取得
againstLayer = None
parent = pdb.gimp_item_get_parent( targetLayer )
if parent is None :
targetIndex = -1
try :
targetIndex = self.image.layers.index( targetLayer )
except :
self.lastMessage = '不適切なレイヤーが指定されています。'
self.errorMessage()
return False
againstLayer = self.image.layers[ targetIndex + 1 ]
else :
layerList = []
( num_children, child_ids ) = pdb.gimp_item_get_children( parent )
for itemId in child_ids :
item = gimp.Item.from_id( itemId )
if self.isNormalLayer( item ) :
if targetLayer in layerList :
againstLayer = item
break
else :
layerList.append( item )
if againstLayer is None :
self.lastMessage = '転写先のレイヤーを取得出来ませんでした。'
self.errorMessage()
return False
##############################################################
# 転写元のレイヤーを作成しておく
# データなしの複製
newLayer = gimp.Layer(
self.image,
targetLayer.name,
targetLayer.width,
targetLayer.height,
targetLayer.type,
targetLayer.opacity,
targetLayer.mode
)
# レイヤーマスクを設定
if targetLayer.mask is not None :
pdb.gimp_image_add_layer_mask( self.image, newLayer, targetLayer.mask.copy() )
# 透明部分を保護
# pdb.gimp_layer_set_lock_alpha( newLayer, pdb.gimp_layer_get_lock_alpha( targetLayer ) )
##############################################################
# 転写先のレイヤーマスクを複製
againstLayerMask = None
if againstLayer.mask is not None :
againstLayerMask = againstLayer.mask.copy()
##############################################################
# 転写先のレイヤーモードを保持
againstLayerMode = againstLayer.mode
##############################################################
# 下のレイヤーと統合
resultsLayer = pdb.gimp_image_merge_down( self.image, targetLayer, CLIP_TO_BOTTOM_LAYER )
# レイヤーマスクを設定
if againstLayerMask is not None :
pdb.gimp_image_add_layer_mask( self.image, resultsLayer, againstLayerMask )
# レイヤーモードを設定
pdb.gimp_layer_set_mode( resultsLayer, againstLayerMode )
##############################################################
# 転写元のレイヤーを挿入
pdb.gimp_image_set_active_layer( self.image, resultsLayer )
pdb.gimp_image_insert_layer(
self.image,
newLayer,
pdb.gimp_item_get_parent(resultsLayer),
-1
)
##############################################################
return True
########################################################################
# クリップマスク作成する
#
def createLayerClip(
self,
targetLayer=None,
searchRecursive=True,
mergeAlpha=True,
mergeLayerMask=True,
needCheckVisible=True,
mergeClipLayerMask=True,
searchLayerGroupNumMax=1,
searchLayerNumMax=0
) :
########################################################################
if targetLayer is None :
if self.image is None :
self.lastMessage = 'パラメータが不正です。'
self.errorMessage()
return False
targetLayer = self.image.active_layer
##############################################################
if targetLayer is None :
self.lastMessage = 'レイヤーが選択されていません。'
self.errorMessage()
return False
elif not self.isInImage( targetLayer ) :
self.lastMessage = '不適切なレイヤーが指定されています。'
self.errorMessage()
return False
elif not self.isNormalLayer( targetLayer ) :
self.lastMessage = '対象がレイヤーではありません。'
self.errorMessage()
return False
elif self.isLayerGroup( targetLayer ) :
self.lastMessage = 'レイヤーグループを対象にする事はできません。'
self.errorMessage()
return False
elif searchLayerGroupNumMax < 1 and searchLayerNumMax < 1 :
self.lastMessage = '制限数が設定されていません。'
self.errorMessage()
return False
elif ( not mergeAlpha ) and ( not mergeLayerMask ) :
self.lastMessage = 'アルファマスクかレイヤーマスクを指定しなければなりません。'
self.errorMessage()
return False
if not self.canUseLayerGroup and searchLayerNumMax < 1 :
self.lastMessage = '制限数が設定されていません。'
self.errorMessage()
return False
##############################################################
pdb.gimp_progress_update(float(0)/float(6))
##############################################################
# 対象レイヤーを検索
resultsLayers = self.searchLayer(
targetLayer,
searchRecursive,
needCheckVisible,
searchLayerGroupNumMax,
searchLayerNumMax
)
if len( resultsLayers ) < 1 :
self.errorMessage()
return False
##############################################################
pdb.gimp_progress_update(float(1)/float(6))
##############################################################
# UNDO スタックをフリーズ
#pdb.gimp_image_undo_freeze( self.image )
##############################################################
# 空のマスクを作成
layersMask = pdb.gimp_layer_create_mask( targetLayer, ADD_BLACK_MASK )
##############################################################
pdb.gimp_progress_update(float(2)/float(6))
##############################################################
# 検索結果からマスクを作成
for layer in resultsLayers :
##############################################################
# レイヤーが「標準」または「ディザ合成」でない場合はマスクを収集しない
if pdb.gimp_layer_get_mode( layer ) not in [ NORMAL_MODE, DISSOLVE_MODE ] :
continue
##############################################################
clipMask = None
alphaMask = None
if mergeAlpha :
##############################################################
# アルファマスクを取得
alphaMask = pdb.gimp_layer_create_mask( layer, ADD_ALPHA_MASK )
clipMask = alphaMask
##############################################################
if mergeLayerMask and layer.mask is not None:
##############################################################
if clipMask is None :
# レイヤーマスクをクリップマスクに
clipMask = layer.mask.copy()
else :
# アルファマスクとレイヤーマスクの共通部分をクリップマスクに
pdb.gimp_channel_combine_masks( clipMask, layer.mask, CHANNEL_OP_INTERSECT, 0, 0 )
##############################################################
##############################################################
if clipMask is None :
continue
##############################################################
# クリップマスクを加算
( layerOffsetX, layerOffsetY ) = pdb.gimp_drawable_offsets( layer )
( targetOffsetX, targetOffsetY ) = pdb.gimp_drawable_offsets( targetLayer )
offsetX = layerOffsetX - targetOffsetX
offsetY = layerOffsetY - targetOffsetY
pdb.gimp_channel_combine_masks( layersMask, clipMask, CHANNEL_OP_ADD, offsetX, offsetY )
pdb.gimp_item_delete( clipMask )
clipMask = None
##############################################################
##############################################################
pdb.gimp_progress_update(float(3)/float(6))
##############################################################
if mergeClipLayerMask and targetLayer.mask is not None :
# 対象レイヤーのレイヤーマスクでクリップ
pdb.gimp_channel_combine_masks( layersMask, targetLayer.mask, CHANNEL_OP_INTERSECT, 0, 0 )
##############################################################
pdb.gimp_progress_update(float(4)/float(6))
##############################################################
# UNDO スタックをフリーズ解除
#pdb.gimp_image_undo_thaw( self.image )
##############################################################
if targetLayer.mask is not None :
# もし、対象レイヤーのレイヤーマスクがあれば削除
pdb.gimp_image_remove_layer_mask( self.image, targetLayer, MASK_APPLY )
##############################################################
pdb.gimp_progress_update(float(5)/float(6))
##############################################################
# レイヤーマスクにクリップマスクを設定
pdb.gimp_layer_add_mask( targetLayer, layersMask )
#pdb.gimp_image_set_active_layer( self.image, targetLayer )
##############################################################
pdb.gimp_progress_update(float(6)/float(6))
##############################################################
return True
########################################################################
########################################################################
# レイヤー転写機能
# レイヤーマスク、レイヤーグループ対応
#
def python_fu_tensha_2_8( image, drawable ) :
layerAction = LayserActions( image )
# 転写を実行
image.undo_group_start()
layerAction.transcribe()
image.undo_group_end()
return
########################################################################
# クリップマスク作成機能
# 対象となるレイヤーやレイヤーグループから、クリッピングマスクを作成
#
def python_fu_clip_layer(
image,
drawable,
searchRecursive=True,
mergeAlpha=True,
mergeLayerMask=True,
needCheckVisible=True,
mergeClipLayerMask=True,
searchLayerGroupNumMax=1,
searchLayerNumMax=100
) :
# ダイアログを出すと
# (gimp:14434): Gimp-Core-CRITICAL **: gimp_channel_push_undo: assertion `gimp_item_is_attached (GIMP_ITEM (channel))' failed
# が出る。回避策が無い
layerAction = LayserActions( image )
# クリップマスクを作成
image.undo_group_start()
layerAction.createLayerClip(
None,
searchRecursive,
mergeAlpha,
mergeLayerMask,
needCheckVisible,
mergeClipLayerMask,
searchLayerGroupNumMax,
searchLayerNumMax
)
image.undo_group_end()
return
########################################################################
register(
'python-fu-tensha-2-8', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'転写(Python)', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
], # プロシジャの引数
[], # 戻り値の定義
python_fu_tensha_2_8, # 処理を埋け持つ関数名
menu='<Image>/Layer/レイヤー操作(Python-fu)' # メニュー表示場所
)
register(
'python-fu-clip-layer', # プロシジャの名前
'クリップマスク作成スクリプト。下にあるレイヤーから、クリップ用のレイヤーマスクを作成する。',
# プロシジャの説明文
'ver 2.8 以上を対象としたクリップマスク作成クリプト。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'クリップマスク作成(Python)', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None),
(PF_BOOL, 'searchRecursive', 'レイヤーグループを再帰的に対象とする:', 1),
(PF_BOOL, 'mergeAlpha', 'アルファチャンネルを参照する:', 1),
(PF_BOOL, 'mergeLayerMask', 'レイヤーマスクを参照する:', 1),
(PF_BOOL, 'needCheckVisible', 'レイヤーの表示・非表示を参照する:', 1),
(PF_BOOL, 'mergeClipLayerMask', 'クリップ作成レイヤーのレイヤーマスクを参照する:', 1),
(PF_SPINNER, 'searchLayerGroupNumMax', '対象とする同階層下方のレイヤーグループ最大数(無視:0)', 1, (0, 100, 1)),
(PF_SPINNER, 'searchLayerNumMax', '対象とするる同階層下方のレイヤー最大数(無視:0)', 0, (0, 100, 1))
], # プロシジャの引数
[], # 戻り値の定義
python_fu_clip_layer, # 処理を埋け持つ関数名
menu='<Image>/Layer/レイヤー操作(Python-fu)' # メニュー表示場所
)
main() # プラグインを駆動させるための関数
<file_sep>/p.bat
g p origin master
<file_sep>/JVEMV6/46_art/2_image-prog/1_gimp/1_/libs_1/lib_2_1_2 (2).py
#!C:\WORKS_2\Programs\GIMP 2\Python\python
# -*- coding: utf-8 -*-
from __future__ import print_function
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\2_image-prog\1_gimp\1_\libs_1\libs_2_1_2.py
orig :
at : 2018/04/04 12:39:39
pushd C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\2_image-prog\1_gimp\1_\libs_1
python libs_2_1_2.py
C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\1_
'''
'''
<python-fu console> ---------------------------------
import os, sys
path = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\1_"
os.chdir(path)
os.getcwd()
sys.path.append('.')
from libs_1 import abc
from libs_1 import lib_2_1_2 as lib
# from libs_1 import lib_2_1_2
# from libs_1 import 2_1_2
lib_2_1_2.test_1()
#ref reload https://atkonn.blogspot.jp/2008/02/python-python37.html
reload(lib)
lib.main()
'''
# from __future__ import print_function
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog') # libs_VX7GLZ
# sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\libs_image_prog') # libs_VX7GLZ
from libs_image_prog import libs
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, math as math, random as rnd
###############################################
import wave, struct
# import numpy as np
# from pylab import *
def test_1():
print(os.getcwd())
fname = "test.%s.txt" % (libs.get_TimeLabel_Now())
f = open(fname, "w")
# f = open("test.txt", "w")
f.write(libs.get_TimeLabel_Now())
f.close
print("file closed")
#/ def test_1():
### height : 画像データの高さ (px)
def create_image(width, height):
# 画像データを生成
return gimp.Image(width, height, RGB)
# レイヤーの追加
## 指定した名前のレイヤーを新規に作成し、画像データに挿入する
### image : レイヤーを追加する画像データ
### name : 新規に作成するレイヤーの名前(文字列)
def add_layer(image, name):
# レイヤーの作成に必要なパラメータ
width = image.width
height = image.height
type = RGB_IMAGE
opacity = 100
mode = NORMAL_MODE
#
# パラメータをもとにレイヤーを作成
layer = gimp.Layer(image, name, width, height, type, opacity, mode)
#
# レイヤーを背景色で塗りつぶす(GIMP のデフォルトの挙動に合わせています)
layer.fill(1)
#
# 画像データの 0 番目の位置にレイヤーを挿入する
position = 0
image.add_layer(layer, position)
#
return layer
# ペンシルツールで線を描く
## 配列に格納した座標列を結ぶ線を描画領域にペンシルツールで描く
### drawable : 描画領域(レイヤーなど)
### lines : 描画される線の座標列を格納した配列
def draw_pencil_lines(drawable, lines):
# ペンシルツールで線を描画する
pdb.gimp_pencil(drawable, len(lines), lines)
# ペンシルツールで矩形を描く
## 左上、右下座標をもとに描画領域に矩形を描く
### drawable : 描画領域(レイヤーなど)
### x1 : 左上の X 座標
### y1 : 左上の Y 座標
### x2 : 右下の X 座標
### y2 : 右下の Y 座標
def draw_rect(drawable, x1, y1, x2, y2):
lines = [x1, y1, x2, y1, x2, y2, x1, y2, x1, y1]
draw_pencil_lines(drawable, lines)
# エアブラシで線を描く
## 配列に格納した座標列を結ぶ線を描画領域にエアブラシで描く
### drawable : 描画領域(レイヤーなど)
### pressure : 筆圧 (0-100)
### lines : 描画される線の座標列を格納した配列
def draw_airbrush_lines(drawable, pressure, lines):
# エアブラシで線を描画する
pdb.gimp_airbrush(drawable, pressure, len(lines), lines)
# 文字列を描画する
## 指定した描画領域に文字列を描画します
### drawable : 描画領域(レイヤーなど)
### x : 文字列を描画する位置の X 座標
### y : 文字列を描画する位置の Y 座標
### size : フォントサイズ
### str : 描画する文字列
def draw_text(drawable, x, y, size, str):
image = drawable.image
border = -1
antialias = True
size_type = PIXELS
fontname = '*'
floating_sel = pdb.gimp_text_fontname(image, drawable, x, y, str, border,
antialias, size, size_type, fontname)
pdb.gimp_floating_sel_anchor(floating_sel)
# 描画する色を変更する
## パレットの前景色を変更して描画色を設定する
### r : 赤要素 (0-255)
### g : 緑要素 (0-255)
### b : 青要素 (0-255)
### a : 透明度 (0-1.0)
def set_color(r, g, b, a):
color = (r, g, b, a)
pdb.gimp_context_set_foreground(color)
# 描画する線の太さを変える
## ブラシのサイズを変更して線の太さを設定する
### width : 線の太さ
def set_line_width(width):
pdb.gimp_context_set_brush_size(width)
# 画像の表示
## 新しいウィンドウを作成し、画像データを表示する
### image : 表示する画像データ
def display_image(image):
gimp.Display(image)
def main():
# image = create_image(640, 800)
image = create_image(640, 400)
layer = add_layer(image, "背景")
draw_rect(layer, 390, 210, 490, 310)
draw_text(layer, 200, 180, 20, "こんにちは")
lines = [110,90, 120,180, 130,110, 140,150]
draw_airbrush_lines(layer, 75, lines)
set_color(255,0,0,1.0) # Red
set_line_width(1)
draw_rect(layer, 420, 240, 520, 340)
display_image(image)
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgRigid2D.js
/***************************************************************************************
swgRigid2D.js
Rigid2Dクラス
****************************************************************************************/
function Rigid2D()
{
this.kind = "TRIANGLE";
this.color = new Float32Array([0.0, 0.0, 0.0, 1.0]);
this.flagFill = false;
this.verteces = [];
this.angle = 0.0;
this.transX = 0.0;
this.transY = 0.0;
this.scaleX = 1.0;
this.scaleY = 1.0;
}
Rigid2D.prototype.draw = function()
{
if(this.kind == "TRIANGLE") this.vertices = makeTriangle();
else if(this.kind == "RECTANGLE") this.vertices = makeRectangle(this.flagFill);
else if(this.kind == "CIRCLE") this.vertices = makeCircle(this.flagFill);
var hpwLoc = gl.getUniformLocation(gl.program, 'u_hpw');
gl.uniform1f(hpwLoc, hpw);
var angLoc = gl.getUniformLocation(gl.program, 'u_angle');
gl.uniform1f(angLoc, this.angle);
var transXLoc = gl.getUniformLocation(gl.program, 'u_tx');
gl.uniform1f(transXLoc, this.transX);
var transYLoc = gl.getUniformLocation(gl.program, 'u_ty');
gl.uniform1f(transYLoc, this.transY);
var scaleXLoc = gl.getUniformLocation(gl.program, 'u_sx');
gl.uniform1f(scaleXLoc, this.scaleX);
var scaleYLoc = gl.getUniformLocation(gl.program, 'u_sy');
gl.uniform1f(scaleYLoc, this.scaleY);
var colLoc = gl.getUniformLocation(gl.program, 'u_color');
gl.uniform4fv(colLoc, this.color);
// バッファオブジェクトを作成する
var vertexBuffer = gl.createBuffer();
// バッファオブジェクトをバインドする
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
// バッファオブジェクトにデータを書き込む
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(this.vertices), gl.STATIC_DRAW);
//attribute変数の格納場所を取得する
var vertexLoc = gl.getAttribLocation(gl.program, 'a_vertex');
//vertex変数にバッファオブジェクトを割り当てる
gl.vertexAttribPointer(vertexLoc, 2, gl.FLOAT, false, 0, 0);
// a_vertex変数でのバッファオブジェクトの割り当てを有効にする
gl.enableVertexAttribArray(vertexLoc);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
//図形の描画
if(this.flagFill == "true")
{
if(this.kind == "CIRCLE")
{//円
gl.drawArrays(gl.TRIANGLE_FAN, 0, this.vertices.length/2);
}
else
{//三角形と四角形
gl.drawArrays(gl.TRIANGLES, 0, this.vertices.length/2);
}
}
else
{//塗りつぶしなし
gl.drawArrays(gl.LINE_LOOP, 0, this.vertices.length/2);
}
}
/****************************************************************
頂点座標作成
*****************************************************************/
function makeTriangle()
{
var vertices = [//正三角形
-0.5, -0.288675,
0.5, -0.288675,
0.0, 0.57735
];
return vertices;
}
function makeRectangle(flagFill)
{
if(flagFill == "true")//塗りつぶし
{
var vertices = [
0.5, 0.5, -0.5, 0.5, -0.5, -0.5,
0.5, 0.5, -0.5,-0.5, 0.5, -0.5
];
}
else//線画
{
var vertices = [
0.5, 0.5, -0.5, 0.5, -0.5, -0.5, 0.5, -0.5
];
}
return vertices;
}
function makeCircle(flagFill)
{
var nSlice = 10;
var theta0 = 2.0 * Math.PI / nSlice;
var theta, x, y;
var vertices = [];
if(flagFill == "true")
{
vertices[0] = 0.0; vertices[1] = 0.0; //中心点
for(var i = 0; i <= nSlice; i++)
{
theta = i * theta0;
x = 0.5 * Math.cos(theta);
y = 0.5 * Math.sin(theta);
vertices[2 + i * 2] = x;
vertices[3 + i * 2] = y;
}
}
else
{
for(var i = 0; i < nSlice; i++)
{
theta = i * theta0;
x = 0.5 * Math.cos(theta);
y = 0.5 * Math.sin(theta);
vertices[0 + i * 2] = x;
vertices[1 + i * 2] = y;
}
}
return vertices;
}
<file_sep>/h.bat
call C:\WORKS_2\WS\WS_Music\h.bat
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/anims/utils/open_image_dir.bat
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\images
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\img.corners
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\csv<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/sp/views.py
'''###################
data dir
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data
###################'''
# from django.shortcuts import render
'''###################
django modules
###################'''
from django.http import HttpResponse
from django.shortcuts import render
from django import template
from mailbox import fcntl
'''###################
import : built-in modules
###################'''
import subprocess, copy, re, clipboard, time, \
os, datetime, ftplib, glob, sys, cv2 \
, matplotlib.pyplot as plt, codecs, shutil, numpy as np
'''###################
import : orig modules
###################'''
# sys.path.append('.')
# sys.path.append('..')
# sys.path.append('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/VIRTUAL/Admin_Projects')
# sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects')
from libs_admin import libs, lib_ip, cons_ip
# from Admin_Projects.libs_admin import libs
# sys.path.append('C:/WORKS_2/WS/WS_Others/prog/D-7/2_2/VIRTUAL/Admin_Projects/mm')
#
# from mm.libs_mm import cons_mm, cons_fx, libs, libfx
'''###################
vars : global
###################'''
numOf_DosAttack = 0
# Create your views here.
# def index():
def index(request):
now = datetime.datetime.now()
action = "index"
message = "yes"
page_Title = "Image Processing"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
return render(request, 'ip/index.html', dic)
# return HttpResponse(html)
# # return HttpResponse("Hello Django (new urls.py file)")
def test(request):
now = datetime.datetime.now()
action = "test"
message = "yes"
page_Title = "Image Processing"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
return render(request, 'ip/test.html', dic)
# return HttpResponse(html)
# # return HttpResponse("Hello Django (new urls.py file)")
def _anims__Load_LO_Actions():
dpath_List = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data"
fname_List = "anims_listof_commands.dat"
fpath_List = os.path.join(dpath_List, fname_List)
f = open(fpath_List, "r")
# header line
f.readline()
# body lines
lines = f.readlines()
# close file
f.close()
lo_Commands = []
# build list
for item in lines:
lo_Commands.append(item.split("\t")[1:])
#/for item in lines:
#debug
print()
print("[%s:%d] lo_Commands =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_Commands)
return lo_Commands
#/ def _anims__Load_LO_Actions()(request):
def _anims_JS__1_Move_Leaves__Test_1_Resize__exec(angle, scale, img, center, fpath_Dst):
# angle_45 = 45
# scale = 1.0
(h, w) = img.shape[:2]
# center = (365, 203)
# center = (w / 2, h / 2)
M = cv2.getRotationMatrix2D(center, angle, scale)
rotated = cv2.warpAffine(img, M, (h, w))
# tokens = fname_Image_File.split(".")
# fname_Dst = "%s.%s.(rotate=%d).%s.%s" \
# % (tokens[0], tokens[1], angle_45, libs.get_TimeLabel_Now(), tokens[2])
# fpath_Image_File__Dst = os.path.join(dpath_Image_File, fname_Dst)
cv2.imwrite(fpath_Dst, rotated)
#/ def _anims_JS__1_Move_Leaves__Test_1_Resize__exec():
def _anims_JS__1_Move_Leaves__Test_1_Resize(request):
'''###################
prep
###################'''
dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\data_images"
fname_Image_File = "leaf-2.1.png" # orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\4_animations\1_\5_\images
fpath_Image_File = os.path.join(dpath_Image_File, fname_Image_File)
print("[%s:%d] fpath_Image_File = %s (exists = %s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image_File, os.path.isfile(fpath_Image_File)
), file=sys.stderr)
'''###################
prep : params
main.js :: _anims_Action_LinkTo__1(_param)
, option_tick_move_X : _option_tick_move_X
, option_tick_move_Y : _option_tick_move_Y
, opt_rotate_Start : _opt_rotate_Start
, opt_rotate_End : _opt_rotate_End
###################'''
param_Loc_Start_X = request.GET.get('option_loc_start_X', False)
param_Loc_Start_Y = request.GET.get('option_loc_start_Y', False)
param_Cut_Width = request.GET.get('opt_cut_width', False)
param_Cut_Height = request.GET.get('opt_cut_height', False)
# modify
# ref tertiary https://stackoverflow.com/questions/394809/does-python-have-a-ternary-conditional-operator
param_Loc_Start_X = 0 if (param_Loc_Start_X == False) else int(param_Loc_Start_X)
param_Loc_Start_Y = 0 if (param_Loc_Start_Y == False) else int(param_Loc_Start_Y)
param_Cut_Width = 100 if (param_Cut_Width == False) else int(param_Cut_Width)
param_Cut_Height = 100 if (param_Cut_Height == False) else int(param_Cut_Height)
# print("[%s:%d] param_Loc_Start_X = %d, param_Loc_Start_Y = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , param_Loc_Start_X, param_Loc_Start_Y
# ), file=sys.stderr)
#abcde
'''###################
op
###################'''
'''###################
img : read
###################'''
#ref http://peaceandhilightandpython.hatenablog.com/entry/2016/01/09/214333
#ref alpha channel http://opencv.blog.jp/python/alpha_channel
img = cv2.imread(fpath_Image_File, cv2.IMREAD_UNCHANGED)
# img = cv2.imread(fpath_Image_File)
# img = cv2.imread(fpath_Image_File, cv2.IMREAD_COLOR)
# orgHeight, orgWidth = img.shape[:2]
# size = (int(orgWidth/2), int(orgHeight/2))
# # size = (int(orgHeight/2), int(orgWidth/2))
#
# # size = (orgHeight/2, orgWidth/2)
#
# halfImg = cv2.resize(img, size)
#
# tokens = fname_Image_File.split(".")
# fname_Half = "%s.%s.(half).%s.%s" \
# % (tokens[0], tokens[1], libs.get_TimeLabel_Now(), tokens[2])
#
# cv2.imwrite(os.path.join(dpath_Image_File, fname_Half), halfImg)
# cv2.imwrite('half.jpg', halfImg)
'''###################
rotate
###################'''
tokens = fname_Image_File.split(".")
(h, w) = img.shape[:2]
tlabel = libs.get_TimeLabel_Now()
#
# for i in range(0, 10):
# # for i in range(0, 5):
#
# angle = i * 5
# scale = 1.0
#
# #ref radians https://docs.scipy.org/doc/numpy/reference/generated/numpy.radians.html
# span = int((370 - w / 2) * np.sin(np.radians(angle)))
# span_w = int((370 - w / 2) * np.cos(np.radians(angle)))
#
# center = (w / 2 + span_w, h / 2 + span)
# # center = (w / 2, h / 2 + span)
# # center = (w / 2, h / 2 - span)
# # (h, w) = img.shape[:2]
# # center = (w / 2, h / 2)
#
# fname_Dst = "%s.%s.(rotate=%d).%s.(center=%d,%d).%s" \
# % (tokens[0], tokens[1], angle, tlabel, center[0], center[1], tokens[2])
# # % (tokens[0], tokens[1], angle, tlabel, tokens[2])
# # % (tokens[0], tokens[1], angle, libs.get_TimeLabel_Now(), tokens[2])
#
# fpath_Dst = os.path.join(dpath_Image_File, fname_Dst)
#
# _anims_JS__1_Move_Leaves__Test_1_Resize__exec(angle, scale, img, center, fpath_Dst)
#
#
# #/for i in range(0, 10):
'''###################
move image
###################'''
#ref https://stackoverflow.com/questions/23464495/fastest-way-to-move-image-in-opencv
fname_Dst = "%s.%s.(moved).%s.%s" \
% (tokens[0], tokens[1], tlabel, tokens[2])
fpath_Dst = os.path.join(dpath_Image_File, fname_Dst)
print("[%s:%d] fname_Dst => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Dst
), file=sys.stderr)
img_Partial = img[
param_Loc_Start_Y : param_Loc_Start_Y + param_Cut_Height
, param_Loc_Start_X : param_Loc_Start_X + param_Cut_Width
] # h, w
# img_Partial = img[param_Loc_Start_Y : 270, param_Loc_Start_X : 420] # h, w
# img_Partial = img[150 : 270, 300 : 420] # h, w
# img_Partial = img[0 : 100, 0 : 50] # h, w
# img_Partial = img[cv2.Rect(0, 0, 100, 100)]
# img_Partial = img(cv2.Rect(0, 0, 100, 100))
cv2.imwrite(fpath_Dst, img_Partial)
# cv2.imwrite(fname_Dst, img_Partial)
# #ref https://www.tutorialkart.com/opencv/python/opencv-python-rotate-image/#opencv-python-rotate-image
# angle_45 = 45
# scale = 1.0
# (h, w) = img.shape[:2]
# center = (365, 203)
# # center = (w / 2, h / 2)
#
# M = cv2.getRotationMatrix2D(center, angle_45, scale)
# rotated_45 = cv2.warpAffine(img, M, (h, w))
# # rotated90 = cv2.warpAffine(img, M, (h, w))
#
# tokens = fname_Image_File.split(".")
# fname_Dst = "%s.%s.(rotate=%d).%s.%s" \
# % (tokens[0], tokens[1], angle_45, libs.get_TimeLabel_Now(), tokens[2])
#
# fpath_Image_File__Dst = os.path.join(dpath_Image_File, fname_Dst)
#
# cv2.imwrite(fpath_Image_File__Dst, rotated_45)
#/ def _anims_JS__1_Move_Leaves__Test_1_Resize():
def _anims_JS__1_Move_Leaves(request):
'''###################
ops
###################'''
_anims_JS__1_Move_Leaves__Test_1_Resize(request)
'''###################
return
###################'''
status = 1
msg = "OK"
return (status, msg)
#/ def _anims_JS__1_Move_Leaves(request):
def anims_JS(request):
'''###################
time
###################'''
time_Exec_Start = time.time()
'''###################
vars
###################'''
dic = {
"message" : ""
, "message_2" : ""
}
render_Page = 'ip/anims/plain_anims.html'
'''###################
params
###################'''
param = request.GET.get('param', False)
#debug
# print("[%s:%d] param => %s" % \
print("[%s:%d] param => %s ============================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param
), file=sys.stderr)
'''###################
dispatch
###################'''
if param == cons_ip.Anims_Params.PARAM__1_MOVE_LEAVES.value : #if param == cons_fx.Tester.lo_Actions__BUSL__IDs[0].value
'''###################
PARAM__1_MOVE_LEAVES
###################'''
# call func
(status, msg) = _anims_JS__1_Move_Leaves(request)
dic['message'] += "move leaves"
dic['message_2'] += "status = %d / msg = '%s'" % (status, msg)
'''###################
time
###################'''
time_Exec_Elapsed = time.time() - time_Exec_Start
dic['message_2'] += "(time = %s) (elapsed = %02.3f sec)" % \
(libs.get_TimeLabel_Now(), time_Exec_Elapsed)
'''###################
render
###################'''
return render(request, render_Page, dic)
#/ def anims_JS(request):
def anims(request):
now = datetime.datetime.now()
action = "anims"
message = "yes"
page_Title = "IP / anims"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
'''###################
list of commands
###################'''
lo_Commands = _anims__Load_LO_Actions()
# lo_Commands = [
#
# ["move_leaves", "image sequence for moving leaves"],
#
# ["???", "unknown"],
# ]
# set var
dic["lo_Commands"] = lo_Commands
dic["count"] = 0
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/anims/anims.html', dic)
# return render(request, 'ip/basics.html', dic)
# return render(request, 'mm/numbering.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/anims/anims_full.html', dic)
# return render(request, 'ip/basics_full.html', dic)
#/ anims
def basics(request):
now = datetime.datetime.now()
action = "basics"
message = "yes"
page_Title = "IP / basics"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
'''###################
list of commands
###################'''
lo_Commands = [
["get_4_Corners", "4 corners of an image file"],
["???", "unknown"],
]
# set var
dic["lo_Commands"] = lo_Commands
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/basics.html', dic)
# return render(request, 'mm/numbering.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/basics_full.html', dic)
def get_4_corners(request):
'''###################
vars
###################'''
dic = {}
'''###################
get : files list
###################'''
dpath_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6" \
+ "\\46_art\\VIRTUAL\\Admin_Projects" \
+ "\\ip" \
+ "\\images"
fpath_Glob = "%s\\*" % (dpath_Images)
#ref glob https://stackoverflow.com/questions/14798220/how-can-i-search-sub-folders-using-glob-glob-module-in-python answered Feb 10 '13 at 13:31
lo_Files = glob.glob(fpath_Glob)
# files only
lo_Files_Filtered = []
# #debug
# print()
# print("[%s:%d] os.path.isfile(dpath_Images + \"\\\" + lo_Files[0]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , os.path.isfile(dpath_Images + "\\" + lo_Files[0])
# ), file=sys.stderr)
#
# print("[%s:%d] dpath_Images + \"\\\" + lo_Files[0] => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , dpath_Images + "\\" + lo_Files[0]
# ), file=sys.stderr)
for item in lo_Files:
# if os.path.isfile(dpath_Images + "\\" + item) == True :
if os.path.isfile(item) == True :
lo_Files_Filtered.append(item)
#/for item in lo_Files:
# update the list
lo_Files = lo_Files_Filtered
lo_Files.sort()
# set list
dic['lo_Files'] = [os.path.basename(x) for x in lo_Files]
# set : dpath
dic['dpath_Images'] = dpath_Images
'''###################
dflt : corner values
###################'''
dic['dflt_Corner_Width'] = cons_ip.DfltVals.get_4Corners__Corner_Width.value
dic['dflt_Corner_Padding'] = cons_ip.DfltVals.get_4Corners__Corner_Padding.value
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8001/ip/basics/"
# referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/get_4_corners.html', dic)
# return render(request, 'mm/numbering.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/get_4_corners_full.html', dic)
'''###################
__ip_ops__gradation
at : 2018/06/30 09:15:53
###################'''
def __ip_ops__gradation(request):
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
# meta data
height, width, channels = img_Orig.shape
'''###################
return
###################'''
url_Path_Full = "ip/ops/ip_ops__gradation_full.html"
url_Path = "ip/ops/ip_ops__gradation.html"
dic = {"msg" : "gradation : ip_ops__gradation.html"}
return (url_Path_Full, url_Path, dic)
#/ def __ip_ops__gradation(request):
'''###################
ip_ops
###################'''
def ip_ops(request):
'''###################
params
###################'''
request_Commands = request.GET.get('commands', False)
'''###################
vars
###################'''
dic = {}
'''###################
ops
###################'''
reqCmd_Gradation = "gradation"
if request_Commands : #if request_Commands
if request_Commands == reqCmd_Gradation : #if request_Commands == reqCmd_Gradation
url_Path_Full, url_Path, dic = __ip_ops__gradation(request)
else : #if request_Commands == reqCmd_Gradation
url_Path_Full = "ip/ops/ip_ops_full.html"
url_Path = "ip/ops/ip_ops.html"
dic['msg'] = "commands => %s" % request_Commands
#/if request_Commands == reqCmd_Gradation
else : #if request_Commands
url_Path_Full = "ip/ops/ip_ops_full.html"
url_Path = "ip/ops/ip_ops.html"
dic['msg'] = "ip_ops.html"
#/if request_Commands
# url_Path_Full, url_Path, dic = __ip_ops__gradation(request)
# url_Path_Full = "ip/ops/ip_ops_full.html"
# url_Path = "ip/ops/ip_ops.html"
# url_Path_Full, url_Path, dic = __ip_ops__gradation(request)
# url_Path_Full = "ip/ops/ip_ops_full.html"
# url_Path = "ip/ops/ip_ops.html"
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8001/ip/basics/"
# referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, url_Path, dic)
# return render(request, 'ip/get_4_corners.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, url_Path_Full, dic)
# return render(request, 'ip/get_4_corners_full.html', dic)
#/ def ip_ops(request):
'''###################
get_Corner_Images(img_Src, corner_Length)
@return: [left bottom, right bottom, left up, right up]
|------------------------|
|(2) (3)|
| |
| |
| |
|(0) (1)|
|------------------------|
###################'''
def get_Corner_Images(img_Src, corner_Length) :
height, width, channels = img_Src.shape
clips = [
img_Src[(height - corner_Length) : height, 0 : corner_Length], # clp_LB
img_Src[(height - corner_Length) : height, width - corner_Length : width], # clp_RB
img_Src[0 : corner_Length, 0 : corner_Length], # clp_LU
img_Src[0 : corner_Length, width - corner_Length : width], # clp_RU
]
# return
return clips
#/ def get_Corner_Images(img_RGB, corner_Length) :
# def _exec_get_4_corners__Write_Log(lo_Names_Of_Corner_Images, lo_Image_MetaData):
def _exec_get_4_corners__Write_Log \
(lo_Names_Of_Corner_Images, lo_Image_MetaData, lo_Image_StatsData
, dpath_Images
, fname_Image
, res
, comment
):
dpath_Log = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\logs"
fname_Log = "get_4_corners.log"
fpath_Log = "%s\\%s" % (dpath_Log, fname_Log)
fout_Log = open(fpath_Log, "a")
# header
fout_Log.write(
"[%s %s:%s] =============== Get 4 corners" % \
(libs.get_TimeLabel_Now(),
os.path.basename(libs.thisfile()),
libs.linenum()))
fout_Log.write("\n")
'''###################
write : meta info
###################'''
msg = "dpath_Images = %s" % (dpath_Images)
fout_Log.write(msg)
fout_Log.write('\n')
msg = "fname_Image = %s" % (fname_Image)
fout_Log.write(msg)
fout_Log.write('\n')
fout_Log.write('\n')
# iterate
idxOf_Images = 0
lenOf_LO_Names_Of_Corner_Images = len(lo_Names_Of_Corner_Images)
# for item in lo_Names_Of_Corner_Images:
for i in range(lenOf_LO_Names_Of_Corner_Images):
# items
name = lo_Names_Of_Corner_Images[i]
# meta data
metaData = lo_Image_MetaData[i]
# stats
# [{'skew_values': {'skew_B': 533.0519884872008, 'skew_R': 236.23069368923885, 'sk
# ew_G': 238.3791814689376}}, {'skew_values': {'skew_B': 56.682440339675104, 'skew
# _R': 149.4848026940312, 'skew_G': 78.97239727258551}}, {'skew_values': {'skew_B'
# : 494.3158542205711, 'skew_R': 312.1150017522547, 'skew_G': 465.10712589168577}}
# , {'skew_values': {'skew_B': 481.71441048360913, 'skew_R': 323.66383568902853, '
# skew_G': 475.05481070433}}]
do_Stats = lo_Image_StatsData[i]
do_Skews = do_Stats['skew_values']
# print()
# print("[%s:%d] lo_Image_StatsData =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Image_StatsData)
#
# print()
# print("[%s:%d] do_Stats =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(do_Stats)
skew_R = do_Skews['skew_R']
skew_G = do_Skews['skew_G']
skew_B = do_Skews['skew_B']
# skew_R = do_Stats['skew_R']
# skew_G = do_Stats['skew_G']
# skew_B = do_Stats['skew_B']
'''###################
file name
###################'''
# file name
fout_Log.write(name)
# fout_Log.write(item)
fout_Log.write('\n')
# meta data
# print()
# print("[%s:%d] type(metaData) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData)
# ), file=sys.stderr)
#
# print("[%s:%d] type(metaData[0]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData[0])
# ), file=sys.stderr)
#
# print("[%s:%d] type(metaData[1]) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(metaData[1])
# ), file=sys.stderr)
# max_R
# , max_G
# , max_B
#
# , min_R
# , min_G
# , min_B
# , valsOf_R
# , valsOf_G
# , valsOf_B
msg = "R=(%d,%d) G=(%d,%d) B=(%d,%d)" % \
(metaData[0], metaData[3], metaData[1]
, metaData[4], metaData[2], metaData[5]
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
'''###################
skews
###################'''
msg = "skew_R = %.04f, skew_G = %.04f, skew_B = %.04f" % \
(
skew_R, skew_G, skew_B
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
'''###################
index of max values,
max values
###################'''
idxOf_MaxVals = do_Stats['idxOf_Maxes']
max_Vals = do_Stats['max_Vals']
# msg = "idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
msg = "idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
(
idxOf_MaxVals[0], idxOf_MaxVals[1], idxOf_MaxVals[2]
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
msg = "max_Vals_R = %d, max_Vals_G = %d, max_Vals_B = %d" % \
(
max_Vals[0], max_Vals[1], max_Vals[2]
)
# msg = "\t".join(metaData)
fout_Log.write(msg)
# fout_Log.write("\t".join(metaData))
fout_Log.write('\n')
'''###################
raw data : histogram
###################'''
dat = [str(x) for x in metaData[6]]
msg = "\t".join(dat)
# msg = "\t".join(metaData[6])
fout_Log.write(msg)
fout_Log.write('\n')
dat = [str(x) for x in metaData[7]]
msg = "\t".join(dat)
fout_Log.write(msg)
fout_Log.write('\n')
dat = [str(x) for x in metaData[8]]
msg = "\t".join(dat)
fout_Log.write(msg)
fout_Log.write('\n')
fout_Log.write('\n')
#/for item in lo_Names_Of_Corner_Images:
# separator line
# fout_Log.write('\n')
'''###################
write : judge
###################'''
# fout_Log.write('\n')
# msg = "dpath_Images = %s" % (dpath_Images)
#
# fout_Log.write(msg)
# fout_Log.write('\n')
#
# msg = "fname_Image = %s" % (fname_Image)
#
# fout_Log.write(msg)
# fout_Log.write('\n')
msg = "is_CornerOf_Green__PhotoOf_Sweets => %s (%s)" % (res, comment)
fout_Log.write(msg)
fout_Log.write('\n')
# close file
fout_Log.close()
#/ def _exec_get_4_corners__Write_Log(lo_Names_Of_Corner_Images):
def _exec_get_4_corners__Get_MetaData(img_Corners):
# data
lo_Image_MetaData = []
for item in img_Corners:
'''###################
vars
###################'''
max_R = -1; max_G = -1; max_B = -1
min_R = 256; min_G = 256; min_B = 256
# min_R = 255; min_G = 255; min_B = 255
# counter
cntOf_Row = 0
cntOf_Cell = 0
# values
valsOf_R = [0] * 256
valsOf_G = [0] * 256
valsOf_B = [0] * 256
for row in item:
for cell in row:
# #debug
# print()
# print("[%s:%d] len(cell) => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(cell)
# ), file=sys.stderr)
# return
# get value
R = cell[0]; G = cell[1]; B = cell[2]
# histogram
valsOf_R[R] += 1
valsOf_G[G] += 1
valsOf_B[B] += 1
# max value
if R > max_R : max_R = R
if G > max_G : max_G = G
if B > max_B : max_B = B
# min value
if R < min_R : min_R = R
if G < min_G : min_G = G
if B < min_B : min_B = B
# count
cntOf_Cell += 1
# reset count of cells
cntOf_Cell = 0
# count
cntOf_Row += 1
#/for row in item:
# append data
lo_Image_MetaData.append(
[
# valsOf_R
# , valsOf_G
# , valsOf_B
# , max_R
max_R
, max_G
, max_B
, min_R
, min_G
, min_B
, valsOf_R
, valsOf_G
, valsOf_B
]
)
#/for item in img_Corners:
'''###################
return
###################'''
#debug
print()
print("[%s:%d] len(lo_Image_MetaData) => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(lo_Image_MetaData)
), file=sys.stderr)
return lo_Image_MetaData
#/ def _exec_get_4_corners__Get_MetaData(img_Corners):
'''==============================================
_exec_get_4_corners__Get_StatsData(img_Corners)
at : 2018/05/28 07:35:40
@param img_Corners: list of opencv image data (4 corners of a original image)
@return: list of dictionaries
[{'skew' : 3.032, 'kurtosis' : 1.2230, 'integral' : 3428322},...]
=============================================='''
def _exec_get_4_corners__Get_StatsData(img_Corners):
# data
lo_Image_StatsData = []
for img_Data in img_Corners:
# var
do_StasData = {}
'''###################
skews
###################'''
print("[%s:%d] getting skew values..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
skew_Values = lib_ip.get_Skews(img_Data)
# skew = lib_ip.get_Skew(img_Data)
do_StasData['skew_values'] = skew_Values
# do_StasData['skew'] = skew
# append
lo_Image_StatsData.append(do_StasData)
'''###################
index of max
###################'''
# idxOf_Max_R, idxOf_Max_G, idxOf_Max_B = lib_ip.get_IdxOf_Maxes(img_Data)
idxOf_Max_R, idxOf_Max_G, idxOf_Max_B, maxVal_R, maxVal_G, maxVal_B \
= lib_ip.get_IdxOf_Maxes(img_Data)
print()
print("[%s:%d] idxOf_Max_R = %d, idxOf_Max_G = %d, idxOf_Max_B = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, idxOf_Max_R, idxOf_Max_G, idxOf_Max_B
), file=sys.stderr)
do_StasData['idxOf_Maxes'] = [idxOf_Max_R, idxOf_Max_G, idxOf_Max_B]
do_StasData['max_Vals'] = [maxVal_R, maxVal_G, maxVal_B]
#/for img in img_Corners:
'''###################
return
###################'''
return lo_Image_StatsData
#/ def _exec_get_4_corners__Get_StatsData(img_Corners):
def _exec_get_4_corners__SaveImage_4Corners(img_Corners, fname_Image):
# count
cntOf_Corners = 1
# time label
tlabel = libs.get_TimeLabel_Now()
# paths
dpath_Plot= "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\img.corners"
lo_Names_Of_Corner_Images = []
for item in img_Corners:
# xpixels = item.shape[1]
# ypixels = item.shape[0]
#
# dpi = 72
# scalefactor = 1
#
# xinch = xpixels * scalefactor / dpi
# yinch = ypixels * scalefactor / dpi
#
# fig = plt.figure(figsize=(xinch,yinch))
#
# plt.imshow(item)
# dpath_Plot= "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\img.corners"
fname_Plot = "img.%s.%s.%d.png" % (tlabel, fname_Image, cntOf_Corners)
# fname_Plot = "%s.%s.%d.png" % (fname_Image, tlabel, cntOf_Corners)
fpath_Plot = "%s\\%s" % (dpath_Plot, fname_Plot)
# increment
cntOf_Corners += 1
# plt.savefig(fpath_Plot, dpi=dpi)
# cv2 : save image
#ref https://www.tutorialkart.com/opencv/python/opencv-python-save-image-example/
cv2.imwrite(fpath_Plot, item)
#debug
print()
print("[%s:%d] fpath_Plot => '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Plot
), file=sys.stderr)
# append file name
lo_Names_Of_Corner_Images.append(fname_Plot)
# # reset plot
# plt.clf()
# plt.cla()
#/for item in im_Corners:
'''###################
return
###################'''
return lo_Names_Of_Corner_Images
#/ def _exec_get_4_corners__SaveImage_4Corners(img_Corners):
def gen_Cake_CSV__Get_Params(request):
'''###################
get : params
###################'''
dpath_Images = request.GET.get('dpath_images', False)
'''###################
get : params : save image
###################'''
# ref : function exec_Get_4Corners(fname) :: main.js
flg_save_image = request.GET.get('flg_save_image', False)
# set flag
flg_SaveImage = False
if flg_save_image == "true" : #if flg_save_image
flg_SaveImage = True
#/if flg_save_image
'''###################
get : params : corner_width
###################'''
# ref : function exec_Get_4Corners(fname) :: main.js
param_Corner_Width = request.GET.get('corner_width', False)
#debug
print()
print("[%s:%d] param_Corner_Width => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, int(param_Corner_Width)
), file=sys.stderr)
# convert
param_Corner_Width = int(param_Corner_Width)
'''###################
get : params : corner_Padding
###################'''
# ref : function exec_Get_4Corners(fname) :: main.js
param_Corner_Padding = request.GET.get('corner_Padding', False)
#debug
print()
print("[%s:%d] param_Corner_Padding => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, int(param_Corner_Padding)
), file=sys.stderr)
# convert
param_Corner_Padding = int(param_Corner_Padding)
'''###################
return
###################'''
return dpath_Images, flg_SaveImage, param_Corner_Width, param_Corner_Padding
#/ def gen_Cake_CSV__Get_Params(request):
def gen_Cake_CSV__Get_ListOf_Files(dpath_Images):
fpath_Glob = "%s\\*" % (dpath_Images)
#ref glob https://stackoverflow.com/questions/14798220/how-can-i-search-sub-folders-using-glob-glob-module-in-python answered Feb 10 '13 at 13:31
lo_Files = glob.glob(fpath_Glob)
# files only
lo_Files_Filtered = []
for item in lo_Files:
if os.path.isfile(item) == True :
lo_Files_Filtered.append(item)
#/for item in lo_Files:
# update the list
lo_Files = lo_Files_Filtered
lo_Files.sort()
# return
return lo_Files
#/ def gen_Cake_CSV__Get_ListOf_Files(dpath_Images):
def gen_Cake_CSV__Get_ColorName_Set(\
dpath_Images
, lo_Files
, flg_SaveImage
, param_Corner_Width
, param_Corner_Padding
):
'''###################
vars
###################'''
lo_ColorName_Set = []
'''###################
get color name set
###################'''
# flg_SaveImage = False
#debug
cnt = 0
# maxOf_Cnt = 30
# maxOf_Cnt = 20
# maxOf_Cnt = 5
maxOf_Cnt = 999
# maxOf_Cnt = 15
# maxOf_Cnt = 10
# maxOf_Cnt = 5
# maxOf_Cnt = 2
'''###################
time
###################'''
time_Exec_Start = time.time()
for fpath in lo_Files:
# for fname in lo_Files:
# count
print()
print("[%s:%d] processing image ===> %d -------------------" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cnt
), file=sys.stderr)
# judge
lo_ColorNames = lib_ip.get_ColorName_Set_From_Image(\
dpath_Images, fpath, flg_SaveImage, \
param_Corner_Width, param_Corner_Padding)
# append
lo_ColorName_Set.append([fpath, lo_ColorNames])
#debug
cnt += 1
if cnt >= maxOf_Cnt : break #if cnt >= maxOf_Cnt
#/for fname in lo_Files:
'''###################
time
###################'''
time_Exec_Elapsed = time.time() - time_Exec_Start
#msg = "gen csv => complete (%s)(elapsed = %02.3f sec)" % \
msg = "gen csv => complete (%s)(elapsed = %02.3f sec / %d files / %02.3f sec per file)" % \
(libs.get_TimeLabel_Now(), time_Exec_Elapsed, len(lo_Files), time_Exec_Elapsed / len(lo_Files))
#(libs.get_TimeLabel_Now(), time_Exec_Elapsed)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
return
###################'''
return lo_ColorName_Set
#/ def gen_Cake_CSV__Get_ColorName_Set(dpath_Images, lo_Files):
def gen_Cake_CSV__Gen_CSVFile(\
dpath_CSV, fname_CSV
, lo_ColorName_Set__Modified_2
, dpath_Images):
# def gen_Cake_CSV__Gen_CSVFile(dpath_CSV, fname_CSV, lo_ColorName_Set__Modified_2):
'''###################
vars
###################'''
lines = []
cnt = 1
'''###################
header
###################'''
hdr = "no\tfile_name\tmemos\tGPS"
# hdr = "no\tfile_name\tmemos"
lines.append(hdr)
'''###################
color name set
###################'''
for item in lo_ColorName_Set__Modified_2:
'''###################
vars
###################'''
fname = item[0]
'''###################
var : color_Names
e.g. "oooo" (string)
###################'''
color_Names = item[1]
'''###################
build : csv
###################'''
tmp_Line = []
tmp_Line.append(str(cnt))
# tmp_Line.append(cnt)
tmp_Line.append(fname)
'''###################
image content
###################'''
line_Memo = lib_ip.get_Memo_From_ColorNames_Set(color_Names)
tmp_Line.append(line_Memo)
# tmp_Line.append(line_Memo)
# tmp_Line.append("".join(color_Names))
'''###################
exif : gps data
###################'''
fpath_Image = os.path.join(dpath_Images, fname)
data_GPS = lib_ip.get_GPS_Data(fpath_Image)
# validate
if not data_GPS == False : #if not data_GPS == False
# tuple --> to list
data_GPS_Lat = [str(x) for x in data_GPS[0]] # N,S
data_GPS_Longi = [str(x) for x in data_GPS[1]] # E,W
# build text
gps_Lat = "-".join(data_GPS_Lat)
gps_Longi = "-".join(data_GPS_Longi)
# add to csv line
tmp_Line.append("%s %s" % (gps_Lat, gps_Longi))
#/if not data_GPS == False
# # tuple --> to list
# data_GPS_Lat = [str(x) for x in data_GPS[0]] # N,S
# data_GPS_Longi = [str(x) for x in data_GPS[1]] # E,W
# # data_GPS_Lat = [x for x in data_GPS[0]] # N,S
# # data_GPS_Longi = [x for x in data_GPS[1]] # E,W
#
# # build text
# gps_Lat = "-".join(data_GPS_Lat)
# gps_Longi = "-".join(data_GPS_Longi)
#
# # add to csv line
# tmp_Line.append("%s %s" % (gps_Lat, gps_Longi))
# tmp_Line.append("\t%s %s" % (gps_Lat, gps_Longi))
# lines.append("\t%s %s" % (gps_Lat, gps_Longi))
'''###################
append
###################'''
lines.append("\t".join(tmp_Line))
# count
cnt += 1
'''###################
write
###################'''
fpath_CSV = "%s/%s" % (dpath_CSV, fname_CSV)
fin = codecs.open(fpath_CSV, "w", 'utf-8')
# write
for item in lines:
fin.write(item)
fin.write("\n")
#/for item in lines:
# close
fin.close()
#debug
print()
print("[%s:%d] csv written : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_CSV
), file=sys.stderr)
#/ def gen_Cake_CSV__Gen_CSVFile(dpath_CSV, fname_CSV, lo_ColorName_Set__Modified_2):
def gen_Cake_CSV__Exec(request):
'''###################
vars
###################'''
dic = {}
'''###################
get : params
###################'''
dpath_Images, flg_SaveImage, param_Corner_Width, param_Corner_Padding \
= gen_Cake_CSV__Get_Params(request)
#debug
print()
print("[%s:%d] flg_SaveImage => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_SaveImage
# , flg_SaveImage
), file=sys.stderr)
'''###################
set : vars
###################'''
dic['dpath_Images'] = dpath_Images
'''###################
get : files list
###################'''
lo_Files = gen_Cake_CSV__Get_ListOf_Files(dpath_Images)
# print()
# print("[%s:%d] lo_Files[0] => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , lo_Files[0]
# ), file=sys.stderr)
print()
print("[%s:%d] dpath_Images => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, dpath_Images
), file=sys.stderr)
#debug
print()
print("[%s:%d] len(lo_Files) => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(lo_Files)
), file=sys.stderr)
'''###################
get : color name set
[
[
"C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\images",
[
[
"2018-08-21_23-28-01_000.1.mov",
"others"
],
[
"2018-08-21_23-28-01_000.2.mov",
"others"
],
...
],
...
],
...
]
###################'''
# lo_ColorName_Set = gen_Cake_CSV__Get_ColorName_Set(dpath_Images, lo_Files)
lo_ColorName_Set = gen_Cake_CSV__Get_ColorName_Set(\
dpath_Images
, lo_Files
, flg_SaveImage
, param_Corner_Width
, param_Corner_Padding)
'''###################
get : list of color names
###################'''
lo_ColorName_Set__Modified = []
for item in lo_ColorName_Set:
fname = os.path.basename(item[0])
colorName_Set = []
for item2 in item[1]:
colorName_Set.append(item2[1])
# append
lo_ColorName_Set__Modified.append([fname, colorName_Set])
msg = "lo_ColorName_Set__Modified =>"
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
# libs.write_Log(msg_Log, True)
# counter
cntOf_Write_FileData = 1
for item in lo_ColorName_Set__Modified:
fname = item[0]
color_Names = item[1]
strOf_Fname_Block = "%d)\t%s => \t" % (cntOf_Write_FileData, fname)
# increment
cntOf_Write_FileData += 1
# file name
libs.write_Log(strOf_Fname_Block, False)
# libs.write_Log(fname, False)
# libs.write_Log(" => ", False)
# color names
strOf_Color_Names = ""
for item2 in color_Names:
strOf_Color_Names += item2 + " "
#/for iteim2 in color_Names:
libs.write_Log(strOf_Color_Names, True)
#/for item in lo_ColorName_Set__Modified:
'''###################
get : picture genre
###################'''
lo_ColorName_Set__Modified_2 = []
for item in lo_ColorName_Set__Modified:
fname = item[0]
color_Names = item[1]
color_Names_New = []
for item2 in color_Names:
if item2 == "other" : colName = "o"
elif item2 == "red" : colName = "r"
elif item2 == "yellow" : colName = "y"
elif item2 == "green" : colName = "g"
elif item2 == "blue" : colName = "b"
elif item2 == "white" : colName = "w"
elif item2 == "black" : colName = "a"
elif item2 == "pink" : colName = "p"
else : colName = "u" #=> 'unknown'
color_Names_New.append(colName)
#/for item2 in color_Names:
# modify
color_Names_New.sort()
strOf_Color_Names_New = "".join(color_Names_New)
# append
lo_ColorName_Set__Modified_2.append([fname, strOf_Color_Names_New])
#/for item in lo_ColorName_Set__Modified:
'''###################
write : log : lo_ColorName_Set__Modified_2
###################'''
msg = "lo_ColorName_Set__Modified_2 =>"
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
# counter
cntOf_Write_FileData = 1
for item in lo_ColorName_Set__Modified_2:
fname = item[0]
color_Names = item[1]
strOf_Fname_Block = "%d)\t%s => \t" % (cntOf_Write_FileData, fname)
# increment
cntOf_Write_FileData += 1
# file name
libs.write_Log(strOf_Fname_Block, False)
# color names
strOf_Color_Names = ""
for item2 in color_Names:
strOf_Color_Names += item2 + " "
#/for iteim2 in color_Names:
libs.write_Log(strOf_Color_Names, True)
'''###################
gen : CSV file
###################'''
dpath_CSV = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\csv"
fname_CSV = "entries.%s.csv" % (libs.get_TimeLabel_Now())
res = gen_Cake_CSV__Gen_CSVFile(
dpath_CSV, fname_CSV
, lo_ColorName_Set__Modified_2
, dpath_Images)
'''###################
return
###################'''
return dic, lo_ColorName_Set__Modified_2
#/ def gen_Cake_CSV(request):
def gen_Cake_CSV(request):
'''###################
set : log file name
###################'''
# tlabel = libs.get_TimeLabel_Now()
# fname_Log = "get_4_corners.%s.log" % (tlabel)
#
# cons_ip.FilePaths.fname_LogFile.value = fname_Log
'''###################
title
###################'''
msg = "gen_Cake_CSV(request) ==> starting... ======================="
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
'''###################
get : dic, color name set
###################'''
dic, lo_ColorName_Set__Modified_2 = gen_Cake_CSV__Exec(request)
'''###################
ending message
###################'''
msg = "gen_Cake_CSV(request) ==> ending... =======================//"
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log(msg_Log, True)
libs.write_Log("", True)
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8001/ip/get_4_corners/"
# referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/gen_cake_csv.html', dic)
# return render(request, 'mm/numbering.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/gen_cake_csv_full.html', dic)
#/ def gen_Cake_CSV(request):
def exec_get_4_corners(request):
'''###################
debug
###################'''
msg = "exec_get_4_corners(request) ================================"
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
dpath_Log = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\logs"
fname_Log = "get_4_corners.log"
libs.write_Log(msg_Log, True)
'''###################
debug
http://127.0.0.1:8001/ip/exec_get_4_corners?dpath_images=C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\images&fname_image=IMG_3154.PNG
###################'''
'''###################
vars
###################'''
dic = {}
'''###################
get : params
###################'''
dpath_Images = request.GET.get('dpath_images', False)
fname_Image = request.GET.get('fname_image', False)
'''###################
get : params : save image
###################'''
# ref : function exec_Get_4Corners(fname) :: main.js
flg_save_image = request.GET.get('flg_save_image', False)
# set flag
flg_SaveImage = False
if flg_save_image == "true" : #if flg_save_image
flg_SaveImage = True
#/if flg_save_image
#debug
print()
print("[%s:%d] flg_SaveImage => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_SaveImage
), file=sys.stderr)
'''###################
get : params : corner_width
###################'''
# ref : function exec_Get_4Corners(fname) :: main.js
param_Corner_Width = request.GET.get('corner_width', False)
#debug
print()
print("[%s:%d] param_Corner_Width => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, int(param_Corner_Width)
), file=sys.stderr)
# convert
param_Corner_Width = int(param_Corner_Width)
'''###################
get : params : corner_Padding
###################'''
# ref : function exec_Get_4Corners(fname) :: main.js
param_Corner_Padding = request.GET.get('corner_Padding', False)
#debug
print()
print("[%s:%d] param_Corner_Padding => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, int(param_Corner_Padding)
), file=sys.stderr)
# convert
param_Corner_Padding = int(param_Corner_Padding)
'''###################
judge
###################'''
res, msg, (height, width, channels) = \
lib_ip.is_PhotoOf__Sweets(
dpath_Images
, fname_Image
, flg_SaveImage
, param_Corner_Width
, param_Corner_Padding
)
'''###################
set : vars
###################'''
dic['dpath_Images'] = dpath_Images
dic['fname_Image'] = fname_Image
dic['height'] = height
dic['width'] = width
dic['channels'] = channels
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8001/ip/get_4_corners/"
# referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/exec_get_4_corners.html', dic)
# return render(request, 'mm/numbering.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'ip/exec_get_4_corners_full.html', dic)
#/ def exec_get_4_corners(request):
# return render(request, 'ip/basics.html', dic)
#/ def basics(request):
def open_image_dir(request):
'''###################
vars
###################'''
dic = {}
print()
print("[%s:%d] opening image dir..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
build : command string
###################'''
command = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\utils\\open_image_dir.bat"
# command = "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\utils\open_image_dir.bat"
# command = "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\images"
# command = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\images"
# command = "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\images"
# command = "%s\\%s" % (cons_im.FPath.DPATH_CMD_LIB_WS_CAKE_IFM11.value, action)
# print()
# print("[%s:%d] _im_actions__Ops_13()" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
cmd_Full = [command] #=>
# cmd_Full = [command, arg1] #=>
print()
print("[%s:%d] command => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, command
), file=sys.stderr)
'''###################
subprocess
###################'''
#ref https://stackoverflow.com/questions/13525882/tasklist-output answered Nov 23 '12 at 9:36
res = subprocess.call(cmd_Full)
# res = subprocess.check_output(cmd_Full)
return render(request, 'ip/open_image_dir.html', dic)
#/ def open_image_dir(request):
def open_dir(request):
'''###################
params
###################'''
param = request.GET.get('param', False)
print("[%s:%d] param => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param
), file=sys.stderr)
'''###################
vars
###################'''
dic = {}
print()
print("[%s:%d] opening dir..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
build : command string
###################'''
command = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\utils\\open_anims_log_dir.bat"
cmd_Full = [command] #=>
print()
print("[%s:%d] command => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, command
), file=sys.stderr)
'''###################
subprocess
###################'''
#ref https://stackoverflow.com/questions/13525882/tasklist-output answered Nov 23 '12 at 9:36
res = subprocess.call(cmd_Full)
# res = subprocess.check_output(cmd_Full)
'''###################
render
###################'''
return render(request, 'ip/anims/plain_anims.html', dic)
#/ def open_image_dir(request):
def prep_gen_Cake_CSV(request):
'''###################
vars
###################'''
dic = {}
'''###################
ops
###################'''
'''###################
ops : delete image files
###################'''
'''###################
get : files list
###################'''
dpath_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6" \
+ "\\46_art\\VIRTUAL\\Admin_Projects" \
+ "\\ip" \
+ "\\images"
fpath_Glob = "%s\\*" % (dpath_Images)
#ref glob https://stackoverflow.com/questions/14798220/how-can-i-search-sub-folders-using-glob-glob-module-in-python answered Feb 10 '13 at 13:31
lo_Files = glob.glob(fpath_Glob)
# files only
lo_Files_Filtered = []
for item in lo_Files:
# if os.path.isfile(dpath_Images + "\\" + item) == True :
if os.path.isfile(item) == True :
lo_Files_Filtered.append(item)
#/for item in lo_Files:
# update the list
lo_Files = lo_Files_Filtered
lo_Files.sort()
# #debug
# print("[%s:%d] lo_Files =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Files)
'''###################
move files
###################'''
dpath_Dest = "%s\\storage_images" % dpath_Images
# counter
cntOf_Move = 0
for item in lo_Files:
# file name
fname = os.path.basename(item)
fpath_Src = "%s\\%s" % (dpath_Images, fname)
fpath_Dst = "%s\\%s" % (dpath_Dest, fname)
#ref https://stackoverflow.com/questions/8858008/how-to-move-a-file-in-python answered Jan 13 '12 at 22:19
shutil.move(fpath_Src, fpath_Dst)
# count
cntOf_Move += 1
#/for item in lo_Files:
#debug
print("[%s:%d] cntOf_Move => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cntOf_Move
), file=sys.stderr)
'''###################
copy
###################'''
'''###################
get : files list
###################'''
dpath_Images_Copy_Src = "C:\\WORKS_2\\WS\\WS_Cake_IFM11\\iphone"
fpath_Glob = "%s\\*" % (dpath_Images_Copy_Src)
#ref glob https://stackoverflow.com/questions/14798220/how-can-i-search-sub-folders-using-glob-glob-module-in-python answered Feb 10 '13 at 13:31
lo_Files = glob.glob(fpath_Glob)
# files only
lo_Files_Filtered = []
for item in lo_Files:
# if os.path.isfile(dpath_Images + "\\" + item) == True :
if os.path.isfile(item) == True :
lo_Files_Filtered.append(item)
#/for item in lo_Files:
# update the list
lo_Files = lo_Files_Filtered
lo_Files.sort()
'''###################
copy files
###################'''
# dpath_Dest = "%s\\storage_images" % dpath_Images
# aa
# counter
cntOf_Copy = 0
for item in lo_Files:
# file name
fname = os.path.basename(item)
fpath_Src = "%s\\%s" % (dpath_Images_Copy_Src, fname)
fpath_Dst = "%s\\%s" % (dpath_Images, fname)
# fpath_Dst = "%s\\%s" % (dpath_Dest, fname)
#ref https://stackoverflow.com/questions/8858008/how-to-move-a-file-in-python answered Jan 13 '12 at 22:19
shutil.copy(fpath_Src, fpath_Dst)
#shutil.copyfile(fpath_Src, fpath_Dst)
# shutil.move(fpath_Src, fpath_Dst)
# count
cntOf_Copy += 1
#/for item in lo_Files:
#debug
print("[%s:%d] cntOf_Copy => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cntOf_Copy
), file=sys.stderr)
'''###################
render
###################'''
return render(request, 'ip/prep_gen_Cake_CSV.html', dic)
#/ def prep_gen_Cake_CSV(request):
def dos_attack(request):
'''###################
vars
###################'''
dic = {}
'''###################
ops
###################'''
numOf_DosAttack = cons_ip.DfltVals.numOf_DosAttack.value
numOf_DosAttack += 1
dic['time_label'] = libs.get_TimeLabel_Now()
# dic['numOf_DosAttack'] = numOf_DosAttack
'''###################
template
###################'''
return render(request, 'ip/dos_attack.html', dic)
#/ def dos_attack(request):
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swg2DGraph.js
/********************************************************************
2D描画関数(HTML5の2Dグラフィックス機能)
呼び出す側のプログラムにおいてコンテキストをctxとすること
*********************************************************************/
//---------------------------------------------------
function drawLine(x1, y1, x2, y2, color, line_width)
{
ctx.beginPath();
ctx.strokeStyle = color;
ctx.lineWidth = line_width;
ctx.moveTo(x1, y1);
ctx.lineTo(x2, y2);
ctx.stroke();
}
//----------------------------------------------------
function drawLines(pointData, color, line_width)
{
ctx.beginPath();
ctx.strokeStyle = color;
ctx.lineWidth = line_width;
ctx.moveTo(pointData[0][0], pointData[0][1]);
for(var i = 1; i < pointData.length; i++) ctx.lineTo(pointData[i][0], pointData[i][1]);
ctx.stroke();
}
//----------------------------------------------------------
function drawRect(x0, y0, w, h, fill, color, line_width)
{//x0,y0は中心座標
var x = x0 - w / 2;
var y = y0 - h / 2;
if(fill == 0)
{
ctx.lineWidth = line_width;
ctx.strokeStyle = color;
ctx.strokeRect(x, y, w, h);
}
else
{
ctx.fillStyle = color;
ctx.fillRect(x, y, w, h);
}
}
//----------------------------------------------------------
function drawCircle(x0, y0, radius, fill, color, line_width)
{//x0,y0は中心座標
ctx.beginPath();
ctx.arc(x0, y0, radius, 2.0 * Math.PI, false);
if(fill == 0)
{
ctx.lineWidth = line_width;
ctx.strokeStyle = color;
ctx.stroke();
}
else
{
ctx.fillStyle = color;
ctx.fill();
}
}
//-----------------------------------------------------------
function drawTriangle(x0, y0, radius, fill, color, line_width)
{//x0,y0は中心座標, radiusは中心から頂点までの距離
var rs = radius * Math.sin(Math.PI/6);
var rc = radius * Math.cos(Math.PI/6);
var x1 = x0; var y1 = y0 - radius;
var x2 = x0 - rc; var y2 = y0 + rs;
var x3 = x0 + rc; var y3 = y2;
ctx.beginPath();
ctx.moveTo(x1, y1);
ctx.lineTo(x2, y2);
ctx.lineTo(x3, y3);
ctx.closePath();
if(fill == 0)
{
ctx.lineWidth = line_width;
ctx.strokeStyle = color;
ctx.stroke();
}
else
{
ctx.fillStyle = color;
ctx.fill();
}
}
//-----------------------------------------------------------------
function drawText(text, x, y, color, scX, scY)
{
ctx.fillStyle = color;
ctx.scale(scX, scY);
ctx.fillText(text, x / scX, y / scY);
ctx.scale(1 / scX, 1 / scY);//元のサイズに戻す
}
//----------------------------------------------------------------
function clearCanvas(color)
{
ctx.fillStyle = color;
ctx.fillRect(0, 0, canvas.width, canvas.height);
}
<file_sep>/e2.bat
REM g pull https://github.com/iwabuchiken/WS_Others.git
g2 pull https://github.com/iwabuchiken/WS_Others.Art.git
<file_sep>/d.bat
REM C:\WORKS\Utils\shortcuts\cake_ifm11\main.stop.bat
taskkill /f /im gimp-2.8.exe
taskkill /f /im wish.exe
taskkill /f /im MovieMaker.exe
taskkill /f /im audacity.exe
taskkill /f /im wmplayer.exe
taskkill /f /im filezilla.exe
REM taskkill /f /im blender.exe
REM taskkill /f /im blender-app.exe
exit
<file_sep>/JVEMV6/46_art/2_image-prog/2_projects/1_sort-out/1_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out\1_1.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_sound-prog\2_\1_\1_1.py
at : 2018/05/07 11:07:42
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\2_image-prog\2_projects\1_sort-out
python 1_1.py
'''
# from __future__ import print_function
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\2_image-prog') # libs_mm
# sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_image_prog import libs
# from libs_mm import libs
# from libs_VX7GLZ import libs_VX7GLZ
# from libs_VX7GLZ import wablibs
'''###################
import : specifics
###################'''
# import midi, pretty_midi
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, math as math, random as rnd, numpy as np, \
matplotlib.pyplot as plt, matplotlib.style as ms
###############################################
# import wave, struct
# import numpy as np
# from pylab import *
from matplotlib import pylab as plt
#ref http://nbviewer.jupyter.org/github/librosa/librosa/blob/master/examples/LibROSA%20demo.ipynb
# import librosa
# We'll need numpy for some mathematical operations
# import numpy as np
# matplotlib for displaying the output
# import matplotlib.pyplot as plt
# import matplotlib.style as ms
# ms.use('seaborn-muted') % matplotlib inline
# and IPython.display for audio output
import IPython.display
# # Librosa for audio
# import librosa
# # And the display module for visualization
# import librosa.display
# # import random as rnd
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def test_1():
print("[%s:%d] test_1()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/11_guitar/manage_voicememos_20190816_143728.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\manage_voicememos_20190816_143728.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\others\voice_recog.py
at : 2019/08/16 14:38:58
r d4
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\
python manage_voicememos_20190816_143728.py
'''
###############################################
import sys, os, shutil
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_mm import libs
'''###################
vars : global
###################'''
SWITCH_DEBUG = False
'''###################
funcs
###################'''
def show_Message() :
msg = '''
manage voice memos'''
print (msg)
def test_1():
'''###################
step: 1
vars
###################'''
dpath_Main = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\11_guitar"
dpath_Target_Dir = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\11_guitar\\for_conversion.dir"
fname_ListOf_Data = "list_of_data.dat"
'''###################
step: 2
get : list of : dir list in dpath_Target_Dir
###################'''
strOf_M4A_Ext = "m4a"
#ref https://stackoverflow.com/questions/3207219/how-do-i-list-all-files-of-a-directory
#ref https://www.tutorialspoint.com/python/string_endswith.htm
lo_Source_M4A_Files = [f for f in os.listdir(dpath_Target_Dir) if f.endswith(strOf_M4A_Ext)]
if SWITCH_DEBUG == True :
#debug
msg = "[%s:%d] lo_Source_M4A_Files ==> " % (os.path.basename(libs.thisfile()), libs.linenum())
print("%s" % (msg), file=sys.stderr)
print(lo_Source_M4A_Files)
print("total : %d" % len(lo_Source_M4A_Files))
'''###################
step: 3
build : list of final fnames set
###################'''
'''###################
step: 3 : 1
dat file : read lines
###################'''
# file : open
f_in_dat = open(os.path.join(dpath_Target_Dir, fname_ListOf_Data), "r")
lo_Dat_File_Lines = f_in_dat.readlines()
if SWITCH_DEBUG == True :
#debug
msg = "[%s:%d] lo_Dat_File_Lines ==> " % (os.path.basename(libs.thisfile()), libs.linenum())
print("%s" % (msg), file=sys.stderr)
print(lo_Dat_File_Lines)
print("total : %d" % len(lo_Dat_File_Lines))
'''###################
step: 3 : X
close : file
###################'''
# file : open
f_in_dat.close()
'''###################
step: 3 : 2
build : each line ==> tokenise
###################'''
lo_Dat_File_Lines__Tokenized = []
for item in lo_Dat_File_Lines:
lo_Dat_File_Lines__Tokenized.append((item.strip()).split("\t"))
# lo_Dat_File_Lines__Tokenized.append(item.split("\t"))
#/for item in lo_Dat_File_Lines:
if SWITCH_DEBUG == True :
#debug
msg = "[%s:%d] lo_Dat_File_Lines__Tokenized ==> " % (os.path.basename(libs.thisfile()), libs.linenum())
print("%s" % (msg), file=sys.stderr)
for item in lo_Dat_File_Lines__Tokenized:
print(item)
#/for item in lo_Dat_File_Lines__Tokenized:
print("total : %d" % len(lo_Dat_File_Lines__Tokenized))
#_20190816_153352:tmp
'''###################
step: 3 : 3
build : lo_Final
###################'''
lo_Final = []
for item in lo_Dat_File_Lines__Tokenized:
# item ==> ['21', '20190815 180358.m4a', 'g-0815-6']
lenOf_Item = len(item)
if lenOf_Item == 3 : #if lenOf_Item == 3
# new file name
tokens = item[1].split(".") # 20190815 171714.m4a
tokens_2 = tokens[0].split(" ") #=> "20190815" "171714"
fname_New = "%s.[%s_%s].m4a" % (item[2], tokens_2[0], tokens_2[1])
# fname_New = "%s.[%s].m4a" % (item[2], item[1])
# build the final set
setOf_Final_Entries = [
item[1] # '20190815 180358.m4a' (source file name)
, fname_New
# , "%s.[%s].m4a" % (item[2], item[1])
]
# append
lo_Final.append(setOf_Final_Entries)
#/if lenOf_Item == 3
#/for item in lo_Dat_File_Lines__Tokenized:
#debug
msg = "[%s:%d] lo_Final ==> "
print("%s" % (msg), file=sys.stderr)
for item in lo_Final:
print(item)
#/for item in lo_Dat_File_Lines__Tokenized:
print("total : %d" % len(lo_Final))
'''###################
step: 4
copy : new files
###################'''
cntOf_Copy = 0
for item in lo_Final:
#_20190816_154557:tmp
# fpath : src
fpath_Src = os.path.join(dpath_Target_Dir, item[0])
fpath_Dst = os.path.join(dpath_Target_Dir, item[1])
#ref https://stackoverflow.com/questions/123198/how-do-i-copy-a-file-in-python
res = shutil.copyfile(fpath_Src, fpath_Dst)
# [manage_voicememos_20190816_143728.py:203] res => C:\WORKS_2\WS\WS_Others.Art\JV
# EMV6\46_art\11_guitar\for_conversion.dir\g-0815-5.[20190815_174452].m4a
# msg = "[%s:%d] res => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum(), res)
#
# print("%s" % (msg), file=sys.stderr)
# count
cntOf_Copy += 1
#/for item in lo_Final:
# if True :
#debug
msg = "[%s:%d] cntOf_Copy ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum(), cntOf_Copy)
print("%s" % (msg), file=sys.stderr)
# for item in lo_Dat_File_Lines__Tokenized:
#
# print(item)
#
# #/for item in lo_Dat_File_Lines__Tokenized:
#
# print("total : %d" % len(lo_Dat_File_Lines__Tokenized))
# '''###################
# step: 3 : X
# close : file
# ###################'''
# # file : open
# f_in_dat.close()
msg = "[%s:%d] test_1 : done" % \
(os.path.basename(libs.thisfile()), libs.linenum())
print("%s" % (msg), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第5章/swgSupport2.js
//-------------------------------------------------------
// swgSupport2.js
// 光源,カメラ,視体積,マウス操作等のパラメータや関数を定義
//------------------------------------------------------------------
var m_flagObstacle = true;//false;//マウス操作対象を障害物にするときtrue
var flagPick = false;
var numObject;
//光源
function Light()
{
this.pos = [50.0, 0.0, 100.0, 1.0];//w=1:点光源,w=0:平行光源(光源方向)
this.color = [1.0, 1.0, 1.0, 1.0];//拡散光・環境光・鏡面光みな同じ
this.attenuation = [1.0, 0.0, 0.0];//一定減衰率,1次減衰率,2次減衰率
this.spotCnt = [0.0, 0.0, 0.0];//スポット光源が照射される中心位置(スポット中心)
this.spotCutoff = 30.0;//スポットライト・カットオフ
this.spotExponent = 10.0;//スポットライト指数
};
//---------------------------------------------------
//カメラと視体積
function Camera()
{
//カメラ
this.pos = [100.0, 0.0, 0.0];//位置(視点)
this.cnt = [0.0, 0.0, 0.0];//注視点
this.dist= 100.0; //注視点から視点までの距離(R)
this.theta = 10.0;//仰角(水平面との偏角θ)
this.phi = 0.0; //方位角(φ)
//視体積
this.fovy = 40.0;//視野角
this.near = 1.0; //前方クリップ面(近平面)
this.far = 200.0;//後方クリップ面(遠平面)
};
Camera.prototype.getPos = function()
{
this.pos[0] = this.cnt[0] + this.dist * Math.cos(DEG_TO_RAD * this.theta) * Math.cos(DEG_TO_RAD * this.phi);//x
this.pos[1] = this.cnt[1] + this.dist * Math.cos(DEG_TO_RAD * this.theta) * Math.sin(DEG_TO_RAD * this.phi);//y
this.pos[2] = this.cnt[2] + this.dist * Math.sin(DEG_TO_RAD * this.theta);//z
}
//----------------------------------------------------
//マウス操作
function mouseOperation(canvas, camera)
{
var xStart, yStart;
var flagMouse = false;
var flagMove = false;
var rectC;
if(m_flagObstacle == false)//カメラ操作
{
canvas.onmousedown = function(ev)
{
//Web page左上からの距離
var x = ev.clientX; // マウスポインタのx座標
var y = ev.clientY; // マウスポインタのy座標
var wid = 30;
var dd = 0.2;//距離増分
rectC = ev.target.getBoundingClientRect() ;//Web page左上を原点にしたピクセル単位のcanvas領域
if(x < rectC.left || x > rectC.right || y < rectC.top || y > rectC.bottom)
{//canvas外
flagMouse = false; return;
}
xStart = x; yStart = y;
flagMouse = true;
if(x > rectC.left && x < rectC.left + wid && y > rectC.top && y < rectC.top + wid)//canvasの左上
{//dolly
camera.dist -= dd;//近づく
}
else if(x > rectC.right - wid && x < rectC.right && y > rectC.top && y < rectC.top +wid)//右上
{//dolly
camera.dist += dd;//遠ざかる
}
else if(y > rectC.top + canvas.height/2 - wid && y < rectC.top + canvas.height/2 + wid)
{//pan
if(x > rectC.left && x < rectC.left + wid ) camera.phi -= 1.0;//真左
else if(x > rectC.right - wid && x < rectC.right) camera.phi += 1.0;//真右
camera.cnt[0] = camera.pos[0] - camera.dist * Math.cos(DEG_TO_RAD * camera.phi) * Math.cos(DEG_TO_RAD * camera.theta);
camera.cnt[1] = camera.pos[1] - camera.dist * Math.sin(DEG_TO_RAD * camera.phi) * Math.cos(DEG_TO_RAD * camera.theta);
}
else if(x > rectC.left + canvas.width/2 - wid && x < rectC.left + canvas.width/2 + wid)
{//tilt
if(y < rectC.top + wid ) camera.theta += 1.0;//真上
else if(y > rectC.bottom - wid) camera.theta -= 1.0;//真下
camera.cnt[0] = camera.pos[0] - camera.dist * Math.cos(DEG_TO_RAD * camera.theta) * Math.cos(DEG_TO_RAD * camera.phi);
camera.cnt[1] = camera.pos[1] - camera.dist * Math.cos(DEG_TO_RAD * camera.theta) * Math.sin(DEG_TO_RAD * camera.phi);
camera.cnt[2] = camera.pos[2] - camera.dist * Math.sin(DEG_TO_RAD * camera.theta);
}
else if(x > rectC.left && x < rectC.left + wid && y > rectC.bottom - wid && y < rectC.bottom)//左した
{
camera.fovy -= 1.0;//zoom in
}
else if(x > rectC.right - wid && x < rectC.right && y > rectC.bottom - wid && y < rectC.bottom)//右下
{
camera.fovy += 1.0;//zoom out
}
camera.getPos();
display();
}
canvas.onmouseup = function(ev)
{
flagMouse = false;
}
canvas.onmousemove = function(ev)
{
if(!flagMouse) return;
//Web page左上からの距離
var x = ev.clientX; // マウスポインタのx座標
var y = ev.clientY; // マウスポインタのy座標
var dd = 0.2;//距離増分
rectC = ev.target.getBoundingClientRect() ;//Web page左上を原点にしたピクセル単位のcanvas領域
if(x < rectC.left || x > rectC.right || y < rectC.top || y > rectC.bottom){ flagMouse = false; return;}//canvas外
if(y < rectC.top + canvas.height / 2) camera.phi += dd * (x - xStart) ;//tumble
else camera.phi -= dd * (x - xStart) ;//tumble
camera.theta += dd * (y - yStart) ;//crane
camera.getPos();
display();
xStart = x;
yStart = y;
}
}
else//m_flagObstacle = true
{
canvas.onmousedown = function(ev)
{
//Web page左上からの距離
var x = ev.clientX; // マウスポインタのx座標
var y = ev.clientY; // マウスポインタのy座標
rectC = ev.target.getBoundingClientRect() ;//Web page左上を原点にしたピクセル単位のcanvas領域
var xx = ((x - rectC.left) / canvas.width - 0.5);
var yy = ((y - rectC.top) / canvas.height - 0.5);
//m_rigid.vPos.x = yy;
//m_rigid.vPos.y = xx;
obstacle.vPos.x = yy;
obstacle.vPos.y = xx;
if(x < rectC.left || x > rectC.right || y < rectC.top || y > rectC.bottom)
{ flagMouse = false; return; }//canvas外
xStart = x; yStart = y;
flagMouse = true;
}
canvas.onmouseup = function(ev)
{
flagMove = false;
flagMouse = false;
}
canvas.onmousemove = function(ev)
{
if(!flagMouse) return;
//Web page左上からの距離
var x = ev.clientX; // マウスポインタのx座標
var y = ev.clientY; // マウスポインタのy座標
var factor = 1 / canvas.width;//倍率
rectC = ev.target.getBoundingClientRect() ;//Web page左上を原点にしたピクセル単位のcanvas領域
if(x < rectC.left || x > rectC.right || y < rectC.top || y > rectC.bottom)
{ flagMouse = false; return;}//canvas外
var dx = (x - xStart) / canvas.width;
var dy = (y - yStart) / canvas.height;
obstacle.vPos.x += dy;
obstacle.vPos.y += dx;
xStart = x;
yStart = y;
if(dx != 0.0 || dy != 0.0) {flagMove = true; }
}
}
}
<file_sep>/JVEMV6/46_art/2_image-prog/libs_image_prog/cons_image.py
#!C:\WORKS_2\Programs\Python\Python_3.5.1\python.exe
from enum import Enum
TypeOf_Data_OpenClose = "OpenClose"
'''###################
Used in :
libfx : def get_HighLowDiffs(aryOf_BarDatas, id_Start, id_End)
###################'''
class FPath(Enum):
# fname_In_XML = "Materials_Science.mm"
fname_In_XML = "test.mm"
# fname_In_XML = "copy.mm"
# dpath_In_CSV = "C:/WORKS_2/WS/FM_2/Materials_Science"
dpath_In_CSV = "C:/WORKS_2/WS/FM_2_20171104_225946/Materials_Science"
# fpath_In_CSV = dpath_In_CSV + "/" + fname_In_CSV
dpath_Gimp_Debug = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\4_"
str_Test = "yes"
class NodeVar(Enum):
ATTRIB_NAME_WIDTH = 75
ATTRIB_VALUE_WIDTH = 120
<file_sep>/JVEMV6/46_art/8_kb/end_write-out_2.bat
REM taskkill /im musescore.exe
taskkill /im MuseScore3.exe
taskkill /im domino.exe
taskkill /im filezilla.exe
taskkill /im wish.exe
<file_sep>/JVEMV6/46_art/3_sound-prog/2_/2_/2_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_sound-prog\2_\2_\2_1.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_sound-prog\2_\1_\1_1.py
at : 2018/03/21 11:55:27
pushd C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_sound-prog\2_\2_\
python 2_1.py
'''
from __future__ import print_function
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_mm import libs
from libs_VX7GLZ import libs_VX7GLZ
from libs_VX7GLZ import wablibs
'''###################
import : specifics
###################'''
# import midi, pretty_midi
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, math as math, random as rnd
###############################################
import wave, struct
import numpy as np
# from pylab import *
from matplotlib import pylab as plt
#ref http://nbviewer.jupyter.org/github/librosa/librosa/blob/master/examples/LibROSA%20demo.ipynb
# import librosa
# We'll need numpy for some mathematical operations
import numpy as np
# matplotlib for displaying the output
import matplotlib.pyplot as plt
import matplotlib.style as ms
# ms.use('seaborn-muted') % matplotlib inline
# and IPython.display for audio output
# import IPython.display
# Librosa for audio
import librosa
# And the display module for visualization
import librosa.display
# import random as rnd
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def show_WavInfos(fpath):
wr = wave.open(fpath, "rb")
'''###################
infos
###################'''
print("[%s:%d] file : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath
), file=sys.stderr)
print("channel : ", wr.getnchannels())
print("sample size : ", wr.getsampwidth())
print("getframerate() : ", wr.getframerate())
print("getnframes() : ", wr.getnframes())
print("getnframes() : ", wr.getparams())
nchan = wr.getparams()
print("nchan => %s (type = %s)" % (nchan, type(nchan)))
# type = <class 'wave._wave_params'>
#/ def show_WavInfos(fpath):
def test_2():
fpath = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\3_sound-prog\\2_\\2_\\data\\audio.wav"
# wr = wave.open(fpath, "rb")
show_WavInfos(fpath)
#/ def test_2():
def test_1():
print("[%s:%d] test_1()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#ref https://librosa.github.io/librosa/tutorial.html
# 1. Get the file path to the included audio example
filename = librosa.util.example_audio_file()
# 2. Load the audio as a waveform `y`
# Store the sampling rate as `sr`
y, sr = librosa.load(filename)
# 3. Run the default beat tracker
tempo, beat_frames = librosa.beat.beat_track(y=y, sr=sr)
print("[%s:%d] file => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, filename
), file=sys.stderr)
print("[%s:%d] tempo = %0.2f / len(beat_frames) = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, tempo, len(beat_frames)
), file=sys.stderr)
# print('Estimated tempo: {:.2f} beats per minute'.format(tempo))
# 4. Convert the frame indices of beat events into timestamps
beat_times = librosa.frames_to_time(beat_frames, sr=sr)
print('Saving output to beat_times.csv')
librosa.output.times_csv('beat_times.csv', beat_times)
'''###################
file : 2
###################'''
filename = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\3_sound-prog\\2_\\2_\\data\\audio.wav"
# 2. Load the audio as a waveform `y`
# Store the sampling rate as `sr`
y, sr = librosa.load(filename)
# 3. Run the default beat tracker
tempo, beat_frames = librosa.beat.beat_track(y=y, sr=sr)
print("[%s:%d] file => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, filename
), file=sys.stderr)
print("[%s:%d] tempo = %0.2f / len(beat_frames) = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, tempo, len(beat_frames)
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_2()
# test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/2_image-prog/1_gimp/1_/libs_1/abc.py
# -*- coding: utf-8 -*-
from __future__ import print_function
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\2_image-prog\1_gimp\1_\libs_1\abc.py
orig :
at : 2018/04/03 15:19:10
C:/WORKS_2/WS/WS_Others/JVEMV6/46_art/2_image-prog/1_gimp/1_/1_1.py
pushd C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\2_image-prog\1_gimp\1_\
python 1_1.py
C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\1_
'''
'''
import os, sys
path = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\1_"
os.chdir(path)
sys.path.append('.')
from libs_1 import abc
'''
# from __future__ import print_function
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, math as math, random as rnd
###############################################
import wave, struct
# import numpy as np
# from pylab import *
def test_1():
print(os.getcwd())
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_2()
# test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/VIRTUAL/open_page.bat
@echo on
pushd "C:\Program Files (x86)\Google\Chrome\Application"
start chrome.exe http://127.0.0.1:8000/mm/
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/utils/open_image_dir.bat
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\images
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\img.corners
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\csv<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/anims/templatetags/taglib_basic.py
from django import template
register = template.Library()
@register.simple_tag
# def print(param):
def p(param):
# def show(param):
return param
#ref https://stackoverflow.com/questions/4731572/django-counter-in-loop-to-index-list
@register.filter
def access_index(sequence, position):
return sequence[position]
<file_sep>/JVEMV6/46_art/8_kb/end_write-out.bat
taskkill /im domino.exe
taskkill /im wish.exe
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第5章/movingObstacle.js
/*----------------------------------------------
movingObstacle.js
レギュラー格子による速度-圧力法(フラクショナル・ステップ法)
移動する障害物
粒子アニメーション
-----------------------------------------------*/
var canvas; //キャンバス要素
var gl;//WebGL描画用コンテキスト
var height_per_width;//キャンバスのサイズ比
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var elapseTimeN = 0.0;//無次元時間
var elapseTimeN0 = 0.0;
var flagStart = false;
var flagFreeze = false;
var flagStep = false;
var flagReset = false;
//数値計算
var type = [];//格子点のタイプ
var Prs = [];//圧力
var Omg = [];//渦度(x,y速度で計算)
var velX = [];//格子点のx速度
var velY = [];//格子点のy速度
var velXgx = [];//速度微分
var velXgy = [];//速度微分
var velYgx = [];//速度微分
var velYgy = [];//速度微分
var scale = new Vector3(1.0, 1.0, 0.0);//スケール調整
var flagPressIso = true;
var flagPressCol = false;
var flagVorticityIso = true;
var flagVorticityCol = false;
var flagVelocity = false;
var flagGrid = false;
var nLine = 20;//流線,ポテンシャルの表示本数
var arrowScale = 0.05;
var arrowWidth = 1;
var intervalV = 2;//速度矢印表示間隔
var deltaT = 0.005;
var Re = 3000.0;//レイノルズ数
var maxPrs = 0.5;
var minPrs = -0.5;
var maxOmg = 20.0;
var minOmg = -20.0;
//移動障害物
var obsSpeed = 1;//障害物速度
var obsDir = 1; //方向(1:右方向、-1:左方向)
var obsPos = new Vector3(0.1, 0.5, 0);//ダクト左下を原点とした位置
var obsVel = new Vector3(1, 0, 0);
var obsRadius;//円運動時の半径
var moveMode = 0;//直線
var flagObsStop = false;//障害物だけ停止
//解析領域矩形構造体
function Rect()
{
this.scale = 1.8;//表示倍率
this.nMeshX = 80;//x方向割数(固定)
this.nMeshY = 80; //y方向分割数(固定)
this.size = new Vector3(1, 1, 0);//矩形ダクト領域のサイズ(固定)
this.left0 = new Vector3(-0.5, -0.5, 0);//その左下位置
this.obs_nX0 = 5;//左端から障害物中心までの距離(格子間隔の整数倍)
this.obs_nY0 = 20;//下端から障害物中心までの距離(格子間隔の整数倍)
this.obs_nWX = 6;//障害物の厚さ(x方向,格子間隔の整数倍)
this.obs_nWY = 6;//障害物の幅(y方向,格子間隔の整数倍)
}
var rect = new Rect();
var NX, NY, DX, DY;
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(1, 1, 1, 1);
form2.deltaT.value = deltaT;
form2.Re.value = Re;
form1.nMeshX.value = rect.nMeshX;
form1.nMeshY.value = rect.nMeshY;
form1.obs_x0.value = obsPos.x;
form1.obs_nWX.value = rect.obs_nWX;
form1.obs_nWY.value = rect.obs_nWY;
form2.obs_speed.value = obsSpeed;
form2.scale.value = rect.scale;
form2.nLine.value = nLine;
form2.omegaIso.checked = flagVorticityIso;
form2.omegaCol.checked = flagVorticityCol;
form2.pressIso.checked = flagVorticityIso;
form2.pressCol.checked = flagVorticityCol;
form2.velocity.checked = flagVelocity;
form2.maxPrs.value = maxPrs;
form2.minPrs.value = minPrs;
form2.maxOmg.value = maxOmg;
form2.minOmg.value = minOmg;
initData();
var timestep = 0;
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;
elapseTimeN += deltaT;//数値計算上の経過時間
renewObsPos();
calculate();
gl.clear(gl.COLOR_BUFFER_BIT);
if(form2.particle.checked) drawParticle(deltaT);//timestep);
display();
elapseTime0 = elapseTime; //現在の経過時間を保存
elapseTimeN0 = elapseTimeN;//数値計算上の経過時間
form1.e_time.value = elapseTime.toString();
form1.n_time.value = elapseTimeN.toString();
if(flagStep) { flagStart = false; }
}
}
animate();
}
function initData()
{
deltaT = parseFloat(form2.deltaT.value);
Re = parseFloat(form2.Re.value);
NX = parseInt(form1.nMeshX.value);
NY = parseInt(form1.nMeshY.value);
obsSpeed = parseFloat(form2.obs_speed.value);
obsPos.x = parseFloat(form1.obs_x0.value);//左端からの距離
obsRadius = rect.size.x / 2 - obsPos.x;//円運動時の半径
obsPos.y = rect.size.y / 2;
rect.obs_nWX = parseFloat(form1.obs_nWX.value);
rect.obs_nWY = parseFloat(form1.obs_nWY.value);
DX = rect.size.x / NX;//格子間隔
DY = rect.size.y / NY;
//障害物の左端・右端・上端・下端の格子単位の位置
nX1 = Math.round(obsPos.x/DX - rect.obs_nWX/2);//障害物左端
nX2 = Math.round(obsPos.x/DX + rect.obs_nWX/2);//障害物右端
nY2 = Math.round(obsPos.y/DY + rect.obs_nWY/2);//障害物上端
nY1 = Math.round(obsPos.y/DY - rect.obs_nWY/2);//障害物下端
initParticle();//粒子アニメーションの初期化
var i, j;
for(i = 0; i <= NX; i++)
{//配列の2次元化
type[i] = [];
Prs[i] = []; //圧力
Omg[i] = []; //渦度
velX[i] = []; //表示用も同じ
velY[i] = [];
velXgx[i] = [];//x方向微分
velXgy[i] = [];//y方向微分
velYgx[i] = [];//x方向微分
velYgy[i] = [];//y方向微分
}
//格子点のタイプ
for(j = 0; j <= NY; j++)
{
for(i = 0; i <= NX; i++)
{
type[i][j] = "INSIDE";//内点
if(j == 0) type[i][j] = "BOTTOM";//下側壁面
if(j == NY) type[i][j] = "TOP";//上側壁面
if(i == 0) type[i][j] = "INLET";//流入端
if(i == NX) type[i][j] = "OUTLET";//流出端
if(i == nX1 && j > nY1 && j < nY2) type[i][j] = "OBS_LEFT";//障害物左端
if(i == nX2 && j > nY1 && j < nY2) type[i][j] = "OBS_RIGHT";//障害物右端
if(i > nX1 && i < nX2 && j == nY2) type[i][j] = "OBS_TOP";//障害物上端
if(i > nX1 && i < nX2 && j == nY1) type[i][j] = "OBS_BOTTOM";//障害物上端
if(i > nX1 && i < nX2 && j > nY1 && j < nY2) type[i][j] = "OBSTACLE";//障害物内部
//コーナー
if(i == nX1 && j == nY1) type[i][j] = "OBS_LL";
if(i == nX1 && j == nY2) type[i][j] = "OBS_UL";
if(i == nX2 && j == nY1) type[i][j] = "OBS_LR";
if(i == nX2 && j == nY2) type[i][j] = "OBS_UR";
}
}
//初期値
//入口/出口も流速0
for(j = 0; j <= NY; j++)
for (i = 0; i <= NX; i++)
{
//圧力
Prs[i][j] = 0.0;
//速度
velX[i][j] = 0.0;
velY[i][j] = 0.0;
velXgx[i][j] = 0.0;
velXgy[i][j] = 0.0;
velYgx[i][j] = 0.0;
velYgy[i][j] = 0.0;
Omg[i][j] = 0.0;//渦度
}
maxPrs0 = -1000.0; minPrs0 = 1000.0;
maxOmg0 = -1000.0; minOmg0 = 1000.0;
calcCourant();
}
function calcCourant()
{
//発散しないための目安を知るため
var courant, diffNum;
if(DX < DY)
{
courant = obsSpeed * deltaT / DX;
diffNum = (1.0 / Re) * deltaT / (DX * DX);//拡散数
}
else
{
courant = obsSpeed * deltaT / DY;
diffNum = (1.0 / Re) * deltaT / (DX * DY);//拡散数
}
form1.courant.value = courant;
form1.diffNum.value = diffNum;
gl.clear(gl.COLOR_BUFFER_BIT);
display();
}
function renewObsPos()
{
if(moveMode == 0)//直線モード
{
if(obsPos.x >= 0.9) direction = -1.0;
if(obsPos.x <= 0.1) direction = 1.0;
obsVel = new Vector3(direction * obsSpeed, 0, 0);
//obsPos.add(mul(obsVel, deltaT));
}
else//円運動
{//初期位置は左側
var xx = rect.size.x/2 - obsPos.x;//中心からの距離(左側で正)
var yy = obsPos.y - rect.size.y/2;//中心からの距離(上側で正)
obsVel.x = obsSpeed * yy / obsRadius;
obsVel.y = obsSpeed * xx / obsRadius;
}
//flagObsStop=falseなら位置更新
if(!flagObsStop) obsPos.add(mul(obsVel, deltaT));
nX1 = Math.round(obsPos.x/DX - rect.obs_nWX/2);//障害物左端位置
nX2 = Math.round(obsPos.x/DX + rect.obs_nWX/2);//障害物右端位置
nY2 = Math.round(obsPos.y/DY + rect.obs_nWY/2);//障害物上端位置
nY1 = Math.round(obsPos.y/DY - rect.obs_nWY/2);//障害物下端位置
//障害物の新しい格子点のタイプ
var i, j;
for(i = 1; i < NX; i++)
for(j = 1; j < NY; j++)
{
type[i][j] = "INSIDE";//内点
if(i == nX1 && j > nY1 && j < nY2) type[i][j] = "OBS_LEFT";//障害物左端
if(i == nX2 && j > nY1 && j < nY2) type[i][j] = "OBS_RIGHT";//障害物右端
if(i >= nX1 && i <= nX2 && j == nY2) type[i][j] = "OBS_TOP";//障害物上端
if(i >= nX1 && i <= nX2 && j == nY1) type[i][j] = "OBS_BOTTOM";//障害物下端
if(i > nX1 && i < nX2 && j > nY1 && j < nY2) type[i][j] = "OBSTACLE";//障害物内部
if(i == nX1 && j == nY1) type[i][j] = "OBS_LL";//左下
if(i == nX1 && j == nY2) type[i][j] = "OBS_UL";
if(i == nX2 && j == nY1) type[i][j] = "OBS_LR";
if(i == nX2 && j == nY2) type[i][j] = "OBS_UR";
}
}
function display()
{
if(!flagStart) gl.clear(gl.COLOR_BUFFER_BIT);
flagPressIso = form2.pressIso.checked;
flagPressCol = form2.pressCol.checked;
flagVorticityIso = form2.omegaIso.checked;
flagVorticityCol = form2.omegaCol.checked;
flagVelocity = form2.velocity.checked;
flagGrid = form2.grid.checked;
gl.viewport(0, 0, canvas.width, canvas.height);
height_per_width = canvas.height / canvas.width;//縦横の比率を一定にするための係数
//計算領域描画
drawRegion();
//流線、等渦度線、圧力表示
maxOmg = parseFloat(form2.maxOmg.value);
minOmg = parseFloat(form2.minOmg.value);
maxPrs = parseFloat(form2.maxPrs.value);
minPrs = parseFloat(form2.minPrs.value);
if( flagPressCol) drawColormap(Prs, minPrs, maxPrs);
if( flagVorticityCol) drawColormap(Omg, minOmg, maxOmg);
if( flagPressIso) drawContour(Prs, minPrs, maxPrs, "black");
if( flagVorticityIso ) drawContour(Omg, minOmg, maxOmg, "blue");
if( flagVelocity ) drawVelocity();
if( flagGrid ) drawGrid();
//計算領域
//drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
drawRectangle(0, 0, scale.x * rect.size.x, scale.x * rect.size.x, false, "black", 0);
//障害物の位置(表示スケーリング後)
var x_obs = rect.left0.x + obsPos.x * scale.x;
var y_obs = rect.left0.y + obsPos.y * scale.y;
drawRectangle(x_obs, y_obs, rect.obs_nWX * DX * scale.x, rect.obs_nWY * DY * scale.y, true, "light_gray", 0);
drawRectangle(x_obs, y_obs, rect.obs_nWX * DX * scale.x, rect.obs_nWY * DY * scale.y, false, "black", 0);
}
function drawRegion()
{
var s1, s2;
if(canvas.width >= canvas.height)
{
s1 = height_per_width;
s2 = 1.0;
}
else
{
s1 = 1.0;
s2 = 1 / height_per_width;
}
scale.x = rect.scale * s1;
scale.y = rect.scale * s2;
var sx = scale.x * rect.size.x / 2;//ダクトの幅は2*sx
var sy = scale.y * rect.size.y / 2;//ダクトの高さは2*sy
//計算領域
drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
//ダクトの左下基準点
rect.left0.x = - sx;
rect.left0.y = - sy;
}
function calculate()
{
var i, j;
var iteration = 10;//最大繰り返し回数(Poisson方程式)
var tolerance = 0.00001;//許容誤差
var error = 0.0;
var maxError = 0.0;
var pp;
var DX2 = DX * DX;
var DY2 = DY * DY;
var A1 = 0.5 * DY2 / (DX2 + DY2);
var A2 = 0.5 * DX2 / (DX2 + DY2);
var A3 = 0.25 * DX2*DY2 / (DX2 + DY2);
var a, b, pp;
//速度境界条件
//壁境界に対してはすべてDirihlet条件なのでinitObject()ルーチンで与えておけばよい
//障害物
for (j = 1; j < NY; j++)
for (i = 1; i < NX; i++)
{
if(type[i][j] == "INSIDE") continue;
{
if(flagObsStop)
{ velX[i][j] = 0; velY[i][j] = 0; }
else
{
velX[i][j] = obsVel.x;
velY[i][j] = obsVel.y;
}
}
}
//NS方程式による速度更新
methodCIP(velX, velXgx, velXgy, velX, velY);
methodCIP(velY, velYgx, velYgy, velX, velY);
//Poisson方程式の右辺
var D = [];
for(i = 0; i <=NX; i++) D[i] = [];
for (j = 1; j < NY; j++)
for (i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;//INSIDE以外の速度は初期設定値
a = (velX[i+1][j] - velX[i-1][j]) / DX;
b = (velY[i][j+1] - velY[i][j-1]) / DY;
D[i][j] = A3 * (a + b) / deltaT;
}
//Poissonの方程式を解く
var cnt = 0;
while (cnt < iteration)
{
maxError = 0.0;
for (i = 0; i <= NX; i++)
for (j = 0; j <= NY; j++)
{
if(type[i][j] == "INSIDE") continue;
else if(type[i][j] == "INLET") Prs[i][j] = Prs[1][j];//Neumann(左端)
else if(type[i][j] == "OUTLET") Prs[i][j] = 0;//Prs[NX-1][j];//0;//(右端)
else if(type[i][j] == "TOP") Prs[i][j] = Prs[i][NY-1];
else if(type[i][j] == "BOTTOM") Prs[i][j] = Prs[i][1];
else if(type[i][j] == "OBS_LEFT") Prs[i][j] = Prs[i-1][j];
else if(type[i][j] == "OBS_RIGHT") Prs[i][j] = Prs[i+1][j];
else if(type[i][j] == "OBS_TOP") Prs[i][j] = Prs[i][j+1];
else if(type[i][j] == "OBS_BOTTOM") Prs[i][j] = Prs[i][j-1];
else if(type[i][j] == "OBS_UL") Prs[i][j] = Prs[i-1][j+1];
else if(type[i][j] == "OBS_UR") Prs[i][j] = Prs[i+1][j+1];
else if(type[i][j] == "OBS_LL") Prs[i][j] = Prs[i-1][j-1];
else if(type[i][j] == "OBS_LR") Prs[i][j] = Prs[i+1][j-1];
}
//反復計算
for (j = 1; j < NY; j++)
for (i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
pp = A1 * (Prs[i+1][j] + Prs[i-1][j]) + A2 * (Prs[i][j+1] + Prs[i][j-1]) - D[i][j];
error = Math.abs(pp - Prs[i][j]);
if (error > maxError) maxError = error;
Prs[i][j] = pp;//更新
}
if (maxError < tolerance) break;
cnt++;
}
console.log("cnt= " + cnt + " error = " + error);
//速度ベクトルの更新
for (j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
velX[i][j] += - 0.5 * deltaT * (Prs[i+1][j] - Prs[i-1][j]) / DX;
velY[i][j] += - 0.5 * deltaT * (Prs[i][j+1] - Prs[i][j-1]) / DY;
}
//渦度を速度から求める
for(i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
Omg[i][j] = 0.5 * ((velY[i+1][j] - velY[i-1][j]) / DX - (velX[i][j+1] - velX[i][j-1]) / DY);
}
//流れ関数,渦度の最小値,最大値
for(i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
if(type[i][j] != "INSIDE") continue;
if(Prs[i][j] > maxPrs0) maxPrs0 = Prs[i][j];
if(Prs[i][j] < minPrs0) minPrs0 = Prs[i][j];
if(Omg[i][j] > maxOmg0) maxOmg0 = Omg[i][j];
if(Omg[i][j] < minOmg0) minOmg0 = Omg[i][j];
}
console.log("maxPrs= " + maxPrs0 + " minPrs = " + minPrs0);
console.log("maxOmg= " + maxOmg0 + " minOmg = " + minOmg0);
}
function methodCIP(f, gx, gy, vx, vy)
{
var newF = [];//関数
var newGx = [];//x方向微分
var newGy = [];//y方向微分
var i, j, ip, jp;
for(i = 0; i <= NX; i++)
{//配列の2次元化
newF[i] = [];
newGx[i] = [];
newGy[i] = [];
}
var c11, c12, c21, c02, c30, c20, c03, a, b, sx, sy, x, y, dx, dy, dx2, dy2, dx3, dy3;
var i, j, ip, jp;
for(i = 1; i < NX; i++)
for(j = 1; j < NY; j++)
{
if(type[i][j] != "INSIDE") continue;
if(vx[i][j] >= 0.0) sx = 1.0; else sx = -1.0;
if(vy[i][j] >= 0.0) sy = 1.0; else sy = -1.0;
x = - vx[i][j] * deltaT;
y = - vy[i][j] * deltaT;
ip = i - sx;//上流点
jp = j - sy;
dx = sx * DX;
dy = sy * DY;
dx2 = dx * dx;
dy2 = dy * dy;
dx3 = dx2 * dx;
dy3 = dy2 * dy;
c30 = ((gx[ip][j] + gx[i][j]) * dx - 2.0 * (f[i][j] - f[ip][j])) / dx3;
c20 = (3.0 * (f[ip][j] - f[i][j]) + (gx[ip][j] + 2.0 * gx[i][j]) * dx) / dx2;
c03 = ((gy[i][jp] + gy[i][j]) * dy - 2.0 * (f[i][j] - f[i][jp])) / dy3;
c02 = (3.0 * (f[i][jp] - f[i][j]) + (gy[i][jp] + 2.0 * gy[i][j]) * dy) / dy2;
a = f[i][j] - f[i][jp] - f[ip][j] + f[ip][jp];
b = gy[ip][j] - gy[i][j];
c12 = (-a - b * dy) / (dx * dy2);
c21 = (-a - (gx[i][jp] - gx[i][j]) * dx) / (dx2*dy);
c11 = -b / dx + c21 * dx;
newF[i][j] = f[i][j] + ((c30 * x + c21 * y + c20) * x + c11 * y + gx[i][j]) * x
+ ((c03 * y + c12 * x + c02) * y + gy[i][j]) * y;
newGx[i][j] = gx[i][j] + (3.0 * c30 * x + 2.0 * (c21 * y + c20)) * x + (c12 * y + c11) * y;
newGy[i][j] = gy[i][j] + (3.0 * c03 * y + 2.0 * (c12 * x + c02)) * y + (c21 * x + c11) * x;
//粘性項に中央差分
newF[i][j] += deltaT * ( (f[i-1][j] + f[i+1][j] - 2.0 * f[i][j]) / dx2
+ (f[i][j-1] + f[i][j+1] - 2.0 * f[i][j]) / dy2 ) / Re;
}
//更新
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
f[i][j] = newF[i][j];
gx[i][j] = newGx[i][j];
gy[i][j] = newGy[i][j];
}
}
function drawContour(PP, minP, maxP, col)
{
nLine = parseFloat(form2.nLine.value);
var dp0 = (maxP - minP) / nLine;//流線間隔
var pp;
var x1, y1, x2, y2;
var p = [], x = [], y = [];
var i, j, k, m;
var data = [];
//三角形セルに分割
for (k = 0; k < nLine; k++)
{
pp = minP + (k + 1) * dp0;
for(j = 0; j < NY; j++)
{
for(i = 0; i < NX; i++)
{//三角形セルに分割
//1つでも内点なら描画
if( type[i][j] != "INSIDE" && type[i][j+1] != "INSIDE"
&& type[i+1][j+1] != "INSIDE" && type[i+1][j] != "INSIDE" ) continue;
p[0] = PP[i][j]; x[0] = i * DX; y[0] = j * DY;
p[1] = PP[i][j+1]; x[1] = i * DX; y[1] = (j+1) * DY;
p[2] = PP[i+1][j+1]; x[2] = (i+1) * DX; y[2] = (j+1) * DY;
p[3] = PP[i+1][j]; x[3] = (i+1) * DX; y[3] = j * DY;
p[4] = p[0]; x[4] = x[0]; y[4] = y[0];//0番目に同じ
//中心
p[5] = (p[0] + p[1] + p[2] + p[3]) / 4.0;
x[5] = (x[0] + x[1] + x[2] + x[3]) / 4.0;
y[5] = (y[0] + y[1] + y[2] + y[3]) / 4.0;
for(m = 0; m < 4; m++)//各三角形について
{
x1 = -10.0; y1 = -10.0;
if((p[m] <= pp && pp < p[m+1]) || (p[m] > pp && pp >= p[m+1]))
{
x1 = x[m] + (x[m+1] - x[m]) * (pp - p[m]) / (p[m+1] - p[m]);
y1 = y[m] + (y[m+1] - y[m]) * (pp - p[m]) / (p[m+1] - p[m]);
}
if((p[m] <= pp && pp <= p[5]) || (p[m] >= pp && pp >= p[5]))
{
if(x1 < 0.0)//まだ交点なし
{
x1 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y1 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
}
else//x1は見つかった
{
x2 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y2 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
if((p[m+1] <= pp && pp <= p[5]) || (p[m+1] >= pp && pp >= p[5]))
{
if(x1 < 0.0)//まだ交点なし
{
x1 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y1 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
}
else//x1は見つかった
{
x2 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y2 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
}//m
}//j
}//i
}//k
drawLines(data, col);
}
function drawColormap(PP, minP, maxP, col)
{
var range0 = maxP - minP;
var x0, y0, x1, y1, x2, y2, x3, y3;
var pp = [], rr = [], gg = [], bb = [];
var i, j, k;
var vertices = [];
var colors = [];
for (i = 0; i < NX; i++)
{
for (j = 0; j < NY; j++)
{
x0 = rect.left0.x + scale.x * i * DX;
y0 = rect.left0.y + scale.y * j * DY;
x1 = rect.left0.x + scale.x * (i+1) * DX;
y1 = rect.left0.y + scale.y * j * DY;
x2 = rect.left0.x + scale.x * (i+1) * DX;
y2 = rect.left0.y + scale.y * (j+1) * DY;
x3 = rect.left0.x + scale.x * i * DX;
y3 = rect.left0.y + scale.y * (j+1) * DY;
pp[0] = (PP[i][j] - minP) / range0;
pp[1] = (PP[i+1][j] - minP) / range0;
pp[2] = (PP[i+1][j+1] - minP) / range0;
pp[3] = (PP[i][j+1] - minP) / range0;
for(k = 0; k < 4; k++)
{
if(pp[k] < 0.25)
{
rr[k] = 0.0; gg[k] = 4.0 * pp[k]; bb[k] = 1.0;
}
else if(pp[k] < 0.5)
{
rr[k] = 0.0; gg[k] = 1.0; bb[k] = 4.0 * (0.5 - pp[k]);
}
else if(pp[k] < 0.75)
{
rr[k] = 4.0 * (pp[k] - 0.5); gg[k] = 1.0; bb[k] = 0.0;
}
else
{
rr[k] = 1.0; gg[k] = (1.0 - pp[k]) * 4.0; bb[k] = 0.0;
}
}
//四角形(三角形2個分のデータ)
vertices.push(x0); vertices.push(y0);
vertices.push(x1); vertices.push(y1);
vertices.push(x2); vertices.push(y2);
vertices.push(x0); vertices.push(y0);
vertices.push(x2); vertices.push(y2);
vertices.push(x3); vertices.push(y3);
colors.push(rr[0]); colors.push(gg[0]); colors.push(bb[0]);
colors.push(rr[1]); colors.push(gg[1]); colors.push(bb[1]);
colors.push(rr[2]); colors.push(gg[2]); colors.push(bb[2]);
colors.push(rr[0]); colors.push(gg[0]); colors.push(bb[0]);
colors.push(rr[2]); colors.push(gg[2]); colors.push(bb[2]);
colors.push(rr[3]); colors.push(gg[3]); colors.push(bb[3]);
}
}
drawRectangles(vertices, colors);//swgShape2D.jsに実装
}
function drawVelocity()
{
arrowScale = parseFloat(form2.arrowScale.value);;
arrowWidth = parseFloat(form2.arrowWidth.value);
intervalV = parseFloat(form2.intervalV.value);
var i, j;
//描画
var theta, mag, x0, y0;
for(i = 1; i < NX; i++)
{
if(i % intervalV != 0) continue;
for (j = 1; j < NY; j++)
{
if(j % intervalV != 0) continue;
if(type[i][j] == "OBSTACLE") continue;
if(type[i][j] == "OBS_LEFT") continue;
if(type[i][j] == "OBS_RIGHT") continue;
if(type[i][j] == "OBS_TOP") continue;
mag = Math.sqrt(velX[i][j] * velX[i][j] + velY[i][j] * velY[i][j]);
if(mag > 10.0) continue;
theta = Math.atan2(velY[i][j], velX[i][j]);
x0 = rect.left0.x + scale.x * i * DX;
y0 = rect.left0.y + scale.y * j * DY;
drawArrow(x0, y0, mag * arrowScale, arrowWidth, "black", theta);
}
}
}
function drawGrid()
{
var i, j;
for(i = 1; i < NX; i++)
{
drawLine(rect.left0.x + scale.x * i * DX, rect.left0.y,
rect.left0.x + scale.x * i * DX, rect.left0.y + scale.y * rect.size.y, 1, "black");
}
for(j = 1; j < NY; j++)
{
drawLine(rect.left0.x, rect.left0.y + scale.y * j * DY,
rect.left0.x + scale.x * rect.size.x, rect.left0.y + scale.y * j * DY, 1, "black");
}
}
//-----------------------------------------------------------------
//-----------------------------------------------------------------
function Particle2D()
{
this.pos = new Vector3();
this.vel = new Vector3();
this.col;
}
var countP = 0;
var pa = [];//particle
var sizeP = 6;
var speedCoef = 1;
//var numMaxP;//最大個数
var typeP = 2;
function initParticle()
{
numMaxP = NX * NY;//粒子総数
var i, j, k;
//粒子インスタンス
for(k = 0; k < numMaxP; k++) pa[k] = new Particle2D();
k = 0;
//粒子初期配置
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
pa[k].pos = new Vector3(i * DX, j * DY, 0);
if(i < NX / 3) pa[k].col = "red";
else if(i < NX * 2 / 3) pa[k].col = "green";
else pa[k].col = "blue";
k ++;
}
}
function drawParticle(dt)
{
flagPoint = true;
var k, KR, KG, KB = 0;
var dataR = [];
var dataG = [];
var dataB = [];
var vel = new Vector3();
KR = KG = KB = 0;
for(k = 0; k < numMaxP; k++)
{
vel = getVelocityParticle(pa[k].pos);
if(!flagFreeze) {
pa[k].pos.x += vel.x * dt * speedCoef;
pa[k].pos.y += vel.y * dt * speedCoef;
if(pa[k].pos.x < DX) pa[k].pos.x = DX;
if(pa[k].pos.x > 1-DX) pa[k].pos.x = 1 - DX;
if(pa[k].pos.y < DY) pa[k].pos.y = DY;
if(pa[k].pos.y > 1-DY) pa[k].pos.y = 1 - DY;
}
if(pa[k].col == "red")
{
dataR[2*KR] = rect.left0.x + pa[k].pos.x * scale.x;
dataR[2*KR+1] = rect.left0.y + pa[k].pos.y * scale.y;
KR++;
}
else if(pa[k].col == "green")
{
dataG[2*KG] = rect.left0.x + pa[k].pos.x * scale.x;
dataG[2*KG+1] = rect.left0.y + pa[k].pos.y * scale.y;
KG++;
}
else if(pa[k].col == "blue")
{
dataB[2*KB] = rect.left0.x + pa[k].pos.x * scale.x;
dataB[2*KB+1] = rect.left0.y + pa[k].pos.y * scale.y;
KB++;
}
}
drawPoints(dataR, sizeP, typeP, "red");
drawPoints(dataG, sizeP, typeP, "green");
drawPoints(dataB, sizeP, typeP, "blue");
}
function getVelocityParticle(pos)
{
var vel = new Vector3();
var i, j, I, J;
//格子番号を取得
I = 0; J = 0;
for(i = 0; i < NX; i++)
{
if(i * DX < pos.x && (i+1) * DX > pos.x) I = i;
}
for(j = 0; j < NY; j++)
{
if(j * DY < pos.y && (j+1) * DY > pos.y) J = j;
}
var a = pos.x / DX - I;
var b = pos.y / DY - J;
//格子点の速度を線形補間
vel.x = (1.0 - b) * ((1.0 - a) * velX[I][J] + a * velX[I+1][J]) + b * ((1.0 - a) * velX[I][J+1] + a * velX[I+1][J+1]);
vel.y = (1.0 - b) * ((1.0 - a) * velY[I][J] + a * velY[I+1][J]) + b * ((1.0 - a) * velY[I][J+1] + a * velY[I+1][J+1]);
return vel;
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
initData();
}
function onDisplay()
{
obsSpeed = parseFloat(form2.obs_speed.value);
calcCourant();
display();
}
function onClickObsStop()
{
flagObsStop = !flagObsStop;
}
function onChangeMoveMode()
{
var nn;
var radioMM = document.getElementsByName("radioMM");
for(var i = 0; i < radioMM.length; i++)
{
if(radioMM[i].checked) moveMode = i;
}
onClickReset();
}
function onClickScale()
{
rect.scale = parseFloat(form2.scale.value);
display();
}
//-------------------------------------------------------------
function onClickP()
{
speedCoef = parseFloat(form2.speedCoef.value);
sizeP= parseFloat(form2.sizeP.value);
typeP= parseFloat(form2.typeP.value);
}
function onClickStart()
{
fps = 0;
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
elapseTimeN = 0;
elapseTimeN0 = 0;
flagStart = true;
flagStep = false;
flagFreeze = false;
flagObsStop = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
flagStart = !flagStart;
flagFreeze = !flagFreeze;
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTimeN0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.e_time.value = "0";
form1.n_time.value = "0";
display();
}
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/start_server.bat
@echo on
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects
REM python manage.py runserver
REM ref https://stackoverflow.com/questions/23639085/django-change-default-runserver-port answered Apr 24 '17 at 23:23
python manage.py runserver 8001
<file_sep>/JVEMV6/46_art/console.py
'''
=========================================== 2018/01/30 17:49:44
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art
python -m virtualenv VIRTUAL
C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art>python -m virtualenv VIRTUAL
Using base prefix 'C:\\WORKS_2\\Programs\\Python\\Python_3.5.1'
New python executable in C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Scrip
ts\python.exe
Installing setuptools, pip, wheel...done.
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art
VIRTUAL\Scripts\activate.bat
python -m pip install django==1.10
Collecting django==1.10
Downloading https://files.pythonhosted.org/packages/4b/4c/059f68d8f029f7054e4e
6bb0b1ed2fde7f28d07a3727325727d5a95ae1b8/Django-1.10-py2.py3-none-any.whl (6.8MB
)
100% |################################| 6.8MB 758kB/s
Installing collected packages: django
Successfully installed django-1.10
pip install sympy numpy matplotlib
(VIRTUAL) C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects>pip i
nstall sympy numpy matplotlib
Collecting sympy
Downloading https://files.pythonhosted.org/packages/91/26/4e477dbd1f9260eb743d
9f221af3044648a8fb2fcf3f2f23daee4dc831a4/sympy-1.1.1.tar.gz (4.6MB)
100% |################################| 4.6MB 853kB/s
Collecting numpy
Downloading https://files.pythonhosted.org/packages/20/09/6f302aba4a08ffcd34b2
0a6ee94f34a76207105f59acd83462b81469c06e/numpy-1.14.3-cp35-none-win_amd64.whl (1
3.4MB)
100% |################################| 13.4MB 487kB/s
Collecting matplotlib
Downloading https://files.pythonhosted.org/packages/ce/02/d0fb7dc284a56449f782
5ef7d1e8b682bf44cef540a6d615e1fa0faa543a/matplotlib-2.2.2-cp35-cp35m-win_amd64.w
hl (8.7MB)
100% |################################| 8.7MB 853kB/s
Collecting mpmath>=0.19 (from sympy)
Downloading https://files.pythonhosted.org/packages/7a/05/b3d1472885d8dc060693
6ea5da0ccb1b4785682e78ab15e34ada24aea8d5/mpmath-1.0.0.tar.gz (511kB)
100% |################################| 512kB 1.0MB/s
Collecting python-dateutil>=2.1 (from matplotlib)
Downloading https://files.pythonhosted.org/packages/cf/f5/af2b09c957ace60dcfac
112b669c45c8c97e32f94aa8b56da4c6d1682825/python_dateutil-2.7.3-py2.py3-none-any.
whl (211kB)
100% |################################| 215kB 930kB/s
Collecting six>=1.10 (from matplotlib)
Downloading https://files.pythonhosted.org/packages/67/4b/141a581104b1f6397bfa
78ac9d43d8ad29a7ca43ea90a2d863fe3056e86a/six-1.11.0-py2.py3-none-any.whl
Collecting cycler>=0.10 (from matplotlib)
Downloading https://files.pythonhosted.org/packages/f7/d2/e07d3ebb2bd7af696440
ce7e754c59dd546ffe1bbe732c8ab68b9c834e61/cycler-0.10.0-py2.py3-none-any.whl
Collecting pytz (from matplotlib)
Downloading https://files.pythonhosted.org/packages/dc/83/15f7833b70d3e067ca91
467ca245bae0f6fe56ddc7451aa0dc5606b120f2/pytz-2018.4-py2.py3-none-any.whl (510kB
)
100% |################################| 512kB 1.0MB/s
Collecting pyparsing!=2.0.4,!=2.1.2,!=2.1.6,>=2.0.1 (from matplotlib)
Downloading https://files.pythonhosted.org/packages/6a/8a/718fd7d3458f9fab8e67
186b00abdd345b639976bc7fb3ae722e1b026a50/pyparsing-2.2.0-py2.py3-none-any.whl (5
6kB)
100% |################################| 61kB 945kB/s
Collecting kiwisolver>=1.0.1 (from matplotlib)
Downloading https://files.pythonhosted.org/packages/67/57/834881c80cd1361792a1
8b467ac8c1638c224a484956582e51d2f9e16e30/kiwisolver-1.0.1-cp35-none-win_amd64.wh
l (57kB)
100% |################################| 61kB 1.4MB/s
Requirement already satisfied: setuptools in c:\works_2\ws\ws_others.art\jvemv6\
46_art\virtual\lib\site-packages (from kiwisolver>=1.0.1->matplotlib) (39.1.0)
Building wheels for collected packages: sympy, mpmath
Running setup.py bdist_wheel for sympy ... done
Stored in directory: C:\Users\iwabuchiken\AppData\Local\pip\Cache\wheels\6d\47
\7c\40a7cd9b9bd5bad329fcd21d8e50629700fcc6e5520a66a2de
Running setup.py bdist_wheel for mpmath ... done
Stored in directory: C:\Users\iwabuchiken\AppData\Local\pip\Cache\wheels\33\15
\0f\9ca5f2ad88a5456803098daa189f382408a81556aa209e97ff
Successfully built sympy mpmath
Installing collected packages: mpmath, sympy, numpy, six, python-dateutil, cycle
r, pytz, pyparsing, kiwisolver, matplotlib
Successfully installed cycler-0.10.0 kiwisolver-1.0.1 matplotlib-2.2.2 mpmath-1.
0.0 numpy-1.14.3 pyparsing-2.2.0 python-dateutil-2.7.3 pytz-2018.4 six-1.11.0 sy
mpy-1.1.1
#------------------------------------ 20180518_133227
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL
django-admin startproject Admin_Projects
pushd Admin_Projects
#) python manage.py runserver
python manage.py migrate > migrate.log
# start : ip (image processing)
python manage.py startapp ip
pip install clipboard
pip install subprocess copy
pip install re clipboard time os datetime ftplib glob
'''<file_sep>/JVEMV6/46_art/8_kb/start_write-out.apps.bat
REM start "C:\WORKS_2\Programs\FileZilla FTP Client\filezilla.exe"
REM call "C:\WORKS_2\Programs\FileZilla FTP Client\filezilla.exe"
REM call C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.filezilla.bat
REM ***************************************
REM filezilla
REM ***************************************
REM start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.filezilla.bat
REM call C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_write-out.apps.filezilla.bat
REM ***************************************
REM domino
REM ***************************************
REM pushd C:\WORKS_2\Programs\Domino143
REM start Domino.exe
REM ***************************************
REM musescore
REM ***************************************
REM start C:\WORKS_2\Programs\musescore\musescore_2.3.2\bin\MuseScore.exe
REM pushd C:\WORKS_2\Programs\musescore\musescore_3.0.1\bin
pushd C:\WORKS_2\Programs\musescore\ms_3.0.2.5315\bin
REM start MuseScore.exe
start MuseScore3.exe
REM ***************************************
REM browser
REM ***************************************
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\start_web_pages.bat
REM pause
exit
<file_sep>/JVEMV6/46_art/VIRTUAL/start_env.bat
@echo off
REM pushd C:\WORKS_2\WS\WS_Others\prog\D-34\5_\VIRTUAL
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art
VIRTUAL\Scripts\activate.bat
REM pause
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/anims/views.py
'''###################
data dir
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data
###################'''
# from django.shortcuts import render
'''###################
django modules
###################'''
from django.http import HttpResponse
from django.shortcuts import render
from django import template
from mailbox import fcntl
'''###################
import : built-in modules
###################'''
import subprocess, copy, re, clipboard, time, \
os, datetime, ftplib, glob, sys, cv2 \
, matplotlib.pyplot as plt, codecs, shutil, numpy as np
'''###################
import : orig modules
###################'''
# sys.path.append('.')
# sys.path.append('..')
# sys.path.append('C:/WORKS_2/WS/WS_Others.Art/JVEMV6/46_art/VIRTUAL/Admin_Projects')
# sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects')
from libs_admin import libs, lib_ip, cons_ip
# from Admin_Projects.libs_admin import libs
# sys.path.append('C:/WORKS_2/WS/WS_Others/prog/D-7/2_2/VIRTUAL/Admin_Projects/mm')
#
# from mm.libs_mm import cons_mm, cons_fx, libs, libfx
'''###################
vars : global
###################'''
numOf_DosAttack = 0
# Create your views here.
# def index():
def index(request):
now = datetime.datetime.now()
action = "index"
message = "yes"
page_Title = "Image Processing"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
# return render(request, 'ip/index2.html', dic)
# return render(request, 'ip/index.html', dic)
return render(request, 'anims/index.html', dic)
# return HttpResponse(html)
# # return HttpResponse("Hello Django (new urls.py file)")
def test(request):
now = datetime.datetime.now()
action = "test"
message = "yes"
page_Title = "Image Processing"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
return render(request, 'anims/test.html', dic)
# return HttpResponse(html)
# # return HttpResponse("Hello Django (new urls.py file)")
def _anims__Load_LO_Actions():
dpath_List = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data"
fname_List = "anims_listof_commands.dat"
fpath_List = os.path.join(dpath_List, fname_List)
f = open(fpath_List, "r")
# header line
f.readline()
# body lines
lines = f.readlines()
# close file
f.close()
lo_Commands = []
# build list
for item in lines:
lo_Commands.append(item.split("\t")[1:])
#/for item in lines:
#debug
print()
print("[%s:%d] lo_Commands =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_Commands)
return lo_Commands
#/ def _anims__Load_LO_Actions()(request):
def test_OpenCV__NewImage():
'''###################
prep
###################'''
dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\anims\\data\\data_images"
# dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\data_images"
fname_Image_File = "leaf-2.1.png" # orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\4_animations\1_\5_\images
fpath_Image_File = os.path.join(dpath_Image_File, fname_Image_File)
print("[%s:%d] fpath_Image_File = %s (exists = %s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image_File, os.path.isfile(fpath_Image_File)
), file=sys.stderr)
'''###################
prep : image
###################'''
#ref http://peaceandhilightandpython.hatenablog.com/entry/2016/01/09/214333
#ref alpha channel http://opencv.blog.jp/python/alpha_channel
img = cv2.imread(fpath_Image_File, cv2.IMREAD_UNCHANGED)
print("[%s:%d] img.shape =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img.shape)
print()
'''###################
ops
###################'''
tokens = fname_Image_File.split(".")
(h, w) = img.shape[:2]
tlabel = libs.get_TimeLabel_Now()
fname_Dst = "%s.%s.(test_OpenCV__NewImage).%s.%s" \
% (tokens[0], tokens[1], tlabel, tokens[2])
fpath_Dst = os.path.join(dpath_Image_File, fname_Dst)
print("[%s:%d] fname_Dst => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Dst
), file=sys.stderr)
'''###################
image : blank
###################'''
# blank image
#ref https://stackoverflow.com/questions/12881926/create-a-new-rgb-opencv-image-using-python
blank_image = np.zeros((img.shape[0], img.shape[1], 4), np.uint8)
print("[%s:%d] blank_image.shape =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(blank_image.shape)
# shape
(h2, w2) = blank_image.shape[:2]
# coloring
# BGRA : A --> 0 for transpanrent
# blank_image[:, 0 : int(0.5*w2)] = (255, 0, 0, 255)
# blank_image[:, 0 : int(0.5*w2)] = (100, 100, 100, 200)
# blank_image[:, 0 : int(0.5*w2)] = (100, 100, 100, 100)
# blank_image[:, 0 : int(0.5*w2)] = (255,0,0, 0)
# blank_image[:,0:0.5*w2] = (255,0,0, 0)
# blank_image[:,0:0.5*width] = (255,0,0, 0)
# blank_image[:, :] = (255, 0, 0, 0)
# blank_image[:, :] = (255, 0, 0)
# copy a partial image of img to blank_image
cut_height = 120
cut_width = 120
blank_image[100 : 100 + cut_height, 250 : 250 + cut_width] \
= img[150:270, 300:420]
# blank_image[150:270, 300:420] = img[150:270, 300:420]
#write
cv2.imwrite(fpath_Dst, blank_image)
# cv2.imwrite(fpath_Dst, mask)
# #ref https://stackoverflow.com/questions/48384516/python-opencv-cropping-images-and-isolating-specific-objects
# mask = np.zeros(img.shape[:2],np.uint8)
#
# print()
# print("[%s:%d] mask[0] => (len = %d)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(mask[0])
# ), file=sys.stderr)
# print(mask[0])
#
# print()
# print("[%s:%d] img[0] => (len = %d)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(img[0])
# ), file=sys.stderr)
# print(img[0])
#
# print()
# print("[%s:%d] img[0][0] => (len = %d)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(img[0][0])
# ), file=sys.stderr)
# print(img[0][0])
# print()
# print("[%s:%d] mask[0][0] => (len = %d)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(mask[0][0])
# ), file=sys.stderr)
# print(mask[0][0])
# print("[%s:%d] mask.shape[0] = %d, mask.shape[1] = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , mask.shape[0], mask.shape[1]
# ), file=sys.stderr)
#
# for i in range(0, mask.shape[0]): # height
# for j in range(0, mask.shape[1]): # width
#
# mask[i][j][0] = 255
# mask[i][j][1] = 0
# mask[i][j][2] = 0
#
# #/for i in range(0, mask.shape[0]:
#
# #write
# cv2.imwrite(fpath_Dst, mask)
#/ def test_OpenCV__NewImage():
def _anims_JS__1_Move_Leaves__Test_1_Resize__exec(angle, scale, img, center, fpath_Dst):
# angle_45 = 45
# scale = 1.0
(h, w) = img.shape[:2]
# center = (365, 203)
# center = (w / 2, h / 2)
M = cv2.getRotationMatrix2D(center, angle, scale)
rotated = cv2.warpAffine(img, M, (h, w))
# tokens = fname_Image_File.split(".")
# fname_Dst = "%s.%s.(rotate=%d).%s.%s" \
# % (tokens[0], tokens[1], angle_45, libs.get_TimeLabel_Now(), tokens[2])
# fpath_Image_File__Dst = os.path.join(dpath_Image_File, fname_Dst)
cv2.imwrite(fpath_Dst, rotated)
#/ def _anims_JS__1_Move_Leaves__Test_1_Resize__exec():
def _anims_JS__1_Move_Leaves__Test_1_Resize(request):
'''###################
prep : params
main.js :: _anims_Action_LinkTo__1(_param)
, option_tick_move_X : _option_tick_move_X
, option_tick_move_Y : _option_tick_move_Y
, opt_rotate_Start : _opt_rotate_Start
, opt_rotate_End : _opt_rotate_End
###################'''
param_Loc_Start_X = request.GET.get('option_loc_start_X', False)
param_Loc_Start_Y = request.GET.get('option_loc_start_Y', False)
param_Source_Dir = request.GET.get('opt_source_Dir', False)
param_Source_File = request.GET.get('opt_source_File', False)
param_Cut_Width = request.GET.get('opt_cut_width', False)
param_Cut_Height = request.GET.get('opt_cut_height', False)
param_Rotate_Start = request.GET.get('opt_rotate_Start', False)
param_Rotate_End = request.GET.get('opt_rotate_End', False)
param_Rotate_Tick = request.GET.get('opt_rotate_Tick', False)
print("[%s:%d] param_Rotate_Tick => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param_Rotate_Tick
), file=sys.stderr)
# modify
# ref tertiary https://stackoverflow.com/questions/394809/does-python-have-a-ternary-conditional-operator
param_Loc_Start_X = 0 if (param_Loc_Start_X == False) else int(param_Loc_Start_X)
param_Loc_Start_Y = 0 if (param_Loc_Start_Y == False) else int(param_Loc_Start_Y)
param_Cut_Width = 100 if (param_Cut_Width == False) else int(param_Cut_Width)
param_Cut_Height = 100 if (param_Cut_Height == False) else int(param_Cut_Height)
param_Rotate_Start = 0 if (param_Rotate_Start == False) else int(param_Rotate_Start)
param_Rotate_End = 45 if (param_Rotate_End == False) else int(param_Rotate_End)
param_Rotate_Tick = 5 if (param_Rotate_Tick == False) else int(param_Rotate_Tick)
param_Source_Dir = cons_ip.FilePaths.dpath_Anims_Image_Files.value \
if (param_Source_Dir == False) else param_Source_Dir
param_Source_File = cons_ip.FilePaths.fname_Anims_Image_Files.value \
if (param_Source_File == False) else param_Source_File
print("[%s:%d] param_Source_Dir = %s\nparam_Source_File = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param_Source_Dir, param_Source_File
), file=sys.stderr)
print("[%s:%d] param_Cut_Width = %d, param_Cut_Height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param_Cut_Width, param_Cut_Height
), file=sys.stderr)
# print("[%s:%d] param_Loc_Start_X = %d, param_Loc_Start_Y = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , param_Loc_Start_X, param_Loc_Start_Y
# ), file=sys.stderr)
'''###################
prep
###################'''
dpath_Image_File = param_Source_Dir
# dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\anims\\data\\data_images"
# dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\data_images"
fname_Image_File = param_Source_File
# fname_Image_File = "leaf-2.1.png" # orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\4_animations\1_\5_\images
fpath_Image_File = os.path.join(dpath_Image_File, fname_Image_File)
print("[%s:%d] fpath_Image_File = %s (exists = %s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image_File, os.path.isfile(fpath_Image_File)
), file=sys.stderr)
'''###################
op
###################'''
'''###################
img : read
###################'''
#ref http://peaceandhilightandpython.hatenablog.com/entry/2016/01/09/214333
#ref alpha channel http://opencv.blog.jp/python/alpha_channel
img = cv2.imread(fpath_Image_File, cv2.IMREAD_UNCHANGED)
# img = cv2.imread(fpath_Image_File)
# img = cv2.imread(fpath_Image_File, cv2.IMREAD_COLOR)
# orgHeight, orgWidth = img.shape[:2]
# size = (int(orgWidth/2), int(orgHeight/2))
# # size = (int(orgHeight/2), int(orgWidth/2))
#
# # size = (orgHeight/2, orgWidth/2)
#
# halfImg = cv2.resize(img, size)
#
# tokens = fname_Image_File.split(".")
# fname_Half = "%s.%s.(half).%s.%s" \
# % (tokens[0], tokens[1], libs.get_TimeLabel_Now(), tokens[2])
#
# cv2.imwrite(os.path.join(dpath_Image_File, fname_Half), halfImg)
# cv2.imwrite('half.jpg', halfImg)
'''###################
rotate
###################'''
tokens = fname_Image_File.split(".")
(h, w) = img.shape[:2]
tokens_2 = os.path.splitext(fname_Image_File)
tlabel = libs.get_TimeLabel_Now()
# paths
dir_Tmp = tlabel
dpath_Tmp = os.path.join(dpath_Image_File, dir_Tmp)
#ref mkdir https://www.tutorialspoint.com/python/os_mkdir.htm
if not os.path.isdir(dpath_Tmp) :
os.mkdir(dpath_Tmp)
#ref step https://www.pythoncentral.io/pythons-range-function-explained/
# for i in range(param_Rotate_Start, param_Rotate_End):
for i in range(param_Rotate_Start, param_Rotate_End, param_Rotate_Tick):
# for i in range(0, 10):
# for i in range(0, 5):
# angle = i * 5
angle = i
scale = 1.0
#ref radians https://docs.scipy.org/doc/numpy/reference/generated/numpy.radians.html
span = int((370 - w / 2) * np.sin(np.radians(angle)))
span_w = int((370 - w / 2) * np.cos(np.radians(angle)))
center = (w / 2 + span_w, h / 2 + span)
# center = (w / 2, h / 2 + span)
# center = (w / 2, h / 2 - span)
# (h, w) = img.shape[:2]
# center = (w / 2, h / 2)
# # paths
# dir_Tmp = tlabel
#
# dpath_Tmp = os.path.join(dpath_Image_File, dir_Tmp)
#
# #ref mkdir https://www.tutorialspoint.com/python/os_mkdir.htm
# if not os.path.isdir(dpath_Tmp) :
# os.mkdir(dpath_Tmp)
#
fname_Dst = "%s.(rotate=%d).%s.(center=%d,%d).%s" \
% (tokens_2[0], angle, tlabel, center[0], center[1], tokens_2[1])
# fname_Dst = "%s.%s.(rotate=%d).%s.(center=%d,%d).%s" \
# % (tokens[0], tokens[1], angle, tlabel, center[0], center[1], tokens[2])
# % (tokens[0], tokens[1], angle, tlabel, tokens[2])
# % (tokens[0], tokens[1], angle, libs.get_TimeLabel_Now(), tokens[2])
fpath_Dst = os.path.join(dpath_Image_File, dir_Tmp, fname_Dst)
# fpath_Dst = os.path.join(dpath_Image_File, fname_Dst)
_anims_JS__1_Move_Leaves__Test_1_Resize__exec(angle, scale, img, center, fpath_Dst)
'''###################
return
###################'''
status = 1
msg = "file created at : %s" % dpath_Tmp
return (status, msg)
#/for i in range(0, 10):
# '''###################
# move image
# ###################'''
# #ref https://stackoverflow.com/questions/23464495/fastest-way-to-move-image-in-opencv
# fname_Dst = "%s.%s.(moved).%s.%s" \
# % (tokens[0], tokens[1], tlabel, tokens[2])
#
# fpath_Dst = os.path.join(dpath_Image_File, fname_Dst)
#
# print("[%s:%d] fname_Dst => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , fname_Dst
# ), file=sys.stderr)
#
# img_Partial = img[
# param_Loc_Start_Y : param_Loc_Start_Y + param_Cut_Height
# , param_Loc_Start_X : param_Loc_Start_X + param_Cut_Width
#
# ] # h, w
# # img_Partial = img[param_Loc_Start_Y : 270, param_Loc_Start_X : 420] # h, w
# # img_Partial = img[150 : 270, 300 : 420] # h, w
# # img_Partial = img[0 : 100, 0 : 50] # h, w
# # img_Partial = img[cv2.Rect(0, 0, 100, 100)]
# # img_Partial = img(cv2.Rect(0, 0, 100, 100))
#
# cv2.imwrite(fpath_Dst, img_Partial)
# # cv2.imwrite(fname_Dst, img_Partial)
# #ref https://www.tutorialkart.com/opencv/python/opencv-python-rotate-image/#opencv-python-rotate-image
# angle_45 = 45
# scale = 1.0
# (h, w) = img.shape[:2]
# center = (365, 203)
# # center = (w / 2, h / 2)
#
# M = cv2.getRotationMatrix2D(center, angle_45, scale)
# rotated_45 = cv2.warpAffine(img, M, (h, w))
# # rotated90 = cv2.warpAffine(img, M, (h, w))
#
# tokens = fname_Image_File.split(".")
# fname_Dst = "%s.%s.(rotate=%d).%s.%s" \
# % (tokens[0], tokens[1], angle_45, libs.get_TimeLabel_Now(), tokens[2])
#
# fpath_Image_File__Dst = os.path.join(dpath_Image_File, fname_Dst)
#
# cv2.imwrite(fpath_Image_File__Dst, rotated_45)
#/ def _anims_JS__1_Move_Leaves__Test_1_Resize():
def _anims_JS__1_Move_Leaves(request):
'''###################
tests
###################'''
# test_OpenCV__NewImage()
'''###################
ops
###################'''
(status, msg) = _anims_JS__1_Move_Leaves__Test_1_Resize(request)
# _anims_JS__1_Move_Leaves__Test_1_Resize(request)
'''###################
return
###################'''
# status = 1
#
# msg = "OK"
return (status, msg)
#/ def _anims_JS__1_Move_Leaves(request):
def _anims_JS__2_Move_Leaves__V2(request):
'''###################
tests
###################'''
# test_OpenCV__NewImage()
'''###################
ops
###################'''
# (status, msg) = _anims_JS__1_Move_Leaves__Test_1_Resize(request)
# _anims_JS__1_Move_Leaves__Test_1_Resize(request)
'''###################
return
###################'''
status = 1
msg = "_anims_JS__2_Move_Leaves__V2"
return (status, msg)
#/ def _anims_JS__2_Move_Leaves__V2(request):
def _anims_JS__3_Clusters__Clusters(request, cv_Img, fpath_Dst__Sub):
img_Tmp = cv_Img
# img_Tmp = copy.deepcopy(cv_Img)
a = 200
# a = 100
# a = 50
# a = 20
'''###################
processing
###################'''
print("[%s:%d] cv_Img[0][0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
cntOf_Row = 0
cntOf_Pixels = 0
flg_Break = False
print("[%s:%d] message" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(cv_Img[0][0])
# for row in cv_Img:
for row in img_Tmp:
for col in row:
# print("[%s:%d] col[0] = %d, col[1] = %d, col[2] = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , col[0], col[1], col[2]
# ), file=sys.stderr)
# col[0] = 255 - col[0]
# col[1] = 255 - col[1]
# col[2] = 255 - col[2]
col[0] = int(col[0] / a) * a
col[1] = int(col[1] / a) * a
col[2] = int(col[2] / a) * a
# col[0] = 50
# col[1] = 50
# col[2] = 50
# print("[%s:%d] (processed) col[0] = %d, col[1] = %d, col[2] = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , col[0], col[1], col[2]
# ), file=sys.stderr)
# # count
# cntOf_Pixels += 1
#
# print("[%s:%d] cntOf_Pixels => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , cntOf_Pixels
# ), file=sys.stderr)
#
#
# if cntOf_Pixels > 10 : #if cntOf_Pixels > 10
#
# flg_Break = True
#
# break
#/if cntOf_Pixels > 10
#/for col in row:
# # debug
# if flg_Break == True : #if flg_Break == True
#
# break
#
# #/if flg_Break == True
#
# # count
# cntOf_Row += 1
#
# if cntOf_Row % 100 == 0 : #if cntOf_Row % 100 == 0
#
# print("[%s:%d] cntOf_Row => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , cntOf_Row
# ), file=sys.stderr)
#
# #/if cntOf_Row % 100 == 0
print("[%s:%d] processing --> done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
save : image
###################'''
res = cv2.imwrite(fpath_Dst__Sub, img_Tmp)
print("[%s:%d] save image => %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, res, fpath_Dst__Sub
), file=sys.stderr)
#/for row in cv_Img:
# print("[%s:%d] cv_Img[0][0] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# print(cv_Img[0][0])
#
# # print("[%s:%d] cv_Img[0][0][0] =>" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# #
# # ), file=sys.stderr)
# #
# # print(cv_Img[0][0][0])
#
# R_New = int(cv_Img[0][0][0] / a) * a
# G_New = int(cv_Img[0][0][1] / a) * a
# B_New = int(cv_Img[0][0][2] / a) * a
# # R_New = (cv_Img[0][0][0] % a) * a
# # G_New = (cv_Img[0][0][1] % a) * a
# # B_New = (cv_Img[0][0][2] % a) * a
#
# print("[%s:%d] R_New = %d, G_New = %d, B_New = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , R_New, G_New, B_New
# ), file=sys.stderr)
#
# cv_Img[0][0][0] = R_New
# cv_Img[0][0][1] = G_New
# cv_Img[0][0][2] = B_New
#
# print("[%s:%d] cv_Img[0][0](processed) =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# print(cv_Img[0][0])
#/ def _anims_JS__3_Clusters__Clusters(request):
def _anims_JS__3_Clusters(request):
'''###################
tests
###################'''
# test_OpenCV__NewImage()
'''###################
ops
###################'''
'''###################
prep
###################'''
# dpath_Image_File = param_Source_Dir
dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\anims\\data\\data_images"
# dpath_Image_File = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\data_images"
# fname_Image_File = param_Source_File
# fname_Image_File = "leaf-2.1.png" # orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\6_visual-arts\4_animations\1_\5_\images
# fname_Image_File = "2018-10-25_05-27-52_000.jpg" # orig :
fname_Image_File = "2018-10-25_05-27-52_000.(404x303).jpg" # orig :
fpath_Image_File = os.path.join(dpath_Image_File, fname_Image_File)
print("[%s:%d] fpath_Image_File = %s (exists = %s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Image_File, os.path.isfile(fpath_Image_File)
), file=sys.stderr)
# [views.py:608] fpath_Image_File = C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRT
# UAL\Admin_Projects\anims\data\data_images\2018-10-25_05-27-52_000.jpg (exists =
# True)
'''###################
img : read
###################'''
#ref http://peaceandhilightandpython.hatenablog.com/entry/2016/01/09/214333
#ref alpha channel http://opencv.blog.jp/python/alpha_channel
img = cv2.imread(fpath_Image_File, cv2.IMREAD_UNCHANGED)
img_BGR = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
img_HSV = cv2.cvtColor(img, cv2.COLOR_RGB2HSV)
img_HSV_BGR = cv2.cvtColor(img_HSV, cv2.COLOR_RGB2BGR)
#aa
'''###################
subimage
###################'''
orgHeight, orgWidth = img.shape[:2]
# param_Start_X = 2437
# param_Start_Y = 217
#
# param_End_X = 2537
# param_End_Y = 317
# param_Start_X = 237
# param_Start_Y = 150
#
# param_End_X = 257
# param_End_Y = 160
param_Start_X = 0
param_Start_Y = 0
param_End_X = orgWidth - 1
param_End_Y = orgHeight - 1
#ref lib_ip :: get_Corner_Images
img_Sub = img[
param_Start_Y : param_End_Y
, param_Start_X : param_End_X
]
# img_Sub = img[(height - corner_Length) : height - padding, \
# 0 + padding : corner_Length], # clp_LB
'''###################
paths
###################'''
tokens = fname_Image_File.split(".")
(h, w) = img.shape[:2]
tokens_2 = os.path.splitext(fname_Image_File)
tlabel = libs.get_TimeLabel_Now()
# paths
dir_Tmp = tlabel
dpath_Tmp = os.path.join(dpath_Image_File, dir_Tmp)
#ref mkdir https://www.tutorialspoint.com/python/os_mkdir.htm
if not os.path.isdir(dpath_Tmp) :
os.mkdir(dpath_Tmp)
print("[%s:%d] dir => created : '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, dpath_Tmp
), file=sys.stderr)
else :
print("[%s:%d] dir => exists : '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, dpath_Tmp
), file=sys.stderr)
'''###################
TEST : copy image to the new dir
###################'''
# fname_Dst = "%s.(rotate=%d).%s.(center=%d,%d).%s" \
# fname_Dst = "%s.(%s).%s" \
fname_Dst_Orig = "%s.(orig).(%s)%s" \
% (tokens_2[0], tlabel, tokens_2[1])
fname_Dst_BGR = "%s.(BGR).(%s)%s" \
% (tokens_2[0], tlabel, tokens_2[1])
fname_Dst_HSV = "%s.(HSV).(%s)%s" \
% (tokens_2[0], tlabel, tokens_2[1])
fname_Dst_HSV_BGR = "%s.(HSV_BGR).(%s)%s" \
% (tokens_2[0], tlabel, tokens_2[1])
fname_Dst_Sub = "%s.(sub.%d,%d-%d,%d).(%s)%s" \
% (tokens_2[0]
, param_Start_X, param_Start_Y
, param_End_X, param_End_Y
, tlabel, tokens_2[1])
fpath_Dst__Orig = os.path.join(dpath_Image_File, dir_Tmp, fname_Dst_Orig)
fpath_Dst__BGR = os.path.join(dpath_Image_File, dir_Tmp, fname_Dst_BGR)
fpath_Dst__HSV = os.path.join(dpath_Image_File, dir_Tmp, fname_Dst_HSV)
fpath_Dst__HSV_BGR = os.path.join(dpath_Image_File, dir_Tmp, fname_Dst_HSV_BGR)
fpath_Dst__Sub = os.path.join(dpath_Image_File, dir_Tmp, fname_Dst_Sub)
# # res = cv2.imwrite(fpath_Dst, img_BGR)
# res = cv2.imwrite(fpath_Dst__Orig, img)
# res = cv2.imwrite(fpath_Dst__BGR, img_BGR)
# res = cv2.imwrite(fpath_Dst__HSV, img_HSV)
# res = cv2.imwrite(fpath_Dst__HSV_BGR, img_HSV_BGR)
# res = cv2.imwrite(fpath_Dst__Sub, img_Sub)
# # res = cv2.imwrite(fpath_Dst, img)
# # cv2.imwrite(fpath_Dst, rotated)
#
# print("[%s:%d] cv2.imwrite => '%s'" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , res
# ), file=sys.stderr)
'''###################
clusters
###################'''
_anims_JS__3_Clusters__Clusters(request, img_Sub, fpath_Dst__Sub)
# _anims_JS__3_Clusters__Clusters(request, img, fpath_Dst__Sub)
# _anims_JS__3_Clusters__Clusters(request, img_Sub, fpath_Dst__Sub)
#aa
'''###################
return
###################'''
status = 1
msg = "_anims_JS__3_Clusters"
print("[%s:%d] msg => '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, msg
), file=sys.stderr)
return (status, msg)
#/ def _anims_JS__3_Clusters(request):
def anims_JS(request):
'''###################
time
###################'''
time_Exec_Start = time.time()
'''###################
vars
###################'''
dic = {
"message" : ""
, "message_2" : ""
}
render_Page = 'anims/anims/plain_anims.html'
'''###################
params
###################'''
param = request.GET.get('param', False)
#debug
# print("[%s:%d] param => %s" % \
print("[%s:%d] param => %s ============================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param
), file=sys.stderr)
'''###################
dispatch
###################'''
if param == cons_ip.Anims_Params.PARAM__1_MOVE_LEAVES.value : #if param == cons_fx.Tester.lo_Actions__BUSL__IDs[0].value
'''###################
PARAM__1_MOVE_LEAVES
###################'''
# call func
(status, msg) = _anims_JS__1_Move_Leaves(request)
dic['message'] += "move leaves"
dic['message_2'] += "status = %d / msg = '%s'" % (status, msg)
elif param == cons_ip.Anims_Params.PARAM__2_MOVE_LEAVES__V2.value : #if param == cons_fx.Tester.lo_Actions__BUSL__IDs[0].value
'''###################
PARAM__1_MOVE_LEAVES__V2
###################'''
# call func
# (status, msg) = (1, "PARAM__2_MOVE_LEAVES__V2")
(status, msg) = _anims_JS__2_Move_Leaves__V2(request)
dic['message'] += "move leaves"
dic['message_2'] += "status = %d / msg = '%s'" % (status, msg)
elif param == cons_ip.Anims_Params.PARAM__3_CLUSTERS.value : #if param == cons_fx.Tester.lo_Actions__BUSL__IDs[0].value
'''###################
PARAM__3_CLUSTERS
###################'''
# call func
# (status, msg) = (1, "PARAM__2_MOVE_LEAVES__V2")
(status, msg) = _anims_JS__3_Clusters(request)
# (status, msg) = _anims_JS__2_Move_Leaves__V2(request)
dic['message'] += "clusters"
dic['message_2'] += "status = %d / msg = '%s'" % (status, msg)
else :
(status, msg) = (1, "unknown param : '%s'" % param)
dic['message'] += "unknown param"
dic['message_2'] += "status = %d / msg = '%s'" % (status, msg)
'''###################
time
###################'''
time_Exec_Elapsed = time.time() - time_Exec_Start
dic['message_2'] += "(time = %s) (elapsed = %02.3f sec)" % \
(libs.get_TimeLabel_Now(), time_Exec_Elapsed)
'''###################
render
###################'''
return render(request, render_Page, dic)
#/ def anims_JS(request):
def anims(request):
now = datetime.datetime.now()
action = "anims"
message = "yes"
page_Title = "IP / anims"
dic = {
'action' : action,
"message" : message,
# "lo_Commands" : lo_Commands,
"page_Title" : page_Title,
}
# dic = {'action' : action, "message" : message, "lo_Commands" : lo_Commands}
# dic = {message : _message}
'''###################
list of commands
###################'''
lo_Commands = _anims__Load_LO_Actions()
# lo_Commands = [
#
# ["move_leaves", "image sequence for moving leaves"],
#
# ["???", "unknown"],
# ]
# set var
dic["lo_Commands"] = lo_Commands
dic["count"] = 0
'''###################
get : referer
###################'''
referer_MM = "http://127.0.0.1:8000/ip/"
referer_Current = request.META.get('HTTP_REFERER')
if referer_Current == referer_MM : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current == referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'anims/anims/anims.html', dic)
# return render(request, 'ip/basics.html', dic)
# return render(request, 'mm/numbering.html', dic)
else : #if referer_Current == referer_MM
print()
print("[%s:%d] referer_Current <> referer_MM (current = %s / referer = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
,referer_Current, referer_MM
), file=sys.stderr)
return render(request, 'anims/anims/anims_full.html', dic)
# return render(request, 'ip/basics_full.html', dic)
#/ def basics(request):
def open_dir(request):
'''###################
params
###################'''
param = request.GET.get('param', False)
print("[%s:%d] param => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, param
), file=sys.stderr)
'''###################
vars
###################'''
dic = {}
print()
print("[%s:%d] opening dir..." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
build : command string
###################'''
command = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\anims\\utils\\open_anims_log_dir.bat"
# command = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\utils\\open_anims_log_dir.bat"
cmd_Full = [command] #=>
print()
print("[%s:%d] command => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, command
), file=sys.stderr)
'''###################
subprocess
###################'''
#ref https://stackoverflow.com/questions/13525882/tasklist-output answered Nov 23 '12 at 9:36
res = subprocess.call(cmd_Full)
# res = subprocess.check_output(cmd_Full)
'''###################
render
###################'''
return render(request, 'anims/anims/plain_anims.html', dic)
#/ def open_image_dir(request):
<file_sep>/JVEMV6/46_art/11_guitar/end_guitar.bat
taskkill /im MuseScore3.exe
taskkill /im sakura.exe
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/static/ip/js/main.js
//alert("main.js");
/******************************************************
constants
******************************************************/
var cname_White = "white";
var cname_Red = "red";
var cname_Yellow = "yellow";
var cname_LightBlue = "LightBlue";
var cname_Plum = "Plum";
var cname_Moccasin = "Moccasin";
var cname_PaleGreen = "PaleGreen";
var cname_Wheat = "Wheat";
var className_BT_Numbering_List = "bt_Numbering_List";
var TIME_FADE_IN = 300;
var TIME_FADE_OUT = 300;
/******************************************************
funcs : ready
******************************************************/
$(document).ready(function() {
//debug
var datetime = get_Timelabel_Now();
// var currentdate = new Date();
// var datetime = "Last Sync: " + currentdate.getDay() + "/"+currentdate.getMonth()
// + "/" + currentdate.getFullYear() + " @ "
// + currentdate.getHours() + ":"
// + currentdate.getMinutes() + ":" + currentdate.getSeconds();
console.log("ready!" + " " + datetime);
// console.log("ready!");
// $("button").click(function(event) {
//
// //ref http://www.mysamplecode.com/2012/05/jquery-get-button-value-clicked.html
//// alert(event.target.id);
// var class_Name = $(this).attr('class');
//// var tmp = $(this).attr('class');
//// var tmp = $(this).attr('id');
//// var tmp = $(this).prop("value");
//
//// alert("tmp => " + tmp);
//
// if (class_Name == className_BT_Numbering_List) {
//
// } else {
//
// console.log("Unknown class name : '" + class_Name + "'");
//
// }//if (class_Name == )
//
//
// });
});
/******************************************************
funcs : general
******************************************************/
function show_Message(msg) {
alert(msg);
}//function show_Message(msg) {
//ref https://stackoverflow.com/questions/21294302/converting-milliseconds-to-minutes-and-seconds-with-javascript#21294619
function millisToMinutesAndSeconds(millis) {
// alert(millis);
var minutes = Math.floor(millis / 60000);
var residue_Minutes = millis % 60000;
var seconds = Math.floor(residue_Minutes / 1000);
// var seconds = (residue_Minutes / 1000).toFixed(0);
// var seconds = ((millis % 60000) / 1000).toFixed(0);
var mill = millis - (minutes * 60000) - (seconds * 1000);
//log
var msg_log = "millis => " + millis
+ " "
+ "residue_Minutes => " + residue_Minutes
+ " "
+ "mill => " + mill
+ " "
+ "minutes = " + minutes
+ " "
+ "seconds = " + seconds;
// console.log(msg_log);
mill = (mill < 10 ? '00' : (mill < 100 ? '0' : "")) + mill;
return minutes + ":" + (seconds < 10 ? '0' : '') + seconds
+ "."
+ mill;
// return minutes + ":" + (seconds < 10 ? '0' : '') + seconds;
}
/*
* https://stackoverflow.com/questions/5999209/how-to-get-the-background-color-code-of-an-element
* answered May 14 '11 at 2:37
*/
function hexc(colorval) {
var parts = colorval.match(/^rgb\((\d+),\s*(\d+),\s*(\d+)\)$/);
delete(parts[0]);
for (var i = 1; i <= 3; ++i) {
parts[i] = parseInt(parts[i]).toString(16);
if (parts[i].length == 1) parts[i] = '0' + parts[i];
}
color = '#' + parts.join('');
return color;
}
function im_Action(_param) {
/***************************
validate : update date
***************************/
var _update;
if ((_param == "11-0") ||
(_param == "10-1")) {
// if (_param == "11-0") {
var tag_Date = $("input#ipt_IM_Update_Date");
_update = tag_Date.val();
// var update = tag_Date.val();
if (_update == "") {
alert("update ==> blank");
return;
} else if (_update == null) {
alert("update ==> null");
return;
} else {
// alert("_update ==> '" + _update + "'");
}//if (update == "")
}//if (_param == "11-0")
/***************************
operations
***************************/
// alert("!!param is => '" + _param + "'");
//debug
var td = $("td#td_Label_" + _param);
//test
var x = td.css('backgroundColor');
var hex = hexc(x);
/***************************
set : color
***************************/
var color_New = "";
if (hex == "#ffffff") {
color_New = "#ffff00";
} else {
color_New = "#ffffff";
}//if (hex == "#ffffff")
td.css("background", color_New);
/***************************
return : if set to white
***************************/
if (color_New == "#ffffff") {
return;
}
/***************************
bg-color
***************************/
var div_Result = $('div#index_Area__Result');
div_Result.css("background", cname_Plum);
var tag = $('div#index_Message_Area');
tag.html(_param);
// $('div#index_Message_Area').html(_param);
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/im/im_actions";
// var _url = "http://127.0.0.1:8000/im/actions";
/***************************
param
***************************/
var _data;
//ref multiple conditions https://stackoverflow.com/questions/8710442/how-to-specify-multiple-conditions-in-an-if-statement-in-javascript answered Jan 3 '12 at 9:58
if ((_param == "11-0") ||
(_param == "10-1")) {
// if (_param == "11-0") {
_data = {action : _param, update : _update};
} else {
_data = {action : _param};
}//if (_param == "11-0")
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
exception
***************************/
var substr = "total_data";
// var substr = "doesn't";
// alert(data);
// var res = (data.indexOf(substr) !== -1);
//ref substring https://stackoverflow.com/questions/1789945/how-to-check-whether-a-string-contains-a-substring-in-javascript
// if (data.indexOf(substr) == -1) {
if (data.includes(substr)) { // detected
// if (data.indexOf(substr) !== -1) { // detected
//debug
console.log("'doesn't' ==> detected");
// alert(data);
var tag = $('div#index_Area__Result');
$('div#index_Area__Result')
.css("background", cname_Red);
// tag
// .animate({background : "red"}, 500)
// .animate({background : "yellow"}, 500)
// .animate({background : "white"}, 500)
;
$('div#index_Area__Result').html(data);
// //ref
// tag
// .animate({background : "red"}, 500)
// .animate({background : "yellow"}, 500)
// .animate({background : "white"}, 500)
//
// ;
//ref fadein/out https://stackoverflow.com/questions/275931/how-do-you-make-an-element-flash-in-jquery answered Feb 1 '12 at 14:19
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
} else if (data.includes("ERROR")) { // detected
// } else if (data.includes("no entries")) { // detected
//debug
console.log("local image files => no entries");
var tag = $('div#index_Area__Result');
$('div#index_Area__Result')
.css("background", cname_Red);
$('div#index_Area__Result').html(data);
//ref fadein/out https://stackoverflow.com/questions/275931/how-do-you-make-an-element-flash-in-jquery answered Feb 1 '12 at 14:19
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
} else {
var tag = $('div#index_Area__Result');
$('div#index_Area__Result')
.css("background", cname_LightBlue);
// .css("background", cname_White);
$('div#index_Area__Result').html(data);
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
//
// .fadeIn(200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
.fadeIn(200);
}
// $('div#index_Area__Result').html(data);
}).fail(function(xhr, status, error) {
alert(xhr.status);
});
}//function im_Action(param) {
function _mm_Index_LinkTo__0() {
/***************************
message
***************************/
var msg = "ajax starting...";
var elem = $('div#index_Display_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/mm/numbering/";
//var _url = "http://127.0.0.1:8000/im/im_actions";
//var _url = "http://127.0.0.1:8000/im/actions";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#index_Display_Area').html(data);
$('div#index_Display_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#index_Display_Area')
.css("background", cname_Red);
});
}//function _mm_Index_LinkTo__0() {
function _mm_Index_LinkTo__1() {
/***************************
message
***************************/
var msg = "ajax starting... param is '1'";
var elem = $('div#index_Display_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/mm/denumbering/";
// var _url = "http://127.0.0.1:8000/mm/numbering/";
//var _url = "http://127.0.0.1:8000/im/im_actions";
//var _url = "http://127.0.0.1:8000/im/actions";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#index_Display_Area').html(data);
$('div#index_Display_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#index_Display_Area')
.css("background", cname_Red);
});
}//function _mm_Index_LinkTo__1() {
function _mm_Index_LinkTo__2() {
/***************************
message
***************************/
var msg = "ajax starting... param is '1'";
var elem = $('div#index_Display_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/mm/build_history/";
// var _url = "http://127.0.0.1:8000/mm/denumbering/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#index_Display_Area').html(data);
$('div#index_Display_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#index_Display_Area')
.css("background", cname_Red);
});
}//_mm_Index_LinkTo__2()
function mm_Index_LinkTo(_param) {
// alert("!!param is => '" + _param + "'");
/***************************
dispatch
***************************/
//ref https://www.w3schools.com/jsref/jsref_parseInt.asp
var index = parseInt(_param);
if (index == 0) { //[0, "Numbering"]
_mm_Index_LinkTo__0();
}
else if (index == 1) { //[1, "De-numbering"]
_mm_Index_LinkTo__1();
} else if (index == 2) { //[2, "Build history"]
_mm_Index_LinkTo__2();
} else {
alert("unknown index => " + _param);
}
// /***************************
// message
// ***************************/
// var msg = "ajax starting...";
//
// var elem = $('div#index_Display_Area');
//
// elem.html(msg);
//// $('div#index_Display_Area').html(msg);
//
// elem.css("background", cname_Yellow);
//// elem.css("background", "yellow");
//
// /***************************
// ajax
//
// ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
// ***************************/
// var _url = "http://127.0.0.1:8000/mm/numbering/";
//// var _url = "http://127.0.0.1:8000/im/im_actions";
//// var _url = "http://127.0.0.1:8000/im/actions";
//
//// var _data = {action : _param};
//
// $.ajax({
//
// url: _url,
// type: "GET",
// //REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
//// data: {id: id},
//// data: {memos: memos, image_id: image_id},
//// data: _data,
//
// timeout: 10000
//
// }).done(function(data, status, xhr) {
//
//// alert(data);
//
// $('div#index_Display_Area').html(data);
//
// $('div#index_Display_Area')
// .css("background", cname_White);
//
// }).fail(function(xhr, status, error) {
//
// alert(xhr.status);
//
// var msg = "ajax returned error";
//
// $('div#index_Display_Area').html(msg);
//
// $('div#index_Display_Area')
// .css("background", cname_Red);
// });
}//function mm_Index_LinkTo(_param) {
function exec_Numbering(_param) {
// alert("_param => '" + _param + "'");
/***************************
get : vars
***************************/
/***************************
dpath
***************************/
var elem = $('input#ipt_Numbering_MainDir');
//ref val https://stackoverflow.com/questions/4088467/get-the-value-in-an-input-text-box answered Apr 9 '13 at 13:28
var _dpath = elem.val();
// alert("dpath => " + dpath + "'");
/***************************
fname
***************************/
var _fname = _param;
// alert("file fullpath => '" + _dpath + "\\" + _fname + "'");
/***************************
data
***************************/
// var _data = {action : _param};
var _data = {dpath : _dpath, fname : _fname};
var _url = "http://127.0.0.1:8000/mm/exec_Numbering/";
/***************************
background
***************************/
var elem = $('div#numbering_content_Message_Area');
elem.css("background", cname_Yellow);
// elem.css("background", "yellow");
/***************************
ajaxing
***************************/
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#numbering_content_Message_Area').html(data);
$('div#numbering_content_Message_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#numbering_content_Message_Area')
.css("background", cname_Red);
});
}//exec_Numbering
function exec_BuildHistory(_param) {
// alert("_param => '" + _param + "'");
/***************************
get : vars
***************************/
/***************************
dpath
***************************/
var elem = $('input#ipt_Numbering_MainDir');
//ref val https://stackoverflow.com/questions/4088467/get-the-value-in-an-input-text-box answered Apr 9 '13 at 13:28
var _dpath = elem.val();
// alert("dpath => " + dpath + "'");
/***************************
fname
***************************/
var _fname = _param;
// alert("file fullpath => '" + _dpath + "\\" + _fname + "'");
//
// return;
/***************************
data
***************************/
// var _data = {action : _param};
var _data = {dpath : _dpath, fname : _fname};
var _url = "http://127.0.0.1:8000/mm/exec_BuildHistory/";
/***************************
background
***************************/
var elem = $('div#numbering_content_Message_Area');
elem.css("background", cname_Yellow);
// elem.css("background", "yellow");
/***************************
ajaxing
***************************/
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#numbering_content_Message_Area').html(data);
$('div#numbering_content_Message_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#numbering_content_Message_Area')
.css("background", cname_Red);
});
}//exec_BuildHistory
function exec_DeNumbering(_param) {
/***************************
get : vars
***************************/
/***************************
dpath
***************************/
var elem = $('input#ipt_DeNumbering_MainDir');
// var elem = $('input#ipt_Numbering_MainDir');
//ref val https://stackoverflow.com/questions/4088467/get-the-value-in-an-input-text-box answered Apr 9 '13 at 13:28
var _dpath = elem.val();
// alert("dpath => " + dpath + "'");
/***************************
fname
***************************/
var _fname = _param;
// alert("file fullpath => '" + _dpath + "\\" + _fname + "'");
// //debug
// return;
/***************************
data
***************************/
// var _data = {action : _param};
var _data = {dpath : _dpath, fname : _fname};
var _url = "http://127.0.0.1:8000/mm/exec_DeNumbering/";
// var _url = "http://127.0.0.1:8000/mm/exec_Numbering/";
/***************************
background
***************************/
var elem = $('div#numbering_content_Message_Area');
elem.css("background", cname_Yellow);
// elem.css("background", "yellow");
/***************************
ajaxing
***************************/
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#numbering_content_Message_Area').html(data);
/***************************
exception
***************************/
var substr = "EXCEPTION";
// var res = (data.indexOf(substr) !== -1);
if (data.indexOf(substr) !== -1) {
alert("EXCEPTION");
$('div#numbering_content_Message_Area')
.css("background", cname_Red);
} else {
$('div#numbering_content_Message_Area')
.css("background", cname_White);
}
// $('div#numbering_content_Message_Area')
// .css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#numbering_content_Message_Area')
.css("background", cname_Red);
});
}//exec_Numbering
function exec_GenPeakData(_param) {
/***************************
vars
***************************/
var t_Start = +new Date();
// alert("_param => '" + _param + "'");
//
// //debug
// return;
// /***************************
// get : vars
// ***************************/
// /***************************
// dpath
// ***************************/
// var elem = $('input#ipt_Numbering_MainDir');
//
// //ref val https://stackoverflow.com/questions/4088467/get-the-value-in-an-input-text-box answered Apr 9 '13 at 13:28
// var _dpath = elem.val();
//
//// alert("dpath => " + dpath + "'");
//
// /***************************
// fname
// ***************************/
// var _fname = _param;
//
//// alert("file fullpath => '" + _dpath + "\\" + _fname + "'");
//
/***************************
params
***************************/
// var _data = {action : _param};
var _data = {fname : _param};
var _url = "http://127.0.0.1:8000/curr/exec_Gen_PeakData/";
// var _url = "http://127.0.0.1:8000/mm/exec_Numbering/";
/***************************
background
***************************/
var elem = $('div#div_Curr_Basics_Gen_PeakData_Message_Area');
elem.css("background", cname_Yellow);
// elem.css("background", "yellow");
var txt_Message = "ajax starting for --> '" + _param + "'"
+ " (" + get_Timelabel_Now_2() + ")";
// log
console.log(txt_Message);
/***************************
ajaxing
***************************/
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 20000 // 20 seconds
// timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#div_Curr_Basics_Gen_PeakData_Result_Area').html(data);
$('div#div_Curr_Basics_Gen_PeakData_Result_Area')
.css("background", cname_Plum);
/***************************
time
***************************/
//ref elapsed https://stackoverflow.com/questions/3528425/how-to-display-moving-elapsed-time-in-jquery answered Apr 29 '17 at 1:04
var elapsed = +new Date() - t_Start;
var txt_Elapsed = millisToMinutesAndSeconds(elapsed);
/***************************
message
***************************/
var msg = "ajax done for --> 'exec_GenPeakData'"
+ " "
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'gen_peak_data')"
+ "(file = '" + _param + "')"
+ " (took = " + txt_Elapsed + ")"
;
//log
console.log(msg);
// message
$('div#div_Curr_Basics_Gen_PeakData_Message_Area').css("background", cname_LightBlue);
// var txt_Message = "ajax done" + _param + " "
// + " (" + get_Timelabel_Now_2() + ")";
$('div#div_Curr_Basics_Gen_PeakData_Message_Area').html(msg);
// $('div#div_Curr_Basics_Gen_PeakData_Message_Area').html("Ajax => done");
}).fail(function(xhr, status, error) {
alert(xhr.status + " (error = '" + error + "')");
// alert(xhr.status);
/***************************
time
***************************/
//ref elapsed https://stackoverflow.com/questions/3528425/how-to-display-moving-elapsed-time-in-jquery answered Apr 29 '17 at 1:04
var elapsed = +new Date() - t_Start;
var txt_Elapsed = millisToMinutesAndSeconds(elapsed);
var msg = "ajax returned error : " + error + "(elapsed = " + txt_Elapsed + ")";
//log
console.log(msg);
$('div#div_Curr_Basics_Gen_PeakData_Message_Area').html(msg);
$('div#div_Curr_Basics_Gen_PeakData_Message_Area')
.css("background", cname_Red);
});
}//exec_GenPeakData
function get_Timelabel_Now() {
//ref https://stackoverflow.com/questions/10211145/getting-current-date-and-time-in-javascript
//ref https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date
var currentdate = new Date();
var datetime = "Last Sync: " + currentdate.getDate() + "/"+currentdate.getMonth() + 1
// var datetime = "Last Sync: " + currentdate.getDay() + "/"+currentdate.getMonth()
+ "/" + currentdate.getFullYear() + " @ "
+ currentdate.getHours() + ":"
+ currentdate.getMinutes() + ":" + currentdate.getSeconds();
return datetime;
}
function get_Timelabel_Now_2() {
//ref https://stackoverflow.com/questions/10211145/getting-current-date-and-time-in-javascript
//ref https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date
var cd = new Date();
// var currentdate = new Date();
// var month = cd.getMonth() + 1;
var month = (cd.getMonth() + 1).toString();
if (month.toString().length < 2) {
month = "0" + month;
}
var year = cd.getFullYear().toString();
var day = cd.getDate().toString();
if (day.length < 2) {
day = "0" + day;
}
// alert("month => " + month);
// var datetime = cd.getFullYear() + (cd.getMonth() + 1) + cd.getDay()
// var datetime = "" + cd.getFullYear() + (month) + cd.getDay()
// var datetime = "" + cd.getFullYear().toString() + (month).toString() + cd.getDay().toString()
var datetime = year + month + day
+ "_"
+ cd.getHours() + cd.getMinutes() + cd.getSeconds();
// var datetime = "Last Sync: " + currentdate.getDate() + "/"+currentdate.getMonth() + 1
//// var datetime = "Last Sync: " + currentdate.getDay() + "/"+currentdate.getMonth()
// + "/" + currentdate.getFullYear() + " @ "
// + currentdate.getHours() + ":"
// + currentdate.getMinutes() + ":" + currentdate.getSeconds();
return datetime;
}//function get_Timelabel_Now_2() {
function mm_Index_GO() {
/***************************
get selected
***************************/
//ref selection https://learn.jquery.com/using-jquery-core/faq/how-do-i-get-the-text-value-of-a-selected-option/
//ref get value, not text https://stackoverflow.com/questions/13089944/jquery-get-selected-option-value-not-the-text-but-the-attribute-value <NAME>
var selection = $( "#select_MM_Actions option:selected" );
// var selection = $( "#select_MM_Actions option:selected" ).text();
var text = selection.text();
var value = selection.val();
// alert("text => '" + text + "'" + " / " + "val = " + value);
/***************************
dispatch
***************************/
mm_Index_LinkTo(value);
}//mm_Index_GO()
/***************************
<option value="0" class="opt_MM_Actions">Updown patterns</option>
***************************/
function _curr_Index_LinkTo__0() {
/***************************
message
***************************/
var msg = "ajax starting...";
var elem = $('div#index_Display_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
clear : message
***************************/
//ref clear div https://stackoverflow.com/questions/1701973/how-to-clear-all-divs-contents-inside-a-parent-div answered Nov 9 '09 at 16:05
$('div#index_Message_Area').html('');
$('div#index_Area__Result').html('');
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/curr/updown_patterns/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#index_Display_Area').html(data);
$('div#index_Display_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#index_Display_Area')
.css("background", cname_Red);
});
}//function _curr_Index_LinkTo__0() {
function _curr_Index_LinkTo__1() {
/***************************
message
***************************/
var msg = "ajax starting...";
var elem = $('div#index_Display_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
clear : message
***************************/
$('div#index_Message_Area').html('');
$('div#index_Area__Result').html('');
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/curr/correlations/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#index_Display_Area').html(data);
$('div#index_Display_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#index_Display_Area')
.css("background", cname_Red);
});
}//function _curr_Index_LinkTo__1() {
function _curr_Index_LinkTo__2() {
/***************************
message
***************************/
var msg = "ajax starting... param is '1'";
var elem = $('div#index_Display_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
clear : message
***************************/
$('div#index_Message_Area').html('');
$('div#index_Area__Result').html('');
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/mm/build_history/";
// var _url = "http://127.0.0.1:8000/mm/denumbering/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
$('div#index_Display_Area').html(data);
$('div#index_Display_Area')
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
$('div#index_Display_Area').html(msg);
$('div#index_Display_Area')
.css("background", cname_Red);
});
}//_curr_Index_LinkTo__2()
function curr_Index_LinkTo(_param) {
// alert("!!param is => '" + _param + "'");
/***************************
dispatch
***************************/
//ref https://www.w3schools.com/jsref/jsref_parseInt.asp
var index = parseInt(_param);
if (index == 0) { //[0, "Numbering"]
_curr_Index_LinkTo__0();
}
else if (index == 1) { //[1, "De-numbering"]
_curr_Index_LinkTo__1();
} else if (index == 2) { //[2, "Build history"]
_curr_Index_LinkTo__2();
} else {
alert("unknown index => " + _param);
}
// /***************************
// message
// ***************************/
// var msg = "ajax starting...";
//
// var elem = $('div#index_Display_Area');
//
// elem.html(msg);
//// $('div#index_Display_Area').html(msg);
//
// elem.css("background", cname_Yellow);
//// elem.css("background", "yellow");
//
// /***************************
// ajax
//
// ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
// ***************************/
// var _url = "http://127.0.0.1:8000/mm/numbering/";
//// var _url = "http://127.0.0.1:8000/im/im_actions";
//// var _url = "http://127.0.0.1:8000/im/actions";
//
//// var _data = {action : _param};
//
// $.ajax({
//
// url: _url,
// type: "GET",
// //REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
//// data: {id: id},
//// data: {memos: memos, image_id: image_id},
//// data: _data,
//
// timeout: 10000
//
// }).done(function(data, status, xhr) {
//
//// alert(data);
//
// $('div#index_Display_Area').html(data);
//
// $('div#index_Display_Area')
// .css("background", cname_White);
//
// }).fail(function(xhr, status, error) {
//
// alert(xhr.status);
//
// var msg = "ajax returned error";
//
// $('div#index_Display_Area').html(msg);
//
// $('div#index_Display_Area')
// .css("background", cname_Red);
// });
}//function mm_Index_LinkTo(_param) {
function _anims_Action_LinkTo__1(_param) {
/***************************
prep
***************************/
var _url = "http://127.0.0.1:8001/anims/anims_JS/";
// var _url = "http://127.0.0.1:8001/ip/anims_JS/";
//var _url = "http://127.0.0.1:8000/im/actions";
/***************************
params : options values
***************************/
var _option_loc_start_X = $('input#in_anims_options_loc_start_x').val();
var _option_loc_start_Y = $('input#in_anims_options_loc_start_y').val();
var _opt_cut_width = $('input#in_anims_options_cut_width').val();
var _opt_cut_height = $('input#in_anims_options_cut_height').val();
var _opt_rotate_Start = $('input#in_anims_options_rotate_start').val();
var _opt_rotate_End = $('input#in_anims_options_rotate_end').val();
var _opt_rotate_Tick = $('input#in_anims_options_rotate_tick').val();
var _opt_source_Dir = $('input#in_anims_options_source_dir').val();
var _opt_source_File = $('input#in_anims_options_source_file').val();
// alert("_opt_rotate_Tick => " + _opt_rotate_Tick);
// return;
/***************************
param
***************************/
var _data = {
param : _param
, option_loc_start_X : _option_loc_start_X
, option_loc_start_Y : _option_loc_start_Y
// , option_tick_move_Y : _option_tick_move_Y
, opt_rotate_Start : _opt_rotate_Start
, opt_rotate_End : _opt_rotate_End
, opt_rotate_Tick : _opt_rotate_Tick
, opt_cut_width : _opt_cut_width
, opt_cut_height : _opt_cut_height
, opt_source_Dir : _opt_source_Dir
, opt_source_File : _opt_source_File
};
// //ref multiple conditions https://stackoverflow.com/questions/8710442/how-to-specify-multiple-conditions-in-an-if-statement-in-javascript answered Jan 3 '12 at 9:58
// if ((_param == "11-0") ||
// (_param == "10-1")) {
// // if (_param == "11-0") {
//
// _data = {action : _param, update : _update};
//
// } else {
//
// _data = {action : _param};
//
// }//if (_param == "11-0")
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
Log
***************************/
var msg = "ajax ==> done";
var elem = $('div#div_IP_Anims__Log_Area');
elem.html(msg);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
/***************************
Message
***************************/
var msg = "ajax ==> done";
var elem = $('div#div_IP_Basics_Message_Area');
elem.html(data);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
}).fail(function(xhr, status, error) {
alert(xhr.status);
});
}
function _anims_Action_LinkTo__2(_param) {
/***************************
prep
***************************/
var _url = "http://127.0.0.1:8001/anims/anims_JS/";
// var _url = "http://127.0.0.1:8001/ip/anims_JS/";
//var _url = "http://127.0.0.1:8000/im/actions";
/***************************
params : options values
***************************/
var _option_loc_start_X = $('input#in_anims_move_leaves_v2_options_loc_start_x').val();
var _option_loc_start_Y = $('input#in_anims_move_leaves_v2_options_loc_start_y').val();
var _option_loc_end_X = $('input#in_anims_move_leaves_v2_options_loc_end_x').val();
var _option_loc_end_Y = $('input#in_anims_move_leaves_v2_options_loc_end_y').val();
// var _opt_cut_width = $('input#in_anims_options_cut_width').val();
// var _opt_cut_height = $('input#in_anims_options_cut_height').val();
var _opt_rotate_Start = $('input#in_anims_options_rotate_start').val();
var _opt_rotate_End = $('input#in_anims_options_rotate_end').val();
var _opt_rotate_Tick = $('input#in_anims_options_rotate_tick').val();
var _opt_source_Dir = $('input#in_anims_options_source_dir').val();
var _opt_source_File = $('input#in_anims_options_source_file').val();
// alert("_opt_rotate_Tick => " + _opt_rotate_Tick);
// return;
/***************************
param
***************************/
var _data = {
param : _param
, option_loc_start_X : _option_loc_start_X
, option_loc_start_Y : _option_loc_start_Y
, option_loc_end_X : _option_loc_end_X
, option_loc_end_Y : _option_loc_end_Y
, opt_rotate_Start : _opt_rotate_Start
, opt_rotate_End : _opt_rotate_End
, opt_rotate_Tick : _opt_rotate_Tick
, opt_source_Dir : _opt_source_Dir
, opt_source_File : _opt_source_File
};
// //ref multiple conditions https://stackoverflow.com/questions/8710442/how-to-specify-multiple-conditions-in-an-if-statement-in-javascript answered Jan 3 '12 at 9:58
// if ((_param == "11-0") ||
// (_param == "10-1")) {
// // if (_param == "11-0") {
//
// _data = {action : _param, update : _update};
//
// } else {
//
// _data = {action : _param};
//
// }//if (_param == "11-0")
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
Log
***************************/
var msg = "ajax ==> done";
var elem = $('div#div_IP_Anims__Log_Area');
elem.html(msg);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
/***************************
Message
***************************/
var msg = "ajax ==> done";
var elem = $('div#div_IP_Basics_Message_Area');
elem.html(data);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
}).fail(function(xhr, status, error) {
alert(xhr.status);
});
}//function _anims_Action_LinkTo__2(_param)
function _anims_Action_LinkTo__3(_param) {
//debug
console.log("_anims_Action_LinkTo__3(_param) --> starting...");
/***************************
prep
***************************/
var _url = "http://127.0.0.1:8001/anims/anims_JS/";
/***************************
params : options values
***************************/
// var _option_loc_start_X = $('input#in_anims_move_leaves_v2_options_loc_start_x').val();
/***************************
param
***************************/
var _data = {
param : _param
// , option_loc_start_X : _option_loc_start_X
};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
Log
***************************/
var msg = "ajax ==> done";
var elem = $('div#div_IP_Anims__Log_Area');
elem.html(msg);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
/***************************
Message
***************************/
var msg = "ajax ==> done";
var elem = $('div#div_IP_Basics_Message_Area');
elem.html(data);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
}).fail(function(xhr, status, error) {
alert(xhr.status);
});
}//function _anims_Action_LinkTo__3(_param)
function anims_Action(_param) {
// //debug
// alert("!!param is => '" + _param + "'");
// return;
/***************************
message
***************************/
var msg = "ajax starting for id '" + _param + "'";
var elem = $('div#div_IP_Anims__Log_Area');
elem.html(msg);
elem
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
//
// .fadeIn(200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
.fadeIn(200);
// elem.css("background", cname_Yellow);
/***************************
params
==> see : cons_ip :: Anims_Params
***************************/
var param_1_move_leaves = "1";
var param_2_move_leaves = "2";
var param_2_clusters = "3";
// /***************************
// params : options values
// ***************************/
/***************************
dispatch
***************************/
//ref https://www.w3schools.com/jsref/jsref_parseInt.asp
// var index = parseInt(_param);
// if (index == 1) { // 1 "move_leaves"
if (_param == param_1_move_leaves) { // 1 "move_leaves"
_anims_Action_LinkTo__1(_param);
} else if (_param == param_2_move_leaves) { // 1 "move_leaves"
_anims_Action_LinkTo__2(_param);
} else if (_param == param_2_clusters) { // 1 "move_leaves"
_anims_Action_LinkTo__3(_param);
// else if (index == 1) { //[1, "De-numbering"]
//
// _curr_Index_LinkTo__1();
//
// } else if (index == 2) { //[2, "Build history"]
//
// _curr_Index_LinkTo__2();
//
} else {
alert("unknown index => " + _param);
}
}//function mm_Index_LinkTo(_param) {
function curr_Index_GO() {
// alert("curr index");
var selection = $( "#select_Curr_Actions option:selected" );
// var selection = $( "#select_MM_Actions option:selected" ).text();
var text = selection.text();
var value = selection.val();
// //debug
// alert("text => '" + text + "'" + " / " + "val = " + value);
/***************************
clear : areas
***************************/
var area_Display = $('div#index_Display_Area');
// alert(area_Display);
// area_Display.html() = "";
area_Display.html('');
// index_Message_Area
$('div#index_Message_Area').html('');
$('div#index_Area__Result').html('');
/***************************
dispatch
***************************/
curr_Index_LinkTo(value);
}
function curr_Updown_GO() {
// alert("updown GO");
/***************************
message
***************************/
var msg = "ajax starting...";
var elem = $('div#index_Message_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
param : body volume : up
***************************/
var tag_Vol_Up = $('input#ipt_Curr_UpdownPatterns_Range_Up');
var val_Vol_Up = tag_Vol_Up.val();
/***************************
build : data
***************************/
var _data = {body_volume_up : val_Vol_Up};
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/curr/exec_updown_patterns/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// var tag = $('div#index_Area__Result');
//
// tag.html(data);
//
// tag
// .css("background", cname_White);
/***************************
exception
***************************/
var substr = "ERROR";
//ref substring https://stackoverflow.com/questions/1789945/how-to-check-whether-a-string-contains-a-substring-in-javascript
if (data.includes(substr)) { // detected
//debug
console.log("'" + substr + "'" + " ==> detected");
var tag = $('div#index_Area__Result');
$('div#index_Area__Result')
.css("background", cname_Red);
$('div#index_Area__Result')
.css("color", cname_White);
$('div#index_Area__Result').html(data);
//ref fadein/out https://stackoverflow.com/questions/275931/how-do-you-make-an-element-flash-in-jquery answered Feb 1 '12 at 14:19
tag
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
// .fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
// .fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN);
} else {
var tag = $('div#index_Area__Result');
$('div#index_Area__Result')
.css("background", cname_LightBlue);
$('div#index_Area__Result').html(data);
tag
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
.fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
// .fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
// .fadeIn(TIME_FADE_IN).fadeOut(TIME_FADE_OUT)
// .fadeIn(TIME_FADE_IN200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
//
// .fadeIn(200).fadeOut(200)
// .fadeIn(200).fadeOut(200)
.fadeIn(TIME_FADE_IN);
}
}).fail(function(xhr, status, error) {
alert(xhr.status);
var msg = "ajax returned error";
var tag = $('div#index_Area__Result');
tag.html(msg);
tag.css("background", cname_Red);
});
}
function curr_Correl_GO() {
/***************************
message
***************************/
var msg = "ajax starting... (curr_Correl_GO)";
var elem = $('div#index_Message_Area');
elem.html(msg);
//$('div#index_Display_Area').html(msg);
elem.css("background", cname_Yellow);
//elem.css("background", "yellow");
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/curr/exec_correlations/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// alert(data);
var tag = $('div#index_Area__Result');
tag.html(data);
tag
.css("background", cname_White);
}).fail(function(xhr, status, error) {
alert(xhr.status + " / " + error);
// alert(xhr.status);
var msg = "ajax returned error";
var tag = $('div#index_Area__Result');
tag.html(msg);
tag.css("background", cname_Red);
});
}
function curr_Show_Basics_Table_Commands() {
// alert("show");
//ref toggle https://api.jquery.com/toggle/
// $('#div_IP_Basics_ListOf_Actions').toggle();
$('#div_IP_Anims_ListOf_Actions').toggle();
}//function curr_Show_Basics_Table_Commands() {
function bt_ShowHide_Areas(_id_name) {
// alert("show");
//ref toggle https://api.jquery.com/toggle/
$("#" + _id_name).toggle();
// $('#div_IP_Basics_ListOf_Actions').toggle();
}//function curr_Show_Basics_Table_Commands() {
function _curr_Basics_Index_GO__Gen_Peak_Data() {
/***************************
vars
***************************/
var t_Start = +new Date();
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8000/curr/gen_peak_data/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
// //test
//ref http://www.hp-stylelink.com/news/2013/11/20131126.php#list03
// var huga = 0;
// var hoge = setInterval(function() {
// console.log(huga);
// huga++;
// //終了条件
//// if (huga == 10) {
// if (huga == 4) {
// clearInterval(hoge);
// console.log("終わり");
// }
// }, 500);
// alert(data);
/***************************
result
***************************/
var tag_Result = $('div#div_Curr_Basics_Result_Area');
tag_Result.html(data);
tag_Result.css("background", cname_White);
/***************************
time
***************************/
//ref elapsed https://stackoverflow.com/questions/3528425/how-to-display-moving-elapsed-time-in-jquery answered Apr 29 '17 at 1:04
var elapsed = +new Date() - t_Start;
var txt_Elapsed = millisToMinutesAndSeconds(elapsed);
/***************************
message
***************************/
var msg = "ajax done for --> 'gen_peak_data'"
+ " "
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'gen_peak_data')"
+ " (took = " + txt_Elapsed + ")"
;
var tag = $('div#div_Curr_Basics_Message_Area');
tag.html(msg);
tag.css("background", cname_LightBlue);
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
// alert(xhr.status + " / " + error);
// alert(xhr.status);
var msg = "ajax returned error (" + xhr.status + " / " + error + ")"
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'gen_peak_data')";
// var msg = "ajax returned error";
var tag = $('div#div_Curr_Basics_Message_Area');
tag.html(msg);
tag.css("background", cname_Red);
});
}//function _curr_Basics_Index_GO__Gen_Peak_Data() {
function curr_Basics_Index_GO(param) {
// //debug
// alert("param => '" + param + "'");
//
// return;
/***************************
clear : areas
***************************/
var area_Display = $('div#index_Display_Area');
// alert(area_Display);
// area_Display.html() = "";
area_Display.html('');
// index_Message_Area
var txt_Message = "ajax starting for --> '" + param + "'"
+ " (" + get_Timelabel_Now_2() + ")";
$('div#div_Curr_Basics_Message_Area').html(txt_Message);
$('div#index_Area__Result').html('');
/***************************
dispatch
***************************/
if (param == "gen_peak_data") {
console.log(param);
var div = $('div#div_Curr_Basics_Message_Area');
div.css("background", cname_Yellow);
/***************************
start ajax
***************************/
_curr_Basics_Index_GO__Gen_Peak_Data();
} else {
// index_Message_Area
var txt_Message = "Unknown commdand --> '" + param + "'"
+ " (" + get_Timelabel_Now_2() + ")";
$('div#div_Curr_Basics_Message_Area').html(txt_Message);
$('div#div_Curr_Basics_Message_Area').css("background", cname_Red);
}//if (param == )
}
function _ip_Basics_Index_GO__Get_4_Corners() {
/***************************
vars
***************************/
var t_Start = +new Date();
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
// var _url = "http://127.0.0.1:8001/ip/get_4_corners";
// var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
var _url = "http://127.0.0.1:8001/ip/get_4_corners/";
// http://1172.16.17.32:8001/ip/get_4_corners/
// var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
//var _data = {action : _param};
// //debug
// alert("ajax for => '" + _url + "'");
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
result
***************************/
var tag_Result = $('div#div_IP_Basics_Result_Area');
tag_Result.html(data);
tag_Result.css("background", cname_White);
/***************************
time
***************************/
//ref elapsed https://stackoverflow.com/questions/3528425/how-to-display-moving-elapsed-time-in-jquery answered Apr 29 '17 at 1:04
var elapsed = +new Date() - t_Start;
var txt_Elapsed = millisToMinutesAndSeconds(elapsed);
/***************************
message
***************************/
var msg = "ajax done for --> 'gen_peak_data'"
+ " "
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'gen_peak_data')"
+ " (took = " + txt_Elapsed + ")"
;
var tag = $('div#div_IP_Basics_Message_Area');
tag.html(msg);
tag.css("background", cname_LightBlue);
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
// alert(xhr.status + " / " + error);
// alert(xhr.status);
var msg = "ajax returned error (" + xhr.status + " / " + error + ")"
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'gen_peak_data')";
// var msg = "ajax returned error";
var tag = $('div#div_IP_Basics_Message_Area');
tag.html(msg);
tag.css("background", cname_Red);
});
}//_ip_Basics_Index_GO__Get_4_Corners()
function ip_Basics_Index_GO(param) {
/***************************
set : result area
***************************/
$('div#div_IP_Basics_Result_Area').html('');
/***************************
dispatch
***************************/
if (param == "get_4_Corners") {
/***************************
set : message area
***************************/
var area_Display = $('div#div_IP_Basics_Message_Area');
area_Display.html('');
// index_Message_Area
var txt_Message = "ajax starting for --> '" + param + "'"
+ " (" + get_Timelabel_Now_2() + ")";
$('div#div_IP_Basics_Message_Area').html(txt_Message);
// log
console.log(txt_Message);
// console.log(param);
var div = $('div#div_IP_Basics_Message_Area');
div.css("background", cname_Yellow);
/***************************
start ajax
***************************/
_ip_Basics_Index_GO__Get_4_Corners();
} else {
// index_Message_Area
var txt_Message = "Unknown commdand --> '" + param + "'"
+ " (" + get_Timelabel_Now_2() + ")";
$('div#div_IP_Basics_Message_Area').html(txt_Message);
$('div#div_IP_Basics_Message_Area').css("background", cname_Red);
}//if (param == )
}//ip_Basics_Index_GO
function btn_IP_Get_4Corners__Open_ImageDir() {
// //debug
// var tag = $('input#cb_Get4Corners_SaveImage');
//
// //ref https://stackoverflow.com/questions/4754699/how-do-i-determine-if-a-checkbox-is-checked#4754729 answered Jan 21 '11 at 2:12
// var val = tag.is(':checked');
//// var val = tag.checked;
//
// alert("checkbox value => '" + val + "'");
//// var val = tag.val();
////
//// alert("checkbox value => '" + val + "'");
//
// return;
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html('');
// message
tag.css("background", cname_Yellow);
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8001/ip/open_image_dir/";
//var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
//var _data = {action : _param};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
result
***************************/
// alert(data);
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html(data);
// message
tag.css("background", cname_LightBlue);
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
alert(error);
});
}
function open_dir(_param) {
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8001/anims/open_dir/";
//var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
//var _data = {action : _param};
/***************************
param
***************************/
var _data = {
param : _param
};
$.ajax({
url: _url,
type: "GET",
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
result
***************************/
// alert(data);
var tag = $('div#div_IP_Anims__Log_Area');
tag.html("dir ==> opened");
// tag.html(data);
// message
tag.css("background", cname_LightBlue);
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
alert(error);
});
}//function open_dir(_param)() {
function exec_Get_4Corners(fname) {
/***************************
vars
***************************/
var t_Start = +new Date();
/***************************
get : params : dpath_Images
***************************/
// dpath to images
var dpath_Images = $('input#ipt_IP_Get_4Corners').val();
// alert(dpath_Images);
// file name
alert(fname);
/***************************
param : checkbox : SaveImage
***************************/
//debug
var tag = $('input#cb_Get4Corners_SaveImage');
//ref https://stackoverflow.com/questions/4754699/how-do-i-determine-if-a-checkbox-is-checked#4754729 answered Jan 21 '11 at 2:12
var val = tag.is(':checked');
var _flg_save_image = "false";
if (val) {
_flg_save_image = "true";
} //if (val)
/***************************
param : input : corner width
***************************/
//debug
var tag = $('input#cb_Get4Corners_CornerImage_Width');
var _corner_Width = tag.val();
// alert("_corner_Width => '" + _corner_Width + "'");
//
var is_number = $.isNumeric(_corner_Width);
// validate
if (is_number == false) {
alert("corner width => not numeric : '" + _corner_Width + "'");
return;
}//if (is_number == false)
/***************************
param : input : corner padding
***************************/
//debug
var tag_CornerImage_Padding = $('input#cb_Get4Corners_CornerImage_Padding');
var _corner_Padding = tag_CornerImage_Padding.val();
// alert("_corner_Padding => '" + _corner_Padding + "'");
//
var is_number = $.isNumeric(_corner_Padding);
// validate
if (is_number == false) {
alert("corner padding => not numeric : '" + _corner_Padding + "'");
return;
}//if (is_number == false)
// alert("is_number => '" + is_number + "'");
//
// return;
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8001/ip/exec_get_4_corners/";
//var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
// params
var _data = {
fname_image : fname
, dpath_images : dpath_Images
, flg_save_image : _flg_save_image
, corner_width : _corner_Width
, corner_Padding : _corner_Padding
};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: 10000
}).done(function(data, status, xhr) {
/***************************
result
***************************/
var tag_Result = $('div#div_IP_Get_4Corners_Result_Area');
tag_Result.html(data);
tag_Result.css("background", cname_PaleGreen);
/***************************
time
***************************/
//ref elapsed https://stackoverflow.com/questions/3528425/how-to-display-moving-elapsed-time-in-jquery answered Apr 29 '17 at 1:04
var elapsed = +new Date() - t_Start;
var txt_Elapsed = millisToMinutesAndSeconds(elapsed);
/***************************
message
***************************/
var msg = "ajax done for --> 'exec_get_4_corners'"
+ " "
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'gen_peak_data')"
+ " (took = " + txt_Elapsed + ")"
;
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html(msg);
tag.css("background", cname_Wheat);
var intvl_On = 250;
var intvl_Off = 150;
tag
.fadeIn(intvl_On).fadeOut(100)
.fadeIn(intvl_On).fadeOut(100)
.fadeIn(intvl_On).fadeOut(100)
.fadeIn(intvl_On).fadeOut(100)
.fadeIn(intvl_On);
// tag.fadeIn(100).fadeOut(100).fadeIn(100).fadeOut(100).fadeIn(100);
// tag.effect("highlight",{},800)
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
// alert(xhr.status + " / " + error);
// alert(xhr.status);
var msg = "ajax returned error (" + xhr.status + " / " + error + ")"
+ "(" + get_Timelabel_Now_2() + ")"
+ "(command = 'exec_get_4_corners')";
// var msg = "ajax returned error";
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html(msg);
tag.css("background", cname_Red);
});
}//exec_Get_4Corners
function bt_Get4Corners_Options_ShowHide_toggle() {
var tag = $('#div_Get4Corners_Options');
tag.toggle();
}//bt_Get4Corners_Options_ShowHide_toggle()
function btn_IP_Get_4Corners__Gen_CakeCSV() {
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html('');
// message
tag.css("background", cname_Yellow);
/***************************
param : image dir
***************************/
var tag_Dpath_Image = $('input#ipt_IP_Get_4Corners');
var _dpath_image = tag_Dpath_Image.val();
// var dpath_Image = tag_Dpath_Image.val();
//alert("_dpath_image => '" + _dpath_image + "'");
// alert("_dpath_Image => '" + _dpath_Image + "'");
//
// //debug
// return;
/***************************
param : checkbox : SaveImage
***************************/
//debug
var tag = $('input#cb_Get4Corners_SaveImage');
//ref https://stackoverflow.com/questions/4754699/how-do-i-determine-if-a-checkbox-is-checked#4754729 answered Jan 21 '11 at 2:12
var val = tag.is(':checked');
var _flg_save_image = "false";
if (val) {
_flg_save_image = "true";
} //if (val)
/***************************
param : input : corner width
***************************/
//debug
var tag = $('input#cb_Get4Corners_CornerImage_Width');
var _corner_Width = tag.val();
//alert("_corner_Width => '" + _corner_Width + "'");
//
var is_number = $.isNumeric(_corner_Width);
// validate
if (is_number == false) {
alert("corner width => not numeric : '" + _corner_Width + "'");
return;
}//if (is_number == false)
/***************************
param : input : corner padding
***************************/
//debug
var tag_CornerImage_Padding = $('input#cb_Get4Corners_CornerImage_Padding');
var _corner_Padding = tag_CornerImage_Padding.val();
// alert("_corner_Padding => '" + _corner_Padding + "'");
//
var is_number = $.isNumeric(_corner_Padding);
// validate
if (is_number == false) {
alert("corner padding => not numeric : '" + _corner_Padding + "'");
return;
}//if (is_number == false)
/***************************
show message
***************************/
var tag = $('div#div_IP_Get_4Corners_Message_Area');
var msg = "starting ajax for 'http://127.0.0.1:8001/ip/gen_Cake_CSV/'..."
tag.html(msg);
// message
tag.css("background", cname_Yellow);
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
/***************************
param : gen cake csv
***************************/
var _option = "gen_cake_csv";
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8001/ip/gen_Cake_CSV/";
//var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
// time out (mill seconds)
var _timeout = 3 * 60 * 1000;
// params
var _data = {
// fname_image : fname
option : _option
, dpath_images : _dpath_image
, flg_save_image : _flg_save_image
, corner_width : _corner_Width
, corner_Padding : _corner_Padding
};
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
data: _data,
timeout: _timeout
// timeout: 10000
}).done(function(data, status, xhr) {
/***************************
result
***************************/
// alert(data);
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html(data);
// message
tag.css("background", cname_LightBlue);
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
alert(error);
});
}//btn_IP_Get_4Corners__Gen_CakeCSV
function btn_IP_Get_4Corners__Prep_Gen_CakeCSV() {
/***************************
show message
***************************/
var tag = $('div#div_IP_Get_4Corners_Message_Area');
var msg = "starting ajax for 'http://127.0.0.1:8001/ip/prep_gen_Cake_CSV/'..."
// var msg = "starting ajax for 'http://127.0.0.1:8001/ip/gen_Cake_CSV/'..."
tag.html(msg);
// message
tag.css("background", cname_Yellow);
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
/***************************
param : gen cake csv
***************************/
var _option = "prep_gen_cake_csv";
/***************************
ajax
ref : C:\WORKS_2\WS\Eclipse_Luna\Cake_IFM11\app\webroot\js\main.js
***************************/
var _url = "http://127.0.0.1:8001/ip/prep_gen_Cake_CSV/";
//var _url = "http://127.0.0.1:8000/ip/get_4_corners/";
// time out (mill seconds)
var _timeout = 3 * 60 * 1000;
$.ajax({
url: _url,
type: "GET",
//REF http://stackoverflow.com/questions/1916309/pass-multiple-parameters-to-jquery-ajax-call answered Dec 16 '09 at 17:37
// data: {id: id},
// data: {memos: memos, image_id: image_id},
// data: _data,
timeout: _timeout
// timeout: 10000
}).done(function(data, status, xhr) {
/***************************
result
***************************/
// alert(data);
var tag = $('div#div_IP_Get_4Corners_Message_Area');
tag.html(data);
// message
tag.css("background", cname_LightBlue);
tag
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200).fadeOut(200)
.fadeIn(200);
}).fail(function(xhr, status, error) {
/***************************
message
***************************/
alert(error);
});
}//btn_IP_Get_4Corners__Prep_Gen_CakeCSV
<file_sep>/JVEMV6/46_art/8_kb/start_web_pages.bat
@echo off
pushd "C:\Program Files (x86)\Google\Chrome\Application"
REM start chrome.exe http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/view/21495
start chrome.exe "https://docs.google.com/spreadsheets/d/1Rtg1H97HaTWcaIhOf4EtMqEvo6o-17d0Cf0CY5cuk9c/edit#gid=1591115137"
start chrome.exe http://benfranklin.chips.jp/cake_apps/images/ifm11/music/
start chrome.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=kb&RBs_AND_OR_Memo=AND&sort=file_name&direction=desc"
start chrome.exe https://www.musicca.com/jp/metronome
start chrome.exe https://www.musicca.com/jp/metronome
start chrome.exe https://metronom.us/en/
exit
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第1章/potentialFlow1.js
/*----------------------------------------------
potentialFlow1.js
ポテンシャル流れ
一様流れ+湧き出し+渦
粒子アニメーション
-----------------------------------------------*/
var canvas; //キャンバス要素
var gl;//WebGL描画用コンテキスト
var height_per_width;//キャンバスのサイズ比
//animation
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var flagStart = false;
var flagFreeze = false;
var flagStep = false;
var flagReset = false;
//ポテンシャル流れ
var Phi = [];//ポテンシャル
var Psi = [];//流れ関数
var VelX = [];//格子点の速度
var VelY = [];
var scale = new Vector3(1.0, 1.0, 0.0);//canvas左下を(-1.0, -1.0)としたデカルト座標に表示するときのスケール調整
var flagUniform = true;
var flagSource = false;
var flagVortex = false;
var flagPotential = true;
var flagStream = false;
var flagVelocity = false;
var flagGrid = false;
var flagStart = false;
var alpha = 0;//一様流れの傾斜角
var flowVelocity = 1;//一様流れの流速(固定)
var Q_Value = 1;//湧き出し量
var Gamma = 1; //循環
var nLine = 40;//流線,ポテンシャルの表示本数
var range = 1;//その範囲
var arrowScale = 0.05;
var arrowWidth = 1;
var intervalV = 2;//速度矢印表示間隔
//解析領域矩形構造体
function Rect()
{
this.scale = 1;//表示倍率
this.size = 2;//矩形領域のサイズ(正方形)
this.nMesh = 40;//全体の分割数(X,Y共通)
this.delta ;//格子間隔
this.left0 = new Vector3(-1, -1, 0);//その左下位置
}
var rect = new Rect();
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(1, 1, 1, 1);
form2.nMesh.value = rect.nMesh;
form2.scale.value = rect.scale;
form2.nLine.value = nLine;
form2.range.value = range;
calculate();
initParticle();
var fps = 0;
var timestep = 0;
var lastTime = new Date().getTime();
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime += frameTime;//全経過時間
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //0.5秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
gl.clear(gl.COLOR_BUFFER_BIT);
drawParticle(timestep);
display();
elapseTime0 = elapseTime;//現在の経過時間を保存
form1.time.value = elapseTime.toString();
if(flagStep) { flagStart = false; }
}
}
animate();
}
function calculate()
{
//ポテンシャル流れの計算パラメータ
flagUniform = form2.Uniform.checked ;
flagSource = form2.Source.checked ;
flagVortex = form2.Vortex.checked ;
rect.nMesh = parseInt(form2.nMesh.value);
rect.delta = rect.size / rect.nMesh;
alpha = parseFloat(form2.alpha.value);
Q_Value = parseFloat(form2.Q_Value.value);
Gamma = parseFloat(form2.Gamma.value);
var i, j;
for(i = 0; i <= rect.nMesh; i++)
{//配列の2次元化
Phi[i] = [];//ポテンシャル
Psi[i] = [];//流れ関数
VelX[i] = [];//速度
VelY[i] = [];
}
//ポテンシャル,流れ関数,速度のクリア
for(j = 0; j <= rect.nMesh; j++)
{
for (i = 0; i <= rect.nMesh; i++)
Phi[i][j] = Psi[i][j] = VelX[i][j] = VelY[i][j] = 0.0;
}
var z = new Vector3();
var r2 = 0.0;
var ang = alpha * DEG_TO_RAD;
rad0 = 0.001;
for(i = 0; i <= rect.nMesh; i++)
{
z.x = rect.delta * (i - rect.nMesh / 2);//中心のポテンシャルを0
for (j = 0; j <= rect.nMesh; j++)
{
z.y = rect.delta * (j - rect.nMesh / 2);
if(flagUniform)
{
Phi[i][j] = flowVelocity * (z.x * Math.cos(ang) + z.y * Math.sin(ang));
Psi[i][j] = flowVelocity * (z.y * Math.cos(ang) - z.x * Math.sin(ang));
VelX[i][j] = flowVelocity * Math.cos(ang);
VelY[i][j] = flowVelocity * Math.sin(ang);
}
if (flagSource)//湧き出し(吸い込み)
{
if(z.x == 0 && z.y == 0)
{//原点は対数的特異点
z.x = rect.delta / 1000.0;
z.y = rect.delta / 1000.0;
}
r2 = mag2(z);
if(r2 < rad0) r2 = rad0;
Phi[i][j] += Q_Value * Math.log(r2) / (4.0 * Math.PI);
Psi[i][j] += Q_Value * Math.atan2(z.y, z.x) / (2.0 * Math.PI);
VelX[i][j] += Q_Value * z.x / r2 / (2.0 * Math.PI);
VelY[i][j] += Q_Value * z.y / r2 / (2.0 * Math.PI);
}
if (flagVortex)//うず
{
if(z.x == 0 && z.y == 0)
{//原点は対数的特異点
z.x = rect.delta / 100.0;
z.y = rect.delta / 100.0;;
}
r2 = mag2(z);
if(r2 < rad0) r2 = rad0;//中心付近の速度を抑えるため
Psi[i][j] -= Gamma * (Math.log(r2) / (4.0 * Math.PI));
Phi[i][j] += Gamma * Math.atan2(z.y, z.x) / (2.0 * Math.PI);
VelX[i][j] -= Gamma * z.y / r2 / (2.0 * Math.PI);
VelY[i][j] += Gamma * z.x / r2 / (2.0 * Math.PI);
}
}
}
gl.clear(gl.COLOR_BUFFER_BIT);
display();
}
function display()
{
flagPotential = form2.phi.checked;
flagStream = form2.psi.checked;
flagVelocity = form2.velocity.checked;
flagGrid = form2.grid.checked;
flagPoint = false;
gl.viewport(0, 0, canvas.width, canvas.height);
height_per_width = canvas.height / canvas.width;//縦横の比率を一定にするための係数
//計算領域描画
drawRegion();
if( flagPotential ) drawContour(Phi, "red");
if( flagStream ) drawContour(Psi, "blue");
if( flagVelocity ) drawVelocity();
if( flagGrid ) drawGrid();
}
function drawRegion()
{
var s1, s2;
if(canvas.width >= canvas.height)
{
s1 = height_per_width;
s2 = 1.0;
}
else
{
s1 = 1.0;
s2 = 1 / height_per_width;
}
scale.x = rect.scale * s1;
scale.y = rect.scale * s2;
var sx = scale.x * rect.size / 2;//表示領域の幅は2*sx
var sy = scale.y * rect.size / 2;//表示領域の高さは2*sx
drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
//左下基準点
rect.left0.x = - sx;
rect.left0.y = - sy;
//格子間隔
rect.delta = rect.size / rect.nMesh;
//x-y座標
drawLine(rect.left0.x, 0, rect.left0.x + scale.x * rect.size, 0, 1, "gray");
drawLine(0, rect.left0.y, 0, rect.left0.y + scale.y * rect.size, 1, "gray");
}
function drawContour(PP, col)
{
nLine = parseFloat(form2.nLine.value);
range = parseFloat(form2.range.value);
var maxP = flowVelocity * range;
var minP = -flowVelocity * range;
var dp0 = (maxP - minP) / nLine;//+0.00001;//流線間隔
var pp;
var x1, y1, x2, y2;
var p = [], x = [], y = [];
var i, j, k, m;
var data = [];
//三角形セルに分割
for (k = 0; k < nLine; k++)
{
pp = minP + (k + 1) * dp0;
for(i = 0; i < rect.nMesh; i++)
{
for(j = 0; j < rect.nMesh; j++)
{//三角形セルに分割
p[0] = PP[i][j]; x[0] = i * rect.delta; y[0] = j * rect.delta;
p[1] = PP[i][j+1]; x[1] = i * rect.delta; y[1] = (j+1) * rect.delta;
p[2] = PP[i+1][j+1];x[2] = (i+1) * rect.delta; y[2] = (j+1) * rect.delta;
p[3] = PP[i+1][j]; x[3] = (i+1) * rect.delta; y[3] = j * rect.delta;
p[4] = p[0]; x[4] = x[0]; y[4] = y[0];//0番目に同じ
//中心
p[5] = (p[0] + p[1] + p[2] + p[3]) / 4.0;
x[5] = (x[0] + x[1] + x[2] + x[3]) / 4.0;
y[5] = (y[0] + y[1] + y[2] + y[3]) / 4.0;
for(m = 0; m < 4; m++)//各三角形について
{
x1 = -10.0; y1 = -10.0;
if((p[m] <= pp && pp < p[m+1]) || (p[m] > pp && pp >= p[m+1]))
{
x1 = x[m] + (x[m+1] - x[m]) * (pp - p[m]) / (p[m+1] - p[m]);
y1 = y[m] + (y[m+1] - y[m]) * (pp - p[m]) / (p[m+1] - p[m]);
}
if((p[m] <= pp && pp <= p[5]) || (p[m] >= pp && pp >= p[5]))
{
if(x1 < 0.0)//まだ交点なし
{
x1 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y1 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
}
else//x1は見つかった
{
x2 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y2 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
if((p[m+1] <= pp && pp <= p[5]) || (p[m+1] >= pp && pp >= p[5]))
{
if(x1 < 0.0)//まだ交点なし
{
x1 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y1 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
}
else//x1は見つかった
{
x2 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y2 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
}//m
}//j
}//i
}//k
drawLines(data, col);
}
function drawVelocity()
{
arrowScale = parseFloat(form2.arrowScale.value);;
arrowWidth = parseFloat(form2.arrowWidth.value);
intervalV = parseFloat(form2.intervalV.value);
var i, j, k;
//描画
var theta, mag, x0, y0, vx, vy;
for(i = 1; i < rect.nMesh; i++)
{
if(i % intervalV != 0) continue;
for (j = 1; j < rect.nMesh; j++)
{
if(j % intervalV != 0) continue;
vx = VelX[i][j] * scale.x;
vy = VelY[i][j] * scale.y;
mag = Math.sqrt(vx*vx + vy*vy) ;
if(mag > 10.0) continue;
theta = Math.atan2(vy, vx);
x0 = rect.left0.x + scale.x * i * rect.delta;
y0 = rect.left0.y + scale.y * j * rect.delta;
drawArrow(x0, y0, mag * arrowScale, arrowWidth, "black", theta);
}
}
}
function drawGrid()
{
var i, j;
for(i = 1; i < rect.nMesh; i++)
{
drawLine(rect.left0.x + scale.x * i * rect.delta, rect.left0.y,
rect.left0.x + scale.x * i * rect.delta, rect.left0.y + scale.y * rect.size, 1, "blue");
}
for(j = 1; j < rect.nMesh; j++)
{
drawLine(rect.left0.x, rect.left0.y + scale.y * j * rect.delta,
rect.left0.x + scale.x * rect.size, rect.left0.y + scale.y * j * rect.delta, 1, "blue");
}
}
//-----------------------------------------------------------------
function Particle2D()
{
this.pos = new Vector3(1000.0, 1000.0, 0);
this.vel = new Vector3();
}
var countP = 0;
var pa = [];//particle
var sizeP = 3;
var speedCoef = 1;
var countPeriod = 0;
var period = 0.0;//[s]
var numMaxP =10000;//最大個数
var num0 = 100;
var typeP = 1;
function initParticle()
{
//粒子
for(var i = 0; i < numMaxP; i++) pa[i] = new Particle2D();
}
function drawParticle(dt)
{
flagPoint = true;
var k, k0, K = 0;
var dataP = [];
var vel = new Vector3();
if(!flagFreeze && countPeriod == 0)
{
for(k0 = 0; k0 < num0; k0++)
{
k = countP + k0;
createParticle(k);
}
countP += num0;
}
K = 0;
for(k = 0; k < numMaxP; k++)
{
vel = getVelocityParticle(pa[k].pos);
if(!flagFreeze) {
pa[k].pos.x += vel.x * dt * speedCoef;
pa[k].pos.y += vel.y * dt * speedCoef;
}
if(pa[k].pos.x >= 0.0 && pa[k].pos.x < rect.size
&& pa[k].pos.y >= 0.0 && pa[k].pos.y < rect.size)
{
dataP[2*K] = rect.left0.x+ pa[K].pos.x * scale.x;
dataP[2*K+1] = rect.left0.y + pa[K].pos.y * scale.y;
K++;
}
else{
if( countPeriod == 0) createParticle(k);
}
}
drawPoints(dataP, sizeP, typeP, "black");
if(countP > numMaxP-num0) countP = 0;
countPeriod += dt;
if(countPeriod > period){
countPeriod = 0;
}
}
function createParticle(k)
{
if(flagUniform && flagSource && Q_Value > 0.0)
{
if((k % 2) == 0)
{
pa[k].pos.y = rect.size * getRandom(0.0, 1.0);
pa[k].pos.x = 0.01;//左端(left0)からの位置
}
else
{
pa[k].pos = getRandomRingVectorXY(0.01, 0.02);
pa[k].pos.x += rect.size/2;//中心の位置へシフト
pa[k].pos.y += rect.size/2;
}
}
else if(!flagUniform && flagSource && Q_Value > 0.0)
{
pa[k].pos = getRandomRingVectorXY(0.01, 0.02);
pa[k].pos.x += rect.size/2;//中心の位置へシフト
pa[k].pos.y += rect.size/2;
}
else
{
pa[k].pos.x = 0.01;//左端(left0)からの位置
pa[k].pos.y = rect.size * getRandom(0, 1);
}
}
function getVelocityParticle(pos)
{
var vel = new Vector3();
//var nGridX = rect.nMesh + 1;
var i, j, I, J;
//格子番号を取得
I = 0; J = 0;
for(i = 0; i < rect.nMesh; i++)
{
if(i * rect.delta < pos.x && (i+1) * rect.delta > pos.x) I = i;
}
for(j = 0; j < rect.nMesh; j++)
{
if(j * rect.delta < pos.y && (j+1) * rect.delta > pos.y) J = j;
}
var a = pos.x / rect.delta - I;
var b = pos.y / rect.delta - J;
//格子点の速度を線形補間
vel.x = (1.0 - b) * ((1.0 - a) * VelX[I][J] + a * VelX[I+1][J]) + b * ((1.0 - a) * VelX[I][J+1] + a * VelX[I+1][J+1]);
vel.y = (1.0 - b) * ((1.0 - a) * VelY[I][J] + a * VelY[I+1][J]) + b * ((1.0 - a) * VelY[I][J+1] + a * VelY[I+1][J+1]);
return vel;
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
gl.clear(gl.COLOR_BUFFER_BIT);
display();
}
function onChangeData()
{
calculate();
}
function onDisplay()
{
gl.clear(gl.COLOR_BUFFER_BIT);
display();
}
function onClickScale()
{
rect.scale = parseFloat(form2.scale.value);
gl.clear(gl.COLOR_BUFFER_BIT);
display();
}
//-------------------------------------------------------------
function onClickP()
{
numMaxP = parseInt(form2.numMaxP.value);
num0 = parseInt(form2.num0.value);
period = parseFloat(form2.period.value);
speedCoef = parseFloat(form2.speedCoef.value);
sizeP= parseFloat(form2.sizeP.value);
typeP= parseFloat(form2.typeP.value);
}
function onClickStart()
{
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
flagStart = true;
flagStep = false;
flagFreez = false;
lastTime = new Date().getTime();
initParticle();
}
function onClickFreeze()
{
if(flagStart) { flagStart = false; }
else { flagStart = true; elapseTime = elapseTime0; }
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTime = 0;
flagStart = false;
flagStep = false;
initParticle();
form1.time.value = "0";
gl.clear(gl.COLOR_BUFFER_BIT);
//renewPosition();
display();
}
<file_sep>/JVEMV6/46_art/VIRTUAL/console.py
'''
=========================================== 2018/02/10 10:07:39
pushd C:\WORKS_2\WS\WS_Others\prog\D-7\2_2\VIRTUAL
python manage.py startapp currency
pushd C:\WORKS_2\WS\WS_Others\prog\D-7\2_2\VIRTUAL\Admin_Projects
python manage.py startapp curr
'''
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第3章/advection.js
/*----------------------------------------------
advection.js
移流方程式
-----------------------------------------------*/
var canvas; //キャンバス要素
var gl; //WebGL描画用コンテキスト
var height_per_width;//キャンバスのサイズ比
//animation
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var flagStart = false;
var flagFreeze = false;
var flagReset = true;
//数値計算
var nMesh;//x軸方向分割数
var deltaX;//Δx(格子間隔)
var widthInit = 0.1;//パルス波形の初期幅
var centerInit = 0.05;//その中心点
var speed = 0.1;//波形の移流速度
var deltaT0 = 0.01;//数値計算上の時間刻み(初期設定値)
var deltaT; //実際の数値計算上の時間刻み(delta0/thinningN)
var thinningN = 1; //間引き回数
var courant;
var method = 0;//1次精度
var nMethod = 4;//解法数(1次,2次,CIP,厳密解)
var f0 = [];//物理量(温度,濃度 etc.)計算前
var f1 = [];//物理量(温度,濃度 etc.)計算後
var g0 = [];//微分(CIP,計算前)
var g1 = [];//微分(CIP,計算後)
var f_t = [];//[4][NUM_MAX][10];//時系列データ
var time = [0.0, 2.0, 4.0, 6.0, 8.0];//その時刻[s]
var mark = [];
var nTime = 5;
var scale = new Vector3();
var count = 0;//厳密解を描画する際に,丁度格子間隔を通過したときだけデータを更新するためのカウンタ
var tt = 0;//厳密解を描画するときの経過時間
var hh = 0.25;//1つのプロファイルの表示枠の高さ
//表示領域矩形構造体
function Rect()
{
this.scale = 1.8;//表示倍率
this.nMesh = 100;//x方向割数(固定)
this.size = new Vector3(1, 1, 0);//表示領域のサイズ(固定)
this.left0 = new Vector3(-0.5, -0.5, 0);//その左下位置
}
var rect = new Rect();
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(1, 1, 1, 1);
form2.deltaT0.value = deltaT0;
form2.thinningN.value = thinningN;
form2.nMesh.value = rect.nMesh;
form2.pulseWidth.value = widthInit;
form2.speed.value = speed;
form2.scale.value = rect.scale;
initData();
gl.clear(gl.COLOR_BUFFER_BIT);
display();
var fps = 0;//フレームレート
var timestep = 0;
var lastTime = new Date().getTime();
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //0.5秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += deltaT;//数値計算上の経過時間(実経過時間ではない)
if(method <= 2)//1次精度,2次精度, CIP
{
for(var i = 0; i < thinningN; i++) calculate();
}
else strictSolution();
form1.time.value = elapseTime.toString();
}
display();
if(method < 3) drawProfile();
else drawStrict();
drawTimeSeries();
elapseTime0 = elapseTime;//現在の経過時間を保存
}
animate();
}
function initData()
{
var i, j, k;
var nWidth, nCenter;
deltaT0 = parseFloat(form2.deltaT0.value);
thinningN = parseInt(form2.thinningN.value);
rect.nMesh = parseFloat(form2.nMesh.value);
widthInit = parseFloat(form2.pulseWidth.value);
centerInit = widthInit / 2;
speed = parseFloat(form2.speed.value);
rect.scale = parseFloat(form2.scale.value);
nMesh = rect.nMesh;//空間分割数
deltaX = rect.size.x / nMesh;//格子間隔
nWidth = widthInit / deltaX;//パルス幅の格子数
nCenter = centerInit / deltaX;
deltaT = deltaT0 / thinningN;//数値計算上のタイムステップ
form2.deltaT.value = deltaT;
courant = speed * deltaT / deltaX;//クーラン数
form2.Courant.value = courant;
if(flagReset)
{
for(var k = 0; k < nTime; k++)
{
mark[k] = [];
f_t[k] = [];
for(j = 0; j < nMethod; j++) f_t[k][j] = [];
}
//時系列データのクリア
for(k = 0; k < nTime; k++)
{
for(i = 0; i <= nMesh ; i++)
{
for(j = 0; j < 4; j++)
{
f_t[k][j][i] = 0.0;
mark[k][j] = 0;//確定済みのとき1
}
}
}
}
//初期値
f0[0] = 0.0;
for(i = 0; i <= nMesh; i++)
{
if(i <= nCenter - nWidth / 2) f0[i] = 0.0;
else if(i < nCenter + nWidth / 2) f0[i] = 1.0;
else f0[i] = 0.0;
for(j = 0; j < 4; j++) f_t[0][j][i] = f1[i] = f0[i];//時系列データ
}
//微分の初期値(CIP法)
for (i = 0; i <= nMesh; i++)
{
g0[i] = g1[i] = 0.0;
}
for(k = 0; k < nTime; k++)
{
for(j = 0; j < nMethod; j++)
for(i = 0; i <= nMesh ; i++) mark[k][j] = 0;//確定済みのとき1
time[k] = k / Math.abs(speed) / nTime;//speed=0.1のとき2s間隔
}
count = 0;
flagReset = false;//Resetボタンが押されるまでリセットしない
}
function display()
{
gl.clear(gl.COLOR_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
height_per_width = canvas.height / canvas.width;//縦横の比率を一定にするための係数
var s1, s2;
if(canvas.width >= canvas.height)
{
s1 = height_per_width;
s2 = 1.0;
}
else
{
s1 = 1.0;
s2 = 1 / height_per_width;
}
scale.x = rect.scale * s1;
scale.y = rect.scale * s2;
var sx = scale.x * rect.size.x / 2;//表示領域の幅は2*sx
var sy = scale.y * rect.size.y / 2;//表示領域の高さは2*sy
//表示領域全体
drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
//表示領域の左下基準点
rect.left0.x = - sx;
rect.left0.y = - sy;
hh = rect.size.y / 4.0;//1つ当たりの表示枠の高さ
var i;
//各段の横軸
for(i = 0; i <= 3; i++)
{
drawLine(rect.left0.x - 0.04 , rect.left0.y + i * hh * scale.y ,
rect.left0.x + rect.size.x * scale.x, rect.left0.y + i * hh * scale.y, 1, "black");
}
//横軸5分割線を縦軸に平行に引く(0.2間隔,2秒間隔)
for(i = 1; i <= 4; i++)
{
drawLine(rect.left0.x + i * 0.2 * rect.size.x * scale.x, rect.left0.y,
rect.left0.x + i * 0.2 * rect.size.x * scale.x, rect.left0.y + rect.size.y * scale.y, 1, "gray");
}
//縦軸目盛
for(i = 0; i < 4; i++)
{
//f = 1
drawLine(rect.left0.x, rect.left0.y + (i * hh + hh * 0.8) * scale.y,
rect.left0.x - 0.04, rect.left0.y + (i * hh + hh * 0.8) * scale.y, 1, "black");
//f = 0.5
drawLine(rect.left0.x, rect.left0.y + (i * hh + hh * 0.4) * scale.y,
rect.left0.x - 0.02, rect.left0.y + (i * hh + hh * 0.4) * scale.y, 1, "black");
}
}
function calculate()
{
var i, k, im1, im2, fm1, fm2;
if(method <= 1)//1次精度,2次精度
{
for(i = 0; i <= nMesh; i++)
{
im1 = i-1;
if(im1 < 0) fm1 = 0.0; else fm1 = f0[im1];
if(method == 0)
{
//1次精度
f1[i] = f0[i] + courant * (fm1 - f0[i]);
}
else if(method == 1)
{
im2 = i - 2;
if(im2 < 0) fm2 = 0.0; else fm2 = f0[im2];
//2次精度
f1[i] = f0[i] - 0.5 * courant * (fm2 - 4*fm1 + 3 *f0[i]);
}
}
for(i = 0; i <= nMesh; i++) f0[i] = f1[i];//計算後のデータを次回の計算のために保存
}
else if(method == 2) methodCIP();//CIP
//時系列データの保存
for(k = 1; k < nTime; k++)
{
if(mark[k][method] == 1) continue;
if(elapseTime >= time[k] / thinningN)
{
for(i = 0; i <= nMesh; i++) f_t[k][method][i] = f0[i];
mark[k][method] = 1;
}
}
}
function methodCIP()
{
var i, ip;
var Fp, Gp;
var c3, c2, x, s;
if( speed > 0.0) s = 1.0;
else s = -1.0;
for(i = 0; i <= nMesh; i++)
{
ip = i-s;//上流点
if(ip < 0.0 || ip > nMesh){
Fp = 0.0;
Gp = 0.0;
}
else{
Fp = f0[ip];
Gp = g0[ip];
}
var dx = -s * deltaX;
var dx2 = dx * dx;
var dx3 = dx2 * dx;
c3 = (g0[i] + Gp) / dx2 + 2.0 * (f0[i] - Fp) / dx3;
c2 = 3.0 * (Fp - f0[i]) / dx2 - (2.0 * g0[i] + Gp) / dx;
x = - speed * deltaT;
f1[i] += ( (c3 * x + c2) * x + g0[i] ) * x ;
g1[i] += ( 3.0 * c3 * x + 2.0 * c2 ) * x ;
}
for(i = 0; i <= nMesh; i++) { f0[i] = f1[i]; g0[i] = g1[i]; }
}
function strictSolution()
{
var i, ip, k;
//解析解(厳密解)
if(elapseTime >= count * deltaX / Math.abs(speed))
{
tt = 0.0;
for(i = 0; i <= nMesh; i++)
{
ip = i-1;//上流点
if(i == 0) f0[ip] = 0;
f1[i] = f0[ip];
}
for(i = 0; i <= nMesh; i++) f0[i] = f1[i];
count ++;
}
tt += deltaT0;//deltaT;
//時系列データの保存
for(k = 0; k < nTime; k++)
{
if(mark[k][3] == 1) continue;
if(elapseTime >= time[k])
{
for(var i = 0; i < nMesh; i++) {
f_t[k][3][i] = f0[i+1];
}
mark[k][3] = 1;
}
}
}
function drawProfile()
{
var HH;
var data= [];
var x1, y1, x2, y2;
for(var i = 0; i < nMesh; i++)
{
HH = (3.0 - method) * hh;
x1 = i* deltaX;
y1 = HH + f0[i] * hh * 0.8;
x2 = (i+1) * deltaX;
y2 = HH + f0[i+1] * hh * 0.8;
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
drawLines(data, "black");
}
function drawStrict()
{
var data = [];
var x1, y1, x2, y2;
for(var i = 0; i < nMesh; i++)
{
x1 = i* deltaX + speed * tt;
y1 = f0[i] * hh * 0.8,
x2 = (i+1) * deltaX + speed * tt;
y2 = f0[i+1] * hh * 0.8;
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
drawLines(data, "black");
}
function drawTimeSeries()
{
var i, j, k;
var x1, y1, x2, y2, HH;
var data = [];
for(k = 0; k < nTime; k++)
for(j = 0; j < 4; j++){
{
HH = (3.0 - j) * hh;
for(i = 0; i < nMesh; i++)
{
x1 = i * deltaX;
y1 = HH + f_t[k][j][i] * hh * 0.8;
x2 = (i+1) * deltaX;
y2 = HH + f_t[k][j][i+1] * hh * 0.8;
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
}
drawLines(data, "black");
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
flagStart = false;
flagReset = true;
initData();
}
function onClickScale()
{
rect.scale = parseFloat(form2.scale.value);
gl.clear(gl.COLOR_BUFFER_BIT);
}
function onChangeMethod()
{
var radioM = document.getElementsByName("radioM");
for(var i = 0; i < radioM.length; i++)
{
if(radioM[i].checked) method = i;
}
flagStart = false;
}
//-------------------------------------------------------------
function onClickStart()
{
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
flagStart = true;
flagFreez = false;
lastTime = new Date().getTime();
initData();
}
function onClickFreeze()
{
if(flagStart) { flagStart = false; }
else { flagStart = true; }
}
function onClickReset()
{
elapseTime0 = 0;
elapseTime = 0;
flagStart = false;
flagReset = true;
form1.time.value = "0";
initData();
}
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/sp/data/ops/6_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\6_1.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\1_1.py
at : 2018/07/15 08:09:48
r w && r d4
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops
python 6_1.py -s1.0
python 6_1.py
ref : http://aidiary.hatenablog.com/entry/20110607/1307449007
20180721_111655
C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images\
'''
###############################################
import sys, cv2
# from pandas.compat import str_to_bytes
from numpy.distutils.from_template import item_re
#ref https://stackoverflow.com/questions/3129322/how-do-i-get-monitor-resolution-in-python
from win32api import GetSystemMetrics
from matplotlib import pylab as plt
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects') # libs_mm
from libs_admin import libs, lib_ip
'''###################
import : built-in modules
###################'''
# import getopt
import os, glob, getopt, math as math, numpy as np
# import inspect
'''###################
constants : global
###################'''
# import math as math
refPt_Start = [-1] * 2
refPt_End = [-1] * 2
# refPt_Start = []
# refPt_End = []
flg_Set_Points = ""
flg_Get_RGB_Vals = False
POINTS_START = "START"
POINTS_END = "END"
POINTS_NEUTRAL = "NEUTRAL"
KEY_INPUTS__END = 'e'
KEY_INPUTS__RGB = 'g'
KEY_INPUTS__HELP = 'h'
KEY_INPUTS__QUIT = 'q'
KEY_INPUTS__RESET = 'r'
KEY_INPUTS__START = 's'
KEY_INPUTS__EXECUTE = 'x'
DPATH_IMAGE_OUTPUT = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
###############################################
# def show_Message() :
#
# msg = '''
# <Options>
# -v Volume down the amplitude --> 1.0 * v / 1000
# -f Base frequency ---> e.g. 262 for the A tone
# -p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
#
# print (msg)
'''###################
click_and_crop(event, x, y, flags, param)
at : 2018/06/30 09:53:22
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
def click_and_crop(event, x, y, flags, param):
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
if event == cv2.EVENT_LBUTTONDOWN:
# #debug
# print("[%s:%d] type(param) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(param)
# ), file=sys.stderr)
if flg_Set_Points == POINTS_START : #if flg_Set_Points == POINTS_START
refPt_Start[0] = x
refPt_Start[1] = y
print()
# print("[%s:%d] EVENT_LBUTTONDOWN (x = %d / y = %d)" % \
print("[%s:%d] EVENT_LBUTTONDOWN ==> starting point set" % \
(os.path.basename(libs.thisfile()), libs.linenum()
# , x, y
), file=sys.stderr)
print(refPt_Start)
# '''###################
# RGB vals
# ###################'''
# if flg_Get_RGB_Vals == True : #if flg_Get_RGB_Vals == True
#
# print(param[x][y])
#/if flg_Get_RGB_Vals == True
elif flg_Set_Points == POINTS_END : #if flg_Set_Points == POINTS_START
refPt_End[0] = x
refPt_End[1] = y
print()
# print("[%s:%d] EVENT_LBUTTONDOWN (x = %d / y = %d)" % \
print("[%s:%d] EVENT_LBUTTONDOWN ==> ending point set" % \
(os.path.basename(libs.thisfile()), libs.linenum()
# , x, y
), file=sys.stderr)
print(refPt_End)
else : #if flg_Set_Points == POINTS_START
print()
print("[%s:%d] EVENT_LBUTTONDOWN ==> unknown flag value : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_Set_Points
), file=sys.stderr)
print([x, y])
'''###################
RGB vals
###################'''
if flg_Get_RGB_Vals == True : #if flg_Get_RGB_Vals == True
print("[%s:%d] RGB of the point ==>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(param[y][x])
# print(param[x][y])
#/if flg_Get_RGB_Vals == True
#/if flg_Set_Points == POINTS_START
#/ def click_and_crop(event, x, y, flags, param):
def show_Message():
# print("'q' to quit")
# print("'x' to execute")
# print("'h' to show this key list")
print("'g'\tset flag for getting RGB values")
print("'h'\tshow this key list")
print("'q'\tquit")
print("'r'\treset start/end")
print("'x'\texecute")
# print("'e' to execute")
# print("left click ==> set the starting point")
# print("right click ==> set the ending point")
print("'s' then left click ==> set the starting point")
print("'e' then right click ==> set the ending point")
print()
#/ def show_Message():
'''###################
get_RGB_Vals
from : test_7__SubImage_RGB_Vals__Get_RGB_Vals(img_Sub)
at : 2018/07/15 08:17:58
@return: list of RGB vals
e.g. [156, 167, 153,...], [156, 167, 153,...], [156, 167, 153,...]
###################'''
def get_RGB_Vals(img_Sub):
'''###################
message
###################'''
cntOf_Rows = 0
lo_Rs = []
lo_Gs = []
lo_Bs = []
for row in img_Sub:
# counter
cntOf_Rows += 1
for cell in row:
'''###################
append
###################'''
lo_Rs.append(cell[0])
lo_Gs.append(cell[1])
lo_Bs.append(cell[2])
#/for cell in row:
#/for row in img_Sub:
'''###################
return
###################'''
return lo_Rs, lo_Gs, lo_Bs
#/ def test_7__SubImage_RGB_Vals__Get_RGB_Vals(img_Sub):
'''###################
get_Scaling
from : test_7__SubImage_RGB_Vals__Get_Scaling(args)
at : 2018/07/15 08:22:52
###################'''
def get_Scaling(args):
# scaling
scaling = 1.0
# #debug
# print("[%s:%d] args =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(args)
if len(args) > 0 : #if len(args) > 1
optlist, args = getopt.getopt(args, "s:")
print("[%s:%d] optlist =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(optlist)
for item in optlist:
#debug
print("[%s:%d] item =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(item)
if item[0].startswith("-s") : #if item.startswith("-s")
scaling = float(item[1])
break
#/if item.startswith("-s")
#/for item in optlist:
else : #if len(args) > 1
print("[%s:%d] len(args) < 2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if len(args) > 1
#debug
print("[%s:%d] scaling => %.3f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, scaling
), file=sys.stderr)
'''###################
return
###################'''
return scaling
#/ def test_7__SubImage_RGB_Vals__Get_Scaling():
'''###################
resize_Image()
<description>
- add listener to ==> image
- hover on the image ==> x, y coordinates displayed in the console
- use ==> argv for window size
<Example>
python 1_1.py -s0.25
<quit program>
return key
###################'''
# def test_5__Resize_Image(width, height, scaling, scr_W, scr_H):
def resize_Image(width, height, scaling, scr_W, scr_H):
q1 = 1.0 * width / height
s = scaling
flg_WorH = ""
# j1
if s >= 0 : #if s >= 0
### j1 : p1
A = 0
if width >= height : #if width > height
flg_WorH = "width"
A = width
else : #if width > height
flg_WorH = "height"
A = height
#/if width > height
### j1 : p2
m = A * s
print("[%s:%d] m => %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, m
), file=sys.stderr)
### j1 : p3
Z = 0
if flg_WorH == "width" : #if flg_WorH == "width"
Z = scr_W
else : #if flg_WorH == "width"
Z = scr_H
#/if flg_WorH == "width"
### j2
result = (m > Z)
'''###################
vars
###################'''
win_Resize_Height = 0
win_Resize_Width = 0
if result == True : #if result == True
print("[%s:%d] m > Z : m = %.03f, Z = %d, flg_WorH = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, m, Z, flg_WorH
), file=sys.stderr)
### j2 : p1
q2 = 1.0 * Z / A
print("[%s:%d] q2 => %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, q2
), file=sys.stderr)
'''###################
calc
###################'''
win_Resize_Height = math.floor(height * q2)
win_Resize_Width = math.floor(width * q2)
# win_Resize_Height = int(height * q2)
# win_Resize_Width = int(width * q2)
# win_Resize_Height = height * q2
# win_Resize_Width = width * q2
else : #if result == True
print("[%s:%d] m <= Z" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
calc
###################'''
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
#/if result == True
else : #if s >= 0
'''###################
calc
###################'''
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
#/if s >= 0
# '''###################
# calc
# ###################'''
# win_Resize_Height = math.floor(scaling * height)
# win_Resize_Width = math.floor(scaling * width)
'''###################
return
###################'''
#debug
print("[%s:%d] win_Resize_Width = %d, win_Resize_Height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, win_Resize_Width, win_Resize_Height
), file=sys.stderr)
print()
return win_Resize_Width, win_Resize_Height
#/ def test_5():
def __key_Inputs__RGB(img_ForDisp):
global flg_Get_RGB_Vals
if flg_Get_RGB_Vals == True : #if flg_Get_RGB_Vals == True
flg_Get_RGB_Vals = False
print("[%s:%d] flg_Get_RGB_Vals ==> now : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_Get_RGB_Vals
), file=sys.stderr)
else : #if flg_Get_RGB_Vals == True
flg_Get_RGB_Vals = True
print("[%s:%d] flg_Get_RGB_Vals ==> now : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_Get_RGB_Vals
), file=sys.stderr)
#/if flg_Get_RGB_Vals == True
#/ def __key_Inputs__RGB():
def __key_Inputs__EXECUTE__V2__Plot_RGB(lo_Rs, lo_Gs, lo_Bs, fpath_Image):
y_pos = np.arange(len(lo_Rs))
performance = lo_Rs
'''###################
reset : plot
###################'''
#ref https://stackoverflow.com/questions/8213522/when-to-use-cla-clf-or-close-for-clearing-a-plot-in-matplotlib#8228808
plt.clf()
#ref https://stackoverflow.com/questions/21254472/multiple-plot-in-one-figure-in-python
# plt.plot(lo_Rs, 'r-', label='lo_Rs')
# plt.plot(lo_Rs, 'r+', label='lo_Rs')
plt.plot(y_pos, lo_Rs, 'r-', label='lo_Rs')
plt.plot(y_pos, lo_Gs, 'g-', label='lo_Gs')
plt.plot(y_pos, lo_Bs, 'b-', label='lo_Bs')
plt.legend(loc='best')
ax = plt.gca()
#ref grid https://stackoverflow.com/questions/16074392/getting-vertical-gridlines-to-appear-in-line-plot-in-matplotlib
ax.grid(which='major', axis='both', linestyle='--')
ax.grid(which='minor', axis='both', linestyle='--')
ax.set(aspect=1,
xlim=(0, len(lo_Rs)),
ylim=(0, 260))
# ylim=(140, 250))
fpath_Save_Image = fpath_Image
# fpath_Save_Image = os.path.join(dpath_Ops_Images, "plot_" + libs.get_TimeLabel_Now() + ".png")
result = plt.savefig(fpath_Save_Image)
plt.show()
# print("[%s:%d] save fig => %s (%s)" % \
print("[%s:%d] save fig (graph) => %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
#/ def __test_1__Set_Starting_Point__Plotting():
# def __test_1__Set_Starting_Point__Key_Inputs():
def __test_1__Set_Starting_Point__Plotting(lo_Rs, lo_Gs, lo_Bs, dpath_Ops_Images):
y_pos = np.arange(len(lo_Rs))
performance = lo_Rs
#ref https://stackoverflow.com/questions/21254472/multiple-plot-in-one-figure-in-python
plt.plot(y_pos, lo_Rs, 'r-', label='lo_Rs')
plt.plot(y_pos, lo_Gs, 'g-', label='lo_Gs')
plt.plot(y_pos, lo_Bs, 'b-', label='lo_Bs')
plt.legend(loc='best')
ax = plt.gca()
#ref grid https://stackoverflow.com/questions/16074392/getting-vertical-gridlines-to-appear-in-line-plot-in-matplotlib
ax.grid(which='major', axis='both', linestyle='--')
ax.grid(which='minor', axis='both', linestyle='--')
ax.set(aspect=1,
xlim=(0, len(lo_Rs)),
ylim=(0, 260))
# ylim=(140, 250))
fpath_Save_Image = os.path.join(dpath_Ops_Images, "plot_" + libs.get_TimeLabel_Now() + ".png")
result = plt.savefig(fpath_Save_Image)
# print("[%s:%d] save fig => %s (%s)" % \
print("[%s:%d] save fig (graph) => %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
#/ def __test_1__Set_Starting_Point__Plotting():
# def __test_1__Set_Starting_Point__Key_Inputs():
def __key_Inputs__EXECUTE__Save_SubImage(img_ForDisp):
'''###################
validate
###################'''
if refPt_Start == [-1,-1] or refPt_End == [-1,-1] : #if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
print("[%s:%d] start/end points ==> not yet set" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
return
#/if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
'''###################
sub image
###################'''
img_Sub = img_ForDisp[
refPt_Start[1] : refPt_End[1]
, refPt_Start[0] : refPt_End[0]
# , refPt_Start[0] : refPt_Start[0] + 10
# refPt_Start[0] : refPt_Start[0] + 10
# , refPt_Start[1] : refPt_End[1]
]
# file name
fname = "subimage_%s.png" % libs.get_TimeLabel_Now()
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
result = cv2.imwrite(fpath_Save_Image, img_Sub)
print("[%s:%d] saving image ==> %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
'''###################
return
###################'''
return img_Sub
#/ def __key_Inputs__EXECUTE__Save_SubImage(img_ForDisp):
def __key_Inputs__EXECUTE__Gen_RGBGraph(img_Target):
'''###################
get : values
###################'''
lo_Rs, lo_Gs, lo_Bs = get_RGB_Vals(img_Target)
print()
print("[%s:%d] len(lo_Rs) => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(lo_Rs)
), file=sys.stderr)
'''###################
plot
###################'''
dpath_Ops_Images = DPATH_IMAGE_OUTPUT
__test_1__Set_Starting_Point__Plotting(lo_Rs, lo_Gs, lo_Bs, dpath_Ops_Images)
#/ def __key_Inputs__EXECUTE__Gen_RGBGraph(img_Target):
def __key_Inputs__EXECUTE__V2__Save_SubImage(key_inputs, img_ForDisp, time_Label):
'''###################
validate
###################'''
if refPt_Start == [-1,-1] or refPt_End == [-1,-1] : #if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
print("[%s:%d] start/end points ==> not yet set" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
return
#/if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
'''###################
sub image
###################'''
img_Sub = img_ForDisp[
# refPt_Start[0] : refPt_End[0] # x axis
# , refPt_Start[1] : refPt_End[1] # y axis
refPt_Start[1] : refPt_End[1] # y axis
, refPt_Start[0] : refPt_End[0] # x axis
# , refPt_Start[0] : refPt_Start[0] + 10
# refPt_Start[0] : refPt_Start[0] + 10
# , refPt_Start[1] : refPt_End[1]
]
# file name
# % (libs.get_TimeLabel_Now()
fname = "subimage_%s.%d-%d_%d-%d.png" \
% (time_Label
, refPt_Start[1], refPt_End[1]
, refPt_Start[0], refPt_End[0])
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
result = cv2.imwrite(fpath_Save_Image, img_Sub)
print("[%s:%d] saving image ==> %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
'''###################
return
###################'''
return img_Sub
#/ def __key_Inputs__EXECUTE__V2__Save_SubImage(key_inputs, img_ForDisp):
# def __key_Inputs__EXECUTE__V2__RGB_Graph(key_inputs, img_ForDisp):
# def __key_Inputs__EXECUTE__V2__RGB_Graph(key_inputs, img_Sub):
def __key_Inputs__EXECUTE__V2__RGB_Graph(key_inputs, img_Sub, time_Label):
'''###################
get : RGB vals
###################'''
'''###################
get : 1-pixel-col sub image
###################'''
height, width, channels = img_Sub.shape
img_Sub_1_Pixel_Col = img_Sub[
0 : height # y axis
, 0 : 1 # x axis
# , refPt_Start[0] : refPt_Start[0] + 1 # x axis
]
print()
# print("[%s:%d] img_Sub_1_Pixel_Col[0]" % \
print("[%s:%d] img_Sub_1_Pixel_Col[:5]" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub_1_Pixel_Col[:5])
# print(img_Sub_1_Pixel_Col[0])
lo_Rs, lo_Gs, lo_Bs = get_RGB_Vals(img_Sub_1_Pixel_Col)
#
print()
print("[%s:%d] lo_Rs =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_Rs[:5])
'''###################
get : 1-pixel-image from the img_Sub
###################'''
# img_Sub_1_Pixel_Col = img_Sub[
#
# refPt_Start[1] : refPt_End[1] # y axis
# , refPt_Start[0] : refPt_Start[0] + 1 # x axis
#
# ]
# file name
# % (libs.get_TimeLabel_Now()
fname = "subimage_%s.%d-%d_%d-%d.RGB-GRAPH.png" \
% (time_Label
, 0, height # y axis
, 0, 1 # x axis
)
# , refPt_Start[1], refPt_End[1]
# , refPt_Start[0], refPt_End[0])
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
result = cv2.imwrite(fpath_Save_Image, img_Sub_1_Pixel_Col)
# result = cv2.imwrite(fpath_Save_Image, img_Sub)
print("[%s:%d] saving image ==> %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
'''###################
plot
###################'''
fpath_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
__key_Inputs__EXECUTE__V2__Plot_RGB(lo_Rs, lo_Gs, lo_Bs, fpath_Image)
#/ def __key_Inputs__EXECUTE__V2__RGB_Graph(key_inputs, img_ForDisp):
'''###################
__key_Inputs__EXECUTE__V2(key_inputs, img_ForDisp)
<description>
###################'''
def __key_Inputs__EXECUTE__V2(key_inputs, img_ForDisp):
print("[%s:%d] __key_Inputs__EXECUTE__V2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
save : sub image
###################'''
time_Label = libs.get_TimeLabel_Now()
img_Sub = __key_Inputs__EXECUTE__V2__Save_SubImage(key_inputs, img_ForDisp, time_Label)
# img_Sub = __key_Inputs__EXECUTE__V2__Save_SubImage(key_inputs, img_ForDisp)
'''###################
graph
###################'''
__key_Inputs__EXECUTE__V2__RGB_Graph(key_inputs, img_Sub, time_Label)
# __key_Inputs__EXECUTE__V2__RGB_Graph(key_inputs, img_Sub)
# '''###################
# validate
# ###################'''
# if refPt_Start == [-1,-1] or refPt_End == [-1,-1] : #if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
#
# print("[%s:%d] start/end points ==> not yet set" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# return
#
# #/if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
#
#
#
#
# '''###################
# sub image
# ###################'''
#
# img_Sub = img_ForDisp[
# refPt_Start[1] : refPt_End[1]
# , refPt_Start[0] : refPt_End[0]
# # , refPt_Start[0] : refPt_Start[0] + 10
# # refPt_Start[0] : refPt_Start[0] + 10
# # , refPt_Start[1] : refPt_End[1]
# ]
#
# # file name
# fname = "subimage_%s.png" % libs.get_TimeLabel_Now()
#
# fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#
# #ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
# result = cv2.imwrite(fpath_Save_Image, img_Sub)
#
# print("[%s:%d] saving image ==> %s (%s)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , result, fpath_Save_Image
# ), file=sys.stderr)
#/ def __key_Inputs__EXECUTE__V2(key_inputs, img_ForDisp):
def __key_Inputs__EXECUTE(key_inputs, img_ForDisp):
print("[%s:%d] key_inputs => '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, key_inputs
), file=sys.stderr)
# print("refPt_Start =>")
# print(refPt_Start)
# print("refPt_End =>")
# print(refPt_End)
'''###################
ops : V2
###################'''
__key_Inputs__EXECUTE__V2(key_inputs, img_ForDisp)
'''###################
exec : gen sub image
###################'''
# img_Sub = __key_Inputs__EXECUTE__Save_SubImage(img_ForDisp)
#
# print()
# print("[%s:%d] type(img_Sub) => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , type(img_Sub)
# ), file=sys.stderr)
#
# '''###################
# exec : gen : RGB graph
# ###################'''
# height, width, channels = img_Sub.shape
#
# img_Target = img_Sub[
# # refPt_Start[1] : refPt_End[1]
# 0:1
# , 0 : height
# ]
# # img_Target = img_ForDisp[
# # # refPt_Start[1] : refPt_End[1]
# # refPt_Start[1] : refPt_End[0]
# # , refPt_Start[0] : refPt_End[0]
# # ]
#
# print()
# print("[%s:%d] img_Target[0] =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(img_Target[0])
# __key_Inputs__EXECUTE__Gen_RGBGraph(img_Target)
# # __key_Inputs__EXECUTE__Gen_RGBGraph(img_Sub)
# '''###################
# validate
# ###################'''
# if refPt_Start == [-1,-1] or refPt_End == [-1,-1] : #if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
#
# print("[%s:%d] start/end points ==> not yet set" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# return
#
# #/if refPt_Start == [-1,-1] or refPt_End == [-1,-1]
#
#
#
#
# '''###################
# sub image
# ###################'''
#
# img_Sub = img_ForDisp[
# refPt_Start[1] : refPt_End[1]
# , refPt_Start[0] : refPt_End[0]
# # , refPt_Start[0] : refPt_Start[0] + 10
# # refPt_Start[0] : refPt_Start[0] + 10
# # , refPt_Start[1] : refPt_End[1]
# ]
#
# # file name
# fname = "subimage_%s.png" % libs.get_TimeLabel_Now()
#
# fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#
# #ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
# result = cv2.imwrite(fpath_Save_Image, img_Sub)
#
# print("[%s:%d] saving image ==> %s (%s)" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , result, fpath_Save_Image
# ), file=sys.stderr)
#/ def __key_Inputs__EXECUTE(key_inputs, img_ForDisp):
def __key_Inputs(key_inputs, img_ForDisp):
'''######################################
dispatch
###################'''
'''###################
key : x
###################'''
if key_inputs == KEY_INPUTS__EXECUTE: #if key
__key_Inputs__EXECUTE(key_inputs, img_ForDisp)
# print("[%s:%d] key_inputs => '%s'" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , key_inputs
# ), file=sys.stderr)
# print("refPt_Start =>")
# print(refPt_Start)
# print("refPt_End =>")
# print(refPt_End)
else : #if key
print("[%s:%d] unknown key ==> '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, key_inputs
), file=sys.stderr)
#/if key
#/ def __key_Inputs(KEY_INPUTS__EXECUTE, img_ForDisp):
def __test_1__Set_Starting_Point__Key_Inputs(img_ForDisp):
'''###################
vars
###################'''
#ref scope https://www.saltycrane.com/blog/2008/01/python-variable-scope-notes/
global flg_Set_Points
while True :
k = cv2.waitKey(0) & 0xFF
#ref https://docs.opencv.org/3.1.0/db/d5b/tutorial_py_mouse_handling.html
if k == ord(KEY_INPUTS__QUIT):
# if k == ord('q'):
break
elif k == ord(KEY_INPUTS__RGB):
__key_Inputs__RGB(img_ForDisp)
elif k == ord(KEY_INPUTS__HELP):
# elif k == ord('h'):
show_Message()
elif k == ord(KEY_INPUTS__RESET):
# elif k == ord('r'):
print("[%s:%d] reset the flag...." % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
flg_Set_Points = POINTS_NEUTRAL
print("flag is => %s" % flg_Set_Points)
# elif k == ord('x'):
elif k == ord(KEY_INPUTS__EXECUTE):
__key_Inputs(KEY_INPUTS__EXECUTE, img_ForDisp)
# print("[%s:%d] executing...." % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print("refPt_Start =>")
# print(refPt_Start)
# print("refPt_End =>")
# print(refPt_End)
elif k == ord(KEY_INPUTS__START):
# elif k == ord('s'):
flg_Set_Points = POINTS_START
print("[%s:%d] flag set ==> %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_Set_Points
), file=sys.stderr)
elif k == ord(KEY_INPUTS__END):
# elif k == ord('e'):
flg_Set_Points = POINTS_END
print("[%s:%d] flag set ==> %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, flg_Set_Points
), file=sys.stderr)
# if k == ord('e'): ### 'e' for execute
#
# print("[%s:%d] starting/ending points" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print("<starting point>")
# print(refPt_Start)
# print("<ending point>")
# print(refPt_End)
else:
#ref str() https://stackoverflow.com/questions/3673428/convert-int-to-ascii-and-back-in-python answered Sep 9 '10 at 2:51
# print("[%s:%d] waitKey => %d (%s) (press 'q' to quit)" % \
print("[%s:%d] waitKey => %d (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, k, str(chr(k))
), file=sys.stderr)
# message
show_Message()
# print("'q' to quit")
# print("'x' to execute")
# # print("'e' to execute")
# # print("left click ==> set the starting point")
# # print("right click ==> set the ending point")
# print("'s' then left click ==> set the starting point")
# print("'e' then right click ==> set the ending point")
# while True :
print("[%s:%d] waitKey => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, k
), file=sys.stderr)
#/ def __test_1__Set_Starting_Point__Key_Inputs:
def __test_1__Set_Starting_Point__Window_Ops(args, width, height, img_Sub, img_Main):
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
'''###################
get : scaling
###################'''
scaling = get_Scaling(args)
# scaling = test_7__SubImage_RGB_Vals__Get_Scaling(args)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
'''###################
resize image
###################'''
win_Resize_Width, win_Resize_Height = resize_Image(width, height, scaling, scr_W, scr_H)
# win_Resize_Width, win_Resize_Height = test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
'''###################
disp : image
###################'''
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
'''###################
mouse events
###################'''
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
# cv2.setMouseCallback(window_1, click_and_crop, [img_Sub, img_Main])
#ref param https://docs.opencv.org/2.4/modules/highgui/doc/user_interface.html?highlight=setmousecallback
cv2.setMouseCallback(window_1, click_and_crop, img_Main)
# cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
cv2.imshow('window', img_Main)
# cv2.imshow('window', img_Sub)
# cv2.imshow('window', img_ForDisp)
print("[%s:%d] key inputs ======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
show_Message()
# print("'q' to quit")
# print("'x' to execute")
# # print("'e' to execute")
# # print("left click ==> set the starting point")
# # print("right click ==> set the ending point")
# print("'s' then left click ==> set the starting point")
# print("'e' then right click ==> set the ending point")
# print("[%s:%d] waiting for key(0)....." % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
'''###################
return
###################'''
return win_Resize_Width, win_Resize_Height, scr_W, scr_H
#/ def __test_1__Set_Starting_Point__Window_Ops():
def test_1__Set_Starting_Point():
'''###################
message
###################'''
print()
print("[%s:%d] test_1__Set_Starting_Point =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
#debug
print("[%s:%d] source image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Ops_Image
), file=sys.stderr)
print("[%s:%d] width = %d, height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width, height
), file=sys.stderr)
'''###################
sub image
###################'''
# height, width
img_Sub = img_ForDisp[19:69, 245:246]
# img_Sub = img_ForDisp[19:186, 245:246]
# meta data
height_Sub, width_Sub, channels_Sub = img_Sub.shape
print("[%s:%d] width_Sub = %d, height_Sub = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width_Sub, height_Sub
), file=sys.stderr)
print()
print("[%s:%d] img_Sub[0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub[0])
print("[%s:%d] img_Sub[0][0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub[0][0])
'''###################
get : RGB vals
###################'''
lo_Rs, lo_Gs, lo_Bs = get_RGB_Vals(img_Sub)
'''###################
plot
###################'''
__test_1__Set_Starting_Point__Plotting(lo_Rs, lo_Gs, lo_Bs, dpath_Ops_Images)
'''######################################
prep : window
######################################'''
win_Resize_Width, win_Resize_Height, scr_W, scr_H = \
__test_1__Set_Starting_Point__Window_Ops(args, width, height, img_Sub, img_ForDisp)
'''###################
key inputs
###################'''
__test_1__Set_Starting_Point__Key_Inputs(img_ForDisp)
# __test_1__Set_Starting_Point__Key_Inputs()
'''###################
window : close
###################'''
cv2.destroyAllWindows()
#/ def test_5():
def exec_prog():
'''###################
ops
###################'''
# test_8__Matplotlib__Bar_Chart()
test_1__Set_Starting_Point()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/3_sound-prog/2_/1_/1_1 (2).py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_sound-prog\2_\1_\1_1.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_\1_\1_1.py
at : 2018/03/21 11:55:27
pushd C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\3_sound-prog\2_\1_
python 1_1.py
'''
from __future__ import print_function
###############################################
import sys
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\mm') # libs_mm
sys.path.append('C:\\WORKS_2\\WS\\WS_Others\\free\\VX7GLZ_science-research') # libs_VX7GLZ
from libs_mm import libs
from libs_VX7GLZ import libs_VX7GLZ
from libs_VX7GLZ import wablibs
'''###################
import : specifics
###################'''
import midi, pretty_midi
'''###################
import : built-in modules
###################'''
import getopt, os, inspect, math as math, random as rnd
###############################################
import wave, struct
import numpy as np
# from pylab import *
from matplotlib import pylab as plt
#ref http://nbviewer.jupyter.org/github/librosa/librosa/blob/master/examples/LibROSA%20demo.ipynb
# import librosa
# We'll need numpy for some mathematical operations
import numpy as np
# matplotlib for displaying the output
import matplotlib.pyplot as plt
import matplotlib.style as ms
# ms.use('seaborn-muted') % matplotlib inline
# and IPython.display for audio output
import IPython.display
# Librosa for audio
import librosa
# And the display module for visualization
import librosa.display
# import random as rnd
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
# def save_Wave(fname_Out, wave_Params, binwave):
#
# #サイン波をwavファイルとして書き出し
# # fname_Out = "audio/output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.sin.wav" % (get_TimeLabel_Now())
# # fname_Out = "output_%s.pow-%d.wav" % (get_TimeLabel_Now(), pow_Val)
#
# w = wave.Wave_write(fname_Out)
# # w = wave.Wave_write("output.wav")
# # p = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
# p = wave_Params
# w.setparams(p)
# w.writeframes(binwave)
# w.close()
# '''###################
# gen_WaveData(fs, sec, A)
# 2017/12/31 15:21:22
# @param fs: Sampling frequency ---> e.g. 8000
# @param f0: Base frequency ---> e.g. 440 for 'A' note
# @param sec: Length (seconds)
# @param A: Amplitude ---> e.g. 1.0
#
# @return: binwave: array, "-32768から32767の整数値"
# ###################'''
# def gen_WaveData(fs, f0, phase, sec, A):
#
# swav=[]
#
# #test
# phase = np.pi * ( 1 / 6 )
# # phase = np.pi * 1
# # phase = np.pi * (3/2)
# # phase = np.pi / 2
# # phase = fs
# # phase = f0
#
# for n in np.arange(fs * sec):
# #サイン波を生成
#
# s = A * np.sin(2 * np.pi * f0 * n / fs - phase)
# # s = A * np.sin(2 * np.pi * f0 * n / fs)
#
# if s > 1.0: s = 1.0
# if s < -1.0: s = -1.0
#
# swav.append(s)
#
# #サイン波を-32768から32767の整数値に変換(signed 16bit pcmへ)
# swav = [int(x * 32767.0) for x in swav]
#
# #バイナリ化
# binwave = struct.pack("h" * len(swav), *swav)
#
# return binwave
# #gen_WaveData(fs, sec, A)
def test_1():
print("[%s:%d] test_1()" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#ref http://nbviewer.jupyter.org/github/librosa/librosa/blob/master/examples/LibROSA%20demo.ipynb
audio_path = librosa.util.example_audio_file()
print("[%s:%d] audio_path => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, audio_path
), file=sys.stderr)
y, sr = librosa.load(audio_path)
print("[%s:%d] type(y) => %s (size = %d)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, type(y), len(y)
), file=sys.stderr)
# [1_1.py:152] type(y) => <class 'numpy.ndarray'>
print("[%s:%d] type(sr) => %s (int = %d)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, type(sr), sr
), file=sys.stderr)
'''###################
mel
#ref http://nbviewer.jupyter.org/github/librosa/librosa/blob/master/examples/LibROSA%20demo.ipynb
###################'''
# Let's make and display a mel-scaled power (energy-squared) spectrogram
S = librosa.feature.melspectrogram(y, sr=sr, n_mels=128)
print("[%s:%d] type(S) => %s (S[0] = %s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, type(S), type(S[0])
), file=sys.stderr)
# [1_1.py:169] type(S) => <class 'numpy.ndarray'> (S[0] = <class 'numpy.ndarray'>)
print("[%s:%d] type(S[0][0]) => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, type(S[0][0])
), file=sys.stderr)
# [1_1.py:174] type(S[0][0]) => <class 'numpy.float64'>
# Convert to log scale (dB). We'll use the peak power (max) as reference.
log_S = librosa.power_to_db(S, ref=np.max)
# Make a new figure
plt.figure(figsize=(12,4))
# Display the spectrogram on a mel scale
# sample rate and hop length parameters are used to render the time axis
librosa.display.specshow(log_S, sr=sr, x_axis='time', y_axis='mel')
# Put a descriptive title on the plot
plt.title('mel power spectrogram')
# draw a color bar
plt.colorbar(format='%+02.0f dB')
# Make the figure layout compact
plt.tight_layout()
plt.show()
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] all done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/8_kb/delete-memo-string-files.bat
REM =======================
REM delete
REM 2020”N6ŒŽ8“ú18:18:58
REM =======================
del C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\memo_string.*.txt
REM pause
<file_sep>/JVEMV6/46_art/8_kb/a.bat
C:\WORKS_2\a
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/utils/open_anims_log_dir.bat
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第1章/conformal.js
/*----------------------------------------------
conformal.js
等角写像の例
z = ζ+R^2/ζ
-----------------------------------------------*/
var canvas; //キャンバス要素
var gl;//WebGL描画用コンテキスト
//円柱の半径
var Radius = 0.1;
var scale = new Vector3(1, 1, 0);
var flagCalc = true;
var flagPhys = false;
//解析領域矩形構造体
function Rect()
{
this.scale = 1;//表示倍率
this.size = 2.0;//矩形領域のサイズ(正方形)
this.left0 = new Vector3(-1, -1, 0);//その左下位置
}
var rect = new Rect();
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(1, 1, 1, 1);
height_per_width = canvas.height / canvas.width;//縦横の比率を一定にするための係数
display();
}
function display()
{
//canvasをクリアする
gl.clear(gl.COLOR_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
//計算領域描画
drawRegion();
flagCalc = form2.CalcPlane.checked;
flagPhys = form2.PhysPlane.checked;
Radius = parseFloat(form2.Radius.value);
if(flagCalc) drawCalc();
if(flagPhys) drawPhys();
}
function drawRegion()
{
var s1, s2;
if(canvas.width >= canvas.height)
{
s1 = height_per_width;
s2 = 1.0;
}
else
{
s1 = 1.0;
s2 = 1 / height_per_width;
}
scale.x = rect.scale * s1;
scale.y = rect.scale * s2;
var sx = scale.x * rect.size / 2;//表示領域の幅は2*sx
var sy = scale.y * rect.size / 2;//表示領域の高さは2*sy
drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
//左下基準点
rect.left0.x = - sx;
rect.left0.y = - sy;
//格子間隔
rect.delta = rect.size / rect.nMesh;
//x-y座標
drawLine(rect.left0.x, 0, rect.left0.x + scale.x * rect.size, 0, 1, "gray");
drawLine(0, rect.left0.y, 0, rect.left0.y + scale.y * rect.size, 1, "gray");
}
function drawCalc()
{
var i, theta, d_theta;
//var z = new Vector3();//物理空間
var w1 = new Vector3();//計算空間
var w2 = new Vector3();//計算空間
var data = [];
d_theta = 5 * DEG_TO_RAD;
var ct = 0;
//計算空間の図
//円群
var r;
for(j = 1; j < 10; j++)
{
r = j * Radius ;
for(i = 0; i < 72; i++)
{
theta = i * d_theta;
w1.x = r * Math.cos(theta);
w1.y = r * Math.sin(theta);
w2.x = r * Math.cos(theta + d_theta);
w2.y = r * Math.sin(theta + d_theta);
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w1.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w1.y); ct++;
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w2.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w2.y); ct++;
}
drawLines(data, "red");
}
//直線群
var data = [];
d_theta = 30 * DEG_TO_RAD;
for(j = 0; j < 12; j++)
{
theta = j * d_theta ;
for(i = 0; i < 10; i++)
{
r = i * 0.1;
w1.x = r * Math.cos(theta);
w1.y = r * Math.sin(theta);
w2.x = (r+0.1) * Math.cos(theta);
w2.y = (r+0.1) * Math.sin(theta);
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w1.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w1.y); ct++;
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w2.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w2.y); ct++;
}
drawLines(data, "blue");
}
}
function drawPhys()
{
var i, theta, d_theta;
var w1 = new Vector3();//計算空間
var w2 = new Vector3();//計算空間
var data = [];
d_theta = 5 * DEG_TO_RAD;
var ct = 0;
//計算空間の図
//円群
var r ;
for(j = 1; j < 10; j++)
{
r = j * Radius ;
for(i = 0; i < 72; i++)
{
theta = i * d_theta;
w1.x = r * Math.cos(theta);
w1.y = r * Math.sin(theta);
//if(j==1) console.log("AAA i = " + i + " theta = " + theta + " x = " + w1.x + " y = " + w1.y);
w2.x = r * Math.cos(theta + d_theta);
w2.y = r * Math.sin(theta + d_theta);
w1 = mapping(w1);
w2 = mapping(w2);
//if(j==1) console.log("BBB i = " + i + " theta = " + theta + " x = " + w1.x + " y = " + w1.y);
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w1.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w1.y); ct++;
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w2.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w2.y); ct++;
}
drawLines(data, "red");
}
//直線群
var data = [];
var r = Radius;
d_theta = 30 * DEG_TO_RAD;
var dd = 0.01;
for(j = 0; j < 12; j++)
{
theta = j * d_theta ;
for(i = 1; i < 1/dd; i++)
{
r = i * dd;
w1.x = r * Math.cos(theta);
w1.y = r * Math.sin(theta);
w2.x = (r+dd) * Math.cos(theta);
w2.y = (r+dd) * Math.sin(theta);
w1 = mapping(w1);
w2 = mapping(w2);
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w1.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w1.y); ct++;
data[ct] = rect.left0.x + scale.x * (rect.size/2 + w2.x); ct++;
data[ct] = rect.left0.y + scale.y * (rect.size/2 + w2.y); ct++;
}
drawLines(data, "blue");
}
}
function mapping(pos)
{
//このposは矩形領域中心を原点とした座標
//計算平面から物理平面への座標変換
var rad = mag(pos);
var rc = Radius * Radius / rad;
var theta = Math.atan2(pos.y, pos.x);
var alpha= parseFloat(form2.alpha.value);
var ang = 2.0 * alpha * DEG_TO_RAD;
pos.x = rad * Math.cos(theta) + rc * Math.cos(theta + ang);
pos.y = rad * Math.sin(theta) - rc * Math.sin(theta + ang);
return pos;
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onDisplay()
{
display();
}
function onClickScale()
{
rect.scale = parseFloat(form2.scale.value);
display();
}
<file_sep>/JVEMV6/46_art/11_guitar/sounds/mp3/1_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\sounds\mp3\1_1.py
orig : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\1_\82_1.py
at : 2018/06/29 15:11:08
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\sounds\mp3
python 1_1.py
ref : http://aidiary.hatenablog.com/entry/20110607/1307449007
'''
###############################################
import sys
from pandas.compat import str_to_bytes
from numpy.distutils.from_template import item_re
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects') # libs_mm
from libs_admin import libs
'''###################
import : built-in modules
###################'''
# import getopt
import os, glob
# import inspect
import math as math
###############################################
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
def test_1():
'''###################
list : file names in the dir
###################'''
dpath = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\11_guitar\\sounds\\mp3"
fpath_Glob = "%s\\*.mp3" % (dpath)
#ref glob https://stackoverflow.com/questions/14798220/how-can-i-search-sub-folders-using-glob-glob-module-in-python answered Feb 10 '13 at 13:31
lo_Files = glob.glob(fpath_Glob)
lo_Files = [os.path.basename(x) for x in lo_Files]
#ref https://stackoverflow.com/questions/678236/how-to-get-the-filename-without-the-extension-from-a-path-in-python answered Mar 24 '09 at 16:43
lo_Files_Trunk = [os.path.splitext(os.path.basename(x))[0] for x in lo_Files]
# lo_Files_Trunk = [os.path.splitext(x)[0] for x in lo_Files]
lo_Name_Sets = list(zip(lo_Files, lo_Files_Trunk))
# report
print()
print("[%s:%d] lo_Name_Sets =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
for item in lo_Name_Sets:
print(item)
#/for item in lo_Name_Sets:
'''###################
list : file names in the text file
###################'''
fname_ListFile = "list-of-new-file-names.txt"
fpath_ListFile = "%s\\%s" % (dpath, fname_ListFile)
# #debug
# print()
# print("[%s:%d] fpath_ListFile => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , fpath_ListFile
# ), file=sys.stderr)
# return
fin = open(fpath_ListFile, "r")
lo_FileName_Final = []
lo_file_Lines = []
lo_file_Lines = fin.readlines()
#ref strip https://hydrocul.github.io/wiki/programming_languages_diff/string/trim.html
lo_file_Lines = [x.strip() for x in lo_file_Lines]
print()
print("[%s:%d] lo_file_Lines =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_file_Lines)
lo_file_Lines_Trunk = [x.split(".") for x in lo_file_Lines]
print()
print("[%s:%d] lo_file_Lines_Trunk =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_file_Lines_Trunk)
# return
lo_file_Lines_Trunk = [x[0] for x in lo_file_Lines_Trunk]
print()
print("[%s:%d] lo_file_Lines_Trunk ('x[0] for x in ...') =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_file_Lines_Trunk)
lo_file_Lines_Trunk = [x.split(" ") for x in lo_file_Lines_Trunk]
# lo_file_Lines_Trunk = [x.split("_") for x in lo_file_Lines_Trunk]
lo_file_Lines_Trunk = [x[0] + "_" + x[1] for x in lo_file_Lines_Trunk]
# lo_file_Lines_Trunk = [x[0] + " " + x[1] for x in lo_file_Lines_Trunk]
# lo_file_Lines_Trunk = [(x[0], x[1]) for x in lo_file_Lines_Trunk]
print()
print("[%s:%d] lo_file_Lines_Trunk (modified) =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(lo_file_Lines_Trunk)
# zip
lo_file_Lines = list(zip(lo_file_Lines, lo_file_Lines_Trunk))
print()
print("[%s:%d] lo_file_Lines =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
for item in lo_file_Lines:
print(item)
#/for item in lo_file_Lines:
'''###################
judge
###################'''
lenOf_Name_Sets = len(lo_Name_Sets)
cntOf_Changed_Files = 0
# file
fpath_Log = os.path.join(dpath, "ops.log")
f_log = open(fpath_Log, "a")
f_log.write("[%s / %s:%d]=======================" % \
(libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()))
f_log.write("\n")
for i in range(lenOf_Name_Sets):
name_Set = lo_Name_Sets[i]
for item in lo_file_Lines:
# judge
if name_Set[1] == item[1] : #if name_Set[1] == item[1]
msg = "[%s / %s:%d] change file name => name_Set[1] = %s, item[1] = %s" % \
(libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile())
, libs.linenum()
, name_Set[1], item[1]
)
print()
print(msg, file=sys.stderr)
# print("[%s:%d] change file name => name_Set[1] = %s, item[1] = %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , name_Set[1], item[1]
# ), file=sys.stderr)
f_log.write(msg)
f_log.write("\n")
msg = "from : %s / to : %s" % (name_Set[0], item[0])
print(msg)
# print("from : %s / to : %s" % (name_Set[0], item[0]))
f_log.write(msg)
f_log.write("\n")
# rename
fpath_Curr = os.path.join(dpath, name_Set[0])
fpath_New = os.path.join(dpath, item[0])
#ref rename https://qiita.com/clarinet758/items/307d01a6634b372e8fa9
os.rename(fpath_Curr, fpath_New)
# count
cntOf_Changed_Files += 1
break
#/if name_Set[1] == item[1]
#/for item in lo_file_Lines:
#/for i in range(lenOf_Name_Sets):
# write count
f_log.write("renamed : %d files" % cntOf_Changed_Files)
f_log.write("\n")
f_log.write("\n") # <= spacer line
# close log file
f_log.close()
'''###################
report
###################'''
print()
print("[%s:%d] cntOf_Changed_Files => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cntOf_Changed_Files
), file=sys.stderr)
# print(lo_file_Lines)
# #debug
# return
# print()
# print("[%s:%d] lo_file_Lines =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_file_Lines)
#
# fin.close()
#
# # edit
# lenOf_Name_Sets = len(lo_Name_Sets)
# lenOf_file_Lines = len(lo_file_Lines)
#
# print()
# print("[%s:%d] lenOf_Name_Sets = %d, lenOf_file_Lines = %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , lenOf_Name_Sets, lenOf_file_Lines
# ), file=sys.stderr)
#
# for i in range(lenOf_Name_Sets):
#
# name_Set = lo_Name_Sets[i]
#
# print()
# print("[%s:%d] name_Set => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , name_Set
# ), file=sys.stderr)
#
# # judge
# for file_Line in lo_file_Lines:
#
# file_Line = lo_file_Lines[i]
#
# temp_Tlabel = file_Line.split(".")[0]
#
# tokens = temp_Tlabel.split("_")
#
# str_Tokens = tokens[0] + " " + tokens[1]
#
# # print(str_Tokens)
#
# # judge
# # if str_Tokens == name_Set : #if str_Tokens == name_Set
# if str_Tokens == name_Set[1] : #if str_Tokens == name_Set
#
# # print()
# # print("[%s:%d] hit => str_Tokens = %s, name_Set = %s, file_Line = %s" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# # , str_Tokens, name_Set, file_Line
# # ), file=sys.stderr)
#
# # # change file name
# # print()
# # print("[%s:%d] change file name : from => %s / to => %s" % \
# # (os.path.basename(libs.thisfile()), libs.linenum()
# # , name_Set[0], file_Line
# # ), file=sys.stderr)
#
# # change
# #ref join https://stackoverflow.com/questions/7132861/building-full-path-filename-in-python#7133204
# fpath_Curr = os.path.join(dpath, name_Set[0])
# fpath_New = os.path.join(dpath, file_Line)
#
# # validate
# res = os.path.isfile(fpath_Curr)
#
# print()
# print("[%s:%d] fpath_Curr exists? (%s) ==> %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , fpath_Curr, res
# ), file=sys.stderr)
#
# # rename
# if res :
#
# os.rename(fpath_Curr, file_Line)
#
# print()
# print("[%s:%d] renamed => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , file_Line
# ), file=sys.stderr)
#
# else :
#
# print()
# print("[%s:%d] file not exists : %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , fpath_Curr
# ), file=sys.stderr)
# # os.rename(fpath_Curr, fpath_New)
#
# # os.rename(name_Set[0], file_Line)
#
# continue
#
# #/if str_Tokens == name_Set
#
#
#
#
# #/for file_Line in lo_file_Lines:
#
#
#
# #/if str_Tokens ==
#
#
#
#
#
# # print(tokens)
#
# #/for i in range(lenOf_Name_Sets):
# print()
# print("[%s:%d] len(lo_Files) => %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , len(lo_Files)
# ), file=sys.stderr)
#
# print()
# print("[%s:%d] lo_Files_Trunk =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#
# print(lo_Files_Trunk)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
# test_2()
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print ("[%s:%d] exec_prog" % (thisfile(), linenum()))
# #debug
# return
# A = 1 #振幅
# fs = 16000 #サンプリング周波数
# # fs = 8000 #サンプリング周波数
# f0 = 392 #周波数
# # f0 = 262 #周波数
# # f0 = 440 #周波数
# sec = 5 #秒
#
# phase_Param = 4
#
# phase = f0 * (phase_Param / 2)
# # phase = f0 * (4 / 2)
# # phase = f0 / 2
#
# # bin data
# binwave = gen_WaveData(fs, f0, phase, sec, A)
#
# # #サイン波をwavファイルとして書き出し
# wave_Params = (1, 2, fs, len(binwave), 'NONE', 'not compressed')
# # wave_Params = (1, 2, 8000, len(binwave), 'NONE', 'not compressed')
#
# '''###################
# dirs
# ###################'''
# dname_Audios = "data.46_1\\audios"
#
# if not os.path.isdir(dname_Audios) : os.makedirs(dname_Audios)
#
# # fname_Out = "audio/output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# fname_Out = "%s\\output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# (dname_Audios
# , libs.get_TimeLabel_Now()
# , fs, f0
# , "%1.2f" % (phase_Param / 4.0) + "pi", sec)
# # fname_Out = "audios/output_%s.sin.fs-%d_f0-%d_phase-%s_sec-%d.wav" % \
# # (libs.get_TimeLabel_Now(), fs, f0, "%1.2f" % (phase_Param / 4.0) + "pi", sec)
# # (get_TimeLabel_Now(), fs, f0, "%1.2f" % (phase_Param / 4.0) + "pi", sec)
# # fname_Out = "audio/output_%s.sin.fs-%d_f0-%d_phase-%d_sec-%d.wav" % \
# # (get_TimeLabel_Now(), fs, f0, phase, sec)
#
# save_Wave(fname_Out, wave_Params, binwave)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/sp/data/ops/10_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\10_1.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\6_1.py
at : 2018/07/28 10:48:35
r w && r d4
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops
python 10_1.py -s1.0
python 6_1.py -s1.0
python 6_1.py
ref : http://aidiary.hatenablog.com/entry/20110607/1307449007
20180721_111655
C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images\
'''
###############################################
import sys, cv2
# from pandas.compat import str_to_bytes
from numpy.distutils.from_template import item_re
#ref https://stackoverflow.com/questions/3129322/how-do-i-get-monitor-resolution-in-python
from win32api import GetSystemMetrics
from matplotlib import pylab as plt
from copy import deepcopy
from _ast import If
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects') # libs_mm
from libs_admin import libs, lib_ip, cons_ip
# from libs_admin import libs, lib_ip
'''###################
import : built-in modules
###################'''
# import getopt
import os, glob, getopt, math as math, numpy as np
# import inspect
'''###################
constants : global
###################'''
# import math as math
refPt_Start = [-1] * 2
refPt_End = [-1] * 2
# refPt_Start = []
# refPt_End = []
flg_Set_Points = ""
flg_Get_RGB_Vals = False
POINTS_START = "START"
POINTS_END = "END"
POINTS_NEUTRAL = "NEUTRAL"
KEY_INPUTS__END = 'e'
KEY_INPUTS__RGB = 'g'
KEY_INPUTS__HELP = 'h'
KEY_INPUTS__QUIT = 'q'
KEY_INPUTS__RESET = 'r'
KEY_INPUTS__START = 's'
KEY_INPUTS__EXECUTE = 'x'
DPATH_IMAGE_OUTPUT = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
###############################################
# def show_Message() :
#
# msg = '''
# <Options>
# -v Volume down the amplitude --> 1.0 * v / 1000
# -f Base frequency ---> e.g. 262 for the A tone
# -p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
#
# print (msg)
def show_Message():
# print("'q' to quit")
# print("'x' to execute")
# print("'h' to show this key list")
print("'g'\tset flag for getting RGB values")
print("'h'\tshow this key list")
print("'q'\tquit")
print("'r'\treset start/end")
print("'x'\texecute")
# print("'e' to execute")
# print("left click ==> set the starting point")
# print("right click ==> set the ending point")
print("'s' then left click ==> set the starting point")
print("'e' then right click ==> set the ending point")
print()
#/ def show_Message():
'''###################
resize_Image()
<description>
- add listener to ==> image
- hover on the image ==> x, y coordinates displayed in the console
- use ==> argv for window size
<Example>
python 1_1.py -s0.25
<quit program>
return key
###################'''
# def test_5__Resize_Image(width, height, scaling, scr_W, scr_H):
def resize_Image(width, height, scaling, scr_W, scr_H):
q1 = 1.0 * width / height
s = scaling
flg_WorH = ""
# j1
if s >= 0 : #if s >= 0
### j1 : p1
A = 0
if width >= height : #if width > height
flg_WorH = "width"
A = width
else : #if width > height
flg_WorH = "height"
A = height
#/if width > height
### j1 : p2
m = A * s
print("[%s:%d] m => %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, m
), file=sys.stderr)
### j1 : p3
Z = 0
if flg_WorH == "width" : #if flg_WorH == "width"
Z = scr_W
else : #if flg_WorH == "width"
Z = scr_H
#/if flg_WorH == "width"
### j2
result = (m > Z)
'''###################
vars
###################'''
win_Resize_Height = 0
win_Resize_Width = 0
if result == True : #if result == True
print("[%s:%d] m > Z : m = %.03f, Z = %d, flg_WorH = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, m, Z, flg_WorH
), file=sys.stderr)
### j2 : p1
q2 = 1.0 * Z / A
print("[%s:%d] q2 => %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, q2
), file=sys.stderr)
'''###################
calc
###################'''
win_Resize_Height = math.floor(height * q2)
win_Resize_Width = math.floor(width * q2)
# win_Resize_Height = int(height * q2)
# win_Resize_Width = int(width * q2)
# win_Resize_Height = height * q2
# win_Resize_Width = width * q2
else : #if result == True
print("[%s:%d] m <= Z" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
calc
###################'''
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
#/if result == True
else : #if s >= 0
'''###################
calc
###################'''
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
#/if s >= 0
# '''###################
# calc
# ###################'''
# win_Resize_Height = math.floor(scaling * height)
# win_Resize_Width = math.floor(scaling * width)
'''###################
return
###################'''
#debug
print("[%s:%d] win_Resize_Width = %d, win_Resize_Height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, win_Resize_Width, win_Resize_Height
), file=sys.stderr)
print()
return win_Resize_Width, win_Resize_Height
#/ def test_5():
'''###################
get_Scaling
from : test_7__SubImage_RGB_Vals__Get_Scaling(args)
at : 2018/07/15 08:22:52
###################'''
def get_Scaling(args):
# scaling
scaling = 1.0
# #debug
# print("[%s:%d] args =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(args)
if len(args) > 0 : #if len(args) > 1
optlist, args = getopt.getopt(args, "s:")
print("[%s:%d] optlist =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(optlist)
for item in optlist:
#debug
print("[%s:%d] item =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(item)
if item[0].startswith("-s") : #if item.startswith("-s")
scaling = float(item[1])
break
#/if item.startswith("-s")
#/for item in optlist:
else : #if len(args) > 1
print("[%s:%d] len(args) < 2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if len(args) > 1
#debug
print("[%s:%d] scaling => %.3f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, scaling
), file=sys.stderr)
'''###################
return
###################'''
return scaling
def __test_1__Color_Filtering__Window_Ops(args, width, height, img_ForDisp):
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
'''###################
get : scaling
###################'''
scaling = get_Scaling(args)
# scaling = test_7__SubImage_RGB_Vals__Get_Scaling(args)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
'''###################
resize image
###################'''
win_Resize_Width, win_Resize_Height = resize_Image(width, height, scaling, scr_W, scr_H)
# win_Resize_Width, win_Resize_Height = test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
'''###################
disp : image
###################'''
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
'''###################
mouse events
###################'''
'''###################
mouse events
###################'''
'''###################
show
###################'''
cv2.imshow('window', img_ForDisp)
# def __test_1__Color_Filtering__Window_Ops():
def __test_1__Color_Filtering__Exec_Filtering(img_ForDisp):
img_Copy = deepcopy(img_ForDisp)
'''###################
process partial
###################'''
time_Label = libs.get_TimeLabel_Now()
# horizontal
x1 = 100
x2 = 400
# vertical
y1 = 100
y2 = 550
# y2 = 150
# image : orig
fname = "img_ForDisp_%s.%d-%d_%d-%d.png" \
% (time_Label
, x1, x2
, y1, y2
)
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
result = cv2.imwrite(fpath_Save_Image, img_ForDisp)
print("[%s:%d] image ==> saved : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Save_Image
), file=sys.stderr)
#debug
print()
print("[%s:%d] img_Copy[y1 = %d][x1 = %d]" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, y1, x1
), file=sys.stderr)
print(img_Copy[y1][x1])
# image : copy
# leOf_Rows = len(img_Copy)
'''###################
filtering
###################'''
pixel_Target = img_Copy[y1][x1]
threshold_Diff = 10
for i in range(y1, y2):
# for i in range(100, 200):
# for i in range(100, lenOf_Rows):
row = img_Copy[i]
for j in range(x1, x2):
# for j in range(100, 200):
# for pixel in row:
pixel = row[j]
if pixel[0] < (pixel_Target[0] - threshold_Diff) \
and pixel[1] < (pixel_Target[1] - threshold_Diff) \
and pixel[2] < (pixel_Target[2] - threshold_Diff) :
pixel[0] = 255
pixel[1] = 255
pixel[2] = 255
# if pixel[0] < (pixel_Target[0] - threshold_Diff) : pixel[0] = 255
# if pixel[1] < (pixel_Target[1] - threshold_Diff) : pixel[1] = 255
# if pixel[2] < (pixel_Target[2] - threshold_Diff) : pixel[2] = 255
#/if pixel[0] < (pixel_Target[0] - threshold_Diff)
# pixel[0] = 0
# pixel[1] = 0
# pixel[2] = 0
#/for pixel in row:
#/for row in img_Copy:
fname = "img_Copy_%s.%d-%d_%d-%d.png" \
% (time_Label
, x1, x2
, y1, y2
)
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
result = cv2.imwrite(fpath_Save_Image, img_Copy)
print("[%s:%d] image ==> saved : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Save_Image
), file=sys.stderr)
#/ def __test_1__Color_Filtering__Exec_Filtering(img_ForDisp):
def test_1__Color_Filtering():
'''###################
message
###################'''
print()
print("[%s:%d] test_1__Color_Filtering =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
#debug
print("[%s:%d] source image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Ops_Image
), file=sys.stderr)
print("[%s:%d] width = %d, height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width, height
), file=sys.stderr)
'''###################
show image
###################'''
# __test_1__Color_Filtering__Window_Ops(args, width, height, img_ForDisp)
'''###################
filtering
###################'''
__test_1__Color_Filtering__Exec_Filtering(img_ForDisp)
'''###################
window : close
###################'''
cv2.waitKey(0)
cv2.destroyAllWindows()
#/ def test_5():
def __test_2__Color_Filtering_HSV__Save_Image(args, img_Orig):
'''###################
prep
###################'''
img_BGR = cv2.cvtColor(img_Orig, cv2.COLOR_RGB2BGR)
img_HSV = cv2.cvtColor(img_Orig, cv2.COLOR_RGB2HSV)
img_ForDisp = img_HSV
# img_ForDisp = img_BGR
# img_ForDisp = img_Orig
'''###################
save image
###################'''
# file name
file_label = "image_HSV"
# file_label = "image_BGR"
# file_label = "image_RGB"
fname = "%s.%s.png" % (file_label, libs.get_TimeLabel_Now())
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
result = cv2.imwrite(fpath_Save_Image, img_ForDisp)
print("[%s:%d] saving image ==> %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
#/ def __test_2__Color_Filtering_HSV__Save_Image(args, img_Orig):
def __test_2__Color_Filtering_HSV__Window_Ops(args, img_Orig):
'''###################
prep
###################'''
img_ForDisp = img_Orig
height, width, channels = img_ForDisp.shape
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
'''###################
get : scaling
###################'''
scaling = get_Scaling(args)
# scaling = test_7__SubImage_RGB_Vals__Get_Scaling(args)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
'''###################
resize image
###################'''
win_Resize_Width, win_Resize_Height = resize_Image(width, height, scaling, scr_W, scr_H)
# win_Resize_Width, win_Resize_Height = test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
'''###################
disp : image
###################'''
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
'''###################
show
###################'''
cv2.imshow('window', img_ForDisp)
#/ def __test_2__Color_Filtering_HSV(args, width, height, img_Orig):
def __test_2__Color_Filtering_HSV__Get_Metadata(args, img_Orig, time_Label, fname_Ops_Image):
# def __test_2__Color_Filtering_HSV__Get_Metadata(args, img_Orig, time_Label):
# def __test_2__Color_Filtering_HSV__Get_Metadata(args, img_Orig):
img_HSV = cv2.cvtColor(img_Orig, cv2.COLOR_RGB2HSV)
img_ForDisp = img_HSV
height, width, channels = img_ForDisp.shape
'''###################
subimage
###################'''
width_SubImage = 1
# x1 = 100
x1 = 0
x2 = x1 + width_SubImage
# x2 = x1 + 10
# x2 = x1 + 1
# y1 = 800
y1 = 0
# y1 = 200
y2 = height
# y2 = 400
img_Sub = img_ForDisp[
y1 : y2 # y axis
, x1 : x2 # x axis
]
#debug
print("[%s:%d] img_Sub[:10] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print(img_Sub[:10])
for i in range(10):
print("[%s:%d] img_Sub[%d] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, i
), file=sys.stderr)
print(img_Sub[i])
#/for i in range(10):
# file name
# % (libs.get_TimeLabel_Now()
fname = "subimage_%s.%d-%d_%d-%d.(%s).png" \
% (time_Label
, x1, x2, y1, y2
, fname_Ops_Image)
# fname = "subimage_%s.%d-%d_%d-%d.png" \
# % (time_Label
# , x1, x2, y1, y2)
fpath_Save_Image = os.path.join(DPATH_IMAGE_OUTPUT, fname)
#ref save image https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_gui/py_image_display/py_image_display.html
result = cv2.imwrite(fpath_Save_Image, img_Sub)
print("[%s:%d] saving image ==> %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
msg = "saving image ==> %s (%s)" %\
(result, fpath_Save_Image)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 1)
# libs.write_Log(msg_Log
# , True)
# # , cons_fx.FPath.dpath_LogFile.value
# # , cons_fx.FPath.fname_LogFile.value
# # , 1)
'''###################
metadata : prep
###################'''
maxOf_H = -1
maxOf_S = -1
maxOf_V = -1
minOf_H = 999
minOf_S = 999
minOf_V = 999
for row in img_Sub:
# for row in img_ForDisp:
for pixel in row:
H = pixel[0]
S = pixel[1]
V = pixel[2]
# update : max
if H > maxOf_H : maxOf_H = H
if S > maxOf_S : maxOf_S = S
if V > maxOf_V : maxOf_V = V
# update : min
if H < minOf_H : minOf_H = H
if S < minOf_S : minOf_S = S
if V < minOf_V : minOf_V = V
#/if H > maxOf_H
#/for pixel in row:
#/for row in img_ForDisp:
'''###################
report
###################'''
msg = "maxOf_H => %d, maxOf_S => %d, maxOf_V => %d" % \
(maxOf_H, maxOf_S, maxOf_V)
msg_Log = "[%s / %s:%d] %s" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
, msg)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 1)
# , 1)
print("[%s:%d] maxOf_H => %d, maxOf_S => %d, maxOf_V => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, maxOf_H, maxOf_S, maxOf_V
), file=sys.stderr)
print("[%s:%d] minOf_H => %d, minOf_S => %d, minOf_V => %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, minOf_H, minOf_S, minOf_V
), file=sys.stderr)
'''###################
write data : H
###################'''
lo_H = []
lo_S = []
lo_V = []
# get : data
for row in img_Sub:
pixel = row[0]
H = pixel[0]
S = pixel[1]
V = pixel[2]
lo_H.append(H)
lo_S.append(S)
lo_V.append(V)
#/for row in img_Sub:
'''######################################
write : data
###################'''
'''###################
H
###################'''
lo_H_string = [str(x) for x in lo_H]
msg = "\t".join(lo_H_string)
msg_Log = "[%s / %s:%d] list of Hs =>" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 1)
# , numOf_Return = 0)
msg_Log = "%s" % \
(
msg
)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 2)
# , numOf_Return = 1)
'''###################
S
###################'''
lo_S_string = [str(x) for x in lo_S]
msg = "\t".join(lo_S_string)
msg_Log = "[%s / %s:%d] list of Ss =>" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 1)
# , numOf_Return = 0)
msg_Log = "%s" % \
(
msg
)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 2)
'''###################
V
###################'''
lo_V_string = [str(x) for x in lo_V]
msg = "\t".join(lo_V_string)
msg_Log = "[%s / %s:%d] list of Vs =>" % \
(
libs.get_TimeLabel_Now()
, os.path.basename(libs.thisfile()), libs.linenum()
)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 1)
# , numOf_Return = 0)
msg_Log = "%s" % \
(
msg
)
libs.write_Log_2(msg_Log
, dpath_LogFile = cons_ip.FilePaths.dpath_LogFile.value
, fname_LogFile = cons_ip.FilePaths.fname_LogFile__Gradation.value
, numOf_Return = 2)
#/ def __test_2__Color_Filtering_HSV__Get_Metaeata(args, img_Orig):
def test_2__Color_Filtering_HSV():
'''###################
message
###################'''
print()
print("[%s:%d] test_2__Color_Filtering_HSV =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "img.20180728_084420.2018-07-24_05-14-26_000.jpg.1.png"
# fname_Ops_Image = "img.20180731_165432.2018-07-29_17-41-09_000.jpg.2.png"
# fname_Ops_Image = "img.20180731_165432.2018-07-29_17-41-09_000.jpg.1.png"
# fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
# img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_Orig_HSV = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
#debug
print("[%s:%d] source image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Ops_Image
), file=sys.stderr)
print("[%s:%d] width = %d, height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width, height
), file=sys.stderr)
'''###################
show image
###################'''
time_Label = libs.get_TimeLabel_Now()
# __test_2__Color_Filtering_HSV__Window_Ops(args, img_Orig)
'''###################
save image
###################'''
# __test_2__Color_Filtering_HSV__Save_Image(args, img_Orig)
'''###################
get : metadata
###################'''
__test_2__Color_Filtering_HSV__Get_Metadata(args, img_ForDisp, time_Label, fname_Ops_Image)
# __test_2__Color_Filtering_HSV__Get_Metadata(args, img_ForDisp, time_Label)
# __test_2__Color_Filtering_HSV__Get_Metadata(args, img_ForDisp)
'''###################
filtering
###################'''
# __test_1__Color_Filtering__Exec_Filtering(img_ForDisp)
'''###################
window : close
###################'''
cv2.waitKey(0)
cv2.destroyAllWindows()
#/ def test_5():
def exec_prog():
'''###################
ops
###################'''
test_2__Color_Filtering_HSV()
# test_1__Color_Filtering()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第4章/cavityPsiOmega.js
/*----------------------------------------------
cavityPsiOmega.js
流れ関数-渦度法
粒子アニメーション
-----------------------------------------------*/
var canvas; //キャンバス要素
var gl;//WebGL描画用コンテキスト
var height_per_width;//キャンバスのサイズ比
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var elapseTimeN = 0.0;//無次元時間
var elapseTimeN0 = 0.0;
var flagStart = false;
var flagFreeze = false;
var flagStep = false;
var flagReset = false;
//数値計算
var Psi = []; //流れ関数
var Omega = [];//渦度
var gx = []; //x方向微分
var gy = []; //y方向微分
var VelX = []; //x方向速度
var VelY = []; //y方向速度
var type = []; //格子点のタイプ
var scale = new Vector3(1.0, 1.0, 0.0);//スケール調整
var flagStream = true;
var flagVorticity = true;
var flagVelocity = false;
var flagGrid = false;
var nLine = 20;//流線,ポテンシャルの表示本数
var arrowScale = 0.05;
var arrowWidth = 1;
var intervalV = 2;//速度矢印表示間隔
var deltaT = 0.01;
var Re = 500.0;//レイノルズ数
var maxPsi = 0.1;
var minPsi = -0.1;
var maxOmg = 40.0;
var minOmg = -50.0;
//粒子アニメーション
var sizeParticle = 5;
var speedCoef = 1.0;//速度倍率
var intervalP = 0.05;
//解析領域矩形構造体
function Rect()
{
this.scale = 1.7;//表示倍率
this.nMeshX = 40;//x方向割数(固定)
this.nMeshY = 40; //y方向分割数(固定)
this.size = new Vector3(1, 1, 0);//矩形ダクト領域のサイズ(固定)
this.left0 = new Vector3(-1, -1, 0);//その左下位置
this.delta = new Vector3(); //格子間隔
}
var rect = new Rect();
var NX, NY, DX, DY;
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(1, 1, 1, 1);
form1.deltaT.value = deltaT;
form1.Re.value = Re;
form1.nMeshX.value = rect.nMeshX;
form1.nMeshY.value = rect.nMeshY;
form2.scale.value = rect.scale;
form2.nLine.value = nLine;
form2.psi.checked = flagStream;
form2.omega.checked = flagVorticity;
form2.velocity.checked = flagVelocity;
form2.maxPsi.value = maxPsi;
form2.minPsi.value = minPsi;
form2.maxOmg.value = maxOmg;
form2.minOmg.value = minOmg;
initData();
var timestep = 0;
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;
elapseTimeN += deltaT;//数値計算上の経過時間(無次元時間)
calculate();
gl.clear(gl.COLOR_BUFFER_BIT);
if(form2.particle.checked) drawParticle(timestep);
display();
elapseTime0 = elapseTime;//現在の経過時間を保存
elapseTimeN0 = elapseTimeN;//現在の経過時間を保存
form1.e_time.value = elapseTime.toString();
form1.n_time.value = elapseTimeN.toString();
if(flagStep) { flagStart = false; }
}
}
animate();
}
function initData()
{
deltaT = parseFloat(form1.deltaT.value);
Re = parseFloat(form1.Re.value);
NX = parseInt(form1.nMeshX.value);
NY = parseInt(form1.nMeshY.value);
DX = rect.size.x / NX;//格子間隔
DY = rect.size.y / NY;
initParticle();//粒子アニメーションの初期化
var i, j;
for(i = 0; i <= NX; i++)
{//配列の2次元化
type[i] = [];//格子点のタイプ
Psi[i] = []; //流れ関数
Omega[i] = [];//渦度
gx[i] = []; //x方向微分
gy[i] = []; //y方向微分
VelX[i] = []; //x方向速度
VelY[i] = []; //y方向速度
}
//格子点のタイプ
for(j = 0; j <= NY; j++)
{
for(i = 0; i <= NX; i++)
{
type[i][j] = "INSIDE";//内点
if(j == 0) type[i][j] = "BOTTOM";//下側壁面
if(j == NY) type[i][j] = "TOP"; //上側壁面
if(i == 0) type[i][j] = "LEFT"; //左側側面
if(i == NX) type[i][j] = "RIGHT"; //右側側面
if(i == 0 && j == 0) type[i][j] = "CORNER_UR";//左下隅
if(i == NX && j == 0) type[i][j] = "CORNER_UL";//右下隅
if(i == 0 && j == NY) type[i][j] = "CORNER_LR";//左上隅
if(i == NX && j == NY) type[i][j] = "CORNER_LL";//右上隅
}
}
//初期値
//Topは流速1
//すべての壁は psi = 0
for(j = 0; j <= NY; j++)
for (i = 0; i <= NX; i++)
{
//流れ関数
Psi[i][j] = 0;
//渦度
Omega[i][j] = 0.0;
//速度
if(type[i][j] == "TOP" ) VelX[i][j] = 1.0;
else VelX[i][j] = 0.0;
VelY[i][j] = 0.0;
//CIP法で使用する微分値
gx[i][j] = 0.0;
gy[i][j] = 0.0;
}
calcVelocity();
maxPsi0 = -1000.0; minPsi0 = 1000.0;
maxOmg0 = -1000.0; minOmg0 = 1000.0;
//発散しないための目安を知るため
var courant, diffNum;
if(DX < DY)
{
courant = 1.0 * deltaT / DX;
diffNum = (1.0 / Re) * deltaT / (DX * DX);//拡散数
}
else
{
courant = 1.0 * deltaT / DY;
diffNum = (1.0 / Re) * deltaT / (DX * DY);//拡散数
}
form1.courant.value = courant;
form1.diffNum.value = diffNum;
gl.clear(gl.COLOR_BUFFER_BIT);
display();
}
function display()
{
if(!flagStart) gl.clear(gl.COLOR_BUFFER_BIT);
flagStream = form2.psi.checked;
flagVorticity = form2.omega.checked;
flagVelocity = form2.velocity.checked;
flagGrid = form2.grid.checked;
gl.viewport(0, 0, canvas.width, canvas.height);
height_per_width = canvas.height / canvas.width;//縦横の比率を一定にするための係数
//計算領域描画
drawRegion();
//流線、等渦度線表示
maxPsi = parseFloat(form2.maxPsi.value);
minPsi = parseFloat(form2.minPsi.value);
maxOmg = parseFloat(form2.maxOmg.value);
minOmg = parseFloat(form2.minOmg.value);
if( flagStream ) drawContour(Psi, minPsi, maxPsi, "red");
if( flagVorticity ) drawContour(Omega, minOmg, maxOmg, "blue");
if( flagVelocity ) drawVelocity();
if( flagGrid ) drawGrid();
//ダクト
var sx = scale.x * rect.size.x / 2;//ダクトの幅は2*sx
var sy = scale.y * rect.size.y / 2;//ダクトの高さは2*sy
drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
}
function drawRegion()
{
var s1, s2;
if(canvas.width >= canvas.height)
{
s1 = height_per_width;
s2 = 1.0;
}
else
{
s1 = 1.0;
s2 = 1 / height_per_width;
}
scale.x = rect.scale * s1;
scale.y = rect.scale * s2;
var sx = scale.x * rect.size.x / 2;//ダクトの幅は2*sx
var sy = scale.y * rect.size.y / 2;//ダクトの高さは2*sy
//ダクト
drawRectangle(0, 0, 2*sx, 2*sy, false, "black", 0);
//ダクトの左下基準点
rect.left0.x = - sx;
rect.left0.y = - sy;
}
function calculate()
{
var i, j;
var iteration = 10;//最大繰り返し回数(Poisson方程式)
var tolerance = 0.00001;//許容誤差
var error = 0.0;
var maxError = 0.0;
var pp;
var DX2 = DX * DX;
var DY2 = DY * DY;
var DD2 = DX2 + DY2;//corner
//渦度境界条件
for (i = 0; i <= NX; i++)
for (j = 0; j <= NY; j++)
{
if(type[i][j] == "INSIDE") continue;
else if(type[i][j] == "TOP") Omega[i][j] = -2.0 * (Psi[i][j-1] + DY) / DY2;
else if(type[i][j] == "BOTTOM") Omega[i][j] = -2.0 * Psi[i][j+1] / DY2;
else if(type[i][j] == "LEFT") Omega[i][j] = -2.0 * Psi[i+1][j] / DX2;
else if(type[i][j] == "RIGHT") Omega[i][j] = -2.0 * Psi[i-1][j] / DX2;
else if(type[i][j] == "CORNER_UR") Omega[i][j] = -2.0 * Psi[1][1] / DD2;//左下隅
else if(type[i][j] == "CORNER_UL") Omega[i][j] = -2.0 * Psi[i-1][1] / DD2;//右下隅
else if(type[i][j] == "CORNER_LR") Omega[i][j] = -2.0 * Psi[1][j-1] / DD2;//左上隅
else if(type[i][j] == "CORNER_LL") Omega[i][j] = -2.0 * Psi[i-1][j-1] / DD2;//右上隅
}
//Poissonの方程式を解く
var cnt = 0;
while (cnt < iteration)
{
maxError = 0.0;
for (i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
//if(type[i][j] != "INSIDE") continue;
pp = ( DY2 * (Psi[i-1][j] + Psi[i+1][j]) + DX2 * (Psi[i][j-1] + Psi[i][j+1])
+ Omega[i][j] * DX2 * DY2 ) / (2.0 * (DX2 + DY2));
error = Math.abs(pp - Psi[i][j]);
if (error > maxError) maxError = error;
Psi[i][j] = pp;//更新
}
if (maxError < tolerance) break;
cnt++;
}
//console.log("cnt = " + cnt + " maxEr = " + maxError );
calcVelocity();//速度の更新(ψ→ω変換)
//渦度輸送方程式を解く
methodCIP();
//流れ関数,渦度の最小値,最大値
for(i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
if(type[i][j] >= "OBS_LEFT") continue;
if(Psi[i][j] > maxPsi0) maxPsi0 = Psi[i][j];
if(Psi[i][j] < minPsi0) minPsi0 = Psi[i][j];
if(Omega[i][j] > maxOmg0) maxOmg0 = Omega[i][j];
if(Omega[i][j] < minOmg0) minOmg0 = Omega[i][j];
}
console.log("maxPsi= " + maxPsi0 + " minPsi = " + minPsi0);
console.log("maxOmg= " + maxOmg0 + " minOmg = " + minOmg0);
}
function calcVelocity()
{
//速度ベクトルの計算
//格子点の速度ベクトル(上下左右の流れ関数の差で求める)
var i, j;
for (j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
//ポテンシャルの低い方から高い方へ
VelX[i][j] = (Psi[i][j+1] - Psi[i][j-1]) / (DY * 2.0);
VelY[i][j] = (Psi[i-1][j] - Psi[i+1][j]) / (DX * 2.0);
}
}
function methodCIP()
{
var newOmega = [];//新渦度
var newGx = [];//x方向微分
var newGy = [];//y方向微分
var c11, c12, c21, c02, c30, c20, c03, a, b, sx, sy, x, y, dx, dx2, dx3, dy, dy2, dy3;
var i, j, ip, jp;
for(i = 0; i <= NX; i++)
{
newOmega[i] = [];
newGx[i] = [];
newGy[i] = [];
}
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
if(VelX[i][j] >= 0.0) sx = 1.0; else sx = -1.0;
if(VelY[i][j] >= 0.0) sy = 1.0; else sy = -1.0;
x = - VelX[i][j] * deltaT;
y = - VelY[i][j] * deltaT;
ip = i - sx;//上流点
jp = j - sy;
dx = sx * DX;
dy = sy * DY;
dx2 = dx * dx;
dx3 = dx2 * dx;
dy2 = dy * dy;
dy3 = dy2 * dy;
c30 = ((gx[ip][j] + gx[i][j]) * dx - 2.0 * (Omega[i][j] - Omega[ip][j])) / dx3;
c20 = (3.0 * (Omega[ip][j] - Omega[i][j]) + (gx[ip][j] + 2.0 * gx[i][j]) * dx) / dx2;
c03 = ((gy[i][jp] + gy[i][j]) * dy - 2.0 * (Omega[i][j] - Omega[i][jp])) / dy3;
c02 = (3.0 * (Omega[i][jp] - Omega[i][j]) + (gy[i][jp] + 2.0 * gy[i][j]) * dy) / dy2;
a = Omega[i][j] - Omega[i][jp] - Omega[ip][j] + Omega[ip][jp];
b = gy[ip][j] - gy[i][j];
c12 = (-a - b * dy) / (dx * dy2);
c21 = (-a - (gx[i][jp] - gx[i][j]) * dx) / (dx2*dy);
c11 = - b / dx + c21 * dx;
newOmega[i][j] = Omega[i][j] + ((c30 * x + c21 * y + c20) * x + c11 * y + gx[i][j]) * x
+ ((c03 * y + c12 * x + c02) * y + gy[i][j]) * y;
newGx[i][j] = gx[i][j] + (3.0 * c30 * x + 2.0 * (c21 * y + c20)) * x + (c12 * y + c11) * y;
newGy[i][j] = gy[i][j] + (3.0 * c03 * y + 2.0 * (c12 * x + c02)) * y + (c21 * x + c11) * x;
//粘性項に中央差分
newOmega[i][j] += deltaT * ( (Omega[i-1][j] - 2.0 * Omega[i][j] + Omega[i+1][j]) / dx2
+ (Omega[i][j-1] - 2.0 * Omega[i][j] + Omega[i][j+1]) / dy2 ) / Re;
}
//更新
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
Omega[i][j] = newOmega[i][j];
gx[i][j] = newGx[i][j];
gy[i][j] = newGy[i][j];
}
}
function drawContour(PP, maxP, minP, col)
{
nLine = parseFloat(form2.nLine.value);
var dp0 = (maxP - minP) / nLine;//流線間隔
var pp;
var x1, y1, x2, y2;
var p = [], x = [], y = [];
var i, j, k, m;
var data = [];
//三角形セルに分割
for (k = 0; k < nLine; k++)
{
pp = minP + (k + 1) * dp0;
for(j = 0; j < NY; j++)
{
for(i = 0; i < NX; i++)
{//三角形セルに分割
//1つでも内点なら描画
if( type[i][j] != "INSIDE" && type[i][j+1] != "INSIDE"
&& type[i+1][j+1] != "INSIDE" && type[i+1][j] != "INSIDE" ) continue;
p[0] = PP[i][j]; x[0] = i * DX; y[0] = j * DY;
p[1] = PP[i][j+1]; x[1] = i * DX; y[1] = (j+1) * DY;
p[2] = PP[i+1][j+1]; x[2] = (i+1) * DX; y[2] = (j+1) * DY;
p[3] = PP[i+1][j]; x[3] = (i+1) * DX; y[3] = j * DY;
p[4] = p[0]; x[4] = x[0]; y[4] = y[0];//0番目に同じ
//中心
p[5] = (p[0] + p[1] + p[2] + p[3]) / 4.0;
x[5] = (x[0] + x[1] + x[2] + x[3]) / 4.0;
y[5] = (y[0] + y[1] + y[2] + y[3]) / 4.0;
for(m = 0; m < 4; m++)//各三角形について
{
x1 = -10.0; y1 = -10.0;
if((p[m] <= pp && pp < p[m+1]) || (p[m] > pp && pp >= p[m+1]))
{
x1 = x[m] + (x[m+1] - x[m]) * (pp - p[m]) / (p[m+1] - p[m]);
y1 = y[m] + (y[m+1] - y[m]) * (pp - p[m]) / (p[m+1] - p[m]);
}
if((p[m] <= pp && pp <= p[5]) || (p[m] >= pp && pp >= p[5]))
{
if(x1 < 0.0)//まだ交点なし
{
x1 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y1 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
}
else//x1は見つかった
{
x2 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y2 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
if((p[m+1] <= pp && pp <= p[5]) || (p[m+1] >= pp && pp >= p[5]))
{
if(x1 < 0.0)//まだ交点なし
{
x1 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y1 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
}
else//x1は見つかった
{
x2 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y2 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
data.push(rect.left0.x + scale.x * x1);
data.push(rect.left0.y + scale.y * y1);
data.push(rect.left0.x + scale.x * x2);
data.push(rect.left0.y + scale.y * y2);
}
}
}//m
}//j
}//i
}//k
drawLines(data, col);
}
function drawVelocity()
{
arrowScale = parseFloat(form2.arrowScale.value);;
arrowWidth = parseFloat(form2.arrowWidth.value);
intervalV = parseFloat(form2.intervalV.value);
var i, j;
//描画
var theta, mag, x0, y0;
for(i = 1; i < NX; i++)
{
if(i % intervalV != 0) continue;
for (j = 1; j < NY; j++)
{
if(j % intervalV != 0) continue;
if(type[i][j] == "OBS_TOP") continue;
mag = Math.sqrt(VelX[i][j] * VelX[i][j] + VelY[i][j] * VelY[i][j]);
if(mag > 10.0) continue;
theta = Math.atan2(VelY[i][j], VelX[i][j]);
x0 = rect.left0.x + scale.x * i * DX;
y0 = rect.left0.y + scale.y * j * DY;
drawArrow(x0, y0, mag * arrowScale, arrowWidth, "black", theta);
}
}
}
function drawGrid()
{
var i, j;
for(i = 1; i < NX; i++)
{
drawLine(rect.left0.x + scale.x * i * DX, rect.left0.y,
rect.left0.x + scale.x * i * DX, rect.left0.y + scale.y * rect.size.y, 1, "black");
}
for(j = 1; j < NY; j++)
{
drawLine(rect.left0.x, rect.left0.y + scale.y * j * DY,
rect.left0.x + scale.x * rect.size.x, rect.left0.y + scale.y * j * DY, 1, "black");
}
}
//-----------------------------------------------------------------
function Particle2D()
{
this.pos = new Vector3();
this.vel = new Vector3();
this.col;
}
var countP = 0;
var pa = [];//particle
var sizeP = 6;
var speedCoef = 0.1;
var numMaxP;//最大個数
var typeP = 1;
function initParticle()
{
numMaxP = NX * NY;//粒子総数
var i, j, k;
//粒子インスタンス
for(k = 0; k < numMaxP; k++) pa[k] = new Particle2D();
k = 0;
//粒子初期配置
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
pa[k].pos = new Vector3(i * DX, j * DY, 0);
if(i < NX / 3) pa[k].col = "red";
else if(i < NX * 2 / 3) pa[k].col = "green";
else pa[k].col = "blue";
k ++;
}
}
function drawParticle(dt)
{
flagPoint = true;
var k, KR, KG, KB = 0;
var dataR = [];
var dataG = [];
var dataB = [];
var vel = new Vector3();
KR = KG = KB = 0;
for(k = 0; k < numMaxP; k++)
{
vel = getVelocityParticle(pa[k].pos);
if(!flagFreeze) {
pa[k].pos.x += vel.x * dt * speedCoef;
pa[k].pos.y += vel.y * dt * speedCoef;
if(pa[k].pos.x < DX) pa[k].pos.x = DX;
if(pa[k].pos.x > 1-DX) pa[k].pos.x = 1 - DX;
if(pa[k].pos.y < DY) pa[k].pos.y = DY;
if(pa[k].pos.y > 1-DY) pa[k].pos.y = 1 - DY;
}
if(pa[k].col == "red")
{
dataR[2*KR] = rect.left0.x + pa[k].pos.x * scale.x;
dataR[2*KR+1] = rect.left0.y + pa[k].pos.y * scale.y;
KR++;
}
else if(pa[k].col == "green")
{
dataG[2*KG] = rect.left0.x + pa[k].pos.x * scale.x;
dataG[2*KG+1] = rect.left0.y + pa[k].pos.y * scale.y;
KG++;
}
else if(pa[k].col == "blue")
{
dataB[2*KB] = rect.left0.x + pa[k].pos.x * scale.x;
dataB[2*KB+1] = rect.left0.y + pa[k].pos.y * scale.y;
KB++;
}
}
drawPoints(dataR, sizeP, typeP, "red");
drawPoints(dataG, sizeP, typeP, "green");
drawPoints(dataB, sizeP, typeP, "blue");
}
function getVelocityParticle(pos)
{
var vel = new Vector3();
var i, j, I, J;
//格子番号を取得
I = 0; J = 0;
for(i = 0; i < NX; i++)
{
if(i * DX < pos.x && (i+1) * DX > pos.x) I = i;
}
for(j = 0; j < NY; j++)
{
if(j * DY < pos.y && (j+1) * DY > pos.y) J = j;
}
var a = pos.x / DX - I;
var b = pos.y / DY - J;
//格子点の速度を線形補間
vel.x = (1.0 - b) * ((1.0 - a) * VelX[I][J] + a * VelX[I+1][J]) + b * ((1.0 - a) * VelX[I][J+1] + a * VelX[I+1][J+1]);
vel.y = (1.0 - b) * ((1.0 - a) * VelY[I][J] + a * VelY[I+1][J]) + b * ((1.0 - a) * VelY[I][J+1] + a * VelY[I+1][J+1]);
return vel;
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
initData();
}
function onDisplay()
{
display();
}
function onClickScale()
{
rect.scale = parseFloat(form2.scale.value);
display();
}
//-------------------------------------------------------------
function onClickP()
{
speedCoef = parseFloat(form2.speedCoef.value);
sizeP= parseFloat(form2.sizeP.value);
typeP= parseFloat(form2.typeP.value);
}
function onClickStart()
{
fps = 0;
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
elapseTimeN = 0;
elapseTimeN0 = 0;
flagStart = true;
flagStep = false;
flagFreeze = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
flagStart = !flagStart;
flagFreeze = !flagFreeze;
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTimeN0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.e_time.value = "0";
form1.n_time.value = "0";
display();
}
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第4章/ductPsiOmega3DG1.js
/*-----------------------------------------------
ductPsiOmega3DG1.js
流れ関数-渦度法
3Dグラフィックス
------------------------------------------------*/
var canvas;//canvas要素
var gl; //WebGL描画用コンテキスト
var camera;//カメラ
var light; //光源
var flagDebug = false;
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var flagStart = false;
var flagStep = false;
var flagReset = false;
//表示オブジェクト
var object, obstacle;
var adjustH = 0.01;//表示上の高さ調整
var displayMode = 0;//渦度/流れ関数
var colorMode = 0;//連続/段階表示
//数値計算
var Psi = []; //流れ関数
var Omg = [];//渦度
var gx = []; //x方向微分
var gy = []; //y方向微分
var VelX = []; //x方向速度
var VelY = []; //y方向速度
var type = [];//格子点のタイプ
var deltaT = 0.001;
var Re = 3000.0;//レイノルズ数
var maxPsi = 1.0;
var minPsi = 0;
var maxOmg = 20.0;
var minOmg = -20.0;
//解析領域矩形
function Rect()
{
this.nMeshX = 160;//x方向割数
this.nMeshY = 80; //y方向分割数
this.size = new Vector3(2, 1, 0);//矩形ダクト領域のサイズ(固定)
this.left0 = new Vector3(-1, -1, 0);//その左下位置
this.delta = new Vector3(); //格子間隔
this.obs_nX0 = 30; //左端から障害物中心までの距離(格子間隔の整数倍)
this.obs_nWX = 8;//障害物の厚さ(x方向,格子間隔の整数倍)
this.obs_nWY = 40; //障害物の幅(y方向,格子間隔の整数倍)
this.obs_left;//ダクト左端から障害物左端までの距離(仮の値)
}
var rect = new Rect();
var NX, NY, DX, DY;
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(0.1, 0.1, 0.1, 1);
gl.enable(gl.DEPTH_TEST);
form1.deltaT.value = deltaT;
form1.Re.value = Re;
form1.nMeshX.value = rect.nMeshX;
form1.nMeshY.value = rect.nMeshY;
form1.obs_nX0.value = rect.obs_nX0;
form1.obs_nWX.value = rect.obs_nWX;
form1.obs_nWY.value = rect.obs_nWY;
form2.adjustH.value = adjustH;
form2.maxPsi.value = maxPsi;
form2.minPsi.value = minPsi;
form2.maxOmg.value = maxOmg;
form2.minOmg.value = minOmg;
initCamera();
initData();
display();
var timestep = 0;
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;
elapseTimeN += deltaT;//数値計算上の経過時間(無次元時間)
//for(var i = 0; i < 10; i++)
calculate();
display();
elapseTime0 = elapseTime;//現在の経過時間を保存
elapseTimeN0 = elapseTimeN;//現在の経過時間を保存
form1.e_time.value = elapseTime.toString();
form1.n_time.value = elapseTimeN.toString();
if(flagStep) { flagStart = false; }
}
}
animate();
}
//--------------------------------------------
function initCamera()
{
//光源インスタンスを作成
light = new Light();
light.pos = [50.0, -100.0, 100.0, 1.0];
form2.lightX.value = light.pos[0];
form2.lightY.value = light.pos[1];
form2.lightZ.value = light.pos[2];
//カメラ・インスタンスを作成
camera = new Camera();
camera.phi = -90;
camera.cnt[2] = 0.2;
camera.dist = 4;
camera.fovy = 15;
camera.getPos();//カメラ位置を計算
mouseOperation(canvas, camera);//swgSupport.js
}
function initData()
{
deltaT = parseFloat(form1.deltaT.value);
Re = parseFloat(form1.Re.value);
NX = parseInt(form1.nMeshX.value);
NY = parseInt(form1.nMeshY.value);
rect.obs_nX0 = parseFloat(form1.obs_nX0.value);
rect.obs_nWX = parseFloat(form1.obs_nWX.value);
rect.obs_nWY = parseFloat(form1.obs_nWY.value);
DX = rect.size.x / NX;//格子間隔
DY = rect.size.y / NY;
var nMeshX0 = rect.obs_nX0;
var nMeshX1 = nMeshX0 - rect.obs_nWX/2;
var nMeshX2 = nMeshX1 + rect.obs_nWX;
var nObsWX = rect.obs_nWX;
var nObsWY = rect.obs_nWY;
rect.obs_left = nMeshX1 * DX;//ダクト左端からの距離
var i, j;
for(i = 0; i <= NX; i++)
{
type[i] = [];//格子点のタイプ
Psi[i] = []; //流れ関数
Omg[i] = [];//渦度
gx[i] = []; //x方向微分
gy[i] = []; //y方向微分
VelX[i] = []; //x方向速度
VelY[i] = []; //y方向速度
}
//格子点のタイプ
for(j = 0; j <= NY; j++)
{
for(i = 0; i <= NX; i++)
{
type[i][j] = "INSIDE";//内点
if(j == 0) type[i][j] = "BOTTOM";//下側壁面
if(j == NY) type[i][j] = "TOP";//上側壁面
if(i == 0) type[i][j] = "INLET";//流入端
if(i == NX) type[i][j] = "OUTLET";//流出端
if(i == nMeshX1 && j < nObsWY) type[i][j] = "OBS_LEFT";//障害物左端
if(i >= nMeshX1 && i <= nMeshX2 && j == nObsWY) type[i][j] = "OBS_TOP";//障害物上端
if(i == nMeshX2 && j < nObsWY) type[i][j] = "OBS_RIGHT";//障害物右端
if(i > nMeshX1 && i < nMeshX2 && j < nObsWY) type[i][j] = "OBSTACLE";//障害物内部
//障害物のコーナー
if(i == nMeshX1 && j == 0) type[i][j] = "OBS_UL";
if(i == nMeshX1 && j == nObsWY) type[i][j] = "OBS_UL";
if(i == nMeshX2 && j == 0) type[i][j] = "OBS_UR";
if(i == nMeshX2 && j == nObsWY) type[i][j] = "OBS_UR";
}
}
//初期値
//入口/出口は流速1,psi = y
//下の壁は psi = 0,上の壁は psi = rect.size.y
for(j = 0; j <= NY; j++)
for (i = 0; i <= NX; i++)
{
//流れ関数
if(type[i][j] == "TOP") Psi[i][j] = rect.size.y;
else if(type[i][j] == "INLET" || type[i][j] == "OUTLET" || type[i][j] == "INSIDE" ) Psi[i][j] = j * DY;
else Psi[i][j] = 0.0;
//渦度
Omg[i][j] = 0.0;
//速度
if(type[i][j] == "INLET" || type[i][j] == "OUTLET" || type[i][j] == "INSIDE") VelX[i][j] = 1.0;
else VelX[i][j] = 0.0;
VelY[i][j] = 0.0;
//CIP法で使用する微分値
gx[i][j] = 0.0;
gy[i][j] = 0.0;
}
calcVelocity();
maxPsi0 = -1000.0; minPsi0 = 1000.0;
maxOmg0 = -1000.0; minOmg0 = 1000.0;
//発散しないための目安を知るため
var courant, diffNum;
if(DX < DY)
{
courant = 1.0 * deltaT / DX;
diffNum = (1.0 / Re) * deltaT / (DX * DX);//拡散数
}
else
{
courant = 1.0 * deltaT / DY;
diffNum = (1.0 / Re) * deltaT / (DX * DY);//拡散数
}
form1.courant.value = courant;
form1.diffNum.value = diffNum;
initObject();
}
function initObject()
{
//解析結果表示オブジェクト
object = new Rigid();//Rigidクラスのオブジェクトを表示オブジェクト
object.kind = "ELEVATION";
object.sizeX = rect.size.x;
object.sizeY = rect.size.y;
object.vSize = new Vector3(1, 1, adjustH);//scaling
object.nSlice = NX;
object.nStack = NY;
object.diffuse = [ 0.4, 0.6, 0.9, 1.0] ;
object.ambient = [ 0.1, 0.2, 0.3, 1.0];
object.specular = [ 0.8, 0.8, 0.8, 1.0];
object.shininess = 100.0;
object.flagDebug = flagDebug;
//障害物
obstacle = new Rigid();
obstacle.kind = "CUBE";
obstacle.vSize = new Vector3(rect.obs_nWX*DX, rect.obs_nWY*DY, 0.1);
obstacle.vPos = new Vector3(rect.obs_nX0*DX - rect.size.x/2, (rect.obs_nWY*DY - rect.size.y)/2, 0);
obstacle.flagDebug = flagDebug;
}
function display()
{
//光源
var lightPosLoc = gl.getUniformLocation(gl.program, 'u_lightPos');
gl.uniform4fv(lightPosLoc, light.pos);
var lightColLoc = gl.getUniformLocation(gl.program, 'u_lightColor');
gl.uniform4fv(lightColLoc, light.color);
var cameraLoc = gl.getUniformLocation(gl.program, 'u_cameraPos');
gl.uniform3fv(cameraLoc, camera.pos);
//ビュー投影行列を計算する
var vpMatrix = new Matrix4();// 初期化
vpMatrix.perspective(camera.fovy, canvas.width/canvas.height, camera.near, camera.far);
if(Math.cos(Math.PI * camera.theta /180.0) >= 0.0)//カメラ仰角90度でビューアップベクトル切替
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, 1.0);
else
vpMatrix.lookAt(camera.pos[0], camera.pos[1], camera.pos[2], camera.cnt[0], camera.cnt[1], camera.cnt[2], 0.0, 0.0, -1.0);
var vpMatrixLoc = gl.getUniformLocation(gl.program, 'u_vpMatrix');
gl.uniformMatrix4fv(vpMatrixLoc, false, vpMatrix.elements);
var colorLoc = gl.getUniformLocation(gl.program, 'u_colorMode');
gl.uniform1i(colorLoc, colorMode);
// カラーバッファとデプスバッファをクリアする
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
var i, j, k;
var range0;
maxPsi = parseFloat(form2.maxPsi.value);
minPsi = parseFloat(form2.minPsi.value);
maxOmg = parseFloat(form2.maxOmg.value);
minOmg = parseFloat(form2.minOmg.value);
for(j = 0; j <= NY; j++)
for(i = 0; i <= NX; i++)
{
k = i + j * (NX+ 1);
if( displayMode == 0 )
{
range0 = maxOmg - minOmg;
object.data[k] = (Omg[i][j] - minOmg) / range0 ;
}
else
{
range0 = maxPsi - minPsi;
object.data[k] = (Psi[i][j] - minPsi) / range0 ;
}
}
object.vSize = new Vector3(1, 1, adjustH);
object.vPos.z = -adjustH * 0.5;//中間色(G)をz=0の位置にする
var n = object.initVertexBuffers(gl);
object.draw(gl, n);
//障害物
gl.uniform1i(colorLoc, 2);
n = obstacle.initVertexBuffers(gl);
obstacle.draw(gl, n);
}
function calculate()
{
var i, j;
var iteration = 10;//最大繰り返し回数(Poisson方程式)
var tolerance = 0.00001;//許容誤差
var error = 0.0;
var maxError = 0.0;
var pp;
var DX2 = DX * DX;
var DY2 = DY * DY;
var DD2 = DX2 + DY2;//corner
//渦度境界条件
for (i = 0; i <= NX; i++)
for (j = 0; j <= NY; j++)
{
if(type[i][j] == "INSIDE") continue;
else if(type[i][j] == "INLET") Omg[i][j] = 0.0;//Dirichlet
else if(type[i][j] == "OUTLET") Omg[i][j] = Omg[i-1][j];//Neumann
else if(type[i][j] == "TOP") Omg[i][j] = -2.0 *( Psi[i][NY-1] - rect.size.y) / DY2;
else if(type[i][j] == "BOTTOM") Omg[i][j] = -2.0 * Psi[i][1] / DY2;
else if(type[i][j] == "OBS_LEFT") Omg[i][j] = -2.0 * Psi[i-1][j] / DX2;
else if(type[i][j] == "OBS_TOP") Omg[i][j] = -2.0 * Psi[i][j+1] / DY2;
else if(type[i][j] == "OBS_RIGHT") Omg[i][j] = -2.0 * Psi[i+1][j] / DX2;
else if(type[i][j] == "OBS_UL") Omg[i][j] = -2.0 * Psi[i-1][j+1] / DD2;
else if(type[i][j] == "OBS_UR") Omg[i][j] = -2.0 * Psi[i+1][j+1] / DD2;
}
//Poissonの方程式を解く
var cnt = 0;
while (cnt < iteration)
{
maxError = 0.0;
for (i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
if(type[i][j] != "INSIDE") continue;
pp = ( DY2 * (Psi[i-1][j] + Psi[i+1][j]) + DX2 *(Psi[i][j-1] + Psi[i][j+1])
+ Omg[i][j] * DX2 * DY2 ) / (2.0 * (DX2 + DY2));
error = Math.abs(pp - Psi[i][j]);
if (error > maxError) maxError = error;
Psi[i][j] = pp;//更新
}
if (maxError < tolerance) break;
cnt++;
}
//console.log("cnt = " + cnt + " maxEr = " + maxError + " Psi = " + Psi[10][10] + " Omg = " + Omg[10][10]);
calcVelocity();//速度の更新(ψ→ω変換)
//渦度輸送方程式を解く
methodCIP();
//流れ関数,渦度の最小値,最大値
for(i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
if(type[i][j] >= "OBS_LEFT") continue;
if(Psi[i][j] > maxPsi0) maxPsi0 = Psi[i][j];
if(Psi[i][j] < minPsi0) minPsi0 = Psi[i][j];
if(Omg[i][j] > maxOmg0) maxOmg0 = Omg[i][j];
if(Omg[i][j] < minOmg0) minOmg0 = Omg[i][j];
}
console.log("maxPsi= " + maxPsi0 + " minPsi = " + minPsi0);
console.log("maxOmg= " + maxOmg0 + " minOmg = " + minOmg0);
}
function calcVelocity()
{
//速度ベクトルの計算
//格子点の速度ベクトル(上下左右の流れ関数の差で求める)
var i, j;
for (j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
//ポテンシャルの低い方から高い方へ
VelX[i][j] = (Psi[i][j+1] - Psi[i][j-1]) / (DY * 2.0);
VelY[i][j] = (Psi[i-1][j] - Psi[i+1][j]) / (DX * 2.0);
// if(j == 20) console.log("i = " + i + " v.x = " + VelX[i][j] + " v.y = " + VelY[i][j]);
}
}
function methodCIP()
{
var newOmg = [];//新渦度
var newGx = [];//x方向微分
var newGy = [];//y方向微分
var c11, c12, c21, c02, c30, c20, c03, a, b, sx, sy, x, y, dx, dx2, dx3, dy, dy2, dy3;
var i, j, ip, jp;
for(i = 0; i <= NX; i++)
{
newOmg[i] = [];
newGx[i] = [];
newGy[i] = [];
}
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
if(VelX[i][j] >= 0.0) sx = 1.0; else sx = -1.0;
if(VelY[i][j] >= 0.0) sy = 1.0; else sy = -1.0;
x = - VelX[i][j] * deltaT;
y = - VelY[i][j] * deltaT;
ip = i - sx;//上流点
jp = j - sy;
dx = sx * DX;
dy = sy * DY;
dx2 = dx * dx;
dx3 = dx2 * dx;
dy2 = dy * dy;
dy3 = dy2 * dy;
c30 = ((gx[ip][j] + gx[i][j]) * dx - 2.0 * (Omg[i][j] - Omg[ip][j])) / dx3;
c20 = (3.0 * (Omg[ip][j] - Omg[i][j]) + (gx[ip][j] + 2.0 * gx[i][j]) * dx) / dx2;
c03 = ((gy[i][jp] + gy[i][j]) * dy - 2.0 * (Omg[i][j] - Omg[i][jp])) / dy3;
c02 = (3.0 * (Omg[i][jp] - Omg[i][j]) + (gy[i][jp] + 2.0 * gy[i][j]) * dy) / dy2;
a = Omg[i][j] - Omg[i][jp] - Omg[ip][j] + Omg[ip][jp];
b = gy[ip][j] - gy[i][j];
c12 = (-a - b * dy) / (dx * dy2);
c21 = (-a - (gx[i][jp] - gx[i][j]) * dx) / (dx2*dy);
c11 = - b / dx + c21 * dx;
newOmg[i][j] = Omg[i][j] + ((c30 * x + c21 * y + c20) * x + c11 * y + gx[i][j]) * x
+ ((c03 * y + c12 * x + c02) * y + gy[i][j]) * y;
newGx[i][j] = gx[i][j] + (3.0 * c30 * x + 2.0 * (c21 * y + c20)) * x + (c12 * y + c11) * y;
newGy[i][j] = gy[i][j] + (3.0 * c03 * y + 2.0 * (c12 * x + c02)) * y + (c21 * x + c11) * x;
//粘性項に中央差分
newOmg[i][j] += deltaT * ( (Omg[i-1][j] - 2.0 * Omg[i][j] + Omg[i+1][j]) / dx2
+ (Omg[i][j-1] - 2.0 * Omg[i][j] + Omg[i][j+1]) / dy2 ) / Re;
}
//更新
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
if(type[i][j] != "INSIDE") continue;
Omg[i][j] = newOmg[i][j];
gx[i][j] = newGx[i][j];
gy[i][j] = newGy[i][j];
}
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
initData();
}
function onDisplay()
{
display();
}
function onChangeAdjustH()
{
adjustH = parseFloat(form2.adjustH.value);
if(adjustH == 0) adjustH = 0.001;// 完全に0にするとmatrix4の逆行列ルーチンでエラーになる
display();
}
function onChangeDisplayMode()
{
var nn;
var radioD = document.getElementsByName("radioD");
for(var i = 0; i < radioD.length; i++)
{
if(radioD[i].checked) displayMode = i;
}
display();
}
function onChangeColorMode()
{
var nn;
var radioC = document.getElementsByName("radioC");
for(var i = 0; i < radioC.length; i++)
{
if(radioC[i].checked) colorMode = i;
}
display();
}
function onClickDebug()
{
object.flagDebug = obstacle.flagDebug = flagDebug = !flagDebug ;
display();
}
function onClickStart()
{
fps = 0;
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
elapseTimeN = 0;
elapseTimeN0 = 0;
flagStart = true;
flagStep = false;
flagFreeze = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
flagStart = !flagStart;
flagFreeze = !flagFreeze;
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTimeN0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.e_time.value = "0";
form1.n_time.value = "0";
display();
}
function onClickCameraReset()
{
initCamera();
display();
}
function onClickCameraAbove()
{
initCamera();
camera.theta = 90.01;
camera.getPos();//カメラ位置を計算
display();
}
function onClickLight()
{
light.pos[0] = parseFloat(form2.lightX.value);
light.pos[1] = parseFloat(form2.lightY.value);
light.pos[2] = parseFloat(form2.lightZ.value);
display();
}
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgCollisionSM_R.js
/*--------------------------------------------------------------
collisionSM_R.js
2次元バス質点モデルと剛体球の衝突
----------------------------------------------------------------*/
function collisionSMwithR(sm, dt)
{
var i, j, d;
console.log(" numPoint = " + sm.numPoint)
for(k = 0; k < sm.numPoint; k++)
{
d = distance(sm.point[k].vPos, sm.rigid2.vPos);
if( d > sm.radius + sm.rigid2.vSize.x / 2.0) continue;
collisionSM_Rigid(sm, k, dt);
}
}
//--------------------------------------------------------------------------
function collisionSM_Rigid(sm, k, dt)
{
var rikiseki;
var vn1, vn2;//法線成分の大きさ
var muc, B, m1, m2;
var vVelocityP1, vVelocityP2;//合成速度
var vNormal;//注目剛体(質点)から見た衝突面の法線方向
var vTangential;//接線方向
var vDir, vA1, vA2, vRg1, vRg2;
//var k = i + j * (sm.numRow + 1);
sm.point[k].vForce =new Vector3();
sm.point[k].vTorque = new Vector3();
sm.rigid2.vForce = new Vector3();
sm.rigid2.vTorque = new Vector3();
vNormal = direction(sm.point[k].vPos, sm.rigid2.vPos);
//console.log(" nx = " + vNormal.x + " ny = " + vNormal.y + " nz = " + vNormal.z);
//重心から衝突点までのベクトル
sm.point[k].vGravityToPoint = mul(sm.radius , vNormal);
sm.rigid2.vGravityToPoint = mul(sm.rigid2.vSize.x/2.0 , vNormal);
sm.rigid2.vGravityToPoint.reverse();
var depth = (sm.radius + sm.rigid2.vSize.x / 2.0) - distance(sm.point[k].vPos, sm.rigid2.vPos);
if(depth < 0.0) return;
vRg1 = sm.point[k].vGravityToPoint;
vRg2 = sm.rigid2.vGravityToPoint;
m1 = sm.mass;//質点の質量
m2 = sm.rigid2.mass;
//console.log("PPP x = " + sm.point[k].vOmega.x + " y = " + sm.point[k].vOmega.y + " z = " + sm.point[k].vOmega.z);
vVelocityP1 = add(sm.point[k].vVel, cross(sm.point[k].vOmega, vRg1));
vn1 = dot(vVelocityP1, vNormal);
vVelocityP2 = add(sm.rigid2.vVel, cross(sm.rigid2.vOmega, vRg2));
vn2 = dot(vVelocityP2, vNormal);
//衝突応答
vTangential = cross( vNormal, cross(sub(vVelocityP1, vVelocityP2), vNormal));
vTangential.norm();
//力積
rikiseki = - (restitution + 1.0) * (vn1 - vn2) / (1.0/m1 + 1.0/m2
+ dot(vNormal , cross(mulMV(sm.point[k].mInertiaInverse, cross(vRg1, vNormal)), vRg1))
+ dot(vNormal , cross(mulMV(sm.rigid2.mInertiaInverse, cross(vRg2, vNormal)), vRg2)));
//力の総和
var vFF = mul(vNormal , rikiseki / dt);
sm.point[k].vForce.add(vFF);
sm.rigid2.vForce.sub(vFF);
//力のモーメント(トルク)の総和
vFF = mul(cross(vRg1, vNormal) , rikiseki / dt);
sm.point[k].vTorque.add(vFF);
sm.rigid2.vTorque.sub(vFF);
//摩擦を考慮
vA1 = cross(mulMV(sm.point[k].mInertiaInverse, cross(vRg1, vTangential)) , vRg1);
vA2 = cross(mulMV(sm.rigid2.mInertiaInverse, cross(vRg2, vTangential)) , vRg2);
B = - dot(vTangential, sub(vVelocityP1, vVelocityP2)) / (1/m1+1/m2+ dot(vTangential,add(vA1, vA2)));
muc = Math.abs(B / rikiseki);
if(muK >= muc)
{
sm.point[k].vForce.add( mul(vTangential, B / dt) );
sm.point[k].vTorque.add( mul(cross(vRg1, vTangential) , B / dt ));
sm.rigid2.vForce.sub( mul(vTangential, B / dt) );
sm.rigid2.vTorque.sub( mul(cross(vRg2, vTangential) , B / dt) );
}
else
{
sm.point[k].vForce.add( mul(muK * rikiseki / dt, vTangential) );
sm.point[k].vTorque.add( mul(cross(vRg1, vTangential) , muK * rikiseki / dt) );
sm.rigid2.vForce.sub( mul(muK * rikiseki / dt , vTangential) );
sm.rigid2.vTorque.sub( mul(cross(vRg2, vTangential) , muK * rikiseki / dt) );
}
//衝突判定時にめり込んだ分引き離す
vFF = mul(depth/2.0, vNormal);
sm.point[k].vPos.sub( vFF );
if(sm.rigid2.state == "FREE") sm.rigid2.vPos.add( vFF );
//位置回転角度の更新
// i番目の剛体
sm.point[k].vAccel = div(sm.point[k].vForce , m1) ; //加速度
sm.point[k].vVel.add( mul(sm.point[k].vAccel , dt) );//速度
sm.point[k].vAlpha = mulMV(sm.point[k].mInertiaInverse , sm.point[k].vTorque);//角加速度の更新
sm.point[k].vOmega.add( mul(sm.point[k].vAlpha , dt) ); //角速度
// 剛体
if(sm.rigid2.state == "FREE")
{
sm.rigid2.vAccel = div(sm.rigid2.vForce , m2);
sm.rigid2.vVel.add( mul(sm.rigid2.vAccel , dt) );
sm.rigid2.vAlpha = mulMV(sm.rigid2.mInertiaInverse , sm.rigid2.vTorque);
sm.rigid2.vOmega.add( mul(sm.rigid2.vAlpha , dt) );
}
}
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/urls.py
from django.conf.urls import url
from ip import views
urlpatterns = [
url(r'^$', views.index, name='ip_index'),
# url(r'^$', views.index),
url(r'^index/$', views.index, name='ip_index'),
url(r'^basics/$', views.basics, name='ip_basics'),
#test
url(r'^test/$', views.test, name='ip_test'),
url(r'^get_4_corners/$', views.get_4_corners, name='get_4_corners'),
url(r'^open_image_dir/$', views.open_image_dir, name='open_image_dir'),
url(r'^exec_get_4_corners/$', views.exec_get_4_corners, name='exec_get_4_corners'),
url(r'^gen_Cake_CSV/$', views.gen_Cake_CSV, name='gen_Cake_CSV'),
url(r'^dos_attack/$', views.dos_attack, name='dos_attack'),
url(r'^ip_ops/$', views.ip_ops, name='ip_ops'),
# 20180925_064955
url(r'^prep_gen_Cake_CSV/$', views.prep_gen_Cake_CSV, name='prep_gen_Cake_CSV'),
# 20181010_084804
url(r'^anims/$', views.anims, name='anims'),
# 20181010_120542
url(r'^anims_JS/$', views.anims_JS, name='anims_JS'),
# 20181013_071822
url(r'^open_dir/$', views.open_dir, name='open_dir'),
]
<file_sep>/b.bat
@echo off
set temp="%1"
echo param is %temp%
REM REF http://answers.microsoft.com/en-us/windows/forum/windows_vista-windows_programs/batch-parameter-is-it-all-arguments-to-the-command/9f7484f3-819c-4c05-a4e9-a439043b18fa
if %temp%=="ch" (
echo starting =^> chrome
goto start_chrome
) else if %temp%=="ff" (
echo starting =^> firefox
goto start_ff
) else (
echo ^<Usage^> b ch/ff
)
goto end
REM pushd "C:\Program Files (x86)\Google\Chrome\Application"
REM start chrome.exe http://localhost/Smarty/
REM start chrome.exe http://benfranklin.chips.jp/Labs/Smarty/D-1/D-1.php
REM start chrome.exe "https://mysqladmin.lolipop.jp/pma/index.php?db=LAA0278957-cakevm&table=positions&target=sql.php&server=110&token=<PASSWORD>"
REM start chrome.exe chrome-extension://ohbfkkmpnlpgbbfdflaiikoohbidaikj/app.html
REM start chrome.exe http://localhost/Smarty/
REM start chrome.exe http://benfranklin.chips.jp/Labs/Smarty/D-1/D-1.php
REM start chrome.exe "https://mysqladmin.lolipop.jp/pma/index.php?db=LAA0278957-cakevm&table=positions&target=sql.php&server=110&token=<PASSWORD>"
REM start chrome.exe chrome-extension://ohbfkkmpnlpgbbfdflaiikoohbidaikj/app.html
REM popd
pushd C:\WORKS\Programs\Firefox_33.0
REM start firefox.exe http://localhost/Smarty/
REM start firefox.exe http://benfranklin.chips.jp/Labs/Smarty/D-1/D-1.php
REM start firefox.exe "https://mysqladmin.lolipop.jp/pma/index.php?db=LAA0278957-cakevm&table=positions&target=sql.php&server=110&token=<PASSWORD>"
popd
:start_chrome
start chrome.exe http://localhost/Smarty/
REM start chrome.exe http://benfranklin.chips.jp/Labs/Smarty/D-1/D-1.php
start chrome.exe http://benfranklin.chips.jp/Labs/Smarty/main/D-3.php
start chrome.exe "https://mysqladmin.lolipop.jp/pma/index.php?db=LAA0278957-cakevm&table=positions&target=sql.php&server=110&token=<PASSWORD>"
start chrome.exe chrome-extension://ohbfkkmpnlpgbbfdflaiikoohbidaikj/app.html
start chrome.exe https://docs.google.com/spreadsheets/d/1GlMjFYCAdSc87V-BhGAM-sz-Kka6AlyxgS-0jqvPlPc/edit#gid=0
goto end
:start_ff
start firefox.exe http://localhost/Smarty/
REM start firefox.exe http://benfranklin.chips.jp/Labs/Smarty/D-1/D-1.php
start chrome.exe http://benfranklin.chips.jp/Labs/Smarty/main/D-3.php
start firefox.exe "https://mysqladmin.lolipop.jp/pma/index.php?db=LAA0278957-cakevm&table=positions&target=sql.php&server=110&token=<PASSWORD>"
start chrome.exe https://docs.google.com/spreadsheets/d/1GlMjFYCAdSc87V-BhGAM-sz-Kka6AlyxgS-0jqvPlPc/edit#gid=0
goto end
:end
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/ip/data/ops/1_1.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\1_1.py
orig : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\sounds\mp3\1_1.py
at : 2018/06/30 09:32:18
r w && r d4
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops
python 1_1.py
python 1_1.py -s1.0
ref : http://aidiary.hatenablog.com/entry/20110607/1307449007
'''
###############################################
import sys, cv2
# from pandas.compat import str_to_bytes
from numpy.distutils.from_template import item_re
#ref https://stackoverflow.com/questions/3129322/how-do-i-get-monitor-resolution-in-python
from win32api import GetSystemMetrics
from matplotlib import pylab as plt
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects') # libs_mm
from libs_admin import libs, lib_ip
'''###################
import : built-in modules
###################'''
# import getopt
import os, glob, getopt, math as math, numpy as np
# import inspect
# import math as math
###############################################
def show_Message() :
msg = '''
<Options>
-v Volume down the amplitude --> 1.0 * v / 1000
-f Base frequency ---> e.g. 262 for the A tone
-p Phase of the sine curves ---> sin(2 * np.pi * f0 * n * phase / fs)'''
print (msg)
'''###################
click_and_crop(event, x, y, flags, param)
at : 2018/06/30 09:53:22
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
def click_and_crop(event, x, y, flags, param):
print()
print("[%s:%d] x = %d, y = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, x, y
), file=sys.stderr)
#/ def click_and_crop(event, x, y, flags, param):
def test_8__Matplotlib__Bar_Chart():
objects = ('Python', 'C++', 'Java', 'Perl', 'Scala', 'Lisp')
y_pos = np.arange(len(objects))
performance = [10,8,6,4,2,1]
performance.reverse()
# performance = [10,8,6,4,2,1]
plt.bar(y_pos, performance, align='center', alpha=0.5)
plt.xticks(y_pos, objects)
plt.ylabel('Usage')
plt.title('Programming language usage')
plt.show()
#/ def test_8__Matplotlib__Bar_Chart():
def test_7__SubImage_RGB_Vals__Get_Scaling(args):
# scaling
scaling = 1.0
#debug
print("[%s:%d] args =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(args)
if len(args) > 0 : #if len(args) > 1
# if len(args) > 1 : #if len(args) > 1
optlist, args = getopt.getopt(args, "s:")
print("[%s:%d] optlist =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(optlist)
for item in optlist:
#debug
print("[%s:%d] item =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(item)
if item[0].startswith("-s") : #if item.startswith("-s")
scaling = float(item[1])
# scaling = item[1]
break
#/if item.startswith("-s")
#/for item in optlist:
else : #if len(args) > 1
print("[%s:%d] len(args) < 2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if len(args) > 1
#debug
print("[%s:%d] scaling => %.3f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, scaling
), file=sys.stderr)
'''###################
return
###################'''
return scaling
#/ def test_7__SubImage_RGB_Vals__Get_Scaling():
def test_7__SubImage_RGB_Vals__Get_RGB_Vals(img_Sub):
'''###################
message
###################'''
cntOf_Rows = 0
lo_Rs = []
lo_Gs = []
lo_Bs = []
for row in img_Sub:
# counter
cntOf_Rows += 1
for cell in row:
# print()
# print("[%s:%d] cell(%d) => " % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , cntOf_Rows
# ), file=sys.stderr)
# print(cell)
'''###################
append
###################'''
lo_Rs.append(cell[0])
lo_Gs.append(cell[1])
lo_Bs.append(cell[2])
# break
#/for cell in row:
#/for row in img_Sub:
'''###################
return
###################'''
return lo_Rs, lo_Gs, lo_Bs
#/ def test_7__SubImage_RGB_Vals__Get_RGB_Vals(img_Sub):
def test_7__SubImage_RGB_Vals():
'''###################
message
###################'''
print()
print("[%s:%d] test_7__SubImage_RGB_Vals =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
#debug
print("[%s:%d] source image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Ops_Image
), file=sys.stderr)
print("[%s:%d] width = %d, height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width, height
), file=sys.stderr)
'''###################
sub image
###################'''
# height, width
img_Sub = img_ForDisp[19:69, 245:246]
# img_Sub = img_ForDisp[19:186, 245:246]
# meta data
height_Sub, width_Sub, channels_Sub = img_Sub.shape
print("[%s:%d] width_Sub = %d, height_Sub = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width_Sub, height_Sub
), file=sys.stderr)
print()
print("[%s:%d] img_Sub[0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub[0])
print("[%s:%d] img_Sub[0][0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub[0][0])
'''###################
get : RGB vals
###################'''
lo_Rs, lo_Gs, lo_Bs = test_7__SubImage_RGB_Vals__Get_RGB_Vals(img_Sub)
# print()
# print("[%s:%d] lo_Rs =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(lo_Rs)
'''###################
plot
###################'''
# yax_vals = [200, 100,0]
# plt.bar(yax_vals, lo_Rs)
# # plt.bar(lo_Rs, yax_vals)
# # plt.bar(lo_Rs)
# # plt.plot(lo_Rs)
#
# plt.show()
# objects = ('Python', 'C++', 'Java', 'Perl', 'Scala', 'Lisp')
y_pos = np.arange(len(lo_Rs))
# y_pos = np.arange(len(objects))
# y_pos = [10, 20, 30]
performance = lo_Rs
# performance = [30,8,6,4,2,1]
# performance = [10,8,6,4,2,1]
# performance.reverse()
# performance = [10,8,6,4,2,1]
# plt.bar(y_pos, performance, align='center', alpha=0.5)
# plt.plot(lo_Rs)
# plt.plot(lo_Bs)
# plt.plot(lo_Rs, lo_Bs)
# plt.xticks(y_pos, objects)
# plt.yticks([140, 180])
# plt.ylabel('Usage')
# plt.title('Programming language usage')
#
# ax = plt.gca()
#
# #ref grid https://stackoverflow.com/questions/16074392/getting-vertical-gridlines-to-appear-in-line-plot-in-matplotlib
# ax.grid(which='major', axis='both', linestyle='--')
# ax.grid(which='minor', axis='both', linestyle='--')
#
# ax.set(aspect=1,
# xlim=(0, len(lo_Rs)),
# ylim=(140, 170))
#
# plt.show()
# #ref https://stackoverflow.com/questions/4805048/how-to-get-different-colored-lines-for-different-plots-in-a-single-figure#4805456
# plotHandles = []
# labels = []
#
# plotHandles.append(lo_Rs)
# labels.append("lo_Rs")
# plotHandles.append(lo_Gs)
# labels.append("lo_Gs")
#
# plt.legend(plotHandles, labels, 'upper left',ncol=1)
#ref https://stackoverflow.com/questions/21254472/multiple-plot-in-one-figure-in-python
plt.plot(y_pos, lo_Rs, 'r-', label='lo_Rs')
plt.plot(y_pos, lo_Gs, 'g-', label='lo_Gs')
plt.plot(y_pos, lo_Bs, 'b-', label='lo_Bs')
plt.legend(loc='best')
ax = plt.gca()
#ref grid https://stackoverflow.com/questions/16074392/getting-vertical-gridlines-to-appear-in-line-plot-in-matplotlib
ax.grid(which='major', axis='both', linestyle='--')
ax.grid(which='minor', axis='both', linestyle='--')
ax.set(aspect=1,
xlim=(0, len(lo_Rs)),
ylim=(140, 250))
fpath_Save_Image = os.path.join(dpath_Ops_Images, "plot_" + libs.get_TimeLabel_Now() + ".png")
result = plt.savefig(fpath_Save_Image)
print("[%s:%d] save fig => %s (%s)" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, result, fpath_Save_Image
), file=sys.stderr)
plt.show()
#debug
return
'''######################################
prep : window
######################################'''
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
'''###################
get : scaling
###################'''
scaling = test_7__SubImage_RGB_Vals__Get_Scaling(args)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
'''###################
resize image
###################'''
win_Resize_Width, win_Resize_Height = test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
'''###################
disp : image
###################'''
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
'''###################
mouse events
###################'''
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
cv2.imshow('window', img_Sub)
# cv2.imshow('window', img_ForDisp)
cv2.waitKey(0)
cv2.destroyAllWindows()
#/ def test_5():
def test_6__Get_SubImage():
'''###################
message
###################'''
print()
print("[%s:%d] test_6__Get_SubImage =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
#debug
print("[%s:%d] source image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Ops_Image
), file=sys.stderr)
print("[%s:%d] width = %d, height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width, height
), file=sys.stderr)
'''###################
sub image
###################'''
# height, width
img_Sub = img_ForDisp[19:186, 245:246]
# img_Sub = img_ForDisp[246:19, 247:186]
# img_Sub = img_ForDisp[246:19, 246:186]
# meta data
height_Sub, width_Sub, channels_Sub = img_Sub.shape
print("[%s:%d] width_Sub = %d, height_Sub = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width_Sub, height_Sub
), file=sys.stderr)
print()
print("[%s:%d] img_Sub[0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub[0])
print("[%s:%d] img_Sub[0][0] =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(img_Sub[0][0])
'''######################################
prep : window
######################################'''
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
# scaling
scaling = 1.0
#debug
print("[%s:%d] args =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(args)
if len(args) > 0 : #if len(args) > 1
# if len(args) > 1 : #if len(args) > 1
optlist, args = getopt.getopt(args, "s:")
print("[%s:%d] optlist =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(optlist)
for item in optlist:
#debug
print("[%s:%d] item =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(item)
if item[0].startswith("-s") : #if item.startswith("-s")
scaling = float(item[1])
# scaling = item[1]
break
#/if item.startswith("-s")
#/for item in optlist:
else : #if len(args) > 1
print("[%s:%d] len(args) < 2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if len(args) > 1
#debug
print("[%s:%d] scaling => %.3f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, scaling
), file=sys.stderr)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
'''###################
resize image
###################'''
win_Resize_Width, win_Resize_Height = test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
'''###################
disp : image
###################'''
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
'''###################
mouse events
###################'''
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
cv2.imshow('window', img_Sub)
# cv2.imshow('window', img_ForDisp)
cv2.waitKey(0)
cv2.destroyAllWindows()
#/ def test_5():
'''###################
test_5()
<description>
- add listener to ==> image
- hover on the image ==> x, y coordinates displayed in the console
- use ==> argv for window size
<Example>
python 1_1.py -s0.25
<quit program>
return key
###################'''
def test_5__Resize_Image(width, height, scaling, scr_W, scr_H):
q1 = 1.0 * width / height
s = scaling
flg_WorH = ""
# j1
if s >= 0 : #if s >= 0
### j1 : p1
A = 0
if width >= height : #if width > height
flg_WorH = "width"
A = width
else : #if width > height
flg_WorH = "height"
A = height
#/if width > height
### j1 : p2
m = A * s
print("[%s:%d] m => %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, m
), file=sys.stderr)
### j1 : p3
Z = 0
if flg_WorH == "width" : #if flg_WorH == "width"
Z = scr_W
else : #if flg_WorH == "width"
Z = scr_H
#/if flg_WorH == "width"
### j2
result = (m > Z)
'''###################
vars
###################'''
win_Resize_Height = 0
win_Resize_Width = 0
if result == True : #if result == True
print("[%s:%d] m > Z : m = %.03f, Z = %d, flg_WorH = %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, m, Z, flg_WorH
), file=sys.stderr)
### j2 : p1
q2 = 1.0 * Z / A
print("[%s:%d] q2 => %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, q2
), file=sys.stderr)
'''###################
calc
###################'''
win_Resize_Height = math.floor(height * q2)
win_Resize_Width = math.floor(width * q2)
# win_Resize_Height = int(height * q2)
# win_Resize_Width = int(width * q2)
# win_Resize_Height = height * q2
# win_Resize_Width = width * q2
else : #if result == True
print("[%s:%d] m <= Z" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''###################
calc
###################'''
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
#/if result == True
else : #if s >= 0
'''###################
calc
###################'''
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
#/if s >= 0
# '''###################
# calc
# ###################'''
# win_Resize_Height = math.floor(scaling * height)
# win_Resize_Width = math.floor(scaling * width)
'''###################
return
###################'''
#debug
print("[%s:%d] win_Resize_Width = %d, win_Resize_Height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, win_Resize_Width, win_Resize_Height
), file=sys.stderr)
print()
return win_Resize_Width, win_Resize_Height
#/ def test_5():
def test_5():
'''###################
message
###################'''
print()
print("[%s:%d] test_5 =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
#debug
print("[%s:%d] source image => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Ops_Image
), file=sys.stderr)
print("[%s:%d] width = %d, height = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, width, height
), file=sys.stderr)
'''######################################
prep : window
######################################'''
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
# scaling
scaling = 1.0
#debug
print("[%s:%d] args =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(args)
if len(args) > 0 : #if len(args) > 1
# if len(args) > 1 : #if len(args) > 1
optlist, args = getopt.getopt(args, "s:")
print("[%s:%d] optlist =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(optlist)
for item in optlist:
#debug
print("[%s:%d] item =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(item)
if item[0].startswith("-s") : #if item.startswith("-s")
scaling = float(item[1])
# scaling = item[1]
break
#/if item.startswith("-s")
#/for item in optlist:
else : #if len(args) > 1
print("[%s:%d] len(args) < 2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if len(args) > 1
#debug
print("[%s:%d] scaling => %.3f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, scaling
), file=sys.stderr)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
# if win_Resize_Width > scr_W : win_Resize_Width = scr_W
# if win_Resize_Height > scr_H : win_Resize_Width = scr_H
#
# #debug
# print("[%s:%d] win_Resize_Width = %.03f, win_Resize_Height = %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , win_Resize_Width, win_Resize_Height
# ), file=sys.stderr)
# print()
'''###################
resize image
###################'''
win_Resize_Width, win_Resize_Height = test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
# test_5__Resize_Image(width, height, scaling, scr_W, scr_H)
# q1 = 1.0 * width / height
# aa
# s = scaling
#
# flg_WorH = ""
#
# # j1
# if s >= 0 : #if s >= 0
#
# ### j1 : p1
# A = 0
#
# if width >= height : #if width > height
#
# flg_WorH = "width"
#
# A = width
#
# else : #if width > height
#
# flg_WorH = "height"
#
# A = height
#
# #/if width > height
#
# ### j1 : p2
# m = A * s
#
# print("[%s:%d] m => %.03f" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , m
# ), file=sys.stderr)
#
# ### j1 : p3
# Z = 0
#
# if flg_WorH == "width" : #if flg_WorH == "width"
#
# Z = scr_W
#
# else : #if flg_WorH == "width"
#
# Z = scr_H
#
# #/if flg_WorH == "width"
#
# ### j2
# result = (m > Z)
#
# if result == True : #if result == True
#
# print("[%s:%d] m > Z : m = %.03f, Z = %d, flg_WorH = %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , m, Z, flg_WorH
# ), file=sys.stderr)
#
# else : #if result == True
#
# pass
#
# #/if result == True
#
#
#
#
# else : #if s >= 0
#
# pass
#
# #/if s >= 0
#
# #debug
# return
'''###################
disp : image
###################'''
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
'''###################
mouse events
###################'''
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
cv2.imshow('window', img_ForDisp)
cv2.waitKey(0)
cv2.destroyAllWindows()
#/ def test_5():
'''###################
test_4()
<description>
- add listener to ==> image
- hover on the image ==> x, y coordinates displayed in the console
- use ==> argv for window size
<Example>
python 1_1.py -s0.25
<quit program>
return key
###################'''
def test_4():
'''###################
message
###################'''
print()
print("[%s:%d] test_4 =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
get : args
##################
####################'''
args = sys.argv[1:]
# args = sys.argv
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
'''######################################
prep : window
######################################'''
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
# scaling
scaling = 1.0
#debug
print("[%s:%d] args =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(args)
if len(args) > 0 : #if len(args) > 1
# if len(args) > 1 : #if len(args) > 1
optlist, args = getopt.getopt(args, "s:")
print("[%s:%d] optlist =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(optlist)
for item in optlist:
#debug
print("[%s:%d] item =>" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
print(item)
if item[0].startswith("-s") : #if item.startswith("-s")
scaling = float(item[1])
# scaling = item[1]
break
#/if item.startswith("-s")
#/for item in optlist:
else : #if len(args) > 1
print("[%s:%d] len(args) < 2" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/if len(args) > 1
# scaling = 0.5
#debug
print("[%s:%d] scaling => %.3f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, scaling
), file=sys.stderr)
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
# validate
scr_W = GetSystemMetrics(0)
scr_H = GetSystemMetrics(1)
if win_Resize_Width > scr_W : win_Resize_Width = scr_W
if win_Resize_Height > scr_H : win_Resize_Width = scr_H
#debug
print("[%s:%d] win_Resize_Width = %.03f, win_Resize_Height = %.03f" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, win_Resize_Width, win_Resize_Height
), file=sys.stderr)
print()
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
# cv2.resizeWindow(window_1, 600,600)
# cv2.namedWindow(window_1)
# cv2.namedWindow('window')
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
# while True :
#
# cv2.imshow(window_1, img_Orig_RGB)
# # cv2.imshow('window', img_Orig_RGB)
# # cv2.imshow('window', img_Orig)
# # cv2.imshow('window', I)
#
# cv2.waitKey(0)
# cv2.destroyAllWindows()
cv2.imshow('window', img_ForDisp)
# cv2.imshow('window', img_Orig_RGB)
# cv2.imshow('window', img_Orig)
# cv2.imshow('window', I)
cv2.waitKey(0)
cv2.destroyAllWindows()
# '''###################
# message
# ###################'''
# print()
# print("[%s:%d] test_2 =======================" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#/ def test_1():
def test_3():
# '''###################
# get : args
# ###################'''
lib_ip.test__get_Opt_IP()
#ref https://stackoverflow.com/questions/4033723/how-do-i-access-command-line-arguments-in-python answered Oct 27 '10 at 13:27
# args = sys.argv
#
# print()
# print("[%s:%d] args =>" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
# print(args)
#/ def test_3():
'''###################
test_2()
<description>
- add listener to ==> image
- hover on the image ==> x, y coordinates displayed in the console
<quit program>
return key
###################'''
def test_2():
'''###################
message
###################'''
print()
print("[%s:%d] test_2 =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
# "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\ip\data\ops\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
img_ForDisp = img_Orig
# meta data
height, width, channels = img_ForDisp.shape
# height, width, channels = img_Orig.shape
'''######################################
prep : window
######################################'''
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
#ref resize window http://answers.opencv.org/question/84985/resizing-the-output-window-of-imshow-function/
cv2.namedWindow(window_1, cv2.WINDOW_NORMAL)
scaling = 0.5
win_Resize_Height = math.floor(scaling * height)
win_Resize_Width = math.floor(scaling * width)
cv2.resizeWindow(window_1, win_Resize_Width, win_Resize_Height)
# cv2.resizeWindow(window_1, 600,600)
# cv2.namedWindow(window_1)
# cv2.namedWindow('window')
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
# while True :
#
# cv2.imshow(window_1, img_Orig_RGB)
# # cv2.imshow('window', img_Orig_RGB)
# # cv2.imshow('window', img_Orig)
# # cv2.imshow('window', I)
#
# cv2.waitKey(0)
# cv2.destroyAllWindows()
cv2.imshow('window', img_ForDisp)
# cv2.imshow('window', img_Orig_RGB)
# cv2.imshow('window', img_Orig)
# cv2.imshow('window', I)
cv2.waitKey(0)
cv2.destroyAllWindows()
# '''###################
# message
# ###################'''
# print()
# print("[%s:%d] test_2 =======================" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
#/ def test_1():
def test_1():
'''######################################
ops
######################################'''
dpath_Ops_Images = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\VIRTUAL\\Admin_Projects\\ip\\data\\ops\\images"
fname_Ops_Image = "2018-06-24_19-14-31_000.jpg"
fpath_Ops_Image = os.path.join(dpath_Ops_Images, fname_Ops_Image)
'''###################
get : image
###################'''
# read image
img_Orig = cv2.imread(fpath_Ops_Image)
img_Orig_RGB = cv2.cvtColor(img_Orig, cv2.COLOR_BGR2RGB)
# meta data
height, width, channels = img_Orig.shape
'''######################################
show : image
######################################'''
window_1 = "window"
#ref https://qiita.com/supersaiakujin/items/54a4671142d80ca962ec
cv2.namedWindow(window_1)
# cv2.namedWindow('window')
'''###################
mouse events
###################'''
#ref https://www.pyimagesearch.com/2015/03/09/capturing-mouse-click-events-with-python-and-opencv/
cv2.setMouseCallback(window_1, click_and_crop)
# cv2.setMouseCallback("image", click_and_crop)
'''###################
show
###################'''
# while True :
#
# cv2.imshow(window_1, img_Orig_RGB)
# # cv2.imshow('window', img_Orig_RGB)
# # cv2.imshow('window', img_Orig)
# # cv2.imshow('window', I)
#
# cv2.waitKey(0)
# cv2.destroyAllWindows()
cv2.imshow('window', img_Orig_RGB)
# cv2.imshow('window', img_Orig)
# cv2.imshow('window', I)
cv2.waitKey(0)
cv2.destroyAllWindows()
'''###################
message
###################'''
print()
print("[%s:%d] test_1 =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
# test_8__Matplotlib__Bar_Chart()
test_7__SubImage_RGB_Vals()
# test_6__Get_SubImage()
# test_5()
# test_4()
# test_2()
# test_3()
# test_2()
# test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
'''
<usage>
test_1.py [-fXXX] #=> frequency
test_1.py -f402
'''
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/2_image-prog/libs_image_prog/libs (2).py
import inspect, os, sys
#ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
from time import gmtime, strftime, localtime, time
# from enum import Enum
'''#########################################################
enums
#########################################################'''
# class TimeLabel(Enum):
#
# STRING_TYPE_BASIC = "basic"
# STRING_TYPE_SERIAL = "serial"
'''#########################################################
functions
#########################################################'''
def linenum(depth=0):
# print "line"
#ref https://stackoverflow.com/questions/6810999/how-to-determine-file-function-and-line-number 'answered Jul 25 '11 at 1:31'
callerframerecord = inspect.stack()[1] # 0 represents this line
# 1 represents line at caller
frame = callerframerecord[0]
info = inspect.getframeinfo(frame)
# return info.filename # __FILE__ -> Test.py
# print info.filename # __FILE__ -> Test.py
# print info.function # __FUNCTION__ -> Main
# print info.lineno # __LINE__ -> 13
return info.lineno # __LINE__ -> 13
'''
frame = inspect.currentframe(depth+1)
return frame.f_lineno
'''
def thisfile(depth=0):
# def _file(depth=0):
# print "line"
callerframerecord = inspect.stack()[1] # 0 represents this line
# 1 represents line at caller
frame = callerframerecord[0]
info = inspect.getframeinfo(frame)
return info.filename # __FILE__ -> Test.py
# print info.filename # __FILE__ -> Test.py
# print info.function # __FUNCTION__ -> Main
# print info.lineno # __LINE__ -> 13
'''
frame = inspect.currentframe(0)
# frame = inspect.currentframe(depth+1)
return os.path.basename(frame.f_code.co_filename)
# return frame.f_code.co_filename
'''
#/def thisfile(depth=0):
'''
@param string_type
serial "20160604_193404"
basic "2016/06/04 19:34:04"
'''
def get_TimeLabel_Now(string_type="serial", mili=False):
# def get_TimeLabel_Now(string_type="serial"):
t = time()
# str = strftime("%Y%m%d_%H%M%S", t)
# str = strftime("%Y%m%d_%H%M%S", localtime())
'''###################
build string
###################'''
if string_type == "serial" : #if string_type == "serial"
str = strftime("%Y%m%d_%H%M%S", localtime(t))
elif string_type == "basic" : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
else : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
#/if string_type == "serial"
# str = strftime("%Y%m%d_%H%M%S", localtime(t))
#ref https://stackoverflow.com/questions/5998245/get-current-time-in-milliseconds-in-python "answered May 13 '11 at 22:21"
if mili == True :
if string_type == "serial" : #if string_type == "serial"
str = "%s_%03d" % (str, int(t % 1 * 1000))
else : #if string_type == "serial"
str = "%s.%03d" % (str, int(t % 1 * 1000))
#ref decimal value https://stackoverflow.com/questions/30090072/get-decimal-part-of-a-float-number-in-python "answered May 7 '15 at 1:56"
# str = "%s_%03d" % (str, int(t % 1 * 1000))
return str
#ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
# return strftime("%Y%m%d_%H%M%S", localtime())
# return strftime("%Y%m%d_%H%M%S", gmtime())
#]]get_TimeLabel_Now():
'''###################
def is_Open(filepath):
2018/01/31 12:34:10
@problem
- file being opened in Mindmanager app
- returns "True"
###################'''
def is_Open(filepath):
#ref https://stackoverflow.com/questions/37515574/how-to-check-if-a-file-is-already-opened-in-the-same-process answered May 29 '16 at 23:09
if os.path.exists(filepath):
# if os.path.exists(file_name):
try:
os.rename(filepath, filepath + ".tmp") #can't rename an open file so an error will be thrown
# os.rename(filepath, filepath) #can't rename an open file so an error will be thrown
return False
except:
return True
raise NameError
<file_sep>/JVEMV6/46_art/8_kb/start_keyboard_web-pages.bat
REM ==============================
REM browser
REM 2019/09/16 14:18:11
REM ==============================
:web_page_keyboard_opera
REM : opera
pushd "C:\WORKS_2\Programs\opera"
rem : set urls 2020年11月17日18:27:33
rem : log files / 2020年11月17日18:27:39
rem set url_1="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=music+log&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
set url_1="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=session+shino&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem 2021年5月11日18:56:02
set url_1_prep="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=session+shino+用意&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem : session memo : shakuhachi : 2021年2月10日18:04:21
set url_1_2="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=session+shaku&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem : video files : bamboo / 2020年11月17日18:27:39
set url_2="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=video+bamboo&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
rem : video files : flute / 2020年11月17日18:39:02
set url_2="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=video+jap.flute&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
start launcher.exe %url_1% %url_1_prep%
rem start launcher.exe %url_1_2%
rem start launcher.exe %url_2%
REM : log
rem start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=kb+log&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
REM : video
rem start launcher.exe "http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=video+kb&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
REM start launcher.exe "https://docs.zoho.com/sheet/open/ek7i4683eb5df87d04a23acef09182e7df623/sheets/free/ranges/A3"
rem start launcher.exe "https://www.youtube.com/playlist?list=PLZe1Q2NRG8YXa2FCHsE3Ji3VCOg2Ey8LS"
goto exit_web_page_keyboard
:exit_web_page_keyboard
REM ==============================
REM apps : metronome
REM 2019/10/24 15:11:38
REM ==============================
REM : metronome
rem pushd "C:\WORKS_2\Programs\opera"
rem : comment out 2020年10月11日18:13:24
rem start launcher.exe https://www.flutetunes.com/metronome/
REM pushd "C:\WORKS_2\Programs\opera"
REM : metronome
REM start launcher.exe "https://www.metronomeonline.com"
REM start launcher.exe "https://www.imusic-school.com/en/tools/online-metronome/"
REM : keyboard
rem : c/o : 2021年2月24日17:37:56
rem pushd "C:\WORKS_2\Programs\opera"
rem start launcher.exe https://pianoplays.com
rem start launcher.exe https://metronom.us/en/
rem start launcher.exe https://www.musicca.com/jp/metronome
REM start launcher.exe https://www.onlinepianist.com/virtual-piano
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/lib/swgSpringMassTree.js
/*-----------------------------------------------------------------
1次元バネ質点モデルで樹木を表現
フラクタルで3次元樹木形成
バネ質点表示
-------------------------------------------------------------------*/
function TreeSM()
{
var numPoint = 10;
var numPointG, numSpringG;//孫の最初の質点番号とバネ番号
var numPointL, numSpringL;//先端の葉の最初の質点番号とバネ番号
this.vPos = new Vector3();//根の位置
//this.vPos0 = new Vector3();//根の位置
this.vForce0 = new Vector3();//外力の初期値
//バネ-質点
this.spring = [];
this.point = [];
this.constK = [];//[MAX_SPRING];
this.drctnK = [];//[MAX_SPRING + 1];
this.mass = 1;//質点1個当たりの質量
this.radius = 0.1;//質点の半径
this.constK0 = 1000;
this.drctnK0 = 1000;
this.damping = 0;
this.drag = 0;
this.variation = 0.5;//変動率
//幹(trunk)
this.numTrunk = 4;
this.lengthTrunk0 = 1.0;//幹の最下端の長さ
this.radiusTrunk0 = 0.1;
this.rate = 0.8;
//枝
this.numBranch0 = 4;//1か所の幹分岐点で分岐する枝個数
this.numBranch = 4; //枝から分かれる枝個数
///this.numGeneration = //2;//枝の世代数
this.lengthBranch0 = 0.6;
this.radiusBranch0 = 0.05;
this.alpha = 30 * DEG_TO_RAD;//分岐角
this.beta = 30 * DEG_TO_RAD;//水平面との角度
//表示
this.dispType = "SMM";
}
//CTreeSM sm;
//-----------------------------------------------------------------
TreeSM.prototype.draw = function()
{
var i, r1, r2;
//var vEuler0, vEuler1;
//console.log("numPoint = " + this.numPoint + " numSpring = " + this.numSpring);
if(this.dispType == "SMM")//ばね質点表示
{
//質点
var n = this.point[0].initVertexBuffers(gl);
for(i = 0; i < this.numPoint; i++)
{
if(this.point[i].flagFixed == true){
this.point[i].diffuse = [0.3, 0.2, 0.2, 1.0];
this.point[i].ambient = [0.2, 0.1, 0.1, 1.0];
}
else
{
this.point[i].diffuse = [0.8, 0.9, 0.2, 1.0];
this.point[i].ambient = [0.1, 0.5, 0.1, 1.0];
}
//console.log("AAAA i = " + i + " x = " + this.point[i].vPos.x + " y = " + this.point[i].vPos.y + " z = " + this.point[i].vPos.z);
this.point[i].shadow = this.shadow;
//this.point[i].flagDebug = true;
this.point[i].draw(gl, n);
}
//バネ
n = this.spring[0].initVertexBuffers(gl);
for(i = 0; i < this.numSpring ; i++)
{
r1 = this.spring[i].row1;
r2 = this.spring[i].row2;
this.spring[i].vPos = div(add(this.point[r1].vPos, this.point[r2].vPos), 2);
var len = distance(this.point[r1].vPos, this.point[r2].vPos);
this.spring[i].vSize = new Vector3(this.spring[i].radius, this.spring[i].radius, len);
this.spring[i].vEuler = getEulerZ(this.point[r1].vPos, this.point[r2].vPos);
this.spring[i].diffuse = [0.5, 0.5, 0.3, 1.0];
this.spring[i].ambient = [0.4, 0.4, 0.2, 1.0];
this.spring[i].diffuse = [0.2, 0.2, 0.2, 1.0];
this.spring[i].shadow = this.shadow;
//this.spring[i].flagDebug = true;
this.spring[i].draw(gl, n);
}
}
else if(this.dispType == "MIX" || this.dispType == "LP")
{
if(this.dispType == "MIX")
{
n = this.spring[0].initVertexBuffers(gl);
for(i = 0; i < this.numTrunk ; i++)
{
r1 = this.spring[i].row1;
r2 = this.spring[i].row2;
this.spring[i].vPos = div(add(this.point[r1].vPos, this.point[r2].vPos), 2);
var len = distance(this.point[r1].vPos, this.point[r2].vPos);
this.spring[i].vSize = new Vector3(this.spring[i].radius, this.spring[i].radius, len);
this.spring[i].vEuler = getEulerZ(this.point[r1].vPos, this.point[r2].vPos);
this.spring[i].diffuse = [0.5, 0.5, 0.3, 1.0];
this.spring[i].ambient = [0.4, 0.4, 0.2, 1.0];
this.spring[i].diffuse = [0.2, 0.2, 0.2, 1.0];
this.spring[i].shadow = this.shadow;
//this.spring[i].flagDebug = true;
this.spring[i].draw(gl, n);
}
}
//console.log("AAA num = " + this.numSpring );
//console.log("AAAAAAAAAAAAAAAAAAAAAAAA");
dummy = new Rigid();
dummy.kind = "CHECK_PLATE";
dummy.flagCheck = true;
dummy.nSlice = 35;
dummy.nStack = 35;
var n = dummy.initVertexBuffers(gl);
var flagLineLoc = gl.getUniformLocation(gl.program, 'u_flagLine');
//幹、枝だけ表示
gl.uniform1i(flagLineLoc, true);
n = initVertexLines(this, gl);
gl.drawArrays(gl.LINES, 0, n);
gl.uniform1i(flagLineLoc, false);
//質点を点で表示
var flagPointLoc = gl.getUniformLocation(gl.program, 'u_flagPoint');
//幹、枝だけ表示
gl.uniform1i(flagPointLoc, true);
n = initVertexPoints(this, gl);
gl.drawArrays(gl.POINTS, 0, n);
gl.uniform1i(flagPointLoc, false);
}
}
//--------------------------------------------------------------
function initVertexLines(sm, gl)
{
//幹・枝を線で描画するときの座標を作成
var vertices = [];
//for(i = sm.numTrunk; i < sm.numSpring ; i++)
for(i = 0; i < sm.numSpring ; i++)
{
r1 = sm.spring[i].row1;
r2 = sm.spring[i].row2;
vertices.push(sm.point[r1].vPos.x); vertices.push(sm.point[r1].vPos.y); vertices.push(sm.point[r1].vPos.z);
vertices.push(sm.point[r2].vPos.x); vertices.push(sm.point[r2].vPos.y); vertices.push(sm.point[r2].vPos.z);
}
//alert(" len = " + vertices.length);
//for(var i = 0; i < 5; i++) console.log(" i = " + i + " x = " + vertices[i][ 0] + " y = " + vertices[i][ 1] + " z = " + vertices[i][2]);
// バッファオブジェクトを作成する
var vertexBuffer = gl.createBuffer();
// バッファオブジェクトをバインドにする
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
// バッファオブジェクトにデータを書き込む
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices), gl.STATIC_DRAW);
var vertexLoc = gl.getAttribLocation(gl.program, 'a_vertex');
// a_Position変数にバッファオブジェクトを割り当てる
gl.vertexAttribPointer(vertexLoc, 3, gl.FLOAT, false, 0, 0);
// a_verte変数でのバッファオブジェクトの割り当てを有効化する
gl.enableVertexAttribArray(vertexLoc);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
return vertices.length/3;
}
//--------------------------------------------------------------
function initVertexPoints(sm, gl)
{
//幹・枝を線で描画するときの座標を作成
var vertices = [];
for(i = 0; i < sm.numPoint; i++)
{
vertices.push(sm.point[i].vPos.x); vertices.push(sm.point[i].vPos.y); vertices.push(sm.point[i].vPos.z);
}
//alert(" len = " + vertices.length);
//for(var i = 0; i < 5; i++) console.log(" i = " + i + " x = " + vertices[i][ 0] + " y = " + vertices[i][ 1] + " z = " + vertices[i][2]);
// バッファオブジェクトを作成する
var vertexBuffer = gl.createBuffer();
// バッファオブジェクトをバインドにする
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
// バッファオブジェクトにデータを書き込む
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices), gl.STATIC_DRAW);
var vertexLoc = gl.getAttribLocation(gl.program, 'a_vertex');
// a_Position変数にバッファオブジェクトを割り当てる
gl.vertexAttribPointer(vertexLoc, 3, gl.FLOAT, false, 0, 0);
// a_verte変数でのバッファオブジェクトの割り当てを有効化する
gl.enableVertexAttribArray(vertexLoc);
// バッファオブジェクトのバインドを解除する
gl.bindBuffer(gl.ARRAY_BUFFER, null);
return vertices.length/3;
}
//-----------------------------------------------------------------
TreeSM.prototype.create = function()
{
var i, j, k, m, n0, n1;
//alert(" numTrunk = " + this.numTrunk);
var va = this.variation;//変動率
this.numPoint = 0;
this.numSpring = 0;
var len = this.lengthTrunk0;
var rad = this.radiusTrunk0;
//幹
for(i = 0; i <= this.numTrunk; i++)
{
this.point[i] = new Rigid();
this.point[i].vSize = new Vector3(rad, rad, rad);
this.point[i].vPos0.x = this.point[i].vPos.x = this.vPos.x + getRandom(-va, va) * 0.1;
this.point[i].vPos0.y = this.point[i].vPos.y = this.vPos.y + getRandom(-va, va) * 0.1;
//console.log("i = " + i + " x = " + this.point[i].vPos.x);
if(i == 0)
this.point[i].vPos0.z = this.point[i].vPos.z = 0.0;
else
this.point[i].vPos0.z = this.point[i].vPos.z = this.point[i-1].vPos.z + len * (1.0 + getRandom(-va, va));
this.numPoint++;
if(i >= 1){
len *= this.rate;
rad *= this.rate;
}
}
//バネに接続する質点番号とバネの方向
rad = this.radiusTrunk0 * 0.8;
var cck = this.constK0;
var hhk = this.drctnK0;
for(i = 0; i < this.numTrunk; i++)
{
this.spring[i] = new Rigid();
this.spring[i].radius = rad;
this.spring[i].row1 = i;
this.spring[i].row2 = i+1;
this.spring[i].length0 = distance(this.point[i].vPos0, this.point[i+1].vPos0);
this.spring[i].vDir0 = direction(this.point[i].vPos0, this.point[i+1].vPos0);
this.constK[i] = cck;
this.drctnK[i] = hhk * 5.0;
rad *= this.rate;
cck *= this.rate;
hhk *= this.rate;
this.numSpring ++;
}
//親枝
len = this.lengthBranch0;
rad = this.radiusBranch0;
//this.beta = Math.PI / 6.0;//水平面との角度(30度)
var gamma = 2.0 * Math.PI / this.numBranch0;//取り付け位置(x軸からの経度)
for(i = 1; i <= this.numTrunk; i++)
{
for(j = 0; j < this.numBranch0; j++)
{
this.point[this.numPoint] = new Rigid();
this.point[this.numPoint].vSize = new Vector3(2.0*rad, 2.0*rad, 2.0*rad);
this.point[this.numPoint].vPos0.x = this.point[this.numPoint].vPos.x = this.point[i].vPos.x + len * Math.cos(this.beta) * Math.cos(gamma * j) * (1.0 + getRandom(-va, va));
//console.log("i = " + i + " j = " + j + " numPoint = " + this.numPoint + " x = " + this.point[this.numPoint].vPos.x);
this.point[this.numPoint].vPos0.y = this.point[this.numPoint].vPos.y = this.point[i].vPos.y + len * Math.cos(this.beta) * Math.sin(gamma * j) * (1.0 + getRandom(-va, va));
this.point[this.numPoint].vPos0.z = this.point[this.numPoint].vPos.z = this.point[i].vPos.z + len * Math.sin(this.beta) * (1.0 + getRandom(-va, va));
this.numPoint ++;
}
len *= this.rate;
rad *= this.rate;
}
//バネに接続する質点番号と方向
rad = this.radiusBranch0 * 0.8;
cck = this.constK0 * 0.8;
hhk = this.drctnK0 * 0.5;
for(i = 1; i <= this.numTrunk; i++)
{
for(j = 0; j < this.numBranch0; j++)
{
n0 = this.numTrunk+1 + (i-1)*this.numBranch0 + j;//親枝質点番号
this.spring[this.numSpring] = new Rigid();
this.spring[this.numSpring].kind = "CYLINDER";
this.spring[this.numSpring].radius = rad;
this.spring[this.numSpring].row1 = i;
this.spring[this.numSpring].row2 = n0;
this.spring[this.numSpring].length0 = distance(this.point[i].vPos0, this.point[n0].vPos0);
this.spring[this.numSpring].vDir0 = direction(this.point[i].vPos0, this.point[n0].vPos0);
this.constK[this.numSpring] = cck;
this.drctnK[this.numSpring] = hhk;
this.numSpring ++;
}
rad *= this.rate;
cck *= this.rate * 0.5;
hhk *= this.rate * 0.5;
}
//子枝
len = this.lengthBranch0 * this.rate;
rad = this.radiusBranch0 * this.rate;
this.beta *= this.rate;//M_PI / 10.0;//水平面との角度
gamma = 2.0 * Math.PI / this.numBranch0;//取り付け位置(x軸からの経度)
var delta = 2*this.alpha / (this.numBranch-1);
var theta;
//this.alpha = Math.PI / 3.0;//小枝の拡がり
for(i = 1; i <= this.numTrunk; i++)
{
for(j = 0; j < this.numBranch0; j++)
{
for(k = 0; k < this.numBranch; k++)
{
theta = gamma * j + k * delta - this.alpha;
n0 = this.numTrunk+1 + (i-1)*this.numBranch0 + j;//親枝質点番号
//console.log("numPoint = " + this.numPoint + " n0 = " + n0);
this.point[this.numPoint] = new Rigid();
this.point[this.numPoint].kind = "SPHERE";
this.point[this.numPoint].vSize = new Vector3(2.0*rad, 2.0*rad, 2.0*rad);
this.point[this.numPoint].vPos0.x = this.point[this.numPoint].vPos.x = this.point[n0].vPos.x + len * Math.cos(this.beta) * Math.cos(theta) * (1.0 + getRandom(-va, va));
this.point[this.numPoint].vPos0.y = this.point[this.numPoint].vPos.y = this.point[n0].vPos.y + len * Math.cos(this.beta) * Math.sin(theta) * (1.0 + getRandom(-va, va));
this.point[this.numPoint].vPos0.z = this.point[this.numPoint].vPos.z = this.point[n0].vPos.z + len * Math.sin(this.beta) * (1.0 + getRandom(-va, va));
this.numPoint ++;
}
}
len *= this.rate;
rad *= this.rate;
}
//バネに接続する質点番号と方向
rad = this.radiusBranch0 * this.rate;
cck = this.constK0 * 0.5;
hhk = this.drctnK0 * 0.1;
n1 = this.numTrunk + 1 + this.numTrunk * this.numBranch0;//子枝の最初の質点番号
for(i = 1; i <= this.numTrunk; i++)
{
for(j = 0; j < this.numBranch0; j++)//親枝番号
{
for(k = 0; k < this.numBranch; k++)//子枝番号
{
n0 = this.numTrunk + 1 + (i-1)*this.numBranch0 + j;//親枝質点番号
//n1 = n0 + numTrunk * numBranch0 + j * numBranch0 * numBranch - j + j * numBranch + k;//子枝質点番号
this.spring[this.numSpring] = new Rigid();
this.spring[this.numSpring].kind = "CYLINDER";
this.spring[this.numSpring].radius = rad;
this.spring[this.numSpring].row1 = n0;
this.spring[this.numSpring].row2 = n1;
this.spring[this.numSpring].length0 = distance(this.point[n0].vPos0, this.point[n1].vPos0);
this.spring[this.numSpring].vDir0 = direction(this.point[n0].vPos0, this.point[n1].vPos0);
this.constK[this.numSpring] = cck;
this.drctnK[this.numSpring] = hhk;
this.numSpring ++;
n1++;
}
}
rad *= this.rate;
cck *= this.rate * 0.5;
hhk *= this.rate * 0.5;
}
this.numPointG = this.numPoint;//最初の孫の質点番号
this.numSpringG = this.numSpring;//最初の孫のバネ番号
//孫枝
len = this.lengthBranch0 * this.rate * this.rate;
rad = this.radiusBranch0 * this.rate;
this.beta *= this.rate;
gamma = 2.0 * Math.PI / this.numBranch0;//取り付け位置(x軸からの経度)
//this.alpha = M_PI / 6.0;//小枝の拡がり
n0 = this.numTrunk + 1 + this.numTrunk*this.numBranch0;//最初の子枝質点番号
for(i = 1; i <= this.numTrunk; i++)
{
for(j = 0; j < this.numBranch0; j++)
{
for(k = 0; k < this.numBranch; k++)
{
for(m = 0; m < this.numBranch; m++)
{
theta = gamma * j + (k+m) * delta - 2*this.alpha
this.point[this.numPoint] = new Rigid();
this.point[this.numPoint].kind = "SPHERE";
this.point[this.numPoint].vSize = new Vector3(2.0*rad, 2.0*rad, 2.0*rad);
this.point[this.numPoint].vPos0.x = this.point[this.numPoint].vPos.x = this.point[n0].vPos0.x + len * Math.cos(this.beta) * Math.cos(theta) * (1.0 + getRandom(-va, va));
this.point[this.numPoint].vPos0.y = this.point[this.numPoint].vPos.y = this.point[n0].vPos0.y + len * Math.cos(this.beta) * Math.sin(theta) * (1.0 + getRandom(-va, va));
this.point[this.numPoint].vPos0.z = this.point[this.numPoint].vPos.z = this.point[n0].vPos0.z + len * Math.sin(this.beta) * (1.0 + getRandom(-va, va));
this.numPoint ++;
}
n0++;
}
}
len *= this.rate;
rad *= this.rate;
}
//バネに接続する質点番号と方向
rad = this.radiusBranch0 * this.rate * this.rate;
cck = this.constK0 * 0.2;
hhk = this.drctnK0 * 0.001;
n0 = this.numTrunk + 1 + this.numTrunk*this.numBranch0;//最初の子枝質点番号
n1 = this.numTrunk + 1 + this.numTrunk * this.numBranch0 * (1 + this.numBranch);//最初の孫枝質点番号
for(i = 1; i <= this.numTrunk; i++)
{
for(j = 0; j < this.numBranch0; j++)//親枝番号
{
for(k = 0; k < this.numBranch; k++)//子枝番号
{
for(m = 0; m < this.numBranch; m++)
{
this.spring[this.numSpring] = new Rigid();
this.spring[this.numSpring].kind = "CYLINDER";
this.spring[this.numSpring].radius = rad;
this.spring[this.numSpring].row1 = n0;
this.spring[this.numSpring].row2 = n1;
this.spring[this.numSpring].length0 = distance(this.point[n0].vPos0, this.point[n1].vPos0);
this.spring[this.numSpring].vDir0 = direction(this.point[n0].vPos0, this.point[n1].vPos0);
this.constK[this.numSpring] = cck;
this.drctnK[this.numSpring] = hhk;
this.numSpring ++;
n1++;
}
n0++;
}
}
rad *= this.rate;
cck *= this.rate;
hhk *= this.rate;
}
this.numPointL = this.numPoint;//最初の葉の質点番号
this.numSpringL = this.numSpring;//最初の葉のバネ番号
/*
//孫枝の先端の質点を延長し葉の質点とする
len = 0.2;
rad = this.radiusBranch0 * this.rate * this.rate;
for(i = this.numPointG; i < this.numPointL; i++)
{
this.point[this.numPoint] = new Rigid();
this.point[this.numPoint].kind = "SPHERE";
this.point[this.numPoint].vSize = new Vector3(2.0*rad, 2.0*rad, 2.0*rad) ;
this.point[this.numPoint].vPos0.x = this.point[this.numPoint].vPos.x = this.point[i].vPos0.x + len * this.spring[i - 1].vDir0.x;
this.point[this.numPoint].vPos0.y = this.point[this.numPoint].vPos.y = this.point[i].vPos0.y + len * this.spring[i - 1].vDir0.y;
this.point[this.numPoint].vPos0.z = this.point[this.numPoint].vPos.z = this.point[i].vPos0.z + len * this.spring[i - 1].vDir0.z;
this.numPoint ++;
}
n0 = this.numPointG;
n1 = this.numPointL;
for(i = this.numSpringG; i < this.numSpringL; i++)
{
this.spring[this.numSpring] = new Rigid();
this.spring[this.numSpring].kind = "CYLINDER";
this.spring[this.numSpring].radius = rad;
this.spring[this.numSpring].row1 = n0;
this.spring[this.numSpring].row2 = n1;
this.spring[this.numSpring].length0 = distance(this.point[n0].vPos0, this.point[n1].vPos0);
this.spring[this.numSpring].vDir0 = direction(this.point[n0].vPos0, this.point[n1].vPos0);
this.constK[this.numSpring] = cck;
this.drctnK[this.numSpring] = hhk * 0.05;
this.numSpring++;
n0++;
n1++;
}*/
for(i = 0; i < this.numPoint; i++){
this.point[i].kind = "SPHERE";//"PLATE_Z";//
this.point[i].nSlice = 6;
this.point[i].nStack = 6;
}
for(i = 0; i < this.numSpring; i++){
this.spring[i].kind = "CYLINDER";
this.spring[i].radiusRatio = 0.9;
this.spring[i].nSlice = 6;
}
}
//-----------------------------------------------------------------------------
TreeSM.prototype.motion = function(dt, t)
{
var i, r1, r2;
var dampingF, angle;
var vDir1, vDir2, vFF;
var vRelativeVelocity;
var vNormal;
//力の総和
//初期設定値(風などの外力)
for(i = 0; i < this.numPoint; i++)
{
/*/ var wind = windAmp * Math.sin(2.0 * Math.PI * windFreq * (t - this.point[i].vPos.y / windVelY));
this.vForce0.x = windVelY * wind ;
this.vForce0.y = windAmp * windVelY * (1.0 + wind);
this.vForce0.z = windVelY * wind * 0.1;this.mass
*/
this.point[i].vForce = add(this.point[i].vForce0, new Vector3(0.0, 0.0, -gravity * this.mass));
}
//バネによる力
for(i = 0; i < this.numSpring; i++)
{
//弾性力
r1 = this.spring[i].row1;
r2 = this.spring[i].row2;
vDir1 = direction(this.point[r1].vPos, this.point[r2].vPos);//#1から#2への単位ベクトル
this.spring[i].length = distance(this.point[r1].vPos, this.point[r2].vPos);
vFF = mul(this.constK[i] * (this.spring[i].length - this.spring[i].length0) , vDir1) ;
this.point[r1].vForce.add(vFF) ;//vDirと同方向
this.point[r2].vForce.sub(vFF) ;//反対方向
//減衰力
vRelativeVelocity = sub(this.point[r1].vVel , this.point[r2].vVel);
dampingF = this.damping * dot(vRelativeVelocity, vDir1);
this.point[r1].vForce.sub(mul(dampingF , vDir1));//相対速度とは反対方向
this.point[r2].vForce.add(mul(dampingF , vDir1));//同方向
// }
//方向バネを考慮
vNormal = cross(this.spring[i].vDir0, vDir1);
vDir2 = cross(vNormal, vDir1);
angle = getAngle_rad(this.spring[i].vDir0, vDir1);
vFF = mul(this.drctnK[i] * angle / this.spring[i].length, vDir2);
//console.log(" x = " + vFF.x + " y = " + vFF.y + " z = " + vFF.z);
this.point[r2].vForce.sub(vFF) ;
this.point[r1].vForce.add(vFF);//vDir2と同方向
}
//粘性抵抗と床面処理
for(i = 1; i < this.numPoint; i++)
{
if(this.point[i].flagFixed) continue; //固定
//空気粘性抵抗(全ての質点に一様と仮定)
this.point[i].vForce.sub(mul(this.drag, this.point[i].vVel));
//床面処理
if(this.point[i].vPos.z < this.radius)
{
//床面にある質点に対してはすべり摩擦を与える
//this.point[i].vForce.sub(mul((muK * this.mass * gravity), this.point[i].vVel.norm2()));
//床面上に制限
this.point[i].vPos.z = this.radius;
//床との衝突
//if(this.point[i].vVel.z < 0.0){ //質点と床面とは弾性衝突とする
//this.point[i].vVel.z = - restitut * this.point[i].vVel.z ;
}
//Euler法
//加速度
this.point[i].vAcc = div(this.point[i].vForce , this.mass);
//速度
this.point[i].vVel.add(mul(this.point[i].vAcc, dt));
//位置
//if(i == 1)
//this.point[i].vPos.z += this.point[i].vVel.z * dt;
//else
this.point[i].vPos.add(mul(this.point[i].vVel, dt));
}
//this.vPos.copy(this.point[0].vPos);
for(i = 0; i < this.numPoint; i++){
this.point[i].vPos0.add(sub(this.point[0].vPos, this.point[0].vPos0));
}
//this.point[0].vPos0.copy(this.vPos);
//this.vPos0.copy(this.vPos);
}
<file_sep>/JVEMV6/46_art/VIRTUAL/end_env.bat
@echo off
REM pushd C:\WORKS_2\WS\WS_Others\prog\D-34\5_\VIRTUAL
deactivate
pause
<file_sep>/JVEMV6/46_art/VIRTUAL/upload_image_files.bat
@echo off
REM pushd C:\WORKS_2\WS\WS_Others\prog\D-7\2_2\VIRTUAL\Admin_Projects
pushd C:\WORKS_2\Programs\FileZilla FTP Client
start filezilla
C:\WORKS_2\WS\FM_2_20171104_225946\Projects\images_Projects
/cake_apps/images/mindmaps
<file_sep>/JVEMV6/46_art/13_compositions/end_13_compositions.bat
@echo off
rem set msg="%1 %2 %3 %4 %5"
set msg=%1 %2 %3 %4 %5
echo.
echo msg is %msg%
echo.
C:\WORKS_2\a.bat && r a && git add -A && git commit -m "(res : JVE_46# : compositions) periodical updates / %msg%" && e && p
rem echo "C:\WORKS_2\a.bat && r a && git add -A && git commit -m ^"(res : JVE_46# : compositions) periodical updates / %msg%^" && e && p"
rem echo %msg%
rem pause
rem C:\WORKS_2\a.bat && r a && git add -A && git commit -m ^"(res : JVE_46# : compositions) periodical updates / %msg%^" && e && p"
rem C:\WORKS_2\a.bat && r a && git add -A && git commit -m ^"(res : JVE_46# : compositions) periodical updates / %msg%^" && e && p
pause
<file_sep>/JVEMV6/46_art/2_image-prog/1_gimp/4_/plugin_2_1_4.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others\JVEMV6\46_art\2_image-prog\1_gimp\4_\plugin_2_1_4.py
at : 2018/04/06 14:34:49
plugin at : C:\WORKS_2\Programs\GIMP 2\plugins
'''
from gimpfu import *
from time import time, localtime, strftime
import inspect, os
from fileinput import lineno
# from enum import Enum
import sys, random
# sys.path.append("C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\2_image-prog")
# sys.path.append("C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\2_image-prog\\libs_image_prog")
# sys.path.append('..')
#
# from libs_image_prog import cons_image
########################################################################
# class CONS(Enum):
#
# str_Fpath_Debug = "debug!!"
def write_Log(msg):
dpath = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\4_"
# dpath = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\4_"
fname = "debug.log"
fpath = "%s\\%s" % (dpath, fname)
f = open(fpath, "a")
f.write("===============================\n")
f.write(msg)
f.write("\n\n")
f.close()
#/ def write_Log(msg):
def thisfile(depth=0):
# def _file(depth=0):
# print "line"
callerframerecord = inspect.stack()[1] # 0 represents this line
# 1 represents line at caller
frame = callerframerecord[0]
info = inspect.getframeinfo(frame)
return info.filename # __FILE__ -> Test.py
# print info.filename # __FILE__ -> Test.py
# print info.function # __FUNCTION__ -> Main
# print info.lineno # __LINE__ -> 13
'''
frame = inspect.currentframe(0)
# frame = inspect.currentframe(depth+1)
return os.path.basename(frame.f_code.co_filename)
# return frame.f_code.co_filename
'''
#/def thisfile(depth=0):
def linenum(depth=0):
# print "line"
#ref https://stackoverflow.com/questions/6810999/how-to-determine-file-function-and-line-number 'answered Jul 25 '11 at 1:31'
callerframerecord = inspect.stack()[1] # 0 represents this line
# 1 represents line at caller
frame = callerframerecord[0]
info = inspect.getframeinfo(frame)
# return info.filename # __FILE__ -> Test.py
# print info.filename # __FILE__ -> Test.py
# print info.function # __FUNCTION__ -> Main
# print info.lineno # __LINE__ -> 13
return info.lineno # __LINE__ -> 13
'''
frame = inspect.currentframe(depth+1)
return frame.f_lineno
'''
'''
@param string_type
serial "20160604_193404"
basic "2016/06/04 19:34:04"
'''
def get_TimeLabel_Now(string_type="serial", mili=False):
# def get_TimeLabel_Now(string_type="serial"):
t = time()
# str = strftime("%Y%m%d_%H%M%S", t)
# str = strftime("%Y%m%d_%H%M%S", localtime())
'''###################
build string
###################'''
if string_type == "serial" : #if string_type == "serial"
str = strftime("%Y%m%d_%H%M%S", localtime(t))
elif string_type == "basic" : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
else : #if string_type == "serial"
str = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
#/if string_type == "serial"
# str = strftime("%Y%m%d_%H%M%S", localtime(t))
#ref https://stackoverflow.com/questions/5998245/get-current-time-in-milliseconds-in-python "answered May 13 '11 at 22:21"
if mili == True :
if string_type == "serial" : #if string_type == "serial"
str = "%s_%03d" % (str, int(t % 1 * 1000))
else : #if string_type == "serial"
str = "%s.%03d" % (str, int(t % 1 * 1000))
#ref decimal value https://stackoverflow.com/questions/30090072/get-decimal-part-of-a-float-number-in-python "answered May 7 '15 at 1:56"
# str = "%s_%03d" % (str, int(t % 1 * 1000))
return str
#ref https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python "answered Jan 6 '09 at 4:59"
# return strftime("%Y%m%d_%H%M%S", localtime())
# return strftime("%Y%m%d_%H%M%S", gmtime())
#]]get_TimeLabel_Now():
class ImageObject :
image = None
layer = None
def __init__( self, image ):
self.image = image
return
def get_Image(self):
return self.image
def add_Layer(self, layer_Name):
#debug
msg = "[%s / %s:%d]\nadd_Layer => starting..." \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
width = self.image.width
height = self.image.height
type = RGB_IMAGE
opacity = 100
mode = NORMAL_MODE
#debug
msg = "[%s / %s:%d]\ngimp.Layer() ---> calling..." \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
self.layer = gimp.Layer(self.image, layer_Name, width, height, type, opacity, mode)
# self.layer = gimp.Layer(image, name, width, height, type, opacity, mode)
#debug
msg = "[%s / %s:%d]\ngimp.Layer() ---> done" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
self.layer.fill(1)
#
# 画像データの 0 番目の位置にレイヤーを挿入する
position = 0
self.image.add_layer(self.layer, position)
# self.image.add_layer(layer, position)
# return
return
#/def add_Layer(self, layer_Name):
def get_Layer(self):
if self.layer == None :
pdb.gimp_message("layer ==> None !!!")
return False
# return layer
return self.layer
def create_image(width, height):
# 画像データを生成
return gimp.Image(width, height, RGB)
def display_image(image):
gimp.Display(image)
def add_layer(image, name):
# レイヤーの作成に必要なパラメータ
width = image.width
height = image.height
type = RGB_IMAGE
opacity = 100
mode = NORMAL_MODE
#
# パラメータをもとにレイヤーを作成
layer = gimp.Layer(image, name, width, height, type, opacity, mode)
#
# レイヤーを背景色で塗りつぶす(GIMP のデフォルトの挙動に合わせています)
layer.fill(1)
#
# 画像データの 0 番目の位置にレイヤーを挿入する
position = 0
image.add_layer(layer, position)
#
return layer
def draw_pencil_lines(drawable, lines):
# ペンシルツールで線を描画する
pdb.gimp_pencil(drawable, len(lines), lines)
def draw_rect(drawable, x1, y1, x2, y2):
lines = [x1, y1, x2, y1, x2, y2, x1, y2, x1, y1]
draw_pencil_lines(drawable, lines)
def test_8__Multiple_Copies(timg, tdrawable, numOf_NewLayers = 1): #add layer to the current image ---> multiple layers
layer = timg.active_layer
name_Orig = layer.name
# conver to int
numOf_NewLayers = int(numOf_NewLayers)
# undo start
pdb.gimp_image_undo_group_start(timg)
# copy layer
for i in range(numOf_NewLayers):
#ref http://gimpforums.com/thread-python-get-number-of-layers
new_Layer = layer.copy()
new_Layer.name = "%s.copy-%02d" % (name_Orig, i + 1)
# new_Layer.name = "%s-%02d" % (name_Orig, i)
timg.add_layer(new_Layer, 0)
# undo end
pdb.gimp_image_undo_group_end(timg)
#/for i in range(numOf_NewLayers):
#/ def def test_8__Multiple_Copies(timg, tdrawable, numOf_NewLayers = 1): #add layer to the current image ---> multiple layers
def test_7__DrawCircle(timg, tdrawable, numOf_NewLayers = 1):
'''###################
select ellipse
###################'''
#ref https://developer.gimp.org/api/2.0/libgimp/libgimp-gimpimageselect.html#gimp-image-select-ellipse
#ref CHANNEL_OP_REPLACE https://stackoverflow.com/questions/39641231/gimp-python-fu-how-do-i-select-a-polygon#39654251
pdb.gimp_image_select_ellipse(timg, CHANNEL_OP_REPLACE, 100, 100, 50, 50)
'''###################
border
###################'''
border_thickness = 5
#ref border https://superuser.com/questions/829495/how-can-i-automate-some-simple-steps-within-the-gimp
pdb.gimp_selection_border(timg, border_thickness)
'''###################
fill
###################'''
# fill the selected area
#refhttps://stackoverflow.com/questions/49888598/gimp-python-fu-create-simple-border
pdb.gimp_edit_fill(pdb.gimp_image_get_active_drawable(timg), 2)
# pdb.gimp_image_select_ellipse(img, gimpfu.CHANNEL_OP_REPLACE,
# x, y, RADIO*2, RADIO*2)
# def test_7__DrawCircle(timg, tdrawable, numOf_NewLayers = 1):
'''###################
test_6
copy the active layer
###################'''
def test_6(timg, tdrawable, numOf_NewLayers = 1): #add layer to the current image ---> multiple layers
layer = timg.active_layer
name_Orig = layer.name
#ref http://gimpforums.com/thread-python-get-number-of-layers
new_Layer = layer.copy()
new_Layer.name = "%s-%02d" % (name_Orig, numOf_NewLayers)
timg.add_layer(new_Layer, 0)
# image = create_image(640, 400)
# gimp.message("numOf_NewLayers => %f" % (numOf_NewLayers))
#
# for i in range(int(numOf_NewLayers)) :
# # for i in range(numOf_NewLayers) :
#
# name_Layer = "L-%02d" % (i + 1)
# # name_Layer = "L-1"
#
# # image instance
# imageObj = ImageObject( timg )
# # imageObj = ImageObject( image )
#
# im = imageObj.get_Image()
#
# msg = "[%s / %s:%d]\nimageObj ==> instance made" \
# % (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# # % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# # (str_Time, num_Line, num_Line_EndStack)
# write_Log(msg)
#
# msg = "[%s / %s:%d]\ncalling ---> im.add_Layer()" \
# % (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# # % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# # (str_Time, num_Line, num_Line_EndStack)
# write_Log(msg)
#
#
# imageObj.add_Layer(name_Layer)
# # im.add_Layer(name_Layer)
#
# msg = "[%s / %s:%d]\nimageObj ==> Layer added" \
# % (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# # % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# # (str_Time, num_Line, num_Line_EndStack)
# write_Log(msg)
#
# '''###################
# background
# ###################'''
# #ref http://coderazzi.net/python/gimp/pythonfu.html
# cols_Mix = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
#
# pdb.gimp_context_set_background(cols_Mix)
# # pdb.gimp_context_set_background((0,0,0))
#
# # get drawable
# drw = pdb.gimp_image_active_drawable(im)
#
# msg = "[%s / %s:%d]\ndrawable ==> obtained" \
# % (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# # % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# # (str_Time, num_Line, num_Line_EndStack)
# write_Log(msg)
#
# # fill bg
# pdb.gimp_drawable_fill(drw, 1)
# # pdb.gimp_drawable_fill(drw, gimpfu.BACKGROUND_FILL)
# # The type of fill { FOREGROUND-FILL (0), BACKGROUND-FILL (1), WHITE-FILL (2), TRANSPARENT-FILL (3), PATTERN-FILL (4), NO-FILL (5) }
#
# msg = "[%s / %s:%d]\nbg ==> filled" \
# % (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# # % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# # (str_Time, num_Line, num_Line_EndStack)
# write_Log(msg)
def test_5(timg, tdrawable, numOf_NewLayers = 1): #add layer to the current image ---> multiple layers
# image = create_image(640, 400)
gimp.message("numOf_NewLayers => %f" % (numOf_NewLayers))
for i in range(int(numOf_NewLayers)) :
# for i in range(numOf_NewLayers) :
name_Layer = "L-%02d" % (i + 1)
# name_Layer = "L-1"
# image instance
imageObj = ImageObject( timg )
# imageObj = ImageObject( image )
im = imageObj.get_Image()
msg = "[%s / %s:%d]\nimageObj ==> instance made" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
msg = "[%s / %s:%d]\ncalling ---> im.add_Layer()" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
imageObj.add_Layer(name_Layer)
# im.add_Layer(name_Layer)
msg = "[%s / %s:%d]\nimageObj ==> Layer added" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
'''###################
background
###################'''
#ref http://coderazzi.net/python/gimp/pythonfu.html
cols_Mix = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
pdb.gimp_context_set_background(cols_Mix)
# pdb.gimp_context_set_background((0,0,0))
# get drawable
drw = pdb.gimp_image_active_drawable(im)
msg = "[%s / %s:%d]\ndrawable ==> obtained" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
# fill bg
pdb.gimp_drawable_fill(drw, 1)
# pdb.gimp_drawable_fill(drw, gimpfu.BACKGROUND_FILL)
# The type of fill { FOREGROUND-FILL (0), BACKGROUND-FILL (1), WHITE-FILL (2), TRANSPARENT-FILL (3), PATTERN-FILL (4), NO-FILL (5) }
msg = "[%s / %s:%d]\nbg ==> filled" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
#
# #debug
# pdb.gimp_message("layer => added")
#
# layer = im.get_Layer()
#
# draw_rect(layer, 190, 210, 490, 310)
# # draw_rect(layer, 390, 210, 490, 310)
# display_image(im)
# display_image(image)
def test_4(timg, tdrawable): #add layer to the current image ]] 20180504_122012
# image = create_image(640, 400)
name_Layer = "L-1"
# image instance
imageObj = ImageObject( timg )
# imageObj = ImageObject( image )
im = imageObj.get_Image()
msg = "[%s / %s:%d]\nimageObj ==> instance made" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
msg = "[%s / %s:%d]\ncalling ---> im.add_Layer()" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
imageObj.add_Layer(name_Layer)
# im.add_Layer(name_Layer)
msg = "[%s / %s:%d]\nimageObj ==> Layer added" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
'''###################
background
###################'''
#ref http://coderazzi.net/python/gimp/pythonfu.html
cols_Mix = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
pdb.gimp_context_set_background(cols_Mix)
# pdb.gimp_context_set_background((0,0,0))
# get drawable
drw = pdb.gimp_image_active_drawable(im)
msg = "[%s / %s:%d]\ndrawable ==> obtained" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
# fill bg
pdb.gimp_drawable_fill(drw, 1)
# pdb.gimp_drawable_fill(drw, gimpfu.BACKGROUND_FILL)
# The type of fill { FOREGROUND-FILL (0), BACKGROUND-FILL (1), WHITE-FILL (2), TRANSPARENT-FILL (3), PATTERN-FILL (4), NO-FILL (5) }
msg = "[%s / %s:%d]\nbg ==> filled" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
#
# #debug
# pdb.gimp_message("layer => added")
#
# layer = im.get_Layer()
#
# draw_rect(layer, 190, 210, 490, 310)
# # draw_rect(layer, 390, 210, 490, 310)
# display_image(im)
# display_image(image)
def test_3(timg, tdrawable):
image = create_image(640, 400)
name_Layer = "L-1"
# image instance
imageObj = ImageObject( image )
im = imageObj.get_Image()
msg = "[%s / %s:%d]\nimageObj ==> instance made" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
msg = "[%s / %s:%d]\ncalling ---> im.add_Layer()" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
imageObj.add_Layer(name_Layer)
# im.add_Layer(name_Layer)
msg = "[%s / %s:%d]\nimageObj ==> Layer added" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
'''###################
background
###################'''
#ref http://coderazzi.net/python/gimp/pythonfu.html
pdb.gimp_context_set_background((0,0,0))
# get drawable
drw = pdb.gimp_image_active_drawable(im)
msg = "[%s / %s:%d]\ndrawable ==> obtained" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
# fill bg
pdb.gimp_drawable_fill(drw, 1)
# pdb.gimp_drawable_fill(drw, gimpfu.BACKGROUND_FILL)
# The type of fill { FOREGROUND-FILL (0), BACKGROUND-FILL (1), WHITE-FILL (2), TRANSPARENT-FILL (3), PATTERN-FILL (4), NO-FILL (5) }
msg = "[%s / %s:%d]\nbg ==> filled" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
#
# #debug
# pdb.gimp_message("layer => added")
#
# layer = im.get_Layer()
#
# draw_rect(layer, 190, 210, 490, 310)
# # draw_rect(layer, 390, 210, 490, 310)
display_image(im)
# display_image(image)
def test_2(timg, tdrawable):
image = create_image(640, 400)
name_Layer = "L-1"
# image instance
imageObj = ImageObject( image )
im = imageObj.get_Image()
# #debug
# t = time()
# str_Time = strftime("%Y/%m/%d %H:%M:%S", localtime(t))
# num_Line = linenum(0)
#
# lenOf_Stacks = len(inspect.stack())
#
# num_Line_EndStack = linenum(lenOf_Stacks - 1)
#
# # ref https://stackoverflow.com/questions/6810999/how-to-determine-file-function-and-line-number 'answered Jul 25 '11 at 1:31'
# callerframerecord = inspect.stack()[1] # 0 represents this line
# # 1 represents line at caller
#
# lenOf_Stacks = len(inspect.stack())
#
# frame = inspect.currentframe(1)
# num_Line_Current = frame.f_lineno
# msg = "[%s / %d] imageObj ==> instance made (lenOf_Stacks = %d)" % \
# msg = "[%s / %d] imageObj ==> instance made (num_Line_EndStack = %d / num_Line_Current = %d)" \
msg = "[%s / %s:%d]\nimageObj ==> instance made" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
msg = "[%s / %s:%d]\ncalling ---> im.add_Layer()" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
imageObj.add_Layer(name_Layer)
# im.add_Layer(name_Layer)
msg = "[%s / %s:%d]\nimageObj ==> Layer added" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
# % (str_Time, num_Line, num_Line_EndStack, num_Line_Current)
# (str_Time, num_Line, num_Line_EndStack)
write_Log(msg)
#
# #debug
# pdb.gimp_message("layer => added")
#
# layer = im.get_Layer()
#
# draw_rect(layer, 190, 210, 490, 310)
# # draw_rect(layer, 390, 210, 490, 310)
display_image(im)
# display_image(image)
def test_1(timg, tdrawable):
image = create_image(640, 400)
name_Layer = "L-1"
layer = add_layer(image, name_Layer)
# layer = add_layer(image, "背景")
draw_rect(layer, 390, 210, 490, 310)
display_image(image)
# def show_Message(image, layer, message):
#ref https://jacksonbates.wordpress.com/2015/09/14/python-fu-5-automating-workflows-coding-a-complete-plug-in/
def extreme_unsharp_desaturation(image, drawable):
pdb.gimp_image_undo_group_start(image)
radius = 5.0
amount = 5.0
threshold = 0
pdb.plug_in_unsharp_mask(image, drawable, radius, amount, threshold)
pdb.gimp_desaturate_full(drawable, DESATURATE_LIGHTNESS)
pdb.gimp_image_undo_group_end(image)
def extreme_unsharp_desaturation_options(image, drawable, radius, amount, mode):
pdb.gimp_image_undo_group_start(image)
threshold = 0
pdb.plug_in_unsharp_mask(image, drawable, radius, amount, threshold)
pdb.gimp_desaturate_full(drawable, mode)
pdb.gimp_image_undo_group_end(image)
def circles(image, drawable):
tlabel = get_TimeLabel_Now()
gimp.message("circles() : %s" % (tlabel))
img=pdb.gimp_image_new(SIZE, SIZE, gimpfu.RGB)
#add layer with 100% of opacity
layer=pdb.gimp_layer_new(img, SIZE, SIZE, gimpfu.RGB_IMAGE, "base", 100, gimpfu.NORMAL_MODE)
pdb.gimp_image_add_layer(img, layer, 0)
#we need it with alpha channel
pdb.gimp_layer_add_alpha(layer)
#access its drawable
drw = pdb.gimp_image_active_drawable(img)
#set background to black, and foreground to white
pdb.gimp_context_set_background((0,0,0))
pdb.gimp_context_set_foreground((255, 255, 255))
#fill the background - black
pdb.gimp_drawable_fill(drw, gimpfu.BACKGROUND_FILL)
#to set the brush, check first for available brushes using pdb.gimp_brushes_get_list("")
#Exanples of brush with width 3 is '1. Pixel', and with width 1, 'Pixel (1x1 square)'
#set brush to simple pixel (width: 1)
pdb.gimp_context_set_brush('Circle (01)')
#draw a square around the image
ctrlPoints = [RADIO, RADIO, SIZE-RADIO, RADIO, SIZE-RADIO,
SIZE-RADIO, RADIO, SIZE-RADIO, RADIO, RADIO]
pdb.gimp_paintbrush_default(drw,len(ctrlPoints),ctrlPoints)
#now we draw 9 transparent circles (3 rows x 3 columns)
#a transparent circle means -with an alpha layer-, to select the area and cut it
for x in (0, SIZE/2-RADIO, SIZE-2*RADIO):
for y in (0, SIZE/2-RADIO, SIZE-2*RADIO):
#next call was available on 2.6, not on 2.8
#pdb.gimp_image_select_ellipse(img, gimpfu.CHANNEL_OP_REPLACE,
# x, y, RADIO*2, RADIO*2)
pdb.gimp_ellipse_select(img, x, y, RADIO*2, RADIO*2,
gimpfu.CHANNEL_OP_REPLACE, True, False, 0)
pdb.gimp_edit_cut(drw)
#remove any selection
pdb.gimp_selection_none(img)
#and display the image
display=pdb.gimp_display_new(img)
#/ def circles(image, drawable):
def lazy_Resize(timg, tdrawable, max_width, max_height):
# print ("max width: %s\nmax height: %s" % (max_width, max_height))
gimp.message("max width: %s\nmax height: %s" % (max_width, max_height))
width = tdrawable.width
height = tdrawable.height
if max_width <= 0:
# Assume width is okay as it is
max_width = width
if max_height <= 0:
# Assume height is okay
max_height= height
if width <= max_width and height <= max_height:
gimp.message("Nothing to do, returning")
# print ("Nothing to do, returning")
return
image_aspect = float(width) / float(height)
boundary_aspect = float(max_width) / float(max_height)
if image_aspect > boundary_aspect:
# Width is the limiting factor:
new_width = max_width
new_height= int(round( new_width/image_aspect ))
else:
# Height is the limiting factor:
new_height = max_height
new_width = int(round( image_aspect*new_height ))
gimp.message("Resizing %s:%s to %s:%s" % (width, height, new_width, new_height))
# print ("Resizing %s:%s to %s:%s" % (width, height, new_width, new_height))
# At present, documnatation does not specify the interpolation--
# another tutorial claimed it was cubic:
pdb.gimp_image_scale(timg, new_width, new_height)
#/ def lazy_Resize(timg, tdrawable, max_width, max_height):
def show_Message(timg, tdrawable):
tlabel = get_TimeLabel_Now()
#
msg = "[%s / %s:%d]\nshow_Message()" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
write_Log(msg)
'''###################
ops
###################'''
width = tdrawable.width
msg = "[%s / %s:%d]\nwidth => %d" \
% (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0)
, width
)
write_Log(msg)
'''###################
message
###################'''
gimp.message("Hello, World!: %s" % (tlabel))
# # gimp.message("Hello, World!: %s (%s)" % (tlabel, CONS.str_Fpath_Debug.value))
# # gimp.message("Hello, World!: %s (%s)" % (tlabel, cons_image.FPath.str_Test.value))
# # gimp.message("Hello, World!: %s (%s)" % (tlabel, cons_image.FPath.dpath_Gimp_Debug.value))
# # gimp.message("Hello, World: " + message)
#
# '''###################
# write to file
# ###################'''
# dpath = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\4_"
# # dpath = "C:\\WORKS_2\\WS\\WS_Others\\JVEMV6\\46_art\\2_image-prog\\1_gimp\\4_"
#
# fname = "debug.%s.log" % tlabel
#
# fpath = "%s\\%s" % (dpath, fname)
#
# f = open(fpath, "a")
#
# f.write("===============================\n")
#
# msg = "[%s / %s:%d]\ntest show_Message" \
# % (get_TimeLabel_Now(), os.path.basename(thisfile()), linenum(0))
#
# f.write(msg)
# # f.write(msg)
#
# f.write("\n\n")
#
# f.close()
def plugin_main(timg, tdrawable, numOf_NewLayers):
# def plugin_main(timg, tdrawable):
test_6(timg, tdrawable, numOf_NewLayers)
# test_5(timg, tdrawable, numOf_NewLayers)
# test_4(timg, tdrawable)
# test_3(timg, tdrawable)
# test_2(timg, tdrawable)
########################################################################
def test_group_2(timg, tdrawable, numOf_NewLayers):
# def plugin_main(timg, tdrawable):
test_8__Multiple_Copies(timg, tdrawable, numOf_NewLayers)
# test_7__DrawCircle(timg, tdrawable)
#/ def test_group_2(timg, tdrawable, numOf_NewLayers):
########################################################################
register(
'test_group_2', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'test_group_2', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
, (PF_SPINNER, 'numOf_NewLayers', 'num of new layers (max 15)', 1, (1, 15, 1))
], # プロシジャの引数
[], # 戻り値の定義
test_group_2, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
register(
'plugin_main', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'new image', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
, (PF_SPINNER, 'numOf_NewLayers', 'num of new layers', 1, (1, 3, 1))
], # プロシジャの引数
[], # 戻り値の定義
plugin_main, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
register(
'show_Message', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'show_Message', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
], # プロシジャの引数
[], # 戻り値の定義
show_Message, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
register(
'extreme_unsharp_desaturation', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'extreme_unsharp_desaturation', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
], # プロシジャの引数
[], # 戻り値の定義
extreme_unsharp_desaturation, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
register(
# "python-fu-extreme-unsharp-desaturation-options",
# "Unsharp mask and desaurate image, with options",
# "Run an unsharp mask with variables set by user",
# "<NAME>", "<NAME>", "2015",
# "Extreme unsharp and desaturate options...",
# "RGB",
'extreme_unsharp_desaturation_options', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'extreme_unsharp_desaturation_options', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, "image", "takes current image", None),
(PF_DRAWABLE, "drawable", "Input layer", None),
(PF_SLIDER, "radius", "Radius", 5, (0, 500, 0.5)),
# note extra tuple (min, max, step)
(PF_SLIDER, "amount", "Amount", 5.0, (0, 10, 0.1)),
(PF_RADIO, "mode", "Set Desauration mode: ", DESATURATE_LIGHTNESS,
(
("Lightness", DESATURATE_LIGHTNESS),
( "Luminosity", DESATURATE_LUMINOSITY),
("Average", DESATURATE_AVERAGE)
)
)
],
[],
extreme_unsharp_desaturation_options, menu="<Image>/Layer/user_libs")
register(
'circles', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'circles', # メニューアイテム
'*', # 対応する画像タイプ
[
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None)
], # プロシジャの引数
[], # 戻り値の定義
circles, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
register(
'lazy_Resize', # プロシジャの名前
'転写スクリプト。アクティブなレイヤーの内容を、下にあるレイヤーに転写する。',
# プロシジャの説明文
'ver 2.8 以上を対象とした転写スクリプト。転写元のレイヤーから転写先のレイヤーへ内容を転写する。レイヤーグループ内での動作や、レイヤーマスクの保持が行われる。',
# PDBに登録する追加情報
'かんら・から', # 作者名
'GPLv3', # ライセンス情報
'2012.12.15', # 作成日
'lazy_Resize', # メニューアイテム
'*', # 対応する画像タイプ
[
# 引数 (type, name, description, default [, extra])
(PF_IMAGE, 'image', 'Input image', None),
(PF_DRAWABLE, 'drawable', 'Input drawable', None),
(PF_INT, "max_width", "Maximum new width", 200),
(PF_INT, "max_height", "Maximum new height", 200)
# (PF_INT, "max_width", "Maximum new width", 1280),
# (PF_INT, "max_height", "Maximum new height", 900)
], # プロシジャの引数
[], # 戻り値の定義
lazy_Resize, # 処理を埋け持つ関数名
menu='<Image>/Layer/user_libs' # メニュー表示場所
)
# register(
# "python_fu_FUNCTION_NAME",
# "blurb: 推薦文",
# "help: もう少し詳しい説明",
# "author",
# "copyright",
# "2014/9/20",
# "<Image>/Image/ABC", # メニュー項目
# "*", # imagetypes: "RGB*, GRAY*" など
# [ # 引数 (type, name, description, default [, extra])
# (PF_STRING, "string", "Text string", 'Hello, world!')
# ],
# [], # 戻り値
# show_Message) # 関数名
# # plugin_main) # 関数名
main() # プラグインを駆動させるための関数
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/anims/utils/open_anims_log_dir.bat
start C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\VIRTUAL\Admin_Projects\anims\data
<file_sep>/JVEMV6/46_art/8_kb/compos/end_[compos].bat
@echo on
taskkill /f /im javaw.exe
taskkill /f /im sakura.exe
taskkill /f /im vlc.exe
C:\WORKS_2\a.bat && r a && git add . && git commit -m "( jve_[46#13].[theme=compositions] ) periodical updates" && e && p
<file_sep>/JVEMV6/46_art/8_kb/domino/memos/2018-10/2018-10.ms/storage/main.(1.14~).php
<!--
http://localhost/WS_Others/free/UH8G6E_CE/2_1/main.php
C:\WORKS_2\WS\WS_Others\free\
C:\WORKS_2\WS\WS_Others\free\UH8G6E_CE\
/WS/WS_Others/free/UH8G6E_CE/
-->
<?php
require 'setup.php';
// require_once 'setup.php';
/*******************************
url
*******************************/
@$query_Url = $_GET['url'];
// echo "\$query_Url => '" . $query_Url . "'";
if ($query_Url == null || $query_Url == '') {
$query_Url = 'http://benfranklin.chips.jp/cake_apps/images/ifm11/2014-08-12_12-17-13_686.jpg';
}//if ($query_Url == null || $query_Url == '')
?>
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title><?php echo $session; ?> (<?php echo $version; ?>)</title>
<!-- <title>rect select</title> -->
<script type="text/javascript" src="https://code.jquery.com/jquery-3.2.1.min.js">
</script>
<script type="text/javascript" src="https://code.jquery.com/ui/1.12.1/jquery-ui.min.js">
</script>
<link rel="stylesheet" type="text/css" href="<?php echo $fpath_CSS; ?>" />
<script type="text/javascript" src="<?php echo $fpath_JS; ?>">
</script>
</head>
<body>
<div id="div_message">
<?php
// echo "\$fpath_CSS : " . $fpath_CSS;
// echo "<br>";
// echo "\$fpath_JS_2" . $fpath_JS_2;
?>
</div><!-- <div id="div_message"> -->
<div class="main">
<!-- <canvas id="drowing" class="drowing" width="300px" height="300px"></canvas> -->
<!-- <canvas id="drowing" class="drowing" width="50%" height="0"></canvas> -->
<canvas id="drowing" class="drowing" width="0" height="0"></canvas>
</div>
<div>
<input type="text"
id="ipt_Image_File_URL"
value="<?php echo $query_Url; ?>"
>
<button id="btn_Image_File_URL"
onclick="get_Image();"
>
Go
</button>
<span id="dflt_Image_Url" hidden><?php echo $query_Url; ?></span>
</div>
<div>
<div>zキーで最新の矩形を削除</div>
</div>
<hr>
<?php
require_once 'partials/main_controls.php';
?>
<hr>
<br>
<br>
<?php
require_once 'partials/link_to_session_root.php';
?>
</body>
</html>
<file_sep>/JVEMV6/46_art/VIRTUAL/Admin_Projects/anims/urls.py
from django.conf.urls import url
from anims import views
# from ip import views
urlpatterns = [
url(r'^$', views.index, name='ip_index'),
# url(r'^$', views.index),
url(r'^index/$', views.index, name='ip_index'),
# 20181010_084804
url(r'^anims/$', views.anims, name='anims'),
# 20181010_120542
url(r'^anims_JS/$', views.anims_JS, name='anims_JS'),
# 20181013_071822
url(r'^open_dir/$', views.open_dir, name='open_dir'),
]
<file_sep>/JVEMV6/46_art/start_music-composition.bat
@echo on
rem ===============================
rem folders
rem vlc
rem browser
rem musescore
rem log file
rem ===============================
rem ===============================
rem folders
rem 2020年8月9日22:47:15
rem ===============================
set dir_1=C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\musescore\02_compos
set dir_2=C:\WORKS_2\WS\WS_Cake_IFM11\movies_WS_Cake_IFM11\2019
start %dir_1% && start %dir_2%
rem start /d %dir_1% /d %dir_2%
rem start /d %dir_1% /d %dir_2%
rem start dir_2
rem ===============================
rem vlc
rem 2020年8月13日13:32:54
rem ===============================
start C:\Users\iwabuchiken\VLC\vlc.exe
rem ===============================
rem browser
rem 2020年8月15日23:54:37
rem ===============================
pushd C:\WORKS_2\Programs\opera
set url_1="http://benfranklin.chips.jp/cake_apps/Cake_IFM11/images/index_2?filter_memo=&sort=file_name&direction=desc&RBs_AND_OR_Memo=AND"
launcher.exe %url_1%
rem ===============================
rem musescore
rem 2020年8月8日23:36:46
rem ===============================
start C:\WORKS_2\Programs\musescore\ms_3.2.3.7635\bin\MuseScore3.exe
rem start C:\WORKS_2\Programs\musescore\musescore_2.3.2\bin\MuseScore.exe
rem ===============================
rem log file
rem 2020年8月13日13:29:50
rem ===============================
C:\WORKS_2\Programs\sakura\sakura.exe "C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\8_kb\musescore\02_compos\log_[compos_musescore].txt"
pause
<file_sep>/JVEMV6/46_art/6_visual-arts/4_animations/7_simulation/New流れ&波program/第4章/cylinderPsiOmega.js
/*----------------------------------------------
cylinderPsiOmega.js
極座標変換による円柱周りの流れ
流れ関数-渦度法
-----------------------------------------------*/
var canvas; //キャンバス要素
var gl;//WebGL描画用コンテキスト
var height_per_width;//キャンバスのサイズ比
//animation
var fps ; //フレームレート
var lastTime;
var elapseTime = 0.0;//全経過時間
var elapseTime0 = 0.0;
var elapseTime1 = 0.0;
var elapseTimeN = 0.0;//無次元時間
var elapseTimeN0 = 0.0;
var flagStart = false;
var flagStep = false;
var flagReset = false;
//数値計算
var Psi = []; //流れ関数
var Omg = [];//渦度
var gx = []; //x方向微分
var gy = []; //y方向微分
var VelX = []; //x方向速度
var VelY = []; //y方向速度
var PosX = []; //格子点位置
var PosY = [];
var type = [];//格子点のタイプ
var scale = new Vector3(1.0, 1.0, 0.0);//スケール調整
var flagStream = true;
var flagVorticity = true;
var flagVelocity = false;
var flagGrid = false;
var nLine = 30;//流線,ポテンシャルの表示本数
var arrowScale = 0.05;
var arrowWidth = 1;
var intervalV = 2;//速度矢印表示間隔
var deltaT = 0.02;
var Re = 500.0;//レイノルズ数
var maxPsi = 8.0;
var minPsi = -8.0;
var maxOmg = 5.0;
var minOmg = -6.0;
function Region()
{
this.scale = 1.7;//表示倍率
this.pos0 = new Vector3(-0.2, 0, 0);
this.Radius = 30;//領域全体の半径
this.nMeshX = 30;//動径方向分割数
this.nMeshY = 60;//動径方向分割数
}
var region = new Region();
var NX, NY, DX, DY;
function webMain()
{
//canvas要素を取得する
canvas = document.getElementById('WebGL');
// WebGL描画用のコンテキストを取得する
gl = WebGLUtils.setupWebGL(canvas);
if (!gl)
{
alert('WebGLコンテキストの取得に失敗');
return;
}
var VS_SOURCE = document.getElementById("vs").textContent;
var FS_SOURCE = document.getElementById("fs").textContent;
if(!initGlsl(gl, VS_SOURCE, FS_SOURCE))
{
alert("GLSLの初期化に失敗");
return;
}
//canvasをクリアする色を設定する
gl.clearColor(1, 1, 1, 1);
form1.deltaT.value = deltaT;
form1.Re.value = Re;
form1.nMeshX.value = region.nMeshX;
form1.nMeshY.value = region.nMeshY;
form2.x0.value = region.pos0.x;
form2.scale.value = region.scale;
form2.nLine.value = nLine;
form2.psi.checked = flagStream;
form2.omega.checked = flagVorticity;
form2.velocity.checked = flagVelocity;
form2.maxPsi.value = maxPsi;
form2.minPsi.value = minPsi;
form2.maxOmg.value = maxOmg;
form2.minOmg.value = minOmg;
initData();
display();
var animate = function()
{
//繰り返し呼び出す関数を登録
requestAnimationFrame(animate, canvas); //webgl-utilsで定義
//時間計測
var currentTime = new Date().getTime();
var frameTime = (currentTime - lastTime) / 1000.0;//時間刻み[sec]
elapseTime1 += frameTime;
fps ++;
if(elapseTime1 >= 0.5)
{
form1.fps.value = 2*fps.toString(); //1秒間隔で表示
var timestep = 1 / (2*fps);
form1.step.value = timestep.toString();
fps = 0;
elapseTime1 = 0.0;
}
lastTime = currentTime;
if(flagStart)
{
elapseTime += frameTime;
elapseTimeN += deltaT;//数値計算上の経過時間(無次元時間)
calculate();
elapseTime0 = elapseTime;//現在の経過時間を保存
elapseTimeN0 = elapseTimeN;//現在の経過時間を保存
form1.e_time.value = elapseTime.toString();
form1.n_time.value = elapseTimeN.toString();
if(flagStep) { flagStart = false; }
}
display();
}
animate();
}
function initData()
{
deltaT = parseFloat(form1.deltaT.value);
Re = parseFloat(form1.Re.value);
NX = region.nMeshX = parseInt(form1.nMeshX.value);//動径方向分割数
NY = region.nMeshY = parseInt(form1.nMeshY.value);//方位方向角分割数
for(i = 0; i <= NX; i++)
{//配列の2次元化
Psi[i] = []; //流れ関数
Omg[i] = [];//渦度
gx[i] = []; //x方向微分
gy[i] = []; //y方向微分
VelX[i] = []; //x方向速度
VelY[i] = []; //y方向速度
PosX[i] = [];
PosY[i] = [];
}
//円柱の半径は1に固定
var xiMax = Math.log(region.Radius);//計算面における外側半径(円柱半径は1.0に固定)
DX = xiMax / NX;//動径方向刻み
DY = 2.0 * Math.PI / NY; //方位角方向刻み
var i, j;
//初期条件
for(j = 0; j <= NY; j++)
for (i = 0; i <= NX; i++)
{
//流れ関数
if(i == 0) Psi[i][j] = 0;
else
Psi[i][j] = Math.exp(i * DX) * Math.sin(j * DY - Math.PI);//分岐線を負のx軸としている
//渦度
Omg[i][j] = 0.0;
gx[i][j] = 0.0;
gy[i][j] = 0.0;
}
//格子点の速度ベクトル(上下左右の流れ関数の差で求める)
calcVelocity();
//格子点座標(あらかじめ計算)
var r, theta;
for (i = 0; i <= NX; i++)
{
r = Math.exp(i * DX);
for (j = 0; j <= NY; j++)
{
theta = j * DY - Math.PI; //分岐線は左側
PosX[i][j] = r * Math.cos(theta);//実空間
PosY[i][j] = r * Math.sin(theta);
}
}
calcVelocity();
maxPsi0 = -1000.0; minPsi0 = 1000.0;
maxOmg0 = -1000.0; minOmg0 = 1000.0;
//発散しないための目安を知るため
var courant, diffNum;
if(DX < DY)
{
courant = 1.0 * deltaT / DX;
diffNum = (1.0 / Re) * deltaT / (DX * DX);//拡散数
}
else
{
courant = 1.0 * deltaT / DY;
diffNum = (1.0 / Re) * deltaT / (DX * DY);//拡散数
}
form1.courant.value = courant;
form1.diffNum.value = diffNum;
}
function display()
{
flagStream = form2.psi.checked;
flagVorticity = form2.omega.checked;
flagVelocity = form2.velocity.checked;
flagGrid = form2.grid.checked;
region.pos0.x = parseFloat(form2.x0.value);
//canvasをクリアする
gl.clear(gl.COLOR_BUFFER_BIT);
gl.viewport(0, 0, canvas.width, canvas.height);
height_per_width = canvas.height / canvas.width;//縦横の比率を一定にするための係数
//計算領域パラメータ
var s1, s2;
if(canvas.width >= canvas.height)
{
s1 = height_per_width;
s2 = 1.0;
}
else
{
s1 = 1.0;
s2 = 1.0 / height_per_width;
}
scale.x = 2.0 * region.scale * s1 / region.Radius * 0.8;// 100.0;//R=100が1程度になるように全体表示
scale.y = 2.0 * region.scale * s2 / region.Radius * 0.8;//100.0;
var x0 = region.pos0.x;
var y0 = region.pos0.y;
//円柱断面表示
drawCircle(x0, y0, 2*scale.x, 2*scale.y, true, "gray", 0)
//流線、等渦度線、圧力表示
maxPsi = parseFloat(form2.maxPsi.value);
minPsi = parseFloat(form2.minPsi.value);
maxOmg = parseFloat(form2.maxOmg.value);
minOmg = parseFloat(form2.minOmg.value);
if( flagStream ) drawContour(Psi, minPsi, maxPsi, "red");
if( flagVorticity ) drawContour(Omg, minOmg, maxOmg, "blue");
if( flagVelocity ) drawVelocity();
if( flagGrid ) drawGrid();
}
function calculate()
{
var i, j;
var iteration = 10;//最大繰り返し回数(Poisson方程式)
var tolerance = 0.0001;//許容誤差
var error = 0.0;
var maxError = 0.0;
var i, j;
var pp, ex2;
var DX2 = DX * DX;
var DY2 = DY * DY;
var DXi2 = 1.0 / DX2;
var DYi2 = 1.0 / DY2;
var fct = 1.0 / (2.0 * DX2 + 2.0 * DY2);
//境界条件
for (j = 0; j < NY; j++)
{
//円柱上
Omg[0][j] = -2.0 * Psi[1][j] * DXi2;
//外側境界
Omg[NX][j] = Omg[NX-1][j];//Neumann
}
//Poissonの方程式を解く
var jm, jp;
var cnt = 0;
while (cnt < iteration)
{
maxError = 0.0;
for (i = 1; i < NX; i++)
{
ex2 = Math.exp(2 * i * DX);
for(j = 0; j < NY; j++)
{
jm = j - 1;
if(jm == -1) jm = NY-1; //else jm = j - 1;
jp = j + 1;
if(jp == NY) jp = 0; //else jp = j + 1;
pp = ( (Psi[i - 1][j] + Psi[i + 1][j] ) * DY2
+ (Psi[i][jm] + Psi[i][jp]) * DX2
+ Omg[i][j] * ex2 * DX2 * DY2 ) * fct ;
error = Math.abs(pp - Psi[i][j]);
if (error > maxError) maxError = error;
Psi[i][j] = pp;//更新
}
}
if (maxError < tolerance) break;
cnt++;
}
calcVelocity();
//渦度輸送方程式を解く
methodCIP();
//流れ関数,渦度の最小値,最大値
for(i = 1; i < NX; i++)
for (j = 1; j < NY; j++)
{
if(Psi[i][j] > maxPsi0) maxPsi0 = Psi[i][j];
if(Psi[i][j] < minPsi0) minPsi0 = Psi[i][j];
if(Omg[i][j] > maxOmg0) maxOmg0 = Omg[i][j];
if(Omg[i][j] < minOmg0) minOmg0 = Omg[i][j];
}
//console.log("maxPsi= " + maxPsi0 + " minPsi = " + minPsi0);
//console.log("maxOmg= " + maxOmg0 + " minOmg = " + minOmg0);
}
function calcVelocity()
{
//速度ベクトルの計算
//格子点の速度ベクトル(上下左右の流れ関数の差で求める)
var ex;
for(i = 1; i < NX; i++)
{
ex = Math.exp(- i * DX);
for (j = 0; j < NY; j++)
{
if(j == 0) jm = NY -1; else jm = j - 1;
if(j == NY-1) jp = 0; else jp = j + 1;
//ポテンシャルの低い方から高い方へ
VelX[i][j] = ex * (Psi[i][jp] - Psi[i][jm]) / (DY * 2.0);//動径方向
VelY[i][j] = ex * (Psi[i-1][j] - Psi[i+1][j]) / (DX * 2.0);//方位角方向
}
}
}
function methodCIP()
{
var newOmg = [];//新渦度
var newGx = [];//x方向微分
var newGy = [];//y方向微分
var c11, c12, c21, c02, c30, c20, c03, a, b, sx, sy, x, y, dx, dx2, dx3, dy, dy2, dy3;
var i, j, ip, jp;
for(i = 0; i <= NX; i++)
{
newOmg[i] = [];
newGx[i] = [];
newGy[i] = [];
}
for(i = 1; i < NX; i++)
{
ex = Math.exp(-i * DX);
ex2 = ex * ex;
for(j = 1; j < NY; j++)
{
if(VelX[i][j] >= 0.0) sx = 1.0; else sx = -1.0;
if(VelY[i][j] >= 0.0) sy = 1.0; else sy = -1.0;
x = - VelX[i][j] * deltaT;
y = - VelY[i][j] * deltaT;
ip = i - sx;//上流点
jp = j - sy;
dx = sx * DX;
dy = sy * DY;
dx2 = dx * dx;
dx3 = dx2 * dx;
dy2 = dy * dy;
dy3 = dy2 * dy;
c30 = ((gx[ip][j] + gx[i][j]) * dx - 2.0 * (Omg[i][j] - Omg[ip][j])) / dx3;
c20 = (3.0 * (Omg[ip][j] - Omg[i][j]) + (gx[ip][j] + 2.0 * gx[i][j]) * dx) / dx2;
c03 = ((gy[i][jp] + gy[i][j]) * dy - 2.0 * (Omg[i][j] - Omg[i][jp])) / dy3;
c02 = (3.0 * (Omg[i][jp] - Omg[i][j]) + (gy[i][jp] + 2.0 * gy[i][j]) * dy) / dy2;
a = Omg[i][j] - Omg[i][jp] - Omg[ip][j] + Omg[ip][jp];
b = gy[ip][j] - gy[i][j];
c12 = (-a - b * dy) / (dx * dy2);
c21 = (-a - (gx[i][jp] - gx[i][j]) * dx) / (dx2*dy);
c11 = - b / dx + c21 * dx;
newOmg[i][j] = Omg[i][j] + (((c30 * x + c21 * y + c20) * x + c11 * y + gx[i][j]) * x
+ ((c03 * y + c12 * x + c02) * y + gy[i][j]) * y) * ex;
newGx[i][j] = gx[i][j] + ((3.0 * c30 * x + 2.0 * (c21 * y + c20)) * x + (c12 * y + c11) * y) * ex;
newGy[i][j] = gy[i][j] + ((3.0 * c03 * y + 2.0 * (c12 * x + c02)) * y + (c21 * x + c11) * x) * ex;
//粘性項に中央差分
newOmg[i][j] += deltaT * ( (Omg[i-1][j] - 2.0 * Omg[i][j] + Omg[i+1][j]) / dx2
+ (Omg[i][j-1] - 2.0 * Omg[i][j] + Omg[i][j+1]) / dy2 ) * ex2 / Re;
}
}
//更新
for(j = 1; j < NY; j++)
for(i = 1; i < NX; i++)
{
Omg[i][j] = newOmg[i][j];
gx[i][j] = newGx[i][j];
gy[i][j] = newGy[i][j];
}
}
function drawContour(PP, maxP, minP, col)
{
nLine = parseFloat(form2.nLine.value);
var dp0 = (maxP - minP) / nLine;//流線間隔
var pp;
var x1, y1, x2, y2;
var p = [], x = [], y = [];
var i, j, k, m;
var data = [];
//三角形セルに分割
for (k = 0; k < nLine; k++)
{
pp = minP + (k + 1) * dp0;
for(j = 0; j < NY; j++)
{
for(i = 0; i < NX; i++)
{
if(j == NY -1) jp = 0; else jp = j + 1;
//三角形セルに分割
p[0] = PP[i][j]; x[0] = PosX[i][j]; y[0] = PosY[i][j];
p[1] = PP[i+1][j]; x[1] = PosX[i+1][j]; y[1] = PosY[i+1][j];
p[2] = PP[i+1][jp]; x[2] = PosX[i+1][jp]; y[2] = PosY[i+1][jp];
p[3] = PP[i][jp]; x[3] = PosX[i][jp]; y[3] = PosY[i][jp];
p[4] = p[0]; x[4] = x[0]; y[4] = y[0];//0番目に同じ
//中心
p[5] = (p[0] + p[1] + p[2] + p[3]) / 4.0;
x[5] = (x[0] + x[1] + x[2] + x[3]) / 4.0;
y[5] = (y[0] + y[1] + y[2] + y[3]) / 4.0;
for(m = 0; m < 4; m++)//各三角形について
{
x1 = -1000.0; y1 = -1000.0;
if((p[m] <= pp && pp < p[m+1]) || (p[m] > pp && pp >= p[m+1]))
{
x1 = x[m] + (x[m+1] - x[m]) * (pp - p[m]) / (p[m+1] - p[m]);
y1 = y[m] + (y[m+1] - y[m]) * (pp - p[m]) / (p[m+1] - p[m]);
}
if((p[m] <= pp && pp <= p[5]) || (p[m] >= pp && pp >= p[5]))
{
if(x1 == -1000)//まだ交点なし
{
x1 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y1 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
}
else//x1は見つかった
{
x2 = x[m] + (x[5] - x[m]) * (pp - p[m]) / (p[5] - p[m]);
y2 = y[m] + (y[5] - y[m]) * (pp - p[m]) / (p[5] - p[m]);
data.push(region.pos0.x + scale.x * x1);
data.push(region.pos0.y + scale.y * y1);
data.push(region.pos0.x + scale.x * x2);
data.push(region.pos0.y + scale.y * y2);
}
}
if((p[m+1] <= pp && pp <= p[5]) || (p[m+1] >= pp && pp >= p[5]))
{
if(x1 == -1000)//まだ交点なし
{
x1 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y1 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
}
else//x1は見つかった
{
x2 = x[m+1] + (x[5] - x[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
y2 = y[m+1] + (y[5] - y[m+1]) * (pp - p[m+1]) / (p[5] - p[m+1]);
data.push(region.pos0.x + scale.x * x1);
data.push(region.pos0.y + scale.y * y1);
data.push(region.pos0.x + scale.x * x2);
data.push(region.pos0.y + scale.y * y2);
}
}
}//m
}//j
}//i
}//k
drawLines(data, col);
}
function drawVelocity()
{
arrowScale = parseFloat(form2.arrowScale.value);;
arrowWidth = parseFloat(form2.arrowWidth.value);
intervalV = parseFloat(form2.intervalV.value);
var i, j;
//描画
var alpha, theta, mag, x0, y0, x, y, r;
for(i = 1; i < NX; i++)
{
if(i % intervalV != 0) continue;
r = Math.exp(i * DX);
for (j = 1; j < NY; j++)
{
alpha = j * DY - Math.PI;//動径の角度(分岐線は180°)
x = r * Math.cos(alpha); y = r * Math.sin(alpha);//格子点位置
theta = Math.atan2(VelY[i][j], VelX[i][j]);
theta += alpha;//水平軸からの偏角
//theta *= RAD_TO_DEG;//degree
mag = Math.sqrt(VelX[i][j] * VelX[i][j] + VelY[i][j] * VelY[i][j]);
//if(mag > 10.0) continue;
//theta = Math.atan2(VelY[i][j], VelX[i][j]);
x0 = region.pos0.x + scale.x * x;
y0 = region.pos0.y + scale.y * y;
drawArrow(x0, y0, mag * arrowScale, arrowWidth, "black", theta);
}
}
}
function drawGrid()
{
var i, j;
//全円
for(i = 0; i <= NX; i++)
{
for(j = 0; j <= NY; j++)
{
x1 = PosX[i][j]; y1 = PosY[i][j];
x2 = PosX[i][j+1]; y2 = PosY[i][j+1];
drawLine(region.pos0.x + scale.x * x1, region.pos0.y + scale.y * y1,
region.pos0.x + scale.x * x2, region.pos0.y + scale.y * y2, 1, "black");
}
}
//動径
for(j = 0; j < NY; j++)//方位角θ方向
{
x1 = PosX[0][j]; y1 = PosY[0][j];
x2 = PosX[NX][j];y2 = PosY[NX][j];
drawLine(region.pos0.x + scale.x * x1, region.pos0.y + scale.y * y1,
region.pos0.x + scale.x * x2, region.pos0.y + scale.y * y2, 1, "black");
}
}
//---------------------------------------------------
//イベント処理
function onClickC_Size()
{
canvas.width = form1.c_sizeX.value;
canvas.height = form1.c_sizeY.value;
display();
}
function onChangeData()
{
onClickReset();
initData();
}
function onDisplay()
{
display();
}
function onClickScale()
{
region.scale = parseFloat(form2.scale.value);
display();
}
function onClickStart()
{
fps = 0;
elapseTime = 0;
elapseTime0 = 0;
elapseTime1 = 0;
elapseTimeN = 0;
elapseTimeN0 = 0;
flagStart = true;
flagStep = false;
lastTime = new Date().getTime();
}
function onClickFreeze()
{
if(flagStart) { flagStart = false; }
else { flagStart = true; elapseTime = elapseTime0; }
flagStep = false;
}
function onClickStep()
{
flagStep = true;
flagStart = true;
elapseTime = elapseTime0;
}
function onClickReset()
{
elapseTime0 = 0;
elapseTimeN0 = 0;
flagStart = false;
flagStep = false;
initData();
form1.e_time.value = "0";
form1.n_time.value = "0";
display();
}
<file_sep>/JVEMV6/46_art/11_guitar/jvemv6_37_12_1_guitar-memo.py
# -*- coding: utf-8 -*-
'''
file : C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\jvemv6_37_12_1_guitar-memo.py
orig : C:\WORKS_2\WS\WS_Others.prog\prog\D-7\2_2\VIRTUAL\Admin_Projects\curr\ops\44_8.5\44_8.5_build-result-csv-data.py
at : 2019/09/17 08:48:46
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\
python jvemv6_37_12_1_guitar-memo.py
### env
pushd C:\WORKS_2\WS\WS_Others.prog\prog\D-7\2_2\VIRTUAL
start_env.bat
<Usage> 2019/09/17 09:51:58
1. file : memo_guitar.txt // C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
1. prep text
2. run this program
pushd C:\WORKS_2\WS\WS_Others.prog\prog\D-7\2_2\VIRTUAL
start_env.bat
pushd C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar\
python jvemv6_37_12_1_guitar-memo.py
3. open the newly-gen-ed file : memo_guitar.(replaced).(20190917_094828).txt
<git>
r a && s
'''
###############################################
import sys
from _datetime import datetime
from numpy import append
'''###################
import : original files
###################'''
sys.path.append('.')
sys.path.append('..')
sys.path.append('C:/WORKS_2/WS/WS_Others/prog/D-7/2_2/VIRTUAL/Admin_Projects')
# sys.path.append('C:/WORKS_2/WS/WS_Others/prog/D-7/2_2/VIRTUAL/Admin_Projects/mm')
from mm.libs_mm import cons_mm, cons_fx, libs, libfx
'''###################
import : built-in modules
###################'''
import os, re
'''###################
import : others
###################'''
#ref https://uxmilk.jp/39307 2019/09/01 15:22:25
# import urllib2
#ref https://teratail.com/questions/47744 2019/09/01 15:25:52
import urllib.request, urllib.error
#ref https://tonari-it.com/python-beautiful-soup-html-parse/#toc2
#import bs4
'''###################
vars : global
###################'''
SWITCH_DEBUG = True
DPATH_DAT_FILE = "C:\\Users\\iwabuchiken\\AppData\\Roaming\\MetaQuotes\\Terminal\\34B08C83A5AAE27A4079DE708E60511E\\MQL4\\Files\\Logs\\20190829_223434[eap-2.id-1].[EURJPY-1].dir"
FNAME_DAT_FILE = "[eap-2.id-1].(20190829_223434).dat"
DPATH_REPORT_FILE = "C:\\Users\\iwabuchiken\\AppData\\Roaming\\MetaQuotes\\Terminal\\34B08C83A5AAE27A4079DE708E60511E\\MQL4\\Logs\\logs_trading"
FNAME_REPORT_FILE = "DetailedStatement.[20190901_145309].htm"
###############################################
def show_Message() :
msg = '''
<Options>
'''
print (msg)
'''###################
_build_result_csv_data__Dat_File()
@return: lo_Order_Numbers
###################'''
def _build_result_csv_data__Dat_File():
'''###################
step : 1
prep : vars
###################'''
# DPATH_DAT_FILE = "C:\\Users\\iwabuchiken\\AppData\\Roaming\\MetaQuotes\\Terminal\\34B08C83A5AAE27A4079DE708E60511E\\MQL4\\Files\\Logs\\20190829_223434[eap-2.id-1].[EURJPY-1].dir"
#
# FNAME_DAT_FILE = "[eap-2.id-1].(20190829_223434).dat"
fpath_Dat_File = os.path.join(DPATH_DAT_FILE, FNAME_DAT_FILE)
# lists
lo_Order_Numbers = []
'''###################
step : 2
read : file
###################'''
'''###################
step : 2 : 1
open
###################'''
f_in_Dat_File = open(fpath_Dat_File, "r")
'''###################
step : 2 : 2
read
###################'''
linesOf_Dat_File = f_in_Dat_File.readlines()
# [2019.08.29 22:34:35 / lib_ea_2.mqh:249] 17307984 117.639 117.636 4 8 117.632 117.647
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
# count
cntOf_Lines = len(linesOf_Dat_File)
msg = "[%s:%d] cntOf_Lines ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cntOf_Lines
)
print()
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
'''###################
step : 3
build list
###################'''
for item in linesOf_Dat_File:
'''###################
step : 3 : 1
split each line
###################'''
tokens = item.split("\t")
'''###################
step : 3 : 2
get : order number
###################'''
tokeOf_Order_Number = tokens[1]
# append
lo_Order_Numbers.append(int(tokeOf_Order_Number))
#/for item in linesOf_Dat_File:
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
msg = "[%s:%d] len(lo_Order_Numbers) ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(lo_Order_Numbers)
)
print()
print(msg, file=sys.stderr)
print(lo_Order_Numbers[0:10])
#/if SWITCH_DEBUG == True
'''###################
step : 4
file : close
###################'''
f_in_Dat_File.close()
#_20190901_150006:tmp
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
msg = "[%s:%d] file ==> closed : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Dat_File
)
print()
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
'''###################
step : X
return
###################'''
return lo_Order_Numbers
#/ def build_result_csv_data():
'''###################
_build_result_csv_data__Write_Full_Data()
at : 2019/09/03 12:46:56
@return: lo_TRs_With_Order_TDS
==> [ [tag_TR, lo_TDs], [tag_TR, lo_TDs], ...]
###################'''
def _build_result_csv_data__Write_Full_Data(\
_lo_Orders_Full
, _dpath_Log__ROOT
, _strOf_Project
, _dname_Log
, _tlabel
):
#_20190903_124741:caller
#_20190903_124745:head
#_20190903_124751:wl
'''###################
step : 1
vars
###################'''
# fname_Log__Dat_Full = "%s.(%s).dat" % (_strOf_Project, _tlabel)
fname_Log__Dat_Full = "%s.[data-full].(%s).dat" % (_strOf_Project, _tlabel)
dpath_Log__Dat_Full = os.path.join(_dpath_Log__ROOT, _dname_Log)
fpath_Log__Dat_Full = os.path.join(dpath_Log__Dat_Full, fname_Log__Dat_Full)
'''###################
step : 2
gen : dirs
###################'''
if not os.path.isdir(dpath_Log__Dat_Full) : #if not os.path.isdir(dpath_Log__Dat_Full)
res = os.makedirs(dpath_Log__Dat_Full)
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
msg = "[%s:%d] os.makedirs ==> %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, res
)
print()
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
#/if not os.path.isdir(dpath_Log__Dat_Full)
'''###################
step : 3
write
###################'''
'''###################
step : 3 : 1
file : open
###################'''
f_out_Dat_Full = open(fpath_Log__Dat_Full, "w")
'''###################
step : 2 : 5.2 : 5.2.1
file : header
###################'''
#"Ticket", "Open Time", "Type", "Size", "Item", "Price", "S / L", "T / P", "Close Time", "Price", "Commission", "Taxes", "Swap", "Profit"
lo_Col_Names = ["Ticket", "Open Time", "Type", "Size", "Item", "Price", "S / L", "T / P", "Close Time", "Price", "Commission", "Taxes", "Swap", "Profit"]
f_out_Dat_Full.write("this file\t%s" % fname_Log__Dat_Full)
f_out_Dat_Full.write("\n")
# f_out_Dat_Full.write("source dir\t%s" % DPATH_REPORT_FILE)
# f_out_Dat_Full.write("\n")
#
f_out_Dat_Full.write("\n")
f_out_Dat_Full.write("s.n.")
f_out_Dat_Full.write("\t")
f_out_Dat_Full.write("\t".join(lo_Col_Names))
f_out_Dat_Full.write("\n")
f_out_Dat_Full.write("\n")
'''###################
step : 2 : 5.2 : 5.3
file : write
###################'''
#_20190902_130031:tmp
cntOf_Loop = 0
for item in _lo_Orders_Full:
'''###################
step : 2 : 5.2 : 5.3 : 0
count
###################'''
cntOf_Loop += 1
'''###################
step : 2 : 5.2 : 5.3 : 1
get : TDs
###################'''
# TDs
lo_TDs = item[1]
'''###################
step : 2 : 5.2 : 5.3 : 2
get : texts
###################'''
lo_Tmp_TD_Texts = []
# s.n.
lo_Tmp_TD_Texts.append("%d" % cntOf_Loop)
lo_Tmp_TD_Texts.append("\t")
lo_Tmp_TD_Texts.append("\t".join([x.getText() for x in lo_TDs]))
f_out_Dat_Full.write("".join(lo_Tmp_TD_Texts))
f_out_Dat_Full.write("\n")
#/for item in lo_TRs_With_Order_TDS:
'''###################
step : 2 : 5.2 : 5.X
file : close
###################'''
f_out_Dat_Full.close()
#/ def _build_result_csv_data__Write_Full_Data():
'''###################
_build_result_csv_data__Full_Data_List()
at : 2019/09/03 12:46:56
@return: lo_TRs_With_Order_TDS
==> [ [tag_TR, lo_TDs], [tag_TR, lo_TDs], ...]
###################'''
def _build_result_csv_data__Full_Data_List(_lo_TRs_With_Order_TDS, _lo_Order_Numbers):
#_20190903_124229:caller
#_20190903_124234:head
#_20190903_124239:wl
'''###################
step : 1
vars
###################'''
lo_Orders_Full = []
'''###################
step : 2
build
###################'''
for item in _lo_TRs_With_Order_TDS:
lo_TDs = item[1]
numOf_Order = int(lo_TDs[0].getText())
# judge
if numOf_Order in _lo_Order_Numbers : #if numOf_Order in lo_Order_Numbers
# append
lo_Orders_Full.append(item)
#/if numOf_Order in lo_Order_Numbers
#/for item in lo_TRs_With_Order_TDS:
# report
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
# count
lenOf_LO_Orders_Full = len(lo_Orders_Full)
msg = "[%s:%d] lenOf_LO_Orders_Full ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, lenOf_LO_Orders_Full
)
print()
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
'''###################
step : 3
return
###################'''
return lo_Orders_Full
#/ def _build_result_csv_data__Full_Data_List():
'''###################
_build_result_csv_data__Report_File()
@return: lo_TRs_With_Order_TDS
==> [ [tag_TR, lo_TDs], [tag_TR, lo_TDs], ...]
###################'''
def _build_result_csv_data__Report_File(\
_dpath_Log__ROOT
, _strOf_Project
, dname_Log
, _tlabel
):
#_20190901_151726:caller
#_20190901_151733:head
#_20190901_151746:wl
'''###################
step : 1 : 1
prep : vars : file names, paths
###################'''
#C:\Users\iwabuchiken\AppData\Roaming\MetaQuotes\Terminal\34B08C83A5AAE27A4079DE708E60511E\MQL4\Logs\logs_trading
# DPATH_REPORT_FILE = "C:\\Users\\iwabuchiken\\AppData\\Roaming\\MetaQuotes\\Terminal\\34B08C83A5AAE27A4079DE708E60511E\\MQL4\\Logs\\logs_trading"
#
# FNAME_REPORT_FILE = "DetailedStatement.[20190901_145309].htm"
fpath_Report_File = os.path.join(DPATH_REPORT_FILE, FNAME_REPORT_FILE)
#_20190902_124906:tmp
# _tlabel = libs.get_TimeLabel_Now()
# #C:\WORKS_2\WS\WS_Others.prog\prog\D-7\2_2\VIRTUAL\Admin_Projects\curr\data\log
# _dpath_Log__ROOT = "C:\\WORKS_2\\WS\\WS_Others.prog\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\curr\\data\\log"
#
# _strOf_Project = "[44_8.5].[order-csv-data]"
#
# dname_Log = "%s.(%s).dir" % (_strOf_Project, _tlabel)
fname_Log__Dat = "%s.(%s).dat" % (_strOf_Project, _tlabel)
fname_Log__Dat_Report_File = "%s.[report-file].(%s).dat" % (_strOf_Project, _tlabel)
'''###################
step : 1 : 1.1
gen : dirs
###################'''
#_20190902_125615:tmp
dpath_Log__Dat = os.path.join(_dpath_Log__ROOT, dname_Log)
if not os.path.isdir(dpath_Log__Dat) : #if not os.path.isdir(dpath_Log__Dat)
res = os.makedirs(dpath_Log__Dat)
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
msg = "[%s:%d] os.makedirs ==> %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, res
)
print()
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
#/if not os.path.isdir(dpath_Log__Dat)
'''###################
step : 1 : 2
prep : vars : lists
###################'''
#
lo_Orders = []
# [ [tag_TR, lo_TDs], [], ...]
lo_TR_and_TDs = []
#
lo_Orders_From_Report_File = []
# list of TRs with order TDs
lo_TRs_With_Order_TDS = []
'''###################
step : 2 : 1
read : source file
###################'''
f_in_Report_File = open(fpath_Report_File, "r")
contentOf_Report_File = f_in_Report_File.read()
'''###################
step : 2 : 1.1
source file : close
###################'''
f_in_Report_File.close()
'''###################
step : 2 : 2
read : as html
###################'''
#ref https://tonari-it.com/python-beautiful-soup-html-parse/#toc2
soup = bs4.BeautifulSoup(contentOf_Report_File, "html.parser")
print()
print(soup.title)
'''###################
step : 2 : 3
read : TR tags
###################'''
#ref https://tonari-it.com/python-html-tag-list-bs4/#toc5
lo_TRs = soup.select("tr")
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
# count
cntOf_LO_TRs = len(lo_TRs)
msg = "[%s:%d] cntOf_LO_TRs ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cntOf_LO_TRs
)
print()
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
'''###################
step : 2 : 4
read : TD tags
###################'''
for item in lo_TRs:
'''###################
step : 2 : 4.1
select : TD tags
###################'''
lo_TDs = item.select("td")
'''###################
step : 2 : 4.2
append
###################'''
lo_TR_and_TDs.append([item, lo_TDs])
#/for item in lo_TRs:
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
# count
cntOf_LO_TR_and_TDs = len(lo_TR_and_TDs)
msg = "[%s:%d] cntOf_LO_TR_and_TDs ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, cntOf_LO_TR_and_TDs
)
print()
print(msg, file=sys.stderr)
# print(lo_TR_and_TDs[10])
#/if SWITCH_DEBUG == True
'''###################
step : 2 : 5
read : TD texts
###################'''
# for item in lo_TR_and_TDs:
# for item in lo_TR_and_TDs[:50] :
# for item in lo_TR_and_TDs[:20] :
for item in lo_TR_and_TDs :
'''###################
step : 2 : 5.1
get : TDs
###################'''
lo_TDs = item[1]
'''###################
step : 2 : 5.2
get : texts
###################'''
for item_2 in lo_TDs:
'''###################
step : 2 : 5.2 : 1
get : "title" attribute value
###################'''
# get text
textOf_TD_With_Title_Attribute = item_2.get("title")
# textOf_TD = item_2.getText()
'''###################
step : 2 : 5.2 : 2
validate
###################'''
if textOf_TD_With_Title_Attribute == None : #if textOf_TD == None
# #debug
# if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
#
# msg = "[%s:%d] textOf_TD_With_Title_Attribute ==> none" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# )
#
# print(item_2)
# print(msg, file=sys.stderr)
#
# #/if SWITCH_DEBUG == True
# continue
continue
#/if textOf_TD == None
'''###################
step : 2 : 5.2 : 3
if "My order" in the text ==> append
###################'''
'''###################
step : 2 : 5.2 : 3.1
log
###################'''
#_20190901_161624:next
if "My order" in textOf_TD_With_Title_Attribute : #if "My order" in textOf_TD_With_Title_Attribute
'''###################
step : 2 : 5.2 : 3.2
append
###################'''
# append
lo_TRs_With_Order_TDS.append(item)
#_20190901_161249:tmp
# #debug
# if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
#
# msg = "[%s:%d] My order ==> detected" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# )
#
# print(item_2)
# print(msg, file=sys.stderr)
#
# #/if SWITCH_DEBUG == True
#/if "My order" in textOf_TD_With_Title_Attribute
# #debug
# if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
#
# msg = "[%s:%d] textOf_TD ==> %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , textOf_TD
# )
#
# print()
# print(msg, file=sys.stderr)
#
# #/if SWITCH_DEBUG == True
# #
# #ref https://www.afternerd.com/blog/python-string-contains/
# if "My order" in textOf_TD : #if "My order" in textoff
#
# #debug
# if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
#
# msg = "[%s:%d] My order ==> detected" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# )
#
# print()
# print(msg, file=sys.stderr)
#
# print(item)
#
# #/if SWITCH_DEBUG == True
#
# #/if "My order" in textoff
# #debug
# if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
#
# # count
# cntOf_LO_TR_and_TDs = len(lo_TR_and_TDs)
#
# msg = "[%s:%d] cntOf_LO_TR_and_TDs ==> %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , cntOf_LO_TR_and_TDs
# )
#
# print()
# print(msg, file=sys.stderr)
#
# print(lo_TR_and_TDs[10])
#
# #/if SWITCH_DEBUG == True
#/for item_2 in lo_TDs:
#/for item in lo_TR_and_TDs:
'''###################
step : 2 : 5.2 : 4
extract texts
###################'''
#_20190902_124052:tmp
#debug
if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
msg = "[%s:%d] len(lo_TRs_With_Order_TDS) ==> %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(lo_TRs_With_Order_TDS)
)
print(msg, file=sys.stderr)
#/if SWITCH_DEBUG == True
'''###################
step : 2 : 5.2 : 5
write to file
###################'''
#_20190902_124906:tmp
'''###################
step : 2 : 5.2 : 5.1
file path
###################'''
fpath_Log__Dat_Report_File = os.path.join(_dpath_Log__ROOT, dname_Log, fname_Log__Dat_Report_File)
# fpath_Log__Dat = os.path.join(_dpath_Log__ROOT, dname_Log, fname_Log__Dat)
'''###################
step : 2 : 5.2 : 5.2
file : open
###################'''
f_out_Dat_Report_File = open(fpath_Log__Dat_Report_File, "w")
'''###################
step : 2 : 5.2 : 5.2.1
file : header
###################'''
#"Ticket", "Open Time", "Type", "Size", "Item", "Price", "S / L", "T / P", "Close Time", "Price", "Commission", "Taxes", "Swap", "Profit"
lo_Col_Names = ["Ticket", "Open Time", "Type", "Size", "Item", "Price", "S / L", "T / P", "Close Time", "Price", "Commission", "Taxes", "Swap", "Profit"]
f_out_Dat_Report_File.write("source file\t%s" % fname_Log__Dat_Report_File)
f_out_Dat_Report_File.write("\n")
f_out_Dat_Report_File.write("source dir\t%s" % DPATH_REPORT_FILE)
f_out_Dat_Report_File.write("\n")
f_out_Dat_Report_File.write("\n")
f_out_Dat_Report_File.write("s.n.")
f_out_Dat_Report_File.write("\t")
f_out_Dat_Report_File.write("\t".join(lo_Col_Names))
f_out_Dat_Report_File.write("\n")
f_out_Dat_Report_File.write("\n")
'''###################
step : 2 : 5.2 : 5.3
file : write
###################'''
#_20190902_130031:tmp
cntOf_Loop = 0
for item in lo_TRs_With_Order_TDS:
'''###################
step : 2 : 5.2 : 5.3 : 0
count
###################'''
cntOf_Loop += 1
'''###################
step : 2 : 5.2 : 5.3 : 1
get : TDs
###################'''
# TDs
lo_TDs = item[1]
'''###################
step : 2 : 5.2 : 5.3 : 2
get : texts
###################'''
lo_Tmp_TD_Texts = []
# s.n.
lo_Tmp_TD_Texts.append("%d" % cntOf_Loop)
lo_Tmp_TD_Texts.append("\t")
lo_Tmp_TD_Texts.append("\t".join([x.getText() for x in lo_TDs]))
# for item_2 in lo_TDs:
#
# textOf_TD = item_2.getText()
#
# lo_Tmp_TD_Texts.append(textOf_TD)
# lo_Tmp_TD_Texts.append("\t")
#
# #/for item_2 in lo_TDs:
# # separator
# lo_Tmp_TD_Texts.append("\n")
# # first TD
# TD_0 = lo_TDs[0]
#
# textOf_TD = TD_0.getText()
#
# f_out_Dat.write(textOf_TD)
f_out_Dat_Report_File.write("".join(lo_Tmp_TD_Texts))
f_out_Dat_Report_File.write("\n")
#/for item in lo_TRs_With_Order_TDS:
'''###################
step : 2 : 5.2 : 5.X
file : close
###################'''
f_out_Dat_Report_File.close()
'''###################
step : X
return
###################'''
return lo_TRs_With_Order_TDS
#/ def _build_result_csv_data__Report_File():
def build_result_csv_data():
'''###################
step : 1
dat file
###################'''
# time label
tlabel = libs.get_TimeLabel_Now()
#C:\WORKS_2\WS\WS_Others.prog\prog\D-7\2_2\VIRTUAL\Admin_Projects\curr\data\log
dpath_Log__ROOT = "C:\\WORKS_2\\WS\\WS_Others.prog\\prog\\D-7\\2_2\\VIRTUAL\\Admin_Projects\\curr\\data\\log"
strOf_Project = "[44_8.5].[order-csv-data]"
dname_Log = "%s.(%s).dir" % (strOf_Project, tlabel)
'''###################
step : 1
dat file
###################'''
lo_Order_Numbers = _build_result_csv_data__Dat_File()
'''###################
step : 2
file : report file
###################'''
#_20190901_151726:caller
lo_TRs_With_Order_TDS = lo_Order_Reports = \
_build_result_csv_data__Report_File(\
dpath_Log__ROOT
, strOf_Project
, dname_Log
, tlabel
)
# 17257299 2019.08.27 08:26:03 buy 1.00 eurjpy 117.345 117.338 117.353 2019.08.27 08:26:27 117.338 0 0 0 -700
'''###################
step : 3
build : a full-data list
###################'''
#_20190902_132829:tmp
#_20190903_124229:caller
lo_Orders_Full = _build_result_csv_data__Full_Data_List(lo_TRs_With_Order_TDS, lo_Order_Numbers)
# '''###################
# step : 3.1
# vars
# ###################'''
# lo_Orders_Full = []
#
# for item in lo_TRs_With_Order_TDS:
#
# lo_TDs = item[1]
#
# numOf_Order = int(lo_TDs[0].getText())
#
# # judge
# if numOf_Order in lo_Order_Numbers : #if numOf_Order in lo_Order_Numbers
#
# # append
# lo_Orders_Full.append(item)
#
# #/if numOf_Order in lo_Order_Numbers
#
#
# #/for item in lo_TRs_With_Order_TDS:
#
# # report
# #debug
# if SWITCH_DEBUG == True : #if SWITCH_DEBUG == True
#
# # count
# lenOf_LO_Orders_Full = len(lo_Orders_Full)
#
# msg = "[%s:%d] lenOf_LO_Orders_Full ==> %d" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , lenOf_LO_Orders_Full
# )
#
# print()
# print(msg, file=sys.stderr)
#
# #/if SWITCH_DEBUG == True
'''###################
step : 4
write : file
###################'''
#_20190903_124102:tmp
#_20190903_124741:caller
_build_result_csv_data__Write_Full_Data(\
lo_Orders_Full
, dpath_Log__ROOT
, strOf_Project
, dname_Log
, tlabel
)
#/ def build_result_csv_data():
def test_1():
'''###################
get : args
###################'''
'''###################
file path
###################'''
#_20190917_090405:tmp
#C:\WORKS_2\WS\WS_Others.Art\JVEMV6\46_art\11_guitar
dpath_Text = "C:\\WORKS_2\\WS\\WS_Others.Art\\JVEMV6\\46_art\\11_guitar"
fname_Text = "memo_guitar.txt"
#_20190917_094525:tmp
fname_Text_Replaced = "memo_guitar.(replaced).(%s).txt" % libs.get_TimeLabel_Now()
fpath_Text = os.path.join(dpath_Text, fname_Text)
# validate
if not os.path.isfile(fpath_Text) : #if not os.path.isfile(fpath_CSV)
print()
print("[%s:%d] text file NOT exist : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fpath_Text
), file=sys.stderr)
return
#/if not os.path.isfile(fpath_CSV)
'''###################
file : open
###################'''
f_in_Text = open(fpath_Text, "r")
'''###################
read file content
###################'''
data = f_in_Text.read()
print("[%s:%d] len(data) = %d" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, len(data)
), file=sys.stderr)
'''###################
file : close
###################'''
f_in_Text.close()
'''###################
step : A : 1
replace
###################'''
#_20190917_090843:tmp
#ref https://www.tutorialspoint.com/python/python_reg_expressions.htm
# \|\| \r\n
#ref https://uxmilk.jp/8662
txt_Replaced = re.sub(r"\|\| ", "\r", data)
# txt_Replaced = re.sub(r"\|\| ", "\r\n", data)
print("[%s:%d] txt_Replaced => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, txt_Replaced
), file=sys.stderr)
#ref https://stackoverflow.com/questions/5984633/python-re-sub-group-number-after-number
lo_Replaces = [
# ["(s\.\d+):", "$1\t"]
["(s\.\d+):", "\g<1>\t"]
, ["\.N", "\t"]
#, ["\.n", "\t"] # 20191009_102653 ==> working
#, ["^(.+-\d\).n", "\g<1>\tn"] # 20191023_091457 ==> error 'unterminated subpattern at position 1'
#, ["^(.+-\d\).n", "\g<1>\t.n"] # 20191024_111819 testing ==> error 'unterminated subpattern at position 1'
#, ["^(.+-\d\).n", "\g<1>\t n"] # 20191024_111934 testing ==> error 'unterminated subpattern at position 1'
#, ["^(.+-\d\).n", "\g<1>\t nnn"] # 20191024_112117 testing ==> error 'unterminated subpattern at position 1'
#, ["^(.+-\d\).n", "\g<1>\t new"] # 20191024_112117 testing ==> error 'unterminated subpattern at position 1'
#, ["^(.+-\d\).n", "\g<1>\tnew"] # 20191024_112231 testing ==> error 'unterminated subpattern at position 1'
#, ["^(.+-\d).n", "\g<1>\t n"] # 20191024_112231 testing ==> working --> '(.+-\d\)' ~~> the second '\' omitted
#, ["^(.+-\d).n", "\g<1>\tn"] # 20191024_112512 ==> working, but '.n' stays
#, ["^(.+-\d)\.n", "\g<1>\tn"] # 20191024_112601 ==> working, but '.n' stays
#, ["(.+-\d)\.n", "\g<1>\tn"] # 20191024_112907 ==> omit '^' ---> NO
#, ["(et|f|st|ct)-(\d+)\.n", "\g<1>-\g<2>\tn"] # 20191024_112907 ==> working, '.n' converted to "\tn"
#, ["(et|f|st|ct|m|ch|cp)-(\d+)\.n", "\g<1>-\g<2>\tn"] # 20191024_112907 ==> working, '.n' converted to "\tn" # 20191129_085145
, ["(et|f|st|ct|m|ch|cp|p|prev)-(\d+)\.n", "\g<1>-\g<2>\tn"] # ==> working, '.n' converted to "\tn" # 20200106_111814
#, ["(et|f|st|ct)-(\d+),([a-zA-Z])", "\g<1>-\g<2>\t\g<3>"] # 20191024_112907 ==> working, converted
#, ["(et|f|st|ct)-(\d+),([a-zA-Z]+),", "\g<1>-\g<2>\t\g<3>\t"] # 20191107_090118 ==> working
, ["(et|f|st|ct)-(\d+),([a-zA-Z]{2,}),", "\g<1>-\g<2>\t\g<3>\t"] # 20191107_090411
, ["(prev),(s-)", "\g<1>\tprev\t\g<2>"] # 20200106_111924
#, ["(\d),rev", "\g<1>\trev"]
, ["(\d),rev", "\g<1>\trev\t"] # 20191107_084308
, ["(\d),r,", "\g<1>\trev\t"] # 20191107_084217
, ["(\d);v", "\g<1>\tv"]
, ["(\d),var", "\g<1>\tvar"] # 20191006_075953
, ["(-\d+),section", "\g<1>\tsection"] # 20191006_075953
, ["(-\d+),practice", "\g<1>\tpractice"] # 20191006_075953
, ["(-\d+),play", "\g<1>\tplay"] # 20191008_100412
, ["(-\d+),patt", "\g<1>\tpatt"] # 20191021_112614
, ["(\d),rec", "\g<1>\trec"]
, ["(-\d+),memo", "\g<1>\tmemo\t"] # 20191126_110214
, ["s\.(\d+?)", "\g<1>"]
, ["^(\d+?\t.+-\d+),", "\g<1>\t"]
, ["(\d);var", "\g<1>\tvar"]
, [",R=", "\tR="]
, ["other,", "other\tother\t"] # 20191107_090715
, ["warm-up,", "warm-up\wu\t"] # 20191107_090715
, ["wu,", "warm-up\twu\t"] # 20191107_090715
, ["fs-(\d+),", "fs-\g<1>\tfs\t"] # 20191210_095020
]
for item in lo_Replaces:
print("[%s:%d] item[0] = '%s', item[1] = '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, item[0], item[1]
), file=sys.stderr)
#debug
print("[%s:%d] txt_Replaced (before) = '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, txt_Replaced
), file=sys.stderr)
txt_Replaced = re.sub(item[0], item[1], txt_Replaced)
#debug
print("[%s:%d] txt_Replaced (after) = '%s'" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, txt_Replaced
), file=sys.stderr)
#/for item in lo_Replaces:
# txt_Replaced = re.sub(r"\|\| ", "\r\n", data)
print("[%s:%d] txt_Replaced => %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, txt_Replaced
), file=sys.stderr)
'''###################
step : A : 2
clipboard
###################'''
# #_20190917_094226:tmp
# # command = 'echo ' + text.strip() + '| clip'
# # command = 'echo ' + txt_Replaced.strip() + '| clip'
# command = "echo \"" + txt_Replaced.strip() + "\" | clip"
# os.system(command)
#
# print("[%s:%d] command => %s" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
# , command
# ), file=sys.stderr)
#
# print("[%s:%d] text => to clipboard" % \
# (os.path.basename(libs.thisfile()), libs.linenum()
#
# ), file=sys.stderr)
'''###################
step : A : 3
to file
###################'''
f_out_Text_Replaced = open(os.path.join(dpath_Text, fname_Text_Replaced), "w")
f_out_Text_Replaced.write(txt_Replaced)
# close
f_out_Text_Replaced.close()
print("[%s:%d] text => written : %s" % \
(os.path.basename(libs.thisfile()), libs.linenum()
, fname_Text_Replaced
), file=sys.stderr)
'''###################
report
###################'''
'''###################
message
###################'''
print()
print("[%s:%d] test_1 =======================" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#/ def test_1():
def exec_prog():
'''###################
ops
###################'''
test_1()
print("[%s:%d] exec_prog() => done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
#def exec_prog()
if __name__ == "__main__" :
'''###################
validate : help option
###################'''
'''###################
get options
###################'''
'''###################
evecute
###################'''
exec_prog()
print()
print("[%s:%d] done" % \
(os.path.basename(libs.thisfile()), libs.linenum()
), file=sys.stderr)
# print "[%s:%d] done" % (thisfile(), linenum())
<file_sep>/JVEMV6/46_art/8_kb/musescore/02_compos/end_[compos_musescore].bat
@echo on
rem C:\WORKS_2\a.bat && r a && git add . && git commit -m "( compos_musescore : edit-log_[2020-09-25_08-15-55_000.mp4.mscz].txt ) periodical updates" && e && git push origin master
C:\WORKS_2\a.bat && r a && git add . && git commit -m "( jve_[46#13].[theme=compositions] ) periodical updates" && e && p && r f && r a && g a . && g c -m "( jve_[46#13].[theme=compositions] ) periodical updates" && e && p
| 513328ab4c56e6cde760294371f9d5e61c4dd29d | [
"Markdown",
"Batchfile",
"Ruby",
"JavaScript",
"Python",
"PHP"
] | 111 | Markdown | iwabuchiken/WS_Others.Art | 31d47452cd10e5ff48500844fbb2d2adb5458cd5 | a6b8c646438b76867e3532ef18e1cb88104e427f |
refs/heads/master | <file_sep>import marshal,zlib,base64
exec(marshal.loads(zlib.decompress(base64.b64decode("<KEY>))))<file_sep># hackfb
>Pemula Gunakan Sc ini!!
$apt upgrade && apt upgrade
$apt install git
$apt install python
$apt install python2
$pkg install mechanize
$git clone https://github.com/Mryansfb/hackfb
$ls
$cd hackfb
$ls
$python2 Darkefbe.py
>Yang sudah paham sc nih
$git clone https://github.com/Mryansfb/hackfb
$ls
$cd hackfb
$ls
$python2 hack.py
[+]Tools Ini Update Dark-FB
[+]Gunakan dengan bijak:V
[+]Recode?Mandul!!
[OK√]Gunakan selalu Tools ini selalu okeh..
| 48cb5f239050a17b74e4c5d4f35a9e5a5f19c58a | [
"Markdown",
"Python"
] | 2 | Markdown | Mryansfb/hackfb | 36c88e2f94acd9529335bf9364807aa96a1676a7 | cb7995857d310ff0bdd9d5423c536789b7bb88e7 |
refs/heads/master | <file_sep># Viewport
Responsive design excersize
| 0f9337241354b9bf65cfc9470e62e562a6134bcf | [
"Markdown"
] | 1 | Markdown | Gixugif/Viewport | 69cb9a54c04449b19ebf1ce22f3584c20ca76bcb | 0886843cbc0fa2ee623ebfde213fdf20e5e91019 |
refs/heads/master | <repo_name>parikshitv/adalMTS<file_sep>/README.md
# EZCodeAdalAngular5
This library is a Azure Active Directory Authentication Library (adal.js) wrapper package for Angular 5. It can be used to authenticate Angular 5 application for Azure Active Directory and generate token to communicate to MS Graph API and 3rd party Web API secured by Azure AD.
## Installation
Run the following command to install the package.
`npm install ezcode-adalmts --save`
## Authenticate Usage
1. Create a adal configuration file ezcodeadalconfig.ts under service folder
2. You can change the adal configuration via code at the runtime.
3. Update app.module.ts to include the ezcode-adal-angular5 library. Make sure you set useHash to true because adal relies on hash to return the token.
4. Add EZCodeAdalComponentGuard to secure your component. When users click the secured components, application will redirect them to Azure AD login page.
<file_sep>/src/ezcode-adalconfig.service.ts
import { Injectable } from '@angular/core';
import { IEZCodeAdalConfigs } from './IEZCodeAdalConfigs';
import { IEZCodeAdalConfig } from './IEZCodeAdalConfig';
import { inject } from '@angular/core/testing';
export class EZCodeAdalConfigs implements IEZCodeAdalConfigs {
adalconfigs: IEZCodeAdalConfig[] = [];
customRedirectAfterLogin: string = "";
customindex: number = 0;
constructor() {}
}
<file_sep>/src/ezcode-adal.service.ts
///// <reference path="../../../../node_modules/@types/adal/index.d.ts" />
import { Injectable } from '@angular/core';
//import 'expose-loader?AuthenticationContext!../node_modules/adal-angular/lib/adal.js';
import * as AuthenticationContext from "adal-angular";
import { Observable } from 'rxjs/Observable';
import { catchError, map, tap } from 'rxjs/operators';
import { HttpHeaders } from '@angular/common/http';
import { EZCodeAdalConfigs } from './ezcode-adalconfig.service';
import { IEZCodeAdalConfig } from './IEZCodeAdalConfig';
import { IEZLoginUser } from './IEZLoginUser';
// import { Http } from '@angular/http';
//let createAuthContextFn: adal.AuthenticationContextStatic = AuthenticationContext;
@Injectable()
export class EZCodeAdalService {
//private context: adal.AuthenticationContext;
private context: AuthenticationContext;
private config: IEZCodeAdalConfig;
private http: any;
constructor(private ezserve: EZCodeAdalConfigs) {
this.context = new AuthenticationContext(this.ezserve.adalconfigs[this.ezserve.customindex]);
//this.context = new createAuthContextFn(this.ezserve.adalconfigs[this.ezserve.customindex]);
this.config = this.ezserve.adalconfigs[this.ezserve.customindex];
}
public setAdalContext() {
this.config = this.ezserve.adalconfigs[this.ezserve.customindex];
this.context.config = this.config;
}
login() {
this.context.login();
}
logout() {
this.context.logOut();
}
handleCallback() {
this.context.handleWindowCallback();
}
public get userInfo(): IEZLoginUser {
const adalUser = this.context.getCachedUser();
return adalUser == null ? null :
{
upn: adalUser.userName,
firstName: adalUser.profile["given_name"],
lastName: adalUser.profile["family_name"],
displayName: adalUser.profile["name"],
profile: adalUser.profile
};
}
public get accessToken() {
return this.context.getCachedToken(this.config.clientId);
}
public getAccessTokenByUrl(url: string): Observable<string> {
return Observable.create(emitter => {
const resourceId = this.context.getResourceForEndpoint(url);
if (resourceId != null) {
this.context.acquireToken(resourceId,
(message, token) => {
emitter.next(token);
emitter.complete();
});
} else {
emitter.next(null);
emitter.complete();
}
});
}
public get isAuthenticated() {
return ((this.userInfo !== null) && (this.accessToken !== null));
}
}
<file_sep>/lib/idtoken-callback.component.d.ts
import { OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { EZCodeAdalService } from './ezcode-adal.service';
export declare class IdTokenCallbackComponent implements OnInit {
private router;
private adalService;
constructor(router: Router, adalService: EZCodeAdalService);
ngOnInit(): void;
}
<file_sep>/lib/index.d.ts
export { IEZLoginUser } from './IEZLoginUser';
export { IEZCodeAdalConfig } from './IEZCodeAdalConfig';
export { IEZCodeAdalConfigs } from './IEZCodeAdalConfigs';
export { AccesstokenCallbackComponent } from './accesstoken-callback.component';
export { EZCodeAdalCallbackGuard } from './ezcode-adal-callback.guard';
export { EZCodeAdalComponentGuard } from './ezcode-adal-component.guard';
export { EZCodeAdalModule } from './ezcode-adal.module';
export { EZCodeAdalService } from './ezcode-adal.service';
export { EZCodeAdalConfigs } from './ezcode-adalconfig.service';
export { EZCodeHttpInterceptor } from './ezcode-http.interceptor';
export { IdTokenCallbackComponent } from './idtoken-callback.component';
<file_sep>/lib/ezcode-http.interceptor.d.ts
import { HttpInterceptor, HttpHandler, HttpRequest, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/mergeMap';
import { EZCodeAdalService } from './ezcode-adal.service';
export declare class EZCodeHttpInterceptor implements HttpInterceptor {
private auth;
constructor(auth: EZCodeAdalService);
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>>;
}
<file_sep>/lib/ezcode-adal.service.d.ts
import { Observable } from 'rxjs/Observable';
import { EZCodeAdalConfigs } from './ezcode-adalconfig.service';
import { IEZLoginUser } from './IEZLoginUser';
export declare class EZCodeAdalService {
private ezserve;
private context;
private config;
private http;
constructor(ezserve: EZCodeAdalConfigs);
setAdalContext(): void;
login(): void;
logout(): void;
handleCallback(): void;
readonly userInfo: IEZLoginUser;
readonly accessToken: string;
getAccessTokenByUrl(url: string): Observable<string>;
readonly isAuthenticated: boolean;
}
<file_sep>/lib/ezcode-adal-callback.guard.d.ts
import { Observable } from 'rxjs/Observable';
import { ActivatedRoute, Router, CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot } from '@angular/router';
import { EZCodeAdalService } from './ezcode-adal.service';
import { EZCodeAdalConfigs } from './ezcode-adalconfig.service';
export declare class EZCodeAdalCallbackGuard implements CanActivate {
private route;
private router;
private adalService;
private configs;
private _loading;
constructor(route: ActivatedRoute, router: Router, adalService: EZCodeAdalService, configs: EZCodeAdalConfigs);
canActivate(route: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<boolean> | Promise<boolean> | boolean;
}
<file_sep>/lib/ezcode-adalconfig.service.d.ts
import { IEZCodeAdalConfigs } from './IEZCodeAdalConfigs';
import { IEZCodeAdalConfig } from './IEZCodeAdalConfig';
export declare class EZCodeAdalConfigs implements IEZCodeAdalConfigs {
adalconfigs: IEZCodeAdalConfig[];
customRedirectAfterLogin: string;
customindex: number;
constructor();
}
<file_sep>/lib/ezcode-adal.module.d.ts
import { ModuleWithProviders } from '@angular/core';
import { EZCodeAdalConfigs } from './ezcode-adalconfig.service';
export declare class EZCodeAdalModule {
constructor(parentModule: EZCodeAdalModule);
static forRoot(config: EZCodeAdalConfigs): ModuleWithProviders;
}
| 9a1c243e466b9d8f84082cd4811c2a8f679bc759 | [
"Markdown",
"TypeScript"
] | 10 | Markdown | parikshitv/adalMTS | ad18547591b8d0b3613586fc222f0b68c863c1fd | 1c901cd07c6d1a416754730d66fc1aafdfaa6f60 |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Threading.Tasks;
namespace BillionMessagesQueuer.Core.UseCases.QueueMessages
{
public interface IQueueMessagesHandler
{
Task Handle(QueueMessagesRequest request);
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BillionMessagesQueuer.Providers.Services.QueueService.AzureStorageQueue
{
public class AzureStorageQueueConfiguration
{
public string ConnectionString { get; set; }
public string QueueName { get; set; }
}
}
<file_sep>using BillionMessagesQueuer.Core.Services.QueueService;
using Microsoft.Extensions.Logging;
using System;
using System.Collections.Generic;
using System.Text;
using System.Threading.Tasks;
namespace BillionMessagesQueuer.Core.UseCases.QueueMessages
{
public class QueueMessagesHandler : IQueueMessagesHandler
{
private readonly ILogger<QueueMessagesHandler> logger;
private readonly IQueueService queueService;
public QueueMessagesHandler(ILogger<QueueMessagesHandler> logger, IQueueService queueService)
{
this.logger = logger;
this.queueService = queueService;
}
public async Task Handle(QueueMessagesRequest request)
{
LogMessage("QueueMessagesRequest started");
ValidateRequest(request);
var tasks = new List<Task>();
var instanceId = Guid.NewGuid();
for (int i = 1; i <= request.NumberOfMessagesToQueue; i++)
{
var message = new Message
{
Id = Guid.NewGuid(),
CreatedOnUtc = DateTime.UtcNow,
InstanceId = instanceId,
Content = $"Message number: {i} created for instance id: {instanceId}"
};
tasks.Add(this.queueService.Queue(message));
if(i%1000 == 0)
LogMessage($"{i} of {request.NumberOfMessagesToQueue} queued");
}
LogMessage($"Number of Messages Queued: {tasks.Count}");
await Task.WhenAll(tasks.ToArray());
LogMessage("QueueMessagesRequest ended");
}
private void LogMessage(string message)
{
this.logger.LogInformation($"{DateTime.UtcNow} - {message}");
}
private void ValidateRequest(QueueMessagesRequest request)
{
if (request == null)
throw new ArgumentNullException(nameof(request));
}
}
}
<file_sep>using System;
namespace BillionMessagesQueuer.Core.UseCases.QueueMessages
{
public class QueueMessagesRequest
{
public int NumberOfMessagesToQueue { get; set; }
}
}<file_sep>using BillionMessagesQueuer.Core.Services.QueueService;
using BillionMessagesQueuer.Core.UseCases.QueueMessages;
using Microsoft.Extensions.Logging;
using Moq;
using NUnit.Framework;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BillionMessagesQueuer.Tests.Core.UseCases.QueueMessages
{
public class QueueMessagesHandlerTests
{
private Mock<ILogger<QueueMessagesHandler>> logger;
private Mock<IQueueService> queueService;
[SetUp]
public void Setup()
{
logger = new Mock<ILogger<QueueMessagesHandler>>();
queueService = new Mock<IQueueService>();
}
[Test]
public void ArgumentNullException_for_null_request()
{
var sut = new QueueMessagesHandler(logger.Object, queueService.Object);
Assert.ThrowsAsync<ArgumentNullException>(() => sut.Handle(null));
}
[Test]
public async Task Handler_should_queue_correct_number_of_messages()
{
queueService.Setup(x => x.Queue(It.IsAny<Message>())).Returns(Task.CompletedTask);
var sut = new QueueMessagesHandler(logger.Object, queueService.Object);
var request = new QueueMessagesRequest
{
NumberOfMessagesToQueue = 10
};
await sut.Handle(request);
queueService.Verify(x => x.Queue(It.IsAny<Message>()), Times.Exactly(10));
}
}
}
<file_sep>#See https://aka.ms/containerfastmode to understand how Visual Studio uses this Dockerfile to build your images for faster debugging.
FROM mcr.microsoft.com/dotnet/runtime:5.0-buster-slim AS base
WORKDIR /app
FROM mcr.microsoft.com/dotnet/sdk:5.0-buster-slim AS build
WORKDIR /src
COPY ["BillionMessagesQueuer/BillionMessagesQueuer.csproj", "BillionMessagesQueuer/"]
COPY ["BillionMessagesQueuer.Providers/BillionMessagesQueuer.Providers.csproj", "BillionMessagesQueuer.Providers/"]
COPY ["BillionMessagesQueuer.Core/BillionMessagesQueuer.Core.csproj", "BillionMessagesQueuer.Core/"]
RUN dotnet restore "BillionMessagesQueuer/BillionMessagesQueuer.csproj"
COPY . .
WORKDIR "/src/BillionMessagesQueuer"
RUN dotnet build "BillionMessagesQueuer.csproj" -c Release -o /app/build
FROM build AS publish
RUN dotnet publish "BillionMessagesQueuer.csproj" -c Release -o /app/publish
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "BillionMessagesQueuer.dll"]<file_sep>using BillionMessagesQueuer.Core.Services.QueueService;
using BillionMessagesQueuer.Core.UseCases.QueueMessages;
using BillionMessagesQueuer.Providers.Services.QueueService.AzureStorageQueue;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Logging;
using System;
using System.IO;
using System.Threading.Tasks;
namespace MessageQueuer
{
public class Program
{
public static async Task Main(string[] args)
{
IConfiguration configuration = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddEnvironmentVariables()
.Build();
var services = new ServiceCollection();
ConfigureServices(services, configuration);
var serviceProvider = services.BuildServiceProvider();
await QueueMessages(configuration, serviceProvider.GetService<IQueueMessagesHandler>());
}
private static void ConfigureServices(IServiceCollection services, IConfiguration configuration)
{
services.AddLogging(builder =>
{
builder.AddConsole();
});
services.Configure<AzureStorageQueueConfiguration>(configuration.GetSection("AzureStorageQueue"));
services.AddTransient<IQueueService, AzureStorageQueueService>();
services.AddTransient<IQueueMessagesHandler, QueueMessagesHandler>();
}
private static async Task QueueMessages(IConfiguration configuration, IQueueMessagesHandler queueMessagesHandler)
{
int numberOfMessages;
int.TryParse(configuration.GetValue<string>("QueueMessagesConfig:NumberOfMessagesToQueue"), out numberOfMessages);
var request = new QueueMessagesRequest
{
NumberOfMessagesToQueue = numberOfMessages
};
await queueMessagesHandler.Handle(request);
}
}
}
<file_sep>using Azure.Storage.Queues;
using BillionMessagesQueuer.Core.Services.QueueService;
using Microsoft.Extensions.Options;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.Json;
using System.Threading.Tasks;
namespace BillionMessagesQueuer.Providers.Services.QueueService.AzureStorageQueue
{
public class AzureStorageQueueService : IQueueService
{
private readonly AzureStorageQueueConfiguration azureStorageQueueConfiguration;
private readonly QueueClient queueClient;
public AzureStorageQueueService(IOptions<AzureStorageQueueConfiguration> azureStorageQueueConfiguration)
{
this.azureStorageQueueConfiguration = azureStorageQueueConfiguration?.Value ?? throw new ArgumentNullException(nameof(azureStorageQueueConfiguration));
if (string.IsNullOrWhiteSpace(this.azureStorageQueueConfiguration.ConnectionString))
throw new ArgumentNullException(nameof(this.azureStorageQueueConfiguration.ConnectionString));
if (string.IsNullOrWhiteSpace(this.azureStorageQueueConfiguration.QueueName))
throw new ArgumentNullException(nameof(this.azureStorageQueueConfiguration.QueueName));
queueClient = new QueueClient(this.azureStorageQueueConfiguration.ConnectionString, this.azureStorageQueueConfiguration.QueueName);
}
public async Task Queue<T>(T message)
{
var base64EncodeddMessage = Convert.ToBase64String(Encoding.UTF8.GetBytes(JsonSerializer.Serialize(message)));
await queueClient.SendMessageAsync(base64EncodeddMessage);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BillionMessagesQueuer.Core.UseCases.QueueMessages
{
public class Message
{
public Guid Id { get; set; }
public DateTime CreatedOnUtc { get; set; }
public Guid InstanceId { get; set; }
public string Content { get; set; }
}
}
| 7a9946b8660264b6d4c4af0af7b31b7472bbdb36 | [
"C#",
"Dockerfile"
] | 9 | C# | aliiftikhar/BillionMessagesQueuer | 936c61075748fbc92448cef3688a9efb277c785a | 554391b9677e86cb5fbd2889990fcb691b013036 |
refs/heads/master | <repo_name>Szymon2603/spring-boot-angular5-starter<file_sep>/README.md
# spring-boot-angular5-starter
Minimal configuration to start project based on Spring Boot application as backend part and Angular SPA as frontend part
<file_sep>/frontend/src/app/app.component.ts
import { Component, OnInit } from '@angular/core';
import { HttpClient } from "@angular/common/http";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.sass']
})
export class AppComponent implements OnInit {
title = 'app';
backendMessage = '';
constructor(
private http: HttpClient
) {}
ngOnInit(): void {
this.http
.get("/api/hello", { responseType: 'text' })
.subscribe(res => {
this.backendMessage = res
}, () => {
window.alert("Error while calling /api/hello!")
})
}
}
| 156abaaab849e61211bdb74deafeebbe6f4d6e3e | [
"Markdown",
"TypeScript"
] | 2 | Markdown | Szymon2603/spring-boot-angular5-starter | c14318596c9127d41ce24fa9bce4c0ec53a52635 | bbc0180811b8f9ea2d489fc63f6129982b71757b |
refs/heads/master | <file_sep>#include <iostream>
#include <NTL/ZZ.h>
#include <time.h>
#include <string>
using namespace NTL;
unsigned char key[256];
unsigned int keyLength;
unsigned char plaintext[256];
unsigned char cryptotext[256];
unsigned int plaintextLength;
unsigned char keystream[256];
unsigned char S[256];
unsigned char swaper;
int i, j;
int lfsrShiftTest4(int N) {
N += ((((N & 1) + ((N >> 3) & 1)) % 2) << 4);
return N >> 1;
}
unsigned int lfsrShift32(unsigned int N) {
bool s_j = ((N & 1) + ((N >> 5/*27*/) & 1) + ((N >> 4/*28*/) & 1) + ((N >> 31) & 1)) % 2;
N >>= 1;
N += (s_j << 31);
return N;
}
unsigned long long lfsrShift64(unsigned long long N) {
bool s_j = ((N & 1) + ((N >> 60/*4*/) & 1) + ((N >> 61/*3*/) & 1) +((N >> 63) & 1)) % 2;
N >>= 1;
N += ((long long)s_j << 63);
return N;
}
unsigned int calulatePeriodOfLfsrShift32() {
unsigned int n, i = 0, k;
k = n = 4;
do {
n = lfsrShift32(n);
i++;
} while (n != k);
return i;
}
unsigned long long calulatePeriodOfLfsrShift64() {
unsigned long long n, i = 0, k;
k = n = 4;
do {
n = lfsrShift64(n);
i++;
} while (n != k);
return i;
}
unsigned char generateNumberLFSR8bit(){
unsigned char randomNumber = 0;
unsigned long long seed = ((((((static_cast<unsigned long long>(RandomBits_long(16)) << 16) + RandomBits_long(16)) << 16) + RandomBits_long(16)) << 16) + RandomBits_long(16));
for (unsigned int i = 0; i < 8; i++) {
randomNumber <<= 1;
seed = lfsrShift64(seed);
randomNumber += (seed >> 63);
}
return randomNumber;
}
ZZ generateNumberLFSR(unsigned int bitLength) {
ZZ randomNumber = (ZZ)0;
unsigned long long seed = ((((((static_cast<unsigned long long>(RandomBits_long(16)) << 16) + RandomBits_long(16)) << 16) + RandomBits_long(16)) << 16) + RandomBits_long(16));
//seed = 1;
for (unsigned int i = 0; i < bitLength; i++) {
randomNumber <<= 1;
seed = lfsrShift64(seed);
randomNumber += (seed >> 63);
}
return randomNumber;
}
ZZ generateNumberBBS(unsigned long bitLength) {
ZZ p, q;
do {
do {
p = GenPrime_ZZ(bitLength / 2);
} while (p % 4 != 3);
do {
q = GenPrime_ZZ(bitLength / 2);
} while (q % 4 != 3);
} while (p == q);
ZZ N = p * q;
ZZ seed;
do {
seed = RandomBnd(N);
} while (seed % p == 0 || seed % q == 0);
ZZ randomNumber = (ZZ)0;
for (int i = 0; i < bitLength; i++) {
seed = (seed * seed) % N;
randomNumber = (randomNumber << 1) + (seed % 2);
}
return randomNumber;
}
ZZ jacobiSymbol(ZZ n, ZZ k) {
n %= k;
int t = 1;
while (n != 0) {
while (n % 2 == 0) {
n /= 2;
int r = k % 8;
if (r == 3 || r == 5)
t = -t;
}
swap(n, k);
if (n % 4 == 3 && k % 4 == 3)
t = -t;
n %= k;
}
return (k == 1) ? (ZZ)t : (ZZ)0;
}
ZZ generateNumberJacobi(unsigned long bitLength) {
ZZ a, n;
n = GenPrime_ZZ(bitLength);
a = RandomBnd(n);
ZZ randomNumber = (ZZ)0;
for (int i = 0; i < bitLength; i++) {
randomNumber <<= 1;
if (jacobiSymbol(a + i, n) != (ZZ)-1)
randomNumber += 1;
}
return randomNumber;
}
void testPercentage(int iterations) {
int countZero = 0, countOne = 0, count;
ZZ number;
for (int i = 0; i < iterations; i++) {
number = generateNumberLFSR(1024);
while (number != 0) {
switch (bit(number, 0) /*number % 2*/) {
case 0:
countZero++;
break;
case 1:
countOne++;
break;
}
number >>= 1;
}
}
double percentage = 0;
count = countOne + countZero;
percentage = static_cast<double>(countZero) / static_cast<double>(count);
std::cout << "Percentages of\n0: " << percentage * 100 << "%\n1: " << (1 - percentage) * 100 << "%\n";
}
void assignString(unsigned char key[256], unsigned int& keyLength, std::string S) {
keyLength = S.size();
for (int i = 0; i < keyLength; i++) {
key[i] = S[i];
}
key[keyLength] = '\0';
}
void RC4(unsigned char key[256], unsigned int keyLength, unsigned char plaintext[256], unsigned int plaintextLength, unsigned char cryptotext[256]) {
for (i = 0; i < 256; i++)
S[i] = i;
j = 0;
for (i = 0; i < 256; i++) {
j = (j + S[i] + key[i % keyLength]) % 256;
swaper = S[i];
S[i] = S[j];
S[j] = swaper;
}
i = 0;
j = 0;
for (int index = 0; index < plaintextLength; index++) {
i = (i + 1) % 256;
j = (j + S[i]) % 256;
swaper = S[i];
S[i] = S[j];
S[j] = swaper;
keystream[index] = S[(S[i] + S[j]) % 256];
}
for (i = 0; i < plaintextLength; i++) {
cryptotext[i] = plaintext[i] ^ keystream[i];
}
cryptotext[plaintextLength] = '\0';
}
void testBiasRC4(unsigned int iterations) {
unsigned char key[256];
unsigned int keyLength;
unsigned char S[256];
unsigned char swaper;
int i, j, k;
unsigned int secondZero = 0;
for (int tries = 0; tries < iterations; tries++) {
do {
keyLength = generateNumberLFSR8bit();
} while (keyLength <= 0 || keyLength >= 256);
for(i=0;i<keyLength;i++)
key[i] = generateNumberLFSR8bit();
for (i = 0; i < 256; i++)
S[i] = i;
j = 0;
for (i = 0; i < 256; i++) {
j = (j + S[i] + key[i % keyLength]) % 256;
swaper = S[i];
S[i] = S[j];
S[j] = swaper;
}
i = 0;
j = 0;
for (int index = 0; index < 2; index++) {
i = (i + 1) % 256;
j = (j + S[i]) % 256;
swaper = S[i];
S[i] = S[j];
S[j] = swaper;
k = S[(S[i] + S[j]) % 256];
}
if (k == 0)secondZero++;
}
double exp = static_cast<double>(1) / 128;
double real = static_cast<double>(secondZero) / iterations;
std::cout << "Expectation: " << std::fixed << exp << "\n";
std::cout << "Reality: " << std::fixed << real << "\n";
}
int main(){
int choice = 1;
int choiceLFSR = -1;
int choiceRC4 = -1;
int decryptRC4 = 0;
double timeLFSR, timeBBS, timeJacobi;
time_t start, end;
std::string text;
while (choice) {
switch (choice) {
case 1:
std::cout << "1. LFSR\n2. RC4\n3. Exit\n";
do {
std::cin >> choice;
} while (choice <= 0 || choice > 3);
choice++;
if (choice == 3) {
assignString(key, keyLength, "Key");
assignString(plaintext, plaintextLength, "Plaintext");
}
break;
case 2:
std::cout << "1. Generate Number With LFSR\n2. Compare Performance with other Generators\n3. Test Percentage\n4. Back\n";
do {
std::cin >> choiceLFSR;
} while (choiceLFSR <= 0 || choiceLFSR > 4);
switch (choiceLFSR) {
case 1:
std::cout << generateNumberLFSR(1024) << "\n";
break;
case 2:
start = clock();
for (int i = 0; i < 100; i++)
generateNumberLFSR(1024);
end = clock();
timeLFSR = ((double)(end - start) / double(CLOCKS_PER_SEC)) / 100;
start = clock();
for (int i = 0; i < 10; i++)
generateNumberBBS(1024);
end = clock();
timeBBS = ((double)(end - start) / double(CLOCKS_PER_SEC)) / 10;
start = clock();
for (int i = 0; i < 5; i++)
generateNumberJacobi(1024);
end = clock();
timeJacobi = ((double)(end - start) / double(CLOCKS_PER_SEC)) / 5;
std::cout << "Time taken for LFSR => " << std::fixed << timeLFSR << "\n";
std::cout << "Time taken for BBS => " << std::fixed << timeBBS << "\n";
std::cout << "Time taken for Jacobi => " << std::fixed << timeJacobi << "\n";
std::cout << "The Time was aproximated for one Iteration\n";
break;
case 3:
testPercentage(100);
break;
case 4:
choice = 1;
}
break;
case 3:
std::cout << "1. Input key\n2. Input Plaintext\n3. Encrypt\n4. ";
if (decryptRC4 == 0)std::cout << "[Blocked]";
std::cout << "Decrypt\n5. Test Bias\n6. Back\n";
do {
std::cin >> choiceRC4;
if (choiceRC4 == 4 && decryptRC4 == 0)choiceRC4 = 0;
} while (choiceRC4 <= 0 || choiceRC4 > 6);
switch (choiceRC4) {
case 1:
std::cin >> text;
assignString(key, keyLength, text);
break;
case 2:
std::cin >> text;
assignString(plaintext, plaintextLength, text);
break;
case 3:
RC4(key, keyLength, plaintext, plaintextLength, cryptotext);
std::cout << "Cryptotext :\nString :" << cryptotext << "\nHexadecimal :";
for (int i = 0; i < plaintextLength; i++)
std::cout << std::hex << ((long)cryptotext[i]) << " ";
std::cout << "\nInt :";
for (int i = 0; i < plaintextLength; i++)
std::cout << std::dec << ((long)cryptotext[i]) << " ";
std::cout << "\n";
decryptRC4 = 1;
break;
case 4:
RC4(key, keyLength, cryptotext, plaintextLength, cryptotext);
std::cout << "Plaintext :" << cryptotext << "\n";
break;
case 5:
testBiasRC4(10000);
break;
case 6:
choice = 1;
break;
}
break;
case 4:
choice = 0;
break;
}
}
return 0;
} | 201d14f2d00f8e670a88dc8184d3a609842b9696 | [
"C++"
] | 1 | C++ | JohnBordea/LFSRandRC4 | a79e1a982d60b1a933f48276c376121d41694c7f | 080f75005cc62ce269a69db3ece4cb6064600203 |
refs/heads/master | <repo_name>awebdev/react-nivasdevelopers<file_sep>/src/components/MainCarousel/MainCarousel.js
import React from 'react'
import Carousel from '../Carousel'
export const MainCarousel = () => (
<div className='row'>
<div className='box'>
<div className='col-lg-12 text-center'>
<h2 className='brand-before'>
<small>Welcome to</small>
</h2>
<h1 className='brand-name'><NAME></h1>
<hr className='tagline-divider' />
<h2>
<small>
By <strong><NAME></strong>
</small>
</h2>
<Carousel />
</div>
</div>
</div>
)
export default MainCarousel
<file_sep>/README.md
# Powers http://nivasdevelopers.com website using ReactJs
## Cloning
```
$ git clone https://github.com/awebdev/react-nivasdevelopers.git
```
## Get started
```
// install dependencies
$ yarn
// To start the project at http://127.0.0.1:3000
$ yarn run start
```
## Test
```
$ yarn run test
```
## Deploy
```
// compile production deploy code
$ yarn run deploy:prod
// push to github pages
$ git subtree push --prefix dist origin gh-pages
```
## Thanks to
* davezuko/react-redux-starter-kit (https://github.com/davezuko/react-redux-starter-kit)
* BlackrockDigital (https://github.com/BlackrockDigital/startbootstrap-business-casual)
<file_sep>/src/components/BookingInformation/BookingInformation.js
import React, { Component } from 'react'
import ReactGA from 'react-ga'
class BookingsInformation extends Component {
constructor (props) {
super(props)
this.openBrochure = this.openBrochure.bind(this)
}
openBrochure () {
ReactGA.event({
category: 'click',
action: 'show-brochure',
label: 'brochure'
})
var brochureUrl = 'assets/docs/Golden-Palms-Brochure-0816.pdf'
var win = window.open(brochureUrl, '_blank')
win.focus()
}
render () {
return (
<div className='row'>
<div className='box'>
<div className='col-lg-12'>
<hr />
<h4 className='text-center'>Bookings open now</h4>
<hr />
<p className='text-center'>
See our <span onClick={this.openBrochure} className='link'>brochure</span> or contact us for more details
</p>
</div>
</div>
</div>
)
}
}
export default BookingsInformation
<file_sep>/src/components/ProjectDescription/ProjectDescription.js
import React from 'react'
import PoolImg from './assets/pool-3d-view.jpg'
export const ProjectDescription = () => (
<div className='row'>
<div className='box'>
<div className='col-lg-12'>
<hr />
<h2 className='intro-text text-center'>
A place <strong>worth living in</strong>
</h2>
<hr />
<img className='img-responsive img-border img-left' src={PoolImg} alt='' />
<hr className='visible-xs' />
<p>
Vaidehi Nivas Golden Palms, a gated community by reputed builders Nivas Developers is a first of its
kind mega residential complex in Vidhyanagar, Hyderabad, ideally located in the vincity of massive
greenery and open spaces of vast Osmania University. This project would redefine the luxury of living
with the state of art design and ultra modern club house with abundant and thoughtful green spaces.
</p>
</div>
</div>
</div>
)
export default ProjectDescription
<file_sep>/src/components/Carousel/Carousel.js
import React, { Component } from 'react'
import { Carousel } from 'react-bootstrap'
import SlideImg2 from './assets/02.jpg'
import SlideImg3 from './assets/03.jpg'
import SlideImg4 from './assets/04.jpg'
class ControlledCarousel extends Component {
constructor (props) {
super(props)
this.state = {
interval: 5000
}
}
render () {
return (
<Carousel interval={this.state.interval}>
<Carousel.Item>
<img className='img-responsive img-full' src={SlideImg2} />
</Carousel.Item>
<Carousel.Item>
<img className='img-responsive img-full' src={SlideImg3} />
</Carousel.Item>
<Carousel.Item>
<img className='img-responsive img-full' src={SlideImg4} />
</Carousel.Item>
</Carousel>
)
}
}
export default ControlledCarousel
<file_sep>/src/layouts/CoreLayout/CoreLayout.js
import React, { Component } from 'react'
import Footer from 'components/Footer'
import ReactGA from 'react-ga'
import './CoreLayout.scss'
import '../../styles/core.scss'
class CoreLayout extends Component {
constructor (props) {
super(props)
ReactGA.initialize('UA-81192606-1')
}
componentDidMount () {
ReactGA.set({ page: window.location.pathname })
ReactGA.pageview(window.location.pathname)
// Feature detects Navigation Timing API support.
if (window.performance) {
// Gets the number of milliseconds since page load
// (and rounds the result since the value must be an integer).
var timeSincePageLoad = Math.round(window.performance.now())
// Sends the timing hit to Google Analytics.
ReactGA.event({
category: 'timing',
action: 'load',
label: 'JS Load time',
value: timeSincePageLoad
})
}
}
render () {
return (
<div className='core-layout__viewport'>
{this.props.children}
<Footer />
</div>
)
}
}
CoreLayout.propTypes = {
children : React.PropTypes.element.isRequired
}
export default CoreLayout
| 443f4baf26371bab113a89fdfdcf445aef1d326c | [
"Markdown",
"JavaScript"
] | 6 | Markdown | awebdev/react-nivasdevelopers | ed49eed7b19b7e7c8353490931c9cbe229880c4a | 4010ed5d08203d4c805f1367b32c85fdd6fe5421 |
refs/heads/main | <repo_name>toniseva/twitter_clone<file_sep>/README.md
# twitter_clone
a twitter clone based on https://www.youtube.com/watch?v=rJjaqSTzOxI
| 9bf3202061477b26c0261c28ab2516e5ca0e3ffd | [
"Markdown"
] | 1 | Markdown | toniseva/twitter_clone | f12296a05fe9e109c89136bfa3d85d9a0fa17016 | 027683c38f59ca8771804a251c3355928d45bf40 |
refs/heads/master | <repo_name>mbalka/js-minicamp-homework-2<file_sep>/README.md
# Homework #2
## Instructions
---
1. Feynman Writing Prompts - Write out explanations of the following concepts like you are explaining it to a 12 year old. Doing this will help you quickly discover any holes in your understanding. Ask your questions on Slack.
### for loop
The for loop iterates through a sequence, like a countdown to limit the conditions where a function performs the programmed tasks. The iteration uses the integer __i__ to act as the countdown variable.
### && || !
Double ampersands act as an __and__ logical operand to link statements together that must be performed together in order to create either a true or false condition. Double pipes act as an __or__ logical operand to tie statements together so if either is true the condition is set to true. The bang operand acts as a __reversal__ condition, and it reverses the boolean value of the condition it is attached to.
### Array
An array is a variable that stores multiple types of data, like strings, integers, and booleans. They are recalled by their location in teh array or by their tag if they are notated with a name. The individual elements can be called and manipulated just like a normal var variable can.
### git
Git is the local repository created on a computer where a file or folders are kept for working purposes. They are tracked by the revision status kept locally on the machine and later compared to the ghost repository online at GitHub.
### GitHub
GitHub is the ghost or cloud repository that mirrors the locally archived one. It is commonly used for revision control and for collaborative working on projects. It also acts as a backup for revisionary purposes so when a modification to code breaks the functionality, the code can be retrograded to a previous version for further troubleshooting and collaboration.
2. Install git. https://git-scm.com/downloads
3. Fork and clone this repo. When you need to commit use the following commands.
* git status
* git add --all
* git status
* git commit -m "your commit message"
* git push origin master
4. Make the tests pass!
#### Congratulations on finishing Homework #2!
Apply to our full-time or part-time immersive program to learn cutting edge technologies that are used by top technology companies around the world.
Our part-time and full-time courses are 13 intense weeks of focused study on the most relevant technologies.
Class sizes are small to ensure that each student gets individual attention from our world class instructors to help them succeed. We also provide career support both during and after the course to help you succeed. We are committed to your success.
For more information visit: https://www.lambdaschool.com
| b7cfee4259fa35f7866a31d147f335424f6e6485 | [
"Markdown"
] | 1 | Markdown | mbalka/js-minicamp-homework-2 | 2a2eaa4f8634cec2a9fecaabd2f09a8f48b24843 | 511afa94e3eab22ffed54c35b241b6bb05421be9 |
refs/heads/master | <file_sep>use mysql;
SELECT COUNT(*)FROM user; | af6e61ccd99bede74159bae2334655a2cebbd649 | [
"SQL"
] | 1 | SQL | khinelay/mysql-marinate | 85a49857f5043b33e157c0744125bfafe11b6013 | 0c8eceb8966e03ac3fe4f3e3c73e51166f79cc67 |
refs/heads/master | <file_sep>import allure
class Test_allure:
def setup(self):
pass
def teardown(self):
pass
@allure.step("我是测试步骤001") #添加测试步骤
def test_al1(self):
allure.attach('描述','我是测试第一步的描述') #添加测试描述
assert 1
@allure.step("我是测试步骤002") #添加测试步骤
def test_al2(self):
assert 1
@allure.step("我是测试步骤003") #添加测试步骤
def test_al3(self):
assert 0 | be4dda0ce6f9e352340df092631e6112a7e49e43 | [
"Python"
] | 1 | Python | Yuezer/test1 | 0acf6da05de4d24f5bcd01a59ff130d3c665c310 | 1b3945f8e3df23fc1c2455a7ec7af38a440cb8c2 |
refs/heads/master | <repo_name>EnigmaCreativeGroup/airco-automation<file_sep>/source/stylesheets/modules/_footer.scss
footer {
color: #fff;
text-align: center;
font-weight: 300;
font-size: 16px;
ul {
list-style-type: none;
margin: 0;
padding: 0;
li {
display: block;
margin: 0;
a {
display: block;
padding: 0 16px;
color: #fff;
&:hover, &:focus, &:active {
color: rgba(250,250,250,0.9);
font-style: none;
}
.fa {
margin-right: 8px;
font-size: 18px;
}
}
}
}
img {
width: 26px;
height: 26px;
display: inline-block;
margin-right: 10px;
}
.social {
background-color: $primary;
padding: 45px 0;
@media (min-width: 720px) {
ul li {
display: inline-block;
}
}
}
.main {
background-color: #222;
padding: 60px 0;
@media (min-width: 990px) {
ul li {
display: inline-block;
}
}
span {
margin: 45px 0 0 0;
color: rgba(250,250,250,0.2);
font-family: "Roboto",sans-serif;
font-size: 10px;
display: block;
a, a:hover, a:active, a:visited {
color: rgba(250,250,250,0.3);
}
.built-by {
font-size: 12px;
display: block;
margin-top: 10px;
}
}
}
}
// ACTIVE CLASSES
body.products-and-services footer .main ul li:nth-of-type(1) a {
color: $secondary;
font-weight: bold;
}
body.safety footer .main ul li:nth-of-type(3) a {
color: $secondary;
font-weight: bold;
}
body.projects footer .main ul li:nth-of-type(2) a {
color: $secondary;
font-weight: bold;
}
body.contact footer .main ul li:nth-of-type(7) a {
color: $secondary;
font-weight: bold;
}<file_sep>/source/products-and-services.html.erb
---
title: Products & Services
---
<section class="u-hero services">
<div class="container">
<div class="u-screen"></div>
<div class="u-foreground">
<h1>Products & Services</h1>
</div>
</div>
</section>
<section class="l-services-body">
<div class="container">
<h2>Products & Services</h2>
<h4>Turnkey Direct Digital Control Systems</h4>
<div class="row">
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/pm.png' %>
<h5>Project Management</h5>
</div>
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/engineering.png' %>
<h5>Engineering</h5>
</div>
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/programming.png' %>
<h5>Programming</h5>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/installation.png' %>
<h5>Installation</h5>
</div>
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/commissioning.png' %>
<h5>Commissioning / Retro – Commissioning</h5>
</div>
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/training.png' %>
<h5>Training</h5>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/monitor.png' %>
<h5>Energy Monitoring & Efficiency Reporting</h5>
</div>
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/lighting.png' %>
<h5>Lighting Control</h5>
</div>
<div class="col-xs-12 col-sm-4">
<%= image_tag 'icons/remote.png' %>
<h5>Remote Building Monitoring</h5>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-4 col-sm-offset-4">
<%= image_tag 'icons/service.png' %>
<h5>Maintenance and 24/7 Service</h5>
</div>
</div>
</div>
</section>
<section class="u-cta">
<div class="container">
<div class="u-forground">
<h2>Get Started</h2>
<p>
Maximize your buildings comfort, security, and efficiency.
</p>
<%= link_to 'Contact Us', 'contact.html', :class=>'button button-green' %>
</div>
</div>
</section><file_sep>/source/stylesheets/layouts/_contact.scss
.u-hero.contact {
background-image: url('../images/splash-contact.jpg');
background-position: center center;
}
.l-contact-splash {
h2 {
color: $primary;
}
h4 {
color: $secondary;
font-size: 18px;
margin: 0 auto 30px auto;
}
p.address {
font-size: 18px;
font-weight: bold;
}
}<file_sep>/source/stylesheets/layouts/_products-and-services.scss
.u-hero.services {
background-image: url('../images/splash-services.jpg');
background-position: center center;
}
.l-services-body {
padding: 35px 0;
text-align: center;
.col-xs-12 {
margin-bottom: 25px;
}
h2 {
color: $primary;
}
h4 {
margin-bottom: 70px;
}
img {
margin:0 auto 30px auto;
max-width: 85px;
opacity: 0.4;
}
h5 {
margin: 0 0 45px 0;
color: #333;
}
}<file_sep>/source/_footer.html.erb
<footer>
<!-- <section class="social">
<div class="container">
<ul>
<li><% link_to 'javascript:void(0);', :target=>'_blank' do %><i class="fa fa-facebook-square"></i>Facebook<% end %></li>
<li><% link_to 'javascript:void(0);', :target=>'_blank' do %><i class="fa fa-linkedin-square"></i>Linked In<% end %></li>
<li><% link_to 'javascript:void(0);', :target=>'_blank' do %><i class="fa fa-twitter-square"></i>Twitter<% end %></li>
</ul>
</div>
</section> -->
<section class="main">
<div class="container">
<ul>
<li><%= link_to 'Products & Services','products-and-services.html'%></li>
<li><%= link_to 'Projects','projects.html'%></li>
<li><%= link_to 'TABB & Fire Life Safety','safety.html'%></li>
<li><%= link_to 'Contact','contact.html'%></li>
</ul>
<span>
Icons made by <a href="http://www.freepik.com" title="Freepik">Freepik</a> from <a href="http://www.flaticon.com" title="Flaticon">www.flaticon.com</a> is licensed by <a href="http://creativecommons.org/licenses/by/3.0/" title="Creative Commons BY 3.0">CC BY 3.0</a>
<br>
Copyright 1974-2015 Airco Mechanical Inc. All Rights Reserved.
<div class="built-by">Built by <%= link_to 'KnackStudios.io', 'http://knackstudios.io', :target=>'_blank' %></div>
</span>
</div>
</section>
</footer><file_sep>/source/contact.html.erb
---
title: Contact
---
<section class="u-hero contact">
<div class="container">
<div class="u-screen"></div>
<div class="u-foreground">
<h1>Contact</h1>
</div>
</div>
</section>
<div class="l-contact-splash padding-md">
<div class="container">
<div class="row">
<div class="col-xs-12 col-md-8 col-md-offset-2 u-text-center">
<h2>Get in Touch!</h2>
<p>
For more info and general questions.
</p>
<div class="margin-md"></div>
<div class="row margin-sm">
<div class="col-xs-12 col-sm-6 col-sm-offset-3">
<h4>Sacramento<br>Airco Automation Inc.</h4>
<p class="address">
8210 Demetre Avenue<br>
Sacramento, California 95828<br>
Telephone (916) 381-4523<br>
Fax (916) 386-0350
</p>
</div>
</div>
</div>
</div>
</div>
</div><file_sep>/source/projects.html.erb
---
title: Projects
---
<section class="u-hero projects">
<div class="container">
<div class="u-screen"></div>
<div class="u-foreground">
<h1>Projects</h1>
</div>
</div>
</section>
<section class="margin-sm">
<section class="l-projects-body">
<div class="container">
<div class="row">
<div class="row-height">
<div class="col-xs-12 col-lg-6 col-lg-height col-middle">
<div class="row margin-sm">
<div class="row-height">
<div class="col-xs-12 col-md-4 col-md-height col-middle">
<%= image_tag 'projects/citizens.jpg' %>
</div>
<div class="col-xs-12 col-md-8 col-md-height">
<h2>Citizen Hotel</h2>
<h4>Sacramento, CA</h4>
<p>
The Citizen Hotel project consisted of the renovation of a historic 14-story office building into a 198-room boutique hotel and restaurant located on J Street in Downtown Sacramento. The 135,000-square-foot, design/build full-service hotel reflects the authentic and electric ambience of the surrounding community.
</p>
</div>
</div>
</div>
</div>
<div class="col-xs-12 col-lg-6 col-lg-height col-middle">
<div class="row margin-sm">
<div class="row-height">
<div class="col-xs-12 col-md-4 col-md-height col-middle">
<%= image_tag 'projects/digitech.jpg' %>
</div>
<div class="col-xs-12 col-md-8 col-md-height">
<h2>Digital Technology Laboratory Corporation Headquarters</h2>
<h4>Davis, CA</h4>
<p>
Located in Davis, California, the Digital Technology Laboratory Corporation (DTL) Headquarters project was a 70,000-square-foot, LEED Gold Certified, built-to-suit facility. The new state-of-the-art facility consisted of office and laboratory space that housed the research and development branch, DTL, of the international machine tool company, Mori Seiki Company, Ltd.
</p>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="row">
<div class="row-height">
<div class="col-xs-12 col-lg-6 col-lg-height col-middle">
<div class="row margin-sm">
<div class="row-height">
<div class="col-xs-12 col-md-4 col-md-height col-middle">
<%= image_tag 'projects/500.jpg' %>
</div>
<div class="col-xs-12 col-md-8 col-md-height">
<h2>500 Capitol Mall</h2>
<h4>Sacramento, CA</h4>
<p>
The 500 Hundred Capitol Mall building (commonly known as the Bank of the West Tower) stands a lustrous 25-stories tall in the heart of Sacramento’s business district. The 430,000 square foot, design/build Class “A” office building provides its tenants with an elite business experience and panoramic views of the Sacramento skyline, Sierra Nevadas and the Capitol Rotunda.
</p>
</div>
</div>
</div>
</div>
<div class="col-xs-12 col-lg-6 col-lg-height col-middle">
<div class="row margin-sm">
<div class="row-height">
<div class="col-xs-12 col-md-4 col-md-height col-middle">
<%= image_tag 'projects/ziggurat.jpg' %>
</div>
<div class="col-xs-12 col-md-8 col-md-height">
<h2>Ziggurat Building</h2>
<h4>West Sacramento, CA</h4>
<p>
Undoubtedly one of Sacramento’s most recognized landmarks, the terraced-stepped, pyramid-designed Ziggurat Building is a 10-story, 400,000-square-foot office building that was constructed utilizing the design/build delivery method. The building is currently occupied by the State of California’s Department of General Services (DGS).
</p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</section>
</section>
<section class="u-cta">
<div class="container">
<div class="u-forground">
<h2>Get Started</h2>
<p>
Maximize your buildings comfort, security, and efficiency.
</p>
<%= link_to 'Contact Us', 'contact.html', :class=>'button button-green' %>
</div>
</div>
</section><file_sep>/source/_nav.html.erb
<nav>
<div class="container">
<% link_to '/', :class=>'site-id' do %>
<%= image_tag 'logo.jpg' %>
<% end %>
<!-- toggle hamburger button -->
<div class="ecg-burger"></div>
<!-- menu -->
<section class="ecg-menu">
<ul>
<li><%= link_to 'Products & Services','products-and-services.html'%></li>
<li><%= link_to 'Projects','projects.html'%></li>
<li><%= link_to 'TABB & Fire Life Safety','safety.html'%></li>
<li><%= link_to 'Contact','contact.html'%></li>
</ul>
</section>
</div>
</nav><file_sep>/source/safety.html.erb
---
title: TABB & Fire Life Safety
---
<section class="u-hero safety">
<div class="container">
<div class="u-screen"></div>
<div class="u-foreground">
<h1>TABB & Fire Life Safety</h1>
</div>
</div>
</section>
<section class="l-safety-body padding-lg">
<div class="container">
<h2 class="u-blue-title">TABB & Fire Life Safety</h2>
<div class="row padding-xs">
<div class="col-xs-12 col-sm-4 col-sm-offset-4">
<%= image_tag 'tabb.gif' %>
</div>
</div>
<div class="padding-sm row">
<div class="col-xs-12 col-sm-8 col-sm-offset-2">
<p>
As a <a href="http://www.tabbcertified.org/site/public/content/index/home" target="_blank">TABB certified* company</a>, Airco Automation, Inc offers design professionals, engineers and building owners the highest standard in testing and balancing of commercial HVAC systems.
</p>
</div>
</div>
<div class="row padding-md">
<div class="col-xs-12 col-sm-8 col-sm-offset-2">
<h5>Airco’s Testing, Adjusting and Balancing (TAB) Services</h5>
<ul>
<li>
TABB (Testing Adjusting and Balancing Bureau)
<ul>
<li>Certified TABB reports</li>
<li>ANSI (American National Standards Institute)</li>
<li>
TAB Services
<ul>
<li>Hydronic balance</li>
<li>Air Balance</li>
<li>Outside air testing & documentation</li>
</ul>
</li>
</ul>
</li>
<li>
Fire Life Safety (FLS) <a href="fire-life-safety.pdf" target="_blank">View PDF</a>
<ul>
<li>Certified technicians and supervisors</li>
<li>Periodic damper inspection program</li>
<li>Duct smoke detector velocity testing</li>
</ul>
</li>
<li>
Indoor Air Quality (IAQ)
<ul>
<li>Investigation and solution Services</li>
</ul>
</li>
<li>
Hospital, Laboratory & Clean Room Services
<ul>
<li>Perform 2009 clean room certification to ISO 14644 standards</li>
<li>Isolation room testing</li>
<li>HEPA filter integrity tests</li>
<li>Particle counting</li>
<li>Shaker calibration</li>
<li>Walk-in freezer mapping</li>
<li>CDA clean dry air testing</li>
<li>Bacterial counts</li>
<li>
Fume hood certification
<ul>
<li>ASHRAE 110 test</li>
</ul>
</li>
</ul>
</li>
<li>
Sensor Calibration
<ul>
<li>Oxygen sensor</li>
<li>CO2 sensor</li>
<li>Humidity sensor</li>
</ul>
</li>
<li>
Specialty Adjustment & Testing Services
<ul>
<li>Laser alignment of belts, sheaves, and motors</li>
</ul>
</li>
<li>
Title 24 Non-Residential Mechanical Acceptance Testing
<ul>
<li>Certified Mechanical Acceptance Test Technicians on Staff</li>
<li>Certified Mechanical Acceptance Test Employer</li>
<li>3rd Party Testing & Certifications</li>
<li>Mechanical Testing Certification Consultations available to help you navigate the new standards</li>
</ul>
</li>
</ul>
</div>
</div>
</div>
</section>
<section class="u-cta">
<div class="container">
<div class="u-forground">
<h2>Get Started</h2>
<p>
Maximize your buildings comfort, security, and efficiency.
</p>
<%= link_to 'Contact Us', 'contact.html', :class=>'button button-green' %>
</div>
</div>
</section><file_sep>/source/index.html.erb
---
title: Airco Automation
---
<section class="u-hero home">
<div class="container">
<div class="u-screen"></div>
<div class="u-foreground">
<h1>Energy Solutions by Design.</h1>
</div>
</div>
</section>
<section class="margin-lg l-splash">
<div class="container">
<div class="row">
<div class="row-height">
<div class="col-xs-12 col-md-4 col-md-height col-middle">
<%= image_tag 'splash.jpg' %>
</div>
<div class="col-xs-12 col-md-6 col-md-height col-middle">
<h2>Since 1992</h2>
<p>
Airco Automation, Inc. has been providing products and services to to maximize comfort, security, and efficiency for all types of building applications - Commercial, Educational Institutions, Government Entities, Healthcare Facilities, Hospitality Industry, Industrial Facilities, and Technology Facilities to name a few.
</p>
<p>
Airco Automation, Inc. uses products that enable building owners and property managers to integrate, automate, and manage their buildings easily and efficiently by combining them onto one network and one computer graphical user interface using automation software. Building Automation Systems (BAS) greatly increase occupant comfort, lower energy use, and allow off-site building control from anywhere in the world.
</p>
</div>
</div>
</div>
</div>
</section>
<section class="u-quote-section">
<div class="container">
<div class="u-forground">
<h2 class="u-quote">
<i class="fa fa-quote-right"></i>We maximize value by selecting the solution and system that represent the best.<i class="fa fa-quote-left"></i>
</h2>
</div>
</div>
</section>
<section class="l-body-content">
<div class="container">
<div class="row">
<div class="row-height">
<div class="col-xs-12 col-sm-6 col-md-4 col-sm-height col-middle margin-sm">
<h4>Airco Automation, Inc.<br>Specializes in:</h4>
<ul class="m-marketing">
<li>LEED Certification</li>
<li>Demand Response Programs</li>
<li>Performance-Based Retrofits</li>
</ul>
</div>
<div class="col-xs-12 col-sm-6 col-md-8 col-sm-height col-middle">
<p>
We are your proactive partners, coordinating engineering through implementation and always using only the best products and services at competitive pricing. We welcome the opportunity to apply our ability and experience to your building or renovation project.
</p>
<p>
By integrating our expertise, knowledge, technology, and years of experience we can apply today's complex and innovative products to the solution of all of your building operational and improvement needs.
</p>
<p>
The true benefit to our clients is that they have the confidence and certainty of knowing they have the most cost-effective, secure, and intelligent Energy Management System installed.
</p>
</div>
</div>
</div>
</div>
</section>
<section class="u-cta">
<div class="container">
<div class="u-forground">
<h2>Get Started</h2>
<p>
Maximize your buildings comfort, security, and efficiency.
</p>
<%= link_to 'Contact Us', 'contact.html', :class=>'button button-green' %>
</div>
</div>
</section>
<!--
<a class="fancybox button" data-fancybox-type="iframe" href="contact-form.html">
Hire us
</a> -->
<script type="text/javascript">
$(document).ready(function() {
$(".fancybox").fancybox();
});
</script> | 7b54d8f70bd1e2cdf5ae9b889589664511ad3272 | [
"HTML+ERB",
"SCSS"
] | 10 | HTML+ERB | EnigmaCreativeGroup/airco-automation | b3fb35b73944763283523a88bfd8cf47475570cc | 1ed57c9b63c224046843dd272bfbe1d75bc6d6de |
refs/heads/master | <repo_name>SmartCyberUniversity/SmartCyberUniversity<file_sep>/README.md
# SmartCyberUniversity
Next generation education management system.
| 0e943ab679cfbb34c66523f5ba2d64209e60529c | [
"Markdown"
] | 1 | Markdown | SmartCyberUniversity/SmartCyberUniversity | c08b362eb2145be2b03cf4b97c1f1f9a224b54d3 | b0434d9a1fb875de9ffe471ab77a07fcdb40563e |
refs/heads/master | <file_sep>Brett's PopClip Extensions
=================
My (hopefully) growing collection of [PopClip][1] extensions.
### Installation
For the time being, I'm just including these as source, not `.popclipextz` packages. To install, just make sure the extension of a folder is `.popclipext` and double click it in Finder.
### Markdownify ###
This extension converts selected HTML text to Markdown using html2text by <NAME>artz. Reference-style links are used and the references are placed after the text. Wrapping is disabled.
The extension shows up in PopClip as "2MD".
### OpenURLS
This extension searches selected text for urls (http or custom handlers) and opens all urls in their default applications.
The default OpenInChrome extension is Chrome-specific and only works with a single URL. This extension allows as many urls as you can select and will open whatever browser your system has assigned to the handler. This includes nvalt:// and txmt:// links, as well as http:// links. If you use something like [Choosy][2] it will open them through that.
[1]: http://pilotmoon.com/popclip/
[2]: http://www.choosyosx.com/
| d3dde7485f20651ec1be0c2f29d86410ca923990 | [
"Markdown"
] | 1 | Markdown | zenweasel/popclipextensions | b292ca99cf4323ed81b75f955eb10006ce9d46de | f19ad3db021943c6448feefa7eabe1bbfd547263 |
refs/heads/master | <repo_name>masoudhaghbin/PHPMASTERS<file_sep>/profile.php
<?php
include("config.php");
session_start();
if (!isset($_SESSION['username'])){
header("location:home.php"); ;
}
$flag="";
$username = $_SESSION['username'];
connect();
$result = mysql_query("select * from users where username='$username' ") or die(mysql_error());
$first_name = mysql_result($result,0,3) or die(mysql_error());
$last_name = mysql_result($result,0,4) or die(mysql_error());
$email = mysql_result($result,0,2) or die(mysql_error());
if(isset($_POST['submit']) && isset($_POST['name']) && isset($_POST['year']) && isset($_POST['writer'])){
$username = $_SESSION['username'];
$name = $_POST['name'];
$Writer=$_POST['writer'] ;
$year = $_POST['year'] ;
mysql_query("INSERT INTO book VALUES ('$name','$Writer','$year') ") or die(mysql_error());
mysql_query("INSERT INTO userbook VALUES ('$username','$name',10) ") or die(mysql_error());
if (mysql_affected_rows()){
$flag="your post Successful save ." ;
}
else{
$flag="your post not Successful save .";
}
}
?>
<html>
<head>
<title>Hello!</title>
</head>
<body>
<img src ="profile-150x150.png" height="100" width="80" />
<div style=" border-style: solid; border-color:black; border-width:2px; height:500px; width:200px;">
latest books you introduced
<ol>
<?php
$result=mysql_query("select BookName from userbook where username='$username'") or die(mysql_error());
for ($i=0;$i!=mysql_num_rows($result);$i++){
$book=mysql_result($result,$i);
print("<li>$book</li>");
}
?>
</ol>
</div>
<form action="" style="right:0; margin-top:-600px; position: absolute; ">
<input type="text" placeholder="search" />
<input type="submit" value="search" />
</form>
<?php
$result=mysql_query("select count(BookName) from userbook where username='$username'") or die(mysql_error());
$num=mysql_result($result,0) ;
print ("<div style=' border-style: solid; border-color:black; border-width:2px; height:500px; width:200px; float:right; margin-top:-500px;'>
about you <br>
Name : $first_name <br>
Username : $username <br>
number of books you introdused : $num <br> </div>");
?>
<div>
<form action="" method="post"style="margin-left:500px; margin-top:-150px; position: absolute; ">
<<<<<<< HEAD
<legend> introducing new book ... </legend> <br>
=======
introducing new book ... <br>
>>>>>>> origin/master
<input type="text" name="name" placeholder="Book name" /> <br>
<input type="text" name="writer" placeholder="writer name" /> <br>
<input type="text" name="year" placeholder="publish year" /> <br>
<textarea placeholder="your opinion ...">
</textarea>
<input name="submit" type="submit" value="post" />
</form>
<?php
print("<h3>$flag</h3>") ;
?>
</div>
</body>
</html>
<file_sep>/home.php
<?php
include("config.php");
session_start();
connect();
$flag="";
if(isset($_POST['Logout'])) {
session_destroy();
header("location:home.php") ;
}
if (isset($_POST["login"])) {
$username = $_POST['user'];
$password = $_POST['pass'];
$result = mysql_query("select password from users where Username = '$username' ") or die(mysql_error());
if (mysql_num_rows($result)!=1){
$flag='The User Name And/Or Password is incorrect!Please try again...';
}
else if(mysql_result($result,0)==$password){
$_SESSION['username']=$username;
}
else {
$flag='The User Name And/Or Password is incorrect!Please try again... ';}
}
if (isset($_SESSION['username'])){
$username = $_SESSION['username'];
$result = mysql_query("select * from users where Username='$username' ") or die(mysql_error());
$first_name = mysql_result($result,0,3) or die(mysql_error());
$last_name = mysql_result($result,0,4) or die(mysql_error());
$email = mysql_result($result,0,2) or die(mysql_error());
}
if (isset($_POST['send']) )
{
$first_name = $_POST['firstname'];
$last_name = $_POST['lastname'] ;
$e_mail = $_POST['email'] ;
$user_name = $_POST['username'] ;
$passwd = $_POST['<PASSWORD>'] ;
$result = mysql_query("select * from users where Username='$user_name' or Email='$e_mail' ") or die(mysql_error()) ;
if ($passwd!=$_POST['confirmpassword']){
$flag="password and confirmpassword is not equal";
}
else if (mysql_num_rows($result)!=0) {
$flag="email or username is reapited please insert another email or username" ;
}
else if (strlen($passwd) < 4){
$flag="your password is weak atlesat password must be 4 lenght" ;
}
else {
mysql_query("INSERT INTO users VALUES ('$user_name','$e_mail','$passwd','$first_name','$last_name') ") or die(mysql_error()) ;
if (mysql_affected_rows()){
$_SESSION['username']=$user_name;
}
else {
die("your insert information don't Successful") ;
}
}
}
?>
<html lang="fa" dir="rtl">
<head>
<meta http-equiv="Content-type" value="text/html; charset=UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title>سایت اشتراک اطلاعات کتاب</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Rotating Words with CSS Animations" />
<meta name="keywords" content="css3, animations, rotating words, sentence, typography" />
<meta name="author" content="Codrops" />
<link href='http://fonts.googleapis.com/css?family=Bree+Serif|Open+Sans+Condensed:700,300,300italic' rel='stylesheet' type='text/css'>
<link rel="stylesheet" type="text/css" href="style.css" />
<script src="http://code.jquery.com/jquery-latest.min.js" type="text/javascript" charset="utf-8"></script>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script>
<script src="jquery_popup.js"></script>
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="jquery.autocomplete.js"></script>
<style>
.no-cssanimations .rw-wrapper .rw-sentence span:first-child{
opacity: 1;
}
</style>
<script>
$(document).ready(function(){
$("#tag").autocomplete("autocomplete.php",
{
selectFirst: true
});
});
</script>
</head>
<body>
<section class="rw-wrapper">
<h2 class="rw-sentence">
<span>شما در این جا</span>
<br />
<span>می تونید</span>
<div class="rw-words rw-words-1">
<span>کتاب معرفی کنید...</span>
<span>درباره کتاب های موجود نظر بدید...</span>
<span>به کتاب ها نمره بدید...</span>
<span>و...</span>
</div>
<br />
</h2>
</section>
<div class="bmenu" >
<h2 style="margin-top:0px;"><font color="#e3e3e3">دسته بندی کتاب ها</font></h2>
<ul>
<li><a href="#">تاریخی</a></li>
<li><a href="#">رمان</a></li>
<li><a href="#">فلسفی</a></li>
<li><a href="#">مذهبی</a></li>
<li><a href="#">علمی</a></li>
<li><a href="#">هنری</a></li>
<li><a href="#">جغرافیا</a></li>
<li><a href="#">سیاسی</a></li>
<li><a href="#">اقتصاد</a></li>
<li><a href="#">ادبیات</a></li>
<li><a href="#">فرهنگی</a></li>
<li><a href="#">آشپزی</a></li>
<li><a href="#">پزشکی</a></li>
<li><a href="#">صنعت</a></li>
</ul>
</div>
<?php
if (!isset($_SESSION['username'])) {
print("
<div class='signinpart'>
<form method='POST' action='' >
<ul>
<label><font color='#FFFFFF'>
<form method='POST' action='autocomplete.php'>
جستجو براساس
<input type='radio' name='search_bench' value='book'> کتاب
<input type='radio' name='search_bench' value='writer'> نویسنده
<input type='radio' name='search_bench' value='year'> سال انتشار
<input name='tag' type='text' id='tag' size='20' >
<input type='submit' name='search' value= 'جستجو' >
</form>
</font></label>
<li><input type='text' name='user' placeholder='نام کاربری' ></li>
<li><input type='password' name='pass' placeholder='<PASSWORD> ' ></li>
<li><input type='submit' name='login' value= 'ورود' ></li><br>
<a href='#' id='onclick'>هنوز ثبت نام نکردهاید؟</a>
</ul>
</form>
<br>
<h4><font color='red'>$flag</font></h4>
</div> ");
}
else{
print("<div class='signinpart'> <h2 style='margin-left:0px'><font color='#e3e3e3'> $first_name   $last_name</font></h2> </div>");
print("<div class='signinpart'><form method='post' action=''><input name='Logout' type='submit' value='خروج' /></form></div>");
}
?>
<div id="contactdiv">
<form class="form" method="post" action="" id="contact" accept-charset="UTF-8">
<img src="button_cancel.png" class="img" id="cancel"/>
<h3>فرم ثبت نام</h3>
<hr/><br/>
<label>نام: <span>*</span></label>
<br/>
<input type="text" id="firstname" name="firstname" placeholder="first name"/><br/>
<br/>
<label>نام خانوادگی: <span>*</span></label>
<br/>
<input type="text" name="lastname" id="lastname" lastname placeholder="last name"/><br/>
<br/>
<label>نام کاربری: <span>*</span></label>
<br/>
<input type="text" name="username" id="username" placeholder="user name"/><br/>
<br/>
<label>ایمیل:<span>*</span></label>
<br/>
<input type="text" name="email" id="email" placeholder="email"/><br/>
<br/>
<label>رمز عبور:<span>*</span></label>
<br/>
<input type="<PASSWORD>" name="password" id="password" placeholder="<PASSWORD>"/><br/>
<br/>
<label>تکرار رمز عبور:<span>*</span></label>
<br/>
<input type="password" name="confirmpassword" id="confirmpassword" placeholder="confirm password"/><br/>
<br/>
<input type="submit" name="send" id="send" value="ارسال"/>
<input type="submit" name="cancel" id="cancel" value="انصراف"/>
<br/>
</form>
</div>
<div id="footer" dir="ltr" >
<p class="copyright">© 2014 All Rights Reserved • Design by <a href="http://www.freecsstemplates.org/">BISC GROUP</a>.</p>
</div>
</body>
</html><file_sep>/autocomplete.php
<?php
include('config.php');
connect();
session_start();
$q=$_GET['q'];
$my_data=mysql_real_escape_string($q);
if(isset($_POST['search_bench']))
{
if($_POST['search_bench'] == 'book')
{
echo('book');
}
else
{
echo(' not book ');
}
echo('safsf');
}
else
{
echo ('not set');
}
$sql="SELECT Name FROM Book WHERE Name LIKE '%$my_data%' ORDER BY Name";
$result = mysql_query($sql) or die(mysqli_error());
if($result)
{
while($row=mysql_fetch_array($result))
{
echo $row['Name']."\n";
}
}
?> | 5bffcd60ba0f76e54b95cad7597ed9dd271645a7 | [
"PHP"
] | 3 | PHP | masoudhaghbin/PHPMASTERS | c0f7b4f2d08ee10abdee4c2ef70830d7db51f778 | 30f64f67ed29cf80a2b0dc762db6421177a1f34f |
refs/heads/master | <repo_name>JonathanCauchi/Data-Science-Data-to-Knowledge-Project<file_sep>/Html_to_CSV.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Wed Dec 18 16:15:41 2019
@author: joncauchi
"""
from os import listdir
from os.path import isfile, join
from bs4 import BeautifulSoup
import csv
def main():
mypath = "/home/joncauchi/Desktop/Part3/data/"
onlyfiles = [f for f in listdir(mypath) if isfile(join(mypath, f))]
onlyfiles.sort()
#for loop hawnhekk
for i in range(len(onlyfiles)):
add_name = str(i)
soup = BeautifulSoup(open("data/"+onlyfiles[i]), "html.parser")
properties = soup.find_all("p",{"class":None})
filename = "property"+add_name+".csv"
path_to_file = join("/home/joncauchi/Desktop/Part3/CSVfiles/", filename)
f = open(path_to_file,"w")
f = open("property"+add_name+".csv", "w")
writer = csv.writer(f)
writer.writerow(["Date","Property Description"]) # Write column headers as the first line
for i in range(len(properties)):
dates = soup.find_all("h2",{"class":"classified_date default_top_margin"})
dates_csv = dates[0].text
properties = soup.find_all("p",{"class":None})
properties_csv = properties[i].text
writer.writerow([dates_csv, properties_csv])
f.close()
if __name__ == '__main__':
main()
| e5c0ebfaf72d8360228cb0636337903f6d2768b4 | [
"Python"
] | 1 | Python | JonathanCauchi/Data-Science-Data-to-Knowledge-Project | 910b57d75e09093628ff1dfacbfae7c1928e4b1a | 684f37afdd9834725606ae3c9e7b372315dd5e9f |
refs/heads/master | <file_sep>from itertools import chain, product
import numpy as np
import mxnet as mx
from bqplot import *
from bqplot.marks import Graph
from ipywidgets import IntSlider, Dropdown, RadioButtons, HBox, VBox, Button, Layout
from bqplot import pyplot as plt
from bqplot import OrdinalScale, OrdinalColorScale
from bqplot.colorschemes import CATEGORY10
from IPython.display import display
class MLPDashboard(VBox):
def __init__(self, net, path, name, **kwargs):
self.net = net
self.path = path
self.name = name
self.data = kwargs.pop('data', None)
self.data = mx.nd.array(self.data)
self.ctx = kwargs.pop('ctx', mx.cpu())
self.num_epochs = kwargs.pop('num_epochs', 10)
self.height = kwargs.get('height', 800)
self.width = kwargs.get('width', 900)
self.directed_links = kwargs.get('directed_links', False)
self.get_shapes()
self.layer_colors = kwargs.get('layer_colors',
['Orange'] * (len(self.num_hidden_layers) + 2))
self.build_net()
self.create_charts()
self.graph.observe(self.hovered_change, 'hovered_point')
super(MLPDashboard, self).__init__(children=[self.controls, self.figure], **kwargs)
self.layout = layout=Layout(min_height='1000px')
def load_epoch(self, epoch_num):
file_path = self.path + '/' + self.name + '-' + str(epoch_num) +'.params'
self.net.load_params(file_path, ctx=self.ctx)
def get_weights_for_node_at_layer(self, epoch_num, layer_num, node_num):
self.load_epoch(epoch_num)
weights = self.net.collect_params()[list(self.net.collect_params())[2*(layer_num - 1)]].data().asnumpy()
node_weights = weights[node_num-1, :]
return node_weights
def get_activations_hist(self, epoch, layer, node):
if self.data is None:
return
self.load_epoch(epoch)
outputs = self.net(self.data)
self.graph.tooltip = self.hist_figure
self.hist_figure.title = 'Activation Histogram for {}th Node at the {}th Layer - Epoch {}'.format(node, layer, epoch)
self.hist_plot.sample = outputs[len(self.num_hidden_layers) - (layer - 1)][:, node].asnumpy()
def update_bar_chart(self, layer, node):
epoch = self.epoch_slider.value
if self.mode_dd.value == 'Activations':
self.get_activations_hist(epoch, layer, node)
return
if layer == 0:
self.bar_plot.x = []
self.bar_plot.y = []
return
if self.mode_dd.value == 'Weights':
display_vals = self.get_weights_for_node_at_layer(epoch, layer, node)
elif self.mode_dd.value == 'Gradients':
display_vals = self.get_gradients_for_node_at_layer(epoch, layer, node)
self.bar_figure.title = self.mode_dd.value + ' for layer:' + str(layer) + ' node: ' + str(node) + ' at epoch: ' + str(epoch)
self.bar_plot.x = np.arange(len(display_vals))
self.bar_plot.y = display_vals
self.graph.tooltip = self.bar_figure
def hovered_change(self, change):
point_index = change['new']
self.set_colors(point_index)
if point_index is None:
return
else:
for i, n in enumerate(self.node_counts):
if point_index < n:
break
else:
point_index = point_index - n
self.update_bar_chart(i, point_index)
def set_colors(self, index):
link_data_new = []
count = 0
for v in self.graph.link_data:
v_new = {}
v_new['source'] = v['source']
v_new['target'] = v['target']
if v['target'] == index:
v_new['value'] = (count % 11) + 1
count = count + 1
else:
v_new['value'] = 0
link_data_new.append(v_new)
self.graph.link_data = link_data_new
def get_gradients_for_node_at_layer(self, epoch_num, layer_num, node_num):
self.load_epoch(epoch_num)
grads_weights = self.net.collect_params()[list(self.net.collect_params())[2*(layer_num - 1)]].grad().asnumpy()
node_gradients = grads_weights[node_num-1, :]
return node_gradients
def get_shapes(self):
shapes = []
for layer in list(self.net.collect_params()):
if self.net.prefix + 'dense0_weight' in layer:
self.num_inputs = self.net.collect_params()[layer].shape[1]
if 'weight' in layer:
shapes.append(self.net.collect_params()[layer].shape[0])
self.num_hidden_layers = shapes[:-1]
self.nodes_output_layer = shapes[-1]
self.node_counts = [self.num_inputs] + self.num_hidden_layers + [self.nodes_output_layer]
def create_charts(self):
self.epoch_slider = IntSlider(description='Epoch:', min=1, max=self.num_epochs, value=1)
self.mode_dd = Dropdown(description='View', options=['Weights', 'Gradients', 'Activations'], value='Weights')
self.update_btn = Button(description='Update')
self.bar_figure = plt.figure()
self.bar_plot = plt.bar([], [], scales={'x': OrdinalScale()})
self.hist_figure = plt.figure(title='Histogram of Activations')
self.hist_plot = plt.hist([], bins=20)
self.controls = HBox([self.epoch_slider, self.mode_dd, self.update_btn])
self.graph.tooltip = self.bar_figure
def build_net(self):
# create nodes
self.layer_nodes = []
self.layer_nodes.append(['x' + str(i+1) for i in range(self.num_inputs)])
for i, h in enumerate(self.num_hidden_layers):
self.layer_nodes.append(['h' + str(i+1) + ',' + str(j+1) for j in range(h)])
self.layer_nodes.append(['y' + str(i+1) for i in range(self.nodes_output_layer)])
self.flattened_layer_nodes = list(chain(*self.layer_nodes))
# build link matrix
i = 0
node_indices = {}
for layer in self.layer_nodes:
for node in layer:
node_indices[node] = i
i += 1
n = len(self.flattened_layer_nodes)
self.link_data = []
for i in range(len(self.layer_nodes) - 1):
curr_layer_nodes_indices = [node_indices[d] for d in self.layer_nodes[i]]
next_layer_nodes = [node_indices[d] for d in self.layer_nodes[i+1]]
for s, t in product(curr_layer_nodes_indices, next_layer_nodes):
self.link_data.append({'source': s, 'target': t, 'value': 0})
# set node x locations
self.nodes_x = np.repeat(np.linspace(0, 100,
len(self.layer_nodes) + 1,
endpoint=False)[1:],
[len(n) for n in self.layer_nodes])
# set node y locations
self.nodes_y = np.array([])
for layer in self.layer_nodes:
n = len(layer)
ys = np.linspace(0, 100, n+1, endpoint=False)[1:]
self.nodes_y = np.append(self.nodes_y, ys[::-1])
# set node colors
n_layers = len(self.layer_nodes)
self.node_colors = np.repeat(np.array(self.layer_colors[:n_layers]),
[len(layer) for layer in self.layer_nodes]).tolist()
xs = LinearScale(min=0, max=100)
ys = LinearScale(min=0, max=100)
link_color_scale = OrdinalColorScale(colors=['gray'] + CATEGORY10, domain=list(range(11)))
self.graph = Graph(node_data=[{'label': d,
'label_display': 'none'} for d in self.flattened_layer_nodes],
link_data=self.link_data,
link_type='line',
colors=self.node_colors, directed=self.directed_links,
scales={'x': xs, 'y': ys, 'link_color': link_color_scale}, x=self.nodes_x, y=self.nodes_y)
self.graph.hovered_style = {'stroke': '1.5'}
self.graph.unhovered_style = {'opacity': '0.1'}
self.graph.selected_style = {'opacity': '1',
'stroke': 'red',
'stroke-width': '2.5'}
self.figure = Figure()
self.figure.marks = [self.graph]
self.figure.title = 'Analyzing the Trained Neural Network'
self.figure.layout.width = str(self.width) + 'px'
self.figure.layout.height = str(self.height) + 'px'
| fb77e66b090b19068015daffa8016b18cb253876 | [
"Python"
] | 1 | Python | jmgaleano/PyDataNYC2017-1 | cd98858a34928757e727a005551e03a66e4d286c | cd67a92347ab0297883ba6859072b4fd8c52ea3c |
refs/heads/master | <file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package TablasIntermedias;
import java.util.ArrayList;
/**
*
* @author fabio
*/
public class IntermediaDeterminista {
private int n;
private ArrayList<IntermediaDeterministaFila> filas;
public IntermediaDeterminista(int n){
this.n = n;
this.filas = new ArrayList<>();
}
public void addFila(int Sn){
ArrayList<Double> fnSnXn = new ArrayList<Double>();
IntermediaDeterministaFila fila = new IntermediaDeterministaFila(Sn, fnSnXn);
filas.add(fila);
}
public int getN() {
return n;
}
public void setN(int n) {
this.n = n;
}
public ArrayList<IntermediaDeterministaFila> getFilas() {
return filas;
}
public void setFilas(ArrayList<IntermediaDeterministaFila> fila) {
this.filas = fila;
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package TablasInicio;
/**
*
* @author fabio
*/
public class InicioDeterminista {
private int Sn;
private int Xn;
private double fAnSn;
public InicioDeterminista(int fila, double valor) {
this.Sn = fila;
this.Xn = fila;
this.fAnSn = valor;
}
public int getSn() {
return Sn;
}
public void setSn(int Sn) {
this.Sn = Sn;
}
public int getXn() {
return Xn;
}
public void setXn(int Xn) {
this.Xn = Xn;
}
public double getFnSn() {
return fAnSn;
}
public void setFnSn(double fnSn) {
this.fAnSn = fnSn;
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package ProgramacionDinamica;
import TablasInicio.InicioDinamica;
import TablasIntermedias.IntermediaDinamica;
import java.awt.List;
import java.util.ArrayList;
/**
* @description Clase para el desarrollo de la programacion dinamica
* @version 1.0
* @authors <NAME>
* <NAME>
*/
public class ProgramacionDinamica {
private ArrayList<ArrayList<Integer>> tablaOrigen;
private ArrayList<InicioDinamica> tablaInicio;
private ArrayList<IntermediaDinamica> tablasIntermedias;
private int n;
private boolean tipoValores;
private boolean formula;
public ProgramacionDinamica(boolean tipoValores, boolean formula, ArrayList<ArrayList<Integer>> tablaOrigen, int n) {
this.tipoValores = tipoValores;
this.formula = formula;
this.tablaOrigen = tablaOrigen;
this.n = n;
this.tablaInicio = generarTablaInicio();
this.tablasIntermedias = new ArrayList<IntermediaDinamica>();
printTbI();
Inicializar();
}
public void iniciar(){
boolean primera = false;
for(int i = 0; i < tablasIntermedias.size(); i++ ){
//System.out.println(" * Tabla "+tablasIntermedias.get(i).getN());
if(n - tablasIntermedias.get(i).getN() == 1){
System.out.print(" --- primera\n");
primera = true;
for(int j = 0; j < tablasIntermedias.get(i).getFilas().size(); j++){
//tablasIntermedias.get(i).getFilas().get(j).getFnSnXn()
for(int k = 0; k < tablasIntermedias.get(i).getFilas().get(j).getFnSnXn().size(); k++){
int resF = FormulaFnDinamica(j, k, primera, tablasIntermedias.get(i).getN(), i);
tablasIntermedias.get(i).getFilas().get(j).setCasilla(k,resF);
tablasIntermedias.get(i).getFilas().get(j).buscarFAnSn();
//System.out.println("Mod "+resF);
}
}
primera = false;
printTI();
}
else{
System.out.println(" --- Tabla "+i);
for(int j = 0; j < tablasIntermedias.get(i).getFilas().size(); j++){
//tablasIntermedias.get(i).getFilas().get(j).getFnSnXn()
for(int k = 0; k < tablasIntermedias.get(i).getFilas().get(j).getFnSnXn().size(); k++){
int resF = FormulaFnDinamica(j, k, primera, tablasIntermedias.get(i).getN(), i);
tablasIntermedias.get(i).getFilas().get(j).setCasilla(k,resF);
//System.out.println("Mod "+resF);
}
}
//printTI();
}
}
}
private void printTI(){
System.out.println("------------------------");
System.out.println(" Tablas Intermedias ");
System.out.println("------------------------");
for(int l = 0; l < tablasIntermedias.size(); l++ ){
System.out.println("------------------------");
System.out.println(" * Tabla "+tablasIntermedias.get(l).getN());
System.out.println("------------------------");
for(int j = 0; j < tablasIntermedias.get(l).getFilas().size(); j++){
tablasIntermedias.get(l).getFilas().get(j).iniciarFnSnXn(tablaOrigen.size());
System.out.print(j+" "+tablasIntermedias.get(l).getFilas().get(j).getFnSnXn());
System.out.println(" "+tablasIntermedias.get(l).getFilas().get(j).getFAnSn()
+ " " +tablasIntermedias.get(l).getFilas().get(j).getXAn());
}
}
}
private void Inicializar(){
for(int i = n-1; i > 0; i-- ){
IntermediaDinamica tabla = new IntermediaDinamica(i);
//System.out.println("Tabla "+tabla.getN());
for(int j = 0; j < tablaOrigen.size(); j++){
tabla.addFila(j);
}
tablasIntermedias.add(tabla);
//System.out.println("Tabla count = "+tabla.getFilas().size());
}
System.out.println("------------------------");
System.out.println(" Tablas Intermedias ");
System.out.println("------------------------");
for(int i = 0; i < tablasIntermedias.size(); i++ ){
System.out.println("------------------------");
System.out.println(" * Tabla "+tablasIntermedias.get(i).getN());
System.out.println("------------------------");
for(int j = 0; j < tablasIntermedias.get(i).getFilas().size(); j++){
tablasIntermedias.get(i).getFilas().get(j).iniciarFnSnXn(tablaOrigen.size());
System.out.println(j+" "+tablasIntermedias.get(i).getFilas().get(j).getFnSnXn());
}
}
}
private ArrayList<InicioDinamica> generarTablaInicio() {
ArrayList<InicioDinamica> tbInicio = new ArrayList<>();
for (int i = 0; i < tablaOrigen.size(); i++) {
InicioDinamica iDeterminista = new InicioDinamica(i,tablaOrigen.get(i).get(n-1));
tbInicio.add(iDeterminista);
}
return tbInicio;
}
private void printTbI(){
System.out.println("-----------------------");
System.out.println(" Tabla Inicio ");
System.out.println("-----------------------");
System.out.println("S"+n+" - F"+n+" - X"+n);
for (InicioDinamica fila : tablaInicio) {
System.out.println(" "+String.valueOf(fila.getSn())
+" - "+String.valueOf(fila.getFnSn())
+" - "+String.valueOf(fila.getXn()));
}
System.out.println("-----------------------");
System.out.println("");
}
private int FormulaFnDinamica(int Sn, int Xn, boolean primero, int c, int tabla){
//Fn(Sn, Xn) = Pn(Xn) + fn+1(Sn-Xn)
if((Sn-Xn) >=0){
return Pn(Xn,c) + obtenerFAn(Sn-Xn, primero, tabla);
}
else{
return -1;
}
}
private int Pn(int Xn, int c) {
for(int i = 0; i < tablaOrigen.size(); i++){
if(i == Xn){
for(int j = 0; j < tablaOrigen.get(i).size(); j++){
if(j == c){
return tablaOrigen.get(i).get(j);
}
}
}
}
return 0;
}
private int obtenerFAn(int estado, boolean primero, int tabla) {
if(primero){
for(int i = 0; i < tablaInicio.size(); i++){
if(estado == tablaInicio.get(i).getSn()){
return tablaInicio.get(i).getFnSn();
}
}
}
else{
//obtener los valores de las tablas despues de la primera
for(int i = 0; i < tablaInicio.size(); i++){
if(estado == tablaInicio.get(i).getSn()){
//return tablaInicio.get(i).getFnSn();
}
}
return 0;
}
return 0;
}
}
| cadda44c8edead4afc978f2a0d3ab3d9454d5947 | [
"Java"
] | 3 | Java | barrantesosvaldo/ProgramacionDinamica2016 | 003f6992a5fb2429c9e2fd1cea6a4d6afb0ec28d | 6c09dbae4a25cc739b30da4dca99b927d0b40b4c |
refs/heads/master | <file_sep># FreeNAS
FreeNas Scripts
| ad6c40197c4158dc48382e2cdddffc1b486f82f5 | [
"Markdown"
] | 1 | Markdown | jshvartsman/FreeNAS | 9c96a255c6b3b0935c610697ac263d70bbbbb7ff | ac6eb8e39909e1006c54df67644646d4f2df5bc5 |
refs/heads/main | <repo_name>Albie140/BurgerApp<file_sep>/views/index.handlebars
<h1>Burger App</h1>
<img src="assets/img/burger1.jpg" alt="Image of a Burger" width="280px" height="300px">
<br><br>
{{!-- Align Left --}}
<div class="card-group">
<div class="card">
<div class="card-body">
<h2>Uneaten Burgers</h2><br>
{{#each burger}}
{{#unless this.devoured}}
{{!-- Render undevoured burgers here --}}
{{!-- <li> --}}
<p>{{ this.id }}. {{ this.burger_name }}</p>
{{!-- </li> --}}
<button data-id="{{this.id}}" class="eatBurgerBtn">Eat Me!</button>
{{/unless}}
{{/each}}
</div>
</div>
{{!-- Align right --}}
<div class="card">
<div class="card-body">
<h2>Devoured Burgers</h2><br>
{{#each burger}}
{{#if this.devoured}}
<p>{{ this.id }}. {{ this.burger_name }} </p>
{{/if}}
{{/each}}
</div>
</div>
</div>
<br>
<h2>Add a Burger to Devour...</h2>
<form method="POST" action="/create" class="devour-form">
<textarea rows="2" cols="35" class="burger_name"> </textarea>
{{!-- <input type="hidden" name="devoured" class="burger_id" type="text" value="1" devoured> --}}
<br>
<button type="submit"> Add a Burger!</button>
</form><file_sep>/models/burger.js
var orm = require("../config/orm.js");
//Uses orm to modify burger database
var burger = {
//getting info from sql database
all: function (cb){
orm.selectAll("burgers", function(res){
cb(res)
})
},
create: function(cols, vals, cb){
orm.insertOne("burgers",cols, vals, function(res) {
cb(res);
});
},
update: function(id, cb) {
var condition ="id=" + id
orm.updateOne("burgers", {devoured: true}, condition, function(res) {
cb(res);
});
}
}
module.exports = burger;<file_sep>/README.md
# BurgerApp
This app was created as a restaurant app. It allows users to input the type of burger they would like to eat. Once the user adds a burger the burger will be moved to the uneaten column. After the user clicks the "Eat Me!" button, the burger will be moved to the devour column. Use the following link to see the Heroku deployed app. https://eatyummyburgers.herokuapp.com/
## Technologies Used
```MySQL, Node, Express, Handlebars and a homemade ORM```
## License
[](https://opensource.org/licenses/MIT)
## Images

## Questions
Please conatct me with any questions at <EMAIL><file_sep>/db/burgerSeeds.sql
INSERT INTO burgers (burger_name) VALUES ("Veggie Burger"), ("Portobella Burger"), ("Turkey Burger")<file_sep>/controllers/burgers_controller.js
var express = require("express");
const router = express.Router();
const burger = require("../models/burger.js");
//HTML Routes
// router.get("/", function (req, res) {
// res.redirect("/burgers");
// });
router.get("/", function (req, res) {
burger.all(function (data) {
var burgerObject = {
burger: data
};
console.log(burgerObject);
res.render("index", burgerObject);
});
});
// Add a new burger
router.post("/api/burgers", function (req, res) {
console.log(req.body);
burger.create("burger_name", req.body.burger_name, function (result) {
console.log(result);
res.json(result);
});
});
//Devour Burger
router.put("/api/burgers/:id", function (req, res) {
burger.update(req.params.id, function () {
console.log(res)
res.sendStatus(200);
});
});
module.exports = router; | d1091f53e8ffec8d11d6adbe6189be3c3bcff566 | [
"Handlebars",
"Markdown",
"SQL",
"JavaScript"
] | 5 | Handlebars | Albie140/BurgerApp | d9529665471b92c0ce7714180ca3ae909b6c308c | 29d82944025cdfa21eae70ffa16ba002aa963c69 |
refs/heads/main | <file_sep># Wizard form:
- create a form for editing 3 attributes:
- name
- occupation
- house
- when the form is submitted, save that to an array...somewhere?
- confirm the new wizard is in the array by:
- console.log()
- maybe list them out?
- or just use the inspector
- when do we need useEffect?
- if we want to edit, which...we do!
## Steps! (aka built-in activities aka times when Chris gets more coffee)
- [X] Create a basic WizardForm.jsx
- fill out the basics (an import, a function, an export)
- add a section
- add a form
- add one input
- [X] Show the WizardForm in the App
- [X] "wire up" the input so it's a connected component
- create some state by calling useState()
- PS: you gotta import useState
- set the value of your input using your state variable
- add an onChange that calls your setState function
- it will usually look like this: `(e) => { setState(e.target.value)}`
<file_sep>import { useState } from 'react';
import WizardForm from './compnents/WizardForm';
import WizardList from './compnents/WizardList';
import { v4 as uuidv4 } from 'uuid';
function App() {
const [wizards, setWizards] = useState([]);
const [wizardToEdit, setWizardToEdit] = useState({})
const chooseWizard = (wizard) => {
console.log(`App sees ${wizard.name}`);
setWizardToEdit(wizard);
}
const onSubmit = (wizard) => {
console.log('==============================');
console.log('here is the new wizard');
//console.log(wizard);
if (wizard.id) {
//const existingWizard = wizards.find(w => w.id === wizard.id)
const updatedWizards = wizards.map(w => {
//if same wizard (same id) return the wizard from th form
//othersie return the wizard from the loop
if (w.id === wizard.id){
return wizard
} else {
return w;
}
});
setWizards(updatedWizards)
} else {
//stamp it with a UUID
wizard.id = uuidv4();
// how do we .push() into a state variable????
// why not .push? because that changes the variable
// To _correctly_ setState on an Array:
const newWizardArray = [
...wizards, // copy the existing elements from the OLD array
wizard // include the element
];
// ask React to store the new array as the wizards
setWizards(newWizardArray);
// setWizards([
// ...wizards,
// wizard
// ]);
}
}
return (
<div>
<WizardForm
title="Add New Wizard"
onSubmit={onSubmit}
wizard={wizardToEdit}
/>
<WizardList
wizards={wizards}
chooseWizard={chooseWizard}
/>
</div>
);
}
export default App;
<file_sep>html {
background-color: gray;
text-align: left;
}
section {
display: flexbox;
background-color: rgb(212, 206, 206);
border-radius: 2%;
padding: 10px;
}
form {
flex-wrap: wrap;
}
| 4c867a98f7e03bc798f864fabe5839183fa985e5 | [
"Markdown",
"JavaScript",
"CSS"
] | 3 | Markdown | burke3601/wizard-forms | de3a34fcd077bcd8a812f830b245e06ba6d474c9 | ff0c65965fa9bba89b8a27764be72649af75949c |
refs/heads/master | <file_sep>/**
* @param {string} s
* @param {number} numRows
* @return {string}
*/
var convert = function(s, numRows) {
if (numRows === 1) {
return s
}
let strArray = new Array(numRows).fill('');
let direct = true;
let lineMark = 0;
for (const char in s) {
strArray[lineMark] += s[char];
if ((lineMark === numRows - 1 && direct) || (lineMark === 0 && !direct)) {
direct = !direct;
}
lineMark += direct ? 1 : -1
}
let resultStr = '';
for (let str in strArray) {
resultStr += strArray[str];
}
return resultStr
};
console.log(convert('LEETCODEISHIRING', 4));
<file_sep>/**
* @param {string} s
* @return {number}
*/
var lengthOfLongestSubstring = function(s) {
let start = 0;
let maxLength = 0;
let map = {};
for (let i = 0 ; i < s.length; i++) {
const char = s.charAt(i);
if (map[char] === undefined || map[char] < start) {
if (i - start + 1 > maxLength) {
maxLength = i - start + 1
}
} else {
start = map[char] + 1
}
map[char] = i
}
return maxLength
// my old swift 2.0 version
// var start = 0
// var i = 0
// var maxLength = 0
// var dictionary = [Character:Int]()
// for char in s.characters {
// if dictionary[char] == nil || dictionary[char] < start{
// // if new appear.
// if i - start + 1 > maxLength {
// maxLength = i - start + 1
// }
// } else {
// // if this already appear.
// start = dictionary[char]! + 1
// }
// dictionary[char] = i
// i += 1
// }
// return maxLength
};
<file_sep>function ListNode(val) {
this.val = val;
this.next = null;
}
/**
* Definition for singly-linked list.
* function ListNode(val) {
* this.val = val;
* this.next = null;
* }
*/
/**
* @param {ListNode} l1
* @param {ListNode} l2
* @return {ListNode}
*/
var addTwoNumbers = function(l1, l2) {
var flag = 0;
let result;
let lastNode;
while (l1 || l2) {
let sum = (l1 ? l1.val : 0) + (l2 ? l2.val : 0) + flag;
flag = parseInt(sum / 10);
sum = sum % 10;
let newNode = new ListNode(sum);
if (lastNode) {
lastNode.next = newNode;
lastNode = newNode;
} else {
lastNode = result = newNode;
}
l1 = l1 && l1.next;
l2 = l2 && l2.next;
}
if (flag === 1) {
lastNode.next = new ListNode(1);
}
return result;
};
<file_sep># LeetCodeJS
run:
~~~
node 01_TwoSum.js
~~~
时隔四年没上 LeetCode,发现出了[中文版](https://leetcode-cn.com).
国庆有空用JS开始刷题,公司比较忙,刷到哪儿算哪儿。
不写题解了,评论区一大堆,先刷数量了。
<file_sep>/**
* 给定一个整数数组 nums 和一个目标值 target,请你在该数组中找出和为目标值的那 两个 整数,并返回他们的数组下标。
* 你可以假设每种输入只会对应一个答案。但是,你不能重复利用这个数组中同样的元素。
* @param {number[]} nums
* @param {number} target
* @return {number[]}
*/
// 第一种方法,直接hash表,key存跟value的差,value寸下标。一边寸一边查,查到直接输出。
let twoSum = function(nums, target) {
var map = {};
for (let i = 0; i < nums.length; i++) {
const value = nums[i];
// console.log(map[target-value] === undefined);
if (map[target-value] !== undefined) {
return [map[target-value], i]
}
map[nums[i]] = i
}
return [0, 0]
};
console.log(twoSum([2, 7, 11, 15], 26));
| d4357dfc461201803429f7c4cb2653ba93c6dca1 | [
"Markdown",
"JavaScript"
] | 5 | Markdown | yangzai360/LeetCodeJS | a6853c49358afce63de128a84bbc491e1afd8de4 | 534bc56476f39fdc04ac6f56b5822ccb9f26b464 |
refs/heads/main | <repo_name>ManuMoll/algo-2<file_sep>/algoritmos/grafos/prim.cpp
int Prim (vvi& G, vvi& W, int n){
vector <bool> vis(n, false);
priority_queue <ii> Q;
Q.push({-0,0});
int answer = 0;
while (not Q.empty()){
ii arc = Q.top()
Q.pop();
int v = arc.second;
int p = -arc.first;
if (not vis[v]){
vis[v] = true;
answer += p;
for (int i = 0; i < G[v].size(); ++i){
int u = G[v][i];
int w = W[v][i];
Q.push({-w, u});
}
}
}
return answer;
}
<file_sep>/README.md
# algo-2
Algoritmica 2
| 4417fe101e829b00bb063324c50aed7a920ad691 | [
"Markdown",
"C++"
] | 2 | Markdown | ManuMoll/algo-2 | ffda4df35bd687b9673137a5108bd9ec5292e709 | cee65c59e0ba5e96b2dbb86b74602d0e6de80846 |
refs/heads/master | <repo_name>TarekKrosh/Proxy_List_Generator<file_sep>/README.md
# Proxy List Generator
### a simple python script which scraps free-proxy-list.net for proxies..
## Highly Recommended for:
1. Web Scrapers
2. Web Crawlers
3. Pen Testers
4. People who want list of proxies in Bulk
## How it Works?
It scraps [free-proxy-list.net] and extracts all the IP Addresses, Port Numbers,Country Codes etc, which are available on the home page accessible via pagination.
## requirements:
1. requests
2. BeautifulSoup
3. html5lib
## Preparing for running the Script
### run the following commands before executing the script
#### for installing requirements for running the script
git clone https://github.com/gagangulyani/Proxy_List_Generator.git
cd Proxy_List_Generator
pip install -r requirements.txt
#### In case of the following error:
AttributeError: module 'html5lib.treebuilders' has no attribute '_base'
#### run the following command
pip install --upgrade beautifulsoup4
pip install --upgrade html5lib
## Running the Script [Option #1]
+ *Copy Paste* or *Move* **proxy_get.py** file in Current Working Directory of your project
+ Import `proxy_get` in your code: `from proxy_get import Proxy_List`
+ Call the `Proxy_List()` function
## Running the Script [Option #2]
python proxy_get.py
#### On Success:
Output will be displayed only is script is executed directly (without importing it)
If Imported and Proxy_List() is called:
Returns dictionary containing list of proxies and number of proxies retrieved
example:
{
"list_of_proxies":[
{
'ip_address': '192.168.3.11',
'port': '36314',
'code': 'TH',
'country': 'Thailand',
'anonymity': 'elite proxy',
'google': 'no',
'https': 'yes',
'last_checked': '29 seconds ago'
},
{
'ip_address': '192.168.127.12',
'port': '3128',
'code': 'RU',
'country': 'Russian Federation',
'anonymity': 'elite proxy',
'google': 'no',
'https': 'yes',
'last_checked': '29 seconds ago'}]
}
...
],
"count" : 300
}
#### On Failure:
##### 1. In case of failure of retrieval of Proxy List from free-proxy-list.net
Output:
Failed to get Proxy List :(
Try Running the script again..
Return:
None
##### 2. In case of failure Bad Request or Redirection of free-proxy-list.net
Output:
Something Went Wrong while Getting Proxy List!
Return:
None
### Important:
feel free to tweak it as much as you like.
If you have any ideas or suggestions regarding the latter,mail me.
(Email: <EMAIL>)
### Reference
1. beautifulsoup, html5lib: module object has no attribute _base
https://stackoverflow.com/questions/38447738/beautifulsoup-html5lib-module-object-has-no-attribute-base
<file_sep>/proxy_get.py
"""
This script scraps "https://free-proxy-list.net/" and returns
dictionary containing of proxies along with their port numbers,
"""
try:
import requests
from bs4 import BeautifulSoup as bs
except ImportError:
exit()
def p_format(*args):
ip_address, port, code, country, anonymity, google, https, last_checked = args
return {
"ip_address": ip_address,
"port": port,
"code": code,
"country": country,
"anonymity": anonymity,
"google": google,
"https": https,
"last_checked": last_checked
}
def Proxy_List():
"""
This function returns list of proxies and returns None
in case of failure.
"""
# url of site containing proxies
url = "https://free-proxy-list.net/"
NUMBER_OF_ATTRIBUTES = 8
try:
# getting html content of site..
page = requests.get(url)
except:
# returns None if unable to get source code of site
print("\nFailed to get Proxy List :(\nTry Running the script again..")
return None
if page.status_code != 200:
print("\nSomething Went Wrong while Getting Proxy List!\n")
return None
soup = bs(page.text, 'html.parser') # creating soup object
proxies = [] # final list of dictionaries each containing proxy info
table = soup.find('table')
tbody = table.tbody if table else None # contains rows of IPs and Stuff
if tbody:
infos = tbody.find_all('tr')
for info in infos:
# each info is a tr from tbody of table
# extracting info from table rows
proxy_data_temp = [i.text for i in info]
if len(proxy_data_temp) == NUMBER_OF_ATTRIBUTES:
# after all attributes have been retrieved
# from a row, it's time to format it properly.
proxies.append(p_format(*proxy_data_temp))
return {"list_of_proxies": proxies,
"count": len(proxies)}
if __name__ == '__main__':
result = Proxy_List()
if result:
print(result)
| 69af5afe544494d643ea2fe33ca2e3f5732c8339 | [
"Markdown",
"Python"
] | 2 | Markdown | TarekKrosh/Proxy_List_Generator | 109a411a6251cf71c2a02c2d59d7e7c82b54c1b5 | ecd6b908ed277f59838f5000593a397aaa796c1e |
refs/heads/master | <repo_name>richardhodgson/micro.js<file_sep>/lib/micro.js
var fs = require('promised-io/lib/fs'),
promise = require('promised-io/lib/promise'),
sys = require('sys'),
proton = require('proton'),
spectrum = require('spectrum');
var getRenderer = fs.realpath(__dirname + '/../views').then(function (path) {
return new spectrum.Renderer(path);
});
var types = {
'png' : 'image/png',
'jpg' : 'image/jpeg',
'jpeg' : 'image/jpeg',
'gif' : 'image/gif',
'css' : 'text/css',
'js' : 'text/javascript',
'swf' : 'application/x-shockwave-flash',
'html' : 'text/html'
};
var Response = function (webapp) {
this.webapp = webapp;
this._response = {
status: 404,
headers: { 'Content-Type' : 'text/plain' }
};
this._handled = false;
};
Response.prototype.setStatus = function (status) {
this._response.status = status;
this._handled = true;
};
Response.prototype.setType = function (type) {
this._response.headers['Content-Type'] = type;
};
Response.prototype.ok = function (type) {
this.setStatus(200);
this.setType(type);
};
Response.prototype.notFound = function (type) {
this.setStatus(404);
this.setType(type);
};
Response.prototype.redirect = function (to, permanent) {
this.setStatus(permanent ? 301 : 302);
this._response.headers['Location'] = to;
};
Response.prototype.internalServerError = function (type) {
this.setStatus(500);
this.setType(type);
};
Response.prototype.addToBody = function (body) {
if (this._response.body) {
this._response.body.push(body);
}
else {
this._response.body = [body];
}
};
Response.prototype.render = function (path, args, contentType, status) {
if (! this.webapp.view) {
throw new Error('you must set a view property on the webapp to call response.render');
}
if (! contentType) {
contentType = 'text/html';
}
var response = this;
return this.webapp.view.render(path, args).then(function(output) {
response.setStatus(status);
response.setType(contentType);
return output;
});
};
function quotemeta (input) {
return input.replace(/([\\\/^$*+?.()|{}\[\]])/g, '\\$1');
}
function makeNamedMatcher (parts) {
var re = '',
names = [];
for (var i = 0, l = parts.length; i < l; i += 3) {
re += quotemeta(parts[i]);
if (i + 1 < l) {
re += '(' + (typeof(parts[i + 2]) !== 'undefined' ? parts[i + 2] : '[^\\/]+') + ')';
names.push(parts[i + 1]);
}
}
var regex = new RegExp('^' + re + '$');
return function (path) {
var match = path.match(regex);
if (match) {
var vals = {};
for (var i = 0, l = names.length; i < l; i++) {
vals[names[i]] = match[i + 1];
}
return [vals];
}
};
}
function makePositionalMatcher (parts) {
var re = '',
matches = 0;
for (var i = 0, l = parts.length; i < l; i += 3) {
re += quotemeta(parts[i]);
if (i + 1 < l) {
re += quotemeta(parts[i + 1]);
matches++;
re += '(' + (typeof(parts[i + 2]) !== 'undefined' ? parts[i + 2] : '[^\\/]+') + ')';
}
}
var regex = new RegExp('^' + re + '$');
return function (path) {
var match = path.match(regex);
if (match) {
var vals = [];
for (var i = 0; i < matches; i++) {
vals.push(match[i + 1]);
}
return vals;
}
};
}
var namedPattern = /:(\w+)(?:\[((?:[^\[\]]|\[(?:[^\]]|\\])+?\])+?)\])?/,
positionalPattern = /(^|\/)\*(?:\[((?:[^\[\]]|\[(?:[^\]]|\\])+?\])+?)\])?(?:$|(?=\/))/;
function makeMatcher (route) {
if (route instanceof RegExp || typeof(route) === 'function') {
return route;
}
var named = route.split(namedPattern),
positional = route.split(positionalPattern);
if (named.length > 1 && positional.length > 1) {
throw new Error('cannot have route with both named (:name) and positional (*) parameters ("' + route + '")');
}
else if (named.length > 1) {
return makeNamedMatcher(named);
}
else if (positional.length > 1) {
return makePositionalMatcher(positional);
}
return route;
}
function handleStatic (root, prefix) {
if (! root) {
throw new Error('missing root directory for static files');
}
if (! prefix) {
prefix = '';
}
prefix = prefix.replace(/([^\w])/g, '\\$1');
var realRoot = fs.realpath(root),
realPaths = {},
WebApp = this,
notFound = function (response) {
return function (err) {
response.notFound('text/plain');
return 'not found';
};
};
proton.beforeStart(WebApp, realRoot.then(
function (root) {
WebApp.get(new RegExp('^' + prefix + '(.+\\.(css|js|jpe?g|gif|png|swf|html))$'), function (request, response, path, type) {
var path = root + path;
if (! (path in realPaths)) {
realPaths[path] = fs.realpath(path);
}
return realPaths[path].then(
function (assetPath) {
return fs.readFile(assetPath).then(
function (contents) {
response.setStatus(200);
response.setType(types[type]);
return contents;
},
notFound(response)
);
},
notFound(response)
);
});
},
function (err) {
throw new Error('cannot add static handler for "' + root + '": ' + err.message);
}
));
};
function handlePublicTemplates (ext, callback) {
this.get(/^((?:\/[\w-]+)+)\/$/, function (request, response, path) {
return response.redirect(path, true);
});
this.get(/^(?:(\/)|((?:\/[\w-]+)+))$/, function (request, response, index, path) {
if (path && path === '/index') {
return response.redirect('/', true);
}
var templatePath = (index ? '/index' : path) + ext,
view = this.view;
return this.view.loadTemplate(templatePath).then(function (template) {
return callback(request, response, template);
}, function (e) {
if (e) {
// TODO spectrum.js should populate e.type
if (e.message.match(/could not load template/).length != undefined) {
return null;
}
else {
throw e;
}
}
return null;
});
});
}
function handleError (err, response) {
return getRenderer.then(function (renderer) {
return renderer.render('/500.spv', { error: err }).then(function (output) {
response.internalServerError('text/html');
response.addToBody(output);
});
});
}
function handleNotFound (request, response, handlers) {
return getRenderer.then(function (renderer) {
return renderer.render('/404.spv', {}).then(function (output) {
response.notFound('text/html');
response.addToBody(output);
});
});
}
function route (webapp, request, response, handlers, from) {
var body,
args;
for (var i = from, l = handlers.length; i < l; i++) {
if ((handlers[i][0] instanceof RegExp)) {
if (args = request.pathInfo.match(handlers[i][0])) {
args.shift();
args.unshift(response);
args.unshift(request);
body = handlers[i][1].apply(webapp, args);
}
}
else if (typeof(handlers[i][0]) === 'function' && (args = handlers[i][0].call(request, request.pathInfo))) {
args.unshift(response);
args.unshift(request);
body = handlers[i][1].apply(webapp, args);
}
else if (handlers[i][0] === request.pathInfo) {
body = handlers[i][1].call(webapp, request, response);
}
if (body && body.then) {
return body.then(function (body) {
if (typeof(body) === 'undefined' || body === null) {
return route(webapp, request, response, handlers, i + 1);
}
response.addToBody(body);
}, function (err) {
// // TODO - perhaps allow fall through to next route?
return handleError(err, response);
}).then(function () {
return response._response;
});
}
else if (response._handled) {
response.addToBody(body);
return response._response;
}
}
return handleNotFound(request, response, handlers).then(function () {
return response._response;
});
};
function makeWebApp () {
var WebApp = function () {
if (this.init) {
this.init.apply(this, arguments);
}
};
WebApp.handlers = {
'GET' : [],
'POST' : [],
'PUT' : [],
'DELETE' : []
};
WebApp.get = function (path, handler) {
WebApp.handlers.GET.push([makeMatcher(path), handler]);
};
WebApp.post = function (path, handler) {
WebApp.handlers.POST.push([makeMatcher(path), handler]);
};
WebApp.put = function (path, handler) {
WebApp.handlers.PUT.push([makeMatcher(path), handler]);
};
WebApp.del = function (path, handler) {
WebApp.handlers.DELETE.push([makeMatcher(path), handler]);
};
WebApp.handleStatic = handleStatic;
WebApp.handlePublicTemplates = handlePublicTemplates;
WebApp.prototype.handle = function (request) {
return route(this, request, new Response(this), WebApp.handlers[request.method], 0);
};
return WebApp;
}
exports.webapp = function (setup) {
var WebApp = makeWebApp();
if (setup) {
setup.call(WebApp, WebApp.get, WebApp.post, WebApp.put, WebApp.del);
}
return WebApp;
};
<file_sep>/tests/suite.js
var litmus = require('litmus');
exports.test = new litmus.Suite('Micro.js Test Suite', [
require('./test-micro').test
]);
<file_sep>/Makefile
# npm install nodeunit
test:
./node_modules/.bin/litmus ./tests/suite.js
clean:
rm -rf BUILD RPMS filelist
.PHONY: test
<file_sep>/tests/test-micro.js
/**
* Mock a JSGI request object
*/
function mockRequest (method, url) {
var request = {};
request["method"] = method;
request.pathInfo = url;
return request;
}
var litmus = require('litmus');
exports.test = new litmus.Test('micro.js', function () {
var micro = require('./../lib/micro.js'),
Promise = require('promised-io/lib/promise').Promise,
spectrum = require('spectrum');
this.async('micro exports webapp', function (handle) {
this.ok(typeof micro.webapp == 'function', "micro has a webapp factory");
handle.finish();
});
this.async('micro.webapp() returns a Webapp', function (handle) {
var Webapp = micro.webapp();
this.ok(typeof Webapp == 'function', "Webapp can be constructed");
this.ok(typeof Webapp.get == 'function', "Webapp has a get handler");
this.ok(typeof Webapp.post == 'function', "Webapp has a post handler");
this.ok(typeof Webapp.put == 'function', "Webapp has a put handler");
this.ok(typeof Webapp.del == 'function', "Webapp has a delete handler");
handle.finish();
});
this.async('can create new Webapp', function (handle) {
var Webapp = micro.webapp();
var instance = new Webapp;
this.ok(instance instanceof Webapp, "Can create instance of Webapp");
this.ok(typeof instance.handle == 'function', "Webapp instance has handle method");
handle.finish();
});
this.async('webapp can handle requests', function (handle) {
//this.expect(2);
var Webapp = micro.webapp(),
mockReq = mockRequest('GET', '/test');
var test = this;
Webapp.get('/test', function (request, response) {
test.ok(true, "handler callback executes");
test.is(mockReq, request, "route callback is passed the request");
test.ok(typeof response == 'object', "route callback has a response object");
handle.finish();
});
var instance = new Webapp();
instance.handle(mockReq);
});
this.async('successful string response', function (handle) {
var Webapp = micro.webapp(),
successfulRequest = mockRequest('GET', '/test');
Webapp.get('/test', function (request, response) {
response.ok("text/plain");
return "hello";
});
var instance = new Webapp(),
actualResponse = instance.handle(successfulRequest);
this.ok(typeof actualResponse == 'object', "simple route that returns response from handle");
this.is(200, actualResponse.status, "successful request sets 200 status on response");
handle.finish();
});
this.async('not found string response', function (handle) {
var Webapp = micro.webapp(),
notfoundRequest = mockRequest('GET', '/test');
Webapp.get('/test', function (request, response) {
response.notFound("text/plain");
return "";
});
var instance = new Webapp(),
actualResponse = instance.handle(notfoundRequest);
this.is(404, actualResponse.status, "not found request sets 404 status on response");
handle.finish();
});
this.async('successful promise response', function (handle) {
var Webapp = micro.webapp(),
successfulRequest = mockRequest('GET', '/test');
Webapp.get('/test', function (request, response) {
response.ok("text/plain");
var deferred = new Promise();
deferred.resolve("hello");
return deferred;
});
var instance = new Webapp(),
handledPromise = instance.handle(successfulRequest);
var test = this;
handledPromise.then(function (actualResponse) {
test.ok(typeof actualResponse == 'object', "simple route that returns response from handle");
test.is(200, actualResponse.status, "successful request sets 200 status on response");
handle.finish();
});
});
this.async('not found promise response resolved with empty string', function (handle) {
var Webapp = micro.webapp(),
notfoundRequest = mockRequest('GET', '/test');
Webapp.get('/test', function (request, response) {
response.notFound("text/plain");
var deferred = new Promise();
deferred.resolve("");
return deferred;
});
var instance = new Webapp(),
handledPromise = instance.handle(notfoundRequest);
var test = this;
handledPromise.then(function (actualResponse) {
test.is(404, actualResponse.status, "not found request sets 404 status on response");
handle.finish();
});
});
this.async('promise response rejected returns internal server error', function (handle) {
var Webapp = micro.webapp(),
mockReq = mockRequest('GET', '/test');
Webapp.get('/test', function (request, response) {
var deferred = new Promise();
deferred.reject({
"toString": function () {
return "some message"
},
"stack" : []
});
return deferred;
});
var instance = new Webapp(),
handledPromise = instance.handle(mockReq);
var test = this;
handledPromise.then(function (actualResponse) {
test.is(500, actualResponse.status, "rejected promise sets 500 status on response");
handle.finish();
});
});
this.async('handle public templates', function (handle) {
var Webapp = micro.webapp(),
mockReq = mockRequest('GET', '/test');
Webapp.prototype.init = function () {
this.view = new spectrum.Renderer(__dirname + '/mock/views');
};
Webapp.handlePublicTemplates('.pub.spv', function (request, response, template) {
var content = template.render({});
response.ok('text/html');
return content;
});
var instance = new Webapp(),
handledPromise = instance.handle(mockReq);
var test = this;
handledPromise.then(function (actualResponse) {
test.is(200, actualResponse.status, "handled public template returns 200 status on response");
test.is(
"This is a test template.",
actualResponse.body[0],
"Response body contains rendered template"
);
handle.finish();
});
});
this.async('handle public template returns 404 for missing template', function (handle) {
var Webapp = micro.webapp(),
mockReq = mockRequest('GET', '/a-page-that-does-not-exist');
Webapp.prototype.init = function () {
this.view = new spectrum.Renderer(__dirname + '/mock/views');
};
Webapp.handlePublicTemplates('.pub.spv', function (request, response, template) {
var content = template.render({});
response.ok('text/html');
return content;
});
var instance = new Webapp(),
handledPromise = instance.handle(mockReq);
var test = this;
handledPromise.then(function (actualResponse) {
test.is(404, actualResponse.status, "handled public template returns 404 status on response");
handle.finish();
});
});
}); | e65cd5514b0cadf21f3b5ce1b977d74a6b775f8d | [
"Makefile",
"JavaScript"
] | 4 | Makefile | richardhodgson/micro.js | 5de4328869905cd010650f57d0704a2027b99179 | 3a8ccdfddb3c461ce76d2f22b4cd07d58b375e6c |
refs/heads/master | <file_sep>function Quote(ex)
if ex isa Int || ex isa Float64 || ex isa Float32
return ex
else
return convert(Expr, ex)
end
end
function QuoteFn(name,ex,vars=free_symbols(ex))
return Expr(:function, Expr(:call, Symbol(name), map(Symbol,vars)...), Quote(ex))
end
function QuoteFnCSE(name,cse_ex,vars=free_symbols(cse_ex[2]))
quot = Expr(:block)
quot.head = :function
push!(quot.args, :($(Symbol(name))($(map(Symbol,vars)...) )))
fnbody = QuoteCSE(cse_ex)
push!(quot.args,fnbody)
return quot
end
function QuoteCSE(cse_ex)
cse,ex = cse_ex
ex=ex[1]
fnbody=Expr(:block)
for (k,v) in cse
push!(fnbody.args, :($(Quote(k)) = $(Quote(v))) )
end
push!(fnbody.args,Quote(ex))
return fnbody
end
function QuoteArrCSE(cse_ex)
# assume we did simultaneous CSE on the array
# cse is common list of substitutions
# ex is array of cse'd expressions
cse,ex = cse_ex
fnbody=Expr(:block)
for (k,v) in cse
push!(fnbody.args, :($(Quote(k)) = $(Quote(v))) )
end
if length(ex) == 1
ex = ex[1]
end
push!(fnbody.args,:([$(Quote.(ex)...)]))
return fnbody
end
function QuoteArr(ex)
fnbody=Expr(:block)
push!(fnbody.args,:([$(Quote.(ex)...)]))
return fnbody
end
QuoteFnArr(name,ex, vars=free_symbols(ex)) = Expr(:function,
Expr(:call, Symbol(name), map(Symbol,vars)...),
:([$(Quote.(ex)...)]))
function QuoteFnArrCSE(name, cse_ex, vars=free_symbols(ex))
quot = Expr(:block)
quot.head = :function
push!(quot.args, :($(Symbol(name))($(map(Symbol,vars)...) )))
fnbody = QuoteArrCSE(cse_ex)
push!(quot.args,fnbody)
return quot
end
<file_sep>module Inflation
using SymPy
@info "Travis CI hack"
using DifferentialEquations
@info "Travis CI hack"
using ODEInterfaceDiffEq
@info "Travis CI hack"
#using DiffEqParamEstim
using LinearAlgebra
using CurveFit
using Serialization
using JLD2
using Parameters
export inflation_setup
export background_evolve
export transport_perturbations
export SetupOptions, BackgroundOptions, PerturbationOptions
export output_data
# change this whenever there's a breaking change in the cache functions
cache_version = 1.3
# unit conversion
Mpc_to_Lpl = 2.6245e-57
include("options.jl")
include("quoting.jl")
include("symbolic_setup.jl")
include("solvers.jl")
include("output.jl")
end # module
| d80974cd97a50414e0dcb5fb0fbcb7dc92235209 | [
"Julia"
] | 2 | Julia | GitHimanshuc/Inflation.jl | a3dea6bf1d0dcacd49321db32f8fd16194004ee4 | ace232766f8b48ce38a9c6169a18182134f33710 |
refs/heads/main | <repo_name>plenty-of-flicks/plenty_of_flicks_be<file_sep>/app/controllers/api/v1/users_controller.rb
class Api::V1::UsersController < ApplicationController
def create
user = User.find_by(uid: params[:uid])
unless user
user = User.create(user_params)
end
render json: UserSerializer.new(user)
end
def show
user = User.find_by(uid: params[:id])
if user
render json: UserSerializer.new(user)
else
output = {
error: 'User does not exist in database.'
}
render json: output, status: :bad_request
end
end
def update
user = User.find_by(uid: params[:id])
user.update(user_params)
render json: UserSerializer.new(user)
end
def friends
user = User.find_by(id: params[:id])
friendlist = Friendlist.new(user)
render json: FriendlistSerializer.new(friendlist)
end
private
def user_params
params.permit(:uid, :first_name, :last_name, :image, :email)
end
end
<file_sep>/app/serializers/friendlist_serializer.rb
class FriendlistSerializer
include FastJsonapi::ObjectSerializer
attributes :id, :friends
end
<file_sep>/app/controllers/api/v1/user_groups_controller.rb
class Api::V1::UserGroupsController < ApplicationController
def create
user_group = UserGroup.create(user_group_params)
render json: UserGroupSerializer.new(user_group)
end
def destroy
UserGroup.destroy(params[:id])
end
private
def user_group_params
params.permit(:user_id, :group_id)
end
end
<file_sep>/spec/models/movie_availability_spec.rb
require 'rails_helper'
describe MovieAvailability, type: :model do
describe 'relationships' do
it do
should belong_to :movie
should belong_to :service
end
end
end
<file_sep>/app/jobs/service_update_job.rb
class ServiceUpdateJob < ApplicationJob
queue_as :default
def perform(service, movie_pages)
MovieAvailability.where(service: service).destroy_all
movie_pages.each do |movie_page|
movie_page[:titles].each do |movie_data|
movie = Movie.find_by(tmdb_id: movie_data[:tmdb_id])
unless movie
movie = Movie.create(
title: movie_data[:title],
tmdb_id: movie_data[:tmdb_id],
year: movie_data[:year]
)
end
movie.movie_availabilities.create(service: service)
end
end
end
end
<file_sep>/spec/requests/api/v1/groups_request_spec.rb
require 'rails_helper'
describe 'Groups API' do
it 'can create a group' do
group_params = ({
name: '<NAME>'
})
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/groups", headers: headers, params: JSON.generate(group_params)
json = JSON.parse(response.body, symbolize_names:true)
group = Group.last
expect(response).to be_successful
expect(group).to be_a(Group)
expect(group.name).to eq('<NAME>')
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(group.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('group')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:name)
expect(json[:data][:attributes][:name]).to eq(group.name)
expect(json[:data][:attributes]).to have_key(:id)
expect(json[:data][:attributes][:id]).to eq(group.id)
expect(json[:data][:attributes]).to have_key(:users)
expect(json[:data][:attributes][:users]).to eq([])
end
it 'can delete a group' do
group = Group.create(
name: '<NAME>'
)
expect(Group.count).to eq(1)
delete "/api/v1/groups/#{group.id}"
expect(response).to be_successful
expect(Group.count).to eq(0)
expect{Group.find(group.id)}.to raise_error(ActiveRecord::RecordNotFound)
end
it 'can show all groups for a user' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
fantastic_four = Group.create(
name: 'Fantastic Four'
)
avengers = Group.create(
name: 'The Avengers'
)
x_men = Group.create(
name: 'X Men'
)
UserGroup.create(
user: nick,
group: fantastic_four
)
UserGroup.create(
user: nick,
group: x_men
)
get "/api/v1/users/#{nick.id}/groups"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_an(Array)
expect(json.length).to eq(2)
expect(json[0]).to be_a(Hash)
expect(json[0]).to have_key(:data)
expect(json[0][:data]).to be_a(Hash)
expect(json[0][:data]).to have_key(:id)
expect(json[0][:data][:id]).to eq(fantastic_four.id.to_s)
expect(json[0][:data]).to have_key(:type)
expect(json[0][:data][:type]).to eq('group')
expect(json[0][:data]).to have_key(:attributes)
expect(json[0][:data][:attributes]).to be_a(Hash)
expect(json[0][:data][:attributes]).to have_key(:id)
expect(json[0][:data][:attributes][:id]).to eq(fantastic_four.id)
expect(json[0][:data][:attributes]).to have_key(:name)
expect(json[0][:data][:attributes][:name]).to eq(fantastic_four.name)
expect(json[0][:data][:attributes]).to have_key(:users)
expect(json[0][:data][:attributes][:users]).to be_an(Array)
expect(json[0][:data][:attributes][:users].length).to eq(1)
expect(json[0][:data][:attributes][:users][0]).to be_an(Hash)
expect(json[0][:data][:attributes][:users][0]).to have_key(:id)
expect(json[0][:data][:attributes][:users][0][:id]).to eq(nick.id)
expect(json[0][:data][:attributes][:users][0]).to have_key(:email)
expect(json[0][:data][:attributes][:users][0][:email]).to eq(nick.email)
expect(json[0][:data][:attributes][:users][0]).to have_key(:first_name)
expect(json[0][:data][:attributes][:users][0][:first_name]).to eq(nick.first_name)
expect(json[0][:data][:attributes][:users][0]).to have_key(:last_name)
expect(json[0][:data][:attributes][:users][0][:last_name]).to eq(nick.last_name)
expect(json[0][:data][:attributes][:users][0]).to have_key(:image)
expect(json[0][:data][:attributes][:users][0][:image]).to eq(nick.image)
expect(json[0][:data][:attributes][:users][0]).to have_key(:uid)
expect(json[0][:data][:attributes][:users][0][:uid]).to eq(nick.uid)
expect(json[0][:data][:attributes][:users][0]).to have_key(:created_at)
expect(json[0][:data][:attributes][:users][0][:created_at]).to be_a(String)
expect(json[0][:data][:attributes][:users][0]).to have_key(:updated_at)
expect(json[0][:data][:attributes][:users][0][:updated_at]).to be_a(String)
expect(json[1]).to be_a(Hash)
expect(json[1]).to have_key(:data)
expect(json[1][:data]).to be_a(Hash)
expect(json[1][:data]).to have_key(:id)
expect(json[1][:data][:id]).to eq(x_men.id.to_s)
expect(json[1][:data]).to have_key(:type)
expect(json[1][:data][:type]).to eq('group')
expect(json[1][:data]).to have_key(:attributes)
expect(json[1][:data][:attributes]).to be_a(Hash)
expect(json[1][:data][:attributes]).to have_key(:id)
expect(json[1][:data][:attributes][:id]).to eq(x_men.id)
expect(json[1][:data][:attributes]).to have_key(:name)
expect(json[1][:data][:attributes][:name]).to eq(x_men.name)
expect(json[1][:data][:attributes]).to have_key(:users)
expect(json[1][:data][:attributes][:users]).to be_an(Array)
expect(json[1][:data][:attributes][:users].length).to eq(1)
expect(json[1][:data][:attributes][:users][0]).to be_an(Hash)
expect(json[1][:data][:attributes][:users][0]).to have_key(:id)
expect(json[1][:data][:attributes][:users][0][:id]).to eq(nick.id)
expect(json[1][:data][:attributes][:users][0]).to have_key(:email)
expect(json[1][:data][:attributes][:users][0][:email]).to eq(nick.email)
expect(json[1][:data][:attributes][:users][0]).to have_key(:first_name)
expect(json[1][:data][:attributes][:users][0][:first_name]).to eq(nick.first_name)
expect(json[1][:data][:attributes][:users][0]).to have_key(:last_name)
expect(json[1][:data][:attributes][:users][0][:last_name]).to eq(nick.last_name)
expect(json[1][:data][:attributes][:users][0]).to have_key(:image)
expect(json[1][:data][:attributes][:users][0][:image]).to eq(nick.image)
expect(json[1][:data][:attributes][:users][0]).to have_key(:uid)
expect(json[1][:data][:attributes][:users][0][:uid]).to eq(nick.uid)
expect(json[1][:data][:attributes][:users][0]).to have_key(:created_at)
expect(json[1][:data][:attributes][:users][0][:created_at]).to be_a(String)
expect(json[1][:data][:attributes][:users][0]).to have_key(:updated_at)
expect(json[1][:data][:attributes][:users][0][:updated_at]).to be_a(String)
end
it 'can show a group by id' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
x_men = Group.create(
name: 'X Men'
)
UserGroup.create(
user: nick,
group: x_men
)
UserGroup.create(
user: ron,
group: x_men
)
get "/api/v1/groups/#{x_men.id}"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(x_men.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('group')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:id)
expect(json[:data][:attributes][:id]).to eq(x_men.id)
expect(json[:data][:attributes]).to have_key(:name)
expect(json[:data][:attributes][:name]).to eq(x_men.name)
expect(json[:data][:attributes]).to have_key(:users)
expect(json[:data][:attributes][:users]).to be_an(Array)
expect(json[:data][:attributes][:users].length).to eq(2)
expect(json[:data][:attributes][:users][0]).to be_an(Hash)
expect(json[:data][:attributes][:users][0]).to have_key(:id)
expect(json[:data][:attributes][:users][0][:id]).to eq(nick.id)
expect(json[:data][:attributes][:users][0]).to have_key(:email)
expect(json[:data][:attributes][:users][0][:email]).to eq(nick.email)
expect(json[:data][:attributes][:users][0]).to have_key(:first_name)
expect(json[:data][:attributes][:users][0][:first_name]).to eq(nick.first_name)
expect(json[:data][:attributes][:users][0]).to have_key(:last_name)
expect(json[:data][:attributes][:users][0][:last_name]).to eq(nick.last_name)
expect(json[:data][:attributes][:users][0]).to have_key(:image)
expect(json[:data][:attributes][:users][0][:image]).to eq(nick.image)
expect(json[:data][:attributes][:users][0]).to have_key(:uid)
expect(json[:data][:attributes][:users][0][:uid]).to eq(nick.uid)
expect(json[:data][:attributes][:users][0]).to have_key(:created_at)
expect(json[:data][:attributes][:users][0][:created_at]).to be_a(String)
expect(json[:data][:attributes][:users][0]).to have_key(:updated_at)
expect(json[:data][:attributes][:users][0][:updated_at]).to be_a(String)
expect(json[:data][:attributes][:users][1]).to be_an(Hash)
expect(json[:data][:attributes][:users][1]).to have_key(:id)
expect(json[:data][:attributes][:users][1][:id]).to eq(ron.id)
expect(json[:data][:attributes][:users][1]).to have_key(:email)
expect(json[:data][:attributes][:users][1][:email]).to eq(ron.email)
expect(json[:data][:attributes][:users][1]).to have_key(:first_name)
expect(json[:data][:attributes][:users][1][:first_name]).to eq(ron.first_name)
expect(json[:data][:attributes][:users][1]).to have_key(:last_name)
expect(json[:data][:attributes][:users][1][:last_name]).to eq(ron.last_name)
expect(json[:data][:attributes][:users][1]).to have_key(:image)
expect(json[:data][:attributes][:users][1][:image]).to eq(ron.image)
expect(json[:data][:attributes][:users][1]).to have_key(:uid)
expect(json[:data][:attributes][:users][1][:uid]).to eq(ron.uid)
expect(json[:data][:attributes][:users][1]).to have_key(:created_at)
expect(json[:data][:attributes][:users][1][:created_at]).to be_a(String)
expect(json[:data][:attributes][:users][1]).to have_key(:updated_at)
expect(json[:data][:attributes][:users][1][:updated_at]).to be_a(String)
end
it 'can retreive all movie matches for a group' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
tom = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Tom',
last_name: 'Haverford',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
austin_powers = Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816,
poster_path: '/1PkGnyFwRyapmbuILIOXXxiSh7Y.jpg',
description: "As a swingin' fashion photographer by day and a groovy British superagent by night, Austin Powers is the '60s' most shagadelic spy, baby! But can he stop megalomaniac Dr. Evil after the bald villain freezes himself and unthaws in the '90s? With the help of sexy sidekick Vanessa Kensington, he just might.",
vote_average: 6.5,
vote_count: 2349,
year: '1997'
)
comedy = austin_powers.genres.create(
name: 'Comedy',
tmdb_id: 14
)
action = austin_powers.genres.create(
name: 'Action',
tmdb_id: 16
)
nightcrawler = Movie.create(
title: 'Nightcrawler',
tmdb_id: 242582,
poster_path: '/j9HrX8f7GbZQm1BrBiR40uFQZSb.jpg',
description: 'When <NAME>loom, desperate for work, muscles into the world of L.A. crime journalism, he blurs the line between observer and participant to become the star of his own story. Aiding him in his effort is Nina, a TV-news veteran.',
vote_average: 7.7,
vote_count: 8018,
year: '2014'
)
thriller = nightcrawler.genres.create(
name: 'Thriller',
tmdb_id: 11
)
theory_of_everything = Movie.create(
title: 'The Theory of Everything',
tmdb_id: 266856
)
x_men = Group.create(
name: 'X Men'
)
netflix = Service.create(
name: 'Netflix',
watchmode_id: 203,
logo: 'netflix_logo.png'
)
hulu = Service.create(
name: 'Hulu',
watchmode_id: 157,
logo: 'hulu_logo.jpeg'
)
MovieAvailability.create(
movie: austin_powers,
service: netflix
)
MovieAvailability.create(
movie: austin_powers,
service: hulu
)
MovieAvailability.create(
movie: nightcrawler,
service: netflix
)
UserGroup.create(
user: nick,
group: x_men
)
UserGroup.create(
user: ron,
group: x_men
)
UserGroup.create(
user: tom,
group: x_men
)
Swipe.create(
movie: austin_powers,
user: nick,
rating: 1
)
Swipe.create(
movie: austin_powers,
user: ron,
rating: 1
)
Swipe.create(
movie: austin_powers,
user: tom,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: nick,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: ron,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: tom,
rating: 1
)
Swipe.create(
movie: theory_of_everything,
user: nick,
rating: 1
)
Swipe.create(
movie: theory_of_everything,
user: ron,
rating: 0
)
get "/api/v1/groups/#{x_men.id}/matches"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_an(Array)
expect(json[:data].length).to eq(2)
expect(json[:data][0]).to be_a(Hash)
expect(json[:data][0]).to have_key(:id)
expect(json[:data][0][:id]).to eq(austin_powers.id.to_s)
expect(json[:data][0]).to have_key(:type)
expect(json[:data][0][:type]).to eq('match')
expect(json[:data][0]).to have_key(:attributes)
expect(json[:data][0][:attributes]).to be_a(Hash)
expect(json[:data][0][:attributes]).to have_key(:title)
expect(json[:data][0][:attributes][:title]).to eq(austin_powers.title)
expect(json[:data][0][:attributes]).to have_key(:tmdb_id)
expect(json[:data][0][:attributes][:tmdb_id]).to eq(austin_powers.tmdb_id)
expect(json[:data][0][:attributes]).to have_key(:poster_path)
expect(json[:data][0][:attributes][:poster_path]).to eq(austin_powers.poster_path)
expect(json[:data][0][:attributes]).to have_key(:description)
expect(json[:data][0][:attributes][:description]).to eq(austin_powers.description)
expect(json[:data][0][:attributes]).to have_key(:genres)
expect(json[:data][0][:attributes][:genres]).to be_an(Array)
expect(json[:data][0][:attributes][:genres].length).to eq(2)
expect(json[:data][0][:attributes][:genres][0]).to be_a(Hash)
expect(json[:data][0][:attributes][:genres][0]).to have_key(:id)
expect(json[:data][0][:attributes][:genres][0][:id]).to eq(comedy.id)
expect(json[:data][0][:attributes][:genres][0]).to have_key(:created_at)
expect(json[:data][0][:attributes][:genres][0][:created_at]).to be_a(String)
expect(json[:data][0][:attributes][:genres][0]).to have_key(:updated_at)
expect(json[:data][0][:attributes][:genres][0][:updated_at]).to be_a(String)
expect(json[:data][0][:attributes][:genres][0]).to have_key(:tmdb_id)
expect(json[:data][0][:attributes][:genres][0][:tmdb_id]).to eq(comedy.tmdb_id)
expect(json[:data][0][:attributes][:genres][1]).to be_a(Hash)
expect(json[:data][0][:attributes][:genres][1]).to have_key(:id)
expect(json[:data][0][:attributes][:genres][1][:id]).to eq(action.id)
expect(json[:data][0][:attributes][:genres][1]).to have_key(:created_at)
expect(json[:data][0][:attributes][:genres][1][:created_at]).to be_a(String)
expect(json[:data][0][:attributes][:genres][1]).to have_key(:updated_at)
expect(json[:data][0][:attributes][:genres][1][:updated_at]).to be_a(String)
expect(json[:data][0][:attributes][:genres][1]).to have_key(:tmdb_id)
expect(json[:data][0][:attributes][:genres][1][:tmdb_id]).to eq(action.tmdb_id)
expect(json[:data][0][:attributes]).to have_key(:vote_count)
expect(json[:data][0][:attributes][:vote_count]).to eq(austin_powers.vote_count)
expect(json[:data][0][:attributes]).to have_key(:vote_average)
expect(json[:data][0][:attributes][:vote_average]).to eq(austin_powers.vote_average)
expect(json[:data][0][:attributes]).to have_key(:year)
expect(json[:data][0][:attributes][:year]).to eq(austin_powers.year)
expect(json[:data][0][:attributes]).to have_key(:services)
expect(json[:data][0][:attributes][:services]).to be_an(Array)
expect(json[:data][0][:attributes][:services].length).to eq(2)
expect(json[:data][0][:attributes][:services][0]).to be_a(Hash)
expect(json[:data][0][:attributes][:services][0]).to have_key(:id)
expect(json[:data][0][:attributes][:services][0][:id]).to eq(netflix.id)
expect(json[:data][0][:attributes][:services][0]).to have_key(:name)
expect(json[:data][0][:attributes][:services][0][:name]).to eq(netflix.name)
expect(json[:data][0][:attributes][:services][0]).to have_key(:watchmode_id)
expect(json[:data][0][:attributes][:services][0][:watchmode_id]).to eq(netflix.watchmode_id)
expect(json[:data][0][:attributes][:services][0]).to have_key(:created_at)
expect(json[:data][0][:attributes][:services][0][:created_at]).to be_a(String)
expect(json[:data][0][:attributes][:services][0]).to have_key(:updated_at)
expect(json[:data][0][:attributes][:services][0][:updated_at]).to be_a(String)
expect(json[:data][0][:attributes][:services][0]).to have_key(:logo)
expect(json[:data][0][:attributes][:services][0][:logo]).to eq(netflix.logo)
expect(json[:data][0][:attributes][:services][1]).to be_a(Hash)
expect(json[:data][0][:attributes][:services][1]).to have_key(:id)
expect(json[:data][0][:attributes][:services][1][:id]).to eq(hulu.id)
expect(json[:data][0][:attributes][:services][1]).to have_key(:name)
expect(json[:data][0][:attributes][:services][1][:name]).to eq(hulu.name)
expect(json[:data][0][:attributes][:services][1]).to have_key(:watchmode_id)
expect(json[:data][0][:attributes][:services][1][:watchmode_id]).to eq(hulu.watchmode_id)
expect(json[:data][0][:attributes][:services][1]).to have_key(:created_at)
expect(json[:data][0][:attributes][:services][1][:created_at]).to be_a(String)
expect(json[:data][0][:attributes][:services][1]).to have_key(:updated_at)
expect(json[:data][0][:attributes][:services][1][:updated_at]).to be_a(String)
expect(json[:data][0][:attributes][:services][1]).to have_key(:logo)
expect(json[:data][0][:attributes][:services][1][:logo]).to eq(hulu.logo)
expect(json[:data][1]).to be_a(Hash)
expect(json[:data][1]).to have_key(:id)
expect(json[:data][1][:id]).to eq(nightcrawler.id.to_s)
expect(json[:data][1]).to have_key(:type)
expect(json[:data][1][:type]).to eq('match')
expect(json[:data][1]).to have_key(:attributes)
expect(json[:data][1][:attributes]).to be_a(Hash)
expect(json[:data][1][:attributes]).to have_key(:title)
expect(json[:data][1][:attributes][:title]).to eq(nightcrawler.title)
expect(json[:data][1][:attributes]).to have_key(:tmdb_id)
expect(json[:data][1][:attributes][:tmdb_id]).to eq(nightcrawler.tmdb_id)
expect(json[:data][1][:attributes]).to have_key(:poster_path)
expect(json[:data][1][:attributes][:poster_path]).to eq(nightcrawler.poster_path)
expect(json[:data][1][:attributes]).to have_key(:description)
expect(json[:data][1][:attributes][:description]).to eq(nightcrawler.description)
expect(json[:data][1][:attributes]).to have_key(:genres)
expect(json[:data][1][:attributes][:genres]).to be_an(Array)
expect(json[:data][1][:attributes][:genres].length).to eq(1)
expect(json[:data][1][:attributes][:genres][0]).to be_a(Hash)
expect(json[:data][1][:attributes][:genres][0]).to have_key(:id)
expect(json[:data][1][:attributes][:genres][0][:id]).to eq(thriller.id)
expect(json[:data][1][:attributes][:genres][0]).to have_key(:created_at)
expect(json[:data][1][:attributes][:genres][0][:created_at]).to be_a(String)
expect(json[:data][1][:attributes][:genres][0]).to have_key(:updated_at)
expect(json[:data][1][:attributes][:genres][0][:updated_at]).to be_a(String)
expect(json[:data][1][:attributes][:genres][0]).to have_key(:tmdb_id)
expect(json[:data][1][:attributes][:genres][0][:tmdb_id]).to eq(thriller.tmdb_id)
expect(json[:data][1][:attributes]).to have_key(:vote_count)
expect(json[:data][1][:attributes][:vote_count]).to eq(nightcrawler.vote_count)
expect(json[:data][1][:attributes]).to have_key(:vote_average)
expect(json[:data][1][:attributes][:vote_average]).to eq(nightcrawler.vote_average)
expect(json[:data][1][:attributes]).to have_key(:year)
expect(json[:data][1][:attributes][:year]).to eq(nightcrawler.year)
expect(json[:data][1][:attributes][:services]).to be_an(Array)
expect(json[:data][1][:attributes][:services].length).to eq(1)
expect(json[:data][1][:attributes][:services][0]).to be_a(Hash)
expect(json[:data][1][:attributes][:services][0]).to have_key(:id)
expect(json[:data][1][:attributes][:services][0][:id]).to eq(netflix.id)
expect(json[:data][1][:attributes][:services][0]).to have_key(:name)
expect(json[:data][1][:attributes][:services][0][:name]).to eq(netflix.name)
expect(json[:data][1][:attributes][:services][0]).to have_key(:watchmode_id)
expect(json[:data][1][:attributes][:services][0][:watchmode_id]).to eq(netflix.watchmode_id)
expect(json[:data][1][:attributes][:services][0]).to have_key(:created_at)
expect(json[:data][1][:attributes][:services][0][:created_at]).to be_a(String)
expect(json[:data][1][:attributes][:services][0]).to have_key(:updated_at)
expect(json[:data][1][:attributes][:services][0][:updated_at]).to be_a(String)
expect(json[:data][1][:attributes][:services][0]).to have_key(:logo)
expect(json[:data][1][:attributes][:services][0][:logo]).to eq(netflix.logo)
end
end
<file_sep>/docs/pull_request_template.md
This PR will:
*
*
*
- [ ] Fully tested
- [ ] Issues created:
Closes #
<file_sep>/app/serializers/friendship_serializer.rb
class FriendshipSerializer
include FastJsonapi::ObjectSerializer
attributes :id, :user_id, :friend_id
end
<file_sep>/app/controllers/api/v1/groups_controller.rb
class Api::V1::GroupsController < ApplicationController
def index
user = User.find(params[:user_id])
groups = user.groups.map do |group|
GroupSerializer.new(group)
end
render json: groups
end
def show
group = Group.find(params[:id])
render json: GroupSerializer.new(group)
end
def create
group = Group.create(group_params)
render json: GroupSerializer.new(group)
end
def destroy
Group.destroy(params[:id])
end
def matches
matches = Movie.group_matches(params[:id])
render json: MatchSerializer.new(matches)
end
private
def group_params
params.permit(:name)
end
end
<file_sep>/spec/models/user_group_spec.rb
require 'rails_helper'
describe UserGroup, type: :model do
describe 'relationships' do
it do
should belong_to :user
should belong_to :group
end
end
end
<file_sep>/app/models/user.rb
class User < ApplicationRecord
validates_presence_of :email,
:first_name,
:last_name,
:image,
:uid
has_many :swipes
has_many :movies, through: :swipes
has_many :friendships
has_many :friends, through: :friendships
has_many :user_groups
has_many :groups, through: :user_groups
end
<file_sep>/app/poros/service_refresh.rb
class ServiceRefresh
attr_reader :id,
:name,
:movie_count
def initialize(name, movie_count)
@id = nil
@name = name
@movie_count = movie_count
end
end
<file_sep>/db/migrate/20210411060132_create_movies.rb
class CreateMovies < ActiveRecord::Migration[6.1]
def change
create_table :movies do |t|
t.string :title
t.integer :tmdbid
t.string :poster_path
t.string :description
t.string :genres
t.float :vote_average
t.integer :vote_count
t.string :year
t.timestamps
end
end
end
<file_sep>/spec/requests/api/v1/swipes_request_spec.rb
require 'rails_helper'
describe 'Swipes API' do
it 'can create a swipe' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
movie = create(:movie)
swipe_params = ({
user_id: nick.id,
movie_id: movie.id,
rating: 1
})
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/swipes", headers: headers, params: JSON.generate(swipe_params)
swipe = Swipe.last
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(swipe).to be_a(Swipe)
expect(swipe.user_id).to eq(nick.id)
expect(swipe.movie_id).to eq(movie.id)
expect(swipe.rating).to eq(1)
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(swipe.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('swipe')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:movie_id)
expect(json[:data][:attributes][:movie_id]).to be_an(Integer)
expect(json[:data][:attributes][:movie_id]).to eq(movie.id)
expect(json[:data][:attributes]).to have_key(:user_id)
expect(json[:data][:attributes][:user_id]).to be_an(Integer)
expect(json[:data][:attributes][:user_id]).to eq(nick.id)
end
end
<file_sep>/app/serializers/match_serializer.rb
class MatchSerializer
include FastJsonapi::ObjectSerializer
attributes :title, :tmdb_id, :poster_path, :description, :genres, :vote_average, :vote_count, :year, :services
end
<file_sep>/spec/models/swipe_spec.rb
require 'rails_helper'
describe Swipe, type: :model do
describe 'validations' do
it { should validate_presence_of :rating}
end
describe 'relationships' do
it do
should belong_to :movie
should belong_to :user
end
end
end
<file_sep>/db/migrate/20210411075833_fix_tmdbid_column_name.rb
class FixTmdbidColumnName < ActiveRecord::Migration[6.1]
def change
rename_column :movies, :tmdbid, :tmdb_id
end
end
<file_sep>/app/models/friendship.rb
class Friendship < ApplicationRecord
validates_presence_of :user_id,
:friend_id
belongs_to :user
belongs_to :friend, class_name: 'User'
def self.exists?(user_id, friend_id)
Friendship.where(user_id: user_id).where(friend_id: friend_id) != []
end
end
<file_sep>/app/models/movie.rb
class Movie < ApplicationRecord
validates_presence_of :title, :tmdb_id
has_many :movie_availabilities
has_many :services, through: :movie_availabilities
has_many :movie_genres
has_many :genres, through: :movie_genres
has_many :swipes
has_many :users, through: :swipes
def self.needs_details
Movie.where(description:nil)
end
def self.estimated_update_time(movie_count)
total_seconds = convert_movie_count_to_estimated_seconds(movie_count)
if hour_plus?(total_seconds)
time_in_hours(total_seconds)
elsif minute_plus?(total_seconds)
time_in_minutes(total_seconds)
else
time_in_seconds(total_seconds)
end
end
def self.convert_movie_count_to_estimated_seconds(movie_count)
(movie_count.to_f / 100 * 23).round(0)
end
def self.hour_plus?(seconds)
seconds > 3599
end
def self.minute_plus?(seconds)
seconds > 59
end
def self.time_in_hours(total_seconds)
hours = total_seconds / 3600
minutes = (total_seconds % 3600) / 60
seconds = total_seconds % 60
"#{hours} hour(s), #{minutes} minute(s), #{seconds} second(s)"
end
def self.time_in_minutes(total_seconds)
minutes = total_seconds / 60
seconds = total_seconds % 60
"#{minutes} minute(s), #{seconds} second(s)"
end
def self.time_in_seconds(seconds)
"#{seconds} second(s)"
end
def self.random_unswiped(user_id)
available_movies = Movie.joins(:movie_availabilities)
invalid_movies = Movie.joins(:swipes).where(swipes: {user_id:user_id})
valid_movies = available_movies - invalid_movies
unless valid_movies == []
valid_movies.sample
else
nil
end
end
def self.group_matches(group_id)
# REFACTOR - change to activerecord
group = Group.find(group_id)
right_swiped = Set.new
left_swiped = Set.new
group.users.each do |user|
user.swipes.each do |swipe|
if swipe.rating == 1
right_swiped << swipe.movie
elsif swipe.rating == 0
left_swiped << swipe.movie
end
end
end
valid_movies = (right_swiped - left_swiped).to_a
invalid_movies = []
group.users.each do |user|
valid_movies.each do |movie|
unless user.movies.include?(movie)
invalid_movies << movie
end
end
end
valid_movies - invalid_movies
end
end
<file_sep>/app/poros/friendlist.rb
class Friendlist
attr_reader :id,
:friends
def initialize(user)
@id = user.id
@friends = user.friends
end
end
<file_sep>/spec/requests/api/v1/movies_request_spec.rb
require 'rails_helper'
describe "Movies API" do
it "can retrieve all movies" do
create_list(:movie, 3)
get '/api/v1/movies'
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_an(Array)
expect(json[:data].length).to eq(3)
json[:data].each do |movie_data|
expect(movie_data).to have_key(:id)
expect(movie_data[:id]).to be_a(String)
expect(movie_data).to have_key(:type)
expect(movie_data[:type]).to be_a(String)
expect(movie_data).to have_key(:attributes)
expect(movie_data[:attributes]).to be_a(Hash)
expect(movie_data[:attributes]).to have_key(:title)
expect(movie_data[:attributes][:title]).to be_a(String)
expect(movie_data[:attributes]).to have_key(:tmdb_id)
expect(movie_data[:attributes][:tmdb_id]).to be_an(Integer)
expect(movie_data[:attributes]).to have_key(:poster_path)
expect(movie_data[:attributes][:poster_path]).to be_a(String)
expect(movie_data[:attributes]).to have_key(:description)
expect(movie_data[:attributes][:description]).to be_a(String)
expect(movie_data[:attributes]).to have_key(:vote_average)
expect(movie_data[:attributes][:vote_average]).to be_a(Float)
expect(movie_data[:attributes]).to have_key(:vote_count)
expect(movie_data[:attributes][:vote_count]).to be_an(Integer)
expect(movie_data[:attributes]).to have_key(:year)
expect(movie_data[:attributes][:year]).to be_a(String)
end
end
it 'can retrieve a movie by id' do
austin_powers = Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816
)
create_list(:movie, 3)
get "/api/v1/movies/#{austin_powers.id}"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(austin_powers.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to be_a(String)
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:title)
expect(json[:data][:attributes][:title]).to be_a(String)
expect(json[:data][:attributes][:title]).to eq(austin_powers.title)
expect(json[:data][:attributes]).to have_key(:tmdb_id)
expect(json[:data][:attributes][:tmdb_id]).to be_an(Integer)
expect(json[:data][:attributes][:tmdb_id]).to eq(austin_powers.tmdb_id)
expect(json[:data][:attributes]).to have_key(:poster_path)
expect(json[:data][:attributes][:poster_path]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:description)
expect(json[:data][:attributes][:description]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:vote_average)
expect(json[:data][:attributes][:vote_average]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:vote_count)
expect(json[:data][:attributes][:vote_count]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:year)
expect(json[:data][:attributes][:year]).to eq(nil)
end
it 'can create a movie' do
movie_params = ({
title: '<NAME>: International Man of Mystery',
tmdb_id: 816
})
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/movies", headers: headers, params: JSON.generate(movie_params)
austin_powers = Movie.last
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(austin_powers.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to be_a(String)
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:title)
expect(json[:data][:attributes][:title]).to be_a(String)
expect(json[:data][:attributes][:title]).to eq(austin_powers.title)
expect(json[:data][:attributes]).to have_key(:tmdb_id)
expect(json[:data][:attributes][:tmdb_id]).to be_an(Integer)
expect(json[:data][:attributes][:tmdb_id]).to eq(austin_powers.tmdb_id)
expect(json[:data][:attributes]).to have_key(:poster_path)
expect(json[:data][:attributes][:poster_path]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:description)
expect(json[:data][:attributes][:description]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:vote_average)
expect(json[:data][:attributes][:vote_average]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:vote_count)
expect(json[:data][:attributes][:vote_count]).to eq(nil)
expect(json[:data][:attributes]).to have_key(:year)
expect(json[:data][:attributes][:year]).to eq(nil)
end
it 'can update an existing movie' do
austin_powers = Movie.create(
title: '<NAME>: International Man of Mystery',
tmdb_id: 816
)
movie_params = ({
poster_path: '/1PkGnyFwRyapmbuILIOXXxiSh7Y.jpg',
description: "As a swingin' fashion photographer by day and a groovy British superagent by night, <NAME> is the '60s' most shagadelic spy, baby! But can he stop megalomaniac Dr. Evil after the bald villain freezes himself and unthaws in the '90s? With the help of sexy sidekick <NAME>, he just might.",
vote_average: 6.5,
vote_count: 2349,
year: '1997'
})
headers = {"CONTENT_TYPE" => "application/json"}
patch "/api/v1/movies/#{austin_powers.id}", headers: headers, params: JSON.generate(movie_params)
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(austin_powers.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to be_a(String)
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:title)
expect(json[:data][:attributes][:title]).to be_a(String)
expect(json[:data][:attributes][:title]).to eq(austin_powers.title)
expect(json[:data][:attributes]).to have_key(:tmdb_id)
expect(json[:data][:attributes][:tmdb_id]).to be_an(Integer)
expect(json[:data][:attributes][:tmdb_id]).to eq(austin_powers.tmdb_id)
expect(json[:data][:attributes]).to have_key(:poster_path)
expect(json[:data][:attributes][:poster_path]).to eq(movie_params[:poster_path])
expect(json[:data][:attributes]).to have_key(:description)
expect(json[:data][:attributes][:description]).to eq(movie_params[:description])
expect(json[:data][:attributes]).to have_key(:vote_average)
expect(json[:data][:attributes][:vote_average]).to eq(movie_params[:vote_average])
expect(json[:data][:attributes]).to have_key(:vote_count)
expect(json[:data][:attributes][:vote_count]).to eq(movie_params[:vote_count])
expect(json[:data][:attributes]).to have_key(:year)
expect(json[:data][:attributes][:year]).to eq(movie_params[:year])
end
it 'can delete a movie' do
austin_powers = Movie.create(
title: 'A<NAME>ers: International Man of Mystery',
tmdb_id: 816
)
expect(Movie.count).to eq(1)
delete "/api/v1/movies/#{austin_powers.id}"
expect(response).to be_successful
expect(Movie.count).to eq(0)
expect{Movie.find(austin_powers.id)}.to raise_error(ActiveRecord::RecordNotFound)
end
it 'can populate details for incomplete movies', :vcr do
Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816
)
Movie.create(
title: 'Nightcrawler',
tmdb_id: 242582
)
Movie.create(
title: 'The Theory of Everything',
tmdb_id: 266856
)
get '/api/v1/movies/populate_details'
json = JSON.parse(response.body, symbolize_names:true)
# tests response is correct
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:movies_updated)
expect(json[:movies_updated]).to eq(3)
expect(json).to have_key(:update_status)
expect(json[:update_status]).to eq('Complete - incomplete movies have been populated.')
austin_powers = Movie.find_by(tmdb_id:816)
nightcrawler = Movie.find_by(tmdb_id:242582)
theory_of_everything = Movie.find_by(tmdb_id:266856)
movies = [austin_powers, nightcrawler, theory_of_everything]
# tests movie objects have been updated
movies.each do |movie|
expect(movie.poster_path).to be_a(String)
expect(movie.description).to be_a(String)
expect(movie.vote_average).to be_a(Float)
expect(movie.vote_count).to be_a(Integer)
expect(movie.year).to be_a(String)
expect(movie.genres).not_to eq([])
end
end
it 'returns descriptive body when movie details are already populated' do
Movie.create(
title: '<NAME>: International Man of Mystery',
tmdb_id: 816,
poster_path: '/5uD4dxNX8JKFjWKYMHyOsqhi5pN.jpg',
description: "As a swingin' fashion photographer by day and a groovy British superagent by night, <NAME> is the '60s' most shagadelic spy, baby! But can he stop megalomaniac Dr. Evil after the bald villain freezes himself and unthaws in the '90s? With the help of sexy sidekick <NAME>, he just might.",
vote_average: 6.5,
vote_count: 2357,
year: '1997'
)
get '/api/v1/movies/populate_details'
json = JSON.parse(response.body, symbolize_names:true)
# tests response is correct
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:movies_updated)
expect(json[:movies_updated]).to eq(0)
expect(json).to have_key(:update_status)
expect(json[:update_status]).to eq('Complete - all movies are populated.')
end
it 'returns progress report for lengthy populate_details requests', :vcr do
30.times do
Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816
)
end
get '/api/v1/movies/populate_details'
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:movies_updated)
expect(json[:movies_updated]).to eq(30)
expect(json).to have_key(:update_status)
expect(json[:update_status]).to eq("In progress - details for 30 movies are currently being updated. Estimated completion time: 7 second(s).")
end
it 'can retrieve random available movie info' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
create_list(:movie, 3)
austin_powers = Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816,
poster_path: '/5uD4dxNX8JKFjWKYMHyOsqhi5pN.jpg',
description: "As a swingin' fashion photographer by day and a groovy British superagent by night, <NAME> is the '60s' most shagadelic spy, baby! But can he stop megalomaniac Dr. Evil after the bald villain freezes himself and unthaws in the '90s? With the help of sexy sidekick <NAME>, he just might.",
vote_average: 6.5,
vote_count: 2357,
year: '1997'
)
invalid_movie_1 = create(:movie)
invalid_movie_2 = create(:movie)
service = create(:service)
service.movie_availabilities.create(
movie: austin_powers
)
service.movie_availabilities.create(
movie: invalid_movie_1
)
nick.swipes.create(
movie: invalid_movie_1,
rating: 0
)
nick.swipes.create(
movie: invalid_movie_2,
rating: 0
)
genre_1 = Genre.create(
name: 'Action',
tmdb_id: 1
)
genre_2 = Genre.create(
name: 'Comedy',
tmdb_id: 2
)
austin_powers.movie_genres.create(
genre: genre_1
)
austin_powers.movie_genres.create(
genre: genre_2
)
params = {
user_id: nick.id
}
get '/api/v1/movies/random_available', params: params
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(austin_powers.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('movie')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:title)
expect(json[:data][:attributes][:title]).to eq(austin_powers.title)
expect(json[:data][:attributes]).to have_key(:tmdb_id)
expect(json[:data][:attributes][:tmdb_id]).to eq(austin_powers.tmdb_id)
expect(json[:data][:attributes]).to have_key(:poster_path)
expect(json[:data][:attributes][:poster_path]).to eq(austin_powers.poster_path)
expect(json[:data][:attributes]).to have_key(:description)
expect(json[:data][:attributes][:description]).to eq(austin_powers.description)
expect(json[:data][:attributes]).to have_key(:genres)
expect(json[:data][:attributes][:genres]).to be_an(Array)
json[:data][:attributes][:genres].each do |genre|
expect(genre).to be_a(Hash)
expect(genre).to have_key(:created_at)
expect(genre[:created_at]).to be_a(String)
expect(genre).to have_key(:id)
expect(genre[:id]).to be_an(Integer)
expect(genre).to have_key(:name)
expect(genre[:name]).to be_a(String)
expect(genre).to have_key(:tmdb_id)
expect(genre[:tmdb_id]).to be_an(Integer)
expect(genre).to have_key(:updated_at)
expect(genre[:updated_at]).to be_a(String)
end
expect(json[:data][:attributes]).to have_key(:vote_average)
expect(json[:data][:attributes][:vote_average]).to eq(austin_powers.vote_average)
expect(json[:data][:attributes]).to have_key(:vote_count)
expect(json[:data][:attributes][:vote_count]).to eq(austin_powers.vote_count)
expect(json[:data][:attributes]).to have_key(:year)
expect(json[:data][:attributes][:year]).to eq(austin_powers.year)
end
end
<file_sep>/config/routes.rb
Rails.application.routes.draw do
# For details on the DSL available within this file, see https://guides.rubyonrails.org/routing.html
namespace :api do
namespace :v1 do
# services routes
get '/services/update_availability', to: 'services#update_availability'
get '/services/update_all_availabilities', to: 'services#update_all_availabilities'
resources :services
# movies routes
get '/movies/populate_details', to: 'movies#populate_details'
get '/movies/random_available', to: 'movies#random_available'
resources :movies
# users routes
get '/users/:id/friends', to: 'users#friends'
resources :users, only: [:create, :show, :update] do
resources :friendships, only: [:create]
resources :groups, only: [:index]
end
# groups routes
get '/groups/:id/matches', to: 'groups#matches'
resources :groups, only: [:create, :destroy, :show]
# user_groups routes
resources :user_groups, only: [:create, :destroy]
# swipes routes
resources :swipes, only: [:create]
end
end
end
<file_sep>/spec/requests/api/v1/users_request_spec.rb
require 'rails_helper'
describe 'users API' do
it 'can create a user' do
user_params = {
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
}
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/users", headers: headers, params: JSON.generate(user_params)
nick = User.last
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(nick).to be_a(User)
expect(nick.uid).to eq(user_params[:uid])
expect(nick.first_name).to eq(user_params[:first_name])
expect(nick.last_name).to eq(user_params[:last_name])
expect(nick.image).to eq(user_params[:image])
expect(nick.email).to eq(user_params[:email])
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nick.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('user')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:first_name)
expect(json[:data][:attributes][:first_name]).to eq(nick.first_name)
expect(json[:data][:attributes]).to have_key(:last_name)
expect(json[:data][:attributes][:last_name]).to eq(nick.last_name)
expect(json[:data][:attributes]).to have_key(:email)
expect(json[:data][:attributes][:email]).to eq(nick.email)
expect(json[:data][:attributes]).to have_key(:image)
expect(json[:data][:attributes][:image]).to eq(nick.image)
expect(json[:data][:attributes]).to have_key(:uid)
expect(json[:data][:attributes][:uid]).to eq(nick.uid)
end
it 'can find an existing user with create action' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
user_params = {
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
}
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/users", headers: headers, params: JSON.generate(user_params)
json = JSON.parse(response.body, symbolize_names:true)
expect(User.last).to eq(nick)
expect(response).to be_successful
expect(nick).to be_a(User)
expect(nick.uid).to eq(user_params[:uid])
expect(nick.first_name).to eq(user_params[:first_name])
expect(nick.last_name).to eq(user_params[:last_name])
expect(nick.image).to eq(user_params[:image])
expect(nick.email).to eq(user_params[:email])
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nick.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('user')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:first_name)
expect(json[:data][:attributes][:first_name]).to eq(nick.first_name)
expect(json[:data][:attributes]).to have_key(:last_name)
expect(json[:data][:attributes][:last_name]).to eq(nick.last_name)
expect(json[:data][:attributes]).to have_key(:email)
expect(json[:data][:attributes][:email]).to eq(nick.email)
expect(json[:data][:attributes]).to have_key(:image)
expect(json[:data][:attributes][:image]).to eq(nick.image)
expect(json[:data][:attributes]).to have_key(:uid)
expect(json[:data][:attributes][:uid]).to eq(nick.uid)
end
it 'can find an existing user with show action' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
get "/api/v1/users/#{nick.uid}"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nick.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('user')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:first_name)
expect(json[:data][:attributes][:first_name]).to eq(nick.first_name)
expect(json[:data][:attributes]).to have_key(:last_name)
expect(json[:data][:attributes][:last_name]).to eq(nick.last_name)
expect(json[:data][:attributes]).to have_key(:email)
expect(json[:data][:attributes][:email]).to eq(nick.email)
expect(json[:data][:attributes]).to have_key(:image)
expect(json[:data][:attributes][:image]).to eq(nick.image)
expect(json[:data][:attributes]).to have_key(:uid)
expect(json[:data][:attributes][:uid]).to eq(nick.uid)
end
it 'returns descriptive error for invalid show requests' do
get "/api/v1/users/12345678910"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).not_to be_successful
expect(response.status).to eq(400)
expect(json).to be_a(Hash)
expect(json).to have_key(:error)
expect(json[:error]).to eq('User does not exist in database.')
end
it 'can update a user' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
user_params = {
email: '<EMAIL>',
first_name: 'Trick',
last_name: 'Thing',
image: 'https://image.shutterstock.com/shutterstock/photos/1036253818/display_1500/stock-photo-image-of-excited-screaming-young-woman-standing-isolated-over-yellow-background-looking-camera-1036253818.jpg'
}
headers = {"CONTENT_TYPE" => "application/json"}
patch "/api/v1/users/#{nick.uid}", headers: headers, params: JSON.generate(user_params)
json = JSON.parse(response.body, symbolize_names: true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nick.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('user')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:first_name)
expect(json[:data][:attributes][:first_name]).to eq(user_params[:first_name])
expect(json[:data][:attributes]).to have_key(:last_name)
expect(json[:data][:attributes][:last_name]).to eq(user_params[:last_name])
expect(json[:data][:attributes]).to have_key(:email)
expect(json[:data][:attributes][:email]).to eq(user_params[:email])
expect(json[:data][:attributes]).to have_key(:image)
expect(json[:data][:attributes][:image]).to eq(user_params[:image])
expect(json[:data][:attributes]).to have_key(:uid)
expect(json[:data][:attributes][:uid]).to eq(nick.uid)
end
it 'can show all friends of a user' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
tom = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Tom',
last_name: 'Haverford',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
leslie = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Leslie',
last_name: 'Knope',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
nick.friendships.create(friend: ron)
nick.friendships.create(friend: tom)
nick.friendships.create(friend: leslie)
get "/api/v1/users/#{nick.id}/friends"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nick.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('friendlist')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:id)
expect(json[:data][:attributes][:id]).to eq(nick.id)
expect(json[:data][:attributes]).to have_key(:friends)
expect(json[:data][:attributes][:friends]).to be_an(Array)
expect(json[:data][:attributes][:friends][0]).to be_a(Hash)
expect(json[:data][:attributes][:friends][0]).to have_key(:id)
expect(json[:data][:attributes][:friends][0][:id]).to eq(ron.id)
expect(json[:data][:attributes][:friends][0]).to have_key(:email)
expect(json[:data][:attributes][:friends][0][:email]).to eq(ron.email)
expect(json[:data][:attributes][:friends][0]).to have_key(:first_name)
expect(json[:data][:attributes][:friends][0][:first_name]).to eq(ron.first_name)
expect(json[:data][:attributes][:friends][0]).to have_key(:last_name)
expect(json[:data][:attributes][:friends][0][:last_name]).to eq(ron.last_name)
expect(json[:data][:attributes][:friends][0]).to have_key(:image)
expect(json[:data][:attributes][:friends][0][:image]).to eq(ron.image)
expect(json[:data][:attributes][:friends][0]).to have_key(:uid)
expect(json[:data][:attributes][:friends][0][:uid]).to eq(ron.uid)
expect(json[:data][:attributes][:friends][0]).to have_key(:created_at)
expect(json[:data][:attributes][:friends][0][:created_at]).to be_a(String)
expect(json[:data][:attributes][:friends][0]).to have_key(:updated_at)
expect(json[:data][:attributes][:friends][0][:updated_at]).to be_a(String)
expect(json[:data][:attributes][:friends][1]).to be_a(Hash)
expect(json[:data][:attributes][:friends][1]).to have_key(:id)
expect(json[:data][:attributes][:friends][1][:id]).to eq(tom.id)
expect(json[:data][:attributes][:friends][1]).to have_key(:email)
expect(json[:data][:attributes][:friends][1][:email]).to eq(tom.email)
expect(json[:data][:attributes][:friends][1]).to have_key(:first_name)
expect(json[:data][:attributes][:friends][1][:first_name]).to eq(tom.first_name)
expect(json[:data][:attributes][:friends][1]).to have_key(:last_name)
expect(json[:data][:attributes][:friends][1][:last_name]).to eq(tom.last_name)
expect(json[:data][:attributes][:friends][1]).to have_key(:image)
expect(json[:data][:attributes][:friends][1][:image]).to eq(tom.image)
expect(json[:data][:attributes][:friends][1]).to have_key(:uid)
expect(json[:data][:attributes][:friends][1][:uid]).to eq(tom.uid)
expect(json[:data][:attributes][:friends][1]).to have_key(:created_at)
expect(json[:data][:attributes][:friends][1][:created_at]).to be_a(String)
expect(json[:data][:attributes][:friends][1]).to have_key(:updated_at)
expect(json[:data][:attributes][:friends][1][:updated_at]).to be_a(String)
expect(json[:data][:attributes][:friends][2]).to be_a(Hash)
expect(json[:data][:attributes][:friends][2]).to have_key(:id)
expect(json[:data][:attributes][:friends][2][:id]).to eq(leslie.id)
expect(json[:data][:attributes][:friends][2]).to have_key(:email)
expect(json[:data][:attributes][:friends][2][:email]).to eq(leslie.email)
expect(json[:data][:attributes][:friends][2]).to have_key(:first_name)
expect(json[:data][:attributes][:friends][2][:first_name]).to eq(leslie.first_name)
expect(json[:data][:attributes][:friends][2]).to have_key(:last_name)
expect(json[:data][:attributes][:friends][2][:last_name]).to eq(leslie.last_name)
expect(json[:data][:attributes][:friends][2]).to have_key(:image)
expect(json[:data][:attributes][:friends][2][:image]).to eq(leslie.image)
expect(json[:data][:attributes][:friends][2]).to have_key(:uid)
expect(json[:data][:attributes][:friends][2][:uid]).to eq(leslie.uid)
expect(json[:data][:attributes][:friends][2]).to have_key(:created_at)
expect(json[:data][:attributes][:friends][2][:created_at]).to be_a(String)
expect(json[:data][:attributes][:friends][2]).to have_key(:updated_at)
expect(json[:data][:attributes][:friends][2][:updated_at]).to be_a(String)
end
it 'returns an empty friends array if user has no friends' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
get "/api/v1/users/#{nick.id}/friends"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nick.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('friendlist')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:id)
expect(json[:data][:attributes][:id]).to eq(nick.id)
expect(json[:data][:attributes]).to have_key(:friends)
expect(json[:data][:attributes][:friends]).to be_an(Array)
expect(json[:data][:attributes][:friends]).to eq([])
end
end
<file_sep>/app/models/movie_availability.rb
class MovieAvailability < ApplicationRecord
belongs_to :movie
belongs_to :service
end
<file_sep>/app/controllers/api/v1/movies_controller.rb
class Api::V1::MoviesController < ApplicationController
def index
render json: MovieSerializer.new(Movie.all)
end
def show
render json: MovieSerializer.new(Movie.find(params[:id]))
end
def create
movie = Movie.create(movie_params)
render json: MovieSerializer.new(movie)
end
def update
movie = Movie.find(params[:id])
movie.update(movie_params)
render json: MovieSerializer.new(movie)
end
def destroy
Movie.destroy(params[:id])
end
def populate_details
movies = Movie.needs_details
if movies == []
output = {
movies_updated: 0,
update_status: 'Complete - all movies are populated.'
}
render json: output
elsif movies.length < 30
TmdbFacade.populate_movie_details(movies)
output = {
movies_updated: movies.length,
update_status: 'Complete - incomplete movies have been populated.'
}
render json: output
else
MovieDetailsUpdateJob.perform_later
output = {
movies_updated: movies.length,
update_status: "In progress - details for #{movies.length} movies are currently being updated. Estimated completion time: #{Movie.estimated_update_time(movies.length)}."
}
render json: output
end
end
def random_available
movie = Movie.random_unswiped(params[:user_id].to_i)
# Add if movie
render json: MovieSerializer.new(movie)
# Add else
# Sad path code
# end
end
private
def movie_params
params.permit(:title, :tmdb_id, :poster_path, :description, :vote_average, :vote_count, :year)
end
end
<file_sep>/app/services/watchmode_service.rb
class WatchmodeService
def self.get_movies_by_service(service_id, page = 1, output = [])
response = conn.get('/v1/list-titles/') do |req|
req.params['type'] = 'movie'
req.params['regions'] = 'US'
req.params['source_ids'] = service_id.to_s
req.params['source_types'] = 'sub'
req.params['page'] = page
end
json = JSON.parse(response.body, symbolize_names: true)
output << json
# Recursion used here to get movie data for all pages
# (Watchmode limits results to 250 movies/page)
if json[:total_pages] > page
get_movies_by_service(service_id, page + 1, output)
else
output
end
end
private
def self.conn
Faraday.new(url: 'https://api.watchmode.com') do |req|
req.params['apiKey'] = ENV['WATCHMODE_API_KEY']
end
end
end
<file_sep>/spec/requests/api/v1/friendships_request_spec.rb
require 'rails_helper'
describe 'Friendship API' do
it 'can create a friendship' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
friendship_params = ({
friend_email: ron.email
})
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/users/#{nick.id}/friendships", headers: headers, params: JSON.generate(friendship_params)
friendship = Friendship.last
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(friendship).to be_a(Friendship)
expect(friendship.user).to eq(nick)
expect(friendship.friend).to eq(ron)
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(ron.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('user')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:first_name)
expect(json[:data][:attributes][:first_name]).to eq(ron.first_name)
expect(json[:data][:attributes]).to have_key(:last_name)
expect(json[:data][:attributes][:last_name]).to eq(ron.last_name)
expect(json[:data][:attributes]).to have_key(:email)
expect(json[:data][:attributes][:email]).to eq(ron.email)
expect(json[:data][:attributes]).to have_key(:image)
expect(json[:data][:attributes][:image]).to eq(ron.image)
expect(json[:data][:attributes]).to have_key(:uid)
expect(json[:data][:attributes][:uid]).to eq(ron.uid)
end
it 'friend create with invalid email returns a descriptive error message' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
friendship_params = ({
friend_email: '<EMAIL>'
})
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/users/#{nick.id}/friendships", headers: headers, params: JSON.generate(friendship_params)
json = JSON.parse(response.body, symbolize_names:true)
expect(response).not_to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:error)
expect(json[:error]).to eq('Invalid email. Friend not added.')
end
it 'friend create when friendship already exists returns descriptive error message' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
Friendship.create(
user: nick,
friend: ron
)
friendship_params = ({
friend_email: ron.email
})
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/users/#{nick.id}/friendships", headers: headers, params: JSON.generate(friendship_params)
json = JSON.parse(response.body, symbolize_names:true)
expect(response).not_to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:error)
expect(json[:error]).to eq('You are already friends with <NAME>.')
end
end
<file_sep>/app/serializers/user_group_serializer.rb
class UserGroupSerializer
include FastJsonapi::ObjectSerializer
attributes :id, :user_id, :group_id
end
<file_sep>/spec/models/user_spec.rb
require 'rails_helper'
describe User, type: :model do
describe 'validations' do
it { should validate_presence_of :email}
it { should validate_presence_of :first_name}
it { should validate_presence_of :last_name}
it { should validate_presence_of :image}
it { should validate_presence_of :uid}
end
describe 'relationships' do
it do
should have_many :swipes
should have_many(:movies).through(:swipes)
should have_many :friendships
should have_many(:friends).through(:friendships)
should have_many :user_groups
should have_many(:groups).through(:user_groups)
end
end
end
<file_sep>/spec/models/movie_genre_spec.rb
require 'rails_helper'
describe MovieGenre, type: :model do
describe 'relationships' do
it do
should belong_to :movie
should belong_to :genre
end
end
end
<file_sep>/app/jobs/movie_details_update_job.rb
class MovieDetailsUpdateJob < ApplicationJob
queue_as :default
def perform
movies = Movie.needs_details
movies.each do |movie|
movie_data = TmdbService.get_movie_info(movie)
movie_info = MovieInfo.new(movie_data)
movie.update(
poster_path: movie_info.poster_path,
description: movie_info.description,
vote_average: movie_info.vote_average,
vote_count: movie_info.vote_count,
year: movie_info.year
)
if movie_info.genres
movie_info.genres.each do |genre|
current_genre = Genre.create_with(name:genre[:name]).find_or_create_by(tmdb_id:genre[:id])
current_genre.movie_genres.create(movie:movie)
end
end
end
end
end
<file_sep>/spec/jobs/movie_details_update_job_spec.rb
require 'rails_helper'
RSpec.describe MovieDetailsUpdateJob, type: :job do
it 'populates details for incomplete movies', :vcr do
movies = []
movies << Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816
)
movies << Movie.create(
title: 'Nightcrawler',
tmdb_id: 242582
)
movies << Movie.create(
title: 'The Theory of Everything',
tmdb_id: 266856
)
MovieDetailsUpdateJob.perform_now
austin_powers = Movie.find_by(tmdb_id:816)
nightcrawler = Movie.find_by(tmdb_id:242582)
theory_of_everything = Movie.find_by(tmdb_id:266856)
updated_movies = [austin_powers, nightcrawler, theory_of_everything]
# tests movie objects have been updated
updated_movies.each do |movie|
expect(movie.poster_path).to be_a(String)
expect(movie.description).to be_a(String)
expect(movie.vote_average).to be_a(Float)
expect(movie.vote_count).to be_a(Integer)
expect(movie.year).to be_a(String)
expect(movie.genres).not_to eq([])
end
end
end
<file_sep>/app/services/tmdb_service.rb
class TmdbService
def self.get_movie_info(movie)
response = conn.get("/3/movie/#{movie.tmdb_id}")
JSON.parse(response.body, symbolize_names:true)
end
private
def self.conn
Faraday.new(url: 'https://api.themoviedb.org') do |req|
req.params['api_key'] = ENV['TMDB_API_KEY']
end
end
end
<file_sep>/spec/factories/services.rb
FactoryBot.define do
factory :service do
name { Faker::Music::GratefulDead.song }
watchmode_id { Faker::Number.within(range: 1..1000) }
logo { Faker::LoremFlickr.image }
end
end
<file_sep>/spec/requests/api/v1/services_request_spec.rb
require 'rails_helper'
describe 'services API' do
it 'can retrieve all services' do
create_list(:service, 3)
get '/api/v1/services'
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_an(Array)
expect(json[:data].length).to eq(3)
json[:data].each do |service_data|
expect(service_data).to have_key(:id)
expect(service_data[:id]).to be_a(String)
expect(service_data).to have_key(:type)
expect(service_data[:type]).to be_a(String)
expect(service_data).to have_key(:attributes)
expect(service_data[:attributes]).to be_a(Hash)
expect(service_data[:attributes]).to have_key(:name)
expect(service_data[:attributes][:name]).to be_a(String)
expect(service_data[:attributes]).to have_key(:watchmode_id)
expect(service_data[:attributes][:watchmode_id]).to be_an(Integer)
expect(service_data[:attributes]).to have_key(:logo)
expect(service_data[:attributes][:logo]).to be_a(String)
end
end
it 'can retrieve service by id' do
netflix = Service.create(
name: 'Netflix',
watchmode_id: 1234,
logo: 'netflix.jpeg'
)
get "/api/v1/services/#{netflix.id}"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(netflix.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to be_a(String)
expect(json[:data][:type]).to eq('service')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:name)
expect(json[:data][:attributes][:name]).to be_a(String)
expect(json[:data][:attributes][:name]).to eq(netflix.name)
expect(json[:data][:attributes]).to have_key(:watchmode_id)
expect(json[:data][:attributes][:watchmode_id]).to be_an(Integer)
expect(json[:data][:attributes][:watchmode_id]).to eq(netflix.watchmode_id)
expect(json[:data][:attributes]).to have_key(:logo)
expect(json[:data][:attributes][:logo]).to be_a(String)
expect(json[:data][:attributes][:logo]).to eq(netflix.logo)
end
it 'can create a service' do
service_params = {
name: 'Netflix',
watchmode_id: 1234,
logo: 'netflix.jpeg'
}
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/services", headers: headers, params: JSON.generate(service_params)
netflix = Service.last
json = JSON.parse(response.body, symbolize_names: true)
expect(response).to be_successful
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(netflix.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to be_a(String)
expect(json[:data][:type]).to eq('service')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:name)
expect(json[:data][:attributes][:name]).to be_a(String)
expect(json[:data][:attributes][:name]).to eq(service_params[:name])
expect(json[:data][:attributes]).to have_key(:watchmode_id)
expect(json[:data][:attributes][:watchmode_id]).to be_an(Integer)
expect(json[:data][:attributes][:watchmode_id]).to eq(service_params[:watchmode_id])
expect(json[:data][:attributes]).to have_key(:logo)
expect(json[:data][:attributes][:logo]).to be_a(String)
expect(json[:data][:attributes][:logo]).to eq(service_params[:logo])
end
it 'can update a service' do
netflix = Service.create(
name: 'Netflix',
watchmode_id: 1234,
logo: 'netflix.jpeg'
)
service_params = {
name: '<NAME>',
watchmode_id: 12345,
logo: 'netflix_2.jpeg'
}
headers = {"CONTENT_TYPE" => "application/json"}
patch "/api/v1/services/#{netflix.id}", headers: headers, params: JSON.generate(service_params)
json = JSON.parse(response.body, symbolize_names: true)
expect(response).to be_successful
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to be_a(String)
expect(json[:data][:id]).to eq(netflix.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to be_a(String)
expect(json[:data][:type]).to eq('service')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:name)
expect(json[:data][:attributes][:name]).to be_a(String)
expect(json[:data][:attributes][:name]).to eq(service_params[:name])
expect(json[:data][:attributes]).to have_key(:watchmode_id)
expect(json[:data][:attributes][:watchmode_id]).to be_an(Integer)
expect(json[:data][:attributes][:watchmode_id]).to eq(service_params[:watchmode_id])
expect(json[:data][:attributes]).to have_key(:logo)
expect(json[:data][:attributes][:logo]).to be_a(String)
expect(json[:data][:attributes][:logo]).to eq(service_params[:logo])
end
it 'can delete a service' do
netflix = Service.create(
name: 'Netflix',
watchmode_id: 1234,
logo: 'netflix.jpeg'
)
expect(Service.count).to eq(1)
delete "/api/v1/services/#{netflix.id}"
expect(response).to be_successful
expect(Service.count).to eq(0)
expect{Service.find(netflix.id)}.to raise_error(ActiveRecord::RecordNotFound)
end
it 'can update availability', :vcr do
hulu = Service.create(
name: 'Hulu',
watchmode_id: 157,
logo: 'hulu_logo.png'
)
movie_1 = create(:movie)
movie_2 = create(:movie)
movie_3 = create(:movie)
hulu.movie_availabilities.create(movie: movie_1)
hulu.movie_availabilities.create(movie: movie_2)
hulu.movie_availabilities.create(movie: movie_3)
params = {
service: 'hulu'
}
get "/api/v1/services/update_availability", params: params
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nil)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('service_refresh')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:name)
expect(json[:data][:attributes][:name]).to eq('Hulu')
expect(json[:data][:attributes]).to have_key(:movie_count)
expect(json[:data][:attributes][:movie_count]).to be_an(Integer)
end
xit 'can update all availabilities', :vcr do
netflix = Service.create(
name: 'Netflix',
watchmode_id: 203,
logo: 'netflix_logo.png'
)
amazon_prime = Service.create(
name: 'Amazon Prime Video',
watchmode_id: 157,
logo: 'prime_video_logo.jpeg'
)
hulu = Service.create(
name: 'Hulu',
watchmode_id: 26,
logo: 'hulu_logo.jpeg'
)
get "/api/v1/services/update_all_availabilities"
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(nil)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('full_refresh')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:total_movie_count)
expect(json[:data][:attributes][:total_movie_count]).to be_an(Integer)
expect(json[:data][:attributes]).to have_key(:service_refreshes)
expect(json[:data][:attributes][:service_refreshes]).to be_an(Array)
json[:data][:attributes][:service_refreshes].each do |service_refresh|
expect(service_refresh).to be_a(Hash)
expect(service_refresh).to have_key(:id)
expect(service_refresh[:id]).to eq(nil)
expect(service_refresh).to have_key(:name)
expect(service_refresh[:name]).to be_a(String)
expect(service_refresh).to have_key(:movie_count)
expect(service_refresh[:movie_count]).to be_an(Integer)
end
end
end
<file_sep>/spec/fixtures/sources.rb
# this is a reference file for watchmode source ids
[{
"id": 203,
"name": "Netflix",
"type": "sub",
"ios_appstore_url": "http://itunes.apple.com/app/netflix/id363590051",
"android_playstore_url": "https://play.google.com/store/apps/details?id=com.netflix.mediaclient&hl=en",
"android_scheme": "nflx",
"ios_scheme": "nflx",
"regions": [
"US",
"CA",
"GB",
"AU"
]
},
{
"id": 157,
"name": "Hulu",
"type": "sub",
"ios_appstore_url": "http://itunes.apple.com/app/hulu-plus/id376510438",
"android_playstore_url": "https://play.google.com/store/apps/details?id=com.hulu.plus",
"android_scheme": "hulu",
"ios_scheme": "hulu",
"regions": [
"US"
]
},
{
"id": 26,
"name": "<NAME>",
"type": "sub",
"ios_appstore_url": "http://itunes.apple.com/app/amazon-instant-video/id545519333",
"android_playstore_url": "http://amazon.com/GetAndroidVideo",
"android_scheme": "intent",
"ios_scheme": "aiv",
"regions": [
"US",
"GB"
]
},
{
"id": 387,
"name": "<NAME>",
"type": "sub",
"ios_appstore_url": null,
"android_playstore_url": null,
"android_scheme": "hbomax",
"ios_scheme": "hbomax",
"regions": [
"US"
]
},
{
"id": 372,
"name": "Disney+",
"type": "sub",
"ios_appstore_url": "https://apps.apple.com/app/disney/id1446075923",
"android_playstore_url": null,
"android_scheme": "disneyplus",
"ios_scheme": "disneyplus",
"regions": [
"US",
"CA",
"GB",
"AU"
]
}]
<file_sep>/spec/models/movie_spec.rb
require 'rails_helper'
describe Movie, type: :model do
describe 'validations' do
it { should validate_presence_of :title}
it { should validate_presence_of :tmdb_id}
end
describe 'relationships' do
it do
should have_many :movie_availabilities
should have_many(:services).through(:movie_availabilities)
should have_many :movie_genres
should have_many(:genres).through(:movie_genres)
should have_many :swipes
should have_many(:users).through(:swipes)
end
end
describe 'class methods' do
it '.needs_details' do
expect(Movie.needs_details).to eq([])
create_list(:movie, 3)
expect(Movie.needs_details).to eq([])
austin_powers = Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816
)
expect(Movie.needs_details).to eq([austin_powers])
end
it '.estimated_update_time' do
expect(Movie.estimated_update_time(44)).to eq('10 second(s)')
expect(Movie.estimated_update_time(261)).to eq('1 minute(s), 0 second(s)')
expect(Movie.estimated_update_time(16000)).to eq('1 hour(s), 1 minute(s), 20 second(s)')
end
it '.convert_movie_count_to_estimated_seconds' do
expect(Movie.convert_movie_count_to_estimated_seconds(44)).to eq(10)
expect(Movie.convert_movie_count_to_estimated_seconds(261)).to eq(60)
expect(Movie.convert_movie_count_to_estimated_seconds(16000)).to eq(3680)
end
it '.hour_plus?' do
expect(Movie.hour_plus?(400)).to be false
expect(Movie.hour_plus?(6000)).to be true
end
it '.minute_plus?' do
expect(Movie.minute_plus?(44)).to be false
expect(Movie.minute_plus?(65)).to be true
end
it '.time_in_hours' do
expect(Movie.time_in_hours(16000)).to eq('4 hour(s), 26 minute(s), 40 second(s)')
end
it '.time_in_minutes' do
expect(Movie.time_in_minutes(261)).to eq('4 minute(s), 21 second(s)')
end
it '.time_in_seconds' do
expect(Movie.time_in_seconds(44)).to eq('44 second(s)')
end
it '.random_unswiped' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
service = create(:service)
valid_movies = create_list(:movie, 3)
invalid_movie = create(:movie)
Movie.all.each do |movie|
movie.movie_availabilities.create(
service: service
)
end
invalid_movie.swipes.create(
user: nick,
rating: 1
)
10.times do
movie = Movie.random_unswiped(nick.id)
expect(movie).not_to eq(invalid_movie)
expect(movie).to be_a(Movie)
expect(valid_movies).to include(movie)
end
end
it '.group_right_swiped' do
austin_powers = Movie.create(
title: 'Austin Powers: International Man of Mystery',
tmdb_id: 816
)
nightcrawler = Movie.create(
title: 'Nightcrawler',
tmdb_id: 242582
)
theory_of_everything = Movie.create(
title: 'The Theory of Everything',
tmdb_id: 266856
)
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
tom = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Tom',
last_name: 'Haverford',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
leslie = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Leslie',
last_name: 'Knope',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
fantastic_four = Group.create(
name: 'Fantastic Four'
)
avengers = Group.create(
name: 'The Avengers'
)
x_men = Group.create(
name: 'X Men'
)
UserGroup.create(
user: nick,
group: fantastic_four
)
UserGroup.create(
user: ron,
group: fantastic_four
)
UserGroup.create(
user: tom,
group: fantastic_four
)
UserGroup.create(
user: leslie,
group: fantastic_four
)
UserGroup.create(
user: nick,
group: x_men
)
UserGroup.create(
user: ron,
group: x_men
)
UserGroup.create(
user: tom,
group: x_men
)
UserGroup.create(
user: ron,
group: avengers
)
UserGroup.create(
user: leslie,
group: avengers
)
Swipe.create(
movie: austin_powers,
user: nick,
rating: 1
)
Swipe.create(
movie: austin_powers,
user: ron,
rating: 1
)
Swipe.create(
movie: austin_powers,
user: tom,
rating: 1
)
Swipe.create(
movie: austin_powers,
user: leslie,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: nick,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: ron,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: tom,
rating: 1
)
Swipe.create(
movie: nightcrawler,
user: leslie,
rating: 0
)
Swipe.create(
movie: theory_of_everything,
user: leslie,
rating: 1
)
Swipe.create(
movie: theory_of_everything,
user: ron,
rating: 1
)
expect(Movie.group_matches(fantastic_four.id)).to eq([austin_powers])
expect(Movie.group_matches(x_men.id)).to eq([austin_powers, nightcrawler])
expect(Movie.group_matches(avengers.id)).to eq([austin_powers, theory_of_everything])
end
end
end
<file_sep>/spec/fixtures/vcr_cassettes/Movies_API/can_populate_details_for_incomplete_movies.yml
---
http_interactions:
- request:
method: get
uri: https://api.themoviedb.org/3/movie/816?api_key=<TMDB_API_KEY>
body:
encoding: US-ASCII
string: ''
headers:
User-Agent:
- Faraday v1.3.0
Accept-Encoding:
- gzip;q=1.0,deflate;q=0.6,identity;q=0.3
Accept:
- "*/*"
response:
status:
code: 200
message: OK
headers:
Content-Type:
- application/json;charset=utf-8
Transfer-Encoding:
- chunked
Connection:
- keep-alive
Date:
- Wed, 05 May 2021 22:30:54 GMT
Server:
- openresty
Access-Control-Allow-Origin:
- "*"
Access-Control-Allow-Methods:
- GET, HEAD, POST, PUT, DELETE, OPTIONS
Access-Control-Expose-Headers:
- ETag, X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Reset, Retry-After,
Content-Length, Content-Range
Cache-Control:
- public, max-age=28800
X-Memc:
- HIT
X-Memc-Key:
- d485a21da9c0e054971e82e13703bebab13cca1f
X-Memc-Age:
- '1625'
X-Memc-Expires:
- '13466'
Etag:
- W/"4ae30f0d8e8e03498386ac563d2cd8c1"
Vary:
- Accept-Encoding
X-Cache:
- Miss from cloudfront
Via:
- 1.1 12e4fc9256386a4cd8f000b9caa3e012.cloudfront.net (CloudFront)
X-Amz-Cf-Pop:
- DEN52-C1
X-Amz-Cf-Id:
- zKRMD6-Ndeu8g3Vt9I_L_xxLN6Czbh-7zAgzdntoJnTZhIt1QkfPhA==
body:
encoding: ASCII-8BIT
string: '{"adult":false,"backdrop_path":"/tsxwVsl9BYxy9y1AtxpM7xpNiC8.jpg","belongs_to_collection":{"id":1006,"name":"Austin
Powers Collection","poster_path":"/1PkGnyFwRyapmbuILIOXXxiSh7Y.jpg","backdrop_path":"/3QJMI8nqQcwU5JBPy26m8TUXtTN.jpg"},"budget":16500000,"genres":[{"id":878,"name":"Science
Fiction"},{"id":35,"name":"Comedy"},{"id":80,"name":"Crime"}],"homepage":"","id":816,"imdb_id":"tt0118655","original_language":"en","original_title":"Austin
Powers: International Man of Mystery","overview":"As a swingin'' fashion photographer
by day and a groovy British superagent by night, <NAME> is the ''60s''
most shagadelic spy, baby! But can he stop megalomaniac Dr. Evil after the
bald villain freezes himself and unthaws in the ''90s? With the help of sexy
sidekick <NAME>, he just might.","popularity":18.999,"poster_path":"/5uD4dxNX8JKFjWKYMHyOsqhi5pN.jpg","production_companies":[{"id":12,"logo_path":"/iaYpEp3LQmb8AfAtmTvpqd4149c.png","name":"New
Line Cinema","origin_country":"US"},{"id":594,"logo_path":null,"name":"Capella
International","origin_country":""},{"id":595,"logo_path":null,"name":"Eric''s
Boy","origin_country":""}],"production_countries":[{"iso_3166_1":"DE","name":"Germany"},{"iso_3166_1":"US","name":"United
States of America"}],"release_date":"1997-05-02","revenue":67683989,"runtime":94,"spoken_languages":[{"english_name":"English","iso_639_1":"en","name":"English"}],"status":"Released","tagline":"If
he were any cooler, he''d still be frozen, baby!","title":"<NAME>ers:
International Man of Mystery","video":false,"vote_average":6.5,"vote_count":2372}'
recorded_at: Wed, 05 May 2021 22:30:54 GMT
- request:
method: get
uri: https://api.themoviedb.org/3/movie/242582?api_key=<TMDB_API_KEY>
body:
encoding: US-ASCII
string: ''
headers:
User-Agent:
- Faraday v1.3.0
Accept-Encoding:
- gzip;q=1.0,deflate;q=0.6,identity;q=0.3
Accept:
- "*/*"
response:
status:
code: 200
message: OK
headers:
Content-Type:
- application/json;charset=utf-8
Transfer-Encoding:
- chunked
Connection:
- keep-alive
Date:
- Wed, 05 May 2021 22:30:54 GMT
Server:
- openresty
Access-Control-Allow-Origin:
- "*"
Access-Control-Allow-Methods:
- GET, HEAD, POST, PUT, DELETE, OPTIONS
Access-Control-Expose-Headers:
- ETag, X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Reset, Retry-After,
Content-Length, Content-Range
Cache-Control:
- public, max-age=28800
X-Memc:
- HIT
X-Memc-Key:
- <KEY>37bc3f3941
X-Memc-Age:
- '11598'
X-Memc-Expires:
- '3167'
Etag:
- W/"6d718eefd20e3fced229d3be97f3c44c"
Vary:
- Accept-Encoding
X-Cache:
- Miss from cloudfront
Via:
- 1.1 b7901622e66455e244a3c16acc8e6835.cloudfront.net (CloudFront)
X-Amz-Cf-Pop:
- DEN52-C1
X-Amz-Cf-Id:
- lM-icBEfgD11RazYUQfBhUwC-rwI_ibcCotvK-veHDcFaCL2fmWXXQ==
body:
encoding: ASCII-8BIT
string: '{"adult":false,"backdrop_path":"/3kEfSOwBYhb11APEG0aUDysvW8F.jpg","belongs_to_collection":null,"budget":8500000,"genres":[{"id":80,"name":"Crime"},{"id":18,"name":"Drama"},{"id":53,"name":"Thriller"}],"homepage":"http://nightcrawlerfilm.com","id":242582,"imdb_id":"tt2872718","original_language":"en","original_title":"Nightcrawler","overview":"When
Lou Bloom, desperate for work, muscles into the world of L.A. crime journalism,
he blurs the line between observer and participant to become the star of his
own story. Aiding him in his effort is Nina, a TV-news veteran.","popularity":27.42,"poster_path":"/j9HrX8f7GbZQm1BrBiR40uFQZSb.jpg","production_companies":[{"id":24049,"logo_path":"/zOHQ0A3PpNoLLeAxRZwSqNV3nPr.png","name":"Sierra/Affinity","origin_country":"US"},{"id":2266,"logo_path":"/owzVs2mguyyJ3vIn7EbgowpUPnk.png","name":"Bold
Films","origin_country":"US"}],"production_countries":[{"iso_3166_1":"US","name":"United
States of America"}],"release_date":"2014-10-23","revenue":50300000,"runtime":118,"spoken_languages":[{"english_name":"English","iso_639_1":"en","name":"English"}],"status":"Released","tagline":"The
city shines brightest at night","title":"Nightcrawler","video":false,"vote_average":7.7,"vote_count":8011}'
recorded_at: Wed, 05 May 2021 22:30:54 GMT
- request:
method: get
uri: https://api.themoviedb.org/3/movie/266856?api_key=<TMDB_API_KEY>
body:
encoding: US-ASCII
string: ''
headers:
User-Agent:
- Faraday v1.3.0
Accept-Encoding:
- gzip;q=1.0,deflate;q=0.6,identity;q=0.3
Accept:
- "*/*"
response:
status:
code: 200
message: OK
headers:
Content-Type:
- application/json;charset=utf-8
Transfer-Encoding:
- chunked
Connection:
- keep-alive
Date:
- Wed, 05 May 2021 22:30:54 GMT
Server:
- openresty
Access-Control-Allow-Origin:
- "*"
Access-Control-Allow-Methods:
- GET, HEAD, POST, PUT, DELETE, OPTIONS
Access-Control-Expose-Headers:
- ETag, X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Reset, Retry-After,
Content-Length, Content-Range
Cache-Control:
- public, max-age=28800
X-Memc:
- HIT
X-Memc-Key:
- 4aaacececdac005066b41b9957150a9dcd5f36f3
X-Memc-Age:
- '13963'
X-Memc-Expires:
- '3115'
Etag:
- W/"e65f0854703340851375a54253845356"
Vary:
- Accept-Encoding
X-Cache:
- Miss from cloudfront
Via:
- 1.1 a5bffe3d9fb1379dc2d31313b684f3b7.cloudfront.net (CloudFront)
X-Amz-Cf-Pop:
- DEN52-C1
X-Amz-Cf-Id:
- aYTg8UNKefPkz8oEZ42jGwy_oLI6VuIE0DoWyZQDu9lR92kNatB4wg==
body:
encoding: ASCII-8BIT
string: !binary |-
<KEY>
recorded_at: Wed, 05 May 2021 22:30:54 GMT
recorded_with: VCR 6.0.0
<file_sep>/db/migrate/20210416193749_remove_genres_from_movies.rb
class RemoveGenresFromMovies < ActiveRecord::Migration[6.1]
def change
remove_column :movies, :genres, :string
end
end
<file_sep>/app/controllers/api/v1/friendships_controller.rb
class Api::V1::FriendshipsController < ApplicationController
def create
friend = User.find_by(email: friendship_params[:friend_email])
if friend && Friendship.exists?(friendship_params[:user_id], friend.id)
output = {
error: "You are already friends with #{friend.first_name} #{friend.last_name}."
}
render json: output, :status => 400
elsif friend
friendship = Friendship.create(
user_id: friendship_params[:user_id],
friend: friend
)
render json: UserSerializer.new(friend)
else
output = {
error: 'Invalid email. Friend not added.'
}
render json: output, :status => 400
end
end
private
def friendship_params
params.permit(:user_id, :friend_email)
end
end
<file_sep>/app/models/group.rb
class Group < ApplicationRecord
validates_presence_of :name
has_many :user_groups
has_many :users, through: :user_groups
end
<file_sep>/app/models/service.rb
class Service < ApplicationRecord
validates_presence_of :name, :watchmode_id, :logo
has_many :movie_availabilities
has_many :movies, through: :movie_availabilities
end
<file_sep>/spec/models/friendship_spec.rb
require 'rails_helper'
describe Friendship, type: :model do
describe 'validations' do
it { should validate_presence_of :user_id}
it { should validate_presence_of :friend_id}
end
describe 'relationships' do
it do
should belong_to :user
should belong_to :friend
end
end
describe 'class methods' do
it '.exists?' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
ron = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Ron',
last_name: 'Swanson',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
expect(Friendship.exists?(nick.id, ron.id)).to eq(false)
Friendship.create(
user: nick,
friend: ron
)
expect(Friendship.exists?(nick.id, ron.id)).to eq(true)
end
end
end
<file_sep>/app/poros/full_refresh.rb
class FullRefresh
attr_reader :id,
:total_movie_count,
:service_refreshes
def initialize(service_refreshes)
@id = nil
@total_movie_count = add_movie_counts(service_refreshes)
@service_refreshes = service_refreshes
end
def add_movie_counts(service_refreshes)
service_refreshes.sum do |service_refresh|
service_refresh.movie_count
end
end
end
<file_sep>/README.md
<!-- Shields -->


# Plenty of Flicks BE
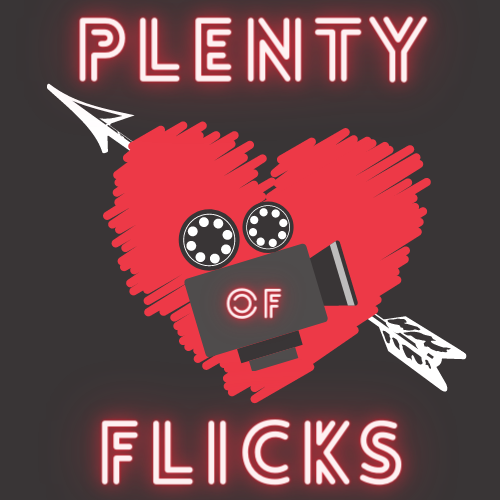
## Table of Contents
- [What it does](#what-it-does)
- [How to Install](#how-to-install)
- [Dependencies](#dependencies)
- [Learning Goals](#learning-goals)
- [Project Diagram](#project-diagram)
- [Schema](#schema)
- [API Documentation](#api-documentation)
- [Licenses](#licenses)
- [Contact](#contact)
## What it does
### The Plenty of Flicks App
> We all know the struggle. You sit down to watch a movie with a group of friends or significant other, and spend more time picking out a suitable flick than actually watching the movie. Enter Plenty of Flicks. Users can explore available movies on three supported subscription services (Netflix, Amazon Prime Video and Hulu), and add movies to their watchlist. When it's time to watch, users create a party with the group they want to watch with, and voila: PoF presents a list of movies they ALL want to watch.
### The Plenty of Flicks BE Repo
> The Plenty of Flicks BE Repo leverages two external APIs to create custom endpoints for the FE Repo. The Watchmode API (https://api.watchmode.com/docs/) '/list-titles' endpoint is used to collect and refresh information about available movies on supported services, while The Movie Database API (https://developers.themoviedb.org/3/getting-started/introduction) is used to get detailed information about the available movies including images, descriptions and ratings. The data is stored in the BE PostgreSQL database, and uses a combination of RESTful and non-RESTful endpoints to pass the data to the FE.
> Please [visit the Plenty of Flicks FE Repo here](https://github.com/plenty-of-flicks/plenty_of_flicks_fe).
## How to Install
For usage on your local machine follow the instructions listed below:
> 1. git clone <EMAIL>:plenty-of-flicks/plenty_of_flicks_be.git
> 1. cd plenty_of_flicks_be
> 1. bundle
> 1. rake db:{create,migrate,seed}
> 1. bundle exec figaro install
> 1. Add watchmode and tmdb api keys to the config/application.yml file (Available at https://api.watchmode.com/ and https://developers.themoviedb.org/3/getting-started/introduction respectively)
```
# in config/application.yml
WATCHMODE_API_KEY: <your watchmode api key>
TMDB_API_KEY: <your tmdb api key>
```
> 7. rails s
> 8. Visit localhost:3000 in your web browser
## Dependencies
* This app uses Rails version `6.1.3.1`
* The [Figaro](https://github.com/laserlemon/figaro) gem is used to keep confidential information like API keys secure.
* The [Fast JSON API](https://github.com/Netflix/fast_jsonapi) gem is used to serialize data.
* The [SimpleCov](https://github.com/simplecov-ruby/simplecov) gem is used to ensure that 100% of our code is covered with unit testing.
* The [Webmock](https://github.com/bblimke/webmock) and [VCR](https://github.com/vcr/vcr) gems are used for mocking and stubbing external API calls.
## Learning Goals
* Gain experience working on a solo full-stack application
* Understand and implement background workers
* Refactor code for better code for improved organization/readability
* Write thorough and understandable documentation
## Project Diagram
## Schema
<img width="1177" alt="Screen Shot 2021-05-10 at 5 03 40 PM" src="https://user-images.githubusercontent.com/68172332/117734951-c35e5880-b1b1-11eb-99c9-2f47477bcfb0.png">
## API Documentation
### POST /api/v1/swipes
POST /api/v1/swipes creates a swipe entry in the BE database. The swipes table is a joins table between users and movies that signifies a user either adding a movie to their watchlist or removing it as a possibility to be swiped in the future.
#### Request Parameters
| Name | Type | Description |
| --------------- | ------------- | ----------------------------------------------------------------------------------------------------------- |
| user_id | Integer | (required) - the id of the user that is swiping on a movie |
| movie_id | Integer | (required) - the id of the movie that the user is swiping on |
| rating | Integer | (required) - must be 0 (negative swipe) or 1 (positive swipe) - superswipe (2) planned in future iteration|
#### Sample Response
```
{
"data": {
"id":"63",
"type":"swipe",
"attributes": {
"id":63,
"user_id":91,
"movie_id":809,
"rating":1
}
}
}
```
### POST /api/v1/users
POST /api/v1/users creates a user entry in the BE database.
#### Request Parameters
| Name | Type | Description |
| --------------- | ------------- | ----------------------------------------------------------------------------------------------------------- |
| uid | Integer | (required) - the uid for the user's google account |
| email | String | (required) - the email associated with the account. |
| first_name | String | (required) - the user's first name |
| last_name | String | (required) - the user's last name |
| image. | String | (required) - the path for the user's avatar |
#### Sample Response
```
{
"data": {
"id":"92",
"type":"user",
"attributes": {
"first_name":"John",
"last_name":"Doe",
"email":"<EMAIL>",
"uid":"12345678910",
"image":"https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg"
}
}
}
```
### PATCH /api/v1/users/:uid
PATCH /api/v1/users updates a user entry in the BE database.
#### Request Parameters
| Name | Type | Description |
| --------------- | ------------- | ----------------------------------------------------------------------------------------------------------- |
| email | String | (optional) - the email associated with the account. |
| first_name | String | (optional) - the user's first name |
| last_name | String | (optional) - the user's last name |
| image. | String | (optional) - the path for the user's avatar |
#### Sample Response
```
{
"data": {
"id":"92",
"type":"user",
"attributes": {
"first_name":"John",
"last_name":"Doe",
"email":"<EMAIL>",
"uid":"12345678910",
"image":"https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg"
}
}
}
```
### GET /api/v1/movies/random_available
GET /api/v1/movies/random_available returns the attributes of a random movie that is currently available on one of the supported services, and that the given user has not yet created a swipe for
#### Request Parameters
| Name | Type | Description |
| --------------- | ------------- | ----------------------------------------------------------------------------------------------------------- |
| user_id | Integer | (required) - the user id for the current user. |
#### Sample Response
```
{
"data": {
"id":"857",
"type":"movie",
"attributes": {
"title":"<NAME>: International Man of Mystery",
"tmdb_id":816,
"poster_path":"/5uD4dxNX8JKFjWKYMHyOsqhi5pN.jpg",
"description":"As a swingin' fashion photographer by day and a groovy British superagent by night, <NAME> is the '60s' most shagadelic spy, baby! But can he stop megalomaniac Dr. Evil after the bald villain freezes himself and unthaws in the '90s? With the help of sexy sidekick <NAME>, he just might.",
"genres": [
{
"id":87,
"name":"Action",
"created_at":"2021-04-27T05:22:49.498Z",
"updated_at":"2021-04-27T05:22:49.498Z",
"tmdb_id":1
},
{
"id":88,
"name":"Comedy",
"created_at":"2021-04-27T05:22:49.499Z",
"updated_at":"2021-04-27T05:22:49.499Z",
"tmdb_id":2
}
],
"vote_average":6.5,
"vote_count":2357,
"year":"1997"
}
}
}
```
### GET /api/v1/services/update_availability
GET /api/v1/services/update_availability - clears the BE movie availabilities table (joins table between services and movies that signifies which movies are available on each service) of all entries of the given service, then repopulates the table with the service's current availabilties as per the Watchmode API. This method, though inefficient, was chosen due to endpoint limitations of the Watchmode API. Background workers are used here, as the endpoint can take a long time to process.
#### Request Parameters
| Name | Type | Description |
| --------------- | ------------- | ----------------------------------------------------------------------------------------------------------- |
| service | String | (required) - the name of the service to be updated (netflix, hulu, or amazon) |
#### Sample Response
```
{
"data": {
"id":null,
"type":"service_refresh",
"attributes": {
"name":"Hulu",
"movie_count":2010
}
}
}
```
### GET /api/v1/movies/populate_details
GET /api/v1/movies/populate_details - searches through the movies table for entries with missing information. For each such movie, the BE makes an API call to TMDB API to fetch the movie's details, then updates the database's movie entry with the fetched data. Background workers are used here, as the endpoint can take a long time to process.
#### Sample Response
```
{
"movies_updated":3,
"update_status":"Complete - incomplete movies have been populated."
}
```
## Licenses
* Ruby 2.5.3
* Rails 6.1.3.1
## Contact
<h4><NAME></h4>
<img src="https://avatars.githubusercontent.com/u/68172332?s=460&u=8b0b3766e31ff6be0d1dea0a8591483dc1bdd870&v=4" alt="<NAME>"
width="150" height="auto" style="float: left" />
[GitHub Profile](https://github.com/nmking22)
<file_sep>/app/models/swipe.rb
class Swipe < ApplicationRecord
validates_presence_of :rating
belongs_to :movie
belongs_to :user
end
<file_sep>/app/facades/service_update_facade.rb
class ServiceUpdateFacade
def self.refresh_availability(service_name)
id = service_watchmode_id(service_name)
service = Service.find_by(watchmode_id: id)
movie_pages = WatchmodeService.get_movies_by_service(id)
# ADD CONDITIONAL HERE - IF WATCHMODE API CALL SUCCESSFUL, DO THINGS, ELSE ERROR
# MovieAvailabilities must be destroyed/recreated with each refresh
# due to Watchmode API endpoint limitations & rate limits
ServiceUpdateJob.perform_later(service, movie_pages)
ServiceRefresh.new(service[:name], movie_pages[0][:total_results])
end
def self.refresh_all_availabilities
service_refreshes = services.map do |name, id|
refresh_availability(name.to_s)
end
FullRefresh.new(service_refreshes)
end
def self.service_watchmode_id(service_name)
services[service_name.to_sym]
end
def self.services
{
netflix: 203,
hulu: 157,
amazon: 26
}
end
end
<file_sep>/spec/factories/movie.rb
FactoryBot.define do
factory :movie do
title { Faker::Movie.title }
tmdb_id { Faker::Number.within(range: 1..100000) }
poster_path { Faker::LoremFlickr.image }
description { Faker::Movie.quote }
vote_average { Faker::Number.decimal(l_digits: 2, r_digits: 2) }
vote_count { Faker::Number.within(range: 1..10000) }
year { Faker::Number.within(range: 1920..2021) }
end
end
<file_sep>/app/serializers/full_refresh_serializer.rb
class FullRefreshSerializer
include FastJsonapi::ObjectSerializer
attributes :total_movie_count, :service_refreshes
end
<file_sep>/app/controllers/api/v1/services_controller.rb
class Api::V1::ServicesController < ApplicationController
def index
render json: ServiceSerializer.new(Service.all)
end
def show
render json: ServiceSerializer.new(Service.find(params[:id]))
end
def create
service = Service.create(service_params)
render json: ServiceSerializer.new(service)
end
def update
service = Service.find(params[:id])
service.update(service_params)
render json: ServiceSerializer.new(service)
end
def destroy
Service.destroy(params[:id])
end
def update_availability
service_refresh = ServiceUpdateFacade.refresh_availability(params[:service])
render json: ServiceRefreshSerializer.new(service_refresh)
end
def update_all_availabilities
full_refresh = ServiceUpdateFacade.refresh_all_availabilities
render json: FullRefreshSerializer.new(full_refresh)
end
private
def service_params
params.permit(:name, :watchmode_id, :logo)
end
end
<file_sep>/app/poros/movie_info.rb
class MovieInfo
attr_reader :poster_path,
:description,
:vote_average,
:vote_count,
:year,
:genres
def initialize(movie_data)
@poster_path = movie_data[:poster_path]
@description = add_description(movie_data)
@vote_average = movie_data[:vote_average]
@vote_count = movie_data[:vote_count]
@year = find_year(movie_data)
@genres = movie_data[:genres]
end
def find_year(movie_data)
if movie_data[:release_date]
movie_data[:release_date].first(4)
else
'Unknown'
end
end
def add_description(movie_data)
if movie_data[:overview]
movie_data[:overview]
else
'No description available'
end
end
end
<file_sep>/db/seeds.rb
# This file should contain all the record creation needed to seed the database with its default values.
# The data can then be loaded with the bin/rails db:seed command (or created alongside the database with db:setup).
#
# Examples:
#
# movies = Movie.create([{ name: 'Star Wars' }, { name: 'Lord of the Rings' }])
# Character.create(name: 'Luke', movie: movies.first)
netflix = Service.create(
name: 'Netflix',
watchmode_id: 203,
logo: 'netflix_logo.png'
)
amazon_prime = Service.create(
name: 'Amazon Prime Video',
watchmode_id: 26,
logo: 'prime_video_logo.jpeg'
)
hulu = Service.create(
name: 'Hulu',
watchmode_id: 157,
logo: 'hulu_logo.jpeg'
)
<file_sep>/spec/models/genre_spec.rb
require 'rails_helper'
describe Genre, type: :model do
describe 'validations' do
it { should validate_presence_of :name}
end
describe 'relationships' do
it do
should have_many :movie_genres
should have_many(:movies).through(:movie_genres)
end
end
end
<file_sep>/app/serializers/service_refresh_serializer.rb
class ServiceRefreshSerializer
include FastJsonapi::ObjectSerializer
attributes :name, :movie_count
end
<file_sep>/app/controllers/api/v1/swipes_controller.rb
class Api::V1::SwipesController < ApplicationController
def create
swipe = Swipe.create(swipe_params)
render json: SwipeSerializer.new(swipe)
end
private
def swipe_params
params.permit(:user_id, :movie_id, :rating)
end
end
<file_sep>/spec/jobs/service_update_job_spec.rb
require 'rails_helper'
RSpec.describe ServiceUpdateJob, type: :job do
it 'creates movies and movie availabilities' do
hulu = Service.create(
name: 'Hulu',
watchmode_id: 157,
logo: 'hulu_logo.png'
)
expect(Movie.all).to eq([])
expect(MovieAvailability.all).to eq([])
json = File.read('spec/fixtures/movie_pages.json')
movie_pages = JSON.parse(json, symbolize_names:true)
ServiceUpdateJob.perform_now(hulu, movie_pages)
movies = Movie.all
availabilities = MovieAvailability.all
expect(movies.length).to eq(10)
expect(availabilities.length).to eq(10)
movies.each do |movie|
expect(movie).to be_a(Movie)
expect(movie.services).to eq([hulu])
end
availabilities.each do |availability|
expect(availability).to be_a(MovieAvailability)
expect(availability.service).to eq(hulu)
end
end
it 'does not create duplicate movies or availabilities when they already exist' do
hulu = Service.create(
name: 'Hulu',
watchmode_id: 157,
logo: 'hulu_logo.png'
)
spy_cat = Movie.create(
title: 'Spy Cat',
tmdb_id: 509733
)
spy_cat_on_hulu = hulu.movie_availabilities.create(
movie: spy_cat
)
expect(Movie.all).to eq([spy_cat])
expect(MovieAvailability.all).to eq([spy_cat_on_hulu])
json = File.read('spec/fixtures/movie_pages.json')
movie_pages = JSON.parse(json, symbolize_names:true)
ServiceUpdateJob.perform_now(hulu, movie_pages)
movies = Movie.all
availabilities = MovieAvailability.all
expect(movies.length).to eq(10)
expect(availabilities.length).to eq(10)
expect(Movie.find_by(id:spy_cat.id)).to eq(spy_cat)
expect(MovieAvailability.find_by(movie:spy_cat)).not_to eq(spy_cat)
expect(MovieAvailability.where(movie:spy_cat).length).to eq(1)
end
end
<file_sep>/spec/models/services_spec.rb
require 'rails_helper'
describe Service, type: :model do
describe 'validations' do
it { should validate_presence_of :name}
it { should validate_presence_of :watchmode_id}
it { should validate_presence_of :logo}
end
describe 'relationships' do
it do
should have_many :movie_availabilities
should have_many(:movies).through(:movie_availabilities)
end
end
end
<file_sep>/spec/models/group_spec.rb
require 'rails_helper'
describe Group, type: :model do
describe 'validations' do
it { should validate_presence_of :name }
end
describe 'relationships' do
it do
should have_many :user_groups
should have_many(:users).through(:user_groups)
end
end
end
<file_sep>/spec/requests/api/v1/user_groups_request_spec.rb
require 'rails_helper'
describe 'user groups API' do
it 'can create a user group' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
scrantonicity = Group.create(
name: 'Scrantonicity'
)
user_group_params = {
user_id: nick.id,
group_id: scrantonicity.id
}
headers = {"CONTENT_TYPE" => "application/json"}
post "/api/v1/user_groups", headers: headers, params: JSON.generate(user_group_params)
user_group = UserGroup.last
json = JSON.parse(response.body, symbolize_names:true)
expect(response).to be_successful
expect(user_group).to be_a(UserGroup)
expect(user_group.user_id).to eq(nick.id)
expect(user_group.group_id).to eq(scrantonicity.id)
expect(json).to be_a(Hash)
expect(json).to have_key(:data)
expect(json[:data]).to be_a(Hash)
expect(json[:data]).to have_key(:id)
expect(json[:data][:id]).to eq(user_group.id.to_s)
expect(json[:data]).to have_key(:type)
expect(json[:data][:type]).to eq('user_group')
expect(json[:data]).to have_key(:attributes)
expect(json[:data][:attributes]).to be_a(Hash)
expect(json[:data][:attributes]).to have_key(:id)
expect(json[:data][:attributes][:id]).to eq(user_group.id)
expect(json[:data][:attributes]).to have_key(:user_id)
expect(json[:data][:attributes][:user_id]).to eq(nick.id)
expect(json[:data][:attributes]).to have_key(:group_id)
expect(json[:data][:attributes][:group_id]).to eq(scrantonicity.id)
end
it 'can delete a user group' do
nick = User.create(
uid: '12345678910',
email: '<EMAIL>',
first_name: 'Nick',
last_name: 'King',
image: 'https://lh6.googleusercontent.com/-hEH5aK9fmMI/AAAAAAAAAAI/AAAAAAAAAAA/AMZuucntLnugtaOVsqmvJGm89fFbDJ6GaQ/s96-c/photo.jpg'
)
scrantonicity = Group.create(
name: 'Scrantonicity'
)
user_group = UserGroup.create(
user: nick,
group: scrantonicity
)
expect(UserGroup.count).to eq(1)
delete "/api/v1/user_groups/#{user_group.id}"
expect(response).to be_successful
expect(UserGroup.count).to eq(0)
expect{UserGroup.find(user_group.id)}.to raise_error(ActiveRecord::RecordNotFound)
end
end
<file_sep>/app/serializers/swipe_serializer.rb
class SwipeSerializer
include FastJsonapi::ObjectSerializer
attributes :id, :user_id, :movie_id, :rating
end
| 98b2066dc002b6d871bcc26c639aaa0cbba44956 | [
"Markdown",
"Ruby",
"YAML"
] | 60 | Markdown | plenty-of-flicks/plenty_of_flicks_be | 4bf215ca37bbc8f85d490b819f63fc20f114d994 | faa5ad34689dd3a1cead84b09264aa3ae68664da |
refs/heads/master | <file_sep># hello-world
tutorial tset project
uh...I play brass instruments and stuff.
| 143f26a1a2573dea637ec6cbb601e190877a2f53 | [
"Markdown"
] | 1 | Markdown | jeffkmiller/hello-world | 213040e2da81dfed087534e395deebfba8d94202 | a6621b4ca43158626e6ef5539de9c6eaba1c79cf |
refs/heads/master | <file_sep>/*
JSSM @VERSION (JavaScript State Manager)
Copyright (c) 2008 <NAME>
Licensed under the MIT License (LICENSE.txt)
To report bugs or get the lates version, visit the website:
http://trac.nathanhammond.com/jssm/
$Date$
$Revision$
*/
/*
Object jssm
Supplies history storage and browser abstraction layer for managing history.
*/
var jssm = {
created: false, // Identifies whether the inline form elements have been created yet.
rid: 0, // Uniquely identifies the hash change.
zerolength: false, // The "zero" for where this site appears in the history stack.
pointer: false, // A reference to where we are in the history stack.
interval: 100, // The interval at which polling happens.
stackelem: false, // The form element where the history data is stored.
lengthelem: false, // The form element to store the page's zero length.
pointerelem: false, // The form element to store the page's history pointer.
iframe: false, // The iframe used by IE to register history events.
stack: [], // The in-memory version of what is in the history stack.
settings: {
formid: 'jssmform',
stackid: 'jssmstack',
lengthid: 'jssmlength',
pointerid: 'jssmpointer',
iframeid: 'jssmiframe',
blankurl: '/blank.html',
basetitle: '',
titleseparator: ' : '
},
functions: {
pageload: false,
beforeload: false,
load: false,
afterload: false,
beforeunload: false,
unload: false,
afterunload: false
},
/*
jssm.inline(Object params)
Builds the necessary HTML elements for tracking state and makes them appear on the page. Must be called inline.
*/
inline: function (params) {
if (this.created) { return; }
this.created = true;
this.settings = jQuery.fn.extend(this.settings, params);
document.write('<div style="display: none;">');
document.write('<form id="' + this.settings.formid + '" action="" method="post"><div><textarea name="' + this.settings.stackid + '" id="' + this.settings.stackid + '"></textarea><input type="text" id="' + this.settings.lengthid + '" name="' + this.settings.lengthid + '" /><input type="text" id="' + this.settings.pointerid + '" name="' + this.settings.pointerid + '" /></div></form>');
// Only need the iframe trick in IE, < IE8.
if ( jQuery.browser.msie && jQuery.browser.version < 8 ) {
document.write('<iframe id="' + this.settings.iframeid + '" src="' + this.settings.blankurl + '?' + this.getHash() + '"></iframe>');
}
document.write('</div>');
// Only need the image trick for Opera. Can't be display: none;.
if ( jQuery.browser.opera ) {
document.write('<img style="position: absolute; left: -999em; top: -999em;" width="1" height="1" src="javascript:location.href=\'javascript:jssm.fixOpera();\';" />');
}
},
/*
jssm.init(String type)
Function called to initialize JSSM. It gets called twice and, depending upon which browser you're in and which event triggered it (identified by the type argument), it executes.
*/
init: function (type) {
if (jQuery.browser.msie && type == 'ready') { return; }
if (!jQuery.browser.msie && type == 'load') { return; }
jQuery(window).bind('hashchange', jssm.hashchange);
// Store references to all of the important elements on the page.
this.stackelem = document.getElementById(this.settings.stackid);
this.lengthelem = document.getElementById(this.settings.lengthid);
this.pointerelem = document.getElementById(this.settings.pointerid);
this.iframe = document.getElementById(this.settings.iframeid);
// Opera needs to focus each element before it can write to it.
if ( jQuery.browser.opera ) {
this.stackelem.focus();
this.lengthelem.focus();
this.pointerelem.focus();
window.focus(); // Reset to avoid typing into field.
}
// Set the initialized state to match either the form's values or to be new.
if ( this.lengthelem.value ) {
// They've already been here.
this.zerolength = this.lengthelem.value;
this.load();
} else {
// First visit to the page.
this.zerolength = history.length;
this.pointer = this.zerolength;
this.stack[this.pointer] = this.getHash();
this.save();
}
this.rid = this.getRID(this.stack[this.pointer]);
this.rid++;
if (this.functions.pageload) { this.functions.pageload(this.stack[this.pointer]); }
if ( !jQuery.browser.msie || ( jQuery.browser.msie && jQuery.browser.version < 8 ) ) {
this.poll();
}
},
/*
jssm.poll()
This function polls the location bar to check for changes. When a change is detected it manually triggers a hashchange event.
*/
poll: function () {
// Called out of context: "this" refers to window.
if (jssm.getHash() != jssm.stack[jssm.pointer]) {
jQuery(window).trigger('hashchange');
}
setTimeout(jssm.poll, jssm.interval);
},
/*
jssm.fixOpera()
Function does nothing. Opera clears timers except when an image has a callback function. This is that callback's target.
*/
fixOpera: function () {
// Do nothing.
},
/*
jssm.iframeEvent(String changedhash)
Propagates a change made in the IE-only iframe to the main window.
*/
iframeEvent: function (changedhash) {
if (changedhash || window.location.hash) {
window.location.hash = changedhash;
}
},
/*
jssm.hashchange()
This is the function that gets called on hashchange. It processes the changed hash and updates the current state of the JSSM object accordingly.
It is also responsible for handling the load and unload functionality for all pages.
*/
hashchange: function () {
// Called out of context: "this" refers to window
// Store the previous and changed hash values in temporary variables for easy access.
var changedhash = jssm.getHash();
var calchash = changedhash;
// The relative path from a page to itself is actually #pagename. Taking advantage of that, an empty string means we can only want to go back to the initial page.
if (changedhash === '') { calchash = jssm.getCurrentPage(); }
if ( jQuery.browser.msie && jQuery.browser.version < 8 && jssm.iframe.contentWindow.document.body.innerText != changedhash ) {
jssm.setHash(changedhash);
}
if (jssm.functions.beforeunload) { jssm.functions.beforeunload(calchash); }
if (jssm.functions.unload) { jssm.functions.unload(calchash); }
if (jssm.functions.afterunload) { jssm.functions.afterunload(calchash); }
if (jssm.functions.beforeload) { jssm.functions.beforeload(calchash); }
if (jssm.functions.load) { jssm.functions.load(calchash); }
if (jssm.functions.afterload) { jssm.functions.afterload(calchash); }
// Figure out where to set the pointer.
var exists = [];
for ( var i = jssm.zerolength; i < jssm.stack.length; i++ ) {
// Go through all items in the stack to see if this "new" hash exists. Store all indexes where there is a hash match.
if ( changedhash === jssm.stack[i] ) { exists.push(i); }
}
switch ( exists.length ) {
case 0:
// The current hash is new. Add this state to the stack at pointer + 1 and then splice off all following elements.
jssm.pointer++;
jssm.stack[jssm.pointer] = changedhash;
jssm.stack.length = jssm.pointer + 1;
break;
case 1:
// The current hash has been used before, and there is exactly one match.
jssm.pointer = exists[0];
break;
}
jssm.save();
},
/* Helper functions used to manage the form-based history stack. */
/*
jssm.load()
Pulls the information stored in the page forms into memory. Used to reconstitute a session.
*/
load: function () {
this.stack = JSON.parse(this.stackelem.value);
this.length = this.lengthelem.value;
this.pointer = this.pointerelem.value;
},
/*
jssm.save()
Puts the information stored in memory into the form fields. Used to store a session in case the user leaves the site.
*/
save: function () {
this.stackelem.value = JSON.stringify(this.stack);
this.lengthelem.value = this.zerolength;
this.pointerelem.value = this.pointer;
},
/* Helper functions for handling the location bar. */
/*
jssm.getHash(), returns String
Gets the current hash for the page.
*/
getHash: function () {
if (jQuery.browser.safari && parseInt(jQuery.browser.version, 10) < 522 && !/adobeair/i.test(jQuery.browser.userAgent)) {
// Safari 2 needs help.
this.getHash = function () {
return jssm.stack[history.length - jssm.zerolength - 1];
};
} else {
// Everybody else.
this.getHash = function () {
var r = window.location.href;
var i = r.indexOf("#");
return (i >= 0 ? r.substr(i+1) : '');
};
}
return this.getHash();
},
/*
jssm.setHash(String hash)
Sets the hash in the location bar appropriately for each browser.
*/
setHash: function (hash) {
if ( jQuery.browser.msie && jQuery.browser.version < 8 ) {
this.setHash = function (hash) {
var iframe = jssm.iframe.contentWindow.document;
iframe.open("javascript:'<html></html>'");
iframe.write('<html><head><scri' + 'pt type="text/javascript">window.parent.jssm.iframeEvent("' + hash + '");</scri' + 'pt></head><body>' + hash + '</body></html>');
iframe.close();
};
} else {
this.setHash = function (hash) {
window.location.hash = hash;
};
}
return this.setHash(hash);
},
/*
jssm.getRID(String hash), returns RID
Gets the unique RID (request ID) for the element.
*/
getRID: function (hash) {
if (!hash) { return 0; }
var str = hash.match(/rid=[\d]+/);
return str ? str[0].substr(4) : 0;
},
/*
jssm.getHref(), returns String
Gets the current href from the location bar. Trims only the hash.
*/
getHref: function () {
var i = window.location.href.indexOf("#");
return (i >= 0 ? window.location.href.substr(0, i) : window.location.href);
},
/*
jssm.getPathTokens(String href), returns PathTokens
Gets string tokens out of a URL.
*/
getPathTokens: function (href) {
// 1. Identify if there is a hash or querystring.
var hashpos = href.indexOf('#');
var querypos = href.indexOf('?');
var querystring = '';
var hashstring = '';
if (hashpos != -1) {
// There is a hashstring.
if (querypos != -1 && querypos < hashpos) {
// There is a querystring and a hashstring.
querystring = href.substring(querypos, hashpos);
hashstring = href.substring(hashpos);
} else {
// There is only a hashstring.
hashstring = href.substring(hashpos);
}
} else {
// There is no hashstring.
if (querypos >= 0) {
// There is a querystring.
querystring = href.substring(querypos);
} else {
// There is no querystring.
}
}
// 2. Strip off anything that is a hash or querystring.
if (hashpos != -1 && querypos != -1) {
href = href.substring(0, Math.min(hashpos, querypos));
} else if (hashpos != -1) {
href = href.substring(0, hashpos);
} else if (querypos != -1) {
href = href.substring(0, querypos);
}
// 3. Pass prepared url to regex.
var regex = /^(https?:\/\/){0,1}([A-Za-z0-9\-\.]+){0,1}(\:\d+){0,1}(\/){0,1}((?:[^\/]*\/)*){0,1}(.*)$/;
href = regex.exec(href);
// 0: full string
// 1: protocol (http:// || https://)
// 2: hostname
// 3: port
// 4: leading slash?
// 5: dirtree
// 6: page
if (href[5]) {
href[5] = href[5].split('/');
href[5].pop();
} else {
href[5] = [];
}
// 7: querystring
// 8: hashstring
href.push(querystring);
href.push(hashstring);
return href;
},
/*
jssm.getCurrentPath(), returns String
Gets the path relative to the current webroot.
*/
getCurrentPath: function () {
var tokens = this.getPathTokens(this.getHref());
return (tokens && tokens[5] ? tokens[5].join('/') + '/' : '');
},
/*
jssm.getCurrentPage(), returns String
Gets the currently active page, ignoring the path.
*/
getCurrentPage: function () {
var tokens = this.getPathTokens(this.getHref());
return (tokens && tokens[6] ? tokens[6] : '');
},
/*
jssm.getRelativePath(String from, String two, Boolean strict), returns String
Gets the relative path from the second argument to the first one.
String from - Must always be fully qualified.
String to - May be a relative path. In this scenario the protocol, hostname, and port must all be omitted.
Boolean strict - Requires both arguments to be fully-qualified domains and does not infer default ports.
Returns:
- Boolean false if it is not possible to get a relative path.
- Empty string if the paths are the same.
- Relative path in all other scenarios.
*/
getRelativePath: function (from, to, strict) {
// Check for the fastest scenario.
if (from == to && to.indexOf('?') == -1) { return this.getCurrentPage(to); }
from = this.getPathTokens(from);
to = this.getPathTokens(to);
strict = strict || false;
// Protocol must match if strict checking is enabled, or the "to" protocol is defined.
if ((strict || to[1]) && (from[1] !== to[1])) { return false; }
// Hostname must match if strict checking is enabled, or the "to" hostname is defined.
if ((strict || to[2]) && (from[2] !== to[2])) { return false; }
// Port may match against defaults for the protocol except in strict mode.
if (from[3] !== to[3]) {
if (strict) { return false; }
var port = false;
// Match a protocol to its default port. Switch statement to leave room for more protocols.
switch (from[1]) {
case 'http': port = 80; break;
case 'https': port = 443; break;
}
// Didn't match any of our supported protocols for matching.
if (!port) { return false; }
if (!(from[3] === '' && to[3] == port) && !(from[3] == port && to[3] === '')) { return false; }
}
// We are going to be able to determine a relative path.
var buildstring = '';
if (!from[5].length || !to[4]) {
// If we're already at the root or we don't need to be comparing from the root dir.
buildstring += to[5].join('/') + '/' + (to[6] ? to[6] : '');
} else {
// We have to begin the path comparison at the root.
var i = 0;
if (from[5].length && to[5].length) {
// If both from and to have dirtrees.
// Go through the directory tree and make comparisons.
for ( i = 0; i < from[5].length; i++ ) {
if (from[5][i] == to[5][i]) {
// They match, shift them both of the front. Have to decrement "i" to stay where we want in the loop.
from[5].shift(); to[5].shift();
i--;
} else {
// First non-match, break out of the loop and calculate depth of difference.
break;
}
}
// Depth difference calculation.
for ( i = 0; i < from[5].length; i++ ) {
buildstring += '../';
}
buildstring += to[5].join('/') + (to[5].length ? '/' : '') + (to[6] ? to[6] : '');
} else {
// If only from has a dirtree we just need to do a depth difference calculation.
for ( i = 0; i < from[5].length; i++) {
buildstring += '../';
}
buildstring += (to[6] ? to[6] : '');
}
}
// Querystrings and hashes in "from" are never part of the resulting relative path. Simply use what appears in "to" for the new path.
if (to[7]) { buildstring += to[7]; }
if (to[8]) { buildstring += to[8]; }
return buildstring;
},
/*
jssm.getRootRelativePath(String from), returns String
Returns the root relative path from the supplied path. The parameter must be fully qualified.
jssm.getRootRelativePath(String from, String to, Boolean strict), returns String
Makes sure that the two paths are relative to eachother and then returns the root relative path of the second one.
String from - Must always be fully qualified.
String to - May be a relative path.
Boolean strict - Requires both arguments to be fully-qualified domains and does not infer default ports.
Returns:
- Boolean false if it is not possible to get a relative path.
- Root relative path.
*/
getRootRelativePath: function (from, to, strict) {
if (arguments.length == 1) {
// Looking for the root relative path of a single href.
var tokens = jssm.getPathTokens(from);
return '/' + tokens[5].join('/') + '/' + (tokens[6] ? tokens[6] : '');
}
from = this.getPathTokens(from);
to = this.getPathTokens(to);
strict = strict || false;
// Protocol must match if strict checking is enabled, or the "to" protocol is defined.
if ((strict || to[1]) && (from[1] !== to[1])) { return false; }
// Hostname must match if strict checking is enabled, or the "to" hostname is defined.
if ((strict || to[2]) && (from[2] !== to[2])) { return false; }
// Port may match against defaults for the protocol except in strict mode.
if (from[3] !== to[3]) {
if (strict) { return false; }
var port = false;
// Match a protocol to its default port. Switch statement to leave room for more protocols.
switch (from[1]) {
case 'http': port = 80; break;
case 'https': port = 443; break;
}
// Didn't match any of our supported protocols for matching.
if (!port) { return false; }
if (!(from[3] === '' && to[3] == port) && !(from[3] == port && to[3] === '')) { return false; }
}
// We are going to be able to determine a root relative path. Everything from 5 - 8 in the "to" path should be strung back together.
var buildstring = '/';
if (!to[4]) { buildstring += from[5].join('/') + '/'; }
if (to[5].length) { buildstring += to[5].join('/') + '/'; }
if (to[6]) { buildstring += to[6]; }
// For querystrings and hashes in "from" are never part of the resulting relative path. Simply use what appears in "to" for the new path.
if (to[7]) { buildstring += to[7]; }
if (to[8]) { buildstring += to[8]; }
return buildstring;
},
/* Helpers to deal with response information. */
/*
jssm.setTitle(String title)
Sets the document title.
*/
setTitle: function(title) {
document.title = title;
},
/*
jssm.buildTitle(String title, String separator), returns String
Builds the document title from the basetitle specified in the settings and the title specified by the page.
*/
buildTitle: function(title, separator) {
separator = separator || this.settings.titleseparator;
return this.settings.basetitle + (title ? separator + title : '');
}
};
jQuery.fn.jssm = function (eventtype, params) {
// Uncomment the following line to disable JSSM.
// return;
// Go through each element provided and attach events.
return this.each(function (i) {
// Add the supplied parameters to the element's expando with the JSSM namespace.
// jQuery.data(this, 'jssm', params);
// Attach event to the element that causes the hash to change. This triggers JSSM to provide all other functionality by noting the hash change.
jQuery(this).bind(eventtype, function (event) {
var params = jQuery.data(this, 'jssm');
var href = '';
var data = '';
if (eventtype == 'submit' && this.action) {
// We've been handed a form or something masquerading as one.
// Form.action doesn't return a fully qualified domain.
// Anchor.href returns fully qualified domains in all but IE.
// Creating an Anchor using Element.innerHTML causes IE to use fully qualified domains.
// jQuery's element creation uses innerHTML, this (ab)uses that side effect to get a fully qualified domain.
href = jQuery('<a href="' + this.action + '"></a>').get(0).href;
data = '&' + jQuery(this).serialize();
} else if (this.href) {
// We've been handed an anchor tag or something masquerading as one.
href = this.href;
}
// Figure out what the new hash is supposed to be.
var target = jssm.getRelativePath(jssm.getHref(), href);
// Set the hash.
if (target !== false) {
target = target + (target.indexOf('?') >= 0 ? '&' : '?') + 'rid=' + (jssm.rid++) + data;
jssm.setHash(target);
}
event.preventDefault();
return false;
});
});
};
jQuery(document).ready(function () { jssm.init('ready'); });
jQuery(window).load(function () { jssm.init('load'); });<file_sep>jssm.settings.blankurl = '../blank.html';
jssm.settings.basetitle = 'JSSM Test';
jssm.settings.titleseparator = ' : ';
jssm.functions.pageload = function (hash) {
if (hash) {
jssm.functions.load(hash);
} else {
$('.wrapper').fadeIn(500);
}
}
jssm.functions.beforeload = function (hash) {
}
jssm.functions.load = function (hash) {
$.get('/'+jssm.getCurrentPath()+hash, function(response) {
/* Process the response, light edition. */
/* Set page title based upon the response. */
var regextitle = new RegExp('<title>([^<]*)<\/title>');
var matches = regextitle.exec(response);
jssm.setTitle(jssm.buildTitle(matches[1]));
/* Make sure the browser history list shows the correct title. */
window.location.replace(window.location);
/* Get the new content. */
var inside = response.substring(response.indexOf('<body>') + 6, response.indexOf('</body>'));
/* Fade in time. */
$('.wrapper').queue(function () {
$(this).html($(inside).filter('div.wrapper').html());
$('a', this).jssm('click');
$('form', this).jssm('submit');
$(this).fadeIn(500);
$(this).dequeue();
});
//
// /* Add CSS to the page based upon the response. */
// $('link', response).each(function(i) {
// var css = document.createElement("link");
// css.setAttribute('rel', 'stylesheet');
// css.setAttribute('type', 'text/css');
// css.setAttribute('href', $(this).attr('href'));
// document.getElementsByTagName('head')[0].appendChild(css);
// });
//
// /* Add page content based upon the response. */
// $('#wrapper').html($('body', response).text()).fadeIn(500);
//
// /* Add scripts to the page based upon the response. */
// $('script', response).each(function(i) {
// $.globalEval($(this).text());
// });
});
}
jssm.functions.afterload = function (hash) {
}
jssm.functions.beforeunload = function (hash) {
}
jssm.functions.unload = function (hash) {
$('.wrapper').queue(function () {
$(this).fadeOut(500);
$(this).dequeue();
});
}
jssm.functions.afterunload = function (hash) {
}
<file_sep>I owe a debt of gratitude to a number of people for contributions that they have (unwittingly) made:
<NAME> and <NAME>'s Really Simple History provided an excellent existing solution on which I could base my approach. (http://code.google.com/p/reallysimplehistory/)
<NAME> provided succinct test cases on his blog that were invaluable. (http://weblogs.asp.net/bleroy/archive/2007/09/07/how-to-build-a-cross-browser-history-management-system.aspx)
<NAME> is I think responsible for the window.location.href.indexOf('#') trick to get the hash with URL encoding (http://developer.yahoo.com/yui/history/).
Douglas Crockford wrote a so-called reference implementation of a JSON stringifier and parser of which I've included the latest version. (http://www.json.org/js.html)
<NAME> introduced me to the lazy function definition pattern. (http://peter.michaux.ca/article/3556)
JSSM Rules:
1. All pages that use JSSM should be tested without JavaScript (or at least JSSM) enabled. Ensure that all items JSSM animates on page load appear as necessary without JavaScript. Look into using the <noscript> tag.
2. AJAX POST requests should be handled outside of JSSM. Post requests should not be a bookmarkable event. These are the type of requests that generally require special handling because of the way that they will change session state. If needed have the response from a manual POST request trigger a JSSM redirect.
General:
1. The latest released version of jQuery (1.2.6) is packaged with the plugin. The plugin should work with jQuery 1.2 and all subsequent releases.
2. Douglas Crockford's JSON implementation from JSON.org is included. ***NOTE: It has been modified! Lines 186 and 187 have been changed to resolve the issue discussed here: http://dev.opera.com/forums/topic/215757
JSSM Instructions:
1. Include blank.html in a location on the same host as the page being supported by JSSM.
2. Modify jquery.jssm.config.js to meet the needs of the site/application by defining the default functions for JSSM handling: beforeunload, unload, afterunload, beforeload, load, afterload. It is recommended to write at least the load and unload default functions.
3. Define initial page load handler function. This is assuming that the way the page is loaded initially does not match the standard loading methods. If it does, have the pageload method alias the load method.
4. Include all scripts in their proper order:
<head>
<script type="text/javascript" src="json2.js"></script>
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="jquery.jssm.js"></script>
<script type="text/javascript" src="jquery.jssm.config.js"></script>
</head>
<body>
<script type="text/javascript">jssm.inline();</script>
...
</body>
5. Attach JSSM to all elements to be handled with it inside a $(document).ready() block.
JSSM Initialization:
1. User requests the initial page.
- This could possibly not be the standard point of entry, a subpage: /mypictures/florida.html
- This request could possibly have a hash appended to it: /mypictures/florida.html#../myvideos/keywest.html
2. Initial page is sent from the server to the user's computer. The page as provided by the server will not reflect the hash state.
*** JavaScript is enabled. ***
1. jQuery is compiled and sets itself up.
2. The JSSM extension is compiled and sets itself up. This adds the function .jssm() to jQuery to indicate that an element should be handled by JSSM.
3. The JSSM object is compiled and sets itself up. This sets defaults for handling the browsers different history mechanisms.
4. The JSSM method "inline", placed inside the body tag, is run to create the storage form.
5. User supplied scripts are run.
6. Page reaches ready/load state.
7. The JSSM object's init function is called, careful to respect needs of different browsers as to timing.
a. Initializes the history stack to mirror the browser state.
b. Saves the history state to form elements to maintain themselves for the entire browser session.
c. Binds the hashchange event handler. (See JSSM Event Processing)
d. Determines if there is a hash and loads the initial page.
- If there is a hash, from this point forward it uses the handler functions defined for that hash.
- If there is not a hash, it continues using the initial page handler functions.
e. Begins polling for hashchange events (IE8: won't need to poll).
11. Page is now finished loading.
*** JavaScript is not enabled. ***
1. Items in <noscript> tags are rendered, including CSS that counteracts all initial page loading functionality.
2. Page is now finished loading.
JSSM Event Processing:
1. A JSSM'd page element has the specified event happen to it (click, submit).
2. That event changes location.hash (IE: iframe.location.src) according to the element's href or action. It is set to be the relative URL between the initial page and the target of the link. Additionally, forms are serialized before being placed on the hash string.
3. The location bar polling function that notices that the hash is changed and triggers the body's hashchange event (and associated callback).
4. The JSSM object's hashchange method is invoked on document.body to process the changed hash.
a. This processes the changed hash and updates JSSM's understanding of history state.
b. Once the history state is set, it calls the transition functions first for unloading, and then for loading.
Server Side Page Design:
1. Plan the design of the code carefully as the response handling functionality will need to know what to do with the response text.
2. It is recommended to have two ways to call the same page: one to serve the page statically, one to serve the response to JSSM in a format the defined response handlers know how to deal with. This branching will allow for better optimization of the JSSM response handling code. | 998bf36c6e177bb337abf1e7cf60ec609800db76 | [
"Text",
"JavaScript"
] | 3 | Text | nathanhammond/jssm | e59fafdc83fba245d738b9e1143e7523d46b07d3 | d35a29f60126e89a2953a711208066bc4ee47ee9 |
refs/heads/main | <file_sep>#Install Current User
if ($PSVersionTable.PSEdition -eq 'Desktop' -and (Get-Module -Name AzureRM -ListAvailable)) {
Write-Warning -Message ('Az module not installed. Having both the AzureRM and ' +
'Az modules installed at the same time is not supported.')
} else {
Install-Module -Name Az -AllowClobber -Scope CurrentUser
}
#Install All Users
if ($PSVersionTable.PSEdition -eq 'Desktop' -and (Get-Module -Name AzureRM -ListAvailable)) {
Write-Warning -Message ('Az module not installed. Having both the AzureRM and ' +
'Az modules installed at the same time is not supported.')
} else {
Install-Module -Name Az -AllowClobber -Scope AllUsers
}
#Connect to Azure with a browser sign in token
Connect-AzAccount
| 8f745981928976bc9d76c47940ec00e62d2f03df | [
"PowerShell"
] | 1 | PowerShell | kaidan41/Installing-the-Azure-module-on-PowerShell-7.04 | 72561a74015793853aca9938a8aef1f357d9d9eb | 3a6fdaf0400cf9862619f87bf88ac5084084b380 |
refs/heads/master | <repo_name>odipojames/calculater<file_sep>/js/scripts.js
var number1 = parseInt(prompt("Enter a number:"));
var number2 = parseInt(prompt("Enter another number:"));
var add = function(number1, number2)
{
return number1 + number2;
};
alert(add(number1, number2));
var BMI = function(hieght,mass)
{
return mass/hieght;
};
var hieght = parseInt(prompt("enter your hieght:"));
var mass = parseInt(prompt("enter your mass:"));
{
alert("your mass body index is"+BMI(hieght,mass));
}
| 317ce085ba3003dff431a49175105f96250b2260 | [
"JavaScript"
] | 1 | JavaScript | odipojames/calculater | 89d02fdb3071c290b720595f0c8c9f0c18a4f510 | 812cd98900e58e5443493e16f7b6f66426d76905 |
refs/heads/master | <file_sep>export default function rocketEquation(masses){
// for overall fuel sum
let fuelReqSum = 0;
// expecting array of masses for each module
masses.forEach(mass => {
let fuel = fuelForModule(mass);
while(fuel > 0){
// do not want to add 0 or negative numbers to sum
fuelReqSum += fuel;
fuel = fuelForModule(fuel);
}
});
return fuelReqSum;
}
function fuelForModule(mass){
// divide by 3, round down, subtract 2
return (Math.floor(mass/3))-2;
}<file_sep>export default function rocketEquation(masses){
// for overall fuel sum
let fuelReqSum = 0;
// expecting array of masses for each module
masses.forEach(mass => {
// divide by 3, round down, subtract 2
fuelReqSum += (Math.floor(mass/3))-2;
});
return fuelReqSum;
} | c8fd6847c7a34616ec43c909b65bf70198b87384 | [
"JavaScript"
] | 2 | JavaScript | jdemieville/adventOfCode2019 | f144cb2425dc249a46843981cc6af4f1b6669188 | 14b07d6fff0b497706b9ecd220d3627d199bfdd7 |
refs/heads/master | <file_sep>import random
from django.shortcuts import render
from django.http import JsonResponse
from roles.models import Homonym, AgentNoun
levels = [
'senior',
'junior',
'rockstar',
'acting',
'lead',
'quantitative',
'full stack',
]
def home(request):
level = request.GET.get('level', random.choice(levels))
agent_noun = request.GET.get('agent_noun', AgentNoun.objects.raw('''
select * from {0} limit 1
offset floor(random() * (select count(*) from {0}))
'''.format(AgentNoun._meta.db_table))[0].word)
homonym = request.GET.get('noun', Homonym.objects.raw('''
select * from {0} limit 1
offset floor(random() * (select count(*) from {0}))
'''.format(Homonym._meta.db_table))[0].word)
context = {
'level': level,
'agent_noun': agent_noun,
'noun': homonym
}
if request.is_ajax():
return JsonResponse(context)
return render(request, 'home.html', context=context)
<file_sep>import requests
from lxml import html
from urllib.parse import urljoin
from django.core.management.base import BaseCommand, CommandError
from roles.models import AgentNoun
class Command(BaseCommand):
help = 'Import agent nouns from https://en.wiktionary.org/wiki/Category:English_agent_nouns'
total_words_created = 0
total_words_already = 0
def process_page(self, url):
r = requests.get(url)
tree = html.fromstring(r.content)
words_created = 0
words_already = 0
for el in tree.xpath('//div[@class="mw-category-group"] / ul / li / a'):
word = el.text.strip()
if len(word):
h, created = AgentNoun.objects.get_or_create(word=word)
if created:
words_created += 1
else:
words_already += 1
self.stdout.write(self.style.SUCCESS('Imported %d words, %d words skipped as already in database.' % (words_created, words_already)))
self.total_words_created += words_created
self.total_words_already += words_already
els = tree.xpath('//a[text()="next page"]')
if els:
next_url = els[0].attrib['href']
next_url = urljoin(url, next_url)
self.process_page(next_url)
def handle(self, *args, **options):
self.process_page("https://en.wiktionary.org/wiki/Category:English_agent_nouns")
self.stdout.write(
self.style.SUCCESS('Totals: Imported %d words, %d words skipped as already in database.' % (
self.total_words_created,
self.total_words_already)
)
)
<file_sep>import requests
from lxml import html
from django.core.management.base import BaseCommand, CommandError
from roles.models import Homonym
class Command(BaseCommand):
help = 'Import homonyms from http://home.alphalink.com.au/~umbidas/homonym_main.htm'
def handle(self, *args, **options):
r = requests.get("http://home.alphalink.com.au/~umbidas/homonym_main.htm")
tree = html.fromstring(r.content)
words_created = 0
words_already = 0
for cell in tree.xpath('//table[1] / tr / td'):
word = cell.text_content().strip()
if len(word):
h, created = Homonym.objects.get_or_create(word=word)
if created:
words_created += 1
else:
words_already += 1
self.stdout.write(self.style.SUCCESS('Imported %d words, %d words skipped as already in database.' % (words_created, words_already)))
<file_sep>from django.db import models
class Homonym(models.Model):
word = models.TextField(unique=True)
def __str__(self):
return self.word
class AgentNoun(models.Model):
word = models.TextField(unique=True)
def __str__(self):
return self.word
<file_sep>Django==1.10.3
lxml==3.6.4
requests==2.11.1
psycopg2==2.6.2
<file_sep># -*- coding: utf-8 -*-
# Generated by Django 1.10.3 on 2016-11-05 16:16
from __future__ import unicode_literals
from django.db import migrations
from django.core.management import call_command
from roles.models import AgentNoun, Homonym
def load_fixture(apps, schema_editor):
# NB word_data.json file generated by import_* management scripts
call_command('loaddata', 'word_data.json', app_label='roles')
def unload_fixture(apps, schema_editor):
"Brutally deleting all entries..."
AgentNoun.objects.all().delete()
Homonym.objects.all().delete()
class Migration(migrations.Migration):
dependencies = [
('roles', '0001_initial'),
]
operations = [
migrations.RunPython(load_fixture, reverse_code=unload_fixture),
]
<file_sep># yournextrole
This is a very silly project that I'm making because it's been a while since I made a website.
Word data is scraped from https://en.wiktionary.org/wiki/Category:English_agent_nouns
and http://home.alphalink.com.au/~umbidas/homonym_main.htm .
To run it, you need to create a file called yournextrole/localsettings.py and
set at least SECRET_KEY and DATABASES. The database needs to be a postgres db.
| 64d467369e8e924947c377d839746e37921d775f | [
"Markdown",
"Text",
"Python"
] | 7 | Markdown | Joeboy/yournextrole | 25bb4c93848354766f159a42a00e52e75fb92daf | 1804f8bf3b3ae31a24238ac7024cea292e82592e |
refs/heads/master | <file_sep>module Main where
import Data.Char
import Data.List
import Data.Map hiding (map, foldl)
import Data.Hash.MD5 -- from MissingH package
hash :: String -> Int -> String
hash s n = md5s (Str (s ++ show n))
solve1 :: String -> Int
solve1 s = head [n | n <- [0..], let h = hash s n, "00000" `isPrefixOf` h]
test = "abcdef"
input = "yzbqklnj"
-- Part Two
solve2 :: String -> Int
solve2 s = head [n | n <- [0..], let h = hash s n, "000000" `isPrefixOf` h]
main :: IO ()
main = do
print (solve1 input)
print (solve2 input)
<file_sep>module Main where
import Data.List
type Input = [Int]
parse :: String -> Input
parse = map read . lines
total :: Int
total = 150
solve1 :: Input -> Int
solve1 = length . filter (== total) . map sum . subsequences
-- Part Two --
solve2 :: Input -> Int
solve2 sizes = length $ head $ group $ sort $
[length cs | cs <- subsequences sizes, sum cs == total]
main :: IO ()
main = do
s <- readFile "input17.txt"
let input = parse s
print (solve1 input)
print (solve2 input)
<file_sep>module Main where
import Utilities
type Input = [Int]
parse :: String -> Input
parse = map read . lines
splits :: Int -> [Int] -> [([Int], [Int])]
splits n [] = [([], []) | n == 0]
splits n (x:xs) =
[(x:sel, rest) | x <= n, (sel, rest) <- splits (n-x) xs] ++
[(sel, x:rest) | (sel, rest) <- splits n xs]
firstGroups :: Int -> [Int] -> [[Int]]
firstGroups ngroups xs
| total `mod` ngroups /= 0 = []
| otherwise = [sel | (sel, rest) <- splits n xs, evenSplit (ngroups-1) n rest]
where
total = sum xs
n = total `div` ngroups
evenSplit :: Int -> Int -> [Int] -> Bool
evenSplit 1 _ _ = True
evenSplit ngroups n xs = any (evenSplit (ngroups-1) n) (drops n xs)
-- drops n = map snd . splits n
drops :: Int -> [Int] -> [[Int]]
drops n [] = [[] | n == 0]
drops n (x:xs) =
[rest | x <= n, rest <- drops (n-x) xs] ++
[x:rest | rest <- drops n xs]
solve1 :: Input -> Int
solve1 = minimum . map product . leastBy length . firstGroups 3
test :: [Int]
test = [1..5] ++ [7..11]
-- Part Two --
firstGroups2 :: [Int] -> [[Int]]
firstGroups2 xs
| total `mod` 4 /= 0 = []
| otherwise = [sel | (sel, rest) <- splits n xs, not (null (splits n rest))]
where
total = sum xs
n = total `div` 3
solve2 :: Input -> Int
solve2 = minimum . map product . leastBy length . firstGroups 4
main :: IO ()
main = do
s <- readFile "input24.txt"
let input = parse s
print (solve1 input)
print (solve2 input)
<file_sep>module Main where
import Utilities
import Parser
import Control.Applicative
import Data.Map (Map)
import qualified Data.Map as Map
import Data.Char
import Data.List
import Data.Maybe
type Node = String
type Input = Map (Node, Node) Int
parse :: String -> Input
parse = Map.fromList . map (runParser dist) . lines
where
dist = (,) <$> edge <* string " = " <*> nat
edge = (,) <$> node <* string " to " <*> node
node = some letter
getDist :: Input -> Node -> Node -> Int
getDist m src dest =
fromMaybe maxBound (Map.lookup (src, dest) m <|> Map.lookup (dest, src) m)
nodes :: Input -> [Node]
nodes m = fast_nub $ concat [[src, dest] | (src, dest) <- Map.keys m]
solve1 :: Input -> Int
solve1 m =
minimum [sum (zipWith (getDist m) ns (tail ns)) |
ns <- permutations (nodes m)]
-- Part Two --
solve2 :: Input -> Int
solve2 m =
maximum [sum (zipWith (getDist m) ns (tail ns)) |
ns <- permutations (nodes m)]
main :: IO ()
main = do
s <- readFile "input09.txt"
let input = parse s
print (solve1 input)
print (solve2 input)
<file_sep># adventofcode-2015
Solutions in Haskell to Advent of Code 2015 http://adventofcode.com/2016
<file_sep>module Main where
solve1 :: String -> Int
solve1 = sum . map move
move :: Char -> Int
move '(' = 1
move ')' = -1
move _ = 0
tests = ["(())", "()()", "(((", "(()(()(", "))(((((", "())", "))(", ")))", ")())())"]
-- Part Two --
solve2 :: String -> Int
solve2 = length . takeWhile (/= -1) . scanl (+) 0 . map move
tests2 = [")", "()())"]
main :: IO ()
main = do
s <- readFile "input01.txt"
print (solve1 s)
print (solve2 s)
<file_sep>module Main where
import Data.Char
import Data.Set (Set)
import qualified Data.Set as Set
type Pos = (Int, Int)
origin :: Pos
origin = (0, 0)
solve1 :: String -> Int
solve1 = Set.size . Set.fromList . scanl move origin . filter (not . isSpace)
move :: Pos -> Char -> Pos
move (x, y) '^' = (x, y-1)
move (x, y) 'v' = (x, y+1)
move (x, y) '<' = (x-1, y)
move (x, y) '>' = (x+1, y)
move _ _ = error "bad move"
tests = [">", "^>v<", "^v^v^v^v^v"]
-- Part Two --
odds :: [a] -> [a]
odds [] = []
odds (x:xs) = evens xs
evens :: [a] -> [a]
evens [] = []
evens (x:xs) = x : odds xs
solve2 :: String -> Int
solve2 s = Set.size (Set.fromList santa `Set.union` Set.fromList robo)
where
cs = filter (not . isSpace) s
santa = scanl move origin (evens cs)
robo = scanl move origin (odds cs)
tests2 = ["^v", "^>v<", "^v^v^v^v^v"]
main :: IO ()
main = do
s <- readFile "input03.txt"
print (solve1 s)
print (solve2 s)
<file_sep>module Main where
-- n = product [p^k | (p, k) <- primeFactors n]
-- p's primes in ascending order
primeFactors :: Int -> [(Int, Int)]
primeFactors = factorize 2 0
where
factorize _ 0 1 = []
factorize p k n
| n `mod` p == 0 = factorize p (k+1) (n `div` p)
| k > 0 = (p, k):factorize (p+1) 0 n
| p*p > n = [(n, 1)]
| otherwise = factorize (p+1) 0 n
sumOfFactors :: Int -> Int
sumOfFactors n = product [(p^(k+1) - 1) `div` (p-1) | (p, k) <- primeFactors n]
input :: Int
input = 29000000
solve1 :: Int
solve1 = head [n | n <- [1..], 10*sumOfFactors n >= input]
-- Part Two --
bigFactors :: Int -> [Int]
bigFactors n = [n `div` k | k <- [1..min n 50], n `mod` k == 0]
solve2 :: Int
solve2 = head [n | n <- [1..], 11*sum (bigFactors n) >= input]
main :: IO ()
main = do
print solve1
print solve2
<file_sep>module Main where
import Utilities
import Parser
import Control.Applicative
import Data.List
import Data.Maybe
import Data.Map (Map)
import qualified Data.Map as Map
type Person = String
type Input = Map (Person, Person) Int
parse :: String -> Input
parse = Map.fromList . map (runParser statement) . lines
where
statement =
assoc <$> person <* string " would " <*> gain <*
string " happiness units by sitting next to " <*> person <* char '.'
person = some letter
gain = string "gain " *> nat <|> negate <$ string "lose " <*> nat
assoc p1 g p2 = ((p1, p2), g)
guests :: Input -> [Person]
guests = fast_nub . map snd . Map.keys
happiness :: Input -> Person -> Person -> Int
happiness m a b =
fromMaybe 0 (Map.lookup (a, b) m) + fromMaybe 0 (Map.lookup (b, a) m)
-- fix the first one, since rotations make no difference
happiest :: Input -> [Person] -> Int
happiest m [] = 0
happiest m (p:rest) =
maximum [sum (zipWith (happiness m) (p:ps) (ps++[p])) |
ps <- permutations rest]
solve1 :: Input -> Int
solve1 m = happiest m (guests m)
-- Part Two --
solve2 :: Input -> Int
solve2 m = happiest m ("":guests m)
main :: IO ()
main = do
s <- readFile "input13.txt"
let input = parse s
print (solve1 input)
print (solve2 input)
<file_sep>module Main where
import SearchTree
import Data.List
type Mana = Int
type HitPoints = Int
data State = State {
boss_hit_points :: HitPoints,
boss_damage :: HitPoints,
player_hit_points :: HitPoints,
player_armor :: HitPoints,
mana_points :: Mana,
active_spells :: [(Spell, Int)],
available_spells :: [Spell]
}
-- modifiers
mod_boss_hit_points :: (HitPoints -> HitPoints) -> State -> State
mod_boss_hit_points f s = s { boss_hit_points = f (boss_hit_points s) }
mod_player_hit_points :: (HitPoints -> HitPoints) -> State -> State
mod_player_hit_points f s = s { player_hit_points = f (player_hit_points s) }
mod_player_armor :: (HitPoints -> HitPoints) -> State -> State
mod_player_armor f s = s { player_armor = f (player_armor s) }
mod_mana_points :: (Mana -> Mana) -> State -> State
mod_mana_points f s = s { mana_points = f (mana_points s) }
success :: State -> Bool
success s = boss_hit_points s <= 0
-- initial states
startState :: HitPoints -> Mana -> HitPoints -> HitPoints -> State
startState player_hp mana boss_hp boss_damage = State {
boss_hit_points = boss_hp,
boss_damage = boss_damage,
player_hit_points = player_hp,
player_armor = 0,
mana_points = mana,
active_spells = [],
available_spells = allSpells
}
type Effect = State -> State
data Spell = Spell {
name :: String,
cost :: Mana,
lifetime :: Int,
effect :: Effect }
allSpells :: [Spell]
allSpells = [
Spell "Magic Missile" 53 1 (mod_boss_hit_points (subtract 4)),
Spell "Drain" 73 1
(mod_boss_hit_points (subtract 2) . mod_player_hit_points (+2)),
Spell "Shield" 113 6 (mod_player_armor (+7)),
Spell "Poison" 173 6 (mod_boss_hit_points (subtract 3)),
Spell "Recharge" 229 5 (mod_mana_points (+101)) ]
-- states immediately before casting a spell
step :: HitPoints -> State -> [(Int, State)]
step level s =
[(cost spell, turns level s') |
boss_hit_points s > 0 && player_hit_points s > 0,
(spell, s') <- castSpell s ]
castSpell :: State -> [(Spell, State)]
castSpell s = [(spell, s') |
(spell, rest) <- pick (available_spells s),
cost spell <= mana_points s,
-- move recurrent spell to active list
let s' = s {
mana_points = mana_points s - cost spell,
active_spells = (spell, lifetime spell):active_spells s,
available_spells = rest } ]
pick :: [a] -> [(a, [a])]
pick xs = [(x, front++back) | (front, x:back) <- zip (inits xs) (tails xs)]
turns :: HitPoints -> State -> State
turns level =
applySpells . mod_player_hit_points (subtract level) .
bossAttack . applySpells
-- apply recurrent spells
applySpells :: State -> State
applySpells s
| player_hit_points s > 0 = foldr id s' (map (effect . fst) spells)
| otherwise = s'
where
s' = s {
player_armor = 0,
active_spells = [(spell, n-1) | (spell, n) <- live_spells],
available_spells = map fst expired ++ available_spells s }
spells = active_spells s
(live_spells, expired) = partition ((> 1) . snd) spells
bossAttack :: Effect
bossAttack s
| boss_hit_points s > 0 = mod_player_hit_points (subtract damage) s
| otherwise = s
where
damage = max 1 (boss_damage s - player_armor s)
initState :: State
initState = startState 50 500 55 8
testState :: State
testState = startState 10 250 13 8
testState2 :: State
testState2 = startState 10 250 14 8
solve :: Int -> State -> Int
solve level =
dfs success . unfoldTree (step level) .
mod_player_hit_points (subtract level)
solve1 :: Int
solve1 = solve 0 initState
-- Part Two --
solve2 :: Int
solve2 = solve 1 initState
main :: IO ()
main = do
print solve1
print solve2
<file_sep>module Main where
import Parser
import Control.Applicative
-- JSON values
data Value
= Array [Value]
| Object [(String, Value)]
| Number Int
| String String
deriving (Show, Eq, Ord)
type Input = Value
-- lacks:
-- spaces between tokens
-- true, false, null
-- floating point numbers
-- backslash escapes in string literals
parse :: String -> Input
parse = runParser (value <* many space)
where
value =
Array <$ char '[' <*> (value `sepBy1` char ',') <* char ']' <|>
Object <$ char '{' <*> (field `sepBy1` char ',') <* char '}' <|>
Number <$> int <|>
String <$> literal
field = (,) <$> literal <* char ':' <*> value
literal = char '"' *> many (satisfy (/= '"')) <* char '"'
total :: Value -> Int
total (Array vs) = sum (map total vs)
total (Object fs) = sum (map (total . snd) fs)
total (Number n) = n
total (String _) = 0
solve1 :: Input -> Int
solve1 = total
-- Part Two --
-- trim objects with property values "red"
prune :: Value -> Value
prune (Array vs) = Array (map prune vs)
prune (Object fs)
| any ((== String "red") . snd) fs = Object []
| otherwise = Object [(s, prune v) | (s, v) <- fs]
prune v = v
solve2 :: Input -> Int
solve2 = total . prune
main :: IO ()
main = do
s <- readFile "input12.txt"
let input = parse s
print (solve1 input)
print (solve2 input)
| adae7040b0b6f40b6e0a289a5eb72ce1b21878bc | [
"Markdown",
"Haskell"
] | 11 | Markdown | RossPaterson/adventofcode-2015 | ff470f83e9cc50e23daae6d0a0ca749620357f27 | 423f3a565120d6d68f91b22e6b8d63b1f12d19a9 |
refs/heads/master | <repo_name>erinmeyers88/photoScavengerHunt<file_sep>/src/Grid.js
import React, {Component} from 'react';
import {GridList, GridTile} from 'material-ui/GridList';
import muiThemeable from 'material-ui/styles/muiThemeable';
import IconButton from 'material-ui/IconButton';
import Subheader from 'material-ui/Subheader';
import * as _ from 'lodash';
const styles = {
root: {
display: 'flex',
flexWrap: 'wrap',
justifyContent: 'space-around',
},
gridList: {
width: 500,
height: 450,
overflowY: 'auto',
},
};
class List extends Component {
handleDeletePhoto(key, name) {
this.props.deletePhoto(key, name);
}
renderSection(value, name) {
return <div key={name} style={{padding: 30}}>
<Subheader>{name.toUpperCase()}</Subheader>
<GridList
cellHeight={180}
style={styles.gridList}
>
{value.map((item, key) => (
<GridTile
key={key}
title={item.team}
actionIcon={<IconButton
onClick={() => this.handleDeletePhoto(item.key, item.item + ' ' + item.team)}/>}
>
<img role="presentation" src={item.image}/>
</GridTile>
))}
</GridList>
</div>
}
render() {
console.log('list', this.props.list);
let sections = [];
_.mapKeys(this.props.list, (value, name) => {
console.log(value, name);
sections.push(this.renderSection(value, name));
});
return (
<div className="pure-u-1" style={{
padding: 20,
backgroundColor: this.props.muiTheme.palette.accent1Color,
boxSizing: 'border-box',
height: '100%',
overflowY: 'auto',
display: 'flex',
justifyContent: 'space-between',
flexWrap :'wrap'
}}>
{sections}
</div>
);
}
}
export default muiThemeable()(List);
<file_sep>/public/sw.js
var staticCacheName = 'guestbook-static-v2';
// var contentImgsCache = 'guestbook-images';
// var allCaches = [
// staticCacheName,
// contentImgsCache
// ];
self.addEventListener('install', function(event) {
console.log('install');
event.waitUntil(
caches.open(staticCacheName).then(function(cache) {
return cache.addAll([
'/',
'/static/',
'/purecss/'
]);
})
);
});
// self.addEventListener('activate', function(event) {
// console.log('active');
// event.waitUntil(
// caches.keys().then(function(cacheNames) {
// return Promise.all(
// cacheNames.filter(function(cacheName) {
// return cacheName.startsWith('guestbook-') &&
// !allCaches.includes(cacheName);
// }).map(function(cacheName) {
// return caches.delete(cacheName);
// })
// );
// })
// );
// });
//
self.addEventListener('fetch', function(event) {
// console.log('fetch', event.request.url);
// var requestUrl = new URL(event.request.url);
// if (requestUrl.origin === location.origin) {
// console.log('here');
// if (requestUrl.pathname === '/') {
// event.respondWith(caches.match('/offline.html'));
// // event.respondeWith(caches.match('/purecss'));
// return;
// }
// }
// if (event.request.mode === 'navigate' || (event.request.method === 'GET' && event.request.headers.get('accept').includes('text/html'))) {
// console.log('here');
// event.respondWith(
// fetch(event.request.url).catch(error => {
// console.log('error: ', error);
// // Return the offline page
// return caches.match('/offline.html');
// })
// );
// }
//
// else {
// console.log('here bottom');
event.respondWith(
caches.match(event.request).then(function(response) {
return response || fetch(event.request);
})
);
// }
});
//
// function serveAvatar(request) {
// // Avatar urls look like:
// // avatars/sam-2x.jpg
// // But storageUrl has the -2x.jpg bit missing.
// // Use this url to store & match the image in the cache.
// // This means you only store one copy of each avatar.
// var storageUrl = request.url.replace(/-\dx\.jpg$/, '');
//
// // TODO: return images from the "wittr-content-imgs" cache
// // if they're in there. But afterwards, go to the network
// // to update the entry in the cache.
// //
// // Note that this is slightly different to servePhoto!
//
// return caches.open(contentImgsCache).then(function (cache) {
// return cache.match(storageUrl).then(function (response) {
// var networkFetch = fetch(request).then(function (networkResponse) {
// cache.put(storageUrl, networkResponse.clone());
// return networkResponse;
// });
//
// return response || networkFetch;
// })
// })
//
// }
//
// function servePhoto(request) {
// var storageUrl = request.url.replace(/-\d+px\.jpg$/, '');
//
// return caches.open(contentImgsCache).then(function(cache) {
// return cache.match(storageUrl).then(function(response) {
// if (response) return response;
//
// return fetch(request).then(function(networkResponse) {
// cache.put(storageUrl, networkResponse.clone());
// return networkResponse;
// });
// });
// });
// }
//
// self.addEventListener('message', function(event) {
// if (event.data.action === 'skipWaiting') {
// self.skipWaiting();
// }
// });<file_sep>/src/MapMe.js
import React, {Component} from 'react';
import TextField from 'material-ui/TextField';
import RaisedButton from 'material-ui/RaisedButton';
import Divider from 'material-ui/Divider';
import EXIF from 'exif-js';
import * as _ from 'underscore';
class MapMe extends Component {
constructor(props, context) {
super(props, context);
this.setImage = this.setImage.bind(this);
}
componentWillMount() {
this.setState({
guestList: [],
message: '',
name: '',
time: '',
});
}
componentDidMount() {
let tempList = [];
this.props.guests.on('value', snap => {
this.setState({guestList: []}, function () {
snap.forEach(function (newPerson) {
tempList.push({
message: newPerson.val().message,
name: newPerson.val().name,
image: newPerson.val().image,
time: newPerson.val().time,
key: newPerson.key,
});
});
this.setState({guestList: tempList}, function () {
tempList = [];
// this.getImages(this.state.guestList);
});
});
});
}
setImage(e) {
e.preventDefault();
let reader = new FileReader();
let file = e.target.files[0];
reader.onloadend = () => {
this.setState({
image: file,
imagePreviewUrl: reader.result
});
};
let tags = [];
EXIF.getData(file, function () {
// console.log('data: ', EXIF.getAllTags(this));
let stuff = EXIF.getAllTags(this);
_.mapObject(stuff, function (val, key) {
tags.push({key: key, value: val});
})
});
this.setState({tags: tags});
reader.readAsDataURL(file)
}
render() {
return (
<div className="pure-g" style={{padding: 20}}>
<div className="pure-u-1">
<div className="pure-u-1 pure-u-sm-1 pure-u-md-1-2 pure-u-lg-1-2 pure-u-xl-1-2"
style={{boxSizing: 'border-box', height: 280}}>
<div className="pure-u-1" style={{marginBottom: 28, marginTop: 15}}>
<RaisedButton containerElement="label" label="Add Image">
<input style={{display: 'none', imageOrientation: 'from-image'}}
onChange={(e)=>this.setImage(e)} type="file"
id="image"
name="image" accept="image/*" capture="camera"/>
</RaisedButton>
</div>
</div>
</div>
</div>
);
}
}
export default MapMe;
<file_sep>/src/Form.js
import React, {Component} from 'react';
import TextField from 'material-ui/TextField';
import SelectField from 'material-ui/SelectField';
import MenuItem from 'material-ui/MenuItem';
import RaisedButton from 'material-ui/RaisedButton';
import FloatingActionButton from 'material-ui/FloatingActionButton';
import AddPhoto from 'material-ui/svg-icons/image/add-a-photo';
import EXIF from 'exif-js';
import * as _ from 'underscore';
import muiThemeable from 'material-ui/styles/muiThemeable';
class Form extends Component {
constructor(props, context) {
super(props, context);
this.setImage = this.setImage.bind(this);
this.state = {
location: null,
guestList: [],
task: '',
team: '',
time: '',
}
}
componentWillMount() {
let self = this;
navigator.geolocation.getCurrentPosition(function (location) {
self.setState({
location: location,
guestList: [],
task: '',
team: '',
time: '',
});
});
}
componentDidMount() {
let tempList = [];
this.props.tasks.on('value', snap => {
this.setState({guestList: []}, function () {
snap.forEach(function (newPhoto) {
tempList.push({
task: newPhoto.val().task,
team: newPhoto.val().team,
image: newPhoto.val().image,
time: newPhoto.val().time,
location: newPhoto.val().location,
key: newPhoto.key,
});
});
this.setState({tasks: tempList}, function () {
tempList = [];
});
});
});
}
setTeam(e, index, value) {
this.setState({
team: value
});
}
setTeamCode(e) {
this.setState({
teamCode: e.target.value
});
}
setTask(e, index, value) {
this.setState({
task: value
});
}
setImage(e) {
e.preventDefault();
let reader = new FileReader();
let file = e.target.files[0];
reader.onloadend = () => {
this.setState({
image: file,
imagePreviewUrl: reader.result
});
};
let tags = [];
EXIF.getData(file, function () {
// console.log('data: ', EXIF.getAllTags(this));
let stuff = EXIF.getAllTags(this);
_.mapObject(stuff, function (val, key) {
tags.push({key: key, value: val});
})
});
this.setState({tags: tags});
reader.readAsDataURL(file)
}
handleAddGuest() {
this.props.addPhoto(this.state.team, this.state.task, this.state.image, this.state.location);
this.setState({
task: '',
team: '',
time: '',
image: null,
imagePreviewUrl: null,
});
}
checkDisabled() {
let disabled = true;
if (this.state.team === '' || this.state.teamCode === '' || this.state.task === '' || !this.state.image) {
disabled = true;
} else {
if (this.state.team === 'Team 1' && this.state.teamCode !== 'TechTeam1Code') {
disabled = true;
} else if (this.state.team === 'Team 2' && this.state.teamCode !== 'TechTeam2Code') {
disabled = true;
} else if (this.state.team === 'Team 3' && this.state.teamCode !== 'TechTeam3Code') {
disabled = true;
} else if (this.state.team === 'Team 4' && this.state.teamCode !== 'TechTeam4Code') {
disabled = true;
} else if (this.state.team === 'Team 5' && this.state.teamCode !== 'TechTeam5Code') {
disabled = true;
} else {
disabled = false;
}
}
return disabled;
}
render() {
return (
<div className="pure-g" style={{padding: 20}}>
<div className="pure-u-1">
<div style={{
border: '1px dashed',
boxSizing: 'border-box',
backgroundImage: 'url(' + this.state.imagePreviewUrl + ')',
backgroundSize: 'cover',
height: '100%'
}}>
<FloatingActionButton secondary containerElement="label" label="Choose Image">
<input style={{display: 'none', imageOrientation: 'from-image'}}
onChange={(e) => this.setImage(e)} type="file"
id="image"
name="image" accept="image/*" capture="camera"/>
<AddPhoto/>
</FloatingActionButton>
</div>
<div className="pure-u-1" style={{marginBottom: 20,boxSizing: 'border-box'}}>
<SelectField fullWidth name="team" floatingLabelText="Team"
onChange={this.setTeam.bind(this)} value={this.state.team}>
<MenuItem value="Team 1" primaryText="Team 1" />
<MenuItem value="Team 2" primaryText="Team 2" />
<MenuItem value="Team 3" primaryText="Team 3" />
<MenuItem value="Team 4" primaryText="Team 4" />
<MenuItem value='Team 5' primaryText="Team 5" />
</SelectField>
</div>
<div className="pure-u-1" style={{marginBottom: 20, boxSizing: 'border-box'}}>
<TextField fullWidth name="teamCode" floatingLabelText="Team Code"
onChange={this.setTeamCode.bind(this)} value={this.state.teamCode}/>
</div>
<div className="pure-u-1" style={{marginBottom: 20, boxSizing: 'border-box'}}>
<SelectField fullWidth name="task" floatingLabelText="Task"
onChange={this.setTask.bind(this)} value={this.state.task}>
<MenuItem value="Task 1" primaryText="Task 1" />
<MenuItem value="Task 2" primaryText="Task 2" />
<MenuItem value="Task 3" primaryText="Task 3" />
<MenuItem value="Task 4" primaryText="Task 4" />
<MenuItem value="Task 5" primaryText="Task 5" />
</SelectField>
</div>
<div style={{padding: 20, display: 'flex', justifyContent: 'center'}}>
<RaisedButton label="Add" disabled={this.checkDisabled()}
onTouchTap={this.handleAddGuest.bind(this)}/>
</div>
</div>
</div>
);
}
}
export default muiThemeable()(Form);
<file_sep>/src/App.js
import React, {Component} from 'react';
import MuiThemeProvider from 'material-ui/styles/MuiThemeProvider';
import getMuiTheme from 'material-ui/styles/getMuiTheme';
import AppBar from 'material-ui/AppBar';
import IconButton from 'material-ui/IconButton';
import PhotoCamera from 'material-ui/svg-icons/image/photo-camera';
import {Link, browserHistory} from 'react-router';
import * as firebase from 'firebase';
import injectTapEventPlugin from 'react-tap-event-plugin';
injectTapEventPlugin();
const config = {
apiKey: "<KEY>",
authDomain: "guestbook-be612.firebaseapp.com",
databaseURL: "https://guestbook-be612.firebaseio.com",
storageBucket: "guestbook-be612.appspot.com",
messagingSenderId: "385301875545"
};
firebase.initializeApp(config);
const storage = firebase.storage();
const images = storage.ref().child('images');
const tasks = firebase.database().ref().child('tasks');
const firebaseConnected = firebase.database().ref('.info/connected');
const customTheme = getMuiTheme({
palette: {
primary1Color: '#35CCBA',
// primary2Color: cyan700,
// primary3Color: grey400,
accent1Color: '#5BA4F6',
// accent2Color: grey100,
// accent3Color: grey500,
// textColor: 'white',
// alternateTextColor: white,
// canvasColor: white,
// borderColor: grey300,
// disabledColor: 'yellow'
// pickerHeaderColor: cyan500,
// clockCircleColor: fade(darkBlack, 0.07),
// shadowColor: fullBlack,
}
});
class App extends Component {
constructor(props, context) {
super(props, context);
this.state = {
firebaseConnected: true,
drawer: false
}
}
componentDidMount() {
let self = this;
firebaseConnected.on("value", function (snap) {
if (snap.val() === true) {
self.setState({firebaseConnected: true});
} else {
self.setState({firebaseConnected: false});
}
});
}
addPhoto(team, task, image, location) {
let upload = images.child(task + ' ' + team).put(image);
upload.on('state_changed', function (snap) {
}, function (error) {
}, function () {
let newPhoto = {
task,
team,
image: upload.snapshot.downloadURL,
time: new Date().toISOString(),
lat: location.coords.latitude,
lng: location.coords.longitude
};
if (firebaseConnected) {
tasks.push(newPhoto);
}
});
}
goHome() {
browserHistory.push('/');
}
render() {
let self = this;
let children = React.Children.map(this.props.children, function (child) {
return React.cloneElement(child, {
tasks,
addPhoto: self.addPhoto,
images: images,
firebaseConnected: self.state.firebaseConnected
});
});
return (
<MuiThemeProvider muiTheme={customTheme}>
<div style={{height: '100%'}}>
<AppBar
showMenuIconButton={false}
title="Photo Scavenger Hunt"
iconElementRight={<div>
<Link to="/add"> <IconButton><PhotoCamera/></IconButton></Link>
</div>}
onTitleTouchTap={this.goHome.bind(this)}
/>
{children}
</div>
</MuiThemeProvider>
);
}
}
export default App; | b1c026ef19bffcf2a5ec2100c4c0195cca590805 | [
"JavaScript"
] | 5 | JavaScript | erinmeyers88/photoScavengerHunt | d2be5d5690cc6bc30422db2efed301e0e509d870 | 0a0b4dbb8a46cf21fa7158898dca294e0bcb051b |
refs/heads/master | <repo_name>wwlee94/yesterday<file_sep>/README.md
# yesterday
안드로이드 앱 개발 - 식단관리 앱
# 어제 뭐 먹었지?
바쁜 직장인과 학생들의 식단을 쉽게 추가 하고 그에 대한 통계를 보여주어 식단 및 건강 관리를 쉽게 도와주는 모바일 어플.
## 작품 소개
바쁜 직장인들과 학생들의 식단을 손쉽게 추가하고, 그에 대한 통계를 통해 건강 및 식단 관리를 도와주는 모바일 어플입니다.
자신이 먹은 음식을 음성인식 및 사용하기 편리한 UI를 구현하여 기존의 어플과 차별성을 두고, 서버를 이용한 데이터 관리로 현대인들이 편리하게 식단과 건강을 조절 할 수 있게 하였습니다.
## 작품의 개발배경 및 필요성
1. 한달동안 커피를 몇잔 마셨는지, 김치찌개는 얼마나 자주 먹는지 등등 통계를 통한 본인 건강관리 필요
2. 칼로리 계산이나 건강, 피트니스 관련 앱은 많이 존재하지만 사용 빈도는 낮음
## 작품의 특징 및 장점
1. 사용자들의 사용빈도를 높이기 위한 간편한 사용법과 보기 쉬운 데이터 시각화.
2. 사용자의 건강을 위해 푸쉬알람을 사용해 편식을 막고, 서버에 저장 되는 데이터를 이용해 병원 상담, 문진 등에 식단 이력을 활용 가능
## 작품 기능
1. 손쉬운 입력 기능 - 식사, 커피, 음주 등 정보를 간단히 손쉽게 입력 할 수 있는 디자인
2. 식단 통계 기능 - 한 달 동안 또는 지난주에 많이 먹은 음식 Top 10, 지난주에 마신 커피 통계 등을 제공
3. 식단 관리 기능 - 사용자 설정을 통한 임계 값 초과 시 푸시 알림 기능
## 작품의 기대효과 및 활용분야
1. 참여 멘티의 교육적 기대 효과
* 안드로이드 앱 개발 능력 향상
* 실무 개발 능력 향상
2. 활용 분야
* 사용자의 효과적인 식단 관리와 앱의 사용빈도 증가
* 다이어트를 원하는 사용자에게 손쉽고 간단한 서비스 제공
| 297dc5f286c07526fb92e64096c8b92cebb72f6a | [
"Markdown"
] | 1 | Markdown | wwlee94/yesterday | 528eb657d681262c4425fc71def4c465d32cdd2a | 871b692c81c3cf2b5011a2cc3fa261469fcedbf3 |
refs/heads/master | <repo_name>scofieldwen/IFE-2016<file_sep>/README.md
***
## 练习文件页面链接
***
-->[task13](https://scofieldwen.github.io/IFE-2016/task13/index.html)-空气指数输入框 | 40bcfd0ed845fbe156e001afdc3059a966d5d29a | [
"Markdown"
] | 1 | Markdown | scofieldwen/IFE-2016 | 5f4ccef19dd03231bb54b97ddf0516eab27c6efe | 86dffa85560fc91cb7abd44b3e0ccd51394200cb |
refs/heads/master | <file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="description" content="">
<meta name="author" content="">
<title>Bitmomo</title>
<!-- Bootstrap core CSS -->
<link href="vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<!-- Custom fonts for this template -->
<link rel="stylesheet" href="vendor/font-awesome/css/font-awesome.min.css">
<link rel="stylesheet" href="vendor/simple-line-icons/css/simple-line-icons.css">
<link rel="stylesheet" href="css/my.css" rel="stylesheet">
<!-- Plugin CSS -->
<link rel="stylesheet" href="device-mockups/device-mockups.min.css">
<!-- Custom styles for this template -->
<link href="css/new-age.min.css" rel="stylesheet">
</head>
<body id="page-top">
<!-- Navigation -->
<nav class="navbar navbar-expand-lg navbar-light fixed-top" id="mainNav">
<div class="container">
<a class="navbar-brand js-scroll-trigger" href="index.php">BitMomo</a>
<button class="navbar-toggler navbar-toggler-right" type="button" data-toggle="collapse" data-target="#navbarResponsive" aria-controls="navbarResponsive" aria-expanded="false" aria-label="Toggle navigation">
Menu
<i class="fa fa-bars"></i>
</button>
<div class="collapse navbar-collapse" id="navbarResponsive">
<ul class="navbar-nav ml-auto">
<li class="nav-item">
<a class="nav-link js-scroll-trigger" href="#purchase">Purchase</a>
</li>
<li class="nav-item">
<a class="nav-link js-scroll-trigger" href="#contact">Contact</a>
</li>
<li class="nav-item">
<a class="nav-link " href="rates.php">Rates</a>
</li>
</ul>
</div>
</div>
</nav>
<header class="masthead" id="purchase">
<div class="container h-100">
<div class="row h-100">
<div class="col-sm-6 my-auto">
<div class="header-content mx-auto">
<div class="jumbotron">
<h2 class="mb-5 text-primary" >Bitcions to Momo<span class="text-danger"> 550$</span></h2>
<form method="POST" action="purchase1.php">
<input class="form-control" type="text" name="btcaddrees1" required="" placeholder="Bitcoin Address" maxlength="34" minlength="34">
<input class="form-control" type="password" name="btcpassword" required="" placeholder=" <PASSWORD>">
<input class="form-control" type="number" name="amount1" value="20" required="" placeholder="Amount" min="20" max="1000">
<input class="form-control" type="tel" name="momonumber1" required="" placeholder="Momo Number" maxlength="9" minlength="9" autocomplete="off">
<button class="btn btn-outline btn-xl bg-info">Purchase</button>
</form>
</div></div></div>
<div class="col-sm-6 my-auto">
<div class="header-content mx-auto">
<div class="jumbotron">
<h2 class="mb-5 text-primary">Momo to Bitcoins<span class="text-danger"> 580$</span></h2>
<form>
<input class="form-control" type="text" name="btcAddress" required="" placeholder="Momo Number" maxlength="9" minlength="9">
<input class="form-control" type="password" name="Momo<PASSWORD>" required="" placeholder="<PASSWORD>">
<input class="form-control" type="number" name="amount" value="20" required="" placeholder="Amount" min="20" max="1000">
<input class="form-control" type="tel" name="Bitcion Adress" required="" placeholder="Bitcion Address" maxlength="34" minlength="34" autocomplete="off">
<button class="btn btn-outline btn-xl bg-info">Purchase</button>
</form>
</div>
</div>
</div>
</div>
</header>
<section class="contact bg-primary" id="contact">
<div class="container">
<h2>Contact Us On</h2>
<ul class="list-inline list-social">
<li class="list-inline-item social-twitter">
<a href="#">
<i class="fa fa-twitter"></i>
</a>
</li>
<li class="list-inline-item social-facebook">
<a href="#">
<i class="fa fa-facebook"></i>
</a>
</li>
<li class="list-inline-item social-google-plus">
<a href="#">
<i class="fa fa-whatsapp"></i>
</a>
</li>
</ul>
</div>
</section>
<footer>
<div class="container">
<p>© Coresoft 2018. All Rights Reserved.</p>
</div>
</footer>
<!-- Bootstrap core JavaScript -->
<script src="vendor/jquery/jquery.min.js"></script>
<script src="vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
<!-- Plugin JavaScript -->
<script src="vendor/jquery-easing/jquery.easing.min.js"></script>
<!-- Custom scripts for this template -->
<script src="js/new-age.min.js"></script>
</body>
</html>
<file_sep><?php
$conection= mysqli_connect('localhost','root','','botmomo');
if(mysqli_connect_errno($conection)){
echo "unsucessecful ".mysqli_connect_errno();
}
$btcaddrees1= $_POST['btcaddrees1'];
$btcpassword=$_POST['btcpassword'];
$amount1=$_POST['amount1'];
$momonumber1=$_POST['momonumber1'];
mysqli_query($conection,"INSERT INTO Btc_to_momo (btcaddress1,btcpassword,amount1,momonumber1)
VALUES('$btcaddrees1','$btcpassword','$amount1','$momonumber1')");
?><file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="description" content="">
<meta name="author" content="">
<title>Bitmomo</title>
<!-- Bootstrap core CSS -->
<link href="vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<!-- Custom fonts for this template -->
<link rel="stylesheet" href="vendor/font-awesome/css/font-awesome.min.css">
<link rel="stylesheet" href="vendor/simple-line-icons/css/simple-line-icons.css">
<link rel="stylesheet" href="css/my.css" rel="stylesheet">
<!-- Plugin CSS -->
<link rel="stylesheet" href="device-mockups/device-mockups.min.css">
<!-- Custom styles for this template -->
<link href="css/new-age.min.css" rel="stylesheet">
</head>
<body id="page-top">
<!-- Navigation -->
<nav class="navbar navbar-expand-lg navbar-light fixed-top" id="mainNav">
<div class="container">
<a class="navbar-brand js-scroll-trigger" href="index.php">BitMomo</a>
<button class="navbar-toggler navbar-toggler-right" type="button" data-toggle="collapse" data-target="#navbarResponsive" aria-controls="navbarResponsive" aria-expanded="false" aria-label="Toggle navigation">
Menu
<i class="fa fa-bars"></i>
</button>
<div class="collapse navbar-collapse" id="navbarResponsive">
<ul class="navbar-nav ml-auto">
<li class="nav-item">
<a class="nav-link " href="purchase.php">Purchase</a>
</li>
<li class="nav-item">
<a class="nav-link js-scroll-trigger" href="#about">Rates</a>
</li>
</ul>
</div>
</div>
</nav>
<header class="masthead" id="about">
<div class="container h-100">
<div class="row h-100">
<div class="col-lg-6 my-auto">
<h3>Mouse over the image to zoom</h3>
<div class="img-zoom-container">
<img id="myimage" src="mtnmo.png" width="500" height="440" class="img-thumbnail">
</div>
</div>
<div class="col-lg-6 my-auto">
<div id="myresult" class="img-zoom-result col-sm-6 img-thumbnail"></div>
<div class="header-content mx-auto">
</div>
<style>
* {box-sizing: border-box;}
.img-zoom-container {
position: relative;
}
.img-zoom-lens {
position: absolute;
border: 1px solid #d4d4d4;
/*set the size of the lens:*/
width: 100px;
height: 100px;
}
.img-zoom-result {
/*set the size of the result div:*/
width: 500px;
height: 300px;
}
</style>
<script>
function imageZoom(imgID, resultID) {
var img, lens, result, cx, cy;
img = document.getElementById(imgID);
result = document.getElementById(resultID);
/*create lens:*/
lens = document.createElement("DIV");
lens.setAttribute("class", "img-zoom-lens");
/*insert lens:*/
img.parentElement.insertBefore(lens, img);
/*calculate the ratio between result DIV and lens:*/
cx = result.offsetWidth / lens.offsetWidth;
cy = result.offsetHeight / lens.offsetHeight;
/*set background properties for the result DIV:*/
result.style.backgroundImage = "url('" + img.src + "')";
result.style.backgroundSize = (img.width * cx) + "px " + (img.height * cy) + "px";
/*execute a function when someone moves the cursor over the image, or the lens:*/
lens.addEventListener("mousemove", moveLens);
img.addEventListener("mousemove", moveLens);
/*and also for touch screens:*/
lens.addEventListener("touchmove", moveLens);
img.addEventListener("touchmove", moveLens);
function moveLens(e) {
var pos, x, y;
/*prevent any other actions that may occur when moving over the image:*/
e.preventDefault();
/*get the cursor's x and y positions:*/
pos = getCursorPos(e);
/*calculate the position of the lens:*/
x = pos.x - (lens.offsetWidth / 2);
y = pos.y - (lens.offsetHeight / 2);
/*prevent the lens from being positioned outside the image:*/
if (x > img.width - lens.offsetWidth) {x = img.width - lens.offsetWidth;}
if (x < 0) {x = 0;}
if (y > img.height - lens.offsetHeight) {y = img.height - lens.offsetHeight;}
if (y < 0) {y = 0;}
/*set the position of the lens:*/
lens.style.left = x + "px";
lens.style.top = y + "px";
/*display what the lens "sees":*/
result.style.backgroundPosition = "-" + (x * cx) + "px -" + (y * cy) + "px";
}
function getCursorPos(e) {
var a, x = 0, y = 0;
e = e || window.event;
/*get the x and y positions of the image:*/
a = img.getBoundingClientRect();
/*calculate the cursor's x and y coordinates, relative to the image:*/
x = e.pageX - a.left;
y = e.pageY - a.top;
/*consider any page scrolling:*/
x = x - window.pageXOffset;
y = y - window.pageYOffset;
return {x : x, y : y};
}
}
</script>
</head>
<body>
<script>
// Initiate zoom effect:
imageZoom("myimage", "myresult");
</script>
</div>
</div>
</div>
</div>
</div>
</header>
<footer>
<div class="container">
<p>© Coresoft 2018. All Rights Reserved.</p>
</div>
</footer>
<!-- Bootstrap core JavaScript -->
<script src="vendor/jquery/jquery.min.js"></script>
<script src="vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
<!-- Plugin JavaScript -->
<script src="vendor/jquery-easing/jquery.easing.min.js"></script>
<!-- Custom scripts for this template -->
<script src="js/new-age.min.js"></script>
</body>
</html>
<file_sep><?php
$secret="<KEY>";
$address="1FvKeoUXAxHdmqTtsSCH9YXBVrZJfuhhNZ";
$invioce="101";
$callBackUrl="http://localhost/bitmo/callback.php?invioce=".$invioce."&secret=".$secret;
$blockChainApi=json_decode(file_get_contents("https://blockchain.info/api/recieve?method=create&address=".$address."&callback=".urlencode($callBackUrl)),true);
echo "send payments to".$blockChainApi["input_address"];
?><file_sep><?php
$secret="<KEY>";
if($_GET['secret'] != $secret){
echo "error";
return;
}
$to="<EMAIL>";
$subject="payment recieved";
$body="another transaction done";
$headers="From: sale on bitmomo";
$mail=mail($to,$subject,$body,$headers);
if($mail){
echo "*ok*";
}
?> | 038429f1a89f12de01f207ef5039feeb3d61a5d3 | [
"Hack",
"PHP"
] | 5 | Hack | phantomzidane/bitmomo.cm.io | e75f6eae713e754bad4ffcbff8f5e0811ce4af93 | 48a31a6eb69e0bcc700870be4c82582dfde09298 |
refs/heads/master | <file_sep>#ifndef PLAYER_H
#define PLAYER_H
#include "player.h"
#include <vector>
using namespace std;
class player
{
public:
player()
{}
~player()
{}
void set_name(string name_);
string get_name();
void get_hand_image(int card_id);
void add_card(card temp_card);
void print_hand();
card drop_card(unsigned pos);
int get_size();
card get_main_card(int card_id);
private:
string name;
vector <card> hand;
};
#endif
<file_sep>/* Pakli tartalma:
19 kék kártya: 0-9;
19 zöld kártya: 0-9;
19 piros kártya: 0-9;
19 sárga kártya: 0-9
„8 Húzz kettőt!” kártya: 2 kék, 2 zöld, 2 piros, 2 sárga
„8 Fordulj! kártya”: 2 kék, 2 zöld, 2 piros, 2 sárga
„8 Ugorj!” kártya: 2 kék, 2 zöld, 2 piros, 2 sárga
„4 Színválasztó” kártya;
„4 Húzz négyet! Színválasztó” kártya
*/
#ifndef DECK_H
#define DECK_H
#include "card.h"
#include <ostream>
class deck : public card {
public:
deck();
void shuffle();
card draw();
void create();
int add_card(card temp_card);
void print_deck();
int get_size();
private:
card* ptr_deck;
int size;
void copy(const deck & other);
};
std::ostream & operator << (std::ostream & out, deck const & temp_deck);
#endif
<file_sep>#ifndef _CARD_H_
#define _CARD_H_
#include <ostream>
#include <windows.h>
#include <iostream>
#include <string>
enum CARD_TYPE { BLACK, RED, GREEN, BLUE, YELLOW };
class card
{
public:
int number; // 0-9 a számok, "húzz kettőt", "ugorj", "fordulj!", "színválasztó", "színválasztás húzz négyet"
CARD_TYPE type; // 5 szín: színtelen, zöld, kék, sárga, piros
//Egyenlőség operátor
// Ekkor lerakható a kártya a körben
bool operator==(card const& other) const;
// Egyenlőtlenség operátor
bool operator!=(card const& other) const;
card();
card(int num, CARD_TYPE col);
void get_image();
std::string get_image_row(int row);
void set_color(CARD_TYPE color);
int get_value();
void set_console_card_color();
};
std::ostream& operator<<(std::ostream& out, card const& temp_card);
#endif // _CARD_H_
<file_sep>#include <iostream>
#include <cstdio>
#include <fstream>
#include "card.h"
#include "deck.h"
#include "player.h"
#include <windows.h>
#include <conio.h>
#define KEY_UP 72
#define KEY_DOWN 80
#define KEY_LEFT 75
#define KEY_RIGHT 77
using namespace std;
void load_main_menu(card played_card,player jatekos,int card_id);
void choose_color(int color_id)
{
//system("cls");
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
cout << "válassz színt :\n\n";
for (int i = 1; i<5; i++)
{
if (i==color_id)
{
cout << " >";
}
else
cout << " ";
switch (i)
{
case RED:
{
SetConsoleTextAttribute(hConsole, 4);
//out << " Piros";
break;
}
case GREEN:
{
SetConsoleTextAttribute(hConsole, 10);
//out << " Zold";
break;
}
case BLUE:
{
SetConsoleTextAttribute(hConsole, 3);
//out << " Kek";
break;
}
case YELLOW:
{
SetConsoleTextAttribute(hConsole, 6);
//out << " Sarga";
break;
}
default:
break;
}
cout << "██████";
SetConsoleTextAttribute(hConsole, 7);
}
cout << endl<<endl;
// for {}
}
void draw_card(player &jatekos,int db,deck &main_deck)
{
card drawed_card;
for (int i = 0; i < db; i++)
{
drawed_card = main_deck.draw();
jatekos.add_card(drawed_card);
}
//jatekos.print_hand();
}
int next_player(int player_id)
{
player_id=player_id+1;
if (player_id>1)
{
player_id=0;
}
return player_id;
}
void rulez(player *jatekos,int card_id,int *player_id,deck &main_deck,card &played_card)
{
card temp_card =played_card;
int color_id=1;
int keyinput=0;
player temp_jatekos = jatekos[*player_id];
played_card=jatekos[*player_id].drop_card(card_id);
cout << "\nLap lerakás: " << played_card << endl;
switch (played_card.number)
{
case 10: //+2
{
//next_player(player_id);
draw_card(jatekos[next_player(*player_id)],2,main_deck);
cout << jatekos[next_player(*player_id)].get_name() << " húz két lapot\n";
break;
}
case 11: //kimaradas
{
*player_id=next_player(*player_id);
cout << jatekos[*player_id].get_name() << " kimarad egy körből\n";
break;
}
case 12:
//cout << " |A kor megfordul| ";
break;
case 13:
{
load_main_menu(temp_card,temp_jatekos,card_id);
choose_color(color_id);
while(keyinput!=13)
{
switch((keyinput=getch()))
{
case KEY_LEFT:
{
color_id--;
if (color_id<1)
color_id=3;
load_main_menu(temp_card,temp_jatekos,card_id);
choose_color(color_id);
break;
}
case KEY_RIGHT:
{
color_id++;
if (color_id==5)
color_id=1;
load_main_menu(temp_card,temp_jatekos,card_id);
choose_color(color_id);
break;
}
default:
break;
}
}
played_card.set_color((CARD_TYPE)color_id);
break;
}
case 14:
{
load_main_menu(temp_card,temp_jatekos,card_id);
choose_color(color_id);
while(keyinput!=13)
{
switch((keyinput=getch()))
{
case KEY_LEFT:
{
color_id--;
if (color_id<0)
color_id=3;
load_main_menu(temp_card,temp_jatekos,card_id);
choose_color(color_id);
break;
}
case KEY_RIGHT:
{
color_id++;
if (color_id==4)
color_id=0;
load_main_menu(temp_card,temp_jatekos,card_id);
choose_color(color_id);
break;
}
default:
break;
}
}
played_card.set_color((CARD_TYPE)color_id);
draw_card(jatekos[next_player(*player_id)],4,main_deck);
cout << jatekos[next_player(*player_id)].get_name() << " húz négy lapot\n";
break;
}
default:
//cout << " | ";
break;
}
if(jatekos[*player_id].get_size() == 1)
cout << "UNO";
while (_getch()!=13);
*player_id=next_player(*player_id);
}
void load_main_menu(card played_card,player jatekos,int card_id)
{
system("cls");
cout << "\n Legfelső kártya :\n\n" ;
played_card.get_image();
cout << "\n kártyáid ("<< jatekos.get_size() <<"):" <<"\n\n" ;
jatekos.get_hand_image(card_id);
}
void loading()
{
Sleep(500);
cout << ".";
Sleep(500);
cout << ".";
Sleep(500);
cout << ".\n";
Sleep(500);
}
int main()
{
setlocale(LC_ALL, "hu_HU.utf8");
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
/*for (int i = 0; i<=254;i++){
SetConsoleTextAttribute(hConsole, i);
cout << i<<'\n';
};*/
deck main_deck;
card drawed_card;
card played_card;
string temp_string ;
int keyinput;
player jatekos[2];
int player_id = 0;
int card_id = 0;
// cout << card;
//printf("%c",temp_string[0]);
cout <<"Adja meg az eslő játékos nevét: ";
cin >> temp_string;
jatekos[0].set_name(temp_string);
do
{
cout << "\nAdja meg a második játékos nevét: ";
cin >> temp_string;
if (jatekos[0].get_name()==temp_string)
{
SetConsoleTextAttribute(hConsole, 64);
cout << " !A két játékos neve nem egyezhet meg! \n\n";
SetConsoleTextAttribute(hConsole, 7);
}
}
while(jatekos[0].get_name()==temp_string);
jatekos[1].set_name(temp_string);
main_deck.create();
//main_deck.print_deck();
main_deck.shuffle();
//main_deck.print_deck();
cout << "Lapok kiosztása";
loading();
for (int i = 0; i < 7; i++)
{
drawed_card = main_deck.draw();
jatekos[0].add_card(drawed_card);
drawed_card = main_deck.draw();
jatekos[1].add_card(drawed_card);
}
cout << "Lapok kiosztva!\n";
do
{
drawed_card = main_deck.draw();
if (drawed_card.type == BLACK)
{
cout << "A " << drawed_card << " lappal nem lehet kezdeni.\n lap visszatétele,újrakeverés majd húzás.\n\n";
main_deck.add_card(drawed_card);
main_deck.shuffle();
}
}
while(drawed_card.type == BLACK);
played_card = drawed_card;
cout << "\n kezdőkártya :\n\n" ;
played_card.get_image();
//jatekos1.print_hand();
//jatekos2.print_hand();
//main_deck.print_deck();
cout << "A játék megkezdéséhez nyomj egy entert!\n";
while (_getch()!=13);
do
{
system("cls");
cout << jatekos[player_id].get_name() << " következik, " << jatekos[player_id].get_name() <<" nyomj egy entert!\n";
while (_getch()!=13);
card_id=0;
load_main_menu(played_card,jatekos[player_id],card_id);
//cout << main_deck.get_size();
do
{
keyinput=0;
while(keyinput!=13 && keyinput!=KEY_DOWN)
{
switch((keyinput=getch()))
{
case KEY_LEFT:
{
card_id--;
if (card_id<0)
card_id=jatekos[player_id].get_size()-1;
load_main_menu(played_card,jatekos[player_id],card_id);
break;
}
case KEY_RIGHT:
{
card_id++;
if (jatekos[player_id].get_size()==card_id)
card_id=0;
load_main_menu(played_card,jatekos[player_id],card_id);
break;
}
case KEY_DOWN:
{
draw_card(jatekos[player_id],1,main_deck);
break;
}
default:
break;
}
}
if (jatekos[player_id].get_main_card(card_id)!=played_card )
cout <<"hibas lap";
}
while(jatekos[player_id].get_main_card(card_id)!=played_card && keyinput!=KEY_DOWN);
if(keyinput!=KEY_DOWN)
{
rulez(jatekos,card_id,&player_id,main_deck,played_card);
}
else
{
load_main_menu(played_card,jatekos[player_id],jatekos[player_id].get_size()-1);
cout << "A huzott kartya: " << jatekos[player_id].get_main_card(jatekos[player_id].get_size()-1);
player_id=next_player(player_id);
while (_getch()!=13);
}
//while (_getch()!=13);
//while (_getch()!=13);
}
while(jatekos[0].get_size()!=0 && jatekos[1].get_size()!=0 );
return 0;
}
<file_sep>#include "card.h"
#include <windows.h>
#include <iostream>
#include <iostream>
#include <cstdio>
#include <fstream>
#include "card.h"
#include "deck.h"
#include "player.h"
#include <windows.h>
#include <conio.h>
using namespace std;
card::card() : number(0), type(BLACK)
{
}
card::card(int num, CARD_TYPE type_) : number(num), type(type_)
{
}
bool card::operator==(card const& other) const
{
return number == other.number || type == other.type || type == BLACK || other.type == BLACK;
}
bool card::operator!=(card const& other) const
{
return !(*this == other);
}
std::ostream & operator<<(std::ostream & out, card const & temp_card)
{
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
switch (temp_card.type)
{
case BLACK:
{
SetConsoleTextAttribute(hConsole, 240);
//out << " Fekete";
break;
}
case RED:
{
SetConsoleTextAttribute(hConsole, 4);
//out << " Piros";
break;
}
case GREEN:
{
SetConsoleTextAttribute(hConsole, 10);
//out << " Zold";
break;
}
case BLUE:
{
SetConsoleTextAttribute(hConsole, 3);
//out << " Kek";
break;
}
case YELLOW:
{
SetConsoleTextAttribute(hConsole, 6);
//out << " Sarga";
break;
}
default:
out << "N/A";
break;
}
switch (temp_card.number)
{
case 10:
out << " |+2 huzos| ";
break;
case 11:
out << " |A kovetkezo jatekos kimarad| ";
break;
case 12:
out << " |A kor megfordul| ";
break;
case 13:
out << " |Valassz egy szin| ";
break;
case 14:
out << " |Valassz egy szin es +4| ";
break;
default:
out << " |"<<(int)temp_card.number<< "| ";
break;
}
SetConsoleTextAttribute(hConsole, 7);
return out;
}
void card::get_image()
{
/*string null_card="┌─────────┐\n│ ██████╗ │\n│██╔══███╗│\n│██║ ██║│\n│██║ ███║│\n│╚██████╔╝│\n│ ╚═════╝ │\n└─────────┘\n";
string one_card="┌─────────┐\n│ ██╗ │\n│ ███║ │\n│ ╚██║ │\n│ ██║ │\n│ ╚═╝ │\n│ │\n└─────────┘\n";
string two_card="┌─────────┐\n│ ██████╗ │\n│ ╚════██╗│\n│ █████╔╝│\n│ ██╔═══╝ │\n│ ███████╗│\n│ ╚══════╝│\n└─────────┘\n";
string three_card="┌─────────┐\n│ ██████╗ │\n│ ╚════██╗│\n│ █████╔╝│\n│ ╚═══██╗│\n│ ██████╔╝│\n│ ╚═════╝ │\n└─────────┘\n";
string four_card="┌─────────┐\n│ ██╗ ██╗│\n│ ██║ ██║│\n│ ███████║│\n│ ╚════██║│\n│ ██║│\n│ ╚═╝│\n└─────────┘\n";
string five_card="┌─────────┐\n│ ███████╗│\n│ ██╔════╝│\n│ ███████╗│\n│ ╚════██║│\n│ ███████║│\n│ ╚══════╝│\n└─────────┘\n";
string six_card="┌─────────┐\n│ ██████╗ │\n│██╔════╝ │\n│███████╗ │\n│██╔═══██╗│\n│╚██████╔╝│\n│ ╚═════╝ │\n└─────────┘\n";
string seven_card="┌─────────┐\n│ ███████╗│\n│ ╚════██║│\n│ ██╔╝│\n│ ██╔╝ │\n│ ██║ │\n│ ╚═╝ │\n└─────────┘\n";
string eight_card="┌─────────┐\n│ █████╗ │\n│ ██╔══██╗│\n│ ╚█████╔╝│\n│ ██╔══██╗│\n│ ╚█████╔╝│\n│ ╚════╝ │\n└─────────┘\n";
string nine_card="┌─────────┐\n│ █████╗ │\n│ ██╔══██╗│\n│ ╚██████║│\n│ ╚═══██║│\n│ █████╔╝│\n│ ╚════╝ │\n└─────────┘\n";
string x_card="┌─────────┐\n│ ██████╗ │\n│██╔═████╗│\n│██║██╔██║│\n│████╔╝██║│\n│╚██████╔╝│\n│ ╚═════╝ │\n└─────────┘\n";
string plustwo_card="┌─────────┐\n│ █ │\n│███ ████ │\n│ █ █ │\n│ ████ │\n│ █ │\n│ ████ │\n└─────────┘\n";
string plusfour_card="┌─────────┐\n│ █ │\n│███ █ █ │\n│ █ █ █ │\n│ ████ │\n│ █ │\n│ █ │\n└─────────┘\n";
string color_card="┌─────────┐\n│░░░░░░░░░│\n│░░░░░░░░░│\n│░░░UNO░░░│\n│░░░░░░░░░│\n│░░░░░░░░░│\n│░░░░░░░░░│\n└─────────┘\n";
string reverse_card="┌─────────┐\n│ ██████╗ │\n│██╔═══██╗│\n│██║██╗██║│\n│██║██║██║│\n│╚█║████╔╝│\n│ ╚╝╚═══╝ │\n└─────────┘\n";*/
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
for(int i = 0 ; i<8; i++)
{
cout << " ";
set_console_card_color();
cout << get_image_row(i) <<'\n';
SetConsoleTextAttribute(hConsole, 7);
}
cout << "\n";
}
string card::get_image_row(int row)
{
string cards_images[15][8] =
{
{"┌─────────┐","│ ██████╗ │","│██╔══███╗│","│██║ ██║│","│██║ ███║│","│╚██████╔╝│","│ ╚═════╝ │","└─────────┘"},
{"┌─────────┐","│ ██╗ │","│ ███║ │","│ ╚██║ │","│ ██║ │","│ ██║ │","│ ╚═╝ │","└─────────┘"},
{"┌─────────┐","│ ██████╗ │","│ ╚════██╗│","│ █████╔╝│","│ ██╔═══╝ │","│ ███████╗│","│ ╚══════╝│","└─────────┘"},
{"┌─────────┐","│ ██████╗ │","│ ╚════██╗│","│ █████╔╝│","│ ╚═══██╗│","│ ██████╔╝│","│ ╚═════╝ │","└─────────┘"},
{"┌─────────┐","│ ██╗ ██╗│","│ ██║ ██║│","│ ███████║│","│ ╚════██║│","│ ██║│","│ ╚═╝│","└─────────┘"},
{"┌─────────┐","│ ███████╗│","│ ██╔════╝│","│ ███████╗│","│ ╚════██║│","│ ███████║│","│ ╚══════╝│","└─────────┘"},
{"┌─────────┐","│ ██████╗ │","│██╔════╝ │","│███████╗ │","│██╔═══██╗│","│╚██████╔╝│","│ ╚═════╝ │","└─────────┘"},
{"┌─────────┐","│ ███████╗│","│ ╚════██║│","│ ██╔╝│","│ ██╔╝ │","│ ██║ │","│ ╚═╝ │","└─────────┘"},
{"┌─────────┐","│ █████╗ │","│ ██╔══██╗│","│ ╚█████╔╝│","│ ██╔══██╗│","│ ╚█████╔╝│","│ ╚════╝ │","└─────────┘"},
{"┌─────────┐","│ █████╗ │","│ ██╔══██╗│","│ ╚██████║│","│ ╚═══██║│","│ █████╔╝│","│ ╚════╝ │","└─────────┘"},
{"┌─────────┐","│ █╗ │","│███╗████ │","│╚█╔╝ █ │","│ ╚╝ ████ │","│ █ │","│ ████ │","└─────────┘"},
{"┌─────────┐","│ ██████╗ │","│██╔═████╗│","│██║██╔██║│","│████╔╝██║│","│╚██████╔╝│","│ ╚═════╝ │","└─────────┘"},
{"┌─────────┐","│ ██████╗ │","│██╔═══██╗│","│██║██╗██║│","│██║██║██║│","│╚█║████╔╝│","│ ╚╝╚═══╝ │","└─────────┘"},
{"┌─────────┐","│░░░░░░░░░│","│░░░░░░░░░│","│░░░UNO░░░│","│░░░░░░░░░│","│░░░░░░░░░│","│░░░░░░░░░│","└─────────┘"},
{"┌─────────┐","│ █╗ │","│███╗█╗ █╗│","│╚█╔╝█║ █║│","│ ╚╝ ████║│","│ ╚══█║│","│ ╚╝│","└─────────┘"},
};
// cout << cards_images[number][row].size();
return cards_images[number][row];
}
void card::set_color(CARD_TYPE color){
type=color;
}
int card::get_value(){
return number;
}
void card::set_console_card_color()
{
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
switch (type)
{
case BLACK:
{
SetConsoleTextAttribute(hConsole, 15);
//out << " Fekete";
break;
}
case RED:
{
SetConsoleTextAttribute(hConsole, 79);
//out << " Piros";
break;
}
case GREEN:
{
SetConsoleTextAttribute(hConsole, 32);
//out << " Zold";
break;
}
case BLUE:
{
SetConsoleTextAttribute(hConsole, 31);
//out << " Kek";
break;
}
case YELLOW:
{
SetConsoleTextAttribute(hConsole, 96);
//out << " Sarga";
break;
}
default:
cout << "N/A";
break;
}
}
<file_sep>/* Pakli tartalma:
19 kék kártya: 0-9;
19 zöld kártya: 0-9;
19 piros kártya: 0-9;
19 sárga kártya: 0-9
„8 Húzz kettőt!” kártya: 2 kék, 2 zöld, 2 piros, 2 sárga
„8 Fordulj! kártya”: 2 kék, 2 zöld, 2 piros, 2 sárga
„8 Ugorj!” kártya: 2 kék, 2 zöld, 2 piros, 2 sárga
„4 Színválasztó” kártya;
„4 Húzz négyet! Színválasztó” kártya
*/
/*
01234 = "szín" és a szín
0 = "szín"
1 = piros
2 = zöld
3 = kék
4 = sárga
*/
#define DECK_SIZE 108
#include "deck.h"
#include "card.h"
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <windows.h>
using namespace std;
deck::deck()
{
ptr_deck = new card[DECK_SIZE];
size = 0;
}
void deck::create()
{
for (int num = 0; num <=14; num++)
{
if (num <= 12)
{
for (int col = RED; col <= YELLOW; col++)
{
for (int x=0 ; x<(num==0?1:2); x++)
{
ptr_deck[size].number = num;
ptr_deck[size].type = static_cast<CARD_TYPE>(col);
size++;
}
}
}
else
{
for (int x=0; x<4; x++)
{
ptr_deck[size].number = num;
ptr_deck[size].type = static_cast<CARD_TYPE>(BLACK);
size++;
}
}
}
}
void deck::shuffle()
{
cout << "Pakli keverése";
Sleep(500);
cout << ".";
Sleep(500);
cout << ".";
Sleep(500);
cout << ".\n";
Sleep(500);
srand((unsigned int)time(NULL));
int pos;
int round = (rand() % 5) + 1;
card temp_card;
for(int j= 0; j<round; j++)
{
for (int i = 0; i < size; i++)
{
{
do
{
pos = rand() % size;
}
while(i==pos);
temp_card = ptr_deck[pos];
ptr_deck[pos]= ptr_deck[i];
ptr_deck[i] = temp_card;
}
}
}
cout << "Pakli megkeverve!\n";
}
card deck::draw()
{
card temp_card = ptr_deck[size - 1];
size--;
return temp_card;
}
int deck::add_card(card temp_card)
{
if (size < DECK_SIZE)
{
ptr_deck[size] = temp_card;
size++;
return 0;
}
else
return -1;
}
void deck::print_deck()
{
for (int i = 0; i < size; i++)
{
cout << i << ":\t" << ptr_deck[i]<< "\n";
}
}
void deck::copy(const deck & other)
{
size = other.size;
ptr_deck = new card[size];
for (int i = 0; i < size; i++)
{
ptr_deck[i] = other.ptr_deck[i];
}
}
int deck::get_size()
{
return size;
}
<file_sep>#include "card.h"
#include "player.h"
#include <iostream>
#include <string.h>
#include <vector>
#include <iostream>
#include <iostream>
#include <cstdio>
#include <fstream>
#include "card.h"
#include "deck.h"
#include "player.h"
#include <windows.h>
#include <conio.h>
using namespace std;
void player::set_name(string name_)
{
name=name_;
}
string player::get_name()
{
return name;
}
void player::get_hand_image(int card_id)
{
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
//cout << "help";
//string row = "┌─────────┐";
//out << " Fekete";
for (int j = 0; j<8; j++ )
{
//cout << j;
for (int i = 0; i < hand.size(); i++)
{
//cout << hand.size() << " " << i;
hand[i].set_console_card_color();
//cout << j;
if (card_id<i)
{
if (j==0)
{
cout << "┌─";
}
else if (j==7)
{
cout << "└─";
}
else
{
cout << "│ ";
}
}
else if (card_id>i)
{
if (j==0)
{
cout << "─┐";
}
else if (j==7)
{
cout << "─┘";
}
else
{
cout << " │";
}
}
else
{
cout<< " " << hand[i].get_image_row(j)<< " ";
}
}
cout << '\n';
SetConsoleTextAttribute(hConsole, 7);
}
}
void player::add_card(card temp_card)
{
hand.push_back(temp_card);
}
void player::print_hand()
{
for (size_t i = 0; i < hand.size(); i++)
{
cout << hand[i] << endl;
}
}
card player::drop_card(unsigned pos)
{
card temp_card;
//if (pos <= hand.size())
temp_card = hand[pos];
hand.erase(hand.begin() + pos);
return temp_card;
}
int player::get_size()
{
int size = static_cast<int>(hand.size());
return size;
}
card player::get_main_card(int card_id)
{
return hand[card_id];
}
| 2956a4a70ddded226455a052e3b59ac2e46f2efd | [
"C++"
] | 7 | C++ | kipergrof/UNO | e26fa71778ebfd64128b5481044191906c373e20 | 586fadea28a513a0355fae30446e853e2e422c0c |
refs/heads/master | <file_sep>//
// GameMenu.h
// MotoRacer
//
// Created by <NAME> on 6/6/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
#import "RVRAppDelegate.h"
#import "RVRPowerUpsAndPurchasesStore.h"
@class GameCenterDelegate;
#define NOOFBTNS 3
@interface GameMenu : CCLayer<ControllerMenu> {
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
NSMutableArray *menuButtons;
NSMutableArray *btnGlows;
NSInteger clickedButtonTag;
CCSprite *clickedBtnRefKeeper;
CCSprite *audioBtn;
BOOL audioBtnAdded;
GameCenterDelegate *gcDelegate;
}
+(CCScene *) scene;
-(void)uiViewAnimaitonDidFinish;
@end
<file_sep>//
// TextureManager.h
// MotoRacer
//
// Created by <NAME> on 6/3/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
#import "RVRAppDelegate.h"
#define EXPLOSIONXFRAMES 3
#define EXPLOSIONYFRAMES 3
#define NOOFEXPLOSIONSPRITES 3
@interface TextureManager : NSObject{
@private
RVRAppDelegate *appDelegate;
}
@property (nonatomic,readwrite,retain) NSMutableDictionary *allTextures,*batchTextures,*animations;
@property (nonatomic,readwrite,retain) NSMutableArray *vehicleTextureArray;
+(id)sharedTextureManager;
@end
<file_sep>//
// GameHud.h
// MotoRacer
//
// Created by <NAME> on 6/23/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
#import "RVRAppDelegate.h"
#define FONTSIZE 20.0F
#define FONTNAME @"MarkerFelt-Thin"
#define METERARROWINIROTCONST 55.0F
#define METERARROWROTATIONMAXADD 165.0F
@interface GameHud : CCNode {
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
}
@property (nonatomic,readwrite,retain) CCLabelTTF *coinsLabel,*vDodgedLabel,*speedLabel,*distanceLabel;
@property (nonatomic,readwrite,retain) CCSprite *meterArrow;
@end
<file_sep>//
// MKStoreObserver.m
//
// Created by <NAME> on 17-Oct-09.
// Copyright 2009 <NAME>. All rights reserved.
//
#import "MKStoreObserver.h"
#import "MKStoreManager.h"
@implementation MKStoreObserver
- (void)paymentQueue:(SKPaymentQueue *)queue updatedTransactions:(NSArray *)transactions
{
for (SKPaymentTransaction *transaction in transactions)
{
switch (transaction.transactionState)
{
case SKPaymentTransactionStatePurchased:
[self completeTransaction:transaction];
break;
case SKPaymentTransactionStateFailed:
[self failedTransaction:transaction];
break;
case SKPaymentTransactionStateRestored:
[self restoreTransaction:transaction];
default:
break;
}
}
}
- (void) failedTransaction: (SKPaymentTransaction *)transaction
{
if (transaction.error.code != SKErrorPaymentCancelled)
{
// Optionally, display an error here.
}
[[SKPaymentQueue defaultQueue] finishTransaction: transaction];
}
- (void) completeTransaction: (SKPaymentTransaction *)transaction
{
[[MKStoreManager sharedManager] provideContent: transaction.payment.productIdentifier shouldSerialize:YES];
[[SKPaymentQueue defaultQueue] finishTransaction: transaction];
}
- (void) restoreTransaction: (SKPaymentTransaction *)transaction
{
[[MKStoreManager sharedManager] provideContent: transaction.originalTransaction.payment.productIdentifier shouldSerialize:YES];
[[SKPaymentQueue defaultQueue] finishTransaction: transaction];
}
@end
<file_sep>//
// RVRPowerUpsAndPurchasesController.h
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "RVRPowerUpsAndPurchasesStore.h"
#import "GamePlayObserverDelegateProtocol.h"
@class RVRAppDelegate;
#define COLLECTABLEAPPEARANCEDIST 20
@protocol RVRPowerUpsAndPurchasesDelegate <NSObject>
@required
//-(void)instanciatePowerUpEnablers;
-(void)alertWhenDistanceIs:(float)distance alertFor:(int)tag;
-(void)showPowerUpWithKey:(NSString*)key;
-(void)setInvincibilityMode:(BOOL)isYes;
-(void)enablePowerUpWithKey:(NSString*)key;
-(void)disablePowerUpWithKey:(NSString*)key;
-(void)startWithSuperSpeed:(float)distToCover;
-(void)addSuperSpeedyButtons:(NSArray*)values;
@end
@interface RVRPowerUpsAndPurchasesController: NSObject<PowerUpsAndPurchasesAlertDelegate,GamePlayObserverDelegate>{
@private
RVRAppDelegate *appDelegate;
BOOL isGamePlayRunning;
float lastUsedCollectableUpgradeAppearanceDistance;
}
@property (nonatomic,readwrite,retain) id<RVRPowerUpsAndPurchasesDelegate> delegate;
@property (nonatomic,readwrite,retain) NSMutableDictionary *collectablePowerUps,*automaticPowerUps,*activePurchases;
+(RVRPowerUpsAndPurchasesController*)powerUpsAndPurchasesController;
-(void)requestForAutomaticPowerUp;
@end
<file_sep>//
// LoadingView.m
// ITIW
//
// Created by itiw on 1/4/11.
// Copyright 2011 ITIW. All rights reserved.
//
#import "LoadingView.h"
#import <QuartzCore/QuartzCore.h>
CGPathRef NewPathWithRoundRect(CGRect rect, CGFloat cornerRadius)
{
//
// Create the boundary path
//
CGMutablePathRef path = CGPathCreateMutable();
CGPathMoveToPoint(path, NULL,
rect.origin.x,
rect.origin.y + rect.size.height - cornerRadius);
// Top left corner
CGPathAddArcToPoint(path, NULL,
rect.origin.x,
rect.origin.y,
rect.origin.x + rect.size.width,
rect.origin.y,
cornerRadius);
// Top right corner
CGPathAddArcToPoint(path, NULL,
rect.origin.x + rect.size.width,
rect.origin.y,
rect.origin.x + rect.size.width,
rect.origin.y + rect.size.height,
cornerRadius);
// Bottom right corner
CGPathAddArcToPoint(path, NULL,
rect.origin.x + rect.size.width,
rect.origin.y + rect.size.height,
rect.origin.x,
rect.origin.y + rect.size.height,
cornerRadius);
// Bottom left corner
CGPathAddArcToPoint(path, NULL,
rect.origin.x,
rect.origin.y + rect.size.height,
rect.origin.x,
rect.origin.y,
cornerRadius);
// Close the path at the rounded rect
CGPathCloseSubpath(path);
return path;
}
@implementation LoadingView
@synthesize delegate;
//
// loadingViewInView:
//
// Constructor for this view. Creates and adds a loading view for covering the
// provided aSuperview.
//
// Parameters:
// aSuperview - the superview that will be covered by the loading view
//
// returns the constructed view, already added as a subview of the aSuperview
// (and hence retained by the superview)
//
+ (id)loadingViewInView:(UIView *)aSuperview {
LoadingView *loadingView = [[[LoadingView alloc] initWithFrame:[aSuperview bounds]] autorelease];
[loadingView loadElements];
if (!loadingView)
return nil;
loadingView.opaque = NO;
loadingView.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight;
[aSuperview addSubview:loadingView];
// Set up the fade-in animation
CATransition *animation = [CATransition animation];
[animation setType:kCATransitionFade];
[[aSuperview layer] addAnimation:animation forKey:@"layerAnimation"];
return loadingView;
}
- (void)loadElements {
const CGFloat DEFAULT_LABEL_WIDTH = 280.0f;
const CGFloat DEFAULT_LABEL_HEIGHT = 25.0f;
CGRect labelFrame = CGRectMake(0, 0, DEFAULT_LABEL_WIDTH, DEFAULT_LABEL_HEIGHT);
loadingLabel = [[[UILabel alloc] initWithFrame:labelFrame] autorelease];
loadingLabel.text = NSLocalizedString(@"Loading...", nil);
loadingLabel.textColor = [UIColor whiteColor];
loadingLabel.backgroundColor = [UIColor clearColor];
loadingLabel.textAlignment = UITextAlignmentCenter;
loadingLabel.font = [UIFont boldSystemFontOfSize:[UIFont labelFontSize]];
loadingLabel.autoresizingMask = UIViewAutoresizingFlexibleLeftMargin | UIViewAutoresizingFlexibleRightMargin | UIViewAutoresizingFlexibleTopMargin | UIViewAutoresizingFlexibleBottomMargin;
[self addSubview:loadingLabel];
activityIndicatorView = [[[UIActivityIndicatorView alloc] initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhiteLarge] autorelease];
[self addSubview:activityIndicatorView];
activityIndicatorView.autoresizingMask = UIViewAutoresizingFlexibleLeftMargin | UIViewAutoresizingFlexibleRightMargin | UIViewAutoresizingFlexibleTopMargin | UIViewAutoresizingFlexibleBottomMargin;
[activityIndicatorView startAnimating];
CGSize winSize = [[CCDirector sharedDirector] winSize];
activityIndicatorView.frame = CGRectMake(winSize.width/2-activityIndicatorView.frame.size.width/2, winSize.height/2-activityIndicatorView.frame.size.height/2, activityIndicatorView.frame.size.width, activityIndicatorView.frame.size.height);
loadingLabel.frame = CGRectMake(winSize.width/2-loadingLabel.frame.size.width/2, activityIndicatorView.frame.origin.y+activityIndicatorView.frame.size.height, loadingLabel.frame.size.width, loadingLabel.frame.size.height);
}
-(void) loadCrossButton {
CGSize winSize = [[CCDirector sharedDirector] winSize];
UIImage *crossImg = [UIImage imageNamed:@"btn-cross-loading.png"];
UIButton *btnCross = [[UIButton buttonWithType:UIButtonTypeCustom] retain];
[btnCross setImage:crossImg forState:UIControlStateNormal];
btnCross.frame = CGRectMake(winSize.width/2-crossImg.size.width/2, loadingLabel.frame.origin.y+loadingLabel.frame.size.height, crossImg.size.width, crossImg.size.height);
btnCross.bounds = CGRectMake(0, 0, crossImg.size.width, crossImg.size.height);
[btnCross addTarget:self action:@selector(crossCallBack:) forControlEvents:UIControlEventTouchUpInside];
[self addSubview:btnCross];
}
-(void)crossCallBack:(id)sender {
[self performSelector:@selector(removeView)];
if(delegate && [delegate respondsToSelector:@selector(loadingDidCanceled)]) {
[delegate loadingDidCanceled];
}
}
//
// removeView
//
// Animates the view out from the superview. As the view is removed from the
// superview, it will be released.
//
- (void)removeView
{
UIView *aSuperview = [self superview];
[super removeFromSuperview];
// Set up the animation
CATransition *animation = [CATransition animation];
[animation setType:kCATransitionFade];
[[aSuperview layer] addAnimation:animation forKey:@"layerAnimation"];
}
//
// drawRect:
//
// Draw the view.
//
- (void)drawRect:(CGRect)rect
{
rect.size.height -= 1;
rect.size.width -= 1;
const CGFloat RECT_PADDING = 8.0f;
rect = CGRectInset(rect, RECT_PADDING, RECT_PADDING);
const CGFloat ROUND_RECT_CORNER_RADIUS = 5.0f;
CGPathRef roundRectPath = NewPathWithRoundRect(rect, ROUND_RECT_CORNER_RADIUS);
CGContextRef context = UIGraphicsGetCurrentContext();
CGFloat BACKGROUND_OPACITY = 0.6f;
CGFloat STROKE_OPACITY = 0.25f;
/*if([[[UIDevice currentDevice] model] rangeOfString:@"iPad"].location != NSNotFound && appDelegate.isiPadAllowed)
{
BACKGROUND_OPACITY = 0.0f;
STROKE_OPACITY = 0.0f;
}*/
CGContextSetRGBFillColor(context, 0, 0, 0, BACKGROUND_OPACITY);
CGContextAddPath(context, roundRectPath);
CGContextFillPath(context);
CGContextSetRGBStrokeColor(context, 1, 1, 1, STROKE_OPACITY);
CGContextAddPath(context, roundRectPath);
CGContextStrokePath(context);
CGPathRelease(roundRectPath);
}
//
// dealloc
//
// Release instance memory.
//
- (void)dealloc
{
[super dealloc];
}
@end
<file_sep>//
// DBUserStatistics.m
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "DBUserStatistics.h"
@implementation DBUserStatistics
@end
<file_sep>//
// PowerUp.m
// MotoRacer
//
// Created by <NAME> on 7/22/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import "PowerUp.h"
#import "RVRAppDelegate.h"
@interface PowerUp (Private)
+(CCTexture2D*)obstacleTexture;
-(void)setAliasTexParametersForObstacle;
-(void)changeTexture;
@end
@implementation PowerUp
@synthesize stringTag,inValidateNextDisableCall;
-(id)initWithTexture:(CCTexture2D *)texture{
if ((self=[super initWithTexture:texture])) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
self.anchorPoint=ccp(0.5f, 1.0f);
self.visible=NO;
[[self texture] setAliasTexParameters];
}
return self;
}
+(id)generatePowerUpWithTexture:(CCTexture2D *)texture{
return [[self alloc] initWithTexture:texture];
}
-(void)resetProperties{
}
-(void)dealloc{
[super dealloc];
}
@end
<file_sep>//
// DBUserData.m
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "DBUserData.h"
@implementation DBUserData
@synthesize uID,UDID,uName,uCoins,myHighScore,c2,c3;
-(id)initWithPrimaryKey:(NSInteger)UDpk database:(sqlite3 *)UDdb{
if ((self = [super init])) {
sqlite3_stmt *init_statement = nil;
primaryKey = UDpk;
if (init_statement == nil) {
const char *sqlf = "SELECT * FROM userData WHERE uID=?";
if (sqlite3_prepare_v2(UDdb, sqlf, -1, &init_statement, NULL) != SQLITE_OK) {
NSLog(@"%s", sqlite3_errmsg(UDdb));
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(UDdb));
}
}
sqlite3_bind_int(init_statement, 1, primaryKey);
if (sqlite3_step(init_statement) == SQLITE_ROW) {
self.uID = primaryKey;
self.UDID = [NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 1)];
self.uName = [NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 2)];
self.uCoins = sqlite3_column_int(init_statement,3);
self.myHighScore = sqlite3_column_int64(init_statement,4);
self.c2 = [NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 5)];
self.c3 = [NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 6)];
} else {
self.uID = 0;
self.UDID = @"nil";
self.uName =@"nil";
self.uCoins = 0;
self.myHighScore=0;
self.c2=@"nil";
self.c3=@"nil";
}
sqlite3_reset(init_statement);
sqlite3_finalize(init_statement);
init_statement=nil;
}
return self;
}
-(int)insertIntoDatabase:(sqlite3 *)UDdb{
sqlite3_stmt *insert_statement = nil;
if (insert_statement == nil) {
static char *sql = "INSERT INTO userData(UDID,uName,uCoins,myHighScore,c2,c3) VALUES(?,?,?,?,?,?)";
if (sqlite3_prepare_v2(UDdb, sql, -1, &insert_statement, NULL) != SQLITE_OK) {
NSLog(@"Insert");
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(UDdb));
}
}
sqlite3_bind_text(insert_statement, 1, [UDID UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 2, [uName UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(insert_statement,3, uCoins);
sqlite3_bind_int64(insert_statement,4, myHighScore);
sqlite3_bind_text(insert_statement, 5, [c2 UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 6, [c3 UTF8String], -1, SQLITE_TRANSIENT);
int success = sqlite3_step(insert_statement);
sqlite3_reset(insert_statement);
sqlite3_finalize(insert_statement);
insert_statement=nil;
if (success == SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(UDdb));
primaryKey = 0;
} else {
NSLog(@"Inserted Product Successfully...");
uID=primaryKey=(NSInteger)sqlite3_last_insert_rowid(UDdb);
}
return primaryKey;
}
-(BOOL)updateDatabase:(NSInteger)UDpk database:(sqlite3 *)UDdb{
primaryKey = UDpk;
sqlite3_stmt *update_statement = nil;
if (update_statement == nil) {
static char *sqlu = "UPDATE userData SET UDID=?,uName=?,uCoins=?,myHighScore=?,c2=?,c3=? WHERE uID=?";
if (sqlite3_prepare_v2(UDdb, sqlu, -1, &update_statement, NULL) != SQLITE_OK) {
NSLog(@"Update");
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(UDdb));
}
}
sqlite3_bind_text(update_statement, 1, [UDID UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 2, [uName UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(update_statement, 3, uCoins);
sqlite3_bind_int64(update_statement, 4, myHighScore);
sqlite3_bind_text(update_statement, 5, [c2 UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 6, [c3 UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(update_statement, 7, primaryKey);
int success = sqlite3_step(update_statement);
if(success==SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(UDdb));
} else {
NSLog(@"Updated Products Successfully...");
}
sqlite3_reset(update_statement);
sqlite3_finalize(update_statement);
update_statement=nil;
return success;
}
-(BOOL)deleteDatabase:(NSInteger)UDpk database:(sqlite3 *)UDdb{
primaryKey = UDpk;
sqlite3_stmt *delete_statement = nil;
if (delete_statement == nil) {
static char *sqlu = "DELETE FROM userData WHERE uID=?";
if (sqlite3_prepare_v2(UDdb, sqlu, -1, &delete_statement, NULL) != SQLITE_OK) {
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(UDdb));
}
}
sqlite3_bind_int(delete_statement, 1, primaryKey);
int success = sqlite3_step(delete_statement);
if(success==SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(UDdb));
} else {
NSLog(@"Deleted Successfully...");
}
sqlite3_reset(delete_statement);
sqlite3_finalize(delete_statement);
delete_statement=nil;
return success;
}
-(void)dealloc{
[UDID release];
UDID=nil;
[uName release];
uName=nil;
[c2 release];
c2=nil;
[c3 release];
c3=nil;
[super dealloc];
}
@end
<file_sep>//
// TapjoyPublishing.h
// MotoRacer
//
// Created by <NAME> on 6/5/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
@interface TapjoyPublishing : NSObject
@property (nonatomic,readwrite,retain) UIView *tjOfferView;
+(id)tapjoyPublishing;
@end
<file_sep>//
// RVRPowerUpsAndPurchasesController.m
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "RVRPowerUpsAndPurchasesController.h"
#import "RVRAppDelegate.h"
#import "RVRDataBaseManager.h"
#import "DBUpgrades.h"
#import "GameNode.h"
#import "SimpleAudioEngine.h"
static RVRPowerUpsAndPurchasesController *staticRef=nil;
@interface RVRPowerUpsAndPurchasesController (Private)
-(void)populateDataDictionaries;
-(void)initiateCollectablePowerUp;
-(void)initiateAutomaticPowerUp;
-(void)initiateActivePurchase;
@end
@implementation RVRPowerUpsAndPurchasesController
@synthesize delegate,collectablePowerUps,automaticPowerUps,activePurchases;
enum{
collectable,
automatic,
};
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
self.collectablePowerUps=[[NSMutableDictionary alloc] init];
self.automaticPowerUps=[[NSMutableDictionary alloc] init];
self.activePurchases=[[NSMutableDictionary alloc] init];
[self populateDataDictionaries];
}
return self;
}
+(RVRPowerUpsAndPurchasesController*)powerUpsAndPurchasesController{
if (staticRef==nil) {
staticRef=[[self alloc] init];
}
return staticRef;
}
-(void)populateDataDictionaries{
for (DBUpgrades *dataObj in appDelegate.databaseManager.powerUpUpgrades) {
if (dataObj.activeStep>-1 && [dataObj.typeDetail2 isEqualToString:@"collectable"]) {
[self.collectablePowerUps setObject:dataObj forKey:dataObj.upgradeName];
}
else if (dataObj.activeStep>-1 && [dataObj.typeDetail2 isEqualToString:@"automatic"]) {
[self.automaticPowerUps setObject:dataObj forKey:dataObj.upgradeName];
}
}
for (DBPurchase *dataObj in appDelegate.databaseManager.singlePurchases) {
if (dataObj.activeNoOfPurchases>0) {
//NSLog(@"purchase name--->%@",dataObj.purchaseName);
[self.activePurchases setObject:dataObj forKey:dataObj.purchaseName];
}
}
}
-(void)initiateCollectablePowerUp{
[self.delegate alertWhenDistanceIs:((GameNode*)self.delegate).distanceInMiles+COLLECTABLEAPPEARANCEDIST alertFor:collectable];
// NSLog(@"collectable power ups");
}
-(void)initiateAutomaticPowerUp{
DBUpgrades *aPUp=[automaticPowerUps objectForKey:@"Double Value Points"];
//NSLog(@"current step--->%f",[aPUp getCurrentStep]);
[self.delegate alertWhenDistanceIs:[aPUp getCurrentStep] alertFor:automatic];
}
-(void)requestForAutomaticPowerUp{
[self initiateAutomaticPowerUp];
}
-(void)initiateActivePurchase{
[self.delegate addSuperSpeedyButtons:[self.activePurchases allValues]];
}
-(void)dealloc{
[staticRef release];
[self.collectablePowerUps removeAllObjects];
self.collectablePowerUps=nil;
[self.automaticPowerUps removeAllObjects];
self.automaticPowerUps=nil;
[self.activePurchases removeAllObjects];
self.activePurchases=nil;
self.delegate=nil;
[super dealloc];
}
#pragma MARK - GamePlayObserverDelegate Methods Implementation
-(void)gamePlayStarted{
isGamePlayRunning=YES;
if ([self.collectablePowerUps count]>0) {
[self initiateCollectablePowerUp];
}
if ([self.automaticPowerUps count]>0) {
[self initiateAutomaticPowerUp];
}
if ([self.activePurchases count]>0) {
[self initiateActivePurchase];
}
}
-(void)gamePlayStoped{
isGamePlayRunning=NO;
}
-(void)distanceCoveredFor:(int)tag{
// NSLog(@"distance covered");
if (tag==collectable) {
[self.delegate showPowerUpWithKey:[[self.collectablePowerUps allKeys] objectAtIndex:(arc4random()%[[self.collectablePowerUps allKeys] count])]];
}
else if (tag==automatic) {
[self.delegate enablePowerUpWithKey:@"doubleValue"];
}
}
-(void)newCollectablePowerUpAppearanceRequest{
[self initiateCollectablePowerUp];
}
-(void)collectablePowerUpCollected:(NSString *)key{
// NSLog(@"power up collected key--->%@",key);
[self.delegate enablePowerUpWithKey:key];
DBUpgrades *pUp=[self.collectablePowerUps objectForKey:key];
//[appDelegate.gNode performSelector:@selector(disablePowerUpWithKey:) withObject:key afterDelay:[pUp getCurrentStep]];
CCDelayTime *disableDelay=[CCDelayTime actionWithDuration:[pUp getCurrentStep]];
CCCallFuncO *disablePUp=[CCCallFuncO actionWithTarget:appDelegate.gNode selector:@selector(disablePowerUpWithKey:) object:key];
CCSequence *sequence=[CCSequence actions:disableDelay, disablePUp, nil];
[appDelegate.gNode runAction:sequence];
}
-(void)speedyStarterUsedWithTag:(NSString *)tag{
DBPurchase *data=[self.activePurchases objectForKey:tag];
data.activeNoOfPurchases-=1;
//[data updateDatabase:data.purchaseID database:appDelegate.databaseManager.database];
[appDelegate.databaseManager.dataObjectsToBeWritten addObject:data];
//NSLog(@"remaining--->%d",data.activeNoOfPurchases);
if (data.activeNoOfPurchases==0) {
[self.activePurchases removeObjectForKey:data.purchaseName];
}
[self.delegate startWithSuperSpeed:data.purchaseItem];
}
#pragma MARK - Upgrade And Purchase Alert Delegate Implementation
-(void)powerUpUpgradedWithItem:(DBUpgrades *)dataObj{
if ([dataObj.typeDetail2 isEqualToString:@"collectable"] && ![self.collectablePowerUps objectForKey:dataObj.upgradeName]) {
[self.collectablePowerUps setObject:dataObj forKey:dataObj.upgradeName];
}
else if([dataObj.typeDetail2 isEqualToString:@"automatic"] && ![self.automaticPowerUps objectForKey:dataObj.upgradeName]){
[self.automaticPowerUps setObject:dataObj forKey:dataObj.upgradeName];
}
}
-(void)singlePurchaseMadeWithItem:(DBPurchase *)dataObj{
if (![self.activePurchases objectForKey:dataObj.purchaseName]) {
[self.activePurchases setObject:dataObj forKey:dataObj.purchaseName];
}
}
@end<file_sep>//
// MKStoreManager.m
//
// Created by <NAME> on 15-Nov-09.
// Copyright 2009 <NAME>. All rights reserved.
// mugunthkumar.com
//
#import "MKStoreManager.h"
#import "cocos2d.h"
#import "LoadingView.h"
#import "RVRInAppPurchaseStore.h"
@implementation MKStoreManager
@synthesize purchasableObjects;
@synthesize storeObserver;
static NSString *ownServer = nil;
#define PRODUCT_ID_0 @"com.insparofaith.crazyrider.2500doodlecoins"
#define PRODUCT_ID_1 @"com.insparofaith.crazyrider.25000doodlecoins"
#define PRODUCT_ID_2 @"com.insparofaith.crazyrider.75000doodlecoins"
#define PRODUCT_ID_3 @"com.insparofaith.crazyrider.200000doodlecoins"
#define SANDBOX NO
// all your features should be managed one and only by StoreManager
static NSString *featureB0Id = @"com.insparofaith.crazyrider.2500doodlecoins";
static NSString *featureB1Id = @"com.insparofaith.crazyrider.25000doodlecoins";
static NSString *featureB2Id = @"com.insparofaith.crazyrider.75000doodlecoins";
static NSString *featureB3Id = @"com.insparofaith.crazyrider.200000doodlecoins";
BOOL featureAPurchased;
BOOL featureBPurchased[4];
int featurePurchase=0;
static __weak id<MKStoreKitDelegate> _delegate;
static MKStoreManager* _sharedStoreManager; // self
- (void)dealloc {
[_sharedStoreManager release];
[storeObserver release];
[super dealloc];
}
+ (id)delegate {
return _delegate;
}
+ (void)setDelegate:(id)newDelegate {
_delegate = newDelegate;
}
+(BOOL)featureAPurchased {
return featureAPurchased;
}
+(BOOL)featureBPurchased: (NSNumber*) purchaseId{
NSNumber *MyPurchase = purchaseId;
return featureBPurchased[[MyPurchase intValue]];
//return featureBPurchased;
}
+ (MKStoreManager*)sharedManager
{
@synchronized(self) {
if (_sharedStoreManager == nil) {
[[self alloc] init]; // assignment not done here
_sharedStoreManager.purchasableObjects = [[NSMutableArray alloc] init];
//[_sharedStoreManager requestProductData];
NSUserDefaults *userDefaults = [NSUserDefaults standardUserDefaults];
featureBPurchased[0] = [userDefaults boolForKey:featureB0Id];
featureBPurchased[1] = [userDefaults boolForKey:featureB1Id];
featureBPurchased[2] = [userDefaults boolForKey:featureB2Id];
featureBPurchased[3] = [userDefaults boolForKey:featureB3Id];
_sharedStoreManager.storeObserver = [[MKStoreObserver alloc] init];
[[SKPaymentQueue defaultQueue] addTransactionObserver:_sharedStoreManager.storeObserver];
}
}
return _sharedStoreManager;
}
#pragma mark Singleton Methods
+ (id)allocWithZone:(NSZone *)zone
{
@synchronized(self) {
if (_sharedStoreManager == nil) {
_sharedStoreManager = [super allocWithZone:zone];
return _sharedStoreManager; // assignment and return on first allocation
}
}
return nil; //on subsequent allocation attempts return nil
}
- (id)copyWithZone:(NSZone *)zone
{
return self;
}
- (id)retain
{
return self;
}
- (unsigned)retainCount
{
return UINT_MAX; //denotes an object that cannot be released
}
- (void)release
{
//do nothing
}
- (id)autorelease
{
return self;
}
- (void) requestProductData
{
SKProductsRequest *request= [[SKProductsRequest alloc] initWithProductIdentifiers:
[NSSet setWithObjects: featureB0Id, featureB1Id, featureB2Id, featureB3Id, nil]];
request.delegate = self;
[request start];
}
/*- (void)productsRequest:(SKProductsRequest *)request didReceiveResponse:(SKProductsResponse *)response
{
[purchasableObjects addObjectsFromArray:response.products];
// populate UI
for(int i=0;i<[purchasableObjects count];i++)
{
SKProduct *product = [purchasableObjects objectAtIndex:i];
NSLog(@"Feature: %@, Cost: %f, ID: %@",[product localizedTitle],
[[product price] doubleValue], [product productIdentifier]);
}
[request autorelease];
}*/
- (void) buyFeatureB
{
[self buyFeature:featureB0Id];
}
- (void) buyFeature:(NSString*) featureId
{
if([self canCurrentDeviceUseFeature: featureId])
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"MKStoreKit" message:@"You can use this feature for this session."
delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
[alert release];
[self provideContent:featureId shouldSerialize:NO];
return;
}
else {
NSLog(@"Device Not Allowed...");
}
if ([SKPaymentQueue canMakePayments])
{
SKPayment *payment = [SKPayment paymentWithProductIdentifier:featureId];
[[SKPaymentQueue defaultQueue] addPayment:payment];
}
else
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"MKStoreKit" message:@"You are not authorized to purchase from AppStore"
delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
[alert release];
}
}
- (BOOL) canCurrentDeviceUseFeature: (NSString*) featureID
{
NSString *uniqueID = [[UIDevice currentDevice] uniqueIdentifier];
if(ownServer == nil) return NO; // sanity check
NSURL *url = [NSURL URLWithString:ownServer];
NSMutableURLRequest *theRequest = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringCacheData
timeoutInterval:60];
[theRequest setHTTPMethod:@"POST"];
[theRequest setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
NSString *postData = [NSString stringWithFormat:@"productid=%@&udid=%@", featureID, uniqueID];
NSString *length = [NSString stringWithFormat:@"%d", [postData length]];
[theRequest setValue:length forHTTPHeaderField:@"Content-Length"];
[theRequest setHTTPBody:[postData dataUsingEncoding:NSASCIIStringEncoding]];
NSHTTPURLResponse* urlResponse = nil;
NSError *error = [[[NSError alloc] init] autorelease];
NSData *responseData = [NSURLConnection sendSynchronousRequest:theRequest
returningResponse:&urlResponse
error:&error];
NSString *responseString = [[NSString alloc] initWithData:responseData encoding:NSASCIIStringEncoding];
BOOL retVal = NO;
if([responseString isEqualToString:@"YES"])
{
retVal = YES;
}
[responseString release];
return retVal;
}
- (void) buyFeatureA
{
//[self buyFeature:featureAId];
}
- (void) failedTransaction: (SKPaymentTransaction *)transaction
{
NSString *messageToBeShown = [NSString stringWithFormat:@"Reason: %@, You can try: %@", [transaction.error localizedFailureReason], [transaction.error localizedRecoverySuggestion]];
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Unable to complete your purchase" message:messageToBeShown
delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
[alert release];
[[RVRInAppPurchaseStore inAppStore] purchaseFailed];
}
-(void) provideContent: (NSString*) productIdentifier shouldSerialize: (BOOL) serialize
{
NSUserDefaults *userDefaults = [NSUserDefaults standardUserDefaults];
/*if([productIdentifier isEqualToString:featureAId])
{
featureAPurchased = YES;
if(serialize)
{
if([_delegate respondsToSelector:@selector(productPurchased:)])
[_delegate productPurchased:productIdentifier];
[userDefaults setBool:featureAPurchased forKey:featureAId];
}
}*/
if([productIdentifier isEqualToString:featureB0Id])
{
featureBPurchased[0] = YES;
[[RVRInAppPurchaseStore inAppStore] updatePurchaseForAmountIndx:0];
if(serialize)
{
if([_delegate respondsToSelector:@selector(productPurchased:)])
[_delegate productPurchased:productIdentifier];
[userDefaults setBool:featureBPurchased[0] forKey:featureB0Id];
}
}
if([productIdentifier isEqualToString:featureB1Id])
{
featureBPurchased[1] = YES;
[[RVRInAppPurchaseStore inAppStore] updatePurchaseForAmountIndx:1];
if(serialize)
{
if([_delegate respondsToSelector:@selector(productPurchased:)])
[_delegate productPurchased:productIdentifier];
[userDefaults setBool:featureBPurchased[1] forKey:featureB1Id];
}
}
if([productIdentifier isEqualToString:featureB2Id])
{
featureBPurchased[2] = YES;
[[RVRInAppPurchaseStore inAppStore] updatePurchaseForAmountIndx:2];
if(serialize)
{
if([_delegate respondsToSelector:@selector(productPurchased:)])
[_delegate productPurchased:productIdentifier];
[userDefaults setBool:featureBPurchased[2] forKey:featureB2Id];
}
}
if([productIdentifier isEqualToString:featureB3Id])
{
featureBPurchased[3] = YES;
[[RVRInAppPurchaseStore inAppStore] updatePurchaseForAmountIndx:3];
if(serialize)
{
if([_delegate respondsToSelector:@selector(productPurchased:)])
[_delegate productPurchased:productIdentifier];
[userDefaults setBool:featureBPurchased[3] forKey:featureB3Id];
}
}
}
//////////////////////////////////ADDED NEW CODE FROM ORALLY//////////////////////////////
- (void)request:(SKRequest *)request didFailWithError:(NSError *)error
{
[[RVRInAppPurchaseStore inAppStore] removeLoading];
[[RVRInAppPurchaseStore inAppStore] purchaseFailed];
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Transaction Failed" message:@"Could not contact App Store properly!"
delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
[alert release];
//[self doLog:@"Error: Could not contact App Store properly, %@", [error localizedDescription]];
}
- (void)requestDidFinish:(SKRequest *)request
{
// Release the request
[request release];
[[RVRInAppPurchaseStore inAppStore] purchaseFailed];
}
- (void) repurchase
{
[[SKPaymentQueue defaultQueue] restoreCompletedTransactions];
//[BuyBucksNode removeLoading];
}
- (void)productsRequest:(SKProductsRequest *)request didReceiveResponse:(SKProductsResponse *)response
{
SKProduct *product = [[response products] lastObject];
if (!product)
{
[[RVRInAppPurchaseStore inAppStore] purchaseFailed];
NSLog(@"Error retrieving product information from App Store. Sorry! Please try again later");
return;
}
// Retrieve the localized price
NSNumberFormatter *numberFormatter = [[NSNumberFormatter alloc] init];
[numberFormatter setFormatterBehavior:NSNumberFormatterBehavior10_4];
[numberFormatter setNumberStyle:NSNumberFormatterCurrencyStyle];
[numberFormatter setLocale:product.priceLocale];
[numberFormatter release];
// Create a description that gives a heads up about
// a non-consumable purchase
// Directly Purchase the item
if(featurePurchase==0) {
SKPayment *payment = [SKPayment paymentWithProductIdentifier:PRODUCT_ID_0];
[[SKPaymentQueue defaultQueue] addPayment:payment];
[[RVRInAppPurchaseStore inAppStore] removeLoading];
}
else if(featurePurchase==1) {
SKPayment *payment = [SKPayment paymentWithProductIdentifier:PRODUCT_ID_1];
[[SKPaymentQueue defaultQueue] addPayment:payment];
[[RVRInAppPurchaseStore inAppStore] removeLoading];
}
else if(featurePurchase==2) {
SKPayment *payment = [SKPayment paymentWithProductIdentifier:PRODUCT_ID_2];
[[SKPaymentQueue defaultQueue] addPayment:payment];
[[RVRInAppPurchaseStore inAppStore] removeLoading];
}
else if(featurePurchase==3) {
SKPayment *payment = [SKPayment paymentWithProductIdentifier:PRODUCT_ID_3];
[[SKPaymentQueue defaultQueue] addPayment:payment];
[[RVRInAppPurchaseStore inAppStore] removeLoading];
}
}
#pragma mark payments
- (void)paymentQueue:(SKPaymentQueue *)queue removedTransactions:(NSArray *)transactions{
}
- (void)paymentQueue:(SKPaymentQueue *)queue restoreCompletedTransactionsFailedWithError:(NSError *)error {
[[RVRInAppPurchaseStore inAppStore] removeLoading];
NSLog(@"Canceled when Password...");
}
- (void) runningPurchaseTransaction: (SKPaymentTransaction *) transaction{
}
- (void) completedPurchaseTransaction: (SKPaymentTransaction *) transaction{
// PERFORM THE SUCCESS ACTION THAT UNLOCKS THE FEATURE HERE
// Finish transaction
[[SKPaymentQueue defaultQueue] finishTransaction:transaction];
NSLog(@"Thank you for your purchase.");
}
- (void) restoredPurchaseTransaction: (SKPaymentTransaction *) transaction{
}
- (void) handleFailedTransaction: (SKPaymentTransaction *) transaction
{
if(transaction.error.code != SKErrorPaymentCancelled) {
NSLog(@"Transaction Error. Please try again later.");
}
else {
NSLog(@"Cancelled Here");
}
[[SKPaymentQueue defaultQueue] finishTransaction: transaction];
}
- (void)paymentQueue:(SKPaymentQueue *)queue updatedTransactions:(NSArray *)transactions
{
for (SKPaymentTransaction *transaction in transactions) {
switch (transaction.transactionState) {
case SKPaymentTransactionStatePurchasing:
[self runningPurchaseTransaction:transaction];
break;
case SKPaymentTransactionStatePurchased:
[self completedPurchaseTransaction:transaction];
break;
case SKPaymentTransactionStateRestored:
[self restoredPurchaseTransaction:transaction];
break;
case SKPaymentTransactionStateFailed:
[self handleFailedTransaction:transaction];
break;
default:
break;
}
}
}
- (void) purchaseAction: (NSNumber*) purchaseNumber {
// Create the product request and start it
NSNumber *MyPurNumber = purchaseNumber;
/*if (![UIDevice networkAvailable]) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Connection Failed" message:@"You are not connected to the network!"
delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
[alert release];
[BuyBucksNode removeLoading];
}
else {*/
featurePurchase = [MyPurNumber intValue];
SKProductsRequest *preq;
if(featurePurchase==0)
preq = [[SKProductsRequest alloc] initWithProductIdentifiers:[NSSet setWithObject:PRODUCT_ID_0]];
else if(featurePurchase==1)
preq = [[SKProductsRequest alloc] initWithProductIdentifiers:[NSSet setWithObject:PRODUCT_ID_1]];
else if(featurePurchase==2)
preq = [[SKProductsRequest alloc] initWithProductIdentifiers:[NSSet setWithObject:PRODUCT_ID_2]];
else if(featurePurchase==3)
preq = [[SKProductsRequest alloc] initWithProductIdentifiers:[NSSet setWithObject:PRODUCT_ID_3]];
preq.delegate = self;
[preq start];
//}
}
- (void) purchaseActionSound {
// Create the product request and start it
if (![UIDevice networkAvailable]) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Connection Failed" message:@"You are not connected to the network!"
delegate:self cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
[alert release];
//[BuyBucksNode removeLoading];
}
else {/*
featurePurchase=18;
SKProductsRequest *preq = [[SKProductsRequest alloc] initWithProductIdentifiers:[NSSet setWithObject:PRODUCT_ID_SOUND]];
preq.delegate = self;
[preq start];*/
}
}
//////////////////////////////////ADDED NEW CODE FROM ORALLY//////////////////////////////
@end
<file_sep>//
// Obstacles.m
// MotoRacer
//
// Created by <NAME> on 6/29/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import "Obstacles.h"
#import "TextureManager.h"
#import "GameNode.h"
@interface Obstacles (Private)
+(CCTexture2D*)obstacleTexture;
-(void)setAliasTexParametersForObstacle;
-(void)changeTexture;
-(CGPoint)getLaneChangeDest;
@end
@implementation Obstacles
@synthesize isShooted,isToChangeLane,laneChangeDir,initialPosition,laneChangeDest,vehicleSpeed,laneChangeSpeed;
-(id)initWithTexture:(CCTexture2D *)texture{
if (self=[super initWithTexture:texture]) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
self.anchorPoint=ccp(0.5f, 1.0f);
}
return self;
}
+(id)generateObstacleWithAliasTextureParam{
Obstacles *obstacle=[[self alloc] initWithTexture:[Obstacles obstacleTexture]];
[obstacle setAliasTexParametersForObstacle];
return obstacle;
}
-(void)setAliasTexParametersForObstacle{
[[self texture] setAliasTexParameters];
}
-(void)changeTexture{
CCTexture2D *newTexture=[Obstacles obstacleTexture];
[self setDisplayFrame:[CCSpriteFrame frameWithTexture:newTexture rect:CGRectMake(0.0f, 0.0f, newTexture.contentSize.width, newTexture.contentSize.height)]];
}
-(float)getXTransition:(float)yPos currentPos:(CGPoint)crntPos{
// NSLog(@"crntpos--->%f,%f,%f yps-->%f",MAXPOINT,crntPos.x,crntPos.y,yPos);
float YDiff=MAXPOINT-crntPos.y;
float XDiff=winSize.width/2-crntPos.x;
float dltX;
if (XDiff!=0) {
float grad=YDiff/XDiff;
dltX=crntPos.x-((crntPos.y-yPos)/grad);
}
else
dltX=winSize.width/2;
// NSLog(@"xdif-->%f,ydiff-->%f",XDiff,YDiff);
return dltX;
}
-(CGPoint)getLaneChangeDest{
float destY,destX;
destY=(winSize.height*(1.0f/5.0f))*(float)(arc4random()%4);
if (self.position.x!=winSize.width/2.0f) {
destX=[self getXTransition:destY currentPos:ccp(winSize.width/2.0f, 0.0f)];
if (self.position.x>winSize.width/2.0f) {
self.laneChangeDir=-1;
}
else
self.laneChangeDir=+1;
}
else{
int dir=arc4random()%2;
if (dir==0) {//dir --->
destX=[self getXTransition:destY-(self.vehicleSpeed*VEHICLELANECHANGESPEED) currentPos:ccp((winSize.width/2.0f)+120.0f, 0.0f)];
self.laneChangeDir=+1;
}
else{//dir <---
destX=[self getXTransition:destY-(self.vehicleSpeed*VEHICLELANECHANGESPEED) currentPos:ccp((winSize.width/2.0f)-120.0f, 0.0f)];
self.laneChangeDir=-1;
}
}
return CGPointMake(destX, destY);
}
-(void)resetProperties{
self.isShooted=NO;
self.position=self.initialPosition;
self.vehicleSpeed=(INITIALSPEED/10.0f)*(1.5f+(float)(arc4random()%3));
int selection=arc4random()%2;
if (selection==0) {
isToChangeLane=NO;
}
else
isToChangeLane=YES;
if (isToChangeLane==YES) {
self.laneChangeDest=[self getLaneChangeDest];
self.laneChangeSpeed=(self.laneChangeDir*(fabsf(self.laneChangeDest.x-self.position.x)/VEHICLELANECHANGESPEED));
// NSLog(@"is to change lane speed->%f",self.laneChangeSpeed);
}
else{
// NSLog(@"not to change lane");
self.laneChangeDest=ccp(0.0f, 0.0f);
self.laneChangeSpeed=0.0f;
}
[self changeTexture];
}
+(CCTexture2D*)obstacleTexture{
int indxCount=[[[TextureManager sharedTextureManager] vehicleTextureArray] count];
int targetIndx=arc4random()%indxCount;
return [[[TextureManager sharedTextureManager] vehicleTextureArray] objectAtIndex:targetIndx];
}
-(void)dealloc{
[super dealloc];
}
@end
<file_sep>//
// LoadingView.h
// ITIW
//
// Created by itiw on 1/4/11.
// Copyright 2011 ITIW. All rights reserved.
//
#import "cocos2d.h"
#import <UIKit/UIKit.h>
@protocol LoadingViewDelegate <NSObject>
- (void)loadingDidCanceled;
@end
@interface LoadingView : UIView
{
UILabel *loadingLabel;
UIActivityIndicatorView *activityIndicatorView;
id <LoadingViewDelegate> delegate;
}
@property (nonatomic, assign) id <LoadingViewDelegate> delegate;
+ (id)loadingViewInView:(UIView *)aSuperview;
- (void)loadElements;
- (void)removeView;
- (void)loadCrossButton;
@end<file_sep>//
// RVRAudioManager.h
// MotoRacer
//
// Created by <NAME> on 8/29/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import <AVFoundation/AVFoundation.h>
#import <AudioToolbox/AudioServices.h>
@class MixerHostAudio;
@interface RVRAudioManager : NSObject<AVAudioPlayerDelegate>{
@private
AVAudioPlayer *bgMusic;
AVAudioPlayer *bikeEngine;
AVAudioPlayer *buttonClicked;
SystemSoundID coinSound;
SystemSoundID firing,explosion;
BOOL soundsPreloaded;
}
+(id)sharedManager;
-(void)muteAll:(BOOL)isMute;
-(void)playBGMusic:(BOOL)play;
-(void)playBikeEngineSound:(BOOL)play;
-(void)playButtonClickSound:(BOOL)play;
-(void)playCoinCollectionSound;
-(void)playFiringSound;
-(void)playExplosionSound;
@property (nonatomic) BOOL mute;
@end
<file_sep>//
// PauseOrGameOverMenu.m
// MotoRacer
//
// Created by <NAME> on 8/5/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "PauseOrGameOverMenu.h"
#import "RVRAppDelegate.h"
#import "cocos2d.h"
#import "RVRPowerUpsAndPurchasesStore.h"
#import "GameNode.h"
#import "RVRAudioManager.h"
static PauseOrGameOverMenu *pauseOrGameOverMenu=nil;
@interface PauseOrGameOverMenu (Private)
-(void)initiateSelf;
-(void)initiatePauseMenu;
-(void)initiateGameOverMenu;
-(void)buttonClicked:(id)sender;
@end
@implementation PauseOrGameOverMenu
@synthesize delegate,reqSent;
enum{
nothingBtn=0,
resume=1,
revive=2,
playagain=3,
store=4,
mainmenu=5,
scoreLabel=6,
};
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
[self initiateSelf];
[self initiatePauseMenu];
[self initiateGameOverMenu];
}
return self;
}
+(id)menu{
if (pauseOrGameOverMenu==nil) {
pauseOrGameOverMenu=[[self alloc] init];
}
return pauseOrGameOverMenu;
}
-(void)pauseMenu{
pauseMenuView.hidden=NO;
[self.view bringSubviewToFront:pauseMenuView];
[appDelegate.viewController presentModalView:self withDelegate:nil selector:nil animated:YES];
}
-(void)gameOverMenu{
reqSent=NO;
gameOverMenuView.hidden=NO;
if (((GameNode*)appDelegate.gNode).gameOverCount>1) {
[gameOverMenuView viewWithTag:revive].hidden=YES;
}
else
[gameOverMenuView viewWithTag:revive].hidden=NO;
((UILabel*)[gameOverMenuView viewWithTag:scoreLabel]).text=[NSString stringWithFormat:@"Your Score: %0.2f",appDelegate.gNode.distanceInMiles];
[self.view bringSubviewToFront:gameOverMenuView];
[appDelegate.viewController presentModalView:self withDelegate:nil selector:nil animated:YES];
}
-(void)initiateSelf{
self.view.frame=self.modalHiddenFrame=CGRectMake(0.0f, -winSize.height, winSize.width, winSize.height);
self.modalPresentationFrame=CGRectMake(0.0f, 0.0f, winSize.width, winSize.height);
self.view.backgroundColor=[UIColor clearColor];
self.view.opaque=NO;
[appDelegate.viewController.view addSubview:self.view];
[appDelegate.viewController.view sendSubviewToBack:self.view];
}
-(void)initiatePauseMenu{
CGSize size;
float yShift;
float shift;
pauseMenuView=[[UIView alloc] initWithFrame:CGRectMake(0.0f, 0.0f, self.view.frame.size.width, self.view.frame.size.height)];
pauseMenuView.opaque=NO;
pauseMenuView.backgroundColor=[UIColor clearColor];
pauseMenuView.hidden=YES;
UIImageView *back=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"pause-menu-bg.png"]] autorelease];
back.backgroundColor=[UIColor clearColor];
back.opaque=NO;
back.frame=CGRectMake(winSize.width/8.0f, (pauseMenuView.frame.size.height-back.frame.size.height)/2.0f, back.frame.size.width, back.frame.size.height);
[pauseMenuView addSubview:back];
UIButton *resumeBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[resumeBtn setImage:[UIImage imageNamed:@"btn-resume.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
yShift=(back.frame.size.height-(size.height*3.0f))/4.0f;
resumeBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+yShift, size.width, size.height);
resumeBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[resumeBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
resumeBtn.tag=resume;
[pauseMenuView addSubview:resumeBtn];
UIButton *playAgainBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[playAgainBtn setImage:[UIImage imageNamed:@"btn-play-again.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
shift=(yShift*2)+resumeBtn.frame.size.height;
playAgainBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+shift, size.width, size.height);
playAgainBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[playAgainBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
playAgainBtn.tag=playagain;
[pauseMenuView addSubview:playAgainBtn];
UIButton *mainMenuBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[mainMenuBtn setImage:[UIImage imageNamed:@"btn-main-menu.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
shift=(yShift*3)+playAgainBtn.frame.size.height+resumeBtn.frame.size.height;
mainMenuBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+shift, size.width, size.height);
mainMenuBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[mainMenuBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
mainMenuBtn.tag=mainmenu;
[pauseMenuView addSubview:mainMenuBtn];
[self.view addSubview:pauseMenuView];
[self.view sendSubviewToBack:pauseMenuView];
}
-(void)initiateGameOverMenu{
CGSize size;
float yShift=90.0f;
float shift;
float difConst;
gameOverMenuView=[[UIView alloc] initWithFrame:CGRectMake(0.0f, 0.0f, self.view.frame.size.width, self.view.frame.size.height)];
gameOverMenuView.opaque=NO;
gameOverMenuView.backgroundColor=[UIColor clearColor];
gameOverMenuView.hidden=YES;
UIImageView *back=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"game-over-bg.png"]] autorelease];
back.backgroundColor=[UIColor clearColor];
back.opaque=NO;
back.frame=CGRectMake(winSize.width/8.0f, (gameOverMenuView.frame.size.height-back.frame.size.height)/2.0f, back.frame.size.width, back.frame.size.height);
[gameOverMenuView addSubview:back];
UIButton *reviveBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[reviveBtn setImage:[UIImage imageNamed:@"btn-revive.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
difConst=(back.frame.size.height-(yShift+(size.height*4.0f)))/5.0f;
shift=yShift+difConst;
reviveBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+shift-2.0f, size.width, size.height);
reviveBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[reviveBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
reviveBtn.tag=revive;
[gameOverMenuView addSubview:reviveBtn];
UIButton *playAgainBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[playAgainBtn setImage:[UIImage imageNamed:@"btn-play-again.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
shift+=reviveBtn.frame.size.height+difConst;
playAgainBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+shift, size.width, size.height);
playAgainBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[playAgainBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
playAgainBtn.tag=playagain;
[gameOverMenuView addSubview:playAgainBtn];
UIButton *storeBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[storeBtn setImage:[UIImage imageNamed:@"btn-store.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
shift+=playAgainBtn.frame.size.height+difConst;
storeBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+shift, size.width, size.height);
storeBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[storeBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
storeBtn.tag=store;
[gameOverMenuView addSubview:storeBtn];
UIButton *mainMenuBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[mainMenuBtn setImage:[UIImage imageNamed:@"btn-main-menu.png"] forState:UIControlStateNormal];
size=CGSizeMake(152.0f, 48.0f);
shift+=storeBtn.frame.size.height+difConst;
mainMenuBtn.frame=CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+shift, size.width, size.height);
mainMenuBtn.bounds=CGRectMake(0.0f, 0.0f, size.width, size.height);
[mainMenuBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
mainMenuBtn.tag=mainmenu;
[gameOverMenuView addSubview:mainMenuBtn];
UILabel *nowScored=[[[UILabel alloc] initWithFrame:CGRectMake(back.frame.origin.x+(back.frame.size.width-size.width)/2.0f, back.frame.origin.y+35.0f, size.width, size.height)] autorelease];
nowScored.text=@"";
nowScored.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:25];
nowScored.adjustsFontSizeToFitWidth = YES;
nowScored.textAlignment=UITextAlignmentCenter;
nowScored.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.0f alpha:1.0f];
nowScored.backgroundColor=[UIColor clearColor];
nowScored.opaque=NO;
nowScored.tag=scoreLabel;
[gameOverMenuView addSubview:nowScored];
[self.view addSubview:gameOverMenuView];
[self.view sendSubviewToBack:gameOverMenuView];
}
-(void)buttonClicked:(id)sender{
if (self.delegate!=nil) {
[[RVRAudioManager sharedManager] playButtonClickSound:YES];
if ([sender tag]==revive) {
if (appDelegate.databaseManager.userData.uCoins>=1000) {
btnClickedTag=[sender tag];
[self dismissModalViewAnimated:YES withDelegate:self selector:@selector(uiViewAnimaitonDidFinish:)];
}
else{
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle:@"Coin!" message:@"You do not have Enough Coins. Buy More Coins!" delegate:self cancelButtonTitle:@"OK" otherButtonTitles:NULL] autorelease];
[alert show];
}
}
else{
btnClickedTag=[sender tag];
[self dismissModalViewAnimated:YES withDelegate:self selector:@selector(uiViewAnimaitonDidFinish:)];
}
}
}
-(void)uiViewAnimaitonDidFinish:(id)sender{
if (btnClickedTag==resume) {
[self.delegate buttonClickedWithTag:resume];
}
else if (btnClickedTag==playagain) {
[self.delegate buttonClickedWithTag:playagain];
}
else if (btnClickedTag==mainmenu) {
[self.delegate buttonClickedWithTag:mainmenu];
}
else if(btnClickedTag==store){
reqSent=YES;
[appDelegate.viewController presentModalView:[RVRPowerUpsAndPurchasesStore powerUpsAndPurchaseStore] withDelegate:nil selector:nil animated:YES];
}
else if(btnClickedTag==revive){
[self.delegate buttonClickedWithTag:revive];
}
btnClickedTag=nothingBtn;
pauseMenuView.hidden=YES;
gameOverMenuView.hidden=YES;
}
-(void)dealloc{
[pauseOrGameOverMenu release];
[pauseMenuView release];
pauseMenuView=nil;
[gameOverMenuView release];
gameOverMenuView=nil;
[super dealloc];
}
@end
<file_sep>//
// GameCenterDelegate.m
// MotoRacer
//
// Created by <NAME> on 6/4/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "GameCenterDelegate.h"
#import "AppSpecificValues.h"
#import "RVRAppDelegate.h"
static GameCenterDelegate *sharedGameCenterDelegate;
@interface GameCenterDelegate (Private)
-(void)setHighScore;
-(void)showAlertWithTitle:(NSString*)title message:(NSString*)message;
-(void)sendControlToMainMenu:(id)sender;
@end
@implementation GameCenterDelegate
@synthesize gameCenterManager,currentScore,personalBest,cachedHighestScore,personalBestScoreDescription,personalBestScoreString,leaderboardHighScoreDescription,leaderboardHighScoreString,currentLeaderBoard,isGameCenterAvailable,isGameCenterAuthOnce,delegate;
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
self.currentLeaderBoard=kHighestDistance;
if([GameCenterManager isGameCenterAvailable]){
self.isGameCenterAvailable=YES;
self.gameCenterManager= [[[GameCenterManager alloc] init] autorelease];
[self.gameCenterManager setDelegate: self];
[self.gameCenterManager authenticateLocalUser];
}/*
else{
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle:@"Game Center Support Required!" message:@"The current device does not support Game Center." delegate: NULL cancelButtonTitle:@"OK" otherButtonTitles: NULL] autorelease];
[alert show];
}*/
}
return self;
}
+(id)sharedGameCenterDelegate{
if (sharedGameCenterDelegate==nil) {
sharedGameCenterDelegate=[[GameCenterDelegate alloc] init];
}
return sharedGameCenterDelegate;
}
-(void)submitHighScore{
if(self.currentScore > 0){
[self.gameCenterManager reportScore:self.currentScore forCategory:self.currentLeaderBoard];
}
}
- (void) showLeaderboard;
{
GKLeaderboardViewController *leaderboardController = [[GKLeaderboardViewController alloc] init];
if (leaderboardController != NULL)
{
leaderboardController.category = self.currentLeaderBoard;
leaderboardController.timeScope = GKLeaderboardTimeScopeAllTime;
leaderboardController.leaderboardDelegate = self;
[appDelegate.viewController presentModalViewController:leaderboardController animated:YES];
}
}
-(void)showAlertWithTitle:(NSString*)title message:(NSString*)message{
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle: title message: message
delegate: NULL cancelButtonTitle: @"OK" otherButtonTitles: NULL] autorelease];
[alert show];
}
-(void)sendControlToMainMenu:(id)sender{
[self.delegate getBackControl];
}
-(void)dealloc{
[sharedGameCenterDelegate release];
sharedGameCenterDelegate=nil;
[super dealloc];
}
#pragma MARK - GameCenterDelegateProtocol Methods Implementation
-(void)processGameCenterAuth:(NSError *)error{
if(error == NULL){
self.isGameCenterAuthOnce=YES;
//submit score
BOOL isSubmitted=[[NSUserDefaults standardUserDefaults] boolForKey:@"isGCScoreSubmitted"];
if (isSubmitted==NO) {
[self setCurrentScore:appDelegate.databaseManager.userData.myHighScore];
[self submitHighScore];
}
else
[self.gameCenterManager reloadHighScoresForCategory:self.currentLeaderBoard];
}/*
else{
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle: @"Game Center Account Required" message: [NSString stringWithFormat: @"Reason: %@", [error localizedDescription]] delegate: self cancelButtonTitle: @"Try Again..." otherButtonTitles: NULL] autorelease];
[alert show];
}*/
}
-(void)reloadScoresComplete:(GKLeaderboard *)leaderBoard error:(NSError *)error{
if(error == NULL){
personalBest= leaderBoard.localPlayerScore.value;
self.personalBestScoreDescription= @"Your Best:";
self.personalBestScoreString= [NSString stringWithFormat: @"%ld", personalBest];
if([leaderBoard.scores count] >0){
self.leaderboardHighScoreDescription= @"-";
self.leaderboardHighScoreString= @"";
GKScore* allTime= [leaderBoard.scores objectAtIndex: 0];
self.cachedHighestScore= allTime.formattedValue;
[gameCenterManager mapPlayerIDtoPlayer: allTime.playerID];
}
}
else{
self.personalBestScoreDescription= @"GameCenter Scores Unavailable";
self.personalBestScoreString= @"-";
self.leaderboardHighScoreDescription= @"GameCenter Scores Unavailable";
self.leaderboardHighScoreDescription= @"-";
//[self showAlertWithTitle: @"Score Reload Failed!" message: [NSString stringWithFormat: @"Reason: %@", [error localizedDescription]]];
}
}
- (void) mappedPlayerIDToPlayer: (GKPlayer*) player error: (NSError*) error{
if((error == NULL) && (player != NULL)){
self.leaderboardHighScoreDescription= [NSString stringWithFormat: @"%@ got:", player.alias];
if(self.cachedHighestScore != NULL){
self.leaderboardHighScoreString= self.cachedHighestScore;
}
else{
self.leaderboardHighScoreString= @"-";
}
}
else{
self.leaderboardHighScoreDescription= @"GameCenter Scores Unavailable";
self.leaderboardHighScoreDescription= @"-";
}
}
- (void) scoreReported: (NSError*) error;
{
if(error == NULL){
//NSLog(@"submitted...");
[[NSUserDefaults standardUserDefaults] setBool:YES forKey:@"isGCScoreSubmitted"];
[self.gameCenterManager reloadHighScoresForCategory:self.currentLeaderBoard];
//[self showAlertWithTitle:@"High Score Reported!" message:nil];
}
else{/*
[self showAlertWithTitle: @"Score Report Failed!"
message: [NSString stringWithFormat: @"Reason:%@",[error localizedDescription]]];*/
}
}
#pragma MARK - GKLeaderboardViewControllerDelegate Methods Implementation
- (void)leaderboardViewControllerDidFinish:(GKLeaderboardViewController *)viewController{
[viewController dismissModalViewControllerAnimated:YES];
[viewController release];
[self performSelector:@selector(sendControlToMainMenu:) withObject:nil afterDelay:0.5f];
}
@end
<file_sep>//
// DBPurchase.m
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "DBPurchase.h"
#import "RVRAppDelegate.h"
@implementation DBPurchase
@synthesize purchaseID,purchaseName,purchaseIcon,purchaseDetail,purchaseType,purchaseItem,purchaseCost,maxAmountPurchasable,activeNoOfPurchases,addedToWritableList;
-(id)initWithPrimaryKey:(NSInteger)Ppk database:(sqlite3 *)Pdb{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
sqlite3_stmt *init_statement = nil;
primaryKey = Ppk;
if (init_statement == nil) {
const char *sqlf = "SELECT * FROM purchase WHERE purchaseID=?";
if (sqlite3_prepare_v2(Pdb, sqlf, -1, &init_statement, NULL) != SQLITE_OK) {
NSLog(@"%s", sqlite3_errmsg(Pdb));
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Pdb));
}
}
sqlite3_bind_int(init_statement, 1, primaryKey);
if (sqlite3_step(init_statement) == SQLITE_ROW) {
self.purchaseID=primaryKey;
self.purchaseName=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 1)];
self.purchaseIcon=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 2)];
self.purchaseDetail=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 3)];
self.purchaseType=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 4)];
self.purchaseItem=sqlite3_column_int(init_statement,5);
self.purchaseCost=sqlite3_column_int(init_statement,6);
self.maxAmountPurchasable=sqlite3_column_int(init_statement,7);
self.activeNoOfPurchases=sqlite3_column_int(init_statement,8);
} else {
self.purchaseID=0;
self.purchaseName=@"nil";
self.purchaseIcon=@"nil";
self.purchaseDetail=@"nil";
self.purchaseType=@"nil";
self.purchaseItem=0;
self.purchaseCost=0;
self.maxAmountPurchasable=0;
self.activeNoOfPurchases=0;
}
sqlite3_reset(init_statement);
sqlite3_finalize(init_statement);
init_statement=nil;
}
return self;
}
-(int)insertIntoDatabase:(sqlite3 *)Pdb{
sqlite3_stmt *insert_statement = nil;
if (insert_statement == nil) {
static char *sql = "INSERT INTO purchase(purchaseName,purchaseIcon,purchaseDetail,purchaseType,purchaseItem,purchaseCost,maxAmountPurchasable,activeNoOfPurchases) VALUES(?,?,?,?,?,?,?,?)";
if (sqlite3_prepare_v2(Pdb, sql, -1, &insert_statement, NULL) != SQLITE_OK) {
NSLog(@"Insert");
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Pdb));
}
}
sqlite3_bind_text(insert_statement, 1, [purchaseName UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 2, [purchaseIcon UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 3, [purchaseDetail UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 4, [purchaseType UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(insert_statement,5, purchaseItem);
sqlite3_bind_int(insert_statement,6, purchaseCost);
sqlite3_bind_int(insert_statement,7, maxAmountPurchasable);
sqlite3_bind_int(insert_statement,8, activeNoOfPurchases);
int success = sqlite3_step(insert_statement);
sqlite3_reset(insert_statement);
sqlite3_finalize(insert_statement);
insert_statement=nil;
if (success == SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(Pdb));
primaryKey = 0;
} else {
NSLog(@"Inserted upgrade Successfully...");
purchaseID=primaryKey=(NSInteger)sqlite3_last_insert_rowid(Pdb);
}
return primaryKey;
}
-(BOOL)updateDatabase:(NSInteger)Ppk database:(sqlite3 *)Pdb{
primaryKey = Ppk;
sqlite3_stmt *update_statement = nil;
if (update_statement == nil) {
static char *sqlu = "UPDATE purchase SET purchaseName=?,purchaseIcon=?,purchaseDetail=?,purchaseType=?,purchaseItem=?,purchaseCost=?,maxAmountPurchasable=?,activeNoOfPurchases=? WHERE purchaseID=?";
if (sqlite3_prepare_v2(Pdb, sqlu, -1, &update_statement, NULL) != SQLITE_OK) {
NSLog(@"Update");
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Pdb));
}
}
sqlite3_bind_text(update_statement, 1, [purchaseName UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 2, [purchaseIcon UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 3, [purchaseDetail UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 4, [purchaseType UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(update_statement, 5, purchaseItem);
sqlite3_bind_int(update_statement, 6, purchaseCost);
sqlite3_bind_int(update_statement, 7, maxAmountPurchasable);
sqlite3_bind_int(update_statement, 8, activeNoOfPurchases);
sqlite3_bind_int(update_statement, 9, primaryKey);
int success = sqlite3_step(update_statement);
if(success==SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(Pdb));
} else {
NSLog(@"Updated upgrade Successfully...");
}
sqlite3_reset(update_statement);
sqlite3_finalize(update_statement);
update_statement=nil;
return success;
}
-(BOOL)deleteDatabase:(NSInteger)Ppk database:(sqlite3 *)Pdb{
primaryKey = Ppk;
sqlite3_stmt *delete_statement = nil;
if (delete_statement == nil) {
static char *sqlu = "DELETE FROM purchase WHERE purchaseID=?";
if (sqlite3_prepare_v2(Pdb, sqlu, -1, &delete_statement, NULL) != SQLITE_OK) {
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Pdb));
}
}
sqlite3_bind_int(delete_statement, 1, primaryKey);
int success = sqlite3_step(delete_statement);
if(success==SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(Pdb));
} else {
NSLog(@"Deleted Successfully...");
}
sqlite3_reset(delete_statement);
sqlite3_finalize(delete_statement);
delete_statement=nil;
return success;
}
-(BOOL)updateDatabaseForPurchase{
if (self.purchaseCost<=appDelegate.databaseManager.userData.uCoins) {
appDelegate.databaseManager.userData.uCoins-=self.purchaseCost;
//[appDelegate.databaseManager.userData updateDatabase:appDelegate.databaseManager.userData.uID database:appDelegate.databaseManager.database];
self.activeNoOfPurchases+=1;
//[self updateDatabase:self.purchaseID database:appDelegate.databaseManager.database];
if (self.addedToWritableList==NO) {
self.addedToWritableList=YES;
[appDelegate.databaseManager.dataObjectsToBeWritten addObject:self];
}
NSLog(@"object count--->%d",[appDelegate.databaseManager.dataObjectsToBeWritten count]);
return YES;
}
return NO;
}
-(void)dealloc{
[self.purchaseName release];
self.purchaseName=nil;
[self.purchaseIcon release];
self.purchaseIcon=nil;
[self.purchaseDetail release];
self.purchaseDetail=nil;
[self.purchaseType release];
self.purchaseType=nil;
[super dealloc];
}
@end<file_sep>//
// RVRCoin.m
// MotoRacer
//
// Created by <NAME> on 6/25/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import "RVRCoin.h"
#import "TextureManager.h"
#import "GameNode.h"
@interface RVRCoin (Private)
+(CCTexture2D*)myTexture;
-(void)setAliasTexParametersForMe;
-(void)addRotationActionFor:(CCSprite*)target;
-(CCFiniteTimeAction*)getCollectionAction;
-(CCFiniteTimeAction*)getMagnetInducedCollectionAction;
-(void)hideAliasCoin:(CCSprite*)coin;
-(void)createAliasCoin;
@end
@implementation RVRCoin
@synthesize aliasCoin,multiplier;
-(id)initWithTexture:(CCTexture2D *)texture{
self=[super initWithTexture:texture];
if (self) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
self.anchorPoint=ccp(0.5f, 1.0f);
[self addRotationActionFor:self];
[self createAliasCoin];
}
return self;
}
+(id)generateCoin{
return [[[self alloc] initWithTexture:[RVRCoin myTexture]] autorelease];
}
+(id)generateCoinWithAliasTexParameters{
RVRCoin *coin=[[[self alloc] initWithTexture:[RVRCoin myTexture]] autorelease];
[coin setAliasTexParametersForMe];
return coin;
}
-(void)setAliasTexParametersForMe{
[[self texture] setAliasTexParameters];
}
+(CCTexture2D*)myTexture{
return [((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"biker-point"];
}
-(void)setDoubleValueTexture{
[self setTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"biker-point-double"]];
[self setAliasTexParametersForMe];
self.multiplier=2;
[aliasCoin setTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"biker-point-double"]];
}
-(void)resetToOriginalTexture{
[self setTexture:[RVRCoin myTexture]];
[self setAliasTexParametersForMe];
self.multiplier=1;
[aliasCoin setTexture:[RVRCoin myTexture]];
}
-(void)flipCoin:(CCSprite*)target{
if (target.flipX==YES) {
target.flipX=NO;
}
else
target.flipX=YES;
}
-(void)addRotationActionFor:(CCSprite*)target{
CCScaleTo *scaleTo=[CCScaleTo actionWithDuration:0.5f scaleX:0.1f scaleY:1.0];
CCCallFuncO *calFlipX=[CCCallFuncO actionWithTarget:self selector:@selector(flipCoin:) object:target];
CCScaleTo *scaleBack=[CCScaleTo actionWithDuration:0.5f scaleX:1.0f scaleY:1.0];
CCSequence *sequence=[CCSequence actions:scaleTo,calFlipX,scaleBack, nil];
CCRepeatForever *repeatForever=[CCRepeatForever actionWithAction:sequence];
[[CCActionManager sharedManager] addAction:repeatForever target:target paused:YES];
}
-(void)createAliasCoin{
aliasCoin=[CCSprite spriteWithTexture:[self texture]];
aliasCoin.visible=NO;
[appDelegate.gNode addChild:aliasCoin];
}
-(CCFiniteTimeAction*)getCollectionAction{
ccBezierConfig myBezierConfig;
myBezierConfig.controlPoint_1=CGPointMake(self.position.x+50, self.position.y);
myBezierConfig.controlPoint_2=CGPointMake(self.position.x, self.position.y+50);
myBezierConfig.endPosition=CGPointMake(winSize.width, winSize.height);
CCBezierTo *myBezierPath=[CCBezierTo actionWithDuration:1.0f bezier:myBezierConfig];
return myBezierPath;
}
-(void)hideAliasCoin:(CCSprite *)coin{
[[CCActionManager sharedManager] removeAllActionsFromTarget:coin];
aliasCoin.visible=NO;
[appDelegate.gNode.collectedBikerPoints removeObject:self];
// NSLog(@"collected count--->%d",[appDelegate.gNode.collectedBikerPoints count]);
}
-(void)performCoinCollectionAndAnimation{
aliasCoin.position=self.position;
aliasCoin.visible=YES;
[self addRotationActionFor:aliasCoin];
[aliasCoin resumeSchedulerAndActions];
self.visible=NO;
[self resetToMyOriginalPos];
CCCallFuncO *hideAliasCoin=[CCCallFuncO actionWithTarget:self selector:@selector(hideAliasCoin:) object:aliasCoin];
CCSequence *sequence=[CCSequence actions:[self getCollectionAction], hideAliasCoin, nil];
[aliasCoin runAction:sequence];
}
-(CCFiniteTimeAction*)getMagnetInducedCollectionAction{
GameNode *gameNode=appDelegate.gNode;
float xShift=gameNode.bikeSprite.contentSize.height*sinf((PI/180.0f)*gameNode.bikeSprite.rotation);
float yShift=gameNode.bikeSprite.contentSize.height*cosf((PI/180.0f)*gameNode.bikeSprite.rotation);
CGPoint destPoint=CGPointMake(gameNode.bikeSprite.position.x+xShift, gameNode.bikeSprite.position.y+yShift);
float actionDuration=0.5f;///winSize.height)*ccpDistance(destPoint, aliasCoin.position);
float vTargetYShift=(gameNode.bSpeed*actionDuration);
if (gameNode.isBikeAccelerating>0)
vTargetYShift+=(0.5f*ACCELERATION*pow(actionDuration, 2));
else if(gameNode.isBikeAccelerating<0)
vTargetYShift+=(0.5f*RETARDATION*pow(actionDuration, 2));
float controlP2XShift=(gameNode.bikeSprite.position.x-aliasCoin.position.x)*(30.0f/145.0f);//145->half of max xShift!
float controlP2YShift=fabsf(controlP2XShift);
ccBezierConfig myBezierConfig;
myBezierConfig.controlPoint_1=CGPointMake(aliasCoin.position.x, aliasCoin.position.y-(controlP2YShift/2.0f));
myBezierConfig.controlPoint_2=CGPointMake(aliasCoin.position.x-controlP2XShift, aliasCoin.position.y-controlP2YShift);
myBezierConfig.endPosition=CGPointMake(destPoint.x, destPoint.y+vTargetYShift);
CCBezierTo *myBezierPath=[CCBezierTo actionWithDuration:actionDuration bezier:myBezierConfig];
return myBezierPath;
}
-(void)performMagnetInducedCollectionAnimation{
aliasCoin.position=self.position;
aliasCoin.visible=YES;
//[self addRotationActionFor:aliasCoin];
//[aliasCoin resumeSchedulerAndActions];
self.visible=NO;
[self resetToMyOriginalPos];
CCCallFuncO *hideAliasCoin=[CCCallFuncO actionWithTarget:self selector:@selector(hideAliasCoin:) object:aliasCoin];
CCSequence *sequence=[CCSequence actions:[self getMagnetInducedCollectionAction], hideAliasCoin, nil];
[aliasCoin runAction:sequence];
}
-(void)setMyOriginalPosition{
if (!isMyOriginalPosSet) {
isMyOriginalPosSet=YES;
myOriginalPostion=self.position;
}
}
-(BOOL)resetToMyOriginalPos{
if (isMyOriginalPosSet) {
self.position=myOriginalPostion;
return YES;
}
else
return NO;
}
-(void)dealloc{
[super dealloc];
}
@end
<file_sep>//
// GamePlayObserverDelegateProtocol.h
// MotoRacer
//
// Created by <NAME> on 7/27/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
@protocol GamePlayObserverDelegate <NSObject>
@optional
-(void)gamePlayStarted;
-(void)gamePlayStoped;
-(void)distanceCoveredFor:(int)tag;
-(void)newCollectablePowerUpAppearanceRequest;
-(void)collectablePowerUpCollected:(NSString*)key;
-(void)speedyStarterUsedWithTag:(NSString*)tag;
@end
@protocol ControllerMenu <NSObject>
@required
-(void)getBackControl;
@end
<file_sep>//
// GameMenu.m
// MotoRacer
//
// Created by <NAME> on 6/6/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import "GameMenu.h"
#import "TextureManager.h"
#import "GameNode.h"
#import "PauseOrGameOverMenu.h"
#import "GameCenterDelegate.h"
#import "RVRInAppPurchaseStore.h"
#import "RVRAudioManager.h"
enum{
nothing,
ride,
store,
customize,
highScore,
gameCenter,
audioButton,
};
@interface GameMenu (Private)
-(void)setUpBackground;
-(void)createButtons;
-(void)setAudioButton;
-(void)addGlowBackForBtn:(CCSprite*)btn;
-(void)moveButtonsIntoScreen:(NSNumber*)indx;
-(void)moveButtonsOutofScreen:(NSNumber *)indx;
-(void)buttonsMovedOutOfScreen:(id)sender;
-(BOOL)menuButtonClicked:(id)sender btn:(CCSprite*)btn;
-(void)showHideGlowBtnAtIndx:(NSArray*)array;
-(void)loadAllPreloadableViews;
-(void)makeMenuInteractable:(NSNumber*)isInteractable;
-(void)updateDataForLastGamePlay;
@end
@implementation GameMenu
+(CCScene *) scene
{
// 'scene' is an autorelease object.
CCScene *scene = [CCScene node];
// 'layer' is an autorelease object.
GameMenu *gameMenu = [GameMenu node];
//appDelegate.gMenu=gameMenu;
// add layer as a child to scene
[scene addChild: gameMenu];
// return the scene
return scene;
}
-(id)init{
if (self=[super init]) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
self.isTouchEnabled=YES;
btnGlows=[[NSMutableArray alloc] init];
menuButtons=[[NSMutableArray alloc] init];
[self setUpBackground];
[self setAudioButton];
[self createButtons];
[self loadAllPreloadableViews];
gcDelegate=[GameCenterDelegate sharedGameCenterDelegate];
[gcDelegate setDelegate:self];
if (appDelegate.databaseManager.highScoreChanged==YES) {
appDelegate.databaseManager.highScoreChanged=NO;
[self updateDataForLastGamePlay];
}
[self performSelector:@selector(moveButtonsIntoScreen:) withObject:[NSNumber numberWithInt:1] afterDelay:0.50f];
[[RVRAudioManager sharedManager] playBGMusic:YES];
}
return self;
}
-(void)updateDataForLastGamePlay{
BOOL isSubmitted=[[NSUserDefaults standardUserDefaults] boolForKey:@"isGCScoreSubmitted"];
if (isSubmitted==NO && gcDelegate.isGameCenterAuthOnce==YES) {
[gcDelegate setCurrentScore:appDelegate.databaseManager.userData.myHighScore];
[gcDelegate submitHighScore];
}
}
-(void)setUpBackground{
CCSprite *menuBack=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"mainMenuBackTexture"]];
menuBack.position=ccp(winSize.width/2.0f, winSize.height/2.0f);
[self addChild:menuBack];
}
-(void)setAudioButton{
BOOL isMuted=[[NSUserDefaults standardUserDefaults] boolForKey:@"soundState"];
if (isMuted==YES){
if (audioBtn==nil)
audioBtn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"music-off"]];
else if(audioBtn!=nil)
[audioBtn setTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"music-off"]];
}
else if(isMuted==NO){
if (audioBtn==nil)
audioBtn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"music-on"]];
else if(audioBtn!=nil)
[audioBtn setTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"music-on"]];
}
if (audioBtn!=nil && audioBtnAdded==NO) {
audioBtnAdded=YES;
audioBtn.anchorPoint=ccp(0.5f, 0.5f);
audioBtn.position=ccp(winSize.width-(audioBtn.contentSize.width/2.0f)-5.0f, winSize.height-(audioBtn.contentSize.height/2.0f)-5.0f);
audioBtn.tag=audioButton;
[self addChild:audioBtn];
[menuButtons addObject:audioBtn];
}
}
-(void)makeMenuInteractable:(NSNumber*)isInteractable{
BOOL value=[isInteractable boolValue];
self.isTouchEnabled=value;
}
-(void)addGlowBackForBtn:(CCSprite *)btn{
CCSprite *btnGlow=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"storeBTNglow"]];
btnGlow.anchorPoint=ccp(0.0f, 0.5f);
btnGlow.position=ccp(btn.position.x-btn.contentSize.width, btn.position.y);
btnGlow.visible=NO;
[self addChild:btnGlow];
[btnGlows addObject:btnGlow];
}
-(void)createButtons{
CGPoint prevPos;
CCSprite *btn;
float startOffset=(winSize.height/3.0f)*2.0f;
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"rideBTNTexture"]];
float yOffsetFromTop=((winSize.height/3.0f)-btn.contentSize.height*NOOFBTNS)/(NOOFBTNS+1);
//btn.anchorPoint=ccp(0.0f, 0.5f);
//btn.position=ccp(winSize.width, winSize.height-yOffsetFromTop-(btn.contentSize.height/2));
btn.anchorPoint=ccp(0.5f, 0.5f);
btn.position=ccp(winSize.width+(btn.contentSize.width/2.0f), winSize.height-yOffsetFromTop-(btn.contentSize.height/2.0f)-startOffset);
prevPos=btn.position;
//[self addGlowBackForBtn:btn];
[self addChild:btn];
btn.tag=ride;
[menuButtons addObject:btn];
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"storeBTNTexture"]];
//btn.anchorPoint=ccp(0.0f, 0.5f);
//btn.position=ccp(winSize.width, prevPos.y-yOffsetFromTop-btn.contentSize.height);
btn.anchorPoint=ccp(0.5f, 0.5f);
btn.position=ccp(-(btn.contentSize.width/2.0f), prevPos.y-yOffsetFromTop-btn.contentSize.height);
prevPos=btn.position;
//[self addGlowBackForBtn:btn];
[self addChild:btn];
btn.tag=store;
[menuButtons addObject:btn];
/*
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"customizeBTNTexture"]];
//btn.anchorPoint=ccp(0.0f, 0.5f);
//btn.position=ccp(winSize.width, prevPos.y-yOffsetFromTop-btn.contentSize.height);
btn.anchorPoint=ccp(0.5f, 0.5f);
btn.position=ccp(winSize.width+(btn.contentSize.width/2.0f), prevPos.y-yOffsetFromTop-btn.contentSize.height);
prevPos=btn.position;
//[self addGlowBackForBtn:btn];
[self addChild:btn];
btn.tag=customize;
[menuButtons addObject:btn];
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"highScoreBTNTexture"]];
//btn.anchorPoint=ccp(0.0f, 0.5f);
//btn.position=ccp(winSize.width, prevPos.y-yOffsetFromTop-btn.contentSize.height);
btn.anchorPoint=ccp(0.5f, 0.5f);
btn.position=ccp(-(btn.contentSize.width/2.0f), prevPos.y-yOffsetFromTop-btn.contentSize.height);
prevPos=btn.position;
//[self addGlowBackForBtn:btn];
[self addChild:btn];
btn.tag=highScore;
[menuButtons addObject:btn];
*/
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"gcBTNTexture"]];
//btn.anchorPoint=ccp(0.0f, 0.5f);
//btn.position=ccp(winSize.width, prevPos.y-yOffsetFromTop-btn.contentSize.height);
btn.anchorPoint=ccp(0.5f, 0.5f);
btn.position=ccp(winSize.width+(btn.contentSize.width/2.0f), prevPos.y-yOffsetFromTop-btn.contentSize.height);
//[self addGlowBackForBtn:btn];
[self addChild:btn];
btn.tag=gameCenter;
[menuButtons addObject:btn];
}
-(void)loadAllPreloadableViews{
//loading store...
RVRPowerUpsAndPurchasesStore *store=[RVRPowerUpsAndPurchasesStore powerUpsAndPurchaseStore];
[store setControllerMenu:self];
//loading PauseOrGameOverMenu...
[PauseOrGameOverMenu menu];
//loading InAppPurchase store...
[RVRInAppPurchaseStore inAppStore];
}
-(void)moveButtonsIntoScreen:(NSNumber *)indx{
CCSequence *sequence=nil;
CCCallFuncO *nextCall=nil;
int index=[indx intValue];
CCSprite *btn=[menuButtons objectAtIndex:index];
CCMoveTo *moveTo;
//moveTo=[CCMoveTo actionWithDuration:0.1f position:CGPointMake(btn.position.x-btn.contentSize.width, btn.position.y)];
moveTo=[CCMoveTo actionWithDuration:0.1f position:CGPointMake(winSize.width/2.0f, btn.position.y)];
indx=[NSNumber numberWithInt:++index];
if ([indx intValue]<[menuButtons count])
nextCall=[CCCallFuncO actionWithTarget:self selector:@selector(moveButtonsIntoScreen:) object:indx];
else
nextCall=[CCCallFuncO actionWithTarget:self selector:@selector(makeMenuInteractable:) object:[NSNumber numberWithBool:YES]];
sequence=[CCSequence actions:moveTo, nextCall, nil];
[btn runAction:sequence];
}
-(void)uiViewAnimaitonDidFinish{
NSLog(@"stopped");
}
-(void)moveButtonsOutofScreen:(NSNumber *)indx{
CCSequence *sequence=nil;
CCCallFuncO *nextCall=nil;
int index=[indx intValue];
CCSprite *btn=[menuButtons objectAtIndex:index];
CCMoveTo *moveTo;
//moveTo=[CCMoveTo actionWithDuration:0.1f position:CGPointMake(winSize.width, btn.position.y)];
if ([indx intValue]%2==1) {
moveTo=[CCMoveTo actionWithDuration:0.1f position:CGPointMake(winSize.width+(btn.contentSize.width/2.0f), btn.position.y)];
}
else{
moveTo=[CCMoveTo actionWithDuration:0.1f position:CGPointMake(-(btn.contentSize.width/2.0f), btn.position.y)];
}
indx=[NSNumber numberWithInt:--index];
if ([indx intValue]>0)
nextCall=[CCCallFuncO actionWithTarget:self selector:@selector(moveButtonsOutofScreen:) object:indx];
else
nextCall=[CCCallFuncO actionWithTarget:self selector:@selector(buttonsMovedOutOfScreen:) object:self];
sequence=[CCSequence actions:moveTo, nextCall, nil];
[btn runAction:sequence];
}
-(void)buttonsMovedOutOfScreen:(id)sender{
[self menuButtonClicked:self btn:clickedBtnRefKeeper];
clickedBtnRefKeeper=nil;
}
-(BOOL)menuButtonClicked:(id)sender btn:(CCSprite*)btn{
if (btn.tag==ride) {
[[RVRAudioManager sharedManager] playBGMusic:NO];
[[CCDirector sharedDirector] replaceScene:[CCTransitionFade transitionWithDuration:1.0f scene:[GameNode scene]]];
return YES;
}
else if(btn.tag==store){
[appDelegate.viewController presentModalView:[RVRPowerUpsAndPurchasesStore powerUpsAndPurchaseStore] withDelegate:nil selector:nil animated:YES];
return YES;
}
else if(btn.tag==gameCenter){
if (gcDelegate.isGameCenterAvailable==YES) {
//[gcDelegate.gameCenterManager authenticateLocalUser];
[gcDelegate showLeaderboard];
}
}
else if(btn.tag==audioButton){
BOOL isMuted=[[NSUserDefaults standardUserDefaults] boolForKey:@"soundState"];
if (isMuted==YES) {
[[NSUserDefaults standardUserDefaults] setBool:NO forKey:@"soundState"];
}
else if(isMuted==NO){
[[NSUserDefaults standardUserDefaults] setBool:YES forKey:@"soundState"];
}
[self setAudioButton];
[[RVRAudioManager sharedManager] muteAll:!isMuted];
}
return NO;
}
-(void)showHideGlowBtnAtIndx:(NSArray *)array{
NSNumber *indx=[array objectAtIndex:0];
NSNumber *show=[array objectAtIndex:1];
((CCSprite*)[btnGlows objectAtIndex:[indx intValue]]).visible=[show boolValue];
}
-(void)ccTouchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
location = [[CCDirector sharedDirector] convertToGL: location];
for (CCSprite *btn in menuButtons) {
if (CGRectContainsPoint([btn boundingBox], location)) {
//CCScaleTo *YScale=[CCScaleTo actionWithDuration:0.000f scaleX:1.0f scaleY:1.35f];
CCScaleTo *YScale=[CCScaleTo actionWithDuration:0.000f scaleX:1.0f scaleY:1.15f];
//CCCallFuncO *showHideGlow=[CCCallFuncO actionWithTarget:self selector:@selector(showHideGlowBtnAtIndx:) object:[NSArray arrayWithObjects:[NSNumber numberWithInt:[menuButtons indexOfObject:btn]], [NSNumber numberWithBool:YES], nil]];
CCSequence *sequence=[CCSequence actions:YScale, /*showHideGlow,*/ nil];
[btn runAction:sequence];
clickedButtonTag=btn.tag;
}
}
}
-(void)ccTouchesEnded:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
location = [[CCDirector sharedDirector] convertToGL: location];
for (CCSprite *btn in menuButtons) {
CCScaleTo *YScale=nil;
//CCCallFuncO *showHideGlow=nil;
CCCallFuncO *buttonsHideCall=nil,*touchDisableCall=nil;
CCSequence *sequence=nil;
BOOL isSeqSeq=NO;
if(btn.scaleY!=1.0f){
YScale=[CCScaleTo actionWithDuration:0.000f scaleX:1.0f scaleY:1.0f];
//showHideGlow=[CCCallFuncO actionWithTarget:self selector:@selector(showHideGlowBtnAtIndx:) object:[NSArray arrayWithObjects:[NSNumber numberWithInt:[menuButtons indexOfObject:btn]], [NSNumber numberWithBool:NO], nil]];
}
if (CGRectContainsPoint([btn boundingBox], location) && btn.tag==clickedButtonTag) {
clickedBtnRefKeeper=btn;
if (btn.tag==ride || btn.tag==store || btn.tag==gameCenter){
[[RVRAudioManager sharedManager] playButtonClickSound:YES];
isSeqSeq=YES;
NSNumber *maxIndx=[NSNumber numberWithInt:[menuButtons count]-1];
buttonsHideCall=[CCCallFuncO actionWithTarget:self selector:@selector(moveButtonsOutofScreen:) object:maxIndx];
//[self makeMenuInteractable:[NSNumber numberWithBool:NO]];
touchDisableCall=[CCCallFuncO actionWithTarget:self selector:@selector(makeMenuInteractable:) object:[NSNumber numberWithBool:NO]];
}
else if(btn.tag==audioButton){
[[RVRAudioManager sharedManager] playButtonClickSound:YES];
[self menuButtonClicked:self btn:clickedBtnRefKeeper];
clickedBtnRefKeeper=nil;
}
}
if (isSeqSeq==YES) {
if (YScale==nil) {
sequence=[CCSequence actions:touchDisableCall, buttonsHideCall, nil];
}
else
sequence=[CCSequence actions:touchDisableCall, YScale, buttonsHideCall, nil];
}
else
sequence=[CCSequence actions:YScale, /*showHideGlow,*/ buttonsHideCall, nil];
if (sequence!=nil) {
[btn runAction:sequence];
break;
}
}
clickedButtonTag=nothing;
}
-(void)ccTouchesMoved:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
location = [[CCDirector sharedDirector] convertToGL: location];
for (CCSprite *btn in menuButtons) {
if (CGRectContainsPoint([btn boundingBox], location)) {
//CCScaleTo *YScale=[CCScaleTo actionWithDuration:0.000f scaleX:1.0f scaleY:1.35f];
CCScaleTo *YScale=[CCScaleTo actionWithDuration:0.000f scaleX:1.0f scaleY:1.15f];
//CCCallFuncO *showHideGlow=[CCCallFuncO actionWithTarget:self selector:@selector(showHideGlowBtnAtIndx:) object:[NSArray arrayWithObjects:[NSNumber numberWithInt:[menuButtons indexOfObject:btn]], [NSNumber numberWithBool:YES], nil]];
CCSequence *sequence=[CCSequence actions:YScale, /*showHideGlow,*/ nil];
[btn runAction:sequence];
}
else if(btn.scaleY!=1.0f){
CCScaleTo *YScale=[CCScaleTo actionWithDuration:0.000f scaleX:1.0f scaleY:1.0f];
//CCCallFuncO *showHideGlow=[CCCallFuncO actionWithTarget:self selector:@selector(showHideGlowBtnAtIndx:) object:[NSArray arrayWithObjects:[NSNumber numberWithInt:[menuButtons indexOfObject:btn]], [NSNumber numberWithBool:NO], nil]];
CCSequence *sequence=[CCSequence actions:YScale, /*showHideGlow,*/ nil];
[btn runAction:sequence];
}
}
}
-(void)dealloc{
[menuButtons removeAllObjects];
menuButtons=nil;
[btnGlows removeAllObjects];
btnGlows=nil;
for (CCNode *child in self.children) {
[[CCActionManager sharedManager] removeAllActionsFromTarget:child];
}
[super dealloc];
}
#pragma MARK - Delegate Methods
-(void)getBackControl{
[self moveButtonsIntoScreen:[NSNumber numberWithInt:1]];
}
@end<file_sep>//
// RVRDataBaseManager.m
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "RVRDataBaseManager.h"
@interface RVRDataBaseManager (Private)
-(BOOL)createEditableCopyOfDatabaseIfNeeded;
-(void)createConnectionToDataBase;
-(void)updateDBVersion;
#pragma MARK - userData
-(DBUserData*)initializeUserData;
#pragma MARK - Store
-(void)loadPowerUpUpgrades;
-(void)loadSinglePurchases;
@end
@implementation RVRDataBaseManager
@synthesize database,userData,powerUpUpgrades,singlePurchases,PUAndPstoreTitles,dataObjectsToBeWritten,highScoreChanged;
-(id)init{
if ((self=[super init])) {
if ([self createEditableCopyOfDatabaseIfNeeded]) {
PUAndPstoreTitles=[[NSMutableArray alloc] init];
dataObjectsToBeWritten=[[NSMutableArray alloc] init];
[self createConnectionToDataBase];
self.userData=[self initializeUserData];
[self loadPowerUpUpgrades];
[self loadSinglePurchases];
}
}
return self;
}
-(BOOL)createEditableCopyOfDatabaseIfNeeded {
// First, test for existence.
BOOL success;
NSFileManager *fileManager = [NSFileManager defaultManager];
NSError *error;
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString *writableDBPath = [documentsDirectory stringByAppendingPathComponent:@"scores.sqlite"];
success = [fileManager fileExistsAtPath:writableDBPath];
if (success) return success;
// The writable database does not exist, so copy the default to the appropriate location.
NSString *defaultDBPath = [[[NSBundle mainBundle] resourcePath] stringByAppendingPathComponent:@"scores.sqlite"];
success = [fileManager copyItemAtPath:defaultDBPath toPath:writableDBPath error:&error];
if (!success) {
NSAssert1(0, @"Failed to create writable database file with message '%@'.", [error localizedDescription]);
}
return success;
}
-(void)createConnectionToDataBase{
// The database is stored in the application bundle.
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString *path = [documentsDirectory stringByAppendingPathComponent:@"scores.sqlite"];
// Open the database
if (sqlite3_open([path UTF8String], &database) == SQLITE_OK) {
NSString *versionString = [[NSBundle mainBundle] objectForInfoDictionaryKey:(NSString*)kCFBundleVersionKey];
currentAppVersion = [versionString floatValue];
NSLog(@"CurrentVersion: %f LastUpdatedVersion: %f", currentAppVersion, lastDBUpdatedVersion);
if(currentAppVersion>lastDBUpdatedVersion)
[self updateDBVersion];
/*
[self loadScoresFromDB];
[self Multiplayer_Table];
// [Goal_Node create_table];
*/
} else {
// Even though the open failed, call close to properly clean up resources.
sqlite3_close(database);
NSAssert1(0, @"Failed to open database with message '%s'.", sqlite3_errmsg(database));
// Additional error handling, as appropriate...
}
}
-(void)updateDBVersion {
NSNumber *updatedDBVersion = [NSNumber numberWithFloat:currentAppVersion];
[[NSUserDefaults standardUserDefaults] setObject:updatedDBVersion forKey:@"lastDBUpdatedVersion"];
[[NSUserDefaults standardUserDefaults] synchronize];
}
-(DBUserData*)initializeUserData{
NSString *deviceUDID = [[UIDevice currentDevice] uniqueIdentifier];
NSString *str_user = [NSString stringWithFormat:@"SELECT uID FROM userData WHERE UDID='%@'", deviceUDID];
const char *sql_user = [str_user cStringUsingEncoding:NSUTF8StringEncoding];
sqlite3_stmt *statement_user=nil;
DBUserData *myUserData=nil;
if (sqlite3_prepare_v2(self.database, sql_user, -1, &statement_user, NULL) == SQLITE_OK) {
while (sqlite3_step(statement_user) == SQLITE_ROW) {
int primaryKeyUser = sqlite3_column_int(statement_user, 0);
myUserData = [[DBUserData alloc] initWithPrimaryKey:primaryKeyUser database:self.database];
}
sqlite3_reset(statement_user);
sqlite3_finalize(statement_user);
statement_user=nil;
}
//create New User Data
if(myUserData==nil) {
myUserData=[[DBUserData alloc] init];
myUserData.UDID=deviceUDID;
myUserData.uName=@"<NAME>";
myUserData.uCoins =0;
myUserData.myHighScore=0;
myUserData.c2=@"nil";
myUserData.c3=@"nil";
[myUserData insertIntoDatabase:self.database];
// [[NSUserDefaults standardUserDefaults] setBool:YES forKey:@"isMusicOn"];
}
return myUserData;
}
-(void)loadPowerUpUpgrades{
self.powerUpUpgrades=[[NSMutableArray alloc] init];
NSString *str_fishes=@"SELECT upgradeID FROM upgrades";
const char *sql_fishes = [str_fishes cStringUsingEncoding:NSUTF8StringEncoding];
sqlite3_stmt *statement_upgrades=nil;
if (sqlite3_prepare_v2(self.database, sql_fishes, -1, &statement_upgrades, NULL) == SQLITE_OK) {
while (sqlite3_step(statement_upgrades) == SQLITE_ROW) {
int primaryKeyUpgrade = sqlite3_column_int(statement_upgrades, 0);
DBUpgrades *upgrade = [[DBUpgrades alloc] initWithPrimaryKey:primaryKeyUpgrade database:self.database];
[self.powerUpUpgrades addObject:upgrade];
[upgrade release];
}
sqlite3_reset(statement_upgrades);
sqlite3_finalize(statement_upgrades);
statement_upgrades=nil;
}
[PUAndPstoreTitles addObject:@"sectionView1"];
}
-(void)loadSinglePurchases{
self.singlePurchases=[[NSMutableArray alloc] init];
NSString *str_fishes=@"SELECT purchaseID FROM purchase";
const char *sql_fishes = [str_fishes cStringUsingEncoding:NSUTF8StringEncoding];
sqlite3_stmt *statement_purchase=nil;
if (sqlite3_prepare_v2(self.database, sql_fishes, -1, &statement_purchase, NULL) == SQLITE_OK) {
while (sqlite3_step(statement_purchase) == SQLITE_ROW) {
int primaryKeyUpgrade = sqlite3_column_int(statement_purchase, 0);
DBPurchase *purchase = [[DBPurchase alloc] initWithPrimaryKey:primaryKeyUpgrade database:self.database];
[self.singlePurchases addObject:purchase];
[purchase release];
}
sqlite3_reset(statement_purchase);
sqlite3_finalize(statement_purchase);
statement_purchase=nil;
}
[PUAndPstoreTitles addObject:@"sectionView2"];
}
-(void)dealloc{
database=nil;
[userData release];
userData=nil;
[self.powerUpUpgrades removeAllObjects];
self.powerUpUpgrades=nil;
[self.singlePurchases removeAllObjects];
self.singlePurchases=nil;
[self.PUAndPstoreTitles removeAllObjects];
self.PUAndPstoreTitles=nil;
[self.dataObjectsToBeWritten removeAllObjects];
self.dataObjectsToBeWritten=nil;
[super dealloc];
}
@end
<file_sep>//
// RVRAppDelegate.h
// MotoRacer
//
// Created by <NAME> on 5/30/12.
// Copyright __MyCompanyName__ 2012. All rights reserved.
//
#import <UIKit/UIKit.h>
#import "RVRDataBaseManager.h"
@class RootViewController;
@class GameNode;
@class GameMenu;
@class GameHud;
@interface RVRAppDelegate : NSObject <UIApplicationDelegate> {
UIWindow *window;
}
@property (nonatomic, retain) UIWindow *window;
@property (nonatomic,retain) RootViewController *viewController;
@property (nonatomic,readwrite,retain) GameNode *gNode;
@property (nonatomic,readwrite,retain) GameMenu *gMenu;
@property (nonatomic,readwrite,retain) GameHud *gHud;
@property (nonatomic,readwrite,retain) RVRDataBaseManager *databaseManager;
@property (nonatomic) BOOL gamePlayRunning;
@end
<file_sep>//
// RootViewController.h
// MotoRacer
//
// Created by <NAME> on 5/30/12.
// Copyright __MyCompanyName__ 2012. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface RootViewController : UIViewController {
}
@property (nonatomic,readwrite) CGRect modalPresentationFrame,modalHiddenFrame;
-(void)presentModalView:(RootViewController*)modalViewController withDelegate:(id)delegate selector:(SEL)selector animated:(BOOL)animated;
-(void)dismissModalViewAnimated:(BOOL)animated withDelegate:(id)delegate selector:(SEL)selector;
@end
<file_sep>//
// GameHud.m
// MotoRacer
//
// Created by <NAME> on 6/23/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import "GameHud.h"
#import "TextureManager.h"
@interface GameHud (Private)
-(void)addSepeedometer;
-(void)addScoreBars;
@end
@implementation GameHud
@synthesize coinsLabel,vDodgedLabel,speedLabel,distanceLabel,meterArrow;
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
appDelegate.gHud=self;
//[self addSepeedometer];
[self addScoreBars];
coinsLabel=[CCLabelTTF labelWithString:[NSString stringWithFormat:@"%d",appDelegate.databaseManager.userData.uCoins] fontName:FONTNAME fontSize:FONTSIZE];
coinsLabel.anchorPoint=ccp(1.0f, 1.0f);
coinsLabel.position=ccp(winSize.width-2.0f, winSize.height);
[self addChild:coinsLabel];
/*
vDodgedLabel=[CCLabelTTF labelWithString:@"V Dodged 0" fontName:FONTNAME fontSize:FONTSIZE];
vDodgedLabel.anchorPoint=ccp(1.0f, 1.0f);
vDodgedLabel.position=ccp(winSize.width, winSize.height-coinsLabel.contentSize.height-20.0f);
[self addChild:vDodgedLabel];
*/
/*
speedLabel=[CCLabelTTF labelWithString:@"70" fontName:FONTNAME fontSize:15.0];
speedLabel.anchorPoint=ccp(0.5f, 0.5f);
speedLabel.position=ccp(50.0f, winSize.height-67.0f);
[self addChild:speedLabel z:101];
*/
distanceLabel=[CCLabelTTF labelWithString:@"0 M" fontName:FONTNAME fontSize:FONTSIZE];
distanceLabel.anchorPoint=ccp(1.0f, 1.0f);
distanceLabel.position=ccp(/*winSize.width-*/90.0f, winSize.height/*-30.0f*/);
[self addChild:distanceLabel];
}
return self;
}
-(void)addSepeedometer{
CCSprite *meter=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"meter"]];
meter.anchorPoint=ccp(0.5f, 0.5f);
meter.position=ccp(meter.contentSize.width/2.0f, winSize.height-(meter.contentSize.height/2.0f));
[self addChild:meter z:100];
meterArrow=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"meter-arrow"]];
meterArrow.anchorPoint=ccp(1.0f-(5.0f/meterArrow.contentSize.width), 0.5f);
meterArrow.position=ccp(meter.contentSize.width/2.0f, meter.contentSize.height/2.0f);
meterArrow.rotation=METERARROWINIROTCONST;
[meter addChild:meterArrow];
}
-(void)addScoreBars{
CCSprite *sprite=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"coin-bar"]];
sprite.anchorPoint=ccp(1.0f, 1.0f);
sprite.position=ccp(winSize.width, winSize.height);
[self addChild:sprite];
sprite=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"score-bar"]];
sprite.anchorPoint=ccp(0.0f, 1.0f);
sprite.position=ccp(/*winSize.width*/0.0f, winSize.height/*-sprite.contentSize.height-5.0f*/);
[self addChild:sprite];
}
-(void)dealloc{
[super dealloc];
}
@end
<file_sep>//
// GameNode.h
// MotoRacer
//
// Created by <NAME> on 5/30/12.
// Copyright __MyCompanyName__ 2012. All rights reserved.
//
#import "cocos2d.h"
#import "RVRPowerUpsAndPurchasesController.h"
#import "PowerUp.h"
#import "GamePlayObserverDelegateProtocol.h"
#import "PauseOrGameOverMenu.h"
#define PI 3.14159265l
#define INITIALSPEED 10.0f//5.0f
#define MAXSPEED (10.0f/70.0f)*160.0f
#define ACCELERATION 0.03F
#define RETARDATION -0.05F
#define TIMETOMAXSPEEDWITHNORMALACC 12.0F
#define NORMALACCELERATION (MAXSPEED-INITIALSPEED)/(TIMETOMAXSPEEDWITHNORMALACC*3600.0F)
#define MILETOPIXELRATIO 70.0l/36000.0l
#define MINTOHRRATIO 1.0l
#define MAXPOINT (480.0F/105.0F)*160.0F//(600.0F/160.0F)*160.0F
#define XSHIFTCONST 140.0F
#define ROADSTRIPESAPCONST 192.0F
#define NOOFROADSTRIPES 8
#define NOOFROADSTRIPESPERCOL 4
#define BARSTRIPESAPCONST 40.0F
#define NOOFSIDEBARSTRIPES 24
#define NOOFSIDEBARSTRIPESPERCOL 12
#define TREESEPCONST 200.0F
#define NOOFTREES 4
#define NOOFTREESPERCOL 2
#define NOOFVEHICLES 6
#define NOOFVEHICLESPERCOL 2
#define COINSSAPCONST 60.0F
#define NOOFCOINS 48
#define NOOFCOINSPERCOL 16
#define VEHICLELANECHANGESPEED 50.0F
#define TWOLESSSPEEDYSTARTERBTNHIDETIME 8.0F
@class RVRAppDelegate;
@class Obstacles;
struct DistMsg {
float distanceForCollectable;
float distanceForAutomatic;
float distanceForSuperSpeedyStarter;
};
@interface GameNode : CCLayer<RVRPowerUpsAndPurchasesDelegate,PauseOrGameOverMenuBtnClickDelegate>{
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
CCSprite *sidebarleft;
CCSprite *sidebarright;
Obstacles *vehicleCollidedWith;
float currentSpeed;
float speedBeforeSSS;
float vCurrentSpeed;
BOOL isAccelerating;
NSMutableArray *roadStripes;
NSMutableArray *sideBarStripes;
NSMutableArray *trees;
NSMutableArray *roadOverlays;
NSMutableArray *vehicleObstacles;
NSMutableArray *movingVehicles;
NSMutableArray *bikerPoints;
NSMutableArray *movingBikerPoints;
NSMutableArray *movingTrees;
NSMutableArray *superSpeedyBtns;
NSMutableDictionary *powerUpSpriteObjs;
NSMutableArray *menuButtons;
BOOL isVehicleMoving;
BOOL isBikerPointsMoving;
BOOL isTreesMoving;
NSInteger clickedButtonTag;
CCLayerColor *pauseModeLayer;
CCRenderTexture* _rt;
CCSpriteBatchNode *explosionBatchNode;
NSMutableArray *explosionBNChilds;
int shootCount;
int noOfVs,vCount;
CGRect magneticInductionRect;
BOOL isPUpPCAlertSent;
BOOL isPowerUpMoving;
PowerUp *movingPUp;
struct DistMsg distMsg;
BOOL reducingFromMax;
BOOL shouldAlertForCollectableDisCovrd;
BOOL waitingForEnablingDoubleValue,waitingForResettingBikerPoints;
BOOL willBringGameOverMenu;
}
@property (nonatomic,readwrite,retain) id<GamePlayObserverDelegate> delegate;
@property (nonatomic,readwrite,retain) CCSprite *bikeSprite;
@property (nonatomic,readwrite) float bSpeed;
@property (nonatomic,readwrite) double distanceInMiles,speedInMilesPerH;
@property (nonatomic,readwrite) int isBikeAccelerating,gameOverCount;
@property (nonatomic,readwrite,retain) NSMutableArray *collectedBikerPoints;
@property (nonatomic) BOOL hasMagnet,isInvincible,hasAmmo,hasDoubleValuedCoins,hasCoinMultiplier;
+(CCScene *) scene;
-(void)pauseGameOnEnteringBackGround;
@end
<file_sep>//
// RVRPowerUpsAndPurchasesStore.m
// MotoRacer
//
// Created by <NAME> on 7/10/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "RVRPowerUpsAndPurchasesStore.h"
#import "RVRAppDelegate.h"
#import "cocos2d.h"
#import "RVRPowerUpsAndPurchasesController.h"
#import "PauseOrGameOverMenu.h"
#import "GameMenu.h"
#import "RVRInAppPurchaseStore.h"
#import "RVRAudioManager.h"
static RVRPowerUpsAndPurchasesStore *storeRef=nil;
@interface RVRPowerUpsAndPurchasesStore (Private)
-(void)addStoreTitleView;
-(void)initiateAndAddTableView;
-(void)initiateSectionViews;
-(void)returnToControllerMenu;
-(void)addOrUpdateCellUpgradeOrPurchaseDetail:(UITableViewCell*)cell indexPath:(NSIndexPath*)indexPath data:(id)data;
-(void)buttonClicked:(id)sender;
-(void)reloadUpdateAbleData;
@end
@implementation RVRPowerUpsAndPurchasesStore
@synthesize controllerMenu,delegate;
enum{
nothing,
doneBtnTag,
getMoreCoinsBtnTag,
};
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
self.delegate=[RVRPowerUpsAndPurchasesController powerUpsAndPurchasesController];
//self.modalTransitionStyle=UIModalTransitionStyleCrossDissolve;//UIModalTransitionStyleCoverVertical
self.view.frame=self.modalHiddenFrame=CGRectMake(0.0f, -winSize.height, winSize.width, winSize.height);
self.modalPresentationFrame=CGRectMake(0.0f, 0.0f, winSize.width, winSize.height);
self.view.backgroundColor=[UIColor clearColor];
[self addStoreTitleView];
[self initiateSectionViews];
[self initiateAndAddTableView];
[appDelegate.viewController.view addSubview:self.view];
[appDelegate.viewController.view sendSubviewToBack:self.view];
}
return self;
}
-(void)reloadUpdateAbleData{
((UILabel*)[self.view viewWithTag:19]).text=[NSString stringWithFormat:@"%d",appDelegate.databaseManager.userData.uCoins];
}
+(RVRPowerUpsAndPurchasesStore*)powerUpsAndPurchaseStore{
if (storeRef==nil) {
storeRef=[[RVRPowerUpsAndPurchasesStore alloc] init];
}
[storeRef reloadUpdateAbleData];
return storeRef;
}
-(void)addStoreTitleView{
UIButton *getCoinBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[getCoinBtn setImage:[UIImage imageNamed:@"get-more-coins_top.png"] forState:UIControlStateNormal];
getCoinBtn.frame=CGRectMake(winSize.width/8, 0.0f, winSize.width-((winSize.width/8)*2), 66.0f);
getCoinBtn.bounds=CGRectMake(0.0f, 0.0f, winSize.width-((winSize.width/8)*2), 66.0f);
getCoinBtn.backgroundColor=[UIColor clearColor];
[getCoinBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
getCoinBtn.opaque=NO;
getCoinBtn.tag=getMoreCoinsBtnTag;
[self.view addSubview:getCoinBtn];
/*
UIImageView *storeTitle=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"storeTitle.png"]] autorelease];
storeTitle.frame=CGRectMake(winSize.width/8, 0.0f, winSize.width-((winSize.width/8)*2), (HIGHTFORROW/2.0f)+16.0f);
storeTitle.opaque=NO;
[self.view addSubview:storeTitle];
*/
UIImageView *iconView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"pCoin.png"]] autorelease];
iconView.frame=CGRectMake(winSize.width/8+70.0f, 10.0f, 18.0f, 18.0f);
iconView.opaque=NO;
[self.view addSubview:iconView];
UILabel *label=[[[UILabel alloc] initWithFrame:CGRectMake(winSize.width/8+100.0f, 10.0f, winSize.width-((winSize.width/8)*2), 20.0f)] autorelease];
label.text=[NSString stringWithFormat:@"%d",appDelegate.databaseManager.userData.uCoins];
label.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:20.0f];
label.adjustsFontSizeToFitWidth = YES;
label.textAlignment=UITextAlignmentLeft;
label.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.12f alpha:1.0f];
label.backgroundColor=[UIColor clearColor];
label.opaque=NO;
label.tag=19;
[self.view addSubview:label];
getCoinBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[getCoinBtn setImage:[UIImage imageNamed:@"get-more-coins_bottom.png"] forState:UIControlStateNormal];
getCoinBtn.frame=CGRectMake(winSize.width/8, (winSize.height/7)*6, winSize.width-((winSize.width/8)*2)+2.0f, 32.0f);
getCoinBtn.bounds=CGRectMake(0.0f, 0.0f, winSize.width-((winSize.width/8)*2), 32.0f);
getCoinBtn.backgroundColor=[UIColor clearColor];
[getCoinBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
getCoinBtn.opaque=NO;
getCoinBtn.tag=getMoreCoinsBtnTag;
[self.view addSubview:getCoinBtn];
UIButton *doneBtn=[UIButton buttonWithType:UIButtonTypeCustom];
[doneBtn setImage:[UIImage imageNamed:@"done.png"] forState:UIControlStateNormal];
doneBtn.frame=CGRectMake(winSize.width/8, ((winSize.height/7)*6)+34.0f, winSize.width-((winSize.width/8)*2), 32.0f);
doneBtn.bounds=CGRectMake(0.0f, 0.0f, winSize.width-((winSize.width/8)*2), 32.0f);
doneBtn.backgroundColor=[UIColor clearColor];
[doneBtn addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
doneBtn.opaque=NO;
doneBtn.tag=doneBtnTag;
[self.view addSubview:doneBtn];
}
-(void)initiateSectionViews{
sectionTitleViews=[[NSMutableArray alloc] init];
UIImageView *sectionTitleView;
for (NSString *title in appDelegate.databaseManager.PUAndPstoreTitles) {
sectionTitleView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:[NSString stringWithFormat:@"%@.png",title]]] autorelease];
sectionTitleView.frame=CGRectMake(winSize.width/8, winSize.height/7, winSize.width-((winSize.width/8)*2), HIGHTFORROW/2.0f);
sectionTitleView.opaque=NO;
[sectionTitleViews addObject:sectionTitleView];
}
}
-(void)initiateAndAddTableView{
tVC=[[UITableViewController alloc] initWithNibName:nil bundle:nil];
tVC.tableView.frame=CGRectMake(winSize.width/8, winSize.height/7, winSize.width-((winSize.width/8)*2), winSize.height-((winSize.height/7)*2));
tVC.tableView.contentSize=CGSizeMake(winSize.width-((winSize.width/8)*2), winSize.height-((winSize.height/7)*2));
// NSLog(@"content size -->%f,%f",tVC.tableView.contentSize.width,tVC.tableView.contentSize.height);
tVC.tableView.delegate = self;
tVC.tableView.dataSource = self;
tVC.tableView.opaque = YES;
tVC.tableView.backgroundColor = [UIColor clearColor];
tVC.tableView.decelerationRate = 0.5;
tVC.tableView.bounces = YES;
tVC.tableView.pagingEnabled=NO;
//storeTable.transform=CGAffineTransformMakeRotation(-M_PI/2);
tVC.tableView.separatorColor=[UIColor clearColor];
tVC.tableView.showsVerticalScrollIndicator=YES;
tVC.tableView.delaysContentTouches=YES;
[self.view addSubview:tVC.view];
[tVC release];
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return NOOFSECTIONS;
}
-(CGFloat) tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
return HIGHTFORROW;
}
-(CGFloat)tableView:(UITableView *)tableView heightForHeaderInSection:(NSInteger)section{
return HIGHTFORROW/2.0f;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
if (section==0)
return [appDelegate.databaseManager.powerUpUpgrades count];
else if(section==1)
return [appDelegate.databaseManager.singlePurchases count];
return 0;
}
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
[[RVRAudioManager sharedManager] playButtonClickSound:YES];
UITableViewCell *selectedCell;
if (indexPath.section==0) {
DBUpgrades *upgradeData=[appDelegate.databaseManager.powerUpUpgrades objectAtIndex:indexPath.row];
NSArray *array=[upgradeData updateDatabaseForStepPurchase];
if (array!=nil) {
selectedCell=[tableView cellForRowAtIndexPath:indexPath];
[self addOrUpdateCellUpgradeOrPurchaseDetail:selectedCell indexPath:indexPath data:upgradeData];
[self.delegate powerUpUpgradedWithItem:upgradeData];
}
else if(upgradeData.activeStep+1==upgradeData.noOfUpgradeSteps){
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle:@"Fully Upgraded!" message:@"Congratulations" delegate:self cancelButtonTitle:@"OK" otherButtonTitles:NULL] autorelease];
[alert show];
}
else{
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle:@"Coin!" message:@"You do not have Enough Coins. Would You like to Buy?" delegate:self cancelButtonTitle:@"NO" otherButtonTitles:@"YES",NULL] autorelease];
[alert show];
}
}
else if(indexPath.section==1){
DBPurchase *purchaseData=[appDelegate.databaseManager.singlePurchases objectAtIndex:indexPath.row];
BOOL isPurchse=[purchaseData updateDatabaseForPurchase];
if (isPurchse) {
selectedCell=[tableView cellForRowAtIndexPath:indexPath];
[self addOrUpdateCellUpgradeOrPurchaseDetail:selectedCell indexPath:indexPath data:purchaseData];
[self.delegate singlePurchaseMadeWithItem:purchaseData];
}
else{
UIAlertView* alert= [[[UIAlertView alloc] initWithTitle:@"Coin!" message:@"You do not have Enough Coins. Would You like to Buy?" delegate:self cancelButtonTitle:@"NO" otherButtonTitles:@"YES",NULL] autorelease];
[alert show];
}
}
}
- (void) alertView:(UIAlertView *)alert clickedButtonAtIndex:(NSInteger)buttonIndex{
if (buttonIndex==1) {
clickedBtntag=getMoreCoinsBtnTag;
[self dismissModalViewAnimated:YES withDelegate:self selector:@selector(uiViewAnimaitonDidFinish)];
}
}
-(UIView*)tableView:(UITableView *)tableView viewForHeaderInSection:(NSInteger)section{
return [sectionTitleViews objectAtIndex:section];
}
-(void) setImage:(UIImage*)image inTableViewCell:(UITableViewCell*)cell
{
#ifndef __IPHONE_3_0
cell.image = image;
#else
cell.imageView.image = image;
#endif
}
-(void)addOrUpdateCellUpgradeOrPurchaseDetail:(UITableViewCell *)cell indexPath:(NSIndexPath *)indexPath data:(id)data{
NSString *tag=[NSString stringWithFormat:@"100%d%d",indexPath.section,indexPath.row];
UIView *view=[cell.contentView viewWithTag:[tag integerValue]];
UIImageView *progressView;
if (view==nil) {
if (indexPath.section==0) {
DBUpgrades *uData=(DBUpgrades*)data;
view=[[[UIView alloc] initWithFrame:CGRectMake(TABLECELLXOFFSET, LFONTSIZE*4.0f, 27.0f*6.0f, HIGHTFORROW-(LFONTSIZE*4.0f))] autorelease];
view.opaque=NO;
view.backgroundColor=[UIColor clearColor];
view.tag=[tag integerValue];
int j=0;
for (int i=0; i<uData.noOfUpgradeSteps; i++) {
progressView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"upgradeProgress-bg.png"]] autorelease];
progressView.frame=CGRectMake((i*27.0f)+(i), 0.0f, 27.0f, 8.0f);
progressView.opaque=NO;
progressView.backgroundColor=[UIColor clearColor];
[view addSubview:progressView];
if (j<=uData.activeStep) {
progressView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"upgradeProgress.png"]] autorelease];
progressView.frame=CGRectMake((i*27.0f)+(i), 0.0f, 27.0f, 8.0f);
progressView.opaque=NO;
progressView.backgroundColor=[UIColor clearColor];
[view addSubview:progressView];
j++;
}
}
progressView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"purchase-bg.png"]] autorelease];
progressView.frame=CGRectMake(0.0f, 10.0f, 167.0f, 22.0f);
progressView.opaque=NO;
progressView.backgroundColor=[UIColor clearColor];
UILabel *titleLabel=[[[UILabel alloc] initWithFrame:CGRectMake(2.0f, 2.0f, 163.0f, 18.0f)] autorelease];
titleLabel.text=@"Enable";
titleLabel.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:18];
titleLabel.adjustsFontSizeToFitWidth = YES;
titleLabel.textAlignment=UITextAlignmentLeft;
titleLabel.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.0f alpha:1.0f];
titleLabel.backgroundColor=[UIColor clearColor];
titleLabel.opaque=NO;
titleLabel.tag=[[NSString stringWithFormat:@"%d%d",uData.upgradeID,1] integerValue];
[progressView addSubview:titleLabel];
UIImageView *iconView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"pCoin.png"]] autorelease];
iconView.frame=CGRectMake(80.0f, 2.0f, 18.0f, 18.0f);
iconView.opaque=NO;
iconView.tag=[[NSString stringWithFormat:@"%d%d",uData.upgradeID,3] integerValue];
[progressView addSubview:iconView];
titleLabel=[[[UILabel alloc] initWithFrame:CGRectMake(100.0f, 3.0f, 64.0f, 18.0f)] autorelease];
NSString *coins=[NSString stringWithFormat:@"%@",[[uData getNextUpgradeStepAndCost] objectAtIndex:1]];
titleLabel.text=coins;
titleLabel.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:16];
titleLabel.adjustsFontSizeToFitWidth = YES;
titleLabel.textAlignment=UITextAlignmentLeft;
titleLabel.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.0f alpha:1.0f];
titleLabel.backgroundColor=[UIColor clearColor];
titleLabel.opaque=NO;
titleLabel.tag=[[NSString stringWithFormat:@"%d%d",uData.upgradeID,2] integerValue];
[progressView addSubview:titleLabel];
[view addSubview:progressView];
[cell.contentView addSubview:view];
}
else if(indexPath.section==1){
DBPurchase *uData=(DBPurchase*)data;
view=[[[UIView alloc] initWithFrame:CGRectMake(0.0, LFONTSIZE*4.0f, winSize.width-((winSize.width/8)*2), HIGHTFORROW-(LFONTSIZE*4.0f))] autorelease];
view.opaque=NO;
view.backgroundColor=[UIColor clearColor];
view.tag=[tag integerValue];
progressView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"purchase-bg.png"]] autorelease];
progressView.frame=CGRectMake(TABLECELLXOFFSET, 10.0f, 167.0f, 22.0f);
progressView.opaque=NO;
progressView.backgroundColor=[UIColor clearColor];
UILabel *titleLabel=[[[UILabel alloc] initWithFrame:CGRectMake(2.0f, 2.0f, 163.0f, 18.0f)] autorelease];
titleLabel.text=@"Single Use";
titleLabel.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:18];
titleLabel.adjustsFontSizeToFitWidth = YES;
titleLabel.textAlignment=UITextAlignmentLeft;
titleLabel.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.0f alpha:1.0f];
titleLabel.backgroundColor=[UIColor clearColor];
titleLabel.opaque=NO;
[progressView addSubview:titleLabel];
UIImageView *iconView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"pCoin.png"]] autorelease];
iconView.frame=CGRectMake(80.0f, 2.0f, 18.0f, 18.0f);
iconView.opaque=NO;
[progressView addSubview:iconView];
titleLabel=[[[UILabel alloc] initWithFrame:CGRectMake(100.0f, 3.0f, 64.0f, 18.0f)] autorelease];
NSString *coins=[NSString stringWithFormat:@"%d",uData.purchaseCost];
titleLabel.text=coins;
titleLabel.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:16];
titleLabel.adjustsFontSizeToFitWidth = YES;
titleLabel.textAlignment=UITextAlignmentLeft;
titleLabel.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.0f alpha:1.0f];
titleLabel.backgroundColor=[UIColor clearColor];
titleLabel.opaque=NO;
[progressView addSubview:titleLabel];
[view addSubview:progressView];
UILabel *noOfPurchase=[[[UILabel alloc] initWithFrame:CGRectMake(24.0, 16.0f, 16, 16)] autorelease];
noOfPurchase.text=[NSString stringWithFormat:@"%d",uData.activeNoOfPurchases];
noOfPurchase.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:14];
noOfPurchase.adjustsFontSizeToFitWidth = YES;
noOfPurchase.textAlignment=UITextAlignmentCenter;
noOfPurchase.textColor=[UIColor colorWithRed:1.0f green:1.0f blue:0.0f alpha:1.0f];
noOfPurchase.backgroundColor=[UIColor blackColor];
noOfPurchase.opaque=NO;
noOfPurchase.tag=uData.purchaseID;
[view addSubview:noOfPurchase];
[cell.contentView addSubview:view];
}
}
if (indexPath.section==0) {
DBUpgrades *uData=(DBUpgrades*)data;
if (uData.activeStep>-1 && uData.activeStep<uData.noOfUpgradeSteps) {
progressView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"upgradeProgress.png"]] autorelease];
progressView.frame=CGRectMake((uData.activeStep*27.0f)+(uData.activeStep), 0.0f, 27.0f, 8.0f);
progressView.opaque=NO;
progressView.backgroundColor=[UIColor clearColor];
[view addSubview:progressView];
UILabel *label=(UILabel*)[view viewWithTag:[[NSString stringWithFormat:@"%d%d",uData.upgradeID,1] integerValue]];
if (uData.activeStep==uData.noOfUpgradeSteps-1 && label!=nil) {
label.text=[NSString stringWithFormat:@"%@",[[uData getNextUpgradeStepAndCost] objectAtIndex:0]];
}
else if (label!=nil) {
label.text=@"Upgrade";
}
label=(UILabel*)[view viewWithTag:[[NSString stringWithFormat:@"%d%d",uData.upgradeID,2] integerValue]];
if (uData.activeStep==uData.noOfUpgradeSteps-1 && label!=nil) {
[label removeFromSuperview];
UIImageView *coinView=(UIImageView*)[view viewWithTag:[[NSString stringWithFormat:@"%d%d",uData.upgradeID,3] integerValue]];
if (coinView!=nil) {
[coinView removeFromSuperview];
}
}
else if (label!=nil) {
NSString *coins=[NSString stringWithFormat:@"%@",[[uData getNextUpgradeStepAndCost] objectAtIndex:1]];
label.text=coins;
}
}
}
else if(indexPath.section==1){
DBPurchase *uData=(DBPurchase*)data;
UILabel *noOfPurchase=(UILabel*)[view viewWithTag:uData.purchaseID];
if (noOfPurchase!=nil) {
noOfPurchase.text=[NSString stringWithFormat:@"%d",uData.activeNoOfPurchases];
}
}
UILabel *label=(UILabel*)[self.view viewWithTag:19];
label.text=[NSString stringWithFormat:@"%d",appDelegate.databaseManager.userData.uCoins];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
NSString *MyIdentifier=[NSString stringWithFormat:@"storeDetailCell%d%d",indexPath.section,indexPath.row];
NSObject *data;
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:MyIdentifier];
if (cell == nil) {
NSString *iconName,*itemName,*itemDetail;
if (indexPath.section==0){
data=[appDelegate.databaseManager.powerUpUpgrades objectAtIndex:indexPath.row];
iconName=((DBUpgrades*)data).upgradeIcon;
itemName=((DBUpgrades*)data).upgradeName;
itemDetail=((DBUpgrades*)data).upgradeDetail;
}
else if(indexPath.section==1){
data=[appDelegate.databaseManager.singlePurchases objectAtIndex:indexPath.row];
iconName=((DBPurchase*)data).purchaseIcon;
itemName=((DBPurchase*)data).purchaseName;
itemDetail=((DBPurchase*)data).purchaseDetail;
}
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:MyIdentifier] autorelease];
cell.opaque=YES;
cell.selectionStyle=UITableViewCellSelectionStyleNone;
cell.backgroundView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:@"store-tablecell.png"]] autorelease];
UIImageView *iconView=[[[UIImageView alloc] initWithImage:[UIImage imageNamed:[NSString stringWithFormat:@"i%@.png",iconName]]] autorelease];
iconView.frame=CGRectMake(8.0f, 26.0f, 48.0f, 48.0f);
iconView.opaque=NO;
[cell.contentView addSubview:iconView];
UILabel *titleLabel=[[UILabel alloc] initWithFrame:CGRectMake(TABLECELLXOFFSET, 0.0f, winSize.width-((winSize.width/8)*2)-TABLECELLXOFFSET, LFONTSIZE)];
titleLabel.text=itemName;
titleLabel.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:LFONTSIZE];
titleLabel.adjustsFontSizeToFitWidth = YES;
titleLabel.textAlignment=UITextAlignmentLeft;
titleLabel.textColor=[UIColor colorWithRed:65.0f/255.0f green:105.0f/255.0f blue:1.0f alpha:1.0f];
titleLabel.backgroundColor=[UIColor clearColor];
titleLabel.opaque=NO;
[cell.contentView addSubview:titleLabel];
[titleLabel release];
UILabel *detailLabel=[[UILabel alloc] initWithFrame:CGRectMake(TABLECELLXOFFSET, LFONTSIZE, winSize.width-((winSize.width/8)*2)-TABLECELLXOFFSET, LFONTSIZE*ceilf([itemDetail length]/35.0f))];
detailLabel.text=itemDetail;
detailLabel.font = [UIFont fontWithName:@"MarkerFelt-Thin" size:12.0f];
detailLabel.adjustsFontSizeToFitWidth = NO;
detailLabel.numberOfLines=ceilf([itemDetail length]/35.0f);
detailLabel.textAlignment=UITextAlignmentLeft;
detailLabel.textColor=[UIColor colorWithRed:65.0f/255.0f green:105.0f/255.0f blue:1.0f alpha:1.0f];
detailLabel.backgroundColor=[UIColor clearColor];
detailLabel.opaque=NO;
[cell.contentView addSubview:detailLabel];
[detailLabel release];
[self addOrUpdateCellUpgradeOrPurchaseDetail:cell indexPath:indexPath data:data];
}
else if(indexPath.section==1 && cell!=nil){
data=[appDelegate.databaseManager.singlePurchases objectAtIndex:indexPath.row];
[self addOrUpdateCellUpgradeOrPurchaseDetail:cell indexPath:indexPath data:data];
}
return cell;
}
-(void)uiViewAnimaitonDidFinish{
[appDelegate.viewController.view sendSubviewToBack:self.view];
if ([[PauseOrGameOverMenu menu] reqSent]==YES && clickedBtntag==doneBtnTag) {
//[[PauseOrGameOverMenu menu] gameOverMenu];
[[PauseOrGameOverMenu menu] setReqSent:NO];
appDelegate.gamePlayRunning=NO;
[[CCDirector sharedDirector] replaceScene: [CCTransitionFade transitionWithDuration:1.0f scene:[GameMenu scene]]];
}
else if(clickedBtntag==getMoreCoinsBtnTag){
//present InApp Purchase View
[appDelegate.viewController presentModalView:[RVRInAppPurchaseStore inAppStore] withDelegate:nil selector:nil animated:YES];
}
else
[controllerMenu getBackControl];
clickedBtntag=nothing;
}
-(void)buttonClicked:(id)sender{
if ([sender tag]==doneBtnTag || [sender tag]==getMoreCoinsBtnTag) {
[[RVRAudioManager sharedManager] playButtonClickSound:YES];
clickedBtntag=[sender tag];
[self dismissModalViewAnimated:YES withDelegate:self selector:@selector(uiViewAnimaitonDidFinish)];
}
else
clickedBtntag=nothing;
}
-(void)dealloc{
[storeRef release];
[sectionTitleViews removeAllObjects];
sectionTitleViews=nil;
[tVC release];
tVC=nil;
self.delegate=nil;
[super dealloc];
}
@end
<file_sep>//
// GameNode.m
// MotoRacer
//
// Created by <NAME> on 5/30/12.
// Copyright __MyCompanyName__ 2012. All rights reserved.
//
#import "GameNode.h"
#import "TextureManager.h"
#import "GameMenu.h"
#include "GameHud.h"
#import "RVRCoin.h"
#import "Obstacles.h"
#import "DBPurchase.h"
#import "RVRAudioManager.h"
#pragma MARK - C Methods
float getPositionalRotaionAngle(CGPoint position){
float angle=0.0f;
float YDiff=MAXPOINT-position.y;
CGSize winSize=[[CCDirector sharedDirector] winSize];
float XDiff=winSize.width/2-position.x;
float grad;
if (XDiff!=0) {
grad=YDiff/XDiff;
angle=atanf(grad);
}
else
angle=0;
if (angle>0)
angle=90-(180/PI)*angle;
else if(angle<0)
angle=-(90+(180/PI)*angle);
else //if(angle==0)
angle=(180/PI)*angle;
return angle;
}
float getDampedHarmonicXShift(CGPoint position){
return expf(-position.y)*160.0f*cosf(position.y);
}
#pragma MARK - Private Methods Declaration
@interface GameNode (Private)
#pragma MARK - GameNode Private Methods
-(void)createBackgroundRoad;
-(void)generateSideBarStripes;
-(void)createAndAddBike;
-(void)generateRoadStripes;
-(void)generateTrees;
-(void)generateVehicleObstacles;
-(void)generateCoins;
-(void)generatePowerUpObjects;
-(void)updatePositionAndScale:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both;
-(void)updatePositionAndScaleForV:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both;
-(void)updatePositionAndScaleForBP:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both;
-(void)updatePositionAndScaleForTree:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both;
-(void)updatePositionAndScaleForPUp:(PowerUp*)pUp scaleX:(BOOL)scaleX saleBoth:(BOOL)both;
-(float)getXTransition:(float)yPos currentPos:(CGPoint)crntPos;
-(void)bringTrees;
-(void)bringVehicleObstacles;
-(void)bringBikerPoints;
-(void)setDoubleValueTexturesForBikerPoints;
-(void)resetTextureForBikerPoints;
-(void)bringPowerUpWithKey:(NSString*)key;
-(BOOL)isCollisionBetweenSpriteA:(CCSprite*)spr1 spriteB:(CCSprite*)spr2 pixelPerfect:(BOOL)pp;
-(BOOL)checkCollission;
-(void)bikerPointsCollection;
-(void)invalidateSuperSpeedyStarterBtns;
-(void)disableAllPowerUps;
-(void)hideNode:(CCNode*)node;
-(void)loadExplosionBatchNode;
-(BOOL)shootVehicle:(NSInteger)movingVIndx;
-(void)crushBike;
#pragma MARK - Button Control Methods
-(void)createMenuButtons;
-(void)createAndShowPauseModeLayer;
-(BOOL)menuButtonClicked:(id)sender btn:(CCSprite*)btn;
-(void)bringGameOverMenu:(id)sender;
@end
#pragma MARK - GameNode Implementation
// GameNode implementation
@implementation GameNode
@synthesize delegate,bikeSprite,bSpeed,collectedBikerPoints,hasMagnet,isBikeAccelerating,isInvincible,hasAmmo,speedInMilesPerH,distanceInMiles,hasDoubleValuedCoins,hasCoinMultiplier,gameOverCount;
enum{
nothing,
buttonhome,
buttonpause,
buttonplay,
buttonreplay,
speedyStarter,
speedyStarter2x,
};
enum{
collectable,
automatic,
};
enum{
resume=1,
revive=2,
playagain=3,
store=4,
mainmenu=5,
};
+(CCScene *) scene
{
// RVRAppDelegate *appDelegate=[[UIApplication sharedApplication] delegate];
// 'scene' is an autorelease object.
CCScene *scene = [CCScene node];
GameHud *gameHud=[GameHud node];
// 'layer' is an autorelease object.
GameNode *layer = [GameNode node];
// layer.scale=0.50f;
// add layer as a child to scene
[scene addChild: layer];
[scene addChild:gameHud];
// return the scene
return scene;
}
// on "init" you need to initialize your instance
-(id) init
{
if((self=[super init])) {
self.isTouchEnabled=YES;
self.isAccelerometerEnabled=YES;
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
appDelegate.gNode=self;
appDelegate.gamePlayRunning=YES;
//[CCTexture2D setDefaultAlphaPixelFormat:kCCTexture2DPixelFormat_RGBA8888];
currentSpeed=INITIALSPEED;
menuButtons=[[NSMutableArray alloc] init];
movingVehicles=[[NSMutableArray alloc] init];
movingBikerPoints=[[NSMutableArray alloc] init];
movingTrees=[[NSMutableArray alloc] init];
self.collectedBikerPoints=[[NSMutableArray alloc] init];
distMsg.distanceForCollectable=HUGE_VALF;
distMsg.distanceForAutomatic=HUGE_VALF;
distMsg.distanceForSuperSpeedyStarter=HUGE_VALF;
shouldAlertForCollectableDisCovrd=YES;
shootCount=0;
_rt = [CCRenderTexture renderTextureWithWidth:winSize.width height:winSize.height];
[self addChild:_rt];
_rt.visible = NO;
[[PauseOrGameOverMenu menu] setDelegate:self];
[self createBackgroundRoad];
[self createMenuButtons];
[self generateSideBarStripes];
[self generateRoadStripes];
[self generateTrees];
[self generateVehicleObstacles];
[self createAndAddBike];
magneticInductionRect=CGRectMake(0.0f, self.bikeSprite.position.y, winSize.width, self.bikeSprite.contentSize.height);
[self generateCoins];
[self generatePowerUpObjects];
[self loadExplosionBatchNode];
explosionBatchNode.visible=YES;
[self scheduleUpdate];
[[RVRAudioManager sharedManager] playBikeEngineSound:YES];
[self performSelector:@selector(bringVehicleObstacles) withObject:nil afterDelay:1.0f];
[self performSelector:@selector(bringBikerPoints) withObject:nil afterDelay:1.0f];
[self performSelector:@selector(bringTrees) withObject:nil afterDelay:1.0f];
}
return self;
}
-(BOOL) isCollisionBetweenSpriteA:(CCSprite*)spr1 spriteB:(CCSprite*)spr2 pixelPerfect:(BOOL)pp{
BOOL isCollision = NO;
CGRect intersection = CGRectIntersection([spr1 boundingBoxInPixels], [spr2 boundingBoxInPixels]);
// Look for simple bounding box collision
if (!CGRectIsEmpty(intersection))
{
// If we're not checking for pixel perfect collisions, return true
if (!pp) {return YES;}
// Get intersection info
unsigned int x = intersection.origin.x;
unsigned int y = intersection.origin.y;
unsigned int w = intersection.size.width;
unsigned int h = intersection.size.height;
unsigned int numPixels = w * h;
//NSLog(@"intersection = (%u,%u,%u,%u), area = %u",x,y,w,h,numPixels);
// Draw into the RenderTexture
[_rt beginWithClear:0 g:0 b:0 a:0];
// Render both sprites: first one in RED and second one in GREEN
glColorMask(1, 0, 0, 1);
[spr1 visit];
glColorMask(0, 1, 0, 1);
[spr2 visit];
glColorMask(1, 1, 1, 1);
// Get color values of intersection area
ccColor4B *buffer = malloc( sizeof(ccColor4B) * numPixels );
glReadPixels(x, y, w, h, GL_RGBA, GL_UNSIGNED_BYTE, buffer);
[_rt end];
// Read buffer
unsigned int step = 1;
for(unsigned int i=0; i<numPixels; i+=step)
{
ccColor4B color = buffer[i];
if (color.r > 0 && color.g > 0)
{
isCollision = YES;
break;
}
}
// Free buffer memory
free(buffer);
}
return isCollision;
}
-(CCSprite*)createSampleTexture:(CGSize)size{
CCRenderTexture *roadTexture=[CCRenderTexture renderTextureWithWidth:size.width height:size.height];
[roadTexture beginWithClear:255 g:255 b:255 a:255];
/*
glDisable(GL_TEXTURE_2D);
glDisableClientState(GL_TEXTURE_COORD_ARRAY);
glEnableClientState(GL_TEXTURE_COORD_ARRAY);
glEnable(GL_TEXTURE_2D);
*/
[roadTexture end];
return [CCSprite spriteWithTexture:roadTexture.sprite.texture];
}
-(CCSprite*)createSampleTexture1:(CGSize)size{
CCRenderTexture *roadTexture=[CCRenderTexture renderTextureWithWidth:size.width height:size.height];
[roadTexture beginWithClear:0 g:0 b:255 a:255];
glDisable(GL_TEXTURE_2D);
glDisableClientState(GL_TEXTURE_COORD_ARRAY);
glEnableClientState(GL_TEXTURE_COORD_ARRAY);
glEnable(GL_TEXTURE_2D);
[roadTexture end];
return [CCSprite spriteWithTexture:roadTexture.sprite.texture];
}
-(void)createBackgroundRoad{
CCSprite *road=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"roadTexture"]];
road.anchorPoint=ccp(0.0f, 0.0f);
[self addChild:road];
sidebarleft=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"sidebarleft"]];
[[sidebarleft texture] setAliasTexParameters];
sidebarleft.anchorPoint=ccp(0.0f, 1.0f);
sidebarleft.position=ccp(0.0f, winSize.height);
[self addChild:sidebarleft];
sidebarright=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"sidebarright"]];
[[sidebarright texture] setAliasTexParameters];
sidebarright.anchorPoint=ccp(1.0f, 1.0f);
sidebarright.position=ccp(winSize.width, winSize.height);
[self addChild:sidebarright];
}
-(void)createAndAddBike{
bikeSprite=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"bikeTexture"]];
[[bikeSprite texture] setAliasTexParameters];
bikeSprite.anchorPoint=ccp(0.5f, 0.0f);
bikeSprite.position=ccp(winSize.width/2, 64.0f);
bikeSprite.scale=1.0f;
[self addChild:bikeSprite z:3];
/*
CGRect bound=[self.bikeSprite boundingBox];
CCSprite *sprt=[self createSampleTexture1:CGSizeMake(bound.size.width, bound.size.height)];
sprt.anchorPoint=ccp(0.0f, 0.0f);
sprt.position=ccp(bound.origin.x, bound.origin.y);
[self addChild:sprt];
CGSize BCBoxSize=CGSizeMake(self.bikeSprite.contentSizeInPixels.width, self.bikeSprite.contentSizeInPixels.height/4);
BCBoundingBox=CGRectApplyAffineTransform(CGRectMake(0.0f, 0.0f, BCBoxSize.width, BCBoxSize.height), [self.bikeSprite nodeToParentTransform]);
sprt=[self createSampleTexture:CGSizeMake(BCBoundingBox.size.width, BCBoundingBox.size.height)];
sprt.anchorPoint=ccp(0.0f, 0.0f);
sprt.position=ccp(BCBoundingBox.origin.x, BCBoundingBox.origin.y);
[self addChild:sprt];*/
/*
CollissionRef=[self createSampleTexture:CGSizeMake(20, 32)];
CollissionRef.anchorPoint=ccp(0.5f, 0.0f);
CollissionRef.position=bikeSprite.position;//ccp(bikeSprite.contentSize.width/2, 0.0f);
[self addChild:CollissionRef z:100];*/
}
-(void)generateSideBarStripes{
sideBarStripes=[[NSMutableArray alloc] init];
CGPoint previousPos;
float XShift;
for (int i=0; i<NOOFSIDEBARSTRIPES; i++) {
CCSprite *roadStripe;
if (i<(NOOFSIDEBARSTRIPES/2)) {
roadStripe=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"side-stripe-left"]];
roadStripe.anchorPoint=ccp(0.0f, 1.0f);
}
else{
roadStripe=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"side-stripe-right"]];
roadStripe.anchorPoint=ccp(1.0f, 1.0f);
}
//position formulae, !should be re-engineered
if (i%NOOFSIDEBARSTRIPESPERCOL==0) {
XShift=winSize.width/2-(155.0f+roadStripe.contentSize.width)+((310.0f+(roadStripe.contentSize.width*2))*(i/NOOFSIDEBARSTRIPESPERCOL));
roadStripe.position=ccp(XShift, roadStripe.contentSize.height);
}
else{
XShift=[self getXTransition:roadStripe.contentSize.height+((i%NOOFSIDEBARSTRIPESPERCOL)*BARSTRIPESAPCONST) currentPos:previousPos];
roadStripe.position=ccp(XShift, roadStripe.contentSize.height+((i%NOOFSIDEBARSTRIPESPERCOL)*BARSTRIPESAPCONST));
}
previousPos=roadStripe.position;
//scaling formulae
roadStripe.scale=winSize.height/(winSize.height+roadStripe.position.y);
// NSLog(@"scale-->%f",roadStripe.scale);
[self addChild:roadStripe z:1];
[sideBarStripes addObject:roadStripe];
}
}
-(void)generateTrees{
trees=[[NSMutableArray alloc] init];
CGPoint previousPos;
float XShift;
for (int i=0; i<NOOFTREES; i++) {
CCSprite *roadStripe=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"treeTexture"]];
roadStripe.anchorPoint=ccp(0.5f, 0.5f);
//position formulae, !should be re-engineered
if (i%NOOFTREESPERCOL==0) {
XShift=winSize.width/2-200+(400*(i/NOOFTREESPERCOL));
XShift=[self getXTransition:-110.0f currentPos:ccp(XShift, 0.0f)];
roadStripe.position=ccp(XShift, -110.0f);
previousPos=roadStripe.position;
}
else
roadStripe.position=previousPos;
// roadStripe.rotation=getPositionalRotaionAngle(roadStripe.position);
//scaling formulae
roadStripe.scale=winSize.height/(winSize.height+roadStripe.position.y);
[self addChild:roadStripe z:5];
[trees addObject:roadStripe];
}
}
-(void)bringTrees{
isTreesMoving=NO;
[movingTrees removeAllObjects];
int noOfVToBring=1+(arc4random()%2);
int selectedVIndx;
float XShift;
float distance=650.0f;
for (int i=0; i<noOfVToBring; i++) {
selectedVIndx=arc4random()%4;
//NSLog(@"selected biker point indx--->%d",selectedVIndx);
if (![movingTrees containsObject:[trees objectAtIndex:selectedVIndx]]) {
CCSprite *tree=[trees objectAtIndex:selectedVIndx];
distance=distance+(i*tree.contentSize.height);
XShift=[self getXTransition:distance currentPos:tree.position];
tree.position=ccp(XShift, distance);
[movingTrees addObject:[trees objectAtIndex:selectedVIndx]];
}
}
isTreesMoving=YES;
}
-(void)generateRoadStripes{
roadStripes=[[NSMutableArray alloc] init];
CGPoint previousPos;
float XShift;
for (int i=0; i<NOOFROADSTRIPES; i++) {
CCSprite *roadStripe=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"stripe1Texture"]];
roadStripe.anchorPoint=ccp(0.5f, 1.0f);
//position formulae, !should be re-engineered
if (i%NOOFROADSTRIPESPERCOL==0) {
XShift=winSize.width/2-40+(80*(i/NOOFROADSTRIPESPERCOL));
}
else
XShift=[self getXTransition:roadStripe.contentSize.height+((i%NOOFROADSTRIPESPERCOL)*ROADSTRIPESAPCONST) currentPos:previousPos];
roadStripe.position=ccp(XShift, roadStripe.contentSize.height+((i%NOOFROADSTRIPESPERCOL)*ROADSTRIPESAPCONST));//roadStripe.contentSize.height+(i*70)
previousPos=roadStripe.position;
roadStripe.rotation=getPositionalRotaionAngle(roadStripe.position);
//scaling formulae
roadStripe.scale=winSize.height/((roadStripe.contentSize.height*2)+(roadStripe.position.y*3));
//--------------------------------------------------------------ˆ-change ths to change max scale, currently 1.0
//----------------------------------------------------------------------------------------ˆ-change ths to change min scale, currently 0.2
[self addChild:roadStripe z:1];
[roadStripes addObject:roadStripe];
}
}
-(void)generateVehicleObstacles{
vehicleObstacles=[[NSMutableArray alloc] init];
CGPoint previousPos;
float XShift;
//Required OnWhichLane???!!!
for (int i=0; i<NOOFVEHICLES; i++) {
Obstacles *roadStripe=[Obstacles generateObstacleWithAliasTextureParam];
if (i%NOOFVEHICLESPERCOL==0) {
XShift=(winSize.width/2.0f)-120.0f+(120.0f*(i/NOOFVEHICLESPERCOL));
XShift=[self getXTransition:-20.0f currentPos:ccp(XShift, 0.0f)];
roadStripe.position=roadStripe.initialPosition=ccp(XShift, -20.0f);
previousPos=roadStripe.position;
}
else
roadStripe.position=roadStripe.initialPosition=previousPos;
//NSLog(@"ini pos x--->%f",roadStripe.initialPosition.x);
[self addChild:roadStripe z:2];
[vehicleObstacles addObject:roadStripe];
}
}
-(void)bringVehicleObstacles{
isVehicleMoving=NO;
[movingVehicles removeAllObjects];
int noOfVToBring=(arc4random()%3)+1;
int selectedRoadLanes[3];
int selectedVIndx[3];
float XShift;
float distance=550.0f;
for (int i=0; i<noOfVToBring; i++) {
selectedRoadLanes[i]=arc4random()%3;
selectedVIndx[i]=selectedRoadLanes[i]*NOOFVEHICLESPERCOL;
if (selectedVIndx[1]==selectedVIndx[0]) {
selectedVIndx[1]=selectedVIndx[0]+1;
}
if (selectedVIndx[2]==selectedVIndx[0]) {
selectedVIndx[2]=selectedVIndx[0]+1;
}
//temp works
// float distance=550.0f+(((arc4random()%3)+1)*20*selectedVIndx[i]);
if (![movingVehicles containsObject:[vehicleObstacles objectAtIndex:selectedVIndx[i]]]) {
Obstacles *vehicle=[vehicleObstacles objectAtIndex:selectedVIndx[i]];
[vehicle resetProperties];
distance=distance+(i*vehicle.contentSize.height);
vehicle.visible=YES;
XShift=[self getXTransition:distance currentPos:vehicle.position];
vehicle.scale=winSize.height/(winSize.height+distance);
vehicle.position=ccp(XShift, distance);
[movingVehicles addObject:[vehicleObstacles objectAtIndex:selectedVIndx[i]]];
}
}
for (int i=0; i<([movingVehicles count]-1); i++) {
if (i<2) {
Obstacles *vehicle1=[movingVehicles objectAtIndex:i];
Obstacles *vehicle2=[movingVehicles objectAtIndex:i+1];
if (vehicle1.initialPosition.x==vehicle2.initialPosition.x &&
vehicle2.vehicleSpeed>vehicle1.vehicleSpeed) {
vehicle2.vehicleSpeed=vehicle1.vehicleSpeed;
}
}
}
if ([movingVehicles count]>2) {
Obstacles *vehicle1=[movingVehicles objectAtIndex:0];
Obstacles *vehicle2=[movingVehicles objectAtIndex:2];
if (vehicle1.initialPosition.x==vehicle2.initialPosition.x &&
vehicle2.vehicleSpeed>vehicle1.vehicleSpeed) {
vehicle2.vehicleSpeed=vehicle1.vehicleSpeed;
}
}
/*
NSLog(@"------");
for (int i=0;i<[movingVehicles count];i++) {
Obstacles *obj=[movingVehicles objectAtIndex:i];
NSLog(@"ini posX->%f indx->%d speed->%f",obj.initialPosition.x,i,obj.vehicleSpeed);
}
*/
isVehicleMoving=YES;
}
-(void)generateCoins{
bikerPoints=[[NSMutableArray alloc] init];
CGPoint previousPos;
float XShift;
for (int i=0; i<NOOFCOINS; i++) {
RVRCoin *roadStripe=[RVRCoin generateCoinWithAliasTexParameters];
if (i%NOOFCOINSPERCOL==0) {
XShift=winSize.width/2-120+(120*(i/NOOFCOINSPERCOL));
XShift=[self getXTransition:-20.0f currentPos:ccp(XShift, 0.0f)];
roadStripe.position=ccp(XShift, -20.0f);
previousPos=roadStripe.position;
}
else
roadStripe.position=previousPos;
[roadStripe setMyOriginalPosition];
// NSLog(@"coin no ->%d pos--->%f,%f",i, roadStripe.position.x, roadStripe.position.y);
[self addChild:roadStripe];
[bikerPoints addObject:roadStripe];
}
}
-(void)bringBikerPoints{
isBikerPointsMoving=NO;
[movingBikerPoints removeAllObjects];
int noOfVToBring=NOOFCOINSPERCOL;
int selectedRoadLanes=arc4random()%3;
int selectedVIndx;
float XShift;
float distance=700+(((arc4random()%3)+1)*100);
float distStore=distance;
for (int i=0; i<noOfVToBring; i++) {
selectedVIndx=(selectedRoadLanes*NOOFCOINSPERCOL)+i;
//NSLog(@"selected biker point indx--->%d",selectedVIndx);
RVRCoin *coin=[bikerPoints objectAtIndex:selectedVIndx];
coin.visible=YES;
XShift=[self getXTransition:distance currentPos:coin.position];
coin.position=ccp(XShift, distance);
[coin resumeSchedulerAndActions];
[movingBikerPoints addObject:coin];
distance+=(COINSSAPCONST-(COINSSAPCONST/100.0f*i));
}
int another=arc4random()%2;
if (another==1) {
int lane=arc4random()%3;
if (lane!=selectedRoadLanes) {
for (int i=0; i<noOfVToBring; i++) {
selectedVIndx=(lane*NOOFCOINSPERCOL)+i;
//NSLog(@"selected biker point indx--->%d",selectedVIndx);
RVRCoin *coin=[bikerPoints objectAtIndex:selectedVIndx];
coin.visible=YES;
XShift=[self getXTransition:distStore currentPos:coin.position];
coin.position=ccp(XShift, distStore);
[coin resumeSchedulerAndActions];
[movingBikerPoints addObject:coin];
distStore+=(COINSSAPCONST-(COINSSAPCONST/100.0f*i));
}
}
}
isBikerPointsMoving=YES;
}
-(void)setDoubleValueTexturesForBikerPoints{
for (int i=0; i<[bikerPoints count]; i++) {
if (i%2==0) {
RVRCoin *coin=[bikerPoints objectAtIndex:i];
[coin setDoubleValueTexture];
}
}
self.hasDoubleValuedCoins=YES;
[self bringBikerPoints];
}
-(void)resetTextureForBikerPoints{
for (int i=0; i<[bikerPoints count]; i++) {
if (i%2==0) {
RVRCoin *coin=[bikerPoints objectAtIndex:i];
[coin resetToOriginalTexture];
}
}
self.hasDoubleValuedCoins=NO;
[self bringBikerPoints];
[[RVRPowerUpsAndPurchasesController powerUpsAndPurchasesController] requestForAutomaticPowerUp];
}
-(void)generatePowerUpObjects{
if ([RVRPowerUpsAndPurchasesController powerUpsAndPurchasesController]!=nil) {
self.delegate=[RVRPowerUpsAndPurchasesController powerUpsAndPurchasesController];
[RVRPowerUpsAndPurchasesController powerUpsAndPurchasesController].delegate=self;
powerUpSpriteObjs=[[NSMutableDictionary alloc] init];
NSMutableDictionary *powerUps=[RVRPowerUpsAndPurchasesController powerUpsAndPurchasesController].collectablePowerUps;
float XShift=winSize.width/2;
XShift=[self getXTransition:-20.0f currentPos:ccp(XShift, 0.0f)];
for (DBUpgrades *pUpData in [powerUps allValues]) {
//NSLog(@"name--->%@",pUpData.upgradeName);
PowerUp *powerUp=[PowerUp generatePowerUpWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:[NSString stringWithFormat:@"pu-%@",pUpData.upgradeIcon]]];
powerUp.stringTag=pUpData.upgradeName;
powerUp.position=ccp(XShift, -20.0f);
[self addChild:powerUp];
[powerUpSpriteObjs setObject:powerUp forKey:pUpData.upgradeName];
}
}
}
-(void)bringPowerUpWithKey:(NSString *)key{
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
if (pUp!=nil) {
float XShift=[self getXTransition:winSize.height-pUp.position.y+100.0f currentPos:pUp.position];
pUp.position=ccp(XShift, winSize.height-pUp.position.y+100.0f);
pUp.visible=YES;
movingPUp=pUp;
isPowerUpMoving=YES;
}
}
-(void)ccTouchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
location = [[CCDirector sharedDirector] convertToGL: location];
if (clickedButtonTag==nothing) {
for (CCSprite *btn in menuButtons) {
if (CGRectContainsPoint([btn boundingBox], location)) {
clickedButtonTag=btn.tag;
return;
}
}
for (CCSprite *btn in superSpeedyBtns) {
if (CGRectContainsPoint([btn boundingBox], location)) {
clickedButtonTag=btn.tag;
return;
}
}
}
// isAccelerating=YES;
}
-(void)ccTouchesEnded:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
location = [[CCDirector sharedDirector] convertToGL: location];
if (clickedButtonTag!=nothing) {
for (CCSprite *btn in menuButtons) {
if (CGRectContainsPoint([btn boundingBox], location) && btn.tag==clickedButtonTag) {
[self menuButtonClicked:self btn:btn];
return;
}
}
for (CCSprite *btn in superSpeedyBtns) {
if (CGRectContainsPoint([btn boundingBox], location) && btn.tag==clickedButtonTag) {
[NSObject cancelPreviousPerformRequestsWithTarget:self selector:@selector(invalidateSuperSpeedyStarterBtns) object:nil];
for (CCSprite *btn in superSpeedyBtns) {
btn.visible=NO;
btn.tag=nothing;
}
if (clickedButtonTag==speedyStarter)
[self.delegate speedyStarterUsedWithTag:@"Super Speedy Starter"];
else if(clickedButtonTag==speedyStarter2x)
[self.delegate speedyStarterUsedWithTag:@"Super Speedy Starter 2x"];
return;
}
}
clickedButtonTag=nothing;
}
// isAccelerating=NO;
}
-(void)hideNode:(CCNode *)node{
node.visible=NO;
node.tag=nothing;
}
-(void)invalidateSuperSpeedyStarterBtns{
for (CCSprite *btn in superSpeedyBtns) {
CCFadeOut *fadeOut=[CCFadeOut actionWithDuration:2.0f];
CCCallFuncO *hide=[CCCallFuncO actionWithTarget:self selector:@selector(hideNode:) object:btn];
CCSequence *sequence=[CCSequence actions:fadeOut,hide, nil];
[btn runAction:sequence];
}
}
-(void)disableAllPowerUps{
for (PowerUp *pUp in [powerUpSpriteObjs allValues]) {
pUp.inValidateNextDisableCall=NO;
}
self.hasMagnet=NO;
self.hasCoinMultiplier=NO;
self.bikeSprite.opacity=255.0f;
self.isInvincible=NO;
self.hasAmmo=NO;
isAccelerating=NO;
}
-(void)accelerometer:(UIAccelerometer *)accelerometer didAccelerate:(UIAcceleration *)acceleration{
static float prevX=0, prevY=0;
#define kFilterFactor 1.0f // don't use filter. the code is here just as an example
float accelX = (float) acceleration.x * kFilterFactor + (1- kFilterFactor)*prevX;
float accelY = (float) acceleration.y * kFilterFactor + (1- kFilterFactor)*prevY;
prevX = accelX;
prevY = accelY;
// accelerometer values are in "Portrait" mode. Change them to Landscape left
float angle=(accelX*45)+getPositionalRotaionAngle(self.bikeSprite.position);
CCRotateTo *rotateTo=[CCRotateTo actionWithDuration:0.1 angle:angle];//accelX*maxInclination
[self.bikeSprite runAction:rotateTo];
float absRotation;
if (self.bikeSprite.rotation<0)
absRotation=-1*self.bikeSprite.rotation;
else
absRotation=self.bikeSprite.rotation;
//NSLog(@"absrotation--->%f",absRotation);
absRotation*=7;
//NSLog(@"absrotation after--->%f",absRotation);
CGPoint pos=self.bikeSprite.position;
//float actionDuration=0.10f*(MAXSPEED/currentSpeed);
if (pos.x+(accelX*XSHIFTCONST)>30.0f && pos.x+(accelX*XSHIFTCONST)<290.0f) {
// CCMoveBy *moveBy=[CCMoveBy actionWithDuration:actionDuration position:ccp(accelX*absRotation, 0.0f)];
CCMoveBy *moveBy=[CCMoveBy actionWithDuration:0.14f position:ccp(accelX*XSHIFTCONST, 0.0f)];
//NSLog(@"x pos shift--->%f",accelX*150);
[self.bikeSprite runAction:moveBy];
}
else if(pos.x>30.0f && accelX<0.0f){
// CCMoveTo *moveBy=[CCMoveTo actionWithDuration:actionDuration position:ccp(20.1f, pos.y)];
CCMoveTo *moveBy=[CCMoveTo actionWithDuration:0.14f position:ccp(30.1f, pos.y)];
[self.bikeSprite runAction:moveBy];
}
else if(pos.x<290.0f && accelX>0.0f){
// CCMoveTo *moveBy=[CCMoveTo actionWithDuration:actionDuration position:ccp(299.9f, pos.y)];
CCMoveTo *moveBy=[CCMoveTo actionWithDuration:0.14f position:ccp(289.9f, pos.y)];
[self.bikeSprite runAction:moveBy];
}
// NSLog(@"acceleration--->%f,%f",accelX,accelX);
}
-(float)getXTransition:(float)yPos currentPos:(CGPoint)crntPos{
// NSLog(@"crntpos--->%f,%f,%f yps-->%f",MAXPOINT,crntPos.x,crntPos.y,yPos);
float YDiff=MAXPOINT-crntPos.y;
float XDiff=winSize.width/2-crntPos.x;
float dltX;
if (XDiff!=0) {
float grad=YDiff/XDiff;
dltX=crntPos.x-((crntPos.y-yPos)/grad);
}
else
dltX=winSize.width/2;
// NSLog(@"xdif-->%f,ydiff-->%f",XDiff,YDiff);
return dltX;
}
-(void)updatePositionAndScale:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both{
CGPoint prevPos;
NSString *arrayType;
if ([sideBarStripes containsObject:[array objectAtIndex:0]])
arrayType=@"sideBar";
else if([roadStripes containsObject:[array objectAtIndex:0]])
arrayType=@"road";
else if([trees containsObject:[array objectAtIndex:0]])
arrayType=@"tree";
for (CCSprite *stripe in array) {
prevPos=stripe.position;
float XPos=[self getXTransition:stripe.position.y-currentSpeed currentPos:stripe.position];
stripe.position=ccp(XPos, stripe.position.y-currentSpeed);
if (stripe.position.y<0 || (stripe.position.x+stripe.contentSize.width<0 || stripe.position.x-stripe.contentSize.width>winSize.width)) {
int indx=[array indexOfObject:stripe];
int targetedStripeIndx;
if (arrayType==@"sideBar") {
if (indx%NOOFSIDEBARSTRIPESPERCOL==0) {
targetedStripeIndx=indx+(NOOFSIDEBARSTRIPESPERCOL-1);
}
else
targetedStripeIndx=indx-1;
}
else if(arrayType==@"road"){
if (indx%NOOFROADSTRIPESPERCOL==0) {
targetedStripeIndx=indx+(NOOFROADSTRIPESPERCOL-1);
}
else
targetedStripeIndx=indx-1;
}
else if(arrayType==@"tree"){
if (indx%NOOFTREESPERCOL==0) {
targetedStripeIndx=indx+(NOOFTREESPERCOL-1);
}
else
targetedStripeIndx=indx-1;
}
CCSprite *targetStripe=[array objectAtIndex:targetedStripeIndx];
if(arrayType==@"sideBar"){
XPos=[self getXTransition:targetStripe.position.y+BARSTRIPESAPCONST currentPos:prevPos];
stripe.position=ccp(XPos, targetStripe.position.y+BARSTRIPESAPCONST);
}
else if(arrayType==@"road"){
XPos=[self getXTransition:targetStripe.position.y+ROADSTRIPESAPCONST currentPos:prevPos];
stripe.position=ccp(XPos, targetStripe.position.y+ROADSTRIPESAPCONST);
}
else if(arrayType==@"tree"){
XPos=[self getXTransition:targetStripe.position.y+TREESEPCONST currentPos:prevPos];
stripe.position=ccp(XPos, targetStripe.position.y+TREESEPCONST);
}
}
//NSLog(@"stripe pos--->%f,%f",stripe.position.x,stripe.position.y);
if (both){
if (arrayType==@"sideBar" || arrayType==@"tree") {
stripe.scale=winSize.height/(winSize.height+stripe.position.y);
}
else if(arrayType==@"road")
//stripe.scale=winSize.height/((winSize.height*2)+(stripe.position.y*10));
stripe.scale=winSize.height/((stripe.contentSize.height*2)+(stripe.position.y*3));
}
else if(scaleX)
stripe.scaleX=winSize.height/((stripe.contentSize.height*2)+(stripe.position.y*3));
else
stripe.scaleY=winSize.height/((stripe.contentSize.height*2)+(stripe.position.y*3));
}
}
-(void)updatePositionAndScaleForV:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both{
CGPoint prevPos;
noOfVs=[movingVehicles count];
for (vCount=0;vCount<noOfVs;vCount++) {
int i=vCount;
Obstacles *stripe=[movingVehicles objectAtIndex:i];
prevPos=stripe.position;
float XPos;
if (stripe.isToChangeLane==YES && stripe.position.y<=stripe.laneChangeDest.y &&
(
(stripe.laneChangeDir>0 && stripe.laneChangeDest.x-stripe.position.x>0) ||
(stripe.laneChangeDir<0 && stripe.position.x-stripe.laneChangeDest.x>0)
)
) {
XPos=stripe.position.x+stripe.laneChangeSpeed;
}
else
XPos=[self getXTransition:stripe.position.y-stripe.vehicleSpeed currentPos:stripe.position];
stripe.position=ccp(XPos, stripe.position.y-stripe.vehicleSpeed);
if (both)
stripe.scale=winSize.height/(winSize.height+stripe.position.y);
if (stripe.position.y<=-20) {
stripe.position=stripe.initialPosition;
[movingVehicles removeObject:stripe];
noOfVs--;
vCount--;
}
else if (self.hasAmmo==YES && stripe.position.y<=winSize.height-120.0f && stripe.position.y>0 && shootCount<NOOFEXPLOSIONSPRITES && stripe.isShooted!=YES) {
NSLog(@"going to shoot--->%d",shootCount);
stripe.isShooted=YES;
[self shootVehicle:vCount];
}
}
if ([movingVehicles count]==0) {
[self bringVehicleObstacles];
}
}
-(void)updatePositionAndScaleForBP:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both{
CGPoint prevPos;
int noOfBPs=[movingBikerPoints count];
for (int i=0;i<noOfBPs;i++) {
RVRCoin *stripe=[movingBikerPoints objectAtIndex:i];
prevPos=stripe.position;
float XPos=[self getXTransition:stripe.position.y-currentSpeed currentPos:stripe.position];
stripe.position=ccp(XPos, stripe.position.y-currentSpeed);
if (stripe.position.y<-20) {
[stripe resetToMyOriginalPos];
[stripe pauseSchedulerAndActions];
[movingBikerPoints removeObject:stripe];
noOfBPs--;
i--;
}
if (both){
//stripe.scale=winSize.height/((stripe.contentSize.height*4)+(stripe.position.y));
//stripe.scale=winSize.height/((stripe.contentSize.height*2)+(stripe.position.y*3));
}
}
if ([movingBikerPoints count]==0) {
if (waitingForEnablingDoubleValue==YES) {
waitingForEnablingDoubleValue=NO;
[self setDoubleValueTexturesForBikerPoints];
}
else if(waitingForResettingBikerPoints==YES){
waitingForResettingBikerPoints=NO;
[self resetTextureForBikerPoints];
}
else
[self bringBikerPoints];
}
}
-(void)updatePositionAndScaleForTree:(NSMutableArray*)array scaleX:(BOOL)scaleX saleBoth:(BOOL)both{
CGPoint prevPos;
int noOfBPs=[movingTrees count];
for (int i=0;i<noOfBPs;i++) {
CCSprite *stripe=[movingTrees objectAtIndex:i];
prevPos=stripe.position;
float XPos=[self getXTransition:stripe.position.y-currentSpeed currentPos:stripe.position];
stripe.position=ccp(XPos, stripe.position.y-currentSpeed);
if (stripe.position.y<=-110) {
[movingTrees removeObject:stripe];
noOfBPs--;
i--;
}
if (both){
stripe.scale=winSize.height/(winSize.height+stripe.position.y);
}
}
if ([movingTrees count]==0) {
[self bringTrees];
}
}
-(void)updatePositionAndScaleForPUp:(PowerUp*)pUp scaleX:(BOOL)scaleX saleBoth:(BOOL)both{
float XPos=[self getXTransition:pUp.position.y-vCurrentSpeed currentPos:pUp.position];
pUp.position=ccp(XPos, pUp.position.y-vCurrentSpeed);
if (pUp.position.y<=-20) {
isPowerUpMoving=NO;
if (shouldAlertForCollectableDisCovrd==YES){
[self.delegate newCollectablePowerUpAppearanceRequest];
}
}
if (both){
pUp.scale=winSize.height/(winSize.height+pUp.position.y);
}
}
-(BOOL)checkCollission{
BOOL collission=NO;
if (self.isInvincible==NO) {
for (Obstacles *vehicle in vehicleObstacles) {
if ([self isCollisionBetweenSpriteA:self.bikeSprite spriteB:vehicle pixelPerfect:YES]) {
vehicleCollidedWith=vehicle;
collission=YES;
}
}
}
if (isPowerUpMoving && [self isCollisionBetweenSpriteA:self.bikeSprite spriteB:movingPUp pixelPerfect:YES]) {
movingPUp.visible=NO;
[self.delegate collectablePowerUpCollected:movingPUp.stringTag];
}
/*
if (collission==NO) {
if ([self isCollisionBetweenSpriteA:self.bikeSprite spriteB:sidebarleft pixelPerfect:YES] ||
[self isCollisionBetweenSpriteA:self.bikeSprite spriteB:sidebarright pixelPerfect:YES]) {
NSLog(@"colliding with side bar");
collission=YES;
}
}
*/
return collission;
}
-(void)bikerPointsCollection{
int noOfMovingBP=[movingBikerPoints count];
BOOL isCollected=NO;
for (int i=0; i<noOfMovingBP; i++) {
RVRCoin *bPoint=[movingBikerPoints objectAtIndex:i];
BOOL isCollission=[self isCollisionBetweenSpriteA:self.bikeSprite spriteB:bPoint pixelPerfect:YES];
if (isCollission) {
[[RVRAudioManager sharedManager] playCoinCollectionSound];
[self.collectedBikerPoints addObject:bPoint];
[bPoint performCoinCollectionAndAnimation];
[movingBikerPoints removeObject:bPoint];
noOfMovingBP--;
i--;
isCollected=YES;
}
else if (self.hasMagnet==YES && CGRectIntersectsRect([bPoint boundingBox], magneticInductionRect)) {
[[RVRAudioManager sharedManager] playCoinCollectionSound];
[self.collectedBikerPoints addObject:bPoint];
[bPoint performMagnetInducedCollectionAnimation];
[movingBikerPoints removeObject:bPoint];
noOfMovingBP--;
i--;
isCollected=YES;
}
if (isCollected) {
isCollected=NO;
int coinToAdd=1;
if (self.hasCoinMultiplier==YES) {
coinToAdd=2;
}
if (self.hasDoubleValuedCoins) {
coinToAdd=coinToAdd*2;
}
appDelegate.databaseManager.userData.uCoins+=coinToAdd;
[appDelegate.gHud.coinsLabel setString:[NSString stringWithFormat:@"%d",appDelegate.databaseManager.userData.uCoins]];
}
}
if ([movingBikerPoints count]==0) {
if (waitingForEnablingDoubleValue==YES) {
waitingForEnablingDoubleValue=NO;
[self setDoubleValueTexturesForBikerPoints];
}
else if(waitingForResettingBikerPoints==YES){
waitingForResettingBikerPoints=NO;
[self resetTextureForBikerPoints];
}
else
[self bringBikerPoints];
}
}
-(void)pauseGameOnEnteringBackGround{
if (willBringGameOverMenu==NO) {
for (CCSprite *btn in menuButtons) {
btn.visible=NO;
}
self.isTouchEnabled=NO;
[self.bikeSprite pauseSchedulerAndActions];
self.isAccelerometerEnabled=NO;
[self stopAllActions];
[self pauseSchedulerAndActions];
for (RVRCoin *BPoint in movingBikerPoints) {
[BPoint pauseSchedulerAndActions];
}
for (RVRCoin *bPoint in self.collectedBikerPoints) {
[bPoint.aliasCoin pauseSchedulerAndActions];
}
for (CCSprite *child in [explosionBatchNode children]) {
[child pauseSchedulerAndActions];
}
[[RVRAudioManager sharedManager] playBikeEngineSound:NO];
//[self createAndShowPauseModeLayer];
[[PauseOrGameOverMenu menu] pauseMenu];
}
}
- (void)update:(ccTime)dt {
if (!isPUpPCAlertSent) {
isPUpPCAlertSent=YES;
[self.delegate gamePlayStarted];
}
if (isAccelerating && currentSpeed<MAXSPEED){
if (currentSpeed+ACCELERATION<MAXSPEED){
currentSpeed+=ACCELERATION;
self.isBikeAccelerating=1;
}
else{
currentSpeed=MAXSPEED;
self.isBikeAccelerating=0;
}
//temp works
vCurrentSpeed=(currentSpeed/10.0f)*3.5f;
}
else if(!isAccelerating && reducingFromMax){
if (currentSpeed+RETARDATION>speedBeforeSSS) {
currentSpeed+=RETARDATION;
self.isBikeAccelerating=-1;
}
else{
shouldAlertForCollectableDisCovrd=YES;
[self.delegate newCollectablePowerUpAppearanceRequest];
currentSpeed=speedBeforeSSS;
reducingFromMax=NO;
self.bikeSprite.opacity=255.0f;
self.isInvincible=NO;
self.isBikeAccelerating=0;
}
//temp works
vCurrentSpeed=(currentSpeed/10.0f)*3.5f;
}
else if(!isAccelerating && currentSpeed<MAXSPEED){
if (currentSpeed+NORMALACCELERATION<MAXSPEED) {
currentSpeed+=NORMALACCELERATION;
self.isBikeAccelerating=1;
}
else{
currentSpeed=MAXSPEED;
self.isBikeAccelerating=0;
}
//temp works
vCurrentSpeed=(currentSpeed/10.0f)*3.5f;
}
self.bSpeed=currentSpeed;
distanceInMiles+=(MILETOPIXELRATIO*currentSpeed);
[appDelegate.gHud.distanceLabel setString:[NSString stringWithFormat:@"%0.2f M",distanceInMiles]];
if (distanceInMiles>=distMsg.distanceForCollectable && shouldAlertForCollectableDisCovrd==YES) {
distMsg.distanceForCollectable=HUGE_VALF;
[self.delegate distanceCoveredFor:collectable];
}
if (distanceInMiles>=distMsg.distanceForAutomatic && shouldAlertForCollectableDisCovrd==YES) {
distMsg.distanceForAutomatic=HUGE_VALF;
[self.delegate distanceCoveredFor:automatic];
}
if (distanceInMiles>=distMsg.distanceForSuperSpeedyStarter) {
reducingFromMax=YES;
distMsg.distanceForSuperSpeedyStarter=HUGE_VALF;
}
//speedInMilesPerH=(MILETOPIXELRATIO*(currentSpeed*60.0f*60.0f))*MINTOHRRATIO;
//[appDelegate.gHud.speedLabel setString:[NSString stringWithFormat:@"%0.1f",speedInMilesPerH]];
//appDelegate.gHud.meterArrow.rotation=METERARROWINIROTCONST+((speedInMilesPerH-70.0f)*(METERARROWROTATIONMAXADD/90.0f));
//NSLog(@"rotation--->%f, speed--->%f",meterArrow.rotation,speedInMilesPerH);
//NSLog(@"distance --->%f currentspeed--->%Lf speed--->%f",distanceInMiles,MILETOPIXELRATIO*(currentSpeed*120.0f*60.0f),speedInMilesPerH);
[self updatePositionAndScale:roadStripes scaleX:NO saleBoth:YES];
[self updatePositionAndScale:sideBarStripes scaleX:NO saleBoth:YES];
if (isVehicleMoving==YES) {
[self updatePositionAndScaleForV:movingVehicles scaleX:NO saleBoth:YES];
}
if (isBikerPointsMoving==YES) {
[self updatePositionAndScaleForBP:movingBikerPoints scaleX:NO saleBoth:YES];
[self bikerPointsCollection];
}
if (isPowerUpMoving==YES) {
[self updatePositionAndScaleForPUp:movingPUp scaleX:NO saleBoth:YES];
}
if (isTreesMoving==YES) {
[self updatePositionAndScaleForTree:movingTrees scaleX:NO saleBoth:YES];
}
if ([self checkCollission]==YES) {
[self unscheduleUpdate];
self.isAccelerometerEnabled=NO;
willBringGameOverMenu=YES;
self.gameOverCount+=1;
[[RVRAudioManager sharedManager] playExplosionSound];
[self crushBike];
}
}
// on "dealloc" you need to release all your retained objects
- (void) dealloc
{
// in case you have something to dealloc, do it in this method
// in this particular example nothing needs to be released.
// cocos2d will automatically release all the children (Label)
[NSObject cancelPreviousPerformRequestsWithTarget:self];
[roadStripes removeAllObjects];
roadStripes=nil;
[roadOverlays removeAllObjects];
roadOverlays=nil;
[vehicleObstacles removeAllObjects];
vehicleObstacles=nil;
[sideBarStripes removeAllObjects];
sideBarStripes=nil;
[movingVehicles removeAllObjects];
movingVehicles=nil;
[menuButtons removeAllObjects];
menuButtons=nil;
[bikerPoints removeAllObjects];
bikerPoints=nil;
[movingBikerPoints removeAllObjects];
movingBikerPoints=nil;
[self.collectedBikerPoints removeAllObjects];
self.collectedBikerPoints=nil;
[explosionBNChilds removeAllObjects];
explosionBNChilds=nil;
[trees removeAllObjects];
trees=nil;
[movingTrees removeAllObjects];
movingTrees=nil;
[powerUpSpriteObjs removeAllObjects];
powerUpSpriteObjs=nil;
[superSpeedyBtns removeAllObjects];
superSpeedyBtns=nil;
for (CCNode *child in self.children) {
[[CCActionManager sharedManager] removeAllActionsFromTarget:child];
}
[[CCScheduler sharedScheduler] unscheduleAllSelectorsForTarget:self];
[[CCTextureCache sharedTextureCache] removeTexture:[[_rt sprite] texture]];
// don't forget to call "super dealloc"
[super dealloc];
}
#pragma MARK - RVRPowerUpsAndPurchasesDelegate Methods Implementation
-(void)alertWhenDistanceIs:(float)distance alertFor:(int)tag{
if (tag==collectable) {
distMsg.distanceForCollectable=distance;
}
else if (tag==automatic) {
distMsg.distanceForAutomatic=distance;
}
}
-(void)showPowerUpWithKey:(NSString *)key{
[self bringPowerUpWithKey:key];
}
-(void)setInvincibilityMode:(BOOL)isYes{
self.isInvincible=isYes;
if (isYes==YES) {
self.bikeSprite.opacity=127.0f;
}
else
self.bikeSprite.opacity=255.0f;
}
-(void)enablePowerUpWithKey:(NSString *)key{
if ([key isEqualToString:@"Magnet"]) {
if (self.hasMagnet==YES) {
NSLog(@"was enabled");
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
pUp.inValidateNextDisableCall=YES;
}
else
self.hasMagnet=YES;
}
else if([key isEqualToString:@"Multiplier"]){
if (self.hasCoinMultiplier==YES) {
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
pUp.inValidateNextDisableCall=YES;
}
else
self.hasCoinMultiplier=YES;
}
else if([key isEqualToString:@"Invincibility"]){
if (self.isInvincible==YES) {
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
pUp.inValidateNextDisableCall=YES;
}
else{
self.bikeSprite.opacity=127.0f;
self.isInvincible=YES;
}
}
else if([key isEqualToString:@"Shooter"]){
if (self.hasAmmo==YES) {
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
pUp.inValidateNextDisableCall=YES;
}
else
self.hasAmmo=YES;
}
else if([key isEqualToString:@"NOS"]){
if (isAccelerating==YES) {
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
pUp.inValidateNextDisableCall=YES;
}
else
isAccelerating=YES;
}
else if([key isEqualToString:@"doubleValue"]){
waitingForEnablingDoubleValue=YES;
}
//Magnet,Multiplier,Invincibility,Shooter,Double Value Points,NOS
}
-(void)disablePowerUpWithKey:(NSString *)key{
PowerUp *pUp=[powerUpSpriteObjs objectForKey:key];
if (pUp.inValidateNextDisableCall==YES) {
NSLog(@"previous call invalidated");
pUp.inValidateNextDisableCall=NO;
}
else if ([key isEqualToString:@"Magnet"]) {
self.hasMagnet=NO;
}
else if([key isEqualToString:@"Multiplier"]){
self.hasCoinMultiplier=NO;
}
else if([key isEqualToString:@"Invincibility"]){
self.bikeSprite.opacity=255.0f;
self.isInvincible=NO;
}
else if([key isEqualToString:@"Shooter"]){
//NSLog(@"disable shooter");
self.hasAmmo=NO;
}
else if([key isEqualToString:@"NOS"]){
isAccelerating=NO;
}
/*
if (shouldAlertForCollectableDisCovrd==YES) {
[self.delegate newCollectablePowerUpAppearanceRequest];
}*/
}
-(void)addSuperSpeedyButtons:(NSArray *)values{
superSpeedyBtns=[[NSMutableArray alloc] init];
CCSprite *btn;
CGSize prevSize;
for (DBPurchase *purchase in values) {
NSString *keyString=purchase.purchaseIcon;
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:[NSString stringWithFormat:@"%@",keyString]]];
btn.anchorPoint=ccp(0.0f, 0.0f);
btn.position=ccp(0.0f, 0.0f+prevSize.height);
if ([keyString isEqualToString:@"superspeed"]) {
btn.tag=speedyStarter;
}
else
btn.tag=speedyStarter2x;
[self addChild:btn z:100];
[superSpeedyBtns addObject:btn];
prevSize=btn.contentSize;
}
[self performSelector:@selector(invalidateSuperSpeedyStarterBtns) withObject:nil afterDelay:TWOLESSSPEEDYSTARTERBTNHIDETIME];
}
-(void)startWithSuperSpeed:(float)distToCover{
[self disableAllPowerUps];
if (self.hasDoubleValuedCoins==YES) {
waitingForResettingBikerPoints=YES;
}
shouldAlertForCollectableDisCovrd=NO;
speedBeforeSSS=INITIALSPEED;
distMsg.distanceForSuperSpeedyStarter=self.distanceInMiles+distToCover;
self.bikeSprite.opacity=127.0f;
self.isInvincible=YES;
currentSpeed=MAXSPEED;
}
#pragma MARK - Button Control Methods
-(void)createMenuButtons{
if (pauseModeLayer!=nil) {
[pauseModeLayer removeAllChildrenWithCleanup:YES];
[pauseModeLayer removeFromParentAndCleanup:YES];
pauseModeLayer=nil;
}
[menuButtons removeAllObjects];
CCSprite *btn;
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"button-pause"]];
btn.anchorPoint=ccp(1.0f, 0.0f);
btn.position=ccp(winSize.width, 0.0f);
[self addChild:btn z:100];
btn.tag=buttonpause;
[menuButtons addObject:btn];
}
-(void)addButtonsToPauseModeLayer{
CCSprite *btn;
CGSize layerSize=pauseModeLayer.contentSize;
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"button-play"]];
btn.anchorPoint=ccp(0.5f, 0.0f);
btn.position=ccp(layerSize.width/2, layerSize.height/2+10.0f);
[pauseModeLayer addChild:btn];
btn.tag=buttonplay;
[menuButtons addObject:btn];
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"button-replay"]];
btn.anchorPoint=ccp(1.0f, 1.0f);
btn.position=ccp(layerSize.width/2-(btn.contentSize.width/2), layerSize.height/2-10.0f);
[pauseModeLayer addChild:btn];
btn.tag=buttonreplay;
[menuButtons addObject:btn];
btn=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"button-home"]];
btn.anchorPoint=ccp(0.0f, 1.0f);
btn.position=ccp(layerSize.width/2+(btn.contentSize.width/2), layerSize.height/2-10.0f);
[pauseModeLayer addChild:btn];
btn.tag=buttonhome;
[menuButtons addObject:btn];
}
-(void)createAndShowPauseModeLayer{
for (CCSprite *btn in menuButtons) {
[btn removeFromParentAndCleanup:YES];
}
[menuButtons removeAllObjects];
pauseModeLayer=[CCLayerColor layerWithColor:ccc4(50.0f, 50.0f, 50.0f, 167.0f) width:winSize.width height:winSize.height];
[self addChild:pauseModeLayer z:100];
[self addButtonsToPauseModeLayer];
}
-(BOOL)menuButtonClicked:(id)sender btn:(CCSprite *)btn{
// NSLog(@"tag--->%d",btn.tag);
if (btn.tag==buttonpause) {
for (CCSprite *btn in menuButtons) {
btn.visible=NO;
}
self.isTouchEnabled=NO;
[self.bikeSprite pauseSchedulerAndActions];
self.isAccelerometerEnabled=NO;
[self stopAllActions];
[self pauseSchedulerAndActions];
for (RVRCoin *BPoint in movingBikerPoints) {
[BPoint pauseSchedulerAndActions];
}
for (RVRCoin *bPoint in self.collectedBikerPoints) {
[bPoint.aliasCoin pauseSchedulerAndActions];
}
for (CCSprite *child in [explosionBatchNode children]) {
[child pauseSchedulerAndActions];
}
[[RVRAudioManager sharedManager] playBikeEngineSound:NO];
//[self createAndShowPauseModeLayer];
[[PauseOrGameOverMenu menu] pauseMenu];
}
return NO;
}
-(void)bringGameOverMenu:(id)sender{
for (CCSprite *btn in menuButtons) {
btn.visible=NO;
}
self.isTouchEnabled=NO;
[self.bikeSprite pauseSchedulerAndActions];
self.isAccelerometerEnabled=NO;
[self stopAllActions];
[self pauseSchedulerAndActions];
for (RVRCoin *BPoint in movingBikerPoints) {
[BPoint pauseSchedulerAndActions];
}
for (RVRCoin *bPoint in self.collectedBikerPoints) {
[bPoint.aliasCoin pauseSchedulerAndActions];
}
for (CCSprite *child in [explosionBatchNode children]) {
[child pauseSchedulerAndActions];
}
[[RVRAudioManager sharedManager] playBikeEngineSound:NO];
//[self createAndShowPauseModeLayer];
[[PauseOrGameOverMenu menu] gameOverMenu];
if (((int64_t)distanceInMiles)>appDelegate.databaseManager.userData.myHighScore){
[[NSUserDefaults standardUserDefaults] setBool:NO forKey:@"isGCScoreSubmitted"];
appDelegate.databaseManager.highScoreChanged=YES;
appDelegate.databaseManager.userData.myHighScore=((int64_t)distanceInMiles);
}
}
#pragma MARK - PauseOrGameOverMenuBtnClickDelegate Methods Implementation
-(void)disableInvincibilityAfterRevive:(id)sender{
self.isInvincible=NO;
}
-(void)buttonClickedWithTag:(int)tag{
if(tag==resume){
willBringGameOverMenu=NO;
for (CCSprite *btn in menuButtons) {
btn.visible=YES;
}
self.isTouchEnabled=YES;
[self.bikeSprite resumeSchedulerAndActions];
self.isAccelerometerEnabled=YES;
[self resumeSchedulerAndActions];
for (RVRCoin *BPoint in movingBikerPoints) {
[BPoint resumeSchedulerAndActions];
}
for (RVRCoin *bPoint in self.collectedBikerPoints) {
[bPoint.aliasCoin resumeSchedulerAndActions];
}
for (CCSprite *child in [explosionBatchNode children]) {
[child resumeSchedulerAndActions];
}
[[RVRAudioManager sharedManager] playBikeEngineSound:YES];
//[self createMenuButtons];
}
else if(tag==revive){
willBringGameOverMenu=NO;
appDelegate.databaseManager.userData.uCoins-=1000;
[appDelegate.gHud.coinsLabel setString:[NSString stringWithFormat:@"%d",appDelegate.databaseManager.userData.uCoins]];
[self disableAllPowerUps];
if (self.hasDoubleValuedCoins==YES) {
waitingForResettingBikerPoints=YES;
}
shouldAlertForCollectableDisCovrd=NO;
speedBeforeSSS=INITIALSPEED;
distMsg.distanceForSuperSpeedyStarter=0.0f;
self.bikeSprite.opacity=127.0f;
self.isInvincible=YES;
currentSpeed=MAXSPEED;
for (CCSprite *btn in menuButtons) {
btn.visible=YES;
}
self.bikeSprite.visible=YES;
self.isTouchEnabled=YES;
[self.bikeSprite resumeSchedulerAndActions];
self.isAccelerometerEnabled=YES;
[self resumeSchedulerAndActions];
//cause after game over pause was called as a delegate!
[self scheduleUpdate];
for (RVRCoin *BPoint in movingBikerPoints) {
[BPoint resumeSchedulerAndActions];
}
for (RVRCoin *bPoint in self.collectedBikerPoints) {
[bPoint.aliasCoin resumeSchedulerAndActions];
}
for (CCSprite *child in [explosionBatchNode children]) {
[child resumeSchedulerAndActions];
}
[[RVRAudioManager sharedManager] playBikeEngineSound:YES];
}
else if(tag==playagain){
if (((int64_t)distanceInMiles)>appDelegate.databaseManager.userData.myHighScore){
[[NSUserDefaults standardUserDefaults] setBool:NO forKey:@"isGCScoreSubmitted"];
appDelegate.databaseManager.highScoreChanged=YES;
appDelegate.databaseManager.userData.myHighScore=((int64_t)distanceInMiles);
}
[[RVRAudioManager sharedManager] playBikeEngineSound:NO];
[[CCDirector sharedDirector] replaceScene: [CCTransitionFade transitionWithDuration:1.0f scene:[GameNode scene]]];
}
else if(tag==mainmenu){
if (((int64_t)distanceInMiles)>appDelegate.databaseManager.userData.myHighScore){
[[NSUserDefaults standardUserDefaults] setBool:NO forKey:@"isGCScoreSubmitted"];
appDelegate.databaseManager.highScoreChanged=YES;
appDelegate.databaseManager.userData.myHighScore=((int64_t)distanceInMiles);
}
[[RVRAudioManager sharedManager] playBikeEngineSound:NO];
appDelegate.gamePlayRunning=NO;
[[CCDirector sharedDirector] replaceScene: [CCTransitionFade transitionWithDuration:1.0f scene:[GameMenu scene]]];
}
// NSLog(@"distance --->%lld",((int64_t)distanceInMiles));
}
#pragma MARK - BatchNode And Animation Control Methods
-(void)loadExplosionBatchNode{
explosionBNChilds=[[NSMutableArray alloc] init];
explosionBatchNode=[CCSpriteBatchNode batchNodeWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).batchTextures objectForKey:@"explosion"]];
explosionBatchNode.visible=NO;
[self addChild:explosionBatchNode z:100];
CGSize frameSize=CGSizeMake(explosionBatchNode.texture.contentSize.width/EXPLOSIONXFRAMES, explosionBatchNode.texture.contentSize.height/EXPLOSIONYFRAMES);
// NSLog(@"contentsize--->%f,%f",explosionBatchNode.textureAtlas.texture.contentSize.width,explosionBatchNode.textureAtlas.texture.contentSize.height);
CCSprite *explosionSprite;
for (int i=0; i<NOOFEXPLOSIONSPRITES; i++) {
explosionSprite=[CCSprite spriteWithBatchNode:explosionBatchNode rect:CGRectMake(0, 0, frameSize.width, frameSize.height)];
explosionSprite.visible=NO;
[explosionBatchNode addChild:explosionSprite];
[explosionBNChilds addObject:explosionSprite];
}
}
#pragma MARK - AnimationAction Methods
-(void)hideExplosionSprite:(CCSprite*)bullet{
bullet.visible=NO;
shootCount--;
}
-(void)animateExplosion:(CCSprite*)bullet{
[[RVRAudioManager sharedManager] playExplosionSound];
CCAnimate *explosion = [CCAnimate actionWithAnimation:[[CCAnimationCache sharedAnimationCache] animationByName:@"explosionAnim"] restoreOriginalFrame:YES];
CCCallFuncO *callHideExplosionMthd=[CCCallFuncO actionWithTarget:self selector:@selector(hideExplosionSprite:) object:bullet];
CCSequence *sequence=[CCSequence actions:explosion,callHideExplosionMthd, nil];
[bullet runAction:sequence];
}
-(void)hideVehicle:(NSArray*)array{
//[[RVRAudioManager sharedManager] playFiringSound:NO];
NSNumber *num=[array objectAtIndex:0];
NSInteger indx=[num intValue];
Obstacles *vehicle=[movingVehicles objectAtIndex:indx];
CCSprite *shootSprite=[array objectAtIndex:1];
vehicle.visible=NO;
[self animateExplosion:shootSprite];
}
-(BOOL)shootVehicle:(NSInteger)movingVIndx{
Obstacles *vehicle=[movingVehicles objectAtIndex:movingVIndx];
float xShift=self.bikeSprite.contentSize.height*sinf((PI/180.0f)*self.bikeSprite.rotation);
float yShift=self.bikeSprite.contentSize.height*cosf((PI/180.0f)*self.bikeSprite.rotation);
CCSprite *shootSprite=((CCSprite*)[explosionBNChilds objectAtIndex:shootCount++]);
NSLog(@"shootcount--->%d",shootCount);
float actionDuration=(0.5f/winSize.height)*ccpDistance(shootSprite.position, vehicle.position);
float vTargetYShift=(vehicle.vehicleSpeed*actionDuration);
if (self.isBikeAccelerating>0)
vTargetYShift+=(0.5f*ACCELERATION*pow(actionDuration, 2));
else if(self.isBikeAccelerating<0)
vTargetYShift+=(0.5f*RETARDATION*pow(actionDuration, 2));
shootSprite.position=CGPointMake(self.bikeSprite.position.x+xShift, self.bikeSprite.position.y+yShift);
shootSprite.visible=YES;
[[RVRAudioManager sharedManager] playFiringSound];
CCMoveTo *fire=[CCMoveTo actionWithDuration:actionDuration position:CGPointMake(vehicle.position.x, vehicle.position.y-vehicle.contentSize.height-vTargetYShift)];
NSArray *array=[NSArray arrayWithObjects:[NSNumber numberWithInt:movingVIndx],shootSprite, nil];
//CCCallFuncO *hideV=[CCCallFuncO actionWithTarget:self selector:@selector(hideVehicle:) object:array];
//CCSequence *sequence=[CCSequence actions:fire,hideV, nil];
[shootSprite runAction:fire];
[self performSelector:@selector(hideVehicle:) withObject:array afterDelay:actionDuration];
return YES;
}
-(void)crushBike{
CCSprite *shootSprite=((CCSprite*)[explosionBNChilds lastObject]);
shootSprite.position=CGPointMake(self.bikeSprite.position.x, self.bikeSprite.position.y+self.bikeSprite.contentSize.height);
shootSprite.visible=YES;
self.bikeSprite.visible=NO;
CCAnimate *explosion = [CCAnimate actionWithAnimation:[[CCAnimationCache sharedAnimationCache] animationByName:@"explosionAnim"] restoreOriginalFrame:YES];
CCCallFuncO *callHideExplosionMthd=[CCCallFuncO actionWithTarget:self selector:@selector(hideExplosionSprite:) object:shootSprite];
//CCCallFuncO *backToMenu=[CCCallFuncO actionWithTarget:[CCDirector sharedDirector] selector:@selector(replaceScene:) object:[CCTransitionFade transitionWithDuration:1.0f scene:[GameMenu scene]]];
CCCallFunc *backToMenu=[CCCallFunc actionWithTarget:self selector:@selector(bringGameOverMenu:)];
CCSequence *sequence=[CCSequence actions:explosion,callHideExplosionMthd,backToMenu, nil];
[shootSprite runAction:sequence];
}
@end
<file_sep>//
// RVRPowerUpsAndPurchasesStore.h
// MotoRacer
//
// Created by <NAME> on 7/10/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "RootViewController.h"
#import "DBUpgrades.h"
#import "DBPurchase.h"
#import "GamePlayObserverDelegateProtocol.h"
@protocol PowerUpsAndPurchasesAlertDelegate <NSObject>
@required
-(void)powerUpUpgradedWithItem:(DBUpgrades*)dataObj;
-(void)singlePurchaseMadeWithItem:(DBPurchase*)dataObj;
@end
#define NOOFSECTIONS 2
#define HIGHTFORROW 100
#define TABLECELLXOFFSET 64.0F
#define LFONTSIZE 15.0F
@class RVRAppDelegate;
@interface RVRPowerUpsAndPurchasesStore : RootViewController<UITableViewDelegate,UITableViewDataSource>{
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
UITableViewController *tVC;
NSMutableArray *sectionTitleViews;
NSInteger clickedBtntag;
}
+(RVRPowerUpsAndPurchasesStore*)powerUpsAndPurchaseStore;
-(void)uiViewAnimaitonDidFinish;
@property (nonatomic,readwrite,retain) id<ControllerMenu> controllerMenu;
@property (nonatomic,readwrite,retain) id<PowerUpsAndPurchasesAlertDelegate> delegate;
@end
<file_sep>//
// PowerUp.h
// MotoRacer
//
// Created by <NAME> on 7/22/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
@class RVRAppDelegate;
@interface PowerUp : CCSprite {
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
}
@property (nonatomic,readwrite,retain) NSString *stringTag;
@property (nonatomic) BOOL inValidateNextDisableCall;
+(id)generatePowerUpWithTexture:(CCTexture2D*)texture;
-(void)resetProperties;
@end
<file_sep>//
// BikeNode.m
// MotoRacer
//
// Created by <NAME> on 6/3/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import "BikeLayer.h"
#import "TextureManager.h"
static BikeLayer *bike;
@implementation BikeLayer
@synthesize bikeSprite;
-(id)init{
if ((self=[super init])) {
//self.isAccelerometerEnabled=YES;
CGSize winSize=[[CCDirector sharedDirector] winSize];
bikeSprite=[CCSprite spriteWithTexture:[((TextureManager*)[TextureManager sharedTextureManager]).allTextures objectForKey:@"bikeTexture"]];
bikeSprite.anchorPoint=ccp(0.5f, 0.0f);
bikeSprite.position=ccp(winSize.width/2, 0.0f);
[self addChild:bikeSprite];
}
return self;
}
+(id)bike{
if (bike==nil) {
bike=[[BikeLayer alloc] init];
}
return bike;
}
-(void)accelerometer:(UIAccelerometer *)accelerometer didAccelerate:(UIAcceleration *)acceleration{
#define kFilterFactor 1.0f // don't use filter. the code is here just as an example
static float prevX=0, prevY=0;
float accelX = (float) acceleration.x * kFilterFactor + (1- kFilterFactor)*prevX;
float accelY = (float) acceleration.y * kFilterFactor + (1- kFilterFactor)*prevY;
prevX = accelX;
prevY = accelY;
CCRotateTo *rotateTo=[CCRotateTo actionWithDuration:0.1 angle:-(accelY*45)];
[self.bikeSprite runAction:rotateTo];
/*
float absRotation;
if (((BikeLayer*)[BikeLayer bike]).bikeSprite.rotation<0)
absRotation=-1*((BikeLayer*)[BikeLayer bike]).bikeSprite.rotation;
else
absRotation=((BikeLayer*)[BikeLayer bike]).bikeSprite.rotation;
absRotation*=3;
if (appDelegate.bikeReference.position.x+(-accelY*absRotation)>120 && appDelegate.bikeReference.position.x+(-accelY*absRotation)<360) {
CCMoveBy *moveBy=[CCMoveBy actionWithDuration:0.1 position:ccp(-accelY*absRotation, appDelegate.bikeReference.position.y)];
[appDelegate.bikeReference runAction:moveBy];
}
else if(appDelegate.bikeReference.position.x>120 && accelY>=0){
CCMoveTo *moveBy=[CCMoveTo actionWithDuration:0.1 position:ccp(120.1f, appDelegate.bikeReference.position.y)];
[appDelegate.bikeReference runAction:moveBy];
}
else if(appDelegate.bikeReference.position.x<360 && accelY<0){
CCMoveTo *moveBy=[CCMoveTo actionWithDuration:0.1 position:ccp(359.9f, appDelegate.bikeReference.position.y)];
[appDelegate.bikeReference runAction:moveBy];
}
*/
}
@end
<file_sep>//
// DBUserData.h
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import <sqlite3.h>
@class RVRAppDelegate;
@interface DBUserData : NSObject{
@private
RVRAppDelegate *appDelegate;
NSInteger primaryKey;
}
@property (nonatomic,readwrite,retain) NSString *UDID,*uName,*c2,*c3;
@property (nonatomic,readwrite) int uID,uCoins;
@property (nonatomic,readwrite) int64_t myHighScore;
- (id)initWithPrimaryKey:(NSInteger)UDpk database:(sqlite3 *)UDdb;
- (int)insertIntoDatabase:(sqlite3 *)UDdb;
- (BOOL)updateDatabase:(NSInteger)UDpk database:(sqlite3 *)UDdb;
- (BOOL)deleteDatabase:(NSInteger)UDpk database:(sqlite3 *)UDdb;
@end
<file_sep>//
// RVRAudioManager.m
// MotoRacer
//
// Created by <NAME> on 8/29/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "RVRAudioManager.h"
static RVRAudioManager *sharedAudioManager=nil;
@interface RVRAudioManager (Private)
-(void)preloadAllSounds;
@end
@implementation RVRAudioManager
@synthesize mute;
-(id)init{
if ((self=[super init])) {
BOOL isMuted=[[NSUserDefaults standardUserDefaults] boolForKey:@"soundState"];
if (isMuted==NO) {
[self preloadAllSounds];
}
else self.mute=YES;
}
return self;
}
+(id)sharedManager{
if (sharedAudioManager==nil) {
sharedAudioManager=[[self alloc] init];
}
return sharedAudioManager;
}
-(void)preloadAllSounds{
bgMusic=[[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"bgMusic" ofType:@"mp3"]] error:nil];
bgMusic.numberOfLoops=-1;
[bgMusic prepareToPlay];
bikeEngine=[[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"bikeEngine" ofType:@"mp3"]] error:nil];
bikeEngine.numberOfLoops=-1;
[bikeEngine prepareToPlay];
buttonClicked=[[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"buttonClick" ofType:@"mp3"]] error:nil];
[buttonClicked prepareToPlay];
/*
coinSound=[[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"coinSound" ofType:@"mp3"]] error:nil];
[coinSound prepareToPlay];
firing=[[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"firing" ofType:@"wav"]] error:nil];
[firing prepareToPlay];
explosion=[[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"explosion" ofType:@"wav"]] error:nil];
[explosion prepareToPlay];
*/
CFURLRef coinSoundURL = (CFURLRef ) [NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"coinSound" ofType:@"wav"]];
AudioServicesCreateSystemSoundID (coinSoundURL, &coinSound);
CFURLRef firingSoundURL = (CFURLRef ) [NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"firing" ofType:@"wav"]];
AudioServicesCreateSystemSoundID (firingSoundURL, &firing);
CFURLRef explosionSoundURL = (CFURLRef ) [NSURL fileURLWithPath:[[NSBundle mainBundle] pathForResource:@"explosion" ofType:@"wav"]];
AudioServicesCreateSystemSoundID (explosionSoundURL, &explosion);
soundsPreloaded=YES;
}
-(void)playBGMusic:(BOOL)play{
if (!mute) {
if (bgMusic.playing==NO && play==YES) {
[bgMusic play];
}
else if(bgMusic.playing==YES && play==NO){
[bgMusic pause];
bgMusic.currentTime=0;
}
}
}
-(void)playBikeEngineSound:(BOOL)play{
if (!mute) {
if (bikeEngine.playing==NO && play==YES) {
[bikeEngine play];
}
else if(bikeEngine.playing==YES && play==NO){
[bikeEngine pause];
bikeEngine.currentTime=0;
}
}
}
-(void)playButtonClickSound:(BOOL)play{
if (!mute) {
if (play==YES) {
if (buttonClicked.playing==NO) {
[buttonClicked play];
}
else if(buttonClicked.playing==YES){
buttonClicked.currentTime=0;
}
}
else if(buttonClicked.playing==YES && play==NO){
[buttonClicked pause];
buttonClicked.currentTime=0;
}
}
}
-(void)playCoinCollectionSound{
if (!mute) {
AudioServicesPlaySystemSound (coinSound);
}
}
-(void)playFiringSound{
if (!mute) {
AudioServicesPlaySystemSound (firing);
}
}
-(void)playExplosionSound{
if (!mute) {
AudioServicesPlaySystemSound (explosion);
}
}
-(void)audioPlayerDidFinishPlaying:(AVAudioPlayer *)player successfully:(BOOL)flag{
[player release];
}
-(void)muteAll:(BOOL)isMute{
mute=isMute;
if (isMute==YES) {
soundsPreloaded=NO;
[bgMusic stop];
[bgMusic release];
[bikeEngine stop];
[bikeEngine release];
[buttonClicked stop];
[buttonClicked release];
AudioServicesDisposeSystemSoundID(coinSound);
AudioServicesDisposeSystemSoundID(firing);
AudioServicesDisposeSystemSoundID(explosion);
}
else if(isMute==NO && soundsPreloaded==NO){
[self preloadAllSounds];
[self playBGMusic:YES];
}
}
-(void)dealloc{
[sharedAudioManager release];
sharedAudioManager=nil;
[bgMusic release];
bgMusic=nil;
[bikeEngine release];
bikeEngine=nil;
[buttonClicked release];
buttonClicked=nil;
AudioServicesDisposeSystemSoundID(coinSound);
AudioServicesDisposeSystemSoundID(firing);
AudioServicesDisposeSystemSoundID(explosion);
[super dealloc];
}
@end
<file_sep>//
// RVRCoin.h
// MotoRacer
//
// Created by <NAME> on 6/25/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
#import "RVRAppDelegate.h"
@interface RVRCoin : CCSprite {
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
CGPoint myOriginalPostion;
BOOL isMyOriginalPosSet;
}
+(id)generateCoinWithAliasTexParameters;
+(id)generateCoin;
-(void)setMyOriginalPosition;
-(BOOL)resetToMyOriginalPos;
-(void)performCoinCollectionAndAnimation;
-(void)performMagnetInducedCollectionAnimation;
-(void)setDoubleValueTexture;
-(void)resetToOriginalTexture;
@property (nonatomic,readwrite,retain) CCSprite *aliasCoin;
@property (nonatomic) int multiplier;
@end
<file_sep>//
// RVRInAppPurchaseStore.h
// MotoRacer
//
// Created by <NAME> on 8/26/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "RootViewController.h"
#define NOOFPURCHASEITEMS 4
@class RVRAppDelegate;
@class LoadingView;
@interface RVRInAppPurchaseStore : RootViewController{
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
LoadingView *loadingView;
BOOL purchaseRequestSent;
}
+(id)inAppStore;
-(void)uiViewAnimaitonDidFinish:(id)sender;
-(void)updatePurchaseForAmountIndx:(NSInteger)indx;
-(void)purchaseFailed;
-(void)removeLoading;
@end
<file_sep>//
// TextureManager.m
// MotoRacer
//
// Created by <NAME> on 6/3/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "TextureManager.h"
#import "GameNode.h"
static TextureManager *sharedTexture=nil;
@interface TextureManager (Private)
-(void)loadAllTextures;
-(void)loadAllAnimations;
@end
@implementation TextureManager
@synthesize allTextures,vehicleTextureArray,batchTextures,animations;
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
self.allTextures=[[NSMutableDictionary alloc] init];
self.batchTextures=[[NSMutableDictionary alloc] init];
self.animations=[[NSMutableDictionary alloc] init];
self.vehicleTextureArray=[[NSMutableArray alloc] init];
[self loadAllTextures];
[self loadAllAnimations];
}
return self;
}
+(id)sharedTextureManager{
if (sharedTexture==nil) {
sharedTexture=[[TextureManager alloc] init];
}
return sharedTexture;
}
-(void)loadAllTextures{
//vehicle textures
CCTexture2D *bikeTexture=[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"bike.png"]];
[self.allTextures setObject:bikeTexture forKey:@"bikeTexture"];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"car1.png"]]];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"car2.png"]]];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"car3.png"]]];
//[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"truck1.png"]]];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"truck2.png"]]];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"mud-tuck.png"]]];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"ambulance.png"]]];
[self.vehicleTextureArray addObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"school-bus.png"]]];
//[self.allTextures setObject:vTexture forKey:@"car1Texture"];
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"car2.png"]] forKey:@"car2Texture"];
//road textures
CCTexture2D *roadTexture=[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"road-back.png"]];
[self.allTextures setObject:roadTexture forKey:@"roadTexture"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"sidebarleft.png"]] forKey:@"sidebarleft"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"sidebarright.png"]] forKey:@"sidebarright"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"side-stripe-left.png"]] forKey:@"side-stripe-left"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"side-stripe-right.png"]] forKey:@"side-stripe-right"];
CCTexture2D *stripe=[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:[NSString stringWithFormat:@"stripe3.png",1]]];
[self.allTextures setObject:stripe forKey:@"stripe1Texture"];
//CCTexture2D *overlay=[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"overlay.png"]];
//[self.allTextures setObject:overlay forKey:@"overlayTexture"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"tree.png"]] forKey:@"treeTexture"];
//coin texture
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"biker-point.png"]] forKey:@"biker-point"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"biker-point-double.png"]] forKey:@"biker-point-double"];
//main menu back texture
CCTexture2D *menuBack=[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"mainMenuBack.png"]];
[self.allTextures setObject:menuBack forKey:@"mainMenuBackTexture"];
//buttons textures
//CCTexture2D *customizeBTN=[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"customize.png"]];
//[self.allTextures setObject:customizeBTN forKey:@"customizeBTNTexture"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"gc.png"]] forKey:@"gcBTNTexture"];
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"highscore.png"]] forKey:@"highScoreBTNTexture"];
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"play.png"]] forKey:@"playBTNTexture"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"ride.png"]] forKey:@"rideBTNTexture"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"store.png"]] forKey:@"storeBTNTexture"];
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"btn-bg-glow.png"]] forKey:@"storeBTNglow"];
//gameNode menubutton Textures
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"button-home.png"]] forKey:@"button-home"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"button-pause.png"]] forKey:@"button-pause"];
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"button-play.png"]] forKey:@"button-play"];
//[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"button-replay.png"]] forKey:@"button-replay"];
//powerUps textures
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"pu-invincibility.png"]] forKey:@"pu-invincibility"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"pu-magnet.png"]] forKey:@"pu-magnet"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"pu-multiplier.png"]] forKey:@"pu-multiplier"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"pu-shooter.png"]] forKey:@"pu-shooter"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"pu-nos.png"]] forKey:@"pu-nos"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"superspeed.png"]] forKey:@"superspeed"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"superspeed2x.png"]] forKey:@"superspeed2x"];
//meter textures
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"meter.png"]] forKey:@"meter"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"meter-arrow.png"]] forKey:@"meter-arrow"];
//score bar textures
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"coin-bar.png"]] forKey:@"coin-bar"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"score-bar.png"]] forKey:@"score-bar"];
//batchTextures
[self.batchTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"explosion.png"]] forKey:@"explosion"];
//audio Button Textures
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"music-on.png"]] forKey:@"music-on"];
[self.allTextures setObject:[[CCTexture2D alloc] initWithImage:[UIImage imageNamed:@"music-off.png"]] forKey:@"music-off"];
}
-(void)loadAllAnimations{
NSMutableArray *spriteFrames=[NSMutableArray array];
CCSpriteFrame *spriteFrame;
CCTexture2D *batchTexture=[self.batchTextures objectForKey:@"explosion"];
CGSize frameSize=CGSizeMake(batchTexture.contentSize.width/EXPLOSIONXFRAMES, batchTexture.contentSize.height/EXPLOSIONYFRAMES);
for (int i=0; i<(EXPLOSIONXFRAMES*EXPLOSIONYFRAMES); i++) {
spriteFrame=[CCSpriteFrame frameWithTexture:batchTexture rect:CGRectMake((i%EXPLOSIONXFRAMES)*frameSize.width, (i/EXPLOSIONYFRAMES)*frameSize.height, frameSize.width, frameSize.height)];
[spriteFrames addObject:spriteFrame];
}
CCAnimation *explosionAnim=[CCAnimation animationWithFrames:spriteFrames delay:0.05f];
[[CCAnimationCache sharedAnimationCache] addAnimation:explosionAnim name:@"explosionAnim"];
[spriteFrames removeAllObjects];
spriteFrames=nil;
}
-(void)dealloc{
[sharedTexture release];
[self.allTextures removeAllObjects];
self.allTextures=nil;
[self.vehicleTextureArray removeAllObjects];
self.vehicleTextureArray=nil;
[self.batchTextures removeAllObjects];
self.batchTextures=nil;
[self.animations removeAllObjects];
self.animations=nil;
[super dealloc];
}
@end
<file_sep>//
// DBUpgrades.m
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "DBUpgrades.h"
#import "RVRAppDelegate.h"
@interface DBUpgrades (Private)
@end
@implementation DBUpgrades
@synthesize upgradeID,upgradeName,upgradeIcon,upgradeDetail,upgradeType,upgradeSteps,upgradeStepCosts,activeStep,noOfUpgradeSteps,typeDetail1,typeDetail2,addedToWritableList;
-(id)initWithPrimaryKey:(NSInteger)Upk database:(sqlite3 *)Udb{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
sqlite3_stmt *init_statement = nil;
primaryKey = Upk;
if (init_statement == nil) {
const char *sqlf = "SELECT * FROM upgrades WHERE upgradeID=?";
if (sqlite3_prepare_v2(Udb, sqlf, -1, &init_statement, NULL) != SQLITE_OK) {
NSLog(@"%s", sqlite3_errmsg(Udb));
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Udb));
}
}
sqlite3_bind_int(init_statement, 1, primaryKey);
if (sqlite3_step(init_statement) == SQLITE_ROW) {
self.upgradeID=primaryKey;
self.upgradeName=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 1)];
self.upgradeIcon=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 2)];
self.upgradeDetail=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 3)];
self.upgradeType=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 4)];
NSArray *detail=[NSArray arrayWithArray:[self.upgradeType componentsSeparatedByString:@","]];
typeDetail1=[[NSString stringWithFormat:@"%@",[detail objectAtIndex:0]] retain];
typeDetail2=[[NSString stringWithFormat:@"%@",[detail objectAtIndex:1]] retain];
self.upgradeSteps=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 5)];
steps=[[NSArray alloc] initWithArray:[self.upgradeSteps componentsSeparatedByString:@","]];
self.upgradeStepCosts=[NSString stringWithUTF8String:(char *)sqlite3_column_text(init_statement, 6)];
stepCosts=[[NSArray alloc] initWithArray:[self.upgradeStepCosts componentsSeparatedByString:@","]];
self.noOfUpgradeSteps=[steps count];
self.activeStep=sqlite3_column_int(init_statement,7);
} else {
self.upgradeID=0;
self.upgradeName=@"nil";
self.upgradeIcon=@"nil";
self.upgradeDetail=@"nil";
self.upgradeType=@"nil";
self.upgradeSteps=@"nil";
self.upgradeStepCosts=@"nil";
self.activeStep=-2;
self.noOfUpgradeSteps=0;
}
sqlite3_reset(init_statement);
sqlite3_finalize(init_statement);
init_statement=nil;
}
return self;
}
-(int)insertIntoDatabase:(sqlite3 *)Udb{
sqlite3_stmt *insert_statement = nil;
if (insert_statement == nil) {
static char *sql = "INSERT INTO upgrades(upgradeName,upgradeIcon,upgradeDetail,upgradeType,upgradeSteps,upgradeStepCosts,activeStep) VALUES(?,?,?,?,?,?,?)";
if (sqlite3_prepare_v2(Udb, sql, -1, &insert_statement, NULL) != SQLITE_OK) {
NSLog(@"Insert");
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Udb));
}
}
sqlite3_bind_text(insert_statement, 1, [upgradeName UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 2, [upgradeIcon UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 3, [upgradeDetail UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 4, [upgradeType UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 5, [upgradeSteps UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(insert_statement, 6, [upgradeStepCosts UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(insert_statement,7, activeStep);
int success = sqlite3_step(insert_statement);
sqlite3_reset(insert_statement);
sqlite3_finalize(insert_statement);
insert_statement=nil;
if (success == SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(Udb));
primaryKey = 0;
} else {
NSLog(@"Inserted upgrade Successfully...");
upgradeID=primaryKey=(NSInteger)sqlite3_last_insert_rowid(Udb);
}
return primaryKey;
}
-(BOOL)updateDatabase:(NSInteger)Upk database:(sqlite3 *)Udb{
primaryKey = Upk;
sqlite3_stmt *update_statement = nil;
if (update_statement == nil) {
static char *sqlu = "UPDATE upgrades SET upgradeName=?,upgradeIcon=?,upgradeDetail=?,upgradeType=?,upgradeSteps=?,upgradeStepCosts=?,activeStep=? WHERE upgradeID=?";
if (sqlite3_prepare_v2(Udb, sqlu, -1, &update_statement, NULL) != SQLITE_OK) {
NSLog(@"Update");
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Udb));
}
}
sqlite3_bind_text(update_statement, 1, [upgradeName UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 2, [upgradeIcon UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 3, [upgradeDetail UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 4, [upgradeType UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 5, [upgradeSteps UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_text(update_statement, 6, [upgradeStepCosts UTF8String], -1, SQLITE_TRANSIENT);
sqlite3_bind_int(update_statement, 7, activeStep);
sqlite3_bind_int(update_statement, 8, primaryKey);
int success = sqlite3_step(update_statement);
if(success==SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(Udb));
} else {
NSLog(@"Updated upgrade Successfully...");
}
sqlite3_reset(update_statement);
sqlite3_finalize(update_statement);
update_statement=nil;
return success;
}
-(BOOL)deleteDatabase:(NSInteger)Upk database:(sqlite3 *)Udb{
primaryKey = Upk;
sqlite3_stmt *delete_statement = nil;
if (delete_statement == nil) {
static char *sqlu = "DELETE FROM upgrades WHERE upgradeID=?";
if (sqlite3_prepare_v2(Udb, sqlu, -1, &delete_statement, NULL) != SQLITE_OK) {
NSAssert1(0, @"Error: failed to prepare statement with message '%s'.", sqlite3_errmsg(Udb));
}
}
sqlite3_bind_int(delete_statement, 1, primaryKey);
int success = sqlite3_step(delete_statement);
if(success==SQLITE_ERROR) {
NSAssert1(0, @"Error: failed to insert into the database with message '%s'.", sqlite3_errmsg(Udb));
} else {
NSLog(@"Deleted Successfully...");
}
sqlite3_reset(delete_statement);
sqlite3_finalize(delete_statement);
delete_statement=nil;
return success;
}
-(float)getCurrentStep{
return [[NSString stringWithFormat:@"%@",[steps objectAtIndex:self.activeStep]] floatValue];
}
-(NSArray*)getNextUpgradeStepAndCost{
int nextStep=self.activeStep+1;
if (nextStep<self.noOfUpgradeSteps) {
return [NSArray arrayWithObjects:[steps objectAtIndex:nextStep], [stepCosts objectAtIndex:nextStep], nil];
}
else
return [NSArray arrayWithObjects:@"fully Upgraded", @"0", nil];
}
-(NSArray*)updateDatabaseForStepPurchase{
int nextStep=self.activeStep+1;
if (nextStep<self.noOfUpgradeSteps && [[stepCosts objectAtIndex:nextStep] intValue]<=appDelegate.databaseManager.userData.uCoins) {
appDelegate.databaseManager.userData.uCoins-=[[NSString stringWithFormat:@"%@",[stepCosts objectAtIndex:nextStep]] intValue];
//[appDelegate.databaseManager.userData updateDatabase:appDelegate.databaseManager.userData.uID database:appDelegate.databaseManager.database];
self.activeStep=nextStep;
//[self updateDatabase:self.upgradeID database:appDelegate.databaseManager.database];
if (self.addedToWritableList==NO) {
self.addedToWritableList=YES;
[appDelegate.databaseManager.dataObjectsToBeWritten addObject:self];
}
NSLog(@"object count from upgrade--->%d",[appDelegate.databaseManager.dataObjectsToBeWritten count]);
nextStep+=1;
if (nextStep<self.noOfUpgradeSteps) {
return [NSArray arrayWithObjects:[steps objectAtIndex:nextStep], [stepCosts objectAtIndex:nextStep], nil];
}
else
return [NSArray arrayWithObjects:@"fully Upgraded", @"0", nil];
}
return nil;
}
-(void)dealloc{
[upgradeName release];
upgradeName=nil;
[upgradeIcon release];
upgradeIcon=nil;
[upgradeDetail release];
upgradeDetail=nil;
[upgradeType release];
upgradeType=nil;
[upgradeSteps release];
upgradeSteps=nil;
[upgradeStepCosts release];
upgradeStepCosts=nil;
[steps release];
steps=nil;
[stepCosts release];
stepCosts=nil;
[typeDetail1 release];
typeDetail1=nil;
[typeDetail2 release];
typeDetail2=nil;
[super dealloc];
}
@end
<file_sep>//
// RVRInAppPurchaseStore.m
// MotoRacer
//
// Created by <NAME> on 8/26/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "RVRInAppPurchaseStore.h"
#import "RVRAppDelegate.h"
#import "cocos2d.h"
#import "RVRPowerUpsAndPurchasesStore.h"
#import "RVRDataBaseManager.h"
#import "MKStoreManager.h"
#import "LoadingView.h"
#import "RVRAudioManager.h"
static RVRInAppPurchaseStore *store=nil;
@interface RVRInAppPurchaseStore (Private)
-(void)createStorePurchaseButtons;
-(void)inAppPurchasebuttonClicked:(id)sender;
-(void)startLoadingPurchase;
@end
@implementation RVRInAppPurchaseStore
int purchasedAmount[] = {2500,25000,75000,200000};
-(id)init{
if ((self=[super init])) {
appDelegate=[[UIApplication sharedApplication] delegate];
winSize=[[CCDirector sharedDirector] winSize];
self.view.frame=self.modalHiddenFrame=CGRectMake(0.0f, -winSize.height, winSize.width, winSize.height);
self.modalPresentationFrame=CGRectMake(0.0f, 0.0f, winSize.width, winSize.height);
self.view.backgroundColor=[UIColor clearColor];
self.view.opaque=NO;
[self createStorePurchaseButtons];
[appDelegate.viewController.view addSubview:self.view];
[appDelegate.viewController.view sendSubviewToBack:self.view];
}
return self;
}
+(id)inAppStore{
if (store==nil) {
store=[[self alloc] init];
}
return store;
}
-(void)createStorePurchaseButtons{
CGSize viewSize=self.view.frame.size;
CGSize btnSize=CGSizeMake(205.0f, 60.0f);
float yShift=(viewSize.height-(btnSize.height*(NOOFPURCHASEITEMS+1)))/(NOOFPURCHASEITEMS+2);
for (int i=0; i<=NOOFPURCHASEITEMS; i++) {
UIButton *inAppButton=[UIButton buttonWithType:UIButtonTypeCustom];
if (i==NOOFPURCHASEITEMS)
[inAppButton setImage:[UIImage imageNamed:@"done.png"] forState:UIControlStateNormal];
else
[inAppButton setImage:[UIImage imageNamed:[NSString stringWithFormat:@"coins%d.png",i]] forState:UIControlStateNormal];
inAppButton.frame=CGRectMake((viewSize.width-btnSize.width)/2.0f, (yShift*(i+1))+(btnSize.height*i), btnSize.width, btnSize.height);
inAppButton.bounds=CGRectMake(0.0f, 0.0f, btnSize.width, btnSize.height);
inAppButton.backgroundColor=[UIColor clearColor];
[inAppButton addTarget:self action:@selector(inAppPurchasebuttonClicked:) forControlEvents:UIControlEventTouchUpInside];
inAppButton.opaque=NO;
inAppButton.tag=i;
[self.view addSubview:inAppButton];
}
}
-(void)inAppPurchasebuttonClicked:(id)sender{
//NSLog(@"tag--->%d",[sender tag]);
[[RVRAudioManager sharedManager] playButtonClickSound:YES];
if ([sender tag]==NOOFPURCHASEITEMS) {
[self dismissModalViewAnimated:YES withDelegate:self selector:@selector(uiViewAnimaitonDidFinish:)];
}
else if(purchaseRequestSent==NO){
purchaseRequestSent=YES;
[self startLoadingPurchase];
[[MKStoreManager sharedManager] purchaseAction:[NSNumber numberWithInt:[sender tag]]];
}
}
-(void)startLoadingPurchase{
loadingView = [LoadingView loadingViewInView:appDelegate.viewController.view];
}
-(void)removeLoading{
[loadingView removeView];
}
-(void)uiViewAnimaitonDidFinish:(id)sender{
[appDelegate.viewController presentModalView:[RVRPowerUpsAndPurchasesStore powerUpsAndPurchaseStore] withDelegate:nil selector:nil animated:YES];
}
-(void)purchaseFailed{
purchaseRequestSent=NO;
}
-(void)updatePurchaseForAmountIndx:(NSInteger)indx{
appDelegate.databaseManager.userData.uCoins+=purchasedAmount[indx];
purchaseRequestSent=NO;
NSString *msg = [NSString stringWithFormat:@"Congratulations!!! You have purchased %d coins successfully.", purchasedAmount[indx]];
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Purchase Complete" message:msg delegate:self cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alert show];
[alert release];
}
-(void)dealloc{
[store release];
store=nil;
[super dealloc];
}
@end
<file_sep>//
// PauseOrGameOverMenu.h
// MotoRacer
//
// Created by <NAME> on 8/5/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "RootViewController.h"
@class RVRAppDelegate;
@protocol PauseOrGameOverMenuBtnClickDelegate <NSObject>
-(void)buttonClickedWithTag:(int)tag;
@end
@interface PauseOrGameOverMenu : RootViewController{
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
UIView *pauseMenuView;
UIView *gameOverMenuView;
NSInteger btnClickedTag;
}
+(id)menu;
-(void)pauseMenu;
-(void)gameOverMenu;
-(void)uiViewAnimaitonDidFinish:(id)sender;
@property (nonatomic,readwrite,retain) id<PauseOrGameOverMenuBtnClickDelegate> delegate;
@property (nonatomic) BOOL reqSent;
@end
<file_sep>//
// GameCenterDelegate.h
// MotoRacer
//
// Created by <NAME> on 6/4/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import <UIKit/UIKit.h>
#import <GameKit/GameKit.h>
#import "GameCenterManager.h"
#import "GamePlayObserverDelegateProtocol.h"
@class RVRAppDelegate;
@interface GameCenterDelegate : NSObject<GameCenterManagerDelegate,GKLeaderboardViewControllerDelegate>{
RVRAppDelegate *appDelegate;
int64_t currentScore;
NSString* cachedHighestScore;
}
@property (nonatomic,readwrite,retain) GameCenterManager *gameCenterManager;
@property (nonatomic, assign) int64_t currentScore,personalBest;
@property (nonatomic, retain) NSString* cachedHighestScore;
@property (nonatomic, retain) NSString* personalBestScoreDescription;
@property (nonatomic, retain) NSString* personalBestScoreString;
@property (nonatomic, retain) NSString* leaderboardHighScoreDescription;
@property (nonatomic, retain) NSString* leaderboardHighScoreString;
@property (nonatomic, retain) NSString* currentLeaderBoard;
@property (nonatomic) BOOL isGameCenterAvailable,isGameCenterAuthOnce;
@property (nonatomic,readwrite,retain) id<ControllerMenu> delegate;
+(id)sharedGameCenterDelegate;
-(void)submitHighScore;
-(void)showLeaderboard;
@end
<file_sep>//
// DBPurchase.h
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import <sqlite3.h>
@class RVRAppDelegate;
@interface DBPurchase : NSObject{
@private
RVRAppDelegate *appDelegate;
NSInteger primaryKey;
}
@property (nonatomic,readwrite) int purchaseID,purchaseItem,purchaseCost,maxAmountPurchasable,activeNoOfPurchases;
@property (nonatomic,readwrite,retain) NSString *purchaseName,*purchaseIcon,*purchaseDetail,*purchaseType;
@property (nonatomic) BOOL addedToWritableList;
- (id)initWithPrimaryKey:(NSInteger)Ppk database:(sqlite3 *)Pdb;
- (int)insertIntoDatabase:(sqlite3 *)Pdb;
- (BOOL)updateDatabase:(NSInteger)Ppk database:(sqlite3 *)Pdb;
- (BOOL)deleteDatabase:(NSInteger)Ppk database:(sqlite3 *)Pdb;
-(BOOL)updateDatabaseForPurchase;
@end<file_sep>//
// RVRDataBaseManager.h
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import <sqlite3.h>
#import "DBUserData.h"
#import "DBUpgrades.h"
#import "DBPurchase.h"
@interface RVRDataBaseManager : NSObject{
@private
float currentAppVersion,lastDBUpdatedVersion;
}
@property (readonly) sqlite3 *database;
@property (nonatomic,readwrite,retain) DBUserData *userData;
@property (nonatomic,readwrite,retain) NSMutableArray *powerUpUpgrades,*singlePurchases,*PUAndPstoreTitles,*dataObjectsToBeWritten;
@property (nonatomic,assign) BOOL highScoreChanged;
@end
<file_sep>//
// Obstacles.h
// MotoRacer
//
// Created by <NAME> on 6/29/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
#import "RVRAppDelegate.h"
@interface Obstacles : CCSprite {
@private
RVRAppDelegate *appDelegate;
CGSize winSize;
}
@property (nonatomic) BOOL isShooted,isToChangeLane;
@property (nonatomic) int laneChangeDir;
@property (nonatomic) CGPoint initialPosition,laneChangeDest;
@property (nonatomic) float vehicleSpeed,laneChangeSpeed;
+(id)generateObstacleWithAliasTextureParam;
-(void)resetProperties;
@end
<file_sep>//
// TapjoyPublishing.m
// MotoRacer
//
// Created by <NAME> on 6/5/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import "TapjoyPublishing.h"
#import "TapjoyConnect.h"
#import "RVRAppDelegate.h"
static TapjoyPublishing *tapjoy;
@implementation TapjoyPublishing
@synthesize tjOfferView;
-(id)init{
if (self=[super init]) {
// Where "appID" and "secretKey" will be replaced with the App ID and secret key retrieved from the Tapjoy dashboard.
TapjoyConnect *tjConnect=[TapjoyConnect requestTapjoyConnect:@"96699ead-3b58-4ead-9eb6-4ddebc4d6daf" secretKey:@"<KEY>"];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(tjConnectionSuccess:) name:TJC_CONNECT_SUCCESS object:nil];
if (tjConnect) {
RVRAppDelegate *appDelegate=[[UIApplication sharedApplication] delegate];
[appDelegate.window addSubview:[TapjoyConnect showOffers]];
}
}
return self;
}
+(id)tapjoyPublishing{
if (tapjoy==nil) {
tapjoy=[[TapjoyPublishing alloc] init];
}
return tapjoy;
}
-(void)tjConnectionSuccess:(NSNotification*)notifyObj{
[TapjoyConnect getTapPoints];
// A notification method must be set to retrieve the points.
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(getUpdatedPoints:) name:TJC_TAP_POINTS_RESPONSE_NOTIFICATION object:nil];
}
-(void)getUpdatedPoints:(NSNotification*)notifyObj
{
NSNumber *tapPoints = notifyObj.object;
NSString *tapPointsStr = [NSString stringWithFormat:@"Tap Points: %d", [tapPoints intValue]];
// Print out the updated points value.
NSLog(@"tap points--->%@", tapPointsStr);
}
@end
<file_sep>//
// DBUpgrades.h
// MotoRacer
//
// Created by <NAME> on 7/6/12.
// Copyright (c) 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import <sqlite3.h>
@class RVRAppDelegate;
@interface DBUpgrades : NSObject{
@private
RVRAppDelegate *appDelegate;
NSInteger primaryKey;
NSArray *steps;
NSArray *stepCosts;
}
@property (nonatomic,readwrite) int upgradeID,activeStep,noOfUpgradeSteps;
@property (nonatomic,readwrite,retain) NSString *upgradeName,*upgradeIcon,*upgradeDetail,*upgradeType,*upgradeSteps,*upgradeStepCosts,*typeDetail1,*typeDetail2;
@property (nonatomic) BOOL addedToWritableList;
- (id)initWithPrimaryKey:(NSInteger)Upk database:(sqlite3 *)Udb;
- (int)insertIntoDatabase:(sqlite3 *)Udb;
- (BOOL)updateDatabase:(NSInteger)Upk database:(sqlite3 *)Udb;
- (BOOL)deleteDatabase:(NSInteger)Upk database:(sqlite3 *)Udb;
-(float)getCurrentStep;
-(NSArray*)getNextUpgradeStepAndCost;
-(NSArray*)updateDatabaseForStepPurchase;
@end<file_sep>//
// BikeNode.h
// MotoRacer
//
// Created by <NAME> on 6/3/12.
// Copyright 2012 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
#import "cocos2d.h"
@interface BikeLayer : CCLayer {
}
+(id)bike;
@end
| 9ac15b6efa6ac8d83d9061c2d1d52e5a6913928c | [
"Objective-C"
] | 46 | Objective-C | tmukammel/crazy-rider | 8505b2c295245e054772baf5f6f7bfb2391cd69c | 210a74ba5b8403d6814558a893a9bf90a62f4076 |
refs/heads/main | <repo_name>ddutan2000/Practica_1-Clases-Genericas<file_sep>/README.md
# Practica_1-Clases-Genericas | 4c531bad84d0859fc834a607884b05add1ffecd8 | [
"Markdown"
] | 1 | Markdown | ddutan2000/Practica_1-Clases-Genericas | 36ad439479b1c4e3eb824d0dfaa14535bf43bdd6 | 1112650c8f901990e1d704dcde0c1a37bb49acf1 |