text
stringlengths 184
4.48M
|
---|
require 'benchmark'
CYCLES = 1_000_000_000
def slide(original_platform, direction)
platform = case direction
when :north, :south
original_platform.transpose
when :east, :west
original_platform.map(&:dup)
end
swap_order = case direction
when :north, :west
%w[. O]
when :east, :south
%w[O .]
end
result = platform.each_with_index.map do |row, row_i|
loop do
swapped = false
(1..row.size).each do |i|
next unless row[i - 1] == swap_order[0] && row[i] == swap_order[1]
row[i - 1], row[i] = row[i], row[i - 1]
swapped = true
end
break unless swapped
end
row
end
case direction
when :north, :south
result.transpose
else
result
end
end
def count_weight(platform)
platform.each_with_index.map do |row, index|
boulders_count = row.count('O')
distance = platform.size - index
boulders_count * distance
end.sum
end
@cache = {}
def calculate(text)
platform = text.split("\n").map(&:chars)
result = nil
time = Benchmark.realtime do
new_platform = platform
iterations = 0
loop do
puts "#{iterations}"
if @cache.key?(new_platform)
loop_offset = @cache[new_platform]
period = iterations - loop_offset + 1
target_iteration = (CYCLES - iterations) % period + loop_offset
new_platform = @cache.key(target_iteration)
break
end
iterations += 1
unless @cache.key?(new_platform)
@cache[new_platform] = iterations
end
new_platform = %i[north west south east].inject(new_platform) do |platform_result, direction|
slide(platform_result, direction)
end
end
puts new_platform.map(&:join).join("\n")
result = count_weight(new_platform)
end
puts "Time: #{time} seconds"
result
end
pp calculate(File.read(File.expand_path('./day14/input.txt'))) |
//
// Copyright (c) 1995-2020 Mort Bay Consulting Pty Ltd and others.
// ------------------------------------------------------------------------
// All rights reserved. This program and the accompanying materials
// are made available under the terms of the Eclipse Public License v1.0
// and Apache License v2.0 which accompanies this distribution.
//
// The Eclipse Public License is available at
// http://www.eclipse.org/legal/epl-v10.html
//
// The Apache License v2.0 is available at
// http://www.opensource.org/licenses/apache2.0.php
//
// You may elect to redistribute this code under either of these licenses.
//
package org.eclipse.jetty.util.ssl;
import java.io.File;
import java.io.IOException;
import java.util.Collections;
import java.util.function.Consumer;
import org.eclipse.jetty.util.Scanner;
import org.eclipse.jetty.util.annotation.ManagedAttribute;
import org.eclipse.jetty.util.annotation.ManagedOperation;
import org.eclipse.jetty.util.component.ContainerLifeCycle;
import org.eclipse.jetty.util.log.Log;
import org.eclipse.jetty.util.log.Logger;
/**
* <p>The {@link KeyStoreScanner} is used to monitor the KeyStore file used by the {@link SslContextFactory}.
* It will reload the {@link SslContextFactory} if it detects that the KeyStore file has been modified.</p>
* <p>If the TrustStore file needs to be changed, then this should be done before touching the KeyStore file,
* the {@link SslContextFactory#reload(Consumer)} will only occur after the KeyStore file has been modified.</p>
*/
public class KeyStoreScanner extends ContainerLifeCycle implements Scanner.DiscreteListener
{
private static final Logger LOG = Log.getLogger(KeyStoreScanner.class);
private final SslContextFactory sslContextFactory;
private final File keystoreFile;
private final Scanner _scanner;
public KeyStoreScanner(SslContextFactory sslContextFactory)
{
this.sslContextFactory = sslContextFactory;
try
{
keystoreFile = sslContextFactory.getKeyStoreResource().getFile();
if (keystoreFile == null || !keystoreFile.exists())
throw new IllegalArgumentException("keystore file does not exist");
if (keystoreFile.isDirectory())
throw new IllegalArgumentException("expected keystore file not directory");
}
catch (IOException e)
{
throw new IllegalArgumentException("could not obtain keystore file", e);
}
File parentFile = keystoreFile.getParentFile();
if (!parentFile.exists() || !parentFile.isDirectory())
throw new IllegalArgumentException("error obtaining keystore dir");
_scanner = new Scanner();
_scanner.setScanDirs(Collections.singletonList(parentFile));
_scanner.setScanInterval(1);
_scanner.setReportDirs(false);
_scanner.setReportExistingFilesOnStartup(false);
_scanner.setScanDepth(1);
_scanner.addListener(this);
addBean(_scanner);
}
@Override
public void fileAdded(String filename)
{
if (LOG.isDebugEnabled())
LOG.debug("added {}", filename);
if (keystoreFile.toPath().toString().equals(filename))
reload();
}
@Override
public void fileChanged(String filename)
{
if (LOG.isDebugEnabled())
LOG.debug("changed {}", filename);
if (keystoreFile.toPath().toString().equals(filename))
reload();
}
@Override
public void fileRemoved(String filename)
{
if (LOG.isDebugEnabled())
LOG.debug("removed {}", filename);
if (keystoreFile.toPath().toString().equals(filename))
reload();
}
@ManagedOperation(value = "Scan for changes in the SSL Keystore", impact = "ACTION")
public void scan()
{
if (LOG.isDebugEnabled())
LOG.debug("scanning");
_scanner.scan();
_scanner.scan();
}
@ManagedOperation(value = "Reload the SSL Keystore", impact = "ACTION")
public void reload()
{
if (LOG.isDebugEnabled())
LOG.debug("reloading keystore file {}", keystoreFile);
try
{
sslContextFactory.reload(scf -> {});
}
catch (Throwable t)
{
LOG.warn("Keystore Reload Failed", t);
}
}
@ManagedAttribute("scanning interval to detect changes which need reloaded")
public int getScanInterval()
{
return _scanner.getScanInterval();
}
public void setScanInterval(int scanInterval)
{
_scanner.setScanInterval(scanInterval);
}
} |
using Harmony.Application.Contracts.Repositories;
using Harmony.Shared.Wrapper;
using MediatR;
using Microsoft.Extensions.Localization;
using Harmony.Application.Contracts.Services;
using AutoMapper;
using Harmony.Application.Contracts.Services.Management;
using Harmony.Application.Constants;
using Harmony.Application.Contracts.Messaging;
using Harmony.Application.Notifications;
using Harmony.Domain.Enums;
using static Harmony.Application.Notifications.BoardListArchivedMessage;
using Harmony.Application.Models;
using Harmony.Domain.Entities;
using Harmony.Domain.Extensions;
namespace Harmony.Application.Features.Lists.Commands.ArchiveList
{
public class ArchiveListCommandHandler : IRequestHandler<UpdateListStatusCommand, Result<bool>>
{
private readonly IBoardListRepository _boardListRepository;
private readonly ICurrentUserService _currentUserService;
private readonly IStringLocalizer<ArchiveListCommandHandler> _localizer;
private readonly INotificationsPublisher _notificationsPublisher;
private readonly IListService _listService;
private readonly ICacheService _cacheService;
private readonly IMapper _mapper;
public ArchiveListCommandHandler(IBoardListRepository boardListRepository,
ICurrentUserService currentUserService,
IStringLocalizer<ArchiveListCommandHandler> localizer,
INotificationsPublisher notificationsPublisher,
IListService listService,
ICacheService cacheService,
IMapper mapper)
{
_boardListRepository = boardListRepository;
_currentUserService = currentUserService;
_localizer = localizer;
_notificationsPublisher = notificationsPublisher;
_listService = listService;
_cacheService = cacheService;
_mapper = mapper;
}
public async Task<Result<bool>> Handle(UpdateListStatusCommand request, CancellationToken cancellationToken)
{
var userId = _currentUserService.UserId;
List<BoardListOrder> newPositions = null;
if (string.IsNullOrEmpty(userId))
{
return await Result<bool>.FailAsync(_localizer["Login required to complete this operator"]);
}
var list = await _boardListRepository.Get(request.ListId);
list.Status = request.Status;
if(request.Status == BoardListStatus.Archived)
{
newPositions = await _listService.ReorderAfterArchive(list);
}
var dbResult = await _boardListRepository.Update(list);
if (dbResult > 0)
{
if(request.Status == BoardListStatus.Archived)
{
var lists = await _cacheService.HashGetAsync<List<BoardList>>(
CacheKeys.Board(request.BoardId), CacheKeys.BoardLists(request.BoardId));
var archivedList = lists.FirstOrDefault(l => l.Id == request.ListId);
if (archivedList != null)
{
lists.Remove(archivedList);
await _cacheService.HashHSetAsync(CacheKeys.Board(request.BoardId),
CacheKeys.BoardLists(request.BoardId), lists.SerializeLists());
}
var message = new BoardListArchivedMessage(list.BoardId, list.Id, newPositions);
_notificationsPublisher.PublishMessage(message,
NotificationType.BoardListArchived, routingKey: BrokerConstants.RoutingKeys.SignalR);
}
return await Result<bool>.SuccessAsync(true, _localizer["List status updated"]);
}
return await Result<bool>.FailAsync(_localizer["Operation failed"]);
}
}
} |
import React from 'react';
import { useState } from 'react/cjs/react.development';
import { Button } from '../../core';
import { TextArea } from '../../core/inputs/TextArea/TextArea';
import { TextInput } from '../../core/inputs/TextInput';
import constants from '../../../utils/constants'
import { postProject, validateForm } from './NewProjectFormService';
import { useNavigate } from 'react-router-dom';
export const NewProjectForm = (props) => {
const {
values,
errors,
setValues,
setErrors
} = props;
const navigate = useNavigate()
const [edittedTagName, setEdittedTagName] = useState(false);
const [apiError, setApiError] = useState(false);
const handleInputChange = (e) => {
const { id, value } = e.target;
if (id === 'tag' && !edittedTagName) {
setEdittedTagName(true);
}
setValues((prev) => ({ ...prev, [id]: value }))
}
const handleNameInputBlur = (e) => {
if (!edittedTagName) {
const { value } = e.target;
const valueArr = value.split(' ');
const valueInitialsArr = valueArr.map(x => x[0]);
const valueInitials = valueInitialsArr.join('');
const valueInitialsUpper = valueInitials.toUpperCase();
const predicted = valueInitialsUpper;
setValues((prev) => ({ ...prev, tag: predicted }))
}
}
const handleCreateButtonClick = () => {
const localErrors = validateForm(values);
const formIsValid = Object.keys(localErrors).length === 0;
if (formIsValid) {
postProject(values, setApiError, navigate)
} else {
setErrors(localErrors)
}
}
return (
<div>
{apiError && <p>{apiError}</p>}
<TextInput
id='name'
label='Name'
value={values?.name}
error={errors?.name}
onChange={handleInputChange}
onBlur={handleNameInputBlur}
/>
<TextInput
id='tag'
label='Tag'
value={values?.tag}
error={errors?.tag}
onChange={handleInputChange}
/>
<TextArea
id='description'
label='Description'
value={values?.description}
error={errors?.description}
onChange={handleInputChange}
/>
<Button
label='Create Project'
onClick={handleCreateButtonClick}
/>
</div>
)
} |
module Main exposing (..)
import Browser
import Html exposing (Html, button, div, text)
import Html.Events exposing (onClick)
import Svg
import Svg.Attributes as Attr
import Time
-- MAIN
main =
Browser.element
{ init = init, view = view, update = update, subscriptions = subscriptions }
-- MODEL
type Direction
= Up
| Down
| Right
| Left
type alias Pixel =
{ x : Int
, y : Int
}
type alias Snake =
List Pixel
maxLength =
20
nextPixel : Direction -> Pixel -> Pixel
nextPixel direction pixel =
case direction of
Up ->
{ x = pixel.x, y = min (pixel.y + 1) maxLength }
Down ->
{ x = pixel.x, y = max (pixel.y - 1) 0 }
Right ->
{ x = min (pixel.x + 1) maxLength, y = pixel.y }
Left ->
{ x = max (pixel.x - 1) 0, y = pixel.y }
type alias Model =
{ snake : Snake
, direction : Direction
}
init : () -> ( Model, Cmd Msg )
init _ =
( { snake =
[ { x = 5, y = 5 }
, { x = 6, y = 6 }
, { x = 7, y = 5 }
]
, direction = Right
}
, Cmd.none
)
-- STYLE
background : Html msg
background =
Svg.svg
[ Attr.viewBox "0 0 400 400"
, Attr.width "400"
, Attr.height "400"
]
[ Svg.rect
[ Attr.x "0"
, Attr.y "0"
, Attr.width "400"
, Attr.height "400"
, Attr.fill "green"
, Attr.stroke "black"
, Attr.strokeWidth "2"
]
[]
]
-- UPDATE
type Msg
= Key Direction
| Apple
| Tick Time.Posix
addOnePixel : Snake -> Direction -> Snake
addOnePixel snake direction =
let
tailRecord =
List.head (List.reverse snake)
in
case tailRecord of
Nothing ->
[]
Just tailPixel ->
List.append snake [ nextPixel direction tailPixel ]
updateSnake : Model -> Snake
updateSnake model =
case model.snake of
[] ->
[]
_ :: tail ->
addOnePixel tail model.direction
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Key newDirection ->
case ( newDirection, model.direction ) of
( Up, Down ) ->
( model, Cmd.none )
( Down, Up ) ->
( model, Cmd.none )
( Left, Right ) ->
( model, Cmd.none )
( Right, Left ) ->
( model, Cmd.none )
( _, _ ) ->
( { model | direction = newDirection }
, Cmd.none
)
Apple ->
( { model | snake = addOnePixel model.snake model.direction }, Cmd.none )
Tick _ ->
( { model | snake = updateSnake model }
, Cmd.none
)
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions _ =
Time.every 1000 Tick
-- VIEW
view : Model -> Html Msg
view model =
div []
[ background
, div [] [ text (List.foldr (\x a -> "{ " ++ String.fromInt x.x ++ ", " ++ String.fromInt x.y ++ "}, " ++ a) "" model.snake) ]
, button [ onClick (Key Up) ] [ text "⬆️" ]
, div [] []
, button [ onClick (Key Left) ] [ text "⬅️" ]
, button [ onClick (Key Down) ] [ text "⬇️" ]
, button [ onClick (Key Right) ] [ text "➡️" ]
, button [ onClick Apple ] [ text "🍎️" ]
] |
import { readFileSync } from "fs";
import solc from "solc";
import { JsonRpcProvider, ContractFactory } from "ethers";
const provider = new JsonRpcProvider("http://127.0.0.1:8545");
const signer = await provider.getSigner();
console.log("Address:", signer.address);
const balance = await provider.getBalance(signer.address);
console.log("Balance:", balance);
const network = await provider.getNetwork();
console.log("Chain ID:", network.chainId);
let latestBlock = await provider.getBlockNumber();
console.log("Latest Block:", latestBlock);
const CONTRACT_FILE = "contracts/Storage.sol";
const content = readFileSync(CONTRACT_FILE).toString();
const input = {
language: "Solidity",
sources: {
[CONTRACT_FILE]: {
content: content,
},
},
settings: {
outputSelection: {
"*": {
"*": ["*"],
},
},
},
};
const compiled = solc.compile(JSON.stringify(input));
const output = JSON.parse(compiled);
const abi = output.contracts[CONTRACT_FILE].Storage.abi;
const bytecode = output.contracts[CONTRACT_FILE].Storage.evm.bytecode.object;
const factory = new ContractFactory(abi, bytecode, signer);
const contract = await factory.deploy();
const trx = await contract.store("Hello, KBA!");
console.log("Transaction Hash:", trx.hash);
const message = await contract.retrieve();
console.log("Message:", message);
latestBlock = await provider.getBlockNumber();
console.log("Latest Block:", latestBlock); |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Element类型</title>
<style>
.red{
background: red;
}
.blue{
background: blue;
}
</style>
<script>
window.onload = function(){
var div = document.body.children[0];
// console.log(div);
// console.log(document.body.firstElementChild);
// 获取属性值
console.log(div.className); //red
console.log(div.title); //我是div
console.log(div.getAttribute('class')); //red
console.log(div.getAttribute('data-flag')); //1001
console.log(div.style);
console.log(div.getAttribute('style'));
div.style.color = 'skyblue';
// div.style.backgroundColor = 'red';
console.log(div.style);
console.log(div.onclick); //函数
console.log(div.getAttribute('onclick')); //字符串
//更改属性值
div.title = 'hello';
div.setAttribute('title','world')
//移除属性
div.removeAttribute('title');
// 打印所有的属性
console.log(div.attributes); //属性节点组成的类数组
console.log(div.attributes[2]); //属性节点对象 nodeType 2
// 修改属性值
div.attributes[2].nodeValue = 'blue';
//获取属性对应的属性节点
console.log(div.attributes.getNamedItem('class'));
//获取内部内容
console.log(div.innerHTML);
// 获取内部文本 没有回车换行
console.log(div.innerText);
// 获取内部文本 有回车换行
console.log(div.textContent);
//修改内部文本
// div.innerText = 'hello';
// div.innerHTML = 'hello'
// div.innerText = '<span>span</span>';
// div.innerHTML = '<span>span</span>';
div.innerHTML = `
<div>
<span>hello<span>
</div>
`;
}
function test(){
var div = document.getElementById('one');
// class的切换
/*var str = 'red';
if(div.className==='red'){
str = 'blue';
}
div.className = str;*/
div.className = (div.className==='red')?'blue':'red';
}
</script>
</head>
<body>
hello
<div id="one" style="color:coral" class="red" title="我是div" onclick="test()" data-flag='1001'>
one
two
three
<span>four</span>
</div>
</body>
</html> |
import pandas as pd
import seaborn as sns
from matplotlib import pyplot as plt
from utils import read_a_multilist, read_list
def load_generation_data(PATH):
"""Load the generation counts for different mutation and crossover rates experiments."""
mutation_rates = [0.05, 0.1, 0.15, 0.2]
crossover_rates = [0.1, 0.2, 0.3, 0.4]
gens_data = {}
for mut_rate in mutation_rates:
for cross_rate in crossover_rates:
key = f'mut_{mut_rate}_cross_{cross_rate}'
file_name = f'mut_{mut_rate}_cross_{cross_rate}'
gens_data[key] = read_list(PATH, 'medium_1', 'generation_counts', file_name)
return gens_data
def prepare_data(gens_data):
"""Prepare the data for the boxplot."""
data = []
for key, values in gens_data.items():
mutation_rate, crossover_rate = key.split('_')[1], key.split('_')[3]
for value in values:
data.append({'Mutation Rate': mutation_rate, 'Crossover Rate': crossover_rate, 'Generations': value})
return pd.DataFrame(data)
def plot_boxplot(df):
plt.figure(figsize=(10, 6))
sns.boxplot(x='Mutation Rate', y='Generations', hue='Crossover Rate', data=df)
plt.xlabel('Mutation Rate')
plt.ylabel('Generations')
plt.title('Generation Counts over Different Mutation and Crossover Rates')
plt.legend(title='Crossover Rate')
plt.show()
def plot_boxplot_generations_per_rates():
PATH = 'results/rates/'
gens_data = load_generation_data(PATH)
df = prepare_data(gens_data)
plot_boxplot(df)
def calculate_average_fitness_per_generation(all_runs):
"""Calculate the average fitness per generation for all runs."""
max_generations = max(len(run) for run in all_runs)
averages = []
for i in range(max_generations):
gen_values = [float(run[i]) for run in all_runs if i < len(run)]
if gen_values:
averages.append(sum(gen_values) / len(gen_values))
else:
averages.append(None)
return averages
def plot_fitness(PATH, name):
"""
Plot the average mean fitness per generation for the different levels puzzles.
"""
elite = [True, False]
local_search = [False, True]
plt.figure(figsize=(6, 4))
for elite_flag in elite:
for local_search_flag in local_search:
if not(local_search_flag and elite_flag):
fitnesses = read_a_multilist(f'{PATH}{name}/', name, 'fitness_mean_histories',
f'local_search_{local_search_flag}_elite_{elite_flag}')
average_fitnesses = calculate_average_fitness_per_generation(fitnesses)
if local_search_flag:
label = 'Local Search'
elif elite_flag:
label = 'Elite Population Learning'
else:
label = 'Baseline'
plt.plot(average_fitnesses, label=label)
plt.legend()
plt.xlabel('Generation')
plt.ylabel('Average Mean Population Fitness')
plt.ylim(0, 30)
# plt.title(f'Average Mean Fitness Population per Generation for {name} Twodoku')
plt.savefig(f'./results/comparison/{name}_plot.png')
plt.show()
#save the plot
def plot_fitness_lines_per_puzzle():
"""
Plot the average mean fitness per generation for the different level puzzles
"""
PATH = './results/comparison/'
easy_puzzle_names = ['easy_1', 'easy_2', 'easy_3']
medium_puzzle_names = ['medium_1', 'medium_2']
hard_puzzle_names = ['hard_1', 'hard_2']
for name in easy_puzzle_names:
plot_fitness(PATH, name)
for name in medium_puzzle_names:
plot_fitness(PATH, name)
for name in hard_puzzle_names:
plot_fitness(PATH, name)
if __name__ == "__main__":
#plot_boxplot_generations_per_rates()
plot_fitness_lines_per_puzzle() |
import React from "react";
import { render } from "@testing-library/react-native";
import { AppWrapper } from "../../../../tests/AppWrapper";
import { LiveTvMakesItBetter } from "../LiveTvMakesItBetter";
const MockComponent: React.FC = () => {
return (
<AppWrapper>
<LiveTvMakesItBetter />
</AppWrapper>
);
};
describe("LiveTvMakesItBetter Component", () => {
it("should renders the component", () => {
const { getByTestId, getByText, debug } = render(<MockComponent />);
expect(getByTestId("liveTvMakesItBetter")).toBeTruthy();
debug();
expect(
getByText("Hulu + live tv, maintenant avec disney+ and espn+")
).toBeTruthy();
expect(getByText("Live TV Makes It Better")).toBeTruthy();
expect(
getByText(
/Abandonnez le câble. Obtenez plus de 75 chaînes de premier plan sur/i
)
).toBeTruthy();
expect(getByText(/Voir les chaînes dans votre région/i)).toBeTruthy();
});
it("should renders 2 icons", () => {
const { getAllByTestId } = render(<MockComponent />);
expect(getAllByTestId("icon")).toHaveLength(2);
});
}); |
import { useEffect } from 'react';
import { useDispatch, useSelector } from 'react-redux';
import CircularProgress from '@mui/material/CircularProgress';
import Typography from '@mui/material/Typography';
import Link from '@mui/material/Link';
import Stack from '@mui/material/Stack';
import Avatar from '@mui/material/Avatar';
import Button from '@mui/material/Button';
import Card from '@mui/material/Card';
import CardActionArea from '@mui/material/CardActionArea';
import CardContent from '@mui/material/CardContent';
import CardActions from '@mui/material/CardActions';
import NoPerson from '@mui/icons-material/VoiceOverOff';
import Alert from '../common/Alert';
import {
getHighestBid,
getWinnerSellerDetails,
resetWinnerSellerDetails,
selectWinnerSellerDetails,
selectAuctionData,
} from '../features/auctionSlice';
import { formatCurrency } from '../utils/utilityFunctions';
const AuctionWinner = () => {
const dispatch = useDispatch();
const { _id, seller, winner } = useSelector(selectAuctionData);
const { loading, error, data } = useSelector(selectWinnerSellerDetails);
const highestBid = useSelector(getHighestBid);
const { user } = useSelector((state) => state.auth.userLogin);
const requestContactDetails = () => {
dispatch(getWinnerSellerDetails({ auctionId: _id }));
};
useEffect(() => {
return () => {
dispatch(resetWinnerSellerDetails());
};
}, []);
return (
<Card sx={{ p: 2 }}>
<CardActionArea>
{winner ? (
<Avatar
sx={{ mx: 'auto', width: '60px', height: '60px' }}
src={winner.avatar}
/>
) : (
<Avatar
sx={{
mx: 'auto',
width: '60px',
height: '60px',
bgcolor: 'error.dark',
}}
>
<NoPerson fontSize='large' />
</Avatar>
)}
<CardContent>
<Typography
gutterBottom
variant='h5'
component='p'
color='success.dark'
textAlign='center'
>
{!winner
? 'Nobody Won'
: winner._id === user.id
? 'Hooray! 🎉'
: 'Winner'}
</Typography>
<Typography
gutterBottom
variant='h5'
component='p'
color='error.dark'
textAlign='center'
>
Highest Bid {formatCurrency({ amount: highestBid })}
</Typography>
<Typography variant='body2' color='text.secondary' textAlign='center'>
{winner
? `${
winner._id === user.id ? 'You' : winner.name
} won this auction. Stay tuned for more such auction!`
: 'Nobody attended this auction. Hence nobody bid on this auction'}
</Typography>
</CardContent>
</CardActionArea>
<CardActions sx={{ flexDirection: 'column' }}>
{winner && winner._id === user.id ? (
<Button
onClick={requestContactDetails}
color='error'
variant='contained'
sx={{ mx: 'auto' }}
disabled={loading || !!error || !!data}
>
{loading ? 'Please wait...' : 'Show Seller Contact Detail'}
</Button>
) : winner && seller._id === user.id ? (
<Button
onClick={requestContactDetails}
color='error'
variant='contained'
sx={{ mx: 'auto' }}
disabled={loading || !!error || !!data}
>
{loading ? 'Please wait...' : 'Show Winner Contact Detail'}
</Button>
) : null}
<Stack mt={winner ? 3 : 0}>
{loading ? (
<CircularProgress color='secondary' />
) : error ? (
<Alert message={error} variant='outlined' />
) : data ? (
<>
<Typography gutterBottom variant='subtitle1' textAlign='center'>
Name: {data.contactDetails.name}
</Typography>
<Typography gutterBottom variant='subtitle1' textAlign='center'>
E-mail:{' '}
<Link
href={`mailto:${data.contactDetails.email}`}
rel='noopener noreferrer'
underline='hover'
>
{data.contactDetails.email}
</Link>
</Typography>
<Typography gutterBottom variant='subtitle1' textAlign='center'>
Mobile:{' '}
<Link
href={`tel:+91-${data.contactDetails.mobile}`}
rel='noopener noreferrer'
underline='hover'
>
+91-{data.contactDetails.mobile}
</Link>
</Typography>
</>
) : null}
</Stack>
</CardActions>
</Card>
);
};
export default AuctionWinner; |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Cynthia's Porfolio</title>
<!-- Satoshi from fontshare -->
<link
href="https://api.fontshare.com/v2/css?f[]=satoshi@700,400&display=swap"
rel="stylesheet"
/>
<!-- Linking to CSS -->
<link rel="stylesheet" href="style.css" />
<!-- font awesome logos -->
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css"
integrity="sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA=="
crossorigin="anonymous"
referrerpolicy="no-referrer"
/>
<!-- attempt aos -->
<script defer src="app.js"></script>
</head>
<body>
<!-- Navigation menu -->
<nav id="nav-bar">
<a id="nav-logo" href="#hero-section"><p>Cynthia Lam</p></a>
<!-- Navigation items -->
<ul class="nav-list">
<li><a class="nav-links" href="#about-section">About</a></li>
<li><a class="nav-links" href="#projects-section">Projects</a></li>
<li><a class="nav-links" href="#contact-section">Contact</a></li>
</ul>
</nav>
<section id="hero-section">
<h1>Hi I'm Cynthia 😊</h1>
<p>I'm an aspiring developer from Toronto!</p>
<p>View my resume <a href="Cynthia Lam - Health Tech Ed Resume.pdf" target="_blank"><em>here</em></a>!</p>
</section>
<section id="about-section">
<!-- about info: left text boxes + pic w/o background -->
<div class="about-info">
<!-- left text boxes -->
<div class="about-text">
<div><h2>About me:</h2></div>
<div class="fade-lefttoright">
<p>
Hello World! My name is Cynthia, a proud member of IDRF's WiT's 6th cohort! I'm currently an AI Education Intern at Vector Institute (it sounds fancier than it is, I promise!)
My professional interests include improving patient care, applying technology, and bridging
the gap between the two industries through technical education and workshop facilitation. My favourite saying that I picked up recently is <em>"Never stop learning!"</em> -
I strive to be both an amazing educator as well as a lifelong learner; I'm always picking up new skills and so thankful to also be meeting amazing people along the way.
</p>
<p>
In my free time, I like to go rock climbing or hit the gym, sketch up stick-and-poke ideas, and try new experiences with my friends (potentially trying pottery and tufting next!)
</p>
</div>
</div>
<!-- pic -->
<div class="image-container">
<img class="fade-righttoleft rounded-image"
src="C:\Users\cynth\OneDrive\Desktop\Jobs\Cynthia Lam Photo.jpg"
alt="In case you can't see, it's me!"
style="width: 270px; height: 370px"
/>
</div>
</div>
</section>
<section id="projects-section">
<div class="project-content">
<div><h2><br><br><br>Projects</h2></div>
<!-- timeline -->
<div class="timeline">
<div class="container left fade-lefttoright">
<div class="project-box">
<br> <!-- room for the image to expand -->
<a href="file:///c%3A/Users/cynth/Documents/wit6/Calculator-Project/index.html" target="_blank">
<img src="Calculator.PNG" alt="Click here for spookiness" width="300" height="300" class="image-shadow">
</a>
<br> <!-- room for the image to expand -->
<h3>Project 1: Spooky Calculator</h3>
<p class="small-p">Made using purely HTML and CSS with the guidance of our amazing instructors. It's almost spooky season so I figured I'd jazz it up with some decorations!
You can check it out by clicking the photo or for more info, head to my <a href="https://github.com/cynthia-lam/Calculator-Project" target="_blank"> repo</a>.
</p>
</div>
</div>
<div class="container right fade-righttoleft">
<div class="project-box">
<br> <!-- room for the image to expand -->
<a href="https://docs.google.com/presentation/d/1Vo10Z7AV2igm2AAMFAHlo3YNz1524qer/edit#slide=id.p1" target="_blank">
<img src="BT PPG.PNG" alt="Click here for my capstone project!" width="300" height="300" class="image-shadow" >
</a>
<br> <!-- room for the image to expand -->
<h3>Project 2: Bluetooth Photoplethysmography Sensor</h3>
<p class="small-p">Using Arduino, C++, and way too many online guides, I made a heart rate sensor for my Mech Eng Capstone Project at UBC.</p>
</div>
</div>
<div class="container left fade-lefttoright">
<div class="project-box">
<br> <!-- room for the image to expand -->
<a href="https://docs.google.com/document/d/1dANJaybaZrVs12NpgMmlbnapEuEPxgbA/edit" target="_blank">
<img src="Fickle Cat.PNG" alt="Click here for a mean cat!" width="300" height="300" class="image-shadow" >
</a>
<br> <!-- room for the image to expand -->
<h3>Project 3: Fickle Cat</h3>
<p class="small-p">Round two of Arduino! As a final project for my one of my prototyping class, we had to create a toy with a cardboard exterior, and an Arduino and at least 3 moving parts for the interior.
This cat is fickle and every 5 seconds will change its mind, displaying a blue light for "Please pet - come closer!", red for "Don't touch me - move further!", and green for "That's a good distance". The 3 moving parts
are a proximity sensor, a motor arm for displaying its mood, and a RGB LED.
</p>
</div>
</div>
<div class="container right fade-righttoleft">
<div class="project-box">
<h3>More to come!</h3>
<p class="small-p">👀</p>
</div>
</div>
</div>
</div>
</section>
<section id="contact-section">
<div class="contact-info">
<!-- lefty text boxes -->
<div class="contact-text">
<div><h2>Let's connect!</h2></div>
<div>
<p>
I'd be happy to connect to chat about anything and everything! Feel free to check out my LinkedIn and GitHub, linked below.
</p>
</div>
<div>
<p>
For specific inquiries, you can send me an email using the form on the right and I'll get back to you as soon as I can!
</p>
</div>
<br>
<div id="logo-container">
<!-- link to email, LI, github -->
<a href="https://www.linkedin.com/in/lam-cynthia/"
><i class="fa-brands fa-linkedin fa-2xl" style="color: #153243"></i
></a>
<a href="https://github.com/cynthia-lam"
><i class="fa-brands fa-github fa-2xl" style="color: #153243"></i
></a>
</div>
</div>
<!-- form -->
<div id="contact-form">
<p>Reach me here:</p>
<form action="/submit" method="post">
<label for="name">Name:</label> <br />
<input
class="text-input"
type="text"
id="name"
name="name"
placeholder="Your name"
/>
<br />
<label for="email">Email:</label> <br />
<input
class="text-input"
type="email"
id="email"
name="email"
placeholder="Your email"
/>
<br />
<label for="message"> Your Message:</label> <br />
<textarea
id="message"
name="contact-me"
placeholder="Your message here"
></textarea>
<br />
<input type="submit" value="Submit" />
</form>
</div>
</section>
<footer>
<p id="footer-text">Made with 💖 by Cynthia Lam</p>
</footer>
</body>
</html> |
import React, {useState, useEffect, useMemo} from 'react';
import {makeStyles} from '@material-ui/core/styles';
import Grid from '@material-ui/core/Grid';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import Dialog from '@material-ui/core/Dialog';
import DialogActions from '@material-ui/core/DialogActions';
import DialogContent from '@material-ui/core/DialogContent';
import DialogTitle from '@material-ui/core/DialogTitle';
import Collapse from '@material-ui/core/Collapse';
import {createTwoFilesPatch} from 'diff';
import {basicSetup, EditorView} from 'codemirror';
import {javascript} from '@codemirror/lang-javascript';
import {defaultHighlightStyle, syntaxHighlighting} from '@codemirror/language';
import {EditorState} from '@codemirror/state';
import {gutter, lineNumbers} from '@codemirror/view';
import CodeMirror from 'rodemirror';
import {lintGutter} from '@codemirror/lint';
import ErrorBoundary from './error_boundary';
const formatTime = (time) =>
Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'short',
day: 'numeric',
hour: 'numeric',
minute: 'numeric'
}).format(new Date(time));
const useStyles = makeStyles((theme) => ({
revision_header: {
borderBottom: '1px solid #ccc',
padding: '5px 0px'
},
diff: {
height: '200px',
border: '1px solid #ccc',
'& .cm-editor': {
height: '100%',
width: '100%'
},
'& div': {
height: '100%',
width: '100%'
},
'& .cm-editor div': {
height: 'auto',
width: 'auto'
}
},
actions: {
paddingRight: '25px'
}
}));
const toJson = (doc) => JSON.stringify(doc, null, 2);
const diff = ({revision, current, prev, revisionDoc, diffType}) => {
if (diffType == 'text') return revisionDoc(revision);
if (diffType == 'curr') {
return createTwoFilesPatch(
'revision',
'curr',
revisionDoc(revision),
revisionDoc(current)
);
} else {
return createTwoFilesPatch(
'prev',
'revision',
prev ? revisionDoc(prev) : revisionDoc({}),
revisionDoc(revision)
);
}
};
const RevisionHistory = ({
getRevisions,
revisionDoc,
dateField='updated_at',
update,
open,
onClose
}) => {
const [revisions, setRevisions] = useState(null);
const [selectedRevision, setSelectedRevision] = useState(null);
const [diffType, setDiffType] = useState('text');
const setSelected = (revision, newDiffType) => {
if (selectedRevision == revision && diffType == newDiffType) {
setSelectedRevision(null);
return;
}
setSelectedRevision(revision);
setDiffType(newDiffType);
};
const classes = useStyles();
useEffect(() => {
getRevisions().then((revisions) => setRevisions(revisions));
}, []);
const extensions = useMemo(
() => [
basicSetup,
syntaxHighlighting(defaultHighlightStyle, {fallback: true}),
javascript(),
EditorView.editable.of(false),
EditorState.readOnly.of(true),
EditorView.lineWrapping,
EditorState.tabSize.of(2),
gutter({class: 'CodeMirror-lint-markers'}),
lineNumbers(),
lintGutter()
],
[]
);
return (
<Dialog
maxWidth='md'
fullWidth
scroll='paper'
open={open}
onClose={onClose}
aria-labelledby='form-dialog-title'
>
<DialogTitle id='form-dialog-title'>
Revision History
<Grid container className={classes.revision_header}>
<Grid xs={6} item>
Comment
</Grid>
<Grid xs={2} item>
Diff
</Grid>
<Grid xs={4} item>
Updated
</Grid>
</Grid>
</DialogTitle>
<DialogContent>
{revisions &&
revisions.map((revision, i) => (
<React.Fragment key={i}>
<Grid container alignItems='center'>
<Grid xs={6} item>
<Button variant='text' onClick={() => setSelected(i, 'text')}>
{revision.comment || 'No comment'}
</Button>
</Grid>
<Grid xs={2} container item>
{i != 0 ? (
<Button
onClick={() => setSelected(i, 'curr')}
variant='text'
size='small'
>
curr
</Button>
) : (
<div style={{width: '64px', display: 'inline'}} />
)}
{i < revisions.length - 1 && (
<Button
onClick={() => setSelected(i, 'prev')}
variant='text'
size='small'
>
prev
</Button>
)}
</Grid>
<Grid xs={4} item>
<Typography>{formatTime(revision[dateField])}</Typography>
</Grid>
</Grid>
<Collapse in={selectedRevision == i} timeout='auto' unmountOnExit>
<Grid container className={classes.diff}>
{selectedRevision == i && (
<ErrorBoundary>
<CodeMirror
extensions={extensions}
value={diff({
revision,
diffType,
revisionDoc,
current: revisions[0],
prev: revisions[i + 1]
})}
/>
</ErrorBoundary>
)}
</Grid>
</Collapse>
</React.Fragment>
))}
</DialogContent>
<DialogActions className={classes.actions}>
{revisions && selectedRevision != null && (
<Button onClick={() => update(revisions[selectedRevision])}>
Load
</Button>
)}
</DialogActions>
</Dialog>
);
};
export default RevisionHistory; |
import React, { useEffect, useState } from 'react';
import { Table, Form, Input, Space, Button, message, Modal, Tag, Select } from 'antd';
import PageLayout from '../../layout/PageLayout';
import { doGet, doPost, doDelete, doPut } from '../../service'; // Adjust the path based on your project structure
import TextEditor from '../../components/TextEditor';
import './styles.css';
const ReplyFormComponent = ({ record, callback }) => {
const [form] = Form.useForm();
const handleReply = async (facebookId, replyContent) => {
try {
await doPost(`/facebooks-reply/${facebookId}`, { content: replyContent });
message.success('Reply thành công');
await callback();
} catch (error) {
message.error('Reply lỗi ' + error.message);
}
};
const onFinish = (values) => {
handleReply(record._id, values.content);
form.resetFields(); // Reset the form fields after submission if needed
};
return (
<Form form={form} onFinish={onFinish}>
<Form.Item name="content">
<TextEditor placeholder="Nhập phản hồi" />
</Form.Item>
<Form.Item>
<Button type="primary" htmlType="submit">
Phản hồi
</Button>
</Form.Item>
</Form>
);
};
const Facebook = () => {
const [facebooks, setFacebooks] = useState([]);
const [currentPage, setCurrentPage] = useState(1);
const [status, setStatus] = useState('iddle');
const [type, setType] = useState('post');
async function getFacebooks(page = 1, status = 'iddle', type = 'post', pageSize = 50) {
try {
const response = await doGet('/facebooks', { status, page, type, pageSize });
setFacebooks(response.data);
} catch (error) {
// Handle error
console.error('Error fetching Facebooks:', error);
}
}
useEffect(() => {
getFacebooks(currentPage, status, type);
}, [currentPage, status, type]);
const [isModalVisible, setIsModalVisible] = useState(false);
const [formAdd] = Form.useForm();
const handleOk = async () => {
try {
const values = await formAdd.validateFields();
await doPost('/facebooks', values);
await getFacebooks(currentPage, status, type);
formAdd.resetFields();
setIsModalVisible(false);
} catch (error) {
message.error('Lưu lỗi ' + error.message);
}
};
const handleStatusChange = (value) => {
setStatus(value);
};
const handleTypeChange = (value) => {
setType(value);
};
const handleCancel = () => {
setIsModalVisible(false);
};
return (
<PageLayout title="Facebook List">
<>
<Select
value={status}
placeholder="Lọc trạng thái"
style={{ width: 200 }}
onChange={handleStatusChange}
>
<Option value="iddle">Chưa xử lý</Option>
<Option value="replied">Đã phản hồi</Option>
</Select>
<Select
value={type}
placeholder="Lọc loại bài viết"
style={{ width: 200 }}
onChange={handleTypeChange}
>
<Option value="post">Bài viết trên page</Option>
<Option value="group-post">Bài viết trong nhóm</Option>
</Select>
<Table
bordered
pagination={{
pageSize: 50,
current: currentPage,
total: 5000,
onChange: (page, pageSize) => {
setCurrentPage(page);
},
}}
columns={[
{
title: 'Từ khóa',
dataIndex: 'keyword',
width: 100,
key: 'keyword',
},
// {
// title: 'Số lượng comment',
// dataIndex: 'number_of_comment',
// width: 100,
// key: 'number_of_comment',
// },
{
title: 'Tag',
width: 100,
key: 'tag',
render: (value, record, index) => {
return record?.tags.map((tag) => (
<Tag style={{ background: tag?.color ? tag?.color : 'black', color: 'white' }}>
{tag?.name}
</Tag>
));
},
},
{
title: 'Nội dung câu hỏi',
key: 'title',
render: (value, record, index) => {
return (
<div
className="show-fb-content"
dangerouslySetInnerHTML={{
__html: record?.title,
}}
/>
);
},
},
{
title: 'Link',
width: 90,
key: 'url',
render: (value, record, index) => {
return (
<a href={record?.url} target="_blank">
Link
</a>
);
},
},
{
title: 'Trạng thái',
width: 100,
key: 'status',
render: (value, record, index) => {
return (
<div>
{record?.status == 'replied' ? (
<>
<Tag style={{ background: 'green', color: 'white' }}>Đã phản hồi</Tag>
</>
) : (
<>
<Tag style={{ background: 'grey', color: 'white' }}>Chưa xử lý</Tag>
</>
)}
</div>
);
},
},
{
title: 'Reply',
width: 600,
render: (value, record) => {
return record?.reply ? (
<Space direction="vertical">
<Space direction="horizontal">
<a href={record?.answer_url}>Link câu trả lời</a>
</Space>
<div>{record?.reply}</div>
<div>{record?.answer_url}</div>
</Space>
) : (
<ReplyFormComponent
record={record}
callback={() => getFacebooks(currentPage, status, type)}
/>
);
},
},
// Add more columns as needed
{
title: 'Thao tác',
width: 100,
render: (value, record) => (
<Space>
<Button
type="primary"
onClick={async () => {
await doPut(`/facebooks/${record._id}`, { visible: false });
await getFacebooks(currentPage, status, type);
}}
>
Ẩn
</Button>
<Button
type="ghost"
danger
onClick={async () => {
await doDelete(`/facebooks/${record._id}`);
await getFacebooks(currentPage, status, type);
}}
>
Xóa
</Button>
</Space>
),
},
]}
dataSource={facebooks}
rowKey="_id"
/>
<Modal
title="Add Facebook"
visible={isModalVisible}
onOk={handleOk}
onCancel={handleCancel}
>
<Form form={formAdd}>
<Form.Item
label="Từ khóa"
name="keyword"
rules={[{ required: true, message: 'Please enter the keyword' }]}
>
<Input placeholder="Enter the keyword" />
</Form.Item>
<Form.Item
label="Nội dung câu hỏi"
name="title"
rules={[{ required: true, message: 'Please enter the title' }]}
>
<Input placeholder="Enter the title" />
</Form.Item>
<Form.Item
label="URL"
name="url"
rules={[{ required: true, message: 'Please enter the URL' }]}
>
<Input placeholder="Enter the URL" />
</Form.Item>
<Form.Item
label="Trạng thái"
name="status"
rules={[{ required: true, message: 'Please enter the status' }]}
>
<Input placeholder="Enter the status" />
</Form.Item>
<Form.Item label="Upvotes" name="number_of_upvote">
<Input placeholder="Enter the number of upvotes" defaultValue={'0'} />
</Form.Item>
<Form.Item label="Comments" name="number_of_comment">
<Input placeholder="Enter the number of comments" defaultValue={'0'} />
</Form.Item>
<Form.Item label="Client ID" name="client_id">
<Input placeholder="Enter the client ID" />
</Form.Item>
<Form.Item label="Client Name" name="client_name">
<Input placeholder="Enter the client name" />
</Form.Item>
</Form>
</Modal>
</>
</PageLayout>
);
};
export default Facebook; |
import 'package:emlak/screens/home_screen.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class BottomNavBarRiverpod extends ChangeNotifier {
BuildContext? context;
List<BottomNavigationBarItem> items = const [
BottomNavigationBarItem(
icon: Icon(
CupertinoIcons.home,
color: Colors.black54,
),
label: "Anasayfa",
),
BottomNavigationBarItem(
icon: Icon(
CupertinoIcons.cart,
color: Colors.black54,
),
label: "Sepetim",
),
BottomNavigationBarItem(
icon: Icon(
CupertinoIcons.person,
color: Colors.black54,
),
label: "Profil",
),
];
int currentIndex = 0;
void setCurrentIndex(int index) {
currentIndex = index;
notifyListeners();
}
String appbarTitle() {
switch (currentIndex) {
case 1:
return "Sepetim";
case 2:
return "Hesabım";
case 0:
default:
return "Menü";
}
}
Widget appbarIcon() {
switch (currentIndex) {
case 1:
return const SizedBox();
case 2:
return IconButton(
icon: const Icon(Icons.notifications),
onPressed: () {},
);
case 0:
default:
return IconButton(
icon: const Icon(Icons.notifications),
onPressed: () {},
);
}
}
Widget body() {
switch (currentIndex) {
case 1:
return const HomeScreen();
case 2:
return const HomeScreen();
case 0:
default:
return HomeScreen();
}
}
} |
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.nn.utils.rnn import pad_sequence, pack_padded_sequence, pad_packed_sequence
import torch
from utils import CustomError
class LSTM_attention(nn.Module):
def __init__(self,
vocab_size,
embedding_dim,
hidden_dim,
num_layers=1,
drop_keep_prob=0.2,
n_class=2,
bidirectional=True,
pretrained_weight=None,
update_w2v=True,
):
super(LSTM_attention, self).__init__()
self.hidden_dim = hidden_dim
self.num_layers = num_layers
self.n_class = n_class
self.bidirectional = bidirectional
self.embedding = nn.Embedding(vocab_size, embedding_dim)
if pretrained_weight is not None:
self.embedding.weight = nn.Parameter(torch.tensor(pretrained_weight, dtype=torch.float32))
self.embedding.weight.requires_grad = update_w2v
self.encoder = nn.LSTM(
input_size=embedding_dim,
hidden_size=self.hidden_dim,
num_layers=num_layers,
bidirectional=self.bidirectional,
dropout=drop_keep_prob,
batch_first=True
)
self.attention_W = nn.Linear(2 * hidden_dim if bidirectional else hidden_dim, 2 * hidden_dim if bidirectional else hidden_dim, bias=False)
self.attention_proj = nn.Linear(2 * hidden_dim if bidirectional else hidden_dim, 1, bias=False)
if self.bidirectional:
self.dense = nn.Linear(2 * hidden_dim, n_class)
else:
self.dense = nn.Linear(hidden_dim, n_class)
def forward(self, inputs):
lengths = torch.sum(inputs != 0, dim=1)
# lengths = torch.clamp(lengths, min=1)
embeddings = self.embedding(inputs.data)
packed_embeddings = pack_padded_sequence(embeddings, lengths.cpu(), batch_first=True, enforce_sorted=False)
packed_output, (hidden, cell) = self.encoder(packed_embeddings)
output, _ = pad_packed_sequence(packed_output, batch_first=True)
u = torch.tanh(self.attention_W(output))
att = self.attention_proj(u)
att_score = F.softmax(att, dim=1)
scored_x = output * att_score
encoding = torch.sum(scored_x, dim=1)
outputs = self.dense(encoding)
return outputs
class LSTM_Model(nn.Module):
def __init__(self,
vocab_size,
embedding_dim,
hidden_dim,
num_layers=1,
drop_keep_prob=0.2,
n_class=2,
bidirectional=True,
pretrained_weight=None,
update_w2v=True,
):
super(LSTM_Model, self).__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
if pretrained_weight is not None:
self.embedding.weight = nn.Parameter(torch.tensor(pretrained_weight, dtype=torch.float32))
self.embedding.weight.requires_grad = update_w2v
self.lstm = nn.LSTM(embedding_dim, hidden_dim, num_layers=num_layers, dropout=drop_keep_prob, bidirectional = bidirectional)
lstm_output_dim = hidden_dim * 2 if bidirectional else hidden_dim
self.dense = nn.Linear(lstm_output_dim, n_class)
def forward(self, inputs):
lengths = torch.sum(inputs != 0, dim=1)
# lengths = torch.clamp(lengths, min=1)
embedded = self.embedding(inputs)
packed_embedded = pack_padded_sequence(embedded, lengths.cpu(), batch_first=True, enforce_sorted=False)
packed_output, (hidden, cell) = self.lstm(packed_embedded)
output, _ = pad_packed_sequence(packed_output, batch_first=True)
# final_hidden = output[torch.arange(output.size(0)), lengths-1]
if self.lstm.bidirectional:
final_hidden = torch.cat((hidden[-2], hidden[-1]), dim=1)
else:
final_hidden = hidden[-1]
outputs = self.dense(final_hidden)
return outputs |
/**
* Works like the python zip function, but for only two arguments
*
* @param a the first array
* @param b the second array
* @returns an array of tuples of the corresponding elements in a and b
*/
export function zip<T, U>(a: Array<T>, b: Array<U>): Array<[T, U]> {
if (a.length <= b.length)
return a.map((element, index) => [element, b[index]]);
else
return b.map((element, index) => [a[index], element]);
} |
#
# 3D Spherical Gap Heat Transfer Test.
#
# This test exercises 3D gap heat transfer for a constant conductivity gap.
#
# The mesh consists of an inner solid sphere of radius = 1 unit, and outer
# hollow sphere with an inner radius of 2. In other words, the gap between
# them is 1 radial unit in length.
#
# The conductivity of both spheres is set very large to achieve a uniform
# temperature in each sphere. The temperature of the center node of the
# inner sphere is ramped from 100 to 200 over one time unit. The
# temperature of the outside of the outer, hollow sphere is held fixed
# at 100.
#
# A simple analytical solution is possible for the integrated heat flux
# between the inner and outer spheres:
#
# Integrated Flux = (T_left - T_right) * (gapK/(r^2*((1/r1)-(1/r2)))) * Area
#
# For gapK = 1 (default value)
#
# The area is taken as the area of the secondary (inner) surface:
#
# Area = 4 * pi * 1^2 (4*pi*r^2)
#
# The integrated heat flux across the gap at time 1 is then:
#
# 4*pi*k*delta_T/((1/r1)-(1/r2))
# 4*pi*1*100/((1/1) - (1/2)) = 2513.3 watts
#
# For comparison, see results from the integrated flux post processors.
# This simulation makes use of symmetry, so only 1/8 of the spheres is meshed
# As such, the integrated flux from the post processors is 1/8 of the total,
# or 314.159 watts... i.e. 100*pi.
# The value coming from the post processor is slightly less than this
# but converges as mesh refinement increases.
#
# Simulating contact is challenging. Regression tests that exercise
# contact features can be difficult to solve consistently across multiple
# platforms. While designing these tests, we felt it worth while to note
# some aspects of these tests. The following applies to:
# sphere3D.i, sphere2DRZ.i, cyl2D.i, and cyl3D.i.
# 1. We decided that to perform consistently across multiple platforms we
# would use very small convergence tolerance. In this test we chose an
# nl_rel_tol of 1e-12.
# 2. Due to such a high value for thermal conductivity (used here so that the
# domains come to a uniform temperature) the integrated flux at time = 0
# was relatively large (the value coming from SideIntegralFlux =
# -_diffusion_coef[_qp]*_grad_u[_qp]*_normals[_qp] where the diffusion coefficient
# here is thermal conductivity).
# Even though _grad_u[_qp] is small, in this case the diffusion coefficient
# is large. The result is a number that isn't exactly zero and tends to
# fail exodiff. For this reason the parameter execute_on = initial should not
# be used. That parameter is left to default settings in these regression tests.
#
[GlobalParams]
order = SECOND
family = LAGRANGE
[]
[Mesh]
file = sphere3D.e
[]
[Functions]
[./temp]
type = PiecewiseLinear
x = '0 1'
y = '100 200'
[../]
[]
[Variables]
[./temp]
initial_condition = 100
[../]
[]
[AuxVariables]
[./gap_conductance]
order = CONSTANT
family = MONOMIAL
[../]
[]
[Kernels]
[./heat_conduction]
type = HeatConduction
variable = temp
[../]
[]
[AuxKernels]
[./gap_cond]
type = MaterialRealAux
property = gap_conductance
variable = gap_conductance
boundary = 2
[../]
[]
[Materials]
[./heat1]
type = HeatConductionMaterial
block = '1 2'
specific_heat = 1.0
thermal_conductivity = 100000000.0
[../]
[]
[ThermalContact]
[./thermal_contact]
type = GapHeatTransfer
variable = temp
primary = 3
secondary = 2
emissivity_primary = 0
emissivity_secondary = 0
gap_conductivity = 1
quadrature = true
gap_geometry_type = SPHERE
sphere_origin = '0 0 0'
[../]
[]
[BCs]
[./mid]
type = FunctionDirichletBC
boundary = 5
variable = temp
function = temp
[../]
[./temp_far_right]
type = DirichletBC
boundary = 4
variable = temp
value = 100
[../]
[]
[Executioner]
type = Transient
solve_type = 'PJFNK'
petsc_options_iname = '-pc_type -pc_factor_mat_solver_package'
petsc_options_value = 'lu superlu_dist'
dt = 1
dtmin = 0.01
end_time = 1
nl_rel_tol = 1e-12
nl_abs_tol = 1e-7
[./Quadrature]
order = fifth
side_order = seventh
[../]
[]
[Outputs]
exodus = true
[./Console]
type = Console
[../]
[]
[Postprocessors]
[./temp_left]
type = SideAverageValue
boundary = 2
variable = temp
[../]
[./temp_right]
type = SideAverageValue
boundary = 3
variable = temp
[../]
[./flux_left]
type = SideDiffusiveFluxIntegral
variable = temp
boundary = 2
diffusivity = thermal_conductivity
[../]
[./flux_right]
type = SideDiffusiveFluxIntegral
variable = temp
boundary = 3
diffusivity = thermal_conductivity
[../]
[] |
# To pivot a weekly file coming in, where the last two columns are replaced each week
# Structure of the file:
# Store Nbr | Store Type Descr | City | State | Zip Code | 201938 POS Sales | 201938 POS Qty
# Required format:
# Week (2019xx) | Total Sales (Sum of POS Sales) | Total Qty (Sum of POS Qty)
# At the top of the report, there's a header which will be truncated
#Set working directory, where the file resides
setwd('C:/Users/pushpindersingh/Desktop/')
getwd()
#Call library readxl to read XL file
#install.packages("readxl")
library(readxl)
#Form the master dataset by reading the file
pi_walmart <- read_excel('sample report.xls', range = "A15:G40000")
#Need these variables later for grouping (storing header names)
var_week = read_excel('sample report.xls')
var_week = data.frame(var_week)
#for later use
week_sales <- var_week$...6[[14]]
week_qty <- var_week$...7[[14]]
b <- gsub(" ", '.',week_sales)
b <- paste("X",b, sep = "")
c <- gsub(" ", ".", week_qty)
c <- paste("X", c, sep = "")
#Call dplyr package
#install.packages("dplyr")
library(dplyr)
pi_walmart_df = data.frame(pi_walmart)
#new_data <- pi_walmart[-(1:13),]
# file_headers <- new_data[,1]
# file_headers
# var_week = as.character(var_week)
# var_week
# the "YYYYWW" combination, which will be added as a constant column to the df
week <- strsplit(week_sales, " ")[[1]][1]
# Adding the constant year-week, which will be unique for each file
pi_walmart_df_2 <- cbind(pi_walmart_df, week)
# Grouping & Summarising data
final <-
select(pi_walmart_df_2, one_of(b,c),week) %>%
group_by(week) %>%
summarise_all(list(sum), na.rm = TRUE)
# Rename columns
colnames(final) <- c("Week", "Total_Sales", "Total_Qty")
# Write the file
write.csv(final,
'C:/Users/DT-045/Desktop//PI_Walmart_Weekly_Pivoted.csv',
row.names = FALSE) |
// SPDX-FileCopyrightText: 2017-2022 City of Espoo
//
// SPDX-License-Identifier: LGPL-2.1-or-later
import classNames from 'classnames'
import React from 'react'
import ReactSelect, { Props } from 'react-select'
import styled, { useTheme } from 'styled-components'
import { scrollIntoViewSoftKeyboard } from 'lib-common/utils/scrolling'
import { tabletMin } from 'lib-components/breakpoints'
import { FixedSpaceColumn, FixedSpaceRow } from '../../layout/flex-helpers'
import { fontWeights } from '../../typography'
import { defaultMargins } from '../../white-space'
import { StaticCheckBox } from './Checkbox'
interface MultiSelectProps<T> {
value: readonly T[]
options: readonly T[]
getOptionId: (value: T) => string
getOptionLabel: (value: T) => string
getOptionSecondaryText?: (value: T) => string
onChange: (selected: T[]) => void
maxSelected?: number
placeholder: string
noOptionsMessage?: string
showValuesInInput?: boolean
closeMenuOnSelect?: Props<never>['closeMenuOnSelect']
isClearable?: Props<never>['isClearable']
inputId?: Props<never>['inputId']
'data-qa'?: string
autoFocus?: boolean
}
function MultiSelect<T>({
value,
options,
getOptionId,
getOptionLabel,
getOptionSecondaryText,
onChange,
closeMenuOnSelect,
noOptionsMessage,
maxSelected,
showValuesInInput: showSelectedValues,
autoFocus,
...props
}: MultiSelectProps<T>) {
const { colors } = useTheme()
return (
<div data-qa={props['data-qa']} className="multi-select">
<ReactSelect
styles={{
menu: (base) => ({
...base,
zIndex: 15
}),
clearIndicator: (base) => ({
...base,
[`@media (max-width: ${tabletMin})`]: {
display: 'none'
}
}),
group: (base) => ({
...base,
position: 'relative',
':not(:last-child):after': {
position: 'absolute',
content: '""',
bottom: '-2px',
left: defaultMargins.s,
right: defaultMargins.s,
borderBottom: `1px solid ${colors.grayscale.g15}`
}
})
}}
autoFocus={autoFocus}
isMulti
isSearchable={true}
hideSelectedOptions={false}
backspaceRemovesValue={false}
closeMenuOnSelect={closeMenuOnSelect ?? false}
noOptionsMessage={() => noOptionsMessage ?? 'Ei tuloksia'}
getOptionLabel={getOptionLabel}
getOptionValue={getOptionId}
value={value}
tabSelectsValue={false}
onFocus={(ev) => {
const parentContainer = ev.target.closest('.multi-select')
if (parentContainer) {
scrollIntoViewSoftKeyboard(parentContainer, 'start')
}
}}
options={[
{
options: value
},
{
options:
maxSelected === undefined || value.length < maxSelected
? options.filter(
(o) =>
!value
.map((o2) => getOptionId(o2))
.includes(getOptionId(o))
)
: []
}
]}
onChange={(selected) => {
const selectionsArray =
selected && 'length' in selected ? (selected as T[]) : []
onChange(selectionsArray)
}}
filterOption={({ data }, q) =>
getOptionLabel(data).toLowerCase().includes(q.toLowerCase())
}
controlShouldRenderValue={showSelectedValues ?? true}
components={{
Option: function Option({ innerRef, innerProps, ...props }) {
const data = props.data
return (
<OptionWrapper
{...innerProps}
data-qa="option"
data-id={getOptionId(data)}
ref={innerRef}
key={getOptionId(data)}
className={classNames({ focused: props.isFocused })}
>
<OptionContents
label={getOptionLabel(data)}
secondaryText={
getOptionSecondaryText && getOptionSecondaryText(data)
}
selected={props.isSelected}
/>
</OptionWrapper>
)
}
}}
{...props}
/>
</div>
)
}
export default React.memo(MultiSelect) as typeof MultiSelect
const OptionWrapper = styled.div`
cursor: pointer;
&:hover,
&.focused {
background-color: ${(p) => p.theme.colors.main.m4};
}
padding: ${defaultMargins.xxs} ${defaultMargins.s};
`
const OptionContents = React.memo(function Option({
label,
secondaryText,
selected
}: {
label: string
secondaryText?: string
selected: boolean
}) {
return (
<FixedSpaceRow alignItems="center">
<StaticCheckBox checked={selected} />
<FixedSpaceColumn spacing="zero">
<span>{label}</span>
{!!secondaryText && <SecondaryText>{secondaryText}</SecondaryText>}
</FixedSpaceColumn>
</FixedSpaceRow>
)
})
const SecondaryText = styled.span`
font-size: 14px;
line-height: 21px;
font-weight: ${fontWeights.semibold};
color: ${(p) => p.theme.colors.grayscale.g70};
` |
OCA objective Map
1. Java Basics
1.1 Define scope of variables
1.2 Define structure of Java class
1.3 Create executable Java application with main()
1.4 Import Java classes and make them accessible in your app
1.5 Compare and contrast features of Java
2. Working with Java data types
2.1 Declare and initialize variables
2.2 Differentiate between object references and primitives
2.3 Know how to read and write objet fields
2.4 Explain Object lifecycle
2.5 Use wrapper classes
3. Using operators and decision constructs
4. Creating and using Arrays
4.1 Declare, instantiate, initialize, and use a one-dimensional array
4.2 Declare, instantiate, initialize, and use multidimensional array. (sic)
5. Using loop constructs
6. Working with methods and encapsulation
6.2 Apply the static keyword to methods and fields.
6.3 Create and overload constructors; including impact on default constructors. (sic)
6.4 Apply access modifiers.
7. Working with inheritence
7.5 Use abstract classes and interfaces.
8. Exception handling
9. Working with selected classes from the Java API
Features and Benefits of Java (OCA Objective 1.5)
Object oriented
OO design makes software easy to test and enhance.
OO design also makes it easy to think of software components as object with behaviour and state.
Encapsulation
Encapsulation allows a software component to hide its data from other components, protecting the data from being updated without the component’s approval or knowledge.
Memory managed Language
Java provides automatic memory management which is quiet complex and error prone if done programattically.
Further, tracking down bugs related to memory management (often called memory leaks) is a common, error-prone, and time-consuming process.
Huge library
Java has an enormous library of prewritten, well-tested, and well-supported code, which is easy to include and consume.
Secure by design
When compiled Java code is executed, it runs inside the Java Virtual Machine (JVM).
The JVM provides a secure “sandbox” for your Java code to run in, and the JVM makes sure that nefarious programmers cannot write Java code that will cause trouble on other people’s machines when it runs.
Cross platform execution
One of the goals (largely, but not perfectly achieved) of Java is that much of the Java code you write can run on many platforms.
Strongly typed Language
A strongly typed language usually requires the programmer to explicitly declare the types of the data and objects being used in the program.
Strong typing allows the Java compiler to catch many potential programming errors before your code even compiles.
Strong typing allows programmers to develop reliable, testable and production-quality code.
Multithreaded Language
Java provides built-in language features and APIs that allow programs to use many operating-system processes (hence, many “cores”) at the same time.
Multithreaded programming is never simple, but Java provides a rich toolkit to make it as easy as possible.
Distributed computing
Another way to tackle big programming problems is to distribute the workload across many machines.
The Java API provides several ways to simplify tasks related to distributed computing.
One such example is serialization, a process in which a Java object is converted to a portable form. Serialized objects can be sent to other machines, deserialized, and then used as a normal Java object.
Identifiers and Keywords (OCA Objectives 1.2 and 2.1)
Legal Identifiers
Technically, legal identifiers must be composed of only Unicode characters, numbers, currency symbols, and connecting characters (such as underscores).
Identifiers must start with a letter, a currency character ($), or a connecting character such as the underscore (_).
Identifiers cannot start with a digit!
After the first character, identifiers can contain any combination of letters, currency characters, connecting characters, or numbers.
In practice, there is no limit to the number of characters an identifier can contain.
You can’t use a Java keyword as an identifier.
Oracle code conventions
Classes and interfaces
The first letter should be capitalized, and if several words are linked together to form the name, the first letter of the inner words should be uppercase (a format that’s sometimes called “CamelCase”).
For interfaces, the names should typically be adjectives.
Methods
The first letter should be lowercase, and then normal CamelCase rules should be used. In addition, the names should typically be verb-noun pairs
Variables
Like methods, the CamelCase format should be used, but starting with a lowercase letter.
Constants
Java constants are created by marking variables static and final. They should be named using uppercase letters with underscore characters as separators:
Define Classes (OCA Objectives 1.2, 1.3, 1.4, 6.4, and 7.5)
Source file declaration rules
There can be only one public class per source code file.
Comments can appear at the beginning or end of any line in the source code file.
There can be only one public class per source code file.
If the class is part of a package, the package statement must be the first line in the source code file.
If there are import statements, they must go between the package statement (if there is one) and the class declaration.
If there isn’t a package statement, then the import statement(s) must be the first line(s) in the source code file. If there are no package or import statements, the class declaration must be the first line in the source code file.
import and package statements apply to all classes within a source code file.
A file can have more than one non-public class.
Files with no public classes can have a name that does not match any of the classes in the file.
Using the javac and java Commands
The javac command is used to invoke Java’s compiler. You can specify many options when running javac.
General syntax => javac [options] [source files] => Both the [options] and the [source files] are optional parts of the command, and both allow multiple entries.
The java command is used to invoke the Java Virtual Machine (JVM).
General syntax => java [options] class [args] => The [options] and [args] parts of the java command are optional, and they can both have multiple values.
You must specify exactly one class file to execute, and the java command assumes you’re talking about a .class file, so you don’t specify the .class extension on the command line.
Consider => java -showversion MyClass x 1 => This command can be interpreted as “Show me the version of the JVM being used, and then launch the file named MyClass.class and send it two String arguments whose values are x and 1.”
Using public static void main(String[ ] args)
main() is the method that the JVM uses to start execution of a Java program.
As far as the compiler and the JVM are concerned, the main that is started by JVM is the main() with this signature => public static void main(String[] args)
Other versions of main() with other signatures are perfectly legal, but they’re treated as normal methods.
There is some flexibility in the declaration of the main() method: the order of its modifiers can be altered a little; the String array doesn’t have to be named args; and it can be declared using var-args syntax.
The valid declarations of main() are as follows
static public void main(String[] args)
public static void main(String... x)
static public void main(String[] someArray)
main() can be overloaded.
Import Statements and the Java API
Instead of using fully qualified names for classes, import statements are used in order to save some typing.
The fully qualified names consists of the package name followed by the class name. Import statements can be on the class level or package level.
If we want to import all the classes in a package, we need to append the import statement with a * symbol instead of the class name.
Static import statements
Sometimes classes will contain static members. Static class members can exist in the classes you write and in a lot of the classes in the Java API.
Static imports can be used when you want to “save typing” while using a class’s static members.
Consider
public class Test {
public static void main(String[] args) {
System.out.print(Integer.MAX_VALUE);
}
}
import static java.lang.Integer.MAX_VALUE;
import static java.lang.System.out;
public class Test {
public static void main(String[] args) {
out.print(MAX_VALUE);
}
}
Rules:
You must say import static; you can’t say static import.
If you do a static import for both the Integer class and the Long class, referring to MAX_VALUE will cause a compiler error, because both Integer and Long have a MAX_VALUE constant and Java won’t know which MAX_VALUE you’re referring to.
You can do a static import on static object references, constants, and static methods.
Using the * is valid only inside a specific package and class. It doesn’t work between directory. => import java.*; wont search the entire java package.
Class modifiers
Access modifiers => public, private, protected
Non access modifiers => strictfp, final, abstract
Class access
When we say code from one class (class A) has access to another class (class B), it means class A can do one of three things:
Create an instance of class B
Extend class b or become subclass of class B.
Use or consume certain methods and members of class B.
In effect, access means visibility.
Default class access
A class with default access has no modifier preceding it in the declaration!
Think of default access as package-level access, because a class with default access can be seen/accessed only by classes within the same package.
When you see a question with complex logic, be sure to look at the access modifiers first.
Public class access
All classes in the Java Universe (JU) have access to a public class.
If a public class you’re trying to use is in a different package from the class you’re writing, you’ll still need to import the public class.
Non access class modifiers
You can modify a class declaration using the keyword final, abstract, or strictfp. These modifiers are in addition to whatever access control is on the class, so you could, for example, declare a class as both public and final.
strictfp
Marking a class as strictfp means that any method code in the class will conform strictly to the IEEE 754 standard rules for floating points.
final
When used in a class declaration, the final keyword means the class can’t be subclassed. In other words, no other class can ever extend (inherit from) a final class, and any attempts to do so will result in a compiler error.
You should make a final class only if you need an absolute guarantee that none of the methods in that class will ever be overridden.
Marking a class final finished off any scope there is for the improvement of the class.
A final class obliterates a key benefit of OO—extensibility.
abstract
Marking a class abstract means that no instances of that particular class can be created. If we try to instatiate, we will get compile time error.
The sole purpose of an abstract class is to be subclassed by other classes.
An abstract class is mostly used to provide a base for subclasses to extend and implement the abstract methods and override or use the implemented methods in abstract class.
Non abstract classes have methods that end with curly braces.
In an abstract class, methods end with a semi-colon.
If one method is made abstract in a class, then the whole class must be made abstract.
Non abstract methods can be put in an abstract class and all subclasses will get the same implementation of the method.
The concrete subclasses get to inherit functionality and need to implement only the methods that define subclass-specific behavior.
You can’t mark a class as both abstract and final.
Use Interfaces (OCA Objective 7.5)
Declaring an interface
When you create an interface, you’re defining a contract for what a class can do, without saying anything about how the class will do it.
Any concrete class type that implements an interface must agree to write the code for all the methods declared in the interface.
Interfaces can be implemented by any class, from any inheritance tree. This lets you take radically different classes and give them a common characteristic.
Think of a traditional interface as a 100 percent abstract class.
An abstract class can define both abstract and nonabstract methods, but an interface generally has only abstract methods.
Rules for declaring interfaces
Interface methods are implicitly public and abstract, unless declared as default or static.
All variables defined in an interface must be public, static, and final—in other words, interfaces can declare only constants, not instance variables.
Interface methods cannot be marked final, strictfp, or native.
An interface can extend one or more other interfaces.
An interface cannot extend anything but another interface.
An interface cannot implement another interface or class.
An interface must be declared with the keyword interface.
Interface types can be used polymorphically
The abstract modifier can be used while declaring an interface.
Rules for declaring interface methods.
All interface methods are implicitly public and abstract. Typing those two modifiers on interface methods is redundant.
All interface methods not declared default or static are public and abstract regardless of what you see in the interface definition.
Using final, private and protected modifiers on interface methods will result in compile time error.
Declaring Interface Constants
You’re allowed to put constants in an interface. By doing so, you guarantee that any class implementing the interface will have access to the same constant.
Interface constants must always be declared as => public static final, as interface constants are no different from any publically accessed constants.
Just because interface constants are defined in an interface, they don’t have to be declared as public, static, or final. They must be public, static, and final, but you don’t actually have to declare them that way.
Once the interface constant has been declared, its value cant be changed by any of the implementing classes.
Declaring default Interface Methods
A default method in an interface is one which can have an implementation in the interface itself and the same implementation will be inherited by the implementing classes.
default methods are declared by using the default keyword.
The default keyword can be used only with interface method signatures, not class method signatures.
default methods are public by definition, and the public modifier is optional.
default methods cannot be marked as private, protected, static, final, or abstract.
default methods must have a concrete method body.
Declaring static Interface Methods
A static method is one which can be invoked directly without creating an instance. Interfaces can include static methods with concrete implementation.
static interface methods are declared by using the static keyword.
static interface methods are public by default, and the public modifier is optional.
static interface methods cannot be marked as private, protected, final, or abstract.
static interface methods must have a concrete method body.
When invoking a static interface method, the method’s type (interface name) MUST be included in the invocation.
Declare Class Members (OCA Objectives 2.1, 2.2, 2.3, 4.1, 4.2, 6.2, 6.3, and 6.4)
Access Modifiers
Methods and non local variables can also be given access using the access and non access modifiers.
A class can use only public and default modifiers where as members can use protected and private in addition to public and default modifiers.
The class members get default access if no access modifier is specified.
If class A has access to a member of class B, it means that class B’s member is visible to class A. This implies that
Whether method code in one class can access a member of another class => this type of access is achieved using the dot operator.
Whether a subclass can inherit a member of its superclass => This type of access revolves around which, if any, members of a superclass a subclass can access through inheritance. If a subclass inherits a member, it’s exactly as if the subclass actually declared the member itself.
If class A itself can’t be accessed by class B, then no members within class A can be accessed by class B.
Public members
When a method or variable member is declared public, it means all other classes, regardless of the package they belong to, can access the member (assuming the class itself is visible).
For a subclass, if a member of its superclass is declared public, the subclass inherits that member regardless of whether both classes are in the same package.
If you see a method invoked (or a variable accessed) without the dot operator (.), it means the method or variable belongs to the class where you see that code. It also means that the method or variable is implicitly being accessed using the this reference.
The reference this always refers to the currently executing object—in other words, the object running the code where you see the this reference.
Private Members
Members marked as private can be seen only from code inside the same class. Code outside the class can't access private members.
In case of inheritence, the subclass can't inherit a private member of a superclass. The private members of superclass stay hidden to the subclass.
A same member can be declared in a subclass. However, this is not equivalent to overriding a method. It is just declaring a new method.
Protected and Default Members
A default member may be accessed only if the class accessing the member belongs to the same package.
A protected member can be accessed (through inheritance) by a subclass even if the subclass is in a different package.
If the protected keyword is used to define a member, any subclass of the class declaring the member can access it through inheritance. It doesn’t matter if the superclass and subclass are in different packages; the protected superclass member is still visible to the subclass
The default behavior, which doesn’t allow a subclass to access a superclass member unless the subclass is in the same package as the superclass.
Whereas default access doesn’t extend any special consideration to subclasses, the protected modifier respects the parent-child relationship, even when the child class moves away into a different package.
So when you think of default access, think package restriction. But when you think protected, think package + kids.
A class with a protected member is marking that member as having package-level access for all classes, but with a special exception for subclasses outside the package.
Consider a subclass in a different package. The subclass can inherit the protected member of a superclass but not access the same protected member via dot operator. example
package cert;
public class Parent {
protected int x = 9;
}
package other;
public class Child extend Parent {
public void test() {
System.out.print("x = "+x); //prints 9
}
}
package cert;
public class Parent {
protected int x = 9;
}
package other;
public class Child extend Parent {
public void test() {
Parent p = new Parent();
System.out.print("x = "+p.x); //compile time error
}
}
Once the subclass-outside-the-package inherits the protected member, that member becomes private to any code outside the subclass, with the exception of subclasses of the subclass.
Default details
Consider
package cert;
public class Parent {
int x = 9; // default/package level access.
}
package other;
public class Child extend Parent {
public void test() {
System.out.print("x = "+x); // compile time error.
}
}
Also consider
package cert;
public class Parent {
int x = 9; // default/package level access.
}
package cert;
public class Child extend Parent {
public void test() {
System.out.print("x = "+x); // prints 9
}
}
Local Variables and Access Modifiers
Access modifiers cannot be applied to local variables. Any code with access modifiers on local variables will not compile.
The only modifier that can be applied to a local variable is final.
Non access modifiers applied to methods.
final
The final keyword prevents a method from being overridden in a subclass and is often used to enforce the API functionality of a method.
Preventing a subclass from overriding a method stifles many of the benefits of OO, including extensibility through polymorphism.
Classes marked final can't be subclassed where are methods marked final in non final superclasses can't be overridden in the subclass.
final arguments
Method arguments are the variable declarations that appear in between the parentheses in a method declaration. Method arguments are essentially local variables to the method where they are declared.
Method arguments marked final cannot be assigned a new variable inside the method.
abstract
An abstract method is a method that’s been declared (as abstract) but not implemented.
Abstract method contains no functional code.
An abstract method declaration doesn’t even have curly braces for where the implementation code goes, but instead closes with a semicolon.
You mark a method abstract when you want to force subclasses to provide the implementation.
It is illegal to have even a single abstract method in a class that is not explicitly declared abstract. You can have an abstract class with no abstract methods.
Clues to identify a non-abstract method
The method is not marked abstract.
The method declaration includes curly braces, as opposed to ending in a semicolon.
The method might provide actual implementation code inside the curly braces.
The first concrete subclass of an abstract class must implement all abstract methods of the superclass. Concrete means not abstract.
If you have an abstract class extending another abstract class, the abstract subclass doesn’t need to provide implementations for the inherited abstract methods. Only concrete subclasses need to implement the abstract methods.
Let us assume there are n-1 abstract classes in an inheritance tree and nth class is a concrete one. The concrete subclass must implement all the abstract methods defined in n-1 ancestors.
If the concrete subclass doesn’t implement all the abstract methods from its ancestors' superclasses, then we get compile time error.
A method can never, ever, ever be marked as both abstract and final, or both abstract and private.
abstract methods must be implemented whereas final method must not be overridden and private methods aren't even visible in the subclass.
The abstract modifier can never be combined with the static modifier as static methods are called without instance object and abstract methods dont have an implementation.
synchronized
The synchronized keyword indicates that a method can be accessed by only one thread at a time.
The synchronized modifier can be applied only to methods—not variables, not classes, just methods.
The synchronized modifier can be matched with any of the four access control levels.
native
The native modifier indicates that a method is implemented in platform-dependent code.
native can be applied only to methods.
Note that a native method’s body must be a semicolon (;) indicating that the implementation is omitted.
strictfp
strictfp forces floating points (and any floating-point operations) to adhere to the IEEE 754 standard.
If you don’t declare a class as strictfp, you can still declare an individual method as strictfp.
A variable can never be declared strictfp. Only classes and methods can be declared strictfp.
Methods with Variable Argument Lists (var-args)
Java allows you to create methods that can take a variable number of arguments.
Declare and Use enums (OCA Objective 1.2) |
## Vending Machine Capstone
This was a capstone project completed for [Merit America's](https://meritamerica.org/) Fullstack Java Web Developer Bootcamp - A 31-week intensive program focused on Full Stack Web Application Development, including hands-on
coursework in Java Development, Client-Server Programming (SQL + Spring), and Frontend Development (Classic Web + React).
Program Capstone Project 1 of 3.
## Project Description
Virtual vending machine application with command-line interface allowing users to deposit money, choose a product, and return the correct change. Inventory is loaded via a text file and transactions are also logged to a text file.
## Table of Contents
- [Vending Machine Capstone](#vending-machine-capstone)
- [Project Description](#project-description)
- [Table of Contents](#table-of-contents)
- [Project Requirements](#project-requirements)
- [Running the Application](#running-the-application)
- [Requirements](#requirements)
- [Getting Started](#getting-started)
- [Team Members](#team-members)
## Project Requirements
1. Each vending machine item has a name and a price
2. A main menu must display when the software runs, presenting the following options:
> ```
> (1) Display Vending Machine Items
> (2) Purchase
> (3) Exit
> ```
3. Vending machine inventory is stocked via an input file when the vending machine starts
4. The vending machine is automatically restocked each time the application runs.
When the customer selects "(1) Display Vending Machine Items", they're presented
with a list of all items in the vending machine with its quantity remaining:
- Each vending machine product has a slot identifier and a purchase price.
- Each slot in the vending machine has enough room for 5 of that product.
- Every product is initially stocked to the maximum amount.
- A product that has run out must indicate that it is SOLD OUT.
6. When the customer selects "(2) Purchase", they are guided through the purchasing
process menu:
>```
>(1) Feed Money
>(2) Select Product
>(3) Finish Transaction
>
> Current Money Provided: $2.00
>```
7. The purchase process flow is as follows:
1. Selecting "(1) Feed Money" allows the customer to repeatedly feed money into the
machine in valid, whole dollar amounts—for example, $1, $2, $5, or $10.
- The "Current Money Provided" indicates how much money the customer
has fed into the machine.
2. Selecting "(2) Select Product" allows the customer to select a product to
purchase.
- Show the list of products available and allow the customer to enter
a code to select an item.
- If the product code does not exist, the customer is informed and returned
to the Purchase menu.
- If a product is sold out, the customer is informed and returned to the
Purchase menu.
- If a valid product is selected, it is dispensed to the customer.
- Dispensing an item prints the item name, cost, and the money
remaining. Dispensing also returns a message:
- All chip items print "Crunch Crunch, Yum!"
- All candy items print "Munch Munch, Yum!"
- All drink items print "Glug Glug, Yum!"
- All gum items print "Chew Chew, Yum!"
- After the product is dispensed, the machine must update its balance
accordingly and return the customer to the Purchase menu.
3. Selecting "(3) Finish Transaction" allows the customer to complete the
transaction and receive any remaining change.
- The customer's money is returned using nickels, dimes, and quarters
(using the smallest amount of coins possible).
- The machine's current balance must be updated to $0 remaining.
4. After completing their purchase, the user is returned to the "Main" menu to
continue using the vending machine.
8. All purchases must be audited to prevent theft from the vending machine:
- Each purchase must generate a line in a file called `Log.txt`.
- The audit entry must be in the format:
>```
> 01/01/2016 12:00:00 PM FEED MONEY: $5.00 $5.00
>01/01/2016 12:00:15 PM FEED MONEY: $5.00 $10.00
>01/01/2016 12:00:20 PM Crunchie B4 $10.00 $8.50
>01/01/2016 12:01:25 PM Cowtales B2 $8.50 $7.50
>01/01/2016 12:01:35 PM GIVE CHANGE: $7.50 $0.00
>```
9. Create as many of your classes as possible to be "testable" classes. Limit console
input and output to as few classes as possible.
10. Optional - Sales Report
- Provide a "Hidden" menu option on the main menu ("4") that writes to a sales
report that shows the total sales since the machine was started. The name of the
file must include the date and time so each sales report is uniquely named.
- An example of the output format is provided below.
11. Provide unit tests demonstrating that your code works correctly.
---
## Running the Application
### Requirements
- [JDK 11](https://www.oracle.com/java/technologies/javase/jdk11-archive-downloads.html)
- [Maven](https://maven.apache.org/download.cgi)
- IDE - The program built with [IntelliJ](https://www.jetbrains.com/idea/)
### Getting Started
1. Download code and open with IDE capable of running Java programs
2. Load the Maven file in the root folder
3. To begin the application, run the VendingMachineCLI.java in an IDE.
- The program requires at least Java 10 to be run.
- If running in an IDE, ensure a resource folder is included with all files in the same location they are set in this zip file
---
## Team Members
- Erin Czarnecki - <a href="https://www.linkedin.com/in/erin-czarnecki" target="_blank">LinkedIn</a> - <a href="https://github.com/erinczarnecki" target="_blank">GitHub</a>
- Patrick Tucker - <a href="https://www.linkedin.com/in/PatrickETucker/" target="_blank">LinkedIn</a> - <a href="https://github.com/PatrickETucker" target="_blank">GitHub</a> |
mysql> CREATE TABLE testing_table(
-> name Char(20),
-> contact_name Char(20),
-> roll_no Char(20)
-> );
Query OK, 0 rows affected (0.12 sec)
mysql> INSERT INTO testing_table VALUES("Dilpreet","dp",103);
Query OK, 1 row affected (0.00 sec)
mysql> SELECT * FROM testing_table;
+----------+--------------+---------+
| name | contact_name | roll_no |
+----------+--------------+---------+
| Dilpreet | dp | 103 |
+----------+--------------+---------+
1 row in set (0.00 sec)
mysql> ALTER table testing_table
-> DROP COLUMN name;
Query OK, 1 row affected (0.43 sec)
Records: 1 Duplicates: 0 Warnings: 0
mysql> SELECT * FROM testing_table;
+--------------+---------+
| contact_name | roll_no |
+--------------+---------+
| dp | 103 |
+--------------+---------+
1 row in set (0.01 sec)
mysql> ALTER TABLE testing_table
-> CHANGE contact_name username char(20);
Query OK, 1 row affected (0.38 sec)
Records: 1 Duplicates: 0 Warnings: 0
mysql> SELECT * FROM testing_table;
+----------+---------+
| username | roll_no |
+----------+---------+
| dp | 103 |
+----------+---------+
1 row in set (0.00 sec)
mysql> ALTER TABLE testing_table ADD first_name char(20);
Query OK, 1 row affected (0.09 sec)
Records: 1 Duplicates: 0 Warnings: 0
mysql> ALTER TABLE testing_table ADD last_name char(20);
Query OK, 1 row affected (0.14 sec)
Records: 1 Duplicates: 0 Warnings: 0
mysql> SELECT * FROM testing_table;
+----------+---------+------------+-----------+
| username | roll_no | first_name | last_name |
+----------+---------+------------+-----------+
| dp | 103 | NULL | NULL |
+----------+---------+------------+-----------+
1 row in set (0.00 sec)
mysql> ALTER TABLE testing_table
-> MODIFY roll_no INT(20);
Query OK, 1 row affected (0.06 sec)
Records: 1 Duplicates: 0 Warnings: 0
mysql> SELECT * FROM testing_table;
+----------+---------+------------+-----------+
| username | roll_no | first_name | last_name |
+----------+---------+------------+-----------+
| dp | 103 | NULL | NULL |
+----------+---------+------------+-----------+
1 row in set (0.00 sec)
mysql> DESC testing_table;
+------------+----------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+------------+----------+------+-----+---------+-------+
| username | char(20) | YES | | NULL | |
| roll_no | int(20) | YES | | NULL | |
| first_name | char(20) | YES | | NULL | |
| last_name | char(20) | YES | | NULL | |
+------------+----------+------+-----+---------+-------+
4 rows in set (0.04 sec) |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<script src="https://unpkg.com/vue"></script>
</head>
<body>
<div id="app">
<h1 style="background-color: skyblue">일반 스타일</h1>
<h1 v-bind:style="{backgroundColor: 'orange', color: 'white'}">
Vue 스타일
</h1>
<h1 :style="variableStyle">Vue 변수 스타일</h1>
</div>
</body>
<script>
const vueApp = Vue.createApp({
name: '인라인 스타일',
data() {
return {
variableStyle: { backgroundColor: 'dodgerblue' },
};
},
}).mount('#app');
</script>
</html> |
import React from 'react';
import { standardTransitions } from '@/theme/constants';
import { Typography, useTheme } from '@mui/material';
import RouterLink from '@components/routerLink/RouterLink';
const homeBreadcrumb: Breadcrumb = {
name: 'Home',
path: '/',
};
const Breadcrumb: React.FC<BreadcrumbProps> = ({ items }) => {
const theme = useTheme();
return (
<Typography sx={{ mb: 1, transition: standardTransitions(theme) }} variant="body1">
{[homeBreadcrumb, ...items].map((item: Breadcrumb, i: number, arr: Breadcrumb[]) => {
return (
<React.Fragment key={`breadcrumb-${i}`}>
{!item.path ? (
<>{item.name}</>
) : (
<RouterLink underlinedText to={item.path}>
{item.name}
</RouterLink>
)}
{i < arr.length - 1 && <>{' > '}</>}
</React.Fragment>
);
})}
</Typography>
);
};
type Breadcrumb = {
name: string;
path?: string;
};
interface BreadcrumbProps {
items: Breadcrumb[];
}
export default Breadcrumb; |
package br.com.sysmap.bootcamp.domain.service;
import br.com.sysmap.bootcamp.domain.entities.Users;
import br.com.sysmap.bootcamp.domain.entities.Wallet;
import br.com.sysmap.bootcamp.domain.exception.EntityNotFoundException;
import br.com.sysmap.bootcamp.domain.repository.WalletRepository;
import br.com.sysmap.bootcamp.dto.WalletDto;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import java.math.BigDecimal;
import java.time.DayOfWeek;
import java.time.LocalDateTime;
import java.util.HashMap;
import java.util.Map;
@RequiredArgsConstructor
@Service
public class WalletService {
private final UsersService usersService;
private final WalletRepository walletRepository;
private static final Map<DayOfWeek, Long> POINTS_PER_DAY = new HashMap<>();
static {
POINTS_PER_DAY.put(DayOfWeek.SUNDAY, 25L);
POINTS_PER_DAY.put(DayOfWeek.MONDAY, 7L);
POINTS_PER_DAY.put(DayOfWeek.TUESDAY, 6L);
POINTS_PER_DAY.put(DayOfWeek.WEDNESDAY, 2L);
POINTS_PER_DAY.put(DayOfWeek.THURSDAY, 10L);
POINTS_PER_DAY.put(DayOfWeek.FRIDAY, 15L);
POINTS_PER_DAY.put(DayOfWeek.SATURDAY, 20L);
}
public void debit(WalletDto walletDto) {
Users users = usersService.findByEmail(walletDto.getEmail());
Wallet wallet = walletRepository.findByUsers(users).orElseThrow(
() -> new EntityNotFoundException("User not found"));
wallet.setBalance(wallet.getBalance().subtract(walletDto.getValue()));
wallet.setPoints(wallet.getPoints() + calculatePoints());
wallet.setLastUpdate(LocalDateTime.now());
walletRepository.save(wallet);
}
@Transactional(propagation = Propagation.REQUIRED)
public void creditWallet(BigDecimal value, Users user) {
Wallet wallet = this.walletRepository.findByUsers(user).orElseThrow(
() -> new EntityNotFoundException("User not found"));
wallet.setBalance(wallet.getBalance().add(value));
walletRepository.save(wallet);
}
@Transactional(readOnly = true)
public Wallet getWallet(Users user){
return this.walletRepository.findByUsers(user).orElseThrow(
() -> new EntityNotFoundException("User not found"));
}
public Long calculatePoints() {
DayOfWeek day = LocalDateTime.now().getDayOfWeek();
Long points = POINTS_PER_DAY.getOrDefault(day, 0L);
return points;
}
} |
import { type tCalcPercentFromBasisPoint } from '../../interfaces';
/**
* @alpha
* @category Calculations
* @name calcPercentFromBasisPoint
* @param {tCalcPercentFromBasisPoint} args
* @returns `number` - calculated percentage value
* @description
* Calculates the percentage value for a given basis point and number value.
* @example
* ```typescript
* import { calcPercentFromBasisPoint } from '@ultra-alliance/ultra-sdk';
*
* const percent = calcPercentFromBasisPoint({
* basis_point: 600,
* value: 100
* });
*
* // percent = 6
* ```
*/
function calcPercentFromBasisPoint({
basis_point,
value,
}: tCalcPercentFromBasisPoint): number {
return (basis_point / 10000) * value;
}
export default calcPercentFromBasisPoint; |
import React from 'react'
import { Formik, Form } from 'formik';
import * as Yup from 'yup';
import FormikControl from './FormikControl';
const FormikContainer = () => {
const dropdownOptins = [
{ key: 'Select an option', value: '' },
{ key: 'Option 1', value: 'option1' },
{ key: 'Option 2', value: 'option2' },
{ key: 'Option 3', value: 'option3' },
]
const radioOptions = [
{ key: 'Radio 1', value: 'radioOption1' },
{ key: 'Radio 2', value: 'radioOption2' },
{ key: 'Radio 3', value: 'radioOption3' }
]
const checkboxOptions = [
{ key: 'CheckKey 1', value: 'checkValue1' },
{ key: 'CheckKey 2', value: 'checkValue2' },
{ key: 'CheckKey 3', value: 'checkValue3' }
]
const initialValues = {
email: '',
description: '',
selectOption: '',
radioOption: '',
checkboxOption: [],
birthDate:null
}
const validationSchema = Yup.object({
email: Yup.string().required('Email is Required'),
description: Yup.string().required('Description is Required'),
selectOption: Yup.string().required('Option is Required'),
radioOption: Yup.string().required('Radio Option is Required'),
checkboxOption: Yup.array().required('Checkbox Required'),
birthDate: Yup.date().required('Date of Birth Required').nullable(),
})
const onSubmit = values => {
console.log('Form data', values)
console.log('Save data', JSON.parse(JSON.stringify(values)))
}
return (
<Formik
initialValues={initialValues}
validationSchema={validationSchema}
onSubmit={onSubmit}
>
{formik => (
<Form>
<FormikControl
control='input'
type='email'
label='Email'
name='email'
/>
<FormikControl
control='textarea'
label='Description'
name='description'
/>
<FormikControl
control='select'
label='Select a topic'
name='selectOption'
options={dropdownOptins}
/>
<FormikControl
control='radio'
label='Choose Radio topic'
name='radioOption'
options={radioOptions}
/>
<FormikControl
control='checkbox'
label='Select checkbox topic'
name='checkboxOption'
options={checkboxOptions}
/>
<FormikControl
control='date'
label='Date of Birth'
name='birthDate'
/>
<button type='reset'>Reset</button>
<button type='submit'>Submit</button>
</Form>
)}
</Formik>
)
}
export default FormikContainer |
import { IsBoolean, IsNumber, IsString } from 'class-validator';
import { Transform } from 'class-transformer';
import { mapEnvironmentKeys } from 'src/env/utils';
export class Environment {
@IsString()
ENV: string;
@Transform(({ value }) => Number(value))
@IsNumber()
PORT: number;
@IsString()
DB_URI: string;
@Transform(({ value }) => value === 'true')
@IsBoolean()
SHOW_DOCS: boolean;
@IsString()
AUTH0_ISSUER: string;
@IsString()
AUTH0_AUDIENCE: string;
}
export const ENV = mapEnvironmentKeys<Environment>(Environment); |
import React from "react";
import PropTypes from "prop-types";
import Row from "react-bootstrap/Row";
import SelectionCardColumn from "temp-library-components/cards/SelectionCardColumn";
import CenterLoadingIndicator from "components/common/loading/CenterLoadingIndicator";
import CenteredContentWrapper from "components/common/wrapper/CenteredContentWrapper";
export default function VerticalCardViewBase(
{
data,
getCardFunction,
noDataMessage,
isLoading,
minHeight,
}) {
if (!Array.isArray(data) || data.length === 0) {
if (isLoading === true) {
return (
<CenterLoadingIndicator minHeight={minHeight} />
);
}
return (
<CenteredContentWrapper minHeight={minHeight}>
{noDataMessage}
</CenteredContentWrapper>
);
}
return (
<Row className={"mx-0 p-2"}>
{data.map((toolData, index) => (
<SelectionCardColumn
key={index}
>
{getCardFunction(toolData)}
</SelectionCardColumn>
))}
</Row>
);
}
VerticalCardViewBase.propTypes = {
data: PropTypes.array,
getCardFunction: PropTypes.func,
noDataMessage: PropTypes.string,
isLoading: PropTypes.bool,
minHeight: PropTypes.string,
};
VerticalCardViewBase.defaultProps = {
noDataMessage: "No data is currently available",
}; |
<template>
<div class="container">
<div class="chat-content">
<template v-if="chatList && chatList.length">
<div
v-for="(chat, index) in chatList"
class="message-box"
:class="{'right-message': chat.user.id === userInfo.user.id}"
:key="index"
>
<div v-if="chat.user.id !== userInfo.user.id" class="user">
<el-avatar class="avatar" :src="chat.user.avatar" ></el-avatar>
<div class="info">
<div class="name">{{chat.user.name}}</div>
<div class="time">{{chat.createTime}}</div>
</div>
</div>
<div v-else class="user">
<div class="info">
<div class="time">{{chat.createTime}}</div>
<div class="name">{{chat.user.name}}</div>
</div>
<el-avatar class="avatar" :src="chat.user.avatar" ></el-avatar>
</div>
<div class="message"><div class="block">{{chat.message}}</div></div>
</div>
</template>
<div v-else class="empty">
没有消息
</div>
</div>
<div class="chat-bottom">
<el-input v-model="chatMsg" class="chat-input" placeholder="请输入内容" />
<el-button class="chat-btn" type="primary" @click="sendMsg">发送</el-button>
</div>
<div style="margin-top: 10px;">当前用户:
<el-select v-model="userInfo.user" value-key="id" @change="selectUser" placeholder="Select">
<el-option
v-for="item in userList"
:key="item.id"
:label="item.name"
:value="item"
>
</el-option>
</el-select>
</div>
</div>
</template>
<script>
import { onMounted, ref, reactive } from 'vue';
import io from 'socket.io-client';
const avatar = 'https://cube.elemecdn.com/0/88/03b0d39583f48206768a7534e55bcpng.png';
export default {
name: 'HomePage',
setup() {
const chatList = ref([]);
const chatMsg = ref('');
const userList = [{ name: '舔狗', id: 0, avatar }, { name: '女神', id: 1, avatar }];
const userInfo = reactive({ user: userList[0] });
let socket;
onMounted(() => {
socket = io('http://localhost:3001');
socket.on('connect', () => {
console.log(socket.id, '监听客户端连接成功-connect');
});
socket.on('fresh-message', (data) => {
chatList.value = data;
});
});
const selectUser = (user) => {
userInfo.user = user;
};
const sendMsg = () => {
socket.emit('send-message', userInfo.user, chatMsg.value);
chatMsg.value = '';
};
return {
chatMsg,
chatList,
userList,
userInfo,
sendMsg,
selectUser,
};
},
};
</script>
<style lang="scss" scoped>
@mixin flex($align) {
display: flex;
align-items: $align;
}
.container {
padding: 24px;
}
.chat-bottom {
@include flex(center)
}
.chat-content {
width: 100%;
padding: 10px;
border: 1px solid #ddd;
border-bottom: none;
box-sizing: border-box;
.message-box {
margin-bottom: 10px;
.message {
margin-left: 42px;
border-radius: 4px;
box-sizing: border-box;
margin-top: 5px;
width: 100%;
.block {
display: inline-block;
font-size: 14px;
line-height: 1.5;
border-radius: 4px;
padding: 8px;
background-color: #eee;
}
}
.user {
.avatar {
width: 32px;
height: 32px;
margin-right: 10px;
}
@include flex(center);
}
.info {
@include flex(center);
font-size: 12px;
color: #999;
.name {
margin-right: 10px;
}
}
&.right-message {
text-align: right;
.message {
padding-right: 42px;
margin-left: auto;
}
.user {
.name { margin-right: 0px; margin-left: 10px; }
justify-content: flex-end
}
.avatar {
margin-right: 0px;
margin-left: 10px;
}
}
}
}
.empty {
font-size: 14px;
padding: 50px 0;
text-align: center;
}
.chat-input {
&:deep(.el-input__inner) {
border-top-right-radius: 0;
border-top-left-radius: 0;
border-bottom-right-radius: 0;
}
}
.chat-btn {
border-top-left-radius: 0;
border-top-right-radius: 0;
border-bottom-left-radius: 0;
}
</style> |
import React from 'react';
import PropTypes from 'prop-types';
import s from './ContList.module.css';
export default function ContactList({ onRemoveContact, contacts, filter }) {
const filterName = contacts.filter(contact => {
if (contact.name === undefined) {
// eslint-disable-next-line array-callback-return
return;
}
return contact.name.toLowerCase().includes(filter.toLowerCase());
});
return (
<ol className={s.ul}>
{filterName &&
filterName.map(({ id, name, number }) => {
return (
<li className={s.li} key={id}>
{name}: {number}
<button
className={s.btn}
name="delete"
onClick={() => onRemoveContact({ id, name })}
type="button"
>
Delete
</button>
</li>
);
})}
</ol>
);
}
ContactList.propTypes = {
onRemoveContact: PropTypes.func,
}; |
//
// ViewController.swift
// test_auth
//
// Created by User on 02.01.2023.
//
import UIKit
import SnapKit
class AuthenticationViewController: UIViewController {
// MARK: - Properties
let signUpViewController = SignUpViewController()
let loginViewController = LoginViewController()
let forgotPasswordViewController = ForgotPasswordViewController()
// MARK: - Outlets
let logoImageView = UIImageView(
image: Images.logoImage,
contentMode: .scaleAspectFit
)
let signUpLabel = UILabel(
text: Locale.signUpLabel.string,
font: .avenir20()
)
let alreadyOnboardLabel = UILabel(
text: Locale.alreadyHaveAccountLabel.string,
font: .avenir20()
)
let emailButton = UIButton(
title: Locale.emailLabel.string,
titleColor: .white,
backgroundColor: .buttonBlack()
)
let loginButton = UIButton(
title: Locale.loginButtonTitle.string,
titleColor: .buttonRed(),
backgroundColor: .mainWhite(),
isShadow: true
)
let mainStackView = UIStackView(
axis: .vertical,
spacing: 40
)
// MARK: - Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .white
setupHierachy()
setupLayout()
configureTargetsForButtons()
setupDelegates()
}
// MARK: - Setups
func setupDelegates() {
loginViewController.delegate = self
signUpViewController.delegate = self
forgotPasswordViewController.delegate = self
}
func setupHierachy() {
view.addSubview(logoImageView)
let email = ButtonView(
label: signUpLabel,
button: emailButton
)
let alreadyOnboard = ButtonView(
label: alreadyOnboardLabel,
button: loginButton
)
mainStackView.addArrangedSubview(email)
mainStackView.addArrangedSubview(alreadyOnboard)
view.addSubview(mainStackView)
}
func setupLayout() {
logoImageView.snp.makeConstraints { make in
make.centerX.equalTo(view)
make.centerY.equalTo(view).multipliedBy(0.45)
make.width.equalTo(view.snp.width).multipliedBy(0.9)
}
mainStackView.snp.makeConstraints { make in
make.top.equalTo(logoImageView.snp.bottom).multipliedBy(0.75)
make.leading.equalTo(view.snp.leading).offset(40)
make.trailing.equalTo(view.snp.trailing).offset(-40)
}
}
private func configureTargetsForButtons() {
emailButton.addTarget(
self,
action: #selector(emailButtonPressed),
for: .touchUpInside
)
loginButton.addTarget(
self,
action: #selector(loginButtonPressed),
for: .touchUpInside
)
}
}
// MARK: - Actions For Buttons Extension
extension AuthenticationViewController {
@objc private func emailButtonPressed() {
present(signUpViewController, animated: true)
}
@objc private func loginButtonPressed() {
present(loginViewController, animated: true)
}
}
// MARK: - AuthenticationNavigtionDelegate Extension
extension AuthenticationViewController: AuthenticationNavigtionDelegate {
func toLoginVC() {
present(loginViewController, animated: true)
}
func toSignUpVC() {
present(signUpViewController, animated: true)
}
func toForgotPasswordVC() {
present(forgotPasswordViewController, animated: true)
}
func goBackToLoginVC() {
dismiss(animated: true)
}
} |
import { Component, OnInit } from '@angular/core';
import { FetchServicesService } from 'src/app/services/fetch-services.service';
import { ActivatedRoute } from '@angular/router';
import { IRecipe } from 'src/app/shared/interfaces/recipe';
@Component({
selector: 'app-main',
templateUrl: './main.component.html',
styleUrls: ['./main.component.css'],
})
export class MainComponent implements OnInit {
fetchedMeals = [];
shoppingListIngredients: Array<any> = [];
loggedIn: boolean = false;
searchTerm: string = '';
searchedRecipes: IRecipe[] = [];
constructor(private fetchServices: FetchServicesService, private activatedRoute: ActivatedRoute) {
}
ngOnInit(): void {
this.activatedRoute.params.subscribe((params) => {
this.searchTerm = params.searchTerm + '*'; // allows for partial search in the DB
if (params.searchTerm) {
this.fetchServices.getAll(undefined, this.searchTerm) // searches all text fields in across the collection
.then((res: any) => {
const searchTermWithoutWildcard = this.searchTerm.slice(0, this.searchTerm.length - 1)
const filteredByName = res.documents.filter((x: any) => x.name.toLowerCase().includes(searchTermWithoutWildcard.toLowerCase()))
this.fetchedMeals = filteredByName;
});
} else {
this.fetchServices.getAll()
.then((res: any) => {
this.fetchedMeals = res.documents;
});
}
})
localStorage.getItem('user') ? this.loggedIn = true : this.loggedIn = false;
}
ngDoCheck(): void {
if (this.shoppingListIngredients.length > 0) {
localStorage.setItem('cachedShoppingList', JSON.stringify(this.shoppingListIngredients));
} else if (this.shoppingListIngredients.length === 0 && localStorage.getItem('cachedShoppingList')) {
const cache: any = localStorage.getItem('cachedShoppingList')
this.shoppingListIngredients = JSON.parse(cache);
}
}
addToShoppingListHandler(ingredients: any): void {
ingredients.forEach((item: any) => {
if (this.shoppingListIngredients.find((x: any) => x.ingredient === item.ingredient)) {
let existingItem: any = this.shoppingListIngredients.find((x: any) => x.ingredient === item.ingredient);
let newItem = existingItem.quantity += Number(item.quantity);
} else {
this.shoppingListIngredients.push({ ingredient: item.ingredient, quantity: Number(item.quantity), measurement: item.measurement })
}
})
}
removeAllHandler(): void {
localStorage.removeItem('cachedShoppingList');
this.shoppingListIngredients = [];
}
removeFromShoppingListHandler(ingredient: any) {
this.shoppingListIngredients = this.shoppingListIngredients.filter(x => x.ingredient !== ingredient);
if (this.shoppingListIngredients.length === 0) {
localStorage.removeItem('cachedShoppingList');
}
}
} |
/*
* MIT License
*
* Copyright (c) 2022 Nauman Khaliq
*/
package com.nk.we1.model.response.user
import androidx.room.Embedded
import com.squareup.moshi.Json
import com.squareup.moshi.JsonClass
@JsonClass(generateAdapter = true)
data class Location (
@Embedded(prefix = "st")
@Json(name = "street") var street: Street?,
@Json(name = "city") var city: String?,
@Json(name = "state") var state: String?,
@Json(name = "country") var country: String?,
@Json(name = "postcode") var postCode: String?,
@Embedded(prefix = "cr")
@Json(name = "coordinates") var coordinates: Coordinate?,
@Embedded(prefix = "tz")
@Json(name = "timezone") var timeZone: TimeZone?,
) |
@smoketest @catalogManagement @catalog
Feature: Catalog Brand Management
Background:
Given I sign in to CM as admin user
And I go to Catalog Management
@cleanupCatalog
Scenario: Update, Edit, Delete new brand for an existing catalog
Given I create a new catalog with following details
| catalogName | language |
| ATest Catalog | English |
And I go to Catalog Management
And I open the newly created catalog editor
When I add a brand testBrand
Then the brand testBrand is displayed in the brands table
When I edit brand name to newBrandName for the newly added brand
Then the brand newBrandName is displayed in the brands table
When I delete brand newBrandName
Then The brand newBrandName is deleted
Scenario: Unable to add duplicate brand if it already exists
When I select catalog Mobile Catalog in the list
And I open the selected catalog
And I add an existing brand Disney
Then an error message of A brand with the code you provided already exists. It cannot be added. is displayed in the add dialog
Scenario: Unable to delete a brand already used by a product
When I select catalog Mobile Catalog in the list
And I open the selected catalog
And I attempt to delete an existing brand Samsung used by product
Then an error message of The following brand is currently in use. It cannot be removed. is displayed |
import React from 'react'
import { Button, Row, Col, Modal, Form } from 'react-bootstrap'
import { showBlockModalChanged, blockTypeChanged, showBlockSampleChanged } from 'redux/webpageSlice'
import { useSelector, useDispatch } from 'react-redux'
import { RootState } from 'redux/store'
import SelectHeadingAtomModal from 'components/molecules/SelectHeadingAtomModal'
import SelectTextAtomModal from 'components/molecules/SelectTextAtomModal'
import SelectImageAtomModal from 'components/molecules/SelectImageAtomModal'
import SelectImageSlideAtomModal from 'components/molecules/SelectImageSlideAtomModal'
import SelectExternalLinkAtomModal from 'components/molecules/SelectExternalLinkAtomModal'
import SelectIframeAtomModal from 'components/molecules/SelectIframeAtomModal'
import SelectHTMLAtomModal from 'components/molecules/SelectHTMLAtomModal'
const SelectWebPageAtomModal = (): JSX.Element => {
const dispatch = useDispatch()
const selectedAtomType = useSelector((state: RootState) => state.webpage.selectedAtomType)
const showBlockSample = useSelector((state: RootState) => state.webpage.showBlockSample)
return (
<>
<Modal.Header>
<Modal.Title>
<span>追加したい要素を選択してください</span>
</Modal.Title>
</Modal.Header>
<Modal.Body>
<Form className='mb20'>
<Form.Check
type='switch'
id='show-sample'
label='サンプルを表示'
checked={showBlockSample}
onChange={() => dispatch(showBlockSampleChanged(!showBlockSample))}
/>
</Form>
<Row>
<Col>
<SelectHeadingAtomModal></SelectHeadingAtomModal>
<br />
<SelectTextAtomModal></SelectTextAtomModal>
<br />
<SelectExternalLinkAtomModal></SelectExternalLinkAtomModal>
<br />
<SelectIframeAtomModal></SelectIframeAtomModal>
<br />
<SelectHTMLAtomModal></SelectHTMLAtomModal>
<br />
<SelectImageAtomModal></SelectImageAtomModal>
<br />
<SelectImageSlideAtomModal></SelectImageSlideAtomModal>
</Col>
</Row>
</Modal.Body>
<Modal.Footer>
<Button variant='secondary' onClick={() => { dispatch(showBlockModalChanged(false)); dispatch(blockTypeChanged(''))}}>閉じる</Button>
</Modal.Footer>
</>
)
}
export default SelectWebPageAtomModal |
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.buildinit.plugins.internal;
import org.apache.commons.lang.StringUtils;
import org.gradle.api.internal.DocumentationRegistry;
import org.gradle.api.internal.file.FileResolver;
import static org.gradle.buildinit.plugins.internal.BuildInitTestFramework.SPOCK;
import static org.gradle.buildinit.plugins.internal.BuildInitTestFramework.TESTNG;
public abstract class JavaProjectInitDescriptor extends LanguageLibraryProjectInitDescriptor {
private final static Description DESCRIPTION = new Description(
"Java",
"Java Quickstart",
"tutorial_java_projects",
"java"
);
private final DocumentationRegistry documentationRegistry;
public JavaProjectInitDescriptor(TemplateOperationFactory templateOperationFactory,
FileResolver fileResolver,
TemplateLibraryVersionProvider libraryVersionProvider,
ProjectInitDescriptor globalSettingsDescriptor,
DocumentationRegistry documentationRegistry) {
super("java", templateOperationFactory, fileResolver, libraryVersionProvider, globalSettingsDescriptor);
this.documentationRegistry = documentationRegistry;
}
@Override
public void generate(BuildInitTestFramework testFramework) {
globalSettingsDescriptor.generate(testFramework);
Description desc = getDescription();
BuildScriptBuilder buildScriptBuilder = new BuildScriptBuilder(fileResolver.resolve("build.gradle"))
.fileComment("This generated file contains a sample " + desc.projectType + " project to get you started.")
.fileComment("For more details take a look at the " + desc.chapterName + " chapter in the Gradle")
.fileComment("user guide available at " + documentationRegistry.getDocumentationFor(desc.userguideId))
.plugin("Apply the " + desc.pluginName +" plugin to add support for " + desc.projectType, desc.pluginName);
configureBuildScript(buildScriptBuilder);
addTestFramework(testFramework, buildScriptBuilder);
buildScriptBuilder.create().generate();
TemplateOperation javaSourceTemplate = sourceTemplateOperation();
whenNoSourcesAvailable(javaSourceTemplate, testTemplateOperation(testFramework)).generate();
}
protected Description getDescription() {
return DESCRIPTION;
}
protected String getImplementationConfigurationName() {
return "compile";
}
protected String getTestImplementationConfigurationName() {
return "test" + StringUtils.capitalize(getImplementationConfigurationName());
}
private void addTestFramework(BuildInitTestFramework testFramework, BuildScriptBuilder buildScriptBuilder) {
switch (testFramework) {
case SPOCK:
buildScriptBuilder
.plugin("Apply the groovy plugin to also add support for Groovy (needed for Spock)", "groovy")
.dependency(getTestImplementationConfigurationName(), "Use the latest Groovy version for Spock testing",
"org.codehaus.groovy:groovy-all:" + libraryVersionProvider.getVersion("groovy"))
.dependency(getTestImplementationConfigurationName(), "Use the awesome Spock testing and specification framework even with Java",
"org.spockframework:spock-core:" + libraryVersionProvider.getVersion("spock"),
"junit:junit:" + libraryVersionProvider.getVersion("junit"));
break;
case TESTNG:
buildScriptBuilder
.dependency(getTestImplementationConfigurationName(), "Use TestNG framework, also requires calling test.useTestNG() below",
"org.testng:testng:" + libraryVersionProvider.getVersion("testng"))
.configuration("Use TestNG for unit tests", "test.useTestNG()");
break;
default:
buildScriptBuilder
.dependency(getTestImplementationConfigurationName(), "Use JUnit test framework", "junit:junit:" + libraryVersionProvider.getVersion("junit"));
break;
}
}
protected void configureBuildScript(BuildScriptBuilder buildScriptBuilder) {
// todo: once we use "implementation" for Java projects too, we need to change the comment
buildScriptBuilder.dependency(getImplementationConfigurationName(),
"This dependency is found on compile classpath of this component and consumers.",
"com.google.guava:guava:" + libraryVersionProvider.getVersion("guava"));
}
protected abstract TemplateOperation sourceTemplateOperation();
protected abstract TemplateOperation testTemplateOperation(BuildInitTestFramework testFramework);
@Override
public boolean supports(BuildInitTestFramework testFramework) {
return testFramework == SPOCK || testFramework == TESTNG;
}
protected static class Description {
private final String projectType;
private final String chapterName;
private final String userguideId;
private final String pluginName;
public Description(String projectType, String chapterName, String userguideId, String pluginName) {
this.projectType = projectType;
this.chapterName = chapterName;
this.userguideId = userguideId;
this.pluginName = pluginName;
}
}
} |
import React, { useState, useEffect } from "react"
import { v4 } from "uuid"
import { db } from "../../../../firebase.config"
import {
doc,
arrayUnion,
updateDoc,
addDoc,
collection,
getDoc,
serverTimestamp,
Timestamp,
} from "firebase/firestore"
import Spinner from "../../../../components/Spinner/Spinner"
import { useLocation, useNavigate } from "react-router-dom"
import { toast } from "react-toastify"
import Required from "../../../../components/Required Icon/Required"
function CreateScrollingTextForm() {
const navigate = useNavigate()
const [loading, setLoading] = useState(false)
const [formData, setFormData] = useState({
title: "", // data type changed
hyperlinkText: "",
hyperlink: "",
})
const { title, hyperlink, hyperlinkText } = formData
const canSave = Boolean(title) && Boolean(hyperlink) && Boolean(hyperlinkText)
const onMutate = (e) => {
// Text/Booleans
if (!e.target.files) {
setFormData((prevState) => ({
...prevState,
[e.target.id]: e.target.value,
}))
}
}
const onSubmit = async (e) => {
window.scroll(0, 0)
e.preventDefault()
setLoading(true)
const docRef = doc(db, "current", "doc")
const formDataCopy = {
...formData,
createdAt: Timestamp.fromDate(new Date()),
id: v4(),
}
try {
await updateDoc(docRef, {
scrollingText: arrayUnion(formDataCopy),
})
toast.success(" Created Successfully")
setLoading(false)
navigate("/admin")
} catch (error) {
toast.error(error.message)
setLoading(false)
}
console.log(formDataCopy)
setLoading(false)
}
return (
<div className="">
{loading ? (
<>
<Spinner />
</>
) : (
<form className="w-full">
<div className="mb-6">
<div className="pb-2">
<label htmlFor="title" className=" left-0 text-gray-600 ">
Title
<Required />
</label>
</div>
<input
type="text"
placeholder="title"
className="
w-full
rounded
p-3
text-gray-800
border-gray-500
outline-none
focus-visible:shadow-none
focus:border-primary
"
name="title"
id="title"
onChange={onMutate}
value={title}
/>
</div>
<div className="mb-6">
<div className="pb-2">
<label
htmlFor="HyperlinkText"
className=" left-0 text-gray-600 "
>
Hyperlink Text
<Required />
</label>
</div>
<input
type="text"
placeholder="Event HyperlinkText"
className="
w-full
rounded
p-3
text-gray-800
border-gray-500
outline-none
focus-visible:shadow-none
focus:border-primary
"
name="hyperlinkText"
id="hyperlinkText"
onChange={onMutate}
value={hyperlinkText}
/>
</div>
<div className="mb-6">
<div className="pb-2">
<label htmlFor="hyperlink" className=" left-0 text-gray-600 ">
Hyperlink
<Required />
</label>
</div>
<input
type="text"
placeholder="Hyperlink"
className="
w-full
rounded
p-3
text-gray-800
border-gray-500
outline-none
focus-visible:shadow-none
focus:border-primary
"
name="hyperlink"
id="hyperlink"
onChange={onMutate}
value={hyperlink}
/>
</div>
<div>
<button
disabled={!canSave}
type="submit"
onClick={onSubmit}
className={
canSave
? "w-full text-gray-900 bg-green-400 rounded-lg border border-primary text-lg p-3 transition ease-in-out duration-500"
: "w-full text-gray-400 bg-green-200 rounded-lg border border-primary text-lg p-3 transition ease-in-out duration-500"
}
>
Create Scrolling text
</button>
</div>
</form>
)}
</div>
)
}
export default CreateScrollingTextForm |
#include "TranslatorPluginTest.h"
#include "generator/plugins/ClassPlugin.h"
#include "generator/plugins/lua/LuaFunctionPlugin.h"
class LuaFunctionPluginTest : public TranslatorPluginTest {
protected:
static void Run(TranslatedProject &project) {
ClassPlugin(project, {}).Run();
LuaFunctionPlugin(project, {}).Run();
}
};
TEST_F(LuaFunctionPluginTest, VoidFunctionNoArgs) {
auto project = Parse(R"R(
struct TestData {
@luaFunc
func TestFunction();
}
)R");
Run(project);
auto cls = project.GetClass("TestData");
ASSERT_NE(cls, nullptr);
ASSERT_NE(cls->GetField("mLuaFuncHandle_TestFunction"), nullptr);
helpers::ExpectEqual(*cls->GetField("mLuaFuncHandle_TestFunction"),
ClassField{"mLuaFuncHandle_TestFunction", Type{"std::string"}});
ASSERT_NE(cls->GetMethod("TestFunction", Constness::Const), nullptr);
auto method = ClassMethod{"TestFunction", Type{"void"}, Visibility::Public, Constness::Const};
method.mFunction = cls->mStruct->GetFunction("TestFunction");
method.mArguments.emplace_back("luaState", Type{"lua_State", PassByType::Pointer});
method.mExposeToLua = true;
helpers::ExpectEqual(*cls->GetMethod("TestFunction", Constness::Const), method, R"R(
HOLGEN_WARN_AND_RETURN_IF(mLuaFuncHandle_TestFunction.empty(), void(), "Calling unset TestFunction function");
lua_getglobal(luaState, mLuaFuncHandle_TestFunction.c_str());
if (lua_isnil(luaState, -1)) {
HOLGEN_WARN("Calling undefined TestFunction function {}", mLuaFuncHandle_TestFunction);
lua_pop(luaState, 1);
return void();
}
LuaHelper::Push(*this, luaState);
lua_call(luaState, 1, 0);
)R");
}
TEST_F(LuaFunctionPluginTest, VoidFunctionInTableNoArgs) {
auto project = Parse(R"R(
struct TestData {
@luaFunc(sourceTable=Table)
func TestFunction() -> void;
}
)R");
Run(project);
auto cls = project.GetClass("TestData");
ASSERT_NE(cls, nullptr);
ASSERT_NE(cls->GetMethod("TestFunction", Constness::Const), nullptr);
auto method = ClassMethod{"TestFunction", Type{"void"}, Visibility::Public, Constness::Const};
method.mFunction = cls->mStruct->GetFunction("TestFunction");
method.mArguments.emplace_back("luaState", Type{"lua_State", PassByType::Pointer});
method.mExposeToLua = true;
helpers::ExpectEqual(*cls->GetMethod("TestFunction", Constness::Const), method, R"R(
HOLGEN_WARN_AND_RETURN_IF(mLuaFuncHandle_TestFunction.empty(), void(), "Calling unset TestFunction function");
lua_getglobal(luaState, "Table");
lua_pushstring(luaState, mLuaFuncHandle_TestFunction.c_str());
lua_gettable(luaState, -2);
if (lua_isnil(luaState, -1)) {
HOLGEN_WARN("Calling undefined TestFunction function Table.{}", mLuaFuncHandle_TestFunction);
lua_pop(luaState, 1);
return void();
}
LuaHelper::Push(*this, luaState);
lua_call(luaState, 1, 0);
lua_pop(luaState, 1);
)R");
}
TEST_F(LuaFunctionPluginTest, FunctionNoArgs) {
auto project = Parse(R"R(
struct TestData {
@luaFunc
func TestFunction() -> u32;
}
)R");
Run(project);
auto cls = project.GetClass("TestData");
ASSERT_NE(cls, nullptr);
ASSERT_NE(cls->GetMethod("TestFunction", Constness::Const), nullptr);
auto method = ClassMethod{"TestFunction", Type{"uint32_t"}, Visibility::Public, Constness::Const};
method.mFunction = cls->mStruct->GetFunction("TestFunction");
method.mArguments.emplace_back("luaState", Type{"lua_State", PassByType::Pointer});
method.mExposeToLua = true;
helpers::ExpectEqual(*cls->GetMethod("TestFunction", Constness::Const), method, R"R(
HOLGEN_WARN_AND_RETURN_IF(mLuaFuncHandle_TestFunction.empty(), {}, "Calling unset TestFunction function");
lua_getglobal(luaState, mLuaFuncHandle_TestFunction.c_str());
if (lua_isnil(luaState, -1)) {
HOLGEN_WARN("Calling undefined TestFunction function {}", mLuaFuncHandle_TestFunction);
lua_pop(luaState, 1);
return {};
}
LuaHelper::Push(*this, luaState);
lua_call(luaState, 1, 1);
uint32_t result;
LuaHelper::Read(result, luaState, -1);
lua_pop(luaState, 1);
return result;
)R");
}
TEST_F(LuaFunctionPluginTest, FunctionInTableNoArgs) {
auto project = Parse(R"R(
struct TestData {
@luaFunc(sourceTable=Table)
func TestFunction() -> u32;
}
)R");
Run(project);
auto cls = project.GetClass("TestData");
ASSERT_NE(cls, nullptr);
ASSERT_NE(cls->GetMethod("TestFunction", Constness::Const), nullptr);
auto method = ClassMethod{"TestFunction", Type{"uint32_t"}, Visibility::Public, Constness::Const};
method.mFunction = cls->mStruct->GetFunction("TestFunction");
method.mArguments.emplace_back("luaState", Type{"lua_State", PassByType::Pointer});
method.mExposeToLua = true;
helpers::ExpectEqual(*cls->GetMethod("TestFunction", Constness::Const), method, R"R(
HOLGEN_WARN_AND_RETURN_IF(mLuaFuncHandle_TestFunction.empty(), {}, "Calling unset TestFunction function");
lua_getglobal(luaState, "Table");
lua_pushstring(luaState, mLuaFuncHandle_TestFunction.c_str());
lua_gettable(luaState, -2);
if (lua_isnil(luaState, -1)) {
HOLGEN_WARN("Calling undefined TestFunction function Table.{}", mLuaFuncHandle_TestFunction);
lua_pop(luaState, 1);
return {};
}
LuaHelper::Push(*this, luaState);
lua_call(luaState, 1, 1);
uint32_t result;
LuaHelper::Read(result, luaState, -1);
lua_pop(luaState, 2);
return result;
)R");
}
TEST_F(LuaFunctionPluginTest, FunctionWithArgs) {
auto project = Parse(R"R(
@managed(by=DataManager, field=inners)
struct InnerStruct{
@id u32 id;
}
struct DataManager {
@container(elemName=inner)
vector<InnerStruct> inners;
}
struct TestData {
@luaFunc
func TestFunction(s32 a1, string a2, InnerStruct a3) -> string;
}
)R");
Run(project);
auto cls = project.GetClass("TestData");
ASSERT_NE(cls, nullptr);
ASSERT_NE(cls->GetMethod("TestFunction", Constness::Const), nullptr);
auto method =
ClassMethod{"TestFunction", Type{"std::string"}, Visibility::Public, Constness::Const};
method.mFunction = cls->mStruct->GetFunction("TestFunction");
method.mArguments.emplace_back("luaState", Type{"lua_State", PassByType::Pointer});
method.mArguments.emplace_back("a1", Type{"int32_t", PassByType::Value, Constness::Const});
method.mArguments.emplace_back("a2",
Type{"std::string", PassByType::Reference, Constness::Const});
method.mArguments.emplace_back("a3",
Type{"InnerStruct", PassByType::Reference, Constness::Const});
method.mExposeToLua = true;
helpers::ExpectEqual(*cls->GetMethod("TestFunction", Constness::Const), method, R"R(
HOLGEN_WARN_AND_RETURN_IF(mLuaFuncHandle_TestFunction.empty(), {}, "Calling unset TestFunction function");
lua_getglobal(luaState, mLuaFuncHandle_TestFunction.c_str());
if (lua_isnil(luaState, -1)) {
HOLGEN_WARN("Calling undefined TestFunction function {}", mLuaFuncHandle_TestFunction);
lua_pop(luaState, 1);
return {};
}
LuaHelper::Push(*this, luaState);
LuaHelper::Push(a1, luaState);
LuaHelper::Push(a2, luaState);
LuaHelper::Push(a3, luaState);
lua_call(luaState, 4, 1);
std::string result;
LuaHelper::Read(result, luaState, -1);
lua_pop(luaState, 1);
return result;
)R");
}
TEST_F(LuaFunctionPluginTest, FunctionReturningStruct) {
// TODO: in blackbox, test this with managed, unmanaged with id and without.
auto project = Parse(R"R(
struct InnerStruct{
@id u32 id;
}
struct TestData {
@luaFunc
func TestFunction() -> InnerStruct;
}
)R");
Run(project);
auto cls = project.GetClass("TestData");
ASSERT_NE(cls, nullptr);
ASSERT_NE(cls->GetMethod("TestFunction", Constness::Const), nullptr);
auto method = ClassMethod{"TestFunction", Type{"InnerStruct", PassByType::Value},
Visibility::Public, Constness::Const};
method.mFunction = cls->mStruct->GetFunction("TestFunction");
method.mArguments.emplace_back("luaState", Type{"lua_State", PassByType::Pointer});
method.mExposeToLua = true;
helpers::ExpectEqual(*cls->GetMethod("TestFunction", Constness::Const), method, R"R(
HOLGEN_WARN_AND_RETURN_IF(mLuaFuncHandle_TestFunction.empty(), {}, "Calling unset TestFunction function");
lua_getglobal(luaState, mLuaFuncHandle_TestFunction.c_str());
if (lua_isnil(luaState, -1)) {
HOLGEN_WARN("Calling undefined TestFunction function {}", mLuaFuncHandle_TestFunction);
lua_pop(luaState, 1);
return {};
}
LuaHelper::Push(*this, luaState);
lua_call(luaState, 1, 1);
InnerStruct resultMirror;
InnerStruct *result;
if (lua_getmetatable(luaState, -1)) {
lua_pop(luaState, 1);
result = InnerStruct::ReadProxyFromLua(luaState, -1);
} else {
resultMirror = InnerStruct::ReadMirrorFromLua(luaState, -1);
result = &resultMirror;
}
lua_pop(luaState, 1);
return *result;
)R");
}
TEST_F(LuaFunctionPluginTest, DuplicateFunction) {
ExpectErrorMessage(R"R(
struct A {
@luaFunc
func TestFunction() -> u32;
@luaFunc
func TestFunction() -> u32;
}
)R",
Run, "Duplicate method: A.TestFunction ({0}:3:3) and A.TestFunction ({0}:5:3)",
Source);
ExpectErrorMessage(R"R(
struct A {
@luaFunc
func TestFunction(string arg) -> u32;
@luaFunc
func TestFunction(string arg) -> u32;
}
)R",
Run, "Duplicate method: A.TestFunction ({0}:3:3) and A.TestFunction ({0}:5:3)",
Source);
}
TEST_F(LuaFunctionPluginTest, InvalidType) {
ExpectErrorMessage("struct A { @luaFunc func TestFunction() -> u63; }", Run,
"Unknown type u63 used by A.TestFunction ({0}:1:21)", Source);
ExpectErrorMessage("struct A { @luaFunc func TestFunction(u33 arg) -> u64; }", Run,
"Unknown type u33 used by A.TestFunction ({0}:1:21)", Source);
ExpectErrorMessage("struct A { @luaFunc func TestFunction(void arg) -> u64; }", Run,
"Invalid void usage in A.TestFunction ({0}:1:21)", Source);
ExpectErrorMessage("struct A { @luaFunc func TestFunction() -> void<u32>; }", Run,
"Void cannot have template parameters in A.TestFunction ({0}:1:21)", Source);
} |
import { Command, CommandRunner, Option } from 'nest-commander';
import { UserService } from 'src/services/user.service';
interface CreateUserCommandOptions {
username: string;
password: string;
}
@Command({ name: 'user:create' })
export class CreateUserCommand extends CommandRunner {
constructor(private readonly userService: UserService) {
super();
}
async run(
passedParam: string[],
options: CreateUserCommandOptions,
): Promise<void> {
const user = await this.userService.createUser(options as any);
console.log(`User ${user.username} has been created.`);
}
@Option({
flags: '-u, --username [string]',
required: true,
})
parseUsername(value: string) {
return value;
}
@Option({
flags: '-p, --password [string]',
required: true,
})
parsePassword(value: string): string {
return value;
}
} |
package com.greatlearning.student.services;
import java.util.List;
import javax.transaction.Transactional;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import com.greatlearning.student.models.Student;
@Repository
public class StudentServiceImpl implements StudentService {
private SessionFactory sessionFactory;
private Session session;
@Autowired
public StudentServiceImpl(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
try {
this.session = sessionFactory.getCurrentSession();
} catch (HibernateException e) {
this.session = sessionFactory.openSession();
}
}
@Override
public List<Student> findAll() {
List<Student> student = session.createQuery("from Student").list();
return student;
}
@Override
public Student findById(int theId) {
Student student = new Student();
Transaction tx = session.beginTransaction();
// find record with Id from the database table
student = session.get(Student.class, theId);
tx.commit();
return student;
}
@Transactional
public void save(Student thestudent) {
Transaction tx = session.beginTransaction();
// save transaction
session.saveOrUpdate(thestudent);
tx.commit();
}
@Transactional
public void deleteById(int theId) {
Transaction tx = session.beginTransaction();
// get transaction
Student student = session.get(Student.class, theId);
// delete record
session.delete(student);
tx.commit();
}
} |
import os
from typing import Dict, Optional
import google.generativeai as genai
import pandas as pd
from mindsdb.integrations.libs.base import BaseMLEngine
from mindsdb.utilities import log
from mindsdb.utilities.config import Config
logger = log.getLogger(__name__)
class GoogleGeminiHandler(BaseMLEngine):
"""
Integration with the Google generative AI Python Library
"""
name = "google_gemini"
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.default_chat_model = "gemini-pro"
self.supported_chat_models = ["gemini-pro"]
self.generative = True
self.connection = None
def create(
self,
target: str,
df: Optional[pd.DataFrame] = None,
args: Optional[Dict] = None,
) -> None:
if "model" not in args["using"]:
args["using"]["model"] = self.default_chat_model
elif args["using"]["model"] not in self.supported_chat_models:
raise Exception(
f"Invalid chat model. Please use one of {self.supported_chat_models}"
)
api_key = self._get_google_gemini_api_key(args)
try:
genai.configure(api_key=api_key)
model = genai.GenerativeModel(args["using"]["model"])
model.generate_content("test")
except Exception as e:
raise Exception(
f"{e}: Invalid api key please check your api key"
)
args["using"]["api_key"] = api_key
self.model_storage.json_set("args", args)
def predict(
self, df: Optional[pd.DataFrame] = None, args: Optional[Dict] = None
) -> pd.DataFrame:
args = self.model_storage.json_get("args")
api_key = args["using"]["api_key"]
genai.configure(api_key=api_key)
input_column = args["using"]["column"]
if input_column not in df.columns:
raise RuntimeError(f'Column "{input_column}" not found in input data')
self.connection = genai.GenerativeModel(args["using"]["model"])
result_df = pd.DataFrame()
result_df["predictions"] = df[input_column].apply(self.predict_answer)
result_df = result_df.rename(columns={"predictions": args["target"]})
return result_df
def _get_google_gemini_api_key(self, args, strict=True):
"""
API_KEY preference order:
1. provided at model creation
2. provided at engine creation
3. GOOGLE_GENAI_API_KEY env variable
4. google_gemini.api_key setting in config.json
"""
if "api_key" in args["using"]:
return args["using"]["api_key"]
# 2
connection_args = self.engine_storage.get_connection_args()
if "api_key" in connection_args:
return connection_args["api_key"]
# 3
api_key = os.getenv("GOOGLE_GENAI_API_KEY")
if api_key is not None:
return api_key
# 4
config = Config()
google_gemini_config = config.get("google_gemini", {})
if "api_key" in google_gemini_config:
return google_gemini_config["api_key"]
if strict:
raise Exception(
'Missing API key "api_key". Either re-create this ML_ENGINE specifying the `api_key` parameter,\
or re-create this model and pass the API key with `USING` syntax.'
)
def predict_answer(self, text):
"""
connects with google generative AI api to predict the answer for the particular question
"""
completion = self.connection.generate_content(
text
)
return completion.text |
#ifndef HEADER_H
#define HEADER_H
#include <iostream>
class Rectangle
{
int length, width;
public:
Rectangle();
Rectangle(int, int);
Rectangle operator+(Rectangle &);
Rectangle operator+(int);
friend Rectangle operator+(int n,Rectangle&);
// friend void operator<<(std::ostream os,Rectangle&);
friend std::ostream& operator<<(std::ostream &os,const Rectangle&);
Rectangle operator-(Rectangle &);
Rectangle operator-();
Rectangle operator-(int );
Rectangle& operator++();
Rectangle operator++(int );
// Rectangle operator<<(Rectangle& r4 );
bool operator==(Rectangle &);
bool operator!=(Rectangle &);
int calculateArea();
int calculatePerimeter();
void display();
~Rectangle();
};
#endif // HEADER_H |
import {
WS_CONNECTION_CLOSED,
WS_CONNECTION_ERROR,
WS_CONNECTION_SUCCESS,
WS_GET_ORDERS,
} from "../actions/wsActions";
import { wsReducer } from "./wsReducers";
describe("Лента заказов", () => {
const initialState = {
wsConnected: false,
wsError: false,
orders: [],
total: 0,
totalToday: 0,
};
it("Начальное состояние", () => {
expect(wsReducer(undefined, {})).toEqual(initialState);
});
it("WS соединение успешно", () => {
const action = {
type: WS_CONNECTION_SUCCESS,
};
expect(wsReducer(initialState, action)).toEqual({
...initialState,
wsConnected: true,
});
});
it("WS соединение завершилось с ошибкой", () => {
const action = {
type: WS_CONNECTION_ERROR,
message: "",
};
expect(wsReducer(initialState, action)).toEqual({
...initialState,
wsConnected: false,
wsError: action.message,
});
});
it("WS соединение закрыто", () => {
const action = {
type: WS_CONNECTION_CLOSED,
};
expect(wsReducer(initialState, action)).toEqual({
...initialState,
wsConnected: false,
orders: null,
});
});
it("WS заказы получены", () => {
const action = {
type: WS_GET_ORDERS,
payload: {
orders: 0,
total: 0,
totalToday: 0,
},
};
expect(wsReducer(initialState, action)).toEqual({
...initialState,
orders: action.payload.orders,
total: action.payload.total,
totalToday: action.payload.totalToday,
});
});
}); |
var baseExtremum = require('./internal/baseExtremum'),
identity = require('./identity'),
lt = require('./lt');
/**
* Computes the minimum value of `array`. If `array` is empty or falsey
* `undefined` is returned.
*
* @static
* @memberOf _
* @category Math
* @param {Array} array The array to iterate over.
* @returns {*} Returns the minimum value.
* @example
*
* _.min([4, 2, 8, 6]);
* // => 2
*
* _.min([]);
* // => undefined
*/
function min(array) {
return (array && array.length)
? baseExtremum(array, identity, lt)
: undefined;
}
module.exports = min; |
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Newsletter form</title>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/style.css">
<link href="https://fonts.googleapis.com/css?family=Poppins:700" rel="stylesheet">
<link href="https://fonts.googleapis.com/css?family=Open+Sans" rel="stylesheet">
</head>
<body>
<header>
<h1>THE CODE REVIEW</h1>
</header>
<main>
<div id="intro">
<h2>Sign up for our newsletter</h2>
<p>Get the latest news on how your code is doing right in your inbox.</p>
</div>
<form>
<fieldset id="contact_info">
<legend>Contact Information</legend>
<div class="input_wrapper">
<label for="full_name">Full Name</label>
<input type="text" id="full_name" name="user_name" placeholder="Required" required>
</div>
<diV class="input_wrapper">
<label for="user_email">Email Address</label>
<input type="email" id="user_email" name="user_email" placeholder="Required" required>
</diV>
<div class="input_wrapper">
<label for="phone_number">Phone number</label>
<input type="tel" id="phone_number" name="phone_number" placeholder="e.g. +56 8434 543 23">
</div>
<div class="input_wrapper">
<label for="address">Street Address</label>
<input type="text" id="address" name="address" placeholder="Street and house number are required">
</div>
<div class="input_wrapper">
<label for="city">City</label>
<input type="text" id="city" name="city" placeholder="e.g. Los Angeles">
</div>
<div class="input_wrapper">
<label for="state">State</label>
<select id="state">
<option value="">Choose State</option>
<option value="california">California</option>
<option value="luisiana">Luisiana</option>
<option value="nevada">Nevada</option>
<option value="new_mexico">New Mexico</option>
</select>
</div>
<div class="input_wrapper">
<label for="zip_code">Zip Code</label>
<input type="text" id="zip_code" name="zip_code" placeholder="e.g. 04677">
</div>
</fieldset>
<fieldset id="newsletter">
<legend>Newsletter</legend>
<label id="news_topic">Select the newsletters you would like to receive:</label><br>
<input type="checkbox" id="ht_news" value="html_news" name="type_of_newsletter"><label for="ht_news">HTML News</label><br>
<input type="checkbox" id="cs_news" value="css_news" name="type_of_newsletter"><label for="cs_news">CSS News</label><br>
<input type="checkbox" id="js_news" value="js_news" name="type_of_newsletter"><label for="js_news">Javascript News</label><br>
<label id="news_format">Newsletter format</label>
<input type="radio" id="ht_format" value="html_format" name="newsletter_format"><label for="ht_format">HTML</label><br>
<input type="radio" id="text_format" value="text_format" name="newsletter_format"><label for="text_format">Plain text</label>
<label for="topics" id="ll_topics">Other topics you´d would like to hear about</label>
<textarea id="topics" name="other_topics"></textarea>
</fieldset>
<button type="submit" id="submit">Sign Up</button>
</form>
</main>
<footer>
<p>Copyright The Code Review</p>
</footer>
</body>
</html> |
---
title: REST API
type:
tags:
- API
- Database
DateStarted: 2023-12-27
DateModified: 2024-04-17
status:
---
### REST API
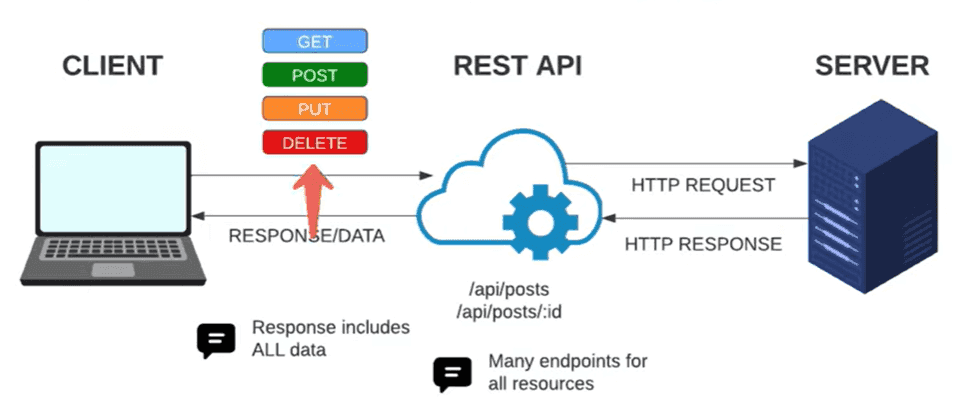
- Representational State Transfer
- Architecture style for designing network application
- Rely on a stateless, **client-server** protocol, almost always HTTP
- Use specific HTTP methods and endpoints
- Fetches all or nothing
- Treat server objects as resources that can be created or destroyed.
- Can be used by virtually any programming language
- JSON as the response type of REST APIs
- Good for CRUD
#### 1. Pros
- Standard method names, arguments and status code
- Utilized HTTP features
- Easy to maintain
#### 2. Cons
- Big payloads
- Multiple HTTP roundtrips (To get the resource and the sub-resource, you have to get each individually, no way to combine the results together)
#### 3. CRUD
- POST
- PUT
- PATCH
- GET
- DELETE
#### 4. NON-CRUD
- Archive
- Deactivate
- Search
- 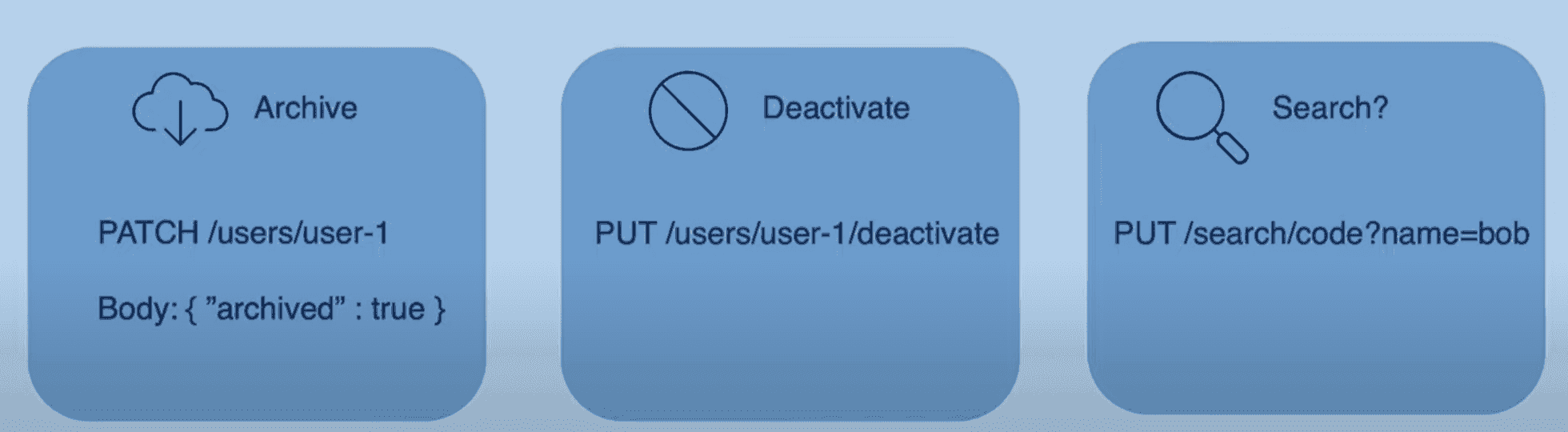 |
import React from "react";
import { Link } from "react-router-dom";
import { Button, Icon, Item, Label, Segment } from "semantic-ui-react";
import { Book } from "../../../app/models/book";
import { format } from "date-fns";
import BookListItemAttendee from "./BookListItemAttendee";
interface Props {
book: Book;
}
export default function BookListItem({ book }: Props) {
return (
<Segment.Group>
<Segment>
{book.isCancelled && (
<Label
attached="top"
color="red"
content="Cancelled"
style={{ textAlign: "center" }}
/>
)}
<Item.Group>
<Item>
<Item.Image
style={{ marginBottom: 3 }}
size="tiny"
circular
src={book.host?.image || "/assets/user.png"}
/>
<Item.Content>
<Item.Header as={Link} to={`/books/${book.id}`}>
{book.title}
</Item.Header>
<Item.Description>
Added by{" "}
<Link to={`/profiles/${book.hostUsername}`}>
{book.host?.displayName}
</Link>
</Item.Description>
{book.isHost && (
<Item.Description>
<Label basic color="orange">
You are hosting this book
</Label>
</Item.Description>
)}
{book.isReading && !book.isHost && (
<Item.Description>
<Label basic color="green">
You are going to read this book
</Label>
</Item.Description>
)}
</Item.Content>
</Item>
</Item.Group>
</Segment>
<Segment>
<span>
<Icon name="clock" />
{format(book.date!, "dd MMM yyyy h:mm aa")}
<Icon name="book" />
{book.category}
</span>
</Segment>
<Segment secondary>
<BookListItemAttendee attendees={book.attendees!} />
</Segment>
<Segment clearing>
<span>{book.description}</span>
<Button
as={Link}
to={`/books/${book.id}`}
color="teal"
floated="right"
content="View"
/>
</Segment>
</Segment.Group>
);
} |
// SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/token/ERC20/utils/SafeERC20.sol";
//import {console2} from "forge-std/console2.sol";
contract BulkSender {
using SafeERC20 for IERC20;
error InvalidRecipient();
error InvalidRecipients();
error InvalidAmount();
error InvalidToken();
error NotEnoughBalance();
error NotEnoughApproval();
error FailedToSendValue();
/**
* @dev Use this function to send specific amount of kava to a list of users.
* @param recipients: the list of users addresses that will receive the token.
* @param amount: the amount to be sent to each user.
*/
function sendKavaToMany(address[] memory recipients, uint[] memory amount) external payable{
if( recipients.length == 0 || recipients.length != amount.length )
revert InvalidRecipients();
uint total = recipients.length;
for( uint i = 0 ; i < total; i++ ){
address recipient = recipients[i];
uint value = amount[i];
if( recipient == address(0) )
revert InvalidRecipient();
if( value == 0 )
revert InvalidAmount();
(bool sent,) = recipient.call{value: value}("");
if( ! sent )
revert FailedToSendValue();
}
}
/**
* @dev Use this function to send same amount of an erc20 token to a list of users.
* @param recipients: the list of users addresses that will receive the token.
* @param amount: the amount to be sent to each user.
*/
function sendSameAmountToManyInFee(address[] memory recipients, uint amount) external payable{
if( recipients.length == 0 )
revert InvalidRecipients();
if( amount == 0 )
revert InvalidAmount();
if( recipients.length * amount > msg.value ){
revert NotEnoughBalance();
}
uint total = recipients.length;
for( uint i = 0 ; i < total; i++ ){
address recipient = recipients[i];
if( recipient == address(0) )
revert InvalidRecipient();
(bool sent,) = recipient.call{value: amount}("");
if( ! sent )
revert FailedToSendValue();
}
}
/**
* @dev Use this function to send same amount of an erc20 token to a list of users.
* @param token: the token you want to send to users, ex an USDC token.
* @param recipients: the list of users addresses that will receive the token.
* @param amount: the amount to be sent to each user.
*/
function sendSameAmountToMany(IERC20 token, address[] memory recipients, uint amount) external{
if( address(token) == address(0) )
revert InvalidToken();
if( recipients.length == 0 )
revert InvalidRecipients();
if( amount == 0 )
revert InvalidAmount();
if( recipients.length * amount > token.balanceOf(msg.sender) )
revert NotEnoughBalance();
if( token.allowance(msg.sender, address(this) ) < recipients.length * amount )
revert NotEnoughApproval();
uint total = recipients.length;
for( uint i = 0 ; i < total; i++ ){
address recipient = recipients[i];
if( recipient == address(0) )
revert InvalidRecipient();
token.safeTransferFrom(msg.sender, recipient, amount);
}
}
} |
'use strict';
module.exports = {
async up (queryInterface, Sequelize) {
await queryInterface.createTable('Books', {
id: {
allowNull: false,
autoIncrement: true,
primaryKey: true,
type: Sequelize.INTEGER,
},
title: {
allowNull: false,
type: Sequelize.STRING,
},
author: {
allowNull: true,
type: Sequelize.STRING,
},
pageQuantity: {
allowNull: false,
type: Sequelize.INTEGER,
field: 'page_quantity',
},
createdAt: {
allowNull: false,
type: Sequelize.DATE,
field: 'created_at',
},
updatedAt: {
allowNull: false,
type: Sequelize.DATE,
field: 'updated_at',
}
})
},
async down (queryInterface) {
await queryInterface.dropTable('Books');
}
}; |
interface EventListener {
(...args: any[]): void;
}
interface EventMap {
[eventName: string]: EventListener[];
}
class EventEmitter {
private events: EventMap = {};
on(eventName: string, listener: EventListener): void {
if (!this.events[eventName]) {
this.events[eventName] = [];
}
this.events[eventName].push(listener);
}
off(eventName: string, listener: EventListener): void {
if (!this.events[eventName]) return;
this.events[eventName] = this.events[eventName].filter(
(l) => l !== listener
);
}
emit(eventName: string, ...args: any[]): void {
if (!this.events[eventName]) return;
this.events[eventName].forEach((listener) => listener(...args));
}
}
export const eventEmitter = new EventEmitter(); |
package com.baomidou.mybatisplus.extension;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.core.metadata.OrderItem;
import com.baomidou.mybatisplus.core.toolkit.StringPool;
import com.baomidou.mybatisplus.extension.mapper.MysqlBaseMapper;
import com.baomidou.mybatisplus.toolkit.MybatisUtil;
import com.baomidou.mybatisplus.toolkit.ReflectUtils;
import org.apache.ibatis.type.TypeReference;
import java.lang.reflect.Type;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public abstract class MybatisQueryableStream<T, R, Children extends MybatisQueryableStream<T, R, Children>> extends MybatisStream<T, R, ExQueryWrapper<T>, Children> {
protected final Type[] renameClass;
abstract Function<Object[], R> getReturnMapper();
public MybatisQueryableStream(Class<T> entityClass, MysqlBaseMapper<T> baseMapper, Type... renameClass) {
this(null, entityClass, baseMapper, renameClass);
}
public MybatisQueryableStream(ExQueryWrapper<T> queryWrapper, Class<T> entityClass, MysqlBaseMapper<T> baseMapper, Type... renameClass) {
super(queryWrapper == null ? new ExQueryWrapper<T>() {{
setFromTable(MybatisUtil.getTableInfo(entityClass), null);
}} : queryWrapper, entityClass, baseMapper);
this.renameClass = renameClass;
}
@SafeVarargs
public MybatisQueryableStream(Class<T> entityClass, MysqlBaseMapper<T> baseMapper, TypeReference<?>... renameType) {
this(null, entityClass, baseMapper, renameType);
}
@SafeVarargs
public MybatisQueryableStream(ExQueryWrapper<T> queryWrapper, Class<T> entityClass, MysqlBaseMapper<T> baseMapper, TypeReference<?>... renameType) {
super(queryWrapper == null ? new ExQueryWrapper<T>() {{
setFromTable(MybatisUtil.getTableInfo(entityClass), null);
}} : queryWrapper, entityClass, baseMapper);
this.renameClass = Arrays.stream(renameType).map(item -> ReflectUtils.invokeMethod(item, "getSuperclassTypeParameter")).toArray(Type[]::new);
}
// /**
// * 获取子查询sql并传入注入参数
// *
// * @param queryWrapper 待传入注入参数的queryWrapper
// * @return 子查询sql
// */
// @Override
// public String getSqlSegment(ExQueryWrapper<?> queryWrapper) {
// String sqlSelect = getQueryWrapper().getSqlSelect();
// if (StringUtils.isEmpty(sqlSelect)) {
// sqlSelect = "*";
// }
//
// String customSqlSegment = getSqlSegmentWithParam(getQueryWrapper().getCustomSqlSegment(), getQueryWrapper(), queryWrapper);
// return "SELECT " + sqlSelect + " FROM " + getQueryWrapper().getSqlFrom() + " " + customSqlSegment;
// }
/**
* 分组
*
* @param group 分组表达式
* @return 实例本身
*/
public Children group(Consumer<GroupLambdaQueryWrapper> group) {
GroupLambdaQueryWrapper groupLambda = new GroupLambdaQueryWrapper(queryWrapper);
group.accept(groupLambda);
return typedThis;
}
/**
* 去重
*
* @return 实例本身
*/
@Override
public Children distinct() {
this.queryWrapper.setDistinct(true);
return typedThis;
}
/**
* 排序
*
* @param order 排序表达式
* @return 实例本身
*/
@Override
public Children sorted(Consumer<OrderLambdaQueryWrapper> order) {
OrderLambdaQueryWrapper orderLambda = new OrderLambdaQueryWrapper(queryWrapper);
if (order != null) {
order.accept(orderLambda);
}
return typedThis;
}
/**
* 限制条数
*
* @param maxSize 限制条数
* @return 实例本身
*/
@Override
public Children limit(long maxSize) {
queryWrapper.setLimit(maxSize);
queryWrapper.last("LIMIT " + queryWrapper.getSkip() + ", " + queryWrapper.getLimit() + (queryWrapper.isForUpdate() ? " FOR UPDATE" : ""));
return typedThis;
}
/**
* 跳过条数
*
* @param n 跳过条数
* @return 实例本身
*/
@Override
public Children skip(long n) {
queryWrapper.setSkip(n);
queryWrapper.last("LIMIT " + queryWrapper.getSkip() + ", " + queryWrapper.getLimit() + (queryWrapper.isForUpdate() ? " FOR UPDATE" : ""));
return typedThis;
}
@Override
public boolean exist() {
ExQueryWrapper<T> existQueryWrapper = this.queryWrapper.toExistWrapper();
boolean exist = baseMapper.list(existQueryWrapper).size() > 0;
this.queryWrapper.reset();
return exist;
}
@Override
public long count() {
ExQueryWrapper<T> countQueryWrapper = this.queryWrapper.toCountQueryWrapper("C");
Long count = (Long) baseMapper.list(countQueryWrapper).get(0).get("C");
this.queryWrapper.reset();
return count;
}
@SuppressWarnings("unchecked")
@Override
protected Stream<R> toStream() {
String sqlSelect = queryWrapper.getSqlSelect();
if (renameClass.length == 1 && renameClass[0].equals(Object.class)) {
sqlSelect = sqlSelect.split(",(?![^()]*+\\))")[0].split("(?i) as ")[0] + " AS V";
}
if (queryWrapper.isDistinct()) {
sqlSelect = "DISTINCT " + sqlSelect.trim().replaceAll("^(?i)distinct\\s+", "");
}
queryWrapper.select(sqlSelect);
List<Map<String, Object>> result = baseMapper.list(queryWrapper);
this.queryWrapper.reset();
if (renameClass.length == 1 && renameClass[0].equals(Object.class)) {
// 单值类型
return result.stream().filter(Objects::nonNull).map(x -> (R) x.get("V")).filter(Objects::nonNull);
} else {
// 实体类型
return MybatisUtil.mapStream(result, renameClass).map(getReturnMapper());
}
}
public Children forUpdate() {
queryWrapper.setForUpdate(true);
queryWrapper.last("LIMIT " + queryWrapper.getSkip() + ", " + queryWrapper.getLimit() + (queryWrapper.isForUpdate() ? " FOR UPDATE" : ""));
return typedThis;
}
/**
* 分页查询
*
* @param page 分页参数
* @param <P> 分页实体类
* @return 分页
*/
public <P extends IPage<R>> P page(P page) {
return page(page, Function.identity());
}
/**
* 分页查询
*
* @param page 分页参数
* @param mapper 转换方法
* @param <V> 值类型
* @param <P> 分页实体类
* @return 分页
*/
@SuppressWarnings("unchecked")
public <V, P extends IPage<V>> P page(P page, Function<R, V> mapper) {
// Page<Map<String, Object>> p = new Page<>(page.getCurrent(), page.getSize());
// String sqlSelect = queryWrapper.getSqlSelect();
// if (renameClass == null) {
// sqlSelect = sqlSelect.split(",")[0].split("(?i) as ")[0] + " AS V";
// }
// if (queryWrapper.isDistinct()) {
// sqlSelect = "DISTINCT " + sqlSelect.trim().replaceAll("^(?i)distinct\\s+", "");
// }
// queryWrapper.select(sqlSelect);
// if (!CollectionUtils.isEmpty(page.orders())) {
// for (OrderItem orderItem : page.orders()) {
// queryWrapper.orderBy(true, orderItem.isAsc(), orderItem.getColumn());
// }
// }
// IPage<Map<String, Object>> page1 = baseMapper.page(new Page<>(page.getCurrent(), page.getSize()), queryWrapper);
ExQueryWrapper<T> countQueryWrapper = queryWrapper.toCountQueryWrapper("C");
Long total = (Long) baseMapper.list(countQueryWrapper).get(0).get("C");
if (total > 0) {
List<ReflectUtils.Property[]> propertiesArr = Arrays.stream(this.renameClass).map(ReflectUtils::getDeclaredProperties).collect(Collectors.toList());
List<OrderItem> orders = page.orders().stream().map(
x -> {
OrderItem orderItem = new OrderItem();
String column = x.getColumn().replaceAll(StringPool.BACKTICK, "");
if (x instanceof LambdaOrderItem) {
for (int i = 0; i < this.renameClass.length; i++) {
if (ReflectUtils.getGenericClass(this.renameClass[i]).equals(((LambdaOrderItem<?>) x).getClazz())) {
column = StringPool.BACKTICK + column + StringPool.SLASH + (i + 1) + StringPool.BACKTICK;
break;
}
}
} else {
boolean b = false;
for (int i = 0; i < this.renameClass.length; i++) {
if (!Object.class.equals(this.renameClass[i]) && !ReflectUtils.isPrimitive(ReflectUtils.getGenericClass(this.renameClass[i]))) {
for (ReflectUtils.Property property : propertiesArr.get(i)) {
if (property.getName().equals(column)) {
column = StringPool.BACKTICK + column + StringPool.SLASH + (i + 1) + StringPool.BACKTICK;
b = true;
break;
}
}
if (b) {
break;
}
} else {
column = StringPool.BACKTICK + column + StringPool.SLASH + (i + 1) + StringPool.BACKTICK;
}
}
}
orderItem.setColumn(column);
orderItem.setAsc(x.isAsc());
return orderItem;
}
).collect(Collectors.toList());
ExQueryWrapper<T> pageQueryWrapper = queryWrapper.toPageQueryWrapper(page.getCurrent(), page.getSize(), orders);
List<Map<String, Object>> pageResult = baseMapper.list(pageQueryWrapper);
List<V> result;
if (renameClass.length == 1 && renameClass[0].equals(SingleValue.class)) {
// 单值类型
result = pageResult.stream().filter(Objects::nonNull).map(x -> (V) x.entrySet().iterator().next().getValue()).filter(Objects::nonNull).collect(Collectors.toList());
} else {
// 实体类型
result = MybatisUtil.mapStream(pageResult, renameClass).map(getReturnMapper()).map(mapper).collect(Collectors.toList());
}
page.setRecords(result);
}
page.setTotal(total);
queryWrapper.reset();
return page;
}
} |
import { useLoaderData } from 'react-router-dom';
import ProductsGrid from './ProductsGrid';
import ProductsList from './ProductsList';
import { useState } from 'react';
import { BsFillGridFill, BsList } from 'react-icons/bs'
const ProductsContainer = () => {
const {meta} = useLoaderData();
const totalProducts = meta.pagination.total;
const [layout, setLayout] = useState('grid');
const setActiveStyles = (pattern) => {
return `text-xl btn btn-circle btn-sm
${pattern === layout ? 'btn-primary text-primary-content' : 'btn-ghost text-base-content'}`
}
return (
<>
{/* HEADER */}
<div className='flex justify-between items-center mt-8 border-b border-base-300 pb-5'>
<h4 className='font-medium text-md'>{totalProducts} product{totalProducts > 1 && 's'}</h4>
<div className='flex gap-x-2'>
<button type="button" onClick={() => setLayout('grid')} className={setActiveStyles('grid')}>
<BsFillGridFill />
</button>
<button type="button" onClick={() => setLayout('list')} className={setActiveStyles('list')}>
<BsList />
</button>
</div>
</div>
{/* PRODUCTS */}
{totalProducts === 0 ? (
<h5 className='mt-16 text-2xl'>Sorry, no products matched your search...</h5>
) : layout === 'grid' ? <ProductsGrid /> : <ProductsList />
}
</>
)
}
export default ProductsContainer |
import { Component } from 'react'
import { withRouter } from 'react-router-dom'
import { bitcoinService } from "../services/bitcoinService"
import { userService } from "../services/userService"
class _HomePage extends Component {
state = {
userName: null,
rate: null,
}
componentDidMount() {
if (this.props.user) this.loadRate()
}
loadRate = async () => {
try {
const rate = await bitcoinService.getRate(this.props.user.coins)
this.setState({ rate })
} catch (err) {
console.log('err:', err)
}
}
handleChange = (ev) => {
this.setState({userName: ev.target.value})
}
signup = () => {
userService.signup(this.state.userName)
this.props.history.push('/contact')
this.props.updateUser()
}
render() {
const { rate } = this.state
const { user } = this.props
return (
<section className='home-page'>
{
!user ?
<div className='signup'>
<h1 className='title'>Bitcoin is an innovative payment network and a new kind of money.</h1>
<div className="form__group field">
<input type="text" className="form__field" placeholder="Name" name="name" id='name' onChange={this.handleChange} />
<label htmlFor="name" className="form__label">Name</label>
</div>
<button className='signup-btn' onClick={this.signup}>
<img className='icon' src={require('../assets/imgs/signup.png')} alt="signup" />
</button>
</div> :
<div>
<h4>Home {user?.name}!</h4>
<p>Coins: {user?.coins}</p>
<p>BTC: {rate}</p>
</div>
}
{/* <div>
<h4>Home {user?.name}!</h4>
<p>Coins: {user?.coins}</p>
<p>BTC: {rate}</p>
</div> */}
</section>
)
}
}
export const HomePage = withRouter(_HomePage) |
# String concatenation and f strings
## Key ideas
- Using formatted strings
- Concatenating strings
## String Concatenation and f-strings
When there’s a variable we want to combine with a string to print out a message, so far we’ve added the strings with `+`.
```python
name = "Mercy"
print("Hello, " + name) # Hello, Mercy
```
When the message is longer, this becomes more confusing and harder to type.
```python
name = "Mercy"
print("The alarm went off at exactly 6:00 AM as it had every morning for the past five years. " + name + " began her morning and was ready to eat breakfast by 7:00 AM. The day appeared to be as normal as any other, and " + name + " was not expecting anything to change.")
```
There’s another way to format long text that uses variables, called **f-strings.**
```python
name = "Mercy"
print(f"Hello, {name}") # Hello, Mercy
print(f"The alarm went off at exactly 6:00 AM as it had every morning for the past five years. {name} began her morning and was ready to eat breakfast by 7:00 AM. The day appeared to be as normal as any other, and {name} was not expecting anything to change.")
```
Instead of using `+` to combine the variable and the string, we start the string with `f` and we use `{}` to insert the variable right inside the string. That way, there’s less confusion about quote marks and spaces.
### Other f-string uses
We can also use f-strings for rounding.
```python
one_third = 1/3
print(one_third)# 0.3333333333333333
print(f"{one_third:.2}") # 0.33
```
f-strings have other formatting powers, but we’ll leave it at rounding floats for now.
## Practice: f-strings
<aside>
👩🏿💻 Practice using f-strings to format results.
</aside>
<div style="position: relative; padding-bottom: 56.25%; height: 0;"><iframe src="https://replit.com/team/kibo-fpwp6/W15-F-strings-Practice" frameborder="0" webkitallowfullscreen mozallowfullscreen allowfullscreen style="position: absolute; top: 0; left: 0; width: 100%; height: 100%;"></iframe></div>
<details><summary>Solution: F-strings practice</summary>
```python
first_num = float(input("enter first number: "))
second_num = float(input("enter second number: "))
# change the line below
result = first_num / second_num
print(f"the result is {result:.3} ")
```
</details> |
//
// MER.swift
// MarsImagesIOS
//
// Created by Mark Powell on 7/28/17.
// Copyright © 2017 Mark Powell. All rights reserved.
//
import Foundation
class MER: Mission {
let SOL = "Sol"
let LTST = "LTST"
let RMC = "RMC"
let COURSE = "Course"
enum TitleState {
case START,
SOL_NUMBER,
IMAGESET_ID,
INSTRUMENT_NAME,
MARS_LOCAL_TIME,
DISTANCE,
YAW,
PITCH,
ROLL,
TILT,
ROVER_MOTION_COUNTER
}
override init() {
super.init()
self.eyeIndex = 23
self.instrumentIndex = 1
self.sampleTypeIndex = 12
self.cameraFOVs["N"] = 0.78539816
self.cameraFOVs["P"] = 0.27925268
}
override func getSortableImageFilename(url: String) -> String {
let tokens = url.components(separatedBy: "/")
if tokens.count > 0 {
let filename = tokens[tokens.count-1]
if filename.hasPrefix("Sol") {
return "0" //sort Cornell Pancam images first
}
else if (filename.hasPrefix("1") || filename.hasPrefix("2")) && filename.count == 31 {
let index = filename.index(filename.startIndex, offsetBy: 23)
return filename.substring(from: index)
}
return filename
}
return url
}
override func rowTitle(_ title: String) -> String {
let merTitle = tokenize(title) as! MERTitle
if merTitle.instrumentName == "Course Plot" {
let distanceFormatted = String.localizedStringWithFormat("%.2f", merTitle.distance)
return "Drive for \(distanceFormatted) meters"
}
return merTitle.instrumentName
}
override func caption(_ title: String) -> String {
if let t = tokenize(title) as? MERTitle {
if (t.instrumentName == "Course Plot") {
return String(format:"Drive for %.2f meters on Sol %d", t.distance, t.sol)
}
else {
return "\(t.instrumentName) image taken on Sol \(t.sol)."
}
}
else {
return super.caption(title)
}
}
override func tokenize(_ title: String) -> Title {
var mer = MERTitle()
let tokens = title.components(separatedBy: " ")
var state = TitleState.START
for word in tokens {
if word == SOL {
state = TitleState.SOL_NUMBER
continue
}
else if word == LTST {
state = TitleState.MARS_LOCAL_TIME
continue
}
else if word == RMC {
state = TitleState.ROVER_MOTION_COUNTER
continue
}
var indices:[Int] = []
switch (state) {
case .START:
break
case .SOL_NUMBER:
mer.sol = Int(word)!
state = TitleState.IMAGESET_ID
break
case .IMAGESET_ID:
if word == COURSE {
mer = parseCoursePlotTitle(title: title, mer: mer)
return mer
} else {
mer.imageSetID = word
}
state = TitleState.INSTRUMENT_NAME
break
case .INSTRUMENT_NAME:
if mer.instrumentName.isEmpty {
mer.instrumentName = String(word)
} else {
mer.instrumentName.append(" \(word)")
}
break
case .MARS_LOCAL_TIME:
mer.marsLocalTime = word
break
case .ROVER_MOTION_COUNTER:
indices = word.components(separatedBy: "-").map { Int($0)! }
mer.siteIndex = indices[0]
mer.driveIndex = indices[1]
break
default:
print("Unexpected state in parsing image title: \(state)")
break
}
}
return mer
}
func parseCoursePlotTitle(title:String, mer: MERTitle) -> MERTitle {
let tokens = title.components(separatedBy: " ")
var state = TitleState.START
for word in tokens {
if word == COURSE {
mer.instrumentName = "Course Plot"
} else if word == "Distance" {
state = TitleState.DISTANCE
continue
} else if word == "yaw" {
state = TitleState.YAW
continue
} else if word == "pitch" {
state = TitleState.PITCH
continue
} else if word == "roll" {
state = TitleState.ROLL
continue
} else if word == "tilt" {
state = TitleState.TILT
continue
} else if word == "RMC" {
state = TitleState.ROVER_MOTION_COUNTER
continue
}
var indices:[Int] = []
switch (state) {
case .START:
break
case .DISTANCE:
mer.distance = Double(word)!
break
case .YAW:
mer.yaw = Double(word)!
break
case .PITCH:
mer.pitch = Double(word)!
break
case .ROLL:
mer.roll = Double(word)!
break
case .TILT:
mer.tilt = Double(word)!
break
case .ROVER_MOTION_COUNTER:
indices = word.components(separatedBy: "-").map { Int($0)! }
mer.siteIndex = indices[0]
mer.driveIndex = indices[1]
break
default:
print("Unexpected state in parsing course plot title: \(state)")
}
}
return mer
}
override func imageName(imageId: String) -> String {
if imageId.range(of:"False") != nil {
return "Color"
}
let irange = imageId.index(imageId.startIndex, offsetBy: instrumentIndex)..<imageId.index(imageId.startIndex, offsetBy: instrumentIndex+1)
let instrument = imageId[irange]
if instrument == "N" || instrument == "F" || instrument == "R" {
let erange = imageId.index(imageId.startIndex, offsetBy: eyeIndex)..<imageId.index(imageId.startIndex, offsetBy:eyeIndex+1)
let eye = imageId[erange]
if eye == "L" {
return "Left"
} else {
return "Right"
}
} else if instrument == "P" {
let prange = imageId.index(imageId.startIndex, offsetBy: eyeIndex)..<imageId.index(imageId.startIndex, offsetBy:eyeIndex+2)
return String(imageId[prange])
}
return ""
}
override func stereoImageIndices(imageIDs: [String]) -> (Int,Int)? {
let imageid = imageIDs[0]
let instrument = getInstrument(imageId: imageid)
if !isStereo(instrument: instrument) {
return nil
}
var leftImageIndex = -1;
var rightImageIndex = -1;
var index = 0;
for imageId in imageIDs {
let eye = getEye(imageId: imageId)
if leftImageIndex == -1 && eye=="L" && !imageId.hasPrefix("Sol") {
leftImageIndex = index;
}
if rightImageIndex == -1 && eye=="R" {
rightImageIndex = index;
}
index += 1;
}
if (leftImageIndex >= 0 && rightImageIndex >= 0) {
return (Int(leftImageIndex), Int(rightImageIndex))
}
return nil
}
func isStereo(instrument:String) -> Bool {
return instrument == "F" || instrument == "R" || instrument == "N" || instrument == "P"
}
override func getCameraId(imageId: String) -> String {
if imageId.range(of:"Sol") != nil {
return "P";
} else {
return imageId[1]
}
}
override func mastPosition() -> [Double] {
return [0.456,0.026,-1.0969]
}
}
class MERTitle: Title {
var distance = 0.0
var yaw = 0.0
var pitch = 0.0
var roll = 0.0
var tilt = 0.0
} |
import React, { Component } from 'react'
import './Menu.css'
import escapeRegExp from 'escape-string-regexp'
// Places Data
class Menu extends Component {
state = {
query: '',
places: this.props.places
}
updateQuery = (query) => {
this.setState({ query })
let allPlaces = this.props.places
let newPlaces
if(this.state.query && (this.state.query !== '')) {
const match = new RegExp(escapeRegExp(query), 'i');
newPlaces = allPlaces.filter((place) => match.test(place.venue.name))
this.setState({places: newPlaces})
this.props.updatePlaces(newPlaces)
} else {
this.setState({places: allPlaces})
}
}
triggerMarkerClick = (placeTitle) => {
this.props.markers.map((marker) => {
if(marker.title === placeTitle) {
window.google.maps.event.trigger(marker, 'click');
}
})
}
render() {
return (
<aside>
<div className="search-form">
<label htmlFor="searchQuery">Find A Place!</label>
<input
id="searchQuery"
type="text"
placeholder="Search Here"
onChange={(e) => this.updateQuery(e.target.value)}
value={this.state.query}
/>
</div>
{this.state.places.length !== 0 && (
<ul className="search-result">
{this.state.places.map((place, index) => (
<li
key={index}
tabindex={index}
className="item"
onClick={() => this.triggerMarkerClick(place.venue.name)}
>
{place.venue.name}
</li>
))}
</ul>
)}
{this.state.places === 0 && (
<ul className="search-result">
<li className="item">No Places Found..</li>
</ul>
)}
</aside>
)
}
}
export default Menu |
# FluxIO
## What is this?
This library was created to support access to instance fields without the use of reflection.
It includes the following features:
- Fast search for instance fields
- Direct access to instance fields without boxing/unboxing
- Cost-free high-speed access to instance fields
**And, this project is being developed targeting 64-bit processors.**
This is a project still under development.
Please refer to [this table](./docs/Compatibility.md) to check compatibility with specific systems.
## Performance
### Benchmark Result Summary
- `Direct`: Access private fields through public properties. This is a scenario where performance loss is completely non-existent.
- `Reflection`: Access private fields using `System.Reflection`.
- `FluxIO`: Access private fields using `fluxiolib`.
| Method | N | Mean | Error | StdDev |
|------------------------------------------- |----- |---------------:|--------------:|------------:|
| **Direct** | **1** | **2.067 ns** | **0.0081 ns** | **0.0076 ns** |
| Reflection | 1 | 181.778 ns | 0.9892 ns | 0.9253 ns |
| FluxIO | 1 | 2.092 ns | 0.0137 ns | 0.0128 ns |
| **Direct** | **10** | **31.265 ns** | **0.1310 ns** | **0.1225 ns** |
| Reflection | 10 | 1,965.770 ns | 12.2738 ns | 11.4810 ns |
| FluxIO | 10 | 31.368 ns | 0.1340 ns | 0.1254 ns |
| **Direct** | **100** | **325.373 ns** | **1.4319 ns** | **1.2693 ns** |
| Reflection | 100 | 19,270.552 ns | 60.8898 ns | 56.9564 ns |
| FluxIO | 100 | 325.419 ns | 1.1952 ns | 1.0595 ns |
| **Direct** | **1000** | **3,269.418 ns** | **16.7019 ns** | **15.6230 ns** |
| Reflection | 1000 | 190,516.761 ns | 859.9003 ns | 718.0557 ns |
| FluxIO | 1000 | 3,267.093 ns | 10.0056 ns | 9.3593 ns |
Benchmark source code: [Zip Archive](./.benchmark/FluxIOLib.Benchmark.zip)
Full benchmark results can be viewed [here](./docs/Benchmark.Result.md).
## Obtaining Build Artifacts
- [한국어](./docs/ko/GetBuildArtifacts.md)
- [English](./docs/en/GetBuildArtifacts.md)
## How to Use
This is the recommended way to use it for a single field:
```csharp
// FILE: Foo.cs
class Foo
{
public int age;
protected float score;
// Property to retrieve the score
public float MyScore
{
get
{
return this.score;
}
}
}
```
```csharp
// FILE: Program.cs
using fluxiolib;
using System.Reflection; // for 'FieldInfo' and 'BindingFlags'
FieldInfo? fieldInfo = typeof(Foo).GetField("score", BindingFlags.NonPublic | BindingFlags.Instance);
if (fieldInfo == null) {
Console.WriteLine("Error!");
return;
}
FluxRuntimeFieldDesc fd_score = FluxTool.ToRuntimeFieldDesc(fieldInfo);
Foo foo = new Foo();
Console.Write("Before: ");
Console.WriteLine(foo.MyScore);
fd_score.FieldAccessor.Value<float>(foo) = 250;
Console.Write("After: ");
Console.WriteLine(foo.MyScore);
Console.Write("FieldOffset: ");
Console.WriteLine(fd_score.FieldOffset);
// Print:
// Before: 0
// After: 250
// FieldOffset: 4
```
Although there is a built-in field search feature, the performance variability is much greater than searching fields using Reflection.
Therefore, while the basic guide will include instructions on using the built-in field search feature, using it as shown in the example above is best for a single field.
More information on how to use it can be found [here](#usage-guide).
## Usage Guide
- [한국어](./docs/ko/HowToUse_Basic.md)
- [English](./docs/en/HowToUse_Basic.md)
## API Document
## .NET 8.0
- [한국어](./docs/ko/API/net8.0/fluxiolib.md)
- English (**Not ready yet**)
## .NET Standard 2.0
- [한국어](./docs/ko/API/netstandard2.0/fluxiolib.md)
- English (**Not ready yet**) |
package com.backend.IntegradorFinal.service.imp;
import com.backend.IntegradorFinal.dto.DomicilioDto;
import com.backend.IntegradorFinal.dto.PacienteDto;
import com.backend.IntegradorFinal.entity.Paciente;
import com.backend.IntegradorFinal.exceptions.BadRequestException;
import com.backend.IntegradorFinal.exceptions.ResourceNotFoundException;
import com.backend.IntegradorFinal.repository.PacienteRepository;
import com.backend.IntegradorFinal.service.IPacienteService;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class PacienteService implements IPacienteService {
private static final Logger LOGGER = LoggerFactory.getLogger(PacienteService.class);
private final PacienteRepository pacienteRepository;
private final ObjectMapper objectMapper;
@Autowired
public PacienteService(PacienteRepository pacienteRepository, ObjectMapper objectMapper) {
this.pacienteRepository = pacienteRepository;
this.objectMapper = objectMapper;
}
@Override
public List<PacienteDto> listarPacientes() {
List<Paciente> pacientes = pacienteRepository.findAll();
List<PacienteDto> pacienteDtos = pacientes.stream()
.map(paciente -> {
PacienteDto pacienteDto = null;
DomicilioDto domicilioDto = objectMapper.convertValue(paciente.getDomicilio(), DomicilioDto.class);
pacienteDto = objectMapper.convertValue(paciente, PacienteDto.class);
pacienteDto.setDomicilioDto(domicilioDto);
return pacienteDto;
}).toList();
LOGGER.info("Lista de todos los pacientes: {}", pacienteDtos);
return pacienteDtos;
}
@Override
public PacienteDto buscarPacientePorId(Long id) {
Paciente pacienteBuscado = pacienteRepository.findById(id).orElse(null);
PacienteDto pacienteDto = null;
if(pacienteBuscado != null){
DomicilioDto domicilioDto = objectMapper.convertValue(pacienteBuscado.getDomicilio(), DomicilioDto.class);
pacienteDto = objectMapper.convertValue(pacienteBuscado, PacienteDto.class);
pacienteDto.setDomicilioDto(domicilioDto);
LOGGER.info("Paciente encontrado: {}", pacienteDto);
}else LOGGER.info("El id no se encuentra registrado en la base de datos");
return pacienteDto;
}
@Override
public PacienteDto guardarPaciente(Paciente paciente) {
Paciente pacienteNuevo = pacienteRepository.save(paciente);
DomicilioDto domicilioDto = objectMapper.convertValue(pacienteNuevo.getDomicilio(), DomicilioDto.class);
PacienteDto pacienteDtoNuevo = objectMapper.convertValue(pacienteNuevo, PacienteDto.class);
pacienteDtoNuevo.setDomicilioDto(domicilioDto);
LOGGER.info("Nuevo paciente registrado con exito: {}", pacienteDtoNuevo);
return pacienteDtoNuevo;
}
@Override
public PacienteDto actualizarPaciente(Paciente paciente) {
Paciente pacienteAActualizar = pacienteRepository.findById(paciente.getId()).orElse(null);
PacienteDto pacienteDtoActualizado = null;
if(pacienteAActualizar != null){
pacienteAActualizar = paciente;
pacienteRepository.save(pacienteAActualizar);
DomicilioDto domicilioDto = objectMapper.convertValue(pacienteAActualizar.getDomicilio(), DomicilioDto.class);
pacienteDtoActualizado = objectMapper.convertValue(pacienteAActualizar, PacienteDto.class);
pacienteDtoActualizado.setDomicilioDto(domicilioDto);
LOGGER.info("Paciente actualizado con exito: {}", pacienteDtoActualizado);
}else LOGGER.error("No fue posible actualizar los datos ya que el paciente no se encuentra registrado");
return pacienteDtoActualizado;
}
@Override
public void eliminarPaciente(Long id) throws ResourceNotFoundException {
if(buscarPacientePorId(id) != null){
pacienteRepository.deleteById(id);
LOGGER.warn("Se ha eliminado el paciente con id {}", id);
}
else{
LOGGER.error("No se ha encontrado el paciente con id {}", id);
throw new ResourceNotFoundException("No se ha encontrado el paciente con id " + id);
}
}
} |
Listas
lista1 = ["Multimídia", 100, True]
lista2 = [42, "Python", 3.14159, lista1]
lista2.append(1.88) // Ultima posição add 1.88
lista2.insert(2,False) // Add false na posição 2
print("Elemento na posição 2 e 3:", lista2[2:4]) // 2 excluso, 4 incluso
print("Último elemento:", lista2[-1]) // Pega o último elemento.
print("Penúltimo elemento:", lista2[-2]) // Pega o penúltimo elemento.
lista2.reverse()
lista3 = [8, 4, 3, 10, -20]
lista3.sort()
lista3.sort(reverse=True)
numpy
import numpy as np
v = [2, 3, 5, 7, 11]
vetor = np.array(v) // Cria um vetor com os valores passados
print(vetor.shape) // Mostra a quantidade de elementos do vetor
print(vetor[0]) // Mostra o primeiro elemento do vetor
print(vetor.dtype) // Mostra o tipo de dado do vetor
m = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
matriz = np.array(m) // Cria uma matriz com os valores passados
print(matriz.shape) // Mostra a quantidade de elementos da matriz
print(matriz[0, 0]) // Mostra o primeiro elemento da matriz
print(matriz.dtype) // Mostra o tipo de dado da matriz
Array tamanho 2x4 preenchido com 1:
print(np.ones((2, 4)))
Array tamanho 2x4x3 preenchido com 0:
print(np.zeros((3, 2, 4))) # Ordem invertida: [página, linha, coluna].
Array 3x4 com valores aleatórios em [0, 10):
print(np.random.randint(10, size=(3, 4)))
Array com valores em [0, 1) e passo 0.1:
print(np.arange(0, 1, 0.1))
matriz_2x3 = np.array([[0, 1, 2], [3, 4, 5]])
Transposta:
print(matriz_2x3.T)
matriz_2x2 = np.array([[6, 7], [8, 9]])
matriz_3x3 = np.array([[6, 7, 8], [9, 10, 11], [12, 13, 14]])
Matriz 2x3 e Matriz 3x3 - Concatenação no eixo das linhas (eixo 0)
print(np.concatenate([matriz_2x3, matriz_3x3], axis=0))
Matriz 2x3 e Matriz 2x2 - Concatenação no eixo das colunas (eixo 1)
print(np.concatenate([matriz_2x3, matriz_2x2], axis=1))
Multiplicação com escalar:
print(np.ones((2, 3)) * 2)
Soma com escalar:
print(np.zeros(6) + 3)
Soma ponto-a-ponto:
# Equivalente a [[1, 1], [1, 1]] + [[10, 10], [10, 10]]:
print(np.ones((2, 2)) + 10 * np.ones((2, 2)))
Multiplicação ponto-a-ponto:
# Equivalente a [2, 2, 2, 2] * [5, 5, 5, 5]:
print((2 * np.ones(4)) * (5 * np.ones(4)))
Multiplicação matricial:
print(
np.matmul(
np.array([[1, 2],
[3, 4]]),
np.array([[5, 6],
[7, 8]])))
Ponto a ponto Escalar:
#Equivalente a 1 * (-1) + 2 * 0 + 3 * 1:
print(np.dot(np.array([1, 2, 3]), np.array([-1, 0, 1])))
Imagens Matriciais
Importar bibliotecas:
from PIL import Image
import matplotlib.pyplot as plt
import numpy as np
Ler/gravar/converter imagens:
img = Image.open('imagem.extensao') //Abir imagem
plt.imshow(img) //Plot imagem
img_np = np.array(img) //Transformar em Array
img_pil = Image.fromarray(img_np) //Transofrmar em iamgem
img.save('imagem.extensao') //Salvar imagem
img_np2 = img_np[:,:,:3] #tirar o canal alfa
ex = np.zeros(shape=(8, 8 * 3, 3), dtype=np.uint8)
ex[:, :8] = (255, 0, 0) # pintar todas as linhas das colunas 0:8 de vermelho
ex[:, 8:16] = (0, 255, 0) # todas as linhas, colunas 8:16 de verde
ex[:, 16:] = (0, 0, 255) # todas as linhas, colunas 16:24 de azul
ex[0, 0] = (0, 0, 0) # linha 0, coluna 0, colocar preto]
ex[0, 8] = (255,255,255) # linha 0, coluna 8,colocar branco) |
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package org.apache.shindig.gadgets.rewrite.image;
import org.junit.Before;
import org.junit.Test;
import org.junit.Assert;
import java.io.*;
/**
* Tests for {@code JpegImageUtils}
*/
public class JpegImageUtilsTest extends Assert {
@Before
public void setUp() throws Exception {
}
@Test
public void testGetJpegImageData_huffmanOptimized() throws Exception {
String resource = "org/apache/shindig/gadgets/rewrite/image/testImage420.jpg";
InputStream is = getClass().getClassLoader().getResourceAsStream(resource);
JpegImageUtils.JpegImageParams imageParams = JpegImageUtils.getJpegImageData(is, resource);
assertEquals(true, imageParams.isHuffmanOptimized());
}
@Test
public void testGetJpegImageData_420Sampling() throws Exception {
String resource = "org/apache/shindig/gadgets/rewrite/image/testImage420.jpg";
InputStream is = getClass().getClassLoader().getResourceAsStream(resource);
JpegImageUtils.JpegImageParams imageParams = JpegImageUtils.getJpegImageData(is, resource);
assertEquals(JpegImageUtils.SamplingModes.YUV420, imageParams.getSamplingMode());
}
@Test
public void testGetJpegImageData_444Sampling() throws Exception {
String resource = "org/apache/shindig/gadgets/rewrite/image/testImage444.jpg";
InputStream is = getClass().getClassLoader().getResourceAsStream(resource);
JpegImageUtils.JpegImageParams imageParams = JpegImageUtils.getJpegImageData(is, resource);
assertEquals(JpegImageUtils.SamplingModes.YUV444, imageParams.getSamplingMode());
assertEquals(0.90F, imageParams.getChromaQualityFactor(), 0.01F);
assertEquals(0.90F, imageParams.getLumaQualityFactor(), 0.01F);
assertEquals(0.90F, imageParams.getApproxQualityFactor(), 0.01F);
}
@Test
public void testGetJpegImageData_notHuffmanOptimized() throws Exception {
String resource = "org/apache/shindig/gadgets/rewrite/image/testImageNotHuffmanOptimized.jpg";
InputStream is = getClass().getClassLoader().getResourceAsStream(resource);
JpegImageUtils.JpegImageParams imageParams = JpegImageUtils.getJpegImageData(is, resource);
assertEquals(false, imageParams.isHuffmanOptimized());
}
} |
import { it, describe, expect, beforeAll, beforeEach, afterAll } from "vitest"
import { render, screen, waitFor, fireEvent } from '@testing-library/react'
import { http, HttpResponse } from 'msw'
import { stages } from '../../mocks/db'
import { server } from '../../mocks/server'
import Board from "."
import { QueryClient, QueryClientProvider } from '@tanstack/react-query'
import { queryClient } from "../../App"
beforeAll(() => server.listen())
beforeEach(() => server.resetHandlers())
afterAll(() => server.close())
describe('Stage', () => {
it('should render loading', async () => {
render(
<QueryClientProvider client={queryClient}>
<Board />
</QueryClientProvider>
)
await waitFor(() => {
expect(screen.getByRole('heading')).toHaveTextContent('Loading...')
})
})
it('should be able to call api and render stages', async () => {
render(
<QueryClientProvider client={queryClient}>
<Board />
</QueryClientProvider>
)
await waitFor(() => {
const stagesComponents = screen.getAllByTestId("stage-component")
expect(stagesComponents).toHaveLength(stages.length)
stages.forEach((stage, index) => {
expect(stagesComponents[index]).toHaveTextContent(stage.title)
})
})
})
it('should be able to show a error message when API returns a error', async () => {
const queryClient = new QueryClient({
defaultOptions: {
queries: {
retry: false
}
}
})
server.use(
http.get('http://localhost:3001/api/v1/stages', () => {
return HttpResponse.error()
})
)
render(
<QueryClientProvider client={queryClient}>
<Board />
</QueryClientProvider>
)
await waitFor(() => {
expect(screen.getByRole('heading')).toHaveTextContent('Error...')
})
})
it('should be able to add a card to a stage', async () => {
render(
<QueryClientProvider client={queryClient}>
<Board />
</QueryClientProvider>
)
const addButton = screen.getAllByRole('button', { name: 'Criar' })
fireEvent.click(addButton[0])
const input = screen.getByRole('textbox')
fireEvent.change(input, { target: { value: 'New Card Title' } });
const createButton = screen.getByRole('button', { name: 'Create' })
fireEvent.click(createButton)
await waitFor(() => {
expect(screen.getByText('New Card Title')).toBeInTheDocument()
})
})
}) |
from django.contrib.auth.models import Permission
from django.contrib.auth import get_user_model
from django.db.models.signals import post_save, post_delete
from django.dispatch import receiver
from repair_core.models import RepairMan
from repair_core.permissions import REPAIRMAN_DEFAULT_PERMISSIONS
User = get_user_model()
@receiver(post_save, sender=RepairMan)
def add_repairman_default_permissions(sender, instance, created, **kwargs):
"""Assign a set of default permissions to a RepairMan object on its creation"""
if created:
try:
repairman_user = User.objects.get(pk=instance.user_id)
except User.DoesNotExist:
# TODO: A simple logging might be nice here?
return False
permissions = Permission.objects.filter(
codename__in=REPAIRMAN_DEFAULT_PERMISSIONS
)
# Assign the new permissions.
repairman_user.user_permissions.set(permissions)
# Mark the Repairman's user profile as a staff.
User.objects.filter(pk=repairman_user.pk).update(is_staff=True)
@receiver(post_delete, sender=RepairMan)
def remove_repairman_permissions(sender, instance, **kwargs):
"""Remove permissions and revoke staff status when deleting a RepairMan object"""
try:
repairman_user = User.objects.get(pk=instance.user_id)
except User.DoesNotExist:
return False
# Remove permissions assigned to the user
repairman_user.user_permissions.clear()
# Revoke staff status
User.objects.filter(pk=repairman_user.pk).update(is_staff=False) |
/*
* Copyright (c) 2015, University of Oslo
* * All rights reserved.
* *
* * Redistribution and use in source and binary forms, with or without
* * modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright notice, this
* * list of conditions and the following disclaimer.
* *
* * Redistributions in binary form must reproduce the above copyright notice,
* * this list of conditions and the following disclaimer in the documentation
* * and/or other materials provided with the distribution.
* * Neither the name of the HISP project nor the names of its contributors may
* * be used to endorse or promote products derived from this software without
* * specific prior written permission.
* *
* * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
* * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON
* * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
*/
package org.hisp.dhis.android.sdk.network.managers;
import com.squareup.okhttp.OkHttpClient;
import java.net.InetAddress;
import java.util.concurrent.TimeUnit;
public class NetworkManager {
private static final String CLASS_TAG = "NetworkManager";
private static NetworkManager mNetworkManager;
private String serverUrl;
private String credentials;
private Base64Manager base64Manager;
private IHttpManager httpManager;
private NetworkManager() {
// no instances through
// constructor for client code
OkHttpClient okHttpClient = new OkHttpClient();
okHttpClient.setFollowSslRedirects(true);
okHttpClient.setConnectTimeout(HttpManager.TIME_OUT,
TimeUnit.MILLISECONDS);
httpManager = new HttpManager(okHttpClient);
base64Manager = new Base64Manager();
}
public static NetworkManager getInstance() {
if (mNetworkManager == null) {
mNetworkManager = new NetworkManager();
}
return mNetworkManager;
}
/**
* Returns true if able to connect to the internet.
* Will return false if run on the main ui thread
* @return
*/
public static boolean hasInternetConnection() {
try {
String url = getInstance().getServerUrl();
if(url == null) {
return false;
}
InetAddress ipAddr = InetAddress.getByName(url); //You can replace it with your name
if (ipAddr.equals("")) {
return false;
} else {
return true;
}
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
public String getServerUrl() {
return serverUrl;
}
public void setServerUrl(String serverUrl) {
this.serverUrl = serverUrl;
}
public String getCredentials() {
return credentials;
}
public void setCredentials(String credentials) {
this.credentials = credentials;
}
public Base64Manager getBase64Manager() {
return base64Manager;
}
public IHttpManager getHttpManager() {
return httpManager;
}
} |
/*
* This file is part of the ct-Bot teensy framework.
* Copyright (c) 2019 Timo Sandmann
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, version 3.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
/**
* @file tft_display.h
* @brief TFT display driver
* @author Timo Sandmann
* @date 10.04.2019
*/
#pragma once
#include "ctbot_config.h"
#include "logger.h"
#include "leds_i2c.h"
#define FOONATHAN_HAS_EXCEPTION_SUPPORT 0
#include "memory_pool.hpp"
#include "namespace_alias.hpp"
#include "static_allocator.hpp"
#undef FOONATHAN_HAS_EXCEPTION_SUPPORT
#include "avr/pgmspace.h"
#include <cstdint>
#include <memory>
#include <string>
#include <atomic>
#include <mutex>
#include <array>
#ifndef EXTMEM
#define EXTMEM
#endif
class TFT_SPI;
class Adafruit_GFX;
class Adafruit_GFX_Button;
class GFXcanvas16;
class XPT2046_Touchscreen;
class TS_Point;
namespace ctbot {
class TFTColorHelper {
public:
/**
* @brief Given 8-bit red, green and blue values, return a 'packed' 16-bit color value
* in '565' RGB format (5 bits red, 6 bits green, 5 bits blue)
* @param[in] red: 8-bit red brightnesss (0 = off, 255 = max)
* @param[in] green: 8-bit green brightnesss (0 = off, 255 = max)
* @param[in] blue: 8-bit blue brightnesss (0 = off, 255 = max)
* @return 'Packed' 16-bit color value (565 format)
*/
static constexpr uint16_t color565(const uint8_t red, const uint8_t green, const uint8_t blue) {
return ((red & 0xf8) << 8) | ((green & 0xfc) << 3) | (blue >> 3);
}
};
class TFTColors : public TFTColorHelper {
public:
static constexpr uint16_t BLACK { color565(0, 0, 0) };
static constexpr uint16_t NAVY { color565(0, 0, 123) };
static constexpr uint16_t DARKGREEN { color565(0, 125, 0) };
static constexpr uint16_t DARKCYAN { color565(0, 125, 123) };
static constexpr uint16_t MAROON { color565(123, 0, 0) };
static constexpr uint16_t PURPLE { color565(123, 0, 123) };
static constexpr uint16_t OLIVE { color565(123, 125, 0) };
static constexpr uint16_t LIGHTGREY { color565(198, 195, 198) };
static constexpr uint16_t DARKGREY { color565(123, 125, 123) };
static constexpr uint16_t BLUE { color565(0, 0, 255) };
static constexpr uint16_t GREEN { color565(0, 255, 0) };
static constexpr uint16_t CYAN { color565(0, 255, 255) };
static constexpr uint16_t RED { color565(255, 0, 0) };
static constexpr uint16_t MAGENTA { color565(255, 0, 255) };
static constexpr uint16_t YELLOW { color565(255, 255, 0) };
static constexpr uint16_t WHITE { color565(255, 255, 255) };
static constexpr uint16_t ORANGE { color565(255, 165, 0) };
static constexpr uint16_t GREENYELLOW { color565(173, 255, 41) };
static constexpr uint16_t PINK { color565(255, 130, 198) };
};
class TFTDisplay {
static constexpr bool DEBUG_ { false };
protected:
static constexpr uint32_t FRAMES_PER_SEC_ { 10 };
static constexpr int16_t WIDTH_ { CtBotConfig::TFT_CONTROLLER_TYPE == 9486 ? 480 : 320 };
static constexpr int16_t HEIGHT_ { CtBotConfig::TFT_CONTROLLER_TYPE == 9486 ? 320 : 240 };
static constexpr uint16_t TOUCH_THRESHOLD_ { 800 };
static constexpr size_t FB_CHUNK_SIZE_ { WIDTH_ };
using static_pool_t = memory::memory_pool<memory::array_pool, memory::static_allocator>;
EXTMEM static memory::static_allocator_storage<WIDTH_ * HEIGHT_ * sizeof(uint16_t) + 16> framebuffer_pool_;
static_pool_t mem_pool_ { FB_CHUNK_SIZE_, sizeof(framebuffer_pool_), framebuffer_pool_ };
std::array<uint16_t, WIDTH_ * HEIGHT_>* framebuffer_mem_;
TFT_SPI* p_display_;
GFXcanvas16* p_framebuffer_;
XPT2046_Touchscreen* p_touch_;
TaskHandle_t task_;
std::atomic<bool> service_running_;
mutable std::atomic<bool> updated_;
mutable std::mutex fb_mutex_;
LedsI2cEna<>* p_backl_pwm_;
uint32_t touch_counter_;
TS_Point* p_touch_point_;
mutable std::mutex touch_mutex_;
decltype(framebuffer_mem_) init_memory();
void run();
/**
* @brief Write a message out to a SerialConnection
* @param[in] p_str: Message as pointer to std::string
* @return Number of characters written
*/
FLASHMEM uint8_t print(const std::string* p_str, bool clear = false) const;
public:
FLASHMEM TFTDisplay(LedsI2cEna<>& backl_pwm);
FLASHMEM ~TFTDisplay();
auto get_width() const {
return WIDTH_;
}
auto get_height() const {
return HEIGHT_;
}
/**
* @brief Clear entire display, set cursor to home position
*/
void clear() const;
void clear(uint8_t row) const;
void flush() const;
/**
* @brief Set the cursor to a position
* @param[in] row: New row of cursor [1; 20]
* @param[in] column: New column of cursor [1; 4]
*/
void set_cursor_line(uint8_t row, uint8_t column) const;
void set_cursor(int16_t x, int16_t y) const;
void set_text_size(uint8_t size) const;
void set_text_color(uint16_t color) const;
void set_text_wrap(bool wrap) const;
/**
* @brief Set the display backlight
* @param[in] brightness: new brightness in percentage
*/
void set_backlight(float brightness) const;
/**
* @brief Write a char to the display, starting at the current position
* @param[in] c: Character to write
* @return Number of written chars (1)
*/
FLASHMEM uint8_t print(char c, bool clear = false) const;
/**
* @brief Write a string to the display, starting at the current position
* @param[in] str: Reference to the string
* @return Number of written chars
*/
FLASHMEM uint8_t print(const std::string& str, bool clear = false) const;
/**
* @brief Write an formatted string (like std::printf) out to display
* @tparam Args: Values to print
* @param[in] format: Format string
* @return Number of characters written
*/
template <typename... Args>
FLASHMEM uint8_t printf(const char* format, const Args&... args) const {
if (const auto str { LoggerTarget::string_format(format, args...) }; str.has_value()) {
return print(*str);
} else {
return 0;
}
}
int16_t get_cursor_x() const;
int16_t get_cursor_y() const;
void get_text_bounds(const std::string& str, int16_t x, int16_t y, int16_t* p_x, int16_t* p_y, uint16_t* p_w, uint16_t* p_h) const;
void fill_screen(uint16_t color) const;
void fill_rect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color) const;
void draw_line(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color) const;
void draw_rect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color) const;
void draw_triangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) const;
void fill_triangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) const;
void draw_circle(int16_t x, int16_t y, int16_t r, uint16_t color) const;
void fill_circle(int16_t x, int16_t y, int16_t r, uint16_t color) const;
void draw_button(Adafruit_GFX_Button* button, bool invert = false) const;
auto get_touch_counter() const {
std::lock_guard<std::mutex> lock { touch_mutex_ };
return touch_counter_;
}
bool get_touch_point(int16_t& x, int16_t& y, int16_t& z) const;
Adafruit_GFX* get_context() const {
return reinterpret_cast<Adafruit_GFX*>(p_framebuffer_);
}
};
} // namespace ctbot |
// sidebar
const menuItems = document.querySelectorAll(".menu-item");
// messages
const messageNotification = document.querySelector("#messages-notification");
const messages = document.querySelector(".messages");
const message = messages.querySelectorAll(".message");
const messageSearch = document.querySelector("#message-search");
// theme
const theme = document.querySelector("#theme");
const themeModal = document.querySelector(".customize-theme");
const fontSizes = document.querySelectorAll(".choose-size span");
var root = document.querySelector(":root");
const colorPalette = document.querySelectorAll(".choose-color span");
const Bg1 = document.querySelector(".bg-1");
const Bg2 = document.querySelector(".bg-2");
const Bg3 = document.querySelector(".bg-3");
// ****** Sidebar ******
// remove active class from all menu items
const changeActiveItem = () => {
menuItems.forEach((item) => {
item.classList.remove("active");
});
};
menuItems.forEach((item) => {
item.addEventListener("click", () => {
changeActiveItem();
item.classList.add("active");
if (item.id != "notifications") {
document.querySelector(".notifications-popup").style.display = "none";
} else {
document.querySelector(".notifications-popup").style.display = "block";
document.querySelector(
"#notifications .notification-count"
).style.display = "none";
}
});
});
// ******* Messages *******
// searches chats
const searchMessage = () => {
const val = messageSearch.value.toLowerCase();
console.log(val);
message.forEach((user) => {
let name = user.querySelectorAll("h5").textContent.toLowerCase();
if (name.indexOf(val) != -1) {
user.style.display = "flex";
} else {
user.style.display = "none";
}
});
};
// searches chat
messageSearch.addEventListener("keyup", searchMessage);
// highlight messages card when message notification menu item is clicked
messageNotification.addEventListener("click", () => {
messages.style.boxShadow = "0 0.5rem 2.5rem var(--color-primary)";
messageNotification.querySelector(".notification-count").style.display =
"none";
setTimeout(() => {
messages.style.boxShadow = "none";
}, 3000);
});
// theme / display customization
// open madal
const openThemeModal = () => {
themeModal.style.display = "grid";
};
// close modal when clicked outside
const closeThemeModal = (e) => {
if (e.target.classList.contains("customize-theme")) {
themeModal.style.display = "none";
}
};
// close modal
themeModal.addEventListener("click", closeThemeModal);
theme.addEventListener("click", openThemeModal);
// **** font size ****
// remove active class from spans or fonts size selectors
const removeSizeSelector = () => {
fontSizes.forEach((size) => {
size.classList.remove("active");
});
};
fontSizes.forEach((size) => {
size.addEventListener("click", () => {
removeSizeSelector();
let fontSize;
size.classList.toggle("active");
if (size.classList.contains("font-size-1")) {
fontSize = "10px";
root.style.setProperty("--sticky-top-left", "5.4rem");
root.style.setProperty("--sticky-top-right", "5.4rem");
} else if (size.classList.contains("font-size-2")) {
fontSize = "12px";
root.style.setProperty("--sticky-top-left", "5.4rem");
root.style.setProperty("--sticky-top-right", "-7rem");
} else if (size.classList.contains("font-size-3")) {
fontSize = "16px";
root.style.setProperty("--sticky-top-left", "-2rem");
root.style.setProperty("--sticky-top-right", "-17rem");
} else if (size.classList.contains("font-size-4")) {
fontSize = "19px";
root.style.setProperty("--sticky-top-left", "-5rem");
root.style.setProperty("--sticky-top-right", "-25rem");
} else if (size.classList.contains("font-size-5")) {
fontSize = "22px";
root.style.setProperty("--sticky-top-left", "-12rem");
root.style.setProperty("--sticky-top-right", "-35rem");
}
// change font size of the root html element
document.querySelector("html").style.fontSize = fontSize;
});
});
// remove active class from colors
const changeActiveColorClass = () => {
colorPalette.forEach((colorPicker) => {
colorPicker.classList.remove("active");
});
};
// change primary colors
colorPalette.forEach((color) => {
color.addEventListener("click", () => {
let primaryHue;
changeActiveColorClass();
if (color.classList.contains("color-1")) {
primaryHue = 252;
} else if (color.classList.contains("color-2")) {
primaryHue = 52;
} else if (color.classList.contains("color-3")) {
primaryHue = 352;
} else if (color.classList.contains("color-4")) {
primaryHue = 152;
} else if (color.classList.contains("color-5")) {
primaryHue = 202;
}
color.classList.add("active");
root.style.setProperty("--primary-color-hue", primaryHue);
});
});
// theme background values
let lightColorLightness;
let whiteColorLightness;
let darkColorLightness;
// change background color
const changeBG = () => {
root.style.setProperty("--light-color-lightness", lightColorLightness);
root.style.setProperty("--white-color-lightness", whiteColorLightness);
root.style.setProperty("--dark-color-lightness", darkColorLightness);
};
Bg1.addEventListener("click", () => {
// add active class
Bg1.classList.add("active");
// remove active class from the other BGs
Bg2.classList.remove("active");
Bg3.classList.remove("active");
// remove customized changes fron local storage
window.location.reload();
});
Bg2.addEventListener("click", () => {
darkColorLightness = "95%";
whiteColorLightness = "30%"; //20
lightColorLightness = "15%";
// add active class
Bg2.classList.add("active");
// remove active class from the other BGs
Bg1.classList.remove("active");
Bg3.classList.remove("active");
changeBG();
});
Bg3.addEventListener("click", () => {
darkColorLightness = "95%";
whiteColorLightness = "5%";
lightColorLightness = "0%";
// add active class
Bg3.classList.add("active");
// remove active class from the other BGs
Bg1.classList.remove("active");
Bg2.classList.remove("active");
changeBG();
}); |
<!DOCTYPE html>
<html lang="es">
<head>
<meta charset="UTF-8">
<title>Vercrops</title>
<!-- meta viewport sirve para que la pagina se adapte a cualquier dispositivo -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- link para importar imagen de icono -->
<link rel="shortcut icon" type="x-icon" href="https://verqor.com/favicon/256.png">
<link rel="stylesheet" href="styles.css">
<link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css">
<!-- Estas líneas establecen una preconexión con los dominios mencionados.
Esto puede hacer que la carga de los recursos de estos dominios sea más rápida, ya que
la conexión se establece antes de que se soliciten los recursos.-->
<link rel="preconnect" href="https://fonts.gstatic.com">
<link rel="preconnect" href="https://fonts.googleapis.com">
<!-- Estas líneas importan fuentes de Google Fonts. Las fuentes importadas son "Outfit" y "Micro 5".-->
<link href="https://fonts.googleapis.com/css2?family=Outfit:wght@300&display=swap" rel="stylesheet">
<link href="https://fonts.googleapis.com/css2?family=Micro+5&display=swap" rel="stylesheet">
<!-- Esta línea importa la fuente Ubuntu de Google Fonts.-->
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Outfit:wght@100..900&family=Ubuntu:ital,wght@0,300;0,400;0,500;0,700;1,300;1,400;1,500;1,700&display=swap" rel="stylesheet">
</head>
<body>
<!-- Encabezado movil estatico siempre esta en la parte de arriba sin importar que scrolles
en toda la pagina -->
<header class="my-header">
<a href="#" class="logo" style="text-decoration: none;">
<img src="https://verqor.com/favicon/256.png " alt="logo_vercrops">
<h2 class="nombre_proyecto color-header">Vercrops</h2>
</a>
<nav>
<a href="" class="nav-link color-header">Bienvenida</a>
<a href="" class="nav-link color-header">Reglas</a>
<a href="#instTit" class="nav-link color-header">Instrucciones</a>
<a href="#aprendiendo" class="nav-link color-header">Aprende</a>
<!--<a href="LogIn.html" class="nav-link color-header">Iniciar</a>
<a href="SignUp.html" class="nav-link color-header">Registrar</a>-->
<a href="/admin" class="nav-link color-header invisible">Dashboard</a>
</nav>
</header>
<!-- Seccion de bienvenida y de boton del juego -->
<section id="seccionInicial">
<div id="PrimerContenedor">
<div id="bienvenida" class="bienvenidaYboton w3-half">
<div id="text-bienvenida">
<h1 class="titulo">BIENVENIDO A VERCROPS</h1>
<p class="descripcion">Bienvenidos al vercrops, una plataforma donde podrás enriquecer tus conocimientos de
agricultura asi como tener acceso a nuestro videojuego, este te permitirá divertirte mientras aprendes.
</p>
</div>
</div>
<div class="w3-half contenedorBoton bienvenidaYboton">
<div class="divImagenJuego">
<div class="contenedor-padre" id="imagen_juego">
<img src="vercrops_full.jpeg" alt="imagen_juego" class="imagen-padre">
<a id="imagen_boton" class="contenedor-hijo" href="indexGAMe.html">
<img src="play_img.png" alt="play" class="imagen-hijo"/>
</a>
</div>
</div>
<p class="click">Click para jugar</p>
</div>
</div>
</section>
<!-- Seccion Instrucciones -->
<section id="sectInstruc" style="text-align: center;">
<div id="instTit" style="margin-top: 5%;">
<!--
<div class="estilo-div-titulo" > -->
<h2 class="titulo secTit">INSTRUCCIONES</h2>
<!-- </div> -->
<div class="container">
<div class="fade">
<img src="jueguito.jpg" alt="Imagen">
<div class="gray-box">
<!--<p id="huevo">"SI TE ESCONDES EN LAS SOMBRAS, SOLO ME ESTÁS ARRASTRANDO A CASA"</p>-->
<div class="instruccion-elemento">
<img src="wheat.png">
</div>
<div class="instruccion-elemento">
<img src="cucaracha.jpeg">
</div>
<div class="instruccion-elemento">
<img src="hoe.jpeg">
</div>
<div class="instruccion-elemento">
<img src="shield.jpeg">
</div>
</div>
<div class="text-inst">
<!--<p id="huevo">"SI TE ESCONDES EN LAS SOMBRAS, SOLO ME ESTÁS ARRASTRANDO A CASA"</p>-->
<p>Inicio de Partida: Al comenzar el juego, cada jugador recibe tres cartas. Durante su turno, cada jugador debe jugar una carta y luego tomar otra del mazo para siempre mantener tres cartas en mano. </p>
</div>
</div>
</div>
</div>
</div>
</section>
<section id="seccionAprendizaje" style="text-align: center;">
<div id="aprendiendo">
<h2 class="titulo secTit">¡Más sobre agricultura!</h2>
<div class="AprendMainDiv">
<div class="card">
<div class="face front">
<img src="pumpking.png" alt="">
<h3>Calabaza</h3>
</div>
<div class="face back">
<h3>Calabaza</h3>
<p>Las calabazas son una de las plantas domesticadas más antiguas de América, cultivadas desde hace más de 7,500 años.</p>
</div>
</div>
<div class="card">
<div class="face front">
<img src="maiz.png" alt="">
<h3>Maíz</h3>
</div>
<div class="face back">
<h3>Maíz</h3>
<p>El maíz es originario de México y fue domesticado por primera vez por pueblos indígenas en la región hace al menos 9,000 años.</p>
</div>
</div>
<div class="card">
<div class="face front">
<img src="beans.png" alt="">
<h3>Frijoles</h3>
</div>
<div class="face back">
<h3>Frijoles</h3>
<p>Los frijoles han sido un alimento básico en muchas culturas desde hace miles de años; evidencias arqueológicas sugieren su cultivo desde hace al menos 7,000 años en América.</p>
</div>
</div>
<div class="card">
<div class="face front">
<img src="carrot.png" alt="">
<h3>Zanahoria</h3>
</div>
<div class="face back">
<h3>Zanahoria</h3>
<p>Originalmente, las zanahorias no eran naranjas; las primeras cultivadas en Asia Central y Persia hace miles de años eran de colores púrpura y amarillo.</p>
</div>
</div>
<div class="card">
<div class="face front">
<img src="pumpking.png" alt="">
<h3>Calabaza</h3>
</div>
<div class="face back">
<h3>Calabaza</h3>
<p>La calabaza no solo es un alimento versátil, también fue utilizada como contenedor y material para utensilios por las culturas indígenas.</p>
</div>
</div>
<div class="card">
<div class="face front">
<img src="beans.png" alt="">
<h3>Frijoles</h3>
</div>
<div class="face back">
<h3>Frijoles</h3>
<p>Los frijoles desempeñan un papel crucial en la agricultura sostenible debido a su habilidad para fijar nitrógeno en el suelo, mejorando así la fertilidad del mismo.</p>
</div>
</div>
<div class="card">
<div class="face front">
<img src="carta--azul-zanahoria-Photoroom-recortado.png" alt="">
<h3>Zanahoria</h3>
</div>
<div class="face back">
<h3>Zanahoria</h3>
<p>Durante la Segunda Guerra Mundial, el gobierno británico propagó el mito de que comer zanahorias mejoraba la visión nocturna, como parte de una campaña de desinformación para encubrir el uso de tecnología de radar.</p>
</div>
</div>
</div>
</div>
</section>
<section>
<h2>reglas</h2>
</section>
</body>
</html> |
package co.com.jorge.springboot.webflux.app.models.services;
import co.com.jorge.springboot.webflux.app.models.documents.Category;
import co.com.jorge.springboot.webflux.app.models.repository.CategoryRepository;
import co.com.jorge.springboot.webflux.app.models.repository.ProductRepository;
import co.com.jorge.springboot.webflux.app.models.documents.Product;
import org.springframework.stereotype.Service;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
@Service
public class ProductServicesImpl implements ProductService{
private ProductRepository productRepository;
private CategoryRepository categoryRepository;
public ProductServicesImpl(ProductRepository productRepository, CategoryRepository categoryRepository) {
this.productRepository = productRepository;
this.categoryRepository = categoryRepository;
}
@Override
public Flux<Product> findAll() {
return productRepository.findAll();
}
@Override
public Flux<Product> findAllWithNameUpperCase() {
return productRepository.findAll().map(product -> {
product.setName(product.getName().toUpperCase());
return product;
});
}
@Override
public Flux<Product> findAllWithNameUpperCaseRepeat() {
return findAllWithNameUpperCase().repeat(100);
}
@Override
public Mono<Product> findById(String id) {
return productRepository.findById(id);
}
@Override
public Mono<Product> save(Product product) {
return productRepository.save(product);
}
@Override
public Mono<Void> delete(Product product) {
return productRepository.delete(product);
}
@Override
public Mono<Product> findProductByName(String name) {
return productRepository.findByName(name);
}
@Override
public Flux<Category> findAllCategory() {
return categoryRepository.findAll();
}
@Override
public Mono<Category> findCategoryById(String id) {
return categoryRepository.findById(id);
}
@Override
public Mono<Category> saveCategory(Category category) {
return categoryRepository.save(category);
}
@Override
public Mono<Void> deleteCategory(Category category) {
return categoryRepository.delete(category);
}
@Override
public Mono<Category> findCategoryByName(String name) {
return categoryRepository.findByName(name);
}
} |
import { Injectable } from '@angular/core';
import {HttpClient} from '@angular/common/http';
import {catchError, map, retry} from 'rxjs/operators';
import {UtilityService} from '../../services/utility.service';
import {Observable} from 'rxjs';
import {PagedDataModel} from '../../models/paged-data.model';
import {Constants} from '../../../config/index';
import {Banner} from '../../models/banner/banner.model';
export interface PagedBannersData extends PagedDataModel {
data: Array<Banner>;
}
@Injectable({
providedIn: 'root'
})
export class BannerService {
bannerUrl = Constants.serviceUrl + 'banner';
constructor(private http: HttpClient) {
}
createBanner(bannerPayload: any) {
console.log('****', bannerPayload);
return this.http.post(this.bannerUrl, bannerPayload, Constants.TokenHttpHeaders).pipe(
retry(1)
);
}
getBanners(bannerStatus = 'ALL'): Observable<PagedBannersData> {
return this.http.get(this.bannerUrl + '?bannerStatus=' + bannerStatus, Constants.TokenHttpHeaders).pipe(
retry(3),
map((res: any) => res.data)
);
}
// TODO implement de-activate banner
deactivateBanner(bannerId: number) {
return this.http.put(this.bannerUrl + '/' + bannerId + '/deactivate', {}, Constants.TokenHttpHeaders).pipe(
retry(3),
map(data => data)
);
}
// TODO implement activate banner
activateBanner(bannerId: number) {
return this.http.put(this.bannerUrl + '/' + bannerId + '/activate', {}, Constants.TokenHttpHeaders).pipe(
retry(3),
map(data => data)
);
}
// TODO implement delete banner
deleteBanner(bannerId: number) {
return this.http.delete(this.bannerUrl + '/' + bannerId, Constants.TokenHttpHeaders).pipe(
retry(1)
);
}
updateBanner(bannerData: any, bannerId: number ) {
return this.http.put(this.bannerUrl + '/' + bannerId, bannerData, Constants.TokenHttpHeaders).pipe(
retry(1)
);
}
uploadBannerImage(fd: FormData, bannerId: number ): any {
return this.http.put(this.bannerUrl + '/' + bannerId + '/add-image', fd, {
reportProgress: true, observe: 'events'
}).pipe(
retry(2)
);
}
getBannerById(bannerId: number): Observable<Banner> {
return this.http.get(this.bannerUrl + '/' + bannerId, Constants.TokenHttpHeaders).pipe(
retry(1),
map((res: any) => new Banner(res.data))
);
}
} |
import React, { useState, useEffect } from 'react';
import { getAllEvents } from '../api/events.api';
import { EventCard } from './EventCard';
export function EventList() {
const [events, setEvents] = useState([]);
const loadEvents = async () => {
try {
const res = await getAllEvents();
setEvents(res.data);
} catch (error) {
console.error('Error loading events:', error);
}
};
useEffect(() => {
loadEvents();
}, []);
return (
<div className="grid grid-cols-1 sm:grid-cols-2 md:grid-cols-3 lg:grid-cols-4 gap-4 p-4">
{events.map((event) => (
<EventCard key={event.id} event={event} />
))}
</div>
);
} |
import React, { useState } from 'react';
import './App.css';
function App() {
const [point, setPoint] = useState(0);
let increasePoint = () => {
setPoint(point + 1);
}
let decreasePoint = () => {
setPoint(point - 1);
}
let resetPoint = () => {
setPoint(0);
}
return (
<div className="App">
<nav className="navbar navbar-dark bg-dark">
<span className="navbar-brand mb-0 h1">React Counter App</span>
</nav>
<div className="container-fluid mt-5">
<div className='row d-flex justify-content-center'>
<div className="card">
<div className="card-body">
<h5 className="card-title">Counter App</h5>
<h6 className="card-subtitle mt-2 mb-2 text-muted">Point: {point}</h6>
<div className="btn-group mt-3" role="group" aria-label="Button Group">
<button type="button" className="btn btn-primary" onClick={increasePoint}>+</button>
<button type="button" className="btn btn-danger" onClick={decreasePoint}>-</button>
<button type="button" className="btn btn-success" onClick={resetPoint}>Reset</button>
</div>
</div>
</div>
</div>
</div>
</div>
);
}
export default App; |
## 描述
引用[[@express-sessionExpressjsSessionSimple]]所描述的resave功能
> Forces the session to be saved back to the session store, even if the session was never modified during the request. Depending on your store this may be necessary, but it can also create race conditions where a client makes two parallel requests to your server and changes made to the session in one request may get overwritten when the other request ends, even if it made no changes (this behavior also depends on what store you're using).
> The default value is `true`, but using the default has been deprecated, as the default will change in the future. Please research into this setting and choose what is appropriate to your use-case. Typically, you'll want `false`.
> How do I know if this is necessary for my store? The best way to know is to check with your store if it implements the `touch` method. If it does, then you can safely set `resave: false`. If it does not implement the `touch` method and your store sets an expiration date on stored sessions, then you likely need `resave: true`.
重點:
- session store本身會為了釋放空間,而清除掉沒在使用的session,通常做法會是替每個session設定過期時間
- resave功能是只要與client進行連線,那伺服器就會根據對應client的session內容重新寫進另一份session至store,最新的session會綁定新的過期時間,舊的session則會因為舊的過期時間而被釋放或者是直接被刪除
- 若resave為true就啟用該功能;反之,若為false就關閉
### 如何釋放閒置sesion
由於express-session與session-store進行分割,express-session管理好自己的業務-管理每個請求下的session和cookie,同時也委託session-store負責管理儲存/釋放session存在session,所以具體來說會依賴著store本身是否具有機制能夠移除閒置不用的session
## 複習
#🧠 session store 如何釋放沒在使用的session?(提示:跟時間有關)->->-> `session store本身會為了釋放空間,而清除掉沒在使用的session,通常做法會是替每個session設定過期時間`
<!--SR:!2023-10-01,295,250-->
#🧠 express-session上的resave機制上目的是什麼 ->->-> `session的resave功能是盡可能讓潛在可用的session繼續留在session store,繼而延續先前處理結果來增加效率`
<!--SR:!2024-08-23,466,230-->
#🧠 express-session上的resave功能是什麼?->->-> ` resave功能是只要與client進行連線,那伺服器就會根據對應client的session內容重新寫進另一份session至store,最新的session會綁定新的過期時間,舊的session則會因為舊的過期時間而被釋放或者是直接被刪除`
<!--SR:!2024-05-30,443,250-->
#🧠 express-session:如何釋放閒置sesion(提示:express-session 和session store是獨立的)->->-> `由於express-session與session-store進行分割,express-session管理好自己的業務-管理整體的session和cookie,同時也委託session-store負責管理儲存/釋放session存在session,所以具體來說會依賴著store本身是否具有機制能夠移除閒置不用的session`
<!--SR:!2023-08-29,106,228-->
---
Status: #🌱
Tags:
[[Session]] - [[Express]]
Links:
[[Express-Session 讓Express 伺服器能產生和管理對應的cookie和session]]
[[若session的resave關閉後,會依賴著store本身是否具有機制能夠刷新閒置不用的session之過期時間]]
References:
[[@express-sessionExpressjsSessionSimple]] |
package day05ternarystringmanipulations;
import java.util.Scanner;
public class Switch01 {
public static void main(String[] args) {
/*
Ask user to enter country name among "America, England, Germany, Turkey, India, Peru, Spain, Bulgaria, Albania, France"
Type code to print abbreviation of the countries. "US, UK, DE, TR, IN, PE, ES, BG, AL, FR"
*/
Scanner input = new Scanner(System.in);
System.out.println("Enter the country name, please!");
String country = input.nextLine().toLowerCase();
switch(country){//Note:Inside the switch parenthesis you can use just "int", "byte", "short", "char", "String" data types
//Note: "boolean", "long", "float", "double" cannot be used in switch statement
//Note: Switch is a tool, and every tool has a limitation
case "america":
System.out.println("US");
break;
case "england":
System.out.println("UK");
break;
case "germany":
System.out.println("DE");
break;
case "turkey":
System.out.println("TR");
break;
case "india":
System.out.println("IN");
break;
case "peru":
System.out.println("PE");
break;
case "spain":
System.out.println("ES");
break;
case "bulgaria":
System.out.println("BG");
break;
case "albania":
System.out.println("AL");
break;
case "france":
System.out.println("FR");
break;
default:
System.out.println("No information about the country");
}
}
} |
from sqlalchemy import create_engine, Column, Integer, String, Float
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
DATABASE_URL = "sqlite:///movies.db"
engine = create_engine(DATABASE_URL)
Base = declarative_base()
Session = sessionmaker(bind=engine)
session = Session()
class Movie(Base):
__tablename__ = 'movies'
id = Column(Integer, primary_key=True)
title = Column(String)
genre = Column(String)
class Rating(Base):
__tablename__ = 'ratings'
id = Column(Integer, primary_key=True)
user_id = Column(Integer)
movie_id = Column(Integer)
rating = Column(Float)
Base.metadata.create_all(engine) |
import { useEffect } from "react";
import { useMsal } from "@azure/msal-react";
import { useNavigate } from "react-router-dom";
import Button from "@mui/material/Button";
import Paper from "@mui/material/Paper";
import Box from "@mui/material/Box";
import Grid from "@mui/material/Grid";
import logo from "../../assets/imgs/logo.svg";
import bg from "../../assets/imgs/bg.jpg";
import {
CssBaseline,
Link,
ThemeProvider,
Typography,
createTheme,
} from "@mui/material";
import { CustomCircularProgressFullScreen } from "../../components/CustomCircularProgress";
import { usePageTitle } from "../../components/PageTitleProvider";
import React from "react";
function Copyright(props: any) {
return (
<Typography
variant="body2"
color="text.secondary"
align="center"
{...props}
>
{"Copyright © "}
<Link color="inherit" href="https://github.com/WillyPestana">
Portal RH
</Link>{" "}
{new Date().getFullYear()}
{"."}
</Typography>
);
}
function AuthPage() {
const { setPageTitle } = usePageTitle();
React.useEffect(() => {
setPageTitle("Autenticação");
}, [setPageTitle]);
const navigate = useNavigate();
const { accounts, instance } = useMsal();
const handleLogin = () => {
const loginRequest = {
scopes: process.env.REACT_APP_AZURE_AD_SCOPES!.split(",") || [],
prompt: "select_account",
redirectUri: window.location.origin,
};
instance.loginRedirect(loginRequest).catch((error) => {
console.error("Erro durante o redirecionamento:", error);
});
};
useEffect(() => {
if (accounts.length > 0) {
navigate("/home");
} else {
localStorage.removeItem("profile");
}
}, [accounts, instance, navigate]);
if (accounts.length > 0) return <CustomCircularProgressFullScreen />;
const defaultTheme = createTheme();
return (
<ThemeProvider theme={defaultTheme}>
<Grid container component="main" sx={{ height: "100vh" }}>
<CssBaseline />
<Grid
item
xs={false}
sm={4}
md={7}
sx={{
backgroundImage: `url(${bg})`,
backgroundRepeat: "no-repeat",
backgroundColor: (t) =>
t.palette.mode === "light"
? t.palette.grey[50]
: t.palette.grey[900],
backgroundSize: "cover",
backgroundPosition: "center",
}}
/>
<Grid item xs={12} sm={8} md={5} component={Paper} elevation={6} square>
<Box
sx={{
my: 8,
mx: 4,
display: "flex",
flexDirection: "column",
alignItems: "center",
}}
>
<img src={logo} srcSet={logo} alt="Portal" height="150px" />
<Typography variant="overline" display="block" gutterBottom>
BEM-VINDO AO{" "}
<Typography
variant="overline"
display="inline-block"
color={"#ff6600"}
gutterBottom
>
PORTAL
</Typography>{" "}
<Typography
variant="overline"
display="inline-block"
color={"#003366"}
gutterBottom
>
PORTAL
</Typography>
</Typography>
<Button
type="button"
fullWidth
variant="contained"
onClick={handleLogin}
sx={{ mt: 3, mb: 2 }}
>
Entrar
</Button>
<Copyright sx={{ mt: 5 }} />
</Box>
</Grid>
</Grid>
</ThemeProvider>
);
}
export default AuthPage; |
import Task from "./Task";
import {useState} from "react";
import {Observer, observer} from "mobx-react-lite";
import {store} from "../store/store";
const Column = ({
item,
dragStartHandler,
dropHandler,
dropCardHandler,
dragOverHandler,
changeNameColumn,
dragOverCardHandler,
dragLeaveHandler
}) => {
const [isCreating, setIsCreating] = useState(false);
const {taskValue, setTaskValue, addTaskToColumn, keyDownHandler} = store;
const newTask = () => {
setIsCreating(!isCreating);
}
return (
<div key={item.id}
className="board bg-blue-100 text-gray-900 w-80 p-2 pl-4 mx-1 rounded-xl"
onDrop={event => dropCardHandler(event, item)} onDragOver={event => dragOverCardHandler(event)}>
<input
className="mb-2 p-1 rounded bg-transparent border-0 cursor-pointer focus:bg-amber-50 focus:cursor-auto"
value={item.name}
onChange={(e) => changeNameColumn(e, item.id)}/>
<ul>
{item.tasks.map((task) => (
<Task key={task.id}
task={task}
item={item}
dragStartHandler={dragStartHandler}
dragOverHandler={dragOverHandler}
dragLeaveHandler={dragLeaveHandler}
dropHandler={dropHandler}/>
))}
</ul>
{isCreating
? <Observer>
{() => (<textarea className="w-full rounded-md p-2" value={taskValue} placeholder="Enter a new task..."
onChange={(e) => setTaskValue(e)}
onKeyDown={(e) => keyDownHandler(e, item.id)}></textarea>)}
</Observer>
: null}
{!isCreating
? <button className="bg-gray-900 text-amber-200 w-full rounded-xl mt-2 hover:bg-gray-800"
onClick={newTask}>+ Add task
</button>
: <div className="flex">
<button className="bg-gray-900 text-amber-200 w-full rounded-xl mt-2 hover:bg-gray-800 mr-1"
onClick={(e) => addTaskToColumn(e, item.id)}>Add
</button>
<button className="bg-gray-900 text-amber-200 w-full rounded-xl mt-2 hover:bg-gray-800"
onClick={newTask}>
Close
</button>
</div>}
</div>
)
}
export default observer(Column); |
package com.personal.project.service;
import com.personal.project.common.exception.CustomException;
import com.personal.project.common.exception.ErrorCode;
import com.personal.project.controller.studyboard.dto.request.StudyBoardRequest;
import com.personal.project.controller.studyboard.dto.response.StudyBoardResponse;
import com.personal.project.entity.StudyBoardEntity;
import com.personal.project.entity.UserEntity;
import com.personal.project.repository.StudyBoardRepository;
import com.personal.project.repository.UserRepository;
import jakarta.servlet.http.Cookie;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
import lombok.RequiredArgsConstructor;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Collectors;
@Service
@RequiredArgsConstructor
public class StudyBoardService {
private final StudyBoardRepository studyBoardRepository;
private final UserRepository userRepository;
// 스터디 게시판 만들기
@Transactional
public StudyBoardResponse createStudyBoard(String username, StudyBoardRequest studyBoardRequest) {
UserEntity user = userRepository.findByUsername(username)
.orElseThrow(() -> new CustomException(ErrorCode.USER_NOT_FOUND));
StudyBoardEntity studyBoard = studyBoardRepository.save(
StudyBoardEntity.builder()
.title(studyBoardRequest.getTitle())
.content(studyBoardRequest.getContent())
.address(studyBoardRequest.getAddress())
.detailAddress(studyBoardRequest.getDetailAddress())
.mainCategory(studyBoardRequest.getMainCategory())
.subCategory(studyBoardRequest.getSubCategory())
.user(user)
.build());
return StudyBoardResponse.fromEntity(studyBoard);
}
// 스터디 게시판 클릭시 조회
@Transactional
public StudyBoardResponse getOneStudyBoard(Long studyBoardId, HttpServletRequest request, HttpServletResponse response) {
// 쿠키를 통해서 중복방지 조회수 올리기
viewCountUp(studyBoardId, request, response);
return StudyBoardResponse.fromEntity(studyBoardRepository.findById(studyBoardId)
.orElseThrow(() -> new CustomException(ErrorCode.STUDY_BOARD_NOT_FOUND)));
}
// 스터디 게시판 전체 조회
@Transactional(readOnly = true)
public List<StudyBoardResponse> getAllStudyBoard(Integer page, Integer pageSize) {
Pageable pageable = PageRequest.of(page, pageSize);
return studyBoardRepository.findAll(pageable)
.stream()
.map(StudyBoardEntity::toResponse)
.collect(Collectors.toList());
}
// 스터디게시판 삭제
@Transactional
public void deleteOneStudyBoard(UserDetails userDetails, Long studyBoardId) {
UserEntity user = userRepository.findByUsername(userDetails.getUsername())
.orElseThrow(() -> new CustomException(ErrorCode.USER_NOT_FOUND));
StudyBoardEntity studyBoard = studyBoardRepository.findById(studyBoardId)
.orElseThrow(() -> new CustomException(ErrorCode.STUDY_BOARD_NOT_FOUND));
if (!Objects.equals(user.getId(), studyBoard.getUser().getId())) {
throw new CustomException(ErrorCode.USER_INFO_NOT_MATCH);
}
studyBoardRepository.deleteByIdAndUser(studyBoardId, user);
}
// 스터게시판 수정
public StudyBoardResponse updateStudyBoard(UserDetails userDetails, Long studyBoardId, StudyBoardRequest studyBoardRequest) {
UserEntity user = userRepository.findByUsername(userDetails.getUsername())
.orElseThrow(() -> new CustomException(ErrorCode.USER_NOT_FOUND));
StudyBoardEntity studyBoard = studyBoardRepository.findById(studyBoardId)
.orElseThrow(() -> new CustomException(ErrorCode.STUDY_BOARD_NOT_FOUND));
if (!Objects.equals(user.getId(), studyBoard.getUser().getId())) {
throw new CustomException(ErrorCode.USER_INFO_NOT_MATCH);
}
studyBoard.setTitle(studyBoardRequest.getTitle());
studyBoard.setContent(studyBoardRequest.getContent());
studyBoard.setAddress(studyBoardRequest.getAddress());
studyBoard.setDetailAddress(studyBoardRequest.getDetailAddress());
studyBoard.setMainCategory(studyBoardRequest.getMainCategory());
studyBoard.setSubCategory(studyBoardRequest.getMainCategory());
return StudyBoardResponse.fromEntity(studyBoardRepository.save(studyBoard));
}
// studyBoardRepository로 조회수 올리는 로직
@Transactional
public int updateViews(Long id) {
return studyBoardRepository.updateView(id);
}
// 쿠키를 통해서 중복방지 조회수 올리는 로직
public void viewCountUp(Long id, HttpServletRequest request, HttpServletResponse response) {
Cookie oldCookie = null;
// postView 이름으로 된 쿠키 찾기
Cookie[] cookies = request.getCookies();
if(cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals("postView")) {
oldCookie = cookie;
}
}
}
// postView의 쿠키가 있을 때 같은 storyBoard 아이디 값이 없을때 조회수 올리고 _[]으로 번호 넣기
if (oldCookie != null) {
if(!oldCookie.getValue().contains("[" + id.toString() + "]")) {
updateViews(id);
oldCookie.setValue(oldCookie.getValue() + "_[" + id + "]");
oldCookie.setPath("/"); // 경로를 /로 설정하면 해당 쿠키는 웹 애플리케이션 내의 모든 경로에서 사용할 수 있습니다.
oldCookie.setMaxAge(60 * 60 * 24);
response.addCookie(oldCookie);
System.out.println(oldCookie);
}
} else {
updateViews(id);
Cookie newCookie = new Cookie("postView", "[" + id + "]");
newCookie.setPath("/");
newCookie.setMaxAge(60 * 50 * 24);
response.addCookie(newCookie);
System.out.println(newCookie);
}
}
} |
O ENVIO DE REQUESTS FUNCIONA, EM UM GERAL, EM NOSSO PROJETO
AGORA DEVEMOS linkar ESSE ENVIO À NOSSA UI, porque isso é um pouco mais realista, algo
que geralmente acontece em sites...
PARA ISSO, TAMBÉM PRECISAMOS DE ACESSO À 'FORM'....
à form e ao bottão 'fetch posts'....
PARA ISSO VAMOS USAR OS CLÁSSICOS QUERYSELECTORS...
ex:
const form = document.querySelector('#new-post form'); //////OU: const form = document.querySelector('form');
const fetchButton = document.getElementById('available-posts');
--------------------------
AGORA, PORTANTO, TEMOS ACESSO A ESSES 2 ELEMENTOS....
Certo.
Para o fetching, nós vamos lá para
o fetchBUtton
E ADICIONAMOS UM EVENTLISTENER A ELE....
ex:
fetchButton.addEventListener('click', ...);
-----------------------------------------
aí eu vou lá e digito como segundo parâmetro (coisa que será ativada, a função que será ativada)
UMA FUNÇÃO ANÔNIMA (() => {}) EM QUE
CHAMAMOS
'fetchPosts();'
ex:
fetchButton.addEventListener('click', () => {
fetchPosts();
});
--------------------------------------
PROFESSOR ADMITE, NO ENTANTO, QUE PODERÍAMOS SIMPLIFICAR
TODA ESSA EXPRESSÃO como :
fetchButton.addEventListener('click', fetchPosts);
-------------------------------------------------
PARA A 'FORM',
eu vou adicionar um addEventListener ao
EVENTO 'submit'... AÍ EU VOU
RECEBER COMO PARÂMETRO O OBJETO 'EVENT'...
dentro desse 'event' eu vou querer EXECUTAR O MÉTODO
'preventDefault'....
ex:
form.addEventListener('submit', event => {
event.preventDefault();
})
----------------------------------------
ESSE 'preventDefault' VAI FAZER COM QUE EVITEMOS
O COMPORTAMENTO PADRÃO DO BROWSER NOS BOTÕES EM FORM (ou seja,
o browser não vai 'enviar/submittar' a form e então recarregar a página,
como geralmente faz.)
AGORA NÓS PODERÍAMOS VALIDAR A FORM AQUI, se assim o desejarmos...
Professor não fará isso, mas é uma boa ideia.
Se você quisesse isso, você checaria os inputs, checaria
OS VALORES que o usuário inputtaria,
usaria if checks e tal..., retornando erros e etc...
-------------------------------------
EM VEZ DISSO, PROFESSOR QUER SIMPLESMENTE PEGAR O input do field de tipo 'text'
dentro da form....
tbm o field de id 'content'....
PARA GANHAR ACESSO A ELES,
o professor vai criar uma constante de
nome
'enteredTitle'......
ela terá o valor
de
'event.currentTarget' (target é uma propriedade dentro de 'event', é uma propriedade
que SELECIONA O VALOR DO LUGAR EM QUE
O USUÁRIO/EVENTO OBJETIVOU... nesse caso, é o botão...).....
ELE VAI DIGITAR
event.currentTarget.querySelector('#title'); (ISSO FARÁ COM QUE O QUERYSELECTOR PROCURE ALGO COM ESSA ID DENTRO DESSE TARGET... você pode estar se perguntando: ok, mas o currentTarget não seria o 'button'...? NÃO! O CURRENTTARGET É, NA VERDADE, A SECTION/ELEMENTO PAI QUE CONTÉM O BOTÃO, PQ QUANDO O USUÁRIO CLICA, ACONTECE AQUELE NEGÓCIO DE 'bubbling' (eu acho)... enfim, o que interessa é que o ELEMENTO MAIS 'de baixo', ou seja, o QUE CONTÉM OS OUTROS ELEMENTOS, O QUE É O 'PARENT' acaba sendo o currentTarget do click nesse elemento 'button'... )
depois ele vai lá e coloca '.value', pois é nisso que ele está interessado
desse elemento 'title'..
ex:
const enteredTitle = event.currentTarget.querySelector('#title').value;
----------------------------------
ELE FAZ A MESMA COISA PARA O 'CONTENT' dos posts....
ex:
event.currentTarget.querySelector('#content').value;
Feito isso, professor pode finalmente chamar 'createPost()' dentro dessa função
interna do eventListener de
'fetchbutton'....
Dentro do 'createPost()', professor passa os parâmetros
'enteredTitle' e 'enteredContent'...
ex:
fetchButton.addEventListener('click', event => {
event.preventDefault();
const enteredTitle = event.currentTarget.querySelector('#title').value;
const enteredContent = event.currentTarget.querySelector('#content').value;
createPost();
}
)
------------------------------------------------------
ex:
async function createPost(title, content) {
const userId = Math.random();
const post = {
title: title,
body: content,
userId: userId
};
sendHttpRequest('POST', 'https://jsonplaceholder.typicode.com/posts', post);
}
fetchButton.addEventListener('click', event => {
event.preventDefault();
const enteredTitle = event.currentTarget.querySelector('#title').value;
const enteredContent = event.currentTarget.querySelector('#content').value;
createPost(enteredTitle, enteredContent);
}
)
----------------------------------------------
COM ISSO, PROFESSOR SALVA E RECARREGA NOSSA PÁGINA.
(OOOPS.. ENCONTRAMOS UM ERRO NO NOSSO CÓDIGO:
havíamos colocado toda essas linhas de lógica,
' event.preventDefault();
const enteredTitle = event.currentTarget.querySelector('#title').value;
const enteredContent = event.currentTarget.querySelector('#content').value;
createPost(enteredTitle, enteredContent);' DENTRO
DO LISTENER DE 'fetchBUtton', mas deveríamos ter colocado tudo
ISSO DENTRO DO LISTENER de 'form' (que nem mesmo tinha sido criado).)
RESULTADO:
fetchButton.addEventListener('click', fetchPosts);
form.addEventListener('submit', event => {
event.preventDefault();
const enteredTitle = event.currentTarget.querySelector('#title').value;
const enteredContent = event.currentTarget.querySelector('#content').value;
createPost();
});
----------------------------------------
CERTO.
AGORA o botão 'fetch' funciona... quando clicamos nele, carregamos a lista de posts...
PODEMOS DIGITAR COISAS NA FORM E ENTÃO CLICAR 'submit'... (ADD)....
SE O FIZERMOS, A INTERFACE NÃO SERÁ ATUALIZADA (a lista de posts não crescerá em 1 item, por exemplo),
MAS MESMO ASSIM, SE ABRIRMOS A ABA 'NETWORK' DO DEVTOOLS e então
olharmos as fontes da página,
veremos QUE UM
'posts' É ADICIONADO QUANDO CLICAMOS EM 'ADD'(o botão de submit....)
isso SIGNIFICA QUE ESSE
REQUEST DO TIPO 'POST' É, DE FATO, ENVIADO....
e esse post terá OS DADOS QUE RECÉM INPUTTAMOS...
PORTANTO, CONSEGUIMOS CONECTAR ESSES 2 REQUESTS À UI... (único problema é que a UI não atualiza quando
criamos/enviamos um post....)
AGORA DEVEMOS VER COMO ENVIAR UM ___REQUEST ___ DE TIPO ____ 'DELETE'...
Just a quick note to avoid confusion: In the demo app, the "Fetch" button always appends data without clearing existing data first. That means that pressing the button multiple times will add more and more items.
You can of course adjust the code to clear the content first. (para fazer 'fetch' de verdade...). |
#include<stdio.h>
#include<iostream>
#include<vector>
#include<algorithm>
#include<string>
#include<string.h>
#ifdef LOCAL
#define eprintf(...) fprintf(stderr, __VA_ARGS__)
#else
#define NDEBUG
#define eprintf(...) do {} while (0)
#endif
#include<cassert>
using namespace std;
typedef long long LL;
typedef vector<int> VI;
#define REP(i,n) for(int i=0, i##_len=(n); i<i##_len; ++i)
#define EACH(i,c) for(__typeof((c).begin()) i=(c).begin(),i##_end=(c).end();i!=i##_end;++i)
template<class T> inline void amin(T &x, const T &y) { if (y<x) x=y; }
template<class T> inline void amax(T &x, const T &y) { if (x<y) x=y; }
template<class Iter> void rprintf(const char *fmt, Iter begin, Iter end) {
for (bool sp=0; begin!=end; ++begin) { if (sp) putchar(' '); else sp = true; printf(fmt, *begin); }
putchar('\n');
}
template<unsigned MOD> struct ModInt {
static const unsigned static_MOD = MOD;
unsigned x;
void undef() { x = (unsigned)-1; }
bool isnan() const { return x == (unsigned)-1; }
inline int geti() const { return (int)x; }
ModInt() { x = 0; }
ModInt(const ModInt &y) { x = y.x; }
ModInt(int y) { if (y<0 || (int)MOD<=y) y %= (int)MOD; if (y<0) y += MOD; x=y; }
ModInt(unsigned y) { if (MOD<=y) x = y % MOD; else x = y; }
ModInt(long long y) { if (y<0 || MOD<=y) y %= MOD; if (y<0) y += MOD; x=y; }
ModInt(unsigned long long y) { if (MOD<=y) x = y % MOD; else x = y; }
ModInt &operator+=(const ModInt y) { if ((x += y.x) >= MOD) x -= MOD; return *this; }
ModInt &operator-=(const ModInt y) { if ((x -= y.x) & (1u<<31)) x += MOD; return *this; }
ModInt &operator*=(const ModInt y) { x = (unsigned long long)x * y.x % MOD; return *this; }
ModInt &operator/=(const ModInt y) { x = (unsigned long long)x * y.inv().x % MOD; return *this; }
ModInt operator-() const { return (x ? MOD-x: 0); }
ModInt inv() const {
unsigned a = MOD, b = x; int u = 0, v = 1;
while (b) {
int t = a / b;
a -= t * b; swap(a, b);
u -= t * v; swap(u, v);
}
if (u < 0) u += MOD;
return ModInt(u);
}
ModInt pow(long long y) const {
ModInt b = *this, r = 1;
if (y < 0) { b = b.inv(); y = -y; }
for (; y; y>>=1) {
if (y&1) r *= b;
b *= b;
}
return r;
}
friend ModInt operator+(ModInt x, const ModInt y) { return x += y; }
friend ModInt operator-(ModInt x, const ModInt y) { return x -= y; }
friend ModInt operator*(ModInt x, const ModInt y) { return x *= y; }
friend ModInt operator/(ModInt x, const ModInt y) { return x *= y.inv(); }
friend bool operator<(const ModInt x, const ModInt y) { return x.x < y.x; }
friend bool operator==(const ModInt x, const ModInt y) { return x.x == y.x; }
friend bool operator!=(const ModInt x, const ModInt y) { return x.x != y.x; }
};
const LL MOD = 1000000007;
//const LL MOD = 998244353;
typedef ModInt<MOD> Mint;
const int MAX = 200000;
int min_factor[MAX+1];
vector<int>prime;
void make_prime() {
for (int i=2; i<=MAX; i+=2) min_factor[i] = 2;
for (int i=3; i<=MAX; i+=3) if (!min_factor[i]) min_factor[i] = 3;
for (int i=5, d=2; i*i<=MAX; ) {
if (!min_factor[i]) {
min_factor[i] = i;
for (int j=i*i; j<=MAX; j+=i)
if (!min_factor[j]) min_factor[j] = i;
}
i += d; d = 6 - d;
}
for (int i=2; i<=MAX; i++) {
if (min_factor[i]==0) min_factor[i] = i;
if (min_factor[i]==i) prime.push_back(i);
}
}
int mu[MAX+1];
void make_moebius() {
for (int i=1; i<=MAX; i++) mu[i] = 1;
for (int i=0; i<(int)prime.size(); i++) {
LL d = prime[i];
for (LL k=d; k<=MAX; k+=d) mu[k] = -mu[k];
d *= d;
for (LL k=d; k<=MAX; k+=d) mu[k] = 0;
}
}
int N;
void MAIN() {
make_prime();
make_moebius();
scanf("%d", &N);
Mint ans = 1;
for (int i=2; i<=N; i++) if (mu[i]) {
int t = N/i;
ans -= (Mint(N) / (N-t) - 1) * mu[i];
}
printf("%d\n", ans.geti());
}
int main() {
int TC = 1;
// scanf("%d", &TC);
REP (tc, TC) MAIN();
return 0;
} |
package com.dwsolutions.projectSPRING1.resources;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.dwsolutions.projectSPRING1.entities.User;
import com.dwsolutions.projectSPRING1.services.UserService;
@RestController
@RequestMapping(value = "/users")
public class UserResource {
@Autowired
private UserService service;
@GetMapping
public ResponseEntity<List<User>> findAll(){
List<User> list = service.findAll();
return ResponseEntity.ok().body(list);
}
@GetMapping(value = "/{id}")
public ResponseEntity<User> findById(@PathVariable Long id){
User u = service.findById(id);
return ResponseEntity.ok().body(u);
}
} |
import css from './SearchBar.module.css';
import { Component } from 'react';
import PropTypes from 'prop-types';
export class SearchBar extends Component {
state = {
searchValue: '',
images: [],
};
handleSubmitForm = e => {
e.preventDefault();
const search = this.state.searchValue;
this.props.onSubmit(search);
};
handleChange = e => {
this.setState({ searchValue: e.target.value });
};
render() {
const { searchValue } = this.state;
return (
<header className={css.searchbar}>
<form onSubmit={this.handleSubmitForm} className={css.searchForm}>
<button type="submit" className={css.searchForm_button}>
<span className={css.searchForm_button_label}>Search</span>
</button>
<input
className={css.searchForm_input}
type="text"
autoComplete="off"
autoFocus
placeholder="Search images and photos"
onChange={this.handleChange}
value={searchValue}
/>
</form>
</header>
);
}
}
SearchBar.propTypes = {
onSubmit: PropTypes.func,
}; |
//首页的样式文件
@import "common";
// @import导入的意思 可以把一个样式文件导入到另外一个样式文件里面
// link是把一个样式文件引入到html页面里面
a{
text-decoration: none;
}
body{
min-width: 320px;
width: 15rem;
margin: 0px auto;
margin: 0 auto;
line-height: 1.15;
font-family: Arial, Helvetica;
background: #F2F2F2;
}
// 页面元素rem计算公式 页面元素的px / html 字体大小 50
// <!-- 顶部收缩框 -->
@baseFont: 50;
.search-content{
display: flex;
position: fixed;
top: 0;
left: 50%;
transform: translateX(-50%);
width: 15rem;
height: (88rem/50);
background-color: #FFC001;
.classify{
width: (44rem / @baseFont);
height: (70rem / @baseFont);
background: url(../images/classify.png) no-repeat;
// 背景缩放
background-size: (44rem / @baseFont) (70rem / @baseFont);
margin: (11rem/@baseFont) (25rem/@baseFont) (7rem/@baseFont) (24rem/@baseFont);
}
.search{
flex: 1;
input{
outline: none;
width: 100%;
border: 0;
height: (66rem / @baseFont);
border-radius: (33rem / @baseFont);
background-color: #FFF2CC;
margin-top: (12rem / @baseFont);
font-size: (25rem / @baseFont);
padding-left: (55rem / @baseFont);
color: #757575;
}
}
.login{
width: (75rem / @baseFont);
height: (70rem / @baseFont);
margin: (10rem / @baseFont);
font-size: (25rem / @baseFont);
text-align: center;
line-height: (70rem / @baseFont);
color: #fff;
}
}
// <!-- banner模块 -->
.banner{
width: (750rem / @baseFont);
height: (368rem / @baseFont);
img{
width: 100%;
height: 100%;
}
}
// <!-- 广告部分 -->
.ad{
display: flex;
a {
flex: 1;
img{
width: 100%;
}
}
}
// <!-- nav模块 -->
nav{
width: (750rem / @baseFont);
a{
float: left;
width: (150rem / @baseFont);
height: (140rem / @baseFont);
text-align: center;
img{
display: block;
width: (82rem / @baseFont);
height: (82rem / @baseFont);
margin: (10rem / @baseFont) auto 0;
}
span{
font-size: (25rem / @baseFont);
color: #333;
}
}
} |
import React from 'react';
import PropTypes from 'prop-types';
import { makeStyles } from '@material-ui/core/styles';
import Tabs from '@material-ui/core/Tabs';
import Tab from '@material-ui/core/Tab';
import Typography from '@material-ui/core/Typography';
import Box from '@material-ui/core/Box';
import Paper from '@material-ui/core/Paper'
import Transaction from './Transaction'
import Subscription from './Subscription';
import ActiveUser from './ActiveUser';
function TabPanel(props) {
const { children, value, index, ...other } = props;
return (
<div
role="tabpanel"
hidden={value !== index}
id={`scrollable-auto-tabpanel-${index}`}
aria-labelledby={`scrollable-auto-tab-${index}`}
{...other}
>
{value === index && (
<Box p={3}>
<Typography>{children}</Typography>
</Box>
)}
</div>
);
}
TabPanel.propTypes = {
children: PropTypes.node,
index: PropTypes.any.isRequired,
value: PropTypes.any.isRequired,
};
function a11yProps(index) {
return {
id: `scrollable-auto-tab-${index}`,
'aria-controls': `scrollable-auto-tabpanel-${index}`,
};
}
const useStyles = makeStyles((theme) => ({
root: {
marginTop: 60,
width: "100%",
paddingLeft: "15px"
},
}));
export default function TabBar() {
const classes = useStyles();
const [value, setValue] = React.useState(0);
const handleChange = (event, newValue) => {
setValue(newValue);
};
return (
<>
<div className={classes.root}>
<Paper elevation={3} square>
<Tabs
value={value}
onChange={handleChange}
indicatorColor="primary"
textColor="primary"
>
<Tab label="Subscriptions" {...a11yProps(0)}/>
<Tab label="Transaction" {...a11yProps(1)}/>
<Tab label="ActiveUser" {...a11yProps(2)}/>
</Tabs>
</Paper>
<TabPanel value={value} index={0}>
<Subscription/>
</TabPanel>
<TabPanel value={value} index={1}>
<Transaction/>
</TabPanel>
<TabPanel value={value} index={2}>
<ActiveUser/>
</TabPanel>
</div>
</>
);
} |
import React, { useEffect } from "react";
import { HashRouter as Router, Route, Switch } from "react-router-dom";
import "bootstrap/dist/css/bootstrap.min.css";
import { Navigation } from "./components";
import {
HomeView,
HashView,
BlockView,
BlockchainView,
DistributedView,
TokensView,
CoinbaseView,
KeysView,
SignaturesView,
TransactionsView,
SignedBlockchainView,
ErrorView,
} from "./views";
import { fetch_data } from "./javascript/fetch_api";
import "./App.css";
/* Main App component. Display Navigation on top and use React Router to switch between Views */
const App = () => {
/* When app first load, poke to API Server to wake him up */
useEffect(() => {
fetch_data("");
}, []);
return (
<>
<Navigation />
<Router>
<Switch>
<Route exact path="/" component={HomeView} />
<Route path="/hash" component={HashView} />
<Route path="/block" component={BlockView} />
<Route path="/blockchain" component={BlockchainView} />
<Route path="/distributed" component={DistributedView} />
<Route path="/tokens" component={TokensView} />
<Route path="/coinbase" component={CoinbaseView} />
<Route path="/keys" component={KeysView} />
<Route path="/signatures" component={SignaturesView} />
<Route path="/transactions" component={TransactionsView} />
<Route path="/signedblockchain" component={SignedBlockchainView} />
<Route path="*" component={ErrorView} />
</Switch>
</Router>
</>
);
};
export default App; |
<div class="sidebar" ngClass.gt-sm="desktop-height" ngClass.lt-md="mobile-height">
<div fxLayout="column">
<div class="sidebarHeader">
<app-story-component></app-story-component>
</div>
<div fxLayout="row" fxLayoutAlign="space-between center">
<div class="searchBox">
<img class="search icon" width="19" alt="search" src="../../../assets/images/searchIcon.svg"/>
<img *ngIf="filter.searchText" (click)="clearSearchText()" class="close icon" width="19"
alt="close" src="../../../assets/images/closeIcon.svg"/>
<input
[(ngModel)]="filter.searchText"
type="text"
placeholder="Pesquisar..."
(ngModelChange)="onChangeSearchText()"
/>
</div>
<div fxLayoutAlign="center center" (click)="changeListType()"
style="cursor: pointer; height: 100%; background-color: #fbfbfb; border-bottom: 1px solid #e1e4e5;">
<span class="chatListType" style="background-color: #137f36; padding: 5px 25px; color: white; border-radius: 25px; margin: 10px;" >
{{chatListType === CHAT_LIST_TYPE.LAW ? 'Votações' : 'Parlamentares'}}
<img src="../../../assets/images/chevron-down.png" alt="chevron-down">
</span>
</div>
</div>
<div class="filter" fxLayout="row" fxLayoutAlign="space-between center" fxLayoutGap="10px">
<div fxLayout="column" style="color: #137f36">
<select style="border: 2px solid #137f36; padding: 3px; border-radius: 20px; color: #137f36 "
[(ngModel)]="filter.state"
(ngModelChange)="onChangeState()">
<option value="">Todos Estados</option>
<ng-container *ngFor="let option of states">
<option [value]="option.sigla">{{option.sigla}}</option>
</ng-container>
</select>
<ng-container *ngIf="chatListType === CHAT_LIST_TYPE.VOTE">
<div style="padding-top: 10px; cursor: pointer" (click)="changeBestWorst()">
<ng-container *ngIf="filter.fromBestToWorse else fromWorst">
<span style="color: green">
Do <strong>MELHOR</strong> Para Pior ⬇
</span>
</ng-container>
<ng-template #fromWorst>
<span style="color: #d10202">
Do <strong>PIOR</strong> Para Melhor ⬆
</span>
</ng-template>
</div>
</ng-container>
</div>
<div fxLayout="column" fxLayoutGap="5px">
<div class="radio-option" (click)="selectRadioFilter(RadioFilterEnum.CHAMBER)">
<div class="radio" [ngClass]="{selected: filter.chamber}"></div>
<div>CÂMARA</div>
</div>
<div class="radio-option" (click)="selectRadioFilter(RadioFilterEnum.SENATE)">
<div class="radio" [ngClass]="{selected: filter.senate}"></div>
<div>SENADO</div>
</div>
</div>
</div>
</div>
<div id="sidebarContent"
infiniteScroll
(scrolled)="onScroll()"
(scrolledUp)="onScroll()"
[infiniteScrollDistance]="2"
[infiniteScrollThrottle]="50"
[scrollWindow]="false"
fxLayout="column"
>
<ng-container *ngIf="chatListType === CHAT_LIST_TYPE.LAW; else parliamentarianList">
<app-skeleton-user-list *ngIf="!laws?.length || loading; else lawContent"></app-skeleton-user-list>
<ng-template #lawContent>
<ng-container *ngFor="let law of filteredLaws">
<div class="conversation"
(click)="handleLawClicked(law)">
<div class="picture">
{{law.house === 'Senado' ? 'S' : 'C'}}
</div>
<div class="content">
<div class="conversationHeader">
<div fxLayout="column" class="w-100">
<div class="name"> {{ law.myRankingTitle }} </div>
<div fxLayout="column" class="w-100">
<div fxLayout="row"
class="progress">
<ng-container *ngFor="let resume of getProgressBar(law?.lawResumes)">
<div style="height: 100%;"
[ngStyle]="{'width': resume.percent + '%', 'background-color': resume.color}">
</div>
</ng-container>
</div>
<div fxLayout="row" style="padding-top: 10px">
<ng-container
*ngFor="let resume of getProgressBar(law?.lawResumes); let last = last">
<div class="" fxLayoutAlign="start center">
<div style="height: 12px; width: 12px; border-radius: 12px"
[ngStyle]="{'background-color': resume.color}">
</div>
<span style="margin: 0 10px 0 5px; font-size: 12px">
{{resume.percent + '% ' + resume.vote}}
</span>
</div>
<ng-container *ngIf="last">
<div class="" fxLayoutAlign="end end"
style="font-size: 14px; flex: 1">
{{resume.total + ' votos'}}
</div>
</ng-container>
</ng-container>
</div>
</div>
</div>
</div>
</div>
</div>
</ng-container>
</ng-template>
</ng-container>
<ng-template #parliamentarianList>
<app-skeleton-user-list
*ngIf="!filteredConversations?.length || loading; else conversationContent"></app-skeleton-user-list>
<ng-template #conversationContent>
<ng-container *ngFor="let conversation of filteredConversations">
<div class="conversation"
(click)="handleConversationClicked(conversation)">
<div class="picture">
<img alt="photo" src="{{ getThumbnail(conversation.parliamentarianId)}}"/>
<div class="score"
[ngClass]="{'good': conversation.scoreRanking <= 150, 'regular': conversation.scoreRanking > 150 && conversation.scoreRanking <= 350, 'bad': conversation.scoreRanking > 350 }">
<h2>{{conversation.scoreRanking}}º</h2>
</div>
</div>
<div class="content">
<div class="conversationHeader">
<div fxLayout="column">
<div class="name"> {{ conversation.parliamentarian.nickname }} </div>
<div *ngIf="conversation.parliamentarian.isReelection" class="chip">CANDIDATO A
REELEIÇÃO
</div>
</div>
<div class="time" fxLayoutAlign="end end">{{conversation.scoreTotal}} pts</div>
</div>
<div class="message">
<img alt="unread"
*ngIf="!conversation.parliamentarian.latestMessageRead"
src="../../../assets/images/doubleTick.svg"
/>
<img alt="read"
*ngIf="conversation.parliamentarian.latestMessageRead"
src="../../../assets/images/doubleTickBlue.svg"
/>
<span *ngIf="conversation.parliamentarian.latestLawStatusId === 1"
style="font-weight: bold;color: green">SIM</span>
<span *ngIf="conversation.parliamentarian.latestLawStatusId === 2"
style="font-weight: bold;color: red">NÃO</span>
{{ conversation.parliamentarian.position }} {{ conversation.parliamentarian.party.prefix }}
- {{conversation.parliamentarian.state.prefix}}
</div>
</div>
</div>
</ng-container>
</ng-template>
</ng-template>
</div>
<app-share-button fxHide.gt-sm [bottom]="'60px'"></app-share-button>
</div> |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Meta</title>
<link rel="icon" href="./images/logo.jpg">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="./css/style.css">
</head>
<body>
<!-- navbar -->
<div id="nav" >
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<a href="#" class="navbar-brand" style="display: flex;" ><span style="align-self: center;"><img src="./images/logo.jpg" alt="logo" style="width: 40px;"></span> <span style="font-size: 16pt; font-weight: bolder; padding-left: 10px;"> Meta</span></a>
</div>
<ul class="nav navbar-nav" style="float: right; padding-right: 10vw;">
<li><a href="#">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact-us">Contact Us</a></li>
</ul>
</div>
</nav>
</div>
<!-- login -->
<div class="login">
<!-- Trigger the modal with a button -->
<button type="button" class="btn btn1 btn-success btn-sm" data-toggle="modal" data-target="#myModal">Login</button>
<!-- Modal -->
<div class="modal fade" id="myModal" role="dialog">
<div class="modal-dialog">
<!-- Modal content-->
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title login-h4">Login</h4>
</div>
<div class="modal-body modalform">
<div class="modal-form-1">
<form action="#">
<div class="form-body">
<div>
<div class="form-group">
<label for="">Email </label>
<input type="email" class="form-control" id="name">
</div>
<div class="form-group">
<label for="">Password</label>
<input type="password" class="form-control" id="psw">
</div>
</div>
<button type="submit" class="btn btn-default btn11">Login</button>
</div>
</form>
<div class="forgot">
<small><a href="#" >Forget your Password?</a></small>
</div>
</div>
</div>
<div class="modal-footer">
<div class="signup">
<p>Don't have an Account <span><a href="./pages/signup.html" target="_blank"> Sign Up</a></span></p>
</div>
<!-- <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> -->
</div>
</div>
</div>
</div>
</div>
<!-- image slider -->
<div id="myCarousel" class="carousel slide" data-ride="carousel">
<!-- Indicators -->
<ol class="carousel-indicators">
<li data-target="#myCarousel" data-slide-to="0" class="active"></li>
<li data-target="#myCarousel" data-slide-to="1"></li>
<li data-target="#myCarousel" data-slide-to="2"></li>
</ol>
<!-- Wrapper for slides -->
<div class="carousel-inner">
<div class="item active">
<img src="./images/header-1.jpg" alt="+" style="width:100%; height: 50vh; object-fit: cover;">
</div>
<div class="item">
<img src="./images/header-2.jpg" alt="+" style="width:100%; height: 50vh; object-fit: cover;">
</div>
<div class="item">
<img src="./images/header-3.jpg" alt="+" style="width:100%; height: 50vh; object-fit: cover;">
</div>
</div>
<!-- Left and right controls -->
<a class="left carousel-control" href="#myCarousel" data-slide="prev">
<span class="glyphicon glyphicon-chevron-left"></span>
<span class="sr-only">Previous</span>
</a>
<a class="right carousel-control" href="#myCarousel" data-slide="next">
<span class="glyphicon glyphicon-chevron-right"></span>
<span class="sr-only">Next</span>
</a>
</div>
<!-- body -->
<div class="intro">
<h1>Meta</h1>
<p>
Meta is an one stop solution for all your online needs focused on brand development. We offer solutions, with a view to promote and establish your business into a brand. We entertain an infinite realm of imagination that articulates into creative solutions.</p>
</div>
<!-- about -->
<div id="about" class="about">
<div class="about-flex">
<div class="about-heading">
<h4>About</h4>
</div>
<div class="container">
<div class="row">
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/workplace.jpg" style="width:100%">
<!-- <div class="caption">
</div> -->
</a>
</div>
</div>
<div class="col-md-8">
<p style="font-size: 16pt; font-weight: bolder; padding-top: 83px; color: white;">What we do?</p>
<p style="font-size: 12pt; color: white; ">Meta is an exclusive company with a vested focus into Brand Development. Our priority extends to produce the best-in-class creative subjects which instils a unique identity of a brands among consumers in our society. We believe in nurturing creativity at its optimum with brief yet powerful contents, themes, designs and online marketing strategy for our Clients at its best.</p>
</div>
</div>
</div>
<!-- <div class="container">
<div class="row">
<div class="col-md-8">
<p style="font-size: 16pt; font-weight: bolder; ">Our Team</p>
<p style="font-size: 12pt;">Meta inhabits highly skilled individuals in our team. We devote the concept of “Dynamism”, which indeed gives us the strength to work with young talents that stimulates out-of box thinking. Our team of qualified professionals are up to date on the changing trends and demand expectations of consumers in the market. Team Odozi Technologies aspires to meet your brand expectations at various levels through continuous brand engagements with the consumers through online medium, by keeping a regular check on new updates without compromising the existing delivery standards. </p>
<p style="font-size: 12pt;">
We prioritise in offering personalized service to our Clients, as a result we have dedicated Relationship Managers for each of our Clients to address any queries related to our services 24×7.</p>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/about-img.jpg" style="width:100%">
<div class="caption">
</div>
</a>
</div>
</div>
</div> -->
</div>
</div>
</div>
<div class="gallery">
<div class="container">
<div class="gal-heading">
<h4>Gallery</h4>
</div>
<div class="row">
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g1.jpg" style="width:100%">
<div class="caption">
7 <p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g2.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g3.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
</div>
<div>
<button class="seeMore active-cbtn" id="collapse-btn1" data-toggle="collapse" data-target="#gal-colapse">see more...</button>
<button class="seeLess" id="collapse-btn1" data-toggle="collapse" data-target="#gal-colapse">see less...</button>
</div>
<div id="gal-colapse" class="collapse ">
<div class="row">
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g1.jpg" style="width:100%">
<div class="caption">
7 <p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g2.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g3.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
</div>
<div class="row">
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/header-2.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g5.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/header-2.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
</div>
<div class="row">
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g7.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g8.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
<div class="col-md-4">
<div class="thumbnail">
<a href="#" target="_blank">
<img src="./images/g9.jpg" style="width:100%">
<div class="caption">
<p>Lorem ipsum donec id elit non mi porta gravida at eget metus.</p>
</div>
</a>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- contact-us -->
<div id="contact-us">
<div class="form-div">
<form action="#">
<h4>Contact Us</h4>
<div class="form-body" style="box-shadow: 0px 5px 10px 10px rgba(0,0,0,0);">
<div>
<div class="form-group">
<label for="">Name :</label>
<input type="text" class="form-control" id="email">
</div>
<div class="form-group">
<label for="">Email Id:</label>
<input type="email" class="form-control" id="email">
</div>
<div class="form-group">
<label for="">Message :</label>
<textarea name="" id="comment" rows="5" class="form-control"></textarea>
</div>
</div>
<button type="submit" class="btn btn-default" style="width: 100%;">Submit</button>
</div>
</form>
</div>
</div>
<div class="footer">
<div class="footer-content">
<div class="address">
<h4>Address</h4>
<address>
North Screet,<br>
Main Road, Marthandam.
</address>
</div>
<div class="branches">
<h4>Branches</h4>
<address>
Kanniyakumari <br>
Chennai
</address>
</div>
</div>
<footer>
<span>Meta © 2022 all rights reserved</span>
</footer><br>
</div>
</div>
<script src="./script/app.js"></script>
</body>
</html> |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
{% if title %}
<title>
{{ title }} - Моя соц сеть
</title>
{% else %}
<title> Моя соц сеть </title>
{% endif %}
</head>
<body>
<div>Моя соц сеть:
<a href="{{ url_for('index') }}">Домашняя страница </a>
<a href="{{ url_for('news') }}">Новости </a>
{% if current_user.is_anonymous %} <!--Если пользователь анонимный-->
<a href="{{ url_for('login') }}">Вход </a>
{% else %}
<a href="{{ url_for('user', username=current_user.username) }}">Профиль пользователя</a>
<a href="{{ url_for('logout') }}">Выйти </a>
{% endif %}
</div>
<hr>
{% with messages = get_flashed_messages() %}
{% if messages %}
<ul>
{% for message in messages %}
<li> {{ message }} </li>
{% endfor %}
</ul>
{% endif %}
{% endwith %}
{% block content %}
{% endblock %}
</body>
</html> |
import React from 'react'
import { graphql } from 'gatsby'
import Img from 'gatsby-image'
import styled from 'styled-components'
import { animated, useSpring, config } from 'react-spring'
import Layout from '../components/layout'
import GridItem from '../components/grid-item'
import SEO from '../components/SEO'
import { ChildImageSharp } from '../types'
import InfoPages from '../components/infoPages'
type PageProps = {
data: {
firstProject: {
title: string
slug: string
cover: ChildImageSharp
}
threeProjects: {
nodes: {
title: string
slug: string
cover: ChildImageSharp
}[]
}
posts: {
edges: {
node: {
id: string
title: string
slug: string
featuredImage: {
sourceUrl: string
}
}
}
}
aboutUs: ChildImageSharp
instagram: ChildImageSharp
}
}
const Area = styled(animated.div)`
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: 35vw 40vw 25vw;
grid-template-areas:
'first-project about-us about-us'
'three-projects three-projects three-projects'
'instagram instagram instagram';
@media (max-width: ${(props) => props.theme.breakpoints[3]}) {
grid-template-columns: repeat(4, 1fr);
grid-template-rows: 35vw 30vw 30vw 25vw;
grid-template-areas:
'first-project first-project about-us about-us'
'three-projects three-projects three-projects three-projects'
'three-projects three-projects three-projects three-projects'
'instagram instagram instagram instagram';
}
@media (max-width: ${(props) => props.theme.breakpoints[1]}) {
grid-template-columns: repeat(2, 1fr);
grid-template-rows: repeat(5, 38vw);
grid-template-areas:
'first-project about-us'
'three-projects three-projects'
'three-projects three-projects'
'three-projects three-projects'
'instagram instagram';
}
@media (max-width: ${(props) => props.theme.breakpoints[0]}) {
grid-template-columns: 1fr;
grid-template-rows: repeat(6, 50vw);
grid-template-areas:
'first-project'
'about-us'
'three-projects'
'three-projects'
'three-projects'
'instagram';
}
`
const FirstProject = styled(GridItem)`
grid-area: first-project;
`
const AboutUs = styled(GridItem)`
grid-area: about-us;
`
const ThreeProjects = styled.div`
grid-area: three-projects;
display: grid;
grid-template-columns: repeat(3, 1fr);
@media (max-width: ${(props) => props.theme.breakpoints[1]}) {
grid-template-columns: 1fr;
grid-template-rows: 1fr 1fr 1fr;
}
`
const Instagram = styled(GridItem)`
grid-area: instagram;
`
const Index: React.FunctionComponent<PageProps> = () => {
const pageAnimation = useSpring({
config: config.slow,
from: { opacity: 0 },
to: { opacity: 1 },
})
return (
<Layout>
<SEO />
<InfoPages />
{/**<Area style={pageAnimation}>
<FirstProject to={firstProject.slug} aria-label={`View project "${firstProject.title}"`}>
<Img fluid={firstProject.cover.childImageSharp.fluid} />
<span>{firstProject.title}</span>
</FirstProject>
<AboutUs to="/about" aria-label="Visit my about page">
<Img fluid={aboutUs.childImageSharp.fluid} />
<span>About</span>
</AboutUs>
<ThreeProjects>
{posts.edges.node.map((project) => (
<GridItem to={project.slug} key={project.slug} aria-label={`View project "${project.title}"`}>
<Img fluid={firstProject.cover.childImageSharp.fluid} />
<span>{project.title}</span>
</GridItem>
))}
</ThreeProjects>
<Instagram to="/instagram" aria-label="See my Instagram pictures">
<Img fluid={instagram.childImageSharp.fluid} />
<span>Instagram</span>
</Instagram>
</Area>*/}
</Layout>
)
}
export default Index
// export const query = graphql`
// query Index {
// firstProject: projectsYaml {
// title
// slug
// cover {
// childImageSharp {
// fluid(quality: 95, maxWidth: 1200) {
// ...GatsbyImageSharpFluid_withWebp
// }
// }
// }
// }
// threeProjects: allProjectsYaml(limit: 3, skip: 1) {
// nodes {
// title
// slug
// cover {
// childImageSharp {
// fluid(quality: 95, maxWidth: 1200) {
// ...GatsbyImageSharpFluid_withWebp
// }
// }
// }
// }
// }
// aboutUs: file(sourceInstanceName: { eq: "images" }, name: { eq: "about-us" }) {
// childImageSharp {
// fluid(quality: 95, maxWidth: 1200) {
// ...GatsbyImageSharpFluid_withWebp
// }
// }
// }
// instagram: file(sourceInstanceName: { eq: "images" }, name: { eq: "instagram" }) {
// childImageSharp {
// fluid(quality: 95, maxWidth: 1920) {
// ...GatsbyImageSharpFluid_withWebp
// }
// }
// }
// }
// `
// wpgraphql{
// posts{
// edges{
// node{
// id
// title
// slug
// featuredImage{
// sourceUrl
// }
// }
// }
// }
// }
//
// allFile {
// edges {
// node {
// name
// parent{
// id
// }
// childImageSharp {
// fluid (maxWidth: 500){
// srcSet
// ...GatsbyImageSharpFluid
//
// }
// }
// }
// }
// }
// } |
import React, { useState } from 'react';
import { Button, TextField } from '@material-ui/core';
import './CreateFundraiser.css';
import { useNavigate } from 'react-router-dom';
const CreateFundraiser = () => {
const [containerState, setContainerState] = useState({
title: '',
description: '',
profileImage: '',
QNA: [],
goal: 0,
currentStep: 0,
});
const navigate=useNavigate();
const onSubmit = () => {
navigate('/');
}
const resetState = () => {
setContainerState({
title: '',
description: '',
profileImage: '',
QNA: [],
goal: 0,
currentStep: 0,
});
};
const backState = () => {
setContainerState(prev => {
return {
...prev,
currentStep: prev.currentStep - 1,
};
});
};
const nextStep = () => {
setContainerState(prev => {
return {
...prev,
currentStep: prev.currentStep + 1,
};
});
};
const addQNA = () => {
setContainerState(prev => {
return {
...prev,
QNA: [
...prev.QNA,
{ question: '', answer: '' },
],
};
});
};
const [question, setQuestion] = useState('');
const [answer, setAnswer] = useState('');
const handleChange = e => {
setContainerState(prev => {
return {
...prev,
[e.target.name]: e.target.value,
};
});
};
return (
<div className="fundraiser-container">
<div className='fundraiser-container-contents'>
<div className='container-title'>
<h1>Create Fundraiser</h1>
</div>
<div className='fundraiser-content'>
<div className='fundraiser-left-content'>
<div className='container-form-controller'>
<TextField
name='title'
label='Title'
variant='outlined'
value={containerState.title}
onChange={handleChange}
/>
<TextField
name='description'
label='Description'
variant='outlined'
value={containerState.description}
onChange={handleChange}
rows={10}
multiline
/>
<h4> Create QnA </h4>
{
containerState.QNA.map((item, index) => {
return (
<div key={index + "QNA_chip"} className='QnA-chip'>
<TextField
name='question'
label='Question'
variant='outlined'
value={item.question}
onChange={(e) => {
setContainerState(prev => {
return {
...prev,
QNA: prev.QNA.map((item, i) => {
if (i === index) {
return {
...item,
question: e.target.value,
};
}
return item;
}),
};
});
}}
/>
<TextField
name='answer'
label='Answer'
variant='outlined'
value={item.answer}
onChange={(e) => {
setContainerState(prev => {
return {
...prev,
QNA: prev.QNA.map((item, i) => {
if (i === index) {
return {
...item,
answer: e.target.value,
};
}
return item;
}),
};
});
}}
/>
</div>
);
})
}
<div
//className='createQuestionButton'
>
<Button
variant='contained'
color='primary'
onClick={addQNA}
>
+ Add QnA
</Button>
</div>
</div>
</div>
<div className='fundraiser-right-content'>
<div className='upload-container'>
<div className='upload-image'>
<img src="/upload.svg" alt="" />
<p>Upload Image</p>
</div>
</div>
<div className='goal-container'>
<h5>How much you would like to raise?</h5>
<TextField
name='goal'
label='Goal'
variant='outlined'
value={containerState.goal}
onChange={handleChange}
/>
</div>
<div className="submit-button">
<Button
variant='contained'
color='primary'
onClick={onSubmit}
>
Submit
</Button>
</div>
</div>
</div>
</div>
</div>
);
};
export default CreateFundraiser; |
import { HardhatRuntimeEnvironment } from "hardhat/types";
import { ABI, DeployFunction } from "hardhat-deploy/types";
import { deployments, ethers } from "hardhat";
import {
OptionsFactory,
Token,
OptionsPool,
SemiFungiblePositionManager,
MockUniswapV3Pool,
} from "../types";
import { grantTokens } from "../test/utils";
import * as OptionEncoding from "../test/libraries/OptionEncoding";
import { token } from "../types/@openzeppelin/contracts";
const WETH_ADDRESS = "0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2";
const USDC_ADDRESS = "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48";
const USDC_SLOT = 9;
const testOptionsPool: DeployFunction = async function (hre: HardhatRuntimeEnvironment) {
const { deployments, getNamedAccounts } = hre;
const { deployer, seller, buyer } = await getNamedAccounts();
const { address: token1Address } = await deployments.get("Token1");
const token1 = (await ethers.getContractAt("Token", token1Address)) as Token;
const amount1 = ethers.utils.parseEther("10");
const { address: poolAddress } = await deployments.get("OptionsPool-ETH-USDC");
const pool = (await ethers.getContractAt("OptionsPool", poolAddress)) as OptionsPool;
//granting USDC and WETH (forked mainnet)
// await grantTokens(WETH_ADDRESS, deployer, WETH_SLOT, wethBalance);
// await grantTokens(USDC_ADDRESS, deployer, USDC_SLOT, usdcBalance);
// await grantTokens(WETH_ADDRESS, seller, WETH_SLOT, wethBalance);
// await grantTokens(USDC_ADDRESS, seller, USDC_SLOT, usdcBalance);
// await grantTokens(WETH_ADDRESS, buyer, WETH_SLOT, wethBalance);
// await grantTokens(USDC_ADDRESS, buyer, USDC_SLOT, usdcBalance);
// to comment for proper deployment
const tick = 1190;
const width = 10;
let strike = tick - 1100;
strike = strike - (strike % 10);
//initialize pool
const SFPMdeployment = await deployments.get("SemiFungiblePositionManager");
const sfpm = (await ethers.getContractAt(
"SemiFungiblePositionManager",
SFPMdeployment.address
)) as SemiFungiblePositionManager;
await sfpm.initializePool({ token0: USDC_ADDRESS, token1: WETH_ADDRESS, fee: 500 });
// //deposit WETH_
let deployPoolTx = await token1.mint(deployer, amount1);
let receipt = await deployPoolTx.wait();
deployPoolTx = await token1.approve(pool.address, amount1);
receipt = await deployPoolTx.wait();
deployPoolTx = await pool.deposit(amount1, token1.address);
receipt = await deployPoolTx.wait();
deployPoolTx = await pool.withdraw(amount1, token1.address, []);
receipt = await deployPoolTx.wait();
// //mint option
const numberOfContracts = ethers.utils.parseEther("1");
const uniPoolAddress = await pool.pool();
const poolId = BigInt(uniPoolAddress.slice(0, 22).toLowerCase());
const tokenId = OptionEncoding.encodeID(poolId, [
{
width,
ratio: 3,
strike,
long: false,
tokenType: 1,
riskPartner: 0,
},
]);
// await pool.mintOptions([tokenId], numberOfContracts);
// events
// let eventFilter = sfpm.filters["TokenizedPositionMinted(address,uint256,uint128)"]();
// console.log("TokenizedPositionMinted", await sfpm.queryFilter(eventFilter));
// let eventFilterTransfer = token1.filters["Transfer(address,address,uint256)"]();
// const transferEvent = await token1.queryFilter(eventFilterTransfer);
// console.log("Transfer", transferEvent[0].args);
// let eventFilterDeposited = pool.filters["Deposited(address,address,uint256)"]();
// const depositedEvent = await pool.queryFilter(eventFilterDeposited);
// console.log("Deposited", depositedEvent[0].args);
// let eventFilterWithdrawn = pool.filters["Withdrawn(address,address,uint256)"]();
// const withdrawnEvent = await pool.queryFilter(eventFilterWithdrawn);
// console.log("Withdrawn", withdrawnEvent[0]);
// let eventFilterPool = pool.filters["PoolStarted(address,address)"]();
// const poolStartedEvent = await pool.queryFilter(eventFilterPool);
// let eventFilterMint = sfpm.filters["TokenizedPositionMinted(address,uint256,uint128,address)"]();
// const mintEvent = await pool.queryFilter(eventFilterMint);
// console.log("mintEvent", mintEvent[0]);
// console.log("blockNumber", poolStartedEvent[0].blockNumber);
};
export default testOptionsPool;
testOptionsPool.tags = ["testOptionsPool"]; |
import axios from "axios";
const API_URL = "http://localhost:8000";
//------------------------------------------------------------------------
const updateTask = async (data) => {
try {
const res = await axios.put(`${API_URL}/update/${data._id}`, data);
if (res.status === 200) {
return { isSuccess: true, data: res.data };
} else {
return { isFailure: true, msg: "Unexpected response from server" };
}
} catch (error) {
console.error("Error while updating task", error);
return { isFailure: true, msg: "Error while updating task" };
}
};
const getTaskById = async (id) => {
try {
const res = await axios.get(`${API_URL}/task/${id}`);
if (res.status === 200) {
return { isSuccess: true, data: res.data };
} else {
return { isFailure: true, msg: "Unexpected response from server" };
}
} catch (error) {
console.error("Error while getting task", error);
return { isFailure: true, msg: "Error while getting task" };
}
};
const getAllTask = async () => {
try {
const res = await axios.get(`${API_URL}/tasks`);
if (res.status === 200) {
return { isSuccess: true, data: res.data };
} else {
return { isFailure: true, msg: "Unexpected response from server" };
}
} catch (error) {
console.error("Error while getting all task", error);
return { isFailure: true, msg: "Error while getting all task" };
}
};
const createTask = async (data) => {
try {
const res = await axios.post(`${API_URL}/create`, data);
if (res.status === 200) {
return { isSuccess: true, data: res.data };
} else {
return { isFailure: true, msg: "Unexpected response from server" };
}
} catch (error) {
console.error("Error while creating task:", error);
return { isFailure: true, msg: "Error while creating task" };
}
};
//-------------------------------------------------------------------------
const userLogin = async (data) => {
try {
const response = await axios.post(`${API_URL}/login`, data);
if (response.status === 200) {
return { isSuccess: true, data: response.data };
} else {
return { isFailure: true, msg: "Unexpected response from server" };
}
} catch (error) {
console.error("Error while logging in:", error);
return { isFailure: true, msg: "Error while login" };
}
};
const userSignup = async (data) => {
try {
let response = await axios.post(`${API_URL}/signup`, data);
if (response.status === 200) {
return { isSuccess: true, data: response.data };
}
} catch (error) {
return { isFailure: true, msg: "Error while signup" };
}
};
/*-------------------------------------------------------------------*/
const deleteTask = async (id) => {
try {
let response = await axios.delete(`${API_URL}/delete/${id}`, {});
if (response?.status === 200) {
return { isSuccess: true, data: response.data };
} else {
return {
isFailure: true,
status: response?.status,
msg: response?.msg,
code: response?.code,
};
}
} catch (error) {
return { isFailure: true, msg: "Error deleting post" };
}
};
export {
userLogin,
userSignup,
createTask,
getTaskById,
getAllTask,
updateTask,
deleteTask,
}; |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Web Studio</title>
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Raleway:wght@800&family=Roboto:wght@400;500;700;900&display=swap"
rel="stylesheet"
/>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/modern-normalize/1.1.0/modern-normalize.css"
integrity="sha512-Y0MM+V6ymRKN2zStTqzQ227DWhhp4Mzdo6gnlRnuaNCbc+YN/ZH0sBCLTM4M0oSy9c9VKiCvd4Jk44aCLrNS5Q=="
crossorigin="anonymous"
referrerpolicy="no-referrer"
/>
<link rel="stylesheet" href="./css/styles.css" />
</head>
<!-- body -->
<body>
<!-- <header> -->
<header class="header">
<div class="container cont-nav">
<nav>
<a class="logo-link link" href="./index.html"
>Web<span class="logo-part">Studio</span></a
>
<!-- navigation -->
<ul class="menu list">
<li class="menu-item">
<a class="menu-link menu-link-active link" href="./index.html"
>Studio
</a>
</li>
<li class="menu-item">
<a class="menu-link link" href="./portfolio.html">Portfolio </a>
</li>
<li class="menu-item">
<a class="menu-link link" href="">Contacts </a>
</li>
</ul>
</nav>
<!-- contacts -->
<address class="contacts">
<ul class="address-list list">
<li class="address-menu-item">
<a class="contact-unit link" href="mailto:info@devstudio.com"
>info@devstudio.com
</a>
</li>
<li class="address-menu-item">
<a class="contact-unit link" href="tel:+110001111111"
>+11 (000) 111-11-11
</a>
</li>
</ul>
</address>
<!---- hamburger button ---->
<button type="button" class="hamburger-button" data-menu-open>
<svg class="hamburger-icon">
<use
class="svg-hamburger"
href="./images/symbol-defs.svg#icon-menu-hamburger"
></use>
</svg>
</button>
</div>
<!---- mobile menu ---->
<div class="mobile-menu is-hidden" data-menu>
<div class="container mobile-menu_container">
<div>
<button
type="button"
class="modal-close-btn mobile-menu-close-btn"
data-menu-close
>
<svg class="modal-close-btn-icon">
<use href="./images/symbol-defs.svg#icon-close-modal"></use>
</svg>
</button>
<nav>
<ul class="list">
<li class="mobile-menu-item">
<a class="link mobile-menu-link" href="./index.html"
>Studio
</a>
</li>
<li class="mobile-menu-item">
<a class="link mobile-menu-link" href="./portfolio.html"
>Portfolio
</a>
</li>
<li class="mobile-menu-item">
<a class="link mobile-menu-link" href="">Contacts </a>
</li>
</ul>
</nav>
</div>
<div>
<address class="">
<ul class="list">
<li class="modal-menu-phone">
<a class="link modal-menu-phone-link" href="tel:+110001111111"
>+11 (000) 111-11-11
</a>
</li>
<li class="modal-menu-email">
<a
class="link modal-menu-email-link"
href="mailto:info@devstudio.com"
>info@devstudio.com
</a>
</li>
</ul>
</address>
<ul class="member-social-links mobile-menu-social-item list">
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-instagram"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-twitter"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-facebook"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-linkedin"></use>
</svg>
</a>
</li>
</ul>
</div>
</div>
</div>
</header>
<!-- main -->
<main>
<!-- section 1 -->
<section class="hero-section">
<div class="container content">
<h1 class="main-title">
Effective Solutions <br />
for Your Business
</h1>
<button class="hero-button" type="button" data-modal-open>
Order Service
</button>
</div>
</section>
<!-- section 2 -->
<section class="second-section">
<div class="container second-section-cont">
<h2 class="secondary-title visually-hidden">Our advances</h2>
<ul class="list second-section-list">
<li class="second-section-item">
<div class="icon-wrapper">
<svg class="advances-icon">
<use href="./images/symbol-defs.svg#icon-strategy"></use>
</svg>
</div>
<h3 class="suptitle">Strategy</h3>
<p class="title-description">
Our goal is to identify the business problem to walk away with
the perfect and creative solution.
</p>
</li>
<li class="second-section-item">
<div class="icon-wrapper">
<svg class="advances-icon">
<use href="./images/symbol-defs.svg#icon-punctuality"></use>
</svg>
</div>
<h3 class="suptitle">Punctuality</h3>
<p class="title-description">
Bring the key message to the brand's audience for the best price
within the shortest possible time.
</p>
</li>
<li class="second-section-item">
<div class="icon-wrapper">
<svg class="advances-icon">
<use href="./images/symbol-defs.svg#icon-diligence"></use>
</svg>
</div>
<h3 class="suptitle">Diligence</h3>
<p class="title-description">
Research and confirm brands that present the strongest digital
growth opportunities and minimize risk.
</p>
</li>
<li class="second-section-item">
<div class="icon-wrapper">
<svg class="advances-icon">
<use href="./images/symbol-defs.svg#icon-technologies"></use>
</svg>
</div>
<h3 class="suptitle">Technologies</h3>
<p class="title-description">
Design practice focused on digital experiences. We bring forth a
deep passion for problem-solving.
</p>
</li>
</ul>
</div>
</section>
<!-- section 3 -->
<section class="third-section">
<div class="container">
<h2 class="subtitle">What are we doing</h2>
<ul class="list third-section-list">
<li>
<img
srcset="./images/img-1.jpg 1x, ./images/img-1@2.jpg 2x"
src="./images/img-1.jpg"
alt="personal computer"
width="360"
height="300"
/>
</li>
<li>
<img
srcset="./images/img-2.jpg 1x, ./images/img-2@2.jpg 2x"
src="./images/img-2.jpg"
alt="smarphone, mobile application"
width="360"
height="300"
/>
</li>
<li>
<img
srcset="./images/img-3.jpg 1x, ./images/img-3@2.jpg 2x"
src="./images/img-3.jpg"
alt="smarphone, laptop"
width="360"
height="300"
/>
</li>
</ul>
</div>
</section>
<!-- section 4 -->
<section class="fourth-section">
<div class="container">
<h2 class="subtitle">Our Team</h2>
<ul class="list fourth-section-list">
<li class="fourth-section-item">
<img
srcset="./images/img-4.jpg 1x, ./images/img-4@2.jpg 2x"
src="./images/img-4.jpg"
alt="team member"
width="264"
height="260"
/>
<div class="team-member">
<h3 class="suptitle">Mark Guerrero</h3>
<p class="title-description">Product Designer</p>
<ul class="member-social-links list">
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-instagram"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-twitter"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-facebook"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-linkedin"
></use>
</svg>
</a>
</li>
</ul>
</div>
</li>
<li class="fourth-section-item">
<img
srcset="./images/img-5.jpg 1x, ./images/img-5@2.jpg 2x"
src="./images/img-5.jpg"
alt="team member"
width="264"
height="260"
/>
<div class="team-member">
<h3 class="suptitle">Tom Ford</h3>
<p class="title-description">Frontend Developer</p>
<ul class="member-social-links list">
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-instagram"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-twitter"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-facebook"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-linkedin"
></use>
</svg>
</a>
</li>
</ul>
</div>
</li>
<li class="fourth-section-item">
<img
srcset="./images/img-6.jpg 1x, ./images/img-6@2.jpg 2x"
src="./images/img-6.jpg"
alt="team member"
width="264"
height="260"
/>
<div class="team-member">
<h3 class="suptitle">Camila Garcia</h3>
<p class="title-description">Marketing</p>
<ul class="member-social-links list">
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-instagram"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-twitter"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-facebook"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-linkedin"
></use>
</svg>
</a>
</li>
</ul>
</div>
</li>
<li class="fourth-section-item">
<img
srcset="./images/img-7.jpg 1x, ./images/img-7@2.jpg 2x"
src="./images/img-7.jpg"
alt="team member"
width="264"
height="260"
/>
<div class="team-member">
<h3 class="suptitle">Daniel Wilson</h3>
<p class="title-description">UI Designer</p>
<ul class="member-social-links list">
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-instagram"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-twitter"></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-facebook"
></use>
</svg>
</a>
</li>
<li class="social-link-item">
<a class="social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use
href="./images/symbol-defs.svg#icon-linkedin"
></use>
</svg>
</a>
</li>
</ul>
</div>
</li>
</ul>
</div>
</section>
<!-- section 5 -->
<section class="fifth-section">
<div class="container">
<h2 class="subtitle">Customers</h2>
<ul class="company-list list">
<li class="company-list-item">
<a href="" class="company-logo link">
<svg class="company-svg" width="104" height="56">
<use
href="./images/symbol-defs.svg#icon-company-tagline"
></use>
</svg>
</a>
</li>
<li class="company-list-item">
<a href="" class="company-logo link">
<svg class="company-svg" width="104" height="56">
<use href="./images/symbol-defs.svg#icon-brand"></use>
</svg>
</a>
</li>
<li class="company-list-item">
<a href="" class="company-logo link">
<svg class="company-svg" width="104" height="56">
<use href="./images/symbol-defs.svg#icon-foster-peters"></use>
</svg>
</a>
</li>
<li class="company-list-item">
<a href="" class="company-logo link">
<svg class="company-svg" width="104" height="56">
<use href="./images/symbol-defs.svg#icon-company"></use>
</svg>
</a>
</li>
<li class="company-list-item">
<a href="" class="company-logo link">
<svg class="company-svg" width="104" height="56">
<use href="./images/symbol-defs.svg#icon-tagline-here"></use>
</svg>
</a>
</li>
<li class="company-list-item">
<a href="" class="company-logo link">
<svg class="company-svg" width="104" height="56">
<use href="./images/symbol-defs.svg#icon-yago"></use>
</svg>
</a>
</li>
</ul>
</div>
</section>
</main>
<!-- footer -->
<footer class="footer">
<div class="container tablet-footer">
<div class="footer-content">
<a class="logo-link link" href="./index.html"
>Web<span class="logo-footer">Studio</span>
</a>
<p class="footer-title">
Increase the flow of customers and sales for your business with
digital marketing & growth solutions.
</p>
</div>
<div class="footer-soc-links">
<p class="footer-second-tittle">Social media</p>
<ul class="member-social-links list">
<li class="footer-social-link-item">
<a class="footer-social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-instagram"></use>
</svg>
</a>
</li>
<li class="footer-social-link-item">
<a class="footer-social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-twitter"></use>
</svg>
</a>
</li>
<li class="footer-social-link-item">
<a class="footer-social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-facebook"></use>
</svg>
</a>
</li>
<li class="footer-social-link-item">
<a class="footer-social-link link" href="">
<svg class="social-link-icon" width="16" height="16">
<use href="./images/symbol-defs.svg#icon-linkedin"></use>
</svg>
</a>
</li>
</ul>
</div>
<div class="footer-form-wrapper">
<form class="footer-email-form" name="user-email-form">
<div class="footer-input-wrapper">
<label class="footer-form-title" for="footer_email_input"
>Subscribe
</label>
<input
class="input-footer-email"
type="email"
name="user-email"
placeholder="E-mail"
id="footer_email_input"
/>
</div>
<button class="footer-email-form-btn" type="submit">
Subscribe
<svg class="footer-email-svg">
<use href="./images/symbol-defs.svg#icon-telegraph"></use>
</svg>
</button>
</form>
</div>
</div>
</footer>
<div class="backdrop is-hidden" data-modal>
<div class="modal">
<button type="button" class="modal-close-btn" data-modal-close>
<svg class="modal-close-btn-icon">
<use href="./images/symbol-defs.svg#icon-close-modal"></use>
</svg>
</button>
<form class="modal-form">
<p class="modal-title">
Leave your contacts and we will call you back
</p>
<label class="modal-form-field">
<span class="modal-form-input-desc">Name</span>
<span class="modal-form-wrapper">
<input
type="text"
name="user-name"
class="modal-form-input"
required
pattern="^[a-zA-Zа-яА-Я]+"
minlength="2"
/>
<svg class="modal-icon">
<use href="./images/symbol-defs.svg#icon-person"></use>
</svg>
</span>
</label>
<label class="modal-form-field">
<span class="modal-form-input-desc">Phone</span>
<span class="modal-form-wrapper">
<input
type="tel"
name="user-phone"
class="modal-form-input"
required
pattern="[0-9]{3}-[0-9]{3}-[0-9]{2}-[0-9]{2}"
title="xxx-xxx-xx-xx"
/>
<svg class="modal-icon">
<use href="./images/symbol-defs.svg#icon-phone"></use>
</svg>
</span>
</label>
<label class="modal-form-field">
<span class="modal-form-input-desc">Email</span>
<span class="modal-form-wrapper">
<input
type="text"
name="user-email"
class="modal-form-input"
required
/>
<svg class="modal-icon">
<use href="./images/symbol-defs.svg#icon-email"></use>
</svg>
</span>
</label>
<label class="modal-form-field">
<span class="modal-form-input-desc">Comment</span>
<textarea
name="user_message"
class="modal-form-message"
placeholder="Text input"
></textarea>
</label>
<input
id="user-policy"
type="checkbox"
name="user_policy"
class="modal-form-check visually-hidden"
/>
<label class="modal-form-check-desc" for="user-policy">
<span class="modal-form-fake-checkbox">
<svg class="fake-checkbox">
<use href="./images/symbol-defs.svg#icon-check-on"></use>
</svg>
</span>
<span>
I accept the terms and conditions of the
<a href="">Privacy Policy</a>
</span>
</label>
<button type="submit" class="modal-form-submit">Send</button>
</form>
</div>
</div>
<script src="./js/mobile-menu.js"></script>
<script src="./js/modal.js"></script>
</body>
</html> |
class Solution(object):
def maxArea(self, height):
"""
:type height: List[int]
:rtype: int
Input: height = [1,8,6,2,5,4,8,3,7]
Output: 49
"""
area = 0
start = 0
end = len(height) - 1
while start < end:
area = max(area, min(height[start], height[end]) * (end - start))
if height[start] < height[end]:
start += 1
else:
end -= 1
return area |
// Copyright 2024 The PipeCD Authors.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package main
import (
"bufio"
"context"
"encoding/json"
"fmt"
"github.com/shurcooL/githubv4"
"log"
"os"
"os/exec"
"path/filepath"
"strings"
"time"
"unicode/utf8"
)
type PlanPreviewResult struct {
Applications []ApplicationResult
FailureApplications []FailureApplication
FailurePipeds []FailurePiped
}
func (r *PlanPreviewResult) HasError() bool {
return len(r.FailureApplications)+len(r.FailurePipeds) > 0
}
func (r *PlanPreviewResult) NoChange() bool {
return len(r.Applications)+len(r.FailureApplications)+len(r.FailurePipeds) == 0
}
type ApplicationResult struct {
ApplicationInfo
SyncStrategy string // QUICK_SYNC, PIPELINE
PlanSummary string
PlanDetails string
NoChange bool
}
type FailurePiped struct {
PipedInfo
Reason string
}
type FailureApplication struct {
ApplicationInfo
Reason string
PlanDetails string
}
type PipedInfo struct {
PipedID string
PipedURL string
}
type ApplicationInfo struct {
ApplicationID string
ApplicationName string
ApplicationURL string
Env string
ApplicationKind string // KUBERNETES, TERRAFORM, CLOUDRUN, LAMBDA, ECS
ApplicationDirectory string
}
func retrievePlanPreview(
ctx context.Context,
remoteURL,
baseBranch,
headBranch,
headCommit,
address,
apiKey string,
timeout time.Duration,
pipedHandleTimeout time.Duration,
) (*PlanPreviewResult, error) {
dir, err := os.MkdirTemp("", "")
if err != nil {
return nil, fmt.Errorf("failed to create a temporary directory (%w)", err)
}
outPath := filepath.Join(dir, "result.json")
args := []string{
"plan-preview",
"--repo-remote-url", remoteURL,
"--base-branch", baseBranch,
"--head-branch", headBranch,
"--head-commit", headCommit,
"--address", address,
"--api-key", apiKey,
"--timeout", timeout.String(),
"--piped-handle-timeout", pipedHandleTimeout.String(),
"--out", outPath,
}
cmd := exec.CommandContext(ctx, "pipectl", args...)
out, err := cmd.CombinedOutput()
if err != nil {
return nil, fmt.Errorf("failed to execute pipectl command (%w) (%s)", err, string(out))
}
log.Println(string(out))
data, err := os.ReadFile(outPath)
if err != nil {
return nil, fmt.Errorf("failed to read result file (%w)", err)
}
var r PlanPreviewResult
if err := json.Unmarshal(data, &r); err != nil {
return nil, fmt.Errorf("failed to parse result file (%w)", err)
}
return &r, nil
}
const (
successBadgeURL = `<!-- pipecd-plan-preview-->
[](https://pipecd.dev/docs/user-guide/plan-preview/)`
failureBadgeURL = `<!-- pipecd-plan-preview-->
[](https://pipecd.dev/docs/user-guide/plan-preview/)`
actionBadgeURLFormat = "[](%s)"
noChangeTitleFormat = "Ran plan-preview against head commit %s of this pull request. PipeCD detected `0` updated application. It means no deployment will be triggered once this pull request got merged.\n"
hasChangeTitleFormat = "Ran plan-preview against head commit %s of this pull request. PipeCD detected `%d` updated applications and here are their plan results. Once this pull request got merged their deployments will be triggered to run as these estimations.\n"
detailsFormat = "<details>\n<summary>Details (Click me)</summary>\n<p>\n\n``` %s\n%s\n```\n</p>\n</details>\n\n"
detailsOmittedMessage = "The details are too long to display. Please check the actions log to see full details."
appInfoWithEnvFormat = "app: [%s](%s), env: %s, kind: %s"
appInfoWithoutEnvFormat = "app: [%s](%s), kind: %s"
ghMessageLenLimit = 65536
// Limit of details
detailsLenLimit = ghMessageLenLimit - 5000 // 5000 characters could be used for other parts in the comment message.
githubServerURLEnv = "GITHUB_SERVER_URL"
githubRepositoryEnv = "GITHUB_REPOSITORY"
githubRunIDEnv = "GITHUB_RUN_ID"
// Terraform plan format
prefixTerraformPlan = "Terraform will perform the following actions:"
)
func makeCommentBody(event *githubEvent, r *PlanPreviewResult) string {
var b strings.Builder
if !r.HasError() {
b.WriteString(successBadgeURL)
} else {
b.WriteString(failureBadgeURL)
}
if actionLogURL := makeActionLogURL(); actionLogURL != "" {
fmt.Fprintf(&b, " ")
fmt.Fprintf(&b, actionBadgeURLFormat, actionLogURL)
}
b.WriteString("\n\n")
if event.IsComment {
b.WriteString(fmt.Sprintf("@%s ", event.SenderLogin))
}
if r.NoChange() {
fmt.Fprintf(&b, noChangeTitleFormat, event.HeadCommit)
return b.String()
}
b.WriteString(fmt.Sprintf(hasChangeTitleFormat, event.HeadCommit, len(r.Applications)))
changedApps, pipelineApps, quickSyncApps := groupApplicationResults(r.Applications)
if len(changedApps)+len(pipelineApps)+len(quickSyncApps) > 0 {
b.WriteString("\n## Plans\n\n")
}
var detailLen int
for _, app := range changedApps {
fmt.Fprintf(&b, "### %s\n", makeTitleText(&app.ApplicationInfo))
fmt.Fprintf(&b, "Sync strategy: %s\n", app.SyncStrategy)
fmt.Fprintf(&b, "Summary: %s\n\n", app.PlanSummary)
var (
lang = "diff"
details = app.PlanDetails
)
if app.ApplicationKind == "TERRAFORM" {
lang = "hcl"
if shortened, err := generateTerraformShortPlanDetails(details); err == nil {
details = shortened
}
}
l := utf8.RuneCountInString(details)
if detailLen+l > detailsLenLimit {
fmt.Fprintf(&b, detailsFormat, lang, detailsOmittedMessage)
detailLen += utf8.RuneCountInString(detailsOmittedMessage)
continue
}
if l > 0 {
detailLen += l
fmt.Fprintf(&b, detailsFormat, lang, details)
}
}
if len(pipelineApps)+len(quickSyncApps) > 0 {
b.WriteString("### No resource changes were detected but the following apps will also be triggered\n")
if len(pipelineApps) > 0 {
b.WriteString("\n###### `PIPELINE`\n")
for _, app := range pipelineApps {
fmt.Fprintf(&b, "\n- %s\n", makeTitleText(&app.ApplicationInfo))
}
}
if len(quickSyncApps) > 0 {
b.WriteString("\n###### `QUICK_SYNC`\n")
for _, app := range quickSyncApps {
fmt.Fprintf(&b, "\n- %s\n", makeTitleText(&app.ApplicationInfo))
}
}
}
if !r.HasError() {
return b.String()
}
fmt.Fprintf(&b, "\n## NOTE\n\n")
if len(r.FailureApplications) > 0 {
fmt.Fprintf(&b, "**An error occurred while building plan-preview for the following applications**\n")
for _, app := range r.FailureApplications {
fmt.Fprintf(&b, "\n### %s\n", makeTitleText(&app.ApplicationInfo))
fmt.Fprintf(&b, "Reason: %s\n\n", app.Reason)
var lang = "diff"
if app.ApplicationKind == "TERRAFORM" {
lang = "hcl"
}
if len(app.PlanDetails) > 0 {
fmt.Fprintf(&b, detailsFormat, lang, app.PlanDetails)
}
}
}
if len(r.FailurePipeds) > 0 {
fmt.Fprintf(&b, "**An error occurred while building plan-preview for applications of the following Pipeds**\n")
for _, piped := range r.FailurePipeds {
fmt.Fprintf(&b, "\n### piped: [%s](%s)\n", piped.PipedID, piped.PipedURL)
fmt.Fprintf(&b, "Reason: %s\n\n", piped.Reason)
}
}
return b.String()
}
func groupApplicationResults(apps []ApplicationResult) (changes, pipelines, quicks []ApplicationResult) {
for _, app := range apps {
if !app.NoChange {
changes = append(changes, app)
continue
}
if app.SyncStrategy == "PIPELINE" {
pipelines = append(pipelines, app)
continue
}
quicks = append(quicks, app)
}
return
}
func makeActionLogURL() string {
serverURL := os.Getenv(githubServerURLEnv)
if serverURL == "" {
return ""
}
repoURL := os.Getenv(githubRepositoryEnv)
if repoURL == "" {
return ""
}
runID := os.Getenv(githubRunIDEnv)
if runID == "" {
return ""
}
return fmt.Sprintf("%s/%s/actions/runs/%s", serverURL, repoURL, runID)
}
func makeTitleText(app *ApplicationInfo) string {
if app.Env == "" {
return fmt.Sprintf(appInfoWithoutEnvFormat, app.ApplicationName, app.ApplicationURL, strings.ToLower(app.ApplicationKind))
}
return fmt.Sprintf(appInfoWithEnvFormat, app.ApplicationName, app.ApplicationURL, app.Env, strings.ToLower(app.ApplicationKind))
}
func generateTerraformShortPlanDetails(details string) (string, error) {
r := strings.NewReader(details)
scanner := bufio.NewScanner(r)
var (
start, length int
newLine = len([]byte("\n"))
)
// NOTE: scanner.Scan() return false if the buffer size of one line exceed bufio.MaxScanTokenSize(65536 byte).
for scanner.Scan() {
line := scanner.Text()
if strings.Contains(line, prefixTerraformPlan) {
start = length
break
}
length += len(scanner.Bytes())
length += newLine
}
if err := scanner.Err(); err != nil {
return "", err
}
return details[start:], nil
}
func minimizePreviousComment(ctx context.Context, ghGraphQLClient *githubv4.Client, event *githubEvent) {
// Find comments we sent before
comment, err := findLatestPlanPreviewComment(ctx, ghGraphQLClient, event.Owner, event.Repo, event.PRNumber)
if err != nil {
log.Printf("Unable to find the previous comment to minimize (%v)\n", err)
}
if comment == nil {
return
}
if bool(comment.IsMinimized) {
log.Println("Previous plan-preview comment has already minimized. So don't minimize anything")
return
}
if err := minimizeComment(ctx, ghGraphQLClient, comment.ID, "OUTDATED"); err != nil {
log.Printf("warning: cannot minimize comment: %s\n", err.Error())
return
}
log.Println("Successfully minimized last plan-preview result on pull request")
} |
package storagemanager;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNull;
import java.security.PublicKey;
import java.util.ArrayList;
import java.util.List;
import javax.crypto.SecretKey;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import crypto.Crypto;
import types.Bark;
import types.Conversation;
import types.DawgIdentifier;
import types.Message;
import types.TestUtils;
public class MapStorageManagerTest {
// runtime-defined test objects
private Bark b;
private DawgIdentifier d;
private Conversation c;
private Message m;
private MapStorageManager mapStorageManager;
@BeforeEach
public void setup() {
b = TestUtils.generateRandomizedBark();
d = TestUtils.generateRandomizedDawgIdentifier();
c = TestUtils.generateRandomizedConversation();
m = TestUtils.generateRandomizedMessage();
this.mapStorageManager = new MapStorageManager();
}
@Test
public void testBarkStorageLifecycle() {
// create the object in the storage manager.
this.mapStorageManager.storeBark(b);
// lookup the object in the storage manager.
final Bark obtainedBark = this.mapStorageManager.lookupBark(b.getUniqueId());
assertEquals(b, obtainedBark);
// successfully delete the object.
this.mapStorageManager.deleteBark(b.getUniqueId());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupBark(b.getUniqueId()));
}
@Test
public void testDawgIdentifierStorageLifecycleUuidMethods() {
// create the object in the storage manager.
this.mapStorageManager.storeDawgIdentifier(d);
// lookup the object in the storage manager.
final DawgIdentifier obtainedDawgIdentifier = this.mapStorageManager.lookupDawgIdentifierForUuid(d.getUUID());
assertEquals(d, obtainedDawgIdentifier);
// successfully delete the object.
this.mapStorageManager.deleteDawgIdentifierByUuid(d.getUUID());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupDawgIdentifierForUuid(d.getUUID()));
}
@Test
public void testDawgIdentifierStorageLifecycleUsernameMethods() {
// create the object in the storage manager.
this.mapStorageManager.storeDawgIdentifier(d);
// lookup the object in the storage manager.
final DawgIdentifier obtainedDawgIdentifier = this.mapStorageManager.lookupDawgIdentifierForUsername(d.getUsername());
assertEquals(d, obtainedDawgIdentifier);
// successfully delete the object.
this.mapStorageManager.deleteDawgIdentifierByUsername(d.getUsername());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupDawgIdentifierForUsername(d.getUsername()));
}
@Test
public void testConversationStorageLifecycle() {
// create the object in the storage manager.
this.mapStorageManager.storeConversation(c);
// lookup the object in the storage manager.
final Conversation obtainedConversation = this.mapStorageManager
.lookupConversation(c.getOtherPerson().getUUID());
assertEquals(c, obtainedConversation);
// successfully delete the object.
this.mapStorageManager.deleteConversation(c.getOtherPerson().getUUID());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupConversation(c.getOtherPerson().getUUID()));
}
@Test
public void testSecretKeyStorageLifecycle() {
// create a List of Key objects to store in the storage manager.
// we want to store the maximum allowed number of Keys.
final List<SecretKey> keyList = new ArrayList<>();
for (int i = 0; i < StorageManager.MAX_NUM_HISTORICAL_KEYS_TO_STORE; i++) {
keyList.add(Crypto.generateSecretKey());
}
// store the Key objects one-by-one in the storage manager and verify that the
// stored List<Key> is updated along the way.
for (int i = 0; i < StorageManager.MAX_NUM_HISTORICAL_KEYS_TO_STORE; i++) {
final SecretKey currentKey = keyList.get(i);
// store the currentKey.
this.mapStorageManager.storeSecretKeyForUUID(d.getUUID(), currentKey);
// verify that the Key was successfully stored in the List.
final List<SecretKey> obtainedKeys = this.mapStorageManager.lookupSecretKeysForUUID(d.getUUID());
assertEquals(currentKey, obtainedKeys.get(obtainedKeys.size() - 1));
assertEquals(i + 1, obtainedKeys.size()); // assert the List contains all Keys added so far.
}
// verify that the oldest key is removed from the stored List when we add Keys
// after hitting the size limit.
final SecretKey extraKey = Crypto.generateSecretKey();
this.mapStorageManager.storeSecretKeyForUUID(d.getUUID(), extraKey);
final List<SecretKey> obtainedKeys = this.mapStorageManager.lookupSecretKeysForUUID(d.getUUID());
// assert that obtainedKeys == the maximum number of Keys we allow to be stored.
assertEquals(StorageManager.MAX_NUM_HISTORICAL_KEYS_TO_STORE, obtainedKeys.size());
// assert that the returned List is as follows:
// - contains all contents of the original List except the first element
// - each element should be shifted down one index
// - the extraKey is appended to the end of the List
final List<SecretKey> expectedKeyList = new ArrayList<>(keyList);
expectedKeyList.remove(0);
expectedKeyList.add(extraKey);
assertEquals(expectedKeyList, obtainedKeys);
// successfully delete the object.
this.mapStorageManager.deleteSecretKeysForUUID(d.getUUID());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupSecretKeysForUUID(d.getUUID()));
}
@Test
public void testPublicKeyStorageLifecycle() {
final PublicKey publicKey = Crypto.ALICE_KEYPAIR.getPublic();
// store the key
this.mapStorageManager.storePublicKeyForUUID(d.getUUID(), publicKey);
// verify that the Key was successfully stored in the List.
final PublicKey obtainedKey = this.mapStorageManager.lookupPublicKeyForUUID(d.getUUID());
assertEquals(publicKey, obtainedKey);
// delete the key.
this.mapStorageManager.deleteSecretKeysForUUID(d.getUUID());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupSecretKeysForUUID(d.getUUID()));
}
@Test
public void testMessageStorageLifecycle() {
// create the object in the storage manager.
this.mapStorageManager.storeMessage(m);
// lookup the object in the storage manager.
final Message obtainedMessage = this.mapStorageManager.lookupMessage(m.getUniqueId());
assertEquals(m, obtainedMessage);
// successfully delete the object.
this.mapStorageManager.deleteMessage(m.getUniqueId());
// verify that the object was deleted.
assertNull(this.mapStorageManager.lookupMessage(m.getUniqueId()));
}
@Test
public void testListConversations_allConversationsAreListed() {
// create the object in the storage manager.
this.mapStorageManager.storeConversation(c);
// lookup the object in the storage manager.
final Conversation obtainedConversation = this.mapStorageManager.listAllConversations().get(0);
assertEquals(c, obtainedConversation);
}
} |
<!DOCTYPE html>
<html lang="pt-br">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="/Estilizando_Paginas_com_CSS/aula1.css">
<title>Páginas Web com HTML.</title>
</head>
<body>
<header>
<h1>Páginas Web com HTML.</h1>
<p>Básico - 2 Horas</p>
</header>
<main>
<h2>Tags Básicas</h2>
<ul>
<li>
<h1>, <h2>, <h3>, <h4>, <h5>, <h6> são: TAGS para definir um titulo e subtitulos, variando de 1 a 6, sendo h1 o título mais importante e h6 o de menos importância. <br>
</li>
<li>
<code><p></code> - Tags para definir paragrafo. <br>
</li>
<li>
<code><Header></code> - define cabeçalho. <br>
<section> - Define uma seção.
</li>
<li>
<code><article></code> - define um artigo.
</li>
<li>
<code><a></code> - links servem para nossa página ou acessar uma página externa. <br>
<p>Exemplo: <br>
<a href="https://www.google.com/" target="_blank">Clique aqui para acessar a página do google</a>, usar atributo <code><i>target</i>="_blank"</code> para abrir a página em outra aba. </p>
</li>
<li>
<code><div></code> define uma divisão.</li>
<li>
<code><footer></code> - define um rodapé
</li>
<li>
<code><nav></code> - define uma área de navegacão (como menus);
</li>
<li>
<code><table></code> - define uma tabela;
</li>
<li>
<code><ol></code> - define uma lista ordenada;
</li>
<li>
<code><ul></code> - define uma lista não ordenada;
</li>
<li>
<code><li></code> - define o item de uma lista;
</li>
<li>
<code><form></code> - define um formulário;
</li>
<li>
<code><input></code> - define os campos do formulário.
</li>
<li><code><textarea></code> - define uma área para o usuário digitar um texto. <br> Exemplo:</li>
<textarea name="text" id="text" cols="30" rows="10"></textarea>
<li>
<code><button></code> - define um botão. <br>
<button>Esse e um Botão</button>
</li>
<li>
<code><img></code> - permite inserir uma imagem no seu documento.
</li>
<p>
Para saber mais sobre todas as tags disponiveis você deve consultar o site oficial do <a href="https://www.w3schools.com" target="_blank"><img src="https://internet-salmagundi.com/wp-content/uploads/2019/03/W3-MatFrame-895x493px-Qual100.jpg" alt="logow3" width="250" height="150" ></a>
</p>
</ul>
<h2>
O que são MetaTags
</h2>
<p>
As meta tags em HTML são elementos usados para fornecer informações adicionais sobre uma página da web. Elas são colocadas no cabeçalho (head) do documento HTML e não são visíveis na página quando ela é exibida no navegador. Em vez disso, as meta tags fornecem metadados que descrevem características da página ou fornecem informações adicionais para os navegadores, motores de busca e outros serviços web. <br><br>
Alguns exemplos comuns de meta tags incluem:
</p>
<ol>
<li><strong>Meta Tag de Charset:</strong></li>
<code style="background-color: black; color: white;"><meta charset="UTF-8">
</code> --
Define a codificação de caracteres da página.
</ol>
<h2>
Listas
</h2>
<p>
As listas são muito importantes quando queremos listas alguns itens no site e também para a criação de menu de navegação. <br><br>
<h3>- Listas Ordenadas </h3>
<div>
<ol>
<li>Primeiro item</li>
<li>Segundo Item</li>
<li> Terceiro item</li>
</ol>
</div>
<hr>
<h3> - Listas não ordenadas</h3>
<div>
<ul>
<li>Item A</li>
<li>Item B</li>
<li>Item C</li>
</ul>
</div>
<hr>
<h3>- Listas por aninhadas </h3>
<div>
<ol>
<li> Item 1 </li>
<li>Segundo item
<ol>
<li>segundo item primeiro subitem</li>
<li>segundo item segundo subitem</li>
<li>segundo item terceiro subitem</li>
</li>
</ol>
</li>
<li>Terceiro item</li>
</ol>
<hr>
</div>
<h3>- Listas por definição</h3>
<div>
<dl>
<dt>sapo</dt>
<dd>Criatura pegajosa.</dd>
</dl>
</div>
</p> <br><br>
<h2>Tabelas</h2>
<p>
Tabelas são listas de dados em duas dimensões e são compostas por linhas e colunas. Portanto, são muito utilizadas para apresentar dados de uma forma organizada.
</p>
<h3>Exemplo de Tabela</h3>
<div>
<table border="2">
<thead>
<tr>
<th>Código</th>
<th>Nome</th>
<th>Idade</th>
</tr>
</thead>
<tbody>
<tr>
<td>01</td>
<td>Jõao</td>
<td>25</td>
</tr>
<tr>
<td>02</td>
<td>José</td>
<td>33</td>
</tr>
<tfoot>
<tr>
<td colspan="3">Soma da idade = 58</td>
</tr>
</tfoot>
</tbody>
</table>
</div>
<h2>Formularios</h2>
<p>O formulário em HTMl é um documento de preenchimento de dados ou que resulta em uma ação desejada utilizando a linguagem de marcação HTML. É formado por um ou mais campos. Esses campos podem ser de textos, caixas de seleção botões, radio buttons e checkboxes utilizando tags do próprio html. Dessa forma, o usuário pode interagir com a página ao executar ações através desses formulários.</p>
<h3>Exemplos.</h3>
<h4>Deixei seus dados Abaixo</h4>
<div>
<form action="##" method="get">
<div>
<label for="nome">Nome</label>
<br>
<input type="text" id="nome" name="nome">
</div>
<div>
<label for="email">E-mail</label>
<br>
<input type="email" name="email" id="email">
</div>
<div>
<label for="CPF">CPF</label>
<br>
<input type="number" name="number" id="number">
</div>
<p> Para conhecer todas os inputs consulte o site oficial da <a href="https://www.w3schools.com/html/html_forms.asp" target="_blank">w3schools.</a></p>
</form>
</div>
<h2>Html Semântico</h2>
<p>O HTML semântico é a forma de deixar o site com suas informações bem explicadas e compreensíveis para o computador, ajudando até mesmo a sua busca no google e facilitando o entendimento de leitores de acessibilidade.</p>
<h3>Porque Utilizar o HTML semântico?</h3>
<p>Além de facilitar a busca de forma orgânica e rankear sua página em mecanismos de busca, o HTML semântico ajuda os leitores de tela para deficientes visuais, facilitando seu compreendimento, Ele também, deixa seu código mais limpo e mais compeensivel, tanto em organização como em facilidade de vizualizar uma tag específica só de passar o olho pela tela.</p>
<img id="antes" src="/paginas_web_com_HTML/img/semantica.png" alt="foto antes e depois">
<br><br>
<h2>Parabéns pela conclusão do curso de HTML!</h2>
<p>Ao longo dessas duas horas de aprendizado, você adquiriu conhecimentos fundamentais que são a base para a construção de páginas web. As tags básicas, como <h1> a <h6>, <p>, <header>, <section>, <article>, <a>, <div>, <footer>, <nav>, <table>, <ol>, <ul>, <li>, <form>, <input>, <textarea>, <button>, <img>, e muitas outras, agora fazem parte do seu repertório, permitindo criar estruturas e layouts para suas páginas.</p>
<p>A compreensão das MetaTags adiciona uma camada importante ao seu entendimento, proporcionando a capacidade de fornecer informações adicionais sobre suas páginas para navegadores e motores de busca.</p>
<p>Você também explorou a importância das listas na organização de conteúdo, as tabelas para apresentação de dados estruturados, e os formulários, que são essenciais para interação do usuário.</p>
<p>Além disso, a incorporação de conceitos de HTML Semântico destaca a relevância de estruturar o conteúdo de forma clara e compreensível não apenas para os motores de busca, mas também para leitores de tela e pessoas com deficiência visual. Essa prática não só contribui para uma melhor experiência do usuário, mas também para a otimização nos resultados de busca.</p>
<p>Ao comparar o "antes e depois" de suas habilidades, certamente notou uma evolução significativa na forma como concebe e desenvolve suas páginas web. Continue explorando e praticando, e esteja sempre atento às novas possibilidades e atualizações na área. O conhecimento em HTML é uma base sólida para construir sua jornada no mundo do desenvolvimento web. Parabéns novamente pelo esforço e dedicação!</p>
<br>
<br>
<h2 id="curse">Outros cursos</h2>
<div class="cursos">
<div class="module">
<a href="/Estilizando_Paginas_com_CSS/css.html" target="_blank"><img class="logos" src="/paginas_web_com_HTML/img/css_logo.webp" alt="logocss"></a>
</div>
<div class="module">
<a href="/paginas_web_com_HTML/teste.html" target="_blank"><img class="logos" src="/paginas_web_com_HTML/img/js_logo.png" alt="logoJavaScript"></a>
</div>
</div>
<br><br>
</main>
<footer>
<div>
Desenvolvido por Jaelson Freitas - 2023
</div>
</footer>
</body>
</html> |
import { BrowserRouter, Routes, Route } from "react-router-dom"
import Footer from "./components/Footer"
import Navbar from "./components/Navbar"
import About from "./pages/about/About"
import Contact from "./pages/contact/Contact"
import Gallery from "./pages/gallery/Gallery"
import Home from "./pages/home/Home"
import NotFound from "./pages/notFound/NotFound"
import Plans from "./pages/plans/Plans"
import Trainers from "./pages/trainers/Trainers"
import ScrollTop from "./components/ScrollTop"
const App = () => {
return (
<BrowserRouter>
<ScrollTop/>
<Navbar />
<Routes>
<Route index element={<Home/>}/>
<Route path="about" element={<About/>}/>
<Route path="contact" element={<Contact/>}/>
<Route path="gallery" element={<Gallery/>}/>
<Route path="plans" element={<Plans/>}/>
<Route path="trainers" element={<Trainers/>}/>
<Route path="*" element={<NotFound/>}/>
</Routes>
<Footer/>
</BrowserRouter>
)
}
export default App |
import React, { Component } from "react";
import { connect } from "react-redux";
import {
StyleSheet,
Text,
View,
TextInput,
TouchableOpacity,
KeyboardAvoidingView
} from "react-native";
import { addDeck } from "../../actions/decks";
import { saveDeck } from "../../utils/api";
import { globalStyles } from "../../utils/styles";
class DeckNew extends Component {
state = {
title: ""
};
handleChange = deckTitle => {
this.setState(() => ({ title: deckTitle }));
};
handleSubmit = () => {
const { title } = this.state;
const { dispatch, navigation } = this.props;
const deck = {
title,
questions: []
};
saveDeck(deck)
.then(dispatch(addDeck(deck)))
.then(navigation.pop());
};
render() {
return (
<KeyboardAvoidingView behavior="padding" style={{ paddingBottom: 100 }}>
<Text style={globalStyles.title}>New Deck</Text>
<View style={globalStyles.deck}>
<TextInput
style={globalStyles.input}
underlineColorAndroid="transparent"
onChangeText={this.handleChange}
value={this.state.title}
placeholder="Deck Name"
/>
<TouchableOpacity
style={[globalStyles.button, globalStyles.submitButton]}
disabled={this.state.title.length > 1 ? false : true}
onPress={this.handleSubmit}
>
<Text style={globalStyles.submitButtonText}>Submit</Text>
</TouchableOpacity>
</View>
</KeyboardAvoidingView>
);
}
}
export default connect()(DeckNew); |
import time
from copy import deepcopy
from dataclasses import dataclass
from enum import Enum
from random import randint
from pygame import constants
from pygame.event import Event
from game.settings import (
IMAGE_PLAYER_WIDTH,
SCREEN_WIDTH,
WALL_WIDTH,
IMAGE_PLAYER_HEIGHT,
SCREEN_HEIGHT,
MAX_Y_PLAYER_VELOCITY,
)
from game.sprites.player import IcyTowerSprite
from game.states.base import State
@dataclass
class Coordinates:
x: float
y: float
class DirectionEnum(str, Enum):
LEFT = "left"
RIGHT = "right"
NONE = "none"
@dataclass
class MovementState:
direction: DirectionEnum = DirectionEnum.NONE
blocked_direction: DirectionEnum = DirectionEnum.NONE
speed: int = 0.5
velocity: int = 0
max_velocity: int = 7
jump: bool = False
jump_count: int = 0
coordinates: Coordinates = Coordinates(0.0, 0.0)
gravity: float = 0.5
y_velocity: float = 0
max_y_velocity: int = MAX_Y_PLAYER_VELOCITY
def reset_direction(self):
self.direction = DirectionEnum.NONE
def reset_velocity(self):
self.velocity = 0
def bump_velocity(self):
if self.velocity < self.max_velocity:
self.velocity += self.speed
else:
print("Max speed....")
class PlayerState(State):
def __init__(self, screen, image_store, platforms, walls):
super().__init__(screen, image_store)
self.sprite = IcyTowerSprite(
screen=self.screen.screen,
image=self.image_store.icy_tower_image.image,
x=randint(
int(WALL_WIDTH) + 10,
int(SCREEN_WIDTH - IMAGE_PLAYER_WIDTH - WALL_WIDTH),
),
y=SCREEN_HEIGHT - IMAGE_PLAYER_HEIGHT * 2,
)
self._movement_state = MovementState(
coordinates=Coordinates(self.sprite.rect.x, self.sprite.rect.y)
)
self._platforms = platforms
self._walls = walls
def handle_event(self, event: Event):
if hasattr(event, "key"):
self.handle_key(event.key, event.type)
def handle_key(self, key, event_type):
reset = event_type == constants.KEYUP
match key:
case constants.K_LEFT:
self._set_direction_or_reset(DirectionEnum.LEFT, reset)
self._movement_state.jump = False
case constants.K_RIGHT:
self._set_direction_or_reset(DirectionEnum.RIGHT, reset)
self._movement_state.jump = False
case constants.K_SPACE | constants.K_UP:
if not reset and not self._movement_state.jump:
self._movement_state.jump = True
self._movement_state.jump_time = time.time()
else:
self._movement_state.jump = False
def _set_direction_or_reset(self, direction: DirectionEnum, reset=False):
if reset:
self._movement_state.reset_direction()
self._movement_state.reset_velocity()
self._movement_state.blocked_direction = DirectionEnum.NONE
elif self._movement_state.direction == direction:
self._movement_state.bump_velocity()
else:
self._movement_state.direction = direction
self._movement_state.bump_velocity()
def _set_x_coordinate(self, new_value):
if self.sprite.collide_with_any_wall(new_value, self._walls):
self._movement_state.reset_velocity()
else:
self._movement_state.coordinates.x = new_value
def update(self):
if self._movement_state.direction == DirectionEnum.LEFT:
self._set_x_coordinate(
deepcopy(self._movement_state.coordinates.x)
- self._movement_state.velocity
)
else:
self._set_x_coordinate(
deepcopy(self._movement_state.coordinates.x)
+ self._movement_state.velocity
)
if (
self._movement_state.jump
and self._movement_state.y_velocity <= self._movement_state.max_y_velocity
):
self._movement_state.y_velocity += self._movement_state.gravity
self._movement_state.coordinates.y -= self._movement_state.gravity
elif (
not self.sprite.collide_any(self._platforms, "collide_platform")
and not self._movement_state.jump
):
self._movement_state.coordinates.y += self._movement_state.y_velocity
self._movement_state.y_velocity = 0
self.sprite.rect.x = round(self._movement_state.coordinates.x)
self.sprite.rect.y = round(self._movement_state.coordinates.y)
def render(self):
self.sprite.draw() |
package entities;
import javax.persistence.*;
import java.util.List;
@Entity
@Table(name = "curso")
public class Curso {
@Id
@GeneratedValue
private Long id;
private String nome;
private String sigla;
@OneToOne(cascade = CascadeType.ALL)
private MaterialCurso materialCurso;
@ManyToMany
@JoinTable(name="curso_aluno",
joinColumns={@JoinColumn(name="curso_id")},
inverseJoinColumns={@JoinColumn(name="aluno_id")})
private List<Aluno> alunos;
@OneToOne(cascade = CascadeType.ALL)
private Professor professor;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getNome() {
return nome;
}
public void setNome(String nome) {
this.nome = nome;
}
public String getSigla() {
return sigla;
}
public void setSigla(String sigla) {
this.sigla = sigla;
}
public MaterialCurso getMaterialCurso() {
return materialCurso;
}
public void setMaterialCurso(MaterialCurso materialCurso) {
this.materialCurso = materialCurso;
}
public List<Aluno> getAlunos() {
return alunos;
}
public void setAlunos(List<Aluno> alunos) {
this.alunos = alunos;
}
public Professor getProfessor() {
return professor;
}
public void setProfessor(Professor professor) {
this.professor = professor;
}
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.