files
dict |
---|
{
"content": "Title: renderToStaticMarkup – React\n\nURL Source: https://react.dev/reference/react-dom/server/renderToStaticMarkup\n\nMarkdown Content:\nrenderToStaticMarkup – React\n===============\n\nrenderToStaticMarkup[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#undefined \"Link for this heading\")\n===========================================================================================================================\n\n`renderToStaticMarkup` renders a non-interactive React tree to an HTML string.\n\n```\nconst html = renderToStaticMarkup(reactNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/server/renderToStaticMarkup#reference)\n * [`renderToStaticMarkup(reactNode, options?)`](https://react.dev/reference/react-dom/server/renderToStaticMarkup#rendertostaticmarkup)\n* [Usage](https://react.dev/reference/react-dom/server/renderToStaticMarkup#usage)\n * [Rendering a non-interactive React tree as HTML to a string](https://react.dev/reference/react-dom/server/renderToStaticMarkup#rendering-a-non-interactive-react-tree-as-html-to-a-string)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------------------------\n\n### `renderToStaticMarkup(reactNode, options?)`[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#rendertostaticmarkup \"Link for this heading\")\n\nOn the server, call `renderToStaticMarkup` to render your app to HTML.\n\n```\nimport { renderToStaticMarkup } from 'react-dom/server';const html = renderToStaticMarkup(<Page />);\n```\n\nIt will produce non-interactive HTML output of your React components.\n\n[See more examples below.](https://react.dev/reference/react-dom/server/renderToStaticMarkup#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#parameters \"Link for Parameters \")\n\n* `reactNode`: A React node you want to render to HTML. For example, a JSX node like `<Page />`.\n* **optional** `options`: An object for server render.\n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page.\n\n#### Returns[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#returns \"Link for Returns \")\n\nAn HTML string.\n\n#### Caveats[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#caveats \"Link for Caveats \")\n\n* `renderToStaticMarkup` output cannot be hydrated.\n \n* `renderToStaticMarkup` has limited Suspense support. If a component suspends, `renderToStaticMarkup` immediately sends its fallback as HTML.\n \n* `renderToStaticMarkup` works in the browser, but using it in the client code is not recommended. If you need to render a component to HTML in the browser, [get the HTML by rendering it into a DOM node.](https://react.dev/reference/react-dom/server/renderToString#removing-rendertostring-from-the-client-code)\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#usage \"Link for Usage \")\n--------------------------------------------------------------------------------------------------\n\n### Rendering a non-interactive React tree as HTML to a string[](https://react.dev/reference/react-dom/server/renderToStaticMarkup#rendering-a-non-interactive-react-tree-as-html-to-a-string \"Link for Rendering a non-interactive React tree as HTML to a string \")\n\nCall `renderToStaticMarkup` to render your app to an HTML string which you can send with your server response:\n\n```\nimport { renderToStaticMarkup } from 'react-dom/server';// The route handler syntax depends on your backend frameworkapp.use('/', (request, response) => { const html = renderToStaticMarkup(<Page />); response.send(html);});\n```\n\nThis will produce the initial non-interactive HTML output of your React components.\n\n### Pitfall\n\nThis method renders **non-interactive HTML that cannot be hydrated.** This is useful if you want to use React as a simple static page generator, or if you’re rendering completely static content like emails.\n\nInteractive apps should use [`renderToString`](https://react.dev/reference/react-dom/server/renderToString) on the server and [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) on the client.\n\n[PreviousrenderToReadableStream](https://react.dev/reference/react-dom/server/renderToReadableStream)[NextrenderToStaticNodeStream](https://react.dev/reference/react-dom/server/renderToStaticNodeStream)\n",
"filename": "renderToStaticMarkup.md",
"package": "react"
} |
{
"content": "Title: renderToStaticNodeStream – React\n\nURL Source: https://react.dev/reference/react-dom/server/renderToStaticNodeStream\n\nMarkdown Content:\nrenderToStaticNodeStream – React\n===============\n\nrenderToStaticNodeStream[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#undefined \"Link for this heading\")\n===================================================================================================================================\n\n`renderToStaticNodeStream` renders a non-interactive React tree to a [Node.js Readable Stream.](https://nodejs.org/api/stream.html#readable-streams)\n\n```\nconst stream = renderToStaticNodeStream(reactNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#reference)\n * [`renderToStaticNodeStream(reactNode, options?)`](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#rendertostaticnodestream)\n* [Usage](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#usage)\n * [Rendering a React tree as static HTML to a Node.js Readable Stream](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#rendering-a-react-tree-as-static-html-to-a-nodejs-readable-stream)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------------------------\n\n### `renderToStaticNodeStream(reactNode, options?)`[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#rendertostaticnodestream \"Link for this heading\")\n\nOn the server, call `renderToStaticNodeStream` to get a [Node.js Readable Stream](https://nodejs.org/api/stream.html#readable-streams).\n\n```\nimport { renderToStaticNodeStream } from 'react-dom/server';const stream = renderToStaticNodeStream(<Page />);stream.pipe(response);\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#usage)\n\nThe stream will produce non-interactive HTML output of your React components.\n\n#### Parameters[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#parameters \"Link for Parameters \")\n\n* `reactNode`: A React node you want to render to HTML. For example, a JSX element like `<Page />`.\n \n* **optional** `options`: An object for server render.\n \n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page.\n\n#### Returns[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#returns \"Link for Returns \")\n\nA [Node.js Readable Stream](https://nodejs.org/api/stream.html#readable-streams) that outputs an HTML string. The resulting HTML can’t be hydrated on the client.\n\n#### Caveats[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#caveats \"Link for Caveats \")\n\n* `renderToStaticNodeStream` output cannot be hydrated.\n \n* This method will wait for all [Suspense boundaries](https://react.dev/reference/react/Suspense) to complete before returning any output.\n \n* As of React 18, this method buffers all of its output, so it doesn’t actually provide any streaming benefits.\n \n* The returned stream is a byte stream encoded in utf-8. If you need a stream in another encoding, take a look at a project like [iconv-lite](https://www.npmjs.com/package/iconv-lite), which provides transform streams for transcoding text.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#usage \"Link for Usage \")\n------------------------------------------------------------------------------------------------------\n\n### Rendering a React tree as static HTML to a Node.js Readable Stream[](https://react.dev/reference/react-dom/server/renderToStaticNodeStream#rendering-a-react-tree-as-static-html-to-a-nodejs-readable-stream \"Link for Rendering a React tree as static HTML to a Node.js Readable Stream \")\n\nCall `renderToStaticNodeStream` to get a [Node.js Readable Stream](https://nodejs.org/api/stream.html#readable-streams) which you can pipe to your server response:\n\n```\nimport { renderToStaticNodeStream } from 'react-dom/server';// The route handler syntax depends on your backend frameworkapp.use('/', (request, response) => { const stream = renderToStaticNodeStream(<Page />); stream.pipe(response);});\n```\n\nThe stream will produce the initial non-interactive HTML output of your React components.\n\n### Pitfall\n\nThis method renders **non-interactive HTML that cannot be hydrated.** This is useful if you want to use React as a simple static page generator, or if you’re rendering completely static content like emails.\n\nInteractive apps should use [`renderToPipeableStream`](https://react.dev/reference/react-dom/server/renderToPipeableStream) on the server and [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) on the client.\n\n[PreviousrenderToStaticMarkup](https://react.dev/reference/react-dom/server/renderToStaticMarkup)[NextrenderToString](https://react.dev/reference/react-dom/server/renderToString)\n",
"filename": "renderToStaticNodeStream.md",
"package": "react"
} |
{
"content": "Title: renderToString – React\n\nURL Source: https://react.dev/reference/react-dom/server/renderToString\n\nMarkdown Content:\n### Pitfall\n\n`renderToString` does not support streaming or waiting for data. [See the alternatives.](https://react.dev/reference/react-dom/server/renderToString#alternatives)\n\n`renderToString` renders a React tree to an HTML string.\n\n```\nconst html = renderToString(reactNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/server/renderToString#reference)\n * [`renderToString(reactNode, options?)`](https://react.dev/reference/react-dom/server/renderToString#rendertostring)\n* [Usage](https://react.dev/reference/react-dom/server/renderToString#usage)\n * [Rendering a React tree as HTML to a string](https://react.dev/reference/react-dom/server/renderToString#rendering-a-react-tree-as-html-to-a-string)\n* [Alternatives](https://react.dev/reference/react-dom/server/renderToString#alternatives)\n * [Migrating from `renderToString` to a streaming method on the server](https://react.dev/reference/react-dom/server/renderToString#migrating-from-rendertostring-to-a-streaming-method-on-the-server)\n * [Removing `renderToString` from the client code](https://react.dev/reference/react-dom/server/renderToString#removing-rendertostring-from-the-client-code)\n* [Troubleshooting](https://react.dev/reference/react-dom/server/renderToString#troubleshooting)\n * [When a component suspends, the HTML always contains a fallback](https://react.dev/reference/react-dom/server/renderToString#when-a-component-suspends-the-html-always-contains-a-fallback)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/server/renderToString#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------------------\n\n### `renderToString(reactNode, options?)`[](https://react.dev/reference/react-dom/server/renderToString#rendertostring \"Link for this heading\")\n\nOn the server, call `renderToString` to render your app to HTML.\n\n```\nimport { renderToString } from 'react-dom/server';const html = renderToString(<App />);\n```\n\nOn the client, call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to make the server-generated HTML interactive.\n\n[See more examples below.](https://react.dev/reference/react-dom/server/renderToString#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/server/renderToString#parameters \"Link for Parameters \")\n\n* `reactNode`: A React node you want to render to HTML. For example, a JSX node like `<App />`.\n \n* **optional** `options`: An object for server render.\n \n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as passed to [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot#parameters)\n\n#### Returns[](https://react.dev/reference/react-dom/server/renderToString#returns \"Link for Returns \")\n\nAn HTML string.\n\n#### Caveats[](https://react.dev/reference/react-dom/server/renderToString#caveats \"Link for Caveats \")\n\n* `renderToString` has limited Suspense support. If a component suspends, `renderToString` immediately sends its fallback as HTML.\n \n* `renderToString` works in the browser, but using it in the client code is [not recommended.](https://react.dev/reference/react-dom/server/renderToString#removing-rendertostring-from-the-client-code)\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/server/renderToString#usage \"Link for Usage \")\n--------------------------------------------------------------------------------------------\n\n### Rendering a React tree as HTML to a string[](https://react.dev/reference/react-dom/server/renderToString#rendering-a-react-tree-as-html-to-a-string \"Link for Rendering a React tree as HTML to a string \")\n\nCall `renderToString` to render your app to an HTML string which you can send with your server response:\n\n```\nimport { renderToString } from 'react-dom/server';// The route handler syntax depends on your backend frameworkapp.use('/', (request, response) => {const html = renderToString(<App />);response.send(html);});\n```\n\nThis will produce the initial non-interactive HTML output of your React components. On the client, you will need to call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to _hydrate_ that server-generated HTML and make it interactive.\n\n### Pitfall\n\n`renderToString` does not support streaming or waiting for data. [See the alternatives.](https://react.dev/reference/react-dom/server/renderToString#alternatives)\n\n* * *\n\nAlternatives[](https://react.dev/reference/react-dom/server/renderToString#alternatives \"Link for Alternatives \")\n-----------------------------------------------------------------------------------------------------------------\n\n### Migrating from `renderToString` to a streaming method on the server[](https://react.dev/reference/react-dom/server/renderToString#migrating-from-rendertostring-to-a-streaming-method-on-the-server \"Link for this heading\")\n\n`renderToString` returns a string immediately, so it does not support streaming or waiting for data.\n\nWhen possible, we recommend using these fully-featured alternatives:\n\n* If you use Node.js, use [`renderToPipeableStream`.](https://react.dev/reference/react-dom/server/renderToPipeableStream)\n* If you use Deno or a modern edge runtime with [Web Streams](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API), use [`renderToReadableStream`.](https://react.dev/reference/react-dom/server/renderToReadableStream)\n\nYou can continue using `renderToString` if your server environment does not support streams.\n\n* * *\n\n### Removing `renderToString` from the client code[](https://react.dev/reference/react-dom/server/renderToString#removing-rendertostring-from-the-client-code \"Link for this heading\")\n\nSometimes, `renderToString` is used on the client to convert some component to HTML.\n\n```\n// 🚩 Unnecessary: using renderToString on the clientimport { renderToString } from 'react-dom/server';const html = renderToString(<MyIcon />);console.log(html); // For example, \"<svg>...</svg>\"\n```\n\nImporting `react-dom/server` **on the client** unnecessarily increases your bundle size and should be avoided. If you need to render some component to HTML in the browser, use [`createRoot`](https://react.dev/reference/react-dom/client/createRoot) and read HTML from the DOM:\n\n```\nimport { createRoot } from 'react-dom/client';import { flushSync } from 'react-dom';const div = document.createElement('div');const root = createRoot(div);flushSync(() => {root.render(<MyIcon />);});console.log(div.innerHTML); // For example, \"<svg>...</svg>\"\n```\n\nThe [`flushSync`](https://react.dev/reference/react-dom/flushSync) call is necessary so that the DOM is updated before reading its [`innerHTML`](https://developer.mozilla.org/en-US/docs/Web/API/Element/innerHTML) property.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react-dom/server/renderToString#troubleshooting \"Link for Troubleshooting \")\n--------------------------------------------------------------------------------------------------------------------------\n\n### When a component suspends, the HTML always contains a fallback[](https://react.dev/reference/react-dom/server/renderToString#when-a-component-suspends-the-html-always-contains-a-fallback \"Link for When a component suspends, the HTML always contains a fallback \")\n\n`renderToString` does not fully support Suspense.\n\nIf some component suspends (for example, because it’s defined with [`lazy`](https://react.dev/reference/react/lazy) or fetches data), `renderToString` will not wait for its content to resolve. Instead, `renderToString` will find the closest [`<Suspense>`](https://react.dev/reference/react/Suspense) boundary above it and render its `fallback` prop in the HTML. The content will not appear until the client code loads.\n\nTo solve this, use one of the [recommended streaming solutions.](https://react.dev/reference/react-dom/server/renderToString#migrating-from-rendertostring-to-a-streaming-method-on-the-server) They can stream content in chunks as it resolves on the server so that the user sees the page being progressively filled in before the client code loads.\n",
"filename": "renderToString.md",
"package": "react"
} |
{
"content": "Title: Rendering Lists – React\n\nURL Source: https://react.dev/learn/rendering-lists\n\nMarkdown Content:\nYou will often want to display multiple similar components from a collection of data. You can use the [JavaScript array methods](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array#) to manipulate an array of data. On this page, you’ll use [`filter()`](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) and [`map()`](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/map) with React to filter and transform your array of data into an array of components.\n\n### You will learn\n\n* How to render components from an array using JavaScript’s `map()`\n* How to render only specific components using JavaScript’s `filter()`\n* When and why to use React keys\n\nRendering data from arrays[](https://react.dev/learn/rendering-lists#rendering-data-from-arrays \"Link for Rendering data from arrays \")\n---------------------------------------------------------------------------------------------------------------------------------------\n\nSay that you have a list of content.\n\n```\n<ul><li>Creola Katherine Johnson: mathematician</li><li>Mario José Molina-Pasquel Henríquez: chemist</li><li>Mohammad Abdus Salam: physicist</li><li>Percy Lavon Julian: chemist</li><li>Subrahmanyan Chandrasekhar: astrophysicist</li></ul>\n```\n\nThe only difference among those list items is their contents, their data. You will often need to show several instances of the same component using different data when building interfaces: from lists of comments to galleries of profile images. In these situations, you can store that data in JavaScript objects and arrays and use methods like [`map()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) and [`filter()`](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) to render lists of components from them.\n\nHere’s a short example of how to generate a list of items from an array:\n\n1. **Move** the data into an array:\n\n```\nconst people = ['Creola Katherine Johnson: mathematician','Mario José Molina-Pasquel Henríquez: chemist','Mohammad Abdus Salam: physicist','Percy Lavon Julian: chemist','Subrahmanyan Chandrasekhar: astrophysicist'];\n```\n\n2. **Map** the `people` members into a new array of JSX nodes, `listItems`:\n\n```\nconst listItems = people.map(person => <li>{person}</li>);\n```\n\n3. **Return** `listItems` from your component wrapped in a `<ul>`:\n\n```\nreturn <ul>{listItems}</ul>;\n```\n\nHere is the result:\n\nNotice the sandbox above displays a console error:\n\nWarning: Each child in a list should have a unique “key” prop.\n\nYou’ll learn how to fix this error later on this page. Before we get to that, let’s add some structure to your data.\n\nFiltering arrays of items[](https://react.dev/learn/rendering-lists#filtering-arrays-of-items \"Link for Filtering arrays of items \")\n------------------------------------------------------------------------------------------------------------------------------------\n\nThis data can be structured even more.\n\n```\nconst people = [{id: 0,name: 'Creola Katherine Johnson',profession: 'mathematician',}, {id: 1,name: 'Mario José Molina-Pasquel Henríquez',profession: 'chemist',}, {id: 2,name: 'Mohammad Abdus Salam',profession: 'physicist',}, {id: 3,name: 'Percy Lavon Julian',profession: 'chemist', }, {id: 4,name: 'Subrahmanyan Chandrasekhar',profession: 'astrophysicist',}];\n```\n\nLet’s say you want a way to only show people whose profession is `'chemist'`. You can use JavaScript’s `filter()` method to return just those people. This method takes an array of items, passes them through a “test” (a function that returns `true` or `false`), and returns a new array of only those items that passed the test (returned `true`).\n\nYou only want the items where `profession` is `'chemist'`. The “test” function for this looks like `(person) => person.profession === 'chemist'`. Here’s how to put it together:\n\n1. **Create** a new array of just “chemist” people, `chemists`, by calling `filter()` on the `people` filtering by `person.profession === 'chemist'`:\n\n```\nconst chemists = people.filter(person =>person.profession === 'chemist');\n```\n\n2. Now **map** over `chemists`:\n\n```\nconst listItems = chemists.map(person =><li><imgsrc={getImageUrl(person)}alt={person.name}/><p><b>{person.name}:</b>{' ' + person.profession + ' '} known for {person.accomplishment}</p></li>);\n```\n\n3. Lastly, **return** the `listItems` from your component:\n\n```\nreturn <ul>{listItems}</ul>;\n```\n\nimport { people } from './data.js';\nimport { getImageUrl } from './utils.js';\n\nexport default function List() {\n const chemists = people.filter(person \\=>\n person.profession === 'chemist'\n );\n const listItems = chemists.map(person \\=>\n <li\\>\n <img\n src\\={getImageUrl(person)}\n alt\\={person.name}\n />\n <p\\>\n <b\\>{person.name}:</b\\>\n {' ' + person.profession + ' '}\n known for {person.accomplishment}\n </p\\>\n </li\\>\n );\n return <ul\\>{listItems}</ul\\>;\n}\n\n### Pitfall\n\nArrow functions implicitly return the expression right after `=>`, so you didn’t need a `return` statement:\n\n```\nconst listItems = chemists.map(person =><li>...</li> // Implicit return!);\n```\n\nHowever, **you must write `return` explicitly if your `=>` is followed by a `{` curly brace!**\n\n```\nconst listItems = chemists.map(person => { // Curly bracereturn <li>...</li>;});\n```\n\nArrow functions containing `=> {` are said to have a [“block body”.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions#function_body) They let you write more than a single line of code, but you _have to_ write a `return` statement yourself. If you forget it, nothing gets returned!\n\nKeeping list items in order with `key`[](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key \"Link for this heading\")\n----------------------------------------------------------------------------------------------------------------------------------------------\n\nNotice that all the sandboxes above show an error in the console:\n\nWarning: Each child in a list should have a unique “key” prop.\n\nYou need to give each array item a `key` — a string or a number that uniquely identifies it among other items in that array:\n\n```\n<li key={person.id}>...</li>\n```\n\n### Note\n\nJSX elements directly inside a `map()` call always need keys!\n\nKeys tell React which array item each component corresponds to, so that it can match them up later. This becomes important if your array items can move (e.g. due to sorting), get inserted, or get deleted. A well-chosen `key` helps React infer what exactly has happened, and make the correct updates to the DOM tree.\n\nRather than generating keys on the fly, you should include them in your data:\n\nexport const people = \\[{\n id: 0, \n name: 'Creola Katherine Johnson',\n profession: 'mathematician',\n accomplishment: 'spaceflight calculations',\n imageId: 'MK3eW3A'\n}, {\n id: 1, \n name: 'Mario José Molina-Pasquel Henríquez',\n profession: 'chemist',\n accomplishment: 'discovery of Arctic ozone hole',\n imageId: 'mynHUSa'\n}, {\n id: 2, \n name: 'Mohammad Abdus Salam',\n profession: 'physicist',\n accomplishment: 'electromagnetism theory',\n imageId: 'bE7W1ji'\n}, {\n id: 3, \n name: 'Percy Lavon Julian',\n profession: 'chemist',\n accomplishment: 'pioneering cortisone drugs, steroids and birth control pills',\n imageId: 'IOjWm71'\n}, {\n id: 4, \n name: 'Subrahmanyan Chandrasekhar',\n profession: 'astrophysicist',\n accomplishment: 'white dwarf star mass calculations',\n imageId: 'lrWQx8l'\n}\\];\n\n##### Deep Dive\n\n#### Displaying several DOM nodes for each list item[](https://react.dev/learn/rendering-lists#displaying-several-dom-nodes-for-each-list-item \"Link for Displaying several DOM nodes for each list item \")\n\nWhat do you do when each item needs to render not one, but several DOM nodes?\n\nThe short [`<>...</>` Fragment](https://react.dev/reference/react/Fragment) syntax won’t let you pass a key, so you need to either group them into a single `<div>`, or use the slightly longer and [more explicit `<Fragment>` syntax:](https://react.dev/reference/react/Fragment#rendering-a-list-of-fragments)\n\n```\nimport { Fragment } from 'react';// ...const listItems = people.map(person =><Fragment key={person.id}><h1>{person.name}</h1><p>{person.bio}</p></Fragment>);\n```\n\nFragments disappear from the DOM, so this will produce a flat list of `<h1>`, `<p>`, `<h1>`, `<p>`, and so on.\n\n### Where to get your `key`[](https://react.dev/learn/rendering-lists#where-to-get-your-key \"Link for this heading\")\n\nDifferent sources of data provide different sources of keys:\n\n* **Data from a database:** If your data is coming from a database, you can use the database keys/IDs, which are unique by nature.\n* **Locally generated data:** If your data is generated and persisted locally (e.g. notes in a note-taking app), use an incrementing counter, [`crypto.randomUUID()`](https://developer.mozilla.org/en-US/docs/Web/API/Crypto/randomUUID) or a package like [`uuid`](https://www.npmjs.com/package/uuid) when creating items.\n\n### Rules of keys[](https://react.dev/learn/rendering-lists#rules-of-keys \"Link for Rules of keys \")\n\n* **Keys must be unique among siblings.** However, it’s okay to use the same keys for JSX nodes in _different_ arrays.\n* **Keys must not change** or that defeats their purpose! Don’t generate them while rendering.\n\n### Why does React need keys?[](https://react.dev/learn/rendering-lists#why-does-react-need-keys \"Link for Why does React need keys? \")\n\nImagine that files on your desktop didn’t have names. Instead, you’d refer to them by their order — the first file, the second file, and so on. You could get used to it, but once you delete a file, it would get confusing. The second file would become the first file, the third file would be the second file, and so on.\n\nFile names in a folder and JSX keys in an array serve a similar purpose. They let us uniquely identify an item between its siblings. A well-chosen key provides more information than the position within the array. Even if the _position_ changes due to reordering, the `key` lets React identify the item throughout its lifetime.\n\n### Pitfall\n\nYou might be tempted to use an item’s index in the array as its key. In fact, that’s what React will use if you don’t specify a `key` at all. But the order in which you render items will change over time if an item is inserted, deleted, or if the array gets reordered. Index as a key often leads to subtle and confusing bugs.\n\nSimilarly, do not generate keys on the fly, e.g. with `key={Math.random()}`. This will cause keys to never match up between renders, leading to all your components and DOM being recreated every time. Not only is this slow, but it will also lose any user input inside the list items. Instead, use a stable ID based on the data.\n\nNote that your components won’t receive `key` as a prop. It’s only used as a hint by React itself. If your component needs an ID, you have to pass it as a separate prop: `<Profile key={id} userId={id} />`.\n\nRecap[](https://react.dev/learn/rendering-lists#recap \"Link for Recap\")\n-----------------------------------------------------------------------\n\nOn this page you learned:\n\n* How to move data out of components and into data structures like arrays and objects.\n* How to generate sets of similar components with JavaScript’s `map()`.\n* How to create arrays of filtered items with JavaScript’s `filter()`.\n* Why and how to set `key` on each component in a collection so React can keep track of each of them even if their position or data changes.\n\nTry out some challenges[](https://react.dev/learn/rendering-lists#challenges \"Link for Try out some challenges\")\n----------------------------------------------------------------------------------------------------------------\n\n#### Splitting a list in two[](https://react.dev/learn/rendering-lists#splitting-a-list-in-two \"Link for this heading\")\n\nThis example shows a list of all people.\n\nChange it to show two separate lists one after another: **Chemists** and **Everyone Else.** Like previously, you can determine whether a person is a chemist by checking if `person.profession === 'chemist'`.\n\nimport { people } from './data.js';\nimport { getImageUrl } from './utils.js';\n\nexport default function List() {\n const listItems = people.map(person \\=>\n <li key\\={person.id}\\>\n <img\n src\\={getImageUrl(person)}\n alt\\={person.name}\n />\n <p\\>\n <b\\>{person.name}:</b\\>\n {' ' + person.profession + ' '}\n known for {person.accomplishment}\n </p\\>\n </li\\>\n );\n return (\n <article\\>\n <h1\\>Scientists</h1\\>\n <ul\\>{listItems}</ul\\>\n </article\\>\n );\n}\n",
"filename": "rendering-lists.md",
"package": "react"
} |
{
"content": "Title: Responding to Events – React\n\nURL Source: https://react.dev/learn/responding-to-events\n\nMarkdown Content:\nReact lets you add _event handlers_ to your JSX. Event handlers are your own functions that will be triggered in response to interactions like clicking, hovering, focusing form inputs, and so on.\n\n### You will learn\n\n* Different ways to write an event handler\n* How to pass event handling logic from a parent component\n* How events propagate and how to stop them\n\nAdding event handlers[](https://react.dev/learn/responding-to-events#adding-event-handlers \"Link for Adding event handlers \")\n-----------------------------------------------------------------------------------------------------------------------------\n\nTo add an event handler, you will first define a function and then [pass it as a prop](https://react.dev/learn/passing-props-to-a-component) to the appropriate JSX tag. For example, here is a button that doesn’t do anything yet:\n\nYou can make it show a message when a user clicks by following these three steps:\n\n1. Declare a function called `handleClick` _inside_ your `Button` component.\n2. Implement the logic inside that function (use `alert` to show the message).\n3. Add `onClick={handleClick}` to the `<button>` JSX.\n\nYou defined the `handleClick` function and then [passed it as a prop](https://react.dev/learn/passing-props-to-a-component) to `<button>`. `handleClick` is an **event handler.** Event handler functions:\n\n* Are usually defined _inside_ your components.\n* Have names that start with `handle`, followed by the name of the event.\n\nBy convention, it is common to name event handlers as `handle` followed by the event name. You’ll often see `onClick={handleClick}`, `onMouseEnter={handleMouseEnter}`, and so on.\n\nAlternatively, you can define an event handler inline in the JSX:\n\n```\n<button onClick={function handleClick() {alert('You clicked me!');}}>\n```\n\nOr, more concisely, using an arrow function:\n\n```\n<button onClick={() => {alert('You clicked me!');}}>\n```\n\nAll of these styles are equivalent. Inline event handlers are convenient for short functions.\n\n### Pitfall\n\nFunctions passed to event handlers must be passed, not called. For example:\n\n| passing a function (correct) | calling a function (incorrect) |\n| --- | --- |\n| `<button onClick={handleClick}>` | `<button onClick={handleClick()}>` |\n\nThe difference is subtle. In the first example, the `handleClick` function is passed as an `onClick` event handler. This tells React to remember it and only call your function when the user clicks the button.\n\nIn the second example, the `()` at the end of `handleClick()` fires the function _immediately_ during [rendering](https://react.dev/learn/render-and-commit), without any clicks. This is because JavaScript inside the [JSX `{` and `}`](https://react.dev/learn/javascript-in-jsx-with-curly-braces) executes right away.\n\nWhen you write code inline, the same pitfall presents itself in a different way:\n\n| passing a function (correct) | calling a function (incorrect) |\n| --- | --- |\n| `<button onClick={() => alert('...')}>` | `<button onClick={alert('...')}>` |\n\nPassing inline code like this won’t fire on click—it fires every time the component renders:\n\n```\n// This alert fires when the component renders, not when clicked!<button onClick={alert('You clicked me!')}>\n```\n\nIf you want to define your event handler inline, wrap it in an anonymous function like so:\n\n```\n<button onClick={() => alert('You clicked me!')}>\n```\n\nRather than executing the code inside with every render, this creates a function to be called later.\n\nIn both cases, what you want to pass is a function:\n\n* `<button onClick={handleClick}>` passes the `handleClick` function.\n* `<button onClick={() => alert('...')}>` passes the `() => alert('...')` function.\n\n[Read more about arrow functions.](https://javascript.info/arrow-functions-basics)\n\n### Reading props in event handlers[](https://react.dev/learn/responding-to-events#reading-props-in-event-handlers \"Link for Reading props in event handlers \")\n\nBecause event handlers are declared inside of a component, they have access to the component’s props. Here is a button that, when clicked, shows an alert with its `message` prop:\n\nfunction AlertButton({ message, children }) {\n return (\n <button onClick\\={() \\=> alert(message)}\\>\n {children}\n </button\\>\n );\n}\n\nexport default function Toolbar() {\n return (\n <div\\>\n <AlertButton message\\=\"Playing!\"\\>\n Play Movie\n </AlertButton\\>\n <AlertButton message\\=\"Uploading!\"\\>\n Upload Image\n </AlertButton\\>\n </div\\>\n );\n}\n\nThis lets these two buttons show different messages. Try changing the messages passed to them.\n\n### Passing event handlers as props[](https://react.dev/learn/responding-to-events#passing-event-handlers-as-props \"Link for Passing event handlers as props \")\n\nOften you’ll want the parent component to specify a child’s event handler. Consider buttons: depending on where you’re using a `Button` component, you might want to execute a different function—perhaps one plays a movie and another uploads an image.\n\nTo do this, pass a prop the component receives from its parent as the event handler like so:\n\nfunction Button({ onClick, children }) {\n return (\n <button onClick\\={onClick}\\>\n {children}\n </button\\>\n );\n}\n\nfunction PlayButton({ movieName }) {\n function handlePlayClick() {\n alert(\\`Playing ${movieName}!\\`);\n }\n\n return (\n <Button onClick\\={handlePlayClick}\\>\n Play \"{movieName}\"\n </Button\\>\n );\n}\n\nfunction UploadButton() {\n return (\n <Button onClick\\={() \\=> alert('Uploading!')}\\>\n Upload Image\n </Button\\>\n );\n}\n\nexport default function Toolbar() {\n return (\n <div\\>\n <PlayButton movieName\\=\"Kiki's Delivery Service\" />\n <UploadButton />\n </div\\>\n );\n}\n\nHere, the `Toolbar` component renders a `PlayButton` and an `UploadButton`:\n\n* `PlayButton` passes `handlePlayClick` as the `onClick` prop to the `Button` inside.\n* `UploadButton` passes `() => alert('Uploading!')` as the `onClick` prop to the `Button` inside.\n\nFinally, your `Button` component accepts a prop called `onClick`. It passes that prop directly to the built-in browser `<button>` with `onClick={onClick}`. This tells React to call the passed function on click.\n\nIf you use a [design system](https://uxdesign.cc/everything-you-need-to-know-about-design-systems-54b109851969), it’s common for components like buttons to contain styling but not specify behavior. Instead, components like `PlayButton` and `UploadButton` will pass event handlers down.\n\n### Naming event handler props[](https://react.dev/learn/responding-to-events#naming-event-handler-props \"Link for Naming event handler props \")\n\nBuilt-in components like `<button>` and `<div>` only support [browser event names](https://react.dev/reference/react-dom/components/common#common-props) like `onClick`. However, when you’re building your own components, you can name their event handler props any way that you like.\n\nBy convention, event handler props should start with `on`, followed by a capital letter.\n\nFor example, the `Button` component’s `onClick` prop could have been called `onSmash`:\n\nfunction Button({ onSmash, children }) {\n return (\n <button onClick\\={onSmash}\\>\n {children}\n </button\\>\n );\n}\n\nexport default function App() {\n return (\n <div\\>\n <Button onSmash\\={() \\=> alert('Playing!')}\\>\n Play Movie\n </Button\\>\n <Button onSmash\\={() \\=> alert('Uploading!')}\\>\n Upload Image\n </Button\\>\n </div\\>\n );\n}\n\nIn this example, `<button onClick={onSmash}>` shows that the browser `<button>` (lowercase) still needs a prop called `onClick`, but the prop name received by your custom `Button` component is up to you!\n\nWhen your component supports multiple interactions, you might name event handler props for app-specific concepts. For example, this `Toolbar` component receives `onPlayMovie` and `onUploadImage` event handlers:\n\nexport default function App() {\n return (\n <Toolbar\n onPlayMovie\\={() \\=> alert('Playing!')}\n onUploadImage\\={() \\=> alert('Uploading!')}\n />\n );\n}\n\nfunction Toolbar({ onPlayMovie, onUploadImage }) {\n return (\n <div\\>\n <Button onClick\\={onPlayMovie}\\>\n Play Movie\n </Button\\>\n <Button onClick\\={onUploadImage}\\>\n Upload Image\n </Button\\>\n </div\\>\n );\n}\n\nfunction Button({ onClick, children }) {\n return (\n <button onClick\\={onClick}\\>\n {children}\n </button\\>\n );\n}\n\nNotice how the `App` component does not need to know _what_ `Toolbar` will do with `onPlayMovie` or `onUploadImage`. That’s an implementation detail of the `Toolbar`. Here, `Toolbar` passes them down as `onClick` handlers to its `Button`s, but it could later also trigger them on a keyboard shortcut. Naming props after app-specific interactions like `onPlayMovie` gives you the flexibility to change how they’re used later.\n\n### Note\n\nMake sure that you use the appropriate HTML tags for your event handlers. For example, to handle clicks, use [`<button onClick={handleClick}>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/button) instead of `<div onClick={handleClick}>`. Using a real browser `<button>` enables built-in browser behaviors like keyboard navigation. If you don’t like the default browser styling of a button and want to make it look more like a link or a different UI element, you can achieve it with CSS. [Learn more about writing accessible markup.](https://developer.mozilla.org/en-US/docs/Learn/Accessibility/HTML)\n\nEvent propagation[](https://react.dev/learn/responding-to-events#event-propagation \"Link for Event propagation \")\n-----------------------------------------------------------------------------------------------------------------\n\nEvent handlers will also catch events from any children your component might have. We say that an event “bubbles” or “propagates” up the tree: it starts with where the event happened, and then goes up the tree.\n\nThis `<div>` contains two buttons. Both the `<div>` _and_ each button have their own `onClick` handlers. Which handlers do you think will fire when you click a button?\n\nIf you click on either button, its `onClick` will run first, followed by the parent `<div>`’s `onClick`. So two messages will appear. If you click the toolbar itself, only the parent `<div>`’s `onClick` will run.\n\n### Pitfall\n\nAll events propagate in React except `onScroll`, which only works on the JSX tag you attach it to.\n\n### Stopping propagation[](https://react.dev/learn/responding-to-events#stopping-propagation \"Link for Stopping propagation \")\n\nEvent handlers receive an **event object** as their only argument. By convention, it’s usually called `e`, which stands for “event”. You can use this object to read information about the event.\n\nThat event object also lets you stop the propagation. If you want to prevent an event from reaching parent components, you need to call `e.stopPropagation()` like this `Button` component does:\n\nfunction Button({ onClick, children }) {\n return (\n <button onClick\\={e \\=> {\n e.stopPropagation();\n onClick();\n }}\\>\n {children}\n </button\\>\n );\n}\n\nexport default function Toolbar() {\n return (\n <div className\\=\"Toolbar\" onClick\\={() \\=> {\n alert('You clicked on the toolbar!');\n }}\\>\n <Button onClick\\={() \\=> alert('Playing!')}\\>\n Play Movie\n </Button\\>\n <Button onClick\\={() \\=> alert('Uploading!')}\\>\n Upload Image\n </Button\\>\n </div\\>\n );\n}\n\nWhen you click on a button:\n\n1. React calls the `onClick` handler passed to `<button>`.\n2. That handler, defined in `Button`, does the following:\n * Calls `e.stopPropagation()`, preventing the event from bubbling further.\n * Calls the `onClick` function, which is a prop passed from the `Toolbar` component.\n3. That function, defined in the `Toolbar` component, displays the button’s own alert.\n4. Since the propagation was stopped, the parent `<div>`’s `onClick` handler does _not_ run.\n\nAs a result of `e.stopPropagation()`, clicking on the buttons now only shows a single alert (from the `<button>`) rather than the two of them (from the `<button>` and the parent toolbar `<div>`). Clicking a button is not the same thing as clicking the surrounding toolbar, so stopping the propagation makes sense for this UI.\n\n##### Deep Dive\n\n#### Capture phase events[](https://react.dev/learn/responding-to-events#capture-phase-events \"Link for Capture phase events \")\n\nIn rare cases, you might need to catch all events on child elements, _even if they stopped propagation_. For example, maybe you want to log every click to analytics, regardless of the propagation logic. You can do this by adding `Capture` at the end of the event name:\n\n```\n<div onClickCapture={() => { /* this runs first */ }}><button onClick={e => e.stopPropagation()} /><button onClick={e => e.stopPropagation()} /></div>\n```\n\nEach event propagates in three phases:\n\n1. It travels down, calling all `onClickCapture` handlers.\n2. It runs the clicked element’s `onClick` handler.\n3. It travels upwards, calling all `onClick` handlers.\n\nCapture events are useful for code like routers or analytics, but you probably won’t use them in app code.\n\n### Passing handlers as alternative to propagation[](https://react.dev/learn/responding-to-events#passing-handlers-as-alternative-to-propagation \"Link for Passing handlers as alternative to propagation \")\n\nNotice how this click handler runs a line of code _and then_ calls the `onClick` prop passed by the parent:\n\n```\nfunction Button({ onClick, children }) {return (<button onClick={e => {e.stopPropagation();onClick();}}>{children}</button>);}\n```\n\nYou could add more code to this handler before calling the parent `onClick` event handler, too. This pattern provides an _alternative_ to propagation. It lets the child component handle the event, while also letting the parent component specify some additional behavior. Unlike propagation, it’s not automatic. But the benefit of this pattern is that you can clearly follow the whole chain of code that executes as a result of some event.\n\nIf you rely on propagation and it’s difficult to trace which handlers execute and why, try this approach instead.\n\n### Preventing default behavior[](https://react.dev/learn/responding-to-events#preventing-default-behavior \"Link for Preventing default behavior \")\n\nSome browser events have default behavior associated with them. For example, a `<form>` submit event, which happens when a button inside of it is clicked, will reload the whole page by default:\n\nYou can call `e.preventDefault()` on the event object to stop this from happening:\n\nDon’t confuse `e.stopPropagation()` and `e.preventDefault()`. They are both useful, but are unrelated:\n\n* [`e.stopPropagation()`](https://developer.mozilla.org/docs/Web/API/Event/stopPropagation) stops the event handlers attached to the tags above from firing.\n* [`e.preventDefault()`](https://developer.mozilla.org/docs/Web/API/Event/preventDefault) prevents the default browser behavior for the few events that have it.\n\nCan event handlers have side effects?[](https://react.dev/learn/responding-to-events#can-event-handlers-have-side-effects \"Link for Can event handlers have side effects? \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAbsolutely! Event handlers are the best place for side effects.\n\nUnlike rendering functions, event handlers don’t need to be [pure](https://react.dev/learn/keeping-components-pure), so it’s a great place to _change_ something—for example, change an input’s value in response to typing, or change a list in response to a button press. However, in order to change some information, you first need some way to store it. In React, this is done by using [state, a component’s memory.](https://react.dev/learn/state-a-components-memory) You will learn all about it on the next page.\n\nRecap[](https://react.dev/learn/responding-to-events#recap \"Link for Recap\")\n----------------------------------------------------------------------------\n\n* You can handle events by passing a function as a prop to an element like `<button>`.\n* Event handlers must be passed, **not called!** `onClick={handleClick}`, not `onClick={handleClick()}`.\n* You can define an event handler function separately or inline.\n* Event handlers are defined inside a component, so they can access props.\n* You can declare an event handler in a parent and pass it as a prop to a child.\n* You can define your own event handler props with application-specific names.\n* Events propagate upwards. Call `e.stopPropagation()` on the first argument to prevent that.\n* Events may have unwanted default browser behavior. Call `e.preventDefault()` to prevent that.\n* Explicitly calling an event handler prop from a child handler is a good alternative to propagation.\n\nTry out some challenges[](https://react.dev/learn/responding-to-events#challenges \"Link for Try out some challenges\")\n---------------------------------------------------------------------------------------------------------------------\n\n#### Fix an event handler[](https://react.dev/learn/responding-to-events#fix-an-event-handler \"Link for this heading\")\n\nClicking this button is supposed to switch the page background between white and black. However, nothing happens when you click it. Fix the problem. (Don’t worry about the logic inside `handleClick`—that part is fine.)\n\nexport default function LightSwitch() {\n function handleClick() {\n let bodyStyle = document.body.style;\n if (bodyStyle.backgroundColor === 'black') {\n bodyStyle.backgroundColor = 'white';\n } else {\n bodyStyle.backgroundColor = 'black';\n }\n }\n\n return (\n <button onClick\\={handleClick()}\\>\n Toggle the lights\n </button\\>\n );\n}\n",
"filename": "responding-to-events.md",
"package": "react"
} |
{
"content": "Title: Reusing Logic with Custom Hooks – React\n\nURL Source: https://react.dev/learn/reusing-logic-with-custom-hooks\n\nMarkdown Content:\nReact comes with several built-in Hooks like `useState`, `useContext`, and `useEffect`. Sometimes, you’ll wish that there was a Hook for some more specific purpose: for example, to fetch data, to keep track of whether the user is online, or to connect to a chat room. You might not find these Hooks in React, but you can create your own Hooks for your application’s needs.\n\n### You will learn\n\n* What custom Hooks are, and how to write your own\n* How to reuse logic between components\n* How to name and structure your custom Hooks\n* When and why to extract custom Hooks\n\nCustom Hooks: Sharing logic between components[](https://react.dev/learn/reusing-logic-with-custom-hooks#custom-hooks-sharing-logic-between-components \"Link for Custom Hooks: Sharing logic between components \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nImagine you’re developing an app that heavily relies on the network (as most apps do). You want to warn the user if their network connection has accidentally gone off while they were using your app. How would you go about it? It seems like you’ll need two things in your component:\n\n1. A piece of state that tracks whether the network is online.\n2. An Effect that subscribes to the global [`online`](https://developer.mozilla.org/en-US/docs/Web/API/Window/online_event) and [`offline`](https://developer.mozilla.org/en-US/docs/Web/API/Window/offline_event) events, and updates that state.\n\nThis will keep your component [synchronized](https://react.dev/learn/synchronizing-with-effects) with the network status. You might start with something like this:\n\nTry turning your network on and off, and notice how this `StatusBar` updates in response to your actions.\n\nNow imagine you _also_ want to use the same logic in a different component. You want to implement a Save button that will become disabled and show “Reconnecting…” instead of “Save” while the network is off.\n\nTo start, you can copy and paste the `isOnline` state and the Effect into `SaveButton`:\n\nimport { useState, useEffect } from 'react';\n\nexport default function SaveButton() {\n const \\[isOnline, setIsOnline\\] = useState(true);\n useEffect(() \\=> {\n function handleOnline() {\n setIsOnline(true);\n }\n function handleOffline() {\n setIsOnline(false);\n }\n window.addEventListener('online', handleOnline);\n window.addEventListener('offline', handleOffline);\n return () \\=> {\n window.removeEventListener('online', handleOnline);\n window.removeEventListener('offline', handleOffline);\n };\n }, \\[\\]);\n\n function handleSaveClick() {\n console.log('✅ Progress saved');\n }\n\n return (\n <button disabled\\={!isOnline} onClick\\={handleSaveClick}\\>\n {isOnline ? 'Save progress' : 'Reconnecting...'}\n </button\\>\n );\n}\n\nVerify that, if you turn off the network, the button will change its appearance.\n\nThese two components work fine, but the duplication in logic between them is unfortunate. It seems like even though they have different _visual appearance,_ you want to reuse the logic between them.\n\nImagine for a moment that, similar to [`useState`](https://react.dev/reference/react/useState) and [`useEffect`](https://react.dev/reference/react/useEffect), there was a built-in `useOnlineStatus` Hook. Then both of these components could be simplified and you could remove the duplication between them:\n\n```\nfunction StatusBar() {const isOnline = useOnlineStatus();return <h1>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1>;}function SaveButton() {const isOnline = useOnlineStatus();function handleSaveClick() {console.log('✅ Progress saved');}return (<button disabled={!isOnline} onClick={handleSaveClick}>{isOnline ? 'Save progress' : 'Reconnecting...'}</button>);}\n```\n\nAlthough there is no such built-in Hook, you can write it yourself. Declare a function called `useOnlineStatus` and move all the duplicated code into it from the components you wrote earlier:\n\n```\nfunction useOnlineStatus() {const [isOnline, setIsOnline] = useState(true);useEffect(() => {function handleOnline() {setIsOnline(true);}function handleOffline() {setIsOnline(false);}window.addEventListener('online', handleOnline);window.addEventListener('offline', handleOffline);return () => {window.removeEventListener('online', handleOnline);window.removeEventListener('offline', handleOffline);};}, []);return isOnline;}\n```\n\nAt the end of the function, return `isOnline`. This lets your components read that value:\n\nimport { useOnlineStatus } from './useOnlineStatus.js';\n\nfunction StatusBar() {\n const isOnline = useOnlineStatus();\n return <h1\\>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1\\>;\n}\n\nfunction SaveButton() {\n const isOnline = useOnlineStatus();\n\n function handleSaveClick() {\n console.log('✅ Progress saved');\n }\n\n return (\n <button disabled\\={!isOnline} onClick\\={handleSaveClick}\\>\n {isOnline ? 'Save progress' : 'Reconnecting...'}\n </button\\>\n );\n}\n\nexport default function App() {\n return (\n <\\>\n <SaveButton />\n <StatusBar />\n </\\>\n );\n}\n\nVerify that switching the network on and off updates both components.\n\nNow your components don’t have as much repetitive logic. **More importantly, the code inside them describes _what they want to do_ (use the online status!) rather than _how to do it_ (by subscribing to the browser events).**\n\nWhen you extract logic into custom Hooks, you can hide the gnarly details of how you deal with some external system or a browser API. The code of your components expresses your intent, not the implementation.\n\n### Hook names always start with `use`[](https://react.dev/learn/reusing-logic-with-custom-hooks#hook-names-always-start-with-use \"Link for this heading\")\n\nReact applications are built from components. Components are built from Hooks, whether built-in or custom. You’ll likely often use custom Hooks created by others, but occasionally you might write one yourself!\n\nYou must follow these naming conventions:\n\n1. **React component names must start with a capital letter,** like `StatusBar` and `SaveButton`. React components also need to return something that React knows how to display, like a piece of JSX.\n2. **Hook names must start with `use` followed by a capital letter,** like [`useState`](https://react.dev/reference/react/useState) (built-in) or `useOnlineStatus` (custom, like earlier on the page). Hooks may return arbitrary values.\n\nThis convention guarantees that you can always look at a component and know where its state, Effects, and other React features might “hide”. For example, if you see a `getColor()` function call inside your component, you can be sure that it can’t possibly contain React state inside because its name doesn’t start with `use`. However, a function call like `useOnlineStatus()` will most likely contain calls to other Hooks inside!\n\n### Note\n\nIf your linter is [configured for React,](https://react.dev/learn/editor-setup#linting) it will enforce this naming convention. Scroll up to the sandbox above and rename `useOnlineStatus` to `getOnlineStatus`. Notice that the linter won’t allow you to call `useState` or `useEffect` inside of it anymore. Only Hooks and components can call other Hooks!\n\n##### Deep Dive\n\n#### Should all functions called during rendering start with the use prefix?[](https://react.dev/learn/reusing-logic-with-custom-hooks#should-all-functions-called-during-rendering-start-with-the-use-prefix \"Link for Should all functions called during rendering start with the use prefix? \")\n\nNo. Functions that don’t _call_ Hooks don’t need to _be_ Hooks.\n\nIf your function doesn’t call any Hooks, avoid the `use` prefix. Instead, write it as a regular function _without_ the `use` prefix. For example, `useSorted` below doesn’t call Hooks, so call it `getSorted` instead:\n\n```\n// 🔴 Avoid: A Hook that doesn't use Hooksfunction useSorted(items) {return items.slice().sort();}// ✅ Good: A regular function that doesn't use Hooksfunction getSorted(items) {return items.slice().sort();}\n```\n\nThis ensures that your code can call this regular function anywhere, including conditions:\n\n```\nfunction List({ items, shouldSort }) {let displayedItems = items;if (shouldSort) {// ✅ It's ok to call getSorted() conditionally because it's not a HookdisplayedItems = getSorted(items);}// ...}\n```\n\nYou should give `use` prefix to a function (and thus make it a Hook) if it uses at least one Hook inside of it:\n\n```\n// ✅ Good: A Hook that uses other Hooksfunction useAuth() {return useContext(Auth);}\n```\n\nTechnically, this isn’t enforced by React. In principle, you could make a Hook that doesn’t call other Hooks. This is often confusing and limiting so it’s best to avoid that pattern. However, there may be rare cases where it is helpful. For example, maybe your function doesn’t use any Hooks right now, but you plan to add some Hook calls to it in the future. Then it makes sense to name it with the `use` prefix:\n\n```\n// ✅ Good: A Hook that will likely use some other Hooks laterfunction useAuth() {// TODO: Replace with this line when authentication is implemented:// return useContext(Auth);return TEST_USER;}\n```\n\nThen components won’t be able to call it conditionally. This will become important when you actually add Hook calls inside. If you don’t plan to use Hooks inside it (now or later), don’t make it a Hook.\n\nIn the earlier example, when you turned the network on and off, both components updated together. However, it’s wrong to think that a single `isOnline` state variable is shared between them. Look at this code:\n\n```\nfunction StatusBar() {const isOnline = useOnlineStatus();// ...}function SaveButton() {const isOnline = useOnlineStatus();// ...}\n```\n\nIt works the same way as before you extracted the duplication:\n\n```\nfunction StatusBar() {const [isOnline, setIsOnline] = useState(true);useEffect(() => {// ...}, []);// ...}function SaveButton() {const [isOnline, setIsOnline] = useState(true);useEffect(() => {// ...}, []);// ...}\n```\n\nThese are two completely independent state variables and Effects! They happened to have the same value at the same time because you synchronized them with the same external value (whether the network is on).\n\nTo better illustrate this, we’ll need a different example. Consider this `Form` component:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[firstName, setFirstName\\] = useState('Mary');\n const \\[lastName, setLastName\\] = useState('Poppins');\n\n function handleFirstNameChange(e) {\n setFirstName(e.target.value);\n }\n\n function handleLastNameChange(e) {\n setLastName(e.target.value);\n }\n\n return (\n <\\>\n <label\\>\n First name:\n <input value\\={firstName} onChange\\={handleFirstNameChange} />\n </label\\>\n <label\\>\n Last name:\n <input value\\={lastName} onChange\\={handleLastNameChange} />\n </label\\>\n <p\\><b\\>Good morning, {firstName} {lastName}.</b\\></p\\>\n </\\>\n );\n}\n\nThere’s some repetitive logic for each form field:\n\n1. There’s a piece of state (`firstName` and `lastName`).\n2. There’s a change handler (`handleFirstNameChange` and `handleLastNameChange`).\n3. There’s a piece of JSX that specifies the `value` and `onChange` attributes for that input.\n\nYou can extract the repetitive logic into this `useFormInput` custom Hook:\n\nimport { useState } from 'react';\n\nexport function useFormInput(initialValue) {\n const \\[value, setValue\\] = useState(initialValue);\n\n function handleChange(e) {\n setValue(e.target.value);\n }\n\n const inputProps = {\n value: value,\n onChange: handleChange\n };\n\n return inputProps;\n}\n\nNotice that it only declares _one_ state variable called `value`.\n\nHowever, the `Form` component calls `useFormInput` _two times:_\n\n```\nfunction Form() {const firstNameProps = useFormInput('Mary');const lastNameProps = useFormInput('Poppins');// ...\n```\n\nThis is why it works like declaring two separate state variables!\n\n**Custom Hooks let you share _stateful logic_ but not _state itself._ Each call to a Hook is completely independent from every other call to the same Hook.** This is why the two sandboxes above are completely equivalent. If you’d like, scroll back up and compare them. The behavior before and after extracting a custom Hook is identical.\n\nWhen you need to share the state itself between multiple components, [lift it up and pass it down](https://react.dev/learn/sharing-state-between-components) instead.\n\nPassing reactive values between Hooks[](https://react.dev/learn/reusing-logic-with-custom-hooks#passing-reactive-values-between-hooks \"Link for Passing reactive values between Hooks \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThe code inside your custom Hooks will re-run during every re-render of your component. This is why, like components, custom Hooks [need to be pure.](https://react.dev/learn/keeping-components-pure) Think of custom Hooks’ code as part of your component’s body!\n\nBecause custom Hooks re-render together with your component, they always receive the latest props and state. To see what this means, consider this chat room example. Change the server URL or the chat room:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\nimport { showNotification } from './notifications.js';\n\nexport default function ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n\n useEffect(() \\=> {\n const options = {\n serverUrl: serverUrl,\n roomId: roomId\n };\n const connection = createConnection(options);\n connection.on('message', (msg) \\=> {\n showNotification('New message: ' + msg);\n });\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId, serverUrl\\]);\n\n return (\n <\\>\n <label\\>\n Server URL:\n <input value\\={serverUrl} onChange\\={e \\=> setServerUrl(e.target.value)} />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nWhen you change `serverUrl` or `roomId`, the Effect [“reacts” to your changes](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values) and re-synchronizes. You can tell by the console messages that the chat re-connects every time that you change your Effect’s dependencies.\n\nNow move the Effect’s code into a custom Hook:\n\n```\nexport function useChatRoom({ serverUrl, roomId }) {useEffect(() => {const options = {serverUrl: serverUrl,roomId: roomId};const connection = createConnection(options);connection.connect();connection.on('message', (msg) => {showNotification('New message: ' + msg);});return () => connection.disconnect();}, [roomId, serverUrl]);}\n```\n\nThis lets your `ChatRoom` component call your custom Hook without worrying about how it works inside:\n\n```\nexport default function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useChatRoom({roomId: roomId,serverUrl: serverUrl});return (<><label> Server URL:<input value={serverUrl} onChange={e => setServerUrl(e.target.value)} /></label><h1>Welcome to the {roomId} room!</h1></>);}\n```\n\nThis looks much simpler! (But it does the same thing.)\n\nNotice that the logic _still responds_ to prop and state changes. Try editing the server URL or the selected room:\n\nimport { useState } from 'react';\nimport { useChatRoom } from './useChatRoom.js';\n\nexport default function ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n\n useChatRoom({\n roomId: roomId,\n serverUrl: serverUrl\n });\n\n return (\n <\\>\n <label\\>\n Server URL:\n <input value\\={serverUrl} onChange\\={e \\=> setServerUrl(e.target.value)} />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nNotice how you’re taking the return value of one Hook:\n\n```\nexport default function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useChatRoom({roomId: roomId,serverUrl: serverUrl});// ...\n```\n\nand pass it as an input to another Hook:\n\n```\nexport default function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useChatRoom({roomId: roomId,serverUrl: serverUrl});// ...\n```\n\nEvery time your `ChatRoom` component re-renders, it passes the latest `roomId` and `serverUrl` to your Hook. This is why your Effect re-connects to the chat whenever their values are different after a re-render. (If you ever worked with audio or video processing software, chaining Hooks like this might remind you of chaining visual or audio effects. It’s as if the output of `useState` “feeds into” the input of the `useChatRoom`.)\n\n### Passing event handlers to custom Hooks[](https://react.dev/learn/reusing-logic-with-custom-hooks#passing-event-handlers-to-custom-hooks \"Link for Passing event handlers to custom Hooks \")\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nAs you start using `useChatRoom` in more components, you might want to let components customize its behavior. For example, currently, the logic for what to do when a message arrives is hardcoded inside the Hook:\n\n```\nexport function useChatRoom({ serverUrl, roomId }) {useEffect(() => {const options = {serverUrl: serverUrl,roomId: roomId};const connection = createConnection(options);connection.connect();connection.on('message', (msg) => {showNotification('New message: ' + msg);});return () => connection.disconnect();}, [roomId, serverUrl]);}\n```\n\nLet’s say you want to move this logic back to your component:\n\n```\nexport default function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useChatRoom({roomId: roomId,serverUrl: serverUrl,onReceiveMessage(msg) {showNotification('New message: ' + msg);}});// ...\n```\n\nTo make this work, change your custom Hook to take `onReceiveMessage` as one of its named options:\n\n```\nexport function useChatRoom({ serverUrl, roomId, onReceiveMessage }) {useEffect(() => {const options = {serverUrl: serverUrl,roomId: roomId};const connection = createConnection(options);connection.connect();connection.on('message', (msg) => {onReceiveMessage(msg);});return () => connection.disconnect();}, [roomId, serverUrl, onReceiveMessage]); // ✅ All dependencies declared}\n```\n\nThis will work, but there’s one more improvement you can do when your custom Hook accepts event handlers.\n\nAdding a dependency on `onReceiveMessage` is not ideal because it will cause the chat to re-connect every time the component re-renders. [Wrap this event handler into an Effect Event to remove it from the dependencies:](https://react.dev/learn/removing-effect-dependencies#wrapping-an-event-handler-from-the-props)\n\n```\nimport { useEffect, useEffectEvent } from 'react';// ...export function useChatRoom({ serverUrl, roomId, onReceiveMessage }) {const onMessage = useEffectEvent(onReceiveMessage);useEffect(() => {const options = {serverUrl: serverUrl,roomId: roomId};const connection = createConnection(options);connection.connect();connection.on('message', (msg) => {onMessage(msg);});return () => connection.disconnect();}, [roomId, serverUrl]); // ✅ All dependencies declared}\n```\n\nNow the chat won’t re-connect every time that the `ChatRoom` component re-renders. Here is a fully working demo of passing an event handler to a custom Hook that you can play with:\n\nimport { useState } from 'react';\nimport { useChatRoom } from './useChatRoom.js';\nimport { showNotification } from './notifications.js';\n\nexport default function ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n\n useChatRoom({\n roomId: roomId,\n serverUrl: serverUrl,\n onReceiveMessage(msg) {\n showNotification('New message: ' + msg);\n }\n });\n\n return (\n <\\>\n <label\\>\n Server URL:\n <input value\\={serverUrl} onChange\\={e \\=> setServerUrl(e.target.value)} />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nNotice how you no longer need to know _how_ `useChatRoom` works in order to use it. You could add it to any other component, pass any other options, and it would work the same way. That’s the power of custom Hooks.\n\nWhen to use custom Hooks[](https://react.dev/learn/reusing-logic-with-custom-hooks#when-to-use-custom-hooks \"Link for When to use custom Hooks \")\n-------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou don’t need to extract a custom Hook for every little duplicated bit of code. Some duplication is fine. For example, extracting a `useFormInput` Hook to wrap a single `useState` call like earlier is probably unnecessary.\n\nHowever, whenever you write an Effect, consider whether it would be clearer to also wrap it in a custom Hook. [You shouldn’t need Effects very often,](https://react.dev/learn/you-might-not-need-an-effect) so if you’re writing one, it means that you need to “step outside React” to synchronize with some external system or to do something that React doesn’t have a built-in API for. Wrapping it into a custom Hook lets you precisely communicate your intent and how the data flows through it.\n\nFor example, consider a `ShippingForm` component that displays two dropdowns: one shows the list of cities, and another shows the list of areas in the selected city. You might start with some code that looks like this:\n\n```\nfunction ShippingForm({ country }) {const [cities, setCities] = useState(null);// This Effect fetches cities for a countryuseEffect(() => {let ignore = false;fetch(`/api/cities?country=${country}`) .then(response => response.json()) .then(json => {if (!ignore) {setCities(json);}});return () => {ignore = true;};}, [country]);const [city, setCity] = useState(null);const [areas, setAreas] = useState(null);// This Effect fetches areas for the selected cityuseEffect(() => {if (city) {let ignore = false;fetch(`/api/areas?city=${city}`) .then(response => response.json()) .then(json => {if (!ignore) {setAreas(json);}});return () => {ignore = true;};}}, [city]);// ...\n```\n\nAlthough this code is quite repetitive, [it’s correct to keep these Effects separate from each other.](https://react.dev/learn/removing-effect-dependencies#is-your-effect-doing-several-unrelated-things) They synchronize two different things, so you shouldn’t merge them into one Effect. Instead, you can simplify the `ShippingForm` component above by extracting the common logic between them into your own `useData` Hook:\n\n```\nfunction useData(url) {const [data, setData] = useState(null);useEffect(() => {if (url) {let ignore = false;fetch(url) .then(response => response.json()) .then(json => {if (!ignore) {setData(json);}});return () => {ignore = true;};}}, [url]);return data;}\n```\n\nNow you can replace both Effects in the `ShippingForm` components with calls to `useData`:\n\n```\nfunction ShippingForm({ country }) {const cities = useData(`/api/cities?country=${country}`);const [city, setCity] = useState(null);const areas = useData(city ? `/api/areas?city=${city}` : null);// ...\n```\n\nExtracting a custom Hook makes the data flow explicit. You feed the `url` in and you get the `data` out. By “hiding” your Effect inside `useData`, you also prevent someone working on the `ShippingForm` component from adding [unnecessary dependencies](https://react.dev/learn/removing-effect-dependencies) to it. With time, most of your app’s Effects will be in custom Hooks.\n\n##### Deep Dive\n\n#### Keep your custom Hooks focused on concrete high-level use cases[](https://react.dev/learn/reusing-logic-with-custom-hooks#keep-your-custom-hooks-focused-on-concrete-high-level-use-cases \"Link for Keep your custom Hooks focused on concrete high-level use cases \")\n\nStart by choosing your custom Hook’s name. If you struggle to pick a clear name, it might mean that your Effect is too coupled to the rest of your component’s logic, and is not yet ready to be extracted.\n\nIdeally, your custom Hook’s name should be clear enough that even a person who doesn’t write code often could have a good guess about what your custom Hook does, what it takes, and what it returns:\n\n* ✅ `useData(url)`\n* ✅ `useImpressionLog(eventName, extraData)`\n* ✅ `useChatRoom(options)`\n\nWhen you synchronize with an external system, your custom Hook name may be more technical and use jargon specific to that system. It’s good as long as it would be clear to a person familiar with that system:\n\n* ✅ `useMediaQuery(query)`\n* ✅ `useSocket(url)`\n* ✅ `useIntersectionObserver(ref, options)`\n\n**Keep custom Hooks focused on concrete high-level use cases.** Avoid creating and using custom “lifecycle” Hooks that act as alternatives and convenience wrappers for the `useEffect` API itself:\n\n* 🔴 `useMount(fn)`\n* 🔴 `useEffectOnce(fn)`\n* 🔴 `useUpdateEffect(fn)`\n\nFor example, this `useMount` Hook tries to ensure some code only runs “on mount”:\n\n```\nfunction ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');// 🔴 Avoid: using custom \"lifecycle\" HooksuseMount(() => {const connection = createConnection({ roomId, serverUrl });connection.connect();post('/analytics/event', { eventName: 'visit_chat' });});// ...}// 🔴 Avoid: creating custom \"lifecycle\" Hooksfunction useMount(fn) {useEffect(() => {fn();}, []); // 🔴 React Hook useEffect has a missing dependency: 'fn'}\n```\n\n**Custom “lifecycle” Hooks like `useMount` don’t fit well into the React paradigm.** For example, this code example has a mistake (it doesn’t “react” to `roomId` or `serverUrl` changes), but the linter won’t warn you about it because the linter only checks direct `useEffect` calls. It won’t know about your Hook.\n\nIf you’re writing an Effect, start by using the React API directly:\n\n```\nfunction ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');// ✅ Good: two raw Effects separated by purposeuseEffect(() => {const connection = createConnection({ serverUrl, roomId });connection.connect();return () => connection.disconnect();}, [serverUrl, roomId]);useEffect(() => {post('/analytics/event', { eventName: 'visit_chat', roomId });}, [roomId]);// ...}\n```\n\nThen, you can (but don’t have to) extract custom Hooks for different high-level use cases:\n\n```\nfunction ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');// ✅ Great: custom Hooks named after their purposeuseChatRoom({ serverUrl, roomId });useImpressionLog('visit_chat', { roomId });// ...}\n```\n\n**A good custom Hook makes the calling code more declarative by constraining what it does.** For example, `useChatRoom(options)` can only connect to the chat room, while `useImpressionLog(eventName, extraData)` can only send an impression log to the analytics. If your custom Hook API doesn’t constrain the use cases and is very abstract, in the long run it’s likely to introduce more problems than it solves.\n\n### Custom Hooks help you migrate to better patterns[](https://react.dev/learn/reusing-logic-with-custom-hooks#custom-hooks-help-you-migrate-to-better-patterns \"Link for Custom Hooks help you migrate to better patterns \")\n\nEffects are an [“escape hatch”](https://react.dev/learn/escape-hatches): you use them when you need to “step outside React” and when there is no better built-in solution for your use case. With time, the React team’s goal is to reduce the number of the Effects in your app to the minimum by providing more specific solutions to more specific problems. Wrapping your Effects in custom Hooks makes it easier to upgrade your code when these solutions become available.\n\nLet’s return to this example:\n\nimport { useState, useEffect } from 'react';\n\nexport function useOnlineStatus() {\n const \\[isOnline, setIsOnline\\] = useState(true);\n useEffect(() \\=> {\n function handleOnline() {\n setIsOnline(true);\n }\n function handleOffline() {\n setIsOnline(false);\n }\n window.addEventListener('online', handleOnline);\n window.addEventListener('offline', handleOffline);\n return () \\=> {\n window.removeEventListener('online', handleOnline);\n window.removeEventListener('offline', handleOffline);\n };\n }, \\[\\]);\n return isOnline;\n}\n\nIn the above example, `useOnlineStatus` is implemented with a pair of [`useState`](https://react.dev/reference/react/useState) and [`useEffect`.](https://react.dev/reference/react/useEffect) However, this isn’t the best possible solution. There is a number of edge cases it doesn’t consider. For example, it assumes that when the component mounts, `isOnline` is already `true`, but this may be wrong if the network already went offline. You can use the browser [`navigator.onLine`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/onLine) API to check for that, but using it directly would not work on the server for generating the initial HTML. In short, this code could be improved.\n\nLuckily, React 18 includes a dedicated API called [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore) which takes care of all of these problems for you. Here is how your `useOnlineStatus` Hook, rewritten to take advantage of this new API:\n\nimport { useSyncExternalStore } from 'react';\n\nfunction subscribe(callback) {\n window.addEventListener('online', callback);\n window.addEventListener('offline', callback);\n return () \\=> {\n window.removeEventListener('online', callback);\n window.removeEventListener('offline', callback);\n };\n}\n\nexport function useOnlineStatus() {\n return useSyncExternalStore(\n subscribe,\n () \\=> navigator.onLine, \n () \\=> true \n );\n}\n\nNotice how **you didn’t need to change any of the components** to make this migration:\n\n```\nfunction StatusBar() {const isOnline = useOnlineStatus();// ...}function SaveButton() {const isOnline = useOnlineStatus();// ...}\n```\n\nThis is another reason for why wrapping Effects in custom Hooks is often beneficial:\n\n1. You make the data flow to and from your Effects very explicit.\n2. You let your components focus on the intent rather than on the exact implementation of your Effects.\n3. When React adds new features, you can remove those Effects without changing any of your components.\n\nSimilar to a [design system,](https://uxdesign.cc/everything-you-need-to-know-about-design-systems-54b109851969) you might find it helpful to start extracting common idioms from your app’s components into custom Hooks. This will keep your components’ code focused on the intent, and let you avoid writing raw Effects very often. Many excellent custom Hooks are maintained by the React community.\n\n##### Deep Dive\n\n#### Will React provide any built-in solution for data fetching?[](https://react.dev/learn/reusing-logic-with-custom-hooks#will-react-provide-any-built-in-solution-for-data-fetching \"Link for Will React provide any built-in solution for data fetching? \")\n\nWe’re still working out the details, but we expect that in the future, you’ll write data fetching like this:\n\n```\nimport { use } from 'react'; // Not available yet!function ShippingForm({ country }) {const cities = use(fetch(`/api/cities?country=${country}`));const [city, setCity] = useState(null);const areas = city ? use(fetch(`/api/areas?city=${city}`)) : null;// ...\n```\n\nIf you use custom Hooks like `useData` above in your app, it will require fewer changes to migrate to the eventually recommended approach than if you write raw Effects in every component manually. However, the old approach will still work fine, so if you feel happy writing raw Effects, you can continue to do that.\n\n### There is more than one way to do it[](https://react.dev/learn/reusing-logic-with-custom-hooks#there-is-more-than-one-way-to-do-it \"Link for There is more than one way to do it \")\n\nLet’s say you want to implement a fade-in animation _from scratch_ using the browser [`requestAnimationFrame`](https://developer.mozilla.org/en-US/docs/Web/API/window/requestAnimationFrame) API. You might start with an Effect that sets up an animation loop. During each frame of the animation, you could change the opacity of the DOM node you [hold in a ref](https://react.dev/learn/manipulating-the-dom-with-refs) until it reaches `1`. Your code might start like this:\n\nimport { useState, useEffect, useRef } from 'react';\n\nfunction Welcome() {\n const ref = useRef(null);\n\n useEffect(() \\=> {\n const duration = 1000;\n const node = ref.current;\n\n let startTime = performance.now();\n let frameId = null;\n\n function onFrame(now) {\n const timePassed = now - startTime;\n const progress = Math.min(timePassed / duration, 1);\n onProgress(progress);\n if (progress < 1) {\n \n frameId = requestAnimationFrame(onFrame);\n }\n }\n\n function onProgress(progress) {\n node.style.opacity = progress;\n }\n\n function start() {\n onProgress(0);\n startTime = performance.now();\n frameId = requestAnimationFrame(onFrame);\n }\n\n function stop() {\n cancelAnimationFrame(frameId);\n startTime = null;\n frameId = null;\n }\n\n start();\n return () \\=> stop();\n }, \\[\\]);\n\n return (\n <h1 className\\=\"welcome\" ref\\={ref}\\>\n Welcome\n </h1\\>\n );\n}\n\nexport default function App() {\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Remove' : 'Show'}\n </button\\>\n <hr />\n {show && <Welcome />}\n </\\>\n );\n}\n\nTo make the component more readable, you might extract the logic into a `useFadeIn` custom Hook:\n\nimport { useState, useEffect, useRef } from 'react';\nimport { useFadeIn } from './useFadeIn.js';\n\nfunction Welcome() {\n const ref = useRef(null);\n\n useFadeIn(ref, 1000);\n\n return (\n <h1 className\\=\"welcome\" ref\\={ref}\\>\n Welcome\n </h1\\>\n );\n}\n\nexport default function App() {\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Remove' : 'Show'}\n </button\\>\n <hr />\n {show && <Welcome />}\n </\\>\n );\n}\n\nYou could keep the `useFadeIn` code as is, but you could also refactor it more. For example, you could extract the logic for setting up the animation loop out of `useFadeIn` into a custom `useAnimationLoop` Hook:\n\nimport { useState, useEffect } from 'react';\nimport { experimental\\_useEffectEvent as useEffectEvent } from 'react';\n\nexport function useFadeIn(ref, duration) {\n const \\[isRunning, setIsRunning\\] = useState(true);\n\n useAnimationLoop(isRunning, (timePassed) \\=> {\n const progress = Math.min(timePassed / duration, 1);\n ref.current.style.opacity = progress;\n if (progress === 1) {\n setIsRunning(false);\n }\n });\n}\n\nfunction useAnimationLoop(isRunning, drawFrame) {\n const onFrame = useEffectEvent(drawFrame);\n\n useEffect(() \\=> {\n if (!isRunning) {\n return;\n }\n\n const startTime = performance.now();\n let frameId = null;\n\n function tick(now) {\n const timePassed = now - startTime;\n onFrame(timePassed);\n frameId = requestAnimationFrame(tick);\n }\n\n tick();\n return () \\=> cancelAnimationFrame(frameId);\n }, \\[isRunning\\]);\n}\n\nHowever, you didn’t _have to_ do that. As with regular functions, ultimately you decide where to draw the boundaries between different parts of your code. You could also take a very different approach. Instead of keeping the logic in the Effect, you could move most of the imperative logic inside a JavaScript [class:](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes)\n\nEffects let you connect React to external systems. The more coordination between Effects is needed (for example, to chain multiple animations), the more it makes sense to extract that logic out of Effects and Hooks _completely_ like in the sandbox above. Then, the code you extracted _becomes_ the “external system”. This lets your Effects stay simple because they only need to send messages to the system you’ve moved outside React.\n\nThe examples above assume that the fade-in logic needs to be written in JavaScript. However, this particular fade-in animation is both simpler and much more efficient to implement with a plain [CSS Animation:](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Animations/Using_CSS_animations)\n\nSometimes, you don’t even need a Hook!\n\nRecap[](https://react.dev/learn/reusing-logic-with-custom-hooks#recap \"Link for Recap\")\n---------------------------------------------------------------------------------------\n\n* Custom Hooks let you share logic between components.\n* Custom Hooks must be named starting with `use` followed by a capital letter.\n* Custom Hooks only share stateful logic, not state itself.\n* You can pass reactive values from one Hook to another, and they stay up-to-date.\n* All Hooks re-run every time your component re-renders.\n* The code of your custom Hooks should be pure, like your component’s code.\n* Wrap event handlers received by custom Hooks into Effect Events.\n* Don’t create custom Hooks like `useMount`. Keep their purpose specific.\n* It’s up to you how and where to choose the boundaries of your code.\n\nTry out some challenges[](https://react.dev/learn/reusing-logic-with-custom-hooks#challenges \"Link for Try out some challenges\")\n--------------------------------------------------------------------------------------------------------------------------------\n\nThis component uses a state variable and an Effect to display a number that increments every second. Extract this logic into a custom Hook called `useCounter`. Your goal is to make the `Counter` component implementation look exactly like this:\n\n```\nexport default function Counter() {const count = useCounter();return <h1>Seconds passed: {count}</h1>;}\n```\n\nYou’ll need to write your custom Hook in `useCounter.js` and import it into the `App.js` file.\n",
"filename": "reusing-logic-with-custom-hooks.md",
"package": "react"
} |
{
"content": "Title: Rules of Hooks – React\n\nURL Source: https://react.dev/reference/rules/rules-of-hooks\n\nMarkdown Content:\nRules of Hooks – React\n===============\n\nRules of Hooks[](https://react.dev/reference/rules/rules-of-hooks#undefined \"Link for this heading\")\n====================================================================================================\n\nHooks are defined using JavaScript functions, but they represent a special type of reusable UI logic with restrictions on where they can be called.\n\n* [Only call Hooks at the top level](https://react.dev/reference/rules/rules-of-hooks#only-call-hooks-at-the-top-level)\n* [Only call Hooks from React functions](https://react.dev/reference/rules/rules-of-hooks#only-call-hooks-from-react-functions)\n\n* * *\n\nOnly call Hooks at the top level[](https://react.dev/reference/rules/rules-of-hooks#only-call-hooks-at-the-top-level \"Link for Only call Hooks at the top level \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nFunctions whose names start with `use` are called [_Hooks_](https://react.dev/reference/react) in React.\n\n**Don’t call Hooks inside loops, conditions, nested functions, or `try`/`catch`/`finally` blocks.** Instead, always use Hooks at the top level of your React function, before any early returns. You can only call Hooks while React is rendering a function component:\n\n* ✅ Call them at the top level in the body of a [function component](https://react.dev/learn/your-first-component).\n* ✅ Call them at the top level in the body of a [custom Hook](https://react.dev/learn/reusing-logic-with-custom-hooks).\n\n```\nfunction Counter() { // ✅ Good: top-level in a function component const [count, setCount] = useState(0); // ...}function useWindowWidth() { // ✅ Good: top-level in a custom Hook const [width, setWidth] = useState(window.innerWidth); // ...}\n```\n\nIt’s **not** supported to call Hooks (functions starting with `use`) in any other cases, for example:\n\n* 🔴 Do not call Hooks inside conditions or loops.\n* 🔴 Do not call Hooks after a conditional `return` statement.\n* 🔴 Do not call Hooks in event handlers.\n* 🔴 Do not call Hooks in class components.\n* 🔴 Do not call Hooks inside functions passed to `useMemo`, `useReducer`, or `useEffect`.\n* 🔴 Do not call Hooks inside `try`/`catch`/`finally` blocks.\n\nIf you break these rules, you might see this error.\n\n```\nfunction Bad({ cond }) { if (cond) { // 🔴 Bad: inside a condition (to fix, move it outside!) const theme = useContext(ThemeContext); } // ...}function Bad() { for (let i = 0; i < 10; i++) { // 🔴 Bad: inside a loop (to fix, move it outside!) const theme = useContext(ThemeContext); } // ...}function Bad({ cond }) { if (cond) { return; } // 🔴 Bad: after a conditional return (to fix, move it before the return!) const theme = useContext(ThemeContext); // ...}function Bad() { function handleClick() { // 🔴 Bad: inside an event handler (to fix, move it outside!) const theme = useContext(ThemeContext); } // ...}function Bad() { const style = useMemo(() => { // 🔴 Bad: inside useMemo (to fix, move it outside!) const theme = useContext(ThemeContext); return createStyle(theme); }); // ...}class Bad extends React.Component { render() { // 🔴 Bad: inside a class component (to fix, write a function component instead of a class!) useEffect(() => {}) // ... }}function Bad() { try { // 🔴 Bad: inside try/catch/finally block (to fix, move it outside!) const [x, setX] = useState(0); } catch { const [x, setX] = useState(1); }}\n```\n\nYou can use the [`eslint-plugin-react-hooks` plugin](https://www.npmjs.com/package/eslint-plugin-react-hooks) to catch these mistakes.\n\n### Note\n\n[Custom Hooks](https://react.dev/learn/reusing-logic-with-custom-hooks) _may_ call other Hooks (that’s their whole purpose). This works because custom Hooks are also supposed to only be called while a function component is rendering.\n\n* * *\n\nOnly call Hooks from React functions[](https://react.dev/reference/rules/rules-of-hooks#only-call-hooks-from-react-functions \"Link for Only call Hooks from React functions \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nDon’t call Hooks from regular JavaScript functions. Instead, you can:\n\n✅ Call Hooks from React function components. ✅ Call Hooks from [custom Hooks](https://react.dev/learn/reusing-logic-with-custom-hooks#extracting-your-own-custom-hook-from-a-component).\n\nBy following this rule, you ensure that all stateful logic in a component is clearly visible from its source code.\n\n```\nfunction FriendList() { const [onlineStatus, setOnlineStatus] = useOnlineStatus(); // ✅}function setOnlineStatus() { // ❌ Not a component or custom Hook! const [onlineStatus, setOnlineStatus] = useOnlineStatus();}\n```\n\n[PreviousReact calls Components and Hooks](https://react.dev/reference/rules/react-calls-components-and-hooks)\n",
"filename": "rules-of-hooks.md",
"package": "react"
} |
{
"content": "Title: Rules of React – React\n\nURL Source: https://react.dev/reference/rules\n\nMarkdown Content:\nJust as different programming languages have their own ways of expressing concepts, React has its own idioms — or rules — for how to express patterns in a way that is easy to understand and yields high-quality applications.\n\n* [Components and Hooks must be pure](https://react.dev/reference/rules#components-and-hooks-must-be-pure)\n* [React calls Components and Hooks](https://react.dev/reference/rules#react-calls-components-and-hooks)\n* [Rules of Hooks](https://react.dev/reference/rules#rules-of-hooks)\n\n* * *\n\n### Note\n\nTo learn more about expressing UIs with React, we recommend reading [Thinking in React](https://react.dev/learn/thinking-in-react).\n\nThis section describes the rules you need to follow to write idiomatic React code. Writing idiomatic React code can help you write well organized, safe, and composable applications. These properties make your app more resilient to changes and makes it easier to work with other developers, libraries, and tools.\n\nThese rules are known as the **Rules of React**. They are rules – and not just guidelines – in the sense that if they are broken, your app likely has bugs. Your code also becomes unidiomatic and harder to understand and reason about.\n\nWe strongly recommend using [Strict Mode](https://react.dev/reference/react/StrictMode) alongside React’s [ESLint plugin](https://www.npmjs.com/package/eslint-plugin-react-hooks) to help your codebase follow the Rules of React. By following the Rules of React, you’ll be able to find and address these bugs and keep your application maintainable.\n\n* * *\n\nComponents and Hooks must be pure[](https://react.dev/reference/rules#components-and-hooks-must-be-pure \"Link for Components and Hooks must be pure \")\n------------------------------------------------------------------------------------------------------------------------------------------------------\n\n[Purity in Components and Hooks](https://react.dev/reference/rules/components-and-hooks-must-be-pure) is a key rule of React that makes your app predictable, easy to debug, and allows React to automatically optimize your code.\n\n* [Components must be idempotent](https://react.dev/reference/rules/components-and-hooks-must-be-pure#components-and-hooks-must-be-idempotent) – React components are assumed to always return the same output with respect to their inputs – props, state, and context.\n* [Side effects must run outside of render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#side-effects-must-run-outside-of-render) – Side effects should not run in render, as React can render components multiple times to create the best possible user experience.\n* [Props and state are immutable](https://react.dev/reference/rules/components-and-hooks-must-be-pure#props-and-state-are-immutable) – A component’s props and state are immutable snapshots with respect to a single render. Never mutate them directly.\n* [Return values and arguments to Hooks are immutable](https://react.dev/reference/rules/components-and-hooks-must-be-pure#return-values-and-arguments-to-hooks-are-immutable) – Once values are passed to a Hook, you should not modify them. Like props in JSX, values become immutable when passed to a Hook.\n* [Values are immutable after being passed to JSX](https://react.dev/reference/rules/components-and-hooks-must-be-pure#values-are-immutable-after-being-passed-to-jsx) – Don’t mutate values after they’ve been used in JSX. Move the mutation before the JSX is created.\n\n* * *\n\nReact calls Components and Hooks[](https://react.dev/reference/rules#react-calls-components-and-hooks \"Link for React calls Components and Hooks \")\n---------------------------------------------------------------------------------------------------------------------------------------------------\n\n[React is responsible for rendering components and hooks when necessary to optimize the user experience.](https://react.dev/reference/rules/react-calls-components-and-hooks) It is declarative: you tell React what to render in your component’s logic, and React will figure out how best to display it to your user.\n\n* [Never call component functions directly](https://react.dev/reference/rules/react-calls-components-and-hooks#never-call-component-functions-directly) – Components should only be used in JSX. Don’t call them as regular functions.\n* [Never pass around hooks as regular values](https://react.dev/reference/rules/react-calls-components-and-hooks#never-pass-around-hooks-as-regular-values) – Hooks should only be called inside of components. Never pass it around as a regular value.\n\n* * *\n\nRules of Hooks[](https://react.dev/reference/rules#rules-of-hooks \"Link for Rules of Hooks \")\n---------------------------------------------------------------------------------------------\n\nHooks are defined using JavaScript functions, but they represent a special type of reusable UI logic with restrictions on where they can be called. You need to follow the [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) when using them.\n\n* [Only call Hooks at the top level](https://react.dev/reference/rules/rules-of-hooks#only-call-hooks-at-the-top-level) – Don’t call Hooks inside loops, conditions, or nested functions. Instead, always use Hooks at the top level of your React function, before any early returns.\n* [Only call Hooks from React functions](https://react.dev/reference/rules/rules-of-hooks#only-call-hooks-from-react-functions) – Don’t call Hooks from regular JavaScript functions.\n",
"filename": "rules.md",
"package": "react"
} |
{
"content": "Title: Scaling Up with Reducer and Context – React\n\nURL Source: https://react.dev/learn/scaling-up-with-reducer-and-context\n\nMarkdown Content:\nReducers let you consolidate a component’s state update logic. Context lets you pass information deep down to other components. You can combine reducers and context together to manage state of a complex screen.\n\n### You will learn\n\n* How to combine a reducer with context\n* How to avoid passing state and dispatch through props\n* How to keep context and state logic in a separate file\n\nCombining a reducer with context[](https://react.dev/learn/scaling-up-with-reducer-and-context#combining-a-reducer-with-context \"Link for Combining a reducer with context \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn this example from [the introduction to reducers](https://react.dev/learn/extracting-state-logic-into-a-reducer), the state is managed by a reducer. The reducer function contains all of the state update logic and is declared at the bottom of this file:\n\nA reducer helps keep the event handlers short and concise. However, as your app grows, you might run into another difficulty. **Currently, the `tasks` state and the `dispatch` function are only available in the top-level `TaskApp` component.** To let other components read the list of tasks or change it, you have to explicitly [pass down](https://react.dev/learn/passing-props-to-a-component) the current state and the event handlers that change it as props.\n\nFor example, `TaskApp` passes a list of tasks and the event handlers to `TaskList`:\n\n```\n<TaskListtasks={tasks}onChangeTask={handleChangeTask}onDeleteTask={handleDeleteTask}/>\n```\n\nAnd `TaskList` passes the event handlers to `Task`:\n\n```\n<Tasktask={task}onChange={onChangeTask}onDelete={onDeleteTask}/>\n```\n\nIn a small example like this, this works well, but if you have tens or hundreds of components in the middle, passing down all state and functions can be quite frustrating!\n\nThis is why, as an alternative to passing them through props, you might want to put both the `tasks` state and the `dispatch` function [into context.](https://react.dev/learn/passing-data-deeply-with-context) **This way, any component below `TaskApp` in the tree can read the tasks and dispatch actions without the repetitive “prop drilling”.**\n\nHere is how you can combine a reducer with context:\n\n1. **Create** the context.\n2. **Put** state and dispatch into context.\n3. **Use** context anywhere in the tree.\n\n### Step 1: Create the context[](https://react.dev/learn/scaling-up-with-reducer-and-context#step-1-create-the-context \"Link for Step 1: Create the context \")\n\nThe `useReducer` Hook returns the current `tasks` and the `dispatch` function that lets you update them:\n\n```\nconst [tasks, dispatch] = useReducer(tasksReducer, initialTasks);\n```\n\nTo pass them down the tree, you will [create](https://react.dev/learn/passing-data-deeply-with-context#step-2-use-the-context) two separate contexts:\n\n* `TasksContext` provides the current list of tasks.\n* `TasksDispatchContext` provides the function that lets components dispatch actions.\n\nExport them from a separate file so that you can later import them from other files:\n\nHere, you’re passing `null` as the default value to both contexts. The actual values will be provided by the `TaskApp` component.\n\n### Step 2: Put state and dispatch into context[](https://react.dev/learn/scaling-up-with-reducer-and-context#step-2-put-state-and-dispatch-into-context \"Link for Step 2: Put state and dispatch into context \")\n\nNow you can import both contexts in your `TaskApp` component. Take the `tasks` and `dispatch` returned by `useReducer()` and [provide them](https://react.dev/learn/passing-data-deeply-with-context#step-3-provide-the-context) to the entire tree below:\n\n```\nimport { TasksContext, TasksDispatchContext } from './TasksContext.js';export default function TaskApp() {const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);// ...return (<TasksContext.Provider value={tasks}><TasksDispatchContext.Provider value={dispatch}> ...</TasksDispatchContext.Provider></TasksContext.Provider>);}\n```\n\nFor now, you pass the information both via props and in context:\n\nimport { useReducer } from 'react';\nimport AddTask from './AddTask.js';\nimport TaskList from './TaskList.js';\nimport { TasksContext, TasksDispatchContext } from './TasksContext.js';\n\nexport default function TaskApp() {\n const \\[tasks, dispatch\\] = useReducer(\n tasksReducer,\n initialTasks\n );\n\n function handleAddTask(text) {\n dispatch({\n type: 'added',\n id: nextId++,\n text: text,\n });\n }\n\n function handleChangeTask(task) {\n dispatch({\n type: 'changed',\n task: task\n });\n }\n\n function handleDeleteTask(taskId) {\n dispatch({\n type: 'deleted',\n id: taskId\n });\n }\n\n return (\n <TasksContext.Provider value\\={tasks}\\>\n <TasksDispatchContext.Provider value\\={dispatch}\\>\n <h1\\>Day off in Kyoto</h1\\>\n <AddTask\n onAddTask\\={handleAddTask}\n />\n <TaskList\n tasks\\={tasks}\n onChangeTask\\={handleChangeTask}\n onDeleteTask\\={handleDeleteTask}\n />\n </TasksDispatchContext.Provider\\>\n </TasksContext.Provider\\>\n );\n}\n\nfunction tasksReducer(tasks, action) {\n switch (action.type) {\n case 'added': {\n return \\[...tasks, {\n id: action.id,\n text: action.text,\n done: false\n }\\];\n }\n case 'changed': {\n return tasks.map(t \\=> {\n if (t.id === action.task.id) {\n return action.task;\n } else {\n return t;\n }\n });\n }\n case 'deleted': {\n return tasks.filter(t \\=> t.id !== action.id);\n }\n default: {\n throw Error('Unknown action: ' + action.type);\n }\n }\n}\n\nlet nextId = 3;\nconst initialTasks = \\[\n { id: 0, text: 'Philosopher’s Path', done: true },\n { id: 1, text: 'Visit the temple', done: false },\n { id: 2, text: 'Drink matcha', done: false }\n\\];\n\nIn the next step, you will remove prop passing.\n\n### Step 3: Use context anywhere in the tree[](https://react.dev/learn/scaling-up-with-reducer-and-context#step-3-use-context-anywhere-in-the-tree \"Link for Step 3: Use context anywhere in the tree \")\n\nNow you don’t need to pass the list of tasks or the event handlers down the tree:\n\n```\n<TasksContext.Provider value={tasks}><TasksDispatchContext.Provider value={dispatch}><h1>Day off in Kyoto</h1><AddTask /><TaskList /></TasksDispatchContext.Provider></TasksContext.Provider>\n```\n\nInstead, any component that needs the task list can read it from the `TaskContext`:\n\n```\nexport default function TaskList() {const tasks = useContext(TasksContext);// ...\n```\n\nTo update the task list, any component can read the `dispatch` function from context and call it:\n\n```\nexport default function AddTask() {const [text, setText] = useState('');const dispatch = useContext(TasksDispatchContext);// ...return (// ...<button onClick={() => {setText('');dispatch({type: 'added',id: nextId++,text: text,});}}>Add</button>// ...\n```\n\n**The `TaskApp` component does not pass any event handlers down, and the `TaskList` does not pass any event handlers to the `Task` component either.** Each component reads the context that it needs:\n\nimport { useState, useContext } from 'react';\nimport { TasksContext, TasksDispatchContext } from './TasksContext.js';\n\nexport default function TaskList() {\n const tasks = useContext(TasksContext);\n return (\n <ul\\>\n {tasks.map(task \\=> (\n <li key\\={task.id}\\>\n <Task task\\={task} />\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nfunction Task({ task }) {\n const \\[isEditing, setIsEditing\\] = useState(false);\n const dispatch = useContext(TasksDispatchContext);\n let taskContent;\n if (isEditing) {\n taskContent = (\n <\\>\n <input\n value\\={task.text}\n onChange\\={e \\=> {\n dispatch({\n type: 'changed',\n task: {\n ...task,\n text: e.target.value\n }\n });\n }} />\n <button onClick\\={() \\=> setIsEditing(false)}\\>\n Save\n </button\\>\n </\\>\n );\n } else {\n taskContent = (\n <\\>\n {task.text}\n <button onClick\\={() \\=> setIsEditing(true)}\\>\n Edit\n </button\\>\n </\\>\n );\n }\n return (\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={task.done}\n onChange\\={e \\=> {\n dispatch({\n type: 'changed',\n task: {\n ...task,\n done: e.target.checked\n }\n });\n }}\n />\n {taskContent}\n <button onClick\\={() \\=> {\n dispatch({\n type: 'deleted',\n id: task.id\n });\n }}\\>\n Delete\n </button\\>\n </label\\>\n );\n}\n\n**The state still “lives” in the top-level `TaskApp` component, managed with `useReducer`.** But its `tasks` and `dispatch` are now available to every component below in the tree by importing and using these contexts.\n\nMoving all wiring into a single file[](https://react.dev/learn/scaling-up-with-reducer-and-context#moving-all-wiring-into-a-single-file \"Link for Moving all wiring into a single file \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou don’t have to do this, but you could further declutter the components by moving both reducer and context into a single file. Currently, `TasksContext.js` contains only two context declarations:\n\n```\nimport { createContext } from 'react';export const TasksContext = createContext(null);export const TasksDispatchContext = createContext(null);\n```\n\nThis file is about to get crowded! You’ll move the reducer into that same file. Then you’ll declare a new `TasksProvider` component in the same file. This component will tie all the pieces together:\n\n1. It will manage the state with a reducer.\n2. It will provide both contexts to components below.\n3. It will [take `children` as a prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) so you can pass JSX to it.\n\n```\nexport function TasksProvider({ children }) {const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);return (<TasksContext.Provider value={tasks}><TasksDispatchContext.Provider value={dispatch}>{children}</TasksDispatchContext.Provider></TasksContext.Provider>);}\n```\n\n**This removes all the complexity and wiring from your `TaskApp` component:**\n\nYou can also export functions that _use_ the context from `TasksContext.js`:\n\n```\nexport function useTasks() {return useContext(TasksContext);}export function useTasksDispatch() {return useContext(TasksDispatchContext);}\n```\n\nWhen a component needs to read context, it can do it through these functions:\n\n```\nconst tasks = useTasks();const dispatch = useTasksDispatch();\n```\n\nThis doesn’t change the behavior in any way, but it lets you later split these contexts further or add some logic to these functions. **Now all of the context and reducer wiring is in `TasksContext.js`. This keeps the components clean and uncluttered, focused on what they display rather than where they get the data:**\n\nimport { useState } from 'react';\nimport { useTasks, useTasksDispatch } from './TasksContext.js';\n\nexport default function TaskList() {\n const tasks = useTasks();\n return (\n <ul\\>\n {tasks.map(task \\=> (\n <li key\\={task.id}\\>\n <Task task\\={task} />\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nfunction Task({ task }) {\n const \\[isEditing, setIsEditing\\] = useState(false);\n const dispatch = useTasksDispatch();\n let taskContent;\n if (isEditing) {\n taskContent = (\n <\\>\n <input\n value\\={task.text}\n onChange\\={e \\=> {\n dispatch({\n type: 'changed',\n task: {\n ...task,\n text: e.target.value\n }\n });\n }} />\n <button onClick\\={() \\=> setIsEditing(false)}\\>\n Save\n </button\\>\n </\\>\n );\n } else {\n taskContent = (\n <\\>\n {task.text}\n <button onClick\\={() \\=> setIsEditing(true)}\\>\n Edit\n </button\\>\n </\\>\n );\n }\n return (\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={task.done}\n onChange\\={e \\=> {\n dispatch({\n type: 'changed',\n task: {\n ...task,\n done: e.target.checked\n }\n });\n }}\n />\n {taskContent}\n <button onClick\\={() \\=> {\n dispatch({\n type: 'deleted',\n id: task.id\n });\n }}\\>\n Delete\n </button\\>\n </label\\>\n );\n}\n\nYou can think of `TasksProvider` as a part of the screen that knows how to deal with tasks, `useTasks` as a way to read them, and `useTasksDispatch` as a way to update them from any component below in the tree.\n\n### Note\n\nFunctions like `useTasks` and `useTasksDispatch` are called _[Custom Hooks.](https://react.dev/learn/reusing-logic-with-custom-hooks)_ Your function is considered a custom Hook if its name starts with `use`. This lets you use other Hooks, like `useContext`, inside it.\n\nAs your app grows, you may have many context-reducer pairs like this. This is a powerful way to scale your app and [lift state up](https://react.dev/learn/sharing-state-between-components) without too much work whenever you want to access the data deep in the tree.\n\nRecap[](https://react.dev/learn/scaling-up-with-reducer-and-context#recap \"Link for Recap\")\n-------------------------------------------------------------------------------------------\n\n* You can combine reducer with context to let any component read and update state above it.\n* To provide state and the dispatch function to components below:\n 1. Create two contexts (for state and for dispatch functions).\n 2. Provide both contexts from the component that uses the reducer.\n 3. Use either context from components that need to read them.\n* You can further declutter the components by moving all wiring into one file.\n * You can export a component like `TasksProvider` that provides context.\n * You can also export custom Hooks like `useTasks` and `useTasksDispatch` to read it.\n* You can have many context-reducer pairs like this in your app.\n",
"filename": "scaling-up-with-reducer-and-context.md",
"package": "react"
} |
{
"content": "Title: <script> – React\n\nURL Source: https://react.dev/reference/react-dom/components/script\n\nMarkdown Content:\n### Canary\n\nThe [built-in browser `<script>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/script) lets you add a script to your document.\n\n```\n<script> alert(\"hi!\") </script>\n```\n\n* [Reference](https://react.dev/reference/react-dom/components/script#reference)\n * [`<script>`](https://react.dev/reference/react-dom/components/script#script)\n* [Usage](https://react.dev/reference/react-dom/components/script#usage)\n * [Rendering an external script](https://react.dev/reference/react-dom/components/script#rendering-an-external-script)\n * [Rendering an inline script](https://react.dev/reference/react-dom/components/script#rendering-an-inline-script)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/script#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------\n\n### `<script>`[](https://react.dev/reference/react-dom/components/script#script \"Link for this heading\")\n\nTo add inline or external scripts to your document, render the [built-in browser `<script>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/script). You can render `<script>` from any component and React will [in certain cases](https://react.dev/reference/react-dom/components/script#special-rendering-behavior) place the corresponding DOM element in the document head and de-duplicate identical scripts.\n\n```\n<script> alert(\"hi!\") </script><script src=\"script.js\" />\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/script#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/script#props \"Link for Props \")\n\n`<script>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\nIt should have _either_ `children` or a `src` prop.\n\n* `children`: a string. The source code of an inline script.\n* `src`: a string. The URL of an external script.\n\nOther supported props:\n\n* `async`: a boolean. Allows the browser to defer execution of the script until the rest of the document has been processed — the preferred behavior for performance.\n* `crossOrigin`: a string. The [CORS policy](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/crossorigin) to use. Its possible values are `anonymous` and `use-credentials`.\n* `fetchPriority`: a string. Lets the browser rank scripts in priority when fetching multiple scripts at the same time. Can be `\"high\"`, `\"low\"`, or `\"auto\"` (the default).\n* `integrity`: a string. A cryptographic hash of the script, to [verify its authenticity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity).\n* `noModule`: a boolean. Disables the script in browsers that support ES modules — allowing for a fallback script for browsers that do not.\n* `nonce`: a string. A cryptographic [nonce to allow the resource](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/nonce) when using a strict Content Security Policy.\n* `referrer`: a string. Says [what Referer header to send](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/script#referrerpolicy) when fetching the script and any resources that the script fetches in turn.\n* `type`: a string. Says whether the script is a [classic script, ES module, or import map](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/script/type).\n\nProps that disable React’s [special treatment of scripts](https://react.dev/reference/react-dom/components/script#special-rendering-behavior):\n\n* `onError`: a function. Called when the script fails to load.\n* `onLoad`: a function. Called when the script finishes being loaded.\n\nProps that are **not recommended** for use with React:\n\n* `blocking`: a string. If set to `\"render\"`, instructs the browser not to render the page until the scriptsheet is loaded. React provides more fine-grained control using Suspense.\n* `defer`: a string. Prevents the browser from executing the script until the document is done loading. Not compatible with streaming server-rendered components. Use the `async` prop instead.\n\n#### Special rendering behavior[](https://react.dev/reference/react-dom/components/script#special-rendering-behavior \"Link for Special rendering behavior \")\n\nReact can move `<script>` components to the document’s `<head>` and de-duplicate identical scripts.\n\nTo opt into this behavior, provide the `src` and `async={true}` props. React will de-duplicate scripts if they have the same `src`. The `async` prop must be true to allow scripts to be safely moved.\n\nThis special treatment comes with two caveats:\n\n* React will ignore changes to props after the script has been rendered. (React will issue a warning in development if this happens.)\n* React may leave the script in the DOM even after the component that rendered it has been unmounted. (This has no effect as scripts just execute once when they are inserted into the DOM.)\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/script#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------\n\n### Rendering an external script[](https://react.dev/reference/react-dom/components/script#rendering-an-external-script \"Link for Rendering an external script \")\n\nIf a component depends on certain scripts in order to be displayed correctly, you can render a `<script>` within the component. However, the component might be committed before the script has finished loading. You can start depending on the script content once the `load` event is fired e.g. by using the `onLoad` prop.\n\nReact will de-duplicate scripts that have the same `src`, inserting only one of them into the DOM even if multiple components render it.\n\nimport ShowRenderedHTML from './ShowRenderedHTML.js';\n\nfunction Map({lat, long}) {\n return (\n <\\>\n <script async src\\=\"map-api.js\" onLoad\\={() \\=> console.log('script loaded')} />\n <div id\\=\"map\" data-lat\\={lat} data-long\\={long} />\n </\\>\n );\n}\n\nexport default function Page() {\n return (\n <ShowRenderedHTML\\>\n <Map />\n </ShowRenderedHTML\\>\n );\n}\n\n### Note\n\nWhen you want to use a script, it can be beneficial to call the [preinit](https://react.dev/reference/react-dom/preinit) function. Calling this function may allow the browser to start fetching the script earlier than if you just render a `<script>` component, for example by sending an [HTTP Early Hints response](https://developer.mozilla.org/en-US/docs/Web/HTTP/Status/103).\n\n### Rendering an inline script[](https://react.dev/reference/react-dom/components/script#rendering-an-inline-script \"Link for Rendering an inline script \")\n\nTo include an inline script, render the `<script>` component with the script source code as its children. Inline scripts are not de-duplicated or moved to the document `<head>`.\n\nimport ShowRenderedHTML from './ShowRenderedHTML.js';\n\nfunction Tracking() {\n return (\n <script\\>\n ga('send', 'pageview');\n </script\\>\n );\n}\n\nexport default function Page() {\n return (\n <ShowRenderedHTML\\>\n <h1\\>My Website</h1\\>\n <Tracking />\n <p\\>Welcome</p\\>\n </ShowRenderedHTML\\>\n );\n}\n",
"filename": "script.md",
"package": "react"
} |
{
"content": "Title: <select> – React\n\nURL Source: https://react.dev/reference/react-dom/components/select\n\nMarkdown Content:\nThe [built-in browser `<select>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select) lets you render a select box with options.\n\n```\n<select><option value=\"someOption\">Some option</option><option value=\"otherOption\">Other option</option></select>\n```\n\n* [Reference](https://react.dev/reference/react-dom/components/select#reference)\n * [`<select>`](https://react.dev/reference/react-dom/components/select#select)\n* [Usage](https://react.dev/reference/react-dom/components/select#usage)\n * [Displaying a select box with options](https://react.dev/reference/react-dom/components/select#displaying-a-select-box-with-options)\n * [Providing a label for a select box](https://react.dev/reference/react-dom/components/select#providing-a-label-for-a-select-box)\n * [Providing an initially selected option](https://react.dev/reference/react-dom/components/select#providing-an-initially-selected-option)\n * [Enabling multiple selection](https://react.dev/reference/react-dom/components/select#enabling-multiple-selection)\n * [Reading the select box value when submitting a form](https://react.dev/reference/react-dom/components/select#reading-the-select-box-value-when-submitting-a-form)\n * [Controlling a select box with a state variable](https://react.dev/reference/react-dom/components/select#controlling-a-select-box-with-a-state-variable)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/select#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------\n\n### `<select>`[](https://react.dev/reference/react-dom/components/select#select \"Link for this heading\")\n\nTo display a select box, render the [built-in browser `<select>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select) component.\n\n```\n<select><option value=\"someOption\">Some option</option><option value=\"otherOption\">Other option</option></select>\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/select#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/select#props \"Link for Props \")\n\n`<select>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\nYou can [make a select box controlled](https://react.dev/reference/react-dom/components/select#controlling-a-select-box-with-a-state-variable) by passing a `value` prop:\n\n* `value`: A string (or an array of strings for [`multiple={true}`](https://react.dev/reference/react-dom/components/select#enabling-multiple-selection)). Controls which option is selected. Every value string match the `value` of some `<option>` nested inside the `<select>`.\n\nWhen you pass `value`, you must also pass an `onChange` handler that updates the passed value.\n\nIf your `<select>` is uncontrolled, you may pass the `defaultValue` prop instead:\n\n* `defaultValue`: A string (or an array of strings for [`multiple={true}`](https://react.dev/reference/react-dom/components/select#enabling-multiple-selection)). Specifies [the initially selected option.](https://react.dev/reference/react-dom/components/select#providing-an-initially-selected-option)\n\nThese `<select>` props are relevant both for uncontrolled and controlled select boxes:\n\n* [`autoComplete`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#autocomplete): A string. Specifies one of the possible [autocomplete behaviors.](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/autocomplete#values)\n* [`autoFocus`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#autofocus): A boolean. If `true`, React will focus the element on mount.\n* `children`: `<select>` accepts [`<option>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/option), [`<optgroup>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/optgroup), and [`<datalist>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/datalist) components as children. You can also pass your own components as long as they eventually render one of the allowed components. If you pass your own components that eventually render `<option>` tags, each `<option>` you render must have a `value`.\n* [`disabled`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#disabled): A boolean. If `true`, the select box will not be interactive and will appear dimmed.\n* [`form`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#form): A string. Specifies the `id` of the `<form>` this select box belongs to. If omitted, it’s the closest parent form.\n* [`multiple`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#multiple): A boolean. If `true`, the browser allows [multiple selection.](https://react.dev/reference/react-dom/components/select#enabling-multiple-selection)\n* [`name`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#name): A string. Specifies the name for this select box that’s [submitted with the form.](https://react.dev/reference/react-dom/components/select#reading-the-select-box-value-when-submitting-a-form)\n* `onChange`: An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Required for [controlled select boxes.](https://react.dev/reference/react-dom/components/select#controlling-a-select-box-with-a-state-variable) Fires immediately when the user picks a different option. Behaves like the browser [`input` event.](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event)\n* `onChangeCapture`: A version of `onChange` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onInput`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires immediately when the value is changed by the user. For historical reasons, in React it is idiomatic to use `onChange` instead which works similarly.\n* `onInputCapture`: A version of `onInput` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onInvalid`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement/invalid_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires if an input fails validation on form submit. Unlike the built-in `invalid` event, the React `onInvalid` event bubbles.\n* `onInvalidCapture`: A version of `onInvalid` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`required`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#required): A boolean. If `true`, the value must be provided for the form to submit.\n* [`size`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select#size): A number. For `multiple={true}` selects, specifies the preferred number of initially visible items.\n\n#### Caveats[](https://react.dev/reference/react-dom/components/select#caveats \"Link for Caveats \")\n\n* Unlike in HTML, passing a `selected` attribute to `<option>` is not supported. Instead, use [`<select defaultValue>`](https://react.dev/reference/react-dom/components/select#providing-an-initially-selected-option) for uncontrolled select boxes and [`<select value>`](https://react.dev/reference/react-dom/components/select#controlling-a-select-box-with-a-state-variable) for controlled select boxes.\n* If a select box receives a `value` prop, it will be [treated as controlled.](https://react.dev/reference/react-dom/components/select#controlling-a-select-box-with-a-state-variable)\n* A select box can’t be both controlled and uncontrolled at the same time.\n* A select box cannot switch between being controlled or uncontrolled over its lifetime.\n* Every controlled select box needs an `onChange` event handler that synchronously updates its backing value.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/select#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------\n\n### Displaying a select box with options[](https://react.dev/reference/react-dom/components/select#displaying-a-select-box-with-options \"Link for Displaying a select box with options \")\n\nRender a `<select>` with a list of `<option>` components inside to display a select box. Give each `<option>` a `value` representing the data to be submitted with the form.\n\n* * *\n\n### Providing a label for a select box[](https://react.dev/reference/react-dom/components/select#providing-a-label-for-a-select-box \"Link for Providing a label for a select box \")\n\nTypically, you will place every `<select>` inside a [`<label>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/label) tag. This tells the browser that this label is associated with that select box. When the user clicks the label, the browser will automatically focus the select box. It’s also essential for accessibility: a screen reader will announce the label caption when the user focuses the select box.\n\nIf you can’t nest `<select>` into a `<label>`, associate them by passing the same ID to `<select id>` and [`<label htmlFor>`.](https://developer.mozilla.org/en-US/docs/Web/API/HTMLLabelElement/htmlFor) To avoid conflicts between multiple instances of one component, generate such an ID with [`useId`.](https://react.dev/reference/react/useId)\n\nimport { useId } from 'react';\n\nexport default function Form() {\n const vegetableSelectId = useId();\n return (\n <\\>\n <label\\>\n Pick a fruit:\n <select name\\=\"selectedFruit\"\\>\n <option value\\=\"apple\"\\>Apple</option\\>\n <option value\\=\"banana\"\\>Banana</option\\>\n <option value\\=\"orange\"\\>Orange</option\\>\n </select\\>\n </label\\>\n <hr />\n <label htmlFor\\={vegetableSelectId}\\>\n Pick a vegetable:\n </label\\>\n <select id\\={vegetableSelectId} name\\=\"selectedVegetable\"\\>\n <option value\\=\"cucumber\"\\>Cucumber</option\\>\n <option value\\=\"corn\"\\>Corn</option\\>\n <option value\\=\"tomato\"\\>Tomato</option\\>\n </select\\>\n </\\>\n );\n}\n\n* * *\n\n### Providing an initially selected option[](https://react.dev/reference/react-dom/components/select#providing-an-initially-selected-option \"Link for Providing an initially selected option \")\n\nBy default, the browser will select the first `<option>` in the list. To select a different option by default, pass that `<option>`’s `value` as the `defaultValue` to the `<select>` element.\n\n### Pitfall\n\nUnlike in HTML, passing a `selected` attribute to an individual `<option>` is not supported.\n\n* * *\n\n### Enabling multiple selection[](https://react.dev/reference/react-dom/components/select#enabling-multiple-selection \"Link for Enabling multiple selection \")\n\nPass `multiple={true}` to the `<select>` to let the user select multiple options. In that case, if you also specify `defaultValue` to choose the initially selected options, it must be an array.\n\nexport default function FruitPicker() {\n return (\n <label\\>\n Pick some fruits:\n <select\n name\\=\"selectedFruit\"\n defaultValue\\={\\['orange', 'banana'\\]}\n multiple\\={true}\n \\>\n <option value\\=\"apple\"\\>Apple</option\\>\n <option value\\=\"banana\"\\>Banana</option\\>\n <option value\\=\"orange\"\\>Orange</option\\>\n </select\\>\n </label\\>\n );\n}\n\n* * *\n\n### Reading the select box value when submitting a form[](https://react.dev/reference/react-dom/components/select#reading-the-select-box-value-when-submitting-a-form \"Link for Reading the select box value when submitting a form \")\n\nAdd a [`<form>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) around your select box with a [`<button type=\"submit\">`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/button) inside. It will call your `<form onSubmit>` event handler. By default, the browser will send the form data to the current URL and refresh the page. You can override that behavior by calling `e.preventDefault()`. Read the form data with [`new FormData(e.target)`](https://developer.mozilla.org/en-US/docs/Web/API/FormData).\n\nexport default function EditPost() {\n function handleSubmit(e) {\n \n e.preventDefault();\n \n const form = e.target;\n const formData = new FormData(form);\n \n fetch('/some-api', { method: form.method, body: formData });\n \n console.log(new URLSearchParams(formData).toString());\n \n const formJson = Object.fromEntries(formData.entries());\n console.log(formJson); \n \n console.log(\\[...formData.entries()\\]);\n }\n\n return (\n <form method\\=\"post\" onSubmit\\={handleSubmit}\\>\n <label\\>\n Pick your favorite fruit:\n <select name\\=\"selectedFruit\" defaultValue\\=\"orange\"\\>\n <option value\\=\"apple\"\\>Apple</option\\>\n <option value\\=\"banana\"\\>Banana</option\\>\n <option value\\=\"orange\"\\>Orange</option\\>\n </select\\>\n </label\\>\n <label\\>\n Pick all your favorite vegetables:\n <select\n name\\=\"selectedVegetables\"\n multiple\\={true}\n defaultValue\\={\\['corn', 'tomato'\\]}\n \\>\n <option value\\=\"cucumber\"\\>Cucumber</option\\>\n <option value\\=\"corn\"\\>Corn</option\\>\n <option value\\=\"tomato\"\\>Tomato</option\\>\n </select\\>\n </label\\>\n <hr />\n <button type\\=\"reset\"\\>Reset</button\\>\n <button type\\=\"submit\"\\>Submit</button\\>\n </form\\>\n );\n}\n\n### Note\n\nGive a `name` to your `<select>`, for example `<select name=\"selectedFruit\" />`. The `name` you specified will be used as a key in the form data, for example `{ selectedFruit: \"orange\" }`.\n\nIf you use `<select multiple={true}>`, the [`FormData`](https://developer.mozilla.org/en-US/docs/Web/API/FormData) you’ll read from the form will include each selected value as a separate name-value pair. Look closely at the console logs in the example above.\n\n### Pitfall\n\nBy default, _any_ `<button>` inside a `<form>` will submit it. This can be surprising! If you have your own custom `Button` React component, consider returning [`<button type=\"button\">`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/button) instead of `<button>`. Then, to be explicit, use `<button type=\"submit\">` for buttons that _are_ supposed to submit the form.\n\n* * *\n\n### Controlling a select box with a state variable[](https://react.dev/reference/react-dom/components/select#controlling-a-select-box-with-a-state-variable \"Link for Controlling a select box with a state variable \")\n\nA select box like `<select />` is _uncontrolled._ Even if you [pass an initially selected value](https://react.dev/reference/react-dom/components/select#providing-an-initially-selected-option) like `<select defaultValue=\"orange\" />`, your JSX only specifies the initial value, not the value right now.\n\n**To render a _controlled_ select box, pass the `value` prop to it.** React will force the select box to always have the `value` you passed. Typically, you will control a select box by declaring a [state variable:](https://react.dev/reference/react/useState)\n\n```\nfunction FruitPicker() {const [selectedFruit, setSelectedFruit] = useState('orange'); // Declare a state variable...// ...return (<selectvalue={selectedFruit} // ...force the select's value to match the state variable...onChange={e => setSelectedFruit(e.target.value)} // ... and update the state variable on any change!><option value=\"apple\">Apple</option><option value=\"banana\">Banana</option><option value=\"orange\">Orange</option></select>);}\n```\n\nThis is useful if you want to re-render some part of the UI in response to every selection.\n\nimport { useState } from 'react';\n\nexport default function FruitPicker() {\n const \\[selectedFruit, setSelectedFruit\\] = useState('orange');\n const \\[selectedVegs, setSelectedVegs\\] = useState(\\['corn', 'tomato'\\]);\n return (\n <\\>\n <label\\>\n Pick a fruit:\n <select\n value\\={selectedFruit}\n onChange\\={e \\=> setSelectedFruit(e.target.value)}\n \\>\n <option value\\=\"apple\"\\>Apple</option\\>\n <option value\\=\"banana\"\\>Banana</option\\>\n <option value\\=\"orange\"\\>Orange</option\\>\n </select\\>\n </label\\>\n <hr />\n <label\\>\n Pick all your favorite vegetables:\n <select\n multiple\\={true}\n value\\={selectedVegs}\n onChange\\={e \\=> {\n const options = \\[...e.target.selectedOptions\\];\n const values = options.map(option \\=> option.value);\n setSelectedVegs(values);\n }}\n \\>\n <option value\\=\"cucumber\"\\>Cucumber</option\\>\n <option value\\=\"corn\"\\>Corn</option\\>\n <option value\\=\"tomato\"\\>Tomato</option\\>\n </select\\>\n </label\\>\n <hr />\n <p\\>Your favorite fruit: {selectedFruit}</p\\>\n <p\\>Your favorite vegetables: {selectedVegs.join(', ')}</p\\>\n </\\>\n );\n}\n\n### Pitfall\n\n**If you pass `value` without `onChange`, it will be impossible to select an option.** When you control a select box by passing some `value` to it, you _force_ it to always have the value you passed. So if you pass a state variable as a `value` but forget to update that state variable synchronously during the `onChange` event handler, React will revert the select box after every keystroke back to the `value` that you specified.\n\nUnlike in HTML, passing a `selected` attribute to an individual `<option>` is not supported.\n",
"filename": "select.md",
"package": "react"
} |
{
"content": "Title: Separating Events from Effects – React\n\nURL Source: https://react.dev/learn/separating-events-from-effects\n\nMarkdown Content:\nEvent handlers only re-run when you perform the same interaction again. Unlike event handlers, Effects re-synchronize if some value they read, like a prop or a state variable, is different from what it was during the last render. Sometimes, you also want a mix of both behaviors: an Effect that re-runs in response to some values but not others. This page will teach you how to do that.\n\n### You will learn\n\n* How to choose between an event handler and an Effect\n* Why Effects are reactive, and event handlers are not\n* What to do when you want a part of your Effect’s code to not be reactive\n* What Effect Events are, and how to extract them from your Effects\n* How to read the latest props and state from Effects using Effect Events\n\nChoosing between event handlers and Effects[](https://react.dev/learn/separating-events-from-effects#choosing-between-event-handlers-and-effects \"Link for Choosing between event handlers and Effects \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nFirst, let’s recap the difference between event handlers and Effects.\n\nImagine you’re implementing a chat room component. Your requirements look like this:\n\n1. Your component should automatically connect to the selected chat room.\n2. When you click the “Send” button, it should send a message to the chat.\n\nLet’s say you’ve already implemented the code for them, but you’re not sure where to put it. Should you use event handlers or Effects? Every time you need to answer this question, consider [_why_ the code needs to run.](https://react.dev/learn/synchronizing-with-effects#what-are-effects-and-how-are-they-different-from-events)\n\n### Event handlers run in response to specific interactions[](https://react.dev/learn/separating-events-from-effects#event-handlers-run-in-response-to-specific-interactions \"Link for Event handlers run in response to specific interactions \")\n\nFrom the user’s perspective, sending a message should happen _because_ the particular “Send” button was clicked. The user will get rather upset if you send their message at any other time or for any other reason. This is why sending a message should be an event handler. Event handlers let you handle specific interactions:\n\n```\nfunction ChatRoom({ roomId }) {const [message, setMessage] = useState('');// ...function handleSendClick() {sendMessage(message);}// ...return (<><input value={message} onChange={e => setMessage(e.target.value)} /><button onClick={handleSendClick}>Send</button></>);}\n```\n\nWith an event handler, you can be sure that `sendMessage(message)` will _only_ run if the user presses the button.\n\n### Effects run whenever synchronization is needed[](https://react.dev/learn/separating-events-from-effects#effects-run-whenever-synchronization-is-needed \"Link for Effects run whenever synchronization is needed \")\n\nRecall that you also need to keep the component connected to the chat room. Where does that code go?\n\nThe _reason_ to run this code is not some particular interaction. It doesn’t matter why or how the user navigated to the chat room screen. Now that they’re looking at it and could interact with it, the component needs to stay connected to the selected chat server. Even if the chat room component was the initial screen of your app, and the user has not performed any interactions at all, you would _still_ need to connect. This is why it’s an Effect:\n\n```\nfunction ChatRoom({ roomId }) {// ...useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [roomId]);// ...}\n```\n\nWith this code, you can be sure that there is always an active connection to the currently selected chat server, _regardless_ of the specific interactions performed by the user. Whether the user has only opened your app, selected a different room, or navigated to another screen and back, your Effect ensures that the component will _remain synchronized_ with the currently selected room, and will [re-connect whenever it’s necessary.](https://react.dev/learn/lifecycle-of-reactive-effects#why-synchronization-may-need-to-happen-more-than-once)\n\nimport { useState, useEffect } from 'react';\nimport { createConnection, sendMessage } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n const \\[message, setMessage\\] = useState('');\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n function handleSendClick() {\n sendMessage(message);\n }\n\n return (\n <\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <input value\\={message} onChange\\={e \\=> setMessage(e.target.value)} />\n <button onClick\\={handleSendClick}\\>Send</button\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Close chat' : 'Open chat'}\n </button\\>\n {show && <hr />}\n {show && <ChatRoom roomId\\={roomId} />}\n </\\>\n );\n}\n\nReactive values and reactive logic[](https://react.dev/learn/separating-events-from-effects#reactive-values-and-reactive-logic \"Link for Reactive values and reactive logic \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIntuitively, you could say that event handlers are always triggered “manually”, for example by clicking a button. Effects, on the other hand, are “automatic”: they run and re-run as often as it’s needed to stay synchronized.\n\nThere is a more precise way to think about this.\n\nProps, state, and variables declared inside your component’s body are called reactive values. In this example, `serverUrl` is not a reactive value, but `roomId` and `message` are. They participate in the rendering data flow:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {const [message, setMessage] = useState('');// ...}\n```\n\nReactive values like these can change due to a re-render. For example, the user may edit the `message` or choose a different `roomId` in a dropdown. Event handlers and Effects respond to changes differently:\n\n* **Logic inside event handlers is _not reactive._** It will not run again unless the user performs the same interaction (e.g. a click) again. Event handlers can read reactive values without “reacting” to their changes.\n* **Logic inside Effects is _reactive._** If your Effect reads a reactive value, [you have to specify it as a dependency.](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values) Then, if a re-render causes that value to change, React will re-run your Effect’s logic with the new value.\n\nLet’s revisit the previous example to illustrate this difference.\n\n### Logic inside event handlers is not reactive[](https://react.dev/learn/separating-events-from-effects#logic-inside-event-handlers-is-not-reactive \"Link for Logic inside event handlers is not reactive \")\n\nTake a look at this line of code. Should this logic be reactive or not?\n\n```\n// ...sendMessage(message);// ...\n```\n\nFrom the user’s perspective, **a change to the `message` does _not_ mean that they want to send a message.** It only means that the user is typing. In other words, the logic that sends a message should not be reactive. It should not run again only because the reactive value has changed. That’s why it belongs in the event handler:\n\n```\nfunction handleSendClick() {sendMessage(message);}\n```\n\nEvent handlers aren’t reactive, so `sendMessage(message)` will only run when the user clicks the Send button.\n\n### Logic inside Effects is reactive[](https://react.dev/learn/separating-events-from-effects#logic-inside-effects-is-reactive \"Link for Logic inside Effects is reactive \")\n\nNow let’s return to these lines:\n\n```\n// ...const connection = createConnection(serverUrl, roomId);connection.connect();// ...\n```\n\nFrom the user’s perspective, **a change to the `roomId` _does_ mean that they want to connect to a different room.** In other words, the logic for connecting to the room should be reactive. You _want_ these lines of code to “keep up” with the reactive value, and to run again if that value is different. That’s why it belongs in an Effect:\n\n```\nuseEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect()};}, [roomId]);\n```\n\nEffects are reactive, so `createConnection(serverUrl, roomId)` and `connection.connect()` will run for every distinct value of `roomId`. Your Effect keeps the chat connection synchronized to the currently selected room.\n\nThings get more tricky when you want to mix reactive logic with non-reactive logic.\n\nFor example, imagine that you want to show a notification when the user connects to the chat. You read the current theme (dark or light) from the props so that you can show the notification in the correct color:\n\n```\nfunction ChatRoom({ roomId, theme }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.on('connected', () => {showNotification('Connected!', theme);});connection.connect();// ...\n```\n\nHowever, `theme` is a reactive value (it can change as a result of re-rendering), and [every reactive value read by an Effect must be declared as its dependency.](https://react.dev/learn/lifecycle-of-reactive-effects#react-verifies-that-you-specified-every-reactive-value-as-a-dependency) Now you have to specify `theme` as a dependency of your Effect:\n\n```\nfunction ChatRoom({ roomId, theme }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.on('connected', () => {showNotification('Connected!', theme);});connection.connect();return () => {connection.disconnect()};}, [roomId, theme]); // ✅ All dependencies declared// ...\n```\n\nPlay with this example and see if you can spot the problem with this user experience:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection, sendMessage } from './chat.js';\nimport { showNotification } from './notifications.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId, theme }) {\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.on('connected', () \\=> {\n showNotification('Connected!', theme);\n });\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId, theme\\]);\n\n return <h1\\>Welcome to the {roomId} room!</h1\\>\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[isDark, setIsDark\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isDark}\n onChange\\={e \\=> setIsDark(e.target.checked)}\n />\n Use dark theme\n </label\\>\n <hr />\n <ChatRoom\n roomId\\={roomId}\n theme\\={isDark ? 'dark' : 'light'}\n />\n </\\>\n );\n}\n\nWhen the `roomId` changes, the chat re-connects as you would expect. But since `theme` is also a dependency, the chat _also_ re-connects every time you switch between the dark and the light theme. That’s not great!\n\nIn other words, you _don’t_ want this line to be reactive, even though it is inside an Effect (which is reactive):\n\n```\n// ...showNotification('Connected!', theme);// ...\n```\n\nYou need a way to separate this non-reactive logic from the reactive Effect around it.\n\n### Declaring an Effect Event[](https://react.dev/learn/separating-events-from-effects#declaring-an-effect-event \"Link for Declaring an Effect Event \")\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nUse a special Hook called [`useEffectEvent`](https://react.dev/reference/react/experimental_useEffectEvent) to extract this non-reactive logic out of your Effect:\n\n```\nimport { useEffect, useEffectEvent } from 'react';function ChatRoom({ roomId, theme }) {const onConnected = useEffectEvent(() => {showNotification('Connected!', theme);});// ...\n```\n\nHere, `onConnected` is called an _Effect Event._ It’s a part of your Effect logic, but it behaves a lot more like an event handler. The logic inside it is not reactive, and it always “sees” the latest values of your props and state.\n\nNow you can call the `onConnected` Effect Event from inside your Effect:\n\n```\nfunction ChatRoom({ roomId, theme }) {const onConnected = useEffectEvent(() => {showNotification('Connected!', theme);});useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.on('connected', () => {onConnected();});connection.connect();return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...\n```\n\nThis solves the problem. Note that you had to _remove_ `onConnected` from the list of your Effect’s dependencies. **Effect Events are not reactive and must be omitted from dependencies.**\n\nVerify that the new behavior works as you would expect:\n\nimport { useState, useEffect } from 'react';\nimport { experimental\\_useEffectEvent as useEffectEvent } from 'react';\nimport { createConnection, sendMessage } from './chat.js';\nimport { showNotification } from './notifications.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId, theme }) {\n const onConnected = useEffectEvent(() \\=> {\n showNotification('Connected!', theme);\n });\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.on('connected', () \\=> {\n onConnected();\n });\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n return <h1\\>Welcome to the {roomId} room!</h1\\>\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[isDark, setIsDark\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isDark}\n onChange\\={e \\=> setIsDark(e.target.checked)}\n />\n Use dark theme\n </label\\>\n <hr />\n <ChatRoom\n roomId\\={roomId}\n theme\\={isDark ? 'dark' : 'light'}\n />\n </\\>\n );\n}\n\nYou can think of Effect Events as being very similar to event handlers. The main difference is that event handlers run in response to a user interactions, whereas Effect Events are triggered by you from Effects. Effect Events let you “break the chain” between the reactivity of Effects and code that should not be reactive.\n\n### Reading latest props and state with Effect Events[](https://react.dev/learn/separating-events-from-effects#reading-latest-props-and-state-with-effect-events \"Link for Reading latest props and state with Effect Events \")\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nEffect Events let you fix many patterns where you might be tempted to suppress the dependency linter.\n\nFor example, say you have an Effect to log the page visits:\n\n```\nfunction Page() {useEffect(() => {logVisit();}, []);// ...}\n```\n\nLater, you add multiple routes to your site. Now your `Page` component receives a `url` prop with the current path. You want to pass the `url` as a part of your `logVisit` call, but the dependency linter complains:\n\n```\nfunction Page({ url }) {useEffect(() => {logVisit(url);}, []); // 🔴 React Hook useEffect has a missing dependency: 'url'// ...}\n```\n\nThink about what you want the code to do. You _want_ to log a separate visit for different URLs since each URL represents a different page. In other words, this `logVisit` call _should_ be reactive with respect to the `url`. This is why, in this case, it makes sense to follow the dependency linter, and add `url` as a dependency:\n\n```\nfunction Page({ url }) {useEffect(() => {logVisit(url);}, [url]); // ✅ All dependencies declared// ...}\n```\n\nNow let’s say you want to include the number of items in the shopping cart together with every page visit:\n\n```\nfunction Page({ url }) {const { items } = useContext(ShoppingCartContext);const numberOfItems = items.length;useEffect(() => {logVisit(url, numberOfItems);}, [url]); // 🔴 React Hook useEffect has a missing dependency: 'numberOfItems'// ...}\n```\n\nYou used `numberOfItems` inside the Effect, so the linter asks you to add it as a dependency. However, you _don’t_ want the `logVisit` call to be reactive with respect to `numberOfItems`. If the user puts something into the shopping cart, and the `numberOfItems` changes, this _does not mean_ that the user visited the page again. In other words, _visiting the page_ is, in some sense, an “event”. It happens at a precise moment in time.\n\nSplit the code in two parts:\n\n```\nfunction Page({ url }) {const { items } = useContext(ShoppingCartContext);const numberOfItems = items.length;const onVisit = useEffectEvent(visitedUrl => {logVisit(visitedUrl, numberOfItems);});useEffect(() => {onVisit(url);}, [url]); // ✅ All dependencies declared// ...}\n```\n\nHere, `onVisit` is an Effect Event. The code inside it isn’t reactive. This is why you can use `numberOfItems` (or any other reactive value!) without worrying that it will cause the surrounding code to re-execute on changes.\n\nOn the other hand, the Effect itself remains reactive. Code inside the Effect uses the `url` prop, so the Effect will re-run after every re-render with a different `url`. This, in turn, will call the `onVisit` Effect Event.\n\nAs a result, you will call `logVisit` for every change to the `url`, and always read the latest `numberOfItems`. However, if `numberOfItems` changes on its own, this will not cause any of the code to re-run.\n\n### Note\n\nYou might be wondering if you could call `onVisit()` with no arguments, and read the `url` inside it:\n\n```\nconst onVisit = useEffectEvent(() => {logVisit(url, numberOfItems);});useEffect(() => {onVisit();}, [url]);\n```\n\nThis would work, but it’s better to pass this `url` to the Effect Event explicitly. **By passing `url` as an argument to your Effect Event, you are saying that visiting a page with a different `url` constitutes a separate “event” from the user’s perspective.** The `visitedUrl` is a _part_ of the “event” that happened:\n\n```\nconst onVisit = useEffectEvent(visitedUrl => {logVisit(visitedUrl, numberOfItems);});useEffect(() => {onVisit(url);}, [url]);\n```\n\nSince your Effect Event explicitly “asks” for the `visitedUrl`, now you can’t accidentally remove `url` from the Effect’s dependencies. If you remove the `url` dependency (causing distinct page visits to be counted as one), the linter will warn you about it. You want `onVisit` to be reactive with regards to the `url`, so instead of reading the `url` inside (where it wouldn’t be reactive), you pass it _from_ your Effect.\n\nThis becomes especially important if there is some asynchronous logic inside the Effect:\n\n```\nconst onVisit = useEffectEvent(visitedUrl => {logVisit(visitedUrl, numberOfItems);});useEffect(() => {setTimeout(() => {onVisit(url);}, 5000); // Delay logging visits}, [url]);\n```\n\nHere, `url` inside `onVisit` corresponds to the _latest_ `url` (which could have already changed), but `visitedUrl` corresponds to the `url` that originally caused this Effect (and this `onVisit` call) to run.\n\n##### Deep Dive\n\n#### Is it okay to suppress the dependency linter instead?[](https://react.dev/learn/separating-events-from-effects#is-it-okay-to-suppress-the-dependency-linter-instead \"Link for Is it okay to suppress the dependency linter instead? \")\n\nIn the existing codebases, you may sometimes see the lint rule suppressed like this:\n\n```\nfunction Page({ url }) {const { items } = useContext(ShoppingCartContext);const numberOfItems = items.length;useEffect(() => {logVisit(url, numberOfItems);// 🔴 Avoid suppressing the linter like this:// eslint-disable-next-line react-hooks/exhaustive-deps}, [url]);// ...}\n```\n\nAfter `useEffectEvent` becomes a stable part of React, we recommend **never suppressing the linter**.\n\nThe first downside of suppressing the rule is that React will no longer warn you when your Effect needs to “react” to a new reactive dependency you’ve introduced to your code. In the earlier example, you added `url` to the dependencies _because_ React reminded you to do it. You will no longer get such reminders for any future edits to that Effect if you disable the linter. This leads to bugs.\n\nHere is an example of a confusing bug caused by suppressing the linter. In this example, the `handleMove` function is supposed to read the current `canMove` state variable value in order to decide whether the dot should follow the cursor. However, `canMove` is always `true` inside `handleMove`.\n\nCan you see why?\n\nimport { useState, useEffect } from 'react';\n\nexport default function App() {\n const \\[position, setPosition\\] = useState({ x: 0, y: 0 });\n const \\[canMove, setCanMove\\] = useState(true);\n\n function handleMove(e) {\n if (canMove) {\n setPosition({ x: e.clientX, y: e.clientY });\n }\n }\n\n useEffect(() \\=> {\n window.addEventListener('pointermove', handleMove);\n return () \\=> window.removeEventListener('pointermove', handleMove);\n \n }, \\[\\]);\n\n return (\n <\\>\n <label\\>\n <input type\\=\"checkbox\"\n checked\\={canMove}\n onChange\\={e \\=> setCanMove(e.target.checked)}\n />\n The dot is allowed to move\n </label\\>\n <hr />\n <div style\\={{\n position: 'absolute',\n backgroundColor: 'pink',\n borderRadius: '50%',\n opacity: 0.6,\n transform: \\`translate(${position.x}px, ${position.y}px)\\`,\n pointerEvents: 'none',\n left: -20,\n top: -20,\n width: 40,\n height: 40,\n }} />\n </\\>\n );\n}\n\nThe problem with this code is in suppressing the dependency linter. If you remove the suppression, you’ll see that this Effect should depend on the `handleMove` function. This makes sense: `handleMove` is declared inside the component body, which makes it a reactive value. Every reactive value must be specified as a dependency, or it can potentially get stale over time!\n\nThe author of the original code has “lied” to React by saying that the Effect does not depend (`[]`) on any reactive values. This is why React did not re-synchronize the Effect after `canMove` has changed (and `handleMove` with it). Because React did not re-synchronize the Effect, the `handleMove` attached as a listener is the `handleMove` function created during the initial render. During the initial render, `canMove` was `true`, which is why `handleMove` from the initial render will forever see that value.\n\n**If you never suppress the linter, you will never see problems with stale values.**\n\nWith `useEffectEvent`, there is no need to “lie” to the linter, and the code works as you would expect:\n\nimport { useState, useEffect } from 'react';\nimport { experimental\\_useEffectEvent as useEffectEvent } from 'react';\n\nexport default function App() {\n const \\[position, setPosition\\] = useState({ x: 0, y: 0 });\n const \\[canMove, setCanMove\\] = useState(true);\n\n const onMove = useEffectEvent(e \\=> {\n if (canMove) {\n setPosition({ x: e.clientX, y: e.clientY });\n }\n });\n\n useEffect(() \\=> {\n window.addEventListener('pointermove', onMove);\n return () \\=> window.removeEventListener('pointermove', onMove);\n }, \\[\\]);\n\n return (\n <\\>\n <label\\>\n <input type\\=\"checkbox\"\n checked\\={canMove}\n onChange\\={e \\=> setCanMove(e.target.checked)}\n />\n The dot is allowed to move\n </label\\>\n <hr />\n <div style\\={{\n position: 'absolute',\n backgroundColor: 'pink',\n borderRadius: '50%',\n opacity: 0.6,\n transform: \\`translate(${position.x}px, ${position.y}px)\\`,\n pointerEvents: 'none',\n left: -20,\n top: -20,\n width: 40,\n height: 40,\n }} />\n </\\>\n );\n}\n\nThis doesn’t mean that `useEffectEvent` is _always_ the correct solution. You should only apply it to the lines of code that you don’t want to be reactive. In the above sandbox, you didn’t want the Effect’s code to be reactive with regards to `canMove`. That’s why it made sense to extract an Effect Event.\n\nRead [Removing Effect Dependencies](https://react.dev/learn/removing-effect-dependencies) for other correct alternatives to suppressing the linter.\n\n### Limitations of Effect Events[](https://react.dev/learn/separating-events-from-effects#limitations-of-effect-events \"Link for Limitations of Effect Events \")\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nEffect Events are very limited in how you can use them:\n\n* **Only call them from inside Effects.**\n* **Never pass them to other components or Hooks.**\n\nFor example, don’t declare and pass an Effect Event like this:\n\n```\nfunction Timer() {const [count, setCount] = useState(0);const onTick = useEffectEvent(() => {setCount(count + 1);});useTimer(onTick, 1000); // 🔴 Avoid: Passing Effect Eventsreturn <h1>{count}</h1>}function useTimer(callback, delay) {useEffect(() => {const id = setInterval(() => {callback();}, delay);return () => {clearInterval(id);};}, [delay, callback]); // Need to specify \"callback\" in dependencies}\n```\n\nInstead, always declare Effect Events directly next to the Effects that use them:\n\n```\nfunction Timer() {const [count, setCount] = useState(0);useTimer(() => {setCount(count + 1);}, 1000);return <h1>{count}</h1>}function useTimer(callback, delay) {const onTick = useEffectEvent(() => {callback();});useEffect(() => {const id = setInterval(() => {onTick(); // ✅ Good: Only called locally inside an Effect}, delay);return () => {clearInterval(id);};}, [delay]); // No need to specify \"onTick\" (an Effect Event) as a dependency}\n```\n\nEffect Events are non-reactive “pieces” of your Effect code. They should be next to the Effect using them.\n\nRecap[](https://react.dev/learn/separating-events-from-effects#recap \"Link for Recap\")\n--------------------------------------------------------------------------------------\n\n* Event handlers run in response to specific interactions.\n* Effects run whenever synchronization is needed.\n* Logic inside event handlers is not reactive.\n* Logic inside Effects is reactive.\n* You can move non-reactive logic from Effects into Effect Events.\n* Only call Effect Events from inside Effects.\n* Don’t pass Effect Events to other components or Hooks.\n\nTry out some challenges[](https://react.dev/learn/separating-events-from-effects#challenges \"Link for Try out some challenges\")\n-------------------------------------------------------------------------------------------------------------------------------\n\n#### Fix a variable that doesn’t update[](https://react.dev/learn/separating-events-from-effects#fix-a-variable-that-doesnt-update \"Link for this heading\")\n\nThis `Timer` component keeps a `count` state variable which increases every second. The value by which it’s increasing is stored in the `increment` state variable. You can control the `increment` variable with the plus and minus buttons.\n\nHowever, no matter how many times you click the plus button, the counter is still incremented by one every second. What’s wrong with this code? Why is `increment` always equal to `1` inside the Effect’s code? Find the mistake and fix it.\n\nimport { useState, useEffect } from 'react';\n\nexport default function Timer() {\n const \\[count, setCount\\] = useState(0);\n const \\[increment, setIncrement\\] = useState(1);\n\n useEffect(() \\=> {\n const id = setInterval(() \\=> {\n setCount(c \\=> c + increment);\n }, 1000);\n return () \\=> {\n clearInterval(id);\n };\n \n }, \\[\\]);\n\n return (\n <\\>\n <h1\\>\n Counter: {count}\n <button onClick\\={() \\=> setCount(0)}\\>Reset</button\\>\n </h1\\>\n <hr />\n <p\\>\n Every second, increment by:\n <button disabled\\={increment === 0} onClick\\={() \\=> {\n setIncrement(i \\=> i - 1);\n }}\\>–</button\\>\n <b\\>{increment}</b\\>\n <button onClick\\={() \\=> {\n setIncrement(i \\=> i + 1);\n }}\\>+</button\\>\n </p\\>\n </\\>\n );\n}\n",
"filename": "separating-events-from-effects.md",
"package": "react"
} |
{
"content": "Title: Server Actions – React\n\nURL Source: https://react.dev/reference/rsc/server-actions\n\nMarkdown Content:\nServer Actions allow Client Components to call async functions executed on the server.\n\n* [Creating a Server Action from a Server Component](https://react.dev/reference/rsc/server-actions#creating-a-server-action-from-a-server-component)\n* [Importing Server Actions from Client Components](https://react.dev/reference/rsc/server-actions#importing-server-actions-from-client-components)\n* [Composing Server Actions with Actions](https://react.dev/reference/rsc/server-actions#composing-server-actions-with-actions)\n* [Form Actions with Server Actions](https://react.dev/reference/rsc/server-actions#form-actions-with-server-actions)\n* [Server Actions with `useActionState`](https://react.dev/reference/rsc/server-actions#server-actions-with-use-action-state)\n* [Progressive enhancement with `useActionState`](https://react.dev/reference/rsc/server-actions#progressive-enhancement-with-useactionstate)\n\n### Note\n\n#### How do I build support for Server Actions?[](https://react.dev/reference/rsc/server-actions#how-do-i-build-support-for-server-actions \"Link for How do I build support for Server Actions? \")\n\nWhile Server Actions in React 19 are stable and will not break between major versions, the underlying APIs used to implement Server Actions in a React Server Components bundler or framework do not follow semver and may break between minors in React 19.x.\n\nTo support Server Actions as a bundler or framework, we recommend pinning to a specific React version, or using the Canary release. We will continue working with bundlers and frameworks to stabilize the APIs used to implement Server Actions in the future.\n\nWhen a Server Action is defined with the `\"use server\"` directive, your framework will automatically create a reference to the server function, and pass that reference to the Client Component. When that function is called on the client, React will send a request to the server to execute the function, and return the result.\n\nServer Actions can be created in Server Components and passed as props to Client Components, or they can be imported and used in Client Components.\n\n### Creating a Server Action from a Server Component[](https://react.dev/reference/rsc/server-actions#creating-a-server-action-from-a-server-component \"Link for Creating a Server Action from a Server Component \")\n\nServer Components can define Server Actions with the `\"use server\"` directive:\n\n```\n// Server Componentimport Button from './Button';function EmptyNote () {async function createNoteAction() {// Server Action'use server';await db.notes.create();}return <Button onClick={createNoteAction}/>;}\n```\n\nWhen React renders the `EmptyNote` Server Component, it will create a reference to the `createNoteAction` function, and pass that reference to the `Button` Client Component. When the button is clicked, React will send a request to the server to execute the `createNoteAction` function with the reference provided:\n\n```\n\"use client\";export default function Button({onClick}) { console.log(onClick); // {$$typeof: Symbol.for(\"react.server.reference\"), $$id: 'createNoteAction'}return <button onClick={onClick}>Create Empty Note</button>}\n```\n\nFor more, see the docs for [`\"use server\"`](https://react.dev/reference/rsc/use-server).\n\n### Importing Server Actions from Client Components[](https://react.dev/reference/rsc/server-actions#importing-server-actions-from-client-components \"Link for Importing Server Actions from Client Components \")\n\nClient Components can import Server Actions from files that use the `\"use server\"` directive:\n\n```\n\"use server\";export async function createNoteAction() {await db.notes.create();}\n```\n\nWhen the bundler builds the `EmptyNote` Client Component, it will create a reference to the `createNoteAction` function in the bundle. When the `button` is clicked, React will send a request to the server to execute the `createNoteAction` function using the reference provided:\n\n```\n\"use client\";import {createNoteAction} from './actions';function EmptyNote() {console.log(createNoteAction);// {$$typeof: Symbol.for(\"react.server.reference\"), $$id: 'createNoteAction'}<button onClick={createNoteAction} />}\n```\n\nFor more, see the docs for [`\"use server\"`](https://react.dev/reference/rsc/use-server).\n\n### Composing Server Actions with Actions[](https://react.dev/reference/rsc/server-actions#composing-server-actions-with-actions \"Link for Composing Server Actions with Actions \")\n\nServer Actions can be composed with Actions on the client:\n\n```\n\"use server\";export async function updateName(name) {if (!name) {return {error: 'Name is required'};}await db.users.updateName(name);}\n```\n\n```\n\"use client\";import {updateName} from './actions';function UpdateName() {const [name, setName] = useState('');const [error, setError] = useState(null);const [isPending, startTransition] = useTransition();const submitAction = async () => {startTransition(async () => {const {error} = await updateName(name);if (!error) {setError(error);} else {setName('');}})}return (<form action={submitAction}><input type=\"text\" name=\"name\" disabled={isPending}/>{state.error && <span>Failed: {state.error}</span>}</form>)}\n```\n\nThis allows you to access the `isPending` state of the Server Action by wrapping it in an Action on the client.\n\nFor more, see the docs for [Calling a Server Action outside of `<form>`](https://react.dev/reference/rsc/use-server#calling-a-server-action-outside-of-form)\n\n### Form Actions with Server Actions[](https://react.dev/reference/rsc/server-actions#form-actions-with-server-actions \"Link for Form Actions with Server Actions \")\n\nServer Actions work with the new Form features in React 19.\n\nYou can pass a Server Action to a Form to automatically submit the form to the server:\n\n```\n\"use client\";import {updateName} from './actions';function UpdateName() {return (<form action={updateName}><input type=\"text\" name=\"name\" /></form>)}\n```\n\nWhen the Form submission succeeds, React will automatically reset the form. You can add `useActionState` to access the pending state, last response, or to support progressive enhancement.\n\nFor more, see the docs for [Server Actions in Forms](https://react.dev/reference/rsc/use-server#server-actions-in-forms).\n\n### Server Actions with `useActionState`[](https://react.dev/reference/rsc/server-actions#server-actions-with-use-action-state \"Link for this heading\")\n\nYou can compose Server Actions with `useActionState` for the common case where you just need access to the action pending state and last returned response:\n\n```\n\"use client\";import {updateName} from './actions';function UpdateName() {const [state, submitAction, isPending] = useActionState(updateName, {error: null});return (<form action={submitAction}><input type=\"text\" name=\"name\" disabled={isPending}/>{state.error && <span>Failed: {state.error}</span>}</form>);}\n```\n\nWhen using `useActionState` with Server Actions, React will also automatically replay form submissions entered before hydration finishes. This means users can interact with your app even before the app has hydrated.\n\nFor more, see the docs for [`useActionState`](https://react.dev/reference/react-dom/hooks/useFormState).\n\n### Progressive enhancement with `useActionState`[](https://react.dev/reference/rsc/server-actions#progressive-enhancement-with-useactionstate \"Link for this heading\")\n\nServer Actions also support progressive enhancement with the third argument of `useActionState`.\n\n```\n\"use client\";import {updateName} from './actions';function UpdateName() {const [, submitAction] = useActionState(updateName, null, `/name/update`);return (<form action={submitAction}> ...</form>);}\n```\n\nWhen the permalink is provided to `useActionState`, React will redirect to the provided URL if the form is submitted before the JavaScript bundle loads.\n\nFor more, see the docs for [`useActionState`](https://react.dev/reference/react-dom/hooks/useFormState).\n",
"filename": "server-actions.md",
"package": "react"
} |
{
"content": "Title: React Server Components – React\n\nURL Source: https://react.dev/reference/rsc/server-components\n\nMarkdown Content:\nServer Components are a new type of Component that renders ahead of time, before bundling, in an environment separate from your client app or SSR server.\n\nThis separate environment is the “server” in React Server Components. Server Components can run once at build time on your CI server, or they can be run for each request using a web server.\n\n* [Server Components without a Server](https://react.dev/reference/rsc/server-components#server-components-without-a-server)\n* [Server Components with a Server](https://react.dev/reference/rsc/server-components#server-components-with-a-server)\n* [Adding interactivity to Server Components](https://react.dev/reference/rsc/server-components#adding-interactivity-to-server-components)\n* [Async components with Server Components](https://react.dev/reference/rsc/server-components#async-components-with-server-components)\n\n### Note\n\n#### How do I build support for Server Components?[](https://react.dev/reference/rsc/server-components#how-do-i-build-support-for-server-components \"Link for How do I build support for Server Components? \")\n\nWhile React Server Components in React 19 are stable and will not break between major versions, the underlying APIs used to implement a React Server Components bundler or framework do not follow semver and may break between minors in React 19.x.\n\nTo support React Server Components as a bundler or framework, we recommend pinning to a specific React version, or using the Canary release. We will continue working with bundlers and frameworks to stabilize the APIs used to implement React Server Components in the future.\n\n### Server Components without a Server[](https://react.dev/reference/rsc/server-components#server-components-without-a-server \"Link for Server Components without a Server \")\n\nServer components can run at build time to read from the filesystem or fetch static content, so a web server is not required. For example, you may want to read static data from a content management system.\n\nWithout Server Components, it’s common to fetch static data on the client with an Effect:\n\n```\n// bundle.jsimport marked from 'marked'; // 35.9K (11.2K gzipped)import sanitizeHtml from 'sanitize-html'; // 206K (63.3K gzipped)function Page({page}) {const [content, setContent] = useState('');// NOTE: loads *after* first page render.useEffect(() => {fetch(`/api/content/${page}`).then((data) => {setContent(data.content);});}, [page]);return <div>{sanitizeHtml(marked(content))}</div>;}\n```\n\n```\n// api.jsapp.get(`/api/content/:page`, async (req, res) => {const page = req.params.page;const content = await file.readFile(`${page}.md`);res.send({content});});\n```\n\nThis pattern means users need to download and parse an additional 75K (gzipped) of libraries, and wait for a second request to fetch the data after the page loads, just to render static content that will not change for the lifetime of the page.\n\nWith Server Components, you can render these components once at build time:\n\n```\nimport marked from 'marked'; // Not included in bundleimport sanitizeHtml from 'sanitize-html'; // Not included in bundleasync function Page({page}) {// NOTE: loads *during* render, when the app is built.const content = await file.readFile(`${page}.md`);return <div>{sanitizeHtml(marked(content))}</div>;}\n```\n\nThe rendered output can then be server-side rendered (SSR) to HTML and uploaded to a CDN. When the app loads, the client will not see the original `Page` component, or the expensive libraries for rendering the markdown. The client will only see the rendered output:\n\n```\n<div><!-- html for markdown --></div>\n```\n\nThis means the content is visible during first page load, and the bundle does not include the expensive libraries needed to render the static content.\n\n### Note\n\nYou may notice that the Server Component above is an async function:\n\n```\nasync function Page({page}) {//...}\n```\n\nAsync Components are a new feature of Server Components that allow you to `await` in render.\n\nSee [Async components with Server Components](https://react.dev/reference/rsc/server-components#async-components-with-server-components) below.\n\n### Server Components with a Server[](https://react.dev/reference/rsc/server-components#server-components-with-a-server \"Link for Server Components with a Server \")\n\nServer Components can also run on a web server during a request for a page, letting you access your data layer without having to build an API. They are rendered before your application is bundled, and can pass data and JSX as props to Client Components.\n\nWithout Server Components, it’s common to fetch dynamic data on the client in an Effect:\n\n```\n// bundle.jsfunction Note({id}) {const [note, setNote] = useState('');// NOTE: loads *after* first render.useEffect(() => {fetch(`/api/notes/${id}`).then(data => {setNote(data.note);});}, [id]);return (<div><Author id={note.authorId} /><p>{note}</p></div>);}function Author({id}) {const [author, setAuthor] = useState('');// NOTE: loads *after* Note renders.// Causing an expensive client-server waterfall.useEffect(() => {fetch(`/api/authors/${id}`).then(data => {setAuthor(data.author);});}, [id]);return <span>By: {author.name}</span>;}\n```\n\n```\n// apiimport db from './database';app.get(`/api/notes/:id`, async (req, res) => {const note = await db.notes.get(id);res.send({note});});app.get(`/api/authors/:id`, async (req, res) => {const author = await db.authors.get(id);res.send({author});});\n```\n\nWith Server Components, you can read the data and render it in the component:\n\n```\nimport db from './database';async function Note({id}) {// NOTE: loads *during* render.const note = await db.notes.get(id);return (<div><Author id={note.authorId} /><p>{note}</p></div>);}async function Author({id}) {// NOTE: loads *after* Note,// but is fast if data is co-located.const author = await db.authors.get(id);return <span>By: {author.name}</span>;}\n```\n\nThe bundler then combines the data, rendered Server Components and dynamic Client Components into a bundle. Optionally, that bundle can then be server-side rendered (SSR) to create the initial HTML for the page. When the page loads, the browser does not see the original `Note` and `Author` components; only the rendered output is sent to the client:\n\n```\n<div><span>By: The React Team</span><p>React 19 is...</p></div>\n```\n\nServer Components can be made dynamic by re-fetching them from a server, where they can access the data and render again. This new application architecture combines the simple “request/response” mental model of server-centric Multi-Page Apps with the seamless interactivity of client-centric Single-Page Apps, giving you the best of both worlds.\n\n### Adding interactivity to Server Components[](https://react.dev/reference/rsc/server-components#adding-interactivity-to-server-components \"Link for Adding interactivity to Server Components \")\n\nServer Components are not sent to the browser, so they cannot use interactive APIs like `useState`. To add interactivity to Server Components, you can compose them with Client Component using the `\"use client\"` directive.\n\n### Note\n\n#### There is no directive for Server Components.[](https://react.dev/reference/rsc/server-components#there-is-no-directive-for-server-components \"Link for There is no directive for Server Components. \")\n\nA common misunderstanding is that Server Components are denoted by `\"use server\"`, but there is no directive for Server Components. The `\"use server\"` directive is used for Server Actions.\n\nFor more info, see the docs for [Directives](https://react.dev/reference/rsc/directives).\n\nIn the following example, the `Notes` Server Component imports an `Expandable` Client Component that uses state to toggle its `expanded` state:\n\n```\n// Server Componentimport Expandable from './Expandable';async function Notes() {const notes = await db.notes.getAll();return (<div>{notes.map(note => (<Expandable key={note.id}><p note={note} /></Expandable>))}</div>)}\n```\n\n```\n// Client Component\"use client\"export default function Expandable({children}) {const [expanded, setExpanded] = useState(false);return (<div><buttononClick={() => setExpanded(!expanded)}> Toggle</button>{expanded && children}</div>)}\n```\n\nThis works by first rendering `Notes` as a Server Component, and then instructing the bundler to create a bundle for the Client Component `Expandable`. In the browser, the Client Components will see output of the Server Components passed as props:\n\n```\n<head><!-- the bundle for Client Components --><script src=\"bundle.js\" /></head><body><div><Expandable key={1}><p>this is the first note</p></Expandable><Expandable key={2}><p>this is the second note</p></Expandable><!--...--></div> </body>\n```\n\n### Async components with Server Components[](https://react.dev/reference/rsc/server-components#async-components-with-server-components \"Link for Async components with Server Components \")\n\nServer Components introduce a new way to write Components using async/await. When you `await` in an async component, React will suspend and wait for the promise to resolve before resuming rendering. This works across server/client boundaries with streaming support for Suspense.\n\nYou can even create a promise on the server, and await it on the client:\n\n```\n// Server Componentimport db from './database';async function Page({id}) {// Will suspend the Server Component.const note = await db.notes.get(id);// NOTE: not awaited, will start here and await on the client. const commentsPromise = db.comments.get(note.id);return (<div>{note}<Suspense fallback={<p>Loading Comments...</p>}><Comments commentsPromise={commentsPromise} /></Suspense></div>);}\n```\n\n```\n// Client Component\"use client\";import {use} from 'react';function Comments({commentsPromise}) {// NOTE: this will resume the promise from the server.// It will suspend until the data is available.const comments = use(commentsPromise);return comments.map(commment => <p>{comment}</p>);}\n```\n\nThe `note` content is important data for the page to render, so we `await` it on the server. The comments are below the fold and lower-priority, so we start the promise on the server, and wait for it on the client with the `use` API. This will Suspend on the client, without blocking the `note` content from rendering.\n\nSince async components are [not supported on the client](https://react.dev/reference/rsc/server-components#why-cant-i-use-async-components-on-the-client), we await the promise with `use`.\n",
"filename": "server-components.md",
"package": "react"
} |
{
"content": "Title: Server React DOM APIs – React\n\nURL Source: https://react.dev/reference/react-dom/server\n\nMarkdown Content:\nServer React DOM APIs – React\n===============\n\nServer React DOM APIs[](https://react.dev/reference/react-dom/server#undefined \"Link for this heading\")\n=======================================================================================================\n\nThe `react-dom/server` APIs let you render React components to HTML on the server. These APIs are only used on the server at the top level of your app to generate the initial HTML. A [framework](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) may call them for you. Most of your components don’t need to import or use them.\n\n* * *\n\nServer APIs for Node.js Streams[](https://react.dev/reference/react-dom/server#server-apis-for-nodejs-streams \"Link for Server APIs for Node.js Streams \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThese methods are only available in the environments with [Node.js Streams:](https://nodejs.org/api/stream.html)\n\n* [`renderToPipeableStream`](https://react.dev/reference/react-dom/server/renderToPipeableStream) renders a React tree to a pipeable [Node.js Stream.](https://nodejs.org/api/stream.html)\n* [`renderToStaticNodeStream`](https://react.dev/reference/react-dom/server/renderToStaticNodeStream) renders a non-interactive React tree to a [Node.js Readable Stream.](https://nodejs.org/api/stream.html#readable-streams)\n\n* * *\n\nServer APIs for Web Streams[](https://react.dev/reference/react-dom/server#server-apis-for-web-streams \"Link for Server APIs for Web Streams \")\n-----------------------------------------------------------------------------------------------------------------------------------------------\n\nThese methods are only available in the environments with [Web Streams](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API), which includes browsers, Deno, and some modern edge runtimes:\n\n* [`renderToReadableStream`](https://react.dev/reference/react-dom/server/renderToReadableStream) renders a React tree to a [Readable Web Stream.](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream)\n\n* * *\n\nServer APIs for non-streaming environments[](https://react.dev/reference/react-dom/server#server-apis-for-non-streaming-environments \"Link for Server APIs for non-streaming environments \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThese methods can be used in the environments that don’t support streams:\n\n* [`renderToString`](https://react.dev/reference/react-dom/server/renderToString) renders a React tree to a string.\n* [`renderToStaticMarkup`](https://react.dev/reference/react-dom/server/renderToStaticMarkup) renders a non-interactive React tree to a string.\n\nThey have limited functionality compared to the streaming APIs.\n\n* * *\n\nDeprecated server APIs[](https://react.dev/reference/react-dom/server#deprecated-server-apis \"Link for Deprecated server APIs \")\n--------------------------------------------------------------------------------------------------------------------------------\n\n### Deprecated\n\nThese APIs will be removed in a future major version of React.\n\n* [`renderToNodeStream`](https://react.dev/reference/react-dom/server/renderToNodeStream) renders a React tree to a [Node.js Readable stream.](https://nodejs.org/api/stream.html#readable-streams) (Deprecated.)\n\n[PrevioushydrateRoot](https://react.dev/reference/react-dom/client/hydrateRoot)[NextrenderToNodeStream](https://react.dev/reference/react-dom/server/renderToNodeStream)\n",
"filename": "server.md",
"package": "react"
} |
{
"content": "Title: Sharing State Between Components – React\n\nURL Source: https://react.dev/learn/sharing-state-between-components\n\nMarkdown Content:\nSometimes, you want the state of two components to always change together. To do it, remove state from both of them, move it to their closest common parent, and then pass it down to them via props. This is known as _lifting state up,_ and it’s one of the most common things you will do writing React code.\n\n### You will learn\n\n* How to share state between components by lifting it up\n* What are controlled and uncontrolled components\n\nLifting state up by example[](https://react.dev/learn/sharing-state-between-components#lifting-state-up-by-example \"Link for Lifting state up by example \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn this example, a parent `Accordion` component renders two separate `Panel`s:\n\n* `Accordion`\n * `Panel`\n * `Panel`\n\nEach `Panel` component has a boolean `isActive` state that determines whether its content is visible.\n\nPress the Show button for both panels:\n\nNotice how pressing one panel’s button does not affect the other panel—they are independent.\n\n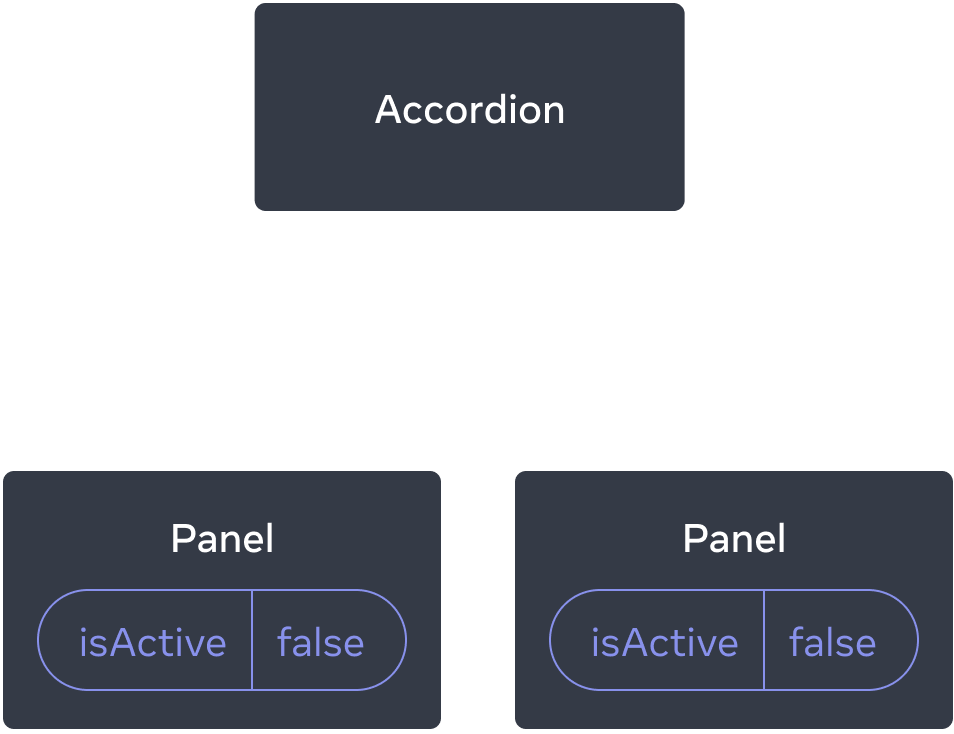\n\n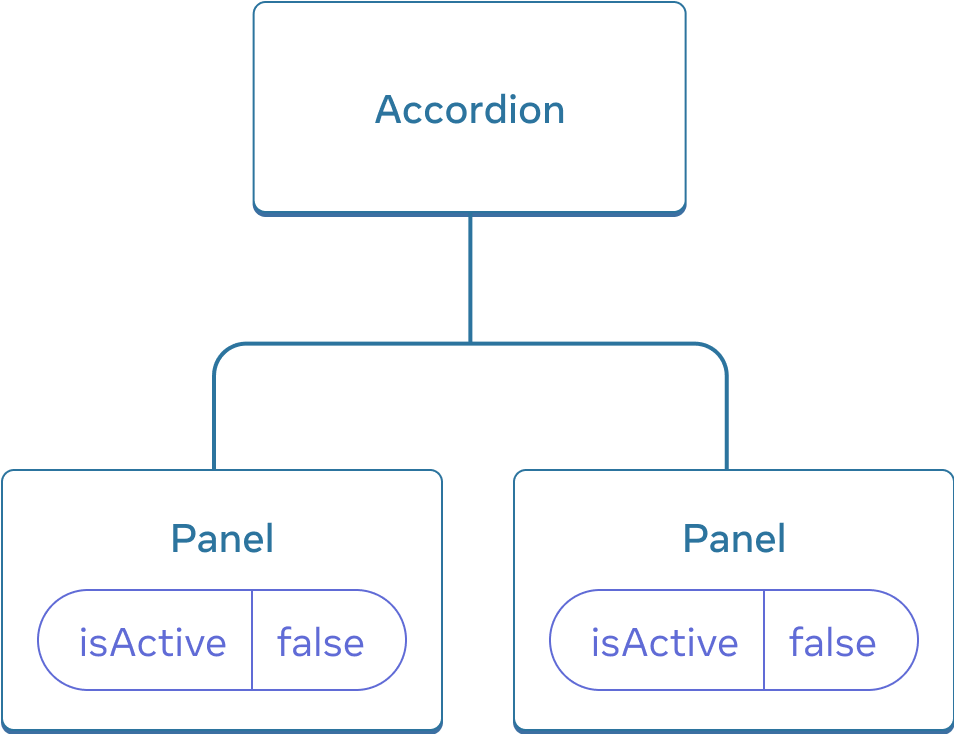\n\nInitially, each `Panel`’s `isActive` state is `false`, so they both appear collapsed\n\n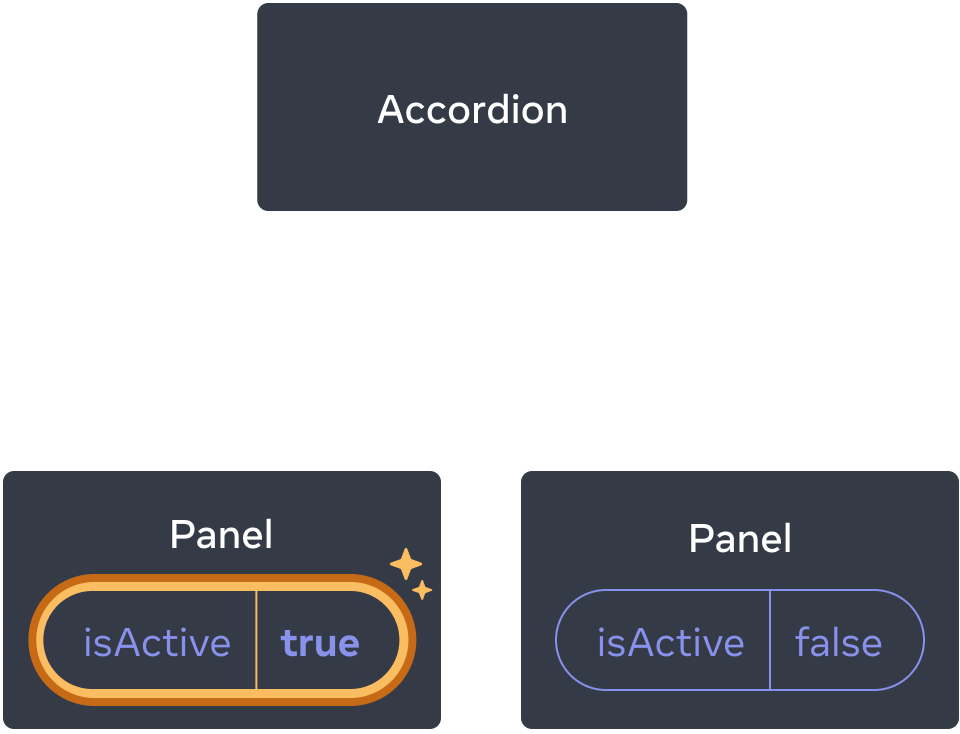\n\n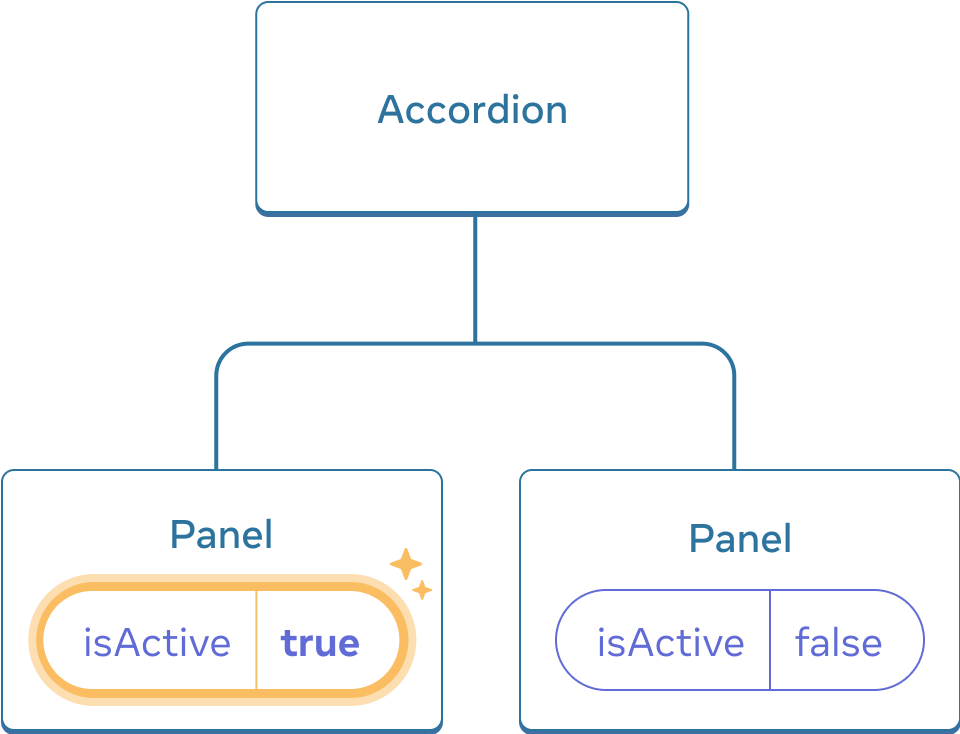\n\nClicking either `Panel`’s button will only update that `Panel`’s `isActive` state alone\n\n**But now let’s say you want to change it so that only one panel is expanded at any given time.** With that design, expanding the second panel should collapse the first one. How would you do that?\n\nTo coordinate these two panels, you need to “lift their state up” to a parent component in three steps:\n\n1. **Remove** state from the child components.\n2. **Pass** hardcoded data from the common parent.\n3. **Add** state to the common parent and pass it down together with the event handlers.\n\nThis will allow the `Accordion` component to coordinate both `Panel`s and only expand one at a time.\n\n### Step 1: Remove state from the child components[](https://react.dev/learn/sharing-state-between-components#step-1-remove-state-from-the-child-components \"Link for Step 1: Remove state from the child components \")\n\nYou will give control of the `Panel`’s `isActive` to its parent component. This means that the parent component will pass `isActive` to `Panel` as a prop instead. Start by **removing this line** from the `Panel` component:\n\n```\nconst [isActive, setIsActive] = useState(false);\n```\n\nAnd instead, add `isActive` to the `Panel`’s list of props:\n\n```\nfunction Panel({ title, children, isActive }) {\n```\n\nNow the `Panel`’s parent component can _control_ `isActive` by [passing it down as a prop.](https://react.dev/learn/passing-props-to-a-component) Conversely, the `Panel` component now has _no control_ over the value of `isActive`—it’s now up to the parent component!\n\n### Step 2: Pass hardcoded data from the common parent[](https://react.dev/learn/sharing-state-between-components#step-2-pass-hardcoded-data-from-the-common-parent \"Link for Step 2: Pass hardcoded data from the common parent \")\n\nTo lift state up, you must locate the closest common parent component of _both_ of the child components that you want to coordinate:\n\n* `Accordion` _(closest common parent)_\n * `Panel`\n * `Panel`\n\nIn this example, it’s the `Accordion` component. Since it’s above both panels and can control their props, it will become the “source of truth” for which panel is currently active. Make the `Accordion` component pass a hardcoded value of `isActive` (for example, `true`) to both panels:\n\nimport { useState } from 'react';\n\nexport default function Accordion() {\n return (\n <\\>\n <h2\\>Almaty, Kazakhstan</h2\\>\n <Panel title\\=\"About\" isActive\\={true}\\>\n With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.\n </Panel\\>\n <Panel title\\=\"Etymology\" isActive\\={true}\\>\n The name comes from <span lang\\=\"kk-KZ\"\\>алма</span\\>, the Kazakh word for \"apple\" and is often translated as \"full of apples\". In fact, the region surrounding Almaty is thought to be the ancestral home of the apple, and the wild <i lang\\=\"la\"\\>Malus sieversii</i\\> is considered a likely candidate for the ancestor of the modern domestic apple.\n </Panel\\>\n </\\>\n );\n}\n\nfunction Panel({ title, children, isActive }) {\n return (\n <section className\\=\"panel\"\\>\n <h3\\>{title}</h3\\>\n {isActive ? (\n <p\\>{children}</p\\>\n ) : (\n <button onClick\\={() \\=> setIsActive(true)}\\>\n Show\n </button\\>\n )}\n </section\\>\n );\n}\n\nTry editing the hardcoded `isActive` values in the `Accordion` component and see the result on the screen.\n\n### Step 3: Add state to the common parent[](https://react.dev/learn/sharing-state-between-components#step-3-add-state-to-the-common-parent \"Link for Step 3: Add state to the common parent \")\n\nLifting state up often changes the nature of what you’re storing as state.\n\nIn this case, only one panel should be active at a time. This means that the `Accordion` common parent component needs to keep track of _which_ panel is the active one. Instead of a `boolean` value, it could use a number as the index of the active `Panel` for the state variable:\n\n```\nconst [activeIndex, setActiveIndex] = useState(0);\n```\n\nWhen the `activeIndex` is `0`, the first panel is active, and when it’s `1`, it’s the second one.\n\nClicking the “Show” button in either `Panel` needs to change the active index in `Accordion`. A `Panel` can’t set the `activeIndex` state directly because it’s defined inside the `Accordion`. The `Accordion` component needs to _explicitly allow_ the `Panel` component to change its state by [passing an event handler down as a prop](https://react.dev/learn/responding-to-events#passing-event-handlers-as-props):\n\n```\n<><PanelisActive={activeIndex === 0}onShow={() => setActiveIndex(0)}> ...</Panel><PanelisActive={activeIndex === 1}onShow={() => setActiveIndex(1)}> ...</Panel></>\n```\n\nThe `<button>` inside the `Panel` will now use the `onShow` prop as its click event handler:\n\nimport { useState } from 'react';\n\nexport default function Accordion() {\n const \\[activeIndex, setActiveIndex\\] = useState(0);\n return (\n <\\>\n <h2\\>Almaty, Kazakhstan</h2\\>\n <Panel\n title\\=\"About\"\n isActive\\={activeIndex === 0}\n onShow\\={() \\=> setActiveIndex(0)}\n \\>\n With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.\n </Panel\\>\n <Panel\n title\\=\"Etymology\"\n isActive\\={activeIndex === 1}\n onShow\\={() \\=> setActiveIndex(1)}\n \\>\n The name comes from <span lang\\=\"kk-KZ\"\\>алма</span\\>, the Kazakh word for \"apple\" and is often translated as \"full of apples\". In fact, the region surrounding Almaty is thought to be the ancestral home of the apple, and the wild <i lang\\=\"la\"\\>Malus sieversii</i\\> is considered a likely candidate for the ancestor of the modern domestic apple.\n </Panel\\>\n </\\>\n );\n}\n\nfunction Panel({\n title,\n children,\n isActive,\n onShow\n}) {\n return (\n <section className\\=\"panel\"\\>\n <h3\\>{title}</h3\\>\n {isActive ? (\n <p\\>{children}</p\\>\n ) : (\n <button onClick\\={onShow}\\>\n Show\n </button\\>\n )}\n </section\\>\n );\n}\n\nThis completes lifting state up! Moving state into the common parent component allowed you to coordinate the two panels. Using the active index instead of two “is shown” flags ensured that only one panel is active at a given time. And passing down the event handler to the child allowed the child to change the parent’s state.\n\n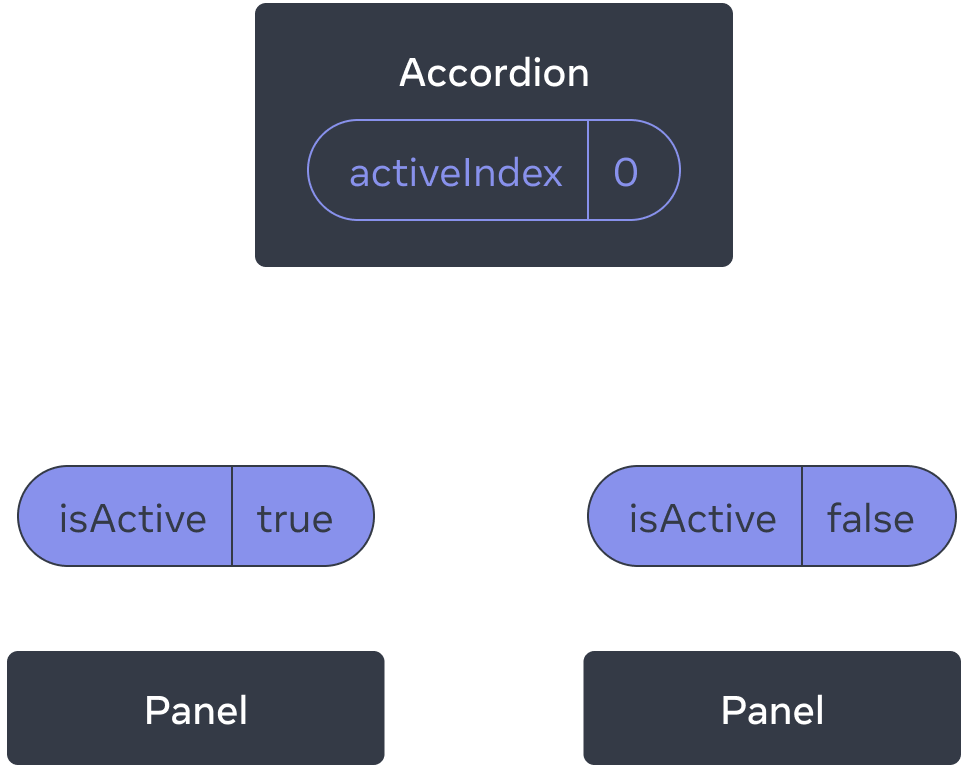\n\n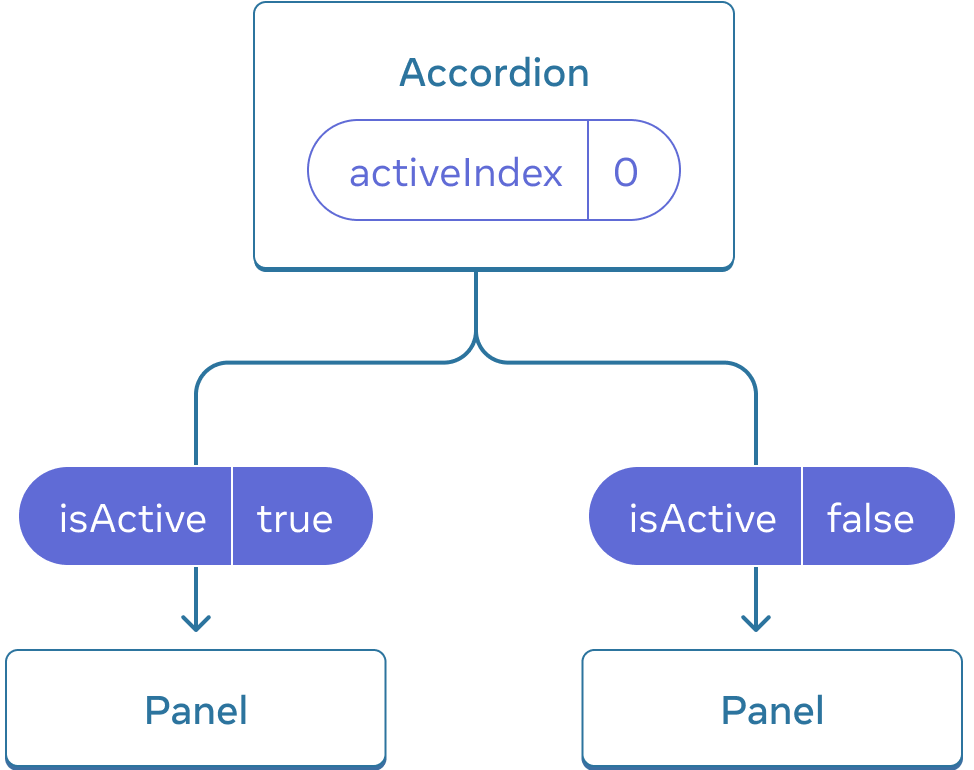\n\nInitially, `Accordion`’s `activeIndex` is `0`, so the first `Panel` receives `isActive = true`\n\n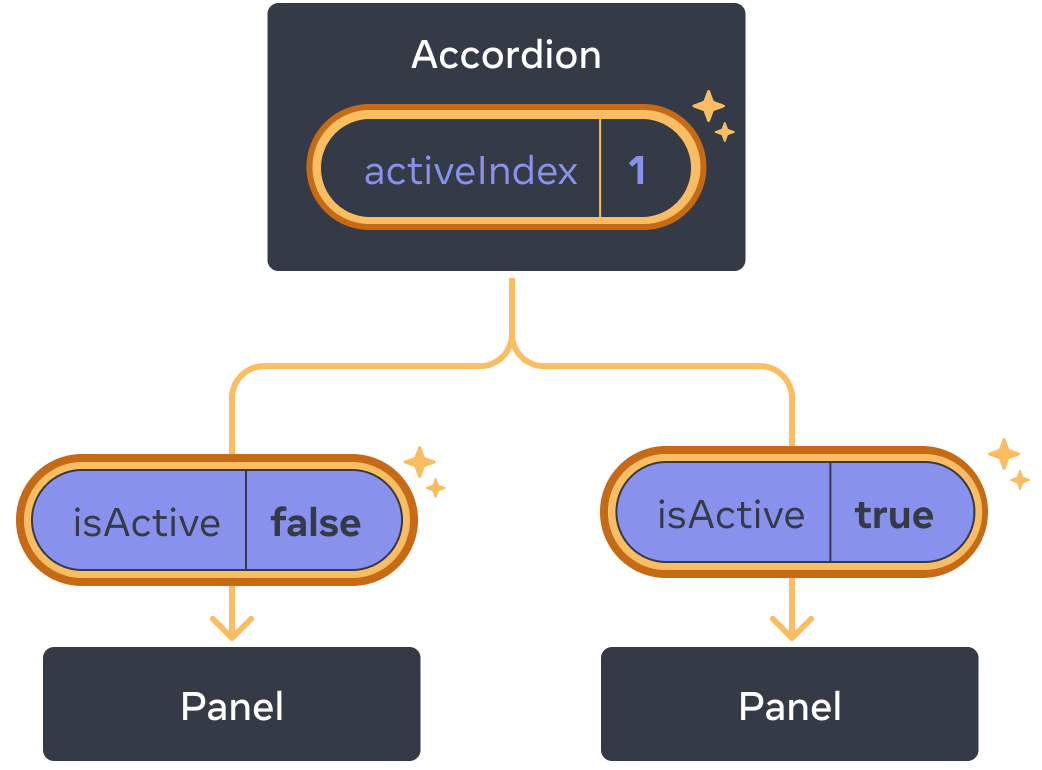\n\n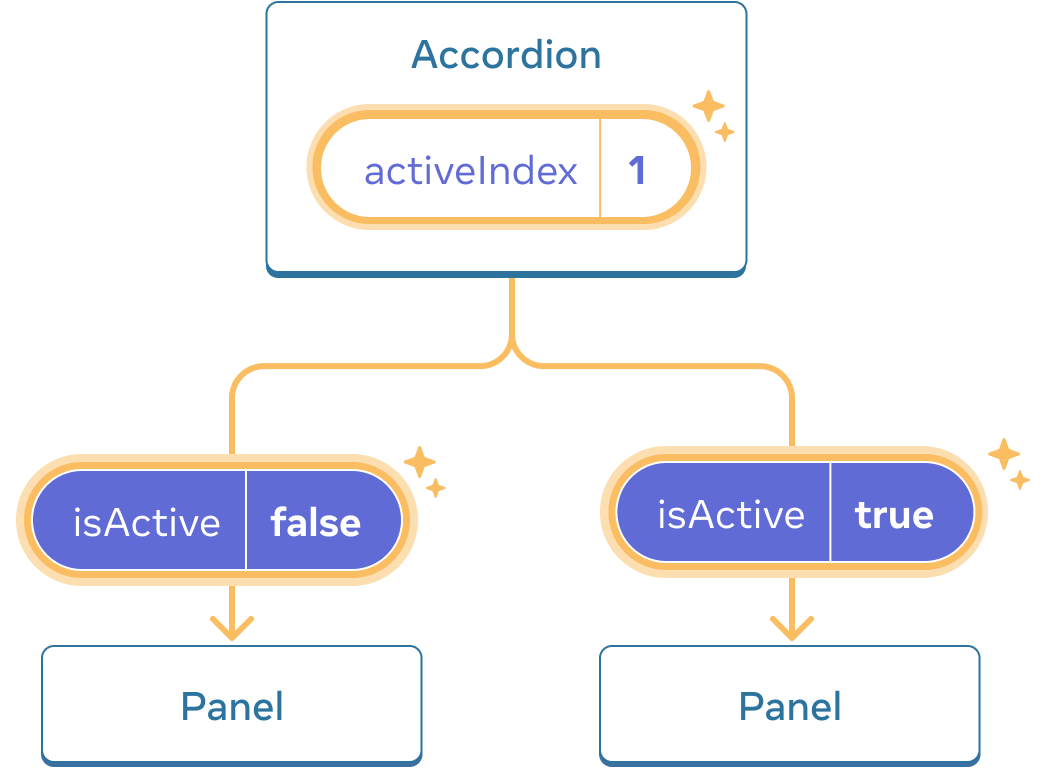\n\nWhen `Accordion`’s `activeIndex` state changes to `1`, the second `Panel` receives `isActive = true` instead\n\n##### Deep Dive\n\n#### Controlled and uncontrolled components[](https://react.dev/learn/sharing-state-between-components#controlled-and-uncontrolled-components \"Link for Controlled and uncontrolled components \")\n\nIt is common to call a component with some local state “uncontrolled”. For example, the original `Panel` component with an `isActive` state variable is uncontrolled because its parent cannot influence whether the panel is active or not.\n\nIn contrast, you might say a component is “controlled” when the important information in it is driven by props rather than its own local state. This lets the parent component fully specify its behavior. The final `Panel` component with the `isActive` prop is controlled by the `Accordion` component.\n\nUncontrolled components are easier to use within their parents because they require less configuration. But they’re less flexible when you want to coordinate them together. Controlled components are maximally flexible, but they require the parent components to fully configure them with props.\n\nIn practice, “controlled” and “uncontrolled” aren’t strict technical terms—each component usually has some mix of both local state and props. However, this is a useful way to talk about how components are designed and what capabilities they offer.\n\nWhen writing a component, consider which information in it should be controlled (via props), and which information should be uncontrolled (via state). But you can always change your mind and refactor later.\n\nA single source of truth for each state[](https://react.dev/learn/sharing-state-between-components#a-single-source-of-truth-for-each-state \"Link for A single source of truth for each state \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn a React application, many components will have their own state. Some state may “live” close to the leaf components (components at the bottom of the tree) like inputs. Other state may “live” closer to the top of the app. For example, even client-side routing libraries are usually implemented by storing the current route in the React state, and passing it down by props!\n\n**For each unique piece of state, you will choose the component that “owns” it.** This principle is also known as having a [“single source of truth”.](https://en.wikipedia.org/wiki/Single_source_of_truth) It doesn’t mean that all state lives in one place—but that for _each_ piece of state, there is a _specific_ component that holds that piece of information. Instead of duplicating shared state between components, _lift it up_ to their common shared parent, and _pass it down_ to the children that need it.\n\nYour app will change as you work on it. It is common that you will move state down or back up while you’re still figuring out where each piece of the state “lives”. This is all part of the process!\n\nTo see what this feels like in practice with a few more components, read [Thinking in React.](https://react.dev/learn/thinking-in-react)\n\nRecap[](https://react.dev/learn/sharing-state-between-components#recap \"Link for Recap\")\n----------------------------------------------------------------------------------------\n\n* When you want to coordinate two components, move their state to their common parent.\n* Then pass the information down through props from their common parent.\n* Finally, pass the event handlers down so that the children can change the parent’s state.\n* It’s useful to consider components as “controlled” (driven by props) or “uncontrolled” (driven by state).\n\nTry out some challenges[](https://react.dev/learn/sharing-state-between-components#challenges \"Link for Try out some challenges\")\n---------------------------------------------------------------------------------------------------------------------------------\n\n#### Synced inputs[](https://react.dev/learn/sharing-state-between-components#synced-inputs \"Link for this heading\")\n\nThese two inputs are independent. Make them stay in sync: editing one input should update the other input with the same text, and vice versa.\n\nimport { useState } from 'react';\n\nexport default function SyncedInputs() {\n return (\n <\\>\n <Input label\\=\"First input\" />\n <Input label\\=\"Second input\" />\n </\\>\n );\n}\n\nfunction Input({ label }) {\n const \\[text, setText\\] = useState('');\n\n function handleChange(e) {\n setText(e.target.value);\n }\n\n return (\n <label\\>\n {label}\n {' '}\n <input\n value\\={text}\n onChange\\={handleChange}\n />\n </label\\>\n );\n}\n",
"filename": "sharing-state-between-components.md",
"package": "react"
} |
{
"content": "Title: Start a New React Project – React\n\nURL Source: https://react.dev/learn/start-a-new-react-project\n\nMarkdown Content:\nIf you want to build a new app or a new website fully with React, we recommend picking one of the React-powered frameworks popular in the community.\n\nYou can use React without a framework, however we’ve found that most apps and sites eventually build solutions to common problems such as code-splitting, routing, data fetching, and generating HTML. These problems are common to all UI libraries, not just React.\n\nBy starting with a framework, you can get started with React quickly, and avoid essentially building your own framework later.\n\n##### Deep Dive\n\n#### Can I use React without a framework?[](https://react.dev/learn/start-a-new-react-project#can-i-use-react-without-a-framework \"Link for Can I use React without a framework? \")\n\nYou can definitely use React without a framework—that’s how you’d [use React for a part of your page.](https://react.dev/learn/add-react-to-an-existing-project#using-react-for-a-part-of-your-existing-page) **However, if you’re building a new app or a site fully with React, we recommend using a framework.**\n\nHere’s why.\n\nEven if you don’t need routing or data fetching at first, you’ll likely want to add some libraries for them. As your JavaScript bundle grows with every new feature, you might have to figure out how to split code for every route individually. As your data fetching needs get more complex, you are likely to encounter server-client network waterfalls that make your app feel very slow. As your audience includes more users with poor network conditions and low-end devices, you might need to generate HTML from your components to display content early—either on the server, or during the build time. Changing your setup to run some of your code on the server or during the build can be very tricky.\n\n**These problems are not React-specific. This is why Svelte has SvelteKit, Vue has Nuxt, and so on.** To solve these problems on your own, you’ll need to integrate your bundler with your router and with your data fetching library. It’s not hard to get an initial setup working, but there are a lot of subtleties involved in making an app that loads quickly even as it grows over time. You’ll want to send down the minimal amount of app code but do so in a single client–server roundtrip, in parallel with any data required for the page. You’ll likely want the page to be interactive before your JavaScript code even runs, to support progressive enhancement. You may want to generate a folder of fully static HTML files for your marketing pages that can be hosted anywhere and still work with JavaScript disabled. Building these capabilities yourself takes real work.\n\n**React frameworks on this page solve problems like these by default, with no extra work from your side.** They let you start very lean and then scale your app with your needs. Each React framework has a community, so finding answers to questions and upgrading tooling is easier. Frameworks also give structure to your code, helping you and others retain context and skills between different projects. Conversely, with a custom setup it’s easier to get stuck on unsupported dependency versions, and you’ll essentially end up creating your own framework—albeit one with no community or upgrade path (and if it’s anything like the ones we’ve made in the past, more haphazardly designed).\n\nIf your app has unusual constraints not served well by these frameworks, or you prefer to solve these problems yourself, you can roll your own custom setup with React. Grab `react` and `react-dom` from npm, set up your custom build process with a bundler like [Vite](https://vitejs.dev/) or [Parcel](https://parceljs.org/), and add other tools as you need them for routing, static generation or server-side rendering, and more.\n\nProduction-grade React frameworks[](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks \"Link for Production-grade React frameworks \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThese frameworks support all the features you need to deploy and scale your app in production and are working towards supporting our [full-stack architecture vision](https://react.dev/learn/start-a-new-react-project#which-features-make-up-the-react-teams-full-stack-architecture-vision). All of the frameworks we recommend are open source with active communities for support, and can be deployed to your own server or a hosting provider. If you’re a framework author interested in being included on this list, [please let us know](https://github.com/reactjs/react.dev/issues/new?assignees=&labels=type%3A+framework&projects=&template=3-framework.yml&title=%5BFramework%5D%3A+).\n\n### Next.js[](https://react.dev/learn/start-a-new-react-project#nextjs-pages-router \"Link for Next.js \")\n\n**[Next.js’ Pages Router](https://nextjs.org/) is a full-stack React framework.** It’s versatile and lets you create React apps of any size—from a mostly static blog to a complex dynamic application. To create a new Next.js project, run in your terminal:\n\nnpx create-next-app@latest\n\nIf you’re new to Next.js, check out the [learn Next.js course.](https://nextjs.org/learn)\n\nNext.js is maintained by [Vercel](https://vercel.com/). You can [deploy a Next.js app](https://nextjs.org/docs/app/building-your-application/deploying) to any Node.js or serverless hosting, or to your own server. Next.js also supports a [static export](https://nextjs.org/docs/pages/building-your-application/deploying/static-exports) which doesn’t require a server.\n\n### Remix[](https://react.dev/learn/start-a-new-react-project#remix \"Link for Remix \")\n\n**[Remix](https://remix.run/) is a full-stack React framework with nested routing.** It lets you break your app into nested parts that can load data in parallel and refresh in response to the user actions. To create a new Remix project, run:\n\nIf you’re new to Remix, check out the Remix [blog tutorial](https://remix.run/docs/en/main/tutorials/blog) (short) and [app tutorial](https://remix.run/docs/en/main/tutorials/jokes) (long).\n\nRemix is maintained by [Shopify](https://www.shopify.com/). When you create a Remix project, you need to [pick your deployment target](https://remix.run/docs/en/main/guides/deployment). You can deploy a Remix app to any Node.js or serverless hosting by using or writing an [adapter](https://remix.run/docs/en/main/other-api/adapter).\n\n### Gatsby[](https://react.dev/learn/start-a-new-react-project#gatsby \"Link for Gatsby \")\n\n**[Gatsby](https://www.gatsbyjs.com/) is a React framework for fast CMS-backed websites.** Its rich plugin ecosystem and its GraphQL data layer simplify integrating content, APIs, and services into one website. To create a new Gatsby project, run:\n\nIf you’re new to Gatsby, check out the [Gatsby tutorial.](https://www.gatsbyjs.com/docs/tutorial/)\n\nGatsby is maintained by [Netlify](https://www.netlify.com/). You can [deploy a fully static Gatsby site](https://www.gatsbyjs.com/docs/how-to/previews-deploys-hosting) to any static hosting. If you opt into using server-only features, make sure your hosting provider supports them for Gatsby.\n\n### Expo (for native apps)[](https://react.dev/learn/start-a-new-react-project#expo \"Link for Expo (for native apps) \")\n\n**[Expo](https://expo.dev/) is a React framework that lets you create universal Android, iOS, and web apps with truly native UIs.** It provides an SDK for [React Native](https://reactnative.dev/) that makes the native parts easier to use. To create a new Expo project, run:\n\nIf you’re new to Expo, check out the [Expo tutorial](https://docs.expo.dev/tutorial/introduction/).\n\nExpo is maintained by [Expo (the company)](https://expo.dev/about). Building apps with Expo is free, and you can submit them to the Google and Apple app stores without restrictions. Expo additionally provides opt-in paid cloud services.\n\nBleeding-edge React frameworks[](https://react.dev/learn/start-a-new-react-project#bleeding-edge-react-frameworks \"Link for Bleeding-edge React frameworks \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAs we’ve explored how to continue improving React, we realized that integrating React more closely with frameworks (specifically, with routing, bundling, and server technologies) is our biggest opportunity to help React users build better apps. The Next.js team has agreed to collaborate with us in researching, developing, integrating, and testing framework-agnostic bleeding-edge React features like [React Server Components.](https://react.dev/blog/2023/03/22/react-labs-what-we-have-been-working-on-march-2023#react-server-components)\n\nThese features are getting closer to being production-ready every day, and we’ve been in talks with other bundler and framework developers about integrating them. Our hope is that in a year or two, all frameworks listed on this page will have full support for these features. (If you’re a framework author interested in partnering with us to experiment with these features, please let us know!)\n\n### Next.js (App Router)[](https://react.dev/learn/start-a-new-react-project#nextjs-app-router \"Link for Next.js (App Router) \")\n\n**[Next.js’s App Router](https://nextjs.org/docs) is a redesign of the Next.js APIs aiming to fulfill the React team’s full-stack architecture vision.** It lets you fetch data in asynchronous components that run on the server or even during the build.\n\nNext.js is maintained by [Vercel](https://vercel.com/). You can [deploy a Next.js app](https://nextjs.org/docs/app/building-your-application/deploying) to any Node.js or serverless hosting, or to your own server. Next.js also supports [static export](https://nextjs.org/docs/app/building-your-application/deploying/static-exports) which doesn’t require a server.\n\n##### Deep Dive\n\n#### Which features make up the React team’s full-stack architecture vision?[](https://react.dev/learn/start-a-new-react-project#which-features-make-up-the-react-teams-full-stack-architecture-vision \"Link for Which features make up the React team’s full-stack architecture vision? \")\n\nNext.js’s App Router bundler fully implements the official [React Server Components specification](https://github.com/reactjs/rfcs/blob/main/text/0188-server-components.md). This lets you mix build-time, server-only, and interactive components in a single React tree.\n\nFor example, you can write a server-only React component as an `async` function that reads from a database or from a file. Then you can pass data down from it to your interactive components:\n\n```\n// This component runs *only* on the server (or during the build).async function Talks({ confId }) {// 1. You're on the server, so you can talk to your data layer. API endpoint not required.const talks = await db.Talks.findAll({ confId });// 2. Add any amount of rendering logic. It won't make your JavaScript bundle larger.const videos = talks.map(talk => talk.video);// 3. Pass the data down to the components that will run in the browser.return <SearchableVideoList videos={videos} />;}\n```\n\nNext.js’s App Router also integrates [data fetching with Suspense](https://react.dev/blog/2022/03/29/react-v18#suspense-in-data-frameworks). This lets you specify a loading state (like a skeleton placeholder) for different parts of your user interface directly in your React tree:\n\n```\n<Suspense fallback={<TalksLoading />}><Talks confId={conf.id} /></Suspense>\n```\n\nServer Components and Suspense are React features rather than Next.js features. However, adopting them at the framework level requires buy-in and non-trivial implementation work. At the moment, the Next.js App Router is the most complete implementation. The React team is working with bundler developers to make these features easier to implement in the next generation of frameworks.\n",
"filename": "start-a-new-react-project.md",
"package": "react"
} |
{
"content": "Title: startTransition – React\n\nURL Source: https://react.dev/reference/react/startTransition\n\nMarkdown Content:\nstartTransition – React\n===============\n\nstartTransition[](https://react.dev/reference/react/startTransition#undefined \"Link for this heading\")\n======================================================================================================\n\n`startTransition` lets you update the state without blocking the UI.\n\n```\nstartTransition(scope)\n```\n\n* [Reference](https://react.dev/reference/react/startTransition#reference)\n * [`startTransition(scope)`](https://react.dev/reference/react/startTransition#starttransitionscope)\n* [Usage](https://react.dev/reference/react/startTransition#usage)\n * [Marking a state update as a non-blocking Transition](https://react.dev/reference/react/startTransition#marking-a-state-update-as-a-non-blocking-transition)\n\n* * *\n\nReference[](https://react.dev/reference/react/startTransition#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------\n\n### `startTransition(scope)`[](https://react.dev/reference/react/startTransition#starttransitionscope \"Link for this heading\")\n\nThe `startTransition` function lets you mark a state update as a Transition.\n\n```\nimport { startTransition } from 'react';function TabContainer() { const [tab, setTab] = useState('about'); function selectTab(nextTab) { startTransition(() => { setTab(nextTab); }); } // ...}\n```\n\n[See more examples below.](https://react.dev/reference/react/startTransition#usage)\n\n#### Parameters[](https://react.dev/reference/react/startTransition#parameters \"Link for Parameters \")\n\n* `scope`: A function that updates some state by calling one or more [`set` functions.](https://react.dev/reference/react/useState#setstate) React immediately calls `scope` with no arguments and marks all state updates scheduled synchronously during the `scope` function call as Transitions. They will be [non-blocking](https://react.dev/reference/react/useTransition#marking-a-state-update-as-a-non-blocking-transition) and [will not display unwanted loading indicators.](https://react.dev/reference/react/useTransition#preventing-unwanted-loading-indicators)\n\n#### Returns[](https://react.dev/reference/react/startTransition#returns \"Link for Returns \")\n\n`startTransition` does not return anything.\n\n#### Caveats[](https://react.dev/reference/react/startTransition#caveats \"Link for Caveats \")\n\n* `startTransition` does not provide a way to track whether a Transition is pending. To show a pending indicator while the Transition is ongoing, you need [`useTransition`](https://react.dev/reference/react/useTransition) instead.\n \n* You can wrap an update into a Transition only if you have access to the `set` function of that state. If you want to start a Transition in response to some prop or a custom Hook return value, try [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue) instead.\n \n* The function you pass to `startTransition` must be synchronous. React immediately executes this function, marking all state updates that happen while it executes as Transitions. If you try to perform more state updates later (for example, in a timeout), they won’t be marked as Transitions.\n \n* A state update marked as a Transition will be interrupted by other state updates. For example, if you update a chart component inside a Transition, but then start typing into an input while the chart is in the middle of a re-render, React will restart the rendering work on the chart component after handling the input state update.\n \n* Transition updates can’t be used to control text inputs.\n \n* If there are multiple ongoing Transitions, React currently batches them together. This is a limitation that will likely be removed in a future release.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/startTransition#usage \"Link for Usage \")\n----------------------------------------------------------------------------------\n\n### Marking a state update as a non-blocking Transition[](https://react.dev/reference/react/startTransition#marking-a-state-update-as-a-non-blocking-transition \"Link for Marking a state update as a non-blocking Transition \")\n\nYou can mark a state update as a _Transition_ by wrapping it in a `startTransition` call:\n\n```\nimport { startTransition } from 'react';function TabContainer() { const [tab, setTab] = useState('about'); function selectTab(nextTab) { startTransition(() => { setTab(nextTab); }); } // ...}\n```\n\nTransitions let you keep the user interface updates responsive even on slow devices.\n\nWith a Transition, your UI stays responsive in the middle of a re-render. For example, if the user clicks a tab but then change their mind and click another tab, they can do that without waiting for the first re-render to finish.\n\n### Note\n\n`startTransition` is very similar to [`useTransition`](https://react.dev/reference/react/useTransition), except that it does not provide the `isPending` flag to track whether a Transition is ongoing. You can call `startTransition` when `useTransition` is not available. For example, `startTransition` works outside components, such as from a data library.\n\n[Learn about Transitions and see examples on the `useTransition` page.](https://react.dev/reference/react/useTransition)\n\n[Previousmemo](https://react.dev/reference/react/memo)[Nextuse](https://react.dev/reference/react/use)\n",
"filename": "startTransition.md",
"package": "react"
} |
{
"content": "Title: State: A Component's Memory – React\n\nURL Source: https://react.dev/learn/state-a-components-memory\n\nMarkdown Content:\nState: A Component's Memory – React\n===============\n\nState: A Component's Memory[](https://react.dev/learn/state-a-components-memory#undefined \"Link for this heading\")\n==================================================================================================================\n\nComponents often need to change what’s on the screen as a result of an interaction. Typing into the form should update the input field, clicking “next” on an image carousel should change which image is displayed, clicking “buy” should put a product in the shopping cart. Components need to “remember” things: the current input value, the current image, the shopping cart. In React, this kind of component-specific memory is called _state_.\n\n### You will learn\n\n* How to add a state variable with the [`useState`](https://react.dev/reference/react/useState) Hook\n* What pair of values the `useState` Hook returns\n* How to add more than one state variable\n* Why state is called local\n\nWhen a regular variable isn’t enough[](https://react.dev/learn/state-a-components-memory#when-a-regular-variable-isnt-enough \"Link for When a regular variable isn’t enough \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nHere’s a component that renders a sculpture image. Clicking the “Next” button should show the next sculpture by changing the `index` to `1`, then `2`, and so on. However, this **won’t work** (you can try it!):\n\nApp.jsdata.js\n\nApp.js\n\nReset\n\nFork\n\n99\n\n1\n\n2\n\n3\n\n4\n\n5\n\n6\n\n7\n\n8\n\n9\n\n10\n\n11\n\n12\n\n13\n\n14\n\n15\n\n16\n\n17\n\n18\n\n19\n\n20\n\n21\n\n22\n\n23\n\n24\n\n25\n\n26\n\n27\n\n28\n\n29\n\n30\n\n31\n\n32\n\n33\n\nimport { sculptureList } from './data.js';\n\n \n\nexport default function Gallery() {\n\nlet index = 0;\n\n \n\nfunction handleClick() {\n\nindex = index + 1;\n\n}\n\n \n\nlet sculpture = sculptureList\\[index\\];\n\nreturn (\n\n<\\>\n\n<button onClick\\={handleClick}\\>\n\nNext\n\n</button\\>\n\n<h2\\>\n\n<i\\>{sculpture.name} </i\\>\n\nby {sculpture.artist}\n\n</h2\\>\n\n<h3\\>\n\n({index + 1} of {sculptureList.length})\n\n</h3\\>\n\n<img\n\nsrc\\={sculpture.url}\n\nalt\\={sculpture.alt}\n\n/>\n\n<p\\>\n\n{sculpture.description}\n\n</p\\>\n\n</\\>\n\n);\n\n}\n\n \n\nOpen Sandbox\n\nShow more\n\nThe `handleClick` event handler is updating a local variable, `index`. But two things prevent that change from being visible:\n\n1. **Local variables don’t persist between renders.** When React renders this component a second time, it renders it from scratch—it doesn’t consider any changes to the local variables.\n2. **Changes to local variables won’t trigger renders.** React doesn’t realize it needs to render the component again with the new data.\n\nTo update a component with new data, two things need to happen:\n\n1. **Retain** the data between renders.\n2. **Trigger** React to render the component with new data (re-rendering).\n\nThe [`useState`](https://react.dev/reference/react/useState) Hook provides those two things:\n\n1. A **state variable** to retain the data between renders.\n2. A **state setter function** to update the variable and trigger React to render the component again.\n\nAdding a state variable[](https://react.dev/learn/state-a-components-memory#adding-a-state-variable \"Link for Adding a state variable \")\n----------------------------------------------------------------------------------------------------------------------------------------\n\nTo add a state variable, import `useState` from React at the top of the file:\n\n```\nimport { useState } from 'react';\n```\n\nThen, replace this line:\n\n```\nlet index = 0;\n```\n\nwith\n\n```\nconst [index, setIndex] = useState(0);\n```\n\n`index` is a state variable and `setIndex` is the setter function.\n\n> The `[` and `]` syntax here is called [array destructuring](https://javascript.info/destructuring-assignment) and it lets you read values from an array. The array returned by `useState` always has exactly two items.\n\nThis is how they work together in `handleClick`:\n\n```\nfunction handleClick() { setIndex(index + 1);}\n```\n\nNow clicking the “Next” button switches the current sculpture:\n\nApp.jsdata.js\n\nApp.js\n\nReset\n\nFork\n\nimport { useState } from 'react';\nimport { sculptureList } from './data.js';\n\nexport default function Gallery() {\n const \\[index, setIndex\\] = useState(0);\n\n function handleClick() {\n setIndex(index + 1);\n }\n\n let sculpture = sculptureList\\[index\\];\n return (\n <\\>\n <button onClick\\={handleClick}\\>\n Next\n </button\\>\n <h2\\>\n <i\\>{sculpture.name} </i\\> \n by {sculpture.artist}\n </h2\\>\n <h3\\> \n ({index + 1} of {sculptureList.length})\n </h3\\>\n <img \n src\\={sculpture.url} \n alt\\={sculpture.alt}\n />\n <p\\>\n {sculpture.description}\n </p\\>\n </\\>\n );\n}\n\nShow more\n\n### Meet your first Hook[](https://react.dev/learn/state-a-components-memory#meet-your-first-hook \"Link for Meet your first Hook \")\n\nIn React, `useState`, as well as any other function starting with “`use`”, is called a Hook.\n\n_Hooks_ are special functions that are only available while React is [rendering](https://react.dev/learn/render-and-commit#step-1-trigger-a-render) (which we’ll get into in more detail on the next page). They let you “hook into” different React features.\n\nState is just one of those features, but you will meet the other Hooks later.\n\n### Pitfall\n\n**Hooks—functions starting with `use`—can only be called at the top level of your components or [your own Hooks.](https://react.dev/learn/reusing-logic-with-custom-hooks)** You can’t call Hooks inside conditions, loops, or other nested functions. Hooks are functions, but it’s helpful to think of them as unconditional declarations about your component’s needs. You “use” React features at the top of your component similar to how you “import” modules at the top of your file.\n\n### Anatomy of `useState`[](https://react.dev/learn/state-a-components-memory#anatomy-of-usestate \"Link for this heading\")\n\nWhen you call [`useState`](https://react.dev/reference/react/useState), you are telling React that you want this component to remember something:\n\n```\nconst [index, setIndex] = useState(0);\n```\n\nIn this case, you want React to remember `index`.\n\n### Note\n\nThe convention is to name this pair like `const [something, setSomething]`. You could name it anything you like, but conventions make things easier to understand across projects.\n\nThe only argument to `useState` is the **initial value** of your state variable. In this example, the `index`’s initial value is set to `0` with `useState(0)`.\n\nEvery time your component renders, `useState` gives you an array containing two values:\n\n1. The **state variable** (`index`) with the value you stored.\n2. The **state setter function** (`setIndex`) which can update the state variable and trigger React to render the component again.\n\nHere’s how that happens in action:\n\n```\nconst [index, setIndex] = useState(0);\n```\n\n1. **Your component renders the first time.** Because you passed `0` to `useState` as the initial value for `index`, it will return `[0, setIndex]`. React remembers `0` is the latest state value.\n2. **You update the state.** When a user clicks the button, it calls `setIndex(index + 1)`. `index` is `0`, so it’s `setIndex(1)`. This tells React to remember `index` is `1` now and triggers another render.\n3. **Your component’s second render.** React still sees `useState(0)`, but because React _remembers_ that you set `index` to `1`, it returns `[1, setIndex]` instead.\n4. And so on!\n\nGiving a component multiple state variables[](https://react.dev/learn/state-a-components-memory#giving-a-component-multiple-state-variables \"Link for Giving a component multiple state variables \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou can have as many state variables of as many types as you like in one component. This component has two state variables, a number `index` and a boolean `showMore` that’s toggled when you click “Show details”:\n\nApp.jsdata.js\n\nApp.js\n\nReset\n\nFork\n\nimport { useState } from 'react';\nimport { sculptureList } from './data.js';\n\nexport default function Gallery() {\n const \\[index, setIndex\\] = useState(0);\n const \\[showMore, setShowMore\\] = useState(false);\n\n function handleNextClick() {\n setIndex(index + 1);\n }\n\n function handleMoreClick() {\n setShowMore(!showMore);\n }\n\n let sculpture = sculptureList\\[index\\];\n return (\n <\\>\n <button onClick\\={handleNextClick}\\>\n Next\n </button\\>\n <h2\\>\n <i\\>{sculpture.name} </i\\> \n by {sculpture.artist}\n </h2\\>\n <h3\\> \n ({index + 1} of {sculptureList.length})\n </h3\\>\n <button onClick\\={handleMoreClick}\\>\n {showMore ? 'Hide' : 'Show'} details\n </button\\>\n {showMore && <p\\>{sculpture.description}</p\\>}\n <img \n src\\={sculpture.url} \n alt\\={sculpture.alt}\n />\n </\\>\n );\n}\n\nShow more\n\nIt is a good idea to have multiple state variables if their state is unrelated, like `index` and `showMore` in this example. But if you find that you often change two state variables together, it might be easier to combine them into one. For example, if you have a form with many fields, it’s more convenient to have a single state variable that holds an object than state variable per field. Read [Choosing the State Structure](https://react.dev/learn/choosing-the-state-structure) for more tips.\n\n##### Deep Dive\n\n#### How does React know which state to return?[](https://react.dev/learn/state-a-components-memory#how-does-react-know-which-state-to-return \"Link for How does React know which state to return? \")\n\nShow Details\n\nYou might have noticed that the `useState` call does not receive any information about _which_ state variable it refers to. There is no “identifier” that is passed to `useState`, so how does it know which of the state variables to return? Does it rely on some magic like parsing your functions? The answer is no.\n\nInstead, to enable their concise syntax, Hooks **rely on a stable call order on every render of the same component.** This works well in practice because if you follow the rule above (“only call Hooks at the top level”), Hooks will always be called in the same order. Additionally, a [linter plugin](https://www.npmjs.com/package/eslint-plugin-react-hooks) catches most mistakes.\n\nInternally, React holds an array of state pairs for every component. It also maintains the current pair index, which is set to `0` before rendering. Each time you call `useState`, React gives you the next state pair and increments the index. You can read more about this mechanism in [React Hooks: Not Magic, Just Arrays.](https://medium.com/@ryardley/react-hooks-not-magic-just-arrays-cd4f1857236e)\n\nThis example **doesn’t use React** but it gives you an idea of how `useState` works internally:\n\nindex.jsindex.html\n\nindex.js\n\nReset\n\nFork\n\nlet componentHooks = \\[\\];\nlet currentHookIndex = 0;\n\n// How useState works inside React (simplified).\nfunction useState(initialState) {\n let pair = componentHooks\\[currentHookIndex\\];\n if (pair) {\n // This is not the first render,\n // so the state pair already exists.\n // Return it and prepare for next Hook call.\n currentHookIndex++;\n return pair;\n }\n\n // This is the first time we're rendering,\n // so create a state pair and store it.\n pair = \\[initialState, setState\\];\n\n function setState(nextState) {\n // When the user requests a state change,\n // put the new value into the pair.\n pair\\[0\\] = nextState;\n updateDOM();\n }\n\n // Store the pair for future renders\n // and prepare for the next Hook call.\n componentHooks\\[currentHookIndex\\] = pair;\n currentHookIndex++;\n return pair;\n}\n\nfunction Gallery() {\n // Each useState() call will get the next pair.\n const \\[index, setIndex\\] = useState(0);\n const \\[showMore, setShowMore\\] = useState(false);\n\n function handleNextClick() {\n setIndex(index + 1);\n }\n\n function handleMoreClick() {\n setShowMore(!showMore);\n }\n\n let sculpture = sculptureList\\[index\\];\n // This example doesn't use React, so\n // return an output object instead of JSX.\n return {\n onNextClick: handleNextClick,\n onMoreClick: handleMoreClick,\n header: \\`${sculpture.name} by ${sculpture.artist}\\`,\n counter: \\`${index + 1} of ${sculptureList.length}\\`,\n more: \\`${showMore ? 'Hide' : 'Show'} details\\`,\n description: showMore ? sculpture.description : null,\n imageSrc: sculpture.url,\n imageAlt: sculpture.alt\n };\n}\n\nfunction updateDOM() {\n // Reset the current Hook index\n // before rendering the component.\n currentHookIndex = 0;\n let output = Gallery();\n\n // Update the DOM to match the output.\n // This is the part React does for you.\n nextButton.onclick = output.onNextClick;\n header.textContent = output.header;\n moreButton.onclick = output.onMoreClick;\n moreButton.textContent = output.more;\n image.src = output.imageSrc;\n image.alt = output.imageAlt;\n if (output.description !== null) {\n description.textContent = output.description;\n description.style.display = '';\n } else {\n description.style.display = 'none';\n }\n}\n\nlet nextButton = document.getElementById('nextButton');\nlet header = document.getElementById('header');\nlet moreButton = document.getElementById('moreButton');\nlet description = document.getElementById('description');\nlet image = document.getElementById('image');\nlet sculptureList = \\[{\n name: 'Homenaje a la Neurocirugía',\n artist: 'Marta Colvin Andrade',\n description: 'Although Colvin is predominantly known for abstract themes that allude to pre-Hispanic symbols, this gigantic sculpture, an homage to neurosurgery, is one of her most recognizable public art pieces.',\n url: 'https://i.imgur.com/Mx7dA2Y.jpg',\n alt: 'A bronze statue of two crossed hands delicately holding a human brain in their fingertips.' \n}, {\n name: 'Floralis Genérica',\n artist: 'Eduardo Catalano',\n description: 'This enormous (75 ft. or 23m) silver flower is located in Buenos Aires. It is designed to move, closing its petals in the evening or when strong winds blow and opening them in the morning.',\n url: 'https://i.imgur.com/ZF6s192m.jpg',\n alt: 'A gigantic metallic flower sculpture with reflective mirror-like petals and strong stamens.'\n}, {\n name: 'Eternal Presence',\n artist: 'John Woodrow Wilson',\n description: 'Wilson was known for his preoccupation with equality, social justice, as well as the essential and spiritual qualities of humankind. This massive (7ft. or 2,13m) bronze represents what he described as \"a symbolic Black presence infused with a sense of universal humanity.\"',\n url: 'https://i.imgur.com/aTtVpES.jpg',\n alt: 'The sculpture depicting a human head seems ever-present and solemn. It radiates calm and serenity.'\n}, {\n name: 'Moai',\n artist: 'Unknown Artist',\n description: 'Located on the Easter Island, there are 1,000 moai, or extant monumental statues, created by the early Rapa Nui people, which some believe represented deified ancestors.',\n url: 'https://i.imgur.com/RCwLEoQm.jpg',\n alt: 'Three monumental stone busts with the heads that are disproportionately large with somber faces.'\n}, {\n name: 'Blue Nana',\n artist: 'Niki de Saint Phalle',\n description: 'The Nanas are triumphant creatures, symbols of femininity and maternity. Initially, Saint Phalle used fabric and found objects for the Nanas, and later on introduced polyester to achieve a more vibrant effect.',\n url: 'https://i.imgur.com/Sd1AgUOm.jpg',\n alt: 'A large mosaic sculpture of a whimsical dancing female figure in a colorful costume emanating joy.'\n}, {\n name: 'Ultimate Form',\n artist: 'Barbara Hepworth',\n description: 'This abstract bronze sculpture is a part of The Family of Man series located at Yorkshire Sculpture Park. Hepworth chose not to create literal representations of the world but developed abstract forms inspired by people and landscapes.',\n url: 'https://i.imgur.com/2heNQDcm.jpg',\n alt: 'A tall sculpture made of three elements stacked on each other reminding of a human figure.'\n}, {\n name: 'Cavaliere',\n artist: 'Lamidi Olonade Fakeye',\n description: \"Descended from four generations of woodcarvers, Fakeye's work blended traditional and contemporary Yoruba themes.\",\n url: 'https://i.imgur.com/wIdGuZwm.png',\n alt: 'An intricate wood sculpture of a warrior with a focused face on a horse adorned with patterns.'\n}, {\n name: 'Big Bellies',\n artist: 'Alina Szapocznikow',\n description: \"Szapocznikow is known for her sculptures of the fragmented body as a metaphor for the fragility and impermanence of youth and beauty. This sculpture depicts two very realistic large bellies stacked on top of each other, each around five feet (1,5m) tall.\",\n url: 'https://i.imgur.com/AlHTAdDm.jpg',\n alt: 'The sculpture reminds a cascade of folds, quite different from bellies in classical sculptures.'\n}, {\n name: 'Terracotta Army',\n artist: 'Unknown Artist',\n description: 'The Terracotta Army is a collection of terracotta sculptures depicting the armies of Qin Shi Huang, the first Emperor of China. The army consisted of more than 8,000 soldiers, 130 chariots with 520 horses, and 150 cavalry horses.',\n url: 'https://i.imgur.com/HMFmH6m.jpg',\n alt: '12 terracotta sculptures of solemn warriors, each with a unique facial expression and armor.'\n}, {\n name: 'Lunar Landscape',\n artist: 'Louise Nevelson',\n description: 'Nevelson was known for scavenging objects from New York City debris, which she would later assemble into monumental constructions. In this one, she used disparate parts like a bedpost, juggling pin, and seat fragment, nailing and gluing them into boxes that reflect the influence of Cubism’s geometric abstraction of space and form.',\n url: 'https://i.imgur.com/rN7hY6om.jpg',\n alt: 'A black matte sculpture where the individual elements are initially indistinguishable.'\n}, {\n name: 'Aureole',\n artist: 'Ranjani Shettar',\n description: 'Shettar merges the traditional and the modern, the natural and the industrial. Her art focuses on the relationship between man and nature. Her work was described as compelling both abstractly and figuratively, gravity defying, and a \"fine synthesis of unlikely materials.\"',\n url: 'https://i.imgur.com/okTpbHhm.jpg',\n alt: 'A pale wire-like sculpture mounted on concrete wall and descending on the floor. It appears light.'\n}, {\n name: 'Hippos',\n artist: 'Taipei Zoo',\n description: 'The Taipei Zoo commissioned a Hippo Square featuring submerged hippos at play.',\n url: 'https://i.imgur.com/6o5Vuyu.jpg',\n alt: 'A group of bronze hippo sculptures emerging from the sett sidewalk as if they were swimming.'\n}\\];\n\n// Make UI match the initial state.\nupdateDOM();\n\nShow more\n\nYou don’t have to understand it to use React, but you might find this a helpful mental model.\n\nState is isolated and private[](https://react.dev/learn/state-a-components-memory#state-is-isolated-and-private \"Link for State is isolated and private \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------\n\nState is local to a component instance on the screen. In other words, **if you render the same component twice, each copy will have completely isolated state!** Changing one of them will not affect the other.\n\nIn this example, the `Gallery` component from earlier is rendered twice with no changes to its logic. Try clicking the buttons inside each of the galleries. Notice that their state is independent:\n\nApp.jsGallery.jsdata.js\n\nApp.js\n\nReset\n\nFork\n\nimport Gallery from './Gallery.js';\n\nexport default function Page() {\n return (\n <div className\\=\"Page\"\\>\n <Gallery />\n <Gallery />\n </div\\>\n );\n}\n\nThis is what makes state different from regular variables that you might declare at the top of your module. State is not tied to a particular function call or a place in the code, but it’s “local” to the specific place on the screen. You rendered two `<Gallery />` components, so their state is stored separately.\n\nAlso notice how the `Page` component doesn’t “know” anything about the `Gallery` state or even whether it has any. Unlike props, **state is fully private to the component declaring it.** The parent component can’t change it. This lets you add state to any component or remove it without impacting the rest of the components.\n\nWhat if you wanted both galleries to keep their states in sync? The right way to do it in React is to _remove_ state from child components and add it to their closest shared parent. The next few pages will focus on organizing state of a single component, but we will return to this topic in [Sharing State Between Components.](https://react.dev/learn/sharing-state-between-components)\n\nRecap[](https://react.dev/learn/state-a-components-memory#recap \"Link for Recap\")\n---------------------------------------------------------------------------------\n\n* Use a state variable when a component needs to “remember” some information between renders.\n* State variables are declared by calling the `useState` Hook.\n* Hooks are special functions that start with `use`. They let you “hook into” React features like state.\n* Hooks might remind you of imports: they need to be called unconditionally. Calling Hooks, including `useState`, is only valid at the top level of a component or another Hook.\n* The `useState` Hook returns a pair of values: the current state and the function to update it.\n* You can have more than one state variable. Internally, React matches them up by their order.\n* State is private to the component. If you render it in two places, each copy gets its own state.\n\nTry out some challenges[](https://react.dev/learn/state-a-components-memory#challenges \"Link for Try out some challenges\")\n--------------------------------------------------------------------------------------------------------------------------\n\n1. Complete the gallery 2. Fix stuck form inputs 3. Fix a crash 4. Remove unnecessary state\n\n#### \n\nChallenge 1 of 4:\n\nComplete the gallery[](https://react.dev/learn/state-a-components-memory#complete-the-gallery \"Link for this heading\")\n\nWhen you press “Next” on the last sculpture, the code crashes. Fix the logic to prevent the crash. You may do this by adding extra logic to event handler or by disabling the button when the action is not possible.\n\nAfter fixing the crash, add a “Previous” button that shows the previous sculpture. It shouldn’t crash on the first sculpture.\n\nApp.jsdata.js\n\nApp.js\n\nReset\n\nFork\n\nimport { useState } from 'react';\nimport { sculptureList } from './data.js';\n\nexport default function Gallery() {\n const \\[index, setIndex\\] = useState(0);\n const \\[showMore, setShowMore\\] = useState(false);\n\n function handleNextClick() {\n setIndex(index + 1);\n }\n\n function handleMoreClick() {\n setShowMore(!showMore);\n }\n\n let sculpture = sculptureList\\[index\\];\n return (\n <\\>\n <button onClick\\={handleNextClick}\\>\n Next\n </button\\>\n <h2\\>\n <i\\>{sculpture.name} </i\\> \n by {sculpture.artist}\n </h2\\>\n <h3\\> \n ({index + 1} of {sculptureList.length})\n </h3\\>\n <button onClick\\={handleMoreClick}\\>\n {showMore ? 'Hide' : 'Show'} details\n </button\\>\n {showMore && <p\\>{sculpture.description}</p\\>}\n <img \n src\\={sculpture.url} \n alt\\={sculpture.alt}\n />\n </\\>\n );\n}\n\nShow more\n\nShow solutionNext Challenge\n\n[PreviousResponding to Events](https://react.dev/learn/responding-to-events)[NextRender and Commit](https://react.dev/learn/render-and-commit)\n",
"filename": "state-a-components-memory.md",
"package": "react"
} |
{
"content": "Title: State as a Snapshot – React\n\nURL Source: https://react.dev/learn/state-as-a-snapshot\n\nMarkdown Content:\nState variables might look like regular JavaScript variables that you can read and write to. However, state behaves more like a snapshot. Setting it does not change the state variable you already have, but instead triggers a re-render.\n\n### You will learn\n\n* How setting state triggers re-renders\n* When and how state updates\n* Why state does not update immediately after you set it\n* How event handlers access a “snapshot” of the state\n\nSetting state triggers renders[](https://react.dev/learn/state-as-a-snapshot#setting-state-triggers-renders \"Link for Setting state triggers renders \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou might think of your user interface as changing directly in response to the user event like a click. In React, it works a little differently from this mental model. On the previous page, you saw that [setting state requests a re-render](https://react.dev/learn/render-and-commit#step-1-trigger-a-render) from React. This means that for an interface to react to the event, you need to _update the state_.\n\nIn this example, when you press “send”, `setIsSent(true)` tells React to re-render the UI:\n\nHere’s what happens when you click the button:\n\n1. The `onSubmit` event handler executes.\n2. `setIsSent(true)` sets `isSent` to `true` and queues a new render.\n3. React re-renders the component according to the new `isSent` value.\n\nLet’s take a closer look at the relationship between state and rendering.\n\nRendering takes a snapshot in time[](https://react.dev/learn/state-as-a-snapshot#rendering-takes-a-snapshot-in-time \"Link for Rendering takes a snapshot in time \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\n[“Rendering”](https://react.dev/learn/render-and-commit#step-2-react-renders-your-components) means that React is calling your component, which is a function. The JSX you return from that function is like a snapshot of the UI in time. Its props, event handlers, and local variables were all calculated **using its state at the time of the render.**\n\nUnlike a photograph or a movie frame, the UI “snapshot” you return is interactive. It includes logic like event handlers that specify what happens in response to inputs. React updates the screen to match this snapshot and connects the event handlers. As a result, pressing a button will trigger the click handler from your JSX.\n\nWhen React re-renders a component:\n\n1. React calls your function again.\n2. Your function returns a new JSX snapshot.\n3. React then updates the screen to match the snapshot your function returned.\n\n1. 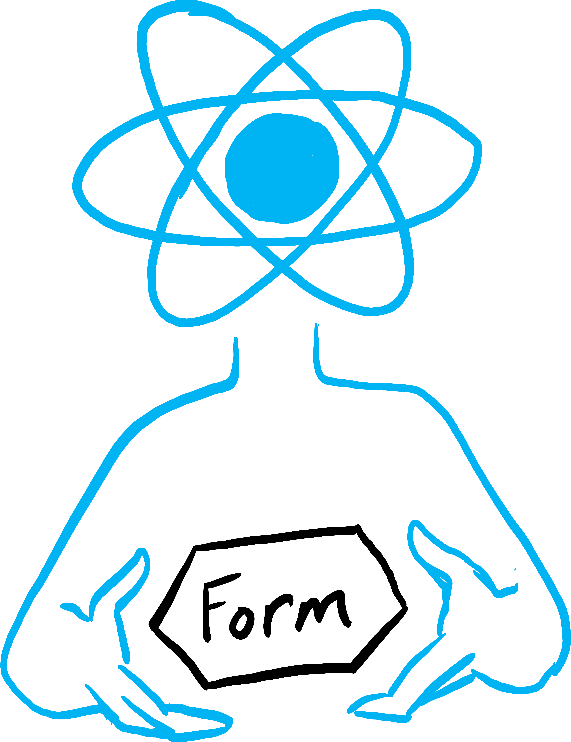\n \n React executing the function\n \n2. 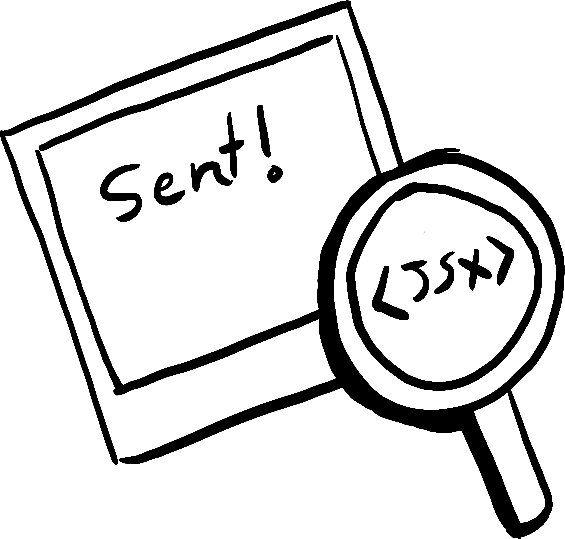\n \n Calculating the snapshot\n \n3. 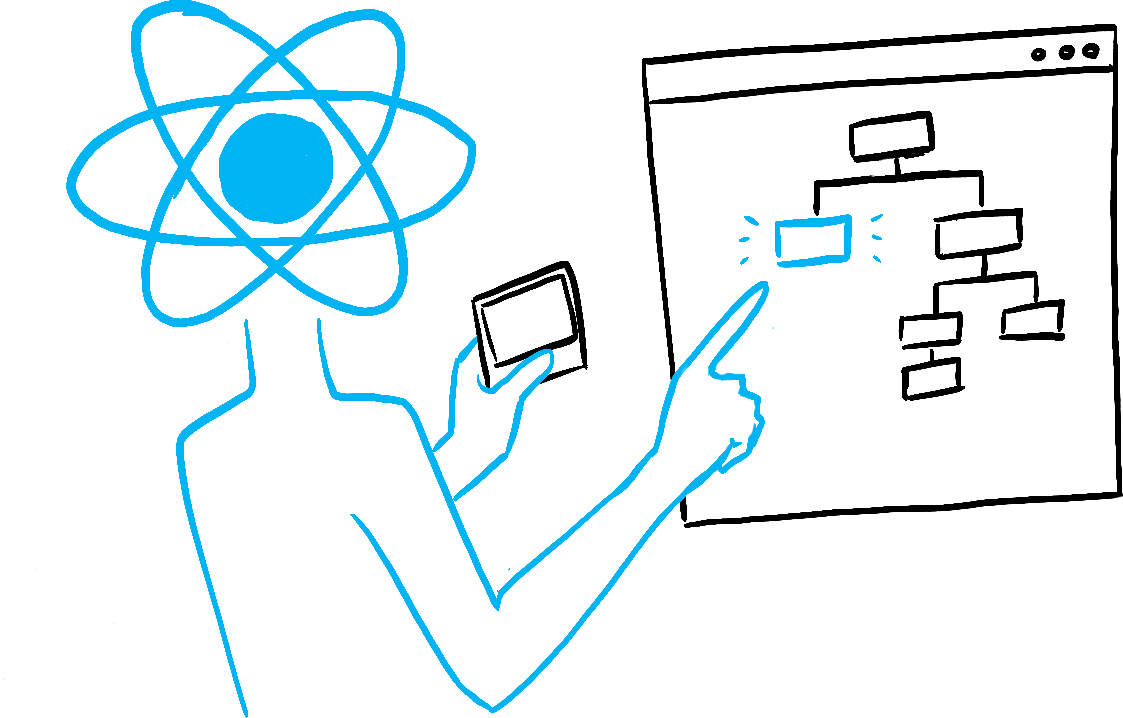\n \n Updating the DOM tree\n \n\nAs a component’s memory, state is not like a regular variable that disappears after your function returns. State actually “lives” in React itself—as if on a shelf!—outside of your function. When React calls your component, it gives you a snapshot of the state for that particular render. Your component returns a snapshot of the UI with a fresh set of props and event handlers in its JSX, all calculated **using the state values from that render!**\n\n1. 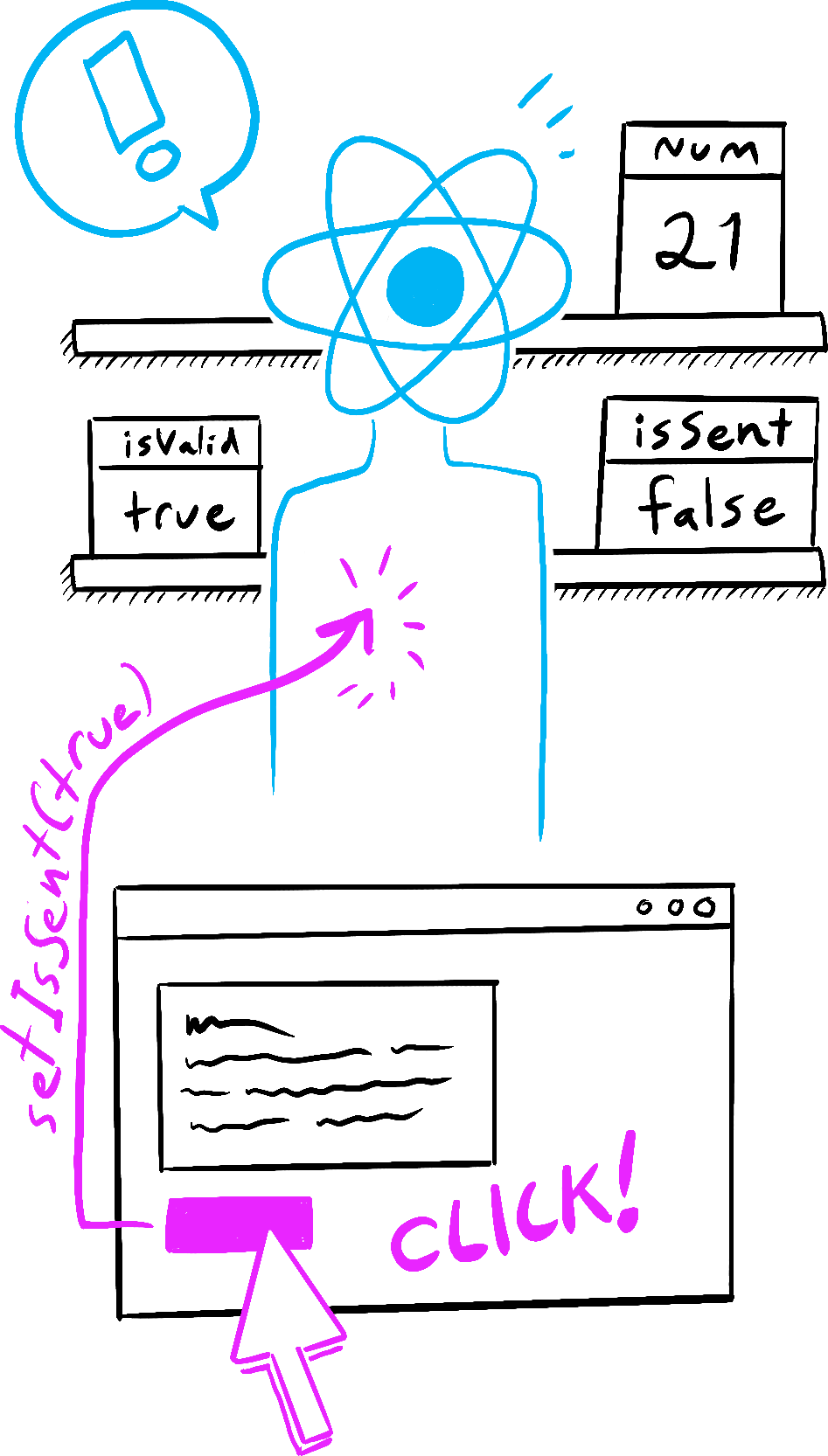\n \n You tell React to update the state\n \n2. 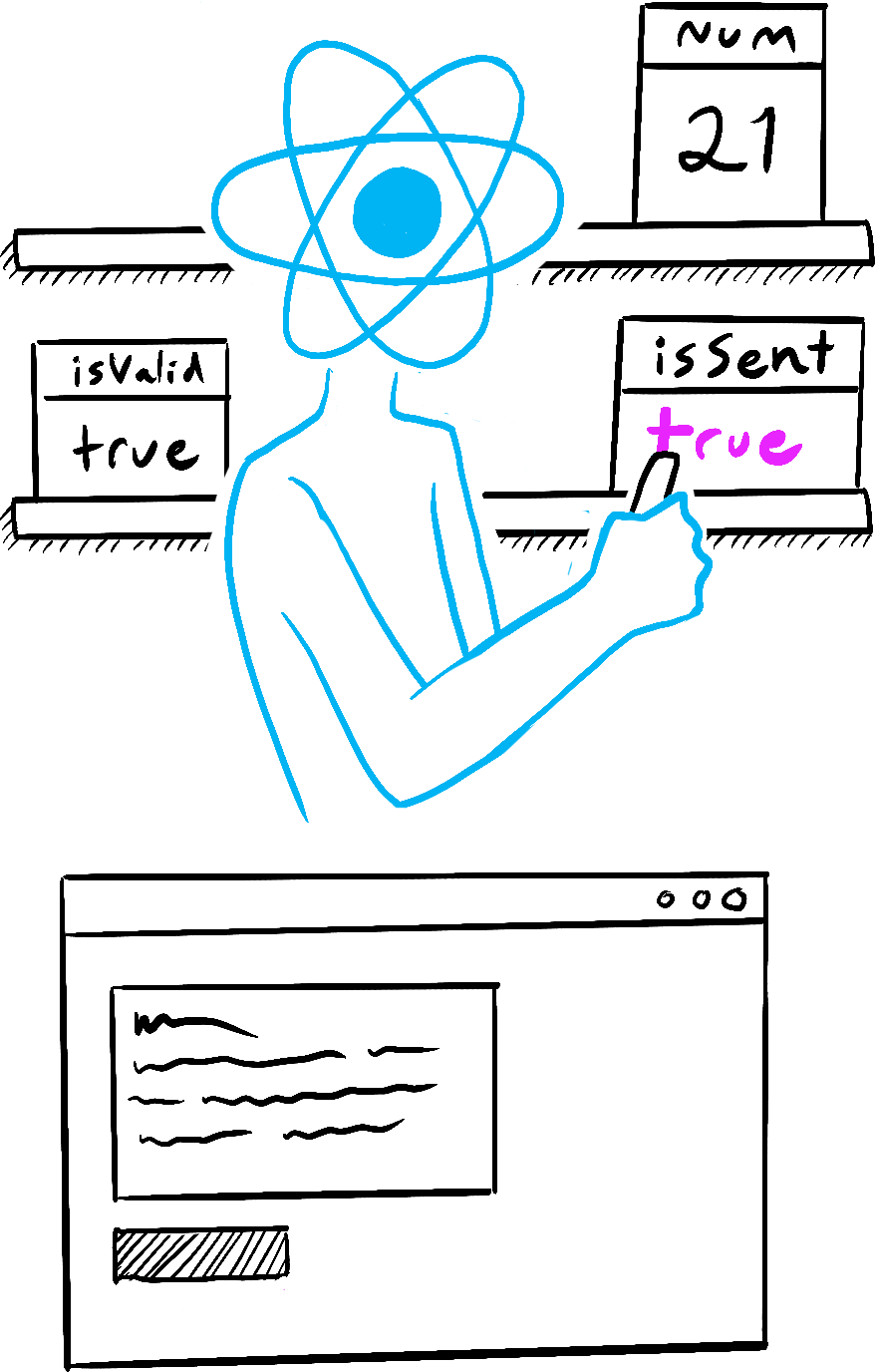\n \n React updates the state value\n \n3. 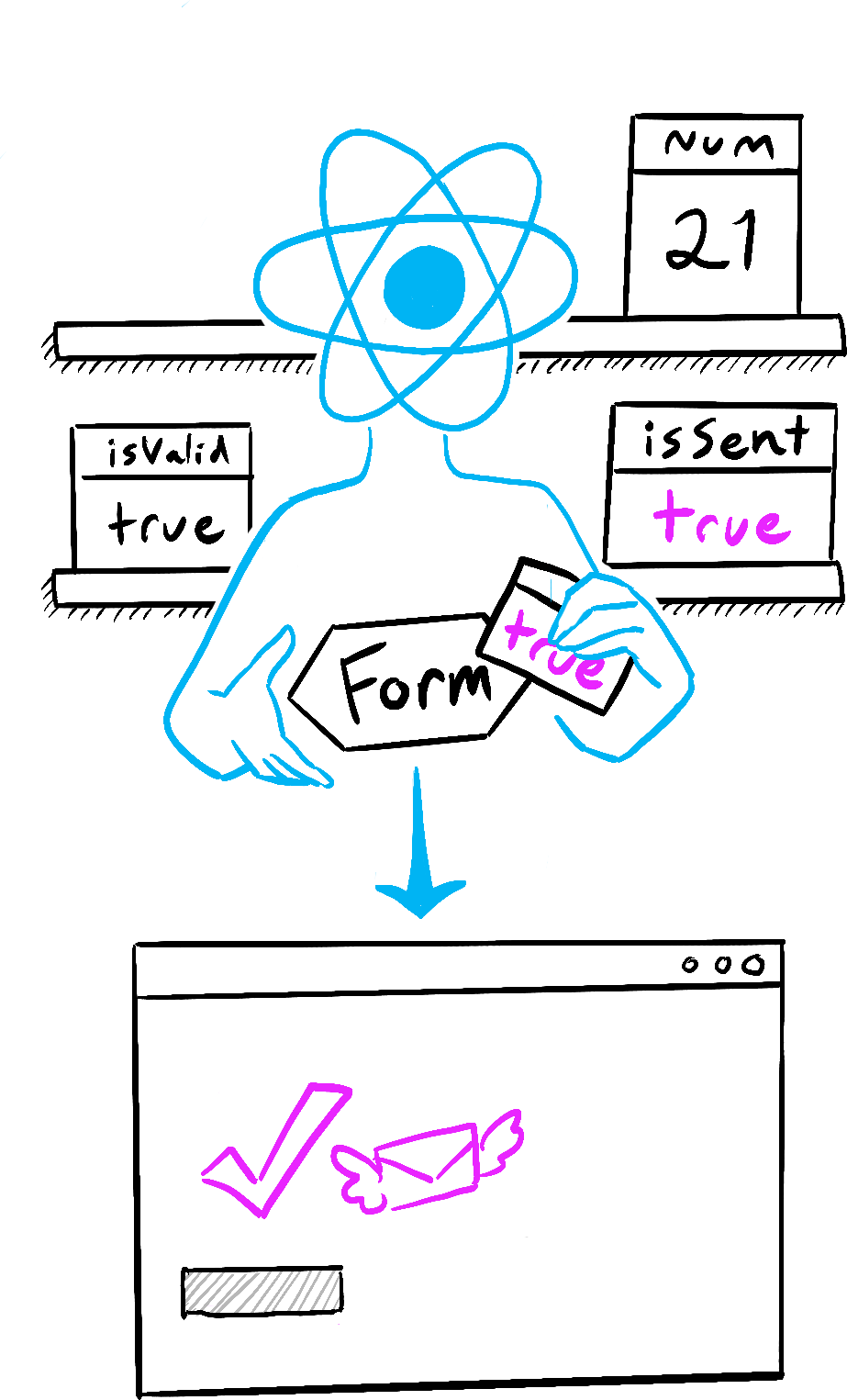\n \n React passes a snapshot of the state value into the component\n \n\nHere’s a little experiment to show you how this works. In this example, you might expect that clicking the “+3” button would increment the counter three times because it calls `setNumber(number + 1)` three times.\n\nSee what happens when you click the “+3” button:\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[number, setNumber\\] = useState(0);\n\n return (\n <\\>\n <h1\\>{number}</h1\\>\n <button onClick\\={() \\=> {\n setNumber(number + 1);\n setNumber(number + 1);\n setNumber(number + 1);\n }}\\>+3</button\\>\n </\\>\n )\n}\n\nNotice that `number` only increments once per click!\n\n**Setting state only changes it for the _next_ render.** During the first render, `number` was `0`. This is why, in _that render’s_ `onClick` handler, the value of `number` is still `0` even after `setNumber(number + 1)` was called:\n\n```\n<button onClick={() => {setNumber(number + 1);setNumber(number + 1);setNumber(number + 1);}}>+3</button>\n```\n\nHere is what this button’s click handler tells React to do:\n\n1. `setNumber(number + 1)`: `number` is `0` so `setNumber(0 + 1)`.\n * React prepares to change `number` to `1` on the next render.\n2. `setNumber(number + 1)`: `number` is `0` so `setNumber(0 + 1)`.\n * React prepares to change `number` to `1` on the next render.\n3. `setNumber(number + 1)`: `number` is `0` so `setNumber(0 + 1)`.\n * React prepares to change `number` to `1` on the next render.\n\nEven though you called `setNumber(number + 1)` three times, in _this render’s_ event handler `number` is always `0`, so you set the state to `1` three times. This is why, after your event handler finishes, React re-renders the component with `number` equal to `1` rather than `3`.\n\nYou can also visualize this by mentally substituting state variables with their values in your code. Since the `number` state variable is `0` for _this render_, its event handler looks like this:\n\n```\n<button onClick={() => {setNumber(0 + 1);setNumber(0 + 1);setNumber(0 + 1);}}>+3</button>\n```\n\nFor the next render, `number` is `1`, so _that render’s_ click handler looks like this:\n\n```\n<button onClick={() => {setNumber(1 + 1);setNumber(1 + 1);setNumber(1 + 1);}}>+3</button>\n```\n\nThis is why clicking the button again will set the counter to `2`, then to `3` on the next click, and so on.\n\nState over time[](https://react.dev/learn/state-as-a-snapshot#state-over-time \"Link for State over time \")\n----------------------------------------------------------------------------------------------------------\n\nWell, that was fun. Try to guess what clicking this button will alert:\n\nIf you use the substitution method from before, you can guess that the alert shows “0”:\n\n```\nsetNumber(0 + 5);alert(0);\n```\n\nBut what if you put a timer on the alert, so it only fires _after_ the component re-rendered? Would it say “0” or “5”? Have a guess!\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[number, setNumber\\] = useState(0);\n\n return (\n <\\>\n <h1\\>{number}</h1\\>\n <button onClick\\={() \\=> {\n setNumber(number + 5);\n setTimeout(() \\=> {\n alert(number);\n }, 3000);\n }}\\>+5</button\\>\n </\\>\n )\n}\n\nSurprised? If you use the substitution method, you can see the “snapshot” of the state passed to the alert.\n\n```\nsetNumber(0 + 5);setTimeout(() => {alert(0);}, 3000);\n```\n\nThe state stored in React may have changed by the time the alert runs, but it was scheduled using a snapshot of the state at the time the user interacted with it!\n\n**A state variable’s value never changes within a render,** even if its event handler’s code is asynchronous. Inside _that render’s_ `onClick`, the value of `number` continues to be `0` even after `setNumber(number + 5)` was called. Its value was “fixed” when React “took the snapshot” of the UI by calling your component.\n\nHere is an example of how that makes your event handlers less prone to timing mistakes. Below is a form that sends a message with a five-second delay. Imagine this scenario:\n\n1. You press the “Send” button, sending “Hello” to Alice.\n2. Before the five-second delay ends, you change the value of the “To” field to “Bob”.\n\nWhat do you expect the `alert` to display? Would it display, “You said Hello to Alice”? Or would it display, “You said Hello to Bob”? Make a guess based on what you know, and then try it:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[to, setTo\\] = useState('Alice');\n const \\[message, setMessage\\] = useState('Hello');\n\n function handleSubmit(e) {\n e.preventDefault();\n setTimeout(() \\=> {\n alert(\\`You said ${message} to ${to}\\`);\n }, 5000);\n }\n\n return (\n <form onSubmit\\={handleSubmit}\\>\n <label\\>\n To:{' '}\n <select\n value\\={to}\n onChange\\={e \\=> setTo(e.target.value)}\\>\n <option value\\=\"Alice\"\\>Alice</option\\>\n <option value\\=\"Bob\"\\>Bob</option\\>\n </select\\>\n </label\\>\n <textarea\n placeholder\\=\"Message\"\n value\\={message}\n onChange\\={e \\=> setMessage(e.target.value)}\n />\n <button type\\=\"submit\"\\>Send</button\\>\n </form\\>\n );\n}\n\n**React keeps the state values “fixed” within one render’s event handlers.** You don’t need to worry whether the state has changed while the code is running.\n\nBut what if you wanted to read the latest state before a re-render? You’ll want to use a [state updater function](https://react.dev/learn/queueing-a-series-of-state-updates), covered on the next page!\n\nRecap[](https://react.dev/learn/state-as-a-snapshot#recap \"Link for Recap\")\n---------------------------------------------------------------------------\n\n* Setting state requests a new render.\n* React stores state outside of your component, as if on a shelf.\n* When you call `useState`, React gives you a snapshot of the state _for that render_.\n* Variables and event handlers don’t “survive” re-renders. Every render has its own event handlers.\n* Every render (and functions inside it) will always “see” the snapshot of the state that React gave to _that_ render.\n* You can mentally substitute state in event handlers, similarly to how you think about the rendered JSX.\n* Event handlers created in the past have the state values from the render in which they were created.\n\nTry out some challenges[](https://react.dev/learn/state-as-a-snapshot#challenges \"Link for Try out some challenges\")\n--------------------------------------------------------------------------------------------------------------------\n\n#### Implement a traffic light[](https://react.dev/learn/state-as-a-snapshot#implement-a-traffic-light \"Link for this heading\")\n\nHere is a crosswalk light component that toggles when the button is pressed:\n\nimport { useState } from 'react';\n\nexport default function TrafficLight() {\n const \\[walk, setWalk\\] = useState(true);\n\n function handleClick() {\n setWalk(!walk);\n }\n\n return (\n <\\>\n <button onClick\\={handleClick}\\>\n Change to {walk ? 'Stop' : 'Walk'}\n </button\\>\n <h1 style\\={{\n color: walk ? 'darkgreen' : 'darkred'\n }}\\>\n {walk ? 'Walk' : 'Stop'}\n </h1\\>\n </\\>\n );\n}\n\nAdd an `alert` to the click handler. When the light is green and says “Walk”, clicking the button should say “Stop is next”. When the light is red and says “Stop”, clicking the button should say “Walk is next”.\n\nDoes it make a difference whether you put the `alert` before or after the `setWalk` call?\n",
"filename": "state-as-a-snapshot.md",
"package": "react"
} |
{
"content": "Title: <style> – React\n\nURL Source: https://react.dev/reference/react-dom/components/style\n\nMarkdown Content:\n### Canary\n\nThe [built-in browser `<style>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/style) lets you add inline CSS stylesheets to your document.\n\n```\n<style>{` p { color: red; } `}</style>\n```\n\n* [Reference](https://react.dev/reference/react-dom/components/style#reference)\n * [`<style>`](https://react.dev/reference/react-dom/components/style#style)\n* [Usage](https://react.dev/reference/react-dom/components/style#usage)\n * [Rendering an inline CSS stylesheet](https://react.dev/reference/react-dom/components/style#rendering-an-inline-css-stylesheet)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/style#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------------\n\n### `<style>`[](https://react.dev/reference/react-dom/components/style#style \"Link for this heading\")\n\nTo add inline styles to your document, render the [built-in browser `<style>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/style). You can render `<style>` from any component and React will [in certain cases](https://react.dev/reference/react-dom/components/style#special-rendering-behavior) place the corresponding DOM element in the document head and de-duplicate identical styles.\n\n```\n<style>{` p { color: red; } `}</style>\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/style#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/style#props \"Link for Props \")\n\n`<style>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\n* `children`: a string, required. The contents of the stylesheet.\n* `precedence`: a string. Tells React where to rank the `<style>` DOM node relative to others in the document `<head>`, which determines which stylesheet can override the other. React will infer that precedence values it discovers first are “lower” and precedence values it discovers later are “higher”. Many style systems can work fine using a single precedence value because style rules are atomic. Stylesheets with the same precedence go together whether they are `<link>` or inline `<style>` tags or loaded using [`preinit`](https://react.dev/reference/react-dom/preinit) functions.\n* `href`: a string. Allows React to [de-duplicate styles](https://react.dev/reference/react-dom/components/style#special-rendering-behavior) that have the same `href`.\n* `media`: a string. Restricts the stylesheet to a certain [media query](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_media_queries/Using_media_queries).\n* `nonce`: a string. A cryptographic [nonce to allow the resource](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/nonce) when using a strict Content Security Policy.\n* `title`: a string. Specifies the name of an [alternative stylesheet](https://developer.mozilla.org/en-US/docs/Web/CSS/Alternative_style_sheets).\n\nProps that are **not recommended** for use with React:\n\n* `blocking`: a string. If set to `\"render\"`, instructs the browser not to render the page until the stylesheet is loaded. React provides more fine-grained control using Suspense.\n\n#### Special rendering behavior[](https://react.dev/reference/react-dom/components/style#special-rendering-behavior \"Link for Special rendering behavior \")\n\nReact can move `<style>` components to the document’s `<head>`, de-duplicate identical stylesheets, and [suspend](https://react.dev/reference/react/Suspense) while the stylesheet is loading.\n\nTo opt into this behavior, provide the `href` and `precedence` props. React will de-duplicate styles if they have the same `href`. The precedence prop tells React where to rank the `<style>` DOM node relative to others in the document `<head>`, which determines which stylesheet can override the other.\n\nThis special treatment comes with two caveats:\n\n* React will ignore changes to props after the style has been rendered. (React will issue a warning in development if this happens.)\n* React may leave the style in the DOM even after the component that rendered it has been unmounted.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/style#usage \"Link for Usage \")\n---------------------------------------------------------------------------------------\n\n### Rendering an inline CSS stylesheet[](https://react.dev/reference/react-dom/components/style#rendering-an-inline-css-stylesheet \"Link for Rendering an inline CSS stylesheet \")\n\nIf a component depends on certain CSS styles in order to be displayed correctly, you can render an inline stylesheet within the component.\n\nIf you supply an `href` and `precedence` prop, your component will suspend while the stylesheet is loading. (Even with inline stylesheets, there may be a loading time due to fonts and images that the stylesheet refers to.) The `href` prop should uniquely identify the stylesheet, because React will de-duplicate stylesheets that have the same `href`.\n\nimport ShowRenderedHTML from './ShowRenderedHTML.js';\nimport { useId } from 'react';\n\nfunction PieChart({data, colors}) {\n const id = useId();\n const stylesheet = colors.map((color, index) \\=>\n \\`#${id} .color-${index}: \\\\{ color: \"${color}\"; \\\\}\\`\n ).join();\n return (\n <\\>\n <style href\\={\"PieChart-\" + JSON.stringify(colors)} precedence\\=\"medium\"\\>\n {stylesheet}\n </style\\>\n <svg id\\={id}\\>\n …\n </svg\\>\n </\\>\n );\n}\n\nexport default function App() {\n return (\n <ShowRenderedHTML\\>\n <PieChart data\\=\"...\" colors\\={\\['red', 'green', 'blue'\\]} />\n </ShowRenderedHTML\\>\n );\n}\n",
"filename": "style.md",
"package": "react"
} |
{
"content": "Title: Synchronizing with Effects – React\n\nURL Source: https://react.dev/learn/synchronizing-with-effects\n\nMarkdown Content:\nSome components need to synchronize with external systems. For example, you might want to control a non-React component based on the React state, set up a server connection, or send an analytics log when a component appears on the screen. _Effects_ let you run some code after rendering so that you can synchronize your component with some system outside of React.\n\n### You will learn\n\n* What Effects are\n* How Effects are different from events\n* How to declare an Effect in your component\n* How to skip re-running an Effect unnecessarily\n* Why Effects run twice in development and how to fix them\n\nWhat are Effects and how are they different from events?[](https://react.dev/learn/synchronizing-with-effects#what-are-effects-and-how-are-they-different-from-events \"Link for What are Effects and how are they different from events? \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nBefore getting to Effects, you need to be familiar with two types of logic inside React components:\n\n* **Rendering code** (introduced in [Describing the UI](https://react.dev/learn/describing-the-ui)) lives at the top level of your component. This is where you take the props and state, transform them, and return the JSX you want to see on the screen. [Rendering code must be pure.](https://react.dev/learn/keeping-components-pure) Like a math formula, it should only _calculate_ the result, but not do anything else.\n \n* **Event handlers** (introduced in [Adding Interactivity](https://react.dev/learn/adding-interactivity)) are nested functions inside your components that _do_ things rather than just calculate them. An event handler might update an input field, submit an HTTP POST request to buy a product, or navigate the user to another screen. Event handlers contain [“side effects”](https://en.wikipedia.org/wiki/Side_effect_/(computer_science/)) (they change the program’s state) caused by a specific user action (for example, a button click or typing).\n \n\nSometimes this isn’t enough. Consider a `ChatRoom` component that must connect to the chat server whenever it’s visible on the screen. Connecting to a server is not a pure calculation (it’s a side effect) so it can’t happen during rendering. However, there is no single particular event like a click that causes `ChatRoom` to be displayed.\n\n**_Effects_ let you specify side effects that are caused by rendering itself, rather than by a particular event.** Sending a message in the chat is an _event_ because it is directly caused by the user clicking a specific button. However, setting up a server connection is an _Effect_ because it should happen no matter which interaction caused the component to appear. Effects run at the end of a [commit](https://react.dev/learn/render-and-commit) after the screen updates. This is a good time to synchronize the React components with some external system (like network or a third-party library).\n\n### Note\n\nHere and later in this text, capitalized “Effect” refers to the React-specific definition above, i.e. a side effect caused by rendering. To refer to the broader programming concept, we’ll say “side effect”.\n\nYou might not need an Effect[](https://react.dev/learn/synchronizing-with-effects#you-might-not-need-an-effect \"Link for You might not need an Effect \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------\n\n**Don’t rush to add Effects to your components.** Keep in mind that Effects are typically used to “step out” of your React code and synchronize with some _external_ system. This includes browser APIs, third-party widgets, network, and so on. If your Effect only adjusts some state based on other state, [you might not need an Effect.](https://react.dev/learn/you-might-not-need-an-effect)\n\nHow to write an Effect[](https://react.dev/learn/synchronizing-with-effects#how-to-write-an-effect \"Link for How to write an Effect \")\n--------------------------------------------------------------------------------------------------------------------------------------\n\nTo write an Effect, follow these three steps:\n\n1. **Declare an Effect.** By default, your Effect will run after every [commit](https://react.dev/learn/render-and-commit).\n2. **Specify the Effect dependencies.** Most Effects should only re-run _when needed_ rather than after every render. For example, a fade-in animation should only trigger when a component appears. Connecting and disconnecting to a chat room should only happen when the component appears and disappears, or when the chat room changes. You will learn how to control this by specifying _dependencies._\n3. **Add cleanup if needed.** Some Effects need to specify how to stop, undo, or clean up whatever they were doing. For example, “connect” needs “disconnect”, “subscribe” needs “unsubscribe”, and “fetch” needs either “cancel” or “ignore”. You will learn how to do this by returning a _cleanup function_.\n\nLet’s look at each of these steps in detail.\n\n### Step 1: Declare an Effect[](https://react.dev/learn/synchronizing-with-effects#step-1-declare-an-effect \"Link for Step 1: Declare an Effect \")\n\nTo declare an Effect in your component, import the [`useEffect` Hook](https://react.dev/reference/react/useEffect) from React:\n\n```\nimport { useEffect } from 'react';\n```\n\nThen, call it at the top level of your component and put some code inside your Effect:\n\n```\nfunction MyComponent() {useEffect(() => {// Code here will run after *every* render});return <div />;}\n```\n\nEvery time your component renders, React will update the screen _and then_ run the code inside `useEffect`. In other words, **`useEffect` “delays” a piece of code from running until that render is reflected on the screen.**\n\nLet’s see how you can use an Effect to synchronize with an external system. Consider a `<VideoPlayer>` React component. It would be nice to control whether it’s playing or paused by passing an `isPlaying` prop to it:\n\n```\n<VideoPlayer isPlaying={isPlaying} />;\n```\n\nYour custom `VideoPlayer` component renders the built-in browser [`<video>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/video) tag:\n\n```\nfunction VideoPlayer({ src, isPlaying }) {// TODO: do something with isPlayingreturn <video src={src} />;}\n```\n\nHowever, the browser `<video>` tag does not have an `isPlaying` prop. The only way to control it is to manually call the [`play()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/play) and [`pause()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/pause) methods on the DOM element. **You need to synchronize the value of `isPlaying` prop, which tells whether the video _should_ currently be playing, with calls like `play()` and `pause()`.**\n\nWe’ll need to first [get a ref](https://react.dev/learn/manipulating-the-dom-with-refs) to the `<video>` DOM node.\n\nYou might be tempted to try to call `play()` or `pause()` during rendering, but that isn’t correct:\n\nimport { useState, useRef, useEffect } from 'react';\n\nfunction VideoPlayer({ src, isPlaying }) {\n const ref = useRef(null);\n\n if (isPlaying) {\n ref.current.play(); \n } else {\n ref.current.pause(); \n }\n\n return <video ref\\={ref} src\\={src} loop playsInline />;\n}\n\nexport default function App() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setIsPlaying(!isPlaying)}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <VideoPlayer\n isPlaying\\={isPlaying}\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n />\n </\\>\n );\n}\n\nThe reason this code isn’t correct is that it tries to do something with the DOM node during rendering. In React, [rendering should be a pure calculation](https://react.dev/learn/keeping-components-pure) of JSX and should not contain side effects like modifying the DOM.\n\nMoreover, when `VideoPlayer` is called for the first time, its DOM does not exist yet! There isn’t a DOM node yet to call `play()` or `pause()` on, because React doesn’t know what DOM to create until you return the JSX.\n\nThe solution here is to **wrap the side effect with `useEffect` to move it out of the rendering calculation:**\n\n```\nimport { useEffect, useRef } from 'react';function VideoPlayer({ src, isPlaying }) {const ref = useRef(null);useEffect(() => {if (isPlaying) {ref.current.play();} else {ref.current.pause();}});return <video ref={ref} src={src} loop playsInline />;}\n```\n\nBy wrapping the DOM update in an Effect, you let React update the screen first. Then your Effect runs.\n\nWhen your `VideoPlayer` component renders (either the first time or if it re-renders), a few things will happen. First, React will update the screen, ensuring the `<video>` tag is in the DOM with the right props. Then React will run your Effect. Finally, your Effect will call `play()` or `pause()` depending on the value of `isPlaying`.\n\nPress Play/Pause multiple times and see how the video player stays synchronized to the `isPlaying` value:\n\nimport { useState, useRef, useEffect } from 'react';\n\nfunction VideoPlayer({ src, isPlaying }) {\n const ref = useRef(null);\n\n useEffect(() \\=> {\n if (isPlaying) {\n ref.current.play();\n } else {\n ref.current.pause();\n }\n });\n\n return <video ref\\={ref} src\\={src} loop playsInline />;\n}\n\nexport default function App() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setIsPlaying(!isPlaying)}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <VideoPlayer\n isPlaying\\={isPlaying}\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n />\n </\\>\n );\n}\n\nIn this example, the “external system” you synchronized to React state was the browser media API. You can use a similar approach to wrap legacy non-React code (like jQuery plugins) into declarative React components.\n\nNote that controlling a video player is much more complex in practice. Calling `play()` may fail, the user might play or pause using the built-in browser controls, and so on. This example is very simplified and incomplete.\n\n### Pitfall\n\nBy default, Effects run after _every_ render. This is why code like this will **produce an infinite loop:**\n\n```\nconst [count, setCount] = useState(0);useEffect(() => {setCount(count + 1);});\n```\n\nEffects run as a _result_ of rendering. Setting state _triggers_ rendering. Setting state immediately in an Effect is like plugging a power outlet into itself. The Effect runs, it sets the state, which causes a re-render, which causes the Effect to run, it sets the state again, this causes another re-render, and so on.\n\nEffects should usually synchronize your components with an _external_ system. If there’s no external system and you only want to adjust some state based on other state, [you might not need an Effect.](https://react.dev/learn/you-might-not-need-an-effect)\n\n### Step 2: Specify the Effect dependencies[](https://react.dev/learn/synchronizing-with-effects#step-2-specify-the-effect-dependencies \"Link for Step 2: Specify the Effect dependencies \")\n\nBy default, Effects run after _every_ render. Often, this is **not what you want:**\n\n* Sometimes, it’s slow. Synchronizing with an external system is not always instant, so you might want to skip doing it unless it’s necessary. For example, you don’t want to reconnect to the chat server on every keystroke.\n* Sometimes, it’s wrong. For example, you don’t want to trigger a component fade-in animation on every keystroke. The animation should only play once when the component appears for the first time.\n\nTo demonstrate the issue, here is the previous example with a few `console.log` calls and a text input that updates the parent component’s state. Notice how typing causes the Effect to re-run:\n\nimport { useState, useRef, useEffect } from 'react';\n\nfunction VideoPlayer({ src, isPlaying }) {\n const ref = useRef(null);\n\n useEffect(() \\=> {\n if (isPlaying) {\n console.log('Calling video.play()');\n ref.current.play();\n } else {\n console.log('Calling video.pause()');\n ref.current.pause();\n }\n });\n\n return <video ref\\={ref} src\\={src} loop playsInline />;\n}\n\nexport default function App() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n const \\[text, setText\\] = useState('');\n return (\n <\\>\n <input value\\={text} onChange\\={e \\=> setText(e.target.value)} />\n <button onClick\\={() \\=> setIsPlaying(!isPlaying)}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <VideoPlayer\n isPlaying\\={isPlaying}\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n />\n </\\>\n );\n}\n\nYou can tell React to **skip unnecessarily re-running the Effect** by specifying an array of _dependencies_ as the second argument to the `useEffect` call. Start by adding an empty `[]` array to the above example on line 14:\n\n```\nuseEffect(() => {// ...}, []);\n```\n\nYou should see an error saying `React Hook useEffect has a missing dependency: 'isPlaying'`:\n\nimport { useState, useRef, useEffect } from 'react';\n\nfunction VideoPlayer({ src, isPlaying }) {\n const ref = useRef(null);\n\n useEffect(() \\=> {\n if (isPlaying) {\n console.log('Calling video.play()');\n ref.current.play();\n } else {\n console.log('Calling video.pause()');\n ref.current.pause();\n }\n }, \\[\\]); \n\n return <video ref\\={ref} src\\={src} loop playsInline />;\n}\n\nexport default function App() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n const \\[text, setText\\] = useState('');\n return (\n <\\>\n <input value\\={text} onChange\\={e \\=> setText(e.target.value)} />\n <button onClick\\={() \\=> setIsPlaying(!isPlaying)}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <VideoPlayer\n isPlaying\\={isPlaying}\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n />\n </\\>\n );\n}\n\nThe problem is that the code inside of your Effect _depends on_ the `isPlaying` prop to decide what to do, but this dependency was not explicitly declared. To fix this issue, add `isPlaying` to the dependency array:\n\n```\nuseEffect(() => {if (isPlaying) { // It's used here...// ...} else {// ...}}, [isPlaying]); // ...so it must be declared here!\n```\n\nNow all dependencies are declared, so there is no error. Specifying `[isPlaying]` as the dependency array tells React that it should skip re-running your Effect if `isPlaying` is the same as it was during the previous render. With this change, typing into the input doesn’t cause the Effect to re-run, but pressing Play/Pause does:\n\nimport { useState, useRef, useEffect } from 'react';\n\nfunction VideoPlayer({ src, isPlaying }) {\n const ref = useRef(null);\n\n useEffect(() \\=> {\n if (isPlaying) {\n console.log('Calling video.play()');\n ref.current.play();\n } else {\n console.log('Calling video.pause()');\n ref.current.pause();\n }\n }, \\[isPlaying\\]);\n\n return <video ref\\={ref} src\\={src} loop playsInline />;\n}\n\nexport default function App() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n const \\[text, setText\\] = useState('');\n return (\n <\\>\n <input value\\={text} onChange\\={e \\=> setText(e.target.value)} />\n <button onClick\\={() \\=> setIsPlaying(!isPlaying)}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <VideoPlayer\n isPlaying\\={isPlaying}\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n />\n </\\>\n );\n}\n\nThe dependency array can contain multiple dependencies. React will only skip re-running the Effect if _all_ of the dependencies you specify have exactly the same values as they had during the previous render. React compares the dependency values using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. See the [`useEffect` reference](https://react.dev/reference/react/useEffect#reference) for details.\n\n**Notice that you can’t “choose” your dependencies.** You will get a lint error if the dependencies you specified don’t match what React expects based on the code inside your Effect. This helps catch many bugs in your code. If you don’t want some code to re-run, [_edit the Effect code itself_ to not “need” that dependency.](https://react.dev/learn/lifecycle-of-reactive-effects#what-to-do-when-you-dont-want-to-re-synchronize)\n\n### Pitfall\n\nThe behaviors without the dependency array and with an _empty_ `[]` dependency array are different:\n\n```\nuseEffect(() => {// This runs after every render});useEffect(() => {// This runs only on mount (when the component appears)}, []);useEffect(() => {// This runs on mount *and also* if either a or b have changed since the last render}, [a, b]);\n```\n\nWe’ll take a close look at what “mount” means in the next step.\n\n##### Deep Dive\n\n#### Why was the ref omitted from the dependency array?[](https://react.dev/learn/synchronizing-with-effects#why-was-the-ref-omitted-from-the-dependency-array \"Link for Why was the ref omitted from the dependency array? \")\n\nThis Effect uses _both_ `ref` and `isPlaying`, but only `isPlaying` is declared as a dependency:\n\n```\nfunction VideoPlayer({ src, isPlaying }) {const ref = useRef(null);useEffect(() => {if (isPlaying) {ref.current.play();} else {ref.current.pause();}}, [isPlaying]);\n```\n\nThis is because the `ref` object has a _stable identity:_ React guarantees [you’ll always get the same object](https://react.dev/reference/react/useRef#returns) from the same `useRef` call on every render. It never changes, so it will never by itself cause the Effect to re-run. Therefore, it does not matter whether you include it or not. Including it is fine too:\n\n```\nfunction VideoPlayer({ src, isPlaying }) {const ref = useRef(null);useEffect(() => {if (isPlaying) {ref.current.play();} else {ref.current.pause();}}, [isPlaying, ref]);\n```\n\nThe [`set` functions](https://react.dev/reference/react/useState#setstate) returned by `useState` also have stable identity, so you will often see them omitted from the dependencies too. If the linter lets you omit a dependency without errors, it is safe to do.\n\nOmitting always-stable dependencies only works when the linter can “see” that the object is stable. For example, if `ref` was passed from a parent component, you would have to specify it in the dependency array. However, this is good because you can’t know whether the parent component always passes the same ref, or passes one of several refs conditionally. So your Effect _would_ depend on which ref is passed.\n\n### Step 3: Add cleanup if needed[](https://react.dev/learn/synchronizing-with-effects#step-3-add-cleanup-if-needed \"Link for Step 3: Add cleanup if needed \")\n\nConsider a different example. You’re writing a `ChatRoom` component that needs to connect to the chat server when it appears. You are given a `createConnection()` API that returns an object with `connect()` and `disconnect()` methods. How do you keep the component connected while it is displayed to the user?\n\nStart by writing the Effect logic:\n\n```\nuseEffect(() => {const connection = createConnection();connection.connect();});\n```\n\nIt would be slow to connect to the chat after every re-render, so you add the dependency array:\n\n```\nuseEffect(() => {const connection = createConnection();connection.connect();}, []);\n```\n\n**The code inside the Effect does not use any props or state, so your dependency array is `[]` (empty). This tells React to only run this code when the component “mounts”, i.e. appears on the screen for the first time.**\n\nLet’s try running this code:\n\nThis Effect only runs on mount, so you might expect `\"✅ Connecting...\"` to be printed once in the console. **However, if you check the console, `\"✅ Connecting...\"` gets printed twice. Why does it happen?**\n\nImagine the `ChatRoom` component is a part of a larger app with many different screens. The user starts their journey on the `ChatRoom` page. The component mounts and calls `connection.connect()`. Then imagine the user navigates to another screen—for example, to the Settings page. The `ChatRoom` component unmounts. Finally, the user clicks Back and `ChatRoom` mounts again. This would set up a second connection—but the first connection was never destroyed! As the user navigates across the app, the connections would keep piling up.\n\nBugs like this are easy to miss without extensive manual testing. To help you spot them quickly, in development React remounts every component once immediately after its initial mount.\n\nSeeing the `\"✅ Connecting...\"` log twice helps you notice the real issue: your code doesn’t close the connection when the component unmounts.\n\nTo fix the issue, return a _cleanup function_ from your Effect:\n\n```\nuseEffect(() => {const connection = createConnection();connection.connect();return () => {connection.disconnect();};}, []);\n```\n\nReact will call your cleanup function each time before the Effect runs again, and one final time when the component unmounts (gets removed). Let’s see what happens when the cleanup function is implemented:\n\nNow you get three console logs in development:\n\n1. `\"✅ Connecting...\"`\n2. `\"❌ Disconnected.\"`\n3. `\"✅ Connecting...\"`\n\n**This is the correct behavior in development.** By remounting your component, React verifies that navigating away and back would not break your code. Disconnecting and then connecting again is exactly what should happen! When you implement the cleanup well, there should be no user-visible difference between running the Effect once vs running it, cleaning it up, and running it again. There’s an extra connect/disconnect call pair because React is probing your code for bugs in development. This is normal—don’t try to make it go away!\n\n**In production, you would only see `\"✅ Connecting...\"` printed once.** Remounting components only happens in development to help you find Effects that need cleanup. You can turn off [Strict Mode](https://react.dev/reference/react/StrictMode) to opt out of the development behavior, but we recommend keeping it on. This lets you find many bugs like the one above.\n\nHow to handle the Effect firing twice in development?[](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development \"Link for How to handle the Effect firing twice in development? \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nReact intentionally remounts your components in development to find bugs like in the last example. **The right question isn’t “how to run an Effect once”, but “how to fix my Effect so that it works after remounting”.**\n\nUsually, the answer is to implement the cleanup function. The cleanup function should stop or undo whatever the Effect was doing. The rule of thumb is that the user shouldn’t be able to distinguish between the Effect running once (as in production) and a _setup → cleanup → setup_ sequence (as you’d see in development).\n\nMost of the Effects you’ll write will fit into one of the common patterns below.\n\n### Pitfall\n\n#### Don’t use refs to prevent Effects from firing[](https://react.dev/learn/synchronizing-with-effects#dont-use-refs-to-prevent-effects-from-firing \"Link for Don’t use refs to prevent Effects from firing \")\n\nA common pitfall for preventing Effects firing twice in development is to use a `ref` to prevent the Effect from running more than once. For example, you could “fix” the above bug with a `useRef`:\n\n```\nconst connectionRef = useRef(null);useEffect(() => {// 🚩 This wont fix the bug!!!if (!connectionRef.current) {connectionRef.current = createConnection();connectionRef.current.connect();}}, []);\n```\n\nThis makes it so you only see `\"✅ Connecting...\"` once in development, but it doesn’t fix the bug.\n\nWhen the user navigates away, the connection still isn’t closed and when they navigate back, a new connection is created. As the user navigates across the app, the connections would keep piling up, the same as it would before the “fix”.\n\nTo fix the bug, it is not enough to just make the Effect run once. The effect needs to work after re-mounting, which means the connection needs to be cleaned up like in the solution above.\n\nSee the examples below for how to handle common patterns.\n\n### Controlling non-React widgets[](https://react.dev/learn/synchronizing-with-effects#controlling-non-react-widgets \"Link for Controlling non-React widgets \")\n\nSometimes you need to add UI widgets that aren’t written to React. For example, let’s say you’re adding a map component to your page. It has a `setZoomLevel()` method, and you’d like to keep the zoom level in sync with a `zoomLevel` state variable in your React code. Your Effect would look similar to this:\n\n```\nuseEffect(() => {const map = mapRef.current;map.setZoomLevel(zoomLevel);}, [zoomLevel]);\n```\n\nNote that there is no cleanup needed in this case. In development, React will call the Effect twice, but this is not a problem because calling `setZoomLevel` twice with the same value does not do anything. It may be slightly slower, but this doesn’t matter because it won’t remount needlessly in production.\n\nSome APIs may not allow you to call them twice in a row. For example, the [`showModal`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDialogElement/showModal) method of the built-in [`<dialog>`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDialogElement) element throws if you call it twice. Implement the cleanup function and make it close the dialog:\n\n```\nuseEffect(() => {const dialog = dialogRef.current;dialog.showModal();return () => dialog.close();}, []);\n```\n\nIn development, your Effect will call `showModal()`, then immediately `close()`, and then `showModal()` again. This has the same user-visible behavior as calling `showModal()` once, as you would see in production.\n\n### Subscribing to events[](https://react.dev/learn/synchronizing-with-effects#subscribing-to-events \"Link for Subscribing to events \")\n\nIf your Effect subscribes to something, the cleanup function should unsubscribe:\n\n```\nuseEffect(() => {function handleScroll(e) {console.log(window.scrollX, window.scrollY);}window.addEventListener('scroll', handleScroll);return () => window.removeEventListener('scroll', handleScroll);}, []);\n```\n\nIn development, your Effect will call `addEventListener()`, then immediately `removeEventListener()`, and then `addEventListener()` again with the same handler. So there would be only one active subscription at a time. This has the same user-visible behavior as calling `addEventListener()` once, as in production.\n\n### Triggering animations[](https://react.dev/learn/synchronizing-with-effects#triggering-animations \"Link for Triggering animations \")\n\nIf your Effect animates something in, the cleanup function should reset the animation to the initial values:\n\n```\nuseEffect(() => {const node = ref.current;node.style.opacity = 1; // Trigger the animationreturn () => {node.style.opacity = 0; // Reset to the initial value};}, []);\n```\n\nIn development, opacity will be set to `1`, then to `0`, and then to `1` again. This should have the same user-visible behavior as setting it to `1` directly, which is what would happen in production. If you use a third-party animation library with support for tweening, your cleanup function should reset the timeline to its initial state.\n\n### Fetching data[](https://react.dev/learn/synchronizing-with-effects#fetching-data \"Link for Fetching data \")\n\nIf your Effect fetches something, the cleanup function should either [abort the fetch](https://developer.mozilla.org/en-US/docs/Web/API/AbortController) or ignore its result:\n\n```\nuseEffect(() => {let ignore = false;async function startFetching() {const json = await fetchTodos(userId);if (!ignore) {setTodos(json);}}startFetching();return () => {ignore = true;};}, [userId]);\n```\n\nYou can’t “undo” a network request that already happened, but your cleanup function should ensure that the fetch that’s _not relevant anymore_ does not keep affecting your application. If the `userId` changes from `'Alice'` to `'Bob'`, cleanup ensures that the `'Alice'` response is ignored even if it arrives after `'Bob'`.\n\n**In development, you will see two fetches in the Network tab.** There is nothing wrong with that. With the approach above, the first Effect will immediately get cleaned up so its copy of the `ignore` variable will be set to `true`. So even though there is an extra request, it won’t affect the state thanks to the `if (!ignore)` check.\n\n**In production, there will only be one request.** If the second request in development is bothering you, the best approach is to use a solution that deduplicates requests and caches their responses between components:\n\n```\nfunction TodoList() {const todos = useSomeDataLibrary(`/api/user/${userId}/todos`);// ...\n```\n\nThis will not only improve the development experience, but also make your application feel faster. For example, the user pressing the Back button won’t have to wait for some data to load again because it will be cached. You can either build such a cache yourself or use one of the many alternatives to manual fetching in Effects.\n\n##### Deep Dive\n\n#### What are good alternatives to data fetching in Effects?[](https://react.dev/learn/synchronizing-with-effects#what-are-good-alternatives-to-data-fetching-in-effects \"Link for What are good alternatives to data fetching in Effects? \")\n\nWriting `fetch` calls inside Effects is a [popular way to fetch data](https://www.robinwieruch.de/react-hooks-fetch-data/), especially in fully client-side apps. This is, however, a very manual approach and it has significant downsides:\n\n* **Effects don’t run on the server.** This means that the initial server-rendered HTML will only include a loading state with no data. The client computer will have to download all JavaScript and render your app only to discover that now it needs to load the data. This is not very efficient.\n* **Fetching directly in Effects makes it easy to create “network waterfalls”.** You render the parent component, it fetches some data, renders the child components, and then they start fetching their data. If the network is not very fast, this is significantly slower than fetching all data in parallel.\n* **Fetching directly in Effects usually means you don’t preload or cache data.** For example, if the component unmounts and then mounts again, it would have to fetch the data again.\n* **It’s not very ergonomic.** There’s quite a bit of boilerplate code involved when writing `fetch` calls in a way that doesn’t suffer from bugs like [race conditions.](https://maxrozen.com/race-conditions-fetching-data-react-with-useeffect)\n\nThis list of downsides is not specific to React. It applies to fetching data on mount with any library. Like with routing, data fetching is not trivial to do well, so we recommend the following approaches:\n\n* **If you use a [framework](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks), use its built-in data fetching mechanism.** Modern React frameworks have integrated data fetching mechanisms that are efficient and don’t suffer from the above pitfalls.\n* **Otherwise, consider using or building a client-side cache.** Popular open source solutions include [React Query](https://tanstack.com/query/latest), [useSWR](https://swr.vercel.app/), and [React Router 6.4+.](https://beta.reactrouter.com/en/main/start/overview) You can build your own solution too, in which case you would use Effects under the hood, but add logic for deduplicating requests, caching responses, and avoiding network waterfalls (by preloading data or hoisting data requirements to routes).\n\nYou can continue fetching data directly in Effects if neither of these approaches suit you.\n\n### Sending analytics[](https://react.dev/learn/synchronizing-with-effects#sending-analytics \"Link for Sending analytics \")\n\nConsider this code that sends an analytics event on the page visit:\n\n```\nuseEffect(() => {logVisit(url); // Sends a POST request}, [url]);\n```\n\nIn development, `logVisit` will be called twice for every URL, so you might be tempted to try to fix that. **We recommend keeping this code as is.** Like with earlier examples, there is no _user-visible_ behavior difference between running it once and running it twice. From a practical point of view, `logVisit` should not do anything in development because you don’t want the logs from the development machines to skew the production metrics. Your component remounts every time you save its file, so it logs extra visits in development anyway.\n\n**In production, there will be no duplicate visit logs.**\n\nTo debug the analytics events you’re sending, you can deploy your app to a staging environment (which runs in production mode) or temporarily opt out of [Strict Mode](https://react.dev/reference/react/StrictMode) and its development-only remounting checks. You may also send analytics from the route change event handlers instead of Effects. For more precise analytics, [intersection observers](https://developer.mozilla.org/en-US/docs/Web/API/Intersection_Observer_API) can help track which components are in the viewport and how long they remain visible.\n\n### Not an Effect: Initializing the application[](https://react.dev/learn/synchronizing-with-effects#not-an-effect-initializing-the-application \"Link for Not an Effect: Initializing the application \")\n\nSome logic should only run once when the application starts. You can put it outside your components:\n\n```\nif (typeof window !== 'undefined') { // Check if we're running in the browser.checkAuthToken();loadDataFromLocalStorage();}function App() {// ...}\n```\n\nThis guarantees that such logic only runs once after the browser loads the page.\n\n### Not an Effect: Buying a product[](https://react.dev/learn/synchronizing-with-effects#not-an-effect-buying-a-product \"Link for Not an Effect: Buying a product \")\n\nSometimes, even if you write a cleanup function, there’s no way to prevent user-visible consequences of running the Effect twice. For example, maybe your Effect sends a POST request like buying a product:\n\n```\nuseEffect(() => {// 🔴 Wrong: This Effect fires twice in development, exposing a problem in the code.fetch('/api/buy', { method: 'POST' });}, []);\n```\n\nYou wouldn’t want to buy the product twice. However, this is also why you shouldn’t put this logic in an Effect. What if the user goes to another page and then presses Back? Your Effect would run again. You don’t want to buy the product when the user _visits_ a page; you want to buy it when the user _clicks_ the Buy button.\n\nBuying is not caused by rendering; it’s caused by a specific interaction. It should run only when the user presses the button. **Delete the Effect and move your `/api/buy` request into the Buy button event handler:**\n\n```\nfunction handleClick() {// ✅ Buying is an event because it is caused by a particular interaction.fetch('/api/buy', { method: 'POST' });}\n```\n\n**This illustrates that if remounting breaks the logic of your application, this usually uncovers existing bugs.** From a user’s perspective, visiting a page shouldn’t be different from visiting it, clicking a link, then pressing Back to view the page again. React verifies that your components abide by this principle by remounting them once in development.\n\nPutting it all together[](https://react.dev/learn/synchronizing-with-effects#putting-it-all-together \"Link for Putting it all together \")\n-----------------------------------------------------------------------------------------------------------------------------------------\n\nThis playground can help you “get a feel” for how Effects work in practice.\n\nThis example uses [`setTimeout`](https://developer.mozilla.org/en-US/docs/Web/API/setTimeout) to schedule a console log with the input text to appear three seconds after the Effect runs. The cleanup function cancels the pending timeout. Start by pressing “Mount the component”:\n\nimport { useState, useEffect } from 'react';\n\nfunction Playground() {\n const \\[text, setText\\] = useState('a');\n\n useEffect(() \\=> {\n function onTimeout() {\n console.log('⏰ ' + text);\n }\n\n console.log('🔵 Schedule \"' + text + '\" log');\n const timeoutId = setTimeout(onTimeout, 3000);\n\n return () \\=> {\n console.log('🟡 Cancel \"' + text + '\" log');\n clearTimeout(timeoutId);\n };\n }, \\[text\\]);\n\n return (\n <\\>\n <label\\>\n What to log:{' '}\n <input\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n </label\\>\n <h1\\>{text}</h1\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Unmount' : 'Mount'} the component\n </button\\>\n {show && <hr />}\n {show && <Playground />}\n </\\>\n );\n}\n\nYou will see three logs at first: `Schedule \"a\" log`, `Cancel \"a\" log`, and `Schedule \"a\" log` again. Three second later there will also be a log saying `a`. As you learned earlier, the extra schedule/cancel pair is because React remounts the component once in development to verify that you’ve implemented cleanup well.\n\nNow edit the input to say `abc`. If you do it fast enough, you’ll see `Schedule \"ab\" log` immediately followed by `Cancel \"ab\" log` and `Schedule \"abc\" log`. **React always cleans up the previous render’s Effect before the next render’s Effect.** This is why even if you type into the input fast, there is at most one timeout scheduled at a time. Edit the input a few times and watch the console to get a feel for how Effects get cleaned up.\n\nType something into the input and then immediately press “Unmount the component”. Notice how unmounting cleans up the last render’s Effect. Here, it clears the last timeout before it has a chance to fire.\n\nFinally, edit the component above and comment out the cleanup function so that the timeouts don’t get cancelled. Try typing `abcde` fast. What do you expect to happen in three seconds? Will `console.log(text)` inside the timeout print the _latest_ `text` and produce five `abcde` logs? Give it a try to check your intuition!\n\nThree seconds later, you should see a sequence of logs (`a`, `ab`, `abc`, `abcd`, and `abcde`) rather than five `abcde` logs. **Each Effect “captures” the `text` value from its corresponding render.** It doesn’t matter that the `text` state changed: an Effect from the render with `text = 'ab'` will always see `'ab'`. In other words, Effects from each render are isolated from each other. If you’re curious how this works, you can read about [closures](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures).\n\n##### Deep Dive\n\n#### Each render has its own Effects[](https://react.dev/learn/synchronizing-with-effects#each-render-has-its-own-effects \"Link for Each render has its own Effects \")\n\nYou can think of `useEffect` as “attaching” a piece of behavior to the render output. Consider this Effect:\n\n```\nexport default function ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(roomId);connection.connect();return () => connection.disconnect();}, [roomId]);return <h1>Welcome to {roomId}!</h1>;}\n```\n\nLet’s see what exactly happens as the user navigates around the app.\n\n#### Initial render[](https://react.dev/learn/synchronizing-with-effects#initial-render \"Link for Initial render \")\n\nThe user visits `<ChatRoom roomId=\"general\" />`. Let’s [mentally substitute](https://react.dev/learn/state-as-a-snapshot#rendering-takes-a-snapshot-in-time) `roomId` with `'general'`:\n\n```\n// JSX for the first render (roomId = \"general\")return <h1>Welcome to general!</h1>;\n```\n\n**The Effect is _also_ a part of the rendering output.** The first render’s Effect becomes:\n\n```\n// Effect for the first render (roomId = \"general\")() => {const connection = createConnection('general');connection.connect();return () => connection.disconnect();},// Dependencies for the first render (roomId = \"general\")['general']\n```\n\nReact runs this Effect, which connects to the `'general'` chat room.\n\n#### Re-render with same dependencies[](https://react.dev/learn/synchronizing-with-effects#re-render-with-same-dependencies \"Link for Re-render with same dependencies \")\n\nLet’s say `<ChatRoom roomId=\"general\" />` re-renders. The JSX output is the same:\n\n```\n// JSX for the second render (roomId = \"general\")return <h1>Welcome to general!</h1>;\n```\n\nReact sees that the rendering output has not changed, so it doesn’t update the DOM.\n\nThe Effect from the second render looks like this:\n\n```\n// Effect for the second render (roomId = \"general\")() => {const connection = createConnection('general');connection.connect();return () => connection.disconnect();},// Dependencies for the second render (roomId = \"general\")['general']\n```\n\nReact compares `['general']` from the second render with `['general']` from the first render. **Because all dependencies are the same, React _ignores_ the Effect from the second render.** It never gets called.\n\n#### Re-render with different dependencies[](https://react.dev/learn/synchronizing-with-effects#re-render-with-different-dependencies \"Link for Re-render with different dependencies \")\n\nThen, the user visits `<ChatRoom roomId=\"travel\" />`. This time, the component returns different JSX:\n\n```\n// JSX for the third render (roomId = \"travel\")return <h1>Welcome to travel!</h1>;\n```\n\nReact updates the DOM to change `\"Welcome to general\"` into `\"Welcome to travel\"`.\n\nThe Effect from the third render looks like this:\n\n```\n// Effect for the third render (roomId = \"travel\")() => {const connection = createConnection('travel');connection.connect();return () => connection.disconnect();},// Dependencies for the third render (roomId = \"travel\")['travel']\n```\n\nReact compares `['travel']` from the third render with `['general']` from the second render. One dependency is different: `Object.is('travel', 'general')` is `false`. The Effect can’t be skipped.\n\n**Before React can apply the Effect from the third render, it needs to clean up the last Effect that _did_ run.** The second render’s Effect was skipped, so React needs to clean up the first render’s Effect. If you scroll up to the first render, you’ll see that its cleanup calls `disconnect()` on the connection that was created with `createConnection('general')`. This disconnects the app from the `'general'` chat room.\n\nAfter that, React runs the third render’s Effect. It connects to the `'travel'` chat room.\n\n#### Unmount[](https://react.dev/learn/synchronizing-with-effects#unmount \"Link for Unmount \")\n\nFinally, let’s say the user navigates away, and the `ChatRoom` component unmounts. React runs the last Effect’s cleanup function. The last Effect was from the third render. The third render’s cleanup destroys the `createConnection('travel')` connection. So the app disconnects from the `'travel'` room.\n\n#### Development-only behaviors[](https://react.dev/learn/synchronizing-with-effects#development-only-behaviors \"Link for Development-only behaviors \")\n\nWhen [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React remounts every component once after mount (state and DOM are preserved). This [helps you find Effects that need cleanup](https://react.dev/learn/synchronizing-with-effects#step-3-add-cleanup-if-needed) and exposes bugs like race conditions early. Additionally, React will remount the Effects whenever you save a file in development. Both of these behaviors are development-only.\n\nRecap[](https://react.dev/learn/synchronizing-with-effects#recap \"Link for Recap\")\n----------------------------------------------------------------------------------\n\n* Unlike events, Effects are caused by rendering itself rather than a particular interaction.\n* Effects let you synchronize a component with some external system (third-party API, network, etc).\n* By default, Effects run after every render (including the initial one).\n* React will skip the Effect if all of its dependencies have the same values as during the last render.\n* You can’t “choose” your dependencies. They are determined by the code inside the Effect.\n* Empty dependency array (`[]`) corresponds to the component “mounting”, i.e. being added to the screen.\n* In Strict Mode, React mounts components twice (in development only!) to stress-test your Effects.\n* If your Effect breaks because of remounting, you need to implement a cleanup function.\n* React will call your cleanup function before the Effect runs next time, and during the unmount.\n\nTry out some challenges[](https://react.dev/learn/synchronizing-with-effects#challenges \"Link for Try out some challenges\")\n---------------------------------------------------------------------------------------------------------------------------\n\n#### Focus a field on mount[](https://react.dev/learn/synchronizing-with-effects#focus-a-field-on-mount \"Link for this heading\")\n\nIn this example, the form renders a `<MyInput />` component.\n\nUse the input’s [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) method to make `MyInput` automatically focus when it appears on the screen. There is already a commented out implementation, but it doesn’t quite work. Figure out why it doesn’t work, and fix it. (If you’re familiar with the `autoFocus` attribute, pretend that it does not exist: we are reimplementing the same functionality from scratch.)\n\nimport { useEffect, useRef } from 'react';\n\nexport default function MyInput({ value, onChange }) {\n const ref = useRef(null);\n\n \n \n\n return (\n <input\n ref\\={ref}\n value\\={value}\n onChange\\={onChange}\n />\n );\n}\n\nTo verify that your solution works, press “Show form” and verify that the input receives focus (becomes highlighted and the cursor is placed inside). Press “Hide form” and “Show form” again. Verify the input is highlighted again.\n\n`MyInput` should only focus _on mount_ rather than after every render. To verify that the behavior is right, press “Show form” and then repeatedly press the “Make it uppercase” checkbox. Clicking the checkbox should _not_ focus the input above it.\n",
"filename": "synchronizing-with-effects.md",
"package": "react"
} |
{
"content": "Title: <textarea> – React\n\nURL Source: https://react.dev/reference/react-dom/components/textarea\n\nMarkdown Content:\nThe [built-in browser `<textarea>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea) lets you render a multiline text input.\n\n* [Reference](https://react.dev/reference/react-dom/components/textarea#reference)\n * [`<textarea>`](https://react.dev/reference/react-dom/components/textarea#textarea)\n* [Usage](https://react.dev/reference/react-dom/components/textarea#usage)\n * [Displaying a text area](https://react.dev/reference/react-dom/components/textarea#displaying-a-text-area)\n * [Providing a label for a text area](https://react.dev/reference/react-dom/components/textarea#providing-a-label-for-a-text-area)\n * [Providing an initial value for a text area](https://react.dev/reference/react-dom/components/textarea#providing-an-initial-value-for-a-text-area)\n * [Reading the text area value when submitting a form](https://react.dev/reference/react-dom/components/textarea#reading-the-text-area-value-when-submitting-a-form)\n * [Controlling a text area with a state variable](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable)\n* [Troubleshooting](https://react.dev/reference/react-dom/components/textarea#troubleshooting)\n * [My text area doesn’t update when I type into it](https://react.dev/reference/react-dom/components/textarea#my-text-area-doesnt-update-when-i-type-into-it)\n * [My text area caret jumps to the beginning on every keystroke](https://react.dev/reference/react-dom/components/textarea#my-text-area-caret-jumps-to-the-beginning-on-every-keystroke)\n * [I’m getting an error: “A component is changing an uncontrolled input to be controlled”](https://react.dev/reference/react-dom/components/textarea#im-getting-an-error-a-component-is-changing-an-uncontrolled-input-to-be-controlled)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/textarea#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------------\n\n### `<textarea>`[](https://react.dev/reference/react-dom/components/textarea#textarea \"Link for this heading\")\n\nTo display a text area, render the [built-in browser `<textarea>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea) component.\n\n```\n<textarea name=\"postContent\" />\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/textarea#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/textarea#props \"Link for Props \")\n\n`<textarea>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\nYou can [make a text area controlled](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable) by passing a `value` prop:\n\n* `value`: A string. Controls the text inside the text area.\n\nWhen you pass `value`, you must also pass an `onChange` handler that updates the passed value.\n\nIf your `<textarea>` is uncontrolled, you may pass the `defaultValue` prop instead:\n\n* `defaultValue`: A string. Specifies [the initial value](https://react.dev/reference/react-dom/components/textarea#providing-an-initial-value-for-a-text-area) for a text area.\n\nThese `<textarea>` props are relevant both for uncontrolled and controlled text areas:\n\n* [`autoComplete`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#autocomplete): Either `'on'` or `'off'`. Specifies the autocomplete behavior.\n* [`autoFocus`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#autofocus): A boolean. If `true`, React will focus the element on mount.\n* `children`: `<textarea>` does not accept children. To set the initial value, use `defaultValue`.\n* [`cols`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#cols): A number. Specifies the default width in average character widths. Defaults to `20`.\n* [`disabled`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#disabled): A boolean. If `true`, the input will not be interactive and will appear dimmed.\n* [`form`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#form): A string. Specifies the `id` of the `<form>` this input belongs to. If omitted, it’s the closest parent form.\n* [`maxLength`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#maxlength): A number. Specifies the maximum length of text.\n* [`minLength`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#minlength): A number. Specifies the minimum length of text.\n* [`name`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#name): A string. Specifies the name for this input that’s [submitted with the form.](https://react.dev/reference/react-dom/components/textarea#reading-the-textarea-value-when-submitting-a-form)\n* `onChange`: An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Required for [controlled text areas.](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable) Fires immediately when the input’s value is changed by the user (for example, it fires on every keystroke). Behaves like the browser [`input` event.](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event)\n* `onChangeCapture`: A version of `onChange` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onInput`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires immediately when the value is changed by the user. For historical reasons, in React it is idiomatic to use `onChange` instead which works similarly.\n* `onInputCapture`: A version of `onInput` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onInvalid`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement/invalid_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires if an input fails validation on form submit. Unlike the built-in `invalid` event, the React `onInvalid` event bubbles.\n* `onInvalidCapture`: A version of `onInvalid` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSelect`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLTextAreaElement/select_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires after the selection inside the `<textarea>` changes. React extends the `onSelect` event to also fire for empty selection and on edits (which may affect the selection).\n* `onSelectCapture`: A version of `onSelect` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`placeholder`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#placeholder): A string. Displayed in a dimmed color when the text area value is empty.\n* [`readOnly`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#readonly): A boolean. If `true`, the text area is not editable by the user.\n* [`required`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#required): A boolean. If `true`, the value must be provided for the form to submit.\n* [`rows`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#rows): A number. Specifies the default height in average character heights. Defaults to `2`.\n* [`wrap`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#wrap): Either `'hard'`, `'soft'`, or `'off'`. Specifies how the text should be wrapped when submitting a form.\n\n#### Caveats[](https://react.dev/reference/react-dom/components/textarea#caveats \"Link for Caveats \")\n\n* Passing children like `<textarea>something</textarea>` is not allowed. [Use `defaultValue` for initial content.](https://react.dev/reference/react-dom/components/textarea#providing-an-initial-value-for-a-text-area)\n* If a text area receives a string `value` prop, it will be [treated as controlled.](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable)\n* A text area can’t be both controlled and uncontrolled at the same time.\n* A text area cannot switch between being controlled or uncontrolled over its lifetime.\n* Every controlled text area needs an `onChange` event handler that synchronously updates its backing value.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/textarea#usage \"Link for Usage \")\n------------------------------------------------------------------------------------------\n\n### Displaying a text area[](https://react.dev/reference/react-dom/components/textarea#displaying-a-text-area \"Link for Displaying a text area \")\n\nRender `<textarea>` to display a text area. You can specify its default size with the [`rows`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#rows) and [`cols`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/textarea#cols) attributes, but by default the user will be able to resize it. To disable resizing, you can specify `resize: none` in the CSS.\n\n* * *\n\n### Providing a label for a text area[](https://react.dev/reference/react-dom/components/textarea#providing-a-label-for-a-text-area \"Link for Providing a label for a text area \")\n\nTypically, you will place every `<textarea>` inside a [`<label>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/label) tag. This tells the browser that this label is associated with that text area. When the user clicks the label, the browser will focus the text area. It’s also essential for accessibility: a screen reader will announce the label caption when the user focuses the text area.\n\nIf you can’t nest `<textarea>` into a `<label>`, associate them by passing the same ID to `<textarea id>` and [`<label htmlFor>`.](https://developer.mozilla.org/en-US/docs/Web/API/HTMLLabelElement/htmlFor) To avoid conflicts between instances of one component, generate such an ID with [`useId`.](https://react.dev/reference/react/useId)\n\nimport { useId } from 'react';\n\nexport default function Form() {\n const postTextAreaId = useId();\n return (\n <\\>\n <label htmlFor\\={postTextAreaId}\\>\n Write your post:\n </label\\>\n <textarea\n id\\={postTextAreaId}\n name\\=\"postContent\"\n rows\\={4}\n cols\\={40}\n />\n </\\>\n );\n}\n\n* * *\n\n### Providing an initial value for a text area[](https://react.dev/reference/react-dom/components/textarea#providing-an-initial-value-for-a-text-area \"Link for Providing an initial value for a text area \")\n\nYou can optionally specify the initial value for the text area. Pass it as the `defaultValue` string.\n\n### Pitfall\n\nUnlike in HTML, passing initial text like `<textarea>Some content</textarea>` is not supported.\n\n* * *\n\n### Reading the text area value when submitting a form[](https://react.dev/reference/react-dom/components/textarea#reading-the-text-area-value-when-submitting-a-form \"Link for Reading the text area value when submitting a form \")\n\nAdd a [`<form>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) around your textarea with a [`<button type=\"submit\">`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/button) inside. It will call your `<form onSubmit>` event handler. By default, the browser will send the form data to the current URL and refresh the page. You can override that behavior by calling `e.preventDefault()`. Read the form data with [`new FormData(e.target)`](https://developer.mozilla.org/en-US/docs/Web/API/FormData).\n\nexport default function EditPost() {\n function handleSubmit(e) {\n \n e.preventDefault();\n\n \n const form = e.target;\n const formData = new FormData(form);\n\n \n fetch('/some-api', { method: form.method, body: formData });\n\n \n const formJson = Object.fromEntries(formData.entries());\n console.log(formJson);\n }\n\n return (\n <form method\\=\"post\" onSubmit\\={handleSubmit}\\>\n <label\\>\n Post title: <input name\\=\"postTitle\" defaultValue\\=\"Biking\" />\n </label\\>\n <label\\>\n Edit your post:\n <textarea\n name\\=\"postContent\"\n defaultValue\\=\"I really enjoyed biking yesterday!\"\n rows\\={4}\n cols\\={40}\n />\n </label\\>\n <hr />\n <button type\\=\"reset\"\\>Reset edits</button\\>\n <button type\\=\"submit\"\\>Save post</button\\>\n </form\\>\n );\n}\n\n### Note\n\nGive a `name` to your `<textarea>`, for example `<textarea name=\"postContent\" />`. The `name` you specified will be used as a key in the form data, for example `{ postContent: \"Your post\" }`.\n\n### Pitfall\n\nBy default, _any_ `<button>` inside a `<form>` will submit it. This can be surprising! If you have your own custom `Button` React component, consider returning [`<button type=\"button\">`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/button) instead of `<button>`. Then, to be explicit, use `<button type=\"submit\">` for buttons that _are_ supposed to submit the form.\n\n* * *\n\n### Controlling a text area with a state variable[](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable \"Link for Controlling a text area with a state variable \")\n\nA text area like `<textarea />` is _uncontrolled._ Even if you [pass an initial value](https://react.dev/reference/react-dom/components/textarea#providing-an-initial-value-for-a-text-area) like `<textarea defaultValue=\"Initial text\" />`, your JSX only specifies the initial value, not the value right now.\n\n**To render a _controlled_ text area, pass the `value` prop to it.** React will force the text area to always have the `value` you passed. Typically, you will control a text area by declaring a [state variable:](https://react.dev/reference/react/useState)\n\n```\nfunction NewPost() {const [postContent, setPostContent] = useState(''); // Declare a state variable...// ...return (<textareavalue={postContent} // ...force the input's value to match the state variable...onChange={e => setPostContent(e.target.value)} // ... and update the state variable on any edits!/>);}\n```\n\nThis is useful if you want to re-render some part of the UI in response to every keystroke.\n\n### Pitfall\n\n**If you pass `value` without `onChange`, it will be impossible to type into the text area.** When you control a text area by passing some `value` to it, you _force_ it to always have the value you passed. So if you pass a state variable as a `value` but forget to update that state variable synchronously during the `onChange` event handler, React will revert the text area after every keystroke back to the `value` that you specified.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react-dom/components/textarea#troubleshooting \"Link for Troubleshooting \")\n------------------------------------------------------------------------------------------------------------------------\n\n### My text area doesn’t update when I type into it[](https://react.dev/reference/react-dom/components/textarea#my-text-area-doesnt-update-when-i-type-into-it \"Link for My text area doesn’t update when I type into it \")\n\nIf you render a text area with `value` but no `onChange`, you will see an error in the console:\n\n```\n// 🔴 Bug: controlled text area with no onChange handler<textarea value={something} />\n```\n\nYou provided a `value` prop to a form field without an `onChange` handler. This will render a read-only field. If the field should be mutable use `defaultValue`. Otherwise, set either `onChange` or `readOnly`.\n\nAs the error message suggests, if you only wanted to [specify the _initial_ value,](https://react.dev/reference/react-dom/components/textarea#providing-an-initial-value-for-a-text-area) pass `defaultValue` instead:\n\n```\n// ✅ Good: uncontrolled text area with an initial value<textarea defaultValue={something} />\n```\n\nIf you want [to control this text area with a state variable,](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable) specify an `onChange` handler:\n\n```\n// ✅ Good: controlled text area with onChange<textarea value={something} onChange={e => setSomething(e.target.value)} />\n```\n\nIf the value is intentionally read-only, add a `readOnly` prop to suppress the error:\n\n```\n// ✅ Good: readonly controlled text area without on change<textarea value={something} readOnly={true} />\n```\n\n* * *\n\n### My text area caret jumps to the beginning on every keystroke[](https://react.dev/reference/react-dom/components/textarea#my-text-area-caret-jumps-to-the-beginning-on-every-keystroke \"Link for My text area caret jumps to the beginning on every keystroke \")\n\nIf you [control a text area,](https://react.dev/reference/react-dom/components/textarea#controlling-a-text-area-with-a-state-variable) you must update its state variable to the text area’s value from the DOM during `onChange`.\n\nYou can’t update it to something other than `e.target.value`:\n\n```\nfunction handleChange(e) {// 🔴 Bug: updating an input to something other than e.target.valuesetFirstName(e.target.value.toUpperCase());}\n```\n\nYou also can’t update it asynchronously:\n\n```\nfunction handleChange(e) {// 🔴 Bug: updating an input asynchronouslysetTimeout(() => {setFirstName(e.target.value);}, 100);}\n```\n\nTo fix your code, update it synchronously to `e.target.value`:\n\n```\nfunction handleChange(e) {// ✅ Updating a controlled input to e.target.value synchronouslysetFirstName(e.target.value);}\n```\n\nIf this doesn’t fix the problem, it’s possible that the text area gets removed and re-added from the DOM on every keystroke. This can happen if you’re accidentally [resetting state](https://react.dev/learn/preserving-and-resetting-state) on every re-render. For example, this can happen if the text area or one of its parents always receives a different `key` attribute, or if you nest component definitions (which is not allowed in React and causes the “inner” component to remount on every render).\n\n* * *\n\n### I’m getting an error: “A component is changing an uncontrolled input to be controlled”[](https://react.dev/reference/react-dom/components/textarea#im-getting-an-error-a-component-is-changing-an-uncontrolled-input-to-be-controlled \"Link for I’m getting an error: “A component is changing an uncontrolled input to be controlled” \")\n\nIf you provide a `value` to the component, it must remain a string throughout its lifetime.\n\nYou cannot pass `value={undefined}` first and later pass `value=\"some string\"` because React won’t know whether you want the component to be uncontrolled or controlled. A controlled component should always receive a string `value`, not `null` or `undefined`.\n\nIf your `value` is coming from an API or a state variable, it might be initialized to `null` or `undefined`. In that case, either set it to an empty string (`''`) initially, or pass `value={someValue ?? ''}` to ensure `value` is a string.\n",
"filename": "textarea.md",
"package": "react"
} |
{
"content": "Title: Thinking in React – React\n\nURL Source: https://react.dev/learn/thinking-in-react\n\nMarkdown Content:\nReact can change how you think about the designs you look at and the apps you build. When you build a user interface with React, you will first break it apart into pieces called _components_. Then, you will describe the different visual states for each of your components. Finally, you will connect your components together so that the data flows through them. In this tutorial, we’ll guide you through the thought process of building a searchable product data table with React.\n\nStart with the mockup[](https://react.dev/learn/thinking-in-react#start-with-the-mockup \"Link for Start with the mockup \")\n--------------------------------------------------------------------------------------------------------------------------\n\nImagine that you already have a JSON API and a mockup from a designer.\n\nThe JSON API returns some data that looks like this:\n\n```\n[{ category: \"Fruits\", price: \"$1\", stocked: true, name: \"Apple\" },{ category: \"Fruits\", price: \"$1\", stocked: true, name: \"Dragonfruit\" },{ category: \"Fruits\", price: \"$2\", stocked: false, name: \"Passionfruit\" },{ category: \"Vegetables\", price: \"$2\", stocked: true, name: \"Spinach\" },{ category: \"Vegetables\", price: \"$4\", stocked: false, name: \"Pumpkin\" },{ category: \"Vegetables\", price: \"$1\", stocked: true, name: \"Peas\" }]\n```\n\nThe mockup looks like this:\n\n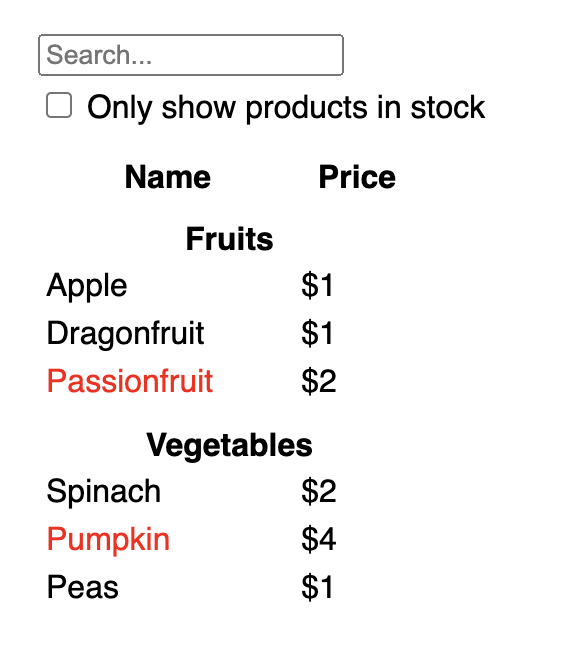\n\nTo implement a UI in React, you will usually follow the same five steps.\n\nStep 1: Break the UI into a component hierarchy[](https://react.dev/learn/thinking-in-react#step-1-break-the-ui-into-a-component-hierarchy \"Link for Step 1: Break the UI into a component hierarchy \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nStart by drawing boxes around every component and subcomponent in the mockup and naming them. If you work with a designer, they may have already named these components in their design tool. Ask them!\n\nDepending on your background, you can think about splitting up a design into components in different ways:\n\n* **Programming**—use the same techniques for deciding if you should create a new function or object. One such technique is the [single responsibility principle](https://en.wikipedia.org/wiki/Single_responsibility_principle), that is, a component should ideally only do one thing. If it ends up growing, it should be decomposed into smaller subcomponents.\n* **CSS**—consider what you would make class selectors for. (However, components are a bit less granular.)\n* **Design**—consider how you would organize the design’s layers.\n\nIf your JSON is well-structured, you’ll often find that it naturally maps to the component structure of your UI. That’s because UI and data models often have the same information architecture—that is, the same shape. Separate your UI into components, where each component matches one piece of your data model.\n\nThere are five components on this screen:\n\n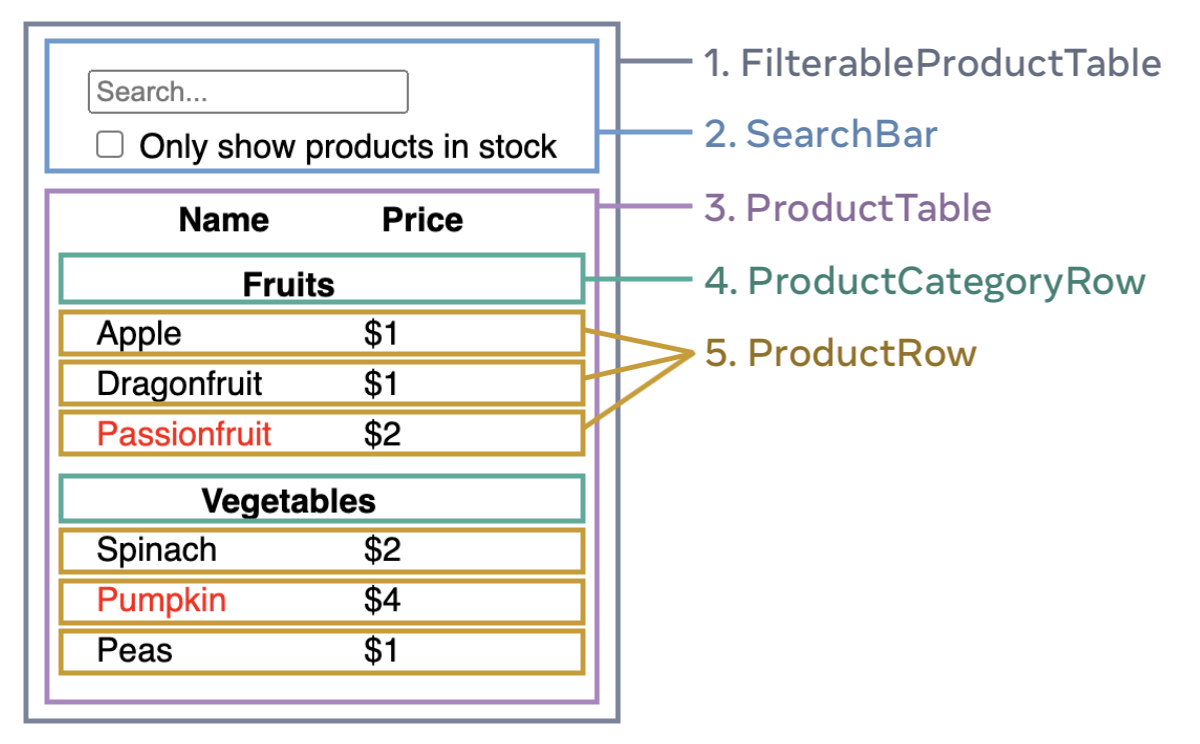\n\n1. `FilterableProductTable` (grey) contains the entire app.\n2. `SearchBar` (blue) receives the user input.\n3. `ProductTable` (lavender) displays and filters the list according to the user input.\n4. `ProductCategoryRow` (green) displays a heading for each category.\n5. `ProductRow` (yellow) displays a row for each product.\n\nIf you look at `ProductTable` (lavender), you’ll see that the table header (containing the “Name” and “Price” labels) isn’t its own component. This is a matter of preference, and you could go either way. For this example, it is a part of `ProductTable` because it appears inside the `ProductTable`’s list. However, if this header grows to be complex (e.g., if you add sorting), you can move it into its own `ProductTableHeader` component.\n\nNow that you’ve identified the components in the mockup, arrange them into a hierarchy. Components that appear within another component in the mockup should appear as a child in the hierarchy:\n\n* `FilterableProductTable`\n * `SearchBar`\n * `ProductTable`\n * `ProductCategoryRow`\n * `ProductRow`\n\nStep 2: Build a static version in React[](https://react.dev/learn/thinking-in-react#step-2-build-a-static-version-in-react \"Link for Step 2: Build a static version in React \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nNow that you have your component hierarchy, it’s time to implement your app. The most straightforward approach is to build a version that renders the UI from your data model without adding any interactivity… yet! It’s often easier to build the static version first and add interactivity later. Building a static version requires a lot of typing and no thinking, but adding interactivity requires a lot of thinking and not a lot of typing.\n\nTo build a static version of your app that renders your data model, you’ll want to build [components](https://react.dev/learn/your-first-component) that reuse other components and pass data using [props.](https://react.dev/learn/passing-props-to-a-component) Props are a way of passing data from parent to child. (If you’re familiar with the concept of [state](https://react.dev/learn/state-a-components-memory), don’t use state at all to build this static version. State is reserved only for interactivity, that is, data that changes over time. Since this is a static version of the app, you don’t need it.)\n\nYou can either build “top down” by starting with building the components higher up in the hierarchy (like `FilterableProductTable`) or “bottom up” by working from components lower down (like `ProductRow`). In simpler examples, it’s usually easier to go top-down, and on larger projects, it’s easier to go bottom-up.\n\nfunction ProductCategoryRow({ category }) {\n return (\n <tr\\>\n <th colSpan\\=\"2\"\\>\n {category}\n </th\\>\n </tr\\>\n );\n}\n\nfunction ProductRow({ product }) {\n const name = product.stocked ? product.name :\n <span style\\={{ color: 'red' }}\\>\n {product.name}\n </span\\>;\n\n return (\n <tr\\>\n <td\\>{name}</td\\>\n <td\\>{product.price}</td\\>\n </tr\\>\n );\n}\n\nfunction ProductTable({ products }) {\n const rows = \\[\\];\n let lastCategory = null;\n\n products.forEach((product) \\=> {\n if (product.category !== lastCategory) {\n rows.push(\n <ProductCategoryRow\n category\\={product.category}\n key\\={product.category} />\n );\n }\n rows.push(\n <ProductRow\n product\\={product}\n key\\={product.name} />\n );\n lastCategory = product.category;\n });\n\n return (\n <table\\>\n <thead\\>\n <tr\\>\n <th\\>Name</th\\>\n <th\\>Price</th\\>\n </tr\\>\n </thead\\>\n <tbody\\>{rows}</tbody\\>\n </table\\>\n );\n}\n\nfunction SearchBar() {\n return (\n <form\\>\n <input type\\=\"text\" placeholder\\=\"Search...\" />\n <label\\>\n <input type\\=\"checkbox\" />\n {' '}\n Only show products in stock\n </label\\>\n </form\\>\n );\n}\n\nfunction FilterableProductTable({ products }) {\n return (\n <div\\>\n <SearchBar />\n <ProductTable products\\={products} />\n </div\\>\n );\n}\n\nconst PRODUCTS = \\[\n {category: \"Fruits\", price: \"$1\", stocked: true, name: \"Apple\"},\n {category: \"Fruits\", price: \"$1\", stocked: true, name: \"Dragonfruit\"},\n {category: \"Fruits\", price: \"$2\", stocked: false, name: \"Passionfruit\"},\n {category: \"Vegetables\", price: \"$2\", stocked: true, name: \"Spinach\"},\n {category: \"Vegetables\", price: \"$4\", stocked: false, name: \"Pumpkin\"},\n {category: \"Vegetables\", price: \"$1\", stocked: true, name: \"Peas\"}\n\\];\n\nexport default function App() {\n return <FilterableProductTable products\\={PRODUCTS} />;\n}\n\n(If this code looks intimidating, go through the [Quick Start](https://react.dev/learn) first!)\n\nAfter building your components, you’ll have a library of reusable components that render your data model. Because this is a static app, the components will only return JSX. The component at the top of the hierarchy (`FilterableProductTable`) will take your data model as a prop. This is called _one-way data flow_ because the data flows down from the top-level component to the ones at the bottom of the tree.\n\n### Pitfall\n\nAt this point, you should not be using any state values. That’s for the next step!\n\nStep 3: Find the minimal but complete representation of UI state[](https://react.dev/learn/thinking-in-react#step-3-find-the-minimal-but-complete-representation-of-ui-state \"Link for Step 3: Find the minimal but complete representation of UI state \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nTo make the UI interactive, you need to let users change your underlying data model. You will use _state_ for this.\n\nThink of state as the minimal set of changing data that your app needs to remember. The most important principle for structuring state is to keep it [DRY (Don’t Repeat Yourself).](https://en.wikipedia.org/wiki/Don%27t_repeat_yourself) Figure out the absolute minimal representation of the state your application needs and compute everything else on-demand. For example, if you’re building a shopping list, you can store the items as an array in state. If you want to also display the number of items in the list, don’t store the number of items as another state value—instead, read the length of your array.\n\nNow think of all of the pieces of data in this example application:\n\n1. The original list of products\n2. The search text the user has entered\n3. The value of the checkbox\n4. The filtered list of products\n\nWhich of these are state? Identify the ones that are not:\n\n* Does it **remain unchanged** over time? If so, it isn’t state.\n* Is it **passed in from a parent** via props? If so, it isn’t state.\n* **Can you compute it** based on existing state or props in your component? If so, it _definitely_ isn’t state!\n\nWhat’s left is probably state.\n\nLet’s go through them one by one again:\n\n1. The original list of products is **passed in as props, so it’s not state.**\n2. The search text seems to be state since it changes over time and can’t be computed from anything.\n3. The value of the checkbox seems to be state since it changes over time and can’t be computed from anything.\n4. The filtered list of products **isn’t state because it can be computed** by taking the original list of products and filtering it according to the search text and value of the checkbox.\n\nThis means only the search text and the value of the checkbox are state! Nicely done!\n\n##### Deep Dive\n\n#### Props vs State[](https://react.dev/learn/thinking-in-react#props-vs-state \"Link for Props vs State \")\n\nThere are two types of “model” data in React: props and state. The two are very different:\n\n* [**Props** are like arguments you pass](https://react.dev/learn/passing-props-to-a-component) to a function. They let a parent component pass data to a child component and customize its appearance. For example, a `Form` can pass a `color` prop to a `Button`.\n* [**State** is like a component’s memory.](https://react.dev/learn/state-a-components-memory) It lets a component keep track of some information and change it in response to interactions. For example, a `Button` might keep track of `isHovered` state.\n\nProps and state are different, but they work together. A parent component will often keep some information in state (so that it can change it), and _pass it down_ to child components as their props. It’s okay if the difference still feels fuzzy on the first read. It takes a bit of practice for it to really stick!\n\nStep 4: Identify where your state should live[](https://react.dev/learn/thinking-in-react#step-4-identify-where-your-state-should-live \"Link for Step 4: Identify where your state should live \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAfter identifying your app’s minimal state data, you need to identify which component is responsible for changing this state, or _owns_ the state. Remember: React uses one-way data flow, passing data down the component hierarchy from parent to child component. It may not be immediately clear which component should own what state. This can be challenging if you’re new to this concept, but you can figure it out by following these steps!\n\nFor each piece of state in your application:\n\n1. Identify _every_ component that renders something based on that state.\n2. Find their closest common parent component—a component above them all in the hierarchy.\n3. Decide where the state should live:\n 1. Often, you can put the state directly into their common parent.\n 2. You can also put the state into some component above their common parent.\n 3. If you can’t find a component where it makes sense to own the state, create a new component solely for holding the state and add it somewhere in the hierarchy above the common parent component.\n\nIn the previous step, you found two pieces of state in this application: the search input text, and the value of the checkbox. In this example, they always appear together, so it makes sense to put them into the same place.\n\nNow let’s run through our strategy for them:\n\n1. **Identify components that use state:**\n * `ProductTable` needs to filter the product list based on that state (search text and checkbox value).\n * `SearchBar` needs to display that state (search text and checkbox value).\n2. **Find their common parent:** The first parent component both components share is `FilterableProductTable`.\n3. **Decide where the state lives**: We’ll keep the filter text and checked state values in `FilterableProductTable`.\n\nSo the state values will live in `FilterableProductTable`.\n\nAdd state to the component with the [`useState()` Hook.](https://react.dev/reference/react/useState) Hooks are special functions that let you “hook into” React. Add two state variables at the top of `FilterableProductTable` and specify their initial state:\n\n```\nfunction FilterableProductTable({ products }) {const [filterText, setFilterText] = useState('');const [inStockOnly, setInStockOnly] = useState(false);\n```\n\nThen, pass `filterText` and `inStockOnly` to `ProductTable` and `SearchBar` as props:\n\n```\n<div><SearchBar filterText={filterText} inStockOnly={inStockOnly} /><ProductTable products={products}filterText={filterText}inStockOnly={inStockOnly} /></div>\n```\n\nYou can start seeing how your application will behave. Edit the `filterText` initial value from `useState('')` to `useState('fruit')` in the sandbox code below. You’ll see both the search input text and the table update:\n\nimport { useState } from 'react';\n\nfunction FilterableProductTable({ products }) {\n const \\[filterText, setFilterText\\] = useState('');\n const \\[inStockOnly, setInStockOnly\\] = useState(false);\n\n return (\n <div\\>\n <SearchBar \n filterText\\={filterText} \n inStockOnly\\={inStockOnly} />\n <ProductTable \n products\\={products}\n filterText\\={filterText}\n inStockOnly\\={inStockOnly} />\n </div\\>\n );\n}\n\nfunction ProductCategoryRow({ category }) {\n return (\n <tr\\>\n <th colSpan\\=\"2\"\\>\n {category}\n </th\\>\n </tr\\>\n );\n}\n\nfunction ProductRow({ product }) {\n const name = product.stocked ? product.name :\n <span style\\={{ color: 'red' }}\\>\n {product.name}\n </span\\>;\n\n return (\n <tr\\>\n <td\\>{name}</td\\>\n <td\\>{product.price}</td\\>\n </tr\\>\n );\n}\n\nfunction ProductTable({ products, filterText, inStockOnly }) {\n const rows = \\[\\];\n let lastCategory = null;\n\n products.forEach((product) \\=> {\n if (\n product.name.toLowerCase().indexOf(\n filterText.toLowerCase()\n ) === -1\n ) {\n return;\n }\n if (inStockOnly && !product.stocked) {\n return;\n }\n if (product.category !== lastCategory) {\n rows.push(\n <ProductCategoryRow\n category\\={product.category}\n key\\={product.category} />\n );\n }\n rows.push(\n <ProductRow\n product\\={product}\n key\\={product.name} />\n );\n lastCategory = product.category;\n });\n\n return (\n <table\\>\n <thead\\>\n <tr\\>\n <th\\>Name</th\\>\n <th\\>Price</th\\>\n </tr\\>\n </thead\\>\n <tbody\\>{rows}</tbody\\>\n </table\\>\n );\n}\n\nfunction SearchBar({ filterText, inStockOnly }) {\n return (\n <form\\>\n <input \n type\\=\"text\" \n value\\={filterText} \n placeholder\\=\"Search...\"/>\n <label\\>\n <input \n type\\=\"checkbox\" \n checked\\={inStockOnly} />\n {' '}\n Only show products in stock\n </label\\>\n </form\\>\n );\n}\n\nconst PRODUCTS = \\[\n {category: \"Fruits\", price: \"$1\", stocked: true, name: \"Apple\"},\n {category: \"Fruits\", price: \"$1\", stocked: true, name: \"Dragonfruit\"},\n {category: \"Fruits\", price: \"$2\", stocked: false, name: \"Passionfruit\"},\n {category: \"Vegetables\", price: \"$2\", stocked: true, name: \"Spinach\"},\n {category: \"Vegetables\", price: \"$4\", stocked: false, name: \"Pumpkin\"},\n {category: \"Vegetables\", price: \"$1\", stocked: true, name: \"Peas\"}\n\\];\n\nexport default function App() {\n return <FilterableProductTable products\\={PRODUCTS} />;\n}\n\nNotice that editing the form doesn’t work yet. There is a console error in the sandbox above explaining why:\n\nYou provided a \\`value\\` prop to a form field without an \\`onChange\\` handler. This will render a read-only field.\n\nIn the sandbox above, `ProductTable` and `SearchBar` read the `filterText` and `inStockOnly` props to render the table, the input, and the checkbox. For example, here is how `SearchBar` populates the input value:\n\n```\nfunction SearchBar({ filterText, inStockOnly }) {return (<form><input type=\"text\" value={filterText} placeholder=\"Search...\"/>\n```\n\nHowever, you haven’t added any code to respond to the user actions like typing yet. This will be your final step.\n\nStep 5: Add inverse data flow[](https://react.dev/learn/thinking-in-react#step-5-add-inverse-data-flow \"Link for Step 5: Add inverse data flow \")\n-------------------------------------------------------------------------------------------------------------------------------------------------\n\nCurrently your app renders correctly with props and state flowing down the hierarchy. But to change the state according to user input, you will need to support data flowing the other way: the form components deep in the hierarchy need to update the state in `FilterableProductTable`.\n\nReact makes this data flow explicit, but it requires a little more typing than two-way data binding. If you try to type or check the box in the example above, you’ll see that React ignores your input. This is intentional. By writing `<input value={filterText} />`, you’ve set the `value` prop of the `input` to always be equal to the `filterText` state passed in from `FilterableProductTable`. Since `filterText` state is never set, the input never changes.\n\nYou want to make it so whenever the user changes the form inputs, the state updates to reflect those changes. The state is owned by `FilterableProductTable`, so only it can call `setFilterText` and `setInStockOnly`. To let `SearchBar` update the `FilterableProductTable`’s state, you need to pass these functions down to `SearchBar`:\n\n```\nfunction FilterableProductTable({ products }) {const [filterText, setFilterText] = useState('');const [inStockOnly, setInStockOnly] = useState(false);return (<div><SearchBar filterText={filterText} inStockOnly={inStockOnly}onFilterTextChange={setFilterText}onInStockOnlyChange={setInStockOnly} />\n```\n\nInside the `SearchBar`, you will add the `onChange` event handlers and set the parent state from them:\n\n```\nfunction SearchBar({filterText,inStockOnly,onFilterTextChange,onInStockOnlyChange}) {return (<form><inputtype=\"text\"value={filterText}placeholder=\"Search...\"onChange={(e) => onFilterTextChange(e.target.value)}/><label><inputtype=\"checkbox\"checked={inStockOnly}onChange={(e) => onInStockOnlyChange(e.target.checked)}\n```\n\nNow the application fully works!\n\nimport { useState } from 'react';\n\nfunction FilterableProductTable({ products }) {\n const \\[filterText, setFilterText\\] = useState('');\n const \\[inStockOnly, setInStockOnly\\] = useState(false);\n\n return (\n <div\\>\n <SearchBar \n filterText\\={filterText} \n inStockOnly\\={inStockOnly} \n onFilterTextChange\\={setFilterText} \n onInStockOnlyChange\\={setInStockOnly} />\n <ProductTable \n products\\={products} \n filterText\\={filterText}\n inStockOnly\\={inStockOnly} />\n </div\\>\n );\n}\n\nfunction ProductCategoryRow({ category }) {\n return (\n <tr\\>\n <th colSpan\\=\"2\"\\>\n {category}\n </th\\>\n </tr\\>\n );\n}\n\nfunction ProductRow({ product }) {\n const name = product.stocked ? product.name :\n <span style\\={{ color: 'red' }}\\>\n {product.name}\n </span\\>;\n\n return (\n <tr\\>\n <td\\>{name}</td\\>\n <td\\>{product.price}</td\\>\n </tr\\>\n );\n}\n\nfunction ProductTable({ products, filterText, inStockOnly }) {\n const rows = \\[\\];\n let lastCategory = null;\n\n products.forEach((product) \\=> {\n if (\n product.name.toLowerCase().indexOf(\n filterText.toLowerCase()\n ) === -1\n ) {\n return;\n }\n if (inStockOnly && !product.stocked) {\n return;\n }\n if (product.category !== lastCategory) {\n rows.push(\n <ProductCategoryRow\n category\\={product.category}\n key\\={product.category} />\n );\n }\n rows.push(\n <ProductRow\n product\\={product}\n key\\={product.name} />\n );\n lastCategory = product.category;\n });\n\n return (\n <table\\>\n <thead\\>\n <tr\\>\n <th\\>Name</th\\>\n <th\\>Price</th\\>\n </tr\\>\n </thead\\>\n <tbody\\>{rows}</tbody\\>\n </table\\>\n );\n}\n\nfunction SearchBar({\n filterText,\n inStockOnly,\n onFilterTextChange,\n onInStockOnlyChange\n}) {\n return (\n <form\\>\n <input \n type\\=\"text\" \n value\\={filterText} placeholder\\=\"Search...\" \n onChange\\={(e) \\=> onFilterTextChange(e.target.value)} />\n <label\\>\n <input \n type\\=\"checkbox\" \n checked\\={inStockOnly} \n onChange\\={(e) \\=> onInStockOnlyChange(e.target.checked)} />\n {' '}\n Only show products in stock\n </label\\>\n </form\\>\n );\n}\n\nconst PRODUCTS = \\[\n {category: \"Fruits\", price: \"$1\", stocked: true, name: \"Apple\"},\n {category: \"Fruits\", price: \"$1\", stocked: true, name: \"Dragonfruit\"},\n {category: \"Fruits\", price: \"$2\", stocked: false, name: \"Passionfruit\"},\n {category: \"Vegetables\", price: \"$2\", stocked: true, name: \"Spinach\"},\n {category: \"Vegetables\", price: \"$4\", stocked: false, name: \"Pumpkin\"},\n {category: \"Vegetables\", price: \"$1\", stocked: true, name: \"Peas\"}\n\\];\n\nexport default function App() {\n return <FilterableProductTable products\\={PRODUCTS} />;\n}\n",
"filename": "thinking-in-react.md",
"package": "react"
} |
{
"content": "Title: <title> – React\n\nURL Source: https://react.dev/reference/react-dom/components/title\n\nMarkdown Content:\nReact\nv18.3.1\nSearch\nCtrl\nK\nLearn\nReference\nCommunity\nBlog\nreact@18.3.1\nOverview\nHooks\nComponents\nAPIs\nreact-dom@18.3.1\nHooks\nComponents\nCommon (e.g. <div>)\n<form> \n<input>\n<option>\n<progress>\n<select>\n<textarea>\n<link> \n<meta> \n<script> \n<style> \n<title> \nAPIs\nClient APIs\nServer APIs\nRules of React\nOverview\nReact Server Components\nServer Components \nServer Actions \nDirectives \nLegacy APIs\nLegacy React APIs\n\nIs this page useful?\n\nAPI REFERENCE\nCOMPONENTS\n<title>\nCanary\n\nReact’s extensions to <title> are currently only available in React’s canary and experimental channels. In stable releases of React <title> works only as a built-in browser HTML component. Learn more about React’s release channels here.\n\nThe built-in browser <title> component lets you specify the title of the document.\n\n<title>My Blog</title>\nReference\n<title>\nUsage\nSet the document title\nUse variables in the title\nReference \n<title> \n\nTo specify the title of the document, render the built-in browser <title> component. You can render <title> from any component and React will always place the corresponding DOM element in the document head.\n\n<title>My Blog</title>\n\nSee more examples below.\n\nProps \n\n<title> supports all common element props.\n\nchildren: <title> accepts only text as a child. This text will become the title of the document. You can also pass your own components as long as they only render text.\nSpecial rendering behavior \n\nReact will always place the DOM element corresponding to the <title> component within the document’s <head>, regardless of where in the React tree it is rendered. The <head> is the only valid place for <title> to exist within the DOM, yet it’s convenient and keeps things composable if a component representing a specific page can render its <title> itself.\n\nThere are two exception to this:\n\nIf <title> is within an <svg> component, then there is no special behavior, because in this context it doesn’t represent the document’s title but rather is an accessibility annotation for that SVG graphic.\nIf the <title> has an itemProp prop, there is no special behavior, because in this case it doesn’t represent the document’s title but rather metadata about a specific part of the page.\nPitfall\n\nOnly render a single <title> at a time. If more than one component renders a <title> tag at the same time, React will place all of those titles in the document head. When this happens, the behavior of browsers and search engines is undefined.\n\nUsage \nSet the document title \n\nRender the <title> component from any component with text as its children. React will put a <title> DOM node in the document <head>.\n\nApp.js\nShowRenderedHTML.js\nReset\nFork\nimport ShowRenderedHTML from './ShowRenderedHTML.js';\n\nexport default function ContactUsPage() {\n return (\n <ShowRenderedHTML>\n <title>My Site: Contact Us</title>\n <h1>Contact Us</h1>\n <p>Email us at support@example.com</p>\n </ShowRenderedHTML>\n );\n}\n\n\nUse variables in the title \n\nThe children of the <title> component must be a single string of text. (Or a single number or a single object with a toString method.) It might not be obvious, but using JSX curly braces like this:\n\n<title>Results page {pageNumber}</title> // 🔴 Problem: This is not a single string\n\n… actually causes the <title> component to get a two-element array as its children (the string \"Results page\" and the value of pageNumber). This will cause an error. Instead, use string interpolation to pass <title> a single string:\n\n<title>{`Results page ${pageNumber}`}</title>\nPREVIOUS\n<style>\nNEXT\nAPIs\n©2024\nuwu?\nLearn React\nQuick Start\nInstallation\nDescribing the UI\nAdding Interactivity\nManaging State\nEscape Hatches\nAPI Reference\nReact APIs\nReact DOM APIs\nCommunity\nCode of Conduct\nMeet the Team\nDocs Contributors\nAcknowledgements\nMore\nBlog\nReact Native\nPrivacy\nTerms\n",
"filename": "title.md",
"package": "react"
} |
{
"content": "Title: Tutorial: Tic-Tac-Toe – React\n\nURL Source: https://react.dev/learn/tutorial-tic-tac-toe\n\nMarkdown Content:\nYou will build a small tic-tac-toe game during this tutorial. This tutorial does not assume any existing React knowledge. The techniques you’ll learn in the tutorial are fundamental to building any React app, and fully understanding it will give you a deep understanding of React.\n\n### Note\n\nThis tutorial is designed for people who prefer to **learn by doing** and want to quickly try making something tangible. If you prefer learning each concept step by step, start with [Describing the UI.](https://react.dev/learn/describing-the-ui)\n\nThe tutorial is divided into several sections:\n\n* [Setup for the tutorial](https://react.dev/learn/tutorial-tic-tac-toe#setup-for-the-tutorial) will give you **a starting point** to follow the tutorial.\n* [Overview](https://react.dev/learn/tutorial-tic-tac-toe#overview) will teach you **the fundamentals** of React: components, props, and state.\n* [Completing the game](https://react.dev/learn/tutorial-tic-tac-toe#completing-the-game) will teach you **the most common techniques** in React development.\n* [Adding time travel](https://react.dev/learn/tutorial-tic-tac-toe#adding-time-travel) will give you **a deeper insight** into the unique strengths of React.\n\n### What are you building?[](https://react.dev/learn/tutorial-tic-tac-toe#what-are-you-building \"Link for What are you building? \")\n\nIn this tutorial, you’ll build an interactive tic-tac-toe game with React.\n\nYou can see what it will look like when you’re finished here:\n\nIf the code doesn’t make sense to you yet, or if you are unfamiliar with the code’s syntax, don’t worry! The goal of this tutorial is to help you understand React and its syntax.\n\nWe recommend that you check out the tic-tac-toe game above before continuing with the tutorial. One of the features that you’ll notice is that there is a numbered list to the right of the game’s board. This list gives you a history of all of the moves that have occurred in the game, and it is updated as the game progresses.\n\nOnce you’ve played around with the finished tic-tac-toe game, keep scrolling. You’ll start with a simpler template in this tutorial. Our next step is to set you up so that you can start building the game.\n\nSetup for the tutorial[](https://react.dev/learn/tutorial-tic-tac-toe#setup-for-the-tutorial \"Link for Setup for the tutorial \")\n--------------------------------------------------------------------------------------------------------------------------------\n\nIn the live code editor below, click **Fork** in the top-right corner to open the editor in a new tab using the website CodeSandbox. CodeSandbox lets you write code in your browser and preview how your users will see the app you’ve created. The new tab should display an empty square and the starter code for this tutorial.\n\n### Note\n\nYou can also follow this tutorial using your local development environment. To do this, you need to:\n\n1. Install [Node.js](https://nodejs.org/en/)\n2. In the CodeSandbox tab you opened earlier, press the top-left corner button to open the menu, and then choose **Download Sandbox** in that menu to download an archive of the files locally\n3. Unzip the archive, then open a terminal and `cd` to the directory you unzipped\n4. Install the dependencies with `npm install`\n5. Run `npm start` to start a local server and follow the prompts to view the code running in a browser\n\nIf you get stuck, don’t let this stop you! Follow along online instead and try a local setup again later.\n\nOverview[](https://react.dev/learn/tutorial-tic-tac-toe#overview \"Link for Overview \")\n--------------------------------------------------------------------------------------\n\nNow that you’re set up, let’s get an overview of React!\n\n### Inspecting the starter code[](https://react.dev/learn/tutorial-tic-tac-toe#inspecting-the-starter-code \"Link for Inspecting the starter code \")\n\nIn CodeSandbox you’ll see three main sections:\n\n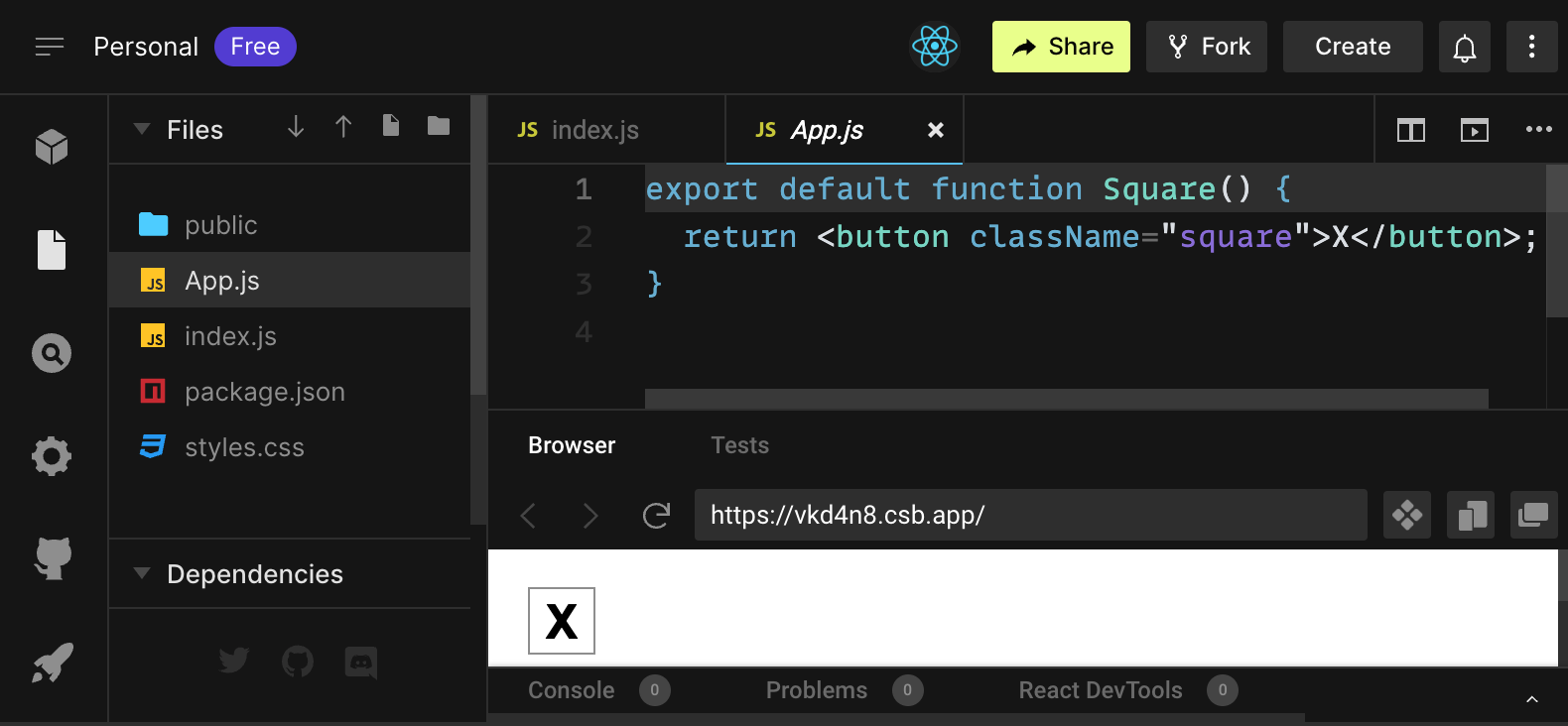\n\n1. The _Files_ section with a list of files like `App.js`, `index.js`, `styles.css` and a folder called `public`\n2. The _code editor_ where you’ll see the source code of your selected file\n3. The _browser_ section where you’ll see how the code you’ve written will be displayed\n\nThe `App.js` file should be selected in the _Files_ section. The contents of that file in the _code editor_ should be:\n\n```\nexport default function Square() {return <button className=\"square\">X</button>;}\n```\n\nThe _browser_ section should be displaying a square with a X in it like this:\n\n\n\nNow let’s have a look at the files in the starter code.\n\n#### `App.js`[](https://react.dev/learn/tutorial-tic-tac-toe#appjs \"Link for this heading\")\n\nThe code in `App.js` creates a _component_. In React, a component is a piece of reusable code that represents a part of a user interface. Components are used to render, manage, and update the UI elements in your application. Let’s look at the component line by line to see what’s going on:\n\n```\nexport default function Square() {return <button className=\"square\">X</button>;}\n```\n\nThe first line defines a function called `Square`. The `export` JavaScript keyword makes this function accessible outside of this file. The `default` keyword tells other files using your code that it’s the main function in your file.\n\n```\nexport default function Square() {return <button className=\"square\">X</button>;}\n```\n\nThe second line returns a button. The `return` JavaScript keyword means whatever comes after is returned as a value to the caller of the function. `<button>` is a _JSX element_. A JSX element is a combination of JavaScript code and HTML tags that describes what you’d like to display. `className=\"square\"` is a button property or _prop_ that tells CSS how to style the button. `X` is the text displayed inside of the button and `</button>` closes the JSX element to indicate that any following content shouldn’t be placed inside the button.\n\n#### `styles.css`[](https://react.dev/learn/tutorial-tic-tac-toe#stylescss \"Link for this heading\")\n\nClick on the file labeled `styles.css` in the _Files_ section of CodeSandbox. This file defines the styles for your React app. The first two _CSS selectors_ (`*` and `body`) define the style of large parts of your app while the `.square` selector defines the style of any component where the `className` property is set to `square`. In your code, that would match the button from your Square component in the `App.js` file.\n\n#### `index.js`[](https://react.dev/learn/tutorial-tic-tac-toe#indexjs \"Link for this heading\")\n\nClick on the file labeled `index.js` in the _Files_ section of CodeSandbox. You won’t be editing this file during the tutorial but it is the bridge between the component you created in the `App.js` file and the web browser.\n\n```\nimport { StrictMode } from 'react';import { createRoot } from 'react-dom/client';import './styles.css';import App from './App';\n```\n\nLines 1-5 bring all the necessary pieces together:\n\n* React\n* React’s library to talk to web browsers (React DOM)\n* the styles for your components\n* the component you created in `App.js`.\n\nThe remainder of the file brings all the pieces together and injects the final product into `index.html` in the `public` folder.\n\n### Building the board[](https://react.dev/learn/tutorial-tic-tac-toe#building-the-board \"Link for Building the board \")\n\nLet’s get back to `App.js`. This is where you’ll spend the rest of the tutorial.\n\nCurrently the board is only a single square, but you need nine! If you just try and copy paste your square to make two squares like this:\n\n```\nexport default function Square() {return <button className=\"square\">X</button><button className=\"square\">X</button>;}\n```\n\nYou’ll get this error:\n\n/src/App.js: Adjacent JSX elements must be wrapped in an enclosing tag. Did you want a JSX Fragment `<>...</>`?\n\nReact components need to return a single JSX element and not multiple adjacent JSX elements like two buttons. To fix this you can use _Fragments_ (`<>` and `</>`) to wrap multiple adjacent JSX elements like this:\n\n```\nexport default function Square() {return (<><button className=\"square\">X</button><button className=\"square\">X</button></>);}\n```\n\nNow you should see:\n\n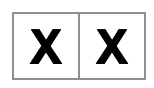\n\nGreat! Now you just need to copy-paste a few times to add nine squares and…\n\n\n\nOh no! The squares are all in a single line, not in a grid like you need for our board. To fix this you’ll need to group your squares into rows with `div`s and add some CSS classes. While you’re at it, you’ll give each square a number to make sure you know where each square is displayed.\n\nIn the `App.js` file, update the `Square` component to look like this:\n\n```\nexport default function Square() {return (<><div className=\"board-row\"><button className=\"square\">1</button><button className=\"square\">2</button><button className=\"square\">3</button></div><div className=\"board-row\"><button className=\"square\">4</button><button className=\"square\">5</button><button className=\"square\">6</button></div><div className=\"board-row\"><button className=\"square\">7</button><button className=\"square\">8</button><button className=\"square\">9</button></div></>);}\n```\n\nThe CSS defined in `styles.css` styles the divs with the `className` of `board-row`. Now that you’ve grouped your components into rows with the styled `div`s you have your tic-tac-toe board:\n\n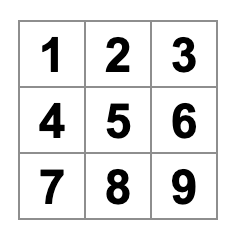\n\nBut you now have a problem. Your component named `Square`, really isn’t a square anymore. Let’s fix that by changing the name to `Board`:\n\n```\nexport default function Board() {//...}\n```\n\nAt this point your code should look something like this:\n\nexport default function Board() {\n return (\n <\\>\n <div className\\=\"board-row\"\\>\n <button className\\=\"square\"\\>1</button\\>\n <button className\\=\"square\"\\>2</button\\>\n <button className\\=\"square\"\\>3</button\\>\n </div\\>\n <div className\\=\"board-row\"\\>\n <button className\\=\"square\"\\>4</button\\>\n <button className\\=\"square\"\\>5</button\\>\n <button className\\=\"square\"\\>6</button\\>\n </div\\>\n <div className\\=\"board-row\"\\>\n <button className\\=\"square\"\\>7</button\\>\n <button className\\=\"square\"\\>8</button\\>\n <button className\\=\"square\"\\>9</button\\>\n </div\\>\n </\\>\n );\n}\n\n### Note\n\nPsssst… That’s a lot to type! It’s okay to copy and paste code from this page. However, if you’re up for a little challenge, we recommend only copying code that you’ve manually typed at least once yourself.\n\n### Passing data through props[](https://react.dev/learn/tutorial-tic-tac-toe#passing-data-through-props \"Link for Passing data through props \")\n\nNext, you’ll want to change the value of a square from empty to “X” when the user clicks on the square. With how you’ve built the board so far you would need to copy-paste the code that updates the square nine times (once for each square you have)! Instead of copy-pasting, React’s component architecture allows you to create a reusable component to avoid messy, duplicated code.\n\nFirst, you are going to copy the line defining your first square (`<button className=\"square\">1</button>`) from your `Board` component into a new `Square` component:\n\n```\nfunction Square() {return <button className=\"square\">1</button>;}export default function Board() {// ...}\n```\n\nThen you’ll update the Board component to render that `Square` component using JSX syntax:\n\n```\n// ...export default function Board() {return (<><div className=\"board-row\"><Square /><Square /><Square /></div><div className=\"board-row\"><Square /><Square /><Square /></div><div className=\"board-row\"><Square /><Square /><Square /></div></>);}\n```\n\nNote how unlike the browser `div`s, your own components `Board` and `Square` must start with a capital letter.\n\nLet’s take a look:\n\n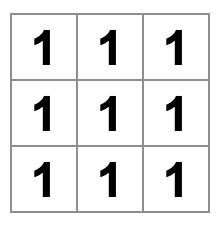\n\nOh no! You lost the numbered squares you had before. Now each square says “1”. To fix this, you will use _props_ to pass the value each square should have from the parent component (`Board`) to its child (`Square`).\n\nUpdate the `Square` component to read the `value` prop that you’ll pass from the `Board`:\n\n```\nfunction Square({ value }) {return <button className=\"square\">1</button>;}\n```\n\n`function Square({ value })` indicates the Square component can be passed a prop called `value`.\n\nNow you want to display that `value` instead of `1` inside every square. Try doing it like this:\n\n```\nfunction Square({ value }) {return <button className=\"square\">value</button>;}\n```\n\nOops, this is not what you wanted:\n\n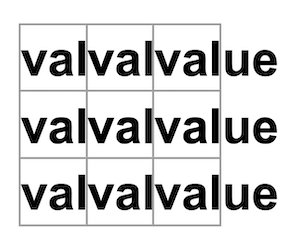\n\nYou wanted to render the JavaScript variable called `value` from your component, not the word “value”. To “escape into JavaScript” from JSX, you need curly braces. Add curly braces around `value` in JSX like so:\n\n```\nfunction Square({ value }) {return <button className=\"square\">{value}</button>;}\n```\n\nFor now, you should see an empty board:\n\n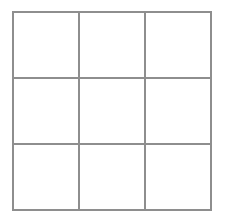\n\nThis is because the `Board` component hasn’t passed the `value` prop to each `Square` component it renders yet. To fix it you’ll add the `value` prop to each `Square` component rendered by the `Board` component:\n\n```\nexport default function Board() {return (<><div className=\"board-row\"><Square value=\"1\" /><Square value=\"2\" /><Square value=\"3\" /></div><div className=\"board-row\"><Square value=\"4\" /><Square value=\"5\" /><Square value=\"6\" /></div><div className=\"board-row\"><Square value=\"7\" /><Square value=\"8\" /><Square value=\"9\" /></div></>);}\n```\n\nNow you should see a grid of numbers again:\n\n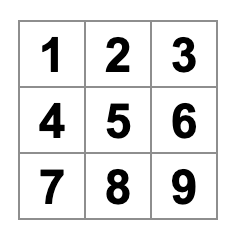\n\nYour updated code should look like this:\n\nfunction Square({ value }) {\n return <button className\\=\"square\"\\>{value}</button\\>;\n}\n\nexport default function Board() {\n return (\n <\\>\n <div className\\=\"board-row\"\\>\n <Square value\\=\"1\" />\n <Square value\\=\"2\" />\n <Square value\\=\"3\" />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\=\"4\" />\n <Square value\\=\"5\" />\n <Square value\\=\"6\" />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\=\"7\" />\n <Square value\\=\"8\" />\n <Square value\\=\"9\" />\n </div\\>\n </\\>\n );\n}\n\n### Making an interactive component[](https://react.dev/learn/tutorial-tic-tac-toe#making-an-interactive-component \"Link for Making an interactive component \")\n\nLet’s fill the `Square` component with an `X` when you click it. Declare a function called `handleClick` inside of the `Square`. Then, add `onClick` to the props of the button JSX element returned from the `Square`:\n\n```\nfunction Square({ value }) {function handleClick() {console.log('clicked!');}return (<buttonclassName=\"square\"onClick={handleClick}>{value}</button>);}\n```\n\nIf you click on a square now, you should see a log saying `\"clicked!\"` in the _Console_ tab at the bottom of the _Browser_ section in CodeSandbox. Clicking the square more than once will log `\"clicked!\"` again. Repeated console logs with the same message will not create more lines in the console. Instead, you will see an incrementing counter next to your first `\"clicked!\"` log.\n\n### Note\n\nIf you are following this tutorial using your local development environment, you need to open your browser’s Console. For example, if you use the Chrome browser, you can view the Console with the keyboard shortcut **Shift + Ctrl + J** (on Windows/Linux) or **Option + ⌘ + J** (on macOS).\n\nAs a next step, you want the Square component to “remember” that it got clicked, and fill it with an “X” mark. To “remember” things, components use _state_.\n\nReact provides a special function called `useState` that you can call from your component to let it “remember” things. Let’s store the current value of the `Square` in state, and change it when the `Square` is clicked.\n\nImport `useState` at the top of the file. Remove the `value` prop from the `Square` component. Instead, add a new line at the start of the `Square` that calls `useState`. Have it return a state variable called `value`:\n\n```\nimport { useState } from 'react';function Square() {const [value, setValue] = useState(null);function handleClick() {//...\n```\n\n`value` stores the value and `setValue` is a function that can be used to change the value. The `null` passed to `useState` is used as the initial value for this state variable, so `value` here starts off equal to `null`.\n\nSince the `Square` component no longer accepts props anymore, you’ll remove the `value` prop from all nine of the Square components created by the Board component:\n\n```\n// ...export default function Board() {return (<><div className=\"board-row\"><Square /><Square /><Square /></div><div className=\"board-row\"><Square /><Square /><Square /></div><div className=\"board-row\"><Square /><Square /><Square /></div></>);}\n```\n\nNow you’ll change `Square` to display an “X” when clicked. Replace the `console.log(\"clicked!\");` event handler with `setValue('X');`. Now your `Square` component looks like this:\n\n```\nfunction Square() {const [value, setValue] = useState(null);function handleClick() {setValue('X');}return (<buttonclassName=\"square\"onClick={handleClick}>{value}</button>);}\n```\n\nBy calling this `set` function from an `onClick` handler, you’re telling React to re-render that `Square` whenever its `<button>` is clicked. After the update, the `Square`’s `value` will be `'X'`, so you’ll see the “X” on the game board. Click on any Square, and “X” should show up:\n\n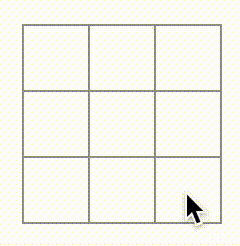\n\nEach Square has its own state: the `value` stored in each Square is completely independent of the others. When you call a `set` function in a component, React automatically updates the child components inside too.\n\nAfter you’ve made the above changes, your code will look like this:\n\nimport { useState } from 'react';\n\nfunction Square() {\n const \\[value, setValue\\] = useState(null);\n\n function handleClick() {\n setValue('X');\n }\n\n return (\n <button\n className\\=\"square\"\n onClick\\={handleClick}\n \\>\n {value}\n </button\\>\n );\n}\n\nexport default function Board() {\n return (\n <\\>\n <div className\\=\"board-row\"\\>\n <Square />\n <Square />\n <Square />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square />\n <Square />\n <Square />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square />\n <Square />\n <Square />\n </div\\>\n </\\>\n );\n}\n\n### React Developer Tools[](https://react.dev/learn/tutorial-tic-tac-toe#react-developer-tools \"Link for React Developer Tools \")\n\nReact DevTools let you check the props and the state of your React components. You can find the React DevTools tab at the bottom of the _browser_ section in CodeSandbox:\n\n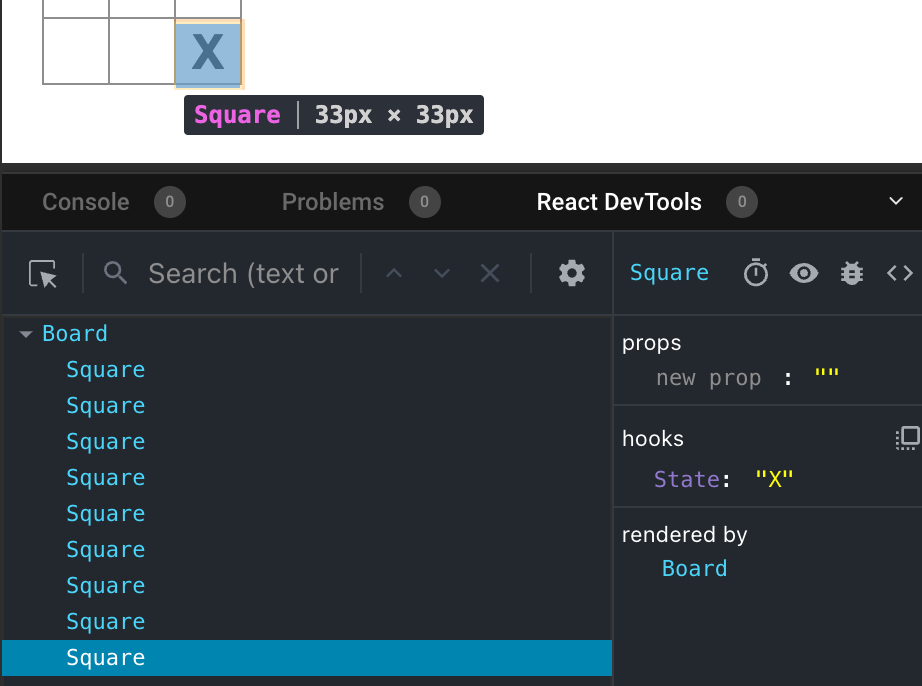\n\nTo inspect a particular component on the screen, use the button in the top left corner of React DevTools:\n\n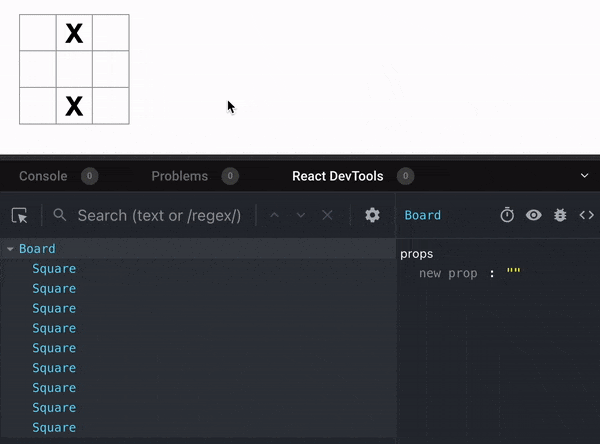\n\n### Note\n\nFor local development, React DevTools is available as a [Chrome](https://chrome.google.com/webstore/detail/react-developer-tools/fmkadmapgofadopljbjfkapdkoienihi?hl=en), [Firefox](https://addons.mozilla.org/en-US/firefox/addon/react-devtools/), and [Edge](https://microsoftedge.microsoft.com/addons/detail/react-developer-tools/gpphkfbcpidddadnkolkpfckpihlkkil) browser extension. Install it, and the _Components_ tab will appear in your browser Developer Tools for sites using React.\n\nCompleting the game[](https://react.dev/learn/tutorial-tic-tac-toe#completing-the-game \"Link for Completing the game \")\n-----------------------------------------------------------------------------------------------------------------------\n\nBy this point, you have all the basic building blocks for your tic-tac-toe game. To have a complete game, you now need to alternate placing “X”s and “O”s on the board, and you need a way to determine a winner.\n\n### Lifting state up[](https://react.dev/learn/tutorial-tic-tac-toe#lifting-state-up \"Link for Lifting state up \")\n\nCurrently, each `Square` component maintains a part of the game’s state. To check for a winner in a tic-tac-toe game, the `Board` would need to somehow know the state of each of the 9 `Square` components.\n\nHow would you approach that? At first, you might guess that the `Board` needs to “ask” each `Square` for that `Square`’s state. Although this approach is technically possible in React, we discourage it because the code becomes difficult to understand, susceptible to bugs, and hard to refactor. Instead, the best approach is to store the game’s state in the parent `Board` component instead of in each `Square`. The `Board` component can tell each `Square` what to display by passing a prop, like you did when you passed a number to each Square.\n\n**To collect data from multiple children, or to have two child components communicate with each other, declare the shared state in their parent component instead. The parent component can pass that state back down to the children via props. This keeps the child components in sync with each other and with their parent.**\n\nLifting state into a parent component is common when React components are refactored.\n\nLet’s take this opportunity to try it out. Edit the `Board` component so that it declares a state variable named `squares` that defaults to an array of 9 nulls corresponding to the 9 squares:\n\n```\n// ...export default function Board() {const [squares, setSquares] = useState(Array(9).fill(null));return (// ...);}\n```\n\n`Array(9).fill(null)` creates an array with nine elements and sets each of them to `null`. The `useState()` call around it declares a `squares` state variable that’s initially set to that array. Each entry in the array corresponds to the value of a square. When you fill the board in later, the `squares` array will look like this:\n\n```\n['O', null, 'X', 'X', 'X', 'O', 'O', null, null]\n```\n\nNow your `Board` component needs to pass the `value` prop down to each `Square` that it renders:\n\n```\nexport default function Board() {const [squares, setSquares] = useState(Array(9).fill(null));return (<><div className=\"board-row\"><Square value={squares[0]} /><Square value={squares[1]} /><Square value={squares[2]} /></div><div className=\"board-row\"><Square value={squares[3]} /><Square value={squares[4]} /><Square value={squares[5]} /></div><div className=\"board-row\"><Square value={squares[6]} /><Square value={squares[7]} /><Square value={squares[8]} /></div></>);}\n```\n\nNext, you’ll edit the `Square` component to receive the `value` prop from the Board component. This will require removing the Square component’s own stateful tracking of `value` and the button’s `onClick` prop:\n\n```\nfunction Square({value}) {return <button className=\"square\">{value}</button>;}\n```\n\nAt this point you should see an empty tic-tac-toe board:\n\n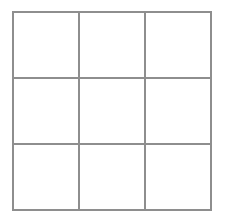\n\nAnd your code should look like this:\n\nimport { useState } from 'react';\n\nfunction Square({ value }) {\n return <button className\\=\"square\"\\>{value}</button\\>;\n}\n\nexport default function Board() {\n const \\[squares, setSquares\\] = useState(Array(9).fill(null));\n return (\n <\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} />\n <Square value\\={squares\\[1\\]} />\n <Square value\\={squares\\[2\\]} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} />\n <Square value\\={squares\\[4\\]} />\n <Square value\\={squares\\[5\\]} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} />\n <Square value\\={squares\\[7\\]} />\n <Square value\\={squares\\[8\\]} />\n </div\\>\n </\\>\n );\n}\n\nEach Square will now receive a `value` prop that will either be `'X'`, `'O'`, or `null` for empty squares.\n\nNext, you need to change what happens when a `Square` is clicked. The `Board` component now maintains which squares are filled. You’ll need to create a way for the `Square` to update the `Board`’s state. Since state is private to a component that defines it, you cannot update the `Board`’s state directly from `Square`.\n\nInstead, you’ll pass down a function from the `Board` component to the `Square` component, and you’ll have `Square` call that function when a square is clicked. You’ll start with the function that the `Square` component will call when it is clicked. You’ll call that function `onSquareClick`:\n\n```\nfunction Square({ value }) {return (<button className=\"square\" onClick={onSquareClick}>{value}</button>);}\n```\n\nNext, you’ll add the `onSquareClick` function to the `Square` component’s props:\n\n```\nfunction Square({ value, onSquareClick }) {return (<button className=\"square\" onClick={onSquareClick}>{value}</button>);}\n```\n\nNow you’ll connect the `onSquareClick` prop to a function in the `Board` component that you’ll name `handleClick`. To connect `onSquareClick` to `handleClick` you’ll pass a function to the `onSquareClick` prop of the first `Square` component:\n\n```\nexport default function Board() {const [squares, setSquares] = useState(Array(9).fill(null));return (<><div className=\"board-row\"><Square value={squares[0]} onSquareClick={handleClick} /> //... );}\n```\n\nLastly, you will define the `handleClick` function inside the Board component to update the `squares` array holding your board’s state:\n\n```\nexport default function Board() {const [squares, setSquares] = useState(Array(9).fill(null));function handleClick() {const nextSquares = squares.slice();nextSquares[0] = \"X\";setSquares(nextSquares);}return (// ...)}\n```\n\nThe `handleClick` function creates a copy of the `squares` array (`nextSquares`) with the JavaScript `slice()` Array method. Then, `handleClick` updates the `nextSquares` array to add `X` to the first (`[0]` index) square.\n\nCalling the `setSquares` function lets React know the state of the component has changed. This will trigger a re-render of the components that use the `squares` state (`Board`) as well as its child components (the `Square` components that make up the board).\n\n### Note\n\nJavaScript supports [closures](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures) which means an inner function (e.g. `handleClick`) has access to variables and functions defined in a outer function (e.g. `Board`). The `handleClick` function can read the `squares` state and call the `setSquares` method because they are both defined inside of the `Board` function.\n\nNow you can add X’s to the board… but only to the upper left square. Your `handleClick` function is hardcoded to update the index for the upper left square (`0`). Let’s update `handleClick` to be able to update any square. Add an argument `i` to the `handleClick` function that takes the index of the square to update:\n\n```\nexport default function Board() {const [squares, setSquares] = useState(Array(9).fill(null));function handleClick(i) {const nextSquares = squares.slice();nextSquares[i] = \"X\";setSquares(nextSquares);}return (// ...)}\n```\n\nNext, you will need to pass that `i` to `handleClick`. You could try to set the `onSquareClick` prop of square to be `handleClick(0)` directly in the JSX like this, but it won’t work:\n\n```\n<Square value={squares[0]} onSquareClick={handleClick(0)} />\n```\n\nHere is why this doesn’t work. The `handleClick(0)` call will be a part of rendering the board component. Because `handleClick(0)` alters the state of the board component by calling `setSquares`, your entire board component will be re-rendered again. But this runs `handleClick(0)` again, leading to an infinite loop:\n\nToo many re-renders. React limits the number of renders to prevent an infinite loop.\n\nWhy didn’t this problem happen earlier?\n\nWhen you were passing `onSquareClick={handleClick}`, you were passing the `handleClick` function down as a prop. You were not calling it! But now you are _calling_ that function right away—notice the parentheses in `handleClick(0)`—and that’s why it runs too early. You don’t _want_ to call `handleClick` until the user clicks!\n\nYou could fix this by creating a function like `handleFirstSquareClick` that calls `handleClick(0)`, a function like `handleSecondSquareClick` that calls `handleClick(1)`, and so on. You would pass (rather than call) these functions down as props like `onSquareClick={handleFirstSquareClick}`. This would solve the infinite loop.\n\nHowever, defining nine different functions and giving each of them a name is too verbose. Instead, let’s do this:\n\n```\nexport default function Board() {// ...return (<><div className=\"board-row\"><Square value={squares[0]} onSquareClick={() => handleClick(0)} /> // ... );}\n```\n\nNotice the new `() =>` syntax. Here, `() => handleClick(0)` is an _arrow function,_ which is a shorter way to define functions. When the square is clicked, the code after the `=>` “arrow” will run, calling `handleClick(0)`.\n\nNow you need to update the other eight squares to call `handleClick` from the arrow functions you pass. Make sure that the argument for each call of the `handleClick` corresponds to the index of the correct square:\n\n```\nexport default function Board() {// ...return (<><div className=\"board-row\"><Square value={squares[0]} onSquareClick={() => handleClick(0)} /><Square value={squares[1]} onSquareClick={() => handleClick(1)} /><Square value={squares[2]} onSquareClick={() => handleClick(2)} /></div><div className=\"board-row\"><Square value={squares[3]} onSquareClick={() => handleClick(3)} /><Square value={squares[4]} onSquareClick={() => handleClick(4)} /><Square value={squares[5]} onSquareClick={() => handleClick(5)} /></div><div className=\"board-row\"><Square value={squares[6]} onSquareClick={() => handleClick(6)} /><Square value={squares[7]} onSquareClick={() => handleClick(7)} /><Square value={squares[8]} onSquareClick={() => handleClick(8)} /></div></>);};\n```\n\nNow you can again add X’s to any square on the board by clicking on them:\n\n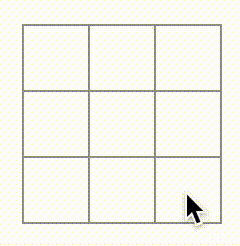\n\nBut this time all the state management is handled by the `Board` component!\n\nThis is what your code should look like:\n\nimport { useState } from 'react';\n\nfunction Square({ value, onSquareClick }) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nexport default function Board() {\n const \\[squares, setSquares\\] = useState(Array(9).fill(null));\n\n function handleClick(i) {\n const nextSquares = squares.slice();\n nextSquares\\[i\\] = 'X';\n setSquares(nextSquares);\n }\n\n return (\n <\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nNow that your state handling is in the `Board` component, the parent `Board` component passes props to the child `Square` components so that they can be displayed correctly. When clicking on a `Square`, the child `Square` component now asks the parent `Board` component to update the state of the board. When the `Board`’s state changes, both the `Board` component and every child `Square` re-renders automatically. Keeping the state of all squares in the `Board` component will allow it to determine the winner in the future.\n\nLet’s recap what happens when a user clicks the top left square on your board to add an `X` to it:\n\n1. Clicking on the upper left square runs the function that the `button` received as its `onClick` prop from the `Square`. The `Square` component received that function as its `onSquareClick` prop from the `Board`. The `Board` component defined that function directly in the JSX. It calls `handleClick` with an argument of `0`.\n2. `handleClick` uses the argument (`0`) to update the first element of the `squares` array from `null` to `X`.\n3. The `squares` state of the `Board` component was updated, so the `Board` and all of its children re-render. This causes the `value` prop of the `Square` component with index `0` to change from `null` to `X`.\n\nIn the end the user sees that the upper left square has changed from empty to having a `X` after clicking it.\n\n### Note\n\nThe DOM `<button>` element’s `onClick` attribute has a special meaning to React because it is a built-in component. For custom components like Square, the naming is up to you. You could give any name to the `Square`’s `onSquareClick` prop or `Board`’s `handleClick` function, and the code would work the same. In React, it’s conventional to use `onSomething` names for props which represent events and `handleSomething` for the function definitions which handle those events.\n\n### Why immutability is important[](https://react.dev/learn/tutorial-tic-tac-toe#why-immutability-is-important \"Link for Why immutability is important \")\n\nNote how in `handleClick`, you call `.slice()` to create a copy of the `squares` array instead of modifying the existing array. To explain why, we need to discuss immutability and why immutability is important to learn.\n\nThere are generally two approaches to changing data. The first approach is to _mutate_ the data by directly changing the data’s values. The second approach is to replace the data with a new copy which has the desired changes. Here is what it would look like if you mutated the `squares` array:\n\n```\nconst squares = [null, null, null, null, null, null, null, null, null];squares[0] = 'X';// Now `squares` is [\"X\", null, null, null, null, null, null, null, null];\n```\n\nAnd here is what it would look like if you changed data without mutating the `squares` array:\n\n```\nconst squares = [null, null, null, null, null, null, null, null, null];const nextSquares = ['X', null, null, null, null, null, null, null, null];// Now `squares` is unchanged, but `nextSquares` first element is 'X' rather than `null`\n```\n\nThe result is the same but by not mutating (changing the underlying data) directly, you gain several benefits.\n\nImmutability makes complex features much easier to implement. Later in this tutorial, you will implement a “time travel” feature that lets you review the game’s history and “jump back” to past moves. This functionality isn’t specific to games—an ability to undo and redo certain actions is a common requirement for apps. Avoiding direct data mutation lets you keep previous versions of the data intact, and reuse them later.\n\nThere is also another benefit of immutability. By default, all child components re-render automatically when the state of a parent component changes. This includes even the child components that weren’t affected by the change. Although re-rendering is not by itself noticeable to the user (you shouldn’t actively try to avoid it!), you might want to skip re-rendering a part of the tree that clearly wasn’t affected by it for performance reasons. Immutability makes it very cheap for components to compare whether their data has changed or not. You can learn more about how React chooses when to re-render a component in [the `memo` API reference](https://react.dev/reference/react/memo).\n\n### Taking turns[](https://react.dev/learn/tutorial-tic-tac-toe#taking-turns \"Link for Taking turns \")\n\nIt’s now time to fix a major defect in this tic-tac-toe game: the “O”s cannot be marked on the board.\n\nYou’ll set the first move to be “X” by default. Let’s keep track of this by adding another piece of state to the Board component:\n\n```\nfunction Board() {const [xIsNext, setXIsNext] = useState(true);const [squares, setSquares] = useState(Array(9).fill(null));// ...}\n```\n\nEach time a player moves, `xIsNext` (a boolean) will be flipped to determine which player goes next and the game’s state will be saved. You’ll update the `Board`’s `handleClick` function to flip the value of `xIsNext`:\n\n```\nexport default function Board() {const [xIsNext, setXIsNext] = useState(true);const [squares, setSquares] = useState(Array(9).fill(null));function handleClick(i) {const nextSquares = squares.slice();if (xIsNext) {nextSquares[i] = \"X\";} else {nextSquares[i] = \"O\";}setSquares(nextSquares);setXIsNext(!xIsNext);}return (//...);}\n```\n\nNow, as you click on different squares, they will alternate between `X` and `O`, as they should!\n\nBut wait, there’s a problem. Try clicking on the same square multiple times:\n\n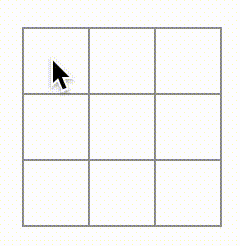\n\nThe `X` is overwritten by an `O`! While this would add a very interesting twist to the game, we’re going to stick to the original rules for now.\n\nWhen you mark a square with a `X` or an `O` you aren’t first checking to see if the square already has a `X` or `O` value. You can fix this by _returning early_. You’ll check to see if the square already has a `X` or an `O`. If the square is already filled, you will `return` in the `handleClick` function early—before it tries to update the board state.\n\n```\nfunction handleClick(i) {if (squares[i]) {return;}const nextSquares = squares.slice();//...}\n```\n\nNow you can only add `X`’s or `O`’s to empty squares! Here is what your code should look like at this point:\n\nimport { useState } from 'react';\n\nfunction Square({value, onSquareClick}) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nexport default function Board() {\n const \\[xIsNext, setXIsNext\\] = useState(true);\n const \\[squares, setSquares\\] = useState(Array(9).fill(null));\n\n function handleClick(i) {\n if (squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n setSquares(nextSquares);\n setXIsNext(!xIsNext);\n }\n\n return (\n <\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\n### Declaring a winner[](https://react.dev/learn/tutorial-tic-tac-toe#declaring-a-winner \"Link for Declaring a winner \")\n\nNow that the players can take turns, you’ll want to show when the game is won and there are no more turns to make. To do this you’ll add a helper function called `calculateWinner` that takes an array of 9 squares, checks for a winner and returns `'X'`, `'O'`, or `null` as appropriate. Don’t worry too much about the `calculateWinner` function; it’s not specific to React:\n\n```\nexport default function Board() {//...}function calculateWinner(squares) {const lines = [[0, 1, 2],[3, 4, 5],[6, 7, 8],[0, 3, 6],[1, 4, 7],[2, 5, 8],[0, 4, 8],[2, 4, 6]];for (let i = 0; i < lines.length; i++) {const [a, b, c] = lines[i];if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {return squares[a];}}return null;}\n```\n\n### Note\n\nIt does not matter whether you define `calculateWinner` before or after the `Board`. Let’s put it at the end so that you don’t have to scroll past it every time you edit your components.\n\nYou will call `calculateWinner(squares)` in the `Board` component’s `handleClick` function to check if a player has won. You can perform this check at the same time you check if a user has clicked a square that already has a `X` or and `O`. We’d like to return early in both cases:\n\n```\nfunction handleClick(i) {if (squares[i] || calculateWinner(squares)) {return;}const nextSquares = squares.slice();//...}\n```\n\nTo let the players know when the game is over, you can display text such as “Winner: X” or “Winner: O”. To do that you’ll add a `status` section to the `Board` component. The status will display the winner if the game is over and if the game is ongoing you’ll display which player’s turn is next:\n\n```\nexport default function Board() {// ...const winner = calculateWinner(squares);let status;if (winner) {status = \"Winner: \" + winner;} else {status = \"Next player: \" + (xIsNext ? \"X\" : \"O\");}return (<><div className=\"status\">{status}</div><div className=\"board-row\"> // ... )}\n```\n\nCongratulations! You now have a working tic-tac-toe game. And you’ve just learned the basics of React too. So _you_ are the real winner here. Here is what the code should look like:\n\nimport { useState } from 'react';\n\nfunction Square({value, onSquareClick}) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nexport default function Board() {\n const \\[xIsNext, setXIsNext\\] = useState(true);\n const \\[squares, setSquares\\] = useState(Array(9).fill(null));\n\n function handleClick(i) {\n if (calculateWinner(squares) || squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n setSquares(nextSquares);\n setXIsNext(!xIsNext);\n }\n\n const winner = calculateWinner(squares);\n let status;\n if (winner) {\n status = 'Winner: ' + winner;\n } else {\n status = 'Next player: ' + (xIsNext ? 'X' : 'O');\n }\n\n return (\n <\\>\n <div className\\=\"status\"\\>{status}</div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nfunction calculateWinner(squares) {\n const lines = \\[\n \\[0, 1, 2\\],\n \\[3, 4, 5\\],\n \\[6, 7, 8\\],\n \\[0, 3, 6\\],\n \\[1, 4, 7\\],\n \\[2, 5, 8\\],\n \\[0, 4, 8\\],\n \\[2, 4, 6\\],\n \\];\n for (let i = 0; i < lines.length; i++) {\n const \\[a, b, c\\] = lines\\[i\\];\n if (squares\\[a\\] && squares\\[a\\] === squares\\[b\\] && squares\\[a\\] === squares\\[c\\]) {\n return squares\\[a\\];\n }\n }\n return null;\n}\n\nAdding time travel[](https://react.dev/learn/tutorial-tic-tac-toe#adding-time-travel \"Link for Adding time travel \")\n--------------------------------------------------------------------------------------------------------------------\n\nAs a final exercise, let’s make it possible to “go back in time” to the previous moves in the game.\n\n### Storing a history of moves[](https://react.dev/learn/tutorial-tic-tac-toe#storing-a-history-of-moves \"Link for Storing a history of moves \")\n\nIf you mutated the `squares` array, implementing time travel would be very difficult.\n\nHowever, you used `slice()` to create a new copy of the `squares` array after every move, and treated it as immutable. This will allow you to store every past version of the `squares` array, and navigate between the turns that have already happened.\n\nYou’ll store the past `squares` arrays in another array called `history`, which you’ll store as a new state variable. The `history` array represents all board states, from the first to the last move, and has a shape like this:\n\n```\n[// Before first move[null, null, null, null, null, null, null, null, null],// After first move[null, null, null, null, 'X', null, null, null, null],// After second move[null, null, null, null, 'X', null, null, null, 'O'],// ...]\n```\n\n### Lifting state up, again[](https://react.dev/learn/tutorial-tic-tac-toe#lifting-state-up-again \"Link for Lifting state up, again \")\n\nYou will now write a new top-level component called `Game` to display a list of past moves. That’s where you will place the `history` state that contains the entire game history.\n\nPlacing the `history` state into the `Game` component will let you remove the `squares` state from its child `Board` component. Just like you “lifted state up” from the `Square` component into the `Board` component, you will now lift it up from the `Board` into the top-level `Game` component. This gives the `Game` component full control over the `Board`’s data and lets it instruct the `Board` to render previous turns from the `history`.\n\nFirst, add a `Game` component with `export default`. Have it render the `Board` component and some markup:\n\n```\nfunction Board() {// ...}export default function Game() {return (<div className=\"game\"><div className=\"game-board\"><Board /></div><div className=\"game-info\"><ol>{/*TODO*/}</ol></div></div>);}\n```\n\nNote that you are removing the `export default` keywords before the `function Board() {` declaration and adding them before the `function Game() {` declaration. This tells your `index.js` file to use the `Game` component as the top-level component instead of your `Board` component. The additional `div`s returned by the `Game` component are making room for the game information you’ll add to the board later.\n\nAdd some state to the `Game` component to track which player is next and the history of moves:\n\n```\nexport default function Game() {const [xIsNext, setXIsNext] = useState(true);const [history, setHistory] = useState([Array(9).fill(null)]);// ...\n```\n\nNotice how `[Array(9).fill(null)]` is an array with a single item, which itself is an array of 9 `null`s.\n\nTo render the squares for the current move, you’ll want to read the last squares array from the `history`. You don’t need `useState` for this—you already have enough information to calculate it during rendering:\n\n```\nexport default function Game() {const [xIsNext, setXIsNext] = useState(true);const [history, setHistory] = useState([Array(9).fill(null)]);const currentSquares = history[history.length - 1];// ...\n```\n\nNext, create a `handlePlay` function inside the `Game` component that will be called by the `Board` component to update the game. Pass `xIsNext`, `currentSquares` and `handlePlay` as props to the `Board` component:\n\n```\nexport default function Game() {const [xIsNext, setXIsNext] = useState(true);const [history, setHistory] = useState([Array(9).fill(null)]);const currentSquares = history[history.length - 1];function handlePlay(nextSquares) {// TODO}return (<div className=\"game\"><div className=\"game-board\"><Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} /> //... )}\n```\n\nLet’s make the `Board` component fully controlled by the props it receives. Change the `Board` component to take three props: `xIsNext`, `squares`, and a new `onPlay` function that `Board` can call with the updated squares array when a player makes a move. Next, remove the first two lines of the `Board` function that call `useState`:\n\n```\nfunction Board({ xIsNext, squares, onPlay }) {function handleClick(i) {//...}// ...}\n```\n\nNow replace the `setSquares` and `setXIsNext` calls in `handleClick` in the `Board` component with a single call to your new `onPlay` function so the `Game` component can update the `Board` when the user clicks a square:\n\n```\nfunction Board({ xIsNext, squares, onPlay }) {function handleClick(i) {if (calculateWinner(squares) || squares[i]) {return;}const nextSquares = squares.slice();if (xIsNext) {nextSquares[i] = \"X\";} else {nextSquares[i] = \"O\";}onPlay(nextSquares);}//...}\n```\n\nThe `Board` component is fully controlled by the props passed to it by the `Game` component. You need to implement the `handlePlay` function in the `Game` component to get the game working again.\n\nWhat should `handlePlay` do when called? Remember that Board used to call `setSquares` with an updated array; now it passes the updated `squares` array to `onPlay`.\n\nThe `handlePlay` function needs to update `Game`’s state to trigger a re-render, but you don’t have a `setSquares` function that you can call any more—you’re now using the `history` state variable to store this information. You’ll want to update `history` by appending the updated `squares` array as a new history entry. You also want to toggle `xIsNext`, just as Board used to do:\n\n```\nexport default function Game() {//...function handlePlay(nextSquares) {setHistory([...history, nextSquares]);setXIsNext(!xIsNext);}//...}\n```\n\nHere, `[...history, nextSquares]` creates a new array that contains all the items in `history`, followed by `nextSquares`. (You can read the `...history` [_spread syntax_](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax) as “enumerate all the items in `history`”.)\n\nFor example, if `history` is `[[null,null,null], [\"X\",null,null]]` and `nextSquares` is `[\"X\",null,\"O\"]`, then the new `[...history, nextSquares]` array will be `[[null,null,null], [\"X\",null,null], [\"X\",null,\"O\"]]`.\n\nAt this point, you’ve moved the state to live in the `Game` component, and the UI should be fully working, just as it was before the refactor. Here is what the code should look like at this point:\n\nimport { useState } from 'react';\n\nfunction Square({ value, onSquareClick }) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nfunction Board({ xIsNext, squares, onPlay }) {\n function handleClick(i) {\n if (calculateWinner(squares) || squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n onPlay(nextSquares);\n }\n\n const winner = calculateWinner(squares);\n let status;\n if (winner) {\n status = 'Winner: ' + winner;\n } else {\n status = 'Next player: ' + (xIsNext ? 'X' : 'O');\n }\n\n return (\n <\\>\n <div className\\=\"status\"\\>{status}</div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nexport default function Game() {\n const \\[xIsNext, setXIsNext\\] = useState(true);\n const \\[history, setHistory\\] = useState(\\[Array(9).fill(null)\\]);\n const currentSquares = history\\[history.length - 1\\];\n\n function handlePlay(nextSquares) {\n setHistory(\\[...history, nextSquares\\]);\n setXIsNext(!xIsNext);\n }\n\n return (\n <div className\\=\"game\"\\>\n <div className\\=\"game-board\"\\>\n <Board xIsNext\\={xIsNext} squares\\={currentSquares} onPlay\\={handlePlay} />\n </div\\>\n <div className\\=\"game-info\"\\>\n <ol\\>{}</ol\\>\n </div\\>\n </div\\>\n );\n}\n\nfunction calculateWinner(squares) {\n const lines = \\[\n \\[0, 1, 2\\],\n \\[3, 4, 5\\],\n \\[6, 7, 8\\],\n \\[0, 3, 6\\],\n \\[1, 4, 7\\],\n \\[2, 5, 8\\],\n \\[0, 4, 8\\],\n \\[2, 4, 6\\],\n \\];\n for (let i = 0; i < lines.length; i++) {\n const \\[a, b, c\\] = lines\\[i\\];\n if (squares\\[a\\] && squares\\[a\\] === squares\\[b\\] && squares\\[a\\] === squares\\[c\\]) {\n return squares\\[a\\];\n }\n }\n return null;\n}\n\n### Showing the past moves[](https://react.dev/learn/tutorial-tic-tac-toe#showing-the-past-moves \"Link for Showing the past moves \")\n\nSince you are recording the tic-tac-toe game’s history, you can now display a list of past moves to the player.\n\nReact elements like `<button>` are regular JavaScript objects; you can pass them around in your application. To render multiple items in React, you can use an array of React elements.\n\nYou already have an array of `history` moves in state, so now you need to transform it to an array of React elements. In JavaScript, to transform one array into another, you can use the [array `map` method:](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map)\n\n```\n[1, 2, 3].map((x) => x * 2) // [2, 4, 6]\n```\n\nYou’ll use `map` to transform your `history` of moves into React elements representing buttons on the screen, and display a list of buttons to “jump” to past moves. Let’s `map` over the `history` in the Game component:\n\n```\nexport default function Game() {const [xIsNext, setXIsNext] = useState(true);const [history, setHistory] = useState([Array(9).fill(null)]);const currentSquares = history[history.length - 1];function handlePlay(nextSquares) {setHistory([...history, nextSquares]);setXIsNext(!xIsNext);}function jumpTo(nextMove) {// TODO}const moves = history.map((squares, move) => {let description;if (move > 0) {description = 'Go to move #' + move;} else {description = 'Go to game start';}return (<li><button onClick={() => jumpTo(move)}>{description}</button></li>);});return (<div className=\"game\"><div className=\"game-board\"><Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} /></div><div className=\"game-info\"><ol>{moves}</ol></div></div>);}\n```\n\nYou can see what your code should look like below. Note that you should see an error in the developer tools console that says:\n\nWarning: Each child in an array or iterator should have a unique “key” prop. Check the render method of \\`Game\\`.\n\nYou’ll fix this error in the next section.\n\nimport { useState } from 'react';\n\nfunction Square({ value, onSquareClick }) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nfunction Board({ xIsNext, squares, onPlay }) {\n function handleClick(i) {\n if (calculateWinner(squares) || squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n onPlay(nextSquares);\n }\n\n const winner = calculateWinner(squares);\n let status;\n if (winner) {\n status = 'Winner: ' + winner;\n } else {\n status = 'Next player: ' + (xIsNext ? 'X' : 'O');\n }\n\n return (\n <\\>\n <div className\\=\"status\"\\>{status}</div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nexport default function Game() {\n const \\[xIsNext, setXIsNext\\] = useState(true);\n const \\[history, setHistory\\] = useState(\\[Array(9).fill(null)\\]);\n const currentSquares = history\\[history.length - 1\\];\n\n function handlePlay(nextSquares) {\n setHistory(\\[...history, nextSquares\\]);\n setXIsNext(!xIsNext);\n }\n\n function jumpTo(nextMove) {\n \n }\n\n const moves = history.map((squares, move) \\=> {\n let description;\n if (move > 0) {\n description = 'Go to move #' + move;\n } else {\n description = 'Go to game start';\n }\n return (\n <li\\>\n <button onClick\\={() \\=> jumpTo(move)}\\>{description}</button\\>\n </li\\>\n );\n });\n\n return (\n <div className\\=\"game\"\\>\n <div className\\=\"game-board\"\\>\n <Board xIsNext\\={xIsNext} squares\\={currentSquares} onPlay\\={handlePlay} />\n </div\\>\n <div className\\=\"game-info\"\\>\n <ol\\>{moves}</ol\\>\n </div\\>\n </div\\>\n );\n}\n\nfunction calculateWinner(squares) {\n const lines = \\[\n \\[0, 1, 2\\],\n \\[3, 4, 5\\],\n \\[6, 7, 8\\],\n \\[0, 3, 6\\],\n \\[1, 4, 7\\],\n \\[2, 5, 8\\],\n \\[0, 4, 8\\],\n \\[2, 4, 6\\],\n \\];\n for (let i = 0; i < lines.length; i++) {\n const \\[a, b, c\\] = lines\\[i\\];\n if (squares\\[a\\] && squares\\[a\\] === squares\\[b\\] && squares\\[a\\] === squares\\[c\\]) {\n return squares\\[a\\];\n }\n }\n return null;\n}\n\nAs you iterate through `history` array inside the function you passed to `map`, the `squares` argument goes through each element of `history`, and the `move` argument goes through each array index: `0`, `1`, `2`, …. (In most cases, you’d need the actual array elements, but to render a list of moves you will only need indexes.)\n\nFor each move in the tic-tac-toe game’s history, you create a list item `<li>` which contains a button `<button>`. The button has an `onClick` handler which calls a function called `jumpTo` (that you haven’t implemented yet).\n\nFor now, you should see a list of the moves that occurred in the game and an error in the developer tools console. Let’s discuss what the “key” error means.\n\n### Picking a key[](https://react.dev/learn/tutorial-tic-tac-toe#picking-a-key \"Link for Picking a key \")\n\nWhen you render a list, React stores some information about each rendered list item. When you update a list, React needs to determine what has changed. You could have added, removed, re-arranged, or updated the list’s items.\n\nImagine transitioning from\n\n```\n<li>Alexa: 7 tasks left</li><li>Ben: 5 tasks left</li>\n```\n\nto\n\n```\n<li>Ben: 9 tasks left</li><li>Claudia: 8 tasks left</li><li>Alexa: 5 tasks left</li>\n```\n\nIn addition to the updated counts, a human reading this would probably say that you swapped Alexa and Ben’s ordering and inserted Claudia between Alexa and Ben. However, React is a computer program and does not know what you intended, so you need to specify a _key_ property for each list item to differentiate each list item from its siblings. If your data was from a database, Alexa, Ben, and Claudia’s database IDs could be used as keys.\n\n```\n<li key={user.id}>{user.name}: {user.taskCount} tasks left</li>\n```\n\nWhen a list is re-rendered, React takes each list item’s key and searches the previous list’s items for a matching key. If the current list has a key that didn’t exist before, React creates a component. If the current list is missing a key that existed in the previous list, React destroys the previous component. If two keys match, the corresponding component is moved.\n\nKeys tell React about the identity of each component, which allows React to maintain state between re-renders. If a component’s key changes, the component will be destroyed and re-created with a new state.\n\n`key` is a special and reserved property in React. When an element is created, React extracts the `key` property and stores the key directly on the returned element. Even though `key` may look like it is passed as props, React automatically uses `key` to decide which components to update. There’s no way for a component to ask what `key` its parent specified.\n\n**It’s strongly recommended that you assign proper keys whenever you build dynamic lists.** If you don’t have an appropriate key, you may want to consider restructuring your data so that you do.\n\nIf no key is specified, React will report an error and use the array index as a key by default. Using the array index as a key is problematic when trying to re-order a list’s items or inserting/removing list items. Explicitly passing `key={i}` silences the error but has the same problems as array indices and is not recommended in most cases.\n\nKeys do not need to be globally unique; they only need to be unique between components and their siblings.\n\n### Implementing time travel[](https://react.dev/learn/tutorial-tic-tac-toe#implementing-time-travel \"Link for Implementing time travel \")\n\nIn the tic-tac-toe game’s history, each past move has a unique ID associated with it: it’s the sequential number of the move. Moves will never be re-ordered, deleted, or inserted in the middle, so it’s safe to use the move index as a key.\n\nIn the `Game` function, you can add the key as `<li key={move}>`, and if you reload the rendered game, React’s “key” error should disappear:\n\n```\nconst moves = history.map((squares, move) => {//...return (<li key={move}><button onClick={() => jumpTo(move)}>{description}</button></li>);});\n```\n\nimport { useState } from 'react';\n\nfunction Square({ value, onSquareClick }) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nfunction Board({ xIsNext, squares, onPlay }) {\n function handleClick(i) {\n if (calculateWinner(squares) || squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n onPlay(nextSquares);\n }\n\n const winner = calculateWinner(squares);\n let status;\n if (winner) {\n status = 'Winner: ' + winner;\n } else {\n status = 'Next player: ' + (xIsNext ? 'X' : 'O');\n }\n\n return (\n <\\>\n <div className\\=\"status\"\\>{status}</div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nexport default function Game() {\n const \\[xIsNext, setXIsNext\\] = useState(true);\n const \\[history, setHistory\\] = useState(\\[Array(9).fill(null)\\]);\n const currentSquares = history\\[history.length - 1\\];\n\n function handlePlay(nextSquares) {\n setHistory(\\[...history, nextSquares\\]);\n setXIsNext(!xIsNext);\n }\n\n function jumpTo(nextMove) {\n \n }\n\n const moves = history.map((squares, move) \\=> {\n let description;\n if (move > 0) {\n description = 'Go to move #' + move;\n } else {\n description = 'Go to game start';\n }\n return (\n <li key\\={move}\\>\n <button onClick\\={() \\=> jumpTo(move)}\\>{description}</button\\>\n </li\\>\n );\n });\n\n return (\n <div className\\=\"game\"\\>\n <div className\\=\"game-board\"\\>\n <Board xIsNext\\={xIsNext} squares\\={currentSquares} onPlay\\={handlePlay} />\n </div\\>\n <div className\\=\"game-info\"\\>\n <ol\\>{moves}</ol\\>\n </div\\>\n </div\\>\n );\n}\n\nfunction calculateWinner(squares) {\n const lines = \\[\n \\[0, 1, 2\\],\n \\[3, 4, 5\\],\n \\[6, 7, 8\\],\n \\[0, 3, 6\\],\n \\[1, 4, 7\\],\n \\[2, 5, 8\\],\n \\[0, 4, 8\\],\n \\[2, 4, 6\\],\n \\];\n for (let i = 0; i < lines.length; i++) {\n const \\[a, b, c\\] = lines\\[i\\];\n if (squares\\[a\\] && squares\\[a\\] === squares\\[b\\] && squares\\[a\\] === squares\\[c\\]) {\n return squares\\[a\\];\n }\n }\n return null;\n}\n\nBefore you can implement `jumpTo`, you need the `Game` component to keep track of which step the user is currently viewing. To do this, define a new state variable called `currentMove`, defaulting to `0`:\n\n```\nexport default function Game() {const [xIsNext, setXIsNext] = useState(true);const [history, setHistory] = useState([Array(9).fill(null)]);const [currentMove, setCurrentMove] = useState(0);const currentSquares = history[history.length - 1];//...}\n```\n\nNext, update the `jumpTo` function inside `Game` to update that `currentMove`. You’ll also set `xIsNext` to `true` if the number that you’re changing `currentMove` to is even.\n\n```\nexport default function Game() {// ...function jumpTo(nextMove) {setCurrentMove(nextMove);setXIsNext(nextMove % 2 === 0);}//...}\n```\n\nYou will now make two changes to the `Game`’s `handlePlay` function which is called when you click on a square.\n\n* If you “go back in time” and then make a new move from that point, you only want to keep the history up to that point. Instead of adding `nextSquares` after all items (`...` spread syntax) in `history`, you’ll add it after all items in `history.slice(0, currentMove + 1)` so that you’re only keeping that portion of the old history.\n* Each time a move is made, you need to update `currentMove` to point to the latest history entry.\n\n```\nfunction handlePlay(nextSquares) {const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];setHistory(nextHistory);setCurrentMove(nextHistory.length - 1);setXIsNext(!xIsNext);}\n```\n\nFinally, you will modify the `Game` component to render the currently selected move, instead of always rendering the final move:\n\n```\nexport default function Game() {const [xIsNext, setXIsNext] = useState(true);const [history, setHistory] = useState([Array(9).fill(null)]);const [currentMove, setCurrentMove] = useState(0);const currentSquares = history[currentMove];// ...}\n```\n\nIf you click on any step in the game’s history, the tic-tac-toe board should immediately update to show what the board looked like after that step occurred.\n\nimport { useState } from 'react';\n\nfunction Square({value, onSquareClick}) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nfunction Board({ xIsNext, squares, onPlay }) {\n function handleClick(i) {\n if (calculateWinner(squares) || squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n onPlay(nextSquares);\n }\n\n const winner = calculateWinner(squares);\n let status;\n if (winner) {\n status = 'Winner: ' + winner;\n } else {\n status = 'Next player: ' + (xIsNext ? 'X' : 'O');\n }\n\n return (\n <\\>\n <div className\\=\"status\"\\>{status}</div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nexport default function Game() {\n const \\[xIsNext, setXIsNext\\] = useState(true);\n const \\[history, setHistory\\] = useState(\\[Array(9).fill(null)\\]);\n const \\[currentMove, setCurrentMove\\] = useState(0);\n const currentSquares = history\\[currentMove\\];\n\n function handlePlay(nextSquares) {\n const nextHistory = \\[...history.slice(0, currentMove + 1), nextSquares\\];\n setHistory(nextHistory);\n setCurrentMove(nextHistory.length - 1);\n setXIsNext(!xIsNext);\n }\n\n function jumpTo(nextMove) {\n setCurrentMove(nextMove);\n setXIsNext(nextMove % 2 === 0);\n }\n\n const moves = history.map((squares, move) \\=> {\n let description;\n if (move > 0) {\n description = 'Go to move #' + move;\n } else {\n description = 'Go to game start';\n }\n return (\n <li key\\={move}\\>\n <button onClick\\={() \\=> jumpTo(move)}\\>{description}</button\\>\n </li\\>\n );\n });\n\n return (\n <div className\\=\"game\"\\>\n <div className\\=\"game-board\"\\>\n <Board xIsNext\\={xIsNext} squares\\={currentSquares} onPlay\\={handlePlay} />\n </div\\>\n <div className\\=\"game-info\"\\>\n <ol\\>{moves}</ol\\>\n </div\\>\n </div\\>\n );\n}\n\nfunction calculateWinner(squares) {\n const lines = \\[\n \\[0, 1, 2\\],\n \\[3, 4, 5\\],\n \\[6, 7, 8\\],\n \\[0, 3, 6\\],\n \\[1, 4, 7\\],\n \\[2, 5, 8\\],\n \\[0, 4, 8\\],\n \\[2, 4, 6\\],\n \\];\n for (let i = 0; i < lines.length; i++) {\n const \\[a, b, c\\] = lines\\[i\\];\n if (squares\\[a\\] && squares\\[a\\] === squares\\[b\\] && squares\\[a\\] === squares\\[c\\]) {\n return squares\\[a\\];\n }\n }\n return null;\n}\n\n### Final cleanup[](https://react.dev/learn/tutorial-tic-tac-toe#final-cleanup \"Link for Final cleanup \")\n\nIf you look at the code very closely, you may notice that `xIsNext === true` when `currentMove` is even and `xIsNext === false` when `currentMove` is odd. In other words, if you know the value of `currentMove`, then you can always figure out what `xIsNext` should be.\n\nThere’s no reason for you to store both of these in state. In fact, always try to avoid redundant state. Simplifying what you store in state reduces bugs and makes your code easier to understand. Change `Game` so that it doesn’t store `xIsNext` as a separate state variable and instead figures it out based on the `currentMove`:\n\n```\nexport default function Game() {const [history, setHistory] = useState([Array(9).fill(null)]);const [currentMove, setCurrentMove] = useState(0);const xIsNext = currentMove % 2 === 0;const currentSquares = history[currentMove];function handlePlay(nextSquares) {const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];setHistory(nextHistory);setCurrentMove(nextHistory.length - 1);}function jumpTo(nextMove) {setCurrentMove(nextMove);}// ...}\n```\n\nYou no longer need the `xIsNext` state declaration or the calls to `setXIsNext`. Now, there’s no chance for `xIsNext` to get out of sync with `currentMove`, even if you make a mistake while coding the components.\n\n### Wrapping up[](https://react.dev/learn/tutorial-tic-tac-toe#wrapping-up \"Link for Wrapping up \")\n\nCongratulations! You’ve created a tic-tac-toe game that:\n\n* Lets you play tic-tac-toe,\n* Indicates when a player has won the game,\n* Stores a game’s history as a game progresses,\n* Allows players to review a game’s history and see previous versions of a game’s board.\n\nNice work! We hope you now feel like you have a decent grasp of how React works.\n\nCheck out the final result here:\n\nimport { useState } from 'react';\n\nfunction Square({ value, onSquareClick }) {\n return (\n <button className\\=\"square\" onClick\\={onSquareClick}\\>\n {value}\n </button\\>\n );\n}\n\nfunction Board({ xIsNext, squares, onPlay }) {\n function handleClick(i) {\n if (calculateWinner(squares) || squares\\[i\\]) {\n return;\n }\n const nextSquares = squares.slice();\n if (xIsNext) {\n nextSquares\\[i\\] = 'X';\n } else {\n nextSquares\\[i\\] = 'O';\n }\n onPlay(nextSquares);\n }\n\n const winner = calculateWinner(squares);\n let status;\n if (winner) {\n status = 'Winner: ' + winner;\n } else {\n status = 'Next player: ' + (xIsNext ? 'X' : 'O');\n }\n\n return (\n <\\>\n <div className\\=\"status\"\\>{status}</div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[0\\]} onSquareClick\\={() \\=> handleClick(0)} />\n <Square value\\={squares\\[1\\]} onSquareClick\\={() \\=> handleClick(1)} />\n <Square value\\={squares\\[2\\]} onSquareClick\\={() \\=> handleClick(2)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[3\\]} onSquareClick\\={() \\=> handleClick(3)} />\n <Square value\\={squares\\[4\\]} onSquareClick\\={() \\=> handleClick(4)} />\n <Square value\\={squares\\[5\\]} onSquareClick\\={() \\=> handleClick(5)} />\n </div\\>\n <div className\\=\"board-row\"\\>\n <Square value\\={squares\\[6\\]} onSquareClick\\={() \\=> handleClick(6)} />\n <Square value\\={squares\\[7\\]} onSquareClick\\={() \\=> handleClick(7)} />\n <Square value\\={squares\\[8\\]} onSquareClick\\={() \\=> handleClick(8)} />\n </div\\>\n </\\>\n );\n}\n\nexport default function Game() {\n const \\[history, setHistory\\] = useState(\\[Array(9).fill(null)\\]);\n const \\[currentMove, setCurrentMove\\] = useState(0);\n const xIsNext = currentMove % 2 === 0;\n const currentSquares = history\\[currentMove\\];\n\n function handlePlay(nextSquares) {\n const nextHistory = \\[...history.slice(0, currentMove + 1), nextSquares\\];\n setHistory(nextHistory);\n setCurrentMove(nextHistory.length - 1);\n }\n\n function jumpTo(nextMove) {\n setCurrentMove(nextMove);\n }\n\n const moves = history.map((squares, move) \\=> {\n let description;\n if (move > 0) {\n description = 'Go to move #' + move;\n } else {\n description = 'Go to game start';\n }\n return (\n <li key\\={move}\\>\n <button onClick\\={() \\=> jumpTo(move)}\\>{description}</button\\>\n </li\\>\n );\n });\n\n return (\n <div className\\=\"game\"\\>\n <div className\\=\"game-board\"\\>\n <Board xIsNext\\={xIsNext} squares\\={currentSquares} onPlay\\={handlePlay} />\n </div\\>\n <div className\\=\"game-info\"\\>\n <ol\\>{moves}</ol\\>\n </div\\>\n </div\\>\n );\n}\n\nfunction calculateWinner(squares) {\n const lines = \\[\n \\[0, 1, 2\\],\n \\[3, 4, 5\\],\n \\[6, 7, 8\\],\n \\[0, 3, 6\\],\n \\[1, 4, 7\\],\n \\[2, 5, 8\\],\n \\[0, 4, 8\\],\n \\[2, 4, 6\\],\n \\];\n for (let i = 0; i < lines.length; i++) {\n const \\[a, b, c\\] = lines\\[i\\];\n if (squares\\[a\\] && squares\\[a\\] === squares\\[b\\] && squares\\[a\\] === squares\\[c\\]) {\n return squares\\[a\\];\n }\n }\n return null;\n}\n\nIf you have extra time or want to practice your new React skills, here are some ideas for improvements that you could make to the tic-tac-toe game, listed in order of increasing difficulty:\n\n1. For the current move only, show “You are at move #…” instead of a button.\n2. Rewrite `Board` to use two loops to make the squares instead of hardcoding them.\n3. Add a toggle button that lets you sort the moves in either ascending or descending order.\n4. When someone wins, highlight the three squares that caused the win (and when no one wins, display a message about the result being a draw).\n5. Display the location for each move in the format (row, col) in the move history list.\n\nThroughout this tutorial, you’ve touched on React concepts including elements, components, props, and state. Now that you’ve seen how these concepts work when building a game, check out [Thinking in React](https://react.dev/learn/thinking-in-react) to see how the same React concepts work when building an app’s UI.\n",
"filename": "tutorial-tic-tac-toe.md",
"package": "react"
} |
{
"content": "Title: Using TypeScript – React\n\nURL Source: https://react.dev/learn/typescript\n\nMarkdown Content:\nTypeScript is a popular way to add type definitions to JavaScript codebases. Out of the box, TypeScript [supports JSX](https://react.dev/learn/writing-markup-with-jsx) and you can get full React Web support by adding [`@types/react`](https://www.npmjs.com/package/@types/react) and [`@types/react-dom`](https://www.npmjs.com/package/@types/react-dom) to your project.\n\nInstallation[](https://react.dev/learn/typescript#installation \"Link for Installation \")\n----------------------------------------------------------------------------------------\n\nAll [production-grade React frameworks](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) offer support for using TypeScript. Follow the framework specific guide for installation:\n\n* [Next.js](https://nextjs.org/docs/app/building-your-application/configuring/typescript)\n* [Remix](https://remix.run/docs/en/1.19.2/guides/typescript)\n* [Gatsby](https://www.gatsbyjs.com/docs/how-to/custom-configuration/typescript/)\n* [Expo](https://docs.expo.dev/guides/typescript/)\n\n### Adding TypeScript to an existing React project[](https://react.dev/learn/typescript#adding-typescript-to-an-existing-react-project \"Link for Adding TypeScript to an existing React project \")\n\nTo install the latest version of React’s type definitions:\n\nnpm install @types/react @types/react-dom\n\nThe following compiler options need to be set in your `tsconfig.json`:\n\n1. `dom` must be included in [`lib`](https://www.typescriptlang.org/tsconfig/#lib) (Note: If no `lib` option is specified, `dom` is included by default).\n2. [`jsx`](https://www.typescriptlang.org/tsconfig/#jsx) must be set to one of the valid options. `preserve` should suffice for most applications. If you’re publishing a library, consult the [`jsx` documentation](https://www.typescriptlang.org/tsconfig/#jsx) on what value to choose.\n\nTypeScript with React Components[](https://react.dev/learn/typescript#typescript-with-react-components \"Link for TypeScript with React Components \")\n----------------------------------------------------------------------------------------------------------------------------------------------------\n\n### Note\n\nEvery file containing JSX must use the `.tsx` file extension. This is a TypeScript-specific extension that tells TypeScript that this file contains JSX.\n\nWriting TypeScript with React is very similar to writing JavaScript with React. The key difference when working with a component is that you can provide types for your component’s props. These types can be used for correctness checking and providing inline documentation in editors.\n\nTaking the [`MyButton` component](https://react.dev/learn#components) from the [Quick Start](https://react.dev/learn) guide, we can add a type describing the `title` for the button:\n\n### Note\n\nThese sandboxes can handle TypeScript code, but they do not run the type-checker. This means you can amend the TypeScript sandboxes to learn, but you won’t get any type errors or warnings. To get type-checking, you can use the [TypeScript Playground](https://www.typescriptlang.org/play) or use a more fully-featured online sandbox.\n\nThis inline syntax is the simplest way to provide types for a component, though once you start to have a few fields to describe it can become unwieldy. Instead, you can use an `interface` or `type` to describe the component’s props:\n\ninterface MyButtonProps {\n \n title: string;\n \n disabled: boolean;\n}\n\nfunction MyButton({ title, disabled }: MyButtonProps) {\n return (\n <button disabled\\={disabled}\\>{title}</button\\>\n );\n}\n\nexport default function MyApp() {\n return (\n <div\\>\n <h1\\>Welcome to my app</h1\\>\n <MyButton title\\=\"I'm a disabled button\" disabled\\={true}/>\n </div\\>\n );\n}\n\nThe type describing your component’s props can be as simple or as complex as you need, though they should be an object type described with either a `type` or `interface`. You can learn about how TypeScript describes objects in [Object Types](https://www.typescriptlang.org/docs/handbook/2/objects.html) but you may also be interested in using [Union Types](https://www.typescriptlang.org/docs/handbook/2/everyday-types.html#union-types) to describe a prop that can be one of a few different types and the [Creating Types from Types](https://www.typescriptlang.org/docs/handbook/2/types-from-types.html) guide for more advanced use cases.\n\nExample Hooks[](https://react.dev/learn/typescript#example-hooks \"Link for Example Hooks \")\n-------------------------------------------------------------------------------------------\n\nThe type definitions from `@types/react` include types for the built-in Hooks, so you can use them in your components without any additional setup. They are built to take into account the code you write in your component, so you will get [inferred types](https://www.typescriptlang.org/docs/handbook/type-inference.html) a lot of the time and ideally do not need to handle the minutiae of providing the types.\n\nHowever, we can look at a few examples of how to provide types for Hooks.\n\n### `useState`[](https://react.dev/learn/typescript#typing-usestate \"Link for this heading\")\n\nThe [`useState` Hook](https://react.dev/reference/react/useState) will re-use the value passed in as the initial state to determine what the type of the value should be. For example:\n\n```\n// Infer the type as \"boolean\"const [enabled, setEnabled] = useState(false);\n```\n\nThis will assign the type of `boolean` to `enabled`, and `setEnabled` will be a function accepting either a `boolean` argument, or a function that returns a `boolean`. If you want to explicitly provide a type for the state, you can do so by providing a type argument to the `useState` call:\n\n```\n// Explicitly set the type to \"boolean\"const [enabled, setEnabled] = useState<boolean>(false);\n```\n\nThis isn’t very useful in this case, but a common case where you may want to provide a type is when you have a union type. For example, `status` here can be one of a few different strings:\n\n```\ntype Status = \"idle\" | \"loading\" | \"success\" | \"error\";const [status, setStatus] = useState<Status>(\"idle\");\n```\n\nOr, as recommended in [Principles for structuring state](https://react.dev/learn/choosing-the-state-structure#principles-for-structuring-state), you can group related state as an object and describe the different possibilities via object types:\n\n```\ntype RequestState = | { status: 'idle' } | { status: 'loading' } | { status: 'success', data: any } | { status: 'error', error: Error };const [requestState, setRequestState] = useState<RequestState>({ status: 'idle' });\n```\n\n### `useReducer`[](https://react.dev/learn/typescript#typing-usereducer \"Link for this heading\")\n\nThe [`useReducer` Hook](https://react.dev/reference/react/useReducer) is a more complex Hook that takes a reducer function and an initial state. The types for the reducer function are inferred from the initial state. You can optionally provide a type argument to the `useReducer` call to provide a type for the state, but it is often better to set the type on the initial state instead:\n\nimport {useReducer} from 'react';\n\ninterface State {\n count: number \n};\n\ntype CounterAction =\n | { type: \"reset\" }\n | { type: \"setCount\"; value: State\\[\"count\"\\] }\n\nconst initialState: State = { count: 0 };\n\nfunction stateReducer(state: State, action: CounterAction): State {\n switch (action.type) {\n case \"reset\":\n return initialState;\n case \"setCount\":\n return { ...state, count: action.value };\n default:\n throw new Error(\"Unknown action\");\n }\n}\n\nexport default function App() {\n const \\[state, dispatch\\] = useReducer(stateReducer, initialState);\n\n const addFive = () \\=> dispatch({ type: \"setCount\", value: state.count + 5 });\n const reset = () \\=> dispatch({ type: \"reset\" });\n\n return (\n <div\\>\n <h1\\>Welcome to my counter</h1\\>\n\n <p\\>Count: {state.count}</p\\>\n <button onClick\\={addFive}\\>Add 5</button\\>\n <button onClick\\={reset}\\>Reset</button\\>\n </div\\>\n );\n}\n\nWe are using TypeScript in a few key places:\n\n* `interface State` describes the shape of the reducer’s state.\n* `type CounterAction` describes the different actions which can be dispatched to the reducer.\n* `const initialState: State` provides a type for the initial state, and also the type which is used by `useReducer` by default.\n* `stateReducer(state: State, action: CounterAction): State` sets the types for the reducer function’s arguments and return value.\n\nA more explicit alternative to setting the type on `initialState` is to provide a type argument to `useReducer`:\n\n```\nimport { stateReducer, State } from './your-reducer-implementation';const initialState = { count: 0 };export default function App() {const [state, dispatch] = useReducer<State>(stateReducer, initialState);}\n```\n\n### `useContext`[](https://react.dev/learn/typescript#typing-usecontext \"Link for this heading\")\n\nThe [`useContext` Hook](https://react.dev/reference/react/useContext) is a technique for passing data down the component tree without having to pass props through components. It is used by creating a provider component and often by creating a Hook to consume the value in a child component.\n\nThe type of the value provided by the context is inferred from the value passed to the `createContext` call:\n\nimport { createContext, useContext, useState } from 'react';\n\ntype Theme = \"light\" | \"dark\" | \"system\";\nconst ThemeContext = createContext<Theme\\>(\"system\");\n\nconst useGetTheme = () \\=> useContext(ThemeContext);\n\nexport default function MyApp() {\n const \\[theme, setTheme\\] = useState<Theme\\>('light');\n\n return (\n <ThemeContext.Provider value\\={theme}\\>\n <MyComponent />\n </ThemeContext.Provider\\>\n )\n}\n\nfunction MyComponent() {\n const theme = useGetTheme();\n\n return (\n <div\\>\n <p\\>Current theme: {theme}</p\\>\n </div\\>\n )\n}\n\nThis technique works when you have a default value which makes sense - but there are occasionally cases when you do not, and in those cases `null` can feel reasonable as a default value. However, to allow the type-system to understand your code, you need to explicitly set `ContextShape | null` on the `createContext`.\n\nThis causes the issue that you need to eliminate the `| null` in the type for context consumers. Our recommendation is to have the Hook do a runtime check for it’s existence and throw an error when not present:\n\n```\nimport { createContext, useContext, useState, useMemo } from 'react';// This is a simpler example, but you can imagine a more complex object heretype ComplexObject = {kind: string};// The context is created with `| null` in the type, to accurately reflect the default value.const Context = createContext<ComplexObject | null>(null);// The `| null` will be removed via the check in the Hook.const useGetComplexObject = () => {const object = useContext(Context);if (!object) { throw new Error(\"useGetComplexObject must be used within a Provider\") }return object;}export default function MyApp() {const object = useMemo(() => ({ kind: \"complex\" }), []);return (<Context.Provider value={object}><MyComponent /></Context.Provider>)}function MyComponent() {const object = useGetComplexObject();return (<div><p>Current object: {object.kind}</p></div>)}\n```\n\n### `useMemo`[](https://react.dev/learn/typescript#typing-usememo \"Link for this heading\")\n\nThe [`useMemo`](https://react.dev/reference/react/useMemo) Hooks will create/re-access a memorized value from a function call, re-running the function only when dependencies passed as the 2nd parameter are changed. The result of calling the Hook is inferred from the return value from the function in the first parameter. You can be more explicit by providing a type argument to the Hook.\n\n```\n// The type of visibleTodos is inferred from the return value of filterTodosconst visibleTodos = useMemo(() => filterTodos(todos, tab), [todos, tab]);\n```\n\n### `useCallback`[](https://react.dev/learn/typescript#typing-usecallback \"Link for this heading\")\n\nThe [`useCallback`](https://react.dev/reference/react/useCallback) provide a stable reference to a function as long as the dependencies passed into the second parameter are the same. Like `useMemo`, the function’s type is inferred from the return value of the function in the first parameter, and you can be more explicit by providing a type argument to the Hook.\n\n```\nconst handleClick = useCallback(() => {// ...}, [todos]);\n```\n\nWhen working in TypeScript strict mode `useCallback` requires adding types for the parameters in your callback. This is because the type of the callback is inferred from the return value of the function, and without parameters the type cannot be fully understood.\n\nDepending on your code-style preferences, you could use the `*EventHandler` functions from the React types to provide the type for the event handler at the same time as defining the callback:\n\n```\nimport { useState, useCallback } from 'react';export default function Form() {const [value, setValue] = useState(\"Change me\");const handleChange = useCallback<React.ChangeEventHandler<HTMLInputElement>>((event) => {setValue(event.currentTarget.value);}, [setValue])return (<><input value={value} onChange={handleChange} /><p>Value: {value}</p></>);}\n```\n\nUseful Types[](https://react.dev/learn/typescript#useful-types \"Link for Useful Types \")\n----------------------------------------------------------------------------------------\n\nThere is quite an expansive set of types which come from the `@types/react` package, it is worth a read when you feel comfortable with how React and TypeScript interact. You can find them [in React’s folder in DefinitelyTyped](https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/types/react/index.d.ts). We will cover a few of the more common types here.\n\n### DOM Events[](https://react.dev/learn/typescript#typing-dom-events \"Link for DOM Events \")\n\nWhen working with DOM events in React, the type of the event can often be inferred from the event handler. However, when you want to extract a function to be passed to an event handler, you will need to explicitly set the type of the event.\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[value, setValue\\] = useState(\"Change me\");\n\n function handleChange(event: React.ChangeEvent<HTMLInputElement>) {\n setValue(event.currentTarget.value);\n }\n\n return (\n <\\>\n <input value\\={value} onChange\\={handleChange} />\n <p\\>Value: {value}</p\\>\n </\\>\n );\n}\n\nThere are many types of events provided in the React types - the full list can be found [here](https://github.com/DefinitelyTyped/DefinitelyTyped/blob/b580df54c0819ec9df62b0835a315dd48b8594a9/types/react/index.d.ts#L1247C1-L1373) which is based on the [most popular events from the DOM](https://developer.mozilla.org/en-US/docs/Web/Events).\n\nWhen determining the type you are looking for you can first look at the hover information for the event handler you are using, which will show the type of the event.\n\nIf you need to use an event that is not included in this list, you can use the `React.SyntheticEvent` type, which is the base type for all events.\n\n### Children[](https://react.dev/learn/typescript#typing-children \"Link for Children \")\n\nThere are two common paths to describing the children of a component. The first is to use the `React.ReactNode` type, which is a union of all the possible types that can be passed as children in JSX:\n\n```\ninterface ModalRendererProps { title: string; children: React.ReactNode;}\n```\n\nThis is a very broad definition of children. The second is to use the `React.ReactElement` type, which is only JSX elements and not JavaScript primitives like strings or numbers:\n\n```\ninterface ModalRendererProps { title: string; children: React.ReactElement;}\n```\n\nNote, that you cannot use TypeScript to describe that the children are a certain type of JSX elements, so you cannot use the type-system to describe a component which only accepts `<li>` children.\n\nYou can see an example of both `React.ReactNode` and `React.ReactElement` with the type-checker in [this TypeScript playground](https://www.typescriptlang.org/play?#code/JYWwDg9gTgLgBAJQKYEMDG8BmUIjgIilQ3wChSB6CxYmAOmXRgDkIATJOdNJMGAZzgwAFpxAR+8YADswAVwGkZMJFEzpOjDKw4AFHGEEBvUnDhphwADZsi0gFw0mDWjqQBuUgF9yaCNMlENzgAXjgACjADfkctFnYkfQhDAEpQgD44AB42YAA3dKMo5P46C2tbJGkvLIpcgt9-QLi3AEEwMFCItJDMrPTTbIQ3dKywdIB5aU4kKyQQKpha8drhhIGzLLWODbNs3b3s8YAxKBQAcwXpAThMaGWDvbH0gFloGbmrgQfBzYpd1YjQZbEYARkB6zMwO2SHSAAlZlYIBCdtCRkZpHIrFYahQYQD8UYYFA5EhcfjyGYqHAXnJAsIUHlOOUbHYhMIIHJzsI0Qk4P9SLUBuRqXEXEwAKKfRZcNA8PiCfxWACecAAUgBlAAacFm80W-CU11U6h4TgwUv11yShjgJjMLMqDnN9Dilq+nh8pD8AXgCHdMrCkWisVoAet0R6fXqhWKhjKllZVVxMcavpd4Zg7U6Qaj+2hmdG4zeRF10uu-Aeq0LBfLMEe-V+T2L7zLVu+FBWLdLeq+lc7DYFf39deFVOotMCACNOCh1dq219a+30uC8YWoZsRyuEdjkevR8uvoVMdjyTWt4WiSSydXD4NqZP4AymeZE072ZzuUeZQKheQgA).\n\n### Style Props[](https://react.dev/learn/typescript#typing-style-props \"Link for Style Props \")\n\nWhen using inline styles in React, you can use `React.CSSProperties` to describe the object passed to the `style` prop. This type is a union of all the possible CSS properties, and is a good way to ensure you are passing valid CSS properties to the `style` prop, and to get auto-complete in your editor.\n\n```\ninterface MyComponentProps { style: React.CSSProperties;}\n```\n\nFurther learning[](https://react.dev/learn/typescript#further-learning \"Link for Further learning \")\n----------------------------------------------------------------------------------------------------\n\nThis guide has covered the basics of using TypeScript with React, but there is a lot more to learn. Individual API pages on the docs may contain more in-depth documentation on how to use them with TypeScript.\n\nWe recommend the following resources:\n\n* [The TypeScript handbook](https://www.typescriptlang.org/docs/handbook/) is the official documentation for TypeScript, and covers most key language features.\n \n* [The TypeScript release notes](https://devblogs.microsoft.com/typescript/) cover new features in depth.\n \n* [React TypeScript Cheatsheet](https://react-typescript-cheatsheet.netlify.app/) is a community-maintained cheatsheet for using TypeScript with React, covering a lot of useful edge cases and providing more breadth than this document.\n \n* [TypeScript Community Discord](https://discord.com/invite/typescript) is a great place to ask questions and get help with TypeScript and React issues.\n",
"filename": "typescript.md",
"package": "react"
} |
{
"content": "Title: Understanding Your UI as a Tree – React\n\nURL Source: https://react.dev/learn/understanding-your-ui-as-a-tree\n\nMarkdown Content:\nYour React app is taking shape with many components being nested within each other. How does React keep track of your app’s component structure?\n\nReact, and many other UI libraries, model UI as a tree. Thinking of your app as a tree is useful for understanding the relationship between components. This understanding will help you debug future concepts like performance and state management.\n\n### You will learn\n\n* How React “sees” component structures\n* What a render tree is and what it is useful for\n* What a module dependency tree is and what it is useful for\n\nYour UI as a tree[](https://react.dev/learn/understanding-your-ui-as-a-tree#your-ui-as-a-tree \"Link for Your UI as a tree \")\n----------------------------------------------------------------------------------------------------------------------------\n\nTrees are a relationship model between items and UI is often represented using tree structures. For example, browsers use tree structures to model HTML ([DOM](https://developer.mozilla.org/docs/Web/API/Document_Object_Model/Introduction)) and CSS ([CSSOM](https://developer.mozilla.org/docs/Web/API/CSS_Object_Model)). Mobile platforms also use trees to represent their view hierarchy.\n\n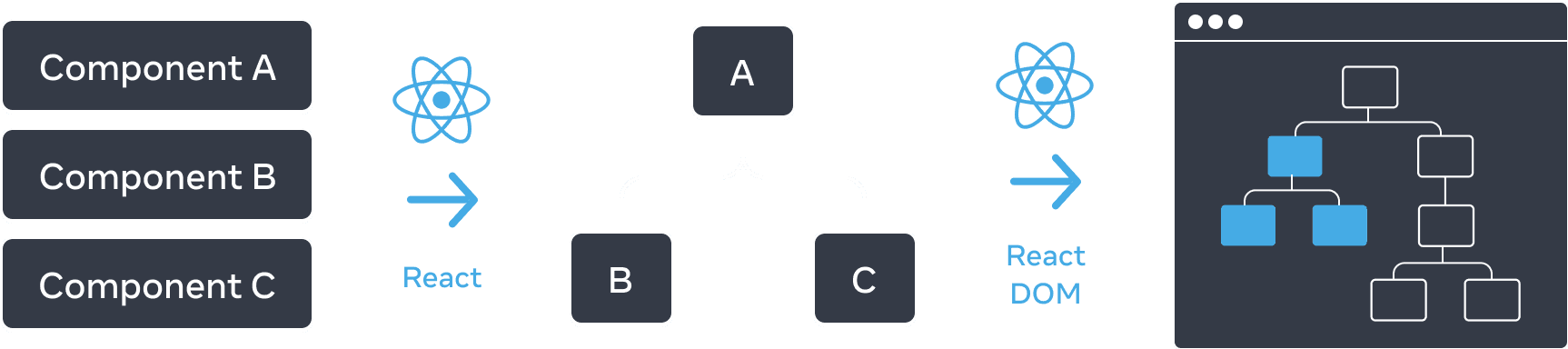\n\n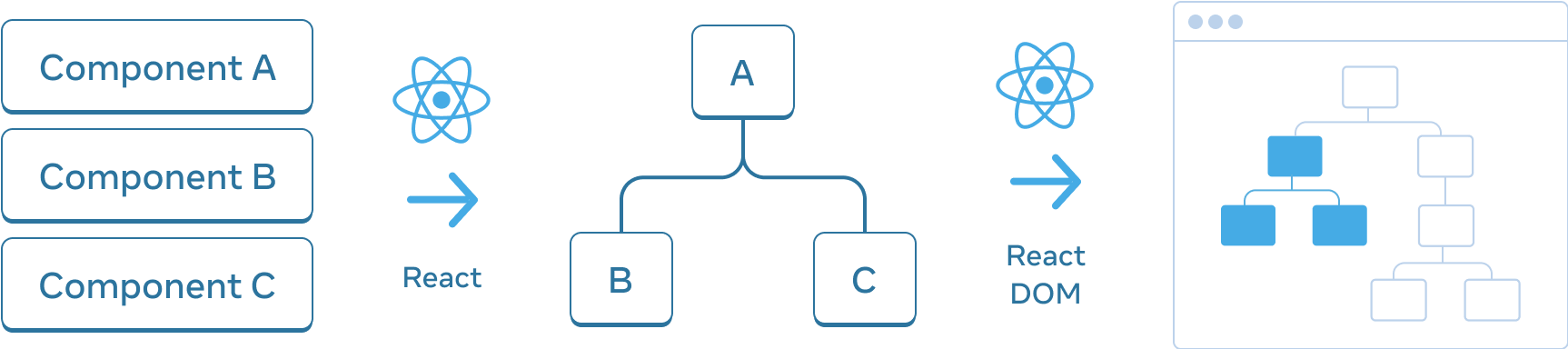\n\nReact creates a UI tree from your components. In this example, the UI tree is then used to render to the DOM.\n\nLike browsers and mobile platforms, React also uses tree structures to manage and model the relationship between components in a React app. These trees are useful tools to understand how data flows through a React app and how to optimize rendering and app size.\n\nThe Render Tree[](https://react.dev/learn/understanding-your-ui-as-a-tree#the-render-tree \"Link for The Render Tree \")\n----------------------------------------------------------------------------------------------------------------------\n\nA major feature of components is the ability to compose components of other components. As we [nest components](https://react.dev/learn/your-first-component#nesting-and-organizing-components), we have the concept of parent and child components, where each parent component may itself be a child of another component.\n\nWhen we render a React app, we can model this relationship in a tree, known as the render tree.\n\nHere is a React app that renders inspirational quotes.\n\n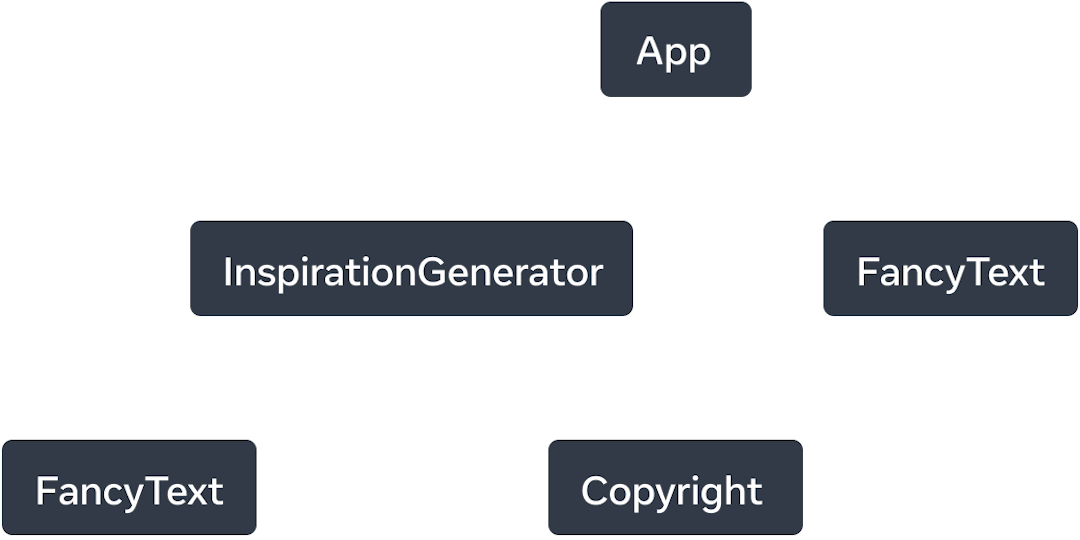\n\n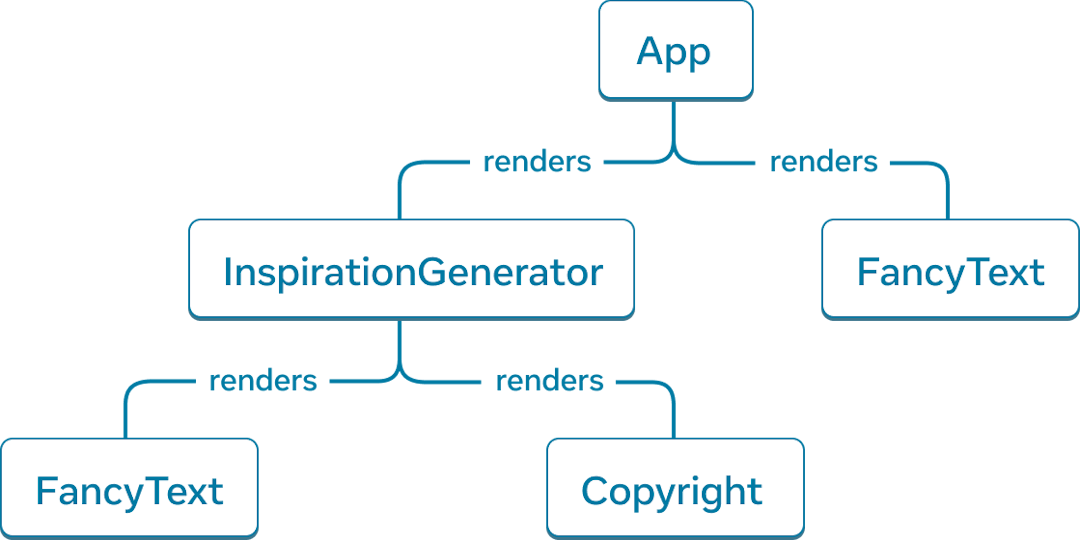\n\nReact creates a _render tree_, a UI tree, composed of the rendered components.\n\nFrom the example app, we can construct the above render tree.\n\nThe tree is composed of nodes, each of which represents a component. `App`, `FancyText`, `Copyright`, to name a few, are all nodes in our tree.\n\nThe root node in a React render tree is the [root component](https://react.dev/learn/importing-and-exporting-components#the-root-component-file) of the app. In this case, the root component is `App` and it is the first component React renders. Each arrow in the tree points from a parent component to a child component.\n\n##### Deep Dive\n\n#### Where are the HTML tags in the render tree?[](https://react.dev/learn/understanding-your-ui-as-a-tree#where-are-the-html-elements-in-the-render-tree \"Link for Where are the HTML tags in the render tree? \")\n\nYou’ll notice in the above render tree, there is no mention of the HTML tags that each component renders. This is because the render tree is only composed of React [components](https://react.dev/learn/your-first-component#components-ui-building-blocks).\n\nReact, as a UI framework, is platform agnostic. On react.dev, we showcase examples that render to the web, which uses HTML markup as its UI primitives. But a React app could just as likely render to a mobile or desktop platform, which may use different UI primitives like [UIView](https://developer.apple.com/documentation/uikit/uiview) or [FrameworkElement](https://learn.microsoft.com/en-us/dotnet/api/system.windows.frameworkelement?view=windowsdesktop-7.0).\n\nThese platform UI primitives are not a part of React. React render trees can provide insight to our React app regardless of what platform your app renders to.\n\nA render tree represents a single render pass of a React application. With [conditional rendering](https://react.dev/learn/conditional-rendering), a parent component may render different children depending on the data passed.\n\nWe can update the app to conditionally render either an inspirational quote or color.\n\n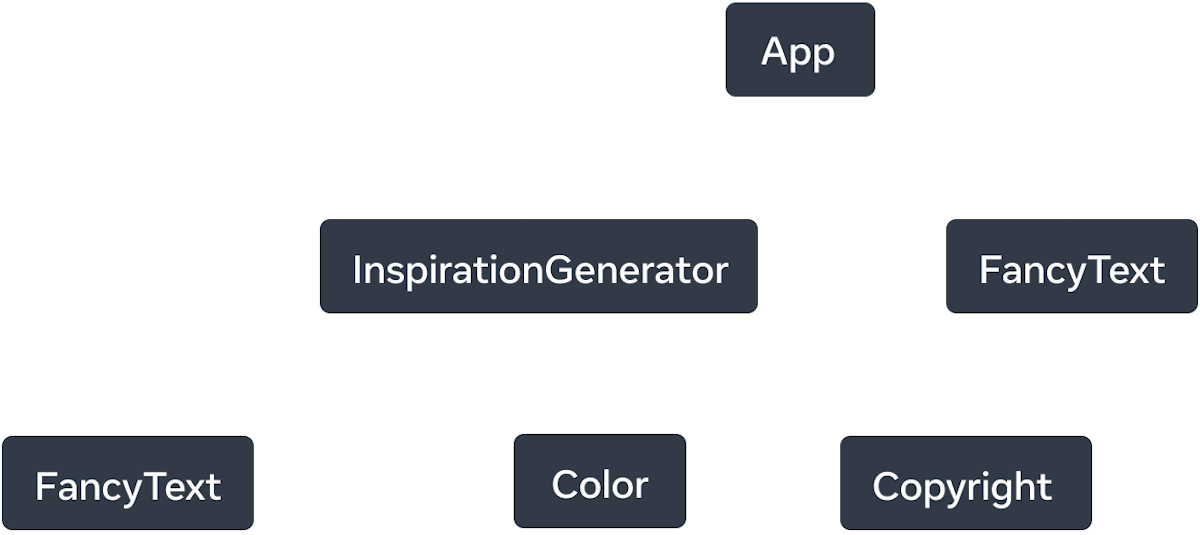\n\n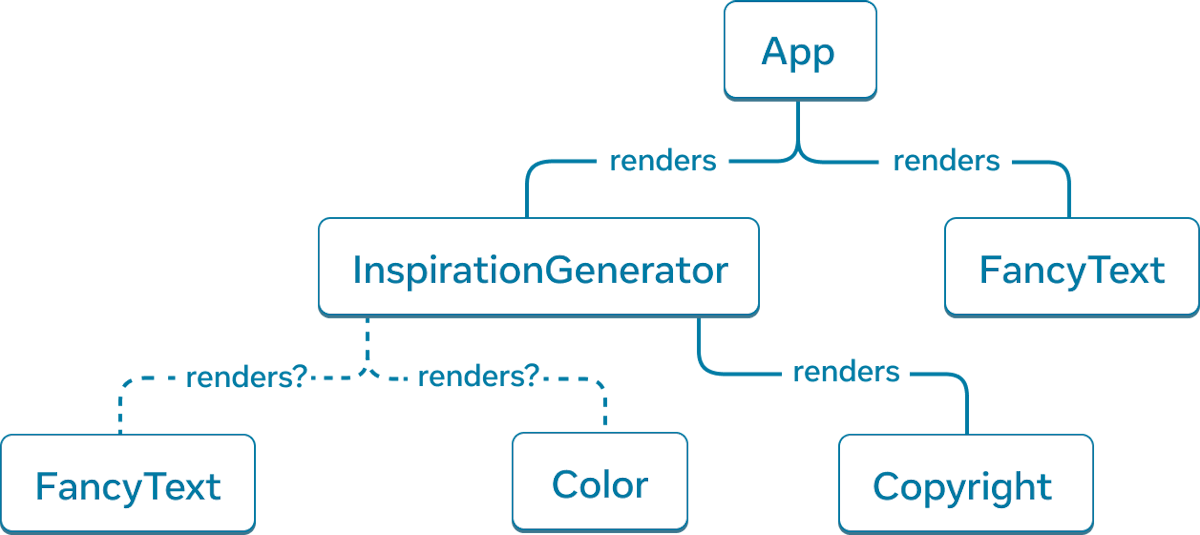\n\nWith conditional rendering, across different renders, the render tree may render different components.\n\nIn this example, depending on what `inspiration.type` is, we may render `<FancyText>` or `<Color>`. The render tree may be different for each render pass.\n\nAlthough render trees may differ across render passes, these trees are generally helpful for identifying what the _top-level_ and _leaf components_ are in a React app. Top-level components are the components nearest to the root component and affect the rendering performance of all the components beneath them and often contain the most complexity. Leaf components are near the bottom of the tree and have no child components and are often frequently re-rendered.\n\nIdentifying these categories of components are useful for understanding data flow and performance of your app.\n\nThe Module Dependency Tree[](https://react.dev/learn/understanding-your-ui-as-a-tree#the-module-dependency-tree \"Link for The Module Dependency Tree \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAnother relationship in a React app that can be modeled with a tree are an app’s module dependencies. As we [break up our components](https://react.dev/learn/importing-and-exporting-components#exporting-and-importing-a-component) and logic into separate files, we create [JS modules](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) where we may export components, functions, or constants.\n\nEach node in a module dependency tree is a module and each branch represents an `import` statement in that module.\n\nIf we take the previous Inspirations app, we can build a module dependency tree, or dependency tree for short.\n\n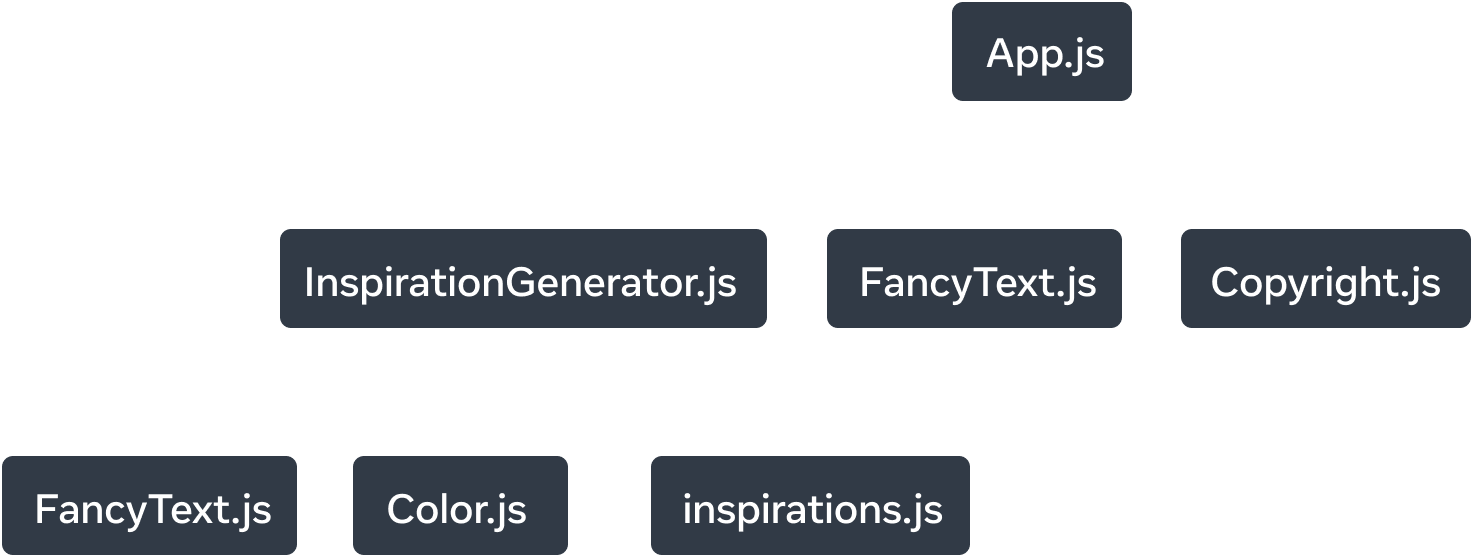\n\n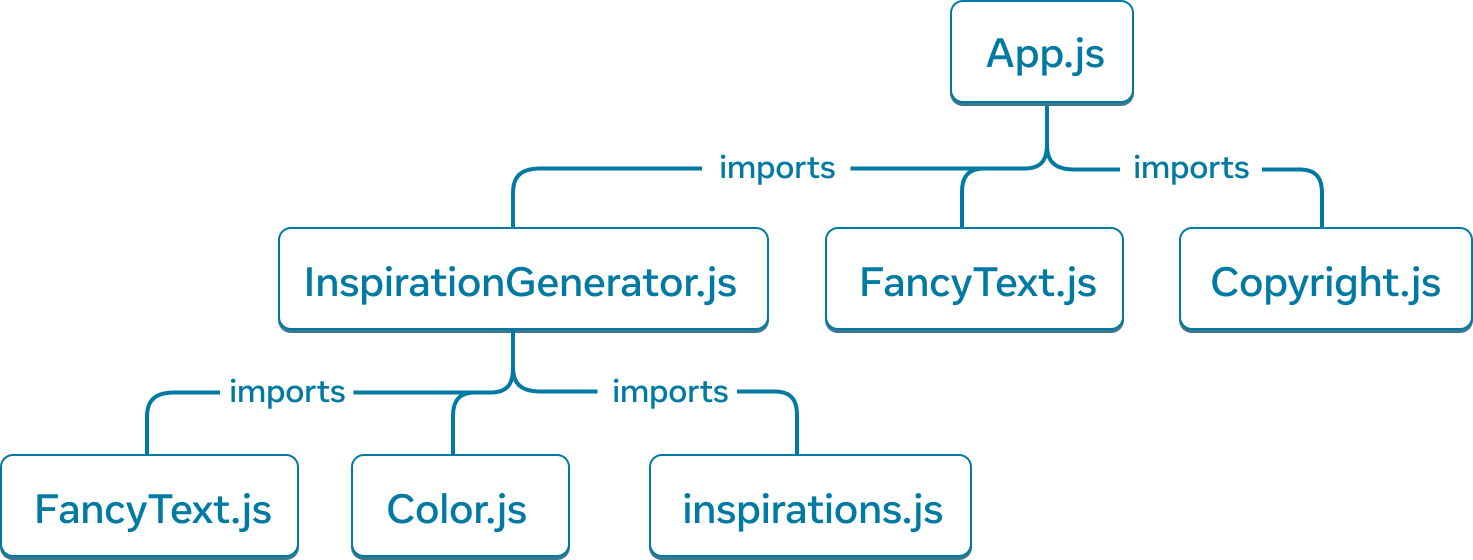\n\nThe module dependency tree for the Inspirations app.\n\nThe root node of the tree is the root module, also known as the entrypoint file. It often is the module that contains the root component.\n\nComparing to the render tree of the same app, there are similar structures but some notable differences:\n\n* The nodes that make-up the tree represent modules, not components.\n* Non-component modules, like `inspirations.js`, are also represented in this tree. The render tree only encapsulates components.\n* `Copyright.js` appears under `App.js` but in the render tree, `Copyright`, the component, appears as a child of `InspirationGenerator`. This is because `InspirationGenerator` accepts JSX as [children props](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children), so it renders `Copyright` as a child component but does not import the module.\n\nDependency trees are useful to determine what modules are necessary to run your React app. When building a React app for production, there is typically a build step that will bundle all the necessary JavaScript to ship to the client. The tool responsible for this is called a [bundler](https://developer.mozilla.org/en-US/docs/Learn/Tools_and_testing/Understanding_client-side_tools/Overview#the_modern_tooling_ecosystem), and bundlers will use the dependency tree to determine what modules should be included.\n\nAs your app grows, often the bundle size does too. Large bundle sizes are expensive for a client to download and run. Large bundle sizes can delay the time for your UI to get drawn. Getting a sense of your app’s dependency tree may help with debugging these issues.\n\nRecap[](https://react.dev/learn/understanding-your-ui-as-a-tree#recap \"Link for Recap\")\n---------------------------------------------------------------------------------------\n\n* Trees are a common way to represent the relationship between entities. They are often used to model UI.\n* Render trees represent the nested relationship between React components across a single render.\n* With conditional rendering, the render tree may change across different renders. With different prop values, components may render different children components.\n* Render trees help identify what the top-level and leaf components are. Top-level components affect the rendering performance of all components beneath them and leaf components are often re-rendered frequently. Identifying them is useful for understanding and debugging rendering performance.\n* Dependency trees represent the module dependencies in a React app.\n* Dependency trees are used by build tools to bundle the necessary code to ship an app.\n* Dependency trees are useful for debugging large bundle sizes that slow time to paint and expose opportunities for optimizing what code is bundled.\n",
"filename": "understanding-your-ui-as-a-tree.md",
"package": "react"
} |
{
"content": "Title: unmountComponentAtNode – React\n\nURL Source: https://react.dev/reference/react-dom/unmountComponentAtNode\n\nMarkdown Content:\nunmountComponentAtNode – React\n===============\n\nunmountComponentAtNode[](https://react.dev/reference/react-dom/unmountComponentAtNode#undefined \"Link for this heading\")\n========================================================================================================================\n\n### Deprecated\n\nThis API will be removed in a future major version of React.\n\nIn React 18, `unmountComponentAtNode` was replaced by [`root.unmount()`](https://react.dev/reference/react-dom/client/createRoot#root-unmount).\n\n`unmountComponentAtNode` removes a mounted React component from the DOM.\n\n```\nunmountComponentAtNode(domNode)\n```\n\n* [Reference](https://react.dev/reference/react-dom/unmountComponentAtNode#reference)\n * [`unmountComponentAtNode(domNode)`](https://react.dev/reference/react-dom/unmountComponentAtNode#unmountcomponentatnode)\n* [Usage](https://react.dev/reference/react-dom/unmountComponentAtNode#usage)\n * [Removing a React app from a DOM element](https://react.dev/reference/react-dom/unmountComponentAtNode#removing-a-react-app-from-a-dom-element)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/unmountComponentAtNode#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------------------\n\n### `unmountComponentAtNode(domNode)`[](https://react.dev/reference/react-dom/unmountComponentAtNode#unmountcomponentatnode \"Link for this heading\")\n\nCall `unmountComponentAtNode` to remove a mounted React component from the DOM and clean up its event handlers and state.\n\n```\nimport { unmountComponentAtNode } from 'react-dom';const domNode = document.getElementById('root');render(<App />, domNode);unmountComponentAtNode(domNode);\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/unmountComponentAtNode#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/unmountComponentAtNode#parameters \"Link for Parameters \")\n\n* `domNode`: A [DOM element.](https://developer.mozilla.org/en-US/docs/Web/API/Element) React will remove a mounted React component from this element.\n\n#### Returns[](https://react.dev/reference/react-dom/unmountComponentAtNode#returns \"Link for Returns \")\n\n`unmountComponentAtNode` returns `true` if a component was unmounted and `false` otherwise.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/unmountComponentAtNode#usage \"Link for Usage \")\n---------------------------------------------------------------------------------------------\n\nCall `unmountComponentAtNode` to remove a mounted React component from a browser DOM node and clean up its event handlers and state.\n\n```\nimport { render, unmountComponentAtNode } from 'react-dom';import App from './App.js';const rootNode = document.getElementById('root');render(<App />, rootNode);// ...unmountComponentAtNode(rootNode);\n```\n\n### Removing a React app from a DOM element[](https://react.dev/reference/react-dom/unmountComponentAtNode#removing-a-react-app-from-a-dom-element \"Link for Removing a React app from a DOM element \")\n\nOccasionally, you may want to “sprinkle” React on an existing page, or a page that is not fully written in React. In those cases, you may need to “stop” the React app, by removing all of the UI, state, and listeners from the DOM node it was rendered to.\n\nIn this example, clicking “Render React App” will render a React app. Click “Unmount React App” to destroy it:\n\nindex.jsindex.htmlApp.js\n\nindex.js\n\nReset\n\nFork\n\nimport './styles.css';\nimport { render, unmountComponentAtNode } from 'react-dom';\nimport App from './App.js';\n\nconst domNode = document.getElementById('root');\n\ndocument.getElementById('render').addEventListener('click', () \\=> {\n render(<App />, domNode);\n});\n\ndocument.getElementById('unmount').addEventListener('click', () \\=> {\n unmountComponentAtNode(domNode);\n});\n\nOpen Sandbox\n\n[Previousrender](https://react.dev/reference/react-dom/render)[NextClient APIs](https://react.dev/reference/react-dom/client)\n",
"filename": "unmountComponentAtNode.md",
"package": "react"
} |
{
"content": "Title: Updating Arrays in State – React\n\nURL Source: https://react.dev/learn/updating-arrays-in-state\n\nMarkdown Content:\nArrays are mutable in JavaScript, but you should treat them as immutable when you store them in state. Just like with objects, when you want to update an array stored in state, you need to create a new one (or make a copy of an existing one), and then set state to use the new array.\n\n### You will learn\n\n* How to add, remove, or change items in an array in React state\n* How to update an object inside of an array\n* How to make array copying less repetitive with Immer\n\nUpdating arrays without mutation[](https://react.dev/learn/updating-arrays-in-state#updating-arrays-without-mutation \"Link for Updating arrays without mutation \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn JavaScript, arrays are just another kind of object. [Like with objects](https://react.dev/learn/updating-objects-in-state), **you should treat arrays in React state as read-only.** This means that you shouldn’t reassign items inside an array like `arr[0] = 'bird'`, and you also shouldn’t use methods that mutate the array, such as `push()` and `pop()`.\n\nInstead, every time you want to update an array, you’ll want to pass a _new_ array to your state setting function. To do that, you can create a new array from the original array in your state by calling its non-mutating methods like `filter()` and `map()`. Then you can set your state to the resulting new array.\n\nHere is a reference table of common array operations. When dealing with arrays inside React state, you will need to avoid the methods in the left column, and instead prefer the methods in the right column:\n\n| | avoid (mutates the array) | prefer (returns a new array) |\n| --- | --- | --- |\n| adding | `push`, `unshift` | `concat`, `[...arr]` spread syntax ([example](https://react.dev/learn/updating-arrays-in-state#adding-to-an-array)) |\n| removing | `pop`, `shift`, `splice` | `filter`, `slice` ([example](https://react.dev/learn/updating-arrays-in-state#removing-from-an-array)) |\n| replacing | `splice`, `arr[i] = ...` assignment | `map` ([example](https://react.dev/learn/updating-arrays-in-state#replacing-items-in-an-array)) |\n| sorting | `reverse`, `sort` | copy the array first ([example](https://react.dev/learn/updating-arrays-in-state#making-other-changes-to-an-array)) |\n\nAlternatively, you can [use Immer](https://react.dev/learn/updating-arrays-in-state#write-concise-update-logic-with-immer) which lets you use methods from both columns.\n\n### Pitfall\n\nUnfortunately, [`slice`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice) and [`splice`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/splice) are named similarly but are very different:\n\n* `slice` lets you copy an array or a part of it.\n* `splice` **mutates** the array (to insert or delete items).\n\nIn React, you will be using `slice` (no `p`!) a lot more often because you don’t want to mutate objects or arrays in state. [Updating Objects](https://react.dev/learn/updating-objects-in-state) explains what mutation is and why it’s not recommended for state.\n\n### Adding to an array[](https://react.dev/learn/updating-arrays-in-state#adding-to-an-array \"Link for Adding to an array \")\n\n`push()` will mutate an array, which you don’t want:\n\nimport { useState } from 'react';\n\nlet nextId = 0;\n\nexport default function List() {\n const \\[name, setName\\] = useState('');\n const \\[artists, setArtists\\] = useState(\\[\\]);\n\n return (\n <\\>\n <h1\\>Inspiring sculptors:</h1\\>\n <input\n value\\={name}\n onChange\\={e \\=> setName(e.target.value)}\n />\n <button onClick\\={() \\=> {\n artists.push({\n id: nextId++,\n name: name,\n });\n }}\\>Add</button\\>\n <ul\\>\n {artists.map(artist \\=> (\n <li key\\={artist.id}\\>{artist.name}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\nUnable to establish connection with the sandpack bundler. Make sure you are online or try again later. If the problem persists, please report it via [email](mailto:hello@codesandbox.io?subject=Sandpack%20Timeout%20Error) or submit an issue on [GitHub.](https://github.com/codesandbox/sandpack/issues)\n\nInstead, create a _new_ array which contains the existing items _and_ a new item at the end. There are multiple ways to do this, but the easiest one is to use the `...` [array spread](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax#spread_in_array_literals) syntax:\n\n```\nsetArtists( // Replace the state[ // with a new array...artists, // that contains all the old items{ id: nextId++, name: name } // and one new item at the end]);\n```\n\nNow it works correctly:\n\nimport { useState } from 'react';\n\nlet nextId = 0;\n\nexport default function List() {\n const \\[name, setName\\] = useState('');\n const \\[artists, setArtists\\] = useState(\\[\\]);\n\n return (\n <\\>\n <h1\\>Inspiring sculptors:</h1\\>\n <input\n value\\={name}\n onChange\\={e \\=> setName(e.target.value)}\n />\n <button onClick\\={() \\=> {\n setArtists(\\[\n ...artists,\n { id: nextId++, name: name }\n \\]);\n }}\\>Add</button\\>\n <ul\\>\n {artists.map(artist \\=> (\n <li key\\={artist.id}\\>{artist.name}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\nThe array spread syntax also lets you prepend an item by placing it _before_ the original `...artists`:\n\n```\nsetArtists([{ id: nextId++, name: name },...artists // Put old items at the end]);\n```\n\nIn this way, spread can do the job of both `push()` by adding to the end of an array and `unshift()` by adding to the beginning of an array. Try it in the sandbox above!\n\n### Removing from an array[](https://react.dev/learn/updating-arrays-in-state#removing-from-an-array \"Link for Removing from an array \")\n\nThe easiest way to remove an item from an array is to _filter it out_. In other words, you will produce a new array that will not contain that item. To do this, use the `filter` method, for example:\n\nimport { useState } from 'react';\n\nlet initialArtists = \\[\n { id: 0, name: 'Marta Colvin Andrade' },\n { id: 1, name: 'Lamidi Olonade Fakeye'},\n { id: 2, name: 'Louise Nevelson'},\n\\];\n\nexport default function List() {\n const \\[artists, setArtists\\] = useState(\n initialArtists\n );\n\n return (\n <\\>\n <h1\\>Inspiring sculptors:</h1\\>\n <ul\\>\n {artists.map(artist \\=> (\n <li key\\={artist.id}\\>\n {artist.name}{' '}\n <button onClick\\={() \\=> {\n setArtists(\n artists.filter(a \\=>\n a.id !== artist.id\n )\n );\n }}\\>\n Delete\n </button\\>\n </li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\nClick the “Delete” button a few times, and look at its click handler.\n\n```\nsetArtists(artists.filter(a => a.id !== artist.id));\n```\n\nHere, `artists.filter(a => a.id !== artist.id)` means “create an array that consists of those `artists` whose IDs are different from `artist.id`”. In other words, each artist’s “Delete” button will filter _that_ artist out of the array, and then request a re-render with the resulting array. Note that `filter` does not modify the original array.\n\n### Transforming an array[](https://react.dev/learn/updating-arrays-in-state#transforming-an-array \"Link for Transforming an array \")\n\nIf you want to change some or all items of the array, you can use `map()` to create a **new** array. The function you will pass to `map` can decide what to do with each item, based on its data or its index (or both).\n\nIn this example, an array holds coordinates of two circles and a square. When you press the button, it moves only the circles down by 50 pixels. It does this by producing a new array of data using `map()`:\n\nimport { useState } from 'react';\n\nlet initialShapes = \\[\n { id: 0, type: 'circle', x: 50, y: 100 },\n { id: 1, type: 'square', x: 150, y: 100 },\n { id: 2, type: 'circle', x: 250, y: 100 },\n\\];\n\nexport default function ShapeEditor() {\n const \\[shapes, setShapes\\] = useState(\n initialShapes\n );\n\n function handleClick() {\n const nextShapes = shapes.map(shape \\=> {\n if (shape.type === 'square') {\n \n return shape;\n } else {\n \n return {\n ...shape,\n y: shape.y + 50,\n };\n }\n });\n \n setShapes(nextShapes);\n }\n\n return (\n <\\>\n <button onClick\\={handleClick}\\>\n Move circles down!\n </button\\>\n {shapes.map(shape \\=> (\n <div\n key\\={shape.id}\n style\\={{\n background: 'purple',\n position: 'absolute',\n left: shape.x,\n top: shape.y,\n borderRadius:\n shape.type === 'circle'\n ? '50%' : '',\n width: 20,\n height: 20,\n }} />\n ))}\n </\\>\n );\n}\n\n### Replacing items in an array[](https://react.dev/learn/updating-arrays-in-state#replacing-items-in-an-array \"Link for Replacing items in an array \")\n\nIt is particularly common to want to replace one or more items in an array. Assignments like `arr[0] = 'bird'` are mutating the original array, so instead you’ll want to use `map` for this as well.\n\nTo replace an item, create a new array with `map`. Inside your `map` call, you will receive the item index as the second argument. Use it to decide whether to return the original item (the first argument) or something else:\n\nimport { useState } from 'react';\n\nlet initialCounters = \\[\n 0, 0, 0\n\\];\n\nexport default function CounterList() {\n const \\[counters, setCounters\\] = useState(\n initialCounters\n );\n\n function handleIncrementClick(index) {\n const nextCounters = counters.map((c, i) \\=> {\n if (i === index) {\n \n return c + 1;\n } else {\n \n return c;\n }\n });\n setCounters(nextCounters);\n }\n\n return (\n <ul\\>\n {counters.map((counter, i) \\=> (\n <li key\\={i}\\>\n {counter}\n <button onClick\\={() \\=> {\n handleIncrementClick(i);\n }}\\>+1</button\\>\n </li\\>\n ))}\n </ul\\>\n );\n}\n\n### Inserting into an array[](https://react.dev/learn/updating-arrays-in-state#inserting-into-an-array \"Link for Inserting into an array \")\n\nSometimes, you may want to insert an item at a particular position that’s neither at the beginning nor at the end. To do this, you can use the `...` array spread syntax together with the `slice()` method. The `slice()` method lets you cut a “slice” of the array. To insert an item, you will create an array that spreads the slice _before_ the insertion point, then the new item, and then the rest of the original array.\n\nIn this example, the Insert button always inserts at the index `1`:\n\nimport { useState } from 'react';\n\nlet nextId = 3;\nconst initialArtists = \\[\n { id: 0, name: 'Marta Colvin Andrade' },\n { id: 1, name: 'Lamidi Olonade Fakeye'},\n { id: 2, name: 'Louise Nevelson'},\n\\];\n\nexport default function List() {\n const \\[name, setName\\] = useState('');\n const \\[artists, setArtists\\] = useState(\n initialArtists\n );\n\n function handleClick() {\n const insertAt = 1; \n const nextArtists = \\[\n \n ...artists.slice(0, insertAt),\n \n { id: nextId++, name: name },\n \n ...artists.slice(insertAt)\n \\];\n setArtists(nextArtists);\n setName('');\n }\n\n return (\n <\\>\n <h1\\>Inspiring sculptors:</h1\\>\n <input\n value\\={name}\n onChange\\={e \\=> setName(e.target.value)}\n />\n <button onClick\\={handleClick}\\>\n Insert\n </button\\>\n <ul\\>\n {artists.map(artist \\=> (\n <li key\\={artist.id}\\>{artist.name}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\n### Making other changes to an array[](https://react.dev/learn/updating-arrays-in-state#making-other-changes-to-an-array \"Link for Making other changes to an array \")\n\nThere are some things you can’t do with the spread syntax and non-mutating methods like `map()` and `filter()` alone. For example, you may want to reverse or sort an array. The JavaScript `reverse()` and `sort()` methods are mutating the original array, so you can’t use them directly.\n\n**However, you can copy the array first, and then make changes to it.**\n\nFor example:\n\nimport { useState } from 'react';\n\nconst initialList = \\[\n { id: 0, title: 'Big Bellies' },\n { id: 1, title: 'Lunar Landscape' },\n { id: 2, title: 'Terracotta Army' },\n\\];\n\nexport default function List() {\n const \\[list, setList\\] = useState(initialList);\n\n function handleClick() {\n const nextList = \\[...list\\];\n nextList.reverse();\n setList(nextList);\n }\n\n return (\n <\\>\n <button onClick\\={handleClick}\\>\n Reverse\n </button\\>\n <ul\\>\n {list.map(artwork \\=> (\n <li key\\={artwork.id}\\>{artwork.title}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\nHere, you use the `[...list]` spread syntax to create a copy of the original array first. Now that you have a copy, you can use mutating methods like `nextList.reverse()` or `nextList.sort()`, or even assign individual items with `nextList[0] = \"something\"`.\n\nHowever, **even if you copy an array, you can’t mutate existing items _inside_ of it directly.** This is because copying is shallow—the new array will contain the same items as the original one. So if you modify an object inside the copied array, you are mutating the existing state. For example, code like this is a problem.\n\n```\nconst nextList = [...list];nextList[0].seen = true; // Problem: mutates list[0]setList(nextList);\n```\n\nAlthough `nextList` and `list` are two different arrays, **`nextList[0]` and `list[0]` point to the same object.** So by changing `nextList[0].seen`, you are also changing `list[0].seen`. This is a state mutation, which you should avoid! You can solve this issue in a similar way to [updating nested JavaScript objects](https://react.dev/learn/updating-objects-in-state#updating-a-nested-object)—by copying individual items you want to change instead of mutating them. Here’s how.\n\nUpdating objects inside arrays[](https://react.dev/learn/updating-arrays-in-state#updating-objects-inside-arrays \"Link for Updating objects inside arrays \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nObjects are not _really_ located “inside” arrays. They might appear to be “inside” in code, but each object in an array is a separate value, to which the array “points”. This is why you need to be careful when changing nested fields like `list[0]`. Another person’s artwork list may point to the same element of the array!\n\n**When updating nested state, you need to create copies from the point where you want to update, and all the way up to the top level.** Let’s see how this works.\n\nIn this example, two separate artwork lists have the same initial state. They are supposed to be isolated, but because of a mutation, their state is accidentally shared, and checking a box in one list affects the other list:\n\nimport { useState } from 'react';\n\nlet nextId = 3;\nconst initialList = \\[\n { id: 0, title: 'Big Bellies', seen: false },\n { id: 1, title: 'Lunar Landscape', seen: false },\n { id: 2, title: 'Terracotta Army', seen: true },\n\\];\n\nexport default function BucketList() {\n const \\[myList, setMyList\\] = useState(initialList);\n const \\[yourList, setYourList\\] = useState(\n initialList\n );\n\n function handleToggleMyList(artworkId, nextSeen) {\n const myNextList = \\[...myList\\];\n const artwork = myNextList.find(\n a \\=> a.id === artworkId\n );\n artwork.seen = nextSeen;\n setMyList(myNextList);\n }\n\n function handleToggleYourList(artworkId, nextSeen) {\n const yourNextList = \\[...yourList\\];\n const artwork = yourNextList.find(\n a \\=> a.id === artworkId\n );\n artwork.seen = nextSeen;\n setYourList(yourNextList);\n }\n\n return (\n <\\>\n <h1\\>Art Bucket List</h1\\>\n <h2\\>My list of art to see:</h2\\>\n <ItemList\n artworks\\={myList}\n onToggle\\={handleToggleMyList} />\n <h2\\>Your list of art to see:</h2\\>\n <ItemList\n artworks\\={yourList}\n onToggle\\={handleToggleYourList} />\n </\\>\n );\n}\n\nfunction ItemList({ artworks, onToggle }) {\n return (\n <ul\\>\n {artworks.map(artwork \\=> (\n <li key\\={artwork.id}\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={artwork.seen}\n onChange\\={e \\=> {\n onToggle(\n artwork.id,\n e.target.checked\n );\n }}\n />\n {artwork.title}\n </label\\>\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nThe problem is in code like this:\n\n```\nconst myNextList = [...myList];const artwork = myNextList.find(a => a.id === artworkId);artwork.seen = nextSeen; // Problem: mutates an existing itemsetMyList(myNextList);\n```\n\nAlthough the `myNextList` array itself is new, the _items themselves_ are the same as in the original `myList` array. So changing `artwork.seen` changes the _original_ artwork item. That artwork item is also in `yourList`, which causes the bug. Bugs like this can be difficult to think about, but thankfully they disappear if you avoid mutating state.\n\n**You can use `map` to substitute an old item with its updated version without mutation.**\n\n```\nsetMyList(myList.map(artwork => {if (artwork.id === artworkId) {// Create a *new* object with changesreturn { ...artwork, seen: nextSeen };} else {// No changesreturn artwork;}}));\n```\n\nHere, `...` is the object spread syntax used to [create a copy of an object.](https://react.dev/learn/updating-objects-in-state#copying-objects-with-the-spread-syntax)\n\nWith this approach, none of the existing state items are being mutated, and the bug is fixed:\n\nimport { useState } from 'react';\n\nlet nextId = 3;\nconst initialList = \\[\n { id: 0, title: 'Big Bellies', seen: false },\n { id: 1, title: 'Lunar Landscape', seen: false },\n { id: 2, title: 'Terracotta Army', seen: true },\n\\];\n\nexport default function BucketList() {\n const \\[myList, setMyList\\] = useState(initialList);\n const \\[yourList, setYourList\\] = useState(\n initialList\n );\n\n function handleToggleMyList(artworkId, nextSeen) {\n setMyList(myList.map(artwork \\=> {\n if (artwork.id === artworkId) {\n \n return { ...artwork, seen: nextSeen };\n } else {\n \n return artwork;\n }\n }));\n }\n\n function handleToggleYourList(artworkId, nextSeen) {\n setYourList(yourList.map(artwork \\=> {\n if (artwork.id === artworkId) {\n \n return { ...artwork, seen: nextSeen };\n } else {\n \n return artwork;\n }\n }));\n }\n\n return (\n <\\>\n <h1\\>Art Bucket List</h1\\>\n <h2\\>My list of art to see:</h2\\>\n <ItemList\n artworks\\={myList}\n onToggle\\={handleToggleMyList} />\n <h2\\>Your list of art to see:</h2\\>\n <ItemList\n artworks\\={yourList}\n onToggle\\={handleToggleYourList} />\n </\\>\n );\n}\n\nfunction ItemList({ artworks, onToggle }) {\n return (\n <ul\\>\n {artworks.map(artwork \\=> (\n <li key\\={artwork.id}\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={artwork.seen}\n onChange\\={e \\=> {\n onToggle(\n artwork.id,\n e.target.checked\n );\n }}\n />\n {artwork.title}\n </label\\>\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nIn general, **you should only mutate objects that you have just created.** If you were inserting a _new_ artwork, you could mutate it, but if you’re dealing with something that’s already in state, you need to make a copy.\n\n### Write concise update logic with Immer[](https://react.dev/learn/updating-arrays-in-state#write-concise-update-logic-with-immer \"Link for Write concise update logic with Immer \")\n\nUpdating nested arrays without mutation can get a little bit repetitive. [Just as with objects](https://react.dev/learn/updating-objects-in-state#write-concise-update-logic-with-immer):\n\n* Generally, you shouldn’t need to update state more than a couple of levels deep. If your state objects are very deep, you might want to [restructure them differently](https://react.dev/learn/choosing-the-state-structure#avoid-deeply-nested-state) so that they are flat.\n* If you don’t want to change your state structure, you might prefer to use [Immer](https://github.com/immerjs/use-immer), which lets you write using the convenient but mutating syntax and takes care of producing the copies for you.\n\nHere is the Art Bucket List example rewritten with Immer:\n\nNote how with Immer, **mutation like `artwork.seen = nextSeen` is now okay:**\n\n```\nupdateMyTodos(draft => {const artwork = draft.find(a => a.id === artworkId);artwork.seen = nextSeen;});\n```\n\nThis is because you’re not mutating the _original_ state, but you’re mutating a special `draft` object provided by Immer. Similarly, you can apply mutating methods like `push()` and `pop()` to the content of the `draft`.\n\nBehind the scenes, Immer always constructs the next state from scratch according to the changes that you’ve done to the `draft`. This keeps your event handlers very concise without ever mutating state.\n\nRecap[](https://react.dev/learn/updating-arrays-in-state#recap \"Link for Recap\")\n--------------------------------------------------------------------------------\n\n* You can put arrays into state, but you can’t change them.\n* Instead of mutating an array, create a _new_ version of it, and update the state to it.\n* You can use the `[...arr, newItem]` array spread syntax to create arrays with new items.\n* You can use `filter()` and `map()` to create new arrays with filtered or transformed items.\n* You can use Immer to keep your code concise.\n\nTry out some challenges[](https://react.dev/learn/updating-arrays-in-state#challenges \"Link for Try out some challenges\")\n-------------------------------------------------------------------------------------------------------------------------\n\n#### Update an item in the shopping cart[](https://react.dev/learn/updating-arrays-in-state#update-an-item-in-the-shopping-cart \"Link for this heading\")\n\nFill in the `handleIncreaseClick` logic so that pressing ”+” increases the corresponding number:\n\nimport { useState } from 'react';\n\nconst initialProducts = \\[{\n id: 0,\n name: 'Baklava',\n count: 1,\n}, {\n id: 1,\n name: 'Cheese',\n count: 5,\n}, {\n id: 2,\n name: 'Spaghetti',\n count: 2,\n}\\];\n\nexport default function ShoppingCart() {\n const \\[\n products,\n setProducts\n \\] = useState(initialProducts)\n\n function handleIncreaseClick(productId) {\n\n }\n\n return (\n <ul\\>\n {products.map(product \\=> (\n <li key\\={product.id}\\>\n {product.name}\n {' '}\n (<b\\>{product.count}</b\\>)\n <button onClick\\={() \\=> {\n handleIncreaseClick(product.id);\n }}\\>\n +\n </button\\>\n </li\\>\n ))}\n </ul\\>\n );\n}\n",
"filename": "updating-arrays-in-state.md",
"package": "react"
} |
{
"content": "Title: Updating Objects in State – React\n\nURL Source: https://react.dev/learn/updating-objects-in-state\n\nMarkdown Content:\nState can hold any kind of JavaScript value, including objects. But you shouldn’t change objects that you hold in the React state directly. Instead, when you want to update an object, you need to create a new one (or make a copy of an existing one), and then set the state to use that copy.\n\n### You will learn\n\n* How to correctly update an object in React state\n* How to update a nested object without mutating it\n* What immutability is, and how not to break it\n* How to make object copying less repetitive with Immer\n\nWhat’s a mutation?[](https://react.dev/learn/updating-objects-in-state#whats-a-mutation \"Link for What’s a mutation? \")\n-----------------------------------------------------------------------------------------------------------------------\n\nYou can store any kind of JavaScript value in state.\n\n```\nconst [x, setX] = useState(0);\n```\n\nSo far you’ve been working with numbers, strings, and booleans. These kinds of JavaScript values are “immutable”, meaning unchangeable or “read-only”. You can trigger a re-render to _replace_ a value:\n\nThe `x` state changed from `0` to `5`, but the _number `0` itself_ did not change. It’s not possible to make any changes to the built-in primitive values like numbers, strings, and booleans in JavaScript.\n\nNow consider an object in state:\n\n```\nconst [position, setPosition] = useState({ x: 0, y: 0 });\n```\n\nTechnically, it is possible to change the contents of _the object itself_. **This is called a mutation:**\n\nHowever, although objects in React state are technically mutable, you should treat them **as if** they were immutable—like numbers, booleans, and strings. Instead of mutating them, you should always replace them.\n\nTreat state as read-only[](https://react.dev/learn/updating-objects-in-state#treat-state-as-read-only \"Link for Treat state as read-only \")\n-------------------------------------------------------------------------------------------------------------------------------------------\n\nIn other words, you should **treat any JavaScript object that you put into state as read-only.**\n\nThis example holds an object in state to represent the current pointer position. The red dot is supposed to move when you touch or move the cursor over the preview area. But the dot stays in the initial position:\n\nimport { useState } from 'react';\nexport default function MovingDot() {\n const \\[position, setPosition\\] = useState({\n x: 0,\n y: 0\n });\n return (\n <div\n onPointerMove\\={e \\=> {\n position.x = e.clientX;\n position.y = e.clientY;\n }}\n style\\={{\n position: 'relative',\n width: '100vw',\n height: '100vh',\n }}\\>\n <div style\\={{\n position: 'absolute',\n backgroundColor: 'red',\n borderRadius: '50%',\n transform: \\`translate(${position.x}px, ${position.y}px)\\`,\n left: -10,\n top: -10,\n width: 20,\n height: 20,\n }} />\n </div\\>\n );\n}\n\nThe problem is with this bit of code.\n\n```\nonPointerMove={e => {position.x = e.clientX;position.y = e.clientY;}}\n```\n\nThis code modifies the object assigned to `position` from [the previous render.](https://react.dev/learn/state-as-a-snapshot#rendering-takes-a-snapshot-in-time) But without using the state setting function, React has no idea that object has changed. So React does not do anything in response. It’s like trying to change the order after you’ve already eaten the meal. While mutating state can work in some cases, we don’t recommend it. You should treat the state value you have access to in a render as read-only.\n\nTo actually [trigger a re-render](https://react.dev/learn/state-as-a-snapshot#setting-state-triggers-renders) in this case, **create a _new_ object and pass it to the state setting function:**\n\n```\nonPointerMove={e => {setPosition({x: e.clientX,y: e.clientY});}}\n```\n\nWith `setPosition`, you’re telling React:\n\n* Replace `position` with this new object\n* And render this component again\n\nNotice how the red dot now follows your pointer when you touch or hover over the preview area:\n\nimport { useState } from 'react';\nexport default function MovingDot() {\n const \\[position, setPosition\\] = useState({\n x: 0,\n y: 0\n });\n return (\n <div\n onPointerMove\\={e \\=> {\n setPosition({\n x: e.clientX,\n y: e.clientY\n });\n }}\n style\\={{\n position: 'relative',\n width: '100vw',\n height: '100vh',\n }}\\>\n <div style\\={{\n position: 'absolute',\n backgroundColor: 'red',\n borderRadius: '50%',\n transform: \\`translate(${position.x}px, ${position.y}px)\\`,\n left: -10,\n top: -10,\n width: 20,\n height: 20,\n }} />\n </div\\>\n );\n}\n\n##### Deep Dive\n\n#### Local mutation is fine[](https://react.dev/learn/updating-objects-in-state#local-mutation-is-fine \"Link for Local mutation is fine \")\n\nCode like this is a problem because it modifies an _existing_ object in state:\n\n```\nposition.x = e.clientX;position.y = e.clientY;\n```\n\nBut code like this is **absolutely fine** because you’re mutating a fresh object you have _just created_:\n\n```\nconst nextPosition = {};nextPosition.x = e.clientX;nextPosition.y = e.clientY;setPosition(nextPosition);\n```\n\nIn fact, it is completely equivalent to writing this:\n\n```\nsetPosition({x: e.clientX,y: e.clientY});\n```\n\nMutation is only a problem when you change _existing_ objects that are already in state. Mutating an object you’ve just created is okay because _no other code references it yet._ Changing it isn’t going to accidentally impact something that depends on it. This is called a “local mutation”. You can even do local mutation [while rendering.](https://react.dev/learn/keeping-components-pure#local-mutation-your-components-little-secret) Very convenient and completely okay!\n\nCopying objects with the spread syntax[](https://react.dev/learn/updating-objects-in-state#copying-objects-with-the-spread-syntax \"Link for Copying objects with the spread syntax \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn the previous example, the `position` object is always created fresh from the current cursor position. But often, you will want to include _existing_ data as a part of the new object you’re creating. For example, you may want to update _only one_ field in a form, but keep the previous values for all other fields.\n\nThese input fields don’t work because the `onChange` handlers mutate the state:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[person, setPerson\\] = useState({\n firstName: 'Barbara',\n lastName: 'Hepworth',\n email: 'bhepworth@sculpture.com'\n });\n\n function handleFirstNameChange(e) {\n person.firstName = e.target.value;\n }\n\n function handleLastNameChange(e) {\n person.lastName = e.target.value;\n }\n\n function handleEmailChange(e) {\n person.email = e.target.value;\n }\n\n return (\n <\\>\n <label\\>\n First name:\n <input\n value\\={person.firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:\n <input\n value\\={person.lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n <label\\>\n Email:\n <input\n value\\={person.email}\n onChange\\={handleEmailChange}\n />\n </label\\>\n <p\\>\n {person.firstName}{' '}\n {person.lastName}{' '}\n ({person.email})\n </p\\>\n </\\>\n );\n}\n\nFor example, this line mutates the state from a past render:\n\n```\nperson.firstName = e.target.value;\n```\n\nThe reliable way to get the behavior you’re looking for is to create a new object and pass it to `setPerson`. But here, you want to also **copy the existing data into it** because only one of the fields has changed:\n\n```\nsetPerson({firstName: e.target.value, // New first name from the inputlastName: person.lastName,email: person.email});\n```\n\nYou can use the `...` [object spread](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax#spread_in_object_literals) syntax so that you don’t need to copy every property separately.\n\n```\nsetPerson({...person, // Copy the old fieldsfirstName: e.target.value // But override this one});\n```\n\nNow the form works!\n\nNotice how you didn’t declare a separate state variable for each input field. For large forms, keeping all data grouped in an object is very convenient—as long as you update it correctly!\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[person, setPerson\\] = useState({\n firstName: 'Barbara',\n lastName: 'Hepworth',\n email: 'bhepworth@sculpture.com'\n });\n\n function handleFirstNameChange(e) {\n setPerson({\n ...person,\n firstName: e.target.value\n });\n }\n\n function handleLastNameChange(e) {\n setPerson({\n ...person,\n lastName: e.target.value\n });\n }\n\n function handleEmailChange(e) {\n setPerson({\n ...person,\n email: e.target.value\n });\n }\n\n return (\n <\\>\n <label\\>\n First name:\n <input\n value\\={person.firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:\n <input\n value\\={person.lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n <label\\>\n Email:\n <input\n value\\={person.email}\n onChange\\={handleEmailChange}\n />\n </label\\>\n <p\\>\n {person.firstName}{' '}\n {person.lastName}{' '}\n ({person.email})\n </p\\>\n </\\>\n );\n}\n\nNote that the `...` spread syntax is “shallow”—it only copies things one level deep. This makes it fast, but it also means that if you want to update a nested property, you’ll have to use it more than once.\n\n##### Deep Dive\n\n#### Using a single event handler for multiple fields[](https://react.dev/learn/updating-objects-in-state#using-a-single-event-handler-for-multiple-fields \"Link for Using a single event handler for multiple fields \")\n\nYou can also use the `[` and `]` braces inside your object definition to specify a property with dynamic name. Here is the same example, but with a single event handler instead of three different ones:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[person, setPerson\\] = useState({\n firstName: 'Barbara',\n lastName: 'Hepworth',\n email: 'bhepworth@sculpture.com'\n });\n\n function handleChange(e) {\n setPerson({\n ...person,\n \\[e.target.name\\]: e.target.value\n });\n }\n\n return (\n <\\>\n <label\\>\n First name:\n <input\n name\\=\"firstName\"\n value\\={person.firstName}\n onChange\\={handleChange}\n />\n </label\\>\n <label\\>\n Last name:\n <input\n name\\=\"lastName\"\n value\\={person.lastName}\n onChange\\={handleChange}\n />\n </label\\>\n <label\\>\n Email:\n <input\n name\\=\"email\"\n value\\={person.email}\n onChange\\={handleChange}\n />\n </label\\>\n <p\\>\n {person.firstName}{' '}\n {person.lastName}{' '}\n ({person.email})\n </p\\>\n </\\>\n );\n}\n\nHere, `e.target.name` refers to the `name` property given to the `<input>` DOM element.\n\nUpdating a nested object[](https://react.dev/learn/updating-objects-in-state#updating-a-nested-object \"Link for Updating a nested object \")\n-------------------------------------------------------------------------------------------------------------------------------------------\n\nConsider a nested object structure like this:\n\n```\nconst [person, setPerson] = useState({name: 'Niki de Saint Phalle',artwork: {title: 'Blue Nana',city: 'Hamburg',image: 'https://i.imgur.com/Sd1AgUOm.jpg',}});\n```\n\nIf you wanted to update `person.artwork.city`, it’s clear how to do it with mutation:\n\n```\nperson.artwork.city = 'New Delhi';\n```\n\nBut in React, you treat state as immutable! In order to change `city`, you would first need to produce the new `artwork` object (pre-populated with data from the previous one), and then produce the new `person` object which points at the new `artwork`:\n\n```\nconst nextArtwork = { ...person.artwork, city: 'New Delhi' };const nextPerson = { ...person, artwork: nextArtwork };setPerson(nextPerson);\n```\n\nOr, written as a single function call:\n\n```\nsetPerson({...person, // Copy other fieldsartwork: { // but replace the artwork...person.artwork, // with the same onecity: 'New Delhi' // but in New Delhi!}});\n```\n\nThis gets a bit wordy, but it works fine for many cases:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[person, setPerson\\] = useState({\n name: 'Niki de Saint Phalle',\n artwork: {\n title: 'Blue Nana',\n city: 'Hamburg',\n image: 'https://i.imgur.com/Sd1AgUOm.jpg',\n }\n });\n\n function handleNameChange(e) {\n setPerson({\n ...person,\n name: e.target.value\n });\n }\n\n function handleTitleChange(e) {\n setPerson({\n ...person,\n artwork: {\n ...person.artwork,\n title: e.target.value\n }\n });\n }\n\n function handleCityChange(e) {\n setPerson({\n ...person,\n artwork: {\n ...person.artwork,\n city: e.target.value\n }\n });\n }\n\n function handleImageChange(e) {\n setPerson({\n ...person,\n artwork: {\n ...person.artwork,\n image: e.target.value\n }\n });\n }\n\n return (\n <\\>\n <label\\>\n Name:\n <input\n value\\={person.name}\n onChange\\={handleNameChange}\n />\n </label\\>\n <label\\>\n Title:\n <input\n value\\={person.artwork.title}\n onChange\\={handleTitleChange}\n />\n </label\\>\n <label\\>\n City:\n <input\n value\\={person.artwork.city}\n onChange\\={handleCityChange}\n />\n </label\\>\n <label\\>\n Image:\n <input\n value\\={person.artwork.image}\n onChange\\={handleImageChange}\n />\n </label\\>\n <p\\>\n <i\\>{person.artwork.title}</i\\>\n {' by '}\n {person.name}\n <br />\n (located in {person.artwork.city})\n </p\\>\n <img \n src\\={person.artwork.image} \n alt\\={person.artwork.title}\n />\n </\\>\n );\n}\n\n##### Deep Dive\n\n#### Objects are not really nested[](https://react.dev/learn/updating-objects-in-state#objects-are-not-really-nested \"Link for Objects are not really nested \")\n\nAn object like this appears “nested” in code:\n\n```\nlet obj = {name: 'Niki de Saint Phalle',artwork: {title: 'Blue Nana',city: 'Hamburg',image: 'https://i.imgur.com/Sd1AgUOm.jpg',}};\n```\n\nHowever, “nesting” is an inaccurate way to think about how objects behave. When the code executes, there is no such thing as a “nested” object. You are really looking at two different objects:\n\n```\nlet obj1 = {title: 'Blue Nana',city: 'Hamburg',image: 'https://i.imgur.com/Sd1AgUOm.jpg',};let obj2 = {name: 'Niki de Saint Phalle',artwork: obj1};\n```\n\nThe `obj1` object is not “inside” `obj2`. For example, `obj3` could “point” at `obj1` too:\n\n```\nlet obj1 = {title: 'Blue Nana',city: 'Hamburg',image: 'https://i.imgur.com/Sd1AgUOm.jpg',};let obj2 = {name: 'Niki de Saint Phalle',artwork: obj1};let obj3 = {name: 'Copycat',artwork: obj1};\n```\n\nIf you were to mutate `obj3.artwork.city`, it would affect both `obj2.artwork.city` and `obj1.city`. This is because `obj3.artwork`, `obj2.artwork`, and `obj1` are the same object. This is difficult to see when you think of objects as “nested”. Instead, they are separate objects “pointing” at each other with properties.\n\n### Write concise update logic with Immer[](https://react.dev/learn/updating-objects-in-state#write-concise-update-logic-with-immer \"Link for Write concise update logic with Immer \")\n\nIf your state is deeply nested, you might want to consider [flattening it.](https://react.dev/learn/choosing-the-state-structure#avoid-deeply-nested-state) But, if you don’t want to change your state structure, you might prefer a shortcut to nested spreads. [Immer](https://github.com/immerjs/use-immer) is a popular library that lets you write using the convenient but mutating syntax and takes care of producing the copies for you. With Immer, the code you write looks like you are “breaking the rules” and mutating an object:\n\n```\nupdatePerson(draft => {draft.artwork.city = 'Lagos';});\n```\n\nBut unlike a regular mutation, it doesn’t overwrite the past state!\n\n##### Deep Dive\n\n#### How does Immer work?[](https://react.dev/learn/updating-objects-in-state#how-does-immer-work \"Link for How does Immer work? \")\n\nThe `draft` provided by Immer is a special type of object, called a [Proxy](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Proxy), that “records” what you do with it. This is why you can mutate it freely as much as you like! Under the hood, Immer figures out which parts of the `draft` have been changed, and produces a completely new object that contains your edits.\n\nTo try Immer:\n\n1. Run `npm install use-immer` to add Immer as a dependency\n2. Then replace `import { useState } from 'react'` with `import { useImmer } from 'use-immer'`\n\nHere is the above example converted to Immer:\n\nNotice how much more concise the event handlers have become. You can mix and match `useState` and `useImmer` in a single component as much as you like. Immer is a great way to keep the update handlers concise, especially if there’s nesting in your state, and copying objects leads to repetitive code.\n\n##### Deep Dive\n\n#### Why is mutating state not recommended in React?[](https://react.dev/learn/updating-objects-in-state#why-is-mutating-state-not-recommended-in-react \"Link for Why is mutating state not recommended in React? \")\n\nThere are a few reasons:\n\n* **Debugging:** If you use `console.log` and don’t mutate state, your past logs won’t get clobbered by the more recent state changes. So you can clearly see how state has changed between renders.\n* **Optimizations:** Common React [optimization strategies](https://react.dev/reference/react/memo) rely on skipping work if previous props or state are the same as the next ones. If you never mutate state, it is very fast to check whether there were any changes. If `prevObj === obj`, you can be sure that nothing could have changed inside of it.\n* **New Features:** The new React features we’re building rely on state being [treated like a snapshot.](https://react.dev/learn/state-as-a-snapshot) If you’re mutating past versions of state, that may prevent you from using the new features.\n* **Requirement Changes:** Some application features, like implementing Undo/Redo, showing a history of changes, or letting the user reset a form to earlier values, are easier to do when nothing is mutated. This is because you can keep past copies of state in memory, and reuse them when appropriate. If you start with a mutative approach, features like this can be difficult to add later on.\n* **Simpler Implementation:** Because React does not rely on mutation, it does not need to do anything special with your objects. It does not need to hijack their properties, always wrap them into Proxies, or do other work at initialization as many “reactive” solutions do. This is also why React lets you put any object into state—no matter how large—without additional performance or correctness pitfalls.\n\nIn practice, you can often “get away” with mutating state in React, but we strongly advise you not to do that so that you can use new React features developed with this approach in mind. Future contributors and perhaps even your future self will thank you!\n\nRecap[](https://react.dev/learn/updating-objects-in-state#recap \"Link for Recap\")\n---------------------------------------------------------------------------------\n\n* Treat all state in React as immutable.\n* When you store objects in state, mutating them will not trigger renders and will change the state in previous render “snapshots”.\n* Instead of mutating an object, create a _new_ version of it, and trigger a re-render by setting state to it.\n* You can use the `{...obj, something: 'newValue'}` object spread syntax to create copies of objects.\n* Spread syntax is shallow: it only copies one level deep.\n* To update a nested object, you need to create copies all the way up from the place you’re updating.\n* To reduce repetitive copying code, use Immer.\n\nTry out some challenges[](https://react.dev/learn/updating-objects-in-state#challenges \"Link for Try out some challenges\")\n--------------------------------------------------------------------------------------------------------------------------\n\n#### Fix incorrect state updates[](https://react.dev/learn/updating-objects-in-state#fix-incorrect-state-updates \"Link for this heading\")\n\nThis form has a few bugs. Click the button that increases the score a few times. Notice that it does not increase. Then edit the first name, and notice that the score has suddenly “caught up” with your changes. Finally, edit the last name, and notice that the score has disappeared completely.\n\nYour task is to fix all of these bugs. As you fix them, explain why each of them happens.\n\nimport { useState } from 'react';\n\nexport default function Scoreboard() {\n const \\[player, setPlayer\\] = useState({\n firstName: 'Ranjani',\n lastName: 'Shettar',\n score: 10,\n });\n\n function handlePlusClick() {\n player.score++;\n }\n\n function handleFirstNameChange(e) {\n setPlayer({\n ...player,\n firstName: e.target.value,\n });\n }\n\n function handleLastNameChange(e) {\n setPlayer({\n lastName: e.target.value\n });\n }\n\n return (\n <\\>\n <label\\>\n Score: <b\\>{player.score}</b\\>\n {' '}\n <button onClick\\={handlePlusClick}\\>\n +1\n </button\\>\n </label\\>\n <label\\>\n First name:\n <input\n value\\={player.firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:\n <input\n value\\={player.lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n </\\>\n );\n}\n",
"filename": "updating-objects-in-state.md",
"package": "react"
} |
{
"content": "Title: 'use client' directive – React\n\nURL Source: https://react.dev/reference/rsc/use-client\n\nMarkdown Content:\n### Canary\n\n`'use client'` lets you mark what code runs on the client.\n\n* [Reference](https://react.dev/reference/rsc/use-client#reference)\n * [`'use client'`](https://react.dev/reference/rsc/use-client#use-client)\n * [How `'use client'` marks client code](https://react.dev/reference/rsc/use-client#how-use-client-marks-client-code)\n * [When to use `'use client'`](https://react.dev/reference/rsc/use-client#when-to-use-use-client)\n * [Serializable types returned by Server Components](https://react.dev/reference/rsc/use-client#serializable-types)\n* [Usage](https://react.dev/reference/rsc/use-client#usage)\n * [Building with interactivity and state](https://react.dev/reference/rsc/use-client#building-with-interactivity-and-state)\n * [Using client APIs](https://react.dev/reference/rsc/use-client#using-client-apis)\n * [Using third-party libraries](https://react.dev/reference/rsc/use-client#using-third-party-libraries)\n\n* * *\n\nReference[](https://react.dev/reference/rsc/use-client#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `'use client'`[](https://react.dev/reference/rsc/use-client#use-client \"Link for this heading\")\n\nAdd `'use client'` at the top of a file to mark the module and its transitive dependencies as client code.\n\n```\n'use client';import { useState } from 'react';import { formatDate } from './formatters';import Button from './button';export default function RichTextEditor({ timestamp, text }) {const date = formatDate(timestamp);// ...const editButton = <Button />;// ...}\n```\n\nWhen a file marked with `'use client'` is imported from a Server Component, [compatible bundlers](https://react.dev/learn/start-a-new-react-project#bleeding-edge-react-frameworks) will treat the module import as a boundary between server-run and client-run code.\n\nAs dependencies of `RichTextEditor`, `formatDate` and `Button` will also be evaluated on the client regardless of whether their modules contain a `'use client'` directive. Note that a single module may be evaluated on the server when imported from server code and on the client when imported from client code.\n\n#### Caveats[](https://react.dev/reference/rsc/use-client#caveats \"Link for Caveats \")\n\n* `'use client'` must be at the very beginning of a file, above any imports or other code (comments are OK). They must be written with single or double quotes, but not backticks.\n* When a `'use client'` module is imported from another client-rendered module, the directive has no effect.\n* When a component module contains a `'use client'` directive, any usage of that component is guaranteed to be a Client Component. However, a component can still be evaluated on the client even if it does not have a `'use client'` directive.\n * A component usage is considered a Client Component if it is defined in module with `'use client'` directive or when it is a transitive dependency of a module that contains a `'use client'` directive. Otherwise, it is a Server Component.\n* Code that is marked for client evaluation is not limited to components. All code that is a part of the Client module sub-tree is sent to and run by the client.\n* When a server evaluated module imports values from a `'use client'` module, the values must either be a React component or [supported serializable prop values](https://react.dev/reference/rsc/use-client#passing-props-from-server-to-client-components) to be passed to a Client Component. Any other use case will throw an exception.\n\n### How `'use client'` marks client code[](https://react.dev/reference/rsc/use-client#how-use-client-marks-client-code \"Link for this heading\")\n\nIn a React app, components are often split into separate files, or [modules](https://react.dev/learn/importing-and-exporting-components#exporting-and-importing-a-component).\n\nFor apps that use React Server Components, the app is server-rendered by default. `'use client'` introduces a server-client boundary in the [module dependency tree](https://react.dev/learn/understanding-your-ui-as-a-tree#the-module-dependency-tree), effectively creating a subtree of Client modules.\n\nTo better illustrate this, consider the following React Server Components app.\n\nIn the module dependency tree of this example app, the `'use client'` directive in `InspirationGenerator.js` marks that module and all of its transitive dependencies as Client modules. The subtree starting at `InspirationGenerator.js` is now marked as Client modules.\n\n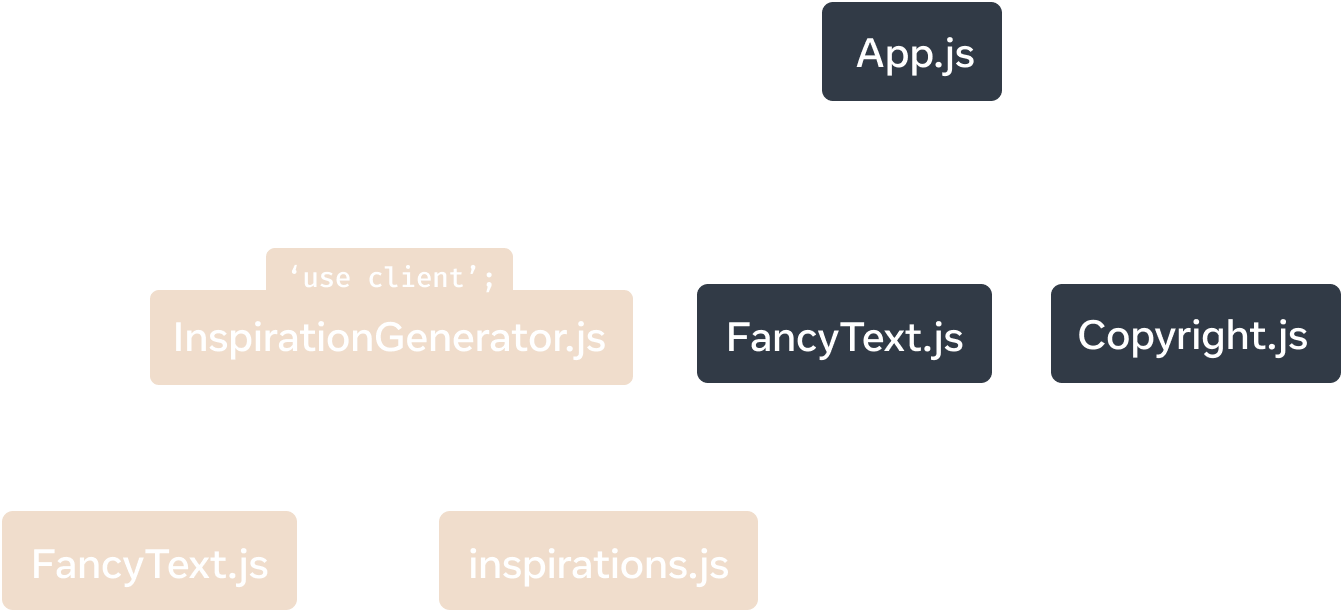\n\n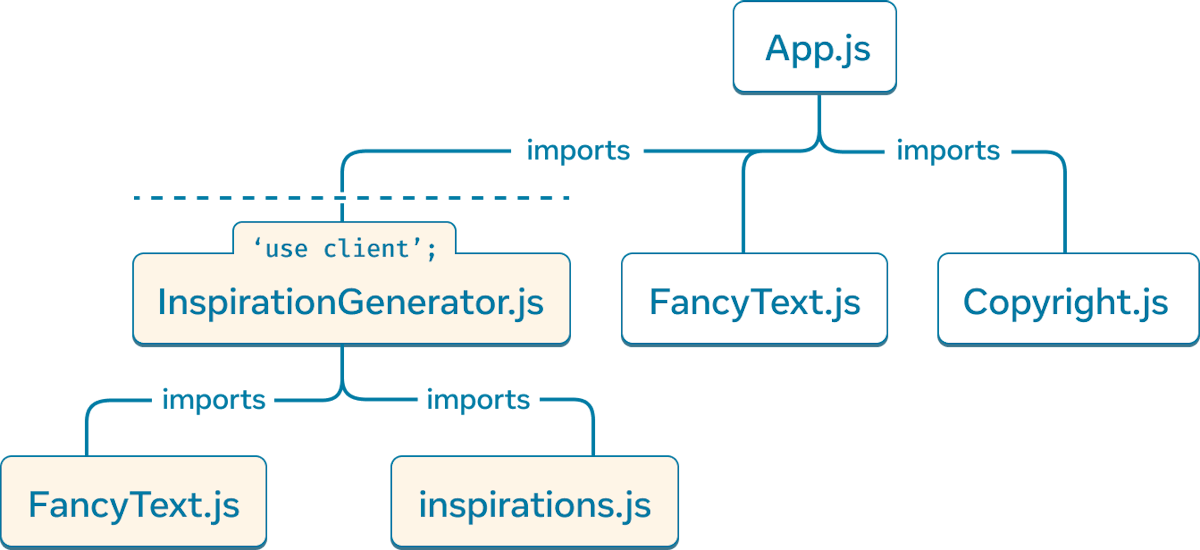\n\n`'use client'` segments the module dependency tree of the React Server Components app, marking `InspirationGenerator.js` and all of its dependencies as client-rendered.\n\nDuring render, the framework will server-render the root component and continue through the [render tree](https://react.dev/learn/understanding-your-ui-as-a-tree#the-render-tree), opting-out of evaluating any code imported from client-marked code.\n\nThe server-rendered portion of the render tree is then sent to the client. The client, with its client code downloaded, then completes rendering the rest of the tree.\n\n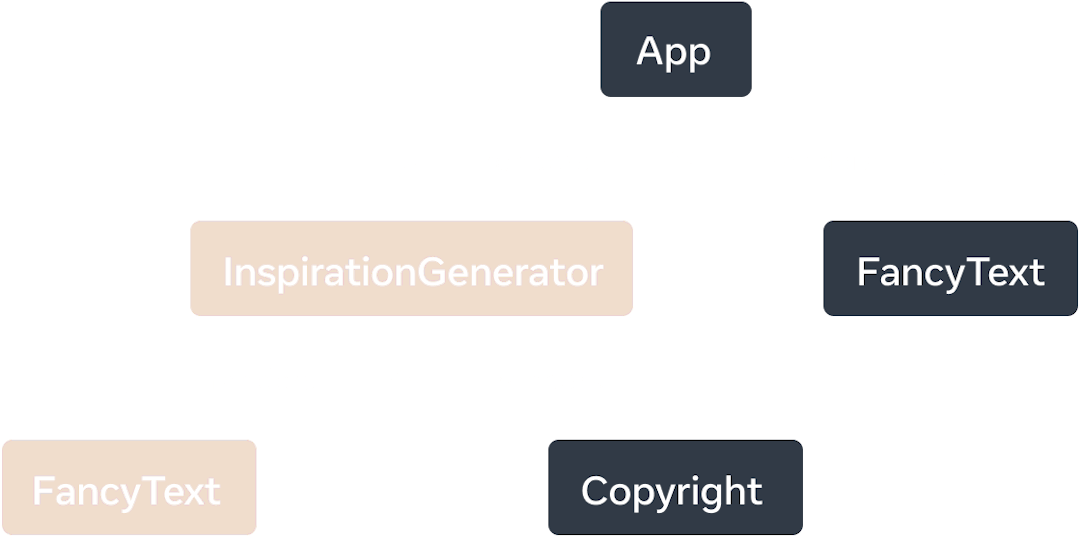\n\n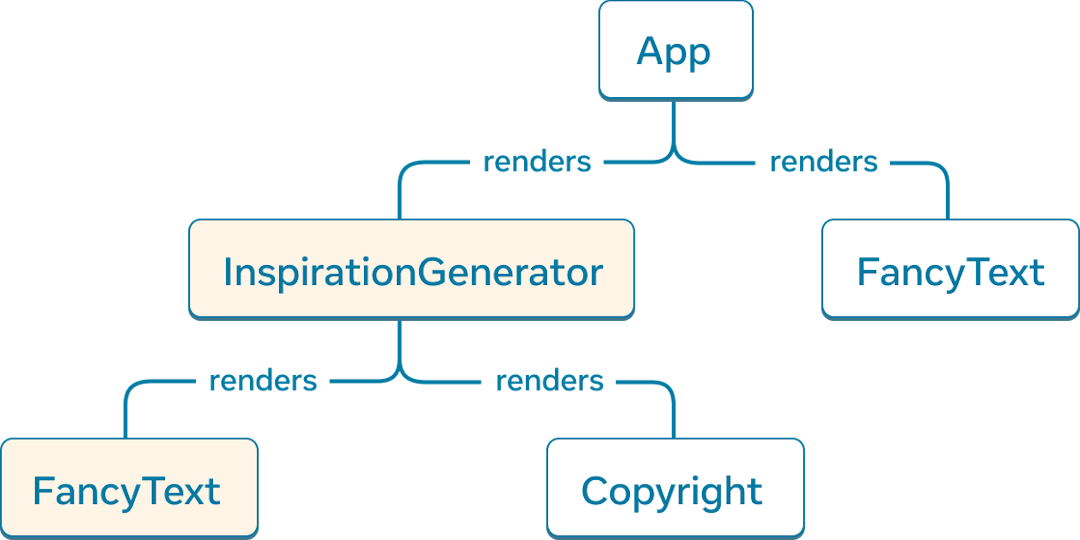\n\nThe render tree for the React Server Components app. `InspirationGenerator` and its child component `FancyText` are components exported from client-marked code and considered Client Components.\n\nWe introduce the following definitions:\n\n* **Client Components** are components in a render tree that are rendered on the client.\n* **Server Components** are components in a render tree that are rendered on the server.\n\nWorking through the example app, `App`, `FancyText` and `Copyright` are all server-rendered and considered Server Components. As `InspirationGenerator.js` and its transitive dependencies are marked as client code, the component `InspirationGenerator` and its child component `FancyText` are Client Components.\n\n##### Deep Dive\n\n#### How is `FancyText` both a Server and a Client Component?[](https://react.dev/reference/rsc/use-client#how-is-fancytext-both-a-server-and-a-client-component \"Link for this heading\")\n\nBy the above definitions, the component `FancyText` is both a Server and Client Component, how can that be?\n\nFirst, let’s clarify that the term “component” is not very precise. Here are just two ways “component” can be understood:\n\n1. A “component” can refer to a **component definition**. In most cases this will be a function.\n\n```\n// This is a definition of a componentfunction MyComponent() {return <p>My Component</p>}\n```\n\n2. A “component” can also refer to a **component usage** of its definition.\n\n```\nimport MyComponent from './MyComponent';function App() {// This is a usage of a componentreturn <MyComponent />;}\n```\n\nOften, the imprecision is not important when explaining concepts, but in this case it is.\n\nWhen we talk about Server or Client Components, we are referring to component usages.\n\n* If the component is defined in a module with a `'use client'` directive, or the component is imported and called in a Client Component, then the component usage is a Client Component.\n* Otherwise, the component usage is a Server Component.\n\n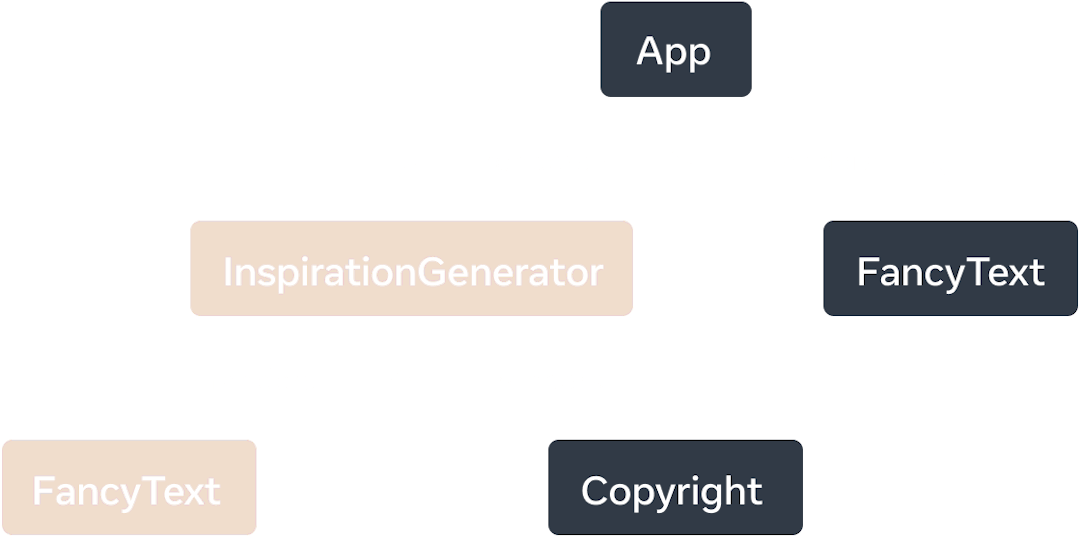\n\n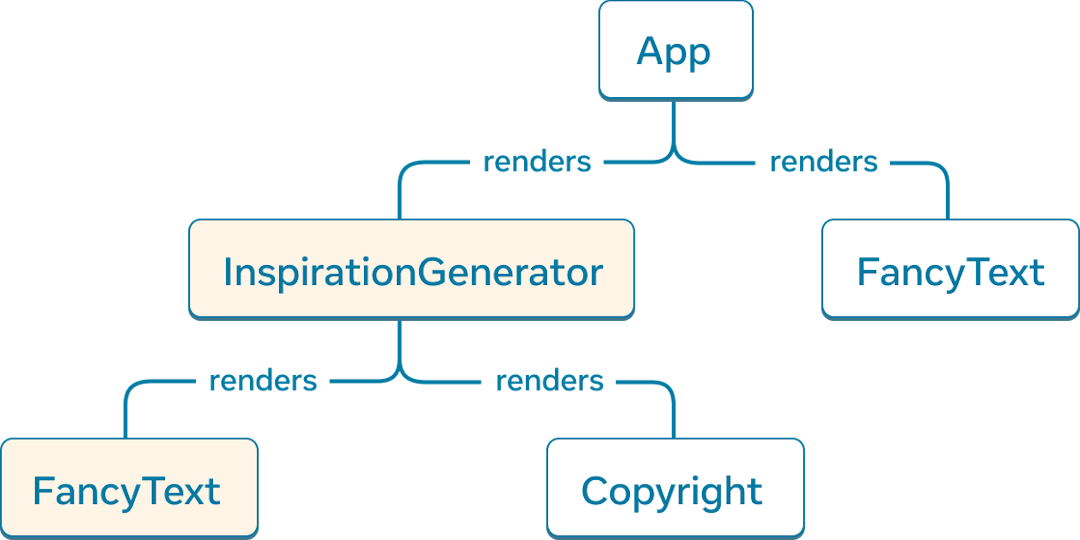\n\nA render tree illustrates component usages.\n\nBack to the question of `FancyText`, we see that the component definition does _not_ have a `'use client'` directive and it has two usages.\n\nThe usage of `FancyText` as a child of `App`, marks that usage as a Server Component. When `FancyText` is imported and called under `InspirationGenerator`, that usage of `FancyText` is a Client Component as `InspirationGenerator` contains a `'use client'` directive.\n\nThis means that the component definition for `FancyText` will both be evaluated on the server and also downloaded by the client to render its Client Component usage.\n\n##### Deep Dive\n\n#### Why is `Copyright` a Server Component?[](https://react.dev/reference/rsc/use-client#why-is-copyright-a-server-component \"Link for this heading\")\n\nBecause `Copyright` is rendered as a child of the Client Component `InspirationGenerator`, you might be surprised that it is a Server Component.\n\nRecall that `'use client'` defines the boundary between server and client code on the _module dependency tree_, not the render tree.\n\n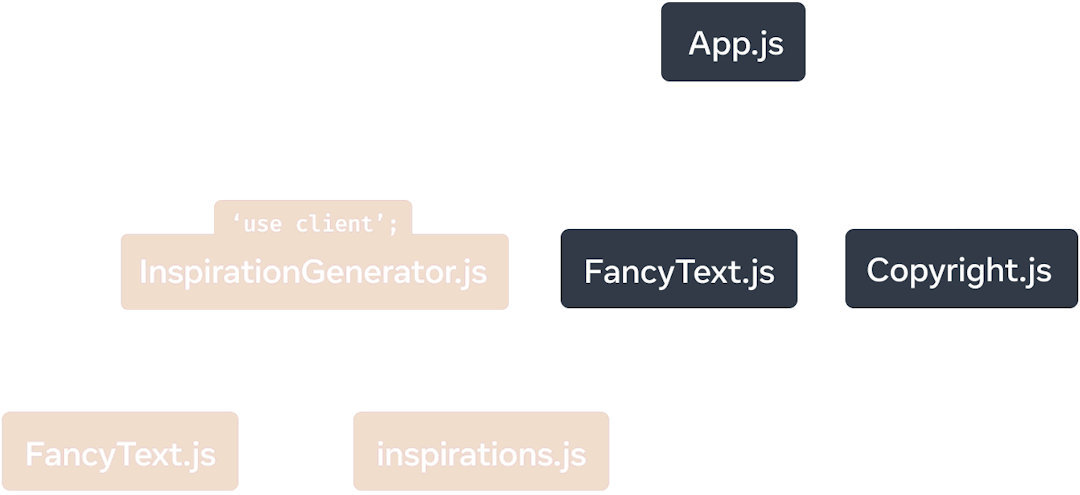\n\n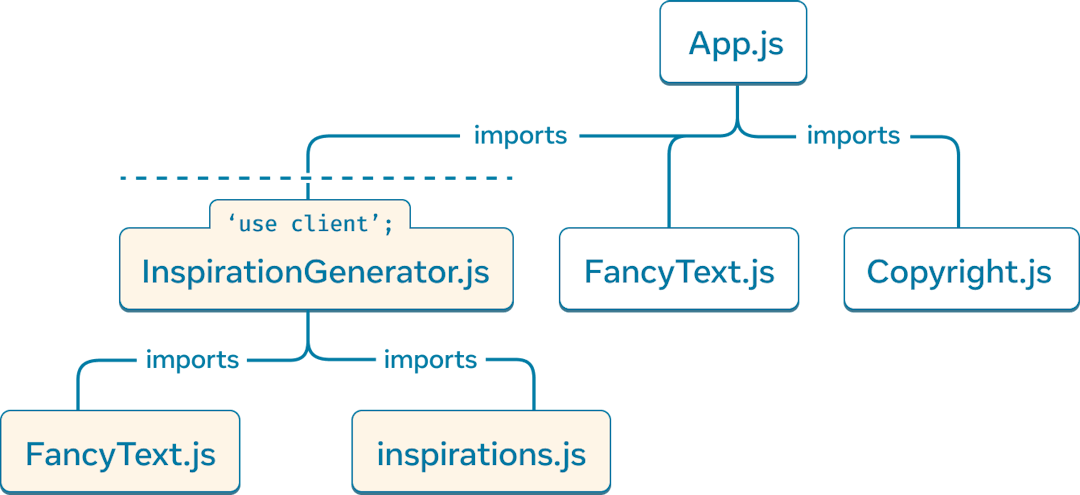\n\n`'use client'` defines the boundary between server and client code on the module dependency tree.\n\nIn the module dependency tree, we see that `App.js` imports and calls `Copyright` from the `Copyright.js` module. As `Copyright.js` does not contain a `'use client'` directive, the component usage is rendered on the server. `App` is rendered on the server as it is the root component.\n\nClient Components can render Server Components because you can pass JSX as props. In this case, `InspirationGenerator` receives `Copyright` as [children](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children). However, the `InspirationGenerator` module never directly imports the `Copyright` module nor calls the component, all of that is done by `App`. In fact, the `Copyright` component is fully executed before `InspirationGenerator` starts rendering.\n\nThe takeaway is that a parent-child render relationship between components does not guarantee the same render environment.\n\n### When to use `'use client'`[](https://react.dev/reference/rsc/use-client#when-to-use-use-client \"Link for this heading\")\n\nWith `'use client'`, you can determine when components are Client Components. As Server Components are default, here is a brief overview of the advantages and limitations to Server Components to determine when you need to mark something as client rendered.\n\nFor simplicity, we talk about Server Components, but the same principles apply to all code in your app that is server run.\n\n#### Advantages of Server Components[](https://react.dev/reference/rsc/use-client#advantages \"Link for Advantages of Server Components \")\n\n* Server Components can reduce the amount of code sent and run by the client. Only Client modules are bundled and evaluated by the client.\n* Server Components benefit from running on the server. They can access the local filesystem and may experience low latency for data fetches and network requests.\n\n#### Limitations of Server Components[](https://react.dev/reference/rsc/use-client#limitations \"Link for Limitations of Server Components \")\n\n* Server Components cannot support interaction as event handlers must be registered and triggered by a client.\n * For example, event handlers like `onClick` can only be defined in Client Components.\n* Server Components cannot use most Hooks.\n * When Server Components are rendered, their output is essentially a list of components for the client to render. Server Components do not persist in memory after render and cannot have their own state.\n\n### Serializable types returned by Server Components[](https://react.dev/reference/rsc/use-client#serializable-types \"Link for Serializable types returned by Server Components \")\n\nAs in any React app, parent components pass data to child components. As they are rendered in different environments, passing data from a Server Component to a Client Component requires extra consideration.\n\nProp values passed from a Server Component to Client Component must be serializable.\n\nSerializable props include:\n\n* Primitives\n * [string](https://developer.mozilla.org/en-US/docs/Glossary/String)\n * [number](https://developer.mozilla.org/en-US/docs/Glossary/Number)\n * [bigint](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/BigInt)\n * [boolean](https://developer.mozilla.org/en-US/docs/Glossary/Boolean)\n * [undefined](https://developer.mozilla.org/en-US/docs/Glossary/Undefined)\n * [null](https://developer.mozilla.org/en-US/docs/Glossary/Null)\n * [symbol](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol), only symbols registered in the global Symbol registry via [`Symbol.for`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol/for)\n* Iterables containing serializable values\n * [String](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String)\n * [Array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array)\n * [Map](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map)\n * [Set](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Set)\n * [TypedArray](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray) and [ArrayBuffer](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/ArrayBuffer)\n* [Date](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date)\n* Plain [objects](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object): those created with [object initializers](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Object_initializer), with serializable properties\n* Functions that are [Server Actions](https://react.dev/reference/rsc/use-server)\n* Client or Server Component elements (JSX)\n* [Promises](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise)\n\nNotably, these are not supported:\n\n* [Functions](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function) that are not exported from client-marked modules or marked with [`'use server'`](https://react.dev/reference/rsc/use-server)\n* [Classes](https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Classes_in_JavaScript)\n* Objects that are instances of any class (other than the built-ins mentioned) or objects with [a null prototype](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object#null-prototype_objects)\n* Symbols not registered globally, ex. `Symbol('my new symbol')`\n\nUsage[](https://react.dev/reference/rsc/use-client#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Building with interactivity and state[](https://react.dev/reference/rsc/use-client#building-with-interactivity-and-state \"Link for Building with interactivity and state \")\n\n'use client';\n\nimport { useState } from 'react';\n\nexport default function Counter({initialValue = 0}) {\n const \\[countValue, setCountValue\\] = useState(initialValue);\n const increment = () \\=> setCountValue(countValue + 1);\n const decrement = () \\=> setCountValue(countValue - 1);\n return (\n <\\>\n <h2\\>Count Value: {countValue}</h2\\>\n <button onClick\\={increment}\\>+1</button\\>\n <button onClick\\={decrement}\\>\\-1</button\\>\n </\\>\n );\n}\n\nAs `Counter` requires both the `useState` Hook and event handlers to increment or decrement the value, this component must be a Client Component and will require a `'use client'` directive at the top.\n\nIn contrast, a component that renders UI without interaction will not need to be a Client Component.\n\n```\nimport { readFile } from 'node:fs/promises';import Counter from './Counter';export default async function CounterContainer() {const initialValue = await readFile('/path/to/counter_value');return <Counter initialValue={initialValue} />}\n```\n\nFor example, `Counter`’s parent component, `CounterContainer`, does not require `'use client'` as it is not interactive and does not use state. In addition, `CounterContainer` must be a Server Component as it reads from the local file system on the server, which is possible only in a Server Component.\n\nThere are also components that don’t use any server or client-only features and can be agnostic to where they render. In our earlier example, `FancyText` is one such component.\n\n```\nexport default function FancyText({title, text}) {return title ? <h1 className='fancy title'>{text}</h1> : <h3 className='fancy cursive'>{text}</h3>}\n```\n\nIn this case, we don’t add the `'use client'` directive, resulting in `FancyText`’s _output_ (rather than its source code) to be sent to the browser when referenced from a Server Component. As demonstrated in the earlier Inspirations app example, `FancyText` is used as both a Server or Client Component, depending on where it is imported and used.\n\nBut if `FancyText`’s HTML output was large relative to its source code (including dependencies), it might be more efficient to force it to always be a Client Component. Components that return a long SVG path string are one case where it may be more efficient to force a component to be a Client Component.\n\n### Using client APIs[](https://react.dev/reference/rsc/use-client#using-client-apis \"Link for Using client APIs \")\n\nYour React app may use client-specific APIs, such as the browser’s APIs for web storage, audio and video manipulation, and device hardware, among [others](https://developer.mozilla.org/en-US/docs/Web/API).\n\nIn this example, the component uses [DOM APIs](https://developer.mozilla.org/en-US/docs/Glossary/DOM) to manipulate a [`canvas`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/canvas) element. Since those APIs are only available in the browser, it must be marked as a Client Component.\n\n```\n'use client';import {useRef, useEffect} from 'react';export default function Circle() {const ref = useRef(null);useLayoutEffect(() => {const canvas = ref.current;const context = canvas.getContext('2d');context.reset();context.beginPath();context.arc(100, 75, 50, 0, 2 * Math.PI);context.stroke();});return <canvas ref={ref} />;}\n```\n\n### Using third-party libraries[](https://react.dev/reference/rsc/use-client#using-third-party-libraries \"Link for Using third-party libraries \")\n\nOften in a React app, you’ll leverage third-party libraries to handle common UI patterns or logic.\n\nThese libraries may rely on component Hooks or client APIs. Third-party components that use any of the following React APIs must run on the client:\n\n* [createContext](https://react.dev/reference/react/createContext)\n* [`react`](https://react.dev/reference/react/hooks) and [`react-dom`](https://react.dev/reference/react-dom/hooks) Hooks, excluding [`use`](https://react.dev/reference/react/use) and [`useId`](https://react.dev/reference/react/useId)\n* [forwardRef](https://react.dev/reference/react/forwardRef)\n* [memo](https://react.dev/reference/react/memo)\n* [startTransition](https://react.dev/reference/react/startTransition)\n* If they use client APIs, ex. DOM insertion or native platform views\n\nIf these libraries have been updated to be compatible with React Server Components, then they will already include `'use client'` markers of their own, allowing you to use them directly from your Server Components. If a library hasn’t been updated, or if a component needs props like event handlers that can only be specified on the client, you may need to add your own Client Component file in between the third-party Client Component and your Server Component where you’d like to use it.\n",
"filename": "use-client.md",
"package": "react"
} |
{
"content": "Title: 'use server' directive – React\n\nURL Source: https://react.dev/reference/rsc/use-server\n\nMarkdown Content:\n### Canary\n\n`'use server'` marks server-side functions that can be called from client-side code.\n\n* [Reference](https://react.dev/reference/rsc/use-server#reference)\n * [`'use server'`](https://react.dev/reference/rsc/use-server#use-server)\n * [Security considerations](https://react.dev/reference/rsc/use-server#security)\n * [Serializable arguments and return values](https://react.dev/reference/rsc/use-server#serializable-parameters-and-return-values)\n* [Usage](https://react.dev/reference/rsc/use-server#usage)\n * [Server Actions in forms](https://react.dev/reference/rsc/use-server#server-actions-in-forms)\n * [Calling a Server Action outside of `<form>`](https://react.dev/reference/rsc/use-server#calling-a-server-action-outside-of-form)\n\n* * *\n\nReference[](https://react.dev/reference/rsc/use-server#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `'use server'`[](https://react.dev/reference/rsc/use-server#use-server \"Link for this heading\")\n\nAdd `'use server'` at the top of an async function body to mark the function as callable by the client. We call these functions _Server Actions_.\n\n```\nasync function addToCart(data) {'use server';// ...}\n```\n\nWhen calling a Server Action on the client, it will make a network request to the server that includes a serialized copy of any arguments passed. If the Server Action returns a value, that value will be serialized and returned to the client.\n\nInstead of individually marking functions with `'use server'`, you can add the directive to the top of a file to mark all exports within that file as Server Actions that can be used anywhere, including imported in client code.\n\n#### Caveats[](https://react.dev/reference/rsc/use-server#caveats \"Link for Caveats \")\n\n* `'use server'` must be at the very beginning of their function or module; above any other code including imports (comments above directives are OK). They must be written with single or double quotes, not backticks.\n* `'use server'` can only be used in server-side files. The resulting Server Actions can be passed to Client Components through props. See supported [types for serialization](https://react.dev/reference/rsc/use-server#serializable-parameters-and-return-values).\n* To import a Server Action from [client code](https://react.dev/reference/rsc/use-client), the directive must be used on a module level.\n* Because the underlying network calls are always asynchronous, `'use server'` can only be used on async functions.\n* Always treat arguments to Server Actions as untrusted input and authorize any mutations. See [security considerations](https://react.dev/reference/rsc/use-server#security).\n* Server Actions should be called in a [Transition](https://react.dev/reference/react/useTransition). Server Actions passed to [`<form action>`](https://react.dev/reference/react-dom/components/form#props) or [`formAction`](https://react.dev/reference/react-dom/components/input#props) will automatically be called in a transition.\n* Server Actions are designed for mutations that update server-side state; they are not recommended for data fetching. Accordingly, frameworks implementing Server Actions typically process one action at a time and do not have a way to cache the return value.\n\n### Security considerations[](https://react.dev/reference/rsc/use-server#security \"Link for Security considerations \")\n\nArguments to Server Actions are fully client-controlled. For security, always treat them as untrusted input, and make sure to validate and escape arguments as appropriate.\n\nIn any Server Action, make sure to validate that the logged-in user is allowed to perform that action.\n\n### Under Construction\n\n### Serializable arguments and return values[](https://react.dev/reference/rsc/use-server#serializable-parameters-and-return-values \"Link for Serializable arguments and return values \")\n\nAs client code calls the Server Action over the network, any arguments passed will need to be serializable.\n\nHere are supported types for Server Action arguments:\n\n* Primitives\n * [string](https://developer.mozilla.org/en-US/docs/Glossary/String)\n * [number](https://developer.mozilla.org/en-US/docs/Glossary/Number)\n * [bigint](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/BigInt)\n * [boolean](https://developer.mozilla.org/en-US/docs/Glossary/Boolean)\n * [undefined](https://developer.mozilla.org/en-US/docs/Glossary/Undefined)\n * [null](https://developer.mozilla.org/en-US/docs/Glossary/Null)\n * [symbol](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol), only symbols registered in the global Symbol registry via [`Symbol.for`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol/for)\n* Iterables containing serializable values\n * [String](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String)\n * [Array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array)\n * [Map](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map)\n * [Set](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Set)\n * [TypedArray](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray) and [ArrayBuffer](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/ArrayBuffer)\n* [Date](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date)\n* [FormData](https://developer.mozilla.org/en-US/docs/Web/API/FormData) instances\n* Plain [objects](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object): those created with [object initializers](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Object_initializer), with serializable properties\n* Functions that are Server Actions\n* [Promises](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise)\n\nNotably, these are not supported:\n\n* React elements, or [JSX](https://react.dev/learn/writing-markup-with-jsx)\n* Functions, including component functions or any other function that is not a Server Action\n* [Classes](https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Classes_in_JavaScript)\n* Objects that are instances of any class (other than the built-ins mentioned) or objects with [a null prototype](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object#null-prototype_objects)\n* Symbols not registered globally, ex. `Symbol('my new symbol')`\n\nSupported serializable return values are the same as [serializable props](https://react.dev/reference/rsc/use-client#passing-props-from-server-to-client-components) for a boundary Client Component.\n\nUsage[](https://react.dev/reference/rsc/use-server#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Server Actions in forms[](https://react.dev/reference/rsc/use-server#server-actions-in-forms \"Link for Server Actions in forms \")\n\nThe most common use case of Server Actions will be calling server functions that mutate data. On the browser, the [HTML form element](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) is the traditional approach for a user to submit a mutation. With React Server Components, React introduces first-class support for Server Actions in [forms](https://react.dev/reference/react-dom/components/form).\n\nHere is a form that allows a user to request a username.\n\n```\n// App.jsasync function requestUsername(formData) {'use server';const username = formData.get('username');// ...}export default function App() {return (<form action={requestUsername}><input type=\"text\" name=\"username\" /><button type=\"submit\">Request</button></form>);}\n```\n\nIn this example `requestUsername` is a Server Action passed to a `<form>`. When a user submits this form, there is a network request to the server function `requestUsername`. When calling a Server Action in a form, React will supply the form’s [FormData](https://developer.mozilla.org/en-US/docs/Web/API/FormData) as the first argument to the Server Action.\n\nBy passing a Server Action to the form `action`, React can [progressively enhance](https://developer.mozilla.org/en-US/docs/Glossary/Progressive_Enhancement) the form. This means that forms can be submitted before the JavaScript bundle is loaded.\n\n#### Handling return values in forms[](https://react.dev/reference/rsc/use-server#handling-return-values \"Link for Handling return values in forms \")\n\nIn the username request form, there might be the chance that a username is not available. `requestUsername` should tell us if it fails or not.\n\nTo update the UI based on the result of a Server Action while supporting progressive enhancement, use [`useActionState`](https://react.dev/reference/react/useActionState).\n\n```\n// requestUsername.js'use server';export default async function requestUsername(formData) {const username = formData.get('username');if (canRequest(username)) {// ...return 'successful';}return 'failed';}\n```\n\n```\n// UsernameForm.js'use client';import { useActionState } from 'react';import requestUsername from './requestUsername';function UsernameForm() {const [state, action] = useActionState(requestUsername, null, 'n/a');return (<><form action={action}><input type=\"text\" name=\"username\" /><button type=\"submit\">Request</button></form><p>Last submission request returned: {state}</p></>);}\n```\n\nNote that like most Hooks, `useActionState` can only be called in [client code](https://react.dev/reference/rsc/use-client).\n\n### Calling a Server Action outside of `<form>`[](https://react.dev/reference/rsc/use-server#calling-a-server-action-outside-of-form \"Link for this heading\")\n\nServer Actions are exposed server endpoints and can be called anywhere in client code.\n\nWhen using a Server Action outside of a [form](https://react.dev/reference/react-dom/components/form), call the Server Action in a [Transition](https://react.dev/reference/react/useTransition), which allows you to display a loading indicator, show [optimistic state updates](https://react.dev/reference/react/useOptimistic), and handle unexpected errors. Forms will automatically wrap Server Actions in transitions.\n\n```\nimport incrementLike from './actions';import { useState, useTransition } from 'react';function LikeButton() {const [isPending, startTransition] = useTransition();const [likeCount, setLikeCount] = useState(0);const onClick = () => {startTransition(async () => {const currentCount = await incrementLike();setLikeCount(currentCount);});};return (<><p>Total Likes: {likeCount}</p><button onClick={onClick} disabled={isPending}>Like</button>;</>);}\n```\n\n```\n// actions.js'use server';let likeCount = 0;export default async function incrementLike() {likeCount++;return likeCount;}\n```\n\nTo read a Server Action return value, you’ll need to `await` the promise returned.\n",
"filename": "use-server.md",
"package": "react"
} |
{
"content": "Title: use – React\n\nURL Source: https://react.dev/reference/react/use\n\nMarkdown Content:\n### Canary\n\n`use` is a React API that lets you read the value of a resource like a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) or [context](https://react.dev/learn/passing-data-deeply-with-context).\n\n```\nconst value = use(resource);\n```\n\n* [Reference](https://react.dev/reference/react/use#reference)\n * [`use(resource)`](https://react.dev/reference/react/use#use)\n* [Usage](https://react.dev/reference/react/use#usage)\n * [Reading context with `use`](https://react.dev/reference/react/use#reading-context-with-use)\n * [Streaming data from the server to the client](https://react.dev/reference/react/use#streaming-data-from-server-to-client)\n * [Dealing with rejected Promises](https://react.dev/reference/react/use#dealing-with-rejected-promises)\n* [Troubleshooting](https://react.dev/reference/react/use#troubleshooting)\n * [“Suspense Exception: This is not a real error!”](https://react.dev/reference/react/use#suspense-exception-error)\n\n* * *\n\nReference[](https://react.dev/reference/react/use#reference \"Link for Reference \")\n----------------------------------------------------------------------------------\n\n### `use(resource)`[](https://react.dev/reference/react/use#use \"Link for this heading\")\n\nCall `use` in your component to read the value of a resource like a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) or [context](https://react.dev/learn/passing-data-deeply-with-context).\n\n```\nimport { use } from 'react';function MessageComponent({ messagePromise }) {const message = use(messagePromise);const theme = use(ThemeContext);// ...\n```\n\nUnlike React Hooks, `use` can be called within loops and conditional statements like `if`. Like React Hooks, the function that calls `use` must be a Component or Hook.\n\nWhen called with a Promise, the `use` API integrates with [`Suspense`](https://react.dev/reference/react/Suspense) and [error boundaries](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary). The component calling `use` _suspends_ while the Promise passed to `use` is pending. If the component that calls `use` is wrapped in a Suspense boundary, the fallback will be displayed. Once the Promise is resolved, the Suspense fallback is replaced by the rendered components using the data returned by the `use` API. If the Promise passed to `use` is rejected, the fallback of the nearest Error Boundary will be displayed.\n\n[See more examples below.](https://react.dev/reference/react/use#usage)\n\n#### Parameters[](https://react.dev/reference/react/use#parameters \"Link for Parameters \")\n\n* `resource`: this is the source of the data you want to read a value from. A resource can be a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) or a [context](https://react.dev/learn/passing-data-deeply-with-context).\n\n#### Returns[](https://react.dev/reference/react/use#returns \"Link for Returns \")\n\nThe `use` API returns the value that was read from the resource like the resolved value of a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) or [context](https://react.dev/learn/passing-data-deeply-with-context).\n\n#### Caveats[](https://react.dev/reference/react/use#caveats \"Link for Caveats \")\n\n* The `use` API must be called inside a Component or a Hook.\n* When fetching data in a [Server Component](https://react.dev/reference/rsc/use-server), prefer `async` and `await` over `use`. `async` and `await` pick up rendering from the point where `await` was invoked, whereas `use` re-renders the component after the data is resolved.\n* Prefer creating Promises in [Server Components](https://react.dev/reference/rsc/use-server) and passing them to [Client Components](https://react.dev/reference/rsc/use-client) over creating Promises in Client Components. Promises created in Client Components are recreated on every render. Promises passed from a Server Component to a Client Component are stable across re-renders. [See this example](https://react.dev/reference/react/use#streaming-data-from-server-to-client).\n\n* * *\n\nUsage[](https://react.dev/reference/react/use#usage \"Link for Usage \")\n----------------------------------------------------------------------\n\n### Reading context with `use`[](https://react.dev/reference/react/use#reading-context-with-use \"Link for this heading\")\n\nWhen a [context](https://react.dev/learn/passing-data-deeply-with-context) is passed to `use`, it works similarly to [`useContext`](https://react.dev/reference/react/useContext). While `useContext` must be called at the top level of your component, `use` can be called inside conditionals like `if` and loops like `for`. `use` is preferred over `useContext` because it is more flexible.\n\n```\nimport { use } from 'react';function Button() {const theme = use(ThemeContext);// ...\n```\n\n`use` returns the context value for the context you passed. To determine the context value, React searches the component tree and finds **the closest context provider above** for that particular context.\n\nTo pass context to a `Button`, wrap it or one of its parent components into the corresponding context provider.\n\n```\nfunction MyPage() {return (<ThemeContext.Provider value=\"dark\"><Form /></ThemeContext.Provider>);}function Form() {// ... renders buttons inside ...}\n```\n\nIt doesn’t matter how many layers of components there are between the provider and the `Button`. When a `Button` _anywhere_ inside of `Form` calls `use(ThemeContext)`, it will receive `\"dark\"` as the value.\n\nUnlike [`useContext`](https://react.dev/reference/react/useContext), `use` can be called in conditionals and loops like `if`.\n\n```\nfunction HorizontalRule({ show }) {if (show) {const theme = use(ThemeContext);return <hr className={theme} />;}return false;}\n```\n\n`use` is called from inside a `if` statement, allowing you to conditionally read values from a Context.\n\n### Pitfall\n\nLike `useContext`, `use(context)` always looks for the closest context provider _above_ the component that calls it. It searches upwards and **does not** consider context providers in the component from which you’re calling `use(context)`.\n\nimport { createContext, use } from 'react';\n\nconst ThemeContext = createContext(null);\n\nexport default function MyApp() {\n return (\n <ThemeContext.Provider value\\=\"dark\"\\>\n <Form />\n </ThemeContext.Provider\\>\n )\n}\n\nfunction Form() {\n return (\n <Panel title\\=\"Welcome\"\\>\n <Button show\\={true}\\>Sign up</Button\\>\n <Button show\\={false}\\>Log in</Button\\>\n </Panel\\>\n );\n}\n\nfunction Panel({ title, children }) {\n const theme = use(ThemeContext);\n const className = 'panel-' + theme;\n return (\n <section className\\={className}\\>\n <h1\\>{title}</h1\\>\n {children}\n </section\\>\n )\n}\n\nfunction Button({ show, children }) {\n if (show) {\n const theme = use(ThemeContext);\n const className = 'button-' + theme;\n return (\n <button className\\={className}\\>\n {children}\n </button\\>\n );\n }\n return false\n}\n\n### Streaming data from the server to the client[](https://react.dev/reference/react/use#streaming-data-from-server-to-client \"Link for Streaming data from the server to the client \")\n\nData can be streamed from the server to the client by passing a Promise as a prop from a Server Component to a Client Component.\n\n```\nimport { fetchMessage } from './lib.js';import { Message } from './message.js';export default function App() {const messagePromise = fetchMessage();return (<Suspense fallback={<p>waiting for message...</p>}><Message messagePromise={messagePromise} /></Suspense>);}\n```\n\nThe Client Component then takes the Promise it received as a prop and passes it to the `use` API. This allows the Client Component to read the value from the Promise that was initially created by the Server Component.\n\n```\n// message.js'use client';import { use } from 'react';export function Message({ messagePromise }) {const messageContent = use(messagePromise);return <p>Here is the message: {messageContent}</p>;}\n```\n\nBecause `Message` is wrapped in [`Suspense`](https://react.dev/reference/react/Suspense), the fallback will be displayed until the Promise is resolved. When the Promise is resolved, the value will be read by the `use` API and the `Message` component will replace the Suspense fallback.\n\n\"use client\";\n\nimport { use, Suspense } from \"react\";\n\nfunction Message({ messagePromise }) {\n const messageContent = use(messagePromise);\n return <p\\>Here is the message: {messageContent}</p\\>;\n}\n\nexport function MessageContainer({ messagePromise }) {\n return (\n <Suspense fallback\\={<p\\>⌛Downloading message...</p\\>}\\>\n <Message messagePromise\\={messagePromise} />\n </Suspense\\>\n );\n}\n\n### Note\n\nWhen passing a Promise from a Server Component to a Client Component, its resolved value must be serializable to pass between server and client. Data types like functions aren’t serializable and cannot be the resolved value of such a Promise.\n\n##### Deep Dive\n\n#### Should I resolve a Promise in a Server or Client Component?[](https://react.dev/reference/react/use#resolve-promise-in-server-or-client-component \"Link for Should I resolve a Promise in a Server or Client Component? \")\n\nA Promise can be passed from a Server Component to a Client Component and resolved in the Client Component with the `use` API. You can also resolve the Promise in a Server Component with `await` and pass the required data to the Client Component as a prop.\n\n```\nexport default async function App() {const messageContent = await fetchMessage();return <Message messageContent={messageContent} />}\n```\n\nBut using `await` in a [Server Component](https://react.dev/reference/react/components#server-components) will block its rendering until the `await` statement is finished. Passing a Promise from a Server Component to a Client Component prevents the Promise from blocking the rendering of the Server Component.\n\n### Dealing with rejected Promises[](https://react.dev/reference/react/use#dealing-with-rejected-promises \"Link for Dealing with rejected Promises \")\n\nIn some cases a Promise passed to `use` could be rejected. You can handle rejected Promises by either:\n\n1. [Displaying an error to users with an error boundary.](https://react.dev/reference/react/use#displaying-an-error-to-users-with-error-boundary)\n2. [Providing an alternative value with `Promise.catch`](https://react.dev/reference/react/use#providing-an-alternative-value-with-promise-catch)\n\n### Pitfall\n\n`use` cannot be called in a try-catch block. Instead of a try-catch block [wrap your component in an Error Boundary](https://react.dev/reference/react/use#displaying-an-error-to-users-with-error-boundary), or [provide an alternative value to use with the Promise’s `.catch` method](https://react.dev/reference/react/use#providing-an-alternative-value-with-promise-catch).\n\n#### Displaying an error to users with an error boundary[](https://react.dev/reference/react/use#displaying-an-error-to-users-with-error-boundary \"Link for Displaying an error to users with an error boundary \")\n\nIf you’d like to display an error to your users when a Promise is rejected, you can use an [error boundary](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary). To use an error boundary, wrap the component where you are calling the `use` API in an error boundary. If the Promise passed to `use` is rejected the fallback for the error boundary will be displayed.\n\n\"use client\";\n\nimport { use, Suspense } from \"react\";\nimport { ErrorBoundary } from \"react-error-boundary\";\n\nexport function MessageContainer({ messagePromise }) {\n return (\n <ErrorBoundary fallback\\={<p\\>⚠️Something went wrong</p\\>}\\>\n <Suspense fallback\\={<p\\>⌛Downloading message...</p\\>}\\>\n <Message messagePromise\\={messagePromise} />\n </Suspense\\>\n </ErrorBoundary\\>\n );\n}\n\nfunction Message({ messagePromise }) {\n const content = use(messagePromise);\n return <p\\>Here is the message: {content}</p\\>;\n}\n\n#### Providing an alternative value with `Promise.catch`[](https://react.dev/reference/react/use#providing-an-alternative-value-with-promise-catch \"Link for this heading\")\n\nIf you’d like to provide an alternative value when the Promise passed to `use` is rejected you can use the Promise’s [`catch`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/catch) method.\n\n```\nimport { Message } from './message.js';export default function App() {const messagePromise = new Promise((resolve, reject) => {reject();}).catch(() => {return \"no new message found.\";});return (<Suspense fallback={<p>waiting for message...</p>}><Message messagePromise={messagePromise} /></Suspense>);}\n```\n\nTo use the Promise’s `catch` method, call `catch` on the Promise object. `catch` takes a single argument: a function that takes an error message as an argument. Whatever is returned by the function passed to `catch` will be used as the resolved value of the Promise.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/use#troubleshooting \"Link for Troubleshooting \")\n----------------------------------------------------------------------------------------------------\n\n### “Suspense Exception: This is not a real error!”[](https://react.dev/reference/react/use#suspense-exception-error \"Link for “Suspense Exception: This is not a real error!” \")\n\nYou are either calling `use` outside of a React Component or Hook function, or calling `use` in a try–catch block. If you are calling `use` inside a try–catch block, wrap your component in an error boundary, or call the Promise’s `catch` to catch the error and resolve the Promise with another value. [See these examples](https://react.dev/reference/react/use#dealing-with-rejected-promises).\n\nIf you are calling `use` outside a React Component or Hook function, move the `use` call to a React Component or Hook function.\n\n```\nfunction MessageComponent({messagePromise}) {function download() {// ❌ the function calling `use` is not a Component or Hookconst message = use(messagePromise);// ...\n```\n\nInstead, call `use` outside any component closures, where the function that calls `use` is a Component or Hook.\n\n```\nfunction MessageComponent({messagePromise}) {// ✅ `use` is being called from a component. const message = use(messagePromise);// ...\n```\n",
"filename": "use.md",
"package": "react"
} |
{
"content": "Title: useActionState – React\n\nURL Source: https://react.dev/reference/react/useActionState\n\nMarkdown Content:\n### Canary\n\nThe `useActionState` Hook is currently only available in React’s Canary and experimental channels. Learn more about [release channels here](https://react.dev/community/versioning-policy#all-release-channels). In addition, you need to use a framework that supports [React Server Components](https://react.dev/reference/rsc/use-client) to get the full benefit of `useActionState`.\n\n### Note\n\nIn earlier React Canary versions, this API was part of React DOM and called `useFormState`.\n\n`useActionState` is a Hook that allows you to update state based on the result of a form action.\n\n```\nconst [state, formAction] = useActionState(fn, initialState, permalink?);\n```\n\n* [Reference](https://react.dev/reference/react/useActionState#reference)\n * [`useActionState(action, initialState, permalink?)`](https://react.dev/reference/react/useActionState#useactionstate)\n* [Usage](https://react.dev/reference/react/useActionState#usage)\n * [Using information returned by a form action](https://react.dev/reference/react/useActionState#using-information-returned-by-a-form-action)\n* [Troubleshooting](https://react.dev/reference/react/useActionState#troubleshooting)\n * [My action can no longer read the submitted form data](https://react.dev/reference/react/useActionState#my-action-can-no-longer-read-the-submitted-form-data)\n\n* * *\n\nReference[](https://react.dev/reference/react/useActionState#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------\n\n### `useActionState(action, initialState, permalink?)`[](https://react.dev/reference/react/useActionState#useactionstate \"Link for this heading\")\n\nCall `useActionState` at the top level of your component to create component state that is updated [when a form action is invoked](https://react.dev/reference/react-dom/components/form). You pass `useActionState` an existing form action function as well as an initial state, and it returns a new action that you use in your form, along with the latest form state. The latest form state is also passed to the function that you provided.\n\n```\nimport { useActionState } from \"react\";async function increment(previousState, formData) {return previousState + 1;}function StatefulForm({}) {const [state, formAction] = useActionState(increment, 0);return (<form>{state}<button formAction={formAction}>Increment</button></form>)}\n```\n\nThe form state is the value returned by the action when the form was last submitted. If the form has not yet been submitted, it is the initial state that you pass.\n\nIf used with a Server Action, `useActionState` allows the server’s response from submitting the form to be shown even before hydration has completed.\n\n[See more examples below.](https://react.dev/reference/react/useActionState#usage)\n\n#### Parameters[](https://react.dev/reference/react/useActionState#parameters \"Link for Parameters \")\n\n* `fn`: The function to be called when the form is submitted or button pressed. When the function is called, it will receive the previous state of the form (initially the `initialState` that you pass, subsequently its previous return value) as its initial argument, followed by the arguments that a form action normally receives.\n* `initialState`: The value you want the state to be initially. It can be any serializable value. This argument is ignored after the action is first invoked.\n* **optional** `permalink`: A string containing the unique page URL that this form modifies. For use on pages with dynamic content (eg: feeds) in conjunction with progressive enhancement: if `fn` is a [server action](https://react.dev/reference/rsc/use-server) and the form is submitted before the JavaScript bundle loads, the browser will navigate to the specified permalink URL, rather than the current page’s URL. Ensure that the same form component is rendered on the destination page (including the same action `fn` and `permalink`) so that React knows how to pass the state through. Once the form has been hydrated, this parameter has no effect.\n\n#### Returns[](https://react.dev/reference/react/useActionState#returns \"Link for Returns \")\n\n`useActionState` returns an array with exactly two values:\n\n1. The current state. During the first render, it will match the `initialState` you have passed. After the action is invoked, it will match the value returned by the action.\n2. A new action that you can pass as the `action` prop to your `form` component or `formAction` prop to any `button` component within the form.\n\n#### Caveats[](https://react.dev/reference/react/useActionState#caveats \"Link for Caveats \")\n\n* When used with a framework that supports React Server Components, `useActionState` lets you make forms interactive before JavaScript has executed on the client. When used without Server Components, it is equivalent to component local state.\n* The function passed to `useActionState` receives an extra argument, the previous or initial state, as its first argument. This makes its signature different than if it were used directly as a form action without using `useActionState`.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useActionState#usage \"Link for Usage \")\n---------------------------------------------------------------------------------\n\n### Using information returned by a form action[](https://react.dev/reference/react/useActionState#using-information-returned-by-a-form-action \"Link for Using information returned by a form action \")\n\nCall `useActionState` at the top level of your component to access the return value of an action from the last time a form was submitted.\n\n```\nimport { useActionState } from 'react';import { action } from './actions.js';function MyComponent() {const [state, formAction] = useActionState(action, null);// ...return (<form action={formAction}>{/* ... */}</form>);}\n```\n\n`useActionState` returns an array with exactly two items:\n\n1. The current state of the form, which is initially set to the initial state you provided, and after the form is submitted is set to the return value of the action you provided.\n2. A new action that you pass to `<form>` as its `action` prop.\n\nWhen the form is submitted, the action function that you provided will be called. Its return value will become the new current state of the form.\n\nThe action that you provide will also receive a new first argument, namely the current state of the form. The first time the form is submitted, this will be the initial state you provided, while with subsequent submissions, it will be the return value from the last time the action was called. The rest of the arguments are the same as if `useActionState` had not been used.\n\n```\nfunction action(currentState, formData) {// ...return 'next state';}\n```\n\nTroubleshooting[](https://react.dev/reference/react/useActionState#troubleshooting \"Link for Troubleshooting \")\n---------------------------------------------------------------------------------------------------------------\n\n### My action can no longer read the submitted form data[](https://react.dev/reference/react/useActionState#my-action-can-no-longer-read-the-submitted-form-data \"Link for My action can no longer read the submitted form data \")\n\nWhen you wrap an action with `useActionState`, it gets an extra argument _as its first argument_. The submitted form data is therefore its _second_ argument instead of its first as it would usually be. The new first argument that gets added is the current state of the form.\n\n```\nfunction action(currentState, formData) {// ...}\n```\n",
"filename": "useActionState.md",
"package": "react"
} |
{
"content": "Title: useCallback – React\n\nURL Source: https://react.dev/reference/react/useCallback\n\nMarkdown Content:\n`useCallback` is a React Hook that lets you cache a function definition between re-renders.\n\n```\nconst cachedFn = useCallback(fn, dependencies)\n```\n\n* [Reference](https://react.dev/reference/react/useCallback#reference)\n * [`useCallback(fn, dependencies)`](https://react.dev/reference/react/useCallback#usecallback)\n* [Usage](https://react.dev/reference/react/useCallback#usage)\n * [Skipping re-rendering of components](https://react.dev/reference/react/useCallback#skipping-re-rendering-of-components)\n * [Updating state from a memoized callback](https://react.dev/reference/react/useCallback#updating-state-from-a-memoized-callback)\n * [Preventing an Effect from firing too often](https://react.dev/reference/react/useCallback#preventing-an-effect-from-firing-too-often)\n * [Optimizing a custom Hook](https://react.dev/reference/react/useCallback#optimizing-a-custom-hook)\n* [Troubleshooting](https://react.dev/reference/react/useCallback#troubleshooting)\n * [Every time my component renders, `useCallback` returns a different function](https://react.dev/reference/react/useCallback#every-time-my-component-renders-usecallback-returns-a-different-function)\n * [I need to call `useCallback` for each list item in a loop, but it’s not allowed](https://react.dev/reference/react/useCallback#i-need-to-call-usememo-for-each-list-item-in-a-loop-but-its-not-allowed)\n\n* * *\n\nReference[](https://react.dev/reference/react/useCallback#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------\n\n### `useCallback(fn, dependencies)`[](https://react.dev/reference/react/useCallback#usecallback \"Link for this heading\")\n\nCall `useCallback` at the top level of your component to cache a function definition between re-renders:\n\n```\nimport { useCallback } from 'react';export default function ProductPage({ productId, referrer, theme }) {const handleSubmit = useCallback((orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails,});}, [productId, referrer]);\n```\n\n[See more examples below.](https://react.dev/reference/react/useCallback#usage)\n\n#### Parameters[](https://react.dev/reference/react/useCallback#parameters \"Link for Parameters \")\n\n* `fn`: The function value that you want to cache. It can take any arguments and return any values. React will return (not call!) your function back to you during the initial render. On next renders, React will give you the same function again if the `dependencies` have not changed since the last render. Otherwise, it will give you the function that you have passed during the current render, and store it in case it can be reused later. React will not call your function. The function is returned to you so you can decide when and whether to call it.\n \n* `dependencies`: The list of all reactive values referenced inside of the `fn` code. Reactive values include props, state, and all the variables and functions declared directly inside your component body. If your linter is [configured for React](https://react.dev/learn/editor-setup#linting), it will verify that every reactive value is correctly specified as a dependency. The list of dependencies must have a constant number of items and be written inline like `[dep1, dep2, dep3]`. React will compare each dependency with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison algorithm.\n \n\n#### Returns[](https://react.dev/reference/react/useCallback#returns \"Link for Returns \")\n\nOn the initial render, `useCallback` returns the `fn` function you have passed.\n\nDuring subsequent renders, it will either return an already stored `fn` function from the last render (if the dependencies haven’t changed), or return the `fn` function you have passed during this render.\n\n#### Caveats[](https://react.dev/reference/react/useCallback#caveats \"Link for Caveats \")\n\n* `useCallback` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a new component and move the state into it.\n* React **will not throw away the cached function unless there is a specific reason to do that.** For example, in development, React throws away the cache when you edit the file of your component. Both in development and in production, React will throw away the cache if your component suspends during the initial mount. In the future, React may add more features that take advantage of throwing away the cache—for example, if React adds built-in support for virtualized lists in the future, it would make sense to throw away the cache for items that scroll out of the virtualized table viewport. This should match your expectations if you rely on `useCallback` as a performance optimization. Otherwise, a [state variable](https://react.dev/reference/react/useState#im-trying-to-set-state-to-a-function-but-it-gets-called-instead) or a [ref](https://react.dev/reference/react/useRef#avoiding-recreating-the-ref-contents) may be more appropriate.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useCallback#usage \"Link for Usage \")\n------------------------------------------------------------------------------\n\n### Skipping re-rendering of components[](https://react.dev/reference/react/useCallback#skipping-re-rendering-of-components \"Link for Skipping re-rendering of components \")\n\nWhen you optimize rendering performance, you will sometimes need to cache the functions that you pass to child components. Let’s first look at the syntax for how to do this, and then see in which cases it’s useful.\n\nTo cache a function between re-renders of your component, wrap its definition into the `useCallback` Hook:\n\n```\nimport { useCallback } from 'react';function ProductPage({ productId, referrer, theme }) {const handleSubmit = useCallback((orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails,});}, [productId, referrer]);// ...\n```\n\nYou need to pass two things to `useCallback`:\n\n1. A function definition that you want to cache between re-renders.\n2. A list of dependencies including every value within your component that’s used inside your function.\n\nOn the initial render, the returned function you’ll get from `useCallback` will be the function you passed.\n\nOn the following renders, React will compare the dependencies with the dependencies you passed during the previous render. If none of the dependencies have changed (compared with [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)), `useCallback` will return the same function as before. Otherwise, `useCallback` will return the function you passed on _this_ render.\n\nIn other words, `useCallback` caches a function between re-renders until its dependencies change.\n\n**Let’s walk through an example to see when this is useful.**\n\nSay you’re passing a `handleSubmit` function down from the `ProductPage` to the `ShippingForm` component:\n\n```\nfunction ProductPage({ productId, referrer, theme }) {// ...return (<div className={theme}><ShippingForm onSubmit={handleSubmit} /></div>);\n```\n\nYou’ve noticed that toggling the `theme` prop freezes the app for a moment, but if you remove `<ShippingForm />` from your JSX, it feels fast. This tells you that it’s worth trying to optimize the `ShippingForm` component.\n\n**By default, when a component re-renders, React re-renders all of its children recursively.** This is why, when `ProductPage` re-renders with a different `theme`, the `ShippingForm` component _also_ re-renders. This is fine for components that don’t require much calculation to re-render. But if you verified a re-render is slow, you can tell `ShippingForm` to skip re-rendering when its props are the same as on last render by wrapping it in [`memo`:](https://react.dev/reference/react/memo)\n\n```\nimport { memo } from 'react';const ShippingForm = memo(function ShippingForm({ onSubmit }) {// ...});\n```\n\n**With this change, `ShippingForm` will skip re-rendering if all of its props are the _same_ as on the last render.** This is when caching a function becomes important! Let’s say you defined `handleSubmit` without `useCallback`:\n\n```\nfunction ProductPage({ productId, referrer, theme }) {// Every time the theme changes, this will be a different function...function handleSubmit(orderDetails) {post('/product/' + productId + '/buy', {referrer,orderDetails,});}return (<div className={theme}>{/* ... so ShippingForm's props will never be the same, and it will re-render every time */}<ShippingForm onSubmit={handleSubmit} /></div>);}\n```\n\n**In JavaScript, a `function () {}` or `() => {}` always creates a _different_ function,** similar to how the `{}` object literal always creates a new object. Normally, this wouldn’t be a problem, but it means that `ShippingForm` props will never be the same, and your [`memo`](https://react.dev/reference/react/memo) optimization won’t work. This is where `useCallback` comes in handy:\n\n```\nfunction ProductPage({ productId, referrer, theme }) {// Tell React to cache your function between re-renders...const handleSubmit = useCallback((orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails,});}, [productId, referrer]); // ...so as long as these dependencies don't change...return (<div className={theme}>{/* ...ShippingForm will receive the same props and can skip re-rendering */}<ShippingForm onSubmit={handleSubmit} /></div>);}\n```\n\n**By wrapping `handleSubmit` in `useCallback`, you ensure that it’s the _same_ function between the re-renders** (until dependencies change). You don’t _have to_ wrap a function in `useCallback` unless you do it for some specific reason. In this example, the reason is that you pass it to a component wrapped in [`memo`,](https://react.dev/reference/react/memo) and this lets it skip re-rendering. There are other reasons you might need `useCallback` which are described further on this page.\n\n### Note\n\n**You should only rely on `useCallback` as a performance optimization.** If your code doesn’t work without it, find the underlying problem and fix it first. Then you may add `useCallback` back.\n\n##### Deep Dive\n\nYou will often see [`useMemo`](https://react.dev/reference/react/useMemo) alongside `useCallback`. They are both useful when you’re trying to optimize a child component. They let you [memoize](https://en.wikipedia.org/wiki/Memoization) (or, in other words, cache) something you’re passing down:\n\n```\nimport { useMemo, useCallback } from 'react';function ProductPage({ productId, referrer }) {const product = useData('/product/' + productId);const requirements = useMemo(() => { // Calls your function and caches its resultreturn computeRequirements(product);}, [product]);const handleSubmit = useCallback((orderDetails) => { // Caches your function itselfpost('/product/' + productId + '/buy', {referrer,orderDetails,});}, [productId, referrer]);return (<div className={theme}><ShippingForm requirements={requirements} onSubmit={handleSubmit} /></div>);}\n```\n\nThe difference is in _what_ they’re letting you cache:\n\n* **[`useMemo`](https://react.dev/reference/react/useMemo) caches the _result_ of calling your function.** In this example, it caches the result of calling `computeRequirements(product)` so that it doesn’t change unless `product` has changed. This lets you pass the `requirements` object down without unnecessarily re-rendering `ShippingForm`. When necessary, React will call the function you’ve passed during rendering to calculate the result.\n* **`useCallback` caches _the function itself._** Unlike `useMemo`, it does not call the function you provide. Instead, it caches the function you provided so that `handleSubmit` _itself_ doesn’t change unless `productId` or `referrer` has changed. This lets you pass the `handleSubmit` function down without unnecessarily re-rendering `ShippingForm`. Your code won’t run until the user submits the form.\n\nIf you’re already familiar with [`useMemo`,](https://react.dev/reference/react/useMemo) you might find it helpful to think of `useCallback` as this:\n\n```\n// Simplified implementation (inside React)function useCallback(fn, dependencies) {return useMemo(() => fn, dependencies);}\n```\n\n[Read more about the difference between `useMemo` and `useCallback`.](https://react.dev/reference/react/useMemo#memoizing-a-function)\n\n##### Deep Dive\n\n#### Should you add useCallback everywhere?[](https://react.dev/reference/react/useCallback#should-you-add-usecallback-everywhere \"Link for Should you add useCallback everywhere? \")\n\nIf your app is like this site, and most interactions are coarse (like replacing a page or an entire section), memoization is usually unnecessary. On the other hand, if your app is more like a drawing editor, and most interactions are granular (like moving shapes), then you might find memoization very helpful.\n\nCaching a function with `useCallback` is only valuable in a few cases:\n\n* You pass it as a prop to a component wrapped in [`memo`.](https://react.dev/reference/react/memo) You want to skip re-rendering if the value hasn’t changed. Memoization lets your component re-render only if dependencies changed.\n* The function you’re passing is later used as a dependency of some Hook. For example, another function wrapped in `useCallback` depends on it, or you depend on this function from [`useEffect.`](https://react.dev/reference/react/useEffect)\n\nThere is no benefit to wrapping a function in `useCallback` in other cases. There is no significant harm to doing that either, so some teams choose to not think about individual cases, and memoize as much as possible. The downside is that code becomes less readable. Also, not all memoization is effective: a single value that’s “always new” is enough to break memoization for an entire component.\n\nNote that `useCallback` does not prevent _creating_ the function. You’re always creating a function (and that’s fine!), but React ignores it and gives you back a cached function if nothing changed.\n\n**In practice, you can make a lot of memoization unnecessary by following a few principles:**\n\n1. When a component visually wraps other components, let it [accept JSX as children.](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) Then, if the wrapper component updates its own state, React knows that its children don’t need to re-render.\n2. Prefer local state and don’t [lift state up](https://react.dev/learn/sharing-state-between-components) any further than necessary. Don’t keep transient state like forms and whether an item is hovered at the top of your tree or in a global state library.\n3. Keep your [rendering logic pure.](https://react.dev/learn/keeping-components-pure) If re-rendering a component causes a problem or produces some noticeable visual artifact, it’s a bug in your component! Fix the bug instead of adding memoization.\n4. Avoid [unnecessary Effects that update state.](https://react.dev/learn/you-might-not-need-an-effect) Most performance problems in React apps are caused by chains of updates originating from Effects that cause your components to render over and over.\n5. Try to [remove unnecessary dependencies from your Effects.](https://react.dev/learn/removing-effect-dependencies) For example, instead of memoization, it’s often simpler to move some object or a function inside an Effect or outside the component.\n\nIf a specific interaction still feels laggy, [use the React Developer Tools profiler](https://legacy.reactjs.org/blog/2018/09/10/introducing-the-react-profiler.html) to see which components benefit the most from memoization, and add memoization where needed. These principles make your components easier to debug and understand, so it’s good to follow them in any case. In long term, we’re researching [doing memoization automatically](https://www.youtube.com/watch?v=lGEMwh32soc) to solve this once and for all.\n\n* * *\n\n### Updating state from a memoized callback[](https://react.dev/reference/react/useCallback#updating-state-from-a-memoized-callback \"Link for Updating state from a memoized callback \")\n\nSometimes, you might need to update state based on previous state from a memoized callback.\n\nThis `handleAddTodo` function specifies `todos` as a dependency because it computes the next todos from it:\n\n```\nfunction TodoList() {const [todos, setTodos] = useState([]);const handleAddTodo = useCallback((text) => {const newTodo = { id: nextId++, text };setTodos([...todos, newTodo]);}, [todos]);// ...\n```\n\nYou’ll usually want memoized functions to have as few dependencies as possible. When you read some state only to calculate the next state, you can remove that dependency by passing an [updater function](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state) instead:\n\n```\nfunction TodoList() {const [todos, setTodos] = useState([]);const handleAddTodo = useCallback((text) => {const newTodo = { id: nextId++, text };setTodos(todos => [...todos, newTodo]);}, []); // ✅ No need for the todos dependency// ...\n```\n\nHere, instead of making `todos` a dependency and reading it inside, you pass an instruction about _how_ to update the state (`todos => [...todos, newTodo]`) to React. [Read more about updater functions.](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state)\n\n* * *\n\n### Preventing an Effect from firing too often[](https://react.dev/reference/react/useCallback#preventing-an-effect-from-firing-too-often \"Link for Preventing an Effect from firing too often \")\n\nSometimes, you might want to call a function from inside an [Effect:](https://react.dev/learn/synchronizing-with-effects)\n\n```\nfunction ChatRoom({ roomId }) {const [message, setMessage] = useState('');function createOptions() {return {serverUrl: 'https://localhost:1234',roomId: roomId};}useEffect(() => {const options = createOptions();const connection = createConnection();connection.connect();// ...\n```\n\nThis creates a problem. [Every reactive value must be declared as a dependency of your Effect.](https://react.dev/learn/lifecycle-of-reactive-effects#react-verifies-that-you-specified-every-reactive-value-as-a-dependency) However, if you declare `createOptions` as a dependency, it will cause your Effect to constantly reconnect to the chat room:\n\n```\nuseEffect(() => {const options = createOptions();const connection = createConnection();connection.connect();return () => connection.disconnect();}, [createOptions]); // 🔴 Problem: This dependency changes on every render// ...\n```\n\nTo solve this, you can wrap the function you need to call from an Effect into `useCallback`:\n\n```\nfunction ChatRoom({ roomId }) {const [message, setMessage] = useState('');const createOptions = useCallback(() => {return {serverUrl: 'https://localhost:1234',roomId: roomId};}, [roomId]); // ✅ Only changes when roomId changesuseEffect(() => {const options = createOptions();const connection = createConnection();connection.connect();return () => connection.disconnect();}, [createOptions]); // ✅ Only changes when createOptions changes// ...\n```\n\nThis ensures that the `createOptions` function is the same between re-renders if the `roomId` is the same. **However, it’s even better to remove the need for a function dependency.** Move your function _inside_ the Effect:\n\n```\nfunction ChatRoom({ roomId }) {const [message, setMessage] = useState('');useEffect(() => {function createOptions() { // ✅ No need for useCallback or function dependencies!return {serverUrl: 'https://localhost:1234',roomId: roomId};}const options = createOptions();const connection = createConnection();connection.connect();return () => connection.disconnect();}, [roomId]); // ✅ Only changes when roomId changes// ...\n```\n\nNow your code is simpler and doesn’t need `useCallback`. [Learn more about removing Effect dependencies.](https://react.dev/learn/removing-effect-dependencies#move-dynamic-objects-and-functions-inside-your-effect)\n\n* * *\n\n### Optimizing a custom Hook[](https://react.dev/reference/react/useCallback#optimizing-a-custom-hook \"Link for Optimizing a custom Hook \")\n\nIf you’re writing a [custom Hook,](https://react.dev/learn/reusing-logic-with-custom-hooks) it’s recommended to wrap any functions that it returns into `useCallback`:\n\n```\nfunction useRouter() {const { dispatch } = useContext(RouterStateContext);const navigate = useCallback((url) => {dispatch({ type: 'navigate', url });}, [dispatch]);const goBack = useCallback(() => {dispatch({ type: 'back' });}, [dispatch]);return {navigate,goBack,};}\n```\n\nThis ensures that the consumers of your Hook can optimize their own code when needed.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useCallback#troubleshooting \"Link for Troubleshooting \")\n------------------------------------------------------------------------------------------------------------\n\n### Every time my component renders, `useCallback` returns a different function[](https://react.dev/reference/react/useCallback#every-time-my-component-renders-usecallback-returns-a-different-function \"Link for this heading\")\n\nMake sure you’ve specified the dependency array as a second argument!\n\nIf you forget the dependency array, `useCallback` will return a new function every time:\n\n```\nfunction ProductPage({ productId, referrer }) {const handleSubmit = useCallback((orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails,});}); // 🔴 Returns a new function every time: no dependency array// ...\n```\n\nThis is the corrected version passing the dependency array as a second argument:\n\n```\nfunction ProductPage({ productId, referrer }) {const handleSubmit = useCallback((orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails,});}, [productId, referrer]); // ✅ Does not return a new function unnecessarily// ...\n```\n\nIf this doesn’t help, then the problem is that at least one of your dependencies is different from the previous render. You can debug this problem by manually logging your dependencies to the console:\n\n```\nconst handleSubmit = useCallback((orderDetails) => {// ..}, [productId, referrer]);console.log([productId, referrer]);\n```\n\nYou can then right-click on the arrays from different re-renders in the console and select “Store as a global variable” for both of them. Assuming the first one got saved as `temp1` and the second one got saved as `temp2`, you can then use the browser console to check whether each dependency in both arrays is the same:\n\n```\nObject.is(temp1[0], temp2[0]); // Is the first dependency the same between the arrays?Object.is(temp1[1], temp2[1]); // Is the second dependency the same between the arrays?Object.is(temp1[2], temp2[2]); // ... and so on for every dependency ...\n```\n\nWhen you find which dependency is breaking memoization, either find a way to remove it, or [memoize it as well.](https://react.dev/reference/react/useMemo#memoizing-a-dependency-of-another-hook)\n\n* * *\n\n### I need to call `useCallback` for each list item in a loop, but it’s not allowed[](https://react.dev/reference/react/useCallback#i-need-to-call-usememo-for-each-list-item-in-a-loop-but-its-not-allowed \"Link for this heading\")\n\nSuppose the `Chart` component is wrapped in [`memo`](https://react.dev/reference/react/memo). You want to skip re-rendering every `Chart` in the list when the `ReportList` component re-renders. However, you can’t call `useCallback` in a loop:\n\n```\nfunction ReportList({ items }) {return (<article>{items.map(item => {// 🔴 You can't call useCallback in a loop like this:const handleClick = useCallback(() => {sendReport(item)}, [item]);return (<figure key={item.id}><Chart onClick={handleClick} /></figure>);})}</article>);}\n```\n\nInstead, extract a component for an individual item, and put `useCallback` there:\n\n```\nfunction ReportList({ items }) {return (<article>{items.map(item =><Report key={item.id} item={item} />)}</article>);}function Report({ item }) {// ✅ Call useCallback at the top level:const handleClick = useCallback(() => {sendReport(item)}, [item]);return (<figure><Chart onClick={handleClick} /></figure>);}\n```\n\nAlternatively, you could remove `useCallback` in the last snippet and instead wrap `Report` itself in [`memo`.](https://react.dev/reference/react/memo) If the `item` prop does not change, `Report` will skip re-rendering, so `Chart` will skip re-rendering too:\n\n```\nfunction ReportList({ items }) {// ...}const Report = memo(function Report({ item }) {function handleClick() {sendReport(item);}return (<figure><Chart onClick={handleClick} /></figure>);});\n```\n",
"filename": "useCallback.md",
"package": "react"
} |
{
"content": "Title: useContext – React\n\nURL Source: https://react.dev/reference/react/useContext\n\nMarkdown Content:\n`useContext` is a React Hook that lets you read and subscribe to [context](https://react.dev/learn/passing-data-deeply-with-context) from your component.\n\n```\nconst value = useContext(SomeContext)\n```\n\n* [Reference](https://react.dev/reference/react/useContext#reference)\n * [`useContext(SomeContext)`](https://react.dev/reference/react/useContext#usecontext)\n* [Usage](https://react.dev/reference/react/useContext#usage)\n * [Passing data deeply into the tree](https://react.dev/reference/react/useContext#passing-data-deeply-into-the-tree)\n * [Updating data passed via context](https://react.dev/reference/react/useContext#updating-data-passed-via-context)\n * [Specifying a fallback default value](https://react.dev/reference/react/useContext#specifying-a-fallback-default-value)\n * [Overriding context for a part of the tree](https://react.dev/reference/react/useContext#overriding-context-for-a-part-of-the-tree)\n * [Optimizing re-renders when passing objects and functions](https://react.dev/reference/react/useContext#optimizing-re-renders-when-passing-objects-and-functions)\n* [Troubleshooting](https://react.dev/reference/react/useContext#troubleshooting)\n * [My component doesn’t see the value from my provider](https://react.dev/reference/react/useContext#my-component-doesnt-see-the-value-from-my-provider)\n * [I am always getting `undefined` from my context although the default value is different](https://react.dev/reference/react/useContext#i-am-always-getting-undefined-from-my-context-although-the-default-value-is-different)\n\n* * *\n\nReference[](https://react.dev/reference/react/useContext#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------\n\n### `useContext(SomeContext)`[](https://react.dev/reference/react/useContext#usecontext \"Link for this heading\")\n\nCall `useContext` at the top level of your component to read and subscribe to [context.](https://react.dev/learn/passing-data-deeply-with-context)\n\n```\nimport { useContext } from 'react';function MyComponent() {const theme = useContext(ThemeContext);// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/useContext#usage)\n\n#### Parameters[](https://react.dev/reference/react/useContext#parameters \"Link for Parameters \")\n\n* `SomeContext`: The context that you’ve previously created with [`createContext`](https://react.dev/reference/react/createContext). The context itself does not hold the information, it only represents the kind of information you can provide or read from components.\n\n#### Returns[](https://react.dev/reference/react/useContext#returns \"Link for Returns \")\n\n`useContext` returns the context value for the calling component. It is determined as the `value` passed to the closest `SomeContext.Provider` above the calling component in the tree. If there is no such provider, then the returned value will be the `defaultValue` you have passed to [`createContext`](https://react.dev/reference/react/createContext) for that context. The returned value is always up-to-date. React automatically re-renders components that read some context if it changes.\n\n#### Caveats[](https://react.dev/reference/react/useContext#caveats \"Link for Caveats \")\n\n* `useContext()` call in a component is not affected by providers returned from the _same_ component. The corresponding `<Context.Provider>` **needs to be _above_** the component doing the `useContext()` call.\n* React **automatically re-renders** all the children that use a particular context starting from the provider that receives a different `value`. The previous and the next values are compared with the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. Skipping re-renders with [`memo`](https://react.dev/reference/react/memo) does not prevent the children receiving fresh context values.\n* If your build system produces duplicates modules in the output (which can happen with symlinks), this can break context. Passing something via context only works if `SomeContext` that you use to provide context and `SomeContext` that you use to read it are **_exactly_ the same object**, as determined by a `===` comparison.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useContext#usage \"Link for Usage \")\n-----------------------------------------------------------------------------\n\n### Passing data deeply into the tree[](https://react.dev/reference/react/useContext#passing-data-deeply-into-the-tree \"Link for Passing data deeply into the tree \")\n\nCall `useContext` at the top level of your component to read and subscribe to [context.](https://react.dev/learn/passing-data-deeply-with-context)\n\n```\nimport { useContext } from 'react';function Button() {const theme = useContext(ThemeContext);// ...\n```\n\n`useContext` returns the context value for the context you passed. To determine the context value, React searches the component tree and finds **the closest context provider above** for that particular context.\n\nTo pass context to a `Button`, wrap it or one of its parent components into the corresponding context provider:\n\n```\nfunction MyPage() {return (<ThemeContext.Provider value=\"dark\"><Form /></ThemeContext.Provider>);}function Form() {// ... renders buttons inside ...}\n```\n\nIt doesn’t matter how many layers of components there are between the provider and the `Button`. When a `Button` _anywhere_ inside of `Form` calls `useContext(ThemeContext)`, it will receive `\"dark\"` as the value.\n\n### Pitfall\n\n`useContext()` always looks for the closest provider _above_ the component that calls it. It searches upwards and **does not** consider providers in the component from which you’re calling `useContext()`.\n\nimport { createContext, useContext } from 'react';\n\nconst ThemeContext = createContext(null);\n\nexport default function MyApp() {\n return (\n <ThemeContext.Provider value\\=\"dark\"\\>\n <Form />\n </ThemeContext.Provider\\>\n )\n}\n\nfunction Form() {\n return (\n <Panel title\\=\"Welcome\"\\>\n <Button\\>Sign up</Button\\>\n <Button\\>Log in</Button\\>\n </Panel\\>\n );\n}\n\nfunction Panel({ title, children }) {\n const theme = useContext(ThemeContext);\n const className = 'panel-' + theme;\n return (\n <section className\\={className}\\>\n <h1\\>{title}</h1\\>\n {children}\n </section\\>\n )\n}\n\nfunction Button({ children }) {\n const theme = useContext(ThemeContext);\n const className = 'button-' + theme;\n return (\n <button className\\={className}\\>\n {children}\n </button\\>\n );\n}\n\n* * *\n\n### Updating data passed via context[](https://react.dev/reference/react/useContext#updating-data-passed-via-context \"Link for Updating data passed via context \")\n\nOften, you’ll want the context to change over time. To update context, combine it with [state.](https://react.dev/reference/react/useState) Declare a state variable in the parent component, and pass the current state down as the context value to the provider.\n\n```\nfunction MyPage() {const [theme, setTheme] = useState('dark');return (<ThemeContext.Provider value={theme}><Form /><Button onClick={() => {setTheme('light');}}> Switch to light theme</Button></ThemeContext.Provider>);}\n```\n\nNow any `Button` inside of the provider will receive the current `theme` value. If you call `setTheme` to update the `theme` value that you pass to the provider, all `Button` components will re-render with the new `'light'` value.\n\n#### Examples of updating context[](https://react.dev/reference/react/useContext#examples-basic \"Link for Examples of updating context\")\n\n#### Updating a value via context[](https://react.dev/reference/react/useContext#updating-a-value-via-context \"Link for this heading\")\n\nIn this example, the `MyApp` component holds a state variable which is then passed to the `ThemeContext` provider. Checking the “Dark mode” checkbox updates the state. Changing the provided value re-renders all the components using that context.\n\nimport { createContext, useContext, useState } from 'react';\n\nconst ThemeContext = createContext(null);\n\nexport default function MyApp() {\n const \\[theme, setTheme\\] = useState('light');\n return (\n <ThemeContext.Provider value\\={theme}\\>\n <Form />\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={theme === 'dark'}\n onChange\\={(e) \\=> {\n setTheme(e.target.checked ? 'dark' : 'light')\n }}\n />\n Use dark mode\n </label\\>\n </ThemeContext.Provider\\>\n )\n}\n\nfunction Form({ children }) {\n return (\n <Panel title\\=\"Welcome\"\\>\n <Button\\>Sign up</Button\\>\n <Button\\>Log in</Button\\>\n </Panel\\>\n );\n}\n\nfunction Panel({ title, children }) {\n const theme = useContext(ThemeContext);\n const className = 'panel-' + theme;\n return (\n <section className\\={className}\\>\n <h1\\>{title}</h1\\>\n {children}\n </section\\>\n )\n}\n\nfunction Button({ children }) {\n const theme = useContext(ThemeContext);\n const className = 'button-' + theme;\n return (\n <button className\\={className}\\>\n {children}\n </button\\>\n );\n}\n\nNote that `value=\"dark\"` passes the `\"dark\"` string, but `value={theme}` passes the value of the JavaScript `theme` variable with [JSX curly braces.](https://react.dev/learn/javascript-in-jsx-with-curly-braces) Curly braces also let you pass context values that aren’t strings.\n\n* * *\n\n### Specifying a fallback default value[](https://react.dev/reference/react/useContext#specifying-a-fallback-default-value \"Link for Specifying a fallback default value \")\n\nIf React can’t find any providers of that particular context in the parent tree, the context value returned by `useContext()` will be equal to the default value that you specified when you [created that context](https://react.dev/reference/react/createContext):\n\n```\nconst ThemeContext = createContext(null);\n```\n\nThe default value **never changes**. If you want to update context, use it with state as [described above.](https://react.dev/reference/react/useContext#updating-data-passed-via-context)\n\nOften, instead of `null`, there is some more meaningful value you can use as a default, for example:\n\n```\nconst ThemeContext = createContext('light');\n```\n\nThis way, if you accidentally render some component without a corresponding provider, it won’t break. This also helps your components work well in a test environment without setting up a lot of providers in the tests.\n\nIn the example below, the “Toggle theme” button is always light because it’s **outside any theme context provider** and the default context theme value is `'light'`. Try editing the default theme to be `'dark'`.\n\nimport { createContext, useContext, useState } from 'react';\n\nconst ThemeContext = createContext('light');\n\nexport default function MyApp() {\n const \\[theme, setTheme\\] = useState('light');\n return (\n <\\>\n <ThemeContext.Provider value\\={theme}\\>\n <Form />\n </ThemeContext.Provider\\>\n <Button onClick\\={() \\=> {\n setTheme(theme === 'dark' ? 'light' : 'dark');\n }}\\>\n Toggle theme\n </Button\\>\n </\\>\n )\n}\n\nfunction Form({ children }) {\n return (\n <Panel title\\=\"Welcome\"\\>\n <Button\\>Sign up</Button\\>\n <Button\\>Log in</Button\\>\n </Panel\\>\n );\n}\n\nfunction Panel({ title, children }) {\n const theme = useContext(ThemeContext);\n const className = 'panel-' + theme;\n return (\n <section className\\={className}\\>\n <h1\\>{title}</h1\\>\n {children}\n </section\\>\n )\n}\n\nfunction Button({ children, onClick }) {\n const theme = useContext(ThemeContext);\n const className = 'button-' + theme;\n return (\n <button className\\={className} onClick\\={onClick}\\>\n {children}\n </button\\>\n );\n}\n\n* * *\n\n### Overriding context for a part of the tree[](https://react.dev/reference/react/useContext#overriding-context-for-a-part-of-the-tree \"Link for Overriding context for a part of the tree \")\n\nYou can override the context for a part of the tree by wrapping that part in a provider with a different value.\n\n```\n<ThemeContext.Provider value=\"dark\"> ...<ThemeContext.Provider value=\"light\"><Footer /></ThemeContext.Provider> ...</ThemeContext.Provider>\n```\n\nYou can nest and override providers as many times as you need.\n\n#### Examples of overriding context[](https://react.dev/reference/react/useContext#examples \"Link for Examples of overriding context\")\n\n#### Overriding a theme[](https://react.dev/reference/react/useContext#overriding-a-theme \"Link for this heading\")\n\nHere, the button _inside_ the `Footer` receives a different context value (`\"light\"`) than the buttons outside (`\"dark\"`).\n\nimport { createContext, useContext } from 'react';\n\nconst ThemeContext = createContext(null);\n\nexport default function MyApp() {\n return (\n <ThemeContext.Provider value\\=\"dark\"\\>\n <Form />\n </ThemeContext.Provider\\>\n )\n}\n\nfunction Form() {\n return (\n <Panel title\\=\"Welcome\"\\>\n <Button\\>Sign up</Button\\>\n <Button\\>Log in</Button\\>\n <ThemeContext.Provider value\\=\"light\"\\>\n <Footer />\n </ThemeContext.Provider\\>\n </Panel\\>\n );\n}\n\nfunction Footer() {\n return (\n <footer\\>\n <Button\\>Settings</Button\\>\n </footer\\>\n );\n}\n\nfunction Panel({ title, children }) {\n const theme = useContext(ThemeContext);\n const className = 'panel-' + theme;\n return (\n <section className\\={className}\\>\n {title && <h1\\>{title}</h1\\>}\n {children}\n </section\\>\n )\n}\n\nfunction Button({ children }) {\n const theme = useContext(ThemeContext);\n const className = 'button-' + theme;\n return (\n <button className\\={className}\\>\n {children}\n </button\\>\n );\n}\n\n* * *\n\n### Optimizing re-renders when passing objects and functions[](https://react.dev/reference/react/useContext#optimizing-re-renders-when-passing-objects-and-functions \"Link for Optimizing re-renders when passing objects and functions \")\n\nYou can pass any values via context, including objects and functions.\n\n```\nfunction MyApp() {const [currentUser, setCurrentUser] = useState(null);function login(response) {storeCredentials(response.credentials);setCurrentUser(response.user);}return (<AuthContext.Provider value={{ currentUser, login }}><Page /></AuthContext.Provider>);}\n```\n\nHere, the context value is a JavaScript object with two properties, one of which is a function. Whenever `MyApp` re-renders (for example, on a route update), this will be a _different_ object pointing at a _different_ function, so React will also have to re-render all components deep in the tree that call `useContext(AuthContext)`.\n\nIn smaller apps, this is not a problem. However, there is no need to re-render them if the underlying data, like `currentUser`, has not changed. To help React take advantage of that fact, you may wrap the `login` function with [`useCallback`](https://react.dev/reference/react/useCallback) and wrap the object creation into [`useMemo`](https://react.dev/reference/react/useMemo). This is a performance optimization:\n\n```\nimport { useCallback, useMemo } from 'react';function MyApp() {const [currentUser, setCurrentUser] = useState(null);const login = useCallback((response) => {storeCredentials(response.credentials);setCurrentUser(response.user);}, []);const contextValue = useMemo(() => ({currentUser,login}), [currentUser, login]);return (<AuthContext.Provider value={contextValue}><Page /></AuthContext.Provider>);}\n```\n\nAs a result of this change, even if `MyApp` needs to re-render, the components calling `useContext(AuthContext)` won’t need to re-render unless `currentUser` has changed.\n\nRead more about [`useMemo`](https://react.dev/reference/react/useMemo#skipping-re-rendering-of-components) and [`useCallback`.](https://react.dev/reference/react/useCallback#skipping-re-rendering-of-components)\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useContext#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------------\n\n### My component doesn’t see the value from my provider[](https://react.dev/reference/react/useContext#my-component-doesnt-see-the-value-from-my-provider \"Link for My component doesn’t see the value from my provider \")\n\nThere are a few common ways that this can happen:\n\n1. You’re rendering `<SomeContext.Provider>` in the same component (or below) as where you’re calling `useContext()`. Move `<SomeContext.Provider>` _above and outside_ the component calling `useContext()`.\n2. You may have forgotten to wrap your component with `<SomeContext.Provider>`, or you might have put it in a different part of the tree than you thought. Check whether the hierarchy is right using [React DevTools.](https://react.dev/learn/react-developer-tools)\n3. You might be running into some build issue with your tooling that causes `SomeContext` as seen from the providing component and `SomeContext` as seen by the reading component to be two different objects. This can happen if you use symlinks, for example. You can verify this by assigning them to globals like `window.SomeContext1` and `window.SomeContext2` and then checking whether `window.SomeContext1 === window.SomeContext2` in the console. If they’re not the same, fix that issue on the build tool level.\n\n### I am always getting `undefined` from my context although the default value is different[](https://react.dev/reference/react/useContext#i-am-always-getting-undefined-from-my-context-although-the-default-value-is-different \"Link for this heading\")\n\nYou might have a provider without a `value` in the tree:\n\n```\n// 🚩 Doesn't work: no value prop<ThemeContext.Provider><Button /></ThemeContext.Provider>\n```\n\nIf you forget to specify `value`, it’s like passing `value={undefined}`.\n\nYou may have also mistakingly used a different prop name by mistake:\n\n```\n// 🚩 Doesn't work: prop should be called \"value\"<ThemeContext.Provider theme={theme}><Button /></ThemeContext.Provider>\n```\n\nIn both of these cases you should see a warning from React in the console. To fix them, call the prop `value`:\n\n```\n// ✅ Passing the value prop<ThemeContext.Provider value={theme}><Button /></ThemeContext.Provider>\n```\n\nNote that the [default value from your `createContext(defaultValue)` call](https://react.dev/reference/react/useContext#specifying-a-fallback-default-value) is only used **if there is no matching provider above at all.** If there is a `<SomeContext.Provider value={undefined}>` component somewhere in the parent tree, the component calling `useContext(SomeContext)` _will_ receive `undefined` as the context value.\n",
"filename": "useContext.md",
"package": "react"
} |
{
"content": "Title: useDebugValue – React\n\nURL Source: https://react.dev/reference/react/useDebugValue\n\nMarkdown Content:\nuseDebugValue – React\n===============\n\n[Hooks](https://react.dev/reference/react/hooks)\n\nuseDebugValue[](https://react.dev/reference/react/useDebugValue#undefined \"Link for this heading\")\n==================================================================================================\n\n`useDebugValue` is a React Hook that lets you add a label to a custom Hook in [React DevTools.](https://react.dev/learn/react-developer-tools)\n\n```\nuseDebugValue(value, format?)\n```\n\n* [Reference](https://react.dev/reference/react/useDebugValue#reference)\n * [`useDebugValue(value, format?)`](https://react.dev/reference/react/useDebugValue#usedebugvalue)\n* [Usage](https://react.dev/reference/react/useDebugValue#usage)\n * [Adding a label to a custom Hook](https://react.dev/reference/react/useDebugValue#adding-a-label-to-a-custom-hook)\n * [Deferring formatting of a debug value](https://react.dev/reference/react/useDebugValue#deferring-formatting-of-a-debug-value)\n\n* * *\n\nReference[](https://react.dev/reference/react/useDebugValue#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `useDebugValue(value, format?)`[](https://react.dev/reference/react/useDebugValue#usedebugvalue \"Link for this heading\")\n\nCall `useDebugValue` at the top level of your [custom Hook](https://react.dev/learn/reusing-logic-with-custom-hooks) to display a readable debug value:\n\n```\nimport { useDebugValue } from 'react';function useOnlineStatus() { // ... useDebugValue(isOnline ? 'Online' : 'Offline'); // ...}\n```\n\n[See more examples below.](https://react.dev/reference/react/useDebugValue#usage)\n\n#### Parameters[](https://react.dev/reference/react/useDebugValue#parameters \"Link for Parameters \")\n\n* `value`: The value you want to display in React DevTools. It can have any type.\n* **optional** `format`: A formatting function. When the component is inspected, React DevTools will call the formatting function with the `value` as the argument, and then display the returned formatted value (which may have any type). If you don’t specify the formatting function, the original `value` itself will be displayed.\n\n#### Returns[](https://react.dev/reference/react/useDebugValue#returns \"Link for Returns \")\n\n`useDebugValue` does not return anything.\n\nUsage[](https://react.dev/reference/react/useDebugValue#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Adding a label to a custom Hook[](https://react.dev/reference/react/useDebugValue#adding-a-label-to-a-custom-hook \"Link for Adding a label to a custom Hook \")\n\nCall `useDebugValue` at the top level of your [custom Hook](https://react.dev/learn/reusing-logic-with-custom-hooks) to display a readable debug value for [React DevTools.](https://react.dev/learn/react-developer-tools)\n\n```\nimport { useDebugValue } from 'react';function useOnlineStatus() { // ... useDebugValue(isOnline ? 'Online' : 'Offline'); // ...}\n```\n\nThis gives components calling `useOnlineStatus` a label like `OnlineStatus: \"Online\"` when you inspect them:\n\n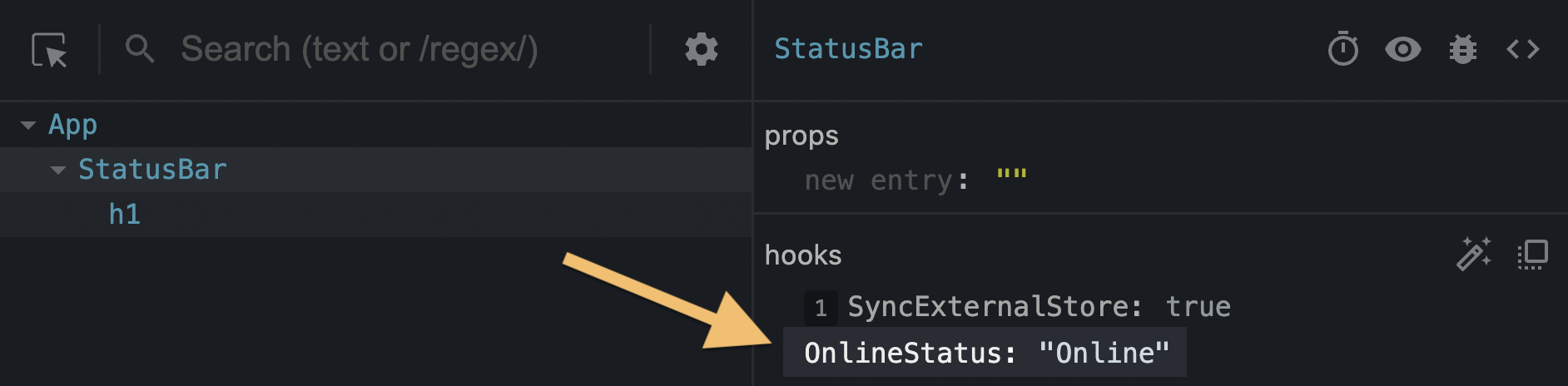Without the `useDebugValue` call, only the underlying data (in this example, `true`) would be displayed.\n\nApp.jsuseOnlineStatus.js\n\nuseOnlineStatus.js\n\nReset\n\nFork\n\nimport { useSyncExternalStore, useDebugValue } from 'react';\n\nexport function useOnlineStatus() {\n const isOnline = useSyncExternalStore(subscribe, () \\=> navigator.onLine, () \\=> true);\n useDebugValue(isOnline ? 'Online' : 'Offline');\n return isOnline;\n}\n\nfunction subscribe(callback) {\n window.addEventListener('online', callback);\n window.addEventListener('offline', callback);\n return () \\=> {\n window.removeEventListener('online', callback);\n window.removeEventListener('offline', callback);\n };\n}\n\nOpen Sandbox\n\nShow more\n\n### Note\n\nDon’t add debug values to every custom Hook. It’s most valuable for custom Hooks that are part of shared libraries and that have a complex internal data structure that’s difficult to inspect.\n\n* * *\n\n### Deferring formatting of a debug value[](https://react.dev/reference/react/useDebugValue#deferring-formatting-of-a-debug-value \"Link for Deferring formatting of a debug value \")\n\nYou can also pass a formatting function as the second argument to `useDebugValue`:\n\n```\nuseDebugValue(date, date => date.toDateString());\n```\n\nYour formatting function will receive the debug value as a parameter and should return a formatted display value. When your component is inspected, React DevTools will call this function and display its result.\n\nThis lets you avoid running potentially expensive formatting logic unless the component is actually inspected. For example, if `date` is a Date value, this avoids calling `toDateString()` on it for every render.\n\n[PrevioususeContext](https://react.dev/reference/react/useContext)[NextuseDeferredValue](https://react.dev/reference/react/useDeferredValue)\n",
"filename": "useDebugValue.md",
"package": "react"
} |
{
"content": "Title: useDeferredValue – React\n\nURL Source: https://react.dev/reference/react/useDeferredValue\n\nMarkdown Content:\n`useDeferredValue` is a React Hook that lets you defer updating a part of the UI.\n\n```\nconst deferredValue = useDeferredValue(value)\n```\n\n* [Reference](https://react.dev/reference/react/useDeferredValue#reference)\n * [`useDeferredValue(value, initialValue?)`](https://react.dev/reference/react/useDeferredValue#usedeferredvalue)\n* [Usage](https://react.dev/reference/react/useDeferredValue#usage)\n * [Showing stale content while fresh content is loading](https://react.dev/reference/react/useDeferredValue#showing-stale-content-while-fresh-content-is-loading)\n * [Indicating that the content is stale](https://react.dev/reference/react/useDeferredValue#indicating-that-the-content-is-stale)\n * [Deferring re-rendering for a part of the UI](https://react.dev/reference/react/useDeferredValue#deferring-re-rendering-for-a-part-of-the-ui)\n\n* * *\n\nReference[](https://react.dev/reference/react/useDeferredValue#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------------\n\n### `useDeferredValue(value, initialValue?)`[](https://react.dev/reference/react/useDeferredValue#usedeferredvalue \"Link for this heading\")\n\nCall `useDeferredValue` at the top level of your component to get a deferred version of that value.\n\n```\nimport { useState, useDeferredValue } from 'react';function SearchPage() {const [query, setQuery] = useState('');const deferredQuery = useDeferredValue(query);// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react/useDeferredValue#usage)\n\n#### Parameters[](https://react.dev/reference/react/useDeferredValue#parameters \"Link for Parameters \")\n\n* `value`: The value you want to defer. It can have any type.\n* Canary only **optional** `initialValue`: A value to use during the initial render of a component. If this option is omitted, `useDeferredValue` will not defer during the initial render, because there’s no previous version of `value` that it can render instead.\n\n#### Returns[](https://react.dev/reference/react/useDeferredValue#returns \"Link for Returns \")\n\n* `currentValue`: During the initial render, the returned deferred value will be the same as the value you provided. During updates, React will first attempt a re-render with the old value (so it will return the old value), and then try another re-render in the background with the new value (so it will return the updated value).\n\n### Canary\n\nIn the latest React Canary versions, `useDeferredValue` returns the `initialValue` on initial render, and schedules a re-render in the background with the `value` returned.\n\n#### Caveats[](https://react.dev/reference/react/useDeferredValue#caveats \"Link for Caveats \")\n\n* When an update is inside a Transition, `useDeferredValue` always returns the new `value` and does not spawn a deferred render, since the update is already deferred.\n \n* The values you pass to `useDeferredValue` should either be primitive values (like strings and numbers) or objects created outside of rendering. If you create a new object during rendering and immediately pass it to `useDeferredValue`, it will be different on every render, causing unnecessary background re-renders.\n \n* When `useDeferredValue` receives a different value (compared with [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)), in addition to the current render (when it still uses the previous value), it schedules a re-render in the background with the new value. The background re-render is interruptible: if there’s another update to the `value`, React will restart the background re-render from scratch. For example, if the user is typing into an input faster than a chart receiving its deferred value can re-render, the chart will only re-render after the user stops typing.\n \n* `useDeferredValue` is integrated with [`<Suspense>`.](https://react.dev/reference/react/Suspense) If the background update caused by a new value suspends the UI, the user will not see the fallback. They will see the old deferred value until the data loads.\n \n* `useDeferredValue` does not by itself prevent extra network requests.\n \n* There is no fixed delay caused by `useDeferredValue` itself. As soon as React finishes the original re-render, React will immediately start working on the background re-render with the new deferred value. Any updates caused by events (like typing) will interrupt the background re-render and get prioritized over it.\n \n* The background re-render caused by `useDeferredValue` does not fire Effects until it’s committed to the screen. If the background re-render suspends, its Effects will run after the data loads and the UI updates.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useDeferredValue#usage \"Link for Usage \")\n-----------------------------------------------------------------------------------\n\n### Showing stale content while fresh content is loading[](https://react.dev/reference/react/useDeferredValue#showing-stale-content-while-fresh-content-is-loading \"Link for Showing stale content while fresh content is loading \")\n\nCall `useDeferredValue` at the top level of your component to defer updating some part of your UI.\n\n```\nimport { useState, useDeferredValue } from 'react';function SearchPage() {const [query, setQuery] = useState('');const deferredQuery = useDeferredValue(query);// ...}\n```\n\nDuring the initial render, the deferred value will be the same as the value you provided.\n\nDuring updates, the deferred value will “lag behind” the latest value. In particular, React will first re-render _without_ updating the deferred value, and then try to re-render with the newly received value in the background.\n\n**Let’s walk through an example to see when this is useful.**\n\n### Note\n\nIn this example, the `SearchResults` component [suspends](https://react.dev/reference/react/Suspense#displaying-a-fallback-while-content-is-loading) while fetching the search results. Try typing `\"a\"`, waiting for the results, and then editing it to `\"ab\"`. The results for `\"a\"` get replaced by the loading fallback.\n\nimport { Suspense, useState } from 'react';\nimport SearchResults from './SearchResults.js';\n\nexport default function App() {\n const \\[query, setQuery\\] = useState('');\n return (\n <\\>\n <label\\>\n Search albums:\n <input value\\={query} onChange\\={e \\=> setQuery(e.target.value)} />\n </label\\>\n <Suspense fallback\\={<h2\\>Loading...</h2\\>}\\>\n <SearchResults query\\={query} />\n </Suspense\\>\n </\\>\n );\n}\n\nA common alternative UI pattern is to _defer_ updating the list of results and to keep showing the previous results until the new results are ready. Call `useDeferredValue` to pass a deferred version of the query down:\n\n```\nexport default function App() {const [query, setQuery] = useState('');const deferredQuery = useDeferredValue(query);return (<><label> Search albums:<input value={query} onChange={e => setQuery(e.target.value)} /></label><Suspense fallback={<h2>Loading...</h2>}><SearchResults query={deferredQuery} /></Suspense></>);}\n```\n\nThe `query` will update immediately, so the input will display the new value. However, the `deferredQuery` will keep its previous value until the data has loaded, so `SearchResults` will show the stale results for a bit.\n\nEnter `\"a\"` in the example below, wait for the results to load, and then edit the input to `\"ab\"`. Notice how instead of the Suspense fallback, you now see the stale result list until the new results have loaded:\n\nimport { Suspense, useState, useDeferredValue } from 'react';\nimport SearchResults from './SearchResults.js';\n\nexport default function App() {\n const \\[query, setQuery\\] = useState('');\n const deferredQuery = useDeferredValue(query);\n return (\n <\\>\n <label\\>\n Search albums:\n <input value\\={query} onChange\\={e \\=> setQuery(e.target.value)} />\n </label\\>\n <Suspense fallback\\={<h2\\>Loading...</h2\\>}\\>\n <SearchResults query\\={deferredQuery} />\n </Suspense\\>\n </\\>\n );\n}\n\n##### Deep Dive\n\n#### How does deferring a value work under the hood?[](https://react.dev/reference/react/useDeferredValue#how-does-deferring-a-value-work-under-the-hood \"Link for How does deferring a value work under the hood? \")\n\nYou can think of it as happening in two steps:\n\n1. **First, React re-renders with the new `query` (`\"ab\"`) but with the old `deferredQuery` (still `\"a\")`.** The `deferredQuery` value, which you pass to the result list, is _deferred:_ it “lags behind” the `query` value.\n \n2. **In the background, React tries to re-render with _both_ `query` and `deferredQuery` updated to `\"ab\"`.** If this re-render completes, React will show it on the screen. However, if it suspends (the results for `\"ab\"` have not loaded yet), React will abandon this rendering attempt, and retry this re-render again after the data has loaded. The user will keep seeing the stale deferred value until the data is ready.\n \n\nThe deferred “background” rendering is interruptible. For example, if you type into the input again, React will abandon it and restart with the new value. React will always use the latest provided value.\n\nNote that there is still a network request per each keystroke. What’s being deferred here is displaying results (until they’re ready), not the network requests themselves. Even if the user continues typing, responses for each keystroke get cached, so pressing Backspace is instant and doesn’t fetch again.\n\n* * *\n\n### Indicating that the content is stale[](https://react.dev/reference/react/useDeferredValue#indicating-that-the-content-is-stale \"Link for Indicating that the content is stale \")\n\nIn the example above, there is no indication that the result list for the latest query is still loading. This can be confusing to the user if the new results take a while to load. To make it more obvious to the user that the result list does not match the latest query, you can add a visual indication when the stale result list is displayed:\n\n```\n<div style={{opacity: query !== deferredQuery ? 0.5 : 1,}}><SearchResults query={deferredQuery} /></div>\n```\n\nWith this change, as soon as you start typing, the stale result list gets slightly dimmed until the new result list loads. You can also add a CSS transition to delay dimming so that it feels gradual, like in the example below:\n\nimport { Suspense, useState, useDeferredValue } from 'react';\nimport SearchResults from './SearchResults.js';\n\nexport default function App() {\n const \\[query, setQuery\\] = useState('');\n const deferredQuery = useDeferredValue(query);\n const isStale = query !== deferredQuery;\n return (\n <\\>\n <label\\>\n Search albums:\n <input value\\={query} onChange\\={e \\=> setQuery(e.target.value)} />\n </label\\>\n <Suspense fallback\\={<h2\\>Loading...</h2\\>}\\>\n <div style\\={{\n opacity: isStale ? 0.5 : 1,\n transition: isStale ? 'opacity 0.2s 0.2s linear' : 'opacity 0s 0s linear'\n }}\\>\n <SearchResults query\\={deferredQuery} />\n </div\\>\n </Suspense\\>\n </\\>\n );\n}\n\n* * *\n\n### Deferring re-rendering for a part of the UI[](https://react.dev/reference/react/useDeferredValue#deferring-re-rendering-for-a-part-of-the-ui \"Link for Deferring re-rendering for a part of the UI \")\n\nYou can also apply `useDeferredValue` as a performance optimization. It is useful when a part of your UI is slow to re-render, there’s no easy way to optimize it, and you want to prevent it from blocking the rest of the UI.\n\nImagine you have a text field and a component (like a chart or a long list) that re-renders on every keystroke:\n\n```\nfunction App() {const [text, setText] = useState('');return (<><input value={text} onChange={e => setText(e.target.value)} /><SlowList text={text} /></>);}\n```\n\nFirst, optimize `SlowList` to skip re-rendering when its props are the same. To do this, [wrap it in `memo`:](https://react.dev/reference/react/memo#skipping-re-rendering-when-props-are-unchanged)\n\n```\nconst SlowList = memo(function SlowList({ text }) {// ...});\n```\n\nHowever, this only helps if the `SlowList` props are _the same_ as during the previous render. The problem you’re facing now is that it’s slow when they’re _different,_ and when you actually need to show different visual output.\n\nConcretely, the main performance problem is that whenever you type into the input, the `SlowList` receives new props, and re-rendering its entire tree makes the typing feel janky. In this case, `useDeferredValue` lets you prioritize updating the input (which must be fast) over updating the result list (which is allowed to be slower):\n\n```\nfunction App() {const [text, setText] = useState('');const deferredText = useDeferredValue(text);return (<><input value={text} onChange={e => setText(e.target.value)} /><SlowList text={deferredText} /></>);}\n```\n\nThis does not make re-rendering of the `SlowList` faster. However, it tells React that re-rendering the list can be deprioritized so that it doesn’t block the keystrokes. The list will “lag behind” the input and then “catch up”. Like before, React will attempt to update the list as soon as possible, but will not block the user from typing.\n\n#### The difference between useDeferredValue and unoptimized re-rendering[](https://react.dev/reference/react/useDeferredValue#examples \"Link for The difference between useDeferredValue and unoptimized re-rendering\")\n\n#### Deferred re-rendering of the list[](https://react.dev/reference/react/useDeferredValue#deferred-re-rendering-of-the-list \"Link for this heading\")\n\nIn this example, each item in the `SlowList` component is **artificially slowed down** so that you can see how `useDeferredValue` lets you keep the input responsive. Type into the input and notice that typing feels snappy while the list “lags behind” it.\n\n### Pitfall\n\nThis optimization requires `SlowList` to be wrapped in [`memo`.](https://react.dev/reference/react/memo) This is because whenever the `text` changes, React needs to be able to re-render the parent component quickly. During that re-render, `deferredText` still has its previous value, so `SlowList` is able to skip re-rendering (its props have not changed). Without [`memo`,](https://react.dev/reference/react/memo) it would have to re-render anyway, defeating the point of the optimization.\n\n##### Deep Dive\n\n#### How is deferring a value different from debouncing and throttling?[](https://react.dev/reference/react/useDeferredValue#how-is-deferring-a-value-different-from-debouncing-and-throttling \"Link for How is deferring a value different from debouncing and throttling? \")\n\nThere are two common optimization techniques you might have used before in this scenario:\n\n* _Debouncing_ means you’d wait for the user to stop typing (e.g. for a second) before updating the list.\n* _Throttling_ means you’d update the list every once in a while (e.g. at most once a second).\n\nWhile these techniques are helpful in some cases, `useDeferredValue` is better suited to optimizing rendering because it is deeply integrated with React itself and adapts to the user’s device.\n\nUnlike debouncing or throttling, it doesn’t require choosing any fixed delay. If the user’s device is fast (e.g. powerful laptop), the deferred re-render would happen almost immediately and wouldn’t be noticeable. If the user’s device is slow, the list would “lag behind” the input proportionally to how slow the device is.\n\nAlso, unlike with debouncing or throttling, deferred re-renders done by `useDeferredValue` are interruptible by default. This means that if React is in the middle of re-rendering a large list, but the user makes another keystroke, React will abandon that re-render, handle the keystroke, and then start rendering in the background again. By contrast, debouncing and throttling still produce a janky experience because they’re _blocking:_ they merely postpone the moment when rendering blocks the keystroke.\n\nIf the work you’re optimizing doesn’t happen during rendering, debouncing and throttling are still useful. For example, they can let you fire fewer network requests. You can also use these techniques together.\n",
"filename": "useDeferredValue.md",
"package": "react"
} |
{
"content": "Title: useEffect – React\n\nURL Source: https://react.dev/reference/react/useEffect\n\nMarkdown Content:\n`useEffect` is a React Hook that lets you [synchronize a component with an external system.](https://react.dev/learn/synchronizing-with-effects)\n\n```\nuseEffect(setup, dependencies?)\n```\n\n* [Reference](https://react.dev/reference/react/useEffect#reference)\n * [`useEffect(setup, dependencies?)`](https://react.dev/reference/react/useEffect#useeffect)\n* [Usage](https://react.dev/reference/react/useEffect#usage)\n * [Connecting to an external system](https://react.dev/reference/react/useEffect#connecting-to-an-external-system)\n * [Wrapping Effects in custom Hooks](https://react.dev/reference/react/useEffect#wrapping-effects-in-custom-hooks)\n * [Controlling a non-React widget](https://react.dev/reference/react/useEffect#controlling-a-non-react-widget)\n * [Fetching data with Effects](https://react.dev/reference/react/useEffect#fetching-data-with-effects)\n * [Specifying reactive dependencies](https://react.dev/reference/react/useEffect#specifying-reactive-dependencies)\n * [Updating state based on previous state from an Effect](https://react.dev/reference/react/useEffect#updating-state-based-on-previous-state-from-an-effect)\n * [Removing unnecessary object dependencies](https://react.dev/reference/react/useEffect#removing-unnecessary-object-dependencies)\n * [Removing unnecessary function dependencies](https://react.dev/reference/react/useEffect#removing-unnecessary-function-dependencies)\n * [Reading the latest props and state from an Effect](https://react.dev/reference/react/useEffect#reading-the-latest-props-and-state-from-an-effect)\n * [Displaying different content on the server and the client](https://react.dev/reference/react/useEffect#displaying-different-content-on-the-server-and-the-client)\n* [Troubleshooting](https://react.dev/reference/react/useEffect#troubleshooting)\n * [My Effect runs twice when the component mounts](https://react.dev/reference/react/useEffect#my-effect-runs-twice-when-the-component-mounts)\n * [My Effect runs after every re-render](https://react.dev/reference/react/useEffect#my-effect-runs-after-every-re-render)\n * [My Effect keeps re-running in an infinite cycle](https://react.dev/reference/react/useEffect#my-effect-keeps-re-running-in-an-infinite-cycle)\n * [My cleanup logic runs even though my component didn’t unmount](https://react.dev/reference/react/useEffect#my-cleanup-logic-runs-even-though-my-component-didnt-unmount)\n * [My Effect does something visual, and I see a flicker before it runs](https://react.dev/reference/react/useEffect#my-effect-does-something-visual-and-i-see-a-flicker-before-it-runs)\n\n* * *\n\nReference[](https://react.dev/reference/react/useEffect#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------\n\n### `useEffect(setup, dependencies?)`[](https://react.dev/reference/react/useEffect#useeffect \"Link for this heading\")\n\nCall `useEffect` at the top level of your component to declare an Effect:\n\n```\nimport { useEffect } from 'react';import { createConnection } from './chat.js';function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [serverUrl, roomId]);// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react/useEffect#usage)\n\n#### Parameters[](https://react.dev/reference/react/useEffect#parameters \"Link for Parameters \")\n\n* `setup`: The function with your Effect’s logic. Your setup function may also optionally return a _cleanup_ function. When your component is added to the DOM, React will run your setup function. After every re-render with changed dependencies, React will first run the cleanup function (if you provided it) with the old values, and then run your setup function with the new values. After your component is removed from the DOM, React will run your cleanup function.\n \n* **optional** `dependencies`: The list of all reactive values referenced inside of the `setup` code. Reactive values include props, state, and all the variables and functions declared directly inside your component body. If your linter is [configured for React](https://react.dev/learn/editor-setup#linting), it will verify that every reactive value is correctly specified as a dependency. The list of dependencies must have a constant number of items and be written inline like `[dep1, dep2, dep3]`. React will compare each dependency with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. If you omit this argument, your Effect will re-run after every re-render of the component. [See the difference between passing an array of dependencies, an empty array, and no dependencies at all.](https://react.dev/reference/react/useEffect#examples-dependencies)\n \n\n#### Returns[](https://react.dev/reference/react/useEffect#returns \"Link for Returns \")\n\n`useEffect` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/useEffect#caveats \"Link for Caveats \")\n\n* `useEffect` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a new component and move the state into it.\n \n* If you’re **not trying to synchronize with some external system,** [you probably don’t need an Effect.](https://react.dev/learn/you-might-not-need-an-effect)\n \n* When Strict Mode is on, React will **run one extra development-only setup+cleanup cycle** before the first real setup. This is a stress-test that ensures that your cleanup logic “mirrors” your setup logic and that it stops or undoes whatever the setup is doing. If this causes a problem, [implement the cleanup function.](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development)\n \n* If some of your dependencies are objects or functions defined inside the component, there is a risk that they will **cause the Effect to re-run more often than needed.** To fix this, remove unnecessary [object](https://react.dev/reference/react/useEffect#removing-unnecessary-object-dependencies) and [function](https://react.dev/reference/react/useEffect#removing-unnecessary-function-dependencies) dependencies. You can also [extract state updates](https://react.dev/reference/react/useEffect#updating-state-based-on-previous-state-from-an-effect) and [non-reactive logic](https://react.dev/reference/react/useEffect#reading-the-latest-props-and-state-from-an-effect) outside of your Effect.\n \n* If your Effect wasn’t caused by an interaction (like a click), React will generally let the browser **paint the updated screen first before running your Effect.** If your Effect is doing something visual (for example, positioning a tooltip), and the delay is noticeable (for example, it flickers), replace `useEffect` with [`useLayoutEffect`.](https://react.dev/reference/react/useLayoutEffect)\n \n* If your Effect is caused by an interaction (like a click), **React may run your Effect before the browser paints the updated screen**. This ensures that the result of the Effect can be observed by the event system. Usually, this works as expected. However, if you must defer the work until after paint, such as an `alert()`, you can use `setTimeout`. See [reactwg/react-18/128](https://github.com/reactwg/react-18/discussions/128) for more information.\n \n* Even if your Effect was caused by an interaction (like a click), **React may allow the browser to repaint the screen before processing the state updates inside your Effect.** Usually, this works as expected. However, if you must block the browser from repainting the screen, you need to replace `useEffect` with [`useLayoutEffect`.](https://react.dev/reference/react/useLayoutEffect)\n \n* Effects **only run on the client.** They don’t run during server rendering.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useEffect#usage \"Link for Usage \")\n----------------------------------------------------------------------------\n\n### Connecting to an external system[](https://react.dev/reference/react/useEffect#connecting-to-an-external-system \"Link for Connecting to an external system \")\n\nSome components need to stay connected to the network, some browser API, or a third-party library, while they are displayed on the page. These systems aren’t controlled by React, so they are called _external._\n\nTo [connect your component to some external system,](https://react.dev/learn/synchronizing-with-effects) call `useEffect` at the top level of your component:\n\n```\nimport { useEffect } from 'react';import { createConnection } from './chat.js';function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [serverUrl, roomId]);// ...}\n```\n\nYou need to pass two arguments to `useEffect`:\n\n1. A _setup function_ with setup code that connects to that system.\n * It should return a _cleanup function_ with cleanup code that disconnects from that system.\n2. A list of dependencies including every value from your component used inside of those functions.\n\n**React calls your setup and cleanup functions whenever it’s necessary, which may happen multiple times:**\n\n1. Your setup code runs when your component is added to the page _(mounts)_.\n2. After every re-render of your component where the dependencies have changed:\n * First, your cleanup code runs with the old props and state.\n * Then, your setup code runs with the new props and state.\n3. Your cleanup code runs one final time after your component is removed from the page _(unmounts)._\n\n**Let’s illustrate this sequence for the example above.**\n\nWhen the `ChatRoom` component above gets added to the page, it will connect to the chat room with the initial `serverUrl` and `roomId`. If either `serverUrl` or `roomId` change as a result of a re-render (say, if the user picks a different chat room in a dropdown), your Effect will _disconnect from the previous room, and connect to the next one._ When the `ChatRoom` component is removed from the page, your Effect will disconnect one last time.\n\n**To [help you find bugs,](https://react.dev/learn/synchronizing-with-effects#step-3-add-cleanup-if-needed) in development React runs setup and cleanup one extra time before the setup.** This is a stress-test that verifies your Effect’s logic is implemented correctly. If this causes visible issues, your cleanup function is missing some logic. The cleanup function should stop or undo whatever the setup function was doing. The rule of thumb is that the user shouldn’t be able to distinguish between the setup being called once (as in production) and a _setup_ → _cleanup_ → _setup_ sequence (as in development). [See common solutions.](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development)\n\n**Try to [write every Effect as an independent process](https://react.dev/learn/lifecycle-of-reactive-effects#each-effect-represents-a-separate-synchronization-process) and [think about a single setup/cleanup cycle at a time.](https://react.dev/learn/lifecycle-of-reactive-effects#thinking-from-the-effects-perspective)** It shouldn’t matter whether your component is mounting, updating, or unmounting. When your cleanup logic correctly “mirrors” the setup logic, your Effect is resilient to running setup and cleanup as often as needed.\n\n### Note\n\n#### Examples of connecting to an external system[](https://react.dev/reference/react/useEffect#examples-connecting \"Link for Examples of connecting to an external system\")\n\n#### Connecting to a chat server[](https://react.dev/reference/react/useEffect#connecting-to-a-chat-server \"Link for this heading\")\n\nIn this example, the `ChatRoom` component uses an Effect to stay connected to an external system defined in `chat.js`. Press “Open chat” to make the `ChatRoom` component appear. This sandbox runs in development mode, so there is an extra connect-and-disconnect cycle, as [explained here.](https://react.dev/learn/synchronizing-with-effects#step-3-add-cleanup-if-needed) Try changing the `roomId` and `serverUrl` using the dropdown and the input, and see how the Effect re-connects to the chat. Press “Close chat” to see the Effect disconnect one last time.\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nfunction ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> {\n connection.disconnect();\n };\n }, \\[roomId, serverUrl\\]);\n\n return (\n <\\>\n <label\\>\n Server URL:{' '}\n <input\n value\\={serverUrl}\n onChange\\={e \\=> setServerUrl(e.target.value)}\n />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Close chat' : 'Open chat'}\n </button\\>\n {show && <hr />}\n {show && <ChatRoom roomId\\={roomId} />}\n </\\>\n );\n}\n\n* * *\n\n### Wrapping Effects in custom Hooks[](https://react.dev/reference/react/useEffect#wrapping-effects-in-custom-hooks \"Link for Wrapping Effects in custom Hooks \")\n\nEffects are an [“escape hatch”:](https://react.dev/learn/escape-hatches) you use them when you need to “step outside React” and when there is no better built-in solution for your use case. If you find yourself often needing to manually write Effects, it’s usually a sign that you need to extract some [custom Hooks](https://react.dev/learn/reusing-logic-with-custom-hooks) for common behaviors your components rely on.\n\nFor example, this `useChatRoom` custom Hook “hides” the logic of your Effect behind a more declarative API:\n\n```\nfunction useChatRoom({ serverUrl, roomId }) {useEffect(() => {const options = {serverUrl: serverUrl,roomId: roomId};const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, [roomId, serverUrl]);}\n```\n\nThen you can use it from any component like this:\n\n```\nfunction ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useChatRoom({roomId: roomId,serverUrl: serverUrl});// ...\n```\n\nThere are also many excellent custom Hooks for every purpose available in the React ecosystem.\n\n[Learn more about wrapping Effects in custom Hooks.](https://react.dev/learn/reusing-logic-with-custom-hooks)\n\n#### Examples of wrapping Effects in custom Hooks[](https://react.dev/reference/react/useEffect#examples-custom-hooks \"Link for Examples of wrapping Effects in custom Hooks\")\n\n#### Custom `useChatRoom` Hook[](https://react.dev/reference/react/useEffect#custom-usechatroom-hook \"Link for this heading\")\n\nThis example is identical to one of the [earlier examples,](https://react.dev/reference/react/useEffect#examples-connecting) but the logic is extracted to a custom Hook.\n\nimport { useState } from 'react';\nimport { useChatRoom } from './useChatRoom.js';\n\nfunction ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n\n useChatRoom({\n roomId: roomId,\n serverUrl: serverUrl\n });\n\n return (\n <\\>\n <label\\>\n Server URL:{' '}\n <input\n value\\={serverUrl}\n onChange\\={e \\=> setServerUrl(e.target.value)}\n />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Close chat' : 'Open chat'}\n </button\\>\n {show && <hr />}\n {show && <ChatRoom roomId\\={roomId} />}\n </\\>\n );\n}\n\n* * *\n\n### Controlling a non-React widget[](https://react.dev/reference/react/useEffect#controlling-a-non-react-widget \"Link for Controlling a non-React widget \")\n\nSometimes, you want to keep an external system synchronized to some prop or state of your component.\n\nFor example, if you have a third-party map widget or a video player component written without React, you can use an Effect to call methods on it that make its state match the current state of your React component. This Effect creates an instance of a `MapWidget` class defined in `map-widget.js`. When you change the `zoomLevel` prop of the `Map` component, the Effect calls the `setZoom()` on the class instance to keep it synchronized:\n\nimport { useRef, useEffect } from 'react';\nimport { MapWidget } from './map-widget.js';\n\nexport default function Map({ zoomLevel }) {\n const containerRef = useRef(null);\n const mapRef = useRef(null);\n\n useEffect(() \\=> {\n if (mapRef.current === null) {\n mapRef.current = new MapWidget(containerRef.current);\n }\n\n const map = mapRef.current;\n map.setZoom(zoomLevel);\n }, \\[zoomLevel\\]);\n\n return (\n <div\n style\\={{ width: 200, height: 200 }}\n ref\\={containerRef}\n />\n );\n}\n\nIn this example, a cleanup function is not needed because the `MapWidget` class manages only the DOM node that was passed to it. After the `Map` React component is removed from the tree, both the DOM node and the `MapWidget` class instance will be automatically garbage-collected by the browser JavaScript engine.\n\n* * *\n\n### Fetching data with Effects[](https://react.dev/reference/react/useEffect#fetching-data-with-effects \"Link for Fetching data with Effects \")\n\nYou can use an Effect to fetch data for your component. Note that [if you use a framework,](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) using your framework’s data fetching mechanism will be a lot more efficient than writing Effects manually.\n\nIf you want to fetch data from an Effect manually, your code might look like this:\n\n```\nimport { useState, useEffect } from 'react';import { fetchBio } from './api.js';export default function Page() {const [person, setPerson] = useState('Alice');const [bio, setBio] = useState(null);useEffect(() => {let ignore = false;setBio(null);fetchBio(person).then(result => {if (!ignore) {setBio(result);}});return () => {ignore = true;};}, [person]);// ...\n```\n\nNote the `ignore` variable which is initialized to `false`, and is set to `true` during cleanup. This ensures [your code doesn’t suffer from “race conditions”:](https://maxrozen.com/race-conditions-fetching-data-react-with-useeffect) network responses may arrive in a different order than you sent them.\n\nimport { useState, useEffect } from 'react';\nimport { fetchBio } from './api.js';\n\nexport default function Page() {\n const \\[person, setPerson\\] = useState('Alice');\n const \\[bio, setBio\\] = useState(null);\n useEffect(() \\=> {\n let ignore = false;\n setBio(null);\n fetchBio(person).then(result \\=> {\n if (!ignore) {\n setBio(result);\n }\n });\n return () \\=> {\n ignore = true;\n }\n }, \\[person\\]);\n\n return (\n <\\>\n <select value\\={person} onChange\\={e \\=> {\n setPerson(e.target.value);\n }}\\>\n <option value\\=\"Alice\"\\>Alice</option\\>\n <option value\\=\"Bob\"\\>Bob</option\\>\n <option value\\=\"Taylor\"\\>Taylor</option\\>\n </select\\>\n <hr />\n <p\\><i\\>{bio ?? 'Loading...'}</i\\></p\\>\n </\\>\n );\n}\n\nYou can also rewrite using the [`async` / `await`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/async_function) syntax, but you still need to provide a cleanup function:\n\nimport { useState, useEffect } from 'react';\nimport { fetchBio } from './api.js';\n\nexport default function Page() {\n const \\[person, setPerson\\] = useState('Alice');\n const \\[bio, setBio\\] = useState(null);\n useEffect(() \\=> {\n async function startFetching() {\n setBio(null);\n const result = await fetchBio(person);\n if (!ignore) {\n setBio(result);\n }\n }\n\n let ignore = false;\n startFetching();\n return () \\=> {\n ignore = true;\n }\n }, \\[person\\]);\n\n return (\n <\\>\n <select value\\={person} onChange\\={e \\=> {\n setPerson(e.target.value);\n }}\\>\n <option value\\=\"Alice\"\\>Alice</option\\>\n <option value\\=\"Bob\"\\>Bob</option\\>\n <option value\\=\"Taylor\"\\>Taylor</option\\>\n </select\\>\n <hr />\n <p\\><i\\>{bio ?? 'Loading...'}</i\\></p\\>\n </\\>\n );\n}\n\nWriting data fetching directly in Effects gets repetitive and makes it difficult to add optimizations like caching and server rendering later. [It’s easier to use a custom Hook—either your own or maintained by the community.](https://react.dev/learn/reusing-logic-with-custom-hooks#when-to-use-custom-hooks)\n\n##### Deep Dive\n\n#### What are good alternatives to data fetching in Effects?[](https://react.dev/reference/react/useEffect#what-are-good-alternatives-to-data-fetching-in-effects \"Link for What are good alternatives to data fetching in Effects? \")\n\nWriting `fetch` calls inside Effects is a [popular way to fetch data](https://www.robinwieruch.de/react-hooks-fetch-data/), especially in fully client-side apps. This is, however, a very manual approach and it has significant downsides:\n\n* **Effects don’t run on the server.** This means that the initial server-rendered HTML will only include a loading state with no data. The client computer will have to download all JavaScript and render your app only to discover that now it needs to load the data. This is not very efficient.\n* **Fetching directly in Effects makes it easy to create “network waterfalls”.** You render the parent component, it fetches some data, renders the child components, and then they start fetching their data. If the network is not very fast, this is significantly slower than fetching all data in parallel.\n* **Fetching directly in Effects usually means you don’t preload or cache data.** For example, if the component unmounts and then mounts again, it would have to fetch the data again.\n* **It’s not very ergonomic.** There’s quite a bit of boilerplate code involved when writing `fetch` calls in a way that doesn’t suffer from bugs like [race conditions.](https://maxrozen.com/race-conditions-fetching-data-react-with-useeffect)\n\nThis list of downsides is not specific to React. It applies to fetching data on mount with any library. Like with routing, data fetching is not trivial to do well, so we recommend the following approaches:\n\n* **If you use a [framework](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks), use its built-in data fetching mechanism.** Modern React frameworks have integrated data fetching mechanisms that are efficient and don’t suffer from the above pitfalls.\n* **Otherwise, consider using or building a client-side cache.** Popular open source solutions include [React Query](https://tanstack.com/query/latest/), [useSWR](https://swr.vercel.app/), and [React Router 6.4+.](https://beta.reactrouter.com/en/main/start/overview) You can build your own solution too, in which case you would use Effects under the hood but also add logic for deduplicating requests, caching responses, and avoiding network waterfalls (by preloading data or hoisting data requirements to routes).\n\nYou can continue fetching data directly in Effects if neither of these approaches suit you.\n\n* * *\n\n### Specifying reactive dependencies[](https://react.dev/reference/react/useEffect#specifying-reactive-dependencies \"Link for Specifying reactive dependencies \")\n\n**Notice that you can’t “choose” the dependencies of your Effect.** Every reactive value used by your Effect’s code must be declared as a dependency. Your Effect’s dependency list is determined by the surrounding code:\n\n```\nfunction ChatRoom({ roomId }) { // This is a reactive valueconst [serverUrl, setServerUrl] = useState('https://localhost:1234'); // This is a reactive value toouseEffect(() => {const connection = createConnection(serverUrl, roomId); // This Effect reads these reactive valuesconnection.connect();return () => connection.disconnect();}, [serverUrl, roomId]); // ✅ So you must specify them as dependencies of your Effect// ...}\n```\n\nIf either `serverUrl` or `roomId` change, your Effect will reconnect to the chat using the new values.\n\n**[Reactive values](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values) include props and all variables and functions declared directly inside of your component.** Since `roomId` and `serverUrl` are reactive values, you can’t remove them from the dependencies. If you try to omit them and [your linter is correctly configured for React,](https://react.dev/learn/editor-setup#linting) the linter will flag this as a mistake you need to fix:\n\n```\nfunction ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, []); // 🔴 React Hook useEffect has missing dependencies: 'roomId' and 'serverUrl'// ...}\n```\n\n**To remove a dependency, you need to [“prove” to the linter that it _doesn’t need_ to be a dependency.](https://react.dev/learn/removing-effect-dependencies#removing-unnecessary-dependencies)** For example, you can move `serverUrl` out of your component to prove that it’s not reactive and won’t change on re-renders:\n\n```\nconst serverUrl = 'https://localhost:1234'; // Not a reactive value anymorefunction ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...}\n```\n\nNow that `serverUrl` is not a reactive value (and can’t change on a re-render), it doesn’t need to be a dependency. **If your Effect’s code doesn’t use any reactive values, its dependency list should be empty (`[]`):**\n\n```\nconst serverUrl = 'https://localhost:1234'; // Not a reactive value anymoreconst roomId = 'music'; // Not a reactive value anymorefunction ChatRoom() {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, []); // ✅ All dependencies declared// ...}\n```\n\n[An Effect with empty dependencies](https://react.dev/learn/lifecycle-of-reactive-effects#what-an-effect-with-empty-dependencies-means) doesn’t re-run when any of your component’s props or state change.\n\n### Pitfall\n\nIf you have an existing codebase, you might have some Effects that suppress the linter like this:\n\n```\nuseEffect(() => {// ...// 🔴 Avoid suppressing the linter like this:// eslint-ignore-next-line react-hooks/exhaustive-deps}, []);\n```\n\n**When dependencies don’t match the code, there is a high risk of introducing bugs.** By suppressing the linter, you “lie” to React about the values your Effect depends on. [Instead, prove they’re unnecessary.](https://react.dev/learn/removing-effect-dependencies#removing-unnecessary-dependencies)\n\n#### Examples of passing reactive dependencies[](https://react.dev/reference/react/useEffect#examples-dependencies \"Link for Examples of passing reactive dependencies\")\n\n#### Passing a dependency array[](https://react.dev/reference/react/useEffect#passing-a-dependency-array \"Link for this heading\")\n\nIf you specify the dependencies, your Effect runs **after the initial render _and_ after re-renders with changed dependencies.**\n\n```\nuseEffect(() => {// ...}, [a, b]); // Runs again if a or b are different\n```\n\nIn the below example, `serverUrl` and `roomId` are [reactive values,](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values) so they both must be specified as dependencies. As a result, selecting a different room in the dropdown or editing the server URL input causes the chat to re-connect. However, since `message` isn’t used in the Effect (and so it isn’t a dependency), editing the message doesn’t re-connect to the chat.\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nfunction ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n const \\[message, setMessage\\] = useState('');\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> {\n connection.disconnect();\n };\n }, \\[serverUrl, roomId\\]);\n\n return (\n <\\>\n <label\\>\n Server URL:{' '}\n <input\n value\\={serverUrl}\n onChange\\={e \\=> setServerUrl(e.target.value)}\n />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <label\\>\n Your message:{' '}\n <input value\\={message} onChange\\={e \\=> setMessage(e.target.value)} />\n </label\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[show, setShow\\] = useState(false);\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Close chat' : 'Open chat'}\n </button\\>\n </label\\>\n {show && <hr />}\n {show && <ChatRoom roomId\\={roomId}/>}\n </\\>\n );\n}\n\n* * *\n\n### Updating state based on previous state from an Effect[](https://react.dev/reference/react/useEffect#updating-state-based-on-previous-state-from-an-effect \"Link for Updating state based on previous state from an Effect \")\n\nWhen you want to update state based on previous state from an Effect, you might run into a problem:\n\n```\nfunction Counter() {const [count, setCount] = useState(0);useEffect(() => {const intervalId = setInterval(() => {setCount(count + 1); // You want to increment the counter every second...}, 1000)return () => clearInterval(intervalId);}, [count]); // 🚩 ... but specifying `count` as a dependency always resets the interval.// ...}\n```\n\nSince `count` is a reactive value, it must be specified in the list of dependencies. However, that causes the Effect to cleanup and setup again every time the `count` changes. This is not ideal.\n\nTo fix this, [pass the `c => c + 1` state updater](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state) to `setCount`:\n\nNow that you’re passing `c => c + 1` instead of `count + 1`, [your Effect no longer needs to depend on `count`.](https://react.dev/learn/removing-effect-dependencies#are-you-reading-some-state-to-calculate-the-next-state) As a result of this fix, it won’t need to cleanup and setup the interval again every time the `count` changes.\n\n* * *\n\n### Removing unnecessary object dependencies[](https://react.dev/reference/react/useEffect#removing-unnecessary-object-dependencies \"Link for Removing unnecessary object dependencies \")\n\nIf your Effect depends on an object or a function created during rendering, it might run too often. For example, this Effect re-connects after every render because the `options` object is [different for every render:](https://react.dev/learn/removing-effect-dependencies#does-some-reactive-value-change-unintentionally)\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {const [message, setMessage] = useState('');const options = { // 🚩 This object is created from scratch on every re-renderserverUrl: serverUrl,roomId: roomId};useEffect(() => {const connection = createConnection(options); // It's used inside the Effectconnection.connect();return () => connection.disconnect();}, [options]); // 🚩 As a result, these dependencies are always different on a re-render// ...\n```\n\nAvoid using an object created during rendering as a dependency. Instead, create the object inside the Effect:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n const \\[message, setMessage\\] = useState('');\n\n useEffect(() \\=> {\n const options = {\n serverUrl: serverUrl,\n roomId: roomId\n };\n const connection = createConnection(options);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n return (\n <\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <input value\\={message} onChange\\={e \\=> setMessage(e.target.value)} />\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nNow that you create the `options` object inside the Effect, the Effect itself only depends on the `roomId` string.\n\nWith this fix, typing into the input doesn’t reconnect the chat. Unlike an object which gets re-created, a string like `roomId` doesn’t change unless you set it to another value. [Read more about removing dependencies.](https://react.dev/learn/removing-effect-dependencies)\n\n* * *\n\n### Removing unnecessary function dependencies[](https://react.dev/reference/react/useEffect#removing-unnecessary-function-dependencies \"Link for Removing unnecessary function dependencies \")\n\nIf your Effect depends on an object or a function created during rendering, it might run too often. For example, this Effect re-connects after every render because the `createOptions` function is [different for every render:](https://react.dev/learn/removing-effect-dependencies#does-some-reactive-value-change-unintentionally)\n\n```\nfunction ChatRoom({ roomId }) {const [message, setMessage] = useState('');function createOptions() { // 🚩 This function is created from scratch on every re-renderreturn {serverUrl: serverUrl,roomId: roomId};}useEffect(() => {const options = createOptions(); // It's used inside the Effectconst connection = createConnection();connection.connect();return () => connection.disconnect();}, [createOptions]); // 🚩 As a result, these dependencies are always different on a re-render// ...\n```\n\nBy itself, creating a function from scratch on every re-render is not a problem. You don’t need to optimize that. However, if you use it as a dependency of your Effect, it will cause your Effect to re-run after every re-render.\n\nAvoid using a function created during rendering as a dependency. Instead, declare it inside the Effect:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n const \\[message, setMessage\\] = useState('');\n\n useEffect(() \\=> {\n function createOptions() {\n return {\n serverUrl: serverUrl,\n roomId: roomId\n };\n }\n\n const options = createOptions();\n const connection = createConnection(options);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n return (\n <\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <input value\\={message} onChange\\={e \\=> setMessage(e.target.value)} />\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nNow that you define the `createOptions` function inside the Effect, the Effect itself only depends on the `roomId` string. With this fix, typing into the input doesn’t reconnect the chat. Unlike a function which gets re-created, a string like `roomId` doesn’t change unless you set it to another value. [Read more about removing dependencies.](https://react.dev/learn/removing-effect-dependencies)\n\n* * *\n\n### Reading the latest props and state from an Effect[](https://react.dev/reference/react/useEffect#reading-the-latest-props-and-state-from-an-effect \"Link for Reading the latest props and state from an Effect \")\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nBy default, when you read a reactive value from an Effect, you have to add it as a dependency. This ensures that your Effect “reacts” to every change of that value. For most dependencies, that’s the behavior you want.\n\n**However, sometimes you’ll want to read the _latest_ props and state from an Effect without “reacting” to them.** For example, imagine you want to log the number of the items in the shopping cart for every page visit:\n\n```\nfunction Page({ url, shoppingCart }) {useEffect(() => {logVisit(url, shoppingCart.length);}, [url, shoppingCart]); // ✅ All dependencies declared// ...}\n```\n\n**What if you want to log a new page visit after every `url` change, but _not_ if only the `shoppingCart` changes?** You can’t exclude `shoppingCart` from dependencies without breaking the [reactivity rules.](https://react.dev/reference/react/useEffect#specifying-reactive-dependencies) However, you can express that you _don’t want_ a piece of code to “react” to changes even though it is called from inside an Effect. [Declare an _Effect Event_](https://react.dev/learn/separating-events-from-effects#declaring-an-effect-event) with the [`useEffectEvent`](https://react.dev/reference/react/experimental_useEffectEvent) Hook, and move the code reading `shoppingCart` inside of it:\n\n```\nfunction Page({ url, shoppingCart }) {const onVisit = useEffectEvent(visitedUrl => {logVisit(visitedUrl, shoppingCart.length)});useEffect(() => {onVisit(url);}, [url]); // ✅ All dependencies declared// ...}\n```\n\n**Effect Events are not reactive and must always be omitted from dependencies of your Effect.** This is what lets you put non-reactive code (where you can read the latest value of some props and state) inside of them. By reading `shoppingCart` inside of `onVisit`, you ensure that `shoppingCart` won’t re-run your Effect.\n\n[Read more about how Effect Events let you separate reactive and non-reactive code.](https://react.dev/learn/separating-events-from-effects#reading-latest-props-and-state-with-effect-events)\n\n* * *\n\n### Displaying different content on the server and the client[](https://react.dev/reference/react/useEffect#displaying-different-content-on-the-server-and-the-client \"Link for Displaying different content on the server and the client \")\n\nIf your app uses server rendering (either [directly](https://react.dev/reference/react-dom/server) or via a [framework](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks)), your component will render in two different environments. On the server, it will render to produce the initial HTML. On the client, React will run the rendering code again so that it can attach your event handlers to that HTML. This is why, for [hydration](https://react.dev/reference/react-dom/client/hydrateRoot#hydrating-server-rendered-html) to work, your initial render output must be identical on the client and the server.\n\nIn rare cases, you might need to display different content on the client. For example, if your app reads some data from [`localStorage`](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage), it can’t possibly do that on the server. Here is how you could implement this:\n\n```\nfunction MyComponent() {const [didMount, setDidMount] = useState(false);useEffect(() => {setDidMount(true);}, []);if (didMount) {// ... return client-only JSX ...} else {// ... return initial JSX ...}}\n```\n\nWhile the app is loading, the user will see the initial render output. Then, when it’s loaded and hydrated, your Effect will run and set `didMount` to `true`, triggering a re-render. This will switch to the client-only render output. Effects don’t run on the server, so this is why `didMount` was `false` during the initial server render.\n\nUse this pattern sparingly. Keep in mind that users with a slow connection will see the initial content for quite a bit of time—potentially, many seconds—so you don’t want to make jarring changes to your component’s appearance. In many cases, you can avoid the need for this by conditionally showing different things with CSS.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useEffect#troubleshooting \"Link for Troubleshooting \")\n----------------------------------------------------------------------------------------------------------\n\n### My Effect runs twice when the component mounts[](https://react.dev/reference/react/useEffect#my-effect-runs-twice-when-the-component-mounts \"Link for My Effect runs twice when the component mounts \")\n\nWhen Strict Mode is on, in development, React runs setup and cleanup one extra time before the actual setup.\n\nThis is a stress-test that verifies your Effect’s logic is implemented correctly. If this causes visible issues, your cleanup function is missing some logic. The cleanup function should stop or undo whatever the setup function was doing. The rule of thumb is that the user shouldn’t be able to distinguish between the setup being called once (as in production) and a setup → cleanup → setup sequence (as in development).\n\nRead more about [how this helps find bugs](https://react.dev/learn/synchronizing-with-effects#step-3-add-cleanup-if-needed) and [how to fix your logic.](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development)\n\n* * *\n\n### My Effect runs after every re-render[](https://react.dev/reference/react/useEffect#my-effect-runs-after-every-re-render \"Link for My Effect runs after every re-render \")\n\nFirst, check that you haven’t forgotten to specify the dependency array:\n\n```\nuseEffect(() => {// ...}); // 🚩 No dependency array: re-runs after every render!\n```\n\nIf you’ve specified the dependency array but your Effect still re-runs in a loop, it’s because one of your dependencies is different on every re-render.\n\nYou can debug this problem by manually logging your dependencies to the console:\n\n```\nuseEffect(() => {// ..}, [serverUrl, roomId]);console.log([serverUrl, roomId]);\n```\n\nYou can then right-click on the arrays from different re-renders in the console and select “Store as a global variable” for both of them. Assuming the first one got saved as `temp1` and the second one got saved as `temp2`, you can then use the browser console to check whether each dependency in both arrays is the same:\n\n```\nObject.is(temp1[0], temp2[0]); // Is the first dependency the same between the arrays?Object.is(temp1[1], temp2[1]); // Is the second dependency the same between the arrays?Object.is(temp1[2], temp2[2]); // ... and so on for every dependency ...\n```\n\nWhen you find the dependency that is different on every re-render, you can usually fix it in one of these ways:\n\n* [Updating state based on previous state from an Effect](https://react.dev/reference/react/useEffect#updating-state-based-on-previous-state-from-an-effect)\n* [Removing unnecessary object dependencies](https://react.dev/reference/react/useEffect#removing-unnecessary-object-dependencies)\n* [Removing unnecessary function dependencies](https://react.dev/reference/react/useEffect#removing-unnecessary-function-dependencies)\n* [Reading the latest props and state from an Effect](https://react.dev/reference/react/useEffect#reading-the-latest-props-and-state-from-an-effect)\n\nAs a last resort (if these methods didn’t help), wrap its creation with [`useMemo`](https://react.dev/reference/react/useMemo#memoizing-a-dependency-of-another-hook) or [`useCallback`](https://react.dev/reference/react/useCallback#preventing-an-effect-from-firing-too-often) (for functions).\n\n* * *\n\n### My Effect keeps re-running in an infinite cycle[](https://react.dev/reference/react/useEffect#my-effect-keeps-re-running-in-an-infinite-cycle \"Link for My Effect keeps re-running in an infinite cycle \")\n\nIf your Effect runs in an infinite cycle, these two things must be true:\n\n* Your Effect is updating some state.\n* That state leads to a re-render, which causes the Effect’s dependencies to change.\n\nBefore you start fixing the problem, ask yourself whether your Effect is connecting to some external system (like DOM, network, a third-party widget, and so on). Why does your Effect need to set state? Does it synchronize with that external system? Or are you trying to manage your application’s data flow with it?\n\nIf there is no external system, consider whether [removing the Effect altogether](https://react.dev/learn/you-might-not-need-an-effect) would simplify your logic.\n\nIf you’re genuinely synchronizing with some external system, think about why and under what conditions your Effect should update the state. Has something changed that affects your component’s visual output? If you need to keep track of some data that isn’t used by rendering, a [ref](https://react.dev/reference/react/useRef#referencing-a-value-with-a-ref) (which doesn’t trigger re-renders) might be more appropriate. Verify your Effect doesn’t update the state (and trigger re-renders) more than needed.\n\nFinally, if your Effect is updating the state at the right time, but there is still a loop, it’s because that state update leads to one of the Effect’s dependencies changing. [Read how to debug dependency changes.](https://react.dev/reference/react/useEffect#my-effect-runs-after-every-re-render)\n\n* * *\n\n### My cleanup logic runs even though my component didn’t unmount[](https://react.dev/reference/react/useEffect#my-cleanup-logic-runs-even-though-my-component-didnt-unmount \"Link for My cleanup logic runs even though my component didn’t unmount \")\n\nThe cleanup function runs not only during unmount, but before every re-render with changed dependencies. Additionally, in development, React [runs setup+cleanup one extra time immediately after component mounts.](https://react.dev/reference/react/useEffect#my-effect-runs-twice-when-the-component-mounts)\n\nIf you have cleanup code without corresponding setup code, it’s usually a code smell:\n\n```\nuseEffect(() => {// 🔴 Avoid: Cleanup logic without corresponding setup logicreturn () => {doSomething();};}, []);\n```\n\nYour cleanup logic should be “symmetrical” to the setup logic, and should stop or undo whatever setup did:\n\n```\nuseEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [serverUrl, roomId]);\n```\n\n[Learn how the Effect lifecycle is different from the component’s lifecycle.](https://react.dev/learn/lifecycle-of-reactive-effects#the-lifecycle-of-an-effect)\n\n* * *\n\n### My Effect does something visual, and I see a flicker before it runs[](https://react.dev/reference/react/useEffect#my-effect-does-something-visual-and-i-see-a-flicker-before-it-runs \"Link for My Effect does something visual, and I see a flicker before it runs \")\n\nIf your Effect must block the browser from [painting the screen,](https://react.dev/learn/render-and-commit#epilogue-browser-paint) replace `useEffect` with [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect). Note that **this shouldn’t be needed for the vast majority of Effects.** You’ll only need this if it’s crucial to run your Effect before the browser paint: for example, to measure and position a tooltip before the user sees it.\n",
"filename": "useEffect.md",
"package": "react"
} |
{
"content": "Title: useFormStatus – React\n\nURL Source: https://react.dev/reference/react-dom/hooks/useFormStatus\n\nMarkdown Content:\nuseFormStatus - This feature is available in the latest Canary[](https://react.dev/reference/react-dom/hooks/useFormStatus#undefined \"Link for this heading\")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------\n\n### Canary\n\n`useFormStatus` is a Hook that gives you status information of the last form submission.\n\n```\nconst { pending, data, method, action } = useFormStatus();\n```\n\n* [Reference](https://react.dev/reference/react-dom/hooks/useFormStatus#reference)\n * [`useFormStatus()`](https://react.dev/reference/react-dom/hooks/useFormStatus#use-form-status)\n* [Usage](https://react.dev/reference/react-dom/hooks/useFormStatus#usage)\n * [Display a pending state during form submission](https://react.dev/reference/react-dom/hooks/useFormStatus#display-a-pending-state-during-form-submission)\n * [Read the form data being submitted](https://react.dev/reference/react-dom/hooks/useFormStatus#read-form-data-being-submitted)\n* [Troubleshooting](https://react.dev/reference/react-dom/hooks/useFormStatus#troubleshooting)\n * [`status.pending` is never `true`](https://react.dev/reference/react-dom/hooks/useFormStatus#pending-is-never-true)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/hooks/useFormStatus#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------------\n\n### `useFormStatus()`[](https://react.dev/reference/react-dom/hooks/useFormStatus#use-form-status \"Link for this heading\")\n\nThe `useFormStatus` Hook provides status information of the last form submission.\n\n```\nimport { useFormStatus } from \"react-dom\";import action from './actions';function Submit() {const status = useFormStatus();return <button disabled={status.pending}>Submit</button>}export default function App() {return (<form action={action}><Submit /></form>);}\n```\n\nTo get status information, the `Submit` component must be rendered within a `<form>`. The Hook returns information like the `pending` property which tells you if the form is actively submitting.\n\nIn the above example, `Submit` uses this information to disable `<button>` presses while the form is submitting.\n\n[See more examples below.](https://react.dev/reference/react-dom/hooks/useFormStatus#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/hooks/useFormStatus#parameters \"Link for Parameters \")\n\n`useFormStatus` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react-dom/hooks/useFormStatus#returns \"Link for Returns \")\n\nA `status` object with the following properties:\n\n* `pending`: A boolean. If `true`, this means the parent `<form>` is pending submission. Otherwise, `false`.\n \n* `data`: An object implementing the [`FormData interface`](https://developer.mozilla.org/en-US/docs/Web/API/FormData) that contains the data the parent `<form>` is submitting. If there is no active submission or no parent `<form>`, it will be `null`.\n \n* `method`: A string value of either `'get'` or `'post'`. This represents whether the parent `<form>` is submitting with either a `GET` or `POST` [HTTP method](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods). By default, a `<form>` will use the `GET` method and can be specified by the [`method`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form#method) property.\n \n\n* `action`: A reference to the function passed to the `action` prop on the parent `<form>`. If there is no parent `<form>`, the property is `null`. If there is a URI value provided to the `action` prop, or no `action` prop specified, `status.action` will be `null`.\n\n#### Caveats[](https://react.dev/reference/react-dom/hooks/useFormStatus#caveats \"Link for Caveats \")\n\n* The `useFormStatus` Hook must be called from a component that is rendered inside a `<form>`.\n* `useFormStatus` will only return status information for a parent `<form>`. It will not return status information for any `<form>` rendered in that same component or children components.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/hooks/useFormStatus#usage \"Link for Usage \")\n------------------------------------------------------------------------------------------\n\n### Display a pending state during form submission[](https://react.dev/reference/react-dom/hooks/useFormStatus#display-a-pending-state-during-form-submission \"Link for Display a pending state during form submission \")\n\nTo display a pending state while a form is submitting, you can call the `useFormStatus` Hook in a component rendered in a `<form>` and read the `pending` property returned.\n\nHere, we use the `pending` property to indicate the form is submitting.\n\nimport { useFormStatus } from \"react-dom\";\nimport { submitForm } from \"./actions.js\";\n\nfunction Submit() {\n const { pending } = useFormStatus();\n return (\n <button type\\=\"submit\" disabled\\={pending}\\>\n {pending ? \"Submitting...\" : \"Submit\"}\n </button\\>\n );\n}\n\nfunction Form({ action }) {\n return (\n <form action\\={action}\\>\n <Submit />\n </form\\>\n );\n}\n\nexport default function App() {\n return <Form action\\={submitForm} />;\n}\n\n### Pitfall\n\n##### `useFormStatus` will not return status information for a `<form>` rendered in the same component.[](https://react.dev/reference/react-dom/hooks/useFormStatus#useformstatus-will-not-return-status-information-for-a-form-rendered-in-the-same-component \"Link for this heading\")\n\nThe `useFormStatus` Hook only returns status information for a parent `<form>` and not for any `<form>` rendered in the same component calling the Hook, or child components.\n\n```\nfunction Form() {// 🚩 `pending` will never be true// useFormStatus does not track the form rendered in this componentconst { pending } = useFormStatus();return <form action={submit}></form>;}\n```\n\nInstead call `useFormStatus` from inside a component that is located inside `<form>`.\n\n```\nfunction Submit() {// ✅ `pending` will be derived from the form that wraps the Submit componentconst { pending } = useFormStatus(); return <button disabled={pending}>...</button>;}function Form() {// This is the <form> `useFormStatus` tracksreturn (<form action={submit}><Submit /></form>);}\n```\n\n### Read the form data being submitted[](https://react.dev/reference/react-dom/hooks/useFormStatus#read-form-data-being-submitted \"Link for Read the form data being submitted \")\n\nYou can use the `data` property of the status information returned from `useFormStatus` to display what data is being submitted by the user.\n\nHere, we have a form where users can request a username. We can use `useFormStatus` to display a temporary status message confirming what username they have requested.\n\nimport {useState, useMemo, useRef} from 'react';\nimport {useFormStatus} from 'react-dom';\n\nexport default function UsernameForm() {\n const {pending, data} = useFormStatus();\n\n return (\n <div\\>\n <h3\\>Request a Username: </h3\\>\n <input type\\=\"text\" name\\=\"username\" disabled\\={pending}/>\n <button type\\=\"submit\" disabled\\={pending}\\>\n Submit\n </button\\>\n <br />\n <p\\>{data ? \\`Requesting ${data?.get(\"username\")}...\\`: ''}</p\\>\n </div\\>\n );\n}\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react-dom/hooks/useFormStatus#troubleshooting \"Link for Troubleshooting \")\n------------------------------------------------------------------------------------------------------------------------\n\n### `status.pending` is never `true`[](https://react.dev/reference/react-dom/hooks/useFormStatus#pending-is-never-true \"Link for this heading\")\n\n`useFormStatus` will only return status information for a parent `<form>`.\n\nIf the component that calls `useFormStatus` is not nested in a `<form>`, `status.pending` will always return `false`. Verify `useFormStatus` is called in a component that is a child of a `<form>` element.\n\n`useFormStatus` will not track the status of a `<form>` rendered in the same component. See [Pitfall](https://react.dev/reference/react-dom/hooks/useFormStatus#useformstatus-will-not-return-status-information-for-a-form-rendered-in-the-same-component) for more details.\n",
"filename": "useFormStatus.md",
"package": "react"
} |
{
"content": "Title: useId – React\n\nURL Source: https://react.dev/reference/react/useId\n\nMarkdown Content:\n`useId` is a React Hook for generating unique IDs that can be passed to accessibility attributes.\n\n* [Reference](https://react.dev/reference/react/useId#reference)\n * [`useId()`](https://react.dev/reference/react/useId#useid)\n* [Usage](https://react.dev/reference/react/useId#usage)\n * [Generating unique IDs for accessibility attributes](https://react.dev/reference/react/useId#generating-unique-ids-for-accessibility-attributes)\n * [Generating IDs for several related elements](https://react.dev/reference/react/useId#generating-ids-for-several-related-elements)\n * [Specifying a shared prefix for all generated IDs](https://react.dev/reference/react/useId#specifying-a-shared-prefix-for-all-generated-ids)\n * [Using the same ID prefix on the client and the server](https://react.dev/reference/react/useId#using-the-same-id-prefix-on-the-client-and-the-server)\n\n* * *\n\nReference[](https://react.dev/reference/react/useId#reference \"Link for Reference \")\n------------------------------------------------------------------------------------\n\n### `useId()`[](https://react.dev/reference/react/useId#useid \"Link for this heading\")\n\nCall `useId` at the top level of your component to generate a unique ID:\n\n```\nimport { useId } from 'react';function PasswordField() {const passwordHintId = useId();// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/useId#usage)\n\n#### Parameters[](https://react.dev/reference/react/useId#parameters \"Link for Parameters \")\n\n`useId` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react/useId#returns \"Link for Returns \")\n\n`useId` returns a unique ID string associated with this particular `useId` call in this particular component.\n\n#### Caveats[](https://react.dev/reference/react/useId#caveats \"Link for Caveats \")\n\n* `useId` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a new component and move the state into it.\n \n* `useId` **should not be used to generate keys** in a list. [Keys should be generated from your data.](https://react.dev/learn/rendering-lists#where-to-get-your-key)\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useId#usage \"Link for Usage \")\n------------------------------------------------------------------------\n\n### Pitfall\n\n### Generating unique IDs for accessibility attributes[](https://react.dev/reference/react/useId#generating-unique-ids-for-accessibility-attributes \"Link for Generating unique IDs for accessibility attributes \")\n\nCall `useId` at the top level of your component to generate a unique ID:\n\n```\nimport { useId } from 'react';function PasswordField() {const passwordHintId = useId();// ...\n```\n\nYou can then pass the generated ID to different attributes:\n\n```\n<><input type=\"password\" aria-describedby={passwordHintId} /><p id={passwordHintId}></>\n```\n\n**Let’s walk through an example to see when this is useful.**\n\n[HTML accessibility attributes](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA) like [`aria-describedby`](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/Attributes/aria-describedby) let you specify that two tags are related to each other. For example, you can specify that an element (like an input) is described by another element (like a paragraph).\n\nIn regular HTML, you would write it like this:\n\n```\n<label> Password:<inputtype=\"password\"aria-describedby=\"password-hint\"/></label><p id=\"password-hint\"> The password should contain at least 18 characters</p>\n```\n\nHowever, hardcoding IDs like this is not a good practice in React. A component may be rendered more than once on the page—but IDs have to be unique! Instead of hardcoding an ID, generate a unique ID with `useId`:\n\n```\nimport { useId } from 'react';function PasswordField() {const passwordHintId = useId();return (<><label> Password:<inputtype=\"password\"aria-describedby={passwordHintId}/></label><p id={passwordHintId}> The password should contain at least 18 characters</p></>);}\n```\n\nNow, even if `PasswordField` appears multiple times on the screen, the generated IDs won’t clash.\n\nimport { useId } from 'react';\n\nfunction PasswordField() {\n const passwordHintId = useId();\n return (\n <\\>\n <label\\>\n Password:\n <input\n type\\=\"password\"\n aria-describedby\\={passwordHintId}\n />\n </label\\>\n <p id\\={passwordHintId}\\>\n The password should contain at least 18 characters\n </p\\>\n </\\>\n );\n}\n\nexport default function App() {\n return (\n <\\>\n <h2\\>Choose password</h2\\>\n <PasswordField />\n <h2\\>Confirm password</h2\\>\n <PasswordField />\n </\\>\n );\n}\n\n[Watch this video](https://www.youtube.com/watch?v=0dNzNcuEuOo) to see the difference in the user experience with assistive technologies.\n\n### Pitfall\n\nWith [server rendering](https://react.dev/reference/react-dom/server), **`useId` requires an identical component tree on the server and the client**. If the trees you render on the server and the client don’t match exactly, the generated IDs won’t match.\n\n##### Deep Dive\n\n#### Why is useId better than an incrementing counter?[](https://react.dev/reference/react/useId#why-is-useid-better-than-an-incrementing-counter \"Link for Why is useId better than an incrementing counter? \")\n\nYou might be wondering why `useId` is better than incrementing a global variable like `nextId++`.\n\nThe primary benefit of `useId` is that React ensures that it works with [server rendering.](https://react.dev/reference/react-dom/server) During server rendering, your components generate HTML output. Later, on the client, [hydration](https://react.dev/reference/react-dom/client/hydrateRoot) attaches your event handlers to the generated HTML. For hydration to work, the client output must match the server HTML.\n\nThis is very difficult to guarantee with an incrementing counter because the order in which the Client Components are hydrated may not match the order in which the server HTML was emitted. By calling `useId`, you ensure that hydration will work, and the output will match between the server and the client.\n\nInside React, `useId` is generated from the “parent path” of the calling component. This is why, if the client and the server tree are the same, the “parent path” will match up regardless of rendering order.\n\n* * *\n\nIf you need to give IDs to multiple related elements, you can call `useId` to generate a shared prefix for them:\n\nThis lets you avoid calling `useId` for every single element that needs a unique ID.\n\n* * *\n\n### Specifying a shared prefix for all generated IDs[](https://react.dev/reference/react/useId#specifying-a-shared-prefix-for-all-generated-ids \"Link for Specifying a shared prefix for all generated IDs \")\n\nIf you render multiple independent React applications on a single page, pass `identifierPrefix` as an option to your [`createRoot`](https://react.dev/reference/react-dom/client/createRoot#parameters) or [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) calls. This ensures that the IDs generated by the two different apps never clash because every identifier generated with `useId` will start with the distinct prefix you’ve specified.\n\n* * *\n\n### Using the same ID prefix on the client and the server[](https://react.dev/reference/react/useId#using-the-same-id-prefix-on-the-client-and-the-server \"Link for Using the same ID prefix on the client and the server \")\n\nIf you [render multiple independent React apps on the same page](https://react.dev/reference/react/useId#specifying-a-shared-prefix-for-all-generated-ids), and some of these apps are server-rendered, make sure that the `identifierPrefix` you pass to the [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) call on the client side is the same as the `identifierPrefix` you pass to the [server APIs](https://react.dev/reference/react-dom/server) such as [`renderToPipeableStream`.](https://react.dev/reference/react-dom/server/renderToPipeableStream)\n\n```\n// Serverimport { renderToPipeableStream } from 'react-dom/server';const { pipe } = renderToPipeableStream(<App />,{ identifierPrefix: 'react-app1' });\n```\n\n```\n// Clientimport { hydrateRoot } from 'react-dom/client';const domNode = document.getElementById('root');const root = hydrateRoot(domNode,reactNode,{ identifierPrefix: 'react-app1' });\n```\n\nYou do not need to pass `identifierPrefix` if you only have one React app on the page.\n",
"filename": "useId.md",
"package": "react"
} |
{
"content": "Title: useImperativeHandle – React\n\nURL Source: https://react.dev/reference/react/useImperativeHandle\n\nMarkdown Content:\n`useImperativeHandle` is a React Hook that lets you customize the handle exposed as a [ref.](https://react.dev/learn/manipulating-the-dom-with-refs)\n\n```\nuseImperativeHandle(ref, createHandle, dependencies?)\n```\n\n* [Reference](https://react.dev/reference/react/useImperativeHandle#reference)\n * [`useImperativeHandle(ref, createHandle, dependencies?)`](https://react.dev/reference/react/useImperativeHandle#useimperativehandle)\n* [Usage](https://react.dev/reference/react/useImperativeHandle#usage)\n * [Exposing a custom ref handle to the parent component](https://react.dev/reference/react/useImperativeHandle#exposing-a-custom-ref-handle-to-the-parent-component)\n * [Exposing your own imperative methods](https://react.dev/reference/react/useImperativeHandle#exposing-your-own-imperative-methods)\n\n* * *\n\nReference[](https://react.dev/reference/react/useImperativeHandle#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------------\n\n### `useImperativeHandle(ref, createHandle, dependencies?)`[](https://react.dev/reference/react/useImperativeHandle#useimperativehandle \"Link for this heading\")\n\nCall `useImperativeHandle` at the top level of your component to customize the ref handle it exposes:\n\n```\nimport { forwardRef, useImperativeHandle } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {useImperativeHandle(ref, () => {return {// ... your methods ...};}, []);// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/useImperativeHandle#usage)\n\n#### Parameters[](https://react.dev/reference/react/useImperativeHandle#parameters \"Link for Parameters \")\n\n* `ref`: The `ref` you received as the second argument from the [`forwardRef` render function.](https://react.dev/reference/react/forwardRef#render-function)\n \n* `createHandle`: A function that takes no arguments and returns the ref handle you want to expose. That ref handle can have any type. Usually, you will return an object with the methods you want to expose.\n \n* **optional** `dependencies`: The list of all reactive values referenced inside of the `createHandle` code. Reactive values include props, state, and all the variables and functions declared directly inside your component body. If your linter is [configured for React](https://react.dev/learn/editor-setup#linting), it will verify that every reactive value is correctly specified as a dependency. The list of dependencies must have a constant number of items and be written inline like `[dep1, dep2, dep3]`. React will compare each dependency with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. If a re-render resulted in a change to some dependency, or if you omitted this argument, your `createHandle` function will re-execute, and the newly created handle will be assigned to the ref.\n \n\n#### Returns[](https://react.dev/reference/react/useImperativeHandle#returns \"Link for Returns \")\n\n`useImperativeHandle` returns `undefined`.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useImperativeHandle#usage \"Link for Usage \")\n--------------------------------------------------------------------------------------\n\n### Exposing a custom ref handle to the parent component[](https://react.dev/reference/react/useImperativeHandle#exposing-a-custom-ref-handle-to-the-parent-component \"Link for Exposing a custom ref handle to the parent component \")\n\nBy default, components don’t expose their DOM nodes to parent components. For example, if you want the parent component of `MyInput` to [have access](https://react.dev/learn/manipulating-the-dom-with-refs) to the `<input>` DOM node, you have to opt in with [`forwardRef`:](https://react.dev/reference/react/forwardRef)\n\n```\nimport { forwardRef } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {return <input {...props} ref={ref} />;});\n```\n\nWith the code above, [a ref to `MyInput` will receive the `<input>` DOM node.](https://react.dev/reference/react/forwardRef#exposing-a-dom-node-to-the-parent-component) However, you can expose a custom value instead. To customize the exposed handle, call `useImperativeHandle` at the top level of your component:\n\n```\nimport { forwardRef, useImperativeHandle } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {useImperativeHandle(ref, () => {return {// ... your methods ...};}, []);return <input {...props} />;});\n```\n\nNote that in the code above, the `ref` is no longer forwarded to the `<input>`.\n\nFor example, suppose you don’t want to expose the entire `<input>` DOM node, but you want to expose two of its methods: `focus` and `scrollIntoView`. To do this, keep the real browser DOM in a separate ref. Then use `useImperativeHandle` to expose a handle with only the methods that you want the parent component to call:\n\n```\nimport { forwardRef, useRef, useImperativeHandle } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {const inputRef = useRef(null);useImperativeHandle(ref, () => {return {focus() {inputRef.current.focus();},scrollIntoView() {inputRef.current.scrollIntoView();},};}, []);return <input {...props} ref={inputRef} />;});\n```\n\nNow, if the parent component gets a ref to `MyInput`, it will be able to call the `focus` and `scrollIntoView` methods on it. However, it will not have full access to the underlying `<input>` DOM node.\n\n* * *\n\n### Exposing your own imperative methods[](https://react.dev/reference/react/useImperativeHandle#exposing-your-own-imperative-methods \"Link for Exposing your own imperative methods \")\n\nThe methods you expose via an imperative handle don’t have to match the DOM methods exactly. For example, this `Post` component exposes a `scrollAndFocusAddComment` method via an imperative handle. This lets the parent `Page` scroll the list of comments _and_ focus the input field when you click the button:\n\n### Pitfall\n\n**Do not overuse refs.** You should only use refs for _imperative_ behaviors that you can’t express as props: for example, scrolling to a node, focusing a node, triggering an animation, selecting text, and so on.\n\n**If you can express something as a prop, you should not use a ref.** For example, instead of exposing an imperative handle like `{ open, close }` from a `Modal` component, it is better to take `isOpen` as a prop like `<Modal isOpen={isOpen} />`. [Effects](https://react.dev/learn/synchronizing-with-effects) can help you expose imperative behaviors via props.\n",
"filename": "useImperativeHandle.md",
"package": "react"
} |
{
"content": "Title: useInsertionEffect – React\n\nURL Source: https://react.dev/reference/react/useInsertionEffect\n\nMarkdown Content:\n### Pitfall\n\n`useInsertionEffect` is for CSS-in-JS library authors. Unless you are working on a CSS-in-JS library and need a place to inject the styles, you probably want [`useEffect`](https://react.dev/reference/react/useEffect) or [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) instead.\n\n`useInsertionEffect` allows inserting elements into the DOM before any layout Effects fire.\n\n```\nuseInsertionEffect(setup, dependencies?)\n```\n\n* [Reference](https://react.dev/reference/react/useInsertionEffect#reference)\n * [`useInsertionEffect(setup, dependencies?)`](https://react.dev/reference/react/useInsertionEffect#useinsertioneffect)\n* [Usage](https://react.dev/reference/react/useInsertionEffect#usage)\n * [Injecting dynamic styles from CSS-in-JS libraries](https://react.dev/reference/react/useInsertionEffect#injecting-dynamic-styles-from-css-in-js-libraries)\n\n* * *\n\nReference[](https://react.dev/reference/react/useInsertionEffect#reference \"Link for Reference \")\n-------------------------------------------------------------------------------------------------\n\n### `useInsertionEffect(setup, dependencies?)`[](https://react.dev/reference/react/useInsertionEffect#useinsertioneffect \"Link for this heading\")\n\nCall `useInsertionEffect` to insert styles before any Effects fire that may need to read layout:\n\n```\nimport { useInsertionEffect } from 'react';// Inside your CSS-in-JS libraryfunction useCSS(rule) {useInsertionEffect(() => {// ... inject <style> tags here ...});return rule;}\n```\n\n[See more examples below.](https://react.dev/reference/react/useInsertionEffect#usage)\n\n#### Parameters[](https://react.dev/reference/react/useInsertionEffect#parameters \"Link for Parameters \")\n\n* `setup`: The function with your Effect’s logic. Your setup function may also optionally return a _cleanup_ function. When your component is added to the DOM, but before any layout Effects fire, React will run your setup function. After every re-render with changed dependencies, React will first run the cleanup function (if you provided it) with the old values, and then run your setup function with the new values. When your component is removed from the DOM, React will run your cleanup function.\n \n* **optional** `dependencies`: The list of all reactive values referenced inside of the `setup` code. Reactive values include props, state, and all the variables and functions declared directly inside your component body. If your linter is [configured for React](https://react.dev/learn/editor-setup#linting), it will verify that every reactive value is correctly specified as a dependency. The list of dependencies must have a constant number of items and be written inline like `[dep1, dep2, dep3]`. React will compare each dependency with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison algorithm. If you don’t specify the dependencies at all, your Effect will re-run after every re-render of the component.\n \n\n#### Returns[](https://react.dev/reference/react/useInsertionEffect#returns \"Link for Returns \")\n\n`useInsertionEffect` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/useInsertionEffect#caveats \"Link for Caveats \")\n\n* Effects only run on the client. They don’t run during server rendering.\n* You can’t update state from inside `useInsertionEffect`.\n* By the time `useInsertionEffect` runs, refs are not attached yet.\n* `useInsertionEffect` may run either before or after the DOM has been updated. You shouldn’t rely on the DOM being updated at any particular time.\n* Unlike other types of Effects, which fire cleanup for every Effect and then setup for every Effect, `useInsertionEffect` will fire both cleanup and setup one component at a time. This results in an “interleaving” of the cleanup and setup functions.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useInsertionEffect#usage \"Link for Usage \")\n-------------------------------------------------------------------------------------\n\n### Injecting dynamic styles from CSS-in-JS libraries[](https://react.dev/reference/react/useInsertionEffect#injecting-dynamic-styles-from-css-in-js-libraries \"Link for Injecting dynamic styles from CSS-in-JS libraries \")\n\nTraditionally, you would style React components using plain CSS.\n\n```\n// In your JS file:<button className=\"success\" />// In your CSS file:.success { color: green; }\n```\n\nSome teams prefer to author styles directly in JavaScript code instead of writing CSS files. This usually requires using a CSS-in-JS library or a tool. There are three common approaches to CSS-in-JS:\n\n1. Static extraction to CSS files with a compiler\n2. Inline styles, e.g. `<div style={{ opacity: 1 }}>`\n3. Runtime injection of `<style>` tags\n\nIf you use CSS-in-JS, we recommend a combination of the first two approaches (CSS files for static styles, inline styles for dynamic styles). **We don’t recommend runtime `<style>` tag injection for two reasons:**\n\n1. Runtime injection forces the browser to recalculate the styles a lot more often.\n2. Runtime injection can be very slow if it happens at the wrong time in the React lifecycle.\n\nThe first problem is not solvable, but `useInsertionEffect` helps you solve the second problem.\n\nCall `useInsertionEffect` to insert the styles before any layout Effects fire:\n\n```\n// Inside your CSS-in-JS librarylet isInserted = new Set();function useCSS(rule) {useInsertionEffect(() => {// As explained earlier, we don't recommend runtime injection of <style> tags.// But if you have to do it, then it's important to do in useInsertionEffect.if (!isInserted.has(rule)) {isInserted.add(rule);document.head.appendChild(getStyleForRule(rule));}});return rule;}function Button() {const className = useCSS('...');return <div className={className} />;}\n```\n\nSimilarly to `useEffect`, `useInsertionEffect` does not run on the server. If you need to collect which CSS rules have been used on the server, you can do it during rendering:\n\n```\nlet collectedRulesSet = new Set();function useCSS(rule) {if (typeof window === 'undefined') {collectedRulesSet.add(rule);}useInsertionEffect(() => {// ...});return rule;}\n```\n\n[Read more about upgrading CSS-in-JS libraries with runtime injection to `useInsertionEffect`.](https://github.com/reactwg/react-18/discussions/110)\n\n##### Deep Dive\n\n#### How is this better than injecting styles during rendering or useLayoutEffect?[](https://react.dev/reference/react/useInsertionEffect#how-is-this-better-than-injecting-styles-during-rendering-or-uselayouteffect \"Link for How is this better than injecting styles during rendering or useLayoutEffect? \")\n\nIf you insert styles during rendering and React is processing a [non-blocking update,](https://react.dev/reference/react/useTransition#marking-a-state-update-as-a-non-blocking-transition) the browser will recalculate the styles every single frame while rendering a component tree, which can be **extremely slow.**\n\n`useInsertionEffect` is better than inserting styles during [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) or [`useEffect`](https://react.dev/reference/react/useEffect) because it ensures that by the time other Effects run in your components, the `<style>` tags have already been inserted. Otherwise, layout calculations in regular Effects would be wrong due to outdated styles.\n",
"filename": "useInsertionEffect.md",
"package": "react"
} |
{
"content": "Title: useLayoutEffect – React\n\nURL Source: https://react.dev/reference/react/useLayoutEffect\n\nMarkdown Content:\n### Pitfall\n\n`useLayoutEffect` can hurt performance. Prefer [`useEffect`](https://react.dev/reference/react/useEffect) when possible.\n\n`useLayoutEffect` is a version of [`useEffect`](https://react.dev/reference/react/useEffect) that fires before the browser repaints the screen.\n\n```\nuseLayoutEffect(setup, dependencies?)\n```\n\n* [Reference](https://react.dev/reference/react/useLayoutEffect#reference)\n * [`useLayoutEffect(setup, dependencies?)`](https://react.dev/reference/react/useLayoutEffect#useinsertioneffect)\n* [Usage](https://react.dev/reference/react/useLayoutEffect#usage)\n * [Measuring layout before the browser repaints the screen](https://react.dev/reference/react/useLayoutEffect#measuring-layout-before-the-browser-repaints-the-screen)\n* [Troubleshooting](https://react.dev/reference/react/useLayoutEffect#troubleshooting)\n * [I’m getting an error: “`useLayoutEffect` does nothing on the server”](https://react.dev/reference/react/useLayoutEffect#im-getting-an-error-uselayouteffect-does-nothing-on-the-server)\n\n* * *\n\nReference[](https://react.dev/reference/react/useLayoutEffect#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------\n\n### `useLayoutEffect(setup, dependencies?)`[](https://react.dev/reference/react/useLayoutEffect#useinsertioneffect \"Link for this heading\")\n\nCall `useLayoutEffect` to perform the layout measurements before the browser repaints the screen:\n\n```\nimport { useState, useRef, useLayoutEffect } from 'react';function Tooltip() {const ref = useRef(null);const [tooltipHeight, setTooltipHeight] = useState(0);useLayoutEffect(() => {const { height } = ref.current.getBoundingClientRect();setTooltipHeight(height);}, []);// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/useLayoutEffect#usage)\n\n#### Parameters[](https://react.dev/reference/react/useLayoutEffect#parameters \"Link for Parameters \")\n\n* `setup`: The function with your Effect’s logic. Your setup function may also optionally return a _cleanup_ function. Before your component is added to the DOM, React will run your setup function. After every re-render with changed dependencies, React will first run the cleanup function (if you provided it) with the old values, and then run your setup function with the new values. Before your component is removed from the DOM, React will run your cleanup function.\n \n* **optional** `dependencies`: The list of all reactive values referenced inside of the `setup` code. Reactive values include props, state, and all the variables and functions declared directly inside your component body. If your linter is [configured for React](https://react.dev/learn/editor-setup#linting), it will verify that every reactive value is correctly specified as a dependency. The list of dependencies must have a constant number of items and be written inline like `[dep1, dep2, dep3]`. React will compare each dependency with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. If you omit this argument, your Effect will re-run after every re-render of the component.\n \n\n#### Returns[](https://react.dev/reference/react/useLayoutEffect#returns \"Link for Returns \")\n\n`useLayoutEffect` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/useLayoutEffect#caveats \"Link for Caveats \")\n\n* `useLayoutEffect` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a component and move the Effect there.\n \n* When Strict Mode is on, React will **run one extra development-only setup+cleanup cycle** before the first real setup. This is a stress-test that ensures that your cleanup logic “mirrors” your setup logic and that it stops or undoes whatever the setup is doing. If this causes a problem, [implement the cleanup function.](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development)\n \n* If some of your dependencies are objects or functions defined inside the component, there is a risk that they will **cause the Effect to re-run more often than needed.** To fix this, remove unnecessary [object](https://react.dev/reference/react/useEffect#removing-unnecessary-object-dependencies) and [function](https://react.dev/reference/react/useEffect#removing-unnecessary-function-dependencies) dependencies. You can also [extract state updates](https://react.dev/reference/react/useEffect#updating-state-based-on-previous-state-from-an-effect) and [non-reactive logic](https://react.dev/reference/react/useEffect#reading-the-latest-props-and-state-from-an-effect) outside of your Effect.\n \n* Effects **only run on the client.** They don’t run during server rendering.\n \n* The code inside `useLayoutEffect` and all state updates scheduled from it **block the browser from repainting the screen.** When used excessively, this makes your app slow. When possible, prefer [`useEffect`.](https://react.dev/reference/react/useEffect)\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useLayoutEffect#usage \"Link for Usage \")\n----------------------------------------------------------------------------------\n\n### Measuring layout before the browser repaints the screen[](https://react.dev/reference/react/useLayoutEffect#measuring-layout-before-the-browser-repaints-the-screen \"Link for Measuring layout before the browser repaints the screen \")\n\nMost components don’t need to know their position and size on the screen to decide what to render. They only return some JSX. Then the browser calculates their _layout_ (position and size) and repaints the screen.\n\nSometimes, that’s not enough. Imagine a tooltip that appears next to some element on hover. If there’s enough space, the tooltip should appear above the element, but if it doesn’t fit, it should appear below. In order to render the tooltip at the right final position, you need to know its height (i.e. whether it fits at the top).\n\nTo do this, you need to render in two passes:\n\n1. Render the tooltip anywhere (even with a wrong position).\n2. Measure its height and decide where to place the tooltip.\n3. Render the tooltip _again_ in the correct place.\n\n**All of this needs to happen before the browser repaints the screen.** You don’t want the user to see the tooltip moving. Call `useLayoutEffect` to perform the layout measurements before the browser repaints the screen:\n\n```\nfunction Tooltip() {const ref = useRef(null);const [tooltipHeight, setTooltipHeight] = useState(0); // You don't know real height yetuseLayoutEffect(() => {const { height } = ref.current.getBoundingClientRect();setTooltipHeight(height); // Re-render now that you know the real height}, []);// ...use tooltipHeight in the rendering logic below...}\n```\n\nHere’s how this works step by step:\n\n1. `Tooltip` renders with the initial `tooltipHeight = 0` (so the tooltip may be wrongly positioned).\n2. React places it in the DOM and runs the code in `useLayoutEffect`.\n3. Your `useLayoutEffect` [measures the height](https://developer.mozilla.org/en-US/docs/Web/API/Element/getBoundingClientRect) of the tooltip content and triggers an immediate re-render.\n4. `Tooltip` renders again with the real `tooltipHeight` (so the tooltip is correctly positioned).\n5. React updates it in the DOM, and the browser finally displays the tooltip.\n\nHover over the buttons below and see how the tooltip adjusts its position depending on whether it fits:\n\nimport { useRef, useLayoutEffect, useState } from 'react';\nimport { createPortal } from 'react-dom';\nimport TooltipContainer from './TooltipContainer.js';\n\nexport default function Tooltip({ children, targetRect }) {\n const ref = useRef(null);\n const \\[tooltipHeight, setTooltipHeight\\] = useState(0);\n\n useLayoutEffect(() \\=> {\n const { height } = ref.current.getBoundingClientRect();\n setTooltipHeight(height);\n console.log('Measured tooltip height: ' + height);\n }, \\[\\]);\n\n let tooltipX = 0;\n let tooltipY = 0;\n if (targetRect !== null) {\n tooltipX = targetRect.left;\n tooltipY = targetRect.top - tooltipHeight;\n if (tooltipY < 0) {\n \n tooltipY = targetRect.bottom;\n }\n }\n\n return createPortal(\n <TooltipContainer x\\={tooltipX} y\\={tooltipY} contentRef\\={ref}\\>\n {children}\n </TooltipContainer\\>,\n document.body\n );\n}\n\nNotice that even though the `Tooltip` component has to render in two passes (first, with `tooltipHeight` initialized to `0` and then with the real measured height), you only see the final result. This is why you need `useLayoutEffect` instead of [`useEffect`](https://react.dev/reference/react/useEffect) for this example. Let’s look at the difference in detail below.\n\n#### useLayoutEffect vs useEffect[](https://react.dev/reference/react/useLayoutEffect#examples \"Link for useLayoutEffect vs useEffect\")\n\n#### `useLayoutEffect` blocks the browser from repainting[](https://react.dev/reference/react/useLayoutEffect#uselayouteffect-blocks-the-browser-from-repainting \"Link for this heading\")\n\nReact guarantees that the code inside `useLayoutEffect` and any state updates scheduled inside it will be processed **before the browser repaints the screen.** This lets you render the tooltip, measure it, and re-render the tooltip again without the user noticing the first extra render. In other words, `useLayoutEffect` blocks the browser from painting.\n\nimport { useRef, useLayoutEffect, useState } from 'react';\nimport { createPortal } from 'react-dom';\nimport TooltipContainer from './TooltipContainer.js';\n\nexport default function Tooltip({ children, targetRect }) {\n const ref = useRef(null);\n const \\[tooltipHeight, setTooltipHeight\\] = useState(0);\n\n useLayoutEffect(() \\=> {\n const { height } = ref.current.getBoundingClientRect();\n setTooltipHeight(height);\n }, \\[\\]);\n\n let tooltipX = 0;\n let tooltipY = 0;\n if (targetRect !== null) {\n tooltipX = targetRect.left;\n tooltipY = targetRect.top - tooltipHeight;\n if (tooltipY < 0) {\n \n tooltipY = targetRect.bottom;\n }\n }\n\n return createPortal(\n <TooltipContainer x\\={tooltipX} y\\={tooltipY} contentRef\\={ref}\\>\n {children}\n </TooltipContainer\\>,\n document.body\n );\n}\n\n### Note\n\nRendering in two passes and blocking the browser hurts performance. Try to avoid this when you can.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useLayoutEffect#troubleshooting \"Link for Troubleshooting \")\n----------------------------------------------------------------------------------------------------------------\n\n### I’m getting an error: “`useLayoutEffect` does nothing on the server”[](https://react.dev/reference/react/useLayoutEffect#im-getting-an-error-uselayouteffect-does-nothing-on-the-server \"Link for this heading\")\n\nThe purpose of `useLayoutEffect` is to let your component [use layout information for rendering:](https://react.dev/reference/react/useLayoutEffect#measuring-layout-before-the-browser-repaints-the-screen)\n\n1. Render the initial content.\n2. Measure the layout _before the browser repaints the screen._\n3. Render the final content using the layout information you’ve read.\n\nWhen you or your framework uses [server rendering](https://react.dev/reference/react-dom/server), your React app renders to HTML on the server for the initial render. This lets you show the initial HTML before the JavaScript code loads.\n\nThe problem is that on the server, there is no layout information.\n\nIn the [earlier example](https://react.dev/reference/react/useLayoutEffect#measuring-layout-before-the-browser-repaints-the-screen), the `useLayoutEffect` call in the `Tooltip` component lets it position itself correctly (either above or below content) depending on the content height. If you tried to render `Tooltip` as a part of the initial server HTML, this would be impossible to determine. On the server, there is no layout yet! So, even if you rendered it on the server, its position would “jump” on the client after the JavaScript loads and runs.\n\nUsually, components that rely on layout information don’t need to render on the server anyway. For example, it probably doesn’t make sense to show a `Tooltip` during the initial render. It is triggered by a client interaction.\n\nHowever, if you’re running into this problem, you have a few different options:\n\n* Replace `useLayoutEffect` with [`useEffect`.](https://react.dev/reference/react/useEffect) This tells React that it’s okay to display the initial render result without blocking the paint (because the original HTML will become visible before your Effect runs).\n \n* Alternatively, [mark your component as client-only.](https://react.dev/reference/react/Suspense#providing-a-fallback-for-server-errors-and-client-only-content) This tells React to replace its content up to the closest [`<Suspense>`](https://react.dev/reference/react/Suspense) boundary with a loading fallback (for example, a spinner or a glimmer) during server rendering.\n \n* Alternatively, you can render a component with `useLayoutEffect` only after hydration. Keep a boolean `isMounted` state that’s initialized to `false`, and set it to `true` inside a `useEffect` call. Your rendering logic can then be like `return isMounted ? <RealContent /> : <FallbackContent />`. On the server and during the hydration, the user will see `FallbackContent` which should not call `useLayoutEffect`. Then React will replace it with `RealContent` which runs on the client only and can include `useLayoutEffect` calls.\n \n* If you synchronize your component with an external data store and rely on `useLayoutEffect` for different reasons than measuring layout, consider [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore) instead which [supports server rendering.](https://react.dev/reference/react/useSyncExternalStore#adding-support-for-server-rendering)\n",
"filename": "useLayoutEffect.md",
"package": "react"
} |
{
"content": "Title: useMemo – React\n\nURL Source: https://react.dev/reference/react/useMemo\n\nMarkdown Content:\n`useMemo` is a React Hook that lets you cache the result of a calculation between re-renders.\n\n```\nconst cachedValue = useMemo(calculateValue, dependencies)\n```\n\n* [Reference](https://react.dev/reference/react/useMemo#reference)\n * [`useMemo(calculateValue, dependencies)`](https://react.dev/reference/react/useMemo#usememo)\n* [Usage](https://react.dev/reference/react/useMemo#usage)\n * [Skipping expensive recalculations](https://react.dev/reference/react/useMemo#skipping-expensive-recalculations)\n * [Skipping re-rendering of components](https://react.dev/reference/react/useMemo#skipping-re-rendering-of-components)\n * [Memoizing a dependency of another Hook](https://react.dev/reference/react/useMemo#memoizing-a-dependency-of-another-hook)\n * [Memoizing a function](https://react.dev/reference/react/useMemo#memoizing-a-function)\n* [Troubleshooting](https://react.dev/reference/react/useMemo#troubleshooting)\n * [My calculation runs twice on every re-render](https://react.dev/reference/react/useMemo#my-calculation-runs-twice-on-every-re-render)\n * [My `useMemo` call is supposed to return an object, but returns undefined](https://react.dev/reference/react/useMemo#my-usememo-call-is-supposed-to-return-an-object-but-returns-undefined)\n * [Every time my component renders, the calculation in `useMemo` re-runs](https://react.dev/reference/react/useMemo#every-time-my-component-renders-the-calculation-in-usememo-re-runs)\n * [I need to call `useMemo` for each list item in a loop, but it’s not allowed](https://react.dev/reference/react/useMemo#i-need-to-call-usememo-for-each-list-item-in-a-loop-but-its-not-allowed)\n\n* * *\n\nReference[](https://react.dev/reference/react/useMemo#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------\n\n### `useMemo(calculateValue, dependencies)`[](https://react.dev/reference/react/useMemo#usememo \"Link for this heading\")\n\nCall `useMemo` at the top level of your component to cache a calculation between re-renders:\n\n```\nimport { useMemo } from 'react';function TodoList({ todos, tab }) {const visibleTodos = useMemo(() => filterTodos(todos, tab),[todos, tab]);// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react/useMemo#usage)\n\n#### Parameters[](https://react.dev/reference/react/useMemo#parameters \"Link for Parameters \")\n\n* `calculateValue`: The function calculating the value that you want to cache. It should be pure, should take no arguments, and should return a value of any type. React will call your function during the initial render. On next renders, React will return the same value again if the `dependencies` have not changed since the last render. Otherwise, it will call `calculateValue`, return its result, and store it so it can be reused later.\n \n* `dependencies`: The list of all reactive values referenced inside of the `calculateValue` code. Reactive values include props, state, and all the variables and functions declared directly inside your component body. If your linter is [configured for React](https://react.dev/learn/editor-setup#linting), it will verify that every reactive value is correctly specified as a dependency. The list of dependencies must have a constant number of items and be written inline like `[dep1, dep2, dep3]`. React will compare each dependency with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison.\n \n\n#### Returns[](https://react.dev/reference/react/useMemo#returns \"Link for Returns \")\n\nOn the initial render, `useMemo` returns the result of calling `calculateValue` with no arguments.\n\nDuring next renders, it will either return an already stored value from the last render (if the dependencies haven’t changed), or call `calculateValue` again, and return the result that `calculateValue` has returned.\n\n#### Caveats[](https://react.dev/reference/react/useMemo#caveats \"Link for Caveats \")\n\n* `useMemo` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a new component and move the state into it.\n* In Strict Mode, React will **call your calculation function twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useMemo#my-calculation-runs-twice-on-every-re-render) This is development-only behavior and does not affect production. If your calculation function is pure (as it should be), this should not affect your logic. The result from one of the calls will be ignored.\n* React **will not throw away the cached value unless there is a specific reason to do that.** For example, in development, React throws away the cache when you edit the file of your component. Both in development and in production, React will throw away the cache if your component suspends during the initial mount. In the future, React may add more features that take advantage of throwing away the cache—for example, if React adds built-in support for virtualized lists in the future, it would make sense to throw away the cache for items that scroll out of the virtualized table viewport. This should be fine if you rely on `useMemo` solely as a performance optimization. Otherwise, a [state variable](https://react.dev/reference/react/useState#avoiding-recreating-the-initial-state) or a [ref](https://react.dev/reference/react/useRef#avoiding-recreating-the-ref-contents) may be more appropriate.\n\n### Note\n\nCaching return values like this is also known as [_memoization_,](https://en.wikipedia.org/wiki/Memoization) which is why this Hook is called `useMemo`.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useMemo#usage \"Link for Usage \")\n--------------------------------------------------------------------------\n\n### Skipping expensive recalculations[](https://react.dev/reference/react/useMemo#skipping-expensive-recalculations \"Link for Skipping expensive recalculations \")\n\nTo cache a calculation between re-renders, wrap it in a `useMemo` call at the top level of your component:\n\n```\nimport { useMemo } from 'react';function TodoList({ todos, tab, theme }) {const visibleTodos = useMemo(() => filterTodos(todos, tab), [todos, tab]);// ...}\n```\n\nYou need to pass two things to `useMemo`:\n\n1. A calculation function that takes no arguments, like `() =>`, and returns what you wanted to calculate.\n2. A list of dependencies including every value within your component that’s used inside your calculation.\n\nOn the initial render, the value you’ll get from `useMemo` will be the result of calling your calculation.\n\nOn every subsequent render, React will compare the dependencies with the dependencies you passed during the last render. If none of the dependencies have changed (compared with [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)), `useMemo` will return the value you already calculated before. Otherwise, React will re-run your calculation and return the new value.\n\nIn other words, `useMemo` caches a calculation result between re-renders until its dependencies change.\n\n**Let’s walk through an example to see when this is useful.**\n\nBy default, React will re-run the entire body of your component every time that it re-renders. For example, if this `TodoList` updates its state or receives new props from its parent, the `filterTodos` function will re-run:\n\n```\nfunction TodoList({ todos, tab, theme }) {const visibleTodos = filterTodos(todos, tab);// ...}\n```\n\nUsually, this isn’t a problem because most calculations are very fast. However, if you’re filtering or transforming a large array, or doing some expensive computation, you might want to skip doing it again if data hasn’t changed. If both `todos` and `tab` are the same as they were during the last render, wrapping the calculation in `useMemo` like earlier lets you reuse `visibleTodos` you’ve already calculated before.\n\nThis type of caching is called _[memoization.](https://en.wikipedia.org/wiki/Memoization)_\n\n### Note\n\n**You should only rely on `useMemo` as a performance optimization.** If your code doesn’t work without it, find the underlying problem and fix it first. Then you may add `useMemo` to improve performance.\n\n##### Deep Dive\n\n#### How to tell if a calculation is expensive?[](https://react.dev/reference/react/useMemo#how-to-tell-if-a-calculation-is-expensive \"Link for How to tell if a calculation is expensive? \")\n\nIn general, unless you’re creating or looping over thousands of objects, it’s probably not expensive. If you want to get more confidence, you can add a console log to measure the time spent in a piece of code:\n\n```\nconsole.time('filter array');const visibleTodos = filterTodos(todos, tab);console.timeEnd('filter array');\n```\n\nPerform the interaction you’re measuring (for example, typing into the input). You will then see logs like `filter array: 0.15ms` in your console. If the overall logged time adds up to a significant amount (say, `1ms` or more), it might make sense to memoize that calculation. As an experiment, you can then wrap the calculation in `useMemo` to verify whether the total logged time has decreased for that interaction or not:\n\n```\nconsole.time('filter array');const visibleTodos = useMemo(() => {return filterTodos(todos, tab); // Skipped if todos and tab haven't changed}, [todos, tab]);console.timeEnd('filter array');\n```\n\n`useMemo` won’t make the _first_ render faster. It only helps you skip unnecessary work on updates.\n\nKeep in mind that your machine is probably faster than your users’ so it’s a good idea to test the performance with an artificial slowdown. For example, Chrome offers a [CPU Throttling](https://developer.chrome.com/blog/new-in-devtools-61/#throttling) option for this.\n\nAlso note that measuring performance in development will not give you the most accurate results. (For example, when [Strict Mode](https://react.dev/reference/react/StrictMode) is on, you will see each component render twice rather than once.) To get the most accurate timings, build your app for production and test it on a device like your users have.\n\n##### Deep Dive\n\n#### Should you add useMemo everywhere?[](https://react.dev/reference/react/useMemo#should-you-add-usememo-everywhere \"Link for Should you add useMemo everywhere? \")\n\nIf your app is like this site, and most interactions are coarse (like replacing a page or an entire section), memoization is usually unnecessary. On the other hand, if your app is more like a drawing editor, and most interactions are granular (like moving shapes), then you might find memoization very helpful.\n\nOptimizing with `useMemo` is only valuable in a few cases:\n\n* The calculation you’re putting in `useMemo` is noticeably slow, and its dependencies rarely change.\n* You pass it as a prop to a component wrapped in [`memo`.](https://react.dev/reference/react/memo) You want to skip re-rendering if the value hasn’t changed. Memoization lets your component re-render only when dependencies aren’t the same.\n* The value you’re passing is later used as a dependency of some Hook. For example, maybe another `useMemo` calculation value depends on it. Or maybe you are depending on this value from [`useEffect.`](https://react.dev/reference/react/useEffect)\n\nThere is no benefit to wrapping a calculation in `useMemo` in other cases. There is no significant harm to doing that either, so some teams choose to not think about individual cases, and memoize as much as possible. The downside of this approach is that code becomes less readable. Also, not all memoization is effective: a single value that’s “always new” is enough to break memoization for an entire component.\n\n**In practice, you can make a lot of memoization unnecessary by following a few principles:**\n\n1. When a component visually wraps other components, let it [accept JSX as children.](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) This way, when the wrapper component updates its own state, React knows that its children don’t need to re-render.\n2. Prefer local state and don’t [lift state up](https://react.dev/learn/sharing-state-between-components) any further than necessary. For example, don’t keep transient state like forms and whether an item is hovered at the top of your tree or in a global state library.\n3. Keep your [rendering logic pure.](https://react.dev/learn/keeping-components-pure) If re-rendering a component causes a problem or produces some noticeable visual artifact, it’s a bug in your component! Fix the bug instead of adding memoization.\n4. Avoid [unnecessary Effects that update state.](https://react.dev/learn/you-might-not-need-an-effect) Most performance problems in React apps are caused by chains of updates originating from Effects that cause your components to render over and over.\n5. Try to [remove unnecessary dependencies from your Effects.](https://react.dev/learn/removing-effect-dependencies) For example, instead of memoization, it’s often simpler to move some object or a function inside an Effect or outside the component.\n\nIf a specific interaction still feels laggy, [use the React Developer Tools profiler](https://legacy.reactjs.org/blog/2018/09/10/introducing-the-react-profiler.html) to see which components would benefit the most from memoization, and add memoization where needed. These principles make your components easier to debug and understand, so it’s good to follow them in any case. In the long term, we’re researching [doing granular memoization automatically](https://www.youtube.com/watch?v=lGEMwh32soc) to solve this once and for all.\n\n* * *\n\n### Skipping re-rendering of components[](https://react.dev/reference/react/useMemo#skipping-re-rendering-of-components \"Link for Skipping re-rendering of components \")\n\nIn some cases, `useMemo` can also help you optimize performance of re-rendering child components. To illustrate this, let’s say this `TodoList` component passes the `visibleTodos` as a prop to the child `List` component:\n\n```\nexport default function TodoList({ todos, tab, theme }) {// ...return (<div className={theme}><List items={visibleTodos} /></div>);}\n```\n\nYou’ve noticed that toggling the `theme` prop freezes the app for a moment, but if you remove `<List />` from your JSX, it feels fast. This tells you that it’s worth trying to optimize the `List` component.\n\n**By default, when a component re-renders, React re-renders all of its children recursively.** This is why, when `TodoList` re-renders with a different `theme`, the `List` component _also_ re-renders. This is fine for components that don’t require much calculation to re-render. But if you’ve verified that a re-render is slow, you can tell `List` to skip re-rendering when its props are the same as on last render by wrapping it in [`memo`:](https://react.dev/reference/react/memo)\n\n```\nimport { memo } from 'react';const List = memo(function List({ items }) {// ...});\n```\n\n**With this change, `List` will skip re-rendering if all of its props are the _same_ as on the last render.** This is where caching the calculation becomes important! Imagine that you calculated `visibleTodos` without `useMemo`:\n\n```\nexport default function TodoList({ todos, tab, theme }) {// Every time the theme changes, this will be a different array...const visibleTodos = filterTodos(todos, tab);return (<div className={theme}>{/* ... so List's props will never be the same, and it will re-render every time */}<List items={visibleTodos} /></div>);}\n```\n\n**In the above example, the `filterTodos` function always creates a _different_ array,** similar to how the `{}` object literal always creates a new object. Normally, this wouldn’t be a problem, but it means that `List` props will never be the same, and your [`memo`](https://react.dev/reference/react/memo) optimization won’t work. This is where `useMemo` comes in handy:\n\n```\nexport default function TodoList({ todos, tab, theme }) {// Tell React to cache your calculation between re-renders...const visibleTodos = useMemo(() => filterTodos(todos, tab),[todos, tab] // ...so as long as these dependencies don't change...);return (<div className={theme}>{/* ...List will receive the same props and can skip re-rendering */}<List items={visibleTodos} /></div>);}\n```\n\n**By wrapping the `visibleTodos` calculation in `useMemo`, you ensure that it has the _same_ value between the re-renders** (until dependencies change). You don’t _have to_ wrap a calculation in `useMemo` unless you do it for some specific reason. In this example, the reason is that you pass it to a component wrapped in [`memo`,](https://react.dev/reference/react/memo) and this lets it skip re-rendering. There are a few other reasons to add `useMemo` which are described further on this page.\n\n##### Deep Dive\n\n#### Memoizing individual JSX nodes[](https://react.dev/reference/react/useMemo#memoizing-individual-jsx-nodes \"Link for Memoizing individual JSX nodes \")\n\nInstead of wrapping `List` in [`memo`](https://react.dev/reference/react/memo), you could wrap the `<List />` JSX node itself in `useMemo`:\n\n```\nexport default function TodoList({ todos, tab, theme }) {const visibleTodos = useMemo(() => filterTodos(todos, tab), [todos, tab]);const children = useMemo(() => <List items={visibleTodos} />, [visibleTodos]);return (<div className={theme}>{children}</div>);}\n```\n\nThe behavior would be the same. If the `visibleTodos` haven’t changed, `List` won’t be re-rendered.\n\nA JSX node like `<List items={visibleTodos} />` is an object like `{ type: List, props: { items: visibleTodos } }`. Creating this object is very cheap, but React doesn’t know whether its contents is the same as last time or not. This is why by default, React will re-render the `List` component.\n\nHowever, if React sees the same exact JSX as during the previous render, it won’t try to re-render your component. This is because JSX nodes are [immutable.](https://en.wikipedia.org/wiki/Immutable_object) A JSX node object could not have changed over time, so React knows it’s safe to skip a re-render. However, for this to work, the node has to _actually be the same object_, not merely look the same in code. This is what `useMemo` does in this example.\n\nManually wrapping JSX nodes into `useMemo` is not convenient. For example, you can’t do this conditionally. This is usually why you would wrap components with [`memo`](https://react.dev/reference/react/memo) instead of wrapping JSX nodes.\n\n* * *\n\n### Memoizing a dependency of another Hook[](https://react.dev/reference/react/useMemo#memoizing-a-dependency-of-another-hook \"Link for Memoizing a dependency of another Hook \")\n\nSuppose you have a calculation that depends on an object created directly in the component body:\n\n```\nfunction Dropdown({ allItems, text }) {const searchOptions = { matchMode: 'whole-word', text };const visibleItems = useMemo(() => {return searchItems(allItems, searchOptions);}, [allItems, searchOptions]); // 🚩 Caution: Dependency on an object created in the component body// ...\n```\n\nDepending on an object like this defeats the point of memoization. When a component re-renders, all of the code directly inside the component body runs again. **The lines of code creating the `searchOptions` object will also run on every re-render.** Since `searchOptions` is a dependency of your `useMemo` call, and it’s different every time, React knows the dependencies are different, and recalculate `searchItems` every time.\n\nTo fix this, you could memoize the `searchOptions` object _itself_ before passing it as a dependency:\n\n```\nfunction Dropdown({ allItems, text }) {const searchOptions = useMemo(() => {return { matchMode: 'whole-word', text };}, [text]); // ✅ Only changes when text changesconst visibleItems = useMemo(() => {return searchItems(allItems, searchOptions);}, [allItems, searchOptions]); // ✅ Only changes when allItems or searchOptions changes// ...\n```\n\nIn the example above, if the `text` did not change, the `searchOptions` object also won’t change. However, an even better fix is to move the `searchOptions` object declaration _inside_ of the `useMemo` calculation function:\n\n```\nfunction Dropdown({ allItems, text }) {const visibleItems = useMemo(() => {const searchOptions = { matchMode: 'whole-word', text };return searchItems(allItems, searchOptions);}, [allItems, text]); // ✅ Only changes when allItems or text changes// ...\n```\n\nNow your calculation depends on `text` directly (which is a string and can’t “accidentally” become different).\n\n* * *\n\n### Memoizing a function[](https://react.dev/reference/react/useMemo#memoizing-a-function \"Link for Memoizing a function \")\n\nSuppose the `Form` component is wrapped in [`memo`.](https://react.dev/reference/react/memo) You want to pass a function to it as a prop:\n\n```\nexport default function ProductPage({ productId, referrer }) {function handleSubmit(orderDetails) {post('/product/' + productId + '/buy', {referrer,orderDetails});}return <Form onSubmit={handleSubmit} />;}\n```\n\nJust as `{}` creates a different object, function declarations like `function() {}` and expressions like `() => {}` produce a _different_ function on every re-render. By itself, creating a new function is not a problem. This is not something to avoid! However, if the `Form` component is memoized, presumably you want to skip re-rendering it when no props have changed. A prop that is _always_ different would defeat the point of memoization.\n\nTo memoize a function with `useMemo`, your calculation function would have to return another function:\n\n```\nexport default function Page({ productId, referrer }) {const handleSubmit = useMemo(() => {return (orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails});};}, [productId, referrer]);return <Form onSubmit={handleSubmit} />;}\n```\n\nThis looks clunky! **Memoizing functions is common enough that React has a built-in Hook specifically for that. Wrap your functions into [`useCallback`](https://react.dev/reference/react/useCallback) instead of `useMemo`** to avoid having to write an extra nested function:\n\n```\nexport default function Page({ productId, referrer }) {const handleSubmit = useCallback((orderDetails) => {post('/product/' + productId + '/buy', {referrer,orderDetails});}, [productId, referrer]);return <Form onSubmit={handleSubmit} />;}\n```\n\nThe two examples above are completely equivalent. The only benefit to `useCallback` is that it lets you avoid writing an extra nested function inside. It doesn’t do anything else. [Read more about `useCallback`.](https://react.dev/reference/react/useCallback)\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useMemo#troubleshooting \"Link for Troubleshooting \")\n--------------------------------------------------------------------------------------------------------\n\n### My calculation runs twice on every re-render[](https://react.dev/reference/react/useMemo#my-calculation-runs-twice-on-every-re-render \"Link for My calculation runs twice on every re-render \")\n\nIn [Strict Mode](https://react.dev/reference/react/StrictMode), React will call some of your functions twice instead of once:\n\n```\nfunction TodoList({ todos, tab }) {// This component function will run twice for every render.const visibleTodos = useMemo(() => {// This calculation will run twice if any of the dependencies change.return filterTodos(todos, tab);}, [todos, tab]);// ...\n```\n\nThis is expected and shouldn’t break your code.\n\nThis **development-only** behavior helps you [keep components pure.](https://react.dev/learn/keeping-components-pure) React uses the result of one of the calls, and ignores the result of the other call. As long as your component and calculation functions are pure, this shouldn’t affect your logic. However, if they are accidentally impure, this helps you notice and fix the mistake.\n\nFor example, this impure calculation function mutates an array you received as a prop:\n\n```\nconst visibleTodos = useMemo(() => {// 🚩 Mistake: mutating a proptodos.push({ id: 'last', text: 'Go for a walk!' });const filtered = filterTodos(todos, tab);return filtered;}, [todos, tab]);\n```\n\nReact calls your function twice, so you’d notice the todo is added twice. Your calculation shouldn’t change any existing objects, but it’s okay to change any _new_ objects you created during the calculation. For example, if the `filterTodos` function always returns a _different_ array, you can mutate _that_ array instead:\n\n```\nconst visibleTodos = useMemo(() => {const filtered = filterTodos(todos, tab);// ✅ Correct: mutating an object you created during the calculationfiltered.push({ id: 'last', text: 'Go for a walk!' });return filtered;}, [todos, tab]);\n```\n\nRead [keeping components pure](https://react.dev/learn/keeping-components-pure) to learn more about purity.\n\nAlso, check out the guides on [updating objects](https://react.dev/learn/updating-objects-in-state) and [updating arrays](https://react.dev/learn/updating-arrays-in-state) without mutation.\n\n* * *\n\n### My `useMemo` call is supposed to return an object, but returns undefined[](https://react.dev/reference/react/useMemo#my-usememo-call-is-supposed-to-return-an-object-but-returns-undefined \"Link for this heading\")\n\nThis code doesn’t work:\n\n```\n// 🔴 You can't return an object from an arrow function with () => {const searchOptions = useMemo(() => { matchMode: 'whole-word',text: text}, [text]);\n```\n\nIn JavaScript, `() => {` starts the arrow function body, so the `{` brace is not a part of your object. This is why it doesn’t return an object, and leads to mistakes. You could fix it by adding parentheses like `({` and `})`:\n\n```\n// This works, but is easy for someone to break againconst searchOptions = useMemo(() => ({matchMode: 'whole-word',text: text}), [text]);\n```\n\nHowever, this is still confusing and too easy for someone to break by removing the parentheses.\n\nTo avoid this mistake, write a `return` statement explicitly:\n\n```\n// ✅ This works and is explicitconst searchOptions = useMemo(() => {return {matchMode: 'whole-word',text: text};}, [text]);\n```\n\n* * *\n\n### Every time my component renders, the calculation in `useMemo` re-runs[](https://react.dev/reference/react/useMemo#every-time-my-component-renders-the-calculation-in-usememo-re-runs \"Link for this heading\")\n\nMake sure you’ve specified the dependency array as a second argument!\n\nIf you forget the dependency array, `useMemo` will re-run the calculation every time:\n\n```\nfunction TodoList({ todos, tab }) {// 🔴 Recalculates every time: no dependency arrayconst visibleTodos = useMemo(() => filterTodos(todos, tab));// ...\n```\n\nThis is the corrected version passing the dependency array as a second argument:\n\n```\nfunction TodoList({ todos, tab }) {// ✅ Does not recalculate unnecessarilyconst visibleTodos = useMemo(() => filterTodos(todos, tab), [todos, tab]);// ...\n```\n\nIf this doesn’t help, then the problem is that at least one of your dependencies is different from the previous render. You can debug this problem by manually logging your dependencies to the console:\n\n```\nconst visibleTodos = useMemo(() => filterTodos(todos, tab), [todos, tab]);console.log([todos, tab]);\n```\n\nYou can then right-click on the arrays from different re-renders in the console and select “Store as a global variable” for both of them. Assuming the first one got saved as `temp1` and the second one got saved as `temp2`, you can then use the browser console to check whether each dependency in both arrays is the same:\n\n```\nObject.is(temp1[0], temp2[0]); // Is the first dependency the same between the arrays?Object.is(temp1[1], temp2[1]); // Is the second dependency the same between the arrays?Object.is(temp1[2], temp2[2]); // ... and so on for every dependency ...\n```\n\nWhen you find which dependency breaks memoization, either find a way to remove it, or [memoize it as well.](https://react.dev/reference/react/useMemo#memoizing-a-dependency-of-another-hook)\n\n* * *\n\n### I need to call `useMemo` for each list item in a loop, but it’s not allowed[](https://react.dev/reference/react/useMemo#i-need-to-call-usememo-for-each-list-item-in-a-loop-but-its-not-allowed \"Link for this heading\")\n\nSuppose the `Chart` component is wrapped in [`memo`](https://react.dev/reference/react/memo). You want to skip re-rendering every `Chart` in the list when the `ReportList` component re-renders. However, you can’t call `useMemo` in a loop:\n\n```\nfunction ReportList({ items }) {return (<article>{items.map(item => {// 🔴 You can't call useMemo in a loop like this:const data = useMemo(() => calculateReport(item), [item]);return (<figure key={item.id}><Chart data={data} /></figure>);})}</article>);}\n```\n\nInstead, extract a component for each item and memoize data for individual items:\n\n```\nfunction ReportList({ items }) {return (<article>{items.map(item =><Report key={item.id} item={item} />)}</article>);}function Report({ item }) {// ✅ Call useMemo at the top level:const data = useMemo(() => calculateReport(item), [item]);return (<figure><Chart data={data} /></figure>);}\n```\n\nAlternatively, you could remove `useMemo` and instead wrap `Report` itself in [`memo`.](https://react.dev/reference/react/memo) If the `item` prop does not change, `Report` will skip re-rendering, so `Chart` will skip re-rendering too:\n\n```\nfunction ReportList({ items }) {// ...}const Report = memo(function Report({ item }) {const data = calculateReport(item);return (<figure><Chart data={data} /></figure>);});\n```\n",
"filename": "useMemo.md",
"package": "react"
} |
{
"content": "Title: useOptimistic – React\n\nURL Source: https://react.dev/reference/react/useOptimistic\n\nMarkdown Content:\nuseOptimistic – React\n===============\n\nuseOptimistic [](https://react.dev/reference/react/useOptimistic#undefined \"Link for this heading\")\n===================================================================================================\n\n### Canary\n\nThe `useOptimistic` Hook is currently only available in React’s Canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n`useOptimistic` is a React Hook that lets you optimistically update the UI.\n\n```\n const [optimisticState, addOptimistic] = useOptimistic(state, updateFn);\n```\n\n* [Reference](https://react.dev/reference/react/useOptimistic#reference)\n * [`useOptimistic(state, updateFn)`](https://react.dev/reference/react/useOptimistic#use)\n* [Usage](https://react.dev/reference/react/useOptimistic#usage)\n * [Optimistically updating forms](https://react.dev/reference/react/useOptimistic#optimistically-updating-with-forms)\n\n* * *\n\nReference[](https://react.dev/reference/react/useOptimistic#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `useOptimistic(state, updateFn)`[](https://react.dev/reference/react/useOptimistic#use \"Link for this heading\")\n\n`useOptimistic` is a React Hook that lets you show a different state while an async action is underway. It accepts some state as an argument and returns a copy of that state that can be different during the duration of an async action such as a network request. You provide a function that takes the current state and the input to the action, and returns the optimistic state to be used while the action is pending.\n\nThis state is called the “optimistic” state because it is usually used to immediately present the user with the result of performing an action, even though the action actually takes time to complete.\n\n```\nimport { useOptimistic } from 'react';function AppContainer() { const [optimisticState, addOptimistic] = useOptimistic( state, // updateFn (currentState, optimisticValue) => { // merge and return new state // with optimistic value } );}\n```\n\n[See more examples below.](https://react.dev/reference/react/useOptimistic#usage)\n\n#### Parameters[](https://react.dev/reference/react/useOptimistic#parameters \"Link for Parameters \")\n\n* `state`: the value to be returned initially and whenever no action is pending.\n* `updateFn(currentState, optimisticValue)`: a function that takes the current state and the optimistic value passed to `addOptimistic` and returns the resulting optimistic state. It must be a pure function. `updateFn` takes in two parameters. The `currentState` and the `optimisticValue`. The return value will be the merged value of the `currentState` and `optimisticValue`.\n\n#### Returns[](https://react.dev/reference/react/useOptimistic#returns \"Link for Returns \")\n\n* `optimisticState`: The resulting optimistic state. It is equal to `state` unless an action is pending, in which case it is equal to the value returned by `updateFn`.\n* `addOptimistic`: `addOptimistic` is the dispatching function to call when you have an optimistic update. It takes one argument, `optimisticValue`, of any type and will call the `updateFn` with `state` and `optimisticValue`.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useOptimistic#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Optimistically updating forms[](https://react.dev/reference/react/useOptimistic#optimistically-updating-with-forms \"Link for Optimistically updating forms \")\n\nThe `useOptimistic` Hook provides a way to optimistically update the user interface before a background operation, like a network request, completes. In the context of forms, this technique helps to make apps feel more responsive. When a user submits a form, instead of waiting for the server’s response to reflect the changes, the interface is immediately updated with the expected outcome.\n\nFor example, when a user types a message into the form and hits the “Send” button, the `useOptimistic` Hook allows the message to immediately appear in the list with a “Sending…” label, even before the message is actually sent to a server. This “optimistic” approach gives the impression of speed and responsiveness. The form then attempts to truly send the message in the background. Once the server confirms the message has been received, the “Sending…” label is removed.\n\nApp.jsactions.js\n\nApp.js\n\nReset\n\nFork\n\nimport { useOptimistic, useState, useRef } from \"react\";\nimport { deliverMessage } from \"./actions.js\";\n\nfunction Thread({ messages, sendMessage }) {\n const formRef = useRef();\n async function formAction(formData) {\n addOptimisticMessage(formData.get(\"message\"));\n formRef.current.reset();\n await sendMessage(formData);\n }\n const \\[optimisticMessages, addOptimisticMessage\\] = useOptimistic(\n messages,\n (state, newMessage) \\=> \\[\n ...state,\n {\n text: newMessage,\n sending: true\n }\n \\]\n );\n\n return (\n <\\>\n {optimisticMessages.map((message, index) \\=> (\n <div key\\={index}\\>\n {message.text}\n {!!message.sending && <small\\> (Sending...)</small\\>}\n </div\\>\n ))}\n <form action\\={formAction} ref\\={formRef}\\>\n <input type\\=\"text\" name\\=\"message\" placeholder\\=\"Hello!\" />\n <button type\\=\"submit\"\\>Send</button\\>\n </form\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[messages, setMessages\\] = useState(\\[\n { text: \"Hello there!\", sending: false, key: 1 }\n \\]);\n async function sendMessage(formData) {\n const sentMessage = await deliverMessage(formData.get(\"message\"));\n setMessages((messages) \\=> \\[...messages, { text: sentMessage }\\]);\n }\n return <Thread messages\\={messages} sendMessage\\={sendMessage} />;\n}\n\nShow more\n\n[PrevioususeMemo](https://react.dev/reference/react/useMemo)[NextuseReducer](https://react.dev/reference/react/useReducer)\n",
"filename": "useOptimistic.md",
"package": "react"
} |
{
"content": "Title: useReducer – React\n\nURL Source: https://react.dev/reference/react/useReducer\n\nMarkdown Content:\n`useReducer` is a React Hook that lets you add a [reducer](https://react.dev/learn/extracting-state-logic-into-a-reducer) to your component.\n\n```\nconst [state, dispatch] = useReducer(reducer, initialArg, init?)\n```\n\n* [Reference](https://react.dev/reference/react/useReducer#reference)\n * [`useReducer(reducer, initialArg, init?)`](https://react.dev/reference/react/useReducer#usereducer)\n * [`dispatch` function](https://react.dev/reference/react/useReducer#dispatch)\n* [Usage](https://react.dev/reference/react/useReducer#usage)\n * [Adding a reducer to a component](https://react.dev/reference/react/useReducer#adding-a-reducer-to-a-component)\n * [Writing the reducer function](https://react.dev/reference/react/useReducer#writing-the-reducer-function)\n * [Avoiding recreating the initial state](https://react.dev/reference/react/useReducer#avoiding-recreating-the-initial-state)\n* [Troubleshooting](https://react.dev/reference/react/useReducer#troubleshooting)\n * [I’ve dispatched an action, but logging gives me the old state value](https://react.dev/reference/react/useReducer#ive-dispatched-an-action-but-logging-gives-me-the-old-state-value)\n * [I’ve dispatched an action, but the screen doesn’t update](https://react.dev/reference/react/useReducer#ive-dispatched-an-action-but-the-screen-doesnt-update)\n * [A part of my reducer state becomes undefined after dispatching](https://react.dev/reference/react/useReducer#a-part-of-my-reducer-state-becomes-undefined-after-dispatching)\n * [My entire reducer state becomes undefined after dispatching](https://react.dev/reference/react/useReducer#my-entire-reducer-state-becomes-undefined-after-dispatching)\n * [I’m getting an error: “Too many re-renders”](https://react.dev/reference/react/useReducer#im-getting-an-error-too-many-re-renders)\n * [My reducer or initializer function runs twice](https://react.dev/reference/react/useReducer#my-reducer-or-initializer-function-runs-twice)\n\n* * *\n\nReference[](https://react.dev/reference/react/useReducer#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------\n\n### `useReducer(reducer, initialArg, init?)`[](https://react.dev/reference/react/useReducer#usereducer \"Link for this heading\")\n\nCall `useReducer` at the top level of your component to manage its state with a [reducer.](https://react.dev/learn/extracting-state-logic-into-a-reducer)\n\n```\nimport { useReducer } from 'react';function reducer(state, action) {// ...}function MyComponent() {const [state, dispatch] = useReducer(reducer, { age: 42 });// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/useReducer#usage)\n\n#### Parameters[](https://react.dev/reference/react/useReducer#parameters \"Link for Parameters \")\n\n* `reducer`: The reducer function that specifies how the state gets updated. It must be pure, should take the state and action as arguments, and should return the next state. State and action can be of any types.\n* `initialArg`: The value from which the initial state is calculated. It can be a value of any type. How the initial state is calculated from it depends on the next `init` argument.\n* **optional** `init`: The initializer function that should return the initial state. If it’s not specified, the initial state is set to `initialArg`. Otherwise, the initial state is set to the result of calling `init(initialArg)`.\n\n#### Returns[](https://react.dev/reference/react/useReducer#returns \"Link for Returns \")\n\n`useReducer` returns an array with exactly two values:\n\n1. The current state. During the first render, it’s set to `init(initialArg)` or `initialArg` (if there’s no `init`).\n2. The [`dispatch` function](https://react.dev/reference/react/useReducer#dispatch) that lets you update the state to a different value and trigger a re-render.\n\n#### Caveats[](https://react.dev/reference/react/useReducer#caveats \"Link for Caveats \")\n\n* `useReducer` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a new component and move the state into it.\n* In Strict Mode, React will **call your reducer and initializer twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useReducer#my-reducer-or-initializer-function-runs-twice) This is development-only behavior and does not affect production. If your reducer and initializer are pure (as they should be), this should not affect your logic. The result from one of the calls is ignored.\n\n* * *\n\n### `dispatch` function[](https://react.dev/reference/react/useReducer#dispatch \"Link for this heading\")\n\nThe `dispatch` function returned by `useReducer` lets you update the state to a different value and trigger a re-render. You need to pass the action as the only argument to the `dispatch` function:\n\n```\nconst [state, dispatch] = useReducer(reducer, { age: 42 });function handleClick() {dispatch({ type: 'incremented_age' });// ...\n```\n\nReact will set the next state to the result of calling the `reducer` function you’ve provided with the current `state` and the action you’ve passed to `dispatch`.\n\n#### Parameters[](https://react.dev/reference/react/useReducer#dispatch-parameters \"Link for Parameters \")\n\n* `action`: The action performed by the user. It can be a value of any type. By convention, an action is usually an object with a `type` property identifying it and, optionally, other properties with additional information.\n\n#### Returns[](https://react.dev/reference/react/useReducer#dispatch-returns \"Link for Returns \")\n\n`dispatch` functions do not have a return value.\n\n#### Caveats[](https://react.dev/reference/react/useReducer#setstate-caveats \"Link for Caveats \")\n\n* The `dispatch` function **only updates the state variable for the _next_ render**. If you read the state variable after calling the `dispatch` function, [you will still get the old value](https://react.dev/reference/react/useReducer#ive-dispatched-an-action-but-logging-gives-me-the-old-state-value) that was on the screen before your call.\n \n* If the new value you provide is identical to the current `state`, as determined by an [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison, React will **skip re-rendering the component and its children.** This is an optimization. React may still need to call your component before ignoring the result, but it shouldn’t affect your code.\n \n* React [batches state updates.](https://react.dev/learn/queueing-a-series-of-state-updates) It updates the screen **after all the event handlers have run** and have called their `set` functions. This prevents multiple re-renders during a single event. In the rare case that you need to force React to update the screen earlier, for example to access the DOM, you can use [`flushSync`.](https://react.dev/reference/react-dom/flushSync)\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useReducer#usage \"Link for Usage \")\n-----------------------------------------------------------------------------\n\n### Adding a reducer to a component[](https://react.dev/reference/react/useReducer#adding-a-reducer-to-a-component \"Link for Adding a reducer to a component \")\n\nCall `useReducer` at the top level of your component to manage state with a [reducer.](https://react.dev/learn/extracting-state-logic-into-a-reducer)\n\n```\nimport { useReducer } from 'react';function reducer(state, action) {// ...}function MyComponent() {const [state, dispatch] = useReducer(reducer, { age: 42 });// ...\n```\n\n`useReducer` returns an array with exactly two items:\n\n1. The current state of this state variable, initially set to the initial state you provided.\n2. The `dispatch` function that lets you change it in response to interaction.\n\nTo update what’s on the screen, call `dispatch` with an object representing what the user did, called an _action_:\n\n```\nfunction handleClick() {dispatch({ type: 'incremented_age' });}\n```\n\nReact will pass the current state and the action to your reducer function. Your reducer will calculate and return the next state. React will store that next state, render your component with it, and update the UI.\n\n`useReducer` is very similar to [`useState`](https://react.dev/reference/react/useState), but it lets you move the state update logic from event handlers into a single function outside of your component. Read more about [choosing between `useState` and `useReducer`.](https://react.dev/learn/extracting-state-logic-into-a-reducer#comparing-usestate-and-usereducer)\n\n* * *\n\n### Writing the reducer function[](https://react.dev/reference/react/useReducer#writing-the-reducer-function \"Link for Writing the reducer function \")\n\nA reducer function is declared like this:\n\n```\nfunction reducer(state, action) {// ...}\n```\n\nThen you need to fill in the code that will calculate and return the next state. By convention, it is common to write it as a [`switch` statement.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/switch) For each `case` in the `switch`, calculate and return some next state.\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {return {name: state.name,age: state.age + 1};}case 'changed_name': {return {name: action.nextName,age: state.age};}}throw Error('Unknown action: ' + action.type);}\n```\n\nActions can have any shape. By convention, it’s common to pass objects with a `type` property identifying the action. It should include the minimal necessary information that the reducer needs to compute the next state.\n\n```\nfunction Form() {const [state, dispatch] = useReducer(reducer, { name: 'Taylor', age: 42 });function handleButtonClick() {dispatch({ type: 'incremented_age' });}function handleInputChange(e) {dispatch({type: 'changed_name',nextName: e.target.value});}// ...\n```\n\nThe action type names are local to your component. [Each action describes a single interaction, even if that leads to multiple changes in data.](https://react.dev/learn/extracting-state-logic-into-a-reducer#writing-reducers-well) The shape of the state is arbitrary, but usually it’ll be an object or an array.\n\nRead [extracting state logic into a reducer](https://react.dev/learn/extracting-state-logic-into-a-reducer) to learn more.\n\n### Pitfall\n\nState is read-only. Don’t modify any objects or arrays in state:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {// 🚩 Don't mutate an object in state like this:state.age = state.age + 1;return state;}\n```\n\nInstead, always return new objects from your reducer:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {// ✅ Instead, return a new objectreturn {...state,age: state.age + 1};}\n```\n\nRead [updating objects in state](https://react.dev/learn/updating-objects-in-state) and [updating arrays in state](https://react.dev/learn/updating-arrays-in-state) to learn more.\n\n* * *\n\n### Avoiding recreating the initial state[](https://react.dev/reference/react/useReducer#avoiding-recreating-the-initial-state \"Link for Avoiding recreating the initial state \")\n\nReact saves the initial state once and ignores it on the next renders.\n\n```\nfunction createInitialState(username) {// ...}function TodoList({ username }) {const [state, dispatch] = useReducer(reducer, createInitialState(username));// ...\n```\n\nAlthough the result of `createInitialState(username)` is only used for the initial render, you’re still calling this function on every render. This can be wasteful if it’s creating large arrays or performing expensive calculations.\n\nTo solve this, you may **pass it as an _initializer_ function** to `useReducer` as the third argument instead:\n\n```\nfunction createInitialState(username) {// ...}function TodoList({ username }) {const [state, dispatch] = useReducer(reducer, username, createInitialState);// ...\n```\n\nNotice that you’re passing `createInitialState`, which is the _function itself_, and not `createInitialState()`, which is the result of calling it. This way, the initial state does not get re-created after initialization.\n\nIn the above example, `createInitialState` takes a `username` argument. If your initializer doesn’t need any information to compute the initial state, you may pass `null` as the second argument to `useReducer`.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useReducer#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------------\n\n### I’ve dispatched an action, but logging gives me the old state value[](https://react.dev/reference/react/useReducer#ive-dispatched-an-action-but-logging-gives-me-the-old-state-value \"Link for I’ve dispatched an action, but logging gives me the old state value \")\n\nCalling the `dispatch` function **does not change state in the running code**:\n\n```\nfunction handleClick() {console.log(state.age); // 42dispatch({ type: 'incremented_age' }); // Request a re-render with 43console.log(state.age); // Still 42!setTimeout(() => {console.log(state.age); // Also 42!}, 5000);}\n```\n\nThis is because [states behaves like a snapshot.](https://react.dev/learn/state-as-a-snapshot) Updating state requests another render with the new state value, but does not affect the `state` JavaScript variable in your already-running event handler.\n\nIf you need to guess the next state value, you can calculate it manually by calling the reducer yourself:\n\n```\nconst action = { type: 'incremented_age' };dispatch(action);const nextState = reducer(state, action);console.log(state); // { age: 42 }console.log(nextState); // { age: 43 }\n```\n\n* * *\n\n### I’ve dispatched an action, but the screen doesn’t update[](https://react.dev/reference/react/useReducer#ive-dispatched-an-action-but-the-screen-doesnt-update \"Link for I’ve dispatched an action, but the screen doesn’t update \")\n\nReact will **ignore your update if the next state is equal to the previous state,** as determined by an [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. This usually happens when you change an object or an array in state directly:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {// 🚩 Wrong: mutating existing objectstate.age++;return state;}case 'changed_name': {// 🚩 Wrong: mutating existing objectstate.name = action.nextName;return state;}// ...}}\n```\n\nYou mutated an existing `state` object and returned it, so React ignored the update. To fix this, you need to ensure that you’re always [updating objects in state](https://react.dev/learn/updating-objects-in-state) and [updating arrays in state](https://react.dev/learn/updating-arrays-in-state) instead of mutating them:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {// ✅ Correct: creating a new objectreturn {...state,age: state.age + 1};}case 'changed_name': {// ✅ Correct: creating a new objectreturn {...state,name: action.nextName};}// ...}}\n```\n\n* * *\n\n### A part of my reducer state becomes undefined after dispatching[](https://react.dev/reference/react/useReducer#a-part-of-my-reducer-state-becomes-undefined-after-dispatching \"Link for A part of my reducer state becomes undefined after dispatching \")\n\nMake sure that every `case` branch **copies all of the existing fields** when returning the new state:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {return {...state, // Don't forget this!age: state.age + 1};}// ...\n```\n\nWithout `...state` above, the returned next state would only contain the `age` field and nothing else.\n\n* * *\n\n### My entire reducer state becomes undefined after dispatching[](https://react.dev/reference/react/useReducer#my-entire-reducer-state-becomes-undefined-after-dispatching \"Link for My entire reducer state becomes undefined after dispatching \")\n\nIf your state unexpectedly becomes `undefined`, you’re likely forgetting to `return` state in one of the cases, or your action type doesn’t match any of the `case` statements. To find why, throw an error outside the `switch`:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'incremented_age': {// ...}case 'edited_name': {// ...}}throw Error('Unknown action: ' + action.type);}\n```\n\nYou can also use a static type checker like TypeScript to catch such mistakes.\n\n* * *\n\n### I’m getting an error: “Too many re-renders”[](https://react.dev/reference/react/useReducer#im-getting-an-error-too-many-re-renders \"Link for I’m getting an error: “Too many re-renders” \")\n\nYou might get an error that says: `Too many re-renders. React limits the number of renders to prevent an infinite loop.` Typically, this means that you’re unconditionally dispatching an action _during render_, so your component enters a loop: render, dispatch (which causes a render), render, dispatch (which causes a render), and so on. Very often, this is caused by a mistake in specifying an event handler:\n\n```\n// 🚩 Wrong: calls the handler during renderreturn <button onClick={handleClick()}>Click me</button>// ✅ Correct: passes down the event handlerreturn <button onClick={handleClick}>Click me</button>// ✅ Correct: passes down an inline functionreturn <button onClick={(e) => handleClick(e)}>Click me</button>\n```\n\nIf you can’t find the cause of this error, click on the arrow next to the error in the console and look through the JavaScript stack to find the specific `dispatch` function call responsible for the error.\n\n* * *\n\n### My reducer or initializer function runs twice[](https://react.dev/reference/react/useReducer#my-reducer-or-initializer-function-runs-twice \"Link for My reducer or initializer function runs twice \")\n\nIn [Strict Mode](https://react.dev/reference/react/StrictMode), React will call your reducer and initializer functions twice. This shouldn’t break your code.\n\nThis **development-only** behavior helps you [keep components pure.](https://react.dev/learn/keeping-components-pure) React uses the result of one of the calls, and ignores the result of the other call. As long as your component, initializer, and reducer functions are pure, this shouldn’t affect your logic. However, if they are accidentally impure, this helps you notice the mistakes.\n\nFor example, this impure reducer function mutates an array in state:\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'added_todo': {// 🚩 Mistake: mutating statestate.todos.push({ id: nextId++, text: action.text });return state;}// ...}}\n```\n\nBecause React calls your reducer function twice, you’ll see the todo was added twice, so you’ll know that there is a mistake. In this example, you can fix the mistake by [replacing the array instead of mutating it](https://react.dev/learn/updating-arrays-in-state#adding-to-an-array):\n\n```\nfunction reducer(state, action) {switch (action.type) {case 'added_todo': {// ✅ Correct: replacing with new statereturn {...state,todos: [...state.todos,{ id: nextId++, text: action.text }]};}// ...}}\n```\n\nNow that this reducer function is pure, calling it an extra time doesn’t make a difference in behavior. This is why React calling it twice helps you find mistakes. **Only component, initializer, and reducer functions need to be pure.** Event handlers don’t need to be pure, so React will never call your event handlers twice.\n\nRead [keeping components pure](https://react.dev/learn/keeping-components-pure) to learn more.\n",
"filename": "useReducer.md",
"package": "react"
} |
{
"content": "Title: useRef – React\n\nURL Source: https://react.dev/reference/react/useRef\n\nMarkdown Content:\n`useRef` is a React Hook that lets you reference a value that’s not needed for rendering.\n\n```\nconst ref = useRef(initialValue)\n```\n\n* [Reference](https://react.dev/reference/react/useRef#reference)\n * [`useRef(initialValue)`](https://react.dev/reference/react/useRef#useref)\n* [Usage](https://react.dev/reference/react/useRef#usage)\n * [Referencing a value with a ref](https://react.dev/reference/react/useRef#referencing-a-value-with-a-ref)\n * [Manipulating the DOM with a ref](https://react.dev/reference/react/useRef#manipulating-the-dom-with-a-ref)\n * [Avoiding recreating the ref contents](https://react.dev/reference/react/useRef#avoiding-recreating-the-ref-contents)\n* [Troubleshooting](https://react.dev/reference/react/useRef#troubleshooting)\n * [I can’t get a ref to a custom component](https://react.dev/reference/react/useRef#i-cant-get-a-ref-to-a-custom-component)\n\n* * *\n\nReference[](https://react.dev/reference/react/useRef#reference \"Link for Reference \")\n-------------------------------------------------------------------------------------\n\n### `useRef(initialValue)`[](https://react.dev/reference/react/useRef#useref \"Link for this heading\")\n\nCall `useRef` at the top level of your component to declare a [ref.](https://react.dev/learn/referencing-values-with-refs)\n\n```\nimport { useRef } from 'react';function MyComponent() {const intervalRef = useRef(0);const inputRef = useRef(null);// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/useRef#usage)\n\n#### Parameters[](https://react.dev/reference/react/useRef#parameters \"Link for Parameters \")\n\n* `initialValue`: The value you want the ref object’s `current` property to be initially. It can be a value of any type. This argument is ignored after the initial render.\n\n#### Returns[](https://react.dev/reference/react/useRef#returns \"Link for Returns \")\n\n`useRef` returns an object with a single property:\n\n* `current`: Initially, it’s set to the `initialValue` you have passed. You can later set it to something else. If you pass the ref object to React as a `ref` attribute to a JSX node, React will set its `current` property.\n\nOn the next renders, `useRef` will return the same object.\n\n#### Caveats[](https://react.dev/reference/react/useRef#caveats \"Link for Caveats \")\n\n* You can mutate the `ref.current` property. Unlike state, it is mutable. However, if it holds an object that is used for rendering (for example, a piece of your state), then you shouldn’t mutate that object.\n* When you change the `ref.current` property, React does not re-render your component. React is not aware of when you change it because a ref is a plain JavaScript object.\n* Do not write _or read_ `ref.current` during rendering, except for [initialization.](https://react.dev/reference/react/useRef#avoiding-recreating-the-ref-contents) This makes your component’s behavior unpredictable.\n* In Strict Mode, React will **call your component function twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice) This is development-only behavior and does not affect production. Each ref object will be created twice, but one of the versions will be discarded. If your component function is pure (as it should be), this should not affect the behavior.\n\n* * *\n\nUsage[](https://react.dev/reference/react/useRef#usage \"Link for Usage \")\n-------------------------------------------------------------------------\n\n### Referencing a value with a ref[](https://react.dev/reference/react/useRef#referencing-a-value-with-a-ref \"Link for Referencing a value with a ref \")\n\nCall `useRef` at the top level of your component to declare one or more [refs.](https://react.dev/learn/referencing-values-with-refs)\n\n```\nimport { useRef } from 'react';function Stopwatch() {const intervalRef = useRef(0);// ...\n```\n\n`useRef` returns a ref object with a single `current` property initially set to the initial value you provided.\n\nOn the next renders, `useRef` will return the same object. You can change its `current` property to store information and read it later. This might remind you of [state](https://react.dev/reference/react/useState), but there is an important difference.\n\n**Changing a ref does not trigger a re-render.** This means refs are perfect for storing information that doesn’t affect the visual output of your component. For example, if you need to store an [interval ID](https://developer.mozilla.org/en-US/docs/Web/API/setInterval) and retrieve it later, you can put it in a ref. To update the value inside the ref, you need to manually change its `current` property:\n\n```\nfunction handleStartClick() {const intervalId = setInterval(() => {// ...}, 1000);intervalRef.current = intervalId;}\n```\n\nLater, you can read that interval ID from the ref so that you can call [clear that interval](https://developer.mozilla.org/en-US/docs/Web/API/clearInterval):\n\n```\nfunction handleStopClick() {const intervalId = intervalRef.current;clearInterval(intervalId);}\n```\n\nBy using a ref, you ensure that:\n\n* You can **store information** between re-renders (unlike regular variables, which reset on every render).\n* Changing it **does not trigger a re-render** (unlike state variables, which trigger a re-render).\n* The **information is local** to each copy of your component (unlike the variables outside, which are shared).\n\nChanging a ref does not trigger a re-render, so refs are not appropriate for storing information you want to display on the screen. Use state for that instead. Read more about [choosing between `useRef` and `useState`.](https://react.dev/learn/referencing-values-with-refs#differences-between-refs-and-state)\n\n#### Examples of referencing a value with useRef[](https://react.dev/reference/react/useRef#examples-value \"Link for Examples of referencing a value with useRef\")\n\n#### Click counter[](https://react.dev/reference/react/useRef#click-counter \"Link for this heading\")\n\nThis component uses a ref to keep track of how many times the button was clicked. Note that it’s okay to use a ref instead of state here because the click count is only read and written in an event handler.\n\nimport { useRef } from 'react';\n\nexport default function Counter() {\n let ref = useRef(0);\n\n function handleClick() {\n ref.current = ref.current + 1;\n alert('You clicked ' + ref.current + ' times!');\n }\n\n return (\n <button onClick\\={handleClick}\\>\n Click me!\n </button\\>\n );\n}\n\nIf you show `{ref.current}` in the JSX, the number won’t update on click. This is because setting `ref.current` does not trigger a re-render. Information that’s used for rendering should be state instead.\n\n### Pitfall\n\n**Do not write _or read_ `ref.current` during rendering.**\n\nReact expects that the body of your component [behaves like a pure function](https://react.dev/learn/keeping-components-pure):\n\n* If the inputs ([props](https://react.dev/learn/passing-props-to-a-component), [state](https://react.dev/learn/state-a-components-memory), and [context](https://react.dev/learn/passing-data-deeply-with-context)) are the same, it should return exactly the same JSX.\n* Calling it in a different order or with different arguments should not affect the results of other calls.\n\nReading or writing a ref **during rendering** breaks these expectations.\n\n```\nfunction MyComponent() {// ...// 🚩 Don't write a ref during renderingmyRef.current = 123;// ...// 🚩 Don't read a ref during renderingreturn <h1>{myOtherRef.current}</h1>;}\n```\n\nYou can read or write refs **from event handlers or effects instead**.\n\n```\nfunction MyComponent() {// ...useEffect(() => {// ✅ You can read or write refs in effectsmyRef.current = 123;});// ...function handleClick() {// ✅ You can read or write refs in event handlersdoSomething(myOtherRef.current);}// ...}\n```\n\nIf you _have to_ read [or write](https://react.dev/reference/react/useState#storing-information-from-previous-renders) something during rendering, [use state](https://react.dev/reference/react/useState) instead.\n\nWhen you break these rules, your component might still work, but most of the newer features we’re adding to React will rely on these expectations. Read more about [keeping your components pure.](https://react.dev/learn/keeping-components-pure#where-you-_can_-cause-side-effects)\n\n* * *\n\n### Manipulating the DOM with a ref[](https://react.dev/reference/react/useRef#manipulating-the-dom-with-a-ref \"Link for Manipulating the DOM with a ref \")\n\nIt’s particularly common to use a ref to manipulate the [DOM.](https://developer.mozilla.org/en-US/docs/Web/API/HTML_DOM_API) React has built-in support for this.\n\nFirst, declare a ref object with an initial value of `null`:\n\n```\nimport { useRef } from 'react';function MyComponent() {const inputRef = useRef(null);// ...\n```\n\nThen pass your ref object as the `ref` attribute to the JSX of the DOM node you want to manipulate:\n\n```\n// ...return <input ref={inputRef} />;\n```\n\nAfter React creates the DOM node and puts it on the screen, React will set the `current` property of your ref object to that DOM node. Now you can access the `<input>`’s DOM node and call methods like [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus):\n\n```\nfunction handleClick() {inputRef.current.focus();}\n```\n\nReact will set the `current` property back to `null` when the node is removed from the screen.\n\nRead more about [manipulating the DOM with refs.](https://react.dev/learn/manipulating-the-dom-with-refs)\n\n#### Examples of manipulating the DOM with useRef[](https://react.dev/reference/react/useRef#examples-dom \"Link for Examples of manipulating the DOM with useRef\")\n\n#### Focusing a text input[](https://react.dev/reference/react/useRef#focusing-a-text-input \"Link for this heading\")\n\nIn this example, clicking the button will focus the input:\n\nimport { useRef } from 'react';\n\nexport default function Form() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <input ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\n* * *\n\n### Avoiding recreating the ref contents[](https://react.dev/reference/react/useRef#avoiding-recreating-the-ref-contents \"Link for Avoiding recreating the ref contents \")\n\nReact saves the initial ref value once and ignores it on the next renders.\n\n```\nfunction Video() {const playerRef = useRef(new VideoPlayer());// ...\n```\n\nAlthough the result of `new VideoPlayer()` is only used for the initial render, you’re still calling this function on every render. This can be wasteful if it’s creating expensive objects.\n\nTo solve it, you may initialize the ref like this instead:\n\n```\nfunction Video() {const playerRef = useRef(null);if (playerRef.current === null) {playerRef.current = new VideoPlayer();}// ...\n```\n\nNormally, writing or reading `ref.current` during render is not allowed. However, it’s fine in this case because the result is always the same, and the condition only executes during initialization so it’s fully predictable.\n\n##### Deep Dive\n\n#### How to avoid null checks when initializing useRef later[](https://react.dev/reference/react/useRef#how-to-avoid-null-checks-when-initializing-use-ref-later \"Link for How to avoid null checks when initializing useRef later \")\n\nIf you use a type checker and don’t want to always check for `null`, you can try a pattern like this instead:\n\n```\nfunction Video() {const playerRef = useRef(null);function getPlayer() {if (playerRef.current !== null) {return playerRef.current;}const player = new VideoPlayer();playerRef.current = player;return player;}// ...\n```\n\nHere, the `playerRef` itself is nullable. However, you should be able to convince your type checker that there is no case in which `getPlayer()` returns `null`. Then use `getPlayer()` in your event handlers.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useRef#troubleshooting \"Link for Troubleshooting \")\n-------------------------------------------------------------------------------------------------------\n\n### I can’t get a ref to a custom component[](https://react.dev/reference/react/useRef#i-cant-get-a-ref-to-a-custom-component \"Link for I can’t get a ref to a custom component \")\n\nIf you try to pass a `ref` to your own component like this:\n\n```\nconst inputRef = useRef(null);return <MyInput ref={inputRef} />;\n```\n\nYou might get an error in the console:\n\nWarning: Function components cannot be given refs. Attempts to access this ref will fail. Did you mean to use React.forwardRef()?\n\nBy default, your own components don’t expose refs to the DOM nodes inside them.\n\nTo fix this, find the component that you want to get a ref to:\n\n```\nexport default function MyInput({ value, onChange }) {return (<inputvalue={value}onChange={onChange}/>);}\n```\n\nAnd then wrap it in [`forwardRef`](https://react.dev/reference/react/forwardRef) like this:\n\n```\nimport { forwardRef } from 'react';const MyInput = forwardRef(({ value, onChange }, ref) => {return (<inputvalue={value}onChange={onChange}ref={ref}/>);});export default MyInput;\n```\n\nThen the parent component can get a ref to it.\n\nRead more about [accessing another component’s DOM nodes.](https://react.dev/learn/manipulating-the-dom-with-refs#accessing-another-components-dom-nodes)\n",
"filename": "useRef.md",
"package": "react"
} |
{
"content": "Title: useState – React\n\nURL Source: https://react.dev/reference/react/useState\n\nMarkdown Content:\n`useState` is a React Hook that lets you add a [state variable](https://react.dev/learn/state-a-components-memory) to your component.\n\n```\nconst [state, setState] = useState(initialState)\n```\n\n* [Reference](https://react.dev/reference/react/useState#reference)\n * [`useState(initialState)`](https://react.dev/reference/react/useState#usestate)\n * [`set` functions, like `setSomething(nextState)`](https://react.dev/reference/react/useState#setstate)\n* [Usage](https://react.dev/reference/react/useState#usage)\n * [Adding state to a component](https://react.dev/reference/react/useState#adding-state-to-a-component)\n * [Updating state based on the previous state](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state)\n * [Updating objects and arrays in state](https://react.dev/reference/react/useState#updating-objects-and-arrays-in-state)\n * [Avoiding recreating the initial state](https://react.dev/reference/react/useState#avoiding-recreating-the-initial-state)\n * [Resetting state with a key](https://react.dev/reference/react/useState#resetting-state-with-a-key)\n * [Storing information from previous renders](https://react.dev/reference/react/useState#storing-information-from-previous-renders)\n* [Troubleshooting](https://react.dev/reference/react/useState#troubleshooting)\n * [I’ve updated the state, but logging gives me the old value](https://react.dev/reference/react/useState#ive-updated-the-state-but-logging-gives-me-the-old-value)\n * [I’ve updated the state, but the screen doesn’t update](https://react.dev/reference/react/useState#ive-updated-the-state-but-the-screen-doesnt-update)\n * [I’m getting an error: “Too many re-renders”](https://react.dev/reference/react/useState#im-getting-an-error-too-many-re-renders)\n * [My initializer or updater function runs twice](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice)\n * [I’m trying to set state to a function, but it gets called instead](https://react.dev/reference/react/useState#im-trying-to-set-state-to-a-function-but-it-gets-called-instead)\n\n* * *\n\nReference[](https://react.dev/reference/react/useState#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `useState(initialState)`[](https://react.dev/reference/react/useState#usestate \"Link for this heading\")\n\nCall `useState` at the top level of your component to declare a [state variable.](https://react.dev/learn/state-a-components-memory)\n\n```\nimport { useState } from 'react';function MyComponent() {const [age, setAge] = useState(28);const [name, setName] = useState('Taylor');const [todos, setTodos] = useState(() => createTodos());// ...\n```\n\nThe convention is to name state variables like `[something, setSomething]` using [array destructuring.](https://javascript.info/destructuring-assignment)\n\n[See more examples below.](https://react.dev/reference/react/useState#usage)\n\n#### Parameters[](https://react.dev/reference/react/useState#parameters \"Link for Parameters \")\n\n* `initialState`: The value you want the state to be initially. It can be a value of any type, but there is a special behavior for functions. This argument is ignored after the initial render.\n * If you pass a function as `initialState`, it will be treated as an _initializer function_. It should be pure, should take no arguments, and should return a value of any type. React will call your initializer function when initializing the component, and store its return value as the initial state. [See an example below.](https://react.dev/reference/react/useState#avoiding-recreating-the-initial-state)\n\n#### Returns[](https://react.dev/reference/react/useState#returns \"Link for Returns \")\n\n`useState` returns an array with exactly two values:\n\n1. The current state. During the first render, it will match the `initialState` you have passed.\n2. The [`set` function](https://react.dev/reference/react/useState#setstate) that lets you update the state to a different value and trigger a re-render.\n\n#### Caveats[](https://react.dev/reference/react/useState#caveats \"Link for Caveats \")\n\n* `useState` is a Hook, so you can only call it **at the top level of your component** or your own Hooks. You can’t call it inside loops or conditions. If you need that, extract a new component and move the state into it.\n* In Strict Mode, React will **call your initializer function twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice) This is development-only behavior and does not affect production. If your initializer function is pure (as it should be), this should not affect the behavior. The result from one of the calls will be ignored.\n\n* * *\n\n### `set` functions, like `setSomething(nextState)`[](https://react.dev/reference/react/useState#setstate \"Link for this heading\")\n\nThe `set` function returned by `useState` lets you update the state to a different value and trigger a re-render. You can pass the next state directly, or a function that calculates it from the previous state:\n\n```\nconst [name, setName] = useState('Edward');function handleClick() {setName('Taylor');setAge(a => a + 1);// ...\n```\n\n#### Parameters[](https://react.dev/reference/react/useState#setstate-parameters \"Link for Parameters \")\n\n* `nextState`: The value that you want the state to be. It can be a value of any type, but there is a special behavior for functions.\n * If you pass a function as `nextState`, it will be treated as an _updater function_. It must be pure, should take the pending state as its only argument, and should return the next state. React will put your updater function in a queue and re-render your component. During the next render, React will calculate the next state by applying all of the queued updaters to the previous state. [See an example below.](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state)\n\n#### Returns[](https://react.dev/reference/react/useState#setstate-returns \"Link for Returns \")\n\n`set` functions do not have a return value.\n\n#### Caveats[](https://react.dev/reference/react/useState#setstate-caveats \"Link for Caveats \")\n\n* The `set` function **only updates the state variable for the _next_ render**. If you read the state variable after calling the `set` function, [you will still get the old value](https://react.dev/reference/react/useState#ive-updated-the-state-but-logging-gives-me-the-old-value) that was on the screen before your call.\n \n* If the new value you provide is identical to the current `state`, as determined by an [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison, React will **skip re-rendering the component and its children.** This is an optimization. Although in some cases React may still need to call your component before skipping the children, it shouldn’t affect your code.\n \n* React [batches state updates.](https://react.dev/learn/queueing-a-series-of-state-updates) It updates the screen **after all the event handlers have run** and have called their `set` functions. This prevents multiple re-renders during a single event. In the rare case that you need to force React to update the screen earlier, for example to access the DOM, you can use [`flushSync`.](https://react.dev/reference/react-dom/flushSync)\n \n* Calling the `set` function _during rendering_ is only allowed from within the currently rendering component. React will discard its output and immediately attempt to render it again with the new state. This pattern is rarely needed, but you can use it to **store information from the previous renders**. [See an example below.](https://react.dev/reference/react/useState#storing-information-from-previous-renders)\n \n* In Strict Mode, React will **call your updater function twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice) This is development-only behavior and does not affect production. If your updater function is pure (as it should be), this should not affect the behavior. The result from one of the calls will be ignored.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useState#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Adding state to a component[](https://react.dev/reference/react/useState#adding-state-to-a-component \"Link for Adding state to a component \")\n\nCall `useState` at the top level of your component to declare one or more [state variables.](https://react.dev/learn/state-a-components-memory)\n\n```\nimport { useState } from 'react';function MyComponent() {const [age, setAge] = useState(42);const [name, setName] = useState('Taylor');// ...\n```\n\nThe convention is to name state variables like `[something, setSomething]` using [array destructuring.](https://javascript.info/destructuring-assignment)\n\n`useState` returns an array with exactly two items:\n\n1. The current state of this state variable, initially set to the initial state you provided.\n2. The `set` function that lets you change it to any other value in response to interaction.\n\nTo update what’s on the screen, call the `set` function with some next state:\n\n```\nfunction handleClick() {setName('Robin');}\n```\n\nReact will store the next state, render your component again with the new values, and update the UI.\n\n### Pitfall\n\nCalling the `set` function [**does not** change the current state in the already executing code](https://react.dev/reference/react/useState#ive-updated-the-state-but-logging-gives-me-the-old-value):\n\n```\nfunction handleClick() {setName('Robin');console.log(name); // Still \"Taylor\"!}\n```\n\nIt only affects what `useState` will return starting from the _next_ render.\n\n#### Basic useState examples[](https://react.dev/reference/react/useState#examples-basic \"Link for Basic useState examples\")\n\n#### Counter (number)[](https://react.dev/reference/react/useState#counter-number \"Link for this heading\")\n\nIn this example, the `count` state variable holds a number. Clicking the button increments it.\n\n* * *\n\n### Updating state based on the previous state[](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state \"Link for Updating state based on the previous state \")\n\nSuppose the `age` is `42`. This handler calls `setAge(age + 1)` three times:\n\n```\nfunction handleClick() {setAge(age + 1); // setAge(42 + 1)setAge(age + 1); // setAge(42 + 1)setAge(age + 1); // setAge(42 + 1)}\n```\n\nHowever, after one click, `age` will only be `43` rather than `45`! This is because calling the `set` function [does not update](https://react.dev/learn/state-as-a-snapshot) the `age` state variable in the already running code. So each `setAge(age + 1)` call becomes `setAge(43)`.\n\nTo solve this problem, **you may pass an _updater function_** to `setAge` instead of the next state:\n\n```\nfunction handleClick() {setAge(a => a + 1); // setAge(42 => 43)setAge(a => a + 1); // setAge(43 => 44)setAge(a => a + 1); // setAge(44 => 45)}\n```\n\nHere, `a => a + 1` is your updater function. It takes the pending state and calculates the next state from it.\n\nReact puts your updater functions in a [queue.](https://react.dev/learn/queueing-a-series-of-state-updates) Then, during the next render, it will call them in the same order:\n\n1. `a => a + 1` will receive `42` as the pending state and return `43` as the next state.\n2. `a => a + 1` will receive `43` as the pending state and return `44` as the next state.\n3. `a => a + 1` will receive `44` as the pending state and return `45` as the next state.\n\nThere are no other queued updates, so React will store `45` as the current state in the end.\n\nBy convention, it’s common to name the pending state argument for the first letter of the state variable name, like `a` for `age`. However, you may also call it like `prevAge` or something else that you find clearer.\n\nReact may [call your updaters twice](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice) in development to verify that they are [pure.](https://react.dev/learn/keeping-components-pure)\n\n##### Deep Dive\n\n#### Is using an updater always preferred?[](https://react.dev/reference/react/useState#is-using-an-updater-always-preferred \"Link for Is using an updater always preferred? \")\n\nYou might hear a recommendation to always write code like `setAge(a => a + 1)` if the state you’re setting is calculated from the previous state. There is no harm in it, but it is also not always necessary.\n\nIn most cases, there is no difference between these two approaches. React always makes sure that for intentional user actions, like clicks, the `age` state variable would be updated before the next click. This means there is no risk of a click handler seeing a “stale” `age` at the beginning of the event handler.\n\nHowever, if you do multiple updates within the same event, updaters can be helpful. They’re also helpful if accessing the state variable itself is inconvenient (you might run into this when optimizing re-renders).\n\nIf you prefer consistency over slightly more verbose syntax, it’s reasonable to always write an updater if the state you’re setting is calculated from the previous state. If it’s calculated from the previous state of some _other_ state variable, you might want to combine them into one object and [use a reducer.](https://react.dev/learn/extracting-state-logic-into-a-reducer)\n\n#### The difference between passing an updater and passing the next state directly[](https://react.dev/reference/react/useState#examples-updater \"Link for The difference between passing an updater and passing the next state directly\")\n\n#### Passing the updater function[](https://react.dev/reference/react/useState#passing-the-updater-function \"Link for this heading\")\n\nThis example passes the updater function, so the “+3” button works.\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[age, setAge\\] = useState(42);\n\n function increment() {\n setAge(a \\=> a + 1);\n }\n\n return (\n <\\>\n <h1\\>Your age: {age}</h1\\>\n <button onClick\\={() \\=> {\n increment();\n increment();\n increment();\n }}\\>+3</button\\>\n <button onClick\\={() \\=> {\n increment();\n }}\\>+1</button\\>\n </\\>\n );\n}\n\n* * *\n\n### Updating objects and arrays in state[](https://react.dev/reference/react/useState#updating-objects-and-arrays-in-state \"Link for Updating objects and arrays in state \")\n\nYou can put objects and arrays into state. In React, state is considered read-only, so **you should _replace_ it rather than _mutate_ your existing objects**. For example, if you have a `form` object in state, don’t mutate it:\n\n```\n// 🚩 Don't mutate an object in state like this:form.firstName = 'Taylor';\n```\n\nInstead, replace the whole object by creating a new one:\n\n```\n// ✅ Replace state with a new objectsetForm({...form,firstName: 'Taylor'});\n```\n\nRead [updating objects in state](https://react.dev/learn/updating-objects-in-state) and [updating arrays in state](https://react.dev/learn/updating-arrays-in-state) to learn more.\n\n#### Examples of objects and arrays in state[](https://react.dev/reference/react/useState#examples-objects \"Link for Examples of objects and arrays in state\")\n\n#### Form (object)[](https://react.dev/reference/react/useState#form-object \"Link for this heading\")\n\nIn this example, the `form` state variable holds an object. Each input has a change handler that calls `setForm` with the next state of the entire form. The `{ ...form }` spread syntax ensures that the state object is replaced rather than mutated.\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[form, setForm\\] = useState({\n firstName: 'Barbara',\n lastName: 'Hepworth',\n email: 'bhepworth@sculpture.com',\n });\n\n return (\n <\\>\n <label\\>\n First name:\n <input\n value\\={form.firstName}\n onChange\\={e \\=> {\n setForm({\n ...form,\n firstName: e.target.value\n });\n }}\n />\n </label\\>\n <label\\>\n Last name:\n <input\n value\\={form.lastName}\n onChange\\={e \\=> {\n setForm({\n ...form,\n lastName: e.target.value\n });\n }}\n />\n </label\\>\n <label\\>\n Email:\n <input\n value\\={form.email}\n onChange\\={e \\=> {\n setForm({\n ...form,\n email: e.target.value\n });\n }}\n />\n </label\\>\n <p\\>\n {form.firstName}{' '}\n {form.lastName}{' '}\n ({form.email})\n </p\\>\n </\\>\n );\n}\n\n* * *\n\n### Avoiding recreating the initial state[](https://react.dev/reference/react/useState#avoiding-recreating-the-initial-state \"Link for Avoiding recreating the initial state \")\n\nReact saves the initial state once and ignores it on the next renders.\n\n```\nfunction TodoList() {const [todos, setTodos] = useState(createInitialTodos());// ...\n```\n\nAlthough the result of `createInitialTodos()` is only used for the initial render, you’re still calling this function on every render. This can be wasteful if it’s creating large arrays or performing expensive calculations.\n\nTo solve this, you may **pass it as an _initializer_ function** to `useState` instead:\n\n```\nfunction TodoList() {const [todos, setTodos] = useState(createInitialTodos);// ...\n```\n\nNotice that you’re passing `createInitialTodos`, which is the _function itself_, and not `createInitialTodos()`, which is the result of calling it. If you pass a function to `useState`, React will only call it during initialization.\n\nReact may [call your initializers twice](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice) in development to verify that they are [pure.](https://react.dev/learn/keeping-components-pure)\n\n#### The difference between passing an initializer and passing the initial state directly[](https://react.dev/reference/react/useState#examples-initializer \"Link for The difference between passing an initializer and passing the initial state directly\")\n\n#### Passing the initializer function[](https://react.dev/reference/react/useState#passing-the-initializer-function \"Link for this heading\")\n\nThis example passes the initializer function, so the `createInitialTodos` function only runs during initialization. It does not run when component re-renders, such as when you type into the input.\n\nimport { useState } from 'react';\n\nfunction createInitialTodos() {\n const initialTodos = \\[\\];\n for (let i = 0; i < 50; i++) {\n initialTodos.push({\n id: i,\n text: 'Item ' + (i + 1)\n });\n }\n return initialTodos;\n}\n\nexport default function TodoList() {\n const \\[todos, setTodos\\] = useState(createInitialTodos);\n const \\[text, setText\\] = useState('');\n\n return (\n <\\>\n <input\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n <button onClick\\={() \\=> {\n setText('');\n setTodos(\\[{\n id: todos.length,\n text: text\n }, ...todos\\]);\n }}\\>Add</button\\>\n <ul\\>\n {todos.map(item \\=> (\n <li key\\={item.id}\\>\n {item.text}\n </li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\n* * *\n\n### Resetting state with a key[](https://react.dev/reference/react/useState#resetting-state-with-a-key \"Link for Resetting state with a key \")\n\nYou’ll often encounter the `key` attribute when [rendering lists.](https://react.dev/learn/rendering-lists) However, it also serves another purpose.\n\nYou can **reset a component’s state by passing a different `key` to a component.** In this example, the Reset button changes the `version` state variable, which we pass as a `key` to the `Form`. When the `key` changes, React re-creates the `Form` component (and all of its children) from scratch, so its state gets reset.\n\nRead [preserving and resetting state](https://react.dev/learn/preserving-and-resetting-state) to learn more.\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[version, setVersion\\] = useState(0);\n\n function handleReset() {\n setVersion(version + 1);\n }\n\n return (\n <\\>\n <button onClick\\={handleReset}\\>Reset</button\\>\n <Form key\\={version} />\n </\\>\n );\n}\n\nfunction Form() {\n const \\[name, setName\\] = useState('Taylor');\n\n return (\n <\\>\n <input\n value\\={name}\n onChange\\={e \\=> setName(e.target.value)}\n />\n <p\\>Hello, {name}.</p\\>\n </\\>\n );\n}\n\n* * *\n\n### Storing information from previous renders[](https://react.dev/reference/react/useState#storing-information-from-previous-renders \"Link for Storing information from previous renders \")\n\nUsually, you will update state in event handlers. However, in rare cases you might want to adjust state in response to rendering — for example, you might want to change a state variable when a prop changes.\n\nIn most cases, you don’t need this:\n\n* **If the value you need can be computed entirely from the current props or other state, [remove that redundant state altogether.](https://react.dev/learn/choosing-the-state-structure#avoid-redundant-state)** If you’re worried about recomputing too often, the [`useMemo` Hook](https://react.dev/reference/react/useMemo) can help.\n* If you want to reset the entire component tree’s state, [pass a different `key` to your component.](https://react.dev/reference/react/useState#resetting-state-with-a-key)\n* If you can, update all the relevant state in the event handlers.\n\nIn the rare case that none of these apply, there is a pattern you can use to update state based on the values that have been rendered so far, by calling a `set` function while your component is rendering.\n\nHere’s an example. This `CountLabel` component displays the `count` prop passed to it:\n\n```\nexport default function CountLabel({ count }) {return <h1>{count}</h1>}\n```\n\nSay you want to show whether the counter has _increased or decreased_ since the last change. The `count` prop doesn’t tell you this — you need to keep track of its previous value. Add the `prevCount` state variable to track it. Add another state variable called `trend` to hold whether the count has increased or decreased. Compare `prevCount` with `count`, and if they’re not equal, update both `prevCount` and `trend`. Now you can show both the current count prop and _how it has changed since the last render_.\n\nimport { useState } from 'react';\n\nexport default function CountLabel({ count }) {\n const \\[prevCount, setPrevCount\\] = useState(count);\n const \\[trend, setTrend\\] = useState(null);\n if (prevCount !== count) {\n setPrevCount(count);\n setTrend(count > prevCount ? 'increasing' : 'decreasing');\n }\n return (\n <\\>\n <h1\\>{count}</h1\\>\n {trend && <p\\>The count is {trend}</p\\>}\n </\\>\n );\n}\n\nNote that if you call a `set` function while rendering, it must be inside a condition like `prevCount !== count`, and there must be a call like `setPrevCount(count)` inside of the condition. Otherwise, your component would re-render in a loop until it crashes. Also, you can only update the state of the _currently rendering_ component like this. Calling the `set` function of _another_ component during rendering is an error. Finally, your `set` call should still [update state without mutation](https://react.dev/reference/react/useState#updating-objects-and-arrays-in-state) — this doesn’t mean you can break other rules of [pure functions.](https://react.dev/learn/keeping-components-pure)\n\nThis pattern can be hard to understand and is usually best avoided. However, it’s better than updating state in an effect. When you call the `set` function during render, React will re-render that component immediately after your component exits with a `return` statement, and before rendering the children. This way, children don’t need to render twice. The rest of your component function will still execute (and the result will be thrown away). If your condition is below all the Hook calls, you may add an early `return;` to restart rendering earlier.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useState#troubleshooting \"Link for Troubleshooting \")\n---------------------------------------------------------------------------------------------------------\n\n### I’ve updated the state, but logging gives me the old value[](https://react.dev/reference/react/useState#ive-updated-the-state-but-logging-gives-me-the-old-value \"Link for I’ve updated the state, but logging gives me the old value \")\n\nCalling the `set` function **does not change state in the running code**:\n\n```\nfunction handleClick() {console.log(count); // 0setCount(count + 1); // Request a re-render with 1console.log(count); // Still 0!setTimeout(() => {console.log(count); // Also 0!}, 5000);}\n```\n\nThis is because [states behaves like a snapshot.](https://react.dev/learn/state-as-a-snapshot) Updating state requests another render with the new state value, but does not affect the `count` JavaScript variable in your already-running event handler.\n\nIf you need to use the next state, you can save it in a variable before passing it to the `set` function:\n\n```\nconst nextCount = count + 1;setCount(nextCount);console.log(count); // 0console.log(nextCount); // 1\n```\n\n* * *\n\n### I’ve updated the state, but the screen doesn’t update[](https://react.dev/reference/react/useState#ive-updated-the-state-but-the-screen-doesnt-update \"Link for I’ve updated the state, but the screen doesn’t update \")\n\nReact will **ignore your update if the next state is equal to the previous state,** as determined by an [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. This usually happens when you change an object or an array in state directly:\n\n```\nobj.x = 10; // 🚩 Wrong: mutating existing objectsetObj(obj); // 🚩 Doesn't do anything\n```\n\nYou mutated an existing `obj` object and passed it back to `setObj`, so React ignored the update. To fix this, you need to ensure that you’re always [_replacing_ objects and arrays in state instead of _mutating_ them](https://react.dev/reference/react/useState#updating-objects-and-arrays-in-state):\n\n```\n// ✅ Correct: creating a new objectsetObj({...obj,x: 10});\n```\n\n* * *\n\n### I’m getting an error: “Too many re-renders”[](https://react.dev/reference/react/useState#im-getting-an-error-too-many-re-renders \"Link for I’m getting an error: “Too many re-renders” \")\n\nYou might get an error that says: `Too many re-renders. React limits the number of renders to prevent an infinite loop.` Typically, this means that you’re unconditionally setting state _during render_, so your component enters a loop: render, set state (which causes a render), render, set state (which causes a render), and so on. Very often, this is caused by a mistake in specifying an event handler:\n\n```\n// 🚩 Wrong: calls the handler during renderreturn <button onClick={handleClick()}>Click me</button>// ✅ Correct: passes down the event handlerreturn <button onClick={handleClick}>Click me</button>// ✅ Correct: passes down an inline functionreturn <button onClick={(e) => handleClick(e)}>Click me</button>\n```\n\nIf you can’t find the cause of this error, click on the arrow next to the error in the console and look through the JavaScript stack to find the specific `set` function call responsible for the error.\n\n* * *\n\n### My initializer or updater function runs twice[](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice \"Link for My initializer or updater function runs twice \")\n\nIn [Strict Mode](https://react.dev/reference/react/StrictMode), React will call some of your functions twice instead of once:\n\n```\nfunction TodoList() {// This component function will run twice for every render.const [todos, setTodos] = useState(() => {// This initializer function will run twice during initialization.return createTodos();});function handleClick() {setTodos(prevTodos => {// This updater function will run twice for every click.return [...prevTodos, createTodo()];});}// ...\n```\n\nThis is expected and shouldn’t break your code.\n\nThis **development-only** behavior helps you [keep components pure.](https://react.dev/learn/keeping-components-pure) React uses the result of one of the calls, and ignores the result of the other call. As long as your component, initializer, and updater functions are pure, this shouldn’t affect your logic. However, if they are accidentally impure, this helps you notice the mistakes.\n\nFor example, this impure updater function mutates an array in state:\n\n```\nsetTodos(prevTodos => {// 🚩 Mistake: mutating stateprevTodos.push(createTodo());});\n```\n\nBecause React calls your updater function twice, you’ll see the todo was added twice, so you’ll know that there is a mistake. In this example, you can fix the mistake by [replacing the array instead of mutating it](https://react.dev/reference/react/useState#updating-objects-and-arrays-in-state):\n\n```\nsetTodos(prevTodos => {// ✅ Correct: replacing with new statereturn [...prevTodos, createTodo()];});\n```\n\nNow that this updater function is pure, calling it an extra time doesn’t make a difference in behavior. This is why React calling it twice helps you find mistakes. **Only component, initializer, and updater functions need to be pure.** Event handlers don’t need to be pure, so React will never call your event handlers twice.\n\nRead [keeping components pure](https://react.dev/learn/keeping-components-pure) to learn more.\n\n* * *\n\n### I’m trying to set state to a function, but it gets called instead[](https://react.dev/reference/react/useState#im-trying-to-set-state-to-a-function-but-it-gets-called-instead \"Link for I’m trying to set state to a function, but it gets called instead \")\n\nYou can’t put a function into state like this:\n\n```\nconst [fn, setFn] = useState(someFunction);function handleClick() {setFn(someOtherFunction);}\n```\n\nBecause you’re passing a function, React assumes that `someFunction` is an [initializer function](https://react.dev/reference/react/useState#avoiding-recreating-the-initial-state), and that `someOtherFunction` is an [updater function](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state), so it tries to call them and store the result. To actually _store_ a function, you have to put `() =>` before them in both cases. Then React will store the functions you pass.\n\n```\nconst [fn, setFn] = useState(() => someFunction);function handleClick() {setFn(() => someOtherFunction);}\n```\n",
"filename": "useState.md",
"package": "react"
} |
{
"content": "Title: useSyncExternalStore – React\n\nURL Source: https://react.dev/reference/react/useSyncExternalStore\n\nMarkdown Content:\n`useSyncExternalStore` is a React Hook that lets you subscribe to an external store.\n\n```\nconst snapshot = useSyncExternalStore(subscribe, getSnapshot, getServerSnapshot?)\n```\n\n* [Reference](https://react.dev/reference/react/useSyncExternalStore#reference)\n * [`useSyncExternalStore(subscribe, getSnapshot, getServerSnapshot?)`](https://react.dev/reference/react/useSyncExternalStore#usesyncexternalstore)\n* [Usage](https://react.dev/reference/react/useSyncExternalStore#usage)\n * [Subscribing to an external store](https://react.dev/reference/react/useSyncExternalStore#subscribing-to-an-external-store)\n * [Subscribing to a browser API](https://react.dev/reference/react/useSyncExternalStore#subscribing-to-a-browser-api)\n * [Extracting the logic to a custom Hook](https://react.dev/reference/react/useSyncExternalStore#extracting-the-logic-to-a-custom-hook)\n * [Adding support for server rendering](https://react.dev/reference/react/useSyncExternalStore#adding-support-for-server-rendering)\n* [Troubleshooting](https://react.dev/reference/react/useSyncExternalStore#troubleshooting)\n * [I’m getting an error: “The result of `getSnapshot` should be cached”](https://react.dev/reference/react/useSyncExternalStore#im-getting-an-error-the-result-of-getsnapshot-should-be-cached)\n * [My `subscribe` function gets called after every re-render](https://react.dev/reference/react/useSyncExternalStore#my-subscribe-function-gets-called-after-every-re-render)\n\n* * *\n\nReference[](https://react.dev/reference/react/useSyncExternalStore#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------------\n\n### `useSyncExternalStore(subscribe, getSnapshot, getServerSnapshot?)`[](https://react.dev/reference/react/useSyncExternalStore#usesyncexternalstore \"Link for this heading\")\n\nCall `useSyncExternalStore` at the top level of your component to read a value from an external data store.\n\n```\nimport { useSyncExternalStore } from 'react';import { todosStore } from './todoStore.js';function TodosApp() {const todos = useSyncExternalStore(todosStore.subscribe, todosStore.getSnapshot);// ...}\n```\n\nIt returns the snapshot of the data in the store. You need to pass two functions as arguments:\n\n1. The `subscribe` function should subscribe to the store and return a function that unsubscribes.\n2. The `getSnapshot` function should read a snapshot of the data from the store.\n\n[See more examples below.](https://react.dev/reference/react/useSyncExternalStore#usage)\n\n#### Parameters[](https://react.dev/reference/react/useSyncExternalStore#parameters \"Link for Parameters \")\n\n* `subscribe`: A function that takes a single `callback` argument and subscribes it to the store. When the store changes, it should invoke the provided `callback`. This will cause the component to re-render. The `subscribe` function should return a function that cleans up the subscription.\n \n* `getSnapshot`: A function that returns a snapshot of the data in the store that’s needed by the component. While the store has not changed, repeated calls to `getSnapshot` must return the same value. If the store changes and the returned value is different (as compared by [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)), React re-renders the component.\n \n* **optional** `getServerSnapshot`: A function that returns the initial snapshot of the data in the store. It will be used only during server rendering and during hydration of server-rendered content on the client. The server snapshot must be the same between the client and the server, and is usually serialized and passed from the server to the client. If you omit this argument, rendering the component on the server will throw an error.\n \n\n#### Returns[](https://react.dev/reference/react/useSyncExternalStore#returns \"Link for Returns \")\n\nThe current snapshot of the store which you can use in your rendering logic.\n\n#### Caveats[](https://react.dev/reference/react/useSyncExternalStore#caveats \"Link for Caveats \")\n\n* The store snapshot returned by `getSnapshot` must be immutable. If the underlying store has mutable data, return a new immutable snapshot if the data has changed. Otherwise, return a cached last snapshot.\n \n* If a different `subscribe` function is passed during a re-render, React will re-subscribe to the store using the newly passed `subscribe` function. You can prevent this by declaring `subscribe` outside the component.\n \n* If the store is mutated during a [non-blocking Transition update](https://react.dev/reference/react/useTransition), React will fall back to performing that update as blocking. Specifically, for every Transition update, React will call `getSnapshot` a second time just before applying changes to the DOM. If it returns a different value than when it was called originally, React will restart the update from scratch, this time applying it as a blocking update, to ensure that every component on screen is reflecting the same version of the store.\n \n* It’s not recommended to _suspend_ a render based on a store value returned by `useSyncExternalStore`. The reason is that mutations to the external store cannot be marked as [non-blocking Transition updates](https://react.dev/reference/react/useTransition), so they will trigger the nearest [`Suspense` fallback](https://react.dev/reference/react/Suspense), replacing already-rendered content on screen with a loading spinner, which typically makes a poor UX.\n \n For example, the following are discouraged:\n \n ```\n const LazyProductDetailPage = lazy(() => import('./ProductDetailPage.js'));function ShoppingApp() {const selectedProductId = useSyncExternalStore(...);// ❌ Calling `use` with a Promise dependent on `selectedProductId`const data = use(fetchItem(selectedProductId))// ❌ Conditionally rendering a lazy component based on `selectedProductId`return selectedProductId != null ? <LazyProductDetailPage /> : <FeaturedProducts />;}\n ```\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useSyncExternalStore#usage \"Link for Usage \")\n---------------------------------------------------------------------------------------\n\n### Subscribing to an external store[](https://react.dev/reference/react/useSyncExternalStore#subscribing-to-an-external-store \"Link for Subscribing to an external store \")\n\nMost of your React components will only read data from their [props,](https://react.dev/learn/passing-props-to-a-component) [state,](https://react.dev/reference/react/useState) and [context.](https://react.dev/reference/react/useContext) However, sometimes a component needs to read some data from some store outside of React that changes over time. This includes:\n\n* Third-party state management libraries that hold state outside of React.\n* Browser APIs that expose a mutable value and events to subscribe to its changes.\n\nCall `useSyncExternalStore` at the top level of your component to read a value from an external data store.\n\n```\nimport { useSyncExternalStore } from 'react';import { todosStore } from './todoStore.js';function TodosApp() {const todos = useSyncExternalStore(todosStore.subscribe, todosStore.getSnapshot);// ...}\n```\n\nIt returns the snapshot of the data in the store. You need to pass two functions as arguments:\n\n1. The `subscribe` function should subscribe to the store and return a function that unsubscribes.\n2. The `getSnapshot` function should read a snapshot of the data from the store.\n\nReact will use these functions to keep your component subscribed to the store and re-render it on changes.\n\nFor example, in the sandbox below, `todosStore` is implemented as an external store that stores data outside of React. The `TodosApp` component connects to that external store with the `useSyncExternalStore` Hook.\n\nimport { useSyncExternalStore } from 'react';\nimport { todosStore } from './todoStore.js';\n\nexport default function TodosApp() {\n const todos = useSyncExternalStore(todosStore.subscribe, todosStore.getSnapshot);\n return (\n <\\>\n <button onClick\\={() \\=> todosStore.addTodo()}\\>Add todo</button\\>\n <hr />\n <ul\\>\n {todos.map(todo \\=> (\n <li key\\={todo.id}\\>{todo.text}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\n### Note\n\nWhen possible, we recommend using built-in React state with [`useState`](https://react.dev/reference/react/useState) and [`useReducer`](https://react.dev/reference/react/useReducer) instead. The `useSyncExternalStore` API is mostly useful if you need to integrate with existing non-React code.\n\n* * *\n\n### Subscribing to a browser API[](https://react.dev/reference/react/useSyncExternalStore#subscribing-to-a-browser-api \"Link for Subscribing to a browser API \")\n\nAnother reason to add `useSyncExternalStore` is when you want to subscribe to some value exposed by the browser that changes over time. For example, suppose that you want your component to display whether the network connection is active. The browser exposes this information via a property called [`navigator.onLine`.](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/onLine)\n\nThis value can change without React’s knowledge, so you should read it with `useSyncExternalStore`.\n\n```\nimport { useSyncExternalStore } from 'react';function ChatIndicator() {const isOnline = useSyncExternalStore(subscribe, getSnapshot);// ...}\n```\n\nTo implement the `getSnapshot` function, read the current value from the browser API:\n\n```\nfunction getSnapshot() {return navigator.onLine;}\n```\n\nNext, you need to implement the `subscribe` function. For example, when `navigator.onLine` changes, the browser fires the [`online`](https://developer.mozilla.org/en-US/docs/Web/API/Window/online_event) and [`offline`](https://developer.mozilla.org/en-US/docs/Web/API/Window/offline_event) events on the `window` object. You need to subscribe the `callback` argument to the corresponding events, and then return a function that cleans up the subscriptions:\n\n```\nfunction subscribe(callback) {window.addEventListener('online', callback);window.addEventListener('offline', callback);return () => {window.removeEventListener('online', callback);window.removeEventListener('offline', callback);};}\n```\n\nNow React knows how to read the value from the external `navigator.onLine` API and how to subscribe to its changes. Disconnect your device from the network and notice that the component re-renders in response:\n\nimport { useSyncExternalStore } from 'react';\n\nexport default function ChatIndicator() {\n const isOnline = useSyncExternalStore(subscribe, getSnapshot);\n return <h1\\>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1\\>;\n}\n\nfunction getSnapshot() {\n return navigator.onLine;\n}\n\nfunction subscribe(callback) {\n window.addEventListener('online', callback);\n window.addEventListener('offline', callback);\n return () \\=> {\n window.removeEventListener('online', callback);\n window.removeEventListener('offline', callback);\n };\n}\n\n* * *\n\nUsually you won’t write `useSyncExternalStore` directly in your components. Instead, you’ll typically call it from your own custom Hook. This lets you use the same external store from different components.\n\nFor example, this custom `useOnlineStatus` Hook tracks whether the network is online:\n\n```\nimport { useSyncExternalStore } from 'react';export function useOnlineStatus() {const isOnline = useSyncExternalStore(subscribe, getSnapshot);return isOnline;}function getSnapshot() {// ...}function subscribe(callback) {// ...}\n```\n\nNow different components can call `useOnlineStatus` without repeating the underlying implementation:\n\nimport { useOnlineStatus } from './useOnlineStatus.js';\n\nfunction StatusBar() {\n const isOnline = useOnlineStatus();\n return <h1\\>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1\\>;\n}\n\nfunction SaveButton() {\n const isOnline = useOnlineStatus();\n\n function handleSaveClick() {\n console.log('✅ Progress saved');\n }\n\n return (\n <button disabled\\={!isOnline} onClick\\={handleSaveClick}\\>\n {isOnline ? 'Save progress' : 'Reconnecting...'}\n </button\\>\n );\n}\n\nexport default function App() {\n return (\n <\\>\n <SaveButton />\n <StatusBar />\n </\\>\n );\n}\n\n* * *\n\n### Adding support for server rendering[](https://react.dev/reference/react/useSyncExternalStore#adding-support-for-server-rendering \"Link for Adding support for server rendering \")\n\nIf your React app uses [server rendering,](https://react.dev/reference/react-dom/server) your React components will also run outside the browser environment to generate the initial HTML. This creates a few challenges when connecting to an external store:\n\n* If you’re connecting to a browser-only API, it won’t work because it does not exist on the server.\n* If you’re connecting to a third-party data store, you’ll need its data to match between the server and client.\n\nTo solve these issues, pass a `getServerSnapshot` function as the third argument to `useSyncExternalStore`:\n\n```\nimport { useSyncExternalStore } from 'react';export function useOnlineStatus() {const isOnline = useSyncExternalStore(subscribe, getSnapshot, getServerSnapshot);return isOnline;}function getSnapshot() {return navigator.onLine;}function getServerSnapshot() {return true; // Always show \"Online\" for server-generated HTML}function subscribe(callback) {// ...}\n```\n\nThe `getServerSnapshot` function is similar to `getSnapshot`, but it runs only in two situations:\n\n* It runs on the server when generating the HTML.\n* It runs on the client during [hydration](https://react.dev/reference/react-dom/client/hydrateRoot), i.e. when React takes the server HTML and makes it interactive.\n\nThis lets you provide the initial snapshot value which will be used before the app becomes interactive. If there is no meaningful initial value for the server rendering, omit this argument to [force rendering on the client.](https://react.dev/reference/react/Suspense#providing-a-fallback-for-server-errors-and-client-only-content)\n\n### Note\n\nMake sure that `getServerSnapshot` returns the same exact data on the initial client render as it returned on the server. For example, if `getServerSnapshot` returned some prepopulated store content on the server, you need to transfer this content to the client. One way to do this is to emit a `<script>` tag during server rendering that sets a global like `window.MY_STORE_DATA`, and read from that global on the client in `getServerSnapshot`. Your external store should provide instructions on how to do that.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useSyncExternalStore#troubleshooting \"Link for Troubleshooting \")\n---------------------------------------------------------------------------------------------------------------------\n\n### I’m getting an error: “The result of `getSnapshot` should be cached”[](https://react.dev/reference/react/useSyncExternalStore#im-getting-an-error-the-result-of-getsnapshot-should-be-cached \"Link for this heading\")\n\nThis error means your `getSnapshot` function returns a new object every time it’s called, for example:\n\n```\nfunction getSnapshot() {// 🔴 Do not return always different objects from getSnapshotreturn {todos: myStore.todos};}\n```\n\nReact will re-render the component if `getSnapshot` return value is different from the last time. This is why, if you always return a different value, you will enter an infinite loop and get this error.\n\nYour `getSnapshot` object should only return a different object if something has actually changed. If your store contains immutable data, you can return that data directly:\n\n```\nfunction getSnapshot() {// ✅ You can return immutable datareturn myStore.todos;}\n```\n\nIf your store data is mutable, your `getSnapshot` function should return an immutable snapshot of it. This means it _does_ need to create new objects, but it shouldn’t do this for every single call. Instead, it should store the last calculated snapshot, and return the same snapshot as the last time if the data in the store has not changed. How you determine whether mutable data has changed depends on your mutable store.\n\n* * *\n\n### My `subscribe` function gets called after every re-render[](https://react.dev/reference/react/useSyncExternalStore#my-subscribe-function-gets-called-after-every-re-render \"Link for this heading\")\n\nThis `subscribe` function is defined _inside_ a component so it is different on every re-render:\n\n```\nfunction ChatIndicator() {const isOnline = useSyncExternalStore(subscribe, getSnapshot);// 🚩 Always a different function, so React will resubscribe on every re-renderfunction subscribe() {// ...}// ...}\n```\n\nReact will resubscribe to your store if you pass a different `subscribe` function between re-renders. If this causes performance issues and you’d like to avoid resubscribing, move the `subscribe` function outside:\n\n```\nfunction ChatIndicator() {const isOnline = useSyncExternalStore(subscribe, getSnapshot);// ...}// ✅ Always the same function, so React won't need to resubscribefunction subscribe() {// ...}\n```\n\nAlternatively, wrap `subscribe` into [`useCallback`](https://react.dev/reference/react/useCallback) to only resubscribe when some argument changes:\n\n```\nfunction ChatIndicator({ userId }) {const isOnline = useSyncExternalStore(subscribe, getSnapshot);// ✅ Same function as long as userId doesn't changeconst subscribe = useCallback(() => {// ...}, [userId]);// ...}\n```\n",
"filename": "useSyncExternalStore.md",
"package": "react"
} |
{
"content": "Title: useTransition – React\n\nURL Source: https://react.dev/reference/react/useTransition\n\nMarkdown Content:\n`useTransition` is a React Hook that lets you update the state without blocking the UI.\n\n```\nconst [isPending, startTransition] = useTransition()\n```\n\n* [Reference](https://react.dev/reference/react/useTransition#reference)\n * [`useTransition()`](https://react.dev/reference/react/useTransition#usetransition)\n * [`startTransition` function](https://react.dev/reference/react/useTransition#starttransition)\n* [Usage](https://react.dev/reference/react/useTransition#usage)\n * [Marking a state update as a non-blocking Transition](https://react.dev/reference/react/useTransition#marking-a-state-update-as-a-non-blocking-transition)\n * [Updating the parent component in a Transition](https://react.dev/reference/react/useTransition#updating-the-parent-component-in-a-transition)\n * [Displaying a pending visual state during the Transition](https://react.dev/reference/react/useTransition#displaying-a-pending-visual-state-during-the-transition)\n * [Preventing unwanted loading indicators](https://react.dev/reference/react/useTransition#preventing-unwanted-loading-indicators)\n * [Building a Suspense-enabled router](https://react.dev/reference/react/useTransition#building-a-suspense-enabled-router)\n * [Displaying an error to users with an error boundary](https://react.dev/reference/react/useTransition#displaying-an-error-to-users-with-error-boundary)\n* [Troubleshooting](https://react.dev/reference/react/useTransition#troubleshooting)\n * [Updating an input in a Transition doesn’t work](https://react.dev/reference/react/useTransition#updating-an-input-in-a-transition-doesnt-work)\n * [React doesn’t treat my state update as a Transition](https://react.dev/reference/react/useTransition#react-doesnt-treat-my-state-update-as-a-transition)\n * [I want to call `useTransition` from outside a component](https://react.dev/reference/react/useTransition#i-want-to-call-usetransition-from-outside-a-component)\n * [The function I pass to `startTransition` executes immediately](https://react.dev/reference/react/useTransition#the-function-i-pass-to-starttransition-executes-immediately)\n\n* * *\n\nReference[](https://react.dev/reference/react/useTransition#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `useTransition()`[](https://react.dev/reference/react/useTransition#usetransition \"Link for this heading\")\n\nCall `useTransition` at the top level of your component to mark some state updates as Transitions.\n\n```\nimport { useTransition } from 'react';function TabContainer() {const [isPending, startTransition] = useTransition();// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react/useTransition#usage)\n\n#### Parameters[](https://react.dev/reference/react/useTransition#parameters \"Link for Parameters \")\n\n`useTransition` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react/useTransition#returns \"Link for Returns \")\n\n`useTransition` returns an array with exactly two items:\n\n1. The `isPending` flag that tells you whether there is a pending Transition.\n2. The [`startTransition` function](https://react.dev/reference/react/useTransition#starttransition) that lets you mark a state update as a Transition.\n\n* * *\n\n### `startTransition` function[](https://react.dev/reference/react/useTransition#starttransition \"Link for this heading\")\n\nThe `startTransition` function returned by `useTransition` lets you mark a state update as a Transition.\n\n```\nfunction TabContainer() {const [isPending, startTransition] = useTransition();const [tab, setTab] = useState('about');function selectTab(nextTab) {startTransition(() => {setTab(nextTab);});}// ...}\n```\n\n#### Parameters[](https://react.dev/reference/react/useTransition#starttransition-parameters \"Link for Parameters \")\n\n* `scope`: A function that updates some state by calling one or more [`set` functions.](https://react.dev/reference/react/useState#setstate) React immediately calls `scope` with no parameters and marks all state updates scheduled synchronously during the `scope` function call as Transitions. They will be [non-blocking](https://react.dev/reference/react/useTransition#marking-a-state-update-as-a-non-blocking-transition) and [will not display unwanted loading indicators.](https://react.dev/reference/react/useTransition#preventing-unwanted-loading-indicators)\n\n#### Returns[](https://react.dev/reference/react/useTransition#starttransition-returns \"Link for Returns \")\n\n`startTransition` does not return anything.\n\n#### Caveats[](https://react.dev/reference/react/useTransition#starttransition-caveats \"Link for Caveats \")\n\n* `useTransition` is a Hook, so it can only be called inside components or custom Hooks. If you need to start a Transition somewhere else (for example, from a data library), call the standalone [`startTransition`](https://react.dev/reference/react/startTransition) instead.\n \n* You can wrap an update into a Transition only if you have access to the `set` function of that state. If you want to start a Transition in response to some prop or a custom Hook value, try [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue) instead.\n \n* The function you pass to `startTransition` must be synchronous. React immediately executes this function, marking all state updates that happen while it executes as Transitions. If you try to perform more state updates later (for example, in a timeout), they won’t be marked as Transitions.\n \n* A state update marked as a Transition will be interrupted by other state updates. For example, if you update a chart component inside a Transition, but then start typing into an input while the chart is in the middle of a re-render, React will restart the rendering work on the chart component after handling the input update.\n \n* Transition updates can’t be used to control text inputs.\n \n* If there are multiple ongoing Transitions, React currently batches them together. This is a limitation that will likely be removed in a future release.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/useTransition#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Marking a state update as a non-blocking Transition[](https://react.dev/reference/react/useTransition#marking-a-state-update-as-a-non-blocking-transition \"Link for Marking a state update as a non-blocking Transition \")\n\nCall `useTransition` at the top level of your component to mark state updates as non-blocking _Transitions_.\n\n```\nimport { useState, useTransition } from 'react';function TabContainer() {const [isPending, startTransition] = useTransition();// ...}\n```\n\n`useTransition` returns an array with exactly two items:\n\n1. The `isPending` flag that tells you whether there is a pending Transition.\n2. The `startTransition` function that lets you mark a state update as a Transition.\n\nYou can then mark a state update as a Transition like this:\n\n```\nfunction TabContainer() {const [isPending, startTransition] = useTransition();const [tab, setTab] = useState('about');function selectTab(nextTab) {startTransition(() => {setTab(nextTab);});}// ...}\n```\n\nTransitions let you keep the user interface updates responsive even on slow devices.\n\nWith a Transition, your UI stays responsive in the middle of a re-render. For example, if the user clicks a tab but then change their mind and click another tab, they can do that without waiting for the first re-render to finish.\n\n#### The difference between useTransition and regular state updates[](https://react.dev/reference/react/useTransition#examples \"Link for The difference between useTransition and regular state updates\")\n\n#### Updating the current tab in a Transition[](https://react.dev/reference/react/useTransition#updating-the-current-tab-in-a-transition \"Link for this heading\")\n\nIn this example, the “Posts” tab is **artificially slowed down** so that it takes at least a second to render.\n\nClick “Posts” and then immediately click “Contact”. Notice that this interrupts the slow render of “Posts”. The “Contact” tab shows immediately. Because this state update is marked as a Transition, a slow re-render did not freeze the user interface.\n\nimport { useState, useTransition } from 'react';\nimport TabButton from './TabButton.js';\nimport AboutTab from './AboutTab.js';\nimport PostsTab from './PostsTab.js';\nimport ContactTab from './ContactTab.js';\n\nexport default function TabContainer() {\n const \\[isPending, startTransition\\] = useTransition();\n const \\[tab, setTab\\] = useState('about');\n\n function selectTab(nextTab) {\n startTransition(() \\=> {\n setTab(nextTab);\n });\n }\n\n return (\n <\\>\n <TabButton\n isActive\\={tab === 'about'}\n onClick\\={() \\=> selectTab('about')}\n \\>\n About\n </TabButton\\>\n <TabButton\n isActive\\={tab === 'posts'}\n onClick\\={() \\=> selectTab('posts')}\n \\>\n Posts (slow)\n </TabButton\\>\n <TabButton\n isActive\\={tab === 'contact'}\n onClick\\={() \\=> selectTab('contact')}\n \\>\n Contact\n </TabButton\\>\n <hr />\n {tab === 'about' && <AboutTab />}\n {tab === 'posts' && <PostsTab />}\n {tab === 'contact' && <ContactTab />}\n </\\>\n );\n}\n\n* * *\n\n### Updating the parent component in a Transition[](https://react.dev/reference/react/useTransition#updating-the-parent-component-in-a-transition \"Link for Updating the parent component in a Transition \")\n\nYou can update a parent component’s state from the `useTransition` call, too. For example, this `TabButton` component wraps its `onClick` logic in a Transition:\n\n```\nexport default function TabButton({ children, isActive, onClick }) {const [isPending, startTransition] = useTransition();if (isActive) {return <b>{children}</b>}return (<button onClick={() => {startTransition(() => {onClick();});}}>{children}</button>);}\n```\n\nBecause the parent component updates its state inside the `onClick` event handler, that state update gets marked as a Transition. This is why, like in the earlier example, you can click on “Posts” and then immediately click “Contact”. Updating the selected tab is marked as a Transition, so it does not block user interactions.\n\nimport { useTransition } from 'react';\n\nexport default function TabButton({ children, isActive, onClick }) {\n const \\[isPending, startTransition\\] = useTransition();\n if (isActive) {\n return <b\\>{children}</b\\>\n }\n return (\n <button onClick\\={() \\=> {\n startTransition(() \\=> {\n onClick();\n });\n }}\\>\n {children}\n </button\\>\n );\n}\n\n* * *\n\n### Displaying a pending visual state during the Transition[](https://react.dev/reference/react/useTransition#displaying-a-pending-visual-state-during-the-transition \"Link for Displaying a pending visual state during the Transition \")\n\nYou can use the `isPending` boolean value returned by `useTransition` to indicate to the user that a Transition is in progress. For example, the tab button can have a special “pending” visual state:\n\n```\nfunction TabButton({ children, isActive, onClick }) {const [isPending, startTransition] = useTransition();// ...if (isPending) {return <b className=\"pending\">{children}</b>;}// ...\n```\n\nNotice how clicking “Posts” now feels more responsive because the tab button itself updates right away:\n\nimport { useTransition } from 'react';\n\nexport default function TabButton({ children, isActive, onClick }) {\n const \\[isPending, startTransition\\] = useTransition();\n if (isActive) {\n return <b\\>{children}</b\\>\n }\n if (isPending) {\n return <b className\\=\"pending\"\\>{children}</b\\>;\n }\n return (\n <button onClick\\={() \\=> {\n startTransition(() \\=> {\n onClick();\n });\n }}\\>\n {children}\n </button\\>\n );\n}\n\n* * *\n\n### Preventing unwanted loading indicators[](https://react.dev/reference/react/useTransition#preventing-unwanted-loading-indicators \"Link for Preventing unwanted loading indicators \")\n\nIn this example, the `PostsTab` component fetches some data using a [Suspense-enabled](https://react.dev/reference/react/Suspense) data source. When you click the “Posts” tab, the `PostsTab` component _suspends_, causing the closest loading fallback to appear:\n\nimport { Suspense, useState } from 'react';\nimport TabButton from './TabButton.js';\nimport AboutTab from './AboutTab.js';\nimport PostsTab from './PostsTab.js';\nimport ContactTab from './ContactTab.js';\n\nexport default function TabContainer() {\n const \\[tab, setTab\\] = useState('about');\n return (\n <Suspense fallback\\={<h1\\>🌀 Loading...</h1\\>}\\>\n <TabButton\n isActive\\={tab === 'about'}\n onClick\\={() \\=> setTab('about')}\n \\>\n About\n </TabButton\\>\n <TabButton\n isActive\\={tab === 'posts'}\n onClick\\={() \\=> setTab('posts')}\n \\>\n Posts\n </TabButton\\>\n <TabButton\n isActive\\={tab === 'contact'}\n onClick\\={() \\=> setTab('contact')}\n \\>\n Contact\n </TabButton\\>\n <hr />\n {tab === 'about' && <AboutTab />}\n {tab === 'posts' && <PostsTab />}\n {tab === 'contact' && <ContactTab />}\n </Suspense\\>\n );\n}\n\nHiding the entire tab container to show a loading indicator leads to a jarring user experience. If you add `useTransition` to `TabButton`, you can instead indicate display the pending state in the tab button instead.\n\nNotice that clicking “Posts” no longer replaces the entire tab container with a spinner:\n\nimport { useTransition } from 'react';\n\nexport default function TabButton({ children, isActive, onClick }) {\n const \\[isPending, startTransition\\] = useTransition();\n if (isActive) {\n return <b\\>{children}</b\\>\n }\n if (isPending) {\n return <b className\\=\"pending\"\\>{children}</b\\>;\n }\n return (\n <button onClick\\={() \\=> {\n startTransition(() \\=> {\n onClick();\n });\n }}\\>\n {children}\n </button\\>\n );\n}\n\n[Read more about using Transitions with Suspense.](https://react.dev/reference/react/Suspense#preventing-already-revealed-content-from-hiding)\n\n### Note\n\nTransitions will only “wait” long enough to avoid hiding _already revealed_ content (like the tab container). If the Posts tab had a [nested `<Suspense>` boundary,](https://react.dev/reference/react/Suspense#revealing-nested-content-as-it-loads) the Transition would not “wait” for it.\n\n* * *\n\n### Building a Suspense-enabled router[](https://react.dev/reference/react/useTransition#building-a-suspense-enabled-router \"Link for Building a Suspense-enabled router \")\n\nIf you’re building a React framework or a router, we recommend marking page navigations as Transitions.\n\n```\nfunction Router() {const [page, setPage] = useState('/');const [isPending, startTransition] = useTransition();function navigate(url) {startTransition(() => {setPage(url);});}// ...\n```\n\nThis is recommended for two reasons:\n\n* [Transitions are interruptible,](https://react.dev/reference/react/useTransition#marking-a-state-update-as-a-non-blocking-transition) which lets the user click away without waiting for the re-render to complete.\n* [Transitions prevent unwanted loading indicators,](https://react.dev/reference/react/useTransition#preventing-unwanted-loading-indicators) which lets the user avoid jarring jumps on navigation.\n\nHere is a tiny simplified router example using Transitions for navigations.\n\nimport { Suspense, useState, useTransition } from 'react';\nimport IndexPage from './IndexPage.js';\nimport ArtistPage from './ArtistPage.js';\nimport Layout from './Layout.js';\n\nexport default function App() {\n return (\n <Suspense fallback\\={<BigSpinner />}\\>\n <Router />\n </Suspense\\>\n );\n}\n\nfunction Router() {\n const \\[page, setPage\\] = useState('/');\n const \\[isPending, startTransition\\] = useTransition();\n\n function navigate(url) {\n startTransition(() \\=> {\n setPage(url);\n });\n }\n\n let content;\n if (page === '/') {\n content = (\n <IndexPage navigate\\={navigate} />\n );\n } else if (page === '/the-beatles') {\n content = (\n <ArtistPage\n artist\\={{\n id: 'the-beatles',\n name: 'The Beatles',\n }}\n />\n );\n }\n return (\n <Layout isPending\\={isPending}\\>\n {content}\n </Layout\\>\n );\n}\n\nfunction BigSpinner() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\n### Note\n\n[Suspense-enabled](https://react.dev/reference/react/Suspense) routers are expected to wrap the navigation updates into Transitions by default.\n\n* * *\n\n### Displaying an error to users with an error boundary[](https://react.dev/reference/react/useTransition#displaying-an-error-to-users-with-error-boundary \"Link for Displaying an error to users with an error boundary \")\n\n### Canary\n\nError Boundary for useTransition is currently only available in React’s canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\nIf a function passed to `startTransition` throws an error, you can display an error to your user with an [error boundary](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary). To use an error boundary, wrap the component where you are calling the `useTransition` in an error boundary. Once the function passed to `startTransition` errors, the fallback for the error boundary will be displayed.\n\nimport { useTransition } from \"react\";\nimport { ErrorBoundary } from \"react-error-boundary\";\n\nexport function AddCommentContainer() {\n return (\n <ErrorBoundary fallback\\={<p\\>⚠️Something went wrong</p\\>}\\>\n <AddCommentButton />\n </ErrorBoundary\\>\n );\n}\n\nfunction addComment(comment) {\n \n if (comment == null) {\n throw new Error(\"Example Error: An error thrown to trigger error boundary\");\n }\n}\n\nfunction AddCommentButton() {\n const \\[pending, startTransition\\] = useTransition();\n\n return (\n <button\n disabled\\={pending}\n onClick\\={() \\=> {\n startTransition(() \\=> {\n \n \n addComment();\n });\n }}\n \\>\n Add comment\n </button\\>\n );\n}\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/useTransition#troubleshooting \"Link for Troubleshooting \")\n--------------------------------------------------------------------------------------------------------------\n\n### Updating an input in a Transition doesn’t work[](https://react.dev/reference/react/useTransition#updating-an-input-in-a-transition-doesnt-work \"Link for Updating an input in a Transition doesn’t work \")\n\nYou can’t use a Transition for a state variable that controls an input:\n\n```\nconst [text, setText] = useState('');// ...function handleChange(e) {// ❌ Can't use Transitions for controlled input statestartTransition(() => {setText(e.target.value);});}// ...return <input value={text} onChange={handleChange} />;\n```\n\nThis is because Transitions are non-blocking, but updating an input in response to the change event should happen synchronously. If you want to run a Transition in response to typing, you have two options:\n\n1. You can declare two separate state variables: one for the input state (which always updates synchronously), and one that you will update in a Transition. This lets you control the input using the synchronous state, and pass the Transition state variable (which will “lag behind” the input) to the rest of your rendering logic.\n2. Alternatively, you can have one state variable, and add [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue) which will “lag behind” the real value. It will trigger non-blocking re-renders to “catch up” with the new value automatically.\n\n* * *\n\n### React doesn’t treat my state update as a Transition[](https://react.dev/reference/react/useTransition#react-doesnt-treat-my-state-update-as-a-transition \"Link for React doesn’t treat my state update as a Transition \")\n\nWhen you wrap a state update in a Transition, make sure that it happens _during_ the `startTransition` call:\n\n```\nstartTransition(() => {// ✅ Setting state *during* startTransition callsetPage('/about');});\n```\n\nThe function you pass to `startTransition` must be synchronous.\n\nYou can’t mark an update as a Transition like this:\n\n```\nstartTransition(() => {// ❌ Setting state *after* startTransition callsetTimeout(() => {setPage('/about');}, 1000);});\n```\n\nInstead, you could do this:\n\n```\nsetTimeout(() => {startTransition(() => {// ✅ Setting state *during* startTransition callsetPage('/about');});}, 1000);\n```\n\nSimilarly, you can’t mark an update as a Transition like this:\n\n```\nstartTransition(async () => {await someAsyncFunction();// ❌ Setting state *after* startTransition callsetPage('/about');});\n```\n\nHowever, this works instead:\n\n```\nawait someAsyncFunction();startTransition(() => {// ✅ Setting state *during* startTransition callsetPage('/about');});\n```\n\n* * *\n\n### I want to call `useTransition` from outside a component[](https://react.dev/reference/react/useTransition#i-want-to-call-usetransition-from-outside-a-component \"Link for this heading\")\n\nYou can’t call `useTransition` outside a component because it’s a Hook. In this case, use the standalone [`startTransition`](https://react.dev/reference/react/startTransition) method instead. It works the same way, but it doesn’t provide the `isPending` indicator.\n\n* * *\n\n### The function I pass to `startTransition` executes immediately[](https://react.dev/reference/react/useTransition#the-function-i-pass-to-starttransition-executes-immediately \"Link for this heading\")\n\nIf you run this code, it will print 1, 2, 3:\n\n```\nconsole.log(1);startTransition(() => {console.log(2);setPage('/about');});console.log(3);\n```\n\n**It is expected to print 1, 2, 3.** The function you pass to `startTransition` does not get delayed. Unlike with the browser `setTimeout`, it does not run the callback later. React executes your function immediately, but any state updates scheduled _while it is running_ are marked as Transitions. You can imagine that it works like this:\n\n```\n// A simplified version of how React workslet isInsideTransition = false;function startTransition(scope) {isInsideTransition = true;scope();isInsideTransition = false;}function setState() {if (isInsideTransition) {// ... schedule a Transition state update ...} else {// ... schedule an urgent state update ...}}\n```\n",
"filename": "useTransition.md",
"package": "react"
} |
{
"content": "Title: Writing Markup with JSX – React\n\nURL Source: https://react.dev/learn/writing-markup-with-jsx\n\nMarkdown Content:\n_JSX_ is a syntax extension for JavaScript that lets you write HTML-like markup inside a JavaScript file. Although there are other ways to write components, most React developers prefer the conciseness of JSX, and most codebases use it.\n\n### You will learn\n\n* Why React mixes markup with rendering logic\n* How JSX is different from HTML\n* How to display information with JSX\n\nJSX: Putting markup into JavaScript[](https://react.dev/learn/writing-markup-with-jsx#jsx-putting-markup-into-javascript \"Link for JSX: Putting markup into JavaScript \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThe Web has been built on HTML, CSS, and JavaScript. For many years, web developers kept content in HTML, design in CSS, and logic in JavaScript—often in separate files! Content was marked up inside HTML while the page’s logic lived separately in JavaScript:\n\nBut as the Web became more interactive, logic increasingly determined content. JavaScript was in charge of the HTML! This is why **in React, rendering logic and markup live together in the same place—components.**\n\n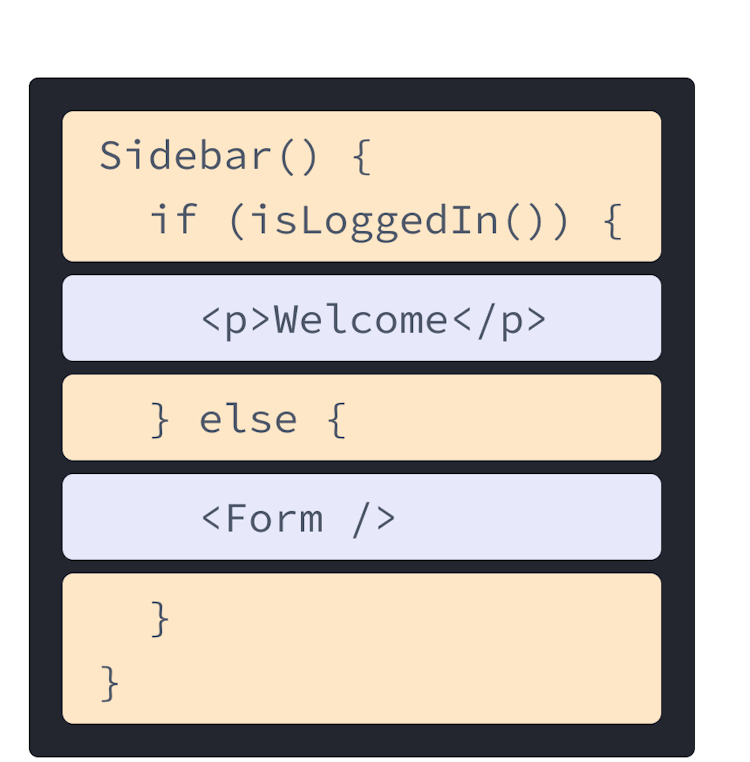\n\n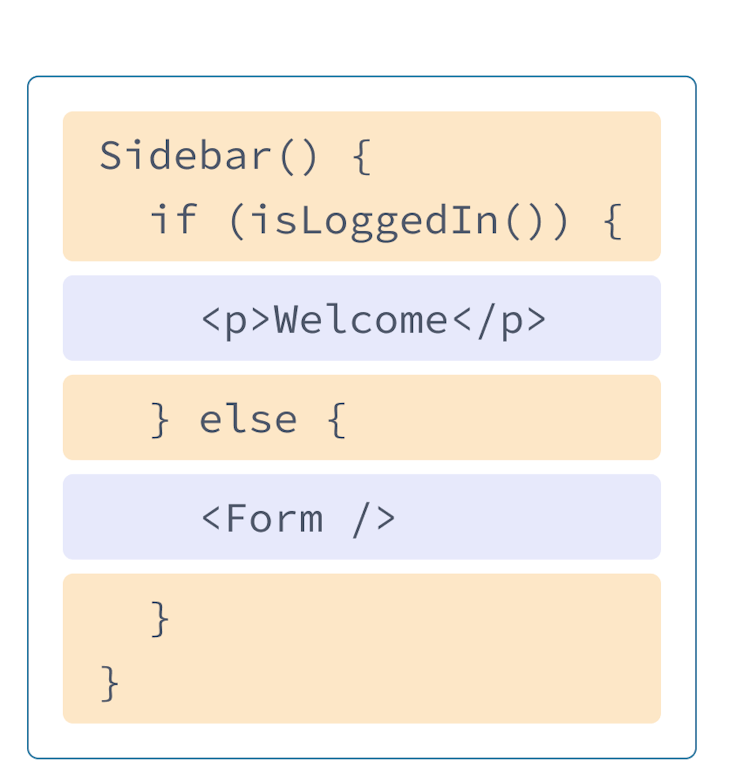\n\n`Sidebar.js` React component\n\n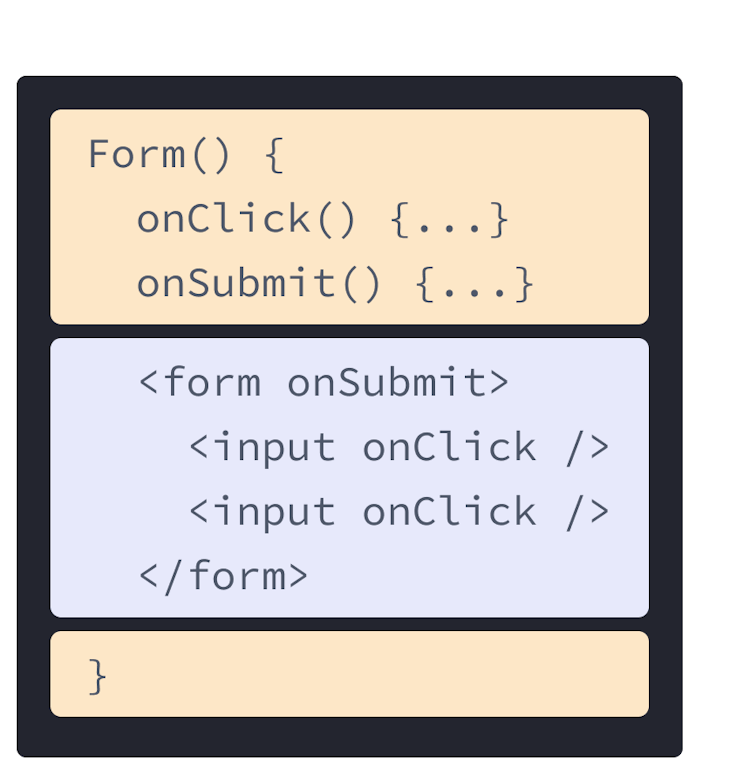\n\n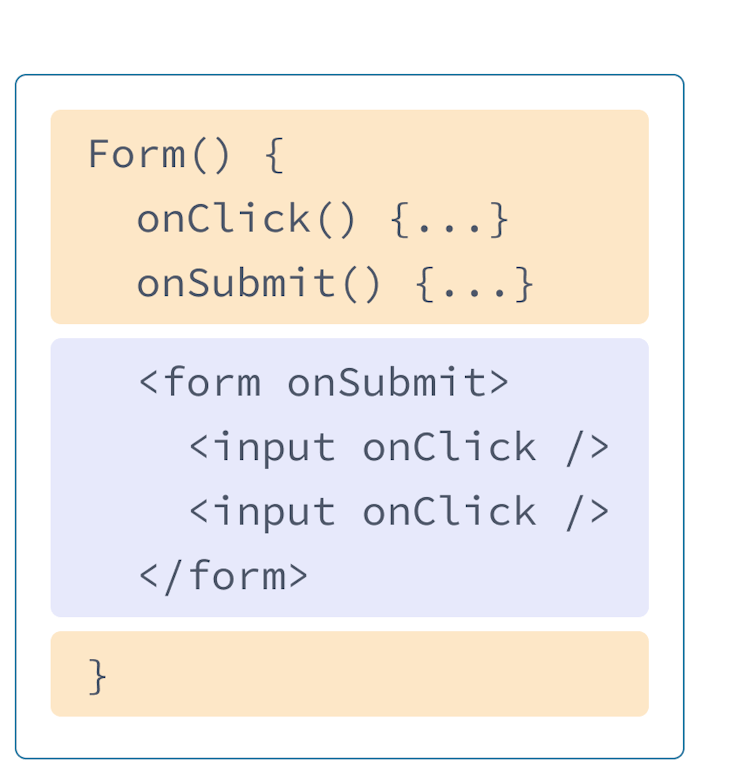\n\n`Form.js` React component\n\nKeeping a button’s rendering logic and markup together ensures that they stay in sync with each other on every edit. Conversely, details that are unrelated, such as the button’s markup and a sidebar’s markup, are isolated from each other, making it safer to change either of them on their own.\n\nEach React component is a JavaScript function that may contain some markup that React renders into the browser. React components use a syntax extension called JSX to represent that markup. JSX looks a lot like HTML, but it is a bit stricter and can display dynamic information. The best way to understand this is to convert some HTML markup to JSX markup.\n\n### Note\n\nJSX and React are two separate things. They’re often used together, but you _can_ [use them independently](https://reactjs.org/blog/2020/09/22/introducing-the-new-jsx-transform.html#whats-a-jsx-transform) of each other. JSX is a syntax extension, while React is a JavaScript library.\n\nConverting HTML to JSX[](https://react.dev/learn/writing-markup-with-jsx#converting-html-to-jsx \"Link for Converting HTML to JSX \")\n-----------------------------------------------------------------------------------------------------------------------------------\n\nSuppose that you have some (perfectly valid) HTML:\n\n```\n<h1>Hedy Lamarr's Todos</h1><img src=\"https://i.imgur.com/yXOvdOSs.jpg\" alt=\"Hedy Lamarr\" class=\"photo\"><ul><li>Invent new traffic lights<li>Rehearse a movie scene<li>Improve the spectrum technology</ul>\n```\n\nAnd you want to put it into your component:\n\n```\nexport default function TodoList() {return (// ???)}\n```\n\nIf you copy and paste it as is, it will not work:\n\nThis is because JSX is stricter and has a few more rules than HTML! If you read the error messages above, they’ll guide you to fix the markup, or you can follow the guide below.\n\n### Note\n\nMost of the time, React’s on-screen error messages will help you find where the problem is. Give them a read if you get stuck!\n\nThe Rules of JSX[](https://react.dev/learn/writing-markup-with-jsx#the-rules-of-jsx \"Link for The Rules of JSX \")\n-----------------------------------------------------------------------------------------------------------------\n\n### 1\\. Return a single root element[](https://react.dev/learn/writing-markup-with-jsx#1-return-a-single-root-element \"Link for 1. Return a single root element \")\n\nTo return multiple elements from a component, **wrap them with a single parent tag.**\n\nFor example, you can use a `<div>`:\n\n```\n<div><h1>Hedy Lamarr's Todos</h1><img src=\"https://i.imgur.com/yXOvdOSs.jpg\" alt=\"Hedy Lamarr\" class=\"photo\"><ul> ...</ul></div>\n```\n\nIf you don’t want to add an extra `<div>` to your markup, you can write `<>` and `</>` instead:\n\n```\n<><h1>Hedy Lamarr's Todos</h1><img src=\"https://i.imgur.com/yXOvdOSs.jpg\" alt=\"Hedy Lamarr\" class=\"photo\"><ul> ...</ul></>\n```\n\nThis empty tag is called a _[Fragment.](https://react.dev/reference/react/Fragment)_ Fragments let you group things without leaving any trace in the browser HTML tree.\n\n##### Deep Dive\n\n#### Why do multiple JSX tags need to be wrapped?[](https://react.dev/learn/writing-markup-with-jsx#why-do-multiple-jsx-tags-need-to-be-wrapped \"Link for Why do multiple JSX tags need to be wrapped? \")\n\nJSX looks like HTML, but under the hood it is transformed into plain JavaScript objects. You can’t return two objects from a function without wrapping them into an array. This explains why you also can’t return two JSX tags without wrapping them into another tag or a Fragment.\n\n### 2\\. Close all the tags[](https://react.dev/learn/writing-markup-with-jsx#2-close-all-the-tags \"Link for 2. Close all the tags \")\n\nJSX requires tags to be explicitly closed: self-closing tags like `<img>` must become `<img />`, and wrapping tags like `<li>oranges` must be written as `<li>oranges</li>`.\n\nThis is how Hedy Lamarr’s image and list items look closed:\n\n```\n<><img src=\"https://i.imgur.com/yXOvdOSs.jpg\" alt=\"Hedy Lamarr\" class=\"photo\"/><ul><li>Invent new traffic lights</li><li>Rehearse a movie scene</li><li>Improve the spectrum technology</li></ul></>\n```\n\n### 3\\. camelCase all most of the things\n\nJSX turns into JavaScript and attributes written in JSX become keys of JavaScript objects. In your own components, you will often want to read those attributes into variables. But JavaScript has limitations on variable names. For example, their names can’t contain dashes or be reserved words like `class`.\n\nThis is why, in React, many HTML and SVG attributes are written in camelCase. For example, instead of `stroke-width` you use `strokeWidth`. Since `class` is a reserved word, in React you write `className` instead, named after the [corresponding DOM property](https://developer.mozilla.org/en-US/docs/Web/API/Element/className):\n\n```\n<img src=\"https://i.imgur.com/yXOvdOSs.jpg\" alt=\"Hedy Lamarr\" className=\"photo\"/>\n```\n\nYou can [find all these attributes in the list of DOM component props.](https://react.dev/reference/react-dom/components/common) If you get one wrong, don’t worry—React will print a message with a possible correction to the [browser console.](https://developer.mozilla.org/docs/Tools/Browser_Console)\n\n### Pitfall\n\nFor historical reasons, [`aria-*`](https://developer.mozilla.org/docs/Web/Accessibility/ARIA) and [`data-*`](https://developer.mozilla.org/docs/Learn/HTML/Howto/Use_data_attributes) attributes are written as in HTML with dashes.\n\n### Pro-tip: Use a JSX Converter[](https://react.dev/learn/writing-markup-with-jsx#pro-tip-use-a-jsx-converter \"Link for Pro-tip: Use a JSX Converter \")\n\nConverting all these attributes in existing markup can be tedious! We recommend using a [converter](https://transform.tools/html-to-jsx) to translate your existing HTML and SVG to JSX. Converters are very useful in practice, but it’s still worth understanding what is going on so that you can comfortably write JSX on your own.\n\nHere is your final result:\n\nRecap[](https://react.dev/learn/writing-markup-with-jsx#recap \"Link for Recap\")\n-------------------------------------------------------------------------------\n\nNow you know why JSX exists and how to use it in components:\n\n* React components group rendering logic together with markup because they are related.\n* JSX is similar to HTML, with a few differences. You can use a [converter](https://transform.tools/html-to-jsx) if you need to.\n* Error messages will often point you in the right direction to fixing your markup.\n",
"filename": "writing-markup-with-jsx.md",
"package": "react"
} |
{
"content": "Title: You Might Not Need an Effect – React\n\nURL Source: https://react.dev/learn/you-might-not-need-an-effect\n\nMarkdown Content:\nEffects are an escape hatch from the React paradigm. They let you “step outside” of React and synchronize your components with some external system like a non-React widget, network, or the browser DOM. If there is no external system involved (for example, if you want to update a component’s state when some props or state change), you shouldn’t need an Effect. Removing unnecessary Effects will make your code easier to follow, faster to run, and less error-prone.\n\n### You will learn\n\n* Why and how to remove unnecessary Effects from your components\n* How to cache expensive computations without Effects\n* How to reset and adjust component state without Effects\n* How to share logic between event handlers\n* Which logic should be moved to event handlers\n* How to notify parent components about changes\n\nHow to remove unnecessary Effects[](https://react.dev/learn/you-might-not-need-an-effect#how-to-remove-unnecessary-effects \"Link for How to remove unnecessary Effects \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThere are two common cases in which you don’t need Effects:\n\n* **You don’t need Effects to transform data for rendering.** For example, let’s say you want to filter a list before displaying it. You might feel tempted to write an Effect that updates a state variable when the list changes. However, this is inefficient. When you update the state, React will first call your component functions to calculate what should be on the screen. Then React will [“commit”](https://react.dev/learn/render-and-commit) these changes to the DOM, updating the screen. Then React will run your Effects. If your Effect _also_ immediately updates the state, this restarts the whole process from scratch! To avoid the unnecessary render passes, transform all the data at the top level of your components. That code will automatically re-run whenever your props or state change.\n* **You don’t need Effects to handle user events.** For example, let’s say you want to send an `/api/buy` POST request and show a notification when the user buys a product. In the Buy button click event handler, you know exactly what happened. By the time an Effect runs, you don’t know _what_ the user did (for example, which button was clicked). This is why you’ll usually handle user events in the corresponding event handlers.\n\nYou _do_ need Effects to [synchronize](https://react.dev/learn/synchronizing-with-effects#what-are-effects-and-how-are-they-different-from-events) with external systems. For example, you can write an Effect that keeps a jQuery widget synchronized with the React state. You can also fetch data with Effects: for example, you can synchronize the search results with the current search query. Keep in mind that modern [frameworks](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) provide more efficient built-in data fetching mechanisms than writing Effects directly in your components.\n\nTo help you gain the right intuition, let’s look at some common concrete examples!\n\n### Updating state based on props or state[](https://react.dev/learn/you-might-not-need-an-effect#updating-state-based-on-props-or-state \"Link for Updating state based on props or state \")\n\nSuppose you have a component with two state variables: `firstName` and `lastName`. You want to calculate a `fullName` from them by concatenating them. Moreover, you’d like `fullName` to update whenever `firstName` or `lastName` change. Your first instinct might be to add a `fullName` state variable and update it in an Effect:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('Taylor');const [lastName, setLastName] = useState('Swift');// 🔴 Avoid: redundant state and unnecessary Effectconst [fullName, setFullName] = useState('');useEffect(() => {setFullName(firstName + ' ' + lastName);}, [firstName, lastName]);// ...}\n```\n\nThis is more complicated than necessary. It is inefficient too: it does an entire render pass with a stale value for `fullName`, then immediately re-renders with the updated value. Remove the state variable and the Effect:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('Taylor');const [lastName, setLastName] = useState('Swift');// ✅ Good: calculated during renderingconst fullName = firstName + ' ' + lastName;// ...}\n```\n\n**When something can be calculated from the existing props or state, [don’t put it in state.](https://react.dev/learn/choosing-the-state-structure#avoid-redundant-state) Instead, calculate it during rendering.** This makes your code faster (you avoid the extra “cascading” updates), simpler (you remove some code), and less error-prone (you avoid bugs caused by different state variables getting out of sync with each other). If this approach feels new to you, [Thinking in React](https://react.dev/learn/thinking-in-react#step-3-find-the-minimal-but-complete-representation-of-ui-state) explains what should go into state.\n\n### Caching expensive calculations[](https://react.dev/learn/you-might-not-need-an-effect#caching-expensive-calculations \"Link for Caching expensive calculations \")\n\nThis component computes `visibleTodos` by taking the `todos` it receives by props and filtering them according to the `filter` prop. You might feel tempted to store the result in state and update it from an Effect:\n\n```\nfunction TodoList({ todos, filter }) {const [newTodo, setNewTodo] = useState('');// 🔴 Avoid: redundant state and unnecessary Effectconst [visibleTodos, setVisibleTodos] = useState([]);useEffect(() => {setVisibleTodos(getFilteredTodos(todos, filter));}, [todos, filter]);// ...}\n```\n\nLike in the earlier example, this is both unnecessary and inefficient. First, remove the state and the Effect:\n\n```\nfunction TodoList({ todos, filter }) {const [newTodo, setNewTodo] = useState('');// ✅ This is fine if getFilteredTodos() is not slow.const visibleTodos = getFilteredTodos(todos, filter);// ...}\n```\n\nUsually, this code is fine! But maybe `getFilteredTodos()` is slow or you have a lot of `todos`. In that case you don’t want to recalculate `getFilteredTodos()` if some unrelated state variable like `newTodo` has changed.\n\nYou can cache (or [“memoize”](https://en.wikipedia.org/wiki/Memoization)) an expensive calculation by wrapping it in a [`useMemo`](https://react.dev/reference/react/useMemo) Hook:\n\n```\nimport { useMemo, useState } from 'react';function TodoList({ todos, filter }) {const [newTodo, setNewTodo] = useState('');const visibleTodos = useMemo(() => {// ✅ Does not re-run unless todos or filter changereturn getFilteredTodos(todos, filter);}, [todos, filter]);// ...}\n```\n\nOr, written as a single line:\n\n```\nimport { useMemo, useState } from 'react';function TodoList({ todos, filter }) {const [newTodo, setNewTodo] = useState('');// ✅ Does not re-run getFilteredTodos() unless todos or filter changeconst visibleTodos = useMemo(() => getFilteredTodos(todos, filter), [todos, filter]);// ...}\n```\n\n**This tells React that you don’t want the inner function to re-run unless either `todos` or `filter` have changed.** React will remember the return value of `getFilteredTodos()` during the initial render. During the next renders, it will check if `todos` or `filter` are different. If they’re the same as last time, `useMemo` will return the last result it has stored. But if they are different, React will call the inner function again (and store its result).\n\nThe function you wrap in [`useMemo`](https://react.dev/reference/react/useMemo) runs during rendering, so this only works for [pure calculations.](https://react.dev/learn/keeping-components-pure)\n\n##### Deep Dive\n\n#### How to tell if a calculation is expensive?[](https://react.dev/learn/you-might-not-need-an-effect#how-to-tell-if-a-calculation-is-expensive \"Link for How to tell if a calculation is expensive? \")\n\nIn general, unless you’re creating or looping over thousands of objects, it’s probably not expensive. If you want to get more confidence, you can add a console log to measure the time spent in a piece of code:\n\n```\nconsole.time('filter array');const visibleTodos = getFilteredTodos(todos, filter);console.timeEnd('filter array');\n```\n\nPerform the interaction you’re measuring (for example, typing into the input). You will then see logs like `filter array: 0.15ms` in your console. If the overall logged time adds up to a significant amount (say, `1ms` or more), it might make sense to memoize that calculation. As an experiment, you can then wrap the calculation in `useMemo` to verify whether the total logged time has decreased for that interaction or not:\n\n```\nconsole.time('filter array');const visibleTodos = useMemo(() => {return getFilteredTodos(todos, filter); // Skipped if todos and filter haven't changed}, [todos, filter]);console.timeEnd('filter array');\n```\n\n`useMemo` won’t make the _first_ render faster. It only helps you skip unnecessary work on updates.\n\nKeep in mind that your machine is probably faster than your users’ so it’s a good idea to test the performance with an artificial slowdown. For example, Chrome offers a [CPU Throttling](https://developer.chrome.com/blog/new-in-devtools-61/#throttling) option for this.\n\nAlso note that measuring performance in development will not give you the most accurate results. (For example, when [Strict Mode](https://react.dev/reference/react/StrictMode) is on, you will see each component render twice rather than once.) To get the most accurate timings, build your app for production and test it on a device like your users have.\n\n### Resetting all state when a prop changes[](https://react.dev/learn/you-might-not-need-an-effect#resetting-all-state-when-a-prop-changes \"Link for Resetting all state when a prop changes \")\n\nThis `ProfilePage` component receives a `userId` prop. The page contains a comment input, and you use a `comment` state variable to hold its value. One day, you notice a problem: when you navigate from one profile to another, the `comment` state does not get reset. As a result, it’s easy to accidentally post a comment on a wrong user’s profile. To fix the issue, you want to clear out the `comment` state variable whenever the `userId` changes:\n\n```\nexport default function ProfilePage({ userId }) {const [comment, setComment] = useState('');// 🔴 Avoid: Resetting state on prop change in an EffectuseEffect(() => {setComment('');}, [userId]);// ...}\n```\n\nThis is inefficient because `ProfilePage` and its children will first render with the stale value, and then render again. It is also complicated because you’d need to do this in _every_ component that has some state inside `ProfilePage`. For example, if the comment UI is nested, you’d want to clear out nested comment state too.\n\nInstead, you can tell React that each user’s profile is conceptually a _different_ profile by giving it an explicit key. Split your component in two and pass a `key` attribute from the outer component to the inner one:\n\n```\nexport default function ProfilePage({ userId }) {return (<ProfileuserId={userId}key={userId}/>);}function Profile({ userId }) {// ✅ This and any other state below will reset on key change automaticallyconst [comment, setComment] = useState('');// ...}\n```\n\nNormally, React preserves the state when the same component is rendered in the same spot. **By passing `userId` as a `key` to the `Profile` component, you’re asking React to treat two `Profile` components with different `userId` as two different components that should not share any state.** Whenever the key (which you’ve set to `userId`) changes, React will recreate the DOM and [reset the state](https://react.dev/learn/preserving-and-resetting-state#option-2-resetting-state-with-a-key) of the `Profile` component and all of its children. Now the `comment` field will clear out automatically when navigating between profiles.\n\nNote that in this example, only the outer `ProfilePage` component is exported and visible to other files in the project. Components rendering `ProfilePage` don’t need to pass the key to it: they pass `userId` as a regular prop. The fact `ProfilePage` passes it as a `key` to the inner `Profile` component is an implementation detail.\n\n### Adjusting some state when a prop changes[](https://react.dev/learn/you-might-not-need-an-effect#adjusting-some-state-when-a-prop-changes \"Link for Adjusting some state when a prop changes \")\n\nSometimes, you might want to reset or adjust a part of the state on a prop change, but not all of it.\n\nThis `List` component receives a list of `items` as a prop, and maintains the selected item in the `selection` state variable. You want to reset the `selection` to `null` whenever the `items` prop receives a different array:\n\n```\nfunction List({ items }) {const [isReverse, setIsReverse] = useState(false);const [selection, setSelection] = useState(null);// 🔴 Avoid: Adjusting state on prop change in an EffectuseEffect(() => {setSelection(null);}, [items]);// ...}\n```\n\nThis, too, is not ideal. Every time the `items` change, the `List` and its child components will render with a stale `selection` value at first. Then React will update the DOM and run the Effects. Finally, the `setSelection(null)` call will cause another re-render of the `List` and its child components, restarting this whole process again.\n\nStart by deleting the Effect. Instead, adjust the state directly during rendering:\n\n```\nfunction List({ items }) {const [isReverse, setIsReverse] = useState(false);const [selection, setSelection] = useState(null);// Better: Adjust the state while renderingconst [prevItems, setPrevItems] = useState(items);if (items !== prevItems) {setPrevItems(items);setSelection(null);}// ...}\n```\n\n[Storing information from previous renders](https://react.dev/reference/react/useState#storing-information-from-previous-renders) like this can be hard to understand, but it’s better than updating the same state in an Effect. In the above example, `setSelection` is called directly during a render. React will re-render the `List` _immediately_ after it exits with a `return` statement. React has not rendered the `List` children or updated the DOM yet, so this lets the `List` children skip rendering the stale `selection` value.\n\nWhen you update a component during rendering, React throws away the returned JSX and immediately retries rendering. To avoid very slow cascading retries, React only lets you update the _same_ component’s state during a render. If you update another component’s state during a render, you’ll see an error. A condition like `items !== prevItems` is necessary to avoid loops. You may adjust state like this, but any other side effects (like changing the DOM or setting timeouts) should stay in event handlers or Effects to [keep components pure.](https://react.dev/learn/keeping-components-pure)\n\n**Although this pattern is more efficient than an Effect, most components shouldn’t need it either.** No matter how you do it, adjusting state based on props or other state makes your data flow more difficult to understand and debug. Always check whether you can [reset all state with a key](https://react.dev/learn/you-might-not-need-an-effect#resetting-all-state-when-a-prop-changes) or [calculate everything during rendering](https://react.dev/learn/you-might-not-need-an-effect#updating-state-based-on-props-or-state) instead. For example, instead of storing (and resetting) the selected _item_, you can store the selected _item ID:_\n\n```\nfunction List({ items }) {const [isReverse, setIsReverse] = useState(false);const [selectedId, setSelectedId] = useState(null);// ✅ Best: Calculate everything during renderingconst selection = items.find(item => item.id === selectedId) ?? null;// ...}\n```\n\nNow there is no need to “adjust” the state at all. If the item with the selected ID is in the list, it remains selected. If it’s not, the `selection` calculated during rendering will be `null` because no matching item was found. This behavior is different, but arguably better because most changes to `items` preserve the selection.\n\n### Sharing logic between event handlers[](https://react.dev/learn/you-might-not-need-an-effect#sharing-logic-between-event-handlers \"Link for Sharing logic between event handlers \")\n\nLet’s say you have a product page with two buttons (Buy and Checkout) that both let you buy that product. You want to show a notification whenever the user puts the product in the cart. Calling `showNotification()` in both buttons’ click handlers feels repetitive so you might be tempted to place this logic in an Effect:\n\n```\nfunction ProductPage({ product, addToCart }) {// 🔴 Avoid: Event-specific logic inside an EffectuseEffect(() => {if (product.isInCart) {showNotification(`Added ${product.name} to the shopping cart!`);}}, [product]);function handleBuyClick() {addToCart(product);}function handleCheckoutClick() {addToCart(product);navigateTo('/checkout');}// ...}\n```\n\nThis Effect is unnecessary. It will also most likely cause bugs. For example, let’s say that your app “remembers” the shopping cart between the page reloads. If you add a product to the cart once and refresh the page, the notification will appear again. It will keep appearing every time you refresh that product’s page. This is because `product.isInCart` will already be `true` on the page load, so the Effect above will call `showNotification()`.\n\n**When you’re not sure whether some code should be in an Effect or in an event handler, ask yourself _why_ this code needs to run. Use Effects only for code that should run _because_ the component was displayed to the user.** In this example, the notification should appear because the user _pressed the button_, not because the page was displayed! Delete the Effect and put the shared logic into a function called from both event handlers:\n\n```\nfunction ProductPage({ product, addToCart }) {// ✅ Good: Event-specific logic is called from event handlersfunction buyProduct() {addToCart(product);showNotification(`Added ${product.name} to the shopping cart!`);}function handleBuyClick() {buyProduct();}function handleCheckoutClick() {buyProduct();navigateTo('/checkout');}// ...}\n```\n\nThis both removes the unnecessary Effect and fixes the bug.\n\n### Sending a POST request[](https://react.dev/learn/you-might-not-need-an-effect#sending-a-post-request \"Link for Sending a POST request \")\n\nThis `Form` component sends two kinds of POST requests. It sends an analytics event when it mounts. When you fill in the form and click the Submit button, it will send a POST request to the `/api/register` endpoint:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('');const [lastName, setLastName] = useState('');// ✅ Good: This logic should run because the component was displayeduseEffect(() => {post('/analytics/event', { eventName: 'visit_form' });}, []);// 🔴 Avoid: Event-specific logic inside an Effectconst [jsonToSubmit, setJsonToSubmit] = useState(null);useEffect(() => {if (jsonToSubmit !== null) {post('/api/register', jsonToSubmit);}}, [jsonToSubmit]);function handleSubmit(e) {e.preventDefault();setJsonToSubmit({ firstName, lastName });}// ...}\n```\n\nLet’s apply the same criteria as in the example before.\n\nThe analytics POST request should remain in an Effect. This is because the _reason_ to send the analytics event is that the form was displayed. (It would fire twice in development, but [see here](https://react.dev/learn/synchronizing-with-effects#sending-analytics) for how to deal with that.)\n\nHowever, the `/api/register` POST request is not caused by the form being _displayed_. You only want to send the request at one specific moment in time: when the user presses the button. It should only ever happen _on that particular interaction_. Delete the second Effect and move that POST request into the event handler:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('');const [lastName, setLastName] = useState('');// ✅ Good: This logic runs because the component was displayeduseEffect(() => {post('/analytics/event', { eventName: 'visit_form' });}, []);function handleSubmit(e) {e.preventDefault();// ✅ Good: Event-specific logic is in the event handlerpost('/api/register', { firstName, lastName });}// ...}\n```\n\nWhen you choose whether to put some logic into an event handler or an Effect, the main question you need to answer is _what kind of logic_ it is from the user’s perspective. If this logic is caused by a particular interaction, keep it in the event handler. If it’s caused by the user _seeing_ the component on the screen, keep it in the Effect.\n\n### Chains of computations[](https://react.dev/learn/you-might-not-need-an-effect#chains-of-computations \"Link for Chains of computations \")\n\nSometimes you might feel tempted to chain Effects that each adjust a piece of state based on other state:\n\n```\nfunction Game() {const [card, setCard] = useState(null);const [goldCardCount, setGoldCardCount] = useState(0);const [round, setRound] = useState(1);const [isGameOver, setIsGameOver] = useState(false);// 🔴 Avoid: Chains of Effects that adjust the state solely to trigger each otheruseEffect(() => {if (card !== null && card.gold) {setGoldCardCount(c => c + 1);}}, [card]);useEffect(() => {if (goldCardCount > 3) {setRound(r => r + 1)setGoldCardCount(0);}}, [goldCardCount]);useEffect(() => {if (round > 5) {setIsGameOver(true);}}, [round]);useEffect(() => {alert('Good game!');}, [isGameOver]);function handlePlaceCard(nextCard) {if (isGameOver) {throw Error('Game already ended.');} else {setCard(nextCard);}}// ...\n```\n\nThere are two problems with this code.\n\nOne problem is that it is very inefficient: the component (and its children) have to re-render between each `set` call in the chain. In the example above, in the worst case (`setCard` → render → `setGoldCardCount` → render → `setRound` → render → `setIsGameOver` → render) there are three unnecessary re-renders of the tree below.\n\nEven if it weren’t slow, as your code evolves, you will run into cases where the “chain” you wrote doesn’t fit the new requirements. Imagine you are adding a way to step through the history of the game moves. You’d do it by updating each state variable to a value from the past. However, setting the `card` state to a value from the past would trigger the Effect chain again and change the data you’re showing. Such code is often rigid and fragile.\n\nIn this case, it’s better to calculate what you can during rendering, and adjust the state in the event handler:\n\n```\nfunction Game() {const [card, setCard] = useState(null);const [goldCardCount, setGoldCardCount] = useState(0);const [round, setRound] = useState(1);// ✅ Calculate what you can during renderingconst isGameOver = round > 5;function handlePlaceCard(nextCard) {if (isGameOver) {throw Error('Game already ended.');}// ✅ Calculate all the next state in the event handlersetCard(nextCard);if (nextCard.gold) {if (goldCardCount <= 3) {setGoldCardCount(goldCardCount + 1);} else {setGoldCardCount(0);setRound(round + 1);if (round === 5) {alert('Good game!');}}}}// ...\n```\n\nThis is a lot more efficient. Also, if you implement a way to view game history, now you will be able to set each state variable to a move from the past without triggering the Effect chain that adjusts every other value. If you need to reuse logic between several event handlers, you can [extract a function](https://react.dev/learn/you-might-not-need-an-effect#sharing-logic-between-event-handlers) and call it from those handlers.\n\nRemember that inside event handlers, [state behaves like a snapshot.](https://react.dev/learn/state-as-a-snapshot) For example, even after you call `setRound(round + 1)`, the `round` variable will reflect the value at the time the user clicked the button. If you need to use the next value for calculations, define it manually like `const nextRound = round + 1`.\n\nIn some cases, you _can’t_ calculate the next state directly in the event handler. For example, imagine a form with multiple dropdowns where the options of the next dropdown depend on the selected value of the previous dropdown. Then, a chain of Effects is appropriate because you are synchronizing with network.\n\n### Initializing the application[](https://react.dev/learn/you-might-not-need-an-effect#initializing-the-application \"Link for Initializing the application \")\n\nSome logic should only run once when the app loads.\n\nYou might be tempted to place it in an Effect in the top-level component:\n\n```\nfunction App() {// 🔴 Avoid: Effects with logic that should only ever run onceuseEffect(() => {loadDataFromLocalStorage();checkAuthToken();}, []);// ...}\n```\n\nHowever, you’ll quickly discover that it [runs twice in development.](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development) This can cause issues—for example, maybe it invalidates the authentication token because the function wasn’t designed to be called twice. In general, your components should be resilient to being remounted. This includes your top-level `App` component.\n\nAlthough it may not ever get remounted in practice in production, following the same constraints in all components makes it easier to move and reuse code. If some logic must run _once per app load_ rather than _once per component mount_, add a top-level variable to track whether it has already executed:\n\n```\nlet didInit = false;function App() {useEffect(() => {if (!didInit) {didInit = true;// ✅ Only runs once per app loadloadDataFromLocalStorage();checkAuthToken();}}, []);// ...}\n```\n\nYou can also run it during module initialization and before the app renders:\n\n```\nif (typeof window !== 'undefined') { // Check if we're running in the browser.// ✅ Only runs once per app loadcheckAuthToken();loadDataFromLocalStorage();}function App() {// ...}\n```\n\nCode at the top level runs once when your component is imported—even if it doesn’t end up being rendered. To avoid slowdown or surprising behavior when importing arbitrary components, don’t overuse this pattern. Keep app-wide initialization logic to root component modules like `App.js` or in your application’s entry point.\n\n### Notifying parent components about state changes[](https://react.dev/learn/you-might-not-need-an-effect#notifying-parent-components-about-state-changes \"Link for Notifying parent components about state changes \")\n\nLet’s say you’re writing a `Toggle` component with an internal `isOn` state which can be either `true` or `false`. There are a few different ways to toggle it (by clicking or dragging). You want to notify the parent component whenever the `Toggle` internal state changes, so you expose an `onChange` event and call it from an Effect:\n\n```\nfunction Toggle({ onChange }) {const [isOn, setIsOn] = useState(false);// 🔴 Avoid: The onChange handler runs too lateuseEffect(() => {onChange(isOn);}, [isOn, onChange])function handleClick() {setIsOn(!isOn);}function handleDragEnd(e) {if (isCloserToRightEdge(e)) {setIsOn(true);} else {setIsOn(false);}}// ...}\n```\n\nLike earlier, this is not ideal. The `Toggle` updates its state first, and React updates the screen. Then React runs the Effect, which calls the `onChange` function passed from a parent component. Now the parent component will update its own state, starting another render pass. It would be better to do everything in a single pass.\n\nDelete the Effect and instead update the state of _both_ components within the same event handler:\n\n```\nfunction Toggle({ onChange }) {const [isOn, setIsOn] = useState(false);function updateToggle(nextIsOn) {// ✅ Good: Perform all updates during the event that caused themsetIsOn(nextIsOn);onChange(nextIsOn);}function handleClick() {updateToggle(!isOn);}function handleDragEnd(e) {if (isCloserToRightEdge(e)) {updateToggle(true);} else {updateToggle(false);}}// ...}\n```\n\nWith this approach, both the `Toggle` component and its parent component update their state during the event. React [batches updates](https://react.dev/learn/queueing-a-series-of-state-updates) from different components together, so there will only be one render pass.\n\nYou might also be able to remove the state altogether, and instead receive `isOn` from the parent component:\n\n```\n// ✅ Also good: the component is fully controlled by its parentfunction Toggle({ isOn, onChange }) {function handleClick() {onChange(!isOn);}function handleDragEnd(e) {if (isCloserToRightEdge(e)) {onChange(true);} else {onChange(false);}}// ...}\n```\n\n[“Lifting state up”](https://react.dev/learn/sharing-state-between-components) lets the parent component fully control the `Toggle` by toggling the parent’s own state. This means the parent component will have to contain more logic, but there will be less state overall to worry about. Whenever you try to keep two different state variables synchronized, try lifting state up instead!\n\n### Passing data to the parent[](https://react.dev/learn/you-might-not-need-an-effect#passing-data-to-the-parent \"Link for Passing data to the parent \")\n\nThis `Child` component fetches some data and then passes it to the `Parent` component in an Effect:\n\n```\nfunction Parent() {const [data, setData] = useState(null);// ...return <Child onFetched={setData} />;}function Child({ onFetched }) {const data = useSomeAPI();// 🔴 Avoid: Passing data to the parent in an EffectuseEffect(() => {if (data) {onFetched(data);}}, [onFetched, data]);// ...}\n```\n\nIn React, data flows from the parent components to their children. When you see something wrong on the screen, you can trace where the information comes from by going up the component chain until you find which component passes the wrong prop or has the wrong state. When child components update the state of their parent components in Effects, the data flow becomes very difficult to trace. Since both the child and the parent need the same data, let the parent component fetch that data, and _pass it down_ to the child instead:\n\n```\nfunction Parent() {const data = useSomeAPI();// ...// ✅ Good: Passing data down to the childreturn <Child data={data} />;}function Child({ data }) {// ...}\n```\n\nThis is simpler and keeps the data flow predictable: the data flows down from the parent to the child.\n\n### Subscribing to an external store[](https://react.dev/learn/you-might-not-need-an-effect#subscribing-to-an-external-store \"Link for Subscribing to an external store \")\n\nSometimes, your components may need to subscribe to some data outside of the React state. This data could be from a third-party library or a built-in browser API. Since this data can change without React’s knowledge, you need to manually subscribe your components to it. This is often done with an Effect, for example:\n\n```\nfunction useOnlineStatus() {// Not ideal: Manual store subscription in an Effectconst [isOnline, setIsOnline] = useState(true);useEffect(() => {function updateState() {setIsOnline(navigator.onLine);}updateState();window.addEventListener('online', updateState);window.addEventListener('offline', updateState);return () => {window.removeEventListener('online', updateState);window.removeEventListener('offline', updateState);};}, []);return isOnline;}function ChatIndicator() {const isOnline = useOnlineStatus();// ...}\n```\n\nHere, the component subscribes to an external data store (in this case, the browser `navigator.onLine` API). Since this API does not exist on the server (so it can’t be used for the initial HTML), initially the state is set to `true`. Whenever the value of that data store changes in the browser, the component updates its state.\n\nAlthough it’s common to use Effects for this, React has a purpose-built Hook for subscribing to an external store that is preferred instead. Delete the Effect and replace it with a call to [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore):\n\n```\nfunction subscribe(callback) {window.addEventListener('online', callback);window.addEventListener('offline', callback);return () => {window.removeEventListener('online', callback);window.removeEventListener('offline', callback);};}function useOnlineStatus() {// ✅ Good: Subscribing to an external store with a built-in Hookreturn useSyncExternalStore(subscribe, // React won't resubscribe for as long as you pass the same function() => navigator.onLine, // How to get the value on the client() => true // How to get the value on the server);}function ChatIndicator() {const isOnline = useOnlineStatus();// ...}\n```\n\nThis approach is less error-prone than manually syncing mutable data to React state with an Effect. Typically, you’ll write a custom Hook like `useOnlineStatus()` above so that you don’t need to repeat this code in the individual components. [Read more about subscribing to external stores from React components.](https://react.dev/reference/react/useSyncExternalStore)\n\n### Fetching data[](https://react.dev/learn/you-might-not-need-an-effect#fetching-data \"Link for Fetching data \")\n\nMany apps use Effects to kick off data fetching. It is quite common to write a data fetching Effect like this:\n\n```\nfunction SearchResults({ query }) {const [results, setResults] = useState([]);const [page, setPage] = useState(1);useEffect(() => {// 🔴 Avoid: Fetching without cleanup logicfetchResults(query, page).then(json => {setResults(json);});}, [query, page]);function handleNextPageClick() {setPage(page + 1);}// ...}\n```\n\nYou _don’t_ need to move this fetch to an event handler.\n\nThis might seem like a contradiction with the earlier examples where you needed to put the logic into the event handlers! However, consider that it’s not _the typing event_ that’s the main reason to fetch. Search inputs are often prepopulated from the URL, and the user might navigate Back and Forward without touching the input.\n\nIt doesn’t matter where `page` and `query` come from. While this component is visible, you want to keep `results` [synchronized](https://react.dev/learn/synchronizing-with-effects) with data from the network for the current `page` and `query`. This is why it’s an Effect.\n\nHowever, the code above has a bug. Imagine you type `\"hello\"` fast. Then the `query` will change from `\"h\"`, to `\"he\"`, `\"hel\"`, `\"hell\"`, and `\"hello\"`. This will kick off separate fetches, but there is no guarantee about which order the responses will arrive in. For example, the `\"hell\"` response may arrive _after_ the `\"hello\"` response. Since it will call `setResults()` last, you will be displaying the wrong search results. This is called a [“race condition”](https://en.wikipedia.org/wiki/Race_condition): two different requests “raced” against each other and came in a different order than you expected.\n\n**To fix the race condition, you need to [add a cleanup function](https://react.dev/learn/synchronizing-with-effects#fetching-data) to ignore stale responses:**\n\n```\nfunction SearchResults({ query }) {const [results, setResults] = useState([]);const [page, setPage] = useState(1);useEffect(() => {let ignore = false;fetchResults(query, page).then(json => {if (!ignore) {setResults(json);}});return () => {ignore = true;};}, [query, page]);function handleNextPageClick() {setPage(page + 1);}// ...}\n```\n\nThis ensures that when your Effect fetches data, all responses except the last requested one will be ignored.\n\nHandling race conditions is not the only difficulty with implementing data fetching. You might also want to think about caching responses (so that the user can click Back and see the previous screen instantly), how to fetch data on the server (so that the initial server-rendered HTML contains the fetched content instead of a spinner), and how to avoid network waterfalls (so that a child can fetch data without waiting for every parent).\n\n**These issues apply to any UI library, not just React. Solving them is not trivial, which is why modern [frameworks](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) provide more efficient built-in data fetching mechanisms than fetching data in Effects.**\n\nIf you don’t use a framework (and don’t want to build your own) but would like to make data fetching from Effects more ergonomic, consider extracting your fetching logic into a custom Hook like in this example:\n\n```\nfunction SearchResults({ query }) {const [page, setPage] = useState(1);const params = new URLSearchParams({ query, page });const results = useData(`/api/search?${params}`);function handleNextPageClick() {setPage(page + 1);}// ...}function useData(url) {const [data, setData] = useState(null);useEffect(() => {let ignore = false;fetch(url) .then(response => response.json()) .then(json => {if (!ignore) {setData(json);}});return () => {ignore = true;};}, [url]);return data;}\n```\n\nYou’ll likely also want to add some logic for error handling and to track whether the content is loading. You can build a Hook like this yourself or use one of the many solutions already available in the React ecosystem. **Although this alone won’t be as efficient as using a framework’s built-in data fetching mechanism, moving the data fetching logic into a custom Hook will make it easier to adopt an efficient data fetching strategy later.**\n\nIn general, whenever you have to resort to writing Effects, keep an eye out for when you can extract a piece of functionality into a custom Hook with a more declarative and purpose-built API like `useData` above. The fewer raw `useEffect` calls you have in your components, the easier you will find to maintain your application.\n\nRecap[](https://react.dev/learn/you-might-not-need-an-effect#recap \"Link for Recap\")\n------------------------------------------------------------------------------------\n\n* If you can calculate something during render, you don’t need an Effect.\n* To cache expensive calculations, add `useMemo` instead of `useEffect`.\n* To reset the state of an entire component tree, pass a different `key` to it.\n* To reset a particular bit of state in response to a prop change, set it during rendering.\n* Code that runs because a component was _displayed_ should be in Effects, the rest should be in events.\n* If you need to update the state of several components, it’s better to do it during a single event.\n* Whenever you try to synchronize state variables in different components, consider lifting state up.\n* You can fetch data with Effects, but you need to implement cleanup to avoid race conditions.\n",
"filename": "you-might-not-need-an-effect.md",
"package": "react"
} |
{
"content": "Title: Your First Component – React\n\nURL Source: https://react.dev/learn/your-first-component\n\nMarkdown Content:\n_Components_ are one of the core concepts of React. They are the foundation upon which you build user interfaces (UI), which makes them the perfect place to start your React journey!\n\n### You will learn\n\n* What a component is\n* What role components play in a React application\n* How to write your first React component\n\nComponents: UI building blocks[](https://react.dev/learn/your-first-component#components-ui-building-blocks \"Link for Components: UI building blocks \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------\n\nOn the Web, HTML lets us create rich structured documents with its built-in set of tags like `<h1>` and `<li>`:\n\n```\n<article><h1>My First Component</h1><ol><li>Components: UI Building Blocks</li><li>Defining a Component</li><li>Using a Component</li></ol></article>\n```\n\nThis markup represents this article `<article>`, its heading `<h1>`, and an (abbreviated) table of contents as an ordered list `<ol>`. Markup like this, combined with CSS for style, and JavaScript for interactivity, lies behind every sidebar, avatar, modal, dropdown—every piece of UI you see on the Web.\n\nReact lets you combine your markup, CSS, and JavaScript into custom “components”, **reusable UI elements for your app.** The table of contents code you saw above could be turned into a `<TableOfContents />` component you could render on every page. Under the hood, it still uses the same HTML tags like `<article>`, `<h1>`, etc.\n\nJust like with HTML tags, you can compose, order and nest components to design whole pages. For example, the documentation page you’re reading is made out of React components:\n\n```\n<PageLayout><NavigationHeader><SearchBar /><Link to=\"/docs\">Docs</Link></NavigationHeader><Sidebar /><PageContent><TableOfContents /><DocumentationText /></PageContent></PageLayout>\n```\n\nAs your project grows, you will notice that many of your designs can be composed by reusing components you already wrote, speeding up your development. Our table of contents above could be added to any screen with `<TableOfContents />`! You can even jumpstart your project with the thousands of components shared by the React open source community like [Chakra UI](https://chakra-ui.com/) and [Material UI.](https://material-ui.com/)\n\nDefining a component[](https://react.dev/learn/your-first-component#defining-a-component \"Link for Defining a component \")\n--------------------------------------------------------------------------------------------------------------------------\n\nTraditionally when creating web pages, web developers marked up their content and then added interaction by sprinkling on some JavaScript. This worked great when interaction was a nice-to-have on the web. Now it is expected for many sites and all apps. React puts interactivity first while still using the same technology: **a React component is a JavaScript function that you can _sprinkle with markup_.** Here’s what that looks like (you can edit the example below):\n\nAnd here’s how to build a component:\n\n### Step 1: Export the component[](https://react.dev/learn/your-first-component#step-1-export-the-component \"Link for Step 1: Export the component \")\n\nThe `export default` prefix is a [standard JavaScript syntax](https://developer.mozilla.org/docs/web/javascript/reference/statements/export) (not specific to React). It lets you mark the main function in a file so that you can later import it from other files. (More on importing in [Importing and Exporting Components](https://react.dev/learn/importing-and-exporting-components)!)\n\n### Step 2: Define the function[](https://react.dev/learn/your-first-component#step-2-define-the-function \"Link for Step 2: Define the function \")\n\nWith `function Profile() { }` you define a JavaScript function with the name `Profile`.\n\n### Pitfall\n\nReact components are regular JavaScript functions, but **their names must start with a capital letter** or they won’t work!\n\n### Step 3: Add markup[](https://react.dev/learn/your-first-component#step-3-add-markup \"Link for Step 3: Add markup \")\n\nThe component returns an `<img />` tag with `src` and `alt` attributes. `<img />` is written like HTML, but it is actually JavaScript under the hood! This syntax is called [JSX](https://react.dev/learn/writing-markup-with-jsx), and it lets you embed markup inside JavaScript.\n\nReturn statements can be written all on one line, as in this component:\n\n```\nreturn <img src=\"https://i.imgur.com/MK3eW3As.jpg\" alt=\"Katherine Johnson\" />;\n```\n\nBut if your markup isn’t all on the same line as the `return` keyword, you must wrap it in a pair of parentheses:\n\n```\nreturn (<div><img src=\"https://i.imgur.com/MK3eW3As.jpg\" alt=\"Katherine Johnson\" /></div>);\n```\n\n### Pitfall\n\nUsing a component[](https://react.dev/learn/your-first-component#using-a-component \"Link for Using a component \")\n-----------------------------------------------------------------------------------------------------------------\n\nNow that you’ve defined your `Profile` component, you can nest it inside other components. For example, you can export a `Gallery` component that uses multiple `Profile` components:\n\nfunction Profile() {\n return (\n <img\n src\\=\"https://i.imgur.com/MK3eW3As.jpg\"\n alt\\=\"Katherine Johnson\"\n />\n );\n}\n\nexport default function Gallery() {\n return (\n <section\\>\n <h1\\>Amazing scientists</h1\\>\n <Profile />\n <Profile />\n <Profile />\n </section\\>\n );\n}\n\n### What the browser sees[](https://react.dev/learn/your-first-component#what-the-browser-sees \"Link for What the browser sees \")\n\nNotice the difference in casing:\n\n* `<section>` is lowercase, so React knows we refer to an HTML tag.\n* `<Profile />` starts with a capital `P`, so React knows that we want to use our component called `Profile`.\n\nAnd `Profile` contains even more HTML: `<img />`. In the end, this is what the browser sees:\n\n```\n<section><h1>Amazing scientists</h1><img src=\"https://i.imgur.com/MK3eW3As.jpg\" alt=\"Katherine Johnson\" /><img src=\"https://i.imgur.com/MK3eW3As.jpg\" alt=\"Katherine Johnson\" /><img src=\"https://i.imgur.com/MK3eW3As.jpg\" alt=\"Katherine Johnson\" /></section>\n```\n\n### Nesting and organizing components[](https://react.dev/learn/your-first-component#nesting-and-organizing-components \"Link for Nesting and organizing components \")\n\nComponents are regular JavaScript functions, so you can keep multiple components in the same file. This is convenient when components are relatively small or tightly related to each other. If this file gets crowded, you can always move `Profile` to a separate file. You will learn how to do this shortly on the [page about imports.](https://react.dev/learn/importing-and-exporting-components)\n\nBecause the `Profile` components are rendered inside `Gallery`—even several times!—we can say that `Gallery` is a **parent component,** rendering each `Profile` as a “child”. This is part of the magic of React: you can define a component once, and then use it in as many places and as many times as you like.\n\n### Pitfall\n\nComponents can render other components, but **you must never nest their definitions:**\n\n```\nexport default function Gallery() {// 🔴 Never define a component inside another component!function Profile() {// ...}// ...}\n```\n\nThe snippet above is [very slow and causes bugs.](https://react.dev/learn/preserving-and-resetting-state#different-components-at-the-same-position-reset-state) Instead, define every component at the top level:\n\n```\nexport default function Gallery() {// ...}// ✅ Declare components at the top levelfunction Profile() {// ...}\n```\n\nWhen a child component needs some data from a parent, [pass it by props](https://react.dev/learn/passing-props-to-a-component) instead of nesting definitions.\n\n##### Deep Dive\n\n#### Components all the way down[](https://react.dev/learn/your-first-component#components-all-the-way-down \"Link for Components all the way down \")\n\nYour React application begins at a “root” component. Usually, it is created automatically when you start a new project. For example, if you use [CodeSandbox](https://codesandbox.io/) or if you use the framework [Next.js](https://nextjs.org/), the root component is defined in `pages/index.js`. In these examples, you’ve been exporting root components.\n\nMost React apps use components all the way down. This means that you won’t only use components for reusable pieces like buttons, but also for larger pieces like sidebars, lists, and ultimately, complete pages! Components are a handy way to organize UI code and markup, even if some of them are only used once.\n\n[React-based frameworks](https://react.dev/learn/start-a-new-react-project) take this a step further. Instead of using an empty HTML file and letting React “take over” managing the page with JavaScript, they _also_ generate the HTML automatically from your React components. This allows your app to show some content before the JavaScript code loads.\n\nStill, many websites only use React to [add interactivity to existing HTML pages.](https://react.dev/learn/add-react-to-an-existing-project#using-react-for-a-part-of-your-existing-page) They have many root components instead of a single one for the entire page. You can use as much—or as little—React as you need.\n\nRecap[](https://react.dev/learn/your-first-component#recap \"Link for Recap\")\n----------------------------------------------------------------------------\n\nYou’ve just gotten your first taste of React! Let’s recap some key points.\n\n* React lets you create components, **reusable UI elements for your app.**\n \n* In a React app, every piece of UI is a component.\n \n* React components are regular JavaScript functions except:\n \n 1. Their names always begin with a capital letter.\n 2. They return JSX markup.\n\nTry out some challenges[](https://react.dev/learn/your-first-component#challenges \"Link for Try out some challenges\")\n---------------------------------------------------------------------------------------------------------------------\n\n#### Export the component[](https://react.dev/learn/your-first-component#export-the-component \"Link for this heading\")\n\nThis sandbox doesn’t work because the root component is not exported:\n\nTry to fix it yourself before looking at the solution!\n",
"filename": "your-first-component.md",
"package": "react"
} |