files
dict |
---|
{
"content": "Title: Express 5.x - API Reference\n\nURL Source: https://expressjs.com/en/5x/api.html\n\nMarkdown Content:\n**Note**: This is early beta documentation that may be incomplete and is still under development.\n\n**Note**: Express 5.0 requires Node.js 18 or higher.\n\nexpress()\n---------\n\nCreates an Express application. The `express()` function is a top-level function exported by the `express` module.\n\n```\nconst express = require('express')\nconst app = express()\n```\n\n### Methods\n\n### express.json(\\[options\\])\n\nThis is a built-in middleware function in Express. It parses incoming requests with JSON payloads and is based on [body-parser](https://expressjs.com/resources/middleware/body-parser.html).\n\nReturns middleware that only parses JSON and only looks at requests where the `Content-Type` header matches the `type` option. This parser accepts any Unicode encoding of the body and supports automatic inflation of `gzip` and `deflate` encodings.\n\nA new `body` object containing the parsed data is populated on the `request` object after the middleware (i.e. `req.body`), or an empty object (`{}`) if there was no body to parse, the `Content-Type` was not matched, or an error occurred.\n\nAs `req.body`’s shape is based on user-controlled input, all properties and values in this object are untrusted and should be validated before trusting. For example, `req.body.foo.toString()` may fail in multiple ways, for example `foo` may not be there or may not be a string, and `toString` may not be a function and instead a string or other user-input.\n\nThe following table describes the properties of the optional `options` object.\n\n| Property | Description | Type | Default |\n| --- | --- | --- | --- |\n| `inflate` | Enables or disables handling deflated (compressed) bodies; when disabled, deflated bodies are rejected. | Boolean | `true` |\n| `limit` | Controls the maximum request body size. If this is a number, then the value specifies the number of bytes; if it is a string, the value is passed to the [bytes](https://www.npmjs.com/package/bytes) library for parsing. | Mixed | `\"100kb\"` |\n| `reviver` | The `reviver` option is passed directly to `JSON.parse` as the second argument. You can find more information on this argument [in the MDN documentation about JSON.parse](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON/parse#Example.3A_Using_the_reviver_parameter). | Function | `null` |\n| `strict` | Enables or disables only accepting arrays and objects; when disabled will accept anything `JSON.parse` accepts. | Boolean | `true` |\n| `type` | This is used to determine what media type the middleware will parse. This option can be a string, array of strings, or a function. If not a function, `type` option is passed directly to the [type-is](https://www.npmjs.org/package/type-is#readme) library and this can be an extension name (like `json`), a mime type (like `application/json`), or a mime type with a wildcard (like `*/*` or `*/json`). If a function, the `type` option is called as `fn(req)` and the request is parsed if it returns a truthy value. | Mixed | `\"application/json\"` |\n| `verify` | This option, if supplied, is called as `verify(req, res, buf, encoding)`, where `buf` is a `Buffer` of the raw request body and `encoding` is the encoding of the request. The parsing can be aborted by throwing an error. | Function | `undefined` |\n\n### express.static(root, \\[options\\])\n\nThis is a built-in middleware function in Express. It serves static files and is based on [serve-static](https://expressjs.com/resources/middleware/serve-static.html).\n\nNOTE: For best results, [use a reverse proxy](https://expressjs.com/en/advanced/best-practice-performance.html#use-a-reverse-proxy) cache to improve performance of serving static assets.\n\nThe `root` argument specifies the root directory from which to serve static assets. The function determines the file to serve by combining `req.url` with the provided `root` directory. When a file is not found, instead of sending a 404 response, it instead calls `next()` to move on to the next middleware, allowing for stacking and fall-backs.\n\nThe following table describes the properties of the `options` object. See also the [example below](https://expressjs.com/en/5x/api.html#example.of.express.static).\n\n| Property | Description | Type | Default |\n| --- | --- | --- | --- |\n| `dotfiles` | Determines how dotfiles (files or directories that begin with a dot “.”) are treated.See [dotfiles](https://expressjs.com/en/5x/api.html#dotfiles) below.\n | String | “ignore” |\n| `etag` | Enable or disable etag generationNOTE: `express.static` always sends weak ETags.\n\n | Boolean | `true` |\n| `extensions` | Sets file extension fallbacks: If a file is not found, search for files with the specified extensions and serve the first one found. Example: `['html', 'htm']`. | Mixed | `false` |\n| `fallthrough` | Let client errors fall-through as unhandled requests, otherwise forward a client error.See [fallthrough](https://expressjs.com/en/5x/api.html#fallthrough) below.\n\n | Boolean | `true` |\n| `immutable` | Enable or disable the `immutable` directive in the `Cache-Control` response header. If enabled, the `maxAge` option should also be specified to enable caching. The `immutable` directive will prevent supported clients from making conditional requests during the life of the `maxAge` option to check if the file has changed. | Boolean | `false` |\n| `index` | Sends the specified directory index file. Set to `false` to disable directory indexing. | Mixed | “index.html” |\n| `lastModified` | Set the `Last-Modified` header to the last modified date of the file on the OS. | Boolean | `true` |\n| `maxAge` | Set the max-age property of the Cache-Control header in milliseconds or a string in [ms format](https://www.npmjs.org/package/ms). | Number | 0 |\n| `redirect` | Redirect to trailing “/” when the pathname is a directory. | Boolean | `true` |\n| `setHeaders` | Function for setting HTTP headers to serve with the file.See [setHeaders](https://expressjs.com/en/5x/api.html#setHeaders) below.\n\n | Function | |\n\nFor more information, see [Serving static files in Express](https://expressjs.com/starter/static-files.html). and [Using middleware - Built-in middleware](https://expressjs.com/en/guide/using-middleware.html#middleware.built-in).\n\n##### dotfiles\n\nPossible values for this option are:\n\n* “allow” - No special treatment for dotfiles.\n* “deny” - Deny a request for a dotfile, respond with `403`, then call `next()`.\n* “ignore” - Act as if the dotfile does not exist, respond with `404`, then call `next()`.\n\n##### fallthrough\n\nWhen this option is `true`, client errors such as a bad request or a request to a non-existent file will cause this middleware to simply call `next()` to invoke the next middleware in the stack. When false, these errors (even 404s), will invoke `next(err)`.\n\nSet this option to `true` so you can map multiple physical directories to the same web address or for routes to fill in non-existent files.\n\nUse `false` if you have mounted this middleware at a path designed to be strictly a single file system directory, which allows for short-circuiting 404s for less overhead. This middleware will also reply to all methods.\n\nFor this option, specify a function to set custom response headers. Alterations to the headers must occur synchronously.\n\nThe signature of the function is:\n\n```\nfn(res, path, stat)\n```\n\nArguments:\n\n* `res`, the [response object](https://expressjs.com/en/5x/api.html#res).\n* `path`, the file path that is being sent.\n* `stat`, the `stat` object of the file that is being sent.\n\n#### Example of express.static\n\nHere is an example of using the `express.static` middleware function with an elaborate options object:\n\n```\nconst options = {\n dotfiles: 'ignore',\n etag: false,\n extensions: ['htm', 'html'],\n index: false,\n maxAge: '1d',\n redirect: false,\n setHeaders (res, path, stat) {\n res.set('x-timestamp', Date.now())\n }\n}\n\napp.use(express.static('public', options))\n```\n\n### express.Router(\\[options\\])\n\nCreates a new [router](https://expressjs.com/en/5x/api.html#router) object.\n\n```\nconst router = express.Router([options])\n```\n\nThe optional `options` parameter specifies the behavior of the router.\n\nYou can add middleware and HTTP method routes (such as `get`, `put`, `post`, and so on) to `router` just like an application.\n\nFor more information, see [Router](https://expressjs.com/en/5x/api.html#router).\n\n### express.urlencoded(\\[options\\])\n\nThis is a built-in middleware function in Express. It parses incoming requests with urlencoded payloads and is based on [body-parser](https://expressjs.com/resources/middleware/body-parser.html).\n\nReturns middleware that only parses urlencoded bodies and only looks at requests where the `Content-Type` header matches the `type` option. This parser accepts only UTF-8 encoding of the body and supports automatic inflation of `gzip` and `deflate` encodings.\n\nA new `body` object containing the parsed data is populated on the `request` object after the middleware (i.e. `req.body`), or an empty object (`{}`) if there was no body to parse, the `Content-Type` was not matched, or an error occurred. This object will contain key-value pairs, where the value can be a string or array (when `extended` is `false`), or any type (when `extended` is `true`).\n\nAs `req.body`’s shape is based on user-controlled input, all properties and values in this object are untrusted and should be validated before trusting. For example, `req.body.foo.toString()` may fail in multiple ways, for example `foo` may not be there or may not be a string, and `toString` may not be a function and instead a string or other user-input.\n\nThe following table describes the properties of the optional `options` object.\n\n| Property | Description | Type | Default |\n| --- | --- | --- | --- |\n| `extended` | This option allows to choose between parsing the URL-encoded data with the `querystring` library (when `false`) or the `qs` library (when `true`). The “extended” syntax allows for rich objects and arrays to be encoded into the URL-encoded format, allowing for a JSON-like experience with URL-encoded. For more information, please [see the qs library](https://www.npmjs.org/package/qs#readme). | Boolean | `false` |\n| `inflate` | Enables or disables handling deflated (compressed) bodies; when disabled, deflated bodies are rejected. | Boolean | `true` |\n| `limit` | Controls the maximum request body size. If this is a number, then the value specifies the number of bytes; if it is a string, the value is passed to the [bytes](https://www.npmjs.com/package/bytes) library for parsing. | Mixed | `\"100kb\"` |\n| `parameterLimit` | This option controls the maximum number of parameters that are allowed in the URL-encoded data. If a request contains more parameters than this value, an error will be raised. | Number | `1000` |\n| `type` | This is used to determine what media type the middleware will parse. This option can be a string, array of strings, or a function. If not a function, `type` option is passed directly to the [type-is](https://www.npmjs.org/package/type-is#readme) library and this can be an extension name (like `urlencoded`), a mime type (like `application/x-www-form-urlencoded`), or a mime type with a wildcard (like `*/x-www-form-urlencoded`). If a function, the `type` option is called as `fn(req)` and the request is parsed if it returns a truthy value. | Mixed | `\"application/x-www-form-urlencoded\"` |\n| `verify` | This option, if supplied, is called as `verify(req, res, buf, encoding)`, where `buf` is a `Buffer` of the raw request body and `encoding` is the encoding of the request. The parsing can be aborted by throwing an error. | Function | `undefined` |\n\nApplication\n-----------\n\nThe `app` object conventionally denotes the Express application. Create it by calling the top-level `express()` function exported by the Express module:\n\n```\nconst express = require('express')\nconst app = express()\n\napp.get('/', (req, res) => {\n res.send('hello world')\n})\n\napp.listen(3000)\n```\n\nThe `app` object has methods for\n\n* Routing HTTP requests; see for example, [app.METHOD](https://expressjs.com/en/5x/api.html#app.METHOD) and [app.param](https://expressjs.com/en/5x/api.html#app.param).\n* Configuring middleware; see [app.route](https://expressjs.com/en/5x/api.html#app.route).\n* Rendering HTML views; see [app.render](https://expressjs.com/en/5x/api.html#app.render).\n* Registering a template engine; see [app.engine](https://expressjs.com/en/5x/api.html#app.engine).\n\nIt also has settings (properties) that affect how the application behaves; for more information, see [Application settings](https://expressjs.com/en/5x/api.html#app.settings.table).\n\nThe Express application object can be referred from the [request object](https://expressjs.com/en/5x/api.html#req) and the [response object](https://expressjs.com/en/5x/api.html#res) as `req.app`, and `res.app`, respectively.\n\n### Properties\n\n### app.locals\n\nThe `app.locals` object has properties that are local variables within the application, and will be available in templates rendered with [res.render](https://expressjs.com/en/5x/api.html#res.render).\n\n```\nconsole.dir(app.locals.title)\n// => 'My App'\n\nconsole.dir(app.locals.email)\n// => 'me@myapp.com'\n```\n\nOnce set, the value of `app.locals` properties persist throughout the life of the application, in contrast with [res.locals](https://expressjs.com/en/5x/api.html#res.locals) properties that are valid only for the lifetime of the request.\n\nYou can access local variables in templates rendered within the application. This is useful for providing helper functions to templates, as well as application-level data. Local variables are available in middleware via `req.app.locals` (see [req.app](https://expressjs.com/en/5x/api.html#req.app))\n\n```\napp.locals.title = 'My App'\napp.locals.strftime = require('strftime')\napp.locals.email = 'me@myapp.com'\n```\n\n### app.mountpath\n\nThe `app.mountpath` property contains one or more path patterns on which a sub-app was mounted.\n\nA sub-app is an instance of `express` that may be used for handling the request to a route.\n\n```\nconst express = require('express')\n\nconst app = express() // the main app\nconst admin = express() // the sub app\n\nadmin.get('/', (req, res) => {\n console.log(admin.mountpath) // /admin\n res.send('Admin Homepage')\n})\n\napp.use('/admin', admin) // mount the sub app\n```\n\nIt is similar to the [baseUrl](https://expressjs.com/en/5x/api.html#req.baseUrl) property of the `req` object, except `req.baseUrl` returns the matched URL path, instead of the matched patterns.\n\nIf a sub-app is mounted on multiple path patterns, `app.mountpath` returns the list of patterns it is mounted on, as shown in the following example.\n\n```\nconst admin = express()\n\nadmin.get('/', (req, res) => {\n console.log(admin.mountpath) // [ '/adm*n', '/manager' ]\n res.send('Admin Homepage')\n})\n\nconst secret = express()\nsecret.get('/', (req, res) => {\n console.log(secret.mountpath) // /secr*t\n res.send('Admin Secret')\n})\n\nadmin.use('/secr*t', secret) // load the 'secret' router on '/secr*t', on the 'admin' sub app\napp.use(['/adm*n', '/manager'], admin) // load the 'admin' router on '/adm*n' and '/manager', on the parent app\n```\n\n### app.router\n\nThe application’s in-built instance of router. This is created lazily, on first access.\n\n```\nconst express = require('express')\nconst app = express()\nconst router = app.router\n\nrouter.get('/', (req, res) => {\n res.send('hello world')\n})\n\napp.listen(3000)\n```\n\nYou can add middleware and HTTP method routes to the `router` just like an application.\n\nFor more information, see [Router](https://expressjs.com/en/5x/api.html#router).\n\n### Events\n\n### app.on('mount', callback(parent))\n\nThe `mount` event is fired on a sub-app, when it is mounted on a parent app. The parent app is passed to the callback function.\n\n**NOTE**\n\nSub-apps will:\n\n* Not inherit the value of settings that have a default value. You must set the value in the sub-app.\n* Inherit the value of settings with no default value.\n\nFor details, see [Application settings](https://expressjs.com/en/5x/api.html#app.settings.table).\n\n```\nconst admin = express()\n\nadmin.on('mount', (parent) => {\n console.log('Admin Mounted')\n console.log(parent) // refers to the parent app\n})\n\nadmin.get('/', (req, res) => {\n res.send('Admin Homepage')\n})\n\napp.use('/admin', admin)\n```\n\n### Methods\n\n### app.all(path, callback \\[, callback ...\\])\n\nThis method is like the standard [app.METHOD()](https://expressjs.com/en/5x/api.html#app.METHOD) methods, except it matches all HTTP verbs.\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\n#### Examples\n\nThe following callback is executed for requests to `/secret` whether using GET, POST, PUT, DELETE, or any other HTTP request method:\n\n```\napp.all('/secret', (req, res, next) => {\n console.log('Accessing the secret section ...')\n next() // pass control to the next handler\n})\n```\n\nThe `app.all()` method is useful for mapping “global” logic for specific path prefixes or arbitrary matches. For example, if you put the following at the top of all other route definitions, it requires that all routes from that point on require authentication, and automatically load a user. Keep in mind that these callbacks do not have to act as end-points: `loadUser` can perform a task, then call `next()` to continue matching subsequent routes.\n\n```\napp.all('*', requireAuthentication, loadUser)\n```\n\nOr the equivalent:\n\n```\napp.all('*', requireAuthentication)\napp.all('*', loadUser)\n```\n\nAnother example is white-listed “global” functionality. The example is similar to the ones above, but it only restricts paths that start with “/api”:\n\n```\napp.all('/api/*', requireAuthentication)\n```\n\n### app.delete(path, callback \\[, callback ...\\])\n\nRoutes HTTP DELETE requests to the specified path with the specified callback functions. For more information, see the [routing guide](https://expressjs.com/en/guide/routing.html).\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\n#### Example\n\n```\napp.delete('/', (req, res) => {\n res.send('DELETE request to homepage')\n})\n```\n\n### app.disable(name)\n\nSets the Boolean setting `name` to `false`, where `name` is one of the properties from the [app settings table](https://expressjs.com/en/5x/api.html#app.settings.table). Calling `app.set('foo', false)` for a Boolean property is the same as calling `app.disable('foo')`.\n\nFor example:\n\n```\napp.disable('trust proxy')\napp.get('trust proxy')\n// => false\n```\n\n### app.disabled(name)\n\nReturns `true` if the Boolean setting `name` is disabled (`false`), where `name` is one of the properties from the [app settings table](https://expressjs.com/en/5x/api.html#app.settings.table).\n\n```\napp.disabled('trust proxy')\n// => true\n\napp.enable('trust proxy')\napp.disabled('trust proxy')\n// => false\n```\n\n### app.enable(name)\n\nSets the Boolean setting `name` to `true`, where `name` is one of the properties from the [app settings table](https://expressjs.com/en/5x/api.html#app.settings.table). Calling `app.set('foo', true)` for a Boolean property is the same as calling `app.enable('foo')`.\n\n```\napp.enable('trust proxy')\napp.get('trust proxy')\n// => true\n```\n\n### app.enabled(name)\n\nReturns `true` if the setting `name` is enabled (`true`), where `name` is one of the properties from the [app settings table](https://expressjs.com/en/5x/api.html#app.settings.table).\n\n```\napp.enabled('trust proxy')\n// => false\n\napp.enable('trust proxy')\napp.enabled('trust proxy')\n// => true\n```\n\n### app.engine(ext, callback)\n\nRegisters the given template engine `callback` as `ext`.\n\nBy default, Express will `require()` the engine based on the file extension. For example, if you try to render a “foo.pug” file, Express invokes the following internally, and caches the `require()` on subsequent calls to increase performance.\n\n```\napp.engine('pug', require('pug').__express)\n```\n\nUse this method for engines that do not provide `.__express` out of the box, or if you wish to “map” a different extension to the template engine.\n\nFor example, to map the EJS template engine to “.html” files:\n\n```\napp.engine('html', require('ejs').renderFile)\n```\n\nIn this case, EJS provides a `.renderFile()` method with the same signature that Express expects: `(path, options, callback)`, though note that it aliases this method as `ejs.__express` internally so if you’re using “.ejs” extensions you don’t need to do anything.\n\nSome template engines do not follow this convention. The [consolidate.js](https://github.com/tj/consolidate.js) library maps Node template engines to follow this convention, so they work seamlessly with Express.\n\n```\nconst engines = require('consolidate')\napp.engine('haml', engines.haml)\napp.engine('html', engines.hogan)\n```\n\n### app.get(name)\n\nReturns the value of `name` app setting, where `name` is one of the strings in the [app settings table](https://expressjs.com/en/5x/api.html#app.settings.table). For example:\n\n```\napp.get('title')\n// => undefined\n\napp.set('title', 'My Site')\napp.get('title')\n// => \"My Site\"\n```\n\n### app.get(path, callback \\[, callback ...\\])\n\nRoutes HTTP GET requests to the specified path with the specified callback functions.\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\nFor more information, see the [routing guide](https://expressjs.com/en/guide/routing.html).\n\n#### Example\n\n```\napp.get('/', (req, res) => {\n res.send('GET request to homepage')\n})\n```\n\n### app.listen(path, \\[callback\\])\n\nStarts a UNIX socket and listens for connections on the given path. This method is identical to Node’s [http.Server.listen()](https://nodejs.org/api/http.html#http_server_listen).\n\n```\nconst express = require('express')\nconst app = express()\napp.listen('/tmp/sock')\n```\n\n### app.listen(\\[port\\[, host\\[, backlog\\]\\]\\]\\[, callback\\])\n\nBinds and listens for connections on the specified host and port. This method is identical to Node’s [http.Server.listen()](https://nodejs.org/api/http.html#http_server_listen).\n\nIf port is omitted or is 0, the operating system will assign an arbitrary unused port, which is useful for cases like automated tasks (tests, etc.).\n\n```\nconst express = require('express')\nconst app = express()\napp.listen(3000)\n```\n\nThe `app` returned by `express()` is in fact a JavaScript `Function`, designed to be passed to Node’s HTTP servers as a callback to handle requests. This makes it easy to provide both HTTP and HTTPS versions of your app with the same code base, as the app does not inherit from these (it is simply a callback):\n\n```\nconst express = require('express')\nconst https = require('https')\nconst http = require('http')\nconst app = express()\n\nhttp.createServer(app).listen(80)\nhttps.createServer(options, app).listen(443)\n```\n\nThe `app.listen()` method returns an [http.Server](https://nodejs.org/api/http.html#http_class_http_server) object and (for HTTP) is a convenience method for the following:\n\n```\napp.listen = function () {\n const server = http.createServer(this)\n return server.listen.apply(server, arguments)\n}\n```\n\nNOTE: All the forms of Node’s [http.Server.listen()](https://nodejs.org/api/http.html#http_server_listen) method are in fact actually supported.\n\n### app.METHOD(path, callback \\[, callback ...\\])\n\nRoutes an HTTP request, where METHOD is the HTTP method of the request, such as GET, PUT, POST, and so on, in lowercase. Thus, the actual methods are `app.get()`, `app.post()`, `app.put()`, and so on. See [Routing methods](https://expressjs.com/en/5x/api.html#routing-methods) below for the complete list.\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\n#### Routing methods\n\nExpress supports the following routing methods corresponding to the HTTP methods of the same names:\n\n<table><tbody><tr><td><ul><li><code>checkout</code></li><li><code>copy</code></li><li><code>delete</code></li><li><code>get</code></li><li><code>head</code></li><li><code>lock</code></li><li><code>merge</code></li><li><code>mkactivity</code></li></ul></td><td><ul><li><code>mkcol</code></li><li><code>move</code></li><li><code>m-search</code></li><li><code>notify</code></li><li><code>options</code></li><li><code>patch</code></li><li><code>post</code></li></ul></td><td><ul><li><code>purge</code></li><li><code>put</code></li><li><code>report</code></li><li><code>search</code></li><li><code>subscribe</code></li><li><code>trace</code></li><li><code>unlock</code></li><li><code>unsubscribe</code></li></ul></td></tr></tbody></table>\n\nThe API documentation has explicit entries only for the most popular HTTP methods `app.get()`, `app.post()`, `app.put()`, and `app.delete()`. However, the other methods listed above work in exactly the same way.\n\nTo route methods that translate to invalid JavaScript variable names, use the bracket notation. For example, `app['m-search']('/', function ...`.\n\nThe `app.get()` function is automatically called for the HTTP `HEAD` method in addition to the `GET` method if `app.head()` was not called for the path before `app.get()`.\n\nThe method, `app.all()`, is not derived from any HTTP method and loads middleware at the specified path for _all_ HTTP request methods. For more information, see [app.all](https://expressjs.com/en/5x/api.html#app.all).\n\nFor more information on routing, see the [routing guide](https://expressjs.com/en/guide/routing.html).\n\n### app.param(name, callback)\n\nAdd callback triggers to [route parameters](https://expressjs.com/en/guide/routing.html#route-parameters), where `name` is the name of the parameter or an array of them, and `callback` is the callback function. The parameters of the callback function are the request object, the response object, the next middleware, the value of the parameter and the name of the parameter, in that order.\n\nIf `name` is an array, the `callback` trigger is registered for each parameter declared in it, in the order in which they are declared. Furthermore, for each declared parameter except the last one, a call to `next` inside the callback will call the callback for the next declared parameter. For the last parameter, a call to `next` will call the next middleware in place for the route currently being processed, just like it would if `name` were just a string.\n\nFor example, when `:user` is present in a route path, you may map user loading logic to automatically provide `req.user` to the route, or perform validations on the parameter input.\n\n```\napp.param('user', (req, res, next, id) => {\n // try to get the user details from the User model and attach it to the request object\n User.find(id, (err, user) => {\n if (err) {\n next(err)\n } else if (user) {\n req.user = user\n next()\n } else {\n next(new Error('failed to load user'))\n }\n })\n})\n```\n\nParam callback functions are local to the router on which they are defined. They are not inherited by mounted apps or routers, nor are they triggered for route parameters inherited from parent routers. Hence, param callbacks defined on `app` will be triggered only by route parameters defined on `app` routes.\n\nAll param callbacks will be called before any handler of any route in which the param occurs, and they will each be called only once in a request-response cycle, even if the parameter is matched in multiple routes, as shown in the following examples.\n\n```\napp.param('id', (req, res, next, id) => {\n console.log('CALLED ONLY ONCE')\n next()\n})\n\napp.get('/user/:id', (req, res, next) => {\n console.log('although this matches')\n next()\n})\n\napp.get('/user/:id', (req, res) => {\n console.log('and this matches too')\n res.end()\n})\n```\n\nOn `GET /user/42`, the following is printed:\n\n```\nCALLED ONLY ONCE\nalthough this matches\nand this matches too\n```\n\n```\napp.param(['id', 'page'], (req, res, next, value) => {\n console.log('CALLED ONLY ONCE with', value)\n next()\n})\n\napp.get('/user/:id/:page', (req, res, next) => {\n console.log('although this matches')\n next()\n})\n\napp.get('/user/:id/:page', (req, res) => {\n console.log('and this matches too')\n res.end()\n})\n```\n\nOn `GET /user/42/3`, the following is printed:\n\n```\nCALLED ONLY ONCE with 42\nCALLED ONLY ONCE with 3\nalthough this matches\nand this matches too\n```\n\n### app.path()\n\nReturns the canonical path of the app, a string.\n\n```\nconst app = express()\nconst blog = express()\nconst blogAdmin = express()\n\napp.use('/blog', blog)\nblog.use('/admin', blogAdmin)\n\nconsole.log(app.path()) // ''\nconsole.log(blog.path()) // '/blog'\nconsole.log(blogAdmin.path()) // '/blog/admin'\n```\n\nThe behavior of this method can become very complicated in complex cases of mounted apps: it is usually better to use [req.baseUrl](https://expressjs.com/en/5x/api.html#req.baseUrl) to get the canonical path of the app.\n\n### app.post(path, callback \\[, callback ...\\])\n\nRoutes HTTP POST requests to the specified path with the specified callback functions. For more information, see the [routing guide](https://expressjs.com/en/guide/routing.html).\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\n#### Example\n\n```\napp.post('/', (req, res) => {\n res.send('POST request to homepage')\n})\n```\n\n### app.put(path, callback \\[, callback ...\\])\n\nRoutes HTTP PUT requests to the specified path with the specified callback functions.\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\n#### Example\n\n```\napp.put('/', (req, res) => {\n res.send('PUT request to homepage')\n})\n```\n\n### app.render(view, \\[locals\\], callback)\n\nReturns the rendered HTML of a view via the `callback` function. It accepts an optional parameter that is an object containing local variables for the view. It is like [res.render()](https://expressjs.com/en/5x/api.html#res.render), except it cannot send the rendered view to the client on its own.\n\nThink of `app.render()` as a utility function for generating rendered view strings. Internally `res.render()` uses `app.render()` to render views.\n\nThe local variable `cache` is reserved for enabling view cache. Set it to `true`, if you want to cache view during development; view caching is enabled in production by default.\n\n```\napp.render('email', (err, html) => {\n // ...\n})\n\napp.render('email', { name: 'Tobi' }, (err, html) => {\n // ...\n})\n```\n\n### app.route(path)\n\nReturns an instance of a single route, which you can then use to handle HTTP verbs with optional middleware. Use `app.route()` to avoid duplicate route names (and thus typo errors).\n\n```\nconst app = express()\n\napp.route('/events')\n .all((req, res, next) => {\n // runs for all HTTP verbs first\n // think of it as route specific middleware!\n })\n .get((req, res, next) => {\n res.json({})\n })\n .post((req, res, next) => {\n // maybe add a new event...\n })\n```\n\n### app.set(name, value)\n\nAssigns setting `name` to `value`. You may store any value that you want, but certain names can be used to configure the behavior of the server. These special names are listed in the [app settings table](https://expressjs.com/en/5x/api.html#app.settings.table).\n\nCalling `app.set('foo', true)` for a Boolean property is the same as calling `app.enable('foo')`. Similarly, calling `app.set('foo', false)` for a Boolean property is the same as calling `app.disable('foo')`.\n\nRetrieve the value of a setting with [`app.get()`](https://expressjs.com/en/5x/api.html#app.get).\n\n```\napp.set('title', 'My Site')\napp.get('title') // \"My Site\"\n```\n\n#### Application Settings\n\nThe following table lists application settings.\n\nNote that sub-apps will:\n\n* Not inherit the value of settings that have a default value. You must set the value in the sub-app.\n* Inherit the value of settings with no default value; these are explicitly noted in the table below.\n\nExceptions: Sub-apps will inherit the value of `trust proxy` even though it has a default value (for backward-compatibility); Sub-apps will not inherit the value of `view cache` in production (when `NODE_ENV` is “production”).\n\n### app.use(\\[path,\\] callback \\[, callback...\\])\n\nMounts the specified [middleware](https://expressjs.com/en/guide/using-middleware.html) function or functions at the specified path: the middleware function is executed when the base of the requested path matches `path`.\n\n#### Arguments\n\n| Argument | Description | Default |\n| --- | --- | --- |\n| `path` | The path for which the middleware function is invoked; can be any of:\n* A string representing a path.\n* A path pattern.\n* A regular expression pattern to match paths.\n* An array of combinations of any of the above.\n\nFor examples, see [Path examples](https://expressjs.com/en/5x/api.html#path-examples). | '/' (root path) |\n| `callback` | Callback functions; can be:\n\n* A middleware function.\n* A series of middleware functions (separated by commas).\n* An array of middleware functions.\n* A combination of all of the above.\n\nYou can provide multiple callback functions that behave just like middleware, except that these callbacks can invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there is no reason to proceed with the current route.\n\nWhen a callback function throws an error or returns a rejected promise, \\`next(err)\\` will be invoked automatically.\n\nSince [router](https://expressjs.com/en/5x/api.html#router) and [app](https://expressjs.com/en/5x/api.html#application) implement the middleware interface, you can use them as you would any other middleware function.\n\nFor examples, see [Middleware callback function examples](https://expressjs.com/en/5x/api.html#middleware-callback-function-examples).\n\n | None |\n\n#### Description\n\nA route will match any path that follows its path immediately with a “`/`”. For example: `app.use('/apple', ...)` will match “/apple”, “/apple/images”, “/apple/images/news”, and so on.\n\nSince `path` defaults to “/”, middleware mounted without a path will be executed for every request to the app. \nFor example, this middleware function will be executed for _every_ request to the app:\n\n```\napp.use((req, res, next) => {\n console.log('Time: %d', Date.now())\n next()\n})\n```\n\n**NOTE**\n\nSub-apps will:\n\n* Not inherit the value of settings that have a default value. You must set the value in the sub-app.\n* Inherit the value of settings with no default value.\n\nFor details, see [Application settings](https://expressjs.com/en/5x/api.html#app.settings.table).\n\nMiddleware functions are executed sequentially, therefore the order of middleware inclusion is important.\n\n```\n// this middleware will not allow the request to go beyond it\napp.use((req, res, next) => {\n res.send('Hello World')\n})\n\n// requests will never reach this route\napp.get('/', (req, res) => {\n res.send('Welcome')\n})\n```\n\n**Error-handling middleware**\n\nError-handling middleware always takes _four_ arguments. You must provide four arguments to identify it as an error-handling middleware function. Even if you don’t need to use the `next` object, you must specify it to maintain the signature. Otherwise, the `next` object will be interpreted as regular middleware and will fail to handle errors. For details about error-handling middleware, see: [Error handling](https://expressjs.com/en/guide/error-handling.html).\n\nDefine error-handling middleware functions in the same way as other middleware functions, except with four arguments instead of three, specifically with the signature `(err, req, res, next)`):\n\n```\napp.use((err, req, res, next) => {\n console.error(err.stack)\n res.status(500).send('Something broke!')\n})\n```\n\n#### Path examples\n\nThe following table provides some simple examples of valid `path` values for mounting middleware.\n\n#### Middleware callback function examples\n\nThe following table provides some simple examples of middleware functions that can be used as the `callback` argument to `app.use()`, `app.METHOD()`, and `app.all()`. Even though the examples are for `app.use()`, they are also valid for `app.use()`, `app.METHOD()`, and `app.all()`.\n\n| Usage | Example |\n| --- | --- |\n| Single Middleware | You can define and mount a middleware function locally.\n```\napp.use((req, res, next) => {\n next()\n})\n```\n\nA router is valid middleware.\n\n```\nconst router = express.Router()\nrouter.get('/', (req, res, next) => {\n next()\n})\napp.use(router)\n```\n\nAn Express app is valid middleware.\n\n```\nconst subApp = express()\nsubApp.get('/', (req, res, next) => {\n next()\n})\napp.use(subApp)\n```\n\n |\n| Series of Middleware | You can specify more than one middleware function at the same mount path.\n\n```\nconst r1 = express.Router()\nr1.get('/', (req, res, next) => {\n next()\n})\n\nconst r2 = express.Router()\nr2.get('/', (req, res, next) => {\n next()\n})\n\napp.use(r1, r2)\n```\n\n |\n| Array | Use an array to group middleware logically.\n\n```\nconst r1 = express.Router()\nr1.get('/', (req, res, next) => {\n next()\n})\n\nconst r2 = express.Router()\nr2.get('/', (req, res, next) => {\n next()\n})\n\napp.use([r1, r2])\n```\n\n |\n| Combination | You can combine all the above ways of mounting middleware.\n\n```\nfunction mw1 (req, res, next) { next() }\nfunction mw2 (req, res, next) { next() }\n\nconst r1 = express.Router()\nr1.get('/', (req, res, next) => { next() })\n\nconst r2 = express.Router()\nr2.get('/', (req, res, next) => { next() })\n\nconst subApp = express()\nsubApp.get('/', (req, res, next) => { next() })\n\napp.use(mw1, [mw2, r1, r2], subApp)\n```\n\n |\n\nFollowing are some examples of using the [express.static](https://expressjs.com/en/guide/using-middleware.html#middleware.built-in) middleware in an Express app.\n\nServe static content for the app from the “public” directory in the application directory:\n\n```\n// GET /style.css etc\napp.use(express.static(path.join(__dirname, 'public')))\n```\n\nMount the middleware at “/static” to serve static content only when their request path is prefixed with “/static”:\n\n```\n// GET /static/style.css etc.\napp.use('/static', express.static(path.join(__dirname, 'public')))\n```\n\nDisable logging for static content requests by loading the logger middleware after the static middleware:\n\n```\napp.use(express.static(path.join(__dirname, 'public')))\napp.use(logger())\n```\n\nServe static files from multiple directories, but give precedence to “./public” over the others:\n\n```\napp.use(express.static(path.join(__dirname, 'public')))\napp.use(express.static(path.join(__dirname, 'files')))\napp.use(express.static(path.join(__dirname, 'uploads')))\n```\n\nRequest\n-------\n\nThe `req` object represents the HTTP request and has properties for the request query string, parameters, body, HTTP headers, and so on. In this documentation and by convention, the object is always referred to as `req` (and the HTTP response is `res`) but its actual name is determined by the parameters to the callback function in which you’re working.\n\nFor example:\n\n```\napp.get('/user/:id', (req, res) => {\n res.send(`user ${req.params.id}`)\n})\n```\n\nBut you could just as well have:\n\n```\napp.get('/user/:id', (request, response) => {\n response.send(`user ${request.params.id}`)\n})\n```\n\nThe `req` object is an enhanced version of Node’s own request object and supports all [built-in fields and methods](https://nodejs.org/api/http.html#http_class_http_incomingmessage).\n\n### Properties\n\nIn Express 4, `req.files` is no longer available on the `req` object by default. To access uploaded files on the `req.files` object, use multipart-handling middleware like [busboy](https://www.npmjs.com/package/busboy), [multer](https://www.npmjs.com/package/multer), [formidable](https://www.npmjs.com/package/formidable), [multiparty](https://www.npmjs.com/package/multiparty), [connect-multiparty](https://www.npmjs.com/package/connect-multiparty), or [pez](https://www.npmjs.com/package/pez).\n\n### req.app\n\nThis property holds a reference to the instance of the Express application that is using the middleware.\n\nIf you follow the pattern in which you create a module that just exports a middleware function and `require()` it in your main file, then the middleware can access the Express instance via `req.app`\n\nFor example:\n\n```\n// index.js\napp.get('/viewdirectory', require('./mymiddleware.js'))\n```\n\n```\n// mymiddleware.js\nmodule.exports = (req, res) => {\n res.send(`The views directory is ${req.app.get('views')}`)\n}\n```\n\n### req.baseUrl\n\nThe URL path on which a router instance was mounted.\n\nThe `req.baseUrl` property is similar to the [mountpath](https://expressjs.com/en/5x/api.html#app.mountpath) property of the `app` object, except `app.mountpath` returns the matched path pattern(s).\n\nFor example:\n\n```\nconst greet = express.Router()\n\ngreet.get('/jp', (req, res) => {\n console.log(req.baseUrl) // /greet\n res.send('Konichiwa!')\n})\n\napp.use('/greet', greet) // load the router on '/greet'\n```\n\nEven if you use a path pattern or a set of path patterns to load the router, the `baseUrl` property returns the matched string, not the pattern(s). In the following example, the `greet` router is loaded on two path patterns.\n\n```\napp.use(['/gre+t', '/hel{2}o'], greet) // load the router on '/gre+t' and '/hel{2}o'\n```\n\nWhen a request is made to `/greet/jp`, `req.baseUrl` is “/greet”. When a request is made to `/hello/jp`, `req.baseUrl` is “/hello”.\n\n### req.body\n\nContains key-value pairs of data submitted in the request body. By default, it is `undefined`, and is populated when you use body-parsing middleware such as [body-parser](https://www.npmjs.org/package/body-parser) and [multer](https://www.npmjs.org/package/multer).\n\nAs `req.body`’s shape is based on user-controlled input, all properties and values in this object are untrusted and should be validated before trusting. For example, `req.body.foo.toString()` may fail in multiple ways, for example `foo` may not be there or may not be a string, and `toString` may not be a function and instead a string or other user-input.\n\nThe following example shows how to use body-parsing middleware to populate `req.body`.\n\n```\nconst app = require('express')()\nconst bodyParser = require('body-parser')\nconst multer = require('multer') // v1.0.5\nconst upload = multer() // for parsing multipart/form-data\n\napp.use(bodyParser.json()) // for parsing application/json\napp.use(bodyParser.urlencoded({ extended: true })) // for parsing application/x-www-form-urlencoded\n\napp.post('/profile', upload.array(), (req, res, next) => {\n console.log(req.body)\n res.json(req.body)\n})\n```\n\n### req.cookies\n\nWhen using [cookie-parser](https://www.npmjs.com/package/cookie-parser) middleware, this property is an object that contains cookies sent by the request. If the request contains no cookies, it defaults to `{}`.\n\n```\n// Cookie: name=tj\nconsole.dir(req.cookies.name)\n// => \"tj\"\n```\n\nIf the cookie has been signed, you have to use [req.signedCookies](https://expressjs.com/en/5x/api.html#req.signedCookies).\n\nFor more information, issues, or concerns, see [cookie-parser](https://github.com/expressjs/cookie-parser).\n\n### req.fresh\n\nWhen the response is still “fresh” in the client’s cache `true` is returned, otherwise `false` is returned to indicate that the client cache is now stale and the full response should be sent.\n\nWhen a client sends the `Cache-Control: no-cache` request header to indicate an end-to-end reload request, this module will return `false` to make handling these requests transparent.\n\nFurther details for how cache validation works can be found in the [HTTP/1.1 Caching Specification](https://tools.ietf.org/html/rfc7234).\n\n```\nconsole.dir(req.fresh)\n// => true\n```\n\n### req.host\n\nContains the host derived from the `Host` HTTP header.\n\nWhen the [`trust proxy` setting](https://expressjs.com/en/5x/api.html#app.settings.table) does not evaluate to `false`, this property will instead get the value from the `X-Forwarded-Host` header field. This header can be set by the client or by the proxy.\n\nIf there is more than one `X-Forwarded-Host` header in the request, the value of the first header is used. This includes a single header with comma-separated values, in which the first value is used.\n\n```\n// Host: \"example.com:3000\"\nconsole.dir(req.host)\n// => 'example.com:3000'\n\n// Host: \"[::1]:3000\"\nconsole.dir(req.host)\n// => '[::1]:3000'\n```\n\n### req.hostname\n\nContains the hostname derived from the `Host` HTTP header.\n\nWhen the [`trust proxy` setting](https://expressjs.com/5x/api.html#trust.proxy.options.table) does not evaluate to `false`, this property will instead get the value from the `X-Forwarded-Host` header field. This header can be set by the client or by the proxy.\n\nIf there is more than one `X-Forwarded-Host` header in the request, the value of the first header is used. This includes a single header with comma-separated values, in which the first value is used.\n\nPrior to Express v4.17.0, the `X-Forwarded-Host` could not contain multiple values or be present more than once.\n\n```\n// Host: \"example.com:3000\"\nconsole.dir(req.hostname)\n// => 'example.com'\n```\n\n### req.ip\n\nContains the remote IP address of the request.\n\nWhen the [`trust proxy` setting](https://expressjs.com/5x/api.html#trust.proxy.options.table) does not evaluate to `false`, the value of this property is derived from the left-most entry in the `X-Forwarded-For` header. This header can be set by the client or by the proxy.\n\n```\nconsole.dir(req.ip)\n// => \"127.0.0.1\"\n```\n\n### req.ips\n\nWhen the [`trust proxy` setting](https://expressjs.com/5x/api.html#trust.proxy.options.table) does not evaluate to `false`, this property contains an array of IP addresses specified in the `X-Forwarded-For` request header. Otherwise, it contains an empty array. This header can be set by the client or by the proxy.\n\nFor example, if `X-Forwarded-For` is `client, proxy1, proxy2`, `req.ips` would be `[\"client\", \"proxy1\", \"proxy2\"]`, where `proxy2` is the furthest downstream.\n\n### req.method\n\nContains a string corresponding to the HTTP method of the request: `GET`, `POST`, `PUT`, and so on.\n\n### req.originalUrl\n\n`req.url` is not a native Express property, it is inherited from Node’s [http module](https://nodejs.org/api/http.html#http_message_url).\n\nThis property is much like `req.url`; however, it retains the original request URL, allowing you to rewrite `req.url` freely for internal routing purposes. For example, the “mounting” feature of [app.use()](https://expressjs.com/en/5x/api.html#app.use) will rewrite `req.url` to strip the mount point.\n\n```\n// GET /search?q=something\nconsole.dir(req.originalUrl)\n// => \"/search?q=something\"\n```\n\n`req.originalUrl` is available both in middleware and router objects, and is a combination of `req.baseUrl` and `req.url`. Consider following example:\n\n```\n// GET 'http://www.example.com/admin/new?sort=desc'\napp.use('/admin', (req, res, next) => {\n console.dir(req.originalUrl) // '/admin/new?sort=desc'\n console.dir(req.baseUrl) // '/admin'\n console.dir(req.path) // '/new'\n next()\n})\n```\n\n### req.params\n\nThis property is an object containing properties mapped to the [named route “parameters”](https://expressjs.com/en/guide/routing.html#route-parameters). For example, if you have the route `/user/:name`, then the “name” property is available as `req.params.name`. This object defaults to `{}`.\n\n```\n// GET /user/tj\nconsole.dir(req.params.name)\n// => \"tj\"\n```\n\nWhen you use a regular expression for the route definition, capture groups are provided in the array using `req.params[n]`, where `n` is the nth capture group. This rule is applied to unnamed wild card matches with string routes such as `/file/*`:\n\n```\n// GET /file/javascripts/jquery.js\nconsole.dir(req.params[0])\n// => \"javascripts/jquery.js\"\n```\n\nIf you need to make changes to a key in `req.params`, use the [app.param](https://expressjs.com/en/5x/api.html#app.param) handler. Changes are applicable only to [parameters](https://expressjs.com/en/guide/routing.html#route-parameters) already defined in the route path.\n\nAny changes made to the `req.params` object in a middleware or route handler will be reset.\n\nNOTE: Express automatically decodes the values in `req.params` (using `decodeURIComponent`).\n\n### req.path\n\nContains the path part of the request URL.\n\n```\n// example.com/users?sort=desc\nconsole.dir(req.path)\n// => \"/users\"\n```\n\nWhen called from a middleware, the mount point is not included in `req.path`. See [app.use()](https://expressjs.com/5x/api.html#app.use) for more details.\n\n### req.protocol\n\nContains the request protocol string: either `http` or (for TLS requests) `https`.\n\nWhen the [`trust proxy` setting](https://expressjs.com/en/5x/api.html#trust.proxy.options.table) does not evaluate to `false`, this property will use the value of the `X-Forwarded-Proto` header field if present. This header can be set by the client or by the proxy.\n\n```\nconsole.dir(req.protocol)\n// => \"http\"\n```\n\n### req.query\n\nThis property is an object containing a property for each query string parameter in the route. When [query parser](https://expressjs.com/en/5x/api.html#app.settings.table) is set to disabled, it is an empty object `{}`, otherwise it is the result of the configured query parser.\n\nAs `req.query`’s shape is based on user-controlled input, all properties and values in this object are untrusted and should be validated before trusting. For example, `req.query.foo.toString()` may fail in multiple ways, for example `foo` may not be there or may not be a string, and `toString` may not be a function and instead a string or other user-input.\n\nThe value of this property can be configured with the [query parser application setting](https://expressjs.com/en/5x/api.html#app.settings.table) to work how your application needs it. A very popular query string parser is the [`qs` module](https://www.npmjs.org/package/qs), and this is used by default. The `qs` module is very configurable with many settings, and it may be desirable to use different settings than the default to populate `req.query`:\n\n```\nconst qs = require('qs')\napp.set('query parser',\n (str) => qs.parse(str, { /* custom options */ }))\n```\n\nCheck out the [query parser application setting](https://expressjs.com/en/5x/api.html#app.settings.table) documentation for other customization options.\n\n### req.res\n\nThis property holds a reference to the [response object](https://expressjs.com/en/5x/api.html#res) that relates to this request object.\n\n### req.route\n\nContains the currently-matched route, a string. For example:\n\n```\napp.get('/user/:id?', (req, res) => {\n console.log(req.route)\n res.send('GET')\n})\n```\n\nExample output from the previous snippet:\n\n```\n{ path: '/user/:id?',\n stack:\n [ { handle: [Function: userIdHandler],\n name: 'userIdHandler',\n params: undefined,\n path: undefined,\n keys: [],\n regexp: /^\\/?$/i,\n method: 'get' } ],\n methods: { get: true }\n}\n```\n\n### req.secure\n\nA Boolean property that is true if a TLS connection is established. Equivalent to the following:\n\n```\nreq.protocol === 'https'\n```\n\n### req.signedCookies\n\nWhen using [cookie-parser](https://www.npmjs.com/package/cookie-parser) middleware, this property contains signed cookies sent by the request, unsigned and ready for use. Signed cookies reside in a different object to show developer intent; otherwise, a malicious attack could be placed on `req.cookie` values (which are easy to spoof). Note that signing a cookie does not make it “hidden” or encrypted; but simply prevents tampering (because the secret used to sign is private).\n\nIf no signed cookies are sent, the property defaults to `{}`.\n\n```\n// Cookie: user=tobi.CP7AWaXDfAKIRfH49dQzKJx7sKzzSoPq7/AcBBRVwlI3\nconsole.dir(req.signedCookies.user)\n// => \"tobi\"\n```\n\nFor more information, issues, or concerns, see [cookie-parser](https://github.com/expressjs/cookie-parser).\n\n### req.stale\n\nIndicates whether the request is “stale,” and is the opposite of `req.fresh`. For more information, see [req.fresh](https://expressjs.com/en/5x/api.html#req.fresh).\n\n```\nconsole.dir(req.stale)\n// => true\n```\n\n### req.subdomains\n\nAn array of subdomains in the domain name of the request.\n\n```\n// Host: \"tobi.ferrets.example.com\"\nconsole.dir(req.subdomains)\n// => [\"ferrets\", \"tobi\"]\n```\n\nThe application property `subdomain offset`, which defaults to 2, is used for determining the beginning of the subdomain segments. To change this behavior, change its value using [app.set](https://expressjs.com/en/5x/api.html#app.set).\n\n### req.xhr\n\nA Boolean property that is `true` if the request’s `X-Requested-With` header field is “XMLHttpRequest”, indicating that the request was issued by a client library such as jQuery.\n\n```\nconsole.dir(req.xhr)\n// => true\n```\n\n### Methods\n\n### req.accepts(types)\n\nChecks if the specified content types are acceptable, based on the request’s `Accept` HTTP header field. The method returns the best match, or if none of the specified content types is acceptable, returns `false` (in which case, the application should respond with `406 \"Not Acceptable\"`).\n\nThe `type` value may be a single MIME type string (such as “application/json”), an extension name such as “json”, a comma-delimited list, or an array. For a list or array, the method returns the _best_ match (if any).\n\n```\n// Accept: text/html\nreq.accepts('html')\n// => \"html\"\n\n// Accept: text/*, application/json\nreq.accepts('html')\n// => \"html\"\nreq.accepts('text/html')\n// => \"text/html\"\nreq.accepts(['json', 'text'])\n// => \"json\"\nreq.accepts('application/json')\n// => \"application/json\"\n\n// Accept: text/*, application/json\nreq.accepts('image/png')\nreq.accepts('png')\n// => false\n\n// Accept: text/*;q=.5, application/json\nreq.accepts(['html', 'json'])\n// => \"json\"\n```\n\nFor more information, or if you have issues or concerns, see [accepts](https://github.com/expressjs/accepts).\n\n### req.acceptsCharsets(charset \\[, ...\\])\n\nReturns the first accepted charset of the specified character sets, based on the request’s `Accept-Charset` HTTP header field. If none of the specified charsets is accepted, returns `false`.\n\nFor more information, or if you have issues or concerns, see [accepts](https://github.com/expressjs/accepts).\n\n### req.acceptsEncodings(encoding \\[, ...\\])\n\nReturns the first accepted encoding of the specified encodings, based on the request’s `Accept-Encoding` HTTP header field. If none of the specified encodings is accepted, returns `false`.\n\nFor more information, or if you have issues or concerns, see [accepts](https://github.com/expressjs/accepts).\n\n### req.acceptsLanguages(lang \\[, ...\\])\n\nReturns the first accepted language of the specified languages, based on the request’s `Accept-Language` HTTP header field. If none of the specified languages is accepted, returns `false`.\n\nFor more information, or if you have issues or concerns, see [accepts](https://github.com/expressjs/accepts).\n\n### req.get(field)\n\nReturns the specified HTTP request header field (case-insensitive match). The `Referrer` and `Referer` fields are interchangeable.\n\n```\nreq.get('Content-Type')\n// => \"text/plain\"\n\nreq.get('content-type')\n// => \"text/plain\"\n\nreq.get('Something')\n// => undefined\n```\n\nAliased as `req.header(field)`.\n\n### req.is(type)\n\nReturns the matching content type if the incoming request’s “Content-Type” HTTP header field matches the MIME type specified by the `type` parameter. If the request has no body, returns `null`. Returns `false` otherwise.\n\n```\n// With Content-Type: text/html; charset=utf-8\nreq.is('html') // => 'html'\nreq.is('text/html') // => 'text/html'\nreq.is('text/*') // => 'text/*'\n\n// When Content-Type is application/json\nreq.is('json') // => 'json'\nreq.is('application/json') // => 'application/json'\nreq.is('application/*') // => 'application/*'\n\nreq.is('html')\n// => false\n```\n\nFor more information, or if you have issues or concerns, see [type-is](https://github.com/expressjs/type-is).\n\n### req.range(size\\[, options\\])\n\n`Range` header parser.\n\nThe `size` parameter is the maximum size of the resource.\n\nThe `options` parameter is an object that can have the following properties.\n\n| Property | Type | Description |\n| --- | --- | --- |\n| `combine` | Boolean | Specify if overlapping & adjacent ranges should be combined, defaults to `false`. When `true`, ranges will be combined and returned as if they were specified that way in the header. |\n\nAn array of ranges will be returned or negative numbers indicating an error parsing.\n\n* `-2` signals a malformed header string\n* `-1` signals an unsatisfiable range\n\n```\n// parse header from request\nconst range = req.range(1000)\n\n// the type of the range\nif (range.type === 'bytes') {\n // the ranges\n range.forEach((r) => {\n // do something with r.start and r.end\n })\n}\n```\n\nResponse\n--------\n\nThe `res` object represents the HTTP response that an Express app sends when it gets an HTTP request.\n\nIn this documentation and by convention, the object is always referred to as `res` (and the HTTP request is `req`) but its actual name is determined by the parameters to the callback function in which you’re working.\n\nFor example:\n\n```\napp.get('/user/:id', (req, res) => {\n res.send(`user ${req.params.id}`)\n})\n```\n\nBut you could just as well have:\n\n```\napp.get('/user/:id', (request, response) => {\n response.send(`user ${request.params.id}`)\n})\n```\n\nThe `res` object is an enhanced version of Node’s own response object and supports all [built-in fields and methods](https://nodejs.org/api/http.html#http_class_http_serverresponse).\n\n### Properties\n\n### res.app\n\nThis property holds a reference to the instance of the Express application that is using the middleware.\n\n`res.app` is identical to the [req.app](https://expressjs.com/en/5x/api.html#req.app) property in the request object.\n\nBoolean property that indicates if the app sent HTTP headers for the response.\n\n```\napp.get('/', (req, res) => {\n console.log(res.headersSent) // false\n res.send('OK')\n console.log(res.headersSent) // true\n})\n```\n\n### res.locals\n\nUse this property to set variables accessible in templates rendered with [res.render](https://expressjs.com/en/5x/api.html#res.render). The variables set on `res.locals` are available within a single request-response cycle, and will not be shared between requests.\n\nIn order to keep local variables for use in template rendering between requests, use [app.locals](https://expressjs.com/en/5x/api.html#app.locals) instead.\n\nThis property is useful for exposing request-level information such as the request path name, authenticated user, user settings, and so on to templates rendered within the application.\n\n```\napp.use((req, res, next) => {\n // Make `user` and `authenticated` available in templates\n res.locals.user = req.user\n res.locals.authenticated = !req.user.anonymous\n next()\n})\n```\n\n### res.req\n\nThis property holds a reference to the [request object](https://expressjs.com/en/5x/api.html#req) that relates to this response object.\n\n### Methods\n\n### res.append(field \\[, value\\])\n\n`res.append()` is supported by Express v4.11.0+\n\nAppends the specified `value` to the HTTP response header `field`. If the header is not already set, it creates the header with the specified value. The `value` parameter can be a string or an array.\n\nNote: calling `res.set()` after `res.append()` will reset the previously-set header value.\n\n```\nres.append('Link', ['<http://localhost/>', '<http://localhost:3000/>'])\nres.append('Set-Cookie', 'foo=bar; Path=/; HttpOnly')\nres.append('Warning', '199 Miscellaneous warning')\n```\n\n### res.attachment(\\[filename\\])\n\nSets the HTTP response `Content-Disposition` header field to “attachment”. If a `filename` is given, then it sets the `Content-Type` based on the extension name via `res.type()`, and sets the `Content-Disposition` “filename=” parameter.\n\n```\nres.attachment()\n// Content-Disposition: attachment\n\nres.attachment('path/to/logo.png')\n// Content-Disposition: attachment; filename=\"logo.png\"\n// Content-Type: image/png\n```\n\n### res.cookie(name, value \\[, options\\])\n\nSets cookie `name` to `value`. The `value` parameter may be a string or object converted to JSON.\n\nThe `options` parameter is an object that can have the following properties.\n\n| Property | Type | Description |\n| --- | --- | --- |\n| `domain` | String | Domain name for the cookie. Defaults to the domain name of the app. |\n| `encode` | Function | A synchronous function used for cookie value encoding. Defaults to `encodeURIComponent`. |\n| `expires` | Date | Expiry date of the cookie in GMT. If not specified or set to 0, creates a session cookie. |\n| `httpOnly` | Boolean | Flags the cookie to be accessible only by the web server. |\n| `maxAge` | Number | Convenient option for setting the expiry time relative to the current time in milliseconds. |\n| `path` | String | Path for the cookie. Defaults to “/”. |\n| `secure` | Boolean | Marks the cookie to be used with HTTPS only. |\n| `signed` | Boolean | Indicates if the cookie should be signed. |\n| `sameSite` | Boolean or String | Value of the “SameSite” **Set-Cookie** attribute. More information at [https://tools.ietf.org/html/draft-ietf-httpbis-cookie-same-site-00#section-4.1.1](https://tools.ietf.org/html/draft-ietf-httpbis-cookie-same-site-00#section-4.1.1). |\n\nAll `res.cookie()` does is set the HTTP `Set-Cookie` header with the options provided. Any option not specified defaults to the value stated in [RFC 6265](http://tools.ietf.org/html/rfc6265).\n\nFor example:\n\n```\nres.cookie('name', 'tobi', { domain: '.example.com', path: '/admin', secure: true })\nres.cookie('rememberme', '1', { expires: new Date(Date.now() + 900000), httpOnly: true })\n```\n\nThe `encode` option allows you to choose the function used for cookie value encoding. Does not support asynchronous functions.\n\nExample use case: You need to set a domain-wide cookie for another site in your organization. This other site (not under your administrative control) does not use URI-encoded cookie values.\n\n```\n// Default encoding\nres.cookie('some_cross_domain_cookie', 'http://mysubdomain.example.com', { domain: 'example.com' })\n// Result: 'some_cross_domain_cookie=http%3A%2F%2Fmysubdomain.example.com; Domain=example.com; Path=/'\n\n// Custom encoding\nres.cookie('some_cross_domain_cookie', 'http://mysubdomain.example.com', { domain: 'example.com', encode: String })\n// Result: 'some_cross_domain_cookie=http://mysubdomain.example.com; Domain=example.com; Path=/;'\n```\n\nThe `maxAge` option is a convenience option for setting “expires” relative to the current time in milliseconds. The following is equivalent to the second example above.\n\n```\nres.cookie('rememberme', '1', { maxAge: 900000, httpOnly: true })\n```\n\nYou can pass an object as the `value` parameter; it is then serialized as JSON and parsed by `bodyParser()` middleware.\n\n```\nres.cookie('cart', { items: [1, 2, 3] })\nres.cookie('cart', { items: [1, 2, 3] }, { maxAge: 900000 })\n```\n\nWhen using [cookie-parser](https://www.npmjs.com/package/cookie-parser) middleware, this method also supports signed cookies. Simply include the `signed` option set to `true`. Then, `res.cookie()` will use the secret passed to `cookieParser(secret)` to sign the value.\n\n```\nres.cookie('name', 'tobi', { signed: true })\n```\n\nLater, you may access this value through the [req.signedCookies](https://expressjs.com/en/5x/api.html#req.signedCookies) object.\n\n### res.clearCookie(name \\[, options\\])\n\nClears the cookie specified by `name`. For details about the `options` object, see [res.cookie()](https://expressjs.com/en/5x/api.html#res.cookie).\n\nWeb browsers and other compliant clients will only clear the cookie if the given `options` is identical to those given to [res.cookie()](https://expressjs.com/en/5x/api.html#res.cookie), excluding `expires` and `maxAge`.\n\n```\nres.cookie('name', 'tobi', { path: '/admin' })\nres.clearCookie('name', { path: '/admin' })\n```\n\n### res.download(path \\[, filename\\] \\[, options\\] \\[, fn\\])\n\nThe optional `options` argument is supported by Express v4.16.0 onwards.\n\nTransfers the file at `path` as an “attachment”. Typically, browsers will prompt the user for download. By default, the `Content-Disposition` header “filename=” parameter is derived from the `path` argument, but can be overridden with the `filename` parameter. If `path` is relative, then it will be based on the current working directory of the process.\n\nThe following table provides details on the `options` parameter.\n\nThe optional `options` argument is supported by Express v4.16.0 onwards.\n\nThe method invokes the callback function `fn(err)` when the transfer is complete or when an error occurs. If the callback function is specified and an error occurs, the callback function must explicitly handle the response process either by ending the request-response cycle, or by passing control to the next route.\n\n```\nres.download('/report-12345.pdf')\n\nres.download('/report-12345.pdf', 'report.pdf')\n\nres.download('/report-12345.pdf', 'report.pdf', (err) => {\n if (err) {\n // Handle error, but keep in mind the response may be partially-sent\n // so check res.headersSent\n } else {\n // decrement a download credit, etc.\n }\n})\n```\n\n### res.end(\\[data\\] \\[, encoding\\])\n\nEnds the response process. This method actually comes from Node core, specifically the [response.end() method of http.ServerResponse](https://nodejs.org/api/http.html#http_response_end_data_encoding_callback).\n\nUse to quickly end the response without any data. If you need to respond with data, instead use methods such as [res.send()](https://expressjs.com/en/5x/api.html#res.send) and [res.json()](https://expressjs.com/en/5x/api.html#res.json).\n\n```\nres.end()\nres.status(404).end()\n```\n\n### res.format(object)\n\nPerforms content-negotiation on the `Accept` HTTP header on the request object, when present. It uses [req.accepts()](https://expressjs.com/en/5x/api.html#req.accepts) to select a handler for the request, based on the acceptable types ordered by their quality values. If the header is not specified, the first callback is invoked. When no match is found, the server responds with 406 “Not Acceptable”, or invokes the `default` callback.\n\nThe `Content-Type` response header is set when a callback is selected. However, you may alter this within the callback using methods such as `res.set()` or `res.type()`.\n\nThe following example would respond with `{ \"message\": \"hey\" }` when the `Accept` header field is set to “application/json” or “\\*/json” (however, if it is “\\*/\\*”, then the response will be “hey”).\n\n```\nres.format({\n 'text/plain' () {\n res.send('hey')\n },\n\n 'text/html' () {\n res.send('<p>hey</p>')\n },\n\n 'application/json' () {\n res.send({ message: 'hey' })\n },\n\n default () {\n // log the request and respond with 406\n res.status(406).send('Not Acceptable')\n }\n})\n```\n\nIn addition to canonicalized MIME types, you may also use extension names mapped to these types for a slightly less verbose implementation:\n\n```\nres.format({\n text () {\n res.send('hey')\n },\n\n html () {\n res.send('<p>hey</p>')\n },\n\n json () {\n res.send({ message: 'hey' })\n }\n})\n```\n\n### res.get(field)\n\nReturns the HTTP response header specified by `field`. The match is case-insensitive.\n\n```\nres.get('Content-Type')\n// => \"text/plain\"\n```\n\n### res.json(\\[body\\])\n\nSends a JSON response. This method sends a response (with the correct content-type) that is the parameter converted to a JSON string using [JSON.stringify()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON/stringify).\n\nThe parameter can be any JSON type, including object, array, string, Boolean, number, or null, and you can also use it to convert other values to JSON.\n\n```\nres.json(null)\nres.json({ user: 'tobi' })\nres.status(500).json({ error: 'message' })\n```\n\n### res.jsonp(\\[body\\])\n\nSends a JSON response with JSONP support. This method is identical to `res.json()`, except that it opts-in to JSONP callback support.\n\n```\nres.jsonp(null)\n// => callback(null)\n\nres.jsonp({ user: 'tobi' })\n// => callback({ \"user\": \"tobi\" })\n\nres.status(500).jsonp({ error: 'message' })\n// => callback({ \"error\": \"message\" })\n```\n\nBy default, the JSONP callback name is simply `callback`. Override this with the [jsonp callback name](https://expressjs.com/en/5x/api.html#app.settings.table) setting.\n\nThe following are some examples of JSONP responses using the same code:\n\n```\n// ?callback=foo\nres.jsonp({ user: 'tobi' })\n// => foo({ \"user\": \"tobi\" })\n\napp.set('jsonp callback name', 'cb')\n\n// ?cb=foo\nres.status(500).jsonp({ error: 'message' })\n// => foo({ \"error\": \"message\" })\n```\n\n### res.links(links)\n\nJoins the `links` provided as properties of the parameter to populate the response’s `Link` HTTP header field.\n\nFor example, the following call:\n\n```\nres.links({\n next: 'http://api.example.com/users?page=2',\n last: 'http://api.example.com/users?page=5'\n})\n```\n\nYields the following results:\n\n```\nLink: <http://api.example.com/users?page=2>; rel=\"next\",\n <http://api.example.com/users?page=5>; rel=\"last\"\n```\n\n### res.location(path)\n\nSets the response `Location` HTTP header to the specified `path` parameter.\n\n```\nres.location('/foo/bar')\nres.location('http://example.com')\nres.location('back')\n```\n\nA `path` value of “back” has a special meaning, it refers to the URL specified in the `Referer` header of the request. If the `Referer` header was not specified, it refers to “/”.\n\nSee also [Security best practices: Prevent open redirect vulnerabilities](http://expressjs.com/en/advanced/best-practice-security.html#prevent-open-redirects).\n\nAfter encoding the URL, if not encoded already, Express passes the specified URL to the browser in the `Location` header, without any validation.\n\nBrowsers take the responsibility of deriving the intended URL from the current URL or the referring URL, and the URL specified in the `Location` header; and redirect the user accordingly.\n\n### res.redirect(\\[status,\\] path)\n\nRedirects to the URL derived from the specified `path`, with specified `status`, a positive integer that corresponds to an [HTTP status code](http://www.w3.org/Protocols/rfc2616/rfc2616-sec10.html). If not specified, `status` defaults to `302 \"Found\"`.\n\n```\nres.redirect('/foo/bar')\nres.redirect('http://example.com')\nres.redirect(301, 'http://example.com')\nres.redirect('../login')\n```\n\nRedirects can be a fully-qualified URL for redirecting to a different site:\n\n```\nres.redirect('http://google.com')\n```\n\nRedirects can be relative to the root of the host name. For example, if the application is on `http://example.com/admin/post/new`, the following would redirect to the URL `http://example.com/admin`:\n\n```\nres.redirect('/admin')\n```\n\nRedirects can be relative to the current URL. For example, from `http://example.com/blog/admin/` (notice the trailing slash), the following would redirect to the URL `http://example.com/blog/admin/post/new`.\n\n```\nres.redirect('post/new')\n```\n\nRedirecting to `post/new` from `http://example.com/blog/admin` (no trailing slash), will redirect to `http://example.com/blog/post/new`.\n\nIf you found the above behavior confusing, think of path segments as directories (with trailing slashes) and files, it will start to make sense.\n\nPath-relative redirects are also possible. If you were on `http://example.com/admin/post/new`, the following would redirect to `http://example.com/admin/post`:\n\n```\nres.redirect('..')\n```\n\nA `back` redirection redirects the request back to the [referer](http://en.wikipedia.org/wiki/HTTP_referer), defaulting to `/` when the referer is missing.\n\n```\nres.redirect('back')\n```\n\nSee also [Security best practices: Prevent open redirect vulnerabilities](http://expressjs.com/en/advanced/best-practice-security.html#prevent-open-redirects).\n\n### res.render(view \\[, locals\\] \\[, callback\\])\n\nRenders a `view` and sends the rendered HTML string to the client. Optional parameters:\n\n* `locals`, an object whose properties define local variables for the view.\n* `callback`, a callback function. If provided, the method returns both the possible error and rendered string, but does not perform an automated response. When an error occurs, the method invokes `next(err)` internally.\n\nThe `view` argument is a string that is the file path of the view file to render. This can be an absolute path, or a path relative to the `views` setting. If the path does not contain a file extension, then the `view engine` setting determines the file extension. If the path does contain a file extension, then Express will load the module for the specified template engine (via `require()`) and render it using the loaded module’s `__express` function.\n\nFor more information, see [Using template engines with Express](https://expressjs.com/en/guide/using-template-engines.html).\n\n**NOTE:** The `view` argument performs file system operations like reading a file from disk and evaluating Node.js modules, and as so for security reasons should not contain input from the end-user.\n\nThe local variable `cache` enables view caching. Set it to `true`, to cache the view during development; view caching is enabled in production by default.\n\n```\n// send the rendered view to the client\nres.render('index')\n\n// if a callback is specified, the rendered HTML string has to be sent explicitly\nres.render('index', (err, html) => {\n res.send(html)\n})\n\n// pass a local variable to the view\nres.render('user', { name: 'Tobi' }, (err, html) => {\n // ...\n})\n```\n\n### res.send(\\[body\\])\n\nSends the HTTP response.\n\nThe `body` parameter can be a `Buffer` object, a `String`, an object, `Boolean`, or an `Array`. For example:\n\n```\nres.send(Buffer.from('whoop'))\nres.send({ some: 'json' })\nres.send('<p>some html</p>')\nres.status(404).send('Sorry, we cannot find that!')\nres.status(500).send({ error: 'something blew up' })\n```\n\nThis method performs many useful tasks for simple non-streaming responses: For example, it automatically assigns the `Content-Length` HTTP response header field and provides automatic HEAD and HTTP cache freshness support.\n\nWhen the parameter is a `Buffer` object, the method sets the `Content-Type` response header field to “application/octet-stream”, unless previously defined as shown below:\n\n```\nres.set('Content-Type', 'text/html')\nres.send(Buffer.from('<p>some html</p>'))\n```\n\nWhen the parameter is a `String`, the method sets the `Content-Type` to “text/html”:\n\n```\nres.send('<p>some html</p>')\n```\n\nWhen the parameter is an `Array` or `Object`, Express responds with the JSON representation:\n\n```\nres.send({ user: 'tobi' })\nres.send([1, 2, 3])\n```\n\n### res.sendFile(path \\[, options\\] \\[, fn\\])\n\n`res.sendFile()` is supported by Express v4.8.0 onwards.\n\nTransfers the file at the given `path`. Sets the `Content-Type` response HTTP header field based on the filename’s extension. Unless the `root` option is set in the options object, `path` must be an absolute path to the file.\n\nThis API provides access to data on the running file system. Ensure that either (a) the way in which the `path` argument was constructed into an absolute path is secure if it contains user input or (b) set the `root` option to the absolute path of a directory to contain access within.\n\nWhen the `root` option is provided, the `path` argument is allowed to be a relative path, including containing `..`. Express will validate that the relative path provided as `path` will resolve within the given `root` option.\n\nThe following table provides details on the `options` parameter.\n\nThe method invokes the callback function `fn(err)` when the transfer is complete or when an error occurs. If the callback function is specified and an error occurs, the callback function must explicitly handle the response process either by ending the request-response cycle, or by passing control to the next route.\n\nHere is an example of using `res.sendFile` with all its arguments.\n\n```\napp.get('/file/:name', (req, res, next) => {\n const options = {\n root: path.join(__dirname, 'public'),\n dotfiles: 'deny',\n headers: {\n 'x-timestamp': Date.now(),\n 'x-sent': true\n }\n }\n\n const fileName = req.params.name\n res.sendFile(fileName, options, (err) => {\n if (err) {\n next(err)\n } else {\n console.log('Sent:', fileName)\n }\n })\n})\n```\n\nThe following example illustrates using `res.sendFile` to provide fine-grained support for serving files:\n\n```\napp.get('/user/:uid/photos/:file', (req, res) => {\n const uid = req.params.uid\n const file = req.params.file\n\n req.user.mayViewFilesFrom(uid, (yes) => {\n if (yes) {\n res.sendFile(`/uploads/${uid}/${file}`)\n } else {\n res.status(403).send(\"Sorry! You can't see that.\")\n }\n })\n})\n```\n\nFor more information, or if you have issues or concerns, see [send](https://github.com/pillarjs/send).\n\n### res.sendStatus(statusCode)\n\nSets the response HTTP status code to `statusCode` and sends the registered status message as the text response body. If an unknown status code is specified, the response body will just be the code number.\n\n```\nres.sendStatus(404)\n```\n\nSome versions of Node.js will throw when `res.statusCode` is set to an invalid HTTP status code (outside of the range `100` to `599`). Consult the HTTP server documentation for the Node.js version being used.\n\n[More about HTTP Status Codes](http://en.wikipedia.org/wiki/List_of_HTTP_status_codes)\n\n### res.set(field \\[, value\\])\n\nSets the response’s HTTP header `field` to `value`. To set multiple fields at once, pass an object as the parameter.\n\n```\nres.set('Content-Type', 'text/plain')\n\nres.set({\n 'Content-Type': 'text/plain',\n 'Content-Length': '123',\n ETag: '12345'\n})\n```\n\nAliased as `res.header(field [, value])`.\n\n### res.status(code)\n\nSets the HTTP status for the response. It is a chainable alias of Node’s [response.statusCode](http://nodejs.org/api/http.html#http_response_statuscode).\n\n```\nres.status(403).end()\nres.status(400).send('Bad Request')\nres.status(404).sendFile('/absolute/path/to/404.png')\n```\n\n### res.type(type)\n\nSets the `Content-Type` HTTP header to the MIME type as determined by the specified `type`. If `type` contains the “/” character, then it sets the `Content-Type` to the exact value of `type`, otherwise it is assumed to be a file extension and the MIME type is looked up in a mapping using the `express.static.mime.lookup()` method.\n\n```\nres.type('.html') // => 'text/html'\nres.type('html') // => 'text/html'\nres.type('json') // => 'application/json'\nres.type('application/json') // => 'application/json'\nres.type('png') // => image/png:\n```\n\n### res.vary(field)\n\nAdds the field to the `Vary` response header, if it is not there already.\n\n```\nres.vary('User-Agent').render('docs')\n```\n\nRouter\n------\n\nA `router` object is an isolated instance of middleware and routes. You can think of it as a “mini-application,” capable only of performing middleware and routing functions. Every Express application has a built-in app router.\n\nA router behaves like middleware itself, so you can use it as an argument to [app.use()](https://expressjs.com/en/5x/api.html#app.use) or as the argument to another router’s [use()](https://expressjs.com/en/5x/api.html#router.use) method.\n\nThe top-level `express` object has a [Router()](https://expressjs.com/en/5x/api.html#express.router) method that creates a new `router` object.\n\nOnce you’ve created a router object, you can add middleware and HTTP method routes (such as `get`, `put`, `post`, and so on) to it just like an application. For example:\n\n```\n// invoked for any requests passed to this router\nrouter.use((req, res, next) => {\n // .. some logic here .. like any other middleware\n next()\n})\n\n// will handle any request that ends in /events\n// depends on where the router is \"use()'d\"\nrouter.get('/events', (req, res, next) => {\n // ..\n})\n```\n\nYou can then use a router for a particular root URL in this way separating your routes into files or even mini-apps.\n\n```\n// only requests to /calendar/* will be sent to our \"router\"\napp.use('/calendar', router)\n```\n\n### Methods\n\n### router.all(path, \\[callback, ...\\] callback)\n\nThis method is just like the `router.METHOD()` methods, except that it matches all HTTP methods (verbs).\n\nThis method is extremely useful for mapping “global” logic for specific path prefixes or arbitrary matches. For example, if you placed the following route at the top of all other route definitions, it would require that all routes from that point on would require authentication, and automatically load a user. Keep in mind that these callbacks do not have to act as end points; `loadUser` can perform a task, then call `next()` to continue matching subsequent routes.\n\n```\nrouter.all('*', requireAuthentication, loadUser)\n```\n\nOr the equivalent:\n\n```\nrouter.all('*', requireAuthentication)\nrouter.all('*', loadUser)\n```\n\nAnother example of this is white-listed “global” functionality. Here, the example is much like before, but it only restricts paths prefixed with “/api”:\n\n```\nrouter.all('/api/*', requireAuthentication)\n```\n\n### router.METHOD(path, \\[callback, ...\\] callback)\n\nThe `router.METHOD()` methods provide the routing functionality in Express, where METHOD is one of the HTTP methods, such as GET, PUT, POST, and so on, in lowercase. Thus, the actual methods are `router.get()`, `router.post()`, `router.put()`, and so on.\n\nThe `router.get()` function is automatically called for the HTTP `HEAD` method in addition to the `GET` method if `router.head()` was not called for the path before `router.get()`.\n\nYou can provide multiple callbacks, and all are treated equally, and behave just like middleware, except that these callbacks may invoke `next('route')` to bypass the remaining route callback(s). You can use this mechanism to perform pre-conditions on a route then pass control to subsequent routes when there is no reason to proceed with the route matched.\n\nThe following snippet illustrates the most simple route definition possible. Express translates the path strings to regular expressions, used internally to match incoming requests. Query strings are _not_ considered when performing these matches, for example “GET /” would match the following route, as would “GET /?name=tobi”.\n\n```\nrouter.get('/', (req, res) => {\n res.send('hello world')\n})\n```\n\nYou can also use regular expressions—useful if you have very specific constraints, for example the following would match “GET /commits/71dbb9c” as well as “GET /commits/71dbb9c..4c084f9”.\n\n```\nrouter.get(/^\\/commits\\/(\\w+)(?:\\.\\.(\\w+))?$/, (req, res) => {\n const from = req.params[0]\n const to = req.params[1] || 'HEAD'\n res.send(`commit range ${from}..${to}`)\n})\n```\n\nYou can use `next` primitive to implement a flow control between different middleware functions, based on a specific program state. Invoking `next` with the string `'router'` will cause all the remaining route callbacks on that router to be bypassed.\n\nThe following example illustrates `next('router')` usage.\n\n```\nfunction fn (req, res, next) {\n console.log('I come here')\n next('router')\n}\nrouter.get('/foo', fn, (req, res, next) => {\n console.log('I dont come here')\n})\nrouter.get('/foo', (req, res, next) => {\n console.log('I dont come here')\n})\napp.get('/foo', (req, res) => {\n console.log(' I come here too')\n res.end('good')\n})\n```\n\n### router.param(name, callback)\n\nAdds callback triggers to route parameters, where `name` is the name of the parameter and `callback` is the callback function. Although `name` is technically optional, using this method without it is deprecated starting with Express v4.11.0 (see below).\n\nThe parameters of the callback function are:\n\n* `req`, the request object.\n* `res`, the response object.\n* `next`, indicating the next middleware function.\n* The value of the `name` parameter.\n* The name of the parameter.\n\nUnlike `app.param()`, `router.param()` does not accept an array of route parameters.\n\nFor example, when `:user` is present in a route path, you may map user loading logic to automatically provide `req.user` to the route, or perform validations on the parameter input.\n\n```\nrouter.param('user', (req, res, next, id) => {\n // try to get the user details from the User model and attach it to the request object\n User.find(id, (err, user) => {\n if (err) {\n next(err)\n } else if (user) {\n req.user = user\n next()\n } else {\n next(new Error('failed to load user'))\n }\n })\n})\n```\n\nParam callback functions are local to the router on which they are defined. They are not inherited by mounted apps or routers, nor are they triggered for route parameters inherited from parent routers. Hence, param callbacks defined on `router` will be triggered only by route parameters defined on `router` routes.\n\nA param callback will be called only once in a request-response cycle, even if the parameter is matched in multiple routes, as shown in the following examples.\n\n```\nrouter.param('id', (req, res, next, id) => {\n console.log('CALLED ONLY ONCE')\n next()\n})\n\nrouter.get('/user/:id', (req, res, next) => {\n console.log('although this matches')\n next()\n})\n\nrouter.get('/user/:id', (req, res) => {\n console.log('and this matches too')\n res.end()\n})\n```\n\nOn `GET /user/42`, the following is printed:\n\n```\nCALLED ONLY ONCE\nalthough this matches\nand this matches too\n```\n\nThe following section describes `router.param(callback)`, which is deprecated as of v4.11.0.\n\nThe behavior of the `router.param(name, callback)` method can be altered entirely by passing only a function to `router.param()`. This function is a custom implementation of how `router.param(name, callback)` should behave - it accepts two parameters and must return a middleware.\n\nThe first parameter of this function is the name of the URL parameter that should be captured, the second parameter can be any JavaScript object which might be used for returning the middleware implementation.\n\nThe middleware returned by the function decides the behavior of what happens when a URL parameter is captured.\n\nIn this example, the `router.param(name, callback)` signature is modified to `router.param(name, accessId)`. Instead of accepting a name and a callback, `router.param()` will now accept a name and a number.\n\n```\nconst express = require('express')\nconst app = express()\nconst router = express.Router()\n\n// customizing the behavior of router.param()\nrouter.param((param, option) => {\n return (req, res, next, val) => {\n if (val === option) {\n next()\n } else {\n res.sendStatus(403)\n }\n }\n})\n\n// using the customized router.param()\nrouter.param('id', 1337)\n\n// route to trigger the capture\nrouter.get('/user/:id', (req, res) => {\n res.send('OK')\n})\n\napp.use(router)\n\napp.listen(3000, () => {\n console.log('Ready')\n})\n```\n\nIn this example, the `router.param(name, callback)` signature remains the same, but instead of a middleware callback, a custom data type checking function has been defined to validate the data type of the user id.\n\n```\nrouter.param((param, validator) => {\n return (req, res, next, val) => {\n if (validator(val)) {\n next()\n } else {\n res.sendStatus(403)\n }\n }\n})\n\nrouter.param('id', (candidate) => {\n return !isNaN(parseFloat(candidate)) && isFinite(candidate)\n})\n```\n\n### router.route(path)\n\nReturns an instance of a single route which you can then use to handle HTTP verbs with optional middleware. Use `router.route()` to avoid duplicate route naming and thus typing errors.\n\nBuilding on the `router.param()` example above, the following code shows how to use `router.route()` to specify various HTTP method handlers.\n\n```\nconst router = express.Router()\n\nrouter.param('user_id', (req, res, next, id) => {\n // sample user, would actually fetch from DB, etc...\n req.user = {\n id,\n name: 'TJ'\n }\n next()\n})\n\nrouter.route('/users/:user_id')\n .all((req, res, next) => {\n // runs for all HTTP verbs first\n // think of it as route specific middleware!\n next()\n })\n .get((req, res, next) => {\n res.json(req.user)\n })\n .put((req, res, next) => {\n // just an example of maybe updating the user\n req.user.name = req.params.name\n // save user ... etc\n res.json(req.user)\n })\n .post((req, res, next) => {\n next(new Error('not implemented'))\n })\n .delete((req, res, next) => {\n next(new Error('not implemented'))\n })\n```\n\nThis approach re-uses the single `/users/:user_id` path and adds handlers for various HTTP methods.\n\nNOTE: When you use `router.route()`, middleware ordering is based on when the _route_ is created, not when method handlers are added to the route. For this purpose, you can consider method handlers to belong to the route to which they were added.\n\n### router.use(\\[path\\], \\[function, ...\\] function)\n\nUses the specified middleware function or functions, with optional mount path `path`, that defaults to “/”.\n\nThis method is similar to [app.use()](https://expressjs.com/en/5x/api.html#app.use). A simple example and use case is described below. See [app.use()](https://expressjs.com/en/5x/api.html#app.use) for more information.\n\nMiddleware is like a plumbing pipe: requests start at the first middleware function defined and work their way “down” the middleware stack processing for each path they match.\n\n```\nconst express = require('express')\nconst app = express()\nconst router = express.Router()\n\n// simple logger for this router's requests\n// all requests to this router will first hit this middleware\nrouter.use((req, res, next) => {\n console.log('%s %s %s', req.method, req.url, req.path)\n next()\n})\n\n// this will only be invoked if the path starts with /bar from the mount point\nrouter.use('/bar', (req, res, next) => {\n // ... maybe some additional /bar logging ...\n next()\n})\n\n// always invoked\nrouter.use((req, res, next) => {\n res.send('Hello World')\n})\n\napp.use('/foo', router)\n\napp.listen(3000)\n```\n\nThe “mount” path is stripped and is _not_ visible to the middleware function. The main effect of this feature is that a mounted middleware function may operate without code changes regardless of its “prefix” pathname.\n\nThe order in which you define middleware with `router.use()` is very important. They are invoked sequentially, thus the order defines middleware precedence. For example, usually a logger is the very first middleware you would use, so that every request gets logged.\n\n```\nconst logger = require('morgan')\n\nrouter.use(logger())\nrouter.use(express.static(path.join(__dirname, 'public')))\nrouter.use((req, res) => {\n res.send('Hello')\n})\n```\n\nNow suppose you wanted to ignore logging requests for static files, but to continue logging routes and middleware defined after `logger()`. You would simply move the call to `express.static()` to the top, before adding the logger middleware:\n\n```\nrouter.use(express.static(path.join(__dirname, 'public')))\nrouter.use(logger())\nrouter.use((req, res) => {\n res.send('Hello')\n})\n```\n\nAnother example is serving files from multiple directories, giving precedence to “./public” over the others:\n\n```\napp.use(express.static(path.join(__dirname, 'public')))\napp.use(express.static(path.join(__dirname, 'files')))\napp.use(express.static(path.join(__dirname, 'uploads')))\n```\n\nThe `router.use()` method also supports named parameters so that your mount points for other routers can benefit from preloading using named parameters.\n\n**NOTE**: Although these middleware functions are added via a particular router, _when_ they run is defined by the path they are attached to (not the router). Therefore, middleware added via one router may run for other routers if its routes match. For example, this code shows two different routers mounted on the same path:\n\n```\nconst authRouter = express.Router()\nconst openRouter = express.Router()\n\nauthRouter.use(require('./authenticate').basic(usersdb))\n\nauthRouter.get('/:user_id/edit', (req, res, next) => {\n // ... Edit user UI ...\n})\nopenRouter.get('/', (req, res, next) => {\n // ... List users ...\n})\nopenRouter.get('/:user_id', (req, res, next) => {\n // ... View user ...\n})\n\napp.use('/users', authRouter)\napp.use('/users', openRouter)\n```\n\nEven though the authentication middleware was added via the `authRouter` it will run on the routes defined by the `openRouter` as well since both routers were mounted on `/users`. To avoid this behavior, use different paths for each router.\n",
"filename": "api.md",
"package": "express"
} |
{
"content": "Title: Express basic routing\n\nURL Source: https://expressjs.com/en/starter/basic-routing.html\n\nMarkdown Content:\nExpress basic routing\n===============\n \nBasic routing\n=============\n\n_Routing_ refers to determining how an application responds to a client request to a particular endpoint, which is a URI (or path) and a specific HTTP request method (GET, POST, and so on).\n\nEach route can have one or more handler functions, which are executed when the route is matched.\n\nRoute definition takes the following structure:\n\n```javascript\napp.METHOD(PATH, HANDLER)\n```\n\nWhere:\n\n* `app` is an instance of `express`.\n* `METHOD` is an [HTTP request method](https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods), in lowercase.\n* `PATH` is a path on the server.\n* `HANDLER` is the function executed when the route is matched.\n\nThis tutorial assumes that an instance of `express` named `app` is created and the server is running. If you are not familiar with creating an app and starting it, see the [Hello world example](https://expressjs.com/en/starter/hello-world.html).\n\nThe following examples illustrate defining simple routes.\n\nRespond with `Hello World!` on the homepage:\n\n```javascript\napp.get('/', (req, res) => {\n res.send('Hello World!')\n})\n```\n\nRespond to POST request on the root route (`/`), the application’s home page:\n\n```javascript\napp.post('/', (req, res) => {\n res.send('Got a POST request')\n})\n```\n\nRespond to a PUT request to the `/user` route:\n\n```javascript\napp.put('/user', (req, res) => {\n res.send('Got a PUT request at /user')\n})\n```\n\nRespond to a DELETE request to the `/user` route:\n\n```javascript\napp.delete('/user', (req, res) => {\n res.send('Got a DELETE request at /user')\n})\n```\n\nFor more details about routing, see the [routing guide](https://expressjs.com/en/guide/routing.html).\n\n### [Previous: Express application generator](https://expressjs.com/en/starter/generator.html) [Next: Serving static files in Express](https://expressjs.com/en/starter/static-files.html)\n\n[](https://expressjs.com/en/starter/basic-routing.html#)\n\nDocumentation translations provided by StrongLoop/IBM: [French](https://expressjs.com/fr/), [German](https://expressjs.com/de/), [Spanish](https://expressjs.com/es/), [Italian](https://expressjs.com/it/), [Japanese](https://expressjs.com/ja/), [Russian](https://expressjs.com/ru/), [Chinese](https://expressjs.com/zh-cn/), [Traditional Chinese](https://expressjs.com/zh-tw/), [Korean](https://expressjs.com/ko/), [Portuguese](https://expressjs.com/pt-br/). \nCommunity translation available for: [Slovak](https://expressjs.com/sk/), [Ukrainian](https://expressjs.com/uk/), [Uzbek](https://expressjs.com/uz/), [Turkish](https://expressjs.com/tr/), [Thai](https://expressjs.com/th/) and [Indonesian](https://expressjs.com/id/).\n\n[](https://www.netlify.com/)\n\n[Express](https://expressjs.com/) is a project of the [OpenJS Foundation](https://openjsf.org/).\n\n[Edit this page on GitHub](https://github.com/expressjs/expressjs.com/blob/gh-pages/en/starter/basic-routing.md).\n\nCopyright © 2017 StrongLoop, IBM, and other expressjs.com contributors.\n\n[](http://creativecommons.org/licenses/by-sa/3.0/us/) This work is licensed under a [Creative Commons Attribution-ShareAlike 3.0 United States License](http://creativecommons.org/licenses/by-sa/3.0/us/).\n",
"filename": "basic-routing.md",
"package": "express"
} |
{
"content": "Title: Express behind proxies\n\nURL Source: https://expressjs.com/en/guide/behind-proxies.html\n\nMarkdown Content:\nWhen running an Express app behind a reverse proxy, some of the Express APIs may return different values than expected. In order to adjust for this, the `trust proxy` application setting may be used to expose information provided by the reverse proxy in the Express APIs. The most common issue is express APIs that expose the client’s IP address may instead show an internal IP address of the reverse proxy.\n\nWhen configuring the `trust proxy` setting, it is important to understand the exact setup of the reverse proxy. Since this setting will trust values provided in the request, it is important that the combination of the setting in Express matches how the reverse proxy operates.\n\nThe application setting `trust proxy` may be set to one of the values listed in the following table.\n\n| Type | Value |\n| --- | --- |\n| Boolean | If `true`, the client’s IP address is understood as the left-most entry in the `X-Forwarded-For` header.\nIf `false`, the app is understood as directly facing the client and the client’s IP address is derived from `req.socket.remoteAddress`. This is the default setting.\n\nWhen setting to `true`, it is important to ensure that the last reverse proxy trusted is removing/overwriting all of the following HTTP headers: `X-Forwarded-For`, `X-Forwarded-Host`, and `X-Forwarded-Proto`, otherwise it may be possible for the client to provide any value.\n\n |\n| IP addresses | An IP address, subnet, or an array of IP addresses and subnets to trust as being a reverse proxy. The following list shows the pre-configured subnet names:\n\n* loopback - `127.0.0.1/8`, `::1/128`\n* linklocal - `169.254.0.0/16`, `fe80::/10`\n* uniquelocal - `10.0.0.0/8`, `172.16.0.0/12`, `192.168.0.0/16`, `fc00::/7`\n\nYou can set IP addresses in any of the following ways:\n\n```\napp.set('trust proxy', 'loopback') // specify a single subnet\napp.set('trust proxy', 'loopback, 123.123.123.123') // specify a subnet and an address\napp.set('trust proxy', 'loopback, linklocal, uniquelocal') // specify multiple subnets as CSV\napp.set('trust proxy', ['loopback', 'linklocal', 'uniquelocal']) // specify multiple subnets as an array\n```\n\nWhen specified, the IP addresses or the subnets are excluded from the address determination process, and the untrusted IP address nearest to the application server is determined as the client’s IP address. This works by checking if `req.socket.remoteAddress` is trusted. If so, then each address in `X-Forwarded-For` is checked from right to left until the first non-trusted address.\n\n |\n| Number | Use the address that is at most `n` number of hops away from the Express application. `req.socket.remoteAddress` is the first hop, and the rest are looked for in the `X-Forwarded-For` header from right to left. A value of `0` means that the first untrusted address would be `req.socket.remoteAddress`, i.e. there is no reverse proxy.\n\nWhen using this setting, it is important to ensure there are not multiple, different-length paths to the Express application such that the client can be less than the configured number of hops away, otherwise it may be possible for the client to provide any value.\n\n |\n| Function | Custom trust implementation.\n\n```\napp.set('trust proxy', (ip) => {\n if (ip === '127.0.0.1' || ip === '123.123.123.123') return true // trusted IPs\n else return false\n})\n```\n\n |\n\nEnabling `trust proxy` will have the following impact:\n\n* The value of [req.hostname](https://expressjs.com/en/api.html#req.hostname) is derived from the value set in the `X-Forwarded-Host` header, which can be set by the client or by the proxy.\n \n* `X-Forwarded-Proto` can be set by the reverse proxy to tell the app whether it is `https` or `http` or even an invalid name. This value is reflected by [req.protocol](https://expressjs.com/en/api.html#req.protocol).\n \n* The [req.ip](https://expressjs.com/en/api.html#req.ip) and [req.ips](https://expressjs.com/en/api.html#req.ips) values are populated based on the socket address and `X-Forwarded-For` header, starting at the first untrusted address.\n \n\nThe `trust proxy` setting is implemented using the [proxy-addr](https://www.npmjs.com/package/proxy-addr) package. For more information, see its documentation.\n",
"filename": "behind-proxies.md",
"package": "express"
} |
{
"content": "Title: Performance Best Practices Using Express in Production\n\nURL Source: https://expressjs.com/en/advanced/best-practice-performance.html\n\nMarkdown Content:\nProduction best practices: performance and reliability\n------------------------------------------------------\n\n### Use gzip compression\n\nGzip compressing can greatly decrease the size of the response body and hence increase the speed of a web app. Use the [compression](https://www.npmjs.com/package/compression) middleware for gzip compression in your Express app. For example:\n\n```\nconst compression = require('compression')\nconst express = require('express')\nconst app = express()\napp.use(compression())\n```\n\nFor a high-traffic website in production, the best way to put compression in place is to implement it at a reverse proxy level (see [Use a reverse proxy](https://expressjs.com/en/advanced/best-practice-performance.html#use-a-reverse-proxy)). In that case, you do not need to use compression middleware. For details on enabling gzip compression in Nginx, see [Module ngx\\_http\\_gzip\\_module](http://nginx.org/en/docs/http/ngx_http_gzip_module.html) in the Nginx documentation.\n\n### Don’t use synchronous functions\n\nSynchronous functions and methods tie up the executing process until they return. A single call to a synchronous function might return in a few microseconds or milliseconds, however in high-traffic websites, these calls add up and reduce the performance of the app. Avoid their use in production.\n\nAlthough Node and many modules provide synchronous and asynchronous versions of their functions, always use the asynchronous version in production. The only time when a synchronous function can be justified is upon initial startup.\n\nIf you are using Node.js 4.0+ or io.js 2.1.0+, you can use the `--trace-sync-io` command-line flag to print a warning and a stack trace whenever your application uses a synchronous API. Of course, you wouldn’t want to use this in production, but rather to ensure that your code is ready for production. See the [node command-line options documentation](https://nodejs.org/api/cli.html#cli_trace_sync_io) for more information.\n\n### Do logging correctly\n\nIn general, there are two reasons for logging from your app: For debugging and for logging app activity (essentially, everything else). Using `console.log()` or `console.error()` to print log messages to the terminal is common practice in development. But [these functions are synchronous](https://nodejs.org/api/console.html#console_console_1) when the destination is a terminal or a file, so they are not suitable for production, unless you pipe the output to another program.\n\n#### For debugging\n\nIf you’re logging for purposes of debugging, then instead of using `console.log()`, use a special debugging module like [debug](https://www.npmjs.com/package/debug). This module enables you to use the DEBUG environment variable to control what debug messages are sent to `console.error()`, if any. To keep your app purely asynchronous, you’d still want to pipe `console.error()` to another program. But then, you’re not really going to debug in production, are you?\n\n#### For app activity\n\nIf you’re logging app activity (for example, tracking traffic or API calls), instead of using `console.log()`, use a logging library like [Winston](https://www.npmjs.com/package/winston) or [Bunyan](https://www.npmjs.com/package/bunyan). For a detailed comparison of these two libraries, see the StrongLoop blog post [Comparing Winston and Bunyan Node.js Logging](https://strongloop.com/strongblog/compare-node-js-logging-winston-bunyan/).\n\n### Handle exceptions properly\n\nNode apps crash when they encounter an uncaught exception. Not handling exceptions and taking appropriate actions will make your Express app crash and go offline. If you follow the advice in [Ensure your app automatically restarts](https://expressjs.com/en/advanced/best-practice-performance.html#ensure-your-app-automatically-restarts) below, then your app will recover from a crash. Fortunately, Express apps typically have a short startup time. Nevertheless, you want to avoid crashing in the first place, and to do that, you need to handle exceptions properly.\n\nTo ensure you handle all exceptions, use the following techniques:\n\n* [Use try-catch](https://expressjs.com/en/advanced/best-practice-performance.html#use-try-catch)\n* [Use promises](https://expressjs.com/en/advanced/best-practice-performance.html#use-promises)\n\nBefore diving into these topics, you should have a basic understanding of Node/Express error handling: using error-first callbacks, and propagating errors in middleware. Node uses an “error-first callback” convention for returning errors from asynchronous functions, where the first parameter to the callback function is the error object, followed by result data in succeeding parameters. To indicate no error, pass null as the first parameter. The callback function must correspondingly follow the error-first callback convention to meaningfully handle the error. And in Express, the best practice is to use the next() function to propagate errors through the middleware chain.\n\nFor more on the fundamentals of error handling, see:\n\n* [Error Handling in Node.js](https://www.tritondatacenter.com/node-js/production/design/errors)\n* [Building Robust Node Applications: Error Handling](https://strongloop.com/strongblog/robust-node-applications-error-handling/) (StrongLoop blog)\n\n#### What not to do\n\nOne thing you should _not_ do is to listen for the `uncaughtException` event, emitted when an exception bubbles all the way back to the event loop. Adding an event listener for `uncaughtException` will change the default behavior of the process that is encountering an exception; the process will continue to run despite the exception. This might sound like a good way of preventing your app from crashing, but continuing to run the app after an uncaught exception is a dangerous practice and is not recommended, because the state of the process becomes unreliable and unpredictable.\n\nAdditionally, using `uncaughtException` is officially recognized as [crude](https://nodejs.org/api/process.html#process_event_uncaughtexception). So listening for `uncaughtException` is just a bad idea. This is why we recommend things like multiple processes and supervisors: crashing and restarting is often the most reliable way to recover from an error.\n\nWe also don’t recommend using [domains](https://nodejs.org/api/domain.html). It generally doesn’t solve the problem and is a deprecated module.\n\n#### Use try-catch\n\nTry-catch is a JavaScript language construct that you can use to catch exceptions in synchronous code. Use try-catch, for example, to handle JSON parsing errors as shown below.\n\nUse a tool such as [JSHint](http://jshint.com/) or [JSLint](http://www.jslint.com/) to help you find implicit exceptions like [reference errors on undefined variables](http://www.jshint.com/docs/options/#undef).\n\nHere is an example of using try-catch to handle a potential process-crashing exception. This middleware function accepts a query field parameter named “params” that is a JSON object.\n\n```\napp.get('/search', (req, res) => {\n // Simulating async operation\n setImmediate(() => {\n const jsonStr = req.query.params\n try {\n const jsonObj = JSON.parse(jsonStr)\n res.send('Success')\n } catch (e) {\n res.status(400).send('Invalid JSON string')\n }\n })\n})\n```\n\nHowever, try-catch works only for synchronous code. Because the Node platform is primarily asynchronous (particularly in a production environment), try-catch won’t catch a lot of exceptions.\n\n#### Use promises\n\nPromises will handle any exceptions (both explicit and implicit) in asynchronous code blocks that use `then()`. Just add `.catch(next)` to the end of promise chains. For example:\n\n```\napp.get('/', (req, res, next) => {\n // do some sync stuff\n queryDb()\n .then((data) => makeCsv(data)) // handle data\n .then((csv) => { /* handle csv */ })\n .catch(next)\n})\n\napp.use((err, req, res, next) => {\n // handle error\n})\n```\n\nNow, all errors asynchronous and synchronous get propagated to the error middleware.\n\nHowever, there are two caveats:\n\n1. All your asynchronous code must return promises (except emitters). If a particular library does not return promises, convert the base object by using a helper function like [Bluebird.promisifyAll()](http://bluebirdjs.com/docs/api/promise.promisifyall.html).\n2. Event emitters (like `streams`) can still cause uncaught exceptions. So make sure you are handling the error event properly; for example:\n\n```\nconst wrap = fn => (...args) => fn(...args).catch(args[2])\n\napp.get('/', wrap(async (req, res, next) => {\n const company = await getCompanyById(req.query.id)\n const stream = getLogoStreamById(company.id)\n stream.on('error', next).pipe(res)\n}))\n```\n\nThe `wrap()` function is a wrapper that catches rejected promises and calls `next()` with the error as the first argument. For details, see [Asynchronous Error Handling in Express with Promises, Generators and ES7](https://strongloop.com/strongblog/async-error-handling-expressjs-es7-promises-generators/#cleaner-code-with-generators).\n\nFor more information about error-handling by using promises, see [Promises in Node.js with Q – An Alternative to Callbacks](https://strongloop.com/strongblog/promises-in-node-js-with-q-an-alternative-to-callbacks/).\n\nThings to do in your environment / setup\n----------------------------------------\n\nHere are some things you can do in your system environment to improve your app’s performance:\n\n* [Set NODE\\_ENV to “production”](https://expressjs.com/en/advanced/best-practice-performance.html#set-node_env-to-production)\n* [Ensure your app automatically restarts](https://expressjs.com/en/advanced/best-practice-performance.html#ensure-your-app-automatically-restarts)\n* [Run your app in a cluster](https://expressjs.com/en/advanced/best-practice-performance.html#run-your-app-in-a-cluster)\n* [Cache request results](https://expressjs.com/en/advanced/best-practice-performance.html#cache-request-results)\n* [Use a load balancer](https://expressjs.com/en/advanced/best-practice-performance.html#use-a-load-balancer)\n* [Use a reverse proxy](https://expressjs.com/en/advanced/best-practice-performance.html#use-a-reverse-proxy)\n\n### Set NODE\\_ENV to “production”\n\nThe NODE\\_ENV environment variable specifies the environment in which an application is running (usually, development or production). One of the simplest things you can do to improve performance is to set NODE\\_ENV to “production.”\n\nSetting NODE\\_ENV to “production” makes Express:\n\n* Cache view templates.\n* Cache CSS files generated from CSS extensions.\n* Generate less verbose error messages.\n\n[Tests indicate](http://apmblog.dynatrace.com/2015/07/22/the-drastic-effects-of-omitting-node_env-in-your-express-js-applications/) that just doing this can improve app performance by a factor of three!\n\nIf you need to write environment-specific code, you can check the value of NODE\\_ENV with `process.env.NODE_ENV`. Be aware that checking the value of any environment variable incurs a performance penalty, and so should be done sparingly.\n\nIn development, you typically set environment variables in your interactive shell, for example by using `export` or your `.bash_profile` file. But in general, you shouldn’t do that on a production server; instead, use your OS’s init system (systemd or Upstart). The next section provides more details about using your init system in general, but setting `NODE_ENV` is so important for performance (and easy to do), that it’s highlighted here.\n\nWith Upstart, use the `env` keyword in your job file. For example:\n\n```\n# /etc/init/env.conf\n env NODE_ENV=production\n```\n\nFor more information, see the [Upstart Intro, Cookbook and Best Practices](http://upstart.ubuntu.com/cookbook/#environment-variables).\n\nWith systemd, use the `Environment` directive in your unit file. For example:\n\n```\n# /etc/systemd/system/myservice.service\nEnvironment=NODE_ENV=production\n```\n\nFor more information, see [Using Environment Variables In systemd Units](https://coreos.com/os/docs/latest/using-environment-variables-in-systemd-units.html).\n\n### Ensure your app automatically restarts\n\nIn production, you don’t want your application to be offline, ever. This means you need to make sure it restarts both if the app crashes and if the server itself crashes. Although you hope that neither of those events occurs, realistically you must account for both eventualities by:\n\n* Using a process manager to restart the app (and Node) when it crashes.\n* Using the init system provided by your OS to restart the process manager when the OS crashes. It’s also possible to use the init system without a process manager.\n\nNode applications crash if they encounter an uncaught exception. The foremost thing you need to do is to ensure your app is well-tested and handles all exceptions (see [handle exceptions properly](https://expressjs.com/en/advanced/best-practice-performance.html#handle-exceptions-properly) for details). But as a fail-safe, put a mechanism in place to ensure that if and when your app crashes, it will automatically restart.\n\n#### Use a process manager\n\nIn development, you started your app simply from the command line with `node server.js` or something similar. But doing this in production is a recipe for disaster. If the app crashes, it will be offline until you restart it. To ensure your app restarts if it crashes, use a process manager. A process manager is a “container” for applications that facilitates deployment, provides high availability, and enables you to manage the application at runtime.\n\nIn addition to restarting your app when it crashes, a process manager can enable you to:\n\n* Gain insights into runtime performance and resource consumption.\n* Modify settings dynamically to improve performance.\n* Control clustering (StrongLoop PM and pm2).\n\nThe most popular process managers for Node are as follows:\n\n* [StrongLoop Process Manager](http://strong-pm.io/)\n* [PM2](https://github.com/Unitech/pm2)\n* [Forever](https://www.npmjs.com/package/forever)\n\nFor a feature-by-feature comparison of the three process managers, see [http://strong-pm.io/compare/](http://strong-pm.io/compare/).\n\nUsing any of these process managers will suffice to keep your application up, even if it does crash from time to time.\n\nHowever, StrongLoop PM has lots of features that specifically target production deployment. You can use it and the related StrongLoop tools to:\n\n* Build and package your app locally, then deploy it securely to your production system.\n* Automatically restart your app if it crashes for any reason.\n* Manage your clusters remotely.\n* View CPU profiles and heap snapshots to optimize performance and diagnose memory leaks.\n* View performance metrics for your application.\n* Easily scale to multiple hosts with integrated control for Nginx load balancer.\n\nAs explained below, when you install StrongLoop PM as an operating system service using your init system, it will automatically restart when the system restarts. Thus, it will keep your application processes and clusters alive forever.\n\n#### Use an init system\n\nThe next layer of reliability is to ensure that your app restarts when the server restarts. Systems can still go down for a variety of reasons. To ensure that your app restarts if the server crashes, use the init system built into your OS. The two main init systems in use today are [systemd](https://wiki.debian.org/systemd) and [Upstart](http://upstart.ubuntu.com/).\n\nThere are two ways to use init systems with your Express app:\n\n* Run your app in a process manager, and install the process manager as a service with the init system. The process manager will restart your app when the app crashes, and the init system will restart the process manager when the OS restarts. This is the recommended approach.\n* Run your app (and Node) directly with the init system. This is somewhat simpler, but you don’t get the additional advantages of using a process manager.\n\n##### Systemd\n\nSystemd is a Linux system and service manager. Most major Linux distributions have adopted systemd as their default init system.\n\nA systemd service configuration file is called a _unit file_, with a filename ending in `.service`. Here’s an example unit file to manage a Node app directly. Replace the values enclosed in `<angle brackets>` for your system and app:\n\n```\n[Unit]\nDescription=<Awesome Express App>\n\n[Service]\nType=simple\nExecStart=/usr/local/bin/node </projects/myapp/index.js>\nWorkingDirectory=</projects/myapp>\n\nUser=nobody\nGroup=nogroup\n\n# Environment variables:\nEnvironment=NODE_ENV=production\n\n# Allow many incoming connections\nLimitNOFILE=infinity\n\n# Allow core dumps for debugging\nLimitCORE=infinity\n\nStandardInput=null\nStandardOutput=syslog\nStandardError=syslog\nRestart=always\n\n[Install]\nWantedBy=multi-user.target\n```\n\nFor more information on systemd, see the [systemd reference (man page)](http://www.freedesktop.org/software/systemd/man/systemd.unit.html).\n\n##### StrongLoop PM as a systemd service\n\nYou can easily install StrongLoop Process Manager as a systemd service. After you do, when the server restarts, it will automatically restart StrongLoop PM, which will then restart all the apps it is managing.\n\nTo install StrongLoop PM as a systemd service:\n\n```\n$ sudo sl-pm-install --systemd\n```\n\nThen start the service with:\n\n```\n$ sudo /usr/bin/systemctl start strong-pm\n```\n\nFor more information, see [Setting up a production host (StrongLoop documentation)](https://docs.strongloop.com/display/SLC/Setting+up+a+production+host#Settingupaproductionhost-RHEL7+,Ubuntu15.04or15.10).\n\n##### Upstart\n\nUpstart is a system tool available on many Linux distributions for starting tasks and services during system startup, stopping them during shutdown, and supervising them. You can configure your Express app or process manager as a service and then Upstart will automatically restart it when it crashes.\n\nAn Upstart service is defined in a job configuration file (also called a “job”) with filename ending in `.conf`. The following example shows how to create a job called “myapp” for an app named “myapp” with the main file located at `/projects/myapp/index.js`.\n\nCreate a file named `myapp.conf` at `/etc/init/` with the following content (replace the bold text with values for your system and app):\n\n```\n# When to start the process\nstart on runlevel [2345]\n\n# When to stop the process\nstop on runlevel [016]\n\n# Increase file descriptor limit to be able to handle more requests\nlimit nofile 50000 50000\n\n# Use production mode\nenv NODE_ENV=production\n\n# Run as www-data\nsetuid www-data\nsetgid www-data\n\n# Run from inside the app dir\nchdir /projects/myapp\n\n# The process to start\nexec /usr/local/bin/node /projects/myapp/index.js\n\n# Restart the process if it is down\nrespawn\n\n# Limit restart attempt to 10 times within 10 seconds\nrespawn limit 10 10\n```\n\nNOTE: This script requires Upstart 1.4 or newer, supported on Ubuntu 12.04-14.10.\n\nSince the job is configured to run when the system starts, your app will be started along with the operating system, and automatically restarted if the app crashes or the system goes down.\n\nApart from automatically restarting the app, Upstart enables you to use these commands:\n\n* `start myapp` – Start the app\n* `restart myapp` – Restart the app\n* `stop myapp` – Stop the app.\n\nFor more information on Upstart, see [Upstart Intro, Cookbook and Best Practises](http://upstart.ubuntu.com/cookbook).\n\n##### StrongLoop PM as an Upstart service\n\nYou can easily install StrongLoop Process Manager as an Upstart service. After you do, when the server restarts, it will automatically restart StrongLoop PM, which will then restart all the apps it is managing.\n\nTo install StrongLoop PM as an Upstart 1.4 service:\n\n```\n$ sudo sl-pm-install\n```\n\nThen run the service with:\n\n```\n$ sudo /sbin/initctl start strong-pm\n```\n\nNOTE: On systems that don’t support Upstart 1.4, the commands are slightly different. See [Setting up a production host (StrongLoop documentation)](https://docs.strongloop.com/display/SLC/Setting+up+a+production+host#Settingupaproductionhost-RHELLinux5and6,Ubuntu10.04-.10,11.04-.10) for more information.\n\n### Run your app in a cluster\n\nIn a multi-core system, you can increase the performance of a Node app by many times by launching a cluster of processes. A cluster runs multiple instances of the app, ideally one instance on each CPU core, thereby distributing the load and tasks among the instances.\n\n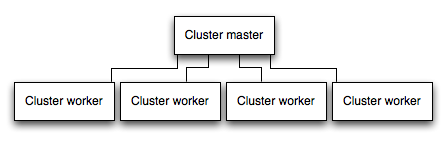\n\nIMPORTANT: Since the app instances run as separate processes, they do not share the same memory space. That is, objects are local to each instance of the app. Therefore, you cannot maintain state in the application code. However, you can use an in-memory datastore like [Redis](http://redis.io/) to store session-related data and state. This caveat applies to essentially all forms of horizontal scaling, whether clustering with multiple processes or multiple physical servers.\n\nIn clustered apps, worker processes can crash individually without affecting the rest of the processes. Apart from performance advantages, failure isolation is another reason to run a cluster of app processes. Whenever a worker process crashes, always make sure to log the event and spawn a new process using cluster.fork().\n\n#### Using Node’s cluster module\n\nClustering is made possible with Node’s [cluster module](https://nodejs.org/dist/latest-v4.x/docs/api/cluster.html). This enables a master process to spawn worker processes and distribute incoming connections among the workers. However, rather than using this module directly, it’s far better to use one of the many tools out there that does it for you automatically; for example [node-pm](https://www.npmjs.com/package/node-pm) or [cluster-service](https://www.npmjs.com/package/cluster-service).\n\n#### Using StrongLoop PM\n\nIf you deploy your application to StrongLoop Process Manager (PM), then you can take advantage of clustering _without_ modifying your application code.\n\nWhen StrongLoop Process Manager (PM) runs an application, it automatically runs it in a cluster with a number of workers equal to the number of CPU cores on the system. You can manually change the number of worker processes in the cluster using the slc command line tool without stopping the app.\n\nFor example, assuming you’ve deployed your app to prod.foo.com and StrongLoop PM is listening on port 8701 (the default), then to set the cluster size to eight using slc:\n\n```\n$ slc ctl -C http://prod.foo.com:8701 set-size my-app 8\n```\n\nFor more information on clustering with StrongLoop PM, see [Clustering](https://docs.strongloop.com/display/SLC/Clustering) in StrongLoop documentation.\n\n#### Using PM2\n\nIf you deploy your application with PM2, then you can take advantage of clustering _without_ modifying your application code. You should ensure your [application is stateless](http://pm2.keymetrics.io/docs/usage/specifics/#stateless-apps) first, meaning no local data is stored in the process (such as sessions, websocket connections and the like).\n\nWhen running an application with PM2, you can enable **cluster mode** to run it in a cluster with a number of instances of your choosing, such as the matching the number of available CPUs on the machine. You can manually change the number of processes in the cluster using the `pm2` command line tool without stopping the app.\n\nTo enable cluster mode, start your application like so:\n\n```\n# Start 4 worker processes\n$ pm2 start npm --name my-app -i 4 -- start\n# Auto-detect number of available CPUs and start that many worker processes\n$ pm2 start npm --name my-app -i max -- start\n```\n\nThis can also be configured within a PM2 process file (`ecosystem.config.js` or similar) by setting `exec_mode` to `cluster` and `instances` to the number of workers to start.\n\nOnce running, the application can be scaled like so:\n\n```\n# Add 3 more workers\n$ pm2 scale my-app +3\n# Scale to a specific number of workers\n$ pm2 scale my-app 2\n```\n\nFor more information on clustering with PM2, see [Cluster Mode](https://pm2.keymetrics.io/docs/usage/cluster-mode/) in the PM2 documentation.\n\n### Cache request results\n\nAnother strategy to improve the performance in production is to cache the result of requests, so that your app does not repeat the operation to serve the same request repeatedly.\n\nUse a caching server like [Varnish](https://www.varnish-cache.org/) or [Nginx](https://www.nginx.com/resources/wiki/start/topics/examples/reverseproxycachingexample/) (see also [Nginx Caching](https://serversforhackers.com/nginx-caching/)) to greatly improve the speed and performance of your app.\n\n### Use a load balancer\n\nNo matter how optimized an app is, a single instance can handle only a limited amount of load and traffic. One way to scale an app is to run multiple instances of it and distribute the traffic via a load balancer. Setting up a load balancer can improve your app’s performance and speed, and enable it to scale more than is possible with a single instance.\n\nA load balancer is usually a reverse proxy that orchestrates traffic to and from multiple application instances and servers. You can easily set up a load balancer for your app by using [Nginx](http://nginx.org/en/docs/http/load_balancing.html) or [HAProxy](https://www.digitalocean.com/community/tutorials/an-introduction-to-haproxy-and-load-balancing-concepts).\n\nWith load balancing, you might have to ensure that requests that are associated with a particular session ID connect to the process that originated them. This is known as _session affinity_, or _sticky sessions_, and may be addressed by the suggestion above to use a data store such as Redis for session data (depending on your application). For a discussion, see [Using multiple nodes](https://socket.io/docs/v4/using-multiple-nodes/).\n\n### Use a reverse proxy\n\nA reverse proxy sits in front of a web app and performs supporting operations on the requests, apart from directing requests to the app. It can handle error pages, compression, caching, serving files, and load balancing among other things.\n\nHanding over tasks that do not require knowledge of application state to a reverse proxy frees up Express to perform specialized application tasks. For this reason, it is recommended to run Express behind a reverse proxy like [Nginx](https://www.nginx.com/) or [HAProxy](http://www.haproxy.org/) in production.\n",
"filename": "best-practice-performance.md",
"package": "express"
} |
{
"content": "Title: Security Best Practices for Express in Production\n\nURL Source: https://expressjs.com/en/advanced/best-practice-security.html\n\nMarkdown Content:\nOverview\n--------\n\nThe term _“production”_ refers to the stage in the software lifecycle when an application or API is generally available to its end-users or consumers. In contrast, in the _“development”_ stage, you’re still actively writing and testing code, and the application is not open to external access. The corresponding system environments are known as _production_ and _development_ environments, respectively.\n\nDevelopment and production environments are usually set up differently and have vastly different requirements. What’s fine in development may not be acceptable in production. For example, in a development environment you may want verbose logging of errors for debugging, while the same behavior can become a security concern in a production environment. And in development, you don’t need to worry about scalability, reliability, and performance, while those concerns become critical in production.\n\nDon’t use deprecated or vulnerable versions of Express\n------------------------------------------------------\n\nExpress 2.x and 3.x are no longer maintained. Security and performance issues in these versions won’t be fixed. Do not use them! If you haven’t moved to version 4, follow the [migration guide](https://expressjs.com/en/guide/migrating-4.html).\n\nAlso ensure you are not using any of the vulnerable Express versions listed on the [Security updates page](https://expressjs.com/en/advanced/security-updates.html). If you are, update to one of the stable releases, preferably the latest.\n\nUse TLS\n-------\n\nIf your app deals with or transmits sensitive data, use [Transport Layer Security](https://en.wikipedia.org/wiki/Transport_Layer_Security) (TLS) to secure the connection and the data. This technology encrypts data before it is sent from the client to the server, thus preventing some common (and easy) hacks. Although Ajax and POST requests might not be visibly obvious and seem “hidden” in browsers, their network traffic is vulnerable to [packet sniffing](https://en.wikipedia.org/wiki/Packet_analyzer) and [man-in-the-middle attacks](https://en.wikipedia.org/wiki/Man-in-the-middle_attack).\n\nYou may be familiar with Secure Socket Layer (SSL) encryption. [TLS is simply the next progression of SSL](https://msdn.microsoft.com/en-us/library/windows/desktop/aa380515/(v=vs.85/).aspx). In other words, if you were using SSL before, consider upgrading to TLS. In general, we recommend Nginx to handle TLS. For a good reference to configure TLS on Nginx (and other servers), see [Recommended Server Configurations (Mozilla Wiki)](https://wiki.mozilla.org/Security/Server_Side_TLS#Recommended_Server_Configurations).\n\nAlso, a handy tool to get a free TLS certificate is [Let’s Encrypt](https://letsencrypt.org/about/), a free, automated, and open certificate authority (CA) provided by the [Internet Security Research Group (ISRG)](https://www.abetterinternet.org/).\n\nDo not trust user input\n-----------------------\n\nFor web applications, one of the most critical security requirements is proper user input validation and handling. This comes in many forms and we will not cover all of them here. Ultimately, the responsibility for validating and correctly handling the types of user input your application accepts. Here are a few examples of validating user input specifically using a few `express` apis.\n\n### Prevent open redirects\n\nAn example of potentially dangerous user input is an _open redirect_, where an application accepts a URL as user input (often in the URL query, for example `?url=https://example.com`) and uses `res.redirect` to set the `location` header and return a 3xx status.\n\nAn application must validate that it supports redirecting to the incoming URL to avoid sending users to malicious links such as phishing websites, among other risks.\n\nHere is an example of checking URLs before using `res.redirect` or `res.location`:\n\n```\napp.use((req, res) => {\n try {\n if (new Url(req.query.url).host !== 'example.com') {\n return res.status(400).end(`Unsupported redirect to host: ${req.query.url}`)\n }\n } catch (e) {\n return res.status(400).end(`Invalid url: ${req.query.url}`)\n }\n res.redirect(req.query.url)\n})\n```\n\nUse Helmet\n----------\n\n[Helmet](https://helmetjs.github.io/) can help protect your app from some well-known web vulnerabilities by setting HTTP headers appropriately.\n\nHelmet is a collection of several smaller middleware functions that set security-related HTTP response headers. Some examples include:\n\n* `helmet.contentSecurityPolicy` which sets the `Content-Security-Policy` header. This helps prevent cross-site scripting attacks among many other things.\n* `helmet.hsts` which sets the `Strict-Transport-Security` header. This helps enforce secure (HTTPS) connections to the server.\n* `helmet.frameguard` which sets the `X-Frame-Options` header. This provides [clickjacking](https://www.owasp.org/index.php/Clickjacking) protection.\n\nHelmet includes several other middleware functions which you can read about [at its documentation website](https://helmetjs.github.io/).\n\nInstall Helmet like any other module:\n\n```\n$ npm install --save helmet\n```\n\nThen to use it in your code:\n\n```\n// ...\n\nconst helmet = require('helmet')\napp.use(helmet())\n\n// ...\n```\n\nReduce fingerprinting\n---------------------\n\nIt can help to provide an extra layer of security to reduce the ability of attackers to determine the software that a server uses, known as “fingerprinting.” Though not a security issue itself, reducing the ability to fingerprint an application improves its overall security posture. Server software can be fingerprinted by quirks in how it responds to specific requests, for example in the HTTP response headers.\n\nBy default, Express sends the `X-Powered-By` response header that you can disable using the `app.disable()` method:\n\n```\napp.disable('x-powered-by')\n```\n\n**Note**: Disabling the `X-Powered-By header` does not prevent a sophisticated attacker from determining that an app is running Express. It may discourage a casual exploit, but there are other ways to determine an app is running Express.\n\nExpress also sends its own formatted “404 Not Found” messages and formatter error response messages. These can be changed by [adding your own not found handler](https://expressjs.com/en/starter/faq.html#how-do-i-handle-404-responses) and [writing your own error handler](https://expressjs.com/en/guide/error-handling.html#writing-error-handlers):\n\n```\n// last app.use calls right before app.listen():\n\n// custom 404\napp.use((req, res, next) => {\n res.status(404).send(\"Sorry can't find that!\")\n})\n\n// custom error handler\napp.use((err, req, res, next) => {\n console.error(err.stack)\n res.status(500).send('Something broke!')\n})\n```\n\nUse cookies securely\n--------------------\n\nTo ensure cookies don’t open your app to exploits, don’t use the default session cookie name and set cookie security options appropriately.\n\nThere are two main middleware cookie session modules:\n\n* [express-session](https://www.npmjs.com/package/express-session) that replaces `express.session` middleware built-in to Express 3.x.\n* [cookie-session](https://www.npmjs.com/package/cookie-session) that replaces `express.cookieSession` middleware built-in to Express 3.x.\n\nThe main difference between these two modules is how they save cookie session data. The [express-session](https://www.npmjs.com/package/express-session) middleware stores session data on the server; it only saves the session ID in the cookie itself, not session data. By default, it uses in-memory storage and is not designed for a production environment. In production, you’ll need to set up a scalable session-store; see the list of [compatible session stores](https://github.com/expressjs/session#compatible-session-stores).\n\nIn contrast, [cookie-session](https://www.npmjs.com/package/cookie-session) middleware implements cookie-backed storage: it serializes the entire session to the cookie, rather than just a session key. Only use it when session data is relatively small and easily encoded as primitive values (rather than objects). Although browsers are supposed to support at least 4096 bytes per cookie, to ensure you don’t exceed the limit, don’t exceed a size of 4093 bytes per domain. Also, be aware that the cookie data will be visible to the client, so if there is any reason to keep it secure or obscure, then `express-session` may be a better choice.\n\n### Don’t use the default session cookie name\n\nUsing the default session cookie name can open your app to attacks. The security issue posed is similar to `X-Powered-By`: a potential attacker can use it to fingerprint the server and target attacks accordingly.\n\nTo avoid this problem, use generic cookie names; for example using [express-session](https://www.npmjs.com/package/express-session) middleware:\n\n```\nconst session = require('express-session')\napp.set('trust proxy', 1) // trust first proxy\napp.use(session({\n secret: 's3Cur3',\n name: 'sessionId'\n}))\n```\n\n### Set cookie security options\n\nSet the following cookie options to enhance security:\n\n* `secure` - Ensures the browser only sends the cookie over HTTPS.\n* `httpOnly` - Ensures the cookie is sent only over HTTP(S), not client JavaScript, helping to protect against cross-site scripting attacks.\n* `domain` - indicates the domain of the cookie; use it to compare against the domain of the server in which the URL is being requested. If they match, then check the path attribute next.\n* `path` - indicates the path of the cookie; use it to compare against the request path. If this and domain match, then send the cookie in the request.\n* `expires` - use to set expiration date for persistent cookies.\n\nHere is an example using [cookie-session](https://www.npmjs.com/package/cookie-session) middleware:\n\n```\nconst session = require('cookie-session')\nconst express = require('express')\nconst app = express()\n\nconst expiryDate = new Date(Date.now() + 60 * 60 * 1000) // 1 hour\napp.use(session({\n name: 'session',\n keys: ['key1', 'key2'],\n cookie: {\n secure: true,\n httpOnly: true,\n domain: 'example.com',\n path: 'foo/bar',\n expires: expiryDate\n }\n}))\n```\n\nMake sure login endpoints are protected to make private data more secure.\n\nA simple and powerful technique is to block authorization attempts using two metrics:\n\n1. The number of consecutive failed attempts by the same user name and IP address.\n2. The number of failed attempts from an IP address over some long period of time. For example, block an IP address if it makes 100 failed attempts in one day.\n\n[rate-limiter-flexible](https://github.com/animir/node-rate-limiter-flexible) package provides tools to make this technique easy and fast. You can find [an example of brute-force protection in the documentation](https://github.com/animir/node-rate-limiter-flexible/wiki/Overall-example#login-endpoint-protection)\n\nEnsure your dependencies are secure\n-----------------------------------\n\nUsing npm to manage your application’s dependencies is powerful and convenient. But the packages that you use may contain critical security vulnerabilities that could also affect your application. The security of your app is only as strong as the “weakest link” in your dependencies.\n\nSince npm@6, npm automatically reviews every install request. Also, you can use `npm audit` to analyze your dependency tree.\n\n```\n$ npm audit\n```\n\nIf you want to stay more secure, consider [Snyk](https://snyk.io/).\n\nSnyk offers both a [command-line tool](https://www.npmjs.com/package/snyk) and a [Github integration](https://snyk.io/docs/github) that checks your application against [Snyk’s open source vulnerability database](https://snyk.io/vuln/) for any known vulnerabilities in your dependencies. Install the CLI as follows:\n\n```\n$ npm install -g snyk\n$ cd your-app\n```\n\nUse this command to test your application for vulnerabilities:\n\n```\n$ snyk test\n```\n\n### Avoid other known vulnerabilities\n\nKeep an eye out for [Node Security Project](https://npmjs.com/advisories) or [Snyk](https://snyk.io/vuln/) advisories that may affect Express or other modules that your app uses. In general, these databases are excellent resources for knowledge and tools about Node security.\n\nFinally, Express apps—like any other web apps—can be vulnerable to a variety of web-based attacks. Familiarize yourself with known [web vulnerabilities](https://www.owasp.org/www-project-top-ten/) and take precautions to avoid them.\n\nAdditional considerations\n-------------------------\n\nHere are some further recommendations from the excellent [Node.js Security Checklist](https://blog.risingstack.com/node-js-security-checklist/). Refer to that blog post for all the details on these recommendations:\n\n* Always filter and sanitize user input to protect against cross-site scripting (XSS) and command injection attacks.\n* Defend against SQL injection attacks by using parameterized queries or prepared statements.\n* Use the open-source [sqlmap](http://sqlmap.org/) tool to detect SQL injection vulnerabilities in your app.\n* Use the [nmap](https://nmap.org/) and [sslyze](https://github.com/nabla-c0d3/sslyze) tools to test the configuration of your SSL ciphers, keys, and renegotiation as well as the validity of your certificate.\n* Use [safe-regex](https://www.npmjs.com/package/safe-regex) to ensure your regular expressions are not susceptible to [regular expression denial of service](https://www.owasp.org/index.php/Regular_expression_Denial_of_Service_-_ReDoS) attacks.\n",
"filename": "best-practice-security.md",
"package": "express"
} |
{
"content": "Title: Express database integration\n\nURL Source: https://expressjs.com/en/guide/database-integration.html\n\nMarkdown Content:\nAdding the capability to connect databases to Express apps is just a matter of loading an appropriate Node.js driver for the database in your app. This document briefly explains how to add and use some of the most popular Node.js modules for database systems in your Express app:\n\n* [Cassandra](https://expressjs.com/en/guide/database-integration.html#cassandra)\n* [Couchbase](https://expressjs.com/en/guide/database-integration.html#couchbase)\n* [CouchDB](https://expressjs.com/en/guide/database-integration.html#couchdb)\n* [LevelDB](https://expressjs.com/en/guide/database-integration.html#leveldb)\n* [MySQL](https://expressjs.com/en/guide/database-integration.html#mysql)\n* [MongoDB](https://expressjs.com/en/guide/database-integration.html#mongodb)\n* [Neo4j](https://expressjs.com/en/guide/database-integration.html#neo4j)\n* [Oracle](https://expressjs.com/en/guide/database-integration.html#oracle)\n* [PostgreSQL](https://expressjs.com/en/guide/database-integration.html#postgresql)\n* [Redis](https://expressjs.com/en/guide/database-integration.html#redis)\n* [SQL Server](https://expressjs.com/en/guide/database-integration.html#sql-server)\n* [SQLite](https://expressjs.com/en/guide/database-integration.html#sqlite)\n* [Elasticsearch](https://expressjs.com/en/guide/database-integration.html#elasticsearch)\n\nThese database drivers are among many that are available. For other options, search on the [npm](https://www.npmjs.com/) site.\n\nCassandra\n---------\n\n**Module**: [cassandra-driver](https://github.com/datastax/nodejs-driver)\n\n### Installation\n\n```\n$ npm install cassandra-driver\n```\n\n### Example\n\n```\nconst cassandra = require('cassandra-driver')\nconst client = new cassandra.Client({ contactPoints: ['localhost'] })\n\nclient.execute('select key from system.local', (err, result) => {\n if (err) throw err\n console.log(result.rows[0])\n})\n```\n\nCouchbase\n---------\n\n**Module**: [couchnode](https://github.com/couchbase/couchnode)\n\n### Installation\n\n```\n$ npm install couchbase\n```\n\n### Example\n\n```\nconst couchbase = require('couchbase')\nconst bucket = (new couchbase.Cluster('http://localhost:8091')).openBucket('bucketName')\n\n// add a document to a bucket\nbucket.insert('document-key', { name: 'Matt', shoeSize: 13 }, (err, result) => {\n if (err) {\n console.log(err)\n } else {\n console.log(result)\n }\n})\n\n// get all documents with shoe size 13\nconst n1ql = 'SELECT d.* FROM `bucketName` d WHERE shoeSize = $1'\nconst query = N1qlQuery.fromString(n1ql)\nbucket.query(query, [13], (err, result) => {\n if (err) {\n console.log(err)\n } else {\n console.log(result)\n }\n})\n```\n\nCouchDB\n-------\n\n**Module**: [nano](https://github.com/dscape/nano)\n\n### Installation\n\n```\n$ npm install nano\n```\n\n### Example\n\n```\nconst nano = require('nano')('http://localhost:5984')\nnano.db.create('books')\nconst books = nano.db.use('books')\n\n// Insert a book document in the books database\nbooks.insert({ name: 'The Art of war' }, null, (err, body) => {\n if (err) {\n console.log(err)\n } else {\n console.log(body)\n }\n})\n\n// Get a list of all books\nbooks.list((err, body) => {\n if (err) {\n console.log(err)\n } else {\n console.log(body.rows)\n }\n})\n```\n\nLevelDB\n-------\n\n**Module**: [levelup](https://github.com/rvagg/node-levelup)\n\n### Installation\n\n```\n$ npm install level levelup leveldown\n```\n\n### Example\n\n```\nconst levelup = require('levelup')\nconst db = levelup('./mydb')\n\ndb.put('name', 'LevelUP', (err) => {\n if (err) return console.log('Ooops!', err)\n\n db.get('name', (err, value) => {\n if (err) return console.log('Ooops!', err)\n\n console.log(`name=${value}`)\n })\n})\n```\n\nMySQL\n-----\n\n**Module**: [mysql](https://github.com/felixge/node-mysql/)\n\n### Installation\n\n```\n$ npm install mysql\n```\n\n### Example\n\n```\nconst mysql = require('mysql')\nconst connection = mysql.createConnection({\n host: 'localhost',\n user: 'dbuser',\n password: 's3kreee7',\n database: 'my_db'\n})\n\nconnection.connect()\n\nconnection.query('SELECT 1 + 1 AS solution', (err, rows, fields) => {\n if (err) throw err\n\n console.log('The solution is: ', rows[0].solution)\n})\n\nconnection.end()\n```\n\nMongoDB\n-------\n\n**Module**: [mongodb](https://github.com/mongodb/node-mongodb-native)\n\n### Installation\n\n```\n$ npm install mongodb\n```\n\n### Example (v2.\\*)\n\n```\nconst MongoClient = require('mongodb').MongoClient\n\nMongoClient.connect('mongodb://localhost:27017/animals', (err, db) => {\n if (err) throw err\n\n db.collection('mammals').find().toArray((err, result) => {\n if (err) throw err\n\n console.log(result)\n })\n})\n```\n\n### Example (v3.\\*)\n\n```\nconst MongoClient = require('mongodb').MongoClient\n\nMongoClient.connect('mongodb://localhost:27017/animals', (err, client) => {\n if (err) throw err\n\n const db = client.db('animals')\n\n db.collection('mammals').find().toArray((err, result) => {\n if (err) throw err\n\n console.log(result)\n })\n})\n```\n\nIf you want an object model driver for MongoDB, look at [Mongoose](https://github.com/LearnBoost/mongoose).\n\nNeo4j\n-----\n\n**Module**: [neo4j-driver](https://github.com/neo4j/neo4j-javascript-driver)\n\n### Installation\n\n```\n$ npm install neo4j-driver\n```\n\n### Example\n\n```\nconst neo4j = require('neo4j-driver')\nconst driver = neo4j.driver('neo4j://localhost:7687', neo4j.auth.basic('neo4j', 'letmein'))\n\nconst session = driver.session()\n\nsession.readTransaction((tx) => {\n return tx.run('MATCH (n) RETURN count(n) AS count')\n .then((res) => {\n console.log(res.records[0].get('count'))\n })\n .catch((error) => {\n console.log(error)\n })\n})\n```\n\nOracle\n------\n\n**Module**: [oracledb](https://github.com/oracle/node-oracledb)\n\n### Installation\n\nNOTE: [See installation prerequisites](https://github.com/oracle/node-oracledb#-installation).\n\n```\n$ npm install oracledb\n```\n\n### Example\n\n```\nconst oracledb = require('oracledb')\nconst config = {\n user: '<your db user>',\n password: '<your db password>',\n connectString: 'localhost:1521/orcl'\n}\n\nasync function getEmployee (empId) {\n let conn\n\n try {\n conn = await oracledb.getConnection(config)\n\n const result = await conn.execute(\n 'select * from employees where employee_id = :id',\n [empId]\n )\n\n console.log(result.rows[0])\n } catch (err) {\n console.log('Ouch!', err)\n } finally {\n if (conn) { // conn assignment worked, need to close\n await conn.close()\n }\n }\n}\n\ngetEmployee(101)\n```\n\nPostgreSQL\n----------\n\n**Module**: [pg-promise](https://github.com/vitaly-t/pg-promise)\n\n### Installation\n\n```\n$ npm install pg-promise\n```\n\n### Example\n\n```\nconst pgp = require('pg-promise')(/* options */)\nconst db = pgp('postgres://username:password@host:port/database')\n\ndb.one('SELECT $1 AS value', 123)\n .then((data) => {\n console.log('DATA:', data.value)\n })\n .catch((error) => {\n console.log('ERROR:', error)\n })\n```\n\nRedis\n-----\n\n**Module**: [redis](https://github.com/mranney/node_redis)\n\n### Installation\n\n```\n$ npm install redis\n```\n\n### Example\n\n```\nconst redis = require('redis')\nconst client = redis.createClient()\n\nclient.on('error', (err) => {\n console.log(`Error ${err}`)\n})\n\nclient.set('string key', 'string val', redis.print)\nclient.hset('hash key', 'hashtest 1', 'some value', redis.print)\nclient.hset(['hash key', 'hashtest 2', 'some other value'], redis.print)\n\nclient.hkeys('hash key', (err, replies) => {\n console.log(`${replies.length} replies:`)\n\n replies.forEach((reply, i) => {\n console.log(` ${i}: ${reply}`)\n })\n\n client.quit()\n})\n```\n\nSQL Server\n----------\n\n**Module**: [tedious](https://github.com/tediousjs/tedious)\n\n### Installation\n\n```\n$ npm install tedious\n```\n\n### Example\n\n```\nconst Connection = require('tedious').Connection\nconst Request = require('tedious').Request\n\nconst config = {\n server: 'localhost',\n authentication: {\n type: 'default',\n options: {\n userName: 'your_username', // update me\n password: 'your_password' // update me\n }\n }\n}\n\nconst connection = new Connection(config)\n\nconnection.on('connect', (err) => {\n if (err) {\n console.log(err)\n } else {\n executeStatement()\n }\n})\n\nfunction executeStatement () {\n request = new Request(\"select 123, 'hello world'\", (err, rowCount) => {\n if (err) {\n console.log(err)\n } else {\n console.log(`${rowCount} rows`)\n }\n connection.close()\n })\n\n request.on('row', (columns) => {\n columns.forEach((column) => {\n if (column.value === null) {\n console.log('NULL')\n } else {\n console.log(column.value)\n }\n })\n })\n\n connection.execSql(request)\n}\n```\n\nSQLite\n------\n\n**Module**: [sqlite3](https://github.com/mapbox/node-sqlite3)\n\n### Installation\n\n```\n$ npm install sqlite3\n```\n\n### Example\n\n```\nconst sqlite3 = require('sqlite3').verbose()\nconst db = new sqlite3.Database(':memory:')\n\ndb.serialize(() => {\n db.run('CREATE TABLE lorem (info TEXT)')\n const stmt = db.prepare('INSERT INTO lorem VALUES (?)')\n\n for (let i = 0; i < 10; i++) {\n stmt.run(`Ipsum ${i}`)\n }\n\n stmt.finalize()\n\n db.each('SELECT rowid AS id, info FROM lorem', (err, row) => {\n console.log(`${row.id}: ${row.info}`)\n })\n})\n\ndb.close()\n```\n\nElasticsearch\n-------------\n\n**Module**: [elasticsearch](https://github.com/elastic/elasticsearch-js)\n\n### Installation\n\n```\n$ npm install elasticsearch\n```\n\n### Example\n\n```\nconst elasticsearch = require('elasticsearch')\nconst client = elasticsearch.Client({\n host: 'localhost:9200'\n})\n\nclient.search({\n index: 'books',\n type: 'book',\n body: {\n query: {\n multi_match: {\n query: 'express js',\n fields: ['title', 'description']\n }\n }\n }\n}).then((response) => {\n const hits = response.hits.hits\n}, (error) => {\n console.trace(error.message)\n})\n```\n",
"filename": "database-integration.md",
"package": "express"
} |
{
"content": "Title: Debugging Express\n\nURL Source: https://expressjs.com/en/guide/debugging.html\n\nMarkdown Content:\nTo see all the internal logs used in Express, set the `DEBUG` environment variable to `express:*` when launching your app.\n\n```\n$ DEBUG=express:* node index.js\n```\n\nOn Windows, use the corresponding command.\n\n```\n> set DEBUG=express:* & node index.js\n```\n\nRunning this command on the default app generated by the [express generator](https://expressjs.com/en/starter/generator.html) prints the following output:\n\n```\n$ DEBUG=express:* node ./bin/www\n express:router:route new / +0ms\n express:router:layer new / +1ms\n express:router:route get / +1ms\n express:router:layer new / +0ms\n express:router:route new / +1ms\n express:router:layer new / +0ms\n express:router:route get / +0ms\n express:router:layer new / +0ms\n express:application compile etag weak +1ms\n express:application compile query parser extended +0ms\n express:application compile trust proxy false +0ms\n express:application booting in development mode +1ms\n express:router use / query +0ms\n express:router:layer new / +0ms\n express:router use / expressInit +0ms\n express:router:layer new / +0ms\n express:router use / favicon +1ms\n express:router:layer new / +0ms\n express:router use / logger +0ms\n express:router:layer new / +0ms\n express:router use / jsonParser +0ms\n express:router:layer new / +1ms\n express:router use / urlencodedParser +0ms\n express:router:layer new / +0ms\n express:router use / cookieParser +0ms\n express:router:layer new / +0ms\n express:router use / stylus +90ms\n express:router:layer new / +0ms\n express:router use / serveStatic +0ms\n express:router:layer new / +0ms\n express:router use / router +0ms\n express:router:layer new / +1ms\n express:router use /users router +0ms\n express:router:layer new /users +0ms\n express:router use / <anonymous> +0ms\n express:router:layer new / +0ms\n express:router use / <anonymous> +0ms\n express:router:layer new / +0ms\n express:router use / <anonymous> +0ms\n express:router:layer new / +0ms\n```\n\nWhen a request is then made to the app, you will see the logs specified in the Express code:\n\n```\n express:router dispatching GET / +4h\n express:router query : / +2ms\n express:router expressInit : / +0ms\n express:router favicon : / +0ms\n express:router logger : / +1ms\n express:router jsonParser : / +0ms\n express:router urlencodedParser : / +1ms\n express:router cookieParser : / +0ms\n express:router stylus : / +0ms\n express:router serveStatic : / +2ms\n express:router router : / +2ms\n express:router dispatching GET / +1ms\n express:view lookup \"index.pug\" +338ms\n express:view stat \"/projects/example/views/index.pug\" +0ms\n express:view render \"/projects/example/views/index.pug\" +1ms\n```\n\nTo see the logs only from the router implementation, set the value of `DEBUG` to `express:router`. Likewise, to see logs only from the application implementation, set the value of `DEBUG` to `express:application`, and so on.\n\nApplications generated by `express`\n-----------------------------------\n\nAn application generated by the `express` command uses the `debug` module and its debug namespace is scoped to the name of the application.\n\nFor example, if you generated the app with `$ express sample-app`, you can enable the debug statements with the following command:\n\n```\n$ DEBUG=sample-app:* node ./bin/www\n```\n\nYou can specify more than one debug namespace by assigning a comma-separated list of names:\n\n```\n$ DEBUG=http,mail,express:* node index.js\n```\n\nAdvanced options\n----------------\n\nWhen running through Node.js, you can set a few environment variables that will change the behavior of the debug logging:\n\n| Name | Purpose |\n| --- | --- |\n| `DEBUG` | Enables/disables specific debugging namespaces. |\n| `DEBUG_COLORS` | Whether or not to use colors in the debug output. |\n| `DEBUG_DEPTH` | Object inspection depth. |\n| `DEBUG_FD` | File descriptor to write debug output to. |\n| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |\n\n**Note:** The environment variables beginning with `DEBUG_` end up being converted into an Options object that gets used with `%o`/`%O` formatters. See the Node.js documentation for [`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options) for the complete list.\n",
"filename": "debugging.md",
"package": "express"
} |
{
"content": "Title: Developing template engines for Express\n\nURL Source: https://expressjs.com/en/advanced/developing-template-engines.html\n\nMarkdown Content:\nDeveloping template engines for Express\n=======================================\n\nUse the `app.engine(ext, callback)` method to create your own template engine. `ext` refers to the file extension, and `callback` is the template engine function, which accepts the following items as parameters: the location of the file, the options object, and the callback function.\n\nThe following code is an example of implementing a very simple template engine for rendering `.ntl` files.\n\n```javascript\nconst fs = require('fs') // this engine requires the fs module\napp.engine('ntl', (filePath, options, callback) => { // define the template engine\n fs.readFile(filePath, (err, content) => {\n if (err) return callback(err)\n // this is an extremely simple template engine\n const rendered = content.toString()\n .replace('#title#', `<title>${options.title}</title>`)\n .replace('#message#', `<h1>${options.message}</h1>`)\n return callback(null, rendered)\n })\n})\napp.set('views', './views') // specify the views directory\napp.set('view engine', 'ntl') // register the template engine\n```\n\nYour app will now be able to render `.ntl` files. Create a file named `index.ntl` in the `views` directory with the following content.\n\n```text\n#title#\n#message#\n```\n\nThen, create the following route in your app.\n\n```javascript\napp.get('/', (req, res) => {\n res.render('index', { title: 'Hey', message: 'Hello there!' })\n})\n```\n\nWhen you make a request to the home page, `index.ntl` will be rendered as HTML.\n\n[](https://expressjs.com/en/advanced/developing-template-engines.html#)\n\nDocumentation translations provided by StrongLoop/IBM: [French](https://expressjs.com/fr/), [German](https://expressjs.com/de/), [Spanish](https://expressjs.com/es/), [Italian](https://expressjs.com/it/), [Japanese](https://expressjs.com/ja/), [Russian](https://expressjs.com/ru/), [Chinese](https://expressjs.com/zh-cn/), [Traditional Chinese](https://expressjs.com/zh-tw/), [Korean](https://expressjs.com/ko/), [Portuguese](https://expressjs.com/pt-br/). \nCommunity translation available for: [Slovak](https://expressjs.com/sk/), [Ukrainian](https://expressjs.com/uk/), [Uzbek](https://expressjs.com/uz/), [Turkish](https://expressjs.com/tr/), [Thai](https://expressjs.com/th/) and [Indonesian](https://expressjs.com/id/).\n\n[](https://www.netlify.com/)\n\n[Express](https://expressjs.com/) is a project of the [OpenJS Foundation](https://openjsf.org/).\n\n[Edit this page on GitHub](https://github.com/expressjs/expressjs.com/blob/gh-pages/en/advanced/developing-template-engines.md).\n\nCopyright © 2017 StrongLoop, IBM, and other expressjs.com contributors.\n\n[](http://creativecommons.org/licenses/by-sa/3.0/us/) This work is licensed under a [Creative Commons Attribution-ShareAlike 3.0 United States License](http://creativecommons.org/licenses/by-sa/3.0/us/).\n",
"filename": "developing-template-engines.md",
"package": "express"
} |
{
"content": "Title: Express error handling\n\nURL Source: https://expressjs.com/en/guide/error-handling.html\n\nMarkdown Content:\n_Error Handling_ refers to how Express catches and processes errors that occur both synchronously and asynchronously. Express comes with a default error handler so you don’t need to write your own to get started.\n\nCatching Errors\n---------------\n\nIt’s important to ensure that Express catches all errors that occur while running route handlers and middleware.\n\nErrors that occur in synchronous code inside route handlers and middleware require no extra work. If synchronous code throws an error, then Express will catch and process it. For example:\n\n```\napp.get('/', (req, res) => {\n throw new Error('BROKEN') // Express will catch this on its own.\n})\n```\n\nFor errors returned from asynchronous functions invoked by route handlers and middleware, you must pass them to the `next()` function, where Express will catch and process them. For example:\n\n```\napp.get('/', (req, res, next) => {\n fs.readFile('/file-does-not-exist', (err, data) => {\n if (err) {\n next(err) // Pass errors to Express.\n } else {\n res.send(data)\n }\n })\n})\n```\n\nStarting with Express 5, route handlers and middleware that return a Promise will call `next(value)` automatically when they reject or throw an error. For example:\n\n```\napp.get('/user/:id', async (req, res, next) => {\n const user = await getUserById(req.params.id)\n res.send(user)\n})\n```\n\nIf `getUserById` throws an error or rejects, `next` will be called with either the thrown error or the rejected value. If no rejected value is provided, `next` will be called with a default Error object provided by the Express router.\n\nIf you pass anything to the `next()` function (except the string `'route'`), Express regards the current request as being an error and will skip any remaining non-error handling routing and middleware functions.\n\nIf the callback in a sequence provides no data, only errors, you can simplify this code as follows:\n\n```\napp.get('/', [\n function (req, res, next) {\n fs.writeFile('/inaccessible-path', 'data', next)\n },\n function (req, res) {\n res.send('OK')\n }\n])\n```\n\nIn the above example, `next` is provided as the callback for `fs.writeFile`, which is called with or without errors. If there is no error, the second handler is executed, otherwise Express catches and processes the error.\n\nYou must catch errors that occur in asynchronous code invoked by route handlers or middleware and pass them to Express for processing. For example:\n\n```\napp.get('/', (req, res, next) => {\n setTimeout(() => {\n try {\n throw new Error('BROKEN')\n } catch (err) {\n next(err)\n }\n }, 100)\n})\n```\n\nThe above example uses a `try...catch` block to catch errors in the asynchronous code and pass them to Express. If the `try...catch` block were omitted, Express would not catch the error since it is not part of the synchronous handler code.\n\nUse promises to avoid the overhead of the `try...catch` block or when using functions that return promises. For example:\n\n```\napp.get('/', (req, res, next) => {\n Promise.resolve().then(() => {\n throw new Error('BROKEN')\n }).catch(next) // Errors will be passed to Express.\n})\n```\n\nSince promises automatically catch both synchronous errors and rejected promises, you can simply provide `next` as the final catch handler and Express will catch errors, because the catch handler is given the error as the first argument.\n\nYou could also use a chain of handlers to rely on synchronous error catching, by reducing the asynchronous code to something trivial. For example:\n\n```\napp.get('/', [\n function (req, res, next) {\n fs.readFile('/maybe-valid-file', 'utf-8', (err, data) => {\n res.locals.data = data\n next(err)\n })\n },\n function (req, res) {\n res.locals.data = res.locals.data.split(',')[1]\n res.send(res.locals.data)\n }\n])\n```\n\nThe above example has a couple of trivial statements from the `readFile` call. If `readFile` causes an error, then it passes the error to Express, otherwise you quickly return to the world of synchronous error handling in the next handler in the chain. Then, the example above tries to process the data. If this fails, then the synchronous error handler will catch it. If you had done this processing inside the `readFile` callback, then the application might exit and the Express error handlers would not run.\n\nWhichever method you use, if you want Express error handlers to be called in and the application to survive, you must ensure that Express receives the error.\n\nThe default error handler\n-------------------------\n\nExpress comes with a built-in error handler that takes care of any errors that might be encountered in the app. This default error-handling middleware function is added at the end of the middleware function stack.\n\nIf you pass an error to `next()` and you do not handle it in a custom error handler, it will be handled by the built-in error handler; the error will be written to the client with the stack trace. The stack trace is not included in the production environment.\n\nSet the environment variable `NODE_ENV` to `production`, to run the app in production mode.\n\nWhen an error is written, the following information is added to the response:\n\n* The `res.statusCode` is set from `err.status` (or `err.statusCode`). If this value is outside the 4xx or 5xx range, it will be set to 500.\n* The `res.statusMessage` is set according to the status code.\n* The body will be the HTML of the status code message when in production environment, otherwise will be `err.stack`.\n* Any headers specified in an `err.headers` object.\n\nIf you call `next()` with an error after you have started writing the response (for example, if you encounter an error while streaming the response to the client), the Express default error handler closes the connection and fails the request.\n\nSo when you add a custom error handler, you must delegate to the default Express error handler, when the headers have already been sent to the client:\n\n```\nfunction errorHandler (err, req, res, next) {\n if (res.headersSent) {\n return next(err)\n }\n res.status(500)\n res.render('error', { error: err })\n}\n```\n\nNote that the default error handler can get triggered if you call `next()` with an error in your code more than once, even if custom error handling middleware is in place.\n\nOther error handling middleware can be found at [Express middleware](https://expressjs.com/en/resources/middleware.html).\n\nWriting error handlers\n----------------------\n\nDefine error-handling middleware functions in the same way as other middleware functions, except error-handling functions have four arguments instead of three: `(err, req, res, next)`. For example:\n\n```\napp.use((err, req, res, next) => {\n console.error(err.stack)\n res.status(500).send('Something broke!')\n})\n```\n\nYou define error-handling middleware last, after other `app.use()` and routes calls; for example:\n\n```\nconst bodyParser = require('body-parser')\nconst methodOverride = require('method-override')\n\napp.use(bodyParser.urlencoded({\n extended: true\n}))\napp.use(bodyParser.json())\napp.use(methodOverride())\napp.use((err, req, res, next) => {\n // logic\n})\n```\n\nResponses from within a middleware function can be in any format, such as an HTML error page, a simple message, or a JSON string.\n\nFor organizational (and higher-level framework) purposes, you can define several error-handling middleware functions, much as you would with regular middleware functions. For example, to define an error-handler for requests made by using `XHR` and those without:\n\n```\nconst bodyParser = require('body-parser')\nconst methodOverride = require('method-override')\n\napp.use(bodyParser.urlencoded({\n extended: true\n}))\napp.use(bodyParser.json())\napp.use(methodOverride())\napp.use(logErrors)\napp.use(clientErrorHandler)\napp.use(errorHandler)\n```\n\nIn this example, the generic `logErrors` might write request and error information to `stderr`, for example:\n\n```\nfunction logErrors (err, req, res, next) {\n console.error(err.stack)\n next(err)\n}\n```\n\nAlso in this example, `clientErrorHandler` is defined as follows; in this case, the error is explicitly passed along to the next one.\n\nNotice that when _not_ calling “next” in an error-handling function, you are responsible for writing (and ending) the response. Otherwise, those requests will “hang” and will not be eligible for garbage collection.\n\n```\nfunction clientErrorHandler (err, req, res, next) {\n if (req.xhr) {\n res.status(500).send({ error: 'Something failed!' })\n } else {\n next(err)\n }\n}\n```\n\nImplement the “catch-all” `errorHandler` function as follows (for example):\n\n```\nfunction errorHandler (err, req, res, next) {\n res.status(500)\n res.render('error', { error: err })\n}\n```\n\nIf you have a route handler with multiple callback functions, you can use the `route` parameter to skip to the next route handler. For example:\n\n```\napp.get('/a_route_behind_paywall',\n (req, res, next) => {\n if (!req.user.hasPaid) {\n // continue handling this request\n next('route')\n } else {\n next()\n }\n }, (req, res, next) => {\n PaidContent.find((err, doc) => {\n if (err) return next(err)\n res.json(doc)\n })\n })\n```\n\nIn this example, the `getPaidContent` handler will be skipped but any remaining handlers in `app` for `/a_route_behind_paywall` would continue to be executed.\n\nCalls to `next()` and `next(err)` indicate that the current handler is complete and in what state. `next(err)` will skip all remaining handlers in the chain except for those that are set up to handle errors as described above.\n",
"filename": "error-handling.md",
"package": "express"
} |
{
"content": "Title: Express FAQ\n\nURL Source: https://expressjs.com/en/starter/faq.html\n\nMarkdown Content:\nHow should I structure my application?\n--------------------------------------\n\nThere is no definitive answer to this question. The answer depends on the scale of your application and the team that is involved. To be as flexible as possible, Express makes no assumptions in terms of structure.\n\nRoutes and other application-specific logic can live in as many files as you wish, in any directory structure you prefer. View the following examples for inspiration:\n\n* [Route listings](https://github.com/expressjs/express/blob/4.13.1/examples/route-separation/index.js#L32-L47)\n* [Route map](https://github.com/expressjs/express/blob/4.13.1/examples/route-map/index.js#L52-L66)\n* [MVC style controllers](https://github.com/expressjs/express/tree/master/examples/mvc)\n\nAlso, there are third-party extensions for Express, which simplify some of these patterns:\n\n* [Resourceful routing](https://github.com/expressjs/express-resource)\n\nHow do I define models?\n-----------------------\n\nExpress has no notion of a database. This concept is left up to third-party Node modules, allowing you to interface with nearly any database.\n\nSee [LoopBack](http://loopback.io/) for an Express-based framework that is centered around models.\n\nHow can I authenticate users?\n-----------------------------\n\nAuthentication is another opinionated area that Express does not venture into. You may use any authentication scheme you wish. For a simple username / password scheme, see [this example](https://github.com/expressjs/express/tree/master/examples/auth).\n\nWhich template engines does Express support?\n--------------------------------------------\n\nExpress supports any template engine that conforms with the `(path, locals, callback)` signature. To normalize template engine interfaces and caching, see the [consolidate.js](https://github.com/visionmedia/consolidate.js) project for support. Unlisted template engines might still support the Express signature.\n\nFor more information, see [Using template engines with Express](https://expressjs.com/en/guide/using-template-engines.html).\n\nHow do I handle 404 responses?\n------------------------------\n\nIn Express, 404 responses are not the result of an error, so the error-handler middleware will not capture them. This behavior is because a 404 response simply indicates the absence of additional work to do; in other words, Express has executed all middleware functions and routes, and found that none of them responded. All you need to do is add a middleware function at the very bottom of the stack (below all other functions) to handle a 404 response:\n\n```\napp.use((req, res, next) => {\n res.status(404).send(\"Sorry can't find that!\")\n})\n```\n\nAdd routes dynamically at runtime on an instance of `express.Router()` so the routes are not superseded by a middleware function.\n\nHow do I setup an error handler?\n--------------------------------\n\nYou define error-handling middleware in the same way as other middleware, except with four arguments instead of three; specifically with the signature `(err, req, res, next)`:\n\n```\napp.use((err, req, res, next) => {\n console.error(err.stack)\n res.status(500).send('Something broke!')\n})\n```\n\nFor more information, see [Error handling](https://expressjs.com/en/guide/error-handling.html).\n\nHow do I render plain HTML?\n---------------------------\n\nYou don’t! There’s no need to “render” HTML with the `res.render()` function. If you have a specific file, use the `res.sendFile()` function. If you are serving many assets from a directory, use the `express.static()` middleware function.\n\nWhat version of Node.js does Express require?\n---------------------------------------------\n\n* [Express 4.x](https://expressjs.com/en/4x/api.html) requires Node.js 0.10 or higher.\n* [Express 5.x](https://expressjs.com/en/5x/api.html) requires Node.js 18 or higher.\n\n### [Previous: More examples](https://expressjs.com/en/starter/examples.html)\n",
"filename": "faq.md",
"package": "express"
} |
{
"content": "Title: Express application generator\n\nURL Source: https://expressjs.com/en/starter/generator.html\n\nMarkdown Content:\nUse the application generator tool, `express-generator`, to quickly create an application skeleton.\n\nYou can run the application generator with the `npx` command (available in Node.js 8.2.0).\n\n```\n$ npx express-generator\n```\n\nFor earlier Node versions, install the application generator as a global npm package and then launch it:\n\n```\n$ npm install -g express-generator\n$ express\n```\n\nDisplay the command options with the `-h` option:\n\n```\n$ express -h\n\n Usage: express [options] [dir]\n\n Options:\n\n -h, --help output usage information\n --version output the version number\n -e, --ejs add ejs engine support\n --hbs add handlebars engine support\n --pug add pug engine support\n -H, --hogan add hogan.js engine support\n --no-view generate without view engine\n -v, --view <engine> add view <engine> support (ejs|hbs|hjs|jade|pug|twig|vash) (defaults to jade)\n -c, --css <engine> add stylesheet <engine> support (less|stylus|compass|sass) (defaults to plain css)\n --git add .gitignore\n -f, --force force on non-empty directory\n```\n\nFor example, the following creates an Express app named _myapp_. The app will be created in a folder named _myapp_ in the current working directory and the view engine will be set to [Pug](https://pugjs.org/ \"Pug documentation\"):\n\n```\n$ express --view=pug myapp\n\n create : myapp\n create : myapp/package.json\n create : myapp/app.js\n create : myapp/public\n create : myapp/public/javascripts\n create : myapp/public/images\n create : myapp/routes\n create : myapp/routes/index.js\n create : myapp/routes/users.js\n create : myapp/public/stylesheets\n create : myapp/public/stylesheets/style.css\n create : myapp/views\n create : myapp/views/index.pug\n create : myapp/views/layout.pug\n create : myapp/views/error.pug\n create : myapp/bin\n create : myapp/bin/www\n```\n\nThen install dependencies:\n\n```\n$ cd myapp\n$ npm install\n```\n\nOn MacOS or Linux, run the app with this command:\n\n```\n$ DEBUG=myapp:* npm start\n```\n\nOn Windows Command Prompt, use this command:\n\n```\n> set DEBUG=myapp:* & npm start\n```\n\nOn Windows PowerShell, use this command:\n\n```\nPS> $env:DEBUG='myapp:*'; npm start\n```\n\nThen, load `http://localhost:3000/` in your browser to access the app.\n\nThe generated app has the following directory structure:\n\n```\n.\n├── app.js\n├── bin\n│ └── www\n├── package.json\n├── public\n│ ├── images\n│ ├── javascripts\n│ └── stylesheets\n│ └── style.css\n├── routes\n│ ├── index.js\n│ └── users.js\n└── views\n ├── error.pug\n ├── index.pug\n └── layout.pug\n\n7 directories, 9 files\n```\n\nThe app structure created by the generator is just one of many ways to structure Express apps. Feel free to use this structure or modify it to best suit your needs.\n\n### [Previous: Hello World](https://expressjs.com/en/starter/hello-world.html) [Next: Basic routing](https://expressjs.com/en/starter/basic-routing.html)\n",
"filename": "generator.md",
"package": "express"
} |
{
"content": "Title: Express glossary\n\nURL Source: https://expressjs.com/en/resources/glossary.html\n\nMarkdown Content:\n### application\n\nIn general, one or more programs that are designed to carry out operations for a specific purpose. In the context of Express, a program that uses the Express API running on the Node.js platform. Might also refer to an [app object](https://expressjs.com/en/api.html#express).\n\n### API\n\nApplication programming interface. Spell out the abbreviation when it is first used.\n\n### Express\n\nA fast, un-opinionated, minimalist web framework for Node.js applications. In general, “Express” is preferred to “Express.js,” though the latter is acceptable.\n\n### libuv\n\nA multi-platform support library which focuses on asynchronous I/O, primarily developed for use by Node.js.\n\n### middleware\n\nA function that is invoked by the Express routing layer before the final request handler, and thus sits in the middle between a raw request and the final intended route. A few fine points of terminology around middleware:\n\n* `var foo = require('middleware')` is called _requiring_ or _using_ a Node.js module. Then the statement `var mw = foo()` typically returns the middleware.\n* `app.use(mw)` is called _adding the middleware to the global processing stack_.\n* `app.get('/foo', mw, function (req, res) { ... })` is called _adding the middleware to the “GET /foo” processing stack_.\n\n### Node.js\n\nA software platform that is used to build scalable network applications. Node.js uses JavaScript as its scripting language, and achieves high throughput via non-blocking I/O and a single-threaded event loop. See [nodejs.org](https://nodejs.org/en/). **Usage note**: Initially, “Node.js,” thereafter “Node”.\n\n### open-source, open source\n\nWhen used as an adjective, hyphenate; for example: “This is open-source software.” See [Open-source software on Wikipedia](http://en.wikipedia.org/wiki/Open-source_software). Note: Although it is common not to hyphenate this term, we are using the standard English rules for hyphenating a compound adjective.\n\n### request\n\nAn HTTP request. A client submits an HTTP request message to a server, which returns a response. The request must use one of several [request methods](https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods) such as GET, POST, and so on.\n\n### response\n\nAn HTTP response. A server returns an HTTP response message to the client. The response contains completion status information about the request and might also contain requested content in its message body.\n\n### route\n\nPart of a URL that identifies a resource. For example, in `http://foo.com/products/id`, “/products/id” is the route.\n\n### router\n\nSee [router](https://expressjs.com/en/api.html#router) in the API reference.\n",
"filename": "glossary.md",
"package": "express"
} |
{
"content": "Title: Health Checks and Graceful Shutdown\n\nURL Source: https://expressjs.com/en/advanced/healthcheck-graceful-shutdown.html\n\nMarkdown Content:\nHealth Checks and Graceful Shutdown\n===============\n \n\n[Express](https://expressjs.com/)\n\n* [Home](https://expressjs.com/)\n* * [Getting started](https://expressjs.com/en/starter/installing.html)\n * [Installing](https://expressjs.com/en/starter/installing.html)\n * [Hello world](https://expressjs.com/en/starter/hello-world.html)\n * [Express generator](https://expressjs.com/en/starter/generator.html)\n * [Basic routing](https://expressjs.com/en/starter/basic-routing.html)\n * [Static files](https://expressjs.com/en/starter/static-files.html)\n * [More examples](https://expressjs.com/en/starter/examples.html)\n * [FAQ](https://expressjs.com/en/starter/faq.html)\n* * [Guide](https://expressjs.com/en/guide/routing.html)\n * [Routing](https://expressjs.com/en/guide/routing.html)\n * [Writing middleware](https://expressjs.com/en/guide/writing-middleware.html)\n * [Using middleware](https://expressjs.com/en/guide/using-middleware.html)\n * [Overriding the Express API](https://expressjs.com/en/guide/overriding-express-api.html)\n * [Using template engines](https://expressjs.com/en/guide/using-template-engines.html)\n * [Error handling](https://expressjs.com/en/guide/error-handling.html)\n * [Debugging](https://expressjs.com/en/guide/debugging.html)\n * [Express behind proxies](https://expressjs.com/en/guide/behind-proxies.html)\n * [Moving to Express 4](https://expressjs.com/en/guide/migrating-4.html)\n * [Moving to Express 5](https://expressjs.com/en/guide/migrating-5.html)\n * [Database integration](https://expressjs.com/en/guide/database-integration.html)\n* * [API reference](https://expressjs.com/en/4x/api.html)\n * [5.x (beta)](https://expressjs.com/en/5x/api.html)\n * [4.x](https://expressjs.com/en/4x/api.html)\n * [3.x (deprecated)](https://expressjs.com/en/3x/api.html)\n * [2.x (deprecated)](https://expressjs.com/2x/)\n* * [Advanced topics](https://expressjs.com/en/advanced/developing-template-engines.html)\n * [Building template engines](https://expressjs.com/en/advanced/developing-template-engines.html)\n * [Security updates](https://expressjs.com/en/advanced/security-updates.html)\n * [Security best practices](https://expressjs.com/en/advanced/best-practice-security.html)\n * [Performance best practices](https://expressjs.com/en/advanced/best-practice-performance.html)\n * [Health checks & shutdown](https://expressjs.com/en/advanced/healthcheck-graceful-shutdown.html)\n* * [Resources](https://expressjs.com/en/resources/glossary.html)\n * [Community](https://expressjs.com/en/resources/community.html)\n * [Glossary](https://expressjs.com/en/resources/glossary.html)\n * [Middleware](https://expressjs.com/en/resources/middleware.html)\n * [Utility modules](https://expressjs.com/en/resources/utils.html)\n * [Contributing to Express](https://expressjs.com/en/resources/contributing.html)\n * [Release Change Log](https://expressjs.com/en/changelog/4x.html)\n\nHealth Checks and Graceful Shutdown\n===================================\n\nGraceful shutdown\n-----------------\n\nWhen you deploy a new version of your application, you must replace the previous version. The process manager you’re using will first send a SIGTERM signal to the application to notify it that it will be killed. Once the application gets this signal, it should stop accepting new requests, finish all the ongoing requests, clean up the resources it used, including database connections and file locks then exit.\n\n### Example\n\n```javascript\nconst server = app.listen(port)\n\nprocess.on('SIGTERM', () => {\n debug('SIGTERM signal received: closing HTTP server')\n server.close(() => {\n debug('HTTP server closed')\n })\n})\n```\n\nHealth checks\n-------------\n\nA load balancer uses health checks to determine if an application instance is healthy and can accept requests. For example, [Kubernetes has two health checks](https://kubernetes.io/docs/tasks/configure-pod-container/configure-liveness-readiness-probes/):\n\n* `liveness`, that determines when to restart a container.\n* `readiness`, that determines when a container is ready to start accepting traffic. When a pod is not ready, it is removed from the service load balancers.\n\n[](https://expressjs.com/en/advanced/healthcheck-graceful-shutdown.html#)\n\nDocumentation translations provided by StrongLoop/IBM: [French](https://expressjs.com/fr/), [German](https://expressjs.com/de/), [Spanish](https://expressjs.com/es/), [Italian](https://expressjs.com/it/), [Japanese](https://expressjs.com/ja/), [Russian](https://expressjs.com/ru/), [Chinese](https://expressjs.com/zh-cn/), [Traditional Chinese](https://expressjs.com/zh-tw/), [Korean](https://expressjs.com/ko/), [Portuguese](https://expressjs.com/pt-br/). \nCommunity translation available for: [Slovak](https://expressjs.com/sk/), [Ukrainian](https://expressjs.com/uk/), [Uzbek](https://expressjs.com/uz/), [Turkish](https://expressjs.com/tr/), [Thai](https://expressjs.com/th/) and [Indonesian](https://expressjs.com/id/).\n\n[](https://www.netlify.com/)\n\n[Express](https://expressjs.com/) is a project of the [OpenJS Foundation](https://openjsf.org/).\n\n[Edit this page on GitHub](https://github.com/expressjs/expressjs.com/blob/gh-pages/en/advanced/healthcheck-graceful-shutdown.md).\n\nCopyright © 2017 StrongLoop, IBM, and other expressjs.com contributors.\n\n[](http://creativecommons.org/licenses/by-sa/3.0/us/) This work is licensed under a [Creative Commons Attribution-ShareAlike 3.0 United States License](http://creativecommons.org/licenses/by-sa/3.0/us/).\n",
"filename": "healthcheck-graceful-shutdown.md",
"package": "express"
} |
{
"content": "Title: Express \"Hello World\" example\n\nURL Source: https://expressjs.com/en/starter/hello-world.html\n\nMarkdown Content:\nEmbedded below is essentially the simplest Express app you can create. It is a single file app — _not_ what you’d get if you use the [Express generator](https://expressjs.com/en/starter/generator.html), which creates the scaffolding for a full app with numerous JavaScript files, Jade templates, and sub-directories for various purposes.\n\n```\n\nconst express = require('express')\nconst app = express()\nconst port = 3000\n\napp.get('/', (req, res) => {\n res.send('Hello World!')\n})\n\napp.listen(port, () => {\n console.log(`Example app listening on port ${port}`)\n})\n```\n\nThis app starts a server and listens on port 3000 for connections. The app responds with “Hello World!” for requests to the root URL (`/`) or _route_. For every other path, it will respond with a **404 Not Found**.\n\nThe example above is actually a working server: Go ahead and click on the URL shown. You’ll get a response, with real-time logs on the page, and any changes you make will be reflected in real time. This is powered by [RunKit](https://runkit.com/), which provides an interactive JavaScript playground connected to a complete Node environment that runs in your web browser. Below are instructions for running the same app on your local machine.\n\nRunKit is a third-party service not affiliated with the Express project.\n\n### Running Locally\n\nFirst create a directory named `myapp`, change to it and run `npm init`. Then, install `express` as a dependency, as per the [installation guide](https://expressjs.com/en/starter/installing.html).\n\nIn the `myapp` directory, create a file named `app.js` and copy the code from the example above.\n\nThe `req` (request) and `res` (response) are the exact same objects that Node provides, so you can invoke `req.pipe()`, `req.on('data', callback)`, and anything else you would do without Express involved.\n\nRun the app with the following command:\n\n```\n$ node app.js\n```\n\nThen, load `http://localhost:3000/` in a browser to see the output.\n\n### [Previous: Installing](https://expressjs.com/en/starter/installing.html) [Next: Express Generator](https://expressjs.com/en/starter/generator.html)\n",
"filename": "hello-world.md",
"package": "express"
} |
{
"content": "Title: Installing Express\n\nURL Source: https://expressjs.com/en/starter/installing.html\n\nMarkdown Content:\nInstalling Express\n===============\n\nInstalling\n==========\n\nAssuming you’ve already installed [Node.js](https://nodejs.org/), create a directory to hold your application, and make that your working directory.\n\n* [Express 4.x](https://expressjs.com/en/4x/api.html) requires Node.js 0.10 or higher.\n* [Express 5.x](https://expressjs.com/en/5x/api.html) requires Node.js 18 or higher.\n\n```console\n$ mkdir myapp\n$ cd myapp\n```\n\nUse the `npm init` command to create a `package.json` file for your application. For more information on how `package.json` works, see [Specifics of npm’s package.json handling](https://docs.npmjs.com/files/package.json).\n\n```console\n$ npm init\n```\n\nThis command prompts you for a number of things, such as the name and version of your application. For now, you can simply hit RETURN to accept the defaults for most of them, with the following exception:\n\n```\nentry point: (index.js)\n```\n\nEnter `app.js`, or whatever you want the name of the main file to be. If you want it to be `index.js`, hit RETURN to accept the suggested default file name.\n\nNow, install Express in the `myapp` directory and save it in the dependencies list. For example:\n\n```console\n$ npm install express\n```\n\nTo install Express temporarily and not add it to the dependencies list:\n\n```console\n$ npm install express --no-save\n```\n\nBy default with version npm 5.0+, `npm install` adds the module to the `dependencies` list in the `package.json` file; with earlier versions of npm, you must specify the `--save` option explicitly. Then, afterwards, running `npm install` in the app directory will automatically install modules in the dependencies list.\n\n### [Next: Hello World](https://expressjs.com/en/starter/hello-world.html)\n\n[](https://expressjs.com/en/starter/installing.html#)\n\nDocumentation translations provided by StrongLoop/IBM: [French](https://expressjs.com/fr/), [German](https://expressjs.com/de/), [Spanish](https://expressjs.com/es/), [Italian](https://expressjs.com/it/), [Japanese](https://expressjs.com/ja/), [Russian](https://expressjs.com/ru/), [Chinese](https://expressjs.com/zh-cn/), [Traditional Chinese](https://expressjs.com/zh-tw/), [Korean](https://expressjs.com/ko/), [Portuguese](https://expressjs.com/pt-br/). \nCommunity translation available for: [Slovak](https://expressjs.com/sk/), [Ukrainian](https://expressjs.com/uk/), [Uzbek](https://expressjs.com/uz/), [Turkish](https://expressjs.com/tr/), [Thai](https://expressjs.com/th/) and [Indonesian](https://expressjs.com/id/).\n\n[](https://www.netlify.com/)\n\n[Express](https://expressjs.com/) is a project of the [OpenJS Foundation](https://openjsf.org/).\n\n[Edit this page on GitHub](https://github.com/expressjs/expressjs.com/blob/gh-pages/en/starter/installing.md).\n\nCopyright © 2017 StrongLoop, IBM, and other expressjs.com contributors.\n\n[](http://creativecommons.org/licenses/by-sa/3.0/us/) This work is licensed under a [Creative Commons Attribution-ShareAlike 3.0 United States License](http://creativecommons.org/licenses/by-sa/3.0/us/).\n",
"filename": "installing.md",
"package": "express"
} |
{
"content": "Title: Express middleware\n\nURL Source: https://expressjs.com/en/resources/middleware.html\n\nMarkdown Content:\nThe Express middleware modules listed here are maintained by the [Expressjs team](https://github.com/orgs/expressjs/people).\n\n| Middleware module | Description | Replaces built-in function (Express 3) |\n| --- | --- | --- |\n| [body-parser](https://expressjs.com/resources/middleware/body-parser.html) | Parse HTTP request body. See also: [body](https://github.com/raynos/body), [co-body](https://github.com/visionmedia/co-body), and [raw-body](https://github.com/stream-utils/raw-body). | express.bodyParser |\n| [compression](https://expressjs.com/resources/middleware/compression.html) | Compress HTTP responses. | express.compress |\n| [connect-rid](https://expressjs.com/resources/middleware/connect-rid.html) | Generate unique request ID. | NA |\n| [cookie-parser](https://expressjs.com/resources/middleware/cookie-parser.html) | Parse cookie header and populate `req.cookies`. See also [cookies](https://github.com/jed/cookies) and [keygrip](https://github.com/jed/keygrip). | express.cookieParser |\n| [cookie-session](https://expressjs.com/resources/middleware/cookie-session.html) | Establish cookie-based sessions. | express.cookieSession |\n| [cors](https://expressjs.com/resources/middleware/cors.html) | Enable cross-origin resource sharing (CORS) with various options. | NA |\n| [errorhandler](https://expressjs.com/resources/middleware/errorhandler.html) | Development error-handling/debugging. | express.errorHandler |\n| [method-override](https://expressjs.com/resources/middleware/method-override.html) | Override HTTP methods using header. | express.methodOverride |\n| [morgan](https://expressjs.com/resources/middleware/morgan.html) | HTTP request logger. | express.logger |\n| [multer](https://expressjs.com/resources/middleware/multer.html) | Handle multi-part form data. | express.bodyParser |\n| [response-time](https://expressjs.com/resources/middleware/response-time.html) | Record HTTP response time. | express.responseTime |\n| [serve-favicon](https://expressjs.com/resources/middleware/serve-favicon.html) | Serve a favicon. | express.favicon |\n| [serve-index](https://expressjs.com/resources/middleware/serve-index.html) | Serve directory listing for a given path. | express.directory |\n| [serve-static](https://expressjs.com/resources/middleware/serve-static.html) | Serve static files. | express.static |\n| [session](https://expressjs.com/resources/middleware/session.html) | Establish server-based sessions (development only). | express.session |\n| [timeout](https://expressjs.com/resources/middleware/timeout.html) | Set a timeout period for HTTP request processing. | express.timeout |\n| [vhost](https://expressjs.com/resources/middleware/vhost.html) | Create virtual domains. | express.vhost |\n\nAdditional middleware modules\n-----------------------------\n\nThese are some additional popular middleware modules.\n\n**Warning**: This information refers to third-party sites, products, or modules that are not maintained by the Expressjs team. Listing here does not constitute an endorsement or recommendation from the Expressjs project team.\n\n| Middleware module | Description |\n| --- | --- |\n| [cls-rtracer](https://github.com/puzpuzpuz/cls-rtracer) | Middleware for CLS-based request id generation. An out-of-the-box solution for adding request ids into your logs. |\n| [connect-image-optimus](https://github.com/msemenistyi/connect-image-optimus) | Optimize image serving. Switches images to `.webp` or `.jxr`, if possible. |\n| [error-handler-json](https://github.com/mifi/error-handler-json) | An error handler for JSON APIs (fork of `api-error-handler`.) |\n| [express-debug](https://github.com/devoidfury/express-debug) | Development tool that adds information about template variables (locals), current session, and so on. |\n| [express-partial-response](https://github.com/nemtsov/express-partial-response) | Filters out parts of JSON responses based on the `fields` query-string; by using Google API’s Partial Response. |\n| [express-simple-cdn](https://github.com/jamiesteven/express-simple-cdn) | Use a CDN for static assets, with multiple host support. |\n| [express-slash](https://github.com/ericf/express-slash) | Handles routes with and without trailing slashes. |\n| [express-uncapitalize](https://github.com/jamiesteven/express-uncapitalize) | Redirects HTTP requests containing uppercase to a canonical lowercase form. |\n| [helmet](https://github.com/helmetjs/helmet) | Helps secure your apps by setting various HTTP headers. |\n| [join-io](https://github.com/coderaiser/join-io) | Joins files on the fly to reduce the requests count. |\n| [passport](https://github.com/jaredhanson/passport) | Authentication using “strategies” such as OAuth, OpenID and many others. See [http://passportjs.org/](http://passportjs.org/) for more information. |\n| [static-expiry](https://github.com/paulwalker/connect-static-expiry) | Fingerprint URLs or caching headers for static assets. |\n| [view-helpers](https://github.com/madhums/node-view-helpers) | Common helper methods for views. |\n| [sriracha-admin](https://github.com/hdngr/siracha) | Dynamically generate an admin site for Mongoose. |\n\nFor more middleware modules, see [http-framework](https://github.com/Raynos/http-framework#modules).\n",
"filename": "middleware.md",
"package": "express"
} |
{
"content": "Title: Migrating to Express 4\n\nURL Source: https://expressjs.com/en/guide/migrating-4.html\n\nMarkdown Content:\nMoving to Express 4\n-------------------\n\nOverview\n--------\n\nExpress 4 is a breaking change from Express 3. That means an existing Express 3 app will _not_ work if you update the Express version in its dependencies.\n\nThis article covers:\n\n* [Changes in Express 4](https://expressjs.com/en/guide/migrating-4.html#changes).\n* [An example](https://expressjs.com/en/guide/migrating-4.html#example-migration) of migrating an Express 3 app to Express 4.\n* [Upgrading to the Express 4 app generator](https://expressjs.com/en/guide/migrating-4.html#app-gen).\n\nChanges in Express 4\n--------------------\n\nThere are several significant changes in Express 4:\n\n* [Changes to Express core and middleware system.](https://expressjs.com/en/guide/migrating-4.html#core-changes) The dependencies on Connect and built-in middleware were removed, so you must add middleware yourself.\n* [Changes to the routing system.](https://expressjs.com/en/guide/migrating-4.html#routing)\n* [Various other changes.](https://expressjs.com/en/guide/migrating-4.html#other-changes)\n\nSee also:\n\n* [New features in 4.x.](https://github.com/expressjs/express/wiki/New-features-in-4.x)\n* [Migrating from 3.x to 4.x.](https://github.com/expressjs/express/wiki/Migrating-from-3.x-to-4.x)\n\n### Changes to Express core and middleware system\n\nExpress 4 no longer depends on Connect, and removes all built-in middleware from its core, except for the `express.static` function. This means that Express is now an independent routing and middleware web framework, and Express versioning and releases are not affected by middleware updates.\n\nWithout built-in middleware, you must explicitly add all the middleware that is required to run your app. Simply follow these steps:\n\n1. Install the module: `npm install --save <module-name>`\n2. In your app, require the module: `require('module-name')`\n3. Use the module according to its documentation: `app.use( ... )`\n\nThe following table lists Express 3 middleware and their counterparts in Express 4.\n\n| Express 3 | Express 4 |\n| --- | --- |\n| `express.bodyParser` | [body-parser](https://github.com/expressjs/body-parser) + [multer](https://github.com/expressjs/multer) |\n| `express.compress` | [compression](https://github.com/expressjs/compression) |\n| `express.cookieSession` | [cookie-session](https://github.com/expressjs/cookie-session) |\n| `express.cookieParser` | [cookie-parser](https://github.com/expressjs/cookie-parser) |\n| `express.logger` | [morgan](https://github.com/expressjs/morgan) |\n| `express.session` | [express-session](https://github.com/expressjs/session) |\n| `express.favicon` | [serve-favicon](https://github.com/expressjs/serve-favicon) |\n| `express.responseTime` | [response-time](https://github.com/expressjs/response-time) |\n| `express.errorHandler` | [errorhandler](https://github.com/expressjs/errorhandler) |\n| `express.methodOverride` | [method-override](https://github.com/expressjs/method-override) |\n| `express.timeout` | [connect-timeout](https://github.com/expressjs/timeout) |\n| `express.vhost` | [vhost](https://github.com/expressjs/vhost) |\n| `express.csrf` | [csurf](https://github.com/expressjs/csurf) |\n| `express.directory` | [serve-index](https://github.com/expressjs/serve-index) |\n| `express.static` | [serve-static](https://github.com/expressjs/serve-static) |\n\nHere is the [complete list](https://github.com/senchalabs/connect#middleware) of Express 4 middleware.\n\nIn most cases, you can simply replace the old version 3 middleware with its Express 4 counterpart. For details, see the module documentation in GitHub.\n\n#### `app.use` accepts parameters\n\nIn version 4 you can use a variable parameter to define the path where middleware functions are loaded, then read the value of the parameter from the route handler. For example:\n\n```\napp.use('/book/:id', (req, res, next) => {\n console.log('ID:', req.params.id)\n next()\n})\n```\n\n### The routing system\n\nApps now implicitly load routing middleware, so you no longer have to worry about the order in which middleware is loaded with respect to the `router` middleware.\n\nThe way you define routes is unchanged, but the routing system has two new features to help organize your routes:\n\n* A new method, `app.route()`, to create chainable route handlers for a route path.\n* A new class, `express.Router`, to create modular mountable route handlers.\n\n#### `app.route()` method\n\nThe new `app.route()` method enables you to create chainable route handlers for a route path. Because the path is specified in a single location, creating modular routes is helpful, as is reducing redundancy and typos. For more information about routes, see [`Router()` documentation](https://expressjs.com/en/4x/api.html#router).\n\nHere is an example of chained route handlers that are defined by using the `app.route()` function.\n\n```\napp.route('/book')\n .get((req, res) => {\n res.send('Get a random book')\n })\n .post((req, res) => {\n res.send('Add a book')\n })\n .put((req, res) => {\n res.send('Update the book')\n })\n```\n\n#### `express.Router` class\n\nThe other feature that helps to organize routes is a new class, `express.Router`, that you can use to create modular mountable route handlers. A `Router` instance is a complete middleware and routing system; for this reason it is often referred to as a “mini-app”.\n\nThe following example creates a router as a module, loads middleware in it, defines some routes, and mounts it on a path on the main app.\n\nFor example, create a router file named `birds.js` in the app directory, with the following content:\n\n```\nvar express = require('express')\nvar router = express.Router()\n\n// middleware specific to this router\nrouter.use((req, res, next) => {\n console.log('Time: ', Date.now())\n next()\n})\n// define the home page route\nrouter.get('/', (req, res) => {\n res.send('Birds home page')\n})\n// define the about route\nrouter.get('/about', (req, res) => {\n res.send('About birds')\n})\n\nmodule.exports = router\n```\n\nThen, load the router module in the app:\n\n```\nvar birds = require('./birds')\n\n// ...\n\napp.use('/birds', birds)\n```\n\nThe app will now be able to handle requests to the `/birds` and `/birds/about` paths, and will call the `timeLog` middleware that is specific to the route.\n\n### Other changes\n\nThe following table lists other small but important changes in Express 4:\n\n| Object | Description |\n| --- | --- |\n| Node.js | Express 4 requires Node.js 0.10.x or later and has dropped support for Node.js 0.8.x. |\n| `http.createServer()`\n | The `http` module is no longer needed, unless you need to directly work with it (socket.io/SPDY/HTTPS). The app can be started by using the `app.listen()` function.\n\n |\n| `app.configure()`\n\n | The `app.configure()` function has been removed. Use the `process.env.NODE_ENV` or `app.get('env')` function to detect the environment and configure the app accordingly.\n\n |\n| `json spaces`\n\n | The `json spaces` application property is disabled by default in Express 4.\n\n |\n| `req.accepted()`\n\n | Use `req.accepts()`, `req.acceptsEncodings()`, `req.acceptsCharsets()`, and `req.acceptsLanguages()`.\n\n |\n| `res.location()`\n\n | No longer resolves relative URLs.\n\n |\n| `req.params`\n\n | Was an array; now an object.\n\n |\n| `res.locals`\n\n | Was a function; now an object.\n\n |\n| `res.headerSent`\n\n | Changed to `res.headersSent`.\n\n |\n| `app.route`\n\n | Now available as `app.mountpath`.\n\n |\n| `res.on('header')`\n\n | Removed.\n\n |\n| `res.charset`\n\n | Removed.\n\n |\n| `res.setHeader('Set-Cookie', val)`\n\n | Functionality is now limited to setting the basic cookie value. Use `res.cookie()` for added functionality.\n\n |\n\nExample app migration\n---------------------\n\nHere is an example of migrating an Express 3 application to Express 4. The files of interest are `app.js` and `package.json`.\n\n### Version 3 app\n\n#### `app.js`\n\nConsider an Express v.3 application with the following `app.js` file:\n\n```\nvar express = require('express')\nvar routes = require('./routes')\nvar user = require('./routes/user')\nvar http = require('http')\nvar path = require('path')\n\nvar app = express()\n\n// all environments\napp.set('port', process.env.PORT || 3000)\napp.set('views', path.join(__dirname, 'views'))\napp.set('view engine', 'pug')\napp.use(express.favicon())\napp.use(express.logger('dev'))\napp.use(express.methodOverride())\napp.use(express.session({ secret: 'your secret here' }))\napp.use(express.bodyParser())\napp.use(app.router)\napp.use(express.static(path.join(__dirname, 'public')))\n\n// development only\nif (app.get('env') === 'development') {\n app.use(express.errorHandler())\n}\n\napp.get('/', routes.index)\napp.get('/users', user.list)\n\nhttp.createServer(app).listen(app.get('port'), () => {\n console.log('Express server listening on port ' + app.get('port'))\n})\n```\n\n#### `package.json`\n\nThe accompanying version 3 `package.json` file might look something like this:\n\n```\n{\n \"name\": \"application-name\",\n \"version\": \"0.0.1\",\n \"private\": true,\n \"scripts\": {\n \"start\": \"node app.js\"\n },\n \"dependencies\": {\n \"express\": \"3.12.0\",\n \"pug\": \"*\"\n }\n}\n```\n\n### Process\n\nBegin the migration process by installing the required middleware for the Express 4 app and updating Express and Pug to their respective latest version with the following command:\n\n```\n$ npm install serve-favicon morgan method-override express-session body-parser multer errorhandler express@latest pug@latest --save\n```\n\nMake the following changes to `app.js`:\n\n1. The built-in Express middleware functions `express.favicon`, `express.logger`, `express.methodOverride`, `express.session`, `express.bodyParser` and `express.errorHandler` are no longer available on the `express` object. You must install their alternatives manually and load them in the app.\n \n2. You no longer need to load the `app.router` function. It is not a valid Express 4 app object, so remove the `app.use(app.router);` code.\n \n3. Make sure that the middleware functions are loaded in the correct order - load `errorHandler` after loading the app routes.\n \n\n### Version 4 app\n\n#### `package.json`\n\nRunning the above `npm` command will update `package.json` as follows:\n\n```\n{\n \"name\": \"application-name\",\n \"version\": \"0.0.1\",\n \"private\": true,\n \"scripts\": {\n \"start\": \"node app.js\"\n },\n \"dependencies\": {\n \"body-parser\": \"^1.5.2\",\n \"errorhandler\": \"^1.1.1\",\n \"express\": \"^4.8.0\",\n \"express-session\": \"^1.7.2\",\n \"pug\": \"^2.0.0\",\n \"method-override\": \"^2.1.2\",\n \"morgan\": \"^1.2.2\",\n \"multer\": \"^0.1.3\",\n \"serve-favicon\": \"^2.0.1\"\n }\n}\n```\n\n#### `app.js`\n\nThen, remove invalid code, load the required middleware, and make other changes as necessary. The `app.js` file will look like this:\n\n```\nvar http = require('http')\nvar express = require('express')\nvar routes = require('./routes')\nvar user = require('./routes/user')\nvar path = require('path')\n\nvar favicon = require('serve-favicon')\nvar logger = require('morgan')\nvar methodOverride = require('method-override')\nvar session = require('express-session')\nvar bodyParser = require('body-parser')\nvar multer = require('multer')\nvar errorHandler = require('errorhandler')\n\nvar app = express()\n\n// all environments\napp.set('port', process.env.PORT || 3000)\napp.set('views', path.join(__dirname, 'views'))\napp.set('view engine', 'pug')\napp.use(favicon(path.join(__dirname, '/public/favicon.ico')))\napp.use(logger('dev'))\napp.use(methodOverride())\napp.use(session({\n resave: true,\n saveUninitialized: true,\n secret: 'uwotm8'\n}))\napp.use(bodyParser.json())\napp.use(bodyParser.urlencoded({ extended: true }))\napp.use(multer())\napp.use(express.static(path.join(__dirname, 'public')))\n\napp.get('/', routes.index)\napp.get('/users', user.list)\n\n// error handling middleware should be loaded after the loading the routes\nif (app.get('env') === 'development') {\n app.use(errorHandler())\n}\n\nvar server = http.createServer(app)\nserver.listen(app.get('port'), () => {\n console.log('Express server listening on port ' + app.get('port'))\n})\n```\n\nUnless you need to work directly with the `http` module (socket.io/SPDY/HTTPS), loading it is not required, and the app can be simply started this way:\n\n```\napp.listen(app.get('port'), () => {\n console.log('Express server listening on port ' + app.get('port'))\n})\n```\n\n### Run the app\n\nThe migration process is complete, and the app is now an Express 4 app. To confirm, start the app by using the following command:\n\n```\n$ node .\n```\n\nLoad [http://localhost:3000](http://localhost:3000/) and see the home page being rendered by Express 4.\n\nUpgrading to the Express 4 app generator\n----------------------------------------\n\nThe command-line tool to generate an Express app is still `express`, but to upgrade to the new version, you must uninstall the Express 3 app generator and then install the new `express-generator`.\n\n### Installing\n\nIf you already have the Express 3 app generator installed on your system, you must uninstall it:\n\n```\n$ npm uninstall -g express\n```\n\nDepending on how your file and directory privileges are configured, you might need to run this command with `sudo`.\n\nNow install the new generator:\n\n```\n$ npm install -g express-generator\n```\n\nDepending on how your file and directory privileges are configured, you might need to run this command with `sudo`.\n\nNow the `express` command on your system is updated to the Express 4 generator.\n\n### Changes to the app generator\n\nCommand options and use largely remain the same, with the following exceptions:\n\n* Removed the `--sessions` option.\n* Removed the `--jshtml` option.\n* Added the `--hogan` option to support [Hogan.js](http://twitter.github.io/hogan.js/).\n\n### Example\n\nExecute the following command to create an Express 4 app:\n\n```\n$ express app4\n```\n\nIf you look at the contents of the `app4/app.js` file, you will notice that all the middleware functions (except `express.static`) that are required for the app are loaded as independent modules, and the `router` middleware is no longer explicitly loaded in the app.\n\nYou will also notice that the `app.js` file is now a Node.js module, in contrast to the standalone app that was generated by the old generator.\n\nAfter installing the dependencies, start the app by using the following command:\n\n```\n$ npm start\n```\n\nIf you look at the `npm start` script in the `package.json` file, you will notice that the actual command that starts the app is `node ./bin/www`, which used to be `node app.js` in Express 3.\n\nBecause the `app.js` file that was generated by the Express 4 generator is now a Node.js module, it can no longer be started independently as an app (unless you modify the code). The module must be loaded in a Node.js file and started via the Node.js file. The Node.js file is `./bin/www` in this case.\n\nNeither the `bin` directory nor the extensionless `www` file is mandatory for creating an Express app or starting the app. They are just suggestions made by the generator, so feel free to modify them to suit your needs.\n\nTo get rid of the `www` directory and keep things the “Express 3 way”, delete the line that says `module.exports = app;` at the end of the `app.js` file, then paste the following code in its place:\n\n```\napp.set('port', process.env.PORT || 3000)\n\nvar server = app.listen(app.get('port'), () => {\n debug('Express server listening on port ' + server.address().port)\n})\n```\n\nEnsure that you load the `debug` module at the top of the `app.js` file by using the following code:\n\n```\nvar debug = require('debug')('app4')\n```\n\nNext, change `\"start\": \"node ./bin/www\"` in the `package.json` file to `\"start\": \"node app.js\"`.\n\nYou have now moved the functionality of `./bin/www` back to `app.js`. This change is not recommended, but the exercise helps you to understand how the `./bin/www` file works, and why the `app.js` file no longer starts on its own.\n",
"filename": "migrating-4.md",
"package": "express"
} |
{
"content": "Title: Migrating to Express 5\n\nURL Source: https://expressjs.com/en/guide/migrating-5.html\n\nMarkdown Content:\nMoving to Express 5\n-------------------\n\nOverview\n--------\n\nExpress 5.0 is still in the beta release stage, but here is a preview of the changes that will be in the release and how to migrate your Express 4 app to Express 5.\n\nTo install the latest beta and to preview Express 5, enter the following command in your application root directory:\n\n```\n$ npm install \"express@>=5.0.0-beta.1\" --save\n```\n\nYou can then run your automated tests to see what fails, and fix problems according to the updates listed below. After addressing test failures, run your app to see what errors occur. You’ll find out right away if the app uses any methods or properties that are not supported.\n\nChanges in Express 5\n--------------------\n\n**Removed methods and properties**\n\n* [app.del()](https://expressjs.com/en/guide/migrating-5.html#app.del)\n* [app.param(fn)](https://expressjs.com/en/guide/migrating-5.html#app.param)\n* [Pluralized method names](https://expressjs.com/en/guide/migrating-5.html#plural)\n* [Leading colon in name argument to app.param(name, fn)](https://expressjs.com/en/guide/migrating-5.html#leading)\n* [req.param(name)](https://expressjs.com/en/guide/migrating-5.html#req.param)\n* [res.json(obj, status)](https://expressjs.com/en/guide/migrating-5.html#res.json)\n* [res.jsonp(obj, status)](https://expressjs.com/en/guide/migrating-5.html#res.jsonp)\n* [res.send(body, status)](https://expressjs.com/en/guide/migrating-5.html#res.send.body)\n* [res.send(status)](https://expressjs.com/en/guide/migrating-5.html#res.send.status)\n* [res.sendfile()](https://expressjs.com/en/guide/migrating-5.html#res.sendfile)\n\n**Changed**\n\n* [Path route matching syntax](https://expressjs.com/en/guide/migrating-5.html#path-syntax)\n* [Rejected promises handled from middleware and handlers](https://expressjs.com/en/guide/migrating-5.html#rejected-promises)\n* [app.router](https://expressjs.com/en/guide/migrating-5.html#app.router)\n* [req.host](https://expressjs.com/en/guide/migrating-5.html#req.host)\n* [req.query](https://expressjs.com/en/guide/migrating-5.html#req.query)\n\n**Improvements**\n\n* [res.render()](https://expressjs.com/en/guide/migrating-5.html#res.render)\n\n### Removed methods and properties\n\nIf you use any of these methods or properties in your app, it will crash. So, you’ll need to change your app after you update to version 5.\n\n#### app.del()\n\nExpress 5 no longer supports the `app.del()` function. If you use this function, an error is thrown. For registering HTTP DELETE routes, use the `app.delete()` function instead.\n\nInitially, `del` was used instead of `delete`, because `delete` is a reserved keyword in JavaScript. However, as of ECMAScript 6, `delete` and other reserved keywords can legally be used as property names.\n\n#### app.param(fn)\n\nThe `app.param(fn)` signature was used for modifying the behavior of the `app.param(name, fn)` function. It has been deprecated since v4.11.0, and Express 5 no longer supports it at all.\n\n#### Pluralized method names\n\nThe following method names have been pluralized. In Express 4, using the old methods resulted in a deprecation warning. Express 5 no longer supports them at all:\n\n`req.acceptsCharset()` is replaced by `req.acceptsCharsets()`.\n\n`req.acceptsEncoding()` is replaced by `req.acceptsEncodings()`.\n\n`req.acceptsLanguage()` is replaced by `req.acceptsLanguages()`.\n\n#### Leading colon (:) in the name for app.param(name, fn)\n\nA leading colon character (:) in the name for the `app.param(name, fn)` function is a remnant of Express 3, and for the sake of backwards compatibility, Express 4 supported it with a deprecation notice. Express 5 will silently ignore it and use the name parameter without prefixing it with a colon.\n\nThis should not affect your code if you follow the Express 4 documentation of [app.param](https://expressjs.com/en/4x/api.html#app.param), as it makes no mention of the leading colon.\n\n#### req.param(name)\n\nThis potentially confusing and dangerous method of retrieving form data has been removed. You will now need to specifically look for the submitted parameter name in the `req.params`, `req.body`, or `req.query` object.\n\n#### res.json(obj, status)\n\nExpress 5 no longer supports the signature `res.json(obj, status)`. Instead, set the status and then chain it to the `res.json()` method like this: `res.status(status).json(obj)`.\n\n#### res.jsonp(obj, status)\n\nExpress 5 no longer supports the signature `res.jsonp(obj, status)`. Instead, set the status and then chain it to the `res.jsonp()` method like this: `res.status(status).jsonp(obj)`.\n\n#### res.send(body, status)\n\nExpress 5 no longer supports the signature `res.send(obj, status)`. Instead, set the status and then chain it to the `res.send()` method like this: `res.status(status).send(obj)`.\n\n#### res.send(status)\n\nExpress 5 no longer supports the signature `res.send(_status_)`, where _`status`_ is a number. Instead, use the `res.sendStatus(statusCode)` function, which sets the HTTP response header status code and sends the text version of the code: “Not Found”, “Internal Server Error”, and so on. If you need to send a number by using the `res.send()` function, quote the number to convert it to a string, so that Express does not interpret it as an attempt to use the unsupported old signature.\n\n#### res.sendfile()\n\nThe `res.sendfile()` function has been replaced by a camel-cased version `res.sendFile()` in Express 5.\n\n### Changed\n\n#### Path route matching syntax\n\nPath route matching syntax is when a string is supplied as the first parameter to the `app.all()`, `app.use()`, `app.METHOD()`, `router.all()`, `router.METHOD()`, and `router.use()` APIs. The following changes have been made to how the path string is matched to an incoming request:\n\n* Add new `?`, `*`, and `+` parameter modifiers.\n* Matching group expressions are only RegExp syntax.\n * `(*)` is no longer valid and must be written as `(.*)`, for example.\n* Named matching groups no longer available by position in `req.params`.\n * `/:foo(.*)` only captures as `req.params.foo` and not available as `req.params[0]`.\n* Regular expressions can only be used in a matching group.\n * `/\\\\d+` is no longer valid and must be written as `/(\\\\d+)`.\n* Special `*` path segment behavior removed.\n * `/foo/*/bar` will match a literal `*` as the middle segment.\n\n#### Rejected promises handled from middleware and handlers\n\nRequest middleware and handlers that return rejected promises are now handled by forwarding the rejected value as an `Error` to the error handling middleware. This means that using `async` functions as middleware and handlers are easier than ever. When an error is thrown in an `async` function or a rejected promise is `await`ed inside an async function, those errors will be passed to the error handler as if calling `next(err)`.\n\nDetails of how Express handles errors is covered in the [error handling documentation](https://expressjs.com/en/guide/error-handling.html).\n\n#### app.router\n\nThe `app.router` object, which was removed in Express 4, has made a comeback in Express 5. In the new version, this object is a just a reference to the base Express router, unlike in Express 3, where an app had to explicitly load it.\n\n#### req.host\n\nIn Express 4, the `req.host` function incorrectly stripped off the port number if it was present. In Express 5, the port number is maintained.\n\n#### req.query\n\nThe `req.query` property is no longer a writable property and is instead a getter. The default query parser has been changed from “extended” to “simple”.\n\n### Improvements\n\n#### res.render()\n\nThis method now enforces asynchronous behavior for all view engines, avoiding bugs caused by view engines that had a synchronous implementation and that violated the recommended interface.\n",
"filename": "migrating-5.md",
"package": "express"
} |
{
"content": "Title: Overriding the Express API\n\nURL Source: https://expressjs.com/en/guide/overriding-express-api.html\n\nMarkdown Content:\nThe Express API consists of various methods and properties on the request and response objects. These are inherited by prototype. There are two extension points for the Express API:\n\n1. The global prototypes at `express.request` and `express.response`.\n2. App-specific prototypes at `app.request` and `app.response`.\n\nAltering the global prototypes will affect all loaded Express apps in the same process. If desired, alterations can be made app-specific by only altering the app-specific prototypes after creating a new app.\n\nMethods\n-------\n\nYou can override the signature and behavior of existing methods with your own, by assigning a custom function.\n\nFollowing is an example of overriding the behavior of [res.sendStatus](https://expressjs.com/4x/api.html#res.sendStatus).\n\n```\napp.response.sendStatus = function (statusCode, type, message) {\n // code is intentionally kept simple for demonstration purpose\n return this.contentType(type)\n .status(statusCode)\n .send(message)\n}\n```\n\nThe above implementation completely changes the original signature of `res.sendStatus`. It now accepts a status code, encoding type, and the message to be sent to the client.\n\nThe overridden method may now be used this way:\n\n```\nres.sendStatus(404, 'application/json', '{\"error\":\"resource not found\"}')\n```\n\nProperties\n----------\n\nProperties in the Express API are either:\n\n1. Assigned properties (ex: `req.baseUrl`, `req.originalUrl`)\n2. Defined as getters (ex: `req.secure`, `req.ip`)\n\nSince properties under category 1 are dynamically assigned on the `request` and `response` objects in the context of the current request-response cycle, their behavior cannot be overridden.\n\nProperties under category 2 can be overwritten using the Express API extensions API.\n\nThe following code rewrites how the value of `req.ip` is to be derived. Now, it simply returns the value of the `Client-IP` request header.\n\n```\nObject.defineProperty(app.request, 'ip', {\n configurable: true,\n enumerable: true,\n get () { return this.get('Client-IP') }\n})\n```\n\nPrototype\n---------\n\nIn order to provide the Express API, the request/response objects passed to Express (via `app(req, res)`, for example) need to inherit from the same prototype chain. By default, this is `http.IncomingRequest.prototype` for the request and `http.ServerResponse.prototype` for the response.\n\nUnless necessary, it is recommended that this be done only at the application level, rather than globally. Also, take care that the prototype that is being used matches the functionality as closely as possible to the default prototypes.\n\n```\n// Use FakeRequest and FakeResponse in place of http.IncomingRequest and http.ServerResponse\n// for the given app reference\nObject.setPrototypeOf(Object.getPrototypeOf(app.request), FakeRequest.prototype)\nObject.setPrototypeOf(Object.getPrototypeOf(app.response), FakeResponse.prototype)\n```\n",
"filename": "overriding-express-api.md",
"package": "express"
} |
{
"content": "Title: Express routing\n\nURL Source: https://expressjs.com/en/guide/routing.html\n\nMarkdown Content:\n_Routing_ refers to how an application’s endpoints (URIs) respond to client requests. For an introduction to routing, see [Basic routing](https://expressjs.com/en/starter/basic-routing.html).\n\nYou define routing using methods of the Express `app` object that correspond to HTTP methods; for example, `app.get()` to handle GET requests and `app.post` to handle POST requests. For a full list, see [app.METHOD](https://expressjs.com/en/4x/api.html#app.METHOD). You can also use [app.all()](https://expressjs.com/en/4x/api.html#app.all) to handle all HTTP methods and [app.use()](https://expressjs.com/en/4x/api.html#app.use) to specify middleware as the callback function (See [Using middleware](https://expressjs.com/en/guide/using-middleware.html) for details).\n\nThese routing methods specify a callback function (sometimes called “handler functions”) called when the application receives a request to the specified route (endpoint) and HTTP method. In other words, the application “listens” for requests that match the specified route(s) and method(s), and when it detects a match, it calls the specified callback function.\n\nIn fact, the routing methods can have more than one callback function as arguments. With multiple callback functions, it is important to provide `next` as an argument to the callback function and then call `next()` within the body of the function to hand off control to the next callback.\n\nThe following code is an example of a very basic route.\n\n```\nconst express = require('express')\nconst app = express()\n\n// respond with \"hello world\" when a GET request is made to the homepage\napp.get('/', (req, res) => {\n res.send('hello world')\n})\n```\n\nRoute methods\n-------------\n\nA route method is derived from one of the HTTP methods, and is attached to an instance of the `express` class.\n\nThe following code is an example of routes that are defined for the GET and the POST methods to the root of the app.\n\n```\n// GET method route\napp.get('/', (req, res) => {\n res.send('GET request to the homepage')\n})\n\n// POST method route\napp.post('/', (req, res) => {\n res.send('POST request to the homepage')\n})\n```\n\nExpress supports methods that correspond to all HTTP request methods: `get`, `post`, and so on. For a full list, see [app.METHOD](https://expressjs.com/en/4x/api.html#app.METHOD).\n\nThere is a special routing method, `app.all()`, used to load middleware functions at a path for _all_ HTTP request methods. For example, the following handler is executed for requests to the route “/secret” whether using GET, POST, PUT, DELETE, or any other HTTP request method supported in the [http module](https://nodejs.org/api/http.html#http_http_methods).\n\n```\napp.all('/secret', (req, res, next) => {\n console.log('Accessing the secret section ...')\n next() // pass control to the next handler\n})\n```\n\nRoute paths\n-----------\n\nRoute paths, in combination with a request method, define the endpoints at which requests can be made. Route paths can be strings, string patterns, or regular expressions.\n\nThe characters `?`, `+`, `*`, and `()` are subsets of their regular expression counterparts. The hyphen (`-`) and the dot (`.`) are interpreted literally by string-based paths.\n\nIf you need to use the dollar character (`$`) in a path string, enclose it escaped within `([` and `])`. For example, the path string for requests at “`/data/$book`”, would be “`/data/([\\$])book`”.\n\nExpress uses [path-to-regexp](https://www.npmjs.com/package/path-to-regexp) for matching the route paths; see the path-to-regexp documentation for all the possibilities in defining route paths. [Express Route Tester](http://forbeslindesay.github.io/express-route-tester/) is a handy tool for testing basic Express routes, although it does not support pattern matching.\n\nQuery strings are not part of the route path.\n\nHere are some examples of route paths based on strings.\n\nThis route path will match requests to the root route, `/`.\n\n```\napp.get('/', (req, res) => {\n res.send('root')\n})\n```\n\nThis route path will match requests to `/about`.\n\n```\napp.get('/about', (req, res) => {\n res.send('about')\n})\n```\n\nThis route path will match requests to `/random.text`.\n\n```\napp.get('/random.text', (req, res) => {\n res.send('random.text')\n})\n```\n\nHere are some examples of route paths based on string patterns.\n\nThis route path will match `acd` and `abcd`.\n\n```\napp.get('/ab?cd', (req, res) => {\n res.send('ab?cd')\n})\n```\n\nThis route path will match `abcd`, `abbcd`, `abbbcd`, and so on.\n\n```\napp.get('/ab+cd', (req, res) => {\n res.send('ab+cd')\n})\n```\n\nThis route path will match `abcd`, `abxcd`, `abRANDOMcd`, `ab123cd`, and so on.\n\n```\napp.get('/ab*cd', (req, res) => {\n res.send('ab*cd')\n})\n```\n\nThis route path will match `/abe` and `/abcde`.\n\n```\napp.get('/ab(cd)?e', (req, res) => {\n res.send('ab(cd)?e')\n})\n```\n\nExamples of route paths based on regular expressions:\n\nThis route path will match anything with an “a” in it.\n\n```\napp.get(/a/, (req, res) => {\n res.send('/a/')\n})\n```\n\nThis route path will match `butterfly` and `dragonfly`, but not `butterflyman`, `dragonflyman`, and so on.\n\n```\napp.get(/.*fly$/, (req, res) => {\n res.send('/.*fly$/')\n})\n```\n\nRoute parameters\n----------------\n\nRoute parameters are named URL segments that are used to capture the values specified at their position in the URL. The captured values are populated in the `req.params` object, with the name of the route parameter specified in the path as their respective keys.\n\n```\nRoute path: /users/:userId/books/:bookId\nRequest URL: http://localhost:3000/users/34/books/8989\nreq.params: { \"userId\": \"34\", \"bookId\": \"8989\" }\n```\n\nTo define routes with route parameters, simply specify the route parameters in the path of the route as shown below.\n\n```\napp.get('/users/:userId/books/:bookId', (req, res) => {\n res.send(req.params)\n})\n```\n\nThe name of route parameters must be made up of “word characters” (\\[A-Za-z0-9\\_\\]).\n\nSince the hyphen (`-`) and the dot (`.`) are interpreted literally, they can be used along with route parameters for useful purposes.\n\n```\nRoute path: /flights/:from-:to\nRequest URL: http://localhost:3000/flights/LAX-SFO\nreq.params: { \"from\": \"LAX\", \"to\": \"SFO\" }\n```\n\n```\nRoute path: /plantae/:genus.:species\nRequest URL: http://localhost:3000/plantae/Prunus.persica\nreq.params: { \"genus\": \"Prunus\", \"species\": \"persica\" }\n```\n\nTo have more control over the exact string that can be matched by a route parameter, you can append a regular expression in parentheses (`()`):\n\n```\nRoute path: /user/:userId(\\d+)\nRequest URL: http://localhost:3000/user/42\nreq.params: {\"userId\": \"42\"}\n```\n\nBecause the regular expression is usually part of a literal string, be sure to escape any `\\` characters with an additional backslash, for example `\\\\d+`.\n\nRoute handlers\n--------------\n\nYou can provide multiple callback functions that behave like [middleware](https://expressjs.com/en/guide/using-middleware.html) to handle a request. The only exception is that these callbacks might invoke `next('route')` to bypass the remaining route callbacks. You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there’s no reason to proceed with the current route.\n\nRoute handlers can be in the form of a function, an array of functions, or combinations of both, as shown in the following examples.\n\nA single callback function can handle a route. For example:\n\n```\napp.get('/example/a', (req, res) => {\n res.send('Hello from A!')\n})\n```\n\nMore than one callback function can handle a route (make sure you specify the `next` object). For example:\n\n```\napp.get('/example/b', (req, res, next) => {\n console.log('the response will be sent by the next function ...')\n next()\n}, (req, res) => {\n res.send('Hello from B!')\n})\n```\n\nAn array of callback functions can handle a route. For example:\n\n```\nconst cb0 = function (req, res, next) {\n console.log('CB0')\n next()\n}\n\nconst cb1 = function (req, res, next) {\n console.log('CB1')\n next()\n}\n\nconst cb2 = function (req, res) {\n res.send('Hello from C!')\n}\n\napp.get('/example/c', [cb0, cb1, cb2])\n```\n\nA combination of independent functions and arrays of functions can handle a route. For example:\n\n```\nconst cb0 = function (req, res, next) {\n console.log('CB0')\n next()\n}\n\nconst cb1 = function (req, res, next) {\n console.log('CB1')\n next()\n}\n\napp.get('/example/d', [cb0, cb1], (req, res, next) => {\n console.log('the response will be sent by the next function ...')\n next()\n}, (req, res) => {\n res.send('Hello from D!')\n})\n```\n\nResponse methods\n----------------\n\nThe methods on the response object (`res`) in the following table can send a response to the client, and terminate the request-response cycle. If none of these methods are called from a route handler, the client request will be left hanging.\n\n| Method | Description |\n| --- | --- |\n| [res.download()](https://expressjs.com/en/4x/api.html#res.download) | Prompt a file to be downloaded. |\n| [res.end()](https://expressjs.com/en/4x/api.html#res.end) | End the response process. |\n| [res.json()](https://expressjs.com/en/4x/api.html#res.json) | Send a JSON response. |\n| [res.jsonp()](https://expressjs.com/en/4x/api.html#res.jsonp) | Send a JSON response with JSONP support. |\n| [res.redirect()](https://expressjs.com/en/4x/api.html#res.redirect) | Redirect a request. |\n| [res.render()](https://expressjs.com/en/4x/api.html#res.render) | Render a view template. |\n| [res.send()](https://expressjs.com/en/4x/api.html#res.send) | Send a response of various types. |\n| [res.sendFile()](https://expressjs.com/en/4x/api.html#res.sendFile) | Send a file as an octet stream. |\n| [res.sendStatus()](https://expressjs.com/en/4x/api.html#res.sendStatus) | Set the response status code and send its string representation as the response body. |\n\napp.route()\n-----------\n\nYou can create chainable route handlers for a route path by using `app.route()`. Because the path is specified at a single location, creating modular routes is helpful, as is reducing redundancy and typos. For more information about routes, see: [Router() documentation](https://expressjs.com/en/4x/api.html#router).\n\nHere is an example of chained route handlers that are defined by using `app.route()`.\n\n```\napp.route('/book')\n .get((req, res) => {\n res.send('Get a random book')\n })\n .post((req, res) => {\n res.send('Add a book')\n })\n .put((req, res) => {\n res.send('Update the book')\n })\n```\n\nexpress.Router\n--------------\n\nUse the `express.Router` class to create modular, mountable route handlers. A `Router` instance is a complete middleware and routing system; for this reason, it is often referred to as a “mini-app”.\n\nThe following example creates a router as a module, loads a middleware function in it, defines some routes, and mounts the router module on a path in the main app.\n\nCreate a router file named `birds.js` in the app directory, with the following content:\n\n```\nconst express = require('express')\nconst router = express.Router()\n\n// middleware that is specific to this router\nconst timeLog = (req, res, next) => {\n console.log('Time: ', Date.now())\n next()\n}\nrouter.use(timeLog)\n\n// define the home page route\nrouter.get('/', (req, res) => {\n res.send('Birds home page')\n})\n// define the about route\nrouter.get('/about', (req, res) => {\n res.send('About birds')\n})\n\nmodule.exports = router\n```\n\nThen, load the router module in the app:\n\n```\nconst birds = require('./birds')\n\n// ...\n\napp.use('/birds', birds)\n```\n\nThe app will now be able to handle requests to `/birds` and `/birds/about`, as well as call the `timeLog` middleware function that is specific to the route.\n",
"filename": "routing.md",
"package": "express"
} |
{
"content": "Title: Serving static files in Express\n\nURL Source: https://expressjs.com/en/starter/static-files.html\n\nMarkdown Content:\nTo serve static files such as images, CSS files, and JavaScript files, use the `express.static` built-in middleware function in Express.\n\nThe function signature is:\n\n```\nexpress.static(root, [options])\n```\n\nThe `root` argument specifies the root directory from which to serve static assets. For more information on the `options` argument, see [express.static](https://expressjs.com/en/4x/api.html#express.static).\n\nFor example, use the following code to serve images, CSS files, and JavaScript files in a directory named `public`:\n\n```\napp.use(express.static('public'))\n```\n\nNow, you can load the files that are in the `public` directory:\n\n```\nhttp://localhost:3000/images/kitten.jpg\nhttp://localhost:3000/css/style.css\nhttp://localhost:3000/js/app.js\nhttp://localhost:3000/images/bg.png\nhttp://localhost:3000/hello.html\n```\n\nExpress looks up the files relative to the static directory, so the name of the static directory is not part of the URL.\n\nTo use multiple static assets directories, call the `express.static` middleware function multiple times:\n\n```\napp.use(express.static('public'))\napp.use(express.static('files'))\n```\n\nExpress looks up the files in the order in which you set the static directories with the `express.static` middleware function.\n\nNOTE: For best results, [use a reverse proxy](https://expressjs.com/en/advanced/best-practice-performance.html#use-a-reverse-proxy) cache to improve performance of serving static assets.\n\nTo create a virtual path prefix (where the path does not actually exist in the file system) for files that are served by the `express.static` function, [specify a mount path](https://expressjs.com/en/4x/api.html#app.use) for the static directory, as shown below:\n\n```\napp.use('/static', express.static('public'))\n```\n\nNow, you can load the files that are in the `public` directory from the `/static` path prefix.\n\n```\nhttp://localhost:3000/static/images/kitten.jpg\nhttp://localhost:3000/static/css/style.css\nhttp://localhost:3000/static/js/app.js\nhttp://localhost:3000/static/images/bg.png\nhttp://localhost:3000/static/hello.html\n```\n\nHowever, the path that you provide to the `express.static` function is relative to the directory from where you launch your `node` process. If you run the express app from another directory, it’s safer to use the absolute path of the directory that you want to serve:\n\n```\nconst path = require('path')\napp.use('/static', express.static(path.join(__dirname, 'public')))\n```\n\nFor more details about the `serve-static` function and its options, see [serve-static](https://expressjs.com/resources/middleware/serve-static.html).\n\n### [Previous: Basic Routing](https://expressjs.com/en/starter/basic-routing.html) [Next: More examples](https://expressjs.com/en/starter/examples.html)\n",
"filename": "static-files.md",
"package": "express"
} |
{
"content": "Title: Using Express middleware\n\nURL Source: https://expressjs.com/en/guide/using-middleware.html\n\nMarkdown Content:\nExpress is a routing and middleware web framework that has minimal functionality of its own: An Express application is essentially a series of middleware function calls.\n\n_Middleware_ functions are functions that have access to the [request object](https://expressjs.com/en/4x/api.html#req) (`req`), the [response object](https://expressjs.com/en/4x/api.html#res) (`res`), and the next middleware function in the application’s request-response cycle. The next middleware function is commonly denoted by a variable named `next`.\n\nMiddleware functions can perform the following tasks:\n\n* Execute any code.\n* Make changes to the request and the response objects.\n* End the request-response cycle.\n* Call the next middleware function in the stack.\n\nIf the current middleware function does not end the request-response cycle, it must call `next()` to pass control to the next middleware function. Otherwise, the request will be left hanging.\n\nAn Express application can use the following types of middleware:\n\n* [Application-level middleware](https://expressjs.com/en/guide/using-middleware.html#middleware.application)\n* [Router-level middleware](https://expressjs.com/en/guide/using-middleware.html#middleware.router)\n* [Error-handling middleware](https://expressjs.com/en/guide/using-middleware.html#middleware.error-handling)\n* [Built-in middleware](https://expressjs.com/en/guide/using-middleware.html#middleware.built-in)\n* [Third-party middleware](https://expressjs.com/en/guide/using-middleware.html#middleware.third-party)\n\nYou can load application-level and router-level middleware with an optional mount path. You can also load a series of middleware functions together, which creates a sub-stack of the middleware system at a mount point.\n\nApplication-level middleware\n----------------------------\n\nBind application-level middleware to an instance of the [app object](https://expressjs.com/en/4x/api.html#app) by using the `app.use()` and `app.METHOD()` functions, where `METHOD` is the HTTP method of the request that the middleware function handles (such as GET, PUT, or POST) in lowercase.\n\nThis example shows a middleware function with no mount path. The function is executed every time the app receives a request.\n\n```\nconst express = require('express')\nconst app = express()\n\napp.use((req, res, next) => {\n console.log('Time:', Date.now())\n next()\n})\n```\n\nThis example shows a middleware function mounted on the `/user/:id` path. The function is executed for any type of HTTP request on the `/user/:id` path.\n\n```\napp.use('/user/:id', (req, res, next) => {\n console.log('Request Type:', req.method)\n next()\n})\n```\n\nThis example shows a route and its handler function (middleware system). The function handles GET requests to the `/user/:id` path.\n\n```\napp.get('/user/:id', (req, res, next) => {\n res.send('USER')\n})\n```\n\nHere is an example of loading a series of middleware functions at a mount point, with a mount path. It illustrates a middleware sub-stack that prints request info for any type of HTTP request to the `/user/:id` path.\n\n```\napp.use('/user/:id', (req, res, next) => {\n console.log('Request URL:', req.originalUrl)\n next()\n}, (req, res, next) => {\n console.log('Request Type:', req.method)\n next()\n})\n```\n\nRoute handlers enable you to define multiple routes for a path. The example below defines two routes for GET requests to the `/user/:id` path. The second route will not cause any problems, but it will never get called because the first route ends the request-response cycle.\n\nThis example shows a middleware sub-stack that handles GET requests to the `/user/:id` path.\n\n```\napp.get('/user/:id', (req, res, next) => {\n console.log('ID:', req.params.id)\n next()\n}, (req, res, next) => {\n res.send('User Info')\n})\n\n// handler for the /user/:id path, which prints the user ID\napp.get('/user/:id', (req, res, next) => {\n res.send(req.params.id)\n})\n```\n\nTo skip the rest of the middleware functions from a router middleware stack, call `next('route')` to pass control to the next route. **NOTE**: `next('route')` will work only in middleware functions that were loaded by using the `app.METHOD()` or `router.METHOD()` functions.\n\nThis example shows a middleware sub-stack that handles GET requests to the `/user/:id` path.\n\n```\napp.get('/user/:id', (req, res, next) => {\n // if the user ID is 0, skip to the next route\n if (req.params.id === '0') next('route')\n // otherwise pass the control to the next middleware function in this stack\n else next()\n}, (req, res, next) => {\n // send a regular response\n res.send('regular')\n})\n\n// handler for the /user/:id path, which sends a special response\napp.get('/user/:id', (req, res, next) => {\n res.send('special')\n})\n```\n\nMiddleware can also be declared in an array for reusability.\n\nThis example shows an array with a middleware sub-stack that handles GET requests to the `/user/:id` path\n\n```\nfunction logOriginalUrl (req, res, next) {\n console.log('Request URL:', req.originalUrl)\n next()\n}\n\nfunction logMethod (req, res, next) {\n console.log('Request Type:', req.method)\n next()\n}\n\nconst logStuff = [logOriginalUrl, logMethod]\napp.get('/user/:id', logStuff, (req, res, next) => {\n res.send('User Info')\n})\n```\n\nRouter-level middleware\n-----------------------\n\nRouter-level middleware works in the same way as application-level middleware, except it is bound to an instance of `express.Router()`.\n\n```\nconst router = express.Router()\n```\n\nLoad router-level middleware by using the `router.use()` and `router.METHOD()` functions.\n\nThe following example code replicates the middleware system that is shown above for application-level middleware, by using router-level middleware:\n\n```\nconst express = require('express')\nconst app = express()\nconst router = express.Router()\n\n// a middleware function with no mount path. This code is executed for every request to the router\nrouter.use((req, res, next) => {\n console.log('Time:', Date.now())\n next()\n})\n\n// a middleware sub-stack shows request info for any type of HTTP request to the /user/:id path\nrouter.use('/user/:id', (req, res, next) => {\n console.log('Request URL:', req.originalUrl)\n next()\n}, (req, res, next) => {\n console.log('Request Type:', req.method)\n next()\n})\n\n// a middleware sub-stack that handles GET requests to the /user/:id path\nrouter.get('/user/:id', (req, res, next) => {\n // if the user ID is 0, skip to the next router\n if (req.params.id === '0') next('route')\n // otherwise pass control to the next middleware function in this stack\n else next()\n}, (req, res, next) => {\n // render a regular page\n res.render('regular')\n})\n\n// handler for the /user/:id path, which renders a special page\nrouter.get('/user/:id', (req, res, next) => {\n console.log(req.params.id)\n res.render('special')\n})\n\n// mount the router on the app\napp.use('/', router)\n```\n\nTo skip the rest of the router’s middleware functions, call `next('router')` to pass control back out of the router instance.\n\nThis example shows a middleware sub-stack that handles GET requests to the `/user/:id` path.\n\n```\nconst express = require('express')\nconst app = express()\nconst router = express.Router()\n\n// predicate the router with a check and bail out when needed\nrouter.use((req, res, next) => {\n if (!req.headers['x-auth']) return next('router')\n next()\n})\n\nrouter.get('/user/:id', (req, res) => {\n res.send('hello, user!')\n})\n\n// use the router and 401 anything falling through\napp.use('/admin', router, (req, res) => {\n res.sendStatus(401)\n})\n```\n\nError-handling middleware\n-------------------------\n\nError-handling middleware always takes _four_ arguments. You must provide four arguments to identify it as an error-handling middleware function. Even if you don’t need to use the `next` object, you must specify it to maintain the signature. Otherwise, the `next` object will be interpreted as regular middleware and will fail to handle errors.\n\nDefine error-handling middleware functions in the same way as other middleware functions, except with four arguments instead of three, specifically with the signature `(err, req, res, next)`:\n\n```\napp.use((err, req, res, next) => {\n console.error(err.stack)\n res.status(500).send('Something broke!')\n})\n```\n\nFor details about error-handling middleware, see: [Error handling](https://expressjs.com/en/guide/error-handling.html).\n\nBuilt-in middleware\n-------------------\n\nStarting with version 4.x, Express no longer depends on [Connect](https://github.com/senchalabs/connect). The middleware functions that were previously included with Express are now in separate modules; see [the list of middleware functions](https://github.com/senchalabs/connect#middleware).\n\nExpress has the following built-in middleware functions:\n\n* [express.static](https://expressjs.com/en/4x/api.html#express.static) serves static assets such as HTML files, images, and so on.\n* [express.json](https://expressjs.com/en/4x/api.html#express.json) parses incoming requests with JSON payloads. **NOTE: Available with Express 4.16.0+**\n* [express.urlencoded](https://expressjs.com/en/4x/api.html#express.urlencoded) parses incoming requests with URL-encoded payloads. **NOTE: Available with Express 4.16.0+**\n\nThird-party middleware\n----------------------\n\nUse third-party middleware to add functionality to Express apps.\n\nInstall the Node.js module for the required functionality, then load it in your app at the application level or at the router level.\n\nThe following example illustrates installing and loading the cookie-parsing middleware function `cookie-parser`.\n\n```\n$ npm install cookie-parser\n```\n\n```\nconst express = require('express')\nconst app = express()\nconst cookieParser = require('cookie-parser')\n\n// load the cookie-parsing middleware\napp.use(cookieParser())\n```\n\nFor a partial list of third-party middleware functions that are commonly used with Express, see: [Third-party middleware](https://expressjs.com/en/resources/middleware.html).\n",
"filename": "using-middleware.md",
"package": "express"
} |
{
"content": "Title: Using template engines with Express\n\nURL Source: https://expressjs.com/en/guide/using-template-engines.html\n\nMarkdown Content:\nA _template engine_ enables you to use static template files in your application. At runtime, the template engine replaces variables in a template file with actual values, and transforms the template into an HTML file sent to the client. This approach makes it easier to design an HTML page.\n\nSome popular template engines that work with Express are [Pug](https://pugjs.org/api/getting-started.html), [Mustache](https://www.npmjs.com/package/mustache), and [EJS](https://www.npmjs.com/package/ejs). The [Express application generator](https://expressjs.com/en/starter/generator.html) uses [Jade](https://www.npmjs.com/package/jade) as its default, but it also supports several others.\n\nSee [Template Engines (Express wiki)](https://github.com/expressjs/express/wiki#template-engines) for a list of template engines you can use with Express. See also [Comparing JavaScript Templating Engines: Jade, Mustache, Dust and More](https://strongloop.com/strongblog/compare-javascript-templates-jade-mustache-dust/).\n\n**Note**: Jade has been renamed to [Pug](https://www.npmjs.com/package/pug). You can continue to use Jade in your app, and it will work just fine. However if you want the latest updates to the template engine, you must replace Jade with Pug in your app.\n\nTo render template files, set the following [application setting properties](https://expressjs.com/en/4x/api.html#app.set), set in `app.js` in the default app created by the generator:\n\n* `views`, the directory where the template files are located. Eg: `app.set('views', './views')`. This defaults to the `views` directory in the application root directory.\n* `view engine`, the template engine to use. For example, to use the Pug template engine: `app.set('view engine', 'pug')`.\n\nThen install the corresponding template engine npm package; for example to install Pug:\n\n```\n$ npm install pug --save\n```\n\nExpress-compliant template engines such as Jade and Pug export a function named `__express(filePath, options, callback)`, which is called by the `res.render()` function to render the template code.\n\nSome template engines do not follow this convention. The [Consolidate.js](https://www.npmjs.org/package/consolidate) library follows this convention by mapping all of the popular Node.js template engines, and therefore works seamlessly within Express.\n\nAfter the view engine is set, you don’t have to specify the engine or load the template engine module in your app; Express loads the module internally, as shown below (for the above example).\n\n```\napp.set('view engine', 'pug')\n```\n\nCreate a Pug template file named `index.pug` in the `views` directory, with the following content:\n\n```\nhtml\n head\n title= title\n body\n h1= message\n```\n\nThen create a route to render the `index.pug` file. If the `view engine` property is not set, you must specify the extension of the `view` file. Otherwise, you can omit it.\n\n```\napp.get('/', (req, res) => {\n res.render('index', { title: 'Hey', message: 'Hello there!' })\n})\n```\n\nWhen you make a request to the home page, the `index.pug` file will be rendered as HTML.\n\nNote: The view engine cache does not cache the contents of the template’s output, only the underlying template itself. The view is still re-rendered with every request even when the cache is on.\n\nTo learn more about how template engines work in Express, see: [“Developing template engines for Express”](https://expressjs.com/en/advanced/developing-template-engines.html).\n",
"filename": "using-template-engines.md",
"package": "express"
} |
{
"content": "Title: Express utilities\n\nURL Source: https://expressjs.com/en/resources/utils.html\n\nMarkdown Content:\nExpress utilities\n===============\n \nExpress utility functions\n-------------------------\n\nThe [pillarjs](https://github.com/pillarjs) GitHub organization contains a number of modules for utility functions that may be generally useful.\n\n| Utility modules | Description |\n| --- | --- |\n| [cookies](https://www.npmjs.com/package/cookies) | Get and set HTTP(S) cookies that can be signed to prevent tampering, using Keygrip. Can be used with the Node.js HTTP library or as Express middleware. |\n| [csrf](https://www.npmjs.com/package/csrf) | Contains the logic behind CSRF token creation and verification. Use this module to create custom CSRF middleware. |\n| [finalhandler](https://www.npmjs.com/package/finalhandler) | Function to invoke as the final step to respond to HTTP request. |\n| [parseurl](https://www.npmjs.com/package/parseurl) | Parse a URL with caching. |\n| [path-match](https://www.npmjs.com/package/path-match) | Thin wrapper around [path-to-regexp](https://github.com/component/path-to-regexp) to make extracting parameter names easier. |\n| [path-to-regexp](https://www.npmjs.com/package/path-to-regexp) | Turn an Express-style path string such as \\`\\`/user/:name\\` into a regular expression. |\n| [resolve-path](https://www.npmjs.com/package/resolve-path) | Resolves a relative path against a root path with validation. |\n| [router](https://www.npmjs.com/package/router) | Simple middleware-style router. |\n| [routington](https://www.npmjs.com/package/routington) | Trie-based URL router for defining and matching URLs. |\n| [send](https://www.npmjs.com/package/send) | Library for streaming files as a HTTP response, with support for partial responses (ranges), conditional-GET negotiation, and granular events. |\n| [templation](https://www.npmjs.com/package/templation) | View system similar to `res.render()` inspired by [co-views](https://github.com/visionmedia/co-views) and [consolidate.js](https://github.com/visionmedia/consolidate.js/). |\n\nFor additional low-level HTTP-related modules, see [jshttp](http://jshttp.github.io/).\n\n[](https://expressjs.com/en/resources/utils.html#)\n\nDocumentation translations provided by StrongLoop/IBM: [French](https://expressjs.com/fr/), [German](https://expressjs.com/de/), [Spanish](https://expressjs.com/es/), [Italian](https://expressjs.com/it/), [Japanese](https://expressjs.com/ja/), [Russian](https://expressjs.com/ru/), [Chinese](https://expressjs.com/zh-cn/), [Traditional Chinese](https://expressjs.com/zh-tw/), [Korean](https://expressjs.com/ko/), [Portuguese](https://expressjs.com/pt-br/). \nCommunity translation available for: [Slovak](https://expressjs.com/sk/), [Ukrainian](https://expressjs.com/uk/), [Uzbek](https://expressjs.com/uz/), [Turkish](https://expressjs.com/tr/), [Thai](https://expressjs.com/th/) and [Indonesian](https://expressjs.com/id/).\n\n[](https://www.netlify.com/)\n\n[Express](https://expressjs.com/) is a project of the [OpenJS Foundation](https://openjsf.org/).\n\n[Edit this page on GitHub](https://github.com/expressjs/expressjs.com/blob/gh-pages/en/resources/utils.md).\n\nCopyright © 2017 StrongLoop, IBM, and other expressjs.com contributors.\n\n[](http://creativecommons.org/licenses/by-sa/3.0/us/) This work is licensed under a [Creative Commons Attribution-ShareAlike 3.0 United States License](http://creativecommons.org/licenses/by-sa/3.0/us/).\n",
"filename": "utils.md",
"package": "express"
} |
{
"content": "Title: Writing middleware for use in Express apps\n\nURL Source: https://expressjs.com/en/guide/writing-middleware.html\n\nMarkdown Content:\nOverview\n--------\n\n_Middleware_ functions are functions that have access to the [request object](https://expressjs.com/en/4x/api.html#req) (`req`), the [response object](https://expressjs.com/en/4x/api.html#res) (`res`), and the `next` function in the application’s request-response cycle. The `next` function is a function in the Express router which, when invoked, executes the middleware succeeding the current middleware.\n\nMiddleware functions can perform the following tasks:\n\n* Execute any code.\n* Make changes to the request and the response objects.\n* End the request-response cycle.\n* Call the next middleware in the stack.\n\nIf the current middleware function does not end the request-response cycle, it must call `next()` to pass control to the next middleware function. Otherwise, the request will be left hanging.\n\nThe following figure shows the elements of a middleware function call:\n\n<table id=\"mw-fig\"><tbody><tr><td id=\"mw-fig-imgcell\"><img src=\"https://expressjs.com/images/express-mw.png\" id=\"mw-fig-img\"></td><td><p>HTTP method for which the middleware function applies.</p><p>Path (route) for which the middleware function applies.</p><p>The middleware function.</p><p>Callback argument to the middleware function, called \"next\" by convention.</p><p>HTTP <a href=\"https://expressjs.com/en/4x/api.html#res\">response</a> argument to the middleware function, called \"res\" by convention.</p><p>HTTP <a href=\"https://expressjs.com/en/4x/api.html#req\">request</a> argument to the middleware function, called \"req\" by convention.</p></td></tr></tbody></table>\n\nStarting with Express 5, middleware functions that return a Promise will call `next(value)` when they reject or throw an error. `next` will be called with either the rejected value or the thrown Error.\n\nExample\n-------\n\nHere is an example of a simple “Hello World” Express application. The remainder of this article will define and add three middleware functions to the application: one called `myLogger` that prints a simple log message, one called `requestTime` that displays the timestamp of the HTTP request, and one called `validateCookies` that validates incoming cookies.\n\n```\nconst express = require('express')\nconst app = express()\n\napp.get('/', (req, res) => {\n res.send('Hello World!')\n})\n\napp.listen(3000)\n```\n\n### Middleware function myLogger\n\nHere is a simple example of a middleware function called “myLogger”. This function just prints “LOGGED” when a request to the app passes through it. The middleware function is assigned to a variable named `myLogger`.\n\n```\nconst myLogger = function (req, res, next) {\n console.log('LOGGED')\n next()\n}\n```\n\nNotice the call above to `next()`. Calling this function invokes the next middleware function in the app. The `next()` function is not a part of the Node.js or Express API, but is the third argument that is passed to the middleware function. The `next()` function could be named anything, but by convention it is always named “next”. To avoid confusion, always use this convention.\n\nTo load the middleware function, call `app.use()`, specifying the middleware function. For example, the following code loads the `myLogger` middleware function before the route to the root path (/).\n\n```\nconst express = require('express')\nconst app = express()\n\nconst myLogger = function (req, res, next) {\n console.log('LOGGED')\n next()\n}\n\napp.use(myLogger)\n\napp.get('/', (req, res) => {\n res.send('Hello World!')\n})\n\napp.listen(3000)\n```\n\nEvery time the app receives a request, it prints the message “LOGGED” to the terminal.\n\nThe order of middleware loading is important: middleware functions that are loaded first are also executed first.\n\nIf `myLogger` is loaded after the route to the root path, the request never reaches it and the app doesn’t print “LOGGED”, because the route handler of the root path terminates the request-response cycle.\n\nThe middleware function `myLogger` simply prints a message, then passes on the request to the next middleware function in the stack by calling the `next()` function.\n\n### Middleware function requestTime\n\nNext, we’ll create a middleware function called “requestTime” and add a property called `requestTime` to the request object.\n\n```\nconst requestTime = function (req, res, next) {\n req.requestTime = Date.now()\n next()\n}\n```\n\nThe app now uses the `requestTime` middleware function. Also, the callback function of the root path route uses the property that the middleware function adds to `req` (the request object).\n\n```\nconst express = require('express')\nconst app = express()\n\nconst requestTime = function (req, res, next) {\n req.requestTime = Date.now()\n next()\n}\n\napp.use(requestTime)\n\napp.get('/', (req, res) => {\n let responseText = 'Hello World!<br>'\n responseText += `<small>Requested at: ${req.requestTime}</small>`\n res.send(responseText)\n})\n\napp.listen(3000)\n```\n\nWhen you make a request to the root of the app, the app now displays the timestamp of your request in the browser.\n\n### Middleware function validateCookies\n\nFinally, we’ll create a middleware function that validates incoming cookies and sends a 400 response if cookies are invalid.\n\nHere’s an example function that validates cookies with an external async service.\n\n```\nasync function cookieValidator (cookies) {\n try {\n await externallyValidateCookie(cookies.testCookie)\n } catch {\n throw new Error('Invalid cookies')\n }\n}\n```\n\nHere, we use the [`cookie-parser`](https://expressjs.com/resources/middleware/cookie-parser.html) middleware to parse incoming cookies off the `req` object and pass them to our `cookieValidator` function. The `validateCookies` middleware returns a Promise that upon rejection will automatically trigger our error handler.\n\n```\nconst express = require('express')\nconst cookieParser = require('cookie-parser')\nconst cookieValidator = require('./cookieValidator')\n\nconst app = express()\n\nasync function validateCookies (req, res, next) {\n await cookieValidator(req.cookies)\n next()\n}\n\napp.use(cookieParser())\n\napp.use(validateCookies)\n\n// error handler\napp.use((err, req, res, next) => {\n res.status(400).send(err.message)\n})\n\napp.listen(3000)\n```\n\nNote how `next()` is called after `await cookieValidator(req.cookies)`. This ensures that if `cookieValidator` resolves, the next middleware in the stack will get called. If you pass anything to the `next()` function (except the string `'route'` or `'router'`), Express regards the current request as being an error and will skip any remaining non-error handling routing and middleware functions.\n\nBecause you have access to the request object, the response object, the next middleware function in the stack, and the whole Node.js API, the possibilities with middleware functions are endless.\n\nFor more information about Express middleware, see: [Using Express middleware](https://expressjs.com/en/guide/using-middleware.html).\n\nConfigurable middleware\n-----------------------\n\nIf you need your middleware to be configurable, export a function which accepts an options object or other parameters, which, then returns the middleware implementation based on the input parameters.\n\nFile: `my-middleware.js`\n\n```\nmodule.exports = function (options) {\n return function (req, res, next) {\n // Implement the middleware function based on the options object\n next()\n }\n}\n```\n\nThe middleware can now be used as shown below.\n\n```\nconst mw = require('./my-middleware.js')\n\napp.use(mw({ option1: '1', option2: '2' }))\n```\n\nRefer to [cookie-session](https://github.com/expressjs/cookie-session) and [compression](https://github.com/expressjs/compression) for examples of configurable middleware.\n",
"filename": "writing-middleware.md",
"package": "express"
} |
{
"content": "Title: Children – React\n\nURL Source: https://react.dev/reference/react/Children\n\nMarkdown Content:\n### Pitfall\n\nUsing `Children` is uncommon and can lead to fragile code. [See common alternatives.](https://react.dev/reference/react/Children#alternatives)\n\n`Children` lets you manipulate and transform the JSX you received as the [`children` prop.](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children)\n\n```\nconst mappedChildren = Children.map(children, child =><div className=\"Row\">{child}</div>);\n```\n\n* [Reference](https://react.dev/reference/react/Children#reference)\n * [`Children.count(children)`](https://react.dev/reference/react/Children#children-count)\n * [`Children.forEach(children, fn, thisArg?)`](https://react.dev/reference/react/Children#children-foreach)\n * [`Children.map(children, fn, thisArg?)`](https://react.dev/reference/react/Children#children-map)\n * [`Children.only(children)`](https://react.dev/reference/react/Children#children-only)\n * [`Children.toArray(children)`](https://react.dev/reference/react/Children#children-toarray)\n* [Usage](https://react.dev/reference/react/Children#usage)\n * [Transforming children](https://react.dev/reference/react/Children#transforming-children)\n * [Running some code for each child](https://react.dev/reference/react/Children#running-some-code-for-each-child)\n * [Counting children](https://react.dev/reference/react/Children#counting-children)\n * [Converting children to an array](https://react.dev/reference/react/Children#converting-children-to-an-array)\n* [Alternatives](https://react.dev/reference/react/Children#alternatives)\n * [Exposing multiple components](https://react.dev/reference/react/Children#exposing-multiple-components)\n * [Accepting an array of objects as a prop](https://react.dev/reference/react/Children#accepting-an-array-of-objects-as-a-prop)\n * [Calling a render prop to customize rendering](https://react.dev/reference/react/Children#calling-a-render-prop-to-customize-rendering)\n* [Troubleshooting](https://react.dev/reference/react/Children#troubleshooting)\n * [I pass a custom component, but the `Children` methods don’t show its render result](https://react.dev/reference/react/Children#i-pass-a-custom-component-but-the-children-methods-dont-show-its-render-result)\n\n* * *\n\nReference[](https://react.dev/reference/react/Children#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `Children.count(children)`[](https://react.dev/reference/react/Children#children-count \"Link for this heading\")\n\nCall `Children.count(children)` to count the number of children in the `children` data structure.\n\n```\nimport { Children } from 'react';function RowList({ children }) {return (<><h1>Total rows: {Children.count(children)}</h1> ...</>);}\n```\n\n[See more examples below.](https://react.dev/reference/react/Children#counting-children)\n\n#### Parameters[](https://react.dev/reference/react/Children#children-count-parameters \"Link for Parameters \")\n\n* `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) received by your component.\n\n#### Returns[](https://react.dev/reference/react/Children#children-count-returns \"Link for Returns \")\n\nThe number of nodes inside these `children`.\n\n#### Caveats[](https://react.dev/reference/react/Children#children-count-caveats \"Link for Caveats \")\n\n* Empty nodes (`null`, `undefined`, and Booleans), strings, numbers, and [React elements](https://react.dev/reference/react/createElement) count as individual nodes. Arrays don’t count as individual nodes, but their children do. **The traversal does not go deeper than React elements:** they don’t get rendered, and their children aren’t traversed. [Fragments](https://react.dev/reference/react/Fragment) don’t get traversed.\n\n* * *\n\n### `Children.forEach(children, fn, thisArg?)`[](https://react.dev/reference/react/Children#children-foreach \"Link for this heading\")\n\nCall `Children.forEach(children, fn, thisArg?)` to run some code for each child in the `children` data structure.\n\n```\nimport { Children } from 'react';function SeparatorList({ children }) {const result = [];Children.forEach(children, (child, index) => {result.push(child);result.push(<hr key={index} />);});// ...\n```\n\n[See more examples below.](https://react.dev/reference/react/Children#running-some-code-for-each-child)\n\n#### Parameters[](https://react.dev/reference/react/Children#children-foreach-parameters \"Link for Parameters \")\n\n* `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) received by your component.\n* `fn`: The function you want to run for each child, similar to the [array `forEach` method](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach) callback. It will be called with the child as the first argument and its index as the second argument. The index starts at `0` and increments on each call.\n* **optional** `thisArg`: The [`this` value](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this) with which the `fn` function should be called. If omitted, it’s `undefined`.\n\n#### Returns[](https://react.dev/reference/react/Children#children-foreach-returns \"Link for Returns \")\n\n`Children.forEach` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/Children#children-foreach-caveats \"Link for Caveats \")\n\n* Empty nodes (`null`, `undefined`, and Booleans), strings, numbers, and [React elements](https://react.dev/reference/react/createElement) count as individual nodes. Arrays don’t count as individual nodes, but their children do. **The traversal does not go deeper than React elements:** they don’t get rendered, and their children aren’t traversed. [Fragments](https://react.dev/reference/react/Fragment) don’t get traversed.\n\n* * *\n\n### `Children.map(children, fn, thisArg?)`[](https://react.dev/reference/react/Children#children-map \"Link for this heading\")\n\nCall `Children.map(children, fn, thisArg?)` to map or transform each child in the `children` data structure.\n\n```\nimport { Children } from 'react';function RowList({ children }) {return (<div className=\"RowList\">{Children.map(children, child =><div className=\"Row\">{child}</div>)}</div>);}\n```\n\n[See more examples below.](https://react.dev/reference/react/Children#transforming-children)\n\n#### Parameters[](https://react.dev/reference/react/Children#children-map-parameters \"Link for Parameters \")\n\n* `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) received by your component.\n* `fn`: The mapping function, similar to the [array `map` method](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) callback. It will be called with the child as the first argument and its index as the second argument. The index starts at `0` and increments on each call. You need to return a React node from this function. This may be an empty node (`null`, `undefined`, or a Boolean), a string, a number, a React element, or an array of other React nodes.\n* **optional** `thisArg`: The [`this` value](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this) with which the `fn` function should be called. If omitted, it’s `undefined`.\n\n#### Returns[](https://react.dev/reference/react/Children#children-map-returns \"Link for Returns \")\n\nIf `children` is `null` or `undefined`, returns the same value.\n\nOtherwise, returns a flat array consisting of the nodes you’ve returned from the `fn` function. The returned array will contain all nodes you returned except for `null` and `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/Children#children-map-caveats \"Link for Caveats \")\n\n* Empty nodes (`null`, `undefined`, and Booleans), strings, numbers, and [React elements](https://react.dev/reference/react/createElement) count as individual nodes. Arrays don’t count as individual nodes, but their children do. **The traversal does not go deeper than React elements:** they don’t get rendered, and their children aren’t traversed. [Fragments](https://react.dev/reference/react/Fragment) don’t get traversed.\n \n* If you return an element or an array of elements with keys from `fn`, **the returned elements’ keys will be automatically combined with the key of the corresponding original item from `children`.** When you return multiple elements from `fn` in an array, their keys only need to be unique locally amongst each other.\n \n\n* * *\n\n### `Children.only(children)`[](https://react.dev/reference/react/Children#children-only \"Link for this heading\")\n\nCall `Children.only(children)` to assert that `children` represent a single React element.\n\n```\nfunction Box({ children }) {const element = Children.only(children);// ...\n```\n\n#### Parameters[](https://react.dev/reference/react/Children#children-only-parameters \"Link for Parameters \")\n\n* `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) received by your component.\n\n#### Returns[](https://react.dev/reference/react/Children#children-only-returns \"Link for Returns \")\n\nIf `children` [is a valid element,](https://react.dev/reference/react/isValidElement) returns that element.\n\nOtherwise, throws an error.\n\n#### Caveats[](https://react.dev/reference/react/Children#children-only-caveats \"Link for Caveats \")\n\n* This method always **throws if you pass an array (such as the return value of `Children.map`) as `children`.** In other words, it enforces that `children` is a single React element, not that it’s an array with a single element.\n\n* * *\n\n### `Children.toArray(children)`[](https://react.dev/reference/react/Children#children-toarray \"Link for this heading\")\n\nCall `Children.toArray(children)` to create an array out of the `children` data structure.\n\n```\nimport { Children } from 'react';export default function ReversedList({ children }) {const result = Children.toArray(children);result.reverse();// ...\n```\n\n#### Parameters[](https://react.dev/reference/react/Children#children-toarray-parameters \"Link for Parameters \")\n\n* `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) received by your component.\n\n#### Returns[](https://react.dev/reference/react/Children#children-toarray-returns \"Link for Returns \")\n\nReturns a flat array of elements in `children`.\n\n#### Caveats[](https://react.dev/reference/react/Children#children-toarray-caveats \"Link for Caveats \")\n\n* Empty nodes (`null`, `undefined`, and Booleans) will be omitted in the returned array. **The returned elements’ keys will be calculated from the original elements’ keys and their level of nesting and position.** This ensures that flattening the array does not introduce changes in behavior.\n\n* * *\n\nUsage[](https://react.dev/reference/react/Children#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Transforming children[](https://react.dev/reference/react/Children#transforming-children \"Link for Transforming children \")\n\nTo transform the children JSX that your component [receives as the `children` prop,](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) call `Children.map`:\n\n```\nimport { Children } from 'react';function RowList({ children }) {return (<div className=\"RowList\">{Children.map(children, child =><div className=\"Row\">{child}</div>)}</div>);}\n```\n\nIn the example above, the `RowList` wraps every child it receives into a `<div className=\"Row\">` container. For example, let’s say the parent component passes three `<p>` tags as the `children` prop to `RowList`:\n\n```\n<RowList><p>This is the first item.</p><p>This is the second item.</p><p>This is the third item.</p></RowList>\n```\n\nThen, with the `RowList` implementation above, the final rendered result will look like this:\n\n```\n<div className=\"RowList\"><div className=\"Row\"><p>This is the first item.</p></div><div className=\"Row\"><p>This is the second item.</p></div><div className=\"Row\"><p>This is the third item.</p></div></div>\n```\n\n`Children.map` is similar to [to transforming arrays with `map()`.](https://react.dev/learn/rendering-lists) The difference is that the `children` data structure is considered _opaque._ This means that even if it’s sometimes an array, you should not assume it’s an array or any other particular data type. This is why you should use `Children.map` if you need to transform it.\n\n##### Deep Dive\n\n#### Why is the children prop not always an array?[](https://react.dev/reference/react/Children#why-is-the-children-prop-not-always-an-array \"Link for Why is the children prop not always an array? \")\n\nIn React, the `children` prop is considered an _opaque_ data structure. This means that you shouldn’t rely on how it is structured. To transform, filter, or count children, you should use the `Children` methods.\n\nIn practice, the `children` data structure is often represented as an array internally. However, if there is only a single child, then React won’t create an extra array since this would lead to unnecessary memory overhead. As long as you use the `Children` methods instead of directly introspecting the `children` prop, your code will not break even if React changes how the data structure is actually implemented.\n\nEven when `children` is an array, `Children.map` has useful special behavior. For example, `Children.map` combines the [keys](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key) on the returned elements with the keys on the `children` you’ve passed to it. This ensures the original JSX children don’t “lose” keys even if they get wrapped like in the example above.\n\n### Pitfall\n\nThe `children` data structure **does not include rendered output** of the components you pass as JSX. In the example below, the `children` received by the `RowList` only contains two items rather than three:\n\n1. `<p>This is the first item.</p>`\n2. `<MoreRows />`\n\nThis is why only two row wrappers are generated in this example:\n\nimport RowList from './RowList.js';\n\nexport default function App() {\n return (\n <RowList\\>\n <p\\>This is the first item.</p\\>\n <MoreRows />\n </RowList\\>\n );\n}\n\nfunction MoreRows() {\n return (\n <\\>\n <p\\>This is the second item.</p\\>\n <p\\>This is the third item.</p\\>\n </\\>\n );\n}\n\n**There is no way to get the rendered output of an inner component** like `<MoreRows />` when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.](https://react.dev/reference/react/Children#alternatives)\n\n* * *\n\n### Running some code for each child[](https://react.dev/reference/react/Children#running-some-code-for-each-child \"Link for Running some code for each child \")\n\nCall `Children.forEach` to iterate over each child in the `children` data structure. It does not return any value and is similar to the [array `forEach` method.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach) You can use it to run custom logic like constructing your own array.\n\n### Pitfall\n\nAs mentioned earlier, there is no way to get the rendered output of an inner component when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.](https://react.dev/reference/react/Children#alternatives)\n\n* * *\n\n### Counting children[](https://react.dev/reference/react/Children#counting-children \"Link for Counting children \")\n\nCall `Children.count(children)` to calculate the number of children.\n\nimport { Children } from 'react';\n\nexport default function RowList({ children }) {\n return (\n <div className\\=\"RowList\"\\>\n <h1 className\\=\"RowListHeader\"\\>\n Total rows: {Children.count(children)}\n </h1\\>\n {Children.map(children, child \\=>\n <div className\\=\"Row\"\\>\n {child}\n </div\\>\n )}\n </div\\>\n );\n}\n\n### Pitfall\n\nAs mentioned earlier, there is no way to get the rendered output of an inner component when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.](https://react.dev/reference/react/Children#alternatives)\n\n* * *\n\n### Converting children to an array[](https://react.dev/reference/react/Children#converting-children-to-an-array \"Link for Converting children to an array \")\n\nCall `Children.toArray(children)` to turn the `children` data structure into a regular JavaScript array. This lets you manipulate the array with built-in array methods like [`filter`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter), [`sort`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort), or [`reverse`.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reverse)\n\n### Pitfall\n\nAs mentioned earlier, there is no way to get the rendered output of an inner component when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.](https://react.dev/reference/react/Children#alternatives)\n\n* * *\n\nAlternatives[](https://react.dev/reference/react/Children#alternatives \"Link for Alternatives \")\n------------------------------------------------------------------------------------------------\n\n### Note\n\nThis section describes alternatives to the `Children` API (with capital `C`) that’s imported like this:\n\n```\nimport { Children } from 'react';\n```\n\nDon’t confuse it with [using the `children` prop](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) (lowercase `c`), which is good and encouraged.\n\n### Exposing multiple components[](https://react.dev/reference/react/Children#exposing-multiple-components \"Link for Exposing multiple components \")\n\nManipulating children with the `Children` methods often leads to fragile code. When you pass children to a component in JSX, you don’t usually expect the component to manipulate or transform the individual children.\n\nWhen you can, try to avoid using the `Children` methods. For example, if you want every child of `RowList` to be wrapped in `<div className=\"Row\">`, export a `Row` component, and manually wrap every row into it like this:\n\nimport { RowList, Row } from './RowList.js';\n\nexport default function App() {\n return (\n <RowList\\>\n <Row\\>\n <p\\>This is the first item.</p\\>\n </Row\\>\n <Row\\>\n <p\\>This is the second item.</p\\>\n </Row\\>\n <Row\\>\n <p\\>This is the third item.</p\\>\n </Row\\>\n </RowList\\>\n );\n}\n\nUnlike using `Children.map`, this approach does not wrap every child automatically. **However, this approach has a significant benefit compared to the [earlier example with `Children.map`](https://react.dev/reference/react/Children#transforming-children) because it works even if you keep extracting more components.** For example, it still works if you extract your own `MoreRows` component:\n\nimport { RowList, Row } from './RowList.js';\n\nexport default function App() {\n return (\n <RowList\\>\n <Row\\>\n <p\\>This is the first item.</p\\>\n </Row\\>\n <MoreRows />\n </RowList\\>\n );\n}\n\nfunction MoreRows() {\n return (\n <\\>\n <Row\\>\n <p\\>This is the second item.</p\\>\n </Row\\>\n <Row\\>\n <p\\>This is the third item.</p\\>\n </Row\\>\n </\\>\n );\n}\n\nThis wouldn’t work with `Children.map` because it would “see” `<MoreRows />` as a single child (and a single row).\n\n* * *\n\n### Accepting an array of objects as a prop[](https://react.dev/reference/react/Children#accepting-an-array-of-objects-as-a-prop \"Link for Accepting an array of objects as a prop \")\n\nYou can also explicitly pass an array as a prop. For example, this `RowList` accepts a `rows` array as a prop:\n\nSince `rows` is a regular JavaScript array, the `RowList` component can use built-in array methods like [`map`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) on it.\n\nThis pattern is especially useful when you want to be able to pass more information as structured data together with children. In the below example, the `TabSwitcher` component receives an array of objects as the `tabs` prop:\n\nimport TabSwitcher from './TabSwitcher.js';\n\nexport default function App() {\n return (\n <TabSwitcher tabs\\={\\[\n {\n id: 'first',\n header: 'First',\n content: <p\\>This is the first item.</p\\>\n },\n {\n id: 'second',\n header: 'Second',\n content: <p\\>This is the second item.</p\\>\n },\n {\n id: 'third',\n header: 'Third',\n content: <p\\>This is the third item.</p\\>\n }\n \\]} />\n );\n}\n\nUnlike passing the children as JSX, this approach lets you associate some extra data like `header` with each item. Because you are working with the `tabs` directly, and it is an array, you do not need the `Children` methods.\n\n* * *\n\n### Calling a render prop to customize rendering[](https://react.dev/reference/react/Children#calling-a-render-prop-to-customize-rendering \"Link for Calling a render prop to customize rendering \")\n\nInstead of producing JSX for every single item, you can also pass a function that returns JSX, and call that function when necessary. In this example, the `App` component passes a `renderContent` function to the `TabSwitcher` component. The `TabSwitcher` component calls `renderContent` only for the selected tab:\n\nA prop like `renderContent` is called a _render prop_ because it is a prop that specifies how to render a piece of the user interface. However, there is nothing special about it: it is a regular prop which happens to be a function.\n\nRender props are functions, so you can pass information to them. For example, this `RowList` component passes the `id` and the `index` of each row to the `renderRow` render prop, which uses `index` to highlight even rows:\n\nimport { RowList, Row } from './RowList.js';\n\nexport default function App() {\n return (\n <RowList\n rowIds\\={\\['first', 'second', 'third'\\]}\n renderRow\\={(id, index) \\=> {\n return (\n <Row isHighlighted\\={index % 2 === 0}\\>\n <p\\>This is the {id} item.</p\\>\n </Row\\> \n );\n }}\n />\n );\n}\n\nThis is another example of how parent and child components can cooperate without manipulating the children.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/Children#troubleshooting \"Link for Troubleshooting \")\n---------------------------------------------------------------------------------------------------------\n\n### I pass a custom component, but the `Children` methods don’t show its render result[](https://react.dev/reference/react/Children#i-pass-a-custom-component-but-the-children-methods-dont-show-its-render-result \"Link for this heading\")\n\nSuppose you pass two children to `RowList` like this:\n\n```\n<RowList><p>First item</p><MoreRows /></RowList>\n```\n\nIf you do `Children.count(children)` inside `RowList`, you will get `2`. Even if `MoreRows` renders 10 different items, or if it returns `null`, `Children.count(children)` will still be `2`. From the `RowList`’s perspective, it only “sees” the JSX it has received. It does not “see” the internals of the `MoreRows` component.\n\nThe limitation makes it hard to extract a component. This is why [alternatives](https://react.dev/reference/react/Children#alternatives) are preferred to using `Children`.\n",
"filename": "Children.md",
"package": "react"
} |
{
"content": "Title: Component – React\n\nURL Source: https://react.dev/reference/react/Component\n\nMarkdown Content:\n### Pitfall\n\nWe recommend defining components as functions instead of classes. [See how to migrate.](https://react.dev/reference/react/Component#alternatives)\n\n`Component` is the base class for the React components defined as [JavaScript classes.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes) Class components are still supported by React, but we don’t recommend using them in new code.\n\n```\nclass Greeting extends Component {render() {return <h1>Hello, {this.props.name}!</h1>;}}\n```\n\nReference[](https://react.dev/reference/react/Component#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------\n\n### `Component`[](https://react.dev/reference/react/Component#component \"Link for this heading\")\n\nTo define a React component as a class, extend the built-in `Component` class and define a [`render` method:](https://react.dev/reference/react/Component#render)\n\n```\nimport { Component } from 'react';class Greeting extends Component {render() {return <h1>Hello, {this.props.name}!</h1>;}}\n```\n\nOnly the `render` method is required, other methods are optional.\n\n[See more examples below.](https://react.dev/reference/react/Component#usage)\n\n* * *\n\n### `context`[](https://react.dev/reference/react/Component#context \"Link for this heading\")\n\nThe [context](https://react.dev/learn/passing-data-deeply-with-context) of a class component is available as `this.context`. It is only available if you specify _which_ context you want to receive using [`static contextType`](https://react.dev/reference/react/Component#static-contexttype) (modern) or [`static contextTypes`](https://react.dev/reference/react/Component#static-contexttypes) (deprecated).\n\nA class component can only read one context at a time.\n\n```\nclass Button extends Component {static contextType = ThemeContext;render() {const theme = this.context;const className = 'button-' + theme;return (<button className={className}>{this.props.children}</button>);}}\n```\n\n### Note\n\nReading `this.context` in class components is equivalent to [`useContext`](https://react.dev/reference/react/useContext) in function components.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-context-from-a-class-to-a-function)\n\n* * *\n\n### `props`[](https://react.dev/reference/react/Component#props \"Link for this heading\")\n\nThe props passed to a class component are available as `this.props`.\n\n```\nclass Greeting extends Component {render() {return <h1>Hello, {this.props.name}!</h1>;}}<Greeting name=\"Taylor\" />\n```\n\n### Note\n\nReading `this.props` in class components is equivalent to [declaring props](https://react.dev/learn/passing-props-to-a-component#step-2-read-props-inside-the-child-component) in function components.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-simple-component-from-a-class-to-a-function)\n\n* * *\n\n### `refs`[](https://react.dev/reference/react/Component#refs \"Link for this heading\")\n\n### Deprecated\n\nLets you access [legacy string refs](https://reactjs.org/docs/refs-and-the-dom.html#legacy-api-string-refs) for this component.\n\n* * *\n\n### `state`[](https://react.dev/reference/react/Component#state \"Link for this heading\")\n\nThe state of a class component is available as `this.state`. The `state` field must be an object. Do not mutate the state directly. If you wish to change the state, call `setState` with the new state.\n\n```\nclass Counter extends Component {state = {age: 42,};handleAgeChange = () => {this.setState({age: this.state.age + 1 });};render() {return (<><button onClick={this.handleAgeChange}> Increment age</button><p>You are {this.state.age}.</p></>);}}\n```\n\n### Note\n\nDefining `state` in class components is equivalent to calling [`useState`](https://react.dev/reference/react/useState) in function components.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-state-from-a-class-to-a-function)\n\n* * *\n\n### `constructor(props)`[](https://react.dev/reference/react/Component#constructor \"Link for this heading\")\n\nThe [constructor](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes/constructor) runs before your class component _mounts_ (gets added to the screen). Typically, a constructor is only used for two purposes in React. It lets you declare state and [bind](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_objects/Function/bind) your class methods to the class instance:\n\n```\nclass Counter extends Component {constructor(props) {super(props);this.state = { counter: 0 };this.handleClick = this.handleClick.bind(this);}handleClick() {// ...}\n```\n\nIf you use modern JavaScript syntax, constructors are rarely needed. Instead, you can rewrite this code above using the [public class field syntax](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes/Public_class_fields) which is supported both by modern browsers and tools like [Babel:](https://babeljs.io/)\n\n```\nclass Counter extends Component {state = { counter: 0 };handleClick = () => {// ...}\n```\n\nA constructor should not contain any side effects or subscriptions.\n\n#### Parameters[](https://react.dev/reference/react/Component#constructor-parameters \"Link for Parameters \")\n\n* `props`: The component’s initial props.\n\n#### Returns[](https://react.dev/reference/react/Component#constructor-returns \"Link for Returns \")\n\n`constructor` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#constructor-caveats \"Link for Caveats \")\n\n* Do not run any side effects or subscriptions in the constructor. Instead, use [`componentDidMount`](https://react.dev/reference/react/Component#componentdidmount) for that.\n \n* Inside a constructor, you need to call `super(props)` before any other statement. If you don’t do that, `this.props` will be `undefined` while the constructor runs, which can be confusing and cause bugs.\n \n* Constructor is the only place where you can assign [`this.state`](https://react.dev/reference/react/Component#state) directly. In all other methods, you need to use [`this.setState()`](https://react.dev/reference/react/Component#setstate) instead. Do not call `setState` in the constructor.\n \n* When you use [server rendering,](https://react.dev/reference/react-dom/server) the constructor will run on the server too, followed by the [`render`](https://react.dev/reference/react/Component#render) method. However, lifecycle methods like `componentDidMount` or `componentWillUnmount` will not run on the server.\n \n* When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React will call `constructor` twice in development and then throw away one of the instances. This helps you notice the accidental side effects that need to be moved out of the `constructor`.\n \n\n### Note\n\nThere is no exact equivalent for `constructor` in function components. To declare state in a function component, call [`useState`.](https://react.dev/reference/react/useState) To avoid recalculating the initial state, [pass a function to `useState`.](https://react.dev/reference/react/useState#avoiding-recreating-the-initial-state)\n\n* * *\n\n### `componentDidCatch(error, info)`[](https://react.dev/reference/react/Component#componentdidcatch \"Link for this heading\")\n\nIf you define `componentDidCatch`, React will call it when some child component (including distant children) throws an error during rendering. This lets you log that error to an error reporting service in production.\n\nTypically, it is used together with [`static getDerivedStateFromError`](https://react.dev/reference/react/Component#static-getderivedstatefromerror) which lets you update state in response to an error and display an error message to the user. A component with these methods is called an _error boundary._\n\n[See an example.](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary)\n\n#### Parameters[](https://react.dev/reference/react/Component#componentdidcatch-parameters \"Link for Parameters \")\n\n* `error`: The error that was thrown. In practice, it will usually be an instance of [`Error`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Error) but this is not guaranteed because JavaScript allows to [`throw`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/throw) any value, including strings or even `null`.\n \n* `info`: An object containing additional information about the error. Its `componentStack` field contains a stack trace with the component that threw, as well as the names and source locations of all its parent components. In production, the component names will be minified. If you set up production error reporting, you can decode the component stack using sourcemaps the same way as you would do for regular JavaScript error stacks.\n \n\n#### Returns[](https://react.dev/reference/react/Component#componentdidcatch-returns \"Link for Returns \")\n\n`componentDidCatch` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#componentdidcatch-caveats \"Link for Caveats \")\n\n* In the past, it was common to call `setState` inside `componentDidCatch` in order to update the UI and display the fallback error message. This is deprecated in favor of defining [`static getDerivedStateFromError`.](https://react.dev/reference/react/Component#static-getderivedstatefromerror)\n \n* Production and development builds of React slightly differ in the way `componentDidCatch` handles errors. In development, the errors will bubble up to `window`, which means that any `window.onerror` or `window.addEventListener('error', callback)` will intercept the errors that have been caught by `componentDidCatch`. In production, instead, the errors will not bubble up, which means any ancestor error handler will only receive errors not explicitly caught by `componentDidCatch`.\n \n\n### Note\n\nThere is no direct equivalent for `componentDidCatch` in function components yet. If you’d like to avoid creating class components, write a single `ErrorBoundary` component like above and use it throughout your app. Alternatively, you can use the [`react-error-boundary`](https://github.com/bvaughn/react-error-boundary) package which does that for you.\n\n* * *\n\n### `componentDidMount()`[](https://react.dev/reference/react/Component#componentdidmount \"Link for this heading\")\n\nIf you define the `componentDidMount` method, React will call it when your component is added _(mounted)_ to the screen. This is a common place to start data fetching, set up subscriptions, or manipulate the DOM nodes.\n\nIf you implement `componentDidMount`, you usually need to implement other lifecycle methods to avoid bugs. For example, if `componentDidMount` reads some state or props, you also have to implement [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) to handle their changes, and [`componentWillUnmount`](https://react.dev/reference/react/Component#componentwillunmount) to clean up whatever `componentDidMount` was doing.\n\n```\nclass ChatRoom extends Component {state = {serverUrl: 'https://localhost:1234'};componentDidMount() {this.setupConnection();}componentDidUpdate(prevProps, prevState) {if (this.props.roomId !== prevProps.roomId ||this.state.serverUrl !== prevState.serverUrl) {this.destroyConnection();this.setupConnection();}}componentWillUnmount() {this.destroyConnection();}// ...}\n```\n\n[See more examples.](https://react.dev/reference/react/Component#adding-lifecycle-methods-to-a-class-component)\n\n#### Parameters[](https://react.dev/reference/react/Component#componentdidmount-parameters \"Link for Parameters \")\n\n`componentDidMount` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react/Component#componentdidmount-returns \"Link for Returns \")\n\n`componentDidMount` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#componentdidmount-caveats \"Link for Caveats \")\n\n* When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, in development React will call `componentDidMount`, then immediately call [`componentWillUnmount`,](https://react.dev/reference/react/Component#componentwillunmount) and then call `componentDidMount` again. This helps you notice if you forgot to implement `componentWillUnmount` or if its logic doesn’t fully “mirror” what `componentDidMount` does.\n \n* Although you may call [`setState`](https://react.dev/reference/react/Component#setstate) immediately in `componentDidMount`, it’s best to avoid that when you can. It will trigger an extra rendering, but it will happen before the browser updates the screen. This guarantees that even though the [`render`](https://react.dev/reference/react/Component#render) will be called twice in this case, the user won’t see the intermediate state. Use this pattern with caution because it often causes performance issues. In most cases, you should be able to assign the initial state in the [`constructor`](https://react.dev/reference/react/Component#constructor) instead. It can, however, be necessary for cases like modals and tooltips when you need to measure a DOM node before rendering something that depends on its size or position.\n \n\n### Note\n\nFor many use cases, defining `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount` together in class components is equivalent to calling [`useEffect`](https://react.dev/reference/react/useEffect) in function components. In the rare cases where it’s important for the code to run before browser paint, [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) is a closer match.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-lifecycle-methods-from-a-class-to-a-function)\n\n* * *\n\n### `componentDidUpdate(prevProps, prevState, snapshot?)`[](https://react.dev/reference/react/Component#componentdidupdate \"Link for this heading\")\n\nIf you define the `componentDidUpdate` method, React will call it immediately after your component has been re-rendered with updated props or state. This method is not called for the initial render.\n\nYou can use it to manipulate the DOM after an update. This is also a common place to do network requests as long as you compare the current props to previous props (e.g. a network request may not be necessary if the props have not changed). Typically, you’d use it together with [`componentDidMount`](https://react.dev/reference/react/Component#componentdidmount) and [`componentWillUnmount`:](https://react.dev/reference/react/Component#componentwillunmount)\n\n```\nclass ChatRoom extends Component {state = {serverUrl: 'https://localhost:1234'};componentDidMount() {this.setupConnection();}componentDidUpdate(prevProps, prevState) {if (this.props.roomId !== prevProps.roomId ||this.state.serverUrl !== prevState.serverUrl) {this.destroyConnection();this.setupConnection();}}componentWillUnmount() {this.destroyConnection();}// ...}\n```\n\n[See more examples.](https://react.dev/reference/react/Component#adding-lifecycle-methods-to-a-class-component)\n\n#### Parameters[](https://react.dev/reference/react/Component#componentdidupdate-parameters \"Link for Parameters \")\n\n* `prevProps`: Props before the update. Compare `prevProps` to [`this.props`](https://react.dev/reference/react/Component#props) to determine what changed.\n \n* `prevState`: State before the update. Compare `prevState` to [`this.state`](https://react.dev/reference/react/Component#state) to determine what changed.\n \n* `snapshot`: If you implemented [`getSnapshotBeforeUpdate`](https://react.dev/reference/react/Component#getsnapshotbeforeupdate), `snapshot` will contain the value you returned from that method. Otherwise, it will be `undefined`.\n \n\n#### Returns[](https://react.dev/reference/react/Component#componentdidupdate-returns \"Link for Returns \")\n\n`componentDidUpdate` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#componentdidupdate-caveats \"Link for Caveats \")\n\n* `componentDidUpdate` will not get called if [`shouldComponentUpdate`](https://react.dev/reference/react/Component#shouldcomponentupdate) is defined and returns `false`.\n \n* The logic inside `componentDidUpdate` should usually be wrapped in conditions comparing `this.props` with `prevProps`, and `this.state` with `prevState`. Otherwise, there’s a risk of creating infinite loops.\n \n* Although you may call [`setState`](https://react.dev/reference/react/Component#setstate) immediately in `componentDidUpdate`, it’s best to avoid that when you can. It will trigger an extra rendering, but it will happen before the browser updates the screen. This guarantees that even though the [`render`](https://react.dev/reference/react/Component#render) will be called twice in this case, the user won’t see the intermediate state. This pattern often causes performance issues, but it may be necessary for rare cases like modals and tooltips when you need to measure a DOM node before rendering something that depends on its size or position.\n \n\n### Note\n\nFor many use cases, defining `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount` together in class components is equivalent to calling [`useEffect`](https://react.dev/reference/react/useEffect) in function components. In the rare cases where it’s important for the code to run before browser paint, [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) is a closer match.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-lifecycle-methods-from-a-class-to-a-function)\n\n* * *\n\n### `componentWillMount()`[](https://react.dev/reference/react/Component#componentwillmount \"Link for this heading\")\n\n### Deprecated\n\nThis API has been renamed from `componentWillMount` to [`UNSAFE_componentWillMount`.](https://react.dev/reference/react/Component#unsafe_componentwillmount) The old name has been deprecated. In a future major version of React, only the new name will work.\n\nRun the [`rename-unsafe-lifecycles` codemod](https://github.com/reactjs/react-codemod#rename-unsafe-lifecycles) to automatically update your components.\n\n* * *\n\n### `componentWillReceiveProps(nextProps)`[](https://react.dev/reference/react/Component#componentwillreceiveprops \"Link for this heading\")\n\n### Deprecated\n\nThis API has been renamed from `componentWillReceiveProps` to [`UNSAFE_componentWillReceiveProps`.](https://react.dev/reference/react/Component#unsafe_componentwillreceiveprops) The old name has been deprecated. In a future major version of React, only the new name will work.\n\nRun the [`rename-unsafe-lifecycles` codemod](https://github.com/reactjs/react-codemod#rename-unsafe-lifecycles) to automatically update your components.\n\n* * *\n\n### `componentWillUpdate(nextProps, nextState)`[](https://react.dev/reference/react/Component#componentwillupdate \"Link for this heading\")\n\n### Deprecated\n\nThis API has been renamed from `componentWillUpdate` to [`UNSAFE_componentWillUpdate`.](https://react.dev/reference/react/Component#unsafe_componentwillupdate) The old name has been deprecated. In a future major version of React, only the new name will work.\n\nRun the [`rename-unsafe-lifecycles` codemod](https://github.com/reactjs/react-codemod#rename-unsafe-lifecycles) to automatically update your components.\n\n* * *\n\n### `componentWillUnmount()`[](https://react.dev/reference/react/Component#componentwillunmount \"Link for this heading\")\n\nIf you define the `componentWillUnmount` method, React will call it before your component is removed _(unmounted)_ from the screen. This is a common place to cancel data fetching or remove subscriptions.\n\nThe logic inside `componentWillUnmount` should “mirror” the logic inside [`componentDidMount`.](https://react.dev/reference/react/Component#componentdidmount) For example, if `componentDidMount` sets up a subscription, `componentWillUnmount` should clean up that subscription. If the cleanup logic in your `componentWillUnmount` reads some props or state, you will usually also need to implement [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) to clean up resources (such as subscriptions) corresponding to the old props and state.\n\n```\nclass ChatRoom extends Component {state = {serverUrl: 'https://localhost:1234'};componentDidMount() {this.setupConnection();}componentDidUpdate(prevProps, prevState) {if (this.props.roomId !== prevProps.roomId ||this.state.serverUrl !== prevState.serverUrl) {this.destroyConnection();this.setupConnection();}}componentWillUnmount() {this.destroyConnection();}// ...}\n```\n\n[See more examples.](https://react.dev/reference/react/Component#adding-lifecycle-methods-to-a-class-component)\n\n#### Parameters[](https://react.dev/reference/react/Component#componentwillunmount-parameters \"Link for Parameters \")\n\n`componentWillUnmount` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react/Component#componentwillunmount-returns \"Link for Returns \")\n\n`componentWillUnmount` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#componentwillunmount-caveats \"Link for Caveats \")\n\n* When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, in development React will call [`componentDidMount`,](https://react.dev/reference/react/Component#componentdidmount) then immediately call `componentWillUnmount`, and then call `componentDidMount` again. This helps you notice if you forgot to implement `componentWillUnmount` or if its logic doesn’t fully “mirror” what `componentDidMount` does.\n\n### Note\n\nFor many use cases, defining `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount` together in class components is equivalent to calling [`useEffect`](https://react.dev/reference/react/useEffect) in function components. In the rare cases where it’s important for the code to run before browser paint, [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) is a closer match.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-lifecycle-methods-from-a-class-to-a-function)\n\n* * *\n\n### `forceUpdate(callback?)`[](https://react.dev/reference/react/Component#forceupdate \"Link for this heading\")\n\nForces a component to re-render.\n\nUsually, this is not necessary. If your component’s [`render`](https://react.dev/reference/react/Component#render) method only reads from [`this.props`](https://react.dev/reference/react/Component#props), [`this.state`](https://react.dev/reference/react/Component#state), or [`this.context`,](https://react.dev/reference/react/Component#context) it will re-render automatically when you call [`setState`](https://react.dev/reference/react/Component#setstate) inside your component or one of its parents. However, if your component’s `render` method reads directly from an external data source, you have to tell React to update the user interface when that data source changes. That’s what `forceUpdate` lets you do.\n\nTry to avoid all uses of `forceUpdate` and only read from `this.props` and `this.state` in `render`.\n\n#### Parameters[](https://react.dev/reference/react/Component#forceupdate-parameters \"Link for Parameters \")\n\n* **optional** `callback` If specified, React will call the `callback` you’ve provided after the update is committed.\n\n#### Returns[](https://react.dev/reference/react/Component#forceupdate-returns \"Link for Returns \")\n\n`forceUpdate` does not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#forceupdate-caveats \"Link for Caveats \")\n\n* If you call `forceUpdate`, React will re-render without calling [`shouldComponentUpdate`.](https://react.dev/reference/react/Component#shouldcomponentupdate)\n\n### Note\n\nReading an external data source and forcing class components to re-render in response to its changes with `forceUpdate` has been superseded by [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore) in function components.\n\n* * *\n\n### `getChildContext()`[](https://react.dev/reference/react/Component#getchildcontext \"Link for this heading\")\n\n### Deprecated\n\nLets you specify the values for the [legacy context](https://reactjs.org/docs/legacy-context.html) is provided by this component.\n\n* * *\n\n### `getSnapshotBeforeUpdate(prevProps, prevState)`[](https://react.dev/reference/react/Component#getsnapshotbeforeupdate \"Link for this heading\")\n\nIf you implement `getSnapshotBeforeUpdate`, React will call it immediately before React updates the DOM. It enables your component to capture some information from the DOM (e.g. scroll position) before it is potentially changed. Any value returned by this lifecycle method will be passed as a parameter to [`componentDidUpdate`.](https://react.dev/reference/react/Component#componentdidupdate)\n\nFor example, you can use it in a UI like a chat thread that needs to preserve its scroll position during updates:\n\n```\nclass ScrollingList extends React.Component {constructor(props) {super(props);this.listRef = React.createRef();}getSnapshotBeforeUpdate(prevProps, prevState) {// Are we adding new items to the list?// Capture the scroll position so we can adjust scroll later.if (prevProps.list.length < this.props.list.length) {const list = this.listRef.current;return list.scrollHeight - list.scrollTop;}return null;}componentDidUpdate(prevProps, prevState, snapshot) {// If we have a snapshot value, we've just added new items.// Adjust scroll so these new items don't push the old ones out of view.// (snapshot here is the value returned from getSnapshotBeforeUpdate)if (snapshot !== null) {const list = this.listRef.current;list.scrollTop = list.scrollHeight - snapshot;}}render() {return (<div ref={this.listRef}>{/* ...contents... */}</div>);}}\n```\n\nIn the above example, it is important to read the `scrollHeight` property directly in `getSnapshotBeforeUpdate`. It is not safe to read it in [`render`](https://react.dev/reference/react/Component#render), [`UNSAFE_componentWillReceiveProps`](https://react.dev/reference/react/Component#unsafe_componentwillreceiveprops), or [`UNSAFE_componentWillUpdate`](https://react.dev/reference/react/Component#unsafe_componentwillupdate) because there is a potential time gap between these methods getting called and React updating the DOM.\n\n#### Parameters[](https://react.dev/reference/react/Component#getsnapshotbeforeupdate-parameters \"Link for Parameters \")\n\n* `prevProps`: Props before the update. Compare `prevProps` to [`this.props`](https://react.dev/reference/react/Component#props) to determine what changed.\n \n* `prevState`: State before the update. Compare `prevState` to [`this.state`](https://react.dev/reference/react/Component#state) to determine what changed.\n \n\n#### Returns[](https://react.dev/reference/react/Component#getsnapshotbeforeupdate-returns \"Link for Returns \")\n\nYou should return a snapshot value of any type that you’d like, or `null`. The value you returned will be passed as the third argument to [`componentDidUpdate`.](https://react.dev/reference/react/Component#componentdidupdate)\n\n#### Caveats[](https://react.dev/reference/react/Component#getsnapshotbeforeupdate-caveats \"Link for Caveats \")\n\n* `getSnapshotBeforeUpdate` will not get called if [`shouldComponentUpdate`](https://react.dev/reference/react/Component#shouldcomponentupdate) is defined and returns `false`.\n\n### Note\n\nAt the moment, there is no equivalent to `getSnapshotBeforeUpdate` for function components. This use case is very uncommon, but if you have the need for it, for now you’ll have to write a class component.\n\n* * *\n\n### `render()`[](https://react.dev/reference/react/Component#render \"Link for this heading\")\n\nThe `render` method is the only required method in a class component.\n\nThe `render` method should specify what you want to appear on the screen, for example:\n\n```\nimport { Component } from 'react';class Greeting extends Component {render() {return <h1>Hello, {this.props.name}!</h1>;}}\n```\n\nReact may call `render` at any moment, so you shouldn’t assume that it runs at a particular time. Usually, the `render` method should return a piece of [JSX](https://react.dev/learn/writing-markup-with-jsx), but a few [other return types](https://react.dev/reference/react/Component#render-returns) (like strings) are supported. To calculate the returned JSX, the `render` method can read [`this.props`](https://react.dev/reference/react/Component#props), [`this.state`](https://react.dev/reference/react/Component#state), and [`this.context`](https://react.dev/reference/react/Component#context).\n\nYou should write the `render` method as a pure function, meaning that it should return the same result if props, state, and context are the same. It also shouldn’t contain side effects (like setting up subscriptions) or interact with the browser APIs. Side effects should happen either in event handlers or methods like [`componentDidMount`.](https://react.dev/reference/react/Component#componentdidmount)\n\n#### Parameters[](https://react.dev/reference/react/Component#render-parameters \"Link for Parameters \")\n\n`render` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react/Component#render-returns \"Link for Returns \")\n\n`render` can return any valid React node. This includes React elements such as `<div />`, strings, numbers, [portals](https://react.dev/reference/react-dom/createPortal), empty nodes (`null`, `undefined`, `true`, and `false`), and arrays of React nodes.\n\n#### Caveats[](https://react.dev/reference/react/Component#render-caveats \"Link for Caveats \")\n\n* `render` should be written as a pure function of props, state, and context. It should not have side effects.\n \n* `render` will not get called if [`shouldComponentUpdate`](https://react.dev/reference/react/Component#shouldcomponentupdate) is defined and returns `false`.\n \n* When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React will call `render` twice in development and then throw away one of the results. This helps you notice the accidental side effects that need to be moved out of the `render` method.\n \n* There is no one-to-one correspondence between the `render` call and the subsequent `componentDidMount` or `componentDidUpdate` call. Some of the `render` call results may be discarded by React when it’s beneficial.\n \n\n* * *\n\n### `setState(nextState, callback?)`[](https://react.dev/reference/react/Component#setstate \"Link for this heading\")\n\nCall `setState` to update the state of your React component.\n\n```\nclass Form extends Component {state = {name: 'Taylor',};handleNameChange = (e) => {const newName = e.target.value;this.setState({name: newName});}render() {return (<><input value={this.state.name} onChange={this.handleNameChange} /><p>Hello, {this.state.name}.</p></>);}}\n```\n\n`setState` enqueues changes to the component state. It tells React that this component and its children need to re-render with the new state. This is the main way you’ll update the user interface in response to interactions.\n\n### Pitfall\n\nCalling `setState` **does not** change the current state in the already executing code:\n\n```\nfunction handleClick() {console.log(this.state.name); // \"Taylor\"this.setState({name: 'Robin'});console.log(this.state.name); // Still \"Taylor\"!}\n```\n\nIt only affects what `this.state` will return starting from the _next_ render.\n\nYou can also pass a function to `setState`. It lets you update state based on the previous state:\n\n```\nhandleIncreaseAge = () => {this.setState(prevState => {return {age: prevState.age + 1};});}\n```\n\nYou don’t have to do this, but it’s handy if you want to update state multiple times during the same event.\n\n#### Parameters[](https://react.dev/reference/react/Component#setstate-parameters \"Link for Parameters \")\n\n* `nextState`: Either an object or a function.\n \n * If you pass an object as `nextState`, it will be shallowly merged into `this.state`.\n * If you pass a function as `nextState`, it will be treated as an _updater function_. It must be pure, should take the pending state and props as arguments, and should return the object to be shallowly merged into `this.state`. React will put your updater function in a queue and re-render your component. During the next render, React will calculate the next state by applying all of the queued updaters to the previous state.\n* **optional** `callback`: If specified, React will call the `callback` you’ve provided after the update is committed.\n \n\n#### Returns[](https://react.dev/reference/react/Component#setstate-returns \"Link for Returns \")\n\n`setState` does not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#setstate-caveats \"Link for Caveats \")\n\n* Think of `setState` as a _request_ rather than an immediate command to update the component. When multiple components update their state in response to an event, React will batch their updates and re-render them together in a single pass at the end of the event. In the rare case that you need to force a particular state update to be applied synchronously, you may wrap it in [`flushSync`,](https://react.dev/reference/react-dom/flushSync) but this may hurt performance.\n \n* `setState` does not update `this.state` immediately. This makes reading `this.state` right after calling `setState` a potential pitfall. Instead, use [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) or the setState `callback` argument, either of which are guaranteed to fire after the update has been applied. If you need to set the state based on the previous state, you can pass a function to `nextState` as described above.\n \n\n### Note\n\nCalling `setState` in class components is similar to calling a [`set` function](https://react.dev/reference/react/useState#setstate) in function components.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-state-from-a-class-to-a-function)\n\n* * *\n\n### `shouldComponentUpdate(nextProps, nextState, nextContext)`[](https://react.dev/reference/react/Component#shouldcomponentupdate \"Link for this heading\")\n\nIf you define `shouldComponentUpdate`, React will call it to determine whether a re-render can be skipped.\n\nIf you are confident you want to write it by hand, you may compare `this.props` with `nextProps` and `this.state` with `nextState` and return `false` to tell React the update can be skipped.\n\n```\nclass Rectangle extends Component {state = {isHovered: false};shouldComponentUpdate(nextProps, nextState) {if (nextProps.position.x === this.props.position.x &&nextProps.position.y === this.props.position.y &&nextProps.size.width === this.props.size.width &&nextProps.size.height === this.props.size.height &&nextState.isHovered === this.state.isHovered) {// Nothing has changed, so a re-render is unnecessaryreturn false;}return true;}// ...}\n```\n\nReact calls `shouldComponentUpdate` before rendering when new props or state are being received. Defaults to `true`. This method is not called for the initial render or when [`forceUpdate`](https://react.dev/reference/react/Component#forceupdate) is used.\n\n#### Parameters[](https://react.dev/reference/react/Component#shouldcomponentupdate-parameters \"Link for Parameters \")\n\n* `nextProps`: The next props that the component is about to render with. Compare `nextProps` to [`this.props`](https://react.dev/reference/react/Component#props) to determine what changed.\n* `nextState`: The next state that the component is about to render with. Compare `nextState` to [`this.state`](https://react.dev/reference/react/Component#props) to determine what changed.\n* `nextContext`: The next context that the component is about to render with. Compare `nextContext` to [`this.context`](https://react.dev/reference/react/Component#context) to determine what changed. Only available if you specify [`static contextType`](https://react.dev/reference/react/Component#static-contexttype) (modern) or [`static contextTypes`](https://react.dev/reference/react/Component#static-contexttypes) (legacy).\n\n#### Returns[](https://react.dev/reference/react/Component#shouldcomponentupdate-returns \"Link for Returns \")\n\nReturn `true` if you want the component to re-render. That’s the default behavior.\n\nReturn `false` to tell React that re-rendering can be skipped.\n\n#### Caveats[](https://react.dev/reference/react/Component#shouldcomponentupdate-caveats \"Link for Caveats \")\n\n* This method _only_ exists as a performance optimization. If your component breaks without it, fix that first.\n \n* Consider using [`PureComponent`](https://react.dev/reference/react/PureComponent) instead of writing `shouldComponentUpdate` by hand. `PureComponent` shallowly compares props and state, and reduces the chance that you’ll skip a necessary update.\n \n* We do not recommend doing deep equality checks or using `JSON.stringify` in `shouldComponentUpdate`. It makes performance unpredictable and dependent on the data structure of every prop and state. In the best case, you risk introducing multi-second stalls to your application, and in the worst case you risk crashing it.\n \n* Returning `false` does not prevent child components from re-rendering when _their_ state changes.\n \n* Returning `false` does not _guarantee_ that the component will not re-render. React will use the return value as a hint but it may still choose to re-render your component if it makes sense to do for other reasons.\n \n\n### Note\n\nOptimizing class components with `shouldComponentUpdate` is similar to optimizing function components with [`memo`.](https://react.dev/reference/react/memo) Function components also offer more granular optimization with [`useMemo`.](https://react.dev/reference/react/useMemo)\n\n* * *\n\n### `UNSAFE_componentWillMount()`[](https://react.dev/reference/react/Component#unsafe_componentwillmount \"Link for this heading\")\n\nIf you define `UNSAFE_componentWillMount`, React will call it immediately after the [`constructor`.](https://react.dev/reference/react/Component#constructor) It only exists for historical reasons and should not be used in any new code. Instead, use one of the alternatives:\n\n* To initialize state, declare [`state`](https://react.dev/reference/react/Component#state) as a class field or set `this.state` inside the [`constructor`.](https://react.dev/reference/react/Component#constructor)\n* If you need to run a side effect or set up a subscription, move that logic to [`componentDidMount`](https://react.dev/reference/react/Component#componentdidmount) instead.\n\n[See examples of migrating away from unsafe lifecycles.](https://legacy.reactjs.org/blog/2018/03/27/update-on-async-rendering.html#examples)\n\n#### Parameters[](https://react.dev/reference/react/Component#unsafe_componentwillmount-parameters \"Link for Parameters \")\n\n`UNSAFE_componentWillMount` does not take any parameters.\n\n#### Returns[](https://react.dev/reference/react/Component#unsafe_componentwillmount-returns \"Link for Returns \")\n\n`UNSAFE_componentWillMount` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#unsafe_componentwillmount-caveats \"Link for Caveats \")\n\n* `UNSAFE_componentWillMount` will not get called if the component implements [`static getDerivedStateFromProps`](https://react.dev/reference/react/Component#static-getderivedstatefromprops) or [`getSnapshotBeforeUpdate`.](https://react.dev/reference/react/Component#getsnapshotbeforeupdate)\n \n* Despite its naming, `UNSAFE_componentWillMount` does not guarantee that the component _will_ get mounted if your app uses modern React features like [`Suspense`.](https://react.dev/reference/react/Suspense) If a render attempt is suspended (for example, because the code for some child component has not loaded yet), React will throw the in-progress tree away and attempt to construct the component from scratch during the next attempt. This is why this method is “unsafe”. Code that relies on mounting (like adding a subscription) should go into [`componentDidMount`.](https://react.dev/reference/react/Component#componentdidmount)\n \n* `UNSAFE_componentWillMount` is the only lifecycle method that runs during [server rendering.](https://react.dev/reference/react-dom/server) For all practical purposes, it is identical to [`constructor`,](https://react.dev/reference/react/Component#constructor) so you should use the `constructor` for this type of logic instead.\n \n\n### Note\n\nCalling [`setState`](https://react.dev/reference/react/Component#setstate) inside `UNSAFE_componentWillMount` in a class component to initialize state is equivalent to passing that state as the initial state to [`useState`](https://react.dev/reference/react/useState) in a function component.\n\n* * *\n\n### `UNSAFE_componentWillReceiveProps(nextProps, nextContext)`[](https://react.dev/reference/react/Component#unsafe_componentwillreceiveprops \"Link for this heading\")\n\nIf you define `UNSAFE_componentWillReceiveProps`, React will call it when the component receives new props. It only exists for historical reasons and should not be used in any new code. Instead, use one of the alternatives:\n\n* If you need to **run a side effect** (for example, fetch data, run an animation, or reinitialize a subscription) in response to prop changes, move that logic to [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) instead.\n* If you need to **avoid re-computing some data only when a prop changes,** use a [memoization helper](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html#what-about-memoization) instead.\n* If you need to **“reset” some state when a prop changes,** consider either making a component [fully controlled](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html#recommendation-fully-controlled-component) or [fully uncontrolled with a key](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html#recommendation-fully-uncontrolled-component-with-a-key) instead.\n* If you need to **“adjust” some state when a prop changes,** check whether you can compute all the necessary information from props alone during rendering. If you can’t, use [`static getDerivedStateFromProps`](https://react.dev/reference/react/Component#static-getderivedstatefromprops) instead.\n\n[See examples of migrating away from unsafe lifecycles.](https://legacy.reactjs.org/blog/2018/03/27/update-on-async-rendering.html#updating-state-based-on-props)\n\n#### Parameters[](https://react.dev/reference/react/Component#unsafe_componentwillreceiveprops-parameters \"Link for Parameters \")\n\n* `nextProps`: The next props that the component is about to receive from its parent component. Compare `nextProps` to [`this.props`](https://react.dev/reference/react/Component#props) to determine what changed.\n* `nextContext`: The next context that the component is about to receive from the closest provider. Compare `nextContext` to [`this.context`](https://react.dev/reference/react/Component#context) to determine what changed. Only available if you specify [`static contextType`](https://react.dev/reference/react/Component#static-contexttype) (modern) or [`static contextTypes`](https://react.dev/reference/react/Component#static-contexttypes) (legacy).\n\n#### Returns[](https://react.dev/reference/react/Component#unsafe_componentwillreceiveprops-returns \"Link for Returns \")\n\n`UNSAFE_componentWillReceiveProps` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#unsafe_componentwillreceiveprops-caveats \"Link for Caveats \")\n\n* `UNSAFE_componentWillReceiveProps` will not get called if the component implements [`static getDerivedStateFromProps`](https://react.dev/reference/react/Component#static-getderivedstatefromprops) or [`getSnapshotBeforeUpdate`.](https://react.dev/reference/react/Component#getsnapshotbeforeupdate)\n \n* Despite its naming, `UNSAFE_componentWillReceiveProps` does not guarantee that the component _will_ receive those props if your app uses modern React features like [`Suspense`.](https://react.dev/reference/react/Suspense) If a render attempt is suspended (for example, because the code for some child component has not loaded yet), React will throw the in-progress tree away and attempt to construct the component from scratch during the next attempt. By the time of the next render attempt, the props might be different. This is why this method is “unsafe”. Code that should run only for committed updates (like resetting a subscription) should go into [`componentDidUpdate`.](https://react.dev/reference/react/Component#componentdidupdate)\n \n* `UNSAFE_componentWillReceiveProps` does not mean that the component has received _different_ props than the last time. You need to compare `nextProps` and `this.props` yourself to check if something changed.\n \n* React doesn’t call `UNSAFE_componentWillReceiveProps` with initial props during mounting. It only calls this method if some of component’s props are going to be updated. For example, calling [`setState`](https://react.dev/reference/react/Component#setstate) doesn’t generally trigger `UNSAFE_componentWillReceiveProps` inside the same component.\n \n\n### Note\n\n* * *\n\n### `UNSAFE_componentWillUpdate(nextProps, nextState)`[](https://react.dev/reference/react/Component#unsafe_componentwillupdate \"Link for this heading\")\n\nIf you define `UNSAFE_componentWillUpdate`, React will call it before rendering with the new props or state. It only exists for historical reasons and should not be used in any new code. Instead, use one of the alternatives:\n\n* If you need to run a side effect (for example, fetch data, run an animation, or reinitialize a subscription) in response to prop or state changes, move that logic to [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) instead.\n* If you need to read some information from the DOM (for example, to save the current scroll position) so that you can use it in [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) later, read it inside [`getSnapshotBeforeUpdate`](https://react.dev/reference/react/Component#getsnapshotbeforeupdate) instead.\n\n[See examples of migrating away from unsafe lifecycles.](https://legacy.reactjs.org/blog/2018/03/27/update-on-async-rendering.html#examples)\n\n#### Parameters[](https://react.dev/reference/react/Component#unsafe_componentwillupdate-parameters \"Link for Parameters \")\n\n* `nextProps`: The next props that the component is about to render with. Compare `nextProps` to [`this.props`](https://react.dev/reference/react/Component#props) to determine what changed.\n* `nextState`: The next state that the component is about to render with. Compare `nextState` to [`this.state`](https://react.dev/reference/react/Component#state) to determine what changed.\n\n#### Returns[](https://react.dev/reference/react/Component#unsafe_componentwillupdate-returns \"Link for Returns \")\n\n`UNSAFE_componentWillUpdate` should not return anything.\n\n#### Caveats[](https://react.dev/reference/react/Component#unsafe_componentwillupdate-caveats \"Link for Caveats \")\n\n* `UNSAFE_componentWillUpdate` will not get called if [`shouldComponentUpdate`](https://react.dev/reference/react/Component#shouldcomponentupdate) is defined and returns `false`.\n \n* `UNSAFE_componentWillUpdate` will not get called if the component implements [`static getDerivedStateFromProps`](https://react.dev/reference/react/Component#static-getderivedstatefromprops) or [`getSnapshotBeforeUpdate`.](https://react.dev/reference/react/Component#getsnapshotbeforeupdate)\n \n* It’s not supported to call [`setState`](https://react.dev/reference/react/Component#setstate) (or any method that leads to `setState` being called, like dispatching a Redux action) during `componentWillUpdate`.\n \n* Despite its naming, `UNSAFE_componentWillUpdate` does not guarantee that the component _will_ update if your app uses modern React features like [`Suspense`.](https://react.dev/reference/react/Suspense) If a render attempt is suspended (for example, because the code for some child component has not loaded yet), React will throw the in-progress tree away and attempt to construct the component from scratch during the next attempt. By the time of the next render attempt, the props and state might be different. This is why this method is “unsafe”. Code that should run only for committed updates (like resetting a subscription) should go into [`componentDidUpdate`.](https://react.dev/reference/react/Component#componentdidupdate)\n \n* `UNSAFE_componentWillUpdate` does not mean that the component has received _different_ props or state than the last time. You need to compare `nextProps` with `this.props` and `nextState` with `this.state` yourself to check if something changed.\n \n* React doesn’t call `UNSAFE_componentWillUpdate` with initial props and state during mounting.\n \n\n### Note\n\nThere is no direct equivalent to `UNSAFE_componentWillUpdate` in function components.\n\n* * *\n\n### `static childContextTypes`[](https://react.dev/reference/react/Component#static-childcontexttypes \"Link for this heading\")\n\n### Deprecated\n\nThis API will be removed in a future major version of React. [Use `static contextType` instead.](https://react.dev/reference/react/Component#static-contexttype)\n\nLets you specify which [legacy context](https://reactjs.org/docs/legacy-context.html) is provided by this component.\n\n* * *\n\n### `static contextTypes`[](https://react.dev/reference/react/Component#static-contexttypes \"Link for this heading\")\n\n### Deprecated\n\nThis API will be removed in a future major version of React. [Use `static contextType` instead.](https://react.dev/reference/react/Component#static-contexttype)\n\nLets you specify which [legacy context](https://reactjs.org/docs/legacy-context.html) is consumed by this component.\n\n* * *\n\n### `static contextType`[](https://react.dev/reference/react/Component#static-contexttype \"Link for this heading\")\n\nIf you want to read [`this.context`](https://react.dev/reference/react/Component#context-instance-field) from your class component, you must specify which context it needs to read. The context you specify as the `static contextType` must be a value previously created by [`createContext`.](https://react.dev/reference/react/createContext)\n\n```\nclass Button extends Component {static contextType = ThemeContext;render() {const theme = this.context;const className = 'button-' + theme;return (<button className={className}>{this.props.children}</button>);}}\n```\n\n### Note\n\nReading `this.context` in class components is equivalent to [`useContext`](https://react.dev/reference/react/useContext) in function components.\n\n[See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-context-from-a-class-to-a-function)\n\n* * *\n\n### `static defaultProps`[](https://react.dev/reference/react/Component#static-defaultprops \"Link for this heading\")\n\nYou can define `static defaultProps` to set the default props for the class. They will be used for `undefined` and missing props, but not for `null` props.\n\nFor example, here is how you define that the `color` prop should default to `'blue'`:\n\n```\nclass Button extends Component {static defaultProps = {color: 'blue'};render() {return <button className={this.props.color}>click me</button>;}}\n```\n\nIf the `color` prop is not provided or is `undefined`, it will be set by default to `'blue'`:\n\n```\n<>{/* this.props.color is \"blue\" */}<Button />{/* this.props.color is \"blue\" */}<Button color={undefined} />{/* this.props.color is null */}<Button color={null} />{/* this.props.color is \"red\" */}<Button color=\"red\" /></>\n```\n\n### Note\n\nDefining `defaultProps` in class components is similar to using [default values](https://react.dev/learn/passing-props-to-a-component#specifying-a-default-value-for-a-prop) in function components.\n\n* * *\n\n### `static propTypes`[](https://react.dev/reference/react/Component#static-proptypes \"Link for this heading\")\n\nYou can define `static propTypes` together with the [`prop-types`](https://www.npmjs.com/package/prop-types) library to declare the types of the props accepted by your component. These types will be checked during rendering and in development only.\n\n```\nimport PropTypes from 'prop-types';class Greeting extends React.Component {static propTypes = {name: PropTypes.string};render() {return (<h1>Hello, {this.props.name}</h1>);}}\n```\n\n### Note\n\nWe recommend using [TypeScript](https://www.typescriptlang.org/) instead of checking prop types at runtime.\n\n* * *\n\n### `static getDerivedStateFromError(error)`[](https://react.dev/reference/react/Component#static-getderivedstatefromerror \"Link for this heading\")\n\nIf you define `static getDerivedStateFromError`, React will call it when a child component (including distant children) throws an error during rendering. This lets you display an error message instead of clearing the UI.\n\nTypically, it is used together with [`componentDidCatch`](https://react.dev/reference/react/Component#componentdidcatch) which lets you send the error report to some analytics service. A component with these methods is called an _error boundary._\n\n[See an example.](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary)\n\n#### Parameters[](https://react.dev/reference/react/Component#static-getderivedstatefromerror-parameters \"Link for Parameters \")\n\n* `error`: The error that was thrown. In practice, it will usually be an instance of [`Error`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Error) but this is not guaranteed because JavaScript allows to [`throw`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/throw) any value, including strings or even `null`.\n\n#### Returns[](https://react.dev/reference/react/Component#static-getderivedstatefromerror-returns \"Link for Returns \")\n\n`static getDerivedStateFromError` should return the state telling the component to display the error message.\n\n#### Caveats[](https://react.dev/reference/react/Component#static-getderivedstatefromerror-caveats \"Link for Caveats \")\n\n* `static getDerivedStateFromError` should be a pure function. If you want to perform a side effect (for example, to call an analytics service), you need to also implement [`componentDidCatch`.](https://react.dev/reference/react/Component#componentdidcatch)\n\n### Note\n\nThere is no direct equivalent for `static getDerivedStateFromError` in function components yet. If you’d like to avoid creating class components, write a single `ErrorBoundary` component like above and use it throughout your app. Alternatively, use the [`react-error-boundary`](https://github.com/bvaughn/react-error-boundary) package which does that.\n\n* * *\n\n### `static getDerivedStateFromProps(props, state)`[](https://react.dev/reference/react/Component#static-getderivedstatefromprops \"Link for this heading\")\n\nIf you define `static getDerivedStateFromProps`, React will call it right before calling [`render`,](https://react.dev/reference/react/Component#render) both on the initial mount and on subsequent updates. It should return an object to update the state, or `null` to update nothing.\n\nThis method exists for [rare use cases](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html#when-to-use-derived-state) where the state depends on changes in props over time. For example, this `Form` component resets the `email` state when the `userID` prop changes:\n\n```\nclass Form extends Component {state = {email: this.props.defaultEmail,prevUserID: this.props.userID};static getDerivedStateFromProps(props, state) {// Any time the current user changes,// Reset any parts of state that are tied to that user.// In this simple example, that's just the email.if (props.userID !== state.prevUserID) {return {prevUserID: props.userID,email: props.defaultEmail};}return null;}// ...}\n```\n\nNote that this pattern requires you to keep a previous value of the prop (like `userID`) in state (like `prevUserID`).\n\n### Pitfall\n\n#### Parameters[](https://react.dev/reference/react/Component#static-getderivedstatefromprops-parameters \"Link for Parameters \")\n\n* `props`: The next props that the component is about to render with.\n* `state`: The next state that the component is about to render with.\n\n#### Returns[](https://react.dev/reference/react/Component#static-getderivedstatefromprops-returns \"Link for Returns \")\n\n`static getDerivedStateFromProps` return an object to update the state, or `null` to update nothing.\n\n#### Caveats[](https://react.dev/reference/react/Component#static-getderivedstatefromprops-caveats \"Link for Caveats \")\n\n* This method is fired on _every_ render, regardless of the cause. This is different from [`UNSAFE_componentWillReceiveProps`](https://react.dev/reference/react/Component#unsafe_cmoponentwillreceiveprops), which only fires when the parent causes a re-render and not as a result of a local `setState`.\n \n* This method doesn’t have access to the component instance. If you’d like, you can reuse some code between `static getDerivedStateFromProps` and the other class methods by extracting pure functions of the component props and state outside the class definition.\n \n\n### Note\n\n* * *\n\nUsage[](https://react.dev/reference/react/Component#usage \"Link for Usage \")\n----------------------------------------------------------------------------\n\n### Defining a class component[](https://react.dev/reference/react/Component#defining-a-class-component \"Link for Defining a class component \")\n\nTo define a React component as a class, extend the built-in `Component` class and define a [`render` method:](https://react.dev/reference/react/Component#render)\n\n```\nimport { Component } from 'react';class Greeting extends Component {render() {return <h1>Hello, {this.props.name}!</h1>;}}\n```\n\nReact will call your [`render`](https://react.dev/reference/react/Component#render) method whenever it needs to figure out what to display on the screen. Usually, you will return some [JSX](https://react.dev/learn/writing-markup-with-jsx) from it. Your `render` method should be a [pure function:](https://en.wikipedia.org/wiki/Pure_function) it should only calculate the JSX.\n\nSimilarly to [function components,](https://react.dev/learn/your-first-component#defining-a-component) a class component can [receive information by props](https://react.dev/learn/your-first-component#defining-a-component) from its parent component. However, the syntax for reading props is different. For example, if the parent component renders `<Greeting name=\"Taylor\" />`, then you can read the `name` prop from [`this.props`](https://react.dev/reference/react/Component#props), like `this.props.name`:\n\nNote that Hooks (functions starting with `use`, like [`useState`](https://react.dev/reference/react/useState)) are not supported inside class components.\n\n### Pitfall\n\nWe recommend defining components as functions instead of classes. [See how to migrate.](https://react.dev/reference/react/Component#migrating-a-simple-component-from-a-class-to-a-function)\n\n* * *\n\n### Adding state to a class component[](https://react.dev/reference/react/Component#adding-state-to-a-class-component \"Link for Adding state to a class component \")\n\nTo add [state](https://react.dev/learn/state-a-components-memory) to a class, assign an object to a property called [`state`](https://react.dev/reference/react/Component#state). To update state, call [`this.setState`](https://react.dev/reference/react/Component#setstate).\n\n### Pitfall\n\nWe recommend defining components as functions instead of classes. [See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-state-from-a-class-to-a-function)\n\n* * *\n\n### Adding lifecycle methods to a class component[](https://react.dev/reference/react/Component#adding-lifecycle-methods-to-a-class-component \"Link for Adding lifecycle methods to a class component \")\n\nThere are a few special methods you can define on your class.\n\nIf you define the [`componentDidMount`](https://react.dev/reference/react/Component#componentdidmount) method, React will call it when your component is added _(mounted)_ to the screen. React will call [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) after your component re-renders due to changed props or state. React will call [`componentWillUnmount`](https://react.dev/reference/react/Component#componentwillunmount) after your component has been removed _(unmounted)_ from the screen.\n\nIf you implement `componentDidMount`, you usually need to implement all three lifecycles to avoid bugs. For example, if `componentDidMount` reads some state or props, you also have to implement `componentDidUpdate` to handle their changes, and `componentWillUnmount` to clean up whatever `componentDidMount` was doing.\n\nFor example, this `ChatRoom` component keeps a chat connection synchronized with props and state:\n\nNote that in development when [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React will call `componentDidMount`, immediately call `componentWillUnmount`, and then call `componentDidMount` again. This helps you notice if you forgot to implement `componentWillUnmount` or if its logic doesn’t fully “mirror” what `componentDidMount` does.\n\n### Pitfall\n\nWe recommend defining components as functions instead of classes. [See how to migrate.](https://react.dev/reference/react/Component#migrating-a-component-with-lifecycle-methods-from-a-class-to-a-function)\n\n* * *\n\n### Catching rendering errors with an error boundary[](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary \"Link for Catching rendering errors with an error boundary \")\n\nBy default, if your application throws an error during rendering, React will remove its UI from the screen. To prevent this, you can wrap a part of your UI into an _error boundary_. An error boundary is a special component that lets you display some fallback UI instead of the part that crashed—for example, an error message.\n\nTo implement an error boundary component, you need to provide [`static getDerivedStateFromError`](https://react.dev/reference/react/Component#static-getderivedstatefromerror) which lets you update state in response to an error and display an error message to the user. You can also optionally implement [`componentDidCatch`](https://react.dev/reference/react/Component#componentdidcatch) to add some extra logic, for example, to log the error to an analytics service.\n\n```\nclass ErrorBoundary extends React.Component {constructor(props) {super(props);this.state = { hasError: false };}static getDerivedStateFromError(error) {// Update state so the next render will show the fallback UI.return { hasError: true };}componentDidCatch(error, info) {// Example \"componentStack\":// in ComponentThatThrows (created by App)// in ErrorBoundary (created by App)// in div (created by App)// in ApplogErrorToMyService(error, info.componentStack);}render() {if (this.state.hasError) {// You can render any custom fallback UIreturn this.props.fallback;}return this.props.children;}}\n```\n\nThen you can wrap a part of your component tree with it:\n\n```\n<ErrorBoundary fallback={<p>Something went wrong</p>}><Profile /></ErrorBoundary>\n```\n\nIf `Profile` or its child component throws an error, `ErrorBoundary` will “catch” that error, display a fallback UI with the error message you’ve provided, and send a production error report to your error reporting service.\n\nYou don’t need to wrap every component into a separate error boundary. When you think about the [granularity of error boundaries,](https://www.brandondail.com/posts/fault-tolerance-react) consider where it makes sense to display an error message. For example, in a messaging app, it makes sense to place an error boundary around the list of conversations. It also makes sense to place one around every individual message. However, it wouldn’t make sense to place a boundary around every avatar.\n\n### Note\n\nThere is currently no way to write an error boundary as a function component. However, you don’t have to write the error boundary class yourself. For example, you can use [`react-error-boundary`](https://github.com/bvaughn/react-error-boundary) instead.\n\n* * *\n\nAlternatives[](https://react.dev/reference/react/Component#alternatives \"Link for Alternatives \")\n-------------------------------------------------------------------------------------------------\n\n### Migrating a simple component from a class to a function[](https://react.dev/reference/react/Component#migrating-a-simple-component-from-a-class-to-a-function \"Link for Migrating a simple component from a class to a function \")\n\nTypically, you will [define components as functions](https://react.dev/learn/your-first-component#defining-a-component) instead.\n\nFor example, suppose you’re converting this `Greeting` class component to a function:\n\nDefine a function called `Greeting`. This is where you will move the body of your `render` function.\n\n```\nfunction Greeting() {// ... move the code from the render method here ...}\n```\n\nInstead of `this.props.name`, define the `name` prop [using the destructuring syntax](https://react.dev/learn/passing-props-to-a-component) and read it directly:\n\n```\nfunction Greeting({ name }) {return <h1>Hello, {name}!</h1>;}\n```\n\nHere is a complete example:\n\n* * *\n\n### Migrating a component with state from a class to a function[](https://react.dev/reference/react/Component#migrating-a-component-with-state-from-a-class-to-a-function \"Link for Migrating a component with state from a class to a function \")\n\nSuppose you’re converting this `Counter` class component to a function:\n\nStart by declaring a function with the necessary [state variables:](https://react.dev/reference/react/useState#adding-state-to-a-component)\n\n```\nimport { useState } from 'react';function Counter() {const [name, setName] = useState('Taylor');const [age, setAge] = useState(42);// ...\n```\n\nNext, convert the event handlers:\n\n```\nfunction Counter() {const [name, setName] = useState('Taylor');const [age, setAge] = useState(42);function handleNameChange(e) {setName(e.target.value);}function handleAgeChange() {setAge(age + 1);}// ...\n```\n\nFinally, replace all references starting with `this` with the variables and functions you defined in your component. For example, replace `this.state.age` with `age`, and replace `this.handleNameChange` with `handleNameChange`.\n\nHere is a fully converted component:\n\n* * *\n\n### Migrating a component with lifecycle methods from a class to a function[](https://react.dev/reference/react/Component#migrating-a-component-with-lifecycle-methods-from-a-class-to-a-function \"Link for Migrating a component with lifecycle methods from a class to a function \")\n\nSuppose you’re converting this `ChatRoom` class component with lifecycle methods to a function:\n\nFirst, verify that your [`componentWillUnmount`](https://react.dev/reference/react/Component#componentwillunmount) does the opposite of [`componentDidMount`.](https://react.dev/reference/react/Component#componentdidmount) In the above example, that’s true: it disconnects the connection that `componentDidMount` sets up. If such logic is missing, add it first.\n\nNext, verify that your [`componentDidUpdate`](https://react.dev/reference/react/Component#componentdidupdate) method handles changes to any props and state you’re using in `componentDidMount`. In the above example, `componentDidMount` calls `setupConnection` which reads `this.state.serverUrl` and `this.props.roomId`. This is why `componentDidUpdate` checks whether `this.state.serverUrl` and `this.props.roomId` have changed, and resets the connection if they did. If your `componentDidUpdate` logic is missing or doesn’t handle changes to all relevant props and state, fix that first.\n\nIn the above example, the logic inside the lifecycle methods connects the component to a system outside of React (a chat server). To connect a component to an external system, [describe this logic as a single Effect:](https://react.dev/reference/react/useEffect#connecting-to-an-external-system)\n\n```\nimport { useState, useEffect } from 'react';function ChatRoom({ roomId }) {const [serverUrl, setServerUrl] = useState('https://localhost:1234');useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [serverUrl, roomId]);// ...}\n```\n\nThis [`useEffect`](https://react.dev/reference/react/useEffect) call is equivalent to the logic in the lifecycle methods above. If your lifecycle methods do multiple unrelated things, [split them into multiple independent Effects.](https://react.dev/learn/removing-effect-dependencies#is-your-effect-doing-several-unrelated-things) Here is a complete example you can play with:\n\n### Note\n\n* * *\n\n### Migrating a component with context from a class to a function[](https://react.dev/reference/react/Component#migrating-a-component-with-context-from-a-class-to-a-function \"Link for Migrating a component with context from a class to a function \")\n\nIn this example, the `Panel` and `Button` class components read [context](https://react.dev/learn/passing-data-deeply-with-context) from [`this.context`:](https://react.dev/reference/react/Component#context)\n\nWhen you convert them to function components, replace `this.context` with [`useContext`](https://react.dev/reference/react/useContext) calls:\n",
"filename": "Component.md",
"package": "react"
} |
{
"content": "Title: <Fragment> (<>...</>) – React\n\nURL Source: https://react.dev/reference/react/Fragment\n\nMarkdown Content:\n`<Fragment>`, often used via `<>...</>` syntax, lets you group elements without a wrapper node.\n\n```\n<><OneChild /><AnotherChild /></>\n```\n\n* [Reference](https://react.dev/reference/react/Fragment#reference)\n * [`<Fragment>`](https://react.dev/reference/react/Fragment#fragment)\n* [Usage](https://react.dev/reference/react/Fragment#usage)\n * [Returning multiple elements](https://react.dev/reference/react/Fragment#returning-multiple-elements)\n * [Assigning multiple elements to a variable](https://react.dev/reference/react/Fragment#assigning-multiple-elements-to-a-variable)\n * [Grouping elements with text](https://react.dev/reference/react/Fragment#grouping-elements-with-text)\n * [Rendering a list of Fragments](https://react.dev/reference/react/Fragment#rendering-a-list-of-fragments)\n\n* * *\n\nReference[](https://react.dev/reference/react/Fragment#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `<Fragment>`[](https://react.dev/reference/react/Fragment#fragment \"Link for this heading\")\n\nWrap elements in `<Fragment>` to group them together in situations where you need a single element. Grouping elements in `Fragment` has no effect on the resulting DOM; it is the same as if the elements were not grouped. The empty JSX tag `<></>` is shorthand for `<Fragment></Fragment>` in most cases.\n\n#### Props[](https://react.dev/reference/react/Fragment#props \"Link for Props \")\n\n* **optional** `key`: Fragments declared with the explicit `<Fragment>` syntax may have [keys.](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key)\n\n#### Caveats[](https://react.dev/reference/react/Fragment#caveats \"Link for Caveats \")\n\n* If you want to pass `key` to a Fragment, you can’t use the `<>...</>` syntax. You have to explicitly import `Fragment` from `'react'` and render `<Fragment key={yourKey}>...</Fragment>`.\n \n* React does not [reset state](https://react.dev/learn/preserving-and-resetting-state) when you go from rendering `<><Child /></>` to `[<Child />]` or back, or when you go from rendering `<><Child /></>` to `<Child />` and back. This only works a single level deep: for example, going from `<><><Child /></></>` to `<Child />` resets the state. See the precise semantics [here.](https://gist.github.com/clemmy/b3ef00f9507909429d8aa0d3ee4f986b)\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/Fragment#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Returning multiple elements[](https://react.dev/reference/react/Fragment#returning-multiple-elements \"Link for Returning multiple elements \")\n\nUse `Fragment`, or the equivalent `<>...</>` syntax, to group multiple elements together. You can use it to put multiple elements in any place where a single element can go. For example, a component can only return one element, but by using a Fragment you can group multiple elements together and then return them as a group:\n\n```\nfunction Post() {return (<><PostTitle /><PostBody /></>);}\n```\n\nFragments are useful because grouping elements with a Fragment has no effect on layout or styles, unlike if you wrapped the elements in another container like a DOM element. If you inspect this example with the browser tools, you’ll see that all `<h1>` and `<article>` DOM nodes appear as siblings without wrappers around them:\n\nexport default function Blog() {\n return (\n <\\>\n <Post title\\=\"An update\" body\\=\"It's been a while since I posted...\" />\n <Post title\\=\"My new blog\" body\\=\"I am starting a new blog!\" />\n </\\>\n )\n}\n\nfunction Post({ title, body }) {\n return (\n <\\>\n <PostTitle title\\={title} />\n <PostBody body\\={body} />\n </\\>\n );\n}\n\nfunction PostTitle({ title }) {\n return <h1\\>{title}</h1\\>\n}\n\nfunction PostBody({ body }) {\n return (\n <article\\>\n <p\\>{body}</p\\>\n </article\\>\n );\n}\n\n##### Deep Dive\n\n#### How to write a Fragment without the special syntax?[](https://react.dev/reference/react/Fragment#how-to-write-a-fragment-without-the-special-syntax \"Link for How to write a Fragment without the special syntax? \")\n\nThe example above is equivalent to importing `Fragment` from React:\n\n```\nimport { Fragment } from 'react';function Post() {return (<Fragment><PostTitle /><PostBody /></Fragment>);}\n```\n\nUsually you won’t need this unless you need to [pass a `key` to your `Fragment`.](https://react.dev/reference/react/Fragment#rendering-a-list-of-fragments)\n\n* * *\n\n### Assigning multiple elements to a variable[](https://react.dev/reference/react/Fragment#assigning-multiple-elements-to-a-variable \"Link for Assigning multiple elements to a variable \")\n\nLike any other element, you can assign Fragment elements to variables, pass them as props, and so on:\n\n```\nfunction CloseDialog() {const buttons = (<><OKButton /><CancelButton /></>);return (<AlertDialog buttons={buttons}> Are you sure you want to leave this page?</AlertDialog>);}\n```\n\n* * *\n\n### Grouping elements with text[](https://react.dev/reference/react/Fragment#grouping-elements-with-text \"Link for Grouping elements with text \")\n\nYou can use `Fragment` to group text together with components:\n\n```\nfunction DateRangePicker({ start, end }) {return (<> From<DatePicker date={start} /> to<DatePicker date={end} /></>);}\n```\n\n* * *\n\n### Rendering a list of Fragments[](https://react.dev/reference/react/Fragment#rendering-a-list-of-fragments \"Link for Rendering a list of Fragments \")\n\nHere’s a situation where you need to write `Fragment` explicitly instead of using the `<></>` syntax. When you [render multiple elements in a loop](https://react.dev/learn/rendering-lists), you need to assign a `key` to each element. If the elements within the loop are Fragments, you need to use the normal JSX element syntax in order to provide the `key` attribute:\n\n```\nfunction Blog() {return posts.map(post =><Fragment key={post.id}><PostTitle title={post.title} /><PostBody body={post.body} /></Fragment>);}\n```\n\nYou can inspect the DOM to verify that there are no wrapper elements around the Fragment children:\n\nimport { Fragment } from 'react';\n\nconst posts = \\[\n { id: 1, title: 'An update', body: \"It's been a while since I posted...\" },\n { id: 2, title: 'My new blog', body: 'I am starting a new blog!' }\n\\];\n\nexport default function Blog() {\n return posts.map(post \\=>\n <Fragment key\\={post.id}\\>\n <PostTitle title\\={post.title} />\n <PostBody body\\={post.body} />\n </Fragment\\>\n );\n}\n\nfunction PostTitle({ title }) {\n return <h1\\>{title}</h1\\>\n}\n\nfunction PostBody({ body }) {\n return (\n <article\\>\n <p\\>{body}</p\\>\n </article\\>\n );\n}\n",
"filename": "Fragment.md",
"package": "react"
} |
{
"content": "Title: <Profiler> – React\n\nURL Source: https://react.dev/reference/react/Profiler\n\nMarkdown Content:\n`<Profiler>` lets you measure rendering performance of a React tree programmatically.\n\n```\n<Profiler id=\"App\" onRender={onRender}><App /></Profiler>\n```\n\n* [Reference](https://react.dev/reference/react/Profiler#reference)\n * [`<Profiler>`](https://react.dev/reference/react/Profiler#profiler)\n * [`onRender` callback](https://react.dev/reference/react/Profiler#onrender-callback)\n* [Usage](https://react.dev/reference/react/Profiler#usage)\n * [Measuring rendering performance programmatically](https://react.dev/reference/react/Profiler#measuring-rendering-performance-programmatically)\n * [Measuring different parts of the application](https://react.dev/reference/react/Profiler#measuring-different-parts-of-the-application)\n\n* * *\n\nReference[](https://react.dev/reference/react/Profiler#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `<Profiler>`[](https://react.dev/reference/react/Profiler#profiler \"Link for this heading\")\n\nWrap a component tree in a `<Profiler>` to measure its rendering performance.\n\n```\n<Profiler id=\"App\" onRender={onRender}><App /></Profiler>\n```\n\n#### Props[](https://react.dev/reference/react/Profiler#props \"Link for Props \")\n\n* `id`: A string identifying the part of the UI you are measuring.\n* `onRender`: An [`onRender` callback](https://react.dev/reference/react/Profiler#onrender-callback) that React calls every time components within the profiled tree update. It receives information about what was rendered and how much time it took.\n\n#### Caveats[](https://react.dev/reference/react/Profiler#caveats \"Link for Caveats \")\n\n* Profiling adds some additional overhead, so **it is disabled in the production build by default.** To opt into production profiling, you need to enable a [special production build with profiling enabled.](https://fb.me/react-profiling)\n\n* * *\n\n### `onRender` callback[](https://react.dev/reference/react/Profiler#onrender-callback \"Link for this heading\")\n\nReact will call your `onRender` callback with information about what was rendered.\n\n```\nfunction onRender(id, phase, actualDuration, baseDuration, startTime, commitTime) {// Aggregate or log render timings...}\n```\n\n#### Parameters[](https://react.dev/reference/react/Profiler#onrender-parameters \"Link for Parameters \")\n\n* `id`: The string `id` prop of the `<Profiler>` tree that has just committed. This lets you identify which part of the tree was committed if you are using multiple profilers.\n* `phase`: `\"mount\"`, `\"update\"` or `\"nested-update\"`. This lets you know whether the tree has just been mounted for the first time or re-rendered due to a change in props, state, or Hooks.\n* `actualDuration`: The number of milliseconds spent rendering the `<Profiler>` and its descendants for the current update. This indicates how well the subtree makes use of memoization (e.g. [`memo`](https://react.dev/reference/react/memo) and [`useMemo`](https://react.dev/reference/react/useMemo)). Ideally this value should decrease significantly after the initial mount as many of the descendants will only need to re-render if their specific props change.\n* `baseDuration`: The number of milliseconds estimating how much time it would take to re-render the entire `<Profiler>` subtree without any optimizations. It is calculated by summing up the most recent render durations of each component in the tree. This value estimates a worst-case cost of rendering (e.g. the initial mount or a tree with no memoization). Compare `actualDuration` against it to see if memoization is working.\n* `startTime`: A numeric timestamp for when React began rendering the current update.\n* `commitTime`: A numeric timestamp for when React committed the current update. This value is shared between all profilers in a commit, enabling them to be grouped if desirable.\n\n* * *\n\nUsage[](https://react.dev/reference/react/Profiler#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Measuring rendering performance programmatically[](https://react.dev/reference/react/Profiler#measuring-rendering-performance-programmatically \"Link for Measuring rendering performance programmatically \")\n\nWrap the `<Profiler>` component around a React tree to measure its rendering performance.\n\n```\n<App><Profiler id=\"Sidebar\" onRender={onRender}><Sidebar /></Profiler><PageContent /></App>\n```\n\nIt requires two props: an `id` (string) and an `onRender` callback (function) which React calls any time a component within the tree “commits” an update.\n\n### Pitfall\n\n### Note\n\n`<Profiler>` lets you gather measurements programmatically. If you’re looking for an interactive profiler, try the Profiler tab in [React Developer Tools](https://react.dev/learn/react-developer-tools). It exposes similar functionality as a browser extension.\n\n* * *\n\n### Measuring different parts of the application[](https://react.dev/reference/react/Profiler#measuring-different-parts-of-the-application \"Link for Measuring different parts of the application \")\n\nYou can use multiple `<Profiler>` components to measure different parts of your application:\n\n```\n<App><Profiler id=\"Sidebar\" onRender={onRender}><Sidebar /></Profiler><Profiler id=\"Content\" onRender={onRender}><Content /></Profiler></App>\n```\n\nYou can also nest `<Profiler>` components:\n\n```\n<App><Profiler id=\"Sidebar\" onRender={onRender}><Sidebar /></Profiler><Profiler id=\"Content\" onRender={onRender}><Content><Profiler id=\"Editor\" onRender={onRender}><Editor /></Profiler><Preview /></Content></Profiler></App>\n```\n\nAlthough `<Profiler>` is a lightweight component, it should be used only when necessary. Each use adds some CPU and memory overhead to an application.\n\n* * *\n",
"filename": "Profiler.md",
"package": "react"
} |
{
"content": "Title: PureComponent – React\n\nURL Source: https://react.dev/reference/react/PureComponent\n\nMarkdown Content:\nPureComponent – React\n===============\n\nPureComponent[](https://react.dev/reference/react/PureComponent#undefined \"Link for this heading\")\n==================================================================================================\n\n### Pitfall\n\nWe recommend defining components as functions instead of classes. [See how to migrate.](https://react.dev/reference/react/PureComponent#alternatives)\n\n`PureComponent` is similar to [`Component`](https://react.dev/reference/react/Component) but it skips re-renders for same props and state. Class components are still supported by React, but we don’t recommend using them in new code.\n\n```\nclass Greeting extends PureComponent { render() { return <h1>Hello, {this.props.name}!</h1>; }}\n```\n\n* [Reference](https://react.dev/reference/react/PureComponent#reference)\n * [`PureComponent`](https://react.dev/reference/react/PureComponent#purecomponent)\n* [Usage](https://react.dev/reference/react/PureComponent#usage)\n * [Skipping unnecessary re-renders for class components](https://react.dev/reference/react/PureComponent#skipping-unnecessary-re-renders-for-class-components)\n* [Alternatives](https://react.dev/reference/react/PureComponent#alternatives)\n * [Migrating from a `PureComponent` class component to a function](https://react.dev/reference/react/PureComponent#migrating-from-a-purecomponent-class-component-to-a-function)\n\n* * *\n\nReference[](https://react.dev/reference/react/PureComponent#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `PureComponent`[](https://react.dev/reference/react/PureComponent#purecomponent \"Link for this heading\")\n\nTo skip re-rendering a class component for same props and state, extend `PureComponent` instead of [`Component`:](https://react.dev/reference/react/Component)\n\n```\nimport { PureComponent } from 'react';class Greeting extends PureComponent { render() { return <h1>Hello, {this.props.name}!</h1>; }}\n```\n\n`PureComponent` is a subclass of `Component` and supports [all the `Component` APIs.](https://react.dev/reference/react/Component#reference) Extending `PureComponent` is equivalent to defining a custom [`shouldComponentUpdate`](https://react.dev/reference/react/Component#shouldcomponentupdate) method that shallowly compares props and state.\n\n[See more examples below.](https://react.dev/reference/react/PureComponent#usage)\n\n* * *\n\nUsage[](https://react.dev/reference/react/PureComponent#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Skipping unnecessary re-renders for class components[](https://react.dev/reference/react/PureComponent#skipping-unnecessary-re-renders-for-class-components \"Link for Skipping unnecessary re-renders for class components \")\n\nReact normally re-renders a component whenever its parent re-renders. As an optimization, you can create a component that React will not re-render when its parent re-renders so long as its new props and state are the same as the old props and state. [Class components](https://react.dev/reference/react/Component) can opt into this behavior by extending `PureComponent`:\n\n```\nclass Greeting extends PureComponent { render() { return <h1>Hello, {this.props.name}!</h1>; }}\n```\n\nA React component should always have [pure rendering logic.](https://react.dev/learn/keeping-components-pure) This means that it must return the same output if its props, state, and context haven’t changed. By using `PureComponent`, you are telling React that your component complies with this requirement, so React doesn’t need to re-render as long as its props and state haven’t changed. However, your component will still re-render if a context that it’s using changes.\n\nIn this example, notice that the `Greeting` component re-renders whenever `name` is changed (because that’s one of its props), but not when `address` is changed (because it’s not passed to `Greeting` as a prop):\n\nApp.js\n\nApp.js\n\nDownload Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app \"Open in CodeSandbox\")\n\nimport { PureComponent, useState } from 'react';\n\nclass Greeting extends PureComponent {\n render() {\n console.log(\"Greeting was rendered at\", new Date().toLocaleTimeString());\n return <h3\\>Hello{this.props.name && ', '}{this.props.name}!</h3\\>;\n }\n}\n\nexport default function MyApp() {\n const \\[name, setName\\] = useState('');\n const \\[address, setAddress\\] = useState('');\n return (\n <\\>\n <label\\>\n Name{': '}\n <input value\\={name} onChange\\={e \\=> setName(e.target.value)} />\n </label\\>\n <label\\>\n Address{': '}\n <input value\\={address} onChange\\={e \\=> setAddress(e.target.value)} />\n </label\\>\n <Greeting name\\={name} />\n </\\>\n );\n}\n\nShow more\n\n### Pitfall\n\nWe recommend defining components as functions instead of classes. [See how to migrate.](https://react.dev/reference/react/PureComponent#alternatives)\n\n* * *\n\nAlternatives[](https://react.dev/reference/react/PureComponent#alternatives \"Link for Alternatives \")\n-----------------------------------------------------------------------------------------------------\n\n### Migrating from a `PureComponent` class component to a function[](https://react.dev/reference/react/PureComponent#migrating-from-a-purecomponent-class-component-to-a-function \"Link for this heading\")\n\nWe recommend using function components instead of [class components](https://react.dev/reference/react/Component) in new code. If you have some existing class components using `PureComponent`, here is how you can convert them. This is the original code:\n\nApp.js\n\nApp.js\n\nDownload Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app \"Open in CodeSandbox\")\n\nimport { PureComponent, useState } from 'react';\n\nclass Greeting extends PureComponent {\n render() {\n console.log(\"Greeting was rendered at\", new Date().toLocaleTimeString());\n return <h3\\>Hello{this.props.name && ', '}{this.props.name}!</h3\\>;\n }\n}\n\nexport default function MyApp() {\n const \\[name, setName\\] = useState('');\n const \\[address, setAddress\\] = useState('');\n return (\n <\\>\n <label\\>\n Name{': '}\n <input value\\={name} onChange\\={e \\=> setName(e.target.value)} />\n </label\\>\n <label\\>\n Address{': '}\n <input value\\={address} onChange\\={e \\=> setAddress(e.target.value)} />\n </label\\>\n <Greeting name\\={name} />\n </\\>\n );\n}\n\nShow more\n\nWhen you [convert this component from a class to a function,](https://react.dev/reference/react/Component#alternatives) wrap it in [`memo`:](https://react.dev/reference/react/memo)\n\nApp.js\n\nApp.js\n\nDownload Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app \"Open in CodeSandbox\")\n\nimport { memo, useState } from 'react';\n\nconst Greeting = memo(function Greeting({ name }) {\n console.log(\"Greeting was rendered at\", new Date().toLocaleTimeString());\n return <h3\\>Hello{name && ', '}{name}!</h3\\>;\n});\n\nexport default function MyApp() {\n const \\[name, setName\\] = useState('');\n const \\[address, setAddress\\] = useState('');\n return (\n <\\>\n <label\\>\n Name{': '}\n <input value\\={name} onChange\\={e \\=> setName(e.target.value)} />\n </label\\>\n <label\\>\n Address{': '}\n <input value\\={address} onChange\\={e \\=> setAddress(e.target.value)} />\n </label\\>\n <Greeting name\\={name} />\n </\\>\n );\n}\n\nShow more\n\n### Note\n\nUnlike `PureComponent`, [`memo`](https://react.dev/reference/react/memo) does not compare the new and the old state. In function components, calling the [`set` function](https://react.dev/reference/react/useState#setstate) with the same state [already prevents re-renders by default,](https://react.dev/reference/react/memo#updating-a-memoized-component-using-state) even without `memo`.\n\n[PreviousisValidElement](https://react.dev/reference/react/isValidElement)\n",
"filename": "PureComponent.md",
"package": "react"
} |
{
"content": "Title: <StrictMode> – React\n\nURL Source: https://react.dev/reference/react/StrictMode\n\nMarkdown Content:\n`<StrictMode>` lets you find common bugs in your components early during development.\n\n```\n<StrictMode><App /></StrictMode>\n```\n\n* [Reference](https://react.dev/reference/react/StrictMode#reference)\n * [`<StrictMode>`](https://react.dev/reference/react/StrictMode#strictmode)\n* [Usage](https://react.dev/reference/react/StrictMode#usage)\n * [Enabling Strict Mode for entire app](https://react.dev/reference/react/StrictMode#enabling-strict-mode-for-entire-app)\n * [Enabling Strict Mode for a part of the app](https://react.dev/reference/react/StrictMode#enabling-strict-mode-for-a-part-of-the-app)\n * [Fixing bugs found by double rendering in development](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-double-rendering-in-development)\n * [Fixing bugs found by re-running Effects in development](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-re-running-effects-in-development)\n * [Fixing deprecation warnings enabled by Strict Mode](https://react.dev/reference/react/StrictMode#fixing-deprecation-warnings-enabled-by-strict-mode)\n\n* * *\n\nReference[](https://react.dev/reference/react/StrictMode#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------\n\n### `<StrictMode>`[](https://react.dev/reference/react/StrictMode#strictmode \"Link for this heading\")\n\nUse `StrictMode` to enable additional development behaviors and warnings for the component tree inside:\n\n```\nimport { StrictMode } from 'react';import { createRoot } from 'react-dom/client';const root = createRoot(document.getElementById('root'));root.render(<StrictMode><App /></StrictMode>);\n```\n\n[See more examples below.](https://react.dev/reference/react/StrictMode#usage)\n\nStrict Mode enables the following development-only behaviors:\n\n* Your components will [re-render an extra time](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-double-rendering-in-development) to find bugs caused by impure rendering.\n* Your components will [re-run Effects an extra time](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-re-running-effects-in-development) to find bugs caused by missing Effect cleanup.\n* Your components will [be checked for usage of deprecated APIs.](https://react.dev/reference/react/StrictMode#fixing-deprecation-warnings-enabled-by-strict-mode)\n\n#### Props[](https://react.dev/reference/react/StrictMode#props \"Link for Props \")\n\n`StrictMode` accepts no props.\n\n#### Caveats[](https://react.dev/reference/react/StrictMode#caveats \"Link for Caveats \")\n\n* There is no way to opt out of Strict Mode inside a tree wrapped in `<StrictMode>`. This gives you confidence that all components inside `<StrictMode>` are checked. If two teams working on a product disagree whether they find the checks valuable, they need to either reach consensus or move `<StrictMode>` down in the tree.\n\n* * *\n\nUsage[](https://react.dev/reference/react/StrictMode#usage \"Link for Usage \")\n-----------------------------------------------------------------------------\n\n### Enabling Strict Mode for entire app[](https://react.dev/reference/react/StrictMode#enabling-strict-mode-for-entire-app \"Link for Enabling Strict Mode for entire app \")\n\nStrict Mode enables extra development-only checks for the entire component tree inside the `<StrictMode>` component. These checks help you find common bugs in your components early in the development process.\n\nTo enable Strict Mode for your entire app, wrap your root component with `<StrictMode>` when you render it:\n\n```\nimport { StrictMode } from 'react';import { createRoot } from 'react-dom/client';const root = createRoot(document.getElementById('root'));root.render(<StrictMode><App /></StrictMode>);\n```\n\nWe recommend wrapping your entire app in Strict Mode, especially for newly created apps. If you use a framework that calls [`createRoot`](https://react.dev/reference/react-dom/client/createRoot) for you, check its documentation for how to enable Strict Mode.\n\nAlthough the Strict Mode checks **only run in development,** they help you find bugs that already exist in your code but can be tricky to reliably reproduce in production. Strict Mode lets you fix bugs before your users report them.\n\n### Note\n\nStrict Mode enables the following checks in development:\n\n* Your components will [re-render an extra time](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-double-rendering-in-development) to find bugs caused by impure rendering.\n* Your components will [re-run Effects an extra time](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-re-running-effects-in-development) to find bugs caused by missing Effect cleanup.\n* Your components will [be checked for usage of deprecated APIs.](https://react.dev/reference/react/StrictMode#fixing-deprecation-warnings-enabled-by-strict-mode)\n\n**All of these checks are development-only and do not impact the production build.**\n\n* * *\n\n### Enabling Strict Mode for a part of the app[](https://react.dev/reference/react/StrictMode#enabling-strict-mode-for-a-part-of-the-app \"Link for Enabling Strict Mode for a part of the app \")\n\nYou can also enable Strict Mode for any part of your application:\n\n```\nimport { StrictMode } from 'react';function App() {return (<><Header /><StrictMode><main><Sidebar /><Content /></main></StrictMode><Footer /></>);}\n```\n\nIn this example, Strict Mode checks will not run against the `Header` and `Footer` components. However, they will run on `Sidebar` and `Content`, as well as all of the components inside them, no matter how deep.\n\n* * *\n\n### Fixing bugs found by double rendering in development[](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-double-rendering-in-development \"Link for Fixing bugs found by double rendering in development \")\n\n[React assumes that every component you write is a pure function.](https://react.dev/learn/keeping-components-pure) This means that React components you write must always return the same JSX given the same inputs (props, state, and context).\n\nComponents breaking this rule behave unpredictably and cause bugs. To help you find accidentally impure code, Strict Mode calls some of your functions (only the ones that should be pure) **twice in development.** This includes:\n\n* Your component function body (only top-level logic, so this doesn’t include code inside event handlers)\n* Functions that you pass to [`useState`](https://react.dev/reference/react/useState), [`set` functions](https://react.dev/reference/react/useState#setstate), [`useMemo`](https://react.dev/reference/react/useMemo), or [`useReducer`](https://react.dev/reference/react/useReducer)\n* Some class component methods like [`constructor`](https://react.dev/reference/react/Component#constructor), [`render`](https://react.dev/reference/react/Component#render), [`shouldComponentUpdate`](https://react.dev/reference/react/Component#shouldcomponentupdate) ([see the whole list](https://reactjs.org/docs/strict-mode.html#detecting-unexpected-side-effects))\n\nIf a function is pure, running it twice does not change its behavior because a pure function produces the same result every time. However, if a function is impure (for example, it mutates the data it receives), running it twice tends to be noticeable (that’s what makes it impure!) This helps you spot and fix the bug early.\n\n**Here is an example to illustrate how double rendering in Strict Mode helps you find bugs early.**\n\nThis `StoryTray` component takes an array of `stories` and adds one last “Create Story” item at the end:\n\nThere is a mistake in the code above. However, it is easy to miss because the initial output appears correct.\n\nThis mistake will become more noticeable if the `StoryTray` component re-renders multiple times. For example, let’s make the `StoryTray` re-render with a different background color whenever you hover over it:\n\nimport { useState } from 'react';\n\nexport default function StoryTray({ stories }) {\n const \\[isHover, setIsHover\\] = useState(false);\n const items = stories;\n items.push({ id: 'create', label: 'Create Story' });\n return (\n <ul\n onPointerEnter\\={() \\=> setIsHover(true)}\n onPointerLeave\\={() \\=> setIsHover(false)}\n style\\={{\n backgroundColor: isHover ? '#ddd' : '#fff'\n }}\n \\>\n {items.map(story \\=> (\n <li key\\={story.id}\\>\n {story.label}\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nNotice how every time you hover over the `StoryTray` component, “Create Story” gets added to the list again. The intention of the code was to add it once at the end. But `StoryTray` directly modifies the `stories` array from the props. Every time `StoryTray` renders, it adds “Create Story” again at the end of the same array. In other words, `StoryTray` is not a pure function—running it multiple times produces different results.\n\nTo fix this problem, you can make a copy of the array, and modify that copy instead of the original one:\n\n```\nexport default function StoryTray({ stories }) {const items = stories.slice(); // Clone the array// ✅ Good: Pushing into a new arrayitems.push({ id: 'create', label: 'Create Story' });\n```\n\nThis would [make the `StoryTray` function pure.](https://react.dev/learn/keeping-components-pure) Each time it is called, it would only modify a new copy of the array, and would not affect any external objects or variables. This solves the bug, but you had to make the component re-render more often before it became obvious that something is wrong with its behavior.\n\n**In the original example, the bug wasn’t obvious. Now let’s wrap the original (buggy) code in `<StrictMode>`:**\n\n**Strict Mode _always_ calls your rendering function twice, so you can see the mistake right away** (“Create Story” appears twice). This lets you notice such mistakes early in the process. When you fix your component to render in Strict Mode, you _also_ fix many possible future production bugs like the hover functionality from before:\n\nimport { useState } from 'react';\n\nexport default function StoryTray({ stories }) {\n const \\[isHover, setIsHover\\] = useState(false);\n const items = stories.slice(); \n items.push({ id: 'create', label: 'Create Story' });\n return (\n <ul\n onPointerEnter\\={() \\=> setIsHover(true)}\n onPointerLeave\\={() \\=> setIsHover(false)}\n style\\={{\n backgroundColor: isHover ? '#ddd' : '#fff'\n }}\n \\>\n {items.map(story \\=> (\n <li key\\={story.id}\\>\n {story.label}\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nWithout Strict Mode, it was easy to miss the bug until you added more re-renders. Strict Mode made the same bug appear right away. Strict Mode helps you find bugs before you push them to your team and to your users.\n\n[Read more about keeping components pure.](https://react.dev/learn/keeping-components-pure)\n\n### Note\n\nIf you have [React DevTools](https://react.dev/learn/react-developer-tools) installed, any `console.log` calls during the second render call will appear slightly dimmed. React DevTools also offers a setting (off by default) to suppress them completely.\n\n* * *\n\n### Fixing bugs found by re-running Effects in development[](https://react.dev/reference/react/StrictMode#fixing-bugs-found-by-re-running-effects-in-development \"Link for Fixing bugs found by re-running Effects in development \")\n\nStrict Mode can also help find bugs in [Effects.](https://react.dev/learn/synchronizing-with-effects)\n\nEvery Effect has some setup code and may have some cleanup code. Normally, React calls setup when the component _mounts_ (is added to the screen) and calls cleanup when the component _unmounts_ (is removed from the screen). React then calls cleanup and setup again if its dependencies changed since the last render.\n\nWhen Strict Mode is on, React will also run **one extra setup+cleanup cycle in development for every Effect.** This may feel surprising, but it helps reveal subtle bugs that are hard to catch manually.\n\n**Here is an example to illustrate how re-running Effects in Strict Mode helps you find bugs early.**\n\nConsider this example that connects a component to a chat:\n\nThere is an issue with this code, but it might not be immediately clear.\n\nTo make the issue more obvious, let’s implement a feature. In the example below, `roomId` is not hardcoded. Instead, the user can select the `roomId` that they want to connect to from a dropdown. Click “Open chat” and then select different chat rooms one by one. Keep track of the number of active connections in the console:\n\nYou’ll notice that the number of open connections always keeps growing. In a real app, this would cause performance and network problems. The issue is that [your Effect is missing a cleanup function:](https://react.dev/learn/synchronizing-with-effects#step-3-add-cleanup-if-needed)\n\n```\nuseEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, [roomId]);\n```\n\nNow that your Effect “cleans up” after itself and destroys the outdated connections, the leak is solved. However, notice that the problem did not become visible until you’ve added more features (the select box).\n\n**In the original example, the bug wasn’t obvious. Now let’s wrap the original (buggy) code in `<StrictMode>`:**\n\n**With Strict Mode, you immediately see that there is a problem** (the number of active connections jumps to 2). Strict Mode runs an extra setup+cleanup cycle for every Effect. This Effect has no cleanup logic, so it creates an extra connection but doesn’t destroy it. This is a hint that you’re missing a cleanup function.\n\nStrict Mode lets you notice such mistakes early in the process. When you fix your Effect by adding a cleanup function in Strict Mode, you _also_ fix many possible future production bugs like the select box from before:\n",
"filename": "StrictMode.md",
"package": "react"
} |
{
"content": "Title: <Suspense> – React\n\nURL Source: https://react.dev/reference/react/Suspense\n\nMarkdown Content:\n`<Suspense>` lets you display a fallback until its children have finished loading.\n\n```\n<Suspense fallback={<Loading />}><SomeComponent /></Suspense>\n```\n\n* [Reference](https://react.dev/reference/react/Suspense#reference)\n * [`<Suspense>`](https://react.dev/reference/react/Suspense#suspense)\n* [Usage](https://react.dev/reference/react/Suspense#usage)\n * [Displaying a fallback while content is loading](https://react.dev/reference/react/Suspense#displaying-a-fallback-while-content-is-loading)\n * [Revealing content together at once](https://react.dev/reference/react/Suspense#revealing-content-together-at-once)\n * [Revealing nested content as it loads](https://react.dev/reference/react/Suspense#revealing-nested-content-as-it-loads)\n * [Showing stale content while fresh content is loading](https://react.dev/reference/react/Suspense#showing-stale-content-while-fresh-content-is-loading)\n * [Preventing already revealed content from hiding](https://react.dev/reference/react/Suspense#preventing-already-revealed-content-from-hiding)\n * [Indicating that a Transition is happening](https://react.dev/reference/react/Suspense#indicating-that-a-transition-is-happening)\n * [Resetting Suspense boundaries on navigation](https://react.dev/reference/react/Suspense#resetting-suspense-boundaries-on-navigation)\n * [Providing a fallback for server errors and client-only content](https://react.dev/reference/react/Suspense#providing-a-fallback-for-server-errors-and-client-only-content)\n* [Troubleshooting](https://react.dev/reference/react/Suspense#troubleshooting)\n * [How do I prevent the UI from being replaced by a fallback during an update?](https://react.dev/reference/react/Suspense#preventing-unwanted-fallbacks)\n\n* * *\n\nReference[](https://react.dev/reference/react/Suspense#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------\n\n### `<Suspense>`[](https://react.dev/reference/react/Suspense#suspense \"Link for this heading\")\n\n#### Props[](https://react.dev/reference/react/Suspense#props \"Link for Props \")\n\n* `children`: The actual UI you intend to render. If `children` suspends while rendering, the Suspense boundary will switch to rendering `fallback`.\n* `fallback`: An alternate UI to render in place of the actual UI if it has not finished loading. Any valid React node is accepted, though in practice, a fallback is a lightweight placeholder view, such as a loading spinner or skeleton. Suspense will automatically switch to `fallback` when `children` suspends, and back to `children` when the data is ready. If `fallback` suspends while rendering, it will activate the closest parent Suspense boundary.\n\n#### Caveats[](https://react.dev/reference/react/Suspense#caveats \"Link for Caveats \")\n\n* React does not preserve any state for renders that got suspended before they were able to mount for the first time. When the component has loaded, React will retry rendering the suspended tree from scratch.\n* If Suspense was displaying content for the tree, but then it suspended again, the `fallback` will be shown again unless the update causing it was caused by [`startTransition`](https://react.dev/reference/react/startTransition) or [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue).\n* If React needs to hide the already visible content because it suspended again, it will clean up [layout Effects](https://react.dev/reference/react/useLayoutEffect) in the content tree. When the content is ready to be shown again, React will fire the layout Effects again. This ensures that Effects measuring the DOM layout don’t try to do this while the content is hidden.\n* React includes under-the-hood optimizations like _Streaming Server Rendering_ and _Selective Hydration_ that are integrated with Suspense. Read [an architectural overview](https://github.com/reactwg/react-18/discussions/37) and watch [a technical talk](https://www.youtube.com/watch?v=pj5N-Khihgc) to learn more.\n\n* * *\n\nUsage[](https://react.dev/reference/react/Suspense#usage \"Link for Usage \")\n---------------------------------------------------------------------------\n\n### Displaying a fallback while content is loading[](https://react.dev/reference/react/Suspense#displaying-a-fallback-while-content-is-loading \"Link for Displaying a fallback while content is loading \")\n\nYou can wrap any part of your application with a Suspense boundary:\n\n```\n<Suspense fallback={<Loading />}><Albums /></Suspense>\n```\n\nReact will display your loading fallback until all the code and data needed by the children has been loaded.\n\nIn the example below, the `Albums` component _suspends_ while fetching the list of albums. Until it’s ready to render, React switches the closest Suspense boundary above to show the fallback—your `Loading` component. Then, when the data loads, React hides the `Loading` fallback and renders the `Albums` component with data.\n\nimport { Suspense } from 'react';\nimport Albums from './Albums.js';\n\nexport default function ArtistPage({ artist }) {\n return (\n <\\>\n <h1\\>{artist.name}</h1\\>\n <Suspense fallback\\={<Loading />}\\>\n <Albums artistId\\={artist.id} />\n </Suspense\\>\n </\\>\n );\n}\n\nfunction Loading() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\n### Note\n\n**Only Suspense-enabled data sources will activate the Suspense component.** They include:\n\n* Data fetching with Suspense-enabled frameworks like [Relay](https://relay.dev/docs/guided-tour/rendering/loading-states/) and [Next.js](https://nextjs.org/docs/getting-started/react-essentials)\n* Lazy-loading component code with [`lazy`](https://react.dev/reference/react/lazy)\n* Reading the value of a Promise with [`use`](https://react.dev/reference/react/use)\n\nSuspense **does not** detect when data is fetched inside an Effect or event handler.\n\nThe exact way you would load data in the `Albums` component above depends on your framework. If you use a Suspense-enabled framework, you’ll find the details in its data fetching documentation.\n\nSuspense-enabled data fetching without the use of an opinionated framework is not yet supported. The requirements for implementing a Suspense-enabled data source are unstable and undocumented. An official API for integrating data sources with Suspense will be released in a future version of React.\n\n* * *\n\n### Revealing content together at once[](https://react.dev/reference/react/Suspense#revealing-content-together-at-once \"Link for Revealing content together at once \")\n\nBy default, the whole tree inside Suspense is treated as a single unit. For example, even if _only one_ of these components suspends waiting for some data, _all_ of them together will be replaced by the loading indicator:\n\n```\n<Suspense fallback={<Loading />}><Biography /><Panel><Albums /></Panel></Suspense>\n```\n\nThen, after all of them are ready to be displayed, they will all appear together at once.\n\nIn the example below, both `Biography` and `Albums` fetch some data. However, because they are grouped under a single Suspense boundary, these components always “pop in” together at the same time.\n\nimport { Suspense } from 'react';\nimport Albums from './Albums.js';\nimport Biography from './Biography.js';\nimport Panel from './Panel.js';\n\nexport default function ArtistPage({ artist }) {\n return (\n <\\>\n <h1\\>{artist.name}</h1\\>\n <Suspense fallback\\={<Loading />}\\>\n <Biography artistId\\={artist.id} />\n <Panel\\>\n <Albums artistId\\={artist.id} />\n </Panel\\>\n </Suspense\\>\n </\\>\n );\n}\n\nfunction Loading() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\nComponents that load data don’t have to be direct children of the Suspense boundary. For example, you can move `Biography` and `Albums` into a new `Details` component. This doesn’t change the behavior. `Biography` and `Albums` share the same closest parent Suspense boundary, so their reveal is coordinated together.\n\n```\n<Suspense fallback={<Loading />}><Details artistId={artist.id} /></Suspense>function Details({ artistId }) {return (<><Biography artistId={artistId} /><Panel><Albums artistId={artistId} /></Panel></>);}\n```\n\n* * *\n\n### Revealing nested content as it loads[](https://react.dev/reference/react/Suspense#revealing-nested-content-as-it-loads \"Link for Revealing nested content as it loads \")\n\nWhen a component suspends, the closest parent Suspense component shows the fallback. This lets you nest multiple Suspense components to create a loading sequence. Each Suspense boundary’s fallback will be filled in as the next level of content becomes available. For example, you can give the album list its own fallback:\n\n```\n<Suspense fallback={<BigSpinner />}><Biography /><Suspense fallback={<AlbumsGlimmer />}><Panel><Albums /></Panel></Suspense></Suspense>\n```\n\nWith this change, displaying the `Biography` doesn’t need to “wait” for the `Albums` to load.\n\nThe sequence will be:\n\n1. If `Biography` hasn’t loaded yet, `BigSpinner` is shown in place of the entire content area.\n2. Once `Biography` finishes loading, `BigSpinner` is replaced by the content.\n3. If `Albums` hasn’t loaded yet, `AlbumsGlimmer` is shown in place of `Albums` and its parent `Panel`.\n4. Finally, once `Albums` finishes loading, it replaces `AlbumsGlimmer`.\n\nimport { Suspense } from 'react';\nimport Albums from './Albums.js';\nimport Biography from './Biography.js';\nimport Panel from './Panel.js';\n\nexport default function ArtistPage({ artist }) {\n return (\n <\\>\n <h1\\>{artist.name}</h1\\>\n <Suspense fallback\\={<BigSpinner />}\\>\n <Biography artistId\\={artist.id} />\n <Suspense fallback\\={<AlbumsGlimmer />}\\>\n <Panel\\>\n <Albums artistId\\={artist.id} />\n </Panel\\>\n </Suspense\\>\n </Suspense\\>\n </\\>\n );\n}\n\nfunction BigSpinner() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\nfunction AlbumsGlimmer() {\n return (\n <div className\\=\"glimmer-panel\"\\>\n <div className\\=\"glimmer-line\" />\n <div className\\=\"glimmer-line\" />\n <div className\\=\"glimmer-line\" />\n </div\\>\n );\n}\n\nSuspense boundaries let you coordinate which parts of your UI should always “pop in” together at the same time, and which parts should progressively reveal more content in a sequence of loading states. You can add, move, or delete Suspense boundaries in any place in the tree without affecting the rest of your app’s behavior.\n\nDon’t put a Suspense boundary around every component. Suspense boundaries should not be more granular than the loading sequence that you want the user to experience. If you work with a designer, ask them where the loading states should be placed—it’s likely that they’ve already included them in their design wireframes.\n\n* * *\n\n### Showing stale content while fresh content is loading[](https://react.dev/reference/react/Suspense#showing-stale-content-while-fresh-content-is-loading \"Link for Showing stale content while fresh content is loading \")\n\nIn this example, the `SearchResults` component suspends while fetching the search results. Type `\"a\"`, wait for the results, and then edit it to `\"ab\"`. The results for `\"a\"` will get replaced by the loading fallback.\n\nimport { Suspense, useState } from 'react';\nimport SearchResults from './SearchResults.js';\n\nexport default function App() {\n const \\[query, setQuery\\] = useState('');\n return (\n <\\>\n <label\\>\n Search albums:\n <input value\\={query} onChange\\={e \\=> setQuery(e.target.value)} />\n </label\\>\n <Suspense fallback\\={<h2\\>Loading...</h2\\>}\\>\n <SearchResults query\\={query} />\n </Suspense\\>\n </\\>\n );\n}\n\nA common alternative UI pattern is to _defer_ updating the list and to keep showing the previous results until the new results are ready. The [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue) Hook lets you pass a deferred version of the query down:\n\n```\nexport default function App() {const [query, setQuery] = useState('');const deferredQuery = useDeferredValue(query);return (<><label> Search albums:<input value={query} onChange={e => setQuery(e.target.value)} /></label><Suspense fallback={<h2>Loading...</h2>}><SearchResults query={deferredQuery} /></Suspense></>);}\n```\n\nThe `query` will update immediately, so the input will display the new value. However, the `deferredQuery` will keep its previous value until the data has loaded, so `SearchResults` will show the stale results for a bit.\n\nTo make it more obvious to the user, you can add a visual indication when the stale result list is displayed:\n\n```\n<div style={{opacity: query !== deferredQuery ? 0.5 : 1 }}><SearchResults query={deferredQuery} /></div>\n```\n\nEnter `\"a\"` in the example below, wait for the results to load, and then edit the input to `\"ab\"`. Notice how instead of the Suspense fallback, you now see the dimmed stale result list until the new results have loaded:\n\nimport { Suspense, useState, useDeferredValue } from 'react';\nimport SearchResults from './SearchResults.js';\n\nexport default function App() {\n const \\[query, setQuery\\] = useState('');\n const deferredQuery = useDeferredValue(query);\n const isStale = query !== deferredQuery;\n return (\n <\\>\n <label\\>\n Search albums:\n <input value\\={query} onChange\\={e \\=> setQuery(e.target.value)} />\n </label\\>\n <Suspense fallback\\={<h2\\>Loading...</h2\\>}\\>\n <div style\\={{ opacity: isStale ? 0.5 : 1 }}\\>\n <SearchResults query\\={deferredQuery} />\n </div\\>\n </Suspense\\>\n </\\>\n );\n}\n\n### Note\n\nBoth deferred values and [Transitions](https://react.dev/reference/react/Suspense#preventing-already-revealed-content-from-hiding) let you avoid showing Suspense fallback in favor of inline indicators. Transitions mark the whole update as non-urgent so they are typically used by frameworks and router libraries for navigation. Deferred values, on the other hand, are mostly useful in application code where you want to mark a part of UI as non-urgent and let it “lag behind” the rest of the UI.\n\n* * *\n\n### Preventing already revealed content from hiding[](https://react.dev/reference/react/Suspense#preventing-already-revealed-content-from-hiding \"Link for Preventing already revealed content from hiding \")\n\nWhen a component suspends, the closest parent Suspense boundary switches to showing the fallback. This can lead to a jarring user experience if it was already displaying some content. Try pressing this button:\n\nimport { Suspense, useState } from 'react';\nimport IndexPage from './IndexPage.js';\nimport ArtistPage from './ArtistPage.js';\nimport Layout from './Layout.js';\n\nexport default function App() {\n return (\n <Suspense fallback\\={<BigSpinner />}\\>\n <Router />\n </Suspense\\>\n );\n}\n\nfunction Router() {\n const \\[page, setPage\\] = useState('/');\n\n function navigate(url) {\n setPage(url);\n }\n\n let content;\n if (page === '/') {\n content = (\n <IndexPage navigate\\={navigate} />\n );\n } else if (page === '/the-beatles') {\n content = (\n <ArtistPage\n artist\\={{\n id: 'the-beatles',\n name: 'The Beatles',\n }}\n />\n );\n }\n return (\n <Layout\\>\n {content}\n </Layout\\>\n );\n}\n\nfunction BigSpinner() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\nWhen you pressed the button, the `Router` component rendered `ArtistPage` instead of `IndexPage`. A component inside `ArtistPage` suspended, so the closest Suspense boundary started showing the fallback. The closest Suspense boundary was near the root, so the whole site layout got replaced by `BigSpinner`.\n\nTo prevent this, you can mark the navigation state update as a _Transition_ with [`startTransition`:](https://react.dev/reference/react/startTransition)\n\n```\nfunction Router() {const [page, setPage] = useState('/');function navigate(url) {startTransition(() => {setPage(url); });}// ...\n```\n\nThis tells React that the state transition is not urgent, and it’s better to keep showing the previous page instead of hiding any already revealed content. Now clicking the button “waits” for the `Biography` to load:\n\nimport { Suspense, startTransition, useState } from 'react';\nimport IndexPage from './IndexPage.js';\nimport ArtistPage from './ArtistPage.js';\nimport Layout from './Layout.js';\n\nexport default function App() {\n return (\n <Suspense fallback\\={<BigSpinner />}\\>\n <Router />\n </Suspense\\>\n );\n}\n\nfunction Router() {\n const \\[page, setPage\\] = useState('/');\n\n function navigate(url) {\n startTransition(() \\=> {\n setPage(url);\n });\n }\n\n let content;\n if (page === '/') {\n content = (\n <IndexPage navigate\\={navigate} />\n );\n } else if (page === '/the-beatles') {\n content = (\n <ArtistPage\n artist\\={{\n id: 'the-beatles',\n name: 'The Beatles',\n }}\n />\n );\n }\n return (\n <Layout\\>\n {content}\n </Layout\\>\n );\n}\n\nfunction BigSpinner() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\nA Transition doesn’t wait for _all_ content to load. It only waits long enough to avoid hiding already revealed content. For example, the website `Layout` was already revealed, so it would be bad to hide it behind a loading spinner. However, the nested `Suspense` boundary around `Albums` is new, so the Transition doesn’t wait for it.\n\n### Note\n\nSuspense-enabled routers are expected to wrap the navigation updates into Transitions by default.\n\n* * *\n\n### Indicating that a Transition is happening[](https://react.dev/reference/react/Suspense#indicating-that-a-transition-is-happening \"Link for Indicating that a Transition is happening \")\n\nIn the above example, once you click the button, there is no visual indication that a navigation is in progress. To add an indicator, you can replace [`startTransition`](https://react.dev/reference/react/startTransition) with [`useTransition`](https://react.dev/reference/react/useTransition) which gives you a boolean `isPending` value. In the example below, it’s used to change the website header styling while a Transition is happening:\n\nimport { Suspense, useState, useTransition } from 'react';\nimport IndexPage from './IndexPage.js';\nimport ArtistPage from './ArtistPage.js';\nimport Layout from './Layout.js';\n\nexport default function App() {\n return (\n <Suspense fallback\\={<BigSpinner />}\\>\n <Router />\n </Suspense\\>\n );\n}\n\nfunction Router() {\n const \\[page, setPage\\] = useState('/');\n const \\[isPending, startTransition\\] = useTransition();\n\n function navigate(url) {\n startTransition(() \\=> {\n setPage(url);\n });\n }\n\n let content;\n if (page === '/') {\n content = (\n <IndexPage navigate\\={navigate} />\n );\n } else if (page === '/the-beatles') {\n content = (\n <ArtistPage\n artist\\={{\n id: 'the-beatles',\n name: 'The Beatles',\n }}\n />\n );\n }\n return (\n <Layout isPending\\={isPending}\\>\n {content}\n </Layout\\>\n );\n}\n\nfunction BigSpinner() {\n return <h2\\>🌀 Loading...</h2\\>;\n}\n\n* * *\n\n### Resetting Suspense boundaries on navigation[](https://react.dev/reference/react/Suspense#resetting-suspense-boundaries-on-navigation \"Link for Resetting Suspense boundaries on navigation \")\n\nDuring a Transition, React will avoid hiding already revealed content. However, if you navigate to a route with different parameters, you might want to tell React it is _different_ content. You can express this with a `key`:\n\n```\n<ProfilePage key={queryParams.id} />\n```\n\nImagine you’re navigating within a user’s profile page, and something suspends. If that update is wrapped in a Transition, it will not trigger the fallback for already visible content. That’s the expected behavior.\n\nHowever, now imagine you’re navigating between two different user profiles. In that case, it makes sense to show the fallback. For example, one user’s timeline is _different content_ from another user’s timeline. By specifying a `key`, you ensure that React treats different users’ profiles as different components, and resets the Suspense boundaries during navigation. Suspense-integrated routers should do this automatically.\n\n* * *\n\n### Providing a fallback for server errors and client-only content[](https://react.dev/reference/react/Suspense#providing-a-fallback-for-server-errors-and-client-only-content \"Link for Providing a fallback for server errors and client-only content \")\n\nIf you use one of the [streaming server rendering APIs](https://react.dev/reference/react-dom/server) (or a framework that relies on them), React will also use your `<Suspense>` boundaries to handle errors on the server. If a component throws an error on the server, React will not abort the server render. Instead, it will find the closest `<Suspense>` component above it and include its fallback (such as a spinner) into the generated server HTML. The user will see a spinner at first.\n\nOn the client, React will attempt to render the same component again. If it errors on the client too, React will throw the error and display the closest [error boundary.](https://react.dev/reference/react/Component#static-getderivedstatefromerror) However, if it does not error on the client, React will not display the error to the user since the content was eventually displayed successfully.\n\nYou can use this to opt out some components from rendering on the server. To do this, throw an error in the server environment and then wrap them in a `<Suspense>` boundary to replace their HTML with fallbacks:\n\n```\n<Suspense fallback={<Loading />}><Chat /></Suspense>function Chat() {if (typeof window === 'undefined') {throw Error('Chat should only render on the client.');}// ...}\n```\n\nThe server HTML will include the loading indicator. It will be replaced by the `Chat` component on the client.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/Suspense#troubleshooting \"Link for Troubleshooting \")\n---------------------------------------------------------------------------------------------------------\n\n### How do I prevent the UI from being replaced by a fallback during an update?[](https://react.dev/reference/react/Suspense#preventing-unwanted-fallbacks \"Link for How do I prevent the UI from being replaced by a fallback during an update? \")\n\nReplacing visible UI with a fallback creates a jarring user experience. This can happen when an update causes a component to suspend, and the nearest Suspense boundary is already showing content to the user.\n\nTo prevent this from happening, [mark the update as non-urgent using `startTransition`](https://react.dev/reference/react/Suspense#preventing-already-revealed-content-from-hiding). During a Transition, React will wait until enough data has loaded to prevent an unwanted fallback from appearing:\n\n```\nfunction handleNextPageClick() {// If this update suspends, don't hide the already displayed contentstartTransition(() => {setCurrentPage(currentPage + 1);});}\n```\n\nThis will avoid hiding existing content. However, any newly rendered `Suspense` boundaries will still immediately display fallbacks to avoid blocking the UI and let the user see the content as it becomes available.\n\n**React will only prevent unwanted fallbacks during non-urgent updates**. It will not delay a render if it’s the result of an urgent update. You must opt in with an API like [`startTransition`](https://react.dev/reference/react/startTransition) or [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue).\n\nIf your router is integrated with Suspense, it should wrap its updates into [`startTransition`](https://react.dev/reference/react/startTransition) automatically.\n",
"filename": "Suspense.md",
"package": "react"
} |
{
"content": "Title: act – React\n\nURL Source: https://react.dev/reference/react/act\n\nMarkdown Content:\n`act` is a test helper to apply pending React updates before making assertions.\n\nTo prepare a component for assertions, wrap the code rendering it and performing updates inside an `await act()` call. This makes your test run closer to how React works in the browser.\n\n### Note\n\nYou might find using `act()` directly a bit too verbose. To avoid some of the boilerplate, you could use a library like [React Testing Library](https://testing-library.com/docs/react-testing-library/intro), whose helpers are wrapped with `act()`.\n\n* [Reference](https://react.dev/reference/react/act#reference)\n * [`await act(async actFn)`](https://react.dev/reference/react/act#await-act-async-actfn)\n* [Usage](https://react.dev/reference/react/act#usage)\n * [Rendering components in tests](https://react.dev/reference/react/act#rendering-components-in-tests)\n * [Dispatching events in tests](https://react.dev/reference/react/act#dispatching-events-in-tests)\n* [Troubleshooting](https://react.dev/reference/react/act#troubleshooting)\n * [I’m getting an error: “The current testing environment is not configured to support act”(…)”](https://react.dev/reference/react/act#error-the-current-testing-environment-is-not-configured-to-support-act)\n\n* * *\n\nReference[](https://react.dev/reference/react/act#reference \"Link for Reference \")\n----------------------------------------------------------------------------------\n\n### `await act(async actFn)`[](https://react.dev/reference/react/act#await-act-async-actfn \"Link for this heading\")\n\nWhen writing UI tests, tasks like rendering, user events, or data fetching can be considered as “units” of interaction with a user interface. React provides a helper called `act()` that makes sure all updates related to these “units” have been processed and applied to the DOM before you make any assertions.\n\nThe name `act` comes from the [Arrange-Act-Assert](https://wiki.c2.com/?ArrangeActAssert) pattern.\n\n```\nit ('renders with button disabled', async () => {await act(async () => {root.render(<TestComponent />)});expect(container.querySelector('button')).toBeDisabled();});\n```\n\n### Note\n\nWe recommend using `act` with `await` and an `async` function. Although the sync version works in many cases, it doesn’t work in all cases and due to the way React schedules updates internally, it’s difficult to predict when you can use the sync version.\n\nWe will deprecate and remove the sync version in the future.\n\n#### Parameters[](https://react.dev/reference/react/act#parameters \"Link for Parameters \")\n\n* `async actFn`: An async function wrapping renders or interactions for components being tested. Any updates triggered within the `actFn`, are added to an internal act queue, which are then flushed together to process and apply any changes to the DOM. Since it is async, React will also run any code that crosses an async boundary, and flush any updates scheduled.\n\n#### Returns[](https://react.dev/reference/react/act#returns \"Link for Returns \")\n\n`act` does not return anything.\n\nUsage[](https://react.dev/reference/react/act#usage \"Link for Usage \")\n----------------------------------------------------------------------\n\nWhen testing a component, you can use `act` to make assertions about its output.\n\nFor example, let’s say we have this `Counter` component, the usage examples below show how to test it:\n\n```\nfunction Counter() {const [count, setCount] = useState(0);const handleClick = () => {setCount(prev => prev + 1);}useEffect(() => {document.title = `You clicked ${this.state.count} times`;}, [count]);return (<div><p>You clicked {this.state.count} times</p><button onClick={this.handleClick}> Click me</button></div>)}\n```\n\n### Rendering components in tests[](https://react.dev/reference/react/act#rendering-components-in-tests \"Link for Rendering components in tests \")\n\nTo test the render output of a component, wrap the render inside `act()`:\n\n```\nimport {act} from 'react';import ReactDOM from 'react-dom/client';import Counter from './Counter';it('can render and update a counter', async () => {container = document.createElement('div');document.body.appendChild(container);// ✅ Render the component inside act().await act(() => {ReactDOM.createRoot(container).render(<Counter />);});const button = container.querySelector('button');const label = container.querySelector('p');expect(label.textContent).toBe('You clicked 0 times');expect(document.title).toBe('You clicked 0 times');});\n```\n\nHere, we create a container, append it to the document, and render the `Counter` component inside `act()`. This ensures that the component is rendered and its effects are applied before making assertions.\n\nUsing `act` ensures that all updates have been applied before we make assertions.\n\n### Dispatching events in tests[](https://react.dev/reference/react/act#dispatching-events-in-tests \"Link for Dispatching events in tests \")\n\nTo test events, wrap the event dispatch inside `act()`:\n\n```\nimport {act} from 'react';import ReactDOM from 'react-dom/client';import Counter from './Counter';it.only('can render and update a counter', async () => {const container = document.createElement('div');document.body.appendChild(container);await act( async () => {ReactDOMClient.createRoot(container).render(<Counter />);});// ✅ Dispatch the event inside act().await act(async () => {button.dispatchEvent(new MouseEvent('click', { bubbles: true }));});const button = container.querySelector('button');const label = container.querySelector('p');expect(label.textContent).toBe('You clicked 1 times');expect(document.title).toBe('You clicked 1 times');});\n```\n\nHere, we render the component with `act`, and then dispatch the event inside another `act()`. This ensures that all updates from the event are applied before making assertions.\n\n### Pitfall\n\nDon’t forget that dispatching DOM events only works when the DOM container is added to the document. You can use a library like [React Testing Library](https://testing-library.com/docs/react-testing-library/intro) to reduce the boilerplate code.\n\nTroubleshooting[](https://react.dev/reference/react/act#troubleshooting \"Link for Troubleshooting \")\n----------------------------------------------------------------------------------------------------\n\n### I’m getting an error: “The current testing environment is not configured to support act”(…)”[](https://react.dev/reference/react/act#error-the-current-testing-environment-is-not-configured-to-support-act \"Link for I’m getting an error: “The current testing environment is not configured to support act”(…)” \")\n\nUsing `act` requires setting `global.IS_REACT_ACT_ENVIRONMENT=true` in your test environment. This is to ensure that `act` is only used in the correct environment.\n\nIf you don’t set the global, you will see an error like this:\n\nWarning: The current testing environment is not configured to support act(…)\n\nTo fix, add this to your global setup file for React tests:\n\n```\nglobal.IS_REACT_ACT_ENVIRONMENT=true\n```\n\n### Note\n",
"filename": "act.md",
"package": "react"
} |
{
"content": "Title: Add React to an Existing Project – React\n\nURL Source: https://react.dev/learn/add-react-to-an-existing-project\n\nMarkdown Content:\nIf you want to add some interactivity to your existing project, you don’t have to rewrite it in React. Add React to your existing stack, and render interactive React components anywhere.\n\n### Note\n\n**You need to install [Node.js](https://nodejs.org/en/) for local development.** Although you can [try React](https://react.dev/learn/installation#try-react) online or with a simple HTML page, realistically most JavaScript tooling you’ll want to use for development requires Node.js.\n\nUsing React for an entire subroute of your existing website[](https://react.dev/learn/add-react-to-an-existing-project#using-react-for-an-entire-subroute-of-your-existing-website \"Link for Using React for an entire subroute of your existing website \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nLet’s say you have an existing web app at `example.com` built with another server technology (like Rails), and you want to implement all routes starting with `example.com/some-app/` fully with React.\n\nHere’s how we recommend to set it up:\n\n1. **Build the React part of your app** using one of the [React-based frameworks](https://react.dev/learn/start-a-new-react-project).\n2. **Specify `/some-app` as the _base path_** in your framework’s configuration (here’s how: [Next.js](https://nextjs.org/docs/api-reference/next.config.js/basepath), [Gatsby](https://www.gatsbyjs.com/docs/how-to/previews-deploys-hosting/path-prefix/)).\n3. **Configure your server or a proxy** so that all requests under `/some-app/` are handled by your React app.\n\nThis ensures the React part of your app can [benefit from the best practices](https://react.dev/learn/start-a-new-react-project#can-i-use-react-without-a-framework) baked into those frameworks.\n\nMany React-based frameworks are full-stack and let your React app take advantage of the server. However, you can use the same approach even if you can’t or don’t want to run JavaScript on the server. In that case, serve the HTML/CSS/JS export ([`next export` output](https://nextjs.org/docs/advanced-features/static-html-export) for Next.js, default for Gatsby) at `/some-app/` instead.\n\nUsing React for a part of your existing page[](https://react.dev/learn/add-react-to-an-existing-project#using-react-for-a-part-of-your-existing-page \"Link for Using React for a part of your existing page \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nLet’s say you have an existing page built with another technology (either a server one like Rails, or a client one like Backbone), and you want to render interactive React components somewhere on that page. That’s a common way to integrate React—in fact, it’s how most React usage looked at Meta for many years!\n\nYou can do this in two steps:\n\n1. **Set up a JavaScript environment** that lets you use the [JSX syntax](https://react.dev/learn/writing-markup-with-jsx), split your code into modules with the [`import`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/import) / [`export`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/export) syntax, and use packages (for example, React) from the [npm](https://www.npmjs.com/) package registry.\n2. **Render your React components** where you want to see them on the page.\n\nThe exact approach depends on your existing page setup, so let’s walk through some details.\n\n### Step 1: Set up a modular JavaScript environment[](https://react.dev/learn/add-react-to-an-existing-project#step-1-set-up-a-modular-javascript-environment \"Link for Step 1: Set up a modular JavaScript environment \")\n\nA modular JavaScript environment lets you write your React components in individual files, as opposed to writing all of your code in a single file. It also lets you use all the wonderful packages published by other developers on the [npm](https://www.npmjs.com/) registry—including React itself! How you do this depends on your existing setup:\n\n* **If your app is already split into files that use `import` statements,** try to use the setup you already have. Check whether writing `<div />` in your JS code causes a syntax error. If it causes a syntax error, you might need to [transform your JavaScript code with Babel](https://babeljs.io/setup), and enable the [Babel React preset](https://babeljs.io/docs/babel-preset-react) to use JSX.\n \n* **If your app doesn’t have an existing setup for compiling JavaScript modules,** set it up with [Vite](https://vitejs.dev/). The Vite community maintains [many integrations with backend frameworks](https://github.com/vitejs/awesome-vite#integrations-with-backends), including Rails, Django, and Laravel. If your backend framework is not listed, [follow this guide](https://vitejs.dev/guide/backend-integration.html) to manually integrate Vite builds with your backend.\n \n\nTo check whether your setup works, run this command in your project folder:\n\nnpm install react react-dom\n\nThen add these lines of code at the top of your main JavaScript file (it might be called `index.js` or `main.js`):\n\nIf the entire content of your page was replaced by a “Hello, world!”, everything worked! Keep reading.\n\n### Note\n\nIntegrating a modular JavaScript environment into an existing project for the first time can feel intimidating, but it’s worth it! If you get stuck, try our [community resources](https://react.dev/community) or the [Vite Chat](https://chat.vitejs.dev/).\n\n### Step 2: Render React components anywhere on the page[](https://react.dev/learn/add-react-to-an-existing-project#step-2-render-react-components-anywhere-on-the-page \"Link for Step 2: Render React components anywhere on the page \")\n\nIn the previous step, you put this code at the top of your main file:\n\n```\nimport { createRoot } from 'react-dom/client';// Clear the existing HTML contentdocument.body.innerHTML = '<div id=\"app\"></div>';// Render your React component insteadconst root = createRoot(document.getElementById('app'));root.render(<h1>Hello, world</h1>);\n```\n\nOf course, you don’t actually want to clear the existing HTML content!\n\nDelete this code.\n\nInstead, you probably want to render your React components in specific places in your HTML. Open your HTML page (or the server templates that generate it) and add a unique [`id`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/id) attribute to any tag, for example:\n\n```\n<!-- ... somewhere in your html ... --><nav id=\"navigation\"></nav><!-- ... more html ... -->\n```\n\nThis lets you find that HTML element with [`document.getElementById`](https://developer.mozilla.org/en-US/docs/Web/API/Document/getElementById) and pass it to [`createRoot`](https://react.dev/reference/react-dom/client/createRoot) so that you can render your own React component inside:\n\nNotice how the original HTML content from `index.html` is preserved, but your own `NavigationBar` React component now appears inside the `<nav id=\"navigation\">` from your HTML. Read the [`createRoot` usage documentation](https://react.dev/reference/react-dom/client/createRoot#rendering-a-page-partially-built-with-react) to learn more about rendering React components inside an existing HTML page.\n\nWhen you adopt React in an existing project, it’s common to start with small interactive components (like buttons), and then gradually keep “moving upwards” until eventually your entire page is built with React. If you ever reach that point, we recommend migrating to [a React framework](https://react.dev/learn/start-a-new-react-project) right after to get the most out of React.\n\nUsing React Native in an existing native mobile app[](https://react.dev/learn/add-react-to-an-existing-project#using-react-native-in-an-existing-native-mobile-app \"Link for Using React Native in an existing native mobile app \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\n[React Native](https://reactnative.dev/) can also be integrated into existing native apps incrementally. If you have an existing native app for Android (Java or Kotlin) or iOS (Objective-C or Swift), [follow this guide](https://reactnative.dev/docs/integration-with-existing-apps) to add a React Native screen to it.\n",
"filename": "add-react-to-an-existing-project.md",
"package": "react"
} |
{
"content": "Title: Adding Interactivity – React\n\nURL Source: https://react.dev/learn/adding-interactivity\n\nMarkdown Content:\nSome things on the screen update in response to user input. For example, clicking an image gallery switches the active image. In React, data that changes over time is called _state._ You can add state to any component, and update it as needed. In this chapter, you’ll learn how to write components that handle interactions, update their state, and display different output over time.\n\nResponding to events[](https://react.dev/learn/adding-interactivity#responding-to-events \"Link for Responding to events \")\n--------------------------------------------------------------------------------------------------------------------------\n\nReact lets you add _event handlers_ to your JSX. Event handlers are your own functions that will be triggered in response to user interactions like clicking, hovering, focusing on form inputs, and so on.\n\nBuilt-in components like `<button>` only support built-in browser events like `onClick`. However, you can also create your own components, and give their event handler props any application-specific names that you like.\n\n* * *\n\nState: a component’s memory[](https://react.dev/learn/adding-interactivity#state-a-components-memory \"Link for State: a component’s memory \")\n---------------------------------------------------------------------------------------------------------------------------------------------\n\nComponents often need to change what’s on the screen as a result of an interaction. Typing into the form should update the input field, clicking “next” on an image carousel should change which image is displayed, clicking “buy” puts a product in the shopping cart. Components need to “remember” things: the current input value, the current image, the shopping cart. In React, this kind of component-specific memory is called _state._\n\nYou can add state to a component with a [`useState`](https://react.dev/reference/react/useState) Hook. _Hooks_ are special functions that let your components use React features (state is one of those features). The `useState` Hook lets you declare a state variable. It takes the initial state and returns a pair of values: the current state, and a state setter function that lets you update it.\n\n```\nconst [index, setIndex] = useState(0);const [showMore, setShowMore] = useState(false);\n```\n\nHere is how an image gallery uses and updates state on click:\n\nimport { useState } from 'react';\nimport { sculptureList } from './data.js';\n\nexport default function Gallery() {\n const \\[index, setIndex\\] = useState(0);\n const \\[showMore, setShowMore\\] = useState(false);\n const hasNext = index < sculptureList.length - 1;\n\n function handleNextClick() {\n if (hasNext) {\n setIndex(index + 1);\n } else {\n setIndex(0);\n }\n }\n\n function handleMoreClick() {\n setShowMore(!showMore);\n }\n\n let sculpture = sculptureList\\[index\\];\n return (\n <\\>\n <button onClick\\={handleNextClick}\\>\n Next\n </button\\>\n <h2\\>\n <i\\>{sculpture.name} </i\\>\n by {sculpture.artist}\n </h2\\>\n <h3\\>\n ({index + 1} of {sculptureList.length})\n </h3\\>\n <button onClick\\={handleMoreClick}\\>\n {showMore ? 'Hide' : 'Show'} details\n </button\\>\n {showMore && <p\\>{sculpture.description}</p\\>}\n <img\n src\\={sculpture.url}\n alt\\={sculpture.alt}\n />\n </\\>\n );\n}\n\n* * *\n\nRender and commit[](https://react.dev/learn/adding-interactivity#render-and-commit \"Link for Render and commit \")\n-----------------------------------------------------------------------------------------------------------------\n\nBefore your components are displayed on the screen, they must be rendered by React. Understanding the steps in this process will help you think about how your code executes and explain its behavior.\n\nImagine that your components are cooks in the kitchen, assembling tasty dishes from ingredients. In this scenario, React is the waiter who puts in requests from customers and brings them their orders. This process of requesting and serving UI has three steps:\n\n1. **Triggering** a render (delivering the diner’s order to the kitchen)\n2. **Rendering** the component (preparing the order in the kitchen)\n3. **Committing** to the DOM (placing the order on the table)\n\n1. 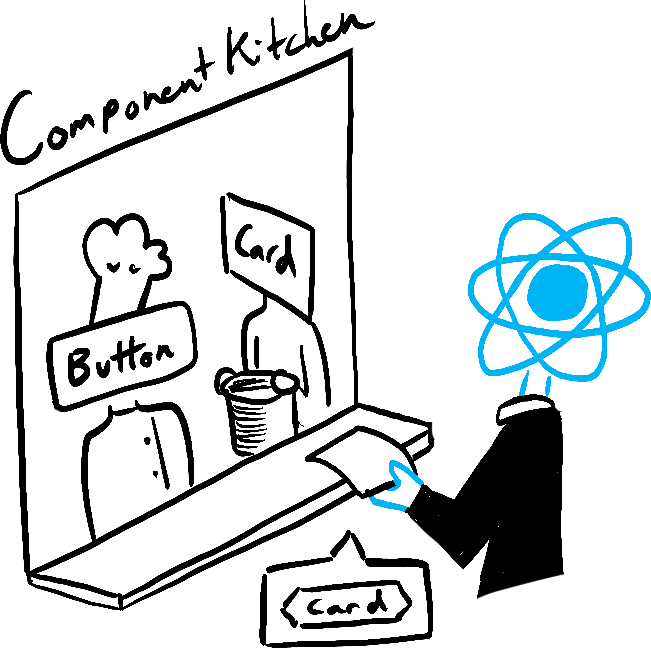\n \n Trigger\n \n2. 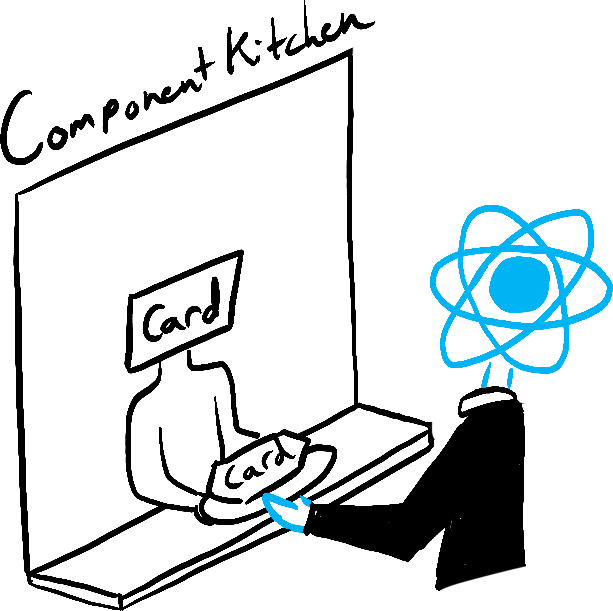\n \n Render\n \n3. 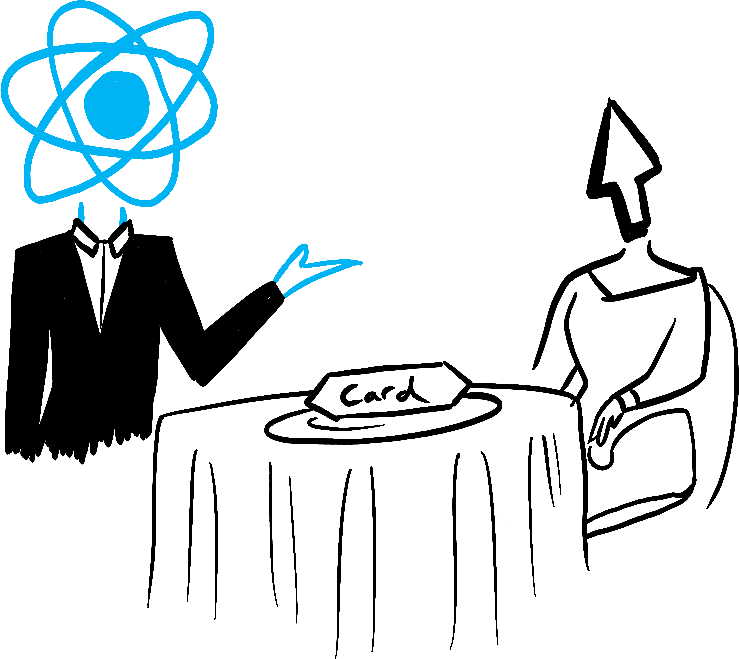\n \n Commit\n \n\n* * *\n\nState as a snapshot[](https://react.dev/learn/adding-interactivity#state-as-a-snapshot \"Link for State as a snapshot \")\n-----------------------------------------------------------------------------------------------------------------------\n\nUnlike regular JavaScript variables, React state behaves more like a snapshot. Setting it does not change the state variable you already have, but instead triggers a re-render. This can be surprising at first!\n\n```\nconsole.log(count); // 0setCount(count + 1); // Request a re-render with 1console.log(count); // Still 0!\n```\n\nThis behavior help you avoid subtle bugs. Here is a little chat app. Try to guess what happens if you press “Send” first and _then_ change the recipient to Bob. Whose name will appear in the `alert` five seconds later?\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[to, setTo\\] = useState('Alice');\n const \\[message, setMessage\\] = useState('Hello');\n\n function handleSubmit(e) {\n e.preventDefault();\n setTimeout(() \\=> {\n alert(\\`You said ${message} to ${to}\\`);\n }, 5000);\n }\n\n return (\n <form onSubmit\\={handleSubmit}\\>\n <label\\>\n To:{' '}\n <select\n value\\={to}\n onChange\\={e \\=> setTo(e.target.value)}\\>\n <option value\\=\"Alice\"\\>Alice</option\\>\n <option value\\=\"Bob\"\\>Bob</option\\>\n </select\\>\n </label\\>\n <textarea\n placeholder\\=\"Message\"\n value\\={message}\n onChange\\={e \\=> setMessage(e.target.value)}\n />\n <button type\\=\"submit\"\\>Send</button\\>\n </form\\>\n );\n}\n\n* * *\n\nQueueing a series of state updates[](https://react.dev/learn/adding-interactivity#queueing-a-series-of-state-updates \"Link for Queueing a series of state updates \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nThis component is buggy: clicking “+3” increments the score only once.\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[score, setScore\\] = useState(0);\n\n function increment() {\n setScore(score + 1);\n }\n\n return (\n <\\>\n <button onClick\\={() \\=> increment()}\\>+1</button\\>\n <button onClick\\={() \\=> {\n increment();\n increment();\n increment();\n }}\\>+3</button\\>\n <h1\\>Score: {score}</h1\\>\n </\\>\n )\n}\n\n[State as a Snapshot](https://react.dev/learn/state-as-a-snapshot) explains why this is happening. Setting state requests a new re-render, but does not change it in the already running code. So `score` continues to be `0` right after you call `setScore(score + 1)`.\n\n```\nconsole.log(score); // 0setScore(score + 1); // setScore(0 + 1);console.log(score); // 0setScore(score + 1); // setScore(0 + 1);console.log(score); // 0setScore(score + 1); // setScore(0 + 1);console.log(score); // 0\n```\n\nYou can fix this by passing an _updater function_ when setting state. Notice how replacing `setScore(score + 1)` with `setScore(s => s + 1)` fixes the “+3” button. This lets you queue multiple state updates.\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[score, setScore\\] = useState(0);\n\n function increment() {\n setScore(s \\=> s + 1);\n }\n\n return (\n <\\>\n <button onClick\\={() \\=> increment()}\\>+1</button\\>\n <button onClick\\={() \\=> {\n increment();\n increment();\n increment();\n }}\\>+3</button\\>\n <h1\\>Score: {score}</h1\\>\n </\\>\n )\n}\n\n* * *\n\nUpdating objects in state[](https://react.dev/learn/adding-interactivity#updating-objects-in-state \"Link for Updating objects in state \")\n-----------------------------------------------------------------------------------------------------------------------------------------\n\nState can hold any kind of JavaScript value, including objects. But you shouldn’t change objects and arrays that you hold in the React state directly. Instead, when you want to update an object and array, you need to create a new one (or make a copy of an existing one), and then update the state to use that copy.\n\nUsually, you will use the `...` spread syntax to copy objects and arrays that you want to change. For example, updating a nested object could look like this:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[person, setPerson\\] = useState({\n name: 'Niki de Saint Phalle',\n artwork: {\n title: 'Blue Nana',\n city: 'Hamburg',\n image: 'https://i.imgur.com/Sd1AgUOm.jpg',\n }\n });\n\n function handleNameChange(e) {\n setPerson({\n ...person,\n name: e.target.value\n });\n }\n\n function handleTitleChange(e) {\n setPerson({\n ...person,\n artwork: {\n ...person.artwork,\n title: e.target.value\n }\n });\n }\n\n function handleCityChange(e) {\n setPerson({\n ...person,\n artwork: {\n ...person.artwork,\n city: e.target.value\n }\n });\n }\n\n function handleImageChange(e) {\n setPerson({\n ...person,\n artwork: {\n ...person.artwork,\n image: e.target.value\n }\n });\n }\n\n return (\n <\\>\n <label\\>\n Name:\n <input\n value\\={person.name}\n onChange\\={handleNameChange}\n />\n </label\\>\n <label\\>\n Title:\n <input\n value\\={person.artwork.title}\n onChange\\={handleTitleChange}\n />\n </label\\>\n <label\\>\n City:\n <input\n value\\={person.artwork.city}\n onChange\\={handleCityChange}\n />\n </label\\>\n <label\\>\n Image:\n <input\n value\\={person.artwork.image}\n onChange\\={handleImageChange}\n />\n </label\\>\n <p\\>\n <i\\>{person.artwork.title}</i\\>\n {' by '}\n {person.name}\n <br />\n (located in {person.artwork.city})\n </p\\>\n <img\n src\\={person.artwork.image}\n alt\\={person.artwork.title}\n />\n </\\>\n );\n}\n\nIf copying objects in code gets tedious, you can use a library like [Immer](https://github.com/immerjs/use-immer) to reduce repetitive code:\n\n* * *\n\nUpdating arrays in state[](https://react.dev/learn/adding-interactivity#updating-arrays-in-state \"Link for Updating arrays in state \")\n--------------------------------------------------------------------------------------------------------------------------------------\n\nArrays are another type of mutable JavaScript objects you can store in state and should treat as read-only. Just like with objects, when you want to update an array stored in state, you need to create a new one (or make a copy of an existing one), and then set state to use the new array:\n\nimport { useState } from 'react';\n\nconst initialList = \\[\n { id: 0, title: 'Big Bellies', seen: false },\n { id: 1, title: 'Lunar Landscape', seen: false },\n { id: 2, title: 'Terracotta Army', seen: true },\n\\];\n\nexport default function BucketList() {\n const \\[list, setList\\] = useState(\n initialList\n );\n\n function handleToggle(artworkId, nextSeen) {\n setList(list.map(artwork \\=> {\n if (artwork.id === artworkId) {\n return { ...artwork, seen: nextSeen };\n } else {\n return artwork;\n }\n }));\n }\n\n return (\n <\\>\n <h1\\>Art Bucket List</h1\\>\n <h2\\>My list of art to see:</h2\\>\n <ItemList\n artworks\\={list}\n onToggle\\={handleToggle} />\n </\\>\n );\n}\n\nfunction ItemList({ artworks, onToggle }) {\n return (\n <ul\\>\n {artworks.map(artwork \\=> (\n <li key\\={artwork.id}\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={artwork.seen}\n onChange\\={e \\=> {\n onToggle(\n artwork.id,\n e.target.checked\n );\n }}\n />\n {artwork.title}\n </label\\>\n </li\\>\n ))}\n </ul\\>\n );\n}\n\nIf copying arrays in code gets tedious, you can use a library like [Immer](https://github.com/immerjs/use-immer) to reduce repetitive code:\n\n* * *\n\nWhat’s next?[](https://react.dev/learn/adding-interactivity#whats-next \"Link for What’s next? \")\n------------------------------------------------------------------------------------------------\n\nHead over to [Responding to Events](https://react.dev/learn/responding-to-events) to start reading this chapter page by page!\n\nOr, if you’re already familiar with these topics, why not read about [Managing State](https://react.dev/learn/managing-state)?\n",
"filename": "adding-interactivity.md",
"package": "react"
} |
{
"content": "Title: cache – React\n\nURL Source: https://react.dev/reference/react/cache\n\nMarkdown Content:\n### Canary\n\n* `cache` is only for use with [React Server Components](https://react.dev/blog/2023/03/22/react-labs-what-we-have-been-working-on-march-2023#react-server-components). See [frameworks](https://react.dev/learn/start-a-new-react-project#bleeding-edge-react-frameworks) that support React Server Components.\n \n* `cache` is only available in React’s [Canary](https://react.dev/community/versioning-policy#canary-channel) and [experimental](https://react.dev/community/versioning-policy#experimental-channel) channels. Please ensure you understand the limitations before using `cache` in production. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n \n\n`cache` lets you cache the result of a data fetch or computation.\n\n```\nconst cachedFn = cache(fn);\n```\n\n* [Reference](https://react.dev/reference/react/cache#reference)\n * [`cache(fn)`](https://react.dev/reference/react/cache#cache)\n* [Usage](https://react.dev/reference/react/cache#usage)\n * [Cache an expensive computation](https://react.dev/reference/react/cache#cache-expensive-computation)\n * [Share a snapshot of data](https://react.dev/reference/react/cache#take-and-share-snapshot-of-data)\n * [Preload data](https://react.dev/reference/react/cache#preload-data)\n* [Troubleshooting](https://react.dev/reference/react/cache#troubleshooting)\n * [My memoized function still runs even though I’ve called it with the same arguments](https://react.dev/reference/react/cache#memoized-function-still-runs)\n\n* * *\n\nReference[](https://react.dev/reference/react/cache#reference \"Link for Reference \")\n------------------------------------------------------------------------------------\n\n### `cache(fn)`[](https://react.dev/reference/react/cache#cache \"Link for this heading\")\n\nCall `cache` outside of any components to create a version of the function with caching.\n\n```\nimport {cache} from 'react';import calculateMetrics from 'lib/metrics';const getMetrics = cache(calculateMetrics);function Chart({data}) {const report = getMetrics(data);// ...}\n```\n\nWhen `getMetrics` is first called with `data`, `getMetrics` will call `calculateMetrics(data)` and store the result in cache. If `getMetrics` is called again with the same `data`, it will return the cached result instead of calling `calculateMetrics(data)` again.\n\n[See more examples below.](https://react.dev/reference/react/cache#usage)\n\n#### Parameters[](https://react.dev/reference/react/cache#parameters \"Link for Parameters \")\n\n* `fn`: The function you want to cache results for. `fn` can take any arguments and return any value.\n\n#### Returns[](https://react.dev/reference/react/cache#returns \"Link for Returns \")\n\n`cache` returns a cached version of `fn` with the same type signature. It does not call `fn` in the process.\n\nWhen calling `cachedFn` with given arguments, it first checks if a cached result exists in the cache. If a cached result exists, it returns the result. If not, it calls `fn` with the arguments, stores the result in the cache, and returns the result. The only time `fn` is called is when there is a cache miss.\n\n### Note\n\nThe optimization of caching return values based on inputs is known as [_memoization_](https://en.wikipedia.org/wiki/Memoization). We refer to the function returned from `cache` as a memoized function.\n\n#### Caveats[](https://react.dev/reference/react/cache#caveats \"Link for Caveats \")\n\n* React will invalidate the cache for all memoized functions for each server request.\n* Each call to `cache` creates a new function. This means that calling `cache` with the same function multiple times will return different memoized functions that do not share the same cache.\n* `cachedFn` will also cache errors. If `fn` throws an error for certain arguments, it will be cached, and the same error is re-thrown when `cachedFn` is called with those same arguments.\n* `cache` is for use in [Server Components](https://react.dev/blog/2023/03/22/react-labs-what-we-have-been-working-on-march-2023#react-server-components) only.\n\n* * *\n\nUsage[](https://react.dev/reference/react/cache#usage \"Link for Usage \")\n------------------------------------------------------------------------\n\n### Cache an expensive computation[](https://react.dev/reference/react/cache#cache-expensive-computation \"Link for Cache an expensive computation \")\n\nUse `cache` to skip duplicate work.\n\n```\nimport {cache} from 'react';import calculateUserMetrics from 'lib/user';const getUserMetrics = cache(calculateUserMetrics);function Profile({user}) {const metrics = getUserMetrics(user);// ...}function TeamReport({users}) {for (let user in users) {const metrics = getUserMetrics(user);// ...}// ...}\n```\n\nIf the same `user` object is rendered in both `Profile` and `TeamReport`, the two components can share work and only call `calculateUserMetrics` once for that `user`.\n\nAssume `Profile` is rendered first. It will call `getUserMetrics`, and check if there is a cached result. Since it is the first time `getUserMetrics` is called with that `user`, there will be a cache miss. `getUserMetrics` will then call `calculateUserMetrics` with that `user` and write the result to cache.\n\nWhen `TeamReport` renders its list of `users` and reaches the same `user` object, it will call `getUserMetrics` and read the result from cache.\n\n### Pitfall\n\n##### Calling different memoized functions will read from different caches.[](https://react.dev/reference/react/cache#pitfall-different-memoized-functions \"Link for Calling different memoized functions will read from different caches. \")\n\nTo access the same cache, components must call the same memoized function.\n\n```\n// Temperature.jsimport {cache} from 'react';import {calculateWeekReport} from './report';export function Temperature({cityData}) {// 🚩 Wrong: Calling `cache` in component creates new `getWeekReport` for each renderconst getWeekReport = cache(calculateWeekReport);const report = getWeekReport(cityData);// ...}\n```\n\n```\n// Precipitation.jsimport {cache} from 'react';import {calculateWeekReport} from './report';// 🚩 Wrong: `getWeekReport` is only accessible for `Precipitation` component.const getWeekReport = cache(calculateWeekReport);export function Precipitation({cityData}) {const report = getWeekReport(cityData);// ...}\n```\n\nIn the above example, `Precipitation` and `Temperature` each call `cache` to create a new memoized function with their own cache look-up. If both components render for the same `cityData`, they will do duplicate work to call `calculateWeekReport`.\n\nIn addition, `Temperature` creates a new memoized function each time the component is rendered which doesn’t allow for any cache sharing.\n\nTo maximize cache hits and reduce work, the two components should call the same memoized function to access the same cache. Instead, define the memoized function in a dedicated module that can be [`import`\\-ed](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/import) across components.\n\n```\n// getWeekReport.jsimport {cache} from 'react';import {calculateWeekReport} from './report';export default cache(calculateWeekReport);\n```\n\n```\n// Temperature.jsimport getWeekReport from './getWeekReport';export default function Temperature({cityData}) {const report = getWeekReport(cityData);// ...}\n```\n\n```\n// Precipitation.jsimport getWeekReport from './getWeekReport';export default function Precipitation({cityData}) {const report = getWeekReport(cityData);// ...}\n```\n\nHere, both components call the same memoized function exported from `./getWeekReport.js` to read and write to the same cache.\n\nTo share a snapshot of data between components, call `cache` with a data-fetching function like `fetch`. When multiple components make the same data fetch, only one request is made and the data returned is cached and shared across components. All components refer to the same snapshot of data across the server render.\n\n```\nimport {cache} from 'react';import {fetchTemperature} from './api.js';const getTemperature = cache(async (city) => {return await fetchTemperature(city);});async function AnimatedWeatherCard({city}) {const temperature = await getTemperature(city);// ...}async function MinimalWeatherCard({city}) {const temperature = await getTemperature(city);// ...}\n```\n\nIf `AnimatedWeatherCard` and `MinimalWeatherCard` both render for the same city, they will receive the same snapshot of data from the memoized function.\n\nIf `AnimatedWeatherCard` and `MinimalWeatherCard` supply different city arguments to `getTemperature`, then `fetchTemperature` will be called twice and each call site will receive different data.\n\nThe city acts as a cache key.\n\n### Note\n\nAsynchronous rendering is only supported for Server Components.\n\n```\nasync function AnimatedWeatherCard({city}) {const temperature = await getTemperature(city);// ...}\n```\n\n### Preload data[](https://react.dev/reference/react/cache#preload-data \"Link for Preload data \")\n\nBy caching a long-running data fetch, you can kick off asynchronous work prior to rendering the component.\n\n```\nconst getUser = cache(async (id) => {return await db.user.query(id);})async function Profile({id}) {const user = await getUser(id);return (<section><img src={user.profilePic} /><h2>{user.name}</h2></section>);}function Page({id}) {// ✅ Good: start fetching the user datagetUser(id);// ... some computational workreturn (<><Profile id={id} /></>);}\n```\n\nWhen rendering `Page`, the component calls `getUser` but note that it doesn’t use the returned data. This early `getUser` call kicks off the asynchronous database query that occurs while `Page` is doing other computational work and rendering children.\n\nWhen rendering `Profile`, we call `getUser` again. If the initial `getUser` call has already returned and cached the user data, when `Profile` asks and waits for this data, it can simply read from the cache without requiring another remote procedure call. If the initial data request hasn’t been completed, preloading data in this pattern reduces delay in data-fetching.\n\n##### Deep Dive\n\n#### Caching asynchronous work[](https://react.dev/reference/react/cache#caching-asynchronous-work \"Link for Caching asynchronous work \")\n\nWhen evaluating an [asynchronous function](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/async_function), you will receive a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) for that work. The promise holds the state of that work (_pending_, _fulfilled_, _failed_) and its eventual settled result.\n\nIn this example, the asynchronous function `fetchData` returns a promise that is awaiting the `fetch`.\n\n```\nasync function fetchData() {return await fetch(`https://...`);}const getData = cache(fetchData);async function MyComponent() {getData();// ... some computational work await getData();// ...}\n```\n\nIn calling `getData` the first time, the promise returned from `fetchData` is cached. Subsequent look-ups will then return the same promise.\n\nNotice that the first `getData` call does not `await` whereas the second does. [`await`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/await) is a JavaScript operator that will wait and return the settled result of the promise. The first `getData` call simply initiates the `fetch` to cache the promise for the second `getData` to look-up.\n\nIf by the second call the promise is still _pending_, then `await` will pause for the result. The optimization is that while we wait on the `fetch`, React can continue with computational work, thus reducing the wait time for the second call.\n\nIf the promise is already settled, either to an error or the _fulfilled_ result, `await` will return that value immediately. In both outcomes, there is a performance benefit.\n\n### Pitfall\n\n##### Calling a memoized function outside of a component will not use the cache.[](https://react.dev/reference/react/cache#pitfall-memoized-call-outside-component \"Link for Calling a memoized function outside of a component will not use the cache. \")\n\n```\nimport {cache} from 'react';const getUser = cache(async (userId) => {return await db.user.query(userId);});// 🚩 Wrong: Calling memoized function outside of component will not memoize.getUser('demo-id');async function DemoProfile() {// ✅ Good: `getUser` will memoize.const user = await getUser('demo-id');return <Profile user={user} />;}\n```\n\nReact only provides cache access to the memoized function in a component. When calling `getUser` outside of a component, it will still evaluate the function but not read or update the cache.\n\nThis is because cache access is provided through a [context](https://react.dev/learn/passing-data-deeply-with-context) which is only accessible from a component.\n\n##### Deep Dive\n\n#### When should I use `cache`, [`memo`](https://react.dev/reference/react/memo), or [`useMemo`](https://react.dev/reference/react/useMemo)?[](https://react.dev/reference/react/cache#cache-memo-usememo \"Link for this heading\")\n\nAll mentioned APIs offer memoization but the difference is what they’re intended to memoize, who can access the cache, and when their cache is invalidated.\n\n#### `useMemo`[](https://react.dev/reference/react/cache#deep-dive-use-memo \"Link for this heading\")\n\nIn general, you should use [`useMemo`](https://react.dev/reference/react/useMemo) for caching a expensive computation in a Client Component across renders. As an example, to memoize a transformation of data within a component.\n\n```\n'use client';function WeatherReport({record}) {const avgTemp = useMemo(() => calculateAvg(record)), record);// ...}function App() {const record = getRecord();return (<><WeatherReport record={record} /><WeatherReport record={record} /></>);}\n```\n\nIn this example, `App` renders two `WeatherReport`s with the same record. Even though both components do the same work, they cannot share work. `useMemo`’s cache is only local to the component.\n\nHowever, `useMemo` does ensure that if `App` re-renders and the `record` object doesn’t change, each component instance would skip work and use the memoized value of `avgTemp`. `useMemo` will only cache the last computation of `avgTemp` with the given dependencies.\n\n#### `cache`[](https://react.dev/reference/react/cache#deep-dive-cache \"Link for this heading\")\n\nIn general, you should use `cache` in Server Components to memoize work that can be shared across components.\n\n```\nconst cachedFetchReport = cache(fetchReport);function WeatherReport({city}) {const report = cachedFetchReport(city);// ...}function App() {const city = \"Los Angeles\";return (<><WeatherReport city={city} /><WeatherReport city={city} /></>);}\n```\n\nRe-writing the previous example to use `cache`, in this case the second instance of `WeatherReport` will be able to skip duplicate work and read from the same cache as the first `WeatherReport`. Another difference from the previous example is that `cache` is also recommended for memoizing data fetches, unlike `useMemo` which should only be used for computations.\n\nAt this time, `cache` should only be used in Server Components and the cache will be invalidated across server requests.\n\n#### `memo`[](https://react.dev/reference/react/cache#deep-dive-memo \"Link for this heading\")\n\nYou should use [`memo`](https://react.dev/reference/react/memo) to prevent a component re-rendering if its props are unchanged.\n\n```\n'use client';function WeatherReport({record}) {const avgTemp = calculateAvg(record); // ...}const MemoWeatherReport = memo(WeatherReport);function App() {const record = getRecord();return (<><MemoWeatherReport record={record} /><MemoWeatherReport record={record} /></>);}\n```\n\nIn this example, both `MemoWeatherReport` components will call `calculateAvg` when first rendered. However, if `App` re-renders, with no changes to `record`, none of the props have changed and `MemoWeatherReport` will not re-render.\n\nCompared to `useMemo`, `memo` memoizes the component render based on props vs. specific computations. Similar to `useMemo`, the memoized component only caches the last render with the last prop values. Once the props change, the cache invalidates and the component re-renders.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/cache#troubleshooting \"Link for Troubleshooting \")\n------------------------------------------------------------------------------------------------------\n\n### My memoized function still runs even though I’ve called it with the same arguments[](https://react.dev/reference/react/cache#memoized-function-still-runs \"Link for My memoized function still runs even though I’ve called it with the same arguments \")\n\nSee prior mentioned pitfalls\n\n* [Calling different memoized functions will read from different caches.](https://react.dev/reference/react/cache#pitfall-different-memoized-functions)\n* [Calling a memoized function outside of a component will not use the cache.](https://react.dev/reference/react/cache#pitfall-memoized-call-outside-component)\n\nIf none of the above apply, it may be a problem with how React checks if something exists in cache.\n\nIf your arguments are not [primitives](https://developer.mozilla.org/en-US/docs/Glossary/Primitive) (ex. objects, functions, arrays), ensure you’re passing the same object reference.\n\nWhen calling a memoized function, React will look up the input arguments to see if a result is already cached. React will use shallow equality of the arguments to determine if there is a cache hit.\n\n```\nimport {cache} from 'react';const calculateNorm = cache((vector) => {// ...});function MapMarker(props) {// 🚩 Wrong: props is an object that changes every render.const length = calculateNorm(props);// ...}function App() {return (<><MapMarker x={10} y={10} z={10} /><MapMarker x={10} y={10} z={10} /></>);}\n```\n\nIn this case the two `MapMarker`s look like they’re doing the same work and calling `calculateNorm` with the same value of `{x: 10, y: 10, z:10}`. Even though the objects contain the same values, they are not the same object reference as each component creates its own `props` object.\n\nReact will call [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) on the input to verify if there is a cache hit.\n\n```\nimport {cache} from 'react';const calculateNorm = cache((x, y, z) => {// ...});function MapMarker(props) {// ✅ Good: Pass primitives to memoized functionconst length = calculateNorm(props.x, props.y, props.z);// ...}function App() {return (<><MapMarker x={10} y={10} z={10} /><MapMarker x={10} y={10} z={10} /></>);}\n```\n\nOne way to address this could be to pass the vector dimensions to `calculateNorm`. This works because the dimensions themselves are primitives.\n\nAnother solution may be to pass the vector object itself as a prop to the component. We’ll need to pass the same object to both component instances.\n\n```\nimport {cache} from 'react';const calculateNorm = cache((vector) => {// ...});function MapMarker(props) {// ✅ Good: Pass the same `vector` objectconst length = calculateNorm(props.vector);// ...}function App() {const vector = [10, 10, 10];return (<><MapMarker vector={vector} /><MapMarker vector={vector} /></>);}\n```\n",
"filename": "cache.md",
"package": "react"
} |
{
"content": "Title: Choosing the State Structure – React\n\nURL Source: https://react.dev/learn/choosing-the-state-structure\n\nMarkdown Content:\nChoosing the State Structure – React\n===============\n\nChoosing the State Structure[](https://react.dev/learn/choosing-the-state-structure#undefined \"Link for this heading\")\n======================================================================================================================\n\nStructuring state well can make a difference between a component that is pleasant to modify and debug, and one that is a constant source of bugs. Here are some tips you should consider when structuring state.\n\n### You will learn\n\n* When to use a single vs multiple state variables\n* What to avoid when organizing state\n* How to fix common issues with the state structure\n\nPrinciples for structuring state[](https://react.dev/learn/choosing-the-state-structure#principles-for-structuring-state \"Link for Principles for structuring state \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you write a component that holds some state, you’ll have to make choices about how many state variables to use and what the shape of their data should be. While it’s possible to write correct programs even with a suboptimal state structure, there are a few principles that can guide you to make better choices:\n\n1. **Group related state.** If you always update two or more state variables at the same time, consider merging them into a single state variable.\n2. **Avoid contradictions in state.** When the state is structured in a way that several pieces of state may contradict and “disagree” with each other, you leave room for mistakes. Try to avoid this.\n3. **Avoid redundant state.** If you can calculate some information from the component’s props or its existing state variables during rendering, you should not put that information into that component’s state.\n4. **Avoid duplication in state.** When the same data is duplicated between multiple state variables, or within nested objects, it is difficult to keep them in sync. Reduce duplication when you can.\n5. **Avoid deeply nested state.** Deeply hierarchical state is not very convenient to update. When possible, prefer to structure state in a flat way.\n\nThe goal behind these principles is to _make state easy to update without introducing mistakes_. Removing redundant and duplicate data from state helps ensure that all its pieces stay in sync. This is similar to how a database engineer might want to [“normalize” the database structure](https://docs.microsoft.com/en-us/office/troubleshoot/access/database-normalization-description) to reduce the chance of bugs. To paraphrase Albert Einstein, **“Make your state as simple as it can be—but no simpler.”**\n\nNow let’s see how these principles apply in action.\n\nGroup related state[](https://react.dev/learn/choosing-the-state-structure#group-related-state \"Link for Group related state \")\n-------------------------------------------------------------------------------------------------------------------------------\n\nYou might sometimes be unsure between using a single or multiple state variables.\n\nShould you do this?\n\n```\nconst [x, setX] = useState(0);const [y, setY] = useState(0);\n```\n\nOr this?\n\n```\nconst [position, setPosition] = useState({ x: 0, y: 0 });\n```\n\nTechnically, you can use either of these approaches. But **if some two state variables always change together, it might be a good idea to unify them into a single state variable.** Then you won’t forget to always keep them in sync, like in this example where moving the cursor updates both coordinates of the red dot:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nexport default function MovingDot() {\n const \\[position, setPosition\\] = useState({\n x: 0,\n y: 0\n });\n return (\n <div\n onPointerMove\\={e \\=> {\n setPosition({\n x: e.clientX,\n y: e.clientY\n });\n }}\n style\\={{\n position: 'relative',\n width: '100vw',\n height: '100vh',\n }}\\>\n <div style\\={{\n position: 'absolute',\n backgroundColor: 'red',\n borderRadius: '50%',\n transform: \\`translate(${position.x}px, ${position.y}px)\\`,\n left: -10,\n top: -10,\n width: 20,\n height: 20,\n }} />\n </div\\>\n )\n}\n\nOpen Sandbox\n\nShow more\n\nAnother case where you’ll group data into an object or an array is when you don’t know how many pieces of state you’ll need. For example, it’s helpful when you have a form where the user can add custom fields.\n\n### Pitfall\n\nIf your state variable is an object, remember that [you can’t update only one field in it](https://react.dev/learn/updating-objects-in-state) without explicitly copying the other fields. For example, you can’t do `setPosition({ x: 100 })` in the above example because it would not have the `y` property at all! Instead, if you wanted to set `x` alone, you would either do `setPosition({ ...position, x: 100 })`, or split them into two state variables and do `setX(100)`.\n\nAvoid contradictions in state[](https://react.dev/learn/choosing-the-state-structure#avoid-contradictions-in-state \"Link for Avoid contradictions in state \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nHere is a hotel feedback form with `isSending` and `isSent` state variables:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nexport default function FeedbackForm() {\n const \\[text, setText\\] = useState('');\n const \\[isSending, setIsSending\\] = useState(false);\n const \\[isSent, setIsSent\\] = useState(false);\n\n async function handleSubmit(e) {\n e.preventDefault();\n setIsSending(true);\n await sendMessage(text);\n setIsSending(false);\n setIsSent(true);\n }\n\n if (isSent) {\n return <h1\\>Thanks for feedback!</h1\\>\n }\n\n return (\n <form onSubmit\\={handleSubmit}\\>\n <p\\>How was your stay at The Prancing Pony?</p\\>\n <textarea\n disabled\\={isSending}\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n <br />\n <button\n disabled\\={isSending}\n type\\=\"submit\"\n \\>\n Send\n </button\\>\n {isSending && <p\\>Sending...</p\\>}\n </form\\>\n );\n}\n\n// Pretend to send a message.\nfunction sendMessage(text) {\n return new Promise(resolve \\=> {\n setTimeout(resolve, 2000);\n });\n}\n\nShow more\n\nWhile this code works, it leaves the door open for “impossible” states. For example, if you forget to call `setIsSent` and `setIsSending` together, you may end up in a situation where both `isSending` and `isSent` are `true` at the same time. The more complex your component is, the harder it is to understand what happened.\n\n**Since `isSending` and `isSent` should never be `true` at the same time, it is better to replace them with one `status` state variable that may take one of _three_ valid states:** `'typing'` (initial), `'sending'`, and `'sent'`:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nexport default function FeedbackForm() {\n const \\[text, setText\\] = useState('');\n const \\[status, setStatus\\] = useState('typing');\n\n async function handleSubmit(e) {\n e.preventDefault();\n setStatus('sending');\n await sendMessage(text);\n setStatus('sent');\n }\n\n const isSending = status === 'sending';\n const isSent = status === 'sent';\n\n if (isSent) {\n return <h1\\>Thanks for feedback!</h1\\>\n }\n\n return (\n <form onSubmit\\={handleSubmit}\\>\n <p\\>How was your stay at The Prancing Pony?</p\\>\n <textarea\n disabled\\={isSending}\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n <br />\n <button\n disabled\\={isSending}\n type\\=\"submit\"\n \\>\n Send\n </button\\>\n {isSending && <p\\>Sending...</p\\>}\n </form\\>\n );\n}\n\n// Pretend to send a message.\nfunction sendMessage(text) {\n return new Promise(resolve \\=> {\n setTimeout(resolve, 2000);\n });\n}\n\nShow more\n\nYou can still declare some constants for readability:\n\n```\nconst isSending = status === 'sending';const isSent = status === 'sent';\n```\n\nBut they’re not state variables, so you don’t need to worry about them getting out of sync with each other.\n\nAvoid redundant state[](https://react.dev/learn/choosing-the-state-structure#avoid-redundant-state \"Link for Avoid redundant state \")\n-------------------------------------------------------------------------------------------------------------------------------------\n\nIf you can calculate some information from the component’s props or its existing state variables during rendering, you **should not** put that information into that component’s state.\n\nFor example, take this form. It works, but can you find any redundant state in it?\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[firstName, setFirstName\\] = useState('');\n const \\[lastName, setLastName\\] = useState('');\n const \\[fullName, setFullName\\] = useState('');\n\n function handleFirstNameChange(e) {\n setFirstName(e.target.value);\n setFullName(e.target.value + ' ' + lastName);\n }\n\n function handleLastNameChange(e) {\n setLastName(e.target.value);\n setFullName(firstName + ' ' + e.target.value);\n }\n\n return (\n <\\>\n <h2\\>Let’s check you in</h2\\>\n <label\\>\n First name:{' '}\n <input\n value\\={firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:{' '}\n <input\n value\\={lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n <p\\>\n Your ticket will be issued to: <b\\>{fullName}</b\\>\n </p\\>\n </\\>\n );\n}\n\nShow more\n\nThis form has three state variables: `firstName`, `lastName`, and `fullName`. However, `fullName` is redundant. **You can always calculate `fullName` from `firstName` and `lastName` during render, so remove it from state.**\n\nThis is how you can do it:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[firstName, setFirstName\\] = useState('');\n const \\[lastName, setLastName\\] = useState('');\n\n const fullName = firstName + ' ' + lastName;\n\n function handleFirstNameChange(e) {\n setFirstName(e.target.value);\n }\n\n function handleLastNameChange(e) {\n setLastName(e.target.value);\n }\n\n return (\n <\\>\n <h2\\>Let’s check you in</h2\\>\n <label\\>\n First name:{' '}\n <input\n value\\={firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:{' '}\n <input\n value\\={lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n <p\\>\n Your ticket will be issued to: <b\\>{fullName}</b\\>\n </p\\>\n </\\>\n );\n}\n\nShow more\n\nHere, `fullName` is _not_ a state variable. Instead, it’s calculated during render:\n\n```\nconst fullName = firstName + ' ' + lastName;\n```\n\nAs a result, the change handlers don’t need to do anything special to update it. When you call `setFirstName` or `setLastName`, you trigger a re-render, and then the next `fullName` will be calculated from the fresh data.\n\n##### Deep Dive\n\n#### Don’t mirror props in state[](https://react.dev/learn/choosing-the-state-structure#don-t-mirror-props-in-state \"Link for Don’t mirror props in state \")\n\nShow Details\n\nA common example of redundant state is code like this:\n\n```\nfunction Message({ messageColor }) { const [color, setColor] = useState(messageColor);\n```\n\nHere, a `color` state variable is initialized to the `messageColor` prop. The problem is that **if the parent component passes a different value of `messageColor` later (for example, `'red'` instead of `'blue'`), the `color` _state variable_ would not be updated!** The state is only initialized during the first render.\n\nThis is why “mirroring” some prop in a state variable can lead to confusion. Instead, use the `messageColor` prop directly in your code. If you want to give it a shorter name, use a constant:\n\n```\nfunction Message({ messageColor }) { const color = messageColor;\n```\n\nThis way it won’t get out of sync with the prop passed from the parent component.\n\n”Mirroring” props into state only makes sense when you _want_ to ignore all updates for a specific prop. By convention, start the prop name with `initial` or `default` to clarify that its new values are ignored:\n\n```\nfunction Message({ initialColor }) { // The `color` state variable holds the *first* value of `initialColor`. // Further changes to the `initialColor` prop are ignored. const [color, setColor] = useState(initialColor);\n```\n\nAvoid duplication in state[](https://react.dev/learn/choosing-the-state-structure#avoid-duplication-in-state \"Link for Avoid duplication in state \")\n----------------------------------------------------------------------------------------------------------------------------------------------------\n\nThis menu list component lets you choose a single travel snack out of several:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nconst initialItems = \\[\n { title: 'pretzels', id: 0 },\n { title: 'crispy seaweed', id: 1 },\n { title: 'granola bar', id: 2 },\n\\];\n\nexport default function Menu() {\n const \\[items, setItems\\] = useState(initialItems);\n const \\[selectedItem, setSelectedItem\\] = useState(\n items\\[0\\]\n );\n\n return (\n <\\>\n <h2\\>What's your travel snack?</h2\\>\n <ul\\>\n {items.map(item \\=> (\n <li key\\={item.id}\\>\n {item.title}\n {' '}\n <button onClick\\={() \\=> {\n setSelectedItem(item);\n }}\\>Choose</button\\>\n </li\\>\n ))}\n </ul\\>\n <p\\>You picked {selectedItem.title}.</p\\>\n </\\>\n );\n}\n\nShow more\n\nCurrently, it stores the selected item as an object in the `selectedItem` state variable. However, this is not great: **the contents of the `selectedItem` is the same object as one of the items inside the `items` list.** This means that the information about the item itself is duplicated in two places.\n\nWhy is this a problem? Let’s make each item editable:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nconst initialItems = \\[\n { title: 'pretzels', id: 0 },\n { title: 'crispy seaweed', id: 1 },\n { title: 'granola bar', id: 2 },\n\\];\n\nexport default function Menu() {\n const \\[items, setItems\\] = useState(initialItems);\n const \\[selectedItem, setSelectedItem\\] = useState(\n items\\[0\\]\n );\n\n function handleItemChange(id, e) {\n setItems(items.map(item \\=> {\n if (item.id === id) {\n return {\n ...item,\n title: e.target.value,\n };\n } else {\n return item;\n }\n }));\n }\n\n return (\n <\\>\n <h2\\>What's your travel snack?</h2\\> \n <ul\\>\n {items.map((item, index) \\=> (\n <li key\\={item.id}\\>\n <input\n value\\={item.title}\n onChange\\={e \\=> {\n handleItemChange(item.id, e)\n }}\n />\n {' '}\n <button onClick\\={() \\=> {\n setSelectedItem(item);\n }}\\>Choose</button\\>\n </li\\>\n ))}\n </ul\\>\n <p\\>You picked {selectedItem.title}.</p\\>\n </\\>\n );\n}\n\nShow more\n\nNotice how if you first click “Choose” on an item and _then_ edit it, **the input updates but the label at the bottom does not reflect the edits.** This is because you have duplicated state, and you forgot to update `selectedItem`.\n\nAlthough you could update `selectedItem` too, an easier fix is to remove duplication. In this example, instead of a `selectedItem` object (which creates a duplication with objects inside `items`), you hold the `selectedId` in state, and _then_ get the `selectedItem` by searching the `items` array for an item with that ID:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState } from 'react';\n\nconst initialItems = \\[\n { title: 'pretzels', id: 0 },\n { title: 'crispy seaweed', id: 1 },\n { title: 'granola bar', id: 2 },\n\\];\n\nexport default function Menu() {\n const \\[items, setItems\\] = useState(initialItems);\n const \\[selectedId, setSelectedId\\] = useState(0);\n\n const selectedItem = items.find(item \\=>\n item.id === selectedId\n );\n\n function handleItemChange(id, e) {\n setItems(items.map(item \\=> {\n if (item.id === id) {\n return {\n ...item,\n title: e.target.value,\n };\n } else {\n return item;\n }\n }));\n }\n\n return (\n <\\>\n <h2\\>What's your travel snack?</h2\\>\n <ul\\>\n {items.map((item, index) \\=> (\n <li key\\={item.id}\\>\n <input\n value\\={item.title}\n onChange\\={e \\=> {\n handleItemChange(item.id, e)\n }}\n />\n {' '}\n <button onClick\\={() \\=> {\n setSelectedId(item.id);\n }}\\>Choose</button\\>\n </li\\>\n ))}\n </ul\\>\n <p\\>You picked {selectedItem.title}.</p\\>\n </\\>\n );\n}\n\nShow more\n\nThe state used to be duplicated like this:\n\n* `items = [{ id: 0, title: 'pretzels'}, ...]`\n* `selectedItem = {id: 0, title: 'pretzels'}`\n\nBut after the change it’s like this:\n\n* `items = [{ id: 0, title: 'pretzels'}, ...]`\n* `selectedId = 0`\n\nThe duplication is gone, and you only keep the essential state!\n\nNow if you edit the _selected_ item, the message below will update immediately. This is because `setItems` triggers a re-render, and `items.find(...)` would find the item with the updated title. You didn’t need to hold _the selected item_ in state, because only the _selected ID_ is essential. The rest could be calculated during render.\n\nAvoid deeply nested state[](https://react.dev/learn/choosing-the-state-structure#avoid-deeply-nested-state \"Link for Avoid deeply nested state \")\n-------------------------------------------------------------------------------------------------------------------------------------------------\n\nImagine a travel plan consisting of planets, continents, and countries. You might be tempted to structure its state using nested objects and arrays, like in this example:\n\nApp.jsplaces.js\n\nplaces.js\n\nReset\n\nFork\n\nexport const initialTravelPlan = {\n id: 0,\n title: '(Root)',\n childPlaces: \\[{\n id: 1,\n title: 'Earth',\n childPlaces: \\[{\n id: 2,\n title: 'Africa',\n childPlaces: \\[{\n id: 3,\n title: 'Botswana',\n childPlaces: \\[\\]\n }, {\n id: 4,\n title: 'Egypt',\n childPlaces: \\[\\]\n }, {\n id: 5,\n title: 'Kenya',\n childPlaces: \\[\\]\n }, {\n id: 6,\n title: 'Madagascar',\n childPlaces: \\[\\]\n }, {\n id: 7,\n title: 'Morocco',\n childPlaces: \\[\\]\n }, {\n id: 8,\n title: 'Nigeria',\n childPlaces: \\[\\]\n }, {\n id: 9,\n title: 'South Africa',\n childPlaces: \\[\\]\n }\\]\n }, {\n id: 10,\n title: 'Americas',\n childPlaces: \\[{\n id: 11,\n title: 'Argentina',\n childPlaces: \\[\\]\n }, {\n id: 12,\n title: 'Brazil',\n childPlaces: \\[\\]\n }, {\n id: 13,\n title: 'Barbados',\n childPlaces: \\[\\]\n }, {\n id: 14,\n title: 'Canada',\n childPlaces: \\[\\]\n }, {\n id: 15,\n title: 'Jamaica',\n childPlaces: \\[\\]\n }, {\n id: 16,\n title: 'Mexico',\n childPlaces: \\[\\]\n }, {\n id: 17,\n title: 'Trinidad and Tobago',\n childPlaces: \\[\\]\n }, {\n id: 18,\n title: 'Venezuela',\n childPlaces: \\[\\]\n }\\]\n }, {\n id: 19,\n title: 'Asia',\n childPlaces: \\[{\n id: 20,\n title: 'China',\n childPlaces: \\[\\]\n }, {\n id: 21,\n title: 'India',\n childPlaces: \\[\\]\n }, {\n id: 22,\n title: 'Singapore',\n childPlaces: \\[\\]\n }, {\n id: 23,\n title: 'South Korea',\n childPlaces: \\[\\]\n }, {\n id: 24,\n title: 'Thailand',\n childPlaces: \\[\\]\n }, {\n id: 25,\n title: 'Vietnam',\n childPlaces: \\[\\]\n }\\]\n }, {\n id: 26,\n title: 'Europe',\n childPlaces: \\[{\n id: 27,\n title: 'Croatia',\n childPlaces: \\[\\],\n }, {\n id: 28,\n title: 'France',\n childPlaces: \\[\\],\n }, {\n id: 29,\n title: 'Germany',\n childPlaces: \\[\\],\n }, {\n id: 30,\n title: 'Italy',\n childPlaces: \\[\\],\n }, {\n id: 31,\n title: 'Portugal',\n childPlaces: \\[\\],\n }, {\n id: 32,\n title: 'Spain',\n childPlaces: \\[\\],\n }, {\n id: 33,\n title: 'Turkey',\n childPlaces: \\[\\],\n }\\]\n }, {\n id: 34,\n title: 'Oceania',\n childPlaces: \\[{\n id: 35,\n title: 'Australia',\n childPlaces: \\[\\],\n }, {\n id: 36,\n title: 'Bora Bora (French Polynesia)',\n childPlaces: \\[\\],\n }, {\n id: 37,\n title: 'Easter Island (Chile)',\n childPlaces: \\[\\],\n }, {\n id: 38,\n title: 'Fiji',\n childPlaces: \\[\\],\n }, {\n id: 39,\n title: 'Hawaii (the USA)',\n childPlaces: \\[\\],\n }, {\n id: 40,\n title: 'New Zealand',\n childPlaces: \\[\\],\n }, {\n id: 41,\n title: 'Vanuatu',\n childPlaces: \\[\\],\n }\\]\n }\\]\n }, {\n id: 42,\n title: 'Moon',\n childPlaces: \\[{\n id: 43,\n title: 'Rheita',\n childPlaces: \\[\\]\n }, {\n id: 44,\n title: 'Piccolomini',\n childPlaces: \\[\\]\n }, {\n id: 45,\n title: 'Tycho',\n childPlaces: \\[\\]\n }\\]\n }, {\n id: 46,\n title: 'Mars',\n childPlaces: \\[{\n id: 47,\n title: 'Corn Town',\n childPlaces: \\[\\]\n }, {\n id: 48,\n title: 'Green Hill',\n childPlaces: \\[\\] \n }\\]\n }\\]\n};\n\nShow more\n\nNow let’s say you want to add a button to delete a place you’ve already visited. How would you go about it? [Updating nested state](https://react.dev/learn/updating-objects-in-state#updating-a-nested-object) involves making copies of objects all the way up from the part that changed. Deleting a deeply nested place would involve copying its entire parent place chain. Such code can be very verbose.\n\n**If the state is too nested to update easily, consider making it “flat”.** Here is one way you can restructure this data. Instead of a tree-like structure where each `place` has an array of _its child places_, you can have each place hold an array of _its child place IDs_. Then store a mapping from each place ID to the corresponding place.\n\nThis data restructuring might remind you of seeing a database table:\n\nApp.jsplaces.js\n\nplaces.js\n\nReset\n\nFork\n\nexport const initialTravelPlan = {\n 0: {\n id: 0,\n title: '(Root)',\n childIds: \\[1, 42, 46\\],\n },\n 1: {\n id: 1,\n title: 'Earth',\n childIds: \\[2, 10, 19, 26, 34\\]\n },\n 2: {\n id: 2,\n title: 'Africa',\n childIds: \\[3, 4, 5, 6 , 7, 8, 9\\]\n }, \n 3: {\n id: 3,\n title: 'Botswana',\n childIds: \\[\\]\n },\n 4: {\n id: 4,\n title: 'Egypt',\n childIds: \\[\\]\n },\n 5: {\n id: 5,\n title: 'Kenya',\n childIds: \\[\\]\n },\n 6: {\n id: 6,\n title: 'Madagascar',\n childIds: \\[\\]\n }, \n 7: {\n id: 7,\n title: 'Morocco',\n childIds: \\[\\]\n },\n 8: {\n id: 8,\n title: 'Nigeria',\n childIds: \\[\\]\n },\n 9: {\n id: 9,\n title: 'South Africa',\n childIds: \\[\\]\n },\n 10: {\n id: 10,\n title: 'Americas',\n childIds: \\[11, 12, 13, 14, 15, 16, 17, 18\\], \n },\n 11: {\n id: 11,\n title: 'Argentina',\n childIds: \\[\\]\n },\n 12: {\n id: 12,\n title: 'Brazil',\n childIds: \\[\\]\n },\n 13: {\n id: 13,\n title: 'Barbados',\n childIds: \\[\\]\n }, \n 14: {\n id: 14,\n title: 'Canada',\n childIds: \\[\\]\n },\n 15: {\n id: 15,\n title: 'Jamaica',\n childIds: \\[\\]\n },\n 16: {\n id: 16,\n title: 'Mexico',\n childIds: \\[\\]\n },\n 17: {\n id: 17,\n title: 'Trinidad and Tobago',\n childIds: \\[\\]\n },\n 18: {\n id: 18,\n title: 'Venezuela',\n childIds: \\[\\]\n },\n 19: {\n id: 19,\n title: 'Asia',\n childIds: \\[20, 21, 22, 23, 24, 25\\], \n },\n 20: {\n id: 20,\n title: 'China',\n childIds: \\[\\]\n },\n 21: {\n id: 21,\n title: 'India',\n childIds: \\[\\]\n },\n 22: {\n id: 22,\n title: 'Singapore',\n childIds: \\[\\]\n },\n 23: {\n id: 23,\n title: 'South Korea',\n childIds: \\[\\]\n },\n 24: {\n id: 24,\n title: 'Thailand',\n childIds: \\[\\]\n },\n 25: {\n id: 25,\n title: 'Vietnam',\n childIds: \\[\\]\n },\n 26: {\n id: 26,\n title: 'Europe',\n childIds: \\[27, 28, 29, 30, 31, 32, 33\\], \n },\n 27: {\n id: 27,\n title: 'Croatia',\n childIds: \\[\\]\n },\n 28: {\n id: 28,\n title: 'France',\n childIds: \\[\\]\n },\n 29: {\n id: 29,\n title: 'Germany',\n childIds: \\[\\]\n },\n 30: {\n id: 30,\n title: 'Italy',\n childIds: \\[\\]\n },\n 31: {\n id: 31,\n title: 'Portugal',\n childIds: \\[\\]\n },\n 32: {\n id: 32,\n title: 'Spain',\n childIds: \\[\\]\n },\n 33: {\n id: 33,\n title: 'Turkey',\n childIds: \\[\\]\n },\n 34: {\n id: 34,\n title: 'Oceania',\n childIds: \\[35, 36, 37, 38, 39, 40, 41\\], \n },\n 35: {\n id: 35,\n title: 'Australia',\n childIds: \\[\\]\n },\n 36: {\n id: 36,\n title: 'Bora Bora (French Polynesia)',\n childIds: \\[\\]\n },\n 37: {\n id: 37,\n title: 'Easter Island (Chile)',\n childIds: \\[\\]\n },\n 38: {\n id: 38,\n title: 'Fiji',\n childIds: \\[\\]\n },\n 39: {\n id: 40,\n title: 'Hawaii (the USA)',\n childIds: \\[\\]\n },\n 40: {\n id: 40,\n title: 'New Zealand',\n childIds: \\[\\]\n },\n 41: {\n id: 41,\n title: 'Vanuatu',\n childIds: \\[\\]\n },\n 42: {\n id: 42,\n title: 'Moon',\n childIds: \\[43, 44, 45\\]\n },\n 43: {\n id: 43,\n title: 'Rheita',\n childIds: \\[\\]\n },\n 44: {\n id: 44,\n title: 'Piccolomini',\n childIds: \\[\\]\n },\n 45: {\n id: 45,\n title: 'Tycho',\n childIds: \\[\\]\n },\n 46: {\n id: 46,\n title: 'Mars',\n childIds: \\[47, 48\\]\n },\n 47: {\n id: 47,\n title: 'Corn Town',\n childIds: \\[\\]\n },\n 48: {\n id: 48,\n title: 'Green Hill',\n childIds: \\[\\]\n }\n};\n\nShow more\n\n**Now that the state is “flat” (also known as “normalized”), updating nested items becomes easier.**\n\nIn order to remove a place now, you only need to update two levels of state:\n\n* The updated version of its _parent_ place should exclude the removed ID from its `childIds` array.\n* The updated version of the root “table” object should include the updated version of the parent place.\n\nHere is an example of how you could go about it:\n\nApp.jsplaces.js\n\nApp.js\n\nReset\n\nFork\n\nimport { useState } from 'react';\nimport { initialTravelPlan } from './places.js';\n\nexport default function TravelPlan() {\n const \\[plan, setPlan\\] = useState(initialTravelPlan);\n\n function handleComplete(parentId, childId) {\n const parent = plan\\[parentId\\];\n // Create a new version of the parent place\n // that doesn't include this child ID.\n const nextParent = {\n ...parent,\n childIds: parent.childIds\n .filter(id \\=> id !== childId)\n };\n // Update the root state object...\n setPlan({\n ...plan,\n // ...so that it has the updated parent.\n \\[parentId\\]: nextParent\n });\n }\n\n const root = plan\\[0\\];\n const planetIds = root.childIds;\n return (\n <\\>\n <h2\\>Places to visit</h2\\>\n <ol\\>\n {planetIds.map(id \\=> (\n <PlaceTree\n key\\={id}\n id\\={id}\n parentId\\={0}\n placesById\\={plan}\n onComplete\\={handleComplete}\n />\n ))}\n </ol\\>\n </\\>\n );\n}\n\nfunction PlaceTree({ id, parentId, placesById, onComplete }) {\n const place = placesById\\[id\\];\n const childIds = place.childIds;\n return (\n <li\\>\n {place.title}\n <button onClick\\={() \\=> {\n onComplete(parentId, id);\n }}\\>\n Complete\n </button\\>\n {childIds.length > 0 &&\n <ol\\>\n {childIds.map(childId \\=> (\n <PlaceTree\n key\\={childId}\n id\\={childId}\n parentId\\={id}\n placesById\\={placesById}\n onComplete\\={onComplete}\n />\n ))}\n </ol\\>\n }\n </li\\>\n );\n}\n\nShow more\n\nYou can nest state as much as you like, but making it “flat” can solve numerous problems. It makes state easier to update, and it helps ensure you don’t have duplication in different parts of a nested object.\n\n##### Deep Dive\n\n#### Improving memory usage[](https://react.dev/learn/choosing-the-state-structure#improving-memory-usage \"Link for Improving memory usage \")\n\nShow Details\n\nIdeally, you would also remove the deleted items (and their children!) from the “table” object to improve memory usage. This version does that. It also [uses Immer](https://react.dev/learn/updating-objects-in-state#write-concise-update-logic-with-immer) to make the update logic more concise.\n\npackage.jsonApp.jsplaces.js\n\npackage.json\n\nReset\n\nFork\n\n{\n \"dependencies\": {\n \"immer\": \"1.7.3\",\n \"react\": \"latest\",\n \"react-dom\": \"latest\",\n \"react-scripts\": \"latest\",\n \"use-immer\": \"0.5.1\"\n },\n \"scripts\": {\n \"start\": \"react-scripts start\",\n \"build\": \"react-scripts build\",\n \"test\": \"react-scripts test --env=jsdom\",\n \"eject\": \"react-scripts eject\"\n },\n \"devDependencies\": {}\n}\n\nSometimes, you can also reduce state nesting by moving some of the nested state into the child components. This works well for ephemeral UI state that doesn’t need to be stored, like whether an item is hovered.\n\nRecap[](https://react.dev/learn/choosing-the-state-structure#recap \"Link for Recap\")\n------------------------------------------------------------------------------------\n\n* If two state variables always update together, consider merging them into one.\n* Choose your state variables carefully to avoid creating “impossible” states.\n* Structure your state in a way that reduces the chances that you’ll make a mistake updating it.\n* Avoid redundant and duplicate state so that you don’t need to keep it in sync.\n* Don’t put props _into_ state unless you specifically want to prevent updates.\n* For UI patterns like selection, keep ID or index in state instead of the object itself.\n* If updating deeply nested state is complicated, try flattening it.\n\nTry out some challenges[](https://react.dev/learn/choosing-the-state-structure#challenges \"Link for Try out some challenges\")\n-----------------------------------------------------------------------------------------------------------------------------\n\n1. Fix a component that’s not updating 2. Fix a broken packing list 3. Fix the disappearing selection 4. Implement multiple selection\n\n#### \n\nChallenge 1 of 4:\n\nFix a component that’s not updating[](https://react.dev/learn/choosing-the-state-structure#fix-a-component-thats-not-updating \"Link for this heading\")\n\nThis `Clock` component receives two props: `color` and `time`. When you select a different color in the select box, the `Clock` component receives a different `color` prop from its parent component. However, for some reason, the displayed color doesn’t update. Why? Fix the problem.\n\nClock.js\n\nClock.js\n\nReset\n\nFork\n\nimport { useState } from 'react';\n\nexport default function Clock(props) {\n const \\[color, setColor\\] = useState(props.color);\n return (\n <h1 style\\={{ color: color }}\\>\n {props.time}\n </h1\\>\n );\n}\n\nShow solutionNext Challenge\n\n[PreviousReacting to Input with State](https://react.dev/learn/reacting-to-input-with-state)[NextSharing State Between Components](https://react.dev/learn/sharing-state-between-components)\n",
"filename": "choosing-the-state-structure.md",
"package": "react"
} |
{
"content": "Title: Client React DOM APIs – React\n\nURL Source: https://react.dev/reference/react-dom/client\n\nMarkdown Content:\nClient React DOM APIs – React\n===============\n\n[API Reference](https://react.dev/reference/react)\n\nClient React DOM APIs[](https://react.dev/reference/react-dom/client#undefined \"Link for this heading\")\n=======================================================================================================\n\nThe `react-dom/client` APIs let you render React components on the client (in the browser). These APIs are typically used at the top level of your app to initialize your React tree. A [framework](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) may call them for you. Most of your components don’t need to import or use them.\n\n* * *\n\nClient APIs[](https://react.dev/reference/react-dom/client#client-apis \"Link for Client APIs \")\n-----------------------------------------------------------------------------------------------\n\n* [`createRoot`](https://react.dev/reference/react-dom/client/createRoot) lets you create a root to display React components inside a browser DOM node.\n* [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) lets you display React components inside a browser DOM node whose HTML content was previously generated by [`react-dom/server`.](https://react.dev/reference/react-dom/server)\n\n* * *\n\nBrowser support[](https://react.dev/reference/react-dom/client#browser-support \"Link for Browser support \")\n-----------------------------------------------------------------------------------------------------------\n\nReact supports all popular browsers, including Internet Explorer 9 and above. Some polyfills are required for older browsers such as IE 9 and IE 10.\n\n[PreviousunmountComponentAtNode](https://react.dev/reference/react-dom/unmountComponentAtNode)[NextcreateRoot](https://react.dev/reference/react-dom/client/createRoot)\n",
"filename": "client.md",
"package": "react"
} |
{
"content": "Title: cloneElement – React\n\nURL Source: https://react.dev/reference/react/cloneElement\n\nMarkdown Content:\n### Pitfall\n\nUsing `cloneElement` is uncommon and can lead to fragile code. [See common alternatives.](https://react.dev/reference/react/cloneElement#alternatives)\n\n`cloneElement` lets you create a new React element using another element as a starting point.\n\n```\nconst clonedElement = cloneElement(element, props, ...children)\n```\n\n* [Reference](https://react.dev/reference/react/cloneElement#reference)\n * [`cloneElement(element, props, ...children)`](https://react.dev/reference/react/cloneElement#cloneelement)\n* [Usage](https://react.dev/reference/react/cloneElement#usage)\n * [Overriding props of an element](https://react.dev/reference/react/cloneElement#overriding-props-of-an-element)\n* [Alternatives](https://react.dev/reference/react/cloneElement#alternatives)\n * [Passing data with a render prop](https://react.dev/reference/react/cloneElement#passing-data-with-a-render-prop)\n * [Passing data through context](https://react.dev/reference/react/cloneElement#passing-data-through-context)\n * [Extracting logic into a custom Hook](https://react.dev/reference/react/cloneElement#extracting-logic-into-a-custom-hook)\n\n* * *\n\nReference[](https://react.dev/reference/react/cloneElement#reference \"Link for Reference \")\n-------------------------------------------------------------------------------------------\n\n### `cloneElement(element, props, ...children)`[](https://react.dev/reference/react/cloneElement#cloneelement \"Link for this heading\")\n\nCall `cloneElement` to create a React element based on the `element`, but with different `props` and `children`:\n\n```\nimport { cloneElement } from 'react';// ...const clonedElement = cloneElement(<Row title=\"Cabbage\"> Hello</Row>,{ isHighlighted: true },'Goodbye');console.log(clonedElement); // <Row title=\"Cabbage\" isHighlighted={true}>Goodbye</Row>\n```\n\n[See more examples below.](https://react.dev/reference/react/cloneElement#usage)\n\n#### Parameters[](https://react.dev/reference/react/cloneElement#parameters \"Link for Parameters \")\n\n* `element`: The `element` argument must be a valid React element. For example, it could be a JSX node like `<Something />`, the result of calling [`createElement`](https://react.dev/reference/react/createElement), or the result of another `cloneElement` call.\n \n* `props`: The `props` argument must either be an object or `null`. If you pass `null`, the cloned element will retain all of the original `element.props`. Otherwise, for every prop in the `props` object, the returned element will “prefer” the value from `props` over the value from `element.props`. The rest of the props will be filled from the original `element.props`. If you pass `props.key` or `props.ref`, they will replace the original ones.\n \n* **optional** `...children`: Zero or more child nodes. They can be any React nodes, including React elements, strings, numbers, [portals](https://react.dev/reference/react-dom/createPortal), empty nodes (`null`, `undefined`, `true`, and `false`), and arrays of React nodes. If you don’t pass any `...children` arguments, the original `element.props.children` will be preserved.\n \n\n#### Returns[](https://react.dev/reference/react/cloneElement#returns \"Link for Returns \")\n\n`cloneElement` returns a React element object with a few properties:\n\n* `type`: Same as `element.type`.\n* `props`: The result of shallowly merging `element.props` with the overriding `props` you have passed.\n* `ref`: The original `element.ref`, unless it was overridden by `props.ref`.\n* `key`: The original `element.key`, unless it was overridden by `props.key`.\n\nUsually, you’ll return the element from your component or make it a child of another element. Although you may read the element’s properties, it’s best to treat every element as opaque after it’s created, and only render it.\n\n#### Caveats[](https://react.dev/reference/react/cloneElement#caveats \"Link for Caveats \")\n\n* Cloning an element **does not modify the original element.**\n \n* You should only **pass children as multiple arguments to `cloneElement` if they are all statically known,** like `cloneElement(element, null, child1, child2, child3)`. If your children are dynamic, pass the entire array as the third argument: `cloneElement(element, null, listItems)`. This ensures that React will [warn you about missing `key`s](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key) for any dynamic lists. For static lists this is not necessary because they never reorder.\n \n* `cloneElement` makes it harder to trace the data flow, so **try the [alternatives](https://react.dev/reference/react/cloneElement#alternatives) instead.**\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/cloneElement#usage \"Link for Usage \")\n-------------------------------------------------------------------------------\n\n### Overriding props of an element[](https://react.dev/reference/react/cloneElement#overriding-props-of-an-element \"Link for Overriding props of an element \")\n\nTo override the props of some React element, pass it to `cloneElement` with the props you want to override:\n\n```\nimport { cloneElement } from 'react';// ...const clonedElement = cloneElement(<Row title=\"Cabbage\" />,{ isHighlighted: true });\n```\n\nHere, the resulting cloned element will be `<Row title=\"Cabbage\" isHighlighted={true} />`.\n\n**Let’s walk through an example to see when it’s useful.**\n\nImagine a `List` component that renders its [`children`](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) as a list of selectable rows with a “Next” button that changes which row is selected. The `List` component needs to render the selected `Row` differently, so it clones every `<Row>` child that it has received, and adds an extra `isHighlighted: true` or `isHighlighted: false` prop:\n\n```\nexport default function List({ children }) {const [selectedIndex, setSelectedIndex] = useState(0);return (<div className=\"List\">{Children.map(children, (child, index) =>cloneElement(child, {isHighlighted: index === selectedIndex }))}\n```\n\nLet’s say the original JSX received by `List` looks like this:\n\n```\n<List><Row title=\"Cabbage\" /><Row title=\"Garlic\" /><Row title=\"Apple\" /></List>\n```\n\nBy cloning its children, the `List` can pass extra information to every `Row` inside. The result looks like this:\n\n```\n<List><Rowtitle=\"Cabbage\"isHighlighted={true} /><Rowtitle=\"Garlic\"isHighlighted={false} /><Rowtitle=\"Apple\"isHighlighted={false} /></List>\n```\n\nNotice how pressing “Next” updates the state of the `List`, and highlights a different row:\n\nimport { Children, cloneElement, useState } from 'react';\n\nexport default function List({ children }) {\n const \\[selectedIndex, setSelectedIndex\\] = useState(0);\n return (\n <div className\\=\"List\"\\>\n {Children.map(children, (child, index) \\=>\n cloneElement(child, {\n isHighlighted: index === selectedIndex \n })\n )}\n <hr />\n <button onClick\\={() \\=> {\n setSelectedIndex(i \\=>\n (i + 1) % Children.count(children)\n );\n }}\\>\n Next\n </button\\>\n </div\\>\n );\n}\n\nTo summarize, the `List` cloned the `<Row />` elements it received and added an extra prop to them.\n\n### Pitfall\n\nCloning children makes it hard to tell how the data flows through your app. Try one of the [alternatives.](https://react.dev/reference/react/cloneElement#alternatives)\n\n* * *\n\nAlternatives[](https://react.dev/reference/react/cloneElement#alternatives \"Link for Alternatives \")\n----------------------------------------------------------------------------------------------------\n\n### Passing data with a render prop[](https://react.dev/reference/react/cloneElement#passing-data-with-a-render-prop \"Link for Passing data with a render prop \")\n\nInstead of using `cloneElement`, consider accepting a _render prop_ like `renderItem`. Here, `List` receives `renderItem` as a prop. `List` calls `renderItem` for every item and passes `isHighlighted` as an argument:\n\n```\nexport default function List({ items, renderItem }) {const [selectedIndex, setSelectedIndex] = useState(0);return (<div className=\"List\">{items.map((item, index) => {const isHighlighted = index === selectedIndex;return renderItem(item, isHighlighted);})}\n```\n\nThe `renderItem` prop is called a “render prop” because it’s a prop that specifies how to render something. For example, you can pass a `renderItem` implementation that renders a `<Row>` with the given `isHighlighted` value:\n\n```\n<Listitems={products}renderItem={(product, isHighlighted) =><Rowkey={product.id}title={product.title}isHighlighted={isHighlighted}/>}/>\n```\n\nThe end result is the same as with `cloneElement`:\n\n```\n<List><Rowtitle=\"Cabbage\"isHighlighted={true} /><Rowtitle=\"Garlic\"isHighlighted={false} /><Rowtitle=\"Apple\"isHighlighted={false} /></List>\n```\n\nHowever, you can clearly trace where the `isHighlighted` value is coming from.\n\nimport { useState } from 'react';\n\nexport default function List({ items, renderItem }) {\n const \\[selectedIndex, setSelectedIndex\\] = useState(0);\n return (\n <div className\\=\"List\"\\>\n {items.map((item, index) \\=> {\n const isHighlighted = index === selectedIndex;\n return renderItem(item, isHighlighted);\n })}\n <hr />\n <button onClick\\={() \\=> {\n setSelectedIndex(i \\=>\n (i + 1) % items.length\n );\n }}\\>\n Next\n </button\\>\n </div\\>\n );\n}\n\nThis pattern is preferred to `cloneElement` because it is more explicit.\n\n* * *\n\n### Passing data through context[](https://react.dev/reference/react/cloneElement#passing-data-through-context \"Link for Passing data through context \")\n\nAnother alternative to `cloneElement` is to [pass data through context.](https://react.dev/learn/passing-data-deeply-with-context)\n\nFor example, you can call [`createContext`](https://react.dev/reference/react/createContext) to define a `HighlightContext`:\n\n```\nexport const HighlightContext = createContext(false);\n```\n\nYour `List` component can wrap every item it renders into a `HighlightContext` provider:\n\n```\nexport default function List({ items, renderItem }) {const [selectedIndex, setSelectedIndex] = useState(0);return (<div className=\"List\">{items.map((item, index) => {const isHighlighted = index === selectedIndex;return (<HighlightContext.Provider key={item.id} value={isHighlighted}>{renderItem(item)}</HighlightContext.Provider>);})}\n```\n\nWith this approach, `Row` does not need to receive an `isHighlighted` prop at all. Instead, it reads the context:\n\n```\nexport default function Row({ title }) {const isHighlighted = useContext(HighlightContext);// ...\n```\n\nThis allows the calling component to not know or worry about passing `isHighlighted` to `<Row>`:\n\n```\n<Listitems={products}renderItem={product =><Row title={product.title} />}/>\n```\n\nInstead, `List` and `Row` coordinate the highlighting logic through context.\n\nimport { useState } from 'react';\nimport { HighlightContext } from './HighlightContext.js';\n\nexport default function List({ items, renderItem }) {\n const \\[selectedIndex, setSelectedIndex\\] = useState(0);\n return (\n <div className\\=\"List\"\\>\n {items.map((item, index) \\=> {\n const isHighlighted = index === selectedIndex;\n return (\n <HighlightContext.Provider\n key\\={item.id}\n value\\={isHighlighted}\n \\>\n {renderItem(item)}\n </HighlightContext.Provider\\>\n );\n })}\n <hr />\n <button onClick\\={() \\=> {\n setSelectedIndex(i \\=>\n (i + 1) % items.length\n );\n }}\\>\n Next\n </button\\>\n </div\\>\n );\n}\n\n[Learn more about passing data through context.](https://react.dev/reference/react/useContext#passing-data-deeply-into-the-tree)\n\n* * *\n\nAnother approach you can try is to extract the “non-visual” logic into your own Hook, and use the information returned by your Hook to decide what to render. For example, you could write a `useList` custom Hook like this:\n\n```\nimport { useState } from 'react';export default function useList(items) {const [selectedIndex, setSelectedIndex] = useState(0);function onNext() {setSelectedIndex(i =>(i + 1) % items.length);}const selected = items[selectedIndex];return [selected, onNext];}\n```\n\nThen you could use it like this:\n\n```\nexport default function App() {const [selected, onNext] = useList(products);return (<div className=\"List\">{products.map(product =><Rowkey={product.id}title={product.title}isHighlighted={selected === product}/>)}<hr /><button onClick={onNext}> Next</button></div>);}\n```\n\nThe data flow is explicit, but the state is inside the `useList` custom Hook that you can use from any component:\n\nimport Row from './Row.js';\nimport useList from './useList.js';\nimport { products } from './data.js';\n\nexport default function App() {\n const \\[selected, onNext\\] = useList(products);\n return (\n <div className\\=\"List\"\\>\n {products.map(product \\=>\n <Row\n key\\={product.id}\n title\\={product.title}\n isHighlighted\\={selected === product}\n />\n )}\n <hr />\n <button onClick\\={onNext}\\>\n Next\n </button\\>\n </div\\>\n );\n}\n\nThis approach is particularly useful if you want to reuse this logic between different components.\n",
"filename": "cloneElement.md",
"package": "react"
} |
{
"content": "Title: Common components (e.g. <div>) – React\n\nURL Source: https://react.dev/reference/react-dom/components/common\n\nMarkdown Content:\n\nReference[](https://react.dev/reference/react-dom/components/common#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------\n\n### Common components (e.g. `<div>`)[](https://react.dev/reference/react-dom/components/common#common \"Link for this heading\")\n\n```\n<div className=\"wrapper\">Some content</div>\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/common#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/common#common-props \"Link for Props \")\n\nThese special React props are supported for all built-in components:\n\n* `children`: A React node (an element, a string, a number, [a portal,](https://react.dev/reference/react-dom/createPortal) an empty node like `null`, `undefined` and booleans, or an array of other React nodes). Specifies the content inside the component. When you use JSX, you will usually specify the `children` prop implicitly by nesting tags like `<div><span /></div>`.\n \n* `dangerouslySetInnerHTML`: An object of the form `{ __html: '<p>some html</p>' }` with a raw HTML string inside. Overrides the [`innerHTML`](https://developer.mozilla.org/en-US/docs/Web/API/Element/innerHTML) property of the DOM node and displays the passed HTML inside. This should be used with extreme caution! If the HTML inside isn’t trusted (for example, if it’s based on user data), you risk introducing an [XSS](https://en.wikipedia.org/wiki/Cross-site_scripting) vulnerability. [Read more about using `dangerouslySetInnerHTML`.](https://react.dev/reference/react-dom/components/common#dangerously-setting-the-inner-html)\n \n* `ref`: A ref object from [`useRef`](https://react.dev/reference/react/useRef) or [`createRef`](https://react.dev/reference/react/createRef), or a [`ref` callback function,](https://react.dev/reference/react-dom/components/common#ref-callback) or a string for [legacy refs.](https://reactjs.org/docs/refs-and-the-dom.html#legacy-api-string-refs) Your ref will be filled with the DOM element for this node. [Read more about manipulating the DOM with refs.](https://react.dev/reference/react-dom/components/common#manipulating-a-dom-node-with-a-ref)\n \n* `suppressContentEditableWarning`: A boolean. If `true`, suppresses the warning that React shows for elements that both have `children` and `contentEditable={true}` (which normally do not work together). Use this if you’re building a text input library that manages the `contentEditable` content manually.\n \n* `suppressHydrationWarning`: A boolean. If you use [server rendering,](https://react.dev/reference/react-dom/server) normally there is a warning when the server and the client render different content. In some rare cases (like timestamps), it is very hard or impossible to guarantee an exact match. If you set `suppressHydrationWarning` to `true`, React will not warn you about mismatches in the attributes and the content of that element. It only works one level deep, and is intended to be used as an escape hatch. Don’t overuse it. [Read about suppressing hydration errors.](https://react.dev/reference/react-dom/client/hydrateRoot#suppressing-unavoidable-hydration-mismatch-errors)\n \n* `style`: An object with CSS styles, for example `{ fontWeight: 'bold', margin: 20 }`. Similarly to the DOM [`style`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/style) property, the CSS property names need to be written as `camelCase`, for example `fontWeight` instead of `font-weight`. You can pass strings or numbers as values. If you pass a number, like `width: 100`, React will automatically append `px` (“pixels”) to the value unless it’s a [unitless property.](https://github.com/facebook/react/blob/81d4ee9ca5c405dce62f64e61506b8e155f38d8d/packages/react-dom-bindings/src/shared/CSSProperty.js#L8-L57) We recommend using `style` only for dynamic styles where you don’t know the style values ahead of time. In other cases, applying plain CSS classes with `className` is more efficient. [Read more about `className` and `style`.](https://react.dev/reference/react-dom/components/common#applying-css-styles)\n \n\nThese standard DOM props are also supported for all built-in components:\n\n* [`accessKey`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/accesskey): A string. Specifies a keyboard shortcut for the element. [Not generally recommended.](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/accesskey#accessibility_concerns)\n* [`aria-*`](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/Attributes): ARIA attributes let you specify the accessibility tree information for this element. See [ARIA attributes](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/Attributes) for a complete reference. In React, all ARIA attribute names are exactly the same as in HTML.\n* [`autoCapitalize`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/autocapitalize): A string. Specifies whether and how the user input should be capitalized.\n* [`className`](https://developer.mozilla.org/en-US/docs/Web/API/Element/className): A string. Specifies the element’s CSS class name. [Read more about applying CSS styles.](https://react.dev/reference/react-dom/components/common#applying-css-styles)\n* [`contentEditable`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/contenteditable): A boolean. If `true`, the browser lets the user edit the rendered element directly. This is used to implement rich text input libraries like [Lexical.](https://lexical.dev/) React warns if you try to pass React children to an element with `contentEditable={true}` because React will not be able to update its content after user edits.\n* [`data-*`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/data-*): Data attributes let you attach some string data to the element, for example `data-fruit=\"banana\"`. In React, they are not commonly used because you would usually read data from props or state instead.\n* [`dir`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/dir): Either `'ltr'` or `'rtl'`. Specifies the text direction of the element.\n* [`draggable`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/draggable): A boolean. Specifies whether the element is draggable. Part of [HTML Drag and Drop API.](https://developer.mozilla.org/en-US/docs/Web/API/HTML_Drag_and_Drop_API)\n* [`enterKeyHint`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/enterKeyHint): A string. Specifies which action to present for the enter key on virtual keyboards.\n* [`htmlFor`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLLabelElement/htmlFor): A string. For [`<label>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/label) and [`<output>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/output), lets you [associate the label with some control.](https://react.dev/reference/react-dom/components/input#providing-a-label-for-an-input) Same as [`for` HTML attribute.](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/for) React uses the standard DOM property names (`htmlFor`) instead of HTML attribute names.\n* [`hidden`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/hidden): A boolean or a string. Specifies whether the element should be hidden.\n* [`id`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/id): A string. Specifies a unique identifier for this element, which can be used to find it later or connect it with other elements. Generate it with [`useId`](https://react.dev/reference/react/useId) to avoid clashes between multiple instances of the same component.\n* [`is`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/is): A string. If specified, the component will behave like a [custom element.](https://react.dev/reference/react-dom/components#custom-html-elements)\n* [`inputMode`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/inputmode): A string. Specifies what kind of keyboard to display (for example, text, number or telephone).\n* [`itemProp`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/itemprop): A string. Specifies which property the element represents for structured data crawlers.\n* [`lang`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/lang): A string. Specifies the language of the element.\n* [`onAnimationEnd`](https://developer.mozilla.org/en-US/docs/Web/API/Element/animationend_event): An [`AnimationEvent` handler](https://react.dev/reference/react-dom/components/common#animationevent-handler) function. Fires when a CSS animation completes.\n* `onAnimationEndCapture`: A version of `onAnimationEnd` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onAnimationIteration`](https://developer.mozilla.org/en-US/docs/Web/API/Element/animationiteration_event): An [`AnimationEvent` handler](https://react.dev/reference/react-dom/components/common#animationevent-handler) function. Fires when an iteration of a CSS animation ends, and another one begins.\n* `onAnimationIterationCapture`: A version of `onAnimationIteration` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onAnimationStart`](https://developer.mozilla.org/en-US/docs/Web/API/Element/animationstart_event): An [`AnimationEvent` handler](https://react.dev/reference/react-dom/components/common#animationevent-handler) function. Fires when a CSS animation starts.\n* `onAnimationStartCapture`: `onAnimationStart`, but fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onAuxClick`](https://developer.mozilla.org/en-US/docs/Web/API/Element/auxclick_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when a non-primary pointer button was clicked.\n* `onAuxClickCapture`: A version of `onAuxClick` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* `onBeforeInput`: An [`InputEvent` handler](https://react.dev/reference/react-dom/components/common#inputevent-handler) function. Fires before the value of an editable element is modified. React does _not_ yet use the native [`beforeinput`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/beforeinput_event) event, and instead attempts to polyfill it using other events.\n* `onBeforeInputCapture`: A version of `onBeforeInput` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* `onBlur`: A [`FocusEvent` handler](https://react.dev/reference/react-dom/components/common#focusevent-handler) function. Fires when an element lost focus. Unlike the built-in browser [`blur`](https://developer.mozilla.org/en-US/docs/Web/API/Element/blur_event) event, in React the `onBlur` event bubbles.\n* `onBlurCapture`: A version of `onBlur` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onClick`](https://developer.mozilla.org/en-US/docs/Web/API/Element/click_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the primary button was clicked on the pointing device.\n* `onClickCapture`: A version of `onClick` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCompositionStart`](https://developer.mozilla.org/en-US/docs/Web/API/Element/compositionstart_event): A [`CompositionEvent` handler](https://react.dev/reference/react-dom/components/common#compositionevent-handler) function. Fires when an [input method editor](https://developer.mozilla.org/en-US/docs/Glossary/Input_method_editor) starts a new composition session.\n* `onCompositionStartCapture`: A version of `onCompositionStart` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCompositionEnd`](https://developer.mozilla.org/en-US/docs/Web/API/Element/compositionend_event): A [`CompositionEvent` handler](https://react.dev/reference/react-dom/components/common#compositionevent-handler) function. Fires when an [input method editor](https://developer.mozilla.org/en-US/docs/Glossary/Input_method_editor) completes or cancels a composition session.\n* `onCompositionEndCapture`: A version of `onCompositionEnd` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCompositionUpdate`](https://developer.mozilla.org/en-US/docs/Web/API/Element/compositionupdate_event): A [`CompositionEvent` handler](https://react.dev/reference/react-dom/components/common#compositionevent-handler) function. Fires when an [input method editor](https://developer.mozilla.org/en-US/docs/Glossary/Input_method_editor) receives a new character.\n* `onCompositionUpdateCapture`: A version of `onCompositionUpdate` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onContextMenu`](https://developer.mozilla.org/en-US/docs/Web/API/Element/contextmenu_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the user tries to open a context menu.\n* `onContextMenuCapture`: A version of `onContextMenu` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCopy`](https://developer.mozilla.org/en-US/docs/Web/API/Element/copy_event): A [`ClipboardEvent` handler](https://react.dev/reference/react-dom/components/common#clipboardevent-handler) function. Fires when the user tries to copy something into the clipboard.\n* `onCopyCapture`: A version of `onCopy` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCut`](https://developer.mozilla.org/en-US/docs/Web/API/Element/cut_event): A [`ClipboardEvent` handler](https://react.dev/reference/react-dom/components/common#clipboardevent-handler) function. Fires when the user tries to cut something into the clipboard.\n* `onCutCapture`: A version of `onCut` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* `onDoubleClick`: A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the user clicks twice. Corresponds to the browser [`dblclick` event.](https://developer.mozilla.org/en-US/docs/Web/API/Element/dblclick_event)\n* `onDoubleClickCapture`: A version of `onDoubleClick` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDrag`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/drag_event): A [`DragEvent` handler](https://react.dev/reference/react-dom/components/common#dragevent-handler) function. Fires while the user is dragging something.\n* `onDragCapture`: A version of `onDrag` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDragEnd`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dragend_event): A [`DragEvent` handler](https://react.dev/reference/react-dom/components/common#dragevent-handler) function. Fires when the user stops dragging something.\n* `onDragEndCapture`: A version of `onDragEnd` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDragEnter`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dragenter_event): A [`DragEvent` handler](https://react.dev/reference/react-dom/components/common#dragevent-handler) function. Fires when the dragged content enters a valid drop target.\n* `onDragEnterCapture`: A version of `onDragEnter` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDragOver`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dragover_event): A [`DragEvent` handler](https://react.dev/reference/react-dom/components/common#dragevent-handler) function. Fires on a valid drop target while the dragged content is dragged over it. You must call `e.preventDefault()` here to allow dropping.\n* `onDragOverCapture`: A version of `onDragOver` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDragStart`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dragstart_event): A [`DragEvent` handler](https://react.dev/reference/react-dom/components/common#dragevent-handler) function. Fires when the user starts dragging an element.\n* `onDragStartCapture`: A version of `onDragStart` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDrop`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/drop_event): A [`DragEvent` handler](https://react.dev/reference/react-dom/components/common#dragevent-handler) function. Fires when something is dropped on a valid drop target.\n* `onDropCapture`: A version of `onDrop` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* `onFocus`: A [`FocusEvent` handler](https://react.dev/reference/react-dom/components/common#focusevent-handler) function. Fires when an element receives focus. Unlike the built-in browser [`focus`](https://developer.mozilla.org/en-US/docs/Web/API/Element/focus_event) event, in React the `onFocus` event bubbles.\n* `onFocusCapture`: A version of `onFocus` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onGotPointerCapture`](https://developer.mozilla.org/en-US/docs/Web/API/Element/gotpointercapture_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when an element programmatically captures a pointer.\n* `onGotPointerCaptureCapture`: A version of `onGotPointerCapture` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onKeyDown`](https://developer.mozilla.org/en-US/docs/Web/API/Element/keydown_event): A [`KeyboardEvent` handler](https://react.dev/reference/react-dom/components/common#keyboardevent-handler) function. Fires when a key is pressed.\n* `onKeyDownCapture`: A version of `onKeyDown` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onKeyPress`](https://developer.mozilla.org/en-US/docs/Web/API/Element/keypress_event): A [`KeyboardEvent` handler](https://react.dev/reference/react-dom/components/common#keyboardevent-handler) function. Deprecated. Use `onKeyDown` or `onBeforeInput` instead.\n* `onKeyPressCapture`: A version of `onKeyPress` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onKeyUp`](https://developer.mozilla.org/en-US/docs/Web/API/Element/keyup_event): A [`KeyboardEvent` handler](https://react.dev/reference/react-dom/components/common#keyboardevent-handler) function. Fires when a key is released.\n* `onKeyUpCapture`: A version of `onKeyUp` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onLostPointerCapture`](https://developer.mozilla.org/en-US/docs/Web/API/Element/lostpointercapture_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when an element stops capturing a pointer.\n* `onLostPointerCaptureCapture`: A version of `onLostPointerCapture` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onMouseDown`](https://developer.mozilla.org/en-US/docs/Web/API/Element/mousedown_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the pointer is pressed down.\n* `onMouseDownCapture`: A version of `onMouseDown` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onMouseEnter`](https://developer.mozilla.org/en-US/docs/Web/API/Element/mouseenter_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the pointer moves inside an element. Does not have a capture phase. Instead, `onMouseLeave` and `onMouseEnter` propagate from the element being left to the one being entered.\n* [`onMouseLeave`](https://developer.mozilla.org/en-US/docs/Web/API/Element/mouseleave_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the pointer moves outside an element. Does not have a capture phase. Instead, `onMouseLeave` and `onMouseEnter` propagate from the element being left to the one being entered.\n* [`onMouseMove`](https://developer.mozilla.org/en-US/docs/Web/API/Element/mousemove_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the pointer changes coordinates.\n* `onMouseMoveCapture`: A version of `onMouseMove` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onMouseOut`](https://developer.mozilla.org/en-US/docs/Web/API/Element/mouseout_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the pointer moves outside an element, or if it moves into a child element.\n* `onMouseOutCapture`: A version of `onMouseOut` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onMouseUp`](https://developer.mozilla.org/en-US/docs/Web/API/Element/mouseup_event): A [`MouseEvent` handler](https://react.dev/reference/react-dom/components/common#mouseevent-handler) function. Fires when the pointer is released.\n* `onMouseUpCapture`: A version of `onMouseUp` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPointerCancel`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointercancel_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when the browser cancels a pointer interaction.\n* `onPointerCancelCapture`: A version of `onPointerCancel` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPointerDown`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointerdown_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when a pointer becomes active.\n* `onPointerDownCapture`: A version of `onPointerDown` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPointerEnter`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointerenter_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when a pointer moves inside an element. Does not have a capture phase. Instead, `onPointerLeave` and `onPointerEnter` propagate from the element being left to the one being entered.\n* [`onPointerLeave`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointerleave_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when a pointer moves outside an element. Does not have a capture phase. Instead, `onPointerLeave` and `onPointerEnter` propagate from the element being left to the one being entered.\n* [`onPointerMove`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointermove_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when a pointer changes coordinates.\n* `onPointerMoveCapture`: A version of `onPointerMove` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPointerOut`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointerout_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when a pointer moves outside an element, if the pointer interaction is cancelled, and [a few other reasons.](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointerout_event)\n* `onPointerOutCapture`: A version of `onPointerOut` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPointerUp`](https://developer.mozilla.org/en-US/docs/Web/API/Element/pointerup_event): A [`PointerEvent` handler](https://react.dev/reference/react-dom/components/common#pointerevent-handler) function. Fires when a pointer is no longer active.\n* `onPointerUpCapture`: A version of `onPointerUp` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPaste`](https://developer.mozilla.org/en-US/docs/Web/API/Element/paste_event): A [`ClipboardEvent` handler](https://react.dev/reference/react-dom/components/common#clipboardevent-handler) function. Fires when the user tries to paste something from the clipboard.\n* `onPasteCapture`: A version of `onPaste` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onScroll`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scroll_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when an element has been scrolled. This event does not bubble.\n* `onScrollCapture`: A version of `onScroll` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSelect`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement/select_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires after the selection inside an editable element like an input changes. React extends the `onSelect` event to work for `contentEditable={true}` elements as well. In addition, React extends it to fire for empty selection and on edits (which may affect the selection).\n* `onSelectCapture`: A version of `onSelect` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onTouchCancel`](https://developer.mozilla.org/en-US/docs/Web/API/Element/touchcancel_event): A [`TouchEvent` handler](https://react.dev/reference/react-dom/components/common#touchevent-handler) function. Fires when the browser cancels a touch interaction.\n* `onTouchCancelCapture`: A version of `onTouchCancel` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onTouchEnd`](https://developer.mozilla.org/en-US/docs/Web/API/Element/touchend_event): A [`TouchEvent` handler](https://react.dev/reference/react-dom/components/common#touchevent-handler) function. Fires when one or more touch points are removed.\n* `onTouchEndCapture`: A version of `onTouchEnd` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onTouchMove`](https://developer.mozilla.org/en-US/docs/Web/API/Element/touchmove_event): A [`TouchEvent` handler](https://react.dev/reference/react-dom/components/common#touchevent-handler) function. Fires one or more touch points are moved.\n* `onTouchMoveCapture`: A version of `onTouchMove` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onTouchStart`](https://developer.mozilla.org/en-US/docs/Web/API/Element/touchstart_event): A [`TouchEvent` handler](https://react.dev/reference/react-dom/components/common#touchevent-handler) function. Fires when one or more touch points are placed.\n* `onTouchStartCapture`: A version of `onTouchStart` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onTransitionEnd`](https://developer.mozilla.org/en-US/docs/Web/API/Element/transitionend_event): A [`TransitionEvent` handler](https://react.dev/reference/react-dom/components/common#transitionevent-handler) function. Fires when a CSS transition completes.\n* `onTransitionEndCapture`: A version of `onTransitionEnd` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onWheel`](https://developer.mozilla.org/en-US/docs/Web/API/Element/wheel_event): A [`WheelEvent` handler](https://react.dev/reference/react-dom/components/common#wheelevent-handler) function. Fires when the user rotates a wheel button.\n* `onWheelCapture`: A version of `onWheel` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`role`](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/Roles): A string. Specifies the element role explicitly for assistive technologies.\n* [`slot`](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/Roles): A string. Specifies the slot name when using shadow DOM. In React, an equivalent pattern is typically achieved by passing JSX as props, for example `<Layout left={<Sidebar />} right={<Content />} />`.\n* [`spellCheck`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/spellcheck): A boolean or null. If explicitly set to `true` or `false`, enables or disables spellchecking.\n* [`tabIndex`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/tabindex): A number. Overrides the default Tab button behavior. [Avoid using values other than `-1` and `0`.](https://www.tpgi.com/using-the-tabindex-attribute/)\n* [`title`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/title): A string. Specifies the tooltip text for the element.\n* [`translate`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/translate): Either `'yes'` or `'no'`. Passing `'no'` excludes the element content from being translated.\n\nYou can also pass custom attributes as props, for example `mycustomprop=\"someValue\"`. This can be useful when integrating with third-party libraries. The custom attribute name must be lowercase and must not start with `on`. The value will be converted to a string. If you pass `null` or `undefined`, the custom attribute will be removed.\n\nThese events fire only for the [`<form>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) elements:\n\n* [`onReset`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLFormElement/reset_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when a form gets reset.\n* `onResetCapture`: A version of `onReset` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSubmit`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLFormElement/submit_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when a form gets submitted.\n* `onSubmitCapture`: A version of `onSubmit` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n\nThese events fire only for the [`<dialog>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/dialog) elements. Unlike browser events, they bubble in React:\n\n* [`onCancel`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDialogElement/cancel_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the user tries to dismiss the dialog.\n* `onCancelCapture`: A version of `onCancel` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onClose`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDialogElement/close_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when a dialog has been closed.\n* `onCloseCapture`: A version of `onClose` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n\nThese events fire only for the [`<details>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/details) elements. Unlike browser events, they bubble in React:\n\n* [`onToggle`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDetailsElement/toggle_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the user toggles the details.\n* `onToggleCapture`: A version of `onToggle` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n\nThese events fire for [`<img>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/img), [`<iframe>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/iframe), [`<object>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/object), [`<embed>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/embed), [`<link>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link), and [SVG `<image>`](https://developer.mozilla.org/en-US/docs/Web/SVG/Tutorial/SVG_Image_Tag) elements. Unlike browser events, they bubble in React:\n\n* `onLoad`: An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the resource has loaded.\n* `onLoadCapture`: A version of `onLoad` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onError`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/error_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the resource could not be loaded.\n* `onErrorCapture`: A version of `onError` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n\nThese events fire for resources like [`<audio>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/audio) and [`<video>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/video). Unlike browser events, they bubble in React:\n\n* [`onAbort`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/abort_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the resource has not fully loaded, but not due to an error.\n* `onAbortCapture`: A version of `onAbort` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCanPlay`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/canplay_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when there’s enough data to start playing, but not enough to play to the end without buffering.\n* `onCanPlayCapture`: A version of `onCanPlay` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onCanPlayThrough`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/canplaythrough_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when there’s enough data that it’s likely possible to start playing without buffering until the end.\n* `onCanPlayThroughCapture`: A version of `onCanPlayThrough` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onDurationChange`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/durationchange_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the media duration has updated.\n* `onDurationChangeCapture`: A version of `onDurationChange` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onEmptied`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/emptied_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the media has become empty.\n* `onEmptiedCapture`: A version of `onEmptied` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onEncrypted`](https://w3c.github.io/encrypted-media/#dom-evt-encrypted): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the browser encounters encrypted media.\n* `onEncryptedCapture`: A version of `onEncrypted` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onEnded`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/ended_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the playback stops because there’s nothing left to play.\n* `onEndedCapture`: A version of `onEnded` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onError`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/error_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the resource could not be loaded.\n* `onErrorCapture`: A version of `onError` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onLoadedData`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/loadeddata_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the current playback frame has loaded.\n* `onLoadedDataCapture`: A version of `onLoadedData` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onLoadedMetadata`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/loadedmetadata_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when metadata has loaded.\n* `onLoadedMetadataCapture`: A version of `onLoadedMetadata` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onLoadStart`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/loadstart_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the browser started loading the resource.\n* `onLoadStartCapture`: A version of `onLoadStart` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPause`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/pause_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the media was paused.\n* `onPauseCapture`: A version of `onPause` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPlay`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/play_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the media is no longer paused.\n* `onPlayCapture`: A version of `onPlay` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onPlaying`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/playing_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the media starts or restarts playing.\n* `onPlayingCapture`: A version of `onPlaying` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onProgress`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/progress_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires periodically while the resource is loading.\n* `onProgressCapture`: A version of `onProgress` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onRateChange`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/ratechange_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when playback rate changes.\n* `onRateChangeCapture`: A version of `onRateChange` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* `onResize`: An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when video changes size.\n* `onResizeCapture`: A version of `onResize` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSeeked`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/seeked_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when a seek operation completes.\n* `onSeekedCapture`: A version of `onSeeked` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSeeking`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/seeking_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when a seek operation starts.\n* `onSeekingCapture`: A version of `onSeeking` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onStalled`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/stalled_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the browser is waiting for data but it keeps not loading.\n* `onStalledCapture`: A version of `onStalled` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSuspend`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/suspend_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when loading the resource was suspended.\n* `onSuspendCapture`: A version of `onSuspend` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onTimeUpdate`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/timeupdate_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the current playback time updates.\n* `onTimeUpdateCapture`: A version of `onTimeUpdate` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onVolumeChange`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/volumechange_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the volume has changed.\n* `onVolumeChangeCapture`: A version of `onVolumeChange` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onWaiting`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/waiting_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires when the playback stopped due to temporary lack of data.\n* `onWaitingCapture`: A version of `onWaiting` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n\n#### Caveats[](https://react.dev/reference/react-dom/components/common#common-caveats \"Link for Caveats \")\n\n* You cannot pass both `children` and `dangerouslySetInnerHTML` at the same time.\n* Some events (like `onAbort` and `onLoad`) don’t bubble in the browser, but bubble in React.\n\n* * *\n\n### `ref` callback function[](https://react.dev/reference/react-dom/components/common#ref-callback \"Link for this heading\")\n\nInstead of a ref object (like the one returned by [`useRef`](https://react.dev/reference/react/useRef#manipulating-the-dom-with-a-ref)), you may pass a function to the `ref` attribute.\n\n```\n<div ref={(node) => console.log(node)} />\n```\n\n[See an example of using the `ref` callback.](https://react.dev/learn/manipulating-the-dom-with-refs#how-to-manage-a-list-of-refs-using-a-ref-callback)\n\nWhen the `<div>` DOM node is added to the screen, React will call your `ref` callback with the DOM `node` as the argument. When that `<div>` DOM node is removed, React will call your `ref` callback with `null`.\n\nReact will also call your `ref` callback whenever you pass a _different_ `ref` callback. In the above example, `(node) => { ... }` is a different function on every render. When your component re-renders, the _previous_ function will be called with `null` as the argument, and the _next_ function will be called with the DOM node.\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#ref-callback-parameters \"Link for Parameters \")\n\n* `node`: A DOM node or `null`. React will pass you the DOM node when the ref gets attached, and `null` when the `ref` gets detached. Unless you pass the same function reference for the `ref` callback on every render, the callback will get temporarily detached and re-attached during every re-render of the component.\n\n### Canary\n\n#### Returns[](https://react.dev/reference/react-dom/components/common#returns \"Link for Returns \")\n\n* **optional** `cleanup function`: When the `ref` is detached, React will call the cleanup function. If a function is not returned by the `ref` callback, React will call the callback again with `null` as the argument when the `ref` gets detached.\n\n```\n<div ref={(node) => {console.log(node);return () => {console.log('Clean up', node)}}}>\n```\n\n#### Caveats[](https://react.dev/reference/react-dom/components/common#caveats \"Link for Caveats \")\n\n* When Strict Mode is on, React will **run one extra development-only setup+cleanup cycle** before the first real setup. This is a stress-test that ensures that your cleanup logic “mirrors” your setup logic and that it stops or undoes whatever the setup is doing. If this causes a problem, implement the cleanup function.\n* When you pass a _different_ `ref` callback, React will call the _previous_ callback’s cleanup function if provided. If not cleanup function is defined, the `ref` callback will be called with `null` as the argument. The _next_ function will be called with the DOM node.\n\n* * *\n\n### React event object[](https://react.dev/reference/react-dom/components/common#react-event-object \"Link for React event object \")\n\nYour event handlers will receive a _React event object._ It is also sometimes known as a “synthetic event”.\n\n```\n<button onClick={e => {console.log(e); // React event object}} />\n```\n\nIt conforms to the same standard as the underlying DOM events, but fixes some browser inconsistencies.\n\nSome React events do not map directly to the browser’s native events. For example in `onMouseLeave`, `e.nativeEvent` will point to a `mouseout` event. The specific mapping is not part of the public API and may change in the future. If you need the underlying browser event for some reason, read it from `e.nativeEvent`.\n\n#### Properties[](https://react.dev/reference/react-dom/components/common#react-event-object-properties \"Link for Properties \")\n\nReact event objects implement some of the standard [`Event`](https://developer.mozilla.org/en-US/docs/Web/API/Event) properties:\n\n* [`bubbles`](https://developer.mozilla.org/en-US/docs/Web/API/Event/bubbles): A boolean. Returns whether the event bubbles through the DOM.\n* [`cancelable`](https://developer.mozilla.org/en-US/docs/Web/API/Event/cancelable): A boolean. Returns whether the event can be canceled.\n* [`currentTarget`](https://developer.mozilla.org/en-US/docs/Web/API/Event/currentTarget): A DOM node. Returns the node to which the current handler is attached in the React tree.\n* [`defaultPrevented`](https://developer.mozilla.org/en-US/docs/Web/API/Event/defaultPrevented): A boolean. Returns whether `preventDefault` was called.\n* [`eventPhase`](https://developer.mozilla.org/en-US/docs/Web/API/Event/eventPhase): A number. Returns which phase the event is currently in.\n* [`isTrusted`](https://developer.mozilla.org/en-US/docs/Web/API/Event/isTrusted): A boolean. Returns whether the event was initiated by user.\n* [`target`](https://developer.mozilla.org/en-US/docs/Web/API/Event/target): A DOM node. Returns the node on which the event has occurred (which could be a distant child).\n* [`timeStamp`](https://developer.mozilla.org/en-US/docs/Web/API/Event/timeStamp): A number. Returns the time when the event occurred.\n\nAdditionally, React event objects provide these properties:\n\n* `nativeEvent`: A DOM [`Event`](https://developer.mozilla.org/en-US/docs/Web/API/Event). The original browser event object.\n\n#### Methods[](https://react.dev/reference/react-dom/components/common#react-event-object-methods \"Link for Methods \")\n\nReact event objects implement some of the standard [`Event`](https://developer.mozilla.org/en-US/docs/Web/API/Event) methods:\n\n* [`preventDefault()`](https://developer.mozilla.org/en-US/docs/Web/API/Event/preventDefault): Prevents the default browser action for the event.\n* [`stopPropagation()`](https://developer.mozilla.org/en-US/docs/Web/API/Event/stopPropagation): Stops the event propagation through the React tree.\n\nAdditionally, React event objects provide these methods:\n\n* `isDefaultPrevented()`: Returns a boolean value indicating whether `preventDefault` was called.\n* `isPropagationStopped()`: Returns a boolean value indicating whether `stopPropagation` was called.\n* `persist()`: Not used with React DOM. With React Native, call this to read event’s properties after the event.\n* `isPersistent()`: Not used with React DOM. With React Native, returns whether `persist` has been called.\n\n#### Caveats[](https://react.dev/reference/react-dom/components/common#react-event-object-caveats \"Link for Caveats \")\n\n* The values of `currentTarget`, `eventPhase`, `target`, and `type` reflect the values your React code expects. Under the hood, React attaches event handlers at the root, but this is not reflected in React event objects. For example, `e.currentTarget` may not be the same as the underlying `e.nativeEvent.currentTarget`. For polyfilled events, `e.type` (React event type) may differ from `e.nativeEvent.type` (underlying type).\n\n* * *\n\n### `AnimationEvent` handler function[](https://react.dev/reference/react-dom/components/common#animationevent-handler \"Link for this heading\")\n\nAn event handler type for the [CSS animation](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Animations/Using_CSS_animations) events.\n\n```\n<divonAnimationStart={e => console.log('onAnimationStart')}onAnimationIteration={e => console.log('onAnimationIteration')}onAnimationEnd={e => console.log('onAnimationEnd')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#animationevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`AnimationEvent`](https://developer.mozilla.org/en-US/docs/Web/API/AnimationEvent) properties:\n * [`animationName`](https://developer.mozilla.org/en-US/docs/Web/API/AnimationEvent/animationName)\n * [`elapsedTime`](https://developer.mozilla.org/en-US/docs/Web/API/AnimationEvent/elapsedTime)\n * [`pseudoElement`](https://developer.mozilla.org/en-US/docs/Web/API/AnimationEvent/pseudoElement)\n\n* * *\n\n### `ClipboardEvent` handler function[](https://react.dev/reference/react-dom/components/common#clipboadevent-handler \"Link for this heading\")\n\nAn event handler type for the [Clipboard API](https://developer.mozilla.org/en-US/docs/Web/API/Clipboard_API) events.\n\n```\n<inputonCopy={e => console.log('onCopy')}onCut={e => console.log('onCut')}onPaste={e => console.log('onPaste')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#clipboadevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`ClipboardEvent`](https://developer.mozilla.org/en-US/docs/Web/API/ClipboardEvent) properties:\n \n * [`clipboardData`](https://developer.mozilla.org/en-US/docs/Web/API/ClipboardEvent/clipboardData)\n\n* * *\n\n### `CompositionEvent` handler function[](https://react.dev/reference/react-dom/components/common#compositionevent-handler \"Link for this heading\")\n\nAn event handler type for the [input method editor (IME)](https://developer.mozilla.org/en-US/docs/Glossary/Input_method_editor) events.\n\n```\n<inputonCompositionStart={e => console.log('onCompositionStart')}onCompositionUpdate={e => console.log('onCompositionUpdate')}onCompositionEnd={e => console.log('onCompositionEnd')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#compositionevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`CompositionEvent`](https://developer.mozilla.org/en-US/docs/Web/API/CompositionEvent) properties:\n * [`data`](https://developer.mozilla.org/en-US/docs/Web/API/CompositionEvent/data)\n\n* * *\n\n### `DragEvent` handler function[](https://react.dev/reference/react-dom/components/common#dragevent-handler \"Link for this heading\")\n\nAn event handler type for the [HTML Drag and Drop API](https://developer.mozilla.org/en-US/docs/Web/API/HTML_Drag_and_Drop_API) events.\n\n```\n<><divdraggable={true}onDragStart={e => console.log('onDragStart')}onDragEnd={e => console.log('onDragEnd')}> Drag source</div><divonDragEnter={e => console.log('onDragEnter')}onDragLeave={e => console.log('onDragLeave')}onDragOver={e => { e.preventDefault(); console.log('onDragOver'); }}onDrop={e => console.log('onDrop')}> Drop target</div></>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#dragevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`DragEvent`](https://developer.mozilla.org/en-US/docs/Web/API/DragEvent) properties:\n \n * [`dataTransfer`](https://developer.mozilla.org/en-US/docs/Web/API/DragEvent/dataTransfer)\n \n It also includes the inherited [`MouseEvent`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent) properties:\n \n * [`altKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/altKey)\n * [`button`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/button)\n * [`buttons`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/buttons)\n * [`ctrlKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/ctrlKey)\n * [`clientX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientX)\n * [`clientY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientY)\n * [`getModifierState(key)`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/getModifierState)\n * [`metaKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/metaKey)\n * [`movementX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementX)\n * [`movementY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementY)\n * [`pageX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageX)\n * [`pageY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageY)\n * [`relatedTarget`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/relatedTarget)\n * [`screenX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenX)\n * [`screenY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenY)\n * [`shiftKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/shiftKey)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `FocusEvent` handler function[](https://react.dev/reference/react-dom/components/common#focusevent-handler \"Link for this heading\")\n\nAn event handler type for the focus events.\n\n```\n<inputonFocus={e => console.log('onFocus')}onBlur={e => console.log('onBlur')}/>\n```\n\n[See an example.](https://react.dev/reference/react-dom/components/common#handling-focus-events)\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#focusevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`FocusEvent`](https://developer.mozilla.org/en-US/docs/Web/API/FocusEvent) properties:\n \n * [`relatedTarget`](https://developer.mozilla.org/en-US/docs/Web/API/FocusEvent/relatedTarget)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `Event` handler function[](https://react.dev/reference/react-dom/components/common#event-handler \"Link for this heading\")\n\nAn event handler type for generic events.\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#event-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with no additional properties.\n\n* * *\n\n### `InputEvent` handler function[](https://react.dev/reference/react-dom/components/common#inputevent-handler \"Link for this heading\")\n\nAn event handler type for the `onBeforeInput` event.\n\n```\n<input onBeforeInput={e => console.log('onBeforeInput')} />\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#inputevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`InputEvent`](https://developer.mozilla.org/en-US/docs/Web/API/InputEvent) properties:\n * [`data`](https://developer.mozilla.org/en-US/docs/Web/API/InputEvent/data)\n\n* * *\n\n### `KeyboardEvent` handler function[](https://react.dev/reference/react-dom/components/common#keyboardevent-handler \"Link for this heading\")\n\nAn event handler type for keyboard events.\n\n```\n<inputonKeyDown={e => console.log('onKeyDown')}onKeyUp={e => console.log('onKeyUp')}/>\n```\n\n[See an example.](https://react.dev/reference/react-dom/components/common#handling-keyboard-events)\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#keyboardevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`KeyboardEvent`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent) properties:\n \n * [`altKey`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/altKey)\n * [`charCode`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/charCode)\n * [`code`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/code)\n * [`ctrlKey`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/ctrlKey)\n * [`getModifierState(key)`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/getModifierState)\n * [`key`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/key)\n * [`keyCode`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/keyCode)\n * [`locale`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/locale)\n * [`metaKey`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/metaKey)\n * [`location`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/location)\n * [`repeat`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/repeat)\n * [`shiftKey`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/shiftKey)\n * [`which`](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/which)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `MouseEvent` handler function[](https://react.dev/reference/react-dom/components/common#mouseevent-handler \"Link for this heading\")\n\nAn event handler type for mouse events.\n\n```\n<divonClick={e => console.log('onClick')}onMouseEnter={e => console.log('onMouseEnter')}onMouseOver={e => console.log('onMouseOver')}onMouseDown={e => console.log('onMouseDown')}onMouseUp={e => console.log('onMouseUp')}onMouseLeave={e => console.log('onMouseLeave')}/>\n```\n\n[See an example.](https://react.dev/reference/react-dom/components/common#handling-mouse-events)\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#mouseevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`MouseEvent`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent) properties:\n \n * [`altKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/altKey)\n * [`button`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/button)\n * [`buttons`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/buttons)\n * [`ctrlKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/ctrlKey)\n * [`clientX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientX)\n * [`clientY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientY)\n * [`getModifierState(key)`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/getModifierState)\n * [`metaKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/metaKey)\n * [`movementX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementX)\n * [`movementY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementY)\n * [`pageX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageX)\n * [`pageY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageY)\n * [`relatedTarget`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/relatedTarget)\n * [`screenX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenX)\n * [`screenY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenY)\n * [`shiftKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/shiftKey)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `PointerEvent` handler function[](https://react.dev/reference/react-dom/components/common#pointerevent-handler \"Link for this heading\")\n\nAn event handler type for [pointer events.](https://developer.mozilla.org/en-US/docs/Web/API/Pointer_events)\n\n```\n<divonPointerEnter={e => console.log('onPointerEnter')}onPointerMove={e => console.log('onPointerMove')}onPointerDown={e => console.log('onPointerDown')}onPointerUp={e => console.log('onPointerUp')}onPointerLeave={e => console.log('onPointerLeave')}/>\n```\n\n[See an example.](https://react.dev/reference/react-dom/components/common#handling-pointer-events)\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#pointerevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`PointerEvent`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent) properties:\n \n * [`height`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/height)\n * [`isPrimary`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/isPrimary)\n * [`pointerId`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/pointerId)\n * [`pointerType`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/pointerType)\n * [`pressure`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/pressure)\n * [`tangentialPressure`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/tangentialPressure)\n * [`tiltX`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/tiltX)\n * [`tiltY`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/tiltY)\n * [`twist`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/twist)\n * [`width`](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent/width)\n \n It also includes the inherited [`MouseEvent`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent) properties:\n \n * [`altKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/altKey)\n * [`button`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/button)\n * [`buttons`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/buttons)\n * [`ctrlKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/ctrlKey)\n * [`clientX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientX)\n * [`clientY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientY)\n * [`getModifierState(key)`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/getModifierState)\n * [`metaKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/metaKey)\n * [`movementX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementX)\n * [`movementY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementY)\n * [`pageX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageX)\n * [`pageY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageY)\n * [`relatedTarget`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/relatedTarget)\n * [`screenX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenX)\n * [`screenY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenY)\n * [`shiftKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/shiftKey)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `TouchEvent` handler function[](https://react.dev/reference/react-dom/components/common#touchevent-handler \"Link for this heading\")\n\nAn event handler type for [touch events.](https://developer.mozilla.org/en-US/docs/Web/API/Touch_events)\n\n```\n<divonTouchStart={e => console.log('onTouchStart')}onTouchMove={e => console.log('onTouchMove')}onTouchEnd={e => console.log('onTouchEnd')}onTouchCancel={e => console.log('onTouchCancel')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#touchevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`TouchEvent`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent) properties:\n \n * [`altKey`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/altKey)\n * [`ctrlKey`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/ctrlKey)\n * [`changedTouches`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/changedTouches)\n * [`getModifierState(key)`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/getModifierState)\n * [`metaKey`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/metaKey)\n * [`shiftKey`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/shiftKey)\n * [`touches`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/touches)\n * [`targetTouches`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent/targetTouches)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `TransitionEvent` handler function[](https://react.dev/reference/react-dom/components/common#transitionevent-handler \"Link for this heading\")\n\nAn event handler type for the CSS transition events.\n\n```\n<divonTransitionEnd={e => console.log('onTransitionEnd')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#transitionevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`TransitionEvent`](https://developer.mozilla.org/en-US/docs/Web/API/TransitionEvent) properties:\n * [`elapsedTime`](https://developer.mozilla.org/en-US/docs/Web/API/TransitionEvent/elapsedTime)\n * [`propertyName`](https://developer.mozilla.org/en-US/docs/Web/API/TransitionEvent/propertyName)\n * [`pseudoElement`](https://developer.mozilla.org/en-US/docs/Web/API/TransitionEvent/pseudoElement)\n\n* * *\n\n### `UIEvent` handler function[](https://react.dev/reference/react-dom/components/common#uievent-handler \"Link for this heading\")\n\nAn event handler type for generic UI events.\n\n```\n<divonScroll={e => console.log('onScroll')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#uievent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\n### `WheelEvent` handler function[](https://react.dev/reference/react-dom/components/common#wheelevent-handler \"Link for this heading\")\n\nAn event handler type for the `onWheel` event.\n\n```\n<divonWheel={e => console.log('onWheel')}/>\n```\n\n#### Parameters[](https://react.dev/reference/react-dom/components/common#wheelevent-handler-parameters \"Link for Parameters \")\n\n* `e`: A [React event object](https://react.dev/reference/react-dom/components/common#react-event-object) with these extra [`WheelEvent`](https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent) properties:\n \n * [`deltaMode`](https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent/deltaMode)\n * [`deltaX`](https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent/deltaX)\n * [`deltaY`](https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent/deltaY)\n * [`deltaZ`](https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent/deltaZ)\n \n It also includes the inherited [`MouseEvent`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent) properties:\n \n * [`altKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/altKey)\n * [`button`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/button)\n * [`buttons`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/buttons)\n * [`ctrlKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/ctrlKey)\n * [`clientX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientX)\n * [`clientY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/clientY)\n * [`getModifierState(key)`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/getModifierState)\n * [`metaKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/metaKey)\n * [`movementX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementX)\n * [`movementY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/movementY)\n * [`pageX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageX)\n * [`pageY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/pageY)\n * [`relatedTarget`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/relatedTarget)\n * [`screenX`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenX)\n * [`screenY`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/screenY)\n * [`shiftKey`](https://developer.mozilla.org/en-US/docs/Web/API/MouseEvent/shiftKey)\n \n It also includes the inherited [`UIEvent`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent) properties:\n \n * [`detail`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/detail)\n * [`view`](https://developer.mozilla.org/en-US/docs/Web/API/UIEvent/view)\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/common#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------\n\n### Applying CSS styles[](https://react.dev/reference/react-dom/components/common#applying-css-styles \"Link for Applying CSS styles \")\n\nIn React, you specify a CSS class with [`className`.](https://developer.mozilla.org/en-US/docs/Web/API/Element/className) It works like the `class` attribute in HTML:\n\n```\n<img className=\"avatar\" />\n```\n\nThen you write the CSS rules for it in a separate CSS file:\n\n```\n/* In your CSS */.avatar {border-radius: 50%;}\n```\n\nReact does not prescribe how you add CSS files. In the simplest case, you’ll add a [`<link>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link) tag to your HTML. If you use a build tool or a framework, consult its documentation to learn how to add a CSS file to your project.\n\nSometimes, the style values depend on data. Use the `style` attribute to pass some styles dynamically:\n\n```\n<imgclassName=\"avatar\"style={{width: user.imageSize,height: user.imageSize}}/>\n```\n\nIn the above example, `style={{}}` is not a special syntax, but a regular `{}` object inside the `style={ }` [JSX curly braces.](https://react.dev/learn/javascript-in-jsx-with-curly-braces) We recommend only using the `style` attribute when your styles depend on JavaScript variables.\n\n##### Deep Dive\n\n#### How to apply multiple CSS classes conditionally?[](https://react.dev/reference/react-dom/components/common#how-to-apply-multiple-css-classes-conditionally \"Link for How to apply multiple CSS classes conditionally? \")\n\nTo apply CSS classes conditionally, you need to produce the `className` string yourself using JavaScript.\n\nFor example, `className={'row ' + (isSelected ? 'selected': '')}` will produce either `className=\"row\"` or `className=\"row selected\"` depending on whether `isSelected` is `true`.\n\nTo make this more readable, you can use a tiny helper library like [`classnames`:](https://github.com/JedWatson/classnames)\n\n```\nimport cn from 'classnames';function Row({ isSelected }) {return (<div className={cn('row', isSelected && 'selected')}> ...</div>);}\n```\n\nIt is especially convenient if you have multiple conditional classes:\n\n```\nimport cn from 'classnames';function Row({ isSelected, size }) {return (<div className={cn('row', {selected: isSelected,large: size === 'large',small: size === 'small',})}> ...</div>);}\n```\n\n* * *\n\n### Manipulating a DOM node with a ref[](https://react.dev/reference/react-dom/components/common#manipulating-a-dom-node-with-a-ref \"Link for Manipulating a DOM node with a ref \")\n\nSometimes, you’ll need to get the browser DOM node associated with a tag in JSX. For example, if you want to focus an `<input>` when a button is clicked, you need to call [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) on the browser `<input>` DOM node.\n\nTo obtain the browser DOM node for a tag, [declare a ref](https://react.dev/reference/react/useRef) and pass it as the `ref` attribute to that tag:\n\n```\nimport { useRef } from 'react';export default function Form() {const inputRef = useRef(null);// ...return (<input ref={inputRef} />// ...\n```\n\nReact will put the DOM node into `inputRef.current` after it’s been rendered to the screen.\n\nRead more about [manipulating DOM with refs](https://react.dev/learn/manipulating-the-dom-with-refs) and [check out more examples.](https://react.dev/reference/react/useRef#examples-dom)\n\nFor more advanced use cases, the `ref` attribute also accepts a [callback function.](https://react.dev/reference/react-dom/components/common#ref-callback)\n\n* * *\n\n### Dangerously setting the inner HTML[](https://react.dev/reference/react-dom/components/common#dangerously-setting-the-inner-html \"Link for Dangerously setting the inner HTML \")\n\nYou can pass a raw HTML string to an element like so:\n\n```\nconst markup = { __html: '<p>some raw html</p>' };return <div dangerouslySetInnerHTML={markup} />;\n```\n\n**This is dangerous. As with the underlying DOM [`innerHTML`](https://developer.mozilla.org/en-US/docs/Web/API/Element/innerHTML) property, you must exercise extreme caution! Unless the markup is coming from a completely trusted source, it is trivial to introduce an [XSS](https://en.wikipedia.org/wiki/Cross-site_scripting) vulnerability this way.**\n\nFor example, if you use a Markdown library that converts Markdown to HTML, you trust that its parser doesn’t contain bugs, and the user only sees their own input, you can display the resulting HTML like this:\n\nThe `{__html}` object should be created as close to where the HTML is generated as possible, like the above example does in the `renderMarkdownToHTML` function. This ensures that all raw HTML being used in your code is explicitly marked as such, and that only variables that you expect to contain HTML are passed to `dangerouslySetInnerHTML`. It is not recommended to create the object inline like `<div dangerouslySetInnerHTML={{__html: markup}} />`.\n\nTo see why rendering arbitrary HTML is dangerous, replace the code above with this:\n\n```\nconst post = {// Imagine this content is stored in the database.content: `<img src=\"\" onerror='alert(\"you were hacked\")'>`};export default function MarkdownPreview() {// 🔴 SECURITY HOLE: passing untrusted input to dangerouslySetInnerHTMLconst markup = { __html: post.content };return <div dangerouslySetInnerHTML={markup} />;}\n```\n\nThe code embedded in the HTML will run. A hacker could use this security hole to steal user information or to perform actions on their behalf. **Only use `dangerouslySetInnerHTML` with trusted and sanitized data.**\n\n* * *\n\n### Handling mouse events[](https://react.dev/reference/react-dom/components/common#handling-mouse-events \"Link for Handling mouse events \")\n\nThis example shows some common [mouse events](https://react.dev/reference/react-dom/components/common#mouseevent-handler) and when they fire.\n\n* * *\n\n### Handling pointer events[](https://react.dev/reference/react-dom/components/common#handling-pointer-events \"Link for Handling pointer events \")\n\nThis example shows some common [pointer events](https://react.dev/reference/react-dom/components/common#pointerevent-handler) and when they fire.\n\n* * *\n\n### Handling focus events[](https://react.dev/reference/react-dom/components/common#handling-focus-events \"Link for Handling focus events \")\n\nIn React, [focus events](https://react.dev/reference/react-dom/components/common#focusevent-handler) bubble. You can use the `currentTarget` and `relatedTarget` to differentiate if the focusing or blurring events originated from outside of the parent element. The example shows how to detect focusing a child, focusing the parent element, and how to detect focus entering or leaving the whole subtree.\n\n* * *\n\n### Handling keyboard events[](https://react.dev/reference/react-dom/components/common#handling-keyboard-events \"Link for Handling keyboard events \")\n\nThis example shows some common [keyboard events](https://react.dev/reference/react-dom/components/common#keyboardevent-handler) and when they fire.\n",
"filename": "common.md",
"package": "react"
} |
{
"content": "Title: Components and Hooks must be pure – React\n\nURL Source: https://react.dev/reference/rules/components-and-hooks-must-be-pure\n\nMarkdown Content:\nPure functions only perform a calculation and nothing more. It makes your code easier to understand, debug, and allows React to automatically optimize your components and Hooks correctly.\n\n### Note\n\nThis reference page covers advanced topics and requires familiarity with the concepts covered in the [Keeping Components Pure](https://react.dev/learn/keeping-components-pure) page.\n\n* [Why does purity matter?](https://react.dev/reference/rules/components-and-hooks-must-be-pure#why-does-purity-matter)\n* [Components and Hooks must be idempotent](https://react.dev/reference/rules/components-and-hooks-must-be-pure#components-and-hooks-must-be-idempotent)\n* [Side effects must run outside of render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#side-effects-must-run-outside-of-render)\n * [When is it okay to have mutation?](https://react.dev/reference/rules/components-and-hooks-must-be-pure#mutation)\n* [Props and state are immutable](https://react.dev/reference/rules/components-and-hooks-must-be-pure#props-and-state-are-immutable)\n * [Don’t mutate Props](https://react.dev/reference/rules/components-and-hooks-must-be-pure#props)\n * [Don’t mutate State](https://react.dev/reference/rules/components-and-hooks-must-be-pure#state)\n* [Return values and arguments to Hooks are immutable](https://react.dev/reference/rules/components-and-hooks-must-be-pure#return-values-and-arguments-to-hooks-are-immutable)\n* [Values are immutable after being passed to JSX](https://react.dev/reference/rules/components-and-hooks-must-be-pure#values-are-immutable-after-being-passed-to-jsx)\n\n### Why does purity matter?[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#why-does-purity-matter \"Link for Why does purity matter? \")\n\nOne of the key concepts that makes React, _React_ is _purity_. A pure component or hook is one that is:\n\n* **Idempotent** – You [always get the same result every time](https://react.dev/learn/keeping-components-pure#purity-components-as-formulas) you run it with the same inputs – props, state, context for component inputs; and arguments for hook inputs.\n* **Has no side effects in render** – Code with side effects should run [**separately from rendering**](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code). For example as an [event handler](https://react.dev/learn/responding-to-events) – where the user interacts with the UI and causes it to update; or as an [Effect](https://react.dev/reference/react/useEffect) – which runs after render.\n* **Does not mutate non-local values**: Components and Hooks should [never modify values that aren’t created locally](https://react.dev/reference/rules/components-and-hooks-must-be-pure#mutation) in render.\n\nWhen render is kept pure, React can understand how to prioritize which updates are most important for the user to see first. This is made possible because of render purity: since components don’t have side effects [in render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code), React can pause rendering components that aren’t as important to update, and only come back to them later when it’s needed.\n\nConcretely, this means that rendering logic can be run multiple times in a way that allows React to give your user a pleasant user experience. However, if your component has an untracked side effect – like modifying the value of a global variable [during render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code) – when React runs your rendering code again, your side effects will be triggered in a way that won’t match what you want. This often leads to unexpected bugs that can degrade how your users experience your app. You can see an [example of this in the Keeping Components Pure page](https://react.dev/learn/keeping-components-pure#side-effects-unintended-consequences).\n\n#### How does React run your code?[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code \"Link for How does React run your code? \")\n\nReact is declarative: you tell React _what_ to render, and React will figure out _how_ best to display it to your user. To do this, React has a few phases where it runs your code. You don’t need to know about all of these phases to use React well. But at a high level, you should know about what code runs in _render_, and what runs outside of it.\n\n_Rendering_ refers to calculating what the next version of your UI should look like. After rendering, [Effects](https://react.dev/reference/react/useEffect) are _flushed_ (meaning they are run until there are no more left) and may update the calculation if the Effects have impacts on layout. React takes this new calculation and compares it to the calculation used to create the previous version of your UI, then _commits_ just the minimum changes needed to the [DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model) (what your user actually sees) to catch it up to the latest version.\n\n##### Deep Dive\n\n#### How to tell if code runs in render[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-to-tell-if-code-runs-in-render \"Link for How to tell if code runs in render \")\n\nOne quick heuristic to tell if code runs during render is to examine where it is: if it’s written at the top level like in the example below, there’s a good chance it runs during render.\n\n```\nfunction Dropdown() {const selectedItems = new Set(); // created during render// ...}\n```\n\nEvent handlers and Effects don’t run in render:\n\n```\nfunction Dropdown() {const selectedItems = new Set();const onSelect = (item) => {// this code is in an event handler, so it's only run when the user triggers thisselectedItems.add(item);}}\n```\n\n```\nfunction Dropdown() {const selectedItems = new Set();useEffect(() => {// this code is inside of an Effect, so it only runs after renderinglogForAnalytics(selectedItems);}, [selectedItems]);}\n```\n\n* * *\n\nComponents and Hooks must be idempotent[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#components-and-hooks-must-be-idempotent \"Link for Components and Hooks must be idempotent \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nComponents must always return the same output with respect to their inputs – props, state, and context. This is known as _idempotency_. [Idempotency](https://en.wikipedia.org/wiki/Idempotence) is a term popularized in functional programming. It refers to the idea that you [always get the same result every time](https://react.dev/learn/keeping-components-pure) you run that piece of code with the same inputs.\n\nThis means that _all_ code that runs [during render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code) must also be idempotent in order for this rule to hold. For example, this line of code is not idempotent (and therefore, neither is the component):\n\n```\nfunction Clock() {const time = new Date(); // 🔴 Bad: always returns a different result!return <span>{time.toLocaleString()}</span>}\n```\n\n`new Date()` is not idempotent as it always returns the current date and changes its result every time it’s called. When you render the above component, the time displayed on the screen will stay stuck on the time that the component was rendered. Similarly, functions like `Math.random()` also aren’t idempotent, because they return different results every time they’re called, even when the inputs are the same.\n\nThis doesn’t mean you shouldn’t use non-idempotent functions like `new Date()` _at all_ – you should just avoid using them [during render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code). In this case, we can _synchronize_ the latest date to this component using an [Effect](https://react.dev/reference/react/useEffect):\n\nBy wrapping the non-idempotent `new Date()` call in an Effect, it moves that calculation [outside of rendering](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code).\n\nIf you don’t need to synchronize some external state with React, you can also consider using an [event handler](https://react.dev/learn/responding-to-events) if it only needs to be updated in response to a user interaction.\n\n* * *\n\nSide effects must run outside of render[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#side-effects-must-run-outside-of-render \"Link for Side effects must run outside of render \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\n[Side effects](https://react.dev/learn/keeping-components-pure#side-effects-unintended-consequences) should not run [in render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code), as React can render components multiple times to create the best possible user experience.\n\n### Note\n\nSide effects are a broader term than Effects. Effects specifically refer to code that’s wrapped in `useEffect`, while a side effect is a general term for code that has any observable effect other than its primary result of returning a value to the caller.\n\nSide effects are typically written inside of [event handlers](https://react.dev/learn/responding-to-events) or Effects. But never during render.\n\nWhile render must be kept pure, side effects are necessary at some point in order for your app to do anything interesting, like showing something on the screen! The key point of this rule is that side effects should not run [in render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code), as React can render components multiple times. In most cases, you’ll use [event handlers](https://react.dev/learn/responding-to-events) to handle side effects. Using an event handler explicitly tells React that this code doesn’t need to run during render, keeping render pure. If you’ve exhausted all options – and only as a last resort – you can also handle side effects using `useEffect`.\n\n### When is it okay to have mutation?[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#mutation \"Link for When is it okay to have mutation? \")\n\n#### Local mutation[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#local-mutation \"Link for Local mutation \")\n\nOne common example of a side effect is mutation, which in JavaScript refers to changing the value of a non-[primitive](https://developer.mozilla.org/en-US/docs/Glossary/Primitive) value. In general, while mutation is not idiomatic in React, _local_ mutation is absolutely fine:\n\n```\nfunction FriendList({ friends }) {const items = []; // ✅ Good: locally createdfor (let i = 0; i < friends.length; i++) {const friend = friends[i];items.push(<Friend key={friend.id} friend={friend} />); // ✅ Good: local mutation is okay}return <section>{items}</section>;}\n```\n\nThere is no need to contort your code to avoid local mutation. [`Array.map`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) could also be used here for brevity, but there is nothing wrong with creating a local array and then pushing items into it [during render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code).\n\nEven though it looks like we are mutating `items`, the key point to note is that this code only does so _locally_ – the mutation isn’t “remembered” when the component is rendered again. In other words, `items` only stays around as long as the component does. Because `items` is always _recreated_ every time `<FriendList />` is rendered, the component will always return the same result.\n\nOn the other hand, if `items` was created outside of the component, it holds on to its previous values and remembers changes:\n\n```\nconst items = []; // 🔴 Bad: created outside of the componentfunction FriendList({ friends }) {for (let i = 0; i < friends.length; i++) {const friend = friends[i];items.push(<Friend key={friend.id} friend={friend} />); // 🔴 Bad: mutates a value created outside of render}return <section>{items}</section>;}\n```\n\nWhen `<FriendList />` runs again, we will continue appending `friends` to `items` every time that component is run, leading to multiple duplicated results. This version of `<FriendList />` has observable side effects [during render](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code) and **breaks the rule**.\n\n#### Lazy initialization[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#lazy-initialization \"Link for Lazy initialization \")\n\nLazy initialization is also fine despite not being fully “pure”:\n\n```\nfunction ExpenseForm() {SuperCalculator.initializeIfNotReady(); // ✅ Good: if it doesn't affect other components// Continue rendering...}\n```\n\n#### Changing the DOM[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#changing-the-dom \"Link for Changing the DOM \")\n\nSide effects that are directly visible to the user are not allowed in the render logic of React components. In other words, merely calling a component function shouldn’t by itself produce a change on the screen.\n\n```\nfunction ProductDetailPage({ product }) {document.window.title = product.title; // 🔴 Bad: Changes the DOM}\n```\n\nOne way to achieve the desired result of updating `window.title` outside of render is to [synchronize the component with `window`](https://react.dev/learn/synchronizing-with-effects).\n\nAs long as calling a component multiple times is safe and doesn’t affect the rendering of other components, React doesn’t care if it’s 100% pure in the strict functional programming sense of the word. It is more important that [components must be idempotent](https://react.dev/reference/rules/components-and-hooks-must-be-pure).\n\n* * *\n\nProps and state are immutable[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#props-and-state-are-immutable \"Link for Props and state are immutable \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nA component’s props and state are immutable [snapshots](https://react.dev/learn/state-as-a-snapshot). Never mutate them directly. Instead, pass new props down, and use the setter function from `useState`.\n\nYou can think of the props and state values as snapshots that are updated after rendering. For this reason, you don’t modify the props or state variables directly: instead you pass new props, or use the setter function provided to you to tell React that state needs to update the next time the component is rendered.\n\n### Don’t mutate Props[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#props \"Link for Don’t mutate Props \")\n\nProps are immutable because if you mutate them, the application will produce inconsistent output, which can be hard to debug since it may or may not work depending on the circumstance.\n\n```\nfunction Post({ item }) {item.url = new Url(item.url, base); // 🔴 Bad: never mutate props directlyreturn <Link url={item.url}>{item.title}</Link>;}\n```\n\n```\nfunction Post({ item }) {const url = new Url(item.url, base); // ✅ Good: make a copy insteadreturn <Link url={url}>{item.title}</Link>;}\n```\n\n### Don’t mutate State[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#state \"Link for Don’t mutate State \")\n\n`useState` returns the state variable and a setter to update that state.\n\n```\nconst [stateVariable, setter] = useState(0);\n```\n\nRather than updating the state variable in-place, we need to update it using the setter function that is returned by `useState`. Changing values on the state variable doesn’t cause the component to update, leaving your users with an outdated UI. Using the setter function informs React that the state has changed, and that we need to queue a re-render to update the UI.\n\n```\nfunction Counter() {const [count, setCount] = useState(0);function handleClick() {count = count + 1; // 🔴 Bad: never mutate state directly}return (<button onClick={handleClick}> You pressed me {count} times</button>);}\n```\n\n```\nfunction Counter() {const [count, setCount] = useState(0);function handleClick() {setCount(count + 1); // ✅ Good: use the setter function returned by useState}return (<button onClick={handleClick}> You pressed me {count} times</button>);}\n```\n\n* * *\n\nReturn values and arguments to Hooks are immutable[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#return-values-and-arguments-to-hooks-are-immutable \"Link for Return values and arguments to Hooks are immutable \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nOnce values are passed to a hook, you should not modify them. Like props in JSX, values become immutable when passed to a hook.\n\n```\nfunction useIconStyle(icon) {const theme = useContext(ThemeContext);if (icon.enabled) {icon.className = computeStyle(icon, theme); // 🔴 Bad: never mutate hook arguments directly}return icon;}\n```\n\n```\nfunction useIconStyle(icon) {const theme = useContext(ThemeContext);const newIcon = { ...icon }; // ✅ Good: make a copy insteadif (icon.enabled) {newIcon.className = computeStyle(icon, theme);}return newIcon;}\n```\n\nOne important principle in React is _local reasoning_: the ability to understand what a component or hook does by looking at its code in isolation. Hooks should be treated like “black boxes” when they are called. For example, a custom hook might have used its arguments as dependencies to memoize values inside it:\n\n```\nfunction useIconStyle(icon) {const theme = useContext(ThemeContext);return useMemo(() => {const newIcon = { ...icon };if (icon.enabled) {newIcon.className = computeStyle(icon, theme);}return newIcon;}, [icon, theme]);}\n```\n\nIf you were to mutate the Hooks arguments, the custom hook’s memoization will become incorrect, so it’s important to avoid doing that.\n\n```\nstyle = useIconStyle(icon); // `style` is memoized based on `icon`icon.enabled = false; // Bad: 🔴 never mutate hook arguments directlystyle = useIconStyle(icon); // previously memoized result is returned\n```\n\n```\nstyle = useIconStyle(icon); // `style` is memoized based on `icon`icon = { ...icon, enabled: false }; // Good: ✅ make a copy insteadstyle = useIconStyle(icon); // new value of `style` is calculated\n```\n\nSimilarly, it’s important to not modify the return values of Hooks, as they may have been memoized.\n\n* * *\n\nValues are immutable after being passed to JSX[](https://react.dev/reference/rules/components-and-hooks-must-be-pure#values-are-immutable-after-being-passed-to-jsx \"Link for Values are immutable after being passed to JSX \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nDon’t mutate values after they’ve been used in JSX. Move the mutation before the JSX is created.\n\nWhen you use JSX in an expression, React may eagerly evaluate the JSX before the component finishes rendering. This means that mutating values after they’ve been passed to JSX can lead to outdated UIs, as React won’t know to update the component’s output.\n\n```\nfunction Page({ colour }) {const styles = { colour, size: \"large\" };const header = <Header styles={styles} />;styles.size = \"small\"; // 🔴 Bad: styles was already used in the JSX aboveconst footer = <Footer styles={styles} />;return (<>{header}<Content />{footer}</>);}\n```\n\n```\nfunction Page({ colour }) {const headerStyles = { colour, size: \"large\" };const header = <Header styles={headerStyles} />;const footerStyles = { colour, size: \"small\" }; // ✅ Good: we created a new valueconst footer = <Footer styles={footerStyles} />;return (<>{header}<Content />{footer}</>);}\n```\n",
"filename": "components-and-hooks-must-be-pure.md",
"package": "react"
} |
{
"content": "Title: Built-in React Components – React\n\nURL Source: https://react.dev/reference/react/components\n\nMarkdown Content:\nBuilt-in React Components – React\n===============\n\nBuilt-in React Components[](https://react.dev/reference/react/components#undefined \"Link for this heading\")\n===========================================================================================================\n\nReact exposes a few built-in components that you can use in your JSX.\n\n* * *\n\nBuilt-in components[](https://react.dev/reference/react/components#built-in-components \"Link for Built-in components \")\n-----------------------------------------------------------------------------------------------------------------------\n\n* [`<Fragment>`](https://react.dev/reference/react/Fragment), alternatively written as `<>...</>`, lets you group multiple JSX nodes together.\n* [`<Profiler>`](https://react.dev/reference/react/Profiler) lets you measure rendering performance of a React tree programmatically.\n* [`<Suspense>`](https://react.dev/reference/react/Suspense) lets you display a fallback while the child components are loading.\n* [`<StrictMode>`](https://react.dev/reference/react/StrictMode) enables extra development-only checks that help you find bugs early.\n\n* * *\n\nYour own components[](https://react.dev/reference/react/components#your-own-components \"Link for Your own components \")\n-----------------------------------------------------------------------------------------------------------------------\n\nYou can also [define your own components](https://react.dev/learn/your-first-component) as JavaScript functions.\n\n[PrevioususeTransition](https://react.dev/reference/react/useTransition)[Next<Fragment> (<>)](https://react.dev/reference/react/Fragment)\n",
"filename": "components.md",
"package": "react"
} |
{
"content": "Title: Conditional Rendering – React\n\nURL Source: https://react.dev/learn/conditional-rendering\n\nMarkdown Content:\nYour components will often need to display different things depending on different conditions. In React, you can conditionally render JSX using JavaScript syntax like `if` statements, `&&`, and `? :` operators.\n\n### You will learn\n\n* How to return different JSX depending on a condition\n* How to conditionally include or exclude a piece of JSX\n* Common conditional syntax shortcuts you’ll encounter in React codebases\n\nConditionally returning JSX[](https://react.dev/learn/conditional-rendering#conditionally-returning-jsx \"Link for Conditionally returning JSX \")\n------------------------------------------------------------------------------------------------------------------------------------------------\n\nLet’s say you have a `PackingList` component rendering several `Item`s, which can be marked as packed or not:\n\nUnable to establish connection with the sandpack bundler. Make sure you are online or try again later. If the problem persists, please report it via [email](mailto:hello@codesandbox.io?subject=Sandpack%20Timeout%20Error) or submit an issue on [GitHub.](https://github.com/codesandbox/sandpack/issues)\n\nNotice that some of the `Item` components have their `isPacked` prop set to `true` instead of `false`. You want to add a checkmark (✔) to packed items if `isPacked={true}`.\n\nYou can write this as an [`if`/`else` statement](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/if...else) like so:\n\n```\nif (isPacked) {return <li className=\"item\">{name} ✔</li>;}return <li className=\"item\">{name}</li>;\n```\n\nIf the `isPacked` prop is `true`, this code **returns a different JSX tree.** With this change, some of the items get a checkmark at the end:\n\nfunction Item({ name, isPacked }) {\n if (isPacked) {\n return <li className\\=\"item\"\\>{name} ✔</li\\>;\n }\n return <li className\\=\"item\"\\>{name}</li\\>;\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n\nUnable to establish connection with the sandpack bundler. Make sure you are online or try again later. If the problem persists, please report it via [email](mailto:hello@codesandbox.io?subject=Sandpack%20Timeout%20Error) or submit an issue on [GitHub.](https://github.com/codesandbox/sandpack/issues)\n\nTry editing what gets returned in either case, and see how the result changes!\n\nNotice how you’re creating branching logic with JavaScript’s `if` and `return` statements. In React, control flow (like conditions) is handled by JavaScript.\n\n### Conditionally returning nothing with `null`[](https://react.dev/learn/conditional-rendering#conditionally-returning-nothing-with-null \"Link for this heading\")\n\nIn some situations, you won’t want to render anything at all. For example, say you don’t want to show packed items at all. A component must return something. In this case, you can return `null`:\n\n```\nif (isPacked) {return null;}return <li className=\"item\">{name}</li>;\n```\n\nIf `isPacked` is true, the component will return nothing, `null`. Otherwise, it will return JSX to render.\n\nfunction Item({ name, isPacked }) {\n if (isPacked) {\n return null;\n }\n return <li className\\=\"item\"\\>{name}</li\\>;\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n\nIn practice, returning `null` from a component isn’t common because it might surprise a developer trying to render it. More often, you would conditionally include or exclude the component in the parent component’s JSX. Here’s how to do that!\n\nConditionally including JSX[](https://react.dev/learn/conditional-rendering#conditionally-including-jsx \"Link for Conditionally including JSX \")\n------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn the previous example, you controlled which (if any!) JSX tree would be returned by the component. You may already have noticed some duplication in the render output:\n\n```\n<li className=\"item\">{name} ✔</li>\n```\n\nis very similar to\n\n```\n<li className=\"item\">{name}</li>\n```\n\nBoth of the conditional branches return `<li className=\"item\">...</li>`:\n\n```\nif (isPacked) {return <li className=\"item\">{name} ✔</li>;}return <li className=\"item\">{name}</li>;\n```\n\nWhile this duplication isn’t harmful, it could make your code harder to maintain. What if you want to change the `className`? You’d have to do it in two places in your code! In such a situation, you could conditionally include a little JSX to make your code more [DRY.](https://en.wikipedia.org/wiki/Don%27t_repeat_yourself)\n\n### Conditional (ternary) operator (`? :`)[](https://react.dev/learn/conditional-rendering#conditional-ternary-operator-- \"Link for this heading\")\n\nJavaScript has a compact syntax for writing a conditional expression — the [conditional operator](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator) or “ternary operator”.\n\nInstead of this:\n\n```\nif (isPacked) {return <li className=\"item\">{name} ✔</li>;}return <li className=\"item\">{name}</li>;\n```\n\nYou can write this:\n\n```\nreturn (<li className=\"item\">{isPacked ? name + ' ✔' : name}</li>);\n```\n\nYou can read it as _“if `isPacked` is true, then (`?`) render `name + ' ✔'`, otherwise (`:`) render `name`”_.\n\n##### Deep Dive\n\n#### Are these two examples fully equivalent?[](https://react.dev/learn/conditional-rendering#are-these-two-examples-fully-equivalent \"Link for Are these two examples fully equivalent? \")\n\nIf you’re coming from an object-oriented programming background, you might assume that the two examples above are subtly different because one of them may create two different “instances” of `<li>`. But JSX elements aren’t “instances” because they don’t hold any internal state and aren’t real DOM nodes. They’re lightweight descriptions, like blueprints. So these two examples, in fact, _are_ completely equivalent. [Preserving and Resetting State](https://react.dev/learn/preserving-and-resetting-state) goes into detail about how this works.\n\nNow let’s say you want to wrap the completed item’s text into another HTML tag, like `<del>` to strike it out. You can add even more newlines and parentheses so that it’s easier to nest more JSX in each of the cases:\n\nfunction Item({ name, isPacked }) {\n return (\n <li className\\=\"item\"\\>\n {isPacked ? (\n <del\\>\n {name + ' ✔'}\n </del\\>\n ) : (\n name\n )}\n </li\\>\n );\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n\nThis style works well for simple conditions, but use it in moderation. If your components get messy with too much nested conditional markup, consider extracting child components to clean things up. In React, markup is a part of your code, so you can use tools like variables and functions to tidy up complex expressions.\n\n### Logical AND operator (`&&`)[](https://react.dev/learn/conditional-rendering#logical-and-operator- \"Link for this heading\")\n\nAnother common shortcut you’ll encounter is the [JavaScript logical AND (`&&`) operator.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_AND#:~:text=The%20logical%20AND%20\\(%20%26%26%20\\)%20operator,it%20returns%20a%20Boolean%20value.) Inside React components, it often comes up when you want to render some JSX when the condition is true, **or render nothing otherwise.** With `&&`, you could conditionally render the checkmark only if `isPacked` is `true`:\n\n```\nreturn (<li className=\"item\">{name} {isPacked && '✔'}</li>);\n```\n\nYou can read this as _“if `isPacked`, then (`&&`) render the checkmark, otherwise, render nothing”_.\n\nHere it is in action:\n\nfunction Item({ name, isPacked }) {\n return (\n <li className\\=\"item\"\\>\n {name} {isPacked && '✔'}\n </li\\>\n );\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n\nA [JavaScript && expression](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_AND) returns the value of its right side (in our case, the checkmark) if the left side (our condition) is `true`. But if the condition is `false`, the whole expression becomes `false`. React considers `false` as a “hole” in the JSX tree, just like `null` or `undefined`, and doesn’t render anything in its place.\n\n### Pitfall\n\n**Don’t put numbers on the left side of `&&`.**\n\nTo test the condition, JavaScript converts the left side to a boolean automatically. However, if the left side is `0`, then the whole expression gets that value (`0`), and React will happily render `0` rather than nothing.\n\nFor example, a common mistake is to write code like `messageCount && <p>New messages</p>`. It’s easy to assume that it renders nothing when `messageCount` is `0`, but it really renders the `0` itself!\n\nTo fix it, make the left side a boolean: `messageCount > 0 && <p>New messages</p>`.\n\n### Conditionally assigning JSX to a variable[](https://react.dev/learn/conditional-rendering#conditionally-assigning-jsx-to-a-variable \"Link for Conditionally assigning JSX to a variable \")\n\nWhen the shortcuts get in the way of writing plain code, try using an `if` statement and a variable. You can reassign variables defined with [`let`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/let), so start by providing the default content you want to display, the name:\n\nUse an `if` statement to reassign a JSX expression to `itemContent` if `isPacked` is `true`:\n\n```\nif (isPacked) {itemContent = name + \" ✔\";}\n```\n\n[Curly braces open the “window into JavaScript”.](https://react.dev/learn/javascript-in-jsx-with-curly-braces#using-curly-braces-a-window-into-the-javascript-world) Embed the variable with curly braces in the returned JSX tree, nesting the previously calculated expression inside of JSX:\n\n```\n<li className=\"item\">{itemContent}</li>\n```\n\nThis style is the most verbose, but it’s also the most flexible. Here it is in action:\n\nfunction Item({ name, isPacked }) {\n let itemContent = name;\n if (isPacked) {\n itemContent = name + \" ✔\";\n }\n return (\n <li className\\=\"item\"\\>\n {itemContent}\n </li\\>\n );\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n\nLike before, this works not only for text, but for arbitrary JSX too:\n\nfunction Item({ name, isPacked }) {\n let itemContent = name;\n if (isPacked) {\n itemContent = (\n <del\\>\n {name + \" ✔\"}\n </del\\>\n );\n }\n return (\n <li className\\=\"item\"\\>\n {itemContent}\n </li\\>\n );\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n\nIf you’re not familiar with JavaScript, this variety of styles might seem overwhelming at first. However, learning them will help you read and write any JavaScript code — and not just React components! Pick the one you prefer for a start, and then consult this reference again if you forget how the other ones work.\n\nRecap[](https://react.dev/learn/conditional-rendering#recap \"Link for Recap\")\n-----------------------------------------------------------------------------\n\n* In React, you control branching logic with JavaScript.\n* You can return a JSX expression conditionally with an `if` statement.\n* You can conditionally save some JSX to a variable and then include it inside other JSX by using the curly braces.\n* In JSX, `{cond ? <A /> : <B />}` means _“if `cond`, render `<A />`, otherwise `<B />`”_.\n* In JSX, `{cond && <A />}` means _“if `cond`, render `<A />`, otherwise nothing”_.\n* The shortcuts are common, but you don’t have to use them if you prefer plain `if`.\n\nTry out some challenges[](https://react.dev/learn/conditional-rendering#challenges \"Link for Try out some challenges\")\n----------------------------------------------------------------------------------------------------------------------\n\n#### Show an icon for incomplete items with `? :`[](https://react.dev/learn/conditional-rendering#show-an-icon-for-incomplete-items-with-- \"Link for this heading\")\n\nUse the conditional operator (`cond ? a : b`) to render a ❌ if `isPacked` isn’t `true`.\n\nfunction Item({ name, isPacked }) {\n return (\n <li className\\=\"item\"\\>\n {name} {isPacked && '✔'}\n </li\\>\n );\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item \n isPacked\\={true} \n name\\=\"Space suit\" \n />\n <Item \n isPacked\\={true} \n name\\=\"Helmet with a golden leaf\" \n />\n <Item \n isPacked\\={false} \n name\\=\"Photo of Tam\" \n />\n </ul\\>\n </section\\>\n );\n}\n",
"filename": "conditional-rendering.md",
"package": "react"
} |
{
"content": "Title: createContext – React\n\nURL Source: https://react.dev/reference/react/createContext\n\nMarkdown Content:\n`createContext` lets you create a [context](https://react.dev/learn/passing-data-deeply-with-context) that components can provide or read.\n\n```\nconst SomeContext = createContext(defaultValue)\n```\n\n* [Reference](https://react.dev/reference/react/createContext#reference)\n * [`createContext(defaultValue)`](https://react.dev/reference/react/createContext#createcontext)\n * [`SomeContext.Provider`](https://react.dev/reference/react/createContext#provider)\n * [`SomeContext.Consumer`](https://react.dev/reference/react/createContext#consumer)\n* [Usage](https://react.dev/reference/react/createContext#usage)\n * [Creating context](https://react.dev/reference/react/createContext#creating-context)\n * [Importing and exporting context from a file](https://react.dev/reference/react/createContext#importing-and-exporting-context-from-a-file)\n* [Troubleshooting](https://react.dev/reference/react/createContext#troubleshooting)\n * [I can’t find a way to change the context value](https://react.dev/reference/react/createContext#i-cant-find-a-way-to-change-the-context-value)\n\n* * *\n\nReference[](https://react.dev/reference/react/createContext#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `createContext(defaultValue)`[](https://react.dev/reference/react/createContext#createcontext \"Link for this heading\")\n\nCall `createContext` outside of any components to create a context.\n\n```\nimport { createContext } from 'react';const ThemeContext = createContext('light');\n```\n\n[See more examples below.](https://react.dev/reference/react/createContext#usage)\n\n#### Parameters[](https://react.dev/reference/react/createContext#parameters \"Link for Parameters \")\n\n* `defaultValue`: The value that you want the context to have when there is no matching context provider in the tree above the component that reads context. If you don’t have any meaningful default value, specify `null`. The default value is meant as a “last resort” fallback. It is static and never changes over time.\n\n#### Returns[](https://react.dev/reference/react/createContext#returns \"Link for Returns \")\n\n`createContext` returns a context object.\n\n**The context object itself does not hold any information.** It represents _which_ context other components read or provide. Typically, you will use [`SomeContext.Provider`](https://react.dev/reference/react/createContext#provider) in components above to specify the context value, and call [`useContext(SomeContext)`](https://react.dev/reference/react/useContext) in components below to read it. The context object has a few properties:\n\n* `SomeContext.Provider` lets you provide the context value to components.\n* `SomeContext.Consumer` is an alternative and rarely used way to read the context value.\n\n* * *\n\n### `SomeContext.Provider`[](https://react.dev/reference/react/createContext#provider \"Link for this heading\")\n\nWrap your components into a context provider to specify the value of this context for all components inside:\n\n```\nfunction App() {const [theme, setTheme] = useState('light');// ...return (<ThemeContext.Provider value={theme}><Page /></ThemeContext.Provider>);}\n```\n\n#### Props[](https://react.dev/reference/react/createContext#provider-props \"Link for Props \")\n\n* `value`: The value that you want to pass to all the components reading this context inside this provider, no matter how deep. The context value can be of any type. A component calling [`useContext(SomeContext)`](https://react.dev/reference/react/useContext) inside of the provider receives the `value` of the innermost corresponding context provider above it.\n\n* * *\n\n### `SomeContext.Consumer`[](https://react.dev/reference/react/createContext#consumer \"Link for this heading\")\n\nBefore `useContext` existed, there was an older way to read context:\n\n```\nfunction Button() {// 🟡 Legacy way (not recommended)return (<ThemeContext.Consumer>{theme => (<button className={theme} />)}</ThemeContext.Consumer>);}\n```\n\nAlthough this older way still works, but **newly written code should read context with [`useContext()`](https://react.dev/reference/react/useContext) instead:**\n\n```\nfunction Button() {// ✅ Recommended wayconst theme = useContext(ThemeContext);return <button className={theme} />;}\n```\n\n#### Props[](https://react.dev/reference/react/createContext#consumer-props \"Link for Props \")\n\n* `children`: A function. React will call the function you pass with the current context value determined by the same algorithm as [`useContext()`](https://react.dev/reference/react/useContext) does, and render the result you return from this function. React will also re-run this function and update the UI whenever the context from the parent components changes.\n\n* * *\n\nUsage[](https://react.dev/reference/react/createContext#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Creating context[](https://react.dev/reference/react/createContext#creating-context \"Link for Creating context \")\n\nContext lets components [pass information deep down](https://react.dev/learn/passing-data-deeply-with-context) without explicitly passing props.\n\nCall `createContext` outside any components to create one or more contexts.\n\n```\nimport { createContext } from 'react';const ThemeContext = createContext('light');const AuthContext = createContext(null);\n```\n\n`createContext` returns a context object. Components can read context by passing it to [`useContext()`](https://react.dev/reference/react/useContext):\n\n```\nfunction Button() {const theme = useContext(ThemeContext);// ...}function Profile() {const currentUser = useContext(AuthContext);// ...}\n```\n\nBy default, the values they receive will be the default values you have specified when creating the contexts. However, by itself this isn’t useful because the default values never change.\n\nContext is useful because you can **provide other, dynamic values from your components:**\n\n```\nfunction App() {const [theme, setTheme] = useState('dark');const [currentUser, setCurrentUser] = useState({ name: 'Taylor' });// ...return (<ThemeContext.Provider value={theme}><AuthContext.Provider value={currentUser}><Page /></AuthContext.Provider></ThemeContext.Provider>);}\n```\n\nNow the `Page` component and any components inside it, no matter how deep, will “see” the passed context values. If the passed context values change, React will re-render the components reading the context as well.\n\n[Read more about reading and providing context and see examples.](https://react.dev/reference/react/useContext)\n\n* * *\n\n### Importing and exporting context from a file[](https://react.dev/reference/react/createContext#importing-and-exporting-context-from-a-file \"Link for Importing and exporting context from a file \")\n\nOften, components in different files will need access to the same context. This is why it’s common to declare contexts in a separate file. Then you can use the [`export` statement](https://developer.mozilla.org/en-US/docs/web/javascript/reference/statements/export) to make context available for other files:\n\n```\n// Contexts.jsimport { createContext } from 'react';export const ThemeContext = createContext('light');export const AuthContext = createContext(null);\n```\n\nComponents declared in other files can then use the [`import`](https://developer.mozilla.org/en-US/docs/web/javascript/reference/statements/import) statement to read or provide this context:\n\n```\n// Button.jsimport { ThemeContext } from './Contexts.js';function Button() {const theme = useContext(ThemeContext);// ...}\n```\n\n```\n// App.jsimport { ThemeContext, AuthContext } from './Contexts.js';function App() {// ...return (<ThemeContext.Provider value={theme}><AuthContext.Provider value={currentUser}><Page /></AuthContext.Provider></ThemeContext.Provider>);}\n```\n\nThis works similar to [importing and exporting components.](https://react.dev/learn/importing-and-exporting-components)\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/createContext#troubleshooting \"Link for Troubleshooting \")\n--------------------------------------------------------------------------------------------------------------\n\n### I can’t find a way to change the context value[](https://react.dev/reference/react/createContext#i-cant-find-a-way-to-change-the-context-value \"Link for I can’t find a way to change the context value \")\n\nCode like this specifies the _default_ context value:\n\n```\nconst ThemeContext = createContext('light');\n```\n\nThis value never changes. React only uses this value as a fallback if it can’t find a matching provider above.\n\nTo make context change over time, [add state and wrap components in a context provider.](https://react.dev/reference/react/useContext#updating-data-passed-via-context)\n",
"filename": "createContext.md",
"package": "react"
} |
{
"content": "Title: createElement – React\n\nURL Source: https://react.dev/reference/react/createElement\n\nMarkdown Content:\n`createElement` lets you create a React element. It serves as an alternative to writing [JSX.](https://react.dev/learn/writing-markup-with-jsx)\n\n```\nconst element = createElement(type, props, ...children)\n```\n\n* [Reference](https://react.dev/reference/react/createElement#reference)\n * [`createElement(type, props, ...children)`](https://react.dev/reference/react/createElement#createelement)\n* [Usage](https://react.dev/reference/react/createElement#usage)\n * [Creating an element without JSX](https://react.dev/reference/react/createElement#creating-an-element-without-jsx)\n\n* * *\n\nReference[](https://react.dev/reference/react/createElement#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `createElement(type, props, ...children)`[](https://react.dev/reference/react/createElement#createelement \"Link for this heading\")\n\nCall `createElement` to create a React element with the given `type`, `props`, and `children`.\n\n```\nimport { createElement } from 'react';function Greeting({ name }) {return createElement('h1',{ className: 'greeting' },'Hello');}\n```\n\n[See more examples below.](https://react.dev/reference/react/createElement#usage)\n\n#### Parameters[](https://react.dev/reference/react/createElement#parameters \"Link for Parameters \")\n\n* `type`: The `type` argument must be a valid React component type. For example, it could be a tag name string (such as `'div'` or `'span'`), or a React component (a function, a class, or a special component like [`Fragment`](https://react.dev/reference/react/Fragment)).\n \n* `props`: The `props` argument must either be an object or `null`. If you pass `null`, it will be treated the same as an empty object. React will create an element with props matching the `props` you have passed. Note that `ref` and `key` from your `props` object are special and will _not_ be available as `element.props.ref` and `element.props.key` on the returned `element`. They will be available as `element.ref` and `element.key`.\n \n* **optional** `...children`: Zero or more child nodes. They can be any React nodes, including React elements, strings, numbers, [portals](https://react.dev/reference/react-dom/createPortal), empty nodes (`null`, `undefined`, `true`, and `false`), and arrays of React nodes.\n \n\n#### Returns[](https://react.dev/reference/react/createElement#returns \"Link for Returns \")\n\n`createElement` returns a React element object with a few properties:\n\n* `type`: The `type` you have passed.\n* `props`: The `props` you have passed except for `ref` and `key`. If the `type` is a component with legacy `type.defaultProps`, then any missing or undefined `props` will get the values from `type.defaultProps`.\n* `ref`: The `ref` you have passed. If missing, `null`.\n* `key`: The `key` you have passed, coerced to a string. If missing, `null`.\n\nUsually, you’ll return the element from your component or make it a child of another element. Although you may read the element’s properties, it’s best to treat every element as opaque after it’s created, and only render it.\n\n#### Caveats[](https://react.dev/reference/react/createElement#caveats \"Link for Caveats \")\n\n* You must **treat React elements and their props as [immutable](https://en.wikipedia.org/wiki/Immutable_object)** and never change their contents after creation. In development, React will [freeze](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/freeze) the returned element and its `props` property shallowly to enforce this.\n \n* When you use JSX, **you must start a tag with a capital letter to render your own custom component.** In other words, `<Something />` is equivalent to `createElement(Something)`, but `<something />` (lowercase) is equivalent to `createElement('something')` (note it’s a string, so it will be treated as a built-in HTML tag).\n \n* You should only **pass children as multiple arguments to `createElement` if they are all statically known,** like `createElement('h1', {}, child1, child2, child3)`. If your children are dynamic, pass the entire array as the third argument: `createElement('ul', {}, listItems)`. This ensures that React will [warn you about missing `key`s](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key) for any dynamic lists. For static lists this is not necessary because they never reorder.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react/createElement#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Creating an element without JSX[](https://react.dev/reference/react/createElement#creating-an-element-without-jsx \"Link for Creating an element without JSX \")\n\nIf you don’t like [JSX](https://react.dev/learn/writing-markup-with-jsx) or can’t use it in your project, you can use `createElement` as an alternative.\n\nTo create an element without JSX, call `createElement` with some type, props, and children:\n\n```\nimport { createElement } from 'react';function Greeting({ name }) {return createElement('h1',{ className: 'greeting' },'Hello ',createElement('i', null, name),'. Welcome!');}\n```\n\nThe children are optional, and you can pass as many as you need (the example above has three children). This code will display a `<h1>` header with a greeting. For comparison, here is the same example rewritten with JSX:\n\n```\nfunction Greeting({ name }) {return (<h1 className=\"greeting\">Hello <i>{name}</i>. Welcome!</h1>);}\n```\n\nTo render your own React component, pass a function like `Greeting` as the type instead of a string like `'h1'`:\n\n```\nexport default function App() {return createElement(Greeting, { name: 'Taylor' });}\n```\n\nWith JSX, it would look like this:\n\n```\nexport default function App() {return <Greeting name=\"Taylor\" />;}\n```\n\nHere is a complete example written with `createElement`:\n\nBoth coding styles are fine, so you can use whichever one you prefer for your project. The main benefit of using JSX compared to `createElement` is that it’s easy to see which closing tag corresponds to which opening tag.\n\n##### Deep Dive\n\n#### What is a React element, exactly?[](https://react.dev/reference/react/createElement#what-is-a-react-element-exactly \"Link for What is a React element, exactly? \")\n\nAn element is a lightweight description of a piece of the user interface. For example, both `<Greeting name=\"Taylor\" />` and `createElement(Greeting, { name: 'Taylor' })` produce an object like this:\n\n```\n// Slightly simplified{ type: Greeting,props: { name: 'Taylor'}, key: null,ref: null,}\n```\n\n**Note that creating this object does not render the `Greeting` component or create any DOM elements.**\n\nA React element is more like a description—an instruction for React to later render the `Greeting` component. By returning this object from your `App` component, you tell React what to do next.\n\nCreating elements is extremely cheap so you don’t need to try to optimize or avoid it.\n",
"filename": "createElement.md",
"package": "react"
} |
{
"content": "Title: createFactory – React\n\nURL Source: https://react.dev/reference/react/createFactory\n\nMarkdown Content:\n### Deprecated\n\nThis API will be removed in a future major version of React. [See the alternatives.](https://react.dev/reference/react/createFactory#alternatives)\n\n`createFactory` lets you create a function that produces React elements of a given type.\n\n```\nconst factory = createFactory(type)\n```\n\n* [Reference](https://react.dev/reference/react/createFactory#reference)\n * [`createFactory(type)`](https://react.dev/reference/react/createFactory#createfactory)\n* [Usage](https://react.dev/reference/react/createFactory#usage)\n * [Creating React elements with a factory](https://react.dev/reference/react/createFactory#creating-react-elements-with-a-factory)\n* [Alternatives](https://react.dev/reference/react/createFactory#alternatives)\n * [Copying `createFactory` into your project](https://react.dev/reference/react/createFactory#copying-createfactory-into-your-project)\n * [Replacing `createFactory` with `createElement`](https://react.dev/reference/react/createFactory#replacing-createfactory-with-createelement)\n * [Replacing `createFactory` with JSX](https://react.dev/reference/react/createFactory#replacing-createfactory-with-jsx)\n\n* * *\n\nReference[](https://react.dev/reference/react/createFactory#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `createFactory(type)`[](https://react.dev/reference/react/createFactory#createfactory \"Link for this heading\")\n\nCall `createFactory(type)` to create a factory function which produces React elements of a given `type`.\n\n```\nimport { createFactory } from 'react';const button = createFactory('button');\n```\n\nThen you can use it to create React elements without JSX:\n\n```\nexport default function App() {return button({onClick: () => {alert('Clicked!')}}, 'Click me');}\n```\n\n[See more examples below.](https://react.dev/reference/react/createFactory#usage)\n\n#### Parameters[](https://react.dev/reference/react/createFactory#parameters \"Link for Parameters \")\n\n* `type`: The `type` argument must be a valid React component type. For example, it could be a tag name string (such as `'div'` or `'span'`), or a React component (a function, a class, or a special component like [`Fragment`](https://react.dev/reference/react/Fragment)).\n\n#### Returns[](https://react.dev/reference/react/createFactory#returns \"Link for Returns \")\n\nReturns a factory function. That factory function receives a `props` object as the first argument, followed by a list of `...children` arguments, and returns a React element with the given `type`, `props` and `children`.\n\n* * *\n\nUsage[](https://react.dev/reference/react/createFactory#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Creating React elements with a factory[](https://react.dev/reference/react/createFactory#creating-react-elements-with-a-factory \"Link for Creating React elements with a factory \")\n\nAlthough most React projects use [JSX](https://react.dev/learn/writing-markup-with-jsx) to describe the user interface, JSX is not required. In the past, `createFactory` used to be one of the ways you could describe the user interface without JSX.\n\nCall `createFactory` to create a _factory function_ for a specific element type like `'button'`:\n\n```\nimport { createFactory } from 'react';const button = createFactory('button');\n```\n\nCalling that factory function will produce React elements with the props and children you have provided:\n\nThis is how `createFactory` was used as an alternative to JSX. However, `createFactory` is deprecated, and you should not call `createFactory` in any new code. See how to migrate away from `createFactory` below.\n\n* * *\n\nAlternatives[](https://react.dev/reference/react/createFactory#alternatives \"Link for Alternatives \")\n-----------------------------------------------------------------------------------------------------\n\n### Copying `createFactory` into your project[](https://react.dev/reference/react/createFactory#copying-createfactory-into-your-project \"Link for this heading\")\n\nIf your project has many `createFactory` calls, copy this `createFactory.js` implementation into your project:\n\nThis lets you keep all of your code unchanged except the imports.\n\n* * *\n\n### Replacing `createFactory` with `createElement`[](https://react.dev/reference/react/createFactory#replacing-createfactory-with-createelement \"Link for this heading\")\n\nIf you have a few `createFactory` calls that you don’t mind porting manually, and you don’t want to use JSX, you can replace every call a factory function with a [`createElement`](https://react.dev/reference/react/createElement) call. For example, you can replace this code:\n\n```\nimport { createFactory } from 'react';const button = createFactory('button');export default function App() {return button({onClick: () => {alert('Clicked!')}}, 'Click me');}\n```\n\nwith this code:\n\n```\nimport { createElement } from 'react';export default function App() {return createElement('button', {onClick: () => {alert('Clicked!')}}, 'Click me');}\n```\n\nHere is a complete example of using React without JSX:\n\n* * *\n\n### Replacing `createFactory` with JSX[](https://react.dev/reference/react/createFactory#replacing-createfactory-with-jsx \"Link for this heading\")\n\nFinally, you can use JSX instead of `createFactory`. This is the most common way to use React:\n\n### Pitfall\n\nSometimes, your existing code might pass some variable as a `type` instead of a constant like `'button'`:\n\n```\nfunction Heading({ isSubheading, ...props }) {const type = isSubheading ? 'h2' : 'h1';const factory = createFactory(type);return factory(props);}\n```\n\nTo do the same in JSX, you need to rename your variable to start with an uppercase letter like `Type`:\n\n```\nfunction Heading({ isSubheading, ...props }) {const Type = isSubheading ? 'h2' : 'h1';return <Type {...props} />;}\n```\n\nOtherwise React will interpret `<type>` as a built-in HTML tag because it is lowercase.\n",
"filename": "createFactory.md",
"package": "react"
} |
{
"content": "Title: createPortal – React\n\nURL Source: https://react.dev/reference/react-dom/createPortal\n\nMarkdown Content:\n`createPortal` lets you render some children into a different part of the DOM.\n\n```\n<div><SomeComponent />{createPortal(children, domNode, key?)}</div>\n```\n\n* [Reference](https://react.dev/reference/react-dom/createPortal#reference)\n * [`createPortal(children, domNode, key?)`](https://react.dev/reference/react-dom/createPortal#createportal)\n* [Usage](https://react.dev/reference/react-dom/createPortal#usage)\n * [Rendering to a different part of the DOM](https://react.dev/reference/react-dom/createPortal#rendering-to-a-different-part-of-the-dom)\n * [Rendering a modal dialog with a portal](https://react.dev/reference/react-dom/createPortal#rendering-a-modal-dialog-with-a-portal)\n * [Rendering React components into non-React server markup](https://react.dev/reference/react-dom/createPortal#rendering-react-components-into-non-react-server-markup)\n * [Rendering React components into non-React DOM nodes](https://react.dev/reference/react-dom/createPortal#rendering-react-components-into-non-react-dom-nodes)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/createPortal#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------------\n\n### `createPortal(children, domNode, key?)`[](https://react.dev/reference/react-dom/createPortal#createportal \"Link for this heading\")\n\nTo create a portal, call `createPortal`, passing some JSX, and the DOM node where it should be rendered:\n\n```\nimport { createPortal } from 'react-dom';// ...<div><p>This child is placed in the parent div.</p>{createPortal(<p>This child is placed in the document body.</p>,document.body)}</div>\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/createPortal#usage)\n\nA portal only changes the physical placement of the DOM node. In every other way, the JSX you render into a portal acts as a child node of the React component that renders it. For example, the child can access the context provided by the parent tree, and events bubble up from children to parents according to the React tree.\n\n#### Parameters[](https://react.dev/reference/react-dom/createPortal#parameters \"Link for Parameters \")\n\n* `children`: Anything that can be rendered with React, such as a piece of JSX (e.g. `<div />` or `<SomeComponent />`), a [Fragment](https://react.dev/reference/react/Fragment) (`<>...</>`), a string or a number, or an array of these.\n \n* `domNode`: Some DOM node, such as those returned by `document.getElementById()`. The node must already exist. Passing a different DOM node during an update will cause the portal content to be recreated.\n \n* **optional** `key`: A unique string or number to be used as the portal’s [key.](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key)\n \n\n#### Returns[](https://react.dev/reference/react-dom/createPortal#returns \"Link for Returns \")\n\n`createPortal` returns a React node that can be included into JSX or returned from a React component. If React encounters it in the render output, it will place the provided `children` inside the provided `domNode`.\n\n#### Caveats[](https://react.dev/reference/react-dom/createPortal#caveats \"Link for Caveats \")\n\n* Events from portals propagate according to the React tree rather than the DOM tree. For example, if you click inside a portal, and the portal is wrapped in `<div onClick>`, that `onClick` handler will fire. If this causes issues, either stop the event propagation from inside the portal, or move the portal itself up in the React tree.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/createPortal#usage \"Link for Usage \")\n-----------------------------------------------------------------------------------\n\n### Rendering to a different part of the DOM[](https://react.dev/reference/react-dom/createPortal#rendering-to-a-different-part-of-the-dom \"Link for Rendering to a different part of the DOM \")\n\n_Portals_ let your components render some of their children into a different place in the DOM. This lets a part of your component “escape” from whatever containers it may be in. For example, a component can display a modal dialog or a tooltip that appears above and outside of the rest of the page.\n\nTo create a portal, render the result of `createPortal` with some JSX and the DOM node where it should go:\n\n```\nimport { createPortal } from 'react-dom';function MyComponent() {return (<div style={{ border: '2px solid black' }}><p>This child is placed in the parent div.</p>{createPortal(<p>This child is placed in the document body.</p>,document.body)}</div>);}\n```\n\nReact will put the DOM nodes for the JSX you passed inside of the DOM node you provided.\n\nWithout a portal, the second `<p>` would be placed inside the parent `<div>`, but the portal “teleported” it into the [`document.body`:](https://developer.mozilla.org/en-US/docs/Web/API/Document/body)\n\nNotice how the second paragraph visually appears outside the parent `<div>` with the border. If you inspect the DOM structure with developer tools, you’ll see that the second `<p>` got placed directly into the `<body>`:\n\n```\n<body><div id=\"root\"> ...<div style=\"border: 2px solid black\"><p>This child is placed inside the parent div.</p></div> ...</div><p>This child is placed in the document body.</p></body>\n```\n\nA portal only changes the physical placement of the DOM node. In every other way, the JSX you render into a portal acts as a child node of the React component that renders it. For example, the child can access the context provided by the parent tree, and events still bubble up from children to parents according to the React tree.\n\n* * *\n\n### Rendering a modal dialog with a portal[](https://react.dev/reference/react-dom/createPortal#rendering-a-modal-dialog-with-a-portal \"Link for Rendering a modal dialog with a portal \")\n\nYou can use a portal to create a modal dialog that floats above the rest of the page, even if the component that summons the dialog is inside a container with `overflow: hidden` or other styles that interfere with the dialog.\n\nIn this example, the two containers have styles that disrupt the modal dialog, but the one rendered into a portal is unaffected because, in the DOM, the modal is not contained within the parent JSX elements.\n\n### Pitfall\n\nIt’s important to make sure that your app is accessible when using portals. For instance, you may need to manage keyboard focus so that the user can move the focus in and out of the portal in a natural way.\n\nFollow the [WAI-ARIA Modal Authoring Practices](https://www.w3.org/WAI/ARIA/apg/#dialog_modal) when creating modals. If you use a community package, ensure that it is accessible and follows these guidelines.\n\n* * *\n\n### Rendering React components into non-React server markup[](https://react.dev/reference/react-dom/createPortal#rendering-react-components-into-non-react-server-markup \"Link for Rendering React components into non-React server markup \")\n\nPortals can be useful if your React root is only part of a static or server-rendered page that isn’t built with React. For example, if your page is built with a server framework like Rails, you can create areas of interactivity within static areas such as sidebars. Compared with having [multiple separate React roots,](https://react.dev/reference/react-dom/client/createRoot#rendering-a-page-partially-built-with-react) portals let you treat the app as a single React tree with shared state even though its parts render to different parts of the DOM.\n\nimport { createPortal } from 'react-dom';\n\nconst sidebarContentEl = document.getElementById('sidebar-content');\n\nexport default function App() {\n return (\n <\\>\n <MainContent />\n {createPortal(\n <SidebarContent />,\n sidebarContentEl\n )}\n </\\>\n );\n}\n\nfunction MainContent() {\n return <p\\>This part is rendered by React</p\\>;\n}\n\nfunction SidebarContent() {\n return <p\\>This part is also rendered by React!</p\\>;\n}\n\n* * *\n\n### Rendering React components into non-React DOM nodes[](https://react.dev/reference/react-dom/createPortal#rendering-react-components-into-non-react-dom-nodes \"Link for Rendering React components into non-React DOM nodes \")\n\nYou can also use a portal to manage the content of a DOM node that’s managed outside of React. For example, suppose you’re integrating with a non-React map widget and you want to render React content inside a popup. To do this, declare a `popupContainer` state variable to store the DOM node you’re going to render into:\n\n```\nconst [popupContainer, setPopupContainer] = useState(null);\n```\n\nWhen you create the third-party widget, store the DOM node returned by the widget so you can render into it:\n\n```\nuseEffect(() => {if (mapRef.current === null) {const map = createMapWidget(containerRef.current);mapRef.current = map;const popupDiv = addPopupToMapWidget(map);setPopupContainer(popupDiv);}}, []);\n```\n\nThis lets you use `createPortal` to render React content into `popupContainer` once it becomes available:\n\n```\nreturn (<div style={{ width: 250, height: 250 }} ref={containerRef}>{popupContainer !== null && createPortal(<p>Hello from React!</p>,popupContainer)}</div>);\n```\n\nHere is a complete example you can play with:\n\nimport { useRef, useEffect, useState } from 'react';\nimport { createPortal } from 'react-dom';\nimport { createMapWidget, addPopupToMapWidget } from './map-widget.js';\n\nexport default function Map() {\n const containerRef = useRef(null);\n const mapRef = useRef(null);\n const \\[popupContainer, setPopupContainer\\] = useState(null);\n\n useEffect(() \\=> {\n if (mapRef.current === null) {\n const map = createMapWidget(containerRef.current);\n mapRef.current = map;\n const popupDiv = addPopupToMapWidget(map);\n setPopupContainer(popupDiv);\n }\n }, \\[\\]);\n\n return (\n <div style\\={{ width: 250, height: 250 }} ref\\={containerRef}\\>\n {popupContainer !== null && createPortal(\n <p\\>Hello from React!</p\\>,\n popupContainer\n )}\n </div\\>\n );\n}\n",
"filename": "createPortal.md",
"package": "react"
} |
{
"content": "Title: createRef – React\n\nURL Source: https://react.dev/reference/react/createRef\n\nMarkdown Content:\ncreateRef – React\n===============\n\ncreateRef[](https://react.dev/reference/react/createRef#undefined \"Link for this heading\")\n==========================================================================================\n\n### Pitfall\n\n`createRef` is mostly used for [class components.](https://react.dev/reference/react/Component) Function components typically rely on [`useRef`](https://react.dev/reference/react/useRef) instead.\n\n`createRef` creates a [ref](https://react.dev/learn/referencing-values-with-refs) object which can contain arbitrary value.\n\n```\nclass MyInput extends Component { inputRef = createRef(); // ...}\n```\n\n* [Reference](https://react.dev/reference/react/createRef#reference)\n * [`createRef()`](https://react.dev/reference/react/createRef#createref)\n* [Usage](https://react.dev/reference/react/createRef#usage)\n * [Declaring a ref in a class component](https://react.dev/reference/react/createRef#declaring-a-ref-in-a-class-component)\n* [Alternatives](https://react.dev/reference/react/createRef#alternatives)\n * [Migrating from a class with `createRef` to a function with `useRef`](https://react.dev/reference/react/createRef#migrating-from-a-class-with-createref-to-a-function-with-useref)\n\n* * *\n\nReference[](https://react.dev/reference/react/createRef#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------\n\n### `createRef()`[](https://react.dev/reference/react/createRef#createref \"Link for this heading\")\n\nCall `createRef` to declare a [ref](https://react.dev/learn/referencing-values-with-refs) inside a [class component.](https://react.dev/reference/react/Component)\n\n```\nimport { createRef, Component } from 'react';class MyComponent extends Component { intervalRef = createRef(); inputRef = createRef(); // ...\n```\n\n[See more examples below.](https://react.dev/reference/react/createRef#usage)\n\n#### Parameters[](https://react.dev/reference/react/createRef#parameters \"Link for Parameters \")\n\n`createRef` takes no parameters.\n\n#### Returns[](https://react.dev/reference/react/createRef#returns \"Link for Returns \")\n\n`createRef` returns an object with a single property:\n\n* `current`: Initially, it’s set to the `null`. You can later set it to something else. If you pass the ref object to React as a `ref` attribute to a JSX node, React will set its `current` property.\n\n#### Caveats[](https://react.dev/reference/react/createRef#caveats \"Link for Caveats \")\n\n* `createRef` always returns a _different_ object. It’s equivalent to writing `{ current: null }` yourself.\n* In a function component, you probably want [`useRef`](https://react.dev/reference/react/useRef) instead which always returns the same object.\n* `const ref = useRef()` is equivalent to `const [ref, _] = useState(() => createRef(null))`.\n\n* * *\n\nUsage[](https://react.dev/reference/react/createRef#usage \"Link for Usage \")\n----------------------------------------------------------------------------\n\n### Declaring a ref in a class component[](https://react.dev/reference/react/createRef#declaring-a-ref-in-a-class-component \"Link for Declaring a ref in a class component \")\n\nTo declare a ref inside a [class component,](https://react.dev/reference/react/Component) call `createRef` and assign its result to a class field:\n\n```\nimport { Component, createRef } from 'react';class Form extends Component { inputRef = createRef(); // ...}\n```\n\nIf you now pass `ref={this.inputRef}` to an `<input>` in your JSX, React will populate `this.inputRef.current` with the input DOM node. For example, here is how you make a button that focuses the input:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { Component, createRef } from 'react';\n\nexport default class Form extends Component {\n inputRef = createRef();\n\n handleClick = () \\=> {\n this.inputRef.current.focus();\n }\n\n render() {\n return (\n <\\>\n <input ref\\={this.inputRef} />\n <button onClick\\={this.handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n }\n}\n\nShow more\n\n### Pitfall\n\n`createRef` is mostly used for [class components.](https://react.dev/reference/react/Component) Function components typically rely on [`useRef`](https://react.dev/reference/react/useRef) instead.\n\n* * *\n\nAlternatives[](https://react.dev/reference/react/createRef#alternatives \"Link for Alternatives \")\n-------------------------------------------------------------------------------------------------\n\n### Migrating from a class with `createRef` to a function with `useRef`[](https://react.dev/reference/react/createRef#migrating-from-a-class-with-createref-to-a-function-with-useref \"Link for this heading\")\n\nWe recommend using function components instead of [class components](https://react.dev/reference/react/Component) in new code. If you have some existing class components using `createRef`, here is how you can convert them. This is the original code:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { Component, createRef } from 'react';\n\nexport default class Form extends Component {\n inputRef = createRef();\n\n handleClick = () \\=> {\n this.inputRef.current.focus();\n }\n\n render() {\n return (\n <\\>\n <input ref\\={this.inputRef} />\n <button onClick\\={this.handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n }\n}\n\nShow more\n\nWhen you [convert this component from a class to a function,](https://react.dev/reference/react/Component#alternatives) replace calls to `createRef` with calls to [`useRef`:](https://react.dev/reference/react/useRef)\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useRef } from 'react';\n\nexport default function Form() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <input ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\nShow more\n\n[PreviouscreateFactory](https://react.dev/reference/react/createFactory)[NextisValidElement](https://react.dev/reference/react/isValidElement)\n",
"filename": "createRef.md",
"package": "react"
} |
{
"content": "Title: createRoot – React\n\nURL Source: https://react.dev/reference/react-dom/client/createRoot\n\nMarkdown Content:\n`createRoot` lets you create a root to display React components inside a browser DOM node.\n\n```\nconst root = createRoot(domNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/client/createRoot#reference)\n * [`createRoot(domNode, options?)`](https://react.dev/reference/react-dom/client/createRoot#createroot)\n * [`root.render(reactNode)`](https://react.dev/reference/react-dom/client/createRoot#root-render)\n * [`root.unmount()`](https://react.dev/reference/react-dom/client/createRoot#root-unmount)\n* [Usage](https://react.dev/reference/react-dom/client/createRoot#usage)\n * [Rendering an app fully built with React](https://react.dev/reference/react-dom/client/createRoot#rendering-an-app-fully-built-with-react)\n * [Rendering a page partially built with React](https://react.dev/reference/react-dom/client/createRoot#rendering-a-page-partially-built-with-react)\n * [Updating a root component](https://react.dev/reference/react-dom/client/createRoot#updating-a-root-component)\n * [Show a dialog for uncaught errors](https://react.dev/reference/react-dom/client/createRoot#show-a-dialog-for-uncaught-errors)\n * [Displaying Error Boundary errors](https://react.dev/reference/react-dom/client/createRoot#displaying-error-boundary-errors)\n * [Displaying a dialog for recoverable errors](https://react.dev/reference/react-dom/client/createRoot#displaying-a-dialog-for-recoverable-errors)\n* [Troubleshooting](https://react.dev/reference/react-dom/client/createRoot#troubleshooting)\n * [I’ve created a root, but nothing is displayed](https://react.dev/reference/react-dom/client/createRoot#ive-created-a-root-but-nothing-is-displayed)\n * [I’m getting an error: “You passed a second argument to root.render”](https://react.dev/reference/react-dom/client/createRoot#im-getting-an-error-you-passed-a-second-argument-to-root-render)\n * [I’m getting an error: “Target container is not a DOM element”](https://react.dev/reference/react-dom/client/createRoot#im-getting-an-error-target-container-is-not-a-dom-element)\n * [I’m getting an error: “Functions are not valid as a React child.”](https://react.dev/reference/react-dom/client/createRoot#im-getting-an-error-functions-are-not-valid-as-a-react-child)\n * [My server-rendered HTML gets re-created from scratch](https://react.dev/reference/react-dom/client/createRoot#my-server-rendered-html-gets-re-created-from-scratch)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/client/createRoot#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------\n\n### `createRoot(domNode, options?)`[](https://react.dev/reference/react-dom/client/createRoot#createroot \"Link for this heading\")\n\nCall `createRoot` to create a React root for displaying content inside a browser DOM element.\n\n```\nimport { createRoot } from 'react-dom/client';const domNode = document.getElementById('root');const root = createRoot(domNode);\n```\n\nReact will create a root for the `domNode`, and take over managing the DOM inside it. After you’ve created a root, you need to call [`root.render`](https://react.dev/reference/react-dom/client/createRoot#root-render) to display a React component inside of it:\n\nAn app fully built with React will usually only have one `createRoot` call for its root component. A page that uses “sprinkles” of React for parts of the page may have as many separate roots as needed.\n\n[See more examples below.](https://react.dev/reference/react-dom/client/createRoot#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/client/createRoot#parameters \"Link for Parameters \")\n\n* `domNode`: A [DOM element.](https://developer.mozilla.org/en-US/docs/Web/API/Element) React will create a root for this DOM element and allow you to call functions on the root, such as `render` to display rendered React content.\n \n* **optional** `options`: An object with options for this React root.\n \n * Canary only **optional** `onCaughtError`: Callback called when React catches an error in an Error Boundary. Called with the `error` caught by the Error Boundary, and an `errorInfo` object containing the `componentStack`.\n * Canary only **optional** `onUncaughtError`: Callback called when an error is thrown and not caught by an Error Boundary. Called with the `error` that was thrown, and an `errorInfo` object containing the `componentStack`.\n * **optional** `onRecoverableError`: Callback called when React automatically recovers from errors. Called with an `error` React throws, and an `errorInfo` object containing the `componentStack`. Some recoverable errors may include the original error cause as `error.cause`.\n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page.\n\n#### Returns[](https://react.dev/reference/react-dom/client/createRoot#returns \"Link for Returns \")\n\n`createRoot` returns an object with two methods: [`render`](https://react.dev/reference/react-dom/client/createRoot#root-render) and [`unmount`.](https://react.dev/reference/react-dom/client/createRoot#root-unmount)\n\n#### Caveats[](https://react.dev/reference/react-dom/client/createRoot#caveats \"Link for Caveats \")\n\n* If your app is server-rendered, using `createRoot()` is not supported. Use [`hydrateRoot()`](https://react.dev/reference/react-dom/client/hydrateRoot) instead.\n* You’ll likely have only one `createRoot` call in your app. If you use a framework, it might do this call for you.\n* When you want to render a piece of JSX in a different part of the DOM tree that isn’t a child of your component (for example, a modal or a tooltip), use [`createPortal`](https://react.dev/reference/react-dom/createPortal) instead of `createRoot`.\n\n* * *\n\n### `root.render(reactNode)`[](https://react.dev/reference/react-dom/client/createRoot#root-render \"Link for this heading\")\n\nCall `root.render` to display a piece of [JSX](https://react.dev/learn/writing-markup-with-jsx) (“React node”) into the React root’s browser DOM node.\n\nReact will display `<App />` in the `root`, and take over managing the DOM inside it.\n\n[See more examples below.](https://react.dev/reference/react-dom/client/createRoot#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/client/createRoot#root-render-parameters \"Link for Parameters \")\n\n* `reactNode`: A _React node_ that you want to display. This will usually be a piece of JSX like `<App />`, but you can also pass a React element constructed with [`createElement()`](https://react.dev/reference/react/createElement), a string, a number, `null`, or `undefined`.\n\n#### Returns[](https://react.dev/reference/react-dom/client/createRoot#root-render-returns \"Link for Returns \")\n\n`root.render` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react-dom/client/createRoot#root-render-caveats \"Link for Caveats \")\n\n* The first time you call `root.render`, React will clear all the existing HTML content inside the React root before rendering the React component into it.\n \n* If your root’s DOM node contains HTML generated by React on the server or during the build, use [`hydrateRoot()`](https://react.dev/reference/react-dom/client/hydrateRoot) instead, which attaches the event handlers to the existing HTML.\n \n* If you call `render` on the same root more than once, React will update the DOM as necessary to reflect the latest JSX you passed. React will decide which parts of the DOM can be reused and which need to be recreated by [“matching it up”](https://react.dev/learn/preserving-and-resetting-state) with the previously rendered tree. Calling `render` on the same root again is similar to calling the [`set` function](https://react.dev/reference/react/useState#setstate) on the root component: React avoids unnecessary DOM updates.\n \n\n* * *\n\n### `root.unmount()`[](https://react.dev/reference/react-dom/client/createRoot#root-unmount \"Link for this heading\")\n\nCall `root.unmount` to destroy a rendered tree inside a React root.\n\nAn app fully built with React will usually not have any calls to `root.unmount`.\n\nThis is mostly useful if your React root’s DOM node (or any of its ancestors) may get removed from the DOM by some other code. For example, imagine a jQuery tab panel that removes inactive tabs from the DOM. If a tab gets removed, everything inside it (including the React roots inside) would get removed from the DOM as well. In that case, you need to tell React to “stop” managing the removed root’s content by calling `root.unmount`. Otherwise, the components inside the removed root won’t know to clean up and free up global resources like subscriptions.\n\nCalling `root.unmount` will unmount all the components in the root and “detach” React from the root DOM node, including removing any event handlers or state in the tree.\n\n#### Parameters[](https://react.dev/reference/react-dom/client/createRoot#root-unmount-parameters \"Link for Parameters \")\n\n`root.unmount` does not accept any parameters.\n\n#### Returns[](https://react.dev/reference/react-dom/client/createRoot#root-unmount-returns \"Link for Returns \")\n\n`root.unmount` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react-dom/client/createRoot#root-unmount-caveats \"Link for Caveats \")\n\n* Calling `root.unmount` will unmount all the components in the tree and “detach” React from the root DOM node.\n \n* Once you call `root.unmount` you cannot call `root.render` again on the same root. Attempting to call `root.render` on an unmounted root will throw a “Cannot update an unmounted root” error. However, you can create a new root for the same DOM node after the previous root for that node has been unmounted.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/client/createRoot#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------\n\n### Rendering an app fully built with React[](https://react.dev/reference/react-dom/client/createRoot#rendering-an-app-fully-built-with-react \"Link for Rendering an app fully built with React \")\n\nIf your app is fully built with React, create a single root for your entire app.\n\n```\nimport { createRoot } from 'react-dom/client';const root = createRoot(document.getElementById('root'));root.render(<App />);\n```\n\nUsually, you only need to run this code once at startup. It will:\n\n1. Find the browser DOM node defined in your HTML.\n2. Display the React component for your app inside.\n\n**If your app is fully built with React, you shouldn’t need to create any more roots, or to call [`root.render`](https://react.dev/reference/react-dom/client/createRoot#root-render) again.**\n\nFrom this point on, React will manage the DOM of your entire app. To add more components, [nest them inside the `App` component.](https://react.dev/learn/importing-and-exporting-components) When you need to update the UI, each of your components can do this by [using state.](https://react.dev/reference/react/useState) When you need to display extra content like a modal or a tooltip outside the DOM node, [render it with a portal.](https://react.dev/reference/react-dom/createPortal)\n\n### Note\n\nWhen your HTML is empty, the user sees a blank page until the app’s JavaScript code loads and runs:\n\nThis can feel very slow! To solve this, you can generate the initial HTML from your components [on the server or during the build.](https://react.dev/reference/react-dom/server) Then your visitors can read text, see images, and click links before any of the JavaScript code loads. We recommend [using a framework](https://react.dev/learn/start-a-new-react-project#production-grade-react-frameworks) that does this optimization out of the box. Depending on when it runs, this is called _server-side rendering (SSR)_ or _static site generation (SSG)._\n\n### Pitfall\n\n**Apps using server rendering or static generation must call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) instead of `createRoot`.** React will then _hydrate_ (reuse) the DOM nodes from your HTML instead of destroying and re-creating them.\n\n* * *\n\n### Rendering a page partially built with React[](https://react.dev/reference/react-dom/client/createRoot#rendering-a-page-partially-built-with-react \"Link for Rendering a page partially built with React \")\n\nIf your page [isn’t fully built with React](https://react.dev/learn/add-react-to-an-existing-project#using-react-for-a-part-of-your-existing-page), you can call `createRoot` multiple times to create a root for each top-level piece of UI managed by React. You can display different content in each root by calling [`root.render`.](https://react.dev/reference/react-dom/client/createRoot#root-render)\n\nHere, two different React components are rendered into two DOM nodes defined in the `index.html` file:\n\nYou could also create a new DOM node with [`document.createElement()`](https://developer.mozilla.org/en-US/docs/Web/API/Document/createElement) and add it to the document manually.\n\n```\nconst domNode = document.createElement('div');const root = createRoot(domNode); root.render(<Comment />);document.body.appendChild(domNode); // You can add it anywhere in the document\n```\n\nTo remove the React tree from the DOM node and clean up all the resources used by it, call [`root.unmount`.](https://react.dev/reference/react-dom/client/createRoot#root-unmount)\n\nThis is mostly useful if your React components are inside an app written in a different framework.\n\n* * *\n\n### Updating a root component[](https://react.dev/reference/react-dom/client/createRoot#updating-a-root-component \"Link for Updating a root component \")\n\nYou can call `render` more than once on the same root. As long as the component tree structure matches up with what was previously rendered, React will [preserve the state.](https://react.dev/learn/preserving-and-resetting-state) Notice how you can type in the input, which means that the updates from repeated `render` calls every second in this example are not destructive:\n\nIt is uncommon to call `render` multiple times. Usually, your components will [update state](https://react.dev/reference/react/useState) instead.\n\n### Show a dialog for uncaught errors[](https://react.dev/reference/react-dom/client/createRoot#show-a-dialog-for-uncaught-errors \"Link for Show a dialog for uncaught errors \")\n\n### Canary\n\n`onUncaughtError` is only available in the latest React Canary release.\n\nBy default, React will log all uncaught errors to the console. To implement your own error reporting, you can provide the optional `onUncaughtError` root option:\n\n```\nimport { createRoot } from 'react-dom/client';const root = createRoot(document.getElementById('root'),{onUncaughtError: (error, errorInfo) => {console.error('Uncaught error',error,errorInfo.componentStack);}});root.render(<App />);\n```\n\nThe onUncaughtError option is a function called with two arguments:\n\n1. The error that was thrown.\n2. An errorInfo object that contains the componentStack of the error.\n\nYou can use the `onUncaughtError` root option to display error dialogs:\n\nimport { createRoot } from \"react-dom/client\";\nimport App from \"./App.js\";\nimport {reportUncaughtError} from \"./reportError\";\nimport \"./styles.css\";\n\nconst container = document.getElementById(\"root\");\nconst root = createRoot(container, {\n onUncaughtError: (error, errorInfo) \\=> {\n if (error.message !== 'Known error') {\n reportUncaughtError({\n error,\n componentStack: errorInfo.componentStack\n });\n }\n }\n});\nroot.render(<App />);\n\n### Displaying Error Boundary errors[](https://react.dev/reference/react-dom/client/createRoot#displaying-error-boundary-errors \"Link for Displaying Error Boundary errors \")\n\n### Canary\n\n`onCaughtError` is only available in the latest React Canary release.\n\nBy default, React will log all errors caught by an Error Boundary to `console.error`. To override this behavior, you can provide the optional `onCaughtError` root option to handle errors caught by an [Error Boundary](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary):\n\n```\nimport { createRoot } from 'react-dom/client';const root = createRoot(document.getElementById('root'),{onCaughtError: (error, errorInfo) => {console.error('Caught error',error,errorInfo.componentStack);}});root.render(<App />);\n```\n\nThe onCaughtError option is a function called with two arguments:\n\n1. The error that was caught by the boundary.\n2. An errorInfo object that contains the componentStack of the error.\n\nYou can use the `onCaughtError` root option to display error dialogs or filter known errors from logging:\n\nimport { createRoot } from \"react-dom/client\";\nimport App from \"./App.js\";\nimport {reportCaughtError} from \"./reportError\";\nimport \"./styles.css\";\n\nconst container = document.getElementById(\"root\");\nconst root = createRoot(container, {\n onCaughtError: (error, errorInfo) \\=> {\n if (error.message !== 'Known error') {\n reportCaughtError({\n error, \n componentStack: errorInfo.componentStack,\n });\n }\n }\n});\nroot.render(<App />);\n\n### Displaying a dialog for recoverable errors[](https://react.dev/reference/react-dom/client/createRoot#displaying-a-dialog-for-recoverable-errors \"Link for Displaying a dialog for recoverable errors \")\n\nReact may automatically render a component a second time to attempt to recover from an error thrown in render. If successful, React will log a recoverable error to the console to notify the developer. To override this behavior, you can provide the optional `onRecoverableError` root option:\n\n```\nimport { createRoot } from 'react-dom/client';const root = createRoot(document.getElementById('root'),{onRecoverableError: (error, errorInfo) => {console.error('Recoverable error',error,error.cause,errorInfo.componentStack,);}});root.render(<App />);\n```\n\nThe onRecoverableError option is a function called with two arguments:\n\n1. The error that React throws. Some errors may include the original cause as error.cause.\n2. An errorInfo object that contains the componentStack of the error.\n\nYou can use the `onRecoverableError` root option to display error dialogs:\n\nimport { createRoot } from \"react-dom/client\";\nimport App from \"./App.js\";\nimport {reportRecoverableError} from \"./reportError\";\nimport \"./styles.css\";\n\nconst container = document.getElementById(\"root\");\nconst root = createRoot(container, {\n onRecoverableError: (error, errorInfo) \\=> {\n reportRecoverableError({\n error,\n cause: error.cause,\n componentStack: errorInfo.componentStack,\n });\n }\n});\nroot.render(<App />);\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react-dom/client/createRoot#troubleshooting \"Link for Troubleshooting \")\n----------------------------------------------------------------------------------------------------------------------\n\n### I’ve created a root, but nothing is displayed[](https://react.dev/reference/react-dom/client/createRoot#ive-created-a-root-but-nothing-is-displayed \"Link for I’ve created a root, but nothing is displayed \")\n\nMake sure you haven’t forgotten to actually _render_ your app into the root:\n\n```\nimport { createRoot } from 'react-dom/client';import App from './App.js';const root = createRoot(document.getElementById('root'));root.render(<App />);\n```\n\nUntil you do that, nothing is displayed.\n\n* * *\n\n### I’m getting an error: “You passed a second argument to root.render”[](https://react.dev/reference/react-dom/client/createRoot#im-getting-an-error-you-passed-a-second-argument-to-root-render \"Link for I’m getting an error: “You passed a second argument to root.render” \")\n\nA common mistake is to pass the options for `createRoot` to `root.render(...)`:\n\nWarning: You passed a second argument to root.render(…) but it only accepts one argument.\n\nTo fix, pass the root options to `createRoot(...)`, not `root.render(...)`:\n\n```\n// 🚩 Wrong: root.render only takes one argument.root.render(App, {onUncaughtError});// ✅ Correct: pass options to createRoot.const root = createRoot(container, {onUncaughtError}); root.render(<App />);\n```\n\n* * *\n\n### I’m getting an error: “Target container is not a DOM element”[](https://react.dev/reference/react-dom/client/createRoot#im-getting-an-error-target-container-is-not-a-dom-element \"Link for I’m getting an error: “Target container is not a DOM element” \")\n\nThis error means that whatever you’re passing to `createRoot` is not a DOM node.\n\nIf you’re not sure what’s happening, try logging it:\n\n```\nconst domNode = document.getElementById('root');console.log(domNode); // ???const root = createRoot(domNode);root.render(<App />);\n```\n\nFor example, if `domNode` is `null`, it means that [`getElementById`](https://developer.mozilla.org/en-US/docs/Web/API/Document/getElementById) returned `null`. This will happen if there is no node in the document with the given ID at the time of your call. There may be a few reasons for it:\n\n1. The ID you’re looking for might differ from the ID you used in the HTML file. Check for typos!\n2. Your bundle’s `<script>` tag cannot “see” any DOM nodes that appear _after_ it in the HTML.\n\nAnother common way to get this error is to write `createRoot(<App />)` instead of `createRoot(domNode)`.\n\n* * *\n\n### I’m getting an error: “Functions are not valid as a React child.”[](https://react.dev/reference/react-dom/client/createRoot#im-getting-an-error-functions-are-not-valid-as-a-react-child \"Link for I’m getting an error: “Functions are not valid as a React child.” \")\n\nThis error means that whatever you’re passing to `root.render` is not a React component.\n\nThis may happen if you call `root.render` with `Component` instead of `<Component />`:\n\n```\n// 🚩 Wrong: App is a function, not a Component.root.render(App);// ✅ Correct: <App /> is a component.root.render(<App />);\n```\n\nOr if you pass a function to `root.render`, instead of the result of calling it:\n\n```\n// 🚩 Wrong: createApp is a function, not a component.root.render(createApp);// ✅ Correct: call createApp to return a component.root.render(createApp());\n```\n\n* * *\n\n### My server-rendered HTML gets re-created from scratch[](https://react.dev/reference/react-dom/client/createRoot#my-server-rendered-html-gets-re-created-from-scratch \"Link for My server-rendered HTML gets re-created from scratch \")\n\nIf your app is server-rendered and includes the initial HTML generated by React, you might notice that creating a root and calling `root.render` deletes all that HTML, and then re-creates all the DOM nodes from scratch. This can be slower, resets focus and scroll positions, and may lose other user input.\n\nServer-rendered apps must use [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) instead of `createRoot`:\n\n```\nimport { hydrateRoot } from 'react-dom/client';import App from './App.js';hydrateRoot(document.getElementById('root'),<App />);\n```\n\nNote that its API is different. In particular, usually there will be no further `root.render` call.\n",
"filename": "createRoot.md",
"package": "react"
} |
{
"content": "Title: Describing the UI – React\n\nURL Source: https://react.dev/learn/describing-the-ui\n\nMarkdown Content:\nReact is a JavaScript library for rendering user interfaces (UI). UI is built from small units like buttons, text, and images. React lets you combine them into reusable, nestable _components._ From web sites to phone apps, everything on the screen can be broken down into components. In this chapter, you’ll learn to create, customize, and conditionally display React components.\n\nYour first component[](https://react.dev/learn/describing-the-ui#your-first-component \"Link for Your first component \")\n-----------------------------------------------------------------------------------------------------------------------\n\nReact applications are built from isolated pieces of UI called _components_. A React component is a JavaScript function that you can sprinkle with markup. Components can be as small as a button, or as large as an entire page. Here is a `Gallery` component rendering three `Profile` components:\n\n* * *\n\nImporting and exporting components[](https://react.dev/learn/describing-the-ui#importing-and-exporting-components \"Link for Importing and exporting components \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou can declare many components in one file, but large files can get difficult to navigate. To solve this, you can _export_ a component into its own file, and then _import_ that component from another file:\n\n* * *\n\nWriting markup with JSX[](https://react.dev/learn/describing-the-ui#writing-markup-with-jsx \"Link for Writing markup with JSX \")\n--------------------------------------------------------------------------------------------------------------------------------\n\nEach React component is a JavaScript function that may contain some markup that React renders into the browser. React components use a syntax extension called JSX to represent that markup. JSX looks a lot like HTML, but it is a bit stricter and can display dynamic information.\n\nIf we paste existing HTML markup into a React component, it won’t always work:\n\nexport default function TodoList() {\n return (\n \n <h1\\>Hedy Lamarr's Todos</h1\\>\n <img\n src\\=\"https://i.imgur.com/yXOvdOSs.jpg\"\n alt\\=\"Hedy Lamarr\"\n class\\=\"photo\"\n >\n <ul\\>\n <li\\>Invent new traffic lights\n <li\\>Rehearse a movie scene\n <li\\>Improve spectrum technology\n </ul\\>\n\nIf you have existing HTML like this, you can fix it using a [converter](https://transform.tools/html-to-jsx):\n\nexport default function TodoList() {\n return (\n <\\>\n <h1\\>Hedy Lamarr's Todos</h1\\>\n <img\n src\\=\"https://i.imgur.com/yXOvdOSs.jpg\"\n alt\\=\"Hedy Lamarr\"\n className\\=\"photo\"\n />\n <ul\\>\n <li\\>Invent new traffic lights</li\\>\n <li\\>Rehearse a movie scene</li\\>\n <li\\>Improve spectrum technology</li\\>\n </ul\\>\n </\\>\n );\n}\n\n* * *\n\nJavaScript in JSX with curly braces[](https://react.dev/learn/describing-the-ui#javascript-in-jsx-with-curly-braces \"Link for JavaScript in JSX with curly braces \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nJSX lets you write HTML-like markup inside a JavaScript file, keeping rendering logic and content in the same place. Sometimes you will want to add a little JavaScript logic or reference a dynamic property inside that markup. In this situation, you can use curly braces in your JSX to “open a window” to JavaScript:\n\nconst person = {\n name: 'Gregorio Y. Zara',\n theme: {\n backgroundColor: 'black',\n color: 'pink'\n }\n};\n\nexport default function TodoList() {\n return (\n <div style\\={person.theme}\\>\n <h1\\>{person.name}'s Todos</h1\\>\n <img\n className\\=\"avatar\"\n src\\=\"https://i.imgur.com/7vQD0fPs.jpg\"\n alt\\=\"Gregorio Y. Zara\"\n />\n <ul\\>\n <li\\>Improve the videophone</li\\>\n <li\\>Prepare aeronautics lectures</li\\>\n <li\\>Work on the alcohol-fuelled engine</li\\>\n </ul\\>\n </div\\>\n );\n}\n\n* * *\n\nPassing props to a component[](https://react.dev/learn/describing-the-ui#passing-props-to-a-component \"Link for Passing props to a component \")\n-----------------------------------------------------------------------------------------------------------------------------------------------\n\nReact components use _props_ to communicate with each other. Every parent component can pass some information to its child components by giving them props. Props might remind you of HTML attributes, but you can pass any JavaScript value through them, including objects, arrays, functions, and even JSX!\n\nimport { getImageUrl } from './utils.js'\n\nexport default function Profile() {\n return (\n <Card\\>\n <Avatar\n size\\={100}\n person\\={{\n name: 'Katsuko Saruhashi',\n imageId: 'YfeOqp2'\n }}\n />\n </Card\\>\n );\n}\n\nfunction Avatar({ person, size }) {\n return (\n <img\n className\\=\"avatar\"\n src\\={getImageUrl(person)}\n alt\\={person.name}\n width\\={size}\n height\\={size}\n />\n );\n}\n\nfunction Card({ children }) {\n return (\n <div className\\=\"card\"\\>\n {children}\n </div\\>\n );\n}\n\n* * *\n\nConditional rendering[](https://react.dev/learn/describing-the-ui#conditional-rendering \"Link for Conditional rendering \")\n--------------------------------------------------------------------------------------------------------------------------\n\nYour components will often need to display different things depending on different conditions. In React, you can conditionally render JSX using JavaScript syntax like `if` statements, `&&`, and `? :` operators.\n\nIn this example, the JavaScript `&&` operator is used to conditionally render a checkmark:\n\nfunction Item({ name, isPacked }) {\n return (\n <li className\\=\"item\"\\>\n {name} {isPacked && '✔'}\n </li\\>\n );\n}\n\nexport default function PackingList() {\n return (\n <section\\>\n <h1\\>Sally Ride's Packing List</h1\\>\n <ul\\>\n <Item\n isPacked\\={true}\n name\\=\"Space suit\"\n />\n <Item\n isPacked\\={true}\n name\\=\"Helmet with a golden leaf\"\n />\n <Item\n isPacked\\={false}\n name\\=\"Photo of Tam\"\n />\n </ul\\>\n </section\\>\n );\n}\n\n* * *\n\nRendering lists[](https://react.dev/learn/describing-the-ui#rendering-lists \"Link for Rendering lists \")\n--------------------------------------------------------------------------------------------------------\n\nYou will often want to display multiple similar components from a collection of data. You can use JavaScript’s `filter()` and `map()` with React to filter and transform your array of data into an array of components.\n\nFor each array item, you will need to specify a `key`. Usually, you will want to use an ID from the database as a `key`. Keys let React keep track of each item’s place in the list even if the list changes.\n\nimport { people } from './data.js';\nimport { getImageUrl } from './utils.js';\n\nexport default function List() {\n const listItems = people.map(person \\=>\n <li key\\={person.id}\\>\n <img\n src\\={getImageUrl(person)}\n alt\\={person.name}\n />\n <p\\>\n <b\\>{person.name}:</b\\>\n {' ' + person.profession + ' '}\n known for {person.accomplishment}\n </p\\>\n </li\\>\n );\n return (\n <article\\>\n <h1\\>Scientists</h1\\>\n <ul\\>{listItems}</ul\\>\n </article\\>\n );\n}\n\nReady to learn this topic?\n--------------------------\n\nRead **[Rendering Lists](https://react.dev/learn/rendering-lists)** to learn how to render a list of components, and how to choose a key.\n\n[Read More](https://react.dev/learn/rendering-lists)\n\n* * *\n\nKeeping components pure[](https://react.dev/learn/describing-the-ui#keeping-components-pure \"Link for Keeping components pure \")\n--------------------------------------------------------------------------------------------------------------------------------\n\nSome JavaScript functions are _pure._ A pure function:\n\n* **Minds its own business.** It does not change any objects or variables that existed before it was called.\n* **Same inputs, same output.** Given the same inputs, a pure function should always return the same result.\n\nBy strictly only writing your components as pure functions, you can avoid an entire class of baffling bugs and unpredictable behavior as your codebase grows. Here is an example of an impure component:\n\nlet guest = 0;\n\nfunction Cup() {\n \n guest = guest + 1;\n return <h2\\>Tea cup for guest #{guest}</h2\\>;\n}\n\nexport default function TeaSet() {\n return (\n <\\>\n <Cup />\n <Cup />\n <Cup />\n </\\>\n );\n}\n\nYou can make this component pure by passing a prop instead of modifying a preexisting variable:\n\n* * *\n\nYour UI as a tree[](https://react.dev/learn/describing-the-ui#your-ui-as-a-tree \"Link for Your UI as a tree \")\n--------------------------------------------------------------------------------------------------------------\n\nReact uses trees to model the relationships between components and modules.\n\nA React render tree is a representation of the parent and child relationship between components.\n\n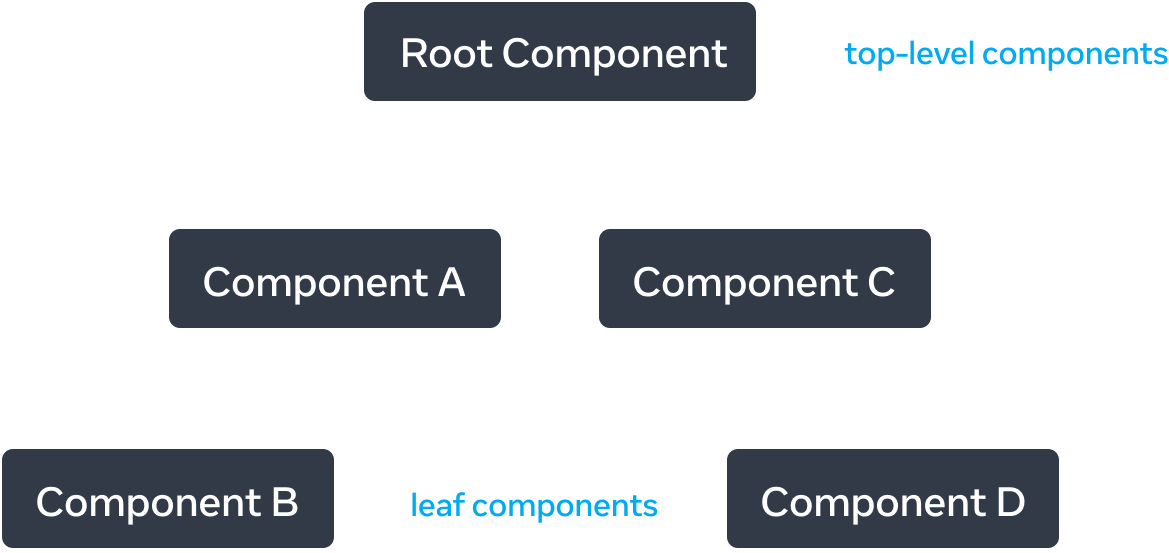\n\n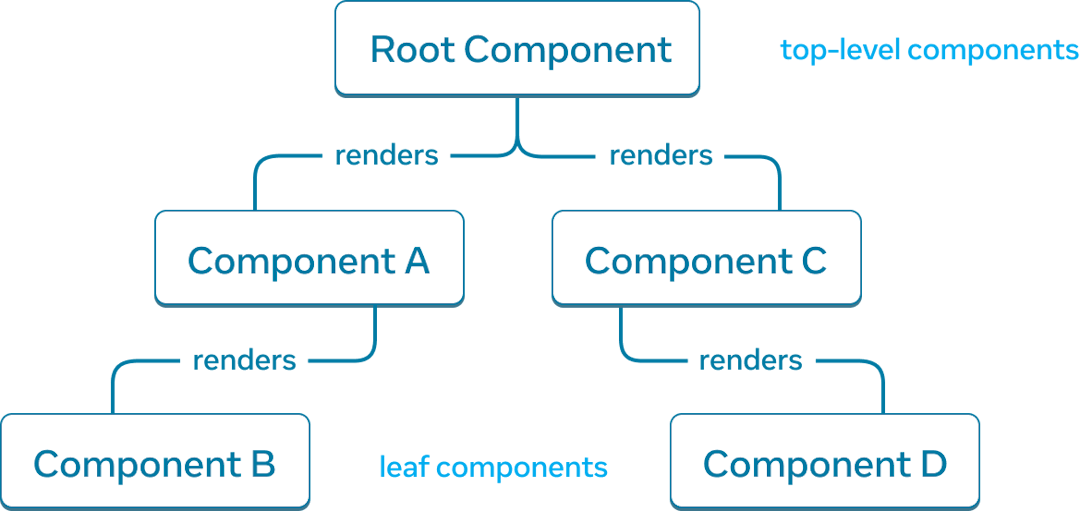\n\nAn example React render tree.\n\nComponents near the top of the tree, near the root component, are considered top-level components. Components with no child components are leaf components. This categorization of components is useful for understanding data flow and rendering performance.\n\nModelling the relationship between JavaScript modules is another useful way to understand your app. We refer to it as a module dependency tree.\n\n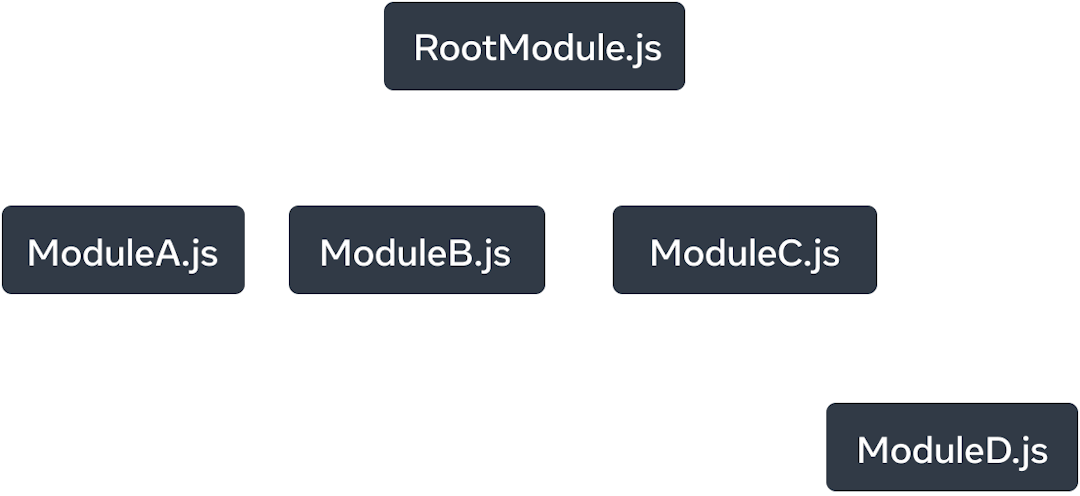\n\n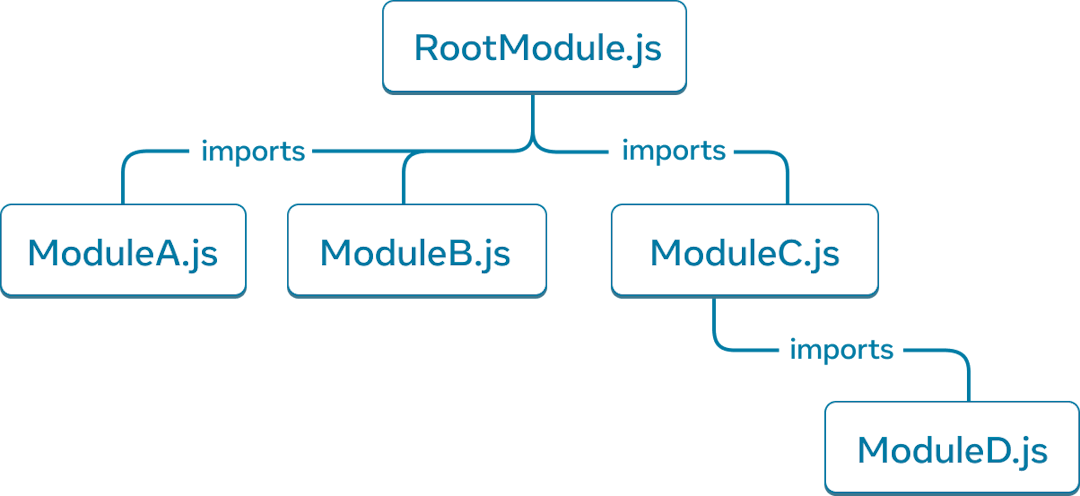\n\nAn example module dependency tree.\n\nA dependency tree is often used by build tools to bundle all the relevant JavaScript code for the client to download and render. A large bundle size regresses user experience for React apps. Understanding the module dependency tree is helpful to debug such issues.\n\nReady to learn this topic?\n--------------------------\n\nRead **[Your UI as a Tree](https://react.dev/learn/understanding-your-ui-as-a-tree)** to learn how to create a render and module dependency trees for a React app and how they’re useful mental models for improving user experience and performance.\n\n[Read More](https://react.dev/learn/understanding-your-ui-as-a-tree)\n\n* * *\n\nWhat’s next?[](https://react.dev/learn/describing-the-ui#whats-next \"Link for What’s next? \")\n---------------------------------------------------------------------------------------------\n\nHead over to [Your First Component](https://react.dev/learn/your-first-component) to start reading this chapter page by page!\n\nOr, if you’re already familiar with these topics, why not read about [Adding Interactivity](https://react.dev/learn/adding-interactivity)?\n",
"filename": "describing-the-ui.md",
"package": "react"
} |
{
"content": "Title: Directives – React\n\nURL Source: https://react.dev/reference/rsc/directives\n\nMarkdown Content:\nDirectives – React\n===============\n\nDirectives [](https://react.dev/reference/rsc/directives#undefined \"Link for this heading\")\n===========================================================================================\n\n### Canary\n\nThese directives are needed only if you’re [using React Server Components](https://react.dev/learn/start-a-new-react-project#bleeding-edge-react-frameworks) or building a library compatible with them.\n\nDirectives provide instructions to [bundlers compatible with React Server Components](https://react.dev/learn/start-a-new-react-project#bleeding-edge-react-frameworks).\n\n* * *\n\nSource code directives[](https://react.dev/reference/rsc/directives#source-code-directives \"Link for Source code directives \")\n------------------------------------------------------------------------------------------------------------------------------\n\n* [`'use client'`](https://react.dev/reference/rsc/use-client) lets you mark what code runs on the client.\n* [`'use server'`](https://react.dev/reference/rsc/use-server) marks server-side functions that can be called from client-side code.\n\n[PreviousServer Actions](https://react.dev/reference/rsc/server-actions)[Next'use client'](https://react.dev/reference/rsc/use-client)\n",
"filename": "directives.md",
"package": "react"
} |
{
"content": "Title: Editor Setup – React\n\nURL Source: https://react.dev/learn/editor-setup\n\nMarkdown Content:\nA properly configured editor can make code clearer to read and faster to write. It can even help you catch bugs as you write them! If this is your first time setting up an editor or you’re looking to tune up your current editor, we have a few recommendations.\n\n### You will learn\n\n* What the most popular editors are\n* How to format your code automatically\n\nYour editor[](https://react.dev/learn/editor-setup#your-editor \"Link for Your editor \")\n---------------------------------------------------------------------------------------\n\n[VS Code](https://code.visualstudio.com/) is one of the most popular editors in use today. It has a large marketplace of extensions and integrates well with popular services like GitHub. Most of the features listed below can be added to VS Code as extensions as well, making it highly configurable!\n\nOther popular text editors used in the React community include:\n\n* [WebStorm](https://www.jetbrains.com/webstorm/) is an integrated development environment designed specifically for JavaScript.\n* [Sublime Text](https://www.sublimetext.com/) has support for JSX and TypeScript, [syntax highlighting](https://stackoverflow.com/a/70960574/458193) and autocomplete built in.\n* [Vim](https://www.vim.org/) is a highly configurable text editor built to make creating and changing any kind of text very efficient. It is included as “vi” with most UNIX systems and with Apple OS X.\n\nRecommended text editor features[](https://react.dev/learn/editor-setup#recommended-text-editor-features \"Link for Recommended text editor features \")\n------------------------------------------------------------------------------------------------------------------------------------------------------\n\nSome editors come with these features built in, but others might require adding an extension. Check to see what support your editor of choice provides to be sure!\n\n### Linting[](https://react.dev/learn/editor-setup#linting \"Link for Linting \")\n\nCode linters find problems in your code as you write, helping you fix them early. [ESLint](https://eslint.org/) is a popular, open source linter for JavaScript.\n\n* [Install ESLint with the recommended configuration for React](https://www.npmjs.com/package/eslint-config-react-app) (be sure you have [Node installed!](https://nodejs.org/en/download/current/))\n* [Integrate ESLint in VSCode with the official extension](https://marketplace.visualstudio.com/items?itemName=dbaeumer.vscode-eslint)\n\n**Make sure that you’ve enabled all the [`eslint-plugin-react-hooks`](https://www.npmjs.com/package/eslint-plugin-react-hooks) rules for your project.** They are essential and catch the most severe bugs early. The recommended [`eslint-config-react-app`](https://www.npmjs.com/package/eslint-config-react-app) preset already includes them.\n\n### Formatting[](https://react.dev/learn/editor-setup#formatting \"Link for Formatting \")\n\nThe last thing you want to do when sharing your code with another contributor is get into a discussion about [tabs vs spaces](https://www.google.com/search?q=tabs+vs+spaces)! Fortunately, [Prettier](https://prettier.io/) will clean up your code by reformatting it to conform to preset, configurable rules. Run Prettier, and all your tabs will be converted to spaces—and your indentation, quotes, etc will also all be changed to conform to the configuration. In the ideal setup, Prettier will run when you save your file, quickly making these edits for you.\n\nYou can install the [Prettier extension in VSCode](https://marketplace.visualstudio.com/items?itemName=esbenp.prettier-vscode) by following these steps:\n\n1. Launch VS Code\n2. Use Quick Open (press Ctrl/Cmd+P)\n3. Paste in `ext install esbenp.prettier-vscode`\n4. Press Enter\n\n#### Formatting on save[](https://react.dev/learn/editor-setup#formatting-on-save \"Link for Formatting on save \")\n\nIdeally, you should format your code on every save. VS Code has settings for this!\n\n1. In VS Code, press `CTRL/CMD + SHIFT + P`.\n2. Type “settings”\n3. Hit Enter\n4. In the search bar, type “format on save”\n5. Be sure the “format on save” option is ticked!\n\n> If your ESLint preset has formatting rules, they may conflict with Prettier. We recommend disabling all formatting rules in your ESLint preset using [`eslint-config-prettier`](https://github.com/prettier/eslint-config-prettier) so that ESLint is _only_ used for catching logical mistakes. If you want to enforce that files are formatted before a pull request is merged, use [`prettier --check`](https://prettier.io/docs/en/cli.html#--check) for your continuous integration.\n",
"filename": "editor-setup.md",
"package": "react"
} |
{
"content": "Title: Escape Hatches – React\n\nURL Source: https://react.dev/learn/escape-hatches\n\nMarkdown Content:\nSome of your components may need to control and synchronize with systems outside of React. For example, you might need to focus an input using the browser API, play and pause a video player implemented without React, or connect and listen to messages from a remote server. In this chapter, you’ll learn the escape hatches that let you “step outside” React and connect to external systems. Most of your application logic and data flow should not rely on these features.\n\nReferencing values with refs[](https://react.dev/learn/escape-hatches#referencing-values-with-refs \"Link for Referencing values with refs \")\n--------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you want a component to “remember” some information, but you don’t want that information to [trigger new renders](https://react.dev/learn/render-and-commit), you can use a _ref_:\n\nLike state, refs are retained by React between re-renders. However, setting state re-renders a component. Changing a ref does not! You can access the current value of that ref through the `ref.current` property.\n\nimport { useRef } from 'react';\n\nexport default function Counter() {\n let ref = useRef(0);\n\n function handleClick() {\n ref.current = ref.current + 1;\n alert('You clicked ' + ref.current + ' times!');\n }\n\n return (\n <button onClick\\={handleClick}\\>\n Click me!\n </button\\>\n );\n}\n\nA ref is like a secret pocket of your component that React doesn’t track. For example, you can use refs to store [timeout IDs](https://developer.mozilla.org/en-US/docs/Web/API/setTimeout#return_value), [DOM elements](https://developer.mozilla.org/en-US/docs/Web/API/Element), and other objects that don’t impact the component’s rendering output.\n\n* * *\n\nManipulating the DOM with refs[](https://react.dev/learn/escape-hatches#manipulating-the-dom-with-refs \"Link for Manipulating the DOM with refs \")\n--------------------------------------------------------------------------------------------------------------------------------------------------\n\nReact automatically updates the DOM to match your render output, so your components won’t often need to manipulate it. However, sometimes you might need access to the DOM elements managed by React—for example, to focus a node, scroll to it, or measure its size and position. There is no built-in way to do those things in React, so you will need a ref to the DOM node. For example, clicking the button will focus the input using a ref:\n\nimport { useRef } from 'react';\n\nexport default function Form() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <input ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\n* * *\n\nSynchronizing with Effects[](https://react.dev/learn/escape-hatches#synchronizing-with-effects \"Link for Synchronizing with Effects \")\n--------------------------------------------------------------------------------------------------------------------------------------\n\nSome components need to synchronize with external systems. For example, you might want to control a non-React component based on the React state, set up a server connection, or send an analytics log when a component appears on the screen. Unlike event handlers, which let you handle particular events, _Effects_ let you run some code after rendering. Use them to synchronize your component with a system outside of React.\n\nPress Play/Pause a few times and see how the video player stays synchronized to the `isPlaying` prop value:\n\nimport { useState, useRef, useEffect } from 'react';\n\nfunction VideoPlayer({ src, isPlaying }) {\n const ref = useRef(null);\n\n useEffect(() \\=> {\n if (isPlaying) {\n ref.current.play();\n } else {\n ref.current.pause();\n }\n }, \\[isPlaying\\]);\n\n return <video ref\\={ref} src\\={src} loop playsInline />;\n}\n\nexport default function App() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setIsPlaying(!isPlaying)}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <VideoPlayer\n isPlaying\\={isPlaying}\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n />\n </\\>\n );\n}\n\nMany Effects also “clean up” after themselves. For example, an Effect that sets up a connection to a chat server should return a _cleanup function_ that tells React how to disconnect your component from that server:\n\nIn development, React will immediately run and clean up your Effect one extra time. This is why you see `\"✅ Connecting...\"` printed twice. This ensures that you don’t forget to implement the cleanup function.\n\n* * *\n\nYou Might Not Need An Effect[](https://react.dev/learn/escape-hatches#you-might-not-need-an-effect \"Link for You Might Not Need An Effect \")\n--------------------------------------------------------------------------------------------------------------------------------------------\n\nEffects are an escape hatch from the React paradigm. They let you “step outside” of React and synchronize your components with some external system. If there is no external system involved (for example, if you want to update a component’s state when some props or state change), you shouldn’t need an Effect. Removing unnecessary Effects will make your code easier to follow, faster to run, and less error-prone.\n\nThere are two common cases in which you don’t need Effects:\n\n* **You don’t need Effects to transform data for rendering.**\n* **You don’t need Effects to handle user events.**\n\nFor example, you don’t need an Effect to adjust some state based on other state:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('Taylor');const [lastName, setLastName] = useState('Swift');// 🔴 Avoid: redundant state and unnecessary Effectconst [fullName, setFullName] = useState('');useEffect(() => {setFullName(firstName + ' ' + lastName);}, [firstName, lastName]);// ...}\n```\n\nInstead, calculate as much as you can while rendering:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('Taylor');const [lastName, setLastName] = useState('Swift');// ✅ Good: calculated during renderingconst fullName = firstName + ' ' + lastName;// ...}\n```\n\nHowever, you _do_ need Effects to synchronize with external systems.\n\n* * *\n\nLifecycle of reactive effects[](https://react.dev/learn/escape-hatches#lifecycle-of-reactive-effects \"Link for Lifecycle of reactive effects \")\n-----------------------------------------------------------------------------------------------------------------------------------------------\n\nEffects have a different lifecycle from components. Components may mount, update, or unmount. An Effect can only do two things: to start synchronizing something, and later to stop synchronizing it. This cycle can happen multiple times if your Effect depends on props and state that change over time.\n\nThis Effect depends on the value of the `roomId` prop. Props are _reactive values,_ which means they can change on a re-render. Notice that the Effect _re-synchronizes_ (and re-connects to the server) if `roomId` changes:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n return <h1\\>Welcome to the {roomId} room!</h1\\>;\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nReact provides a linter rule to check that you’ve specified your Effect’s dependencies correctly. If you forget to specify `roomId` in the list of dependencies in the above example, the linter will find that bug automatically.\n\n* * *\n\nSeparating events from Effects[](https://react.dev/learn/escape-hatches#separating-events-from-effects \"Link for Separating events from Effects \")\n--------------------------------------------------------------------------------------------------------------------------------------------------\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nEvent handlers only re-run when you perform the same interaction again. Unlike event handlers, Effects re-synchronize if any of the values they read, like props or state, are different than during last render. Sometimes, you want a mix of both behaviors: an Effect that re-runs in response to some values but not others.\n\nAll code inside Effects is _reactive._ It will run again if some reactive value it reads has changed due to a re-render. For example, this Effect will re-connect to the chat if either `roomId` or `theme` have changed:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection, sendMessage } from './chat.js';\nimport { showNotification } from './notifications.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId, theme }) {\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.on('connected', () \\=> {\n showNotification('Connected!', theme);\n });\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId, theme\\]);\n\n return <h1\\>Welcome to the {roomId} room!</h1\\>\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[isDark, setIsDark\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isDark}\n onChange\\={e \\=> setIsDark(e.target.checked)}\n />\n Use dark theme\n </label\\>\n <hr />\n <ChatRoom\n roomId\\={roomId}\n theme\\={isDark ? 'dark' : 'light'} \n />\n </\\>\n );\n}\n\nThis is not ideal. You want to re-connect to the chat only if the `roomId` has changed. Switching the `theme` shouldn’t re-connect to the chat! Move the code reading `theme` out of your Effect into an _Effect Event_:\n\nimport { useState, useEffect } from 'react';\nimport { experimental\\_useEffectEvent as useEffectEvent } from 'react';\nimport { createConnection, sendMessage } from './chat.js';\nimport { showNotification } from './notifications.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId, theme }) {\n const onConnected = useEffectEvent(() \\=> {\n showNotification('Connected!', theme);\n });\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.on('connected', () \\=> {\n onConnected();\n });\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n return <h1\\>Welcome to the {roomId} room!</h1\\>\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[isDark, setIsDark\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isDark}\n onChange\\={e \\=> setIsDark(e.target.checked)}\n />\n Use dark theme\n </label\\>\n <hr />\n <ChatRoom\n roomId\\={roomId}\n theme\\={isDark ? 'dark' : 'light'} \n />\n </\\>\n );\n}\n\nCode inside Effect Events isn’t reactive, so changing the `theme` no longer makes your Effect re-connect.\n\n* * *\n\nRemoving Effect dependencies[](https://react.dev/learn/escape-hatches#removing-effect-dependencies \"Link for Removing Effect dependencies \")\n--------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you write an Effect, the linter will verify that you’ve included every reactive value (like props and state) that the Effect reads in the list of your Effect’s dependencies. This ensures that your Effect remains synchronized with the latest props and state of your component. Unnecessary dependencies may cause your Effect to run too often, or even create an infinite loop. The way you remove them depends on the case.\n\nFor example, this Effect depends on the `options` object which gets re-created every time you edit the input:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n const \\[message, setMessage\\] = useState('');\n\n const options = {\n serverUrl: serverUrl,\n roomId: roomId\n };\n\n useEffect(() \\=> {\n const connection = createConnection(options);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[options\\]);\n\n return (\n <\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <input value\\={message} onChange\\={e \\=> setMessage(e.target.value)} />\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nYou don’t want the chat to re-connect every time you start typing a message in that chat. To fix this problem, move creation of the `options` object inside the Effect so that the Effect only depends on the `roomId` string:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n const \\[message, setMessage\\] = useState('');\n\n useEffect(() \\=> {\n const options = {\n serverUrl: serverUrl,\n roomId: roomId\n };\n const connection = createConnection(options);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n\n return (\n <\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <input value\\={message} onChange\\={e \\=> setMessage(e.target.value)} />\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nNotice that you didn’t start by editing the dependency list to remove the `options` dependency. That would be wrong. Instead, you changed the surrounding code so that the dependency became _unnecessary._ Think of the dependency list as a list of all the reactive values used by your Effect’s code. You don’t intentionally choose what to put on that list. The list describes your code. To change the dependency list, change the code.\n\n* * *\n\nReusing logic with custom Hooks[](https://react.dev/learn/escape-hatches#reusing-logic-with-custom-hooks \"Link for Reusing logic with custom Hooks \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------\n\nReact comes with built-in Hooks like `useState`, `useContext`, and `useEffect`. Sometimes, you’ll wish that there was a Hook for some more specific purpose: for example, to fetch data, to keep track of whether the user is online, or to connect to a chat room. To do this, you can create your own Hooks for your application’s needs.\n\nIn this example, the `usePointerPosition` custom Hook tracks the cursor position, while `useDelayedValue` custom Hook returns a value that’s “lagging behind” the value you passed by a certain number of milliseconds. Move the cursor over the sandbox preview area to see a moving trail of dots following the cursor:\n\nimport { usePointerPosition } from './usePointerPosition.js';\nimport { useDelayedValue } from './useDelayedValue.js';\n\nexport default function Canvas() {\n const pos1 = usePointerPosition();\n const pos2 = useDelayedValue(pos1, 100);\n const pos3 = useDelayedValue(pos2, 200);\n const pos4 = useDelayedValue(pos3, 100);\n const pos5 = useDelayedValue(pos4, 50);\n return (\n <\\>\n <Dot position\\={pos1} opacity\\={1} />\n <Dot position\\={pos2} opacity\\={0.8} />\n <Dot position\\={pos3} opacity\\={0.6} />\n <Dot position\\={pos4} opacity\\={0.4} />\n <Dot position\\={pos5} opacity\\={0.2} />\n </\\>\n );\n}\n\nfunction Dot({ position, opacity }) {\n return (\n <div style\\={{\n position: 'absolute',\n backgroundColor: 'pink',\n borderRadius: '50%',\n opacity,\n transform: \\`translate(${position.x}px, ${position.y}px)\\`,\n pointerEvents: 'none',\n left: -20,\n top: -20,\n width: 40,\n height: 40,\n }} />\n );\n}\n\nYou can create custom Hooks, compose them together, pass data between them, and reuse them between components. As your app grows, you will write fewer Effects by hand because you’ll be able to reuse custom Hooks you already wrote. There are also many excellent custom Hooks maintained by the React community.\n\n* * *\n\nWhat’s next?[](https://react.dev/learn/escape-hatches#whats-next \"Link for What’s next? \")\n------------------------------------------------------------------------------------------\n\nHead over to [Referencing Values with Refs](https://react.dev/learn/referencing-values-with-refs) to start reading this chapter page by page!\n",
"filename": "escape-hatches.md",
"package": "react"
} |
{
"content": "Title: experimental_taintObjectReference – React\n\nURL Source: https://react.dev/reference/react/experimental_taintObjectReference\n\nMarkdown Content:\n### Under Construction\n\n**This API is experimental and is not available in a stable version of React yet.**\n\nYou can try it by upgrading React packages to the most recent experimental version:\n\n* `react@experimental`\n* `react-dom@experimental`\n* `eslint-plugin-react-hooks@experimental`\n\nExperimental versions of React may contain bugs. Don’t use them in production.\n\nThis API is only available inside React Server Components.\n\n`taintObjectReference` lets you prevent a specific object instance from being passed to a Client Component like a `user` object.\n\n```\nexperimental_taintObjectReference(message, object);\n```\n\nTo prevent passing a key, hash or token, see [`taintUniqueValue`](https://react.dev/reference/react/experimental_taintUniqueValue).\n\n* [Reference](https://react.dev/reference/react/experimental_taintObjectReference#reference)\n * [`taintObjectReference(message, object)`](https://react.dev/reference/react/experimental_taintObjectReference#taintobjectreference)\n* [Usage](https://react.dev/reference/react/experimental_taintObjectReference#usage)\n * [Prevent user data from unintentionally reaching the client](https://react.dev/reference/react/experimental_taintObjectReference#prevent-user-data-from-unintentionally-reaching-the-client)\n\n* * *\n\nReference[](https://react.dev/reference/react/experimental_taintObjectReference#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------------------\n\n### `taintObjectReference(message, object)`[](https://react.dev/reference/react/experimental_taintObjectReference#taintobjectreference \"Link for this heading\")\n\nCall `taintObjectReference` with an object to register it with React as something that should not be allowed to be passed to the Client as is:\n\n```\nimport {experimental_taintObjectReference} from 'react';experimental_taintObjectReference('Do not pass ALL environment variables to the client.',process.env);\n```\n\n[See more examples below.](https://react.dev/reference/react/experimental_taintObjectReference#usage)\n\n#### Parameters[](https://react.dev/reference/react/experimental_taintObjectReference#parameters \"Link for Parameters \")\n\n* `message`: The message you want to display if the object gets passed to a Client Component. This message will be displayed as a part of the Error that will be thrown if the object gets passed to a Client Component.\n \n* `object`: The object to be tainted. Functions and class instances can be passed to `taintObjectReference` as `object`. Functions and classes are already blocked from being passed to Client Components but the React’s default error message will be replaced by what you defined in `message`. When a specific instance of a Typed Array is passed to `taintObjectReference` as `object`, any other copies of the Typed Array will not be tainted.\n \n\n#### Returns[](https://react.dev/reference/react/experimental_taintObjectReference#returns \"Link for Returns \")\n\n`experimental_taintObjectReference` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/experimental_taintObjectReference#caveats \"Link for Caveats \")\n\n* Recreating or cloning a tainted object creates a new untainted object which may contain sensitive data. For example, if you have a tainted `user` object, `const userInfo = {name: user.name, ssn: user.ssn}` or `{...user}` will create new objects which are not tainted. `taintObjectReference` only protects against simple mistakes when the object is passed through to a Client Component unchanged.\n\n### Pitfall\n\n**Do not rely on just tainting for security.** Tainting an object doesn’t prevent leaking of every possible derived value. For example, the clone of a tainted object will create a new untainted object. Using data from a tainted object (e.g. `{secret: taintedObj.secret}`) will create a new value or object that is not tainted. Tainting is a layer of protection; a secure app will have multiple layers of protection, well designed APIs, and isolation patterns.\n\n* * *\n\nUsage[](https://react.dev/reference/react/experimental_taintObjectReference#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------------------\n\n### Prevent user data from unintentionally reaching the client[](https://react.dev/reference/react/experimental_taintObjectReference#prevent-user-data-from-unintentionally-reaching-the-client \"Link for Prevent user data from unintentionally reaching the client \")\n\nA Client Component should never accept objects that carry sensitive data. Ideally, the data fetching functions should not expose data that the current user should not have access to. Sometimes mistakes happen during refactoring. To protect against these mistakes happening down the line we can “taint” the user object in our data API.\n\n```\nimport {experimental_taintObjectReference} from 'react';export async function getUser(id) {const user = await db`SELECT * FROM users WHERE id = ${id}`;experimental_taintObjectReference('Do not pass the entire user object to the client. ' +'Instead, pick off the specific properties you need for this use case.',user,);return user;}\n```\n\nNow whenever anyone tries to pass this object to a Client Component, an error will be thrown with the passed in error message instead.\n\n##### Deep Dive\n\n#### Protecting against leaks in data fetching[](https://react.dev/reference/react/experimental_taintObjectReference#protecting-against-leaks-in-data-fetching \"Link for Protecting against leaks in data fetching \")\n\nIf you’re running a Server Components environment that has access to sensitive data, you have to be careful not to pass objects straight through:\n\n```\n// api.jsexport async function getUser(id) {const user = await db`SELECT * FROM users WHERE id = ${id}`;return user;}\n```\n\n```\nimport { getUser } from 'api.js';import { InfoCard } from 'components.js';export async function Profile(props) {const user = await getUser(props.userId);// DO NOT DO THISreturn <InfoCard user={user} />;}\n```\n\n```\n// components.js\"use client\";export async function InfoCard({ user }) {return <div>{user.name}</div>;}\n```\n\nIdeally, the `getUser` should not expose data that the current user should not have access to. To prevent passing the `user` object to a Client Component down the line we can “taint” the user object:\n\n```\n// api.jsimport {experimental_taintObjectReference} from 'react';export async function getUser(id) {const user = await db`SELECT * FROM users WHERE id = ${id}`;experimental_taintObjectReference('Do not pass the entire user object to the client. ' +'Instead, pick off the specific properties you need for this use case.',user,);return user;}\n```\n\nNow if anyone tries to pass the `user` object to a Client Component, an error will be thrown with the passed in error message.\n",
"filename": "experimental_taintObjectReference.md",
"package": "react"
} |
{
"content": "Title: experimental_taintUniqueValue – React\n\nURL Source: https://react.dev/reference/react/experimental_taintUniqueValue\n\nMarkdown Content:\n### Under Construction\n\n**This API is experimental and is not available in a stable version of React yet.**\n\nYou can try it by upgrading React packages to the most recent experimental version:\n\n* `react@experimental`\n* `react-dom@experimental`\n* `eslint-plugin-react-hooks@experimental`\n\nExperimental versions of React may contain bugs. Don’t use them in production.\n\nThis API is only available inside [React Server Components](https://react.dev/reference/rsc/use-client).\n\n`taintUniqueValue` lets you prevent unique values from being passed to Client Components like passwords, keys, or tokens.\n\n```\ntaintUniqueValue(errMessage, lifetime, value)\n```\n\nTo prevent passing an object containing sensitive data, see [`taintObjectReference`](https://react.dev/reference/react/experimental_taintObjectReference).\n\n* [Reference](https://react.dev/reference/react/experimental_taintUniqueValue#reference)\n * [`taintUniqueValue(message, lifetime, value)`](https://react.dev/reference/react/experimental_taintUniqueValue#taintuniquevalue)\n* [Usage](https://react.dev/reference/react/experimental_taintUniqueValue#usage)\n * [Prevent a token from being passed to Client Components](https://react.dev/reference/react/experimental_taintUniqueValue#prevent-a-token-from-being-passed-to-client-components)\n\n* * *\n\nReference[](https://react.dev/reference/react/experimental_taintUniqueValue#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------------------\n\n### `taintUniqueValue(message, lifetime, value)`[](https://react.dev/reference/react/experimental_taintUniqueValue#taintuniquevalue \"Link for this heading\")\n\nCall `taintUniqueValue` with a password, token, key or hash to register it with React as something that should not be allowed to be passed to the Client as is:\n\n```\nimport {experimental_taintUniqueValue} from 'react';experimental_taintUniqueValue('Do not pass secret keys to the client.',process,process.env.SECRET_KEY);\n```\n\n[See more examples below.](https://react.dev/reference/react/experimental_taintUniqueValue#usage)\n\n#### Parameters[](https://react.dev/reference/react/experimental_taintUniqueValue#parameters \"Link for Parameters \")\n\n* `message`: The message you want to display if `value` is passed to a Client Component. This message will be displayed as a part of the Error that will be thrown if `value` is passed to a Client Component.\n \n* `lifetime`: Any object that indicates how long `value` should be tainted. `value` will be blocked from being sent to any Client Component while this object still exists. For example, passing `globalThis` blocks the value for the lifetime of an app. `lifetime` is typically an object whose properties contains `value`.\n \n* `value`: A string, bigint or TypedArray. `value` must be a unique sequence of characters or bytes with high entropy such as a cryptographic token, private key, hash, or a long password. `value` will be blocked from being sent to any Client Component.\n \n\n#### Returns[](https://react.dev/reference/react/experimental_taintUniqueValue#returns \"Link for Returns \")\n\n`experimental_taintUniqueValue` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react/experimental_taintUniqueValue#caveats \"Link for Caveats \")\n\n* Deriving new values from tainted values can compromise tainting protection. New values created by uppercasing tainted values, concatenating tainted string values into a larger string, converting tainted values to base64, substringing tainted values, and other similar transformations are not tainted unless you explicitly call `taintUniqueValue` on these newly created values.\n* Do not use `taintUniqueValue` to protect low-entropy values such as PIN codes or phone numbers. If any value in a request is controlled by an attacker, they could infer which value is tainted by enumerating all possible values of the secret.\n\n* * *\n\nUsage[](https://react.dev/reference/react/experimental_taintUniqueValue#usage \"Link for Usage \")\n------------------------------------------------------------------------------------------------\n\n### Prevent a token from being passed to Client Components[](https://react.dev/reference/react/experimental_taintUniqueValue#prevent-a-token-from-being-passed-to-client-components \"Link for Prevent a token from being passed to Client Components \")\n\nTo ensure that sensitive information such as passwords, session tokens, or other unique values do not inadvertently get passed to Client Components, the `taintUniqueValue` function provides a layer of protection. When a value is tainted, any attempt to pass it to a Client Component will result in an error.\n\nThe `lifetime` argument defines the duration for which the value remains tainted. For values that should remain tainted indefinitely, objects like [`globalThis`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/globalThis) or `process` can serve as the `lifetime` argument. These objects have a lifespan that spans the entire duration of your app’s execution.\n\n```\nimport {experimental_taintUniqueValue} from 'react';experimental_taintUniqueValue('Do not pass a user password to the client.',globalThis,process.env.SECRET_KEY);\n```\n\nIf the tainted value’s lifespan is tied to a object, the `lifetime` should be the object that encapsulates the value. This ensures the tainted value remains protected for the lifetime of the encapsulating object.\n\n```\nimport {experimental_taintUniqueValue} from 'react';export async function getUser(id) {const user = await db`SELECT * FROM users WHERE id = ${id}`;experimental_taintUniqueValue('Do not pass a user session token to the client.',user,user.session.token);return user;}\n```\n\nIn this example, the `user` object serves as the `lifetime` argument. If this object gets stored in a global cache or is accessible by another request, the session token remains tainted.\n\n### Pitfall\n\n**Do not rely solely on tainting for security.** Tainting a value doesn’t block every possible derived value. For example, creating a new value by upper casing a tainted string will not taint the new value.\n\n```\nimport {experimental_taintUniqueValue} from 'react';const password = 'correct horse battery staple';experimental_taintUniqueValue('Do not pass the password to the client.',globalThis,password);const uppercasePassword = password.toUpperCase() // `uppercasePassword` is not tainted\n```\n\nIn this example, the constant `password` is tainted. Then `password` is used to create a new value `uppercasePassword` by calling the `toUpperCase` method on `password`. The newly created `uppercasePassword` is not tainted.\n\nOther similar ways of deriving new values from tainted values like concatenating it into a larger string, converting it to base64, or returning a substring create untained values.\n\nTainting only protects against simple mistakes like explicitly passing secret values to the client. Mistakes in calling the `taintUniqueValue` like using a global store outside of React, without the corresponding lifetime object, can cause the tainted value to become untainted. Tainting is a layer of protection; a secure app will have multiple layers of protection, well designed APIs, and isolation patterns.\n\n##### Deep Dive\n\n#### Using `server-only` and `taintUniqueValue` to prevent leaking secrets[](https://react.dev/reference/react/experimental_taintUniqueValue#using-server-only-and-taintuniquevalue-to-prevent-leaking-secrets \"Link for this heading\")\n\nIf you’re running a Server Components environment that has access to private keys or passwords such as database passwords, you have to be careful not to pass that to a Client Component.\n\n```\nexport async function Dashboard(props) {// DO NOT DO THISreturn <Overview password={process.env.API_PASSWORD} />;}\n```\n\n```\n\"use client\";import {useEffect} from '...'export async function Overview({ password }) {useEffect(() => {const headers = { Authorization: password };fetch(url, { headers }).then(...);}, [password]);...}\n```\n\nThis example would leak the secret API token to the client. If this API token can be used to access data this particular user shouldn’t have access to, it could lead to a data breach.\n\nIdeally, secrets like this are abstracted into a single helper file that can only be imported by trusted data utilities on the server. The helper can even be tagged with [`server-only`](https://www.npmjs.com/package/server-only) to ensure that this file isn’t imported on the client.\n\n```\nimport \"server-only\";export function fetchAPI(url) {const headers = { Authorization: process.env.API_PASSWORD };return fetch(url, { headers });}\n```\n\nSometimes mistakes happen during refactoring and not all of your colleagues might know about this. To protect against this mistakes happening down the line we can “taint” the actual password:\n\n```\nimport \"server-only\";import {experimental_taintUniqueValue} from 'react';experimental_taintUniqueValue('Do not pass the API token password to the client. ' +'Instead do all fetches on the server.'process,process.env.API_PASSWORD);\n```\n\nNow whenever anyone tries to pass this password to a Client Component, or send the password to a Client Component with a Server Action, an error will be thrown with message you defined when you called `taintUniqueValue`.\n\n* * *\n",
"filename": "experimental_taintUniqueValue.md",
"package": "react"
} |
{
"content": "Title: Extracting State Logic into a Reducer – React\n\nURL Source: https://react.dev/learn/extracting-state-logic-into-a-reducer\n\nMarkdown Content:\nComponents with many state updates spread across many event handlers can get overwhelming. For these cases, you can consolidate all the state update logic outside your component in a single function, called a _reducer._\n\n### You will learn\n\n* What a reducer function is\n* How to refactor `useState` to `useReducer`\n* When to use a reducer\n* How to write one well\n\nConsolidate state logic with a reducer[](https://react.dev/learn/extracting-state-logic-into-a-reducer#consolidate-state-logic-with-a-reducer \"Link for Consolidate state logic with a reducer \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAs your components grow in complexity, it can get harder to see at a glance all the different ways in which a component’s state gets updated. For example, the `TaskApp` component below holds an array of `tasks` in state and uses three different event handlers to add, remove, and edit tasks:\n\nEach of its event handlers calls `setTasks` in order to update the state. As this component grows, so does the amount of state logic sprinkled throughout it. To reduce this complexity and keep all your logic in one easy-to-access place, you can move that state logic into a single function outside your component, **called a “reducer”.**\n\nReducers are a different way to handle state. You can migrate from `useState` to `useReducer` in three steps:\n\n1. **Move** from setting state to dispatching actions.\n2. **Write** a reducer function.\n3. **Use** the reducer from your component.\n\n### Step 1: Move from setting state to dispatching actions[](https://react.dev/learn/extracting-state-logic-into-a-reducer#step-1-move-from-setting-state-to-dispatching-actions \"Link for Step 1: Move from setting state to dispatching actions \")\n\nYour event handlers currently specify _what to do_ by setting state:\n\n```\nfunction handleAddTask(text) {setTasks([...tasks,{id: nextId++,text: text,done: false,},]);}function handleChangeTask(task) {setTasks(tasks.map((t) => {if (t.id === task.id) {return task;} else {return t;}}));}function handleDeleteTask(taskId) {setTasks(tasks.filter((t) => t.id !== taskId));}\n```\n\nRemove all the state setting logic. What you are left with are three event handlers:\n\n* `handleAddTask(text)` is called when the user presses “Add”.\n* `handleChangeTask(task)` is called when the user toggles a task or presses “Save”.\n* `handleDeleteTask(taskId)` is called when the user presses “Delete”.\n\nManaging state with reducers is slightly different from directly setting state. Instead of telling React “what to do” by setting state, you specify “what the user just did” by dispatching “actions” from your event handlers. (The state update logic will live elsewhere!) So instead of “setting `tasks`” via an event handler, you’re dispatching an “added/changed/deleted a task” action. This is more descriptive of the user’s intent.\n\n```\nfunction handleAddTask(text) {dispatch({type: 'added',id: nextId++,text: text,});}function handleChangeTask(task) {dispatch({type: 'changed',task: task,});}function handleDeleteTask(taskId) {dispatch({type: 'deleted',id: taskId,});}\n```\n\nThe object you pass to `dispatch` is called an “action”:\n\n```\nfunction handleDeleteTask(taskId) {dispatch(// \"action\" object:{type: 'deleted',id: taskId,});}\n```\n\nIt is a regular JavaScript object. You decide what to put in it, but generally it should contain the minimal information about _what happened_. (You will add the `dispatch` function itself in a later step.)\n\n### Note\n\nAn action object can have any shape.\n\nBy convention, it is common to give it a string `type` that describes what happened, and pass any additional information in other fields. The `type` is specific to a component, so in this example either `'added'` or `'added_task'` would be fine. Choose a name that says what happened!\n\n```\ndispatch({// specific to componenttype: 'what_happened',// other fields go here});\n```\n\n### Step 2: Write a reducer function[](https://react.dev/learn/extracting-state-logic-into-a-reducer#step-2-write-a-reducer-function \"Link for Step 2: Write a reducer function \")\n\nA reducer function is where you will put your state logic. It takes two arguments, the current state and the action object, and it returns the next state:\n\n```\nfunction yourReducer(state, action) {// return next state for React to set}\n```\n\nReact will set the state to what you return from the reducer.\n\nTo move your state setting logic from your event handlers to a reducer function in this example, you will:\n\n1. Declare the current state (`tasks`) as the first argument.\n2. Declare the `action` object as the second argument.\n3. Return the _next_ state from the reducer (which React will set the state to).\n\nHere is all the state setting logic migrated to a reducer function:\n\n```\nfunction tasksReducer(tasks, action) {if (action.type === 'added') {return [...tasks,{id: action.id,text: action.text,done: false,},];} else if (action.type === 'changed') {return tasks.map((t) => {if (t.id === action.task.id) {return action.task;} else {return t;}});} else if (action.type === 'deleted') {return tasks.filter((t) => t.id !== action.id);} else {throw Error('Unknown action: ' + action.type);}}\n```\n\nBecause the reducer function takes state (`tasks`) as an argument, you can **declare it outside of your component.** This decreases the indentation level and can make your code easier to read.\n\n### Note\n\nThe code above uses if/else statements, but it’s a convention to use [switch statements](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/switch) inside reducers. The result is the same, but it can be easier to read switch statements at a glance.\n\nWe’ll be using them throughout the rest of this documentation like so:\n\n```\nfunction tasksReducer(tasks, action) {switch (action.type) {case 'added': {return [...tasks,{id: action.id,text: action.text,done: false,},];}case 'changed': {return tasks.map((t) => {if (t.id === action.task.id) {return action.task;} else {return t;}});}case 'deleted': {return tasks.filter((t) => t.id !== action.id);}default: {throw Error('Unknown action: ' + action.type);}}}\n```\n\nWe recommend wrapping each `case` block into the `{` and `}` curly braces so that variables declared inside of different `case`s don’t clash with each other. Also, a `case` should usually end with a `return`. If you forget to `return`, the code will “fall through” to the next `case`, which can lead to mistakes!\n\nIf you’re not yet comfortable with switch statements, using if/else is completely fine.\n\n##### Deep Dive\n\n#### Why are reducers called this way?[](https://react.dev/learn/extracting-state-logic-into-a-reducer#why-are-reducers-called-this-way \"Link for Why are reducers called this way? \")\n\nAlthough reducers can “reduce” the amount of code inside your component, they are actually named after the [`reduce()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce) operation that you can perform on arrays.\n\nThe `reduce()` operation lets you take an array and “accumulate” a single value out of many:\n\n```\nconst arr = [1, 2, 3, 4, 5];const sum = arr.reduce((result, number) => result + number); // 1 + 2 + 3 + 4 + 5\n```\n\nThe function you pass to `reduce` is known as a “reducer”. It takes the _result so far_ and the _current item,_ then it returns the _next result._ React reducers are an example of the same idea: they take the _state so far_ and the _action_, and return the _next state._ In this way, they accumulate actions over time into state.\n\nYou could even use the `reduce()` method with an `initialState` and an array of `actions` to calculate the final state by passing your reducer function to it:\n\nYou probably won’t need to do this yourself, but this is similar to what React does!\n\n### Step 3: Use the reducer from your component[](https://react.dev/learn/extracting-state-logic-into-a-reducer#step-3-use-the-reducer-from-your-component \"Link for Step 3: Use the reducer from your component \")\n\nFinally, you need to hook up the `tasksReducer` to your component. Import the `useReducer` Hook from React:\n\n```\nimport { useReducer } from 'react';\n```\n\nThen you can replace `useState`:\n\n```\nconst [tasks, setTasks] = useState(initialTasks);\n```\n\nwith `useReducer` like so:\n\n```\nconst [tasks, dispatch] = useReducer(tasksReducer, initialTasks);\n```\n\nThe `useReducer` Hook is similar to `useState`—you must pass it an initial state and it returns a stateful value and a way to set state (in this case, the dispatch function). But it’s a little different.\n\nThe `useReducer` Hook takes two arguments:\n\n1. A reducer function\n2. An initial state\n\nAnd it returns:\n\n1. A stateful value\n2. A dispatch function (to “dispatch” user actions to the reducer)\n\nNow it’s fully wired up! Here, the reducer is declared at the bottom of the component file:\n\nComponent logic can be easier to read when you separate concerns like this. Now the event handlers only specify _what happened_ by dispatching actions, and the reducer function determines _how the state updates_ in response to them.\n\nComparing `useState` and `useReducer`[](https://react.dev/learn/extracting-state-logic-into-a-reducer#comparing-usestate-and-usereducer \"Link for this heading\")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nReducers are not without downsides! Here’s a few ways you can compare them:\n\n* **Code size:** Generally, with `useState` you have to write less code upfront. With `useReducer`, you have to write both a reducer function _and_ dispatch actions. However, `useReducer` can help cut down on the code if many event handlers modify state in a similar way.\n* **Readability:** `useState` is very easy to read when the state updates are simple. When they get more complex, they can bloat your component’s code and make it difficult to scan. In this case, `useReducer` lets you cleanly separate the _how_ of update logic from the _what happened_ of event handlers.\n* **Debugging:** When you have a bug with `useState`, it can be difficult to tell _where_ the state was set incorrectly, and _why_. With `useReducer`, you can add a console log into your reducer to see every state update, and _why_ it happened (due to which `action`). If each `action` is correct, you’ll know that the mistake is in the reducer logic itself. However, you have to step through more code than with `useState`.\n* **Testing:** A reducer is a pure function that doesn’t depend on your component. This means that you can export and test it separately in isolation. While generally it’s best to test components in a more realistic environment, for complex state update logic it can be useful to assert that your reducer returns a particular state for a particular initial state and action.\n* **Personal preference:** Some people like reducers, others don’t. That’s okay. It’s a matter of preference. You can always convert between `useState` and `useReducer` back and forth: they are equivalent!\n\nWe recommend using a reducer if you often encounter bugs due to incorrect state updates in some component, and want to introduce more structure to its code. You don’t have to use reducers for everything: feel free to mix and match! You can even `useState` and `useReducer` in the same component.\n\nWriting reducers well[](https://react.dev/learn/extracting-state-logic-into-a-reducer#writing-reducers-well \"Link for Writing reducers well \")\n----------------------------------------------------------------------------------------------------------------------------------------------\n\nKeep these two tips in mind when writing reducers:\n\n* **Reducers must be pure.** Similar to [state updater functions](https://react.dev/learn/queueing-a-series-of-state-updates), reducers run during rendering! (Actions are queued until the next render.) This means that reducers [must be pure](https://react.dev/learn/keeping-components-pure)—same inputs always result in the same output. They should not send requests, schedule timeouts, or perform any side effects (operations that impact things outside the component). They should update [objects](https://react.dev/learn/updating-objects-in-state) and [arrays](https://react.dev/learn/updating-arrays-in-state) without mutations.\n* **Each action describes a single user interaction, even if that leads to multiple changes in the data.** For example, if a user presses “Reset” on a form with five fields managed by a reducer, it makes more sense to dispatch one `reset_form` action rather than five separate `set_field` actions. If you log every action in a reducer, that log should be clear enough for you to reconstruct what interactions or responses happened in what order. This helps with debugging!\n\nWriting concise reducers with Immer[](https://react.dev/learn/extracting-state-logic-into-a-reducer#writing-concise-reducers-with-immer \"Link for Writing concise reducers with Immer \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nJust like with [updating objects](https://react.dev/learn/updating-objects-in-state#write-concise-update-logic-with-immer) and [arrays](https://react.dev/learn/updating-arrays-in-state#write-concise-update-logic-with-immer) in regular state, you can use the Immer library to make reducers more concise. Here, [`useImmerReducer`](https://github.com/immerjs/use-immer#useimmerreducer) lets you mutate the state with `push` or `arr[i] =` assignment:\n\nReducers must be pure, so they shouldn’t mutate state. But Immer provides you with a special `draft` object which is safe to mutate. Under the hood, Immer will create a copy of your state with the changes you made to the `draft`. This is why reducers managed by `useImmerReducer` can mutate their first argument and don’t need to return state.\n\nRecap[](https://react.dev/learn/extracting-state-logic-into-a-reducer#recap \"Link for Recap\")\n---------------------------------------------------------------------------------------------\n\n* To convert from `useState` to `useReducer`:\n 1. Dispatch actions from event handlers.\n 2. Write a reducer function that returns the next state for a given state and action.\n 3. Replace `useState` with `useReducer`.\n* Reducers require you to write a bit more code, but they help with debugging and testing.\n* Reducers must be pure.\n* Each action describes a single user interaction.\n* Use Immer if you want to write reducers in a mutating style.\n",
"filename": "extracting-state-logic-into-a-reducer.md",
"package": "react"
} |
{
"content": "Title: findDOMNode – React\n\nURL Source: https://react.dev/reference/react-dom/findDOMNode\n\nMarkdown Content:\n### Deprecated\n\nThis API will be removed in a future major version of React. [See the alternatives.](https://react.dev/reference/react-dom/findDOMNode#alternatives)\n\n`findDOMNode` finds the browser DOM node for a React [class component](https://react.dev/reference/react/Component) instance.\n\n```\nconst domNode = findDOMNode(componentInstance)\n```\n\n* [Reference](https://react.dev/reference/react-dom/findDOMNode#reference)\n * [`findDOMNode(componentInstance)`](https://react.dev/reference/react-dom/findDOMNode#finddomnode)\n* [Usage](https://react.dev/reference/react-dom/findDOMNode#usage)\n * [Finding the root DOM node of a class component](https://react.dev/reference/react-dom/findDOMNode#finding-the-root-dom-node-of-a-class-component)\n* [Alternatives](https://react.dev/reference/react-dom/findDOMNode#alternatives)\n * [Reading component’s own DOM node from a ref](https://react.dev/reference/react-dom/findDOMNode#reading-components-own-dom-node-from-a-ref)\n * [Reading a child component’s DOM node from a forwarded ref](https://react.dev/reference/react-dom/findDOMNode#reading-a-child-components-dom-node-from-a-forwarded-ref)\n * [Adding a wrapper `<div>` element](https://react.dev/reference/react-dom/findDOMNode#adding-a-wrapper-div-element)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/findDOMNode#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------\n\n### `findDOMNode(componentInstance)`[](https://react.dev/reference/react-dom/findDOMNode#finddomnode \"Link for this heading\")\n\nCall `findDOMNode` to find the browser DOM node for a given React [class component](https://react.dev/reference/react/Component) instance.\n\n```\nimport { findDOMNode } from 'react-dom';const domNode = findDOMNode(componentInstance);\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/findDOMNode#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/findDOMNode#parameters \"Link for Parameters \")\n\n* `componentInstance`: An instance of the [`Component`](https://react.dev/reference/react/Component) subclass. For example, `this` inside a class component.\n\n#### Returns[](https://react.dev/reference/react-dom/findDOMNode#returns \"Link for Returns \")\n\n`findDOMNode` returns the first closest browser DOM node within the given `componentInstance`. When a component renders to `null`, or renders `false`, `findDOMNode` returns `null`. When a component renders to a string, `findDOMNode` returns a text DOM node containing that value.\n\n#### Caveats[](https://react.dev/reference/react-dom/findDOMNode#caveats \"Link for Caveats \")\n\n* A component may return an array or a [Fragment](https://react.dev/reference/react/Fragment) with multiple children. In that case `findDOMNode`, will return the DOM node corresponding to the first non-empty child.\n \n* `findDOMNode` only works on mounted components (that is, components that have been placed in the DOM). If you try to call this on a component that has not been mounted yet (like calling `findDOMNode()` in `render()` on a component that has yet to be created), an exception will be thrown.\n \n* `findDOMNode` only returns the result at the time of your call. If a child component renders a different node later, there is no way for you to be notified of this change.\n \n* `findDOMNode` accepts a class component instance, so it can’t be used with function components.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/findDOMNode#usage \"Link for Usage \")\n----------------------------------------------------------------------------------\n\n### Finding the root DOM node of a class component[](https://react.dev/reference/react-dom/findDOMNode#finding-the-root-dom-node-of-a-class-component \"Link for Finding the root DOM node of a class component \")\n\nCall `findDOMNode` with a [class component](https://react.dev/reference/react/Component) instance (usually, `this`) to find the DOM node it has rendered.\n\n```\nclass AutoselectingInput extends Component {componentDidMount() {const input = findDOMNode(this);input.select()}render() {return <input defaultValue=\"Hello\" />}}\n```\n\nHere, the `input` variable will be set to the `<input>` DOM element. This lets you do something with it. For example, when clicking “Show example” below mounts the input, [`input.select()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement/select) selects all text in the input:\n\n* * *\n\nAlternatives[](https://react.dev/reference/react-dom/findDOMNode#alternatives \"Link for Alternatives \")\n-------------------------------------------------------------------------------------------------------\n\n### Reading component’s own DOM node from a ref[](https://react.dev/reference/react-dom/findDOMNode#reading-components-own-dom-node-from-a-ref \"Link for Reading component’s own DOM node from a ref \")\n\nCode using `findDOMNode` is fragile because the connection between the JSX node and the code manipulating the corresponding DOM node is not explicit. For example, try wrapping this `<input />` into a `<div>`:\n\nThis will break the code because now, `findDOMNode(this)` finds the `<div>` DOM node, but the code expects an `<input>` DOM node. To avoid these kinds of problems, use [`createRef`](https://react.dev/reference/react/createRef) to manage a specific DOM node.\n\nIn this example, `findDOMNode` is no longer used. Instead, `inputRef = createRef(null)` is defined as an instance field on the class. To read the DOM node from it, you can use `this.inputRef.current`. To attach it to the JSX, you render `<input ref={this.inputRef} />`. This connects the code using the DOM node to its JSX:\n\nimport { createRef, Component } from 'react';\n\nclass AutoselectingInput extends Component {\n inputRef = createRef(null);\n\n componentDidMount() {\n const input = this.inputRef.current;\n input.select()\n }\n\n render() {\n return (\n <input ref\\={this.inputRef} defaultValue\\=\"Hello\" />\n );\n }\n}\n\nexport default AutoselectingInput;\n\nIn modern React without class components, the equivalent code would call [`useRef`](https://react.dev/reference/react/useRef) instead:\n\n[Read more about manipulating the DOM with refs.](https://react.dev/learn/manipulating-the-dom-with-refs)\n\n* * *\n\n### Reading a child component’s DOM node from a forwarded ref[](https://react.dev/reference/react-dom/findDOMNode#reading-a-child-components-dom-node-from-a-forwarded-ref \"Link for Reading a child component’s DOM node from a forwarded ref \")\n\nIn this example, `findDOMNode(this)` finds a DOM node that belongs to another component. The `AutoselectingInput` renders `MyInput`, which is your own component that renders a browser `<input>`.\n\nNotice that calling `findDOMNode(this)` inside `AutoselectingInput` still gives you the DOM `<input>`—even though the JSX for this `<input>` is hidden inside the `MyInput` component. This seems convenient for the above example, but it leads to fragile code. Imagine that you wanted to edit `MyInput` later and add a wrapper `<div>` around it. This would break the code of `AutoselectingInput` (which expects to find an `<input>`).\n\nTo replace `findDOMNode` in this example, the two components need to coordinate:\n\n1. `AutoSelectingInput` should declare a ref, like [in the earlier example](https://react.dev/reference/react-dom/findDOMNode#reading-components-own-dom-node-from-a-ref), and pass it to `<MyInput>`.\n2. `MyInput` should be declared with [`forwardRef`](https://react.dev/reference/react/forwardRef) to take that ref and forward it down to the `<input>` node.\n\nThis version does that, so it no longer needs `findDOMNode`:\n\nimport { createRef, Component } from 'react';\nimport MyInput from './MyInput.js';\n\nclass AutoselectingInput extends Component {\n inputRef = createRef(null);\n\n componentDidMount() {\n const input = this.inputRef.current;\n input.select()\n }\n\n render() {\n return (\n <MyInput ref\\={this.inputRef} />\n );\n }\n}\n\nexport default AutoselectingInput;\n\nHere is how this code would look like with function components instead of classes:\n\n* * *\n\n### Adding a wrapper `<div>` element[](https://react.dev/reference/react-dom/findDOMNode#adding-a-wrapper-div-element \"Link for this heading\")\n\nSometimes a component needs to know the position and size of its children. This makes it tempting to find the children with `findDOMNode(this)`, and then use DOM methods like [`getBoundingClientRect`](https://developer.mozilla.org/en-US/docs/Web/API/Element/getBoundingClientRect) for measurements.\n\nThere is currently no direct equivalent for this use case, which is why `findDOMNode` is deprecated but is not yet removed completely from React. In the meantime, you can try rendering a wrapper `<div>` node around the content as a workaround, and getting a ref to that node. However, extra wrappers can break styling.\n\n```\n<div ref={someRef}>{children}</div>\n```\n\nThis also applies to focusing and scrolling to arbitrary children.\n",
"filename": "findDOMNode.md",
"package": "react"
} |
{
"content": "Title: flushSync – React\n\nURL Source: https://react.dev/reference/react-dom/flushSync\n\nMarkdown Content:\nflushSync – React\n===============\n\nflushSync[](https://react.dev/reference/react-dom/flushSync#undefined \"Link for this heading\")\n==============================================================================================\n\n### Pitfall\n\nUsing `flushSync` is uncommon and can hurt the performance of your app.\n\n`flushSync` lets you force React to flush any updates inside the provided callback synchronously. This ensures that the DOM is updated immediately.\n\n```\nflushSync(callback)\n```\n\n* [Reference](https://react.dev/reference/react-dom/flushSync#reference)\n * [`flushSync(callback)`](https://react.dev/reference/react-dom/flushSync#flushsync)\n* [Usage](https://react.dev/reference/react-dom/flushSync#usage)\n * [Flushing updates for third-party integrations](https://react.dev/reference/react-dom/flushSync#flushing-updates-for-third-party-integrations)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/flushSync#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------\n\n### `flushSync(callback)`[](https://react.dev/reference/react-dom/flushSync#flushsync \"Link for this heading\")\n\nCall `flushSync` to force React to flush any pending work and update the DOM synchronously.\n\n```\nimport { flushSync } from 'react-dom';flushSync(() => { setSomething(123);});\n```\n\nMost of the time, `flushSync` can be avoided. Use `flushSync` as last resort.\n\n[See more examples below.](https://react.dev/reference/react-dom/flushSync#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/flushSync#parameters \"Link for Parameters \")\n\n* `callback`: A function. React will immediately call this callback and flush any updates it contains synchronously. It may also flush any pending updates, or Effects, or updates inside of Effects. If an update suspends as a result of this `flushSync` call, the fallbacks may be re-shown.\n\n#### Returns[](https://react.dev/reference/react-dom/flushSync#returns \"Link for Returns \")\n\n`flushSync` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react-dom/flushSync#caveats \"Link for Caveats \")\n\n* `flushSync` can significantly hurt performance. Use sparingly.\n* `flushSync` may force pending Suspense boundaries to show their `fallback` state.\n* `flushSync` may run pending Effects and synchronously apply any updates they contain before returning.\n* `flushSync` may flush updates outside the callback when necessary to flush the updates inside the callback. For example, if there are pending updates from a click, React may flush those before flushing the updates inside the callback.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/flushSync#usage \"Link for Usage \")\n--------------------------------------------------------------------------------\n\n### Flushing updates for third-party integrations[](https://react.dev/reference/react-dom/flushSync#flushing-updates-for-third-party-integrations \"Link for Flushing updates for third-party integrations \")\n\nWhen integrating with third-party code such as browser APIs or UI libraries, it may be necessary to force React to flush updates. Use `flushSync` to force React to flush any state updates inside the callback synchronously:\n\n```\nflushSync(() => { setSomething(123);});// By this line, the DOM is updated.\n```\n\nThis ensures that, by the time the next line of code runs, React has already updated the DOM.\n\n**Using `flushSync` is uncommon, and using it often can significantly hurt the performance of your app.** If your app only uses React APIs, and does not integrate with third-party libraries, `flushSync` should be unnecessary.\n\nHowever, it can be helpful for integrating with third-party code like browser APIs.\n\nSome browser APIs expect results inside of callbacks to be written to the DOM synchronously, by the end of the callback, so the browser can do something with the rendered DOM. In most cases, React handles this for you automatically. But in some cases it may be necessary to force a synchronous update.\n\nFor example, the browser `onbeforeprint` API allows you to change the page immediately before the print dialog opens. This is useful for applying custom print styles that allow the document to display better for printing. In the example below, you use `flushSync` inside of the `onbeforeprint` callback to immediately “flush” the React state to the DOM. Then, by the time the print dialog opens, `isPrinting` displays “yes”:\n\nApp.js\n\nApp.js\n\nDownload Reset\n\nFork\n\nimport { useState, useEffect } from 'react';\nimport { flushSync } from 'react-dom';\n\nexport default function PrintApp() {\n const \\[isPrinting, setIsPrinting\\] = useState(false);\n\n useEffect(() \\=> {\n function handleBeforePrint() {\n flushSync(() \\=> {\n setIsPrinting(true);\n })\n }\n\n function handleAfterPrint() {\n setIsPrinting(false);\n }\n\n window.addEventListener('beforeprint', handleBeforePrint);\n window.addEventListener('afterprint', handleAfterPrint);\n return () \\=> {\n window.removeEventListener('beforeprint', handleBeforePrint);\n window.removeEventListener('afterprint', handleAfterPrint);\n }\n }, \\[\\]);\n\n return (\n <\\>\n <h1\\>isPrinting: {isPrinting ? 'yes' : 'no'}</h1\\>\n <button onClick\\={() \\=> window.print()}\\>\n Print\n </button\\>\n </\\>\n );\n}\n\nShow more\n\nWithout `flushSync`, the print dialog will display `isPrinting` as “no”. This is because React batches the updates asynchronously and the print dialog is displayed before the state is updated.\n\n### Pitfall\n\n`flushSync` can significantly hurt performance, and may unexpectedly force pending Suspense boundaries to show their fallback state.\n\nMost of the time, `flushSync` can be avoided, so use `flushSync` as a last resort.\n\n[PreviouscreatePortal](https://react.dev/reference/react-dom/createPortal)[NextfindDOMNode](https://react.dev/reference/react-dom/findDOMNode)\n",
"filename": "flushSync.md",
"package": "react"
} |
{
"content": "Title: <form> – React\n\nURL Source: https://react.dev/reference/react-dom/components/form\n\nMarkdown Content:\n### Canary\n\nThe [built-in browser `<form>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) lets you create interactive controls for submitting information.\n\n```\n<form action={search}><input name=\"query\" /><button type=\"submit\">Search</button></form>\n```\n\n* [Reference](https://react.dev/reference/react-dom/components/form#reference)\n * [`<form>`](https://react.dev/reference/react-dom/components/form#form)\n* [Usage](https://react.dev/reference/react-dom/components/form#usage)\n * [Handle form submission on the client](https://react.dev/reference/react-dom/components/form#handle-form-submission-on-the-client)\n * [Handle form submission with a Server Action](https://react.dev/reference/react-dom/components/form#handle-form-submission-with-a-server-action)\n * [Display a pending state during form submission](https://react.dev/reference/react-dom/components/form#display-a-pending-state-during-form-submission)\n * [Optimistically updating form data](https://react.dev/reference/react-dom/components/form#optimistically-updating-form-data)\n * [Handling form submission errors](https://react.dev/reference/react-dom/components/form#handling-form-submission-errors)\n * [Display a form submission error without JavaScript](https://react.dev/reference/react-dom/components/form#display-a-form-submission-error-without-javascript)\n * [Handling multiple submission types](https://react.dev/reference/react-dom/components/form#handling-multiple-submission-types)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/form#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------------\n\n### `<form>`[](https://react.dev/reference/react-dom/components/form#form \"Link for this heading\")\n\nTo create interactive controls for submitting information, render the [built-in browser `<form>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form).\n\n```\n<form action={search}><input name=\"query\" /><button type=\"submit\">Search</button></form>\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/form#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/form#props \"Link for Props \")\n\n`<form>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\n[`action`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form#action): a URL or function. When a URL is passed to `action` the form will behave like the HTML form component. When a function is passed to `action` the function will handle the form submission. The function passed to `action` may be async and will be called with a single argument containing the [form data](https://developer.mozilla.org/en-US/docs/Web/API/FormData) of the submitted form. The `action` prop can be overridden by a `formAction` attribute on a `<button>`, `<input type=\"submit\">`, or `<input type=\"image\">` component.\n\n#### Caveats[](https://react.dev/reference/react-dom/components/form#caveats \"Link for Caveats \")\n\n* When a function is passed to `action` or `formAction` the HTTP method will be POST regardless of value of the `method` prop.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/form#usage \"Link for Usage \")\n--------------------------------------------------------------------------------------\n\n### Handle form submission on the client[](https://react.dev/reference/react-dom/components/form#handle-form-submission-on-the-client \"Link for Handle form submission on the client \")\n\nPass a function to the `action` prop of form to run the function when the form is submitted. [`formData`](https://developer.mozilla.org/en-US/docs/Web/API/FormData) will be passed to the function as an argument so you can access the data submitted by the form. This differs from the conventional [HTML action](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form#action), which only accepts URLs.\n\n### Handle form submission with a Server Action[](https://react.dev/reference/react-dom/components/form#handle-form-submission-with-a-server-action \"Link for Handle form submission with a Server Action \")\n\nRender a `<form>` with an input and submit button. Pass a Server Action (a function marked with [`'use server'`](https://react.dev/reference/rsc/use-server)) to the `action` prop of form to run the function when the form is submitted.\n\nPassing a Server Action to `<form action>` allow users to submit forms without JavaScript enabled or before the code has loaded. This is beneficial to users who have a slow connection, device, or have JavaScript disabled and is similar to the way forms work when a URL is passed to the `action` prop.\n\nYou can use hidden form fields to provide data to the `<form>`’s action. The Server Action will be called with the hidden form field data as an instance of [`FormData`](https://developer.mozilla.org/en-US/docs/Web/API/FormData).\n\n```\nimport { updateCart } from './lib.js';function AddToCart({productId}) {async function addToCart(formData) {'use server'const productId = formData.get('productId')await updateCart(productId)}return (<form action={addToCart}><input type=\"hidden\" name=\"productId\" value={productId} /><button type=\"submit\">Add to Cart</button></form>);}\n```\n\nIn lieu of using hidden form fields to provide data to the `<form>`’s action, you can call the `bind` method to supply it with extra arguments. This will bind a new argument (`productId`) to the function in addition to the `formData` that is passed as an argument to the function.\n\n```\nimport { updateCart } from './lib.js';function AddToCart({productId}) {async function addToCart(productId, formData) {\"use server\";await updateCart(productId)}const addProductToCart = addToCart.bind(null, productId);return (<form action={addProductToCart}><button type=\"submit\">Add to Cart</button></form>);}\n```\n\nWhen `<form>` is rendered by a [Server Component](https://react.dev/reference/rsc/use-client), and a [Server Action](https://react.dev/reference/rsc/use-server) is passed to the `<form>`’s `action` prop, the form is [progressively enhanced](https://developer.mozilla.org/en-US/docs/Glossary/Progressive_Enhancement).\n\n### Display a pending state during form submission[](https://react.dev/reference/react-dom/components/form#display-a-pending-state-during-form-submission \"Link for Display a pending state during form submission \")\n\nTo display a pending state when a form is being submitted, you can call the `useFormStatus` Hook in a component rendered in a `<form>` and read the `pending` property returned.\n\nHere, we use the `pending` property to indicate the form is submitting.\n\nimport { useFormStatus } from \"react-dom\";\nimport { submitForm } from \"./actions.js\";\n\nfunction Submit() {\n const { pending } = useFormStatus();\n return (\n <button type\\=\"submit\" disabled\\={pending}\\>\n {pending ? \"Submitting...\" : \"Submit\"}\n </button\\>\n );\n}\n\nfunction Form({ action }) {\n return (\n <form action\\={action}\\>\n <Submit />\n </form\\>\n );\n}\n\nexport default function App() {\n return <Form action\\={submitForm} />;\n}\n\nTo learn more about the `useFormStatus` Hook see the [reference documentation](https://react.dev/reference/react-dom/hooks/useFormStatus).\n\n### Optimistically updating form data[](https://react.dev/reference/react-dom/components/form#optimistically-updating-form-data \"Link for Optimistically updating form data \")\n\nThe `useOptimistic` Hook provides a way to optimistically update the user interface before a background operation, like a network request, completes. In the context of forms, this technique helps to make apps feel more responsive. When a user submits a form, instead of waiting for the server’s response to reflect the changes, the interface is immediately updated with the expected outcome.\n\nFor example, when a user types a message into the form and hits the “Send” button, the `useOptimistic` Hook allows the message to immediately appear in the list with a “Sending…” label, even before the message is actually sent to a server. This “optimistic” approach gives the impression of speed and responsiveness. The form then attempts to truly send the message in the background. Once the server confirms the message has been received, the “Sending…” label is removed.\n\nimport { useOptimistic, useState, useRef } from \"react\";\nimport { deliverMessage } from \"./actions.js\";\n\nfunction Thread({ messages, sendMessage }) {\n const formRef = useRef();\n async function formAction(formData) {\n addOptimisticMessage(formData.get(\"message\"));\n formRef.current.reset();\n await sendMessage(formData);\n }\n const \\[optimisticMessages, addOptimisticMessage\\] = useOptimistic(\n messages,\n (state, newMessage) \\=> \\[\n ...state,\n {\n text: newMessage,\n sending: true\n }\n \\]\n );\n\n return (\n <\\>\n {optimisticMessages.map((message, index) \\=> (\n <div key\\={index}\\>\n {message.text}\n {!!message.sending && <small\\> (Sending...)</small\\>}\n </div\\>\n ))}\n <form action\\={formAction} ref\\={formRef}\\>\n <input type\\=\"text\" name\\=\"message\" placeholder\\=\"Hello!\" />\n <button type\\=\"submit\"\\>Send</button\\>\n </form\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[messages, setMessages\\] = useState(\\[\n { text: \"Hello there!\", sending: false, key: 1 }\n \\]);\n async function sendMessage(formData) {\n const sentMessage = await deliverMessage(formData.get(\"message\"));\n setMessages(\\[...messages, { text: sentMessage }\\]);\n }\n return <Thread messages\\={messages} sendMessage\\={sendMessage} />;\n}\n\n### Handling form submission errors[](https://react.dev/reference/react-dom/components/form#handling-form-submission-errors \"Link for Handling form submission errors \")\n\nIn some cases the function called by a `<form>`’s `action` prop throws an error. You can handle these errors by wrapping `<form>` in an Error Boundary. If the function called by a `<form>`’s `action` prop throws an error, the fallback for the error boundary will be displayed.\n\nimport { ErrorBoundary } from \"react-error-boundary\";\n\nexport default function Search() {\n function search() {\n throw new Error(\"search error\");\n }\n return (\n <ErrorBoundary\n fallback\\={<p\\>There was an error while submitting the form</p\\>}\n \\>\n <form action\\={search}\\>\n <input name\\=\"query\" />\n <button type\\=\"submit\"\\>Search</button\\>\n </form\\>\n </ErrorBoundary\\>\n );\n}\n\n### Display a form submission error without JavaScript[](https://react.dev/reference/react-dom/components/form#display-a-form-submission-error-without-javascript \"Link for Display a form submission error without JavaScript \")\n\nDisplaying a form submission error message before the JavaScript bundle loads for progressive enhancement requires that:\n\n1. `<form>` be rendered by a [Server Component](https://react.dev/reference/rsc/use-client)\n2. the function passed to the `<form>`’s `action` prop be a [Server Action](https://react.dev/reference/rsc/use-server)\n3. the `useActionState` Hook be used to display the error message\n\n`useActionState` takes two parameters: a [Server Action](https://react.dev/reference/rsc/use-server) and an initial state. `useActionState` returns two values, a state variable and an action. The action returned by `useActionState` should be passed to the `action` prop of the form. The state variable returned by `useActionState` can be used to displayed an error message. The value returned by the [Server Action](https://react.dev/reference/rsc/use-server) passed to `useActionState` will be used to update the state variable.\n\nimport { useActionState } from \"react\";\nimport { signUpNewUser } from \"./api\";\n\nexport default function Page() {\n async function signup(prevState, formData) {\n \"use server\";\n const email = formData.get(\"email\");\n try {\n await signUpNewUser(email);\n alert(\\`Added \"${email}\"\\`);\n } catch (err) {\n return err.toString();\n }\n }\n const \\[message, signupAction\\] = useActionState(signup, null);\n return (\n <\\>\n <h1\\>Signup for my newsletter</h1\\>\n <p\\>Signup with the same email twice to see an error</p\\>\n <form action\\={signupAction} id\\=\"signup-form\"\\>\n <label htmlFor\\=\"email\"\\>Email: </label\\>\n <input name\\=\"email\" id\\=\"email\" placeholder\\=\"react@example.com\" />\n <button\\>Sign up</button\\>\n {!!message && <p\\>{message}</p\\>}\n </form\\>\n </\\>\n );\n}\n\nLearn more about updating state from a form action with the [`useActionState`](https://react.dev/reference/react/useActionState) docs\n\n### Handling multiple submission types[](https://react.dev/reference/react-dom/components/form#handling-multiple-submission-types \"Link for Handling multiple submission types \")\n\nForms can be designed to handle multiple submission actions based on the button pressed by the user. Each button inside a form can be associated with a distinct action or behavior by setting the `formAction` prop.\n\nWhen a user taps a specific button, the form is submitted, and a corresponding action, defined by that button’s attributes and action, is executed. For instance, a form might submit an article for review by default but have a separate button with `formAction` set to save the article as a draft.\n\nexport default function Search() {\n function publish(formData) {\n const content = formData.get(\"content\");\n const button = formData.get(\"button\");\n alert(\\`'${content}' was published with the '${button}' button\\`);\n }\n\n function save(formData) {\n const content = formData.get(\"content\");\n alert(\\`Your draft of '${content}' has been saved!\\`);\n }\n\n return (\n <form action\\={publish}\\>\n <textarea name\\=\"content\" rows\\={4} cols\\={40} />\n <br />\n <button type\\=\"submit\" name\\=\"button\" value\\=\"submit\"\\>Publish</button\\>\n <button formAction\\={save}\\>Save draft</button\\>\n </form\\>\n );\n}\n",
"filename": "form.md",
"package": "react"
} |
{
"content": "Title: forwardRef – React\n\nURL Source: https://react.dev/reference/react/forwardRef\n\nMarkdown Content:\n`forwardRef` lets your component expose a DOM node to parent component with a [ref.](https://react.dev/learn/manipulating-the-dom-with-refs)\n\n```\nconst SomeComponent = forwardRef(render)\n```\n\n* [Reference](https://react.dev/reference/react/forwardRef#reference)\n * [`forwardRef(render)`](https://react.dev/reference/react/forwardRef#forwardref)\n * [`render` function](https://react.dev/reference/react/forwardRef#render-function)\n* [Usage](https://react.dev/reference/react/forwardRef#usage)\n * [Exposing a DOM node to the parent component](https://react.dev/reference/react/forwardRef#exposing-a-dom-node-to-the-parent-component)\n * [Forwarding a ref through multiple components](https://react.dev/reference/react/forwardRef#forwarding-a-ref-through-multiple-components)\n * [Exposing an imperative handle instead of a DOM node](https://react.dev/reference/react/forwardRef#exposing-an-imperative-handle-instead-of-a-dom-node)\n* [Troubleshooting](https://react.dev/reference/react/forwardRef#troubleshooting)\n * [My component is wrapped in `forwardRef`, but the `ref` to it is always `null`](https://react.dev/reference/react/forwardRef#my-component-is-wrapped-in-forwardref-but-the-ref-to-it-is-always-null)\n\n* * *\n\nReference[](https://react.dev/reference/react/forwardRef#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------\n\n### `forwardRef(render)`[](https://react.dev/reference/react/forwardRef#forwardref \"Link for this heading\")\n\nCall `forwardRef()` to let your component receive a ref and forward it to a child component:\n\n```\nimport { forwardRef } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {// ...});\n```\n\n[See more examples below.](https://react.dev/reference/react/forwardRef#usage)\n\n#### Parameters[](https://react.dev/reference/react/forwardRef#parameters \"Link for Parameters \")\n\n* `render`: The render function for your component. React calls this function with the props and `ref` that your component received from its parent. The JSX you return will be the output of your component.\n\n#### Returns[](https://react.dev/reference/react/forwardRef#returns \"Link for Returns \")\n\n`forwardRef` returns a React component that you can render in JSX. Unlike React components defined as plain functions, a component returned by `forwardRef` is also able to receive a `ref` prop.\n\n#### Caveats[](https://react.dev/reference/react/forwardRef#caveats \"Link for Caveats \")\n\n* In Strict Mode, React will **call your render function twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useState#my-initializer-or-updater-function-runs-twice) This is development-only behavior and does not affect production. If your render function is pure (as it should be), this should not affect the logic of your component. The result from one of the calls will be ignored.\n\n* * *\n\n### `render` function[](https://react.dev/reference/react/forwardRef#render-function \"Link for this heading\")\n\n`forwardRef` accepts a render function as an argument. React calls this function with `props` and `ref`:\n\n```\nconst MyInput = forwardRef(function MyInput(props, ref) {return (<label>{props.label}<input ref={ref} /></label>);});\n```\n\n#### Parameters[](https://react.dev/reference/react/forwardRef#render-parameters \"Link for Parameters \")\n\n* `props`: The props passed by the parent component.\n \n* `ref`: The `ref` attribute passed by the parent component. The `ref` can be an object or a function. If the parent component has not passed a ref, it will be `null`. You should either pass the `ref` you receive to another component, or pass it to [`useImperativeHandle`.](https://react.dev/reference/react/useImperativeHandle)\n \n\n#### Returns[](https://react.dev/reference/react/forwardRef#render-returns \"Link for Returns \")\n\n`forwardRef` returns a React component that you can render in JSX. Unlike React components defined as plain functions, the component returned by `forwardRef` is able to take a `ref` prop.\n\n* * *\n\nUsage[](https://react.dev/reference/react/forwardRef#usage \"Link for Usage \")\n-----------------------------------------------------------------------------\n\n### Exposing a DOM node to the parent component[](https://react.dev/reference/react/forwardRef#exposing-a-dom-node-to-the-parent-component \"Link for Exposing a DOM node to the parent component \")\n\nBy default, each component’s DOM nodes are private. However, sometimes it’s useful to expose a DOM node to the parent—for example, to allow focusing it. To opt in, wrap your component definition into `forwardRef()`:\n\n```\nimport { forwardRef } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {const { label, ...otherProps } = props;return (<label>{label}<input {...otherProps} /></label>);});\n```\n\nYou will receive a ref as the second argument after props. Pass it to the DOM node that you want to expose:\n\n```\nimport { forwardRef } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {const { label, ...otherProps } = props;return (<label>{label}<input {...otherProps} ref={ref} /></label>);});\n```\n\nThis lets the parent `Form` component access the `<input>` DOM node exposed by `MyInput`:\n\n```\nfunction Form() {const ref = useRef(null);function handleClick() {ref.current.focus();}return (<form><MyInput label=\"Enter your name:\" ref={ref} /><button type=\"button\" onClick={handleClick}> Edit</button></form>);}\n```\n\nThis `Form` component [passes a ref](https://react.dev/reference/react/useRef#manipulating-the-dom-with-a-ref) to `MyInput`. The `MyInput` component _forwards_ that ref to the `<input>` browser tag. As a result, the `Form` component can access that `<input>` DOM node and call [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) on it.\n\nKeep in mind that exposing a ref to the DOM node inside your component makes it harder to change your component’s internals later. You will typically expose DOM nodes from reusable low-level components like buttons or text inputs, but you won’t do it for application-level components like an avatar or a comment.\n\n#### Examples of forwarding a ref[](https://react.dev/reference/react/forwardRef#examples \"Link for Examples of forwarding a ref\")\n\n#### Focusing a text input[](https://react.dev/reference/react/forwardRef#focusing-a-text-input \"Link for this heading\")\n\nClicking the button will focus the input. The `Form` component defines a ref and passes it to the `MyInput` component. The `MyInput` component forwards that ref to the browser `<input>`. This lets the `Form` component focus the `<input>`.\n\nimport { useRef } from 'react';\nimport MyInput from './MyInput.js';\n\nexport default function Form() {\n const ref = useRef(null);\n\n function handleClick() {\n ref.current.focus();\n }\n\n return (\n <form\\>\n <MyInput label\\=\"Enter your name:\" ref\\={ref} />\n <button type\\=\"button\" onClick\\={handleClick}\\>\n Edit\n </button\\>\n </form\\>\n );\n}\n\n* * *\n\n### Forwarding a ref through multiple components[](https://react.dev/reference/react/forwardRef#forwarding-a-ref-through-multiple-components \"Link for Forwarding a ref through multiple components \")\n\nInstead of forwarding a `ref` to a DOM node, you can forward it to your own component like `MyInput`:\n\n```\nconst FormField = forwardRef(function FormField(props, ref) {// ...return (<><MyInput ref={ref} /> ...</>);});\n```\n\nIf that `MyInput` component forwards a ref to its `<input>`, a ref to `FormField` will give you that `<input>`:\n\n```\nfunction Form() {const ref = useRef(null);function handleClick() {ref.current.focus();}return (<form><FormField label=\"Enter your name:\" ref={ref} isRequired={true} /><button type=\"button\" onClick={handleClick}> Edit</button></form>);}\n```\n\nThe `Form` component defines a ref and passes it to `FormField`. The `FormField` component forwards that ref to `MyInput`, which forwards it to a browser `<input>` DOM node. This is how `Form` accesses that DOM node.\n\nimport { useRef } from 'react';\nimport FormField from './FormField.js';\n\nexport default function Form() {\n const ref = useRef(null);\n\n function handleClick() {\n ref.current.focus();\n }\n\n return (\n <form\\>\n <FormField label\\=\"Enter your name:\" ref\\={ref} isRequired\\={true} />\n <button type\\=\"button\" onClick\\={handleClick}\\>\n Edit\n </button\\>\n </form\\>\n );\n}\n\n* * *\n\n### Exposing an imperative handle instead of a DOM node[](https://react.dev/reference/react/forwardRef#exposing-an-imperative-handle-instead-of-a-dom-node \"Link for Exposing an imperative handle instead of a DOM node \")\n\nInstead of exposing an entire DOM node, you can expose a custom object, called an _imperative handle,_ with a more constrained set of methods. To do this, you’d need to define a separate ref to hold the DOM node:\n\n```\nconst MyInput = forwardRef(function MyInput(props, ref) {const inputRef = useRef(null);// ...return <input {...props} ref={inputRef} />;});\n```\n\nPass the `ref` you received to [`useImperativeHandle`](https://react.dev/reference/react/useImperativeHandle) and specify the value you want to expose to the `ref`:\n\n```\nimport { forwardRef, useRef, useImperativeHandle } from 'react';const MyInput = forwardRef(function MyInput(props, ref) {const inputRef = useRef(null);useImperativeHandle(ref, () => {return {focus() {inputRef.current.focus();},scrollIntoView() {inputRef.current.scrollIntoView();},};}, []);return <input {...props} ref={inputRef} />;});\n```\n\nIf some component gets a ref to `MyInput`, it will only receive your `{ focus, scrollIntoView }` object instead of the DOM node. This lets you limit the information you expose about your DOM node to the minimum.\n\nimport { useRef } from 'react';\nimport MyInput from './MyInput.js';\n\nexport default function Form() {\n const ref = useRef(null);\n\n function handleClick() {\n ref.current.focus();\n \n \n }\n\n return (\n <form\\>\n <MyInput placeholder\\=\"Enter your name\" ref\\={ref} />\n <button type\\=\"button\" onClick\\={handleClick}\\>\n Edit\n </button\\>\n </form\\>\n );\n}\n\n[Read more about using imperative handles.](https://react.dev/reference/react/useImperativeHandle)\n\n### Pitfall\n\n**Do not overuse refs.** You should only use refs for _imperative_ behaviors that you can’t express as props: for example, scrolling to a node, focusing a node, triggering an animation, selecting text, and so on.\n\n**If you can express something as a prop, you should not use a ref.** For example, instead of exposing an imperative handle like `{ open, close }` from a `Modal` component, it is better to take `isOpen` as a prop like `<Modal isOpen={isOpen} />`. [Effects](https://react.dev/learn/synchronizing-with-effects) can help you expose imperative behaviors via props.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/forwardRef#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------------\n\n### My component is wrapped in `forwardRef`, but the `ref` to it is always `null`[](https://react.dev/reference/react/forwardRef#my-component-is-wrapped-in-forwardref-but-the-ref-to-it-is-always-null \"Link for this heading\")\n\nThis usually means that you forgot to actually use the `ref` that you received.\n\nFor example, this component doesn’t do anything with its `ref`:\n\n```\nconst MyInput = forwardRef(function MyInput({ label }, ref) {return (<label>{label}<input /></label>);});\n```\n\nTo fix it, pass the `ref` down to a DOM node or another component that can accept a ref:\n\n```\nconst MyInput = forwardRef(function MyInput({ label }, ref) {return (<label>{label}<input ref={ref} /></label>);});\n```\n\nThe `ref` to `MyInput` could also be `null` if some of the logic is conditional:\n\n```\nconst MyInput = forwardRef(function MyInput({ label, showInput }, ref) {return (<label>{label}{showInput && <input ref={ref} />}</label>);});\n```\n\nIf `showInput` is `false`, then the ref won’t be forwarded to any node, and a ref to `MyInput` will remain empty. This is particularly easy to miss if the condition is hidden inside another component, like `Panel` in this example:\n\n```\nconst MyInput = forwardRef(function MyInput({ label, showInput }, ref) {return (<label>{label}<Panel isExpanded={showInput}><input ref={ref} /></Panel></label>);});\n```\n",
"filename": "forwardRef.md",
"package": "react"
} |
{
"content": "Title: Built-in React DOM Hooks – React\n\nURL Source: https://react.dev/reference/react-dom/hooks\n\nMarkdown Content:\nBuilt-in React DOM Hooks – React\n===============\n\nBuilt-in React DOM Hooks[](https://react.dev/reference/react-dom/hooks#undefined \"Link for this heading\")\n=========================================================================================================\n\nThe `react-dom` package contains Hooks that are only supported for web applications (which run in the browser DOM environment). These Hooks are not supported in non-browser environments like iOS, Android, or Windows applications. If you are looking for Hooks that are supported in web browsers _and other environments_ see [the React Hooks page](https://react.dev/reference/react). This page lists all the Hooks in the `react-dom` package.\n\n* * *\n\nForm Hooks[](https://react.dev/reference/react-dom/hooks#form-hooks \"Link for Form Hooks \")\n-------------------------------------------------------------------------------------------\n\n### Canary\n\nForm Hooks are currently only available in React’s canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n_Forms_ let you create interactive controls for submitting information. To manage forms in your components, use one of these Hooks:\n\n* [`useFormStatus`](https://react.dev/reference/react-dom/hooks/useFormStatus) allows you to make updates to the UI based on the status of the a form.\n\n```\nfunction Form({ action }) { async function increment(n) { return n + 1; } const [count, incrementFormAction] = useActionState(increment, 0); return ( <form action={action}> <button formAction={incrementFormAction}>Count: {count}</button> <Button /> </form> );}function Button() { const { pending } = useFormStatus(); return ( <button disabled={pending} type=\"submit\"> Submit </button> );}\n```\n\n[NextuseFormStatus](https://react.dev/reference/react-dom/hooks/useFormStatus)\n",
"filename": "hooks.md",
"package": "react"
} |
{
"content": "Title: hydrate – React\n\nURL Source: https://react.dev/reference/react-dom/hydrate\n\nMarkdown Content:\n### Deprecated\n\nThis API will be removed in a future major version of React.\n\nIn React 18, `hydrate` was replaced by [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot) Using `hydrate` in React 18 will warn that your app will behave as if it’s running React 17. Learn more [here.](https://react.dev/blog/2022/03/08/react-18-upgrade-guide#updates-to-client-rendering-apis)\n\n`hydrate` lets you display React components inside a browser DOM node whose HTML content was previously generated by [`react-dom/server`](https://react.dev/reference/react-dom/server) in React 17 and below.\n\n```\nhydrate(reactNode, domNode, callback?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/hydrate#reference)\n * [`hydrate(reactNode, domNode, callback?)`](https://react.dev/reference/react-dom/hydrate#hydrate)\n* [Usage](https://react.dev/reference/react-dom/hydrate#usage)\n * [Hydrating server-rendered HTML](https://react.dev/reference/react-dom/hydrate#hydrating-server-rendered-html)\n * [Suppressing unavoidable hydration mismatch errors](https://react.dev/reference/react-dom/hydrate#suppressing-unavoidable-hydration-mismatch-errors)\n * [Handling different client and server content](https://react.dev/reference/react-dom/hydrate#handling-different-client-and-server-content)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/hydrate#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------\n\n### `hydrate(reactNode, domNode, callback?)`[](https://react.dev/reference/react-dom/hydrate#hydrate \"Link for this heading\")\n\nCall `hydrate` in React 17 and below to “attach” React to existing HTML that was already rendered by React in a server environment.\n\n```\nimport { hydrate } from 'react-dom';hydrate(reactNode, domNode);\n```\n\nReact will attach to the HTML that exists inside the `domNode`, and take over managing the DOM inside it. An app fully built with React will usually only have one `hydrate` call with its root component.\n\n[See more examples below.](https://react.dev/reference/react-dom/hydrate#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/hydrate#parameters \"Link for Parameters \")\n\n* `reactNode`: The “React node” used to render the existing HTML. This will usually be a piece of JSX like `<App />` which was rendered with a `ReactDOM Server` method such as `renderToString(<App />)` in React 17.\n \n* `domNode`: A [DOM element](https://developer.mozilla.org/en-US/docs/Web/API/Element) that was rendered as the root element on the server.\n \n* **optional**: `callback`: A function. If passed, React will call it after your component is hydrated.\n \n\n#### Returns[](https://react.dev/reference/react-dom/hydrate#returns \"Link for Returns \")\n\n`hydrate` returns null.\n\n#### Caveats[](https://react.dev/reference/react-dom/hydrate#caveats \"Link for Caveats \")\n\n* `hydrate` expects the rendered content to be identical with the server-rendered content. React can patch up differences in text content, but you should treat mismatches as bugs and fix them.\n* In development mode, React warns about mismatches during hydration. There are no guarantees that attribute differences will be patched up in case of mismatches. This is important for performance reasons because in most apps, mismatches are rare, and so validating all markup would be prohibitively expensive.\n* You’ll likely have only one `hydrate` call in your app. If you use a framework, it might do this call for you.\n* If your app is client-rendered with no HTML rendered already, using `hydrate()` is not supported. Use [render()](https://react.dev/reference/react-dom/render) (for React 17 and below) or [createRoot()](https://react.dev/reference/react-dom/client/createRoot) (for React 18+) instead.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/hydrate#usage \"Link for Usage \")\n------------------------------------------------------------------------------\n\nCall `hydrate` to attach a React component into a server-rendered browser DOM node.\n\n```\nimport { hydrate } from 'react-dom';hydrate(<App />, document.getElementById('root'));\n```\n\nUsing `hydrate()` to render a client-only app (an app without server-rendered HTML) is not supported. Use [`render()`](https://react.dev/reference/react-dom/render) (in React 17 and below) or [`createRoot()`](https://react.dev/reference/react-dom/client/createRoot) (in React 18+) instead.\n\n### Hydrating server-rendered HTML[](https://react.dev/reference/react-dom/hydrate#hydrating-server-rendered-html \"Link for Hydrating server-rendered HTML \")\n\nIn React, “hydration” is how React “attaches” to existing HTML that was already rendered by React in a server environment. During hydration, React will attempt to attach event listeners to the existing markup and take over rendering the app on the client.\n\nIn apps fully built with React, **you will usually only hydrate one “root”, once at startup for your entire app**.\n\nUsually you shouldn’t need to call `hydrate` again or to call it in more places. From this point on, React will be managing the DOM of your application. To update the UI, your components will [use state.](https://react.dev/reference/react/useState)\n\nFor more information on hydration, see the docs for [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot)\n\n* * *\n\n### Suppressing unavoidable hydration mismatch errors[](https://react.dev/reference/react-dom/hydrate#suppressing-unavoidable-hydration-mismatch-errors \"Link for Suppressing unavoidable hydration mismatch errors \")\n\nIf a single element’s attribute or text content is unavoidably different between the server and the client (for example, a timestamp), you may silence the hydration mismatch warning.\n\nTo silence hydration warnings on an element, add `suppressHydrationWarning={true}`:\n\nThis only works one level deep, and is intended to be an escape hatch. Don’t overuse it. Unless it’s text content, React still won’t attempt to patch it up, so it may remain inconsistent until future updates.\n\n* * *\n\n### Handling different client and server content[](https://react.dev/reference/react-dom/hydrate#handling-different-client-and-server-content \"Link for Handling different client and server content \")\n\nIf you intentionally need to render something different on the server and the client, you can do a two-pass rendering. Components that render something different on the client can read a [state variable](https://react.dev/reference/react/useState) like `isClient`, which you can set to `true` in an [Effect](https://react.dev/reference/react/useEffect):\n\nThis way the initial render pass will render the same content as the server, avoiding mismatches, but an additional pass will happen synchronously right after hydration.\n\n### Pitfall\n\nThis approach makes hydration slower because your components have to render twice. Be mindful of the user experience on slow connections. The JavaScript code may load significantly later than the initial HTML render, so rendering a different UI immediately after hydration may feel jarring to the user.\n",
"filename": "hydrate.md",
"package": "react"
} |
{
"content": "Title: hydrateRoot – React\n\nURL Source: https://react.dev/reference/react-dom/client/hydrateRoot\n\nMarkdown Content:\n`hydrateRoot` lets you display React components inside a browser DOM node whose HTML content was previously generated by [`react-dom/server`.](https://react.dev/reference/react-dom/server)\n\n```\nconst root = hydrateRoot(domNode, reactNode, options?)\n```\n\nReference[](https://react.dev/reference/react-dom/client/hydrateRoot#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------------------\n\n### `hydrateRoot(domNode, reactNode, options?)`[](https://react.dev/reference/react-dom/client/hydrateRoot#hydrateroot \"Link for this heading\")\n\nCall `hydrateRoot` to “attach” React to existing HTML that was already rendered by React in a server environment.\n\n```\nimport { hydrateRoot } from 'react-dom/client';const domNode = document.getElementById('root');const root = hydrateRoot(domNode, reactNode);\n```\n\nReact will attach to the HTML that exists inside the `domNode`, and take over managing the DOM inside it. An app fully built with React will usually only have one `hydrateRoot` call with its root component.\n\n[See more examples below.](https://react.dev/reference/react-dom/client/hydrateRoot#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/client/hydrateRoot#parameters \"Link for Parameters \")\n\n* `domNode`: A [DOM element](https://developer.mozilla.org/en-US/docs/Web/API/Element) that was rendered as the root element on the server.\n \n* `reactNode`: The “React node” used to render the existing HTML. This will usually be a piece of JSX like `<App />` which was rendered with a `ReactDOM Server` method such as `renderToPipeableStream(<App />)`.\n \n* **optional** `options`: An object with options for this React root.\n \n * Canary only **optional** `onCaughtError`: Callback called when React catches an error in an Error Boundary. Called with the `error` caught by the Error Boundary, and an `errorInfo` object containing the `componentStack`.\n * Canary only **optional** `onUncaughtError`: Callback called when an error is thrown and not caught by an Error Boundary. Called with the `error` that was thrown and an `errorInfo` object containing the `componentStack`.\n * **optional** `onRecoverableError`: Callback called when React automatically recovers from errors. Called with the `error` React throws, and an `errorInfo` object containing the `componentStack`. Some recoverable errors may include the original error cause as `error.cause`.\n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as used on the server.\n\n#### Returns[](https://react.dev/reference/react-dom/client/hydrateRoot#returns \"Link for Returns \")\n\n`hydrateRoot` returns an object with two methods: [`render`](https://react.dev/reference/react-dom/client/hydrateRoot#root-render) and [`unmount`.](https://react.dev/reference/react-dom/client/hydrateRoot#root-unmount)\n\n#### Caveats[](https://react.dev/reference/react-dom/client/hydrateRoot#caveats \"Link for Caveats \")\n\n* `hydrateRoot()` expects the rendered content to be identical with the server-rendered content. You should treat mismatches as bugs and fix them.\n* In development mode, React warns about mismatches during hydration. There are no guarantees that attribute differences will be patched up in case of mismatches. This is important for performance reasons because in most apps, mismatches are rare, and so validating all markup would be prohibitively expensive.\n* You’ll likely have only one `hydrateRoot` call in your app. If you use a framework, it might do this call for you.\n* If your app is client-rendered with no HTML rendered already, using `hydrateRoot()` is not supported. Use [`createRoot()`](https://react.dev/reference/react-dom/client/createRoot) instead.\n\n* * *\n\n### `root.render(reactNode)`[](https://react.dev/reference/react-dom/client/hydrateRoot#root-render \"Link for this heading\")\n\nCall `root.render` to update a React component inside a hydrated React root for a browser DOM element.\n\nReact will update `<App />` in the hydrated `root`.\n\n[See more examples below.](https://react.dev/reference/react-dom/client/hydrateRoot#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/client/hydrateRoot#root-render-parameters \"Link for Parameters \")\n\n* `reactNode`: A “React node” that you want to update. This will usually be a piece of JSX like `<App />`, but you can also pass a React element constructed with [`createElement()`](https://react.dev/reference/react/createElement), a string, a number, `null`, or `undefined`.\n\n#### Returns[](https://react.dev/reference/react-dom/client/hydrateRoot#root-render-returns \"Link for Returns \")\n\n`root.render` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react-dom/client/hydrateRoot#root-render-caveats \"Link for Caveats \")\n\n* If you call `root.render` before the root has finished hydrating, React will clear the existing server-rendered HTML content and switch the entire root to client rendering.\n\n* * *\n\n### `root.unmount()`[](https://react.dev/reference/react-dom/client/hydrateRoot#root-unmount \"Link for this heading\")\n\nCall `root.unmount` to destroy a rendered tree inside a React root.\n\nAn app fully built with React will usually not have any calls to `root.unmount`.\n\nThis is mostly useful if your React root’s DOM node (or any of its ancestors) may get removed from the DOM by some other code. For example, imagine a jQuery tab panel that removes inactive tabs from the DOM. If a tab gets removed, everything inside it (including the React roots inside) would get removed from the DOM as well. You need to tell React to “stop” managing the removed root’s content by calling `root.unmount`. Otherwise, the components inside the removed root won’t clean up and free up resources like subscriptions.\n\nCalling `root.unmount` will unmount all the components in the root and “detach” React from the root DOM node, including removing any event handlers or state in the tree.\n\n#### Parameters[](https://react.dev/reference/react-dom/client/hydrateRoot#root-unmount-parameters \"Link for Parameters \")\n\n`root.unmount` does not accept any parameters.\n\n#### Returns[](https://react.dev/reference/react-dom/client/hydrateRoot#root-unmount-returns \"Link for Returns \")\n\n`root.unmount` returns `undefined`.\n\n#### Caveats[](https://react.dev/reference/react-dom/client/hydrateRoot#root-unmount-caveats \"Link for Caveats \")\n\n* Calling `root.unmount` will unmount all the components in the tree and “detach” React from the root DOM node.\n \n* Once you call `root.unmount` you cannot call `root.render` again on the root. Attempting to call `root.render` on an unmounted root will throw a “Cannot update an unmounted root” error.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/client/hydrateRoot#usage \"Link for Usage \")\n-----------------------------------------------------------------------------------------\n\n### Hydrating server-rendered HTML[](https://react.dev/reference/react-dom/client/hydrateRoot#hydrating-server-rendered-html \"Link for Hydrating server-rendered HTML \")\n\nIf your app’s HTML was generated by [`react-dom/server`](https://react.dev/reference/react-dom/client/createRoot), you need to _hydrate_ it on the client.\n\n```\nimport { hydrateRoot } from 'react-dom/client';hydrateRoot(document.getElementById('root'), <App />);\n```\n\nThis will hydrate the server HTML inside the browser DOM node with the React component for your app. Usually, you will do it once at startup. If you use a framework, it might do this behind the scenes for you.\n\nTo hydrate your app, React will “attach” your components’ logic to the initial generated HTML from the server. Hydration turns the initial HTML snapshot from the server into a fully interactive app that runs in the browser.\n\nYou shouldn’t need to call `hydrateRoot` again or to call it in more places. From this point on, React will be managing the DOM of your application. To update the UI, your components will [use state](https://react.dev/reference/react/useState) instead.\n\n### Pitfall\n\nThe React tree you pass to `hydrateRoot` needs to produce **the same output** as it did on the server.\n\nThis is important for the user experience. The user will spend some time looking at the server-generated HTML before your JavaScript code loads. Server rendering creates an illusion that the app loads faster by showing the HTML snapshot of its output. Suddenly showing different content breaks that illusion. This is why the server render output must match the initial render output on the client.\n\nThe most common causes leading to hydration errors include:\n\n* Extra whitespace (like newlines) around the React-generated HTML inside the root node.\n* Using checks like `typeof window !== 'undefined'` in your rendering logic.\n* Using browser-only APIs like [`window.matchMedia`](https://developer.mozilla.org/en-US/docs/Web/API/Window/matchMedia) in your rendering logic.\n* Rendering different data on the server and the client.\n\nReact recovers from some hydration errors, but **you must fix them like other bugs.** In the best case, they’ll lead to a slowdown; in the worst case, event handlers can get attached to the wrong elements.\n\n* * *\n\n### Hydrating an entire document[](https://react.dev/reference/react-dom/client/hydrateRoot#hydrating-an-entire-document \"Link for Hydrating an entire document \")\n\nApps fully built with React can render the entire document as JSX, including the [`<html>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/html) tag:\n\n```\nfunction App() {return (<html><head><meta charSet=\"utf-8\" /><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\" /><link rel=\"stylesheet\" href=\"/styles.css\"></link><title>My app</title></head><body><Router /></body></html>);}\n```\n\nTo hydrate the entire document, pass the [`document`](https://developer.mozilla.org/en-US/docs/Web/API/Window/document) global as the first argument to `hydrateRoot`:\n\n```\nimport { hydrateRoot } from 'react-dom/client';import App from './App.js';hydrateRoot(document, <App />);\n```\n\n* * *\n\n### Suppressing unavoidable hydration mismatch errors[](https://react.dev/reference/react-dom/client/hydrateRoot#suppressing-unavoidable-hydration-mismatch-errors \"Link for Suppressing unavoidable hydration mismatch errors \")\n\nIf a single element’s attribute or text content is unavoidably different between the server and the client (for example, a timestamp), you may silence the hydration mismatch warning.\n\nTo silence hydration warnings on an element, add `suppressHydrationWarning={true}`:\n\nThis only works one level deep, and is intended to be an escape hatch. Don’t overuse it. Unless it’s text content, React still won’t attempt to patch it up, so it may remain inconsistent until future updates.\n\n* * *\n\n### Handling different client and server content[](https://react.dev/reference/react-dom/client/hydrateRoot#handling-different-client-and-server-content \"Link for Handling different client and server content \")\n\nIf you intentionally need to render something different on the server and the client, you can do a two-pass rendering. Components that render something different on the client can read a [state variable](https://react.dev/reference/react/useState) like `isClient`, which you can set to `true` in an [Effect](https://react.dev/reference/react/useEffect):\n\nThis way the initial render pass will render the same content as the server, avoiding mismatches, but an additional pass will happen synchronously right after hydration.\n\n### Pitfall\n\nThis approach makes hydration slower because your components have to render twice. Be mindful of the user experience on slow connections. The JavaScript code may load significantly later than the initial HTML render, so rendering a different UI immediately after hydration may also feel jarring to the user.\n\n* * *\n\n### Updating a hydrated root component[](https://react.dev/reference/react-dom/client/hydrateRoot#updating-a-hydrated-root-component \"Link for Updating a hydrated root component \")\n\nAfter the root has finished hydrating, you can call [`root.render`](https://react.dev/reference/react-dom/client/hydrateRoot#root-render) to update the root React component. **Unlike with [`createRoot`](https://react.dev/reference/react-dom/client/createRoot), you don’t usually need to do this because the initial content was already rendered as HTML.**\n\nIf you call `root.render` at some point after hydration, and the component tree structure matches up with what was previously rendered, React will [preserve the state.](https://react.dev/learn/preserving-and-resetting-state) Notice how you can type in the input, which means that the updates from repeated `render` calls every second in this example are not destructive:\n\nIt is uncommon to call [`root.render`](https://react.dev/reference/react-dom/client/hydrateRoot#root-render) on a hydrated root. Usually, you’ll [update state](https://react.dev/reference/react/useState) inside one of the components instead.\n\n### Show a dialog for uncaught errors[](https://react.dev/reference/react-dom/client/hydrateRoot#show-a-dialog-for-uncaught-errors \"Link for Show a dialog for uncaught errors \")\n\n### Canary\n\n`onUncaughtError` is only available in the latest React Canary release.\n\nBy default, React will log all uncaught errors to the console. To implement your own error reporting, you can provide the optional `onUncaughtError` root option:\n\n```\nimport { hydrateRoot } from 'react-dom/client';const root = hydrateRoot(document.getElementById('root'),<App />,{onUncaughtError: (error, errorInfo) => {console.error('Uncaught error',error,errorInfo.componentStack);}});root.render(<App />);\n```\n\nThe onUncaughtError option is a function called with two arguments:\n\n1. The error that was thrown.\n2. An errorInfo object that contains the componentStack of the error.\n\nYou can use the `onUncaughtError` root option to display error dialogs:\n\nimport { hydrateRoot } from \"react-dom/client\";\nimport App from \"./App.js\";\nimport {reportUncaughtError} from \"./reportError\";\nimport \"./styles.css\";\nimport {renderToString} from 'react-dom/server';\n\nconst container = document.getElementById(\"root\");\nconst root = hydrateRoot(container, <App />, {\n onUncaughtError: (error, errorInfo) \\=> {\n if (error.message !== 'Known error') {\n reportUncaughtError({\n error,\n componentStack: errorInfo.componentStack\n });\n }\n }\n});\n\n### Displaying Error Boundary errors[](https://react.dev/reference/react-dom/client/hydrateRoot#displaying-error-boundary-errors \"Link for Displaying Error Boundary errors \")\n\n### Canary\n\n`onCaughtError` is only available in the latest React Canary release.\n\nBy default, React will log all errors caught by an Error Boundary to `console.error`. To override this behavior, you can provide the optional `onCaughtError` root option for errors caught by an [Error Boundary](https://react.dev/reference/react/Component#catching-rendering-errors-with-an-error-boundary):\n\n```\nimport { hydrateRoot } from 'react-dom/client';const root = hydrateRoot(document.getElementById('root'),<App />,{onCaughtError: (error, errorInfo) => {console.error('Caught error',error,errorInfo.componentStack);}});root.render(<App />);\n```\n\nThe onCaughtError option is a function called with two arguments:\n\n1. The error that was caught by the boundary.\n2. An errorInfo object that contains the componentStack of the error.\n\nYou can use the `onCaughtError` root option to display error dialogs or filter known errors from logging:\n\nimport { hydrateRoot } from \"react-dom/client\";\nimport App from \"./App.js\";\nimport {reportCaughtError} from \"./reportError\";\nimport \"./styles.css\";\n\nconst container = document.getElementById(\"root\");\nconst root = hydrateRoot(container, <App />, {\n onCaughtError: (error, errorInfo) \\=> {\n if (error.message !== 'Known error') {\n reportCaughtError({\n error,\n componentStack: errorInfo.componentStack\n });\n }\n }\n});\n\n### Show a dialog for recoverable hydration mismatch errors[](https://react.dev/reference/react-dom/client/hydrateRoot#show-a-dialog-for-recoverable-hydration-mismatch-errors \"Link for Show a dialog for recoverable hydration mismatch errors \")\n\nWhen React encounters a hydration mismatch, it will automatically attempt to recover by rendering on the client. By default, React will log hydration mismatch errors to `console.error`. To override this behavior, you can provide the optional `onRecoverableError` root option:\n\n```\nimport { hydrateRoot } from 'react-dom/client';const root = hydrateRoot(document.getElementById('root'),<App />,{onRecoverableError: (error, errorInfo) => {console.error('Caught error',error,error.cause,errorInfo.componentStack);}});\n```\n\nThe onRecoverableError option is a function called with two arguments:\n\n1. The error React throws. Some errors may include the original cause as error.cause.\n2. An errorInfo object that contains the componentStack of the error.\n\nYou can use the `onRecoverableError` root option to display error dialogs for hydration mismatches:\n\nTroubleshooting[](https://react.dev/reference/react-dom/client/hydrateRoot#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------------------------\n\n### I’m getting an error: “You passed a second argument to root.render”[](https://react.dev/reference/react-dom/client/hydrateRoot#im-getting-an-error-you-passed-a-second-argument-to-root-render \"Link for I’m getting an error: “You passed a second argument to root.render” \")\n\nA common mistake is to pass the options for `hydrateRoot` to `root.render(...)`:\n\nWarning: You passed a second argument to root.render(…) but it only accepts one argument.\n\nTo fix, pass the root options to `hydrateRoot(...)`, not `root.render(...)`:\n\n```\n// 🚩 Wrong: root.render only takes one argument.root.render(App, {onUncaughtError});// ✅ Correct: pass options to createRoot.const root = hydrateRoot(container, <App />, {onUncaughtError});\n```\n",
"filename": "hydrateRoot.md",
"package": "react"
} |
{
"content": "Title: Importing and Exporting Components – React\n\nURL Source: https://react.dev/learn/importing-and-exporting-components\n\nMarkdown Content:\nThe magic of components lies in their reusability: you can create components that are composed of other components. But as you nest more and more components, it often makes sense to start splitting them into different files. This lets you keep your files easy to scan and reuse components in more places.\n\n### You will learn\n\n* What a root component file is\n* How to import and export a component\n* When to use default and named imports and exports\n* How to import and export multiple components from one file\n* How to split components into multiple files\n\nThe root component file[](https://react.dev/learn/importing-and-exporting-components#the-root-component-file \"Link for The root component file \")\n-------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn [Your First Component](https://react.dev/learn/your-first-component), you made a `Profile` component and a `Gallery` component that renders it:\n\nThese currently live in a **root component file,** named `App.js` in this example. Depending on your setup, your root component could be in another file, though. If you use a framework with file-based routing, such as Next.js, your root component will be different for every page.\n\nExporting and importing a component[](https://react.dev/learn/importing-and-exporting-components#exporting-and-importing-a-component \"Link for Exporting and importing a component \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhat if you want to change the landing screen in the future and put a list of science books there? Or place all the profiles somewhere else? It makes sense to move `Gallery` and `Profile` out of the root component file. This will make them more modular and reusable in other files. You can move a component in three steps:\n\n1. **Make** a new JS file to put the components in.\n2. **Export** your function component from that file (using either [default](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/export#using_the_default_export) or [named](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/export#using_named_exports) exports).\n3. **Import** it in the file where you’ll use the component (using the corresponding technique for importing [default](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/import#importing_defaults) or [named](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/import#import_a_single_export_from_a_module) exports).\n\nHere both `Profile` and `Gallery` have been moved out of `App.js` into a new file called `Gallery.js`. Now you can change `App.js` to import `Gallery` from `Gallery.js`:\n\nNotice how this example is broken down into two component files now:\n\n1. `Gallery.js`:\n * Defines the `Profile` component which is only used within the same file and is not exported.\n * Exports the `Gallery` component as a **default export.**\n2. `App.js`:\n * Imports `Gallery` as a **default import** from `Gallery.js`.\n * Exports the root `App` component as a **default export.**\n\n### Note\n\nYou may encounter files that leave off the `.js` file extension like so:\n\n```\nimport Gallery from './Gallery';\n```\n\nEither `'./Gallery.js'` or `'./Gallery'` will work with React, though the former is closer to how [native ES Modules](https://developer.mozilla.org/docs/Web/JavaScript/Guide/Modules) work.\n\n##### Deep Dive\n\n#### Default vs named exports[](https://react.dev/learn/importing-and-exporting-components#default-vs-named-exports \"Link for Default vs named exports \")\n\nThere are two primary ways to export values with JavaScript: default exports and named exports. So far, our examples have only used default exports. But you can use one or both of them in the same file. **A file can have no more than one _default_ export, but it can have as many _named_ exports as you like.**\n\n\n\nHow you export your component dictates how you must import it. You will get an error if you try to import a default export the same way you would a named export! This chart can help you keep track:\n\n| Syntax | Export statement | Import statement |\n| --- | --- | --- |\n| Default | `export default function Button() {}` | `import Button from './Button.js';` |\n| Named | `export function Button() {}` | `import { Button } from './Button.js';` |\n\nWhen you write a _default_ import, you can put any name you want after `import`. For example, you could write `import Banana from './Button.js'` instead and it would still provide you with the same default export. In contrast, with named imports, the name has to match on both sides. That’s why they are called _named_ imports!\n\n**People often use default exports if the file exports only one component, and use named exports if it exports multiple components and values.** Regardless of which coding style you prefer, always give meaningful names to your component functions and the files that contain them. Components without names, like `export default () => {}`, are discouraged because they make debugging harder.\n\nExporting and importing multiple components from the same file[](https://react.dev/learn/importing-and-exporting-components#exporting-and-importing-multiple-components-from-the-same-file \"Link for Exporting and importing multiple components from the same file \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhat if you want to show just one `Profile` instead of a gallery? You can export the `Profile` component, too. But `Gallery.js` already has a _default_ export, and you can’t have _two_ default exports. You could create a new file with a default export, or you could add a _named_ export for `Profile`. **A file can only have one default export, but it can have numerous named exports!**\n\n### Note\n\nTo reduce the potential confusion between default and named exports, some teams choose to only stick to one style (default or named), or avoid mixing them in a single file. Do what works best for you!\n\nFirst, **export** `Profile` from `Gallery.js` using a named export (no `default` keyword):\n\n```\nexport function Profile() {// ...}\n```\n\nThen, **import** `Profile` from `Gallery.js` to `App.js` using a named import (with the curly braces):\n\n```\nimport { Profile } from './Gallery.js';\n```\n\nFinally, **render** `<Profile />` from the `App` component:\n\n```\nexport default function App() {return <Profile />;}\n```\n\nNow `Gallery.js` contains two exports: a default `Gallery` export, and a named `Profile` export. `App.js` imports both of them. Try editing `<Profile />` to `<Gallery />` and back in this example:\n\nNow you’re using a mix of default and named exports:\n\n* `Gallery.js`:\n * Exports the `Profile` component as a **named export called `Profile`.**\n * Exports the `Gallery` component as a **default export.**\n* `App.js`:\n * Imports `Profile` as a **named import called `Profile`** from `Gallery.js`.\n * Imports `Gallery` as a **default import** from `Gallery.js`.\n * Exports the root `App` component as a **default export.**\n\nRecap[](https://react.dev/learn/importing-and-exporting-components#recap \"Link for Recap\")\n------------------------------------------------------------------------------------------\n\nOn this page you learned:\n\n* What a root component file is\n* How to import and export a component\n* When and how to use default and named imports and exports\n* How to export multiple components from the same file\n\nTry out some challenges[](https://react.dev/learn/importing-and-exporting-components#challenges \"Link for Try out some challenges\")\n-----------------------------------------------------------------------------------------------------------------------------------\n\n#### Split the components further[](https://react.dev/learn/importing-and-exporting-components#split-the-components-further \"Link for this heading\")\n\nCurrently, `Gallery.js` exports both `Profile` and `Gallery`, which is a bit confusing.\n\nMove the `Profile` component to its own `Profile.js`, and then change the `App` component to render both `<Profile />` and `<Gallery />` one after another.\n\nYou may use either a default or a named export for `Profile`, but make sure that you use the corresponding import syntax in both `App.js` and `Gallery.js`! You can refer to the table from the deep dive above:\n\n| Syntax | Export statement | Import statement |\n| --- | --- | --- |\n| Default | `export default function Button() {}` | `import Button from './Button.js';` |\n| Named | `export function Button() {}` | `import { Button } from './Button.js';` |\n\nexport function Profile() {\n return (\n <img\n src\\=\"https://i.imgur.com/QIrZWGIs.jpg\"\n alt\\=\"Alan L. Hart\"\n />\n );\n}\n\nexport default function Gallery() {\n return (\n <section\\>\n <h1\\>Amazing scientists</h1\\>\n <Profile />\n <Profile />\n <Profile />\n </section\\>\n );\n}\n\nAfter you get it working with one kind of exports, make it work with the other kind.\n",
"filename": "importing-and-exporting-components.md",
"package": "react"
} |
{
"content": "Title: <input> – React\n\nURL Source: https://react.dev/reference/react-dom/components/input\n\nMarkdown Content:\nThe [built-in browser `<input>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input) lets you render different kinds of form inputs.\n\n* [Reference](https://react.dev/reference/react-dom/components/input#reference)\n * [`<input>`](https://react.dev/reference/react-dom/components/input#input)\n* [Usage](https://react.dev/reference/react-dom/components/input#usage)\n * [Displaying inputs of different types](https://react.dev/reference/react-dom/components/input#displaying-inputs-of-different-types)\n * [Providing a label for an input](https://react.dev/reference/react-dom/components/input#providing-a-label-for-an-input)\n * [Providing an initial value for an input](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input)\n * [Reading the input values when submitting a form](https://react.dev/reference/react-dom/components/input#reading-the-input-values-when-submitting-a-form)\n * [Controlling an input with a state variable](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable)\n * [Optimizing re-rendering on every keystroke](https://react.dev/reference/react-dom/components/input#optimizing-re-rendering-on-every-keystroke)\n* [Troubleshooting](https://react.dev/reference/react-dom/components/input#troubleshooting)\n * [My text input doesn’t update when I type into it](https://react.dev/reference/react-dom/components/input#my-text-input-doesnt-update-when-i-type-into-it)\n * [My checkbox doesn’t update when I click on it](https://react.dev/reference/react-dom/components/input#my-checkbox-doesnt-update-when-i-click-on-it)\n * [My input caret jumps to the beginning on every keystroke](https://react.dev/reference/react-dom/components/input#my-input-caret-jumps-to-the-beginning-on-every-keystroke)\n * [I’m getting an error: “A component is changing an uncontrolled input to be controlled”](https://react.dev/reference/react-dom/components/input#im-getting-an-error-a-component-is-changing-an-uncontrolled-input-to-be-controlled)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/input#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------------\n\n### `<input>`[](https://react.dev/reference/react-dom/components/input#input \"Link for this heading\")\n\nTo display an input, render the [built-in browser `<input>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input) component.\n\n[See more examples below.](https://react.dev/reference/react-dom/components/input#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/input#props \"Link for Props \")\n\n`<input>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\n### Canary\n\n[`formAction`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#formaction): A string or function. Overrides the parent `<form action>` for `type=\"submit\"` and `type=\"image\"`. When a URL is passed to `action` the form will behave like a standard HTML form. When a function is passed to `formAction` the function will handle the form submission. See [`<form action>`](https://react.dev/reference/react-dom/components/form#props).\n\nYou can [make an input controlled](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable) by passing one of these props:\n\n* [`checked`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement#checked): A boolean. For a checkbox input or a radio button, controls whether it is selected.\n* [`value`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement#value): A string. For a text input, controls its text. (For a radio button, specifies its form data.)\n\nWhen you pass either of them, you must also pass an `onChange` handler that updates the passed value.\n\nThese `<input>` props are only relevant for uncontrolled inputs:\n\n* [`defaultChecked`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement#defaultChecked): A boolean. Specifies [the initial value](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input) for `type=\"checkbox\"` and `type=\"radio\"` inputs.\n* [`defaultValue`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement#defaultValue): A string. Specifies [the initial value](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input) for a text input.\n\nThese `<input>` props are relevant both for uncontrolled and controlled inputs:\n\n* [`accept`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#accept): A string. Specifies which filetypes are accepted by a `type=\"file\"` input.\n* [`alt`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#alt): A string. Specifies the alternative image text for a `type=\"image\"` input.\n* [`capture`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#capture): A string. Specifies the media (microphone, video, or camera) captured by a `type=\"file\"` input.\n* [`autoComplete`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#autocomplete): A string. Specifies one of the possible [autocomplete behaviors.](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/autocomplete#values)\n* [`autoFocus`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#autofocus): A boolean. If `true`, React will focus the element on mount.\n* [`dirname`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#dirname): A string. Specifies the form field name for the element’s directionality.\n* [`disabled`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#disabled): A boolean. If `true`, the input will not be interactive and will appear dimmed.\n* `children`: `<input>` does not accept children.\n* [`form`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#form): A string. Specifies the `id` of the `<form>` this input belongs to. If omitted, it’s the closest parent form.\n* [`formAction`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#formaction): A string. Overrides the parent `<form action>` for `type=\"submit\"` and `type=\"image\"`.\n* [`formEnctype`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#formenctype): A string. Overrides the parent `<form enctype>` for `type=\"submit\"` and `type=\"image\"`.\n* [`formMethod`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#formmethod): A string. Overrides the parent `<form method>` for `type=\"submit\"` and `type=\"image\"`.\n* [`formNoValidate`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#formnovalidate): A string. Overrides the parent `<form noValidate>` for `type=\"submit\"` and `type=\"image\"`.\n* [`formTarget`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#formtarget): A string. Overrides the parent `<form target>` for `type=\"submit\"` and `type=\"image\"`.\n* [`height`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#height): A string. Specifies the image height for `type=\"image\"`.\n* [`list`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#list): A string. Specifies the `id` of the `<datalist>` with the autocomplete options.\n* [`max`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#max): A number. Specifies the maximum value of numerical and datetime inputs.\n* [`maxLength`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#maxlength): A number. Specifies the maximum length of text and other inputs.\n* [`min`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#min): A number. Specifies the minimum value of numerical and datetime inputs.\n* [`minLength`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#minlength): A number. Specifies the minimum length of text and other inputs.\n* [`multiple`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#multiple): A boolean. Specifies whether multiple values are allowed for `<type=\"file\"` and `type=\"email\"`.\n* [`name`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#name): A string. Specifies the name for this input that’s [submitted with the form.](https://react.dev/reference/react-dom/components/input#reading-the-input-values-when-submitting-a-form)\n* `onChange`: An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Required for [controlled inputs.](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable) Fires immediately when the input’s value is changed by the user (for example, it fires on every keystroke). Behaves like the browser [`input` event.](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event)\n* `onChangeCapture`: A version of `onChange` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onInput`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/input_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires immediately when the value is changed by the user. For historical reasons, in React it is idiomatic to use `onChange` instead which works similarly.\n* `onInputCapture`: A version of `onInput` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onInvalid`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement/invalid_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires if an input fails validation on form submit. Unlike the built-in `invalid` event, the React `onInvalid` event bubbles.\n* `onInvalidCapture`: A version of `onInvalid` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`onSelect`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement/select_event): An [`Event` handler](https://react.dev/reference/react-dom/components/common#event-handler) function. Fires after the selection inside the `<input>` changes. React extends the `onSelect` event to also fire for empty selection and on edits (which may affect the selection).\n* `onSelectCapture`: A version of `onSelect` that fires in the [capture phase.](https://react.dev/learn/responding-to-events#capture-phase-events)\n* [`pattern`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#pattern): A string. Specifies the pattern that the `value` must match.\n* [`placeholder`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#placeholder): A string. Displayed in a dimmed color when the input value is empty.\n* [`readOnly`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#readonly): A boolean. If `true`, the input is not editable by the user.\n* [`required`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#required): A boolean. If `true`, the value must be provided for the form to submit.\n* [`size`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#size): A number. Similar to setting width, but the unit depends on the control.\n* [`src`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#src): A string. Specifies the image source for a `type=\"image\"` input.\n* [`step`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#step): A positive number or an `'any'` string. Specifies the distance between valid values.\n* [`type`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#type): A string. One of the [input types.](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#input_types)\n* [`width`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#width): A string. Specifies the image width for a `type=\"image\"` input.\n\n#### Caveats[](https://react.dev/reference/react-dom/components/input#caveats \"Link for Caveats \")\n\n* Checkboxes need `checked` (or `defaultChecked`), not `value` (or `defaultValue`).\n* If a text input receives a string `value` prop, it will be [treated as controlled.](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable)\n* If a checkbox or a radio button receives a boolean `checked` prop, it will be [treated as controlled.](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable)\n* An input can’t be both controlled and uncontrolled at the same time.\n* An input cannot switch between being controlled or uncontrolled over its lifetime.\n* Every controlled input needs an `onChange` event handler that synchronously updates its backing value.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/input#usage \"Link for Usage \")\n---------------------------------------------------------------------------------------\n\n### Displaying inputs of different types[](https://react.dev/reference/react-dom/components/input#displaying-inputs-of-different-types \"Link for Displaying inputs of different types \")\n\nTo display an input, render an `<input>` component. By default, it will be a text input. You can pass `type=\"checkbox\"` for a checkbox, `type=\"radio\"` for a radio button, [or one of the other input types.](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#input_types)\n\n* * *\n\n### Providing a label for an input[](https://react.dev/reference/react-dom/components/input#providing-a-label-for-an-input \"Link for Providing a label for an input \")\n\nTypically, you will place every `<input>` inside a [`<label>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/label) tag. This tells the browser that this label is associated with that input. When the user clicks the label, the browser will automatically focus the input. It’s also essential for accessibility: a screen reader will announce the label caption when the user focuses the associated input.\n\nIf you can’t nest `<input>` into a `<label>`, associate them by passing the same ID to `<input id>` and [`<label htmlFor>`.](https://developer.mozilla.org/en-US/docs/Web/API/HTMLLabelElement/htmlFor) To avoid conflicts between multiple instances of one component, generate such an ID with [`useId`.](https://react.dev/reference/react/useId)\n\n* * *\n\n### Providing an initial value for an input[](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input \"Link for Providing an initial value for an input \")\n\nYou can optionally specify the initial value for any input. Pass it as the `defaultValue` string for text inputs. Checkboxes and radio buttons should specify the initial value with the `defaultChecked` boolean instead.\n\n* * *\n\n### Reading the input values when submitting a form[](https://react.dev/reference/react-dom/components/input#reading-the-input-values-when-submitting-a-form \"Link for Reading the input values when submitting a form \")\n\nAdd a [`<form>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) around your inputs with a [`<button type=\"submit\">`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/button) inside. It will call your `<form onSubmit>` event handler. By default, the browser will send the form data to the current URL and refresh the page. You can override that behavior by calling `e.preventDefault()`. Read the form data with [`new FormData(e.target)`](https://developer.mozilla.org/en-US/docs/Web/API/FormData).\n\n### Note\n\nGive a `name` to every `<input>`, for example `<input name=\"firstName\" defaultValue=\"Taylor\" />`. The `name` you specified will be used as a key in the form data, for example `{ firstName: \"Taylor\" }`.\n\n### Pitfall\n\nBy default, _any_ `<button>` inside a `<form>` will submit it. This can be surprising! If you have your own custom `Button` React component, consider returning [`<button type=\"button\">`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/button) instead of `<button>`. Then, to be explicit, use `<button type=\"submit\">` for buttons that _are_ supposed to submit the form.\n\n* * *\n\n### Controlling an input with a state variable[](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable \"Link for Controlling an input with a state variable \")\n\nAn input like `<input />` is _uncontrolled._ Even if you [pass an initial value](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input) like `<input defaultValue=\"Initial text\" />`, your JSX only specifies the initial value. It does not control what the value should be right now.\n\n**To render a _controlled_ input, pass the `value` prop to it (or `checked` for checkboxes and radios).** React will force the input to always have the `value` you passed. Usually, you would do this by declaring a [state variable:](https://react.dev/reference/react/useState)\n\n```\nfunction Form() {const [firstName, setFirstName] = useState(''); // Declare a state variable...// ...return (<inputvalue={firstName} // ...force the input's value to match the state variable...onChange={e => setFirstName(e.target.value)} // ... and update the state variable on any edits!/>);}\n```\n\nA controlled input makes sense if you needed state anyway—for example, to re-render your UI on every edit:\n\n```\nfunction Form() {const [firstName, setFirstName] = useState('');return (<><label> First name:<input value={firstName} onChange={e => setFirstName(e.target.value)} /></label>{firstName !== '' && <p>Your name is {firstName}.</p>} ...\n```\n\nIt’s also useful if you want to offer multiple ways to adjust the input state (for example, by clicking a button):\n\n```\nfunction Form() {// ...const [age, setAge] = useState('');const ageAsNumber = Number(age);return (<><label> Age:<inputvalue={age}onChange={e => setAge(e.target.value)}type=\"number\"/><button onClick={() => setAge(ageAsNumber + 10)}> Add 10 years</button>\n```\n\nThe `value` you pass to controlled components should not be `undefined` or `null`. If you need the initial value to be empty (such as with the `firstName` field below), initialize your state variable to an empty string (`''`).\n\n### Pitfall\n\n**If you pass `value` without `onChange`, it will be impossible to type into the input.** When you control an input by passing some `value` to it, you _force_ it to always have the value you passed. So if you pass a state variable as a `value` but forget to update that state variable synchronously during the `onChange` event handler, React will revert the input after every keystroke back to the `value` that you specified.\n\n* * *\n\n### Optimizing re-rendering on every keystroke[](https://react.dev/reference/react-dom/components/input#optimizing-re-rendering-on-every-keystroke \"Link for Optimizing re-rendering on every keystroke \")\n\nWhen you use a controlled input, you set the state on every keystroke. If the component containing your state re-renders a large tree, this can get slow. There’s a few ways you can optimize re-rendering performance.\n\nFor example, suppose you start with a form that re-renders all page content on every keystroke:\n\n```\nfunction App() {const [firstName, setFirstName] = useState('');return (<><form><input value={firstName} onChange={e => setFirstName(e.target.value)} /></form><PageContent /></>);}\n```\n\nSince `<PageContent />` doesn’t rely on the input state, you can move the input state into its own component:\n\n```\nfunction App() {return (<><SignupForm /><PageContent /></>);}function SignupForm() {const [firstName, setFirstName] = useState('');return (<form><input value={firstName} onChange={e => setFirstName(e.target.value)} /></form>);}\n```\n\nThis significantly improves performance because now only `SignupForm` re-renders on every keystroke.\n\nIf there is no way to avoid re-rendering (for example, if `PageContent` depends on the search input’s value), [`useDeferredValue`](https://react.dev/reference/react/useDeferredValue#deferring-re-rendering-for-a-part-of-the-ui) lets you keep the controlled input responsive even in the middle of a large re-render.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react-dom/components/input#troubleshooting \"Link for Troubleshooting \")\n---------------------------------------------------------------------------------------------------------------------\n\n### My text input doesn’t update when I type into it[](https://react.dev/reference/react-dom/components/input#my-text-input-doesnt-update-when-i-type-into-it \"Link for My text input doesn’t update when I type into it \")\n\nIf you render an input with `value` but no `onChange`, you will see an error in the console:\n\n```\n// 🔴 Bug: controlled text input with no onChange handler<input value={something} />\n```\n\nYou provided a `value` prop to a form field without an `onChange` handler. This will render a read-only field. If the field should be mutable use `defaultValue`. Otherwise, set either `onChange` or `readOnly`.\n\nAs the error message suggests, if you only wanted to [specify the _initial_ value,](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input) pass `defaultValue` instead:\n\n```\n// ✅ Good: uncontrolled input with an initial value<input defaultValue={something} />\n```\n\nIf you want [to control this input with a state variable,](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable) specify an `onChange` handler:\n\n```\n// ✅ Good: controlled input with onChange<input value={something} onChange={e => setSomething(e.target.value)} />\n```\n\nIf the value is intentionally read-only, add a `readOnly` prop to suppress the error:\n\n```\n// ✅ Good: readonly controlled input without on change<input value={something} readOnly={true} />\n```\n\n* * *\n\n### My checkbox doesn’t update when I click on it[](https://react.dev/reference/react-dom/components/input#my-checkbox-doesnt-update-when-i-click-on-it \"Link for My checkbox doesn’t update when I click on it \")\n\nIf you render a checkbox with `checked` but no `onChange`, you will see an error in the console:\n\n```\n// 🔴 Bug: controlled checkbox with no onChange handler<input type=\"checkbox\" checked={something} />\n```\n\nYou provided a `checked` prop to a form field without an `onChange` handler. This will render a read-only field. If the field should be mutable use `defaultChecked`. Otherwise, set either `onChange` or `readOnly`.\n\nAs the error message suggests, if you only wanted to [specify the _initial_ value,](https://react.dev/reference/react-dom/components/input#providing-an-initial-value-for-an-input) pass `defaultChecked` instead:\n\n```\n// ✅ Good: uncontrolled checkbox with an initial value<input type=\"checkbox\" defaultChecked={something} />\n```\n\nIf you want [to control this checkbox with a state variable,](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable) specify an `onChange` handler:\n\n```\n// ✅ Good: controlled checkbox with onChange<input type=\"checkbox\" checked={something} onChange={e => setSomething(e.target.checked)} />\n```\n\n### Pitfall\n\nYou need to read `e.target.checked` rather than `e.target.value` for checkboxes.\n\nIf the checkbox is intentionally read-only, add a `readOnly` prop to suppress the error:\n\n```\n// ✅ Good: readonly controlled input without on change<input type=\"checkbox\" checked={something} readOnly={true} />\n```\n\n* * *\n\n### My input caret jumps to the beginning on every keystroke[](https://react.dev/reference/react-dom/components/input#my-input-caret-jumps-to-the-beginning-on-every-keystroke \"Link for My input caret jumps to the beginning on every keystroke \")\n\nIf you [control an input,](https://react.dev/reference/react-dom/components/input#controlling-an-input-with-a-state-variable) you must update its state variable to the input’s value from the DOM during `onChange`.\n\nYou can’t update it to something other than `e.target.value` (or `e.target.checked` for checkboxes):\n\n```\nfunction handleChange(e) {// 🔴 Bug: updating an input to something other than e.target.valuesetFirstName(e.target.value.toUpperCase());}\n```\n\nYou also can’t update it asynchronously:\n\n```\nfunction handleChange(e) {// 🔴 Bug: updating an input asynchronouslysetTimeout(() => {setFirstName(e.target.value);}, 100);}\n```\n\nTo fix your code, update it synchronously to `e.target.value`:\n\n```\nfunction handleChange(e) {// ✅ Updating a controlled input to e.target.value synchronouslysetFirstName(e.target.value);}\n```\n\nIf this doesn’t fix the problem, it’s possible that the input gets removed and re-added from the DOM on every keystroke. This can happen if you’re accidentally [resetting state](https://react.dev/learn/preserving-and-resetting-state) on every re-render, for example if the input or one of its parents always receives a different `key` attribute, or if you nest component function definitions (which is not supported and causes the “inner” component to always be considered a different tree).\n\n* * *\n\n### I’m getting an error: “A component is changing an uncontrolled input to be controlled”[](https://react.dev/reference/react-dom/components/input#im-getting-an-error-a-component-is-changing-an-uncontrolled-input-to-be-controlled \"Link for I’m getting an error: “A component is changing an uncontrolled input to be controlled” \")\n\nIf you provide a `value` to the component, it must remain a string throughout its lifetime.\n\nYou cannot pass `value={undefined}` first and later pass `value=\"some string\"` because React won’t know whether you want the component to be uncontrolled or controlled. A controlled component should always receive a string `value`, not `null` or `undefined`.\n\nIf your `value` is coming from an API or a state variable, it might be initialized to `null` or `undefined`. In that case, either set it to an empty string (`''`) initially, or pass `value={someValue ?? ''}` to ensure `value` is a string.\n\nSimilarly, if you pass `checked` to a checkbox, ensure it’s always a boolean.\n",
"filename": "input.md",
"package": "react"
} |
{
"content": "Title: isValidElement – React\n\nURL Source: https://react.dev/reference/react/isValidElement\n\nMarkdown Content:\n`isValidElement` checks whether a value is a React element.\n\n```\nconst isElement = isValidElement(value)\n```\n\n* [Reference](https://react.dev/reference/react/isValidElement#reference)\n * [`isValidElement(value)`](https://react.dev/reference/react/isValidElement#isvalidelement)\n* [Usage](https://react.dev/reference/react/isValidElement#usage)\n * [Checking if something is a React element](https://react.dev/reference/react/isValidElement#checking-if-something-is-a-react-element)\n\n* * *\n\nReference[](https://react.dev/reference/react/isValidElement#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------\n\n### `isValidElement(value)`[](https://react.dev/reference/react/isValidElement#isvalidelement \"Link for this heading\")\n\nCall `isValidElement(value)` to check whether `value` is a React element.\n\n```\nimport { isValidElement, createElement } from 'react';// ✅ React elementsconsole.log(isValidElement(<p />)); // trueconsole.log(isValidElement(createElement('p'))); // true// ❌ Not React elementsconsole.log(isValidElement(25)); // falseconsole.log(isValidElement('Hello')); // falseconsole.log(isValidElement({ age: 42 })); // false\n```\n\n[See more examples below.](https://react.dev/reference/react/isValidElement#usage)\n\n#### Parameters[](https://react.dev/reference/react/isValidElement#parameters \"Link for Parameters \")\n\n* `value`: The `value` you want to check. It can be any a value of any type.\n\n#### Returns[](https://react.dev/reference/react/isValidElement#returns \"Link for Returns \")\n\n`isValidElement` returns `true` if the `value` is a React element. Otherwise, it returns `false`.\n\n#### Caveats[](https://react.dev/reference/react/isValidElement#caveats \"Link for Caveats \")\n\n* **Only [JSX tags](https://react.dev/learn/writing-markup-with-jsx) and objects returned by [`createElement`](https://react.dev/reference/react/createElement) are considered to be React elements.** For example, even though a number like `42` is a valid React _node_ (and can be returned from a component), it is not a valid React element. Arrays and portals created with [`createPortal`](https://react.dev/reference/react-dom/createPortal) are also _not_ considered to be React elements.\n\n* * *\n\nUsage[](https://react.dev/reference/react/isValidElement#usage \"Link for Usage \")\n---------------------------------------------------------------------------------\n\n### Checking if something is a React element[](https://react.dev/reference/react/isValidElement#checking-if-something-is-a-react-element \"Link for Checking if something is a React element \")\n\nCall `isValidElement` to check if some value is a _React element._\n\nReact elements are:\n\n* Values produced by writing a [JSX tag](https://react.dev/learn/writing-markup-with-jsx)\n* Values produced by calling [`createElement`](https://react.dev/reference/react/createElement)\n\nFor React elements, `isValidElement` returns `true`:\n\n```\nimport { isValidElement, createElement } from 'react';// ✅ JSX tags are React elementsconsole.log(isValidElement(<p />)); // trueconsole.log(isValidElement(<MyComponent />)); // true// ✅ Values returned by createElement are React elementsconsole.log(isValidElement(createElement('p'))); // trueconsole.log(isValidElement(createElement(MyComponent))); // true\n```\n\nAny other values, such as strings, numbers, or arbitrary objects and arrays, are not React elements.\n\nFor them, `isValidElement` returns `false`:\n\n```\n// ❌ These are *not* React elementsconsole.log(isValidElement(null)); // falseconsole.log(isValidElement(25)); // falseconsole.log(isValidElement('Hello')); // falseconsole.log(isValidElement({ age: 42 })); // falseconsole.log(isValidElement([<div />, <div />])); // falseconsole.log(isValidElement(MyComponent)); // false\n```\n\nIt is very uncommon to need `isValidElement`. It’s mostly useful if you’re calling another API that _only_ accepts elements (like [`cloneElement`](https://react.dev/reference/react/cloneElement) does) and you want to avoid an error when your argument is not a React element.\n\nUnless you have some very specific reason to add an `isValidElement` check, you probably don’t need it.\n\n##### Deep Dive\n\n#### React elements vs React nodes[](https://react.dev/reference/react/isValidElement#react-elements-vs-react-nodes \"Link for React elements vs React nodes \")\n\nWhen you write a component, you can return any kind of _React node_ from it:\n\n```\nfunction MyComponent() {// ... you can return any React node ...}\n```\n\nA React node can be:\n\n* A React element created like `<div />` or `createElement('div')`\n* A portal created with [`createPortal`](https://react.dev/reference/react-dom/createPortal)\n* A string\n* A number\n* `true`, `false`, `null`, or `undefined` (which are not displayed)\n* An array of other React nodes\n\n**Note `isValidElement` checks whether the argument is a _React element,_ not whether it’s a React node.** For example, `42` is not a valid React element. However, it is a perfectly valid React node:\n\n```\nfunction MyComponent() {return 42; // It's ok to return a number from component}\n```\n\nThis is why you shouldn’t use `isValidElement` as a way to check whether something can be rendered.\n",
"filename": "isValidElement.md",
"package": "react"
} |
{
"content": "Title: JavaScript in JSX with Curly Braces – React\n\nURL Source: https://react.dev/learn/javascript-in-jsx-with-curly-braces\n\nMarkdown Content:\nJSX lets you write HTML-like markup inside a JavaScript file, keeping rendering logic and content in the same place. Sometimes you will want to add a little JavaScript logic or reference a dynamic property inside that markup. In this situation, you can use curly braces in your JSX to open a window to JavaScript.\n\n### You will learn\n\n* How to pass strings with quotes\n* How to reference a JavaScript variable inside JSX with curly braces\n* How to call a JavaScript function inside JSX with curly braces\n* How to use a JavaScript object inside JSX with curly braces\n\nPassing strings with quotes[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#passing-strings-with-quotes \"Link for Passing strings with quotes \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you want to pass a string attribute to JSX, you put it in single or double quotes:\n\nHere, `\"https://i.imgur.com/7vQD0fPs.jpg\"` and `\"Gregorio Y. Zara\"` are being passed as strings.\n\nBut what if you want to dynamically specify the `src` or `alt` text? You could **use a value from JavaScript by replacing `\"` and `\"` with `{` and `}`**:\n\nNotice the difference between `className=\"avatar\"`, which specifies an `\"avatar\"` CSS class name that makes the image round, and `src={avatar}` that reads the value of the JavaScript variable called `avatar`. That’s because curly braces let you work with JavaScript right there in your markup!\n\nUsing curly braces: A window into the JavaScript world[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#using-curly-braces-a-window-into-the-javascript-world \"Link for Using curly braces: A window into the JavaScript world \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nJSX is a special way of writing JavaScript. That means it’s possible to use JavaScript inside it—with curly braces `{ }`. The example below first declares a name for the scientist, `name`, then embeds it with curly braces inside the `<h1>`:\n\nTry changing the `name`’s value from `'Gregorio Y. Zara'` to `'Hedy Lamarr'`. See how the list title changes?\n\nAny JavaScript expression will work between curly braces, including function calls like `formatDate()`:\n\n### Where to use curly braces[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#where-to-use-curly-braces \"Link for Where to use curly braces \")\n\nYou can only use curly braces in two ways inside JSX:\n\n1. **As text** directly inside a JSX tag: `<h1>{name}'s To Do List</h1>` works, but `<{tag}>Gregorio Y. Zara's To Do List</{tag}>` will not.\n2. **As attributes** immediately following the `=` sign: `src={avatar}` will read the `avatar` variable, but `src=\"{avatar}\"` will pass the string `\"{avatar}\"`.\n\nUsing “double curlies”: CSS and other objects in JSX[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#using-double-curlies-css-and-other-objects-in-jsx \"Link for Using “double curlies”: CSS and other objects in JSX \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn addition to strings, numbers, and other JavaScript expressions, you can even pass objects in JSX. Objects are also denoted with curly braces, like `{ name: \"Hedy Lamarr\", inventions: 5 }`. Therefore, to pass a JS object in JSX, you must wrap the object in another pair of curly braces: `person={{ name: \"Hedy Lamarr\", inventions: 5 }}`.\n\nYou may see this with inline CSS styles in JSX. React does not require you to use inline styles (CSS classes work great for most cases). But when you need an inline style, you pass an object to the `style` attribute:\n\nTry changing the values of `backgroundColor` and `color`.\n\nYou can really see the JavaScript object inside the curly braces when you write it like this:\n\n```\n<ul style={{backgroundColor: 'black',color: 'pink'}}>\n```\n\nThe next time you see `{{` and `}}` in JSX, know that it’s nothing more than an object inside the JSX curlies!\n\n### Pitfall\n\nInline `style` properties are written in camelCase. For example, HTML `<ul style=\"background-color: black\">` would be written as `<ul style={{ backgroundColor: 'black' }}>` in your component.\n\nMore fun with JavaScript objects and curly braces[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#more-fun-with-javascript-objects-and-curly-braces \"Link for More fun with JavaScript objects and curly braces \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou can move several expressions into one object, and reference them in your JSX inside curly braces:\n\nconst person = {\n name: 'Gregorio Y. Zara',\n theme: {\n backgroundColor: 'black',\n color: 'pink'\n }\n};\n\nexport default function TodoList() {\n return (\n <div style\\={person.theme}\\>\n <h1\\>{person.name}'s Todos</h1\\>\n <img\n className\\=\"avatar\"\n src\\=\"https://i.imgur.com/7vQD0fPs.jpg\"\n alt\\=\"Gregorio Y. Zara\"\n />\n <ul\\>\n <li\\>Improve the videophone</li\\>\n <li\\>Prepare aeronautics lectures</li\\>\n <li\\>Work on the alcohol-fuelled engine</li\\>\n </ul\\>\n </div\\>\n );\n}\n\nIn this example, the `person` JavaScript object contains a `name` string and a `theme` object:\n\n```\nconst person = {name: 'Gregorio Y. Zara',theme: {backgroundColor: 'black',color: 'pink'}};\n```\n\nThe component can use these values from `person` like so:\n\n```\n<div style={person.theme}><h1>{person.name}'s Todos</h1>\n```\n\nJSX is very minimal as a templating language because it lets you organize data and logic using JavaScript.\n\nRecap[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#recap \"Link for Recap\")\n-------------------------------------------------------------------------------------------\n\nNow you know almost everything about JSX:\n\n* JSX attributes inside quotes are passed as strings.\n* Curly braces let you bring JavaScript logic and variables into your markup.\n* They work inside the JSX tag content or immediately after `=` in attributes.\n* `{{` and `}}` is not special syntax: it’s a JavaScript object tucked inside JSX curly braces.\n\nTry out some challenges[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#challenges \"Link for Try out some challenges\")\n------------------------------------------------------------------------------------------------------------------------------------\n\n#### Fix the mistake[](https://react.dev/learn/javascript-in-jsx-with-curly-braces#fix-the-mistake \"Link for this heading\")\n\nThis code crashes with an error saying `Objects are not valid as a React child`:\n\nconst person = {\n name: 'Gregorio Y. Zara',\n theme: {\n backgroundColor: 'black',\n color: 'pink'\n }\n};\n\nexport default function TodoList() {\n return (\n <div style\\={person.theme}\\>\n <h1\\>{person}'s Todos</h1\\>\n <img\n className\\=\"avatar\"\n src\\=\"https://i.imgur.com/7vQD0fPs.jpg\"\n alt\\=\"Gregorio Y. Zara\"\n />\n <ul\\>\n <li\\>Improve the videophone</li\\>\n <li\\>Prepare aeronautics lectures</li\\>\n <li\\>Work on the alcohol-fuelled engine</li\\>\n </ul\\>\n </div\\>\n );\n}\n\nCan you find the problem?\n",
"filename": "javascript-in-jsx-with-curly-braces.md",
"package": "react"
} |
{
"content": "Title: Keeping Components Pure – React\n\nURL Source: https://react.dev/learn/keeping-components-pure\n\nMarkdown Content:\nSome JavaScript functions are _pure._ Pure functions only perform a calculation and nothing more. By strictly only writing your components as pure functions, you can avoid an entire class of baffling bugs and unpredictable behavior as your codebase grows. To get these benefits, though, there are a few rules you must follow.\n\n### You will learn\n\n* What purity is and how it helps you avoid bugs\n* How to keep components pure by keeping changes out of the render phase\n* How to use Strict Mode to find mistakes in your components\n\nPurity: Components as formulas[](https://react.dev/learn/keeping-components-pure#purity-components-as-formulas \"Link for Purity: Components as formulas \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn computer science (and especially the world of functional programming), [a pure function](https://wikipedia.org/wiki/Pure_function) is a function with the following characteristics:\n\n* **It minds its own business.** It does not change any objects or variables that existed before it was called.\n* **Same inputs, same output.** Given the same inputs, a pure function should always return the same result.\n\nYou might already be familiar with one example of pure functions: formulas in math.\n\nConsider this math formula: y = 2x.\n\nIf x = 2 then y = 4. Always.\n\nIf x = 3 then y = 6. Always.\n\nIf x = 3, y won’t sometimes be 9 or –1 or 2.5 depending on the time of day or the state of the stock market.\n\nIf y = 2x and x = 3, y will _always_ be 6.\n\nIf we made this into a JavaScript function, it would look like this:\n\n```\nfunction double(number) {return 2 * number;}\n```\n\nIn the above example, `double` is a **pure function.** If you pass it `3`, it will return `6`. Always.\n\nReact is designed around this concept. **React assumes that every component you write is a pure function.** This means that React components you write must always return the same JSX given the same inputs:\n\nfunction Recipe({ drinkers }) {\n return (\n <ol\\> \n <li\\>Boil {drinkers} cups of water.</li\\>\n <li\\>Add {drinkers} spoons of tea and {0.5 \\* drinkers} spoons of spice.</li\\>\n <li\\>Add {0.5 \\* drinkers} cups of milk to boil and sugar to taste.</li\\>\n </ol\\>\n );\n}\n\nexport default function App() {\n return (\n <section\\>\n <h1\\>Spiced Chai Recipe</h1\\>\n <h2\\>For two</h2\\>\n <Recipe drinkers\\={2} />\n <h2\\>For a gathering</h2\\>\n <Recipe drinkers\\={4} />\n </section\\>\n );\n}\n\nWhen you pass `drinkers={2}` to `Recipe`, it will return JSX containing `2 cups of water`. Always.\n\nIf you pass `drinkers={4}`, it will return JSX containing `4 cups of water`. Always.\n\nJust like a math formula.\n\nYou could think of your components as recipes: if you follow them and don’t introduce new ingredients during the cooking process, you will get the same dish every time. That “dish” is the JSX that the component serves to React to [render.](https://react.dev/learn/render-and-commit)\n\n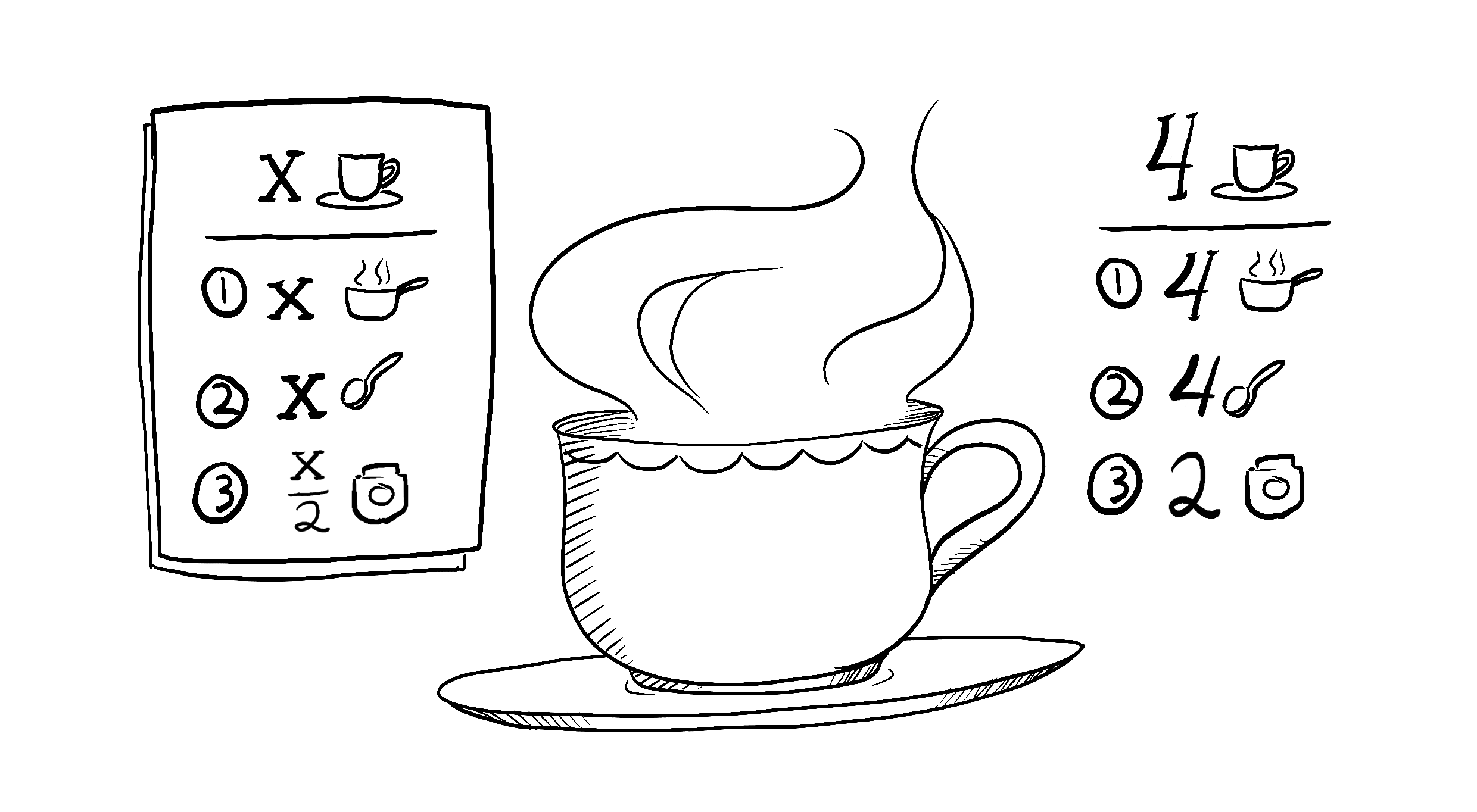\n\nSide Effects: (un)intended consequences[](https://react.dev/learn/keeping-components-pure#side-effects-unintended-consequences \"Link for Side Effects: (un)intended consequences \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nReact’s rendering process must always be pure. Components should only _return_ their JSX, and not _change_ any objects or variables that existed before rendering—that would make them impure!\n\nHere is a component that breaks this rule:\n\nlet guest = 0;\n\nfunction Cup() {\n \n guest = guest + 1;\n return <h2\\>Tea cup for guest #{guest}</h2\\>;\n}\n\nexport default function TeaSet() {\n return (\n <\\>\n <Cup />\n <Cup />\n <Cup />\n </\\>\n );\n}\n\nThis component is reading and writing a `guest` variable declared outside of it. This means that **calling this component multiple times will produce different JSX!** And what’s more, if _other_ components read `guest`, they will produce different JSX, too, depending on when they were rendered! That’s not predictable.\n\nGoing back to our formula y = 2x, now even if x = 2, we cannot trust that y = 4. Our tests could fail, our users would be baffled, planes would fall out of the sky—you can see how this would lead to confusing bugs!\n\nYou can fix this component by [passing `guest` as a prop instead](https://react.dev/learn/passing-props-to-a-component):\n\nNow your component is pure, as the JSX it returns only depends on the `guest` prop.\n\nIn general, you should not expect your components to be rendered in any particular order. It doesn’t matter if you call y = 2x before or after y = 5x: both formulas will resolve independently of each other. In the same way, each component should only “think for itself”, and not attempt to coordinate with or depend upon others during rendering. Rendering is like a school exam: each component should calculate JSX on their own!\n\n##### Deep Dive\n\n#### Detecting impure calculations with StrictMode[](https://react.dev/learn/keeping-components-pure#detecting-impure-calculations-with-strict-mode \"Link for Detecting impure calculations with StrictMode \")\n\nAlthough you might not have used them all yet, in React there are three kinds of inputs that you can read while rendering: [props](https://react.dev/learn/passing-props-to-a-component), [state](https://react.dev/learn/state-a-components-memory), and [context.](https://react.dev/learn/passing-data-deeply-with-context) You should always treat these inputs as read-only.\n\nWhen you want to _change_ something in response to user input, you should [set state](https://react.dev/learn/state-a-components-memory) instead of writing to a variable. You should never change preexisting variables or objects while your component is rendering.\n\nReact offers a “Strict Mode” in which it calls each component’s function twice during development. **By calling the component functions twice, Strict Mode helps find components that break these rules.**\n\nNotice how the original example displayed “Guest #2”, “Guest #4”, and “Guest #6” instead of “Guest #1”, “Guest #2”, and “Guest #3”. The original function was impure, so calling it twice broke it. But the fixed pure version works even if the function is called twice every time. **Pure functions only calculate, so calling them twice won’t change anything**—just like calling `double(2)` twice doesn’t change what’s returned, and solving y = 2x twice doesn’t change what y is. Same inputs, same outputs. Always.\n\nStrict Mode has no effect in production, so it won’t slow down the app for your users. To opt into Strict Mode, you can wrap your root component into `<React.StrictMode>`. Some frameworks do this by default.\n\n### Local mutation: Your component’s little secret[](https://react.dev/learn/keeping-components-pure#local-mutation-your-components-little-secret \"Link for Local mutation: Your component’s little secret \")\n\nIn the above example, the problem was that the component changed a _preexisting_ variable while rendering. This is often called a **“mutation”** to make it sound a bit scarier. Pure functions don’t mutate variables outside of the function’s scope or objects that were created before the call—that makes them impure!\n\nHowever, **it’s completely fine to change variables and objects that you’ve _just_ created while rendering.** In this example, you create an `[]` array, assign it to a `cups` variable, and then `push` a dozen cups into it:\n\nIf the `cups` variable or the `[]` array were created outside the `TeaGathering` function, this would be a huge problem! You would be changing a _preexisting_ object by pushing items into that array.\n\nHowever, it’s fine because you’ve created them _during the same render_, inside `TeaGathering`. No code outside of `TeaGathering` will ever know that this happened. This is called **“local mutation”**—it’s like your component’s little secret.\n\nWhere you _can_ cause side effects[](https://react.dev/learn/keeping-components-pure#where-you-_can_-cause-side-effects \"Link for this heading\")\n------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhile functional programming relies heavily on purity, at some point, somewhere, _something_ has to change. That’s kind of the point of programming! These changes—updating the screen, starting an animation, changing the data—are called **side effects.** They’re things that happen _“on the side”_, not during rendering.\n\nIn React, **side effects usually belong inside [event handlers.](https://react.dev/learn/responding-to-events)** Event handlers are functions that React runs when you perform some action—for example, when you click a button. Even though event handlers are defined _inside_ your component, they don’t run _during_ rendering! **So event handlers don’t need to be pure.**\n\nIf you’ve exhausted all other options and can’t find the right event handler for your side effect, you can still attach it to your returned JSX with a [`useEffect`](https://react.dev/reference/react/useEffect) call in your component. This tells React to execute it later, after rendering, when side effects are allowed. **However, this approach should be your last resort.**\n\nWhen possible, try to express your logic with rendering alone. You’ll be surprised how far this can take you!\n\n##### Deep Dive\n\n#### Why does React care about purity?[](https://react.dev/learn/keeping-components-pure#why-does-react-care-about-purity \"Link for Why does React care about purity? \")\n\nWriting pure functions takes some habit and discipline. But it also unlocks marvelous opportunities:\n\n* Your components could run in a different environment—for example, on the server! Since they return the same result for the same inputs, one component can serve many user requests.\n* You can improve performance by [skipping rendering](https://react.dev/reference/react/memo) components whose inputs have not changed. This is safe because pure functions always return the same results, so they are safe to cache.\n* If some data changes in the middle of rendering a deep component tree, React can restart rendering without wasting time to finish the outdated render. Purity makes it safe to stop calculating at any time.\n\nEvery new React feature we’re building takes advantage of purity. From data fetching to animations to performance, keeping components pure unlocks the power of the React paradigm.\n\nRecap[](https://react.dev/learn/keeping-components-pure#recap \"Link for Recap\")\n-------------------------------------------------------------------------------\n\n* A component must be pure, meaning:\n * **It minds its own business.** It should not change any objects or variables that existed before rendering.\n * **Same inputs, same output.** Given the same inputs, a component should always return the same JSX.\n* Rendering can happen at any time, so components should not depend on each others’ rendering sequence.\n* You should not mutate any of the inputs that your components use for rendering. That includes props, state, and context. To update the screen, [“set” state](https://react.dev/learn/state-a-components-memory) instead of mutating preexisting objects.\n* Strive to express your component’s logic in the JSX you return. When you need to “change things”, you’ll usually want to do it in an event handler. As a last resort, you can `useEffect`.\n* Writing pure functions takes a bit of practice, but it unlocks the power of React’s paradigm.\n\nTry out some challenges[](https://react.dev/learn/keeping-components-pure#challenges \"Link for Try out some challenges\")\n------------------------------------------------------------------------------------------------------------------------\n\n#### Fix a broken clock[](https://react.dev/learn/keeping-components-pure#fix-a-broken-clock \"Link for this heading\")\n\nThis component tries to set the `<h1>`’s CSS class to `\"night\"` during the time from midnight to six hours in the morning, and `\"day\"` at all other times. However, it doesn’t work. Can you fix this component?\n\nYou can verify whether your solution works by temporarily changing the computer’s timezone. When the current time is between midnight and six in the morning, the clock should have inverted colors!\n",
"filename": "keeping-components-pure.md",
"package": "react"
} |
{
"content": "Title: lazy – React\n\nURL Source: https://react.dev/reference/react/lazy\n\nMarkdown Content:\n`lazy` lets you defer loading component’s code until it is rendered for the first time.\n\n```\nconst SomeComponent = lazy(load)\n```\n\n* [Reference](https://react.dev/reference/react/lazy#reference)\n * [`lazy(load)`](https://react.dev/reference/react/lazy#lazy)\n * [`load` function](https://react.dev/reference/react/lazy#load)\n* [Usage](https://react.dev/reference/react/lazy#usage)\n * [Lazy-loading components with Suspense](https://react.dev/reference/react/lazy#suspense-for-code-splitting)\n* [Troubleshooting](https://react.dev/reference/react/lazy#troubleshooting)\n * [My `lazy` component’s state gets reset unexpectedly](https://react.dev/reference/react/lazy#my-lazy-components-state-gets-reset-unexpectedly)\n\n* * *\n\nReference[](https://react.dev/reference/react/lazy#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------\n\n### `lazy(load)`[](https://react.dev/reference/react/lazy#lazy \"Link for this heading\")\n\nCall `lazy` outside your components to declare a lazy-loaded React component:\n\n```\nimport { lazy } from 'react';const MarkdownPreview = lazy(() => import('./MarkdownPreview.js'));\n```\n\n[See more examples below.](https://react.dev/reference/react/lazy#usage)\n\n#### Parameters[](https://react.dev/reference/react/lazy#parameters \"Link for Parameters \")\n\n* `load`: A function that returns a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) or another _thenable_ (a Promise-like object with a `then` method). React will not call `load` until the first time you attempt to render the returned component. After React first calls `load`, it will wait for it to resolve, and then render the resolved value’s `.default` as a React component. Both the returned Promise and the Promise’s resolved value will be cached, so React will not call `load` more than once. If the Promise rejects, React will `throw` the rejection reason for the nearest Error Boundary to handle.\n\n#### Returns[](https://react.dev/reference/react/lazy#returns \"Link for Returns \")\n\n`lazy` returns a React component you can render in your tree. While the code for the lazy component is still loading, attempting to render it will _suspend._ Use [`<Suspense>`](https://react.dev/reference/react/Suspense) to display a loading indicator while it’s loading.\n\n* * *\n\n### `load` function[](https://react.dev/reference/react/lazy#load \"Link for this heading\")\n\n#### Parameters[](https://react.dev/reference/react/lazy#load-parameters \"Link for Parameters \")\n\n`load` receives no parameters.\n\n#### Returns[](https://react.dev/reference/react/lazy#load-returns \"Link for Returns \")\n\nYou need to return a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) or some other _thenable_ (a Promise-like object with a `then` method). It needs to eventually resolve to an object whose `.default` property is a valid React component type, such as a function, [`memo`](https://react.dev/reference/react/memo), or a [`forwardRef`](https://react.dev/reference/react/forwardRef) component.\n\n* * *\n\nUsage[](https://react.dev/reference/react/lazy#usage \"Link for Usage \")\n-----------------------------------------------------------------------\n\n### Lazy-loading components with Suspense[](https://react.dev/reference/react/lazy#suspense-for-code-splitting \"Link for Lazy-loading components with Suspense \")\n\nUsually, you import components with the static [`import`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/import) declaration:\n\n```\nimport MarkdownPreview from './MarkdownPreview.js';\n```\n\nTo defer loading this component’s code until it’s rendered for the first time, replace this import with:\n\n```\nimport { lazy } from 'react';const MarkdownPreview = lazy(() => import('./MarkdownPreview.js'));\n```\n\nThis code relies on [dynamic `import()`,](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/import) which might require support from your bundler or framework. Using this pattern requires that the lazy component you’re importing was exported as the `default` export.\n\nNow that your component’s code loads on demand, you also need to specify what should be displayed while it is loading. You can do this by wrapping the lazy component or any of its parents into a [`<Suspense>`](https://react.dev/reference/react/Suspense) boundary:\n\n```\n<Suspense fallback={<Loading />}><h2>Preview</h2><MarkdownPreview /></Suspense>\n```\n\nIn this example, the code for `MarkdownPreview` won’t be loaded until you attempt to render it. If `MarkdownPreview` hasn’t loaded yet, `Loading` will be shown in its place. Try ticking the checkbox:\n\nThis demo loads with an artificial delay. The next time you untick and tick the checkbox, `Preview` will be cached, so there will be no loading state. To see the loading state again, click “Reset” on the sandbox.\n\n[Learn more about managing loading states with Suspense.](https://react.dev/reference/react/Suspense)\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/lazy#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------\n\n### My `lazy` component’s state gets reset unexpectedly[](https://react.dev/reference/react/lazy#my-lazy-components-state-gets-reset-unexpectedly \"Link for this heading\")\n\nDo not declare `lazy` components _inside_ other components:\n\n```\nimport { lazy } from 'react';function Editor() {// 🔴 Bad: This will cause all state to be reset on re-rendersconst MarkdownPreview = lazy(() => import('./MarkdownPreview.js'));// ...}\n```\n\nInstead, always declare them at the top level of your module:\n\n```\nimport { lazy } from 'react';// ✅ Good: Declare lazy components outside of your componentsconst MarkdownPreview = lazy(() => import('./MarkdownPreview.js'));function Editor() {// ...}\n```\n",
"filename": "lazy.md",
"package": "react"
} |
{
"content": "Title: Quick Start – React\n\nURL Source: https://react.dev/learn\n\nMarkdown Content:\nWelcome to the React documentation! This page will give you an introduction to the 80% of React concepts that you will use on a daily basis.\n\n### You will learn\n\n* How to create and nest components\n* How to add markup and styles\n* How to display data\n* How to render conditions and lists\n* How to respond to events and update the screen\n* How to share data between components\n\nCreating and nesting components[](https://react.dev/learn#components \"Link for Creating and nesting components \")\n-----------------------------------------------------------------------------------------------------------------\n\nReact apps are made out of _components_. A component is a piece of the UI (user interface) that has its own logic and appearance. A component can be as small as a button, or as large as an entire page.\n\nReact components are JavaScript functions that return markup:\n\n```\nfunction MyButton() {return (<button>I'm a button</button>);}\n```\n\nNow that you’ve declared `MyButton`, you can nest it into another component:\n\n```\nexport default function MyApp() {return (<div><h1>Welcome to my app</h1><MyButton /></div>);}\n```\n\nNotice that `<MyButton />` starts with a capital letter. That’s how you know it’s a React component. React component names must always start with a capital letter, while HTML tags must be lowercase.\n\nHave a look at the result:\n\nfunction MyButton() {\n return (\n <button\\>\n I'm a button\n </button\\>\n );\n}\n\nexport default function MyApp() {\n return (\n <div\\>\n <h1\\>Welcome to my app</h1\\>\n <MyButton />\n </div\\>\n );\n}\n\nThe `export default` keywords specify the main component in the file. If you’re not familiar with some piece of JavaScript syntax, [MDN](https://developer.mozilla.org/en-US/docs/web/javascript/reference/statements/export) and [javascript.info](https://javascript.info/import-export) have great references.\n\nWriting markup with JSX[](https://react.dev/learn#writing-markup-with-jsx \"Link for Writing markup with JSX \")\n--------------------------------------------------------------------------------------------------------------\n\nThe markup syntax you’ve seen above is called _JSX_. It is optional, but most React projects use JSX for its convenience. All of the [tools we recommend for local development](https://react.dev/learn/installation) support JSX out of the box.\n\nJSX is stricter than HTML. You have to close tags like `<br />`. Your component also can’t return multiple JSX tags. You have to wrap them into a shared parent, like a `<div>...</div>` or an empty `<>...</>` wrapper:\n\n```\nfunction AboutPage() {return (<><h1>About</h1><p>Hello there.<br />How do you do?</p></>);}\n```\n\nIf you have a lot of HTML to port to JSX, you can use an [online converter.](https://transform.tools/html-to-jsx)\n\nAdding styles[](https://react.dev/learn#adding-styles \"Link for Adding styles \")\n--------------------------------------------------------------------------------\n\nIn React, you specify a CSS class with `className`. It works the same way as the HTML [`class`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/class) attribute:\n\n```\n<img className=\"avatar\" />\n```\n\nThen you write the CSS rules for it in a separate CSS file:\n\n```\n/* In your CSS */.avatar {border-radius: 50%;}\n```\n\nReact does not prescribe how you add CSS files. In the simplest case, you’ll add a [`<link>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link) tag to your HTML. If you use a build tool or a framework, consult its documentation to learn how to add a CSS file to your project.\n\nDisplaying data[](https://react.dev/learn#displaying-data \"Link for Displaying data \")\n--------------------------------------------------------------------------------------\n\nJSX lets you put markup into JavaScript. Curly braces let you “escape back” into JavaScript so that you can embed some variable from your code and display it to the user. For example, this will display `user.name`:\n\n```\nreturn (<h1>{user.name}</h1>);\n```\n\nYou can also “escape into JavaScript” from JSX attributes, but you have to use curly braces _instead of_ quotes. For example, `className=\"avatar\"` passes the `\"avatar\"` string as the CSS class, but `src={user.imageUrl}` reads the JavaScript `user.imageUrl` variable value, and then passes that value as the `src` attribute:\n\n```\nreturn (<imgclassName=\"avatar\"src={user.imageUrl}/>);\n```\n\nYou can put more complex expressions inside the JSX curly braces too, for example, [string concatenation](https://javascript.info/operators#string-concatenation-with-binary):\n\nconst user = {\n name: 'Hedy Lamarr',\n imageUrl: 'https://i.imgur.com/yXOvdOSs.jpg',\n imageSize: 90,\n};\n\nexport default function Profile() {\n return (\n <\\>\n <h1\\>{user.name}</h1\\>\n <img\n className\\=\"avatar\"\n src\\={user.imageUrl}\n alt\\={'Photo of ' + user.name}\n style\\={{\n width: user.imageSize,\n height: user.imageSize\n }}\n />\n </\\>\n );\n}\n\nIn the above example, `style={{}}` is not a special syntax, but a regular `{}` object inside the `style={ }` JSX curly braces. You can use the `style` attribute when your styles depend on JavaScript variables.\n\nConditional rendering[](https://react.dev/learn#conditional-rendering \"Link for Conditional rendering \")\n--------------------------------------------------------------------------------------------------------\n\nIn React, there is no special syntax for writing conditions. Instead, you’ll use the same techniques as you use when writing regular JavaScript code. For example, you can use an [`if`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/if...else) statement to conditionally include JSX:\n\n```\nlet content;if (isLoggedIn) {content = <AdminPanel />;} else {content = <LoginForm />;}return (<div>{content}</div>);\n```\n\nIf you prefer more compact code, you can use the [conditional `?` operator.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator) Unlike `if`, it works inside JSX:\n\n```\n<div>{isLoggedIn ? (<AdminPanel />) : (<LoginForm />)}</div>\n```\n\nWhen you don’t need the `else` branch, you can also use a shorter [logical `&&` syntax](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_AND#short-circuit_evaluation):\n\n```\n<div>{isLoggedIn && <AdminPanel />}</div>\n```\n\nAll of these approaches also work for conditionally specifying attributes. If you’re unfamiliar with some of this JavaScript syntax, you can start by always using `if...else`.\n\nRendering lists[](https://react.dev/learn#rendering-lists \"Link for Rendering lists \")\n--------------------------------------------------------------------------------------\n\nYou will rely on JavaScript features like [`for` loop](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for) and the [array `map()` function](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) to render lists of components.\n\nFor example, let’s say you have an array of products:\n\n```\nconst products = [{ title: 'Cabbage', id: 1 },{ title: 'Garlic', id: 2 },{ title: 'Apple', id: 3 },];\n```\n\nInside your component, use the `map()` function to transform an array of products into an array of `<li>` items:\n\n```\nconst listItems = products.map(product =><li key={product.id}>{product.title}</li>);return (<ul>{listItems}</ul>);\n```\n\nNotice how `<li>` has a `key` attribute. For each item in a list, you should pass a string or a number that uniquely identifies that item among its siblings. Usually, a key should be coming from your data, such as a database ID. React uses your keys to know what happened if you later insert, delete, or reorder the items.\n\nconst products = \\[\n { title: 'Cabbage', isFruit: false, id: 1 },\n { title: 'Garlic', isFruit: false, id: 2 },\n { title: 'Apple', isFruit: true, id: 3 },\n\\];\n\nexport default function ShoppingList() {\n const listItems = products.map(product \\=>\n <li\n key\\={product.id}\n style\\={{\n color: product.isFruit ? 'magenta' : 'darkgreen'\n }}\n \\>\n {product.title}\n </li\\>\n );\n\n return (\n <ul\\>{listItems}</ul\\>\n );\n}\n\nResponding to events[](https://react.dev/learn#responding-to-events \"Link for Responding to events \")\n-----------------------------------------------------------------------------------------------------\n\nYou can respond to events by declaring _event handler_ functions inside your components:\n\n```\nfunction MyButton() {function handleClick() {alert('You clicked me!');}return (<button onClick={handleClick}> Click me</button>);}\n```\n\nNotice how `onClick={handleClick}` has no parentheses at the end! Do not _call_ the event handler function: you only need to _pass it down_. React will call your event handler when the user clicks the button.\n\nUpdating the screen[](https://react.dev/learn#updating-the-screen \"Link for Updating the screen \")\n--------------------------------------------------------------------------------------------------\n\nOften, you’ll want your component to “remember” some information and display it. For example, maybe you want to count the number of times a button is clicked. To do this, add _state_ to your component.\n\nFirst, import [`useState`](https://react.dev/reference/react/useState) from React:\n\n```\nimport { useState } from 'react';\n```\n\nNow you can declare a _state variable_ inside your component:\n\n```\nfunction MyButton() {const [count, setCount] = useState(0);// ...\n```\n\nYou’ll get two things from `useState`: the current state (`count`), and the function that lets you update it (`setCount`). You can give them any names, but the convention is to write `[something, setSomething]`.\n\nThe first time the button is displayed, `count` will be `0` because you passed `0` to `useState()`. When you want to change state, call `setCount()` and pass the new value to it. Clicking this button will increment the counter:\n\n```\nfunction MyButton() {const [count, setCount] = useState(0);function handleClick() {setCount(count + 1);}return (<button onClick={handleClick}> Clicked {count} times</button>);}\n```\n\nReact will call your component function again. This time, `count` will be `1`. Then it will be `2`. And so on.\n\nIf you render the same component multiple times, each will get its own state. Click each button separately:\n\nimport { useState } from 'react';\n\nexport default function MyApp() {\n return (\n <div\\>\n <h1\\>Counters that update separately</h1\\>\n <MyButton />\n <MyButton />\n </div\\>\n );\n}\n\nfunction MyButton() {\n const \\[count, setCount\\] = useState(0);\n\n function handleClick() {\n setCount(count + 1);\n }\n\n return (\n <button onClick\\={handleClick}\\>\n Clicked {count} times\n </button\\>\n );\n}\n\nNotice how each button “remembers” its own `count` state and doesn’t affect other buttons.\n\nUsing Hooks[](https://react.dev/learn#using-hooks \"Link for Using Hooks \")\n--------------------------------------------------------------------------\n\nFunctions starting with `use` are called _Hooks_. `useState` is a built-in Hook provided by React. You can find other built-in Hooks in the [API reference.](https://react.dev/reference/react) You can also write your own Hooks by combining the existing ones.\n\nHooks are more restrictive than other functions. You can only call Hooks _at the top_ of your components (or other Hooks). If you want to use `useState` in a condition or a loop, extract a new component and put it there.\n\nSharing data between components[](https://react.dev/learn#sharing-data-between-components \"Link for Sharing data between components \")\n--------------------------------------------------------------------------------------------------------------------------------------\n\nIn the previous example, each `MyButton` had its own independent `count`, and when each button was clicked, only the `count` for the button clicked changed:\n\n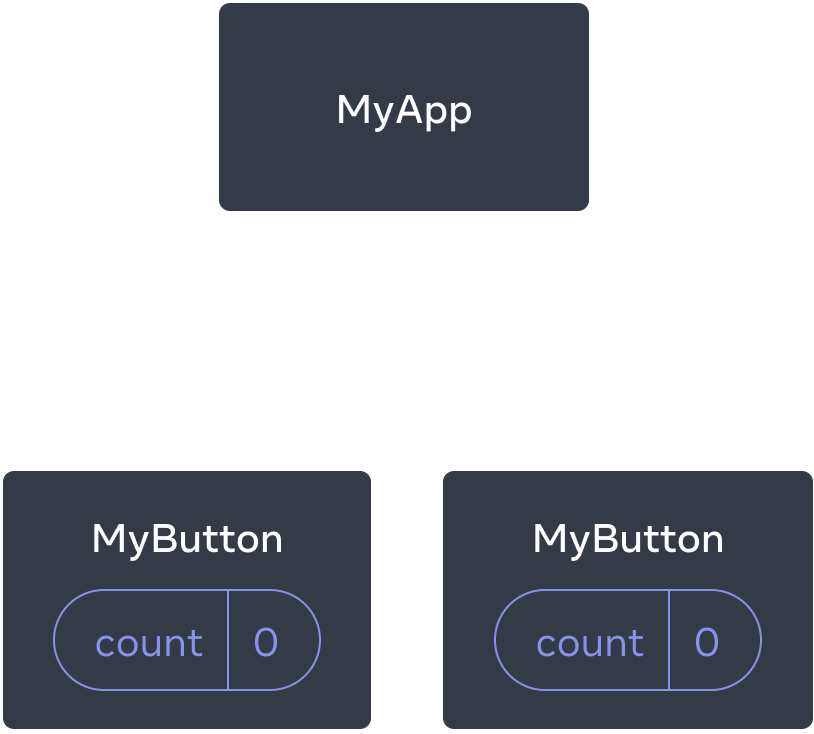\n\n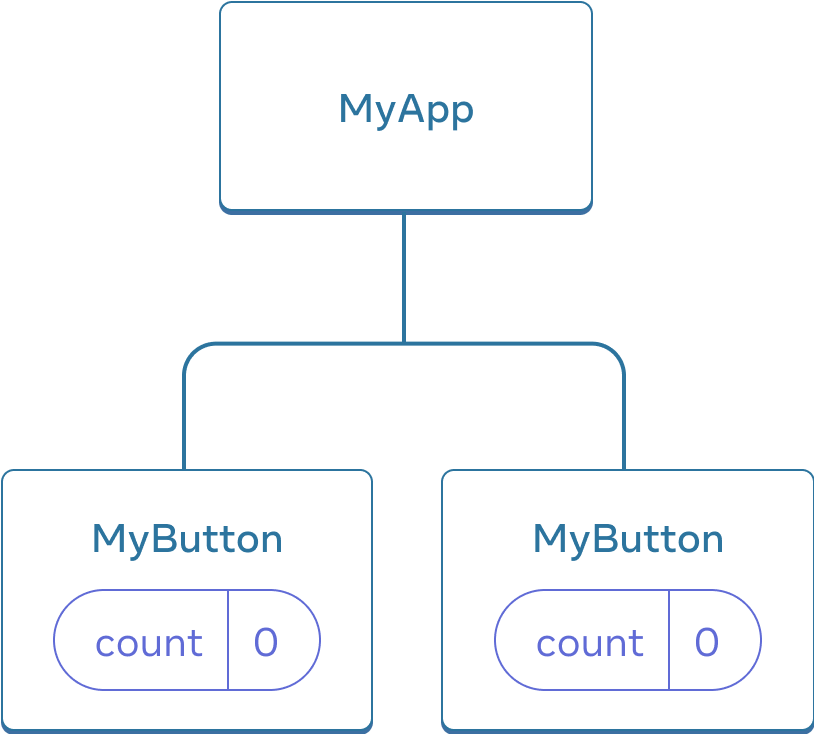\n\nInitially, each `MyButton`’s `count` state is `0`\n\n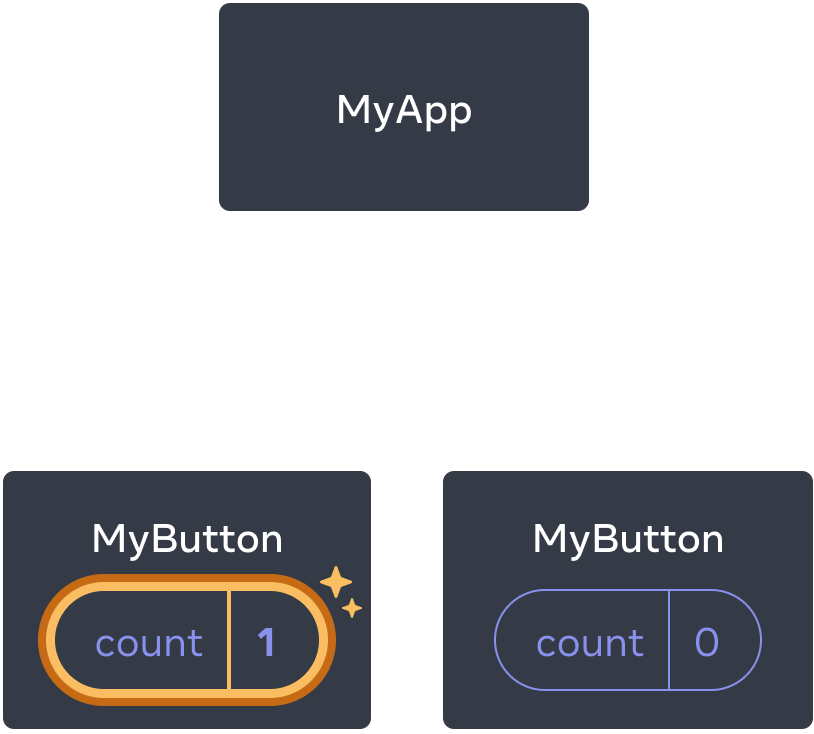\n\n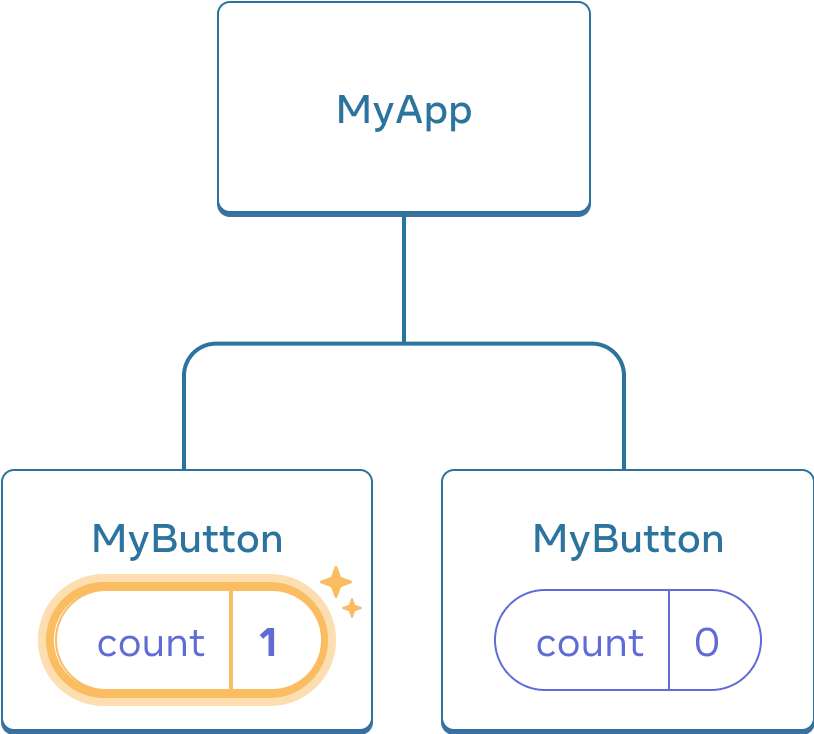\n\nThe first `MyButton` updates its `count` to `1`\n\nHowever, often you’ll need components to _share data and always update together_.\n\nTo make both `MyButton` components display the same `count` and update together, you need to move the state from the individual buttons “upwards” to the closest component containing all of them.\n\nIn this example, it is `MyApp`:\n\n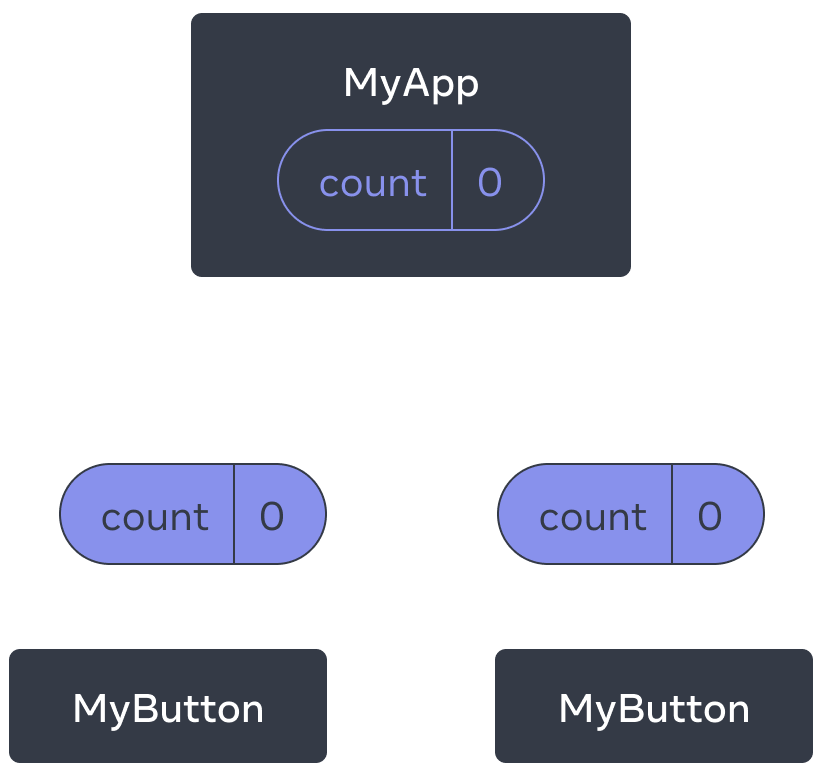\n\n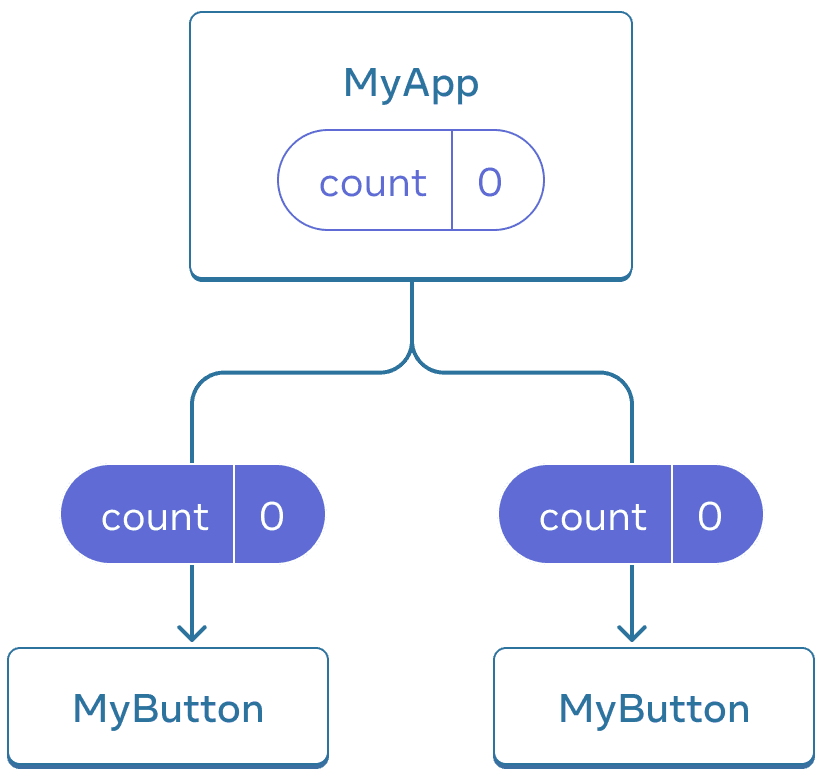\n\nInitially, `MyApp`’s `count` state is `0` and is passed down to both children\n\n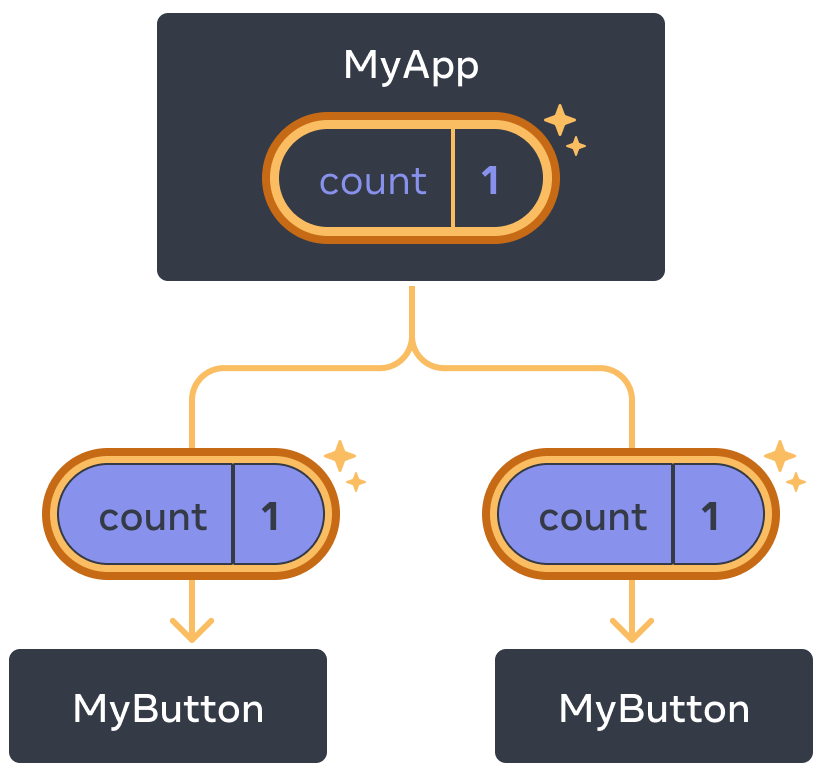\n\n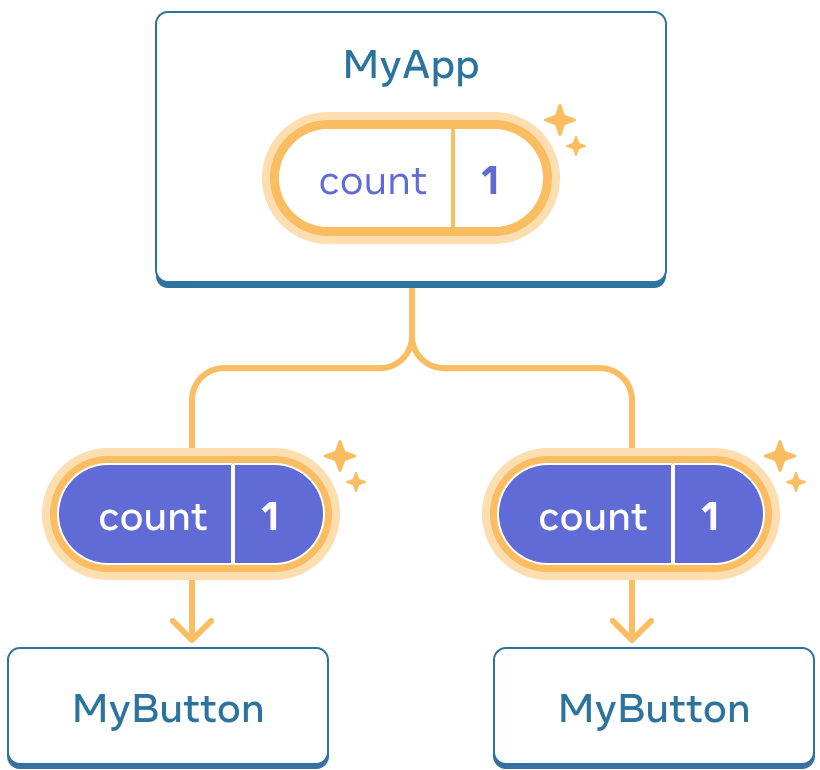\n\nOn click, `MyApp` updates its `count` state to `1` and passes it down to both children\n\nNow when you click either button, the `count` in `MyApp` will change, which will change both of the counts in `MyButton`. Here’s how you can express this in code.\n\nFirst, _move the state up_ from `MyButton` into `MyApp`:\n\n```\nexport default function MyApp() {const [count, setCount] = useState(0);function handleClick() {setCount(count + 1);}return (<div><h1>Counters that update separately</h1><MyButton /><MyButton /></div>);}function MyButton() {// ... we're moving code from here ...}\n```\n\nThen, _pass the state down_ from `MyApp` to each `MyButton`, together with the shared click handler. You can pass information to `MyButton` using the JSX curly braces, just like you previously did with built-in tags like `<img>`:\n\n```\nexport default function MyApp() {const [count, setCount] = useState(0);function handleClick() {setCount(count + 1);}return (<div><h1>Counters that update together</h1><MyButton count={count} onClick={handleClick} /><MyButton count={count} onClick={handleClick} /></div>);}\n```\n\nThe information you pass down like this is called _props_. Now the `MyApp` component contains the `count` state and the `handleClick` event handler, and _passes both of them down as props_ to each of the buttons.\n\nFinally, change `MyButton` to _read_ the props you have passed from its parent component:\n\n```\nfunction MyButton({ count, onClick }) {return (<button onClick={onClick}> Clicked {count} times</button>);}\n```\n\nWhen you click the button, the `onClick` handler fires. Each button’s `onClick` prop was set to the `handleClick` function inside `MyApp`, so the code inside of it runs. That code calls `setCount(count + 1)`, incrementing the `count` state variable. The new `count` value is passed as a prop to each button, so they all show the new value. This is called “lifting state up”. By moving state up, you’ve shared it between components.\n\nimport { useState } from 'react';\n\nexport default function MyApp() {\n const \\[count, setCount\\] = useState(0);\n\n function handleClick() {\n setCount(count + 1);\n }\n\n return (\n <div\\>\n <h1\\>Counters that update together</h1\\>\n <MyButton count\\={count} onClick\\={handleClick} />\n <MyButton count\\={count} onClick\\={handleClick} />\n </div\\>\n );\n}\n\nfunction MyButton({ count, onClick }) {\n return (\n <button onClick\\={onClick}\\>\n Clicked {count} times\n </button\\>\n );\n}\n\nNext Steps[](https://react.dev/learn#next-steps \"Link for Next Steps \")\n-----------------------------------------------------------------------\n\nBy now, you know the basics of how to write React code!\n\nCheck out the [Tutorial](https://react.dev/learn/tutorial-tic-tac-toe) to put them into practice and build your first mini-app with React.\n",
"filename": "learn.md",
"package": "react"
} |
{
"content": "Title: Lifecycle of Reactive Effects – React\n\nURL Source: https://react.dev/learn/lifecycle-of-reactive-effects\n\nMarkdown Content:\nEffects have a different lifecycle from components. Components may mount, update, or unmount. An Effect can only do two things: to start synchronizing something, and later to stop synchronizing it. This cycle can happen multiple times if your Effect depends on props and state that change over time. React provides a linter rule to check that you’ve specified your Effect’s dependencies correctly. This keeps your Effect synchronized to the latest props and state.\n\n### You will learn\n\n* How an Effect’s lifecycle is different from a component’s lifecycle\n* How to think about each individual Effect in isolation\n* When your Effect needs to re-synchronize, and why\n* How your Effect’s dependencies are determined\n* What it means for a value to be reactive\n* What an empty dependency array means\n* How React verifies your dependencies are correct with a linter\n* What to do when you disagree with the linter\n\nThe lifecycle of an Effect[](https://react.dev/learn/lifecycle-of-reactive-effects#the-lifecycle-of-an-effect \"Link for The lifecycle of an Effect \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------\n\nEvery React component goes through the same lifecycle:\n\n* A component _mounts_ when it’s added to the screen.\n* A component _updates_ when it receives new props or state, usually in response to an interaction.\n* A component _unmounts_ when it’s removed from the screen.\n\n**It’s a good way to think about components, but _not_ about Effects.** Instead, try to think about each Effect independently from your component’s lifecycle. An Effect describes how to [synchronize an external system](https://react.dev/learn/synchronizing-with-effects) to the current props and state. As your code changes, synchronization will need to happen more or less often.\n\nTo illustrate this point, consider this Effect connecting your component to a chat server:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [roomId]);// ...}\n```\n\nYour Effect’s body specifies how to **start synchronizing:**\n\n```\n// ...const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};// ...\n```\n\nThe cleanup function returned by your Effect specifies how to **stop synchronizing:**\n\n```\n// ...const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};// ...\n```\n\nIntuitively, you might think that React would **start synchronizing** when your component mounts and **stop synchronizing** when your component unmounts. However, this is not the end of the story! Sometimes, it may also be necessary to **start and stop synchronizing multiple times** while the component remains mounted.\n\nLet’s look at _why_ this is necessary, _when_ it happens, and _how_ you can control this behavior.\n\n### Note\n\nSome Effects don’t return a cleanup function at all. [More often than not,](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development) you’ll want to return one—but if you don’t, React will behave as if you returned an empty cleanup function.\n\n### Why synchronization may need to happen more than once[](https://react.dev/learn/lifecycle-of-reactive-effects#why-synchronization-may-need-to-happen-more-than-once \"Link for Why synchronization may need to happen more than once \")\n\nImagine this `ChatRoom` component receives a `roomId` prop that the user picks in a dropdown. Let’s say that initially the user picks the `\"general\"` room as the `roomId`. Your app displays the `\"general\"` chat room:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId /* \"general\" */ }) {// ...return <h1>Welcome to the {roomId} room!</h1>;}\n```\n\nAfter the UI is displayed, React will run your Effect to **start synchronizing.** It connects to the `\"general\"` room:\n\n```\nfunction ChatRoom({ roomId /* \"general\" */ }) {useEffect(() => {const connection = createConnection(serverUrl, roomId); // Connects to the \"general\" roomconnection.connect();return () => {connection.disconnect(); // Disconnects from the \"general\" room};}, [roomId]);// ...\n```\n\nSo far, so good.\n\nLater, the user picks a different room in the dropdown (for example, `\"travel\"`). First, React will update the UI:\n\n```\nfunction ChatRoom({ roomId /* \"travel\" */ }) {// ...return <h1>Welcome to the {roomId} room!</h1>;}\n```\n\nThink about what should happen next. The user sees that `\"travel\"` is the selected chat room in the UI. However, the Effect that ran the last time is still connected to the `\"general\"` room. **The `roomId` prop has changed, so what your Effect did back then (connecting to the `\"general\"` room) no longer matches the UI.**\n\nAt this point, you want React to do two things:\n\n1. Stop synchronizing with the old `roomId` (disconnect from the `\"general\"` room)\n2. Start synchronizing with the new `roomId` (connect to the `\"travel\"` room)\n\n**Luckily, you’ve already taught React how to do both of these things!** Your Effect’s body specifies how to start synchronizing, and your cleanup function specifies how to stop synchronizing. All that React needs to do now is to call them in the correct order and with the correct props and state. Let’s see how exactly that happens.\n\n### How React re-synchronizes your Effect[](https://react.dev/learn/lifecycle-of-reactive-effects#how-react-re-synchronizes-your-effect \"Link for How React re-synchronizes your Effect \")\n\nRecall that your `ChatRoom` component has received a new value for its `roomId` prop. It used to be `\"general\"`, and now it is `\"travel\"`. React needs to re-synchronize your Effect to re-connect you to a different room.\n\nTo **stop synchronizing,** React will call the cleanup function that your Effect returned after connecting to the `\"general\"` room. Since `roomId` was `\"general\"`, the cleanup function disconnects from the `\"general\"` room:\n\n```\nfunction ChatRoom({ roomId /* \"general\" */ }) {useEffect(() => {const connection = createConnection(serverUrl, roomId); // Connects to the \"general\" roomconnection.connect();return () => {connection.disconnect(); // Disconnects from the \"general\" room};// ...\n```\n\nThen React will run the Effect that you’ve provided during this render. This time, `roomId` is `\"travel\"` so it will **start synchronizing** to the `\"travel\"` chat room (until its cleanup function is eventually called too):\n\n```\nfunction ChatRoom({ roomId /* \"travel\" */ }) {useEffect(() => {const connection = createConnection(serverUrl, roomId); // Connects to the \"travel\" roomconnection.connect();// ...\n```\n\nThanks to this, you’re now connected to the same room that the user chose in the UI. Disaster averted!\n\nEvery time after your component re-renders with a different `roomId`, your Effect will re-synchronize. For example, let’s say the user changes `roomId` from `\"travel\"` to `\"music\"`. React will again **stop synchronizing** your Effect by calling its cleanup function (disconnecting you from the `\"travel\"` room). Then it will **start synchronizing** again by running its body with the new `roomId` prop (connecting you to the `\"music\"` room).\n\nFinally, when the user goes to a different screen, `ChatRoom` unmounts. Now there is no need to stay connected at all. React will **stop synchronizing** your Effect one last time and disconnect you from the `\"music\"` chat room.\n\n### Thinking from the Effect’s perspective[](https://react.dev/learn/lifecycle-of-reactive-effects#thinking-from-the-effects-perspective \"Link for Thinking from the Effect’s perspective \")\n\nLet’s recap everything that’s happened from the `ChatRoom` component’s perspective:\n\n1. `ChatRoom` mounted with `roomId` set to `\"general\"`\n2. `ChatRoom` updated with `roomId` set to `\"travel\"`\n3. `ChatRoom` updated with `roomId` set to `\"music\"`\n4. `ChatRoom` unmounted\n\nDuring each of these points in the component’s lifecycle, your Effect did different things:\n\n1. Your Effect connected to the `\"general\"` room\n2. Your Effect disconnected from the `\"general\"` room and connected to the `\"travel\"` room\n3. Your Effect disconnected from the `\"travel\"` room and connected to the `\"music\"` room\n4. Your Effect disconnected from the `\"music\"` room\n\nNow let’s think about what happened from the perspective of the Effect itself:\n\n```\nuseEffect(() => {// Your Effect connected to the room specified with roomId...const connection = createConnection(serverUrl, roomId);connection.connect();return () => {// ...until it disconnectedconnection.disconnect();};}, [roomId]);\n```\n\nThis code’s structure might inspire you to see what happened as a sequence of non-overlapping time periods:\n\n1. Your Effect connected to the `\"general\"` room (until it disconnected)\n2. Your Effect connected to the `\"travel\"` room (until it disconnected)\n3. Your Effect connected to the `\"music\"` room (until it disconnected)\n\nPreviously, you were thinking from the component’s perspective. When you looked from the component’s perspective, it was tempting to think of Effects as “callbacks” or “lifecycle events” that fire at a specific time like “after a render” or “before unmount”. This way of thinking gets complicated very fast, so it’s best to avoid.\n\n**Instead, always focus on a single start/stop cycle at a time. It shouldn’t matter whether a component is mounting, updating, or unmounting. All you need to do is to describe how to start synchronization and how to stop it. If you do it well, your Effect will be resilient to being started and stopped as many times as it’s needed.**\n\nThis might remind you how you don’t think whether a component is mounting or updating when you write the rendering logic that creates JSX. You describe what should be on the screen, and React [figures out the rest.](https://react.dev/learn/reacting-to-input-with-state)\n\n### How React verifies that your Effect can re-synchronize[](https://react.dev/learn/lifecycle-of-reactive-effects#how-react-verifies-that-your-effect-can-re-synchronize \"Link for How React verifies that your Effect can re-synchronize \")\n\nHere is a live example that you can play with. Press “Open chat” to mount the `ChatRoom` component:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId\\]);\n return <h1\\>Welcome to the {roomId} room!</h1\\>;\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Close chat' : 'Open chat'}\n </button\\>\n {show && <hr />}\n {show && <ChatRoom roomId\\={roomId} />}\n </\\>\n );\n}\n\nNotice that when the component mounts for the first time, you see three logs:\n\n1. `✅ Connecting to \"general\" room at https://localhost:1234...` _(development-only)_\n2. `❌ Disconnected from \"general\" room at https://localhost:1234.` _(development-only)_\n3. `✅ Connecting to \"general\" room at https://localhost:1234...`\n\nThe first two logs are development-only. In development, React always remounts each component once.\n\n**React verifies that your Effect can re-synchronize by forcing it to do that immediately in development.** This might remind you of opening a door and closing it an extra time to check if the door lock works. React starts and stops your Effect one extra time in development to check [you’ve implemented its cleanup well.](https://react.dev/learn/synchronizing-with-effects#how-to-handle-the-effect-firing-twice-in-development)\n\nThe main reason your Effect will re-synchronize in practice is if some data it uses has changed. In the sandbox above, change the selected chat room. Notice how, when the `roomId` changes, your Effect re-synchronizes.\n\nHowever, there are also more unusual cases in which re-synchronization is necessary. For example, try editing the `serverUrl` in the sandbox above while the chat is open. Notice how the Effect re-synchronizes in response to your edits to the code. In the future, React may add more features that rely on re-synchronization.\n\n### How React knows that it needs to re-synchronize the Effect[](https://react.dev/learn/lifecycle-of-reactive-effects#how-react-knows-that-it-needs-to-re-synchronize-the-effect \"Link for How React knows that it needs to re-synchronize the Effect \")\n\nYou might be wondering how React knew that your Effect needed to re-synchronize after `roomId` changes. It’s because _you told React_ that its code depends on `roomId` by including it in the [list of dependencies:](https://react.dev/learn/synchronizing-with-effects#step-2-specify-the-effect-dependencies)\n\n```\nfunction ChatRoom({ roomId }) { // The roomId prop may change over timeuseEffect(() => {const connection = createConnection(serverUrl, roomId); // This Effect reads roomId connection.connect();return () => {connection.disconnect();};}, [roomId]); // So you tell React that this Effect \"depends on\" roomId// ...\n```\n\nHere’s how this works:\n\n1. You knew `roomId` is a prop, which means it can change over time.\n2. You knew that your Effect reads `roomId` (so its logic depends on a value that may change later).\n3. This is why you specified it as your Effect’s dependency (so that it re-synchronizes when `roomId` changes).\n\nEvery time after your component re-renders, React will look at the array of dependencies that you have passed. If any of the values in the array is different from the value at the same spot that you passed during the previous render, React will re-synchronize your Effect.\n\nFor example, if you passed `[\"general\"]` during the initial render, and later you passed `[\"travel\"]` during the next render, React will compare `\"general\"` and `\"travel\"`. These are different values (compared with [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)), so React will re-synchronize your Effect. On the other hand, if your component re-renders but `roomId` has not changed, your Effect will remain connected to the same room.\n\n### Each Effect represents a separate synchronization process[](https://react.dev/learn/lifecycle-of-reactive-effects#each-effect-represents-a-separate-synchronization-process \"Link for Each Effect represents a separate synchronization process \")\n\nResist adding unrelated logic to your Effect only because this logic needs to run at the same time as an Effect you already wrote. For example, let’s say you want to send an analytics event when the user visits the room. You already have an Effect that depends on `roomId`, so you might feel tempted to add the analytics call there:\n\n```\nfunction ChatRoom({ roomId }) {useEffect(() => {logVisit(roomId);const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [roomId]);// ...}\n```\n\nBut imagine you later add another dependency to this Effect that needs to re-establish the connection. If this Effect re-synchronizes, it will also call `logVisit(roomId)` for the same room, which you did not intend. Logging the visit **is a separate process** from connecting. Write them as two separate Effects:\n\n```\nfunction ChatRoom({ roomId }) {useEffect(() => {logVisit(roomId);}, [roomId]);useEffect(() => {const connection = createConnection(serverUrl, roomId);// ...}, [roomId]);// ...}\n```\n\n**Each Effect in your code should represent a separate and independent synchronization process.**\n\nIn the above example, deleting one Effect wouldn’t break the other Effect’s logic. This is a good indication that they synchronize different things, and so it made sense to split them up. On the other hand, if you split up a cohesive piece of logic into separate Effects, the code may look “cleaner” but will be [more difficult to maintain.](https://react.dev/learn/you-might-not-need-an-effect#chains-of-computations) This is why you should think whether the processes are same or separate, not whether the code looks cleaner.\n\nEffects “react” to reactive values[](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values \"Link for Effects “react” to reactive values \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYour Effect reads two variables (`serverUrl` and `roomId`), but you only specified `roomId` as a dependency:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [roomId]);// ...}\n```\n\nWhy doesn’t `serverUrl` need to be a dependency?\n\nThis is because the `serverUrl` never changes due to a re-render. It’s always the same no matter how many times the component re-renders and why. Since `serverUrl` never changes, it wouldn’t make sense to specify it as a dependency. After all, dependencies only do something when they change over time!\n\nOn the other hand, `roomId` may be different on a re-render. **Props, state, and other values declared inside the component are _reactive_ because they’re calculated during rendering and participate in the React data flow.**\n\nIf `serverUrl` was a state variable, it would be reactive. Reactive values must be included in dependencies:\n\n```\nfunction ChatRoom({ roomId }) { // Props change over timeconst [serverUrl, setServerUrl] = useState('https://localhost:1234'); // State may change over timeuseEffect(() => {const connection = createConnection(serverUrl, roomId); // Your Effect reads props and stateconnection.connect();return () => {connection.disconnect();};}, [roomId, serverUrl]); // So you tell React that this Effect \"depends on\" on props and state// ...}\n```\n\nBy including `serverUrl` as a dependency, you ensure that the Effect re-synchronizes after it changes.\n\nTry changing the selected chat room or edit the server URL in this sandbox:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nfunction ChatRoom({ roomId }) {\n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234');\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[roomId, serverUrl\\]);\n\n return (\n <\\>\n <label\\>\n Server URL:{' '}\n <input\n value\\={serverUrl}\n onChange\\={e \\=> setServerUrl(e.target.value)}\n />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nWhenever you change a reactive value like `roomId` or `serverUrl`, the Effect re-connects to the chat server.\n\n### What an Effect with empty dependencies means[](https://react.dev/learn/lifecycle-of-reactive-effects#what-an-effect-with-empty-dependencies-means \"Link for What an Effect with empty dependencies means \")\n\nWhat happens if you move both `serverUrl` and `roomId` outside the component?\n\n```\nconst serverUrl = 'https://localhost:1234';const roomId = 'general';function ChatRoom() {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, []); // ✅ All dependencies declared// ...}\n```\n\nNow your Effect’s code does not use _any_ reactive values, so its dependencies can be empty (`[]`).\n\nThinking from the component’s perspective, the empty `[]` dependency array means this Effect connects to the chat room only when the component mounts, and disconnects only when the component unmounts. (Keep in mind that React would still [re-synchronize it an extra time](https://react.dev/learn/lifecycle-of-reactive-effects#how-react-verifies-that-your-effect-can-re-synchronize) in development to stress-test your logic.)\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\nconst roomId = 'general';\n\nfunction ChatRoom() {\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[\\]);\n return <h1\\>Welcome to the {roomId} room!</h1\\>;\n}\n\nexport default function App() {\n const \\[show, setShow\\] = useState(false);\n return (\n <\\>\n <button onClick\\={() \\=> setShow(!show)}\\>\n {show ? 'Close chat' : 'Open chat'}\n </button\\>\n {show && <hr />}\n {show && <ChatRoom />}\n </\\>\n );\n}\n\nHowever, if you [think from the Effect’s perspective,](https://react.dev/learn/lifecycle-of-reactive-effects#thinking-from-the-effects-perspective) you don’t need to think about mounting and unmounting at all. What’s important is you’ve specified what your Effect does to start and stop synchronizing. Today, it has no reactive dependencies. But if you ever want the user to change `roomId` or `serverUrl` over time (and they would become reactive), your Effect’s code won’t change. You will only need to add them to the dependencies.\n\n### All variables declared in the component body are reactive[](https://react.dev/learn/lifecycle-of-reactive-effects#all-variables-declared-in-the-component-body-are-reactive \"Link for All variables declared in the component body are reactive \")\n\nProps and state aren’t the only reactive values. Values that you calculate from them are also reactive. If the props or state change, your component will re-render, and the values calculated from them will also change. This is why all variables from the component body used by the Effect should be in the Effect dependency list.\n\nLet’s say that the user can pick a chat server in the dropdown, but they can also configure a default server in settings. Suppose you’ve already put the settings state in a [context](https://react.dev/learn/scaling-up-with-reducer-and-context) so you read the `settings` from that context. Now you calculate the `serverUrl` based on the selected server from props and the default server:\n\n```\nfunction ChatRoom({ roomId, selectedServerUrl }) { // roomId is reactiveconst settings = useContext(SettingsContext); // settings is reactiveconst serverUrl = selectedServerUrl ?? settings.defaultServerUrl; // serverUrl is reactiveuseEffect(() => {const connection = createConnection(serverUrl, roomId); // Your Effect reads roomId and serverUrlconnection.connect();return () => {connection.disconnect();};}, [roomId, serverUrl]); // So it needs to re-synchronize when either of them changes!// ...}\n```\n\nIn this example, `serverUrl` is not a prop or a state variable. It’s a regular variable that you calculate during rendering. But it’s calculated during rendering, so it can change due to a re-render. This is why it’s reactive.\n\n**All values inside the component (including props, state, and variables in your component’s body) are reactive. Any reactive value can change on a re-render, so you need to include reactive values as Effect’s dependencies.**\n\nIn other words, Effects “react” to all values from the component body.\n\n##### Deep Dive\n\n#### Can global or mutable values be dependencies?[](https://react.dev/learn/lifecycle-of-reactive-effects#can-global-or-mutable-values-be-dependencies \"Link for Can global or mutable values be dependencies? \")\n\nMutable values (including global variables) aren’t reactive.\n\n**A mutable value like [`location.pathname`](https://developer.mozilla.org/en-US/docs/Web/API/Location/pathname) can’t be a dependency.** It’s mutable, so it can change at any time completely outside of the React rendering data flow. Changing it wouldn’t trigger a re-render of your component. Therefore, even if you specified it in the dependencies, React _wouldn’t know_ to re-synchronize the Effect when it changes. This also breaks the rules of React because reading mutable data during rendering (which is when you calculate the dependencies) breaks [purity of rendering.](https://react.dev/learn/keeping-components-pure) Instead, you should read and subscribe to an external mutable value with [`useSyncExternalStore`.](https://react.dev/learn/you-might-not-need-an-effect#subscribing-to-an-external-store)\n\n**A mutable value like [`ref.current`](https://react.dev/reference/react/useRef#reference) or things you read from it also can’t be a dependency.** The ref object returned by `useRef` itself can be a dependency, but its `current` property is intentionally mutable. It lets you [keep track of something without triggering a re-render.](https://react.dev/learn/referencing-values-with-refs) But since changing it doesn’t trigger a re-render, it’s not a reactive value, and React won’t know to re-run your Effect when it changes.\n\nAs you’ll learn below on this page, a linter will check for these issues automatically.\n\n### React verifies that you specified every reactive value as a dependency[](https://react.dev/learn/lifecycle-of-reactive-effects#react-verifies-that-you-specified-every-reactive-value-as-a-dependency \"Link for React verifies that you specified every reactive value as a dependency \")\n\nIf your linter is [configured for React,](https://react.dev/learn/editor-setup#linting) it will check that every reactive value used by your Effect’s code is declared as its dependency. For example, this is a lint error because both `roomId` and `serverUrl` are reactive:\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nfunction ChatRoom({ roomId }) { \n const \\[serverUrl, setServerUrl\\] = useState('https://localhost:1234'); \n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n }, \\[\\]); \n\n return (\n <\\>\n <label\\>\n Server URL:{' '}\n <input\n value\\={serverUrl}\n onChange\\={e \\=> setServerUrl(e.target.value)}\n />\n </label\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n\nThis may look like a React error, but really React is pointing out a bug in your code. Both `roomId` and `serverUrl` may change over time, but you’re forgetting to re-synchronize your Effect when they change. You will remain connected to the initial `roomId` and `serverUrl` even after the user picks different values in the UI.\n\nTo fix the bug, follow the linter’s suggestion to specify `roomId` and `serverUrl` as dependencies of your Effect:\n\n```\nfunction ChatRoom({ roomId }) { // roomId is reactiveconst [serverUrl, setServerUrl] = useState('https://localhost:1234'); // serverUrl is reactiveuseEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, [serverUrl, roomId]); // ✅ All dependencies declared// ...}\n```\n\nTry this fix in the sandbox above. Verify that the linter error is gone, and the chat re-connects when needed.\n\n### Note\n\nIn some cases, React _knows_ that a value never changes even though it’s declared inside the component. For example, the [`set` function](https://react.dev/reference/react/useState#setstate) returned from `useState` and the ref object returned by [`useRef`](https://react.dev/reference/react/useRef) are _stable_—they are guaranteed to not change on a re-render. Stable values aren’t reactive, so you may omit them from the list. Including them is allowed: they won’t change, so it doesn’t matter.\n\n### What to do when you don’t want to re-synchronize[](https://react.dev/learn/lifecycle-of-reactive-effects#what-to-do-when-you-dont-want-to-re-synchronize \"Link for What to do when you don’t want to re-synchronize \")\n\nIn the previous example, you’ve fixed the lint error by listing `roomId` and `serverUrl` as dependencies.\n\n**However, you could instead “prove” to the linter that these values aren’t reactive values,** i.e. that they _can’t_ change as a result of a re-render. For example, if `serverUrl` and `roomId` don’t depend on rendering and always have the same values, you can move them outside the component. Now they don’t need to be dependencies:\n\n```\nconst serverUrl = 'https://localhost:1234'; // serverUrl is not reactiveconst roomId = 'general'; // roomId is not reactivefunction ChatRoom() {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, []); // ✅ All dependencies declared// ...}\n```\n\nYou can also move them _inside the Effect._ They aren’t calculated during rendering, so they’re not reactive:\n\n```\nfunction ChatRoom() {useEffect(() => {const serverUrl = 'https://localhost:1234'; // serverUrl is not reactiveconst roomId = 'general'; // roomId is not reactiveconst connection = createConnection(serverUrl, roomId);connection.connect();return () => {connection.disconnect();};}, []); // ✅ All dependencies declared// ...}\n```\n\n**Effects are reactive blocks of code.** They re-synchronize when the values you read inside of them change. Unlike event handlers, which only run once per interaction, Effects run whenever synchronization is necessary.\n\n**You can’t “choose” your dependencies.** Your dependencies must include every [reactive value](https://react.dev/learn/lifecycle-of-reactive-effects#all-variables-declared-in-the-component-body-are-reactive) you read in the Effect. The linter enforces this. Sometimes this may lead to problems like infinite loops and to your Effect re-synchronizing too often. Don’t fix these problems by suppressing the linter! Here’s what to try instead:\n\n* **Check that your Effect represents an independent synchronization process.** If your Effect doesn’t synchronize anything, [it might be unnecessary.](https://react.dev/learn/you-might-not-need-an-effect) If it synchronizes several independent things, [split it up.](https://react.dev/learn/lifecycle-of-reactive-effects#each-effect-represents-a-separate-synchronization-process)\n \n* **If you want to read the latest value of props or state without “reacting” to it and re-synchronizing the Effect,** you can split your Effect into a reactive part (which you’ll keep in the Effect) and a non-reactive part (which you’ll extract into something called an _Effect Event_). [Read about separating Events from Effects.](https://react.dev/learn/separating-events-from-effects)\n \n* **Avoid relying on objects and functions as dependencies.** If you create objects and functions during rendering and then read them from an Effect, they will be different on every render. This will cause your Effect to re-synchronize every time. [Read more about removing unnecessary dependencies from Effects.](https://react.dev/learn/removing-effect-dependencies)\n \n\n### Pitfall\n\nThe linter is your friend, but its powers are limited. The linter only knows when the dependencies are _wrong_. It doesn’t know _the best_ way to solve each case. If the linter suggests a dependency, but adding it causes a loop, it doesn’t mean the linter should be ignored. You need to change the code inside (or outside) the Effect so that that value isn’t reactive and doesn’t _need_ to be a dependency.\n\nIf you have an existing codebase, you might have some Effects that suppress the linter like this:\n\n```\nuseEffect(() => {// ...// 🔴 Avoid suppressing the linter like this:// eslint-ignore-next-line react-hooks/exhaustive-deps}, []);\n```\n\nOn the [next](https://react.dev/learn/separating-events-from-effects) [pages](https://react.dev/learn/removing-effect-dependencies), you’ll learn how to fix this code without breaking the rules. It’s always worth fixing!\n\nRecap[](https://react.dev/learn/lifecycle-of-reactive-effects#recap \"Link for Recap\")\n-------------------------------------------------------------------------------------\n\n* Components can mount, update, and unmount.\n* Each Effect has a separate lifecycle from the surrounding component.\n* Each Effect describes a separate synchronization process that can _start_ and _stop_.\n* When you write and read Effects, think from each individual Effect’s perspective (how to start and stop synchronization) rather than from the component’s perspective (how it mounts, updates, or unmounts).\n* Values declared inside the component body are “reactive”.\n* Reactive values should re-synchronize the Effect because they can change over time.\n* The linter verifies that all reactive values used inside the Effect are specified as dependencies.\n* All errors flagged by the linter are legitimate. There’s always a way to fix the code to not break the rules.\n\nTry out some challenges[](https://react.dev/learn/lifecycle-of-reactive-effects#challenges \"Link for Try out some challenges\")\n------------------------------------------------------------------------------------------------------------------------------\n\n#### Fix reconnecting on every keystroke[](https://react.dev/learn/lifecycle-of-reactive-effects#fix-reconnecting-on-every-keystroke \"Link for this heading\")\n\nIn this example, the `ChatRoom` component connects to the chat room when the component mounts, disconnects when it unmounts, and reconnects when you select a different chat room. This behavior is correct, so you need to keep it working.\n\nHowever, there is a problem. Whenever you type into the message box input at the bottom, `ChatRoom` _also_ reconnects to the chat. (You can notice this by clearing the console and typing into the input.) Fix the issue so that this doesn’t happen.\n\nimport { useState, useEffect } from 'react';\nimport { createConnection } from './chat.js';\n\nconst serverUrl = 'https://localhost:1234';\n\nfunction ChatRoom({ roomId }) {\n const \\[message, setMessage\\] = useState('');\n\n useEffect(() \\=> {\n const connection = createConnection(serverUrl, roomId);\n connection.connect();\n return () \\=> connection.disconnect();\n });\n\n return (\n <\\>\n <h1\\>Welcome to the {roomId} room!</h1\\>\n <input\n value\\={message}\n onChange\\={e \\=> setMessage(e.target.value)}\n />\n </\\>\n );\n}\n\nexport default function App() {\n const \\[roomId, setRoomId\\] = useState('general');\n return (\n <\\>\n <label\\>\n Choose the chat room:{' '}\n <select\n value\\={roomId}\n onChange\\={e \\=> setRoomId(e.target.value)}\n \\>\n <option value\\=\"general\"\\>general</option\\>\n <option value\\=\"travel\"\\>travel</option\\>\n <option value\\=\"music\"\\>music</option\\>\n </select\\>\n </label\\>\n <hr />\n <ChatRoom roomId\\={roomId} />\n </\\>\n );\n}\n",
"filename": "lifecycle-of-reactive-effects.md",
"package": "react"
} |
{
"content": "Title: <link> – React\n\nURL Source: https://react.dev/reference/react-dom/components/link\n\nMarkdown Content:\n### Canary\n\nThe [built-in browser `<link>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link) lets you use external resources such as stylesheets or annotate the document with link metadata.\n\n```\n<link rel=\"icon\" href=\"favicon.ico\" />\n```\n\n* [Reference](https://react.dev/reference/react-dom/components/link#reference)\n * [`<link>`](https://react.dev/reference/react-dom/components/link#link)\n* [Usage](https://react.dev/reference/react-dom/components/link#usage)\n * [Linking to related resources](https://react.dev/reference/react-dom/components/link#linking-to-related-resources)\n * [Linking to a stylesheet](https://react.dev/reference/react-dom/components/link#linking-to-a-stylesheet)\n * [Controlling stylesheet precedence](https://react.dev/reference/react-dom/components/link#controlling-stylesheet-precedence)\n * [Deduplicated stylesheet rendering](https://react.dev/reference/react-dom/components/link#deduplicated-stylesheet-rendering)\n * [Annotating specific items within the document with links](https://react.dev/reference/react-dom/components/link#annotating-specific-items-within-the-document-with-links)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/components/link#reference \"Link for Reference \")\n--------------------------------------------------------------------------------------------------\n\n### `<link>`[](https://react.dev/reference/react-dom/components/link#link \"Link for this heading\")\n\nTo link to external resources such as stylesheets, fonts, and icons, or to annotate the document with link metadata, render the [built-in browser `<link>` component](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link). You can render `<link>` from any component and React will [in most cases](https://react.dev/reference/react-dom/components/link#special-rendering-behavior) place the corresponding DOM element in the document head.\n\n```\n<link rel=\"icon\" href=\"favicon.ico\" />\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/components/link#usage)\n\n#### Props[](https://react.dev/reference/react-dom/components/link#props \"Link for Props \")\n\n`<link>` supports all [common element props.](https://react.dev/reference/react-dom/components/common#props)\n\n* `rel`: a string, required. Specifies the [relationship to the resource](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/rel). React [treats links with `rel=\"stylesheet\"` differently](https://react.dev/reference/react-dom/components/link#special-rendering-behavior) from other links.\n\nThese props apply when `rel=\"stylesheet\"`:\n\n* `precedence`: a string. Tells React where to rank the `<link>` DOM node relative to others in the document `<head>`, which determines which stylesheet can override the other. React will infer that precedence values it discovers first are “lower” and precedence values it discovers later are “higher”. Many style systems can work fine using a single precedence value because style rules are atomic. Stylesheets with the same precedence go together whether they are `<link>` or inline `<style>` tags or loaded using [`preinit`](https://react.dev/reference/react-dom/preinit) functions.\n* `media`: a string. Restricts the stylesheet to a certain [media query](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_media_queries/Using_media_queries).\n* `title`: a string. Specifies the name of an [alternative stylesheet](https://developer.mozilla.org/en-US/docs/Web/CSS/Alternative_style_sheets).\n\nThese props apply when `rel=\"stylesheet\"` but disable React’s [special treatment of stylesheets](https://react.dev/reference/react-dom/components/link#special-rendering-behavior):\n\n* `disabled`: a boolean. Disables the stylesheet.\n* `onError`: a function. Called when the stylesheet fails to load.\n* `onLoad`: a function. Called when the stylesheet finishes being loaded.\n\nThese props apply when `rel=\"preload\"` or `rel=\"modulepreload\"`:\n\n* `as`: a string. The type of resource. Its possible values are `audio`, `document`, `embed`, `fetch`, `font`, `image`, `object`, `script`, `style`, `track`, `video`, `worker`.\n* `imageSrcSet`: a string. Applicable only when `as=\"image\"`. Specifies the [source set of the image](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).\n* `imageSizes`: a string. Applicable only when `as=\"image\"`. Specifies the [sizes of the image](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).\n\nThese props apply when `rel=\"icon\"` or `rel=\"apple-touch-icon\"`:\n\n* `sizes`: a string. The [sizes of the icon](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).\n\nThese props apply in all cases:\n\n* `href`: a string. The URL of the linked resource.\n* `crossOrigin`: a string. The [CORS policy](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/crossorigin) to use. Its possible values are `anonymous` and `use-credentials`. It is required when `as` is set to `\"fetch\"`.\n* `referrerPolicy`: a string. The [Referrer header](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link#referrerpolicy) to send when fetching. Its possible values are `no-referrer-when-downgrade` (the default), `no-referrer`, `origin`, `origin-when-cross-origin`, and `unsafe-url`.\n* `fetchPriority`: a string. Suggests a relative priority for fetching the resource. The possible values are `auto` (the default), `high`, and `low`.\n* `hrefLang`: a string. The language of the linked resource.\n* `integrity`: a string. A cryptographic hash of the resource, to [verify its authenticity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity).\n* `type`: a string. The MIME type of the linked resource.\n\nProps that are **not recommended** for use with React:\n\n* `blocking`: a string. If set to `\"render\"`, instructs the browser not to render the page until the stylesheet is loaded. React provides more fine-grained control using Suspense.\n\n#### Special rendering behavior[](https://react.dev/reference/react-dom/components/link#special-rendering-behavior \"Link for Special rendering behavior \")\n\nReact will always place the DOM element corresponding to the `<link>` component within the document’s `<head>`, regardless of where in the React tree it is rendered. The `<head>` is the only valid place for `<link>` to exist within the DOM, yet it’s convenient and keeps things composable if a component representing a specific page can render `<link>` components itself.\n\nThere are a few exceptions to this:\n\n* If the `<link>` has a `rel=\"stylesheet\"` prop, then it has to also have a `precedence` prop to get this special behavior. This is because the order of stylesheets within the document is significant, so React needs to know how to order this stylesheet relative to others, which you specify using the `precedence` prop. If the `precedence` prop is omitted, there is no special behavior.\n* If the `<link>` has an [`itemProp`](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/itemprop) prop, there is no special behavior, because in this case it doesn’t apply to the document but instead represents metadata about a specific part of the page.\n* If the `<link>` has an `onLoad` or `onError` prop, because in that case you are managing the loading of the linked resource manually within your React component.\n\n#### Special behavior for stylesheets[](https://react.dev/reference/react-dom/components/link#special-behavior-for-stylesheets \"Link for Special behavior for stylesheets \")\n\nIn addition, if the `<link>` is to a stylesheet (namely, it has `rel=\"stylesheet\"` in its props), React treats it specially in the following ways:\n\n* The component that renders `<link>` will [suspend](https://react.dev/reference/react/Suspense) while the stylesheet is loading.\n* If multiple components render links to the same stylesheet, React will de-duplicate them and only put a single link into the DOM. Two links are considered the same if they have the same `href` prop.\n\nThere are two exception to this special behavior:\n\n* If the link doesn’t have a `precedence` prop, there is no special behavior, because the order of stylesheets within the document is significant, so React needs to know how to order this stylesheet relative to others, which you specify using the `precedence` prop.\n* If you supply any of the `onLoad`, `onError`, or `disabled` props, there is no special behavior, because these props indicate that you are managing the loading of the stylesheet manually within your component.\n\nThis special treatment comes with two caveats:\n\n* React will ignore changes to props after the link has been rendered. (React will issue a warning in development if this happens.)\n* React may leave the link in the DOM even after the component that rendered it has been unmounted.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/components/link#usage \"Link for Usage \")\n--------------------------------------------------------------------------------------\n\nYou can annotate the document with links to related resources such as an icon, canonical URL, or pingback. React will place this metadata within the document `<head>` regardless of where in the React tree it is rendered.\n\n### Linking to a stylesheet[](https://react.dev/reference/react-dom/components/link#linking-to-a-stylesheet \"Link for Linking to a stylesheet \")\n\nIf a component depends on a certain stylesheet in order to be displayed correctly, you can render a link to that stylesheet within the component. Your component will [suspend](https://react.dev/reference/react/Suspense) while the stylesheet is loading. You must supply the `precedence` prop, which tells React where to place this stylesheet relative to others — stylesheets with higher precedence can override those with lower precedence.\n\n### Note\n\nWhen you want to use a stylesheet, it can be beneficial to call the [preinit](https://react.dev/reference/react-dom/preinit) function. Calling this function may allow the browser to start fetching the stylesheet earlier than if you just render a `<link>` component, for example by sending an [HTTP Early Hints response](https://developer.mozilla.org/en-US/docs/Web/HTTP/Status/103).\n\n### Controlling stylesheet precedence[](https://react.dev/reference/react-dom/components/link#controlling-stylesheet-precedence \"Link for Controlling stylesheet precedence \")\n\nStylesheets can conflict with each other, and when they do, the browser goes with the one that comes later in the document. React lets you control the order of stylesheets with the `precedence` prop. In this example, two components render stylesheets, and the one with the higher precedence goes later in the document even though the component that renders it comes earlier.\n\nimport ShowRenderedHTML from './ShowRenderedHTML.js';\n\nexport default function HomePage() {\n return (\n <ShowRenderedHTML\\>\n <FirstComponent />\n <SecondComponent />\n ...\n </ShowRenderedHTML\\>\n );\n}\n\nfunction FirstComponent() {\n return <link rel\\=\"stylesheet\" href\\=\"first.css\" precedence\\=\"high\" />;\n}\n\nfunction SecondComponent() {\n return <link rel\\=\"stylesheet\" href\\=\"second.css\" precedence\\=\"low\" />;\n}\n\n### Deduplicated stylesheet rendering[](https://react.dev/reference/react-dom/components/link#deduplicated-stylesheet-rendering \"Link for Deduplicated stylesheet rendering \")\n\nIf you render the same stylesheet from multiple components, React will place only a single `<link>` in the document head.\n\n### Annotating specific items within the document with links[](https://react.dev/reference/react-dom/components/link#annotating-specific-items-within-the-document-with-links \"Link for Annotating specific items within the document with links \")\n\nYou can use the `<link>` component with the `itemProp` prop to annotate specific items within the document with links to related resources. In this case, React will _not_ place these annotations within the document `<head>` but will place them like any other React component.\n\n```\n<section itemScope><h3>Annotating specific items</h3><link itemProp=\"author\" href=\"http://example.com/\" /><p>...</p></section>\n```\n",
"filename": "link.md",
"package": "react"
} |
{
"content": "Title: Managing State – React\n\nURL Source: https://react.dev/learn/managing-state\n\nMarkdown Content:\nAs your application grows, it helps to be more intentional about how your state is organized and how the data flows between your components. Redundant or duplicate state is a common source of bugs. In this chapter, you’ll learn how to structure your state well, how to keep your state update logic maintainable, and how to share state between distant components.\n\nReacting to input with state[](https://react.dev/learn/managing-state#reacting-to-input-with-state \"Link for Reacting to input with state \")\n--------------------------------------------------------------------------------------------------------------------------------------------\n\nWith React, you won’t modify the UI from code directly. For example, you won’t write commands like “disable the button”, “enable the button”, “show the success message”, etc. Instead, you will describe the UI you want to see for the different visual states of your component (“initial state”, “typing state”, “success state”), and then trigger the state changes in response to user input. This is similar to how designers think about UI.\n\nHere is a quiz form built using React. Note how it uses the `status` state variable to determine whether to enable or disable the submit button, and whether to show the success message instead.\n\n* * *\n\nChoosing the state structure[](https://react.dev/learn/managing-state#choosing-the-state-structure \"Link for Choosing the state structure \")\n--------------------------------------------------------------------------------------------------------------------------------------------\n\nStructuring state well can make a difference between a component that is pleasant to modify and debug, and one that is a constant source of bugs. The most important principle is that state shouldn’t contain redundant or duplicated information. If there’s unnecessary state, it’s easy to forget to update it, and introduce bugs!\n\nFor example, this form has a **redundant** `fullName` state variable:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[firstName, setFirstName\\] = useState('');\n const \\[lastName, setLastName\\] = useState('');\n const \\[fullName, setFullName\\] = useState('');\n\n function handleFirstNameChange(e) {\n setFirstName(e.target.value);\n setFullName(e.target.value + ' ' + lastName);\n }\n\n function handleLastNameChange(e) {\n setLastName(e.target.value);\n setFullName(firstName + ' ' + e.target.value);\n }\n\n return (\n <\\>\n <h2\\>Let’s check you in</h2\\>\n <label\\>\n First name:{' '}\n <input\n value\\={firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:{' '}\n <input\n value\\={lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n <p\\>\n Your ticket will be issued to: <b\\>{fullName}</b\\>\n </p\\>\n </\\>\n );\n}\n\nYou can remove it and simplify the code by calculating `fullName` while the component is rendering:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[firstName, setFirstName\\] = useState('');\n const \\[lastName, setLastName\\] = useState('');\n\n const fullName = firstName + ' ' + lastName;\n\n function handleFirstNameChange(e) {\n setFirstName(e.target.value);\n }\n\n function handleLastNameChange(e) {\n setLastName(e.target.value);\n }\n\n return (\n <\\>\n <h2\\>Let’s check you in</h2\\>\n <label\\>\n First name:{' '}\n <input\n value\\={firstName}\n onChange\\={handleFirstNameChange}\n />\n </label\\>\n <label\\>\n Last name:{' '}\n <input\n value\\={lastName}\n onChange\\={handleLastNameChange}\n />\n </label\\>\n <p\\>\n Your ticket will be issued to: <b\\>{fullName}</b\\>\n </p\\>\n </\\>\n );\n}\n\nThis might seem like a small change, but many bugs in React apps are fixed this way.\n\n* * *\n\nSharing state between components[](https://react.dev/learn/managing-state#sharing-state-between-components \"Link for Sharing state between components \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------\n\nSometimes, you want the state of two components to always change together. To do it, remove state from both of them, move it to their closest common parent, and then pass it down to them via props. This is known as “lifting state up”, and it’s one of the most common things you will do writing React code.\n\nIn this example, only one panel should be active at a time. To achieve this, instead of keeping the active state inside each individual panel, the parent component holds the state and specifies the props for its children.\n\nimport { useState } from 'react';\n\nexport default function Accordion() {\n const \\[activeIndex, setActiveIndex\\] = useState(0);\n return (\n <\\>\n <h2\\>Almaty, Kazakhstan</h2\\>\n <Panel\n title\\=\"About\"\n isActive\\={activeIndex === 0}\n onShow\\={() \\=> setActiveIndex(0)}\n \\>\n With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.\n </Panel\\>\n <Panel\n title\\=\"Etymology\"\n isActive\\={activeIndex === 1}\n onShow\\={() \\=> setActiveIndex(1)}\n \\>\n The name comes from <span lang\\=\"kk-KZ\"\\>алма</span\\>, the Kazakh word for \"apple\" and is often translated as \"full of apples\". In fact, the region surrounding Almaty is thought to be the ancestral home of the apple, and the wild <i lang\\=\"la\"\\>Malus sieversii</i\\> is considered a likely candidate for the ancestor of the modern domestic apple.\n </Panel\\>\n </\\>\n );\n}\n\nfunction Panel({\n title,\n children,\n isActive,\n onShow\n}) {\n return (\n <section className\\=\"panel\"\\>\n <h3\\>{title}</h3\\>\n {isActive ? (\n <p\\>{children}</p\\>\n ) : (\n <button onClick\\={onShow}\\>\n Show\n </button\\>\n )}\n </section\\>\n );\n}\n\n* * *\n\nPreserving and resetting state[](https://react.dev/learn/managing-state#preserving-and-resetting-state \"Link for Preserving and resetting state \")\n--------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you re-render a component, React needs to decide which parts of the tree to keep (and update), and which parts to discard or re-create from scratch. In most cases, React’s automatic behavior works well enough. By default, React preserves the parts of the tree that “match up” with the previously rendered component tree.\n\nHowever, sometimes this is not what you want. In this chat app, typing a message and then switching the recipient does not reset the input. This can make the user accidentally send a message to the wrong person:\n\nimport { useState } from 'react';\nimport Chat from './Chat.js';\nimport ContactList from './ContactList.js';\n\nexport default function Messenger() {\n const \\[to, setTo\\] = useState(contacts\\[0\\]);\n return (\n <div\\>\n <ContactList\n contacts\\={contacts}\n selectedContact\\={to}\n onSelect\\={contact \\=> setTo(contact)}\n />\n <Chat contact\\={to} />\n </div\\>\n )\n}\n\nconst contacts = \\[\n { name: 'Taylor', email: 'taylor@mail.com' },\n { name: 'Alice', email: 'alice@mail.com' },\n { name: 'Bob', email: 'bob@mail.com' }\n\\];\n\nReact lets you override the default behavior, and _force_ a component to reset its state by passing it a different `key`, like `<Chat key={email} />`. This tells React that if the recipient is different, it should be considered a _different_ `Chat` component that needs to be re-created from scratch with the new data (and UI like inputs). Now switching between the recipients resets the input field—even though you render the same component.\n\nimport { useState } from 'react';\nimport Chat from './Chat.js';\nimport ContactList from './ContactList.js';\n\nexport default function Messenger() {\n const \\[to, setTo\\] = useState(contacts\\[0\\]);\n return (\n <div\\>\n <ContactList\n contacts\\={contacts}\n selectedContact\\={to}\n onSelect\\={contact \\=> setTo(contact)}\n />\n <Chat key\\={to.email} contact\\={to} />\n </div\\>\n )\n}\n\nconst contacts = \\[\n { name: 'Taylor', email: 'taylor@mail.com' },\n { name: 'Alice', email: 'alice@mail.com' },\n { name: 'Bob', email: 'bob@mail.com' }\n\\];\n\n* * *\n\nComponents with many state updates spread across many event handlers can get overwhelming. For these cases, you can consolidate all the state update logic outside your component in a single function, called “reducer”. Your event handlers become concise because they only specify the user “actions”. At the bottom of the file, the reducer function specifies how the state should update in response to each action!\n\nimport { useReducer } from 'react';\nimport AddTask from './AddTask.js';\nimport TaskList from './TaskList.js';\n\nexport default function TaskApp() {\n const \\[tasks, dispatch\\] = useReducer(\n tasksReducer,\n initialTasks\n );\n\n function handleAddTask(text) {\n dispatch({\n type: 'added',\n id: nextId++,\n text: text,\n });\n }\n\n function handleChangeTask(task) {\n dispatch({\n type: 'changed',\n task: task\n });\n }\n\n function handleDeleteTask(taskId) {\n dispatch({\n type: 'deleted',\n id: taskId\n });\n }\n\n return (\n <\\>\n <h1\\>Prague itinerary</h1\\>\n <AddTask\n onAddTask\\={handleAddTask}\n />\n <TaskList\n tasks\\={tasks}\n onChangeTask\\={handleChangeTask}\n onDeleteTask\\={handleDeleteTask}\n />\n </\\>\n );\n}\n\nfunction tasksReducer(tasks, action) {\n switch (action.type) {\n case 'added': {\n return \\[...tasks, {\n id: action.id,\n text: action.text,\n done: false\n }\\];\n }\n case 'changed': {\n return tasks.map(t \\=> {\n if (t.id === action.task.id) {\n return action.task;\n } else {\n return t;\n }\n });\n }\n case 'deleted': {\n return tasks.filter(t \\=> t.id !== action.id);\n }\n default: {\n throw Error('Unknown action: ' + action.type);\n }\n }\n}\n\nlet nextId = 3;\nconst initialTasks = \\[\n { id: 0, text: 'Visit Kafka Museum', done: true },\n { id: 1, text: 'Watch a puppet show', done: false },\n { id: 2, text: 'Lennon Wall pic', done: false }\n\\];\n\n* * *\n\nPassing data deeply with context[](https://react.dev/learn/managing-state#passing-data-deeply-with-context \"Link for Passing data deeply with context \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------\n\nUsually, you will pass information from a parent component to a child component via props. But passing props can become inconvenient if you need to pass some prop through many components, or if many components need the same information. Context lets the parent component make some information available to any component in the tree below it—no matter how deep it is—without passing it explicitly through props.\n\nHere, the `Heading` component determines its heading level by “asking” the closest `Section` for its level. Each `Section` tracks its own level by asking the parent `Section` and adding one to it. Every `Section` provides information to all components below it without passing props—it does that through context.\n\nimport Heading from './Heading.js';\nimport Section from './Section.js';\n\nexport default function Page() {\n return (\n <Section\\>\n <Heading\\>Title</Heading\\>\n <Section\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Section\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Section\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n </Section\\>\n </Section\\>\n </Section\\>\n </Section\\>\n );\n}\n\n* * *\n\nScaling up with reducer and context[](https://react.dev/learn/managing-state#scaling-up-with-reducer-and-context \"Link for Scaling up with reducer and context \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nReducers let you consolidate a component’s state update logic. Context lets you pass information deep down to other components. You can combine reducers and context together to manage state of a complex screen.\n\nWith this approach, a parent component with complex state manages it with a reducer. Other components anywhere deep in the tree can read its state via context. They can also dispatch actions to update that state.\n",
"filename": "managing-state.md",
"package": "react"
} |
{
"content": "Title: Manipulating the DOM with Refs – React\n\nURL Source: https://react.dev/learn/manipulating-the-dom-with-refs\n\nMarkdown Content:\nReact automatically updates the [DOM](https://developer.mozilla.org/docs/Web/API/Document_Object_Model/Introduction) to match your render output, so your components won’t often need to manipulate it. However, sometimes you might need access to the DOM elements managed by React—for example, to focus a node, scroll to it, or measure its size and position. There is no built-in way to do those things in React, so you will need a _ref_ to the DOM node.\n\n### You will learn\n\n* How to access a DOM node managed by React with the `ref` attribute\n* How the `ref` JSX attribute relates to the `useRef` Hook\n* How to access another component’s DOM node\n* In which cases it’s safe to modify the DOM managed by React\n\nGetting a ref to the node[](https://react.dev/learn/manipulating-the-dom-with-refs#getting-a-ref-to-the-node \"Link for Getting a ref to the node \")\n---------------------------------------------------------------------------------------------------------------------------------------------------\n\nTo access a DOM node managed by React, first, import the `useRef` Hook:\n\n```\nimport { useRef } from 'react';\n```\n\nThen, use it to declare a ref inside your component:\n\n```\nconst myRef = useRef(null);\n```\n\nFinally, pass your ref as the `ref` attribute to the JSX tag for which you want to get the DOM node:\n\nThe `useRef` Hook returns an object with a single property called `current`. Initially, `myRef.current` will be `null`. When React creates a DOM node for this `<div>`, React will put a reference to this node into `myRef.current`. You can then access this DOM node from your [event handlers](https://react.dev/learn/responding-to-events) and use the built-in [browser APIs](https://developer.mozilla.org/docs/Web/API/Element) defined on it.\n\n```\n// You can use any browser APIs, for example:myRef.current.scrollIntoView();\n```\n\n### Example: Focusing a text input[](https://react.dev/learn/manipulating-the-dom-with-refs#example-focusing-a-text-input \"Link for Example: Focusing a text input \")\n\nIn this example, clicking the button will focus the input:\n\nimport { useRef } from 'react';\n\nexport default function Form() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <input ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\nTo implement this:\n\n1. Declare `inputRef` with the `useRef` Hook.\n2. Pass it as `<input ref={inputRef}>`. This tells React to **put this `<input>`’s DOM node into `inputRef.current`.**\n3. In the `handleClick` function, read the input DOM node from `inputRef.current` and call [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) on it with `inputRef.current.focus()`.\n4. Pass the `handleClick` event handler to `<button>` with `onClick`.\n\nWhile DOM manipulation is the most common use case for refs, the `useRef` Hook can be used for storing other things outside React, like timer IDs. Similarly to state, refs remain between renders. Refs are like state variables that don’t trigger re-renders when you set them. Read about refs in [Referencing Values with Refs.](https://react.dev/learn/referencing-values-with-refs)\n\n### Example: Scrolling to an element[](https://react.dev/learn/manipulating-the-dom-with-refs#example-scrolling-to-an-element \"Link for Example: Scrolling to an element \")\n\nYou can have more than a single ref in a component. In this example, there is a carousel of three images. Each button centers an image by calling the browser [`scrollIntoView()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollIntoView) method on the corresponding DOM node:\n\nimport { useRef } from 'react';\n\nexport default function CatFriends() {\n const firstCatRef = useRef(null);\n const secondCatRef = useRef(null);\n const thirdCatRef = useRef(null);\n\n function handleScrollToFirstCat() {\n firstCatRef.current.scrollIntoView({\n behavior: 'smooth',\n block: 'nearest',\n inline: 'center'\n });\n }\n\n function handleScrollToSecondCat() {\n secondCatRef.current.scrollIntoView({\n behavior: 'smooth',\n block: 'nearest',\n inline: 'center'\n });\n }\n\n function handleScrollToThirdCat() {\n thirdCatRef.current.scrollIntoView({\n behavior: 'smooth',\n block: 'nearest',\n inline: 'center'\n });\n }\n\n return (\n <\\>\n <nav\\>\n <button onClick\\={handleScrollToFirstCat}\\>\n Tom\n </button\\>\n <button onClick\\={handleScrollToSecondCat}\\>\n Maru\n </button\\>\n <button onClick\\={handleScrollToThirdCat}\\>\n Jellylorum\n </button\\>\n </nav\\>\n <div\\>\n <ul\\>\n <li\\>\n <img\n src\\=\"https://placekitten.com/g/200/200\"\n alt\\=\"Tom\"\n ref\\={firstCatRef}\n />\n </li\\>\n <li\\>\n <img\n src\\=\"https://placekitten.com/g/300/200\"\n alt\\=\"Maru\"\n ref\\={secondCatRef}\n />\n </li\\>\n <li\\>\n <img\n src\\=\"https://placekitten.com/g/250/200\"\n alt\\=\"Jellylorum\"\n ref\\={thirdCatRef}\n />\n </li\\>\n </ul\\>\n </div\\>\n </\\>\n );\n}\n\n##### Deep Dive\n\n#### How to manage a list of refs using a ref callback[](https://react.dev/learn/manipulating-the-dom-with-refs#how-to-manage-a-list-of-refs-using-a-ref-callback \"Link for How to manage a list of refs using a ref callback \")\n\nIn the above examples, there is a predefined number of refs. However, sometimes you might need a ref to each item in the list, and you don’t know how many you will have. Something like this **wouldn’t work**:\n\n```\n<ul>{items.map((item) => {// Doesn't work!const ref = useRef(null);return <li ref={ref} />;})}</ul>\n```\n\nThis is because **Hooks must only be called at the top-level of your component.** You can’t call `useRef` in a loop, in a condition, or inside a `map()` call.\n\nOne possible way around this is to get a single ref to their parent element, and then use DOM manipulation methods like [`querySelectorAll`](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelectorAll) to “find” the individual child nodes from it. However, this is brittle and can break if your DOM structure changes.\n\nAnother solution is to **pass a function to the `ref` attribute.** This is called a [`ref` callback.](https://react.dev/reference/react-dom/components/common#ref-callback) React will call your ref callback with the DOM node when it’s time to set the ref, and with `null` when it’s time to clear it. This lets you maintain your own array or a [Map](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map), and access any ref by its index or some kind of ID.\n\nThis example shows how you can use this approach to scroll to an arbitrary node in a long list:\n\nimport { useRef, useState } from \"react\";\n\nexport default function CatFriends() {\n const itemsRef = useRef(null);\n const \\[catList, setCatList\\] = useState(setupCatList);\n\n function scrollToCat(cat) {\n const map = getMap();\n const node = map.get(cat);\n node.scrollIntoView({\n behavior: \"smooth\",\n block: \"nearest\",\n inline: \"center\",\n });\n }\n\n function getMap() {\n if (!itemsRef.current) {\n \n itemsRef.current = new Map();\n }\n return itemsRef.current;\n }\n\n return (\n <\\>\n <nav\\>\n <button onClick\\={() \\=> scrollToCat(catList\\[0\\])}\\>Tom</button\\>\n <button onClick\\={() \\=> scrollToCat(catList\\[5\\])}\\>Maru</button\\>\n <button onClick\\={() \\=> scrollToCat(catList\\[9\\])}\\>Jellylorum</button\\>\n </nav\\>\n <div\\>\n <ul\\>\n {catList.map((cat) \\=> (\n <li\n key\\={cat}\n ref\\={(node) \\=> {\n const map = getMap();\n if (node) {\n map.set(cat, node);\n } else {\n map.delete(cat);\n }\n }}\n \\>\n <img src\\={cat} />\n </li\\>\n ))}\n </ul\\>\n </div\\>\n </\\>\n );\n}\n\nfunction setupCatList() {\n const catList = \\[\\];\n for (let i = 0; i < 10; i++) {\n catList.push(\"https://loremflickr.com/320/240/cat?lock=\" + i);\n }\n\n return catList;\n}\n\nIn this example, `itemsRef` doesn’t hold a single DOM node. Instead, it holds a [Map](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Map) from item ID to a DOM node. ([Refs can hold any values!](https://react.dev/learn/referencing-values-with-refs)) The [`ref` callback](https://react.dev/reference/react-dom/components/common#ref-callback) on every list item takes care to update the Map:\n\n```\n<likey={cat.id}ref={node => {const map = getMap();if (node) {// Add to the Mapmap.set(cat, node);} else {// Remove from the Mapmap.delete(cat);}}}>\n```\n\nThis lets you read individual DOM nodes from the Map later.\n\n### Canary\n\nThis example shows another approach for managing the Map with a `ref` callback cleanup function.\n\n```\n<likey={cat.id}ref={node => {const map = getMap();// Add to the Mapmap.set(cat, node);return () => {// Remove from the Mapmap.delete(cat);};}}>\n```\n\nAccessing another component’s DOM nodes[](https://react.dev/learn/manipulating-the-dom-with-refs#accessing-another-components-dom-nodes \"Link for Accessing another component’s DOM nodes \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you put a ref on a built-in component that outputs a browser element like `<input />`, React will set that ref’s `current` property to the corresponding DOM node (such as the actual `<input />` in the browser).\n\nHowever, if you try to put a ref on **your own** component, like `<MyInput />`, by default you will get `null`. Here is an example demonstrating it. Notice how clicking the button **does not** focus the input:\n\nimport { useRef } from 'react';\n\nfunction MyInput(props) {\n return <input {...props} />;\n}\n\nexport default function MyForm() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <MyInput ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\nTo help you notice the issue, React also prints an error to the console:\n\nWarning: Function components cannot be given refs. Attempts to access this ref will fail. Did you mean to use React.forwardRef()?\n\nThis happens because by default React does not let a component access the DOM nodes of other components. Not even for its own children! This is intentional. Refs are an escape hatch that should be used sparingly. Manually manipulating _another_ component’s DOM nodes makes your code even more fragile.\n\nInstead, components that _want_ to expose their DOM nodes have to **opt in** to that behavior. A component can specify that it “forwards” its ref to one of its children. Here’s how `MyInput` can use the `forwardRef` API:\n\n```\nconst MyInput = forwardRef((props, ref) => {return <input {...props} ref={ref} />;});\n```\n\nThis is how it works:\n\n1. `<MyInput ref={inputRef} />` tells React to put the corresponding DOM node into `inputRef.current`. However, it’s up to the `MyInput` component to opt into that—by default, it doesn’t.\n2. The `MyInput` component is declared using `forwardRef`. **This opts it into receiving the `inputRef` from above as the second `ref` argument** which is declared after `props`.\n3. `MyInput` itself passes the `ref` it received to the `<input>` inside of it.\n\nNow clicking the button to focus the input works:\n\nimport { forwardRef, useRef } from 'react';\n\nconst MyInput = forwardRef((props, ref) \\=> {\n return <input {...props} ref\\={ref} />;\n});\n\nexport default function Form() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <MyInput ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\nIn design systems, it is a common pattern for low-level components like buttons, inputs, and so on, to forward their refs to their DOM nodes. On the other hand, high-level components like forms, lists, or page sections usually won’t expose their DOM nodes to avoid accidental dependencies on the DOM structure.\n\n##### Deep Dive\n\n#### Exposing a subset of the API with an imperative handle[](https://react.dev/learn/manipulating-the-dom-with-refs#exposing-a-subset-of-the-api-with-an-imperative-handle \"Link for Exposing a subset of the API with an imperative handle \")\n\nIn the above example, `MyInput` exposes the original DOM input element. This lets the parent component call `focus()` on it. However, this also lets the parent component do something else—for example, change its CSS styles. In uncommon cases, you may want to restrict the exposed functionality. You can do that with `useImperativeHandle`:\n\nimport {\n forwardRef, \n useRef, \n useImperativeHandle\n} from 'react';\n\nconst MyInput = forwardRef((props, ref) \\=> {\n const realInputRef = useRef(null);\n useImperativeHandle(ref, () \\=> ({\n \n focus() {\n realInputRef.current.focus();\n },\n }));\n return <input {...props} ref\\={realInputRef} />;\n});\n\nexport default function Form() {\n const inputRef = useRef(null);\n\n function handleClick() {\n inputRef.current.focus();\n }\n\n return (\n <\\>\n <MyInput ref\\={inputRef} />\n <button onClick\\={handleClick}\\>\n Focus the input\n </button\\>\n </\\>\n );\n}\n\nHere, `realInputRef` inside `MyInput` holds the actual input DOM node. However, `useImperativeHandle` instructs React to provide your own special object as the value of a ref to the parent component. So `inputRef.current` inside the `Form` component will only have the `focus` method. In this case, the ref “handle” is not the DOM node, but the custom object you create inside `useImperativeHandle` call.\n\nWhen React attaches the refs[](https://react.dev/learn/manipulating-the-dom-with-refs#when-react-attaches-the-refs \"Link for When React attaches the refs \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn React, every update is split in [two phases](https://react.dev/learn/render-and-commit#step-3-react-commits-changes-to-the-dom):\n\n* During **render,** React calls your components to figure out what should be on the screen.\n* During **commit,** React applies changes to the DOM.\n\nIn general, you [don’t want](https://react.dev/learn/referencing-values-with-refs#best-practices-for-refs) to access refs during rendering. That goes for refs holding DOM nodes as well. During the first render, the DOM nodes have not yet been created, so `ref.current` will be `null`. And during the rendering of updates, the DOM nodes haven’t been updated yet. So it’s too early to read them.\n\nReact sets `ref.current` during the commit. Before updating the DOM, React sets the affected `ref.current` values to `null`. After updating the DOM, React immediately sets them to the corresponding DOM nodes.\n\n**Usually, you will access refs from event handlers.** If you want to do something with a ref, but there is no particular event to do it in, you might need an Effect. We will discuss Effects on the next pages.\n\n##### Deep Dive\n\n#### Flushing state updates synchronously with flushSync[](https://react.dev/learn/manipulating-the-dom-with-refs#flushing-state-updates-synchronously-with-flush-sync \"Link for Flushing state updates synchronously with flushSync \")\n\nConsider code like this, which adds a new todo and scrolls the screen down to the last child of the list. Notice how, for some reason, it always scrolls to the todo that was _just before_ the last added one:\n\nimport { useState, useRef } from 'react';\n\nexport default function TodoList() {\n const listRef = useRef(null);\n const \\[text, setText\\] = useState('');\n const \\[todos, setTodos\\] = useState(\n initialTodos\n );\n\n function handleAdd() {\n const newTodo = { id: nextId++, text: text };\n setText('');\n setTodos(\\[ ...todos, newTodo\\]);\n listRef.current.lastChild.scrollIntoView({\n behavior: 'smooth',\n block: 'nearest'\n });\n }\n\n return (\n <\\>\n <button onClick\\={handleAdd}\\>\n Add\n </button\\>\n <input\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n <ul ref\\={listRef}\\>\n {todos.map(todo \\=> (\n <li key\\={todo.id}\\>{todo.text}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\nlet nextId = 0;\nlet initialTodos = \\[\\];\nfor (let i = 0; i < 20; i++) {\n initialTodos.push({\n id: nextId++,\n text: 'Todo #' + (i + 1)\n });\n}\n\nThe issue is with these two lines:\n\n```\nsetTodos([ ...todos, newTodo]);listRef.current.lastChild.scrollIntoView();\n```\n\nIn React, [state updates are queued.](https://react.dev/learn/queueing-a-series-of-state-updates) Usually, this is what you want. However, here it causes a problem because `setTodos` does not immediately update the DOM. So the time you scroll the list to its last element, the todo has not yet been added. This is why scrolling always “lags behind” by one item.\n\nTo fix this issue, you can force React to update (“flush”) the DOM synchronously. To do this, import `flushSync` from `react-dom` and **wrap the state update** into a `flushSync` call:\n\n```\nflushSync(() => {setTodos([ ...todos, newTodo]);});listRef.current.lastChild.scrollIntoView();\n```\n\nThis will instruct React to update the DOM synchronously right after the code wrapped in `flushSync` executes. As a result, the last todo will already be in the DOM by the time you try to scroll to it:\n\nimport { useState, useRef } from 'react';\nimport { flushSync } from 'react-dom';\n\nexport default function TodoList() {\n const listRef = useRef(null);\n const \\[text, setText\\] = useState('');\n const \\[todos, setTodos\\] = useState(\n initialTodos\n );\n\n function handleAdd() {\n const newTodo = { id: nextId++, text: text };\n flushSync(() \\=> {\n setText('');\n setTodos(\\[ ...todos, newTodo\\]); \n });\n listRef.current.lastChild.scrollIntoView({\n behavior: 'smooth',\n block: 'nearest'\n });\n }\n\n return (\n <\\>\n <button onClick\\={handleAdd}\\>\n Add\n </button\\>\n <input\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n <ul ref\\={listRef}\\>\n {todos.map(todo \\=> (\n <li key\\={todo.id}\\>{todo.text}</li\\>\n ))}\n </ul\\>\n </\\>\n );\n}\n\nlet nextId = 0;\nlet initialTodos = \\[\\];\nfor (let i = 0; i < 20; i++) {\n initialTodos.push({\n id: nextId++,\n text: 'Todo #' + (i + 1)\n });\n}\n\nBest practices for DOM manipulation with refs[](https://react.dev/learn/manipulating-the-dom-with-refs#best-practices-for-dom-manipulation-with-refs \"Link for Best practices for DOM manipulation with refs \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nRefs are an escape hatch. You should only use them when you have to “step outside React”. Common examples of this include managing focus, scroll position, or calling browser APIs that React does not expose.\n\nIf you stick to non-destructive actions like focusing and scrolling, you shouldn’t encounter any problems. However, if you try to **modify** the DOM manually, you can risk conflicting with the changes React is making.\n\nTo illustrate this problem, this example includes a welcome message and two buttons. The first button toggles its presence using [conditional rendering](https://react.dev/learn/conditional-rendering) and [state](https://react.dev/learn/state-a-components-memory), as you would usually do in React. The second button uses the [`remove()` DOM API](https://developer.mozilla.org/en-US/docs/Web/API/Element/remove) to forcefully remove it from the DOM outside of React’s control.\n\nTry pressing “Toggle with setState” a few times. The message should disappear and appear again. Then press “Remove from the DOM”. This will forcefully remove it. Finally, press “Toggle with setState”:\n\nimport { useState, useRef } from 'react';\n\nexport default function Counter() {\n const \\[show, setShow\\] = useState(true);\n const ref = useRef(null);\n\n return (\n <div\\>\n <button\n onClick\\={() \\=> {\n setShow(!show);\n }}\\>\n Toggle with setState\n </button\\>\n <button\n onClick\\={() \\=> {\n ref.current.remove();\n }}\\>\n Remove from the DOM\n </button\\>\n {show && <p ref\\={ref}\\>Hello world</p\\>}\n </div\\>\n );\n}\n\nAfter you’ve manually removed the DOM element, trying to use `setState` to show it again will lead to a crash. This is because you’ve changed the DOM, and React doesn’t know how to continue managing it correctly.\n\n**Avoid changing DOM nodes managed by React.** Modifying, adding children to, or removing children from elements that are managed by React can lead to inconsistent visual results or crashes like above.\n\nHowever, this doesn’t mean that you can’t do it at all. It requires caution. **You can safely modify parts of the DOM that React has _no reason_ to update.** For example, if some `<div>` is always empty in the JSX, React won’t have a reason to touch its children list. Therefore, it is safe to manually add or remove elements there.\n\nRecap[](https://react.dev/learn/manipulating-the-dom-with-refs#recap \"Link for Recap\")\n--------------------------------------------------------------------------------------\n\n* Refs are a generic concept, but most often you’ll use them to hold DOM elements.\n* You instruct React to put a DOM node into `myRef.current` by passing `<div ref={myRef}>`.\n* Usually, you will use refs for non-destructive actions like focusing, scrolling, or measuring DOM elements.\n* A component doesn’t expose its DOM nodes by default. You can opt into exposing a DOM node by using `forwardRef` and passing the second `ref` argument down to a specific node.\n* Avoid changing DOM nodes managed by React.\n* If you do modify DOM nodes managed by React, modify parts that React has no reason to update.\n\nTry out some challenges[](https://react.dev/learn/manipulating-the-dom-with-refs#challenges \"Link for Try out some challenges\")\n-------------------------------------------------------------------------------------------------------------------------------\n\n#### Play and pause the video[](https://react.dev/learn/manipulating-the-dom-with-refs#play-and-pause-the-video \"Link for this heading\")\n\nIn this example, the button toggles a state variable to switch between a playing and a paused state. However, in order to actually play or pause the video, toggling state is not enough. You also need to call [`play()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/play) and [`pause()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/pause) on the DOM element for the `<video>`. Add a ref to it, and make the button work.\n\nimport { useState, useRef } from 'react';\n\nexport default function VideoPlayer() {\n const \\[isPlaying, setIsPlaying\\] = useState(false);\n\n function handleClick() {\n const nextIsPlaying = !isPlaying;\n setIsPlaying(nextIsPlaying);\n }\n\n return (\n <\\>\n <button onClick\\={handleClick}\\>\n {isPlaying ? 'Pause' : 'Play'}\n </button\\>\n <video width\\=\"250\"\\>\n <source\n src\\=\"https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4\"\n type\\=\"video/mp4\"\n />\n </video\\>\n </\\>\n )\n}\n\nFor an extra challenge, keep the “Play” button in sync with whether the video is playing even if the user right-clicks the video and plays it using the built-in browser media controls. You might want to listen to `onPlay` and `onPause` on the video to do that.\n",
"filename": "manipulating-the-dom-with-refs.md",
"package": "react"
} |
{
"content": "Title: memo – React\n\nURL Source: https://react.dev/reference/react/memo\n\nMarkdown Content:\n`memo` lets you skip re-rendering a component when its props are unchanged.\n\n```\nconst MemoizedComponent = memo(SomeComponent, arePropsEqual?)\n```\n\n* [Reference](https://react.dev/reference/react/memo#reference)\n * [`memo(Component, arePropsEqual?)`](https://react.dev/reference/react/memo#memo)\n* [Usage](https://react.dev/reference/react/memo#usage)\n * [Skipping re-rendering when props are unchanged](https://react.dev/reference/react/memo#skipping-re-rendering-when-props-are-unchanged)\n * [Updating a memoized component using state](https://react.dev/reference/react/memo#updating-a-memoized-component-using-state)\n * [Updating a memoized component using a context](https://react.dev/reference/react/memo#updating-a-memoized-component-using-a-context)\n * [Minimizing props changes](https://react.dev/reference/react/memo#minimizing-props-changes)\n * [Specifying a custom comparison function](https://react.dev/reference/react/memo#specifying-a-custom-comparison-function)\n* [Troubleshooting](https://react.dev/reference/react/memo#troubleshooting)\n * [My component re-renders when a prop is an object, array, or function](https://react.dev/reference/react/memo#my-component-rerenders-when-a-prop-is-an-object-or-array)\n\n* * *\n\nReference[](https://react.dev/reference/react/memo#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------\n\n### `memo(Component, arePropsEqual?)`[](https://react.dev/reference/react/memo#memo \"Link for this heading\")\n\nWrap a component in `memo` to get a _memoized_ version of that component. This memoized version of your component will usually not be re-rendered when its parent component is re-rendered as long as its props have not changed. But React may still re-render it: memoization is a performance optimization, not a guarantee.\n\n```\nimport { memo } from 'react';const SomeComponent = memo(function SomeComponent(props) {// ...});\n```\n\n[See more examples below.](https://react.dev/reference/react/memo#usage)\n\n#### Parameters[](https://react.dev/reference/react/memo#parameters \"Link for Parameters \")\n\n* `Component`: The component that you want to memoize. The `memo` does not modify this component, but returns a new, memoized component instead. Any valid React component, including functions and [`forwardRef`](https://react.dev/reference/react/forwardRef) components, is accepted.\n \n* **optional** `arePropsEqual`: A function that accepts two arguments: the component’s previous props, and its new props. It should return `true` if the old and new props are equal: that is, if the component will render the same output and behave in the same way with the new props as with the old. Otherwise it should return `false`. Usually, you will not specify this function. By default, React will compare each prop with [`Object.is`.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)\n \n\n#### Returns[](https://react.dev/reference/react/memo#returns \"Link for Returns \")\n\n`memo` returns a new React component. It behaves the same as the component provided to `memo` except that React will not always re-render it when its parent is being re-rendered unless its props have changed.\n\n* * *\n\nUsage[](https://react.dev/reference/react/memo#usage \"Link for Usage \")\n-----------------------------------------------------------------------\n\n### Skipping re-rendering when props are unchanged[](https://react.dev/reference/react/memo#skipping-re-rendering-when-props-are-unchanged \"Link for Skipping re-rendering when props are unchanged \")\n\nReact normally re-renders a component whenever its parent re-renders. With `memo`, you can create a component that React will not re-render when its parent re-renders so long as its new props are the same as the old props. Such a component is said to be _memoized_.\n\nTo memoize a component, wrap it in `memo` and use the value that it returns in place of your original component:\n\n```\nconst Greeting = memo(function Greeting({ name }) {return <h1>Hello, {name}!</h1>;});export default Greeting;\n```\n\nA React component should always have [pure rendering logic.](https://react.dev/learn/keeping-components-pure) This means that it must return the same output if its props, state, and context haven’t changed. By using `memo`, you are telling React that your component complies with this requirement, so React doesn’t need to re-render as long as its props haven’t changed. Even with `memo`, your component will re-render if its own state changes or if a context that it’s using changes.\n\nIn this example, notice that the `Greeting` component re-renders whenever `name` is changed (because that’s one of its props), but not when `address` is changed (because it’s not passed to `Greeting` as a prop):\n\nimport { memo, useState } from 'react';\n\nexport default function MyApp() {\n const \\[name, setName\\] = useState('');\n const \\[address, setAddress\\] = useState('');\n return (\n <\\>\n <label\\>\n Name{': '}\n <input value\\={name} onChange\\={e \\=> setName(e.target.value)} />\n </label\\>\n <label\\>\n Address{': '}\n <input value\\={address} onChange\\={e \\=> setAddress(e.target.value)} />\n </label\\>\n <Greeting name\\={name} />\n </\\>\n );\n}\n\nconst Greeting = memo(function Greeting({ name }) {\n console.log(\"Greeting was rendered at\", new Date().toLocaleTimeString());\n return <h3\\>Hello{name && ', '}{name}!</h3\\>;\n});\n\n### Note\n\n**You should only rely on `memo` as a performance optimization.** If your code doesn’t work without it, find the underlying problem and fix it first. Then you may add `memo` to improve performance.\n\n##### Deep Dive\n\n#### Should you add memo everywhere?[](https://react.dev/reference/react/memo#should-you-add-memo-everywhere \"Link for Should you add memo everywhere? \")\n\nIf your app is like this site, and most interactions are coarse (like replacing a page or an entire section), memoization is usually unnecessary. On the other hand, if your app is more like a drawing editor, and most interactions are granular (like moving shapes), then you might find memoization very helpful.\n\nOptimizing with `memo` is only valuable when your component re-renders often with the same exact props, and its re-rendering logic is expensive. If there is no perceptible lag when your component re-renders, `memo` is unnecessary. Keep in mind that `memo` is completely useless if the props passed to your component are _always different,_ such as if you pass an object or a plain function defined during rendering. This is why you will often need [`useMemo`](https://react.dev/reference/react/useMemo#skipping-re-rendering-of-components) and [`useCallback`](https://react.dev/reference/react/useCallback#skipping-re-rendering-of-components) together with `memo`.\n\nThere is no benefit to wrapping a component in `memo` in other cases. There is no significant harm to doing that either, so some teams choose to not think about individual cases, and memoize as much as possible. The downside of this approach is that code becomes less readable. Also, not all memoization is effective: a single value that’s “always new” is enough to break memoization for an entire component.\n\n**In practice, you can make a lot of memoization unnecessary by following a few principles:**\n\n1. When a component visually wraps other components, let it [accept JSX as children.](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) This way, when the wrapper component updates its own state, React knows that its children don’t need to re-render.\n2. Prefer local state and don’t [lift state up](https://react.dev/learn/sharing-state-between-components) any further than necessary. For example, don’t keep transient state like forms and whether an item is hovered at the top of your tree or in a global state library.\n3. Keep your [rendering logic pure.](https://react.dev/learn/keeping-components-pure) If re-rendering a component causes a problem or produces some noticeable visual artifact, it’s a bug in your component! Fix the bug instead of adding memoization.\n4. Avoid [unnecessary Effects that update state.](https://react.dev/learn/you-might-not-need-an-effect) Most performance problems in React apps are caused by chains of updates originating from Effects that cause your components to render over and over.\n5. Try to [remove unnecessary dependencies from your Effects.](https://react.dev/learn/removing-effect-dependencies) For example, instead of memoization, it’s often simpler to move some object or a function inside an Effect or outside the component.\n\nIf a specific interaction still feels laggy, [use the React Developer Tools profiler](https://legacy.reactjs.org/blog/2018/09/10/introducing-the-react-profiler.html) to see which components would benefit the most from memoization, and add memoization where needed. These principles make your components easier to debug and understand, so it’s good to follow them in any case. In the long term, we’re researching [doing granular memoization automatically](https://www.youtube.com/watch?v=lGEMwh32soc) to solve this once and for all.\n\n* * *\n\n### Updating a memoized component using state[](https://react.dev/reference/react/memo#updating-a-memoized-component-using-state \"Link for Updating a memoized component using state \")\n\nEven when a component is memoized, it will still re-render when its own state changes. Memoization only has to do with props that are passed to the component from its parent.\n\nimport { memo, useState } from 'react';\n\nexport default function MyApp() {\n const \\[name, setName\\] = useState('');\n const \\[address, setAddress\\] = useState('');\n return (\n <\\>\n <label\\>\n Name{': '}\n <input value\\={name} onChange\\={e \\=> setName(e.target.value)} />\n </label\\>\n <label\\>\n Address{': '}\n <input value\\={address} onChange\\={e \\=> setAddress(e.target.value)} />\n </label\\>\n <Greeting name\\={name} />\n </\\>\n );\n}\n\nconst Greeting = memo(function Greeting({ name }) {\n console.log('Greeting was rendered at', new Date().toLocaleTimeString());\n const \\[greeting, setGreeting\\] = useState('Hello');\n return (\n <\\>\n <h3\\>{greeting}{name && ', '}{name}!</h3\\>\n <GreetingSelector value\\={greeting} onChange\\={setGreeting} />\n </\\>\n );\n});\n\nfunction GreetingSelector({ value, onChange }) {\n return (\n <\\>\n <label\\>\n <input\n type\\=\"radio\"\n checked\\={value === 'Hello'}\n onChange\\={e \\=> onChange('Hello')}\n />\n Regular greeting\n </label\\>\n <label\\>\n <input\n type\\=\"radio\"\n checked\\={value === 'Hello and welcome'}\n onChange\\={e \\=> onChange('Hello and welcome')}\n />\n Enthusiastic greeting\n </label\\>\n </\\>\n );\n}\n\nIf you set a state variable to its current value, React will skip re-rendering your component even without `memo`. You may still see your component function being called an extra time, but the result will be discarded.\n\n* * *\n\n### Updating a memoized component using a context[](https://react.dev/reference/react/memo#updating-a-memoized-component-using-a-context \"Link for Updating a memoized component using a context \")\n\nEven when a component is memoized, it will still re-render when a context that it’s using changes. Memoization only has to do with props that are passed to the component from its parent.\n\nimport { createContext, memo, useContext, useState } from 'react';\n\nconst ThemeContext = createContext(null);\n\nexport default function MyApp() {\n const \\[theme, setTheme\\] = useState('dark');\n\n function handleClick() {\n setTheme(theme === 'dark' ? 'light' : 'dark'); \n }\n\n return (\n <ThemeContext.Provider value\\={theme}\\>\n <button onClick\\={handleClick}\\>\n Switch theme\n </button\\>\n <Greeting name\\=\"Taylor\" />\n </ThemeContext.Provider\\>\n );\n}\n\nconst Greeting = memo(function Greeting({ name }) {\n console.log(\"Greeting was rendered at\", new Date().toLocaleTimeString());\n const theme = useContext(ThemeContext);\n return (\n <h3 className\\={theme}\\>Hello, {name}!</h3\\>\n );\n});\n\nTo make your component re-render only when a _part_ of some context changes, split your component in two. Read what you need from the context in the outer component, and pass it down to a memoized child as a prop.\n\n* * *\n\n### Minimizing props changes[](https://react.dev/reference/react/memo#minimizing-props-changes \"Link for Minimizing props changes \")\n\nWhen you use `memo`, your component re-renders whenever any prop is not _shallowly equal_ to what it was previously. This means that React compares every prop in your component with its previous value using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. Note that `Object.is(3, 3)` is `true`, but `Object.is({}, {})` is `false`.\n\nTo get the most out of `memo`, minimize the times that the props change. For example, if the prop is an object, prevent the parent component from re-creating that object every time by using [`useMemo`:](https://react.dev/reference/react/useMemo)\n\n```\nfunction Page() {const [name, setName] = useState('Taylor');const [age, setAge] = useState(42);const person = useMemo(() => ({ name, age }),[name, age]);return <Profile person={person} />;}const Profile = memo(function Profile({ person }) {// ...});\n```\n\nA better way to minimize props changes is to make sure the component accepts the minimum necessary information in its props. For example, it could accept individual values instead of a whole object:\n\n```\nfunction Page() {const [name, setName] = useState('Taylor');const [age, setAge] = useState(42);return <Profile name={name} age={age} />;}const Profile = memo(function Profile({ name, age }) {// ...});\n```\n\nEven individual values can sometimes be projected to ones that change less frequently. For example, here a component accepts a boolean indicating the presence of a value rather than the value itself:\n\n```\nfunction GroupsLanding({ person }) {const hasGroups = person.groups !== null;return <CallToAction hasGroups={hasGroups} />;}const CallToAction = memo(function CallToAction({ hasGroups }) {// ...});\n```\n\nWhen you need to pass a function to memoized component, either declare it outside your component so that it never changes, or [`useCallback`](https://react.dev/reference/react/useCallback#skipping-re-rendering-of-components) to cache its definition between re-renders.\n\n* * *\n\n### Specifying a custom comparison function[](https://react.dev/reference/react/memo#specifying-a-custom-comparison-function \"Link for Specifying a custom comparison function \")\n\nIn rare cases it may be infeasible to minimize the props changes of a memoized component. In that case, you can provide a custom comparison function, which React will use to compare the old and new props instead of using shallow equality. This function is passed as a second argument to `memo`. It should return `true` only if the new props would result in the same output as the old props; otherwise it should return `false`.\n\n```\nconst Chart = memo(function Chart({ dataPoints }) {// ...}, arePropsEqual);function arePropsEqual(oldProps, newProps) {return (oldProps.dataPoints.length === newProps.dataPoints.length &&oldProps.dataPoints.every((oldPoint, index) => {const newPoint = newProps.dataPoints[index];return oldPoint.x === newPoint.x && oldPoint.y === newPoint.y;}));}\n```\n\nIf you do this, use the Performance panel in your browser developer tools to make sure that your comparison function is actually faster than re-rendering the component. You might be surprised.\n\nWhen you do performance measurements, make sure that React is running in the production mode.\n\n### Pitfall\n\nIf you provide a custom `arePropsEqual` implementation, **you must compare every prop, including functions.** Functions often [close over](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures) the props and state of parent components. If you return `true` when `oldProps.onClick !== newProps.onClick`, your component will keep “seeing” the props and state from a previous render inside its `onClick` handler, leading to very confusing bugs.\n\nAvoid doing deep equality checks inside `arePropsEqual` unless you are 100% sure that the data structure you’re working with has a known limited depth. **Deep equality checks can become incredibly slow** and can freeze your app for many seconds if someone changes the data structure later.\n\n* * *\n\nTroubleshooting[](https://react.dev/reference/react/memo#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------\n\n### My component re-renders when a prop is an object, array, or function[](https://react.dev/reference/react/memo#my-component-rerenders-when-a-prop-is-an-object-or-array \"Link for My component re-renders when a prop is an object, array, or function \")\n\nReact compares old and new props by shallow equality: that is, it considers whether each new prop is reference-equal to the old prop. If you create a new object or array each time the parent is re-rendered, even if the individual elements are each the same, React will still consider it to be changed. Similarly, if you create a new function when rendering the parent component, React will consider it to have changed even if the function has the same definition. To avoid this, [simplify props or memoize props in the parent component](https://react.dev/reference/react/memo#minimizing-props-changes).\n",
"filename": "memo.md",
"package": "react"
} |
{
"content": "Title: <meta> – React\n\nURL Source: https://react.dev/reference/react-dom/components/meta\n\nMarkdown Content:\nReact\nv18.3.1\nSearch\nCtrl\nK\nLearn\nReference\nCommunity\nBlog\nreact@18.3.1\nOverview\nHooks\nComponents\nAPIs\nreact-dom@18.3.1\nHooks\nComponents\nCommon (e.g. <div>)\n<form> \n<input>\n<option>\n<progress>\n<select>\n<textarea>\n<link> \n<meta> \n<script> \n<style> \n<title> \nAPIs\nClient APIs\nServer APIs\nRules of React\nOverview\nReact Server Components\nServer Components \nServer Actions \nDirectives \nLegacy APIs\nLegacy React APIs\n\nIs this page useful?\n\nAPI REFERENCE\nCOMPONENTS\n<meta>\nCanary\n\nReact’s extensions to <meta> are currently only available in React’s canary and experimental channels. In stable releases of React <meta> works only as a built-in browser HTML component. Learn more about React’s release channels here.\n\nThe built-in browser <meta> component lets you add metadata to the document.\n\n<meta name=\"keywords\" content=\"React, JavaScript, semantic markup, html\" />\nReference\n<meta>\nUsage\nAnnotating the document with metadata\nAnnotating specific items within the document with metadata\nReference \n<meta> \n\nTo add document metadata, render the built-in browser <meta> component. You can render <meta> from any component and React will always place the corresponding DOM element in the document head.\n\n<meta name=\"keywords\" content=\"React, JavaScript, semantic markup, html\" />\n\nSee more examples below.\n\nProps \n\n<meta> supports all common element props.\n\nIt should have exactly one of the following props: name, httpEquiv, charset, itemProp. The <meta> component does something different depending on which of these props is specified.\n\nname: a string. Specifies the kind of metadata to be attached to the document.\ncharset: a string. Specifies the character set used by the document. The only valid value is \"utf-8\".\nhttpEquiv: a string. Specifies a directive for processing the document.\nitemProp: a string. Specifies metadata about a particular item within the document rather than the document as a whole.\ncontent: a string. Specifies the metadata to be attached when used with the name or itemProp props or the behavior of the directive when used with the httpEquiv prop.\nSpecial rendering behavior \n\nReact will always place the DOM element corresponding to the <meta> component within the document’s <head>, regardless of where in the React tree it is rendered. The <head> is the only valid place for <meta> to exist within the DOM, yet it’s convenient and keeps things composable if a component representing a specific page can render <meta> components itself.\n\nThere is one exception to this: if <meta> has an itemProp prop, there is no special behavior, because in this case it doesn’t represent metadata about the document but rather metadata about a specific part of the page.\n\nUsage \nAnnotating the document with metadata \n\nYou can annotate the document with metadata such as keywords, a summary, or the author’s name. React will place this metadata within the document <head> regardless of where in the React tree it is rendered.\n\n<meta name=\"author\" content=\"John Smith\" />\n\n<meta name=\"keywords\" content=\"React, JavaScript, semantic markup, html\" />\n\n<meta name=\"description\" content=\"API reference for the <meta> component in React DOM\" />\n\nYou can render the <meta> component from any component. React will put a <meta> DOM node in the document <head>.\n\nApp.js\nShowRenderedHTML.js\nReset\nFork\nimport ShowRenderedHTML from './ShowRenderedHTML.js';\n\nexport default function SiteMapPage() {\n return (\n <ShowRenderedHTML>\n <meta name=\"keywords\" content=\"React\" />\n <meta name=\"description\" content=\"A site map for the React website\" />\n <h1>Site Map</h1>\n <p>...</p>\n </ShowRenderedHTML>\n );\n}\n\n\nAnnotating specific items within the document with metadata \n\nYou can use the <meta> component with the itemProp prop to annotate specific items within the document with metadata. In this case, React will not place these annotations within the document <head> but will place them like any other React component.\n\n<section itemScope>\n\n <h3>Annotating specific items</h3>\n\n <meta itemProp=\"description\" content=\"API reference for using <meta> with itemProp\" />\n\n <p>...</p>\n\n</section>\nPREVIOUS\n<link>\nNEXT\n<script>\n©2024\nuwu?\nLearn React\nQuick Start\nInstallation\nDescribing the UI\nAdding Interactivity\nManaging State\nEscape Hatches\nAPI Reference\nReact APIs\nReact DOM APIs\nCommunity\nCode of Conduct\nMeet the Team\nDocs Contributors\nAcknowledgements\nMore\nBlog\nReact Native\nPrivacy\nTerms\n",
"filename": "meta.md",
"package": "react"
} |
{
"content": "Title: <option> – React\n\nURL Source: https://react.dev/reference/react-dom/components/option\n\nMarkdown Content:\nReact\nv18.3.1\nSearch\nCtrl\nK\nLearn\nReference\nCommunity\nBlog\nreact@18.3.1\nOverview\nHooks\nComponents\nAPIs\nreact-dom@18.3.1\nHooks\nComponents\nCommon (e.g. <div>)\n<form> \n<input>\n<option>\n<progress>\n<select>\n<textarea>\n<link> \n<meta> \n<script> \n<style> \n<title> \nAPIs\nClient APIs\nServer APIs\nRules of React\nOverview\nReact Server Components\nServer Components \nServer Actions \nDirectives \nLegacy APIs\nLegacy React APIs\n\nIs this page useful?\n\nAPI REFERENCE\nCOMPONENTS\n<option>\n\nThe built-in browser <option> component lets you render an option inside a <select> box.\n\n<select>\n\n <option value=\"someOption\">Some option</option>\n\n <option value=\"otherOption\">Other option</option>\n\n</select>\nReference\n<option>\nUsage\nDisplaying a select box with options\nReference \n<option> \n\nThe built-in browser <option> component lets you render an option inside a <select> box.\n\n<select>\n\n <option value=\"someOption\">Some option</option>\n\n <option value=\"otherOption\">Other option</option>\n\n</select>\n\nSee more examples below.\n\nProps \n\n<option> supports all common element props.\n\nAdditionally, <option> supports these props:\n\ndisabled: A boolean. If true, the option will not be selectable and will appear dimmed.\nlabel: A string. Specifies the meaning of the option. If not specified, the text inside the option is used.\nvalue: The value to be used when submitting the parent <select> in a form if this option is selected.\nCaveats \nReact does not support the selected attribute on <option>. Instead, pass this option’s value to the parent <select defaultValue> for an uncontrolled select box, or <select value> for a controlled select.\nUsage \nDisplaying a select box with options \n\nRender a <select> with a list of <option> components inside to display a select box. Give each <option> a value representing the data to be submitted with the form.\n\nRead more about displaying a <select> with a list of <option> components.\n\nApp.js\nDownload\nReset\nFork\nexport default function FruitPicker() {\n return (\n <label>\n Pick a fruit:\n <select name=\"selectedFruit\">\n <option value=\"apple\">Apple</option>\n <option value=\"banana\">Banana</option>\n <option value=\"orange\">Orange</option>\n </select>\n </label>\n );\n}\n\n\nPREVIOUS\n<input>\nNEXT\n<progress>\n©2024\nuwu?\nLearn React\nQuick Start\nInstallation\nDescribing the UI\nAdding Interactivity\nManaging State\nEscape Hatches\nAPI Reference\nReact APIs\nReact DOM APIs\nCommunity\nCode of Conduct\nMeet the Team\nDocs Contributors\nAcknowledgements\nMore\nBlog\nReact Native\nPrivacy\nTerms\n",
"filename": "option.md",
"package": "react"
} |
{
"content": "Title: Passing Data Deeply with Context – React\n\nURL Source: https://react.dev/learn/passing-data-deeply-with-context\n\nMarkdown Content:\nUsually, you will pass information from a parent component to a child component via props. But passing props can become verbose and inconvenient if you have to pass them through many components in the middle, or if many components in your app need the same information. _Context_ lets the parent component make some information available to any component in the tree below it—no matter how deep—without passing it explicitly through props.\n\n### You will learn\n\n* What “prop drilling” is\n* How to replace repetitive prop passing with context\n* Common use cases for context\n* Common alternatives to context\n\nThe problem with passing props[](https://react.dev/learn/passing-data-deeply-with-context#the-problem-with-passing-props \"Link for The problem with passing props \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\n[Passing props](https://react.dev/learn/passing-props-to-a-component) is a great way to explicitly pipe data through your UI tree to the components that use it.\n\nBut passing props can become verbose and inconvenient when you need to pass some prop deeply through the tree, or if many components need the same prop. The nearest common ancestor could be far removed from the components that need data, and [lifting state up](https://react.dev/learn/sharing-state-between-components) that high can lead to a situation called “prop drilling”.\n\nLifting state up\n\n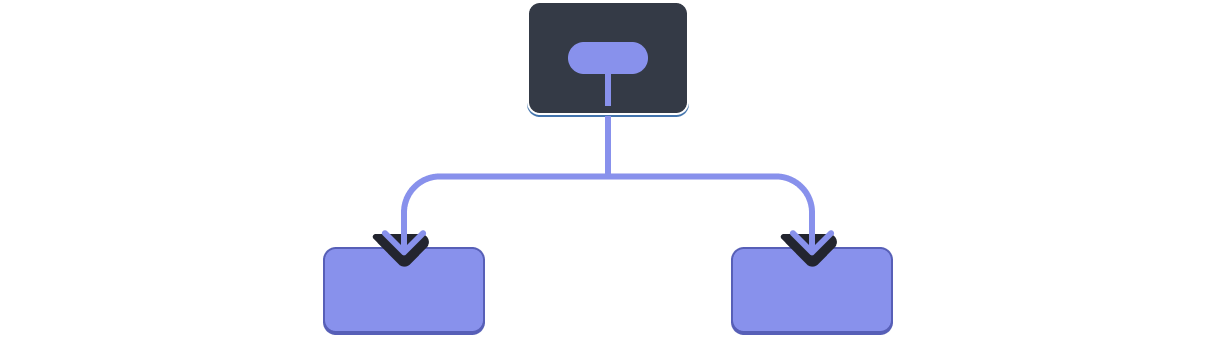\n\n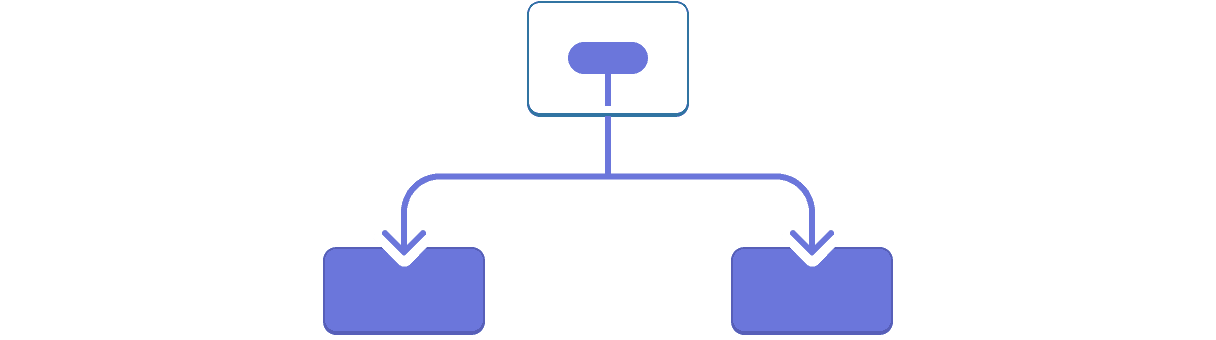\n\nProp drilling\n\n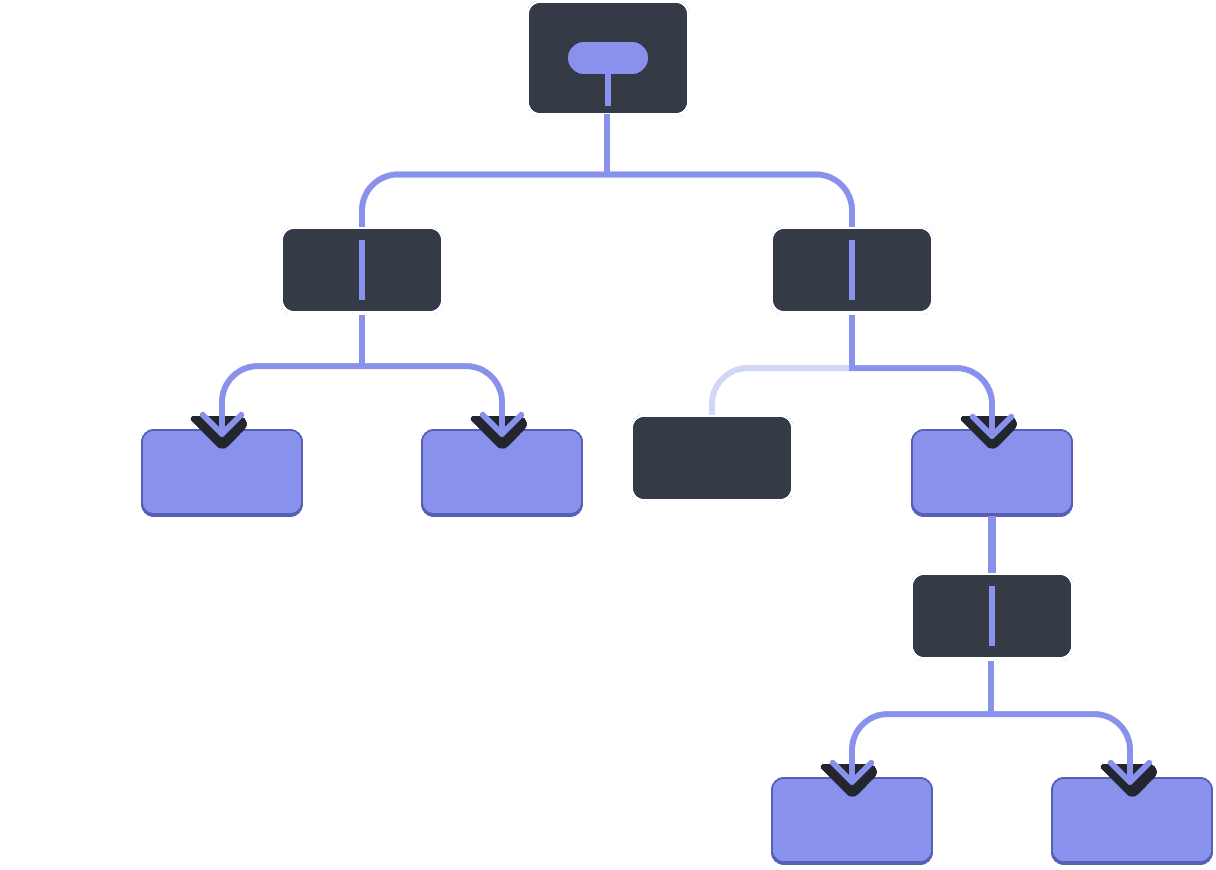\n\n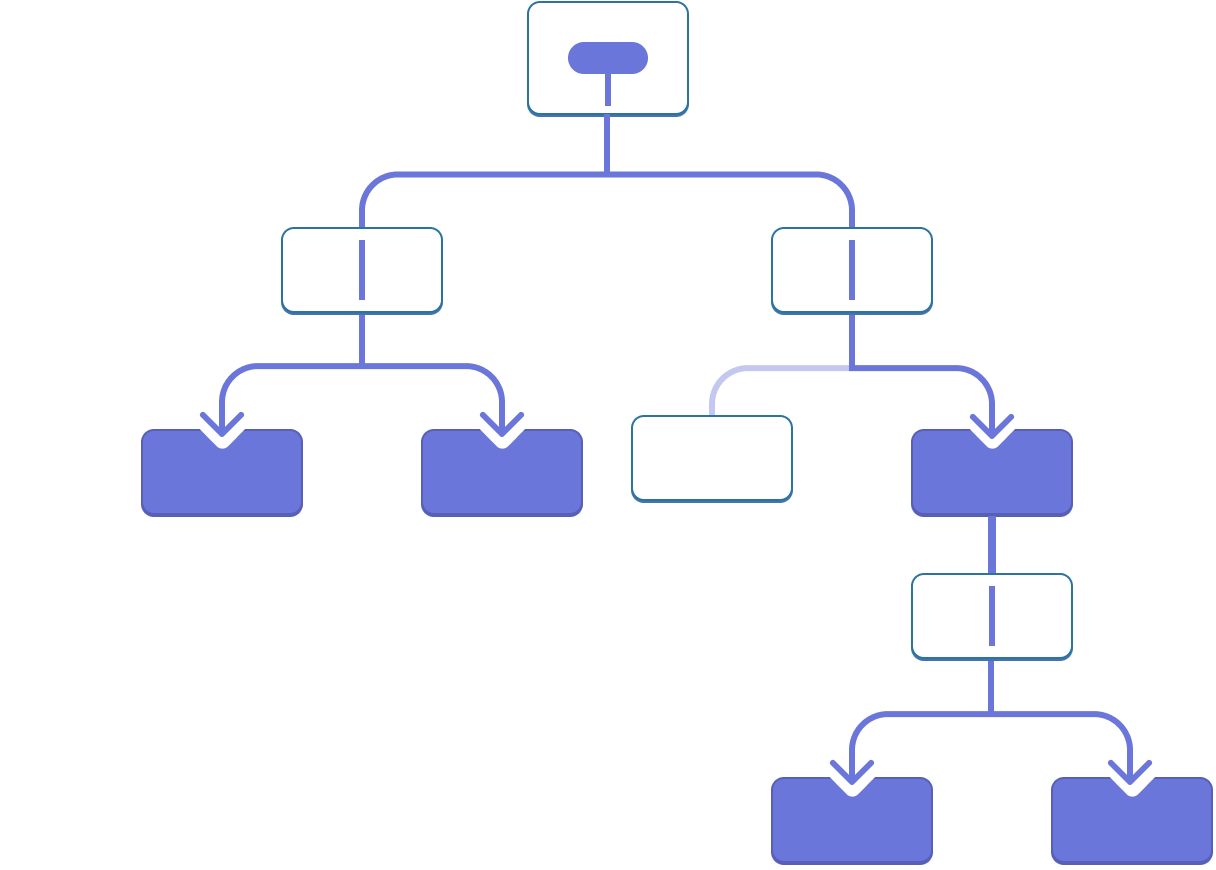\n\nWouldn’t it be great if there were a way to “teleport” data to the components in the tree that need it without passing props? With React’s context feature, there is!\n\nContext: an alternative to passing props[](https://react.dev/learn/passing-data-deeply-with-context#context-an-alternative-to-passing-props \"Link for Context: an alternative to passing props \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nContext lets a parent component provide data to the entire tree below it. There are many uses for context. Here is one example. Consider this `Heading` component that accepts a `level` for its size:\n\nLet’s say you want multiple headings within the same `Section` to always have the same size:\n\nimport Heading from './Heading.js';\nimport Section from './Section.js';\n\nexport default function Page() {\n return (\n <Section\\>\n <Heading level\\={1}\\>Title</Heading\\>\n <Section\\>\n <Heading level\\={2}\\>Heading</Heading\\>\n <Heading level\\={2}\\>Heading</Heading\\>\n <Heading level\\={2}\\>Heading</Heading\\>\n <Section\\>\n <Heading level\\={3}\\>Sub-heading</Heading\\>\n <Heading level\\={3}\\>Sub-heading</Heading\\>\n <Heading level\\={3}\\>Sub-heading</Heading\\>\n <Section\\>\n <Heading level\\={4}\\>Sub-sub-heading</Heading\\>\n <Heading level\\={4}\\>Sub-sub-heading</Heading\\>\n <Heading level\\={4}\\>Sub-sub-heading</Heading\\>\n </Section\\>\n </Section\\>\n </Section\\>\n </Section\\>\n );\n}\n\nCurrently, you pass the `level` prop to each `<Heading>` separately:\n\n```\n<Section><Heading level={3}>About</Heading><Heading level={3}>Photos</Heading><Heading level={3}>Videos</Heading></Section>\n```\n\nIt would be nice if you could pass the `level` prop to the `<Section>` component instead and remove it from the `<Heading>`. This way you could enforce that all headings in the same section have the same size:\n\n```\n<Section level={3}><Heading>About</Heading><Heading>Photos</Heading><Heading>Videos</Heading></Section>\n```\n\nBut how can the `<Heading>` component know the level of its closest `<Section>`? **That would require some way for a child to “ask” for data from somewhere above in the tree.**\n\nYou can’t do it with props alone. This is where context comes into play. You will do it in three steps:\n\n1. **Create** a context. (You can call it `LevelContext`, since it’s for the heading level.)\n2. **Use** that context from the component that needs the data. (`Heading` will use `LevelContext`.)\n3. **Provide** that context from the component that specifies the data. (`Section` will provide `LevelContext`.)\n\nContext lets a parent—even a distant one!—provide some data to the entire tree inside of it.\n\nUsing context in close children\n\n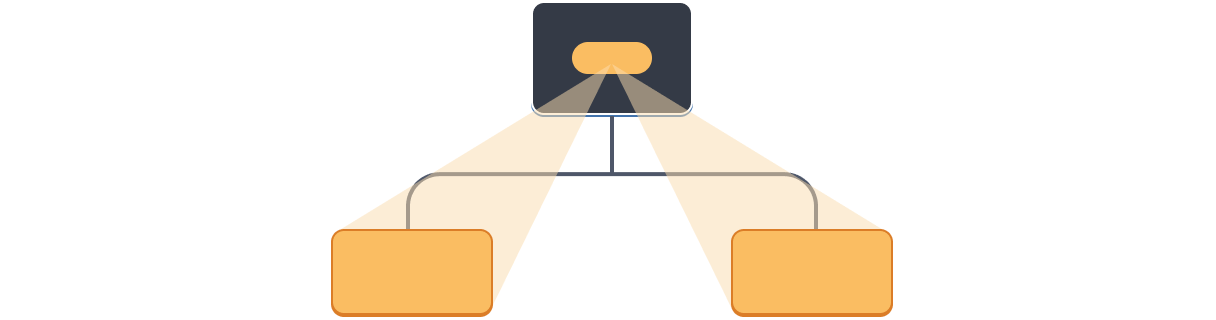\n\n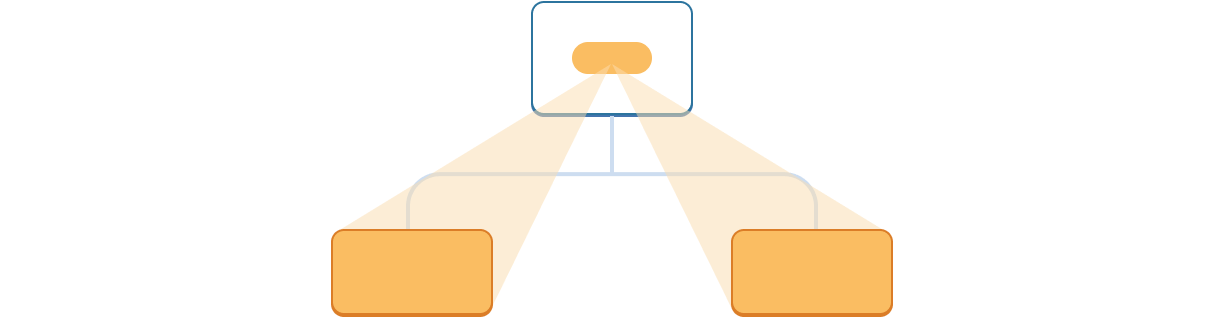\n\nUsing context in distant children\n\n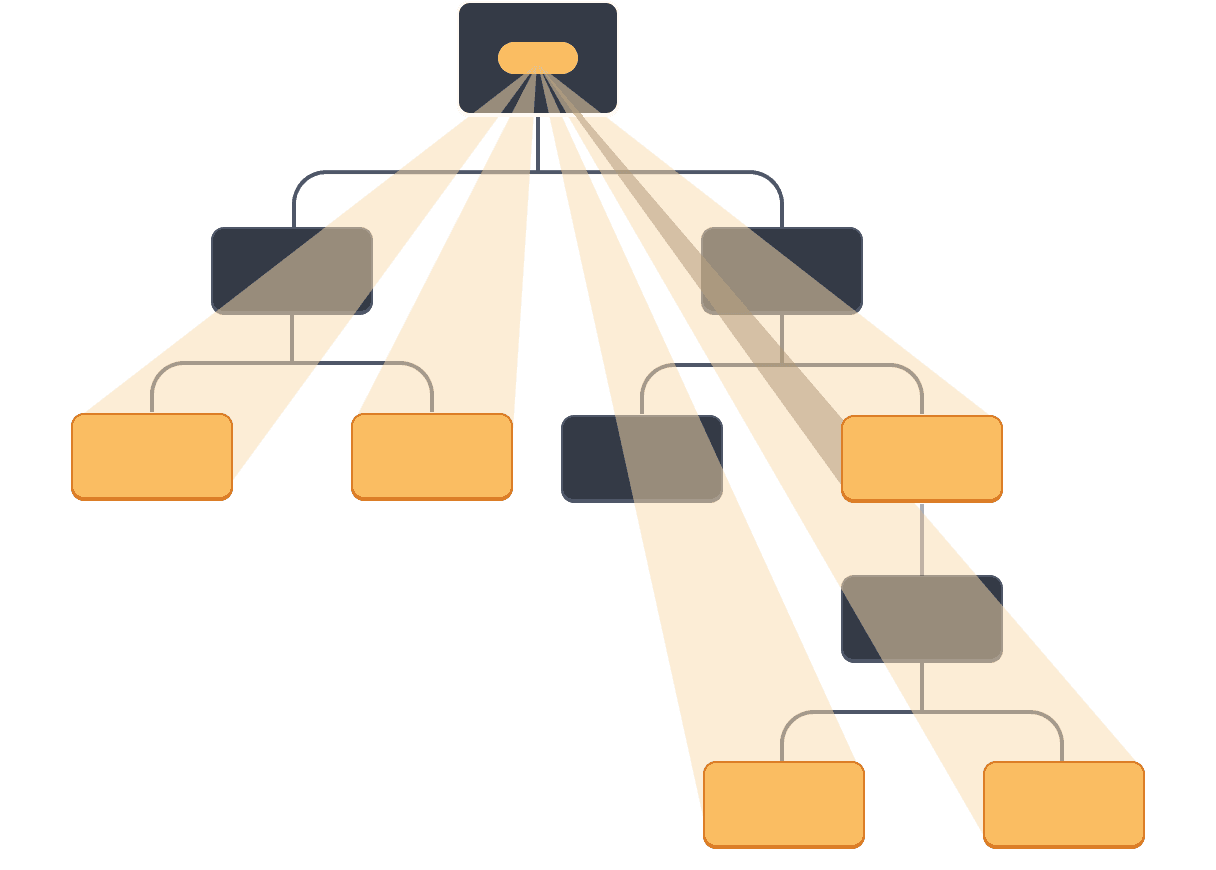\n\n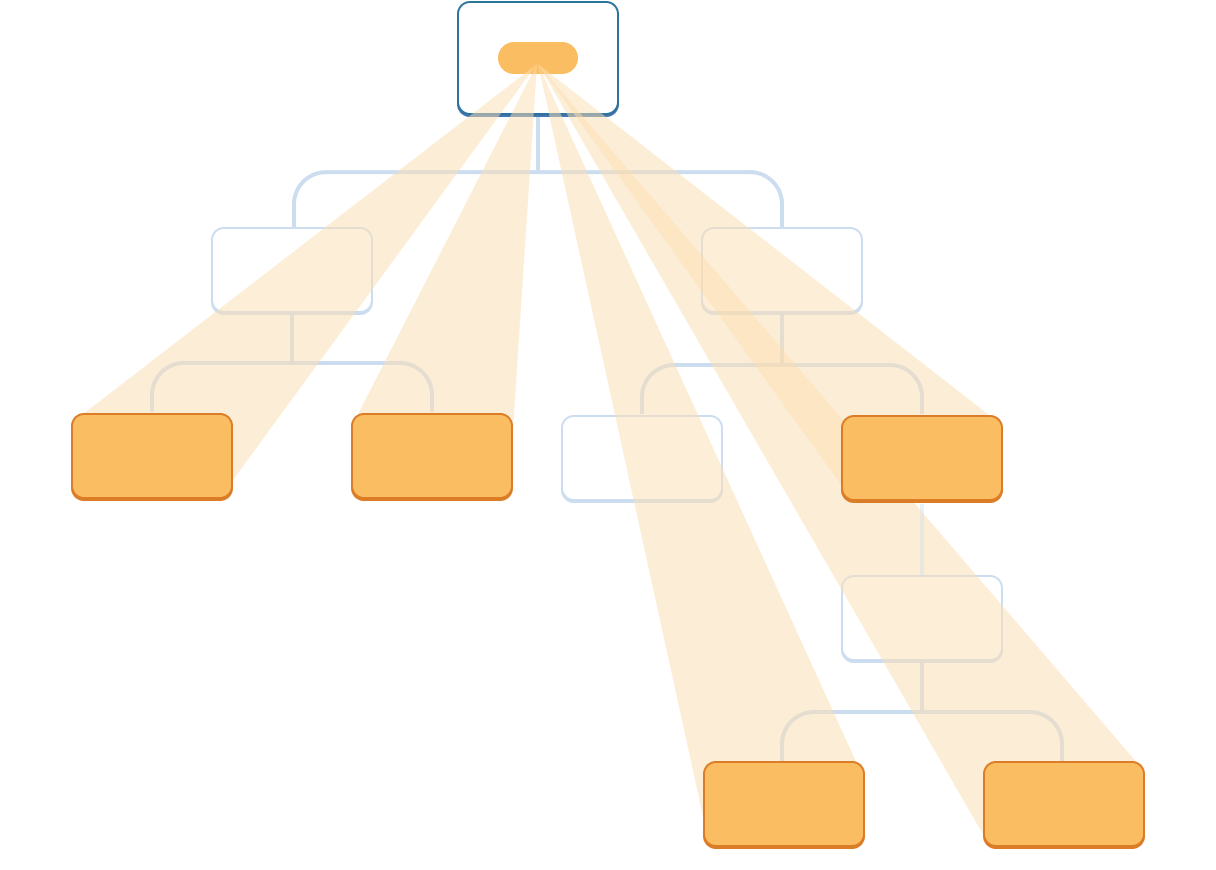\n\n### Step 1: Create the context[](https://react.dev/learn/passing-data-deeply-with-context#step-1-create-the-context \"Link for Step 1: Create the context \")\n\nFirst, you need to create the context. You’ll need to **export it from a file** so that your components can use it:\n\nThe only argument to `createContext` is the _default_ value. Here, `1` refers to the biggest heading level, but you could pass any kind of value (even an object). You will see the significance of the default value in the next step.\n\n### Step 2: Use the context[](https://react.dev/learn/passing-data-deeply-with-context#step-2-use-the-context \"Link for Step 2: Use the context \")\n\nImport the `useContext` Hook from React and your context:\n\n```\nimport { useContext } from 'react';import { LevelContext } from './LevelContext.js';\n```\n\nCurrently, the `Heading` component reads `level` from props:\n\n```\nexport default function Heading({ level, children }) {// ...}\n```\n\nInstead, remove the `level` prop and read the value from the context you just imported, `LevelContext`:\n\n```\nexport default function Heading({ children }) {const level = useContext(LevelContext);// ...}\n```\n\n`useContext` is a Hook. Just like `useState` and `useReducer`, you can only call a Hook immediately inside a React component (not inside loops or conditions). **`useContext` tells React that the `Heading` component wants to read the `LevelContext`.**\n\nNow that the `Heading` component doesn’t have a `level` prop, you don’t need to pass the level prop to `Heading` in your JSX like this anymore:\n\n```\n<Section><Heading level={4}>Sub-sub-heading</Heading><Heading level={4}>Sub-sub-heading</Heading><Heading level={4}>Sub-sub-heading</Heading></Section>\n```\n\nUpdate the JSX so that it’s the `Section` that receives it instead:\n\n```\n<Section level={4}><Heading>Sub-sub-heading</Heading><Heading>Sub-sub-heading</Heading><Heading>Sub-sub-heading</Heading></Section>\n```\n\nAs a reminder, this is the markup that you were trying to get working:\n\nimport Heading from './Heading.js';\nimport Section from './Section.js';\n\nexport default function Page() {\n return (\n <Section level\\={1}\\>\n <Heading\\>Title</Heading\\>\n <Section level\\={2}\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Section level\\={3}\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Section level\\={4}\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n </Section\\>\n </Section\\>\n </Section\\>\n </Section\\>\n );\n}\n\nNotice this example doesn’t quite work, yet! All the headings have the same size because **even though you’re _using_ the context, you have not _provided_ it yet.** React doesn’t know where to get it!\n\nIf you don’t provide the context, React will use the default value you’ve specified in the previous step. In this example, you specified `1` as the argument to `createContext`, so `useContext(LevelContext)` returns `1`, setting all those headings to `<h1>`. Let’s fix this problem by having each `Section` provide its own context.\n\n### Step 3: Provide the context[](https://react.dev/learn/passing-data-deeply-with-context#step-3-provide-the-context \"Link for Step 3: Provide the context \")\n\nThe `Section` component currently renders its children:\n\n```\nexport default function Section({ children }) {return (<section className=\"section\">{children}</section>);}\n```\n\n**Wrap them with a context provider** to provide the `LevelContext` to them:\n\n```\nimport { LevelContext } from './LevelContext.js';export default function Section({ level, children }) {return (<section className=\"section\"><LevelContext.Provider value={level}>{children}</LevelContext.Provider></section>);}\n```\n\nThis tells React: “if any component inside this `<Section>` asks for `LevelContext`, give them this `level`.” The component will use the value of the nearest `<LevelContext.Provider>` in the UI tree above it.\n\nimport Heading from './Heading.js';\nimport Section from './Section.js';\n\nexport default function Page() {\n return (\n <Section level\\={1}\\>\n <Heading\\>Title</Heading\\>\n <Section level\\={2}\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Section level\\={3}\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Section level\\={4}\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n </Section\\>\n </Section\\>\n </Section\\>\n </Section\\>\n );\n}\n\nIt’s the same result as the original code, but you did not need to pass the `level` prop to each `Heading` component! Instead, it “figures out” its heading level by asking the closest `Section` above:\n\n1. You pass a `level` prop to the `<Section>`.\n2. `Section` wraps its children into `<LevelContext.Provider value={level}>`.\n3. `Heading` asks the closest value of `LevelContext` above with `useContext(LevelContext)`.\n\nUsing and providing context from the same component[](https://react.dev/learn/passing-data-deeply-with-context#using-and-providing-context-from-the-same-component \"Link for Using and providing context from the same component \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nCurrently, you still have to specify each section’s `level` manually:\n\n```\nexport default function Page() {return (<Section level={1}> ...<Section level={2}> ...<Section level={3}> ...\n```\n\nSince context lets you read information from a component above, each `Section` could read the `level` from the `Section` above, and pass `level + 1` down automatically. Here is how you could do it:\n\n```\nimport { useContext } from 'react';import { LevelContext } from './LevelContext.js';export default function Section({ children }) {const level = useContext(LevelContext);return (<section className=\"section\"><LevelContext.Provider value={level + 1}>{children}</LevelContext.Provider></section>);}\n```\n\nWith this change, you don’t need to pass the `level` prop _either_ to the `<Section>` or to the `<Heading>`:\n\nimport Heading from './Heading.js';\nimport Section from './Section.js';\n\nexport default function Page() {\n return (\n <Section\\>\n <Heading\\>Title</Heading\\>\n <Section\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Heading\\>Heading</Heading\\>\n <Section\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Heading\\>Sub-heading</Heading\\>\n <Section\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n <Heading\\>Sub-sub-heading</Heading\\>\n </Section\\>\n </Section\\>\n </Section\\>\n </Section\\>\n );\n}\n\nNow both `Heading` and `Section` read the `LevelContext` to figure out how “deep” they are. And the `Section` wraps its children into the `LevelContext` to specify that anything inside of it is at a “deeper” level.\n\n### Note\n\nThis example uses heading levels because they show visually how nested components can override context. But context is useful for many other use cases too. You can pass down any information needed by the entire subtree: the current color theme, the currently logged in user, and so on.\n\nContext passes through intermediate components[](https://react.dev/learn/passing-data-deeply-with-context#context-passes-through-intermediate-components \"Link for Context passes through intermediate components \")\n--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou can insert as many components as you like between the component that provides context and the one that uses it. This includes both built-in components like `<div>` and components you might build yourself.\n\nIn this example, the same `Post` component (with a dashed border) is rendered at two different nesting levels. Notice that the `<Heading>` inside of it gets its level automatically from the closest `<Section>`:\n\nimport Heading from './Heading.js';\nimport Section from './Section.js';\n\nexport default function ProfilePage() {\n return (\n <Section\\>\n <Heading\\>My Profile</Heading\\>\n <Post\n title\\=\"Hello traveller!\"\n body\\=\"Read about my adventures.\"\n />\n <AllPosts />\n </Section\\>\n );\n}\n\nfunction AllPosts() {\n return (\n <Section\\>\n <Heading\\>Posts</Heading\\>\n <RecentPosts />\n </Section\\>\n );\n}\n\nfunction RecentPosts() {\n return (\n <Section\\>\n <Heading\\>Recent Posts</Heading\\>\n <Post\n title\\=\"Flavors of Lisbon\"\n body\\=\"...those pastéis de nata!\"\n />\n <Post\n title\\=\"Buenos Aires in the rhythm of tango\"\n body\\=\"I loved it!\"\n />\n </Section\\>\n );\n}\n\nfunction Post({ title, body }) {\n return (\n <Section isFancy\\={true}\\>\n <Heading\\>\n {title}\n </Heading\\>\n <p\\><i\\>{body}</i\\></p\\>\n </Section\\>\n );\n}\n\nYou didn’t do anything special for this to work. A `Section` specifies the context for the tree inside it, so you can insert a `<Heading>` anywhere, and it will have the correct size. Try it in the sandbox above!\n\n**Context lets you write components that “adapt to their surroundings” and display themselves differently depending on _where_ (or, in other words, _in which context_) they are being rendered.**\n\nHow context works might remind you of [CSS property inheritance.](https://developer.mozilla.org/en-US/docs/Web/CSS/inheritance) In CSS, you can specify `color: blue` for a `<div>`, and any DOM node inside of it, no matter how deep, will inherit that color unless some other DOM node in the middle overrides it with `color: green`. Similarly, in React, the only way to override some context coming from above is to wrap children into a context provider with a different value.\n\nIn CSS, different properties like `color` and `background-color` don’t override each other. You can set all `<div>`’s `color` to red without impacting `background-color`. Similarly, **different React contexts don’t override each other.** Each context that you make with `createContext()` is completely separate from other ones, and ties together components using and providing _that particular_ context. One component may use or provide many different contexts without a problem.\n\nBefore you use context[](https://react.dev/learn/passing-data-deeply-with-context#before-you-use-context \"Link for Before you use context \")\n--------------------------------------------------------------------------------------------------------------------------------------------\n\nContext is very tempting to use! However, this also means it’s too easy to overuse it. **Just because you need to pass some props several levels deep doesn’t mean you should put that information into context.**\n\nHere’s a few alternatives you should consider before using context:\n\n1. **Start by [passing props.](https://react.dev/learn/passing-props-to-a-component)** If your components are not trivial, it’s not unusual to pass a dozen props down through a dozen components. It may feel like a slog, but it makes it very clear which components use which data! The person maintaining your code will be glad you’ve made the data flow explicit with props.\n2. **Extract components and [pass JSX as `children`](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children) to them.** If you pass some data through many layers of intermediate components that don’t use that data (and only pass it further down), this often means that you forgot to extract some components along the way. For example, maybe you pass data props like `posts` to visual components that don’t use them directly, like `<Layout posts={posts} />`. Instead, make `Layout` take `children` as a prop, and render `<Layout><Posts posts={posts} /></Layout>`. This reduces the number of layers between the component specifying the data and the one that needs it.\n\nIf neither of these approaches works well for you, consider context.\n\nUse cases for context[](https://react.dev/learn/passing-data-deeply-with-context#use-cases-for-context \"Link for Use cases for context \")\n-----------------------------------------------------------------------------------------------------------------------------------------\n\n* **Theming:** If your app lets the user change its appearance (e.g. dark mode), you can put a context provider at the top of your app, and use that context in components that need to adjust their visual look.\n* **Current account:** Many components might need to know the currently logged in user. Putting it in context makes it convenient to read it anywhere in the tree. Some apps also let you operate multiple accounts at the same time (e.g. to leave a comment as a different user). In those cases, it can be convenient to wrap a part of the UI into a nested provider with a different current account value.\n* **Routing:** Most routing solutions use context internally to hold the current route. This is how every link “knows” whether it’s active or not. If you build your own router, you might want to do it too.\n* **Managing state:** As your app grows, you might end up with a lot of state closer to the top of your app. Many distant components below may want to change it. It is common to [use a reducer together with context](https://react.dev/learn/scaling-up-with-reducer-and-context) to manage complex state and pass it down to distant components without too much hassle.\n\nContext is not limited to static values. If you pass a different value on the next render, React will update all the components reading it below! This is why context is often used in combination with state.\n\nIn general, if some information is needed by distant components in different parts of the tree, it’s a good indication that context will help you.\n\nRecap[](https://react.dev/learn/passing-data-deeply-with-context#recap \"Link for Recap\")\n----------------------------------------------------------------------------------------\n\n* Context lets a component provide some information to the entire tree below it.\n* To pass context:\n 1. Create and export it with `export const MyContext = createContext(defaultValue)`.\n 2. Pass it to the `useContext(MyContext)` Hook to read it in any child component, no matter how deep.\n 3. Wrap children into `<MyContext.Provider value={...}>` to provide it from a parent.\n* Context passes through any components in the middle.\n* Context lets you write components that “adapt to their surroundings”.\n* Before you use context, try passing props or passing JSX as `children`.\n\nTry out some challenges[](https://react.dev/learn/passing-data-deeply-with-context#challenges \"Link for Try out some challenges\")\n---------------------------------------------------------------------------------------------------------------------------------\n\n#### Replace prop drilling with context[](https://react.dev/learn/passing-data-deeply-with-context#replace-prop-drilling-with-context \"Link for this heading\")\n\nIn this example, toggling the checkbox changes the `imageSize` prop passed to each `<PlaceImage>`. The checkbox state is held in the top-level `App` component, but each `<PlaceImage>` needs to be aware of it.\n\nCurrently, `App` passes `imageSize` to `List`, which passes it to each `Place`, which passes it to the `PlaceImage`. Remove the `imageSize` prop, and instead pass it from the `App` component directly to `PlaceImage`.\n\nYou can declare context in `Context.js`.\n\nimport { useState } from 'react';\nimport { places } from './data.js';\nimport { getImageUrl } from './utils.js';\n\nexport default function App() {\n const \\[isLarge, setIsLarge\\] = useState(false);\n const imageSize = isLarge ? 150 : 100;\n return (\n <\\>\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isLarge}\n onChange\\={e \\=> {\n setIsLarge(e.target.checked);\n }}\n />\n Use large images\n </label\\>\n <hr />\n <List imageSize\\={imageSize} />\n </\\>\n )\n}\n\nfunction List({ imageSize }) {\n const listItems = places.map(place \\=>\n <li key\\={place.id}\\>\n <Place\n place\\={place}\n imageSize\\={imageSize}\n />\n </li\\>\n );\n return <ul\\>{listItems}</ul\\>;\n}\n\nfunction Place({ place, imageSize }) {\n return (\n <\\>\n <PlaceImage\n place\\={place}\n imageSize\\={imageSize}\n />\n <p\\>\n <b\\>{place.name}</b\\>\n {': ' + place.description}\n </p\\>\n </\\>\n );\n}\n\nfunction PlaceImage({ place, imageSize }) {\n return (\n <img\n src\\={getImageUrl(place)}\n alt\\={place.name}\n width\\={imageSize}\n height\\={imageSize}\n />\n );\n}\n",
"filename": "passing-data-deeply-with-context.md",
"package": "react"
} |
{
"content": "Title: Passing Props to a Component – React\n\nURL Source: https://react.dev/learn/passing-props-to-a-component\n\nMarkdown Content:\nReact components use _props_ to communicate with each other. Every parent component can pass some information to its child components by giving them props. Props might remind you of HTML attributes, but you can pass any JavaScript value through them, including objects, arrays, and functions.\n\n### You will learn\n\n* How to pass props to a component\n* How to read props from a component\n* How to specify default values for props\n* How to pass some JSX to a component\n* How props change over time\n\nFamiliar props[](https://react.dev/learn/passing-props-to-a-component#familiar-props \"Link for Familiar props \")\n----------------------------------------------------------------------------------------------------------------\n\nProps are the information that you pass to a JSX tag. For example, `className`, `src`, `alt`, `width`, and `height` are some of the props you can pass to an `<img>`:\n\nThe props you can pass to an `<img>` tag are predefined (ReactDOM conforms to [the HTML standard](https://www.w3.org/TR/html52/semantics-embedded-content.html#the-img-element)). But you can pass any props to _your own_ components, such as `<Avatar>`, to customize them. Here’s how!\n\nPassing props to a component[](https://react.dev/learn/passing-props-to-a-component#passing-props-to-a-component \"Link for Passing props to a component \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn this code, the `Profile` component isn’t passing any props to its child component, `Avatar`:\n\n```\nexport default function Profile() {return (<Avatar />);}\n```\n\nYou can give `Avatar` some props in two steps.\n\n### Step 1: Pass props to the child component[](https://react.dev/learn/passing-props-to-a-component#step-1-pass-props-to-the-child-component \"Link for Step 1: Pass props to the child component \")\n\nFirst, pass some props to `Avatar`. For example, let’s pass two props: `person` (an object), and `size` (a number):\n\n```\nexport default function Profile() {return (<Avatarperson={{ name: 'Lin Lanying', imageId: '1bX5QH6' }}size={100}/>);}\n```\n\n### Note\n\nNow you can read these props inside the `Avatar` component.\n\n### Step 2: Read props inside the child component[](https://react.dev/learn/passing-props-to-a-component#step-2-read-props-inside-the-child-component \"Link for Step 2: Read props inside the child component \")\n\nYou can read these props by listing their names `person, size` separated by the commas inside `({` and `})` directly after `function Avatar`. This lets you use them inside the `Avatar` code, like you would with a variable.\n\n```\nfunction Avatar({ person, size }) {// person and size are available here}\n```\n\nAdd some logic to `Avatar` that uses the `person` and `size` props for rendering, and you’re done.\n\nNow you can configure `Avatar` to render in many different ways with different props. Try tweaking the values!\n\nimport { getImageUrl } from './utils.js';\n\nfunction Avatar({ person, size }) {\n return (\n <img\n className\\=\"avatar\"\n src\\={getImageUrl(person)}\n alt\\={person.name}\n width\\={size}\n height\\={size}\n />\n );\n}\n\nexport default function Profile() {\n return (\n <div\\>\n <Avatar\n size\\={100}\n person\\={{ \n name: 'Katsuko Saruhashi', \n imageId: 'YfeOqp2'\n }}\n />\n <Avatar\n size\\={80}\n person\\={{\n name: 'Aklilu Lemma', \n imageId: 'OKS67lh'\n }}\n />\n <Avatar\n size\\={50}\n person\\={{ \n name: 'Lin Lanying',\n imageId: '1bX5QH6'\n }}\n />\n </div\\>\n );\n}\n\nProps let you think about parent and child components independently. For example, you can change the `person` or the `size` props inside `Profile` without having to think about how `Avatar` uses them. Similarly, you can change how the `Avatar` uses these props, without looking at the `Profile`.\n\nYou can think of props like “knobs” that you can adjust. They serve the same role as arguments serve for functions—in fact, props _are_ the only argument to your component! React component functions accept a single argument, a `props` object:\n\n```\nfunction Avatar(props) {let person = props.person;let size = props.size;// ...}\n```\n\nUsually you don’t need the whole `props` object itself, so you destructure it into individual props.\n\n### Pitfall\n\n**Don’t miss the pair of `{` and `}` curlies** inside of `(` and `)` when declaring props:\n\n```\nfunction Avatar({ person, size }) {// ...}\n```\n\nThis syntax is called [“destructuring”](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment#Unpacking_fields_from_objects_passed_as_a_function_parameter) and is equivalent to reading properties from a function parameter:\n\n```\nfunction Avatar(props) {let person = props.person;let size = props.size;// ...}\n```\n\nSpecifying a default value for a prop[](https://react.dev/learn/passing-props-to-a-component#specifying-a-default-value-for-a-prop \"Link for Specifying a default value for a prop \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIf you want to give a prop a default value to fall back on when no value is specified, you can do it with the destructuring by putting `=` and the default value right after the parameter:\n\n```\nfunction Avatar({ person, size = 100 }) {// ...}\n```\n\nNow, if `<Avatar person={...} />` is rendered with no `size` prop, the `size` will be set to `100`.\n\nThe default value is only used if the `size` prop is missing or if you pass `size={undefined}`. But if you pass `size={null}` or `size={0}`, the default value will **not** be used.\n\nForwarding props with the JSX spread syntax[](https://react.dev/learn/passing-props-to-a-component#forwarding-props-with-the-jsx-spread-syntax \"Link for Forwarding props with the JSX spread syntax \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nSometimes, passing props gets very repetitive:\n\n```\nfunction Profile({ person, size, isSepia, thickBorder }) {return (<div className=\"card\"><Avatarperson={person}size={size}isSepia={isSepia}thickBorder={thickBorder}/></div>);}\n```\n\nThere’s nothing wrong with repetitive code—it can be more legible. But at times you may value conciseness. Some components forward all of their props to their children, like how this `Profile` does with `Avatar`. Because they don’t use any of their props directly, it can make sense to use a more concise “spread” syntax:\n\n```\nfunction Profile(props) {return (<div className=\"card\"><Avatar {...props} /></div>);}\n```\n\nThis forwards all of `Profile`’s props to the `Avatar` without listing each of their names.\n\n**Use spread syntax with restraint.** If you’re using it in every other component, something is wrong. Often, it indicates that you should split your components and pass children as JSX. More on that next!\n\nPassing JSX as children[](https://react.dev/learn/passing-props-to-a-component#passing-jsx-as-children \"Link for Passing JSX as children \")\n-------------------------------------------------------------------------------------------------------------------------------------------\n\nIt is common to nest built-in browser tags:\n\nSometimes you’ll want to nest your own components the same way:\n\nWhen you nest content inside a JSX tag, the parent component will receive that content in a prop called `children`. For example, the `Card` component below will receive a `children` prop set to `<Avatar />` and render it in a wrapper div:\n\nimport Avatar from './Avatar.js';\n\nfunction Card({ children }) {\n return (\n <div className\\=\"card\"\\>\n {children}\n </div\\>\n );\n}\n\nexport default function Profile() {\n return (\n <Card\\>\n <Avatar\n size\\={100}\n person\\={{ \n name: 'Katsuko Saruhashi',\n imageId: 'YfeOqp2'\n }}\n />\n </Card\\>\n );\n}\n\nTry replacing the `<Avatar>` inside `<Card>` with some text to see how the `Card` component can wrap any nested content. It doesn’t need to “know” what’s being rendered inside of it. You will see this flexible pattern in many places.\n\nYou can think of a component with a `children` prop as having a “hole” that can be “filled in” by its parent components with arbitrary JSX. You will often use the `children` prop for visual wrappers: panels, grids, etc.\n\n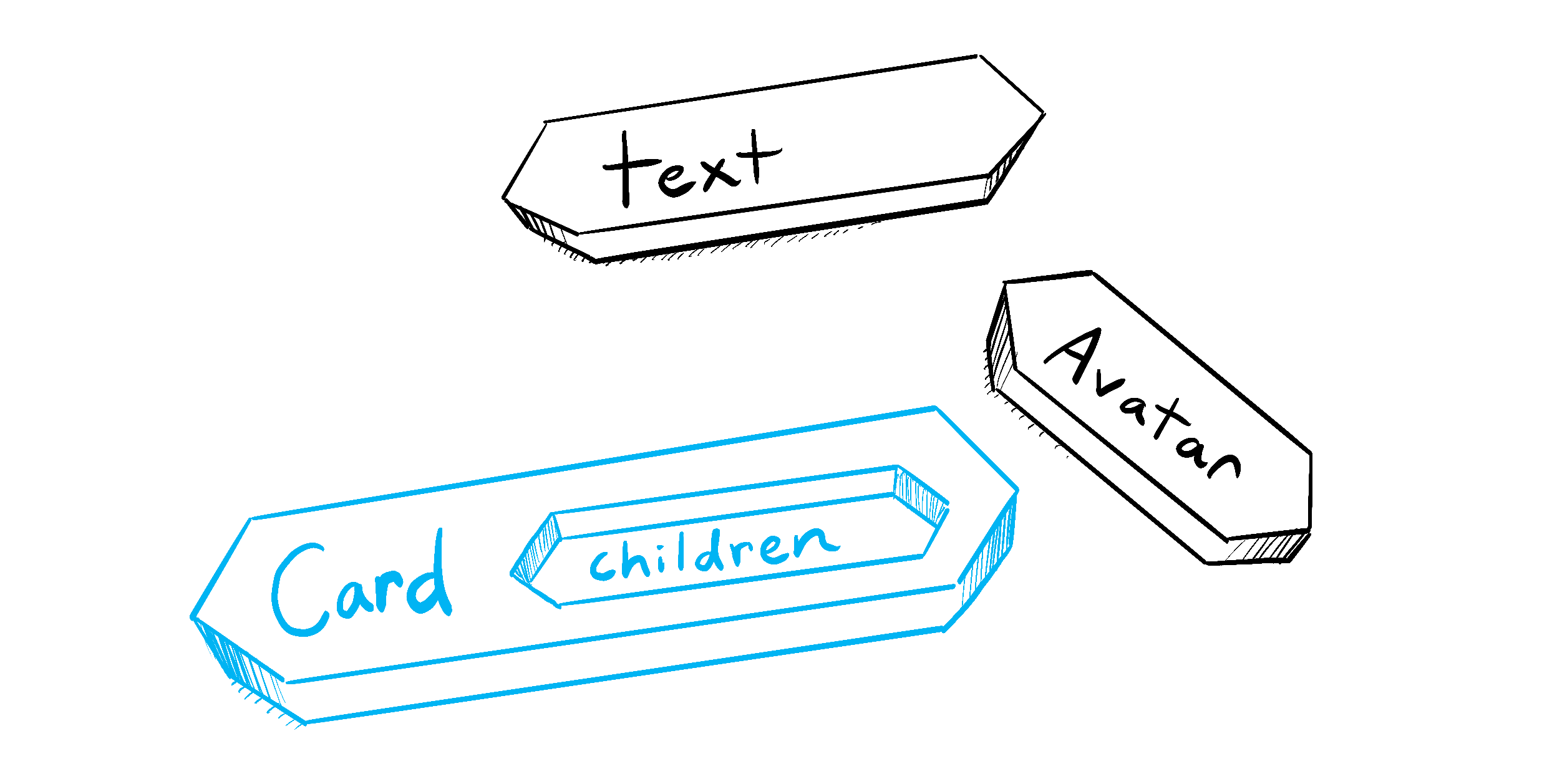\n\nHow props change over time[](https://react.dev/learn/passing-props-to-a-component#how-props-change-over-time \"Link for How props change over time \")\n----------------------------------------------------------------------------------------------------------------------------------------------------\n\nThe `Clock` component below receives two props from its parent component: `color` and `time`. (The parent component’s code is omitted because it uses [state](https://react.dev/learn/state-a-components-memory), which we won’t dive into just yet.)\n\nTry changing the color in the select box below:\n\nThis example illustrates that **a component may receive different props over time.** Props are not always static! Here, the `time` prop changes every second, and the `color` prop changes when you select another color. Props reflect a component’s data at any point in time, rather than only in the beginning.\n\nHowever, props are [immutable](https://en.wikipedia.org/wiki/Immutable_object)—a term from computer science meaning “unchangeable”. When a component needs to change its props (for example, in response to a user interaction or new data), it will have to “ask” its parent component to pass it _different props_—a new object! Its old props will then be cast aside, and eventually the JavaScript engine will reclaim the memory taken by them.\n\n**Don’t try to “change props”.** When you need to respond to the user input (like changing the selected color), you will need to “set state”, which you can learn about in [State: A Component’s Memory.](https://react.dev/learn/state-a-components-memory)\n\nRecap[](https://react.dev/learn/passing-props-to-a-component#recap \"Link for Recap\")\n------------------------------------------------------------------------------------\n\n* To pass props, add them to the JSX, just like you would with HTML attributes.\n* To read props, use the `function Avatar({ person, size })` destructuring syntax.\n* You can specify a default value like `size = 100`, which is used for missing and `undefined` props.\n* You can forward all props with `<Avatar {...props} />` JSX spread syntax, but don’t overuse it!\n* Nested JSX like `<Card><Avatar /></Card>` will appear as `Card` component’s `children` prop.\n* Props are read-only snapshots in time: every render receives a new version of props.\n* You can’t change props. When you need interactivity, you’ll need to set state.\n\nTry out some challenges[](https://react.dev/learn/passing-props-to-a-component#challenges \"Link for Try out some challenges\")\n-----------------------------------------------------------------------------------------------------------------------------\n\nThis `Gallery` component contains some very similar markup for two profiles. Extract a `Profile` component out of it to reduce the duplication. You’ll need to choose what props to pass to it.\n\nimport { getImageUrl } from './utils.js';\n\nexport default function Gallery() {\n return (\n <div\\>\n <h1\\>Notable Scientists</h1\\>\n <section className\\=\"profile\"\\>\n <h2\\>Maria Skłodowska-Curie</h2\\>\n <img\n className\\=\"avatar\"\n src\\={getImageUrl('szV5sdG')}\n alt\\=\"Maria Skłodowska-Curie\"\n width\\={70}\n height\\={70}\n />\n <ul\\>\n <li\\>\n <b\\>Profession: </b\\> \n physicist and chemist\n </li\\>\n <li\\>\n <b\\>Awards: 4 </b\\> \n (Nobel Prize in Physics, Nobel Prize in Chemistry, Davy Medal, Matteucci Medal)\n </li\\>\n <li\\>\n <b\\>Discovered: </b\\>\n polonium (chemical element)\n </li\\>\n </ul\\>\n </section\\>\n <section className\\=\"profile\"\\>\n <h2\\>Katsuko Saruhashi</h2\\>\n <img\n className\\=\"avatar\"\n src\\={getImageUrl('YfeOqp2')}\n alt\\=\"Katsuko Saruhashi\"\n width\\={70}\n height\\={70}\n />\n <ul\\>\n <li\\>\n <b\\>Profession: </b\\> \n geochemist\n </li\\>\n <li\\>\n <b\\>Awards: 2 </b\\> \n (Miyake Prize for geochemistry, Tanaka Prize)\n </li\\>\n <li\\>\n <b\\>Discovered: </b\\>\n a method for measuring carbon dioxide in seawater\n </li\\>\n </ul\\>\n </section\\>\n </div\\>\n );\n}\n",
"filename": "passing-props-to-a-component.md",
"package": "react"
} |
{
"content": "Title: preconnect – React\n\nURL Source: https://react.dev/reference/react-dom/preconnect\n\nMarkdown Content:\npreconnect – React\n===============\n\npreconnect [](https://react.dev/reference/react-dom/preconnect#undefined \"Link for this heading\")\n=================================================================================================\n\n### Canary\n\nThe `preconnect` function is currently only available in React’s Canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n`preconnect` lets you eagerly connect to a server that you expect to load resources from.\n\n```\npreconnect(\"https://example.com\");\n```\n\n* [Reference](https://react.dev/reference/react-dom/preconnect#reference)\n * [`preconnect(href)`](https://react.dev/reference/react-dom/preconnect#preconnect)\n* [Usage](https://react.dev/reference/react-dom/preconnect#usage)\n * [Preconnecting when rendering](https://react.dev/reference/react-dom/preconnect#preconnecting-when-rendering)\n * [Preconnecting in an event handler](https://react.dev/reference/react-dom/preconnect#preconnecting-in-an-event-handler)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/preconnect#reference \"Link for Reference \")\n---------------------------------------------------------------------------------------------\n\n### `preconnect(href)`[](https://react.dev/reference/react-dom/preconnect#preconnect \"Link for this heading\")\n\nTo preconnect to a host, call the `preconnect` function from `react-dom`.\n\n```\nimport { preconnect } from 'react-dom';function AppRoot() { preconnect(\"https://example.com\"); // ...}\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/preconnect#usage)\n\nThe `preconnect` function provides the browser with a hint that it should open a connection to the given server. If the browser chooses to do so, this can speed up the loading of resources from that server.\n\n#### Parameters[](https://react.dev/reference/react-dom/preconnect#parameters \"Link for Parameters \")\n\n* `href`: a string. The URL of the server you want to connect to.\n\n#### Returns[](https://react.dev/reference/react-dom/preconnect#returns \"Link for Returns \")\n\n`preconnect` returns nothing.\n\n#### Caveats[](https://react.dev/reference/react-dom/preconnect#caveats \"Link for Caveats \")\n\n* Multiple calls to `preconnect` with the same server have the same effect as a single call.\n* In the browser, you can call `preconnect` in any situation: while rendering a component, in an Effect, in an event handler, and so on.\n* In server-side rendering or when rendering Server Components, `preconnect` only has an effect if you call it while rendering a component or in an async context originating from rendering a component. Any other calls will be ignored.\n* If you know the specific resources you’ll need, you can call [other functions](https://react.dev/reference/react-dom#resource-preloading-apis) instead that will start loading the resources right away.\n* There is no benefit to preconnecting to the same server the webpage itself is hosted from because it’s already been connected to by the time the hint would be given.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/preconnect#usage \"Link for Usage \")\n---------------------------------------------------------------------------------\n\n### Preconnecting when rendering[](https://react.dev/reference/react-dom/preconnect#preconnecting-when-rendering \"Link for Preconnecting when rendering \")\n\nCall `preconnect` when rendering a component if you know that its children will load external resources from that host.\n\n```\nimport { preconnect } from 'react-dom';function AppRoot() { preconnect(\"https://example.com\"); return ...;}\n```\n\n### Preconnecting in an event handler[](https://react.dev/reference/react-dom/preconnect#preconnecting-in-an-event-handler \"Link for Preconnecting in an event handler \")\n\nCall `preconnect` in an event handler before transitioning to a page or state where external resources will be needed. This gets the process started earlier than if you call it during the rendering of the new page or state.\n\n```\nimport { preconnect } from 'react-dom';function CallToAction() { const onClick = () => { preconnect('http://example.com'); startWizard(); } return ( <button onClick={onClick}>Start Wizard</button> );}\n```\n\n[Previoushydrate](https://react.dev/reference/react-dom/hydrate)[NextprefetchDNS](https://react.dev/reference/react-dom/prefetchDNS)\n",
"filename": "preconnect.md",
"package": "react"
} |
{
"content": "Title: prefetchDNS – React\n\nURL Source: https://react.dev/reference/react-dom/prefetchDNS\n\nMarkdown Content:\nprefetchDNS – React\n===============\n\nprefetchDNS [](https://react.dev/reference/react-dom/prefetchDNS#undefined \"Link for this heading\")\n===================================================================================================\n\n### Canary\n\nThe `prefetchDNS` function is currently only available in React’s Canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n`prefetchDNS` lets you eagerly look up the IP of a server that you expect to load resources from.\n\n```\nprefetchDNS(\"https://example.com\");\n```\n\n* [Reference](https://react.dev/reference/react-dom/prefetchDNS#reference)\n * [`prefetchDNS(href)`](https://react.dev/reference/react-dom/prefetchDNS#prefetchdns)\n* [Usage](https://react.dev/reference/react-dom/prefetchDNS#usage)\n * [Prefetching DNS when rendering](https://react.dev/reference/react-dom/prefetchDNS#prefetching-dns-when-rendering)\n * [Prefetching DNS in an event handler](https://react.dev/reference/react-dom/prefetchDNS#prefetching-dns-in-an-event-handler)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/prefetchDNS#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------\n\n### `prefetchDNS(href)`[](https://react.dev/reference/react-dom/prefetchDNS#prefetchdns \"Link for this heading\")\n\nTo look up a host, call the `prefetchDNS` function from `react-dom`.\n\n```\nimport { prefetchDNS } from 'react-dom';function AppRoot() { prefetchDNS(\"https://example.com\"); // ...}\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/prefetchDNS#usage)\n\nThe prefetchDNS function provides the browser with a hint that it should look up the IP address of a given server. If the browser chooses to do so, this can speed up the loading of resources from that server.\n\n#### Parameters[](https://react.dev/reference/react-dom/prefetchDNS#parameters \"Link for Parameters \")\n\n* `href`: a string. The URL of the server you want to connect to.\n\n#### Returns[](https://react.dev/reference/react-dom/prefetchDNS#returns \"Link for Returns \")\n\n`prefetchDNS` returns nothing.\n\n#### Caveats[](https://react.dev/reference/react-dom/prefetchDNS#caveats \"Link for Caveats \")\n\n* Multiple calls to `prefetchDNS` with the same server have the same effect as a single call.\n* In the browser, you can call `prefetchDNS` in any situation: while rendering a component, in an Effect, in an event handler, and so on.\n* In server-side rendering or when rendering Server Components, `prefetchDNS` only has an effect if you call it while rendering a component or in an async context originating from rendering a component. Any other calls will be ignored.\n* If you know the specific resources you’ll need, you can call [other functions](https://react.dev/reference/react-dom#resource-preloading-apis) instead that will start loading the resources right away.\n* There is no benefit to prefetching the same server the webpage itself is hosted from because it’s already been looked up by the time the hint would be given.\n* Compared with [`preconnect`](https://react.dev/reference/react-dom/preconnect), `prefetchDNS` may be better if you are speculatively connecting to a large number of domains, in which case the overhead of preconnections might outweigh the benefit.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/prefetchDNS#usage \"Link for Usage \")\n----------------------------------------------------------------------------------\n\n### Prefetching DNS when rendering[](https://react.dev/reference/react-dom/prefetchDNS#prefetching-dns-when-rendering \"Link for Prefetching DNS when rendering \")\n\nCall `prefetchDNS` when rendering a component if you know that its children will load external resources from that host.\n\n```\nimport { prefetchDNS } from 'react-dom';function AppRoot() { prefetchDNS(\"https://example.com\"); return ...;}\n```\n\n### Prefetching DNS in an event handler[](https://react.dev/reference/react-dom/prefetchDNS#prefetching-dns-in-an-event-handler \"Link for Prefetching DNS in an event handler \")\n\nCall `prefetchDNS` in an event handler before transitioning to a page or state where external resources will be needed. This gets the process started earlier than if you call it during the rendering of the new page or state.\n\n```\nimport { prefetchDNS } from 'react-dom';function CallToAction() { const onClick = () => { prefetchDNS('http://example.com'); startWizard(); } return ( <button onClick={onClick}>Start Wizard</button> );}\n```\n\n[Previouspreconnect](https://react.dev/reference/react-dom/preconnect)[Nextpreinit](https://react.dev/reference/react-dom/preinit)\n\n* * *\n\n[](https://opensource.fb.com/)\n\n©2024\n\nno uwu plz\n\nuwu?\n\nLogo by[@sawaratsuki1004](https://twitter.com/sawaratsuki1004)\n\n[Learn React](https://react.dev/learn)\n\n[Quick Start](https://react.dev/learn)\n\n[Installation](https://react.dev/learn/installation)\n\n[Describing the UI](https://react.dev/learn/describing-the-ui)\n\n[Adding Interactivity](https://react.dev/learn/adding-interactivity)\n\n[Managing State](https://react.dev/learn/managing-state)\n\n[Escape Hatches](https://react.dev/learn/escape-hatches)\n\n[API Reference](https://react.dev/reference/react)\n\n[React APIs](https://react.dev/reference/react)\n\n[React DOM APIs](https://react.dev/reference/react-dom)\n\n[Community](https://react.dev/community)\n\n[Code of Conduct](https://github.com/facebook/react/blob/main/CODE_OF_CONDUCT.md)\n\n[Meet the Team](https://react.dev/community/team)\n\n[Docs Contributors](https://react.dev/community/docs-contributors)\n\n[Acknowledgements](https://react.dev/community/acknowledgements)\n\nMore\n\n[Blog](https://react.dev/blog)\n\n[React Native](https://reactnative.dev/)\n\n[Privacy](https://opensource.facebook.com/legal/privacy)\n\n[Terms](https://opensource.fb.com/legal/terms/)\n\n[](https://www.facebook.com/react)[](https://twitter.com/reactjs)[](https://github.com/facebook/react)\n\nOn this page\n------------\n\n* [Overview](https://react.dev/reference/react-dom/prefetchDNS#)\n* [Reference](https://react.dev/reference/react-dom/prefetchDNS#reference)\n* [`prefetchDNS(href)`](https://react.dev/reference/react-dom/prefetchDNS#prefetchdns)\n* [Usage](https://react.dev/reference/react-dom/prefetchDNS#usage)\n* [Prefetching DNS when rendering](https://react.dev/reference/react-dom/prefetchDNS#prefetching-dns-when-rendering)\n* [Prefetching DNS in an event handler](https://react.dev/reference/react-dom/prefetchDNS#prefetching-dns-in-an-event-handler)\n",
"filename": "prefetchDNS.md",
"package": "react"
} |
{
"content": "Title: preinit – React\n\nURL Source: https://react.dev/reference/react-dom/preinit\n\nMarkdown Content:\npreinit - This feature is available in the latest Canary[](https://react.dev/reference/react-dom/preinit#undefined \"Link for this heading\")\n-------------------------------------------------------------------------------------------------------------------------------------------\n\n### Canary\n\n### Note\n\n[React-based frameworks](https://react.dev/learn/start-a-new-react-project) frequently handle resource loading for you, so you might not have to call this API yourself. Consult your framework’s documentation for details.\n\n`preinit` lets you eagerly fetch and evaluate a stylesheet or external script.\n\n```\npreinit(\"https://example.com/script.js\", {as: \"script\"});\n```\n\n* [Reference](https://react.dev/reference/react-dom/preinit#reference)\n * [`preinit(href, options)`](https://react.dev/reference/react-dom/preinit#preinit)\n* [Usage](https://react.dev/reference/react-dom/preinit#usage)\n * [Preiniting when rendering](https://react.dev/reference/react-dom/preinit#preiniting-when-rendering)\n * [Preiniting in an event handler](https://react.dev/reference/react-dom/preinit#preiniting-in-an-event-handler)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/preinit#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------\n\n### `preinit(href, options)`[](https://react.dev/reference/react-dom/preinit#preinit \"Link for this heading\")\n\nTo preinit a script or stylesheet, call the `preinit` function from `react-dom`.\n\n```\nimport { preinit } from 'react-dom';function AppRoot() {preinit(\"https://example.com/script.js\", {as: \"script\"});// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/preinit#usage)\n\nThe `preinit` function provides the browser with a hint that it should start downloading and executing the given resource, which can save time. Scripts that you `preinit` are executed when they finish downloading. Stylesheets that you preinit are inserted into the document, which causes them to go into effect right away.\n\n#### Parameters[](https://react.dev/reference/react-dom/preinit#parameters \"Link for Parameters \")\n\n* `href`: a string. The URL of the resource you want to download and execute.\n* `options`: an object. It contains the following properties:\n * `as`: a required string. The type of resource. Its possible values are `script` and `style`.\n * `precedence`: a string. Required with stylesheets. Says where to insert the stylesheet relative to others. Stylesheets with higher precedence can override those with lower precedence. The possible values are `reset`, `low`, `medium`, `high`.\n * `crossOrigin`: a string. The [CORS policy](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/crossorigin) to use. Its possible values are `anonymous` and `use-credentials`. It is required when `as` is set to `\"fetch\"`.\n * `integrity`: a string. A cryptographic hash of the resource, to [verify its authenticity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity).\n * `nonce`: a string. A cryptographic [nonce to allow the resource](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/nonce) when using a strict Content Security Policy.\n * `fetchPriority`: a string. Suggests a relative priority for fetching the resource. The possible values are `auto` (the default), `high`, and `low`.\n\n#### Returns[](https://react.dev/reference/react-dom/preinit#returns \"Link for Returns \")\n\n`preinit` returns nothing.\n\n#### Caveats[](https://react.dev/reference/react-dom/preinit#caveats \"Link for Caveats \")\n\n* Multiple calls to `preinit` with the same `href` have the same effect as a single call.\n* In the browser, you can call `preinit` in any situation: while rendering a component, in an Effect, in an event handler, and so on.\n* In server-side rendering or when rendering Server Components, `preinit` only has an effect if you call it while rendering a component or in an async context originating from rendering a component. Any other calls will be ignored.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/preinit#usage \"Link for Usage \")\n------------------------------------------------------------------------------\n\n### Preiniting when rendering[](https://react.dev/reference/react-dom/preinit#preiniting-when-rendering \"Link for Preiniting when rendering \")\n\nCall `preinit` when rendering a component if you know that it or its children will use a specific resource, and you’re OK with the resource being evaluated and thereby taking effect immediately upon being downloaded.\n\n#### Examples of preiniting[](https://react.dev/reference/react-dom/preinit#examples \"Link for Examples of preiniting\")\n\n#### Preiniting an external script[](https://react.dev/reference/react-dom/preinit#preiniting-an-external-script \"Link for this heading\")\n\n```\nimport { preinit } from 'react-dom';function AppRoot() {preinit(\"https://example.com/script.js\", {as: \"script\"});return ...;}\n```\n\nIf you want the browser to download the script but not to execute it right away, use [`preload`](https://react.dev/reference/react-dom/preload) instead. If you want to load an ESM module, use [`preinitModule`](https://react.dev/reference/react-dom/preinitModule).\n\n### Preiniting in an event handler[](https://react.dev/reference/react-dom/preinit#preiniting-in-an-event-handler \"Link for Preiniting in an event handler \")\n\nCall `preinit` in an event handler before transitioning to a page or state where external resources will be needed. This gets the process started earlier than if you call it during the rendering of the new page or state.\n\n```\nimport { preinit } from 'react-dom';function CallToAction() {const onClick = () => {preinit(\"https://example.com/wizardStyles.css\", {as: \"style\"});startWizard();}return (<button onClick={onClick}>Start Wizard</button>);}\n```\n",
"filename": "preinit.md",
"package": "react"
} |
{
"content": "Title: preinitModule – React\n\nURL Source: https://react.dev/reference/react-dom/preinitModule\n\nMarkdown Content:\n### Canary\n\nThe `preinitModule` function is currently only available in React’s Canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n### Note\n\n[React-based frameworks](https://react.dev/learn/start-a-new-react-project) frequently handle resource loading for you, so you might not have to call this API yourself. Consult your framework’s documentation for details.\n\n`preinitModule` lets you eagerly fetch and evaluate an ESM module.\n\n```\npreinitModule(\"https://example.com/module.js\", {as: \"script\"});\n```\n\n* [Reference](https://react.dev/reference/react-dom/preinitModule#reference)\n * [`preinitModule(href, options)`](https://react.dev/reference/react-dom/preinitModule#preinitmodule)\n* [Usage](https://react.dev/reference/react-dom/preinitModule#usage)\n * [Preloading when rendering](https://react.dev/reference/react-dom/preinitModule#preloading-when-rendering)\n * [Preloading in an event handler](https://react.dev/reference/react-dom/preinitModule#preloading-in-an-event-handler)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/preinitModule#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------\n\n### `preinitModule(href, options)`[](https://react.dev/reference/react-dom/preinitModule#preinitmodule \"Link for this heading\")\n\nTo preinit an ESM module, call the `preinitModule` function from `react-dom`.\n\n```\nimport { preinitModule } from 'react-dom';function AppRoot() {preinitModule(\"https://example.com/module.js\", {as: \"script\"});// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/preinitModule#usage)\n\nThe `preinitModule` function provides the browser with a hint that it should start downloading and executing the given module, which can save time. Modules that you `preinit` are executed when they finish downloading.\n\n#### Parameters[](https://react.dev/reference/react-dom/preinitModule#parameters \"Link for Parameters \")\n\n* `href`: a string. The URL of the module you want to download and exeucute.\n* `options`: an object. It contains the following properties:\n * `as`: a required string. It must be `'script'`.\n * `crossOrigin`: a string. The [CORS policy](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/crossorigin) to use. Its possible values are `anonymous` and `use-credentials`.\n * `integrity`: a string. A cryptographic hash of the module, to [verify its authenticity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity).\n * `nonce`: a string. A cryptographic [nonce to allow the module](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/nonce) when using a strict Content Security Policy.\n\n#### Returns[](https://react.dev/reference/react-dom/preinitModule#returns \"Link for Returns \")\n\n`preinitModule` returns nothing.\n\n#### Caveats[](https://react.dev/reference/react-dom/preinitModule#caveats \"Link for Caveats \")\n\n* Multiple calls to `preinitModule` with the same `href` have the same effect as a single call.\n* In the browser, you can call `preinitModule` in any situation: while rendering a component, in an Effect, in an event handler, and so on.\n* In server-side rendering or when rendering Server Components, `preinitModule` only has an effect if you call it while rendering a component or in an async context originating from rendering a component. Any other calls will be ignored.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/preinitModule#usage \"Link for Usage \")\n------------------------------------------------------------------------------------\n\n### Preloading when rendering[](https://react.dev/reference/react-dom/preinitModule#preloading-when-rendering \"Link for Preloading when rendering \")\n\nCall `preinitModule` when rendering a component if you know that it or its children will use a specific module and you’re OK with the module being evaluated and thereby taking effect immediately upon being downloaded.\n\n```\nimport { preinitModule } from 'react-dom';function AppRoot() {preinitModule(\"https://example.com/module.js\", {as: \"script\"});return ...;}\n```\n\nIf you want the browser to download the module but not to execute it right away, use [`preloadModule`](https://react.dev/reference/react-dom/preloadModule) instead. If you want to preinit a script that isn’t an ESM module, use [`preinit`](https://react.dev/reference/react-dom/preinit).\n\n### Preloading in an event handler[](https://react.dev/reference/react-dom/preinitModule#preloading-in-an-event-handler \"Link for Preloading in an event handler \")\n\nCall `preinitModule` in an event handler before transitioning to a page or state where the module will be needed. This gets the process started earlier than if you call it during the rendering of the new page or state.\n\n```\nimport { preinitModule } from 'react-dom';function CallToAction() {const onClick = () => {preinitModule(\"https://example.com/module.js\", {as: \"script\"});startWizard();}return (<button onClick={onClick}>Start Wizard</button>);}\n```\n",
"filename": "preinitModule.md",
"package": "react"
} |
{
"content": "Title: preload – React\n\nURL Source: https://react.dev/reference/react-dom/preload\n\nMarkdown Content:\npreload – React\n===============\n\npreload [](https://react.dev/reference/react-dom/preload#undefined \"Link for this heading\")\n===========================================================================================\n\n### Canary\n\nThe `preload` function is currently only available in React’s Canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n### Note\n\n[React-based frameworks](https://react.dev/learn/start-a-new-react-project) frequently handle resource loading for you, so you might not have to call this API yourself. Consult your framework’s documentation for details.\n\n`preload` lets you eagerly fetch a resource such as a stylesheet, font, or external script that you expect to use.\n\n```\npreload(\"https://example.com/font.woff2\", {as: \"font\"});\n```\n\n* [Reference](https://react.dev/reference/react-dom/preload#reference)\n * [`preload(href, options)`](https://react.dev/reference/react-dom/preload#preload)\n* [Usage](https://react.dev/reference/react-dom/preload#usage)\n * [Preloading when rendering](https://react.dev/reference/react-dom/preload#preloading-when-rendering)\n * [Preloading in an event handler](https://react.dev/reference/react-dom/preload#preloading-in-an-event-handler)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/preload#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------\n\n### `preload(href, options)`[](https://react.dev/reference/react-dom/preload#preload \"Link for this heading\")\n\nTo preload a resource, call the `preload` function from `react-dom`.\n\n```\nimport { preload } from 'react-dom';function AppRoot() { preload(\"https://example.com/font.woff2\", {as: \"font\"}); // ...}\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/preload#usage)\n\nThe `preload` function provides the browser with a hint that it should start downloading the given resource, which can save time.\n\n#### Parameters[](https://react.dev/reference/react-dom/preload#parameters \"Link for Parameters \")\n\n* `href`: a string. The URL of the resource you want to download.\n* `options`: an object. It contains the following properties:\n * `as`: a required string. The type of resource. Its [possible values](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link#as) are `audio`, `document`, `embed`, `fetch`, `font`, `image`, `object`, `script`, `style`, `track`, `video`, `worker`.\n * `crossOrigin`: a string. The [CORS policy](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/crossorigin) to use. Its possible values are `anonymous` and `use-credentials`. It is required when `as` is set to `\"fetch\"`.\n * `referrerPolicy`: a string. The [Referrer header](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/link#referrerpolicy) to send when fetching. Its possible values are `no-referrer-when-downgrade` (the default), `no-referrer`, `origin`, `origin-when-cross-origin`, and `unsafe-url`.\n * `integrity`: a string. A cryptographic hash of the resource, to [verify its authenticity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity).\n * `type`: a string. The MIME type of the resource.\n * `nonce`: a string. A cryptographic [nonce to allow the resource](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/nonce) when using a strict Content Security Policy.\n * `fetchPriority`: a string. Suggests a relative priority for fetching the resource. The possible values are `auto` (the default), `high`, and `low`.\n * `imageSrcSet`: a string. For use only with `as: \"image\"`. Specifies the [source set of the image](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).\n * `imageSizes`: a string. For use only with `as: \"image\"`. Specifies the [sizes of the image](https://developer.mozilla.org/en-US/docs/Learn/HTML/Multimedia_and_embedding/Responsive_images).\n\n#### Returns[](https://react.dev/reference/react-dom/preload#returns \"Link for Returns \")\n\n`preload` returns nothing.\n\n#### Caveats[](https://react.dev/reference/react-dom/preload#caveats \"Link for Caveats \")\n\n* Multiple equivalent calls to `preload` have the same effect as a single call. Calls to `preload` are considered equivalent according to the following rules:\n * Two calls are equivalent if they have the same `href`, except:\n * If `as` is set to `image`, two calls are equivalent if they have the same `href`, `imageSrcSet`, and `imageSizes`.\n* In the browser, you can call `preload` in any situation: while rendering a component, in an Effect, in an event handler, and so on.\n* In server-side rendering or when rendering Server Components, `preload` only has an effect if you call it while rendering a component or in an async context originating from rendering a component. Any other calls will be ignored.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/preload#usage \"Link for Usage \")\n------------------------------------------------------------------------------\n\n### Preloading when rendering[](https://react.dev/reference/react-dom/preload#preloading-when-rendering \"Link for Preloading when rendering \")\n\nCall `preload` when rendering a component if you know that it or its children will use a specific resource.\n\n#### Examples of preloading[](https://react.dev/reference/react-dom/preload#examples \"Link for Examples of preloading\")\n\n1. Preloading an external script 2. Preloading a stylesheet 3. Preloading a font 4. Preloading an image\n\n#### \n\nExample 1 of 4:\n\nPreloading an external script[](https://react.dev/reference/react-dom/preload#preloading-an-external-script \"Link for this heading\")\n\n```\nimport { preload } from 'react-dom';function AppRoot() { preload(\"https://example.com/script.js\", {as: \"script\"}); return ...;}\n```\n\nIf you want the browser to start executing the script immediately (rather than just downloading it), use [`preinit`](https://react.dev/reference/react-dom/preinit) instead. If you want to load an ESM module, use [`preloadModule`](https://react.dev/reference/react-dom/preloadModule).\n\nNext Example\n\n### Preloading in an event handler[](https://react.dev/reference/react-dom/preload#preloading-in-an-event-handler \"Link for Preloading in an event handler \")\n\nCall `preload` in an event handler before transitioning to a page or state where external resources will be needed. This gets the process started earlier than if you call it during the rendering of the new page or state.\n\n```\nimport { preload } from 'react-dom';function CallToAction() { const onClick = () => { preload(\"https://example.com/wizardStyles.css\", {as: \"style\"}); startWizard(); } return ( <button onClick={onClick}>Start Wizard</button> );}\n```\n\n[PreviouspreinitModule](https://react.dev/reference/react-dom/preinitModule)[NextpreloadModule](https://react.dev/reference/react-dom/preloadModule)\n\n* * *\n\n[](https://opensource.fb.com/)\n\n©2024\n\nno uwu plz\n\nuwu?\n\nLogo by[@sawaratsuki1004](https://twitter.com/sawaratsuki1004)\n\n[Learn React](https://react.dev/learn)\n\n[Quick Start](https://react.dev/learn)\n\n[Installation](https://react.dev/learn/installation)\n\n[Describing the UI](https://react.dev/learn/describing-the-ui)\n\n[Adding Interactivity](https://react.dev/learn/adding-interactivity)\n\n[Managing State](https://react.dev/learn/managing-state)\n\n[Escape Hatches](https://react.dev/learn/escape-hatches)\n\n[API Reference](https://react.dev/reference/react)\n\n[React APIs](https://react.dev/reference/react)\n\n[React DOM APIs](https://react.dev/reference/react-dom)\n\n[Community](https://react.dev/community)\n\n[Code of Conduct](https://github.com/facebook/react/blob/main/CODE_OF_CONDUCT.md)\n\n[Meet the Team](https://react.dev/community/team)\n\n[Docs Contributors](https://react.dev/community/docs-contributors)\n\n[Acknowledgements](https://react.dev/community/acknowledgements)\n\nMore\n\n[Blog](https://react.dev/blog)\n\n[React Native](https://reactnative.dev/)\n\n[Privacy](https://opensource.facebook.com/legal/privacy)\n\n[Terms](https://opensource.fb.com/legal/terms/)\n\n[](https://www.facebook.com/react)[](https://twitter.com/reactjs)[](https://github.com/facebook/react)\n\nOn this page\n------------\n\n* [Overview](https://react.dev/reference/react-dom/preload#)\n* [Reference](https://react.dev/reference/react-dom/preload#reference)\n* [`preload(href, options)`](https://react.dev/reference/react-dom/preload#preload)\n* [Usage](https://react.dev/reference/react-dom/preload#usage)\n* [Preloading when rendering](https://react.dev/reference/react-dom/preload#preloading-when-rendering)\n* [Preloading in an event handler](https://react.dev/reference/react-dom/preload#preloading-in-an-event-handler)\n",
"filename": "preload.md",
"package": "react"
} |
{
"content": "Title: preloadModule – React\n\nURL Source: https://react.dev/reference/react-dom/preloadModule\n\nMarkdown Content:\n### Canary\n\nThe `preloadModule` function is currently only available in React’s Canary and experimental channels. Learn more about [React’s release channels here](https://react.dev/community/versioning-policy#all-release-channels).\n\n### Note\n\n[React-based frameworks](https://react.dev/learn/start-a-new-react-project) frequently handle resource loading for you, so you might not have to call this API yourself. Consult your framework’s documentation for details.\n\n`preloadModule` lets you eagerly fetch an ESM module that you expect to use.\n\n```\npreloadModule(\"https://example.com/module.js\", {as: \"script\"});\n```\n\n* [Reference](https://react.dev/reference/react-dom/preloadModule#reference)\n * [`preloadModule(href, options)`](https://react.dev/reference/react-dom/preloadModule#preloadmodule)\n* [Usage](https://react.dev/reference/react-dom/preloadModule#usage)\n * [Preloading when rendering](https://react.dev/reference/react-dom/preloadModule#preloading-when-rendering)\n * [Preloading in an event handler](https://react.dev/reference/react-dom/preloadModule#preloading-in-an-event-handler)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/preloadModule#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------\n\n### `preloadModule(href, options)`[](https://react.dev/reference/react-dom/preloadModule#preloadmodule \"Link for this heading\")\n\nTo preload an ESM module, call the `preloadModule` function from `react-dom`.\n\n```\nimport { preloadModule } from 'react-dom';function AppRoot() {preloadModule(\"https://example.com/module.js\", {as: \"script\"});// ...}\n```\n\n[See more examples below.](https://react.dev/reference/react-dom/preloadModule#usage)\n\nThe `preloadModule` function provides the browser with a hint that it should start downloading the given module, which can save time.\n\n#### Parameters[](https://react.dev/reference/react-dom/preloadModule#parameters \"Link for Parameters \")\n\n* `href`: a string. The URL of the module you want to download.\n* `options`: an object. It contains the following properties:\n * `as`: a required string. It must be `'script'`.\n * `crossOrigin`: a string. The [CORS policy](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/crossorigin) to use. Its possible values are `anonymous` and `use-credentials`.\n * `integrity`: a string. A cryptographic hash of the module, to [verify its authenticity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity).\n * `nonce`: a string. A cryptographic [nonce to allow the module](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/nonce) when using a strict Content Security Policy.\n\n#### Returns[](https://react.dev/reference/react-dom/preloadModule#returns \"Link for Returns \")\n\n`preloadModule` returns nothing.\n\n#### Caveats[](https://react.dev/reference/react-dom/preloadModule#caveats \"Link for Caveats \")\n\n* Multiple calls to `preloadModule` with the same `href` have the same effect as a single call.\n* In the browser, you can call `preloadModule` in any situation: while rendering a component, in an Effect, in an event handler, and so on.\n* In server-side rendering or when rendering Server Components, `preloadModule` only has an effect if you call it while rendering a component or in an async context originating from rendering a component. Any other calls will be ignored.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/preloadModule#usage \"Link for Usage \")\n------------------------------------------------------------------------------------\n\n### Preloading when rendering[](https://react.dev/reference/react-dom/preloadModule#preloading-when-rendering \"Link for Preloading when rendering \")\n\nCall `preloadModule` when rendering a component if you know that it or its children will use a specific module.\n\n```\nimport { preloadModule } from 'react-dom';function AppRoot() {preloadModule(\"https://example.com/module.js\", {as: \"script\"});return ...;}\n```\n\nIf you want the browser to start executing the module immediately (rather than just downloading it), use [`preinitModule`](https://react.dev/reference/react-dom/preinitModule) instead. If you want to load a script that isn’t an ESM module, use [`preload`](https://react.dev/reference/react-dom/preload).\n\n### Preloading in an event handler[](https://react.dev/reference/react-dom/preloadModule#preloading-in-an-event-handler \"Link for Preloading in an event handler \")\n\nCall `preloadModule` in an event handler before transitioning to a page or state where the module will be needed. This gets the process started earlier than if you call it during the rendering of the new page or state.\n\n```\nimport { preloadModule } from 'react-dom';function CallToAction() {const onClick = () => {preloadModule(\"https://example.com/module.js\", {as: \"script\"});startWizard();}return (<button onClick={onClick}>Start Wizard</button>);}\n```\n",
"filename": "preloadModule.md",
"package": "react"
} |
{
"content": "Title: Preserving and Resetting State – React\n\nURL Source: https://react.dev/learn/preserving-and-resetting-state\n\nMarkdown Content:\nState is isolated between components. React keeps track of which state belongs to which component based on their place in the UI tree. You can control when to preserve state and when to reset it between re-renders.\n\n### You will learn\n\n* When React chooses to preserve or reset the state\n* How to force React to reset component’s state\n* How keys and types affect whether the state is preserved\n\nState is tied to a position in the render tree[](https://react.dev/learn/preserving-and-resetting-state#state-is-tied-to-a-position-in-the-tree \"Link for State is tied to a position in the render tree \")\n-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nReact builds [render trees](https://react.dev/learn/understanding-your-ui-as-a-tree#the-render-tree) for the component structure in your UI.\n\nWhen you give a component state, you might think the state “lives” inside the component. But the state is actually held inside React. React associates each piece of state it’s holding with the correct component by where that component sits in the render tree.\n\nHere, there is only one `<Counter />` JSX tag, but it’s rendered at two different positions:\n\nHere’s how these look as a tree:\n\n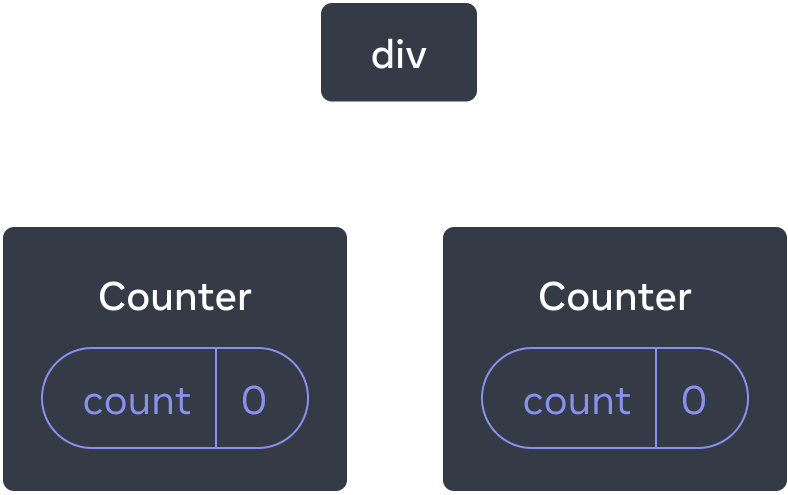\n\n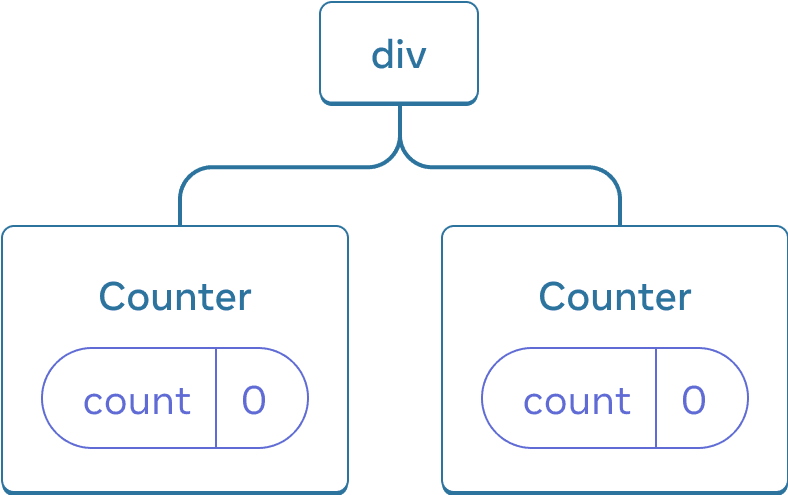\n\nReact tree\n\n**These are two separate counters because each is rendered at its own position in the tree.** You don’t usually have to think about these positions to use React, but it can be useful to understand how it works.\n\nIn React, each component on the screen has fully isolated state. For example, if you render two `Counter` components side by side, each of them will get its own, independent, `score` and `hover` states.\n\nTry clicking both counters and notice they don’t affect each other:\n\nimport { useState } from 'react';\n\nexport default function App() {\n return (\n <div\\>\n <Counter />\n <Counter />\n </div\\>\n );\n}\n\nfunction Counter() {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nAs you can see, when one counter is updated, only the state for that component is updated:\n\n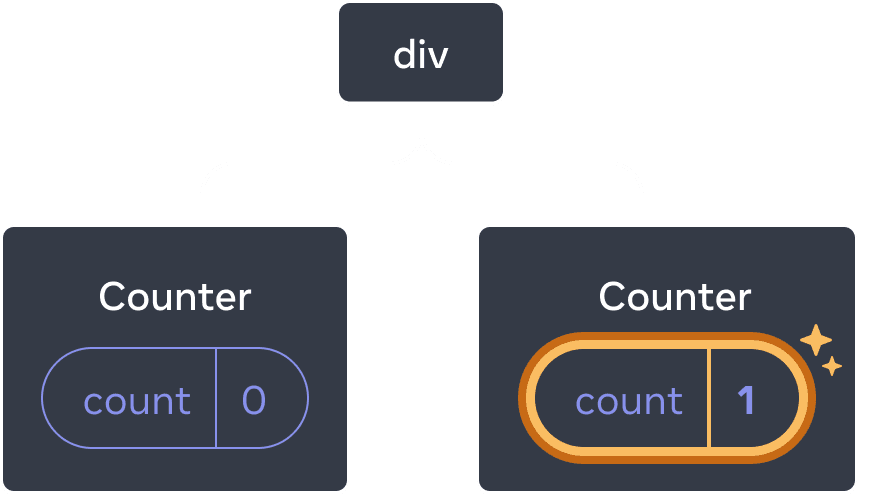\n\n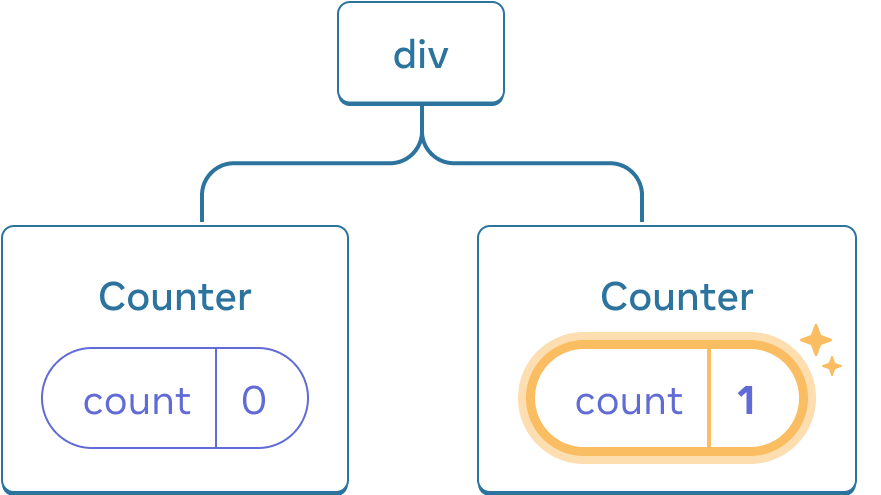\n\nUpdating state\n\nReact will keep the state around for as long as you render the same component at the same position in the tree. To see this, increment both counters, then remove the second component by unchecking “Render the second counter” checkbox, and then add it back by ticking it again:\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[showB, setShowB\\] = useState(true);\n return (\n <div\\>\n <Counter />\n {showB && <Counter />} \n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={showB}\n onChange\\={e \\=> {\n setShowB(e.target.checked)\n }}\n />\n Render the second counter\n </label\\>\n </div\\>\n );\n}\n\nfunction Counter() {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nNotice how the moment you stop rendering the second counter, its state disappears completely. That’s because when React removes a component, it destroys its state.\n\n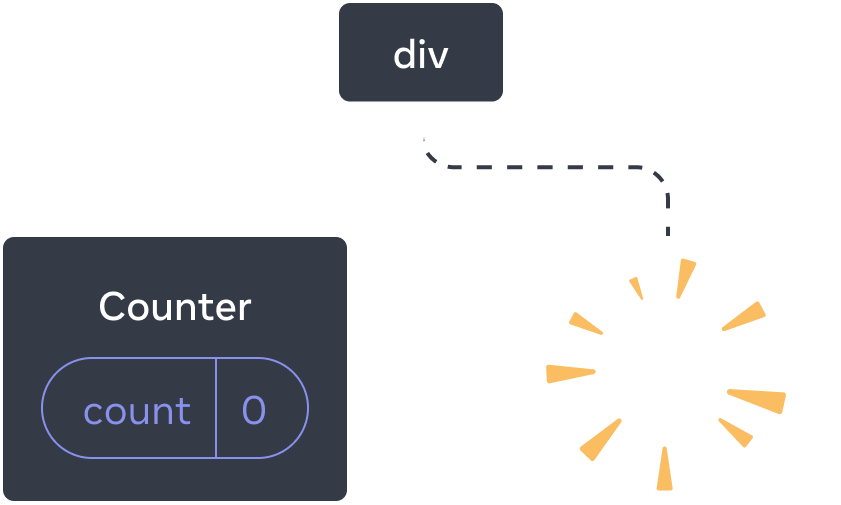\n\n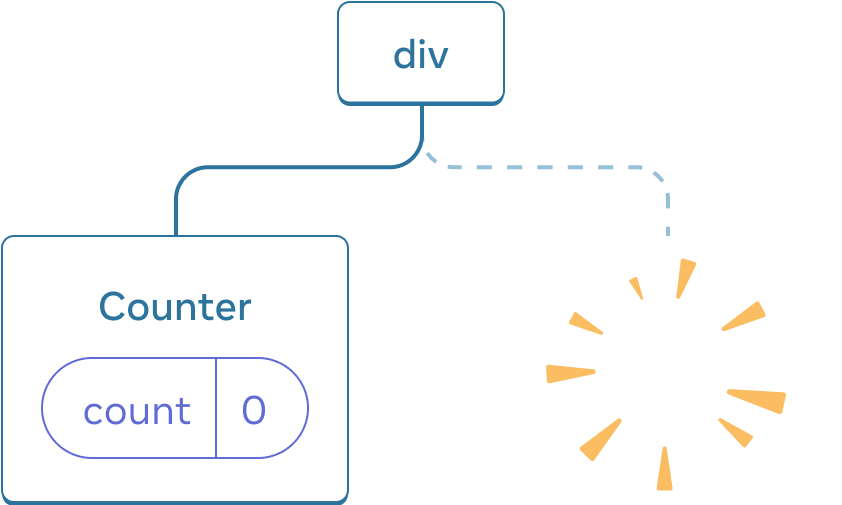\n\nDeleting a component\n\nWhen you tick “Render the second counter”, a second `Counter` and its state are initialized from scratch (`score = 0`) and added to the DOM.\n\n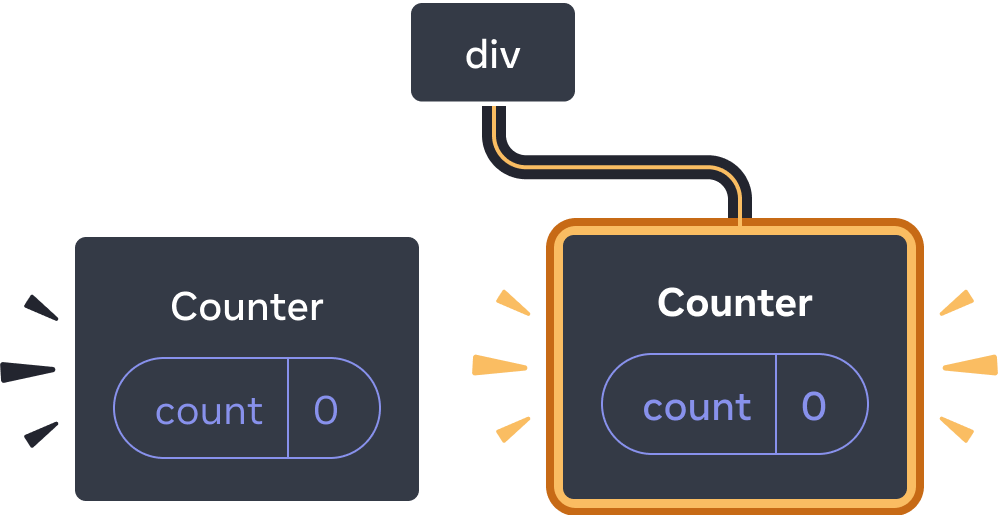\n\n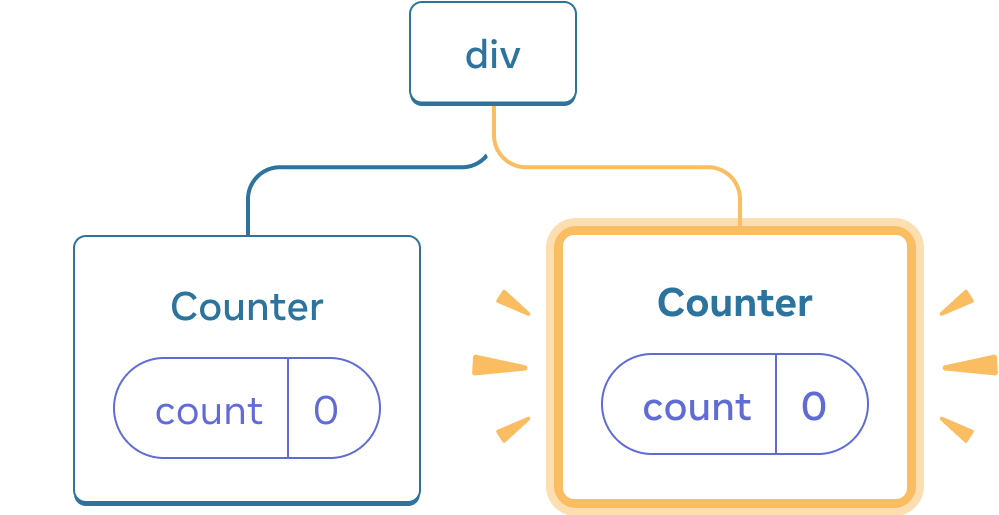\n\nAdding a component\n\n**React preserves a component’s state for as long as it’s being rendered at its position in the UI tree.** If it gets removed, or a different component gets rendered at the same position, React discards its state.\n\nSame component at the same position preserves state[](https://react.dev/learn/preserving-and-resetting-state#same-component-at-the-same-position-preserves-state \"Link for Same component at the same position preserves state \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn this example, there are two different `<Counter />` tags:\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[isFancy, setIsFancy\\] = useState(false);\n return (\n <div\\>\n {isFancy ? (\n <Counter isFancy\\={true} /> \n ) : (\n <Counter isFancy\\={false} /> \n )}\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isFancy}\n onChange\\={e \\=> {\n setIsFancy(e.target.checked)\n }}\n />\n Use fancy styling\n </label\\>\n </div\\>\n );\n}\n\nfunction Counter({ isFancy }) {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n if (isFancy) {\n className += ' fancy';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nWhen you tick or clear the checkbox, the counter state does not get reset. Whether `isFancy` is `true` or `false`, you always have a `<Counter />` as the first child of the `div` returned from the root `App` component:\n\n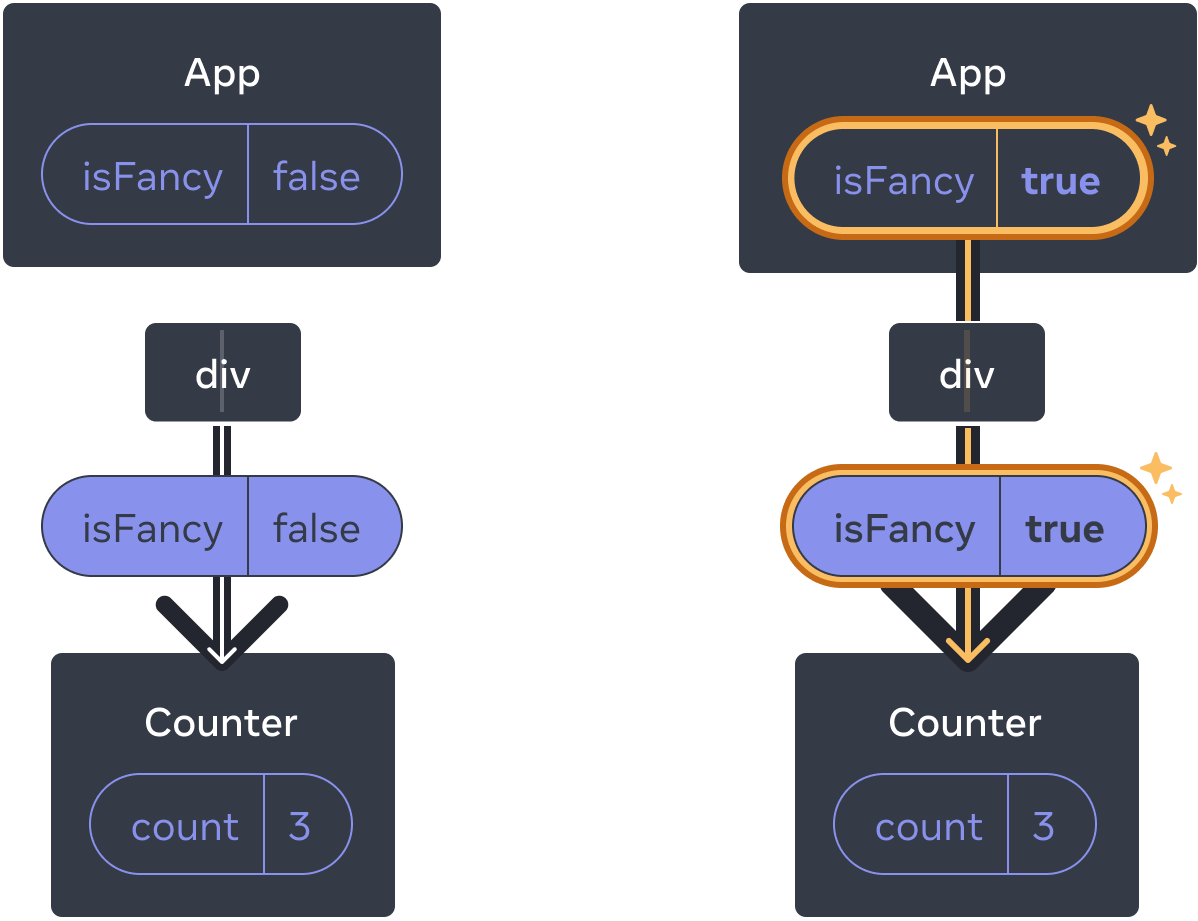\n\n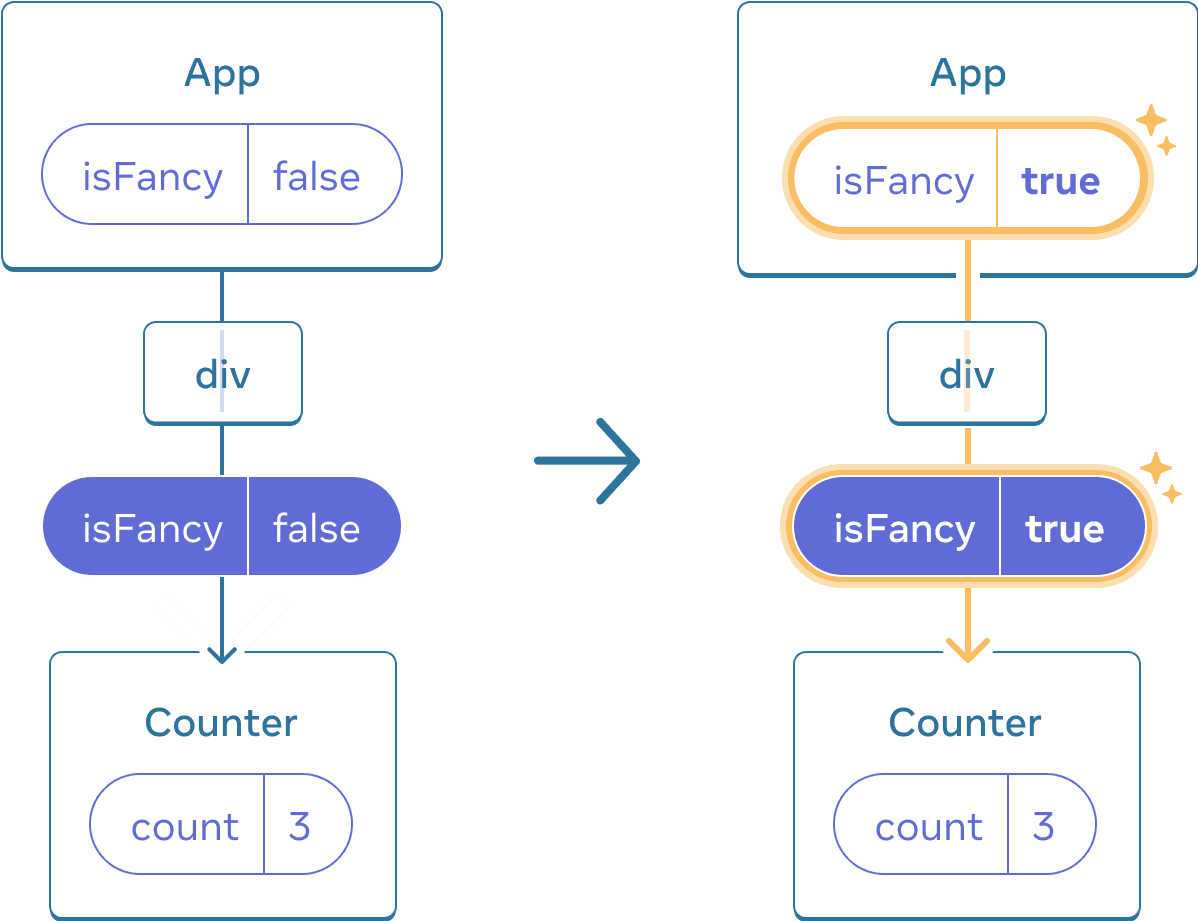\n\nUpdating the `App` state does not reset the `Counter` because `Counter` stays in the same position\n\nIt’s the same component at the same position, so from React’s perspective, it’s the same counter.\n\n### Pitfall\n\nRemember that **it’s the position in the UI tree—not in the JSX markup—that matters to React!** This component has two `return` clauses with different `<Counter />` JSX tags inside and outside the `if`:\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[isFancy, setIsFancy\\] = useState(false);\n if (isFancy) {\n return (\n <div\\>\n <Counter isFancy\\={true} />\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isFancy}\n onChange\\={e \\=> {\n setIsFancy(e.target.checked)\n }}\n />\n Use fancy styling\n </label\\>\n </div\\>\n );\n }\n return (\n <div\\>\n <Counter isFancy\\={false} />\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isFancy}\n onChange\\={e \\=> {\n setIsFancy(e.target.checked)\n }}\n />\n Use fancy styling\n </label\\>\n </div\\>\n );\n}\n\nfunction Counter({ isFancy }) {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n if (isFancy) {\n className += ' fancy';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nYou might expect the state to reset when you tick checkbox, but it doesn’t! This is because **both of these `<Counter />` tags are rendered at the same position.** React doesn’t know where you place the conditions in your function. All it “sees” is the tree you return.\n\nIn both cases, the `App` component returns a `<div>` with `<Counter />` as a first child. To React, these two counters have the same “address”: the first child of the first child of the root. This is how React matches them up between the previous and next renders, regardless of how you structure your logic.\n\nDifferent components at the same position reset state[](https://react.dev/learn/preserving-and-resetting-state#different-components-at-the-same-position-reset-state \"Link for Different components at the same position reset state \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIn this example, ticking the checkbox will replace `<Counter>` with a `<p>`:\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[isPaused, setIsPaused\\] = useState(false);\n return (\n <div\\>\n {isPaused ? (\n <p\\>See you later!</p\\> \n ) : (\n <Counter /> \n )}\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isPaused}\n onChange\\={e \\=> {\n setIsPaused(e.target.checked)\n }}\n />\n Take a break\n </label\\>\n </div\\>\n );\n}\n\nfunction Counter() {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nHere, you switch between _different_ component types at the same position. Initially, the first child of the `<div>` contained a `Counter`. But when you swapped in a `p`, React removed the `Counter` from the UI tree and destroyed its state.\n\n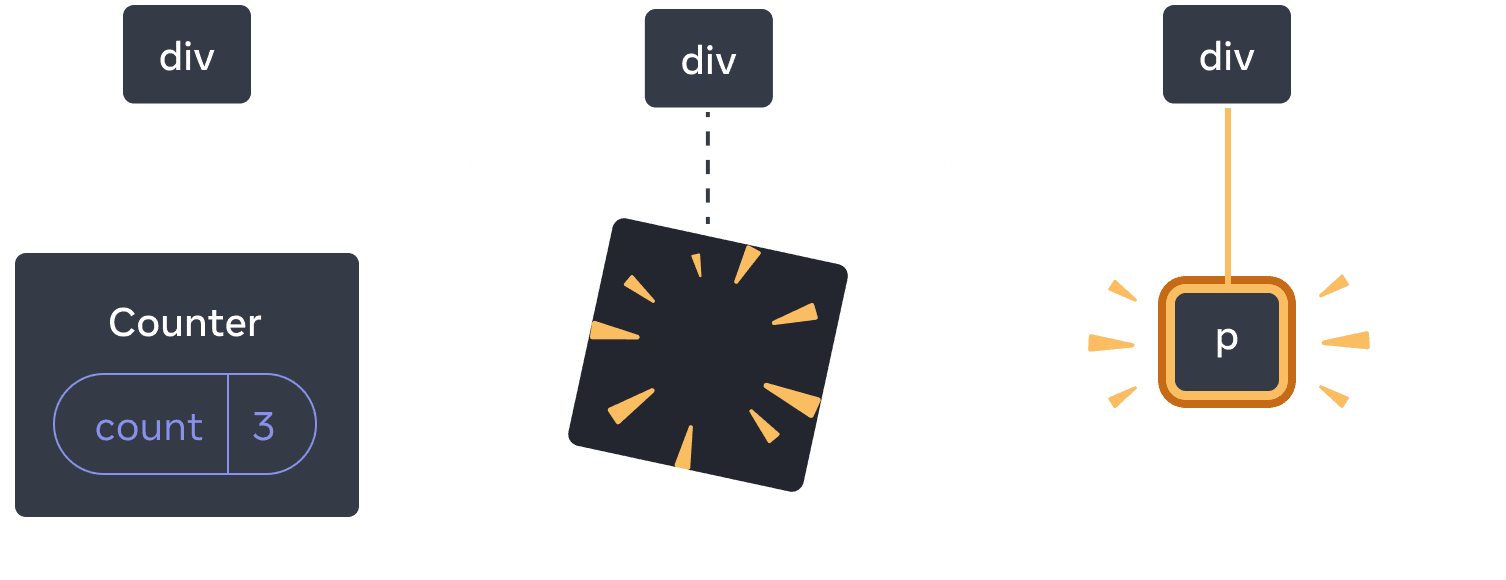\n\n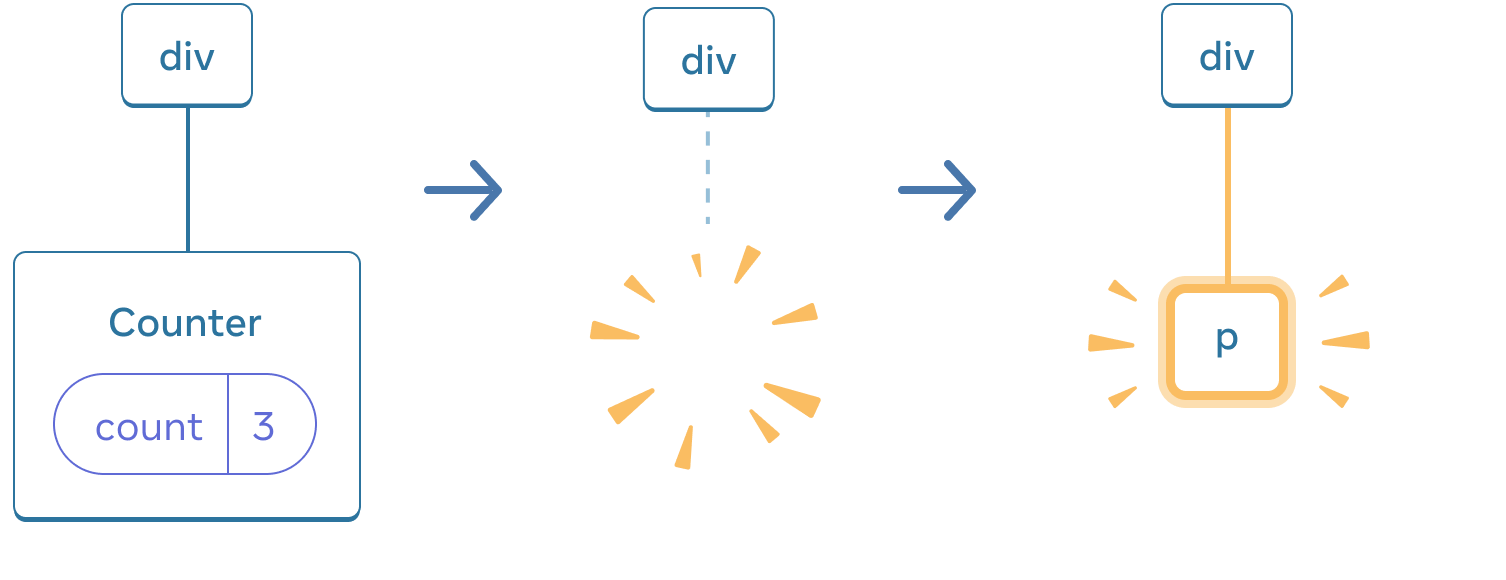\n\nWhen `Counter` changes to `p`, the `Counter` is deleted and the `p` is added\n\n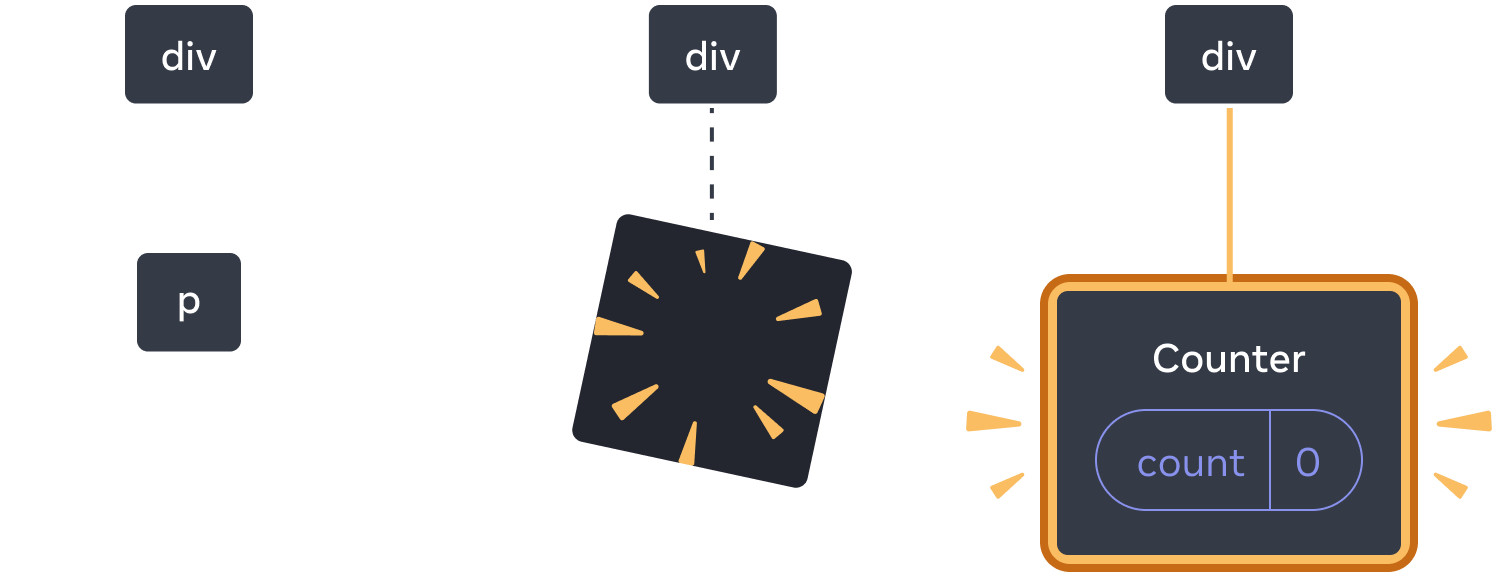\n\n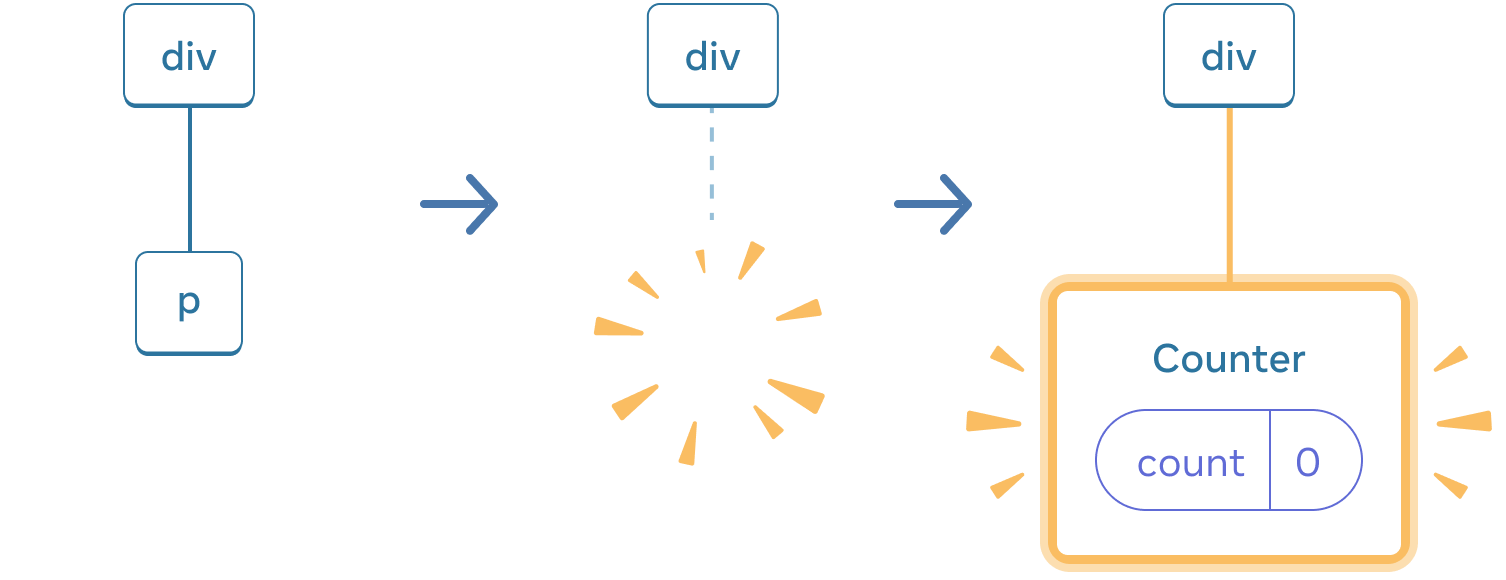\n\nWhen switching back, the `p` is deleted and the `Counter` is added\n\nAlso, **when you render a different component in the same position, it resets the state of its entire subtree.** To see how this works, increment the counter and then tick the checkbox:\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[isFancy, setIsFancy\\] = useState(false);\n return (\n <div\\>\n {isFancy ? (\n <div\\>\n <Counter isFancy\\={true} /> \n </div\\>\n ) : (\n <section\\>\n <Counter isFancy\\={false} />\n </section\\>\n )}\n <label\\>\n <input\n type\\=\"checkbox\"\n checked\\={isFancy}\n onChange\\={e \\=> {\n setIsFancy(e.target.checked)\n }}\n />\n Use fancy styling\n </label\\>\n </div\\>\n );\n}\n\nfunction Counter({ isFancy }) {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n if (isFancy) {\n className += ' fancy';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nThe counter state gets reset when you click the checkbox. Although you render a `Counter`, the first child of the `div` changes from a `div` to a `section`. When the child `div` was removed from the DOM, the whole tree below it (including the `Counter` and its state) was destroyed as well.\n\n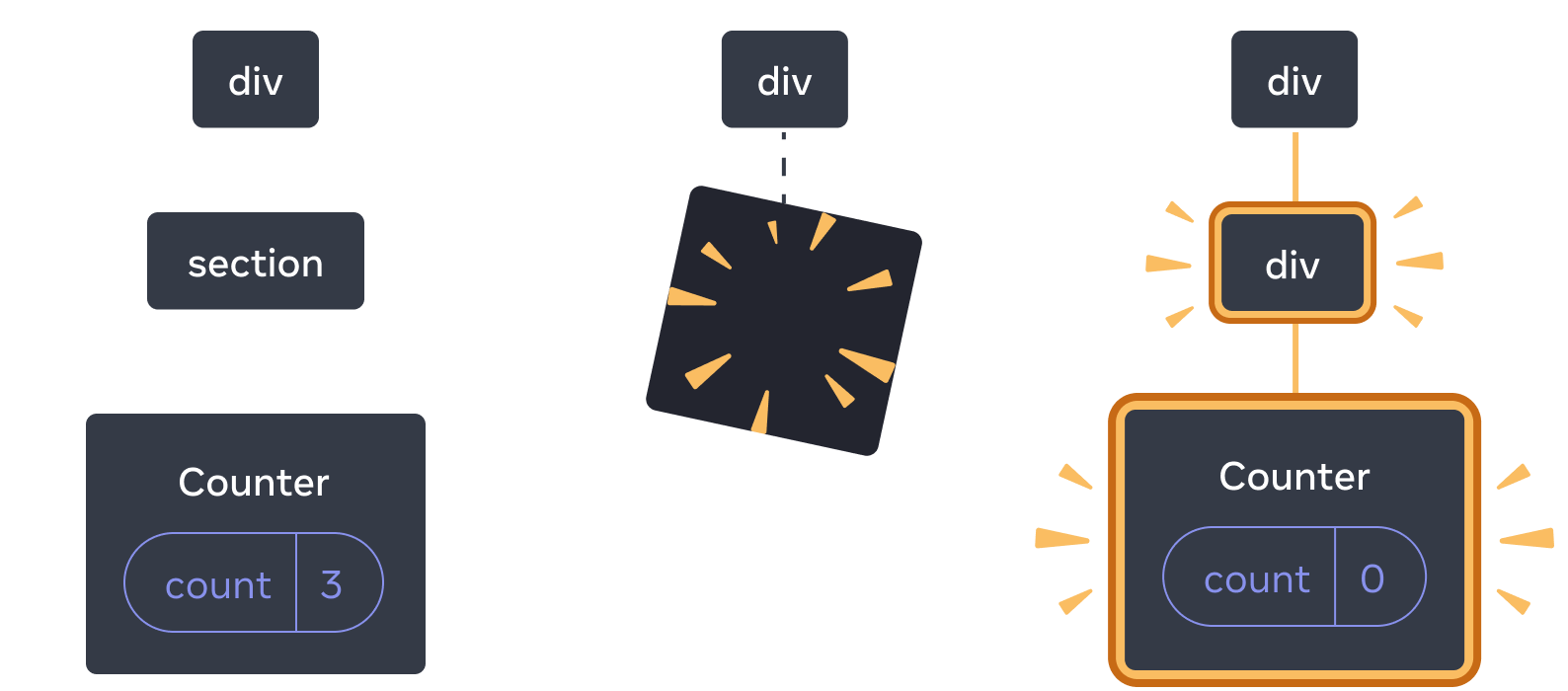\n\n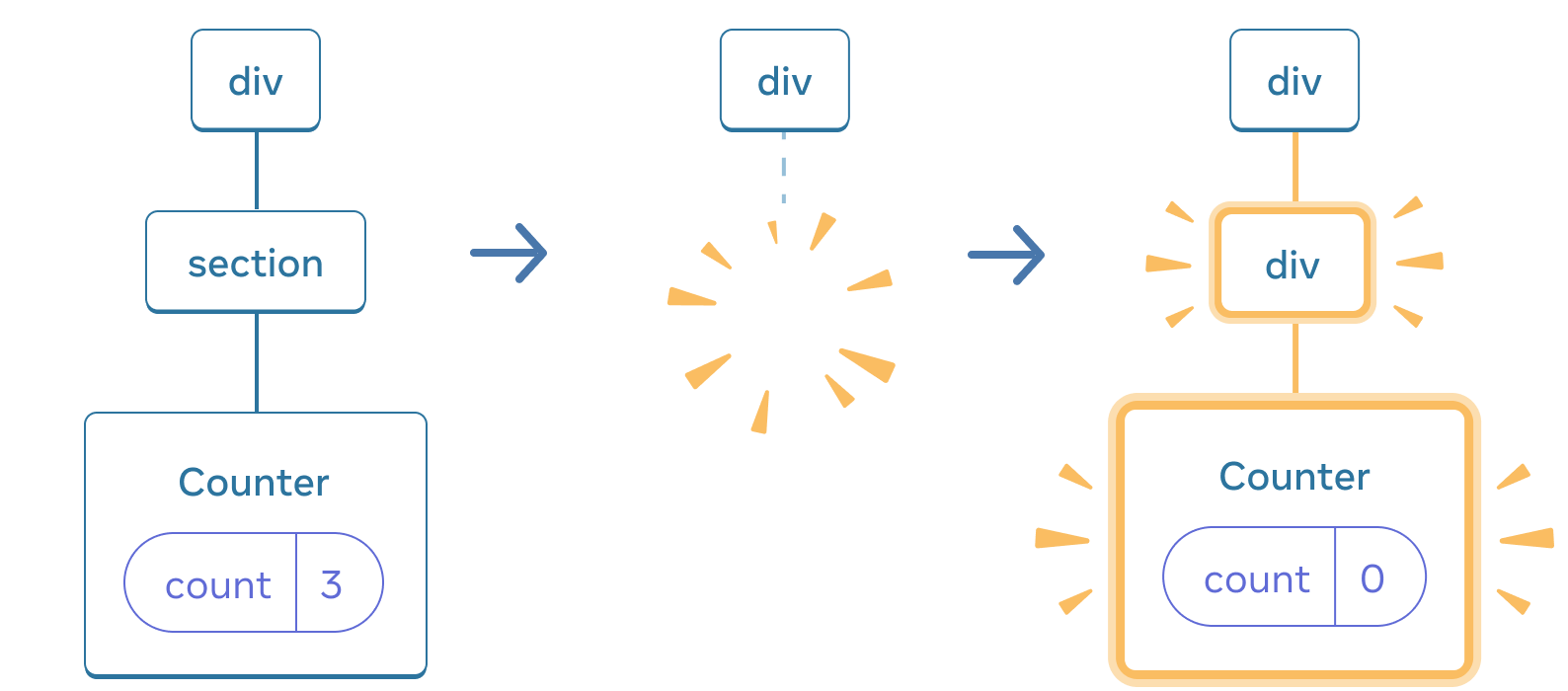\n\nWhen `section` changes to `div`, the `section` is deleted and the new `div` is added\n\n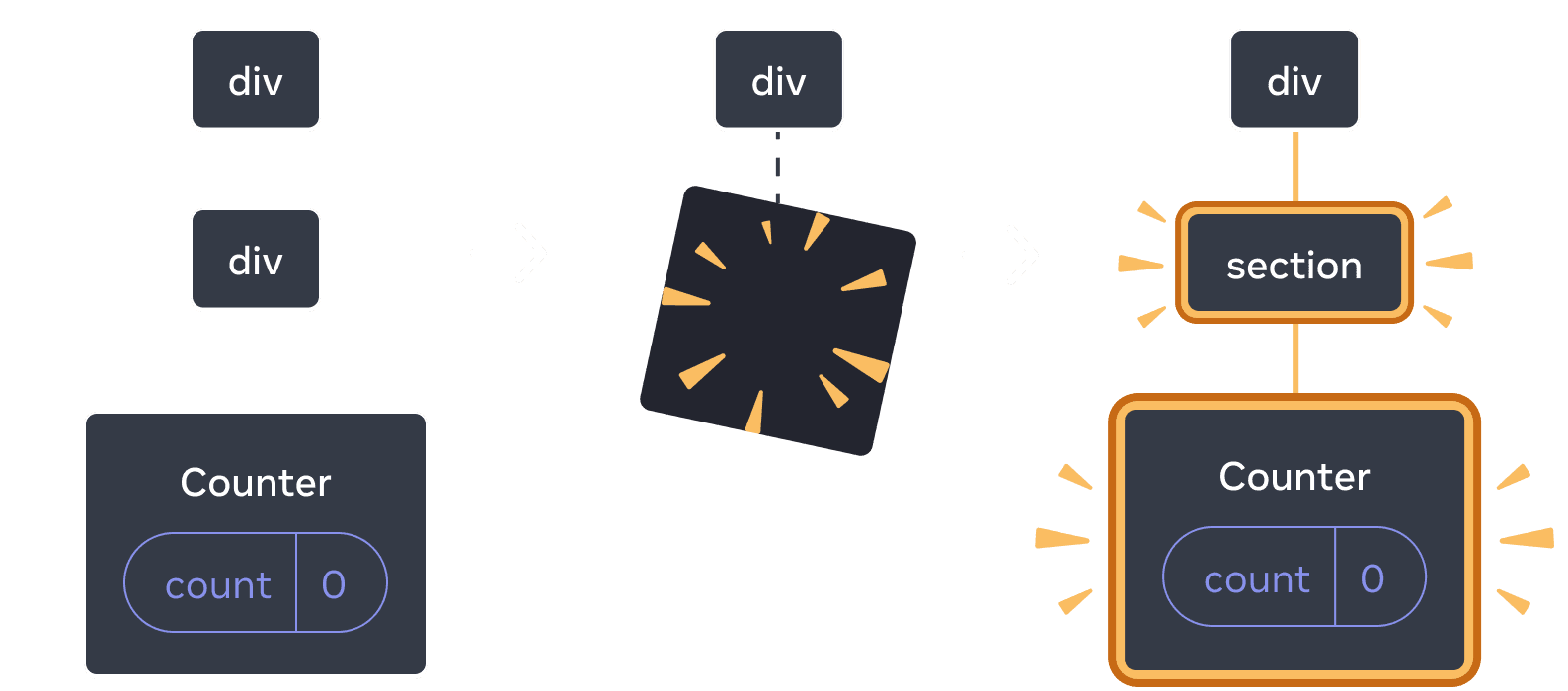\n\n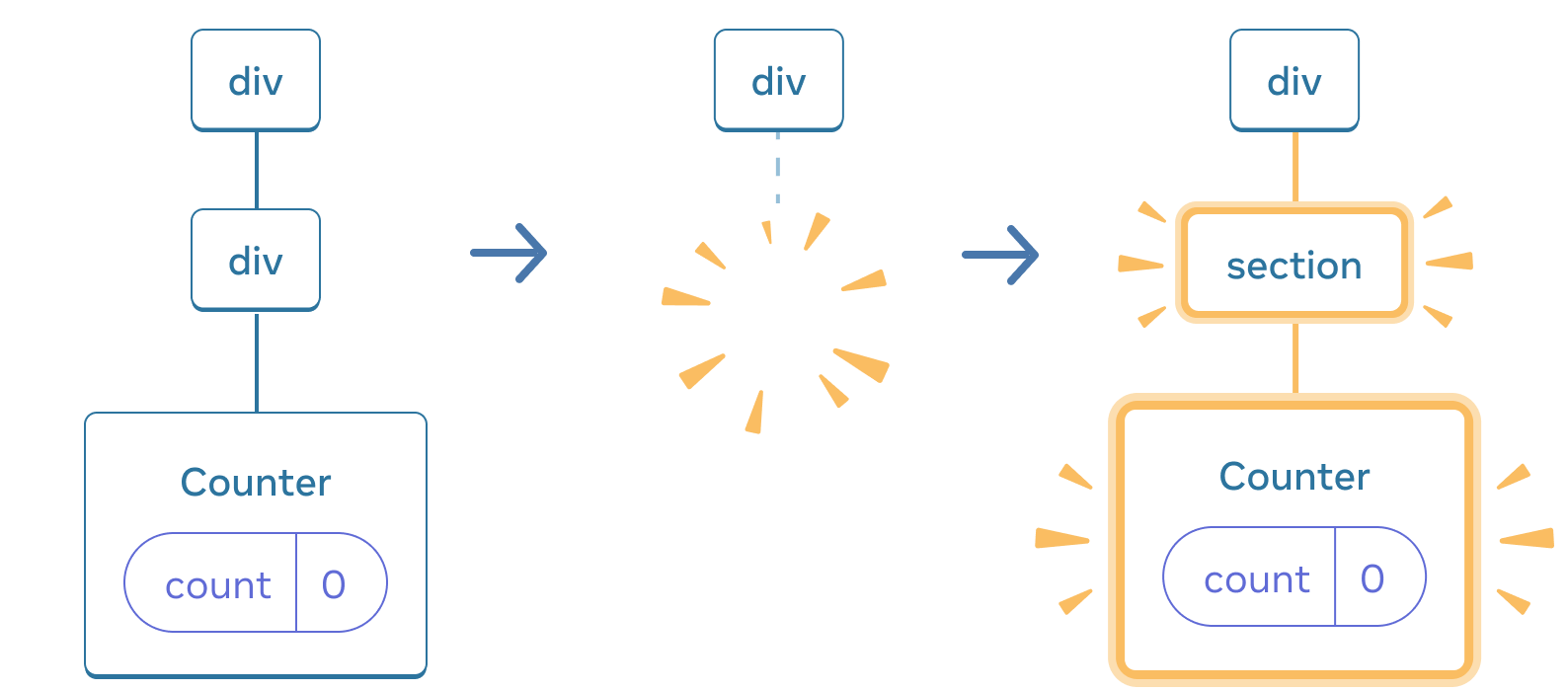\n\nWhen switching back, the `div` is deleted and the new `section` is added\n\nAs a rule of thumb, **if you want to preserve the state between re-renders, the structure of your tree needs to “match up”** from one render to another. If the structure is different, the state gets destroyed because React destroys state when it removes a component from the tree.\n\n### Pitfall\n\nThis is why you should not nest component function definitions.\n\nHere, the `MyTextField` component function is defined _inside_ `MyComponent`:\n\nimport { useState } from 'react';\n\nexport default function MyComponent() {\n const \\[counter, setCounter\\] = useState(0);\n\n function MyTextField() {\n const \\[text, setText\\] = useState('');\n\n return (\n <input\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n );\n }\n\n return (\n <\\>\n <MyTextField />\n <button onClick\\={() \\=> {\n setCounter(counter + 1)\n }}\\>Clicked {counter} times</button\\>\n </\\>\n );\n}\n\nEvery time you click the button, the input state disappears! This is because a _different_ `MyTextField` function is created for every render of `MyComponent`. You’re rendering a _different_ component in the same position, so React resets all state below. This leads to bugs and performance problems. To avoid this problem, **always declare component functions at the top level, and don’t nest their definitions.**\n\nResetting state at the same position[](https://react.dev/learn/preserving-and-resetting-state#resetting-state-at-the-same-position \"Link for Resetting state at the same position \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nBy default, React preserves state of a component while it stays at the same position. Usually, this is exactly what you want, so it makes sense as the default behavior. But sometimes, you may want to reset a component’s state. Consider this app that lets two players keep track of their scores during each turn:\n\nimport { useState } from 'react';\n\nexport default function Scoreboard() {\n const \\[isPlayerA, setIsPlayerA\\] = useState(true);\n return (\n <div\\>\n {isPlayerA ? (\n <Counter person\\=\"Taylor\" />\n ) : (\n <Counter person\\=\"Sarah\" />\n )}\n <button onClick\\={() \\=> {\n setIsPlayerA(!isPlayerA);\n }}\\>\n Next player!\n </button\\>\n </div\\>\n );\n}\n\nfunction Counter({ person }) {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{person}'s score: {score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nCurrently, when you change the player, the score is preserved. The two `Counter`s appear in the same position, so React sees them as _the same_ `Counter` whose `person` prop has changed.\n\nBut conceptually, in this app they should be two separate counters. They might appear in the same place in the UI, but one is a counter for Taylor, and another is a counter for Sarah.\n\nThere are two ways to reset state when switching between them:\n\n1. Render components in different positions\n2. Give each component an explicit identity with `key`\n\n### Option 1: Rendering a component in different positions[](https://react.dev/learn/preserving-and-resetting-state#option-1-rendering-a-component-in-different-positions \"Link for Option 1: Rendering a component in different positions \")\n\nIf you want these two `Counter`s to be independent, you can render them in two different positions:\n\nimport { useState } from 'react';\n\nexport default function Scoreboard() {\n const \\[isPlayerA, setIsPlayerA\\] = useState(true);\n return (\n <div\\>\n {isPlayerA &&\n <Counter person\\=\"Taylor\" />\n }\n {!isPlayerA &&\n <Counter person\\=\"Sarah\" />\n }\n <button onClick\\={() \\=> {\n setIsPlayerA(!isPlayerA);\n }}\\>\n Next player!\n </button\\>\n </div\\>\n );\n}\n\nfunction Counter({ person }) {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{person}'s score: {score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\n* Initially, `isPlayerA` is `true`. So the first position contains `Counter` state, and the second one is empty.\n* When you click the “Next player” button the first position clears but the second one now contains a `Counter`.\n\n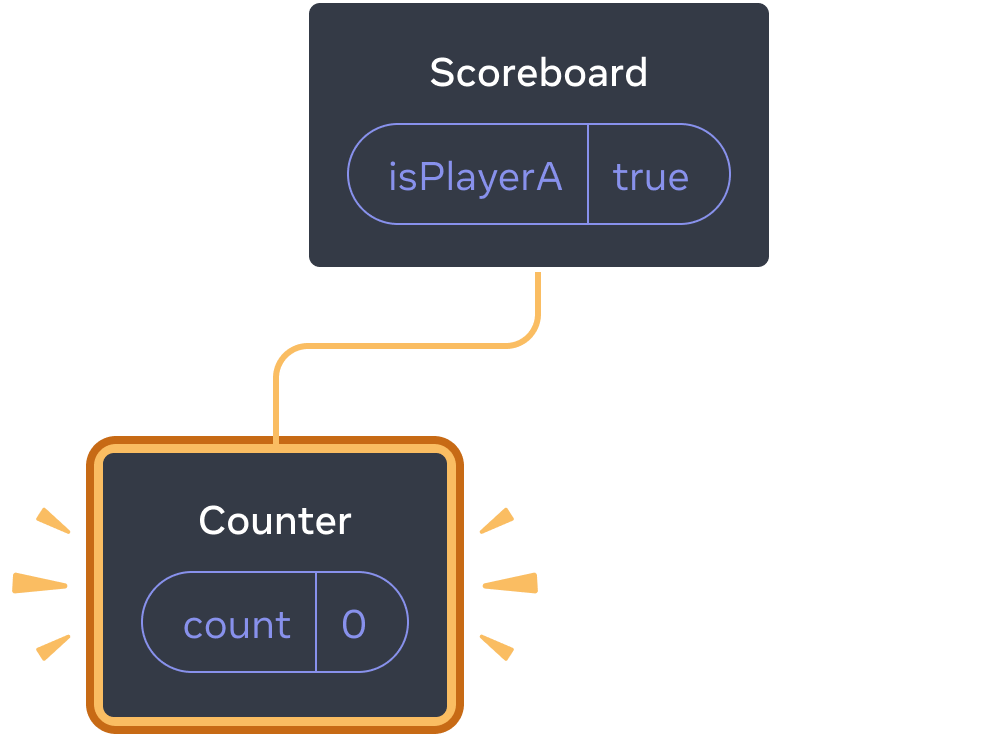\n\n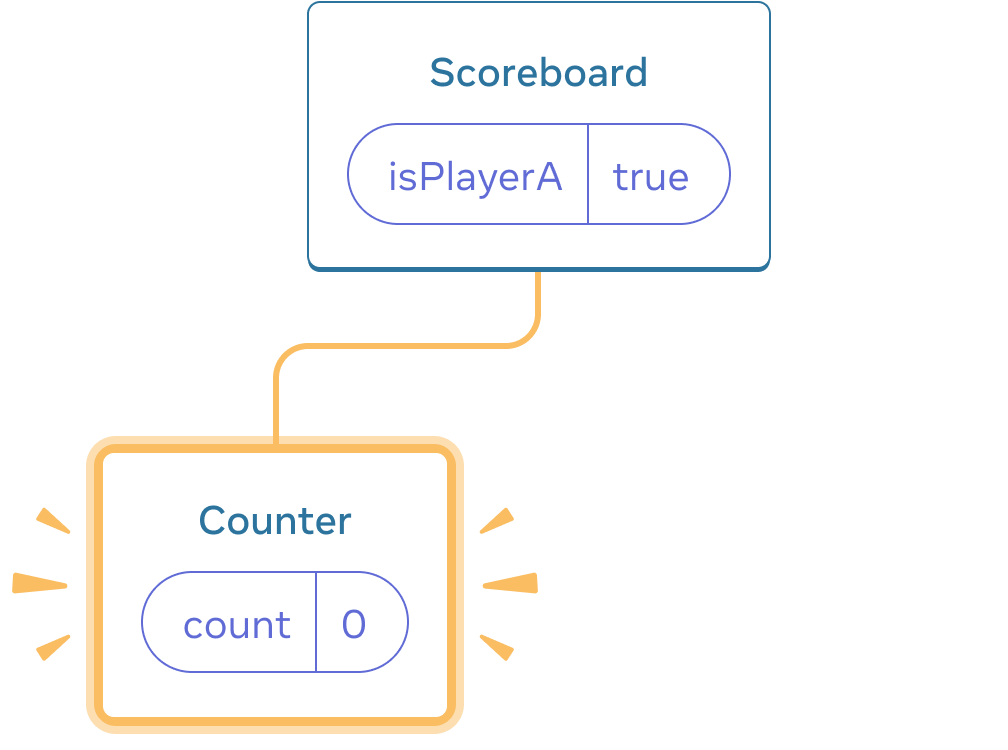\n\nInitial state\n\n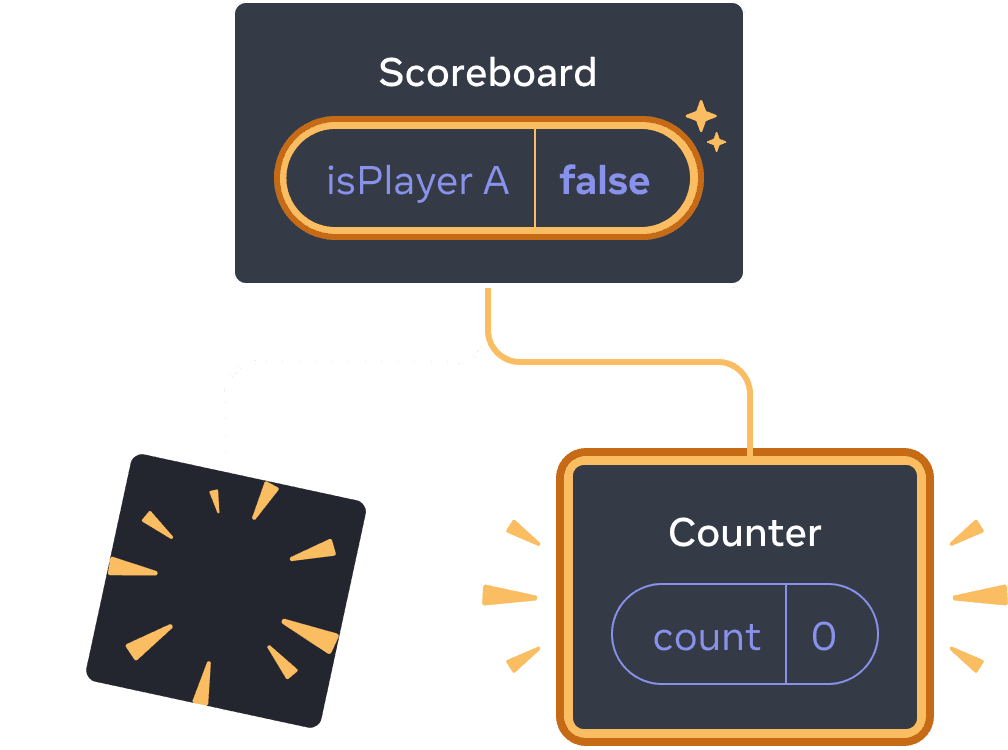\n\n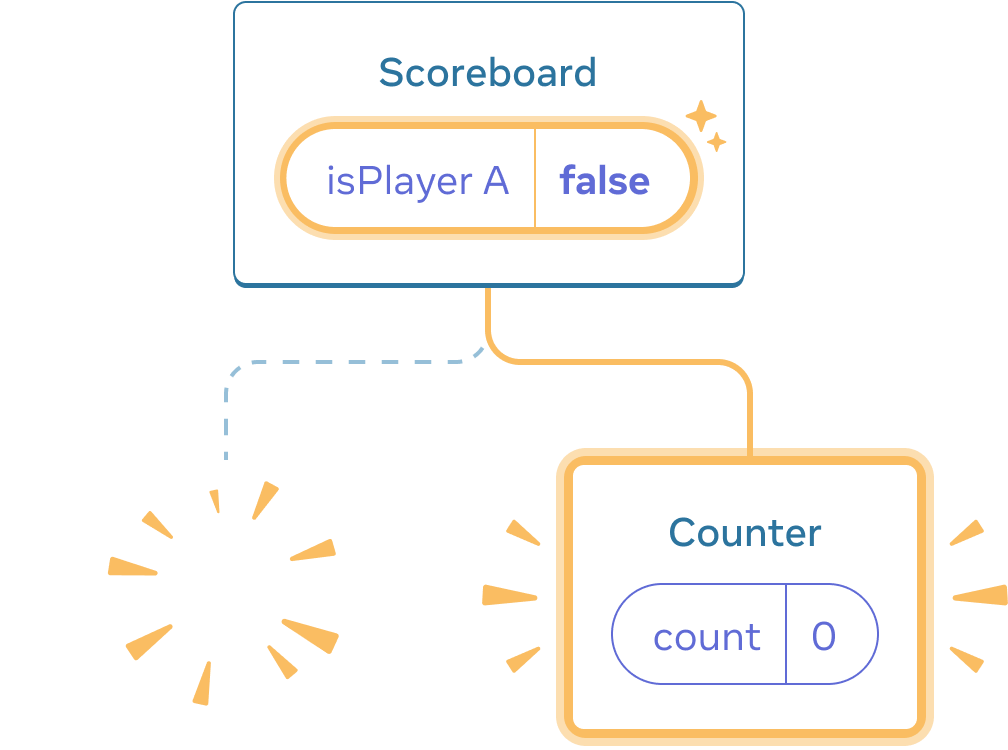\n\nClicking “next”\n\n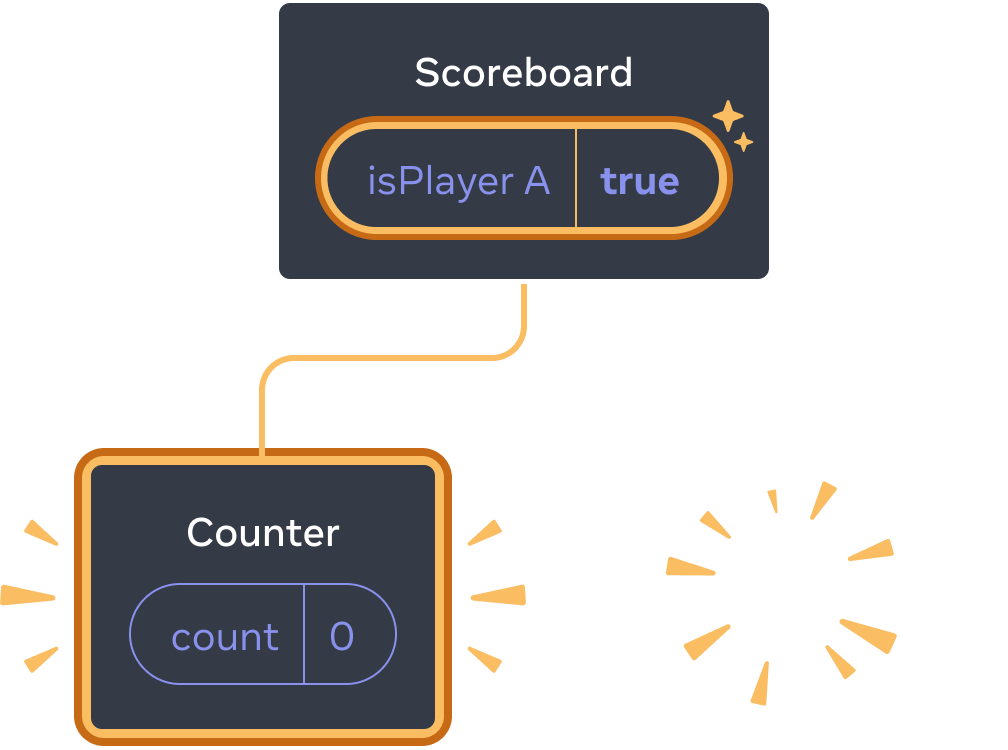\n\n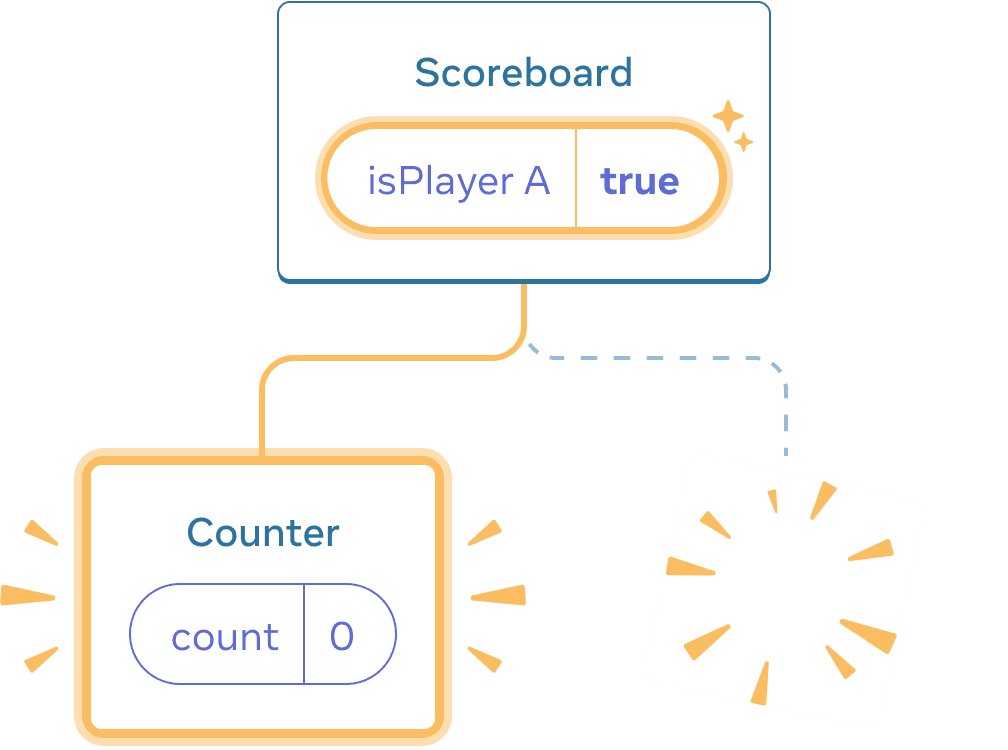\n\nClicking “next” again\n\nEach `Counter`’s state gets destroyed each time it’s removed from the DOM. This is why they reset every time you click the button.\n\nThis solution is convenient when you only have a few independent components rendered in the same place. In this example, you only have two, so it’s not a hassle to render both separately in the JSX.\n\n### Option 2: Resetting state with a key[](https://react.dev/learn/preserving-and-resetting-state#option-2-resetting-state-with-a-key \"Link for Option 2: Resetting state with a key \")\n\nThere is also another, more generic, way to reset a component’s state.\n\nYou might have seen `key`s when [rendering lists.](https://react.dev/learn/rendering-lists#keeping-list-items-in-order-with-key) Keys aren’t just for lists! You can use keys to make React distinguish between any components. By default, React uses order within the parent (“first counter”, “second counter”) to discern between components. But keys let you tell React that this is not just a _first_ counter, or a _second_ counter, but a specific counter—for example, _Taylor’s_ counter. This way, React will know _Taylor’s_ counter wherever it appears in the tree!\n\nIn this example, the two `<Counter />`s don’t share state even though they appear in the same place in JSX:\n\nimport { useState } from 'react';\n\nexport default function Scoreboard() {\n const \\[isPlayerA, setIsPlayerA\\] = useState(true);\n return (\n <div\\>\n {isPlayerA ? (\n <Counter key\\=\"Taylor\" person\\=\"Taylor\" />\n ) : (\n <Counter key\\=\"Sarah\" person\\=\"Sarah\" />\n )}\n <button onClick\\={() \\=> {\n setIsPlayerA(!isPlayerA);\n }}\\>\n Next player!\n </button\\>\n </div\\>\n );\n}\n\nfunction Counter({ person }) {\n const \\[score, setScore\\] = useState(0);\n const \\[hover, setHover\\] = useState(false);\n\n let className = 'counter';\n if (hover) {\n className += ' hover';\n }\n\n return (\n <div\n className\\={className}\n onPointerEnter\\={() \\=> setHover(true)}\n onPointerLeave\\={() \\=> setHover(false)}\n \\>\n <h1\\>{person}'s score: {score}</h1\\>\n <button onClick\\={() \\=> setScore(score + 1)}\\>\n Add one\n </button\\>\n </div\\>\n );\n}\n\nSwitching between Taylor and Sarah does not preserve the state. This is because **you gave them different `key`s:**\n\n```\n{isPlayerA ? (<Counter key=\"Taylor\" person=\"Taylor\" />) : (<Counter key=\"Sarah\" person=\"Sarah\" />)}\n```\n\nSpecifying a `key` tells React to use the `key` itself as part of the position, instead of their order within the parent. This is why, even though you render them in the same place in JSX, React sees them as two different counters, and so they will never share state. Every time a counter appears on the screen, its state is created. Every time it is removed, its state is destroyed. Toggling between them resets their state over and over.\n\n### Note\n\nRemember that keys are not globally unique. They only specify the position _within the parent_.\n\n### Resetting a form with a key[](https://react.dev/learn/preserving-and-resetting-state#resetting-a-form-with-a-key \"Link for Resetting a form with a key \")\n\nResetting state with a key is particularly useful when dealing with forms.\n\nIn this chat app, the `<Chat>` component contains the text input state:\n\nimport { useState } from 'react';\nimport Chat from './Chat.js';\nimport ContactList from './ContactList.js';\n\nexport default function Messenger() {\n const \\[to, setTo\\] = useState(contacts\\[0\\]);\n return (\n <div\\>\n <ContactList\n contacts\\={contacts}\n selectedContact\\={to}\n onSelect\\={contact \\=> setTo(contact)}\n />\n <Chat contact\\={to} />\n </div\\>\n )\n}\n\nconst contacts = \\[\n { id: 0, name: 'Taylor', email: 'taylor@mail.com' },\n { id: 1, name: 'Alice', email: 'alice@mail.com' },\n { id: 2, name: 'Bob', email: 'bob@mail.com' }\n\\];\n\nTry entering something into the input, and then press “Alice” or “Bob” to choose a different recipient. You will notice that the input state is preserved because the `<Chat>` is rendered at the same position in the tree.\n\n**In many apps, this may be the desired behavior, but not in a chat app!** You don’t want to let the user send a message they already typed to a wrong person due to an accidental click. To fix it, add a `key`:\n\n```\n<Chat key={to.id} contact={to} />\n```\n\nThis ensures that when you select a different recipient, the `Chat` component will be recreated from scratch, including any state in the tree below it. React will also re-create the DOM elements instead of reusing them.\n\nNow switching the recipient always clears the text field:\n\nimport { useState } from 'react';\nimport Chat from './Chat.js';\nimport ContactList from './ContactList.js';\n\nexport default function Messenger() {\n const \\[to, setTo\\] = useState(contacts\\[0\\]);\n return (\n <div\\>\n <ContactList\n contacts\\={contacts}\n selectedContact\\={to}\n onSelect\\={contact \\=> setTo(contact)}\n />\n <Chat key\\={to.id} contact\\={to} />\n </div\\>\n )\n}\n\nconst contacts = \\[\n { id: 0, name: 'Taylor', email: 'taylor@mail.com' },\n { id: 1, name: 'Alice', email: 'alice@mail.com' },\n { id: 2, name: 'Bob', email: 'bob@mail.com' }\n\\];\n\n##### Deep Dive\n\n#### Preserving state for removed components[](https://react.dev/learn/preserving-and-resetting-state#preserving-state-for-removed-components \"Link for Preserving state for removed components \")\n\nIn a real chat app, you’d probably want to recover the input state when the user selects the previous recipient again. There are a few ways to keep the state “alive” for a component that’s no longer visible:\n\n* You could render _all_ chats instead of just the current one, but hide all the others with CSS. The chats would not get removed from the tree, so their local state would be preserved. This solution works great for simple UIs. But it can get very slow if the hidden trees are large and contain a lot of DOM nodes.\n* You could [lift the state up](https://react.dev/learn/sharing-state-between-components) and hold the pending message for each recipient in the parent component. This way, when the child components get removed, it doesn’t matter, because it’s the parent that keeps the important information. This is the most common solution.\n* You might also use a different source in addition to React state. For example, you probably want a message draft to persist even if the user accidentally closes the page. To implement this, you could have the `Chat` component initialize its state by reading from the [`localStorage`](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage), and save the drafts there too.\n\nNo matter which strategy you pick, a chat _with Alice_ is conceptually distinct from a chat _with Bob_, so it makes sense to give a `key` to the `<Chat>` tree based on the current recipient.\n\nRecap[](https://react.dev/learn/preserving-and-resetting-state#recap \"Link for Recap\")\n--------------------------------------------------------------------------------------\n\n* React keeps state for as long as the same component is rendered at the same position.\n* State is not kept in JSX tags. It’s associated with the tree position in which you put that JSX.\n* You can force a subtree to reset its state by giving it a different key.\n* Don’t nest component definitions, or you’ll reset state by accident.\n\nTry out some challenges[](https://react.dev/learn/preserving-and-resetting-state#challenges \"Link for Try out some challenges\")\n-------------------------------------------------------------------------------------------------------------------------------\n\n#### Fix disappearing input text[](https://react.dev/learn/preserving-and-resetting-state#fix-disappearing-input-text \"Link for this heading\")\n\nThis example shows a message when you press the button. However, pressing the button also accidentally resets the input. Why does this happen? Fix it so that pressing the button does not reset the input text.\n\nimport { useState } from 'react';\n\nexport default function App() {\n const \\[showHint, setShowHint\\] = useState(false);\n if (showHint) {\n return (\n <div\\>\n <p\\><i\\>Hint: Your favorite city?</i\\></p\\>\n <Form />\n <button onClick\\={() \\=> {\n setShowHint(false);\n }}\\>Hide hint</button\\>\n </div\\>\n );\n }\n return (\n <div\\>\n <Form />\n <button onClick\\={() \\=> {\n setShowHint(true);\n }}\\>Show hint</button\\>\n </div\\>\n );\n}\n\nfunction Form() {\n const \\[text, setText\\] = useState('');\n return (\n <textarea\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n );\n}\n",
"filename": "preserving-and-resetting-state.md",
"package": "react"
} |
{
"content": "Title: <progress> – React\n\nURL Source: https://react.dev/reference/react-dom/components/progress\n\nMarkdown Content:\nReact\nv18.3.1\nSearch\nCtrl\nK\nLearn\nReference\nCommunity\nBlog\nreact@18.3.1\nOverview\nHooks\nComponents\nAPIs\nreact-dom@18.3.1\nHooks\nComponents\nCommon (e.g. <div>)\n<form> \n<input>\n<option>\n<progress>\n<select>\n<textarea>\n<link> \n<meta> \n<script> \n<style> \n<title> \nAPIs\nClient APIs\nServer APIs\nRules of React\nOverview\nReact Server Components\nServer Components \nServer Actions \nDirectives \nLegacy APIs\nLegacy React APIs\n\nIs this page useful?\n\nAPI REFERENCE\nCOMPONENTS\n<progress>\n\nThe built-in browser <progress> component lets you render a progress indicator.\n\n<progress value={0.5} />\nReference\n<progress>\nUsage\nControlling a progress indicator\nReference \n<progress> \n\nTo display a progress indicator, render the built-in browser <progress> component.\n\n<progress value={0.5} />\n\nSee more examples below.\n\nProps \n\n<progress> supports all common element props.\n\nAdditionally, <progress> supports these props:\n\nmax: A number. Specifies the maximum value. Defaults to 1.\nvalue: A number between 0 and max, or null for indeterminate progress. Specifies how much was done.\nUsage \nControlling a progress indicator \n\nTo display a progress indicator, render a <progress> component. You can pass a number value between 0 and the max value you specify. If you don’t pass a max value, it will assumed to be 1 by default.\n\nIf the operation is not ongoing, pass value={null} to put the progress indicator into an indeterminate state.\n\nApp.js\nDownload\nReset\nFork\nexport default function App() {\n return (\n <>\n <progress value={0} />\n <progress value={0.5} />\n <progress value={0.7} />\n <progress value={75} max={100} />\n <progress value={1} />\n <progress value={null} />\n </>\n );\n}\n\n\nPREVIOUS\n<option>\nNEXT\n<select>\n©2024\nuwu?\nLearn React\nQuick Start\nInstallation\nDescribing the UI\nAdding Interactivity\nManaging State\nEscape Hatches\nAPI Reference\nReact APIs\nReact DOM APIs\nCommunity\nCode of Conduct\nMeet the Team\nDocs Contributors\nAcknowledgements\nMore\nBlog\nReact Native\nPrivacy\nTerms\n",
"filename": "progress.md",
"package": "react"
} |
{
"content": "Title: Queueing a Series of State Updates – React\n\nURL Source: https://react.dev/learn/queueing-a-series-of-state-updates\n\nMarkdown Content:\nSetting a state variable will queue another render. But sometimes you might want to perform multiple operations on the value before queueing the next render. To do this, it helps to understand how React batches state updates.\n\n### You will learn\n\n* What “batching” is and how React uses it to process multiple state updates\n* How to apply several updates to the same state variable in a row\n\nReact batches state updates[](https://react.dev/learn/queueing-a-series-of-state-updates#react-batches-state-updates \"Link for React batches state updates \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou might expect that clicking the “+3” button will increment the counter three times because it calls `setNumber(number + 1)` three times:\n\nHowever, as you might recall from the previous section, [each render’s state values are fixed](https://react.dev/learn/state-as-a-snapshot#rendering-takes-a-snapshot-in-time), so the value of `number` inside the first render’s event handler is always `0`, no matter how many times you call `setNumber(1)`:\n\n```\nsetNumber(0 + 1);setNumber(0 + 1);setNumber(0 + 1);\n```\n\nBut there is one other factor at play here. **React waits until _all_ code in the event handlers has run before processing your state updates.** This is why the re-render only happens _after_ all these `setNumber()` calls.\n\nThis might remind you of a waiter taking an order at the restaurant. A waiter doesn’t run to the kitchen at the mention of your first dish! Instead, they let you finish your order, let you make changes to it, and even take orders from other people at the table.\n\n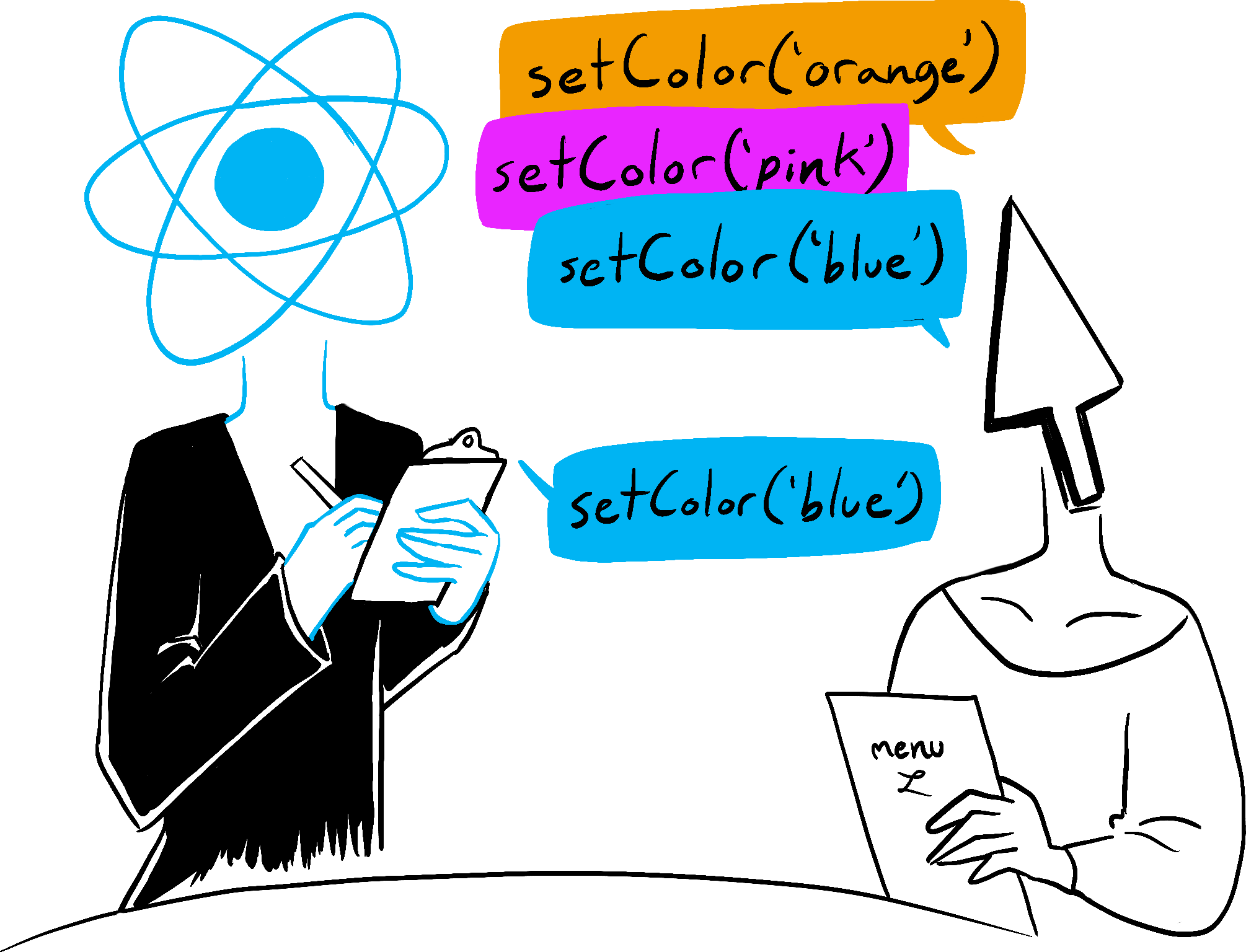\n\nThis lets you update multiple state variables—even from multiple components—without triggering too many [re-renders.](https://react.dev/learn/render-and-commit#re-renders-when-state-updates) But this also means that the UI won’t be updated until _after_ your event handler, and any code in it, completes. This behavior, also known as **batching,** makes your React app run much faster. It also avoids dealing with confusing “half-finished” renders where only some of the variables have been updated.\n\n**React does not batch across _multiple_ intentional events like clicks**—each click is handled separately. Rest assured that React only does batching when it’s generally safe to do. This ensures that, for example, if the first button click disables a form, the second click would not submit it again.\n\nUpdating the same state multiple times before the next render[](https://react.dev/learn/queueing-a-series-of-state-updates#updating-the-same-state-multiple-times-before-the-next-render \"Link for Updating the same state multiple times before the next render \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nIt is an uncommon use case, but if you would like to update the same state variable multiple times before the next render, instead of passing the _next state value_ like `setNumber(number + 1)`, you can pass a _function_ that calculates the next state based on the previous one in the queue, like `setNumber(n => n + 1)`. It is a way to tell React to “do something with the state value” instead of just replacing it.\n\nTry incrementing the counter now:\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[number, setNumber\\] = useState(0);\n\n return (\n <\\>\n <h1\\>{number}</h1\\>\n <button onClick\\={() \\=> {\n setNumber(n \\=> n + 1);\n setNumber(n \\=> n + 1);\n setNumber(n \\=> n + 1);\n }}\\>+3</button\\>\n </\\>\n )\n}\n\nHere, `n => n + 1` is called an **updater function.** When you pass it to a state setter:\n\n1. React queues this function to be processed after all the other code in the event handler has run.\n2. During the next render, React goes through the queue and gives you the final updated state.\n\n```\nsetNumber(n => n + 1);setNumber(n => n + 1);setNumber(n => n + 1);\n```\n\nHere’s how React works through these lines of code while executing the event handler:\n\n1. `setNumber(n => n + 1)`: `n => n + 1` is a function. React adds it to a queue.\n2. `setNumber(n => n + 1)`: `n => n + 1` is a function. React adds it to a queue.\n3. `setNumber(n => n + 1)`: `n => n + 1` is a function. React adds it to a queue.\n\nWhen you call `useState` during the next render, React goes through the queue. The previous `number` state was `0`, so that’s what React passes to the first updater function as the `n` argument. Then React takes the return value of your previous updater function and passes it to the next updater as `n`, and so on:\n\n| queued update | `n` | returns |\n| --- | --- | --- |\n| `n => n + 1` | `0` | `0 + 1 = 1` |\n| `n => n + 1` | `1` | `1 + 1 = 2` |\n| `n => n + 1` | `2` | `2 + 1 = 3` |\n\nReact stores `3` as the final result and returns it from `useState`.\n\nThis is why clicking “+3” in the above example correctly increments the value by 3.\n\n### What happens if you update state after replacing it[](https://react.dev/learn/queueing-a-series-of-state-updates#what-happens-if-you-update-state-after-replacing-it \"Link for What happens if you update state after replacing it \")\n\nWhat about this event handler? What do you think `number` will be in the next render?\n\n```\n<button onClick={() => {setNumber(number + 5);setNumber(n => n + 1);}}>\n```\n\nHere’s what this event handler tells React to do:\n\n1. `setNumber(number + 5)`: `number` is `0`, so `setNumber(0 + 5)`. React adds _“replace with `5`”_ to its queue.\n2. `setNumber(n => n + 1)`: `n => n + 1` is an updater function. React adds _that function_ to its queue.\n\nDuring the next render, React goes through the state queue:\n\n| queued update | `n` | returns |\n| --- | --- | --- |\n| ”replace with `5`” | `0` (unused) | `5` |\n| `n => n + 1` | `5` | `5 + 1 = 6` |\n\nReact stores `6` as the final result and returns it from `useState`.\n\n### Note\n\nYou may have noticed that `setState(5)` actually works like `setState(n => 5)`, but `n` is unused!\n\n### What happens if you replace state after updating it[](https://react.dev/learn/queueing-a-series-of-state-updates#what-happens-if-you-replace-state-after-updating-it \"Link for What happens if you replace state after updating it \")\n\nLet’s try one more example. What do you think `number` will be in the next render?\n\n```\n<button onClick={() => {setNumber(number + 5);setNumber(n => n + 1);setNumber(42);}}>\n```\n\nimport { useState } from 'react';\n\nexport default function Counter() {\n const \\[number, setNumber\\] = useState(0);\n\n return (\n <\\>\n <h1\\>{number}</h1\\>\n <button onClick\\={() \\=> {\n setNumber(number + 5);\n setNumber(n \\=> n + 1);\n setNumber(42);\n }}\\>Increase the number</button\\>\n </\\>\n )\n}\n\nHere’s how React works through these lines of code while executing this event handler:\n\n1. `setNumber(number + 5)`: `number` is `0`, so `setNumber(0 + 5)`. React adds _“replace with `5`”_ to its queue.\n2. `setNumber(n => n + 1)`: `n => n + 1` is an updater function. React adds _that function_ to its queue.\n3. `setNumber(42)`: React adds _“replace with `42`”_ to its queue.\n\nDuring the next render, React goes through the state queue:\n\n| queued update | `n` | returns |\n| --- | --- | --- |\n| ”replace with `5`” | `0` (unused) | `5` |\n| `n => n + 1` | `5` | `5 + 1 = 6` |\n| ”replace with `42`” | `6` (unused) | `42` |\n\nThen React stores `42` as the final result and returns it from `useState`.\n\nTo summarize, here’s how you can think of what you’re passing to the `setNumber` state setter:\n\n* **An updater function** (e.g. `n => n + 1`) gets added to the queue.\n* **Any other value** (e.g. number `5`) adds “replace with `5`” to the queue, ignoring what’s already queued.\n\nAfter the event handler completes, React will trigger a re-render. During the re-render, React will process the queue. Updater functions run during rendering, so **updater functions must be [pure](https://react.dev/learn/keeping-components-pure)** and only _return_ the result. Don’t try to set state from inside of them or run other side effects. In Strict Mode, React will run each updater function twice (but discard the second result) to help you find mistakes.\n\n### Naming conventions[](https://react.dev/learn/queueing-a-series-of-state-updates#naming-conventions \"Link for Naming conventions \")\n\nIt’s common to name the updater function argument by the first letters of the corresponding state variable:\n\n```\nsetEnabled(e => !e);setLastName(ln => ln.reverse());setFriendCount(fc => fc * 2);\n```\n\nIf you prefer more verbose code, another common convention is to repeat the full state variable name, like `setEnabled(enabled => !enabled)`, or to use a prefix like `setEnabled(prevEnabled => !prevEnabled)`.\n\nRecap[](https://react.dev/learn/queueing-a-series-of-state-updates#recap \"Link for Recap\")\n------------------------------------------------------------------------------------------\n\n* Setting state does not change the variable in the existing render, but it requests a new render.\n* React processes state updates after event handlers have finished running. This is called batching.\n* To update some state multiple times in one event, you can use `setNumber(n => n + 1)` updater function.\n\nTry out some challenges[](https://react.dev/learn/queueing-a-series-of-state-updates#challenges \"Link for Try out some challenges\")\n-----------------------------------------------------------------------------------------------------------------------------------\n\n#### Fix a request counter[](https://react.dev/learn/queueing-a-series-of-state-updates#fix-a-request-counter \"Link for this heading\")\n\nYou’re working on an art marketplace app that lets the user submit multiple orders for an art item at the same time. Each time the user presses the “Buy” button, the “Pending” counter should increase by one. After three seconds, the “Pending” counter should decrease, and the “Completed” counter should increase.\n\nHowever, the “Pending” counter does not behave as intended. When you press “Buy”, it decreases to `-1` (which should not be possible!). And if you click fast twice, both counters seem to behave unpredictably.\n\nWhy does this happen? Fix both counters.\n\nimport { useState } from 'react';\n\nexport default function RequestTracker() {\n const \\[pending, setPending\\] = useState(0);\n const \\[completed, setCompleted\\] = useState(0);\n\n async function handleClick() {\n setPending(pending + 1);\n await delay(3000);\n setPending(pending - 1);\n setCompleted(completed + 1);\n }\n\n return (\n <\\>\n <h3\\>\n Pending: {pending}\n </h3\\>\n <h3\\>\n Completed: {completed}\n </h3\\>\n <button onClick\\={handleClick}\\>\n Buy \n </button\\>\n </\\>\n );\n}\n\nfunction delay(ms) {\n return new Promise(resolve \\=> {\n setTimeout(resolve, ms);\n });\n}\n",
"filename": "queueing-a-series-of-state-updates.md",
"package": "react"
} |
{
"content": "Title: React calls Components and Hooks – React\n\nURL Source: https://react.dev/reference/rules/react-calls-components-and-hooks\n\nMarkdown Content:\nReact is responsible for rendering components and Hooks when necessary to optimize the user experience. It is declarative: you tell React what to render in your component’s logic, and React will figure out how best to display it to your user.\n\n* [Never call component functions directly](https://react.dev/reference/rules/react-calls-components-and-hooks#never-call-component-functions-directly)\n* [Never pass around Hooks as regular values](https://react.dev/reference/rules/react-calls-components-and-hooks#never-pass-around-hooks-as-regular-values)\n * [Don’t dynamically mutate a Hook](https://react.dev/reference/rules/react-calls-components-and-hooks#dont-dynamically-mutate-a-hook)\n * [Don’t dynamically use Hooks](https://react.dev/reference/rules/react-calls-components-and-hooks#dont-dynamically-use-hooks)\n\n* * *\n\nNever call component functions directly[](https://react.dev/reference/rules/react-calls-components-and-hooks#never-call-component-functions-directly \"Link for Never call component functions directly \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nComponents should only be used in JSX. Don’t call them as regular functions. React should call it.\n\nReact must decide when your component function is called [during rendering](https://react.dev/reference/rules/components-and-hooks-must-be-pure#how-does-react-run-your-code). In React, you do this using JSX.\n\n```\nfunction BlogPost() {return <Layout><Article /></Layout>; // ✅ Good: Only use components in JSX}\n```\n\n```\nfunction BlogPost() {return <Layout>{Article()}</Layout>; // 🔴 Bad: Never call them directly}\n```\n\nIf a component contains Hooks, it’s easy to violate the [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) when components are called directly in a loop or conditionally.\n\nLetting React orchestrate rendering also allows a number of benefits:\n\n* **Components become more than functions.** React can augment them with features like _local state_ through Hooks that are tied to the component’s identity in the tree.\n* **Component types participate in reconciliation.** By letting React call your components, you also tell it more about the conceptual structure of your tree. For example, when you move from rendering `<Feed>` to the `<Profile>` page, React won’t attempt to re-use them.\n* **React can enhance your user experience.** For example, it can let the browser do some work between component calls so that re-rendering a large component tree doesn’t block the main thread.\n* **A better debugging story.** If components are first-class citizens that the library is aware of, we can build rich developer tools for introspection in development.\n* **More efficient reconciliation.** React can decide exactly which components in the tree need re-rendering and skip over the ones that don’t. That makes your app faster and more snappy.\n\n* * *\n\nNever pass around Hooks as regular values[](https://react.dev/reference/rules/react-calls-components-and-hooks#never-pass-around-hooks-as-regular-values \"Link for Never pass around Hooks as regular values \")\n---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nHooks should only be called inside of components or Hooks. Never pass it around as a regular value.\n\nHooks allow you to augment a component with React features. They should always be called as a function, and never passed around as a regular value. This enables _local reasoning_, or the ability for developers to understand everything a component can do by looking at that component in isolation.\n\nBreaking this rule will cause React to not automatically optimize your component.\n\n### Don’t dynamically mutate a Hook[](https://react.dev/reference/rules/react-calls-components-and-hooks#dont-dynamically-mutate-a-hook \"Link for Don’t dynamically mutate a Hook \")\n\nHooks should be as “static” as possible. This means you shouldn’t dynamically mutate them. For example, this means you shouldn’t write higher order Hooks:\n\n```\nfunction ChatInput() {const useDataWithLogging = withLogging(useData); // 🔴 Bad: don't write higher order Hooksconst data = useDataWithLogging();}\n```\n\nHooks should be immutable and not be mutated. Instead of mutating a Hook dynamically, create a static version of the Hook with the desired functionality.\n\n```\nfunction ChatInput() {const data = useDataWithLogging(); // ✅ Good: Create a new version of the Hook}function useDataWithLogging() {// ... Create a new version of the Hook and inline the logic here}\n```\n\n### Don’t dynamically use Hooks[](https://react.dev/reference/rules/react-calls-components-and-hooks#dont-dynamically-use-hooks \"Link for Don’t dynamically use Hooks \")\n\nHooks should also not be dynamically used: for example, instead of doing dependency injection in a component by passing a Hook as a value:\n\n```\nfunction ChatInput() {return <Button useData={useDataWithLogging} /> // 🔴 Bad: don't pass Hooks as props}\n```\n\nYou should always inline the call of the Hook into that component and handle any logic in there.\n\n```\nfunction ChatInput() {return <Button />}function Button() {const data = useDataWithLogging(); // ✅ Good: Use the Hook directly}function useDataWithLogging() {// If there's any conditional logic to change the Hook's behavior, it should be inlined into// the Hook}\n```\n\nThis way, `<Button />` is much easier to understand and debug. When Hooks are used in dynamic ways, it increases the complexity of your app greatly and inhibits local reasoning, making your team less productive in the long term. It also makes it easier to accidentally break the [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) that Hooks should not be called conditionally. If you find yourself needing to mock components for tests, it’s better to mock the server instead to respond with canned data. If possible, it’s also usually more effective to test your app with end-to-end tests.\n",
"filename": "react-calls-components-and-hooks.md",
"package": "react"
} |
{
"content": "Title: React Compiler – React\n\nURL Source: https://react.dev/learn/react-compiler\n\nMarkdown Content:\nThis page will give you an introduction to the new experimental React Compiler and how to try it out successfully.\n\n### Under Construction\n\nThese docs are still a work in progress. More documentation is available in the [React Compiler Working Group repo](https://github.com/reactwg/react-compiler/discussions), and will be upstreamed into these docs when they are more stable.\n\n### You will learn\n\n* Getting started with the compiler\n* Installing the compiler and eslint plugin\n* Troubleshooting\n\n### Note\n\nReact Compiler is a new experimental compiler that we’ve open sourced to get early feedback from the community. It still has rough edges and is not yet fully ready for production.\n\nReact Compiler requires React 19 RC. If you are unable to upgrade to React 19, you may try a userspace implementation of the cache function as described in the [Working Group](https://github.com/reactwg/react-compiler/discussions/6). However, please note that this is not recommended and you should upgrade to React 19 when possible.\n\nReact Compiler is a new experimental compiler that we’ve open sourced to get early feedback from the community. It is a build-time only tool that automatically optimizes your React app. It works with plain JavaScript, and understands the [Rules of React](https://react.dev/reference/rules), so you don’t need to rewrite any code to use it.\n\nThe compiler also includes an [eslint plugin](https://react.dev/learn/react-compiler#installing-eslint-plugin-react-compiler) that surfaces the analysis from the compiler right in your editor. The plugin runs independently of the compiler and can be used even if you aren’t using the compiler in your app. We recommend all React developers to use this eslint plugin to help improve the quality of your codebase.\n\n### What does the compiler do?[](https://react.dev/learn/react-compiler#what-does-the-compiler-do \"Link for What does the compiler do? \")\n\nIn order to optimize applications, React Compiler automatically memoizes your code. You may be familiar today with memoization through APIs such as `useMemo`, `useCallback`, and `React.memo`. With these APIs you can tell React that certain parts of your application don’t need to recompute if their inputs haven’t changed, reducing work on updates. While powerful, it’s easy to forget to apply memoization or apply them incorrectly. This can lead to inefficient updates as React has to check parts of your UI that don’t have any _meaningful_ changes.\n\nThe compiler uses its knowledge of JavaScript and React’s rules to automatically memoize values or groups of values within your components and hooks. If it detects breakages of the rules, it will automatically skip over just those components or hooks, and continue safely compiling other code.\n\nIf your codebase is already very well-memoized, you might not expect to see major performance improvements with the compiler. However, in practice memoizing the correct dependencies that cause performance issues is tricky to get right by hand.\n\n##### Deep Dive\n\n#### What kind of memoization does React Compiler add?[](https://react.dev/learn/react-compiler#what-kind-of-memoization-does-react-compiler-add \"Link for What kind of memoization does React Compiler add? \")\n\nThe initial release of React Compiler is primarily focused on **improving update performance** (re-rendering existing components), so it focuses on these two use cases:\n\n1. **Skipping cascading re-rendering of components**\n * Re-rendering `<Parent />` causes many components in its component tree to re-render, even though only `<Parent />` has changed\n2. **Skipping expensive calculations from outside of React**\n * For example, calling `expensivelyProcessAReallyLargeArrayOfObjects()` inside of your component or hook that needs that data\n\n#### Optimizing Re-renders[](https://react.dev/learn/react-compiler#optimizing-re-renders \"Link for Optimizing Re-renders \")\n\nReact lets you express your UI as a function of their current state (more concretely: their props, state, and context). In its current implementation, when a component’s state changes, React will re-render that component _and all of its children_ — unless you have applied some form of manual memoization with `useMemo()`, `useCallback()`, or `React.memo()`. For example, in the following example, `<MessageButton>` will re-render whenever `<FriendList>`’s state changes:\n\n```\nfunction FriendList({ friends }) {const onlineCount = useFriendOnlineCount();if (friends.length === 0) {return <NoFriends />;}return (<div><span>{onlineCount} online</span>{friends.map((friend) => (<FriendListCard key={friend.id} friend={friend} />))}<MessageButton /></div>);}\n```\n\n[_See this example in the React Compiler Playground_](https://playground.react.dev/#N4Igzg9grgTgxgUxALhAMygOzgFwJYSYAEAYjHgpgCYAyeYOAFMEWuZVWEQL4CURwADrEicQgyKEANnkwIAwtEw4iAXiJQwCMhWoB5TDLmKsTXgG5hRInjRFGbXZwB0UygHMcACzWr1ABn4hEWsYBBxYYgAeADkIHQ4uAHoAPksRbisiMIiYYkYs6yiqPAA3FMLrIiiwAAcAQ0wU4GlZBSUcbklDNqikusaKkKrgR0TnAFt62sYHdmp+VRT7SqrqhOo6Bnl6mCoiAGsEAE9VUfmqZzwqLrHqM7ubolTVol5eTOGigFkEMDB6u4EAAhKA4HCEZ5DNZ9ErlLIWYTcEDcIA)\n\nReact Compiler automatically applies the equivalent of manual memoization, ensuring that only the relevant parts of an app re-render as state changes, which is sometimes referred to as “fine-grained reactivity”. In the above example, React Compiler determines that the return value of `<FriendListCard />` can be reused even as `friends` changes, and can avoid recreating this JSX _and_ avoid re-rendering `<MessageButton>` as the count changes.\n\n#### Expensive calculations also get memoized[](https://react.dev/learn/react-compiler#expensive-calculations-also-get-memoized \"Link for Expensive calculations also get memoized \")\n\nThe compiler can also automatically memoize for expensive calculations used during rendering:\n\n```\n// **Not** memoized by React Compiler, since this is not a component or hookfunction expensivelyProcessAReallyLargeArrayOfObjects() { /* ... */ }// Memoized by React Compiler since this is a componentfunction TableContainer({ items }) {// This function call would be memoized:const data = expensivelyProcessAReallyLargeArrayOfObjects(items);// ...}\n```\n\n[_See this example in the React Compiler Playground_](https://playground.react.dev/#N4Igzg9grgTgxgUxALhAejQAgFTYHIQAuumAtgqRAJYBeCAJpgEYCemASggIZyGYDCEUgAcqAGwQwANJjBUAdokyEAFlTCZ1meUUxdMcIcIjyE8vhBiYVECAGsAOvIBmURYSonMCAB7CzcgBuCGIsAAowEIhgYACCnFxioQAyXDAA5gixMDBcLADyzvlMAFYIvGAAFACUmMCYaNiYAHStOFgAvk5OGJgAshTUdIysHNy8AkbikrIKSqpaWvqGIiZmhE6u7p7ymAAqXEwSguZcCpKV9VSEFBodtcBOmAYmYHz0XIT6ALzefgFUYKhCJRBAxeLcJIsVIZLI5PKFYplCqVa63aoAbm6u0wMAQhFguwAPPRAQA+YAfL4dIloUmBMlODogDpAA)\n\nHowever, if `expensivelyProcessAReallyLargeArrayOfObjects` is truly an expensive function, you may want to consider implementing its own memoization outside of React, because:\n\n* React Compiler only memoizes React components and hooks, not every function\n* React Compiler’s memoization is not shared across multiple components or hooks\n\nSo if `expensivelyProcessAReallyLargeArrayOfObjects` was used in many different components, even if the same exact items were passed down, that expensive calculation would be run repeatedly. We recommend [profiling](https://react.dev/reference/react/useMemo#how-to-tell-if-a-calculation-is-expensive) first to see if it really is that expensive before making code more complicated.\n\n### What does the compiler assume?[](https://react.dev/learn/react-compiler#what-does-the-compiler-assume \"Link for What does the compiler assume? \")\n\nReact Compiler assumes that your code:\n\n1. Is valid, semantic JavaScript\n2. Tests that nullable/optional values and properties are defined before accessing them (for example, by enabling [`strictNullChecks`](https://www.typescriptlang.org/tsconfig/#strictNullChecks) if using TypeScript), i.e., `if (object.nullableProperty) { object.nullableProperty.foo }` or with optional-chaining `object.nullableProperty?.foo`\n3. Follows the [Rules of React](https://react.dev/reference/rules)\n\nReact Compiler can verify many of the Rules of React statically, and will safely skip compilation when it detects an error. To see the errors we recommend also installing [eslint-plugin-react-compiler](https://www.npmjs.com/package/eslint-plugin-react-compiler).\n\n### Should I try out the compiler?[](https://react.dev/learn/react-compiler#should-i-try-out-the-compiler \"Link for Should I try out the compiler? \")\n\nPlease note that the compiler is still experimental and has many rough edges. While it has been used in production at companies like Meta, rolling out the compiler to production for your app will depend on the health of your codebase and how well you’ve followed the [Rules of React](https://react.dev/reference/rules).\n\n**You don’t have to rush into using the compiler now. It’s okay to wait until it reaches a stable release before adopting it.** However, we do appreciate trying it out in small experiments in your apps so that you can [provide feedback](https://react.dev/learn/react-compiler#reporting-issues) to us to help make the compiler better.\n\nGetting Started[](https://react.dev/learn/react-compiler#getting-started \"Link for Getting Started \")\n-----------------------------------------------------------------------------------------------------\n\nIn addition to these docs, we recommend checking the [React Compiler Working Group](https://github.com/reactwg/react-compiler) for additional information and discussion about the compiler.\n\n### Checking compatibility[](https://react.dev/learn/react-compiler#checking-compatibility \"Link for Checking compatibility \")\n\nPrior to installing the compiler, you can first check to see if your codebase is compatible:\n\nnpx react-compiler-healthcheck@latest\n\nThis script will:\n\n* Check how many components can be successfully optimized: higher is better\n* Check for `<StrictMode>` usage: having this enabled and followed means a higher chance that the [Rules of React](https://react.dev/reference/rules) are followed\n* Check for incompatible library usage: known libraries that are incompatible with the compiler\n\nAs an example:\n\nSuccessfully compiled 8 out of 9 components. StrictMode usage not found. Found no usage of incompatible libraries.\n\n### Installing eslint-plugin-react-compiler[](https://react.dev/learn/react-compiler#installing-eslint-plugin-react-compiler \"Link for Installing eslint-plugin-react-compiler \")\n\nReact Compiler also powers an eslint plugin. The eslint plugin can be used **independently** of the compiler, meaning you can use the eslint plugin even if you don’t use the compiler.\n\nnpm install eslint-plugin-react-compiler\n\nThen, add it to your eslint config:\n\n```\nmodule.exports = {plugins: ['eslint-plugin-react-compiler',],rules: {'react-compiler/react-compiler': \"error\",},}\n```\n\nThe eslint plugin will display any violations of the rules of React in your editor. When it does this, it means that the compiler has skipped over optimizing that component or hook. This is perfectly okay, and the compiler can recover and continue optimizing other components in your codebase.\n\n**You don’t have to fix all eslint violations straight away.** You can address them at your own pace to increase the amount of components and hooks being optimized, but it is not required to fix everything before you can use the compiler.\n\n### Rolling out the compiler to your codebase[](https://react.dev/learn/react-compiler#using-the-compiler-effectively \"Link for Rolling out the compiler to your codebase \")\n\n#### Existing projects[](https://react.dev/learn/react-compiler#existing-projects \"Link for Existing projects \")\n\nThe compiler is designed to compile functional components and hooks that follow the [Rules of React](https://react.dev/reference/rules). It can also handle code that breaks those rules by bailing out (skipping over) those components or hooks. However, due to the flexible nature of JavaScript, the compiler cannot catch every possible violation and may compile with false negatives: that is, the compiler may accidentally compile a component/hook that breaks the Rules of React which can lead to undefined behavior.\n\nFor this reason, to adopt the compiler successfully on existing projects, we recommend running it on a small directory in your product code first. You can do this by configuring the compiler to only run on a specific set of directories:\n\n```\nconst ReactCompilerConfig = {sources: (filename) => {return filename.indexOf('src/path/to/dir') !== -1;},};\n```\n\nIn rare cases, you can also configure the compiler to run in “opt-in” mode using the `compilationMode: \"annotation\"` option. This makes it so the compiler will only compile components and hooks annotated with a `\"use memo\"` directive. Please note that the `annotation` mode is a temporary one to aid early adopters, and that we don’t intend for the `\"use memo\"` directive to be used for the long term.\n\n```\nconst ReactCompilerConfig = {compilationMode: \"annotation\",};// src/app.jsxexport default function App() {\"use memo\";// ...}\n```\n\nWhen you have more confidence with rolling out the compiler, you can expand coverage to other directories as well and slowly roll it out to your whole app.\n\n#### New projects[](https://react.dev/learn/react-compiler#new-projects \"Link for New projects \")\n\nIf you’re starting a new project, you can enable the compiler on your entire codebase, which is the default behavior.\n\nUsage[](https://react.dev/learn/react-compiler#installation \"Link for Usage \")\n------------------------------------------------------------------------------\n\n### Babel[](https://react.dev/learn/react-compiler#usage-with-babel \"Link for Babel \")\n\nnpm install babel-plugin-react-compiler\n\nThe compiler includes a Babel plugin which you can use in your build pipeline to run the compiler.\n\nAfter installing, add it to your Babel config. Please note that it’s critical that the compiler run **first** in the pipeline:\n\n```\n// babel.config.jsconst ReactCompilerConfig = { /* ... */ };module.exports = function () {return {plugins: [['babel-plugin-react-compiler', ReactCompilerConfig], // must run first!// ...],};};\n```\n\n`babel-plugin-react-compiler` should run first before other Babel plugins as the compiler requires the input source information for sound analysis.\n\n### Vite[](https://react.dev/learn/react-compiler#usage-with-vite \"Link for Vite \")\n\nIf you use Vite, you can add the plugin to vite-plugin-react:\n\n```\n// vite.config.jsconst ReactCompilerConfig = { /* ... */ };export default defineConfig(() => {return {plugins: [react({babel: {plugins: [[\"babel-plugin-react-compiler\", ReactCompilerConfig],],},}),],// ...};});\n```\n\n### Next.js[](https://react.dev/learn/react-compiler#usage-with-nextjs \"Link for Next.js \")\n\nNext.js has an experimental configuration to enable the React Compiler. It automatically ensures Babel is set up with `babel-plugin-react-compiler`.\n\n* Install Next.js canary, which uses React 19 Release Candidate\n* Install `babel-plugin-react-compiler`\n\nnpm install next@canary babel-plugin-react-compiler\n\nThen configure the experimental option in `next.config.js`:\n\n```\n// next.config.js/** @type {import('next').NextConfig} */const nextConfig = {experimental: {reactCompiler: true,},};module.exports = nextConfig;\n```\n\nUsing the experimental option ensures support for the React Compiler in:\n\n* App Router\n* Pages Router\n* Webpack (default)\n* Turbopack (opt-in through `--turbo`)\n\n### Remix[](https://react.dev/learn/react-compiler#usage-with-remix \"Link for Remix \")\n\nInstall `vite-plugin-babel`, and add the compiler’s Babel plugin to it:\n\nnpm install vite-plugin-babel\n\n```\n// vite.config.jsimport babel from \"vite-plugin-babel\";const ReactCompilerConfig = { /* ... */ };export default defineConfig({plugins: [remix({ /* ... */}),babel({filter: /\\.[jt]sx?$/,babelConfig: {presets: [\"@babel/preset-typescript\"], // if you use TypeScriptplugins: [[\"babel-plugin-react-compiler\", ReactCompilerConfig],],},}),],});\n```\n\n### Webpack[](https://react.dev/learn/react-compiler#usage-with-webpack \"Link for Webpack \")\n\nYou can create your own loader for React Compiler, like so:\n\n```\nconst ReactCompilerConfig = { /* ... */ };const BabelPluginReactCompiler = require('babel-plugin-react-compiler');function reactCompilerLoader(sourceCode, sourceMap) {// ...const result = transformSync(sourceCode, {// ...plugins: [[BabelPluginReactCompiler, ReactCompilerConfig],],// ...});if (result === null) {this.callback(Error(`Failed to transform \"${options.filename}\"`));return;}this.callback(null,result.code,result.map === null ? undefined : result.map);}module.exports = reactCompilerLoader;\n```\n\n### Expo[](https://react.dev/learn/react-compiler#usage-with-expo \"Link for Expo \")\n\nPlease refer to [Expo’s docs](https://docs.expo.dev/preview/react-compiler/) to enable and use the React Compiler in Expo apps.\n\n### Metro (React Native)[](https://react.dev/learn/react-compiler#usage-with-react-native-metro \"Link for Metro (React Native) \")\n\nReact Native uses Babel via Metro, so refer to the [Usage with Babel](https://react.dev/learn/react-compiler#usage-with-babel) section for installation instructions.\n\n### Rspack[](https://react.dev/learn/react-compiler#usage-with-rspack \"Link for Rspack \")\n\nPlease refer to [Rspack’s docs](https://rspack.dev/guide/tech/react#react-compiler) to enable and use the React Compiler in Rspack apps.\n\n### Rsbuild[](https://react.dev/learn/react-compiler#usage-with-rsbuild \"Link for Rsbuild \")\n\nPlease refer to [Rsbuild’s docs](https://rsbuild.dev/guide/framework/react#react-compiler) to enable and use the React Compiler in Rsbuild apps.\n\nTroubleshooting[](https://react.dev/learn/react-compiler#troubleshooting \"Link for Troubleshooting \")\n-----------------------------------------------------------------------------------------------------\n\nTo report issues, please first create a minimal repro on the [React Compiler Playground](https://playground.react.dev/) and include it in your bug report. You can open issues in the [facebook/react](https://github.com/facebook/react/issues) repo.\n\nYou can also provide feedback in the React Compiler Working Group by applying to be a member. Please see [the README for more details on joining](https://github.com/reactwg/react-compiler).\n\n### `(0 , _c) is not a function` error[](https://react.dev/learn/react-compiler#0--_c-is-not-a-function-error \"Link for this heading\")\n\nThis occurs if you are not using React 19 RC and up. To fix this, [upgrade your app to React 19 RC](https://react.dev/blog/2024/04/25/react-19-upgrade-guide) first.\n\nIf you are unable to upgrade to React 19, you may try a userspace implementation of the cache function as described in the [Working Group](https://github.com/reactwg/react-compiler/discussions/6). However, please note that this is not recommended and you should upgrade to React 19 when possible.\n\n### How do I know my components have been optimized?[](https://react.dev/learn/react-compiler#how-do-i-know-my-components-have-been-optimized \"Link for How do I know my components have been optimized? \")\n\n[React Devtools](https://react.dev/learn/react-developer-tools) (v5.0+) has built-in support for React Compiler and will display a “Memo ✨” badge next to components that have been optimized by the compiler.\n\n### Something is not working after compilation[](https://react.dev/learn/react-compiler#something-is-not-working-after-compilation \"Link for Something is not working after compilation \")\n\nIf you have eslint-plugin-react-compiler installed, the compiler will display any violations of the rules of React in your editor. When it does this, it means that the compiler has skipped over optimizing that component or hook. This is perfectly okay, and the compiler can recover and continue optimizing other components in your codebase. **You don’t have to fix all eslint violations straight away.** You can address them at your own pace to increase the amount of components and hooks being optimized.\n\nDue to the flexible and dynamic nature of JavaScript however, it’s not possible to comprehensively detect all cases. Bugs and undefined behavior such as infinite loops may occur in those cases.\n\nIf your app doesn’t work properly after compilation and you aren’t seeing any eslint errors, the compiler may be incorrectly compiling your code. To confirm this, try to make the issue go away by aggressively opting out any component or hook you think might be related via the [`\"use no memo\"` directive](https://react.dev/learn/react-compiler#opt-out-of-the-compiler-for-a-component).\n\n```\nfunction SuspiciousComponent() {\"use no memo\"; // opts out this component from being compiled by React Compiler// ...}\n```\n\n### Note\n\n#### `\"use no memo\"`[](https://react.dev/learn/react-compiler#use-no-memo \"Link for this heading\")\n\n`\"use no memo\"` is a _temporary_ escape hatch that lets you opt-out components and hooks from being compiled by the React Compiler. This directive is not meant to be long lived the same way as eg [`\"use client\"`](https://react.dev/reference/rsc/use-client) is.\n\nIt is not recommended to reach for this directive unless it’s strictly necessary. Once you opt-out a component or hook, it is opted-out forever until the directive is removed. This means that even if you fix the code, the compiler will still skip over compiling it unless you remove the directive.\n\nWhen you make the error go away, confirm that removing the opt out directive makes the issue come back. Then share a bug report with us (you can try to reduce it to a small repro, or if it’s open source code you can also just paste the entire source) using the [React Compiler Playground](https://playground.react.dev/) so we can identify and help fix the issue.\n\n### Other issues[](https://react.dev/learn/react-compiler#other-issues \"Link for Other issues \")\n\nPlease see [https://github.com/reactwg/react-compiler/discussions/7](https://github.com/reactwg/react-compiler/discussions/7).\n",
"filename": "react-compiler.md",
"package": "react"
} |
{
"content": "Title: React Developer Tools – React\n\nURL Source: https://react.dev/learn/react-developer-tools\n\nMarkdown Content:\nReact Developer Tools – React\n===============\n\nReact Developer Tools[](https://react.dev/learn/react-developer-tools#undefined \"Link for this heading\")\n========================================================================================================\n\nUse React Developer Tools to inspect React [components](https://react.dev/learn/your-first-component), edit [props](https://react.dev/learn/passing-props-to-a-component) and [state](https://react.dev/learn/state-a-components-memory), and identify performance problems.\n\n### You will learn\n\n* How to install React Developer Tools\n\nBrowser extension[](https://react.dev/learn/react-developer-tools#browser-extension \"Link for Browser extension \")\n------------------------------------------------------------------------------------------------------------------\n\nThe easiest way to debug websites built with React is to install the React Developer Tools browser extension. It is available for several popular browsers:\n\n* [Install for **Chrome**](https://chrome.google.com/webstore/detail/react-developer-tools/fmkadmapgofadopljbjfkapdkoienihi?hl=en)\n* [Install for **Firefox**](https://addons.mozilla.org/en-US/firefox/addon/react-devtools/)\n* [Install for **Edge**](https://microsoftedge.microsoft.com/addons/detail/react-developer-tools/gpphkfbcpidddadnkolkpfckpihlkkil)\n\nNow, if you visit a website **built with React,** you will see the _Components_ and _Profiler_ panels.\n\n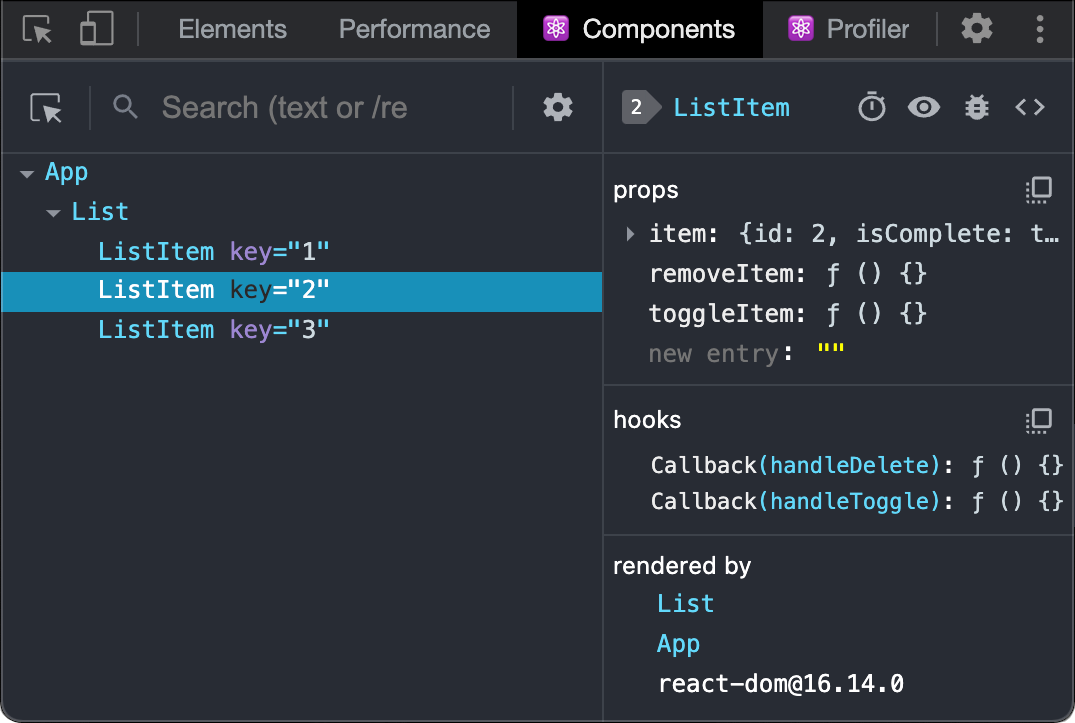\n\n### Safari and other browsers[](https://react.dev/learn/react-developer-tools#safari-and-other-browsers \"Link for Safari and other browsers \")\n\nFor other browsers (for example, Safari), install the [`react-devtools`](https://www.npmjs.com/package/react-devtools) npm package:\n\n```\n# Yarnyarn global add react-devtools# Npmnpm install -g react-devtools\n```\n\nNext open the developer tools from the terminal:\n\n```\nreact-devtools\n```\n\nThen connect your website by adding the following `<script>` tag to the beginning of your website’s `<head>`:\n\n```\n<html> <head> <script src=\"http://localhost:8097\"></script>\n```\n\nReload your website in the browser now to view it in developer tools.\n\n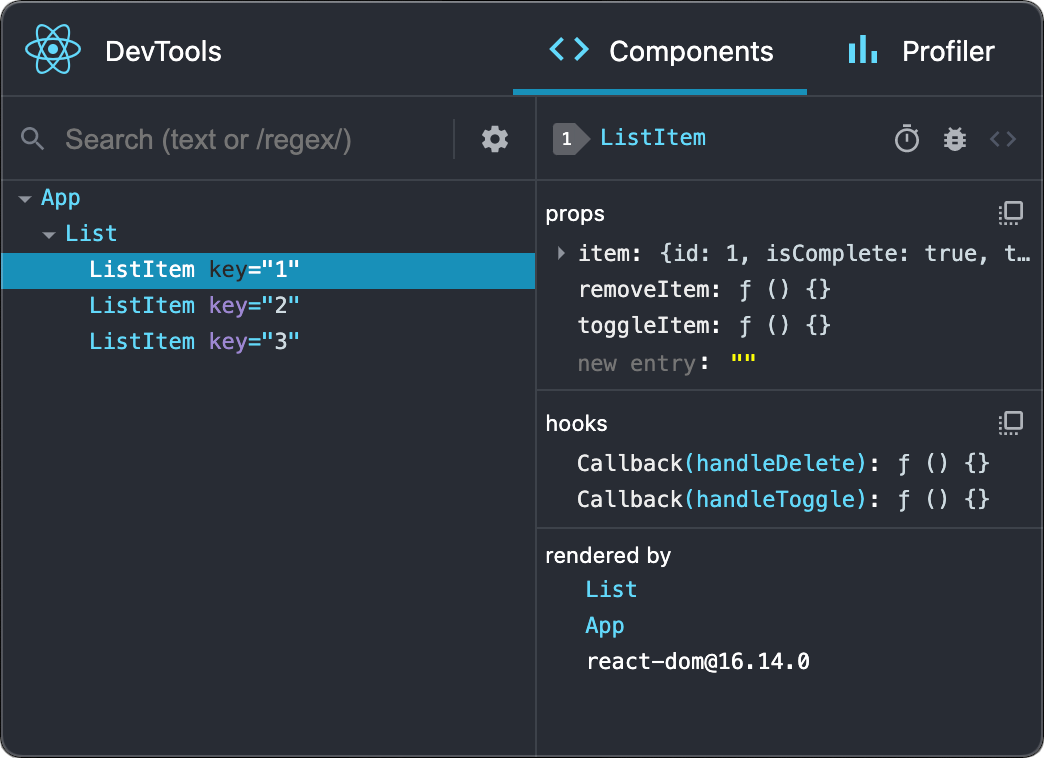\n\nMobile (React Native)[](https://react.dev/learn/react-developer-tools#mobile-react-native \"Link for Mobile (React Native) \")\n----------------------------------------------------------------------------------------------------------------------------\n\nReact Developer Tools can be used to inspect apps built with [React Native](https://reactnative.dev/) as well.\n\nThe easiest way to use React Developer Tools is to install it globally:\n\n```\n# Yarnyarn global add react-devtools# Npmnpm install -g react-devtools\n```\n\nNext open the developer tools from the terminal.\n\n```\nreact-devtools\n```\n\nIt should connect to any local React Native app that’s running.\n\n> Try reloading the app if developer tools doesn’t connect after a few seconds.\n\n[Learn more about debugging React Native.](https://reactnative.dev/docs/debugging)\n\n[PreviousUsing TypeScript](https://react.dev/learn/typescript)[NextReact Compiler](https://react.dev/learn/react-compiler)\n",
"filename": "react-developer-tools.md",
"package": "react"
} |
{
"content": "Title: React DOM APIs – React\n\nURL Source: https://react.dev/reference/react-dom\n\nMarkdown Content:\nReact DOM APIs – React\n===============\n\nReact DOM APIs[](https://react.dev/reference/react-dom#undefined \"Link for this heading\")\n=========================================================================================\n\nThe `react-dom` package contains methods that are only supported for the web applications (which run in the browser DOM environment). They are not supported for React Native.\n\n* * *\n\nAPIs[](https://react.dev/reference/react-dom#apis \"Link for APIs \")\n-------------------------------------------------------------------\n\nThese APIs can be imported from your components. They are rarely used:\n\n* [`createPortal`](https://react.dev/reference/react-dom/createPortal) lets you render child components in a different part of the DOM tree.\n* [`flushSync`](https://react.dev/reference/react-dom/flushSync) lets you force React to flush a state update and update the DOM synchronously.\n\nResource Preloading APIs[](https://react.dev/reference/react-dom#resource-preloading-apis \"Link for Resource Preloading APIs \")\n-------------------------------------------------------------------------------------------------------------------------------\n\nThese APIs can be used to make apps faster by pre-loading resources such as scripts, stylesheets, and fonts as soon as you know you need them, for example before navigating to another page where the resources will be used.\n\n[React-based frameworks](https://react.dev/learn/start-a-new-react-project) frequently handle resource loading for you, so you might not have to call these APIs yourself. Consult your framework’s documentation for details.\n\n* [`prefetchDNS`](https://react.dev/reference/react-dom/prefetchDNS) lets you prefetch the IP address of a DNS domain name that you expect to connect to.\n* [`preconnect`](https://react.dev/reference/react-dom/preconnect) lets you connect to a server you expect to request resources from, even if you don’t know what resources you’ll need yet.\n* [`preload`](https://react.dev/reference/react-dom/preload) lets you fetch a stylesheet, font, image, or external script that you expect to use.\n* [`preloadModule`](https://react.dev/reference/react-dom/preloadModule) lets you fetch an ESM module that you expect to use.\n* [`preinit`](https://react.dev/reference/react-dom/preinit) lets you fetch and evaluate an external script or fetch and insert a stylesheet.\n* [`preinitModule`](https://react.dev/reference/react-dom/preinitModule) lets you fetch and evaluate an ESM module.\n\n* * *\n\nEntry points[](https://react.dev/reference/react-dom#entry-points \"Link for Entry points \")\n-------------------------------------------------------------------------------------------\n\nThe `react-dom` package provides two additional entry points:\n\n* [`react-dom/client`](https://react.dev/reference/react-dom/client) contains APIs to render React components on the client (in the browser).\n* [`react-dom/server`](https://react.dev/reference/react-dom/server) contains APIs to render React components on the server.\n\n* * *\n\nDeprecated APIs[](https://react.dev/reference/react-dom#deprecated-apis \"Link for Deprecated APIs \")\n----------------------------------------------------------------------------------------------------\n\n### Deprecated\n\nThese APIs will be removed in a future major version of React.\n\n* [`findDOMNode`](https://react.dev/reference/react-dom/findDOMNode) finds the closest DOM node corresponding to a class component instance.\n* [`hydrate`](https://react.dev/reference/react-dom/hydrate) mounts a tree into the DOM created from server HTML. Deprecated in favor of [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot).\n* [`render`](https://react.dev/reference/react-dom/render) mounts a tree into the DOM. Deprecated in favor of [`createRoot`](https://react.dev/reference/react-dom/client/createRoot).\n* [`unmountComponentAtNode`](https://react.dev/reference/react-dom/unmountComponentAtNode) unmounts a tree from the DOM. Deprecated in favor of [`root.unmount()`](https://react.dev/reference/react-dom/client/createRoot#root-unmount).\n\n[Previous<title>](https://react.dev/reference/react-dom/components/title)[NextcreatePortal](https://react.dev/reference/react-dom/createPortal)\n",
"filename": "react-dom.md",
"package": "react"
} |
{
"content": "Title: Reacting to Input with State – React\n\nURL Source: https://react.dev/learn/reacting-to-input-with-state\n\nMarkdown Content:\nReact provides a declarative way to manipulate the UI. Instead of manipulating individual pieces of the UI directly, you describe the different states that your component can be in, and switch between them in response to the user input. This is similar to how designers think about the UI.\n\n### You will learn\n\n* How declarative UI programming differs from imperative UI programming\n* How to enumerate the different visual states your component can be in\n* How to trigger the changes between the different visual states from code\n\nHow declarative UI compares to imperative[](https://react.dev/learn/reacting-to-input-with-state#how-declarative-ui-compares-to-imperative \"Link for How declarative UI compares to imperative \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you design UI interactions, you probably think about how the UI _changes_ in response to user actions. Consider a form that lets the user submit an answer:\n\n* When you type something into the form, the “Submit” button **becomes enabled.**\n* When you press “Submit”, both the form and the button **become disabled,** and a spinner **appears.**\n* If the network request succeeds, the form **gets hidden,** and the “Thank you” message **appears.**\n* If the network request fails, an error message **appears,** and the form **becomes enabled** again.\n\nIn **imperative programming,** the above corresponds directly to how you implement interaction. You have to write the exact instructions to manipulate the UI depending on what just happened. Here’s another way to think about this: imagine riding next to someone in a car and telling them turn by turn where to go.\n\n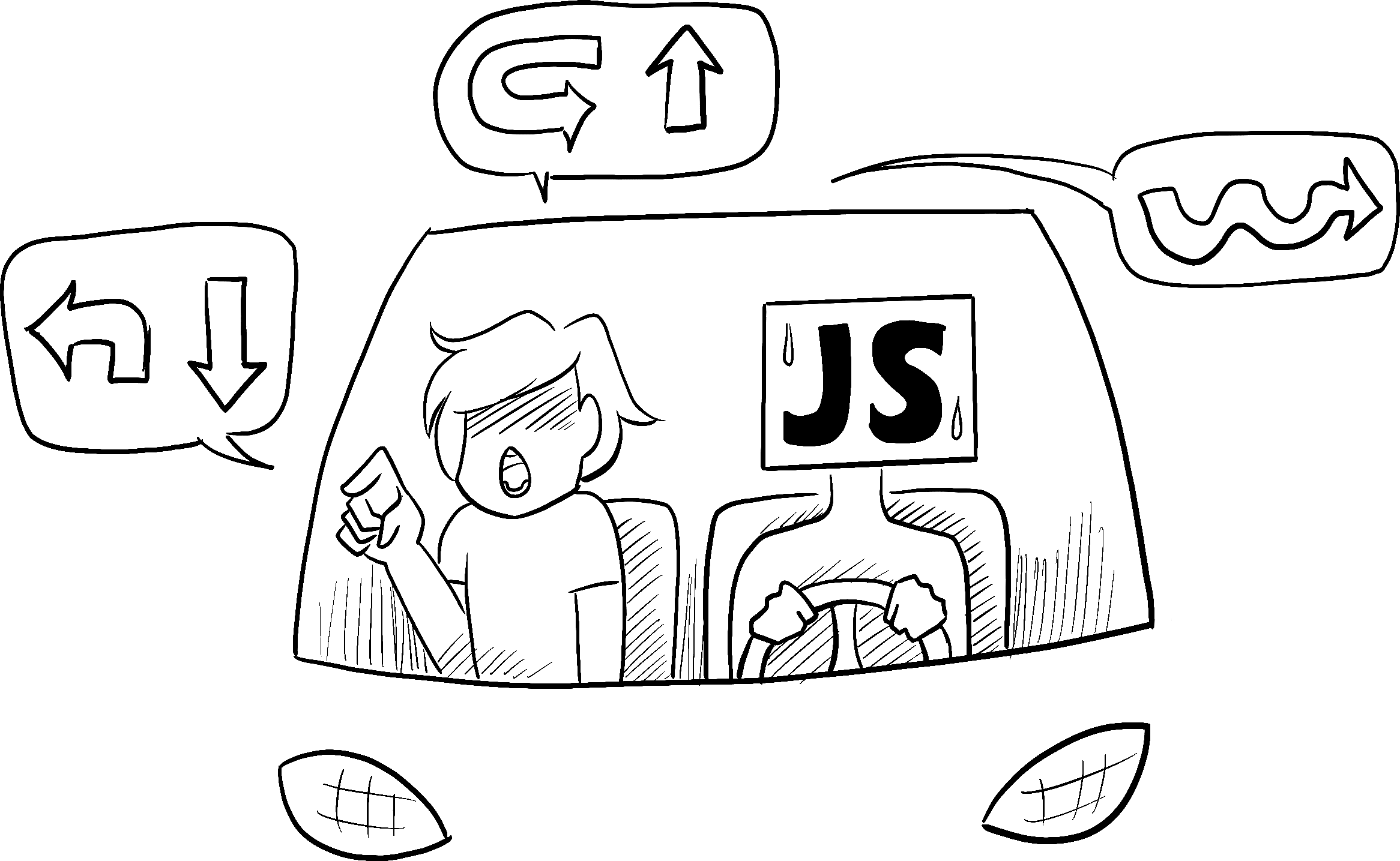\n\nThey don’t know where you want to go, they just follow your commands. (And if you get the directions wrong, you end up in the wrong place!) It’s called _imperative_ because you have to “command” each element, from the spinner to the button, telling the computer _how_ to update the UI.\n\nIn this example of imperative UI programming, the form is built _without_ React. It only uses the browser [DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model):\n\nasync function handleFormSubmit(e) {\n e.preventDefault();\n disable(textarea);\n disable(button);\n show(loadingMessage);\n hide(errorMessage);\n try {\n await submitForm(textarea.value);\n show(successMessage);\n hide(form);\n } catch (err) {\n show(errorMessage);\n errorMessage.textContent = err.message;\n } finally {\n hide(loadingMessage);\n enable(textarea);\n enable(button);\n }\n}\n\nfunction handleTextareaChange() {\n if (textarea.value.length === 0) {\n disable(button);\n } else {\n enable(button);\n }\n}\n\nfunction hide(el) {\n el.style.display = 'none';\n}\n\nfunction show(el) {\n el.style.display = '';\n}\n\nfunction enable(el) {\n el.disabled = false;\n}\n\nfunction disable(el) {\n el.disabled = true;\n}\n\nfunction submitForm(answer) {\n \n return new Promise((resolve, reject) \\=> {\n setTimeout(() \\=> {\n if (answer.toLowerCase() === 'istanbul') {\n resolve();\n } else {\n reject(new Error('Good guess but a wrong answer. Try again!'));\n }\n }, 1500);\n });\n}\n\nlet form = document.getElementById('form');\nlet textarea = document.getElementById('textarea');\nlet button = document.getElementById('button');\nlet loadingMessage = document.getElementById('loading');\nlet errorMessage = document.getElementById('error');\nlet successMessage = document.getElementById('success');\nform.onsubmit = handleFormSubmit;\ntextarea.oninput = handleTextareaChange;\n\nManipulating the UI imperatively works well enough for isolated examples, but it gets exponentially more difficult to manage in more complex systems. Imagine updating a page full of different forms like this one. Adding a new UI element or a new interaction would require carefully checking all existing code to make sure you haven’t introduced a bug (for example, forgetting to show or hide something).\n\nReact was built to solve this problem.\n\nIn React, you don’t directly manipulate the UI—meaning you don’t enable, disable, show, or hide components directly. Instead, you **declare what you want to show,** and React figures out how to update the UI. Think of getting into a taxi and telling the driver where you want to go instead of telling them exactly where to turn. It’s the driver’s job to get you there, and they might even know some shortcuts you haven’t considered!\n\n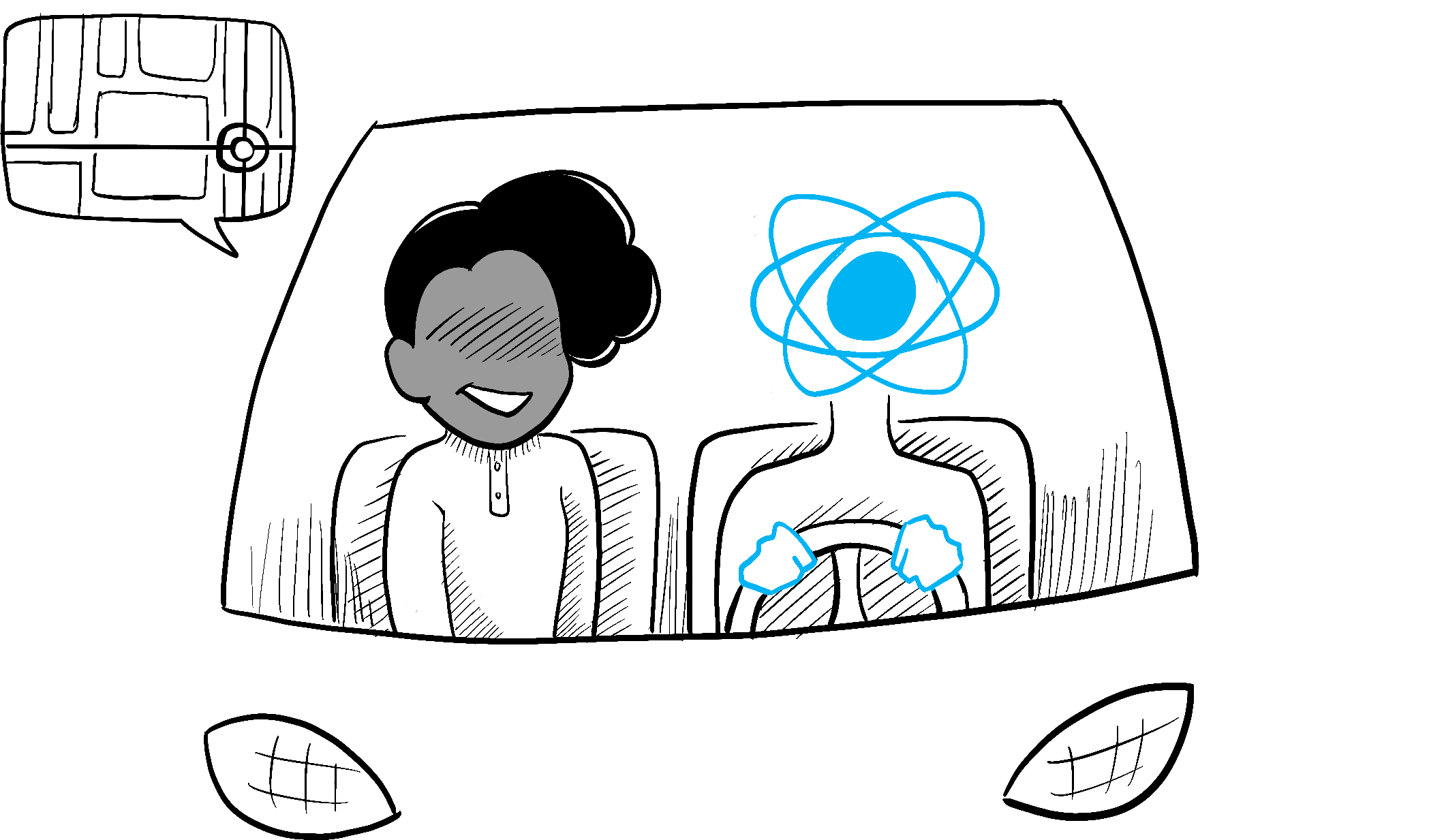\n\nThinking about UI declaratively[](https://react.dev/learn/reacting-to-input-with-state#thinking-about-ui-declaratively \"Link for Thinking about UI declaratively \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou’ve seen how to implement a form imperatively above. To better understand how to think in React, you’ll walk through reimplementing this UI in React below:\n\n1. **Identify** your component’s different visual states\n2. **Determine** what triggers those state changes\n3. **Represent** the state in memory using `useState`\n4. **Remove** any non-essential state variables\n5. **Connect** the event handlers to set the state\n\n### Step 1: Identify your component’s different visual states[](https://react.dev/learn/reacting-to-input-with-state#step-1-identify-your-components-different-visual-states \"Link for Step 1: Identify your component’s different visual states \")\n\nIn computer science, you may hear about a [“state machine”](https://en.wikipedia.org/wiki/Finite-state_machine) being in one of several “states”. If you work with a designer, you may have seen mockups for different “visual states”. React stands at the intersection of design and computer science, so both of these ideas are sources of inspiration.\n\nFirst, you need to visualize all the different “states” of the UI the user might see:\n\n* **Empty**: Form has a disabled “Submit” button.\n* **Typing**: Form has an enabled “Submit” button.\n* **Submitting**: Form is completely disabled. Spinner is shown.\n* **Success**: “Thank you” message is shown instead of a form.\n* **Error**: Same as Typing state, but with an extra error message.\n\nJust like a designer, you’ll want to “mock up” or create “mocks” for the different states before you add logic. For example, here is a mock for just the visual part of the form. This mock is controlled by a prop called `status` with a default value of `'empty'`:\n\nexport default function Form({\n status = 'empty'\n}) {\n if (status === 'success') {\n return <h1\\>That's right!</h1\\>\n }\n return (\n <\\>\n <h2\\>City quiz</h2\\>\n <p\\>\n In which city is there a billboard that turns air into drinkable water?\n </p\\>\n <form\\>\n <textarea />\n <br />\n <button\\>\n Submit\n </button\\>\n </form\\>\n </\\>\n )\n}\n\nYou could call that prop anything you like, the naming is not important. Try editing `status = 'empty'` to `status = 'success'` to see the success message appear. Mocking lets you quickly iterate on the UI before you wire up any logic. Here is a more fleshed out prototype of the same component, still “controlled” by the `status` prop:\n\nexport default function Form({\n \n status = 'empty'\n}) {\n if (status === 'success') {\n return <h1\\>That's right!</h1\\>\n }\n return (\n <\\>\n <h2\\>City quiz</h2\\>\n <p\\>\n In which city is there a billboard that turns air into drinkable water?\n </p\\>\n <form\\>\n <textarea disabled\\={\n status === 'submitting'\n } />\n <br />\n <button disabled\\={\n status === 'empty' ||\n status === 'submitting'\n }\\>\n Submit\n </button\\>\n {status === 'error' &&\n <p className\\=\"Error\"\\>\n Good guess but a wrong answer. Try again!\n </p\\>\n }\n </form\\>\n </\\>\n );\n}\n\n##### Deep Dive\n\n#### Displaying many visual states at once[](https://react.dev/learn/reacting-to-input-with-state#displaying-many-visual-states-at-once \"Link for Displaying many visual states at once \")\n\nIf a component has a lot of visual states, it can be convenient to show them all on one page:\n\nimport Form from './Form.js';\n\nlet statuses = \\[\n 'empty',\n 'typing',\n 'submitting',\n 'success',\n 'error',\n\\];\n\nexport default function App() {\n return (\n <\\>\n {statuses.map(status \\=> (\n <section key\\={status}\\>\n <h4\\>Form ({status}):</h4\\>\n <Form status\\={status} />\n </section\\>\n ))}\n </\\>\n );\n}\n\nPages like this are often called “living styleguides” or “storybooks”.\n\n### Step 2: Determine what triggers those state changes[](https://react.dev/learn/reacting-to-input-with-state#step-2-determine-what-triggers-those-state-changes \"Link for Step 2: Determine what triggers those state changes \")\n\nYou can trigger state updates in response to two kinds of inputs:\n\n* **Human inputs,** like clicking a button, typing in a field, navigating a link.\n* **Computer inputs,** like a network response arriving, a timeout completing, an image loading.\n\n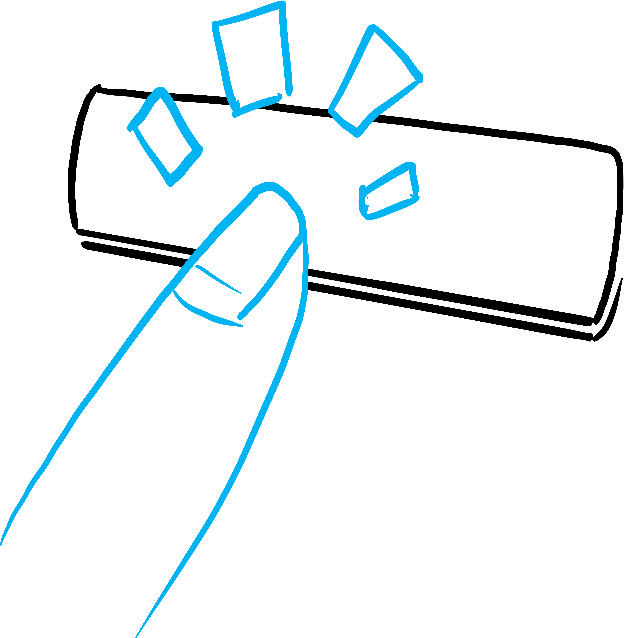\n\nHuman inputs\n\n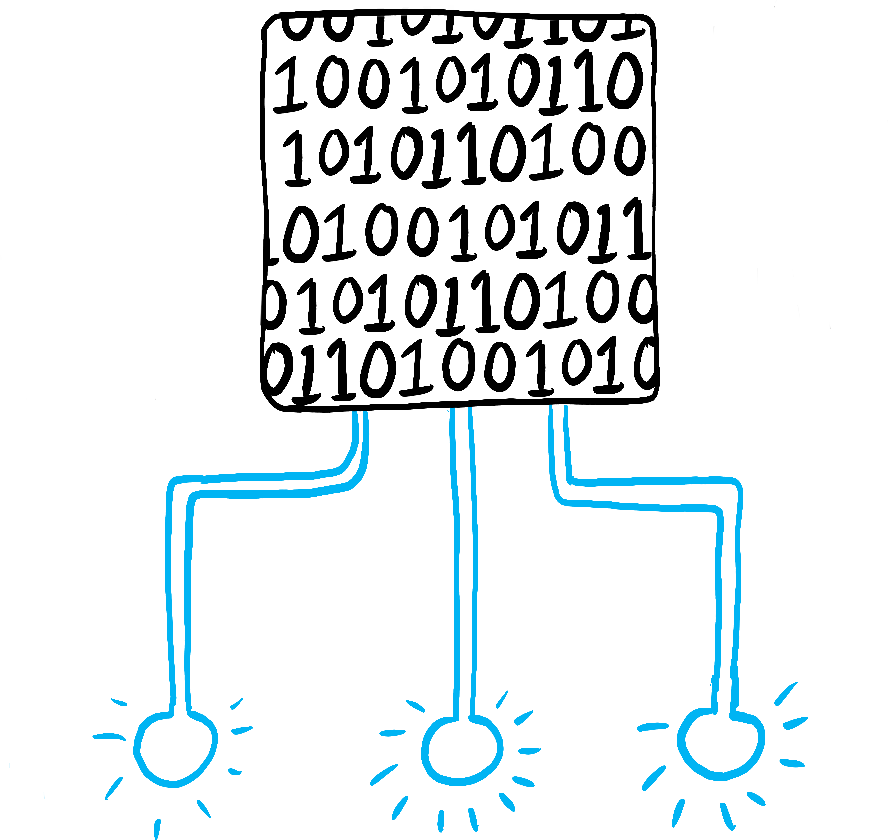\n\nComputer inputs\n\nIn both cases, **you must set [state variables](https://react.dev/learn/state-a-components-memory#anatomy-of-usestate) to update the UI.** For the form you’re developing, you will need to change state in response to a few different inputs:\n\n* **Changing the text input** (human) should switch it from the _Empty_ state to the _Typing_ state or back, depending on whether the text box is empty or not.\n* **Clicking the Submit button** (human) should switch it to the _Submitting_ state.\n* **Successful network response** (computer) should switch it to the _Success_ state.\n* **Failed network response** (computer) should switch it to the _Error_ state with the matching error message.\n\n### Note\n\nTo help visualize this flow, try drawing each state on paper as a labeled circle, and each change between two states as an arrow. You can sketch out many flows this way and sort out bugs long before implementation.\n\n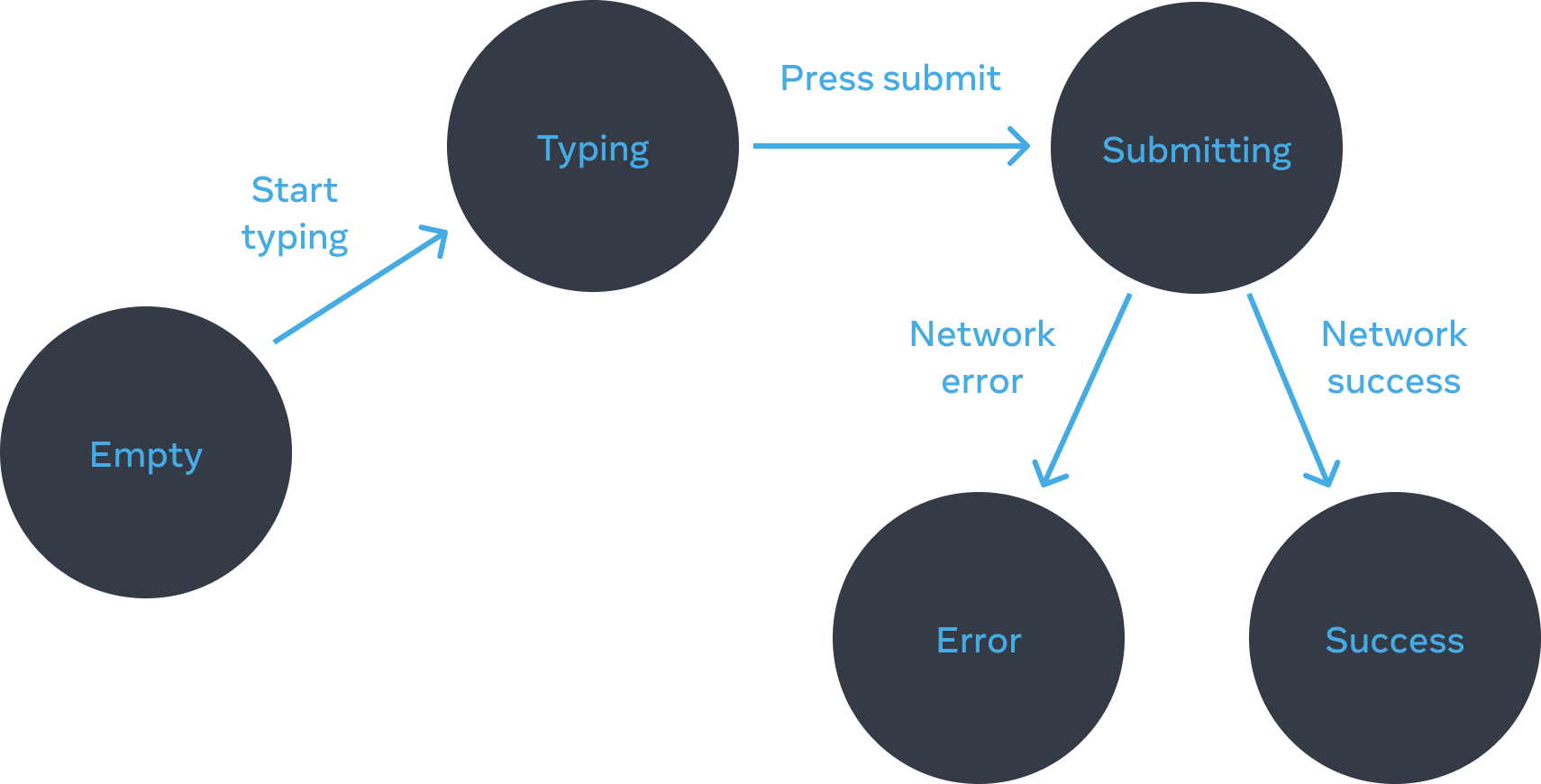\n\n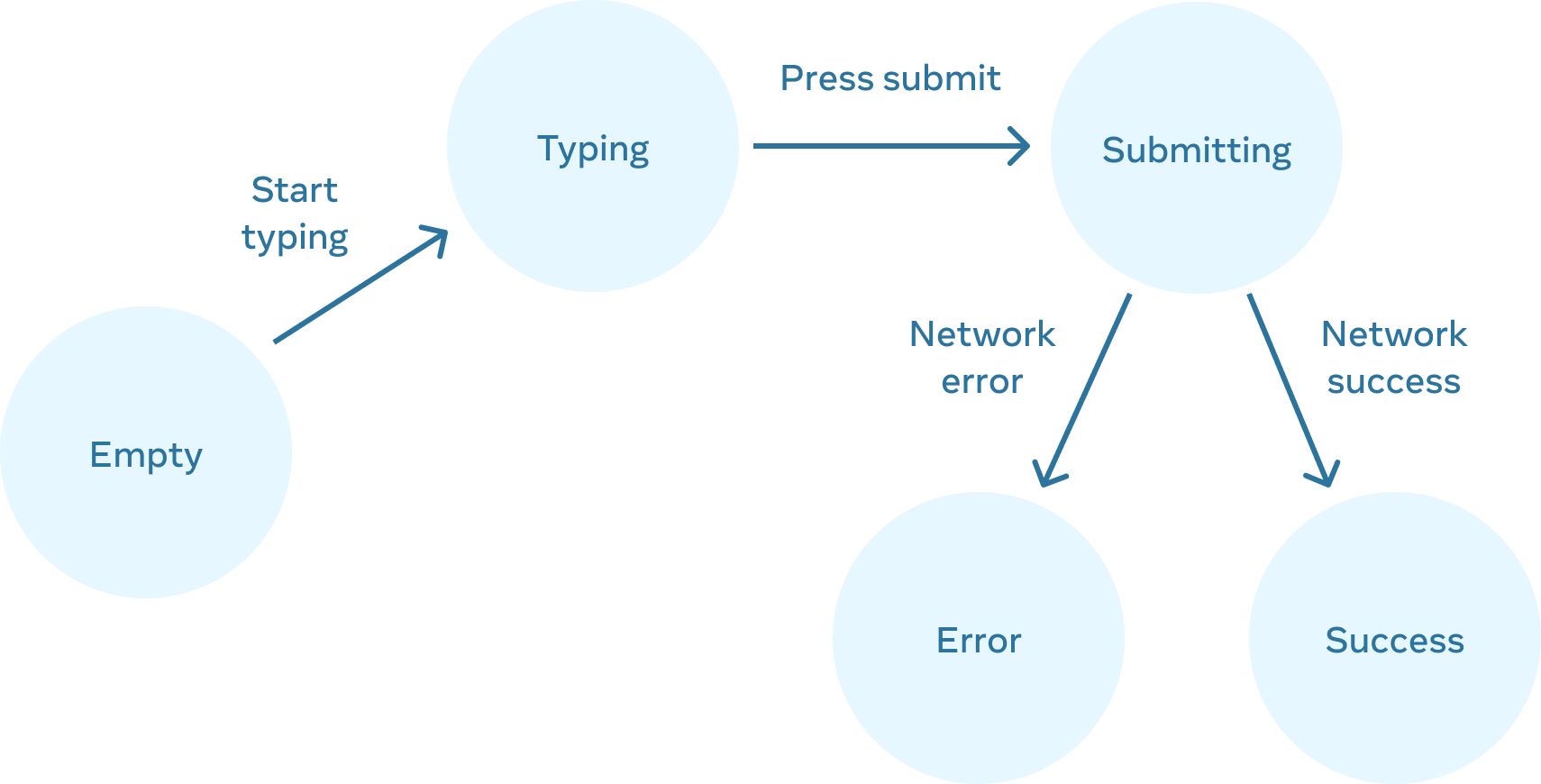\n\nForm states\n\n### Step 3: Represent the state in memory with `useState`[](https://react.dev/learn/reacting-to-input-with-state#step-3-represent-the-state-in-memory-with-usestate \"Link for this heading\")\n\nNext you’ll need to represent the visual states of your component in memory with [`useState`.](https://react.dev/reference/react/useState) Simplicity is key: each piece of state is a “moving piece”, and **you want as few “moving pieces” as possible.** More complexity leads to more bugs!\n\nStart with the state that _absolutely must_ be there. For example, you’ll need to store the `answer` for the input, and the `error` (if it exists) to store the last error:\n\n```\nconst [answer, setAnswer] = useState('');const [error, setError] = useState(null);\n```\n\nThen, you’ll need a state variable representing which one of the visual states that you want to display. There’s usually more than a single way to represent that in memory, so you’ll need to experiment with it.\n\nIf you struggle to think of the best way immediately, start by adding enough state that you’re _definitely_ sure that all the possible visual states are covered:\n\n```\nconst [isEmpty, setIsEmpty] = useState(true);const [isTyping, setIsTyping] = useState(false);const [isSubmitting, setIsSubmitting] = useState(false);const [isSuccess, setIsSuccess] = useState(false);const [isError, setIsError] = useState(false);\n```\n\nYour first idea likely won’t be the best, but that’s ok—refactoring state is a part of the process!\n\n### Step 4: Remove any non-essential state variables[](https://react.dev/learn/reacting-to-input-with-state#step-4-remove-any-non-essential-state-variables \"Link for Step 4: Remove any non-essential state variables \")\n\nYou want to avoid duplication in the state content so you’re only tracking what is essential. Spending a little time on refactoring your state structure will make your components easier to understand, reduce duplication, and avoid unintended meanings. Your goal is to **prevent the cases where the state in memory doesn’t represent any valid UI that you’d want a user to see.** (For example, you never want to show an error message and disable the input at the same time, or the user won’t be able to correct the error!)\n\nHere are some questions you can ask about your state variables:\n\n* **Does this state cause a paradox?** For example, `isTyping` and `isSubmitting` can’t both be `true`. A paradox usually means that the state is not constrained enough. There are four possible combinations of two booleans, but only three correspond to valid states. To remove the “impossible” state, you can combine these into a `status` that must be one of three values: `'typing'`, `'submitting'`, or `'success'`.\n* **Is the same information available in another state variable already?** Another paradox: `isEmpty` and `isTyping` can’t be `true` at the same time. By making them separate state variables, you risk them going out of sync and causing bugs. Fortunately, you can remove `isEmpty` and instead check `answer.length === 0`.\n* **Can you get the same information from the inverse of another state variable?** `isError` is not needed because you can check `error !== null` instead.\n\nAfter this clean-up, you’re left with 3 (down from 7!) _essential_ state variables:\n\n```\nconst [answer, setAnswer] = useState('');const [error, setError] = useState(null);const [status, setStatus] = useState('typing'); // 'typing', 'submitting', or 'success'\n```\n\nYou know they are essential, because you can’t remove any of them without breaking the functionality.\n\n##### Deep Dive\n\n#### Eliminating “impossible” states with a reducer[](https://react.dev/learn/reacting-to-input-with-state#eliminating-impossible-states-with-a-reducer \"Link for Eliminating “impossible” states with a reducer \")\n\nThese three variables are a good enough representation of this form’s state. However, there are still some intermediate states that don’t fully make sense. For example, a non-null `error` doesn’t make sense when `status` is `'success'`. To model the state more precisely, you can [extract it into a reducer.](https://react.dev/learn/extracting-state-logic-into-a-reducer) Reducers let you unify multiple state variables into a single object and consolidate all the related logic!\n\n### Step 5: Connect the event handlers to set state[](https://react.dev/learn/reacting-to-input-with-state#step-5-connect-the-event-handlers-to-set-state \"Link for Step 5: Connect the event handlers to set state \")\n\nLastly, create event handlers that update the state. Below is the final form, with all event handlers wired up:\n\nimport { useState } from 'react';\n\nexport default function Form() {\n const \\[answer, setAnswer\\] = useState('');\n const \\[error, setError\\] = useState(null);\n const \\[status, setStatus\\] = useState('typing');\n\n if (status === 'success') {\n return <h1\\>That's right!</h1\\>\n }\n\n async function handleSubmit(e) {\n e.preventDefault();\n setStatus('submitting');\n try {\n await submitForm(answer);\n setStatus('success');\n } catch (err) {\n setStatus('typing');\n setError(err);\n }\n }\n\n function handleTextareaChange(e) {\n setAnswer(e.target.value);\n }\n\n return (\n <\\>\n <h2\\>City quiz</h2\\>\n <p\\>\n In which city is there a billboard that turns air into drinkable water?\n </p\\>\n <form onSubmit\\={handleSubmit}\\>\n <textarea\n value\\={answer}\n onChange\\={handleTextareaChange}\n disabled\\={status === 'submitting'}\n />\n <br />\n <button disabled\\={\n answer.length === 0 ||\n status === 'submitting'\n }\\>\n Submit\n </button\\>\n {error !== null &&\n <p className\\=\"Error\"\\>\n {error.message}\n </p\\>\n }\n </form\\>\n </\\>\n );\n}\n\nfunction submitForm(answer) {\n \n return new Promise((resolve, reject) \\=> {\n setTimeout(() \\=> {\n let shouldError = answer.toLowerCase() !== 'lima'\n if (shouldError) {\n reject(new Error('Good guess but a wrong answer. Try again!'));\n } else {\n resolve();\n }\n }, 1500);\n });\n}\n\nAlthough this code is longer than the original imperative example, it is much less fragile. Expressing all interactions as state changes lets you later introduce new visual states without breaking existing ones. It also lets you change what should be displayed in each state without changing the logic of the interaction itself.\n\nRecap[](https://react.dev/learn/reacting-to-input-with-state#recap \"Link for Recap\")\n------------------------------------------------------------------------------------\n\n* Declarative programming means describing the UI for each visual state rather than micromanaging the UI (imperative).\n* When developing a component:\n 1. Identify all its visual states.\n 2. Determine the human and computer triggers for state changes.\n 3. Model the state with `useState`.\n 4. Remove non-essential state to avoid bugs and paradoxes.\n 5. Connect the event handlers to set state.\n\nTry out some challenges[](https://react.dev/learn/reacting-to-input-with-state#challenges \"Link for Try out some challenges\")\n-----------------------------------------------------------------------------------------------------------------------------\n\n#### Add and remove a CSS class[](https://react.dev/learn/reacting-to-input-with-state#add-and-remove-a-css-class \"Link for this heading\")\n\nMake it so that clicking on the picture _removes_ the `background--active` CSS class from the outer `<div>`, but _adds_ the `picture--active` class to the `<img>`. Clicking the background again should restore the original CSS classes.\n\nVisually, you should expect that clicking on the picture removes the purple background and highlights the picture border. Clicking outside the picture highlights the background, but removes the picture border highlight.\n",
"filename": "reacting-to-input-with-state.md",
"package": "react"
} |
{
"content": "Title: Referencing Values with Refs – React\n\nURL Source: https://react.dev/learn/referencing-values-with-refs\n\nMarkdown Content:\nWhen you want a component to “remember” some information, but you don’t want that information to [trigger new renders](https://react.dev/learn/render-and-commit), you can use a _ref_.\n\n### You will learn\n\n* How to add a ref to your component\n* How to update a ref’s value\n* How refs are different from state\n* How to use refs safely\n\nAdding a ref to your component[](https://react.dev/learn/referencing-values-with-refs#adding-a-ref-to-your-component \"Link for Adding a ref to your component \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou can add a ref to your component by importing the `useRef` Hook from React:\n\n```\nimport { useRef } from 'react';\n```\n\nInside your component, call the `useRef` Hook and pass the initial value that you want to reference as the only argument. For example, here is a ref to the value `0`:\n\n`useRef` returns an object like this:\n\n```\n{ current: 0 // The value you passed to useRef}\n```\n\n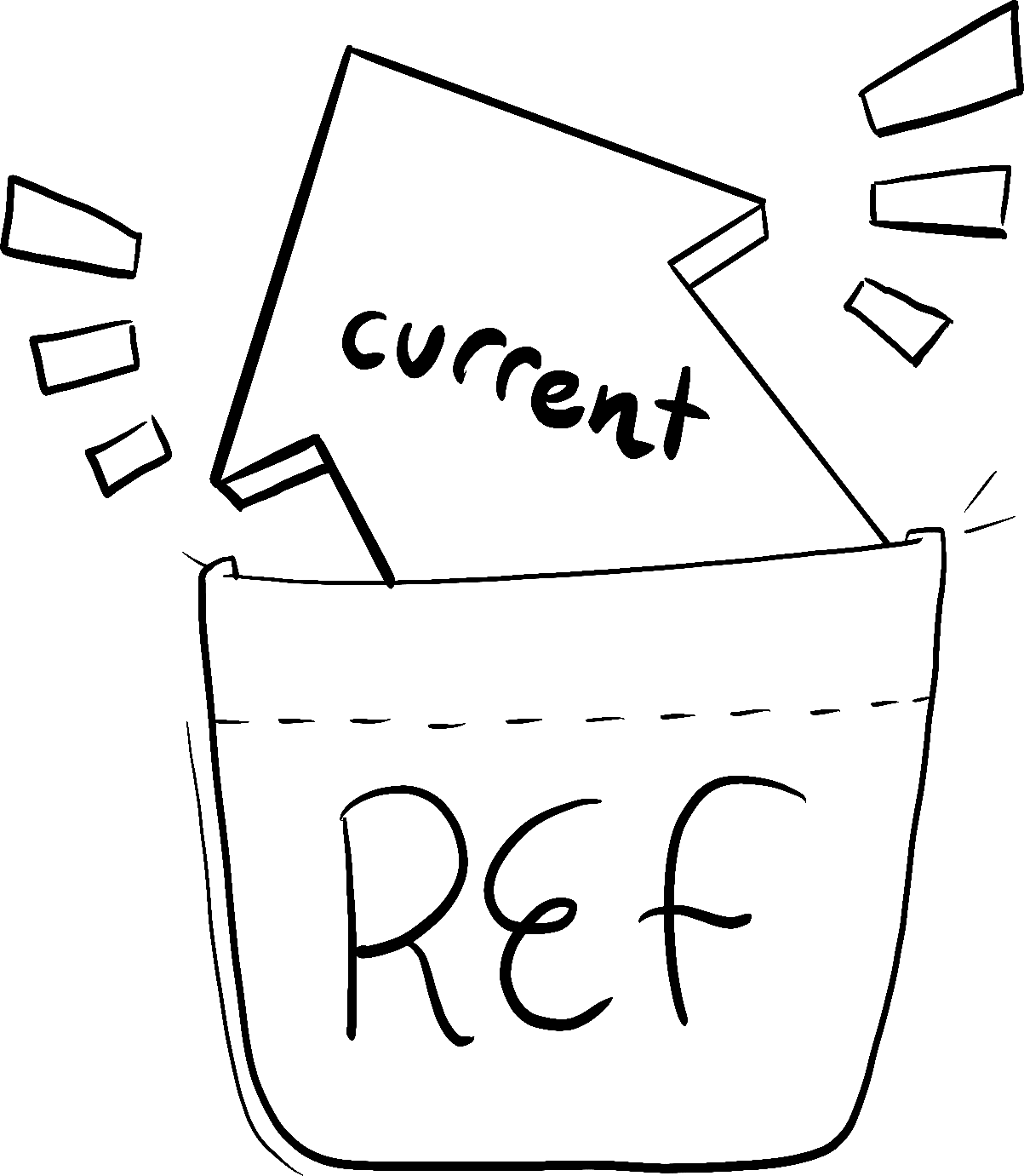\n\nYou can access the current value of that ref through the `ref.current` property. This value is intentionally mutable, meaning you can both read and write to it. It’s like a secret pocket of your component that React doesn’t track. (This is what makes it an “escape hatch” from React’s one-way data flow—more on that below!)\n\nHere, a button will increment `ref.current` on every click:\n\nimport { useRef } from 'react';\n\nexport default function Counter() {\n let ref = useRef(0);\n\n function handleClick() {\n ref.current = ref.current + 1;\n alert('You clicked ' + ref.current + ' times!');\n }\n\n return (\n <button onClick\\={handleClick}\\>\n Click me!\n </button\\>\n );\n}\n\nThe ref points to a number, but, like [state](https://react.dev/learn/state-a-components-memory), you could point to anything: a string, an object, or even a function. Unlike state, ref is a plain JavaScript object with the `current` property that you can read and modify.\n\nNote that **the component doesn’t re-render with every increment.** Like state, refs are retained by React between re-renders. However, setting state re-renders a component. Changing a ref does not!\n\nExample: building a stopwatch[](https://react.dev/learn/referencing-values-with-refs#example-building-a-stopwatch \"Link for Example: building a stopwatch \")\n------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nYou can combine refs and state in a single component. For example, let’s make a stopwatch that the user can start or stop by pressing a button. In order to display how much time has passed since the user pressed “Start”, you will need to keep track of when the Start button was pressed and what the current time is. **This information is used for rendering, so you’ll keep it in state:**\n\n```\nconst [startTime, setStartTime] = useState(null);const [now, setNow] = useState(null);\n```\n\nWhen the user presses “Start”, you’ll use [`setInterval`](https://developer.mozilla.org/docs/Web/API/setInterval) in order to update the time every 10 milliseconds:\n\nimport { useState } from 'react';\n\nexport default function Stopwatch() {\n const \\[startTime, setStartTime\\] = useState(null);\n const \\[now, setNow\\] = useState(null);\n\n function handleStart() {\n \n setStartTime(Date.now());\n setNow(Date.now());\n\n setInterval(() \\=> {\n \n setNow(Date.now());\n }, 10);\n }\n\n let secondsPassed = 0;\n if (startTime != null && now != null) {\n secondsPassed = (now - startTime) / 1000;\n }\n\n return (\n <\\>\n <h1\\>Time passed: {secondsPassed.toFixed(3)}</h1\\>\n <button onClick\\={handleStart}\\>\n Start\n </button\\>\n </\\>\n );\n}\n\nWhen the “Stop” button is pressed, you need to cancel the existing interval so that it stops updating the `now` state variable. You can do this by calling [`clearInterval`](https://developer.mozilla.org/en-US/docs/Web/API/clearInterval), but you need to give it the interval ID that was previously returned by the `setInterval` call when the user pressed Start. You need to keep the interval ID somewhere. **Since the interval ID is not used for rendering, you can keep it in a ref:**\n\nimport { useState, useRef } from 'react';\n\nexport default function Stopwatch() {\n const \\[startTime, setStartTime\\] = useState(null);\n const \\[now, setNow\\] = useState(null);\n const intervalRef = useRef(null);\n\n function handleStart() {\n setStartTime(Date.now());\n setNow(Date.now());\n\n clearInterval(intervalRef.current);\n intervalRef.current = setInterval(() \\=> {\n setNow(Date.now());\n }, 10);\n }\n\n function handleStop() {\n clearInterval(intervalRef.current);\n }\n\n let secondsPassed = 0;\n if (startTime != null && now != null) {\n secondsPassed = (now - startTime) / 1000;\n }\n\n return (\n <\\>\n <h1\\>Time passed: {secondsPassed.toFixed(3)}</h1\\>\n <button onClick\\={handleStart}\\>\n Start\n </button\\>\n <button onClick\\={handleStop}\\>\n Stop\n </button\\>\n </\\>\n );\n}\n\nWhen a piece of information is used for rendering, keep it in state. When a piece of information is only needed by event handlers and changing it doesn’t require a re-render, using a ref may be more efficient.\n\nDifferences between refs and state[](https://react.dev/learn/referencing-values-with-refs#differences-between-refs-and-state \"Link for Differences between refs and state \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nPerhaps you’re thinking refs seem less “strict” than state—you can mutate them instead of always having to use a state setting function, for instance. But in most cases, you’ll want to use state. Refs are an “escape hatch” you won’t need often. Here’s how state and refs compare:\n\n| refs | state |\n| --- | --- |\n| `useRef(initialValue)` returns `{ current: initialValue }` | `useState(initialValue)` returns the current value of a state variable and a state setter function ( `[value, setValue]`) |\n| Doesn’t trigger re-render when you change it. | Triggers re-render when you change it. |\n| Mutable—you can modify and update `current`’s value outside of the rendering process. | ”Immutable”—you must use the state setting function to modify state variables to queue a re-render. |\n| You shouldn’t read (or write) the `current` value during rendering. | You can read state at any time. However, each render has its own [snapshot](https://react.dev/learn/state-as-a-snapshot) of state which does not change. |\n\nHere is a counter button that’s implemented with state:\n\nBecause the `count` value is displayed, it makes sense to use a state value for it. When the counter’s value is set with `setCount()`, React re-renders the component and the screen updates to reflect the new count.\n\nIf you tried to implement this with a ref, React would never re-render the component, so you’d never see the count change! See how clicking this button **does not update its text**:\n\nimport { useRef } from 'react';\n\nexport default function Counter() {\n let countRef = useRef(0);\n\n function handleClick() {\n \n countRef.current = countRef.current + 1;\n }\n\n return (\n <button onClick\\={handleClick}\\>\n You clicked {countRef.current} times\n </button\\>\n );\n}\n\nThis is why reading `ref.current` during render leads to unreliable code. If you need that, use state instead.\n\n##### Deep Dive\n\n#### How does useRef work inside?[](https://react.dev/learn/referencing-values-with-refs#how-does-use-ref-work-inside \"Link for How does useRef work inside? \")\n\nAlthough both `useState` and `useRef` are provided by React, in principle `useRef` could be implemented _on top of_ `useState`. You can imagine that inside of React, `useRef` is implemented like this:\n\n```\n// Inside of Reactfunction useRef(initialValue) {const [ref, unused] = useState({ current: initialValue });return ref;}\n```\n\nDuring the first render, `useRef` returns `{ current: initialValue }`. This object is stored by React, so during the next render the same object will be returned. Note how the state setter is unused in this example. It is unnecessary because `useRef` always needs to return the same object!\n\nReact provides a built-in version of `useRef` because it is common enough in practice. But you can think of it as a regular state variable without a setter. If you’re familiar with object-oriented programming, refs might remind you of instance fields—but instead of `this.something` you write `somethingRef.current`.\n\nWhen to use refs[](https://react.dev/learn/referencing-values-with-refs#when-to-use-refs \"Link for When to use refs \")\n----------------------------------------------------------------------------------------------------------------------\n\nTypically, you will use a ref when your component needs to “step outside” React and communicate with external APIs—often a browser API that won’t impact the appearance of the component. Here are a few of these rare situations:\n\n* Storing [timeout IDs](https://developer.mozilla.org/docs/Web/API/setTimeout)\n* Storing and manipulating [DOM elements](https://developer.mozilla.org/docs/Web/API/Element), which we cover on [the next page](https://react.dev/learn/manipulating-the-dom-with-refs)\n* Storing other objects that aren’t necessary to calculate the JSX.\n\nIf your component needs to store some value, but it doesn’t impact the rendering logic, choose refs.\n\nBest practices for refs[](https://react.dev/learn/referencing-values-with-refs#best-practices-for-refs \"Link for Best practices for refs \")\n-------------------------------------------------------------------------------------------------------------------------------------------\n\nFollowing these principles will make your components more predictable:\n\n* **Treat refs as an escape hatch.** Refs are useful when you work with external systems or browser APIs. If much of your application logic and data flow relies on refs, you might want to rethink your approach.\n* **Don’t read or write `ref.current` during rendering.** If some information is needed during rendering, use [state](https://react.dev/learn/state-a-components-memory) instead. Since React doesn’t know when `ref.current` changes, even reading it while rendering makes your component’s behavior difficult to predict. (The only exception to this is code like `if (!ref.current) ref.current = new Thing()` which only sets the ref once during the first render.)\n\nLimitations of React state don’t apply to refs. For example, state acts like a [snapshot for every render](https://react.dev/learn/state-as-a-snapshot) and [doesn’t update synchronously.](https://react.dev/learn/queueing-a-series-of-state-updates) But when you mutate the current value of a ref, it changes immediately:\n\n```\nref.current = 5;console.log(ref.current); // 5\n```\n\nThis is because **the ref itself is a regular JavaScript object,** and so it behaves like one.\n\nYou also don’t need to worry about [avoiding mutation](https://react.dev/learn/updating-objects-in-state) when you work with a ref. As long as the object you’re mutating isn’t used for rendering, React doesn’t care what you do with the ref or its contents.\n\nRefs and the DOM[](https://react.dev/learn/referencing-values-with-refs#refs-and-the-dom \"Link for Refs and the DOM \")\n----------------------------------------------------------------------------------------------------------------------\n\nYou can point a ref to any value. However, the most common use case for a ref is to access a DOM element. For example, this is handy if you want to focus an input programmatically. When you pass a ref to a `ref` attribute in JSX, like `<div ref={myRef}>`, React will put the corresponding DOM element into `myRef.current`. Once the element is removed from the DOM, React will update `myRef.current` to be `null`. You can read more about this in [Manipulating the DOM with Refs.](https://react.dev/learn/manipulating-the-dom-with-refs)\n\nRecap[](https://react.dev/learn/referencing-values-with-refs#recap \"Link for Recap\")\n------------------------------------------------------------------------------------\n\n* Refs are an escape hatch to hold onto values that aren’t used for rendering. You won’t need them often.\n* A ref is a plain JavaScript object with a single property called `current`, which you can read or set.\n* You can ask React to give you a ref by calling the `useRef` Hook.\n* Like state, refs let you retain information between re-renders of a component.\n* Unlike state, setting the ref’s `current` value does not trigger a re-render.\n* Don’t read or write `ref.current` during rendering. This makes your component hard to predict.\n\nTry out some challenges[](https://react.dev/learn/referencing-values-with-refs#challenges \"Link for Try out some challenges\")\n-----------------------------------------------------------------------------------------------------------------------------\n\n#### Fix a broken chat input[](https://react.dev/learn/referencing-values-with-refs#fix-a-broken-chat-input \"Link for this heading\")\n\nType a message and click “Send”. You will notice there is a three second delay before you see the “Sent!” alert. During this delay, you can see an “Undo” button. Click it. This “Undo” button is supposed to stop the “Sent!” message from appearing. It does this by calling [`clearTimeout`](https://developer.mozilla.org/en-US/docs/Web/API/clearTimeout) for the timeout ID saved during `handleSend`. However, even after “Undo” is clicked, the “Sent!” message still appears. Find why it doesn’t work, and fix it.\n\nimport { useState } from 'react';\n\nexport default function Chat() {\n const \\[text, setText\\] = useState('');\n const \\[isSending, setIsSending\\] = useState(false);\n let timeoutID = null;\n\n function handleSend() {\n setIsSending(true);\n timeoutID = setTimeout(() \\=> {\n alert('Sent!');\n setIsSending(false);\n }, 3000);\n }\n\n function handleUndo() {\n setIsSending(false);\n clearTimeout(timeoutID);\n }\n\n return (\n <\\>\n <input\n disabled\\={isSending}\n value\\={text}\n onChange\\={e \\=> setText(e.target.value)}\n />\n <button\n disabled\\={isSending}\n onClick\\={handleSend}\\>\n {isSending ? 'Sending...' : 'Send'}\n </button\\>\n {isSending &&\n <button onClick\\={handleUndo}\\>\n Undo\n </button\\>\n }\n </\\>\n );\n}\n",
"filename": "referencing-values-with-refs.md",
"package": "react"
} |
{
"content": "Title: Removing Effect Dependencies – React\n\nURL Source: https://react.dev/learn/removing-effect-dependencies\n\nMarkdown Content:\nWhen you write an Effect, the linter will verify that you’ve included every reactive value (like props and state) that the Effect reads in the list of your Effect’s dependencies. This ensures that your Effect remains synchronized with the latest props and state of your component. Unnecessary dependencies may cause your Effect to run too often, or even create an infinite loop. Follow this guide to review and remove unnecessary dependencies from your Effects.\n\n### You will learn\n\n* How to fix infinite Effect dependency loops\n* What to do when you want to remove a dependency\n* How to read a value from your Effect without “reacting” to it\n* How and why to avoid object and function dependencies\n* Why suppressing the dependency linter is dangerous, and what to do instead\n\nDependencies should match the code[](https://react.dev/learn/removing-effect-dependencies#dependencies-should-match-the-code \"Link for Dependencies should match the code \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nWhen you write an Effect, you first specify how to [start and stop](https://react.dev/learn/lifecycle-of-reactive-effects#the-lifecycle-of-an-effect) whatever you want your Effect to be doing:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();// ...}\n```\n\nThen, if you leave the Effect dependencies empty (`[]`), the linter will suggest the correct dependencies:\n\nFill them in according to what the linter says:\n\n```\nfunction ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...}\n```\n\n[Effects “react” to reactive values.](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values) Since `roomId` is a reactive value (it can change due to a re-render), the linter verifies that you’ve specified it as a dependency. If `roomId` receives a different value, React will re-synchronize your Effect. This ensures that the chat stays connected to the selected room and “reacts” to the dropdown:\n\n### To remove a dependency, prove that it’s not a dependency[](https://react.dev/learn/removing-effect-dependencies#to-remove-a-dependency-prove-that-its-not-a-dependency \"Link for To remove a dependency, prove that it’s not a dependency \")\n\nNotice that you can’t “choose” the dependencies of your Effect. Every reactive value used by your Effect’s code must be declared in your dependency list. The dependency list is determined by the surrounding code:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) { // This is a reactive valueuseEffect(() => {const connection = createConnection(serverUrl, roomId); // This Effect reads that reactive valueconnection.connect();return () => connection.disconnect();}, [roomId]); // ✅ So you must specify that reactive value as a dependency of your Effect// ...}\n```\n\n[Reactive values](https://react.dev/learn/lifecycle-of-reactive-effects#all-variables-declared-in-the-component-body-are-reactive) include props and all variables and functions declared directly inside of your component. Since `roomId` is a reactive value, you can’t remove it from the dependency list. The linter wouldn’t allow it:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, []); // 🔴 React Hook useEffect has a missing dependency: 'roomId'// ...}\n```\n\nAnd the linter would be right! Since `roomId` may change over time, this would introduce a bug in your code.\n\n**To remove a dependency, “prove” to the linter that it _doesn’t need_ to be a dependency.** For example, you can move `roomId` out of your component to prove that it’s not reactive and won’t change on re-renders:\n\n```\nconst serverUrl = 'https://localhost:1234';const roomId = 'music'; // Not a reactive value anymorefunction ChatRoom() {useEffect(() => {const connection = createConnection(serverUrl, roomId);connection.connect();return () => connection.disconnect();}, []); // ✅ All dependencies declared// ...}\n```\n\nNow that `roomId` is not a reactive value (and can’t change on a re-render), it doesn’t need to be a dependency:\n\nThis is why you could now specify an [empty (`[]`) dependency list.](https://react.dev/learn/lifecycle-of-reactive-effects#what-an-effect-with-empty-dependencies-means) Your Effect _really doesn’t_ depend on any reactive value anymore, so it _really doesn’t_ need to re-run when any of the component’s props or state change.\n\n### To change the dependencies, change the code[](https://react.dev/learn/removing-effect-dependencies#to-change-the-dependencies-change-the-code \"Link for To change the dependencies, change the code \")\n\nYou might have noticed a pattern in your workflow:\n\n1. First, you **change the code** of your Effect or how your reactive values are declared.\n2. Then, you follow the linter and adjust the dependencies to **match the code you have changed.**\n3. If you’re not happy with the list of dependencies, you **go back to the first step** (and change the code again).\n\nThe last part is important. **If you want to change the dependencies, change the surrounding code first.** You can think of the dependency list as [a list of all the reactive values used by your Effect’s code.](https://react.dev/learn/lifecycle-of-reactive-effects#react-verifies-that-you-specified-every-reactive-value-as-a-dependency) You don’t _choose_ what to put on that list. The list _describes_ your code. To change the dependency list, change the code.\n\nThis might feel like solving an equation. You might start with a goal (for example, to remove a dependency), and you need to “find” the code matching that goal. Not everyone finds solving equations fun, and the same thing could be said about writing Effects! Luckily, there is a list of common recipes that you can try below.\n\n### Pitfall\n\nIf you have an existing codebase, you might have some Effects that suppress the linter like this:\n\n```\nuseEffect(() => {// ...// 🔴 Avoid suppressing the linter like this:// eslint-ignore-next-line react-hooks/exhaustive-deps}, []);\n```\n\n**When dependencies don’t match the code, there is a very high risk of introducing bugs.** By suppressing the linter, you “lie” to React about the values your Effect depends on.\n\nInstead, use the techniques below.\n\n##### Deep Dive\n\n#### Why is suppressing the dependency linter so dangerous?[](https://react.dev/learn/removing-effect-dependencies#why-is-suppressing-the-dependency-linter-so-dangerous \"Link for Why is suppressing the dependency linter so dangerous? \")\n\nSuppressing the linter leads to very unintuitive bugs that are hard to find and fix. Here’s one example:\n\nimport { useState, useEffect } from 'react';\n\nexport default function Timer() {\n const \\[count, setCount\\] = useState(0);\n const \\[increment, setIncrement\\] = useState(1);\n\n function onTick() {\n\tsetCount(count + increment);\n }\n\n useEffect(() \\=> {\n const id = setInterval(onTick, 1000);\n return () \\=> clearInterval(id);\n \n }, \\[\\]);\n\n return (\n <\\>\n <h1\\>\n Counter: {count}\n <button onClick\\={() \\=> setCount(0)}\\>Reset</button\\>\n </h1\\>\n <hr />\n <p\\>\n Every second, increment by:\n <button disabled\\={increment === 0} onClick\\={() \\=> {\n setIncrement(i \\=> i - 1);\n }}\\>–</button\\>\n <b\\>{increment}</b\\>\n <button onClick\\={() \\=> {\n setIncrement(i \\=> i + 1);\n }}\\>+</button\\>\n </p\\>\n </\\>\n );\n}\n\nLet’s say that you wanted to run the Effect “only on mount”. You’ve read that [empty (`[]`) dependencies](https://react.dev/learn/lifecycle-of-reactive-effects#what-an-effect-with-empty-dependencies-means) do that, so you’ve decided to ignore the linter, and forcefully specified `[]` as the dependencies.\n\nThis counter was supposed to increment every second by the amount configurable with the two buttons. However, since you “lied” to React that this Effect doesn’t depend on anything, React forever keeps using the `onTick` function from the initial render. [During that render,](https://react.dev/learn/state-as-a-snapshot#rendering-takes-a-snapshot-in-time) `count` was `0` and `increment` was `1`. This is why `onTick` from that render always calls `setCount(0 + 1)` every second, and you always see `1`. Bugs like this are harder to fix when they’re spread across multiple components.\n\nThere’s always a better solution than ignoring the linter! To fix this code, you need to add `onTick` to the dependency list. (To ensure the interval is only setup once, [make `onTick` an Effect Event.](https://react.dev/learn/separating-events-from-effects#reading-latest-props-and-state-with-effect-events))\n\n**We recommend treating the dependency lint error as a compilation error. If you don’t suppress it, you will never see bugs like this.** The rest of this page documents the alternatives for this and other cases.\n\nRemoving unnecessary dependencies[](https://react.dev/learn/removing-effect-dependencies#removing-unnecessary-dependencies \"Link for Removing unnecessary dependencies \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nEvery time you adjust the Effect’s dependencies to reflect the code, look at the dependency list. Does it make sense for the Effect to re-run when any of these dependencies change? Sometimes, the answer is “no”:\n\n* You might want to re-execute _different parts_ of your Effect under different conditions.\n* You might want to only read the _latest value_ of some dependency instead of “reacting” to its changes.\n* A dependency may change too often _unintentionally_ because it’s an object or a function.\n\nTo find the right solution, you’ll need to answer a few questions about your Effect. Let’s walk through them.\n\n### Should this code move to an event handler?[](https://react.dev/learn/removing-effect-dependencies#should-this-code-move-to-an-event-handler \"Link for Should this code move to an event handler? \")\n\nThe first thing you should think about is whether this code should be an Effect at all.\n\nImagine a form. On submit, you set the `submitted` state variable to `true`. You need to send a POST request and show a notification. You’ve put this logic inside an Effect that “reacts” to `submitted` being `true`:\n\n```\nfunction Form() {const [submitted, setSubmitted] = useState(false);useEffect(() => {if (submitted) {// 🔴 Avoid: Event-specific logic inside an Effectpost('/api/register');showNotification('Successfully registered!');}}, [submitted]);function handleSubmit() {setSubmitted(true);}// ...}\n```\n\nLater, you want to style the notification message according to the current theme, so you read the current theme. Since `theme` is declared in the component body, it is a reactive value, so you add it as a dependency:\n\n```\nfunction Form() {const [submitted, setSubmitted] = useState(false);const theme = useContext(ThemeContext);useEffect(() => {if (submitted) {// 🔴 Avoid: Event-specific logic inside an Effectpost('/api/register');showNotification('Successfully registered!', theme);}}, [submitted, theme]); // ✅ All dependencies declaredfunction handleSubmit() {setSubmitted(true);} // ...}\n```\n\nBy doing this, you’ve introduced a bug. Imagine you submit the form first and then switch between Dark and Light themes. The `theme` will change, the Effect will re-run, and so it will display the same notification again!\n\n**The problem here is that this shouldn’t be an Effect in the first place.** You want to send this POST request and show the notification in response to _submitting the form,_ which is a particular interaction. To run some code in response to particular interaction, put that logic directly into the corresponding event handler:\n\n```\nfunction Form() {const theme = useContext(ThemeContext);function handleSubmit() {// ✅ Good: Event-specific logic is called from event handlerspost('/api/register');showNotification('Successfully registered!', theme);} // ...}\n```\n\nNow that the code is in an event handler, it’s not reactive—so it will only run when the user submits the form. Read more about [choosing between event handlers and Effects](https://react.dev/learn/separating-events-from-effects#reactive-values-and-reactive-logic) and [how to delete unnecessary Effects.](https://react.dev/learn/you-might-not-need-an-effect)\n\nThe next question you should ask yourself is whether your Effect is doing several unrelated things.\n\nImagine you’re creating a shipping form where the user needs to choose their city and area. You fetch the list of `cities` from the server according to the selected `country` to show them in a dropdown:\n\n```\nfunction ShippingForm({ country }) {const [cities, setCities] = useState(null);const [city, setCity] = useState(null);useEffect(() => {let ignore = false;fetch(`/api/cities?country=${country}`) .then(response => response.json()) .then(json => {if (!ignore) {setCities(json);}});return () => {ignore = true;};}, [country]); // ✅ All dependencies declared// ...\n```\n\nThis is a good example of [fetching data in an Effect.](https://react.dev/learn/you-might-not-need-an-effect#fetching-data) You are synchronizing the `cities` state with the network according to the `country` prop. You can’t do this in an event handler because you need to fetch as soon as `ShippingForm` is displayed and whenever the `country` changes (no matter which interaction causes it).\n\nNow let’s say you’re adding a second select box for city areas, which should fetch the `areas` for the currently selected `city`. You might start by adding a second `fetch` call for the list of areas inside the same Effect:\n\n```\nfunction ShippingForm({ country }) {const [cities, setCities] = useState(null);const [city, setCity] = useState(null);const [areas, setAreas] = useState(null);useEffect(() => {let ignore = false;fetch(`/api/cities?country=${country}`) .then(response => response.json()) .then(json => {if (!ignore) {setCities(json);}});// 🔴 Avoid: A single Effect synchronizes two independent processesif (city) {fetch(`/api/areas?city=${city}`) .then(response => response.json()) .then(json => {if (!ignore) {setAreas(json);}});}return () => {ignore = true;};}, [country, city]); // ✅ All dependencies declared// ...\n```\n\nHowever, since the Effect now uses the `city` state variable, you’ve had to add `city` to the list of dependencies. That, in turn, introduced a problem: when the user selects a different city, the Effect will re-run and call `fetchCities(country)`. As a result, you will be unnecessarily refetching the list of cities many times.\n\n**The problem with this code is that you’re synchronizing two different unrelated things:**\n\n1. You want to synchronize the `cities` state to the network based on the `country` prop.\n2. You want to synchronize the `areas` state to the network based on the `city` state.\n\nSplit the logic into two Effects, each of which reacts to the prop that it needs to synchronize with:\n\n```\nfunction ShippingForm({ country }) {const [cities, setCities] = useState(null);useEffect(() => {let ignore = false;fetch(`/api/cities?country=${country}`) .then(response => response.json()) .then(json => {if (!ignore) {setCities(json);}});return () => {ignore = true;};}, [country]); // ✅ All dependencies declaredconst [city, setCity] = useState(null);const [areas, setAreas] = useState(null);useEffect(() => {if (city) {let ignore = false;fetch(`/api/areas?city=${city}`) .then(response => response.json()) .then(json => {if (!ignore) {setAreas(json);}});return () => {ignore = true;};}}, [city]); // ✅ All dependencies declared// ...\n```\n\nNow the first Effect only re-runs if the `country` changes, while the second Effect re-runs when the `city` changes. You’ve separated them by purpose: two different things are synchronized by two separate Effects. Two separate Effects have two separate dependency lists, so they won’t trigger each other unintentionally.\n\nThe final code is longer than the original, but splitting these Effects is still correct. [Each Effect should represent an independent synchronization process.](https://react.dev/learn/lifecycle-of-reactive-effects#each-effect-represents-a-separate-synchronization-process) In this example, deleting one Effect doesn’t break the other Effect’s logic. This means they _synchronize different things,_ and it’s good to split them up. If you’re concerned about duplication, you can improve this code by [extracting repetitive logic into a custom Hook.](https://react.dev/learn/reusing-logic-with-custom-hooks#when-to-use-custom-hooks)\n\n### Are you reading some state to calculate the next state?[](https://react.dev/learn/removing-effect-dependencies#are-you-reading-some-state-to-calculate-the-next-state \"Link for Are you reading some state to calculate the next state? \")\n\nThis Effect updates the `messages` state variable with a newly created array every time a new message arrives:\n\n```\nfunction ChatRoom({ roomId }) {const [messages, setMessages] = useState([]);useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {setMessages([...messages, receivedMessage]);});// ...\n```\n\nIt uses the `messages` variable to [create a new array](https://react.dev/learn/updating-arrays-in-state) starting with all the existing messages and adds the new message at the end. However, since `messages` is a reactive value read by an Effect, it must be a dependency:\n\n```\nfunction ChatRoom({ roomId }) {const [messages, setMessages] = useState([]);useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {setMessages([...messages, receivedMessage]);});return () => connection.disconnect();}, [roomId, messages]); // ✅ All dependencies declared// ...\n```\n\nAnd making `messages` a dependency introduces a problem.\n\nEvery time you receive a message, `setMessages()` causes the component to re-render with a new `messages` array that includes the received message. However, since this Effect now depends on `messages`, this will _also_ re-synchronize the Effect. So every new message will make the chat re-connect. The user would not like that!\n\nTo fix the issue, don’t read `messages` inside the Effect. Instead, pass an [updater function](https://react.dev/reference/react/useState#updating-state-based-on-the-previous-state) to `setMessages`:\n\n```\nfunction ChatRoom({ roomId }) {const [messages, setMessages] = useState([]);useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {setMessages(msgs => [...msgs, receivedMessage]);});return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...\n```\n\n**Notice how your Effect does not read the `messages` variable at all now.** You only need to pass an updater function like `msgs => [...msgs, receivedMessage]`. React [puts your updater function in a queue](https://react.dev/learn/queueing-a-series-of-state-updates) and will provide the `msgs` argument to it during the next render. This is why the Effect itself doesn’t need to depend on `messages` anymore. As a result of this fix, receiving a chat message will no longer make the chat re-connect.\n\n### Do you want to read a value without “reacting” to its changes?[](https://react.dev/learn/removing-effect-dependencies#do-you-want-to-read-a-value-without-reacting-to-its-changes \"Link for Do you want to read a value without “reacting” to its changes? \")\n\n### Under Construction\n\nThis section describes an **experimental API that has not yet been released** in a stable version of React.\n\nSuppose that you want to play a sound when the user receives a new message unless `isMuted` is `true`:\n\n```\nfunction ChatRoom({ roomId }) {const [messages, setMessages] = useState([]);const [isMuted, setIsMuted] = useState(false);useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {setMessages(msgs => [...msgs, receivedMessage]);if (!isMuted) {playSound();}});// ...\n```\n\nSince your Effect now uses `isMuted` in its code, you have to add it to the dependencies:\n\n```\nfunction ChatRoom({ roomId }) {const [messages, setMessages] = useState([]);const [isMuted, setIsMuted] = useState(false);useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {setMessages(msgs => [...msgs, receivedMessage]);if (!isMuted) {playSound();}});return () => connection.disconnect();}, [roomId, isMuted]); // ✅ All dependencies declared// ...\n```\n\nThe problem is that every time `isMuted` changes (for example, when the user presses the “Muted” toggle), the Effect will re-synchronize, and reconnect to the chat. This is not the desired user experience! (In this example, even disabling the linter would not work—if you do that, `isMuted` would get “stuck” with its old value.)\n\nTo solve this problem, you need to extract the logic that shouldn’t be reactive out of the Effect. You don’t want this Effect to “react” to the changes in `isMuted`. [Move this non-reactive piece of logic into an Effect Event:](https://react.dev/learn/separating-events-from-effects#declaring-an-effect-event)\n\n```\nimport { useState, useEffect, useEffectEvent } from 'react';function ChatRoom({ roomId }) {const [messages, setMessages] = useState([]);const [isMuted, setIsMuted] = useState(false);const onMessage = useEffectEvent(receivedMessage => {setMessages(msgs => [...msgs, receivedMessage]);if (!isMuted) {playSound();}});useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {onMessage(receivedMessage);});return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...\n```\n\nEffect Events let you split an Effect into reactive parts (which should “react” to reactive values like `roomId` and their changes) and non-reactive parts (which only read their latest values, like `onMessage` reads `isMuted`). **Now that you read `isMuted` inside an Effect Event, it doesn’t need to be a dependency of your Effect.** As a result, the chat won’t re-connect when you toggle the “Muted” setting on and off, solving the original issue!\n\n#### Wrapping an event handler from the props[](https://react.dev/learn/removing-effect-dependencies#wrapping-an-event-handler-from-the-props \"Link for Wrapping an event handler from the props \")\n\nYou might run into a similar problem when your component receives an event handler as a prop:\n\n```\nfunction ChatRoom({ roomId, onReceiveMessage }) {const [messages, setMessages] = useState([]);useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {onReceiveMessage(receivedMessage);});return () => connection.disconnect();}, [roomId, onReceiveMessage]); // ✅ All dependencies declared// ...\n```\n\nSuppose that the parent component passes a _different_ `onReceiveMessage` function on every render:\n\n```\n<ChatRoomroomId={roomId}onReceiveMessage={receivedMessage => {// ...}}/>\n```\n\nSince `onReceiveMessage` is a dependency, it would cause the Effect to re-synchronize after every parent re-render. This would make it re-connect to the chat. To solve this, wrap the call in an Effect Event:\n\n```\nfunction ChatRoom({ roomId, onReceiveMessage }) {const [messages, setMessages] = useState([]);const onMessage = useEffectEvent(receivedMessage => {onReceiveMessage(receivedMessage);});useEffect(() => {const connection = createConnection();connection.connect();connection.on('message', (receivedMessage) => {onMessage(receivedMessage);});return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...\n```\n\nEffect Events aren’t reactive, so you don’t need to specify them as dependencies. As a result, the chat will no longer re-connect even if the parent component passes a function that’s different on every re-render.\n\n#### Separating reactive and non-reactive code[](https://react.dev/learn/removing-effect-dependencies#separating-reactive-and-non-reactive-code \"Link for Separating reactive and non-reactive code \")\n\nIn this example, you want to log a visit every time `roomId` changes. You want to include the current `notificationCount` with every log, but you _don’t_ want a change to `notificationCount` to trigger a log event.\n\nThe solution is again to split out the non-reactive code into an Effect Event:\n\n```\nfunction Chat({ roomId, notificationCount }) {const onVisit = useEffectEvent(visitedRoomId => {logVisit(visitedRoomId, notificationCount);});useEffect(() => {onVisit(roomId);}, [roomId]); // ✅ All dependencies declared// ...}\n```\n\nYou want your logic to be reactive with regards to `roomId`, so you read `roomId` inside of your Effect. However, you don’t want a change to `notificationCount` to log an extra visit, so you read `notificationCount` inside of the Effect Event. [Learn more about reading the latest props and state from Effects using Effect Events.](https://react.dev/learn/separating-events-from-effects#reading-latest-props-and-state-with-effect-events)\n\n### Does some reactive value change unintentionally?[](https://react.dev/learn/removing-effect-dependencies#does-some-reactive-value-change-unintentionally \"Link for Does some reactive value change unintentionally? \")\n\nSometimes, you _do_ want your Effect to “react” to a certain value, but that value changes more often than you’d like—and might not reflect any actual change from the user’s perspective. For example, let’s say that you create an `options` object in the body of your component, and then read that object from inside of your Effect:\n\n```\nfunction ChatRoom({ roomId }) {// ...const options = {serverUrl: serverUrl,roomId: roomId};useEffect(() => {const connection = createConnection(options);connection.connect();// ...\n```\n\nThis object is declared in the component body, so it’s a [reactive value.](https://react.dev/learn/lifecycle-of-reactive-effects#effects-react-to-reactive-values) When you read a reactive value like this inside an Effect, you declare it as a dependency. This ensures your Effect “reacts” to its changes:\n\n```\n// ...useEffect(() => {const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, [options]); // ✅ All dependencies declared// ...\n```\n\nIt is important to declare it as a dependency! This ensures, for example, that if the `roomId` changes, your Effect will re-connect to the chat with the new `options`. However, there is also a problem with the code above. To see it, try typing into the input in the sandbox below, and watch what happens in the console:\n\nIn the sandbox above, the input only updates the `message` state variable. From the user’s perspective, this should not affect the chat connection. However, every time you update the `message`, your component re-renders. When your component re-renders, the code inside of it runs again from scratch.\n\nA new `options` object is created from scratch on every re-render of the `ChatRoom` component. React sees that the `options` object is a _different object_ from the `options` object created during the last render. This is why it re-synchronizes your Effect (which depends on `options`), and the chat re-connects as you type.\n\n**This problem only affects objects and functions. In JavaScript, each newly created object and function is considered distinct from all the others. It doesn’t matter that the contents inside of them may be the same!**\n\n```\n// During the first renderconst options1 = { serverUrl: 'https://localhost:1234', roomId: 'music' };// During the next renderconst options2 = { serverUrl: 'https://localhost:1234', roomId: 'music' };// These are two different objects!console.log(Object.is(options1, options2)); // false\n```\n\n**Object and function dependencies can make your Effect re-synchronize more often than you need.**\n\nThis is why, whenever possible, you should try to avoid objects and functions as your Effect’s dependencies. Instead, try moving them outside the component, inside the Effect, or extracting primitive values out of them.\n\n#### Move static objects and functions outside your component[](https://react.dev/learn/removing-effect-dependencies#move-static-objects-and-functions-outside-your-component \"Link for Move static objects and functions outside your component \")\n\nIf the object does not depend on any props and state, you can move that object outside your component:\n\n```\nconst options = {serverUrl: 'https://localhost:1234',roomId: 'music'};function ChatRoom() {const [message, setMessage] = useState('');useEffect(() => {const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, []); // ✅ All dependencies declared// ...\n```\n\nThis way, you _prove_ to the linter that it’s not reactive. It can’t change as a result of a re-render, so it doesn’t need to be a dependency. Now re-rendering `ChatRoom` won’t cause your Effect to re-synchronize.\n\nThis works for functions too:\n\n```\nfunction createOptions() {return {serverUrl: 'https://localhost:1234',roomId: 'music'};}function ChatRoom() {const [message, setMessage] = useState('');useEffect(() => {const options = createOptions();const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, []); // ✅ All dependencies declared// ...\n```\n\nSince `createOptions` is declared outside your component, it’s not a reactive value. This is why it doesn’t need to be specified in your Effect’s dependencies, and why it won’t ever cause your Effect to re-synchronize.\n\n#### Move dynamic objects and functions inside your Effect[](https://react.dev/learn/removing-effect-dependencies#move-dynamic-objects-and-functions-inside-your-effect \"Link for Move dynamic objects and functions inside your Effect \")\n\nIf your object depends on some reactive value that may change as a result of a re-render, like a `roomId` prop, you can’t pull it _outside_ your component. You can, however, move its creation _inside_ of your Effect’s code:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {const [message, setMessage] = useState('');useEffect(() => {const options = {serverUrl: serverUrl,roomId: roomId};const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...\n```\n\nNow that `options` is declared inside of your Effect, it is no longer a dependency of your Effect. Instead, the only reactive value used by your Effect is `roomId`. Since `roomId` is not an object or function, you can be sure that it won’t be _unintentionally_ different. In JavaScript, numbers and strings are compared by their content:\n\n```\n// During the first renderconst roomId1 = 'music';// During the next renderconst roomId2 = 'music';// These two strings are the same!console.log(Object.is(roomId1, roomId2)); // true\n```\n\nThanks to this fix, the chat no longer re-connects if you edit the input:\n\nHowever, it _does_ re-connect when you change the `roomId` dropdown, as you would expect.\n\nThis works for functions, too:\n\n```\nconst serverUrl = 'https://localhost:1234';function ChatRoom({ roomId }) {const [message, setMessage] = useState('');useEffect(() => {function createOptions() {return {serverUrl: serverUrl,roomId: roomId};}const options = createOptions();const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, [roomId]); // ✅ All dependencies declared// ...\n```\n\nYou can write your own functions to group pieces of logic inside your Effect. As long as you also declare them _inside_ your Effect, they’re not reactive values, and so they don’t need to be dependencies of your Effect.\n\n#### Read primitive values from objects[](https://react.dev/learn/removing-effect-dependencies#read-primitive-values-from-objects \"Link for Read primitive values from objects \")\n\nSometimes, you may receive an object from props:\n\n```\nfunction ChatRoom({ options }) {const [message, setMessage] = useState('');useEffect(() => {const connection = createConnection(options);connection.connect();return () => connection.disconnect();}, [options]); // ✅ All dependencies declared// ...\n```\n\nThe risk here is that the parent component will create the object during rendering:\n\n```\n<ChatRoomroomId={roomId}options={{serverUrl: serverUrl,roomId: roomId}}/>\n```\n\nThis would cause your Effect to re-connect every time the parent component re-renders. To fix this, read information from the object _outside_ the Effect, and avoid having object and function dependencies:\n\n```\nfunction ChatRoom({ options }) {const [message, setMessage] = useState('');const { roomId, serverUrl } = options;useEffect(() => {const connection = createConnection({roomId: roomId,serverUrl: serverUrl});connection.connect();return () => connection.disconnect();}, [roomId, serverUrl]); // ✅ All dependencies declared// ...\n```\n\nThe logic gets a little repetitive (you read some values from an object outside an Effect, and then create an object with the same values inside the Effect). But it makes it very explicit what information your Effect _actually_ depends on. If an object is re-created unintentionally by the parent component, the chat would not re-connect. However, if `options.roomId` or `options.serverUrl` really are different, the chat would re-connect.\n\n#### Calculate primitive values from functions[](https://react.dev/learn/removing-effect-dependencies#calculate-primitive-values-from-functions \"Link for Calculate primitive values from functions \")\n\nThe same approach can work for functions. For example, suppose the parent component passes a function:\n\n```\n<ChatRoomroomId={roomId}getOptions={() => {return {serverUrl: serverUrl,roomId: roomId};}}/>\n```\n\nTo avoid making it a dependency (and causing it to re-connect on re-renders), call it outside the Effect. This gives you the `roomId` and `serverUrl` values that aren’t objects, and that you can read from inside your Effect:\n\n```\nfunction ChatRoom({ getOptions }) {const [message, setMessage] = useState('');const { roomId, serverUrl } = getOptions();useEffect(() => {const connection = createConnection({roomId: roomId,serverUrl: serverUrl});connection.connect();return () => connection.disconnect();}, [roomId, serverUrl]); // ✅ All dependencies declared// ...\n```\n\nThis only works for [pure](https://react.dev/learn/keeping-components-pure) functions because they are safe to call during rendering. If your function is an event handler, but you don’t want its changes to re-synchronize your Effect, [wrap it into an Effect Event instead.](https://react.dev/learn/removing-effect-dependencies#do-you-want-to-read-a-value-without-reacting-to-its-changes)\n\nRecap[](https://react.dev/learn/removing-effect-dependencies#recap \"Link for Recap\")\n------------------------------------------------------------------------------------\n\n* Dependencies should always match the code.\n* When you’re not happy with your dependencies, what you need to edit is the code.\n* Suppressing the linter leads to very confusing bugs, and you should always avoid it.\n* To remove a dependency, you need to “prove” to the linter that it’s not necessary.\n* If some code should run in response to a specific interaction, move that code to an event handler.\n* If different parts of your Effect should re-run for different reasons, split it into several Effects.\n* If you want to update some state based on the previous state, pass an updater function.\n* If you want to read the latest value without “reacting” it, extract an Effect Event from your Effect.\n* In JavaScript, objects and functions are considered different if they were created at different times.\n* Try to avoid object and function dependencies. Move them outside the component or inside the Effect.\n",
"filename": "removing-effect-dependencies.md",
"package": "react"
} |
{
"content": "Title: Render and Commit – React\n\nURL Source: https://react.dev/learn/render-and-commit\n\nMarkdown Content:\nBefore your components are displayed on screen, they must be rendered by React. Understanding the steps in this process will help you think about how your code executes and explain its behavior.\n\n### You will learn\n\n* What rendering means in React\n* When and why React renders a component\n* The steps involved in displaying a component on screen\n* Why rendering does not always produce a DOM update\n\nImagine that your components are cooks in the kitchen, assembling tasty dishes from ingredients. In this scenario, React is the waiter who puts in requests from customers and brings them their orders. This process of requesting and serving UI has three steps:\n\n1. **Triggering** a render (delivering the guest’s order to the kitchen)\n2. **Rendering** the component (preparing the order in the kitchen)\n3. **Committing** to the DOM (placing the order on the table)\n\n1. 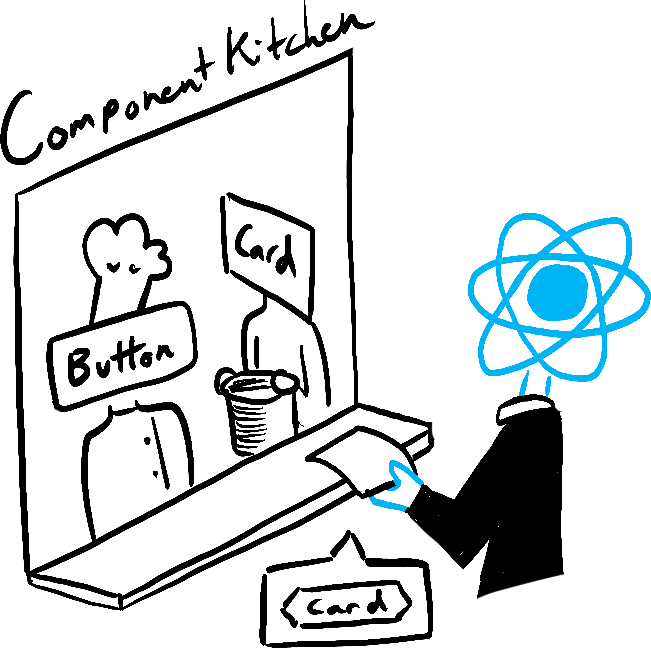\n \n Trigger\n \n2. 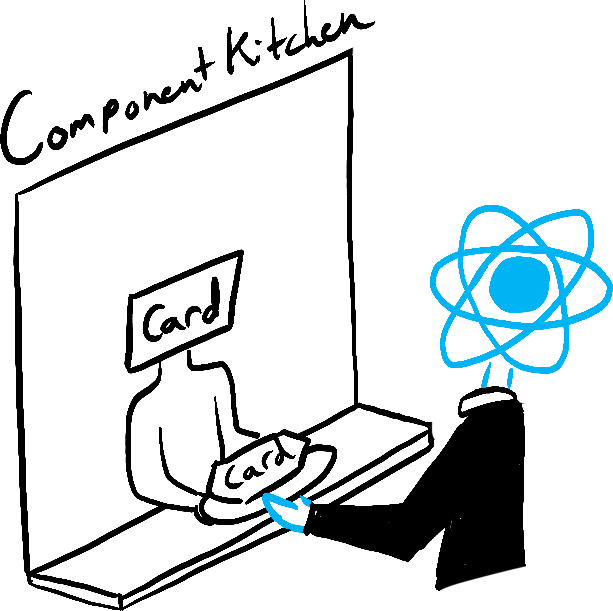\n \n Render\n \n3. 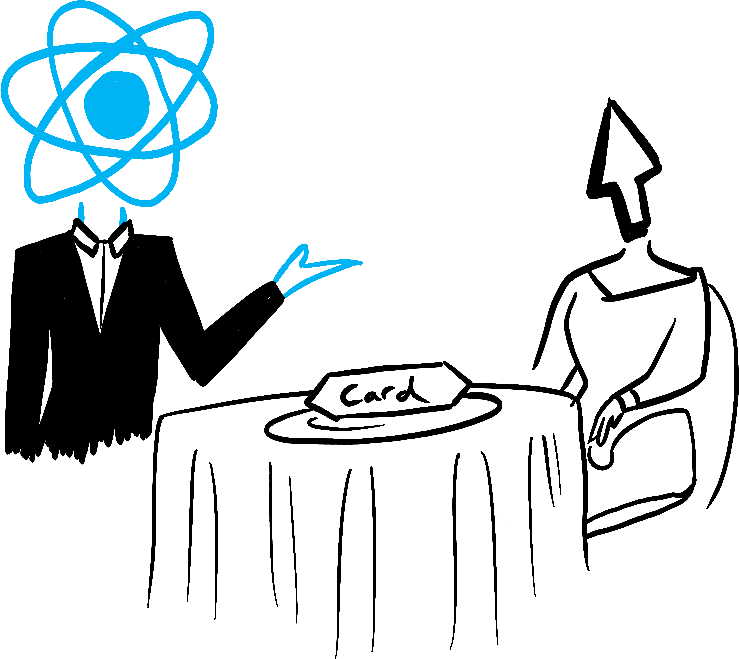\n \n Commit\n \n\nStep 1: Trigger a render[](https://react.dev/learn/render-and-commit#step-1-trigger-a-render \"Link for Step 1: Trigger a render \")\n----------------------------------------------------------------------------------------------------------------------------------\n\nThere are two reasons for a component to render:\n\n1. It’s the component’s **initial render.**\n2. The component’s (or one of its ancestors’) **state has been updated.**\n\n### Initial render[](https://react.dev/learn/render-and-commit#initial-render \"Link for Initial render \")\n\nWhen your app starts, you need to trigger the initial render. Frameworks and sandboxes sometimes hide this code, but it’s done by calling [`createRoot`](https://react.dev/reference/react-dom/client/createRoot) with the target DOM node, and then calling its `render` method with your component:\n\nTry commenting out the `root.render()` call and see the component disappear!\n\n### Re-renders when state updates[](https://react.dev/learn/render-and-commit#re-renders-when-state-updates \"Link for Re-renders when state updates \")\n\nOnce the component has been initially rendered, you can trigger further renders by updating its state with the [`set` function.](https://react.dev/reference/react/useState#setstate) Updating your component’s state automatically queues a render. (You can imagine these as a restaurant guest ordering tea, dessert, and all sorts of things after putting in their first order, depending on the state of their thirst or hunger.)\n\n1. 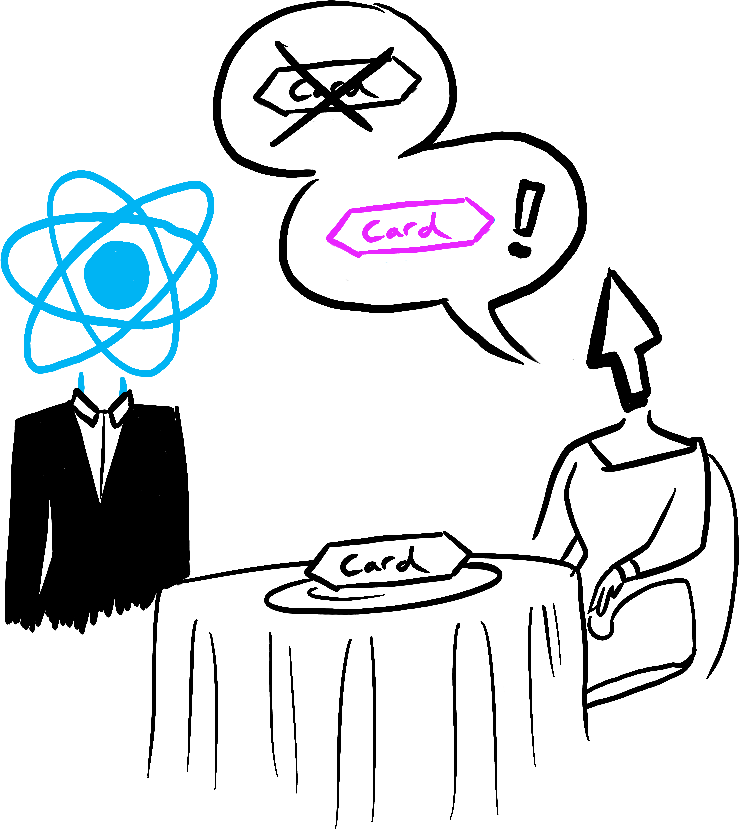\n \n State update...\n \n2. 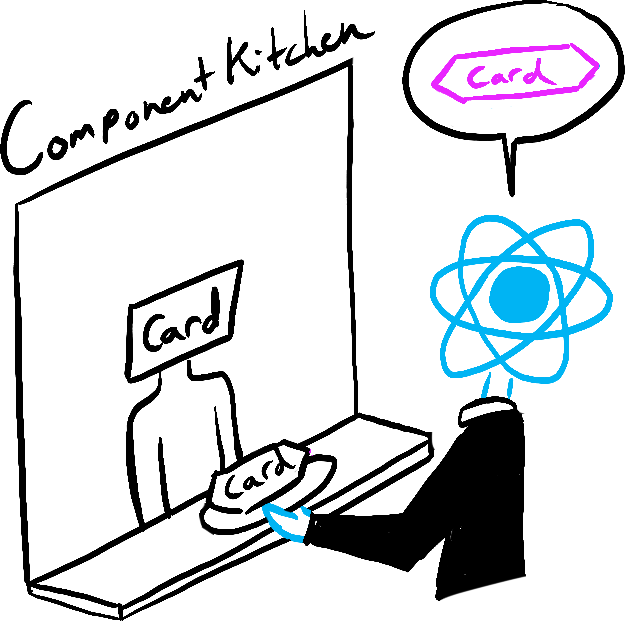\n \n ...triggers...\n \n3. 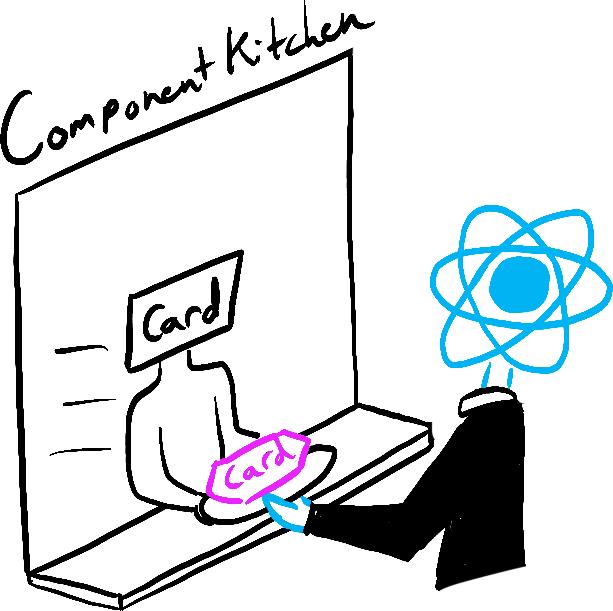\n \n ...render!\n \n\nStep 2: React renders your components[](https://react.dev/learn/render-and-commit#step-2-react-renders-your-components \"Link for Step 2: React renders your components \")\n-------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAfter you trigger a render, React calls your components to figure out what to display on screen. **“Rendering” is React calling your components.**\n\n* **On initial render,** React will call the root component.\n* **For subsequent renders,** React will call the function component whose state update triggered the render.\n\nThis process is recursive: if the updated component returns some other component, React will render _that_ component next, and if that component also returns something, it will render _that_ component next, and so on. The process will continue until there are no more nested components and React knows exactly what should be displayed on screen.\n\nIn the following example, React will call `Gallery()` and `Image()` several times:\n\nexport default function Gallery() {\n return (\n <section\\>\n <h1\\>Inspiring Sculptures</h1\\>\n <Image />\n <Image />\n <Image />\n </section\\>\n );\n}\n\nfunction Image() {\n return (\n <img\n src\\=\"https://i.imgur.com/ZF6s192.jpg\"\n alt\\=\"'Floralis Genérica' by Eduardo Catalano: a gigantic metallic flower sculpture with reflective petals\"\n />\n );\n}\n\n* **During the initial render,** React will [create the DOM nodes](https://developer.mozilla.org/docs/Web/API/Document/createElement) for `<section>`, `<h1>`, and three `<img>` tags.\n* **During a re-render,** React will calculate which of their properties, if any, have changed since the previous render. It won’t do anything with that information until the next step, the commit phase.\n\n### Pitfall\n\nRendering must always be a [pure calculation](https://react.dev/learn/keeping-components-pure):\n\n* **Same inputs, same output.** Given the same inputs, a component should always return the same JSX. (When someone orders a salad with tomatoes, they should not receive a salad with onions!)\n* **It minds its own business.** It should not change any objects or variables that existed before rendering. (One order should not change anyone else’s order.)\n\nOtherwise, you can encounter confusing bugs and unpredictable behavior as your codebase grows in complexity. When developing in “Strict Mode”, React calls each component’s function twice, which can help surface mistakes caused by impure functions.\n\n##### Deep Dive\n\n#### Optimizing performance[](https://react.dev/learn/render-and-commit#optimizing-performance \"Link for Optimizing performance \")\n\nThe default behavior of rendering all components nested within the updated component is not optimal for performance if the updated component is very high in the tree. If you run into a performance issue, there are several opt-in ways to solve it described in the [Performance](https://reactjs.org/docs/optimizing-performance.html) section. **Don’t optimize prematurely!**\n\nStep 3: React commits changes to the DOM[](https://react.dev/learn/render-and-commit#step-3-react-commits-changes-to-the-dom \"Link for Step 3: React commits changes to the DOM \")\n----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------\n\nAfter rendering (calling) your components, React will modify the DOM.\n\n* **For the initial render,** React will use the [`appendChild()`](https://developer.mozilla.org/docs/Web/API/Node/appendChild) DOM API to put all the DOM nodes it has created on screen.\n* **For re-renders,** React will apply the minimal necessary operations (calculated while rendering!) to make the DOM match the latest rendering output.\n\n**React only changes the DOM nodes if there’s a difference between renders.** For example, here is a component that re-renders with different props passed from its parent every second. Notice how you can add some text into the `<input>`, updating its `value`, but the text doesn’t disappear when the component re-renders:\n\nThis works because during this last step, React only updates the content of `<h1>` with the new `time`. It sees that the `<input>` appears in the JSX in the same place as last time, so React doesn’t touch the `<input>`—or its `value`!\n\nEpilogue: Browser paint[](https://react.dev/learn/render-and-commit#epilogue-browser-paint \"Link for Epilogue: Browser paint \")\n-------------------------------------------------------------------------------------------------------------------------------\n\nAfter rendering is done and React updated the DOM, the browser will repaint the screen. Although this process is known as “browser rendering”, we’ll refer to it as “painting” to avoid confusion throughout the docs.\n\n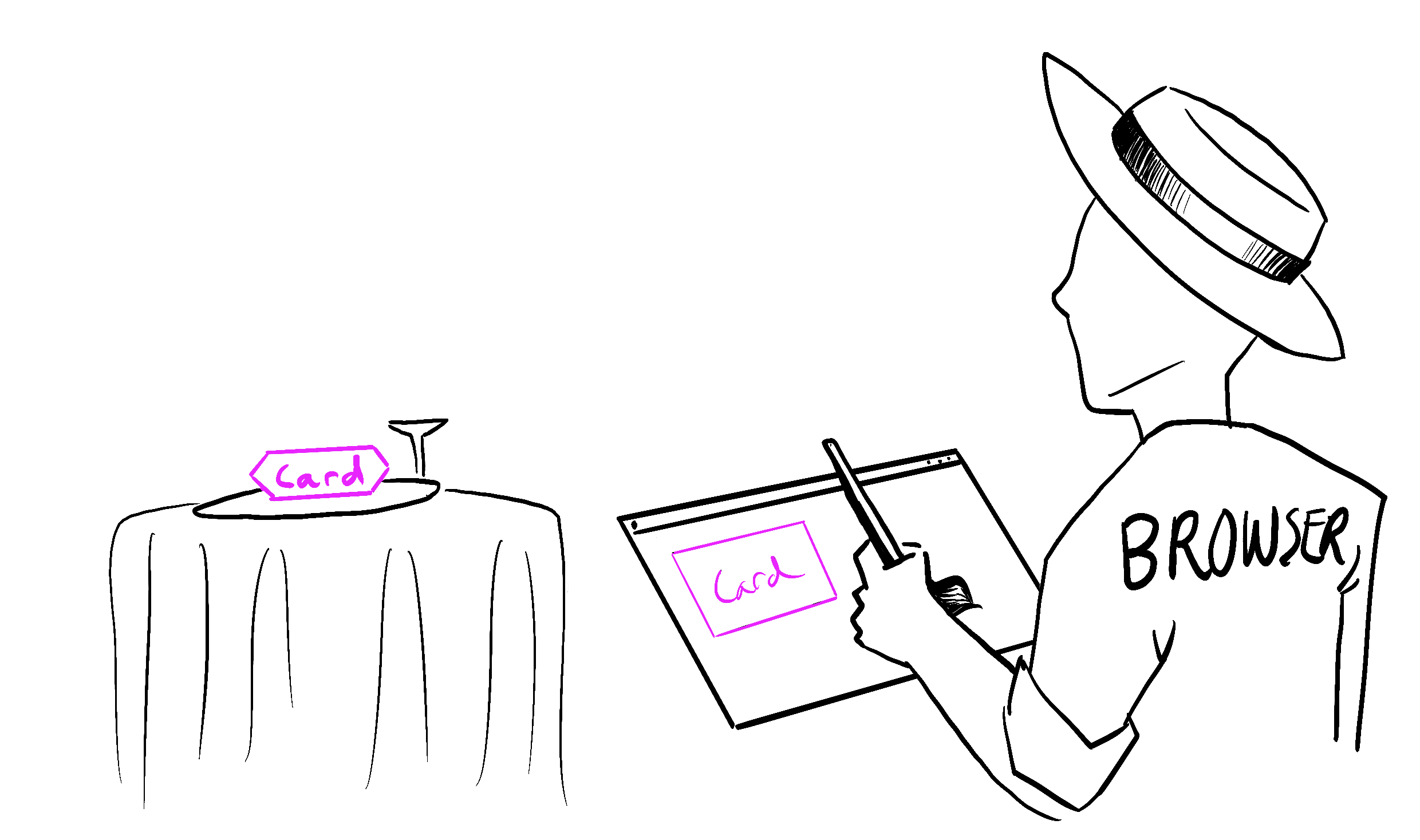\n\nRecap[](https://react.dev/learn/render-and-commit#recap \"Link for Recap\")\n-------------------------------------------------------------------------\n\n* Any screen update in a React app happens in three steps:\n 1. Trigger\n 2. Render\n 3. Commit\n* You can use Strict Mode to find mistakes in your components\n* React does not touch the DOM if the rendering result is the same as last time\n",
"filename": "render-and-commit.md",
"package": "react"
} |
{
"content": "Title: render – React\n\nURL Source: https://react.dev/reference/react-dom/render\n\nMarkdown Content:\n### Deprecated\n\nThis API will be removed in a future major version of React.\n\nIn React 18, `render` was replaced by [`createRoot`.](https://react.dev/reference/react-dom/client/createRoot) Using `render` in React 18 will warn that your app will behave as if it’s running React 17. Learn more [here.](https://react.dev/blog/2022/03/08/react-18-upgrade-guide#updates-to-client-rendering-apis)\n\n`render` renders a piece of [JSX](https://react.dev/learn/writing-markup-with-jsx) (“React node”) into a browser DOM node.\n\n```\nrender(reactNode, domNode, callback?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/render#reference)\n * [`render(reactNode, domNode, callback?)`](https://react.dev/reference/react-dom/render#render)\n* [Usage](https://react.dev/reference/react-dom/render#usage)\n * [Rendering the root component](https://react.dev/reference/react-dom/render#rendering-the-root-component)\n * [Rendering multiple roots](https://react.dev/reference/react-dom/render#rendering-multiple-roots)\n * [Updating the rendered tree](https://react.dev/reference/react-dom/render#updating-the-rendered-tree)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/render#reference \"Link for Reference \")\n-----------------------------------------------------------------------------------------\n\n### `render(reactNode, domNode, callback?)`[](https://react.dev/reference/react-dom/render#render \"Link for this heading\")\n\nCall `render` to display a React component inside a browser DOM element.\n\n```\nimport { render } from 'react-dom';const domNode = document.getElementById('root');render(<App />, domNode);\n```\n\nReact will display `<App />` in the `domNode`, and take over managing the DOM inside it.\n\nAn app fully built with React will usually only have one `render` call with its root component. A page that uses “sprinkles” of React for parts of the page may have as many `render` calls as needed.\n\n[See more examples below.](https://react.dev/reference/react-dom/render#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/render#parameters \"Link for Parameters \")\n\n* `reactNode`: A _React node_ that you want to display. This will usually be a piece of JSX like `<App />`, but you can also pass a React element constructed with [`createElement()`](https://react.dev/reference/react/createElement), a string, a number, `null`, or `undefined`.\n \n* `domNode`: A [DOM element.](https://developer.mozilla.org/en-US/docs/Web/API/Element) React will display the `reactNode` you pass inside this DOM element. From this moment, React will manage the DOM inside the `domNode` and update it when your React tree changes.\n \n* **optional** `callback`: A function. If passed, React will call it after your component is placed into the DOM.\n \n\n#### Returns[](https://react.dev/reference/react-dom/render#returns \"Link for Returns \")\n\n`render` usually returns `null`. However, if the `reactNode` you pass is a _class component_, then it will return an instance of that component.\n\n#### Caveats[](https://react.dev/reference/react-dom/render#caveats \"Link for Caveats \")\n\n* In React 18, `render` was replaced by [`createRoot`.](https://react.dev/reference/react-dom/client/createRoot) Please use `createRoot` for React 18 and beyond.\n \n* The first time you call `render`, React will clear all the existing HTML content inside the `domNode` before rendering the React component into it. If your `domNode` contains HTML generated by React on the server or during the build, use [`hydrate()`](https://react.dev/reference/react-dom/hydrate) instead, which attaches the event handlers to the existing HTML.\n \n* If you call `render` on the same `domNode` more than once, React will update the DOM as necessary to reflect the latest JSX you passed. React will decide which parts of the DOM can be reused and which need to be recreated by [“matching it up”](https://react.dev/learn/preserving-and-resetting-state) with the previously rendered tree. Calling `render` on the same `domNode` again is similar to calling the [`set` function](https://react.dev/reference/react/useState#setstate) on the root component: React avoids unnecessary DOM updates.\n \n* If your app is fully built with React, you’ll likely have only one `render` call in your app. (If you use a framework, it might do this call for you.) When you want to render a piece of JSX in a different part of the DOM tree that isn’t a child of your component (for example, a modal or a tooltip), use [`createPortal`](https://react.dev/reference/react-dom/createPortal) instead of `render`.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/render#usage \"Link for Usage \")\n-----------------------------------------------------------------------------\n\nCall `render` to display a React component inside a browser DOM node.\n\n```\nimport { render } from 'react-dom';import App from './App.js';render(<App />, document.getElementById('root'));\n```\n\n### Rendering the root component[](https://react.dev/reference/react-dom/render#rendering-the-root-component \"Link for Rendering the root component \")\n\nIn apps fully built with React, **you will usually only do this once at startup**—to render the “root” component.\n\nUsually you shouldn’t need to call `render` again or to call it in more places. From this point on, React will be managing the DOM of your application. To update the UI, your components will [use state.](https://react.dev/reference/react/useState)\n\n* * *\n\n### Rendering multiple roots[](https://react.dev/reference/react-dom/render#rendering-multiple-roots \"Link for Rendering multiple roots \")\n\nIf your page [isn’t fully built with React](https://react.dev/learn/add-react-to-an-existing-project#using-react-for-a-part-of-your-existing-page), call `render` for each top-level piece of UI managed by React.\n\nYou can destroy the rendered trees with [`unmountComponentAtNode()`.](https://react.dev/reference/react-dom/unmountComponentAtNode)\n\n* * *\n\n### Updating the rendered tree[](https://react.dev/reference/react-dom/render#updating-the-rendered-tree \"Link for Updating the rendered tree \")\n\nYou can call `render` more than once on the same DOM node. As long as the component tree structure matches up with what was previously rendered, React will [preserve the state.](https://react.dev/learn/preserving-and-resetting-state) Notice how you can type in the input, which means that the updates from repeated `render` calls every second are not destructive:\n\nIt is uncommon to call `render` multiple times. Usually, you’ll [update state](https://react.dev/reference/react/useState) inside your components instead.\n",
"filename": "render.md",
"package": "react"
} |
{
"content": "Title: renderToNodeStream – React\n\nURL Source: https://react.dev/reference/react-dom/server/renderToNodeStream\n\nMarkdown Content:\nrenderToNodeStream – React\n===============\n\nrenderToNodeStream[](https://react.dev/reference/react-dom/server/renderToNodeStream#undefined \"Link for this heading\")\n=======================================================================================================================\n\n### Deprecated\n\nThis API will be removed in a future major version of React. Use [`renderToPipeableStream`](https://react.dev/reference/react-dom/server/renderToPipeableStream) instead.\n\n`renderToNodeStream` renders a React tree to a [Node.js Readable Stream.](https://nodejs.org/api/stream.html#readable-streams)\n\n```\nconst stream = renderToNodeStream(reactNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/server/renderToNodeStream#reference)\n * [`renderToNodeStream(reactNode, options?)`](https://react.dev/reference/react-dom/server/renderToNodeStream#rendertonodestream)\n* [Usage](https://react.dev/reference/react-dom/server/renderToNodeStream#usage)\n * [Rendering a React tree as HTML to a Node.js Readable Stream](https://react.dev/reference/react-dom/server/renderToNodeStream#rendering-a-react-tree-as-html-to-a-nodejs-readable-stream)\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/server/renderToNodeStream#reference \"Link for Reference \")\n------------------------------------------------------------------------------------------------------------\n\n### `renderToNodeStream(reactNode, options?)`[](https://react.dev/reference/react-dom/server/renderToNodeStream#rendertonodestream \"Link for this heading\")\n\nOn the server, call `renderToNodeStream` to get a [Node.js Readable Stream](https://nodejs.org/api/stream.html#readable-streams) which you can pipe into the response.\n\n```\nimport { renderToNodeStream } from 'react-dom/server';const stream = renderToNodeStream(<App />);stream.pipe(response);\n```\n\nOn the client, call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to make the server-generated HTML interactive.\n\n[See more examples below.](https://react.dev/reference/react-dom/server/renderToNodeStream#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/server/renderToNodeStream#parameters \"Link for Parameters \")\n\n* `reactNode`: A React node you want to render to HTML. For example, a JSX element like `<App />`.\n \n* **optional** `options`: An object for server render.\n \n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as passed to [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot#parameters)\n\n#### Returns[](https://react.dev/reference/react-dom/server/renderToNodeStream#returns \"Link for Returns \")\n\nA [Node.js Readable Stream](https://nodejs.org/api/stream.html#readable-streams) that outputs an HTML string.\n\n#### Caveats[](https://react.dev/reference/react-dom/server/renderToNodeStream#caveats \"Link for Caveats \")\n\n* This method will wait for all [Suspense boundaries](https://react.dev/reference/react/Suspense) to complete before returning any output.\n \n* As of React 18, this method buffers all of its output, so it doesn’t actually provide any streaming benefits. This is why it’s recommended that you migrate to [`renderToPipeableStream`](https://react.dev/reference/react-dom/server/renderToPipeableStream) instead.\n \n* The returned stream is a byte stream encoded in utf-8. If you need a stream in another encoding, take a look at a project like [iconv-lite](https://www.npmjs.com/package/iconv-lite), which provides transform streams for transcoding text.\n \n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/server/renderToNodeStream#usage \"Link for Usage \")\n------------------------------------------------------------------------------------------------\n\n### Rendering a React tree as HTML to a Node.js Readable Stream[](https://react.dev/reference/react-dom/server/renderToNodeStream#rendering-a-react-tree-as-html-to-a-nodejs-readable-stream \"Link for Rendering a React tree as HTML to a Node.js Readable Stream \")\n\nCall `renderToNodeStream` to get a [Node.js Readable Stream](https://nodejs.org/api/stream.html#readable-streams) which you can pipe to your server response:\n\n```\nimport { renderToNodeStream } from 'react-dom/server';// The route handler syntax depends on your backend frameworkapp.use('/', (request, response) => { const stream = renderToNodeStream(<App />); stream.pipe(response);});\n```\n\nThe stream will produce the initial non-interactive HTML output of your React components. On the client, you will need to call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to _hydrate_ that server-generated HTML and make it interactive.\n\n[PreviousServer APIs](https://react.dev/reference/react-dom/server)[NextrenderToPipeableStream](https://react.dev/reference/react-dom/server/renderToPipeableStream)\n",
"filename": "renderToNodeStream.md",
"package": "react"
} |
{
"content": "Title: renderToPipeableStream – React\n\nURL Source: https://react.dev/reference/react-dom/server/renderToPipeableStream\n\nMarkdown Content:\n`renderToPipeableStream` renders a React tree to a pipeable [Node.js Stream.](https://nodejs.org/api/stream.html)\n\n```\nconst { pipe, abort } = renderToPipeableStream(reactNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/server/renderToPipeableStream#reference)\n * [`renderToPipeableStream(reactNode, options?)`](https://react.dev/reference/react-dom/server/renderToPipeableStream#rendertopipeablestream)\n* [Usage](https://react.dev/reference/react-dom/server/renderToPipeableStream#usage)\n * [Rendering a React tree as HTML to a Node.js Stream](https://react.dev/reference/react-dom/server/renderToPipeableStream#rendering-a-react-tree-as-html-to-a-nodejs-stream)\n * [Streaming more content as it loads](https://react.dev/reference/react-dom/server/renderToPipeableStream#streaming-more-content-as-it-loads)\n * [Specifying what goes into the shell](https://react.dev/reference/react-dom/server/renderToPipeableStream#specifying-what-goes-into-the-shell)\n * [Logging crashes on the server](https://react.dev/reference/react-dom/server/renderToPipeableStream#logging-crashes-on-the-server)\n * [Recovering from errors inside the shell](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-inside-the-shell)\n * [Recovering from errors outside the shell](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-outside-the-shell)\n * [Setting the status code](https://react.dev/reference/react-dom/server/renderToPipeableStream#setting-the-status-code)\n * [Handling different errors in different ways](https://react.dev/reference/react-dom/server/renderToPipeableStream#handling-different-errors-in-different-ways)\n * [Waiting for all content to load for crawlers and static generation](https://react.dev/reference/react-dom/server/renderToPipeableStream#waiting-for-all-content-to-load-for-crawlers-and-static-generation)\n * [Aborting server rendering](https://react.dev/reference/react-dom/server/renderToPipeableStream#aborting-server-rendering)\n\n### Note\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/server/renderToPipeableStream#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------------------\n\n### `renderToPipeableStream(reactNode, options?)`[](https://react.dev/reference/react-dom/server/renderToPipeableStream#rendertopipeablestream \"Link for this heading\")\n\nCall `renderToPipeableStream` to render your React tree as HTML into a [Node.js Stream.](https://nodejs.org/api/stream.html#writable-streams)\n\n```\nimport { renderToPipeableStream } from 'react-dom/server';const { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);}});\n```\n\nOn the client, call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to make the server-generated HTML interactive.\n\n[See more examples below.](https://react.dev/reference/react-dom/server/renderToPipeableStream#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/server/renderToPipeableStream#parameters \"Link for Parameters \")\n\n* `reactNode`: A React node you want to render to HTML. For example, a JSX element like `<App />`. It is expected to represent the entire document, so the `App` component should render the `<html>` tag.\n \n* **optional** `options`: An object with streaming options.\n \n * **optional** `bootstrapScriptContent`: If specified, this string will be placed in an inline `<script>` tag.\n * **optional** `bootstrapScripts`: An array of string URLs for the `<script>` tags to emit on the page. Use this to include the `<script>` that calls [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot) Omit it if you don’t want to run React on the client at all.\n * **optional** `bootstrapModules`: Like `bootstrapScripts`, but emits [`<script type=\"module\">`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) instead.\n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as passed to [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot#parameters)\n * **optional** `namespaceURI`: A string with the root [namespace URI](https://developer.mozilla.org/en-US/docs/Web/API/Document/createElementNS#important_namespace_uris) for the stream. Defaults to regular HTML. Pass `'http://www.w3.org/2000/svg'` for SVG or `'http://www.w3.org/1998/Math/MathML'` for MathML.\n * **optional** `nonce`: A [`nonce`](http://developer.mozilla.org/en-US/docs/Web/HTML/Element/script#nonce) string to allow scripts for [`script-src` Content-Security-Policy](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Security-Policy/script-src).\n * **optional** `onAllReady`: A callback that fires when all rendering is complete, including both the [shell](https://react.dev/reference/react-dom/server/renderToPipeableStream#specifying-what-goes-into-the-shell) and all additional [content.](https://react.dev/reference/react-dom/server/renderToPipeableStream#streaming-more-content-as-it-loads) You can use this instead of `onShellReady` [for crawlers and static generation.](https://react.dev/reference/react-dom/server/renderToPipeableStream#waiting-for-all-content-to-load-for-crawlers-and-static-generation) If you start streaming here, you won’t get any progressive loading. The stream will contain the final HTML.\n * **optional** `onError`: A callback that fires whenever there is a server error, whether [recoverable](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-outside-the-shell) or [not.](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-inside-the-shell) By default, this only calls `console.error`. If you override it to [log crash reports,](https://react.dev/reference/react-dom/server/renderToPipeableStream#logging-crashes-on-the-server) make sure that you still call `console.error`. You can also use it to [adjust the status code](https://react.dev/reference/react-dom/server/renderToPipeableStream#setting-the-status-code) before the shell is emitted.\n * **optional** `onShellReady`: A callback that fires right after the [initial shell](https://react.dev/reference/react-dom/server/renderToPipeableStream#specifying-what-goes-into-the-shell) has been rendered. You can [set the status code](https://react.dev/reference/react-dom/server/renderToPipeableStream#setting-the-status-code) and call `pipe` here to start streaming. React will [stream the additional content](https://react.dev/reference/react-dom/server/renderToPipeableStream#streaming-more-content-as-it-loads) after the shell along with the inline `<script>` tags that place that replace the HTML loading fallbacks with the content.\n * **optional** `onShellError`: A callback that fires if there was an error rendering the initial shell. It receives the error as an argument. No bytes were emitted from the stream yet, and neither `onShellReady` nor `onAllReady` will get called, so you can [output a fallback HTML shell.](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-inside-the-shell)\n * **optional** `progressiveChunkSize`: The number of bytes in a chunk. [Read more about the default heuristic.](https://github.com/facebook/react/blob/14c2be8dac2d5482fda8a0906a31d239df8551fc/packages/react-server/src/ReactFizzServer.js#L210-L225)\n\n#### Returns[](https://react.dev/reference/react-dom/server/renderToPipeableStream#returns \"Link for Returns \")\n\n`renderToPipeableStream` returns an object with two methods:\n\n* `pipe` outputs the HTML into the provided [Writable Node.js Stream.](https://nodejs.org/api/stream.html#writable-streams) Call `pipe` in `onShellReady` if you want to enable streaming, or in `onAllReady` for crawlers and static generation.\n* `abort` lets you [abort server rendering](https://react.dev/reference/react-dom/server/renderToPipeableStream#aborting-server-rendering) and render the rest on the client.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/server/renderToPipeableStream#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------------------\n\n### Rendering a React tree as HTML to a Node.js Stream[](https://react.dev/reference/react-dom/server/renderToPipeableStream#rendering-a-react-tree-as-html-to-a-nodejs-stream \"Link for Rendering a React tree as HTML to a Node.js Stream \")\n\nCall `renderToPipeableStream` to render your React tree as HTML into a [Node.js Stream:](https://nodejs.org/api/stream.html#writable-streams)\n\n```\nimport { renderToPipeableStream } from 'react-dom/server';// The route handler syntax depends on your backend frameworkapp.use('/', (request, response) => {const { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);}});});\n```\n\nAlong with the root component, you need to provide a list of bootstrap `<script>` paths. Your root component should return **the entire document including the root `<html>` tag.**\n\nFor example, it might look like this:\n\n```\nexport default function App() {return (<html><head><meta charSet=\"utf-8\" /><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\" /><link rel=\"stylesheet\" href=\"/styles.css\"></link><title>My app</title></head><body><Router /></body></html>);}\n```\n\nReact will inject the [doctype](https://developer.mozilla.org/en-US/docs/Glossary/Doctype) and your bootstrap `<script>` tags into the resulting HTML stream:\n\n```\n<!DOCTYPE html><html><!-- ... HTML from your components ... --></html><script src=\"/main.js\" async=\"\"></script>\n```\n\nOn the client, your bootstrap script should [hydrate the entire `document` with a call to `hydrateRoot`:](https://react.dev/reference/react-dom/client/hydrateRoot#hydrating-an-entire-document)\n\n```\nimport { hydrateRoot } from 'react-dom/client';import App from './App.js';hydrateRoot(document, <App />);\n```\n\nThis will attach event listeners to the server-generated HTML and make it interactive.\n\n##### Deep Dive\n\n#### Reading CSS and JS asset paths from the build output[](https://react.dev/reference/react-dom/server/renderToPipeableStream#reading-css-and-js-asset-paths-from-the-build-output \"Link for Reading CSS and JS asset paths from the build output \")\n\nThe final asset URLs (like JavaScript and CSS files) are often hashed after the build. For example, instead of `styles.css` you might end up with `styles.123456.css`. Hashing static asset filenames guarantees that every distinct build of the same asset will have a different filename. This is useful because it lets you safely enable long-term caching for static assets: a file with a certain name would never change content.\n\nHowever, if you don’t know the asset URLs until after the build, there’s no way for you to put them in the source code. For example, hardcoding `\"/styles.css\"` into JSX like earlier wouldn’t work. To keep them out of your source code, your root component can read the real filenames from a map passed as a prop:\n\n```\nexport default function App({ assetMap }) {return (<html><head> ...<link rel=\"stylesheet\" href={assetMap['styles.css']}></link> ...</head> ...</html>);}\n```\n\nOn the server, render `<App assetMap={assetMap} />` and pass your `assetMap` with the asset URLs:\n\n```\n// You'd need to get this JSON from your build tooling, e.g. read it from the build output.const assetMap = {'styles.css': '/styles.123456.css','main.js': '/main.123456.js'};app.use('/', (request, response) => {const { pipe } = renderToPipeableStream(<App assetMap={assetMap} />, {bootstrapScripts: [assetMap['main.js']],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);}});});\n```\n\nSince your server is now rendering `<App assetMap={assetMap} />`, you need to render it with `assetMap` on the client too to avoid hydration errors. You can serialize and pass `assetMap` to the client like this:\n\n```\n// You'd need to get this JSON from your build tooling.const assetMap = {'styles.css': '/styles.123456.css','main.js': '/main.123456.js'};app.use('/', (request, response) => {const { pipe } = renderToPipeableStream(<App assetMap={assetMap} />, {// Careful: It's safe to stringify() this because this data isn't user-generated.bootstrapScriptContent: `window.assetMap = ${JSON.stringify(assetMap)};`,bootstrapScripts: [assetMap['main.js']],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);}});});\n```\n\nIn the example above, the `bootstrapScriptContent` option adds an extra inline `<script>` tag that sets the global `window.assetMap` variable on the client. This lets the client code read the same `assetMap`:\n\n```\nimport { hydrateRoot } from 'react-dom/client';import App from './App.js';hydrateRoot(document, <App assetMap={window.assetMap} />);\n```\n\nBoth client and server render `App` with the same `assetMap` prop, so there are no hydration errors.\n\n* * *\n\n### Streaming more content as it loads[](https://react.dev/reference/react-dom/server/renderToPipeableStream#streaming-more-content-as-it-loads \"Link for Streaming more content as it loads \")\n\nStreaming allows the user to start seeing the content even before all the data has loaded on the server. For example, consider a profile page that shows a cover, a sidebar with friends and photos, and a list of posts:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Sidebar><Friends /><Photos /></Sidebar><Posts /></ProfileLayout>);}\n```\n\nImagine that loading data for `<Posts />` takes some time. Ideally, you’d want to show the rest of the profile page content to the user without waiting for the posts. To do this, [wrap `Posts` in a `<Suspense>` boundary:](https://react.dev/reference/react/Suspense#displaying-a-fallback-while-content-is-loading)\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Sidebar><Friends /><Photos /></Sidebar><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></ProfileLayout>);}\n```\n\nThis tells React to start streaming the HTML before `Posts` loads its data. React will send the HTML for the loading fallback (`PostsGlimmer`) first, and then, when `Posts` finishes loading its data, React will send the remaining HTML along with an inline `<script>` tag that replaces the loading fallback with that HTML. From the user’s perspective, the page will first appear with the `PostsGlimmer`, later replaced by the `Posts`.\n\nYou can further [nest `<Suspense>` boundaries](https://react.dev/reference/react/Suspense#revealing-nested-content-as-it-loads) to create a more granular loading sequence:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<BigSpinner />}><Sidebar><Friends /><Photos /></Sidebar><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></Suspense></ProfileLayout>);}\n```\n\nIn this example, React can start streaming the page even earlier. Only `ProfileLayout` and `ProfileCover` must finish rendering first because they are not wrapped in any `<Suspense>` boundary. However, if `Sidebar`, `Friends`, or `Photos` need to load some data, React will send the HTML for the `BigSpinner` fallback instead. Then, as more data becomes available, more content will continue to be revealed until all of it becomes visible.\n\nStreaming does not need to wait for React itself to load in the browser, or for your app to become interactive. The HTML content from the server will get progressively revealed before any of the `<script>` tags load.\n\n[Read more about how streaming HTML works.](https://github.com/reactwg/react-18/discussions/37)\n\n### Note\n\n**Only Suspense-enabled data sources will activate the Suspense component.** They include:\n\n* Data fetching with Suspense-enabled frameworks like [Relay](https://relay.dev/docs/guided-tour/rendering/loading-states/) and [Next.js](https://nextjs.org/docs/getting-started/react-essentials)\n* Lazy-loading component code with [`lazy`](https://react.dev/reference/react/lazy)\n* Reading the value of a Promise with [`use`](https://react.dev/reference/react/use)\n\nSuspense **does not** detect when data is fetched inside an Effect or event handler.\n\nThe exact way you would load data in the `Posts` component above depends on your framework. If you use a Suspense-enabled framework, you’ll find the details in its data fetching documentation.\n\nSuspense-enabled data fetching without the use of an opinionated framework is not yet supported. The requirements for implementing a Suspense-enabled data source are unstable and undocumented. An official API for integrating data sources with Suspense will be released in a future version of React.\n\n* * *\n\n### Specifying what goes into the shell[](https://react.dev/reference/react-dom/server/renderToPipeableStream#specifying-what-goes-into-the-shell \"Link for Specifying what goes into the shell \")\n\nThe part of your app outside of any `<Suspense>` boundaries is called _the shell:_\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<BigSpinner />}><Sidebar><Friends /><Photos /></Sidebar><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></Suspense></ProfileLayout>);}\n```\n\nIt determines the earliest loading state that the user may see:\n\n```\n<ProfileLayout><ProfileCover /><BigSpinner /></ProfileLayout>\n```\n\nIf you wrap the whole app into a `<Suspense>` boundary at the root, the shell will only contain that spinner. However, that’s not a pleasant user experience because seeing a big spinner on the screen can feel slower and more annoying than waiting a bit more and seeing the real layout. This is why usually you’ll want to place the `<Suspense>` boundaries so that the shell feels _minimal but complete_—like a skeleton of the entire page layout.\n\nThe `onShellReady` callback fires when the entire shell has been rendered. Usually, you’ll start streaming then:\n\n```\nconst { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);}});\n```\n\nBy the time `onShellReady` fires, components in nested `<Suspense>` boundaries might still be loading data.\n\n* * *\n\n### Logging crashes on the server[](https://react.dev/reference/react-dom/server/renderToPipeableStream#logging-crashes-on-the-server \"Link for Logging crashes on the server \")\n\nBy default, all errors on the server are logged to console. You can override this behavior to log crash reports:\n\n```\nconst { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);},onError(error) {console.error(error);logServerCrashReport(error);}});\n```\n\nIf you provide a custom `onError` implementation, don’t forget to also log errors to the console like above.\n\n* * *\n\n### Recovering from errors inside the shell[](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-inside-the-shell \"Link for Recovering from errors inside the shell \")\n\nIn this example, the shell contains `ProfileLayout`, `ProfileCover`, and `PostsGlimmer`:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></ProfileLayout>);}\n```\n\nIf an error occurs while rendering those components, React won’t have any meaningful HTML to send to the client. Override `onShellError` to send a fallback HTML that doesn’t rely on server rendering as the last resort:\n\n```\nconst { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.setHeader('content-type', 'text/html');pipe(response);},onShellError(error) {response.statusCode = 500;response.setHeader('content-type', 'text/html');response.send('<h1>Something went wrong</h1>'); },onError(error) {console.error(error);logServerCrashReport(error);}});\n```\n\nIf there is an error while generating the shell, both `onError` and `onShellError` will fire. Use `onError` for error reporting and use `onShellError` to send the fallback HTML document. Your fallback HTML does not have to be an error page. Instead, you may include an alternative shell that renders your app on the client only.\n\n* * *\n\n### Recovering from errors outside the shell[](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-outside-the-shell \"Link for Recovering from errors outside the shell \")\n\nIn this example, the `<Posts />` component is wrapped in `<Suspense>` so it is _not_ a part of the shell:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></ProfileLayout>);}\n```\n\nIf an error happens in the `Posts` component or somewhere inside it, React will [try to recover from it:](https://react.dev/reference/react/Suspense#providing-a-fallback-for-server-errors-and-client-only-content)\n\n1. It will emit the loading fallback for the closest `<Suspense>` boundary (`PostsGlimmer`) into the HTML.\n2. It will “give up” on trying to render the `Posts` content on the server anymore.\n3. When the JavaScript code loads on the client, React will _retry_ rendering `Posts` on the client.\n\nIf retrying rendering `Posts` on the client _also_ fails, React will throw the error on the client. As with all the errors thrown during rendering, the [closest parent error boundary](https://react.dev/reference/react/Component#static-getderivedstatefromerror) determines how to present the error to the user. In practice, this means that the user will see a loading indicator until it is certain that the error is not recoverable.\n\nIf retrying rendering `Posts` on the client succeeds, the loading fallback from the server will be replaced with the client rendering output. The user will not know that there was a server error. However, the server `onError` callback and the client [`onRecoverableError`](https://react.dev/reference/react-dom/client/hydrateRoot#hydrateroot) callbacks will fire so that you can get notified about the error.\n\n* * *\n\n### Setting the status code[](https://react.dev/reference/react-dom/server/renderToPipeableStream#setting-the-status-code \"Link for Setting the status code \")\n\nStreaming introduces a tradeoff. You want to start streaming the page as early as possible so that the user can see the content sooner. However, once you start streaming, you can no longer set the response status code.\n\nBy [dividing your app](https://react.dev/reference/react-dom/server/renderToPipeableStream#specifying-what-goes-into-the-shell) into the shell (above all `<Suspense>` boundaries) and the rest of the content, you’ve already solved a part of this problem. If the shell errors, you’ll get the `onShellError` callback which lets you set the error status code. Otherwise, you know that the app may recover on the client, so you can send “OK”.\n\n```\nconst { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.statusCode = 200;response.setHeader('content-type', 'text/html');pipe(response);},onShellError(error) {response.statusCode = 500;response.setHeader('content-type', 'text/html');response.send('<h1>Something went wrong</h1>'); },onError(error) {console.error(error);logServerCrashReport(error);}});\n```\n\nIf a component _outside_ the shell (i.e. inside a `<Suspense>` boundary) throws an error, React will not stop rendering. This means that the `onError` callback will fire, but you will still get `onShellReady` instead of `onShellError`. This is because React will try to recover from that error on the client, [as described above.](https://react.dev/reference/react-dom/server/renderToPipeableStream#recovering-from-errors-outside-the-shell)\n\nHowever, if you’d like, you can use the fact that something has errored to set the status code:\n\n```\nlet didError = false;const { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.statusCode = didError ? 500 : 200;response.setHeader('content-type', 'text/html');pipe(response);},onShellError(error) {response.statusCode = 500;response.setHeader('content-type', 'text/html');response.send('<h1>Something went wrong</h1>'); },onError(error) {didError = true;console.error(error);logServerCrashReport(error);}});\n```\n\nThis will only catch errors outside the shell that happened while generating the initial shell content, so it’s not exhaustive. If knowing whether an error occurred for some content is critical, you can move it up into the shell.\n\n* * *\n\n### Handling different errors in different ways[](https://react.dev/reference/react-dom/server/renderToPipeableStream#handling-different-errors-in-different-ways \"Link for Handling different errors in different ways \")\n\nYou can [create your own `Error` subclasses](https://javascript.info/custom-errors) and use the [`instanceof`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/instanceof) operator to check which error is thrown. For example, you can define a custom `NotFoundError` and throw it from your component. Then your `onError`, `onShellReady`, and `onShellError` callbacks can do something different depending on the error type:\n\n```\nlet didError = false;let caughtError = null;function getStatusCode() {if (didError) {if (caughtError instanceof NotFoundError) {return 404;} else {return 500;}} else {return 200;}}const { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {response.statusCode = getStatusCode();response.setHeader('content-type', 'text/html');pipe(response);},onShellError(error) {response.statusCode = getStatusCode();response.setHeader('content-type', 'text/html');response.send('<h1>Something went wrong</h1>'); },onError(error) {didError = true;caughtError = error;console.error(error);logServerCrashReport(error);}});\n```\n\nKeep in mind that once you emit the shell and start streaming, you can’t change the status code.\n\n* * *\n\n### Waiting for all content to load for crawlers and static generation[](https://react.dev/reference/react-dom/server/renderToPipeableStream#waiting-for-all-content-to-load-for-crawlers-and-static-generation \"Link for Waiting for all content to load for crawlers and static generation \")\n\nStreaming offers a better user experience because the user can see the content as it becomes available.\n\nHowever, when a crawler visits your page, or if you’re generating the pages at the build time, you might want to let all of the content load first and then produce the final HTML output instead of revealing it progressively.\n\nYou can wait for all the content to load using the `onAllReady` callback:\n\n```\nlet didError = false;let isCrawler = // ... depends on your bot detection strategy ...const { pipe } = renderToPipeableStream(<App />, {bootstrapScripts: ['/main.js'],onShellReady() {if (!isCrawler) {response.statusCode = didError ? 500 : 200;response.setHeader('content-type', 'text/html');pipe(response);}},onShellError(error) {response.statusCode = 500;response.setHeader('content-type', 'text/html');response.send('<h1>Something went wrong</h1>'); },onAllReady() {if (isCrawler) {response.statusCode = didError ? 500 : 200;response.setHeader('content-type', 'text/html');pipe(response); }},onError(error) {didError = true;console.error(error);logServerCrashReport(error);}});\n```\n\nA regular visitor will get a stream of progressively loaded content. A crawler will receive the final HTML output after all the data loads. However, this also means that the crawler will have to wait for _all_ data, some of which might be slow to load or error. Depending on your app, you could choose to send the shell to the crawlers too.\n\n* * *\n\n### Aborting server rendering[](https://react.dev/reference/react-dom/server/renderToPipeableStream#aborting-server-rendering \"Link for Aborting server rendering \")\n\nYou can force the server rendering to “give up” after a timeout:\n\n```\nconst { pipe, abort } = renderToPipeableStream(<App />, {// ...});setTimeout(() => {abort();}, 10000);\n```\n\nReact will flush the remaining loading fallbacks as HTML, and will attempt to render the rest on the client.\n",
"filename": "renderToPipeableStream.md",
"package": "react"
} |
{
"content": "Title: renderToReadableStream – React\n\nURL Source: https://react.dev/reference/react-dom/server/renderToReadableStream\n\nMarkdown Content:\n`renderToReadableStream` renders a React tree to a [Readable Web Stream.](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream)\n\n```\nconst stream = await renderToReadableStream(reactNode, options?)\n```\n\n* [Reference](https://react.dev/reference/react-dom/server/renderToReadableStream#reference)\n * [`renderToReadableStream(reactNode, options?)`](https://react.dev/reference/react-dom/server/renderToReadableStream#rendertoreadablestream)\n* [Usage](https://react.dev/reference/react-dom/server/renderToReadableStream#usage)\n * [Rendering a React tree as HTML to a Readable Web Stream](https://react.dev/reference/react-dom/server/renderToReadableStream#rendering-a-react-tree-as-html-to-a-readable-web-stream)\n * [Streaming more content as it loads](https://react.dev/reference/react-dom/server/renderToReadableStream#streaming-more-content-as-it-loads)\n * [Specifying what goes into the shell](https://react.dev/reference/react-dom/server/renderToReadableStream#specifying-what-goes-into-the-shell)\n * [Logging crashes on the server](https://react.dev/reference/react-dom/server/renderToReadableStream#logging-crashes-on-the-server)\n * [Recovering from errors inside the shell](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-inside-the-shell)\n * [Recovering from errors outside the shell](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-outside-the-shell)\n * [Setting the status code](https://react.dev/reference/react-dom/server/renderToReadableStream#setting-the-status-code)\n * [Handling different errors in different ways](https://react.dev/reference/react-dom/server/renderToReadableStream#handling-different-errors-in-different-ways)\n * [Waiting for all content to load for crawlers and static generation](https://react.dev/reference/react-dom/server/renderToReadableStream#waiting-for-all-content-to-load-for-crawlers-and-static-generation)\n * [Aborting server rendering](https://react.dev/reference/react-dom/server/renderToReadableStream#aborting-server-rendering)\n\n### Note\n\n* * *\n\nReference[](https://react.dev/reference/react-dom/server/renderToReadableStream#reference \"Link for Reference \")\n----------------------------------------------------------------------------------------------------------------\n\n### `renderToReadableStream(reactNode, options?)`[](https://react.dev/reference/react-dom/server/renderToReadableStream#rendertoreadablestream \"Link for this heading\")\n\nCall `renderToReadableStream` to render your React tree as HTML into a [Readable Web Stream.](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream)\n\n```\nimport { renderToReadableStream } from 'react-dom/server';async function handler(request) {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js']});return new Response(stream, {headers: { 'content-type': 'text/html' },});}\n```\n\nOn the client, call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to make the server-generated HTML interactive.\n\n[See more examples below.](https://react.dev/reference/react-dom/server/renderToReadableStream#usage)\n\n#### Parameters[](https://react.dev/reference/react-dom/server/renderToReadableStream#parameters \"Link for Parameters \")\n\n* `reactNode`: A React node you want to render to HTML. For example, a JSX element like `<App />`. It is expected to represent the entire document, so the `App` component should render the `<html>` tag.\n \n* **optional** `options`: An object with streaming options.\n \n * **optional** `bootstrapScriptContent`: If specified, this string will be placed in an inline `<script>` tag.\n * **optional** `bootstrapScripts`: An array of string URLs for the `<script>` tags to emit on the page. Use this to include the `<script>` that calls [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot) Omit it if you don’t want to run React on the client at all.\n * **optional** `bootstrapModules`: Like `bootstrapScripts`, but emits [`<script type=\"module\">`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) instead.\n * **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as passed to [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot#parameters)\n * **optional** `namespaceURI`: A string with the root [namespace URI](https://developer.mozilla.org/en-US/docs/Web/API/Document/createElementNS#important_namespace_uris) for the stream. Defaults to regular HTML. Pass `'http://www.w3.org/2000/svg'` for SVG or `'http://www.w3.org/1998/Math/MathML'` for MathML.\n * **optional** `nonce`: A [`nonce`](http://developer.mozilla.org/en-US/docs/Web/HTML/Element/script#nonce) string to allow scripts for [`script-src` Content-Security-Policy](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Security-Policy/script-src).\n * **optional** `onError`: A callback that fires whenever there is a server error, whether [recoverable](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-outside-the-shell) or [not.](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-inside-the-shell) By default, this only calls `console.error`. If you override it to [log crash reports,](https://react.dev/reference/react-dom/server/renderToReadableStream#logging-crashes-on-the-server) make sure that you still call `console.error`. You can also use it to [adjust the status code](https://react.dev/reference/react-dom/server/renderToReadableStream#setting-the-status-code) before the shell is emitted.\n * **optional** `progressiveChunkSize`: The number of bytes in a chunk. [Read more about the default heuristic.](https://github.com/facebook/react/blob/14c2be8dac2d5482fda8a0906a31d239df8551fc/packages/react-server/src/ReactFizzServer.js#L210-L225)\n * **optional** `signal`: An [abort signal](https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal) that lets you [abort server rendering](https://react.dev/reference/react-dom/server/renderToReadableStream#aborting-server-rendering) and render the rest on the client.\n\n#### Returns[](https://react.dev/reference/react-dom/server/renderToReadableStream#returns \"Link for Returns \")\n\n`renderToReadableStream` returns a Promise:\n\n* If rendering the [shell](https://react.dev/reference/react-dom/server/renderToReadableStream#specifying-what-goes-into-the-shell) is successful, that Promise will resolve to a [Readable Web Stream.](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream)\n* If rendering the shell fails, the Promise will be rejected. [Use this to output a fallback shell.](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-inside-the-shell)\n\nThe returned stream has an additional property:\n\n* `allReady`: A Promise that resolves when all rendering is complete, including both the [shell](https://react.dev/reference/react-dom/server/renderToReadableStream#specifying-what-goes-into-the-shell) and all additional [content.](https://react.dev/reference/react-dom/server/renderToReadableStream#streaming-more-content-as-it-loads) You can `await stream.allReady` before returning a response [for crawlers and static generation.](https://react.dev/reference/react-dom/server/renderToReadableStream#waiting-for-all-content-to-load-for-crawlers-and-static-generation) If you do that, you won’t get any progressive loading. The stream will contain the final HTML.\n\n* * *\n\nUsage[](https://react.dev/reference/react-dom/server/renderToReadableStream#usage \"Link for Usage \")\n----------------------------------------------------------------------------------------------------\n\n### Rendering a React tree as HTML to a Readable Web Stream[](https://react.dev/reference/react-dom/server/renderToReadableStream#rendering-a-react-tree-as-html-to-a-readable-web-stream \"Link for Rendering a React tree as HTML to a Readable Web Stream \")\n\nCall `renderToReadableStream` to render your React tree as HTML into a [Readable Web Stream:](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream)\n\n```\nimport { renderToReadableStream } from 'react-dom/server';async function handler(request) {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js']});return new Response(stream, {headers: { 'content-type': 'text/html' },});}\n```\n\nAlong with the root component, you need to provide a list of bootstrap `<script>` paths. Your root component should return **the entire document including the root `<html>` tag.**\n\nFor example, it might look like this:\n\n```\nexport default function App() {return (<html><head><meta charSet=\"utf-8\" /><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\" /><link rel=\"stylesheet\" href=\"/styles.css\"></link><title>My app</title></head><body><Router /></body></html>);}\n```\n\nReact will inject the [doctype](https://developer.mozilla.org/en-US/docs/Glossary/Doctype) and your bootstrap `<script>` tags into the resulting HTML stream:\n\n```\n<!DOCTYPE html><html><!-- ... HTML from your components ... --></html><script src=\"/main.js\" async=\"\"></script>\n```\n\nOn the client, your bootstrap script should [hydrate the entire `document` with a call to `hydrateRoot`:](https://react.dev/reference/react-dom/client/hydrateRoot#hydrating-an-entire-document)\n\n```\nimport { hydrateRoot } from 'react-dom/client';import App from './App.js';hydrateRoot(document, <App />);\n```\n\nThis will attach event listeners to the server-generated HTML and make it interactive.\n\n##### Deep Dive\n\n#### Reading CSS and JS asset paths from the build output[](https://react.dev/reference/react-dom/server/renderToReadableStream#reading-css-and-js-asset-paths-from-the-build-output \"Link for Reading CSS and JS asset paths from the build output \")\n\nThe final asset URLs (like JavaScript and CSS files) are often hashed after the build. For example, instead of `styles.css` you might end up with `styles.123456.css`. Hashing static asset filenames guarantees that every distinct build of the same asset will have a different filename. This is useful because it lets you safely enable long-term caching for static assets: a file with a certain name would never change content.\n\nHowever, if you don’t know the asset URLs until after the build, there’s no way for you to put them in the source code. For example, hardcoding `\"/styles.css\"` into JSX like earlier wouldn’t work. To keep them out of your source code, your root component can read the real filenames from a map passed as a prop:\n\n```\nexport default function App({ assetMap }) {return (<html><head><title>My app</title><link rel=\"stylesheet\" href={assetMap['styles.css']}></link></head> ...</html>);}\n```\n\nOn the server, render `<App assetMap={assetMap} />` and pass your `assetMap` with the asset URLs:\n\n```\n// You'd need to get this JSON from your build tooling, e.g. read it from the build output.const assetMap = {'styles.css': '/styles.123456.css','main.js': '/main.123456.js'};async function handler(request) {const stream = await renderToReadableStream(<App assetMap={assetMap} />, {bootstrapScripts: [assetMap['/main.js']]});return new Response(stream, {headers: { 'content-type': 'text/html' },});}\n```\n\nSince your server is now rendering `<App assetMap={assetMap} />`, you need to render it with `assetMap` on the client too to avoid hydration errors. You can serialize and pass `assetMap` to the client like this:\n\n```\n// You'd need to get this JSON from your build tooling.const assetMap = {'styles.css': '/styles.123456.css','main.js': '/main.123456.js'};async function handler(request) {const stream = await renderToReadableStream(<App assetMap={assetMap} />, {// Careful: It's safe to stringify() this because this data isn't user-generated.bootstrapScriptContent: `window.assetMap = ${JSON.stringify(assetMap)};`,bootstrapScripts: [assetMap['/main.js']],});return new Response(stream, {headers: { 'content-type': 'text/html' },});}\n```\n\nIn the example above, the `bootstrapScriptContent` option adds an extra inline `<script>` tag that sets the global `window.assetMap` variable on the client. This lets the client code read the same `assetMap`:\n\n```\nimport { hydrateRoot } from 'react-dom/client';import App from './App.js';hydrateRoot(document, <App assetMap={window.assetMap} />);\n```\n\nBoth client and server render `App` with the same `assetMap` prop, so there are no hydration errors.\n\n* * *\n\n### Streaming more content as it loads[](https://react.dev/reference/react-dom/server/renderToReadableStream#streaming-more-content-as-it-loads \"Link for Streaming more content as it loads \")\n\nStreaming allows the user to start seeing the content even before all the data has loaded on the server. For example, consider a profile page that shows a cover, a sidebar with friends and photos, and a list of posts:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Sidebar><Friends /><Photos /></Sidebar><Posts /></ProfileLayout>);}\n```\n\nImagine that loading data for `<Posts />` takes some time. Ideally, you’d want to show the rest of the profile page content to the user without waiting for the posts. To do this, [wrap `Posts` in a `<Suspense>` boundary:](https://react.dev/reference/react/Suspense#displaying-a-fallback-while-content-is-loading)\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Sidebar><Friends /><Photos /></Sidebar><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></ProfileLayout>);}\n```\n\nThis tells React to start streaming the HTML before `Posts` loads its data. React will send the HTML for the loading fallback (`PostsGlimmer`) first, and then, when `Posts` finishes loading its data, React will send the remaining HTML along with an inline `<script>` tag that replaces the loading fallback with that HTML. From the user’s perspective, the page will first appear with the `PostsGlimmer`, later replaced by the `Posts`.\n\nYou can further [nest `<Suspense>` boundaries](https://react.dev/reference/react/Suspense#revealing-nested-content-as-it-loads) to create a more granular loading sequence:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<BigSpinner />}><Sidebar><Friends /><Photos /></Sidebar><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></Suspense></ProfileLayout>);}\n```\n\nIn this example, React can start streaming the page even earlier. Only `ProfileLayout` and `ProfileCover` must finish rendering first because they are not wrapped in any `<Suspense>` boundary. However, if `Sidebar`, `Friends`, or `Photos` need to load some data, React will send the HTML for the `BigSpinner` fallback instead. Then, as more data becomes available, more content will continue to be revealed until all of it becomes visible.\n\nStreaming does not need to wait for React itself to load in the browser, or for your app to become interactive. The HTML content from the server will get progressively revealed before any of the `<script>` tags load.\n\n[Read more about how streaming HTML works.](https://github.com/reactwg/react-18/discussions/37)\n\n### Note\n\n**Only Suspense-enabled data sources will activate the Suspense component.** They include:\n\n* Data fetching with Suspense-enabled frameworks like [Relay](https://relay.dev/docs/guided-tour/rendering/loading-states/) and [Next.js](https://nextjs.org/docs/getting-started/react-essentials)\n* Lazy-loading component code with [`lazy`](https://react.dev/reference/react/lazy)\n* Reading the value of a Promise with [`use`](https://react.dev/reference/react/use)\n\nSuspense **does not** detect when data is fetched inside an Effect or event handler.\n\nThe exact way you would load data in the `Posts` component above depends on your framework. If you use a Suspense-enabled framework, you’ll find the details in its data fetching documentation.\n\nSuspense-enabled data fetching without the use of an opinionated framework is not yet supported. The requirements for implementing a Suspense-enabled data source are unstable and undocumented. An official API for integrating data sources with Suspense will be released in a future version of React.\n\n* * *\n\n### Specifying what goes into the shell[](https://react.dev/reference/react-dom/server/renderToReadableStream#specifying-what-goes-into-the-shell \"Link for Specifying what goes into the shell \")\n\nThe part of your app outside of any `<Suspense>` boundaries is called _the shell:_\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<BigSpinner />}><Sidebar><Friends /><Photos /></Sidebar><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></Suspense></ProfileLayout>);}\n```\n\nIt determines the earliest loading state that the user may see:\n\n```\n<ProfileLayout><ProfileCover /><BigSpinner /></ProfileLayout>\n```\n\nIf you wrap the whole app into a `<Suspense>` boundary at the root, the shell will only contain that spinner. However, that’s not a pleasant user experience because seeing a big spinner on the screen can feel slower and more annoying than waiting a bit more and seeing the real layout. This is why usually you’ll want to place the `<Suspense>` boundaries so that the shell feels _minimal but complete_—like a skeleton of the entire page layout.\n\nThe async call to `renderToReadableStream` will resolve to a `stream` as soon as the entire shell has been rendered. Usually, you’ll start streaming then by creating and returning a response with that `stream`:\n\n```\nasync function handler(request) {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js']});return new Response(stream, {headers: { 'content-type': 'text/html' },});}\n```\n\nBy the time the `stream` is returned, components in nested `<Suspense>` boundaries might still be loading data.\n\n* * *\n\n### Logging crashes on the server[](https://react.dev/reference/react-dom/server/renderToReadableStream#logging-crashes-on-the-server \"Link for Logging crashes on the server \")\n\nBy default, all errors on the server are logged to console. You can override this behavior to log crash reports:\n\n```\nasync function handler(request) {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js'],onError(error) {console.error(error);logServerCrashReport(error);}});return new Response(stream, {headers: { 'content-type': 'text/html' },});}\n```\n\nIf you provide a custom `onError` implementation, don’t forget to also log errors to the console like above.\n\n* * *\n\n### Recovering from errors inside the shell[](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-inside-the-shell \"Link for Recovering from errors inside the shell \")\n\nIn this example, the shell contains `ProfileLayout`, `ProfileCover`, and `PostsGlimmer`:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></ProfileLayout>);}\n```\n\nIf an error occurs while rendering those components, React won’t have any meaningful HTML to send to the client. Wrap your `renderToReadableStream` call in a `try...catch` to send a fallback HTML that doesn’t rely on server rendering as the last resort:\n\n```\nasync function handler(request) {try {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js'],onError(error) {console.error(error);logServerCrashReport(error);}});return new Response(stream, {headers: { 'content-type': 'text/html' },});} catch (error) {return new Response('<h1>Something went wrong</h1>', {status: 500,headers: { 'content-type': 'text/html' },});}}\n```\n\nIf there is an error while generating the shell, both `onError` and your `catch` block will fire. Use `onError` for error reporting and use the `catch` block to send the fallback HTML document. Your fallback HTML does not have to be an error page. Instead, you may include an alternative shell that renders your app on the client only.\n\n* * *\n\n### Recovering from errors outside the shell[](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-outside-the-shell \"Link for Recovering from errors outside the shell \")\n\nIn this example, the `<Posts />` component is wrapped in `<Suspense>` so it is _not_ a part of the shell:\n\n```\nfunction ProfilePage() {return (<ProfileLayout><ProfileCover /><Suspense fallback={<PostsGlimmer />}><Posts /></Suspense></ProfileLayout>);}\n```\n\nIf an error happens in the `Posts` component or somewhere inside it, React will [try to recover from it:](https://react.dev/reference/react/Suspense#providing-a-fallback-for-server-errors-and-client-only-content)\n\n1. It will emit the loading fallback for the closest `<Suspense>` boundary (`PostsGlimmer`) into the HTML.\n2. It will “give up” on trying to render the `Posts` content on the server anymore.\n3. When the JavaScript code loads on the client, React will _retry_ rendering `Posts` on the client.\n\nIf retrying rendering `Posts` on the client _also_ fails, React will throw the error on the client. As with all the errors thrown during rendering, the [closest parent error boundary](https://react.dev/reference/react/Component#static-getderivedstatefromerror) determines how to present the error to the user. In practice, this means that the user will see a loading indicator until it is certain that the error is not recoverable.\n\nIf retrying rendering `Posts` on the client succeeds, the loading fallback from the server will be replaced with the client rendering output. The user will not know that there was a server error. However, the server `onError` callback and the client [`onRecoverableError`](https://react.dev/reference/react-dom/client/hydrateRoot#hydrateroot) callbacks will fire so that you can get notified about the error.\n\n* * *\n\n### Setting the status code[](https://react.dev/reference/react-dom/server/renderToReadableStream#setting-the-status-code \"Link for Setting the status code \")\n\nStreaming introduces a tradeoff. You want to start streaming the page as early as possible so that the user can see the content sooner. However, once you start streaming, you can no longer set the response status code.\n\nBy [dividing your app](https://react.dev/reference/react-dom/server/renderToReadableStream#specifying-what-goes-into-the-shell) into the shell (above all `<Suspense>` boundaries) and the rest of the content, you’ve already solved a part of this problem. If the shell errors, your `catch` block will run which lets you set the error status code. Otherwise, you know that the app may recover on the client, so you can send “OK”.\n\n```\nasync function handler(request) {try {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js'],onError(error) {console.error(error);logServerCrashReport(error);}});return new Response(stream, {status: 200,headers: { 'content-type': 'text/html' },});} catch (error) {return new Response('<h1>Something went wrong</h1>', {status: 500,headers: { 'content-type': 'text/html' },});}}\n```\n\nIf a component _outside_ the shell (i.e. inside a `<Suspense>` boundary) throws an error, React will not stop rendering. This means that the `onError` callback will fire, but your code will continue running without getting into the `catch` block. This is because React will try to recover from that error on the client, [as described above.](https://react.dev/reference/react-dom/server/renderToReadableStream#recovering-from-errors-outside-the-shell)\n\nHowever, if you’d like, you can use the fact that something has errored to set the status code:\n\n```\nasync function handler(request) {try {let didError = false;const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js'],onError(error) {didError = true;console.error(error);logServerCrashReport(error);}});return new Response(stream, {status: didError ? 500 : 200,headers: { 'content-type': 'text/html' },});} catch (error) {return new Response('<h1>Something went wrong</h1>', {status: 500,headers: { 'content-type': 'text/html' },});}}\n```\n\nThis will only catch errors outside the shell that happened while generating the initial shell content, so it’s not exhaustive. If knowing whether an error occurred for some content is critical, you can move it up into the shell.\n\n* * *\n\n### Handling different errors in different ways[](https://react.dev/reference/react-dom/server/renderToReadableStream#handling-different-errors-in-different-ways \"Link for Handling different errors in different ways \")\n\nYou can [create your own `Error` subclasses](https://javascript.info/custom-errors) and use the [`instanceof`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/instanceof) operator to check which error is thrown. For example, you can define a custom `NotFoundError` and throw it from your component. Then you can save the error in `onError` and do something different before returning the response depending on the error type:\n\n```\nasync function handler(request) {let didError = false;let caughtError = null;function getStatusCode() {if (didError) {if (caughtError instanceof NotFoundError) {return 404;} else {return 500;}} else {return 200;}}try {const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js'],onError(error) {didError = true;caughtError = error;console.error(error);logServerCrashReport(error);}});return new Response(stream, {status: getStatusCode(),headers: { 'content-type': 'text/html' },});} catch (error) {return new Response('<h1>Something went wrong</h1>', {status: getStatusCode(),headers: { 'content-type': 'text/html' },});}}\n```\n\nKeep in mind that once you emit the shell and start streaming, you can’t change the status code.\n\n* * *\n\n### Waiting for all content to load for crawlers and static generation[](https://react.dev/reference/react-dom/server/renderToReadableStream#waiting-for-all-content-to-load-for-crawlers-and-static-generation \"Link for Waiting for all content to load for crawlers and static generation \")\n\nStreaming offers a better user experience because the user can see the content as it becomes available.\n\nHowever, when a crawler visits your page, or if you’re generating the pages at the build time, you might want to let all of the content load first and then produce the final HTML output instead of revealing it progressively.\n\nYou can wait for all the content to load by awaiting the `stream.allReady` Promise:\n\n```\nasync function handler(request) {try {let didError = false;const stream = await renderToReadableStream(<App />, {bootstrapScripts: ['/main.js'],onError(error) {didError = true;console.error(error);logServerCrashReport(error);}});let isCrawler = // ... depends on your bot detection strategy ...if (isCrawler) {await stream.allReady;}return new Response(stream, {status: didError ? 500 : 200,headers: { 'content-type': 'text/html' },});} catch (error) {return new Response('<h1>Something went wrong</h1>', {status: 500,headers: { 'content-type': 'text/html' },});}}\n```\n\nA regular visitor will get a stream of progressively loaded content. A crawler will receive the final HTML output after all the data loads. However, this also means that the crawler will have to wait for _all_ data, some of which might be slow to load or error. Depending on your app, you could choose to send the shell to the crawlers too.\n\n* * *\n\n### Aborting server rendering[](https://react.dev/reference/react-dom/server/renderToReadableStream#aborting-server-rendering \"Link for Aborting server rendering \")\n\nYou can force the server rendering to “give up” after a timeout:\n\n```\nasync function handler(request) {try {const controller = new AbortController();setTimeout(() => {controller.abort();}, 10000);const stream = await renderToReadableStream(<App />, {signal: controller.signal,bootstrapScripts: ['/main.js'],onError(error) {didError = true;console.error(error);logServerCrashReport(error);}});// ...\n```\n\nReact will flush the remaining loading fallbacks as HTML, and will attempt to render the rest on the client.\n",
"filename": "renderToReadableStream.md",
"package": "react"
} |