relative_path
stringclasses 812
values | section
stringclasses 339
values | filename
stringlengths 2
61
| text
stringlengths 6
1.76M
|
---|---|---|---|
PyTorch/SpeechSynthesis/Tacotron2/trtis_cpp/src/trt/plugins/taco2ProjectionPlugin | taco2ProjectionPlugin | taco2ProjectionLayerPlugin | /*
* Copyright (c) 2019-2020, NVIDIA CORPORATION. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the NVIDIA CORPORATION nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL NVIDIA CORPORATION BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef TT2I_PROJECTIONLAYERPLUGIN_H
#define TT2I_PROJECTIONLAYERPLUGIN_H
#include "NvInfer.h"
#include <memory>
#include <string>
#include <vector>
namespace nvinfer1
{
namespace plugin
{
class Taco2ProjectionKernel;
class Taco2ProjectionLayerPlugin : public nvinfer1::IPluginV2DynamicExt
{
public:
using value_type = float;
/**
* @brief Get the name of this plugin.
*
* @return The name.
*/
static const char* getName();
/**
* @brief Get the version of this plugin.
*
* @return The version.
*/
static const char* getVersion();
/**
* @brief Create a new Taco2ProjectionLayerPlugin from serialized data.
*
* @param data The data.
* @param length The length of the data in bytes.
*
* @return The instantiated plugin.
*/
static Taco2ProjectionLayerPlugin deserialize(const void* data, size_t length);
/**
* @brief Create a new Taco2ProjectionLayerPlugin.
*
* @param weightsChannel The weights for channel projection.
* @param weightsGate The weights for gate projection.
* @param biasChannel The bias for channels.
* @param biasGate The bias for the gate.
* @param hiddenIntputLength The hidden input lentgh.
* @param contextIntputLength The context input length.
* @param channelDimension The number of channels.
* @param gateDimension The number of gates.
*/
Taco2ProjectionLayerPlugin(const nvinfer1::Weights& weightsChannel, const nvinfer1::Weights& weightsGate,
const nvinfer1::Weights& biasChannel, const nvinfer1::Weights& biasGate, int hiddenIntputLength,
int contextIntputLength, int channelDimension, int gateDimension);
/**
* @brief The move constructor.
*
* @param other The Taco2ProjectionLayerPlugin to move.
*/
Taco2ProjectionLayerPlugin(Taco2ProjectionLayerPlugin&& other);
/**
* @brief The move assignment operator.
*
* @param other The Taco2ProjectionLayerPlugin to move.
*
* @return This object.
*/
Taco2ProjectionLayerPlugin& operator=(Taco2ProjectionLayerPlugin&& other);
/**
* @brief Destructor.
*/
~Taco2ProjectionLayerPlugin();
// disable copying
Taco2ProjectionLayerPlugin(const Taco2ProjectionLayerPlugin& other) = delete;
Taco2ProjectionLayerPlugin& operator=(const Taco2ProjectionLayerPlugin& other) = delete;
/**
* @brief Return the data type of the plugin output at the requested index.
*
* @param index The output index.
* @param inputTypes The input data types.
* @param nbInputs The number of inputs.
*
* @return The type of output.
*/
nvinfer1::DataType getOutputDataType(int index, const nvinfer1::DataType* inputTypes, int nbInputs) const override;
/**
* @brief Get the plugin type.
*
* @return The plugin type.
*/
const char* getPluginType() const override;
/**
* @brief Get the plugin version.
*
* @return The plugin version.
*/
const char* getPluginVersion() const override;
/**
* @brief Get the number of outputs.
*
* @return The number of outputs.
*/
int getNbOutputs() const override;
/**
* @brief Get the dimensions of an output tensor.
*
* @param outputIndex The index of the output tensor.
* @param inputs Expressions for dimensions of the input tensors.
* @param nbInputs The number of input tensors.
* @param expBuilder Object for generating new expressions.
*
* @return The resulting dimensions.
*/
DimsExprs getOutputDimensions(
int outputIndex, const DimsExprs* inputs, int nbInputs, IExprBuilder& expBuilder) override;
/**
* @brief Check if the given plugin format is supported.
*
* @param pos The format position/index in inOut.format[].
* @param inOut The input and output formats.
* @param nbInputs The number of inputs.
* @param nbOutputs The number of outputs.
*
* @return True if it is supported.
*/
bool supportsFormatCombination(int pos, const PluginTensorDesc* inOut, int nbInputs, int nbOutputs) override;
/**
* @brief Configure this plugin with the given inputs, outputs, and datat
* types.
*
* @param in The input tensor attributes that used for configuration.
* @param nbInputs The number of inputs.
* @param out The output tensor attributes that are used for configuration.
* @param nbOutputs The number of outputs.
*/
void configurePlugin(
const DynamicPluginTensorDesc* in, int nbInputs, const DynamicPluginTensorDesc* out, int nbOutputs) override;
/**
* @brief Initialize the plugin.
*
* @return 0 if initialization was successful. Non-zero otherwise.
*/
int initialize() override;
/**
* @brief Terminate the plugin (deinitialize).
*/
void terminate() override;
/**
* @brief Get workspace size required by this plugin for up to the given
* batch size.
*
* @param in The input tensor descriptors.
* @param nbInputs The number of inputs.
* @param out The output tensor descriptors.
* @param nbOutputs The number of outputs.
*
* @return The workspace size in bytes.
*/
size_t getWorkspaceSize(
const PluginTensorDesc* in, int nbInputs, const PluginTensorDesc* out, int nbOutputs) const override;
/**
* @brief Set this plugin for execution on the stream.
*
* @param inputDesc The input tensor descriptors.
* @param outputDesc The output tensor descriptors.
* @param inputs The input tensors.
* @param outputs The output tensors.
* @param workspace The workspace.
* @param stream The stream to operate on.
*
* @return 0 if successfully queued, non-zero otherwise.
*/
int enqueue(const PluginTensorDesc* inputDesc, const PluginTensorDesc* outputDesc, const void* const* inputs,
void* const* outputs, void* workspace, cudaStream_t stream);
/**
* @brief Get the number of bytes occupied by this plugin if serialized.
*
* @return The size in bytes.
*/
size_t getSerializationSize() const override;
/**
* @brief Serialize this plugin.
*
* @param buffer The buffer to write to.
*/
void serialize(void* buffer) const override;
/**
* @brief Destroy this plugin instance.
*/
void destroy() override;
/**
* @brief Clone this pulgin instance.
*
* @return The cloned plugin.
*/
IPluginV2DynamicExt* clone() const override;
/**
* @brief Set the namespace of this plugin.
*
* @param pluginNamespace The namespace.
*/
void setPluginNamespace(const char* pluginNamespace) override;
/**
* @brief Get the namespace of this plugin.
*
* @return The namespace.
*/
const char* getPluginNamespace() const override;
private:
static const float ONE;
static const float ZERO;
int mHiddenInputLength;
int mContextInputLength;
int mNumChannelDimension;
int mNumGateDimension;
std::vector<value_type> mWeightsChannel;
std::vector<value_type> mWeightsGate;
std::vector<value_type> mBiasChannel;
std::vector<value_type> mBiasGate;
std::unique_ptr<Taco2ProjectionKernel> mKernel;
std::string mNamespace;
int getTotalInputLength() const;
int getTotalDimensions() const;
};
} // namespace plugin
} // namespace nvinfer1
#endif
|
TensorFlow/Classification/ConvNets/dataprep | dataprep | build_imagenet_data | #!/usr/bin/python
# Copyright 2016 Google Inc. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Converts ImageNet data to TFRecords file format with Example protos.
The raw ImageNet data set is expected to reside in JPEG files located in the
following directory structure.
data_dir/n01440764/ILSVRC2012_val_00000293.JPEG
data_dir/n01440764/ILSVRC2012_val_00000543.JPEG
...
where 'n01440764' is the unique synset label associated with
these images.
The training data set consists of 1000 sub-directories (i.e. labels)
each containing 1200 JPEG images for a total of 1.2M JPEG images.
The evaluation data set consists of 1000 sub-directories (i.e. labels)
each containing 50 JPEG images for a total of 50K JPEG images.
This TensorFlow script converts the training and evaluation data into
a sharded data set consisting of 1024 and 128 TFRecord files, respectively.
train_directory/train-00000-of-01024
train_directory/train-00001-of-01024
...
train_directory/train-01023-of-01024
and
validation_directory/validation-00000-of-00128
validation_directory/validation-00001-of-00128
...
validation_directory/validation-00127-of-00128
Each validation TFRecord file contains ~390 records. Each training TFREcord
file contains ~1250 records. Each record within the TFRecord file is a
serialized Example proto. The Example proto contains the following fields:
image/encoded: string containing JPEG encoded image in RGB colorspace
image/height: integer, image height in pixels
image/width: integer, image width in pixels
image/colorspace: string, specifying the colorspace, always 'RGB'
image/channels: integer, specifying the number of channels, always 3
image/format: string, specifying the format, always 'JPEG'
image/filename: string containing the basename of the image file
e.g. 'n01440764_10026.JPEG' or 'ILSVRC2012_val_00000293.JPEG'
image/class/label: integer specifying the index in a classification layer.
The label ranges from [1, 1000] where 0 is not used.
image/class/synset: string specifying the unique ID of the label,
e.g. 'n01440764'
image/class/text: string specifying the human-readable version of the label
e.g. 'red fox, Vulpes vulpes'
image/object/bbox/xmin: list of integers specifying the 0+ human annotated
bounding boxes
image/object/bbox/xmax: list of integers specifying the 0+ human annotated
bounding boxes
image/object/bbox/ymin: list of integers specifying the 0+ human annotated
bounding boxes
image/object/bbox/ymax: list of integers specifying the 0+ human annotated
bounding boxes
image/object/bbox/label: integer specifying the index in a classification
layer. The label ranges from [1, 1000] where 0 is not used. Note this is
always identical to the image label.
Note that the length of xmin is identical to the length of xmax, ymin and ymax
for each example.
Running this script using 16 threads may take around ~2.5 hours on an HP Z420.
"""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from datetime import datetime
import os
import random
import sys
import threading
import numpy as np
import six
import tensorflow as tf
tf.app.flags.DEFINE_string('train_directory', '/tmp/',
'Training data directory')
tf.app.flags.DEFINE_string('validation_directory', '/tmp/',
'Validation data directory')
tf.app.flags.DEFINE_string('output_directory', '/tmp/',
'Output data directory')
tf.app.flags.DEFINE_integer('train_shards', 1024,
'Number of shards in training TFRecord files.')
tf.app.flags.DEFINE_integer('validation_shards', 128,
'Number of shards in validation TFRecord files.')
tf.app.flags.DEFINE_integer('num_threads', 8,
'Number of threads to preprocess the images.')
# The labels file contains a list of valid labels are held in this file.
# Assumes that the file contains entries as such:
# n01440764
# n01443537
# n01484850
# where each line corresponds to a label expressed as a synset. We map
# each synset contained in the file to an integer (based on the alphabetical
# ordering). See below for details.
tf.app.flags.DEFINE_string('labels_file',
'imagenet_lsvrc_2015_synsets.txt',
'Labels file')
# This file containing mapping from synset to human-readable label.
# Assumes each line of the file looks like:
#
# n02119247 black fox
# n02119359 silver fox
# n02119477 red fox, Vulpes fulva
#
# where each line corresponds to a unique mapping. Note that each line is
# formatted as <synset>\t<human readable label>.
tf.app.flags.DEFINE_string('imagenet_metadata_file',
'imagenet_metadata.txt',
'ImageNet metadata file')
# This file is the output of process_bounding_box.py
# Assumes each line of the file looks like:
#
# n00007846_64193.JPEG,0.0060,0.2620,0.7545,0.9940
#
# where each line corresponds to one bounding box annotation associated
# with an image. Each line can be parsed as:
#
# <JPEG file name>, <xmin>, <ymin>, <xmax>, <ymax>
#
# Note that there might exist mulitple bounding box annotations associated
# with an image file.
tf.app.flags.DEFINE_string('bounding_box_file',
'./imagenet_2012_bounding_boxes.csv',
'Bounding box file')
FLAGS = tf.app.flags.FLAGS
def _int64_feature(value):
"""Wrapper for inserting int64 features into Example proto."""
if not isinstance(value, list):
value = [value]
return tf.train.Feature(int64_list=tf.train.Int64List(value=value))
def _float_feature(value):
"""Wrapper for inserting float features into Example proto."""
if not isinstance(value, list):
value = [value]
return tf.train.Feature(float_list=tf.train.FloatList(value=value))
def _bytes_feature(value):
"""Wrapper for inserting bytes features into Example proto."""
if six.PY3 and isinstance(value, six.text_type):
value = six.binary_type(value, encoding='utf-8')
return tf.train.Feature(bytes_list=tf.train.BytesList(value=[value]))
def _convert_to_example(filename, image_buffer, label, synset, human, bbox,
height, width):
"""Build an Example proto for an example.
Args:
filename: string, path to an image file, e.g., '/path/to/example.JPG'
image_buffer: string, JPEG encoding of RGB image
label: integer, identifier for the ground truth for the network
synset: string, unique WordNet ID specifying the label, e.g., 'n02323233'
human: string, human-readable label, e.g., 'red fox, Vulpes vulpes'
bbox: list of bounding boxes; each box is a list of integers
specifying [xmin, ymin, xmax, ymax]. All boxes are assumed to belong to
the same label as the image label.
height: integer, image height in pixels
width: integer, image width in pixels
Returns:
Example proto
"""
xmin = []
ymin = []
xmax = []
ymax = []
for b in bbox:
assert len(b) == 4
# pylint: disable=expression-not-assigned
[l.append(point) for l, point in zip([xmin, ymin, xmax, ymax], b)]
# pylint: enable=expression-not-assigned
colorspace = 'RGB'
channels = 3
image_format = 'JPEG'
example = tf.train.Example(features=tf.train.Features(feature={
'image/height': _int64_feature(height),
'image/width': _int64_feature(width),
'image/colorspace': _bytes_feature(colorspace),
'image/channels': _int64_feature(channels),
'image/class/label': _int64_feature(label),
'image/class/synset': _bytes_feature(synset),
'image/class/text': _bytes_feature(human),
'image/object/bbox/xmin': _float_feature(xmin),
'image/object/bbox/xmax': _float_feature(xmax),
'image/object/bbox/ymin': _float_feature(ymin),
'image/object/bbox/ymax': _float_feature(ymax),
'image/object/bbox/label': _int64_feature([label] * len(xmin)),
'image/format': _bytes_feature(image_format),
'image/filename': _bytes_feature(os.path.basename(filename)),
'image/encoded': _bytes_feature(image_buffer)}))
return example
class ImageCoder(object):
"""Helper class that provides TensorFlow image coding utilities."""
def __init__(self):
# Create a single Session to run all image coding calls.
self._sess = tf.Session()
# Initializes function that converts PNG to JPEG data.
self._png_data = tf.placeholder(dtype=tf.string)
image = tf.image.decode_png(self._png_data, channels=3)
self._png_to_jpeg = tf.image.encode_jpeg(image, format='rgb', quality=100)
# Initializes function that converts CMYK JPEG data to RGB JPEG data.
self._cmyk_data = tf.placeholder(dtype=tf.string)
image = tf.image.decode_jpeg(self._cmyk_data, channels=0)
self._cmyk_to_rgb = tf.image.encode_jpeg(image, format='rgb', quality=100)
# Initializes function that decodes RGB JPEG data.
self._decode_jpeg_data = tf.placeholder(dtype=tf.string)
self._decode_jpeg = tf.image.decode_jpeg(self._decode_jpeg_data, channels=3)
def png_to_jpeg(self, image_data):
return self._sess.run(self._png_to_jpeg,
feed_dict={self._png_data: image_data})
def cmyk_to_rgb(self, image_data):
return self._sess.run(self._cmyk_to_rgb,
feed_dict={self._cmyk_data: image_data})
def decode_jpeg(self, image_data):
image = self._sess.run(self._decode_jpeg,
feed_dict={self._decode_jpeg_data: image_data})
assert len(image.shape) == 3
assert image.shape[2] == 3
return image
def _is_png(filename):
"""Determine if a file contains a PNG format image.
Args:
filename: string, path of the image file.
Returns:
boolean indicating if the image is a PNG.
"""
# File list from:
# https://groups.google.com/forum/embed/?place=forum/torch7#!topic/torch7/fOSTXHIESSU
return 'n02105855_2933.JPEG' in filename
def _is_cmyk(filename):
"""Determine if file contains a CMYK JPEG format image.
Args:
filename: string, path of the image file.
Returns:
boolean indicating if the image is a JPEG encoded with CMYK color space.
"""
# File list from:
# https://github.com/cytsai/ilsvrc-cmyk-image-list
blacklist = ['n01739381_1309.JPEG', 'n02077923_14822.JPEG',
'n02447366_23489.JPEG', 'n02492035_15739.JPEG',
'n02747177_10752.JPEG', 'n03018349_4028.JPEG',
'n03062245_4620.JPEG', 'n03347037_9675.JPEG',
'n03467068_12171.JPEG', 'n03529860_11437.JPEG',
'n03544143_17228.JPEG', 'n03633091_5218.JPEG',
'n03710637_5125.JPEG', 'n03961711_5286.JPEG',
'n04033995_2932.JPEG', 'n04258138_17003.JPEG',
'n04264628_27969.JPEG', 'n04336792_7448.JPEG',
'n04371774_5854.JPEG', 'n04596742_4225.JPEG',
'n07583066_647.JPEG', 'n13037406_4650.JPEG']
return filename.split('/')[-1] in blacklist
def _process_image(filename, coder):
"""Process a single image file.
Args:
filename: string, path to an image file e.g., '/path/to/example.JPG'.
coder: instance of ImageCoder to provide TensorFlow image coding utils.
Returns:
image_buffer: string, JPEG encoding of RGB image.
height: integer, image height in pixels.
width: integer, image width in pixels.
"""
# Read the image file.
with tf.gfile.FastGFile(filename, 'rb') as f:
image_data = f.read()
# Clean the dirty data.
if _is_png(filename):
# 1 image is a PNG.
print('Converting PNG to JPEG for %s' % filename)
image_data = coder.png_to_jpeg(image_data)
elif _is_cmyk(filename):
# 22 JPEG images are in CMYK colorspace.
print('Converting CMYK to RGB for %s' % filename)
image_data = coder.cmyk_to_rgb(image_data)
# Decode the RGB JPEG.
image = coder.decode_jpeg(image_data)
# Check that image converted to RGB
assert len(image.shape) == 3
height = image.shape[0]
width = image.shape[1]
assert image.shape[2] == 3
return image_data, height, width
def _process_image_files_batch(coder, thread_index, ranges, name, filenames,
synsets, labels, humans, bboxes, num_shards):
"""Processes and saves list of images as TFRecord in 1 thread.
Args:
coder: instance of ImageCoder to provide TensorFlow image coding utils.
thread_index: integer, unique batch to run index is within [0, len(ranges)).
ranges: list of pairs of integers specifying ranges of each batches to
analyze in parallel.
name: string, unique identifier specifying the data set
filenames: list of strings; each string is a path to an image file
synsets: list of strings; each string is a unique WordNet ID
labels: list of integer; each integer identifies the ground truth
humans: list of strings; each string is a human-readable label
bboxes: list of bounding boxes for each image. Note that each entry in this
list might contain from 0+ entries corresponding to the number of bounding
box annotations for the image.
num_shards: integer number of shards for this data set.
"""
# Each thread produces N shards where N = int(num_shards / num_threads).
# For instance, if num_shards = 128, and the num_threads = 2, then the first
# thread would produce shards [0, 64).
num_threads = len(ranges)
assert not num_shards % num_threads
num_shards_per_batch = int(num_shards / num_threads)
shard_ranges = np.linspace(ranges[thread_index][0],
ranges[thread_index][1],
num_shards_per_batch + 1).astype(int)
num_files_in_thread = ranges[thread_index][1] - ranges[thread_index][0]
counter = 0
for s in range(num_shards_per_batch):
# Generate a sharded version of the file name, e.g. 'train-00002-of-00010'
shard = thread_index * num_shards_per_batch + s
output_filename = '%s-%.5d-of-%.5d' % (name, shard, num_shards)
output_file = os.path.join(FLAGS.output_directory, output_filename)
writer = tf.python_io.TFRecordWriter(output_file)
shard_counter = 0
files_in_shard = np.arange(shard_ranges[s], shard_ranges[s + 1], dtype=int)
for i in files_in_shard:
filename = filenames[i]
label = labels[i]
synset = synsets[i]
human = humans[i]
bbox = bboxes[i]
image_buffer, height, width = _process_image(filename, coder)
example = _convert_to_example(filename, image_buffer, label,
synset, human, bbox,
height, width)
writer.write(example.SerializeToString())
shard_counter += 1
counter += 1
if not counter % 1000:
print('%s [thread %d]: Processed %d of %d images in thread batch.' %
(datetime.now(), thread_index, counter, num_files_in_thread))
sys.stdout.flush()
writer.close()
print('%s [thread %d]: Wrote %d images to %s' %
(datetime.now(), thread_index, shard_counter, output_file))
sys.stdout.flush()
shard_counter = 0
print('%s [thread %d]: Wrote %d images to %d shards.' %
(datetime.now(), thread_index, counter, num_files_in_thread))
sys.stdout.flush()
def _process_image_files(name, filenames, synsets, labels, humans,
bboxes, num_shards):
"""Process and save list of images as TFRecord of Example protos.
Args:
name: string, unique identifier specifying the data set
filenames: list of strings; each string is a path to an image file
synsets: list of strings; each string is a unique WordNet ID
labels: list of integer; each integer identifies the ground truth
humans: list of strings; each string is a human-readable label
bboxes: list of bounding boxes for each image. Note that each entry in this
list might contain from 0+ entries corresponding to the number of bounding
box annotations for the image.
num_shards: integer number of shards for this data set.
"""
assert len(filenames) == len(synsets)
assert len(filenames) == len(labels)
assert len(filenames) == len(humans)
assert len(filenames) == len(bboxes)
# Break all images into batches with a [ranges[i][0], ranges[i][1]].
spacing = np.linspace(0, len(filenames), FLAGS.num_threads + 1).astype(np.int)
ranges = []
threads = []
for i in range(len(spacing) - 1):
ranges.append([spacing[i], spacing[i + 1]])
# Launch a thread for each batch.
print('Launching %d threads for spacings: %s' % (FLAGS.num_threads, ranges))
sys.stdout.flush()
# Create a mechanism for monitoring when all threads are finished.
coord = tf.train.Coordinator()
# Create a generic TensorFlow-based utility for converting all image codings.
coder = ImageCoder()
threads = []
for thread_index in range(len(ranges)):
args = (coder, thread_index, ranges, name, filenames,
synsets, labels, humans, bboxes, num_shards)
t = threading.Thread(target=_process_image_files_batch, args=args)
t.start()
threads.append(t)
# Wait for all the threads to terminate.
coord.join(threads)
print('%s: Finished writing all %d images in data set.' %
(datetime.now(), len(filenames)))
sys.stdout.flush()
def _find_image_files(data_dir, labels_file):
"""Build a list of all images files and labels in the data set.
Args:
data_dir: string, path to the root directory of images.
Assumes that the ImageNet data set resides in JPEG files located in
the following directory structure.
data_dir/n01440764/ILSVRC2012_val_00000293.JPEG
data_dir/n01440764/ILSVRC2012_val_00000543.JPEG
where 'n01440764' is the unique synset label associated with these images.
labels_file: string, path to the labels file.
The list of valid labels are held in this file. Assumes that the file
contains entries as such:
n01440764
n01443537
n01484850
where each line corresponds to a label expressed as a synset. We map
each synset contained in the file to an integer (based on the alphabetical
ordering) starting with the integer 1 corresponding to the synset
contained in the first line.
The reason we start the integer labels at 1 is to reserve label 0 as an
unused background class.
Returns:
filenames: list of strings; each string is a path to an image file.
synsets: list of strings; each string is a unique WordNet ID.
labels: list of integer; each integer identifies the ground truth.
"""
print('Determining list of input files and labels from %s.' % data_dir)
challenge_synsets = [l.strip() for l in
tf.gfile.FastGFile(labels_file, 'r').readlines()]
labels = []
filenames = []
synsets = []
# Leave label index 0 empty as a background class.
label_index = 1
# Construct the list of JPEG files and labels.
for synset in challenge_synsets:
jpeg_file_path = '%s/%s/*.JPEG' % (data_dir, synset)
matching_files = tf.gfile.Glob(jpeg_file_path)
labels.extend([label_index] * len(matching_files))
synsets.extend([synset] * len(matching_files))
filenames.extend(matching_files)
if not label_index % 100:
print('Finished finding files in %d of %d classes.' % (
label_index, len(challenge_synsets)))
label_index += 1
# Shuffle the ordering of all image files in order to guarantee
# random ordering of the images with respect to label in the
# saved TFRecord files. Make the randomization repeatable.
shuffled_index = list(range(len(filenames)))
random.seed(12345)
random.shuffle(shuffled_index)
filenames = [filenames[i] for i in shuffled_index]
synsets = [synsets[i] for i in shuffled_index]
labels = [labels[i] for i in shuffled_index]
print('Found %d JPEG files across %d labels inside %s.' %
(len(filenames), len(challenge_synsets), data_dir))
return filenames, synsets, labels
def _find_human_readable_labels(synsets, synset_to_human):
"""Build a list of human-readable labels.
Args:
synsets: list of strings; each string is a unique WordNet ID.
synset_to_human: dict of synset to human labels, e.g.,
'n02119022' --> 'red fox, Vulpes vulpes'
Returns:
List of human-readable strings corresponding to each synset.
"""
humans = []
for s in synsets:
assert s in synset_to_human, ('Failed to find: %s' % s)
humans.append(synset_to_human[s])
return humans
def _find_image_bounding_boxes(filenames, image_to_bboxes):
"""Find the bounding boxes for a given image file.
Args:
filenames: list of strings; each string is a path to an image file.
image_to_bboxes: dictionary mapping image file names to a list of
bounding boxes. This list contains 0+ bounding boxes.
Returns:
List of bounding boxes for each image. Note that each entry in this
list might contain from 0+ entries corresponding to the number of bounding
box annotations for the image.
"""
num_image_bbox = 0
bboxes = []
for f in filenames:
basename = os.path.basename(f)
if basename in image_to_bboxes:
bboxes.append(image_to_bboxes[basename])
num_image_bbox += 1
else:
bboxes.append([])
print('Found %d images with bboxes out of %d images' % (
num_image_bbox, len(filenames)))
return bboxes
def _process_dataset(name, directory, num_shards, synset_to_human,
image_to_bboxes):
"""Process a complete data set and save it as a TFRecord.
Args:
name: string, unique identifier specifying the data set.
directory: string, root path to the data set.
num_shards: integer number of shards for this data set.
synset_to_human: dict of synset to human labels, e.g.,
'n02119022' --> 'red fox, Vulpes vulpes'
image_to_bboxes: dictionary mapping image file names to a list of
bounding boxes. This list contains 0+ bounding boxes.
"""
filenames, synsets, labels = _find_image_files(directory, FLAGS.labels_file)
humans = _find_human_readable_labels(synsets, synset_to_human)
bboxes = _find_image_bounding_boxes(filenames, image_to_bboxes)
_process_image_files(name, filenames, synsets, labels,
humans, bboxes, num_shards)
def _build_synset_lookup(imagenet_metadata_file):
"""Build lookup for synset to human-readable label.
Args:
imagenet_metadata_file: string, path to file containing mapping from
synset to human-readable label.
Assumes each line of the file looks like:
n02119247 black fox
n02119359 silver fox
n02119477 red fox, Vulpes fulva
where each line corresponds to a unique mapping. Note that each line is
formatted as <synset>\t<human readable label>.
Returns:
Dictionary of synset to human labels, such as:
'n02119022' --> 'red fox, Vulpes vulpes'
"""
lines = tf.gfile.FastGFile(imagenet_metadata_file, 'r').readlines()
synset_to_human = {}
for l in lines:
if l:
parts = l.strip().split('\t')
assert len(parts) == 2
synset = parts[0]
human = parts[1]
synset_to_human[synset] = human
return synset_to_human
def _build_bounding_box_lookup(bounding_box_file):
"""Build a lookup from image file to bounding boxes.
Args:
bounding_box_file: string, path to file with bounding boxes annotations.
Assumes each line of the file looks like:
n00007846_64193.JPEG,0.0060,0.2620,0.7545,0.9940
where each line corresponds to one bounding box annotation associated
with an image. Each line can be parsed as:
<JPEG file name>, <xmin>, <ymin>, <xmax>, <ymax>
Note that there might exist mulitple bounding box annotations associated
with an image file. This file is the output of process_bounding_boxes.py.
Returns:
Dictionary mapping image file names to a list of bounding boxes. This list
contains 0+ bounding boxes.
"""
lines = tf.gfile.FastGFile(bounding_box_file, 'r').readlines()
images_to_bboxes = {}
num_bbox = 0
num_image = 0
for l in lines:
if l:
parts = l.split(',')
assert len(parts) == 5, ('Failed to parse: %s' % l)
filename = parts[0]
xmin = float(parts[1])
ymin = float(parts[2])
xmax = float(parts[3])
ymax = float(parts[4])
box = [xmin, ymin, xmax, ymax]
if filename not in images_to_bboxes:
images_to_bboxes[filename] = []
num_image += 1
images_to_bboxes[filename].append(box)
num_bbox += 1
print('Successfully read %d bounding boxes '
'across %d images.' % (num_bbox, num_image))
return images_to_bboxes
def main(unused_argv):
assert not FLAGS.train_shards % FLAGS.num_threads, (
'Please make the FLAGS.num_threads commensurate with FLAGS.train_shards')
assert not FLAGS.validation_shards % FLAGS.num_threads, (
'Please make the FLAGS.num_threads commensurate with '
'FLAGS.validation_shards')
print('Saving results to %s' % FLAGS.output_directory)
# Build a map from synset to human-readable label.
synset_to_human = _build_synset_lookup(FLAGS.imagenet_metadata_file)
image_to_bboxes = _build_bounding_box_lookup(FLAGS.bounding_box_file)
# Run it!
_process_dataset('validation', FLAGS.validation_directory,
FLAGS.validation_shards, synset_to_human, image_to_bboxes)
_process_dataset('train', FLAGS.train_directory, FLAGS.train_shards,
synset_to_human, image_to_bboxes)
if __name__ == '__main__':
tf.app.run()
|
TensorFlow/Translation/GNMT | GNMT | gnmt_model | # Copyright 2017 Google Inc. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
#
# Copyright (c) 2019, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
"""GNMT attention sequence-to-sequence model with dynamic RNN support."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import numpy as np
import tensorflow as tf
from tensorflow.contrib.cudnn_rnn.python.layers import cudnn_rnn
import attention_wrapper
import block_lstm
import model
import model_helper
from utils import misc_utils as utils
class GNMTModel(model.BaseModel):
"""Sequence-to-sequence dynamic model with GNMT attention architecture.
"""
def __init__(self,
hparams,
mode,
features,
scope=None,
extra_args=None):
self.is_gnmt_attention = (
hparams.attention_architecture in ["gnmt", "gnmt_v2"])
super(GNMTModel, self).__init__(
hparams=hparams,
mode=mode,
features=features,
scope=scope,
extra_args=extra_args)
def _prepare_beam_search_decoder_inputs(
self, beam_width, memory, source_sequence_length, encoder_state):
memory = tf.contrib.seq2seq.tile_batch(
memory, multiplier=beam_width)
source_sequence_length = tf.contrib.seq2seq.tile_batch(
source_sequence_length, multiplier=beam_width)
encoder_state = tf.contrib.seq2seq.tile_batch(
encoder_state, multiplier=beam_width)
batch_size = self.batch_size * beam_width
return memory, source_sequence_length, encoder_state, batch_size
def _build_encoder(self, hparams):
"""Build a GNMT encoder."""
assert hparams.encoder_type == "gnmt"
# Build GNMT encoder.
num_bi_layers = 1
num_uni_layers = self.num_encoder_layers - num_bi_layers
utils.print_out("# Build a GNMT encoder")
utils.print_out(" num_bi_layers = %d" % num_bi_layers)
utils.print_out(" num_uni_layers = %d" % num_uni_layers)
# source is batch-majored
source = self.features["source"]
import sys
print('source.shape: %s' % source.shape, file=sys.stderr)
if self.time_major:
# Later rnn would use time-majored inputs
source = tf.transpose(source)
with tf.variable_scope("encoder"):
dtype = self.dtype
encoder_emb_inp = tf.cast(
self.encoder_emb_lookup_fn(self.embedding_encoder, source), dtype)
# Build 1st bidi layer.
bi_encoder_outputs, bi_encoder_state = self._build_encoder_layers_bidi(
encoder_emb_inp, self.features["source_sequence_length"], hparams,
dtype)
# Build all the rest unidi layers
encoder_state, encoder_outputs = self._build_encoder_layers_unidi(
bi_encoder_outputs, self.features["source_sequence_length"],
num_uni_layers, hparams, dtype)
# Pass all encoder states to the decoder
# except the first bi-directional layer
encoder_state = (bi_encoder_state[1],) + (
(encoder_state,) if num_uni_layers == 1 else encoder_state)
return encoder_outputs, encoder_state
def _build_encoder_layers_bidi(self, inputs, sequence_length, hparams, dtype):
"""docstring."""
if hparams.use_fused_lstm:
fn = self._build_bidi_rnn_fused
elif hparams.use_cudnn_lstm:
fn = self._build_bidi_rnn_cudnn
else:
fn = self._build_bidi_rnn_base
return fn(inputs, sequence_length, hparams, dtype)
def _build_bidi_rnn_fused(self, inputs, sequence_length, hparams, dtype):
if (not np.isclose(hparams.dropout, 0.) and
self.mode == tf.contrib.learn.ModeKeys.TRAIN):
inputs = tf.nn.dropout(inputs, keep_prob=1-hparams.dropout)
fwd_cell = block_lstm.LSTMBlockFusedCell(
hparams.num_units, hparams.forget_bias, dtype=dtype)
fwd_encoder_outputs, (fwd_final_c, fwd_final_h) = fwd_cell(
inputs,
dtype=dtype,
sequence_length=sequence_length)
inputs_r = tf.reverse_sequence(
inputs, sequence_length, batch_axis=1, seq_axis=0)
bak_cell = block_lstm.LSTMBlockFusedCell(
hparams.num_units, hparams.forget_bias, dtype=dtype)
bak_encoder_outputs, (bak_final_c, bak_final_h) = bak_cell(
inputs_r,
dtype=dtype,
sequence_length=sequence_length)
bak_encoder_outputs = tf.reverse_sequence(
bak_encoder_outputs, sequence_length, batch_axis=1, seq_axis=0)
bi_encoder_outputs = tf.concat(
[fwd_encoder_outputs, bak_encoder_outputs], axis=-1)
fwd_state = tf.nn.rnn_cell.LSTMStateTuple(fwd_final_c, fwd_final_h)
bak_state = tf.nn.rnn_cell.LSTMStateTuple(bak_final_c, bak_final_h)
bi_encoder_state = (fwd_state, bak_state)
# mask aren't applied on outputs, but final states are post-masking.
return bi_encoder_outputs, bi_encoder_state
def _build_unidi_rnn_fused(self, inputs, state,
sequence_length, hparams, dtype):
if (not np.isclose(hparams.dropout, 0.) and
self.mode == tf.contrib.learn.ModeKeys.TRAIN):
inputs = tf.nn.dropout(inputs, keep_prob=1-hparams.dropout)
cell = block_lstm.LSTMBlockFusedCell(
hparams.num_units, hparams.forget_bias, dtype=dtype)
outputs, (final_c, final_h) = cell(
inputs,
state,
dtype=dtype,
sequence_length=sequence_length)
# mask aren't applied on outputs, but final states are post-masking.
return outputs, tf.nn.rnn_cell.LSTMStateTuple(final_c, final_h)
def _build_unidi_rnn_cudnn(self, inputs, state, sequence_length, dtype,
hparams, num_layers, is_fwd):
# cudnn inputs only support time-major
if not self.time_major:
inputs = tf.transpose(inputs, axis=[1, 0, 2])
if num_layers == 1 and not np.isclose(hparams.dropout, 0.):
# Special case when drop is used and only one layer
dropout = 0.
inputs = tf.nn.dropout(inputs, keep_prob=1-dropout)
else:
dropout = hparams.dropout
# the outputs would be in time-majored
sequence_length = tf.transpose(sequence_length)
if not is_fwd:
inputs = tf.reverse_sequence(
inputs, sequence_length, batch_axis=1, seq_axis=0)
cell = tf.contrib.cudnn_rnn.CudnnLSTM(
num_layers=num_layers,
num_units=hparams.num_units,
direction=cudnn_rnn.CUDNN_RNN_UNIDIRECTION,
dtype=self.dtype,
dropout=dropout)
outputs, (h, c) = cell(inputs, initial_state=state)
"""
# Mask outputs
# [batch, time]
mask = tf.sequence_mask(sequence_length, dtype=self.dtype)
# [time, batch]
mask = tf.transpose(mask)
outputs *= mask
"""
if not is_fwd:
outputs = tf.reverse_sequence(
inputs, sequence_length, batch_axis=1, seq_axis=0)
# NOTICE! There's no way to get the "correct" masked cell state in cudnn
# rnn.
if num_layers == 1:
h = tf.squeeze(h, axis=0)
c = tf.squeeze(c, axis=0)
return outputs, tf.nn.rnn_cell.LSTMStateTuple(c=c, h=h)
# Split h and c to form a
h.set_shape((num_layers, None, hparams.num_units))
c.set_shape((num_layers, None, hparams.num_units))
hs = tf.unstack(h)
cs = tf.unstack(c)
# The cell passed to bidi-dyanmic-rnn is a MultiRNNCell consisting 2 regular
# LSTM, the state of each is a simple LSTMStateTuple. Thus the state of the
# MultiRNNCell is a tuple of LSTMStateTuple.
states = tuple(
tf.nn.rnn_cell.LSTMStateTuple(c=c, h=h) for h, c in zip(hs, cs))
# No need to transpose back
return outputs, states
def _build_encoder_cell(self, hparams, num_layers, num_residual_layers,
dtype=None):
"""Build a multi-layer RNN cell that can be used by encoder."""
return model_helper.create_rnn_cell(
unit_type=hparams.unit_type,
num_units=self.num_units,
num_layers=num_layers,
num_residual_layers=num_residual_layers,
forget_bias=hparams.forget_bias,
dropout=hparams.dropout,
mode=self.mode,
dtype=dtype,
single_cell_fn=self.single_cell_fn,
use_block_lstm=hparams.use_block_lstm)
def _build_bidi_rnn_base(self, inputs, sequence_length, hparams, dtype):
"""Create and call biddirectional RNN cells."""
# num_residual_layers: Number of residual layers from top to bottom. For
# example, if `num_bi_layers=4` and `num_residual_layers=2`, the last 2
# RNN layers in each RNN cell will be wrapped with `ResidualWrapper`.
# Construct forward and backward cells
fw_cell = self._build_encoder_cell(hparams,
1, # num_bi_layers,
0, # num_bi_residual_layers,
dtype)
bw_cell = self._build_encoder_cell(hparams,
1, # num_bi_layers,
0, # num_bi_residual_layers,
dtype)
if hparams.use_dynamic_rnn:
bi_outputs, bi_state = tf.nn.bidirectional_dynamic_rnn(
fw_cell,
bw_cell,
inputs,
dtype=dtype,
sequence_length=sequence_length,
time_major=self.time_major,
swap_memory=True)
else:
bi_outputs, bi_state = tf.contrib.recurrent.bidirectional_functional_rnn(
fw_cell,
bw_cell,
inputs,
dtype=dtype,
sequence_length=sequence_length,
time_major=self.time_major,
use_tpu=False)
return tf.concat(bi_outputs, -1), bi_state
def _build_bidi_rnn_cudnn(self, inputs, sequence_length, hparams, dtype):
# Notice cudnn rnn dropout is applied between layers. (if 1 layer only then
# no dropout).
if not np.isclose(hparams.dropout, 0.):
inputs = tf.nn.dropout(inputs, keep_prob=1-hparams.dropout)
if not hparams.use_loose_bidi_cudnn_lstm:
fwd_outputs, fwd_states = self._build_unidi_rnn_cudnn(
inputs, None, # initial_state
sequence_length, dtype, hparams,
1, # num_layer
is_fwd=True)
bak_outputs, bak_states = self._build_unidi_rnn_cudnn(
inputs, None, # initial_state
sequence_length, dtype, hparams,
1, # num_layer
is_fwd=False)
bi_outputs = tf.concat([fwd_outputs, bak_outputs], axis=-1)
return bi_outputs, (fwd_states, bak_states)
else:
# Cudnn only accept time-majored inputs
if not self.time_major:
inputs = tf.transpose(inputs, axis=[1, 0, 2])
bi_outputs, (bi_h, bi_c) = tf.contrib.cudnn_rnn.CudnnLSTM(
num_layers=1, # num_bi_layers,
num_units=hparams.num_units,
direction=cudnn_rnn.CUDNN_RNN_BIDIRECTION,
dropout=0., # one layer, dropout isn't applied anyway,
seed=hparams.random_seed,
dtype=self.dtype,
kernel_initializer=tf.get_variable_scope().initializer,
bias_initializer=tf.zeros_initializer())(inputs)
# state shape is [num_layers * num_dir, batch, dim]
bi_h.set_shape((2, None, hparams.num_units))
bi_c.set_shape((2, None, hparams.num_units))
fwd_h, bak_h = tf.unstack(bi_h)
fwd_c, bak_c = tf.unstack(bi_c)
# No need to transpose back
return bi_outputs, (tf.nn.rnn_cell.LSTMStateTuple(c=fwd_c, h=fwd_h),
tf.nn.rnn_cell.LSTMStateTuple(c=bak_c, h=bak_h))
def _build_encoder_layers_unidi(self, inputs, sequence_length,
num_uni_layers, hparams, dtype):
"""Build encoder layers all at once."""
encoder_outputs = None
encoder_state = tuple()
if hparams.use_fused_lstm:
for i in range(num_uni_layers):
if (not np.isclose(hparams.dropout, 0.) and
self.mode == tf.contrib.learn.ModeKeys.TRAIN):
cell_inputs = tf.nn.dropout(inputs, keep_prob=1-hparams.dropout)
else:
cell_inputs = inputs
cell = block_lstm.LSTMBlockFusedCell(
hparams.num_units, hparams.forget_bias, dtype=dtype)
encoder_outputs, (final_c, final_h) = cell(
cell_inputs,
dtype=dtype,
sequence_length=sequence_length)
encoder_state += (tf.nn.rnn_cell.LSTMStateTuple(final_c, final_h),)
if i >= num_uni_layers - self.num_encoder_residual_layers:
# Add the pre-dropout inputs. Residual wrapper is applied after
# dropout wrapper.
encoder_outputs += inputs
inputs = encoder_outputs
elif hparams.use_cudnn_lstm:
# Single layer cudnn rnn, dropout isnt applied in the kernel
for i in range(num_uni_layers):
if (not np.isclose(hparams.dropout, 0.) and
self.mode == tf.contrib.learn.ModeKeys.TRAIN):
inputs = tf.nn.dropout(inputs, keep_prob=1-hparams.dropout)
encoder_outputs, encoder_states = self._build_unidi_rnn_cudnn(
inputs,
None, # initial_state
sequence_length,
dtype,
hparams,
1, # num_layer
is_fwd=True)
encoder_state += (tf.nn.rnn_cell.LSTMStateTuple(encoder_states.c,
encoder_states.h),)
if i >= num_uni_layers - self.num_encoder_residual_layers:
encoder_outputs += inputs
inputs = encoder_outputs
else:
uni_cell = model_helper.create_rnn_cell(
unit_type=hparams.unit_type,
num_units=hparams.num_units,
num_layers=num_uni_layers,
num_residual_layers=self.num_encoder_residual_layers,
forget_bias=hparams.forget_bias,
dropout=hparams.dropout,
dtype=dtype,
mode=self.mode,
single_cell_fn=self.single_cell_fn,
use_block_lstm=hparams.use_block_lstm)
if hparams.use_dynamic_rnn:
encoder_outputs, encoder_state = tf.nn.dynamic_rnn(
uni_cell,
inputs,
dtype=dtype,
sequence_length=sequence_length,
time_major=self.time_major)
else:
encoder_outputs, encoder_state = tf.contrib.recurrent.functional_rnn(
uni_cell,
inputs,
dtype=dtype,
sequence_length=sequence_length,
time_major=self.time_major,
use_tpu=False)
return encoder_state, encoder_outputs
def _build_decoder_cell(self, hparams, encoder_outputs, encoder_state,
source_sequence_length):
"""Build a RNN cell with GNMT attention architecture."""
# GNMT attention
assert self.is_gnmt_attention
attention_option = hparams.attention
attention_architecture = hparams.attention_architecture
assert attention_option == "normed_bahdanau"
assert attention_architecture == "gnmt_v2"
num_units = hparams.num_units
infer_mode = hparams.infer_mode
dtype = tf.float16 if hparams.use_fp16 else tf.float32
if self.time_major:
memory = tf.transpose(encoder_outputs, [1, 0, 2])
else:
memory = encoder_outputs
if (self.mode == tf.contrib.learn.ModeKeys.INFER and
infer_mode == "beam_search"):
memory, source_sequence_length, encoder_state, batch_size = (
self._prepare_beam_search_decoder_inputs(
hparams.beam_width, memory, source_sequence_length,
encoder_state))
else:
batch_size = self.batch_size
attention_mechanism = model.create_attention_mechanism(
num_units, memory, source_sequence_length, dtype=dtype)
cell_list = model_helper._cell_list( # pylint: disable=protected-access
unit_type=hparams.unit_type,
num_units=num_units,
num_layers=self.num_decoder_layers,
num_residual_layers=self.num_decoder_residual_layers,
forget_bias=hparams.forget_bias,
dropout=hparams.dropout,
mode=self.mode,
dtype=dtype,
single_cell_fn=self.single_cell_fn,
residual_fn=gnmt_residual_fn,
use_block_lstm=hparams.use_block_lstm)
# Only wrap the bottom layer with the attention mechanism.
attention_cell = cell_list.pop(0)
# Only generate alignment in greedy INFER mode.
alignment_history = (self.mode == tf.contrib.learn.ModeKeys.INFER and
infer_mode != "beam_search")
attention_cell = attention_wrapper.AttentionWrapper(
attention_cell,
attention_mechanism,
attention_layer_size=None, # don't use attention layer.
output_attention=False,
alignment_history=alignment_history,
name="attention")
cell = GNMTAttentionMultiCell(attention_cell, cell_list)
if hparams.pass_hidden_state:
decoder_initial_state = tuple(
zs.clone(cell_state=es)
if isinstance(zs, attention_wrapper.AttentionWrapperState) else es
for zs, es in zip(
cell.zero_state(batch_size, dtype), encoder_state))
else:
decoder_initial_state = cell.zero_state(batch_size, dtype)
return cell, decoder_initial_state
def _build_decoder_cudnn(self, encoder_outputs, encoder_state, hparams):
pass
"""
# Training
# Use dynamic_rnn to compute the 1st layer outputs and attention
# GNMT attention
with tf.variable_scope("decoder") as decoder_scope:
assert self.is_gnmt_attention
attention_option = hparams.attention
attention_architecture = hparams.attention_architecture
assert attention_option == "normed_bahdanau"
assert attention_architecture == "gnmt_v2"
num_units = hparams.num_units
infer_mode = hparams.infer_mode
dtype = tf.float16 if hparams.use_fp16 else tf.float32
if self.time_major:
memory = tf.transpose(encoder_outputs, [1, 0, 2])
else:
memory = encoder_outputs
source_sequence_length = self.features["source_sequence_length"]
if (self.mode == tf.contrib.learn.ModeKeys.INFER and
infer_mode == "beam_search"):
memory, source_sequence_length, encoder_state, batch_size = (
self._prepare_beam_search_decoder_inputs(
hparams.beam_width, memory, source_sequence_length,
encoder_state))
else:
batch_size = self.batch_size
attention_mechanism = model.create_attention_mechanism(
num_units, memory, source_sequence_length, dtype=dtype)
attention_cell = model_helper._cell_list( # pylint: disable=protected-access
unit_type=hparams.unit_type,
num_units=num_units,
num_layers=1, # just one layer
num_residual_layers=0, # 1st layer has no residual connection.
forget_bias=hparams.forget_bias,
dropout=hparams.dropout,
mode=self.mode,
dtype=dtype,
single_cell_fn=self.single_cell_fn,
residual_fn=gnmt_residual_fn,
use_block_lstm=False)[0]
# Only generate alignment in greedy INFER mode.
alignment_history = (self.mode == tf.contrib.learn.ModeKeys.INFER and
infer_mode != "beam_search")
attention_cell = attention_wrapper.AttentionWrapper(
attention_cell,
attention_mechanism,
attention_layer_size=None, # don't use attention layer.
output_attention=False,
alignment_history=alignment_history,
name="attention")
decoder_attention_cell_initial_state = attention_cell.zero_state(
batch_size, dtype).clone(cell_state=encoder_state[0])
# TODO(jamesqin): support frnn
# [batch, time]
target_input = self.features["target_input"]
if self.time_major:
# If using time_major mode, then target_input should be [time, batch]
# then the decoder_emb_inp would be [time, batch, dim]
target_input = tf.transpose(target_input)
decoder_emb_inp = tf.cast(
tf.nn.embedding_lookup(self.embedding_decoder, target_input),
self.dtype)
attention_cell_outputs, attention_cell_state = tf.nn.dynamic_rnn(
attention_cell,
decoder_emb_inp,
sequence_length=self.features["target_sequence_length"],
initial_state=decoder_attention_cell_initial_state,
dtype=self.dtype,
scope=decoder_scope,
parallel_iterations=hparams.parallel_iterations,
time_major=self.time_major)
attention = None
inputs = tf.concat([target_input, attention_cell_outputs], axis=-1)
initial_state = encoder_state[1:]
num_bi_layers = 1
num_unidi_decoder_layers = self.num_decoder_layers = num_bi_layers
# 3 layers of uni cudnn
for i in range(num_unidi_decoder_layers):
# Concat input with attention
if (not np.isclose(hparams.dropout, 0.) and
self.mode == tf.contrib.learn.ModeKeys.TRAIN):
inputs = tf.nn.dropout(inputs, keep_prob=1 - hparams.dropout)
outputs, states = self._build_unidi_rnn_cudnn(
inputs,
initial_state[i],
self.features["target_sequence_length"],
self.dtype,
hparams,
1, # num_layer
is_fwd=True)
if i >= num_unidi_decoder_layers - self.num_decoder_residual_layers:
outputs += inputs
inputs = outputs
pass
"""
def _build_decoder_fused_for_training(self, encoder_outputs, initial_state,
decoder_emb_inp, hparams):
assert self.mode == tf.contrib.learn.ModeKeys.TRAIN
num_bi_layers = 1
num_unidi_decoder_layers = self.num_decoder_layers - num_bi_layers
assert num_unidi_decoder_layers == 3
# The 1st LSTM layer
if self.time_major:
batch = tf.shape(encoder_outputs)[1]
tgt_max_len = tf.shape(decoder_emb_inp)[0]
# [batch_size] -> scalar
initial_attention = tf.zeros(
shape=[tgt_max_len, batch, hparams.num_units], dtype=self.dtype)
else:
batch = tf.shape(encoder_outputs)[0]
tgt_max_len = tf.shape(decoder_emb_inp)[1]
initial_attention = tf.zeros(
shape=[batch, tgt_max_len, hparams.num_units], dtype=self.dtype)
# Concat with initial attention
dec_inp = tf.concat([decoder_emb_inp, initial_attention], axis=-1)
# [tgt_time, batch, units]
# var_scope naming chosen to agree with inference graph.
with tf.variable_scope("multi_rnn_cell/cell_0_attention/attention"):
outputs, _ = self._build_unidi_rnn_fused(
dec_inp,
initial_state[0],
self.features["target_sequence_length"],
hparams,
self.dtype)
# Get attention
# Fused attention layer has memory of shape [batch, src_time, ...]
if self.time_major:
memory = tf.transpose(encoder_outputs, [1, 0, 2])
else:
memory = encoder_outputs
fused_attention_layer = attention_wrapper.BahdanauAttentionFusedLayer(
hparams.num_units, memory,
memory_sequence_length=self.features["source_sequence_length"],
dtype=self.dtype)
# [batch, tgt_time, units]
if self.time_major:
queries = tf.transpose(outputs, [1, 0, 2])
else:
queries = outputs
fused_attention = fused_attention_layer(queries)
if self.time_major:
# [tgt_time, batch, units]
fused_attention = tf.transpose(fused_attention, [1, 0, 2])
# 2-4th layer
inputs = outputs
for i in range(num_unidi_decoder_layers):
# [tgt_time, batch, 2 * units]
concat_inputs = tf.concat([inputs, fused_attention], axis=-1)
# var_scope naming chosen to agree with inference graph.
with tf.variable_scope("multi_rnn_cell/cell_%d" % (i+1)):
outputs, _ = self._build_unidi_rnn_fused(
concat_inputs, initial_state[i + 1],
self.features["target_sequence_length"], hparams, self.dtype)
if i >= num_unidi_decoder_layers - self.num_decoder_residual_layers:
# gnmt_v2 attention adds the original inputs.
outputs += inputs
inputs = outputs
return outputs
class GNMTAttentionMultiCell(tf.nn.rnn_cell.MultiRNNCell):
"""A MultiCell with GNMT attention style."""
def __init__(self, attention_cell, cells):
"""Creates a GNMTAttentionMultiCell.
Args:
attention_cell: An instance of AttentionWrapper.
cells: A list of RNNCell wrapped with AttentionInputWrapper.
"""
cells = [attention_cell] + cells
super(GNMTAttentionMultiCell, self).__init__(cells, state_is_tuple=True)
def __call__(self, inputs, state, scope=None):
"""Run the cell with bottom layer's attention copied to all upper layers."""
if not tf.contrib.framework.nest.is_sequence(state):
raise ValueError(
"Expected state to be a tuple of length %d, but received: %s"
% (len(self.state_size), state))
with tf.variable_scope(scope or "multi_rnn_cell"):
new_states = []
with tf.variable_scope("cell_0_attention"):
attention_cell = self._cells[0]
attention_state = state[0]
cur_inp, new_attention_state = attention_cell(inputs, attention_state)
new_states.append(new_attention_state)
for i in range(1, len(self._cells)):
with tf.variable_scope("cell_%d" % i):
cell = self._cells[i]
cur_state = state[i]
cur_inp = tf.concat([cur_inp, new_attention_state.attention], -1)
cur_inp, new_state = cell(cur_inp, cur_state)
new_states.append(new_state)
return cur_inp, tuple(new_states)
def gnmt_residual_fn(inputs, outputs):
"""Residual function that handles different inputs and outputs inner dims.
Args:
inputs: cell inputs, this is actual inputs concatenated with the attention
vector.
outputs: cell outputs
Returns:
outputs + actual inputs
"""
def split_input(inp, out):
inp_dim = inp.get_shape().as_list()[-1]
out_dim = out.get_shape().as_list()[-1]
return tf.split(inp, [out_dim, inp_dim - out_dim], axis=-1)
actual_inputs, _ = tf.contrib.framework.nest.map_structure(
split_input, inputs, outputs)
def assert_shape_match(inp, out):
inp.get_shape().assert_is_compatible_with(out.get_shape())
tf.contrib.framework.nest.assert_same_structure(actual_inputs, outputs)
tf.contrib.framework.nest.map_structure(
assert_shape_match, actual_inputs, outputs)
return tf.contrib.framework.nest.map_structure(
lambda inp, out: inp + out, actual_inputs, outputs)
|
TensorFlow/Segmentation/UNet_Medical/utils/hooks | hooks | training_hook | # Copyright (c) 2019, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import tensorflow as tf
import horovod.tensorflow as hvd
class TrainingHook(tf.estimator.SessionRunHook):
def __init__(self, logger, max_steps, log_every=1):
self._log_every = log_every
self._iter_idx = 0
self.logger = logger
self.max_steps = max_steps
def before_run(self, run_context):
run_args = tf.estimator.SessionRunArgs(
fetches=[
'cross_loss_ref:0',
'dice_loss_ref:0',
'total_loss_ref:0',
]
)
return run_args
def after_run(self,
run_context,
run_values):
cross_loss, dice_loss, total_loss = run_values.results
if (self._iter_idx % self._log_every == 0) and (hvd.rank() == 0):
self.logger.log(step=(self._iter_idx, self.max_steps),
data={'train_ce_loss': float(cross_loss),
'train_dice_loss': float(dice_loss),
'train_total_loss': float(total_loss)})
self._iter_idx += 1
|
TensorFlow2/LanguageModeling/BERT/scripts | scripts | run_inference_benchmark | #!/usr/bin/env bash
# Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
echo "Container nvidia build = " $NVIDIA_BUILD_ID
bert_model=${1:-"large"}
batch_size=${2:-"8"}
precision=${3:-"fp16"}
use_xla=${4:-"true"}
squad_version="1.1"
if [ "$bert_model" = "large" ] ; then
export BERT_DIR=data/download/google_pretrained_weights/uncased_L-24_H-1024_A-16
else
export BERT_DIR=data/download/google_pretrained_weights/uncased_L-12_H-768_A-12
fi
init_checkpoint=$BERT_DIR/bert_model.ckpt
export SQUAD_DIR=data/download/squad/v${squad_version}
export SQUAD_VERSION=v$squad_version
if [ "$squad_version" = "1.1" ] ; then
version_2_with_negative="False"
else
version_2_with_negative="True"
fi
echo "Squad directory set as " $SQUAD_DIR " BERT directory set as " $BERT_DIR
echo "Results directory set as " $RESULTS_DIR
use_fp16=""
if [ "$precision" = "fp16" ] ; then
echo "fp16 activated!"
use_fp16="--use_fp16"
fi
if [ "$use_xla" = "true" ] ; then
use_xla_tag="--enable_xla"
echo "XLA activated"
else
use_xla_tag=""
fi
ckpt_str=${init_checkpoint//\//-}
printf -v TAG "squad_inference_benchmark_%s_%s_bs%d" "$bert_model" "$precision" $batch_size
DATESTAMP=`date +'%y%m%d%H%M%S'`
#Edit to save logs & checkpoints in a different directory
RESULTS_DIR=/tmp/bert_inference_benchmark_${DATESTAMP}
LOGFILE=/results/$TAG.log
printf "Logs written to %s\n" "$LOGFILE"
mkdir -p $RESULTS_DIR
mkdir -p /results
#Check if all necessary files are available before training
for DIR_or_file in $SQUAD_DIR $RESULTS_DIR $BERT_DIR/vocab.txt $BERT_DIR/bert_config.json; do
if [ ! -d "$DIR_or_file" ] && [ ! -f "$DIR_or_file" ]; then
echo "Error! $DIR_or_file directory missing. Please mount correctly"
exit -1
fi
done
python run_squad.py \
--mode=predict \
--input_meta_data_path=${SQUAD_DIR}/squad_${SQUAD_VERSION}_meta_data \
--vocab_file=$BERT_DIR/vocab.txt \
--bert_config_file=$BERT_DIR/bert_config.json \
--init_checkpoint=$init_checkpoint \
--predict_file=$SQUAD_DIR/dev-v${squad_version}.json \
--predict_batch_size=$batch_size \
--model_dir=$RESULTS_DIR \
--benchmark \
$use_fp16 $use_xla_tag |& tee $LOGFILE
rm $RESULTS_DIR -r |
PyTorch/DrugDiscovery/SE3Transformer | SE3Transformer | README | # [SE(3)-Transformers For PyTorch [migrated, click here]](https://github.com/NVIDIA/DeepLearningExamples/blob/master/DGLPyTorch/DrugDiscovery/SE3Transformer)

|
TensorFlow/Detection/SSD/models/research/object_detection/utils | utils | per_image_evaluation_test | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Tests for object_detection.utils.per_image_evaluation."""
import numpy as np
import tensorflow as tf
from object_detection.utils import per_image_evaluation
class SingleClassTpFpWithDifficultBoxesTest(tf.test.TestCase):
def setUp(self):
num_groundtruth_classes = 1
matching_iou_threshold = 0.5
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
self.eval = per_image_evaluation.PerImageEvaluation(
num_groundtruth_classes, matching_iou_threshold, nms_iou_threshold,
nms_max_output_boxes)
self.detected_boxes = np.array([[0, 0, 1, 1], [0, 0, 2, 2], [0, 0, 3, 3]],
dtype=float)
self.detected_scores = np.array([0.6, 0.8, 0.5], dtype=float)
detected_masks_0 = np.array([[0, 1, 1, 0],
[0, 0, 1, 0],
[0, 0, 0, 0]], dtype=np.uint8)
detected_masks_1 = np.array([[1, 0, 0, 0],
[1, 1, 0, 0],
[0, 0, 0, 0]], dtype=np.uint8)
detected_masks_2 = np.array([[0, 0, 0, 0],
[0, 1, 1, 0],
[0, 1, 0, 0]], dtype=np.uint8)
self.detected_masks = np.stack(
[detected_masks_0, detected_masks_1, detected_masks_2], axis=0)
self.groundtruth_boxes = np.array([[0, 0, 1, 1], [0, 0, 10, 10]],
dtype=float)
groundtruth_masks_0 = np.array([[1, 1, 0, 0],
[1, 1, 0, 0],
[0, 0, 0, 0]], dtype=np.uint8)
groundtruth_masks_1 = np.array([[0, 0, 0, 1],
[0, 0, 0, 1],
[0, 0, 0, 1]], dtype=np.uint8)
self.groundtruth_masks = np.stack(
[groundtruth_masks_0, groundtruth_masks_1], axis=0)
def test_match_to_gt_box_0(self):
groundtruth_groundtruth_is_difficult_list = np.array([False, True],
dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, False], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, True, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_match_to_gt_mask_0(self):
groundtruth_groundtruth_is_difficult_list = np.array([False, True],
dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, False], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=self.groundtruth_masks)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([True, False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_match_to_gt_box_1(self):
groundtruth_groundtruth_is_difficult_list = np.array([True, False],
dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, False], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = np.array([0.8, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_match_to_gt_mask_1(self):
groundtruth_groundtruth_is_difficult_list = np.array([True, False],
dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, False], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=self.groundtruth_masks)
expected_scores = np.array([0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
class SingleClassTpFpWithGroupOfBoxesTest(tf.test.TestCase):
def setUp(self):
num_groundtruth_classes = 1
matching_iou_threshold = 0.5
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
self.eval = per_image_evaluation.PerImageEvaluation(
num_groundtruth_classes, matching_iou_threshold, nms_iou_threshold,
nms_max_output_boxes)
self.detected_boxes = np.array(
[[0, 0, 1, 1], [0, 0, 2, 1], [0, 0, 3, 1]], dtype=float)
self.detected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
detected_masks_0 = np.array([[0, 1, 1, 0],
[0, 0, 1, 0],
[0, 0, 0, 0]], dtype=np.uint8)
detected_masks_1 = np.array([[1, 0, 0, 0],
[1, 1, 0, 0],
[0, 0, 0, 0]], dtype=np.uint8)
detected_masks_2 = np.array([[0, 0, 0, 0],
[0, 1, 1, 0],
[0, 1, 0, 0]], dtype=np.uint8)
self.detected_masks = np.stack(
[detected_masks_0, detected_masks_1, detected_masks_2], axis=0)
self.groundtruth_boxes = np.array(
[[0, 0, 1, 1], [0, 0, 5, 5], [10, 10, 20, 20]], dtype=float)
groundtruth_masks_0 = np.array([[1, 0, 0, 0],
[1, 0, 0, 0],
[1, 0, 0, 0]], dtype=np.uint8)
groundtruth_masks_1 = np.array([[0, 0, 1, 0],
[0, 0, 1, 0],
[0, 0, 1, 0]], dtype=np.uint8)
groundtruth_masks_2 = np.array([[0, 1, 0, 0],
[0, 1, 0, 0],
[0, 1, 0, 0]], dtype=np.uint8)
self.groundtruth_masks = np.stack(
[groundtruth_masks_0, groundtruth_masks_1, groundtruth_masks_2], axis=0)
def test_match_to_non_group_of_and_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, True, True], dtype=bool)
expected_scores = np.array([0.8], dtype=float)
expected_tp_fp_labels = np.array([True], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_match_to_non_group_of_and_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, True, True], dtype=bool)
expected_scores = np.array([0.6], dtype=float)
expected_tp_fp_labels = np.array([True], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=self.groundtruth_masks)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_match_two_to_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[True, False, True], dtype=bool)
expected_scores = np.array([0.5], dtype=float)
expected_tp_fp_labels = np.array([False], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_match_two_to_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[True, False, True], dtype=bool)
expected_scores = np.array([0.8], dtype=float)
expected_tp_fp_labels = np.array([True], dtype=bool)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=self.groundtruth_masks)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
class SingleClassTpFpWithGroupOfBoxesTestWeighted(tf.test.TestCase):
def setUp(self):
num_groundtruth_classes = 1
matching_iou_threshold = 0.5
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
self.group_of_weight = 0.5
self.eval = per_image_evaluation.PerImageEvaluation(
num_groundtruth_classes, matching_iou_threshold, nms_iou_threshold,
nms_max_output_boxes, self.group_of_weight)
self.detected_boxes = np.array(
[[0, 0, 1, 1], [0, 0, 2, 1], [0, 0, 3, 1]], dtype=float)
self.detected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
detected_masks_0 = np.array(
[[0, 1, 1, 0], [0, 0, 1, 0], [0, 0, 0, 0]], dtype=np.uint8)
detected_masks_1 = np.array(
[[1, 0, 0, 0], [1, 1, 0, 0], [0, 0, 0, 0]], dtype=np.uint8)
detected_masks_2 = np.array(
[[0, 0, 0, 0], [0, 1, 1, 0], [0, 1, 0, 0]], dtype=np.uint8)
self.detected_masks = np.stack(
[detected_masks_0, detected_masks_1, detected_masks_2], axis=0)
self.groundtruth_boxes = np.array(
[[0, 0, 1, 1], [0, 0, 5, 5], [10, 10, 20, 20]], dtype=float)
groundtruth_masks_0 = np.array(
[[1, 0, 0, 0], [1, 0, 0, 0], [1, 0, 0, 0]], dtype=np.uint8)
groundtruth_masks_1 = np.array(
[[0, 0, 1, 0], [0, 0, 1, 0], [0, 0, 1, 0]], dtype=np.uint8)
groundtruth_masks_2 = np.array(
[[0, 1, 0, 0], [0, 1, 0, 0], [0, 1, 0, 0]], dtype=np.uint8)
self.groundtruth_masks = np.stack(
[groundtruth_masks_0, groundtruth_masks_1, groundtruth_masks_2], axis=0)
def test_match_to_non_group_of_and_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, True, True], dtype=bool)
expected_scores = np.array([0.8, 0.6], dtype=float)
expected_tp_fp_labels = np.array([1.0, self.group_of_weight], dtype=float)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_match_to_non_group_of_and_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, True, True], dtype=bool)
expected_scores = np.array([0.6, 0.8, 0.5], dtype=float)
expected_tp_fp_labels = np.array(
[1.0, self.group_of_weight, self.group_of_weight], dtype=float)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=self.groundtruth_masks)
tf.logging.info(
"test_mask_match_to_non_group_of_and_group_of_box {} {}".format(
tp_fp_labels, expected_tp_fp_labels))
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_match_two_to_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[True, False, True], dtype=bool)
expected_scores = np.array([0.5, 0.8], dtype=float)
expected_tp_fp_labels = np.array([0.0, self.group_of_weight], dtype=float)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
tf.logging.info("test_match_two_to_group_of_box {} {}".format(
tp_fp_labels, expected_tp_fp_labels))
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_match_two_to_group_of_box(self):
groundtruth_groundtruth_is_difficult_list = np.array(
[False, False, False], dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[True, False, True], dtype=bool)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array(
[1.0, self.group_of_weight, self.group_of_weight], dtype=float)
scores, tp_fp_labels = self.eval._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
self.groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=self.groundtruth_masks)
tf.logging.info("test_mask_match_two_to_group_of_box {} {}".format(
tp_fp_labels, expected_tp_fp_labels))
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
class SingleClassTpFpNoDifficultBoxesTest(tf.test.TestCase):
def setUp(self):
num_groundtruth_classes = 1
matching_iou_threshold_high_iou = 0.5
matching_iou_threshold_low_iou = 0.1
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
self.eval_high_iou = per_image_evaluation.PerImageEvaluation(
num_groundtruth_classes, matching_iou_threshold_high_iou,
nms_iou_threshold, nms_max_output_boxes)
self.eval_low_iou = per_image_evaluation.PerImageEvaluation(
num_groundtruth_classes, matching_iou_threshold_low_iou,
nms_iou_threshold, nms_max_output_boxes)
self.detected_boxes = np.array([[0, 0, 1, 1], [0, 0, 2, 2], [0, 0, 3, 3]],
dtype=float)
self.detected_scores = np.array([0.6, 0.8, 0.5], dtype=float)
detected_masks_0 = np.array([[0, 1, 1, 0],
[0, 0, 1, 0],
[0, 0, 0, 0]], dtype=np.uint8)
detected_masks_1 = np.array([[1, 0, 0, 0],
[1, 1, 0, 0],
[0, 0, 0, 0]], dtype=np.uint8)
detected_masks_2 = np.array([[0, 0, 0, 0],
[0, 1, 1, 0],
[0, 1, 0, 0]], dtype=np.uint8)
self.detected_masks = np.stack(
[detected_masks_0, detected_masks_1, detected_masks_2], axis=0)
def test_no_true_positives(self):
groundtruth_boxes = np.array([[100, 100, 105, 105]], dtype=float)
groundtruth_groundtruth_is_difficult_list = np.zeros(1, dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array([False], dtype=bool)
scores, tp_fp_labels = self.eval_high_iou._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_no_true_positives(self):
groundtruth_boxes = np.array([[100, 100, 105, 105]], dtype=float)
groundtruth_masks_0 = np.array([[1, 1, 1, 1],
[1, 1, 1, 1],
[1, 1, 1, 1]], dtype=np.uint8)
groundtruth_masks = np.stack([groundtruth_masks_0], axis=0)
groundtruth_groundtruth_is_difficult_list = np.zeros(1, dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array([False], dtype=bool)
scores, tp_fp_labels = self.eval_high_iou._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=groundtruth_masks)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_one_true_positives_with_large_iou_threshold(self):
groundtruth_boxes = np.array([[0, 0, 1, 1]], dtype=float)
groundtruth_groundtruth_is_difficult_list = np.zeros(1, dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array([False], dtype=bool)
scores, tp_fp_labels = self.eval_high_iou._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, True, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_mask_one_true_positives_with_large_iou_threshold(self):
groundtruth_boxes = np.array([[0, 0, 1, 1]], dtype=float)
groundtruth_masks_0 = np.array([[1, 0, 0, 0],
[1, 1, 0, 0],
[0, 0, 0, 0]], dtype=np.uint8)
groundtruth_masks = np.stack([groundtruth_masks_0], axis=0)
groundtruth_groundtruth_is_difficult_list = np.zeros(1, dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array([False], dtype=bool)
scores, tp_fp_labels = self.eval_high_iou._compute_tp_fp_for_single_class(
self.detected_boxes,
self.detected_scores,
groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list,
detected_masks=self.detected_masks,
groundtruth_masks=groundtruth_masks)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([True, False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_one_true_positives_with_very_small_iou_threshold(self):
groundtruth_boxes = np.array([[0, 0, 1, 1]], dtype=float)
groundtruth_groundtruth_is_difficult_list = np.zeros(1, dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array([False], dtype=bool)
scores, tp_fp_labels = self.eval_low_iou._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([True, False, False], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
def test_two_true_positives_with_large_iou_threshold(self):
groundtruth_boxes = np.array([[0, 0, 1, 1], [0, 0, 3.5, 3.5]], dtype=float)
groundtruth_groundtruth_is_difficult_list = np.zeros(2, dtype=bool)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, False], dtype=bool)
scores, tp_fp_labels = self.eval_high_iou._compute_tp_fp_for_single_class(
self.detected_boxes, self.detected_scores, groundtruth_boxes,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = np.array([0.8, 0.6, 0.5], dtype=float)
expected_tp_fp_labels = np.array([False, True, True], dtype=bool)
self.assertTrue(np.allclose(expected_scores, scores))
self.assertTrue(np.allclose(expected_tp_fp_labels, tp_fp_labels))
class MultiClassesTpFpTest(tf.test.TestCase):
def test_tp_fp(self):
num_groundtruth_classes = 3
matching_iou_threshold = 0.5
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
eval1 = per_image_evaluation.PerImageEvaluation(num_groundtruth_classes,
matching_iou_threshold,
nms_iou_threshold,
nms_max_output_boxes)
detected_boxes = np.array([[0, 0, 1, 1], [10, 10, 5, 5], [0, 0, 2, 2],
[5, 10, 10, 5], [10, 5, 5, 10], [0, 0, 3, 3]],
dtype=float)
detected_scores = np.array([0.8, 0.1, 0.8, 0.9, 0.7, 0.8], dtype=float)
detected_class_labels = np.array([0, 1, 1, 2, 0, 2], dtype=int)
groundtruth_boxes = np.array([[0, 0, 1, 1], [0, 0, 3.5, 3.5]], dtype=float)
groundtruth_class_labels = np.array([0, 2], dtype=int)
groundtruth_groundtruth_is_difficult_list = np.zeros(2, dtype=float)
groundtruth_groundtruth_is_group_of_list = np.array(
[False, False], dtype=bool)
scores, tp_fp_labels, _ = eval1.compute_object_detection_metrics(
detected_boxes, detected_scores, detected_class_labels,
groundtruth_boxes, groundtruth_class_labels,
groundtruth_groundtruth_is_difficult_list,
groundtruth_groundtruth_is_group_of_list)
expected_scores = [np.array([0.8], dtype=float)] * 3
expected_tp_fp_labels = [np.array([True]), np.array([False]), np.array([True
])]
for i in range(len(expected_scores)):
self.assertTrue(np.allclose(expected_scores[i], scores[i]))
self.assertTrue(np.array_equal(expected_tp_fp_labels[i], tp_fp_labels[i]))
class CorLocTest(tf.test.TestCase):
def test_compute_corloc_with_normal_iou_threshold(self):
num_groundtruth_classes = 3
matching_iou_threshold = 0.5
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
eval1 = per_image_evaluation.PerImageEvaluation(num_groundtruth_classes,
matching_iou_threshold,
nms_iou_threshold,
nms_max_output_boxes)
detected_boxes = np.array([[0, 0, 1, 1], [0, 0, 2, 2], [0, 0, 3, 3],
[0, 0, 5, 5]], dtype=float)
detected_scores = np.array([0.9, 0.9, 0.1, 0.9], dtype=float)
detected_class_labels = np.array([0, 1, 0, 2], dtype=int)
groundtruth_boxes = np.array([[0, 0, 1, 1], [0, 0, 3, 3], [0, 0, 6, 6]],
dtype=float)
groundtruth_class_labels = np.array([0, 0, 2], dtype=int)
is_class_correctly_detected_in_image = eval1._compute_cor_loc(
detected_boxes, detected_scores, detected_class_labels,
groundtruth_boxes, groundtruth_class_labels)
expected_result = np.array([1, 0, 1], dtype=int)
self.assertTrue(np.array_equal(expected_result,
is_class_correctly_detected_in_image))
def test_compute_corloc_with_very_large_iou_threshold(self):
num_groundtruth_classes = 3
matching_iou_threshold = 0.9
nms_iou_threshold = 1.0
nms_max_output_boxes = 10000
eval1 = per_image_evaluation.PerImageEvaluation(num_groundtruth_classes,
matching_iou_threshold,
nms_iou_threshold,
nms_max_output_boxes)
detected_boxes = np.array([[0, 0, 1, 1], [0, 0, 2, 2], [0, 0, 3, 3],
[0, 0, 5, 5]], dtype=float)
detected_scores = np.array([0.9, 0.9, 0.1, 0.9], dtype=float)
detected_class_labels = np.array([0, 1, 0, 2], dtype=int)
groundtruth_boxes = np.array([[0, 0, 1, 1], [0, 0, 3, 3], [0, 0, 6, 6]],
dtype=float)
groundtruth_class_labels = np.array([0, 0, 2], dtype=int)
is_class_correctly_detected_in_image = eval1._compute_cor_loc(
detected_boxes, detected_scores, detected_class_labels,
groundtruth_boxes, groundtruth_class_labels)
expected_result = np.array([1, 0, 0], dtype=int)
self.assertTrue(np.array_equal(expected_result,
is_class_correctly_detected_in_image))
if __name__ == "__main__":
tf.test.main()
|
TensorFlow/Detection/SSD/models/research/object_detection/utils | utils | metrics | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Functions for computing metrics like precision, recall, CorLoc and etc."""
from __future__ import division
import numpy as np
def compute_precision_recall(scores, labels, num_gt):
"""Compute precision and recall.
Args:
scores: A float numpy array representing detection score
labels: A float numpy array representing weighted true/false positive labels
num_gt: Number of ground truth instances
Raises:
ValueError: if the input is not of the correct format
Returns:
precision: Fraction of positive instances over detected ones. This value is
None if no ground truth labels are present.
recall: Fraction of detected positive instance over all positive instances.
This value is None if no ground truth labels are present.
"""
if not isinstance(labels, np.ndarray) or len(labels.shape) != 1:
raise ValueError("labels must be single dimension numpy array")
if labels.dtype != np.float and labels.dtype != np.bool:
raise ValueError("labels type must be either bool or float")
if not isinstance(scores, np.ndarray) or len(scores.shape) != 1:
raise ValueError("scores must be single dimension numpy array")
if num_gt < np.sum(labels):
raise ValueError("Number of true positives must be smaller than num_gt.")
if len(scores) != len(labels):
raise ValueError("scores and labels must be of the same size.")
if num_gt == 0:
return None, None
sorted_indices = np.argsort(scores)
sorted_indices = sorted_indices[::-1]
true_positive_labels = labels[sorted_indices]
false_positive_labels = (true_positive_labels <= 0).astype(float)
cum_true_positives = np.cumsum(true_positive_labels)
cum_false_positives = np.cumsum(false_positive_labels)
precision = cum_true_positives.astype(float) / (
cum_true_positives + cum_false_positives)
recall = cum_true_positives.astype(float) / num_gt
return precision, recall
def compute_average_precision(precision, recall):
"""Compute Average Precision according to the definition in VOCdevkit.
Precision is modified to ensure that it does not decrease as recall
decrease.
Args:
precision: A float [N, 1] numpy array of precisions
recall: A float [N, 1] numpy array of recalls
Raises:
ValueError: if the input is not of the correct format
Returns:
average_precison: The area under the precision recall curve. NaN if
precision and recall are None.
"""
if precision is None:
if recall is not None:
raise ValueError("If precision is None, recall must also be None")
return np.NAN
if not isinstance(precision, np.ndarray) or not isinstance(
recall, np.ndarray):
raise ValueError("precision and recall must be numpy array")
if precision.dtype != np.float or recall.dtype != np.float:
raise ValueError("input must be float numpy array.")
if len(precision) != len(recall):
raise ValueError("precision and recall must be of the same size.")
if not precision.size:
return 0.0
if np.amin(precision) < 0 or np.amax(precision) > 1:
raise ValueError("Precision must be in the range of [0, 1].")
if np.amin(recall) < 0 or np.amax(recall) > 1:
raise ValueError("recall must be in the range of [0, 1].")
if not all(recall[i] <= recall[i + 1] for i in range(len(recall) - 1)):
raise ValueError("recall must be a non-decreasing array")
recall = np.concatenate([[0], recall, [1]])
precision = np.concatenate([[0], precision, [0]])
# Preprocess precision to be a non-decreasing array
for i in range(len(precision) - 2, -1, -1):
precision[i] = np.maximum(precision[i], precision[i + 1])
indices = np.where(recall[1:] != recall[:-1])[0] + 1
average_precision = np.sum(
(recall[indices] - recall[indices - 1]) * precision[indices])
return average_precision
def compute_cor_loc(num_gt_imgs_per_class,
num_images_correctly_detected_per_class):
"""Compute CorLoc according to the definition in the following paper.
https://www.robots.ox.ac.uk/~vgg/rg/papers/deselaers-eccv10.pdf
Returns nans if there are no ground truth images for a class.
Args:
num_gt_imgs_per_class: 1D array, representing number of images containing
at least one object instance of a particular class
num_images_correctly_detected_per_class: 1D array, representing number of
images that are correctly detected at least one object instance of a
particular class
Returns:
corloc_per_class: A float numpy array represents the corloc score of each
class
"""
return np.where(
num_gt_imgs_per_class == 0, np.nan,
num_images_correctly_detected_per_class / num_gt_imgs_per_class)
def compute_median_rank_at_k(tp_fp_list, k):
"""Computes MedianRank@k, where k is the top-scoring labels.
Args:
tp_fp_list: a list of numpy arrays; each numpy array corresponds to the all
detection on a single image, where the detections are sorted by score in
descending order. Further, each numpy array element can have boolean or
float values. True positive elements have either value >0.0 or True;
any other value is considered false positive.
k: number of top-scoring proposals to take.
Returns:
median_rank: median rank of all true positive proposals among top k by
score.
"""
ranks = []
for i in range(len(tp_fp_list)):
ranks.append(
np.where(tp_fp_list[i][0:min(k, tp_fp_list[i].shape[0])] > 0)[0])
concatenated_ranks = np.concatenate(ranks)
return np.median(concatenated_ranks)
def compute_recall_at_k(tp_fp_list, num_gt, k):
"""Computes Recall@k, MedianRank@k, where k is the top-scoring labels.
Args:
tp_fp_list: a list of numpy arrays; each numpy array corresponds to the all
detection on a single image, where the detections are sorted by score in
descending order. Further, each numpy array element can have boolean or
float values. True positive elements have either value >0.0 or True;
any other value is considered false positive.
num_gt: number of groundtruth anotations.
k: number of top-scoring proposals to take.
Returns:
recall: recall evaluated on the top k by score detections.
"""
tp_fp_eval = []
for i in range(len(tp_fp_list)):
tp_fp_eval.append(tp_fp_list[i][0:min(k, tp_fp_list[i].shape[0])])
tp_fp_eval = np.concatenate(tp_fp_eval)
return np.sum(tp_fp_eval) / num_gt
|
TensorFlow2/Recommendation/WideAndDeep/scripts | scripts | preproc | #!/bin/bash
# Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
set -e
function usage() {
echo "Usage: bash scripts/preproc.sh"
}
if [ ! -d "scripts" ] || [ ! "$(ls -A 'scripts')" ]; then
echo "You are probably calling this script from wrong directory"
usage
exit 1
fi
time python -m data.outbrain.nvtabular.preproc "$@"
|
TensorFlow/Detection/SSD/models/research/object_detection/utils | utils | dataset_util_test | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Tests for object_detection.utils.dataset_util."""
import os
import tensorflow as tf
from object_detection.utils import dataset_util
class DatasetUtilTest(tf.test.TestCase):
def test_read_examples_list(self):
example_list_data = """example1 1\nexample2 2"""
example_list_path = os.path.join(self.get_temp_dir(), 'examples.txt')
with tf.gfile.Open(example_list_path, 'wb') as f:
f.write(example_list_data)
examples = dataset_util.read_examples_list(example_list_path)
self.assertListEqual(['example1', 'example2'], examples)
if __name__ == '__main__':
tf.test.main()
|
PyTorch/LanguageModeling/Transformer-XL/pytorch/scripts/docker | docker | build | #!/bin/bash
# Copyright (c) 2019-2020, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
docker build . --network=host --rm -t transformer-xl:latest
|
TensorFlow/Segmentation/UNet_3D_Medical/scripts | scripts | unet3d_train_full | # Copyright (c) 2020, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# This script launches 3D-UNet run 5-fold cross-validation FP32 training for 16000 iterations each.
# Usage:
# bash examples/unet3d_train_full.sh <number/of/gpus> <path/to/dataset> <path/to/results/directory> <batch/size>
horovodrun -np $1 python main.py --data_dir $2 --model_dir $3 --log_dir $3/log.json --exec_mode train_and_evaluate --max_steps 16000 --augment --batch_size $4 --fold 0 --use_xla > $3/log_FP32_$1GPU_fold0.txt
horovodrun -np $1 python main.py --data_dir $2 --model_dir $3 --log_dir $3/log.json --exec_mode train_and_evaluate --max_steps 16000 --augment --batch_size $4 --fold 1 --use_xla > $3/log_FP32_$1GPU_fold1.txt
horovodrun -np $1 python main.py --data_dir $2 --model_dir $3 --log_dir $3/log.json --exec_mode train_and_evaluate --max_steps 16000 --augment --batch_size $4 --fold 2 --use_xla > $3/log_FP32_$1GPU_fold2.txt
horovodrun -np $1 python main.py --data_dir $2 --model_dir $3 --log_dir $3/log.json --exec_mode train_and_evaluate --max_steps 16000 --augment --batch_size $4 --fold 3 --use_xla > $3/log_FP32_$1GPU_fold3.txt
horovodrun -np $1 python main.py --data_dir $2 --model_dir $3 --log_dir $3/log.json --exec_mode train_and_evaluate --max_steps 16000 --augment --batch_size $4 --fold 4 --use_xla > $3/log_FP32_$1GPU_fold4.txt
python runtime/parse_results.py --model_dir $3 --env FP32_$1GPU
|
PyTorch/SpeechSynthesis/HiFiGAN | HiFiGAN | inference | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import argparse
import itertools
import sys
import time
import warnings
from pathlib import Path
from tqdm import tqdm
import torch
import numpy as np
from scipy.stats import norm
from scipy.io.wavfile import write
from torch.nn.functional import l1_loss
from torch.nn.utils.rnn import pad_sequence
import dllogger as DLLogger
from dllogger import StdOutBackend, JSONStreamBackend, Verbosity
import models
from common import gpu_affinity
from common.tb_dllogger import stdout_metric_format, unique_log_fpath
from common.text import cmudict
from common.text.text_processing import TextProcessing
from common.utils import l2_promote
from fastpitch.pitch_transform import pitch_transform_custom
from hifigan.data_function import MAX_WAV_VALUE, mel_spectrogram
from hifigan.logging import init_inference_metadata
from hifigan.models import Denoiser
CHECKPOINT_SPECIFIC_ARGS = [
'sampling_rate', 'hop_length', 'win_length', 'p_arpabet', 'text_cleaners',
'symbol_set', 'max_wav_value', 'prepend_space_to_text',
'append_space_to_text']
def parse_args(parser):
"""
Parse commandline arguments.
"""
parser.add_argument('-i', '--input', type=str, required=True,
help='Full path to the input text '
'(phareses separated by newlines)')
parser.add_argument('-o', '--output', default=None,
help='Output folder to save audio (file per phrase)')
parser.add_argument('--log-file', type=str, default=None,
help='Path to a DLLogger log file')
parser.add_argument('--save-mels', action='store_true',
help='Save generator outputs to disk')
parser.add_argument('--cuda', action='store_true',
help='Run inference on a GPU using CUDA')
parser.add_argument('--cudnn-benchmark', action='store_true',
help='Enable cudnn benchmark mode')
parser.add_argument('--l2-promote', action='store_true',
help='Increase max fetch granularity of GPU L2 cache')
parser.add_argument('--fastpitch', type=str, default=None, required=False,
help='Full path to the spectrogram generator .pt file '
'(skip to synthesize from ground truth mels)')
parser.add_argument('--waveglow', type=str, default=None, required=False,
help='Full path to a WaveGlow model .pt file')
parser.add_argument('-s', '--waveglow-sigma-infer', default=0.9, type=float,
help='WaveGlow sigma')
parser.add_argument('--hifigan', type=str, default=None, required=False,
help='Full path to a HiFi-GAN model .pt file')
parser.add_argument('-d', '--denoising-strength', default=0.0, type=float,
help='Capture and subtract model bias to enhance audio')
parser.add_argument('--hop-length', type=int, default=256,
help='STFT hop length for estimating audio length from mel size')
parser.add_argument('--win-length', type=int, default=1024,
help='STFT win length for denoiser and mel loss')
parser.add_argument('-sr', '--sampling-rate', default=22050, type=int,
choices=[22050, 44100], help='Sampling rate')
parser.add_argument('--max_wav_value', default=32768.0, type=float,
help='Maximum audiowave value')
parser.add_argument('--amp', action='store_true',
help='Inference with AMP')
parser.add_argument('-bs', '--batch-size', type=int, default=64)
parser.add_argument('--warmup-steps', type=int, default=0,
help='Warmup iterations before measuring performance')
parser.add_argument('--repeats', type=int, default=1,
help='Repeat inference for benchmarking')
parser.add_argument('--torchscript', action='store_true',
help='Run inference with TorchScript model (convert to TS if needed)')
parser.add_argument('--checkpoint-format', type=str,
choices=['pyt', 'ts'], default='pyt',
help='Input checkpoint format (PyT or TorchScript)')
parser.add_argument('--torch-tensorrt', action='store_true',
help='Run inference with Torch-TensorRT model (compile beforehand)')
parser.add_argument('--report-mel-loss', action='store_true',
help='Report mel loss in metrics')
parser.add_argument('--ema', action='store_true',
help='Use EMA averaged model (if saved in checkpoints)')
parser.add_argument('--dataset-path', type=str,
help='Path to dataset (for loading extra data fields)')
parser.add_argument('--speaker', type=int, default=0,
help='Speaker ID for a multi-speaker model')
parser.add_argument('--affinity', type=str, default='single',
choices=['socket', 'single', 'single_unique',
'socket_unique_interleaved',
'socket_unique_continuous',
'disabled'],
help='type of CPU affinity')
transf = parser.add_argument_group('transform')
transf.add_argument('--fade-out', type=int, default=6,
help='Number of fadeout frames at the end')
transf.add_argument('--pace', type=float, default=1.0,
help='Adjust the pace of speech')
transf.add_argument('--pitch-transform-flatten', action='store_true',
help='Flatten the pitch')
transf.add_argument('--pitch-transform-invert', action='store_true',
help='Invert the pitch wrt mean value')
transf.add_argument('--pitch-transform-amplify', type=float, default=1.0,
help='Multiplicative amplification of pitch variability. '
'Typical values are in the range (1.0, 3.0).')
transf.add_argument('--pitch-transform-shift', type=float, default=0.0,
help='Raise/lower the pitch by <hz>')
transf.add_argument('--pitch-transform-custom', action='store_true',
help='Apply the transform from pitch_transform.py')
txt = parser.add_argument_group('Text processing parameters')
txt.add_argument('--text-cleaners', type=str, nargs='*',
default=['english_cleaners_v2'],
help='Type of text cleaners for input text')
txt.add_argument('--symbol-set', type=str, default='english_basic',
help='Define symbol set for input text')
txt.add_argument('--p-arpabet', type=float, default=0.0, help='')
txt.add_argument('--heteronyms-path', type=str,
default='data/cmudict/heteronyms', help='')
txt.add_argument('--cmudict-path', type=str,
default='data/cmudict/cmudict-0.7b', help='')
return parser
def load_fields(fpath):
lines = [l.strip() for l in open(fpath, encoding='utf-8')]
if fpath.endswith('.tsv'):
columns = lines[0].split('\t')
fields = list(zip(*[t.split('\t') for t in lines[1:]]))
else:
columns = ['text']
fields = [lines]
return {c: f for c, f in zip(columns, fields)}
def prepare_input_sequence(fields, device, symbol_set, text_cleaners,
batch_size=128, dataset=None, load_mels=False,
load_pitch=False, p_arpabet=0.0):
tp = TextProcessing(symbol_set, text_cleaners, p_arpabet=p_arpabet)
fields['text'] = [torch.LongTensor(tp.encode_text(text))
for text in fields['text']]
order = np.argsort([-t.size(0) for t in fields['text']])
fields['text'] = [fields['text'][i] for i in order]
fields['text_lens'] = torch.LongTensor([t.size(0) for t in fields['text']])
for t in fields['text']:
print(tp.sequence_to_text(t.numpy()))
if load_mels:
assert 'mel' in fields
assert dataset is not None
fields['mel'] = [
torch.load(Path(dataset, fields['mel'][i])).t() for i in order]
fields['mel_lens'] = torch.LongTensor([t.size(0) for t in fields['mel']])
if load_pitch:
assert 'pitch' in fields
fields['pitch'] = [
torch.load(Path(dataset, fields['pitch'][i])) for i in order]
fields['pitch_lens'] = torch.LongTensor([t.size(0) for t in fields['pitch']])
if 'output' in fields:
fields['output'] = [fields['output'][i] for i in order]
# cut into batches & pad
batches = []
for b in range(0, len(order), batch_size):
batch = {f: values[b:b+batch_size] for f, values in fields.items()}
for f in batch:
if f == 'text':
batch[f] = pad_sequence(batch[f], batch_first=True)
elif f == 'mel' and load_mels:
batch[f] = pad_sequence(batch[f], batch_first=True).permute(0, 2, 1)
elif f == 'pitch' and load_pitch:
batch[f] = pad_sequence(batch[f], batch_first=True)
if type(batch[f]) is torch.Tensor:
batch[f] = batch[f].to(device)
batches.append(batch)
return batches
def build_pitch_transformation(args):
if args.pitch_transform_custom:
def custom_(pitch, pitch_lens, mean, std):
return (pitch_transform_custom(pitch * std + mean, pitch_lens)
- mean) / std
return custom_
fun = 'pitch'
if args.pitch_transform_flatten:
fun = f'({fun}) * 0.0'
if args.pitch_transform_invert:
fun = f'({fun}) * -1.0'
if args.pitch_transform_amplify != 1.0:
ampl = args.pitch_transform_amplify
fun = f'({fun}) * {ampl}'
if args.pitch_transform_shift != 0.0:
hz = args.pitch_transform_shift
fun = f'({fun}) + {hz} / std'
if fun == 'pitch':
return None
return eval(f'lambda pitch, pitch_lens, mean, std: {fun}')
def setup_mel_loss_reporting(args, voc_train_setup):
if args.denoising_strength > 0.0:
print('WARNING: denoising will be included in vocoder mel loss')
num_mels = voc_train_setup.get('num_mels', 80)
fmin = voc_train_setup.get('mel_fmin', 0)
fmax = voc_train_setup.get('mel_fmax', 8000) # not mel_fmax_loss
def compute_audio_mel_loss(gen_audios, gt_mels, mel_lens):
gen_audios /= MAX_WAV_VALUE
total_loss = 0
for gen_audio, gt_mel, mel_len in zip(gen_audios, gt_mels, mel_lens):
mel_len = mel_len.item()
gen_audio = gen_audio[None, :mel_len * args.hop_length]
gen_mel = mel_spectrogram(gen_audio, args.win_length, num_mels,
args.sampling_rate, args.hop_length,
args.win_length, fmin, fmax)[0]
total_loss += l1_loss(gen_mel, gt_mel[:, :mel_len])
return total_loss.item()
return compute_audio_mel_loss
def compute_mel_loss(mels, lens, gt_mels, gt_lens):
total_loss = 0
for mel, len_, gt_mel, gt_len in zip(mels, lens, gt_mels, gt_lens):
min_len = min(len_, gt_len)
total_loss += l1_loss(gt_mel[:, :min_len], mel[:, :min_len])
return total_loss.item()
class MeasureTime(list):
def __init__(self, *args, cuda=True, **kwargs):
super(MeasureTime, self).__init__(*args, **kwargs)
self.cuda = cuda
def __enter__(self):
if self.cuda:
torch.cuda.synchronize()
self.t0 = time.time()
def __exit__(self, exc_type, exc_value, exc_traceback):
if self.cuda:
torch.cuda.synchronize()
self.append(time.time() - self.t0)
def __add__(self, other):
assert len(self) == len(other)
return MeasureTime((sum(ab) for ab in zip(self, other)), cuda=self.cuda)
def main():
"""
Launches text-to-speech inference on a single GPU.
"""
parser = argparse.ArgumentParser(description='PyTorch FastPitch Inference',
allow_abbrev=False)
parser = parse_args(parser)
args, unk_args = parser.parse_known_args()
if args.affinity != 'disabled':
nproc_per_node = torch.cuda.device_count()
# print(nproc_per_node)
affinity = gpu_affinity.set_affinity(
0,
nproc_per_node,
args.affinity
)
print(f'Thread affinity: {affinity}')
if args.l2_promote:
l2_promote()
torch.backends.cudnn.benchmark = args.cudnn_benchmark
if args.output is not None:
Path(args.output).mkdir(parents=False, exist_ok=True)
log_fpath = args.log_file or str(Path(args.output, 'nvlog_infer.json'))
DLLogger.init(backends=[
JSONStreamBackend(Verbosity.DEFAULT, log_fpath, append=True),
JSONStreamBackend(Verbosity.DEFAULT, unique_log_fpath(log_fpath)),
StdOutBackend(Verbosity.VERBOSE, metric_format=stdout_metric_format)
])
init_inference_metadata(args.batch_size)
[DLLogger.log("PARAMETER", {k: v}) for k, v in vars(args).items()]
device = torch.device('cuda' if args.cuda else 'cpu')
gen_train_setup = {}
voc_train_setup = {}
generator = None
vocoder = None
denoiser = None
is_ts_based_infer = args.torch_tensorrt or args.torchscript
assert args.checkpoint_format == 'pyt' or is_ts_based_infer, \
'TorchScript checkpoint can be used only for TS or Torch-TRT' \
' inference. Please set --torchscript or --torch-tensorrt flag.'
assert args.waveglow is None or args.hifigan is None, \
"Specify a single vocoder model"
def _load_pyt_or_ts_model(model_name, ckpt_path):
if args.checkpoint_format == 'ts':
model = models.load_and_setup_ts_model(model_name, ckpt_path,
args.amp, device)
model_train_setup = {}
return model, model_train_setup
model, _, model_train_setup = models.load_and_setup_model(
model_name, parser, ckpt_path, args.amp, device,
unk_args=unk_args, forward_is_infer=True, jitable=is_ts_based_infer)
if is_ts_based_infer:
model = torch.jit.script(model)
return model, model_train_setup
if args.fastpitch is not None:
gen_name = 'fastpitch'
generator, gen_train_setup = _load_pyt_or_ts_model('FastPitch',
args.fastpitch)
if args.waveglow is not None:
voc_name = 'waveglow'
with warnings.catch_warnings():
warnings.simplefilter("ignore")
vocoder, _, voc_train_setup = models.load_and_setup_model(
'WaveGlow', parser, args.waveglow, args.amp, device,
unk_args=unk_args, forward_is_infer=True, jitable=False)
if args.denoising_strength > 0.0:
denoiser = Denoiser(vocoder, sigma=0.0,
win_length=args.win_length).to(device)
# if args.torchscript:
# vocoder = torch.jit.script(vocoder)
def generate_audio(mel):
audios = vocoder(mel, sigma=args.waveglow_sigma_infer)
if denoiser is not None:
audios = denoiser(audios.float(), args.denoising_strength).squeeze(1)
return audios
elif args.hifigan is not None:
voc_name = 'hifigan'
vocoder, voc_train_setup = _load_pyt_or_ts_model('HiFi-GAN',
args.hifigan)
if args.denoising_strength > 0.0:
denoiser = Denoiser(vocoder, win_length=args.win_length).to(device)
if args.torch_tensorrt:
vocoder = models.convert_ts_to_trt('HiFi-GAN', vocoder, parser,
args.amp, unk_args)
def generate_audio(mel):
audios = vocoder(mel).float()
if denoiser is not None:
audios = denoiser(audios.squeeze(1), args.denoising_strength)
return audios.squeeze(1) * args.max_wav_value
if len(unk_args) > 0:
raise ValueError(f'Invalid options {unk_args}')
for k in CHECKPOINT_SPECIFIC_ARGS:
v1 = gen_train_setup.get(k, None)
v2 = voc_train_setup.get(k, None)
assert v1 is None or v2 is None or v1 == v2, \
f'{k} mismatch in spectrogram generator and vocoder'
val = v1 or v2
if val and getattr(args, k) != val:
src = 'generator' if v2 is None else 'vocoder'
print(f'Overwriting args.{k}={getattr(args, k)} with {val} '
f'from {src} checkpoint.')
setattr(args, k, val)
gen_kw = {'pace': args.pace,
'speaker': args.speaker,
'pitch_tgt': None,
'pitch_transform': build_pitch_transformation(args)}
if is_ts_based_infer and generator is not None:
gen_kw.pop('pitch_transform')
print('Note: --pitch-transform-* args are disabled with TorchScript. '
'To condition on pitch, pass pitch_tgt as input.')
if args.p_arpabet > 0.0:
cmudict.initialize(args.cmudict_path, args.heteronyms_path)
if args.report_mel_loss:
mel_loss_fn = setup_mel_loss_reporting(args, voc_train_setup)
fields = load_fields(args.input)
batches = prepare_input_sequence(
fields, device, args.symbol_set, args.text_cleaners, args.batch_size,
args.dataset_path, load_mels=(generator is None or args.report_mel_loss),
p_arpabet=args.p_arpabet)
cycle = itertools.cycle(batches)
# Use real data rather than synthetic - FastPitch predicts len
for _ in tqdm(range(args.warmup_steps), 'Warmup'):
with torch.no_grad():
b = next(cycle)
if generator is not None:
mel, *_ = generator(b['text'])
else:
mel, mel_lens = b['mel'], b['mel_lens']
if args.amp:
mel = mel.half()
if vocoder is not None:
audios = generate_audio(mel)
gen_measures = MeasureTime(cuda=args.cuda)
vocoder_measures = MeasureTime(cuda=args.cuda)
all_utterances = 0
all_samples = 0
all_batches = 0
all_letters = 0
all_frames = 0
gen_mel_loss_sum = 0
voc_mel_loss_sum = 0
reps = args.repeats
log_enabled = reps == 1
log = lambda s, d: DLLogger.log(step=s, data=d) if log_enabled else None
for rep in (tqdm(range(reps), 'Inference') if reps > 1 else range(reps)):
for b in batches:
if generator is None:
mel, mel_lens = b['mel'], b['mel_lens']
if args.amp:
mel = mel.half()
else:
with torch.no_grad(), gen_measures:
mel, mel_lens, *_ = generator(b['text'], **gen_kw)
if args.report_mel_loss:
gen_mel_loss_sum += compute_mel_loss(
mel, mel_lens, b['mel'], b['mel_lens'])
gen_infer_perf = mel.size(0) * mel.size(2) / gen_measures[-1]
all_letters += b['text_lens'].sum().item()
all_frames += mel.size(0) * mel.size(2)
log(rep, {f"{gen_name}_frames/s": gen_infer_perf})
log(rep, {f"{gen_name}_latency": gen_measures[-1]})
if args.save_mels:
for i, mel_ in enumerate(mel):
m = mel_[:, :mel_lens[i].item()].permute(1, 0)
fname = b['output'][i] if 'output' in b else f'mel_{i}.npy'
mel_path = Path(args.output, Path(fname).stem + '.npy')
np.save(mel_path, m.cpu().numpy())
if vocoder is not None:
with torch.no_grad(), vocoder_measures:
audios = generate_audio(mel)
vocoder_infer_perf = (
audios.size(0) * audios.size(1) / vocoder_measures[-1])
log(rep, {f"{voc_name}_samples/s": vocoder_infer_perf})
log(rep, {f"{voc_name}_latency": vocoder_measures[-1]})
if args.report_mel_loss:
voc_mel_loss_sum += mel_loss_fn(audios, mel, mel_lens)
if args.output is not None and reps == 1:
for i, audio in enumerate(audios):
audio = audio[:mel_lens[i].item() * args.hop_length]
if args.fade_out:
fade_len = args.fade_out * args.hop_length
fade_w = torch.linspace(1.0, 0.0, fade_len)
audio[-fade_len:] *= fade_w.to(audio.device)
audio = audio / torch.max(torch.abs(audio))
fname = b['output'][i] if 'output' in b else f'audio_{i}.wav'
audio_path = Path(args.output, fname)
write(audio_path, args.sampling_rate, audio.cpu().numpy())
if generator is not None:
log(rep, {"latency": (gen_measures[-1] + vocoder_measures[-1])})
all_utterances += mel.size(0)
all_samples += mel_lens.sum().item() * args.hop_length
all_batches += 1
log_enabled = True
if generator is not None:
gm = np.sort(np.asarray(gen_measures))
rtf = all_samples / (all_utterances * gm.mean() * args.sampling_rate)
rtf_at = all_samples / (all_batches * gm.mean() * args.sampling_rate)
log((), {f"avg_{gen_name}_tokens/s": all_letters / gm.sum()})
log((), {f"avg_{gen_name}_frames/s": all_frames / gm.sum()})
log((), {f"avg_{gen_name}_latency": gm.mean()})
log((), {f"avg_{gen_name}_RTF": rtf})
log((), {f"avg_{gen_name}_RTF@{args.batch_size}": rtf_at})
log((), {f"90%_{gen_name}_latency": gm.mean() + norm.ppf((1.0 + 0.90) / 2) * gm.std()})
log((), {f"95%_{gen_name}_latency": gm.mean() + norm.ppf((1.0 + 0.95) / 2) * gm.std()})
log((), {f"99%_{gen_name}_latency": gm.mean() + norm.ppf((1.0 + 0.99) / 2) * gm.std()})
if args.report_mel_loss:
log((), {f"avg_{gen_name}_mel-loss": gen_mel_loss_sum / all_utterances})
if vocoder is not None:
vm = np.sort(np.asarray(vocoder_measures))
rtf = all_samples / (all_utterances * vm.mean() * args.sampling_rate)
rtf_at = all_samples / (all_batches * vm.mean() * args.sampling_rate)
log((), {f"avg_{voc_name}_samples/s": all_samples / vm.sum()})
log((), {f"avg_{voc_name}_latency": vm.mean()})
log((), {f"avg_{voc_name}_RTF": rtf})
log((), {f"avg_{voc_name}_RTF@{args.batch_size}": rtf_at})
log((), {f"90%_{voc_name}_latency": vm.mean() + norm.ppf((1.0 + 0.90) / 2) * vm.std()})
log((), {f"95%_{voc_name}_latency": vm.mean() + norm.ppf((1.0 + 0.95) / 2) * vm.std()})
log((), {f"99%_{voc_name}_latency": vm.mean() + norm.ppf((1.0 + 0.99) / 2) * vm.std()})
if args.report_mel_loss:
log((), {f"avg_{voc_name}_mel-loss": voc_mel_loss_sum / all_utterances})
if generator is not None and vocoder is not None:
m = gm + vm
rtf = all_samples / (all_utterances * m.mean() * args.sampling_rate)
rtf_at = all_samples / (all_batches * m.mean() * args.sampling_rate)
log((), {"avg_samples/s": all_samples / m.sum()})
log((), {"avg_letters/s": all_letters / m.sum()})
log((), {"avg_latency": m.mean()})
log((), {"avg_RTF": rtf})
log((), {f"avg_RTF@{args.batch_size}": rtf_at})
log((), {"90%_latency": m.mean() + norm.ppf((1.0 + 0.90) / 2) * m.std()})
log((), {"95%_latency": m.mean() + norm.ppf((1.0 + 0.95) / 2) * m.std()})
log((), {"99%_latency": m.mean() + norm.ppf((1.0 + 0.99) / 2) * m.std()})
DLLogger.flush()
if __name__ == '__main__':
main()
|
PaddlePaddle/LanguageModeling/BERT/scripts/docker | docker | launch | #!/bin/bash
# Copyright (c) 2022 NVIDIA Corporation. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
CMD=${1:-/bin/bash}
NV_VISIBLE_DEVICES=${2:-"all"}
DOCKER_BRIDGE=${3:-"host"}
docker run -it --rm \
--gpus device=$NV_VISIBLE_DEVICES \
--net=$DOCKER_BRIDGE \
--shm-size=1g \
--ulimit memlock=-1 \
--ulimit stack=67108864 \
-v $PWD:/workspace/bert \
-v $PWD/results:/results \
bert $CMD
|
PyTorch/SpeechSynthesis/FastPitch/waveglow | waveglow | model | # *****************************************************************************
# Copyright (c) 2018, NVIDIA CORPORATION. All rights reserved.
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright
# notice, this list of conditions and the following disclaimer in the
# documentation and/or other materials provided with the distribution.
# * Neither the name of the NVIDIA CORPORATION nor the
# names of its contributors may be used to endorse or promote products
# derived from this software without specific prior written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
# ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
# WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
# DISCLAIMED. IN NO EVENT SHALL NVIDIA CORPORATION BE LIABLE FOR ANY
# DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
# (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
# LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
# ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
# SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
#
# *****************************************************************************
import torch
from torch.autograd import Variable
import torch.nn.functional as F
@torch.jit.script
def fused_add_tanh_sigmoid_multiply(input_a, input_b, n_channels):
n_channels_int = n_channels[0]
in_act = input_a + input_b
t_act = torch.tanh(in_act[:, :n_channels_int, :])
s_act = torch.sigmoid(in_act[:, n_channels_int:, :])
acts = t_act * s_act
return acts
class Invertible1x1Conv(torch.nn.Module):
"""
The layer outputs both the convolution, and the log determinant
of its weight matrix. If reverse=True it does convolution with
inverse
"""
def __init__(self, c):
super(Invertible1x1Conv, self).__init__()
self.conv = torch.nn.Conv1d(c, c, kernel_size=1, stride=1, padding=0,
bias=False)
# Sample a random orthonormal matrix to initialize weights
W = torch.qr(torch.FloatTensor(c, c).normal_())[0]
# Ensure determinant is 1.0 not -1.0
if torch.det(W) < 0:
W[:, 0] = -1 * W[:, 0]
W = W.view(c, c, 1)
W = W.contiguous()
self.conv.weight.data = W
def forward(self, z):
# shape
batch_size, group_size, n_of_groups = z.size()
W = self.conv.weight.squeeze()
# Forward computation
log_det_W = batch_size * n_of_groups * torch.logdet(W.unsqueeze(0).float()).squeeze()
z = self.conv(z)
return z, log_det_W
def infer(self, z):
# shape
batch_size, group_size, n_of_groups = z.size()
W = self.conv.weight.squeeze()
if not hasattr(self, 'W_inverse'):
# Reverse computation
W_inverse = W.float().inverse()
W_inverse = Variable(W_inverse[..., None])
if z.type() == 'torch.cuda.HalfTensor' or z.type() == 'torch.HalfTensor':
W_inverse = W_inverse.half()
self.W_inverse = W_inverse
z = F.conv1d(z, self.W_inverse, bias=None, stride=1, padding=0)
return z
class WN(torch.nn.Module):
"""
This is the WaveNet like layer for the affine coupling. The primary
difference from WaveNet is the convolutions need not be causal. There is
also no dilation size reset. The dilation only doubles on each layer
"""
def __init__(self, n_in_channels, n_mel_channels, n_layers, n_channels,
kernel_size):
super(WN, self).__init__()
assert(kernel_size % 2 == 1)
assert(n_channels % 2 == 0)
self.n_layers = n_layers
self.n_channels = n_channels
self.in_layers = torch.nn.ModuleList()
self.res_skip_layers = torch.nn.ModuleList()
self.cond_layers = torch.nn.ModuleList()
start = torch.nn.Conv1d(n_in_channels, n_channels, 1)
start = torch.nn.utils.weight_norm(start, name='weight')
self.start = start
# Initializing last layer to 0 makes the affine coupling layers
# do nothing at first. This helps with training stability
end = torch.nn.Conv1d(n_channels, 2 * n_in_channels, 1)
end.weight.data.zero_()
end.bias.data.zero_()
self.end = end
for i in range(n_layers):
dilation = 2 ** i
padding = int((kernel_size * dilation - dilation) / 2)
in_layer = torch.nn.Conv1d(n_channels, 2 * n_channels, kernel_size,
dilation=dilation, padding=padding)
in_layer = torch.nn.utils.weight_norm(in_layer, name='weight')
self.in_layers.append(in_layer)
cond_layer = torch.nn.Conv1d(n_mel_channels, 2 * n_channels, 1)
cond_layer = torch.nn.utils.weight_norm(cond_layer, name='weight')
self.cond_layers.append(cond_layer)
# last one is not necessary
if i < n_layers - 1:
res_skip_channels = 2 * n_channels
else:
res_skip_channels = n_channels
res_skip_layer = torch.nn.Conv1d(n_channels, res_skip_channels, 1)
res_skip_layer = torch.nn.utils.weight_norm(
res_skip_layer, name='weight')
self.res_skip_layers.append(res_skip_layer)
def forward(self, forward_input):
audio, spect = forward_input
audio = self.start(audio)
for i in range(self.n_layers):
acts = fused_add_tanh_sigmoid_multiply(
self.in_layers[i](audio),
self.cond_layers[i](spect),
torch.IntTensor([self.n_channels]))
res_skip_acts = self.res_skip_layers[i](acts)
if i < self.n_layers - 1:
audio = res_skip_acts[:, :self.n_channels, :] + audio
skip_acts = res_skip_acts[:, self.n_channels:, :]
else:
skip_acts = res_skip_acts
if i == 0:
output = skip_acts
else:
output = skip_acts + output
return self.end(output)
class WaveGlow(torch.nn.Module):
def __init__(self, n_mel_channels, n_flows, n_group, n_early_every,
n_early_size, WN_config):
super(WaveGlow, self).__init__()
self.upsample = torch.nn.ConvTranspose1d(n_mel_channels,
n_mel_channels,
1024, stride=256)
assert(n_group % 2 == 0)
self.n_flows = n_flows
self.n_group = n_group
self.n_early_every = n_early_every
self.n_early_size = n_early_size
self.WN = torch.nn.ModuleList()
self.convinv = torch.nn.ModuleList()
n_half = int(n_group / 2)
# Set up layers with the right sizes based on how many dimensions
# have been output already
n_remaining_channels = n_group
for k in range(n_flows):
if k % self.n_early_every == 0 and k > 0:
n_half = n_half - int(self.n_early_size / 2)
n_remaining_channels = n_remaining_channels - self.n_early_size
self.convinv.append(Invertible1x1Conv(n_remaining_channels))
self.WN.append(WN(n_half, n_mel_channels * n_group, **WN_config))
self.n_remaining_channels = n_remaining_channels
def forward(self, forward_input):
"""
forward_input[0] = mel_spectrogram: batch x n_mel_channels x frames
forward_input[1] = audio: batch x time
"""
spect, audio = forward_input
# Upsample spectrogram to size of audio
spect = self.upsample(spect)
assert(spect.size(2) >= audio.size(1))
if spect.size(2) > audio.size(1):
spect = spect[:, :, :audio.size(1)]
spect = spect.unfold(2, self.n_group, self.n_group).permute(0, 2, 1, 3)
spect = spect.contiguous().view(spect.size(0), spect.size(1), -1)
spect = spect.permute(0, 2, 1)
audio = audio.unfold(1, self.n_group, self.n_group).permute(0, 2, 1)
output_audio = []
log_s_list = []
log_det_W_list = []
for k in range(self.n_flows):
if k % self.n_early_every == 0 and k > 0:
output_audio.append(audio[:, :self.n_early_size, :])
audio = audio[:, self.n_early_size:, :]
audio, log_det_W = self.convinv[k](audio)
log_det_W_list.append(log_det_W)
n_half = int(audio.size(1) / 2)
audio_0 = audio[:, :n_half, :]
audio_1 = audio[:, n_half:, :]
output = self.WN[k]((audio_0, spect))
log_s = output[:, n_half:, :]
b = output[:, :n_half, :]
audio_1 = torch.exp(log_s) * audio_1 + b
log_s_list.append(log_s)
audio = torch.cat([audio_0, audio_1], 1)
output_audio.append(audio)
return torch.cat(output_audio, 1), log_s_list, log_det_W_list
def infer(self, spect, sigma=1.0):
spect = self.upsample(spect)
# trim conv artifacts. maybe pad spec to kernel multiple
time_cutoff = self.upsample.kernel_size[0] - self.upsample.stride[0]
spect = spect[:, :, :-time_cutoff]
spect = spect.unfold(2, self.n_group, self.n_group).permute(0, 2, 1, 3)
spect = spect.contiguous().view(spect.size(0), spect.size(1), -1)
spect = spect.permute(0, 2, 1)
audio = torch.randn(spect.size(0),
self.n_remaining_channels,
spect.size(2), device=spect.device).to(spect.dtype)
audio = torch.autograd.Variable(sigma * audio)
for k in reversed(range(self.n_flows)):
n_half = int(audio.size(1) / 2)
audio_0 = audio[:, :n_half, :]
audio_1 = audio[:, n_half:, :]
output = self.WN[k]((audio_0, spect))
s = output[:, n_half:, :]
b = output[:, :n_half, :]
audio_1 = (audio_1 - b) / torch.exp(s)
audio = torch.cat([audio_0, audio_1], 1)
audio = self.convinv[k].infer(audio)
if k % self.n_early_every == 0 and k > 0:
z = torch.randn(spect.size(0), self.n_early_size, spect.size(
2), device=spect.device).to(spect.dtype)
audio = torch.cat((sigma * z, audio), 1)
audio = audio.permute(
0, 2, 1).contiguous().view(
audio.size(0), -1).data
return audio
def infer_onnx(self, spect, z, sigma=0.9):
spect = self.upsample(spect)
# trim conv artifacts. maybe pad spec to kernel multiple
time_cutoff = self.upsample.kernel_size[0] - self.upsample.stride[0]
spect = spect[:, :, :-time_cutoff]
length_spect_group = spect.size(2)//8
mel_dim = 80
batch_size = spect.size(0)
spect = spect.view((batch_size, mel_dim, length_spect_group, self.n_group))
spect = spect.permute(0, 2, 1, 3)
spect = spect.contiguous()
spect = spect.view((batch_size, length_spect_group, self.n_group*mel_dim))
spect = spect.permute(0, 2, 1)
spect = spect.contiguous()
audio = z[:, :self.n_remaining_channels, :]
z = z[:, self.n_remaining_channels:self.n_group, :]
audio = sigma*audio
for k in reversed(range(self.n_flows)):
n_half = int(audio.size(1) // 2)
audio_0 = audio[:, :n_half, :]
audio_1 = audio[:, n_half:(n_half+n_half), :]
output = self.WN[k]((audio_0, spect))
s = output[:, n_half:(n_half+n_half), :]
b = output[:, :n_half, :]
audio_1 = (audio_1 - b) / torch.exp(s)
audio = torch.cat([audio_0, audio_1], 1)
audio = self.convinv[k].infer(audio)
if k % self.n_early_every == 0 and k > 0:
audio = torch.cat((z[:, :self.n_early_size, :], audio), 1)
z = z[:, self.n_early_size:self.n_group, :]
audio = audio.permute(0,2,1).contiguous().view(batch_size, (length_spect_group * self.n_group))
return audio
@staticmethod
def remove_weightnorm(model):
waveglow = model
for WN in waveglow.WN:
WN.start = torch.nn.utils.remove_weight_norm(WN.start)
WN.in_layers = remove(WN.in_layers)
WN.cond_layers = remove(WN.cond_layers)
WN.res_skip_layers = remove(WN.res_skip_layers)
return waveglow
def remove(conv_list):
new_conv_list = torch.nn.ModuleList()
for old_conv in conv_list:
old_conv = torch.nn.utils.remove_weight_norm(old_conv)
new_conv_list.append(old_conv)
return new_conv_list
|
PyTorch/SpeechSynthesis/HiFiGAN/common | common | stft | """
BSD 3-Clause License
Copyright (c) 2017, Prem Seetharaman
All rights reserved.
* Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice,
this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this
list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of the copyright holder nor the names of its
contributors may be used to endorse or promote products derived from this
software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR
ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON
ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
"""
import numpy as np
import torch
import torch.nn.functional as F
from librosa.util import pad_center, tiny
from scipy.signal import get_window
from torch.autograd import Variable
from common.audio_processing import window_sumsquare
class STFT(torch.nn.Module):
"""adapted from Prem Seetharaman's https://github.com/pseeth/pytorch-stft"""
def __init__(self, filter_length=800, hop_length=200, win_length=800,
window='hann'):
super(STFT, self).__init__()
self.filter_length = filter_length
self.hop_length = hop_length
self.win_length = win_length
self.window = window
self.forward_transform = None
scale = self.filter_length / self.hop_length
fourier_basis = np.fft.fft(np.eye(self.filter_length))
cutoff = int((self.filter_length / 2 + 1))
fourier_basis = np.vstack([np.real(fourier_basis[:cutoff, :]),
np.imag(fourier_basis[:cutoff, :])])
forward_basis = torch.FloatTensor(fourier_basis[:, None, :])
inverse_basis = torch.FloatTensor(
np.linalg.pinv(scale * fourier_basis).T[:, None, :].copy())
if window is not None:
assert(filter_length >= win_length)
# get window and zero center pad it to filter_length
fft_window = get_window(window, win_length, fftbins=True)
fft_window = pad_center(fft_window, size=filter_length)
fft_window = torch.from_numpy(fft_window).float()
# window the bases
forward_basis *= fft_window
inverse_basis *= fft_window
self.register_buffer('forward_basis', forward_basis.float())
self.register_buffer('inverse_basis', inverse_basis.float())
def transform(self, input_data):
num_batches = input_data.size(0)
num_samples = input_data.size(1)
self.num_samples = num_samples
# similar to librosa, reflect-pad the input
input_data = input_data.view(num_batches, 1, num_samples)
input_data = F.pad(
input_data.unsqueeze(1),
(int(self.filter_length / 2), int(self.filter_length / 2), 0, 0),
mode='reflect')
input_data = input_data.squeeze(1)
forward_transform = F.conv1d(
input_data,
Variable(self.forward_basis, requires_grad=False),
stride=self.hop_length,
padding=0)
cutoff = int((self.filter_length / 2) + 1)
real_part = forward_transform[:, :cutoff, :]
imag_part = forward_transform[:, cutoff:, :]
magnitude = torch.sqrt(real_part**2 + imag_part**2)
phase = torch.autograd.Variable(
torch.atan2(imag_part.data, real_part.data))
return magnitude, phase
def inverse(self, magnitude, phase):
recombine_magnitude_phase = torch.cat(
[magnitude*torch.cos(phase), magnitude*torch.sin(phase)], dim=1)
with torch.no_grad():
inverse_transform = F.conv_transpose1d(
recombine_magnitude_phase, self.inverse_basis,
stride=self.hop_length, padding=0)
if self.window is not None:
window_sum = window_sumsquare(
self.window, magnitude.size(-1), hop_length=self.hop_length,
win_length=self.win_length, n_fft=self.filter_length,
dtype=np.float32)
# remove modulation effects
approx_nonzero_indices = torch.from_numpy(
np.where(window_sum > tiny(window_sum))[0])
window_sum = torch.autograd.Variable(
torch.from_numpy(window_sum), requires_grad=False)
window_sum = window_sum.cuda() if magnitude.is_cuda else window_sum
inverse_transform[:, :, approx_nonzero_indices] /= window_sum[approx_nonzero_indices]
# scale by hop ratio
inverse_transform *= float(self.filter_length) / self.hop_length
inverse_transform = inverse_transform[:, :, int(self.filter_length/2):]
inverse_transform = inverse_transform[:, :, :-int(self.filter_length/2):]
return inverse_transform
def forward(self, input_data):
self.magnitude, self.phase = self.transform(input_data)
reconstruction = self.inverse(self.magnitude, self.phase)
return reconstruction
|
Tools/DGLPyTorch/SyntheticGraphGeneration/syngen/preprocessing/datasets | datasets | ogbn_mag | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import json
import os
import shutil
from typing import Optional
import cudf
import cupy as cp
import numpy as np
import pandas as pd
from ogb.nodeproppred import NodePropPredDataset
from syngen.configuration import SynGenDatasetFeatureSpec
from syngen.preprocessing.base_preprocessing import BasePreprocessing
from syngen.utils.io_utils import dump_dataframe
from syngen.utils.types import MetaData
class OGBN_MAG_Preprocessing(BasePreprocessing):
"""
The OGBN_MAG_Preprocessing class includes the transformation
operation for a subset of the Microsoft Academic Graph (MAG).
It's a heterogeneous network that contains four types of entities—papers
(736,389 nodes), authors (1,134,649 nodes), institutions (8,740 nodes),
and fields of study (59,965 nodes)—as well as four types of directed relations
connecting two types of entities—an author is “affiliated with” an institution,
an author “writes” a paper, a paper “cites” a paper, and a paper “has a topic
of” a field of study. For more information, please check
https://ogb.stanford.edu/docs/nodeprop/
"""
def __init__(
self,
source_path: str,
destination_path: Optional[str] = None,
download: bool = False,
**kwargs,
):
super().__init__(source_path, destination_path, download, **kwargs)
def download(self):
NodePropPredDataset(name="ogbn-mag", root=self.source_path)
def _check_files(self) -> bool:
return True
def transform(self, gpu=False, use_cache=False):
tabular_operator = cudf if gpu else pd
operator = cp if gpu else np
if use_cache and os.path.exists(self.destination_path):
return SynGenDatasetFeatureSpec.instantiate_from_preprocessed(self.destination_path)
shutil.rmtree(self.destination_path, ignore_errors=True)
os.makedirs(self.destination_path)
dataset = NodePropPredDataset(name="ogbn-mag", root=self.source_path)[0]
data = dataset[0]
labels = dataset[1]["paper"]
graph_metadata = {
MetaData.NODES: [],
MetaData.EDGES: [],
}
connections = {}
for e, edges in data["edge_index_dict"].items():
structural_data = pd.DataFrame(edges.T, columns=[MetaData.SRC, MetaData.DST])
connections[e[1]] = tabular_operator.DataFrame({
"src_id": edges[0, :],
"dst_id": edges[1, :],
})
edata = data["edge_reltype"][e]
edge_type = {
MetaData.NAME: e[1],
MetaData.COUNT: len(structural_data),
MetaData.SRC_NODE_TYPE: e[0],
MetaData.DST_NODE_TYPE: e[2],
MetaData.DIRECTED: False,
MetaData.FEATURES: [{
MetaData.NAME: 'feat',
MetaData.DTYPE: str(edata.dtype),
MetaData.FEATURE_TYPE: MetaData.CATEGORICAL,
}],
MetaData.FEATURES_PATH: f"{e[1]}_features.parquet",
MetaData.STRUCTURE_PATH: f"{e[1]}_list.parquet",
}
dump_dataframe(tabular_operator.DataFrame(edata, columns=['feat']),
os.path.join(self.destination_path, edge_type[MetaData.FEATURES_PATH]))
dump_dataframe(structural_data,
os.path.join(self.destination_path, edge_type[MetaData.STRUCTURE_PATH]))
graph_metadata[MetaData.EDGES].append(edge_type)
# paper node type
continuous_column_names = ["feat_" + str(i) for i in range(data["node_feat_dict"]["paper"].shape[1])]
paper_features_dataframe = tabular_operator.DataFrame(
data["node_feat_dict"]["paper"],
columns=continuous_column_names,
).astype("float32")
paper_features_dataframe["year"] = tabular_operator.DataFrame(data["node_year"]["paper"]).astype("int32")
paper_features_dataframe["venue"] = tabular_operator.DataFrame(labels).astype("int32")
paper_node_type = {
MetaData.NAME: "paper",
MetaData.COUNT: data["num_nodes_dict"]['paper'],
MetaData.FEATURES: [
{
MetaData.NAME: name,
MetaData.DTYPE: str(dtype),
MetaData.FEATURE_TYPE:
MetaData.CATEGORICAL if str(dtype).startswith('int') else MetaData.CONTINUOUS,
} for name, dtype in paper_features_dataframe.dtypes.items()
],
MetaData.FEATURES_PATH: "paper.parquet",
}
dump_dataframe(paper_features_dataframe,
os.path.join(self.destination_path, paper_node_type[MetaData.FEATURES_PATH]))
graph_metadata[MetaData.NODES].append(paper_node_type)
# author node type
paper_features_dataframe["paper_id"] = operator.arange(paper_features_dataframe.shape[0])
author_feat = connections["writes"].merge(
paper_features_dataframe,
left_on="dst_id",
right_on="paper_id",
how="left"
).groupby("src_id", sort=True).mean()
author_features_dataframe = author_feat[continuous_column_names]
author_node_type = {
MetaData.NAME: "author",
MetaData.COUNT: data["num_nodes_dict"]['author'],
MetaData.FEATURES: [
{
MetaData.NAME: name,
MetaData.DTYPE: str(dtype),
MetaData.FEATURE_TYPE: MetaData.CONTINUOUS,
} for name, dtype in author_features_dataframe.dtypes.items()
],
MetaData.FEATURES_PATH: "author.parquet",
}
dump_dataframe(author_features_dataframe,
os.path.join(self.destination_path, author_node_type[MetaData.FEATURES_PATH]))
graph_metadata[MetaData.NODES].append(author_node_type)
# institution node type
author_features_dataframe["author_id"] = operator.arange(author_features_dataframe.shape[0])
institution_feat = connections["affiliated_with"].merge(
author_features_dataframe,
left_on="src_id",
right_on="author_id"
).groupby("dst_id", sort=True).mean()
institution_dataframe = institution_feat[continuous_column_names]
institution_node_type = {
MetaData.NAME: "institution",
MetaData.COUNT: data["num_nodes_dict"]['institution'],
MetaData.FEATURES: [
{
MetaData.NAME: name,
MetaData.DTYPE: str(dtype),
MetaData.FEATURE_TYPE: MetaData.CONTINUOUS,
} for name, dtype in institution_dataframe.dtypes.items()
],
MetaData.FEATURES_PATH: "institution.parquet",
}
dump_dataframe(institution_dataframe,
os.path.join(self.destination_path, institution_node_type[MetaData.FEATURES_PATH]))
graph_metadata[MetaData.NODES].append(institution_node_type)
# field_of_study node type
field_of_study_feat = connections["has_topic"].merge(
paper_features_dataframe,
left_on="src_id",
right_on="paper_id"
).groupby("dst_id", sort=True).mean()
field_of_study_dataframe = field_of_study_feat[continuous_column_names]
field_of_study_node_type = {
MetaData.NAME: "field_of_study",
MetaData.COUNT: data["num_nodes_dict"]['field_of_study'],
MetaData.FEATURES: [
{
MetaData.NAME: name,
MetaData.DTYPE: str(dtype),
MetaData.FEATURE_TYPE: MetaData.CONTINUOUS,
} for name, dtype in field_of_study_dataframe.dtypes.items()
],
MetaData.FEATURES_PATH: "field_of_study.parquet",
}
dump_dataframe(field_of_study_dataframe,
os.path.join(self.destination_path, field_of_study_node_type[MetaData.FEATURES_PATH]))
graph_metadata[MetaData.NODES].append(field_of_study_node_type)
with open(os.path.join(self.destination_path, 'graph_metadata.json'), 'w') as f:
json.dump(graph_metadata, f, indent=4)
graph_metadata[MetaData.PATH] = self.destination_path
return SynGenDatasetFeatureSpec(graph_metadata)
|
CUDA-Optimized/FastSpeech/tacotron2 | tacotron2 | layers | # BSD 3-Clause License
# Copyright (c) 2018-2020, NVIDIA Corporation
# All rights reserved.
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
# * Redistributions of source code must retain the above copyright notice, this
# list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright notice,
# this list of conditions and the following disclaimer in the documentation
# and/or other materials provided with the distribution.
# * Neither the name of the copyright holder nor the names of its
# contributors may be used to endorse or promote products derived from
# this software without specific prior written permission.
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
# DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
# FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
# SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
# CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
# OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
# OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
"""https://github.com/NVIDIA/tacotron2"""
import torch
from librosa.filters import mel as librosa_mel_fn
class LinearNorm(torch.nn.Module):
def __init__(self, in_dim, out_dim, bias=True, w_init_gain='linear'):
super(LinearNorm, self).__init__()
self.linear_layer = torch.nn.Linear(in_dim, out_dim, bias=bias)
torch.nn.init.xavier_uniform_(
self.linear_layer.weight,
gain=torch.nn.init.calculate_gain(w_init_gain))
def forward(self, x):
return self.linear_layer(x)
class ConvNorm(torch.nn.Module):
def __init__(self, in_channels, out_channels, kernel_size=1, stride=1,
padding=None, dilation=1, bias=True, w_init_gain='linear'):
super(ConvNorm, self).__init__()
if padding is None:
assert(kernel_size % 2 == 1)
padding = int(dilation * (kernel_size - 1) / 2)
self.conv = torch.nn.Conv1d(in_channels, out_channels,
kernel_size=kernel_size, stride=stride,
padding=padding, dilation=dilation,
bias=bias)
torch.nn.init.xavier_uniform_(
self.conv.weight, gain=torch.nn.init.calculate_gain(w_init_gain))
def forward(self, signal):
conv_signal = self.conv(signal)
return conv_signal
|
TensorFlow/Recommendation/WideAndDeep/utils | utils | schedulers | # Copyright (c) 2020, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import tensorflow as tf
__all__ = ['learning_rate_scheduler']
def learning_rate_scheduler(lr_init, warmup_steps, global_step):
warmup_lr = (lr_init * tf.cast(global_step, tf.float32) / tf.cast(warmup_steps, tf.float32))
return tf.cond(global_step < warmup_steps, lambda: warmup_lr, lambda: lr_init)
|
PyTorch/LanguageModeling/BERT/triton/deployment_toolkit/bermuda | bermuda | pyt | # Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import logging
import typing
from collections import Counter
from pathlib import Path
from typing import Dict, Optional, Union
import numpy as np
import torch # pytype: disable=import-error
import yaml
from model_navigator.model import ModelSignatureConfig
from model_navigator.tensor import TensorSpec
from model_navigator.utils.config import YamlConfigFile
from ..core import (
GET_MODEL_FN_NAME,
BaseLoader,
BaseRunner,
BaseRunnerSession,
BaseSaver,
Format,
Model,
Precision,
load_from_file,
)
from ..extensions import loaders, runners, savers
from .utils import get_dynamic_axes, get_shapes_with_dynamic_axes
LOGGER = logging.getLogger(__name__)
def get_sample_input(dataloader, device):
for batch in dataloader:
_, x, _ = batch
break
if isinstance(x, dict):
sample_input = list(x.values())
elif isinstance(x, list):
sample_input = x
else:
raise TypeError("The first element (x) of batch returned by dataloader must be a list or a dict")
for idx, s in enumerate(sample_input):
sample_input[idx] = torch.from_numpy(s).to(device)
return tuple(sample_input)
def get_model_device(torch_model):
if next(torch_model.parameters()).is_cuda:
return "cuda"
else:
return "cpu"
def infer_model_precision(model):
counter = Counter()
for param in model.parameters():
counter[param.dtype] += 1
if counter[torch.float16] > 0:
return Precision.FP16
else:
return Precision.FP32
def _get_tensor_dtypes(dataloader, precision):
def _get_dtypes(t):
def _get_dtype(v):
dtype = str(v.dtype)
if dtype == "float64":
dtype = "float32"
if precision == Precision.FP16 and dtype == "float32":
dtype = "float16"
return np.dtype(dtype)
return {k: _get_dtype(v) for k, v in t.items()}
batch = next(dataloader)
_, x, y = batch
input_dtypes = _get_dtypes(x)
output_dtypes = _get_dtypes(y)
return input_dtypes, output_dtypes
### TODO assumption: floating point input
### type has same precision as the model
def _get_model_signature(
inputs_names: typing.List[str],
outputs_names: typing.List[str],
precision,
dataloader_fn,
batch_size_dim: typing.Optional[int] = None,
):
dataloader = dataloader_fn()
input_dtypes, output_dtypes = _get_tensor_dtypes(dataloader, precision)
input_shapes, output_shapes = get_shapes_with_dynamic_axes(dataloader, batch_size_dim=batch_size_dim)
inputs = {
name: TensorSpec(name=name, dtype=input_dtypes[name], shape=tuple(input_shapes[name])) for name in inputs_names
}
outputs = {
name: TensorSpec(name=name, dtype=output_dtypes[name], shape=tuple(output_shapes[name]))
for name in outputs_names
}
return ModelSignatureConfig(inputs, outputs)
class PyTorchModelLoader(BaseLoader):
required_fn_name_for_signature_parsing: Optional[str] = GET_MODEL_FN_NAME
def __init__(self, **kwargs):
self._model_args = kwargs
def load(self, model_path: Union[str, Path], **kwargs) -> Model:
if isinstance(model_path, Path):
model_path = model_path.as_posix()
get_model = load_from_file(model_path, "model", GET_MODEL_FN_NAME)
model, io_names_dict = get_model(**self._model_args)
dataloader_fn = kwargs.get("dataloader_fn", None)
output_type = kwargs.get("output_type", None)
precision = infer_model_precision(model)
batch_axis = getattr(model, "bermuda_batch_axis", 0) # by default models supports batching; batch_axis=0
model_signature = _get_model_signature(
inputs_names=io_names_dict["inputs"],
outputs_names=io_names_dict["outputs"],
precision=precision,
dataloader_fn=dataloader_fn,
batch_size_dim=batch_axis,
)
model = Model(handle=model, precision=precision, inputs=model_signature.inputs, outputs=model_signature.outputs)
if output_type == Format.TS_TRACE.value:
return self._trace(model, dataloader_fn)
elif output_type == Format.TS_SCRIPT.value:
return self._script(model)
elif output_type == Format.ONNX.value:
return model
else:
raise ValueError(f"Not supported PyTorch format: {output_type}")
def _trace(self, model: Model, dataloader_fn) -> Model:
device = get_model_device(model.handle)
dummy_input = get_sample_input(dataloader_fn(), device)
traced_model = torch.jit.trace_module(model.handle, {"forward": dummy_input})
return Model(traced_model, precision=model.precision, inputs=model.inputs, outputs=model.outputs)
def _script(self, model: Model) -> Model:
scripted_model = torch.jit.script(model.handle)
return Model(scripted_model, precision=model.precision, inputs=model.inputs, outputs=model.outputs)
class TorchScriptLoader(BaseLoader):
def __init__(self, tensor_names_path: str = None, **kwargs):
self._model_args = kwargs
self._io_spec = None
if tensor_names_path is not None:
with Path(tensor_names_path).open("r") as fh:
tensor_infos = yaml.load(fh, Loader=yaml.SafeLoader)
self._io_spec = ModelSignatureConfig(tensor_infos["inputs"], tensor_infos["outputs"])
def load(self, model_path: Union[str, Path], **_) -> Model:
if not isinstance(model_path, Path):
model_path = Path(model_path)
model = torch.jit.load(model_path.as_posix())
precision = infer_model_precision(model)
io_spec = self._io_spec
if not io_spec:
yaml_path = model_path.parent / f"{model_path.name}.yaml"
if not yaml_path.is_file():
raise ValueError(
f"If `--tensor-names-path is not provided, "
f"TorchScript model loader expects file {yaml_path} with tensor information."
)
with yaml_path.open("r") as fh:
tensor_info = yaml.load(fh, Loader=yaml.SafeLoader)
io_spec = ModelSignatureConfig(tensor_info["inputs"], tensor_info["outputs"])
return Model(handle=model, precision=precision, inputs=io_spec.inputs, outputs=io_spec.outputs)
class PYT2ONNXSaver(BaseSaver):
def __init__(self, onnx_opset: int = None):
self._onnx_opset = onnx_opset
def save(self, model: Model, model_path: Union[str, Path], dataloader_fn) -> Model:
if isinstance(model_path, Path):
model_path = model_path.as_posix()
assert isinstance(model.handle, torch.jit.ScriptModule) or isinstance(
model.handle, torch.nn.Module
), "The model must be of type 'torch.jit.ScriptModule' or 'torch.nn.Module'. Converter aborted."
batch_axis = getattr(model.handle, "bermuda_batch_axis", 0) # by default models supports batching; batch_axis=0
dynamic_axes = get_dynamic_axes(dataloader_fn(), batch_size_dim=batch_axis)
device = get_model_device(model.handle)
dummy_input = get_sample_input(dataloader_fn(), device)
with torch.no_grad():
torch.onnx.export(
model.handle,
dummy_input,
model_path,
do_constant_folding=True,
input_names=list(model.inputs),
output_names=list(model.outputs),
dynamic_axes=dynamic_axes,
opset_version=self._onnx_opset,
enable_onnx_checker=True,
)
class TorchScriptSaver(BaseSaver):
def save(self, model: Model, model_path: Union[str, Path], dataloader_fn) -> None:
if not isinstance(model_path, Path):
model_path = Path(model_path)
if isinstance(model.handle, torch.jit.ScriptModule):
torch.jit.save(model.handle, model_path.as_posix())
else:
raise RuntimeError("The model must be of type 'torch.jit.ScriptModule'. Saving aborted.")
signature_config = ModelSignatureConfig(inputs=model.inputs, outputs=model.outputs)
annotation_path = model_path.parent / f"{model_path.name}.yaml"
with YamlConfigFile(annotation_path) as config_file:
config_file.save_config(signature_config)
class PyTorchRunner(BaseRunner):
def __init__(self):
pass
def init_inference(self, model: Model):
return PyTorchRunnerSession(model=model)
class PyTorchRunnerSession(BaseRunnerSession):
def __init__(self, model: Model):
super().__init__(model)
assert isinstance(model.handle, torch.jit.ScriptModule) or isinstance(
model.handle, torch.nn.Module
), "The model must be of type 'torch.jit.ScriptModule' or 'torch.nn.Module'. Runner aborted."
self._model = model
self._output_names = None
def __enter__(self):
self._output_names = list(self._model.outputs)
return self
def __exit__(self, exc_type, exc_value, traceback):
self._output_names = None
self._model = None
def __call__(self, x: Dict[str, object]):
with torch.no_grad():
feed_list = [torch.from_numpy(v).cuda() for k, v in x.items()]
y_pred = self._model.handle(*feed_list)
if isinstance(y_pred, torch.Tensor):
y_pred = (y_pred,)
y_pred = [t.cpu().numpy() for t in y_pred]
y_pred = dict(zip(self._output_names, y_pred))
return y_pred
loaders.register_extension(Format.PYT.value, PyTorchModelLoader)
loaders.register_extension(Format.TS_TRACE.value, TorchScriptLoader)
loaders.register_extension(Format.TS_SCRIPT.value, TorchScriptLoader)
savers.register_extension(Format.TS_SCRIPT.value, TorchScriptSaver)
savers.register_extension(Format.TS_TRACE.value, TorchScriptSaver)
savers.register_extension(f"{Format.PYT.value}--{Format.ONNX.value}", PYT2ONNXSaver)
runners.register_extension(Format.PYT.value, PyTorchRunner)
runners.register_extension(Format.TS_SCRIPT.value, PyTorchRunner)
runners.register_extension(Format.TS_TRACE.value, PyTorchRunner)
|
PyTorch/SpeechSynthesis/FastPitch/triton/scripts/docker | docker | interactive | #!/usr/bin/env bash
# Copyright (c) 2021 NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
docker run -it --rm \
--gpus "device=all" \
--net=host \
--shm-size=1g \
--ulimit memlock=-1 \
--ulimit stack=67108864 \
--privileged \
-e WORKDIR=$(pwd) \
-v $(pwd):$(pwd) \
-v /var/run/docker.sock:/var/run/docker.sock \
fastpitch:latest bash
|
TensorFlow/Detection/SSD/models/research/object_detection/meta_architectures | meta_architectures | faster_rcnn_meta_arch | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Faster R-CNN meta-architecture definition.
General tensorflow implementation of Faster R-CNN detection models.
See Faster R-CNN: Ren, Shaoqing, et al.
"Faster R-CNN: Towards real-time object detection with region proposal
networks." Advances in neural information processing systems. 2015.
We allow for three modes: number_of_stages={1, 2, 3}. In case of 1 stage,
all of the user facing methods (e.g., predict, postprocess, loss) can be used as
if the model consisted only of the RPN, returning class agnostic proposals
(these can be thought of as approximate detections with no associated class
information). In case of 2 stages, proposals are computed, then passed
through a second stage "box classifier" to yield (multi-class) detections.
Finally, in case of 3 stages which is only used during eval, proposals are
computed, then passed through a second stage "box classifier" that will compute
refined boxes and classes, and then features are pooled from the refined and
non-maximum suppressed boxes and are passed through the box classifier again. If
number of stages is 3 during training it will be reduced to two automatically.
Implementations of Faster R-CNN models must define a new
FasterRCNNFeatureExtractor and override three methods: `preprocess`,
`_extract_proposal_features` (the first stage of the model), and
`_extract_box_classifier_features` (the second stage of the model). Optionally,
the `restore_fn` method can be overridden. See tests for an example.
A few important notes:
+ Batching conventions: We support batched inference and training where
all images within a batch have the same resolution. Batch sizes are determined
dynamically via the shape of the input tensors (rather than being specified
directly as, e.g., a model constructor).
A complication is that due to non-max suppression, we are not guaranteed to get
the same number of proposals from the first stage RPN (region proposal network)
for each image (though in practice, we should often get the same number of
proposals). For this reason we pad to a max number of proposals per image
within a batch. This `self.max_num_proposals` property is set to the
`first_stage_max_proposals` parameter at inference time and the
`second_stage_batch_size` at training time since we subsample the batch to
be sent through the box classifier during training.
For the second stage of the pipeline, we arrange the proposals for all images
within the batch along a single batch dimension. For example, the input to
_extract_box_classifier_features is a tensor of shape
`[total_num_proposals, crop_height, crop_width, depth]` where
total_num_proposals is batch_size * self.max_num_proposals. (And note that per
the above comment, a subset of these entries correspond to zero paddings.)
+ Coordinate representations:
Following the API (see model.DetectionModel definition), our outputs after
postprocessing operations are always normalized boxes however, internally, we
sometimes convert to absolute --- e.g. for loss computation. In particular,
anchors and proposal_boxes are both represented as absolute coordinates.
Images are resized in the `preprocess` method.
The Faster R-CNN meta architecture has two post-processing methods
`_postprocess_rpn` which is applied after first stage and
`_postprocess_box_classifier` which is applied after second stage. There are
three different ways post-processing can happen depending on number_of_stages
configured in the meta architecture:
1. When number_of_stages is 1:
`_postprocess_rpn` is run as part of the `postprocess` method where
true_image_shapes is used to clip proposals, perform non-max suppression and
normalize them.
2. When number of stages is 2:
`_postprocess_rpn` is run as part of the `_predict_second_stage` method where
`resized_image_shapes` is used to clip proposals, perform non-max suppression
and normalize them. In this case `postprocess` method skips `_postprocess_rpn`
and only runs `_postprocess_box_classifier` using `true_image_shapes` to clip
detections, perform non-max suppression and normalize them.
3. When number of stages is 3:
`_postprocess_rpn` is run as part of the `_predict_second_stage` using
`resized_image_shapes` to clip proposals, perform non-max suppression and
normalize them. Subsequently, `_postprocess_box_classifier` is run as part of
`_predict_third_stage` using `true_image_shapes` to clip detections, peform
non-max suppression and normalize them. In this case, the `postprocess` method
skips both `_postprocess_rpn` and `_postprocess_box_classifier`.
"""
from abc import abstractmethod
from functools import partial
import tensorflow as tf
from object_detection.anchor_generators import grid_anchor_generator
from object_detection.builders import box_predictor_builder
from object_detection.core import box_list
from object_detection.core import box_list_ops
from object_detection.core import box_predictor
from object_detection.core import losses
from object_detection.core import model
from object_detection.core import standard_fields as fields
from object_detection.core import target_assigner
from object_detection.utils import ops
from object_detection.utils import shape_utils
slim = tf.contrib.slim
class FasterRCNNFeatureExtractor(object):
"""Faster R-CNN Feature Extractor definition."""
def __init__(self,
is_training,
first_stage_features_stride,
batch_norm_trainable=False,
reuse_weights=None,
weight_decay=0.0):
"""Constructor.
Args:
is_training: A boolean indicating whether the training version of the
computation graph should be constructed.
first_stage_features_stride: Output stride of extracted RPN feature map.
batch_norm_trainable: Whether to update batch norm parameters during
training or not. When training with a relative large batch size
(e.g. 8), it could be desirable to enable batch norm update.
reuse_weights: Whether to reuse variables. Default is None.
weight_decay: float weight decay for feature extractor (default: 0.0).
"""
self._is_training = is_training
self._first_stage_features_stride = first_stage_features_stride
self._train_batch_norm = (batch_norm_trainable and is_training)
self._reuse_weights = reuse_weights
self._weight_decay = weight_decay
@abstractmethod
def preprocess(self, resized_inputs):
"""Feature-extractor specific preprocessing (minus image resizing)."""
pass
def extract_proposal_features(self, preprocessed_inputs, scope):
"""Extracts first stage RPN features.
This function is responsible for extracting feature maps from preprocessed
images. These features are used by the region proposal network (RPN) to
predict proposals.
Args:
preprocessed_inputs: A [batch, height, width, channels] float tensor
representing a batch of images.
scope: A scope name.
Returns:
rpn_feature_map: A tensor with shape [batch, height, width, depth]
activations: A dictionary mapping activation tensor names to tensors.
"""
with tf.variable_scope(scope, values=[preprocessed_inputs]):
return self._extract_proposal_features(preprocessed_inputs, scope)
@abstractmethod
def _extract_proposal_features(self, preprocessed_inputs, scope):
"""Extracts first stage RPN features, to be overridden."""
pass
def extract_box_classifier_features(self, proposal_feature_maps, scope):
"""Extracts second stage box classifier features.
Args:
proposal_feature_maps: A 4-D float tensor with shape
[batch_size * self.max_num_proposals, crop_height, crop_width, depth]
representing the feature map cropped to each proposal.
scope: A scope name.
Returns:
proposal_classifier_features: A 4-D float tensor with shape
[batch_size * self.max_num_proposals, height, width, depth]
representing box classifier features for each proposal.
"""
with tf.variable_scope(
scope, values=[proposal_feature_maps], reuse=tf.AUTO_REUSE):
return self._extract_box_classifier_features(proposal_feature_maps, scope)
@abstractmethod
def _extract_box_classifier_features(self, proposal_feature_maps, scope):
"""Extracts second stage box classifier features, to be overridden."""
pass
def restore_from_classification_checkpoint_fn(
self,
first_stage_feature_extractor_scope,
second_stage_feature_extractor_scope):
"""Returns a map of variables to load from a foreign checkpoint.
Args:
first_stage_feature_extractor_scope: A scope name for the first stage
feature extractor.
second_stage_feature_extractor_scope: A scope name for the second stage
feature extractor.
Returns:
A dict mapping variable names (to load from a checkpoint) to variables in
the model graph.
"""
variables_to_restore = {}
for variable in tf.global_variables():
for scope_name in [first_stage_feature_extractor_scope,
second_stage_feature_extractor_scope]:
if variable.op.name.startswith(scope_name):
var_name = variable.op.name.replace(scope_name + '/', '')
variables_to_restore[var_name] = variable
return variables_to_restore
class FasterRCNNMetaArch(model.DetectionModel):
"""Faster R-CNN Meta-architecture definition."""
def __init__(self,
is_training,
num_classes,
image_resizer_fn,
feature_extractor,
number_of_stages,
first_stage_anchor_generator,
first_stage_target_assigner,
first_stage_atrous_rate,
first_stage_box_predictor_arg_scope_fn,
first_stage_box_predictor_kernel_size,
first_stage_box_predictor_depth,
first_stage_minibatch_size,
first_stage_sampler,
first_stage_non_max_suppression_fn,
first_stage_max_proposals,
first_stage_localization_loss_weight,
first_stage_objectness_loss_weight,
crop_and_resize_fn,
initial_crop_size,
maxpool_kernel_size,
maxpool_stride,
second_stage_target_assigner,
second_stage_mask_rcnn_box_predictor,
second_stage_batch_size,
second_stage_sampler,
second_stage_non_max_suppression_fn,
second_stage_score_conversion_fn,
second_stage_localization_loss_weight,
second_stage_classification_loss_weight,
second_stage_classification_loss,
second_stage_mask_prediction_loss_weight=1.0,
hard_example_miner=None,
parallel_iterations=16,
add_summaries=True,
clip_anchors_to_image=False,
use_static_shapes=False,
resize_masks=True):
"""FasterRCNNMetaArch Constructor.
Args:
is_training: A boolean indicating whether the training version of the
computation graph should be constructed.
num_classes: Number of classes. Note that num_classes *does not*
include the background category, so if groundtruth labels take values
in {0, 1, .., K-1}, num_classes=K (and not K+1, even though the
assigned classification targets can range from {0,... K}).
image_resizer_fn: A callable for image resizing. This callable
takes a rank-3 image tensor of shape [height, width, channels]
(corresponding to a single image), an optional rank-3 instance mask
tensor of shape [num_masks, height, width] and returns a resized rank-3
image tensor, a resized mask tensor if one was provided in the input. In
addition this callable must also return a 1-D tensor of the form
[height, width, channels] containing the size of the true image, as the
image resizer can perform zero padding. See protos/image_resizer.proto.
feature_extractor: A FasterRCNNFeatureExtractor object.
number_of_stages: An integer values taking values in {1, 2, 3}. If
1, the function will construct only the Region Proposal Network (RPN)
part of the model. If 2, the function will perform box refinement and
other auxiliary predictions all in the second stage. If 3, it will
extract features from refined boxes and perform the auxiliary
predictions on the non-maximum suppressed refined boxes.
If is_training is true and the value of number_of_stages is 3, it is
reduced to 2 since all the model heads are trained in parallel in second
stage during training.
first_stage_anchor_generator: An anchor_generator.AnchorGenerator object
(note that currently we only support
grid_anchor_generator.GridAnchorGenerator objects)
first_stage_target_assigner: Target assigner to use for first stage of
Faster R-CNN (RPN).
first_stage_atrous_rate: A single integer indicating the atrous rate for
the single convolution op which is applied to the `rpn_features_to_crop`
tensor to obtain a tensor to be used for box prediction. Some feature
extractors optionally allow for producing feature maps computed at
denser resolutions. The atrous rate is used to compensate for the
denser feature maps by using an effectively larger receptive field.
(This should typically be set to 1).
first_stage_box_predictor_arg_scope_fn: A function to construct tf-slim
arg_scope for conv2d, separable_conv2d and fully_connected ops for the
RPN box predictor.
first_stage_box_predictor_kernel_size: Kernel size to use for the
convolution op just prior to RPN box predictions.
first_stage_box_predictor_depth: Output depth for the convolution op
just prior to RPN box predictions.
first_stage_minibatch_size: The "batch size" to use for computing the
objectness and location loss of the region proposal network. This
"batch size" refers to the number of anchors selected as contributing
to the loss function for any given image within the image batch and is
only called "batch_size" due to terminology from the Faster R-CNN paper.
first_stage_sampler: Sampler to use for first stage loss (RPN loss).
first_stage_non_max_suppression_fn: batch_multiclass_non_max_suppression
callable that takes `boxes`, `scores` and optional `clip_window`(with
all other inputs already set) and returns a dictionary containing
tensors with keys: `detection_boxes`, `detection_scores`,
`detection_classes`, `num_detections`. This is used to perform non max
suppression on the boxes predicted by the Region Proposal Network
(RPN).
See `post_processing.batch_multiclass_non_max_suppression` for the type
and shape of these tensors.
first_stage_max_proposals: Maximum number of boxes to retain after
performing Non-Max Suppression (NMS) on the boxes predicted by the
Region Proposal Network (RPN).
first_stage_localization_loss_weight: A float
first_stage_objectness_loss_weight: A float
crop_and_resize_fn: A differentiable resampler to use for cropping RPN
proposal features.
initial_crop_size: A single integer indicating the output size
(width and height are set to be the same) of the initial bilinear
interpolation based cropping during ROI pooling.
maxpool_kernel_size: A single integer indicating the kernel size of the
max pool op on the cropped feature map during ROI pooling.
maxpool_stride: A single integer indicating the stride of the max pool
op on the cropped feature map during ROI pooling.
second_stage_target_assigner: Target assigner to use for second stage of
Faster R-CNN. If the model is configured with multiple prediction heads,
this target assigner is used to generate targets for all heads (with the
correct `unmatched_class_label`).
second_stage_mask_rcnn_box_predictor: Mask R-CNN box predictor to use for
the second stage.
second_stage_batch_size: The batch size used for computing the
classification and refined location loss of the box classifier. This
"batch size" refers to the number of proposals selected as contributing
to the loss function for any given image within the image batch and is
only called "batch_size" due to terminology from the Faster R-CNN paper.
second_stage_sampler: Sampler to use for second stage loss (box
classifier loss).
second_stage_non_max_suppression_fn: batch_multiclass_non_max_suppression
callable that takes `boxes`, `scores`, optional `clip_window` and
optional (kwarg) `mask` inputs (with all other inputs already set)
and returns a dictionary containing tensors with keys:
`detection_boxes`, `detection_scores`, `detection_classes`,
`num_detections`, and (optionally) `detection_masks`. See
`post_processing.batch_multiclass_non_max_suppression` for the type and
shape of these tensors.
second_stage_score_conversion_fn: Callable elementwise nonlinearity
(that takes tensors as inputs and returns tensors). This is usually
used to convert logits to probabilities.
second_stage_localization_loss_weight: A float indicating the scale factor
for second stage localization loss.
second_stage_classification_loss_weight: A float indicating the scale
factor for second stage classification loss.
second_stage_classification_loss: Classification loss used by the second
stage classifier. Either losses.WeightedSigmoidClassificationLoss or
losses.WeightedSoftmaxClassificationLoss.
second_stage_mask_prediction_loss_weight: A float indicating the scale
factor for second stage mask prediction loss. This is applicable only if
second stage box predictor is configured to predict masks.
hard_example_miner: A losses.HardExampleMiner object (can be None).
parallel_iterations: (Optional) The number of iterations allowed to run
in parallel for calls to tf.map_fn.
add_summaries: boolean (default: True) controlling whether summary ops
should be added to tensorflow graph.
clip_anchors_to_image: Normally, anchors generated for a given image size
are pruned during training if they lie outside the image window. This
option clips the anchors to be within the image instead of pruning.
use_static_shapes: If True, uses implementation of ops with static shape
guarantees.
resize_masks: Indicates whether the masks presend in the groundtruth
should be resized in the model with `image_resizer_fn`
Raises:
ValueError: If `second_stage_batch_size` > `first_stage_max_proposals` at
training time.
ValueError: If first_stage_anchor_generator is not of type
grid_anchor_generator.GridAnchorGenerator.
"""
# TODO(rathodv): add_summaries is currently unused. Respect that directive
# in the future.
super(FasterRCNNMetaArch, self).__init__(num_classes=num_classes)
if not isinstance(first_stage_anchor_generator,
grid_anchor_generator.GridAnchorGenerator):
raise ValueError('first_stage_anchor_generator must be of type '
'grid_anchor_generator.GridAnchorGenerator.')
self._is_training = is_training
self._image_resizer_fn = image_resizer_fn
self._resize_masks = resize_masks
self._feature_extractor = feature_extractor
self._number_of_stages = number_of_stages
self._proposal_target_assigner = first_stage_target_assigner
self._detector_target_assigner = second_stage_target_assigner
# Both proposal and detector target assigners use the same box coder
self._box_coder = self._proposal_target_assigner.box_coder
# (First stage) Region proposal network parameters
self._first_stage_anchor_generator = first_stage_anchor_generator
self._first_stage_atrous_rate = first_stage_atrous_rate
self._first_stage_box_predictor_arg_scope_fn = (
first_stage_box_predictor_arg_scope_fn)
self._first_stage_box_predictor_kernel_size = (
first_stage_box_predictor_kernel_size)
self._first_stage_box_predictor_depth = first_stage_box_predictor_depth
self._first_stage_minibatch_size = first_stage_minibatch_size
self._first_stage_sampler = first_stage_sampler
self._first_stage_box_predictor = (
box_predictor_builder.build_convolutional_box_predictor(
is_training=self._is_training,
num_classes=1,
conv_hyperparams_fn=self._first_stage_box_predictor_arg_scope_fn,
use_dropout=False,
dropout_keep_prob=1.0,
box_code_size=self._box_coder.code_size,
kernel_size=1,
num_layers_before_predictor=0,
min_depth=0,
max_depth=0))
self._first_stage_nms_fn = first_stage_non_max_suppression_fn
self._first_stage_max_proposals = first_stage_max_proposals
self._use_static_shapes = use_static_shapes
self._first_stage_localization_loss = (
losses.WeightedSmoothL1LocalizationLoss())
self._first_stage_objectness_loss = (
losses.WeightedSoftmaxClassificationLoss())
self._first_stage_loc_loss_weight = first_stage_localization_loss_weight
self._first_stage_obj_loss_weight = first_stage_objectness_loss_weight
# Per-region cropping parameters
self._crop_and_resize_fn = crop_and_resize_fn
self._initial_crop_size = initial_crop_size
self._maxpool_kernel_size = maxpool_kernel_size
self._maxpool_stride = maxpool_stride
self._mask_rcnn_box_predictor = second_stage_mask_rcnn_box_predictor
self._second_stage_batch_size = second_stage_batch_size
self._second_stage_sampler = second_stage_sampler
self._second_stage_nms_fn = second_stage_non_max_suppression_fn
self._second_stage_score_conversion_fn = second_stage_score_conversion_fn
self._second_stage_localization_loss = (
losses.WeightedSmoothL1LocalizationLoss())
self._second_stage_classification_loss = second_stage_classification_loss
self._second_stage_mask_loss = (
losses.WeightedSigmoidClassificationLoss())
self._second_stage_loc_loss_weight = second_stage_localization_loss_weight
self._second_stage_cls_loss_weight = second_stage_classification_loss_weight
self._second_stage_mask_loss_weight = (
second_stage_mask_prediction_loss_weight)
self._hard_example_miner = hard_example_miner
self._parallel_iterations = parallel_iterations
self.clip_anchors_to_image = clip_anchors_to_image
if self._number_of_stages <= 0 or self._number_of_stages > 3:
raise ValueError('Number of stages should be a value in {1, 2, 3}.')
@property
def first_stage_feature_extractor_scope(self):
return 'FirstStageFeatureExtractor'
@property
def second_stage_feature_extractor_scope(self):
return 'SecondStageFeatureExtractor'
@property
def first_stage_box_predictor_scope(self):
return 'FirstStageBoxPredictor'
@property
def second_stage_box_predictor_scope(self):
return 'SecondStageBoxPredictor'
@property
def max_num_proposals(self):
"""Max number of proposals (to pad to) for each image in the input batch.
At training time, this is set to be the `second_stage_batch_size` if hard
example miner is not configured, else it is set to
`first_stage_max_proposals`. At inference time, this is always set to
`first_stage_max_proposals`.
Returns:
A positive integer.
"""
if self._is_training and not self._hard_example_miner:
return self._second_stage_batch_size
return self._first_stage_max_proposals
@property
def anchors(self):
if not self._anchors:
raise RuntimeError('anchors have not been constructed yet!')
if not isinstance(self._anchors, box_list.BoxList):
raise RuntimeError('anchors should be a BoxList object, but is not.')
return self._anchors
def preprocess(self, inputs):
"""Feature-extractor specific preprocessing.
See base class.
For Faster R-CNN, we perform image resizing in the base class --- each
class subclassing FasterRCNNMetaArch is responsible for any additional
preprocessing (e.g., scaling pixel values to be in [-1, 1]).
Args:
inputs: a [batch, height_in, width_in, channels] float tensor representing
a batch of images with values between 0 and 255.0.
Returns:
preprocessed_inputs: a [batch, height_out, width_out, channels] float
tensor representing a batch of images.
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
Raises:
ValueError: if inputs tensor does not have type tf.float32
"""
if inputs.dtype is not tf.float32:
raise ValueError('`preprocess` expects a tf.float32 tensor')
with tf.name_scope('Preprocessor'):
outputs = shape_utils.static_or_dynamic_map_fn(
self._image_resizer_fn,
elems=inputs,
dtype=[tf.float32, tf.int32],
parallel_iterations=self._parallel_iterations)
resized_inputs = outputs[0]
true_image_shapes = outputs[1]
return (self._feature_extractor.preprocess(resized_inputs),
true_image_shapes)
def _compute_clip_window(self, image_shapes):
"""Computes clip window for non max suppression based on image shapes.
This function assumes that the clip window's left top corner is at (0, 0).
Args:
image_shapes: A 2-D int32 tensor of shape [batch_size, 3] containing
shapes of images in the batch. Each row represents [height, width,
channels] of an image.
Returns:
A 2-D float32 tensor of shape [batch_size, 4] containing the clip window
for each image in the form [ymin, xmin, ymax, xmax].
"""
clip_heights = image_shapes[:, 0]
clip_widths = image_shapes[:, 1]
clip_window = tf.to_float(tf.stack([tf.zeros_like(clip_heights),
tf.zeros_like(clip_heights),
clip_heights, clip_widths], axis=1))
return clip_window
def predict(self, preprocessed_inputs, true_image_shapes):
"""Predicts unpostprocessed tensors from input tensor.
This function takes an input batch of images and runs it through the
forward pass of the network to yield "raw" un-postprocessed predictions.
If `number_of_stages` is 1, this function only returns first stage
RPN predictions (un-postprocessed). Otherwise it returns both
first stage RPN predictions as well as second stage box classifier
predictions.
Other remarks:
+ Anchor pruning vs. clipping: following the recommendation of the Faster
R-CNN paper, we prune anchors that venture outside the image window at
training time and clip anchors to the image window at inference time.
+ Proposal padding: as described at the top of the file, proposals are
padded to self._max_num_proposals and flattened so that proposals from all
images within the input batch are arranged along the same batch dimension.
Args:
preprocessed_inputs: a [batch, height, width, channels] float tensor
representing a batch of images.
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
Returns:
prediction_dict: a dictionary holding "raw" prediction tensors:
1) rpn_box_predictor_features: A 4-D float32 tensor with shape
[batch_size, height, width, depth] to be used for predicting proposal
boxes and corresponding objectness scores.
2) rpn_features_to_crop: A 4-D float32 tensor with shape
[batch_size, height, width, depth] representing image features to crop
using the proposal boxes predicted by the RPN.
3) image_shape: a 1-D tensor of shape [4] representing the input
image shape.
4) rpn_box_encodings: 3-D float tensor of shape
[batch_size, num_anchors, self._box_coder.code_size] containing
predicted boxes.
5) rpn_objectness_predictions_with_background: 3-D float tensor of shape
[batch_size, num_anchors, 2] containing class
predictions (logits) for each of the anchors. Note that this
tensor *includes* background class predictions (at class index 0).
6) anchors: A 2-D tensor of shape [num_anchors, 4] representing anchors
for the first stage RPN (in absolute coordinates). Note that
`num_anchors` can differ depending on whether the model is created in
training or inference mode.
(and if number_of_stages > 1):
7) refined_box_encodings: a 3-D tensor with shape
[total_num_proposals, num_classes, self._box_coder.code_size]
representing predicted (final) refined box encodings, where
total_num_proposals=batch_size*self._max_num_proposals. If using
a shared box across classes the shape will instead be
[total_num_proposals, 1, self._box_coder.code_size].
8) class_predictions_with_background: a 3-D tensor with shape
[total_num_proposals, num_classes + 1] containing class
predictions (logits) for each of the anchors, where
total_num_proposals=batch_size*self._max_num_proposals.
Note that this tensor *includes* background class predictions
(at class index 0).
9) num_proposals: An int32 tensor of shape [batch_size] representing the
number of proposals generated by the RPN. `num_proposals` allows us
to keep track of which entries are to be treated as zero paddings and
which are not since we always pad the number of proposals to be
`self.max_num_proposals` for each image.
10) proposal_boxes: A float32 tensor of shape
[batch_size, self.max_num_proposals, 4] representing
decoded proposal bounding boxes in absolute coordinates.
11) mask_predictions: (optional) a 4-D tensor with shape
[total_num_padded_proposals, num_classes, mask_height, mask_width]
containing instance mask predictions.
Raises:
ValueError: If `predict` is called before `preprocess`.
"""
(rpn_box_predictor_features, rpn_features_to_crop, anchors_boxlist,
image_shape) = self._extract_rpn_feature_maps(preprocessed_inputs)
(rpn_box_encodings, rpn_objectness_predictions_with_background
) = self._predict_rpn_proposals(rpn_box_predictor_features)
# The Faster R-CNN paper recommends pruning anchors that venture outside
# the image window at training time and clipping at inference time.
clip_window = tf.to_float(tf.stack([0, 0, image_shape[1], image_shape[2]]))
if self._is_training:
if self.clip_anchors_to_image:
anchors_boxlist = box_list_ops.clip_to_window(
anchors_boxlist, clip_window, filter_nonoverlapping=False)
else:
(rpn_box_encodings, rpn_objectness_predictions_with_background,
anchors_boxlist) = self._remove_invalid_anchors_and_predictions(
rpn_box_encodings, rpn_objectness_predictions_with_background,
anchors_boxlist, clip_window)
else:
anchors_boxlist = box_list_ops.clip_to_window(
anchors_boxlist, clip_window,
filter_nonoverlapping=not self._use_static_shapes)
self._anchors = anchors_boxlist
prediction_dict = {
'rpn_box_predictor_features': rpn_box_predictor_features,
'rpn_features_to_crop': rpn_features_to_crop,
'image_shape': image_shape,
'rpn_box_encodings': rpn_box_encodings,
'rpn_objectness_predictions_with_background':
rpn_objectness_predictions_with_background,
'anchors': self._anchors.get()
}
if self._number_of_stages >= 2:
# If mixed-precision training on TPU is enabled, rpn_box_encodings and
# rpn_objectness_predictions_with_background are bfloat16 tensors.
# Considered prediction results, they need to be casted to float32
# tensors for correct postprocess_rpn computation in predict_second_stage.
prediction_dict.update(self._predict_second_stage(
tf.to_float(rpn_box_encodings),
tf.to_float(rpn_objectness_predictions_with_background),
rpn_features_to_crop,
self._anchors.get(), image_shape, true_image_shapes))
if self._number_of_stages == 3:
prediction_dict = self._predict_third_stage(
prediction_dict, true_image_shapes)
return prediction_dict
def _image_batch_shape_2d(self, image_batch_shape_1d):
"""Takes a 1-D image batch shape tensor and converts it to a 2-D tensor.
Example:
If 1-D image batch shape tensor is [2, 300, 300, 3]. The corresponding 2-D
image batch tensor would be [[300, 300, 3], [300, 300, 3]]
Args:
image_batch_shape_1d: 1-D tensor of the form [batch_size, height,
width, channels].
Returns:
image_batch_shape_2d: 2-D tensor of shape [batch_size, 3] were each row is
of the form [height, width, channels].
"""
return tf.tile(tf.expand_dims(image_batch_shape_1d[1:], 0),
[image_batch_shape_1d[0], 1])
def _predict_second_stage(self, rpn_box_encodings,
rpn_objectness_predictions_with_background,
rpn_features_to_crop,
anchors,
image_shape,
true_image_shapes):
"""Predicts the output tensors from second stage of Faster R-CNN.
Args:
rpn_box_encodings: 4-D float tensor of shape
[batch_size, num_valid_anchors, self._box_coder.code_size] containing
predicted boxes.
rpn_objectness_predictions_with_background: 2-D float tensor of shape
[batch_size, num_valid_anchors, 2] containing class
predictions (logits) for each of the anchors. Note that this
tensor *includes* background class predictions (at class index 0).
rpn_features_to_crop: A 4-D float32 or bfloat16 tensor with shape
[batch_size, height, width, depth] representing image features to crop
using the proposal boxes predicted by the RPN.
anchors: 2-D float tensor of shape
[num_anchors, self._box_coder.code_size].
image_shape: A 1D int32 tensors of size [4] containing the image shape.
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
Returns:
prediction_dict: a dictionary holding "raw" prediction tensors:
1) refined_box_encodings: a 3-D tensor with shape
[total_num_proposals, num_classes, self._box_coder.code_size]
representing predicted (final) refined box encodings, where
total_num_proposals=batch_size*self._max_num_proposals. If using a
shared box across classes the shape will instead be
[total_num_proposals, 1, self._box_coder.code_size].
2) class_predictions_with_background: a 3-D tensor with shape
[total_num_proposals, num_classes + 1] containing class
predictions (logits) for each of the anchors, where
total_num_proposals=batch_size*self._max_num_proposals.
Note that this tensor *includes* background class predictions
(at class index 0).
3) num_proposals: An int32 tensor of shape [batch_size] representing the
number of proposals generated by the RPN. `num_proposals` allows us
to keep track of which entries are to be treated as zero paddings and
which are not since we always pad the number of proposals to be
`self.max_num_proposals` for each image.
4) proposal_boxes: A float32 tensor of shape
[batch_size, self.max_num_proposals, 4] representing
decoded proposal bounding boxes in absolute coordinates.
5) proposal_boxes_normalized: A float32 tensor of shape
[batch_size, self.max_num_proposals, 4] representing decoded proposal
bounding boxes in normalized coordinates. Can be used to override the
boxes proposed by the RPN, thus enabling one to extract features and
get box classification and prediction for externally selected areas
of the image.
6) box_classifier_features: a 4-D float32 or bfloat16 tensor
representing the features for each proposal.
"""
image_shape_2d = self._image_batch_shape_2d(image_shape)
proposal_boxes_normalized, _, num_proposals = self._postprocess_rpn(
rpn_box_encodings, rpn_objectness_predictions_with_background,
anchors, image_shape_2d, true_image_shapes)
# If mixed-precision training on TPU is enabled, the dtype of
# rpn_features_to_crop is bfloat16, otherwise it is float32. tf.cast is
# used to match the dtype of proposal_boxes_normalized to that of
# rpn_features_to_crop for further computation.
flattened_proposal_feature_maps = (
self._compute_second_stage_input_feature_maps(
rpn_features_to_crop,
tf.cast(proposal_boxes_normalized, rpn_features_to_crop.dtype)))
box_classifier_features = (
self._feature_extractor.extract_box_classifier_features(
flattened_proposal_feature_maps,
scope=self.second_stage_feature_extractor_scope))
if self._mask_rcnn_box_predictor.is_keras_model:
box_predictions = self._mask_rcnn_box_predictor(
[box_classifier_features],
prediction_stage=2)
else:
box_predictions = self._mask_rcnn_box_predictor.predict(
[box_classifier_features],
num_predictions_per_location=[1],
scope=self.second_stage_box_predictor_scope,
prediction_stage=2)
refined_box_encodings = tf.squeeze(
box_predictions[box_predictor.BOX_ENCODINGS],
axis=1, name='all_refined_box_encodings')
class_predictions_with_background = tf.squeeze(
box_predictions[box_predictor.CLASS_PREDICTIONS_WITH_BACKGROUND],
axis=1, name='all_class_predictions_with_background')
absolute_proposal_boxes = ops.normalized_to_image_coordinates(
proposal_boxes_normalized, image_shape, self._parallel_iterations)
prediction_dict = {
'refined_box_encodings': refined_box_encodings,
'class_predictions_with_background':
class_predictions_with_background,
'num_proposals': num_proposals,
'proposal_boxes': absolute_proposal_boxes,
'box_classifier_features': box_classifier_features,
'proposal_boxes_normalized': proposal_boxes_normalized,
}
return prediction_dict
def _predict_third_stage(self, prediction_dict, image_shapes):
"""Predicts non-box, non-class outputs using refined detections.
For training, masks as predicted directly on the box_classifier_features,
which are region-features from the initial anchor boxes.
For inference, this happens after calling the post-processing stage, such
that masks are only calculated for the top scored boxes.
Args:
prediction_dict: a dictionary holding "raw" prediction tensors:
1) refined_box_encodings: a 3-D tensor with shape
[total_num_proposals, num_classes, self._box_coder.code_size]
representing predicted (final) refined box encodings, where
total_num_proposals=batch_size*self._max_num_proposals. If using a
shared box across classes the shape will instead be
[total_num_proposals, 1, self._box_coder.code_size].
2) class_predictions_with_background: a 3-D tensor with shape
[total_num_proposals, num_classes + 1] containing class
predictions (logits) for each of the anchors, where
total_num_proposals=batch_size*self._max_num_proposals.
Note that this tensor *includes* background class predictions
(at class index 0).
3) num_proposals: An int32 tensor of shape [batch_size] representing the
number of proposals generated by the RPN. `num_proposals` allows us
to keep track of which entries are to be treated as zero paddings and
which are not since we always pad the number of proposals to be
`self.max_num_proposals` for each image.
4) proposal_boxes: A float32 tensor of shape
[batch_size, self.max_num_proposals, 4] representing
decoded proposal bounding boxes in absolute coordinates.
5) box_classifier_features: a 4-D float32 tensor representing the
features for each proposal.
image_shapes: A 2-D int32 tensors of shape [batch_size, 3] containing
shapes of images in the batch.
Returns:
prediction_dict: a dictionary that in addition to the input predictions
does hold the following predictions as well:
1) mask_predictions: a 4-D tensor with shape
[batch_size, max_detection, mask_height, mask_width] containing
instance mask predictions.
"""
if self._is_training:
curr_box_classifier_features = prediction_dict['box_classifier_features']
detection_classes = prediction_dict['class_predictions_with_background']
if self._mask_rcnn_box_predictor.is_keras_model:
mask_predictions = self._mask_rcnn_box_predictor(
[curr_box_classifier_features],
prediction_stage=3)
else:
mask_predictions = self._mask_rcnn_box_predictor.predict(
[curr_box_classifier_features],
num_predictions_per_location=[1],
scope=self.second_stage_box_predictor_scope,
prediction_stage=3)
prediction_dict['mask_predictions'] = tf.squeeze(mask_predictions[
box_predictor.MASK_PREDICTIONS], axis=1)
else:
detections_dict = self._postprocess_box_classifier(
prediction_dict['refined_box_encodings'],
prediction_dict['class_predictions_with_background'],
prediction_dict['proposal_boxes'],
prediction_dict['num_proposals'],
image_shapes)
prediction_dict.update(detections_dict)
detection_boxes = detections_dict[
fields.DetectionResultFields.detection_boxes]
detection_classes = detections_dict[
fields.DetectionResultFields.detection_classes]
rpn_features_to_crop = prediction_dict['rpn_features_to_crop']
batch_size = tf.shape(detection_boxes)[0]
max_detection = tf.shape(detection_boxes)[1]
flattened_detected_feature_maps = (
self._compute_second_stage_input_feature_maps(
rpn_features_to_crop, detection_boxes))
curr_box_classifier_features = (
self._feature_extractor.extract_box_classifier_features(
flattened_detected_feature_maps,
scope=self.second_stage_feature_extractor_scope))
if self._mask_rcnn_box_predictor.is_keras_model:
mask_predictions = self._mask_rcnn_box_predictor(
[curr_box_classifier_features],
prediction_stage=3)
else:
mask_predictions = self._mask_rcnn_box_predictor.predict(
[curr_box_classifier_features],
num_predictions_per_location=[1],
scope=self.second_stage_box_predictor_scope,
prediction_stage=3)
detection_masks = tf.squeeze(mask_predictions[
box_predictor.MASK_PREDICTIONS], axis=1)
_, num_classes, mask_height, mask_width = (
detection_masks.get_shape().as_list())
_, max_detection = detection_classes.get_shape().as_list()
prediction_dict['mask_predictions'] = tf.reshape(
detection_masks, [-1, num_classes, mask_height, mask_width])
if num_classes > 1:
detection_masks = self._gather_instance_masks(
detection_masks, detection_classes)
prediction_dict[fields.DetectionResultFields.detection_masks] = (
tf.reshape(tf.sigmoid(detection_masks),
[batch_size, max_detection, mask_height, mask_width]))
return prediction_dict
def _gather_instance_masks(self, instance_masks, classes):
"""Gathers the masks that correspond to classes.
Args:
instance_masks: A 4-D float32 tensor with shape
[K, num_classes, mask_height, mask_width].
classes: A 2-D int32 tensor with shape [batch_size, max_detection].
Returns:
masks: a 3-D float32 tensor with shape [K, mask_height, mask_width].
"""
_, num_classes, height, width = instance_masks.get_shape().as_list()
k = tf.shape(instance_masks)[0]
instance_masks = tf.reshape(instance_masks, [-1, height, width])
classes = tf.to_int32(tf.reshape(classes, [-1]))
gather_idx = tf.range(k) * num_classes + classes
return tf.gather(instance_masks, gather_idx)
def _extract_rpn_feature_maps(self, preprocessed_inputs):
"""Extracts RPN features.
This function extracts two feature maps: a feature map to be directly
fed to a box predictor (to predict location and objectness scores for
proposals) and a feature map from which to crop regions which will then
be sent to the second stage box classifier.
Args:
preprocessed_inputs: a [batch, height, width, channels] image tensor.
Returns:
rpn_box_predictor_features: A 4-D float32 tensor with shape
[batch, height, width, depth] to be used for predicting proposal boxes
and corresponding objectness scores.
rpn_features_to_crop: A 4-D float32 tensor with shape
[batch, height, width, depth] representing image features to crop using
the proposals boxes.
anchors: A BoxList representing anchors (for the RPN) in
absolute coordinates.
image_shape: A 1-D tensor representing the input image shape.
"""
image_shape = tf.shape(preprocessed_inputs)
rpn_features_to_crop, self.endpoints = (
self._feature_extractor.extract_proposal_features(
preprocessed_inputs,
scope=self.first_stage_feature_extractor_scope))
feature_map_shape = tf.shape(rpn_features_to_crop)
anchors = box_list_ops.concatenate(
self._first_stage_anchor_generator.generate([(feature_map_shape[1],
feature_map_shape[2])]))
with slim.arg_scope(self._first_stage_box_predictor_arg_scope_fn()):
kernel_size = self._first_stage_box_predictor_kernel_size
reuse = tf.get_variable_scope().reuse
rpn_box_predictor_features = slim.conv2d(
rpn_features_to_crop,
self._first_stage_box_predictor_depth,
kernel_size=[kernel_size, kernel_size],
rate=self._first_stage_atrous_rate,
activation_fn=tf.nn.relu6,
scope='Conv',
reuse=reuse)
return (rpn_box_predictor_features, rpn_features_to_crop,
anchors, image_shape)
def _predict_rpn_proposals(self, rpn_box_predictor_features):
"""Adds box predictors to RPN feature map to predict proposals.
Note resulting tensors will not have been postprocessed.
Args:
rpn_box_predictor_features: A 4-D float32 tensor with shape
[batch, height, width, depth] to be used for predicting proposal boxes
and corresponding objectness scores.
Returns:
box_encodings: 3-D float tensor of shape
[batch_size, num_anchors, self._box_coder.code_size] containing
predicted boxes.
objectness_predictions_with_background: 3-D float tensor of shape
[batch_size, num_anchors, 2] containing class
predictions (logits) for each of the anchors. Note that this
tensor *includes* background class predictions (at class index 0).
Raises:
RuntimeError: if the anchor generator generates anchors corresponding to
multiple feature maps. We currently assume that a single feature map
is generated for the RPN.
"""
num_anchors_per_location = (
self._first_stage_anchor_generator.num_anchors_per_location())
if len(num_anchors_per_location) != 1:
raise RuntimeError('anchor_generator is expected to generate anchors '
'corresponding to a single feature map.')
if self._first_stage_box_predictor.is_keras_model:
box_predictions = self._first_stage_box_predictor(
[rpn_box_predictor_features])
else:
box_predictions = self._first_stage_box_predictor.predict(
[rpn_box_predictor_features],
num_anchors_per_location,
scope=self.first_stage_box_predictor_scope)
box_encodings = tf.concat(
box_predictions[box_predictor.BOX_ENCODINGS], axis=1)
objectness_predictions_with_background = tf.concat(
box_predictions[box_predictor.CLASS_PREDICTIONS_WITH_BACKGROUND],
axis=1)
return (tf.squeeze(box_encodings, axis=2),
objectness_predictions_with_background)
def _remove_invalid_anchors_and_predictions(
self,
box_encodings,
objectness_predictions_with_background,
anchors_boxlist,
clip_window):
"""Removes anchors that (partially) fall outside an image.
Also removes associated box encodings and objectness predictions.
Args:
box_encodings: 3-D float tensor of shape
[batch_size, num_anchors, self._box_coder.code_size] containing
predicted boxes.
objectness_predictions_with_background: 3-D float tensor of shape
[batch_size, num_anchors, 2] containing class
predictions (logits) for each of the anchors. Note that this
tensor *includes* background class predictions (at class index 0).
anchors_boxlist: A BoxList representing num_anchors anchors (for the RPN)
in absolute coordinates.
clip_window: a 1-D tensor representing the [ymin, xmin, ymax, xmax]
extent of the window to clip/prune to.
Returns:
box_encodings: 4-D float tensor of shape
[batch_size, num_valid_anchors, self._box_coder.code_size] containing
predicted boxes, where num_valid_anchors <= num_anchors
objectness_predictions_with_background: 2-D float tensor of shape
[batch_size, num_valid_anchors, 2] containing class
predictions (logits) for each of the anchors, where
num_valid_anchors <= num_anchors. Note that this
tensor *includes* background class predictions (at class index 0).
anchors: A BoxList representing num_valid_anchors anchors (for the RPN) in
absolute coordinates.
"""
pruned_anchors_boxlist, keep_indices = box_list_ops.prune_outside_window(
anchors_boxlist, clip_window)
def _batch_gather_kept_indices(predictions_tensor):
return shape_utils.static_or_dynamic_map_fn(
partial(tf.gather, indices=keep_indices),
elems=predictions_tensor,
dtype=tf.float32,
parallel_iterations=self._parallel_iterations,
back_prop=True)
return (_batch_gather_kept_indices(box_encodings),
_batch_gather_kept_indices(objectness_predictions_with_background),
pruned_anchors_boxlist)
def _flatten_first_two_dimensions(self, inputs):
"""Flattens `K-d` tensor along batch dimension to be a `(K-1)-d` tensor.
Converts `inputs` with shape [A, B, ..., depth] into a tensor of shape
[A * B, ..., depth].
Args:
inputs: A float tensor with shape [A, B, ..., depth]. Note that the first
two and last dimensions must be statically defined.
Returns:
A float tensor with shape [A * B, ..., depth] (where the first and last
dimension are statically defined.
"""
combined_shape = shape_utils.combined_static_and_dynamic_shape(inputs)
flattened_shape = tf.stack([combined_shape[0] * combined_shape[1]] +
combined_shape[2:])
return tf.reshape(inputs, flattened_shape)
def postprocess(self, prediction_dict, true_image_shapes):
"""Convert prediction tensors to final detections.
This function converts raw predictions tensors to final detection results.
See base class for output format conventions. Note also that by default,
scores are to be interpreted as logits, but if a score_converter is used,
then scores are remapped (and may thus have a different interpretation).
If number_of_stages=1, the returned results represent proposals from the
first stage RPN and are padded to have self.max_num_proposals for each
image; otherwise, the results can be interpreted as multiclass detections
from the full two-stage model and are padded to self._max_detections.
Args:
prediction_dict: a dictionary holding prediction tensors (see the
documentation for the predict method. If number_of_stages=1, we
expect prediction_dict to contain `rpn_box_encodings`,
`rpn_objectness_predictions_with_background`, `rpn_features_to_crop`,
and `anchors` fields. Otherwise we expect prediction_dict to
additionally contain `refined_box_encodings`,
`class_predictions_with_background`, `num_proposals`,
`proposal_boxes` and, optionally, `mask_predictions` fields.
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
Returns:
detections: a dictionary containing the following fields
detection_boxes: [batch, max_detection, 4]
detection_scores: [batch, max_detections]
detection_classes: [batch, max_detections]
(this entry is only created if rpn_mode=False)
num_detections: [batch]
Raises:
ValueError: If `predict` is called before `preprocess`.
"""
with tf.name_scope('FirstStagePostprocessor'):
if self._number_of_stages == 1:
proposal_boxes, proposal_scores, num_proposals = self._postprocess_rpn(
prediction_dict['rpn_box_encodings'],
prediction_dict['rpn_objectness_predictions_with_background'],
prediction_dict['anchors'],
true_image_shapes,
true_image_shapes)
return {
fields.DetectionResultFields.detection_boxes: proposal_boxes,
fields.DetectionResultFields.detection_scores: proposal_scores,
fields.DetectionResultFields.num_detections:
tf.to_float(num_proposals),
}
# TODO(jrru): Remove mask_predictions from _post_process_box_classifier.
if (self._number_of_stages == 2 or
(self._number_of_stages == 3 and self._is_training)):
with tf.name_scope('SecondStagePostprocessor'):
mask_predictions = prediction_dict.get(box_predictor.MASK_PREDICTIONS)
detections_dict = self._postprocess_box_classifier(
prediction_dict['refined_box_encodings'],
prediction_dict['class_predictions_with_background'],
prediction_dict['proposal_boxes'],
prediction_dict['num_proposals'],
true_image_shapes,
mask_predictions=mask_predictions)
if 'rpn_features_to_crop' in prediction_dict and self._initial_crop_size:
self._add_detection_features_output_node(
detections_dict[fields.DetectionResultFields.detection_boxes],
prediction_dict['rpn_features_to_crop'])
return detections_dict
if self._number_of_stages == 3:
# Post processing is already performed in 3rd stage. We need to transfer
# postprocessed tensors from `prediction_dict` to `detections_dict`.
return prediction_dict
def _add_detection_features_output_node(self, detection_boxes,
rpn_features_to_crop):
"""Add the detection features to the output node.
The detection features are from cropping rpn_features with boxes.
Each bounding box has one feature vector of length depth, which comes from
mean_pooling of the cropped rpn_features.
Args:
detection_boxes: a 3-D float32 tensor of shape
[batch_size, max_detection, 4] which represents the bounding boxes.
rpn_features_to_crop: A 4-D float32 tensor with shape
[batch, height, width, depth] representing image features to crop using
the proposals boxes.
"""
with tf.name_scope('SecondStageDetectionFeaturesExtract'):
flattened_detected_feature_maps = (
self._compute_second_stage_input_feature_maps(
rpn_features_to_crop, detection_boxes))
detection_features_unpooled = (
self._feature_extractor.extract_box_classifier_features(
flattened_detected_feature_maps,
scope=self.second_stage_feature_extractor_scope))
batch_size = tf.shape(detection_boxes)[0]
max_detection = tf.shape(detection_boxes)[1]
detection_features_pool = tf.reduce_mean(
detection_features_unpooled, axis=[1, 2])
detection_features = tf.reshape(
detection_features_pool,
[batch_size, max_detection, tf.shape(detection_features_pool)[-1]])
detection_features = tf.identity(
detection_features, 'detection_features')
def _postprocess_rpn(self,
rpn_box_encodings_batch,
rpn_objectness_predictions_with_background_batch,
anchors,
image_shapes,
true_image_shapes):
"""Converts first stage prediction tensors from the RPN to proposals.
This function decodes the raw RPN predictions, runs non-max suppression
on the result.
Note that the behavior of this function is slightly modified during
training --- specifically, we stop the gradient from passing through the
proposal boxes and we only return a balanced sampled subset of proposals
with size `second_stage_batch_size`.
Args:
rpn_box_encodings_batch: A 3-D float32 tensor of shape
[batch_size, num_anchors, self._box_coder.code_size] containing
predicted proposal box encodings.
rpn_objectness_predictions_with_background_batch: A 3-D float tensor of
shape [batch_size, num_anchors, 2] containing objectness predictions
(logits) for each of the anchors with 0 corresponding to background
and 1 corresponding to object.
anchors: A 2-D tensor of shape [num_anchors, 4] representing anchors
for the first stage RPN. Note that `num_anchors` can differ depending
on whether the model is created in training or inference mode.
image_shapes: A 2-D tensor of shape [batch, 3] containing the shapes of
images in the batch.
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
Returns:
proposal_boxes: A float tensor with shape
[batch_size, max_num_proposals, 4] representing the (potentially zero
padded) proposal boxes for all images in the batch. These boxes are
represented as normalized coordinates.
proposal_scores: A float tensor with shape
[batch_size, max_num_proposals] representing the (potentially zero
padded) proposal objectness scores for all images in the batch.
num_proposals: A Tensor of type `int32`. A 1-D tensor of shape [batch]
representing the number of proposals predicted for each image in
the batch.
"""
rpn_box_encodings_batch = tf.expand_dims(rpn_box_encodings_batch, axis=2)
rpn_encodings_shape = shape_utils.combined_static_and_dynamic_shape(
rpn_box_encodings_batch)
tiled_anchor_boxes = tf.tile(
tf.expand_dims(anchors, 0), [rpn_encodings_shape[0], 1, 1])
proposal_boxes = self._batch_decode_boxes(rpn_box_encodings_batch,
tiled_anchor_boxes)
proposal_boxes = tf.squeeze(proposal_boxes, axis=2)
rpn_objectness_softmax_without_background = tf.nn.softmax(
rpn_objectness_predictions_with_background_batch)[:, :, 1]
clip_window = self._compute_clip_window(image_shapes)
(proposal_boxes, proposal_scores, _, _, _,
num_proposals) = self._first_stage_nms_fn(
tf.expand_dims(proposal_boxes, axis=2),
tf.expand_dims(rpn_objectness_softmax_without_background, axis=2),
clip_window=clip_window)
if self._is_training:
proposal_boxes = tf.stop_gradient(proposal_boxes)
if not self._hard_example_miner:
(groundtruth_boxlists, groundtruth_classes_with_background_list, _,
groundtruth_weights_list
) = self._format_groundtruth_data(true_image_shapes)
(proposal_boxes, proposal_scores,
num_proposals) = self._sample_box_classifier_batch(
proposal_boxes, proposal_scores, num_proposals,
groundtruth_boxlists, groundtruth_classes_with_background_list,
groundtruth_weights_list)
# normalize proposal boxes
def normalize_boxes(args):
proposal_boxes_per_image = args[0]
image_shape = args[1]
normalized_boxes_per_image = box_list_ops.to_normalized_coordinates(
box_list.BoxList(proposal_boxes_per_image), image_shape[0],
image_shape[1], check_range=False).get()
return normalized_boxes_per_image
normalized_proposal_boxes = shape_utils.static_or_dynamic_map_fn(
normalize_boxes, elems=[proposal_boxes, image_shapes], dtype=tf.float32)
return normalized_proposal_boxes, proposal_scores, num_proposals
def _sample_box_classifier_batch(
self,
proposal_boxes,
proposal_scores,
num_proposals,
groundtruth_boxlists,
groundtruth_classes_with_background_list,
groundtruth_weights_list):
"""Samples a minibatch for second stage.
Args:
proposal_boxes: A float tensor with shape
[batch_size, num_proposals, 4] representing the (potentially zero
padded) proposal boxes for all images in the batch. These boxes are
represented in absolute coordinates.
proposal_scores: A float tensor with shape
[batch_size, num_proposals] representing the (potentially zero
padded) proposal objectness scores for all images in the batch.
num_proposals: A Tensor of type `int32`. A 1-D tensor of shape [batch]
representing the number of proposals predicted for each image in
the batch.
groundtruth_boxlists: A list of BoxLists containing (absolute) coordinates
of the groundtruth boxes.
groundtruth_classes_with_background_list: A list of 2-D one-hot
(or k-hot) tensors of shape [num_boxes, num_classes+1] containing the
class targets with the 0th index assumed to map to the background class.
groundtruth_weights_list: A list of 1-D tensors of shape [num_boxes]
indicating the weight associated with the groundtruth boxes.
Returns:
proposal_boxes: A float tensor with shape
[batch_size, second_stage_batch_size, 4] representing the (potentially
zero padded) proposal boxes for all images in the batch. These boxes
are represented in absolute coordinates.
proposal_scores: A float tensor with shape
[batch_size, second_stage_batch_size] representing the (potentially zero
padded) proposal objectness scores for all images in the batch.
num_proposals: A Tensor of type `int32`. A 1-D tensor of shape [batch]
representing the number of proposals predicted for each image in
the batch.
"""
single_image_proposal_box_sample = []
single_image_proposal_score_sample = []
single_image_num_proposals_sample = []
for (single_image_proposal_boxes,
single_image_proposal_scores,
single_image_num_proposals,
single_image_groundtruth_boxlist,
single_image_groundtruth_classes_with_background,
single_image_groundtruth_weights) in zip(
tf.unstack(proposal_boxes),
tf.unstack(proposal_scores),
tf.unstack(num_proposals),
groundtruth_boxlists,
groundtruth_classes_with_background_list,
groundtruth_weights_list):
single_image_boxlist = box_list.BoxList(single_image_proposal_boxes)
single_image_boxlist.add_field(fields.BoxListFields.scores,
single_image_proposal_scores)
sampled_boxlist = self._sample_box_classifier_minibatch_single_image(
single_image_boxlist,
single_image_num_proposals,
single_image_groundtruth_boxlist,
single_image_groundtruth_classes_with_background,
single_image_groundtruth_weights)
sampled_padded_boxlist = box_list_ops.pad_or_clip_box_list(
sampled_boxlist,
num_boxes=self._second_stage_batch_size)
single_image_num_proposals_sample.append(tf.minimum(
sampled_boxlist.num_boxes(),
self._second_stage_batch_size))
bb = sampled_padded_boxlist.get()
single_image_proposal_box_sample.append(bb)
single_image_proposal_score_sample.append(
sampled_padded_boxlist.get_field(fields.BoxListFields.scores))
return (tf.stack(single_image_proposal_box_sample),
tf.stack(single_image_proposal_score_sample),
tf.stack(single_image_num_proposals_sample))
def _format_groundtruth_data(self, true_image_shapes):
"""Helper function for preparing groundtruth data for target assignment.
In order to be consistent with the model.DetectionModel interface,
groundtruth boxes are specified in normalized coordinates and classes are
specified as label indices with no assumed background category. To prepare
for target assignment, we:
1) convert boxes to absolute coordinates,
2) add a background class at class index 0
3) groundtruth instance masks, if available, are resized to match
image_shape.
Args:
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
Returns:
groundtruth_boxlists: A list of BoxLists containing (absolute) coordinates
of the groundtruth boxes.
groundtruth_classes_with_background_list: A list of 2-D one-hot
(or k-hot) tensors of shape [num_boxes, num_classes+1] containing the
class targets with the 0th index assumed to map to the background class.
groundtruth_masks_list: If present, a list of 3-D tf.float32 tensors of
shape [num_boxes, image_height, image_width] containing instance masks.
This is set to None if no masks exist in the provided groundtruth.
"""
groundtruth_boxlists = [
box_list_ops.to_absolute_coordinates(
box_list.BoxList(boxes), true_image_shapes[i, 0],
true_image_shapes[i, 1])
for i, boxes in enumerate(
self.groundtruth_lists(fields.BoxListFields.boxes))
]
groundtruth_classes_with_background_list = [
tf.to_float(
tf.pad(one_hot_encoding, [[0, 0], [1, 0]], mode='CONSTANT'))
for one_hot_encoding in self.groundtruth_lists(
fields.BoxListFields.classes)]
groundtruth_masks_list = self._groundtruth_lists.get(
fields.BoxListFields.masks)
# TODO(rathodv): Remove mask resizing once the legacy pipeline is deleted.
if groundtruth_masks_list is not None and self._resize_masks:
resized_masks_list = []
for mask in groundtruth_masks_list:
_, resized_mask, _ = self._image_resizer_fn(
# Reuse the given `image_resizer_fn` to resize groundtruth masks.
# `mask` tensor for an image is of the shape [num_masks,
# image_height, image_width]. Below we create a dummy image of the
# the shape [image_height, image_width, 1] to use with
# `image_resizer_fn`.
image=tf.zeros(tf.stack([tf.shape(mask)[1],
tf.shape(mask)[2], 1])),
masks=mask)
resized_masks_list.append(resized_mask)
groundtruth_masks_list = resized_masks_list
if self.groundtruth_has_field(fields.BoxListFields.weights):
groundtruth_weights_list = self.groundtruth_lists(
fields.BoxListFields.weights)
else:
# Set weights for all batch elements equally to 1.0
groundtruth_weights_list = []
for groundtruth_classes in groundtruth_classes_with_background_list:
num_gt = tf.shape(groundtruth_classes)[0]
groundtruth_weights = tf.ones(num_gt)
groundtruth_weights_list.append(groundtruth_weights)
return (groundtruth_boxlists, groundtruth_classes_with_background_list,
groundtruth_masks_list, groundtruth_weights_list)
def _sample_box_classifier_minibatch_single_image(
self, proposal_boxlist, num_valid_proposals, groundtruth_boxlist,
groundtruth_classes_with_background, groundtruth_weights):
"""Samples a mini-batch of proposals to be sent to the box classifier.
Helper function for self._postprocess_rpn.
Args:
proposal_boxlist: A BoxList containing K proposal boxes in absolute
coordinates.
num_valid_proposals: Number of valid proposals in the proposal boxlist.
groundtruth_boxlist: A Boxlist containing N groundtruth object boxes in
absolute coordinates.
groundtruth_classes_with_background: A tensor with shape
`[N, self.num_classes + 1]` representing groundtruth classes. The
classes are assumed to be k-hot encoded, and include background as the
zero-th class.
groundtruth_weights: Weights attached to the groundtruth_boxes.
Returns:
a BoxList contained sampled proposals.
"""
(cls_targets, cls_weights, _, _, _) = self._detector_target_assigner.assign(
proposal_boxlist,
groundtruth_boxlist,
groundtruth_classes_with_background,
unmatched_class_label=tf.constant(
[1] + self._num_classes * [0], dtype=tf.float32),
groundtruth_weights=groundtruth_weights)
# Selects all boxes as candidates if none of them is selected according
# to cls_weights. This could happen as boxes within certain IOU ranges
# are ignored. If triggered, the selected boxes will still be ignored
# during loss computation.
cls_weights = tf.reduce_mean(cls_weights, axis=-1)
positive_indicator = tf.greater(tf.argmax(cls_targets, axis=1), 0)
valid_indicator = tf.logical_and(
tf.range(proposal_boxlist.num_boxes()) < num_valid_proposals,
cls_weights > 0
)
selected_positions = self._second_stage_sampler.subsample(
valid_indicator,
self._second_stage_batch_size,
positive_indicator)
return box_list_ops.boolean_mask(
proposal_boxlist,
selected_positions,
use_static_shapes=self._use_static_shapes,
indicator_sum=(self._second_stage_batch_size
if self._use_static_shapes else None))
def _compute_second_stage_input_feature_maps(self, features_to_crop,
proposal_boxes_normalized):
"""Crops to a set of proposals from the feature map for a batch of images.
Helper function for self._postprocess_rpn. This function calls
`tf.image.crop_and_resize` to create the feature map to be passed to the
second stage box classifier for each proposal.
Args:
features_to_crop: A float32 tensor with shape
[batch_size, height, width, depth]
proposal_boxes_normalized: A float32 tensor with shape [batch_size,
num_proposals, box_code_size] containing proposal boxes in
normalized coordinates.
Returns:
A float32 tensor with shape [K, new_height, new_width, depth].
"""
cropped_regions = self._flatten_first_two_dimensions(
self._crop_and_resize_fn(
features_to_crop, proposal_boxes_normalized,
[self._initial_crop_size, self._initial_crop_size]))
return slim.max_pool2d(
cropped_regions,
[self._maxpool_kernel_size, self._maxpool_kernel_size],
stride=self._maxpool_stride)
def _postprocess_box_classifier(self,
refined_box_encodings,
class_predictions_with_background,
proposal_boxes,
num_proposals,
image_shapes,
mask_predictions=None):
"""Converts predictions from the second stage box classifier to detections.
Args:
refined_box_encodings: a 3-D float tensor with shape
[total_num_padded_proposals, num_classes, self._box_coder.code_size]
representing predicted (final) refined box encodings. If using a shared
box across classes the shape will instead be
[total_num_padded_proposals, 1, 4]
class_predictions_with_background: a 3-D tensor float with shape
[total_num_padded_proposals, num_classes + 1] containing class
predictions (logits) for each of the proposals. Note that this tensor
*includes* background class predictions (at class index 0).
proposal_boxes: a 3-D float tensor with shape
[batch_size, self.max_num_proposals, 4] representing decoded proposal
bounding boxes in absolute coordinates.
num_proposals: a 1-D int32 tensor of shape [batch] representing the number
of proposals predicted for each image in the batch.
image_shapes: a 2-D int32 tensor containing shapes of input image in the
batch.
mask_predictions: (optional) a 4-D float tensor with shape
[total_num_padded_proposals, num_classes, mask_height, mask_width]
containing instance mask prediction logits.
Returns:
A dictionary containing:
`detection_boxes`: [batch, max_detection, 4] in normalized co-ordinates.
`detection_scores`: [batch, max_detections]
`detection_classes`: [batch, max_detections]
`num_detections`: [batch]
`detection_masks`:
(optional) [batch, max_detections, mask_height, mask_width]. Note
that a pixel-wise sigmoid score converter is applied to the detection
masks.
"""
refined_box_encodings_batch = tf.reshape(
refined_box_encodings,
[-1,
self.max_num_proposals,
refined_box_encodings.shape[1],
self._box_coder.code_size])
class_predictions_with_background_batch = tf.reshape(
class_predictions_with_background,
[-1, self.max_num_proposals, self.num_classes + 1]
)
refined_decoded_boxes_batch = self._batch_decode_boxes(
refined_box_encodings_batch, proposal_boxes)
class_predictions_with_background_batch = (
self._second_stage_score_conversion_fn(
class_predictions_with_background_batch))
class_predictions_batch = tf.reshape(
tf.slice(class_predictions_with_background_batch,
[0, 0, 1], [-1, -1, -1]),
[-1, self.max_num_proposals, self.num_classes])
clip_window = self._compute_clip_window(image_shapes)
mask_predictions_batch = None
if mask_predictions is not None:
mask_height = mask_predictions.shape[2].value
mask_width = mask_predictions.shape[3].value
mask_predictions = tf.sigmoid(mask_predictions)
mask_predictions_batch = tf.reshape(
mask_predictions, [-1, self.max_num_proposals,
self.num_classes, mask_height, mask_width])
(nmsed_boxes, nmsed_scores, nmsed_classes, nmsed_masks, _,
num_detections) = self._second_stage_nms_fn(
refined_decoded_boxes_batch,
class_predictions_batch,
clip_window=clip_window,
change_coordinate_frame=True,
num_valid_boxes=num_proposals,
masks=mask_predictions_batch)
detections = {
fields.DetectionResultFields.detection_boxes: nmsed_boxes,
fields.DetectionResultFields.detection_scores: nmsed_scores,
fields.DetectionResultFields.detection_classes: nmsed_classes,
fields.DetectionResultFields.num_detections: tf.to_float(num_detections)
}
if nmsed_masks is not None:
detections[fields.DetectionResultFields.detection_masks] = nmsed_masks
return detections
def _batch_decode_boxes(self, box_encodings, anchor_boxes):
"""Decodes box encodings with respect to the anchor boxes.
Args:
box_encodings: a 4-D tensor with shape
[batch_size, num_anchors, num_classes, self._box_coder.code_size]
representing box encodings.
anchor_boxes: [batch_size, num_anchors, self._box_coder.code_size]
representing decoded bounding boxes. If using a shared box across
classes the shape will instead be
[total_num_proposals, 1, self._box_coder.code_size].
Returns:
decoded_boxes: a
[batch_size, num_anchors, num_classes, self._box_coder.code_size]
float tensor representing bounding box predictions (for each image in
batch, proposal and class). If using a shared box across classes the
shape will instead be
[batch_size, num_anchors, 1, self._box_coder.code_size].
"""
combined_shape = shape_utils.combined_static_and_dynamic_shape(
box_encodings)
num_classes = combined_shape[2]
tiled_anchor_boxes = tf.tile(
tf.expand_dims(anchor_boxes, 2), [1, 1, num_classes, 1])
tiled_anchors_boxlist = box_list.BoxList(
tf.reshape(tiled_anchor_boxes, [-1, 4]))
decoded_boxes = self._box_coder.decode(
tf.reshape(box_encodings, [-1, self._box_coder.code_size]),
tiled_anchors_boxlist)
return tf.reshape(decoded_boxes.get(),
tf.stack([combined_shape[0], combined_shape[1],
num_classes, 4]))
def loss(self, prediction_dict, true_image_shapes, scope=None):
"""Compute scalar loss tensors given prediction tensors.
If number_of_stages=1, only RPN related losses are computed (i.e.,
`rpn_localization_loss` and `rpn_objectness_loss`). Otherwise all
losses are computed.
Args:
prediction_dict: a dictionary holding prediction tensors (see the
documentation for the predict method. If number_of_stages=1, we
expect prediction_dict to contain `rpn_box_encodings`,
`rpn_objectness_predictions_with_background`, `rpn_features_to_crop`,
`image_shape`, and `anchors` fields. Otherwise we expect
prediction_dict to additionally contain `refined_box_encodings`,
`class_predictions_with_background`, `num_proposals`, and
`proposal_boxes` fields.
true_image_shapes: int32 tensor of shape [batch, 3] where each row is
of the form [height, width, channels] indicating the shapes
of true images in the resized images, as resized images can be padded
with zeros.
scope: Optional scope name.
Returns:
a dictionary mapping loss keys (`first_stage_localization_loss`,
`first_stage_objectness_loss`, 'second_stage_localization_loss',
'second_stage_classification_loss') to scalar tensors representing
corresponding loss values.
"""
with tf.name_scope(scope, 'Loss', prediction_dict.values()):
(groundtruth_boxlists, groundtruth_classes_with_background_list,
groundtruth_masks_list, groundtruth_weights_list
) = self._format_groundtruth_data(true_image_shapes)
loss_dict = self._loss_rpn(
prediction_dict['rpn_box_encodings'],
prediction_dict['rpn_objectness_predictions_with_background'],
prediction_dict['anchors'], groundtruth_boxlists,
groundtruth_classes_with_background_list, groundtruth_weights_list)
if self._number_of_stages > 1:
loss_dict.update(
self._loss_box_classifier(
prediction_dict['refined_box_encodings'],
prediction_dict['class_predictions_with_background'],
prediction_dict['proposal_boxes'],
prediction_dict['num_proposals'], groundtruth_boxlists,
groundtruth_classes_with_background_list,
groundtruth_weights_list, prediction_dict['image_shape'],
prediction_dict.get('mask_predictions'), groundtruth_masks_list,
prediction_dict.get(
fields.DetectionResultFields.detection_boxes),
prediction_dict.get(
fields.DetectionResultFields.num_detections)))
return loss_dict
def _loss_rpn(self, rpn_box_encodings,
rpn_objectness_predictions_with_background, anchors,
groundtruth_boxlists, groundtruth_classes_with_background_list,
groundtruth_weights_list):
"""Computes scalar RPN loss tensors.
Uses self._proposal_target_assigner to obtain regression and classification
targets for the first stage RPN, samples a "minibatch" of anchors to
participate in the loss computation, and returns the RPN losses.
Args:
rpn_box_encodings: A 4-D float tensor of shape
[batch_size, num_anchors, self._box_coder.code_size] containing
predicted proposal box encodings.
rpn_objectness_predictions_with_background: A 2-D float tensor of shape
[batch_size, num_anchors, 2] containing objectness predictions
(logits) for each of the anchors with 0 corresponding to background
and 1 corresponding to object.
anchors: A 2-D tensor of shape [num_anchors, 4] representing anchors
for the first stage RPN. Note that `num_anchors` can differ depending
on whether the model is created in training or inference mode.
groundtruth_boxlists: A list of BoxLists containing coordinates of the
groundtruth boxes.
groundtruth_classes_with_background_list: A list of 2-D one-hot
(or k-hot) tensors of shape [num_boxes, num_classes+1] containing the
class targets with the 0th index assumed to map to the background class.
groundtruth_weights_list: A list of 1-D tf.float32 tensors of shape
[num_boxes] containing weights for groundtruth boxes.
Returns:
a dictionary mapping loss keys (`first_stage_localization_loss`,
`first_stage_objectness_loss`) to scalar tensors representing
corresponding loss values.
"""
with tf.name_scope('RPNLoss'):
(batch_cls_targets, batch_cls_weights, batch_reg_targets,
batch_reg_weights, _) = target_assigner.batch_assign_targets(
target_assigner=self._proposal_target_assigner,
anchors_batch=box_list.BoxList(anchors),
gt_box_batch=groundtruth_boxlists,
gt_class_targets_batch=(len(groundtruth_boxlists) * [None]),
gt_weights_batch=groundtruth_weights_list)
batch_cls_weights = tf.reduce_mean(batch_cls_weights, axis=2)
batch_cls_targets = tf.squeeze(batch_cls_targets, axis=2)
def _minibatch_subsample_fn(inputs):
cls_targets, cls_weights = inputs
return self._first_stage_sampler.subsample(
tf.cast(cls_weights, tf.bool),
self._first_stage_minibatch_size, tf.cast(cls_targets, tf.bool))
batch_sampled_indices = tf.to_float(shape_utils.static_or_dynamic_map_fn(
_minibatch_subsample_fn,
[batch_cls_targets, batch_cls_weights],
dtype=tf.bool,
parallel_iterations=self._parallel_iterations,
back_prop=True))
# Normalize by number of examples in sampled minibatch
normalizer = tf.reduce_sum(batch_sampled_indices, axis=1)
batch_one_hot_targets = tf.one_hot(
tf.to_int32(batch_cls_targets), depth=2)
sampled_reg_indices = tf.multiply(batch_sampled_indices,
batch_reg_weights)
losses_mask = None
if self.groundtruth_has_field(fields.InputDataFields.is_annotated):
losses_mask = tf.stack(self.groundtruth_lists(
fields.InputDataFields.is_annotated))
localization_losses = self._first_stage_localization_loss(
rpn_box_encodings, batch_reg_targets, weights=sampled_reg_indices,
losses_mask=losses_mask)
objectness_losses = self._first_stage_objectness_loss(
rpn_objectness_predictions_with_background,
batch_one_hot_targets,
weights=tf.expand_dims(batch_sampled_indices, axis=-1),
losses_mask=losses_mask)
localization_loss = tf.reduce_mean(
tf.reduce_sum(localization_losses, axis=1) / normalizer)
objectness_loss = tf.reduce_mean(
tf.reduce_sum(objectness_losses, axis=1) / normalizer)
localization_loss = tf.multiply(self._first_stage_loc_loss_weight,
localization_loss,
name='localization_loss')
objectness_loss = tf.multiply(self._first_stage_obj_loss_weight,
objectness_loss, name='objectness_loss')
loss_dict = {localization_loss.op.name: localization_loss,
objectness_loss.op.name: objectness_loss}
return loss_dict
def _loss_box_classifier(self,
refined_box_encodings,
class_predictions_with_background,
proposal_boxes,
num_proposals,
groundtruth_boxlists,
groundtruth_classes_with_background_list,
groundtruth_weights_list,
image_shape,
prediction_masks=None,
groundtruth_masks_list=None,
detection_boxes=None,
num_detections=None):
"""Computes scalar box classifier loss tensors.
Uses self._detector_target_assigner to obtain regression and classification
targets for the second stage box classifier, optionally performs
hard mining, and returns losses. All losses are computed independently
for each image and then averaged across the batch.
Please note that for boxes and masks with multiple labels, the box
regression and mask prediction losses are only computed for one label.
This function assumes that the proposal boxes in the "padded" regions are
actually zero (and thus should not be matched to).
Args:
refined_box_encodings: a 3-D tensor with shape
[total_num_proposals, num_classes, box_coder.code_size] representing
predicted (final) refined box encodings. If using a shared box across
classes this will instead have shape
[total_num_proposals, 1, box_coder.code_size].
class_predictions_with_background: a 2-D tensor with shape
[total_num_proposals, num_classes + 1] containing class
predictions (logits) for each of the anchors. Note that this tensor
*includes* background class predictions (at class index 0).
proposal_boxes: [batch_size, self.max_num_proposals, 4] representing
decoded proposal bounding boxes.
num_proposals: A Tensor of type `int32`. A 1-D tensor of shape [batch]
representing the number of proposals predicted for each image in
the batch.
groundtruth_boxlists: a list of BoxLists containing coordinates of the
groundtruth boxes.
groundtruth_classes_with_background_list: a list of 2-D one-hot
(or k-hot) tensors of shape [num_boxes, num_classes + 1] containing the
class targets with the 0th index assumed to map to the background class.
groundtruth_weights_list: A list of 1-D tf.float32 tensors of shape
[num_boxes] containing weights for groundtruth boxes.
image_shape: a 1-D tensor of shape [4] representing the image shape.
prediction_masks: an optional 4-D tensor with shape [total_num_proposals,
num_classes, mask_height, mask_width] containing the instance masks for
each box.
groundtruth_masks_list: an optional list of 3-D tensors of shape
[num_boxes, image_height, image_width] containing the instance masks for
each of the boxes.
detection_boxes: 3-D float tensor of shape [batch,
max_total_detections, 4] containing post-processed detection boxes in
normalized co-ordinates.
num_detections: 1-D int32 tensor of shape [batch] containing number of
valid detections in `detection_boxes`.
Returns:
a dictionary mapping loss keys ('second_stage_localization_loss',
'second_stage_classification_loss') to scalar tensors representing
corresponding loss values.
Raises:
ValueError: if `predict_instance_masks` in
second_stage_mask_rcnn_box_predictor is True and
`groundtruth_masks_list` is not provided.
"""
with tf.name_scope('BoxClassifierLoss'):
paddings_indicator = self._padded_batched_proposals_indicator(
num_proposals, proposal_boxes.shape[1])
proposal_boxlists = [
box_list.BoxList(proposal_boxes_single_image)
for proposal_boxes_single_image in tf.unstack(proposal_boxes)]
batch_size = len(proposal_boxlists)
num_proposals_or_one = tf.to_float(tf.expand_dims(
tf.maximum(num_proposals, tf.ones_like(num_proposals)), 1))
normalizer = tf.tile(num_proposals_or_one,
[1, self.max_num_proposals]) * batch_size
(batch_cls_targets_with_background, batch_cls_weights, batch_reg_targets,
batch_reg_weights, _) = target_assigner.batch_assign_targets(
target_assigner=self._detector_target_assigner,
anchors_batch=proposal_boxlists,
gt_box_batch=groundtruth_boxlists,
gt_class_targets_batch=groundtruth_classes_with_background_list,
unmatched_class_label=tf.constant(
[1] + self._num_classes * [0], dtype=tf.float32),
gt_weights_batch=groundtruth_weights_list)
class_predictions_with_background = tf.reshape(
class_predictions_with_background,
[batch_size, self.max_num_proposals, -1])
flat_cls_targets_with_background = tf.reshape(
batch_cls_targets_with_background,
[batch_size * self.max_num_proposals, -1])
one_hot_flat_cls_targets_with_background = tf.argmax(
flat_cls_targets_with_background, axis=1)
one_hot_flat_cls_targets_with_background = tf.one_hot(
one_hot_flat_cls_targets_with_background,
flat_cls_targets_with_background.get_shape()[1])
# If using a shared box across classes use directly
if refined_box_encodings.shape[1] == 1:
reshaped_refined_box_encodings = tf.reshape(
refined_box_encodings,
[batch_size, self.max_num_proposals, self._box_coder.code_size])
# For anchors with multiple labels, picks refined_location_encodings
# for just one class to avoid over-counting for regression loss and
# (optionally) mask loss.
else:
reshaped_refined_box_encodings = (
self._get_refined_encodings_for_postitive_class(
refined_box_encodings,
one_hot_flat_cls_targets_with_background, batch_size))
losses_mask = None
if self.groundtruth_has_field(fields.InputDataFields.is_annotated):
losses_mask = tf.stack(self.groundtruth_lists(
fields.InputDataFields.is_annotated))
second_stage_loc_losses = self._second_stage_localization_loss(
reshaped_refined_box_encodings,
batch_reg_targets,
weights=batch_reg_weights,
losses_mask=losses_mask) / normalizer
second_stage_cls_losses = ops.reduce_sum_trailing_dimensions(
self._second_stage_classification_loss(
class_predictions_with_background,
batch_cls_targets_with_background,
weights=batch_cls_weights,
losses_mask=losses_mask),
ndims=2) / normalizer
second_stage_loc_loss = tf.reduce_sum(
second_stage_loc_losses * tf.to_float(paddings_indicator))
second_stage_cls_loss = tf.reduce_sum(
second_stage_cls_losses * tf.to_float(paddings_indicator))
if self._hard_example_miner:
(second_stage_loc_loss, second_stage_cls_loss
) = self._unpad_proposals_and_apply_hard_mining(
proposal_boxlists, second_stage_loc_losses,
second_stage_cls_losses, num_proposals)
localization_loss = tf.multiply(self._second_stage_loc_loss_weight,
second_stage_loc_loss,
name='localization_loss')
classification_loss = tf.multiply(self._second_stage_cls_loss_weight,
second_stage_cls_loss,
name='classification_loss')
loss_dict = {localization_loss.op.name: localization_loss,
classification_loss.op.name: classification_loss}
second_stage_mask_loss = None
if prediction_masks is not None:
if groundtruth_masks_list is None:
raise ValueError('Groundtruth instance masks not provided. '
'Please configure input reader.')
if not self._is_training:
(proposal_boxes, proposal_boxlists, paddings_indicator,
one_hot_flat_cls_targets_with_background
) = self._get_mask_proposal_boxes_and_classes(
detection_boxes, num_detections, image_shape,
groundtruth_boxlists, groundtruth_classes_with_background_list,
groundtruth_weights_list)
unmatched_mask_label = tf.zeros(image_shape[1:3], dtype=tf.float32)
(batch_mask_targets, _, _, batch_mask_target_weights,
_) = target_assigner.batch_assign_targets(
target_assigner=self._detector_target_assigner,
anchors_batch=proposal_boxlists,
gt_box_batch=groundtruth_boxlists,
gt_class_targets_batch=groundtruth_masks_list,
unmatched_class_label=unmatched_mask_label,
gt_weights_batch=groundtruth_weights_list)
# Pad the prediction_masks with to add zeros for background class to be
# consistent with class predictions.
if prediction_masks.get_shape().as_list()[1] == 1:
# Class agnostic masks or masks for one-class prediction. Logic for
# both cases is the same since background predictions are ignored
# through the batch_mask_target_weights.
prediction_masks_masked_by_class_targets = prediction_masks
else:
prediction_masks_with_background = tf.pad(
prediction_masks, [[0, 0], [1, 0], [0, 0], [0, 0]])
prediction_masks_masked_by_class_targets = tf.boolean_mask(
prediction_masks_with_background,
tf.greater(one_hot_flat_cls_targets_with_background, 0))
mask_height = prediction_masks.shape[2].value
mask_width = prediction_masks.shape[3].value
reshaped_prediction_masks = tf.reshape(
prediction_masks_masked_by_class_targets,
[batch_size, -1, mask_height * mask_width])
batch_mask_targets_shape = tf.shape(batch_mask_targets)
flat_gt_masks = tf.reshape(batch_mask_targets,
[-1, batch_mask_targets_shape[2],
batch_mask_targets_shape[3]])
# Use normalized proposals to crop mask targets from image masks.
flat_normalized_proposals = box_list_ops.to_normalized_coordinates(
box_list.BoxList(tf.reshape(proposal_boxes, [-1, 4])),
image_shape[1], image_shape[2]).get()
flat_cropped_gt_mask = self._crop_and_resize_fn(
tf.expand_dims(flat_gt_masks, -1),
tf.expand_dims(flat_normalized_proposals, axis=1),
[mask_height, mask_width])
# Without stopping gradients into cropped groundtruth masks the
# performance with 100-padded groundtruth masks when batch size > 1 is
# about 4% worse.
# TODO(rathodv): Investigate this since we don't expect any variables
# upstream of flat_cropped_gt_mask.
flat_cropped_gt_mask = tf.stop_gradient(flat_cropped_gt_mask)
batch_cropped_gt_mask = tf.reshape(
flat_cropped_gt_mask,
[batch_size, -1, mask_height * mask_width])
mask_losses_weights = (
batch_mask_target_weights * tf.to_float(paddings_indicator))
mask_losses = self._second_stage_mask_loss(
reshaped_prediction_masks,
batch_cropped_gt_mask,
weights=tf.expand_dims(mask_losses_weights, axis=-1),
losses_mask=losses_mask)
total_mask_loss = tf.reduce_sum(mask_losses)
normalizer = tf.maximum(
tf.reduce_sum(mask_losses_weights * mask_height * mask_width), 1.0)
second_stage_mask_loss = total_mask_loss / normalizer
if second_stage_mask_loss is not None:
mask_loss = tf.multiply(self._second_stage_mask_loss_weight,
second_stage_mask_loss, name='mask_loss')
loss_dict[mask_loss.op.name] = mask_loss
return loss_dict
def _get_mask_proposal_boxes_and_classes(
self, detection_boxes, num_detections, image_shape, groundtruth_boxlists,
groundtruth_classes_with_background_list, groundtruth_weights_list):
"""Returns proposal boxes and class targets to compute evaluation mask loss.
During evaluation, detection boxes are used to extract features for mask
prediction. Therefore, to compute mask loss during evaluation detection
boxes must be used to compute correct class and mask targets. This function
returns boxes and classes in the correct format for computing mask targets
during evaluation.
Args:
detection_boxes: A 3-D float tensor of shape [batch, max_detection_boxes,
4] containing detection boxes in normalized co-ordinates.
num_detections: A 1-D float tensor of shape [batch] containing number of
valid boxes in `detection_boxes`.
image_shape: A 1-D tensor of shape [4] containing image tensor shape.
groundtruth_boxlists: A list of groundtruth boxlists.
groundtruth_classes_with_background_list: A list of groundtruth classes.
groundtruth_weights_list: A list of groundtruth weights.
Return:
mask_proposal_boxes: detection boxes to use for mask proposals in absolute
co-ordinates.
mask_proposal_boxlists: `mask_proposal_boxes` in a list of BoxLists in
absolute co-ordinates.
mask_proposal_paddings_indicator: a tensor indicating valid boxes.
mask_proposal_one_hot_flat_cls_targets_with_background: Class targets
computed using detection boxes.
"""
batch, max_num_detections, _ = detection_boxes.shape.as_list()
proposal_boxes = tf.reshape(box_list_ops.to_absolute_coordinates(
box_list.BoxList(tf.reshape(detection_boxes, [-1, 4])), image_shape[1],
image_shape[2]).get(), [batch, max_num_detections, 4])
proposal_boxlists = [
box_list.BoxList(detection_boxes_single_image)
for detection_boxes_single_image in tf.unstack(proposal_boxes)
]
paddings_indicator = self._padded_batched_proposals_indicator(
tf.to_int32(num_detections), detection_boxes.shape[1])
(batch_cls_targets_with_background, _, _, _,
_) = target_assigner.batch_assign_targets(
target_assigner=self._detector_target_assigner,
anchors_batch=proposal_boxlists,
gt_box_batch=groundtruth_boxlists,
gt_class_targets_batch=groundtruth_classes_with_background_list,
unmatched_class_label=tf.constant(
[1] + self._num_classes * [0], dtype=tf.float32),
gt_weights_batch=groundtruth_weights_list)
flat_cls_targets_with_background = tf.reshape(
batch_cls_targets_with_background, [-1, self._num_classes + 1])
one_hot_flat_cls_targets_with_background = tf.argmax(
flat_cls_targets_with_background, axis=1)
one_hot_flat_cls_targets_with_background = tf.one_hot(
one_hot_flat_cls_targets_with_background,
flat_cls_targets_with_background.get_shape()[1])
return (proposal_boxes, proposal_boxlists, paddings_indicator,
one_hot_flat_cls_targets_with_background)
def _get_refined_encodings_for_postitive_class(
self, refined_box_encodings, flat_cls_targets_with_background,
batch_size):
# We only predict refined location encodings for the non background
# classes, but we now pad it to make it compatible with the class
# predictions
refined_box_encodings_with_background = tf.pad(refined_box_encodings,
[[0, 0], [1, 0], [0, 0]])
refined_box_encodings_masked_by_class_targets = (
box_list_ops.boolean_mask(
box_list.BoxList(
tf.reshape(refined_box_encodings_with_background,
[-1, self._box_coder.code_size])),
tf.reshape(tf.greater(flat_cls_targets_with_background, 0), [-1]),
use_static_shapes=self._use_static_shapes,
indicator_sum=batch_size * self.max_num_proposals
if self._use_static_shapes else None).get())
return tf.reshape(
refined_box_encodings_masked_by_class_targets, [
batch_size, self.max_num_proposals,
self._box_coder.code_size
])
def _padded_batched_proposals_indicator(self,
num_proposals,
max_num_proposals):
"""Creates indicator matrix of non-pad elements of padded batch proposals.
Args:
num_proposals: Tensor of type tf.int32 with shape [batch_size].
max_num_proposals: Maximum number of proposals per image (integer).
Returns:
A Tensor of type tf.bool with shape [batch_size, max_num_proposals].
"""
batch_size = tf.size(num_proposals)
tiled_num_proposals = tf.tile(
tf.expand_dims(num_proposals, 1), [1, max_num_proposals])
tiled_proposal_index = tf.tile(
tf.expand_dims(tf.range(max_num_proposals), 0), [batch_size, 1])
return tf.greater(tiled_num_proposals, tiled_proposal_index)
def _unpad_proposals_and_apply_hard_mining(self,
proposal_boxlists,
second_stage_loc_losses,
second_stage_cls_losses,
num_proposals):
"""Unpads proposals and applies hard mining.
Args:
proposal_boxlists: A list of `batch_size` BoxLists each representing
`self.max_num_proposals` representing decoded proposal bounding boxes
for each image.
second_stage_loc_losses: A Tensor of type `float32`. A tensor of shape
`[batch_size, self.max_num_proposals]` representing per-anchor
second stage localization loss values.
second_stage_cls_losses: A Tensor of type `float32`. A tensor of shape
`[batch_size, self.max_num_proposals]` representing per-anchor
second stage classification loss values.
num_proposals: A Tensor of type `int32`. A 1-D tensor of shape [batch]
representing the number of proposals predicted for each image in
the batch.
Returns:
second_stage_loc_loss: A scalar float32 tensor representing the second
stage localization loss.
second_stage_cls_loss: A scalar float32 tensor representing the second
stage classification loss.
"""
for (proposal_boxlist, single_image_loc_loss, single_image_cls_loss,
single_image_num_proposals) in zip(
proposal_boxlists,
tf.unstack(second_stage_loc_losses),
tf.unstack(second_stage_cls_losses),
tf.unstack(num_proposals)):
proposal_boxlist = box_list.BoxList(
tf.slice(proposal_boxlist.get(),
[0, 0], [single_image_num_proposals, -1]))
single_image_loc_loss = tf.slice(single_image_loc_loss,
[0], [single_image_num_proposals])
single_image_cls_loss = tf.slice(single_image_cls_loss,
[0], [single_image_num_proposals])
return self._hard_example_miner(
location_losses=tf.expand_dims(single_image_loc_loss, 0),
cls_losses=tf.expand_dims(single_image_cls_loss, 0),
decoded_boxlist_list=[proposal_boxlist])
def regularization_losses(self):
"""Returns a list of regularization losses for this model.
Returns a list of regularization losses for this model that the estimator
needs to use during training/optimization.
Returns:
A list of regularization loss tensors.
"""
return tf.get_collection(tf.GraphKeys.REGULARIZATION_LOSSES)
def restore_map(self,
fine_tune_checkpoint_type='detection',
load_all_detection_checkpoint_vars=False):
"""Returns a map of variables to load from a foreign checkpoint.
See parent class for details.
Args:
fine_tune_checkpoint_type: whether to restore from a full detection
checkpoint (with compatible variable names) or to restore from a
classification checkpoint for initialization prior to training.
Valid values: `detection`, `classification`. Default 'detection'.
load_all_detection_checkpoint_vars: whether to load all variables (when
`fine_tune_checkpoint_type` is `detection`). If False, only variables
within the feature extractor scopes are included. Default False.
Returns:
A dict mapping variable names (to load from a checkpoint) to variables in
the model graph.
Raises:
ValueError: if fine_tune_checkpoint_type is neither `classification`
nor `detection`.
"""
if fine_tune_checkpoint_type not in ['detection', 'classification']:
raise ValueError('Not supported fine_tune_checkpoint_type: {}'.format(
fine_tune_checkpoint_type))
if fine_tune_checkpoint_type == 'classification':
return self._feature_extractor.restore_from_classification_checkpoint_fn(
self.first_stage_feature_extractor_scope,
self.second_stage_feature_extractor_scope)
variables_to_restore = tf.global_variables()
variables_to_restore.append(slim.get_or_create_global_step())
# Only load feature extractor variables to be consistent with loading from
# a classification checkpoint.
include_patterns = None
if not load_all_detection_checkpoint_vars:
include_patterns = [
self.first_stage_feature_extractor_scope,
self.second_stage_feature_extractor_scope
]
feature_extractor_variables = tf.contrib.framework.filter_variables(
variables_to_restore, include_patterns=include_patterns)
return {var.op.name: var for var in feature_extractor_variables}
def updates(self):
"""Returns a list of update operators for this model.
Returns a list of update operators for this model that must be executed at
each training step. The estimator's train op needs to have a control
dependency on these updates.
Returns:
A list of update operators.
"""
return tf.get_collection(tf.GraphKeys.UPDATE_OPS)
|
TensorFlow/Classification/ConvNets/model/layers | layers | squeeze_excitation_layer | #!/usr/bin/env python
# -*- coding: utf-8 -*-
# Copyright 2018 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
import tensorflow as tf
from model import layers
from model import blocks
__all__ = ['squeeze_excitation_layer']
def squeeze_excitation_layer(
inputs,
ratio,
training=True,
data_format='NCHW',
kernel_initializer=tf.compat.v1.variance_scaling_initializer(),
bias_initializer=tf.zeros_initializer(),
name="squeeze_excitation_layer"
):
if data_format not in ['NHWC', 'NCHW']:
raise ValueError("Unknown data format: `%s` (accepted: ['NHWC', 'NCHW'])" % data_format)
in_shape = inputs.get_shape()
num_channels = in_shape[1] if data_format == "NCHW" else in_shape[-1]
with tf.variable_scope(name):
net = inputs
# squeeze
squeeze = layers.reduce_mean(
net,
keepdims=False,
data_format=data_format,
name='squeeze_spatial_mean'
)
# fc + relu
excitation = layers.dense(
inputs=squeeze,
units=num_channels // ratio,
use_bias=True,
trainable=training,
kernel_initializer=kernel_initializer,
bias_initializer=bias_initializer
)
excitation = layers.relu(excitation)
# fc + sigmoid
excitation = layers.dense(
inputs=excitation,
units=num_channels,
use_bias=True,
trainable=training,
kernel_initializer=kernel_initializer,
bias_initializer=bias_initializer
)
excitation = layers.sigmoid(excitation)
out_shape = [-1, num_channels, 1, 1] if data_format == "NCHW" else [-1, 1, 1, num_channels]
excitation = tf.reshape(excitation, out_shape)
net = net * excitation
return net
|
PyTorch/Classification/ConvNets/efficientnet/training/FP32 | FP32 | DGX1V-16G_efficientnet-b0_FP32 | python ./multiproc.py --nproc_per_node 8 ./launch.py --model efficientnet-b0 --precision FP32 --mode convergence --platform DGX1V-16G /imagenet --workspace ${1:-./} --raport-file raport.json
|
PyTorch/SpeechSynthesis/FastPitch/hifigan | hifigan | arg_parser | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import argparse
from ast import literal_eval
def parse_hifigan_args(parent, add_help=False):
"""
Parse model specific commandline arguments.
"""
parser = argparse.ArgumentParser(parents=[parent], add_help=add_help,
allow_abbrev=False)
hfg = parser.add_argument_group('HiFi-GAN generator parameters')
hfg.add_argument('--upsample_rates', default=[8, 8, 2, 2],
type=literal_eval_arg,
help='Upsample rates')
hfg.add_argument('--upsample_kernel_sizes', default=[16, 16, 4, 4],
type=literal_eval_arg,
help='Upsample kernel sizes')
hfg.add_argument('--upsample_initial_channel', default=512, type=int,
help='Upsample initial channel')
hfg.add_argument('--resblock', default='1', type=str,
help='Resblock module version')
hfg.add_argument('--resblock_kernel_sizes', default=[3, 7, 11],
type=literal_eval_arg,
help='Resblock kernel sizes')
hfg.add_argument('--resblock_dilation_sizes', type=literal_eval_arg,
default=[[1, 3, 5], [1, 3, 5], [1, 3, 5]],
help='Resblock dilation sizes'),
hfg = parser.add_argument_group('HiFi-GAN discriminator parameters')
hfg.add_argument('--mpd_periods', default=[2, 3, 5, 7, 11],
type=literal_eval_arg,
help='Periods of MultiPeriodDiscriminator')
hfg.add_argument('--concat_fwd', action='store_true',
help='Faster Discriminators (requires more GPU memory)')
hfg.add_argument('--hifigan-config', type=str, default=None, required=False,
help='Path to a HiFi-GAN config .json'
' (if provided, overrides model architecture flags)')
return parser
def literal_eval_arg(val):
try:
return literal_eval(val)
except SyntaxError as e: # Argparse does not handle SyntaxError
raise ValueError(str(e)) from e
|
TensorFlow2/Recommendation/DLRM_and_DCNv2/tensorflow-dot-based-interact/tensorflow_dot_based_interact/cc/kernels/launchers | launchers | dot_based_interact_fp16_launcher | // Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
#ifndef FP16_LAUNCHER_CU
#define FP16_LAUNCHER_CU
#include "../cuda_kernels/dot_based_interact_fp16.cu"
inline void dotBasedInteractFP16Fwd(const void *input,
const void *bottom_mlp_output,
void *output,
uint batch_size,
uint num_rows,
uint num_cols,
cudaStream_t stream) {
// num_rows is num cat variables + 1
// num_cols is embedding dim
const uint kWarpSize = 32;
const uint kWarpSizeLog2 = Log2<kWarpSize>::value;
const uint kTileDim = 16;
const uint kTileDimLog2 = Log2<kTileDim>::value;
const uint warps_per_threadblock = 4;
const uint threadblock_size = warps_per_threadblock * 32;
const uint kRowTilesPerStep = 2;
const uint kColTilesPerStep = 1;
// num tiles - divide rounding up
uint num_row_tiles = (num_rows + kTileDim - 1) >> kTileDimLog2;
uint num_col_tiles = (num_cols + kTileDim - 1) >> kTileDimLog2;
// number of rows and columns after padding
uint num_rows_after_padding = kTileDim << 1; //TODO here we assume that kTileDim*2 >= num_rows.
uint num_cols_after_padding = num_col_tiles << kTileDimLog2; //
uint num_row_steps = num_row_tiles / kRowTilesPerStep;
uint num_col_steps = num_col_tiles / kColTilesPerStep;
const uint K_BLOCKS = 8; //todo what is this? is this 128//16, ie num_col_tiles?
const uint M_BLOCKS = 2; //todo what is this? is this 32//16, ie num_row_tiles?
const uint SKEW_HALF = ((K_BLOCKS % 2) == 0) ? 8 : 0;
const uint SMEM_STRIDE = (K_BLOCKS * 16 + SKEW_HALF); //num_cols padded for tensor cores, then padded again for alignment
// multiple of 2 to guarantee 256-bit alignment for start of the row, at least 16 to safeload a tile
const uint smem_rows_per_warp = M_BLOCKS << 4; //is this m_blocks * tile dim? how many rows we multiply in a single pass
const uint smem_elems_per_warp_mat = smem_rows_per_warp * SMEM_STRIDE;
const uint SKEW_HALF_ACC = ((M_BLOCKS % 2) == 0) ? 8 : 0;
const uint SMEM_STRIDE_ACC = (M_BLOCKS * 16 + SKEW_HALF_ACC);
const uint smem_elems_per_warp_acc = M_BLOCKS * 16 * SMEM_STRIDE_ACC * 2;
const uint smem_elems_per_warp =
(smem_elems_per_warp_mat > smem_elems_per_warp_acc) ? smem_elems_per_warp_mat : smem_elems_per_warp_acc;
uint raw_output_size = ((num_rows * (num_rows - 1)) >> 1) + num_cols;
uint output_size = ((raw_output_size-1)/8 + 1)*8; //round up to multiple of 8
uint padding_size = output_size-raw_output_size;
bool float4_predicate = !((num_cols & 7) || (output_size & 7));
if (float4_predicate) {
//aligned
dotBasedInteractFwdKernelFP16<warps_per_threadblock,
threadblock_size,
M_BLOCKS,
K_BLOCKS,
SMEM_STRIDE,
SMEM_STRIDE_ACC,
kWarpSize,
kWarpSizeLog2,
kTileDim,
kTileDimLog2,
true>
<<<(batch_size + warps_per_threadblock - 1) / warps_per_threadblock,
threadblock_size,
warps_per_threadblock * smem_elems_per_warp * sizeof(__half), stream>>>((const __half *)input,
(half *)output,
batch_size,
num_rows,
num_cols,
num_rows_after_padding,
num_cols_after_padding,
smem_elems_per_warp,
smem_rows_per_warp,
output_size,
num_row_steps,
num_col_steps,
padding_size);
} else {
//not aligned
dotBasedInteractFwdKernelFP16<warps_per_threadblock,
threadblock_size,
M_BLOCKS,
K_BLOCKS,
SMEM_STRIDE,
SMEM_STRIDE_ACC,
kWarpSize,
kWarpSizeLog2,
kTileDim,
kTileDimLog2,
false>
<<<(batch_size + warps_per_threadblock - 1) / warps_per_threadblock,
threadblock_size,
warps_per_threadblock * smem_elems_per_warp * sizeof(__half), stream>>>((const __half *)input,
(half *)output,
batch_size,
num_rows,
num_cols,
num_rows_after_padding,
num_cols_after_padding,
smem_elems_per_warp,
smem_rows_per_warp,
output_size,
num_row_steps,
num_col_steps,
padding_size);
}
}
inline void dotBasedInteractFP16Bwd(const void *input,
const void *upstream_grad,
void *grad,
void *bottom_mlp_grad,
uint batch_size,
uint num_rows,
uint num_cols,
cudaStream_t stream) {
const uint kWarpSize = 32;
const uint kWarpSizeLog2 = Log2<kWarpSize>::value;
const uint kTileDim = 16;
const uint kTileDimLog2 = Log2<kTileDim>::value;
const uint mem_skew_size = 8;
const uint kWarpsPerBlock = 4;
const uint kWarpsPerBlockLog2 = Log2<kWarpsPerBlock>::value;
const uint kNumThreads = kWarpsPerBlock * kWarpSize;
const uint kRowTilesPerStep = 2;
const uint kColTilesPerStep = 1;
uint row_tiles_per_step = num_rows > kTileDim ? kRowTilesPerStep : 1;
// num tiles
uint num_row_tiles = (num_rows + kTileDim - 1) >> kTileDimLog2;
uint num_col_tiles = (num_cols + kTileDim - 1) >> kTileDimLog2;
// number of rows and columns after padding
uint num_rows_after_padding = kTileDim << 1;
uint num_cols_after_padding = num_col_tiles << kTileDimLog2;
// 2D ugrad size and stride
uint interaction_ugrad_2D_stride = num_rows_after_padding + mem_skew_size;
uint interaction_ugrad_2D_size_elems = num_rows_after_padding * interaction_ugrad_2D_stride;
uint interaction_ugrad_2D_size_bytes = interaction_ugrad_2D_size_elems * sizeof(half);
// 1D ugrad size
uint interaction_ugrad_size = num_rows * (num_rows - 1) >> 1;
uint interaction_ugrad_size_with_padding = ((interaction_ugrad_size-1)/8 + 1)*8; //round up to multiple of 8
// in_out place size and stride
uint input_stride = num_cols_after_padding + mem_skew_size;
uint input_size_elems = num_rows_after_padding * input_stride;
uint input_size_bytes = input_size_elems * sizeof(half);
// sample size
uint sample_size = num_rows * num_cols;
// output size
uint output_size_elems = kTileDim * kTileDim * kRowTilesPerStep * kColTilesPerStep;
uint output_size_bytes = output_size_elems * sizeof(float);
// staging area size
uint staging_area_size_bytes =
output_size_bytes > interaction_ugrad_2D_size_bytes ? output_size_bytes : interaction_ugrad_2D_size_bytes;
// Shared memory size
uint shared_mem_per_warp_size_byte = input_size_bytes + staging_area_size_bytes;
uint shared_mem_size_bytes = kWarpsPerBlock * shared_mem_per_warp_size_byte;
uint num_blocks = (batch_size + kWarpsPerBlock - 1) >> kWarpsPerBlockLog2;
uint num_row_steps = num_row_tiles / row_tiles_per_step;
uint num_col_steps = num_col_tiles / kColTilesPerStep;
bool float4_predicate = !((interaction_ugrad_size_with_padding & 7) || (num_cols & 7));
if (float4_predicate) {
//aligned
dotBasedInteractBwdKernelFP16<kWarpsPerBlock,
kNumThreads,
kRowTilesPerStep,
kColTilesPerStep,
kWarpSize,
kWarpSizeLog2,
kTileDim,
kTileDimLog2,
true>
<<<num_blocks, kNumThreads, shared_mem_size_bytes, stream>>>((const half *)input,
(const half *)upstream_grad,
(half *)grad,
(half *)bottom_mlp_grad,
batch_size,
num_rows,
num_cols,
num_rows_after_padding,
num_cols_after_padding,
sample_size,
interaction_ugrad_size,
interaction_ugrad_size_with_padding,
interaction_ugrad_2D_size_elems,
interaction_ugrad_2D_stride,
input_size_elems,
input_stride,
num_row_steps,
num_col_steps,
row_tiles_per_step,
shared_mem_per_warp_size_byte);
} else {
//unaligned
dotBasedInteractBwdKernelFP16<kWarpsPerBlock,
kNumThreads,
kRowTilesPerStep,
kColTilesPerStep,
kWarpSize,
kWarpSizeLog2,
kTileDim,
kTileDimLog2,
false>
<<<num_blocks, kNumThreads, shared_mem_size_bytes, stream>>>((const half *)input,
(const half *)upstream_grad,
(half *)grad,
(half *)bottom_mlp_grad,
batch_size,
num_rows,
num_cols,
num_rows_after_padding,
num_cols_after_padding,
sample_size,
interaction_ugrad_size,
interaction_ugrad_size_with_padding,
interaction_ugrad_2D_size_elems,
interaction_ugrad_2D_stride,
input_size_elems,
input_stride,
num_row_steps,
num_col_steps,
row_tiles_per_step,
shared_mem_per_warp_size_byte);
}
}
#endif /* FP16_LAUNCHER_CU */
|
PaddlePaddle/Classification/RN50v1.5 | RN50v1.5 | program | # Copyright (c) 2022 NVIDIA Corporation. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import logging
import time
from profile import Profiler
import dllogger
import models
import numpy as np
from lr_scheduler import build_lr_scheduler
from optimizer import build_optimizer
from utils.misc import AverageMeter
from utils.mode import Mode, RunScope
from utils.utility import get_num_trainers
import paddle
import paddle.nn.functional as F
from paddle.distributed import fleet
from paddle.distributed.fleet import DistributedStrategy
from paddle.distributed.fleet.meta_optimizers.common import CollectiveHelper
from paddle.incubate import asp as sparsity
def create_feeds(image_shape):
"""
Create feeds mapping for the inputs of Pragrm execution.
Args:
image_shape(list[int]): Model input shape, such as [4, 224, 224].
Returns:
feeds(dict): A dict to map variables'name to their values.
key (string): Name of variable to feed.
Value (tuple): paddle.static.data.
"""
feeds = {}
feeds['data'] = paddle.static.data(
name="data", shape=[None] + image_shape, dtype="float32"
)
feeds['label'] = paddle.static.data(
name="label", shape=[None, 1], dtype="int64"
)
return feeds
def create_fetchs(out, feeds, class_num, label_smoothing=0, mode=Mode.TRAIN):
"""
Create fetchs to obtain specific outputs from Pragrm execution (included loss and measures).
Args:
out(variable): The model output variable.
feeds(dict): A dict of mapping variables'name to their values
(The input of Program execution).
class_num(int): The number of classes.
label_smoothing(float, optional): Epsilon of label smoothing. Default: 0.
mode(utils.Mode, optional): Train or eval mode. Default: Mode.TRAIN
Returns:
fetchs(dict): A dict of outputs from Program execution (included loss and measures).
key (string): Name of variable to fetch.
Value (tuple): (variable, AverageMeter).
"""
fetchs = {}
target = paddle.reshape(feeds['label'], [-1, 1])
if mode == Mode.TRAIN:
if label_smoothing == 0:
loss = F.cross_entropy(out, target)
else:
label_one_hot = F.one_hot(target, class_num)
soft_target = F.label_smooth(label_one_hot, epsilon=label_smoothing)
soft_target = paddle.reshape(soft_target, shape=[-1, class_num])
log_softmax = -F.log_softmax(out, axis=-1)
loss = paddle.sum(log_softmax * soft_target, axis=-1)
else:
loss = F.cross_entropy(out, target)
label = paddle.argmax(out, axis=-1, dtype='int32')
fetchs['label'] = (label, None)
loss = loss.mean()
fetchs['loss'] = (loss, AverageMeter('loss', '7.4f', need_avg=True))
acc_top1 = paddle.metric.accuracy(input=out, label=target, k=1)
acc_top5 = paddle.metric.accuracy(input=out, label=target, k=5)
metric_dict = {}
metric_dict["top1"] = acc_top1
metric_dict["top5"] = acc_top5
for key in metric_dict:
if mode != Mode.TRAIN and paddle.distributed.get_world_size() > 1:
paddle.distributed.all_reduce(
metric_dict[key], op=paddle.distributed.ReduceOp.SUM
)
metric_dict[key] = (
metric_dict[key] / paddle.distributed.get_world_size()
)
fetchs[key] = (
metric_dict[key],
AverageMeter(key, '7.4f', need_avg=True),
)
return fetchs
def create_strategy(args, is_train=True):
"""
Create paddle.static.BuildStrategy and paddle.static.ExecutionStrategy with arguments.
Args:
args(Namespace): Arguments obtained from ArgumentParser.
is_train(bool, optional): Indicate the prupose of strategy is for training
of not. Default is True.
Returns:
build_strategy(paddle.static.BuildStrategy): A instance of BuildStrategy.
exec_strategy(paddle.static.ExecutionStrategy): A instance of ExecutionStrategy.
"""
build_strategy = paddle.static.BuildStrategy()
exec_strategy = paddle.static.ExecutionStrategy()
exec_strategy.num_threads = 1
exec_strategy.num_iteration_per_drop_scope = (
10000 if args.amp and args.use_pure_fp16 else 10
)
paddle.set_flags(
{
'FLAGS_cudnn_exhaustive_search': True,
'FLAGS_conv_workspace_size_limit': 4096,
}
)
if not is_train:
build_strategy.fix_op_run_order = True
if args.amp:
build_strategy.fuse_bn_act_ops = True
build_strategy.fuse_elewise_add_act_ops = True
build_strategy.fuse_bn_add_act_ops = True
build_strategy.enable_addto = True
if args.fuse_resunit and is_train:
build_strategy.fuse_resunit = True
return build_strategy, exec_strategy
def dist_optimizer(args, optimizer):
"""
Create a distributed optimizer based on a given optimizer.
Args:
args(Namespace): Arguments obtained from ArgumentParser.
optimizer(paddle.optimizer): A normal optimizer.
Returns:
optimizer(fleet.distributed_optimizer): A distributed optimizer.
"""
build_strategy, exec_strategy = create_strategy(args)
dist_strategy = DistributedStrategy()
dist_strategy.execution_strategy = exec_strategy
dist_strategy.build_strategy = build_strategy
dist_strategy.fuse_all_reduce_ops = True
all_reduce_size = 16
dist_strategy.fuse_grad_size_in_MB = all_reduce_size
dist_strategy.nccl_comm_num = 1
dist_strategy.sync_nccl_allreduce = True
if args.amp:
dist_strategy.cudnn_batchnorm_spatial_persistent = True
dist_strategy.amp = True
dist_strategy.amp_configs = {
"init_loss_scaling": args.scale_loss,
"use_dynamic_loss_scaling": args.use_dynamic_loss_scaling,
"use_pure_fp16": args.use_pure_fp16,
}
dist_strategy.asp = args.asp
optimizer = fleet.distributed_optimizer(optimizer, strategy=dist_strategy)
return optimizer
def build(args, main_prog, startup_prog, step_each_epoch, is_train=True):
"""
Build a executable paddle.static.Program via following four steps:
1. Create feeds.
2. Create a model.
3. Create fetchs.
4. Create an optimizer if is_train==True.
Args:
args(Namespace): Arguments obtained from ArgumentParser.
main_prog(paddle.static.Program):The main program.
startup_prog(paddle.static.Program):The startup program.
step_each_epoch(int): The number of steps in each epoch.
is_train(bool, optional): Whether the main programe created is for training. Default: True.
Returns:
fetchs(dict): A dict of outputs from Program execution (included loss and measures).
lr_scheduler(paddle.optimizer.lr.LRScheduler): A learning rate scheduler.
feeds(dict): A dict to map variables'name to their values.
optimizer(Optimizer): An optimizer with distributed/AMP/ASP strategy.
"""
with paddle.static.program_guard(main_prog, startup_prog):
with paddle.utils.unique_name.guard():
mode = Mode.TRAIN if is_train else Mode.EVAL
feeds = create_feeds(args.image_shape)
model_name = args.model_arch_name
class_num = args.num_of_class
input_image_channel = args.image_channel
data_format = args.data_layout
use_pure_fp16 = args.use_pure_fp16
bn_weight_decay = args.bn_weight_decay
model = models.__dict__[model_name](
class_num=class_num,
input_image_channel=input_image_channel,
data_format=data_format,
use_pure_fp16=use_pure_fp16,
bn_weight_decay=bn_weight_decay,
)
out = model(feeds["data"])
fetchs = create_fetchs(
out, feeds, class_num, args.label_smoothing, mode=mode
)
if args.asp:
sparsity.set_excluded_layers(main_program=main_prog, param_names=[model.fc.weight.name])
lr_scheduler = None
optimizer = None
if is_train:
lr_scheduler = build_lr_scheduler(args, step_each_epoch)
optimizer = build_optimizer(args, lr_scheduler)
optimizer = dist_optimizer(args, optimizer)
optimizer.minimize(fetchs['loss'][0], startup_prog)
# This is a workaround to "Communicator of ring id 0 has not been initialized.".
# Since Paddle's design, the initialization would be done inside train program,
# eval_only need to manually call initialization.
if (
args.run_scope == RunScope.EVAL_ONLY
and paddle.distributed.get_world_size() > 1
):
collective_helper = CollectiveHelper(
role_maker=fleet.PaddleCloudRoleMaker(is_collective=True)
)
collective_helper.update_startup_program(startup_prog)
return fetchs, lr_scheduler, feeds, optimizer
def compile_prog(args, program, loss_name=None, is_train=True):
"""
Compile the given program, which would fuse computing ops or optimize memory footprint
based building strategy in config.
Args:
args(Namespace): Arguments obtained from ArgumentParser.
program(paddle.static.Program): The main program to be compiled.
loss_name(str, optional): The name of loss variable. Default: None.
is_train(bool, optional): Indicate the prupose of strategy is for
training of not. Default is True.
Returns:
compiled_program(paddle.static.CompiledProgram): A compiled program.
"""
build_strategy, exec_strategy = create_strategy(args, is_train)
compiled_program = paddle.static.CompiledProgram(
program, build_strategy=build_strategy
)
return compiled_program
def run(
args,
dataloader,
exe,
program,
fetchs,
epoch,
mode=Mode.TRAIN,
lr_scheduler=None,
):
"""
Execute program.
Args:
args(Namespace): Arguments obtained from ArgumentParser.
dataloader(nvidia.dali.plugin.paddle.DALIGenericIterator):
Iteratable output of NVIDIA DALI pipeline,
please refer to dali_dataloader in dali.py for details.
exe(paddle.static.Executor): A executor to run program.
program(paddle.static.Program): The program to be executed.
fetchs(dict): A dict of outputs from Program execution (included loss and measures).
epoch(int): Current epoch id to run.
mode(utils.Mode, optional): Train or eval mode. Default: Mode.TRAIN.
lr_scheduler(paddle.optimizer.lr.LRScheduler, optional): A learning rate scheduler.
Default: None.
Returns:
metrics (dict): A dictionary to collect values of metrics.
"""
num_trainers = get_num_trainers()
fetch_list = [f[0] for f in fetchs.values()]
metric_dict = {"lr": AverageMeter('lr', 'f', postfix=",", need_avg=False)}
for k in fetchs:
if fetchs[k][1] is not None:
metric_dict[k] = fetchs[k][1]
metric_dict["batch_time"] = AverageMeter('batch_time', '.5f', postfix=" s,")
metric_dict["data_time"] = AverageMeter('data_time', '.5f', postfix=" s,")
metric_dict["compute_time"] = AverageMeter(
'compute_time', '.5f', postfix=" s,"
)
for m in metric_dict.values():
m.reset()
profiler = Profiler()
tic = time.perf_counter()
idx = 0
batch_size = None
latency = []
total_benchmark_steps = args.benchmark_steps + args.benchmark_warmup_steps
dataloader.reset()
while True:
# profiler.profile_setup return True only when
# profile is enable and idx == stop steps
if profiler.profile_setup(idx):
break
idx += 1
try:
batch = next(dataloader)
except StopIteration:
# Reset dataloader when run benchmark to fill required steps.
if args.benchmark and (idx < total_benchmark_steps):
dataloader.reset()
# Reset tic timestamp to ignore exception handling time.
tic = time.perf_counter()
continue
break
except RuntimeError:
logging.warning(
"Except RuntimeError when reading data from dataloader, try to read once again..."
)
continue
reader_toc = time.perf_counter()
metric_dict['data_time'].update(reader_toc - tic)
batch_size = batch[0]["data"].shape()[0]
feed_dict = batch[0]
with profiler.profile_tag(
idx, "Training" if mode == Mode.TRAIN else "Evaluation"
):
results = exe.run(
program=program, feed=feed_dict, fetch_list=fetch_list
)
for name, m in zip(fetchs.keys(), results):
if name in metric_dict:
metric_dict[name].update(np.mean(m), batch_size)
metric_dict["compute_time"].update(time.perf_counter() - reader_toc)
metric_dict["batch_time"].update(time.perf_counter() - tic)
if mode == Mode.TRAIN:
metric_dict['lr'].update(lr_scheduler.get_lr())
if lr_scheduler is not None:
with profiler.profile_tag(idx, "LR Step"):
lr_scheduler.step()
tic = time.perf_counter()
if idx % args.print_interval == 0:
log_msg = {}
log_msg['loss'] = metric_dict['loss'].val.item()
log_msg['top1'] = metric_dict['top1'].val.item()
log_msg['top5'] = metric_dict['top5'].val.item()
log_msg['data_time'] = metric_dict['data_time'].val
log_msg['compute_time'] = metric_dict['compute_time'].val
log_msg['batch_time'] = metric_dict['batch_time'].val
log_msg['ips'] = (
batch_size * num_trainers / metric_dict['batch_time'].val
)
if mode == Mode.TRAIN:
log_msg['lr'] = metric_dict['lr'].val
log_info((epoch, idx), log_msg, mode)
if args.benchmark:
latency.append(metric_dict['batch_time'].val)
# Ignore the warmup iters
if idx == args.benchmark_warmup_steps:
metric_dict["compute_time"].reset()
metric_dict["data_time"].reset()
metric_dict["batch_time"].reset()
latency.clear()
logging.info("Begin benchmark at step %d", idx + 1)
if idx == total_benchmark_steps:
benchmark_data = {}
benchmark_data['ips'] = (
batch_size * num_trainers / metric_dict['batch_time'].avg
)
if mode == mode.EVAL:
latency = np.array(latency) * 1000
quantile = np.quantile(latency, [0.9, 0.95, 0.99])
benchmark_data['latency_avg'] = np.mean(latency)
benchmark_data['latency_p90'] = quantile[0]
benchmark_data['latency_p95'] = quantile[1]
benchmark_data['latency_p99'] = quantile[2]
logging.info("End benchmark at epoch step %d", idx)
return benchmark_data
epoch_data = {}
epoch_data['loss'] = metric_dict['loss'].avg.item()
epoch_data['epoch_time'] = metric_dict['batch_time'].total
epoch_data['ips'] = (
batch_size
* num_trainers
* metric_dict["batch_time"].count
/ metric_dict["batch_time"].sum
)
if mode == Mode.EVAL:
epoch_data['top1'] = metric_dict['top1'].avg.item()
epoch_data['top5'] = metric_dict['top5'].avg.item()
log_info((epoch,), epoch_data, mode)
return epoch_data
def log_info(step, metrics, mode):
"""
Log metrics with step and mode information.
Args:
step(tuple): Step, coulbe (epoch-id, iter-id). Use tuple() for summary.
metrics(dict): A dictionary collected values of metrics.
mode(utils.Mode): Train or eval mode.
"""
prefix = 'train' if mode == Mode.TRAIN else 'val'
dllogger_iter_data = {}
for key in metrics:
dllogger_iter_data[f"{prefix}.{key}"] = metrics[key]
dllogger.log(step=step, data=dllogger_iter_data)
|
PyTorch/Segmentation/MaskRCNN/pytorch/maskrcnn_benchmark/utils | utils | README | # Utility functions
This folder contain utility functions that are not used in the
core library, but are useful for building models or training
code using the config system.
|
PyTorch/Segmentation/MaskRCNN/pytorch/configs/gn_baselines | gn_baselines | scratch_e2e_mask_rcnn_R_50_FPN_3x_gn | INPUT:
MIN_SIZE_TRAIN: 800
MAX_SIZE_TRAIN: 1333
MIN_SIZE_TEST: 800
MAX_SIZE_TEST: 1333
MODEL:
META_ARCHITECTURE: "GeneralizedRCNN"
WEIGHT: "" # no pretrained model
BACKBONE:
CONV_BODY: "R-50-FPN"
OUT_CHANNELS: 256
FREEZE_CONV_BODY_AT: 0 # finetune all layers
RESNETS: # use GN for backbone
TRANS_FUNC: "BottleneckWithGN"
STEM_FUNC: "StemWithGN"
FPN:
USE_GN: True # use GN for FPN
RPN:
USE_FPN: True
ANCHOR_STRIDE: (4, 8, 16, 32, 64)
PRE_NMS_TOP_N_TRAIN: 2000
PRE_NMS_TOP_N_TEST: 1000
POST_NMS_TOP_N_TEST: 1000
FPN_POST_NMS_TOP_N_TEST: 1000
ROI_HEADS:
USE_FPN: True
BATCH_SIZE_PER_IMAGE: 512
POSITIVE_FRACTION: 0.25
ROI_BOX_HEAD:
USE_GN: True # use GN for bbox head
POOLER_RESOLUTION: 7
POOLER_SCALES: (0.25, 0.125, 0.0625, 0.03125)
POOLER_SAMPLING_RATIO: 2
FEATURE_EXTRACTOR: "FPN2MLPFeatureExtractor"
PREDICTOR: "FPNPredictor"
ROI_MASK_HEAD:
USE_GN: True # use GN for mask head
POOLER_SCALES: (0.25, 0.125, 0.0625, 0.03125)
CONV_LAYERS: (256, 256, 256, 256)
FEATURE_EXTRACTOR: "MaskRCNNFPNFeatureExtractor"
PREDICTOR: "MaskRCNNC4Predictor"
POOLER_RESOLUTION: 14
POOLER_SAMPLING_RATIO: 2
RESOLUTION: 28
SHARE_BOX_FEATURE_EXTRACTOR: False
MASK_ON: True
DATASETS:
TRAIN: ("coco_2014_train", "coco_2014_valminusminival")
TEST: ("coco_2014_minival",)
DATALOADER:
SIZE_DIVISIBILITY: 32
SOLVER:
# Assume 8 gpus
BASE_LR: 0.02
WEIGHT_DECAY: 0.0001
STEPS: (210000, 250000)
MAX_ITER: 270000
IMS_PER_BATCH: 16
TEST:
IMS_PER_BATCH: 8
|
TensorFlow/Detection/SSD/models/research/object_detection/configs | configs | mask_rcnn_resnet50_atrous_coco_8GPU | # Mask R-CNN with Resnet-50 (v1), Atrous version
# Configured for MSCOCO Dataset.
# Users should configure the fine_tune_checkpoint field in the train config as
# well as the label_map_path and input_path fields in the train_input_reader and
# eval_input_reader. Search for "PATH_TO_BE_CONFIGURED" to find the fields that
# should be configured.
#
# Copyright (c) 2019, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
model {
faster_rcnn {
num_classes: 90
image_resizer {
keep_aspect_ratio_resizer {
min_dimension: 800
max_dimension: 1365
}
}
number_of_stages: 3
feature_extractor {
type: 'faster_rcnn_resnet50'
first_stage_features_stride: 8
}
first_stage_anchor_generator {
grid_anchor_generator {
scales: [0.25, 0.5, 1.0, 2.0]
aspect_ratios: [0.5, 1.0, 2.0]
height_stride: 8
width_stride: 8
}
}
first_stage_atrous_rate: 2
first_stage_box_predictor_conv_hyperparams {
op: CONV
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
truncated_normal_initializer {
stddev: 0.01
}
}
}
first_stage_nms_score_threshold: 0.0
first_stage_nms_iou_threshold: 0.7
first_stage_max_proposals: 300
first_stage_localization_loss_weight: 2.0
first_stage_objectness_loss_weight: 1.0
initial_crop_size: 14
maxpool_kernel_size: 2
maxpool_stride: 2
second_stage_box_predictor {
mask_rcnn_box_predictor {
use_dropout: false
dropout_keep_probability: 1.0
predict_instance_masks: true
mask_height: 33
mask_width: 33
mask_prediction_conv_depth: 0
mask_prediction_num_conv_layers: 4
fc_hyperparams {
op: FC
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
variance_scaling_initializer {
factor: 1.0
uniform: true
mode: FAN_AVG
}
}
}
conv_hyperparams {
op: CONV
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
truncated_normal_initializer {
stddev: 0.01
}
}
}
}
}
second_stage_post_processing {
batch_non_max_suppression {
score_threshold: 0.0
iou_threshold: 0.6
max_detections_per_class: 100
max_total_detections: 300
}
score_converter: SOFTMAX
}
second_stage_localization_loss_weight: 2.0
second_stage_classification_loss_weight: 1.0
second_stage_mask_prediction_loss_weight: 4.0
}
}
train_config: {
batch_size: 1
optimizer {
momentum_optimizer: {
learning_rate: {
manual_step_learning_rate {
initial_learning_rate: 0.0024
schedule {
step: 900000
learning_rate: .00024
}
schedule {
step: 1200000
learning_rate: .000024
}
}
}
momentum_optimizer_value: 0.9
}
use_moving_average: false
}
gradient_clipping_by_norm: 10.0
fine_tune_checkpoint: "/checkpoints/resnet_v1_50/model.ckpt"
fine_tune_checkpoint_type: "classification"
# Note: The below line limits the training process to 200K steps, which we
# empirically found to be sufficient enough to train the pets dataset. This
# effectively bypasses the learning rate schedule (the learning rate will
# never decay). Remove the below line to train indefinitely.
num_steps: 200000
data_augmentation_options {
random_horizontal_flip {
}
}
}
train_input_reader: {
tf_record_input_reader {
input_path: "/data/coco_train.record*"
}
label_map_path: "object_detection/data/mscoco_label_map.pbtxt"
}
eval_config: {
metrics_set: "coco_detection_metrics"
use_moving_averages: false
num_examples: 8000
}
eval_input_reader: {
tf_record_input_reader {
input_path: "/data/coco_val.record*"
}
label_map_path: "object_detection/data/mscoco_label_map.pbtxt"
shuffle: false
num_readers: 1
}
|
PyTorch/Translation/GNMT/seq2seq/train | train | table | # Copyright (c) 2018-2020, NVIDIA CORPORATION. All rights reserved.
#
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documentation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in all
# copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
from pytablewriter import MarkdownTableWriter
class TrainingTable:
def __init__(self, acc_unit='BLEU', time_unit='min', perf_unit='tok/s'):
self.data = []
self.acc_unit = acc_unit
self.time_unit = time_unit
self.perf_unit = perf_unit
self.time_unit_convert = {'s': 1, 'min': 1/60, 'h': 1/3600}
def add(self, gpus, batch_size, accuracy, perf, time_to_train):
time_to_train *= self.time_unit_convert[self.time_unit]
if not accuracy:
accuracy = 0.0
accuracy = round(accuracy, 2)
self.data.append([gpus, batch_size, accuracy, perf, time_to_train])
def write(self, title, math):
writer = MarkdownTableWriter()
writer.table_name = f'{title}'
header = [f'**GPUs**',
f'**Batch Size / GPU**',
f'**Accuracy - {math.upper()} ({self.acc_unit})**',
f'**Throughput - {math.upper()} ({self.perf_unit})**',
f'**Time to Train - {math.upper()} ({self.time_unit})**',
]
writer.headers = header
writer.value_matrix = self.data
writer.write_table()
|
PyTorch/SpeechSynthesis/Tacotron2/platform | platform | DGX1_tacotron2_AMP_8NGPU_train | mkdir -p output
python -m multiproc train.py -m Tacotron2 -o output/ --amp -lr 1e-3 --epochs 1501 -bs 104 --weight-decay 1e-6 --grad-clip-thresh 1.0 --cudnn-enabled --load-mel-from-disk --training-files=filelists/ljs_mel_text_train_filelist.txt --validation-files=filelists/ljs_mel_text_val_filelist.txt --log-file nvlog.json --anneal-steps 500 1000 1500 --anneal-factor 0.3
|
TensorFlow2/LanguageModeling/BERT/official/utils/misc | misc | callstack_sampler | """A simple Python callstack sampler."""
import contextlib
import datetime
import signal
import traceback
class CallstackSampler(object):
"""A simple signal-based Python callstack sampler.
"""
def __init__(self, interval=None):
self.stacks = []
self.interval = 0.001 if interval is None else interval
def _sample(self, signum, frame):
"""Samples the current stack."""
del signum
stack = traceback.extract_stack(frame)
formatted_stack = []
formatted_stack.append(datetime.datetime.utcnow())
for filename, lineno, function_name, text in stack:
formatted_frame = '{}:{}({})({})'.format(filename, lineno, function_name,
text)
formatted_stack.append(formatted_frame)
self.stacks.append(formatted_stack)
signal.setitimer(signal.ITIMER_VIRTUAL, self.interval, 0)
@contextlib.contextmanager
def profile(self):
signal.signal(signal.SIGVTALRM, self._sample)
signal.setitimer(signal.ITIMER_VIRTUAL, self.interval, 0)
try:
yield
finally:
signal.setitimer(signal.ITIMER_VIRTUAL, 0)
def save(self, fname):
with open(fname, 'w') as f:
for s in self.stacks:
for l in s:
f.write('%s\n' % l)
f.write('\n')
@contextlib.contextmanager
def callstack_sampling(filename, interval=None):
"""Periodically samples the Python callstack.
Args:
filename: the filename
interval: the sampling interval, in seconds. Defaults to 0.001.
Yields:
nothing
"""
sampler = CallstackSampler(interval=interval)
with sampler.profile():
yield
sampler.save(filename)
|
TensorFlow/Detection/SSD/models/research/object_detection/utils | utils | ops | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""A module for helper tensorflow ops."""
import collections
import math
import numpy as np
import six
import tensorflow as tf
from object_detection.core import standard_fields as fields
from object_detection.utils import shape_utils
from object_detection.utils import static_shape
def expanded_shape(orig_shape, start_dim, num_dims):
"""Inserts multiple ones into a shape vector.
Inserts an all-1 vector of length num_dims at position start_dim into a shape.
Can be combined with tf.reshape to generalize tf.expand_dims.
Args:
orig_shape: the shape into which the all-1 vector is added (int32 vector)
start_dim: insertion position (int scalar)
num_dims: length of the inserted all-1 vector (int scalar)
Returns:
An int32 vector of length tf.size(orig_shape) + num_dims.
"""
with tf.name_scope('ExpandedShape'):
start_dim = tf.expand_dims(start_dim, 0) # scalar to rank-1
before = tf.slice(orig_shape, [0], start_dim)
add_shape = tf.ones(tf.reshape(num_dims, [1]), dtype=tf.int32)
after = tf.slice(orig_shape, start_dim, [-1])
new_shape = tf.concat([before, add_shape, after], 0)
return new_shape
def normalized_to_image_coordinates(normalized_boxes, image_shape,
parallel_iterations=32):
"""Converts a batch of boxes from normal to image coordinates.
Args:
normalized_boxes: a float32 tensor of shape [None, num_boxes, 4] in
normalized coordinates.
image_shape: a float32 tensor of shape [4] containing the image shape.
parallel_iterations: parallelism for the map_fn op.
Returns:
absolute_boxes: a float32 tensor of shape [None, num_boxes, 4] containing
the boxes in image coordinates.
"""
x_scale = tf.cast(image_shape[2], tf.float32)
y_scale = tf.cast(image_shape[1], tf.float32)
def _to_absolute_coordinates(normalized_boxes):
y_min, x_min, y_max, x_max = tf.split(
value=normalized_boxes, num_or_size_splits=4, axis=1)
y_min = y_scale * y_min
y_max = y_scale * y_max
x_min = x_scale * x_min
x_max = x_scale * x_max
scaled_boxes = tf.concat([y_min, x_min, y_max, x_max], 1)
return scaled_boxes
absolute_boxes = shape_utils.static_or_dynamic_map_fn(
_to_absolute_coordinates,
elems=(normalized_boxes),
dtype=tf.float32,
parallel_iterations=parallel_iterations,
back_prop=True)
return absolute_boxes
def meshgrid(x, y):
"""Tiles the contents of x and y into a pair of grids.
Multidimensional analog of numpy.meshgrid, giving the same behavior if x and y
are vectors. Generally, this will give:
xgrid(i1, ..., i_m, j_1, ..., j_n) = x(j_1, ..., j_n)
ygrid(i1, ..., i_m, j_1, ..., j_n) = y(i_1, ..., i_m)
Keep in mind that the order of the arguments and outputs is reverse relative
to the order of the indices they go into, done for compatibility with numpy.
The output tensors have the same shapes. Specifically:
xgrid.get_shape() = y.get_shape().concatenate(x.get_shape())
ygrid.get_shape() = y.get_shape().concatenate(x.get_shape())
Args:
x: A tensor of arbitrary shape and rank. xgrid will contain these values
varying in its last dimensions.
y: A tensor of arbitrary shape and rank. ygrid will contain these values
varying in its first dimensions.
Returns:
A tuple of tensors (xgrid, ygrid).
"""
with tf.name_scope('Meshgrid'):
x = tf.convert_to_tensor(x)
y = tf.convert_to_tensor(y)
x_exp_shape = expanded_shape(tf.shape(x), 0, tf.rank(y))
y_exp_shape = expanded_shape(tf.shape(y), tf.rank(y), tf.rank(x))
xgrid = tf.tile(tf.reshape(x, x_exp_shape), y_exp_shape)
ygrid = tf.tile(tf.reshape(y, y_exp_shape), x_exp_shape)
new_shape = y.get_shape().concatenate(x.get_shape())
xgrid.set_shape(new_shape)
ygrid.set_shape(new_shape)
return xgrid, ygrid
def fixed_padding(inputs, kernel_size, rate=1):
"""Pads the input along the spatial dimensions independently of input size.
Args:
inputs: A tensor of size [batch, height_in, width_in, channels].
kernel_size: The kernel to be used in the conv2d or max_pool2d operation.
Should be a positive integer.
rate: An integer, rate for atrous convolution.
Returns:
output: A tensor of size [batch, height_out, width_out, channels] with the
input, either intact (if kernel_size == 1) or padded (if kernel_size > 1).
"""
kernel_size_effective = kernel_size + (kernel_size - 1) * (rate - 1)
pad_total = kernel_size_effective - 1
pad_beg = pad_total // 2
pad_end = pad_total - pad_beg
padded_inputs = tf.pad(inputs, [[0, 0], [pad_beg, pad_end],
[pad_beg, pad_end], [0, 0]])
return padded_inputs
def pad_to_multiple(tensor, multiple):
"""Returns the tensor zero padded to the specified multiple.
Appends 0s to the end of the first and second dimension (height and width) of
the tensor until both dimensions are a multiple of the input argument
'multiple'. E.g. given an input tensor of shape [1, 3, 5, 1] and an input
multiple of 4, PadToMultiple will append 0s so that the resulting tensor will
be of shape [1, 4, 8, 1].
Args:
tensor: rank 4 float32 tensor, where
tensor -> [batch_size, height, width, channels].
multiple: the multiple to pad to.
Returns:
padded_tensor: the tensor zero padded to the specified multiple.
"""
if multiple == 1:
return tensor
tensor_shape = tensor.get_shape()
batch_size = static_shape.get_batch_size(tensor_shape)
tensor_height = static_shape.get_height(tensor_shape)
tensor_width = static_shape.get_width(tensor_shape)
tensor_depth = static_shape.get_depth(tensor_shape)
if batch_size is None:
batch_size = tf.shape(tensor)[0]
if tensor_height is None:
tensor_height = tf.shape(tensor)[1]
padded_tensor_height = tf.to_int32(
tf.ceil(tf.to_float(tensor_height) / tf.to_float(multiple))) * multiple
else:
padded_tensor_height = int(
math.ceil(float(tensor_height) / multiple) * multiple)
if tensor_width is None:
tensor_width = tf.shape(tensor)[2]
padded_tensor_width = tf.to_int32(
tf.ceil(tf.to_float(tensor_width) / tf.to_float(multiple))) * multiple
else:
padded_tensor_width = int(
math.ceil(float(tensor_width) / multiple) * multiple)
if tensor_depth is None:
tensor_depth = tf.shape(tensor)[3]
# Use tf.concat instead of tf.pad to preserve static shape
if padded_tensor_height != tensor_height:
height_pad = tf.zeros([
batch_size, padded_tensor_height - tensor_height, tensor_width,
tensor_depth
])
tensor = tf.concat([tensor, height_pad], 1)
if padded_tensor_width != tensor_width:
width_pad = tf.zeros([
batch_size, padded_tensor_height, padded_tensor_width - tensor_width,
tensor_depth
])
tensor = tf.concat([tensor, width_pad], 2)
return tensor
def padded_one_hot_encoding(indices, depth, left_pad):
"""Returns a zero padded one-hot tensor.
This function converts a sparse representation of indices (e.g., [4]) to a
zero padded one-hot representation (e.g., [0, 0, 0, 0, 1] with depth = 4 and
left_pad = 1). If `indices` is empty, the result will simply be a tensor of
shape (0, depth + left_pad). If depth = 0, then this function just returns
`None`.
Args:
indices: an integer tensor of shape [num_indices].
depth: depth for the one-hot tensor (integer).
left_pad: number of zeros to left pad the one-hot tensor with (integer).
Returns:
padded_onehot: a tensor with shape (num_indices, depth + left_pad). Returns
`None` if the depth is zero.
Raises:
ValueError: if `indices` does not have rank 1 or if `left_pad` or `depth are
either negative or non-integers.
TODO(rathodv): add runtime checks for depth and indices.
"""
if depth < 0 or not isinstance(depth, six.integer_types):
raise ValueError('`depth` must be a non-negative integer.')
if left_pad < 0 or not isinstance(left_pad, six.integer_types):
raise ValueError('`left_pad` must be a non-negative integer.')
if depth == 0:
return None
rank = len(indices.get_shape().as_list())
if rank != 1:
raise ValueError('`indices` must have rank 1, but has rank=%s' % rank)
def one_hot_and_pad():
one_hot = tf.cast(tf.one_hot(tf.cast(indices, tf.int64), depth,
on_value=1, off_value=0), tf.float32)
return tf.pad(one_hot, [[0, 0], [left_pad, 0]], mode='CONSTANT')
result = tf.cond(tf.greater(tf.size(indices), 0), one_hot_and_pad,
lambda: tf.zeros((depth + left_pad, 0)))
return tf.reshape(result, [-1, depth + left_pad])
def dense_to_sparse_boxes(dense_locations, dense_num_boxes, num_classes):
"""Converts bounding boxes from dense to sparse form.
Args:
dense_locations: a [max_num_boxes, 4] tensor in which only the first k rows
are valid bounding box location coordinates, where k is the sum of
elements in dense_num_boxes.
dense_num_boxes: a [max_num_classes] tensor indicating the counts of
various bounding box classes e.g. [1, 0, 0, 2] means that the first
bounding box is of class 0 and the second and third bounding boxes are
of class 3. The sum of elements in this tensor is the number of valid
bounding boxes.
num_classes: number of classes
Returns:
box_locations: a [num_boxes, 4] tensor containing only valid bounding
boxes (i.e. the first num_boxes rows of dense_locations)
box_classes: a [num_boxes] tensor containing the classes of each bounding
box (e.g. dense_num_boxes = [1, 0, 0, 2] => box_classes = [0, 3, 3]
"""
num_valid_boxes = tf.reduce_sum(dense_num_boxes)
box_locations = tf.slice(dense_locations,
tf.constant([0, 0]), tf.stack([num_valid_boxes, 4]))
tiled_classes = [tf.tile([i], tf.expand_dims(dense_num_boxes[i], 0))
for i in range(num_classes)]
box_classes = tf.concat(tiled_classes, 0)
box_locations.set_shape([None, 4])
return box_locations, box_classes
def indices_to_dense_vector(indices,
size,
indices_value=1.,
default_value=0,
dtype=tf.float32):
"""Creates dense vector with indices set to specific value and rest to zeros.
This function exists because it is unclear if it is safe to use
tf.sparse_to_dense(indices, [size], 1, validate_indices=False)
with indices which are not ordered.
This function accepts a dynamic size (e.g. tf.shape(tensor)[0])
Args:
indices: 1d Tensor with integer indices which are to be set to
indices_values.
size: scalar with size (integer) of output Tensor.
indices_value: values of elements specified by indices in the output vector
default_value: values of other elements in the output vector.
dtype: data type.
Returns:
dense 1D Tensor of shape [size] with indices set to indices_values and the
rest set to default_value.
"""
size = tf.to_int32(size)
zeros = tf.ones([size], dtype=dtype) * default_value
values = tf.ones_like(indices, dtype=dtype) * indices_value
return tf.dynamic_stitch([tf.range(size), tf.to_int32(indices)],
[zeros, values])
def reduce_sum_trailing_dimensions(tensor, ndims):
"""Computes sum across all dimensions following first `ndims` dimensions."""
return tf.reduce_sum(tensor, axis=tuple(range(ndims, tensor.shape.ndims)))
def retain_groundtruth(tensor_dict, valid_indices):
"""Retains groundtruth by valid indices.
Args:
tensor_dict: a dictionary of following groundtruth tensors -
fields.InputDataFields.groundtruth_boxes
fields.InputDataFields.groundtruth_classes
fields.InputDataFields.groundtruth_keypoints
fields.InputDataFields.groundtruth_instance_masks
fields.InputDataFields.groundtruth_is_crowd
fields.InputDataFields.groundtruth_area
fields.InputDataFields.groundtruth_label_types
fields.InputDataFields.groundtruth_difficult
valid_indices: a tensor with valid indices for the box-level groundtruth.
Returns:
a dictionary of tensors containing only the groundtruth for valid_indices.
Raises:
ValueError: If the shape of valid_indices is invalid.
ValueError: field fields.InputDataFields.groundtruth_boxes is
not present in tensor_dict.
"""
input_shape = valid_indices.get_shape().as_list()
if not (len(input_shape) == 1 or
(len(input_shape) == 2 and input_shape[1] == 1)):
raise ValueError('The shape of valid_indices is invalid.')
valid_indices = tf.reshape(valid_indices, [-1])
valid_dict = {}
if fields.InputDataFields.groundtruth_boxes in tensor_dict:
# Prevents reshape failure when num_boxes is 0.
num_boxes = tf.maximum(tf.shape(
tensor_dict[fields.InputDataFields.groundtruth_boxes])[0], 1)
for key in tensor_dict:
if key in [fields.InputDataFields.groundtruth_boxes,
fields.InputDataFields.groundtruth_classes,
fields.InputDataFields.groundtruth_keypoints,
fields.InputDataFields.groundtruth_instance_masks]:
valid_dict[key] = tf.gather(tensor_dict[key], valid_indices)
# Input decoder returns empty tensor when these fields are not provided.
# Needs to reshape into [num_boxes, -1] for tf.gather() to work.
elif key in [fields.InputDataFields.groundtruth_is_crowd,
fields.InputDataFields.groundtruth_area,
fields.InputDataFields.groundtruth_difficult,
fields.InputDataFields.groundtruth_label_types]:
valid_dict[key] = tf.reshape(
tf.gather(tf.reshape(tensor_dict[key], [num_boxes, -1]),
valid_indices), [-1])
# Fields that are not associated with boxes.
else:
valid_dict[key] = tensor_dict[key]
else:
raise ValueError('%s not present in input tensor dict.' % (
fields.InputDataFields.groundtruth_boxes))
return valid_dict
def retain_groundtruth_with_positive_classes(tensor_dict):
"""Retains only groundtruth with positive class ids.
Args:
tensor_dict: a dictionary of following groundtruth tensors -
fields.InputDataFields.groundtruth_boxes
fields.InputDataFields.groundtruth_classes
fields.InputDataFields.groundtruth_keypoints
fields.InputDataFields.groundtruth_instance_masks
fields.InputDataFields.groundtruth_is_crowd
fields.InputDataFields.groundtruth_area
fields.InputDataFields.groundtruth_label_types
fields.InputDataFields.groundtruth_difficult
Returns:
a dictionary of tensors containing only the groundtruth with positive
classes.
Raises:
ValueError: If groundtruth_classes tensor is not in tensor_dict.
"""
if fields.InputDataFields.groundtruth_classes not in tensor_dict:
raise ValueError('`groundtruth classes` not in tensor_dict.')
keep_indices = tf.where(tf.greater(
tensor_dict[fields.InputDataFields.groundtruth_classes], 0))
return retain_groundtruth(tensor_dict, keep_indices)
def replace_nan_groundtruth_label_scores_with_ones(label_scores):
"""Replaces nan label scores with 1.0.
Args:
label_scores: a tensor containing object annoation label scores.
Returns:
a tensor where NaN label scores have been replaced by ones.
"""
return tf.where(
tf.is_nan(label_scores), tf.ones(tf.shape(label_scores)), label_scores)
def filter_groundtruth_with_crowd_boxes(tensor_dict):
"""Filters out groundtruth with boxes corresponding to crowd.
Args:
tensor_dict: a dictionary of following groundtruth tensors -
fields.InputDataFields.groundtruth_boxes
fields.InputDataFields.groundtruth_classes
fields.InputDataFields.groundtruth_keypoints
fields.InputDataFields.groundtruth_instance_masks
fields.InputDataFields.groundtruth_is_crowd
fields.InputDataFields.groundtruth_area
fields.InputDataFields.groundtruth_label_types
Returns:
a dictionary of tensors containing only the groundtruth that have bounding
boxes.
"""
if fields.InputDataFields.groundtruth_is_crowd in tensor_dict:
is_crowd = tensor_dict[fields.InputDataFields.groundtruth_is_crowd]
is_not_crowd = tf.logical_not(is_crowd)
is_not_crowd_indices = tf.where(is_not_crowd)
tensor_dict = retain_groundtruth(tensor_dict, is_not_crowd_indices)
return tensor_dict
def filter_groundtruth_with_nan_box_coordinates(tensor_dict):
"""Filters out groundtruth with no bounding boxes.
Args:
tensor_dict: a dictionary of following groundtruth tensors -
fields.InputDataFields.groundtruth_boxes
fields.InputDataFields.groundtruth_classes
fields.InputDataFields.groundtruth_keypoints
fields.InputDataFields.groundtruth_instance_masks
fields.InputDataFields.groundtruth_is_crowd
fields.InputDataFields.groundtruth_area
fields.InputDataFields.groundtruth_label_types
Returns:
a dictionary of tensors containing only the groundtruth that have bounding
boxes.
"""
groundtruth_boxes = tensor_dict[fields.InputDataFields.groundtruth_boxes]
nan_indicator_vector = tf.greater(tf.reduce_sum(tf.to_int32(
tf.is_nan(groundtruth_boxes)), reduction_indices=[1]), 0)
valid_indicator_vector = tf.logical_not(nan_indicator_vector)
valid_indices = tf.where(valid_indicator_vector)
return retain_groundtruth(tensor_dict, valid_indices)
def normalize_to_target(inputs,
target_norm_value,
dim,
epsilon=1e-7,
trainable=True,
scope='NormalizeToTarget',
summarize=True):
"""L2 normalizes the inputs across the specified dimension to a target norm.
This op implements the L2 Normalization layer introduced in
Liu, Wei, et al. "SSD: Single Shot MultiBox Detector."
and Liu, Wei, Andrew Rabinovich, and Alexander C. Berg.
"Parsenet: Looking wider to see better." and is useful for bringing
activations from multiple layers in a convnet to a standard scale.
Note that the rank of `inputs` must be known and the dimension to which
normalization is to be applied should be statically defined.
TODO(jonathanhuang): Add option to scale by L2 norm of the entire input.
Args:
inputs: A `Tensor` of arbitrary size.
target_norm_value: A float value that specifies an initial target norm or
a list of floats (whose length must be equal to the depth along the
dimension to be normalized) specifying a per-dimension multiplier
after normalization.
dim: The dimension along which the input is normalized.
epsilon: A small value to add to the inputs to avoid dividing by zero.
trainable: Whether the norm is trainable or not
scope: Optional scope for variable_scope.
summarize: Whether or not to add a tensorflow summary for the op.
Returns:
The input tensor normalized to the specified target norm.
Raises:
ValueError: If dim is smaller than the number of dimensions in 'inputs'.
ValueError: If target_norm_value is not a float or a list of floats with
length equal to the depth along the dimension to be normalized.
"""
with tf.variable_scope(scope, 'NormalizeToTarget', [inputs]):
if not inputs.get_shape():
raise ValueError('The input rank must be known.')
input_shape = inputs.get_shape().as_list()
input_rank = len(input_shape)
if dim < 0 or dim >= input_rank:
raise ValueError(
'dim must be non-negative but smaller than the input rank.')
if not input_shape[dim]:
raise ValueError('input shape should be statically defined along '
'the specified dimension.')
depth = input_shape[dim]
if not (isinstance(target_norm_value, float) or
(isinstance(target_norm_value, list) and
len(target_norm_value) == depth) and
all([isinstance(val, float) for val in target_norm_value])):
raise ValueError('target_norm_value must be a float or a list of floats '
'with length equal to the depth along the dimension to '
'be normalized.')
if isinstance(target_norm_value, float):
initial_norm = depth * [target_norm_value]
else:
initial_norm = target_norm_value
target_norm = tf.contrib.framework.model_variable(
name='weights', dtype=tf.float32,
initializer=tf.constant(initial_norm, dtype=tf.float32),
trainable=trainable)
if summarize:
mean = tf.reduce_mean(target_norm)
mean = tf.Print(mean, ['NormalizeToTarget:', mean])
tf.summary.scalar(tf.get_variable_scope().name, mean)
lengths = epsilon + tf.sqrt(tf.reduce_sum(tf.square(inputs), dim, True))
mult_shape = input_rank*[1]
mult_shape[dim] = depth
return tf.reshape(target_norm, mult_shape) * tf.truediv(inputs, lengths)
def batch_position_sensitive_crop_regions(images,
boxes,
crop_size,
num_spatial_bins,
global_pool,
parallel_iterations=64):
"""Position sensitive crop with batches of images and boxes.
This op is exactly like `position_sensitive_crop_regions` below but operates
on batches of images and boxes. See `position_sensitive_crop_regions` function
below for the operation applied per batch element.
Args:
images: A `Tensor`. Must be one of the following types: `uint8`, `int8`,
`int16`, `int32`, `int64`, `half`, `float32`, `float64`.
A 4-D tensor of shape `[batch, image_height, image_width, depth]`.
Both `image_height` and `image_width` need to be positive.
boxes: A `Tensor` of type `float32`.
A 3-D tensor of shape `[batch, num_boxes, 4]`. Each box is specified in
normalized coordinates `[y1, x1, y2, x2]`. A normalized coordinate value
of `y` is mapped to the image coordinate at `y * (image_height - 1)`, so
as the `[0, 1]` interval of normalized image height is mapped to
`[0, image_height - 1] in image height coordinates. We do allow y1 > y2,
in which case the sampled crop is an up-down flipped version of the
original image. The width dimension is treated similarly.
crop_size: See `position_sensitive_crop_regions` below.
num_spatial_bins: See `position_sensitive_crop_regions` below.
global_pool: See `position_sensitive_crop_regions` below.
parallel_iterations: Number of batch items to process in parallel.
Returns:
"""
def _position_sensitive_crop_fn(inputs):
images, boxes = inputs
return position_sensitive_crop_regions(
images,
boxes,
crop_size=crop_size,
num_spatial_bins=num_spatial_bins,
global_pool=global_pool)
return shape_utils.static_or_dynamic_map_fn(
_position_sensitive_crop_fn,
elems=[images, boxes],
dtype=tf.float32,
parallel_iterations=parallel_iterations)
def position_sensitive_crop_regions(image,
boxes,
crop_size,
num_spatial_bins,
global_pool):
"""Position-sensitive crop and pool rectangular regions from a feature grid.
The output crops are split into `spatial_bins_y` vertical bins
and `spatial_bins_x` horizontal bins. For each intersection of a vertical
and a horizontal bin the output values are gathered by performing
`tf.image.crop_and_resize` (bilinear resampling) on a a separate subset of
channels of the image. This reduces `depth` by a factor of
`(spatial_bins_y * spatial_bins_x)`.
When global_pool is True, this function implements a differentiable version
of position-sensitive RoI pooling used in
[R-FCN detection system](https://arxiv.org/abs/1605.06409).
When global_pool is False, this function implements a differentiable version
of position-sensitive assembling operation used in
[instance FCN](https://arxiv.org/abs/1603.08678).
Args:
image: A `Tensor`. Must be one of the following types: `uint8`, `int8`,
`int16`, `int32`, `int64`, `half`, `float32`, `float64`.
A 3-D tensor of shape `[image_height, image_width, depth]`.
Both `image_height` and `image_width` need to be positive.
boxes: A `Tensor` of type `float32`.
A 2-D tensor of shape `[num_boxes, 4]`. Each box is specified in
normalized coordinates `[y1, x1, y2, x2]`. A normalized coordinate value
of `y` is mapped to the image coordinate at `y * (image_height - 1)`, so
as the `[0, 1]` interval of normalized image height is mapped to
`[0, image_height - 1] in image height coordinates. We do allow y1 > y2,
in which case the sampled crop is an up-down flipped version of the
original image. The width dimension is treated similarly.
crop_size: A list of two integers `[crop_height, crop_width]`. All
cropped image patches are resized to this size. The aspect ratio of the
image content is not preserved. Both `crop_height` and `crop_width` need
to be positive.
num_spatial_bins: A list of two integers `[spatial_bins_y, spatial_bins_x]`.
Represents the number of position-sensitive bins in y and x directions.
Both values should be >= 1. `crop_height` should be divisible by
`spatial_bins_y`, and similarly for width.
The number of image channels should be divisible by
(spatial_bins_y * spatial_bins_x).
Suggested value from R-FCN paper: [3, 3].
global_pool: A boolean variable.
If True, we perform average global pooling on the features assembled from
the position-sensitive score maps.
If False, we keep the position-pooled features without global pooling
over the spatial coordinates.
Note that using global_pool=True is equivalent to but more efficient than
running the function with global_pool=False and then performing global
average pooling.
Returns:
position_sensitive_features: A 4-D tensor of shape
`[num_boxes, K, K, crop_channels]`,
where `crop_channels = depth / (spatial_bins_y * spatial_bins_x)`,
where K = 1 when global_pool is True (Average-pooled cropped regions),
and K = crop_size when global_pool is False.
Raises:
ValueError: Raised in four situations:
`num_spatial_bins` is not >= 1;
`num_spatial_bins` does not divide `crop_size`;
`(spatial_bins_y*spatial_bins_x)` does not divide `depth`;
`bin_crop_size` is not square when global_pool=False due to the
constraint in function space_to_depth.
"""
total_bins = 1
bin_crop_size = []
for (num_bins, crop_dim) in zip(num_spatial_bins, crop_size):
if num_bins < 1:
raise ValueError('num_spatial_bins should be >= 1')
if crop_dim % num_bins != 0:
raise ValueError('crop_size should be divisible by num_spatial_bins')
total_bins *= num_bins
bin_crop_size.append(crop_dim // num_bins)
if not global_pool and bin_crop_size[0] != bin_crop_size[1]:
raise ValueError('Only support square bin crop size for now.')
ymin, xmin, ymax, xmax = tf.unstack(boxes, axis=1)
spatial_bins_y, spatial_bins_x = num_spatial_bins
# Split each box into spatial_bins_y * spatial_bins_x bins.
position_sensitive_boxes = []
for bin_y in range(spatial_bins_y):
step_y = (ymax - ymin) / spatial_bins_y
for bin_x in range(spatial_bins_x):
step_x = (xmax - xmin) / spatial_bins_x
box_coordinates = [ymin + bin_y * step_y,
xmin + bin_x * step_x,
ymin + (bin_y + 1) * step_y,
xmin + (bin_x + 1) * step_x,
]
position_sensitive_boxes.append(tf.stack(box_coordinates, axis=1))
image_splits = tf.split(value=image, num_or_size_splits=total_bins, axis=2)
image_crops = []
for (split, box) in zip(image_splits, position_sensitive_boxes):
if split.shape.is_fully_defined() and box.shape.is_fully_defined():
crop = tf.squeeze(
matmul_crop_and_resize(
tf.expand_dims(split, axis=0), tf.expand_dims(box, axis=0),
bin_crop_size),
axis=0)
else:
crop = tf.image.crop_and_resize(
tf.expand_dims(split, 0), box,
tf.zeros(tf.shape(boxes)[0], dtype=tf.int32), bin_crop_size)
image_crops.append(crop)
if global_pool:
# Average over all bins.
position_sensitive_features = tf.add_n(image_crops) / len(image_crops)
# Then average over spatial positions within the bins.
position_sensitive_features = tf.reduce_mean(
position_sensitive_features, [1, 2], keep_dims=True)
else:
# Reorder height/width to depth channel.
block_size = bin_crop_size[0]
if block_size >= 2:
image_crops = [tf.space_to_depth(
crop, block_size=block_size) for crop in image_crops]
# Pack image_crops so that first dimension is for position-senstive boxes.
position_sensitive_features = tf.stack(image_crops, axis=0)
# Unroll the position-sensitive boxes to spatial positions.
position_sensitive_features = tf.squeeze(
tf.batch_to_space_nd(position_sensitive_features,
block_shape=[1] + num_spatial_bins,
crops=tf.zeros((3, 2), dtype=tf.int32)),
squeeze_dims=[0])
# Reorder back the depth channel.
if block_size >= 2:
position_sensitive_features = tf.depth_to_space(
position_sensitive_features, block_size=block_size)
return position_sensitive_features
def reframe_box_masks_to_image_masks(box_masks, boxes, image_height,
image_width):
"""Transforms the box masks back to full image masks.
Embeds masks in bounding boxes of larger masks whose shapes correspond to
image shape.
Args:
box_masks: A tf.float32 tensor of size [num_masks, mask_height, mask_width].
boxes: A tf.float32 tensor of size [num_masks, 4] containing the box
corners. Row i contains [ymin, xmin, ymax, xmax] of the box
corresponding to mask i. Note that the box corners are in
normalized coordinates.
image_height: Image height. The output mask will have the same height as
the image height.
image_width: Image width. The output mask will have the same width as the
image width.
Returns:
A tf.float32 tensor of size [num_masks, image_height, image_width].
"""
# TODO(rathodv): Make this a public function.
def reframe_box_masks_to_image_masks_default():
"""The default function when there are more than 0 box masks."""
def transform_boxes_relative_to_boxes(boxes, reference_boxes):
boxes = tf.reshape(boxes, [-1, 2, 2])
min_corner = tf.expand_dims(reference_boxes[:, 0:2], 1)
max_corner = tf.expand_dims(reference_boxes[:, 2:4], 1)
transformed_boxes = (boxes - min_corner) / (max_corner - min_corner)
return tf.reshape(transformed_boxes, [-1, 4])
box_masks_expanded = tf.expand_dims(box_masks, axis=3)
num_boxes = tf.shape(box_masks_expanded)[0]
unit_boxes = tf.concat(
[tf.zeros([num_boxes, 2]), tf.ones([num_boxes, 2])], axis=1)
reverse_boxes = transform_boxes_relative_to_boxes(unit_boxes, boxes)
return tf.image.crop_and_resize(
image=box_masks_expanded,
boxes=reverse_boxes,
box_ind=tf.range(num_boxes),
crop_size=[image_height, image_width],
extrapolation_value=0.0)
image_masks = tf.cond(
tf.shape(box_masks)[0] > 0,
reframe_box_masks_to_image_masks_default,
lambda: tf.zeros([0, image_height, image_width, 1], dtype=tf.float32))
return tf.squeeze(image_masks, axis=3)
def merge_boxes_with_multiple_labels(boxes,
classes,
confidences,
num_classes,
quantization_bins=10000):
"""Merges boxes with same coordinates and returns K-hot encoded classes.
Args:
boxes: A tf.float32 tensor with shape [N, 4] holding N boxes. Only
normalized coordinates are allowed.
classes: A tf.int32 tensor with shape [N] holding class indices.
The class index starts at 0.
confidences: A tf.float32 tensor with shape [N] holding class confidences.
num_classes: total number of classes to use for K-hot encoding.
quantization_bins: the number of bins used to quantize the box coordinate.
Returns:
merged_boxes: A tf.float32 tensor with shape [N', 4] holding boxes,
where N' <= N.
class_encodings: A tf.int32 tensor with shape [N', num_classes] holding
K-hot encodings for the merged boxes.
confidence_encodings: A tf.float32 tensor with shape [N', num_classes]
holding encodings of confidences for the merged boxes.
merged_box_indices: A tf.int32 tensor with shape [N'] holding original
indices of the boxes.
"""
boxes_shape = tf.shape(boxes)
classes_shape = tf.shape(classes)
confidences_shape = tf.shape(confidences)
box_class_shape_assert = shape_utils.assert_shape_equal_along_first_dimension(
boxes_shape, classes_shape)
box_confidence_shape_assert = (
shape_utils.assert_shape_equal_along_first_dimension(
boxes_shape, confidences_shape))
box_dimension_assert = tf.assert_equal(boxes_shape[1], 4)
box_normalized_assert = shape_utils.assert_box_normalized(boxes)
with tf.control_dependencies(
[box_class_shape_assert, box_confidence_shape_assert,
box_dimension_assert, box_normalized_assert]):
quantized_boxes = tf.to_int64(boxes * (quantization_bins - 1))
ymin, xmin, ymax, xmax = tf.unstack(quantized_boxes, axis=1)
hashcodes = (
ymin +
xmin * quantization_bins +
ymax * quantization_bins * quantization_bins +
xmax * quantization_bins * quantization_bins * quantization_bins)
unique_hashcodes, unique_indices = tf.unique(hashcodes)
num_boxes = tf.shape(boxes)[0]
num_unique_boxes = tf.shape(unique_hashcodes)[0]
merged_box_indices = tf.unsorted_segment_min(
tf.range(num_boxes), unique_indices, num_unique_boxes)
merged_boxes = tf.gather(boxes, merged_box_indices)
def map_box_encodings(i):
"""Produces box K-hot and score encodings for each class index."""
box_mask = tf.equal(
unique_indices, i * tf.ones(num_boxes, dtype=tf.int32))
box_mask = tf.reshape(box_mask, [-1])
box_indices = tf.boolean_mask(classes, box_mask)
box_confidences = tf.boolean_mask(confidences, box_mask)
box_class_encodings = tf.sparse_to_dense(
box_indices, [num_classes], 1, validate_indices=False)
box_confidence_encodings = tf.sparse_to_dense(
box_indices, [num_classes], box_confidences, validate_indices=False)
return box_class_encodings, box_confidence_encodings
class_encodings, confidence_encodings = tf.map_fn(
map_box_encodings,
tf.range(num_unique_boxes),
back_prop=False,
dtype=(tf.int32, tf.float32))
merged_boxes = tf.reshape(merged_boxes, [-1, 4])
class_encodings = tf.reshape(class_encodings, [-1, num_classes])
confidence_encodings = tf.reshape(confidence_encodings, [-1, num_classes])
merged_box_indices = tf.reshape(merged_box_indices, [-1])
return (merged_boxes, class_encodings, confidence_encodings,
merged_box_indices)
def nearest_neighbor_upsampling(input_tensor, scale=None, height_scale=None,
width_scale=None):
"""Nearest neighbor upsampling implementation.
Nearest neighbor upsampling function that maps input tensor with shape
[batch_size, height, width, channels] to [batch_size, height * scale
, width * scale, channels]. This implementation only uses reshape and
broadcasting to make it TPU compatible.
Args:
input_tensor: A float32 tensor of size [batch, height_in, width_in,
channels].
scale: An integer multiple to scale resolution of input data in both height
and width dimensions.
height_scale: An integer multiple to scale the height of input image. This
option when provided overrides `scale` option.
width_scale: An integer multiple to scale the width of input image. This
option when provided overrides `scale` option.
Returns:
data_up: A float32 tensor of size
[batch, height_in*scale, width_in*scale, channels].
Raises:
ValueError: If both scale and height_scale or if both scale and width_scale
are None.
"""
if not scale and (height_scale is None or width_scale is None):
raise ValueError('Provide either `scale` or `height_scale` and'
' `width_scale`.')
with tf.name_scope('nearest_neighbor_upsampling'):
h_scale = scale if height_scale is None else height_scale
w_scale = scale if width_scale is None else width_scale
(batch_size, height, width,
channels) = shape_utils.combined_static_and_dynamic_shape(input_tensor)
output_tensor = tf.reshape(
input_tensor, [batch_size, height, 1, width, 1, channels]) * tf.ones(
[1, 1, h_scale, 1, w_scale, 1], dtype=input_tensor.dtype)
return tf.reshape(output_tensor,
[batch_size, height * h_scale, width * w_scale, channels])
def matmul_gather_on_zeroth_axis(params, indices, scope=None):
"""Matrix multiplication based implementation of tf.gather on zeroth axis.
TODO(rathodv, jonathanhuang): enable sparse matmul option.
Args:
params: A float32 Tensor. The tensor from which to gather values.
Must be at least rank 1.
indices: A Tensor. Must be one of the following types: int32, int64.
Must be in range [0, params.shape[0])
scope: A name for the operation (optional).
Returns:
A Tensor. Has the same type as params. Values from params gathered
from indices given by indices, with shape indices.shape + params.shape[1:].
"""
with tf.name_scope(scope, 'MatMulGather'):
params_shape = shape_utils.combined_static_and_dynamic_shape(params)
indices_shape = shape_utils.combined_static_and_dynamic_shape(indices)
params2d = tf.reshape(params, [params_shape[0], -1])
indicator_matrix = tf.one_hot(indices, params_shape[0])
gathered_result_flattened = tf.matmul(indicator_matrix, params2d)
return tf.reshape(gathered_result_flattened,
tf.stack(indices_shape + params_shape[1:]))
def matmul_crop_and_resize(image, boxes, crop_size, scope=None):
"""Matrix multiplication based implementation of the crop and resize op.
Extracts crops from the input image tensor and bilinearly resizes them
(possibly with aspect ratio change) to a common output size specified by
crop_size. This is more general than the crop_to_bounding_box op which
extracts a fixed size slice from the input image and does not allow
resizing or aspect ratio change.
Returns a tensor with crops from the input image at positions defined at
the bounding box locations in boxes. The cropped boxes are all resized
(with bilinear interpolation) to a fixed size = `[crop_height, crop_width]`.
The result is a 5-D tensor `[batch, num_boxes, crop_height, crop_width,
depth]`.
Running time complexity:
O((# channels) * (# boxes) * (crop_size)^2 * M), where M is the number
of pixels of the longer edge of the image.
Note that this operation is meant to replicate the behavior of the standard
tf.image.crop_and_resize operation but there are a few differences.
Specifically:
1) The extrapolation value (the values that are interpolated from outside
the bounds of the image window) is always zero
2) Only XLA supported operations are used (e.g., matrix multiplication).
3) There is no `box_indices` argument --- to run this op on multiple images,
one must currently call this op independently on each image.
4) All shapes and the `crop_size` parameter are assumed to be statically
defined. Moreover, the number of boxes must be strictly nonzero.
Args:
image: A `Tensor`. Must be one of the following types: `uint8`, `int8`,
`int16`, `int32`, `int64`, `half`, 'bfloat16', `float32`, `float64`.
A 4-D tensor of shape `[batch, image_height, image_width, depth]`.
Both `image_height` and `image_width` need to be positive.
boxes: A `Tensor` of type `float32` or 'bfloat16'.
A 3-D tensor of shape `[batch, num_boxes, 4]`. The boxes are specified in
normalized coordinates and are of the form `[y1, x1, y2, x2]`. A
normalized coordinate value of `y` is mapped to the image coordinate at
`y * (image_height - 1)`, so as the `[0, 1]` interval of normalized image
height is mapped to `[0, image_height - 1] in image height coordinates.
We do allow y1 > y2, in which case the sampled crop is an up-down flipped
version of the original image. The width dimension is treated similarly.
Normalized coordinates outside the `[0, 1]` range are allowed, in which
case we use `extrapolation_value` to extrapolate the input image values.
crop_size: A list of two integers `[crop_height, crop_width]`. All
cropped image patches are resized to this size. The aspect ratio of the
image content is not preserved. Both `crop_height` and `crop_width` need
to be positive.
scope: A name for the operation (optional).
Returns:
A 5-D tensor of shape `[batch, num_boxes, crop_height, crop_width, depth]`
Raises:
ValueError: if image tensor does not have shape
`[batch, image_height, image_width, depth]` and all dimensions statically
defined.
ValueError: if boxes tensor does not have shape `[batch, num_boxes, 4]`
where num_boxes > 0.
ValueError: if crop_size is not a list of two positive integers
"""
img_shape = image.shape.as_list()
boxes_shape = boxes.shape.as_list()
_, img_height, img_width, _ = img_shape
if not isinstance(crop_size, list) or len(crop_size) != 2:
raise ValueError('`crop_size` must be a list of length 2')
dimensions = img_shape + crop_size + boxes_shape
if not all([isinstance(dim, int) for dim in dimensions]):
raise ValueError('all input shapes must be statically defined')
if len(boxes_shape) != 3 or boxes_shape[2] != 4:
raise ValueError('`boxes` should have shape `[batch, num_boxes, 4]`')
if len(img_shape) != 4:
raise ValueError('image should have shape '
'`[batch, image_height, image_width, depth]`')
num_crops = boxes_shape[0]
if not num_crops > 0:
raise ValueError('number of boxes must be > 0')
if not (crop_size[0] > 0 and crop_size[1] > 0):
raise ValueError('`crop_size` must be a list of two positive integers.')
def _lin_space_weights(num, img_size):
if num > 1:
start_weights = tf.linspace(img_size - 1.0, 0.0, num)
stop_weights = img_size - 1 - start_weights
else:
start_weights = tf.constant(num * [.5 * (img_size - 1)], dtype=tf.float32)
stop_weights = tf.constant(num * [.5 * (img_size - 1)], dtype=tf.float32)
return (start_weights, stop_weights)
with tf.name_scope(scope, 'MatMulCropAndResize'):
y1_weights, y2_weights = _lin_space_weights(crop_size[0], img_height)
x1_weights, x2_weights = _lin_space_weights(crop_size[1], img_width)
y1_weights = tf.cast(y1_weights, boxes.dtype)
y2_weights = tf.cast(y2_weights, boxes.dtype)
x1_weights = tf.cast(x1_weights, boxes.dtype)
x2_weights = tf.cast(x2_weights, boxes.dtype)
[y1, x1, y2, x2] = tf.unstack(boxes, axis=2)
# Pixel centers of input image and grid points along height and width
image_idx_h = tf.constant(
np.reshape(np.arange(img_height), (1, 1, 1, img_height)),
dtype=boxes.dtype)
image_idx_w = tf.constant(
np.reshape(np.arange(img_width), (1, 1, 1, img_width)),
dtype=boxes.dtype)
grid_pos_h = tf.expand_dims(
tf.einsum('ab,c->abc', y1, y1_weights) + tf.einsum(
'ab,c->abc', y2, y2_weights),
axis=3)
grid_pos_w = tf.expand_dims(
tf.einsum('ab,c->abc', x1, x1_weights) + tf.einsum(
'ab,c->abc', x2, x2_weights),
axis=3)
# Create kernel matrices of pairwise kernel evaluations between pixel
# centers of image and grid points.
kernel_h = tf.nn.relu(1 - tf.abs(image_idx_h - grid_pos_h))
kernel_w = tf.nn.relu(1 - tf.abs(image_idx_w - grid_pos_w))
# Compute matrix multiplication between the spatial dimensions of the image
# and height-wise kernel using einsum.
intermediate_image = tf.einsum('abci,aiop->abcop', kernel_h, image)
# Compute matrix multiplication between the spatial dimensions of the
# intermediate_image and width-wise kernel using einsum.
return tf.einsum('abno,abcop->abcnp', kernel_w, intermediate_image)
def native_crop_and_resize(image, boxes, crop_size, scope=None):
"""Same as `matmul_crop_and_resize` but uses tf.image.crop_and_resize."""
def get_box_inds(proposals):
proposals_shape = proposals.get_shape().as_list()
if any(dim is None for dim in proposals_shape):
proposals_shape = tf.shape(proposals)
ones_mat = tf.ones(proposals_shape[:2], dtype=tf.int32)
multiplier = tf.expand_dims(
tf.range(start=0, limit=proposals_shape[0]), 1)
return tf.reshape(ones_mat * multiplier, [-1])
with tf.name_scope(scope, 'CropAndResize'):
cropped_regions = tf.image.crop_and_resize(
image, tf.reshape(boxes, [-1] + boxes.shape.as_list()[2:]),
get_box_inds(boxes), crop_size)
final_shape = tf.concat([tf.shape(boxes)[:2],
tf.shape(cropped_regions)[1:]], axis=0)
return tf.reshape(cropped_regions, final_shape)
EqualizationLossConfig = collections.namedtuple('EqualizationLossConfig',
['weight', 'exclude_prefixes'])
|
Tools/DGLPyTorch/SyntheticGraphGeneration/syngen/configuration | configuration | configuration | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import copy
import json
import os
import warnings
from typing import Dict, Optional, Union
from syngen.configuration.utils import optional_comparison, one_field_from_list_of_dicts
from syngen.utils.io_utils import load_dataframe, load_graph
from syngen.utils.types import MetaData, DataSourceInputType
class SynGenDatasetFeatureSpec(dict):
""" SynGenDatasetFeatureSpec is an util class to simply the work with SynGen Dataset Format
Args:
graph_metadata (Dict): dict in SynGen Format
"""
def __init__(self, graph_metadata: Dict):
super().__init__(graph_metadata)
@staticmethod
def instantiate_from_preprocessed(path: str):
""" Creates a SynGenDatasetFeatureSpec and checks all specified files
Args:
path: path to the directory with a dataset in SynGen Format
"""
if os.path.isfile(path):
file_path = path
dir_path = os.path.dirname(file_path)
elif os.path.isdir(path):
file_path = os.path.join(path, 'graph_metadata.json')
dir_path = path
else:
raise ValueError(f"expected path to existing file or directory. got {path}")
with open(file_path, 'r') as f:
graph_metadata = json.load(f)
graph_metadata[MetaData.PATH] = dir_path
config = SynGenDatasetFeatureSpec(graph_metadata)
config.validate()
return config
def get_tabular_data(self, part, name, cache=False, absolute_path=None, return_cat_feats=False):
part_info = self.get_info(part, name)
if MetaData.FEATURES_DATA in part_info:
return part_info[MetaData.FEATURES_DATA]
part_features_info = part_info[MetaData.FEATURES]
part_features_path = part_info[MetaData.FEATURES_PATH]
if part_features_path is None:
raise ValueError()
if MetaData.PATH not in self:
if absolute_path is None:
raise ValueError("Please specify the absolute path for the feature spec: "
"by passing absolute_path argument or specifying MetaData.PATH in the Feature Spec")
else:
self[MetaData.PATH] = absolute_path
features_df = load_dataframe(os.path.join(self[MetaData.PATH], part_features_path),
feature_info=part_features_info)
if cache:
part_info[MetaData.FEATURES_DATA] = features_df
if return_cat_feats:
cat_features = [
feature_info[MetaData.NAME]
for feature_info in part_info[MetaData.FEATURES]
if feature_info[MetaData.FEATURE_TYPE] == MetaData.CATEGORICAL
]
return features_df, cat_features
return features_df
def get_structural_data(self, edge_name, cache=False, absolute_path=None, ):
edge_info = self.get_edge_info(edge_name)
if MetaData.STRUCTURE_DATA in edge_info:
return edge_info[MetaData.STRUCTURE_DATA]
structure_path = edge_info[MetaData.STRUCTURE_PATH]
if structure_path is None:
raise ValueError()
if MetaData.PATH not in self:
if absolute_path is None:
raise ValueError("Please specify the absolute path for the feature spec: "
"by passing absolute_path argument or specifying MetaData.PATH in the Feature Spec")
else:
self[MetaData.PATH] = absolute_path
graph = load_graph(os.path.join(self[MetaData.PATH], structure_path))
if cache:
edge_info[MetaData.STRUCTURE_DATA] = graph
return graph
def get_edge_info(self, name: Union[str, list], src_node_type: Optional[str] = None,
dst_node_type: Optional[str] = None):
if isinstance(name, list):
src_node_type, name, dst_node_type = name
for edge_type in self[MetaData.EDGES]:
if edge_type[MetaData.NAME] == name \
and optional_comparison(src_node_type, edge_type[MetaData.SRC_NODE_TYPE]) \
and optional_comparison(dst_node_type, edge_type[MetaData.DST_NODE_TYPE]):
return edge_type
def get_node_info(self, name: str):
for node_type in self[MetaData.NODES]:
if node_type[MetaData.NAME] == name:
return node_type
def get_info(self, part, name):
if part == MetaData.NODES:
return self.get_node_info(name)
elif part == MetaData.EDGES:
return self.get_edge_info(name)
else:
raise ValueError(f"unsupported FeatureSpec part expected [{MetaData.NODES}, {MetaData.EDGES}], got {part}")
def validate(self):
for part in [MetaData.NODES, MetaData.EDGES]:
for part_info in self[part]:
if part_info[MetaData.FEATURES_PATH]:
tab_path = os.path.join(self[MetaData.PATH], part_info[MetaData.FEATURES_PATH])
assert os.path.exists(tab_path), f"{part}-{part_info[MetaData.NAME]}: {tab_path} does not exist"
assert len(part_info[MetaData.FEATURES]) > 0, \
f"{part}-{part_info[MetaData.NAME]}: tabular features are not specified"
feature_files = one_field_from_list_of_dicts(
part_info[MetaData.FEATURES], MetaData.FEATURE_FILE, res_aggregator=set)
if len(feature_files) > 1:
assert os.path.isdir(tab_path), \
"different feature files are specified MetaData. FEATURES_PATH should be a directory"
for ff in feature_files:
ff_path = os.path.join(tab_path, ff)
assert os.path.exists(ff_path), \
f"{part}-{part_info[MetaData.NAME]}: {ff_path} does not exist"
if part == MetaData.EDGES:
struct_path = os.path.join(self[MetaData.PATH], part_info[MetaData.STRUCTURE_PATH])
assert os.path.exists(struct_path), \
f"{part}-{part_info[MetaData.NAME]}: {struct_path} does not exist"
def copy(self):
res = {}
keys_to_ignore = {MetaData.STRUCTURE_DATA, MetaData.FEATURES_DATA}
for part in (MetaData.EDGES, MetaData.NODES):
res[part] = [
{
k: copy.deepcopy(v)
for k, v in part_info.items() if k not in keys_to_ignore
}
for part_info in self[part]
]
return SynGenDatasetFeatureSpec(res)
class SynGenConfiguration(SynGenDatasetFeatureSpec):
""" SynGen Configuration
"""
def __init__(self, configuration: Dict):
super().__init__(configuration)
self._fill_missing_values()
self.validate()
def validate(self):
if MetaData.ALIGNERS in self:
for aligner_info in self[MetaData.ALIGNERS]:
for edge_name in aligner_info[MetaData.EDGES]:
if not self.get_edge_info(edge_name)[MetaData.FEATURES_PATH].endswith(".parquet"):
raise ValueError("Alignment supports only .parquet files right now")
for node_name in aligner_info[MetaData.NODES]:
if not self.get_node_info(node_name)[MetaData.FEATURES_PATH].endswith(".parquet"):
raise ValueError("Alignment supports only .parquet files right now")
def _process_tabular_generators(self, graph_part_info, part):
if MetaData.TABULAR_GENERATORS not in graph_part_info:
return
if graph_part_info[MetaData.FEATURES] == -1:
assert len(graph_part_info[MetaData.TABULAR_GENERATORS]) == 1
tab_gen_cfg = graph_part_info[MetaData.TABULAR_GENERATORS][0]
assert tab_gen_cfg[MetaData.DATA_SOURCE][MetaData.TYPE] == DataSourceInputType.CONFIGURATION
cfg = SynGenConfiguration.instantiate_from_preprocessed(tab_gen_cfg[MetaData.DATA_SOURCE][MetaData.PATH])
data_source_part_info = cfg.get_info(part, tab_gen_cfg[MetaData.DATA_SOURCE][MetaData.NAME])
graph_part_info[MetaData.FEATURES] = data_source_part_info[MetaData.FEATURES]
for tab_gen_cfg in graph_part_info[MetaData.TABULAR_GENERATORS]:
if tab_gen_cfg[MetaData.FEATURES_LIST] == -1:
assert len(graph_part_info[MetaData.TABULAR_GENERATORS]) == 1, \
"you may use mimic value (-1) only if you specify a single tabular generator"
tab_gen_cfg[MetaData.FEATURES_LIST] = [f[MetaData.NAME] for f in graph_part_info[MetaData.FEATURES]]
if tab_gen_cfg[MetaData.DATA_SOURCE][MetaData.TYPE] == DataSourceInputType.RANDOM:
edge_features = [f[MetaData.NAME] for f in graph_part_info[MetaData.FEATURES]]
for feature_name in tab_gen_cfg[MetaData.FEATURES_LIST]:
if feature_name not in edge_features:
graph_part_info[MetaData.FEATURES].append(
{
MetaData.NAME: feature_name,
MetaData.DTYPE: 'float32',
MetaData.FEATURE_TYPE: MetaData.CONTINUOUS,
# Now random generator supports only continuous features
}
)
def _fill_missing_values(self):
for part in [MetaData.NODES, MetaData.EDGES]:
for part_info in self[part]:
if MetaData.FEATURES not in part_info:
part_info[MetaData.FEATURES] = []
warnings.warn(
f"{part}-{part_info[MetaData.NAME]}: no {MetaData.FEATURES} specified, default is []")
if MetaData.FEATURES_PATH not in part_info:
part_info[MetaData.FEATURES_PATH] = None
warnings.warn(
f"{part}-{part_info[MetaData.NAME]}: no {MetaData.FEATURES_PATH} specified, default is None")
if MetaData.COUNT not in part_info:
part_info[MetaData.COUNT] = -1
warnings.warn(
f"{part}-{part_info[MetaData.NAME]}: no {MetaData.COUNT} specified, "
f"try to mimic based on generators data")
self._process_tabular_generators(part_info, part)
if part == MetaData.EDGES:
if MetaData.DIRECTED not in part_info:
part_info[MetaData.DIRECTED] = False
if part_info[MetaData.COUNT] == -1:
data_source_info = part_info[MetaData.STRUCTURE_GENERATOR][MetaData.DATA_SOURCE]
if data_source_info[MetaData.TYPE] == DataSourceInputType.CONFIGURATION:
cfg = SynGenConfiguration.instantiate_from_preprocessed(data_source_info[MetaData.PATH])
data_source_part_info = cfg.get_info(part, data_source_info[MetaData.NAME])
elif data_source_info[MetaData.TYPE] == DataSourceInputType.RANDOM:
raise ValueError('Can\'t fill the ')
else:
raise ValueError("unsupported structure generator datasource type")
if part_info[MetaData.COUNT] == -1:
part_info[MetaData.COUNT] = data_source_part_info[MetaData.COUNT]
def copy(self):
res = super().copy()
if MetaData.ALIGNERS in self:
res[MetaData.ALIGNERS] = copy.deepcopy(self[MetaData.ALIGNERS])
return SynGenConfiguration(res)
|
PaddlePaddle/LanguageModeling/BERT/vocab | vocab | bert-large-cased-vocab | [PAD]
[unused1]
[unused2]
[unused3]
[unused4]
[unused5]
[unused6]
[unused7]
[unused8]
[unused9]
[unused10]
[unused11]
[unused12]
[unused13]
[unused14]
[unused15]
[unused16]
[unused17]
[unused18]
[unused19]
[unused20]
[unused21]
[unused22]
[unused23]
[unused24]
[unused25]
[unused26]
[unused27]
[unused28]
[unused29]
[unused30]
[unused31]
[unused32]
[unused33]
[unused34]
[unused35]
[unused36]
[unused37]
[unused38]
[unused39]
[unused40]
[unused41]
[unused42]
[unused43]
[unused44]
[unused45]
[unused46]
[unused47]
[unused48]
[unused49]
[unused50]
[unused51]
[unused52]
[unused53]
[unused54]
[unused55]
[unused56]
[unused57]
[unused58]
[unused59]
[unused60]
[unused61]
[unused62]
[unused63]
[unused64]
[unused65]
[unused66]
[unused67]
[unused68]
[unused69]
[unused70]
[unused71]
[unused72]
[unused73]
[unused74]
[unused75]
[unused76]
[unused77]
[unused78]
[unused79]
[unused80]
[unused81]
[unused82]
[unused83]
[unused84]
[unused85]
[unused86]
[unused87]
[unused88]
[unused89]
[unused90]
[unused91]
[unused92]
[unused93]
[unused94]
[unused95]
[unused96]
[unused97]
[unused98]
[unused99]
[UNK]
[CLS]
[SEP]
[MASK]
[unused100]
[unused101]
!
"
#
$
%
&
'
(
)
*
+
,
-
.
/
0
1
2
3
4
5
6
7
8
9
:
;
<
=
>
?
@
A
B
C
D
E
F
G
H
I
J
K
L
M
N
O
P
Q
R
S
T
U
V
W
X
Y
Z
[
\
]
^
_
`
a
b
c
d
e
f
g
h
i
j
k
l
m
n
o
p
q
r
s
t
u
v
w
x
y
z
{
|
}
~
¡
¢
£
¥
§
¨
©
ª
«
¬
®
°
±
²
³
´
µ
¶
·
¹
º
»
¼
½
¾
¿
À
Á
Â
Ä
Å
Æ
Ç
È
É
Í
Î
Ñ
Ó
Ö
×
Ø
Ú
Ü
Þ
ß
à
á
â
ã
ä
å
æ
ç
è
é
ê
ë
ì
í
î
ï
ð
ñ
ò
ó
ô
õ
ö
÷
ø
ù
ú
û
ü
ý
þ
ÿ
Ā
ā
ă
ą
Ć
ć
Č
č
ď
Đ
đ
ē
ė
ę
ě
ğ
ġ
Ħ
ħ
ĩ
Ī
ī
İ
ı
ļ
Ľ
ľ
Ł
ł
ń
ņ
ň
ŋ
Ō
ō
ŏ
ő
Œ
œ
ř
Ś
ś
Ş
ş
Š
š
Ţ
ţ
ť
ũ
ū
ŭ
ů
ű
ų
ŵ
ŷ
ź
Ż
ż
Ž
ž
Ə
ƒ
ơ
ư
ǎ
ǐ
ǒ
ǔ
ǫ
Ș
ș
Ț
ț
ɐ
ɑ
ɔ
ɕ
ə
ɛ
ɡ
ɣ
ɨ
ɪ
ɲ
ɾ
ʀ
ʁ
ʂ
ʃ
ʊ
ʋ
ʌ
ʐ
ʑ
ʒ
ʔ
ʰ
ʲ
ʳ
ʷ
ʻ
ʼ
ʾ
ʿ
ˈ
ː
ˡ
ˢ
ˣ
́
̃
̍
̯
͡
Α
Β
Γ
Δ
Ε
Η
Θ
Ι
Κ
Λ
Μ
Ν
Ο
Π
Σ
Τ
Φ
Χ
Ψ
Ω
ά
έ
ή
ί
α
β
γ
δ
ε
ζ
η
θ
ι
κ
λ
μ
ν
ξ
ο
π
ρ
ς
σ
τ
υ
φ
χ
ψ
ω
ό
ύ
ώ
І
Ј
А
Б
В
Г
Д
Е
Ж
З
И
К
Л
М
Н
О
П
Р
С
Т
У
Ф
Х
Ц
Ч
Ш
Э
Ю
Я
а
б
в
г
д
е
ж
з
и
й
к
л
м
н
о
п
р
с
т
у
ф
х
ц
ч
ш
щ
ъ
ы
ь
э
ю
я
ё
і
ї
ј
њ
ћ
Ա
Հ
ա
ե
ի
կ
մ
յ
ն
ո
ս
տ
ր
ւ
ְ
ִ
ֵ
ֶ
ַ
ָ
ֹ
ּ
א
ב
ג
ד
ה
ו
ז
ח
ט
י
כ
ל
ם
מ
ן
נ
ס
ע
פ
צ
ק
ר
ש
ת
،
ء
آ
أ
إ
ئ
ا
ب
ة
ت
ث
ج
ح
خ
د
ذ
ر
ز
س
ش
ص
ض
ط
ظ
ع
غ
ف
ق
ك
ل
م
ن
ه
و
ى
ي
َ
ِ
ٹ
پ
چ
ک
گ
ہ
ی
ے
ं
आ
क
ग
च
ज
ण
त
द
ध
न
प
ब
भ
म
य
र
ल
व
श
ष
स
ह
ा
ि
ी
ु
े
ो
्
।
॥
আ
ই
এ
ও
ক
খ
গ
চ
ছ
জ
ট
ত
থ
দ
ধ
ন
প
ব
ম
য
র
ল
শ
স
হ
়
া
ি
ী
ু
ে
ো
্
য়
க
த
ப
ம
ய
ர
ல
வ
ா
ி
ு
்
ร
་
ག
ང
ད
ན
བ
མ
ར
ལ
ས
ི
ུ
ེ
ོ
ა
ე
ი
ლ
ნ
ო
რ
ს
ᴬ
ᴵ
ᵀ
ᵃ
ᵇ
ᵈ
ᵉ
ᵍ
ᵏ
ᵐ
ᵒ
ᵖ
ᵗ
ᵘ
ᵢ
ᵣ
ᵤ
ᵥ
ᶜ
ᶠ
ḍ
Ḥ
ḥ
Ḩ
ḩ
ḳ
ṃ
ṅ
ṇ
ṛ
ṣ
ṭ
ạ
ả
ấ
ầ
ẩ
ậ
ắ
ế
ề
ể
ễ
ệ
ị
ọ
ố
ồ
ổ
ộ
ớ
ờ
ợ
ụ
ủ
ứ
ừ
ử
ữ
ự
ỳ
ỹ
ἀ
ἐ
ὁ
ὐ
ὰ
ὶ
ὸ
ῆ
ῖ
ῦ
ῶ
‐
‑
‒
–
—
―
‖
‘
’
‚
“
”
„
†
‡
•
…
‰
′
″
⁄
⁰
ⁱ
⁴
⁵
⁶
⁷
⁸
⁹
⁺
⁻
ⁿ
₀
₁
₂
₃
₄
₅
₆
₇
₈
₉
₊
₍
₎
ₐ
ₑ
ₒ
ₓ
ₕ
ₖ
ₘ
ₙ
ₚ
ₛ
ₜ
₤
€
₱
₹
ℓ
№
ℝ
⅓
←
↑
→
↔
⇌
⇒
∂
∈
−
∗
∘
√
∞
∧
∨
∩
∪
≈
≠
≡
≤
≥
⊂
⊆
⊕
⋅
─
│
■
●
★
☆
☉
♠
♣
♥
♦
♭
♯
⟨
⟩
ⱼ
、
。
《
》
「
」
『
』
〜
い
う
え
お
か
き
く
け
こ
さ
し
す
せ
そ
た
ち
つ
て
と
な
に
の
は
ひ
ま
み
む
め
も
や
ゆ
よ
ら
り
る
れ
ん
ア
ィ
イ
ウ
エ
オ
カ
ガ
キ
ク
グ
コ
サ
シ
ジ
ス
ズ
タ
ダ
ッ
テ
デ
ト
ド
ナ
ニ
ハ
バ
パ
フ
ブ
プ
マ
ミ
ム
ャ
ュ
ラ
リ
ル
レ
ロ
ン
・
ー
一
三
上
下
中
事
二
井
京
人
亻
仁
佐
侍
光
公
力
北
十
南
原
口
史
司
吉
同
和
囗
国
國
土
城
士
大
天
太
夫
女
子
宀
安
宮
宿
小
尚
山
島
川
州
平
年
心
愛
戸
文
新
方
日
明
星
書
月
木
本
李
村
東
松
林
正
武
氏
水
氵
江
河
海
版
犬
王
生
田
白
皇
省
真
石
社
神
竹
美
義
花
藤
西
谷
車
辶
道
郎
郡
部
野
金
長
門
陽
青
食
馬
高
龍
龸
사
씨
의
이
한
fi
fl
!
(
)
,
-
/
:
the
of
and
to
in
was
The
is
for
as
on
with
that
##s
his
by
he
at
from
it
her
He
had
an
were
you
be
In
she
are
but
which
It
not
or
have
my
him
one
this
me
has
also
up
their
first
out
who
been
they
She
into
all
would
its
##ing
time
two
##a
##e
said
about
when
over
more
other
can
after
back
them
then
##ed
there
like
so
only
##n
could
##d
##i
##y
what
no
##o
where
This
made
than
if
You
##ly
through
we
before
##r
just
some
##er
years
do
New
##t
down
between
new
now
will
three
most
On
around
year
used
such
being
well
during
They
know
against
under
later
did
part
known
off
while
His
re
...
##l
people
until
way
American
didn
University
your
both
many
get
United
became
head
There
second
As
work
any
But
still
again
born
even
eyes
After
including
de
took
And
long
team
season
family
see
right
same
called
name
because
film
don
10
found
much
school
##es
going
won
place
away
We
day
left
John
000
hand
since
World
these
how
make
number
each
life
area
man
four
go
No
here
very
National
##m
played
released
never
began
States
album
home
last
too
held
several
May
own
##on
take
end
School
##h
ll
series
What
want
use
another
city
When
2010
side
At
may
That
came
face
June
think
game
those
high
March
early
September
##al
2011
looked
July
state
small
thought
went
January
October
##u
based
August
##us
world
good
April
York
us
12
2012
2008
For
2009
group
along
few
South
little
##k
following
November
something
2013
December
set
2007
old
2006
2014
located
##an
music
County
City
former
##in
room
ve
next
All
##man
got
father
house
##g
body
15
20
18
started
If
2015
town
our
line
War
large
population
named
British
company
member
five
My
single
##en
age
State
moved
February
11
Her
should
century
government
built
come
best
show
However
within
look
men
door
without
need
wasn
2016
water
One
system
knew
every
died
League
turned
asked
North
St
wanted
building
received
song
served
though
felt
##ia
station
band
##ers
local
public
himself
different
death
say
##1
30
##2
2005
16
night
behind
children
English
members
near
saw
together
son
14
voice
village
13
hands
help
##3
due
French
London
top
told
open
published
third
2017
play
across
During
put
final
often
include
25
##le
main
having
2004
once
ever
let
book
led
gave
late
front
find
club
##4
German
included
species
College
form
opened
mother
women
enough
West
must
2000
power
really
17
making
half
##6
order
might
##is
given
million
times
days
point
full
service
With
km
major
##7
original
become
seen
II
north
six
##te
love
##0
national
International
##5
24
So
District
lost
run
couldn
career
always
##9
2003
##th
country
##z
House
air
tell
south
worked
woman
player
##A
almost
war
River
##ic
married
continued
Then
James
close
black
short
##8
##na
using
history
returned
light
car
##ra
sure
William
things
General
##ry
2002
better
support
100
among
From
feet
King
anything
21
19
established
district
2001
feel
great
##ton
level
Cup
These
written
games
others
already
title
story
##p
law
thing
US
record
role
however
By
students
England
white
control
least
inside
land
##C
22
give
community
hard
##ie
non
##c
produced
George
round
period
Park
business
various
##ne
does
present
wife
far
taken
per
reached
David
able
version
working
young
live
created
joined
East
living
appeared
case
High
done
23
important
President
Award
France
position
office
looking
total
general
class
To
production
##S
football
party
brother
keep
mind
free
Street
hair
announced
development
either
nothing
moment
Church
followed
wrote
why
India
San
election
1999
lead
How
##ch
##rs
words
European
course
considered
America
arms
Army
political
##la
28
26
west
east
ground
further
church
less
site
First
Not
Australia
toward
California
##ness
described
works
An
Council
heart
past
military
27
##or
heard
field
human
soon
founded
1998
playing
trying
##x
##ist
##ta
television
mouth
although
taking
win
fire
Division
##ity
Party
Royal
program
Some
Don
Association
According
tried
TV
Paul
outside
daughter
Best
While
someone
match
recorded
Canada
closed
region
Air
above
months
elected
##da
##ian
road
##ar
brought
move
1997
leave
##um
Thomas
1996
am
low
Robert
formed
person
services
points
Mr
miles
##b
stop
rest
doing
needed
international
release
floor
start
sound
call
killed
real
dark
research
finished
language
Michael
professional
change
sent
50
upon
29
track
hit
event
2018
term
example
Germany
similar
return
##ism
fact
pulled
stood
says
ran
information
yet
result
developed
girl
##re
God
1995
areas
signed
decided
##ment
Company
seemed
##el
co
turn
race
common
video
Charles
Indian
##ation
blood
art
red
##able
added
rather
1994
met
director
addition
design
average
minutes
##ies
##ted
available
bed
coming
friend
idea
kind
Union
Road
remained
##ting
everything
##ma
running
care
finally
Chinese
appointed
1992
Australian
##ley
popular
mean
teams
probably
##land
usually
project
social
Championship
possible
word
Russian
instead
mi
herself
##T
Peter
Hall
Center
seat
style
money
1993
else
Department
table
Music
current
31
features
special
events
character
Two
square
sold
debut
##v
process
Although
Since
##ka
40
Central
currently
education
placed
lot
China
quickly
forward
seven
##ling
Europe
arm
performed
Japanese
1991
Henry
Now
Dr
##ion
week
Group
myself
big
UK
Washington
ten
deep
1990
Club
Japan
space
La
directed
smile
episode
hours
whole
##de
##less
Why
wouldn
designed
strong
training
changed
Society
stage
involved
hadn
towards
leading
police
eight
kept
Institute
study
largest
child
eventually
private
modern
Court
throughout
getting
originally
attack
##E
talk
Great
longer
songs
alone
##ine
wide
dead
walked
shot
##ri
Oh
force
##st
Art
today
friends
Island
Richard
1989
center
construction
believe
size
White
ship
completed
##B
gone
Just
rock
sat
##R
radio
below
entire
families
league
includes
type
lived
official
range
hold
featured
Most
##ter
president
passed
means
##f
forces
lips
Mary
Do
guitar
##ce
food
wall
Of
spent
Its
performance
hear
##P
Western
reported
sister
##et
morning
##M
especially
##ive
Minister
itself
post
bit
groups
1988
##tion
Black
##ng
Well
raised
sometimes
Canadian
Paris
Spanish
replaced
schools
Academy
leaving
central
female
Christian
Jack
whose
college
onto
provided
##D
##ville
players
actually
stopped
##son
Museum
doesn
##ts
books
fight
allowed
##ur
beginning
Records
awarded
parents
coach
##os
Red
saying
##ck
Smith
Yes
Lake
##L
aircraft
1987
##ble
previous
ft
action
Italian
African
happened
vocals
Act
future
court
##ge
1986
degree
phone
##ro
Is
countries
winning
breath
Love
river
matter
Lord
Other
list
self
parts
##ate
provide
cut
shows
plan
1st
interest
##ized
Africa
stated
Sir
fell
owned
earlier
ended
competition
attention
1985
lower
nearly
bad
older
stay
Saint
##se
certain
1984
fingers
blue
try
fourth
Grand
##as
king
##nt
makes
chest
movement
states
moving
data
introduced
model
date
section
Los
deal
##I
skin
entered
middle
success
Texas
##w
summer
island
##N
Republic
length
husband
1980
##ey
reason
anyone
forced
via
base
500
job
covered
Festival
Roman
successful
rights
cover
Man
writing
Ireland
##F
related
goal
takes
buildings
true
weeks
1983
Because
opening
novel
ISBN
meet
gold
##ous
mid
km²
standing
Football
Chicago
shook
whom
##ki
1982
Day
feeling
scored
boy
higher
Force
leader
heavy
fall
question
sense
army
Second
energy
meeting
themselves
kill
##am
board
census
##ya
##ns
mine
meant
market
required
battle
campaign
attended
approximately
Kingdom
runs
active
##ha
contract
clear
previously
health
1979
Arts
complete
Catholic
couple
units
##ll
##ty
Committee
shoulder
sea
systems
listed
##O
caught
tournament
##G
northern
author
Film
Your
##men
holding
offered
personal
1981
southern
artist
traditional
studio
200
capital
##ful
regular
ask
giving
organization
month
news
Are
read
managed
helped
studied
student
defeated
natural
industry
Year
noted
decision
Government
quite
##id
smiled
1972
Maybe
tracks
##ke
Mark
al
media
engine
hour
Their
relationship
plays
property
structure
1976
ago
Hill
Martin
1978
ready
Many
Like
Bay
immediately
generally
Italy
Greek
practice
caused
division
significant
Joseph
speed
Let
thinking
completely
1974
primary
mostly
##field
##K
1975
##to
Even
writer
##led
dropped
magazine
collection
understand
route
highest
particular
films
lines
network
Science
loss
carried
direction
green
1977
location
producer
according
Women
Queen
neck
thus
independent
view
1970
Angeles
Soviet
distance
problem
Board
tour
western
income
appearance
access
Mexico
nodded
street
surface
arrived
believed
Old
1968
1973
becoming
whether
1945
figure
singer
stand
Following
issue
window
wrong
pain
everyone
lives
issues
park
slowly
la
act
##va
bring
Lee
operations
key
comes
fine
cold
famous
Navy
1971
Me
additional
individual
##ner
Zealand
goals
county
contains
Service
minute
2nd
reach
talking
particularly
##ham
movie
Director
glass
paper
studies
##co
railway
standard
Education
45
represented
Chief
Louis
launched
Star
terms
60
1969
experience
watched
Another
Press
Tom
staff
starting
subject
break
Virginia
nine
eye
##age
evidence
foot
##est
companies
Prince
##V
gun
create
Big
People
guy
Green
simply
numerous
##line
increased
twenty
##ga
##do
1967
award
officer
stone
Before
material
Northern
grew
male
plant
Life
legs
step
Al
unit
35
except
answer
##U
report
response
Edward
commercial
edition
trade
science
##ca
Irish
Law
shown
rate
failed
##ni
remains
changes
mm
limited
larger
Later
cause
waiting
Time
##wood
cost
Bill
manager
activities
likely
allow
operated
retired
##ping
65
directly
Who
associated
effect
hell
Florida
straight
hot
Valley
management
girls
expected
eastern
Mike
chance
cast
centre
chair
hurt
problems
##li
walk
programs
Team
characters
Battle
edge
pay
maybe
corner
majority
medical
Joe
Summer
##io
attempt
Pacific
command
Radio
##by
names
municipality
1964
train
economic
Brown
feature
sex
source
agreed
remember
Three
1966
1965
Pennsylvania
victory
senior
annual
III
Southern
results
Sam
serving
religious
Jones
appears
##der
despite
claimed
Both
musical
matches
fast
security
selected
Young
double
complex
hospital
chief
Times
##ve
Championships
filled
Public
Despite
beautiful
Research
plans
Province
##ally
Wales
##ko
artists
metal
nearby
Spain
##il
32
houses
supported
piece
##no
stared
recording
nature
legal
Russia
##ization
remaining
looks
##sh
bridge
closer
cases
scene
marriage
Little
##é
uses
Earth
specific
Frank
theory
Good
discovered
referred
bass
culture
university
presented
Congress
##go
metres
continue
1960
isn
Awards
meaning
cell
composed
separate
Series
forms
Blue
cross
##tor
increase
test
computer
slightly
Where
Jewish
Town
tree
status
1944
variety
responsible
pretty
initially
##way
realized
pass
provides
Captain
Alexander
recent
score
broke
Scott
drive
financial
showed
Line
stories
ordered
soldiers
genus
operation
gaze
sitting
society
Only
hope
actor
follow
Empire
Yeah
technology
happy
focus
policy
spread
situation
##ford
##ba
Mrs
watch
Can
1963
Commission
touch
earned
troops
Under
1962
individuals
cannot
19th
##lin
mile
expression
exactly
suddenly
weight
dance
stepped
places
appear
difficult
Railway
anti
numbers
kilometres
star
##ier
department
ice
Britain
removed
Once
##lo
Boston
value
##ant
mission
trees
Order
sports
join
serve
Major
poor
Poland
mainly
Theatre
pushed
Station
##it
Lady
federal
silver
##ler
foreign
##ard
Eastern
##den
box
hall
subsequently
lies
acquired
1942
ancient
CD
History
Jean
beyond
##ger
El
##les
growing
championship
native
Parliament
Williams
watching
direct
overall
offer
Also
80
Secretary
spoke
Latin
ability
##ated
safe
presence
##ial
headed
regional
planned
1961
Johnson
throat
consists
##W
extended
Or
bar
walls
Chris
stations
politician
Olympics
influence
share
fighting
speak
hundred
Carolina
die
stars
##tic
color
Chapter
##ish
fear
sleep
goes
Francisco
oil
Bank
sign
physical
##berg
Dutch
seasons
##rd
Games
Governor
sorry
lack
Centre
memory
baby
smaller
charge
Did
multiple
ships
shirt
Assembly
amount
leaves
3rd
Foundation
conditions
1943
Rock
Democratic
Daniel
##at
winner
products
##ina
store
latter
Professor
civil
prior
host
1956
soft
vote
needs
Each
rules
1958
pressure
letter
normal
proposed
levels
records
1959
paid
intended
Victoria
purpose
okay
historical
issued
1980s
broadcast
rule
simple
picked
firm
Sea
1941
Elizabeth
1940
serious
featuring
highly
graduated
mentioned
choice
1948
replied
percent
Scotland
##hi
females
constructed
1957
settled
Steve
recognized
cities
crew
glanced
kiss
competed
flight
knowledge
editor
More
Conference
##H
fifth
elements
##ee
##tes
function
newspaper
recently
Miss
cultural
brown
twice
Office
1939
truth
Creek
1946
households
USA
1950
quality
##tt
border
seconds
destroyed
pre
wait
ahead
build
image
90
cars
##mi
33
promoted
professor
et
bank
medal
text
broken
Middle
revealed
sides
wing
seems
channel
1970s
Ben
loved
effort
officers
Will
##ff
70
Israel
Jim
upper
fully
label
Jr
assistant
powerful
pair
positive
##ary
gives
1955
20th
races
remain
kitchen
primarily
##ti
Sydney
easy
Tour
whispered
buried
300
News
Polish
1952
Duke
Columbia
produce
accepted
00
approach
minor
1947
Special
44
Asian
basis
visit
Fort
Civil
finish
formerly
beside
leaned
##ite
median
rose
coast
effects
supposed
Cross
##hip
Corps
residents
Jackson
##ir
Bob
basketball
36
Asia
seem
Bishop
Book
##ber
ring
##ze
owner
BBC
##ja
transferred
acting
De
appearances
walking
Le
press
grabbed
1954
officially
1953
##pe
risk
taught
review
##X
lay
##well
council
Avenue
seeing
losing
Ohio
Super
province
ones
travel
##sa
projects
equipment
spot
Berlin
administrative
heat
potential
shut
capacity
elections
growth
fought
Republican
mixed
Andrew
teacher
turning
strength
shoulders
beat
wind
1949
Health
follows
camp
suggested
perhaps
Alex
mountain
contact
divided
candidate
fellow
34
Show
necessary
workers
ball
horse
ways
questions
protect
gas
activity
younger
bottom
founder
Scottish
screen
treatment
easily
com
##house
dedicated
Master
warm
Night
Georgia
Long
von
##me
perfect
website
1960s
piano
efforts
##ide
Tony
sort
offers
Development
Simon
executive
##nd
save
Over
Senate
1951
1990s
draw
master
Police
##ius
renamed
boys
initial
prominent
damage
Co
##ov
##za
online
begin
occurred
captured
youth
Top
account
tells
Justice
conducted
forest
##town
bought
teeth
Jersey
##di
purchased
agreement
Michigan
##ure
campus
prison
becomes
product
secret
guess
Route
huge
types
drums
64
split
defeat
estate
housing
##ot
brothers
Coast
declared
happen
titled
therefore
sun
commonly
alongside
Stadium
library
Home
article
steps
telling
slow
assigned
refused
laughed
wants
Nick
wearing
Rome
Open
##ah
Hospital
pointed
Taylor
lifted
escape
participated
##j
drama
parish
Santa
##per
organized
mass
pick
Airport
gets
Library
unable
pull
Live
##ging
surrounding
##ries
focused
Adam
facilities
##ning
##ny
38
##ring
notable
era
connected
gained
operating
laid
Regiment
branch
defined
Christmas
machine
Four
academic
Iran
adopted
concept
Men
compared
search
traffic
Max
Maria
greater
##ding
widely
##burg
serves
1938
37
Go
hotel
shared
typically
scale
1936
leg
suffered
yards
pieces
Ministry
Wilson
episodes
empty
1918
safety
continues
yellow
historic
settlement
400
Come
Corporation
enemy
content
picture
evening
territory
method
trial
solo
driver
Here
##ls
entrance
Prize
spring
whatever
##ent
75
##ji
reading
Arthur
##cy
Our
clothes
Prime
Illinois
Kong
code
##ria
sit
Harry
Federal
chosen
administration
bodies
begins
stomach
Though
seats
Hong
density
Sun
leaders
Field
museum
chart
platform
languages
##ron
birth
holds
Gold
##un
fish
combined
##ps
4th
1937
largely
captain
trust
Game
van
boat
Oxford
basic
beneath
Islands
painting
nice
Toronto
path
males
sources
block
conference
parties
murder
clubs
crowd
calling
About
Business
peace
knows
lake
speaking
stayed
Brazil
allowing
Born
unique
thick
Technology
##que
receive
des
semi
alive
noticed
format
##ped
coffee
digital
##ned
handed
guard
tall
faced
setting
plants
partner
claim
reduced
temple
animals
determined
classes
##out
estimated
##ad
Olympic
providing
Massachusetts
learned
Inc
Philadelphia
Social
carry
42
possibly
hosted
tonight
respectively
Today
shape
Mount
roles
designated
brain
etc
Korea
thoughts
Brian
Highway
doors
background
drew
models
footballer
tone
turns
1935
quiet
tower
wood
bus
write
software
weapons
flat
marked
1920
newly
tight
Eric
finger
Journal
FC
Van
rise
critical
Atlantic
granted
returning
communities
humans
quick
39
48
ranked
sight
pop
Swedish
Stephen
card
analysis
attacked
##wa
Sunday
identified
Jason
champion
situated
1930
expanded
tears
##nce
reaching
Davis
protection
Emperor
positions
nominated
Bridge
tax
dress
allows
avoid
leadership
killing
actress
guest
steel
knowing
electric
cells
disease
grade
unknown
##ium
resulted
Pakistan
confirmed
##ged
tongue
covers
##Y
roof
entirely
applied
votes
drink
interview
exchange
Township
reasons
##ised
page
calls
dog
agent
nose
teaching
##ds
##ists
advanced
wish
Golden
existing
vehicle
del
1919
develop
attacks
pressed
Sports
planning
resulting
facility
Sarah
notes
1933
Class
Historic
winter
##mo
audience
Community
household
Netherlands
creation
##ize
keeping
1914
claims
dry
guys
opposite
##ak
explained
Ontario
secondary
difference
Francis
actions
organizations
yard
animal
Up
Lewis
titles
Several
1934
Ryan
55
Supreme
rolled
1917
distribution
figures
afraid
rural
yourself
##rt
sets
barely
Instead
passing
awards
41
silence
authority
occupied
environment
windows
engineering
surprised
flying
crime
reports
Mountain
powers
driving
succeeded
reviews
1929
Head
missing
Song
Jesus
opportunity
inspired
ends
albums
conversation
impact
injury
surprise
billion
learning
heavily
oldest
union
creating
##ky
festival
literature
letters
sexual
##tte
apartment
Final
comedy
nation
orders
##sen
contemporary
Power
drawn
existence
connection
##ating
Post
Junior
remembered
message
Medal
castle
note
engineer
sounds
Beach
crossed
##dy
ear
scientific
sales
##ai
theme
starts
clearly
##ut
trouble
##gan
bag
##han
BC
sons
1928
silent
versions
daily
Studies
ending
Rose
guns
1932
headquarters
reference
obtained
Squadron
concert
none
du
Among
##don
prevent
Member
answered
staring
Between
##lla
portion
drug
liked
association
performances
Nations
formation
Castle
lose
learn
scoring
relatively
quarter
47
Premier
##ors
Sweden
baseball
attempted
trip
worth
perform
airport
fields
enter
honor
Medical
rear
commander
officials
condition
supply
materials
52
Anna
volume
threw
Persian
43
interested
Gallery
achieved
visited
laws
relief
Area
Matt
singles
Lieutenant
Country
fans
Cambridge
sky
Miller
effective
tradition
Port
##ana
minister
extra
entitled
System
sites
authorities
acres
committee
racing
1931
desk
trains
ass
weren
Family
farm
##ance
industrial
##head
iron
49
abandoned
Out
Holy
chairman
waited
frequently
display
Light
transport
starring
Patrick
Engineering
eat
FM
judge
reaction
centuries
price
##tive
Korean
defense
Get
arrested
1927
send
urban
##ss
pilot
Okay
Media
reality
arts
soul
thirty
##be
catch
generation
##nes
apart
Anne
drop
See
##ving
sixth
trained
Management
magic
cm
height
Fox
Ian
resources
vampire
principal
Was
haven
##au
Walter
Albert
rich
1922
causing
entry
##ell
shortly
46
worry
doctor
composer
rank
Network
bright
showing
regions
1924
wave
carrying
kissed
finding
missed
Earl
lying
target
vehicles
Military
controlled
dinner
##board
briefly
lyrics
motion
duty
strange
attempts
invited
kg
villages
5th
Land
##mer
Christ
prepared
twelve
check
thousand
earth
copies
en
transfer
citizens
Americans
politics
nor
theatre
Project
##bo
clean
rooms
laugh
##ran
application
contained
anyway
containing
Sciences
1925
rare
speech
exist
1950s
falling
passenger
##im
stands
51
##ol
##ow
phase
governor
kids
details
methods
Vice
employed
performing
counter
Jane
heads
Channel
wine
opposition
aged
1912
Every
1926
highway
##ura
1921
aired
978
permanent
Forest
finds
joint
approved
##pur
brief
doubt
acts
brand
wild
closely
Ford
Kevin
chose
shall
port
sweet
fun
asking
Be
##bury
sought
Dave
Mexican
mom
Right
Howard
Moscow
Charlie
Stone
##mann
admitted
##ver
wooden
1923
Officer
relations
Hot
combat
publication
chain
shop
inhabitants
proved
ideas
address
1915
Memorial
explain
increasing
conflict
Anthony
Melbourne
narrow
temperature
slid
1916
worse
selling
documentary
Ali
Ray
opposed
vision
dad
extensive
Infantry
commissioned
Doctor
offices
programming
core
respect
storm
##pa
##ay
##om
promotion
der
struck
anymore
shit
Region
receiving
DVD
alternative
##ue
ride
maximum
1910
##ious
Third
Affairs
cancer
Executive
##op
dream
18th
Due
##ker
##worth
economy
IV
Billboard
identity
subsequent
statement
skills
##back
funding
##ons
Round
Foreign
truck
Please
lights
wondered
##ms
frame
yes
Still
districts
fiction
Colonel
converted
150
grown
accident
critics
fit
Information
architecture
Point
Five
armed
Billy
poet
functions
consisted
suit
Turkish
Band
object
desire
##ities
sounded
flow
Norwegian
articles
Marie
pulling
thin
singing
Hunter
Human
Battalion
Federation
Kim
origin
represent
dangerous
weather
fuel
ex
##sing
Last
bedroom
aid
knees
Alan
angry
assumed
plane
Something
founding
concerned
global
Fire
di
please
Portuguese
touched
Roger
nuclear
Register
Jeff
fixed
royal
lie
finals
NFL
Manchester
towns
handle
shaped
Chairman
Dean
launch
understanding
Children
violence
failure
sector
Brigade
wrapped
fired
sharp
tiny
developing
expansion
Free
institutions
technical
Nothing
otherwise
Main
inch
Saturday
wore
Senior
attached
cheek
representing
Kansas
##chi
##kin
actual
advantage
Dan
Austria
##dale
hoped
multi
squad
Norway
streets
1913
Services
hired
grow
pp
wear
painted
Minnesota
stuff
Building
54
Philippines
1900
##ties
educational
Khan
Magazine
##port
Cape
signal
Gordon
sword
Anderson
cool
engaged
Commander
images
Upon
tied
Security
cup
rail
Vietnam
successfully
##red
Muslim
gain
bringing
Native
hers
occurs
negative
Philip
Kelly
Colorado
category
##lan
600
Have
supporting
wet
56
stairs
Grace
observed
##ung
funds
restaurant
1911
Jews
##ments
##che
Jake
Back
53
asks
journalist
accept
bands
bronze
helping
##ice
decades
mayor
survived
usual
influenced
Douglas
Hey
##izing
surrounded
retirement
Temple
derived
Pope
registered
producing
##ral
structures
Johnny
contributed
finishing
buy
specifically
##king
patients
Jordan
internal
regarding
Samuel
Clark
##q
afternoon
Finally
scenes
notice
refers
quietly
threat
Water
Those
Hamilton
promise
freedom
Turkey
breaking
maintained
device
lap
ultimately
Champion
Tim
Bureau
expressed
investigation
extremely
capable
qualified
recognition
items
##up
Indiana
adult
rain
greatest
architect
Morgan
dressed
equal
Antonio
collected
drove
occur
Grant
graduate
anger
Sri
worried
standards
##ore
injured
somewhere
damn
Singapore
Jimmy
pocket
homes
stock
religion
aware
regarded
Wisconsin
##tra
passes
fresh
##ea
argued
Ltd
EP
Diego
importance
Census
incident
Egypt
Missouri
domestic
leads
ceremony
Early
camera
Father
challenge
Switzerland
lands
familiar
hearing
spend
educated
Tennessee
Thank
##ram
Thus
concern
putting
inches
map
classical
Allen
crazy
valley
Space
softly
##my
pool
worldwide
climate
experienced
neighborhood
scheduled
neither
fleet
1908
Girl
##J
Part
engines
locations
darkness
Revolution
establishment
lawyer
objects
apparently
Queensland
Entertainment
bill
mark
Television
##ong
pale
demand
Hotel
selection
##rn
##ino
Labour
Liberal
burned
Mom
merged
Arizona
request
##lia
##light
hole
employees
##ical
incorporated
95
independence
Walker
covering
joining
##ica
task
papers
backing
sell
biggest
6th
strike
establish
##ō
gently
59
Orchestra
Winter
protein
Juan
locked
dates
Boy
aren
shooting
Luke
solid
charged
Prior
resigned
interior
garden
spoken
improve
wonder
promote
hidden
##med
combination
Hollywood
Swiss
consider
##ks
Lincoln
literary
drawing
Marine
weapon
Victor
Trust
Maryland
properties
##ara
exhibition
understood
hung
Tell
installed
loud
fashion
affected
junior
landing
flowers
##he
Internet
beach
Heart
tries
Mayor
programme
800
wins
noise
##ster
##ory
58
contain
fair
delivered
##ul
wedding
Square
advance
behavior
Program
Oregon
##rk
residence
realize
certainly
hill
Houston
57
indicated
##water
wounded
Village
massive
Moore
thousands
personnel
dating
opera
poetry
##her
causes
feelings
Frederick
applications
push
approached
foundation
pleasure
sale
fly
gotten
northeast
costs
raise
paintings
##ney
views
horses
formal
Arab
hockey
typical
representative
rising
##des
clock
stadium
shifted
Dad
peak
Fame
vice
disappeared
users
Way
Naval
prize
hoping
values
evil
Bell
consisting
##ón
Regional
##ics
improved
circle
carefully
broad
##ini
Fine
maintain
operate
offering
mention
Death
stupid
Through
Princess
attend
interests
ruled
somewhat
wings
roads
grounds
##ual
Greece
Champions
facing
hide
voted
require
Dark
Matthew
credit
sighed
separated
manner
##ile
Boys
1905
committed
impossible
lip
candidates
7th
Bruce
arranged
Islamic
courses
criminal
##ened
smell
##bed
08
consecutive
##ening
proper
purchase
weak
Prix
1906
aside
introduction
Look
##ku
changing
budget
resistance
factory
Forces
agency
##tone
northwest
user
1907
stating
##one
sport
Design
environmental
cards
concluded
Carl
250
accused
##ology
Girls
sick
intelligence
Margaret
responsibility
Guard
##tus
17th
sq
goods
1909
hate
##ek
capture
stores
Gray
comic
Modern
Silver
Andy
electronic
wheel
##ied
Deputy
##bs
Czech
zone
choose
constant
reserve
##lle
Tokyo
spirit
sub
degrees
flew
pattern
compete
Dance
##ik
secretary
Imperial
99
reduce
Hungarian
confused
##rin
Pierre
describes
regularly
Rachel
85
landed
passengers
##ise
##sis
historian
meters
Youth
##ud
participate
##cing
arrival
tired
Mother
##gy
jumped
Kentucky
faces
feed
Israeli
Ocean
##Q
##án
plus
snow
techniques
plate
sections
falls
jazz
##ris
tank
loan
repeated
opinion
##res
unless
rugby
journal
Lawrence
moments
shock
distributed
##ded
adjacent
Argentina
crossing
uncle
##ric
Detroit
communication
mental
tomorrow
session
Emma
Without
##gen
Miami
charges
Administration
hits
coat
protected
Cole
invasion
priest
09
Gary
enjoyed
plot
measure
bound
friendly
throw
musician
##lon
##ins
Age
knife
damaged
birds
driven
lit
ears
breathing
Arabic
Jan
faster
Jonathan
##gate
Independent
starred
Harris
teachers
Alice
sequence
mph
file
translated
decide
determine
Review
documents
sudden
threatened
##ft
bear
distinct
decade
burning
##sky
1930s
replace
begun
extension
##time
1904
equivalent
accompanied
Christopher
Danish
##ye
Besides
##more
persons
fallen
Rural
roughly
saved
willing
ensure
Belgium
05
musicians
##ang
giant
Six
Retrieved
worst
purposes
##bly
mountains
seventh
slipped
brick
07
##py
somehow
Carter
Iraq
cousin
favor
islands
journey
FIFA
contrast
planet
vs
calm
##ings
concrete
branches
gray
profit
Russell
##ae
##ux
##ens
philosophy
businesses
talked
parking
##ming
owners
Place
##tle
agricultural
Kate
06
southeast
draft
Eddie
earliest
forget
Dallas
Commonwealth
edited
66
inner
ed
operates
16th
Harvard
assistance
##si
designs
Take
bathroom
indicate
CEO
Command
Louisiana
1902
Dublin
Books
1901
tropical
1903
##tors
Places
tie
progress
forming
solution
62
letting
##ery
studying
##jo
duties
Baseball
taste
Reserve
##ru
Ann
##gh
visible
##vi
notably
link
NCAA
southwest
Never
storage
mobile
writers
favorite
Pro
pages
truly
count
##tta
string
kid
98
Ross
row
##idae
Kennedy
##tan
Hockey
hip
waist
grandfather
listen
##ho
feels
busy
72
stream
obvious
cycle
shaking
Knight
##ren
Carlos
painter
trail
web
linked
04
Palace
existed
##ira
responded
closing
End
examples
Marshall
weekend
jaw
Denmark
lady
township
medium
chin
Story
option
fifteen
Moon
represents
makeup
investment
jump
childhood
Oklahoma
roll
normally
Ten
Operation
Graham
Seattle
Atlanta
paused
promised
rejected
treated
returns
flag
##ita
Hungary
danger
glad
movements
visual
subjects
credited
soldier
Norman
ill
translation
José
Quebec
medicine
warning
theater
praised
municipal
01
commune
churches
acid
folk
8th
testing
add
survive
Sound
devices
residential
severe
presidential
Mississippi
Austin
Perhaps
Charlotte
hanging
Montreal
grin
##ten
racial
partnership
shoot
shift
##nie
Les
downtown
Brothers
Garden
matters
restored
mirror
forever
winners
rapidly
poverty
##ible
Until
DC
faith
hundreds
Real
Ukraine
Nelson
balance
Adams
contest
relative
ethnic
Edinburgh
composition
##nts
emergency
##van
marine
reputation
Down
pack
12th
Communist
Mountains
pro
stages
measures
##ld
ABC
Li
victims
benefit
Iowa
Broadway
gathered
rating
Defense
classic
##ily
ceiling
##ions
snapped
Everything
constituency
Franklin
Thompson
Stewart
entering
Judge
forth
##sk
wanting
smiling
moves
tunnel
premiered
grass
unusual
Ukrainian
bird
Friday
tail
Portugal
coal
element
Fred
guards
Senator
collaboration
beauty
Wood
chemical
beer
justice
signs
##Z
sees
##zi
Puerto
##zed
96
smooth
Bowl
gift
limit
97
heading
Source
wake
requires
Ed
Constitution
factor
Lane
factors
adding
Note
cleared
pictures
pink
##ola
Kent
Local
Singh
moth
Ty
##ture
courts
Seven
temporary
involving
Vienna
emerged
fishing
agree
defensive
stuck
secure
Tamil
##ick
bottle
03
Player
instruments
Spring
patient
flesh
contributions
cry
Malaysia
120
Global
da
Alabama
Within
##work
debuted
expect
Cleveland
concerns
retained
horror
10th
spending
Peace
Transport
grand
Crown
instance
institution
acted
Hills
mounted
Campbell
shouldn
1898
##ably
chamber
soil
88
Ethan
sand
cheeks
##gi
marry
61
weekly
classification
DNA
Elementary
Roy
definitely
Soon
Rights
gate
suggests
aspects
imagine
golden
beating
Studios
Warren
differences
significantly
glance
occasionally
##od
clothing
Assistant
depth
sending
possibility
mode
prisoners
requirements
daughters
dated
Representatives
prove
guilty
interesting
smoke
cricket
93
##ates
rescue
Connecticut
underground
Opera
13th
reign
##ski
thanks
leather
equipped
routes
fan
##ans
script
Wright
bishop
Welsh
jobs
faculty
eleven
Railroad
appearing
anniversary
Upper
##down
anywhere
Rugby
Metropolitan
Meanwhile
Nicholas
champions
forehead
mining
drinking
76
Jerry
membership
Brazilian
Wild
Rio
scheme
Unlike
strongly
##bility
fill
##rian
easier
MP
Hell
##sha
Stanley
banks
Baron
##ique
Robinson
67
Gabriel
Austrian
Wayne
exposed
##wan
Alfred
1899
manage
mix
visitors
eating
##rate
Sean
commission
Cemetery
policies
Camp
parallel
traveled
guitarist
02
supplies
couples
poem
blocks
Rick
Training
Energy
achieve
appointment
Wing
Jamie
63
novels
##em
1890
songwriter
Base
Jay
##gar
naval
scared
miss
labor
technique
crisis
Additionally
backed
destroy
seriously
tools
tennis
91
god
##ington
continuing
steam
obviously
Bobby
adapted
fifty
enjoy
Jacob
publishing
column
##ular
Baltimore
Donald
Liverpool
92
drugs
movies
##ock
Heritage
##je
##istic
vocal
strategy
gene
advice
##bi
Ottoman
riding
##side
Agency
Indonesia
11th
laughing
sleeping
und
muttered
listening
deck
tip
77
ownership
grey
Claire
deeply
provincial
popularity
Cooper
##á
Emily
##sed
designer
Murray
describe
Danny
Around
Parker
##dae
68
rates
suffering
considerable
78
nervous
powered
tons
circumstances
wished
belonged
Pittsburgh
flows
9th
##use
belt
81
useful
15th
context
List
Dead
Iron
seek
Season
worn
frequency
legislation
replacement
memories
Tournament
Again
Barry
organisation
copy
Gulf
waters
meets
struggle
Oliver
1895
Susan
protest
kick
Alliance
components
1896
Tower
Windows
demanded
regiment
sentence
Woman
Logan
Referee
hosts
debate
knee
Blood
##oo
universities
practices
Ward
ranking
correct
happening
Vincent
attracted
classified
##stic
processes
immediate
waste
increasingly
Helen
##po
Lucas
Phil
organ
1897
tea
suicide
actors
lb
crash
approval
waves
##ered
hated
grip
700
amongst
69
74
hunting
dying
lasted
illegal
##rum
stare
defeating
##gs
shrugged
°C
Jon
Count
Orleans
94
affairs
formally
##and
##ves
criticized
Disney
Vol
successor
tests
scholars
palace
Would
celebrated
rounds
grant
Schools
Such
commanded
demon
Romania
##all
Karl
71
##yn
84
Daily
totally
Medicine
fruit
Die
upset
Lower
Conservative
14th
Mitchell
escaped
shoes
Morris
##tz
queen
harder
prime
Thanks
indeed
Sky
authors
rocks
definition
Nazi
accounts
printed
experiences
##ters
divisions
Cathedral
denied
depending
Express
##let
73
appeal
loose
colors
filed
##isation
gender
##ew
throne
forests
Finland
domain
boats
Baker
squadron
shore
remove
##ification
careful
wound
railroad
82
seeking
agents
##ved
Blues
##off
customers
ignored
net
##ction
hiding
Originally
declined
##ess
franchise
eliminated
NBA
merely
pure
appropriate
visiting
forty
markets
offensive
coverage
cave
##nia
spell
##lar
Benjamin
##ire
Convention
filmed
Trade
##sy
##ct
Having
palm
1889
Evans
intense
plastic
Julia
document
jeans
vessel
SR
##fully
proposal
Birmingham
le
##ative
assembly
89
fund
lock
1893
AD
meetings
occupation
modified
Years
odd
aimed
reform
Mission
Works
shake
cat
exception
convinced
executed
pushing
dollars
replacing
soccer
manufacturing
##ros
expensive
kicked
minimum
Josh
coastal
Chase
ha
Thailand
publications
deputy
Sometimes
Angel
effectively
##illa
criticism
conduct
Serbian
landscape
NY
absence
passage
##ula
Blake
Indians
1892
admit
Trophy
##ball
Next
##rated
##ians
charts
kW
orchestra
79
heritage
1894
rough
exists
boundary
Bible
Legislative
moon
medieval
##over
cutting
print
##ett
birthday
##hood
destruction
Julian
injuries
influential
sisters
raising
statue
colour
dancing
characteristics
orange
##ok
##aries
Ken
colonial
twin
Larry
surviving
##shi
Barbara
personality
entertainment
assault
##ering
talent
happens
license
86
couch
Century
soundtrack
shower
swimming
cash
Staff
bent
1885
bay
lunch
##lus
dozen
vessels
CBS
greatly
critic
Test
symbol
panel
shell
output
reaches
87
Front
motor
ocean
##era
##ala
maintenance
violent
scent
Limited
Las
Hope
Theater
Which
survey
Robin
recordings
compilation
##ward
bomb
insurance
Authority
sponsored
satellite
Jazz
refer
stronger
blow
whilst
Wrestling
suggest
##rie
climbed
##els
voices
shopping
1891
Neil
discovery
##vo
##ations
burst
Baby
peaked
Brooklyn
knocked
lift
##try
false
nations
Hugh
Catherine
preserved
distinguished
terminal
resolution
ratio
pants
cited
competitions
completion
DJ
bone
uniform
schedule
shouted
83
1920s
rarely
Basketball
Taiwan
artistic
bare
vampires
arrest
Utah
Marcus
assist
gradually
qualifying
Victorian
vast
rival
Warner
Terry
Economic
##cia
losses
boss
versus
audio
runner
apply
surgery
Play
twisted
comfortable
##cs
Everyone
guests
##lt
Harrison
UEFA
lowered
occasions
##lly
##cher
chapter
youngest
eighth
Culture
##room
##stone
1888
Songs
Seth
Digital
involvement
expedition
relationships
signing
1000
fault
annually
circuit
afterwards
meat
creature
##ou
cable
Bush
##net
Hispanic
rapid
gonna
figured
extent
considering
cried
##tin
sigh
dynasty
##ration
cabinet
Richmond
stable
##zo
1864
Admiral
Unit
occasion
shares
badly
longest
##ify
Connor
extreme
wondering
girlfriend
Studio
##tions
1865
tribe
exact
muscles
hat
Luis
Orthodox
decisions
amateur
description
##lis
hips
kingdom
##ute
Portland
whereas
Bachelor
outer
discussion
partly
Arkansas
1880
dreams
perfectly
Lloyd
##bridge
asleep
##tti
Greg
permission
trading
pitch
mill
Stage
liquid
Keith
##tal
wolf
processing
stick
Jerusalem
profile
rushed
spiritual
argument
Ice
Guy
till
Delhi
roots
Section
missions
Glasgow
penalty
NBC
encouraged
identify
keyboards
##zing
##ston
disc
plain
informed
Bernard
thinks
fled
Justin
##day
newspapers
##wick
Ralph
##zer
unlike
Stars
artillery
##ified
recovered
arrangement
searching
##pers
##tory
##rus
deaths
Egyptian
diameter
##í
marketing
corporate
teach
marks
Turner
staying
hallway
Sebastian
chapel
naked
mistake
possession
1887
dominated
jacket
creative
Fellow
Falls
Defence
suspended
employment
##rry
Hebrew
Hudson
Week
Wars
recognize
Natural
controversial
Tommy
thank
Athletic
benefits
decline
intention
##ets
Lost
Wall
participation
elevation
supports
parliament
1861
concentration
Movement
##IS
competing
stops
behalf
##mm
limits
funded
discuss
Collins
departure
obtain
woods
latest
universe
alcohol
Laura
rush
blade
funny
Dennis
forgotten
Amy
Symphony
apparent
graduating
1862
Rob
Grey
collections
Mason
emotions
##ugh
literally
Any
counties
1863
nomination
fighter
habitat
respond
external
Capital
exit
Video
carbon
sharing
Bad
opportunities
Perry
photo
##mus
Orange
posted
remainder
transportation
portrayed
Labor
recommended
percussion
rated
Grade
rivers
partially
suspected
strip
adults
button
struggled
intersection
Canal
##ability
poems
claiming
Madrid
1886
Together
##our
Much
Vancouver
instrument
instrumental
1870
mad
angle
Control
Phoenix
Leo
Communications
mail
##ette
##ev
preferred
adaptation
alleged
discussed
deeper
##ane
Yet
Monday
volumes
thrown
Zane
##logy
displayed
rolling
dogs
Along
Todd
##ivity
withdrew
representation
belief
##sia
crown
Late
Short
hardly
grinned
romantic
Pete
##ken
networks
enemies
Colin
Eventually
Side
donated
##su
steady
grab
guide
Finnish
Milan
pregnant
controversy
reminded
1884
Stuart
##bach
##ade
Race
Belgian
LP
Production
Zone
lieutenant
infantry
Child
confusion
sang
resident
##ez
victim
1881
channels
Ron
businessman
##gle
Dick
colony
pace
producers
##ese
agencies
Craig
Lucy
Very
centers
Yorkshire
photography
##ched
Album
championships
Metro
substantial
Standard
terrible
directors
contribution
advertising
emotional
##its
layer
segment
sir
folded
Roberts
ceased
Hampshire
##ray
detailed
partners
m²
##pt
Beth
genre
commented
generated
remote
aim
Hans
credits
concerts
periods
breakfast
gay
shadow
defence
Too
Had
transition
Afghanistan
##book
eggs
defend
##lli
writes
Systems
bones
mess
seed
scientists
Shortly
Romanian
##zy
Freedom
muscle
hero
parent
agriculture
checked
Islam
Bristol
Freyja
Arena
cabin
Germans
electricity
ranks
viewed
medals
Wolf
associate
Madison
Sorry
fort
Chile
detail
widespread
attorney
boyfriend
##nan
Students
Spencer
##ig
bite
Maine
demolished
Lisa
erected
Someone
operational
Commissioner
NHL
Coach
Bar
forcing
Dream
Rico
cargo
Murphy
##fish
##ase
distant
##master
##ora
Organization
doorway
Steven
traded
electrical
frequent
##wn
Branch
Sure
1882
placing
Manhattan
attending
attributed
excellent
pounds
ruling
principles
component
Mediterranean
Vegas
machines
percentage
infrastructure
throwing
affiliated
Kings
secured
Caribbean
Track
Ted
honour
opponent
Virgin
Construction
grave
produces
Challenge
stretched
paying
murmured
##ata
integrated
waved
Nathan
##ator
transmission
videos
##yan
##hu
Nova
descent
AM
Harold
conservative
Therefore
venue
competitive
##ui
conclusion
funeral
confidence
releases
scholar
##sson
Treaty
stress
mood
##sm
Mac
residing
Action
Fund
##ship
animated
fitted
##kar
defending
voting
tend
##berry
answers
believes
##ci
helps
Aaron
##tis
themes
##lay
populations
Players
stroke
Trinity
electoral
paint
abroad
charity
keys
Fair
##pes
interrupted
participants
murdered
Days
supporters
##ab
expert
borders
mate
##llo
solar
architectural
tension
##bling
Parish
tape
operator
Cultural
Clinton
indicates
publisher
ordinary
sugar
arrive
rifle
acoustic
##uring
assets
##shire
SS
sufficient
options
HMS
Classic
bars
rebuilt
governments
Beijing
reporter
screamed
Abbey
crying
mechanical
instantly
communications
Political
cemetery
Cameron
Stop
representatives
USS
texts
mathematics
innings
civilian
Serbia
##hill
practical
patterns
dust
Faculty
debt
##end
##cus
junction
suppose
experimental
Computer
Food
wrist
abuse
dealing
bigger
cap
principle
##pin
Muhammad
Fleet
Collection
attempting
dismissed
##burn
regime
Herbert
##ua
shadows
1883
Eve
Lanka
1878
Performance
fictional
##lock
Noah
Run
Voivodeship
exercise
broadcasting
##fer
RAF
Magic
Bangladesh
suitable
##low
##del
styles
toured
Code
identical
links
insisted
110
flash
Model
slave
Derek
Rev
fairly
Greater
sole
##lands
connecting
zero
bench
##ome
switched
Fall
Owen
yours
Electric
shocked
convention
##bra
climb
memorial
swept
Racing
decides
belong
##nk
parliamentary
##und
ages
proof
##dan
delivery
1860
##ów
sad
publicly
leaning
Archbishop
dirt
##ose
categories
1876
burn
##bing
requested
Guinea
Historical
rhythm
relation
##heim
ye
pursue
merchant
##mes
lists
continuous
frowned
colored
tool
gods
involves
Duncan
photographs
Cricket
slight
Gregory
atmosphere
wider
Cook
##tar
essential
Being
FA
emperor
wealthy
nights
##bar
licensed
Hawaii
viewers
Language
load
nearest
milk
kilometers
platforms
##ys
territories
Rogers
sheet
Rangers
contested
##lation
isolated
assisted
swallowed
Small
Contemporary
Technical
Edwards
express
Volume
endemic
##ei
tightly
Whatever
indigenous
Colombia
##ulation
hp
characterized
##ida
Nigeria
Professional
duo
Soccer
slaves
Farm
smart
Attorney
Attendance
Common
salt
##vin
tribes
nod
sentenced
bid
sample
Drive
switch
instant
21st
Cuba
drunk
Alaska
proud
awareness
hitting
sessions
Thai
locally
elsewhere
Dragon
gentle
touching
##lee
Springs
Universal
Latino
spin
1871
Chart
recalled
Type
pointing
##ii
lowest
##ser
grandmother
Adelaide
Jacques
spotted
Buffalo
restoration
Son
Joan
farmers
Lily
1879
lucky
##dal
luck
eldest
##rant
Market
drummer
deployed
warned
prince
sing
amazing
sailed
##oon
1875
Primary
traveling
Masters
Sara
cattle
Trail
gang
Further
desert
relocated
##tch
##ord
Flight
illness
Munich
ninth
repair
Singles
##lated
Tyler
tossed
boots
Work
sized
earning
shoved
magazines
housed
dam
researchers
Former
spun
premiere
spaces
organised
wealth
crimes
devoted
stones
Urban
automatic
hop
affect
outstanding
tanks
mechanism
Muslims
Ms
shots
argue
Jeremy
connections
Armenian
increases
rubbed
1867
retail
gear
Pan
bonus
jurisdiction
weird
concerning
whisper
##gal
Microsoft
tenure
hills
www
Gmina
porch
files
reportedly
venture
Storm
##ence
Nature
killer
panic
fate
Secret
Wang
scream
drivers
belongs
Chamber
clan
monument
mixing
Peru
bet
Riley
Friends
Isaac
submarine
1877
130
judges
harm
ranging
affair
prepare
pupils
householder
Policy
decorated
Nation
slammed
activist
implemented
Room
qualify
Publishing
establishing
Baptist
touring
subsidiary
##nal
legend
1872
laughter
PC
Athens
settlers
ties
dual
dear
Draft
strategic
Ivan
reveal
closest
dominant
Ah
##ult
Denver
bond
boundaries
drafted
tables
##TV
eyed
Edition
##ena
1868
belonging
1874
Industrial
cream
Ridge
Hindu
scholarship
Ma
opens
initiated
##ith
yelled
compound
random
Throughout
grades
physics
sank
grows
exclusively
settle
Saints
brings
Amsterdam
Make
Hart
walks
battery
violin
##born
explanation
##ware
1873
##har
provinces
thrust
exclusive
sculpture
shops
##fire
VI
constitution
Barcelona
monster
Devon
Jefferson
Sullivan
bow
##din
desperate
##ć
Julie
##mon
##ising
terminus
Jesse
abilities
golf
##ple
##via
##away
Raymond
measured
jury
firing
revenue
suburb
Bulgarian
1866
##cha
timber
Things
##weight
Morning
spots
Alberta
Data
explains
Kyle
friendship
raw
tube
demonstrated
aboard
immigrants
reply
breathe
Manager
ease
##ban
##dia
Diocese
##vy
##ía
pit
ongoing
##lie
Gilbert
Costa
1940s
Report
voters
cloud
traditions
##MS
gallery
Jennifer
swung
Broadcasting
Does
diverse
reveals
arriving
initiative
##ani
Give
Allied
Pat
Outstanding
monastery
blind
Currently
##war
bloody
stopping
focuses
managing
Florence
Harvey
creatures
900
breast
internet
Artillery
purple
##mate
alliance
excited
fee
Brisbane
lifetime
Private
##aw
##nis
##gue
##ika
phrase
regulations
reflected
manufactured
conventional
pleased
client
##ix
##ncy
Pedro
reduction
##con
welcome
jail
comfort
Iranian
Norfolk
Dakota
##tein
evolution
everywhere
Initially
sensitive
Olivia
Oscar
implementation
sits
stolen
demands
slide
grandson
##ich
merger
##mic
Spirit
##°
ticket
root
difficulty
Nevada
##als
lined
Dylan
Original
Call
biological
EU
dramatic
##hn
Operations
treaty
gap
##list
Am
Romanized
moral
Butler
perspective
Furthermore
Manuel
absolutely
unsuccessful
disaster
dispute
preparation
tested
discover
##ach
shield
squeezed
brushed
battalion
Arnold
##ras
superior
treat
clinical
##so
Apple
Syria
Cincinnati
package
flights
editions
Leader
minority
wonderful
hang
Pop
Philippine
telephone
bell
honorary
##mar
balls
Democrat
dirty
thereafter
collapsed
Inside
slip
wrestling
##ín
listened
regard
bowl
None
Sport
completing
trapped
##view
copper
Wallace
Honor
blame
Peninsula
##ert
##oy
Anglo
bearing
simultaneously
honest
##ias
Mix
Got
speaker
voiced
impressed
prices
error
1869
##feld
trials
Nine
Industry
substitute
Municipal
departed
slept
##ama
Junction
Socialist
flower
dropping
comment
fantasy
##ress
arrangements
travelled
furniture
fist
relieved
##tics
Leonard
linear
earn
expand
Soul
Plan
Leeds
Sierra
accessible
innocent
Winner
Fighter
Range
winds
vertical
Pictures
101
charter
cooperation
prisoner
interviews
recognised
sung
manufacturer
exposure
submitted
Mars
leaf
gauge
screaming
likes
eligible
##ac
gathering
columns
##dra
belly
UN
maps
messages
speakers
##ants
garage
unincorporated
Number
Watson
sixteen
lots
beaten
Could
Municipality
##ano
Horse
talks
Drake
scores
Venice
genetic
##mal
##ère
Cold
Jose
nurse
traditionally
##bus
Territory
Key
Nancy
##win
thumb
São
index
dependent
carries
controls
Comics
coalition
physician
referring
Ruth
Based
restricted
inherited
internationally
stretch
THE
plates
margin
Holland
knock
significance
valuable
Kenya
carved
emotion
conservation
municipalities
overseas
resumed
Finance
graduation
blinked
temperatures
constantly
productions
scientist
ghost
cuts
permitted
##ches
firmly
##bert
patrol
##yo
Croatian
attacking
1850
portrait
promoting
sink
conversion
##kov
locomotives
Guide
##val
nephew
relevant
Marc
drum
originated
Chair
visits
dragged
Price
favour
corridor
properly
respective
Caroline
reporting
inaugural
1848
industries
##ching
edges
Christianity
Maurice
Trent
Economics
carrier
Reed
##gon
tribute
Pradesh
##ale
extend
attitude
Yale
##lu
settlements
glasses
taxes
targets
##ids
quarters
##ological
connect
hence
metre
collapse
underneath
banned
Future
clients
alternate
explosion
kinds
Commons
hungry
dragon
Chapel
Buddhist
lover
depression
pulls
##ges
##uk
origins
computers
crosses
kissing
assume
emphasis
lighting
##ites
personally
crashed
beam
touchdown
lane
comparison
##mont
Hitler
##las
execution
##ene
acre
sum
Pearl
ray
##point
essentially
worker
convicted
tear
Clay
recovery
Literature
Unfortunately
##row
partial
Petersburg
Bulgaria
coaching
evolved
reception
enters
narrowed
elevator
therapy
defended
pairs
##lam
breaks
Bennett
Uncle
cylinder
##ison
passion
bases
Actor
cancelled
battles
extensively
oxygen
Ancient
specialized
negotiations
##rat
acquisition
convince
interpretation
##00
photos
aspect
colleges
Artist
keeps
##wing
Croatia
##ona
Hughes
Otto
comments
##du
Ph
Sweet
adventure
describing
Student
Shakespeare
scattered
objective
Aviation
Phillips
Fourth
athletes
##hal
##tered
Guitar
intensity
née
dining
curve
Obama
topics
legislative
Mill
Cruz
##ars
Members
recipient
Derby
inspiration
corresponding
fed
YouTube
coins
pressing
intent
Karen
cinema
Delta
destination
shorter
Christians
imagined
canal
Newcastle
Shah
Adrian
super
Males
160
liberal
lord
bat
supplied
Claude
meal
worship
##atic
Han
wire
°F
##tha
punishment
thirteen
fighters
##ibility
1859
Ball
gardens
##ari
Ottawa
pole
indicating
Twenty
Higher
Bass
Ivy
farming
##urs
certified
Saudi
plenty
##ces
restaurants
Representative
Miles
payment
##inger
##rit
Confederate
festivals
references
##ić
Mario
PhD
playoffs
witness
rice
mask
saving
opponents
enforcement
automatically
relegated
##oe
radar
whenever
Financial
imperial
uncredited
influences
Abraham
skull
Guardian
Haven
Bengal
impressive
input
mixture
Warsaw
altitude
distinction
1857
collective
Annie
##ean
##bal
directions
Flying
##nic
faded
##ella
contributing
##ó
employee
##lum
##yl
ruler
oriented
conductor
focusing
##die
Giants
Mills
mines
Deep
curled
Jessica
guitars
Louise
procedure
Machine
failing
attendance
Nepal
Brad
Liam
tourist
exhibited
Sophie
depicted
Shaw
Chuck
##can
expecting
challenges
##nda
equally
resignation
##logical
Tigers
loop
pitched
outdoor
reviewed
hopes
True
temporarily
Borough
torn
jerked
collect
Berkeley
Independence
cotton
retreat
campaigns
participating
Intelligence
Heaven
##ked
situations
borough
Democrats
Harbor
##len
Liga
serial
circles
fourteen
##lot
seized
filling
departments
finance
absolute
Roland
Nate
floors
raced
struggling
deliver
protests
##tel
Exchange
efficient
experiments
##dar
faint
3D
binding
Lions
lightly
skill
proteins
difficulties
##cal
monthly
camps
flood
loves
Amanda
Commerce
##oid
##lies
elementary
##tre
organic
##stein
##ph
receives
Tech
enormous
distinctive
Joint
experiment
Circuit
citizen
##hy
shelter
ideal
practically
formula
addressed
Foster
Productions
##ax
variable
punk
Voice
fastest
concentrated
##oma
##yer
stored
surrender
vary
Sergeant
Wells
ward
Wait
##ven
playoff
reducing
cavalry
##dle
Venezuela
tissue
amounts
sweat
##we
Non
##nik
beetle
##bu
##tu
Jared
Hunt
##₂
fat
Sultan
Living
Circle
Secondary
Suddenly
reverse
##min
Travel
##bin
Lebanon
##mas
virus
Wind
dissolved
enrolled
holiday
Keep
helicopter
Clarke
constitutional
technologies
doubles
instructions
##ace
Azerbaijan
##ill
occasional
frozen
trick
wiped
writings
Shanghai
preparing
challenged
mainstream
summit
180
##arian
##rating
designation
##ada
revenge
filming
tightened
Miguel
Montana
reflect
celebration
bitch
flashed
signals
rounded
peoples
##tation
renowned
Google
characteristic
Campaign
sliding
##rman
usage
Record
Using
woke
solutions
holes
theories
logo
Protestant
relaxed
brow
nickname
Reading
marble
##tro
symptoms
Overall
capita
##ila
outbreak
revolution
deemed
Principal
Hannah
approaches
inducted
Wellington
vulnerable
Environmental
Drama
incumbent
Dame
1854
travels
samples
accurate
physically
Sony
Nashville
##sville
##lic
##og
Producer
Lucky
tough
Stanford
resort
repeatedly
eyebrows
Far
choir
commenced
##ep
##ridge
rage
swing
sequel
heir
buses
ad
Grove
##late
##rick
updated
##SA
Delaware
##fa
Athletics
warmth
Off
excitement
verse
Protection
Villa
corruption
intellectual
Jenny
##lyn
mystery
prayer
healthy
##ologist
Bear
lab
Ernest
Remix
register
basement
Montgomery
consistent
tier
1855
Preston
Brooks
##maker
vocalist
laboratory
delayed
wheels
rope
bachelor
pitcher
Block
Nevertheless
suspect
efficiency
Nebraska
siege
FBI
planted
##AC
Newton
breeding
##ain
eighteen
Argentine
encounter
servant
1858
elder
Shadow
Episode
fabric
doctors
survival
removal
chemistry
volunteers
Kane
variant
arrives
Eagle
Left
##fe
Jo
divorce
##ret
yesterday
Bryan
handling
diseases
customer
Sheriff
Tiger
Harper
##oi
resting
Linda
Sheffield
gasped
sexy
economics
alien
tale
footage
Liberty
yeah
fundamental
Ground
flames
Actress
photographer
Maggie
Additional
joke
custom
Survey
Abu
silk
consumption
Ellis
bread
##uous
engagement
puts
Dog
##hr
poured
guilt
CDP
boxes
hardware
clenched
##cio
stem
arena
extending
##com
examination
Steel
encountered
revised
140
picking
Car
hasn
Minor
pride
Roosevelt
boards
##mia
blocked
curious
drag
narrative
brigade
Prefecture
mysterious
namely
connects
Devil
historians
CHAPTER
quit
installation
Golf
empire
elevated
##eo
releasing
Bond
##uri
harsh
ban
##BA
contracts
cloth
presents
stake
chorus
##eau
swear
##mp
allies
generations
Motor
meter
pen
warrior
veteran
##EC
comprehensive
missile
interaction
instruction
Renaissance
rested
Dale
fix
fluid
les
investigate
loaded
widow
exhibit
artificial
select
rushing
tasks
signature
nowhere
Engineer
feared
Prague
bother
extinct
gates
Bird
climbing
heels
striking
artwork
hunt
awake
##hin
Formula
thereby
commitment
imprisoned
Beyond
##MA
transformed
Agriculture
Low
Movie
radical
complicated
Yellow
Auckland
mansion
tenth
Trevor
predecessor
##eer
disbanded
sucked
circular
witch
gaining
lean
Behind
illustrated
rang
celebrate
bike
consist
framework
##cent
Shane
owns
350
comprises
collaborated
colleagues
##cast
engage
fewer
##ave
1856
observation
diplomatic
legislature
improvements
Interstate
craft
MTV
martial
administered
jet
approaching
permanently
attraction
manuscript
numbered
Happy
Andrea
shallow
Gothic
Anti
##bad
improvement
trace
preserve
regardless
rode
dies
achievement
maintaining
Hamburg
spine
##air
flowing
encourage
widened
posts
##bound
125
Southeast
Santiago
##bles
impression
receiver
Single
closure
##unt
communist
honors
Northwest
105
##ulated
cared
un
hug
magnetic
seeds
topic
perceived
prey
prevented
Marvel
Eight
Michel
Transportation
rings
Gate
##gne
Byzantine
accommodate
floating
##dor
equation
ministry
##ito
##gled
Rules
earthquake
revealing
Brother
Celtic
blew
chairs
Panama
Leon
attractive
descendants
Care
Ambassador
tours
breathed
threatening
##cho
smiles
Lt
Beginning
##iness
fake
assists
fame
strings
Mobile
Liu
parks
http
1852
brush
Aunt
bullet
consciousness
##sta
##ther
consequences
gather
dug
1851
bridges
Doug
##sion
Artists
ignore
Carol
brilliant
radiation
temples
basin
clouds
##cted
Stevens
spite
soap
consumer
Damn
Snow
recruited
##craft
Advanced
tournaments
Quinn
undergraduate
questioned
Palmer
Annual
Others
feeding
Spider
printing
##orn
cameras
functional
Chester
readers
Alpha
universal
Faith
Brandon
François
authored
Ring
el
aims
athletic
possessed
Vermont
programmes
##uck
bore
Fisher
statements
shed
saxophone
neighboring
pronounced
barrel
bags
##dge
organisations
pilots
casualties
Kenneth
##brook
silently
Malcolm
span
Essex
anchor
##hl
virtual
lessons
Henri
Trump
Page
pile
locomotive
wounds
uncomfortable
sustained
Diana
Eagles
##pi
2000s
documented
##bel
Cassie
delay
kisses
##ines
variation
##ag
growled
##mark
##ways
Leslie
studios
Friedrich
aunt
actively
armor
eaten
historically
Better
purse
honey
ratings
##ée
naturally
1840
peer
Kenny
Cardinal
database
Looking
runners
handsome
Double
PA
##boat
##sted
protecting
##jan
Diamond
concepts
interface
##aki
Watch
Article
Columbus
dialogue
pause
##rio
extends
blanket
pulse
1853
affiliate
ladies
Ronald
counted
kills
demons
##zation
Airlines
Marco
Cat
companion
mere
Yugoslavia
Forum
Allan
pioneer
Competition
Methodist
patent
nobody
Stockholm
##ien
regulation
##ois
accomplished
##itive
washed
sake
Vladimir
crops
prestigious
humor
Sally
labour
tributary
trap
altered
examined
Mumbai
bombing
Ash
noble
suspension
ruins
##bank
spare
displays
guided
dimensional
Iraqi
##hon
sciences
Franz
relating
fence
followers
Palestine
invented
proceeded
Batman
Bradley
##yard
##ova
crystal
Kerala
##ima
shipping
handled
Want
abolished
Drew
##tter
Powell
Half
##table
##cker
exhibitions
Were
assignment
assured
##rine
Indonesian
Grammy
acknowledged
Kylie
coaches
structural
clearing
stationed
Say
Total
Rail
besides
glow
threats
afford
Tree
Musical
##pp
elite
centered
explore
Engineers
Stakes
Hello
tourism
severely
assessment
##tly
crack
politicians
##rrow
sheets
volunteer
##borough
##hold
announcement
recover
contribute
lungs
##ille
mainland
presentation
Johann
Writing
1849
##bird
Study
Boulevard
coached
fail
airline
Congo
Plus
Syrian
introduce
ridge
Casey
manages
##fi
searched
Support
succession
progressive
coup
cultures
##lessly
sensation
Cork
Elena
Sofia
Philosophy
mini
trunk
academy
Mass
Liz
practiced
Reid
##ule
satisfied
experts
Wilhelm
Woods
invitation
Angels
calendar
joy
Sr
Dam
packed
##uan
bastard
Workers
broadcasts
logic
cooking
backward
##ack
Chen
creates
enzyme
##xi
Davies
aviation
VII
Conservation
fucking
Knights
##kan
requiring
hectares
wars
ate
##box
Mind
desired
oak
absorbed
Really
Vietnamese
Paulo
athlete
##car
##eth
Talk
Wu
##cks
survivors
Yang
Joel
Almost
Holmes
Armed
Joshua
priests
discontinued
##sey
blond
Rolling
suggesting
CA
clay
exterior
Scientific
##sive
Giovanni
Hi
farther
contents
Winners
animation
neutral
mall
Notes
layers
professionals
Armstrong
Against
Piano
involve
monitor
angel
parked
bears
seated
feat
beliefs
##kers
Version
suffer
##ceae
guidance
##eur
honored
raid
alarm
Glen
Ellen
Jamaica
trio
enabled
##ils
procedures
##hus
moderate
upstairs
##ses
torture
Georgian
rebellion
Fernando
Nice
##are
Aires
Campus
beast
##hing
1847
##FA
Isle
##logist
Princeton
cathedral
Oakland
Solomon
##tto
Milwaukee
upcoming
midfielder
Neither
sacred
Eyes
appreciate
Brunswick
secrets
Rice
Somerset
Chancellor
Curtis
##gel
Rich
separation
grid
##los
##bon
urge
##ees
##ree
freight
towers
psychology
requirement
dollar
##fall
##sman
exile
tomb
Salt
Stefan
Buenos
Revival
Porter
tender
diesel
chocolate
Eugene
Legion
Laboratory
sheep
arched
hospitals
orbit
Full
##hall
drinks
ripped
##RS
tense
Hank
leagues
##nberg
PlayStation
fool
Punjab
relatives
Comedy
sur
1846
Tonight
Sox
##if
Rabbi
org
speaks
institute
defender
painful
wishes
Weekly
literacy
portions
snake
item
deals
##tum
autumn
sharply
reforms
thighs
prototype
##ition
argues
disorder
Physics
terror
provisions
refugees
predominantly
independently
march
##graphy
Arabia
Andrews
Bus
Money
drops
##zar
pistol
matrix
revolutionary
##ust
Starting
##ptic
Oak
Monica
##ides
servants
##hed
archaeological
divorced
rocket
enjoying
fires
##nel
assembled
qualification
retiring
##fied
Distinguished
handful
infection
Durham
##itz
fortune
renewed
Chelsea
##sley
curved
gesture
retain
exhausted
##ifying
Perth
jumping
Palestinian
Simpson
colonies
steal
##chy
corners
Finn
arguing
Martha
##var
Betty
emerging
Heights
Hindi
Manila
pianist
founders
regret
Napoleon
elbow
overhead
bold
praise
humanity
##ori
Revolutionary
##ere
fur
##ole
Ashley
Official
##rm
lovely
Architecture
##sch
Baronet
virtually
##OS
descended
immigration
##das
##kes
Holly
Wednesday
maintains
theatrical
Evan
Gardens
citing
##gia
segments
Bailey
Ghost
##city
governing
graphics
##ined
privately
potentially
transformation
Crystal
Cabinet
sacrifice
hesitated
mud
Apollo
Desert
bin
victories
Editor
Railways
Web
Case
tourists
Brussels
Franco
compiled
topped
Gene
engineers
commentary
egg
escort
nerve
arch
necessarily
frustration
Michelle
democracy
genes
Facebook
halfway
##ient
102
flipped
Won
##mit
NASA
Lynn
Provincial
ambassador
Inspector
glared
Change
McDonald
developments
tucked
noting
Gibson
circulation
dubbed
armies
resource
Headquarters
##iest
Mia
Albanian
Oil
Albums
excuse
intervention
Grande
Hugo
integration
civilians
depends
reserves
Dee
compositions
identification
restrictions
quarterback
Miranda
Universe
favourite
ranges
hint
loyal
Op
entity
Manual
quoted
dealt
specialist
Zhang
download
Westminster
Rebecca
streams
Anglican
variations
Mine
detective
Films
reserved
##oke
##key
sailing
##gger
expanding
recall
discovers
particles
behaviour
Gavin
blank
permit
Java
Fraser
Pass
##non
##TA
panels
statistics
notion
courage
dare
venues
##roy
Box
Newport
travelling
Thursday
warriors
Glenn
criteria
360
mutual
restore
varied
bitter
Katherine
##lant
ritual
bits
##à
Henderson
trips
Richardson
Detective
curse
psychological
Il
midnight
streak
facts
Dawn
Indies
Edmund
roster
Gen
##nation
1830
congregation
shaft
##ically
##mination
Indianapolis
Sussex
loving
##bit
sounding
horrible
Continental
Griffin
advised
magical
millions
##date
1845
Safety
lifting
determination
valid
dialect
Penn
Know
triple
avoided
dancer
judgment
sixty
farmer
lakes
blast
aggressive
Abby
tag
chains
inscription
##nn
conducting
Scout
buying
##wich
spreading
##OC
array
hurried
Environment
improving
prompted
fierce
Taking
Away
tune
pissed
Bull
catching
##ying
eyebrow
metropolitan
terrain
##rel
Lodge
manufacturers
creator
##etic
happiness
ports
##ners
Relations
fortress
targeted
##ST
allegedly
blues
##osa
Bosnia
##dom
burial
similarly
stranger
pursued
symbols
rebels
reflection
routine
traced
indoor
eventual
##ska
##ão
##una
MD
##phone
oh
grants
Reynolds
rid
operators
##nus
Joey
vital
siblings
keyboard
br
removing
societies
drives
solely
princess
lighter
Various
Cavalry
believing
SC
underwent
relay
smelled
syndrome
welfare
authorized
seemingly
Hard
chicken
##rina
Ages
Bo
democratic
barn
Eye
shorts
##coming
##hand
disappointed
unexpected
centres
Exhibition
Stories
Site
banking
accidentally
Agent
conjunction
André
Chloe
resist
width
Queens
provision
##art
Melissa
Honorary
Del
prefer
abruptly
duration
##vis
Glass
enlisted
##ado
discipline
Sisters
carriage
##ctor
##sburg
Lancashire
log
fuck
##iz
closet
collecting
holy
rape
trusted
cleaning
inhabited
Rocky
104
editorial
##yu
##ju
succeed
strict
Cuban
##iya
Bronze
outcome
##ifies
##set
corps
Hero
barrier
Kumar
groaned
Nina
Burton
enable
stability
Milton
knots
##ination
slavery
##borg
curriculum
trailer
warfare
Dante
Edgar
revival
Copenhagen
define
advocate
Garrett
Luther
overcome
pipe
750
construct
Scotia
kings
flooding
##hard
Ferdinand
Felix
forgot
Fish
Kurt
elaborate
##BC
graphic
gripped
colonel
Sophia
Advisory
Self
##uff
##lio
monitoring
seal
senses
rises
peaceful
journals
1837
checking
legendary
Ghana
##power
ammunition
Rosa
Richards
nineteenth
ferry
aggregate
Troy
inter
##wall
Triple
steep
tent
Cyprus
1844
##woman
commanding
farms
doi
navy
specified
na
cricketer
transported
Think
comprising
grateful
solve
##core
beings
clerk
grain
vector
discrimination
##TC
Katie
reasonable
drawings
veins
consideration
Monroe
repeat
breed
dried
witnessed
ordained
Current
spirits
remarkable
consultant
urged
Remember
anime
singers
phenomenon
Rhode
Carlo
demanding
findings
manual
varying
Fellowship
generate
safely
heated
withdrawn
##ao
headquartered
##zon
##lav
##ency
Col
Memphis
imposed
rivals
Planet
healing
##hs
ensemble
Warriors
##bone
cult
Frankfurt
##HL
diversity
Gerald
intermediate
##izes
reactions
Sister
##ously
##lica
quantum
awkward
mentions
pursuit
##ography
varies
profession
molecular
consequence
lectures
cracked
103
slowed
##tsu
cheese
upgraded
suite
substance
Kingston
1800
Idaho
Theory
##een
ain
Carson
Molly
##OR
configuration
Whitney
reads
audiences
##tie
Geneva
Outside
##nen
##had
transit
volleyball
Randy
Chad
rubber
motorcycle
respected
eager
Level
coin
##lets
neighbouring
##wski
confident
##cious
poll
uncertain
punch
thesis
Tucker
IATA
Alec
##ographic
##law
1841
desperately
1812
Lithuania
accent
Cox
lightning
skirt
##load
Burns
Dynasty
##ug
chapters
Working
dense
Morocco
##kins
casting
Set
activated
oral
Brien
horn
HIV
dawn
stumbled
altar
tore
considerably
Nicole
interchange
registration
biography
Hull
Stan
bulk
consent
Pierce
##ER
Fifth
marched
terrorist
##piece
##itt
Presidential
Heather
staged
Plant
relegation
sporting
joins
##ced
Pakistani
dynamic
Heat
##lf
ourselves
Except
Elliott
nationally
goddess
investors
Burke
Jackie
##ā
##RA
Tristan
Associate
Tuesday
scope
Near
bunch
##abad
##ben
sunlight
##aire
manga
Willie
trucks
boarding
Lion
lawsuit
Learning
Der
pounding
awful
##mine
IT
Legend
romance
Serie
AC
gut
precious
Robertson
hometown
realm
Guards
Tag
batting
##vre
halt
conscious
1838
acquire
collar
##gg
##ops
Herald
nationwide
citizenship
Aircraft
decrease
em
Fiction
Female
corporation
Located
##ip
fights
unconscious
Tampa
Poetry
lobby
Malta
##sar
##bie
layout
Tate
reader
stained
##bre
##rst
##ulate
loudly
Eva
Cohen
exploded
Merit
Maya
##rable
Rovers
##IC
Morrison
Should
vinyl
##mie
onwards
##gie
vicinity
Wildlife
probability
Mar
Barnes
##ook
spinning
Moses
##vie
Surrey
Planning
conferences
protective
Plaza
deny
Canterbury
manor
Estate
tilted
comics
IBM
destroying
server
Dorothy
##horn
Oslo
lesser
heaven
Marshal
scales
strikes
##ath
firms
attract
##BS
controlling
Bradford
southeastern
Amazon
Travis
Janet
governed
1842
Train
Holden
bleeding
gifts
rent
1839
palms
##ū
judicial
Ho
Finals
conflicts
unlikely
draws
##cies
compensation
adds
elderly
Anton
lasting
Nintendo
codes
ministers
pot
associations
capabilities
##cht
libraries
##sie
chances
performers
runway
##af
##nder
Mid
Vocals
##uch
##eon
interpreted
priority
Uganda
ruined
Mathematics
cook
AFL
Lutheran
AIDS
Capitol
chase
axis
Moreover
María
Saxon
storyline
##ffed
Tears
Kid
cent
colours
Sex
##long
pm
blonde
Edwin
CE
diocese
##ents
##boy
Inn
##ller
Saskatchewan
##kh
stepping
Windsor
##oka
##eri
Xavier
Resources
1843
##top
##rad
##lls
Testament
poorly
1836
drifted
slope
CIA
remix
Lords
mature
hosting
diamond
beds
##ncies
luxury
trigger
##lier
preliminary
hybrid
journalists
Enterprise
proven
expelled
insects
Beautiful
lifestyle
vanished
##ake
##ander
matching
surfaces
Dominican
Kids
referendum
Orlando
Truth
Sandy
privacy
Calgary
Speaker
sts
Nobody
shifting
##gers
Roll
Armenia
Hand
##ES
106
##ont
Guild
larvae
Stock
flame
gravity
enhanced
Marion
surely
##tering
Tales
algorithm
Emmy
darker
VIII
##lash
hamlet
deliberately
occurring
choices
Gage
fees
settling
ridiculous
##ela
Sons
cop
custody
##ID
proclaimed
Cardinals
##pm
Metal
Ana
1835
clue
Cardiff
riders
observations
MA
sometime
##och
performer
intact
Points
allegations
rotation
Tennis
tenor
Directors
##ats
Transit
thigh
Complex
##works
twentieth
Factory
doctrine
Daddy
##ished
pretend
Winston
cigarette
##IA
specimens
hydrogen
smoking
mathematical
arguments
openly
developer
##iro
fists
somebody
##san
Standing
Caleb
intelligent
Stay
Interior
echoed
Valentine
varieties
Brady
cluster
Ever
voyage
##of
deposits
ultimate
Hayes
horizontal
proximity
##ás
estates
exploration
NATO
Classical
##most
bills
condemned
1832
hunger
##ato
planes
deserve
offense
sequences
rendered
acceptance
##ony
manufacture
Plymouth
innovative
predicted
##RC
Fantasy
##une
supporter
absent
Picture
bassist
rescued
##MC
Ahmed
Monte
##sts
##rius
insane
novelist
##és
agrees
Antarctic
Lancaster
Hopkins
calculated
startled
##star
tribal
Amendment
##hoe
invisible
patron
deer
Walk
tracking
Lyon
tickets
##ED
philosopher
compounds
chuckled
##wi
pound
loyalty
Academic
petition
refuses
marking
Mercury
northeastern
dimensions
scandal
Canyon
patch
publish
##oning
Peak
minds
##boro
Presbyterian
Hardy
theoretical
magnitude
bombs
cage
##ders
##kai
measuring
explaining
avoiding
touchdowns
Card
theology
##ured
Popular
export
suspicious
Probably
photograph
Lou
Parks
Arms
compact
Apparently
excess
Banks
lied
stunned
territorial
Filipino
spectrum
learns
wash
imprisonment
ugly
##rose
Albany
Erik
sends
##hara
##rid
consumed
##gling
Belgrade
Da
opposing
Magnus
footsteps
glowing
delicate
Alexandria
Ludwig
gorgeous
Bros
Index
##PA
customs
preservation
bonds
##mond
environments
##nto
instructed
parted
adoption
locality
workshops
goalkeeper
##rik
##uma
Brighton
Slovenia
##ulating
##tical
towel
hugged
stripped
Bears
upright
Wagner
##aux
secretly
Adventures
nest
Course
Lauren
Boeing
Abdul
Lakes
450
##cu
USSR
caps
Chan
##nna
conceived
Actually
Belfast
Lithuanian
concentrate
possess
militia
pine
protagonist
Helena
##PS
##band
Belle
Clara
Reform
currency
pregnancy
1500
##rim
Isabella
hull
Name
trend
journalism
diet
##mel
Recording
acclaimed
Tang
Jace
steering
vacant
suggestion
costume
laser
##š
##ink
##pan
##vić
integral
achievements
wise
classroom
unions
southwestern
##uer
Garcia
toss
Tara
Large
##tate
evident
responsibilities
populated
satisfaction
##bia
casual
Ecuador
##ght
arose
##ović
Cornwall
embrace
refuse
Heavyweight
XI
Eden
activists
##uation
biology
##shan
fraud
Fuck
matched
legacy
Rivers
missionary
extraordinary
Didn
holder
wickets
crucial
Writers
Hurricane
Iceland
gross
trumpet
accordance
hurry
flooded
doctorate
Albania
##yi
united
deceased
jealous
grief
flute
portraits
##а
pleasant
Founded
Face
crowned
Raja
advisor
Salem
##ec
Achievement
admission
freely
minimal
Sudan
developers
estimate
disabled
##lane
downstairs
Bruno
##pus
pinyin
##ude
lecture
deadly
underlying
optical
witnesses
Combat
Julius
tapped
variants
##like
Colonial
Critics
Similarly
mouse
voltage
sculptor
Concert
salary
Frances
##ground
hook
premises
Software
instructor
nominee
##ited
fog
slopes
##zu
vegetation
sail
##rch
Body
Apart
atop
View
utility
ribs
cab
migration
##wyn
bounded
2019
pillow
trails
##ub
Halifax
shade
Rush
##lah
##dian
Notre
interviewed
Alexandra
Springfield
Indeed
rubbing
dozens
amusement
legally
##lers
Jill
Cinema
ignoring
Choice
##ures
pockets
##nell
laying
Blair
tackles
separately
##teen
Criminal
performs
theorem
Communication
suburbs
##iel
competitors
rows
##hai
Manitoba
Eleanor
interactions
nominations
assassination
##dis
Edmonton
diving
##dine
essay
##tas
AFC
Edge
directing
imagination
sunk
implement
Theodore
trembling
sealed
##rock
Nobel
##ancy
##dorf
##chen
genuine
apartments
Nicolas
AA
Bach
Globe
Store
220
##10
Rochester
##ño
alert
107
Beck
##nin
Naples
Basin
Crawford
fears
Tracy
##hen
disk
##pped
seventeen
Lead
backup
reconstruction
##lines
terrified
sleeve
nicknamed
popped
##making
##ern
Holiday
Gospel
ibn
##ime
convert
divine
resolved
##quet
ski
realizing
##RT
Legislature
reservoir
Rain
sinking
rainfall
elimination
challenging
tobacco
##outs
Given
smallest
Commercial
pin
rebel
comedian
exchanged
airing
dish
Salvador
promising
##wl
relax
presenter
toll
aerial
##eh
Fletcher
brass
disappear
zones
adjusted
contacts
##lk
sensed
Walt
mild
toes
flies
shame
considers
wildlife
Hanna
Arsenal
Ladies
naming
##ishing
anxiety
discussions
cute
undertaken
Cash
strain
Wyoming
dishes
precise
Angela
##ided
hostile
twins
115
Built
##pel
Online
tactics
Newman
##bourne
unclear
repairs
embarrassed
listing
tugged
Vale
##gin
Meredith
bout
##cle
velocity
tips
froze
evaluation
demonstrate
##card
criticised
Nash
lineup
Rao
monks
bacteria
lease
##lish
frightened
den
revived
finale
##rance
flee
Letters
decreased
##oh
Sounds
wrap
Sharon
incidents
renovated
everybody
stole
Bath
boxing
1815
withdraw
backs
interim
react
murders
Rhodes
Copa
framed
flown
Estonia
Heavy
explored
##rra
##GA
##ali
Istanbul
1834
##rite
##aging
##ues
Episcopal
arc
orientation
Maxwell
infected
##rot
BCE
Brook
grasp
Roberto
Excellence
108
withdrawal
Marines
rider
Lo
##sin
##run
Subsequently
garrison
hurricane
facade
Prussia
crushed
enterprise
##mber
Twitter
Generation
Physical
Sugar
editing
communicate
Ellie
##hurst
Ernst
wagon
promotional
conquest
Parliamentary
courtyard
lawyers
Superman
email
Prussian
lately
lecturer
Singer
Majesty
Paradise
sooner
Heath
slot
curves
convoy
##vian
induced
synonym
breeze
##plane
##ox
peered
Coalition
##hia
odds
##esh
##lina
Tomorrow
Nadu
##ico
##rah
damp
autonomous
console
Victory
counts
Luxembourg
intimate
Archived
Carroll
spy
Zero
habit
Always
faction
teenager
Johnston
chaos
ruin
commerce
blog
##shed
##the
reliable
Word
Yu
Norton
parade
Catholics
damned
##iling
surgeon
##tia
Allison
Jonas
remarked
##ès
idiot
Making
proposals
Industries
strategies
artifacts
batteries
reward
##vers
Agricultural
distinguish
lengths
Jeffrey
Progressive
kicking
Patricia
##gio
ballot
##ios
skilled
##gation
Colt
limestone
##AS
peninsula
##itis
LA
hotels
shapes
Crime
depicting
northwestern
HD
silly
Das
##²
##ws
##ash
##matic
thermal
Has
forgive
surrendered
Palm
Nacional
drank
haired
Mercedes
##foot
loading
Timothy
##roll
mechanisms
traces
digging
discussing
Natalie
##zhou
Forbes
landmark
Anyway
Manor
conspiracy
gym
knocking
viewing
Formation
Pink
Beauty
limbs
Phillip
sponsor
Joy
granite
Harbour
##ero
payments
Ballet
conviction
##dam
Hood
estimates
lacked
Mad
Jorge
##wen
refuge
##LA
invaded
Kat
suburban
##fold
investigated
Ari
complained
creek
Georges
##uts
powder
accepting
deserved
carpet
Thunder
molecules
Legal
cliff
strictly
enrollment
ranch
##rg
##mba
proportion
renovation
crop
grabbing
##liga
finest
entries
receptor
helmet
blown
Listen
flagship
workshop
resolve
nails
Shannon
portal
jointly
shining
Violet
overwhelming
upward
Mick
proceedings
##dies
##aring
Laurence
Churchill
##rice
commit
170
inclusion
Examples
##verse
##rma
fury
paths
##SC
ankle
nerves
Chemistry
rectangular
sworn
screenplay
cake
Mann
Seoul
Animal
sizes
Speed
vol
Population
Southwest
Hold
continuously
Qualified
wishing
Fighting
Made
disappointment
Portsmouth
Thirty
##beck
Ahmad
teammate
MLB
graph
Charleston
realizes
##dium
exhibits
preventing
##int
fever
rivalry
Male
mentally
dull
##lor
##rich
consistently
##igan
Madame
certificate
suited
Krishna
accuracy
Webb
Budapest
Rex
1831
Cornell
OK
surveillance
##gated
habitats
Adventure
Conrad
Superior
Gay
sofa
aka
boot
Statistics
Jessie
Liberation
##lip
##rier
brands
saint
Heinrich
Christine
bath
Rhine
ballet
Jin
consensus
chess
Arctic
stack
furious
cheap
toy
##yre
##face
##gging
gastropod
##nne
Romans
membrane
answering
25th
architects
sustainable
##yne
Hon
1814
Baldwin
dome
##awa
##zen
celebrity
enclosed
##uit
##mmer
Electronic
locals
##CE
supervision
mineral
Chemical
Slovakia
alley
hub
##az
heroes
Creative
##AM
incredible
politically
ESPN
yanked
halls
Aboriginal
Greatest
yield
##20
congressional
robot
Kiss
welcomed
MS
speeds
proceed
Sherman
eased
Greene
Walsh
Geoffrey
variables
rocky
##print
acclaim
Reverend
Wonder
tonnes
recurring
Dawson
continent
finite
AP
continental
ID
facilitate
essays
Rafael
Neal
1833
ancestors
##met
##gic
Especially
teenage
frustrated
Jules
cock
expense
##oli
##old
blocking
Notable
prohibited
ca
dock
organize
##wald
Burma
Gloria
dimension
aftermath
choosing
Mickey
torpedo
pub
##used
manuscripts
laps
Ulster
staircase
sphere
Insurance
Contest
lens
risks
investigations
ERA
glare
##play
Graduate
auction
Chronicle
##tric
##50
Coming
seating
Wade
seeks
inland
Thames
Rather
butterfly
contracted
positioned
consumers
contestants
fragments
Yankees
Santos
administrator
hypothesis
retire
Denis
agreements
Winnipeg
##rill
1820
trophy
crap
shakes
Jenkins
##rium
ya
twist
labels
Maritime
##lings
##iv
111
##ensis
Cairo
Anything
##fort
opinions
crowded
##nian
abandon
##iff
drained
imported
##rr
tended
##rain
Going
introducing
sculptures
bankruptcy
danced
demonstration
stance
settings
gazed
abstract
pet
Calvin
stiff
strongest
wrestler
##dre
Republicans
grace
allocated
cursed
snail
advancing
Return
errors
Mall
presenting
eliminate
Amateur
Institution
counting
##wind
warehouse
##nde
Ethiopia
trailed
hollow
##press
Literary
capability
nursing
preceding
lamp
Thomson
Morton
##ctic
Crew
Close
composers
boom
Clare
missiles
112
hunter
snap
##oni
##tail
Us
declaration
##cock
rally
huh
lion
straightened
Philippe
Sutton
alpha
valued
maker
navigation
detected
favorable
perception
Charter
##ña
Ricky
rebounds
tunnels
slapped
Emergency
supposedly
##act
deployment
socialist
tubes
anybody
corn
##NA
Seminary
heating
pump
##AA
achieving
souls
##ass
Link
##ele
##smith
greeted
Bates
Americas
Elder
cure
contestant
240
fold
Runner
Uh
licked
Politics
committees
neighbors
fairy
Silva
Leipzig
tipped
correctly
exciting
electronics
foundations
cottage
governmental
##hat
allied
claws
presidency
cruel
Agreement
slender
accompanying
precisely
##pass
driveway
swim
Stand
crews
##mission
rely
everyday
Wings
demo
##hic
recreational
min
nationality
##duction
Easter
##hole
canvas
Kay
Leicester
talented
Discovery
shells
##ech
Kerry
Ferguson
Leave
##place
altogether
adopt
butt
wolves
##nsis
##ania
modest
soprano
Boris
##ught
electron
depicts
hid
cruise
differ
treasure
##nch
Gun
Mama
Bengali
trainer
merchants
innovation
presumably
Shirley
bottles
proceeds
Fear
invested
Pirates
particle
Dominic
blamed
Fight
Daisy
##pper
##graphic
nods
knight
Doyle
tales
Carnegie
Evil
Inter
Shore
Nixon
transform
Savannah
##gas
Baltic
stretching
worlds
protocol
Percy
Toby
Heroes
brave
dancers
##aria
backwards
responses
Chi
Gaelic
Berry
crush
embarked
promises
Madonna
researcher
realised
inaugurated
Cherry
Mikhail
Nottingham
reinforced
subspecies
rapper
##kie
Dreams
Re
Damon
Minneapolis
monsters
suspicion
Tel
surroundings
afterward
complaints
OF
sectors
Algeria
lanes
Sabha
objectives
Donna
bothered
distracted
deciding
##ives
##CA
##onia
bishops
Strange
machinery
Voiced
synthesis
reflects
interference
##TS
##ury
keen
##ign
frown
freestyle
ton
Dixon
Sacred
Ruby
Prison
##ión
1825
outfit
##tain
curiosity
##ight
frames
steadily
emigrated
horizon
##erly
Doc
philosophical
Table
UTC
Marina
##DA
secular
##eed
Zimbabwe
cops
Mack
sheriff
Sanskrit
Francesco
catches
questioning
streaming
Kill
testimony
hissed
tackle
countryside
copyright
##IP
Buddhism
##rator
ladder
##ON
Past
rookie
depths
##yama
##ister
##HS
Samantha
Dana
Educational
brows
Hammond
raids
envelope
##sco
##hart
##ulus
epic
detection
Streets
Potter
statistical
für
ni
accounting
##pot
employer
Sidney
Depression
commands
Tracks
averaged
lets
Ram
longtime
suits
branded
chip
Shield
loans
ought
Said
sip
##rome
requests
Vernon
bordered
veterans
##ament
Marsh
Herzegovina
Pine
##igo
mills
anticipation
reconnaissance
##ef
expectations
protested
arrow
guessed
depot
maternal
weakness
##ap
projected
pour
Carmen
provider
newer
remind
freed
##rily
##wal
##tones
intentions
Fiji
timing
Match
managers
Kosovo
Herman
Wesley
Chang
135
semifinals
shouting
Indo
Janeiro
Chess
Macedonia
Buck
##onies
rulers
Mail
##vas
##sel
MHz
Programme
Task
commercially
subtle
propaganda
spelled
bowling
basically
Raven
1828
Colony
109
##ingham
##wara
anticipated
1829
##iers
graduates
##rton
##fication
endangered
ISO
diagnosed
##tage
exercises
Battery
bolt
poison
cartoon
##ción
hood
bowed
heal
Meyer
Reagan
##wed
subfamily
##gent
momentum
infant
detect
##sse
Chapman
Darwin
mechanics
NSW
Cancer
Brooke
Nuclear
comprised
hire
sanctuary
wingspan
contrary
remembering
surprising
Basic
stealing
OS
hatred
##lled
masters
violation
Rule
##nger
assuming
conquered
louder
robe
Beatles
legitimate
##vation
massacre
Rica
unsuccessfully
poets
##enberg
careers
doubled
premier
battalions
Dubai
Paper
Louisville
gestured
dressing
successive
mumbled
Vic
referee
pupil
##cated
##rre
ceremonies
picks
##IN
diplomat
alike
geographical
rays
##HA
##read
harbour
factories
pastor
playwright
Ultimate
nationalist
uniforms
obtaining
kit
Amber
##pling
screenwriter
ancestry
##cott
Fields
PR
Coleman
rat
Bavaria
squeeze
highlighted
Adult
reflecting
Mel
1824
bicycle
organizing
sided
Previously
Underground
Prof
athletics
coupled
mortal
Hampton
worthy
immune
Ava
##gun
encouraging
simplified
##ssa
##nte
##ann
Providence
entities
Pablo
Strong
Housing
##ista
##ators
kidnapped
mosque
Kirk
whispers
fruits
shattered
fossil
Empress
Johns
Webster
Thing
refusing
differently
specimen
Ha
##EN
##tina
##elle
##night
Horn
neighbourhood
Bolivia
##rth
genres
Pre
##vich
Amelia
swallow
Tribune
Forever
Psychology
Use
##bers
Gazette
ash
##usa
Monster
##cular
delegation
blowing
Oblast
retreated
automobile
##ex
profits
shirts
devil
Treasury
##backs
Drums
Ronnie
gameplay
expertise
Evening
resides
Caesar
unity
Crazy
linking
Vision
donations
Isabel
valve
Sue
WWE
logical
availability
fitting
revolt
##mill
Linux
taxi
Access
pollution
statues
Augustus
##pen
cello
##some
lacking
##ati
Gwen
##aka
##ovich
1821
Wow
initiatives
Uruguay
Cain
stroked
examine
##ī
mentor
moist
disorders
buttons
##tica
##anna
Species
Lynch
museums
scorer
Poor
eligibility
op
unveiled
cats
Title
wheat
critically
Syracuse
##osis
marketed
enhance
Ryder
##NG
##ull
##rna
embedded
throws
foods
happily
##ami
lesson
formats
punched
##rno
expressions
qualities
##sal
Gods
##lity
elect
wives
##lling
jungle
Toyota
reversed
Grammar
Cloud
Agnes
##ules
disputed
verses
Lucien
threshold
##rea
scanned
##bled
##dley
##lice
Kazakhstan
Gardner
Freeman
##rz
inspection
Rita
accommodation
advances
chill
Elliot
thriller
Constantinople
##mos
debris
whoever
1810
Santo
Carey
remnants
Guatemala
##irs
carriers
equations
mandatory
##WA
anxious
measurement
Summit
Terminal
Erin
##zes
LLC
##uo
glancing
sin
##₃
Downtown
flowering
Euro
Leigh
Lance
warn
decent
recommendations
##ote
Quartet
##rrell
Clarence
colleague
guarantee
230
Clayton
Beast
addresses
prospect
destroyer
vegetables
Leadership
fatal
prints
190
##makers
Hyde
persuaded
illustrations
Southampton
Joyce
beats
editors
mount
##grave
Malaysian
Bombay
endorsed
##sian
##bee
applying
Religion
nautical
bomber
Na
airfield
gravel
##rew
Cave
bye
dig
decree
burden
Election
Hawk
Fe
##iled
reunited
##tland
liver
Teams
Put
delegates
Ella
##fect
Cal
invention
Castro
bored
##kawa
##ail
Trinidad
NASCAR
pond
develops
##pton
expenses
Zoe
Released
##rf
organs
beta
parameters
Neill
##lene
lateral
Beat
blades
Either
##hale
Mitch
##ET
##vous
Rod
burnt
phones
Rising
##front
investigating
##dent
Stephanie
##keeper
screening
##uro
Swan
Sinclair
modes
bullets
Nigerian
melody
##ques
Rifle
##12
128
##jin
charm
Venus
##tian
fusion
advocated
visitor
pinned
genera
3000
Ferry
Solo
quantity
regained
platinum
shoots
narrowly
preceded
update
##ichi
equality
unaware
regiments
ally
##tos
transmitter
locks
Seeing
outlets
feast
reopened
##ows
struggles
Buddy
1826
bark
elegant
amused
Pretty
themed
schemes
Lisbon
Te
patted
terrorism
Mystery
##croft
##imo
Madagascar
Journey
dealer
contacted
##quez
ITV
vacation
Wong
Sacramento
organisms
##pts
balcony
coloured
sheer
defines
MC
abortion
forbidden
accredited
Newfoundland
tendency
entrepreneur
Benny
Tanzania
needing
finalist
mythology
weakened
gown
sentences
Guest
websites
Tibetan
UFC
voluntary
annoyed
Welcome
honestly
correspondence
geometry
Deutsche
Biology
Help
##aya
Lines
Hector
##ael
reluctant
##ages
wears
inquiry
##dell
Holocaust
Tourism
Wei
volcanic
##mates
Visual
sorts
neighborhoods
Running
apple
shy
Laws
bend
Northeast
feminist
Speedway
Murder
visa
stuffed
fangs
transmitted
fiscal
Ain
enlarged
##ndi
Cecil
Peterson
Benson
Bedford
acceptable
##CC
##wer
purely
triangle
foster
Alberto
educator
Highland
acute
LGBT
Tina
Mi
adventures
Davidson
Honda
translator
monk
enacted
summoned
##ional
collector
Genesis
Un
liner
Di
Statistical
##CS
filter
Knox
Religious
Stella
Estonian
Turn
##ots
primitive
parishes
##lles
complexity
autobiography
rigid
cannon
pursuing
exploring
##gram
##mme
freshman
caves
Expedition
Traditional
iTunes
certification
cooling
##ort
##gna
##IT
##lman
##VA
Motion
explosive
licence
boxer
shrine
loosely
Brigadier
Savage
Brett
MVP
heavier
##elli
##gged
Buddha
Easy
spells
fails
incredibly
Georg
stern
compatible
Perfect
applies
cognitive
excessive
nightmare
neighbor
Sicily
appealed
static
##₁
Aberdeen
##leigh
slipping
bride
##guard
Um
Clyde
1818
##gible
Hal
Frost
Sanders
interactive
Hour
##vor
hurting
bull
termed
shelf
capturing
##pace
rolls
113
##bor
Chilean
teaches
##rey
exam
shipped
Twin
borrowed
##lift
Shit
##hot
Lindsay
Below
Kiev
Lin
leased
##sto
Eli
Diane
Val
subtropical
shoe
Bolton
Dragons
##rification
Vatican
##pathy
Crisis
dramatically
talents
babies
##ores
surname
##AP
##cology
cubic
opted
Archer
sweep
tends
Karnataka
Judy
stint
Similar
##nut
explicitly
##nga
interact
Mae
portfolio
clinic
abbreviated
Counties
##iko
hearts
##ı
providers
screams
Individual
##etti
Monument
##iana
accessed
encounters
gasp
##rge
defunct
Avery
##rne
nobility
useless
Phase
Vince
senator
##FL
1813
surprisingly
##illo
##chin
Boyd
rumors
equity
Gone
Hearts
chassis
overnight
Trek
wrists
submit
civic
designers
##rity
prominence
decorative
derives
starter
##AF
wisdom
Powers
reluctantly
measurements
doctoral
Noel
Gideon
Baden
Cologne
lawn
Hawaiian
anthology
##rov
Raiders
embassy
Sterling
##pal
Telugu
troubled
##FC
##bian
fountain
observe
ore
##uru
##gence
spelling
Border
grinning
sketch
Benedict
Xbox
dialects
readily
immigrant
Constitutional
aided
nevertheless
SE
tragedy
##ager
##rden
Flash
##MP
Europa
emissions
##ield
panties
Beverly
Homer
curtain
##oto
toilet
Isn
Jerome
Chiefs
Hermann
supernatural
juice
integrity
Scots
auto
Patriots
Strategic
engaging
prosecution
cleaned
Byron
investments
adequate
vacuum
laughs
##inus
##nge
Usually
Roth
Cities
Brand
corpse
##ffy
Gas
rifles
Plains
sponsorship
Levi
tray
owed
della
commanders
##ead
tactical
##rion
García
harbor
discharge
##hausen
gentleman
endless
highways
##itarian
pleaded
##eta
archive
Midnight
exceptions
instances
Gibraltar
cart
##NS
Darren
Bonnie
##yle
##iva
OCLC
bra
Jess
##EA
consulting
Archives
Chance
distances
commissioner
##AR
LL
sailors
##sters
enthusiasm
Lang
##zia
Yugoslav
confirm
possibilities
Suffolk
##eman
banner
1822
Supporting
fingertips
civilization
##gos
technically
1827
Hastings
sidewalk
strained
monuments
Floyd
Chennai
Elvis
villagers
Cumberland
strode
albeit
Believe
planets
combining
Mohammad
container
##mouth
##tures
verb
BA
Tank
Midland
screened
Gang
Democracy
Helsinki
screens
thread
charitable
##version
swiftly
ma
rational
combine
##SS
##antly
dragging
Cliff
Tasmania
quest
professionally
##aj
rap
##lion
livestock
##hua
informal
specially
lonely
Matthews
Dictionary
1816
Observatory
correspondent
constitute
homeless
waving
appreciated
Analysis
Meeting
dagger
##AL
Gandhi
flank
Giant
Choir
##not
glimpse
toe
Writer
teasing
springs
##dt
Glory
healthcare
regulated
complaint
math
Publications
makers
##hips
cement
Need
apologize
disputes
finishes
Partners
boring
ups
gains
1793
Congressional
clergy
Folk
##made
##nza
Waters
stays
encoded
spider
betrayed
Applied
inception
##urt
##zzo
wards
bells
UCLA
Worth
bombers
Mo
trademark
Piper
##vel
incorporates
1801
##cial
dim
Twelve
##word
Appeals
tighter
spacecraft
##tine
coordinates
##iac
mistakes
Zach
laptop
Teresa
##llar
##yr
favored
Nora
sophisticated
Irving
hammer
División
corporations
niece
##rley
Patterson
UNESCO
trafficking
Ming
balanced
plaque
Latvia
broader
##owed
Save
confined
##vable
Dalton
tide
##right
##ural
##num
swords
caring
##eg
IX
Acting
paved
##moto
launching
Antoine
substantially
Pride
Philharmonic
grammar
Indoor
Ensemble
enabling
114
resided
Angelo
publicity
chaired
crawled
Maharashtra
Telegraph
lengthy
preference
differential
anonymous
Honey
##itation
wage
##iki
consecrated
Bryant
regulatory
Carr
##én
functioning
watches
##ú
shifts
diagnosis
Search
app
Peters
##SE
##cat
Andreas
honours
temper
counsel
Urdu
Anniversary
maritime
##uka
harmony
##unk
essence
Lorenzo
choked
Quarter
indie
##oll
loses
##prints
amendment
Adolf
scenario
similarities
##rade
##LC
technological
metric
Russians
thoroughly
##tead
cruiser
1806
##nier
1823
Teddy
##psy
au
progressed
exceptional
broadcaster
partnered
fitness
irregular
placement
mothers
unofficial
Garion
Johannes
1817
regain
Solar
publishes
Gates
Broken
thirds
conversations
dive
Raj
contributor
quantities
Worcester
governance
##flow
generating
pretending
Belarus
##voy
radius
skating
Marathon
1819
affection
undertook
##wright
los
##bro
locate
PS
excluded
recreation
tortured
jewelry
moaned
##logue
##cut
Complete
##rop
117
##II
plantation
whipped
slower
crater
##drome
Volunteer
attributes
celebrations
regards
Publishers
oath
utilized
Robbie
Giuseppe
fiber
indication
melted
archives
Damien
storey
affecting
identifying
dances
alumni
comparable
upgrade
rented
sprint
##kle
Marty
##lous
treating
railways
Lebanese
erupted
occupy
sympathy
Jude
Darling
Qatar
drainage
McCarthy
heel
Klein
computing
wireless
flip
Du
Bella
##ast
##ssen
narrator
mist
sings
alignment
121
2020
securing
##rail
Progress
missionaries
brutal
mercy
##shing
Hip
##ache
##olo
switching
##here
Malay
##ob
constituted
Mohammed
Often
standings
surge
teachings
ink
detached
systematic
Trial
Myanmar
##wo
offs
Reyes
decoration
translations
wherever
reviewer
speculation
Bangkok
terminated
##ester
beard
RCA
Aidan
Associated
Emerson
Charity
1803
generous
Dudley
ATP
##haven
prizes
toxic
gloves
##iles
##dos
Turning
myth
Parade
##building
Hits
##eva
teamed
Above
Duchess
Holt
##oth
Sub
Ace
atomic
inform
Ship
depend
Jun
##bes
Norwich
globe
Baroque
Christina
Cotton
Tunnel
kidding
Concerto
Brittany
tasted
phases
stems
angles
##TE
##nam
##40
charted
Alison
intensive
Willis
glory
##lit
Bergen
est
taller
##dicate
labeled
##ido
commentator
Warrior
Viscount
shortened
aisle
Aria
Spike
spectators
goodbye
overlooking
mammals
##lude
wholly
Barrett
##gus
accompany
seventy
employ
##mb
ambitious
beloved
basket
##mma
##lding
halted
descendant
pad
exclaimed
cloak
##pet
Strait
Bang
Aviv
sadness
##ffer
Donovan
1880s
agenda
swinging
##quin
jerk
Boat
##rist
nervously
Silence
Echo
shout
implies
##iser
##cking
Shiva
Weston
damages
##tist
effectiveness
Horace
cycling
Rey
ache
Photography
PDF
Dear
leans
Lea
##vision
booth
attained
disbelief
##eus
##ution
Hop
pension
toys
Eurovision
faithful
##heads
Andre
owe
default
Atlas
Megan
highlights
lovers
Constantine
Sixth
masses
##garh
emerge
Auto
Slovak
##oa
##vert
Superintendent
flicked
inventor
Chambers
Frankie
Romeo
pottery
companions
Rudolf
##liers
diary
Unless
tap
alter
Randall
##ddle
##eal
limitations
##boards
utterly
knelt
guaranteed
Cowboys
Islander
horns
##ike
Wendy
sexually
Smart
breasts
##cian
compromise
Duchy
AT
Galaxy
analog
Style
##aking
weighed
Nigel
optional
Czechoslovakia
practicing
Ham
##0s
feedback
batted
uprising
operative
applicable
criminals
classrooms
Somehow
##ode
##OM
Naomi
Winchester
##pping
Bart
Regina
competitor
Recorded
Yuan
Vera
lust
Confederation
##test
suck
1809
Lambert
175
Friend
##ppa
Slowly
##⁺
Wake
Dec
##aneous
chambers
Color
Gus
##site
Alternative
##world
Exeter
Omaha
celebrities
striker
210
dwarf
meals
Oriental
Pearson
financing
revenues
underwater
Steele
screw
Feeling
Mt
acids
badge
swore
theaters
Moving
admired
lung
knot
penalties
116
fork
##cribed
Afghan
outskirts
Cambodia
oval
wool
fossils
Ned
Countess
Darkness
delicious
##nica
Evelyn
Recordings
guidelines
##CP
Sandra
meantime
Antarctica
modeling
granddaughter
##rial
Roma
Seventh
Sunshine
Gabe
##nton
Shop
Turks
prolific
soup
parody
##nta
Judith
disciplines
resign
Companies
Libya
Jets
inserted
Mile
retrieve
filmmaker
##rand
realistic
unhappy
##30
sandstone
##nas
##lent
##ush
##rous
Brent
trash
Rescue
##unted
Autumn
disgust
flexible
infinite
sideways
##oss
##vik
trailing
disturbed
50th
Newark
posthumously
##rol
Schmidt
Josef
##eous
determining
menu
Pole
Anita
Luc
peaks
118
Yard
warrant
generic
deserted
Walking
stamp
tracked
##berger
paired
surveyed
sued
Rainbow
##isk
Carpenter
submarines
realization
touches
sweeping
Fritz
module
Whether
resembles
##form
##lop
unsure
hunters
Zagreb
unemployment
Senators
Georgetown
##onic
Barker
foul
commercials
Dresden
Words
collision
Carlton
Fashion
doubted
##ril
precision
MIT
Jacobs
mob
Monk
retaining
gotta
##rod
remake
Fast
chips
##pled
sufficiently
##lights
delivering
##enburg
Dancing
Barton
Officers
metals
##lake
religions
##ré
motivated
differs
dorsal
##birds
##rts
Priest
polished
##aling
Saxony
Wyatt
knockout
##hor
Lopez
RNA
##link
metallic
##kas
daylight
Montenegro
##lining
wrapping
resemble
Jam
Viking
uncertainty
angels
enables
##fy
Stuttgart
tricks
tattoo
127
wicked
asset
breach
##yman
MW
breaths
Jung
im
1798
noon
vowel
##qua
calmly
seasonal
chat
ingredients
cooled
Randolph
ensuring
##ib
##idal
flashing
1808
Macedonian
Cool
councils
##lick
advantages
Immediately
Madras
##cked
Pain
fancy
chronic
Malayalam
begged
##nese
Inner
feathers
##vey
Names
dedication
Sing
pan
Fischer
nurses
Sharp
inning
stamps
Meg
##ello
edged
motioned
Jacksonville
##ffle
##dic
##US
divide
garnered
Ranking
chasing
modifications
##oc
clever
midst
flushed
##DP
void
##sby
ambulance
beaches
groan
isolation
strengthen
prevention
##ffs
Scouts
reformed
geographic
squadrons
Fiona
Kai
Consequently
##uss
overtime
##yas
Fr
##BL
Papua
Mixed
glances
Haiti
Sporting
sandy
confronted
René
Tanner
1811
##IM
advisory
trim
##ibe
González
gambling
Jupiter
##ility
##owski
##nar
122
apology
teased
Pool
feminine
wicket
eagle
shiny
##lator
blend
peaking
nasty
nodding
fraction
tech
Noble
Kuwait
brushing
Italia
Canberra
duet
Johan
1805
Written
cameo
Stalin
pig
cord
##zio
Surely
SA
owing
holidays
123
Ranger
lighthouse
##ige
miners
1804
##ë
##gren
##ried
crashing
##atory
wartime
highlight
inclined
Torres
Tax
##zel
##oud
Own
##corn
Divine
EMI
Relief
Northwestern
ethics
BMW
click
plasma
Christie
coordinator
Shepherd
washing
cooked
##dio
##eat
Cerambycidae
algebra
Engine
costumes
Vampire
vault
submission
virtue
assumption
##rell
Toledo
##oting
##rva
crept
emphasized
##lton
##ood
Greeks
surgical
crest
Patrol
Beta
Tessa
##GS
pizza
traits
rats
Iris
spray
##GC
Lightning
binary
escapes
##take
Clary
crowds
##zong
hauled
maid
##fen
Manning
##yang
Nielsen
aesthetic
sympathetic
affiliation
soaked
Mozart
personalities
begging
##iga
clip
Raphael
yearly
Lima
abundant
##lm
1794
strips
Initiative
reporters
##vsky
consolidated
##itated
Civic
rankings
mandate
symbolic
##ively
1807
rental
duck
nave
complications
##nor
Irene
Nazis
haunted
scholarly
Pratt
Gran
Embassy
Wave
pity
genius
bats
canton
Tropical
marker
##cos
escorted
Climate
##posed
appreciation
freezing
puzzle
Internal
pools
Shawn
pathway
Daniels
Fitzgerald
extant
olive
Vanessa
marriages
cocked
##dging
prone
chemicals
doll
drawer
##HF
Stark
Property
##tai
flowed
Sheridan
##uated
Less
Omar
remarks
catalogue
Seymour
wreck
Carrie
##bby
Mercer
displaced
sovereignty
rip
Flynn
Archie
Quarterfinals
Hassan
##ards
vein
Osaka
pouring
wages
Romance
##cript
##phere
550
##eil
##stown
Documentary
ancestor
CNN
Panthers
publishers
Rise
##mu
biting
Bright
String
succeeding
119
loaned
Warwick
Sheikh
Von
Afterwards
Jax
Camden
helicopters
Hence
Laurel
##ddy
transaction
Corp
clause
##owing
##kel
Investment
cups
Lucia
Moss
Giles
chef
López
decisive
30th
distress
linguistic
surveys
Ready
maiden
Touch
frontier
incorporate
exotic
mollusk
Leopold
Ride
##wain
##ndo
teammates
tones
drift
ordering
Feb
Penny
Normandy
Present
Flag
pipes
##rro
delight
motto
Tibet
leap
Eliza
Produced
teenagers
sitcom
Try
Hansen
Cody
wandered
terrestrial
frog
scare
resisted
employers
coined
##DS
resistant
Fly
captive
dissolution
judged
associates
defining
##court
Hale
##mbo
raises
clusters
twelfth
##metric
Roads
##itude
satisfy
Android
Reds
Gloucester
Category
Valencia
Daemon
stabbed
Luna
Churches
Canton
##eller
Attack
Kashmir
annexed
grabs
asteroid
Hartford
recommendation
Rodriguez
handing
stressed
frequencies
delegate
Bones
Erie
Weber
Hands
Acts
millimetres
24th
Fat
Howe
casually
##SL
convent
1790
IF
##sity
1795
yelling
##ises
drain
addressing
amino
Marcel
Sylvia
Paramount
Gerard
Volleyball
butter
124
Albion
##GB
triggered
1792
folding
accepts
##ße
preparations
Wimbledon
dose
##grass
escaping
##tling
import
charging
##dation
280
Nolan
##fried
Calcutta
##pool
Cove
examining
minded
heartbeat
twisting
domains
bush
Tunisia
Purple
Leone
##code
evacuated
battlefield
tiger
Electrical
##ared
chased
##cre
cultivated
Jet
solved
shrug
ringing
Impact
##iant
kilometre
##log
commemorate
migrated
singular
designing
promptly
Higgins
##own
##aves
freshwater
Marketing
Payne
beg
locker
pray
implied
AAA
corrected
Trans
Europeans
Ashe
acknowledge
Introduction
##writer
##llen
Munster
auxiliary
growl
Hours
Poems
##AT
reduces
Plain
plague
canceled
detention
polite
necklace
Gustav
##gu
##lance
En
Angola
##bb
dwelling
##hea
5000
Qing
Dodgers
rim
##ored
##haus
spilled
Elisabeth
Viktor
backpack
1802
amended
##worthy
Phantom
##ctive
keeper
##loom
Vikings
##gua
employs
Tehran
specialty
##bate
Marx
Mirror
Jenna
rides
needle
prayers
clarinet
forewings
##walk
Midlands
convincing
advocacy
Cao
Birds
cycles
Clement
Gil
bubble
Maximum
humanitarian
Tan
cries
##SI
Parsons
Trio
offshore
Innovation
clutched
260
##mund
##duct
Prairie
relied
Falcon
##ste
Kolkata
Gill
Swift
Negro
Zoo
valleys
##OL
Opening
beams
MPs
outline
Bermuda
Personal
exceed
productive
##MT
republic
forum
##sty
tornado
Known
dipped
Edith
folks
mathematician
watershed
Ricardo
synthetic
##dication
deity
##₄
gaming
subjected
suspects
Foot
swollen
Motors
##tty
##ý
aloud
ceremonial
es
nuts
intend
Carlisle
tasked
hesitation
sponsors
unified
inmates
##ctions
##stan
tiles
jokes
whereby
outcomes
Lights
scary
Stoke
Portrait
Blind
sergeant
violations
cultivation
fuselage
Mister
Alfonso
candy
sticks
teen
agony
Enough
invite
Perkins
Appeal
mapping
undergo
Glacier
Melanie
affects
incomplete
##dd
Colombian
##nate
CBC
purchasing
bypass
Drug
Electronics
Frontier
Coventry
##aan
autonomy
scrambled
Recent
bounced
cow
experiencing
Rouge
cuisine
Elite
disability
Ji
inheritance
wildly
Into
##wig
confrontation
Wheeler
shiver
Performing
aligned
consequently
Alexis
Sin
woodland
executives
Stevenson
Ferrari
inevitable
##cist
##dha
##base
Corner
comeback
León
##eck
##urus
MacDonald
pioneering
breakdown
landscapes
Veterans
Rican
Theological
stirred
participant
Credit
Hyderabad
snails
Claudia
##ocene
compliance
##MI
Flags
Middlesex
storms
winding
asserted
er
##ault
##kal
waking
##rates
abbey
Augusta
tooth
trustees
Commodore
##uded
Cunningham
NC
Witch
marching
Sword
Same
spiral
Harley
##ahan
Zack
Audio
1890s
##fit
Simmons
Kara
Veronica
negotiated
Speaking
FIBA
Conservatory
formations
constituencies
explicit
facial
eleventh
##ilt
villain
##dog
##case
##hol
armored
tin
hairs
##umi
##rai
mattress
Angus
cease
verbal
Recreation
savings
Aurora
peers
Monastery
Airways
drowned
additions
downstream
sticking
Shi
mice
skiing
##CD
Raw
Riverside
warming
hooked
boost
memorable
posed
treatments
320
##dai
celebrating
blink
helpless
circa
Flowers
PM
uncommon
Oct
Hawks
overwhelmed
Sparhawk
repaired
Mercy
pose
counterpart
compare
survives
##½
##eum
coordinate
Lil
grandchildren
notorious
Yi
Judaism
Juliet
accusations
1789
floated
marathon
roar
fortified
reunion
145
Nov
Paula
##fare
##toria
tearing
Cedar
disappearance
Si
gifted
scar
270
PBS
Technologies
Marvin
650
roller
cupped
negotiate
##erman
passport
tram
miracle
styled
##tier
necessity
Des
rehabilitation
Lara
USD
psychic
wipe
##lem
mistaken
##lov
charming
Rider
pageant
dynamics
Cassidy
##icus
defenses
##tadt
##vant
aging
##inal
declare
mistress
supervised
##alis
##rest
Ashton
submerged
sack
Dodge
grocery
ramp
Teacher
lineage
imagery
arrange
inscriptions
Organisation
Siege
combines
pounded
Fleming
legends
columnist
Apostolic
prose
insight
Arabian
expired
##uses
##nos
Alone
elbows
##asis
##adi
##combe
Step
Waterloo
Alternate
interval
Sonny
plains
Goals
incorporating
recruit
adjoining
Cheshire
excluding
marrying
ducked
Cherokee
par
##inate
hiking
Coal
##bow
natives
ribbon
Allies
con
descriptions
positively
##lal
defendant
22nd
Vivian
##beat
Weather
possessions
Date
sweetheart
inability
Salisbury
adviser
ideology
Nordic
##eu
Cubs
IP
Administrative
##nick
facto
liberation
Burnett
Javier
fashioned
Electoral
Turin
theft
unanimous
Per
1799
Clan
Hawkins
Teachers
##wes
Cameroon
Parkway
##gment
demolition
atoms
nucleus
##thi
recovering
##yte
##vice
lifts
Must
deposit
Hancock
Semi
darkened
Declaration
moan
muscular
Myers
attractions
sauce
simulation
##weed
Alps
barriers
##baum
Barack
galleries
Min
holders
Greenwich
donation
Everybody
Wolfgang
sandwich
Kendra
Collegiate
casino
Slavic
ensuing
Porto
##grapher
Jesuit
suppressed
tires
Ibrahim
protesters
Ibn
Amos
1796
phenomena
Hayden
Paraguay
Squad
Reilly
complement
aluminum
##eers
doubts
decay
demise
Practice
patience
fireplace
transparent
monarchy
##person
Rodney
mattered
rotating
Clifford
disposal
Standards
paced
##llie
arise
tallest
tug
documentation
node
freeway
Nikolai
##cite
clicked
imaging
Lorraine
Tactical
Different
Regular
Holding
165
Pilot
guarded
##polis
Classics
Mongolia
Brock
monarch
cellular
receptors
Mini
Chandler
financed
financially
Lives
erection
Fuller
unnamed
Kannada
cc
passive
plateau
##arity
freak
##rde
retrieved
transactions
##sus
23rd
swimmer
beef
fulfill
Arlington
offspring
reasoning
Rhys
saves
pseudonym
centimetres
shivered
shuddered
##ME
Feel
##otic
professors
Blackburn
##eng
##life
##haw
interred
lodge
fragile
Della
guardian
##bbled
catalog
clad
observer
tract
declaring
##headed
Lok
dean
Isabelle
1776
irrigation
spectacular
shuttle
mastering
##aro
Nathaniel
Retired
##lves
Brennan
##kha
dick
##dated
##hler
Rookie
leapt
televised
weekends
Baghdad
Yemen
##fo
factions
ion
Lab
mortality
passionate
Hammer
encompasses
confluence
demonstrations
Ki
derivative
soils
##unch
Ranch
Universities
conventions
outright
aiming
hierarchy
reside
illusion
graves
rituals
126
Antwerp
Dover
##ema
campuses
Hobart
lifelong
aliens
##vity
Memory
coordination
alphabet
##mina
Titans
pushes
Flanders
##holder
Normal
excellence
capped
profound
Taipei
portrayal
sparked
scratch
se
##eas
##hir
Mackenzie
##cation
Neo
Shin
##lined
magnificent
poster
batsman
##rgent
persuade
##ement
Icelandic
miserable
collegiate
Feature
geography
##mura
Comic
Circus
processor
barracks
Tale
##11
Bulls
##rap
strengthened
##bell
injection
miniature
broadly
Letter
fare
hostage
traders
##nium
##mere
Fortune
Rivera
Lu
triumph
Browns
Bangalore
cooperative
Basel
announcing
Sawyer
##him
##cco
##kara
darted
##AD
##nova
sucking
##position
perimeter
flung
Holdings
##NP
Basque
sketches
Augustine
Silk
Elijah
analyst
armour
riots
acquiring
ghosts
##ems
132
Pioneer
Colleges
Simone
Economy
Author
semester
Soldier
il
##unting
##bid
freaking
Vista
tumor
##bat
murderer
##eda
unreleased
##grove
##sser
##té
edit
statute
sovereign
##gawa
Killer
stares
Fury
comply
##lord
##nant
barrels
Andhra
Maple
generator
mascot
unusually
eds
##ante
##runner
rod
##tles
Historically
Jennings
dumped
Established
resemblance
##lium
##cise
##body
##voke
Lydia
##hou
##iring
nonetheless
1797
corrupt
patrons
physicist
sneak
Livingston
Citizens
Architects
Werner
trends
Melody
eighty
markings
brakes
##titled
oversaw
processed
mock
Midwest
intervals
##EF
stretches
werewolf
##MG
Pack
controller
##dition
Honours
cane
Griffith
vague
repertoire
Courtney
orgasm
Abdullah
dominance
occupies
Ya
introduces
Lester
instinct
collaborative
Indigenous
refusal
##rank
outlet
debts
spear
155
##keeping
##ulu
Catalan
##osh
tensions
##OT
bred
crude
Dunn
abdomen
accurately
##fu
##lough
accidents
Row
Audrey
rude
Getting
promotes
replies
Paolo
merge
##nock
trans
Evangelical
automated
Canon
##wear
##ggy
##gma
Broncos
foolish
icy
Voices
knives
Aside
dreamed
generals
molecule
AG
rejection
insufficient
##nagar
deposited
sacked
Landing
arches
helpful
devotion
intake
Flower
PGA
dragons
evolutionary
##mail
330
GM
tissues
##tree
arcade
composite
lid
Across
implications
lacks
theological
assessed
concentrations
Den
##mans
##ulous
Fu
homeland
##stream
Harriet
ecclesiastical
troop
ecological
winked
##xed
eighteenth
Casino
specializing
##sworth
unlocked
supreme
devastated
snatched
trauma
GDP
Nord
saddle
Wes
convenient
competes
##nu
##iss
Marian
subway
##rri
successes
umbrella
##far
##ually
Dundee
##cence
spark
##rix
##я
Quality
Geological
cockpit
rpm
Cam
Bucharest
riot
##PM
Leah
##dad
##pose
Ka
m³
Bundesliga
Wolfe
grim
textile
quartet
expressing
fantastic
destroyers
eternal
picnic
##oro
contractor
1775
spanning
declining
##cating
Lowe
Sutherland
Emirates
downward
nineteen
violently
scout
viral
melting
enterprises
##cer
Crosby
Jubilee
antenna
urgent
Rory
##uin
##sure
wandering
##gler
##vent
Suzuki
Lifetime
Dirty
occupying
##quent
Disc
Guru
mound
Lennon
Humanities
listeners
Walton
uh
Braves
Bologna
##bis
##gra
Dwight
crawl
flags
memoir
Thorne
Archdiocese
dairy
##uz
##tery
roared
adjust
patches
inn
Knowing
##bbed
##zan
scan
Papa
precipitation
angrily
passages
postal
Phi
embraced
blacks
economist
triangular
Sen
shooter
punished
Millennium
Swimming
confessed
Aston
defeats
Era
cousins
Williamson
##rer
daytime
dumb
##rek
underway
specification
Buchanan
prayed
concealed
activation
##issa
canon
awesome
Starr
plural
summers
##fields
Slam
unnecessary
1791
resume
trilogy
compression
##rough
selective
dignity
Yan
##xton
immense
##yun
lone
seeded
hiatus
lightweight
summary
Yo
approve
Galway
rejoined
Elise
garbage
burns
speeches
129
Honduras
##liness
inventory
jersey
FK
assure
slumped
Lionel
Suite
##sbury
Lena
continuation
##AN
brightly
##nti
GT
Knowledge
##park
##lius
lethal
##tribution
##sions
Certificate
Mara
##lby
algorithms
Jade
blows
pirates
fleeing
wheelchair
Stein
sophomore
Alt
Territorial
diploma
snakes
##olic
##tham
Tiffany
Pius
flush
urging
Hanover
Reich
##olate
Unity
Pike
collectively
Theme
ballad
kindergarten
rocked
zoo
##page
whip
Rodríguez
strokes
checks
Becky
Stern
upstream
##uta
Silent
volunteered
Sigma
##ingen
##tract
##ede
Gujarat
screwed
entertaining
##action
##ryn
defenders
innocence
lesbian
que
Richie
nodes
Lie
juvenile
Jakarta
safer
confront
Bert
breakthrough
gospel
Cable
##zie
institutional
Archive
brake
liquor
feeds
##iate
chancellor
Encyclopedia
Animation
scanning
teens
##mother
Core
Rear
Wine
##flower
reactor
Ave
cardinal
sodium
strands
Olivier
crouched
Vaughan
Sammy
Image
scars
Emmanuel
flour
bias
nipple
revelation
##ucci
Denny
##ssy
Form
Runners
admits
Rama
violated
Burmese
feud
underwear
Mohamed
Named
swift
statewide
Door
Recently
comparing
Hundred
##idge
##nity
##rds
Rally
Reginald
Auburn
solving
waitress
Treasurer
##ilization
Halloween
Ministers
Boss
Shut
##listic
Rahman
demonstrating
##pies
Gaza
Yuri
installations
Math
schooling
##bble
Bronx
exiled
gasoline
133
bundle
humid
FCC
proportional
relate
VFL
##dez
continuity
##cene
syndicated
atmospheric
arrows
Wanderers
reinforcements
Willow
Lexington
Rotten
##yon
discovering
Serena
portable
##lysis
targeting
£1
Goodman
Steam
sensors
detachment
Malik
##erie
attitudes
Goes
Kendall
Read
Sleep
beans
Nikki
modification
Jeanne
knuckles
Eleven
##iously
Gross
Jaime
dioxide
moisture
Stones
UCI
displacement
Metacritic
Jury
lace
rendering
elephant
Sergei
##quire
GP
Abbott
##type
projection
Mouse
Bishops
whispering
Kathleen
Rams
##jar
whites
##oran
assess
dispatched
##hire
kin
##mir
Nursing
advocates
tremendous
sweater
assisting
##bil
Farmer
prominently
reddish
Hague
cyclone
##SD
Sage
Lawson
Sanctuary
discharged
retains
##ube
shotgun
wilderness
Reformed
similarity
Entry
Watts
Bahá
Quest
Looks
visions
Reservoir
Arabs
curls
Blu
dripping
accomplish
Verlag
drill
sensor
Dillon
physicians
smashed
##dir
painters
Renault
straw
fading
Directorate
lounge
commissions
Brain
##graph
neo
##urg
plug
coordinated
##houses
Critical
lamps
illustrator
Returning
erosion
Crow
##ciation
blessing
Thought
Wife
medalist
synthesizer
Pam
Thornton
Esther
HBO
fond
Associates
##raz
pirate
permits
Wide
tire
##PC
Ernie
Nassau
transferring
RFC
##ntly
um
spit
AS
##mps
Mining
polar
villa
anchored
##zzi
embarrassment
relates
##ă
Rupert
counterparts
131
Baxter
##18
Igor
recognizes
Clive
##hane
##eries
##ibly
occurrence
##scope
fin
colorful
Rapids
banker
tile
##rative
##dus
delays
destinations
##llis
Pond
Dane
grandparents
rewarded
socially
motorway
##hof
##lying
##human
modeled
Dayton
Forward
conscience
Sharma
whistle
Mayer
Sasha
##pical
circuits
Zhou
##ça
Latvian
finalists
predators
Lafayette
closes
obligations
Resolution
##vier
Trustees
reminiscent
##hos
Highlands
Protected
asylum
evacuation
##acy
Chevrolet
confession
Somalia
emergence
separating
##rica
alright
calcium
Laurent
Welfare
Leonardo
ashes
dental
Deal
minerals
##lump
##mount
accounted
staggered
slogan
photographic
builder
##imes
##raft
tragic
144
SEC
Hit
tailed
##ples
##rring
##rson
ethical
wrestlers
concludes
lunar
##ept
nitrogen
Aid
cyclist
quarterfinals
##ه
harvest
##hem
Pasha
IL
##mis
continually
##forth
Intel
bucket
##ended
witches
pretended
dresses
viewer
peculiar
lowering
volcano
Marilyn
Qualifier
clung
##sher
Cut
modules
Bowie
##lded
onset
transcription
residences
##pie
##itor
scrapped
##bic
Monaco
Mayo
eternity
Strike
uncovered
skeleton
##wicz
Isles
bug
Promoted
##rush
Mechanical
XII
##ivo
gripping
stubborn
velvet
TD
decommissioned
operas
spatial
unstable
Congressman
wasted
##aga
##ume
advertisements
##nya
obliged
Cannes
Conway
bricks
##gnant
##mity
##uise
jumps
Clear
##cine
##sche
chord
utter
Su
podium
spokesman
Royce
assassin
confirmation
licensing
liberty
##rata
Geographic
individually
detained
##ffe
Saturn
crushing
airplane
bushes
knights
##PD
Lilly
hurts
unexpectedly
Conservatives
pumping
Forty
candle
Pérez
peasants
supplement
Sundays
##ggs
##rries
risen
enthusiastic
corresponds
pending
##IF
Owens
floods
Painter
inflation
presumed
inscribed
Chamberlain
bizarre
1200
liability
reacted
tub
Legacy
##eds
##pted
shone
##litz
##NC
Tiny
genome
bays
Eduardo
robbery
stall
hatch
Depot
Variety
Flora
reprinted
trembled
outlined
CR
Theresa
spans
##plication
Jensen
##eering
posting
##rky
pays
##ost
Marcos
fortifications
inferior
##ential
Devi
despair
Talbot
##chus
updates
ego
Booth
Darius
tops
##lau
Scene
##DC
Harlem
Trey
Generally
candles
##α
Neville
Admiralty
##hong
iconic
victorious
1600
Rowan
abundance
miniseries
clutching
sanctioned
##words
obscure
##ision
##rle
##EM
disappearing
Resort
Obviously
##eb
exceeded
1870s
Adults
##cts
Cry
Kerr
ragged
selfish
##lson
circled
pillars
galaxy
##asco
##mental
rebuild
caution
Resistance
Start
bind
splitting
Baba
Hogan
ps
partnerships
slam
Peggy
courthouse
##OD
organizational
packages
Angie
##nds
possesses
##rp
Expressway
Gould
Terror
Him
Geoff
nobles
##ope
shark
##nh
identifies
##oor
testified
Playing
##ump
##isa
stool
Idol
##pice
##tana
Byrne
Gerry
grunted
26th
observing
habits
privilege
immortal
wagons
##thy
dot
Bring
##lian
##witz
newest
##uga
constraints
Screen
Issue
##RNA
##vil
reminder
##gles
addiction
piercing
stunning
var
##rita
Signal
accumulated
##wide
float
devastating
viable
cartoons
Uttar
flared
##encies
Theology
patents
##bahn
privileges
##ava
##CO
137
##oped
##NT
orchestral
medication
225
erect
Nadia
École
fried
Sales
scripts
##rease
airs
Cage
inadequate
structured
countless
Avengers
Kathy
disguise
mirrors
Investigation
reservation
##nson
Legends
humorous
Mona
decorations
attachment
Via
motivation
Browne
strangers
##ński
Shadows
Twins
##pressed
Alma
Nominated
##ott
Sergio
canopy
152
Semifinals
devised
##irk
upwards
Traffic
Goddess
Move
beetles
138
spat
##anne
holdings
##SP
tangled
Whilst
Fowler
anthem
##ING
##ogy
snarled
moonlight
songwriting
tolerance
Worlds
exams
##pia
notices
sensitivity
poetic
Stephens
Boone
insect
reconstructed
Fresh
27th
balloon
##ables
Brendan
mug
##gee
1780
apex
exports
slides
Lahore
hiring
Shell
electorate
sexuality
poker
nonprofit
##imate
cone
##uce
Okinawa
superintendent
##HC
referenced
turret
Sprint
Citizen
equilibrium
Stafford
curb
Driver
Valerie
##rona
aching
impacts
##bol
observers
Downs
Shri
##uth
airports
##uda
assignments
curtains
solitary
icon
patrols
substances
Jasper
mountainous
Published
ached
##ingly
announce
dove
damaging
##tism
Primera
Dexter
limiting
batch
##uli
undergoing
refugee
Ye
admiral
pavement
##WR
##reed
pipeline
desires
Ramsey
Sheila
thickness
Brotherhood
Tea
instituted
Belt
Break
plots
##ais
masculine
##where
Theo
##aged
##mined
Experience
scratched
Ethiopian
Teaching
##nov
Aiden
Abe
Samoa
conditioning
##mous
Otherwise
fade
Jenks
##encing
Nat
##lain
Anyone
##kis
smirk
Riding
##nny
Bavarian
blessed
potatoes
Hook
##wise
likewise
hardened
Merry
amid
persecution
##sten
Elections
Hoffman
Pitt
##vering
distraction
exploitation
infamous
quote
averaging
healed
Rhythm
Germanic
Mormon
illuminated
guides
##ische
interfere
##ilized
rector
perennial
##ival
Everett
courtesy
##nham
Kirby
Mk
##vic
Medieval
##tale
Luigi
limp
##diction
Alive
greeting
shove
##force
##fly
Jasmine
Bend
Capt
Suzanne
ditch
134
##nning
Host
fathers
rebuilding
Vocal
wires
##manship
tan
Factor
fixture
##LS
Māori
Plate
pyramid
##umble
slap
Schneider
yell
##ulture
##tional
Goodbye
sore
##pher
depressed
##dox
pitching
Find
Lotus
##wang
strand
Teen
debates
prevalent
##bilities
exposing
hears
billed
##rse
reorganized
compelled
disturbing
displaying
##tock
Clinical
emotionally
##iah
Derbyshire
grouped
##quel
Bahrain
Journalism
IN
persistent
blankets
Crane
camping
Direct
proving
Lola
##dding
Corporate
birthplace
##boats
##ender
Figure
dared
Assam
precursor
##nched
Tribe
Restoration
slate
Meyrick
hunted
stroking
Earlier
Kind
polls
appeals
monetary
##reate
Kira
Langdon
explores
GPS
extensions
squares
Results
draped
announcer
merit
##ennial
##tral
##roved
##cion
robots
supervisor
snorted
##group
Cannon
procession
monkey
freeze
sleeves
Nile
verdict
ropes
firearms
extraction
tensed
EC
Saunders
##tches
diamonds
Marriage
##amble
curling
Amazing
##haling
unrelated
##roads
Daughter
cum
discarded
kidney
cliffs
forested
Candy
##lap
authentic
tablet
notation
##nburg
Bulldogs
Callum
Meet
mouths
coated
##xe
Truman
combinations
##mation
Steelers
Fan
Than
paternal
##father
##uti
Rebellion
inviting
Fun
theatres
##ي
##rom
curator
##cision
networking
Oz
drought
##ssel
granting
MBA
Shelby
Elaine
jealousy
Kyoto
shores
signaling
tenants
debated
Intermediate
Wise
##hes
##pu
Havana
duke
vicious
exited
servers
Nonetheless
Reports
explode
##beth
Nationals
offerings
Oval
conferred
eponymous
folklore
##NR
Shire
planting
1783
Zeus
accelerated
Constable
consuming
troubles
McCartney
texture
bust
Immigration
excavated
hopefully
##cession
##coe
##name
##ully
lining
Einstein
Venezuelan
reissued
minorities
Beatrice
crystals
##nies
circus
lava
Beirut
extinction
##shu
Becker
##uke
issuing
Zurich
extract
##esta
##rred
regulate
progression
hut
alcoholic
plea
AB
Norse
Hubert
Mansfield
ashamed
##put
Bombardment
stripes
electrons
Denise
horrified
Nor
arranger
Hay
Koch
##ddling
##iner
Birthday
Josie
deliberate
explorer
##jiang
##signed
Arrow
wiping
satellites
baritone
mobility
##rals
Dorset
turbine
Coffee
185
##lder
Cara
Colts
pits
Crossing
coral
##birth
Tai
zombie
smoothly
##hp
mates
##ady
Marguerite
##tary
puzzled
tapes
overly
Sonic
Prayer
Thinking
##uf
IEEE
obligation
##cliffe
Basil
redesignated
##mmy
nostrils
Barney
XIII
##phones
vacated
unused
Berg
##roid
Towards
viola
136
Event
subdivided
rabbit
recruiting
##nery
Namibia
##16
##ilation
recruits
Famous
Francesca
##hari
Goa
##lat
Karachi
haul
biblical
##cible
MGM
##rta
horsepower
profitable
Grandma
importantly
Martinez
incoming
##kill
beneficial
nominal
praying
##isch
gable
nail
noises
##ttle
Polytechnic
rub
##cope
Thor
audition
erotic
##ending
##iano
Ultimately
armoured
##mum
presently
pedestrian
##tled
Ipswich
offence
##ffin
##borne
Flemish
##hman
echo
##cting
auditorium
gentlemen
winged
##tched
Nicaragua
Unknown
prosperity
exhaust
pie
Peruvian
compartment
heights
disabilities
##pole
Harding
Humphrey
postponed
moths
Mathematical
Mets
posters
axe
##nett
Nights
Typically
chuckle
councillors
alternating
141
Norris
##ately
##etus
deficit
dreaming
cooler
oppose
Beethoven
##esis
Marquis
flashlight
headache
investor
responding
appointments
##shore
Elias
ideals
shades
torch
lingering
##real
pier
fertile
Diploma
currents
Snake
##horse
##15
Briggs
##ota
##hima
##romatic
Coastal
Kuala
ankles
Rae
slice
Hilton
locking
Approximately
Workshop
Niagara
strangely
##scence
functionality
advertisement
Rapid
Anders
ho
Soviets
packing
basal
Sunderland
Permanent
##fting
rack
tying
Lowell
##ncing
Wizard
mighty
tertiary
pencil
dismissal
torso
grasped
##yev
Sand
gossip
##nae
Beer
implementing
##19
##riya
Fork
Bee
##eria
Win
##cid
sailor
pressures
##oping
speculated
Freddie
originating
##DF
##SR
##outh
28th
melt
Brenda
lump
Burlington
USC
marginal
##bine
Dogs
swamp
cu
Ex
uranium
metro
spill
Pietro
seize
Chorus
partition
##dock
##media
engineered
##oria
conclusions
subdivision
##uid
Illustrated
Leading
##hora
Berkshire
definite
##books
##cin
##suke
noun
winced
Doris
dissertation
Wilderness
##quest
braced
arbitrary
kidnapping
Kurdish
##but
clearance
excavations
wanna
Allmusic
insult
presided
yacht
##SM
Honour
Tin
attracting
explosives
Gore
Bride
##ience
Packers
Devils
Observer
##course
Loser
##erry
##hardt
##mble
Cyrillic
undefeated
##stra
subordinate
##ame
Wigan
compulsory
Pauline
Cruise
Opposition
##ods
Period
dispersed
expose
##60
##has
Certain
Clerk
Wolves
##hibition
apparatus
allegiance
orbital
justified
thanked
##ević
Biblical
Carolyn
Graves
##tton
Hercules
backgrounds
replica
1788
aquatic
Mega
Stirling
obstacles
filing
Founder
vowels
Deborah
Rotterdam
surpassed
Belarusian
##ologists
Zambia
Ren
Olga
Alpine
bi
councillor
Oaks
Animals
eliminating
digit
Managing
##GE
laundry
##rdo
presses
slamming
Tudor
thief
posterior
##bas
Rodgers
smells
##ining
Hole
SUV
trombone
numbering
representations
Domingo
Paralympics
cartridge
##rash
Combined
shelves
Kraków
revision
##frame
Sánchez
##tracted
##bler
Alain
townships
sic
trousers
Gibbs
anterior
symmetry
vaguely
Castile
IRA
resembling
Penguin
##ulent
infections
##stant
raped
##pressive
worrying
brains
bending
JR
Evidence
Venetian
complexes
Jonah
850
exported
Ambrose
Gap
philanthropist
##atus
Marxist
weighing
##KO
##nath
Soldiers
chiefs
reject
repeating
shaky
Zürich
preserving
##xin
cigarettes
##break
mortar
##fin
Already
reproduction
socks
Waiting
amazed
##aca
dash
##path
Airborne
##harf
##get
descending
OBE
Sant
Tess
Lucius
enjoys
##ttered
##ivation
##ete
Leinster
Phillies
execute
geological
unfinished
Courts
SP
Beaver
Duck
motions
Platinum
friction
##aud
##bet
Parts
Stade
entirety
sprang
Smithsonian
coffin
prolonged
Borneo
##vise
unanimously
##uchi
Cars
Cassandra
Australians
##CT
##rgen
Louisa
spur
Constance
##lities
Patent
racism
tempo
##ssion
##chard
##nology
##claim
Million
Nichols
##dah
Numerous
ing
Pure
plantations
donor
##EP
##rip
convenience
##plate
dots
indirect
##written
Dong
failures
adapt
wizard
unfortunately
##gion
practitioners
economically
Enrique
unchanged
kingdoms
refined
definitions
lazy
worries
railing
##nay
Kaiser
##lug
cracks
sells
ninety
##WC
Directed
denotes
developmental
papal
unfortunate
disappointing
sixteenth
Jen
##urier
NWA
drifting
Horror
##chemical
behaviors
bury
surfaced
foreigners
slick
AND
##rene
##ditions
##teral
scrap
kicks
comprise
buddy
##anda
Mental
##ype
Dom
wines
Limerick
Luca
Rand
##won
Tomatoes
homage
geometric
##nted
telescope
Shelley
poles
##fan
shareholders
Autonomous
cope
intensified
Genoa
Reformation
grazing
##tern
Zhao
provisional
##bies
Con
##riel
Cynthia
Raleigh
vivid
threaten
Length
subscription
roses
Müller
##isms
robin
##tial
Laos
Stanton
nationalism
##clave
##ND
##17
##zz
staging
Busch
Cindy
relieve
##spective
packs
neglected
CBE
alpine
Evolution
uneasy
coastline
Destiny
Barber
Julio
##tted
informs
unprecedented
Pavilion
##bei
##ference
betrayal
awaiting
leaked
V8
puppet
adverse
Bourne
Sunset
collectors
##glass
##sque
copied
Demon
conceded
resembled
Rafe
Levy
prosecutor
##ject
flora
manned
deaf
Mosque
reminds
Lizzie
Products
Funny
cassette
congress
##rong
Rover
tossing
prompting
chooses
Satellite
cautiously
Reese
##UT
Huang
Gloucestershire
giggled
Kitty
##å
Pleasant
Aye
##ond
judging
1860s
intentionally
Hurling
aggression
##xy
transfers
employing
##fies
##oda
Archibald
Blessed
Ski
flavor
Rosie
##burgh
sunset
Scholarship
WC
surround
ranged
##jay
Degree
Houses
squeezing
limb
premium
Leningrad
steals
##inated
##ssie
madness
vacancy
hydraulic
Northampton
##prise
Marks
Boxing
##fying
academics
##lich
##TY
CDs
##lma
hardcore
monitors
paperback
cables
Dimitri
upside
advent
Ra
##clusive
Aug
Christchurch
objected
stalked
Simple
colonists
##laid
CT
discusses
fellowship
Carnival
cares
Miracle
pastoral
rooted
shortage
borne
Quentin
meditation
tapping
Novel
##ades
Alicia
Burn
famed
residency
Fernández
Johannesburg
Zhu
offended
Mao
outward
##inas
XV
denial
noticing
##ís
quarry
##hound
##amo
Bernie
Bentley
Joanna
mortgage
##rdi
##sumption
lenses
extracted
depiction
##RE
Networks
Broad
Revenue
flickered
virgin
flanked
##о
Enterprises
probable
Liberals
Falcons
drowning
phrases
loads
assumes
inhaled
awe
logs
slightest
spiders
waterfall
##pate
rocking
shrub
##uil
roofs
##gard
prehistoric
wary
##rak
TO
clips
sustain
treason
microphone
voter
Lamb
psychologist
wrinkled
##ères
mating
Carrier
340
##lbert
sensing
##rino
destiny
distract
weaker
UC
Nearly
neurons
spends
Apache
##rem
genuinely
wells
##lanted
stereo
##girl
Lois
Leaving
consul
fungi
Pier
Cyril
80s
Jungle
##tani
illustration
Split
##hana
Abigail
##patrick
1787
diminished
Selected
packaging
##EG
Martínez
communal
Manufacturing
sentiment
143
unwilling
praising
Citation
pills
##iti
##rax
muffled
neatly
workforce
Yep
leisure
Tu
##nding
Wakefield
ancestral
##uki
destructive
seas
Passion
showcase
##ceptive
heroic
142
exhaustion
Customs
##aker
Scholar
sliced
##inian
Direction
##OW
Swansea
aluminium
##eep
ceramic
McCoy
Career
Sector
chartered
Damascus
pictured
Interest
stiffened
Plateau
obsolete
##tant
irritated
inappropriate
overs
##nko
bail
Talent
Sur
ours
##nah
barred
legged
sociology
Bud
dictionary
##luk
Cover
obey
##oring
annoying
##dong
apprentice
Cyrus
Role
##GP
##uns
##bag
Greenland
Porsche
Rocket
##32
organism
##ntary
reliability
##vocation
##й
Found
##hine
motors
promoter
unfair
##oms
##note
distribute
eminent
rails
appealing
chiefly
meaningful
Stephan
##rehension
Consumer
psychiatric
bowler
saints
##iful
##н
1777
Pol
Dorian
Townsend
hastily
##jima
Quincy
Sol
fascinated
Scarlet
alto
Avon
certainty
##eding
Keys
##chu
Chu
##VE
ions
tributaries
Thanksgiving
##fusion
astronomer
oxide
pavilion
Supply
Casa
Bollywood
sadly
mutations
Keller
##wave
nationals
##rgo
##ym
predict
Catholicism
Vega
##eration
##ums
Mali
tuned
Lankan
Plans
radial
Bosnian
Lexi
##14
##ü
sacks
unpleasant
Empty
handles
##taking
Bon
switches
intently
tuition
antique
##jk
fraternity
notebook
Desmond
##sei
prostitution
##how
deed
##OP
501
Somewhere
Rocks
##mons
campaigned
frigate
gases
suppress
##hang
Merlin
Northumberland
dominate
expeditions
thunder
##ups
##rical
Cap
thorough
Ariel
##kind
renewable
constructing
pacing
terrorists
Bowen
documentaries
westward
##lass
##nage
Merchant
##ued
Beaumont
Din
##hian
Danube
peasant
Garrison
encourages
gratitude
reminding
stormed
##ouse
pronunciation
##ailed
Weekend
suggestions
##ffing
##DI
Active
Colombo
##logists
Merrill
##cens
Archaeological
Medina
captained
##yk
duel
cracking
Wilkinson
Guam
pickup
renovations
##ël
##izer
delighted
##iri
Weaver
##ctional
tens
##hab
Clint
##usion
##each
petals
Farrell
##sable
caste
##will
Ezra
##qi
##standing
thrilled
ambush
exhaled
##SU
Resource
blur
forearm
specifications
contingent
cafe
##iology
Antony
fundraising
grape
##rgy
turnout
##udi
Clifton
laboratories
Irvine
##opus
##lid
Monthly
Bihar
statutory
Roses
Emil
##rig
lumber
optimal
##DR
pumps
plaster
Mozambique
##aco
nightclub
propelled
##hun
ked
surplus
wax
##urai
pioneered
Sunny
imprint
Forget
Eliot
approximate
patronage
##bek
##ely
##mbe
Partnership
curl
snapping
29th
Patriarch
##jord
seldom
##ature
astronomy
Bremen
XIV
airborne
205
1778
recognizing
stranded
arrogant
bombardment
destined
ensured
146
robust
Davenport
Interactive
Offensive
Fi
prevents
probe
propeller
sorrow
Blade
mounting
automotive
##dged
wallet
201
lashes
Forrest
##ift
Cell
Younger
shouts
##cki
folds
##chet
Epic
yields
homosexual
tunes
##minate
##text
Manny
chemist
hindwings
##urn
pilgrimage
##sfield
##riff
MLS
##rive
Huntington
translates
Path
slim
##ndra
##oz
climax
commuter
desperation
##reet
denying
##rious
daring
seminary
polo
##clamation
Teatro
Torah
Cats
identities
Poles
photographed
fiery
popularly
##cross
winters
Hesse
##vio
Nurse
Senegal
Salon
prescribed
justify
##gues
##и
##orted
HQ
##hiro
evaluated
momentarily
##unts
Debbie
##licity
##TP
Mighty
Rabbit
##chal
Events
Savoy
##ht
Brandenburg
Bordeaux
##laus
Release
##IE
##kowski
1900s
SK
Strauss
##aly
Sonia
Updated
synagogue
McKay
flattened
370
clutch
contests
toast
evaluate
pope
heirs
jam
tutor
reverted
##ading
nonsense
hesitate
Lars
Ceylon
Laurie
##guchi
accordingly
customary
148
Ethics
Multiple
instincts
IGN
##ä
bullshit
##hit
##par
desirable
##ducing
##yam
alias
ashore
licenses
##lification
misery
147
Cola
assassinated
fiercely
##aft
las
goat
substrate
lords
Cass
Bridges
ICC
lasts
sights
reproductive
##asi
Ivory
Clean
fixing
##lace
seeming
aide
1850s
harassment
##FF
##LE
reasonably
##coat
##cano
NYC
1784
Fifty
immunity
Canadians
Cheng
comforting
meanwhile
##tera
##blin
breeds
glowed
##vour
Aden
##verted
##aded
##oral
neat
enforced
poisoning
##ews
##hone
enforce
predecessors
survivor
Month
unfamiliar
pierced
waived
dump
responds
Mai
Declan
angular
Doesn
interpretations
##yar
invest
Dhaka
policeman
Congregation
Eighth
painfully
##este
##vior
Württemberg
##cles
blockade
encouragement
##fie
Caucasus
Malone
Universidad
utilize
Nissan
inherent
151
agreeing
syllable
determines
Protocol
conclude
##gara
40th
Xu
Taiwanese
##ather
boiler
printer
Lacey
titular
Klaus
Fallon
Wembley
fox
Chandra
Governorate
obsessed
##Ps
micro
##25
Cooke
gymnasium
weaving
Shall
Hussein
glaring
softball
Reader
Dominion
Trouble
varsity
Cooperation
Chaos
Kang
Kramer
Eisenhower
proves
Connie
consortium
governors
Bethany
opener
Normally
Willy
linebacker
Regent
Used
AllMusic
Twilight
##shaw
Companion
Tribunal
simpler
##gam
Experimental
Slovenian
cellar
deadline
trout
Hubbard
ads
idol
##hetto
Granada
clues
salmon
1700
Omega
Caldwell
softened
Bills
Honolulu
##gn
Terrace
suitcase
##IL
frantic
##oons
Abbot
Sitting
Fortress
Riders
sickness
enzymes
trustee
Bern
forged
##13
##ruff
##rl
##versity
inspector
champagne
##held
##FI
hereditary
Taliban
handball
##wine
Sioux
##dicated
honoured
139
##tude
Skye
meanings
##rkin
cardiac
analyzed
vegetable
##FS
Royals
dial
freelance
##fest
partisan
petroleum
ridden
Lincolnshire
panting
##comb
presidents
Haley
##chs
contributes
Jew
discoveries
panicked
Woody
eyelids
Fate
Tulsa
mg
whiskey
zombies
Wii
##udge
investigators
##bull
centred
##screen
Bone
Lana
##oise
forts
##ske
Conan
Lyons
##writing
SH
##ride
rhythmic
154
##llah
pioneers
##bright
captivity
Sanchez
Oman
##mith
Flint
Platform
##ioned
emission
packet
Persia
##formed
takeover
tempted
Vance
Few
Toni
receptions
##ن
exchanges
Camille
whale
Chronicles
##rent
##ushing
##rift
Alto
Genus
##asing
onward
foremost
longing
Rockefeller
containers
##cribe
intercepted
##olt
pleading
Bye
bee
##umbling
153
undertake
Izzy
cheaper
Ultra
validity
##pse
Sa
hovering
##pert
vintage
engraved
##rise
farmland
##ever
##ifier
Atlantis
propose
Catalonia
plunged
##edly
demonstrates
gig
##cover
156
Osborne
cowboy
herd
investigator
loops
Burning
rests
Instrumental
embarrassing
focal
install
readings
swirling
Chatham
parameter
##zin
##holders
Mandarin
Moody
converting
Escape
warnings
##chester
incarnation
##ophone
adopting
##lins
Cromwell
##laws
Axis
Verde
Kappa
Schwartz
Serbs
caliber
Wanna
Chung
##ality
nursery
principally
Bulletin
likelihood
logging
##erty
Boyle
supportive
twitched
##usive
builds
Marseille
omitted
motif
Lands
##lusion
##ssed
Barrow
Airfield
Harmony
WWF
endured
merging
convey
branding
examinations
167
Italians
##dh
dude
1781
##teau
crawling
thoughtful
clasped
concluding
brewery
Moldova
Wan
Towers
Heidelberg
202
##ict
Lagos
imposing
##eval
##serve
Bacon
frowning
thirteenth
conception
calculations
##ович
##mile
##ivated
mutation
strap
##lund
demographic
nude
perfection
stocks
##renched
##dit
Alejandro
bites
fragment
##hack
##rchy
GB
Surgery
Berger
punish
boiling
consume
Elle
Sid
Dome
relies
Crescent
treasurer
Bloody
1758
upheld
Guess
Restaurant
signatures
font
millennium
mural
stakes
Abel
hailed
insists
Alumni
Breton
##jun
digits
##FM
##thal
Talking
motive
reigning
babe
masks
##ø
Shaun
potato
sour
whitish
Somali
##derman
##rab
##wy
chancel
telecommunications
Noise
messenger
tidal
grinding
##ogenic
Rebel
constituent
peripheral
recruitment
##ograph
##tler
pumped
Ravi
poked
##gley
Olive
diabetes
discs
liking
sting
fits
stir
Mari
Sega
creativity
weights
Macau
mandated
Bohemia
disastrous
Katrina
Baku
Rajasthan
waiter
##psis
Siberia
verbs
##truction
patented
1782
##ndon
Relegated
Hunters
Greenwood
Shock
accusing
skipped
Sessions
markers
subset
monumental
Viola
comparative
Alright
Barbados
setup
Session
standardized
##ík
##sket
appoint
AFB
Nationalist
##WS
Troop
leaped
Treasure
goodness
weary
originates
100th
compassion
expresses
recommend
168
composing
seventeenth
Tex
Atlético
bald
Finding
Presidency
Sharks
favoured
inactive
##lter
suffix
princes
brighter
##ctus
classics
defendants
culminated
terribly
Strategy
evenings
##ção
##iver
##urance
absorb
##rner
Territories
RBI
soothing
Martín
concurrently
##tr
Nicholson
fibers
swam
##oney
Allie
Algerian
Dartmouth
Mafia
##bos
##tts
Councillor
vocabulary
##bla
##lé
intending
##dler
Guerrero
sunshine
pedal
##TO
administrators
periodic
scholarships
Loop
Madeline
exaggerated
##ressed
Regan
##cellular
Explorer
##oids
Alexandre
vows
Reporter
Unable
Average
absorption
##bedience
Fortunately
Auxiliary
Grandpa
##HP
##ovo
potent
temporal
adrenaline
##udo
confusing
guiding
Dry
qualifications
joking
wherein
heavyweight
##ices
nightmares
pharmaceutical
Commanding
##aled
##ove
Gregor
##UP
censorship
degradation
glorious
Austro
##rench
380
Miriam
sped
##orous
offset
##KA
fined
specialists
Pune
João
##dina
propped
fungus
##ς
frantically
Gabrielle
Hare
committing
##plied
Ask
Wilmington
stunt
numb
warmer
preacher
earnings
##lating
integer
##ija
federation
homosexuality
##cademia
epidemic
grumbled
shoving
Milk
Satan
Tobias
innovations
##dington
geology
memoirs
##IR
spared
culminating
Daphne
Focus
severed
stricken
Paige
Mans
flats
Russo
communes
litigation
strengthening
##powered
Staffordshire
Wiltshire
Painting
Watkins
##د
specializes
Select
##rane
##aver
Fulton
playable
##VN
openings
sampling
##coon
##21
Allah
travelers
allocation
##arily
Loch
##hm
commentators
fulfilled
##troke
Emeritus
Vanderbilt
Vijay
pledged
##tative
diagram
drilling
##MD
##plain
Edison
productivity
31st
##rying
##ption
##gano
##oration
##bara
posture
bothering
platoon
politely
##inating
redevelopment
Job
##vale
stark
incorrect
Mansion
renewal
threatens
Bahamas
fridge
##tata
Uzbekistan
##edia
Sainte
##mio
gaps
neural
##storm
overturned
Preservation
shields
##ngo
##physics
ah
gradual
killings
##anza
consultation
premiership
Felipe
coincidence
##ène
##any
Handbook
##loaded
Edit
Guns
arguably
##ş
compressed
depict
seller
##qui
Kilkenny
##kling
Olympia
librarian
##acles
dramas
JP
Kit
Maj
##lists
proprietary
##nged
##ettes
##tok
exceeding
Lock
induction
numerical
##vist
Straight
foyer
imaginary
##pop
violinist
Carla
bouncing
##ashi
abolition
##uction
restoring
scenic
##č
Doom
overthrow
para
##vid
##ughty
Concord
HC
cocaine
deputies
##aul
visibility
##wart
Kapoor
Hutchinson
##agan
flashes
kn
decreasing
##ronology
quotes
vain
satisfying
##iam
##linger
310
Hanson
fauna
##zawa
##rrel
Trenton
##VB
Employment
vocational
Exactly
bartender
butterflies
tow
##chers
##ocks
pigs
merchandise
##game
##pine
Shea
##gration
Connell
Josephine
monopoly
##dled
Cobb
warships
cancellation
someday
stove
##Cs
candidacy
superhero
unrest
Toulouse
admiration
undergone
whirled
Reconnaissance
costly
##ships
290
Cafe
amber
Tory
##mpt
definitive
##dress
proposes
redesigned
acceleration
##asa
##raphy
Presley
exits
Languages
##cel
Mode
spokesperson
##tius
Ban
forthcoming
grounded
ACC
compelling
logistics
retailers
abused
##gating
soda
##yland
##lution
Landmark
XVI
blush
##tem
hurling
dread
Tobago
Foley
##uad
scenarios
##mentation
##rks
Score
fatigue
hairy
correspond
##iard
defences
confiscated
##rudence
1785
Formerly
Shot
advertised
460
Text
ridges
Promise
Dev
exclusion
NHS
tuberculosis
rockets
##offs
sparkling
256
disappears
mankind
##hore
HP
##omo
taxation
Multi
DS
Virgil
##ams
Dell
stacked
guessing
Jump
Nope
cheer
hates
ballots
overlooked
analyses
Prevention
maturity
dos
##cards
##lect
Mare
##yssa
Petty
##wning
differing
iOS
##ior
Joachim
Sentinel
##nstein
90s
Pamela
480
Asher
##lary
Vicente
landings
portray
##rda
##xley
Virtual
##uary
finances
Jain
Somebody
Tri
behave
Michele
##ider
dwellings
FAA
Gallagher
##lide
Monkey
195
aforementioned
##rism
##bey
##kim
##puted
Mesa
hopped
unopposed
recipients
Reality
Been
gritted
149
playground
pillar
##rone
Guinness
##tad
Théâtre
depended
Tipperary
Reuben
frightening
wooded
Target
globally
##uted
Morales
Baptiste
drunken
Institut
characterised
##chemistry
Strip
discrete
Premiership
##zzling
gazing
Outer
##quisition
Sikh
Booker
##yal
contemporaries
Jericho
##chan
##physical
##witch
Militia
##rez
##zard
dangers
##utter
##₀
Programs
darling
participates
railroads
##ienne
behavioral
bureau
##rook
161
Hicks
##rises
Comes
inflicted
bees
kindness
norm
##ković
generators
##pard
##omy
##ili
methodology
Alvin
façade
latitude
##plified
DE
Morse
##mered
educate
intersects
##MF
##cz
##vated
AL
##graded
##fill
constitutes
artery
feudal
avant
cautious
##ogue
immigrated
##chenko
Saul
Clinic
Fang
choke
Cornelius
flexibility
temperate
pins
##erson
oddly
inequality
157
Natasha
Sal
##uter
215
aft
blinking
##ntino
northward
Exposition
cookies
Wedding
impulse
Overseas
terrifying
##ough
Mortimer
##see
440
https
og
imagining
##cars
Nicola
exceptionally
threads
##cup
Oswald
Provisional
dismantled
deserves
1786
Fairy
discourse
Counsel
departing
Arc
guarding
##orse
420
alterations
vibrant
Em
squinted
terrace
rowing
Led
accessories
SF
Sgt
cheating
Atomic
##raj
Blackpool
##iary
boarded
substituted
bestowed
lime
kernel
##jah
Belmont
shaken
sticky
retrospective
Louie
migrants
weigh
sunglasses
thumbs
##hoff
excavation
##nks
Extra
Polo
motives
Drum
infrared
tastes
berth
verge
##stand
programmed
warmed
Shankar
Titan
chromosome
cafeteria
dividing
pepper
CPU
Stevie
satirical
Nagar
scowled
Died
backyard
##gata
##reath
##bir
Governors
portraying
##yah
Revenge
##acing
1772
margins
Bahn
OH
lowland
##razed
catcher
replay
##yoshi
Seriously
##licit
Aristotle
##ald
Habsburg
weekday
Secretariat
CO
##dly
##joy
##stad
litre
ultra
##cke
Mongol
Tucson
correlation
compose
traps
Groups
Hai
Salvatore
##dea
cents
##eese
concession
clash
Trip
Panzer
Moroccan
cruisers
torque
Ba
grossed
##arate
restriction
concentrating
FDA
##Leod
##ones
Scholars
##esi
throbbing
specialised
##heses
Chicken
##fia
##ificant
Erich
Residence
##trate
manipulation
namesake
##tom
Hoover
cue
Lindsey
Lonely
275
##HT
combustion
subscribers
Punjabi
respects
Jeremiah
penned
##gor
##rilla
suppression
##tration
Crimson
piston
Derry
crimson
lyrical
oversee
portrays
CF
Districts
Lenin
Cora
searches
clans
VHS
##hel
Jacqueline
Redskins
Clubs
desktop
indirectly
alternatives
marijuana
suffrage
##smos
Irwin
##liff
Process
##hawks
Sloane
##bson
Sonata
yielded
Flores
##ares
armament
adaptations
integrate
neighbours
shelters
##tour
Skinner
##jet
##tations
1774
Peterborough
##elles
ripping
Liang
Dickinson
charities
Rwanda
monasteries
crossover
racist
barked
guerrilla
##ivate
Grayson
##iques
##vious
##got
Rolls
denominations
atom
affinity
##delity
Wish
##inted
##inae
interrogation
##cey
##erina
##lifting
192
Sands
1779
mast
Likewise
##hyl
##oft
contempt
##por
assaulted
fills
establishments
Mal
consulted
##omi
##sight
greet
##roma
##egan
Pulitzer
##rried
##dius
##ractical
##voked
Hasan
CB
##zzy
Romanesque
Panic
wheeled
recorder
##tters
##warm
##gly
botanist
Balkan
Lockheed
Polly
farewell
suffers
purchases
Eaton
##80
Quick
commenting
Saga
beasts
hides
motifs
##icks
Alonso
Springer
Wikipedia
circulated
encoding
jurisdictions
snout
UAE
Integrated
unmarried
Heinz
##lein
##figured
deleted
##tley
Zen
Cycling
Fuel
Scandinavian
##rants
Conner
reef
Marino
curiously
lingered
Gina
manners
activism
Mines
Expo
Micah
promotions
Server
booked
derivatives
eastward
detailing
reelection
##chase
182
Campeonato
Po
158
Peel
winger
##itch
canyon
##pit
LDS
A1
##shin
Giorgio
pathetic
##rga
##mist
Aren
##lag
confronts
motel
textbook
shine
turbines
1770
Darcy
##cot
Southeastern
##lessness
Banner
recognise
stray
Kitchen
paperwork
realism
Chrysler
filmmakers
fishermen
##hetic
variously
Vishnu
fiddle
Eddy
Origin
##tec
##ulin
Flames
Rs
bankrupt
Extreme
Pomeranian
##emption
ratified
##iu
jockey
Stratford
##ivating
##oire
Babylon
pardon
AI
affordable
deities
disturbance
Trying
##sai
Ida
Papers
advancement
70s
archbishop
Luftwaffe
announces
tugging
##lphin
##sistence
##eel
##ishes
ambition
aura
##fled
##lected
##vue
Prasad
boiled
clarity
Violin
investigative
routing
Yankee
##uckle
McMahon
bugs
eruption
##rooms
Minutes
relics
##ckle
##nse
sipped
valves
weakly
##ital
Middleton
collided
##quer
bamboo
insignia
Tyne
exercised
Ninth
echoing
polynomial
considerations
lunged
##bius
objections
complain
disguised
plaza
##VC
institutes
Judicial
ascent
imminent
Waterford
hello
Lumpur
Niger
Goldman
vendors
Kensington
Wren
browser
##bner
##tri
##mize
##pis
##lea
Cheyenne
Bold
Settlement
Hollow
Paralympic
axle
##toire
##actic
impose
perched
utilizing
slips
Benz
Michaels
manipulate
Chiang
##mian
Dolphins
prohibition
attacker
ecology
Estadio
##SB
##uild
attracts
recalls
glacier
lad
##rima
Barlow
kHz
melodic
##aby
##iracy
assumptions
Cornish
##aru
DOS
Maddie
##mers
lyric
Luton
nm
##tron
Reno
Fin
YOU
Broadcast
Finch
sensory
##bent
Jeep
##uman
additionally
Buildings
businessmen
treaties
235
Stranger
gateway
Charlton
accomplishments
Diary
apologized
zinc
histories
supplier
##tting
162
asphalt
Treatment
Abbas
##pating
##yres
Bloom
sedan
soloist
##cum
antagonist
denounced
Fairfax
##aving
##enko
noticeable
Budget
Buckingham
Snyder
retreating
Jai
spoon
invading
giggle
woven
gunfire
arrests
##vered
##come
respiratory
violet
##aws
Byrd
shocking
tenant
Jamaican
Ottomans
Seal
theirs
##isse
##48
cooperate
peering
##nius
163
Composer
organist
Mongolian
Bauer
Spy
collects
prophecy
congregations
##moor
Brick
calculation
fixtures
exempt
##dden
Ada
Thousand
##lue
tracing
##achi
bodyguard
vicar
supplying
Łódź
interception
monitored
##heart
Paso
overlap
annoyance
##dice
yellowish
stables
elders
illegally
honesty
##oar
skinny
spinal
##puram
Bourbon
##cor
flourished
Medium
##stics
##aba
Follow
##ckey
stationary
##scription
dresser
scrutiny
Buckley
Clearly
##SF
Lyrics
##heimer
drying
Oracle
internally
rains
##last
Enemy
##oes
McLean
Ole
phosphate
Rosario
Rifles
##mium
battered
Pepper
Presidents
conquer
Château
castles
##aldo
##ulf
Depending
Lesser
Boom
trades
Peyton
164
emphasize
accustomed
SM
Ai
Classification
##mins
##35
##rons
leak
piled
deeds
lush
##self
beginnings
breathless
1660
McGill
##ago
##chaft
##gies
humour
Bomb
securities
Might
##zone
##eves
Matthias
Movies
Levine
vengeance
##ads
Challenger
Misty
Traditionally
constellation
##rass
deepest
workplace
##oof
##vina
impatient
##ML
Mughal
Alessandro
scenery
Slater
postseason
troupe
##ń
Volunteers
Facility
militants
Reggie
sanctions
Expeditionary
Nam
countered
interpret
Basilica
coding
expectation
Duffy
def
Tong
wakes
Bowling
Vehicle
Adler
salad
intricate
stronghold
medley
##uries
##bur
joints
##rac
##yx
##IO
Ordnance
Welch
distributor
Ark
cavern
trench
Weiss
Mauritius
decreases
docks
eagerly
irritation
Matilda
biographer
Visiting
##marked
##iter
##ear
##gong
Moreno
attendant
Bury
instrumentation
theologian
clit
nuns
symphony
translate
375
loser
##user
##VR
##meter
##orious
harmful
##yuki
Commissioners
Mendoza
sniffed
Hulk
##dded
##ulator
##nz
Donnell
##eka
deported
Met
SD
Aerospace
##cultural
##odes
Fantastic
cavity
remark
emblem
fearing
##iance
ICAO
Liberia
stab
##yd
Pac
Gymnasium
IS
Everton
##vanna
mantle
##ief
Ramon
##genic
Shooting
Smoke
Random
Africans
MB
tavern
bargain
voluntarily
Ion
Peoples
Rusty
attackers
Patton
sins
##cake
Hat
moderately
##hala
##alia
requesting
mechanic
##eae
Seine
Robbins
##ulum
susceptible
Bravo
Slade
Strasbourg
rubble
entrusted
Creation
##amp
smoothed
##uintet
evenly
reviewers
skip
Sculpture
177
Rough
##rrie
Reeves
##cede
Administrator
garde
minus
carriages
grenade
Ninja
fuscous
##kley
Punk
contributors
Aragon
Tottenham
##cca
##sir
VA
laced
dealers
##sonic
crisp
harmonica
Artistic
Butch
Andes
Farmers
corridors
unseen
##tium
Countries
Lone
envisioned
Katy
##lang
##cc
Quarterly
##neck
consort
##aceae
bidding
Corey
concurrent
##acts
##gum
Highness
##lient
##rators
arising
##unta
pathways
49ers
bolted
complaining
ecosystem
libretto
Ser
narrated
212
Soft
influx
##dder
incorporation
plagued
tents
##ddled
1750
Risk
citation
Tomas
hostilities
seals
Bruins
Dominique
attic
competent
##UR
##cci
hugging
Breuning
bacterial
Shrewsbury
vowed
eh
elongated
hangs
render
centimeters
##ficient
Mu
turtle
besieged
##gaard
grapes
bravery
collaborations
deprived
##amine
##using
##gins
arid
##uve
coats
hanged
##sting
Pa
prefix
##ranged
Exit
Chain
Flood
Materials
suspicions
##ö
hovered
Hidden
##state
Malawi
##24
Mandy
norms
fascinating
airlines
delivers
##rust
Cretaceous
spanned
pillows
##onomy
jar
##kka
regent
fireworks
morality
discomfort
lure
uneven
##jack
Lucian
171
archaeology
##til
mornings
Billie
Marquess
impending
spilling
tombs
##volved
Celia
Coke
underside
##bation
Vaughn
Daytona
Godfrey
Pascal
Alien
##sign
172
##lage
iPhone
Gonna
genocide
##rber
oven
endure
dashed
simultaneous
##phism
Wally
##rō
ants
predator
reissue
##aper
Speech
funk
Rudy
claw
Hindus
Numbers
Bing
lantern
##aurus
scattering
poisoned
##active
Andrei
algebraic
baseman
##ritz
Gregg
##cola
selections
##putation
lick
Laguna
##IX
Sumatra
Warning
turf
buyers
Burgess
Oldham
exploit
worm
initiate
strapped
tuning
filters
haze
##е
##ledge
##ydro
##culture
amendments
Promotion
##union
Clair
##uria
petty
shutting
##eveloped
Phoebe
Zeke
conducts
grains
clashes
##latter
illegitimate
willingly
Deer
Lakers
Reference
chaplain
commitments
interrupt
salvation
Panther
Qualifying
Assessment
cancel
efficiently
attorneys
Dynamo
impress
accession
clinging
randomly
reviewing
Romero
Cathy
charting
clapped
rebranded
Azerbaijani
coma
indicator
punches
##tons
Sami
monastic
prospects
Pastor
##rville
electrified
##CI
##utical
tumbled
Chef
muzzle
selecting
UP
Wheel
protocols
##tat
Extended
beautifully
nests
##stal
Andersen
##anu
##³
##rini
kneeling
##reis
##xia
anatomy
dusty
Safe
turmoil
Bianca
##elo
analyze
##ر
##eran
podcast
Slovene
Locke
Rue
##retta
##uni
Person
Prophet
crooked
disagreed
Versailles
Sarajevo
Utrecht
##ogen
chewing
##ception
##iidae
Missile
attribute
majors
Arch
intellectuals
##andra
ideological
Cory
Salzburg
##fair
Lot
electromagnetic
Distribution
##oper
##pered
Russ
Terra
repeats
fluttered
Riga
##ific
##gt
cows
Hair
labelled
protects
Gale
Personnel
Düsseldorf
Moran
rematch
##OE
Slow
forgiveness
##ssi
proudly
Macmillan
insist
undoubtedly
Québec
Violence
##yuan
##aine
mourning
linen
accidental
##iol
##arium
grossing
lattice
maneuver
##marine
prestige
petrol
gradient
invasive
militant
Galerie
widening
##aman
##quist
disagreement
##ales
creepy
remembers
buzz
##erial
Exempt
Dirk
mon
Addison
##inen
deposed
##agon
fifteenth
Hang
ornate
slab
##lades
Fountain
contractors
das
Warwickshire
1763
##rc
Carly
Essays
Indy
Ligue
greenhouse
slit
##sea
chewed
wink
##azi
Playhouse
##kon
Gram
Ko
Samson
creators
revive
##rians
spawned
seminars
Craft
Tall
diverted
assistants
computational
enclosure
##acity
Coca
##eve
databases
Drop
##loading
##hage
Greco
Privy
entrances
pork
prospective
Memories
robes
##market
transporting
##lik
Rudolph
Horton
visually
##uay
##nja
Centro
Tor
Howell
##rsey
admitting
postgraduate
herbs
##att
Chin
Rutherford
##bot
##etta
Seasons
explanations
##bery
Friedman
heap
##ryl
##sberg
jaws
##agh
Choi
Killing
Fanny
##suming
##hawk
hopeful
##aid
Monty
gum
remarkably
Secrets
disco
harp
advise
##avia
Marathi
##cycle
Truck
abbot
sincere
urine
##mology
masked
bathing
##tun
Fellows
##TM
##gnetic
owl
##jon
hymn
##leton
208
hostility
##cée
baked
Bottom
##AB
shudder
##ater
##von
##hee
reorganization
Cycle
##phs
Lex
##style
##rms
Translation
##erick
##imeter
##ière
attested
Hillary
##DM
gal
wander
Salle
##laming
Perez
Pit
##LP
USAF
contexts
Disease
blazing
aroused
razor
walled
Danielle
Mont
Funk
royalty
thee
203
donors
##erton
famously
processors
reassigned
welcoming
Goldberg
##quities
undisclosed
Orient
Patty
vaccine
refrigerator
Cypriot
consonant
##waters
176
sober
##lement
Racecourse
##uate
Luckily
Selection
conceptual
vines
Breaking
wa
lions
oversight
sheltered
Dancer
ponds
borrow
##BB
##pulsion
Daly
##eek
fertility
spontaneous
Worldwide
gasping
##tino
169
ABS
Vickers
ambient
energetic
prisons
##eson
Stacy
##roach
GmbH
Afro
Marin
farmhouse
pinched
##cursion
##sp
Sabine
##pire
181
nak
swelling
humble
perfume
##balls
Rai
cannons
##taker
Married
Maltese
canals
interceptions
hats
lever
slowing
##ppy
Nike
Silas
Scarborough
skirts
166
inauguration
Shuttle
alloy
beads
belts
Compton
Cause
battling
critique
surf
Dock
roommate
##ulet
invade
Garland
##slow
nutrition
persona
##zam
Wichita
acquaintance
coincided
##cate
Dracula
clamped
##gau
overhaul
##broken
##rrier
melodies
ventures
Paz
convex
Roots
##holding
Tribute
transgender
##ò
chimney
##riad
Ajax
Thereafter
messed
nowadays
pH
##100
##alog
Pomerania
##yra
Rossi
glove
##TL
Races
##asily
tablets
Jase
##ttes
diner
##rns
Hu
Mohan
anytime
weighted
remixes
Dove
cherry
imports
##urity
GA
##TT
##iated
##sford
Clarkson
evidently
rugged
Dust
siding
##ometer
acquitted
choral
##mite
infants
Domenico
gallons
Atkinson
gestures
slated
##xa
Archaeology
unwanted
##ibes
##duced
premise
Colby
Geelong
disqualified
##pf
##voking
simplicity
Walkover
Qaeda
Warden
##bourg
##ān
Invasion
Babe
harness
183
##tated
maze
Burt
bedrooms
##nsley
Horizon
##oast
minimize
peeked
MLA
Trains
tractor
nudged
##iform
Growth
Benton
separates
##about
##kari
buffer
anthropology
brigades
foil
##wu
Domain
licking
whore
##rage
##sham
Initial
Courthouse
Rutgers
dams
villains
supermarket
##brush
Brunei
Palermo
arises
Passenger
outreach
##gill
Labrador
McLaren
##uy
Lori
##fires
Heads
magistrate
¹⁄₂
Weapons
##wai
##roke
projecting
##ulates
bordering
McKenzie
Pavel
midway
Guangzhou
streamed
racer
##lished
eccentric
spectral
206
##mism
Wilde
Grange
preparatory
lent
##tam
starving
Gertrude
##cea
##ricted
Breakfast
Mira
blurted
derive
##lair
blunt
sob
Cheltenham
Henrik
reinstated
intends
##istan
unite
##ector
playful
sparks
mapped
Cadet
luggage
prosperous
##ein
salon
##utes
Biological
##rland
Tyrone
buyer
##lose
amounted
Saw
smirked
Ronan
Reviews
Adele
trait
##proof
Bhutan
Ginger
##junct
digitally
stirring
##isted
coconut
Hamlet
Dinner
Scale
pledge
##RP
Wrong
Goal
Panel
therapeutic
elevations
infectious
priesthood
##inda
Guyana
diagnostic
##mbre
Blackwell
sails
##arm
literal
periodically
gleaming
Robot
Rector
##abulous
##tres
Reaching
Romantic
CP
Wonderful
##tur
ornamental
##nges
traitor
##zilla
genetics
mentioning
##eim
resonance
Areas
Shopping
##nard
Gail
Solid
##rito
##mara
Willem
Chip
Matches
Volkswagen
obstacle
Organ
invites
Coral
attain
##anus
##dates
Midway
shuffled
Cecilia
dessert
Gateway
Ch
Napoleonic
Petroleum
jets
goose
striped
bowls
vibration
Sims
nickel
Thirteen
problematic
intervene
##grading
##unds
Mum
semifinal
Radical
##izations
refurbished
##sation
##harine
Maximilian
cites
Advocate
Potomac
surged
preserves
Curry
angled
ordination
##pad
Cade
##DE
##sko
researched
torpedoes
Resident
wetlands
hay
applicants
depart
Bernstein
##pic
##ario
##rae
favourable
##wari
##р
metabolism
nobleman
Defaulted
calculate
ignition
Celebrity
Belize
sulfur
Flat
Sc
USB
flicker
Hertfordshire
Sept
CFL
Pasadena
Saturdays
Titus
##nir
Canary
Computing
Isaiah
##mler
formidable
pulp
orchid
Called
Solutions
kilograms
steamer
##hil
Doncaster
successors
Stokes
Holstein
##sius
sperm
API
Rogue
instability
Acoustic
##rag
159
undercover
Wouldn
##pra
##medical
Eliminated
honorable
##chel
denomination
abrupt
Buffy
blouse
fi
Regardless
Subsequent
##rdes
Lover
##tford
bacon
##emia
carving
##cripts
Massacre
Ramos
Latter
##ulp
ballroom
##gement
richest
bruises
Rest
Wiley
##aster
explosions
##lastic
Edo
##LD
Mir
choking
disgusted
faintly
Barracks
blasted
headlights
Tours
ensued
presentations
##cale
wrought
##oat
##coa
Quaker
##sdale
recipe
##gny
corpses
##liance
comfortably
##wat
Landscape
niche
catalyst
##leader
Securities
messy
##RL
Rodrigo
backdrop
##opping
treats
Emilio
Anand
bilateral
meadow
VC
socialism
##grad
clinics
##itating
##ppe
##ymphonic
seniors
Advisor
Armoured
Method
Alley
##orio
Sad
fueled
raided
Axel
NH
rushes
Dixie
Otis
wrecked
##22
capitalism
café
##bbe
##pion
##forcing
Aubrey
Lublin
Whenever
Sears
Scheme
##lana
Meadows
treatise
##RI
##ustic
sacrifices
sustainability
Biography
mystical
Wanted
multiplayer
Applications
disliked
##tisfied
impaired
empirical
forgetting
Fairfield
Sunni
blurred
Growing
Avalon
coil
Camera
Skin
bruised
terminals
##fted
##roving
Commando
##hya
##sper
reservations
needles
dangling
##rsch
##rsten
##spect
##mbs
yoga
regretted
Bliss
Orion
Rufus
glucose
Olsen
autobiographical
##dened
222
humidity
Shan
##ifiable
supper
##rou
flare
##MO
campaigning
descend
socio
declares
Mounted
Gracie
Arte
endurance
##ety
Copper
costa
airplay
##MB
Proceedings
dislike
grimaced
occupants
births
glacial
oblivious
cans
installment
muddy
##ł
captains
pneumonia
Quiet
Sloan
Excuse
##nine
Geography
gymnastics
multimedia
drains
Anthology
Gear
cylindrical
Fry
undertaking
##pler
##tility
Nan
##recht
Dub
philosophers
piss
Atari
##pha
Galicia
México
##nking
Continuing
bump
graveyard
persisted
Shrine
##erapy
defects
Advance
Bomber
##oil
##ffling
cheerful
##lix
scrub
##eto
awkwardly
collaborator
fencing
##alo
prophet
Croix
coughed
##lication
roadway
slaughter
elephants
##erated
Simpsons
vulnerability
ivory
Birth
lizard
scarce
cylinders
fortunes
##NL
Hate
Priory
##lai
McBride
##copy
Lenny
liaison
Triangle
coronation
sampled
savage
amidst
Grady
whatsoever
instinctively
Reconstruction
insides
seizure
Drawing
##rlin
Antioch
Gao
Díaz
1760
Sparks
##tien
##bidae
rehearsal
##bbs
botanical
##hers
compensate
wholesale
Seville
shareholder
prediction
astronomical
Reddy
hardest
circling
whereabouts
termination
Rep
Assistance
Dramatic
Herb
##ghter
climbs
188
Poole
301
##pable
wit
##istice
Walters
relying
Jakob
##redo
proceeding
Langley
affiliates
ou
##allo
##holm
Samsung
##ishi
Missing
Xi
vertices
Claus
foam
restless
##uating
##sso
##ttering
Philips
delta
bombed
Catalogue
coaster
Ling
Willard
satire
410
Composition
Net
Orioles
##ldon
fins
Palatinate
Woodward
tease
tilt
brightness
##70
##bbling
##loss
##dhi
##uilt
Whoever
##yers
hitter
Elton
Extension
ace
Affair
restructuring
##loping
Paterson
hi
##rya
spouse
Shay
Himself
piles
preaching
##gical
bikes
Brave
expulsion
Mirza
stride
Trees
commemorated
famine
masonry
Selena
Watt
Banking
Rancho
Stockton
dip
tattoos
Vlad
acquainted
Flyers
ruthless
fourteenth
illustrate
##akes
EPA
##rows
##uiz
bumped
Designed
Leaders
mastered
Manfred
swirled
McCain
##rout
Artemis
rabbi
flinched
upgrades
penetrate
shipyard
transforming
caretaker
##eiro
Maureen
tightening
##founded
RAM
##icular
##mper
##rung
Fifteen
exploited
consistency
interstate
##ynn
Bridget
contamination
Mistress
##rup
coating
##FP
##jective
Libyan
211
Gemma
dependence
shrubs
##ggled
Germain
retaliation
traction
##PP
Dangerous
terminology
psychiatrist
##garten
hurdles
Natal
wasting
Weir
revolves
stripe
##reased
preferences
##entation
##lde
##áil
##otherapy
Flame
##ologies
viruses
Label
Pandora
veil
##ogical
Coliseum
Cottage
creeping
Jong
lectured
##çaise
shoreline
##fference
##hra
Shade
Clock
Faye
bilingual
Humboldt
Operating
##fter
##was
algae
towed
amphibious
Parma
impacted
smacked
Piedmont
Monsters
##omb
Moor
##lberg
sinister
Postal
178
Drummond
Sign
textbooks
hazardous
Brass
Rosemary
Pick
Sit
Architect
transverse
Centennial
confess
polling
##aia
Julien
##mand
consolidation
Ethel
##ulse
severity
Yorker
choreographer
1840s
##ltry
softer
versa
##geny
##quila
##jō
Caledonia
Friendship
Visa
rogue
##zzle
bait
feather
incidence
Foods
Ships
##uto
##stead
arousal
##rote
Hazel
##bolic
Swing
##ej
##cule
##jana
##metry
##uity
Valuable
##ₙ
Shropshire
##nect
365
Ones
realise
Café
Albuquerque
##grown
##stadt
209
##ᵢ
prefers
withstand
Lillian
MacArthur
Hara
##fulness
domination
##VO
##school
Freddy
ethnicity
##while
adorned
hormone
Calder
Domestic
Freud
Shields
##phus
##rgan
BP
Segunda
Mustang
##GI
Bonn
patiently
remarried
##umbria
Crete
Elephant
Nuremberg
tolerate
Tyson
##evich
Programming
##lander
Bethlehem
segregation
Constituency
quarterly
blushed
photographers
Sheldon
porcelain
Blanche
goddamn
lively
##fused
bumps
##eli
curated
coherent
provoked
##vet
Madeleine
##isco
rainy
Bethel
accusation
ponytail
gag
##lington
quicker
scroll
##vate
Bow
Gender
Ira
crashes
ACT
Maintenance
##aton
##ieu
bitterly
strains
rattled
vectors
##arina
##ishly
173
parole
##nx
amusing
Gonzalez
##erative
Caucus
sensual
Penelope
coefficient
Mateo
##mani
proposition
Duty
lacrosse
proportions
Plato
profiles
Botswana
Brandt
reins
mandolin
encompassing
##gens
Kahn
prop
summon
##MR
##yrian
##zaki
Falling
conditional
thy
##bao
##ych
radioactive
##nics
Newspaper
##people
##nded
Gaming
sunny
##look
Sherwood
crafted
NJ
awoke
187
timeline
giants
possessing
##ycle
Cheryl
ng
Ruiz
polymer
potassium
Ramsay
relocation
##leen
Sociology
##bana
Franciscan
propulsion
denote
##erjee
registers
headline
Tests
emerges
Articles
Mint
livery
breakup
kits
Rap
Browning
Bunny
##mington
##watch
Anastasia
Zachary
arranging
biographical
Erica
Nippon
##membrance
Carmel
##sport
##xes
Paddy
##holes
Issues
Spears
compliment
##stro
##graphs
Castillo
##MU
##space
Corporal
##nent
174
Gentlemen
##ilize
##vage
convinces
Carmine
Crash
##hashi
Files
Doctors
brownish
sweating
goats
##conductor
rendition
##bt
NL
##spiration
generates
##cans
obsession
##noy
Danger
Diaz
heats
Realm
priorities
##phon
1300
initiation
pagan
bursts
archipelago
chloride
Screenplay
Hewitt
Khmer
bang
judgement
negotiating
##ait
Mabel
densely
Boulder
knob
430
Alfredo
##kt
pitches
##ées
##ان
Macdonald
##llum
imply
##mot
Smile
spherical
##tura
Derrick
Kelley
Nico
cortex
launches
differed
parallels
Navigation
##child
##rming
canoe
forestry
reinforce
##mote
confirming
tasting
scaled
##resh
##eting
Understanding
prevailing
Pearce
CW
earnest
Gaius
asserts
denoted
landmarks
Chargers
warns
##flies
Judges
jagged
##dain
tails
Historian
Millie
##sler
221
##uard
absurd
Dion
##ially
makeshift
Specifically
ignorance
Eat
##ieri
comparisons
forensic
186
Giro
skeptical
disciplinary
battleship
##45
Libby
520
Odyssey
ledge
##post
Eternal
Missionary
deficiency
settler
wonders
##gai
raging
##cis
Romney
Ulrich
annexation
boxers
sect
204
ARIA
dei
Hitchcock
te
Varsity
##fic
CC
lending
##nial
##tag
##rdy
##obe
Defensive
##dson
##pore
stellar
Lam
Trials
contention
Sung
##uminous
Poe
superiority
##plicate
325
bitten
conspicuous
##olly
Lila
Pub
Petit
distorted
ISIL
distinctly
##family
Cowboy
mutant
##cats
##week
Changes
Sinatra
epithet
neglect
Innocent
gamma
thrill
reggae
##adia
##ational
##due
landlord
##leaf
visibly
##ì
Darlington
Gomez
##iting
scarf
##lade
Hinduism
Fever
scouts
##roi
convened
##oki
184
Lao
boycott
unemployed
##lore
##ß
##hammer
Curran
disciples
odor
##ygiene
Lighthouse
Played
whales
discretion
Yves
##ceived
pauses
coincide
##nji
dizzy
##scopic
routed
Guardians
Kellan
carnival
nasal
224
##awed
Mitsubishi
640
Cast
silky
Projects
joked
Huddersfield
Rothschild
zu
##olar
Divisions
mildly
##eni
##lge
Appalachian
Sahara
pinch
##roon
wardrobe
##dham
##etal
Bubba
##lini
##rumbling
Communities
Poznań
unification
Beau
Kris
SV
Rowing
Minh
reconciliation
##saki
##sor
taped
##reck
certificates
gubernatorial
rainbow
##uing
litter
##lique
##oted
Butterfly
benefited
Images
induce
Balkans
Velvet
##90
##xon
Bowman
##breaker
penis
##nitz
##oint
##otive
crust
##pps
organizers
Outdoor
nominees
##rika
TX
##ucks
Protestants
##imation
appetite
Baja
awaited
##points
windshield
##igh
##zled
Brody
Buster
stylized
Bryce
##sz
Dollar
vest
mold
ounce
ok
receivers
##uza
Purdue
Harrington
Hodges
captures
##ggio
Reservation
##ssin
##tman
cosmic
straightforward
flipping
remixed
##athed
Gómez
Lim
motorcycles
economies
owning
Dani
##rosis
myths
sire
kindly
1768
Bean
graphs
##mee
##RO
##geon
puppy
Stephenson
notified
##jer
Watching
##rama
Sino
urgency
Islanders
##mash
Plata
fumble
##chev
##stance
##rack
##she
facilitated
swings
akin
enduring
payload
##phine
Deputies
murals
##tooth
610
Jays
eyeing
##quito
transparency
##cote
Timor
negatively
##isan
battled
##fected
thankful
Rage
hospitality
incorrectly
207
entrepreneurs
##cula
##wley
hedge
##cratic
Corpus
Odessa
Whereas
##ln
fetch
happier
Amherst
bullying
graceful
Height
Bartholomew
willingness
qualifier
191
Syed
Wesleyan
Layla
##rrence
Webber
##hum
Rat
##cket
##herence
Monterey
contaminated
Beside
Mustafa
Nana
213
##pruce
Reason
##spense
spike
##gé
AU
disciple
charcoal
##lean
formulated
Diesel
Mariners
accreditation
glossy
1800s
##ih
Mainz
unison
Marianne
shear
overseeing
vernacular
bowled
##lett
unpopular
##ckoned
##monia
Gaston
##TI
##oters
Cups
##bones
##ports
Museo
minors
1773
Dickens
##EL
##NBC
Presents
ambitions
axes
Río
Yukon
bedside
Ribbon
Units
faults
conceal
##lani
prevailed
214
Goodwin
Jaguar
crumpled
Cullen
Wireless
ceded
remotely
Bin
mocking
straps
ceramics
##avi
##uding
##ader
Taft
twenties
##aked
Problem
quasi
Lamar
##ntes
##avan
Barr
##eral
hooks
sa
##ône
194
##ross
Nero
Caine
trance
Homeland
benches
Guthrie
dismiss
##lex
César
foliage
##oot
##alty
Assyrian
Ahead
Murdoch
dictatorship
wraps
##ntal
Corridor
Mackay
respectable
jewels
understands
##pathic
Bryn
##tep
ON
capsule
intrigued
Sleeping
communists
##chayat
##current
##vez
doubling
booklet
##uche
Creed
##NU
spies
##sef
adjusting
197
Imam
heaved
Tanya
canonical
restraint
senators
stainless
##gnate
Matter
cache
restrained
conflicting
stung
##ool
Sustainable
antiquity
193
heavens
inclusive
##ador
fluent
303
911
archaeologist
superseded
##plex
Tammy
inspire
##passing
##lub
Lama
Mixing
##activated
##yote
parlor
tactic
198
Stefano
prostitute
recycling
sorted
banana
Stacey
Musée
aristocratic
cough
##rting
authorised
gangs
runoff
thoughtfully
##nish
Fisheries
Provence
detector
hum
##zhen
pill
##árez
Map
Leaves
Peabody
skater
vent
##color
390
cerebral
hostages
mare
Jurassic
swell
##isans
Knoxville
Naked
Malaya
scowl
Cobra
##anga
Sexual
##dron
##iae
196
##drick
Ravens
Blaine
##throp
Ismail
symmetric
##lossom
Leicestershire
Sylvester
glazed
##tended
Radar
fused
Families
Blacks
Sale
Zion
foothills
microwave
slain
Collingwood
##pants
##dling
killers
routinely
Janice
hearings
##chanted
##ltration
continents
##iving
##yster
##shot
##yna
injected
Guillaume
##ibi
kinda
Confederacy
Barnett
disasters
incapable
##grating
rhythms
betting
draining
##hak
Callie
Glover
##iliated
Sherlock
hearted
punching
Wolverhampton
Leaf
Pi
builders
furnished
knighted
Photo
##zle
Touring
fumbled
pads
##ий
Bartlett
Gunner
eerie
Marius
Bonus
pots
##hino
##pta
Bray
Frey
Ortiz
stalls
belongings
Subway
fascination
metaphor
Bat
Boer
Colchester
sway
##gro
rhetoric
##dheim
Fool
PMID
admire
##hsil
Strand
TNA
##roth
Nottinghamshire
##mat
##yler
Oxfordshire
##nacle
##roner
BS
##nces
stimulus
transports
Sabbath
##postle
Richter
4000
##grim
##shima
##lette
deteriorated
analogous
##ratic
UHF
energies
inspiring
Yiddish
Activities
##quential
##boe
Melville
##ilton
Judd
consonants
labs
smuggling
##fari
avid
##uc
truce
undead
##raith
Mostly
bracelet
Connection
Hussain
awhile
##UC
##vention
liable
genetically
##phic
Important
Wildcats
daddy
transmit
##cas
conserved
Yesterday
##lite
Nicky
Guys
Wilder
Lay
skinned
Communists
Garfield
Nearby
organizer
Loss
crafts
walkway
Chocolate
Sundance
Synod
##enham
modify
swayed
Surface
analysts
brackets
drone
parachute
smelling
Andrés
filthy
frogs
vertically
##OK
localities
marries
AHL
35th
##pian
Palazzo
cube
dismay
relocate
##на
Hear
##digo
##oxide
prefecture
converts
hangar
##oya
##ucking
Spectrum
deepened
spoiled
Keeping
##phobic
Verona
outrage
Improvement
##UI
masterpiece
slung
Calling
chant
Haute
mediated
manipulated
affirmed
##hesis
Hangul
skies
##llan
Worcestershire
##kos
mosaic
##bage
##wned
Putnam
folder
##LM
guts
noteworthy
##rada
AJ
sculpted
##iselle
##rang
recognizable
##pent
dolls
lobbying
impatiently
Se
staple
Serb
tandem
Hiroshima
thieves
##ynx
faculties
Norte
##alle
##trusion
chords
##ylon
Gareth
##lops
##escu
FIA
Levin
auspices
groin
Hui
nun
Listed
Honourable
Larsen
rigorous
##erer
Tonga
##pment
##rave
##track
##aa
##enary
540
clone
sediment
esteem
sighted
cruelty
##boa
inverse
violating
Amtrak
Status
amalgamated
vertex
AR
harmless
Amir
mounts
Coronation
counseling
Audi
CO₂
splits
##eyer
Humans
Salmon
##have
##rado
##čić
216
takeoff
classmates
psychedelic
##gni
Gypsy
231
Anger
GAA
ME
##nist
##tals
Lissa
Odd
baptized
Fiat
fringe
##hren
179
elevators
perspectives
##TF
##ngle
Question
frontal
950
thicker
Molecular
##nological
Sixteen
Baton
Hearing
commemorative
dorm
Architectural
purity
##erse
risky
Georgie
relaxing
##ugs
downed
##rar
Slim
##phy
IUCN
##thorpe
Parkinson
217
Marley
Shipping
sweaty
Jesuits
Sindh
Janata
implying
Armenians
intercept
Ankara
commissioners
ascended
sniper
Grass
Walls
salvage
Dewey
generalized
learnt
PT
##fighter
##tech
DR
##itrus
##zza
mercenaries
slots
##burst
##finger
##nsky
Princes
Rhodesia
##munication
##strom
Fremantle
homework
ins
##Os
##hao
##uffed
Thorpe
Xiao
exquisite
firstly
liberated
technician
Oilers
Phyllis
herb
sharks
MBE
##stock
Product
banjo
##morandum
##than
Visitors
unavailable
unpublished
oxidation
Vogue
##copic
##etics
Yates
##ppard
Leiden
Trading
cottages
Principles
##Millan
##wife
##hiva
Vicar
nouns
strolled
##eorological
##eton
##science
precedent
Armand
Guido
rewards
##ilis
##tise
clipped
chick
##endra
averages
tentatively
1830s
##vos
Certainly
305
Société
Commandant
##crats
##dified
##nka
marsh
angered
ventilation
Hutton
Ritchie
##having
Eclipse
flick
motionless
Amor
Fest
Loire
lays
##icit
##sband
Guggenheim
Luck
disrupted
##ncia
Disco
##vigator
criticisms
grins
##lons
##vial
##ody
salute
Coaches
junk
saxophonist
##eology
Uprising
Diet
##marks
chronicles
robbed
##iet
##ahi
Bohemian
magician
wavelength
Kenyan
augmented
fashionable
##ogies
Luce
F1
Monmouth
##jos
##loop
enjoyment
exemption
Centers
##visor
Soundtrack
blinding
practitioner
solidarity
sacrificed
##oso
##cture
##riated
blended
Abd
Copyright
##nob
34th
##reak
Claudio
hectare
rotor
testify
##ends
##iably
##sume
landowner
##cess
##ckman
Eduard
Silesian
backseat
mutually
##abe
Mallory
bounds
Collective
Poet
Winkler
pertaining
scraped
Phelps
crane
flickering
Proto
bubbles
popularized
removes
##86
Cadillac
Warfare
audible
rites
shivering
##sist
##nst
##biotic
Mon
fascist
Bali
Kathryn
ambiguous
furiously
morale
patio
Sang
inconsistent
topology
Greens
monkeys
Köppen
189
Toy
vow
##ías
bombings
##culus
improvised
lodged
subsidiaries
garment
startling
practised
Hume
Thorn
categorized
Till
Eileen
wedge
##64
Federico
patriotic
unlock
##oshi
badminton
Compared
Vilnius
##KE
Crimean
Kemp
decks
spaced
resolutions
sighs
##mind
Imagine
Cartoon
huddled
policemen
forwards
##rouch
equals
##nter
inspected
Charley
MG
##rte
pamphlet
Arturo
dans
scarcely
##ulton
##rvin
parental
unconstitutional
watts
Susannah
Dare
##sitive
Rowland
Valle
invalid
##ué
Detachment
acronym
Yokohama
verified
##lsson
groove
Liza
clarified
compromised
265
##rgon
##orf
hesitant
Fruit
Application
Mathias
icons
##cell
Qin
interventions
##uron
punt
remnant
##rien
Ames
manifold
spines
floral
##zable
comrades
Fallen
orbits
Annals
hobby
Auditorium
implicated
researching
Pueblo
Ta
terminate
##pella
Rings
approximation
fuzzy
##ús
thriving
##ket
Conor
alarmed
etched
Cary
##rdon
Ally
##rington
Pay
mint
##hasa
##unity
##dman
##itate
Oceania
furrowed
trams
##aq
Wentworth
ventured
choreography
prototypes
Patel
mouthed
trenches
##licing
##yya
Lies
deception
##erve
##vations
Bertrand
earthquakes
##tography
Southwestern
##aja
token
Gupta
##yō
Beckett
initials
ironic
Tsar
subdued
shootout
sobbing
liar
Scandinavia
Souls
ch
therapist
trader
Regulation
Kali
busiest
##pation
32nd
Telephone
Vargas
##moky
##nose
##uge
Favorite
abducted
bonding
219
255
correction
mat
drown
fl
unbeaten
Pocket
Summers
Quite
rods
Percussion
##ndy
buzzing
cadet
Wilkes
attire
directory
utilities
naive
populous
Hendrix
##actor
disadvantage
1400
Landon
Underworld
##ense
Occasionally
mercury
Davey
Morley
spa
wrestled
##vender
eclipse
Sienna
supplemented
thou
Stream
liturgical
##gall
##berries
##piration
1769
Bucks
abandoning
##jutant
##nac
232
venom
##31
Roche
dotted
Currie
Córdoba
Milo
Sharif
divides
justification
prejudice
fortunate
##vide
##ābād
Rowe
inflammatory
##eld
avenue
Sources
##rimal
Messenger
Blanco
advocating
formulation
##pute
emphasizes
nut
Armored
##ented
nutrients
##tment
insistence
Martins
landowners
##RB
comparatively
headlines
snaps
##qing
Celebration
##mad
republican
##NE
Trace
##500
1771
proclamation
NRL
Rubin
Buzz
Weimar
##AG
199
posthumous
##ental
##deacon
Distance
intensely
overheard
Arcade
diagonal
hazard
Giving
weekdays
##ù
Verdi
actresses
##hare
Pulling
##erries
##pores
catering
shortest
##ctors
##cure
##restle
##reta
##runch
##brecht
##uddin
Moments
senate
Feng
Prescott
##thest
218
divisional
Bertie
sparse
surrounds
coupling
gravitational
werewolves
##lax
Rankings
##mated
##tries
Shia
##mart
##23
##vocative
interfaces
morphology
newscast
##bide
inputs
solicitor
Olaf
cabinets
puzzles
##tains
Unified
##firmed
WA
solemn
##opy
Tito
Jaenelle
Neolithic
horseback
##ires
pharmacy
prevalence
##lint
Swami
##bush
##tudes
Philipp
mythical
divers
Scouting
aperture
progressively
##bay
##nio
bounce
Floor
##elf
Lucan
adulthood
helm
Bluff
Passage
Salvation
lemon
napkin
scheduling
##gets
Elements
Mina
Novak
stalled
##llister
Infrastructure
##nky
##tania
##uished
Katz
Norma
sucks
trusting
1765
boilers
Accordingly
##hered
223
Crowley
##fight
##ulo
Henrietta
##hani
pounder
surprises
##chor
##glia
Dukes
##cracy
##zier
##fs
Patriot
silicon
##VP
simulcast
telegraph
Mysore
cardboard
Len
##QL
Auguste
accordion
analytical
specify
ineffective
hunched
abnormal
Transylvania
##dn
##tending
Emilia
glittering
Maddy
##wana
1762
External
Lecture
endorsement
Hernández
Anaheim
Ware
offences
##phorus
Plantation
popping
Bonaparte
disgusting
neared
##notes
Identity
heroin
nicely
##raverse
apron
congestion
##PR
padded
##fts
invaders
##came
freshly
Halle
endowed
fracture
ROM
##max
sediments
diffusion
dryly
##tara
Tam
Draw
Spin
Talon
Anthropology
##lify
nausea
##shirt
insert
Fresno
capitalist
indefinitely
apples
Gift
scooped
60s
Cooperative
mistakenly
##lover
murmur
##iger
Equipment
abusive
orphanage
##9th
##lterweight
##unda
Baird
ant
saloon
33rd
Chesapeake
##chair
##sound
##tend
chaotic
pornography
brace
##aret
heiress
SSR
resentment
Arbor
headmaster
##uren
unlimited
##with
##jn
Bram
Ely
Pokémon
pivotal
##guous
Database
Marta
Shine
stumbling
##ovsky
##skin
Henley
Polk
functioned
##layer
##pas
##udd
##MX
blackness
cadets
feral
Damian
##actions
2D
##yla
Apocalypse
##aic
inactivated
##china
##kovic
##bres
destroys
nap
Macy
sums
Madhya
Wisdom
rejects
##amel
60th
Cho
bandwidth
##sons
##obbing
##orama
Mutual
shafts
##estone
##rsen
accord
replaces
waterfront
##gonal
##rida
convictions
##ays
calmed
suppliers
Cummings
GMA
fearful
Scientist
Sinai
examines
experimented
Netflix
Enforcement
Scarlett
##lasia
Healthcare
##onte
Dude
inverted
##36
##regation
##lidae
Munro
##angay
Airbus
overlapping
Drivers
lawsuits
bodily
##udder
Wanda
Effects
Fathers
##finery
##islav
Ridley
observatory
pod
##utrition
Electricity
landslide
##mable
##zoic
##imator
##uration
Estates
sleepy
Nickelodeon
steaming
irony
schedules
snack
spikes
Hmm
##nesia
##bella
##hibit
Greenville
plucked
Harald
##ono
Gamma
infringement
roaring
deposition
##pol
##orum
660
seminal
passports
engagements
Akbar
rotated
##bina
##gart
Hartley
##lown
##truct
uttered
traumatic
Dex
##ôme
Holloway
MV
apartheid
##nee
Counter
Colton
OR
245
Spaniards
Regency
Schedule
scratching
squads
verify
##alk
keyboardist
rotten
Forestry
aids
commemorating
##yed
##érie
Sting
##elly
Dai
##fers
##berley
##ducted
Melvin
cannabis
glider
##enbach
##rban
Costello
Skating
cartoonist
AN
audit
##pectator
distributing
226
312
interpreter
header
Alternatively
##ases
smug
##kumar
cabins
remastered
Connolly
Kelsey
LED
tentative
Check
Sichuan
shaved
##42
Gerhard
Harvest
inward
##rque
Hopefully
hem
##34
Typical
binds
wrath
Woodstock
forcibly
Fergus
##charged
##tured
prepares
amenities
penetration
##ghan
coarse
##oned
enthusiasts
##av
##twined
fielded
##cky
Kiel
##obia
470
beers
tremble
youths
attendees
##cademies
##sex
Macon
communism
dir
##abi
Lennox
Wen
differentiate
jewel
##SO
activate
assert
laden
unto
Gillespie
Guillermo
accumulation
##GM
NGO
Rosenberg
calculating
drastically
##omorphic
peeled
Liège
insurgents
outdoors
##enia
Aspen
Sep
awakened
##eye
Consul
Maiden
insanity
##brian
furnace
Colours
distributions
longitudinal
syllables
##scent
Martian
accountant
Atkins
husbands
sewage
zur
collaborate
highlighting
##rites
##PI
colonization
nearer
##XT
dunes
positioning
Ku
multitude
luxurious
Volvo
linguistics
plotting
squared
##inder
outstretched
##uds
Fuji
ji
##feit
##ahu
##loat
##gado
##luster
##oku
América
##iza
Residents
vine
Pieces
DD
Vampires
##ová
smoked
harshly
spreads
##turn
##zhi
betray
electors
##settled
Considering
exploits
stamped
Dusty
enraged
Nairobi
##38
intervened
##luck
orchestras
##lda
Hereford
Jarvis
calf
##itzer
##CH
salesman
Lovers
cigar
Angelica
doomed
heroine
##tible
Sanford
offenders
##ulously
articulated
##oam
Emanuel
Gardiner
Edna
Shu
gigantic
##stable
Tallinn
coasts
Maker
ale
stalking
##oga
##smus
lucrative
southbound
##changing
Reg
##lants
Schleswig
discount
grouping
physiological
##OH
##sun
Galen
assurance
reconcile
rib
scarlet
Thatcher
anarchist
##oom
Turnpike
##ceding
cocktail
Sweeney
Allegheny
concessions
oppression
reassuring
##poli
##ticus
##TR
##VI
##uca
##zione
directional
strikeouts
Beneath
Couldn
Kabul
##national
hydroelectric
##jit
Desire
##riot
enhancing
northbound
##PO
Ok
Routledge
volatile
Bernardo
Python
333
ample
chestnut
automobiles
##innamon
##care
##hering
BWF
salaries
Turbo
acquisitions
##stituting
strengths
pilgrims
Ponce
Pig
Actors
Beard
sanitation
##RD
##mett
Telecommunications
worms
##idas
Juno
Larson
Ventura
Northeastern
weighs
Houghton
collaborating
lottery
##rano
Wonderland
gigs
##lmer
##zano
##edd
##nife
mixtape
predominant
tripped
##ruly
Alexei
investing
Belgarath
Brasil
hiss
##crat
##xham
Côte
560
kilometer
##cological
analyzing
##As
engined
listener
##cakes
negotiation
##hisky
Santana
##lemma
IAAF
Seneca
skeletal
Covenant
Steiner
##lev
##uen
Neptune
retention
##upon
Closing
Czechoslovak
chalk
Navarre
NZ
##IG
##hop
##oly
##quatorial
##sad
Brewery
Conflict
Them
renew
turrets
disagree
Petra
Slave
##reole
adjustment
##dela
##regard
##sner
framing
stature
##rca
##sies
##46
##mata
Logic
inadvertently
naturalist
spheres
towering
heightened
Dodd
rink
##fle
Keyboards
bulb
diver
ul
##tsk
Exodus
Deacon
España
Canadiens
oblique
thud
reigned
rug
Whitman
Dash
##iens
Haifa
pets
##arland
manually
dart
##bial
Sven
textiles
subgroup
Napier
graffiti
revolver
humming
Babu
protector
typed
Provinces
Sparta
Wills
subjective
##rella
temptation
##liest
FL
Sadie
manifest
Guangdong
Transfer
entertain
eve
recipes
##33
Benedictine
retailer
##dence
establishes
##cluded
##rked
Ursula
##ltz
##lars
##rena
qualifiers
##curement
colt
depictions
##oit
Spiritual
differentiation
staffed
transitional
##lew
1761
fatalities
##oan
Bayern
Northamptonshire
Weeks
##CU
Fife
capacities
hoarse
##latt
##ة
evidenced
##HD
##ographer
assessing
evolve
hints
42nd
streaked
##lve
Yahoo
##estive
##rned
##zas
baggage
Elected
secrecy
##champ
Character
Pen
Decca
cape
Bernardino
vapor
Dolly
counselor
##isers
Benin
##khar
##CR
notch
##thus
##racy
bounty
lend
grassland
##chtenstein
##dating
pseudo
golfer
simplest
##ceive
Lucivar
Triumph
dinosaur
dinosaurs
##šić
Seahawks
##nco
resorts
reelected
1766
reproduce
universally
##OA
ER
tendencies
Consolidated
Massey
Tasmanian
reckless
##icz
##ricks
1755
questionable
Audience
##lates
preseason
Quran
trivial
Haitian
Freeway
dialed
Appointed
Heard
ecosystems
##bula
hormones
Carbon
Rd
##arney
##working
Christoph
presiding
pu
##athy
Morrow
Dar
ensures
posing
remedy
EA
disclosed
##hui
##rten
rumours
surveying
##ficiency
Aziz
Jewel
Plays
##smatic
Bernhard
Christi
##eanut
##friend
jailed
##dr
govern
neighbour
butler
Acheron
murdering
oils
mac
Editorial
detectives
bolts
##ulon
Guitars
malaria
36th
Pembroke
Opened
##hium
harmonic
serum
##sio
Franks
fingernails
##gli
culturally
evolving
scalp
VP
deploy
uploaded
mater
##evo
Jammu
Spa
##icker
flirting
##cursions
Heidi
Majority
sprawled
##alytic
Zheng
bunker
##lena
ST
##tile
Jiang
ceilings
##ently
##ols
Recovery
dire
##good
Manson
Honestly
Montréal
1764
227
quota
Lakshmi
incentive
Accounting
##cilla
Eureka
Reaper
buzzed
##uh
courtroom
dub
##mberg
KC
Gong
Theodor
Académie
NPR
criticizing
protesting
##pired
##yric
abuses
fisheries
##minated
1767
yd
Gemini
Subcommittee
##fuse
Duff
Wasn
Wight
cleaner
##tite
planetary
Survivor
Zionist
mounds
##rary
landfall
disruption
yielding
##yana
bids
unidentified
Garry
Ellison
Elmer
Fishing
Hayward
demos
modelling
##anche
##stick
caressed
entertained
##hesion
piers
Crimea
##mass
WHO
boulder
trunks
1640
Biennale
Palestinians
Pursuit
##udes
Dora
contender
##dridge
Nanjing
##ezer
##former
##ibel
Whole
proliferation
##tide
##weiler
fuels
predictions
##ente
##onium
Filming
absorbing
Ramón
strangled
conveyed
inhabit
prostitutes
recession
bonded
clinched
##eak
##iji
##edar
Pleasure
Rite
Christy
Therapy
sarcasm
##collegiate
hilt
probation
Sarawak
coefficients
underworld
biodiversity
SBS
groom
brewing
dungeon
##claiming
Hari
turnover
##ntina
##omer
##opped
orthodox
styling
##tars
##ulata
priced
Marjorie
##eley
##abar
Yong
##tically
Crambidae
Hernandez
##ego
##rricular
##ark
##lamour
##llin
##augh
##tens
Advancement
Loyola
##4th
##hh
goin
marshes
Sardinia
##ša
Ljubljana
Singing
suspiciously
##hesive
Félix
Regarding
flap
stimulation
##raught
Apr
Yin
gaping
tighten
skier
##itas
##lad
##rani
264
Ashes
Olson
Problems
Tabitha
##rading
balancing
sunrise
##ease
##iture
##ritic
Fringe
##iciency
Inspired
Linnaeus
PBA
disapproval
##kles
##rka
##tails
##urger
Disaster
Laboratories
apps
paradise
Aero
Came
sneaking
Gee
Beacon
ODI
commodity
Ellington
graphical
Gretchen
spire
##skaya
##trine
RTÉ
efficacy
plc
tribunal
##ytic
downhill
flu
medications
##kaya
widen
Sunrise
##nous
distinguishing
pawn
##BO
##irn
##ssing
##ν
Easton
##vila
Rhineland
##aque
defect
##saurus
Goose
Ju
##classified
Middlesbrough
shaping
preached
1759
##erland
Ein
Hailey
musicals
##altered
Galileo
Hilda
Fighters
Lac
##ometric
295
Leafs
Milano
##lta
##VD
##ivist
penetrated
Mask
Orchard
plaintiff
##icorn
Yvonne
##fred
outfielder
peek
Collier
Caracas
repealed
Bois
dell
restrict
Dolores
Hadley
peacefully
##LL
condom
Granny
Orders
sabotage
##toon
##rings
compass
marshal
gears
brigadier
dye
Yunnan
communicating
donate
emerald
vitamin
administer
Fulham
##classical
##llas
Buckinghamshire
Held
layered
disclosure
Akira
programmer
shrimp
Crusade
##ximal
Luzon
bakery
##cute
Garth
Citadel
uniquely
Curling
info
mum
Para
##ști
sleek
##ione
hey
Lantern
mesh
##lacing
##lizzard
##gade
prosecuted
Alba
Gilles
greedy
twists
##ogged
Viper
##kata
Appearances
Skyla
hymns
##pelled
curving
predictable
Grave
Watford
##dford
##liptic
##vary
Westwood
fluids
Models
statutes
##ynamite
1740
##culate
Framework
Johanna
##gression
Vuelta
imp
##otion
##raga
##thouse
Ciudad
festivities
##love
Beyoncé
italics
##vance
DB
##haman
outs
Singers
##ueva
##urning
##51
##ntiary
##mobile
285
Mimi
emeritus
nesting
Keeper
Ways
##onal
##oux
Edmond
MMA
##bark
##oop
Hampson
##ñez
##rets
Gladstone
wreckage
Pont
Playboy
reluctance
##ná
apprenticeship
preferring
Value
originate
##wei
##olio
Alexia
##rog
Parachute
jammed
stud
Eton
vols
##ganized
1745
straining
creep
indicators
##mán
humiliation
hinted
alma
tanker
##egation
Haynes
Penang
amazement
branched
rumble
##ddington
archaeologists
paranoid
expenditure
Absolutely
Musicians
banished
##fining
baptism
Joker
Persons
hemisphere
##tieth
##ück
flock
##xing
lbs
Kung
crab
##dak
##tinent
Regulations
barrage
parcel
##ós
Tanaka
##rsa
Natalia
Voyage
flaws
stepfather
##aven
##eological
Botanical
Minsk
##ckers
Cinderella
Feast
Loving
Previous
Shark
##took
barrister
collaborators
##nnes
Croydon
Graeme
Juniors
##7th
##formation
##ulos
##ák
£2
##hwa
##rove
##ș
Whig
demeanor
Otago
##TH
##ooster
Faber
instructors
##ahl
##bha
emptied
##schen
saga
##lora
exploding
##rges
Crusaders
##caster
##uations
streaks
CBN
bows
insights
ka
1650
diversion
LSU
Wingspan
##liva
Response
sanity
Producers
imitation
##fine
Lange
Spokane
splash
weed
Siberian
magnet
##rocodile
capitals
##rgus
swelled
Rani
Bells
Silesia
arithmetic
rumor
##hampton
favors
Weird
marketplace
##orm
tsunami
unpredictable
##citation
##ferno
Tradition
postwar
stench
succeeds
##roup
Anya
Users
oversized
totaling
pouch
##nat
Tripoli
leverage
satin
##cline
Bathurst
Lund
Niall
thereof
##quid
Bangor
barge
Animated
##53
##alan
Ballard
utilizes
Done
ballistic
NDP
gatherings
##elin
##vening
Rockets
Sabrina
Tamara
Tribal
WTA
##citing
blinded
flux
Khalid
Una
prescription
##jee
Parents
##otics
##food
Silicon
cured
electro
perpendicular
intimacy
##rified
Lots
##ceiving
##powder
incentives
McKenna
##arma
##ounced
##rinkled
Alzheimer
##tarian
262
Seas
##cam
Novi
##hout
##morphic
##hazar
##hul
##nington
Huron
Bahadur
Pirate
pursed
Griffiths
indicted
swap
refrain
##mulating
Lal
stomped
##Pad
##mamoto
Reef
disposed
plastered
weeping
##rato
Minas
hourly
tumors
##ruising
Lyle
##yper
##sol
Odisha
credibility
##Dowell
Braun
Graphic
lurched
muster
##nex
##ührer
##connected
##iek
##ruba
Carthage
Peck
maple
bursting
##lava
Enrico
rite
##jak
Moment
##skar
Styx
poking
Spartan
##urney
Hepburn
Mart
Titanic
newsletter
waits
Mecklenburg
agitated
eats
##dious
Chow
matrices
Maud
##sexual
sermon
234
##sible
##lung
Qi
cemeteries
mined
sprinter
##ckett
coward
##gable
##hell
##thin
##FB
Contact
##hay
rainforest
238
Hemisphere
boasts
##nders
##verance
##kat
Convent
Dunedin
Lecturer
lyricist
##bject
Iberian
comune
##pphire
chunk
##boo
thrusting
fore
informing
pistols
echoes
Tier
battleships
substitution
##belt
moniker
##charya
##lland
Thoroughbred
38th
##01
##tah
parting
tongues
Cale
##seau
Unionist
modular
celebrates
preview
steamed
Bismarck
302
737
vamp
##finity
##nbridge
weaknesses
husky
##berman
absently
##icide
Craven
tailored
Tokugawa
VIP
syntax
Kazan
captives
doses
filtered
overview
Cleopatra
Conversely
stallion
Burger
Suez
Raoul
th
##reaves
Dickson
Nell
Rate
anal
colder
##sław
Arm
Semitic
##green
reflective
1100
episcopal
journeys
##ours
##pository
##dering
residue
Gunn
##27
##ntial
##crates
##zig
Astros
Renee
Emerald
##vili
connectivity
undrafted
Sampson
treasures
##kura
##theon
##vern
Destroyer
##iable
##ener
Frederic
briefcase
confinement
Bree
##WD
Athena
233
Padres
Thom
speeding
##hali
Dental
ducks
Putin
##rcle
##lou
Asylum
##usk
dusk
pasture
Institutes
ONE
jack
##named
diplomacy
Intercontinental
Leagues
Towns
comedic
premature
##edic
##mona
##ories
trimmed
Charge
Cream
guarantees
Dmitry
splashed
Philosophical
tramway
##cape
Maynard
predatory
redundant
##gratory
##wry
sobs
Burgundy
edible
outfits
Handel
dazed
dangerously
idle
Operational
organizes
##sional
blackish
broker
weddings
##halt
Becca
McGee
##gman
protagonists
##pelling
Keynes
aux
stumble
##ordination
Nokia
reel
sexes
##woods
##pheric
##quished
##voc
##oir
##pathian
##ptus
##sma
##tating
##ê
fulfilling
sheath
##ayne
Mei
Ordinary
Collin
Sharpe
grasses
interdisciplinary
##OX
Background
##ignment
Assault
transforms
Hamas
Serge
ratios
##sik
swaying
##rcia
Rosen
##gant
##versible
cinematographer
curly
penny
Kamal
Mellon
Sailor
Spence
phased
Brewers
amassed
Societies
##ropriations
##buted
mythological
##SN
##byss
##ired
Sovereign
preface
Parry
##ife
altitudes
crossings
##28
Crewe
southernmost
taut
McKinley
##owa
##tore
254
##ckney
compiling
Shelton
##hiko
228
Poll
Shepard
Labs
Pace
Carlson
grasping
##ов
Delaney
Winning
robotic
intentional
shattering
##boarding
##git
##grade
Editions
Reserves
ignorant
proposing
##hanna
cutter
Mongols
NW
##eux
Codex
Cristina
Daughters
Rees
forecast
##hita
NGOs
Stations
Beaux
Erwin
##jected
##EX
##trom
Schumacher
##hrill
##rophe
Maharaja
Oricon
##sul
##dynamic
##fighting
Ce
Ingrid
rumbled
Prospect
stairwell
Barnard
applause
complementary
##uba
grunt
##mented
Bloc
Carleton
loft
noisy
##hey
490
contrasted
##inator
##rief
##centric
##fica
Cantonese
Blanc
Lausanne
License
artifact
##ddin
rot
Amongst
Prakash
RF
##topia
milestone
##vard
Winters
Mead
churchyard
Lulu
estuary
##ind
Cha
Infinity
Meadow
subsidies
##valent
CONCACAF
Ching
medicinal
navigate
Carver
Twice
abdominal
regulating
RB
toilets
Brewer
weakening
ambushed
##aut
##vignon
Lansing
unacceptable
reliance
stabbing
##mpo
##naire
Interview
##ested
##imed
bearings
##lts
Rashid
##iation
authenticity
vigorous
##frey
##uel
biologist
NFC
##rmaid
##wash
Makes
##aunt
##steries
withdrawing
##qa
Buccaneers
bleed
inclination
stain
##ilo
##ppel
Torre
privileged
cereal
trailers
alumnus
neon
Cochrane
Mariana
caress
##47
##ients
experimentation
Window
convict
signaled
##YP
rower
Pharmacy
interacting
241
Strings
dominating
kinase
Dinamo
Wire
pains
sensations
##suse
Twenty20
##39
spotlight
##hend
elemental
##pura
Jameson
Swindon
honoring
pained
##ediatric
##lux
Psychological
assemblies
ingredient
Martial
Penguins
beverage
Monitor
mysteries
##ION
emigration
mused
##sique
crore
AMC
Funding
Chinatown
Establishment
Finalist
enjoyable
1756
##mada
##rams
NO
newborn
CS
comprehend
Invisible
Siemens
##acon
246
contraction
##volving
##moration
##rok
montane
##ntation
Galloway
##llow
Verity
directorial
pearl
Leaning
##rase
Fernandez
swallowing
Automatic
Madness
haunting
paddle
##UE
##rrows
##vies
##zuki
##bolt
##iber
Fender
emails
paste
##lancing
hind
homestead
hopeless
##dles
Rockies
garlic
fatty
shrieked
##ismic
Gillian
Inquiry
Schultz
XML
##cius
##uld
Domesday
grenades
northernmost
##igi
Tbilisi
optimistic
##poon
Refuge
stacks
Bose
smash
surreal
Nah
Straits
Conquest
##roo
##weet
##kell
Gladys
CH
##lim
##vitation
Doctorate
NRHP
knocks
Bey
Romano
##pile
242
Diamonds
strides
eclectic
Betsy
clade
##hady
##leashed
dissolve
moss
Suburban
silvery
##bria
tally
turtles
##uctive
finely
industrialist
##nary
Ernesto
oz
pact
loneliness
##hov
Tomb
multinational
risked
Layne
USL
ne
##quiries
Ad
Message
Kamen
Kristen
reefs
implements
##itative
educators
garments
gunshot
##essed
##rve
Montevideo
vigorously
Stamford
assemble
packaged
##same
état
Viva
paragraph
##eter
##wire
Stick
Navajo
MCA
##pressing
ensembles
ABA
##zor
##llus
Partner
raked
##BI
Iona
thump
Celeste
Kiran
##iscovered
##rith
inflammation
##arel
Features
loosened
##yclic
Deluxe
Speak
economical
Frankenstein
Picasso
showcased
##zad
##eira
##planes
##linear
##overs
monsoon
prosecutors
slack
Horses
##urers
Angry
coughing
##truder
Questions
##tō
##zak
challenger
clocks
##ieving
Newmarket
##acle
cursing
stimuli
##mming
##qualified
slapping
##vasive
narration
##kini
Advertising
CSI
alliances
mixes
##yes
covert
amalgamation
reproduced
##ardt
##gis
1648
id
Annette
Boots
Champagne
Brest
Daryl
##emon
##jou
##llers
Mean
adaptive
technicians
##pair
##usal
Yoga
fronts
leaping
Jul
harvesting
keel
##44
petitioned
##lved
yells
Endowment
proponent
##spur
##tised
##zal
Homes
Includes
##ifer
##oodoo
##rvette
awarding
mirrored
ransom
Flute
outlook
##ganj
DVDs
Sufi
frontman
Goddard
barren
##astic
Suicide
hillside
Harlow
Lau
notions
Amnesty
Homestead
##irt
GE
hooded
umpire
mustered
Catch
Masonic
##erd
Dynamics
Equity
Oro
Charts
Mussolini
populace
muted
accompaniment
##lour
##ndes
ignited
##iferous
##laced
##atch
anguish
registry
##tub
##hards
##neer
251
Hooker
uncomfortably
##6th
##ivers
Catalina
MiG
giggling
1754
Dietrich
Kaladin
pricing
##quence
Sabah
##lving
##nical
Gettysburg
Vita
Telecom
Worst
Palais
Pentagon
##brand
##chichte
Graf
unnatural
1715
bio
##26
Radcliffe
##utt
chatting
spices
##aus
untouched
##eper
Doll
turkey
Syndicate
##rlene
##JP
##roots
Como
clashed
modernization
1757
fantasies
##iating
dissipated
Sicilian
inspect
sensible
reputed
##final
Milford
poised
RC
metabolic
Tobacco
Mecca
optimization
##heat
lobe
rabbits
NAS
geologist
##liner
Kilda
carpenter
nationalists
##brae
summarized
##venge
Designer
misleading
beamed
##meyer
Matrix
excuses
##aines
##biology
401
Moose
drafting
Sai
##ggle
Comprehensive
dripped
skate
##WI
##enan
##ruk
narrower
outgoing
##enter
##nounce
overseen
##structure
travellers
banging
scarred
##thing
##arra
Ebert
Sometime
##nated
BAFTA
Hurricanes
configurations
##MLL
immortality
##heus
gothic
##mpest
clergyman
viewpoint
Maxim
Instituto
emitted
quantitative
1689
Consortium
##rsk
Meat
Tao
swimmers
Shaking
Terence
mainline
##linity
Quantum
##rogate
Nair
banquet
39th
reprised
lagoon
subdivisions
synonymous
incurred
password
sprung
##vere
Credits
Petersen
Faces
##vu
statesman
Zombie
gesturing
##going
Sergey
dormant
possessive
totals
southward
Ángel
##odies
HM
Mariano
Ramirez
Wicked
impressions
##Net
##cap
##ème
Transformers
Poker
RIAA
Redesignated
##chuk
Harcourt
Peña
spacious
tinged
alternatively
narrowing
Brigham
authorization
Membership
Zeppelin
##amed
Handball
steer
##orium
##rnal
##rops
Committees
endings
##MM
##yung
ejected
grams
##relli
Birch
Hilary
Stadion
orphan
clawed
##kner
Motown
Wilkins
ballads
outspoken
##ancipation
##bankment
##cheng
Advances
harvested
novelty
ineligible
oversees
##´s
obeyed
inevitably
Kingdoms
burying
Fabian
relevance
Tatiana
##MCA
sarcastic
##onda
Akron
229
sandwiches
Adobe
Maddox
##azar
Hunting
##onized
Smiling
##tology
Juventus
Leroy
Poets
attach
lo
##rly
##film
Structure
##igate
olds
projections
SMS
outnumbered
##tase
judiciary
paramilitary
playfully
##rsing
##tras
Chico
Vin
informally
abandonment
##russ
Baroness
injuring
octagonal
deciduous
##nea
##olm
Hz
Norwood
poses
Marissa
alerted
willed
##KS
Dino
##ddler
##vani
Barbie
Thankfully
625
bicycles
shimmering
##tinuum
##wolf
Chesterfield
##idy
##urgency
Knowles
sweetly
Ventures
##ponents
##valence
Darryl
Powerplant
RAAF
##pec
Kingsley
Parramatta
penetrating
spectacle
##inia
Marlborough
residual
compatibility
hike
Underwood
depleted
ministries
##odus
##ropriation
rotting
Faso
##inn
Happiness
Lille
Suns
cookie
rift
warmly
##lvin
Bugs
Gotham
Gothenburg
Properties
##seller
##ubi
Created
MAC
Noelle
Requiem
Ulysses
##ails
franchises
##icious
##rwick
celestial
kinetic
720
STS
transmissions
amplitude
forums
freeing
reptiles
tumbling
##continent
##rising
##tropy
physiology
##uster
Loves
bodied
neutrality
Neumann
assessments
Vicky
##hom
hampered
##uku
Custom
timed
##eville
##xious
elastic
##section
rig
stilled
shipment
243
artworks
boulders
Bournemouth
##hly
##LF
##linary
rumored
##bino
##drum
Chun
Freiburg
##dges
Equality
252
Guadalajara
##sors
##taire
Roach
cramped
##ultural
Logistics
Punch
fines
Lai
caravan
##55
lame
Collector
pausing
315
migrant
hawk
signalling
##erham
##oughs
Demons
surfing
Rana
insisting
Wien
adolescent
##jong
##rera
##umba
Regis
brushes
##iman
residues
storytelling
Consider
contrasting
regeneration
##elling
##hlete
afforded
reactors
costing
##biotics
##gat
##евич
chanting
secondly
confesses
##ikos
##uang
##ronological
##−
Giacomo
##eca
vaudeville
weeds
rejecting
revoked
affluent
fullback
progresses
geologic
proprietor
replication
gliding
recounted
##bah
##igma
Flow
ii
newcomer
##lasp
##miya
Candace
fractured
interiors
confidential
Inverness
footing
##robe
Coordinator
Westphalia
jumper
##chism
dormitory
##gno
281
acknowledging
leveled
##éra
Algiers
migrate
Frog
Rare
##iovascular
##urous
DSO
nomadic
##iera
woken
lifeless
##graphical
##ifications
Dot
Sachs
crow
nmi
Tacoma
Weight
mushroom
RS
conditioned
##zine
Tunisian
altering
##mizing
Handicap
Patti
Monsieur
clicking
gorge
interrupting
##powerment
drawers
Serra
##icides
Specialist
##itte
connector
worshipped
##ask
consoles
tags
##iler
glued
##zac
fences
Bratislava
honeymoon
313
A2
disposition
Gentleman
Gilmore
glaciers
##scribed
Calhoun
convergence
Aleppo
shortages
##43
##orax
##worm
##codes
##rmal
neutron
##ossa
Bloomberg
Salford
periodicals
##ryan
Slayer
##ynasties
credentials
##tista
surveyor
File
stinging
unnoticed
Medici
ecstasy
espionage
Jett
Leary
circulating
bargaining
concerto
serviced
37th
HK
##fueling
Delilah
Marcia
graded
##join
Kaplan
feasible
##nale
##yt
Burnley
dreadful
ministerial
Brewster
Judah
##ngled
##rrey
recycled
Iroquois
backstage
parchment
##numbered
Kern
Motorsports
Organizations
##mini
Seems
Warrington
Dunbar
Ezio
##eor
paralyzed
Ara
yeast
##olis
cheated
reappeared
banged
##ymph
##dick
Lyndon
glide
Mat
##natch
Hotels
Household
parasite
irrelevant
youthful
##smic
##tero
##anti
2d
Ignacio
squash
##nets
shale
##اد
Abrams
##oese
assaults
##dier
##otte
Swamp
287
Spurs
##economic
Fargo
auditioned
##mé
Haas
une
abbreviation
Turkic
##tisfaction
favorites
specials
##lial
Enlightenment
Burkina
##vir
Comparative
Lacrosse
elves
##lerical
##pear
Borders
controllers
##villa
excelled
##acher
##varo
camouflage
perpetual
##ffles
devoid
schooner
##bered
##oris
Gibbons
Lia
discouraged
sue
##gnition
Excellent
Layton
noir
smack
##ivable
##evity
##lone
Myra
weaken
weaponry
##azza
Shake
backbone
Certified
clown
occupational
caller
enslaved
soaking
Wexford
perceive
shortlisted
##pid
feminism
Bari
Indie
##avelin
##ldo
Hellenic
Hundreds
Savings
comedies
Honors
Mohawk
Told
coded
Incorporated
hideous
trusts
hose
Calais
Forster
Gabon
Internationale
AK
Colour
##UM
##heist
McGregor
localized
##tronomy
Darrell
##iara
squirrel
freaked
##eking
##manned
##ungen
radiated
##dua
commence
Donaldson
##iddle
MR
SAS
Tavern
Teenage
admissions
Instruments
##ilizer
Konrad
contemplated
##ductor
Jing
Reacher
recalling
Dhabi
emphasizing
illumination
##tony
legitimacy
Goethe
Ritter
McDonnell
Polar
Seconds
aspiring
derby
tunic
##rmed
outlines
Changing
distortion
##cter
Mechanics
##urly
##vana
Egg
Wolverine
Stupid
centralized
knit
##Ms
Saratoga
Ogden
storylines
##vres
lavish
beverages
##grarian
Kyrgyzstan
forcefully
superb
Elm
Thessaloniki
follower
Plants
slang
trajectory
Nowadays
Bengals
Ingram
perch
coloring
carvings
doubtful
##aph
##gratulations
##41
Curse
253
nightstand
Campo
Meiji
decomposition
##giri
McCormick
Yours
##amon
##bang
Texans
injunction
organise
periodical
##peculative
oceans
##aley
Success
Lehigh
##guin
1730
Davy
allowance
obituary
##tov
treasury
##wayne
euros
readiness
systematically
##stered
##igor
##xen
##cliff
##lya
Send
##umatic
Celtics
Judiciary
425
propagation
rebellious
##ims
##lut
Dal
##ayman
##cloth
Boise
pairing
Waltz
torment
Hatch
aspirations
diaspora
##hame
Rank
237
Including
Muir
chained
toxicity
Université
##aroo
Mathews
meadows
##bio
Editing
Khorasan
##them
##ahn
##bari
##umes
evacuate
##sium
gram
kidnap
pinning
##diation
##orms
beacon
organising
McGrath
##ogist
Qur
Tango
##ceptor
##rud
##cend
##cie
##jas
##sided
Tuscany
Venture
creations
exhibiting
##rcerer
##tten
Butcher
Divinity
Pet
Whitehead
falsely
perished
handy
Moines
cyclists
synthesizers
Mortal
notoriety
##ronic
Dialogue
expressive
uk
Nightingale
grimly
vineyards
Driving
relentless
compiler
##district
##tuated
Hades
medicines
objection
Answer
Soap
Chattanooga
##gogue
Haryana
Parties
Turtle
##ferred
explorers
stakeholders
##aar
##rbonne
tempered
conjecture
##tee
##hur
Reeve
bumper
stew
##church
##generate
##ilitating
##chanized
##elier
##enne
translucent
##lows
Publisher
evangelical
inherit
##rted
247
SmackDown
bitterness
lesions
##worked
mosques
wed
##lashes
Ng
Rebels
booking
##nail
Incident
Sailing
yo
confirms
Chaplin
baths
##kled
modernist
pulsing
Cicero
slaughtered
boasted
##losure
zipper
##hales
aristocracy
halftime
jolt
unlawful
Marching
sustaining
Yerevan
bracket
ram
Markus
##zef
butcher
massage
##quisite
Leisure
Pizza
collapsing
##lante
commentaries
scripted
##disciplinary
##sused
eroded
alleging
vase
Chichester
Peacock
commencement
dice
hotter
poisonous
executions
##occo
frost
fielding
vendor
Counts
Troops
maize
Divisional
analogue
shadowy
Nuevo
Ville
radiating
worthless
Adriatic
Buy
blaze
brutally
horizontally
longed
##matical
federally
Rolf
Root
exclude
rag
agitation
Lounge
astonished
##wirl
Impossible
transformations
##IVE
##ceded
##slav
downloaded
fucked
Egyptians
Welles
##ffington
U2
befriended
radios
##jid
archaic
compares
##ccelerator
##imated
##tosis
Hung
Scientists
Thousands
geographically
##LR
Macintosh
fluorescent
##ipur
Wehrmacht
##BR
##firmary
Chao
##ague
Boyer
##grounds
##hism
##mento
##taining
infancy
##cton
510
Boca
##loy
1644
ben
dong
stresses
Sweat
expressway
graders
ochreous
nets
Lawn
thirst
Uruguayan
satisfactory
##tracts
baroque
rusty
##ław
Shen
Gdańsk
chickens
##graving
Hodge
Papal
SAT
bearer
##ogo
##rger
merits
Calendar
Highest
Skills
##ortex
Roberta
paradigm
recounts
frigates
swamps
unitary
##oker
balloons
Hawthorne
Muse
spurred
advisors
reclaimed
stimulate
fibre
pat
repeal
##dgson
##iar
##rana
anthropologist
descends
flinch
reared
##chang
##eric
##lithic
commissioning
##cumenical
##lume
##rchen
Wolff
##tsky
Eurasian
Nepali
Nightmare
ZIP
playback
##latz
##vington
Warm
##75
Martina
Rollins
Saetan
Variations
sorting
##م
530
Joaquin
Ptolemy
thinner
##iator
##pticism
Cebu
Highlanders
Linden
Vanguard
##SV
##mor
##ulge
ISSN
cartridges
repression
Étienne
311
Lauderdale
commodities
null
##rb
1720
gearbox
##reator
Ang
Forgotten
dubious
##rls
##dicative
##phate
Groove
Herrera
##çais
Collections
Maximus
##published
Fell
Qualification
filtering
##tized
Roe
hazards
##37
##lative
##tröm
Guadalupe
Tajikistan
Preliminary
fronted
glands
##paper
##iche
##iding
Cairns
rallies
Location
seduce
##mple
BYU
##itic
##FT
Carmichael
Prentice
songwriters
forefront
Physicians
##rille
##zee
Preparatory
##cherous
UV
##dized
Navarro
misses
##nney
Inland
resisting
##sect
Hurt
##lino
galaxies
##raze
Institutions
devote
##lamp
##ciating
baron
##bracing
Hess
operatic
##CL
##ος
Chevalier
Guiana
##lattered
Fed
##cuted
##smo
Skull
denies
236
Waller
##mah
Sakura
mole
nominate
sermons
##bering
widowed
##röm
Cavendish
##struction
Nehru
Revelation
doom
Gala
baking
Nr
Yourself
banning
Individuals
Sykes
orchestrated
630
Phone
steered
620
specialising
starvation
##AV
##alet
##upation
seductive
##jects
##zure
Tolkien
Benito
Wizards
Submarine
dictator
Duo
Caden
approx
basins
##nc
shrink
##icles
##sponsible
249
mit
outpost
##bayashi
##rouse
##tl
Jana
Lombard
RBIs
finalized
humanities
##function
Honorable
tomato
##iot
Pie
tee
##pect
Beaufort
Ferris
bucks
##graduate
##ocytes
Directory
anxiously
##nating
flanks
##Ds
virtues
##believable
Grades
criterion
manufactures
sourced
##balt
##dance
##tano
Ying
##BF
##sett
adequately
blacksmith
totaled
trapping
expanse
Historia
Worker
Sense
ascending
housekeeper
##oos
Crafts
Resurrection
##verty
encryption
##aris
##vat
##pox
##runk
##iability
gazes
spying
##ths
helmets
wired
##zophrenia
Cheung
WR
downloads
stereotypes
239
Lucknow
bleak
Bragg
hauling
##haft
prohibit
##ermined
##castle
barony
##hta
Typhoon
antibodies
##ascism
Hawthorn
Kurdistan
Minority
Gorge
Herr
appliances
disrupt
Drugs
Lazarus
##ilia
##ryo
##tany
Gotta
Masovian
Roxy
choreographed
##rissa
turbulent
##listed
Anatomy
exiting
##det
##isław
580
Kaufman
sage
##apa
Symposium
##rolls
Kaye
##ptera
##rocław
jerking
##menclature
Guo
M1
resurrected
trophies
##lard
Gathering
nestled
serpent
Dow
reservoirs
Claremont
arbitration
chronicle
eki
##arded
##zers
##mmoth
Congregational
Astronomical
NE
RA
Robson
Scotch
modelled
slashed
##imus
exceeds
##roper
##utile
Laughing
vascular
superficial
##arians
Barclay
Caucasian
classmate
sibling
Kimberly
Shreveport
##ilde
##liche
Cheney
Deportivo
Veracruz
berries
##lase
Bed
MI
Anatolia
Mindanao
broadband
##olia
##arte
##wab
darts
##immer
##uze
believers
ordinance
violate
##wheel
##ynth
Alongside
Coupe
Hobbs
arrondissement
earl
townland
##dote
##lihood
##sla
Ghosts
midfield
pulmonary
##eno
cues
##gol
##zda
322
Siena
Sultanate
Bradshaw
Pieter
##thical
Raceway
bared
competence
##ssent
Bet
##urer
##ła
Alistair
Göttingen
appropriately
forge
##osterone
##ugen
DL
345
convoys
inventions
##resses
##cturnal
Fay
Integration
slash
##roats
Widow
barking
##fant
1A
Hooper
##cona
##runched
unreliable
##emont
##esign
##stabulary
##stop
Journalists
bony
##iba
##trata
##ège
horrific
##bish
Jocelyn
##rmon
##apon
##cier
trainers
##ulatory
1753
BR
corpus
synthesized
##bidden
##rafford
Elgin
##entry
Doherty
clockwise
##played
spins
##ample
##bley
Cope
constructions
seater
warlord
Voyager
documenting
fairies
##viator
Lviv
jewellery
suites
##gold
Maia
NME
##eavor
##kus
Eugène
furnishings
##risto
MCC
Metropolis
Older
Telangana
##mpus
amplifier
supervising
1710
buffalo
cushion
terminating
##powering
steak
Quickly
contracting
dem
sarcastically
Elsa
##hein
bastards
narratives
Takes
304
composure
typing
variance
##ifice
Softball
##rations
McLaughlin
gaped
shrines
##hogany
Glamorgan
##icle
##nai
##ntin
Fleetwood
Woodland
##uxe
fictitious
shrugs
##iper
BWV
conform
##uckled
Launch
##ductory
##mized
Tad
##stituted
##free
Bel
Chávez
messing
quartz
##iculate
##folia
##lynn
ushered
##29
##ailing
dictated
Pony
##opsis
precinct
802
Plastic
##ughter
##uno
##porated
Denton
Matters
SPD
hating
##rogen
Essential
Deck
Dortmund
obscured
##maging
Earle
##bred
##ittle
##ropolis
saturated
##fiction
##ression
Pereira
Vinci
mute
warehouses
##ún
biographies
##icking
sealing
##dered
executing
pendant
##wives
murmurs
##oko
substrates
symmetrical
Susie
##mare
Yusuf
analogy
##urage
Lesley
limitation
##rby
##ío
disagreements
##mise
embroidered
nape
unarmed
Sumner
Stores
dwell
Wilcox
creditors
##rivatization
##shes
##amia
directs
recaptured
scouting
McGuire
cradle
##onnell
Sato
insulin
mercenary
tolerant
Macquarie
transitions
cradled
##berto
##ivism
##yotes
FF
Ke
Reach
##dbury
680
##bill
##oja
##sui
prairie
##ogan
reactive
##icient
##rits
Cyclone
Sirius
Survival
Pak
##coach
##trar
halves
Agatha
Opus
contrasts
##jection
ominous
##iden
Baylor
Woodrow
duct
fortification
intercourse
##rois
Colbert
envy
##isi
Afterward
geared
##flections
accelerate
##lenching
Witness
##rrer
Angelina
Material
assertion
misconduct
Nix
cringed
tingling
##eti
##gned
Everest
disturb
sturdy
##keepers
##vied
Profile
heavenly
##kova
##victed
translating
##sses
316
Invitational
Mention
martyr
##uristic
Barron
hardness
Nakamura
405
Genevieve
reflections
##falls
jurist
##LT
Pyramid
##yme
Shoot
heck
linguist
##tower
Ives
superiors
##leo
Achilles
##phological
Christophe
Padma
precedence
grassy
Oral
resurrection
##itting
clumsy
##lten
##rue
huts
##stars
Equal
##queduct
Devin
Gaga
diocesan
##plating
##upe
##graphers
Patch
Scream
hail
moaning
tracts
##hdi
Examination
outsider
##ergic
##oter
Archipelago
Havilland
greenish
tilting
Aleksandr
Konstantin
warship
##emann
##gelist
##ought
billionaire
##blivion
321
Hungarians
transplant
##jured
##fters
Corbin
autism
pitchers
Garner
thence
Scientology
transitioned
integrating
repetitive
##dant
Rene
vomit
##burne
1661
Researchers
Wallis
insulted
wavy
##wati
Ewing
excitedly
##kor
frescoes
injustice
##achal
##lumber
##úl
novella
##sca
Liv
##enstein
##river
monstrous
topping
downfall
looming
sinks
trillion
##pont
Effect
##phi
##urley
Sites
catchment
##H1
Hopper
##raiser
1642
Maccabi
lance
##chia
##sboro
NSA
branching
retorted
tensor
Immaculate
drumming
feeder
##mony
Dyer
homicide
Temeraire
fishes
protruding
skins
orchards
##nso
inlet
ventral
##finder
Asiatic
Sul
1688
Melinda
assigns
paranormal
gardening
Tau
calming
##inge
##crow
regimental
Nik
fastened
correlated
##gene
##rieve
Sick
##minster
##politan
hardwood
hurled
##ssler
Cinematography
rhyme
Montenegrin
Packard
debating
##itution
Helens
Trick
Museums
defiance
encompassed
##EE
##TU
##nees
##uben
##ünster
##nosis
435
Hagen
cinemas
Corbett
commended
##fines
##oman
bosses
ripe
scraping
##loc
filly
Saddam
pointless
Faust
Orléans
Syriac
##♭
longitude
##ropic
Alfa
bliss
gangster
##ckling
SL
blending
##eptide
##nner
bends
escorting
##bloid
##quis
burials
##sle
##è
Ambulance
insults
##gth
Antrim
unfolded
##missible
splendid
Cure
warily
Saigon
Waste
astonishment
boroughs
##VS
##dalgo
##reshing
##usage
rue
marital
versatile
unpaid
allotted
bacterium
##coil
##cue
Dorothea
IDF
##location
##yke
RPG
##tropical
devotees
liter
##pree
Johnstone
astronaut
attends
pollen
periphery
doctrines
meta
showered
##tyn
GO
Huh
laude
244
Amar
Christensen
Ping
Pontifical
Austen
raiding
realities
##dric
urges
##dek
Cambridgeshire
##otype
Cascade
Greenberg
Pact
##cognition
##aran
##urion
Riot
mimic
Eastwood
##imating
reversal
##blast
##henian
Pitchfork
##sunderstanding
Staten
WCW
lieu
##bard
##sang
experimenting
Aquino
##lums
TNT
Hannibal
catastrophic
##lsive
272
308
##otypic
41st
Highways
aggregator
##fluenza
Featured
Reece
dispatch
simulated
##BE
Communion
Vinnie
hardcover
inexpensive
til
##adores
groundwater
kicker
blogs
frenzy
##wala
dealings
erase
Anglia
##umour
Hapoel
Marquette
##raphic
##tives
consult
atrocities
concussion
##érard
Decree
ethanol
##aen
Rooney
##chemist
##hoot
1620
menacing
Schuster
##bearable
laborers
sultan
Juliana
erased
onstage
##ync
Eastman
##tick
hushed
##yrinth
Lexie
Wharton
Lev
##PL
Testing
Bangladeshi
##bba
##usions
communicated
integers
internship
societal
##odles
Loki
ET
Ghent
broadcasters
Unix
##auer
Kildare
Yamaha
##quencing
##zman
chilled
##rapped
##uant
Duval
sentiments
Oliveira
packets
Horne
##rient
Harlan
Mirage
invariant
##anger
##tensive
flexed
sweetness
##wson
alleviate
insulting
limo
Hahn
##llars
##hesia
##lapping
buys
##oaming
mocked
pursuits
scooted
##conscious
##ilian
Ballad
jackets
##kra
hilly
##cane
Scenic
McGraw
silhouette
whipping
##roduced
##wark
##chess
##rump
Lemon
calculus
demonic
##latine
Bharatiya
Govt
Que
Trilogy
Ducks
Suit
stairway
##ceipt
Isa
regulator
Automobile
flatly
##buster
##lank
Spartans
topography
Tavi
usable
Chartered
Fairchild
##sance
##vyn
Digest
nuclei
typhoon
##llon
Alvarez
DJs
Grimm
authoritative
firearm
##chschule
Origins
lair
unmistakable
##xial
##cribing
Mouth
##genesis
##shū
##gaon
##ulter
Jaya
Neck
##UN
##oing
##static
relativity
##mott
##utive
##esan
##uveau
BT
salts
##roa
Dustin
preoccupied
Novgorod
##asus
Magnum
tempting
##histling
##ilated
Musa
##ghty
Ashland
pubs
routines
##etto
Soto
257
Featuring
Augsburg
##alaya
Bit
loomed
expects
##abby
##ooby
Auschwitz
Pendleton
vodka
##sent
rescuing
systemic
##inet
##leg
Yun
applicant
revered
##nacht
##ndas
Muller
characterization
##patient
##roft
Carole
##asperated
Amiga
disconnected
gel
##cologist
Patriotic
rallied
assign
veterinary
installing
##cedural
258
Jang
Parisian
incarcerated
stalk
##iment
Jamal
McPherson
Palma
##oken
##viation
512
Rourke
irrational
##rippled
Devlin
erratic
##NI
##payers
Ni
engages
Portal
aesthetics
##rrogance
Milne
assassins
##rots
335
385
Cambodian
Females
fellows
si
##block
##otes
Jayne
Toro
flutter
##eera
Burr
##lanche
relaxation
##fra
Fitzroy
##undy
1751
261
comb
conglomerate
ribbons
veto
##Es
casts
##ege
1748
Ares
spears
spirituality
comet
##nado
##yeh
Veterinary
aquarium
yer
Councils
##oked
##ynamic
Malmö
remorse
auditions
drilled
Hoffmann
Moe
Nagoya
Yacht
##hakti
##race
##rrick
Talmud
coordinating
##EI
##bul
##his
##itors
##ligent
##uerra
Narayan
goaltender
taxa
##asures
Det
##mage
Infinite
Maid
bean
intriguing
##cription
gasps
socket
##mentary
##reus
sewing
transmitting
##different
##furbishment
##traction
Grimsby
sprawling
Shipyard
##destine
##hropic
##icked
trolley
##agi
##lesh
Josiah
invasions
Content
firefighters
intro
Lucifer
subunit
Sahib
Myrtle
inhibitor
maneuvers
##teca
Wrath
slippery
##versing
Shoes
##dial
##illiers
##luded
##mmal
##pack
handkerchief
##edestal
##stones
Fusion
cumulative
##mell
##cacia
##rudge
##utz
foe
storing
swiped
##meister
##orra
batter
strung
##venting
##kker
Doo
Taste
immensely
Fairbanks
Jarrett
Boogie
1746
mage
Kick
legislators
medial
##ilon
##logies
##ranton
Hybrid
##uters
Tide
deportation
Metz
##secration
##virus
UFO
##fell
##orage
##raction
##rrigan
1747
fabricated
##BM
##GR
##rter
muttering
theorist
##tamine
BMG
Kincaid
solvent
##azed
Thin
adorable
Wendell
ta
##viour
pulses
##pologies
counters
exposition
sewer
Luciano
Clancy
##angelo
##riars
Showtime
observes
frankly
##oppy
Bergman
lobes
timetable
##bri
##uest
FX
##dust
##genus
Glad
Helmut
Meridian
##besity
##ontaine
Revue
miracles
##titis
PP
bluff
syrup
307
Messiah
##erne
interfering
picturesque
unconventional
dipping
hurriedly
Kerman
248
Ethnic
Toward
acidic
Harrisburg
##65
intimidating
##aal
Jed
Pontiac
munitions
##nchen
growling
mausoleum
##ération
##wami
Cy
aerospace
caucus
Doing
##around
##miring
Cuthbert
##poradic
##rovisation
##wth
evaluating
##scraper
Belinda
owes
##sitic
##thermal
##fast
economists
##lishing
##uerre
##ân
credible
##koto
Fourteen
cones
##ebrates
bookstore
towels
##phony
Appearance
newscasts
##olin
Karin
Bingham
##elves
1680
306
disks
##lston
##secutor
Levant
##vout
Micro
snuck
##ogel
##racker
Exploration
drastic
##kening
Elsie
endowment
##utnant
Blaze
##rrosion
leaking
45th
##rug
##uernsey
760
Shapiro
cakes
##ehan
##mei
##ité
##kla
repetition
successively
Friendly
Île
Koreans
Au
Tirana
flourish
Spirits
Yao
reasoned
##leam
Consort
cater
marred
ordeal
supremacy
##ritable
Paisley
euro
healer
portico
wetland
##kman
restart
##habilitation
##zuka
##Script
emptiness
communion
##CF
##inhabited
##wamy
Casablanca
pulsed
##rrible
##safe
395
Dual
Terrorism
##urge
##found
##gnolia
Courage
patriarch
segregated
intrinsic
##liography
##phe
PD
convection
##icidal
Dharma
Jimmie
texted
constituents
twitch
##calated
##mitage
##ringing
415
milling
##geons
Armagh
Geometridae
evergreen
needy
reflex
template
##pina
Schubert
##bruck
##icted
##scher
##wildered
1749
Joanne
clearer
##narl
278
Print
automation
consciously
flashback
occupations
##ests
Casimir
differentiated
policing
repay
##aks
##gnesium
Evaluation
commotion
##CM
##smopolitan
Clapton
mitochondrial
Kobe
1752
Ignoring
Vincenzo
Wet
bandage
##rassed
##unate
Maris
##eted
##hetical
figuring
##eit
##nap
leopard
strategically
##reer
Fen
Iain
##ggins
##pipe
Matteo
McIntyre
##chord
##feng
Romani
asshole
flopped
reassure
Founding
Styles
Torino
patrolling
##erging
##ibrating
##ructural
sincerity
##ät
##teacher
Juliette
##cé
##hog
##idated
##span
Winfield
##fender
##nast
##pliant
1690
Bai
Je
Saharan
expands
Bolshevik
rotate
##root
Britannia
Severn
##cini
##gering
##say
sly
Steps
insertion
rooftop
Piece
cuffs
plausible
##zai
Provost
semantic
##data
##vade
##cimal
IPA
indictment
Libraries
flaming
highlands
liberties
##pio
Elders
aggressively
##pecific
Decision
pigeon
nominally
descriptive
adjustments
equestrian
heaving
##mour
##dives
##fty
##yton
intermittent
##naming
##sets
Calvert
Casper
Tarzan
##kot
Ramírez
##IB
##erus
Gustavo
Roller
vaulted
##solation
##formatics
##tip
Hunger
colloquially
handwriting
hearth
launcher
##idian
##ilities
##lind
##locating
Magdalena
Soo
clubhouse
##kushima
##ruit
Bogotá
Organic
Worship
##Vs
##wold
upbringing
##kick
groundbreaking
##urable
##ván
repulsed
##dira
##ditional
##ici
melancholy
##bodied
##cchi
404
concurrency
H₂O
bouts
##gami
288
Leto
troll
##lak
advising
bundled
##nden
lipstick
littered
##leading
##mogeneous
Experiment
Nikola
grove
##ogram
Mace
##jure
cheat
Annabelle
Tori
lurking
Emery
Walden
##riz
paints
Markets
brutality
overrun
##agu
##sat
din
ostensibly
Fielding
flees
##eron
Pound
ornaments
tornadoes
##nikov
##organisation
##reen
##Works
##ldred
##olten
##stillery
soluble
Mata
Grimes
Léon
##NF
coldly
permitting
##inga
##reaked
Agents
hostess
##dl
Dyke
Kota
avail
orderly
##saur
##sities
Arroyo
##ceps
##egro
Hawke
Noctuidae
html
seminar
##ggles
##wasaki
Clube
recited
##sace
Ascension
Fitness
dough
##ixel
Nationale
##solidate
pulpit
vassal
570
Annapolis
bladder
phylogenetic
##iname
convertible
##ppan
Comet
paler
##definite
Spot
##dices
frequented
Apostles
slalom
##ivision
##mana
##runcated
Trojan
##agger
##iq
##league
Concept
Controller
##barian
##curate
##spersed
##tring
engulfed
inquired
##hmann
286
##dict
##osy
##raw
MacKenzie
su
##ienced
##iggs
##quitaine
bisexual
##noon
runways
subsp
##!
##"
###
##$
##%
##&
##'
##(
##)
##*
##+
##,
##-
##.
##/
##:
##;
##<
##=
##>
##?
##@
##[
##\
##]
##^
##_
##`
##{
##|
##}
##~
##¡
##¢
##£
##¥
##§
##¨
##©
##ª
##«
##¬
##®
##±
##´
##µ
##¶
##·
##¹
##º
##»
##¼
##¾
##¿
##À
##Á
##Â
##Ä
##Å
##Æ
##Ç
##È
##É
##Í
##Î
##Ñ
##Ó
##Ö
##×
##Ø
##Ú
##Ü
##Þ
##â
##ã
##æ
##ç
##î
##ï
##ð
##ñ
##ô
##õ
##÷
##û
##þ
##ÿ
##Ā
##ą
##Ć
##Č
##ď
##Đ
##đ
##ē
##ė
##ę
##ě
##ğ
##ġ
##Ħ
##ħ
##ĩ
##Ī
##İ
##ļ
##Ľ
##ľ
##Ł
##ņ
##ň
##ŋ
##Ō
##ŏ
##ő
##Œ
##œ
##ř
##Ś
##ś
##Ş
##Š
##Ţ
##ţ
##ť
##ũ
##ŭ
##ů
##ű
##ų
##ŵ
##ŷ
##ź
##Ż
##ż
##Ž
##ž
##Ə
##ƒ
##ơ
##ư
##ǎ
##ǐ
##ǒ
##ǔ
##ǫ
##Ș
##Ț
##ț
##ɐ
##ɑ
##ɔ
##ɕ
##ə
##ɛ
##ɡ
##ɣ
##ɨ
##ɪ
##ɲ
##ɾ
##ʀ
##ʁ
##ʂ
##ʃ
##ʊ
##ʋ
##ʌ
##ʐ
##ʑ
##ʒ
##ʔ
##ʰ
##ʲ
##ʳ
##ʷ
##ʻ
##ʼ
##ʾ
##ʿ
##ˈ
##ː
##ˡ
##ˢ
##ˣ
##́
##̃
##̍
##̯
##͡
##Α
##Β
##Γ
##Δ
##Ε
##Η
##Θ
##Ι
##Κ
##Λ
##Μ
##Ν
##Ο
##Π
##Σ
##Τ
##Φ
##Χ
##Ψ
##Ω
##ά
##έ
##ή
##ί
##β
##γ
##δ
##ε
##ζ
##η
##θ
##ι
##κ
##λ
##μ
##ξ
##ο
##π
##ρ
##σ
##τ
##υ
##φ
##χ
##ψ
##ω
##ό
##ύ
##ώ
##І
##Ј
##А
##Б
##В
##Г
##Д
##Е
##Ж
##З
##И
##К
##Л
##М
##Н
##О
##П
##Р
##С
##Т
##У
##Ф
##Х
##Ц
##Ч
##Ш
##Э
##Ю
##Я
##б
##в
##г
##д
##ж
##з
##к
##л
##м
##п
##с
##т
##у
##ф
##х
##ц
##ч
##ш
##щ
##ъ
##ы
##ь
##э
##ю
##ё
##і
##ї
##ј
##њ
##ћ
##Ա
##Հ
##ա
##ե
##ի
##կ
##մ
##յ
##ն
##ո
##ս
##տ
##ր
##ւ
##ְ
##ִ
##ֵ
##ֶ
##ַ
##ָ
##ֹ
##ּ
##א
##ב
##ג
##ד
##ה
##ו
##ז
##ח
##ט
##י
##כ
##ל
##ם
##מ
##ן
##נ
##ס
##ע
##פ
##צ
##ק
##ר
##ש
##ת
##،
##ء
##آ
##أ
##إ
##ئ
##ا
##ب
##ت
##ث
##ج
##ح
##خ
##ذ
##ز
##س
##ش
##ص
##ض
##ط
##ظ
##ع
##غ
##ف
##ق
##ك
##ل
##و
##ى
##َ
##ِ
##ٹ
##پ
##چ
##ک
##گ
##ہ
##ی
##ے
##ं
##आ
##क
##ग
##च
##ज
##ण
##त
##द
##ध
##न
##प
##ब
##भ
##म
##य
##र
##ल
##व
##श
##ष
##स
##ह
##ा
##ि
##ी
##ु
##े
##ो
##्
##।
##॥
##আ
##ই
##এ
##ও
##ক
##খ
##গ
##চ
##ছ
##জ
##ট
##ত
##থ
##দ
##ধ
##ন
##প
##ব
##ম
##য
##র
##ল
##শ
##স
##হ
##়
##া
##ি
##ী
##ু
##ে
##ো
##্
##য়
##க
##த
##ப
##ம
##ய
##ர
##ல
##வ
##ா
##ி
##ு
##்
##ร
##་
##ག
##ང
##ད
##ན
##བ
##མ
##ར
##ལ
##ས
##ི
##ུ
##ེ
##ོ
##ა
##ე
##ი
##ლ
##ნ
##ო
##რ
##ს
##ᴬ
##ᴵ
##ᵀ
##ᵃ
##ᵇ
##ᵈ
##ᵉ
##ᵍ
##ᵏ
##ᵐ
##ᵒ
##ᵖ
##ᵗ
##ᵘ
##ᵣ
##ᵤ
##ᵥ
##ᶜ
##ᶠ
##ḍ
##Ḥ
##ḥ
##Ḩ
##ḩ
##ḳ
##ṃ
##ṅ
##ṇ
##ṛ
##ṣ
##ṭ
##ạ
##ả
##ấ
##ầ
##ẩ
##ậ
##ắ
##ế
##ề
##ể
##ễ
##ệ
##ị
##ọ
##ố
##ồ
##ổ
##ộ
##ớ
##ờ
##ợ
##ụ
##ủ
##ứ
##ừ
##ử
##ữ
##ự
##ỳ
##ỹ
##ἀ
##ἐ
##ὁ
##ὐ
##ὰ
##ὶ
##ὸ
##ῆ
##ῖ
##ῦ
##ῶ
##‐
##‑
##‒
##–
##—
##―
##‖
##‘
##’
##‚
##“
##”
##„
##†
##‡
##•
##…
##‰
##′
##″
##⁄
##⁰
##ⁱ
##⁴
##⁵
##⁶
##⁷
##⁸
##⁹
##⁻
##ⁿ
##₅
##₆
##₇
##₈
##₉
##₊
##₍
##₎
##ₐ
##ₑ
##ₒ
##ₓ
##ₕ
##ₖ
##ₘ
##ₚ
##ₛ
##ₜ
##₤
##€
##₱
##₹
##ℓ
##№
##ℝ
##⅓
##←
##↑
##→
##↔
##⇌
##⇒
##∂
##∈
##∗
##∘
##√
##∞
##∧
##∨
##∩
##∪
##≈
##≠
##≡
##≤
##≥
##⊂
##⊆
##⊕
##⋅
##─
##│
##■
##●
##★
##☆
##☉
##♠
##♣
##♥
##♦
##♯
##⟨
##⟩
##ⱼ
##、
##。
##《
##》
##「
##」
##『
##』
##〜
##い
##う
##え
##お
##か
##き
##く
##け
##こ
##さ
##し
##す
##せ
##そ
##た
##ち
##つ
##て
##と
##な
##に
##の
##は
##ひ
##ま
##み
##む
##め
##も
##や
##ゆ
##よ
##ら
##り
##る
##れ
##ん
##ア
##ィ
##イ
##ウ
##エ
##オ
##カ
##ガ
##キ
##ク
##グ
##コ
##サ
##シ
##ジ
##ス
##ズ
##タ
##ダ
##ッ
##テ
##デ
##ト
##ド
##ナ
##ニ
##ハ
##バ
##パ
##フ
##ブ
##プ
##マ
##ミ
##ム
##ャ
##ュ
##ラ
##リ
##ル
##レ
##ロ
##ン
##・
##ー
##一
##三
##上
##下
##中
##事
##二
##井
##京
##人
##亻
##仁
##佐
##侍
##光
##公
##力
##北
##十
##南
##原
##口
##史
##司
##吉
##同
##和
##囗
##国
##國
##土
##城
##士
##大
##天
##太
##夫
##女
##子
##宀
##安
##宮
##宿
##小
##尚
##山
##島
##川
##州
##平
##年
##心
##愛
##戸
##文
##新
##方
##日
##明
##星
##書
##月
##木
##本
##李
##村
##東
##松
##林
##正
##武
##氏
##水
##氵
##江
##河
##海
##版
##犬
##王
##生
##田
##白
##皇
##省
##真
##石
##社
##神
##竹
##美
##義
##花
##藤
##西
##谷
##車
##辶
##道
##郎
##郡
##部
##野
##金
##長
##門
##陽
##青
##食
##馬
##高
##龍
##龸
##사
##씨
##의
##이
##한
##fi
##fl
##!
##(
##)
##,
##-
##/
##:
|
TensorFlow/Detection/SSD/models/research/object_detection/samples/configs | configs | faster_rcnn_inception_v2_coco | # Faster R-CNN with Inception v2, configuration for MSCOCO Dataset.
# Users should configure the fine_tune_checkpoint field in the train config as
# well as the label_map_path and input_path fields in the train_input_reader and
# eval_input_reader. Search for "PATH_TO_BE_CONFIGURED" to find the fields that
# should be configured.
model {
faster_rcnn {
num_classes: 90
image_resizer {
keep_aspect_ratio_resizer {
min_dimension: 600
max_dimension: 1024
}
}
feature_extractor {
type: 'faster_rcnn_inception_v2'
first_stage_features_stride: 16
}
first_stage_anchor_generator {
grid_anchor_generator {
scales: [0.25, 0.5, 1.0, 2.0]
aspect_ratios: [0.5, 1.0, 2.0]
height_stride: 16
width_stride: 16
}
}
first_stage_box_predictor_conv_hyperparams {
op: CONV
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
truncated_normal_initializer {
stddev: 0.01
}
}
}
first_stage_nms_score_threshold: 0.0
first_stage_nms_iou_threshold: 0.7
first_stage_max_proposals: 300
first_stage_localization_loss_weight: 2.0
first_stage_objectness_loss_weight: 1.0
initial_crop_size: 14
maxpool_kernel_size: 2
maxpool_stride: 2
second_stage_box_predictor {
mask_rcnn_box_predictor {
use_dropout: false
dropout_keep_probability: 1.0
fc_hyperparams {
op: FC
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
variance_scaling_initializer {
factor: 1.0
uniform: true
mode: FAN_AVG
}
}
}
}
}
second_stage_post_processing {
batch_non_max_suppression {
score_threshold: 0.0
iou_threshold: 0.6
max_detections_per_class: 100
max_total_detections: 300
}
score_converter: SOFTMAX
}
second_stage_localization_loss_weight: 2.0
second_stage_classification_loss_weight: 1.0
}
}
train_config: {
batch_size: 1
optimizer {
momentum_optimizer: {
learning_rate: {
manual_step_learning_rate {
initial_learning_rate: 0.0002
schedule {
step: 900000
learning_rate: .00002
}
schedule {
step: 1200000
learning_rate: .000002
}
}
}
momentum_optimizer_value: 0.9
}
use_moving_average: false
}
gradient_clipping_by_norm: 10.0
fine_tune_checkpoint: "PATH_TO_BE_CONFIGURED/model.ckpt"
from_detection_checkpoint: true
# Note: The below line limits the training process to 200K steps, which we
# empirically found to be sufficient enough to train the COCO dataset. This
# effectively bypasses the learning rate schedule (the learning rate will
# never decay). Remove the below line to train indefinitely.
num_steps: 200000
data_augmentation_options {
random_horizontal_flip {
}
}
}
train_input_reader: {
tf_record_input_reader {
input_path: "PATH_TO_BE_CONFIGURED/mscoco_train.record-?????-of-00100"
}
label_map_path: "PATH_TO_BE_CONFIGURED/mscoco_label_map.pbtxt"
}
eval_config: {
num_examples: 8000
# Note: The below line limits the evaluation process to 10 evaluations.
# Remove the below line to evaluate indefinitely.
max_evals: 10
}
eval_input_reader: {
tf_record_input_reader {
input_path: "PATH_TO_BE_CONFIGURED/mscoco_val.record-?????-of-00010"
}
label_map_path: "PATH_TO_BE_CONFIGURED/mscoco_label_map.pbtxt"
shuffle: false
num_readers: 1
}
|
TensorFlow/Detection/SSD/models/research/object_detection/dataset_tools | dataset_tools | oid_hierarchical_labels_expansion | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
r"""An executable to expand hierarchically image-level labels and boxes.
Example usage:
python models/research/object_detection/dataset_tools/\
oid_hierarchical_labels_expansion.py \
--json_hierarchy_file=<path to JSON hierarchy> \
--input_annotations=<input csv file> \
--output_annotations=<output csv file> \
--annotation_type=<1 (for boxes) or 2 (for image-level labels)>
"""
from __future__ import print_function
import argparse
import json
def _update_dict(initial_dict, update):
"""Updates dictionary with update content.
Args:
initial_dict: initial dictionary.
update: updated dictionary.
"""
for key, value_list in update.iteritems():
if key in initial_dict:
initial_dict[key].extend(value_list)
else:
initial_dict[key] = value_list
def _build_plain_hierarchy(hierarchy, skip_root=False):
"""Expands tree hierarchy representation to parent-child dictionary.
Args:
hierarchy: labels hierarchy as JSON file.
skip_root: if true skips root from the processing (done for the case when all
classes under hierarchy are collected under virtual node).
Returns:
keyed_parent - dictionary of parent - all its children nodes.
keyed_child - dictionary of children - all its parent nodes
children - all children of the current node.
"""
all_children = []
all_keyed_parent = {}
all_keyed_child = {}
if 'Subcategory' in hierarchy:
for node in hierarchy['Subcategory']:
keyed_parent, keyed_child, children = _build_plain_hierarchy(node)
# Update is not done through dict.update() since some children have multi-
# ple parents in the hiearchy.
_update_dict(all_keyed_parent, keyed_parent)
_update_dict(all_keyed_child, keyed_child)
all_children.extend(children)
if not skip_root:
all_keyed_parent[hierarchy['LabelName']] = all_children
all_children = [hierarchy['LabelName']] + all_children
for child, _ in all_keyed_child.iteritems():
all_keyed_child[child].append(hierarchy['LabelName'])
all_keyed_child[hierarchy['LabelName']] = []
return all_keyed_parent, all_keyed_child, all_children
class OIDHierarchicalLabelsExpansion(object):
""" Main class to perform labels hierachical expansion."""
def __init__(self, hierarchy):
"""Constructor.
Args:
hierarchy: labels hierarchy as JSON object.
"""
self._hierarchy_keyed_parent, self._hierarchy_keyed_child, _ = (
_build_plain_hierarchy(hierarchy, skip_root=True))
def expand_boxes_from_csv(self, csv_row):
"""Expands a row containing bounding boxes from CSV file.
Args:
csv_row: a single row of Open Images released groundtruth file.
Returns:
a list of strings (including the initial row) corresponding to the ground
truth expanded to multiple annotation for evaluation with Open Images
Challenge 2018 metric.
"""
# Row header is expected to be exactly:
# ImageID,Source,LabelName,Confidence,XMin,XMax,YMin,YMax,IsOccluded,
# IsTruncated,IsGroupOf,IsDepiction,IsInside
cvs_row_splitted = csv_row.split(',')
assert len(cvs_row_splitted) == 13
result = [csv_row]
assert cvs_row_splitted[2] in self._hierarchy_keyed_child
parent_nodes = self._hierarchy_keyed_child[cvs_row_splitted[2]]
for parent_node in parent_nodes:
cvs_row_splitted[2] = parent_node
result.append(','.join(cvs_row_splitted))
return result
def expand_labels_from_csv(self, csv_row):
"""Expands a row containing bounding boxes from CSV file.
Args:
csv_row: a single row of Open Images released groundtruth file.
Returns:
a list of strings (including the initial row) corresponding to the ground
truth expanded to multiple annotation for evaluation with Open Images
Challenge 2018 metric.
"""
# Row header is expected to be exactly:
# ImageID,Source,LabelName,Confidence
cvs_row_splited = csv_row.split(',')
assert len(cvs_row_splited) == 4
result = [csv_row]
if int(cvs_row_splited[3]) == 1:
assert cvs_row_splited[2] in self._hierarchy_keyed_child
parent_nodes = self._hierarchy_keyed_child[cvs_row_splited[2]]
for parent_node in parent_nodes:
cvs_row_splited[2] = parent_node
result.append(','.join(cvs_row_splited))
else:
assert cvs_row_splited[2] in self._hierarchy_keyed_parent
child_nodes = self._hierarchy_keyed_parent[cvs_row_splited[2]]
for child_node in child_nodes:
cvs_row_splited[2] = child_node
result.append(','.join(cvs_row_splited))
return result
def main(parsed_args):
with open(parsed_args.json_hierarchy_file) as f:
hierarchy = json.load(f)
expansion_generator = OIDHierarchicalLabelsExpansion(hierarchy)
labels_file = False
if parsed_args.annotation_type == 2:
labels_file = True
elif parsed_args.annotation_type != 1:
print('--annotation_type expected value is 1 or 2.')
return -1
with open(parsed_args.input_annotations, 'r') as source:
with open(parsed_args.output_annotations, 'w') as target:
header = None
for line in source:
if not header:
header = line
target.writelines(header)
continue
if labels_file:
expanded_lines = expansion_generator.expand_labels_from_csv(line)
else:
expanded_lines = expansion_generator.expand_boxes_from_csv(line)
target.writelines(expanded_lines)
if __name__ == '__main__':
parser = argparse.ArgumentParser(
description='Hierarchically expand annotations (excluding root node).')
parser.add_argument(
'--json_hierarchy_file',
required=True,
help='Path to the file containing label hierarchy in JSON format.')
parser.add_argument(
'--input_annotations',
required=True,
help="""Path to Open Images annotations file (either bounding boxes or
image-level labels).""")
parser.add_argument(
'--output_annotations',
required=True,
help="""Path to the output file.""")
parser.add_argument(
'--annotation_type',
type=int,
required=True,
help="""Type of the input annotations: 1 - boxes, 2 - image-level
labels"""
)
args = parser.parse_args()
main(args)
|
TensorFlow2/LanguageModeling/BERT/official/nlp/modeling/layers | layers | transformer_scaffold_test | # Copyright 2019 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Tests for Keras-based transformer block layer."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import json
import numpy as np
import tensorflow as tf
from tensorflow.python.keras import keras_parameterized # pylint: disable=g-direct-tensorflow-import
from official.nlp.modeling.layers import attention
from official.nlp.modeling.layers import transformer_scaffold
# Test class that wraps a standard attention layer. If this layer is called
# at any point, the list passed to the config object will be filled with a
# boolean 'True'. We register this class as a Keras serializable so we can
# test serialization below.
# @tf.keras.utils.register_keras_serializable(package='TestOnly')
class ValidatedAttentionLayer(attention.Attention):
def __init__(self, call_list, **kwargs):
super(ValidatedAttentionLayer, self).__init__(**kwargs)
self.list = call_list
def call(self, inputs):
self.list.append(True)
return super(ValidatedAttentionLayer, self).call(inputs)
def get_config(self):
config = super(ValidatedAttentionLayer, self).get_config()
config['call_list'] = []
return config
# This decorator runs the test in V1, V2-Eager, and V2-Functional mode. It
# guarantees forward compatibility of this code for the V2 switchover.
@keras_parameterized.run_all_keras_modes
class TransformerLayerTest(keras_parameterized.TestCase):
def test_layer_creation(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
output_tensor = test_layer(data_tensor)
# The default output of a transformer layer should be the same as the input.
self.assertEqual(data_tensor.shape.as_list(), output_tensor.shape.as_list())
# If call_list[0] exists and is True, the passed layer class was
# instantiated from the given config properly.
self.assertNotEmpty(call_list)
self.assertTrue(call_list[0], "The passed layer class wasn't instantiated.")
def test_layer_creation_with_mask(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
# Create a 2-dimensional input (the first dimension is implicit).
mask_tensor = tf.keras.Input(shape=(sequence_length, sequence_length))
output_tensor = test_layer([data_tensor, mask_tensor])
# The default output of a transformer layer should be the same as the input.
self.assertEqual(data_tensor.shape.as_list(), output_tensor.shape.as_list())
# If call_list[0] exists and is True, the passed layer class was
# instantiated from the given config properly.
self.assertNotEmpty(call_list)
self.assertTrue(call_list[0], "The passed layer class wasn't instantiated.")
def test_layer_creation_with_incorrect_mask_fails(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
# Create a 2-dimensional input (the first dimension is implicit).
mask_tensor = tf.keras.Input(shape=(sequence_length, sequence_length - 3))
with self.assertRaisesRegex(ValueError, 'When passing a mask tensor.*'):
_ = test_layer([data_tensor, mask_tensor])
def test_layer_invocation(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
output_tensor = test_layer(data_tensor)
# Create a model from the test layer.
model = tf.keras.Model(data_tensor, output_tensor)
# Invoke the model on test data. We can't validate the output data itself
# (the NN is too complex) but this will rule out structural runtime errors.
batch_size = 6
input_data = 10 * np.random.random_sample(
(batch_size, sequence_length, width))
_ = model.predict(input_data)
# If call_list[0] exists and is True, the passed layer class was
# instantiated from the given config properly.
self.assertNotEmpty(call_list)
self.assertTrue(call_list[0], "The passed layer class wasn't instantiated.")
def test_layer_invocation_with_mask(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
# Create a 2-dimensional input (the first dimension is implicit).
mask_tensor = tf.keras.Input(shape=(sequence_length, sequence_length))
output_tensor = test_layer([data_tensor, mask_tensor])
# Create a model from the test layer.
model = tf.keras.Model([data_tensor, mask_tensor], output_tensor)
# Invoke the model on test data. We can't validate the output data itself
# (the NN is too complex) but this will rule out structural runtime errors.
batch_size = 6
input_data = 10 * np.random.random_sample(
(batch_size, sequence_length, width))
# The attention mask should be of shape (batch, from_seq_len, to_seq_len),
# which here is (batch, sequence_length, sequence_length)
mask_data = np.random.randint(
2, size=(batch_size, sequence_length, sequence_length))
_ = model.predict([input_data, mask_data])
# If call_list[0] exists and is True, the passed layer class was
# instantiated from the given config properly.
self.assertNotEmpty(call_list)
self.assertTrue(call_list[0], "The passed layer class wasn't instantiated.")
def test_layer_invocation_with_float16_dtype(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu',
dtype='float16')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(
shape=(sequence_length, width), dtype=tf.float16)
# Create a 2-dimensional input (the first dimension is implicit).
mask_tensor = tf.keras.Input(shape=(sequence_length, sequence_length))
output_tensor = test_layer([data_tensor, mask_tensor])
# Create a model from the test layer.
model = tf.keras.Model([data_tensor, mask_tensor], output_tensor)
# Invoke the model on test data. We can't validate the output data itself
# (the NN is too complex) but this will rule out structural runtime errors.
batch_size = 6
input_data = (10 * np.random.random_sample(
(batch_size, sequence_length, width))).astype(np.float16)
# The attention mask should be of shape (batch, from_seq_len, to_seq_len),
# which here is (batch, sequence_length, sequence_length)
mask_data = np.random.randint(
2, size=(batch_size, sequence_length, sequence_length))
_ = model.predict([input_data, mask_data])
# If call_list[0] exists and is True, the passed layer class was
# instantiated from the given config properly.
self.assertNotEmpty(call_list)
self.assertTrue(call_list[0], "The passed layer class wasn't instantiated.")
def test_transform_with_initializer(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu',
kernel_initializer=tf.keras.initializers.TruncatedNormal(stddev=0.02))
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
output = test_layer(data_tensor)
# The default output of a transformer layer should be the same as the input.
self.assertEqual(data_tensor.shape.as_list(), output.shape.as_list())
# If call_list[0] exists and is True, the passed layer class was
# instantiated from the given config properly.
self.assertNotEmpty(call_list)
self.assertTrue(call_list[0])
def test_layer_restoration_from_config(self):
sequence_length = 21
width = 80
call_list = []
attention_layer_cfg = {
'num_heads': 10,
'head_size': 8,
'call_list': call_list,
'name': 'test_layer',
}
test_layer = transformer_scaffold.TransformerScaffold(
attention_cls=ValidatedAttentionLayer,
attention_cfg=attention_layer_cfg,
num_attention_heads=10,
intermediate_size=2048,
intermediate_activation='relu')
# Create a 3-dimensional input (the first dimension is implicit).
data_tensor = tf.keras.Input(shape=(sequence_length, width))
# Create a 2-dimensional input (the first dimension is implicit).
mask_tensor = tf.keras.Input(shape=(sequence_length, sequence_length))
output_tensor = test_layer([data_tensor, mask_tensor])
# Create a model from the test layer.
model = tf.keras.Model([data_tensor, mask_tensor], output_tensor)
# Invoke the model on test data. We can't validate the output data itself
# (the NN is too complex) but this will rule out structural runtime errors.
batch_size = 6
input_data = 10 * np.random.random_sample(
(batch_size, sequence_length, width))
# The attention mask should be of shape (batch, from_seq_len, to_seq_len),
# which here is (batch, sequence_length, sequence_length)
mask_data = np.random.randint(
2, size=(batch_size, sequence_length, sequence_length))
pre_serialization_output = model.predict([input_data, mask_data])
# Serialize the model config. Pass the serialized data through json to
# ensure that we can serialize this layer to disk.
serialized_data = json.dumps(model.get_config())
post_string_serialized_data = json.loads(serialized_data)
# Create a new model from the old config, and copy the weights. These models
# should have identical outputs.
new_model = tf.keras.Model.from_config(post_string_serialized_data)
new_model.set_weights(model.get_weights())
output = new_model.predict([input_data, mask_data])
self.assertAllClose(pre_serialization_output, output)
# If the layer was configured correctly, it should have a list attribute
# (since it should have the custom class and config passed to it).
new_model.summary()
new_call_list = new_model.get_layer(
name='transformer_scaffold')._attention_layer.list
self.assertNotEmpty(new_call_list)
self.assertTrue(new_call_list[0],
"The passed layer class wasn't instantiated.")
if __name__ == '__main__':
tf.test.main()
|
TensorFlow/Detection/SSD/models/research/object_detection/dataset_tools | dataset_tools | create_pascal_tf_record | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
r"""Convert raw PASCAL dataset to TFRecord for object_detection.
Example usage:
python object_detection/dataset_tools/create_pascal_tf_record.py \
--data_dir=/home/user/VOCdevkit \
--year=VOC2012 \
--output_path=/home/user/pascal.record
"""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import hashlib
import io
import logging
import os
from lxml import etree
import PIL.Image
import tensorflow as tf
from object_detection.utils import dataset_util
from object_detection.utils import label_map_util
flags = tf.app.flags
flags.DEFINE_string('data_dir', '', 'Root directory to raw PASCAL VOC dataset.')
flags.DEFINE_string('set', 'train', 'Convert training set, validation set or '
'merged set.')
flags.DEFINE_string('annotations_dir', 'Annotations',
'(Relative) path to annotations directory.')
flags.DEFINE_string('year', 'VOC2007', 'Desired challenge year.')
flags.DEFINE_string('output_path', '', 'Path to output TFRecord')
flags.DEFINE_string('label_map_path', 'data/pascal_label_map.pbtxt',
'Path to label map proto')
flags.DEFINE_boolean('ignore_difficult_instances', False, 'Whether to ignore '
'difficult instances')
FLAGS = flags.FLAGS
SETS = ['train', 'val', 'trainval', 'test']
YEARS = ['VOC2007', 'VOC2012', 'merged']
def dict_to_tf_example(data,
dataset_directory,
label_map_dict,
ignore_difficult_instances=False,
image_subdirectory='JPEGImages'):
"""Convert XML derived dict to tf.Example proto.
Notice that this function normalizes the bounding box coordinates provided
by the raw data.
Args:
data: dict holding PASCAL XML fields for a single image (obtained by
running dataset_util.recursive_parse_xml_to_dict)
dataset_directory: Path to root directory holding PASCAL dataset
label_map_dict: A map from string label names to integers ids.
ignore_difficult_instances: Whether to skip difficult instances in the
dataset (default: False).
image_subdirectory: String specifying subdirectory within the
PASCAL dataset directory holding the actual image data.
Returns:
example: The converted tf.Example.
Raises:
ValueError: if the image pointed to by data['filename'] is not a valid JPEG
"""
img_path = os.path.join(data['folder'], image_subdirectory, data['filename'])
full_path = os.path.join(dataset_directory, img_path)
with tf.gfile.GFile(full_path, 'rb') as fid:
encoded_jpg = fid.read()
encoded_jpg_io = io.BytesIO(encoded_jpg)
image = PIL.Image.open(encoded_jpg_io)
if image.format != 'JPEG':
raise ValueError('Image format not JPEG')
key = hashlib.sha256(encoded_jpg).hexdigest()
width = int(data['size']['width'])
height = int(data['size']['height'])
xmin = []
ymin = []
xmax = []
ymax = []
classes = []
classes_text = []
truncated = []
poses = []
difficult_obj = []
if 'object' in data:
for obj in data['object']:
difficult = bool(int(obj['difficult']))
if ignore_difficult_instances and difficult:
continue
difficult_obj.append(int(difficult))
xmin.append(float(obj['bndbox']['xmin']) / width)
ymin.append(float(obj['bndbox']['ymin']) / height)
xmax.append(float(obj['bndbox']['xmax']) / width)
ymax.append(float(obj['bndbox']['ymax']) / height)
classes_text.append(obj['name'].encode('utf8'))
classes.append(label_map_dict[obj['name']])
truncated.append(int(obj['truncated']))
poses.append(obj['pose'].encode('utf8'))
example = tf.train.Example(features=tf.train.Features(feature={
'image/height': dataset_util.int64_feature(height),
'image/width': dataset_util.int64_feature(width),
'image/filename': dataset_util.bytes_feature(
data['filename'].encode('utf8')),
'image/source_id': dataset_util.bytes_feature(
data['filename'].encode('utf8')),
'image/key/sha256': dataset_util.bytes_feature(key.encode('utf8')),
'image/encoded': dataset_util.bytes_feature(encoded_jpg),
'image/format': dataset_util.bytes_feature('jpeg'.encode('utf8')),
'image/object/bbox/xmin': dataset_util.float_list_feature(xmin),
'image/object/bbox/xmax': dataset_util.float_list_feature(xmax),
'image/object/bbox/ymin': dataset_util.float_list_feature(ymin),
'image/object/bbox/ymax': dataset_util.float_list_feature(ymax),
'image/object/class/text': dataset_util.bytes_list_feature(classes_text),
'image/object/class/label': dataset_util.int64_list_feature(classes),
'image/object/difficult': dataset_util.int64_list_feature(difficult_obj),
'image/object/truncated': dataset_util.int64_list_feature(truncated),
'image/object/view': dataset_util.bytes_list_feature(poses),
}))
return example
def main(_):
if FLAGS.set not in SETS:
raise ValueError('set must be in : {}'.format(SETS))
if FLAGS.year not in YEARS:
raise ValueError('year must be in : {}'.format(YEARS))
data_dir = FLAGS.data_dir
years = ['VOC2007', 'VOC2012']
if FLAGS.year != 'merged':
years = [FLAGS.year]
writer = tf.python_io.TFRecordWriter(FLAGS.output_path)
label_map_dict = label_map_util.get_label_map_dict(FLAGS.label_map_path)
for year in years:
logging.info('Reading from PASCAL %s dataset.', year)
examples_path = os.path.join(data_dir, year, 'ImageSets', 'Main',
'aeroplane_' + FLAGS.set + '.txt')
annotations_dir = os.path.join(data_dir, year, FLAGS.annotations_dir)
examples_list = dataset_util.read_examples_list(examples_path)
for idx, example in enumerate(examples_list):
if idx % 100 == 0:
logging.info('On image %d of %d', idx, len(examples_list))
path = os.path.join(annotations_dir, example + '.xml')
with tf.gfile.GFile(path, 'r') as fid:
xml_str = fid.read()
xml = etree.fromstring(xml_str)
data = dataset_util.recursive_parse_xml_to_dict(xml)['annotation']
tf_example = dict_to_tf_example(data, FLAGS.data_dir, label_map_dict,
FLAGS.ignore_difficult_instances)
writer.write(tf_example.SerializeToString())
writer.close()
if __name__ == '__main__':
tf.app.run()
|
PyTorch/SpeechRecognition/QuartzNet/common | common | optimizers | # Copyright (c) 2019, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import torch
from torch.optim import Optimizer
import math
def lr_policy(step, epoch, initial_lr, optimizer, steps_per_epoch, warmup_epochs,
hold_epochs, num_epochs=None, policy='linear', min_lr=1e-5,
exp_gamma=None):
"""
learning rate decay
Args:
initial_lr: base learning rate
step: current iteration number
N: total number of iterations over which learning rate is decayed
lr_steps: list of steps to apply exp_gamma
"""
warmup_steps = warmup_epochs * steps_per_epoch
hold_steps = hold_epochs * steps_per_epoch
if policy == 'legacy':
assert num_epochs is not None
tot_steps = num_epochs * steps_per_epoch
if step < warmup_steps:
a = (step + 1) / (warmup_steps + 1)
elif step < warmup_steps + hold_steps:
a = 1.0
else:
a = (((tot_steps - step)
/ (tot_steps - warmup_steps - hold_steps)) ** 2)
elif policy == 'exponential':
assert exp_gamma is not None
if step < warmup_steps:
a = (step + 1) / (warmup_steps + 1)
elif step < warmup_steps + hold_steps:
a = 1.0
else:
a = exp_gamma ** (epoch - warmup_epochs - hold_epochs)
else:
raise ValueError
new_lr = max(a * initial_lr, min_lr)
for param_group in optimizer.param_groups:
param_group['lr'] = new_lr
class AdamW(Optimizer):
"""Implements AdamW algorithm.
It has been proposed in `Adam: A Method for Stochastic Optimization`_.
Arguments:
params (iterable): iterable of parameters to optimize or dicts defining
parameter groups
lr (float, optional): learning rate (default: 1e-3)
betas (Tuple[float, float], optional): coefficients used for computing
running averages of gradient and its square (default: (0.9, 0.999))
eps (float, optional): term added to the denominator to improve
numerical stability (default: 1e-8)
weight_decay (float, optional): weight decay (L2 penalty) (default: 0)
amsgrad (boolean, optional): whether to use the AMSGrad variant of this
algorithm from the paper `On the Convergence of Adam and Beyond`_
Adam: A Method for Stochastic Optimization:
https://arxiv.org/abs/1412.6980
On the Convergence of Adam and Beyond:
https://openreview.net/forum?id=ryQu7f-RZ
"""
def __init__(self, params, lr=1e-3, betas=(0.9, 0.999), eps=1e-8,
weight_decay=0, amsgrad=False):
if not 0.0 <= lr:
raise ValueError("Invalid learning rate: {}".format(lr))
if not 0.0 <= eps:
raise ValueError("Invalid epsilon value: {}".format(eps))
if not 0.0 <= betas[0] < 1.0:
raise ValueError("Invalid beta parameter at index 0: {}".format(betas[0]))
if not 0.0 <= betas[1] < 1.0:
raise ValueError("Invalid beta parameter at index 1: {}".format(betas[1]))
defaults = dict(lr=lr, betas=betas, eps=eps,
weight_decay=weight_decay, amsgrad=amsgrad)
super(AdamW, self).__init__(params, defaults)
def __setstate__(self, state):
super(AdamW, self).__setstate__(state)
for group in self.param_groups:
group.setdefault('amsgrad', False)
def step(self, closure=None):
"""Performs a single optimization step.
Arguments:
closure (callable, optional): A closure that reevaluates the model
and returns the loss.
"""
loss = None
if closure is not None:
loss = closure()
for group in self.param_groups:
for p in group['params']:
if p.grad is None:
continue
grad = p.grad.data
if grad.is_sparse:
raise RuntimeError('Adam does not support sparse gradients, please consider SparseAdam instead')
amsgrad = group['amsgrad']
state = self.state[p]
# State initialization
if len(state) == 0:
state['step'] = 0
# Exponential moving average of gradient values
state['exp_avg'] = torch.zeros_like(p.data)
# Exponential moving average of squared gradient values
state['exp_avg_sq'] = torch.zeros_like(p.data)
if amsgrad:
# Maintains max of all exp. moving avg. of sq. grad. values
state['max_exp_avg_sq'] = torch.zeros_like(p.data)
exp_avg, exp_avg_sq = state['exp_avg'], state['exp_avg_sq']
if amsgrad:
max_exp_avg_sq = state['max_exp_avg_sq']
beta1, beta2 = group['betas']
state['step'] += 1
# Decay the first and second moment running average coefficient
exp_avg.mul_(beta1).add_(grad, alpha=1 - beta1)
exp_avg_sq.mul_(beta2).addcmul_(1 - beta2, grad, grad)
if amsgrad:
# Maintains the maximum of all 2nd moment running avg. till now
torch.max(max_exp_avg_sq, exp_avg_sq, out=max_exp_avg_sq)
# Use the max. for normalizing running avg. of gradient
denom = max_exp_avg_sq.sqrt().add_(group['eps'])
else:
denom = exp_avg_sq.sqrt().add_(group['eps'])
bias_correction1 = 1 - beta1 ** state['step']
bias_correction2 = 1 - beta2 ** state['step']
step_size = group['lr'] * math.sqrt(bias_correction2) / bias_correction1
p.data.add_(torch.mul(p.data, group['weight_decay']).addcdiv_(1, exp_avg, denom), alpha=-step_size)
return loss
class Novograd(Optimizer):
"""
Implements Novograd algorithm.
Args:
params (iterable): iterable of parameters to optimize or dicts defining
parameter groups
lr (float, optional): learning rate (default: 1e-3)
betas (Tuple[float, float], optional): coefficients used for computing
running averages of gradient and its square (default: (0.95, 0))
eps (float, optional): term added to the denominator to improve
numerical stability (default: 1e-8)
weight_decay (float, optional): weight decay (L2 penalty) (default: 0)
grad_averaging: gradient averaging
amsgrad (boolean, optional): whether to use the AMSGrad variant of this
algorithm from the paper `On the Convergence of Adam and Beyond`_
(default: False)
"""
def __init__(self, params, lr=1e-3, betas=(0.95, 0), eps=1e-8,
weight_decay=0, grad_averaging=False, amsgrad=False):
if not 0.0 <= lr:
raise ValueError("Invalid learning rate: {}".format(lr))
if not 0.0 <= eps:
raise ValueError("Invalid epsilon value: {}".format(eps))
if not 0.0 <= betas[0] < 1.0:
raise ValueError("Invalid beta parameter at index 0: {}".format(betas[0]))
if not 0.0 <= betas[1] < 1.0:
raise ValueError("Invalid beta parameter at index 1: {}".format(betas[1]))
defaults = dict(lr=lr, betas=betas, eps=eps,
weight_decay=weight_decay,
grad_averaging=grad_averaging,
amsgrad=amsgrad)
super(Novograd, self).__init__(params, defaults)
def __setstate__(self, state):
super(Novograd, self).__setstate__(state)
for group in self.param_groups:
group.setdefault('amsgrad', False)
def step(self, closure=None):
"""Performs a single optimization step.
Arguments:
closure (callable, optional): A closure that reevaluates the model
and returns the loss.
"""
loss = None
if closure is not None:
loss = closure()
for group in self.param_groups:
for p in group['params']:
if p.grad is None:
continue
grad = p.grad.data
if grad.is_sparse:
raise RuntimeError('Sparse gradients are not supported.')
amsgrad = group['amsgrad']
state = self.state[p]
# State initialization
if len(state) == 0:
state['step'] = 0
# Exponential moving average of gradient values
state['exp_avg'] = torch.zeros_like(p.data)
# Exponential moving average of squared gradient values
state['exp_avg_sq'] = torch.zeros([]).to(state['exp_avg'].device)
if amsgrad:
# Maintains max of all exp. moving avg. of sq. grad. values
state['max_exp_avg_sq'] = torch.zeros([]).to(state['exp_avg'].device)
exp_avg, exp_avg_sq = state['exp_avg'], state['exp_avg_sq']
if amsgrad:
max_exp_avg_sq = state['max_exp_avg_sq']
beta1, beta2 = group['betas']
state['step'] += 1
norm = torch.sum(torch.pow(grad, 2))
if exp_avg_sq == 0:
exp_avg_sq.copy_(norm)
else:
exp_avg_sq.mul_(beta2).add_(norm, alpha=1 - beta2)
if amsgrad:
# Maintains the maximum of all 2nd moment running avg. till now
torch.max(max_exp_avg_sq, exp_avg_sq, out=max_exp_avg_sq)
# Use the max. for normalizing running avg. of gradient
denom = max_exp_avg_sq.sqrt().add_(group['eps'])
else:
denom = exp_avg_sq.sqrt().add_(group['eps'])
grad.div_(denom)
if group['weight_decay'] != 0:
grad.add_(p.data, alpha=group['weight_decay'])
if group['grad_averaging']:
grad.mul_(1 - beta1)
exp_avg.mul_(beta1).add_(grad)
p.data.add_(exp_avg, alpha=-group['lr'])
return loss
|
PyTorch/LanguageModeling/BERT/data | data | WikiDownloader | # Copyright (c) 2019-2021 NVIDIA CORPORATION. All rights reserved.
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import bz2
import os
import urllib.request
import subprocess
import sys
import subprocess
class WikiDownloader:
def __init__(self, language, save_path):
self.save_path = save_path + '/wikicorpus_' + language
if not os.path.exists(self.save_path):
os.makedirs(self.save_path)
self.language = language
# Use a mirror from https://dumps.wikimedia.org/mirrors.html if the below links do not work
self.download_urls = {
'en' : 'https://dumps.wikimedia.org/enwiki/latest/enwiki-latest-pages-articles.xml.bz2',
'zh' : 'https://dumps.wikimedia.org/zhwiki/latest/zhwiki-latest-pages-articles.xml.bz2'
}
self.output_files = {
'en' : 'wikicorpus_en.xml.bz2',
'zh' : 'wikicorpus_zh.xml.bz2'
}
def download(self):
if self.language in self.download_urls:
url = self.download_urls[self.language]
filename = self.output_files[self.language]
print('Downloading:', url)
if os.path.isfile(self.save_path + '/' + filename):
print('** Download file already exists, skipping download')
else:
cmd = ['wget', url, '--output-document={}'.format(self.save_path + '/' + filename)]
print('Running:', cmd)
status = subprocess.run(cmd)
if status.returncode != 0:
raise RuntimeError('Wiki download not successful')
# Always unzipping since this is relatively fast and will overwrite
print('Unzipping:', self.output_files[self.language])
subprocess.run('bzip2 -dk ' + self.save_path + '/' + filename, shell=True, check=True)
else:
assert False, 'WikiDownloader not implemented for this language yet.'
|
TensorFlow/Segmentation/UNet_Industrial/scripts/benchmarking | benchmarking | UNet_evalbench | #!/usr/bin/env bash
# Copyright (c) 2018, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# This script launches UNet evaluation benchmark in FP32/TF32 on 1 GPUs using 16 batch size
# Usage ./UNet_evalbench.sh <path to dataset> <dagm classID (1-10)>
BASEDIR="$( cd "$( dirname "${BASH_SOURCE[0]}" )" >/dev/null 2>&1 && pwd )"
export TF_CPP_MIN_LOG_LEVEL=3
# Cleaning up for benchmark
RESULT_DIR="/tmp"
rm -rf "${RESULT_DIR}"
python "${BASEDIR}/../../main.py" \
--unet_variant='tinyUNet' \
--activation_fn='relu' \
--exec_mode='inference_benchmark' \
--iter_unit='batch' \
--num_iter=1500 \
--batch_size=16 \
--warmup_step=500 \
--results_dir="${RESULT_DIR}" \
--data_dir="${1}" \
--dataset_name='DAGM2007' \
--dataset_classID="${2}" \
--data_format='NCHW' \
--use_auto_loss_scaling \
--noamp \
--xla \
--learning_rate=1e-4 \
--learning_rate_decay_factor=0.8 \
--learning_rate_decay_steps=500 \
--rmsprop_decay=0.9 \
--rmsprop_momentum=0.8 \
--loss_fn_name='adaptive_loss' \
--weight_decay=1e-5 \
--weight_init_method='he_uniform' \
--augment_data \
--display_every=250 \
--debug_verbosity=0
|
PyTorch/Detection/SSD/examples | examples | SSD300_A100_FP16_1GPU | # This script launches SSD300 training in FP16 on 1 GPUs using 256 batch size
# Usage bash SSD300_FP16_1GPU.sh <path to this repository> <path to dataset> <additional flags>
python $1/main.py --backbone resnet50 --warmup 300 --bs 256 --data $2 ${@:3}
|
TensorFlow/Detection/SSD/models/research/object_detection/builders | builders | box_coder_builder | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""A function to build an object detection box coder from configuration."""
from object_detection.box_coders import faster_rcnn_box_coder
from object_detection.box_coders import keypoint_box_coder
from object_detection.box_coders import mean_stddev_box_coder
from object_detection.box_coders import square_box_coder
from object_detection.protos import box_coder_pb2
def build(box_coder_config):
"""Builds a box coder object based on the box coder config.
Args:
box_coder_config: A box_coder.proto object containing the config for the
desired box coder.
Returns:
BoxCoder based on the config.
Raises:
ValueError: On empty box coder proto.
"""
if not isinstance(box_coder_config, box_coder_pb2.BoxCoder):
raise ValueError('box_coder_config not of type box_coder_pb2.BoxCoder.')
if box_coder_config.WhichOneof('box_coder_oneof') == 'faster_rcnn_box_coder':
return faster_rcnn_box_coder.FasterRcnnBoxCoder(scale_factors=[
box_coder_config.faster_rcnn_box_coder.y_scale,
box_coder_config.faster_rcnn_box_coder.x_scale,
box_coder_config.faster_rcnn_box_coder.height_scale,
box_coder_config.faster_rcnn_box_coder.width_scale
])
if box_coder_config.WhichOneof('box_coder_oneof') == 'keypoint_box_coder':
return keypoint_box_coder.KeypointBoxCoder(
box_coder_config.keypoint_box_coder.num_keypoints,
scale_factors=[
box_coder_config.keypoint_box_coder.y_scale,
box_coder_config.keypoint_box_coder.x_scale,
box_coder_config.keypoint_box_coder.height_scale,
box_coder_config.keypoint_box_coder.width_scale
])
if (box_coder_config.WhichOneof('box_coder_oneof') ==
'mean_stddev_box_coder'):
return mean_stddev_box_coder.MeanStddevBoxCoder(
stddev=box_coder_config.mean_stddev_box_coder.stddev)
if box_coder_config.WhichOneof('box_coder_oneof') == 'square_box_coder':
return square_box_coder.SquareBoxCoder(scale_factors=[
box_coder_config.square_box_coder.y_scale,
box_coder_config.square_box_coder.x_scale,
box_coder_config.square_box_coder.length_scale
])
raise ValueError('Empty box coder.')
|
PyTorch/SpeechSynthesis/Tacotron2/notebooks/conversationalai/client/speech_ai_demo/utils/tacotron2 | tacotron2 | cleaners | """ from https://github.com/keithito/tacotron """
'''
Cleaners are transformations that run over the input text at both training and eval time.
Cleaners can be selected by passing a comma-delimited list of cleaner names as the "cleaners"
hyperparameter. Some cleaners are English-specific. You'll typically want to use:
1. "english_cleaners" for English text
2. "transliteration_cleaners" for non-English text that can be transliterated to ASCII using
the Unidecode library (https://pypi.python.org/pypi/Unidecode)
3. "basic_cleaners" if you do not want to transliterate (in this case, you should also update
the symbols in symbols.py to match your data).
'''
import re
from .numbers import normalize_numbers
from .unidecoder import unidecoder
# Regular expression matching whitespace:
_whitespace_re = re.compile(r'\s+')
# List of (regular expression, replacement) pairs for abbreviations:
_abbreviations = [(re.compile('\\b%s\\.' % x[0], re.IGNORECASE), x[1]) for x in [
('mrs', 'misess'),
('mr', 'mister'),
('dr', 'doctor'),
('st', 'saint'),
('co', 'company'),
('jr', 'junior'),
('maj', 'major'),
('gen', 'general'),
('drs', 'doctors'),
('rev', 'reverend'),
('lt', 'lieutenant'),
('hon', 'honorable'),
('sgt', 'sergeant'),
('capt', 'captain'),
('esq', 'esquire'),
('ltd', 'limited'),
('col', 'colonel'),
('ft', 'fort'),
]]
def expand_abbreviations(text):
for regex, replacement in _abbreviations:
text = re.sub(regex, replacement, text)
return text
def expand_numbers(text):
return normalize_numbers(text)
def lowercase(text):
return text.lower()
def collapse_whitespace(text):
return re.sub(_whitespace_re, ' ', text)
def convert_to_ascii(text):
return unidecoder(text)
def basic_cleaners(text):
'''Basic pipeline that lowercases and collapses whitespace without transliteration.'''
text = lowercase(text)
text = collapse_whitespace(text)
return text
def transliteration_cleaners(text):
'''Pipeline for non-English text that transliterates to ASCII.'''
text = convert_to_ascii(text)
text = lowercase(text)
text = collapse_whitespace(text)
return text
def english_cleaners(text):
'''Pipeline for English text, including number and abbreviation expansion.'''
text = convert_to_ascii(text)
text = lowercase(text)
text = expand_numbers(text)
text = expand_abbreviations(text)
text = collapse_whitespace(text)
return text
|
PyTorch/Segmentation/MaskRCNN/pytorch | pytorch | CODE_OF_CONDUCT | # Code of Conduct
Facebook has adopted a Code of Conduct that we expect project participants to adhere to.
Please read the [full text](https://code.fb.com/codeofconduct/)
so that you can understand what actions will and will not be tolerated.
|
PyTorch/Detection/Efficientdet | Efficientdet | .gitignore | # Byte-compiled / optimized / DLL files
__pycache__/
*.py[cod]
*$py.class
# C extensions
*.so
# Distribution / packaging
.Python
build/
develop-eggs/
dist/
downloads/
eggs/
.eggs/
lib/
lib64/
parts/
sdist/
var/
wheels/
pip-wheel-metadata/
share/python-wheels/
*.egg-info/
.installed.cfg
*.egg
MANIFEST
# PyInstaller
# Usually these files are written by a python script from a template
# before PyInstaller builds the exe, so as to inject date/other infos into it.
*.manifest
*.spec
# Installer logs
pip-log.txt
pip-delete-this-directory.txt
# Unit test / coverage reports
htmlcov/
.tox/
.nox/
.coverage
.coverage.*
.cache
nosetests.xml
coverage.xml
*.cover
*.py,cover
.hypothesis/
.pytest_cache/
# Translations
*.mo
*.pot
# Django stuff:
*.log
local_settings.py
db.sqlite3
db.sqlite3-journal
# Flask stuff:
instance/
.webassets-cache
# Scrapy stuff:
.scrapy
# Sphinx documentation
docs/_build/
# PyBuilder
target/
# Jupyter Notebook
.ipynb_checkpoints
# IPython
profile_default/
ipython_config.py
# pyenv
.python-version
# pipenv
# According to pypa/pipenv#598, it is recommended to include Pipfile.lock in version control.
# However, in case of collaboration, if having platform-specific dependencies or dependencies
# having no cross-platform support, pipenv may install dependencies that don't work, or not
# install all needed dependencies.
#Pipfile.lock
# PEP 582; used by e.g. github.com/David-OConnor/pyflow
__pypackages__/
# Celery stuff
celerybeat-schedule
celerybeat.pid
# SageMath parsed files
*.sage.py
# Environments
.env
.venv
env/
venv/
ENV/
env.bak/
venv.bak/
# Spyder project settings
.spyderproject
.spyproject
# Rope project settings
.ropeproject
# mkdocs documentation
/site
# mypy
.mypy_cache/
.dmypy.json
dmypy.json
# Pyre type checker
.pyre/
# PyCharm
.idea
# PyTorch weights
*.tar
*.pth
*.gz
|
PyTorch/SpeechRecognition/wav2vec2 | wav2vec2 | requirements | editdistance==0.6.0
librosa==0.10.1
omegaconf==2.0.6 # optional for handling certain Fairseq ckpts
pyarrow==6.0.1
soundfile==0.12.1
sox==1.4.1
tqdm==4.53.0
git+https://github.com/NVIDIA/dllogger@v1.0.0#egg=dllogger
|
TensorFlow/Detection/SSD/models/research/object_detection/anchor_generators | anchor_generators | multiscale_grid_anchor_generator | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Generates grid anchors on the fly corresponding to multiple CNN layers.
Generates grid anchors on the fly corresponding to multiple CNN layers as
described in:
"Focal Loss for Dense Object Detection" (https://arxiv.org/abs/1708.02002)
T.-Y. Lin, P. Goyal, R. Girshick, K. He, P. Dollar
"""
from object_detection.anchor_generators import grid_anchor_generator
from object_detection.core import anchor_generator
from object_detection.core import box_list_ops
class MultiscaleGridAnchorGenerator(anchor_generator.AnchorGenerator):
"""Generate a grid of anchors for multiple CNN layers of different scale."""
def __init__(self, min_level, max_level, anchor_scale, aspect_ratios,
scales_per_octave, normalize_coordinates=True):
"""Constructs a MultiscaleGridAnchorGenerator.
To construct anchors, at multiple scale resolutions, one must provide a
the minimum level and maximum levels on a scale pyramid. To define the size
of anchor, the anchor scale is provided to decide the size relatively to the
stride of the corresponding feature map. The generator allows one pixel
location on feature map maps to multiple anchors, that have different aspect
ratios and intermediate scales.
Args:
min_level: minimum level in feature pyramid.
max_level: maximum level in feature pyramid.
anchor_scale: anchor scale and feature stride define the size of the base
anchor on an image. For example, given a feature pyramid with strides
[2^3, ..., 2^7] and anchor scale 4. The base anchor size is
4 * [2^3, ..., 2^7].
aspect_ratios: list or tuple of (float) aspect ratios to place on each
grid point.
scales_per_octave: integer number of intermediate scales per scale octave.
normalize_coordinates: whether to produce anchors in normalized
coordinates. (defaults to True).
"""
self._anchor_grid_info = []
self._aspect_ratios = aspect_ratios
self._scales_per_octave = scales_per_octave
self._normalize_coordinates = normalize_coordinates
scales = [2**(float(scale) / scales_per_octave)
for scale in range(scales_per_octave)]
aspects = list(aspect_ratios)
for level in range(min_level, max_level + 1):
anchor_stride = [2**level, 2**level]
base_anchor_size = [2**level * anchor_scale, 2**level * anchor_scale]
self._anchor_grid_info.append({
'level': level,
'info': [scales, aspects, base_anchor_size, anchor_stride]
})
def name_scope(self):
return 'MultiscaleGridAnchorGenerator'
def num_anchors_per_location(self):
"""Returns the number of anchors per spatial location.
Returns:
a list of integers, one for each expected feature map to be passed to
the Generate function.
"""
return len(self._anchor_grid_info) * [
len(self._aspect_ratios) * self._scales_per_octave]
def _generate(self, feature_map_shape_list, im_height=1, im_width=1):
"""Generates a collection of bounding boxes to be used as anchors.
Currently we require the input image shape to be statically defined. That
is, im_height and im_width should be integers rather than tensors.
Args:
feature_map_shape_list: list of pairs of convnet layer resolutions in the
format [(height_0, width_0), (height_1, width_1), ...]. For example,
setting feature_map_shape_list=[(8, 8), (7, 7)] asks for anchors that
correspond to an 8x8 layer followed by a 7x7 layer.
im_height: the height of the image to generate the grid for. If both
im_height and im_width are 1, anchors can only be generated in
absolute coordinates.
im_width: the width of the image to generate the grid for. If both
im_height and im_width are 1, anchors can only be generated in
absolute coordinates.
Returns:
boxes_list: a list of BoxLists each holding anchor boxes corresponding to
the input feature map shapes.
Raises:
ValueError: if im_height and im_width are not integers.
ValueError: if im_height and im_width are 1, but normalized coordinates
were requested.
"""
anchor_grid_list = []
for feat_shape, grid_info in zip(feature_map_shape_list,
self._anchor_grid_info):
# TODO(rathodv) check the feature_map_shape_list is consistent with
# self._anchor_grid_info
level = grid_info['level']
stride = 2**level
scales, aspect_ratios, base_anchor_size, anchor_stride = grid_info['info']
feat_h = feat_shape[0]
feat_w = feat_shape[1]
anchor_offset = [0, 0]
if isinstance(im_height, int) and isinstance(im_width, int):
if im_height % 2.0**level == 0 or im_height == 1:
anchor_offset[0] = stride / 2.0
if im_width % 2.0**level == 0 or im_width == 1:
anchor_offset[1] = stride / 2.0
ag = grid_anchor_generator.GridAnchorGenerator(
scales,
aspect_ratios,
base_anchor_size=base_anchor_size,
anchor_stride=anchor_stride,
anchor_offset=anchor_offset)
(anchor_grid,) = ag.generate(feature_map_shape_list=[(feat_h, feat_w)])
if self._normalize_coordinates:
if im_height == 1 or im_width == 1:
raise ValueError(
'Normalized coordinates were requested upon construction of the '
'MultiscaleGridAnchorGenerator, but a subsequent call to '
'generate did not supply dimension information.')
anchor_grid = box_list_ops.to_normalized_coordinates(
anchor_grid, im_height, im_width, check_range=False)
anchor_grid_list.append(anchor_grid)
return anchor_grid_list
|
TensorFlow/Detection/SSD/models/research/object_detection/models | models | faster_rcnn_nas_feature_extractor | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""NASNet Faster R-CNN implementation.
Learning Transferable Architectures for Scalable Image Recognition
Barret Zoph, Vijay Vasudevan, Jonathon Shlens, Quoc V. Le
https://arxiv.org/abs/1707.07012
"""
import tensorflow as tf
from object_detection.meta_architectures import faster_rcnn_meta_arch
from nets.nasnet import nasnet
from nets.nasnet import nasnet_utils
arg_scope = tf.contrib.framework.arg_scope
slim = tf.contrib.slim
def nasnet_large_arg_scope_for_detection(is_batch_norm_training=False):
"""Defines the default arg scope for the NASNet-A Large for object detection.
This provides a small edit to switch batch norm training on and off.
Args:
is_batch_norm_training: Boolean indicating whether to train with batch norm.
Returns:
An `arg_scope` to use for the NASNet Large Model.
"""
imagenet_scope = nasnet.nasnet_large_arg_scope()
with arg_scope(imagenet_scope):
with arg_scope([slim.batch_norm], is_training=is_batch_norm_training) as sc:
return sc
# Note: This is largely a copy of _build_nasnet_base inside nasnet.py but
# with special edits to remove instantiation of the stem and the special
# ability to receive as input a pair of hidden states.
def _build_nasnet_base(hidden_previous,
hidden,
normal_cell,
reduction_cell,
hparams,
true_cell_num,
start_cell_num):
"""Constructs a NASNet image model."""
# Find where to place the reduction cells or stride normal cells
reduction_indices = nasnet_utils.calc_reduction_layers(
hparams.num_cells, hparams.num_reduction_layers)
# Note: The None is prepended to match the behavior of _imagenet_stem()
cell_outputs = [None, hidden_previous, hidden]
net = hidden
# NOTE: In the nasnet.py code, filter_scaling starts at 1.0. We instead
# start at 2.0 because 1 reduction cell has been created which would
# update the filter_scaling to 2.0.
filter_scaling = 2.0
# Run the cells
for cell_num in range(start_cell_num, hparams.num_cells):
stride = 1
if hparams.skip_reduction_layer_input:
prev_layer = cell_outputs[-2]
if cell_num in reduction_indices:
filter_scaling *= hparams.filter_scaling_rate
net = reduction_cell(
net,
scope='reduction_cell_{}'.format(reduction_indices.index(cell_num)),
filter_scaling=filter_scaling,
stride=2,
prev_layer=cell_outputs[-2],
cell_num=true_cell_num)
true_cell_num += 1
cell_outputs.append(net)
if not hparams.skip_reduction_layer_input:
prev_layer = cell_outputs[-2]
net = normal_cell(
net,
scope='cell_{}'.format(cell_num),
filter_scaling=filter_scaling,
stride=stride,
prev_layer=prev_layer,
cell_num=true_cell_num)
true_cell_num += 1
cell_outputs.append(net)
# Final nonlinearity.
# Note that we have dropped the final pooling, dropout and softmax layers
# from the default nasnet version.
with tf.variable_scope('final_layer'):
net = tf.nn.relu(net)
return net
# TODO(shlens): Only fixed_shape_resizer is currently supported for NASNet
# featurization. The reason for this is that nasnet.py only supports
# inputs with fully known shapes. We need to update nasnet.py to handle
# shapes not known at compile time.
class FasterRCNNNASFeatureExtractor(
faster_rcnn_meta_arch.FasterRCNNFeatureExtractor):
"""Faster R-CNN with NASNet-A feature extractor implementation."""
def __init__(self,
is_training,
first_stage_features_stride,
batch_norm_trainable=False,
reuse_weights=None,
weight_decay=0.0):
"""Constructor.
Args:
is_training: See base class.
first_stage_features_stride: See base class.
batch_norm_trainable: See base class.
reuse_weights: See base class.
weight_decay: See base class.
Raises:
ValueError: If `first_stage_features_stride` is not 16.
"""
if first_stage_features_stride != 16:
raise ValueError('`first_stage_features_stride` must be 16.')
super(FasterRCNNNASFeatureExtractor, self).__init__(
is_training, first_stage_features_stride, batch_norm_trainable,
reuse_weights, weight_decay)
def preprocess(self, resized_inputs):
"""Faster R-CNN with NAS preprocessing.
Maps pixel values to the range [-1, 1].
Args:
resized_inputs: A [batch, height_in, width_in, channels] float32 tensor
representing a batch of images with values between 0 and 255.0.
Returns:
preprocessed_inputs: A [batch, height_out, width_out, channels] float32
tensor representing a batch of images.
"""
return (2.0 / 255.0) * resized_inputs - 1.0
def _extract_proposal_features(self, preprocessed_inputs, scope):
"""Extracts first stage RPN features.
Extracts features using the first half of the NASNet network.
We construct the network in `align_feature_maps=True` mode, which means
that all VALID paddings in the network are changed to SAME padding so that
the feature maps are aligned.
Args:
preprocessed_inputs: A [batch, height, width, channels] float32 tensor
representing a batch of images.
scope: A scope name.
Returns:
rpn_feature_map: A tensor with shape [batch, height, width, depth]
end_points: A dictionary mapping feature extractor tensor names to tensors
Raises:
ValueError: If the created network is missing the required activation.
"""
del scope
if len(preprocessed_inputs.get_shape().as_list()) != 4:
raise ValueError('`preprocessed_inputs` must be 4 dimensional, got a '
'tensor of shape %s' % preprocessed_inputs.get_shape())
with slim.arg_scope(nasnet_large_arg_scope_for_detection(
is_batch_norm_training=self._train_batch_norm)):
with arg_scope([slim.conv2d,
slim.batch_norm,
slim.separable_conv2d],
reuse=self._reuse_weights):
_, end_points = nasnet.build_nasnet_large(
preprocessed_inputs, num_classes=None,
is_training=self._is_training,
final_endpoint='Cell_11')
# Note that both 'Cell_10' and 'Cell_11' have equal depth = 2016.
rpn_feature_map = tf.concat([end_points['Cell_10'],
end_points['Cell_11']], 3)
# nasnet.py does not maintain the batch size in the first dimension.
# This work around permits us retaining the batch for below.
batch = preprocessed_inputs.get_shape().as_list()[0]
shape_without_batch = rpn_feature_map.get_shape().as_list()[1:]
rpn_feature_map_shape = [batch] + shape_without_batch
rpn_feature_map.set_shape(rpn_feature_map_shape)
return rpn_feature_map, end_points
def _extract_box_classifier_features(self, proposal_feature_maps, scope):
"""Extracts second stage box classifier features.
This function reconstructs the "second half" of the NASNet-A
network after the part defined in `_extract_proposal_features`.
Args:
proposal_feature_maps: A 4-D float tensor with shape
[batch_size * self.max_num_proposals, crop_height, crop_width, depth]
representing the feature map cropped to each proposal.
scope: A scope name.
Returns:
proposal_classifier_features: A 4-D float tensor with shape
[batch_size * self.max_num_proposals, height, width, depth]
representing box classifier features for each proposal.
"""
del scope
# Note that we always feed into 2 layers of equal depth
# where the first N channels corresponds to previous hidden layer
# and the second N channels correspond to the final hidden layer.
hidden_previous, hidden = tf.split(proposal_feature_maps, 2, axis=3)
# Note that what follows is largely a copy of build_nasnet_large() within
# nasnet.py. We are copying to minimize code pollution in slim.
# TODO(shlens,skornblith): Determine the appropriate drop path schedule.
# For now the schedule is the default (1.0->0.7 over 250,000 train steps).
hparams = nasnet.large_imagenet_config()
if not self._is_training:
hparams.set_hparam('drop_path_keep_prob', 1.0)
# Calculate the total number of cells in the network
# -- Add 2 for the reduction cells.
total_num_cells = hparams.num_cells + 2
# -- And add 2 for the stem cells for ImageNet training.
total_num_cells += 2
normal_cell = nasnet_utils.NasNetANormalCell(
hparams.num_conv_filters, hparams.drop_path_keep_prob,
total_num_cells, hparams.total_training_steps)
reduction_cell = nasnet_utils.NasNetAReductionCell(
hparams.num_conv_filters, hparams.drop_path_keep_prob,
total_num_cells, hparams.total_training_steps)
with arg_scope([slim.dropout, nasnet_utils.drop_path],
is_training=self._is_training):
with arg_scope([slim.batch_norm], is_training=self._train_batch_norm):
with arg_scope([slim.avg_pool2d,
slim.max_pool2d,
slim.conv2d,
slim.batch_norm,
slim.separable_conv2d,
nasnet_utils.factorized_reduction,
nasnet_utils.global_avg_pool,
nasnet_utils.get_channel_index,
nasnet_utils.get_channel_dim],
data_format=hparams.data_format):
# This corresponds to the cell number just past 'Cell_11' used by
# by _extract_proposal_features().
start_cell_num = 12
# Note that this number equals:
# start_cell_num + 2 stem cells + 1 reduction cell
true_cell_num = 15
with slim.arg_scope(nasnet.nasnet_large_arg_scope()):
net = _build_nasnet_base(hidden_previous,
hidden,
normal_cell=normal_cell,
reduction_cell=reduction_cell,
hparams=hparams,
true_cell_num=true_cell_num,
start_cell_num=start_cell_num)
proposal_classifier_features = net
return proposal_classifier_features
def restore_from_classification_checkpoint_fn(
self,
first_stage_feature_extractor_scope,
second_stage_feature_extractor_scope):
"""Returns a map of variables to load from a foreign checkpoint.
Note that this overrides the default implementation in
faster_rcnn_meta_arch.FasterRCNNFeatureExtractor which does not work for
NASNet-A checkpoints.
Args:
first_stage_feature_extractor_scope: A scope name for the first stage
feature extractor.
second_stage_feature_extractor_scope: A scope name for the second stage
feature extractor.
Returns:
A dict mapping variable names (to load from a checkpoint) to variables in
the model graph.
"""
# Note that the NAS checkpoint only contains the moving average version of
# the Variables so we need to generate an appropriate dictionary mapping.
variables_to_restore = {}
for variable in tf.global_variables():
if variable.op.name.startswith(
first_stage_feature_extractor_scope):
var_name = variable.op.name.replace(
first_stage_feature_extractor_scope + '/', '')
var_name += '/ExponentialMovingAverage'
variables_to_restore[var_name] = variable
if variable.op.name.startswith(
second_stage_feature_extractor_scope):
var_name = variable.op.name.replace(
second_stage_feature_extractor_scope + '/', '')
var_name += '/ExponentialMovingAverage'
variables_to_restore[var_name] = variable
return variables_to_restore
|
DGLPyTorch/DrugDiscovery/SE3Transformer/se3_transformer/model | model | fiber | # Copyright (c) 2021-2022, NVIDIA CORPORATION & AFFILIATES. All rights reserved.
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
#
# SPDX-FileCopyrightText: Copyright (c) 2021-2022 NVIDIA CORPORATION & AFFILIATES
# SPDX-License-Identifier: MIT
from collections import namedtuple
from itertools import product
from typing import Dict
import torch
from torch import Tensor
from se3_transformer.runtime.utils import degree_to_dim
FiberEl = namedtuple('FiberEl', ['degree', 'channels'])
class Fiber(dict):
"""
Describes the structure of some set of features.
Features are split into types (0, 1, 2, 3, ...). A feature of type k has a dimension of 2k+1.
Type-0 features: invariant scalars
Type-1 features: equivariant 3D vectors
Type-2 features: equivariant symmetric traceless matrices
...
As inputs to a SE3 layer, there can be many features of the same types, and many features of different types.
The 'multiplicity' or 'number of channels' is the number of features of a given type.
This class puts together all the degrees and their multiplicities in order to describe
the inputs, outputs or hidden features of SE3 layers.
"""
def __init__(self, structure):
if isinstance(structure, dict):
structure = [FiberEl(int(d), int(m)) for d, m in sorted(structure.items(), key=lambda x: x[1])]
elif not isinstance(structure[0], FiberEl):
structure = list(map(lambda t: FiberEl(*t), sorted(structure, key=lambda x: x[1])))
self.structure = structure
super().__init__({d: m for d, m in self.structure})
@property
def degrees(self):
return sorted([t.degree for t in self.structure])
@property
def channels(self):
return [self[d] for d in self.degrees]
@property
def num_features(self):
""" Size of the resulting tensor if all features were concatenated together """
return sum(t.channels * degree_to_dim(t.degree) for t in self.structure)
@staticmethod
def create(num_degrees: int, num_channels: int):
""" Create a Fiber with degrees 0..num_degrees-1, all with the same multiplicity """
return Fiber([(degree, num_channels) for degree in range(num_degrees)])
@staticmethod
def from_features(feats: Dict[str, Tensor]):
""" Infer the Fiber structure from a feature dict """
structure = {}
for k, v in feats.items():
degree = int(k)
assert len(v.shape) == 3, 'Feature shape should be (N, C, 2D+1)'
assert v.shape[-1] == degree_to_dim(degree)
structure[degree] = v.shape[-2]
return Fiber(structure)
def __getitem__(self, degree: int):
""" fiber[degree] returns the multiplicity for this degree """
return dict(self.structure).get(degree, 0)
def __iter__(self):
""" Iterate over namedtuples (degree, channels) """
return iter(self.structure)
def __mul__(self, other):
"""
If other in an int, multiplies all the multiplicities by other.
If other is a fiber, returns the cartesian product.
"""
if isinstance(other, Fiber):
return product(self.structure, other.structure)
elif isinstance(other, int):
return Fiber({t.degree: t.channels * other for t in self.structure})
def __add__(self, other):
"""
If other in an int, add other to all the multiplicities.
If other is a fiber, add the multiplicities of the fibers together.
"""
if isinstance(other, Fiber):
return Fiber({t.degree: t.channels + other[t.degree] for t in self.structure})
elif isinstance(other, int):
return Fiber({t.degree: t.channels + other for t in self.structure})
def __repr__(self):
return str(self.structure)
@staticmethod
def combine_max(f1, f2):
""" Combine two fiber by taking the maximum multiplicity for each degree in both fibers """
new_dict = dict(f1.structure)
for k, m in f2.structure:
new_dict[k] = max(new_dict.get(k, 0), m)
return Fiber(list(new_dict.items()))
@staticmethod
def combine_selectively(f1, f2):
""" Combine two fiber by taking the sum of multiplicities for each degree in the first fiber """
# only use orders which occur in fiber f1
new_dict = dict(f1.structure)
for k in f1.degrees:
if k in f2.degrees:
new_dict[k] += f2[k]
return Fiber(list(new_dict.items()))
def to_attention_heads(self, tensors: Dict[str, Tensor], num_heads: int):
# dict(N, num_channels, 2d+1) -> (N, num_heads, -1)
fibers = [tensors[str(degree)].reshape(*tensors[str(degree)].shape[:-2], num_heads, -1) for degree in
self.degrees]
fibers = torch.cat(fibers, -1)
return fibers
|
Tools/DGLPyTorch/SyntheticGraphGeneration/demos/performance | performance | struct_generator | #!/usr/bin/env python
# coding: utf-8
# Copyright 2023 NVIDIA Corporation. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
# # Graph structure generation demo
# ## Overview
#
# In this notebbok we compare the performance (throughput) of graph structure generators presented in the SynGen tool.
#
# Available generators:
#
# 1. [Exact RMAT generator (GPU)](#1)
# 1. [Exact RMAT generator (CPU)](#2)
# 1. [Approximate RMAT generator (GPU)](#3)
# 1. [Approximate RMAT generator (CPU)](#4)
# In[1]:
# Generator
from syngen.generator.graph import RMATGenerator
# Others
import numpy as np
import pandas as pd
from matplotlib import pyplot as plt
from matplotlib.pyplot import set_loglevel
set_loglevel('warning')
import time
from itertools import product
# In[2]:
def get_xy(data):
x = [edges for edges, _, _ in data]
y = [eps for _, eps, _ in data]
return x, y
# ## Exact generator
# <a id="1"></a>
# ### GPU
# In[3]:
static_graph_generator=RMATGenerator()
static_graph_generator.gpu=True
# In[4]:
static_graph_generator._fit_results = 0.4, 0.25, 0.2, 0.15
# In[5]:
get_ipython().run_cell_magic('time', '', '\nstart = time.perf_counter()\ndata_proper = static_graph_generator.generate(num_nodes=6_797_556, \n num_edges=168_114, \n is_directed=False, \n has_self_loop=False)\nelapsed = time.perf_counter() - start\n\nprint(elapsed)\n')
# In[6]:
n_range = [15, 20, 30, 40]
n_range = [2 ** x for x in n_range]
edges_range = [1e6, 1e7, 1e8]
edges_range = [int(x // 2) for x in edges_range]
gpu_res = {
int(np.log2(n)): [] for n in n_range
}
# Random run
data_proper = static_graph_generator.generate(num_nodes=168_114,
num_edges=6_797_556,
is_directed=False,
has_self_loop=False)
for n, edges in product(n_range, edges_range):
max_edges = (n * (n - 1)) // 2
density = edges / max_edges
if density > 0.75:
res = "FAIL"
else:
res = "GOOD"
f_string = f"{n:<13} | {edges:<13} | {density:>8.3f} | {res}"
print(f_string)
if res == "FAIL":
continue
start = time.perf_counter()
data_proper = static_graph_generator.generate(num_nodes=n,
num_edges=edges,
is_directed=False,
has_self_loop=False)
elapsed = time.perf_counter() - start
gen_edges = data_proper.shape[0]
edges_per_second = data_proper.shape[0] / elapsed
calculated = (gen_edges, edges_per_second, elapsed)
f_string = f"{n:<13} | {edges:<13} | {edges_per_second}"
print(f_string)
l = gpu_res[int(np.log2(n))]
l.append(calculated)
# In[7]:
plt.figure(figsize=(15, 6))
for n, data in gpu_res.items():
x, y = get_xy(data)
plt.plot(x, y, '--x', label=f'{n}')
plt.xlabel('Total edges to generate')
plt.ylabel('Edges per seconds')
plt.legend()
plt.legend()
plt.yscale('log')
plt.xscale('log')
# <a id="2"></a>
# ### CPU
# In[8]:
static_graph_generator=RMATGenerator()
static_graph_generator.gpu=False
# In[9]:
static_graph_generator._fit_results = 0.4, 0.25, 0.2, 0.15
# In[10]:
n_range = [15, 20, 30, 40]
n_range = [2 ** x for x in n_range]
edges_range = [1e6, 1e7, 1e8]
edges_range = [int(x // 2) for x in edges_range]
cpu_res = {
int(np.log2(n)): [] for n in n_range
}
# Random run
data_proper = static_graph_generator.generate(num_nodes=168_114,
num_edges=6_797_556,
is_directed=False,
has_self_loop=False)
for n, edges in product(n_range, edges_range):
max_edges = (n * (n - 1)) // 2
density = edges / max_edges
if density > 0.75:
res = "FAIL"
else:
res = "GOOD"
f_string = f"{n:<13} | {edges:<13} | {density:>8.3f} | {res}"
print(f_string)
if res == "FAIL":
continue
start = time.perf_counter()
data_proper = static_graph_generator.generate(num_nodes=n,
num_edges=edges,
is_directed=False,
has_self_loop=False)
elapsed = time.perf_counter() - start
gen_edges = data_proper.shape[0]
edges_per_second = data_proper.shape[0] / elapsed
calculated = (gen_edges, edges_per_second, elapsed)
f_string = f"{n:<13} | {edges:<13} | {edges_per_second}"
print(f_string)
l = cpu_res[int(np.log2(n))]
l.append(calculated)
# In[11]:
plt.figure(figsize=(15, 6))
for n, data in cpu_res.items():
x, y = get_xy(data)
plt.plot(x, y, '--x', label=f'{n}')
plt.xlabel('Total edges to generate')
plt.ylabel('Edges per seconds')
plt.legend()
plt.legend()
plt.yscale('log')
plt.xscale('log')
# In[12]:
plt.figure(figsize=(15, 6), dpi=800)
for n, data in gpu_res.items():
x, y = get_xy(data)
plt.plot(x, y, '--x', label=f'2^{n} nodes')
for n, data in cpu_res.items():
x, y = get_xy(data)
plt.plot(x, y, '--o', label=f'2^{n} nodes')
plt.annotate('dense \n graph', (cpu_res[15][2][0], cpu_res[15][2][1]))
plt.annotate('gpu generators', (gpu_res[15][1][0], gpu_res[15][1][1]+2e7))
plt.annotate('cpu generators', (cpu_res[15][1][0], cpu_res[15][1][1]+7e4))
plt.title('Exact generator performance')
plt.xlabel('Total edges to generate')
plt.ylabel('Edges per seconds')
plt.legend()
plt.yscale('log')
plt.xscale('log')
# plt.savefig("myImage.png", format="png", dpi=800)
plt.show()
# ## Approximate generator
# <a id="3"></a>
# ### GPU
# In[13]:
import cupy as cp
import numpy as np
from pylibraft.random import rmat
def generate_gpu_rmat_approx(a, b, c, d,
r_scale, c_scale,
n_edges,
noise=0.5):
gen_graph = None
theta_len = max(r_scale, c_scale) * 4
base_theta = [a, b, c, d]
if noise > 0:
full_theta = []
for i in range(theta_len):
noise_uniform = noise * np.random.uniform(-1, 1, size=len(base_theta))
noise_to_add = np.multiply(base_theta, noise_uniform)
theta_n = base_theta + noise_to_add
theta_n = theta_n / np.sum(theta_n)
full_theta.append(theta_n)
else:
full_theta = base_theta * theta_len
theta_cpu = np.array(full_theta, dtype=np.float32)
theta = cp.asarray(theta_cpu)
tmp = cp.empty((n_edges, 2), dtype=cp.int32)
rmat(tmp, theta, r_scale, c_scale)
return tmp
# In[14]:
a, b, c, d = 0.4, 0.25, 0.2, 0.15
# In[15]:
n_range = [15, 20, 30, 40]
edges_range = [1e6, 1e7, 1e8]
edges_range = [int(x) for x in edges_range]
gpu_res_approx = {
n: [] for n in n_range
}
# Random run
data_proper = generate_gpu_rmat_approx(a, b, c, d,
18, 18,
6_797_556)
for n, edges in product(n_range, edges_range):
max_edges = (2**n * (2**n - 1)) // 2
density = edges / max_edges
if density > 0.75:
res = "FAIL"
else:
res = "GOOD"
f_string = f"{n:<13} | {edges:<13} | {density:>8.3f} | {res}"
print(f_string)
if res == "FAIL":
continue
start = time.perf_counter()
data_proper = generate_gpu_rmat_approx(a, b, c, d, n, n, edges)
elapsed = time.perf_counter() - start
gen_edges = data_proper.shape[0]
edges_per_second = data_proper.shape[0] / elapsed
calculated = (gen_edges, edges_per_second, elapsed)
f_string = f"{n:<13} | {edges:<13} | {edges_per_second}"
print(f_string)
l = gpu_res_approx[n]
l.append(calculated)
# In[16]:
plt.figure(figsize=(15, 6))
for n, data in gpu_res_approx.items():
x, y = get_xy(data)
plt.plot(x, y, '--x', label=f'{n}')
plt.xlabel('Total edges to generate')
plt.ylabel('Edges per seconds')
plt.legend()
plt.legend()
plt.yscale('log')
plt.xscale('log')
# <a id="4"></a>
# ### CPU
# In[17]:
from syngen.generator.graph.utils import effective_nonsquare_rmat_approximate
# In[18]:
a, b, c, d = 0.4, 0.25, 0.2, 0.15
theta = np.array([[a, b], [c, d]])
theta /= a + b + c + d
# In[19]:
part, _, _ = effective_nonsquare_rmat_approximate(
theta,
6_797_556,
(18, 18),
noise_scaling=0.5,
batch_size=1_000_000,
dtype=np.int64,
custom_samplers=None,
generate_back_edges=False
)
# In[20]:
part.shape
# In[21]:
n_range = [15, 20, 30, 40]
#n_range = [2 ** x for x in n_range]
edges_range = [1e6, 1e7, 1e8]
edges_range = [int(x) for x in edges_range]
cpu_res_approx = {
n: [] for n in n_range
}
# Random run
part, _, _ = effective_nonsquare_rmat_approximate(
theta,
6_797_556,
(18, 18),
noise_scaling=0.5,
batch_size=1_000_000,
dtype=np.int64,
custom_samplers=None,
generate_back_edges=False
)
for n, edges in product(n_range, edges_range):
max_edges = (2**n * (2**n - 1)) // 2
density = edges / max_edges
if density > 0.75:
res = "FAIL"
else:
res = "GOOD"
f_string = f"{n:<13} | {edges:<13} | {density:>8.3f} | {res}"
print(f_string)
if res == "FAIL":
continue
start = time.perf_counter()
data_proper, _, _ = effective_nonsquare_rmat_approximate(
theta,
edges,
(n, n),
noise_scaling=0.5,
batch_size=1_000_000,
dtype=np.int64,
custom_samplers=None,
generate_back_edges=False
)
elapsed = time.perf_counter() - start
gen_edges = data_proper.shape[0]
edges_per_second = data_proper.shape[0] / elapsed
calculated = (gen_edges, edges_per_second, elapsed)
f_string = f"{n:<13} | {edges:<13} | {edges_per_second}"
print(f_string)
l = cpu_res_approx[n]
l.append(calculated)
# In[22]:
plt.figure(figsize=(15, 6))
for n, data in cpu_res_approx.items():
x, y = get_xy(data)
plt.plot(x, y, '--x', label=f'{n}')
plt.xlabel('Total edges to generate')
plt.ylabel('Edges per seconds')
plt.legend()
plt.legend()
plt.yscale('log')
plt.xscale('log')
# In[24]:
plt.figure(figsize=(15, 6), dpi=800)
for n, data in gpu_res_approx.items():
x, y = get_xy(data)
plt.plot(x, y, '--x', label=f'2^{n} nodes')
for n, data in cpu_res_approx.items():
x, y = get_xy(data)
plt.plot(x, y, '--o', label=f'2^{n} nodes')
plt.annotate('gpu generators', (gpu_res_approx[15][1][0], gpu_res_approx[15][1][1]+5e9))
plt.annotate('cpu generators', (cpu_res_approx[15][1][0], cpu_res_approx[15][1][1]+5e5))
plt.title('Approximate generator performance')
plt.xlabel('Total edges to generate')
plt.ylabel('Edges per seconds')
plt.legend()
plt.yscale('log')
plt.xscale('log')
plt.savefig("/workspace/img/edge_perf.png")
plt.show()
# In[ ]:
|
CUDA-Optimized/FastSpeech/fastspeech/trt/plugins/add_pos_enc | add_pos_enc | test_add_pos_enc_plugin | # Copyright (c) 2020, NVIDIA CORPORATION. All rights reserved.
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright
# notice, this list of conditions and the following disclaimer in the
# documentation and/or other materials provided with the distribution.
# * Neither the name of the NVIDIA CORPORATION nor the
# names of its contributors may be used to endorse or promote products
# derived from this software without specific prior written permission.
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
# ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
# WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
# DISCLAIMED. IN NO EVENT SHALL NVIDIA CORPORATION BE LIABLE FOR ANY
# DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
# (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
# LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
# ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
# SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
from __future__ import print_function
import numpy as np
import tensorrt as trt
import pycuda.autoinit
import pycuda.driver as cuda
import ctypes
import os
import sys
import time
logger = trt.Logger(trt.Logger.INFO)
PLUGIN_PATH = '/home/dahn/git/fastspeech/fastspeech/trt/plugins/add_pos_enc/AddPosEncPlugin.so'
ctypes.cdll.LoadLibrary(PLUGIN_PATH)
def get_plugin_creator(plugin_name):
trt.init_libnvinfer_plugins(logger, '')
plugin_creator_list = trt.get_plugin_registry().plugin_creator_list
plugin_creator = None
for c in plugin_creator_list:
if c.name == plugin_name:
plugin_creator = c
return plugin_creator
def build_engine(shape):
plugin_creator = get_plugin_creator('AddPosEncPlugin')
if plugin_creator == None:
print('Plugin not found. Exiting')
exit()
builder = trt.Builder(logger)
builder.max_batch_size = 1024
builder.max_workspace_size = 1 << 20
builder.fp16_mode = use_fp16
network = builder.create_network()
tensor = network.add_input('data', trt.DataType.FLOAT, shape)
tensor = network.add_plugin_v2(
[tensor],
plugin_creator.create_plugin('AddPosEncPlugin', trt.PluginFieldCollection())
).get_output(0)
network.mark_output(tensor)
return builder.build_cuda_engine(network)
def run_trt(data):
engine = build_engine(data.shape[1:])
context = engine.create_execution_context()
d_data = cuda.mem_alloc(data.nbytes)
output = np.zeros_like(data, dtype=np.float32)
d_output = cuda.mem_alloc(output.nbytes)
cuda.memcpy_htod(d_data, data)
bindings = [int(d_data), int(d_output)]
start = time.time()
context.execute(data.shape[0], bindings)
end = time.time()
time_elapsed = end - start
print("time elapsed: {:06f}".format(time_elapsed))
cuda.memcpy_dtoh(output, d_output)
return output
use_fp16 = len(sys.argv) > 1 and sys.argv[1].isdigit() and int(sys.argv[1]) == 1
print('Use FP16:', use_fp16)
output = run_trt(np.zeros((16, 128, 384), np.float32))
print(output)
print(output.shape) |
TensorFlow2/Recommendation/WideAndDeep/triton/runner | runner | stages | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import pathlib
from typing import List, Optional, Tuple, Union
# method from PEP-366 to support relative import in executed modules
if __name__ == "__main__" and __package__ is None:
__package__ = pathlib.Path(__file__).parent.name
from .core import Command
class ResultsType:
"""
Results types generated by runner
"""
TRITON_PERFORMANCE_OFFLINE = "triton_performance_offline"
TRITON_PERFORMANCE_ONLINE = "triton_performance_online"
class Stage:
"""
Stage definition
"""
label: str
commands: List[Command]
result_path: Optional[str]
result_type: Optional[str]
def __init__(
self,
commands: Union[Tuple[str, ...], List[str]],
result_path: Optional[str] = None,
result_type: Optional[str] = None,
):
"""
Args:
commands: List or Tuple of commands provided as raw string
result_path: Path to results file generated by stage
result_type: Type of results generated by stage
"""
if type(commands) not in [tuple, list]:
raise ValueError("""Incorrect type of commands list. Please, provide list of commands as tuple.""")
self.commands = list(map(lambda command: Command(data=command), commands))
self.result_path = result_path
self.result_type = result_type
class ExportStage(Stage):
label = "Export Model"
class ConversionStage(Stage):
label = "Convert Model"
class DeployStage(Stage):
label = "Deploy Model"
class CorrectnessStage(Stage):
label = "Model Correctness"
class TritonPreparePerformanceProfilingDataStage(Stage):
label = "Prepare Triton Profiling Data"
class TritonPerformanceOfflineStage(Stage):
label = "Triton Performance Offline"
class TritonPerformanceOnlineStage(Stage):
label = "Triton Performance Online"
|
PyTorch/SpeechSynthesis/FastPitch/common/text | text | numerical | """ adapted from https://github.com/keithito/tacotron """
import inflect
import re
_magnitudes = ['trillion', 'billion', 'million', 'thousand', 'hundred', 'm', 'b', 't']
_magnitudes_key = {'m': 'million', 'b': 'billion', 't': 'trillion'}
_measurements = '(f|c|k|d|m)'
_measurements_key = {'f': 'fahrenheit',
'c': 'celsius',
'k': 'thousand',
'm': 'meters'}
_currency_key = {'$': 'dollar', '£': 'pound', '€': 'euro', '₩': 'won'}
_inflect = inflect.engine()
_comma_number_re = re.compile(r'([0-9][0-9\,]+[0-9])')
_decimal_number_re = re.compile(r'([0-9]+\.[0-9]+)')
_currency_re = re.compile(r'([\$€£₩])([0-9\.\,]*[0-9]+)(?:[ ]?({})(?=[^a-zA-Z]|$))?'.format("|".join(_magnitudes)), re.IGNORECASE)
_measurement_re = re.compile(r'([0-9\.\,]*[0-9]+(\s)?{}\b)'.format(_measurements), re.IGNORECASE)
_ordinal_re = re.compile(r'[0-9]+(st|nd|rd|th)')
# _range_re = re.compile(r'(?<=[0-9])+(-)(?=[0-9])+.*?')
_roman_re = re.compile(r'\b(?=[MDCLXVI]+\b)M{0,4}(CM|CD|D?C{0,3})(XC|XL|L?X{0,3})(IX|IV|V?I{2,3})\b') # avoid I
_multiply_re = re.compile(r'(\b[0-9]+)(x)([0-9]+)')
_number_re = re.compile(r"[0-9]+'s|[0-9]+s|[0-9]+")
def _remove_commas(m):
return m.group(1).replace(',', '')
def _expand_decimal_point(m):
return m.group(1).replace('.', ' point ')
def _expand_currency(m):
currency = _currency_key[m.group(1)]
quantity = m.group(2)
magnitude = m.group(3)
# remove commas from quantity to be able to convert to numerical
quantity = quantity.replace(',', '')
# check for million, billion, etc...
if magnitude is not None and magnitude.lower() in _magnitudes:
if len(magnitude) == 1:
magnitude = _magnitudes_key[magnitude.lower()]
return "{} {} {}".format(_expand_hundreds(quantity), magnitude, currency+'s')
parts = quantity.split('.')
if len(parts) > 2:
return quantity + " " + currency + "s" # Unexpected format
dollars = int(parts[0]) if parts[0] else 0
cents = int(parts[1]) if len(parts) > 1 and parts[1] else 0
if dollars and cents:
dollar_unit = currency if dollars == 1 else currency+'s'
cent_unit = 'cent' if cents == 1 else 'cents'
return "{} {}, {} {}".format(
_expand_hundreds(dollars), dollar_unit,
_inflect.number_to_words(cents), cent_unit)
elif dollars:
dollar_unit = currency if dollars == 1 else currency+'s'
return "{} {}".format(_expand_hundreds(dollars), dollar_unit)
elif cents:
cent_unit = 'cent' if cents == 1 else 'cents'
return "{} {}".format(_inflect.number_to_words(cents), cent_unit)
else:
return 'zero' + ' ' + currency + 's'
def _expand_hundreds(text):
number = float(text)
if 1000 < number < 10000 and (number % 100 == 0) and (number % 1000 != 0):
return _inflect.number_to_words(int(number / 100)) + " hundred"
else:
return _inflect.number_to_words(text)
def _expand_ordinal(m):
return _inflect.number_to_words(m.group(0))
def _expand_measurement(m):
_, number, measurement = re.split('(\d+(?:\.\d+)?)', m.group(0))
number = _inflect.number_to_words(number)
measurement = "".join(measurement.split())
measurement = _measurements_key[measurement.lower()]
return "{} {}".format(number, measurement)
def _expand_range(m):
return ' to '
def _expand_multiply(m):
left = m.group(1)
right = m.group(3)
return "{} by {}".format(left, right)
def _expand_roman(m):
# from https://stackoverflow.com/questions/19308177/converting-roman-numerals-to-integers-in-python
roman_numerals = {'I':1, 'V':5, 'X':10, 'L':50, 'C':100, 'D':500, 'M':1000}
result = 0
num = m.group(0)
for i, c in enumerate(num):
if (i+1) == len(num) or roman_numerals[c] >= roman_numerals[num[i+1]]:
result += roman_numerals[c]
else:
result -= roman_numerals[c]
return str(result)
def _expand_number(m):
_, number, suffix = re.split(r"(\d+(?:'?\d+)?)", m.group(0))
number = int(number)
if number > 1000 < 10000 and (number % 100 == 0) and (number % 1000 != 0):
text = _inflect.number_to_words(number // 100) + " hundred"
elif number > 1000 and number < 3000:
if number == 2000:
text = 'two thousand'
elif number > 2000 and number < 2010:
text = 'two thousand ' + _inflect.number_to_words(number % 100)
elif number % 100 == 0:
text = _inflect.number_to_words(number // 100) + ' hundred'
else:
number = _inflect.number_to_words(number, andword='', zero='oh', group=2).replace(', ', ' ')
number = re.sub(r'-', ' ', number)
text = number
else:
number = _inflect.number_to_words(number, andword='and')
number = re.sub(r'-', ' ', number)
number = re.sub(r',', '', number)
text = number
if suffix in ("'s", "s"):
if text[-1] == 'y':
text = text[:-1] + 'ies'
else:
text = text + suffix
return text
def normalize_numbers(text):
text = re.sub(_comma_number_re, _remove_commas, text)
text = re.sub(_currency_re, _expand_currency, text)
text = re.sub(_decimal_number_re, _expand_decimal_point, text)
text = re.sub(_ordinal_re, _expand_ordinal, text)
# text = re.sub(_range_re, _expand_range, text)
# text = re.sub(_measurement_re, _expand_measurement, text)
text = re.sub(_roman_re, _expand_roman, text)
text = re.sub(_multiply_re, _expand_multiply, text)
text = re.sub(_number_re, _expand_number, text)
return text
|
PyTorch/Segmentation/nnUNet/triton | triton | preprocess | # Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import os
import time
from argparse import ArgumentDefaultsHelpFormatter, ArgumentParser
from data_preprocessing.preprocessor import Preprocessor
from utils.utils import get_task_code
parser = ArgumentParser(formatter_class=ArgumentDefaultsHelpFormatter)
parser.add_argument("--data", type=str, default="/data", help="Path to data directory")
parser.add_argument("--results", type=str, default="/data", help="Path for saving results directory")
parser.add_argument(
"--exec_mode",
type=str,
default="training",
choices=["training", "val", "test"],
help="Mode for data preprocessing",
)
parser.add_argument("--dilation", action="store_true", help="Perform morphological label dilation")
parser.add_argument("--task", type=str, help="Number of task to be run. MSD uses numbers 01-10")
parser.add_argument("--dim", type=int, default=3, choices=[2, 3], help="Data dimension to prepare")
parser.add_argument("--n_jobs", type=int, default=-1, help="Number of parallel jobs for data preprocessing")
if __name__ == "__main__":
args = parser.parse_args()
start = time.time()
Preprocessor(args).run()
task_code = get_task_code(args)
path = os.path.join(args.data, task_code)
if args.exec_mode == "test":
path = os.path.join(path, "test")
end = time.time()
print(f"Preprocessing time: {(end - start):.2f}")
|
TensorFlow/Detection/SSD/models/research/object_detection/samples/configs | configs | ssd_mobilenet_v2_quantized_300x300_coco | # Quantized trained SSD with Mobilenet v2 on MSCOCO Dataset.
# Users should configure the fine_tune_checkpoint field in the train config as
# well as the label_map_path and input_path fields in the train_input_reader and
# eval_input_reader. Search for "PATH_TO_BE_CONFIGURED" to find the fields that
# should be configured.
model {
ssd {
num_classes: 90
box_coder {
faster_rcnn_box_coder {
y_scale: 10.0
x_scale: 10.0
height_scale: 5.0
width_scale: 5.0
}
}
matcher {
argmax_matcher {
matched_threshold: 0.5
unmatched_threshold: 0.5
ignore_thresholds: false
negatives_lower_than_unmatched: true
force_match_for_each_row: true
}
}
similarity_calculator {
iou_similarity {
}
}
anchor_generator {
ssd_anchor_generator {
num_layers: 6
min_scale: 0.2
max_scale: 0.95
aspect_ratios: 1.0
aspect_ratios: 2.0
aspect_ratios: 0.5
aspect_ratios: 3.0
aspect_ratios: 0.3333
}
}
image_resizer {
fixed_shape_resizer {
height: 300
width: 300
}
}
box_predictor {
convolutional_box_predictor {
min_depth: 0
max_depth: 0
num_layers_before_predictor: 0
use_dropout: false
dropout_keep_probability: 0.8
kernel_size: 1
box_code_size: 4
apply_sigmoid_to_scores: false
conv_hyperparams {
activation: RELU_6,
regularizer {
l2_regularizer {
weight: 0.00004
}
}
initializer {
truncated_normal_initializer {
stddev: 0.03
mean: 0.0
}
}
batch_norm {
train: true,
scale: true,
center: true,
decay: 0.9997,
epsilon: 0.001,
}
}
}
}
feature_extractor {
type: 'ssd_mobilenet_v2'
min_depth: 16
depth_multiplier: 1.0
conv_hyperparams {
activation: RELU_6,
regularizer {
l2_regularizer {
weight: 0.00004
}
}
initializer {
truncated_normal_initializer {
stddev: 0.03
mean: 0.0
}
}
batch_norm {
train: true,
scale: true,
center: true,
decay: 0.9997,
epsilon: 0.001,
}
}
}
loss {
classification_loss {
weighted_sigmoid {
}
}
localization_loss {
weighted_smooth_l1 {
}
}
hard_example_miner {
num_hard_examples: 3000
iou_threshold: 0.99
loss_type: CLASSIFICATION
max_negatives_per_positive: 3
min_negatives_per_image: 3
}
classification_weight: 1.0
localization_weight: 1.0
}
normalize_loss_by_num_matches: true
post_processing {
batch_non_max_suppression {
score_threshold: 1e-8
iou_threshold: 0.6
max_detections_per_class: 100
max_total_detections: 100
}
score_converter: SIGMOID
}
}
}
train_config: {
batch_size: 24
optimizer {
rms_prop_optimizer: {
learning_rate: {
exponential_decay_learning_rate {
initial_learning_rate: 0.004
decay_steps: 800720
decay_factor: 0.95
}
}
momentum_optimizer_value: 0.9
decay: 0.9
epsilon: 1.0
}
}
fine_tune_checkpoint: "PATH_TO_BE_CONFIGURED/model.ckpt"
fine_tune_checkpoint_type: "detection"
# Note: The below line limits the training process to 200K steps, which we
# empirically found to be sufficient enough to train the pets dataset. This
# effectively bypasses the learning rate schedule (the learning rate will
# never decay). Remove the below line to train indefinitely.
num_steps: 200000
data_augmentation_options {
random_horizontal_flip {
}
}
data_augmentation_options {
ssd_random_crop {
}
}
}
train_input_reader: {
tf_record_input_reader {
input_path: "PATH_TO_BE_CONFIGURED/mscoco_train.record-?????-of-00100"
}
label_map_path: "PATH_TO_BE_CONFIGURED/mscoco_label_map.pbtxt"
}
eval_config: {
num_examples: 8000
# Note: The below line limits the evaluation process to 10 evaluations.
# Remove the below line to evaluate indefinitely.
max_evals: 10
}
eval_input_reader: {
tf_record_input_reader {
input_path: "PATH_TO_BE_CONFIGURED/mscoco_val.record-?????-of-00010"
}
label_map_path: "PATH_TO_BE_CONFIGURED/mscoco_label_map.pbtxt"
shuffle: false
num_readers: 1
}
graph_rewriter {
quantization {
delay: 48000
weight_bits: 8
activation_bits: 8
}
} |
TensorFlow2/Segmentation/Contrib/UNet3P/models | models | model | """
Returns Unet3+ model
"""
import tensorflow as tf
from omegaconf import DictConfig
from .backbones import vgg16_backbone, vgg19_backbone, unet3plus_backbone
from .unet3plus import unet3plus
from .unet3plus_deep_supervision import unet3plus_deepsup
from .unet3plus_deep_supervision_cgm import unet3plus_deepsup_cgm
def prepare_model(cfg: DictConfig, training=False):
"""
Creates and return model object based on given model type.
"""
input_shape = [cfg.INPUT.HEIGHT, cfg.INPUT.WIDTH, cfg.INPUT.CHANNELS]
input_layer = tf.keras.layers.Input(
shape=input_shape,
name="input_layer"
) # 320*320*3
filters = [64, 128, 256, 512, 1024]
# create backbone
if cfg.MODEL.BACKBONE.TYPE == "unet3plus":
backbone_layers = unet3plus_backbone(
input_layer,
filters
)
elif cfg.MODEL.BACKBONE.TYPE == "vgg16":
backbone_layers = vgg16_backbone(input_layer, )
elif cfg.MODEL.BACKBONE.TYPE == "vgg19":
backbone_layers = vgg19_backbone(input_layer, )
else:
raise ValueError(
"Wrong backbone type passed."
"\nPlease check config file for possible options."
)
print(f"Using {cfg.MODEL.BACKBONE.TYPE} as a backbone.")
if cfg.MODEL.TYPE == "unet3plus":
# training parameter does not matter in this case
outputs, model_name = unet3plus(
backbone_layers,
cfg.OUTPUT.CLASSES,
filters
)
elif cfg.MODEL.TYPE == "unet3plus_deepsup":
outputs, model_name = unet3plus_deepsup(
backbone_layers,
cfg.OUTPUT.CLASSES,
filters,
training
)
elif cfg.MODEL.TYPE == "unet3plus_deepsup_cgm":
if cfg.OUTPUT.CLASSES != 1:
raise ValueError(
"UNet3+ with Deep Supervision and Classification Guided Module"
"\nOnly works when model output classes are equal to 1"
)
outputs, model_name = unet3plus_deepsup_cgm(
backbone_layers,
cfg.OUTPUT.CLASSES,
filters,
training
)
else:
raise ValueError(
"Wrong model type passed."
"\nPlease check config file for possible options."
)
return tf.keras.Model(
inputs=input_layer,
outputs=outputs,
name=model_name
)
if __name__ == "__main__":
"""## Test model Compilation,"""
from omegaconf import OmegaConf
cfg = {
"WORK_DIR": "H:\\Projects\\UNet3P",
"INPUT": {"HEIGHT": 320, "WIDTH": 320, "CHANNELS": 3},
"OUTPUT": {"CLASSES": 1},
# available variants are unet3plus, unet3plus_deepsup, unet3plus_deepsup_cgm
"MODEL": {"TYPE": "unet3plus",
# available variants are unet3plus, vgg16, vgg19
"BACKBONE": {"TYPE": "vgg19", }
}
}
unet_3P = prepare_model(OmegaConf.create(cfg), True)
unet_3P.summary()
# tf.keras.utils.plot_model(unet_3P, show_layer_names=True, show_shapes=True)
# unet_3P.save("unet_3P.hdf5")
|
TensorFlow/Detection/SSD/models/research/object_detection/utils | utils | np_box_list_ops_test | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Tests for object_detection.utils.np_box_list_ops."""
import numpy as np
import tensorflow as tf
from object_detection.utils import np_box_list
from object_detection.utils import np_box_list_ops
class AreaRelatedTest(tf.test.TestCase):
def setUp(self):
boxes1 = np.array([[4.0, 3.0, 7.0, 5.0], [5.0, 6.0, 10.0, 7.0]],
dtype=float)
boxes2 = np.array([[3.0, 4.0, 6.0, 8.0], [14.0, 14.0, 15.0, 15.0],
[0.0, 0.0, 20.0, 20.0]],
dtype=float)
self.boxlist1 = np_box_list.BoxList(boxes1)
self.boxlist2 = np_box_list.BoxList(boxes2)
def test_area(self):
areas = np_box_list_ops.area(self.boxlist1)
expected_areas = np.array([6.0, 5.0], dtype=float)
self.assertAllClose(expected_areas, areas)
def test_intersection(self):
intersection = np_box_list_ops.intersection(self.boxlist1, self.boxlist2)
expected_intersection = np.array([[2.0, 0.0, 6.0], [1.0, 0.0, 5.0]],
dtype=float)
self.assertAllClose(intersection, expected_intersection)
def test_iou(self):
iou = np_box_list_ops.iou(self.boxlist1, self.boxlist2)
expected_iou = np.array([[2.0 / 16.0, 0.0, 6.0 / 400.0],
[1.0 / 16.0, 0.0, 5.0 / 400.0]],
dtype=float)
self.assertAllClose(iou, expected_iou)
def test_ioa(self):
boxlist1 = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75]], dtype=
np.float32))
boxlist2 = np_box_list.BoxList(
np.array(
[[0.5, 0.25, 1.0, 1.0], [0.0, 0.0, 1.0, 1.0]], dtype=np.float32))
ioa21 = np_box_list_ops.ioa(boxlist2, boxlist1)
expected_ioa21 = np.array([[0.5, 0.0],
[1.0, 1.0]],
dtype=np.float32)
self.assertAllClose(ioa21, expected_ioa21)
def test_scale(self):
boxlist = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75]], dtype=
np.float32))
boxlist_scaled = np_box_list_ops.scale(boxlist, 2.0, 3.0)
expected_boxlist_scaled = np_box_list.BoxList(
np.array(
[[0.5, 0.75, 1.5, 2.25], [0.0, 0.0, 1.0, 2.25]], dtype=np.float32))
self.assertAllClose(expected_boxlist_scaled.get(), boxlist_scaled.get())
def test_clip_to_window(self):
boxlist = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75],
[-0.2, -0.3, 0.7, 1.5]],
dtype=np.float32))
boxlist_clipped = np_box_list_ops.clip_to_window(boxlist,
[0.0, 0.0, 1.0, 1.0])
expected_boxlist_clipped = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75],
[0.0, 0.0, 0.7, 1.0]],
dtype=np.float32))
self.assertAllClose(expected_boxlist_clipped.get(), boxlist_clipped.get())
def test_prune_outside_window(self):
boxlist = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75],
[-0.2, -0.3, 0.7, 1.5]],
dtype=np.float32))
boxlist_pruned, _ = np_box_list_ops.prune_outside_window(
boxlist, [0.0, 0.0, 1.0, 1.0])
expected_boxlist_pruned = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75]], dtype=
np.float32))
self.assertAllClose(expected_boxlist_pruned.get(), boxlist_pruned.get())
def test_concatenate(self):
boxlist1 = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75]], dtype=
np.float32))
boxlist2 = np_box_list.BoxList(
np.array(
[[0.5, 0.25, 1.0, 1.0], [0.0, 0.0, 1.0, 1.0]], dtype=np.float32))
boxlists = [boxlist1, boxlist2]
boxlist_concatenated = np_box_list_ops.concatenate(boxlists)
boxlist_concatenated_expected = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75],
[0.5, 0.25, 1.0, 1.0], [0.0, 0.0, 1.0, 1.0]],
dtype=np.float32))
self.assertAllClose(boxlist_concatenated_expected.get(),
boxlist_concatenated.get())
def test_change_coordinate_frame(self):
boxlist = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75]], dtype=
np.float32))
boxlist_coord = np_box_list_ops.change_coordinate_frame(
boxlist, np.array([0, 0, 0.5, 0.5], dtype=np.float32))
expected_boxlist_coord = np_box_list.BoxList(
np.array([[0.5, 0.5, 1.5, 1.5], [0, 0, 1.0, 1.5]], dtype=np.float32))
self.assertAllClose(boxlist_coord.get(), expected_boxlist_coord.get())
def test_filter_scores_greater_than(self):
boxlist = np_box_list.BoxList(
np.array(
[[0.25, 0.25, 0.75, 0.75], [0.0, 0.0, 0.5, 0.75]], dtype=
np.float32))
boxlist.add_field('scores', np.array([0.8, 0.2], np.float32))
boxlist_greater = np_box_list_ops.filter_scores_greater_than(boxlist, 0.5)
expected_boxlist_greater = np_box_list.BoxList(
np.array([[0.25, 0.25, 0.75, 0.75]], dtype=np.float32))
self.assertAllClose(boxlist_greater.get(), expected_boxlist_greater.get())
class GatherOpsTest(tf.test.TestCase):
def setUp(self):
boxes = np.array([[3.0, 4.0, 6.0, 8.0], [14.0, 14.0, 15.0, 15.0],
[0.0, 0.0, 20.0, 20.0]],
dtype=float)
self.boxlist = np_box_list.BoxList(boxes)
self.boxlist.add_field('scores', np.array([0.5, 0.7, 0.9], dtype=float))
self.boxlist.add_field('labels',
np.array([[0, 0, 0, 1, 0], [0, 1, 0, 0, 0],
[0, 0, 0, 0, 1]],
dtype=int))
def test_gather_with_out_of_range_indices(self):
indices = np.array([3, 1], dtype=int)
boxlist = self.boxlist
with self.assertRaises(ValueError):
np_box_list_ops.gather(boxlist, indices)
def test_gather_with_invalid_multidimensional_indices(self):
indices = np.array([[0, 1], [1, 2]], dtype=int)
boxlist = self.boxlist
with self.assertRaises(ValueError):
np_box_list_ops.gather(boxlist, indices)
def test_gather_without_fields_specified(self):
indices = np.array([2, 0, 1], dtype=int)
boxlist = self.boxlist
subboxlist = np_box_list_ops.gather(boxlist, indices)
expected_scores = np.array([0.9, 0.5, 0.7], dtype=float)
self.assertAllClose(expected_scores, subboxlist.get_field('scores'))
expected_boxes = np.array([[0.0, 0.0, 20.0, 20.0], [3.0, 4.0, 6.0, 8.0],
[14.0, 14.0, 15.0, 15.0]],
dtype=float)
self.assertAllClose(expected_boxes, subboxlist.get())
expected_labels = np.array([[0, 0, 0, 0, 1], [0, 0, 0, 1, 0],
[0, 1, 0, 0, 0]],
dtype=int)
self.assertAllClose(expected_labels, subboxlist.get_field('labels'))
def test_gather_with_invalid_field_specified(self):
indices = np.array([2, 0, 1], dtype=int)
boxlist = self.boxlist
with self.assertRaises(ValueError):
np_box_list_ops.gather(boxlist, indices, 'labels')
with self.assertRaises(ValueError):
np_box_list_ops.gather(boxlist, indices, ['objectness'])
def test_gather_with_fields_specified(self):
indices = np.array([2, 0, 1], dtype=int)
boxlist = self.boxlist
subboxlist = np_box_list_ops.gather(boxlist, indices, ['labels'])
self.assertFalse(subboxlist.has_field('scores'))
expected_boxes = np.array([[0.0, 0.0, 20.0, 20.0], [3.0, 4.0, 6.0, 8.0],
[14.0, 14.0, 15.0, 15.0]],
dtype=float)
self.assertAllClose(expected_boxes, subboxlist.get())
expected_labels = np.array([[0, 0, 0, 0, 1], [0, 0, 0, 1, 0],
[0, 1, 0, 0, 0]],
dtype=int)
self.assertAllClose(expected_labels, subboxlist.get_field('labels'))
class SortByFieldTest(tf.test.TestCase):
def setUp(self):
boxes = np.array([[3.0, 4.0, 6.0, 8.0], [14.0, 14.0, 15.0, 15.0],
[0.0, 0.0, 20.0, 20.0]],
dtype=float)
self.boxlist = np_box_list.BoxList(boxes)
self.boxlist.add_field('scores', np.array([0.5, 0.9, 0.4], dtype=float))
self.boxlist.add_field('labels',
np.array([[0, 0, 0, 1, 0], [0, 1, 0, 0, 0],
[0, 0, 0, 0, 1]],
dtype=int))
def test_with_invalid_field(self):
with self.assertRaises(ValueError):
np_box_list_ops.sort_by_field(self.boxlist, 'objectness')
with self.assertRaises(ValueError):
np_box_list_ops.sort_by_field(self.boxlist, 'labels')
def test_with_invalid_sorting_order(self):
with self.assertRaises(ValueError):
np_box_list_ops.sort_by_field(self.boxlist, 'scores', 'Descending')
def test_with_descending_sorting(self):
sorted_boxlist = np_box_list_ops.sort_by_field(self.boxlist, 'scores')
expected_boxes = np.array([[14.0, 14.0, 15.0, 15.0], [3.0, 4.0, 6.0, 8.0],
[0.0, 0.0, 20.0, 20.0]],
dtype=float)
self.assertAllClose(expected_boxes, sorted_boxlist.get())
expected_scores = np.array([0.9, 0.5, 0.4], dtype=float)
self.assertAllClose(expected_scores, sorted_boxlist.get_field('scores'))
def test_with_ascending_sorting(self):
sorted_boxlist = np_box_list_ops.sort_by_field(
self.boxlist, 'scores', np_box_list_ops.SortOrder.ASCEND)
expected_boxes = np.array([[0.0, 0.0, 20.0, 20.0],
[3.0, 4.0, 6.0, 8.0],
[14.0, 14.0, 15.0, 15.0],],
dtype=float)
self.assertAllClose(expected_boxes, sorted_boxlist.get())
expected_scores = np.array([0.4, 0.5, 0.9], dtype=float)
self.assertAllClose(expected_scores, sorted_boxlist.get_field('scores'))
class NonMaximumSuppressionTest(tf.test.TestCase):
def setUp(self):
self._boxes = np.array([[0, 0, 1, 1],
[0, 0.1, 1, 1.1],
[0, -0.1, 1, 0.9],
[0, 10, 1, 11],
[0, 10.1, 1, 11.1],
[0, 100, 1, 101]],
dtype=float)
self._boxlist = np_box_list.BoxList(self._boxes)
def test_with_no_scores_field(self):
boxlist = np_box_list.BoxList(self._boxes)
max_output_size = 3
iou_threshold = 0.5
with self.assertRaises(ValueError):
np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
def test_nms_disabled_max_output_size_equals_three(self):
boxlist = np_box_list.BoxList(self._boxes)
boxlist.add_field('scores',
np.array([.9, .75, .6, .95, .2, .3], dtype=float))
max_output_size = 3
iou_threshold = 1. # No NMS
expected_boxes = np.array([[0, 10, 1, 11], [0, 0, 1, 1], [0, 0.1, 1, 1.1]],
dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
def test_select_from_three_clusters(self):
boxlist = np_box_list.BoxList(self._boxes)
boxlist.add_field('scores',
np.array([.9, .75, .6, .95, .2, .3], dtype=float))
max_output_size = 3
iou_threshold = 0.5
expected_boxes = np.array([[0, 10, 1, 11], [0, 0, 1, 1], [0, 100, 1, 101]],
dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
def test_select_at_most_two_from_three_clusters(self):
boxlist = np_box_list.BoxList(self._boxes)
boxlist.add_field('scores',
np.array([.9, .75, .6, .95, .5, .3], dtype=float))
max_output_size = 2
iou_threshold = 0.5
expected_boxes = np.array([[0, 10, 1, 11], [0, 0, 1, 1]], dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
def test_select_at_most_thirty_from_three_clusters(self):
boxlist = np_box_list.BoxList(self._boxes)
boxlist.add_field('scores',
np.array([.9, .75, .6, .95, .5, .3], dtype=float))
max_output_size = 30
iou_threshold = 0.5
expected_boxes = np.array([[0, 10, 1, 11], [0, 0, 1, 1], [0, 100, 1, 101]],
dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
def test_select_from_ten_indentical_boxes(self):
boxes = np.array(10 * [[0, 0, 1, 1]], dtype=float)
boxlist = np_box_list.BoxList(boxes)
boxlist.add_field('scores', np.array(10 * [0.8]))
iou_threshold = .5
max_output_size = 3
expected_boxes = np.array([[0, 0, 1, 1]], dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
def test_different_iou_threshold(self):
boxes = np.array([[0, 0, 20, 100], [0, 0, 20, 80], [200, 200, 210, 300],
[200, 200, 210, 250]],
dtype=float)
boxlist = np_box_list.BoxList(boxes)
boxlist.add_field('scores', np.array([0.9, 0.8, 0.7, 0.6]))
max_output_size = 4
iou_threshold = .4
expected_boxes = np.array([[0, 0, 20, 100],
[200, 200, 210, 300],],
dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
iou_threshold = .5
expected_boxes = np.array([[0, 0, 20, 100], [200, 200, 210, 300],
[200, 200, 210, 250]],
dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
iou_threshold = .8
expected_boxes = np.array([[0, 0, 20, 100], [0, 0, 20, 80],
[200, 200, 210, 300], [200, 200, 210, 250]],
dtype=float)
nms_boxlist = np_box_list_ops.non_max_suppression(
boxlist, max_output_size, iou_threshold)
self.assertAllClose(nms_boxlist.get(), expected_boxes)
def test_multiclass_nms(self):
boxlist = np_box_list.BoxList(
np.array(
[[0.2, 0.4, 0.8, 0.8], [0.4, 0.2, 0.8, 0.8], [0.6, 0.0, 1.0, 1.0]],
dtype=np.float32))
scores = np.array([[-0.2, 0.1, 0.5, -0.4, 0.3],
[0.7, -0.7, 0.6, 0.2, -0.9],
[0.4, 0.34, -0.9, 0.2, 0.31]],
dtype=np.float32)
boxlist.add_field('scores', scores)
boxlist_clean = np_box_list_ops.multi_class_non_max_suppression(
boxlist, score_thresh=0.25, iou_thresh=0.1, max_output_size=3)
scores_clean = boxlist_clean.get_field('scores')
classes_clean = boxlist_clean.get_field('classes')
boxes = boxlist_clean.get()
expected_scores = np.array([0.7, 0.6, 0.34, 0.31])
expected_classes = np.array([0, 2, 1, 4])
expected_boxes = np.array([[0.4, 0.2, 0.8, 0.8],
[0.4, 0.2, 0.8, 0.8],
[0.6, 0.0, 1.0, 1.0],
[0.6, 0.0, 1.0, 1.0]],
dtype=np.float32)
self.assertAllClose(scores_clean, expected_scores)
self.assertAllClose(classes_clean, expected_classes)
self.assertAllClose(boxes, expected_boxes)
if __name__ == '__main__':
tf.test.main()
|
TensorFlow2/Recommendation/DLRM_and_DCNv2/preproc | preproc | prepare_dataset | #! /bin/bash
# Copyright (c) 2021 NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# Examples:
# to run on a DGX2 with a frequency limit of 3 (will need 8xV100-32GB to fit the model in GPU memory)
# ./prepare_dataset.sh DGX2 3
#
# to run on a DGX2 with a frequency limit of 15 (should fit on a single V100-32GB):
# ./prepare_dataset.sh DGX2 15
#
# to run on CPU with a frequency limit of 15:
# ./prepare_dataset.sh CPU 15
set -e
set -x
ls -ltrash
download_dir=${download_dir:-'/data/criteo_orig'}
./verify_criteo_downloaded.sh ${download_dir}
spark_output_path=${spark_output_path:-'/data/spark/output'}
if [ -f ${spark_output_path}/train/_SUCCESS ] \
&& [ -f ${spark_output_path}/validation/_SUCCESS ] \
&& [ -f ${spark_output_path}/test/_SUCCESS ]; then
echo "Spark preprocessing already carried out"
else
echo "Performing spark preprocessing"
./run_spark.sh $1 ${download_dir} ${spark_output_path} $2
fi
conversion_intermediate_dir=${conversion_intermediate_dir:-'/data/intermediate_binary'}
final_output_dir=${final_output_dir:-'/data/preprocessed'}
if [ -d ${final_output_dir}/train ] \
&& [ -d ${final_output_dir}/validation ] \
&& [ -d ${final_output_dir}/test ] \
&& [ -f ${final_output_dir}/feature_spec.yaml ]; then
echo "Final conversion already done"
else
echo "Performing final conversion to a custom data format"
python parquet_to_binary.py --parallel_jobs 40 --src_dir ${spark_output_path} \
--intermediate_dir ${conversion_intermediate_dir} \
--dst_dir ${final_output_dir}
cp "${spark_output_path}/model_size.json" "${final_output_dir}/model_size.json"
python split_dataset.py --dataset "${final_output_dir}" --output "${final_output_dir}/split"
rm ${final_output_dir}/train_data.bin
rm ${final_output_dir}/validation_data.bin
rm ${final_output_dir}/test_data.bin
rm ${final_output_dir}/model_size.json
mv ${final_output_dir}/split/* ${final_output_dir}
rm -rf ${final_output_dir}/split
fi
echo "Done preprocessing the Criteo Kaggle Dataset"
|
TensorFlow/LanguageModeling/BERT/scripts | scripts | run_pretraining_adam | #! /bin/bash
# Copyright (c) 2019 NVIDIA CORPORATION. All rights reserved.
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
echo "Container nvidia build = " $NVIDIA_BUILD_ID
train_batch_size=${1:-16}
eval_batch_size=${2:-8}
learning_rate=${3:-"1e-4"}
precision=${4:-"fp16"}
use_xla=${5:-"true"}
num_gpus=${6:-8}
warmup_steps=${7:-"10000"}
train_steps=${8:-1144000}
save_checkpoints_steps=${9:-5000}
bert_model=${10:-"large"}
num_accumulation_steps=${11:-1}
seq_len=${12:-512}
max_pred_per_seq=${13:-80}
DATA_DIR=data/tfrecord/lower_case_1_seq_len_${seq_len}_max_pred_${max_pred_per_seq}_masked_lm_prob_0.15_random_seed_12345_dupe_factor_5_shard_1472_test_split_10/books_wiki_en_corpus
if [ "$bert_model" = "large" ] ; then
export BERT_DIR=data/download/nvidia_pretrained/bert_tf_pretraining_large_lamb
else
export BERT_DIR=data/download/nvidia_pretrained/bert_tf_squad11_base_128
fi
PREC=""
if [ "$precision" = "fp16" ] ; then
PREC="--amp"
elif [ "$precision" = "fp32" ] ; then
PREC="--noamp"
elif [ "$precision" = "tf32" ] ; then
PREC="--noamp"
elif [ "$precision" = "manual_fp16" ] ; then
PREC="--noamp --manual_fp16"
else
echo "Unknown <precision> argument"
exit -2
fi
if [ "$use_xla" = "true" ] ; then
PREC="$PREC --use_xla"
echo "XLA activated"
else
PREC="$PREC --nouse_xla"
fi
export GBS=$(expr $train_batch_size \* $num_gpus \* $num_accumulation_steps)
printf -v TAG "tf_bert_pretraining_adam_%s_%s_gbs%d" "$bert_model" "$precision" $GBS
DATESTAMP=`date +'%y%m%d%H%M%S'`
#Edit to save logs & checkpoints in a different directory
RESULTS_DIR=${RESULTS_DIR:-/results/${TAG}_${DATESTAMP}}
LOGFILE=$RESULTS_DIR/$TAG.$DATESTAMP.log
mkdir -m 777 -p $RESULTS_DIR
printf "Saving checkpoints to %s\n" "$RESULTS_DIR"
printf "Logs written to %s\n" "$LOGFILE"
INPUT_FILES="$DATA_DIR/training"
EVAL_FILES="$DATA_DIR/test"
horovod_str=""
mpi=""
if [ $num_gpus -gt 1 ] ; then
mpi="mpiexec --allow-run-as-root -np $num_gpus --bind-to socket"
horovod_str="--horovod"
fi
CMD="$mpi python3 /workspace/bert/run_pretraining.py"
CMD+=" --input_files_dir=$INPUT_FILES"
CMD+=" --eval_files_dir=$EVAL_FILES"
CMD+=" --output_dir=$RESULTS_DIR"
CMD+=" --bert_config_file=$BERT_CONFIG"
CMD+=" --do_train=True"
CMD+=" --do_eval=True"
CMD+=" --train_batch_size=$train_batch_size"
CMD+=" --eval_batch_size=$eval_batch_size"
CMD+=" --max_seq_length=$seq_len"
CMD+=" --max_predictions_per_seq=$max_pred_per_seq"
CMD+=" --num_train_steps=$train_steps"
CMD+=" --num_warmup_steps=$warmup_steps"
CMD+=" --num_accumulation_steps=$num_accumulation_steps"
CMD+=" --save_checkpoints_steps=$save_checkpoints_steps"
CMD+=" --learning_rate=$learning_rate"
CMD+=" --optimizer_type=adam"
CMD+=" $horovod_str $PREC"
CMD+=" --allreduce_post_accumulation=True"
#Check if all necessary files are available before training
for DIR_or_file in $DATA_DIR $BERT_CONFIG $RESULTS_DIR; do
if [ ! -d "$DIR_or_file" ] && [ ! -f "$DIR_or_file" ]; then
echo "Error! $DIR_or_file directory missing. Please mount correctly"
exit -1
fi
done
set -x
if [ -z "$LOGFILE" ] ; then
$CMD
else
(
$CMD
) |& tee $LOGFILE
fi
set +x
|
TensorFlow2/Segmentation/Contrib/UNet3P/data_preparation | data_preparation | verify_data | """
Verify for each image corresponding mask exist or not.
Check against both train and val data
"""
import os
import sys
from omegaconf import DictConfig
from tqdm import tqdm
sys.path.append(os.path.abspath("./"))
from utils.general_utils import join_paths
from utils.images_utils import image_to_mask_name
def check_image_and_mask(cfg, mode):
"""
Check and print names of those images whose mask are not found.
"""
images_path = join_paths(
cfg.WORK_DIR,
cfg.DATASET[mode].IMAGES_PATH
)
mask_path = join_paths(
cfg.WORK_DIR,
cfg.DATASET[mode].MASK_PATH
)
all_images = os.listdir(images_path)
both_found = True
for image in tqdm(all_images):
mask_name = image_to_mask_name(image)
if not (
os.path.exists(
join_paths(images_path, image)
) and
os.path.exists(
join_paths(mask_path, mask_name)
)
):
print(f"{mask_name} did not found against {image}")
both_found = False
return both_found
def verify_data(cfg: DictConfig):
"""
For both train and val data, check for each image its
corresponding mask exist or not. If not then stop the program.
"""
assert check_image_and_mask(cfg, "TRAIN"), \
"Train images and mask should be same in length"
assert check_image_and_mask(cfg, "VAL"), \
"Validation images and mask should be same in length"
|
TensorFlow2/Classification/ConvNets/config/efficientnet_v1 | efficientnet_v1 | b4_cfg | import tensorflow as tf
from config.defaults import Config
# NOTE: this confile file can further be overridden by user-defined params provided at the command line
config = dict(
path_to_impl='model.efficientnet_model_v1',
#data-related model params
num_classes=1000, # must be the same as data.num_classes
input_channels= 3,
rescale_input= 1, # binary,
mean_rgb=(0.485 * 255, 0.456 * 255, 0.406 * 255), # used when rescale_input=True
std_rgb=(0.229 * 255, 0.224 * 255, 0.225 * 255), # used when rescale_input=True
dtype= tf.float32, #used for input image normalization/casting, # tf.float32, tf.bfloat16, tf.float16, tf.float32, tf.bfloat16,
# GUIDE
# width depth resolution dropout
# efficientnet_v1-b0 1.0 1.0 224 0.2
# 'efficientnet_v1-b1 1.0 1.1 240 0.2
# 'efficientnet_v1-b2 1.1 1.2 260 0.3
# 'efficientnet_v1-b3 1.2 1.4 300 0.3
# 'efficientnet_v1-b4 1.4 1.8 380 0.4
# 'efficientnet_v1-b5 1.6 2.2 456 0.4
# 'efficientnet_v1-b6 1.8 2.6 528 0.5
# 'efficientnet_v1-b7 2.0 3.1 600 0.5
# 'efficientnet_v1-b8 2.2 3.6 672 0.5
# 'efficientnet_v1-l2 4.3 5.3 800 0.5
width_coefficient= 1.4,
depth_coefficient= 1.8,
dropout_rate= 0.4,
# image resolution must be set in tr/eval/predict configs below
drop_connect_rate= 0.2,
stem_base_filters= 32,
top_base_filters= 1280,
activation= 'swish',
depth_divisor= 8,
min_depth= None,
use_se= 1, # binary
batch_norm= 'syncbn',
bn_momentum= 0.99,
bn_epsilon= 1e-3,
weight_init= 'fan_out',
blocks= (
# (input_filters, output_filters, kernel_size, num_repeat,expand_ratio, strides, se_ratio)
# pylint: disable=bad-whitespace
dict(input_filters=32, output_filters=16, kernel_size=3, num_repeat=1, expand_ratio=1, strides=(1, 1), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
dict(input_filters=16, output_filters=24, kernel_size=3, num_repeat=2, expand_ratio=6, strides=(2, 2), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
dict(input_filters=24, output_filters=40, kernel_size=5, num_repeat=2, expand_ratio=6, strides=(2, 2), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
dict(input_filters=40, output_filters=80, kernel_size=3, num_repeat=3, expand_ratio=6, strides=(2, 2), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
dict(input_filters=80, output_filters=112, kernel_size=5, num_repeat=3, expand_ratio=6, strides=(1, 1), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
dict(input_filters=112, output_filters=192, kernel_size=5, num_repeat=4, expand_ratio=6, strides=(2, 2), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
dict(input_filters=192, output_filters=320, kernel_size=3, num_repeat=1, expand_ratio=6, strides=(1, 1), se_ratio=0.25,id_skip=True,fused_conv=False,conv_type='depthwise'),
# pylint: enable=bad-whitespace
),
)
# train_config = dict(lr_decay='cosine',
#
# max_epochs=500,
# img_size=380,
# batch_size=256,
# save_checkpoint_freq=5,
# lr_init=0.005,
# weight_decay=5e-6,
# epsilon=0.001,
# resume_checkpoint=1,
# enable_tensorboard=0
# )
#
# eval_config = dict(img_size=380,
# batch_size=256)
#
# data_config = dict(
# data_dir='/data/',
# augmenter_name='autoaugment',
# mixup_alpha=0.0,
#
#
# )
# runtime_config = dict(mode='train_and_eval',
# model_dir='./output/',
# use_amp=1,
# use_xla=1,
# log_steps=100
# )
#
# config = dict(model=model_config,
# train=train_config,
# eval=eval_config,
# data=data_config,
# runtime=runtime_config,
# )
|
PyTorch/Classification/GPUNet/triton/085ms/runner | runner | config_NVIDIA-DGX-1-(1x-V100-32GB) | batching: dynamic
checkpoints:
- name: 0.85ms
url: https://api.ngc.nvidia.com/v2/models/nvidia/dle/gpunet_1_pyt_ckpt/versions/21.12.0_amp/zip
configurations:
- checkpoint: 0.85ms
parameters:
backend_accelerator: trt
checkpoint: 0.85ms
device_kind: gpu
export_format: onnx
export_precision: fp16
format: onnx
max_batch_size: 64
number_of_model_instances: 2
precision: fp16
tensorrt_capture_cuda_graph: 0
torch_jit: none
container_version: '21.12'
datasets:
- name: imagenet
datasets_dir: datasets
ensemble_model_name: null
framework: PyTorch
measurement_steps_offline: 8
measurement_steps_online: 32
model_name: GPUnet
performance_tool: model_analyzer
triton_container_image: nvcr.io/nvidia/tritonserver:21.12-py3
triton_custom_operations: null
triton_dockerfile: null
triton_load_model_method: explicit
|
TensorFlow/Detection/SSD/models/research/object_detection/builders | builders | input_reader_builder | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Input reader builder.
Creates data sources for DetectionModels from an InputReader config. See
input_reader.proto for options.
Note: If users wishes to also use their own InputReaders with the Object
Detection configuration framework, they should define their own builder function
that wraps the build function.
"""
import tensorflow as tf
from object_detection.data_decoders import tf_example_decoder
from object_detection.protos import input_reader_pb2
parallel_reader = tf.contrib.slim.parallel_reader
def build(input_reader_config):
"""Builds a tensor dictionary based on the InputReader config.
Args:
input_reader_config: A input_reader_pb2.InputReader object.
Returns:
A tensor dict based on the input_reader_config.
Raises:
ValueError: On invalid input reader proto.
ValueError: If no input paths are specified.
"""
if not isinstance(input_reader_config, input_reader_pb2.InputReader):
raise ValueError('input_reader_config not of type '
'input_reader_pb2.InputReader.')
if input_reader_config.WhichOneof('input_reader') == 'tf_record_input_reader':
config = input_reader_config.tf_record_input_reader
if not config.input_path:
raise ValueError('At least one input path must be specified in '
'`input_reader_config`.')
_, string_tensor = parallel_reader.parallel_read(
config.input_path[:], # Convert `RepeatedScalarContainer` to list.
reader_class=tf.TFRecordReader,
num_epochs=(input_reader_config.num_epochs
if input_reader_config.num_epochs else None),
num_readers=input_reader_config.num_readers,
shuffle=input_reader_config.shuffle,
dtypes=[tf.string, tf.string],
capacity=input_reader_config.queue_capacity,
min_after_dequeue=input_reader_config.min_after_dequeue)
label_map_proto_file = None
if input_reader_config.HasField('label_map_path'):
label_map_proto_file = input_reader_config.label_map_path
decoder = tf_example_decoder.TfExampleDecoder(
load_instance_masks=input_reader_config.load_instance_masks,
instance_mask_type=input_reader_config.mask_type,
label_map_proto_file=label_map_proto_file)
return decoder.decode(string_tensor)
raise ValueError('Unsupported input_reader_config.')
|
PyTorch/SpeechRecognition/QuartzNet/common/dali | dali | iterator | # Copyright (c) 2020, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import numpy as np
import torch
from nvidia.dali.plugin.base_iterator import LastBatchPolicy
from nvidia.dali.plugin.pytorch import DALIGenericIterator
from common.helpers import print_once
from common.text import _clean_text, punctuation_map
def normalize_string(s, symbols, punct_map):
"""
Normalizes string.
Example:
'call me at 8:00 pm!' -> 'call me at eight zero pm'
"""
labels = set(symbols)
try:
text = _clean_text(s, ["english_cleaners"], punct_map).strip()
return ''.join([tok for tok in text if all(t in labels for t in tok)])
except Exception as e:
print_once(f"WARNING: Normalizing failed: {s} {e}")
class DaliIterator(object):
"""Returns batches of data.
Batches are in the form:
(preprocessed_signal, preprocessed_signal_length, transcript,
transcript_length)
This iterator is not meant to be the entry point to a Dali pipeline.
Use DataLoader instead.
"""
def __init__(self, dali_pipelines, transcripts, symbols, batch_size,
reader_name, train_iterator: bool):
self.transcripts = transcripts
self.symbols = symbols
self.batch_size = batch_size
# in train pipeline shard_size is set to divisable by batch_size,
# so PARTIAL policy is safe
self.dali_it = DALIGenericIterator(
dali_pipelines,
["audio", "label", "audio_shape"],
reader_name=reader_name,
dynamic_shape=True,
auto_reset=True,
last_batch_policy=LastBatchPolicy.DROP)
@staticmethod
def _str2list(s: str):
"""
Returns list of floats, that represents given string.
'0.' denotes separator
'1.' denotes 'a'
'27.' denotes "'"
Assumes, that the string is lower case.
"""
list = []
for c in s:
if c == "'":
list.append(27.)
else:
list.append(max(0., ord(c) - 96.))
return list
@staticmethod
def _pad_lists(lists: list, pad_val=0):
"""
Pads lists, so that all have the same size.
Returns list with actual sizes of corresponding input lists
"""
max_length = 0
sizes = []
for li in lists:
sizes.append(len(li))
max_length = max_length if len(li) < max_length else len(li)
for li in lists:
li += [pad_val] * (max_length - len(li))
return sizes
def _gen_transcripts(self, labels, normalize_transcripts: bool = True):
"""
Generate transcripts in format expected by NN
"""
if normalize_transcripts:
lists = [
self._str2list(normalize_string(self.transcripts[lab.item()],
self.symbols, punctuation_map(self.symbols)))
for lab in labels]
else:
lists = [self._str2list(self.transcripts[lab.item()])
for lab in labels]
sizes = self._pad_lists(lists)
return (torch.tensor(lists).cuda(),
torch.tensor(sizes, dtype=torch.int32).cuda())
def __next__(self):
data = self.dali_it.__next__()
transcripts, transcripts_lengths = self._gen_transcripts(
data[0]["label"])
return (data[0]["audio"], data[0]["audio_shape"][:, 1], transcripts,
transcripts_lengths)
def next(self):
return self.__next__()
def __iter__(self):
return self
# TODO: refactor
class SyntheticDataIterator(object):
def __init__(self, batch_size, nfeatures, feat_min=-5., feat_max=0.,
txt_min=0., txt_max=23., feat_lens_max=1760, txt_lens_max=231,
regenerate=False):
"""
Args:
batch_size
nfeatures: number of features for melfbanks
feat_min: minimum value in `feat` tensor, used for randomization
feat_max: maximum value in `feat` tensor, used for randomization
txt_min: minimum value in `txt` tensor, used for randomization
txt_max: maximum value in `txt` tensor, used for randomization
regenerate: If True, regenerate random tensors for every iterator
step. If False, generate them only at start.
"""
self.batch_size = batch_size
self.nfeatures = nfeatures
self.feat_min = feat_min
self.feat_max = feat_max
self.feat_lens_max = feat_lens_max
self.txt_min = txt_min
self.txt_max = txt_max
self.txt_lens_max = txt_lens_max
self.regenerate = regenerate
if not self.regenerate:
(self.feat, self.feat_lens, self.txt, self.txt_lens
) = self._generate_sample()
def _generate_sample(self):
feat = ((self.feat_max - self.feat_min)
* np.random.random_sample(
(self.batch_size, self.nfeatures, self.feat_lens_max))
+ self.feat_min)
feat_lens = np.random.randint(0, int(self.feat_lens_max) - 1,
size=self.batch_size)
txt = (self.txt_max - self.txt_min) * np.random.random_sample(
(self.batch_size, self.txt_lens_max)) + self.txt_min
txt_lens = np.random.randint(0, int(self.txt_lens_max) - 1,
size=self.batch_size)
return (torch.Tensor(feat).cuda(),
torch.Tensor(feat_lens).cuda(),
torch.Tensor(txt).cuda(),
torch.Tensor(txt_lens).cuda())
def __next__(self):
if self.regenerate:
return self._generate_sample()
return self.feat, self.feat_lens, self.txt, self.txt_lens
def next(self):
return self.__next__()
def __iter__(self):
return self
|
PyTorch/SpeechSynthesis/Tacotron2/trtis_cpp/src/trt/util | util | dims1 | /*
* Copyright (c) 2019-2020, NVIDIA CORPORATION. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the NVIDIA CORPORATION nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL NVIDIA CORPORATION BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef TT2I_DIMS1
#define TT2I_DIMS1
#include "NvInfer.h"
namespace tts
{
class Dims1 : public nvinfer1::Dims
{
public:
/**
* @brief Create a new 1-dimension Dims struct.
*
* @param n The size of the single dimension.
*/
Dims1(const int n)
: Dims()
{
nbDims = 1;
d[0] = n;
}
};
} // namespace tts
#endif
|
TensorFlow/LanguageModeling/BERT | BERT | fused_layer_norm | # coding=utf-8
# Copyright 2018 The Google AI Language Team Authors.
# Copyright (c) 2018, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import collections
import copy
import json
import math
import re
import six
import tensorflow as tf
from tensorflow.python.framework import ops
from tensorflow.contrib.layers.python.layers import utils
from tensorflow.contrib.framework.python.ops import variables
from tensorflow.python.ops import init_ops
import numpy
from tensorflow.python.ops import array_ops
from tensorflow.python.framework import dtypes
from tensorflow.python.ops import nn
def fused_layer_norm(inputs,
center=True,
scale=True,
activation_fn=None,
reuse=None,
variables_collections=None,
outputs_collections=None,
trainable=True,
begin_norm_axis=1,
begin_params_axis=-1,
scope=None,
use_fused_batch_norm=False):
with tf.variable_scope(
scope, 'LayerNorm', [inputs], reuse=reuse) as sc:
inputs = ops.convert_to_tensor(inputs)
inputs_shape = inputs.shape
inputs_rank = inputs_shape.ndims
if inputs_rank is None:
raise ValueError('Inputs %s has undefined rank.' % inputs.name)
dtype = inputs.dtype.base_dtype
if begin_norm_axis < 0:
begin_norm_axis = inputs_rank + begin_norm_axis
if begin_params_axis >= inputs_rank or begin_norm_axis >= inputs_rank:
raise ValueError('begin_params_axis (%d) and begin_norm_axis (%d) '
'must be < rank(inputs) (%d)' %
(begin_params_axis, begin_norm_axis, inputs_rank))
params_shape = inputs_shape[begin_params_axis:]
if not params_shape.is_fully_defined():
raise ValueError(
'Inputs %s: shape(inputs)[%s:] is not fully defined: %s' %
(inputs.name, begin_params_axis, inputs_shape))
# Allocate parameters for the beta and gamma of the normalization.
beta, gamma = None, None
if center:
beta_collections = utils.get_variable_collections(variables_collections,
'beta')
beta = variables.model_variable(
'beta',
shape=params_shape,
dtype=dtype,
initializer=init_ops.zeros_initializer(),
collections=beta_collections,
trainable=trainable)
if scale:
gamma_collections = utils.get_variable_collections(
variables_collections, 'gamma')
gamma = variables.model_variable(
'gamma',
shape=params_shape,
dtype=dtype,
initializer=init_ops.ones_initializer(),
collections=gamma_collections,
trainable=trainable)
if use_fused_batch_norm:
# get static TensorShape if fully defined,
# otherwise retrieve shape tensor
norm_shape = inputs.shape[begin_norm_axis:]
if norm_shape.is_fully_defined():
bn_shape = [1, -1, 1, numpy.prod(norm_shape.as_list())]
else:
norm_shape = tf.shape(inputs)[begin_norm_axis:]
bn_shape = [1, -1, 1, tf.reduce_prod(norm_shape)]
if inputs.get_shape().is_fully_defined():
outputs_shape = inputs.get_shape()
else:
outputs_shape = tf.shape(inputs)
inputs = array_ops.reshape(inputs, bn_shape)
if inputs.get_shape().is_fully_defined():
# static inputs TensorShape fully defined after reshape.
ones = array_ops.ones(inputs.get_shape()[1], dtype=dtypes.float32)
zeros = array_ops.zeros(inputs.get_shape()[1], dtype=dtypes.float32)
else:
# static inputs TensorShape NOT fully defined after reshape.
# must use dynamic shape, which means these input tensors
# have to be created at runtime, which causes a slowdown.
scale_shape = tf.shape(inputs)[1]
ones = array_ops.ones(scale_shape, dtype=dtypes.float32)
zeros = array_ops.zeros(scale_shape, dtype=dtypes.float32)
outputs, mean, variance = nn.fused_batch_norm(
inputs,
ones, zeros,
epsilon=1e-4,
data_format="NCHW")
outputs = array_ops.reshape(outputs, outputs_shape)
if center and scale:
outputs = outputs * gamma + beta
elif center:
outputs = outputs + beta
elif scale:
outputs = outputs * gamma
else:
# Calculate the moments on the last axis (layer activations).
norm_axes = list(range(begin_norm_axis, inputs_rank))
mean, variance = nn.moments(inputs, norm_axes, keep_dims=True)
# Compute layer normalization using the batch_normalization function.
variance_epsilon = 1e-4
outputs = nn.batch_normalization(
inputs,
mean,
variance,
offset=beta,
scale=gamma,
variance_epsilon=variance_epsilon)
outputs.set_shape(inputs_shape)
if activation_fn is not None:
outputs = activation_fn(outputs)
return utils.collect_named_outputs(outputs_collections, sc.name, outputs)
|
TensorFlow/Segmentation/UNet_Industrial | UNet_Industrial | export_saved_model | # !/usr/bin/env python
# -*- coding: utf-8 -*-
# ==============================================================================
#
# Copyright (c) 2019, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
# ==============================================================================
"""
Usage:
python export_saved_model.py \
--activation_fn='relu' \
--batch_size=16 \
--data_format='NCHW' \
--input_dtype="fp32" \
--export_dir="exported_models" \
--model_checkpoint_path="path/to/checkpoint/model.ckpt-2500" \
--unet_variant='tinyUNet' \
--xla \
--amp
"""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import os
import argparse
import pprint
os.environ["TF_CPP_MIN_LOG_LEVEL"] = "3"
import tensorflow as tf
from dllogger.logger import LOGGER
from model.unet import UNet_v1
from model.blocks.activation_blck import authorized_activation_fn
from utils.cmdline_helper import _add_bool_argument
def get_export_flags():
parser = argparse.ArgumentParser(description="JoC-UNet_v1-TF-ExportFlags")
parser.add_argument('--export_dir', default=None, required=True, type=str, help='The export directory.')
parser.add_argument('--model_checkpoint_path', default=None, required=True, help='Checkpoint path.')
parser.add_argument(
'--data_format',
choices=['NHWC', 'NCHW'],
type=str,
default="NCHW",
required=False,
help="""Which Tensor format is used for computation inside the mode"""
)
parser.add_argument(
'--input_dtype',
choices=['fp32', 'fp16'],
type=str,
default="fp32",
required=False,
help="""Tensorflow dtype of the input tensor"""
)
parser.add_argument(
'--unet_variant',
default="tinyUNet",
choices=UNet_v1.authorized_models_variants,
type=str,
required=False,
help="""Which model size is used. This parameter control directly the size and the number of parameters"""
)
parser.add_argument(
'--activation_fn',
choices=authorized_activation_fn,
type=str,
default="relu",
required=False,
help="""Which activation function is used after the convolution layers"""
)
_add_bool_argument(
parser=parser,
name="amp",
default=False,
required=False,
help="Enable Automatic Mixed Precision Computation to maximise performance."
)
_add_bool_argument(
parser=parser,
name="xla",
default=False,
required=False,
help="Enable Tensorflow XLA to maximise performance."
)
parser.add_argument('--batch_size', default=16, type=int, help='Evaluation batch size.')
FLAGS, unknown_args = parser.parse_known_args()
if len(unknown_args) > 0:
for bad_arg in unknown_args:
print("ERROR: Unknown command line arg: %s" % bad_arg)
raise ValueError("Invalid command line arg(s)")
return FLAGS
def export_model(RUNNING_CONFIG):
if RUNNING_CONFIG.amp:
os.environ["TF_ENABLE_AUTO_MIXED_PRECISION_GRAPH_REWRITE"] = "1"
model = UNet_v1(
model_name="UNet_v1",
input_format="NHWC",
compute_format=RUNNING_CONFIG.data_format,
n_output_channels=1,
unet_variant=RUNNING_CONFIG.unet_variant,
weight_init_method="he_normal",
activation_fn=RUNNING_CONFIG.activation_fn
)
config_proto = tf.ConfigProto()
config_proto.allow_soft_placement = True
config_proto.log_device_placement = False
config_proto.gpu_options.allow_growth = True
if RUNNING_CONFIG.xla: # Only working on single GPU
LOGGER.log("XLA is activated - Experimental Feature")
config_proto.graph_options.optimizer_options.global_jit_level = tf.OptimizerOptions.ON_1
config_proto.gpu_options.force_gpu_compatible = True # Force pinned memory
run_config = tf.estimator.RunConfig(
model_dir=None,
tf_random_seed=None,
save_summary_steps=1e9, # disabled
save_checkpoints_steps=None,
save_checkpoints_secs=None,
session_config=config_proto,
keep_checkpoint_max=None,
keep_checkpoint_every_n_hours=1e9, # disabled
log_step_count_steps=1e9,
train_distribute=None,
device_fn=None,
protocol=None,
eval_distribute=None,
experimental_distribute=None
)
estimator = tf.estimator.Estimator(
model_fn=model,
model_dir=RUNNING_CONFIG.model_checkpoint_path,
config=run_config,
params={'debug_verbosity': 0}
)
LOGGER.log('[*] Exporting the model ...')
input_type = tf.float32 if RUNNING_CONFIG.input_dtype else tf.float16
def get_serving_input_receiver_fn():
input_shape = [RUNNING_CONFIG.batch_size, 512, 512, 1]
def serving_input_receiver_fn():
features = tf.placeholder(dtype=input_type, shape=input_shape, name='input_tensor')
return tf.estimator.export.TensorServingInputReceiver(features=features, receiver_tensors=features)
return serving_input_receiver_fn
export_path = estimator.export_saved_model(
export_dir_base=RUNNING_CONFIG.export_dir,
serving_input_receiver_fn=get_serving_input_receiver_fn(),
checkpoint_path=RUNNING_CONFIG.model_checkpoint_path
)
LOGGER.log('[*] Done! path: `%s`' % export_path.decode())
if __name__ == '__main__':
tf.logging.set_verbosity(tf.logging.ERROR)
tf.disable_eager_execution()
flags = get_export_flags()
for endpattern in [".index", ".meta"]:
file_to_check = flags.model_checkpoint_path + endpattern
if not os.path.isfile(file_to_check):
raise FileNotFoundError("The checkpoint file `%s` does not exist" % file_to_check)
print(" ========================= Export Flags =========================\n")
pprint.pprint(dict(flags._get_kwargs()))
print("\n %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%")
export_model(flags)
|
PyTorch/SpeechRecognition/wav2vec2/common | common | fairseq_fake_modules | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
'''Fake fairseq.* modules allowing to torch.load fairseq checkpoints.'''
import sys
class Dummy:
pass
class FakeModule:
def __init__(self, classes=["AverageMeter", "TimeMeter", "StopwatchMeter"]):
[setattr(self, cls, Dummy) for cls in classes]
sys.modules["fairseq"] = Dummy()
sys.modules["fairseq.data"] = Dummy()
sys.modules["fairseq.data.dictionary"] = FakeModule(["Dictionary"])
sys.modules["fairseq.logging.meters"] = FakeModule()
sys.modules["fairseq.meters"] = FakeModule()
|
Tools/DGLPyTorch/SyntheticGraphGeneration/syngen/generator/graph | graph | utils | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import os
import logging
import math
import multiprocessing
from datetime import datetime
from functools import partial
from typing import Tuple, Union, Optional
import cupy as cp
import matplotlib.pyplot as plt
import numpy as np
from tqdm import tqdm
from pylibraft.random import rmat
from scipy import stats
from syngen.utils import NDArray, infer_operator
from syngen.utils.utils import infer_operator
from syngen.utils.io_utils import dump_generated_graph
from syngen.utils.memory_manager import MemoryManager
from syngen.utils.types import NDArray
logger = logging.getLogger(__name__)
def move_ndarray_to_host(ndarray: NDArray):
if isinstance(ndarray, np.ndarray):
return ndarray
elif isinstance(ndarray, cp.ndarray):
return cp.asnumpy(ndarray)
else:
raise ValueError('supports only numpy and cupy ndarrays')
def rearrange_graph(
edge_list: NDArray,
src_nodes: NDArray,
dst_nodes: NDArray,
assume_unique: bool = False,
) -> Tuple[NDArray, NDArray]:
"""
Transforms a bipartite graph from edge list format to lower_left and upper_right adjacency matrices.
Returned matrices are in coordinate list format.
"""
operator = infer_operator(edge_list)
if not isinstance(src_nodes, (np.ndarray, cp.ndarray)):
raise ValueError('src_nodes: expected type NDArray, but %s was passed', type(src_nodes))
if not isinstance(dst_nodes, (np.ndarray, cp.ndarray)):
raise ValueError('dst_nodes: expected type NDArray, but %s was passed', type(dst_nodes))
if not assume_unique:
src_nodes = operator.unique(src_nodes)
dst_nodes = operator.unique(dst_nodes)
if operator.intersect1d(src_nodes, dst_nodes, assume_unique=True).size != 0:
raise ValueError('node sets cannot intersect')
edge_list = edge_list.flatten()
node_set = operator.hstack([src_nodes, dst_nodes])
pos_to_new_id = operator.argsort(node_set)
sorted_node_set = node_set[pos_to_new_id]
pos_in_sorted_nodeset = operator.searchsorted(sorted_node_set, edge_list)
# need to validate since errors could be ignored
# https://docs.cupy.dev/en/stable/user_guide/difference.html#out-of-bounds-indices
message = 'all ids in a graph should be in one of the node sets'
if operator.any(pos_in_sorted_nodeset == len(sorted_node_set)):
raise ValueError(message)
if operator.any(sorted_node_set[pos_in_sorted_nodeset] != edge_list):
raise ValueError(message)
edge_list_mapped = pos_to_new_id[pos_in_sorted_nodeset].reshape(-1, 2)
upper_right = edge_list_mapped[edge_list_mapped[:, 0] < len(src_nodes)]
upper_right[:, 1] -= len(src_nodes)
lower_left = edge_list_mapped[edge_list_mapped[:, 0] >= len(src_nodes)]
lower_left[:, 0] -= len(src_nodes)
return lower_left, upper_right
def reindex_graph(
edge_list: NDArray,
return_counts: bool = False,
) -> Union[NDArray, Tuple[NDArray, int, int]]:
"""
Reindexes a graph by assigning node ids starting from 0.
Returns the processed graph and, optionally, number of nodes and number of edges.
"""
operator = infer_operator(edge_list)
nodes, inverse_flat = operator.unique(edge_list, return_inverse=True)
edge_list_reindexed = inverse_flat.reshape(edge_list.shape)
if return_counts:
return edge_list_reindexed, len(nodes), len(edge_list)
else:
return edge_list_reindexed
def get_reversed_part(part, gpu=False, operator=None):
operator = operator or (cp if gpu else np)
new_part = operator.empty_like(part)
new_part[:, 0] = part[:, 1]
new_part[:, 1] = part[:, 0]
return new_part
# Postprocessing
def recreate_graph(lower: NDArray, upper: NDArray, offset: int, gpu=False):
assert (
lower is not None and upper is not None
), "Upper and lower cannot be None"
operator = cp if gpu else np
lower[:, 0] = lower[:, 0] + offset
upper[:, 1] = upper[:, 1] + offset
new_graph = operator.concatenate((lower, upper), axis=0)
return new_graph
def recreate_bipartite_nondirected(graph, row_shape):
upper = [(row, col + row_shape) for row, col in graph]
lower = [(col, row) for row, col in upper]
new_graph = upper + lower
return new_graph
def to_adj_matrix(graph, shape):
matrix = np.zeros(shape=shape, dtype=np.bool)
arr_indicies = np.array(graph)
matrix[arr_indicies[:, 0], arr_indicies[:, 1]] = 1
return matrix
def plot_graph_adj(graph, shape):
graph_adj = to_adj_matrix(graph, shape=shape)
return plt.imshow(graph_adj, cmap="binary", interpolation="nearest")
def graph_to_snap_file(A, filename):
np.savetxt(filename, A, fmt="%i", delimiter="\t")
def effective_nonsquare_rmat_approximate(
theta,
E,
A_shape,
noise_scaling=1.0,
batch_size=1000,
dtype=np.int64,
custom_samplers=None,
generate_back_edges=False,
verbose=False,
):
""" This function generates list of edges using modified RMat approach
Args:
theta (np.array): seeding matrix, needs to be shape 2x2
E (int): number of edges to be generated
A_shape (tuple): shape of resulting adjacency matrix. numbers has to be powers of 2
A_shape should be equal to (ceil(log2(X)),ceil(log2(Y))) X,Y are
dimensions of original adjacency
noise_scaling (float 0..1): noise scaling factor for good degree distribution
batch_size (int): edges are generated in batches of batch_size size
dtype (numpy dtype np.int32/np.int64): dtype of nodes id's
custom_samplers (List[scipy.stats.rv_discrete]): samplers for each step of genration
process
generate_back_edges (bool): if True then generated edges will also have "back" edges. Not
that setting to True for partite graphs makes no sense.
Returns:
A (np.array 2 x E): matrix containing in every row a signle edge. Edge is always directed
0'th column is FROM edge 1st is TO edge
mtx_shape (tuple) - shape of adjecency matrix (A contains list of edges, this is Adjecency
metrix shape)
custom_samplers (List[scipy.stats.rv_discrete]) - list of samplers needed to generate edges
from the same disctribution for multiple runs of the function
Description:
The generation will consist of theta^[n] (x) theta_p^[m] (x) theta_q^[l]
^[n] is kronecker power
(x) is matrix kronecker product
theta_p (2x1) and theta_q(1x2) are marginals of theta
This way we can generate rectangular shape of adjecency matrix e.g. for bipatrite
graphs
"""
def get_row_col_addres(thetas_n):
thetas_r = [t.shape[0] for t in thetas_n]
thetas_c = [t.shape[1] for t in thetas_n]
row_n = np.prod(thetas_r) # theta_r**quadrant_sequence.shape[1]
col_n = np.prod(thetas_c) # theta_c**quadrant_sequence.shape[1]
row_adders = np.array(
[
int(row_n / thetas_r[i] ** (i + 1)) % row_n
for i in range(len(thetas_n))
]
) # there has to be % as we can have thetas_r[i]==1
col_adders = np.array(
[
int(col_n / thetas_c[i] ** (i + 1)) % col_n
for i in range(len(thetas_n))
]
)
return row_adders, col_adders, thetas_r, thetas_c, row_n, col_n
def parse_quadrants(
quadrant_sequence,
thetas_n,
row_adders,
col_addres,
thetas_r,
thetas_c,
row_n,
col_n,
dtype=np.int64,
):
N = len(thetas_n)
new_edges = np.zeros(
shape=(quadrant_sequence.shape[0], 2)
) # 2 because 0 col=rows_addresses, 1st col = columns
row_addr = np.array(quadrant_sequence // thetas_c, dtype=dtype)
col_addr = np.array(quadrant_sequence % thetas_c, dtype=dtype)
row_adders = np.array(
[int(row_n / thetas_r[i] ** (i + 1)) % row_n for i in range(N)]
) # there has to be % as we can have thetas_r[i]==1
col_adders = np.array(
[int(col_n / thetas_c[i] ** (i + 1)) % col_n for i in range(N)]
)
new_edges[:, 0] = np.sum(np.multiply(row_addr, row_adders), axis=1)
new_edges[:, 1] = np.sum(np.multiply(col_addr, col_adders), axis=1)
return new_edges
if batch_size > E: # if bs>E
batch_size = int(E // 2 * 2)
if generate_back_edges:
assert (
batch_size % 2 == 0 and batch_size >= 2
), "batch size has to be odd and >1"
assert (
np.abs((np.sum(theta) - 1.0)) < 1e-6
), "Theta probabilities has to sum to 1.0"
assert (theta.shape[0] == 2) and (
theta.shape[1] == 2
), "Only 2x2 seeding matrixes are acceptable"
assert len(A_shape) == 2, "A_shape needs to be of len 2"
# get appropriate number of n,m,l always m=0 or l=0 (or both for rectangular adjecency)
r = A_shape[0]
c = A_shape[1]
n = min(r, c) # theta^[n] (x) theta_p^[m] (x) theta_q^[l]
m = max(0, r - c)
# flake8: noqa
l = max(0, c - r)
# calc values of marginal theta matrixes
theta_p = theta.sum(axis=1).reshape((2, -1)) # 2x1
theta_q = theta.sum(axis=0).reshape((1, -1)) # 1x2
# get all thetas
thetas_n = [theta] * n + [theta_p] * m + [theta_q] * l
# prepare samplers for each of n+m+l steps
if custom_samplers is None:
custom_samplers = []
for i in range(n + m + l):
theta_n = thetas_n[
i
] # each of n+m+l steps have their own theta_n which can be theta/theta_p or theta_q +
# noise
noise = noise_scaling * np.random.uniform(
-1, 1, size=theta_n.shape
)
noise_to_add = np.multiply(theta_n, noise)
theta_n = theta_n + noise_to_add
theta_n = theta_n / np.sum(theta_n)
cstm_n = "step_" + str(i)
theta_r = theta_n.shape[0]
theta_c = theta_n.shape[1]
xk = tuple(range(theta_r * theta_c))
pk = theta_n.reshape(-1)
cstm_s = stats.rv_discrete(name=cstm_n, values=(xk, pk))
custom_samplers.append(cstm_s)
# Prepare all batch sizes needed for generation
if batch_size == 0:
batch_count = 0 # XXX: why does this happen anyways?
else:
batch_count = E // batch_size
last_batch_size = E - batch_count * batch_size
if last_batch_size % 2 > 0 and generate_back_edges:
last_batch_size -= 1
A = np.zeros((E, 2), dtype=np.int64)
num_sequences = batch_size
last_num_sequences = last_batch_size
if (
generate_back_edges
): # in case of generating back edges we need to sample just E/2
last_num_sequences = last_batch_size // 2
num_sequences = batch_size // 2
new_back_edges = np.zeros(shape=(num_sequences, 2))
quadrant_sequence = np.zeros(shape=(num_sequences, n + m + l), dtype=dtype)
(
row_adders,
col_addres,
thetas_r,
thetas_c,
row_n,
col_n,
) = get_row_col_addres(thetas_n)
# generate sequences of quadrants from previously prepared samplers
batch_itr = range(batch_count)
if verbose:
batch_itr = tqdm(batch_itr)
for e in batch_itr:
for i in range(
n + m + l
): # each steps in generation has its own sampler
smpl = custom_samplers[i].rvs(size=num_sequences)
quadrant_sequence[:, i] = smpl
# produce new edges
new_edges = parse_quadrants(
quadrant_sequence,
thetas_n,
row_adders,
col_addres,
thetas_r,
thetas_c,
row_n,
col_n,
dtype=dtype,
)
if generate_back_edges:
new_back_edges[:, [0, 1]] = new_edges[:, [1, 0]] # swap columns
A[
e * batch_size: (e + 1) * batch_size: 2, :
] = new_edges # we need interleave so that back edges are "right after" normal edges
A[
e * batch_size + 1: (e + 1) * batch_size: 2, :
] = new_back_edges
else:
A[e * batch_size: (e + 1) * batch_size, :] = new_edges
# generate last batch
if last_batch_size > 0:
for i in range(n + m + l):
smpl = custom_samplers[i].rvs(size=last_num_sequences)
quadrant_sequence[:last_num_sequences, i] = smpl
new_edges = parse_quadrants(
quadrant_sequence[:last_num_sequences, :],
thetas_n,
row_adders,
col_addres,
thetas_r,
thetas_c,
row_n,
col_n,
dtype=dtype,
)
if generate_back_edges:
new_back_edges[:last_num_sequences, [0, 1]] = new_edges[
:last_num_sequences, [1, 0]
]
# we need interleave so that back edges are "right after" normal edges
A[
batch_count * batch_size: batch_count * batch_size
+ last_batch_size: 2,
:,
] = new_edges
# np.concatenate((new_edges,new_back_edges[:last_num_sequences,:]),axis=0)
A[
batch_count * batch_size
+ 1: batch_count * batch_size
+ last_batch_size: 2,
:,
] = new_back_edges[:last_num_sequences, :]
else:
A[
batch_count * batch_size: batch_count * batch_size
+ last_batch_size,
:,
] = new_edges
mtx_shape = (
np.prod([t.shape[0] for t in thetas_n]),
np.prod([t.shape[1] for t in thetas_n]),
) # shape of resulting adjacency matrix
return A, mtx_shape, custom_samplers
def effective_nonsquare_rmat_exact(
theta,
E,
A_shape,
noise_scaling=1.0,
batch_size=1000,
dtype=np.int64,
custom_samplers=None,
remove_selfloops=False,
generate_back_edges=False,
return_node_ids=0,
verbose=False,
):
""" This function generates list of edges using modified RMat approach based on effective_nonsuqare_rmat_approximate
Args:
theta (np.array): seeding matrix, needs to be shape 2x2
E (int): number of edges to be generated
A_shape (tuple): shape of resulting adjacency matrix. numbers has to be powers of 2
A_shape should be equal to (ceil(log2(X)),ceil(log2(Y))) X,Y are
dimensions of original adjacency
noise_scaling (float 0..1): noise scaling factor for good degree distribution
batch_size (int): edges are generated in batches of batch_size size
dtype (numpy dtype np.int32/np.int64): dtype of nodes id's
remove_selfloops (bool): If true edges n->n will not be generated. Note that for partite
graphs this makes no sense
generate_back_edges (bool): if True then generated edges will also have "back" edges. Not
that setting to True for partite graphs makes no sense.
Returns:
A (np.array 2 x E) - matrix containing in every row a signle edge. Edge is always directed
0'th column is FROM edge 1st is TO edge
mtx_shape (tuple) - shape of adjecency matrix (A contains list of edges, this is Adjecency
metrix shape)
custom_samplers (List[scipy.stats.rv_discrete]) - list of samplers needed to generate edges
from the same disctribution for multiple runs of the function
Description:
see effective_nonsuqare_rmat_approximate
"""
heuristics = 1.5
if verbose:
print("Getting egdes")
A, mtx_shape, cs = effective_nonsquare_rmat_approximate(
theta,
int(heuristics * E),
A_shape,
noise_scaling=noise_scaling,
batch_size=batch_size,
dtype=dtype,
custom_samplers=custom_samplers,
generate_back_edges=generate_back_edges,
verbose=verbose,
)
if generate_back_edges:
A = A[
np.sort(np.unique(A, return_index=True, axis=0)[1])
] # permutation is not needed here
else:
if verbose:
print("Getting unique egdes")
A = np.unique(A, axis=0)
if verbose:
print("Permuting edges")
perm = np.random.permutation(
A.shape[0]
) # we need to permute it as othervise unique returns edges in order
A = A[perm]
if remove_selfloops:
if verbose:
print("Removing selfloops")
A = np.delete(A, np.where(A[:, 0] == A[:, 1]), axis=0)
E_already_generated = A.shape[0]
if E_already_generated >= E:
if return_node_ids == 2:
return A[:E, :], np.unique(A[:E, :][:, 0]), np.unique(A[:E, :][:, 1]), mtx_shape, cs
if return_node_ids == 1:
return A[:E, :], np.unique(A[:E, :]), mtx_shape, cs
return A[:E, :], mtx_shape, cs
else:
while E_already_generated < E:
if verbose:
print("Generating some additional edges")
E_to_generate = int(heuristics * (E - E_already_generated))
A_next, mtx_shape, cs = effective_nonsquare_rmat_approximate(
theta,
E_to_generate,
A_shape,
noise_scaling=noise_scaling,
batch_size=batch_size,
dtype=dtype,
custom_samplers=cs,
verbose=verbose,
)
if remove_selfloops:
A_next = np.delete(
A_next, np.where(A_next[:, 0] == A_next[:, 1]), axis=0
)
A = np.concatenate((A, A_next), axis=0)
if generate_back_edges:
A = A[np.sort(np.unique(A, return_index=True, axis=0)[1])]
else:
A = np.unique(A, axis=0)
perm = np.random.permutation(A.shape[0])
A = A[perm]
E_already_generated = A.shape[0]
if return_node_ids == 2:
return A[:E, :], np.unique(A[:E, :][:, 0]), np.unique(A[:E, :][:, 1]), mtx_shape, cs
if return_node_ids == 1:
return A[:E, :], np.unique(A[:E, :]), mtx_shape, cs
return A[:E, :], mtx_shape, cs
def cupy_unique_axis0(array):
# https://stackoverflow.com/questions/58662085/is-there-a-cupy-version-supporting-axis-option-in-cupy-unique-function-any
sortarr = array[cp.lexsort(array.T[::-1])]
mask = cp.empty(array.shape[0], dtype=cp.bool_)
mask[0] = True
mask[1:] = cp.any(sortarr[1:] != sortarr[:-1], axis=1)
return sortarr[mask]
def unique_axis0(ar: NDArray) -> NDArray:
"""
Uniform way of calling operator.unique(ar, axis=0).
axis != None is not supported in cupy yet.
This function provides a workaround for one of the cases.
"""
operator = infer_operator(ar)
if operator == cp:
return cupy_unique_axis0(ar)
else:
return np.unique(ar, axis=0)
def generate_gpu_rmat(
a,
b,
c,
d,
r_scale,
c_scale,
n_edges,
noise=0.5,
is_directed=False,
has_self_loop=False,
return_node_ids=0,
):
if not is_directed and r_scale != c_scale:
raise ValueError('undirected generation works only for square adj matrix')
if not is_directed:
n_edges = n_edges // 2
gen_graph = None
HEURISTIC = 1.2
edges_to_generate = int(HEURISTIC * n_edges)
theta_len = max(r_scale, c_scale)
base_theta = [a, b, c, d]
if noise > 0:
full_theta = []
for i in range(theta_len):
noise_uniform = noise * np.random.uniform(
-1, 1, size=len(base_theta)
)
noise_to_add = np.multiply(base_theta, noise_uniform)
theta_n = base_theta + noise_to_add
theta_n = theta_n / np.sum(theta_n)
full_theta.append(theta_n)
else:
full_theta = base_theta * theta_len
theta_cpu = np.array(full_theta, dtype=np.float32)
theta = cp.asarray(theta_cpu)
while gen_graph is None or gen_graph.shape[0] < n_edges:
tmp = cp.empty((edges_to_generate, 2), dtype=cp.int32)
seed = cp.random.randint(0, high=1_000_000, size=None, dtype=int)
rmat(tmp, theta, r_scale, c_scale, seed=seed)
# Remove self loops
if not has_self_loop:
tmp = tmp[tmp[:, 0] != tmp[:, 1]]
# Keep only one sided edges
if not is_directed:
tmp = tmp[tmp[:, 0] <= tmp[:, 1]]
if gen_graph is None:
# Remove duplicates
gen_graph = cupy_unique_axis0(tmp)
else:
gen_graph = cp.concatenate((gen_graph, tmp), axis=0)
# Remove duplicates
gen_graph = cupy_unique_axis0(gen_graph)
gen_graph = gen_graph[:n_edges]
if not is_directed:
gen_graph_backward = cp.empty((n_edges, 2), dtype=cp.int32)
gen_graph_backward[:, 0] = gen_graph[:, 1]
gen_graph_backward[:, 1] = gen_graph[:, 0]
gen_graph = cp.concatenate((gen_graph, gen_graph_backward), axis=0)
gen_graph = cupy_unique_axis0(
gen_graph
)
if not has_self_loop:
gen_graph = gen_graph[gen_graph[:, 0] != gen_graph[:, 1]]
if return_node_ids == 2:
return cp.asnumpy(gen_graph), cp.asnumpy(cp.unique(gen_graph[:, 0])), cp.asnumpy(cp.unique(gen_graph[:, 1]))
if return_node_ids == 1:
return cp.asnumpy(gen_graph), cp.asnumpy(cp.unique(gen_graph))
return cp.asnumpy(gen_graph)
def generate_theta(base_theta, noise, theta_len, is_directed):
if noise > 0:
full_theta = []
for i in range(theta_len):
noise_uniform = noise * np.random.uniform(
-1, 1, size=len(base_theta)
)
noise_to_add = np.multiply(base_theta, noise_uniform)
theta_n = base_theta + noise_to_add
if not is_directed:
theta_n[2] = theta_n[1]
theta_n = theta_n / np.sum(theta_n)
full_theta.append(theta_n)
else:
full_theta = [base_theta] * theta_len
return full_theta
def prepare_chunks(full_theta, r_scale, c_scale, gpu_bytes_to_use, edges_to_generate):
if r_scale > 32 or c_scale > 32:
bytes_per_edge = 8
max_id = 9223372036854775807 # int64 max
else:
bytes_per_edge = 4
max_id = 2147483647 # int32 max
bytes_to_generate = edges_to_generate * 2 * bytes_per_edge
skip_theta = 0
# approximation
while (bytes_to_generate >> 2 * skip_theta) > gpu_bytes_to_use \
or (bytes_to_generate >> 2 * skip_theta) > max_id:
skip_theta += 1
if skip_theta == 0:
return [], np.array([edges_to_generate]), full_theta, 0, r_scale, c_scale
# chunk size is limited by the smaller side of the rectangular graph
while abs(r_scale - c_scale) > skip_theta:
skip_theta += 1
def repeat(a, scale):
if scale == 1:
return a
return np.repeat(np.repeat(a, scale, axis=0), scale, axis=1)
def tile(a, scale):
if scale == 1:
return a
return np.tile(a, (scale, scale))
def prepare_prefixes(skip_theta):
if skip_theta > 0:
prefix_theta = full_theta[:skip_theta]
gen_theta_len = max(r_scale, c_scale) - skip_theta
prefix_edges = np.ones((1 << skip_theta, 1 << skip_theta), dtype=np.float64)
prefixes = np.zeros((2, 1 << skip_theta, 1 << skip_theta), dtype=np.int32)
for theta_idx, theta in enumerate(prefix_theta):
pref_src = np.array([[0, 0], [1, 1]]) << theta_idx
pref_dst = np.array([[0, 1], [0, 1]]) << theta_idx
theta = np.array(theta, dtype=np.float64).reshape(2, 2)
repeat_scale = 1 << (skip_theta - theta_idx - 1)
tile_scale = 1 << theta_idx
prefix_edges = prefix_edges * tile(repeat(theta, repeat_scale), tile_scale)
prefixes[0] = prefixes[0] + tile(repeat(pref_src, repeat_scale), tile_scale)
prefixes[1] = prefixes[1] + tile(repeat(pref_dst, repeat_scale), tile_scale)
if r_scale != c_scale: # probabilities in the rectangular matrix should sum up to 1.0
r_len = 2 ** (r_scale - gen_theta_len)
c_len = 2 ** (c_scale - gen_theta_len)
prefix_edges[:r_len, :c_len] = prefix_edges[:r_len, :c_len] / prefix_edges[:r_len, :c_len].sum()
prefixes[int(r_scale > c_scale), :r_len, :c_len] = \
prefixes[int(r_scale > c_scale), :r_len, :c_len] >> abs(r_scale - c_scale)
prefix_edges = np.ceil(prefix_edges * edges_to_generate).astype(np.int32).reshape(-1)
prefixes = prefixes.reshape(2, -1)
else:
prefixes = []
prefix_edges = np.array([edges_to_generate])
return prefixes, prefix_edges
prefixes, prefix_edges = prepare_prefixes(skip_theta)
while prefix_edges.max() * 2 * bytes_per_edge > gpu_bytes_to_use:
skip_theta += 1
prefixes, prefix_edges = prepare_prefixes(skip_theta)
generation_theta = full_theta[skip_theta:]
return prefixes, prefix_edges, generation_theta, skip_theta, len(generation_theta), len(generation_theta)
def _generate_gpu_chunk_rmat(
chunk_info,
prefixes,
prefix_edges,
has_self_loop,
is_directed,
generation_theta,
r_log2_nodes,
c_log2_nodes,
r_pref_len,
c_pref_len,
row_len,
gpus,
dtype='int32',
return_node_ids=0,
memmap_kwargs: Optional = None,
chunk_save_path_format: Optional[str] = None):
chunk_id, chunk_end = chunk_info
chunk_size = prefix_edges[chunk_id]
if gpus > 1:
gpu_id = int(multiprocessing.current_process()._identity[0]) % gpus
else:
gpu_id = 0
theta_cpu = np.array(generation_theta, dtype=np.float32)
edge_list = None
is_diagonal_chunk = ((chunk_id // row_len) == (chunk_id % row_len))
use_memmap = memmap_kwargs is not None
if use_memmap:
memmap_outfile = np.load(file=memmap_kwargs['filename'], mmap_mode='r+')
with cp.cuda.Device(gpu_id):
theta = cp.asarray(theta_cpu)
while edge_list is None or edge_list.shape[0] < prefix_edges[chunk_id]:
tmp = cp.empty((prefix_edges[chunk_id], 2), dtype=dtype)
seed = cp.random.randint(0, high=1_000_000, size=None, dtype=int)
rmat(tmp, theta, r_log2_nodes, c_log2_nodes, seed=seed)
if not has_self_loop and is_diagonal_chunk:
tmp = tmp[tmp[:, 0] != tmp[:, 1]]
# undirected diagonal_case
if not is_directed and is_diagonal_chunk:
tmp = tmp[tmp[:, 0] <= tmp[:, 1]]
tmp = cupy_unique_axis0(tmp)
if edge_list is None:
edge_list = tmp
else:
edge_list = cp.concatenate((edge_list, tmp), axis=0)
del tmp
edge_list = cupy_unique_axis0(edge_list)
if len(prefix_edges) > 1:
edge_list[:, 0] = (edge_list[:, 0] << r_pref_len) + prefixes[0][chunk_id]
edge_list[:, 1] = (edge_list[:, 1] << c_pref_len) + prefixes[1][chunk_id]
edge_list = edge_list[:prefix_edges[chunk_id]]
if return_node_ids == 2:
src_nodes_ids = cp.asnumpy(cp.unique(edge_list[:, 0]))
dst_nodes_ids = cp.asnumpy(cp.unique(edge_list[:, 1]))
if return_node_ids == 1:
nodes_ids = cp.asnumpy(cp.unique(edge_list))
result = cp.asnumpy(edge_list)
if use_memmap:
memmap_outfile[chunk_end-chunk_size:chunk_end] = result
del edge_list
if chunk_save_path_format is not None:
dump_generated_graph(chunk_save_path_format.format(chunk_id=chunk_id), result)
result = len(result)
if use_memmap:
result = None
if return_node_ids == 2:
return result, src_nodes_ids, dst_nodes_ids
if return_node_ids == 1:
return result, nodes_ids
return result
def generate_gpu_chunked_rmat(
a,
b,
c,
d,
r_scale,
c_scale,
n_edges,
noise=0.5,
is_directed=False,
has_self_loop=False,
gpus=None,
return_node_ids=0,
save_path: Optional[str] = None,
verbose: bool = False,
):
if not is_directed and r_scale != c_scale:
raise ValueError('undirected generation works only for square adj matrix')
base_theta = [a, b, c, d]
theta_len = max(r_scale, c_scale)
full_theta = generate_theta(base_theta, noise, theta_len, is_directed)
if gpus is None:
gpus = MemoryManager().get_available_gpus()
gpu_bytes_to_use = MemoryManager().get_min_available_across_gpus_memory(gpus=gpus)
gpu_bytes_to_use = math.floor(gpu_bytes_to_use * 0.10)
prefixes, prefix_edges, generation_theta, prefix_len, r_log2_nodes, c_log2_nodes = \
prepare_chunks(full_theta, r_scale, c_scale, gpu_bytes_to_use, n_edges)
chunk_ids = list(range(len(prefix_edges)))
row_len = 1 << prefix_len
r_pref_len = r_scale - len(generation_theta)
c_pref_len = c_scale - len(generation_theta)
if not is_directed: # generate a triangular adj matrix
chunk_ids = [i for i in chunk_ids if (i // row_len) <= (i % row_len)]
# reduce the diagonal chunks
for i in range(prefix_len * 2):
prefix_edges[i * row_len + i] = prefix_edges[i * row_len + i] // 2
if r_scale != c_scale:
chunk_ids = [i for i in chunk_ids if (i // row_len) < 2 ** r_pref_len and (i % row_len) < 2 ** c_pref_len]
is_single_chunk = len(chunk_ids) == 1
memmap_kwargs = None
chunk_save_path_format = None
use_memmap = False
if save_path and os.path.isdir(save_path):
chunk_save_path_format = os.path.join(save_path, 'chunk_{chunk_id}.npy')
elif save_path and save_path.endswith('.npy'):
use_memmap = True
memmap_shape = (sum(prefix_edges[chunk_ids]), 2)
memmap_dtype = np.uint64 if theta_len > 32 else np.uint32
memmap_kwargs = dict(
filename=save_path,
)
memmap_outfile = np.lib.format.open_memmap(save_path, dtype=memmap_dtype, shape=memmap_shape, mode='w+')
dtype = cp.int64 if theta_len > 32 else cp.int32
_generate_gpu_chunk_rmat_p = partial(
_generate_gpu_chunk_rmat,
prefixes=prefixes,
prefix_edges=prefix_edges,
has_self_loop=has_self_loop,
is_directed=is_directed,
generation_theta=generation_theta,
r_log2_nodes=r_log2_nodes,
c_log2_nodes=c_log2_nodes,
r_pref_len=r_pref_len,
c_pref_len=c_pref_len,
row_len=row_len,
dtype=dtype,
return_node_ids=return_node_ids,
chunk_save_path_format=chunk_save_path_format,
memmap_kwargs=memmap_kwargs,
gpus=1 if is_single_chunk else gpus,
)
if is_single_chunk:
chunk_res = _generate_gpu_chunk_rmat_p((chunk_ids[0], prefix_edges[chunk_ids[0]]))
if return_node_ids == 2:
result, src_node_ids, dst_node_ids = chunk_res
elif return_node_ids == 1:
result, node_ids = chunk_res
else:
result = chunk_res
if use_memmap:
result = memmap_outfile
else:
multiprocessing.set_start_method('spawn', force=True)
sub_res_lists = []
if return_node_ids == 2:
src_node_ids_presence = np.full(2**r_scale, False)
dst_node_ids_presence = np.full(2**c_scale, False)
elif return_node_ids == 1:
node_ids_presence = np.full(2**theta_len, False)
with multiprocessing.Pool(processes=gpus) as pool:
chunk_res = pool.imap_unordered(_generate_gpu_chunk_rmat_p,
zip(chunk_ids, np.cumsum(prefix_edges[chunk_ids])),
chunksize=(len(chunk_ids)+gpus-1) // gpus )
if verbose:
chunk_res = tqdm(chunk_res, total=len(chunk_ids))
if return_node_ids == 2:
for res, src_n_ids, dst_n_ids in chunk_res:
sub_res_lists.append(res)
src_node_ids_presence[src_n_ids] = True
dst_node_ids_presence[dst_n_ids] = True
elif return_node_ids == 1:
for res, n_ids in chunk_res:
sub_res_lists.append(res)
node_ids_presence[n_ids] = True
else:
sub_res_lists = list(chunk_res)
if use_memmap:
result = memmap_outfile
elif chunk_save_path_format is None:
result = np.concatenate(sub_res_lists)
else:
result = int(np.sum(sub_res_lists))
if return_node_ids == 2:
src_node_ids, = np.where(src_node_ids_presence)
dst_node_ids, = np.where(dst_node_ids_presence)
elif return_node_ids == 1:
node_ids, = np.where(node_ids_presence)
if return_node_ids == 2:
return result, src_node_ids, dst_node_ids
if return_node_ids == 1:
return result, node_ids
return result
def get_degree_distribution(vertices, gpu=False, operator=None):
operator = operator or (cp if gpu else np)
_, degrees = operator.unique(vertices, return_counts=True)
degree_values, degree_counts = operator.unique(degrees, return_counts=True)
return degree_values, degree_counts
class BaseLogger:
""" Base logger class
Args:
logdir (str): path to the logging directory
"""
def __init__(self, logdir: str = "tmp"):
self.logdir = logdir
os.makedirs(self.logdir, exist_ok=True)
currentDateAndTime = datetime.now()
self.logname = (
f'{currentDateAndTime.strftime("%Y_%m_%d_%H_%M_%S")}.txt'
)
self.logpath = os.path.join(self.logdir, self.logname)
self.setup_logger()
self.log("Initialized logger")
def setup_logger(self):
""" This function setups logger """
logging.basicConfig(
filename=self.logpath,
filemode="a",
format="%(asctime)s| %(message)s",
datefmt="%Y/%m/%d %H:%M:%S",
level=logging.DEBUG,
)
def log(self, msg: str):
""" This function logs messages in debug mode
Args:
msg (str): message to be printed
"""
logging.debug(msg)
def _reshuffle(X: NDArray, mask: NDArray, max_node_id: int) -> None:
"""
Shuffles dst nodes of edges specified by idx.
Preserves degree distribution and keeps edge list sorted.
"""
operator = infer_operator(X)
if not operator.any(mask):
return
target = X[mask, 1]
operator.random.shuffle(target)
X[mask, 1] = target
src_node_mask = operator.zeros(max_node_id + 1, dtype=operator.bool_)
src_node_mask[X[mask, 0]] = True
to_sort_mask = operator.zeros(X.shape[0], dtype=operator.bool_)
to_sort_mask = src_node_mask[X[:, 0]]
to_sort = X[to_sort_mask]
to_sort = to_sort[operator.lexsort(to_sort.T[::-1])]
X[to_sort_mask] = to_sort
def _find_correct_edges(
X: NDArray,
self_loops: bool = False,
assume_sorted: bool = False,
) -> Tuple[NDArray, NDArray]:
""" Finds duplicates and self loops in an edge list. """
operator = infer_operator(X)
if not assume_sorted:
X = X[operator.lexsort(X.T[::-1])]
mask = operator.empty(X.shape[0], dtype=operator.bool_)
mask[0] = True
mask[1:] = operator.any(X[1:] != X[:-1], axis=1)
if not self_loops:
mask &= X[:, 0] != X[:, 1]
return X, mask
def postprocess_edge_list(X: NDArray, n_reshuffle: int = 0, self_loops: bool = False) -> NDArray:
"""
Removes multi-edges and (optionally) self-loops.
If n_reshuffle > 0 is specified, edges are shuffled between nodes
so that the degree distribution is preserved and less edges will be removed.
Assumes node set is reindexed from min_id > 0 to max_id ~ N.
"""
max_node_id = X.max().item()
X, mask = _find_correct_edges(X, self_loops=self_loops)
for _ in range(n_reshuffle):
_reshuffle(X, ~mask, max_node_id)
X, mask = _find_correct_edges(X, self_loops=self_loops, assume_sorted=True)
return X[mask]
|
PyTorch/SpeechSynthesis/Tacotron2/trtis_cpp/trtis_client | trtis_client | CMakeLists | cmake_minimum_required(VERSION 3.0 FATAL_ERROR)
project(tacotron2_inference)
if (DEFINED DEVEL AND NOT DEVEL EQUAL 0)
if ("${CMAKE_CXX_COMPILER_ID}" MATCHES "GNU")
# g++ warnings
set(CPP_DEVEL_FLAGS "${CPP_DEVEL_FLAGS} -Wall")
set(CPP_DEVEL_FLAGS "${CPP_DEVEL_FLAGS} -Werror")
set(CPP_DEVEL_FLAGS "${CPP_DEVEL_FLAGS} -Wpedantic")
set(CPP_DEVEL_FLAGS "${CPP_DEVEL_FLAGS} -Weffc++")
set(CPP_DEVEL_FLAGS "${CPP_DEVEL_FLAGS} -Wextra")
set(CPP_DEVEL_FLAGS "${CPP_DEVEL_FLAGS} -DDEVEL=1")
# nvcc warnings
set(CUDA_DEVEL_FLAGS "${CUDA_DEVEL_FLAGS} -Xcompiler -Wall")
set(CUDA_DEVEL_FLAGS "${CUDA_DEVEL_FLAGS} -Xcompiler -Werror")
set(CUDA_DEVEL_FLAGS "${CUDA_DEVEL_FLAGS} -Xcompiler -Weffc++")
set(CUDA_DEVEL_FLAGS "${CUDA_DEVEL_FLAGS} -Xcompiler -Wextra")
set(CUDA_DEVEL_FLAGS "${CUDA_DEVEL_FLAGS} -Xcompiler -DDEVEL=1")
endif()
endif()
set(CMAKE_CXX_FLAGS_DEBUG "-g -O0")
set(CMAKE_CXX_STANDARD 14)
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -fPIC")
separate_arguments(CPP_DEVEL_FLAGS)
separate_arguments(CUDA_DEVEL_FLAGS)
add_subdirectory("src")
|
PyTorch/Recommendation/DLRM/preproc | preproc | parquet_to_binary | # Copyright (c) 2021 NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import numpy as np
import pandas as pd
import os
from joblib import Parallel, delayed
import glob
import argparse
import tqdm
import subprocess
def process_file(f, dst):
label = '_c0'
dense_columns = [f'_c{i}' for i in range(1, 14)]
categorical_columns = [f'_c{i}' for i in range(14, 40)]
all_columns_sorted = [f'_c{i}' for i in range(0, 40)]
data = pd.read_parquet(f)
data = data[all_columns_sorted]
data[label] = data[label].astype(np.int32)
data[dense_columns] = data[dense_columns].astype(np.float32)
data[categorical_columns] = data[categorical_columns].astype(np.int32)
data = data.to_records(index=False)
data = data.tobytes()
dst_file = dst + '/' + f.split('/')[-1] + '.bin'
with open(dst_file, 'wb') as dst_fd:
dst_fd.write(data)
def main():
parser = argparse.ArgumentParser()
parser.add_argument('--src_dir', type=str)
parser.add_argument('--intermediate_dir', type=str)
parser.add_argument('--dst_dir', type=str)
parser.add_argument('--parallel_jobs', default=40, type=int)
args = parser.parse_args()
print('Processing train files...')
train_src_files = glob.glob(args.src_dir + '/train/*.parquet')
train_intermediate_dir = os.path.join(args.intermediate_dir, 'train')
os.makedirs(train_intermediate_dir, exist_ok=True)
Parallel(n_jobs=args.parallel_jobs)(delayed(process_file)(f, train_intermediate_dir) for f in tqdm.tqdm(train_src_files))
print('Train files conversion done')
print('Processing test files...')
test_src_files = glob.glob(args.src_dir + '/test/*.parquet')
test_intermediate_dir = os.path.join(args.intermediate_dir, 'test')
os.makedirs(test_intermediate_dir, exist_ok=True)
Parallel(n_jobs=args.parallel_jobs)(delayed(process_file)(f, test_intermediate_dir) for f in tqdm.tqdm(test_src_files))
print('Test files conversion done')
print('Processing validation files...')
valid_src_files = glob.glob(args.src_dir + '/validation/*.parquet')
valid_intermediate_dir = os.path.join(args.intermediate_dir, 'validation')
os.makedirs(valid_intermediate_dir, exist_ok=True)
Parallel(n_jobs=args.parallel_jobs)(delayed(process_file)(f, valid_intermediate_dir) for f in tqdm.tqdm(valid_src_files))
print('Validation files conversion done')
os.makedirs(args.dst_dir, exist_ok=True)
print('Concatenating train files')
os.system(f'cat {train_intermediate_dir}/*.bin > {args.dst_dir}/train_data.bin')
print('Concatenating test files')
os.system(f'cat {test_intermediate_dir}/*.bin > {args.dst_dir}/test_data.bin')
print('Concatenating validation files')
os.system(f'cat {valid_intermediate_dir}/*.bin > {args.dst_dir}/validation_data.bin')
print('Done')
if __name__ == '__main__':
main()
|
PyTorch/Segmentation/MaskRCNN/pytorch/configs/pascal_voc | pascal_voc | e2e_faster_rcnn_R_50_C4_1x_1_gpu_voc | MODEL:
META_ARCHITECTURE: "GeneralizedRCNN"
WEIGHT: "catalog://ImageNetPretrained/MSRA/R-50"
RPN:
PRE_NMS_TOP_N_TEST: 6000
POST_NMS_TOP_N_TEST: 300
ANCHOR_SIZES: (128, 256, 512)
ROI_BOX_HEAD:
NUM_CLASSES: 21
DATASETS:
TRAIN: ("voc_2007_train", "voc_2007_val")
TEST: ("voc_2007_test",)
SOLVER:
BASE_LR: 0.001
WEIGHT_DECAY: 0.0001
STEPS: (50000, )
MAX_ITER: 70000
IMS_PER_BATCH: 1
TEST:
IMS_PER_BATCH: 1
|
TensorFlow2/Recommendation/WideAndDeep/triton/deployment_toolkit/triton_performance_runner/perf_analyzer | perf_analyzer | perf_config | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
from typing import Any
from .exceptions import PerfAnalyzerException
class PerfAnalyzerConfig:
"""
A config class to set arguments to the perf_analyzer.
An argument set to None will use the perf_analyzer's default.
"""
perf_analyzer_args = [
"async",
"sync",
"measurement-interval",
"measurement-mode",
"measurement-request-count",
"concurrency-range",
"request-rate-range",
"request-distribution",
"request-intervals",
"binary-search",
"num-of-sequence",
"latency-threshold",
"max-threads",
"stability-percentage",
"max-trials",
"percentile",
"input-data",
"shared-memory",
"output-shared-memory-size",
"sequence-length",
"string-length",
"string-data",
]
perf_analyzer_multiple_args = [
"shape",
]
input_to_options = [
"model-name",
"model-version",
"batch-size",
"url",
"protocol",
"latency-report-file",
"streaming",
]
input_to_verbose = ["verbose", "extra-verbose"]
def __init__(self):
"""
Construct a PerfAnalyzerConfig
"""
self._args = {k: None for k in self.perf_analyzer_args}
self._multiple_args = {k: [] for k in self.perf_analyzer_multiple_args}
self._options = {
"-m": None,
"-x": None,
"-b": None,
"-u": None,
"-i": None,
"-f": None,
"-H": None,
"-c": None,
"-t": None,
}
self._verbose = {"-v": None, "-v -v": None}
self._input_to_options = {
"model-name": "-m",
"model-version": "-x",
"batch-size": "-b",
"url": "-u",
"protocol": "-i",
"latency-report-file": "-f",
"streaming": "-H",
"concurrency": "-c",
"threads": "-t",
}
self._input_to_verbose = {"verbose": "-v", "extra-verbose": "-v -v"}
@classmethod
def allowed_keys(cls):
"""
Returns
-------
list of str
The keys that are allowed to be
passed into perf_analyzer
"""
return (
list(cls.perf_analyzer_args)
+ list(cls.perf_analyzer_multiple_args)
+ list(cls.input_to_options)
+ list(cls.input_to_verbose)
)
def update_config(self, params=None):
"""
Allows setting values from a
params dict
Parameters
----------
params: dict
keys are allowed args to perf_analyzer
"""
if params:
for key in params:
self[key] = params[key]
def to_cli_string(self):
"""
Utility function to convert a config into a
string of arguments to the perf_analyzer with CLI.
Returns
-------
str
cli command string consisting of all arguments
to the perf_analyzer set in the config, without
the executable name.
"""
# single dashed options, then verbose flags, then main args
args = [f"{k} {v}" for k, v in self._options.items() if v]
args += [k for k, v in self._verbose.items() if v]
args += [f"--{k}={v}" for k, v in self._args.items() if v]
for k, v in self._multiple_args.items():
for item in v:
args.append(f"--{k}={item}")
return " ".join(args)
def __getitem__(self, key: str):
"""
Gets an arguments value in config
Parameters
----------
key : str
The name of the argument to the perf_analyzer
Returns
-------
The value that the argument is set to in this config
Raises
------
TritonModelAnalyzerException
If argument not found in the config
"""
if key in self._args:
return self._args[key]
elif key in self._multiple_args:
return self._multiple_args[key]
elif key in self._input_to_options:
return self._options[self._input_to_options[key]]
elif key in self._input_to_verbose:
return self._verbose[self._input_to_verbose[key]]
else:
raise PerfAnalyzerException(f"'{key}' Key not found in config")
def __setitem__(self, key: str, value: Any):
"""
Sets an arguments value in config
after checking if defined/supported.
Parameters
----------
key : str
The name of the argument to the perf_analyzer
value : (any)
The value to which the argument is being set
Raises
------
TritonModelAnalyzerException
If key is unsupported or undefined in the
config class
"""
if key in self._args:
self._args[key] = value
elif key in self._multiple_args:
self._multiple_args[key].append(value)
elif key in self._input_to_options:
self._options[self._input_to_options[key]] = value
elif key in self._input_to_verbose:
self._verbose[self._input_to_verbose[key]] = value
else:
raise PerfAnalyzerException(
f"The argument '{key}' to the perf_analyzer " "is not supported by the model analyzer."
)
|
TensorFlow2/Recommendation/DLRM_and_DCNv2/deployment/tf | tf | __init__ | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
# author: Tomasz Grel (tgrel@nvidia.com)
#from constants import dense_model_name, hps_model_name
from deployment.tf.deploy_dense import deploy_dense
from deployment.tf.deploy_ensemble import deploy_ensemble
from deployment.tf.deploy_sparse import deploy_sparse
from deployment.tf.deploy_monolithic import deploy_monolithic
|
TensorFlow2/Recommendation/WideAndDeep/data/outbrain | outbrain | dataloader | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import cupy
import horovod.tensorflow as hvd
import tensorflow as tf
from nvtabular.loader.tensorflow import KerasSequenceLoader
from data.outbrain.defaults import LABEL_CHANNEL, MAP_FEATURE_CHANNEL, NUMERICAL_CHANNEL, ONEHOT_CHANNEL, \
MULTIHOT_CHANNEL
cupy.random.seed(None)
def seed_fn():
min_int, max_int = tf.int32.limits
max_rand = max_int // hvd.size()
# Generate a seed fragment on each worker
seed_fragment = cupy.random.randint(0, max_rand).get()
# Aggregate seed fragments from all Horovod workers
seed_tensor = tf.constant(seed_fragment)
reduced_seed = hvd.allreduce(seed_tensor, name="shuffle_seed", op=hvd.mpi_ops.Sum)
return reduced_seed % max_rand
def get_dataset(feature_spec, mapping, batch_size, buffer_size=0.1, parts_per_chunk=1,
map_channel_enabled=False, shuffle=True):
data_paths = feature_spec.get_paths_by_mapping(mapping)
label_names = feature_spec.get_names_by_channel(LABEL_CHANNEL)
cat_names = feature_spec.get_names_by_channel(ONEHOT_CHANNEL) + feature_spec.get_names_by_channel(MULTIHOT_CHANNEL)
cont_names = feature_spec.get_names_by_channel(NUMERICAL_CHANNEL)
if map_channel_enabled:
cat_names += feature_spec.get_names_by_channel(MAP_FEATURE_CHANNEL)
tf_dataset = KerasSequenceLoader(
data_paths,
batch_size=batch_size,
label_names=label_names,
cat_names=cat_names,
cont_names=cont_names,
engine="parquet",
shuffle=shuffle,
buffer_size=buffer_size,
parts_per_chunk=parts_per_chunk,
global_size=hvd.size(),
global_rank=hvd.rank(),
seed_fn=seed_fn,
)
return tf_dataset
def make_padding_function(multihot_hotness_dict):
@tf.function(experimental_relax_shapes=True)
def pad_batch(batch):
batch = batch.copy()
for feature, hotness in multihot_hotness_dict.items():
multihot_tuple = batch[feature]
values = multihot_tuple[0][:, 0]
row_lengths = multihot_tuple[1][:, 0]
padded = tf.RaggedTensor.from_row_lengths(
values, row_lengths, validate=False
).to_tensor(default_value=-1, shape=[None, hotness])
batch[feature] = padded
return batch
return pad_batch
|
TensorFlow2/Recommendation/WideAndDeep/triton/deployment_toolkit/triton_performance_runner | triton_performance_runner | runner | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# method from PEP-366 to support relative import in executed modules
import logging
import pathlib
from typing import List, Optional
if __package__ is None:
__package__ = pathlib.Path(__file__).parent.name
from ..core import EvaluationMode, MeasurementMode, OfflineMode, PerformanceTool
from .model_analyzer import ModelAnalyzerRunner
from .perf_analyzer import PerfAnalyzerRunner, PerfAnalyzerWarmupRunner
LOGGER = logging.getLogger("triton_performance_runner")
class TritonPerformanceRunner:
def __init__(
self,
server_url: str,
model_name: str,
input_data: str,
input_shapes: List[str],
batch_sizes: List[int],
concurrency: List[int],
measurement_mode: MeasurementMode,
measurement_interval: int,
measurement_request_count: int,
evaluation_mode: EvaluationMode,
offline_mode: OfflineMode,
output_shared_memory_size: int,
performance_tool: PerformanceTool,
model_repository: str,
result_path: pathlib.Path,
warmup: bool,
timeout: Optional[int],
verbose: bool,
):
self._warmup_runner = None
if warmup:
LOGGER.info("Running warmup before the main test")
self._warmup_runner = PerfAnalyzerWarmupRunner(
server_url=server_url,
model_name=model_name,
input_data=input_data,
input_shapes=input_shapes,
batch_sizes=batch_sizes,
concurrency=concurrency,
measurement_mode=measurement_mode,
measurement_interval=measurement_interval,
measurement_request_count=measurement_request_count,
evaluation_mode=evaluation_mode,
offline_mode=offline_mode,
output_shared_memory_size=output_shared_memory_size,
timeout=timeout,
)
if performance_tool == PerformanceTool.MODEL_ANALYZER:
LOGGER.info("Using Model Analyzer for performance evaluation")
self._runner = ModelAnalyzerRunner(
server_url=server_url,
model_name=model_name,
input_data=input_data,
input_shapes=input_shapes,
batch_sizes=batch_sizes,
concurrency=concurrency,
measurement_mode=measurement_mode,
measurement_interval=measurement_interval,
measurement_request_count=measurement_request_count,
evaluation_mode=evaluation_mode,
offline_mode=offline_mode,
output_shared_memory_size=output_shared_memory_size,
model_repository=model_repository,
result_path=result_path,
timeout=timeout,
verbose=verbose,
)
elif performance_tool == PerformanceTool.PERF_ANALYZER:
LOGGER.info("Using Perf Analyzer for performance evaluation")
self._runner = PerfAnalyzerRunner(
server_url=server_url,
model_name=model_name,
input_data=input_data,
input_shapes=input_shapes,
batch_sizes=batch_sizes,
measurement_mode=measurement_mode,
measurement_interval=measurement_interval,
measurement_request_count=measurement_request_count,
concurrency=concurrency,
evaluation_mode=evaluation_mode,
offline_mode=offline_mode,
output_shared_memory_size=output_shared_memory_size,
result_path=result_path,
timeout=timeout,
verbose=verbose,
)
else:
raise ValueError(f"Unsupported performance tool {performance_tool}")
def run(self):
if self._warmup_runner:
self._warmup_runner.run()
self._runner.run()
|
TensorFlow/Detection/SSD/models/research/slim/nets | nets | cyclegan_test | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Tests for tensorflow.contrib.slim.nets.cyclegan."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import tensorflow as tf
from nets import cyclegan
# TODO(joelshor): Add a test to check generator endpoints.
class CycleganTest(tf.test.TestCase):
def test_generator_inference(self):
"""Check one inference step."""
img_batch = tf.zeros([2, 32, 32, 3])
model_output, _ = cyclegan.cyclegan_generator_resnet(img_batch)
with self.test_session() as sess:
sess.run(tf.global_variables_initializer())
sess.run(model_output)
def _test_generator_graph_helper(self, shape):
"""Check that generator can take small and non-square inputs."""
output_imgs, _ = cyclegan.cyclegan_generator_resnet(tf.ones(shape))
self.assertAllEqual(shape, output_imgs.shape.as_list())
def test_generator_graph_small(self):
self._test_generator_graph_helper([4, 32, 32, 3])
def test_generator_graph_medium(self):
self._test_generator_graph_helper([3, 128, 128, 3])
def test_generator_graph_nonsquare(self):
self._test_generator_graph_helper([2, 80, 400, 3])
def test_generator_unknown_batch_dim(self):
"""Check that generator can take unknown batch dimension inputs."""
img = tf.placeholder(tf.float32, shape=[None, 32, None, 3])
output_imgs, _ = cyclegan.cyclegan_generator_resnet(img)
self.assertAllEqual([None, 32, None, 3], output_imgs.shape.as_list())
def _input_and_output_same_shape_helper(self, kernel_size):
img_batch = tf.placeholder(tf.float32, shape=[None, 32, 32, 3])
output_img_batch, _ = cyclegan.cyclegan_generator_resnet(
img_batch, kernel_size=kernel_size)
self.assertAllEqual(img_batch.shape.as_list(),
output_img_batch.shape.as_list())
def input_and_output_same_shape_kernel3(self):
self._input_and_output_same_shape_helper(3)
def input_and_output_same_shape_kernel4(self):
self._input_and_output_same_shape_helper(4)
def input_and_output_same_shape_kernel5(self):
self._input_and_output_same_shape_helper(5)
def input_and_output_same_shape_kernel6(self):
self._input_and_output_same_shape_helper(6)
def _error_if_height_not_multiple_of_four_helper(self, height):
self.assertRaisesRegexp(
ValueError,
'The input height must be a multiple of 4.',
cyclegan.cyclegan_generator_resnet,
tf.placeholder(tf.float32, shape=[None, height, 32, 3]))
def test_error_if_height_not_multiple_of_four_height29(self):
self._error_if_height_not_multiple_of_four_helper(29)
def test_error_if_height_not_multiple_of_four_height30(self):
self._error_if_height_not_multiple_of_four_helper(30)
def test_error_if_height_not_multiple_of_four_height31(self):
self._error_if_height_not_multiple_of_four_helper(31)
def _error_if_width_not_multiple_of_four_helper(self, width):
self.assertRaisesRegexp(
ValueError,
'The input width must be a multiple of 4.',
cyclegan.cyclegan_generator_resnet,
tf.placeholder(tf.float32, shape=[None, 32, width, 3]))
def test_error_if_width_not_multiple_of_four_width29(self):
self._error_if_width_not_multiple_of_four_helper(29)
def test_error_if_width_not_multiple_of_four_width30(self):
self._error_if_width_not_multiple_of_four_helper(30)
def test_error_if_width_not_multiple_of_four_width31(self):
self._error_if_width_not_multiple_of_four_helper(31)
if __name__ == '__main__':
tf.test.main()
|
PaddlePaddle/LanguageModeling/BERT/vocab | vocab | bert-large-uncased-vocab | [PAD]
[unused0]
[unused1]
[unused2]
[unused3]
[unused4]
[unused5]
[unused6]
[unused7]
[unused8]
[unused9]
[unused10]
[unused11]
[unused12]
[unused13]
[unused14]
[unused15]
[unused16]
[unused17]
[unused18]
[unused19]
[unused20]
[unused21]
[unused22]
[unused23]
[unused24]
[unused25]
[unused26]
[unused27]
[unused28]
[unused29]
[unused30]
[unused31]
[unused32]
[unused33]
[unused34]
[unused35]
[unused36]
[unused37]
[unused38]
[unused39]
[unused40]
[unused41]
[unused42]
[unused43]
[unused44]
[unused45]
[unused46]
[unused47]
[unused48]
[unused49]
[unused50]
[unused51]
[unused52]
[unused53]
[unused54]
[unused55]
[unused56]
[unused57]
[unused58]
[unused59]
[unused60]
[unused61]
[unused62]
[unused63]
[unused64]
[unused65]
[unused66]
[unused67]
[unused68]
[unused69]
[unused70]
[unused71]
[unused72]
[unused73]
[unused74]
[unused75]
[unused76]
[unused77]
[unused78]
[unused79]
[unused80]
[unused81]
[unused82]
[unused83]
[unused84]
[unused85]
[unused86]
[unused87]
[unused88]
[unused89]
[unused90]
[unused91]
[unused92]
[unused93]
[unused94]
[unused95]
[unused96]
[unused97]
[unused98]
[UNK]
[CLS]
[SEP]
[MASK]
[unused99]
[unused100]
[unused101]
[unused102]
[unused103]
[unused104]
[unused105]
[unused106]
[unused107]
[unused108]
[unused109]
[unused110]
[unused111]
[unused112]
[unused113]
[unused114]
[unused115]
[unused116]
[unused117]
[unused118]
[unused119]
[unused120]
[unused121]
[unused122]
[unused123]
[unused124]
[unused125]
[unused126]
[unused127]
[unused128]
[unused129]
[unused130]
[unused131]
[unused132]
[unused133]
[unused134]
[unused135]
[unused136]
[unused137]
[unused138]
[unused139]
[unused140]
[unused141]
[unused142]
[unused143]
[unused144]
[unused145]
[unused146]
[unused147]
[unused148]
[unused149]
[unused150]
[unused151]
[unused152]
[unused153]
[unused154]
[unused155]
[unused156]
[unused157]
[unused158]
[unused159]
[unused160]
[unused161]
[unused162]
[unused163]
[unused164]
[unused165]
[unused166]
[unused167]
[unused168]
[unused169]
[unused170]
[unused171]
[unused172]
[unused173]
[unused174]
[unused175]
[unused176]
[unused177]
[unused178]
[unused179]
[unused180]
[unused181]
[unused182]
[unused183]
[unused184]
[unused185]
[unused186]
[unused187]
[unused188]
[unused189]
[unused190]
[unused191]
[unused192]
[unused193]
[unused194]
[unused195]
[unused196]
[unused197]
[unused198]
[unused199]
[unused200]
[unused201]
[unused202]
[unused203]
[unused204]
[unused205]
[unused206]
[unused207]
[unused208]
[unused209]
[unused210]
[unused211]
[unused212]
[unused213]
[unused214]
[unused215]
[unused216]
[unused217]
[unused218]
[unused219]
[unused220]
[unused221]
[unused222]
[unused223]
[unused224]
[unused225]
[unused226]
[unused227]
[unused228]
[unused229]
[unused230]
[unused231]
[unused232]
[unused233]
[unused234]
[unused235]
[unused236]
[unused237]
[unused238]
[unused239]
[unused240]
[unused241]
[unused242]
[unused243]
[unused244]
[unused245]
[unused246]
[unused247]
[unused248]
[unused249]
[unused250]
[unused251]
[unused252]
[unused253]
[unused254]
[unused255]
[unused256]
[unused257]
[unused258]
[unused259]
[unused260]
[unused261]
[unused262]
[unused263]
[unused264]
[unused265]
[unused266]
[unused267]
[unused268]
[unused269]
[unused270]
[unused271]
[unused272]
[unused273]
[unused274]
[unused275]
[unused276]
[unused277]
[unused278]
[unused279]
[unused280]
[unused281]
[unused282]
[unused283]
[unused284]
[unused285]
[unused286]
[unused287]
[unused288]
[unused289]
[unused290]
[unused291]
[unused292]
[unused293]
[unused294]
[unused295]
[unused296]
[unused297]
[unused298]
[unused299]
[unused300]
[unused301]
[unused302]
[unused303]
[unused304]
[unused305]
[unused306]
[unused307]
[unused308]
[unused309]
[unused310]
[unused311]
[unused312]
[unused313]
[unused314]
[unused315]
[unused316]
[unused317]
[unused318]
[unused319]
[unused320]
[unused321]
[unused322]
[unused323]
[unused324]
[unused325]
[unused326]
[unused327]
[unused328]
[unused329]
[unused330]
[unused331]
[unused332]
[unused333]
[unused334]
[unused335]
[unused336]
[unused337]
[unused338]
[unused339]
[unused340]
[unused341]
[unused342]
[unused343]
[unused344]
[unused345]
[unused346]
[unused347]
[unused348]
[unused349]
[unused350]
[unused351]
[unused352]
[unused353]
[unused354]
[unused355]
[unused356]
[unused357]
[unused358]
[unused359]
[unused360]
[unused361]
[unused362]
[unused363]
[unused364]
[unused365]
[unused366]
[unused367]
[unused368]
[unused369]
[unused370]
[unused371]
[unused372]
[unused373]
[unused374]
[unused375]
[unused376]
[unused377]
[unused378]
[unused379]
[unused380]
[unused381]
[unused382]
[unused383]
[unused384]
[unused385]
[unused386]
[unused387]
[unused388]
[unused389]
[unused390]
[unused391]
[unused392]
[unused393]
[unused394]
[unused395]
[unused396]
[unused397]
[unused398]
[unused399]
[unused400]
[unused401]
[unused402]
[unused403]
[unused404]
[unused405]
[unused406]
[unused407]
[unused408]
[unused409]
[unused410]
[unused411]
[unused412]
[unused413]
[unused414]
[unused415]
[unused416]
[unused417]
[unused418]
[unused419]
[unused420]
[unused421]
[unused422]
[unused423]
[unused424]
[unused425]
[unused426]
[unused427]
[unused428]
[unused429]
[unused430]
[unused431]
[unused432]
[unused433]
[unused434]
[unused435]
[unused436]
[unused437]
[unused438]
[unused439]
[unused440]
[unused441]
[unused442]
[unused443]
[unused444]
[unused445]
[unused446]
[unused447]
[unused448]
[unused449]
[unused450]
[unused451]
[unused452]
[unused453]
[unused454]
[unused455]
[unused456]
[unused457]
[unused458]
[unused459]
[unused460]
[unused461]
[unused462]
[unused463]
[unused464]
[unused465]
[unused466]
[unused467]
[unused468]
[unused469]
[unused470]
[unused471]
[unused472]
[unused473]
[unused474]
[unused475]
[unused476]
[unused477]
[unused478]
[unused479]
[unused480]
[unused481]
[unused482]
[unused483]
[unused484]
[unused485]
[unused486]
[unused487]
[unused488]
[unused489]
[unused490]
[unused491]
[unused492]
[unused493]
[unused494]
[unused495]
[unused496]
[unused497]
[unused498]
[unused499]
[unused500]
[unused501]
[unused502]
[unused503]
[unused504]
[unused505]
[unused506]
[unused507]
[unused508]
[unused509]
[unused510]
[unused511]
[unused512]
[unused513]
[unused514]
[unused515]
[unused516]
[unused517]
[unused518]
[unused519]
[unused520]
[unused521]
[unused522]
[unused523]
[unused524]
[unused525]
[unused526]
[unused527]
[unused528]
[unused529]
[unused530]
[unused531]
[unused532]
[unused533]
[unused534]
[unused535]
[unused536]
[unused537]
[unused538]
[unused539]
[unused540]
[unused541]
[unused542]
[unused543]
[unused544]
[unused545]
[unused546]
[unused547]
[unused548]
[unused549]
[unused550]
[unused551]
[unused552]
[unused553]
[unused554]
[unused555]
[unused556]
[unused557]
[unused558]
[unused559]
[unused560]
[unused561]
[unused562]
[unused563]
[unused564]
[unused565]
[unused566]
[unused567]
[unused568]
[unused569]
[unused570]
[unused571]
[unused572]
[unused573]
[unused574]
[unused575]
[unused576]
[unused577]
[unused578]
[unused579]
[unused580]
[unused581]
[unused582]
[unused583]
[unused584]
[unused585]
[unused586]
[unused587]
[unused588]
[unused589]
[unused590]
[unused591]
[unused592]
[unused593]
[unused594]
[unused595]
[unused596]
[unused597]
[unused598]
[unused599]
[unused600]
[unused601]
[unused602]
[unused603]
[unused604]
[unused605]
[unused606]
[unused607]
[unused608]
[unused609]
[unused610]
[unused611]
[unused612]
[unused613]
[unused614]
[unused615]
[unused616]
[unused617]
[unused618]
[unused619]
[unused620]
[unused621]
[unused622]
[unused623]
[unused624]
[unused625]
[unused626]
[unused627]
[unused628]
[unused629]
[unused630]
[unused631]
[unused632]
[unused633]
[unused634]
[unused635]
[unused636]
[unused637]
[unused638]
[unused639]
[unused640]
[unused641]
[unused642]
[unused643]
[unused644]
[unused645]
[unused646]
[unused647]
[unused648]
[unused649]
[unused650]
[unused651]
[unused652]
[unused653]
[unused654]
[unused655]
[unused656]
[unused657]
[unused658]
[unused659]
[unused660]
[unused661]
[unused662]
[unused663]
[unused664]
[unused665]
[unused666]
[unused667]
[unused668]
[unused669]
[unused670]
[unused671]
[unused672]
[unused673]
[unused674]
[unused675]
[unused676]
[unused677]
[unused678]
[unused679]
[unused680]
[unused681]
[unused682]
[unused683]
[unused684]
[unused685]
[unused686]
[unused687]
[unused688]
[unused689]
[unused690]
[unused691]
[unused692]
[unused693]
[unused694]
[unused695]
[unused696]
[unused697]
[unused698]
[unused699]
[unused700]
[unused701]
[unused702]
[unused703]
[unused704]
[unused705]
[unused706]
[unused707]
[unused708]
[unused709]
[unused710]
[unused711]
[unused712]
[unused713]
[unused714]
[unused715]
[unused716]
[unused717]
[unused718]
[unused719]
[unused720]
[unused721]
[unused722]
[unused723]
[unused724]
[unused725]
[unused726]
[unused727]
[unused728]
[unused729]
[unused730]
[unused731]
[unused732]
[unused733]
[unused734]
[unused735]
[unused736]
[unused737]
[unused738]
[unused739]
[unused740]
[unused741]
[unused742]
[unused743]
[unused744]
[unused745]
[unused746]
[unused747]
[unused748]
[unused749]
[unused750]
[unused751]
[unused752]
[unused753]
[unused754]
[unused755]
[unused756]
[unused757]
[unused758]
[unused759]
[unused760]
[unused761]
[unused762]
[unused763]
[unused764]
[unused765]
[unused766]
[unused767]
[unused768]
[unused769]
[unused770]
[unused771]
[unused772]
[unused773]
[unused774]
[unused775]
[unused776]
[unused777]
[unused778]
[unused779]
[unused780]
[unused781]
[unused782]
[unused783]
[unused784]
[unused785]
[unused786]
[unused787]
[unused788]
[unused789]
[unused790]
[unused791]
[unused792]
[unused793]
[unused794]
[unused795]
[unused796]
[unused797]
[unused798]
[unused799]
[unused800]
[unused801]
[unused802]
[unused803]
[unused804]
[unused805]
[unused806]
[unused807]
[unused808]
[unused809]
[unused810]
[unused811]
[unused812]
[unused813]
[unused814]
[unused815]
[unused816]
[unused817]
[unused818]
[unused819]
[unused820]
[unused821]
[unused822]
[unused823]
[unused824]
[unused825]
[unused826]
[unused827]
[unused828]
[unused829]
[unused830]
[unused831]
[unused832]
[unused833]
[unused834]
[unused835]
[unused836]
[unused837]
[unused838]
[unused839]
[unused840]
[unused841]
[unused842]
[unused843]
[unused844]
[unused845]
[unused846]
[unused847]
[unused848]
[unused849]
[unused850]
[unused851]
[unused852]
[unused853]
[unused854]
[unused855]
[unused856]
[unused857]
[unused858]
[unused859]
[unused860]
[unused861]
[unused862]
[unused863]
[unused864]
[unused865]
[unused866]
[unused867]
[unused868]
[unused869]
[unused870]
[unused871]
[unused872]
[unused873]
[unused874]
[unused875]
[unused876]
[unused877]
[unused878]
[unused879]
[unused880]
[unused881]
[unused882]
[unused883]
[unused884]
[unused885]
[unused886]
[unused887]
[unused888]
[unused889]
[unused890]
[unused891]
[unused892]
[unused893]
[unused894]
[unused895]
[unused896]
[unused897]
[unused898]
[unused899]
[unused900]
[unused901]
[unused902]
[unused903]
[unused904]
[unused905]
[unused906]
[unused907]
[unused908]
[unused909]
[unused910]
[unused911]
[unused912]
[unused913]
[unused914]
[unused915]
[unused916]
[unused917]
[unused918]
[unused919]
[unused920]
[unused921]
[unused922]
[unused923]
[unused924]
[unused925]
[unused926]
[unused927]
[unused928]
[unused929]
[unused930]
[unused931]
[unused932]
[unused933]
[unused934]
[unused935]
[unused936]
[unused937]
[unused938]
[unused939]
[unused940]
[unused941]
[unused942]
[unused943]
[unused944]
[unused945]
[unused946]
[unused947]
[unused948]
[unused949]
[unused950]
[unused951]
[unused952]
[unused953]
[unused954]
[unused955]
[unused956]
[unused957]
[unused958]
[unused959]
[unused960]
[unused961]
[unused962]
[unused963]
[unused964]
[unused965]
[unused966]
[unused967]
[unused968]
[unused969]
[unused970]
[unused971]
[unused972]
[unused973]
[unused974]
[unused975]
[unused976]
[unused977]
[unused978]
[unused979]
[unused980]
[unused981]
[unused982]
[unused983]
[unused984]
[unused985]
[unused986]
[unused987]
[unused988]
[unused989]
[unused990]
[unused991]
[unused992]
[unused993]
!
"
#
$
%
&
'
(
)
*
+
,
-
.
/
0
1
2
3
4
5
6
7
8
9
:
;
<
=
>
?
@
[
\
]
^
_
`
a
b
c
d
e
f
g
h
i
j
k
l
m
n
o
p
q
r
s
t
u
v
w
x
y
z
{
|
}
~
¡
¢
£
¤
¥
¦
§
¨
©
ª
«
¬
®
°
±
²
³
´
µ
¶
·
¹
º
»
¼
½
¾
¿
×
ß
æ
ð
÷
ø
þ
đ
ħ
ı
ł
ŋ
œ
ƒ
ɐ
ɑ
ɒ
ɔ
ɕ
ə
ɛ
ɡ
ɣ
ɨ
ɪ
ɫ
ɬ
ɯ
ɲ
ɴ
ɹ
ɾ
ʀ
ʁ
ʂ
ʃ
ʉ
ʊ
ʋ
ʌ
ʎ
ʐ
ʑ
ʒ
ʔ
ʰ
ʲ
ʳ
ʷ
ʸ
ʻ
ʼ
ʾ
ʿ
ˈ
ː
ˡ
ˢ
ˣ
ˤ
α
β
γ
δ
ε
ζ
η
θ
ι
κ
λ
μ
ν
ξ
ο
π
ρ
ς
σ
τ
υ
φ
χ
ψ
ω
а
б
в
г
д
е
ж
з
и
к
л
м
н
о
п
р
с
т
у
ф
х
ц
ч
ш
щ
ъ
ы
ь
э
ю
я
ђ
є
і
ј
љ
њ
ћ
ӏ
ա
բ
գ
դ
ե
թ
ի
լ
կ
հ
մ
յ
ն
ո
պ
ս
վ
տ
ր
ւ
ք
־
א
ב
ג
ד
ה
ו
ז
ח
ט
י
ך
כ
ל
ם
מ
ן
נ
ס
ע
ף
פ
ץ
צ
ק
ר
ש
ת
،
ء
ا
ب
ة
ت
ث
ج
ح
خ
د
ذ
ر
ز
س
ش
ص
ض
ط
ظ
ع
غ
ـ
ف
ق
ك
ل
م
ن
ه
و
ى
ي
ٹ
پ
چ
ک
گ
ں
ھ
ہ
ی
ے
अ
आ
उ
ए
क
ख
ग
च
ज
ट
ड
ण
त
थ
द
ध
न
प
ब
भ
म
य
र
ल
व
श
ष
स
ह
ा
ि
ी
ो
।
॥
ং
অ
আ
ই
উ
এ
ও
ক
খ
গ
চ
ছ
জ
ট
ড
ণ
ত
থ
দ
ধ
ন
প
ব
ভ
ম
য
র
ল
শ
ষ
স
হ
া
ি
ী
ে
க
ச
ட
த
ந
ன
ப
ம
ய
ர
ல
ள
வ
ா
ி
ு
ே
ை
ನ
ರ
ಾ
ක
ය
ර
ල
ව
ා
ก
ง
ต
ท
น
พ
ม
ย
ร
ล
ว
ส
อ
า
เ
་
།
ག
ང
ད
ན
པ
བ
མ
འ
ར
ལ
ས
မ
ა
ბ
გ
დ
ე
ვ
თ
ი
კ
ლ
მ
ნ
ო
რ
ს
ტ
უ
ᄀ
ᄂ
ᄃ
ᄅ
ᄆ
ᄇ
ᄉ
ᄊ
ᄋ
ᄌ
ᄎ
ᄏ
ᄐ
ᄑ
ᄒ
ᅡ
ᅢ
ᅥ
ᅦ
ᅧ
ᅩ
ᅪ
ᅭ
ᅮ
ᅯ
ᅲ
ᅳ
ᅴ
ᅵ
ᆨ
ᆫ
ᆯ
ᆷ
ᆸ
ᆼ
ᴬ
ᴮ
ᴰ
ᴵ
ᴺ
ᵀ
ᵃ
ᵇ
ᵈ
ᵉ
ᵍ
ᵏ
ᵐ
ᵒ
ᵖ
ᵗ
ᵘ
ᵢ
ᵣ
ᵤ
ᵥ
ᶜ
ᶠ
‐
‑
‒
–
—
―
‖
‘
’
‚
“
”
„
†
‡
•
…
‰
′
″
›
‿
⁄
⁰
ⁱ
⁴
⁵
⁶
⁷
⁸
⁹
⁺
⁻
ⁿ
₀
₁
₂
₃
₄
₅
₆
₇
₈
₉
₊
₍
₎
ₐ
ₑ
ₒ
ₓ
ₕ
ₖ
ₗ
ₘ
ₙ
ₚ
ₛ
ₜ
₤
₩
€
₱
₹
ℓ
№
ℝ
™
⅓
⅔
←
↑
→
↓
↔
↦
⇄
⇌
⇒
∂
∅
∆
∇
∈
−
∗
∘
√
∞
∧
∨
∩
∪
≈
≡
≤
≥
⊂
⊆
⊕
⊗
⋅
─
│
■
▪
●
★
☆
☉
♠
♣
♥
♦
♭
♯
⟨
⟩
ⱼ
⺩
⺼
⽥
、
。
〈
〉
《
》
「
」
『
』
〜
あ
い
う
え
お
か
き
く
け
こ
さ
し
す
せ
そ
た
ち
っ
つ
て
と
な
に
ぬ
ね
の
は
ひ
ふ
へ
ほ
ま
み
む
め
も
や
ゆ
よ
ら
り
る
れ
ろ
を
ん
ァ
ア
ィ
イ
ウ
ェ
エ
オ
カ
キ
ク
ケ
コ
サ
シ
ス
セ
タ
チ
ッ
ツ
テ
ト
ナ
ニ
ノ
ハ
ヒ
フ
ヘ
ホ
マ
ミ
ム
メ
モ
ャ
ュ
ョ
ラ
リ
ル
レ
ロ
ワ
ン
・
ー
一
三
上
下
不
世
中
主
久
之
也
事
二
五
井
京
人
亻
仁
介
代
仮
伊
会
佐
侍
保
信
健
元
光
八
公
内
出
分
前
劉
力
加
勝
北
区
十
千
南
博
原
口
古
史
司
合
吉
同
名
和
囗
四
国
國
土
地
坂
城
堂
場
士
夏
外
大
天
太
夫
奈
女
子
学
宀
宇
安
宗
定
宣
宮
家
宿
寺
將
小
尚
山
岡
島
崎
川
州
巿
帝
平
年
幸
广
弘
張
彳
後
御
德
心
忄
志
忠
愛
成
我
戦
戸
手
扌
政
文
新
方
日
明
星
春
昭
智
曲
書
月
有
朝
木
本
李
村
東
松
林
森
楊
樹
橋
歌
止
正
武
比
氏
民
水
氵
氷
永
江
沢
河
治
法
海
清
漢
瀬
火
版
犬
王
生
田
男
疒
発
白
的
皇
目
相
省
真
石
示
社
神
福
禾
秀
秋
空
立
章
竹
糹
美
義
耳
良
艹
花
英
華
葉
藤
行
街
西
見
訁
語
谷
貝
貴
車
軍
辶
道
郎
郡
部
都
里
野
金
鈴
镇
長
門
間
阝
阿
陳
陽
雄
青
面
風
食
香
馬
高
龍
龸
fi
fl
!
(
)
,
-
.
/
:
?
~
the
of
and
in
to
was
he
is
as
for
on
with
that
it
his
by
at
from
her
##s
she
you
had
an
were
but
be
this
are
not
my
they
one
which
or
have
him
me
first
all
also
their
has
up
who
out
been
when
after
there
into
new
two
its
##a
time
would
no
what
about
said
we
over
then
other
so
more
##e
can
if
like
back
them
only
some
could
##i
where
just
##ing
during
before
##n
do
##o
made
school
through
than
now
years
most
world
may
between
down
well
three
##d
year
while
will
##ed
##r
##y
later
##t
city
under
around
did
such
being
used
state
people
part
know
against
your
many
second
university
both
national
##er
these
don
known
off
way
until
re
how
even
get
head
...
didn
##ly
team
american
because
de
##l
born
united
film
since
still
long
work
south
us
became
any
high
again
day
family
see
right
man
eyes
house
season
war
states
including
took
life
north
same
each
called
name
much
place
however
go
four
group
another
found
won
area
here
going
10
away
series
left
home
music
best
make
hand
number
company
several
never
last
john
000
very
album
take
end
good
too
following
released
game
played
little
began
district
##m
old
want
those
side
held
own
early
county
ll
league
use
west
##u
face
think
##es
2010
government
##h
march
came
small
general
town
june
##on
line
based
something
##k
september
thought
looked
along
international
2011
air
july
club
went
january
october
our
august
april
york
12
few
2012
2008
east
show
member
college
2009
father
public
##us
come
men
five
set
station
church
##c
next
former
november
room
party
located
december
2013
age
got
2007
##g
system
let
love
2006
though
every
2014
look
song
water
century
without
body
black
night
within
great
women
single
ve
building
large
population
river
named
band
white
started
##an
once
15
20
should
18
2015
service
top
built
british
open
death
king
moved
local
times
children
february
book
why
11
door
need
president
order
final
road
wasn
although
due
major
died
village
third
knew
2016
asked
turned
st
wanted
say
##p
together
received
main
son
served
different
##en
behind
himself
felt
members
power
football
law
voice
play
##in
near
park
history
30
having
2005
16
##man
saw
mother
##al
army
point
front
help
english
street
art
late
hands
games
award
##ia
young
14
put
published
country
division
across
told
13
often
ever
french
london
center
six
red
2017
led
days
include
light
25
find
tell
among
species
really
according
central
half
2004
form
original
gave
office
making
enough
lost
full
opened
must
included
live
given
german
player
run
business
woman
community
cup
might
million
land
2000
court
development
17
short
round
ii
km
seen
class
story
always
become
sure
research
almost
director
council
la
##2
career
things
using
island
##z
couldn
car
##is
24
close
force
##1
better
free
support
control
field
students
2003
education
married
##b
nothing
worked
others
record
big
inside
level
anything
continued
give
james
##3
military
established
non
returned
feel
does
title
written
thing
feet
william
far
co
association
hard
already
2002
##ra
championship
human
western
100
##na
department
hall
role
various
production
21
19
heart
2001
living
fire
version
##ers
##f
television
royal
##4
produced
working
act
case
society
region
present
radio
period
looking
least
total
keep
england
wife
program
per
brother
mind
special
22
##le
am
works
soon
##6
political
george
services
taken
created
##7
further
able
reached
david
union
joined
upon
done
important
social
information
either
##ic
##x
appeared
position
ground
lead
rock
dark
election
23
board
france
hair
course
arms
site
police
girl
instead
real
sound
##v
words
moment
##te
someone
##8
summer
project
announced
san
less
wrote
past
followed
##5
blue
founded
al
finally
india
taking
records
america
##ne
1999
design
considered
northern
god
stop
battle
toward
european
outside
described
track
today
playing
language
28
call
26
heard
professional
low
australia
miles
california
win
yet
green
##ie
trying
blood
##ton
southern
science
maybe
everything
match
square
27
mouth
video
race
recorded
leave
above
##9
daughter
points
space
1998
museum
change
middle
common
##0
move
tv
post
##ta
lake
seven
tried
elected
closed
ten
paul
minister
##th
months
start
chief
return
canada
person
sea
release
similar
modern
brought
rest
hit
formed
mr
##la
1997
floor
event
doing
thomas
1996
robert
care
killed
training
star
week
needed
turn
finished
railway
rather
news
health
sent
example
ran
term
michael
coming
currently
yes
forces
despite
gold
areas
50
stage
fact
29
dead
says
popular
2018
originally
germany
probably
developed
result
pulled
friend
stood
money
running
mi
signed
word
songs
child
eventually
met
tour
average
teams
minutes
festival
current
deep
kind
1995
decided
usually
eastern
seemed
##ness
episode
bed
added
table
indian
private
charles
route
available
idea
throughout
centre
addition
appointed
style
1994
books
eight
construction
press
mean
wall
friends
remained
schools
study
##ch
##um
institute
oh
chinese
sometimes
events
possible
1992
australian
type
brown
forward
talk
process
food
debut
seat
performance
committee
features
character
arts
herself
else
lot
strong
russian
range
hours
peter
arm
##da
morning
dr
sold
##ry
quickly
directed
1993
guitar
china
##w
31
list
##ma
performed
media
uk
players
smile
##rs
myself
40
placed
coach
province
towards
wouldn
leading
whole
boy
official
designed
grand
census
##el
europe
attack
japanese
henry
1991
##re
##os
cross
getting
alone
action
lower
network
wide
washington
japan
1990
hospital
believe
changed
sister
##ar
hold
gone
sir
hadn
ship
##ka
studies
academy
shot
rights
below
base
bad
involved
kept
largest
##ist
bank
future
especially
beginning
mark
movement
section
female
magazine
plan
professor
lord
longer
##ian
sat
walked
hill
actually
civil
energy
model
families
size
thus
aircraft
completed
includes
data
captain
##or
fight
vocals
featured
richard
bridge
fourth
1989
officer
stone
hear
##ism
means
medical
groups
management
self
lips
competition
entire
lived
technology
leaving
federal
tournament
bit
passed
hot
independent
awards
kingdom
mary
spent
fine
doesn
reported
##ling
jack
fall
raised
itself
stay
true
studio
1988
sports
replaced
paris
systems
saint
leader
theatre
whose
market
capital
parents
spanish
canadian
earth
##ity
cut
degree
writing
bay
christian
awarded
natural
higher
bill
##as
coast
provided
previous
senior
ft
valley
organization
stopped
onto
countries
parts
conference
queen
security
interest
saying
allowed
master
earlier
phone
matter
smith
winning
try
happened
moving
campaign
los
##ley
breath
nearly
mid
1987
certain
girls
date
italian
african
standing
fell
artist
##ted
shows
deal
mine
industry
1986
##ng
everyone
republic
provide
collection
library
student
##ville
primary
owned
older
via
heavy
1st
makes
##able
attention
anyone
africa
##ri
stated
length
ended
fingers
command
staff
skin
foreign
opening
governor
okay
medal
kill
sun
cover
job
1985
introduced
chest
hell
feeling
##ies
success
meet
reason
standard
meeting
novel
1984
trade
source
buildings
##land
rose
guy
goal
##ur
chapter
native
husband
previously
unit
limited
entered
weeks
producer
operations
mountain
takes
covered
forced
related
roman
complete
successful
key
texas
cold
##ya
channel
1980
traditional
films
dance
clear
approximately
500
nine
van
prince
question
active
tracks
ireland
regional
silver
author
personal
sense
operation
##ine
economic
1983
holding
twenty
isbn
additional
speed
hour
edition
regular
historic
places
whom
shook
movie
km²
secretary
prior
report
chicago
read
foundation
view
engine
scored
1982
units
ask
airport
property
ready
immediately
lady
month
listed
contract
##de
manager
themselves
lines
##ki
navy
writer
meant
##ts
runs
##ro
practice
championships
singer
glass
commission
required
forest
starting
culture
generally
giving
access
attended
test
couple
stand
catholic
martin
caught
executive
##less
eye
##ey
thinking
chair
quite
shoulder
1979
hope
decision
plays
defeated
municipality
whether
structure
offered
slowly
pain
ice
direction
##ion
paper
mission
1981
mostly
200
noted
individual
managed
nature
lives
plant
##ha
helped
except
studied
computer
figure
relationship
issue
significant
loss
die
smiled
gun
ago
highest
1972
##am
male
bring
goals
mexico
problem
distance
commercial
completely
location
annual
famous
drive
1976
neck
1978
surface
caused
italy
understand
greek
highway
wrong
hotel
comes
appearance
joseph
double
issues
musical
companies
castle
income
review
assembly
bass
initially
parliament
artists
experience
1974
particular
walk
foot
engineering
talking
window
dropped
##ter
miss
baby
boys
break
1975
stars
edge
remember
policy
carried
train
stadium
bar
sex
angeles
evidence
##ge
becoming
assistant
soviet
1977
upper
step
wing
1970
youth
financial
reach
##ll
actor
numerous
##se
##st
nodded
arrived
##ation
minute
##nt
believed
sorry
complex
beautiful
victory
associated
temple
1968
1973
chance
perhaps
metal
##son
1945
bishop
##et
lee
launched
particularly
tree
le
retired
subject
prize
contains
yeah
theory
empire
##ce
suddenly
waiting
trust
recording
##to
happy
terms
camp
champion
1971
religious
pass
zealand
names
2nd
port
ancient
tom
corner
represented
watch
legal
anti
justice
cause
watched
brothers
45
material
changes
simply
response
louis
fast
##ting
answer
60
historical
1969
stories
straight
create
feature
increased
rate
administration
virginia
el
activities
cultural
overall
winner
programs
basketball
legs
guard
beyond
cast
doctor
mm
flight
results
remains
cost
effect
winter
##ble
larger
islands
problems
chairman
grew
commander
isn
1967
pay
failed
selected
hurt
fort
box
regiment
majority
journal
35
edward
plans
##ke
##ni
shown
pretty
irish
characters
directly
scene
likely
operated
allow
spring
##j
junior
matches
looks
mike
houses
fellow
##tion
beach
marriage
##ham
##ive
rules
oil
65
florida
expected
nearby
congress
sam
peace
recent
iii
wait
subsequently
cell
##do
variety
serving
agreed
please
poor
joe
pacific
attempt
wood
democratic
piece
prime
##ca
rural
mile
touch
appears
township
1964
1966
soldiers
##men
##ized
1965
pennsylvania
closer
fighting
claimed
score
jones
physical
editor
##ous
filled
genus
specific
sitting
super
mom
##va
therefore
supported
status
fear
cases
store
meaning
wales
minor
spain
tower
focus
vice
frank
follow
parish
separate
golden
horse
fifth
remaining
branch
32
presented
stared
##id
uses
secret
forms
##co
baseball
exactly
##ck
choice
note
discovered
travel
composed
truth
russia
ball
color
kiss
dad
wind
continue
ring
referred
numbers
digital
greater
##ns
metres
slightly
direct
increase
1960
responsible
crew
rule
trees
troops
##no
broke
goes
individuals
hundred
weight
creek
sleep
memory
defense
provides
ordered
code
value
jewish
windows
1944
safe
judge
whatever
corps
realized
growing
pre
##ga
cities
alexander
gaze
lies
spread
scott
letter
showed
situation
mayor
transport
watching
workers
extended
##li
expression
normal
##ment
chart
multiple
border
##ba
host
##ner
daily
mrs
walls
piano
##ko
heat
cannot
##ate
earned
products
drama
era
authority
seasons
join
grade
##io
sign
difficult
machine
1963
territory
mainly
##wood
stations
squadron
1962
stepped
iron
19th
##led
serve
appear
sky
speak
broken
charge
knowledge
kilometres
removed
ships
article
campus
simple
##ty
pushed
britain
##ve
leaves
recently
cd
soft
boston
latter
easy
acquired
poland
##sa
quality
officers
presence
planned
nations
mass
broadcast
jean
share
image
influence
wild
offer
emperor
electric
reading
headed
ability
promoted
yellow
ministry
1942
throat
smaller
politician
##by
latin
spoke
cars
williams
males
lack
pop
80
##ier
acting
seeing
consists
##ti
estate
1961
pressure
johnson
newspaper
jr
chris
olympics
online
conditions
beat
elements
walking
vote
##field
needs
carolina
text
featuring
global
block
shirt
levels
francisco
purpose
females
et
dutch
duke
ahead
gas
twice
safety
serious
turning
highly
lieutenant
firm
maria
amount
mixed
daniel
proposed
perfect
agreement
affairs
3rd
seconds
contemporary
paid
1943
prison
save
kitchen
label
administrative
intended
constructed
academic
nice
teacher
races
1956
formerly
corporation
ben
nation
issued
shut
1958
drums
housing
victoria
seems
opera
1959
graduated
function
von
mentioned
picked
build
recognized
shortly
protection
picture
notable
exchange
elections
1980s
loved
percent
racing
fish
elizabeth
garden
volume
hockey
1941
beside
settled
##ford
1940
competed
replied
drew
1948
actress
marine
scotland
steel
glanced
farm
steve
1957
risk
tonight
positive
magic
singles
effects
gray
screen
dog
##ja
residents
bus
sides
none
secondary
literature
polish
destroyed
flying
founder
households
1939
lay
reserve
usa
gallery
##ler
1946
industrial
younger
approach
appearances
urban
ones
1950
finish
avenue
powerful
fully
growth
page
honor
jersey
projects
advanced
revealed
basic
90
infantry
pair
equipment
visit
33
evening
search
grant
effort
solo
treatment
buried
republican
primarily
bottom
owner
1970s
israel
gives
jim
dream
bob
remain
spot
70
notes
produce
champions
contact
ed
soul
accepted
ways
del
##ally
losing
split
price
capacity
basis
trial
questions
##ina
1955
20th
guess
officially
memorial
naval
initial
##ization
whispered
median
engineer
##ful
sydney
##go
columbia
strength
300
1952
tears
senate
00
card
asian
agent
1947
software
44
draw
warm
supposed
com
pro
##il
transferred
leaned
##at
candidate
escape
mountains
asia
potential
activity
entertainment
seem
traffic
jackson
murder
36
slow
product
orchestra
haven
agency
bbc
taught
website
comedy
unable
storm
planning
albums
rugby
environment
scientific
grabbed
protect
##hi
boat
typically
1954
1953
damage
principal
divided
dedicated
mount
ohio
##berg
pick
fought
driver
##der
empty
shoulders
sort
thank
berlin
prominent
account
freedom
necessary
efforts
alex
headquarters
follows
alongside
des
simon
andrew
suggested
operating
learning
steps
1949
sweet
technical
begin
easily
34
teeth
speaking
settlement
scale
##sh
renamed
ray
max
enemy
semi
joint
compared
##rd
scottish
leadership
analysis
offers
georgia
pieces
captured
animal
deputy
guest
organized
##lin
tony
combined
method
challenge
1960s
huge
wants
battalion
sons
rise
crime
types
facilities
telling
path
1951
platform
sit
1990s
##lo
tells
assigned
rich
pull
##ot
commonly
alive
##za
letters
concept
conducted
wearing
happen
bought
becomes
holy
gets
ocean
defeat
languages
purchased
coffee
occurred
titled
##q
declared
applied
sciences
concert
sounds
jazz
brain
##me
painting
fleet
tax
nick
##ius
michigan
count
animals
leaders
episodes
##line
content
##den
birth
##it
clubs
64
palace
critical
refused
fair
leg
laughed
returning
surrounding
participated
formation
lifted
pointed
connected
rome
medicine
laid
taylor
santa
powers
adam
tall
shared
focused
knowing
yards
entrance
falls
##wa
calling
##ad
sources
chosen
beneath
resources
yard
##ite
nominated
silence
zone
defined
##que
gained
thirty
38
bodies
moon
##ard
adopted
christmas
widely
register
apart
iran
premier
serves
du
unknown
parties
##les
generation
##ff
continues
quick
fields
brigade
quiet
teaching
clothes
impact
weapons
partner
flat
theater
supreme
1938
37
relations
##tor
plants
suffered
1936
wilson
kids
begins
##age
1918
seats
armed
internet
models
worth
laws
400
communities
classes
background
knows
thanks
quarter
reaching
humans
carry
killing
format
kong
hong
setting
75
architecture
disease
railroad
inc
possibly
wish
arthur
thoughts
harry
doors
density
##di
crowd
illinois
stomach
tone
unique
reports
anyway
##ir
liberal
der
vehicle
thick
dry
drug
faced
largely
facility
theme
holds
creation
strange
colonel
##mi
revolution
bell
politics
turns
silent
rail
relief
independence
combat
shape
write
determined
sales
learned
4th
finger
oxford
providing
1937
heritage
fiction
situated
designated
allowing
distribution
hosted
##est
sight
interview
estimated
reduced
##ria
toronto
footballer
keeping
guys
damn
claim
motion
sport
sixth
stayed
##ze
en
rear
receive
handed
twelve
dress
audience
granted
brazil
##well
spirit
##ated
noticed
etc
olympic
representative
eric
tight
trouble
reviews
drink
vampire
missing
roles
ranked
newly
household
finals
wave
critics
##ee
phase
massachusetts
pilot
unlike
philadelphia
bright
guns
crown
organizations
roof
42
respectively
clearly
tongue
marked
circle
fox
korea
bronze
brian
expanded
sexual
supply
yourself
inspired
labour
fc
##ah
reference
vision
draft
connection
brand
reasons
1935
classic
driving
trip
jesus
cells
entry
1920
neither
trail
claims
atlantic
orders
labor
nose
afraid
identified
intelligence
calls
cancer
attacked
passing
stephen
positions
imperial
grey
jason
39
sunday
48
swedish
avoid
extra
uncle
message
covers
allows
surprise
materials
fame
hunter
##ji
1930
citizens
figures
davis
environmental
confirmed
shit
titles
di
performing
difference
acts
attacks
##ov
existing
votes
opportunity
nor
shop
entirely
trains
opposite
pakistan
##pa
develop
resulted
representatives
actions
reality
pressed
##ish
barely
wine
conversation
faculty
northwest
ends
documentary
nuclear
stock
grace
sets
eat
alternative
##ps
bag
resulting
creating
surprised
cemetery
1919
drop
finding
sarah
cricket
streets
tradition
ride
1933
exhibition
target
ear
explained
rain
composer
injury
apartment
municipal
educational
occupied
netherlands
clean
billion
constitution
learn
1914
maximum
classical
francis
lose
opposition
jose
ontario
bear
core
hills
rolled
ending
drawn
permanent
fun
##tes
##lla
lewis
sites
chamber
ryan
##way
scoring
height
1934
##house
lyrics
staring
55
officials
1917
snow
oldest
##tic
orange
##ger
qualified
interior
apparently
succeeded
thousand
dinner
lights
existence
fans
heavily
41
greatest
conservative
send
bowl
plus
enter
catch
##un
economy
duty
1929
speech
authorities
princess
performances
versions
shall
graduate
pictures
effective
remembered
poetry
desk
crossed
starring
starts
passenger
sharp
##ant
acres
ass
weather
falling
rank
fund
supporting
check
adult
publishing
heads
cm
southeast
lane
##burg
application
bc
##ura
les
condition
transfer
prevent
display
ex
regions
earl
federation
cool
relatively
answered
besides
1928
obtained
portion
##town
mix
##ding
reaction
liked
dean
express
peak
1932
##tte
counter
religion
chain
rare
miller
convention
aid
lie
vehicles
mobile
perform
squad
wonder
lying
crazy
sword
##ping
attempted
centuries
weren
philosophy
category
##ize
anna
interested
47
sweden
wolf
frequently
abandoned
kg
literary
alliance
task
entitled
##ay
threw
promotion
factory
tiny
soccer
visited
matt
fm
achieved
52
defence
internal
persian
43
methods
##ging
arrested
otherwise
cambridge
programming
villages
elementary
districts
rooms
criminal
conflict
worry
trained
1931
attempts
waited
signal
bird
truck
subsequent
programme
##ol
ad
49
communist
details
faith
sector
patrick
carrying
laugh
##ss
controlled
korean
showing
origin
fuel
evil
1927
##ent
brief
identity
darkness
address
pool
missed
publication
web
planet
ian
anne
wings
invited
##tt
briefly
standards
kissed
##be
ideas
climate
causing
walter
worse
albert
articles
winners
desire
aged
northeast
dangerous
gate
doubt
1922
wooden
multi
##ky
poet
rising
funding
46
communications
communication
violence
copies
prepared
ford
investigation
skills
1924
pulling
electronic
##ak
##ial
##han
containing
ultimately
offices
singing
understanding
restaurant
tomorrow
fashion
christ
ward
da
pope
stands
5th
flow
studios
aired
commissioned
contained
exist
fresh
americans
##per
wrestling
approved
kid
employed
respect
suit
1925
angel
asking
increasing
frame
angry
selling
1950s
thin
finds
##nd
temperature
statement
ali
explain
inhabitants
towns
extensive
narrow
51
jane
flowers
images
promise
somewhere
object
fly
closely
##ls
1912
bureau
cape
1926
weekly
presidential
legislative
1921
##ai
##au
launch
founding
##ny
978
##ring
artillery
strike
un
institutions
roll
writers
landing
chose
kevin
anymore
pp
##ut
attorney
fit
dan
billboard
receiving
agricultural
breaking
sought
dave
admitted
lands
mexican
##bury
charlie
specifically
hole
iv
howard
credit
moscow
roads
accident
1923
proved
wear
struck
hey
guards
stuff
slid
expansion
1915
cat
anthony
##kin
melbourne
opposed
sub
southwest
architect
failure
plane
1916
##ron
map
camera
tank
listen
regarding
wet
introduction
metropolitan
link
ep
fighter
inch
grown
gene
anger
fixed
buy
dvd
khan
domestic
worldwide
chapel
mill
functions
examples
##head
developing
1910
turkey
hits
pocket
antonio
papers
grow
unless
circuit
18th
concerned
attached
journalist
selection
journey
converted
provincial
painted
hearing
aren
bands
negative
aside
wondered
knight
lap
survey
ma
##ow
noise
billy
##ium
shooting
guide
bedroom
priest
resistance
motor
homes
sounded
giant
##mer
150
scenes
equal
comic
patients
hidden
solid
actual
bringing
afternoon
touched
funds
wedding
consisted
marie
canal
sr
kim
treaty
turkish
recognition
residence
cathedral
broad
knees
incident
shaped
fired
norwegian
handle
cheek
contest
represent
##pe
representing
beauty
##sen
birds
advantage
emergency
wrapped
drawing
notice
pink
broadcasting
##ong
somehow
bachelor
seventh
collected
registered
establishment
alan
assumed
chemical
personnel
roger
retirement
jeff
portuguese
wore
tied
device
threat
progress
advance
##ised
banks
hired
manchester
nfl
teachers
structures
forever
##bo
tennis
helping
saturday
sale
applications
junction
hip
incorporated
neighborhood
dressed
ceremony
##ds
influenced
hers
visual
stairs
decades
inner
kansas
hung
hoped
gain
scheduled
downtown
engaged
austria
clock
norway
certainly
pale
protected
1913
victor
employees
plate
putting
surrounded
##ists
finishing
blues
tropical
##ries
minnesota
consider
philippines
accept
54
retrieved
1900
concern
anderson
properties
institution
gordon
successfully
vietnam
##dy
backing
outstanding
muslim
crossing
folk
producing
usual
demand
occurs
observed
lawyer
educated
##ana
kelly
string
pleasure
budget
items
quietly
colorado
philip
typical
##worth
derived
600
survived
asks
mental
##ide
56
jake
jews
distinguished
ltd
1911
sri
extremely
53
athletic
loud
thousands
worried
shadow
transportation
horses
weapon
arena
importance
users
tim
objects
contributed
dragon
douglas
aware
senator
johnny
jordan
sisters
engines
flag
investment
samuel
shock
capable
clark
row
wheel
refers
session
familiar
biggest
wins
hate
maintained
drove
hamilton
request
expressed
injured
underground
churches
walker
wars
tunnel
passes
stupid
agriculture
softly
cabinet
regarded
joining
indiana
##ea
##ms
push
dates
spend
behavior
woods
protein
gently
chase
morgan
mention
burning
wake
combination
occur
mirror
leads
jimmy
indeed
impossible
singapore
paintings
covering
##nes
soldier
locations
attendance
sell
historian
wisconsin
invasion
argued
painter
diego
changing
egypt
##don
experienced
inches
##ku
missouri
vol
grounds
spoken
switzerland
##gan
reform
rolling
ha
forget
massive
resigned
burned
allen
tennessee
locked
values
improved
##mo
wounded
universe
sick
dating
facing
pack
purchase
user
##pur
moments
##ul
merged
anniversary
1908
coal
brick
understood
causes
dynasty
queensland
establish
stores
crisis
promote
hoping
views
cards
referee
extension
##si
raise
arizona
improve
colonial
formal
charged
##rt
palm
lucky
hide
rescue
faces
95
feelings
candidates
juan
##ell
goods
6th
courses
weekend
59
luke
cash
fallen
##om
delivered
affected
installed
carefully
tries
swiss
hollywood
costs
lincoln
responsibility
##he
shore
file
proper
normally
maryland
assistance
jump
constant
offering
friendly
waters
persons
realize
contain
trophy
800
partnership
factor
58
musicians
cry
bound
oregon
indicated
hero
houston
medium
##ure
consisting
somewhat
##ara
57
cycle
##che
beer
moore
frederick
gotten
eleven
worst
weak
approached
arranged
chin
loan
universal
bond
fifteen
pattern
disappeared
##ney
translated
##zed
lip
arab
capture
interests
insurance
##chi
shifted
cave
prix
warning
sections
courts
coat
plot
smell
feed
golf
favorite
maintain
knife
vs
voted
degrees
finance
quebec
opinion
translation
manner
ruled
operate
productions
choose
musician
discovery
confused
tired
separated
stream
techniques
committed
attend
ranking
kings
throw
passengers
measure
horror
fan
mining
sand
danger
salt
calm
decade
dam
require
runner
##ik
rush
associate
greece
##ker
rivers
consecutive
matthew
##ski
sighed
sq
documents
steam
edited
closing
tie
accused
1905
##ini
islamic
distributed
directors
organisation
bruce
7th
breathing
mad
lit
arrival
concrete
taste
08
composition
shaking
faster
amateur
adjacent
stating
1906
twin
flew
##ran
tokyo
publications
##tone
obviously
ridge
storage
1907
carl
pages
concluded
desert
driven
universities
ages
terminal
sequence
borough
250
constituency
creative
cousin
economics
dreams
margaret
notably
reduce
montreal
mode
17th
ears
saved
jan
vocal
##ica
1909
andy
##jo
riding
roughly
threatened
##ise
meters
meanwhile
landed
compete
repeated
grass
czech
regularly
charges
tea
sudden
appeal
##ung
solution
describes
pierre
classification
glad
parking
##ning
belt
physics
99
rachel
add
hungarian
participate
expedition
damaged
gift
childhood
85
fifty
##red
mathematics
jumped
letting
defensive
mph
##ux
##gh
testing
##hip
hundreds
shoot
owners
matters
smoke
israeli
kentucky
dancing
mounted
grandfather
emma
designs
profit
argentina
##gs
truly
li
lawrence
cole
begun
detroit
willing
branches
smiling
decide
miami
enjoyed
recordings
##dale
poverty
ethnic
gay
##bi
gary
arabic
09
accompanied
##one
##ons
fishing
determine
residential
acid
##ary
alice
returns
starred
mail
##ang
jonathan
strategy
##ue
net
forty
cook
businesses
equivalent
commonwealth
distinct
ill
##cy
seriously
##ors
##ped
shift
harris
replace
rio
imagine
formula
ensure
##ber
additionally
scheme
conservation
occasionally
purposes
feels
favor
##and
##ore
1930s
contrast
hanging
hunt
movies
1904
instruments
victims
danish
christopher
busy
demon
sugar
earliest
colony
studying
balance
duties
##ks
belgium
slipped
carter
05
visible
stages
iraq
fifa
##im
commune
forming
zero
07
continuing
talked
counties
legend
bathroom
option
tail
clay
daughters
afterwards
severe
jaw
visitors
##ded
devices
aviation
russell
kate
##vi
entering
subjects
##ino
temporary
swimming
forth
smooth
ghost
audio
bush
operates
rocks
movements
signs
eddie
##tz
ann
voices
honorary
06
memories
dallas
pure
measures
racial
promised
66
harvard
ceo
16th
parliamentary
indicate
benefit
flesh
dublin
louisiana
1902
1901
patient
sleeping
1903
membership
coastal
medieval
wanting
element
scholars
rice
62
limit
survive
makeup
rating
definitely
collaboration
obvious
##tan
boss
ms
baron
birthday
linked
soil
diocese
##lan
ncaa
##mann
offensive
shell
shouldn
waist
##tus
plain
ross
organ
resolution
manufacturing
adding
relative
kennedy
98
whilst
moth
marketing
gardens
crash
72
heading
partners
credited
carlos
moves
cable
##zi
marshall
##out
depending
bottle
represents
rejected
responded
existed
04
jobs
denmark
lock
##ating
treated
graham
routes
talent
commissioner
drugs
secure
tests
reign
restored
photography
##gi
contributions
oklahoma
designer
disc
grin
seattle
robin
paused
atlanta
unusual
##gate
praised
las
laughing
satellite
hungary
visiting
##sky
interesting
factors
deck
poems
norman
##water
stuck
speaker
rifle
domain
premiered
##her
dc
comics
actors
01
reputation
eliminated
8th
ceiling
prisoners
script
##nce
leather
austin
mississippi
rapidly
admiral
parallel
charlotte
guilty
tools
gender
divisions
fruit
##bs
laboratory
nelson
fantasy
marry
rapid
aunt
tribe
requirements
aspects
suicide
amongst
adams
bone
ukraine
abc
kick
sees
edinburgh
clothing
column
rough
gods
hunting
broadway
gathered
concerns
##ek
spending
ty
12th
snapped
requires
solar
bones
cavalry
##tta
iowa
drinking
waste
index
franklin
charity
thompson
stewart
tip
flash
landscape
friday
enjoy
singh
poem
listening
##back
eighth
fred
differences
adapted
bomb
ukrainian
surgery
corporate
masters
anywhere
##more
waves
odd
sean
portugal
orleans
dick
debate
kent
eating
puerto
cleared
96
expect
cinema
97
guitarist
blocks
electrical
agree
involving
depth
dying
panel
struggle
##ged
peninsula
adults
novels
emerged
vienna
metro
debuted
shoes
tamil
songwriter
meets
prove
beating
instance
heaven
scared
sending
marks
artistic
passage
superior
03
significantly
shopping
##tive
retained
##izing
malaysia
technique
cheeks
##ola
warren
maintenance
destroy
extreme
allied
120
appearing
##yn
fill
advice
alabama
qualifying
policies
cleveland
hat
battery
smart
authors
10th
soundtrack
acted
dated
lb
glance
equipped
coalition
funny
outer
ambassador
roy
possibility
couples
campbell
dna
loose
ethan
supplies
1898
gonna
88
monster
##res
shake
agents
frequency
springs
dogs
practices
61
gang
plastic
easier
suggests
gulf
blade
exposed
colors
industries
markets
pan
nervous
electoral
charts
legislation
ownership
##idae
mac
appointment
shield
copy
assault
socialist
abbey
monument
license
throne
employment
jay
93
replacement
charter
cloud
powered
suffering
accounts
oak
connecticut
strongly
wright
colour
crystal
13th
context
welsh
networks
voiced
gabriel
jerry
##cing
forehead
mp
##ens
manage
schedule
totally
remix
##ii
forests
occupation
print
nicholas
brazilian
strategic
vampires
engineers
76
roots
seek
correct
instrumental
und
alfred
backed
hop
##des
stanley
robinson
traveled
wayne
welcome
austrian
achieve
67
exit
rates
1899
strip
whereas
##cs
sing
deeply
adventure
bobby
rick
jamie
careful
components
cap
useful
personality
knee
##shi
pushing
hosts
02
protest
ca
ottoman
symphony
##sis
63
boundary
1890
processes
considering
considerable
tons
##work
##ft
##nia
cooper
trading
dear
conduct
91
illegal
apple
revolutionary
holiday
definition
harder
##van
jacob
circumstances
destruction
##lle
popularity
grip
classified
liverpool
donald
baltimore
flows
seeking
honour
approval
92
mechanical
till
happening
statue
critic
increasingly
immediate
describe
commerce
stare
##ster
indonesia
meat
rounds
boats
baker
orthodox
depression
formally
worn
naked
claire
muttered
sentence
11th
emily
document
77
criticism
wished
vessel
spiritual
bent
virgin
parker
minimum
murray
lunch
danny
printed
compilation
keyboards
false
blow
belonged
68
raising
78
cutting
##board
pittsburgh
##up
9th
shadows
81
hated
indigenous
jon
15th
barry
scholar
ah
##zer
oliver
##gy
stick
susan
meetings
attracted
spell
romantic
##ver
ye
1895
photo
demanded
customers
##ac
1896
logan
revival
keys
modified
commanded
jeans
##ious
upset
raw
phil
detective
hiding
resident
vincent
##bly
experiences
diamond
defeating
coverage
lucas
external
parks
franchise
helen
bible
successor
percussion
celebrated
il
lift
profile
clan
romania
##ied
mills
##su
nobody
achievement
shrugged
fault
1897
rhythm
initiative
breakfast
carbon
700
69
lasted
violent
74
wound
ken
killer
gradually
filmed
°c
dollars
processing
94
remove
criticized
guests
sang
chemistry
##vin
legislature
disney
##bridge
uniform
escaped
integrated
proposal
purple
denied
liquid
karl
influential
morris
nights
stones
intense
experimental
twisted
71
84
##ld
pace
nazi
mitchell
ny
blind
reporter
newspapers
14th
centers
burn
basin
forgotten
surviving
filed
collections
monastery
losses
manual
couch
description
appropriate
merely
tag
missions
sebastian
restoration
replacing
triple
73
elder
julia
warriors
benjamin
julian
convinced
stronger
amazing
declined
versus
merchant
happens
output
finland
bare
barbara
absence
ignored
dawn
injuries
##port
producers
##ram
82
luis
##ities
kw
admit
expensive
electricity
nba
exception
symbol
##ving
ladies
shower
sheriff
characteristics
##je
aimed
button
ratio
effectively
summit
angle
jury
bears
foster
vessels
pants
executed
evans
dozen
advertising
kicked
patrol
1889
competitions
lifetime
principles
athletics
##logy
birmingham
sponsored
89
rob
nomination
1893
acoustic
##sm
creature
longest
##tra
credits
harbor
dust
josh
##so
territories
milk
infrastructure
completion
thailand
indians
leon
archbishop
##sy
assist
pitch
blake
arrangement
girlfriend
serbian
operational
hence
sad
scent
fur
dj
sessions
hp
refer
rarely
##ora
exists
1892
##ten
scientists
dirty
penalty
burst
portrait
seed
79
pole
limits
rival
1894
stable
alpha
grave
constitutional
alcohol
arrest
flower
mystery
devil
architectural
relationships
greatly
habitat
##istic
larry
progressive
remote
cotton
##ics
##ok
preserved
reaches
##ming
cited
86
vast
scholarship
decisions
cbs
joy
teach
1885
editions
knocked
eve
searching
partly
participation
gap
animated
fate
excellent
##ett
na
87
alternate
saints
youngest
##ily
climbed
##ita
##tors
suggest
##ct
discussion
staying
choir
lakes
jacket
revenue
nevertheless
peaked
instrument
wondering
annually
managing
neil
1891
signing
terry
##ice
apply
clinical
brooklyn
aim
catherine
fuck
farmers
figured
ninth
pride
hugh
evolution
ordinary
involvement
comfortable
shouted
tech
encouraged
taiwan
representation
sharing
##lia
##em
panic
exact
cargo
competing
fat
cried
83
1920s
occasions
pa
cabin
borders
utah
marcus
##isation
badly
muscles
##ance
victorian
transition
warner
bet
permission
##rin
slave
terrible
similarly
shares
seth
uefa
possession
medals
benefits
colleges
lowered
perfectly
mall
transit
##ye
##kar
publisher
##ened
harrison
deaths
elevation
##ae
asleep
machines
sigh
ash
hardly
argument
occasion
parent
leo
decline
1888
contribution
##ua
concentration
1000
opportunities
hispanic
guardian
extent
emotions
hips
mason
volumes
bloody
controversy
diameter
steady
mistake
phoenix
identify
violin
##sk
departure
richmond
spin
funeral
enemies
1864
gear
literally
connor
random
sergeant
grab
confusion
1865
transmission
informed
op
leaning
sacred
suspended
thinks
gates
portland
luck
agencies
yours
hull
expert
muscle
layer
practical
sculpture
jerusalem
latest
lloyd
statistics
deeper
recommended
warrior
arkansas
mess
supports
greg
eagle
1880
recovered
rated
concerts
rushed
##ano
stops
eggs
files
premiere
keith
##vo
delhi
turner
pit
affair
belief
paint
##zing
mate
##ach
##ev
victim
##ology
withdrew
bonus
styles
fled
##ud
glasgow
technologies
funded
nbc
adaptation
##ata
portrayed
cooperation
supporters
judges
bernard
justin
hallway
ralph
##ick
graduating
controversial
distant
continental
spider
bite
##ho
recognize
intention
mixing
##ese
egyptian
bow
tourism
suppose
claiming
tiger
dominated
participants
vi
##ru
nurse
partially
tape
##rum
psychology
##rn
essential
touring
duo
voting
civilian
emotional
channels
##king
apparent
hebrew
1887
tommy
carrier
intersection
beast
hudson
##gar
##zo
lab
nova
bench
discuss
costa
##ered
detailed
behalf
drivers
unfortunately
obtain
##lis
rocky
##dae
siege
friendship
honey
##rian
1861
amy
hang
posted
governments
collins
respond
wildlife
preferred
operator
##po
laura
pregnant
videos
dennis
suspected
boots
instantly
weird
automatic
businessman
alleged
placing
throwing
ph
mood
1862
perry
venue
jet
remainder
##lli
##ci
passion
biological
boyfriend
1863
dirt
buffalo
ron
segment
fa
abuse
##era
genre
thrown
stroke
colored
stress
exercise
displayed
##gen
struggled
##tti
abroad
dramatic
wonderful
thereafter
madrid
component
widespread
##sed
tale
citizen
todd
monday
1886
vancouver
overseas
forcing
crying
descent
##ris
discussed
substantial
ranks
regime
1870
provinces
switch
drum
zane
ted
tribes
proof
lp
cream
researchers
volunteer
manor
silk
milan
donated
allies
venture
principle
delivery
enterprise
##ves
##ans
bars
traditionally
witch
reminded
copper
##uk
pete
inter
links
colin
grinned
elsewhere
competitive
frequent
##oy
scream
##hu
tension
texts
submarine
finnish
defending
defend
pat
detail
1884
affiliated
stuart
themes
villa
periods
tool
belgian
ruling
crimes
answers
folded
licensed
resort
demolished
hans
lucy
1881
lion
traded
photographs
writes
craig
##fa
trials
generated
beth
noble
debt
percentage
yorkshire
erected
ss
viewed
grades
confidence
ceased
islam
telephone
retail
##ible
chile
m²
roberts
sixteen
##ich
commented
hampshire
innocent
dual
pounds
checked
regulations
afghanistan
sung
rico
liberty
assets
bigger
options
angels
relegated
tribute
wells
attending
leaf
##yan
butler
romanian
forum
monthly
lisa
patterns
gmina
##tory
madison
hurricane
rev
##ians
bristol
##ula
elite
valuable
disaster
democracy
awareness
germans
freyja
##ins
loop
absolutely
paying
populations
maine
sole
prayer
spencer
releases
doorway
bull
##ani
lover
midnight
conclusion
##sson
thirteen
lily
mediterranean
##lt
nhl
proud
sample
##hill
drummer
guinea
##ova
murphy
climb
##ston
instant
attributed
horn
ain
railways
steven
##ao
autumn
ferry
opponent
root
traveling
secured
corridor
stretched
tales
sheet
trinity
cattle
helps
indicates
manhattan
murdered
fitted
1882
gentle
grandmother
mines
shocked
vegas
produces
##light
caribbean
##ou
belong
continuous
desperate
drunk
historically
trio
waved
raf
dealing
nathan
bat
murmured
interrupted
residing
scientist
pioneer
harold
aaron
##net
delta
attempting
minority
mini
believes
chorus
tend
lots
eyed
indoor
load
shots
updated
jail
##llo
concerning
connecting
wealth
##ved
slaves
arrive
rangers
sufficient
rebuilt
##wick
cardinal
flood
muhammad
whenever
relation
runners
moral
repair
viewers
arriving
revenge
punk
assisted
bath
fairly
breathe
lists
innings
illustrated
whisper
nearest
voters
clinton
ties
ultimate
screamed
beijing
lions
andre
fictional
gathering
comfort
radar
suitable
dismissed
hms
ban
pine
wrist
atmosphere
voivodeship
bid
timber
##ned
##nan
giants
##ane
cameron
recovery
uss
identical
categories
switched
serbia
laughter
noah
ensemble
therapy
peoples
touching
##off
locally
pearl
platforms
everywhere
ballet
tables
lanka
herbert
outdoor
toured
derek
1883
spaces
contested
swept
1878
exclusive
slight
connections
##dra
winds
prisoner
collective
bangladesh
tube
publicly
wealthy
thai
##ys
isolated
select
##ric
insisted
pen
fortune
ticket
spotted
reportedly
animation
enforcement
tanks
110
decides
wider
lowest
owen
##time
nod
hitting
##hn
gregory
furthermore
magazines
fighters
solutions
##ery
pointing
requested
peru
reed
chancellor
knights
mask
worker
eldest
flames
reduction
1860
volunteers
##tis
reporting
##hl
wire
advisory
endemic
origins
settlers
pursue
knock
consumer
1876
eu
compound
creatures
mansion
sentenced
ivan
deployed
guitars
frowned
involves
mechanism
kilometers
perspective
shops
maps
terminus
duncan
alien
fist
bridges
##pers
heroes
fed
derby
swallowed
##ros
patent
sara
illness
characterized
adventures
slide
hawaii
jurisdiction
##op
organised
##side
adelaide
walks
biology
se
##ties
rogers
swing
tightly
boundaries
##rie
prepare
implementation
stolen
##sha
certified
colombia
edwards
garage
##mm
recalled
##ball
rage
harm
nigeria
breast
##ren
furniture
pupils
settle
##lus
cuba
balls
client
alaska
21st
linear
thrust
celebration
latino
genetic
terror
##cia
##ening
lightning
fee
witness
lodge
establishing
skull
##ique
earning
hood
##ei
rebellion
wang
sporting
warned
missile
devoted
activist
porch
worship
fourteen
package
1871
decorated
##shire
housed
##ock
chess
sailed
doctors
oscar
joan
treat
garcia
harbour
jeremy
##ire
traditions
dominant
jacques
##gon
##wan
relocated
1879
amendment
sized
companion
simultaneously
volleyball
spun
acre
increases
stopping
loves
belongs
affect
drafted
tossed
scout
battles
1875
filming
shoved
munich
tenure
vertical
romance
pc
##cher
argue
##ical
craft
ranging
www
opens
honest
tyler
yesterday
virtual
##let
muslims
reveal
snake
immigrants
radical
screaming
speakers
firing
saving
belonging
ease
lighting
prefecture
blame
farmer
hungry
grows
rubbed
beam
sur
subsidiary
##cha
armenian
sao
dropping
conventional
##fer
microsoft
reply
qualify
spots
1867
sweat
festivals
##ken
immigration
physician
discover
exposure
sandy
explanation
isaac
implemented
##fish
hart
initiated
connect
stakes
presents
heights
householder
pleased
tourist
regardless
slip
closest
##ction
surely
sultan
brings
riley
preparation
aboard
slammed
baptist
experiment
ongoing
interstate
organic
playoffs
##ika
1877
130
##tar
hindu
error
tours
tier
plenty
arrangements
talks
trapped
excited
sank
ho
athens
1872
denver
welfare
suburb
athletes
trick
diverse
belly
exclusively
yelled
1868
##med
conversion
##ette
1874
internationally
computers
conductor
abilities
sensitive
hello
dispute
measured
globe
rocket
prices
amsterdam
flights
tigers
inn
municipalities
emotion
references
3d
##mus
explains
airlines
manufactured
pm
archaeological
1873
interpretation
devon
comment
##ites
settlements
kissing
absolute
improvement
suite
impressed
barcelona
sullivan
jefferson
towers
jesse
julie
##tin
##lu
grandson
hi
gauge
regard
rings
interviews
trace
raymond
thumb
departments
burns
serial
bulgarian
scores
demonstrated
##ix
1866
kyle
alberta
underneath
romanized
##ward
relieved
acquisition
phrase
cliff
reveals
han
cuts
merger
custom
##dar
nee
gilbert
graduation
##nts
assessment
cafe
difficulty
demands
swung
democrat
jennifer
commons
1940s
grove
##yo
completing
focuses
sum
substitute
bearing
stretch
reception
##py
reflected
essentially
destination
pairs
##ched
survival
resource
##bach
promoting
doubles
messages
tear
##down
##fully
parade
florence
harvey
incumbent
partial
framework
900
pedro
frozen
procedure
olivia
controls
##mic
shelter
personally
temperatures
##od
brisbane
tested
sits
marble
comprehensive
oxygen
leonard
##kov
inaugural
iranian
referring
quarters
attitude
##ivity
mainstream
lined
mars
dakota
norfolk
unsuccessful
##°
explosion
helicopter
congressional
##sing
inspector
bitch
seal
departed
divine
##ters
coaching
examination
punishment
manufacturer
sink
columns
unincorporated
signals
nevada
squeezed
dylan
dining
photos
martial
manuel
eighteen
elevator
brushed
plates
ministers
ivy
congregation
##len
slept
specialized
taxes
curve
restricted
negotiations
likes
statistical
arnold
inspiration
execution
bold
intermediate
significance
margin
ruler
wheels
gothic
intellectual
dependent
listened
eligible
buses
widow
syria
earn
cincinnati
collapsed
recipient
secrets
accessible
philippine
maritime
goddess
clerk
surrender
breaks
playoff
database
##ified
##lon
ideal
beetle
aspect
soap
regulation
strings
expand
anglo
shorter
crosses
retreat
tough
coins
wallace
directions
pressing
##oon
shipping
locomotives
comparison
topics
nephew
##mes
distinction
honors
travelled
sierra
ibn
##over
fortress
sa
recognised
carved
1869
clients
##dan
intent
##mar
coaches
describing
bread
##ington
beaten
northwestern
##ona
merit
youtube
collapse
challenges
em
historians
objective
submitted
virus
attacking
drake
assume
##ere
diseases
marc
stem
leeds
##cus
##ab
farming
glasses
##lock
visits
nowhere
fellowship
relevant
carries
restaurants
experiments
101
constantly
bases
targets
shah
tenth
opponents
verse
territorial
##ira
writings
corruption
##hs
instruction
inherited
reverse
emphasis
##vic
employee
arch
keeps
rabbi
watson
payment
uh
##ala
nancy
##tre
venice
fastest
sexy
banned
adrian
properly
ruth
touchdown
dollar
boards
metre
circles
edges
favour
comments
ok
travels
liberation
scattered
firmly
##ular
holland
permitted
diesel
kenya
den
originated
##ral
demons
resumed
dragged
rider
##rus
servant
blinked
extend
torn
##ias
##sey
input
meal
everybody
cylinder
kinds
camps
##fe
bullet
logic
##wn
croatian
evolved
healthy
fool
chocolate
wise
preserve
pradesh
##ess
respective
1850
##ew
chicken
artificial
gross
corresponding
convicted
cage
caroline
dialogue
##dor
narrative
stranger
mario
br
christianity
failing
trent
commanding
buddhist
1848
maurice
focusing
yale
bike
altitude
##ering
mouse
revised
##sley
veteran
##ig
pulls
theology
crashed
campaigns
legion
##ability
drag
excellence
customer
cancelled
intensity
excuse
##lar
liga
participating
contributing
printing
##burn
variable
##rk
curious
bin
legacy
renaissance
##my
symptoms
binding
vocalist
dancer
##nie
grammar
gospel
democrats
ya
enters
sc
diplomatic
hitler
##ser
clouds
mathematical
quit
defended
oriented
##heim
fundamental
hardware
impressive
equally
convince
confederate
guilt
chuck
sliding
##ware
magnetic
narrowed
petersburg
bulgaria
otto
phd
skill
##ama
reader
hopes
pitcher
reservoir
hearts
automatically
expecting
mysterious
bennett
extensively
imagined
seeds
monitor
fix
##ative
journalism
struggling
signature
ranch
encounter
photographer
observation
protests
##pin
influences
##hr
calendar
##all
cruz
croatia
locomotive
hughes
naturally
shakespeare
basement
hook
uncredited
faded
theories
approaches
dare
phillips
filling
fury
obama
##ain
efficient
arc
deliver
min
raid
breeding
inducted
leagues
efficiency
axis
montana
eagles
##ked
supplied
instructions
karen
picking
indicating
trap
anchor
practically
christians
tomb
vary
occasional
electronics
lords
readers
newcastle
faint
innovation
collect
situations
engagement
160
claude
mixture
##feld
peer
tissue
logo
lean
##ration
°f
floors
##ven
architects
reducing
##our
##ments
rope
1859
ottawa
##har
samples
banking
declaration
proteins
resignation
francois
saudi
advocate
exhibited
armor
twins
divorce
##ras
abraham
reviewed
jo
temporarily
matrix
physically
pulse
curled
##ena
difficulties
bengal
usage
##ban
annie
riders
certificate
##pi
holes
warsaw
distinctive
jessica
##mon
mutual
1857
customs
circular
eugene
removal
loaded
mere
vulnerable
depicted
generations
dame
heir
enormous
lightly
climbing
pitched
lessons
pilots
nepal
ram
google
preparing
brad
louise
renowned
##₂
liam
##ably
plaza
shaw
sophie
brilliant
bills
##bar
##nik
fucking
mainland
server
pleasant
seized
veterans
jerked
fail
beta
brush
radiation
stored
warmth
southeastern
nate
sin
raced
berkeley
joke
athlete
designation
trunk
##low
roland
qualification
archives
heels
artwork
receives
judicial
reserves
##bed
woke
installation
abu
floating
fake
lesser
excitement
interface
concentrated
addressed
characteristic
amanda
saxophone
monk
auto
##bus
releasing
egg
dies
interaction
defender
ce
outbreak
glory
loving
##bert
sequel
consciousness
http
awake
ski
enrolled
##ress
handling
rookie
brow
somebody
biography
warfare
amounts
contracts
presentation
fabric
dissolved
challenged
meter
psychological
lt
elevated
rally
accurate
##tha
hospitals
undergraduate
specialist
venezuela
exhibit
shed
nursing
protestant
fluid
structural
footage
jared
consistent
prey
##ska
succession
reflect
exile
lebanon
wiped
suspect
shanghai
resting
integration
preservation
marvel
variant
pirates
sheep
rounded
capita
sailing
colonies
manuscript
deemed
variations
clarke
functional
emerging
boxing
relaxed
curse
azerbaijan
heavyweight
nickname
editorial
rang
grid
tightened
earthquake
flashed
miguel
rushing
##ches
improvements
boxes
brooks
180
consumption
molecular
felix
societies
repeatedly
variation
aids
civic
graphics
professionals
realm
autonomous
receiver
delayed
workshop
militia
chairs
trump
canyon
##point
harsh
extending
lovely
happiness
##jan
stake
eyebrows
embassy
wellington
hannah
##ella
sony
corners
bishops
swear
cloth
contents
xi
namely
commenced
1854
stanford
nashville
courage
graphic
commitment
garrison
##bin
hamlet
clearing
rebels
attraction
literacy
cooking
ruins
temples
jenny
humanity
celebrate
hasn
freight
sixty
rebel
bastard
##art
newton
##ada
deer
##ges
##ching
smiles
delaware
singers
##ets
approaching
assists
flame
##ph
boulevard
barrel
planted
##ome
pursuit
##sia
consequences
posts
shallow
invitation
rode
depot
ernest
kane
rod
concepts
preston
topic
chambers
striking
blast
arrives
descendants
montgomery
ranges
worlds
##lay
##ari
span
chaos
praise
##ag
fewer
1855
sanctuary
mud
fbi
##ions
programmes
maintaining
unity
harper
bore
handsome
closure
tournaments
thunder
nebraska
linda
facade
puts
satisfied
argentine
dale
cork
dome
panama
##yl
1858
tasks
experts
##ates
feeding
equation
##las
##ida
##tu
engage
bryan
##ax
um
quartet
melody
disbanded
sheffield
blocked
gasped
delay
kisses
maggie
connects
##non
sts
poured
creator
publishers
##we
guided
ellis
extinct
hug
gaining
##ord
complicated
##bility
poll
clenched
investigate
##use
thereby
quantum
spine
cdp
humor
kills
administered
semifinals
##du
encountered
ignore
##bu
commentary
##maker
bother
roosevelt
140
plains
halfway
flowing
cultures
crack
imprisoned
neighboring
airline
##ses
##view
##mate
##ec
gather
wolves
marathon
transformed
##ill
cruise
organisations
carol
punch
exhibitions
numbered
alarm
ratings
daddy
silently
##stein
queens
colours
impression
guidance
liu
tactical
##rat
marshal
della
arrow
##ings
rested
feared
tender
owns
bitter
advisor
escort
##ides
spare
farms
grants
##ene
dragons
encourage
colleagues
cameras
##und
sucked
pile
spirits
prague
statements
suspension
landmark
fence
torture
recreation
bags
permanently
survivors
pond
spy
predecessor
bombing
coup
##og
protecting
transformation
glow
##lands
##book
dug
priests
andrea
feat
barn
jumping
##chen
##ologist
##con
casualties
stern
auckland
pipe
serie
revealing
ba
##bel
trevor
mercy
spectrum
yang
consist
governing
collaborated
possessed
epic
comprises
blew
shane
##ack
lopez
honored
magical
sacrifice
judgment
perceived
hammer
mtv
baronet
tune
das
missionary
sheets
350
neutral
oral
threatening
attractive
shade
aims
seminary
##master
estates
1856
michel
wounds
refugees
manufacturers
##nic
mercury
syndrome
porter
##iya
##din
hamburg
identification
upstairs
purse
widened
pause
cared
breathed
affiliate
santiago
prevented
celtic
fisher
125
recruited
byzantine
reconstruction
farther
##mp
diet
sake
au
spite
sensation
##ert
blank
separation
105
##hon
vladimir
armies
anime
##lie
accommodate
orbit
cult
sofia
archive
##ify
##box
founders
sustained
disorder
honours
northeastern
mia
crops
violet
threats
blanket
fires
canton
followers
southwestern
prototype
voyage
assignment
altered
moderate
protocol
pistol
##eo
questioned
brass
lifting
1852
math
authored
##ual
doug
dimensional
dynamic
##san
1851
pronounced
grateful
quest
uncomfortable
boom
presidency
stevens
relating
politicians
chen
barrier
quinn
diana
mosque
tribal
cheese
palmer
portions
sometime
chester
treasure
wu
bend
download
millions
reforms
registration
##osa
consequently
monitoring
ate
preliminary
brandon
invented
ps
eaten
exterior
intervention
ports
documented
log
displays
lecture
sally
favourite
##itz
vermont
lo
invisible
isle
breed
##ator
journalists
relay
speaks
backward
explore
midfielder
actively
stefan
procedures
cannon
blond
kenneth
centered
servants
chains
libraries
malcolm
essex
henri
slavery
##hal
facts
fairy
coached
cassie
cats
washed
cop
##fi
announcement
item
2000s
vinyl
activated
marco
frontier
growled
curriculum
##das
loyal
accomplished
leslie
ritual
kenny
##00
vii
napoleon
hollow
hybrid
jungle
stationed
friedrich
counted
##ulated
platinum
theatrical
seated
col
rubber
glen
1840
diversity
healing
extends
id
provisions
administrator
columbus
##oe
tributary
te
assured
org
##uous
prestigious
examined
lectures
grammy
ronald
associations
bailey
allan
essays
flute
believing
consultant
proceedings
travelling
1853
kit
kerala
yugoslavia
buddy
methodist
##ith
burial
centres
batman
##nda
discontinued
bo
dock
stockholm
lungs
severely
##nk
citing
manga
##ugh
steal
mumbai
iraqi
robot
celebrity
bride
broadcasts
abolished
pot
joel
overhead
franz
packed
reconnaissance
johann
acknowledged
introduce
handled
doctorate
developments
drinks
alley
palestine
##nis
##aki
proceeded
recover
bradley
grain
patch
afford
infection
nationalist
legendary
##ath
interchange
virtually
gen
gravity
exploration
amber
vital
wishes
powell
doctrine
elbow
screenplay
##bird
contribute
indonesian
pet
creates
##com
enzyme
kylie
discipline
drops
manila
hunger
##ien
layers
suffer
fever
bits
monica
keyboard
manages
##hood
searched
appeals
##bad
testament
grande
reid
##war
beliefs
congo
##ification
##dia
si
requiring
##via
casey
1849
regret
streak
rape
depends
syrian
sprint
pound
tourists
upcoming
pub
##xi
tense
##els
practiced
echo
nationwide
guild
motorcycle
liz
##zar
chiefs
desired
elena
bye
precious
absorbed
relatives
booth
pianist
##mal
citizenship
exhausted
wilhelm
##ceae
##hed
noting
quarterback
urge
hectares
##gue
ace
holly
##tal
blonde
davies
parked
sustainable
stepping
twentieth
airfield
galaxy
nest
chip
##nell
tan
shaft
paulo
requirement
##zy
paradise
tobacco
trans
renewed
vietnamese
##cker
##ju
suggesting
catching
holmes
enjoying
md
trips
colt
holder
butterfly
nerve
reformed
cherry
bowling
trailer
carriage
goodbye
appreciate
toy
joshua
interactive
enabled
involve
##kan
collar
determination
bunch
facebook
recall
shorts
superintendent
episcopal
frustration
giovanni
nineteenth
laser
privately
array
circulation
##ovic
armstrong
deals
painful
permit
discrimination
##wi
aires
retiring
cottage
ni
##sta
horizon
ellen
jamaica
ripped
fernando
chapters
playstation
patron
lecturer
navigation
behaviour
genes
georgian
export
solomon
rivals
swift
seventeen
rodriguez
princeton
independently
sox
1847
arguing
entity
casting
hank
criteria
oakland
geographic
milwaukee
reflection
expanding
conquest
dubbed
##tv
halt
brave
brunswick
doi
arched
curtis
divorced
predominantly
somerset
streams
ugly
zoo
horrible
curved
buenos
fierce
dictionary
vector
theological
unions
handful
stability
chan
punjab
segments
##lly
altar
ignoring
gesture
monsters
pastor
##stone
thighs
unexpected
operators
abruptly
coin
compiled
associates
improving
migration
pin
##ose
compact
collegiate
reserved
##urs
quarterfinals
roster
restore
assembled
hurry
oval
##cies
1846
flags
martha
##del
victories
sharply
##rated
argues
deadly
neo
drawings
symbols
performer
##iel
griffin
restrictions
editing
andrews
java
journals
arabia
compositions
dee
pierce
removing
hindi
casino
runway
civilians
minds
nasa
hotels
##zation
refuge
rent
retain
potentially
conferences
suburban
conducting
##tto
##tions
##tle
descended
massacre
##cal
ammunition
terrain
fork
souls
counts
chelsea
durham
drives
cab
##bank
perth
realizing
palestinian
finn
simpson
##dal
betty
##ule
moreover
particles
cardinals
tent
evaluation
extraordinary
##oid
inscription
##works
wednesday
chloe
maintains
panels
ashley
trucks
##nation
cluster
sunlight
strikes
zhang
##wing
dialect
canon
##ap
tucked
##ws
collecting
##mas
##can
##sville
maker
quoted
evan
franco
aria
buying
cleaning
eva
closet
provision
apollo
clinic
rat
##ez
necessarily
ac
##gle
##ising
venues
flipped
cent
spreading
trustees
checking
authorized
##sco
disappointed
##ado
notion
duration
trumpet
hesitated
topped
brussels
rolls
theoretical
hint
define
aggressive
repeat
wash
peaceful
optical
width
allegedly
mcdonald
strict
copyright
##illa
investors
mar
jam
witnesses
sounding
miranda
michelle
privacy
hugo
harmony
##pp
valid
lynn
glared
nina
102
headquartered
diving
boarding
gibson
##ncy
albanian
marsh
routine
dealt
enhanced
er
intelligent
substance
targeted
enlisted
discovers
spinning
observations
pissed
smoking
rebecca
capitol
visa
varied
costume
seemingly
indies
compensation
surgeon
thursday
arsenal
westminster
suburbs
rid
anglican
##ridge
knots
foods
alumni
lighter
fraser
whoever
portal
scandal
##ray
gavin
advised
instructor
flooding
terrorist
##ale
teenage
interim
senses
duck
teen
thesis
abby
eager
overcome
##ile
newport
glenn
rises
shame
##cc
prompted
priority
forgot
bomber
nicolas
protective
360
cartoon
katherine
breeze
lonely
trusted
henderson
richardson
relax
banner
candy
palms
remarkable
##rio
legends
cricketer
essay
ordained
edmund
rifles
trigger
##uri
##away
sail
alert
1830
audiences
penn
sussex
siblings
pursued
indianapolis
resist
rosa
consequence
succeed
avoided
1845
##ulation
inland
##tie
##nna
counsel
profession
chronicle
hurried
##una
eyebrow
eventual
bleeding
innovative
cure
##dom
committees
accounting
con
scope
hardy
heather
tenor
gut
herald
codes
tore
scales
wagon
##oo
luxury
tin
prefer
fountain
triangle
bonds
darling
convoy
dried
traced
beings
troy
accidentally
slam
findings
smelled
joey
lawyers
outcome
steep
bosnia
configuration
shifting
toll
brook
performers
lobby
philosophical
construct
shrine
aggregate
boot
cox
phenomenon
savage
insane
solely
reynolds
lifestyle
##ima
nationally
holdings
consideration
enable
edgar
mo
mama
##tein
fights
relegation
chances
atomic
hub
conjunction
awkward
reactions
currency
finale
kumar
underwent
steering
elaborate
gifts
comprising
melissa
veins
reasonable
sunshine
chi
solve
trails
inhabited
elimination
ethics
huh
ana
molly
consent
apartments
layout
marines
##ces
hunters
bulk
##oma
hometown
##wall
##mont
cracked
reads
neighbouring
withdrawn
admission
wingspan
damned
anthology
lancashire
brands
batting
forgive
cuban
awful
##lyn
104
dimensions
imagination
##ade
dante
##ship
tracking
desperately
goalkeeper
##yne
groaned
workshops
confident
burton
gerald
milton
circus
uncertain
slope
copenhagen
sophia
fog
philosopher
portraits
accent
cycling
varying
gripped
larvae
garrett
specified
scotia
mature
luther
kurt
rap
##kes
aerial
750
ferdinand
heated
es
transported
##shan
safely
nonetheless
##orn
##gal
motors
demanding
##sburg
startled
##brook
ally
generate
caps
ghana
stained
demo
mentions
beds
ap
afterward
diary
##bling
utility
##iro
richards
1837
conspiracy
conscious
shining
footsteps
observer
cyprus
urged
loyalty
developer
probability
olive
upgraded
gym
miracle
insects
graves
1844
ourselves
hydrogen
amazon
katie
tickets
poets
##pm
planes
##pan
prevention
witnessed
dense
jin
randy
tang
warehouse
monroe
bang
archived
elderly
investigations
alec
granite
mineral
conflicts
controlling
aboriginal
carlo
##zu
mechanics
stan
stark
rhode
skirt
est
##berry
bombs
respected
##horn
imposed
limestone
deny
nominee
memphis
grabbing
disabled
##als
amusement
aa
frankfurt
corn
referendum
varies
slowed
disk
firms
unconscious
incredible
clue
sue
##zhou
twist
##cio
joins
idaho
chad
developers
computing
destroyer
103
mortal
tucker
kingston
choices
yu
carson
1800
os
whitney
geneva
pretend
dimension
staged
plateau
maya
##une
freestyle
##bc
rovers
hiv
##ids
tristan
classroom
prospect
##hus
honestly
diploma
lied
thermal
auxiliary
feast
unlikely
iata
##tel
morocco
pounding
treasury
lithuania
considerably
1841
dish
1812
geological
matching
stumbled
destroying
marched
brien
advances
cake
nicole
belle
settling
measuring
directing
##mie
tuesday
bassist
capabilities
stunned
fraud
torpedo
##list
##phone
anton
wisdom
surveillance
ruined
##ulate
lawsuit
healthcare
theorem
halls
trend
aka
horizontal
dozens
acquire
lasting
swim
hawk
gorgeous
fees
vicinity
decrease
adoption
tactics
##ography
pakistani
##ole
draws
##hall
willie
burke
heath
algorithm
integral
powder
elliott
brigadier
jackie
tate
varieties
darker
##cho
lately
cigarette
specimens
adds
##ree
##ensis
##inger
exploded
finalist
cia
murders
wilderness
arguments
nicknamed
acceptance
onwards
manufacture
robertson
jets
tampa
enterprises
blog
loudly
composers
nominations
1838
ai
malta
inquiry
automobile
hosting
viii
rays
tilted
grief
museums
strategies
furious
euro
equality
cohen
poison
surrey
wireless
governed
ridiculous
moses
##esh
##room
vanished
##ito
barnes
attract
morrison
istanbul
##iness
absent
rotation
petition
janet
##logical
satisfaction
custody
deliberately
observatory
comedian
surfaces
pinyin
novelist
strictly
canterbury
oslo
monks
embrace
ibm
jealous
photograph
continent
dorothy
marina
doc
excess
holden
allegations
explaining
stack
avoiding
lance
storyline
majesty
poorly
spike
dos
bradford
raven
travis
classics
proven
voltage
pillow
fists
butt
1842
interpreted
##car
1839
gage
telegraph
lens
promising
expelled
casual
collector
zones
##min
silly
nintendo
##kh
##bra
downstairs
chef
suspicious
afl
flies
vacant
uganda
pregnancy
condemned
lutheran
estimates
cheap
decree
saxon
proximity
stripped
idiot
deposits
contrary
presenter
magnus
glacier
im
offense
edwin
##ori
upright
##long
bolt
##ois
toss
geographical
##izes
environments
delicate
marking
abstract
xavier
nails
windsor
plantation
occurring
equity
saskatchewan
fears
drifted
sequences
vegetation
revolt
##stic
1843
sooner
fusion
opposing
nato
skating
1836
secretly
ruin
lease
##oc
edit
##nne
flora
anxiety
ruby
##ological
##mia
tel
bout
taxi
emmy
frost
rainbow
compounds
foundations
rainfall
assassination
nightmare
dominican
##win
achievements
deserve
orlando
intact
armenia
##nte
calgary
valentine
106
marion
proclaimed
theodore
bells
courtyard
thigh
gonzalez
console
troop
minimal
monte
everyday
##ence
##if
supporter
terrorism
buck
openly
presbyterian
activists
carpet
##iers
rubbing
uprising
##yi
cute
conceived
legally
##cht
millennium
cello
velocity
ji
rescued
cardiff
1835
rex
concentrate
senators
beard
rendered
glowing
battalions
scouts
competitors
sculptor
catalogue
arctic
ion
raja
bicycle
wow
glancing
lawn
##woman
gentleman
lighthouse
publish
predicted
calculated
##val
variants
##gne
strain
##ui
winston
deceased
##nus
touchdowns
brady
caleb
sinking
echoed
crush
hon
blessed
protagonist
hayes
endangered
magnitude
editors
##tine
estimate
responsibilities
##mel
backup
laying
consumed
sealed
zurich
lovers
frustrated
##eau
ahmed
kicking
mit
treasurer
1832
biblical
refuse
terrified
pump
agrees
genuine
imprisonment
refuses
plymouth
##hen
lou
##nen
tara
trembling
antarctic
ton
learns
##tas
crap
crucial
faction
atop
##borough
wrap
lancaster
odds
hopkins
erik
lyon
##eon
bros
##ode
snap
locality
tips
empress
crowned
cal
acclaimed
chuckled
##ory
clara
sends
mild
towel
##fl
##day
##а
wishing
assuming
interviewed
##bal
##die
interactions
eden
cups
helena
##lf
indie
beck
##fire
batteries
filipino
wizard
parted
##lam
traces
##born
rows
idol
albany
delegates
##ees
##sar
discussions
##ex
notre
instructed
belgrade
highways
suggestion
lauren
possess
orientation
alexandria
abdul
beats
salary
reunion
ludwig
alright
wagner
intimate
pockets
slovenia
hugged
brighton
merchants
cruel
stole
trek
slopes
repairs
enrollment
politically
underlying
promotional
counting
boeing
##bb
isabella
naming
##и
keen
bacteria
listing
separately
belfast
ussr
450
lithuanian
anybody
ribs
sphere
martinez
cock
embarrassed
proposals
fragments
nationals
##fs
##wski
premises
fin
1500
alpine
matched
freely
bounded
jace
sleeve
##af
gaming
pier
populated
evident
##like
frances
flooded
##dle
frightened
pour
trainer
framed
visitor
challenging
pig
wickets
##fold
infected
email
##pes
arose
##aw
reward
ecuador
oblast
vale
ch
shuttle
##usa
bach
rankings
forbidden
cornwall
accordance
salem
consumers
bruno
fantastic
toes
machinery
resolved
julius
remembering
propaganda
iceland
bombardment
tide
contacts
wives
##rah
concerto
macdonald
albania
implement
daisy
tapped
sudan
helmet
angela
mistress
##lic
crop
sunk
finest
##craft
hostile
##ute
##tsu
boxer
fr
paths
adjusted
habit
ballot
supervision
soprano
##zen
bullets
wicked
sunset
regiments
disappear
lamp
performs
app
##gia
##oa
rabbit
digging
incidents
entries
##cion
dishes
##oi
introducing
##ati
##fied
freshman
slot
jill
tackles
baroque
backs
##iest
lone
sponsor
destiny
altogether
convert
##aro
consensus
shapes
demonstration
basically
feminist
auction
artifacts
##bing
strongest
twitter
halifax
2019
allmusic
mighty
smallest
precise
alexandra
viola
##los
##ille
manuscripts
##illo
dancers
ari
managers
monuments
blades
barracks
springfield
maiden
consolidated
electron
##end
berry
airing
wheat
nobel
inclusion
blair
payments
geography
bee
cc
eleanor
react
##hurst
afc
manitoba
##yu
su
lineup
fitness
recreational
investments
airborne
disappointment
##dis
edmonton
viewing
##row
renovation
##cast
infant
bankruptcy
roses
aftermath
pavilion
##yer
carpenter
withdrawal
ladder
##hy
discussing
popped
reliable
agreements
rochester
##abad
curves
bombers
220
rao
reverend
decreased
choosing
107
stiff
consulting
naples
crawford
tracy
ka
ribbon
cops
##lee
crushed
deciding
unified
teenager
accepting
flagship
explorer
poles
sanchez
inspection
revived
skilled
induced
exchanged
flee
locals
tragedy
swallow
loading
hanna
demonstrate
##ela
salvador
flown
contestants
civilization
##ines
wanna
rhodes
fletcher
hector
knocking
considers
##ough
nash
mechanisms
sensed
mentally
walt
unclear
##eus
renovated
madame
##cks
crews
governmental
##hin
undertaken
monkey
##ben
##ato
fatal
armored
copa
caves
governance
grasp
perception
certification
froze
damp
tugged
wyoming
##rg
##ero
newman
##lor
nerves
curiosity
graph
115
##ami
withdraw
tunnels
dull
meredith
moss
exhibits
neighbors
communicate
accuracy
explored
raiders
republicans
secular
kat
superman
penny
criticised
##tch
freed
update
conviction
wade
ham
likewise
delegation
gotta
doll
promises
technological
myth
nationality
resolve
convent
##mark
sharon
dig
sip
coordinator
entrepreneur
fold
##dine
capability
councillor
synonym
blown
swan
cursed
1815
jonas
haired
sofa
canvas
keeper
rivalry
##hart
rapper
speedway
swords
postal
maxwell
estonia
potter
recurring
##nn
##ave
errors
##oni
cognitive
1834
##²
claws
nadu
roberto
bce
wrestler
ellie
##ations
infinite
ink
##tia
presumably
finite
staircase
108
noel
patricia
nacional
##cation
chill
eternal
tu
preventing
prussia
fossil
limbs
##logist
ernst
frog
perez
rene
##ace
pizza
prussian
##ios
##vy
molecules
regulatory
answering
opinions
sworn
lengths
supposedly
hypothesis
upward
habitats
seating
ancestors
drank
yield
hd
synthesis
researcher
modest
##var
mothers
peered
voluntary
homeland
##the
acclaim
##igan
static
valve
luxembourg
alto
carroll
fe
receptor
norton
ambulance
##tian
johnston
catholics
depicting
jointly
elephant
gloria
mentor
badge
ahmad
distinguish
remarked
councils
precisely
allison
advancing
detection
crowded
##10
cooperative
ankle
mercedes
dagger
surrendered
pollution
commit
subway
jeffrey
lesson
sculptures
provider
##fication
membrane
timothy
rectangular
fiscal
heating
teammate
basket
particle
anonymous
deployment
##ple
missiles
courthouse
proportion
shoe
sec
##ller
complaints
forbes
blacks
abandon
remind
sizes
overwhelming
autobiography
natalie
##awa
risks
contestant
countryside
babies
scorer
invaded
enclosed
proceed
hurling
disorders
##cu
reflecting
continuously
cruiser
graduates
freeway
investigated
ore
deserved
maid
blocking
phillip
jorge
shakes
dove
mann
variables
lacked
burden
accompanying
que
consistently
organizing
provisional
complained
endless
##rm
tubes
juice
georges
krishna
mick
labels
thriller
##uch
laps
arcade
sage
snail
##table
shannon
fi
laurence
seoul
vacation
presenting
hire
churchill
surprisingly
prohibited
savannah
technically
##oli
170
##lessly
testimony
suited
speeds
toys
romans
mlb
flowering
measurement
talented
kay
settings
charleston
expectations
shattered
achieving
triumph
ceremonies
portsmouth
lanes
mandatory
loser
stretching
cologne
realizes
seventy
cornell
careers
webb
##ulating
americas
budapest
ava
suspicion
##ison
yo
conrad
##hai
sterling
jessie
rector
##az
1831
transform
organize
loans
christine
volcanic
warrant
slender
summers
subfamily
newer
danced
dynamics
rhine
proceeds
heinrich
gastropod
commands
sings
facilitate
easter
ra
positioned
responses
expense
fruits
yanked
imported
25th
velvet
vic
primitive
tribune
baldwin
neighbourhood
donna
rip
hay
pr
##uro
1814
espn
welcomed
##aria
qualifier
glare
highland
timing
##cted
shells
eased
geometry
louder
exciting
slovakia
##sion
##iz
##lot
savings
prairie
##ques
marching
rafael
tonnes
##lled
curtain
preceding
shy
heal
greene
worthy
##pot
detachment
bury
sherman
##eck
reinforced
seeks
bottles
contracted
duchess
outfit
walsh
##sc
mickey
##ase
geoffrey
archer
squeeze
dawson
eliminate
invention
##enberg
neal
##eth
stance
dealer
coral
maple
retire
polo
simplified
##ht
1833
hid
watts
backwards
jules
##oke
genesis
mt
frames
rebounds
burma
woodland
moist
santos
whispers
drained
subspecies
##aa
streaming
ulster
burnt
correspondence
maternal
gerard
denis
stealing
##load
genius
duchy
##oria
inaugurated
momentum
suits
placement
sovereign
clause
thames
##hara
confederation
reservation
sketch
yankees
lets
rotten
charm
hal
verses
ultra
commercially
dot
salon
citation
adopt
winnipeg
mist
allocated
cairo
##boy
jenkins
interference
objectives
##wind
1820
portfolio
armoured
sectors
##eh
initiatives
##world
integrity
exercises
robe
tap
ab
gazed
##tones
distracted
rulers
111
favorable
jerome
tended
cart
factories
##eri
diplomat
valued
gravel
charitable
##try
calvin
exploring
chang
shepherd
terrace
pdf
pupil
##ural
reflects
ups
##rch
governors
shelf
depths
##nberg
trailed
crest
tackle
##nian
##ats
hatred
##kai
clare
makers
ethiopia
longtime
detected
embedded
lacking
slapped
rely
thomson
anticipation
iso
morton
successive
agnes
screenwriter
straightened
philippe
playwright
haunted
licence
iris
intentions
sutton
112
logical
correctly
##weight
branded
licked
tipped
silva
ricky
narrator
requests
##ents
greeted
supernatural
cow
##wald
lung
refusing
employer
strait
gaelic
liner
##piece
zoe
sabha
##mba
driveway
harvest
prints
bates
reluctantly
threshold
algebra
ira
wherever
coupled
240
assumption
picks
##air
designers
raids
gentlemen
##ean
roller
blowing
leipzig
locks
screw
dressing
strand
##lings
scar
dwarf
depicts
##nu
nods
##mine
differ
boris
##eur
yuan
flip
##gie
mob
invested
questioning
applying
##ture
shout
##sel
gameplay
blamed
illustrations
bothered
weakness
rehabilitation
##of
##zes
envelope
rumors
miners
leicester
subtle
kerry
##ico
ferguson
##fu
premiership
ne
##cat
bengali
prof
catches
remnants
dana
##rily
shouting
presidents
baltic
ought
ghosts
dances
sailors
shirley
fancy
dominic
##bie
madonna
##rick
bark
buttons
gymnasium
ashes
liver
toby
oath
providence
doyle
evangelical
nixon
cement
carnegie
embarked
hatch
surroundings
guarantee
needing
pirate
essence
##bee
filter
crane
hammond
projected
immune
percy
twelfth
##ult
regent
doctoral
damon
mikhail
##ichi
lu
critically
elect
realised
abortion
acute
screening
mythology
steadily
##fc
frown
nottingham
kirk
wa
minneapolis
##rra
module
algeria
mc
nautical
encounters
surprising
statues
availability
shirts
pie
alma
brows
munster
mack
soup
crater
tornado
sanskrit
cedar
explosive
bordered
dixon
planets
stamp
exam
happily
##bble
carriers
kidnapped
##vis
accommodation
emigrated
##met
knockout
correspondent
violation
profits
peaks
lang
specimen
agenda
ancestry
pottery
spelling
equations
obtaining
ki
linking
1825
debris
asylum
##20
buddhism
teddy
##ants
gazette
##nger
##sse
dental
eligibility
utc
fathers
averaged
zimbabwe
francesco
coloured
hissed
translator
lynch
mandate
humanities
mackenzie
uniforms
lin
##iana
##gio
asset
mhz
fitting
samantha
genera
wei
rim
beloved
shark
riot
entities
expressions
indo
carmen
slipping
owing
abbot
neighbor
sidney
##av
rats
recommendations
encouraging
squadrons
anticipated
commanders
conquered
##oto
donations
diagnosed
##mond
divide
##iva
guessed
decoration
vernon
auditorium
revelation
conversations
##kers
##power
herzegovina
dash
alike
protested
lateral
herman
accredited
mg
##gent
freeman
mel
fiji
crow
crimson
##rine
livestock
##pped
humanitarian
bored
oz
whip
##lene
##ali
legitimate
alter
grinning
spelled
anxious
oriental
wesley
##nin
##hole
carnival
controller
detect
##ssa
bowed
educator
kosovo
macedonia
##sin
occupy
mastering
stephanie
janeiro
para
unaware
nurses
noon
135
cam
hopefully
ranger
combine
sociology
polar
rica
##eer
neill
##sman
holocaust
##ip
doubled
lust
1828
109
decent
cooling
unveiled
##card
1829
nsw
homer
chapman
meyer
##gin
dive
mae
reagan
expertise
##gled
darwin
brooke
sided
prosecution
investigating
comprised
petroleum
genres
reluctant
differently
trilogy
johns
vegetables
corpse
highlighted
lounge
pension
unsuccessfully
elegant
aided
ivory
beatles
amelia
cain
dubai
sunny
immigrant
babe
click
##nder
underwater
pepper
combining
mumbled
atlas
horns
accessed
ballad
physicians
homeless
gestured
rpm
freak
louisville
corporations
patriots
prizes
rational
warn
modes
decorative
overnight
din
troubled
phantom
##ort
monarch
sheer
##dorf
generals
guidelines
organs
addresses
##zon
enhance
curling
parishes
cord
##kie
linux
caesar
deutsche
bavaria
##bia
coleman
cyclone
##eria
bacon
petty
##yama
##old
hampton
diagnosis
1824
throws
complexity
rita
disputed
##₃
pablo
##sch
marketed
trafficking
##ulus
examine
plague
formats
##oh
vault
faithful
##bourne
webster
##ox
highlights
##ient
##ann
phones
vacuum
sandwich
modeling
##gated
bolivia
clergy
qualities
isabel
##nas
##ars
wears
screams
reunited
annoyed
bra
##ancy
##rate
differential
transmitter
tattoo
container
poker
##och
excessive
resides
cowboys
##tum
augustus
trash
providers
statute
retreated
balcony
reversed
void
storey
preceded
masses
leap
laughs
neighborhoods
wards
schemes
falcon
santo
battlefield
pad
ronnie
thread
lesbian
venus
##dian
beg
sandstone
daylight
punched
gwen
analog
stroked
wwe
acceptable
measurements
dec
toxic
##kel
adequate
surgical
economist
parameters
varsity
##sberg
quantity
ella
##chy
##rton
countess
generating
precision
diamonds
expressway
ga
##ı
1821
uruguay
talents
galleries
expenses
scanned
colleague
outlets
ryder
lucien
##ila
paramount
##bon
syracuse
dim
fangs
gown
sweep
##sie
toyota
missionaries
websites
##nsis
sentences
adviser
val
trademark
spells
##plane
patience
starter
slim
##borg
toe
incredibly
shoots
elliot
nobility
##wyn
cowboy
endorsed
gardner
tendency
persuaded
organisms
emissions
kazakhstan
amused
boring
chips
themed
##hand
llc
constantinople
chasing
systematic
guatemala
borrowed
erin
carey
##hard
highlands
struggles
1810
##ifying
##ced
wong
exceptions
develops
enlarged
kindergarten
castro
##ern
##rina
leigh
zombie
juvenile
##most
consul
##nar
sailor
hyde
clarence
intensive
pinned
nasty
useless
jung
clayton
stuffed
exceptional
ix
apostolic
230
transactions
##dge
exempt
swinging
cove
religions
##ash
shields
dairy
bypass
190
pursuing
bug
joyce
bombay
chassis
southampton
chat
interact
redesignated
##pen
nascar
pray
salmon
rigid
regained
malaysian
grim
publicity
constituted
capturing
toilet
delegate
purely
tray
drift
loosely
striker
weakened
trinidad
mitch
itv
defines
transmitted
ming
scarlet
nodding
fitzgerald
fu
narrowly
sp
tooth
standings
virtue
##₁
##wara
##cting
chateau
gloves
lid
##nel
hurting
conservatory
##pel
sinclair
reopened
sympathy
nigerian
strode
advocated
optional
chronic
discharge
##rc
suck
compatible
laurel
stella
shi
fails
wage
dodge
128
informal
sorts
levi
buddha
villagers
##aka
chronicles
heavier
summoned
gateway
3000
eleventh
jewelry
translations
accordingly
seas
##ency
fiber
pyramid
cubic
dragging
##ista
caring
##ops
android
contacted
lunar
##dt
kai
lisbon
patted
1826
sacramento
theft
madagascar
subtropical
disputes
ta
holidays
piper
willow
mare
cane
itunes
newfoundland
benny
companions
dong
raj
observe
roar
charming
plaque
tibetan
fossils
enacted
manning
bubble
tina
tanzania
##eda
##hir
funk
swamp
deputies
cloak
ufc
scenario
par
scratch
metals
anthem
guru
engaging
specially
##boat
dialects
nineteen
cecil
duet
disability
messenger
unofficial
##lies
defunct
eds
moonlight
drainage
surname
puzzle
honda
switching
conservatives
mammals
knox
broadcaster
sidewalk
cope
##ried
benson
princes
peterson
##sal
bedford
sharks
eli
wreck
alberto
gasp
archaeology
lgbt
teaches
securities
madness
compromise
waving
coordination
davidson
visions
leased
possibilities
eighty
jun
fernandez
enthusiasm
assassin
sponsorship
reviewer
kingdoms
estonian
laboratories
##fy
##nal
applies
verb
celebrations
##zzo
rowing
lightweight
sadness
submit
mvp
balanced
dude
##vas
explicitly
metric
magnificent
mound
brett
mohammad
mistakes
irregular
##hing
##ass
sanders
betrayed
shipped
surge
##enburg
reporters
termed
georg
pity
verbal
bulls
abbreviated
enabling
appealed
##are
##atic
sicily
sting
heel
sweetheart
bart
spacecraft
brutal
monarchy
##tter
aberdeen
cameo
diane
##ub
survivor
clyde
##aries
complaint
##makers
clarinet
delicious
chilean
karnataka
coordinates
1818
panties
##rst
pretending
ar
dramatically
kiev
bella
tends
distances
113
catalog
launching
instances
telecommunications
portable
lindsay
vatican
##eim
angles
aliens
marker
stint
screens
bolton
##rne
judy
wool
benedict
plasma
europa
spark
imaging
filmmaker
swiftly
##een
contributor
##nor
opted
stamps
apologize
financing
butter
gideon
sophisticated
alignment
avery
chemicals
yearly
speculation
prominence
professionally
##ils
immortal
institutional
inception
wrists
identifying
tribunal
derives
gains
##wo
papal
preference
linguistic
vince
operative
brewery
##ont
unemployment
boyd
##ured
##outs
albeit
prophet
1813
bi
##rr
##face
##rad
quarterly
asteroid
cleaned
radius
temper
##llen
telugu
jerk
viscount
menu
##ote
glimpse
##aya
yacht
hawaiian
baden
##rl
laptop
readily
##gu
monetary
offshore
scots
watches
##yang
##arian
upgrade
needle
xbox
lea
encyclopedia
flank
fingertips
##pus
delight
teachings
confirm
roth
beaches
midway
winters
##iah
teasing
daytime
beverly
gambling
bonnie
##backs
regulated
clement
hermann
tricks
knot
##shing
##uring
##vre
detached
ecological
owed
specialty
byron
inventor
bats
stays
screened
unesco
midland
trim
affection
##ander
##rry
jess
thoroughly
feedback
##uma
chennai
strained
heartbeat
wrapping
overtime
pleaded
##sworth
mon
leisure
oclc
##tate
##ele
feathers
angelo
thirds
nuts
surveys
clever
gill
commentator
##dos
darren
rides
gibraltar
##nc
##mu
dissolution
dedication
shin
meals
saddle
elvis
reds
chaired
taller
appreciation
functioning
niece
favored
advocacy
robbie
criminals
suffolk
yugoslav
passport
constable
congressman
hastings
vera
##rov
consecrated
sparks
ecclesiastical
confined
##ovich
muller
floyd
nora
1822
paved
1827
cumberland
ned
saga
spiral
##flow
appreciated
yi
collaborative
treating
similarities
feminine
finishes
##ib
jade
import
##nse
##hot
champagne
mice
securing
celebrities
helsinki
attributes
##gos
cousins
phases
ache
lucia
gandhi
submission
vicar
spear
shine
tasmania
biting
detention
constitute
tighter
seasonal
##gus
terrestrial
matthews
##oka
effectiveness
parody
philharmonic
##onic
1816
strangers
encoded
consortium
guaranteed
regards
shifts
tortured
collision
supervisor
inform
broader
insight
theaters
armour
emeritus
blink
incorporates
mapping
##50
##ein
handball
flexible
##nta
substantially
generous
thief
##own
carr
loses
1793
prose
ucla
romeo
generic
metallic
realization
damages
mk
commissioners
zach
default
##ther
helicopters
lengthy
stems
spa
partnered
spectators
rogue
indication
penalties
teresa
1801
sen
##tric
dalton
##wich
irving
photographic
##vey
dell
deaf
peters
excluded
unsure
##vable
patterson
crawled
##zio
resided
whipped
latvia
slower
ecole
pipes
employers
maharashtra
comparable
va
textile
pageant
##gel
alphabet
binary
irrigation
chartered
choked
antoine
offs
waking
supplement
##wen
quantities
demolition
regain
locate
urdu
folks
alt
114
##mc
scary
andreas
whites
##ava
classrooms
mw
aesthetic
publishes
valleys
guides
cubs
johannes
bryant
conventions
affecting
##itt
drain
awesome
isolation
prosecutor
ambitious
apology
captive
downs
atmospheric
lorenzo
aisle
beef
foul
##onia
kidding
composite
disturbed
illusion
natives
##ffer
emi
rockets
riverside
wartime
painters
adolf
melted
##ail
uncertainty
simulation
hawks
progressed
meantime
builder
spray
breach
unhappy
regina
russians
##urg
determining
##tation
tram
1806
##quin
aging
##12
1823
garion
rented
mister
diaz
terminated
clip
1817
depend
nervously
disco
owe
defenders
shiva
notorious
disbelief
shiny
worcester
##gation
##yr
trailing
undertook
islander
belarus
limitations
watershed
fuller
overlooking
utilized
raphael
1819
synthetic
breakdown
klein
##nate
moaned
memoir
lamb
practicing
##erly
cellular
arrows
exotic
##graphy
witches
117
charted
rey
hut
hierarchy
subdivision
freshwater
giuseppe
aloud
reyes
qatar
marty
sideways
utterly
sexually
jude
prayers
mccarthy
softball
blend
damien
##gging
##metric
wholly
erupted
lebanese
negro
revenues
tasted
comparative
teamed
transaction
labeled
maori
sovereignty
parkway
trauma
gran
malay
121
advancement
descendant
2020
buzz
salvation
inventory
symbolic
##making
antarctica
mps
##gas
##bro
mohammed
myanmar
holt
submarines
tones
##lman
locker
patriarch
bangkok
emerson
remarks
predators
kin
afghan
confession
norwich
rental
emerge
advantages
##zel
rca
##hold
shortened
storms
aidan
##matic
autonomy
compliance
##quet
dudley
atp
##osis
1803
motto
documentation
summary
professors
spectacular
christina
archdiocese
flashing
innocence
remake
##dell
psychic
reef
scare
employ
rs
sticks
meg
gus
leans
##ude
accompany
bergen
tomas
##iko
doom
wages
pools
##nch
##bes
breasts
scholarly
alison
outline
brittany
breakthrough
willis
realistic
##cut
##boro
competitor
##stan
pike
picnic
icon
designing
commercials
washing
villain
skiing
micro
costumes
auburn
halted
executives
##hat
logistics
cycles
vowel
applicable
barrett
exclaimed
eurovision
eternity
ramon
##umi
##lls
modifications
sweeping
disgust
##uck
torch
aviv
ensuring
rude
dusty
sonic
donovan
outskirts
cu
pathway
##band
##gun
##lines
disciplines
acids
cadet
paired
##40
sketches
##sive
marriages
##⁺
folding
peers
slovak
implies
admired
##beck
1880s
leopold
instinct
attained
weston
megan
horace
##ination
dorsal
ingredients
evolutionary
##its
complications
deity
lethal
brushing
levy
deserted
institutes
posthumously
delivering
telescope
coronation
motivated
rapids
luc
flicked
pays
volcano
tanner
weighed
##nica
crowds
frankie
gifted
addressing
granddaughter
winding
##rna
constantine
gomez
##front
landscapes
rudolf
anthropology
slate
werewolf
##lio
astronomy
circa
rouge
dreaming
sack
knelt
drowned
naomi
prolific
tracked
freezing
herb
##dium
agony
randall
twisting
wendy
deposit
touches
vein
wheeler
##bbled
##bor
batted
retaining
tire
presently
compare
specification
daemon
nigel
##grave
merry
recommendation
czechoslovakia
sandra
ng
roma
##sts
lambert
inheritance
sheikh
winchester
cries
examining
##yle
comeback
cuisine
nave
##iv
ko
retrieve
tomatoes
barker
polished
defining
irene
lantern
personalities
begging
tract
swore
1809
175
##gic
omaha
brotherhood
##rley
haiti
##ots
exeter
##ete
##zia
steele
dumb
pearson
210
surveyed
elisabeth
trends
##ef
fritz
##rf
premium
bugs
fraction
calmly
viking
##birds
tug
inserted
unusually
##ield
confronted
distress
crashing
brent
turks
resign
##olo
cambodia
gabe
sauce
##kal
evelyn
116
extant
clusters
quarry
teenagers
luna
##lers
##ister
affiliation
drill
##ashi
panthers
scenic
libya
anita
strengthen
inscriptions
##cated
lace
sued
judith
riots
##uted
mint
##eta
preparations
midst
dub
challenger
##vich
mock
cf
displaced
wicket
breaths
enables
schmidt
analyst
##lum
ag
highlight
automotive
axe
josef
newark
sufficiently
resembles
50th
##pal
flushed
mum
traits
##ante
commodore
incomplete
warming
titular
ceremonial
ethical
118
celebrating
eighteenth
cao
lima
medalist
mobility
strips
snakes
##city
miniature
zagreb
barton
escapes
umbrella
automated
doubted
differs
cooled
georgetown
dresden
cooked
fade
wyatt
rna
jacobs
carlton
abundant
stereo
boost
madras
inning
##hia
spur
ip
malayalam
begged
osaka
groan
escaping
charging
dose
vista
##aj
bud
papa
communists
advocates
edged
tri
##cent
resemble
peaking
necklace
fried
montenegro
saxony
goose
glances
stuttgart
curator
recruit
grocery
sympathetic
##tting
##fort
127
lotus
randolph
ancestor
##rand
succeeding
jupiter
1798
macedonian
##heads
hiking
1808
handing
fischer
##itive
garbage
node
##pies
prone
singular
papua
inclined
attractions
italia
pouring
motioned
grandma
garnered
jacksonville
corp
ego
ringing
aluminum
##hausen
ordering
##foot
drawer
traders
synagogue
##play
##kawa
resistant
wandering
fragile
fiona
teased
var
hardcore
soaked
jubilee
decisive
exposition
mercer
poster
valencia
hale
kuwait
1811
##ises
##wr
##eed
tavern
gamma
122
johan
##uer
airways
amino
gil
##ury
vocational
domains
torres
##sp
generator
folklore
outcomes
##keeper
canberra
shooter
fl
beams
confrontation
##lling
##gram
feb
aligned
forestry
pipeline
jax
motorway
conception
decay
##tos
coffin
##cott
stalin
1805
escorted
minded
##nam
sitcom
purchasing
twilight
veronica
additions
passive
tensions
straw
123
frequencies
1804
refugee
cultivation
##iate
christie
clary
bulletin
crept
disposal
##rich
##zong
processor
crescent
##rol
bmw
emphasized
whale
nazis
aurora
##eng
dwelling
hauled
sponsors
toledo
mega
ideology
theatres
tessa
cerambycidae
saves
turtle
cone
suspects
kara
rusty
yelling
greeks
mozart
shades
cocked
participant
##tro
shire
spit
freeze
necessity
##cos
inmates
nielsen
councillors
loaned
uncommon
omar
peasants
botanical
offspring
daniels
formations
jokes
1794
pioneers
sigma
licensing
##sus
wheelchair
polite
1807
liquor
pratt
trustee
##uta
forewings
balloon
##zz
kilometre
camping
explicit
casually
shawn
foolish
teammates
nm
hassan
carrie
judged
satisfy
vanessa
knives
selective
cnn
flowed
##lice
eclipse
stressed
eliza
mathematician
cease
cultivated
##roy
commissions
browns
##ania
destroyers
sheridan
meadow
##rius
minerals
##cial
downstream
clash
gram
memoirs
ventures
baha
seymour
archie
midlands
edith
fare
flynn
invite
canceled
tiles
stabbed
boulder
incorporate
amended
camden
facial
mollusk
unreleased
descriptions
yoga
grabs
550
raises
ramp
shiver
##rose
coined
pioneering
tunes
qing
warwick
tops
119
melanie
giles
##rous
wandered
##inal
annexed
nov
30th
unnamed
##ished
organizational
airplane
normandy
stoke
whistle
blessing
violations
chased
holders
shotgun
##ctic
outlet
reactor
##vik
tires
tearing
shores
fortified
mascot
constituencies
nc
columnist
productive
tibet
##rta
lineage
hooked
oct
tapes
judging
cody
##gger
hansen
kashmir
triggered
##eva
solved
cliffs
##tree
resisted
anatomy
protesters
transparent
implied
##iga
injection
mattress
excluding
##mbo
defenses
helpless
devotion
##elli
growl
liberals
weber
phenomena
atoms
plug
##iff
mortality
apprentice
howe
convincing
aaa
swimmer
barber
leone
promptly
sodium
def
nowadays
arise
##oning
gloucester
corrected
dignity
norm
erie
##ders
elders
evacuated
sylvia
compression
##yar
hartford
pose
backpack
reasoning
accepts
24th
wipe
millimetres
marcel
##oda
dodgers
albion
1790
overwhelmed
aerospace
oaks
1795
showcase
acknowledge
recovering
nolan
ashe
hurts
geology
fashioned
disappearance
farewell
swollen
shrug
marquis
wimbledon
124
rue
1792
commemorate
reduces
experiencing
inevitable
calcutta
intel
##court
murderer
sticking
fisheries
imagery
bloom
280
brake
##inus
gustav
hesitation
memorable
po
viral
beans
accidents
tunisia
antenna
spilled
consort
treatments
aye
perimeter
##gard
donation
hostage
migrated
banker
addiction
apex
lil
trout
##ously
conscience
##nova
rams
sands
genome
passionate
troubles
##lets
##set
amid
##ibility
##ret
higgins
exceed
vikings
##vie
payne
##zan
muscular
##ste
defendant
sucking
##wal
ibrahim
fuselage
claudia
vfl
europeans
snails
interval
##garh
preparatory
statewide
tasked
lacrosse
viktor
##lation
angola
##hra
flint
implications
employs
teens
patrons
stall
weekends
barriers
scrambled
nucleus
tehran
jenna
parsons
lifelong
robots
displacement
5000
##bles
precipitation
##gt
knuckles
clutched
1802
marrying
ecology
marx
accusations
declare
scars
kolkata
mat
meadows
bermuda
skeleton
finalists
vintage
crawl
coordinate
affects
subjected
orchestral
mistaken
##tc
mirrors
dipped
relied
260
arches
candle
##nick
incorporating
wildly
fond
basilica
owl
fringe
rituals
whispering
stirred
feud
tertiary
slick
goat
honorable
whereby
skip
ricardo
stripes
parachute
adjoining
submerged
synthesizer
##gren
intend
positively
ninety
phi
beaver
partition
fellows
alexis
prohibition
carlisle
bizarre
fraternity
##bre
doubts
icy
cbc
aquatic
sneak
sonny
combines
airports
crude
supervised
spatial
merge
alfonso
##bic
corrupt
scan
undergo
##ams
disabilities
colombian
comparing
dolphins
perkins
##lish
reprinted
unanimous
bounced
hairs
underworld
midwest
semester
bucket
paperback
miniseries
coventry
demise
##leigh
demonstrations
sensor
rotating
yan
##hler
arrange
soils
##idge
hyderabad
labs
##dr
brakes
grandchildren
##nde
negotiated
rover
ferrari
continuation
directorate
augusta
stevenson
counterpart
gore
##rda
nursery
rican
ave
collectively
broadly
pastoral
repertoire
asserted
discovering
nordic
styled
fiba
cunningham
harley
middlesex
survives
tumor
tempo
zack
aiming
lok
urgent
##rade
##nto
devils
##ement
contractor
turin
##wl
##ool
bliss
repaired
simmons
moan
astronomical
cr
negotiate
lyric
1890s
lara
bred
clad
angus
pbs
##ience
engineered
posed
##lk
hernandez
possessions
elbows
psychiatric
strokes
confluence
electorate
lifts
campuses
lava
alps
##ep
##ution
##date
physicist
woody
##page
##ographic
##itis
juliet
reformation
sparhawk
320
complement
suppressed
jewel
##½
floated
##kas
continuity
sadly
##ische
inability
melting
scanning
paula
flour
judaism
safer
vague
##lm
solving
curb
##stown
financially
gable
bees
expired
miserable
cassidy
dominion
1789
cupped
145
robbery
facto
amos
warden
resume
tallest
marvin
ing
pounded
usd
declaring
gasoline
##aux
darkened
270
650
sophomore
##mere
erection
gossip
televised
risen
dial
##eu
pillars
##link
passages
profound
##tina
arabian
ashton
silicon
nail
##ead
##lated
##wer
##hardt
fleming
firearms
ducked
circuits
blows
waterloo
titans
##lina
atom
fireplace
cheshire
financed
activation
algorithms
##zzi
constituent
catcher
cherokee
partnerships
sexuality
platoon
tragic
vivian
guarded
whiskey
meditation
poetic
##late
##nga
##ake
porto
listeners
dominance
kendra
mona
chandler
factions
22nd
salisbury
attitudes
derivative
##ido
##haus
intake
paced
javier
illustrator
barrels
bias
cockpit
burnett
dreamed
ensuing
##anda
receptors
someday
hawkins
mattered
##lal
slavic
1799
jesuit
cameroon
wasted
tai
wax
lowering
victorious
freaking
outright
hancock
librarian
sensing
bald
calcium
myers
tablet
announcing
barack
shipyard
pharmaceutical
##uan
greenwich
flush
medley
patches
wolfgang
pt
speeches
acquiring
exams
nikolai
##gg
hayden
kannada
##type
reilly
##pt
waitress
abdomen
devastated
capped
pseudonym
pharmacy
fulfill
paraguay
1796
clicked
##trom
archipelago
syndicated
##hman
lumber
orgasm
rejection
clifford
lorraine
advent
mafia
rodney
brock
##ght
##used
##elia
cassette
chamberlain
despair
mongolia
sensors
developmental
upstream
##eg
##alis
spanning
165
trombone
basque
seeded
interred
renewable
rhys
leapt
revision
molecule
##ages
chord
vicious
nord
shivered
23rd
arlington
debts
corpus
sunrise
bays
blackburn
centimetres
##uded
shuddered
gm
strangely
gripping
cartoons
isabelle
orbital
##ppa
seals
proving
##lton
refusal
strengthened
bust
assisting
baghdad
batsman
portrayal
mara
pushes
spears
og
##cock
reside
nathaniel
brennan
1776
confirmation
caucus
##worthy
markings
yemen
nobles
ku
lazy
viewer
catalan
encompasses
sawyer
##fall
sparked
substances
patents
braves
arranger
evacuation
sergio
persuade
dover
tolerance
penguin
cum
jockey
insufficient
townships
occupying
declining
plural
processed
projection
puppet
flanders
introduces
liability
##yon
gymnastics
antwerp
taipei
hobart
candles
jeep
wes
observers
126
chaplain
bundle
glorious
##hine
hazel
flung
sol
excavations
dumped
stares
sh
bangalore
triangular
icelandic
intervals
expressing
turbine
##vers
songwriting
crafts
##igo
jasmine
ditch
rite
##ways
entertaining
comply
sorrow
wrestlers
basel
emirates
marian
rivera
helpful
##some
caution
downward
networking
##atory
##tered
darted
genocide
emergence
replies
specializing
spokesman
convenient
unlocked
fading
augustine
concentrations
resemblance
elijah
investigator
andhra
##uda
promotes
bean
##rrell
fleeing
wan
simone
announcer
##ame
##bby
lydia
weaver
132
residency
modification
##fest
stretches
##ast
alternatively
nat
lowe
lacks
##ented
pam
tile
concealed
inferior
abdullah
residences
tissues
vengeance
##ided
moisture
peculiar
groove
zip
bologna
jennings
ninja
oversaw
zombies
pumping
batch
livingston
emerald
installations
1797
peel
nitrogen
rama
##fying
##star
schooling
strands
responding
werner
##ost
lime
casa
accurately
targeting
##rod
underway
##uru
hemisphere
lester
##yard
occupies
2d
griffith
angrily
reorganized
##owing
courtney
deposited
##dd
##30
estadio
##ifies
dunn
exiled
##ying
checks
##combe
##о
##fly
successes
unexpectedly
blu
assessed
##flower
##ه
observing
sacked
spiders
kn
##tail
mu
nodes
prosperity
audrey
divisional
155
broncos
tangled
adjust
feeds
erosion
paolo
surf
directory
snatched
humid
admiralty
screwed
gt
reddish
##nese
modules
trench
lamps
bind
leah
bucks
competes
##nz
##form
transcription
##uc
isles
violently
clutching
pga
cyclist
inflation
flats
ragged
unnecessary
##hian
stubborn
coordinated
harriet
baba
disqualified
330
insect
wolfe
##fies
reinforcements
rocked
duel
winked
embraced
bricks
##raj
hiatus
defeats
pending
brightly
jealousy
##xton
##hm
##uki
lena
gdp
colorful
##dley
stein
kidney
##shu
underwear
wanderers
##haw
##icus
guardians
m³
roared
habits
##wise
permits
gp
uranium
punished
disguise
bundesliga
elise
dundee
erotic
partisan
pi
collectors
float
individually
rendering
behavioral
bucharest
ser
hare
valerie
corporal
nutrition
proportional
##isa
immense
##kis
pavement
##zie
##eld
sutherland
crouched
1775
##lp
suzuki
trades
endurance
operas
crosby
prayed
priory
rory
socially
##urn
gujarat
##pu
walton
cube
pasha
privilege
lennon
floods
thorne
waterfall
nipple
scouting
approve
##lov
minorities
voter
dwight
extensions
assure
ballroom
slap
dripping
privileges
rejoined
confessed
demonstrating
patriotic
yell
investor
##uth
pagan
slumped
squares
##cle
##kins
confront
bert
embarrassment
##aid
aston
urging
sweater
starr
yuri
brains
williamson
commuter
mortar
structured
selfish
exports
##jon
cds
##him
unfinished
##rre
mortgage
destinations
##nagar
canoe
solitary
buchanan
delays
magistrate
fk
##pling
motivation
##lier
##vier
recruiting
assess
##mouth
malik
antique
1791
pius
rahman
reich
tub
zhou
smashed
airs
galway
xii
conditioning
honduras
discharged
dexter
##pf
lionel
129
debates
lemon
tiffany
volunteered
dom
dioxide
procession
devi
sic
tremendous
advertisements
colts
transferring
verdict
hanover
decommissioned
utter
relate
pac
racism
##top
beacon
limp
similarity
terra
occurrence
ant
##how
becky
capt
updates
armament
richie
pal
##graph
halloween
mayo
##ssen
##bone
cara
serena
fcc
dolls
obligations
##dling
violated
lafayette
jakarta
exploitation
##ime
infamous
iconic
##lah
##park
kitty
moody
reginald
dread
spill
crystals
olivier
modeled
bluff
equilibrium
separating
notices
ordnance
extinction
onset
cosmic
attachment
sammy
expose
privy
anchored
##bil
abbott
admits
bending
baritone
emmanuel
policeman
vaughan
winged
climax
dresses
denny
polytechnic
mohamed
burmese
authentic
nikki
genetics
grandparents
homestead
gaza
postponed
metacritic
una
##sby
##bat
unstable
dissertation
##rial
##cian
curls
obscure
uncovered
bronx
praying
disappearing
##hoe
prehistoric
coke
turret
mutations
nonprofit
pits
monaco
##ي
##usion
prominently
dispatched
podium
##mir
uci
##uation
133
fortifications
birthplace
kendall
##lby
##oll
preacher
rack
goodman
##rman
persistent
##ott
countless
jaime
recorder
lexington
persecution
jumps
renewal
wagons
##11
crushing
##holder
decorations
##lake
abundance
wrath
laundry
£1
garde
##rp
jeanne
beetles
peasant
##sl
splitting
caste
sergei
##rer
##ema
scripts
##ively
rub
satellites
##vor
inscribed
verlag
scrapped
gale
packages
chick
potato
slogan
kathleen
arabs
##culture
counterparts
reminiscent
choral
##tead
rand
retains
bushes
dane
accomplish
courtesy
closes
##oth
slaughter
hague
krakow
lawson
tailed
elias
ginger
##ttes
canopy
betrayal
rebuilding
turf
##hof
frowning
allegiance
brigades
kicks
rebuild
polls
alias
nationalism
td
rowan
audition
bowie
fortunately
recognizes
harp
dillon
horrified
##oro
renault
##tics
ropes
##α
presumed
rewarded
infrared
wiping
accelerated
illustration
##rid
presses
practitioners
badminton
##iard
detained
##tera
recognizing
relates
misery
##sies
##tly
reproduction
piercing
potatoes
thornton
esther
manners
hbo
##aan
ours
bullshit
ernie
perennial
sensitivity
illuminated
rupert
##jin
##iss
##ear
rfc
nassau
##dock
staggered
socialism
##haven
appointments
nonsense
prestige
sharma
haul
##tical
solidarity
gps
##ook
##rata
igor
pedestrian
##uit
baxter
tenants
wires
medication
unlimited
guiding
impacts
diabetes
##rama
sasha
pas
clive
extraction
131
continually
constraints
##bilities
sonata
hunted
sixteenth
chu
planting
quote
mayer
pretended
abs
spat
##hua
ceramic
##cci
curtains
pigs
pitching
##dad
latvian
sore
dayton
##sted
##qi
patrols
slice
playground
##nted
shone
stool
apparatus
inadequate
mates
treason
##ija
desires
##liga
##croft
somalia
laurent
mir
leonardo
oracle
grape
obliged
chevrolet
thirteenth
stunning
enthusiastic
##ede
accounted
concludes
currents
basil
##kovic
drought
##rica
mai
##aire
shove
posting
##shed
pilgrimage
humorous
packing
fry
pencil
wines
smells
144
marilyn
aching
newest
clung
bon
neighbours
sanctioned
##pie
mug
##stock
drowning
##mma
hydraulic
##vil
hiring
reminder
lilly
investigators
##ncies
sour
##eous
compulsory
packet
##rion
##graphic
##elle
cannes
##inate
depressed
##rit
heroic
importantly
theresa
##tled
conway
saturn
marginal
rae
##xia
corresponds
royce
pact
jasper
explosives
packaging
aluminium
##ttered
denotes
rhythmic
spans
assignments
hereditary
outlined
originating
sundays
lad
reissued
greeting
beatrice
##dic
pillar
marcos
plots
handbook
alcoholic
judiciary
avant
slides
extract
masculine
blur
##eum
##force
homage
trembled
owens
hymn
trey
omega
signaling
socks
accumulated
reacted
attic
theo
lining
angie
distraction
primera
talbot
##key
1200
ti
creativity
billed
##hey
deacon
eduardo
identifies
proposition
dizzy
gunner
hogan
##yam
##pping
##hol
ja
##chan
jensen
reconstructed
##berger
clearance
darius
##nier
abe
harlem
plea
dei
circled
emotionally
notation
fascist
neville
exceeded
upwards
viable
ducks
##fo
workforce
racer
limiting
shri
##lson
possesses
1600
kerr
moths
devastating
laden
disturbing
locking
##cture
gal
fearing
accreditation
flavor
aide
1870s
mountainous
##baum
melt
##ures
motel
texture
servers
soda
##mb
herd
##nium
erect
puzzled
hum
peggy
examinations
gould
testified
geoff
ren
devised
sacks
##law
denial
posters
grunted
cesar
tutor
ec
gerry
offerings
byrne
falcons
combinations
ct
incoming
pardon
rocking
26th
avengers
flared
mankind
seller
uttar
loch
nadia
stroking
exposing
##hd
fertile
ancestral
instituted
##has
noises
prophecy
taxation
eminent
vivid
pol
##bol
dart
indirect
multimedia
notebook
upside
displaying
adrenaline
referenced
geometric
##iving
progression
##ddy
blunt
announce
##far
implementing
##lav
aggression
liaison
cooler
cares
headache
plantations
gorge
dots
impulse
thickness
ashamed
averaging
kathy
obligation
precursor
137
fowler
symmetry
thee
225
hears
##rai
undergoing
ads
butcher
bowler
##lip
cigarettes
subscription
goodness
##ically
browne
##hos
##tech
kyoto
donor
##erty
damaging
friction
drifting
expeditions
hardened
prostitution
152
fauna
blankets
claw
tossing
snarled
butterflies
recruits
investigative
coated
healed
138
communal
hai
xiii
academics
boone
psychologist
restless
lahore
stephens
mba
brendan
foreigners
printer
##pc
ached
explode
27th
deed
scratched
dared
##pole
cardiac
1780
okinawa
proto
commando
compelled
oddly
electrons
##base
replica
thanksgiving
##rist
sheila
deliberate
stafford
tidal
representations
hercules
ou
##path
##iated
kidnapping
lenses
##tling
deficit
samoa
mouths
consuming
computational
maze
granting
smirk
razor
fixture
ideals
inviting
aiden
nominal
##vs
issuing
julio
pitt
ramsey
docks
##oss
exhaust
##owed
bavarian
draped
anterior
mating
ethiopian
explores
noticing
##nton
discarded
convenience
hoffman
endowment
beasts
cartridge
mormon
paternal
probe
sleeves
interfere
lump
deadline
##rail
jenks
bulldogs
scrap
alternating
justified
reproductive
nam
seize
descending
secretariat
kirby
coupe
grouped
smash
panther
sedan
tapping
##18
lola
cheer
germanic
unfortunate
##eter
unrelated
##fan
subordinate
##sdale
suzanne
advertisement
##ility
horsepower
##lda
cautiously
discourse
luigi
##mans
##fields
noun
prevalent
mao
schneider
everett
surround
governorate
kira
##avia
westward
##take
misty
rails
sustainability
134
unused
##rating
packs
toast
unwilling
regulate
thy
suffrage
nile
awe
assam
definitions
travelers
affordable
##rb
conferred
sells
undefeated
beneficial
torso
basal
repeating
remixes
##pass
bahrain
cables
fang
##itated
excavated
numbering
statutory
##rey
deluxe
##lian
forested
ramirez
derbyshire
zeus
slamming
transfers
astronomer
banana
lottery
berg
histories
bamboo
##uchi
resurrection
posterior
bowls
vaguely
##thi
thou
preserving
tensed
offence
##inas
meyrick
callum
ridden
watt
langdon
tying
lowland
snorted
daring
truman
##hale
##girl
aura
overly
filing
weighing
goa
infections
philanthropist
saunders
eponymous
##owski
latitude
perspectives
reviewing
mets
commandant
radial
##kha
flashlight
reliability
koch
vowels
amazed
ada
elaine
supper
##rth
##encies
predator
debated
soviets
cola
##boards
##nah
compartment
crooked
arbitrary
fourteenth
##ctive
havana
majors
steelers
clips
profitable
ambush
exited
packers
##tile
nude
cracks
fungi
##е
limb
trousers
josie
shelby
tens
frederic
##ος
definite
smoothly
constellation
insult
baton
discs
lingering
##nco
conclusions
lent
staging
becker
grandpa
shaky
##tron
einstein
obstacles
sk
adverse
elle
economically
##moto
mccartney
thor
dismissal
motions
readings
nostrils
treatise
##pace
squeezing
evidently
prolonged
1783
venezuelan
je
marguerite
beirut
takeover
shareholders
##vent
denise
digit
airplay
norse
##bbling
imaginary
pills
hubert
blaze
vacated
eliminating
##ello
vine
mansfield
##tty
retrospective
barrow
borne
clutch
bail
forensic
weaving
##nett
##witz
desktop
citadel
promotions
worrying
dorset
ieee
subdivided
##iating
manned
expeditionary
pickup
synod
chuckle
185
barney
##rz
##ffin
functionality
karachi
litigation
meanings
uc
lick
turbo
anders
##ffed
execute
curl
oppose
ankles
typhoon
##د
##ache
##asia
linguistics
compassion
pressures
grazing
perfection
##iting
immunity
monopoly
muddy
backgrounds
136
namibia
francesca
monitors
attracting
stunt
tuition
##ии
vegetable
##mates
##quent
mgm
jen
complexes
forts
##ond
cellar
bites
seventeenth
royals
flemish
failures
mast
charities
##cular
peruvian
capitals
macmillan
ipswich
outward
frigate
postgraduate
folds
employing
##ouse
concurrently
fiery
##tai
contingent
nightmares
monumental
nicaragua
##kowski
lizard
mal
fielding
gig
reject
##pad
harding
##ipe
coastline
##cin
##nos
beethoven
humphrey
innovations
##tam
##nge
norris
doris
solicitor
huang
obey
141
##lc
niagara
##tton
shelves
aug
bourbon
curry
nightclub
specifications
hilton
##ndo
centennial
dispersed
worm
neglected
briggs
sm
font
kuala
uneasy
plc
##nstein
##bound
##aking
##burgh
awaiting
pronunciation
##bbed
##quest
eh
optimal
zhu
raped
greens
presided
brenda
worries
##life
venetian
marxist
turnout
##lius
refined
braced
sins
grasped
sunderland
nickel
speculated
lowell
cyrillic
communism
fundraising
resembling
colonists
mutant
freddie
usc
##mos
gratitude
##run
mural
##lous
chemist
wi
reminds
28th
steals
tess
pietro
##ingen
promoter
ri
microphone
honoured
rai
sant
##qui
feather
##nson
burlington
kurdish
terrorists
deborah
sickness
##wed
##eet
hazard
irritated
desperation
veil
clarity
##rik
jewels
xv
##gged
##ows
##cup
berkshire
unfair
mysteries
orchid
winced
exhaustion
renovations
stranded
obe
infinity
##nies
adapt
redevelopment
thanked
registry
olga
domingo
noir
tudor
ole
##atus
commenting
behaviors
##ais
crisp
pauline
probable
stirling
wigan
##bian
paralympics
panting
surpassed
##rew
luca
barred
pony
famed
##sters
cassandra
waiter
carolyn
exported
##orted
andres
destructive
deeds
jonah
castles
vacancy
suv
##glass
1788
orchard
yep
famine
belarusian
sprang
##forth
skinny
##mis
administrators
rotterdam
zambia
zhao
boiler
discoveries
##ride
##physics
lucius
disappointing
outreach
spoon
##frame
qualifications
unanimously
enjoys
regency
##iidae
stade
realism
veterinary
rodgers
dump
alain
chestnut
castile
censorship
rumble
gibbs
##itor
communion
reggae
inactivated
logs
loads
##houses
homosexual
##iano
ale
informs
##cas
phrases
plaster
linebacker
ambrose
kaiser
fascinated
850
limerick
recruitment
forge
mastered
##nding
leinster
rooted
threaten
##strom
borneo
##hes
suggestions
scholarships
propeller
documentaries
patronage
coats
constructing
invest
neurons
comet
entirety
shouts
identities
annoying
unchanged
wary
##antly
##ogy
neat
oversight
##kos
phillies
replay
constance
##kka
incarnation
humble
skies
minus
##acy
smithsonian
##chel
guerrilla
jar
cadets
##plate
surplus
audit
##aru
cracking
joanna
louisa
pacing
##lights
intentionally
##iri
diner
nwa
imprint
australians
tong
unprecedented
bunker
naive
specialists
ark
nichols
railing
leaked
pedal
##uka
shrub
longing
roofs
v8
captains
neural
tuned
##ntal
##jet
emission
medina
frantic
codex
definitive
sid
abolition
intensified
stocks
enrique
sustain
genoa
oxide
##written
clues
cha
##gers
tributaries
fragment
venom
##rity
##ente
##sca
muffled
vain
sire
laos
##ingly
##hana
hastily
snapping
surfaced
sentiment
motive
##oft
contests
approximate
mesa
luckily
dinosaur
exchanges
propelled
accord
bourne
relieve
tow
masks
offended
##ues
cynthia
##mmer
rains
bartender
zinc
reviewers
lois
##sai
legged
arrogant
rafe
rosie
comprise
handicap
blockade
inlet
lagoon
copied
drilling
shelley
petals
##inian
mandarin
obsolete
##inated
onward
arguably
productivity
cindy
praising
seldom
busch
discusses
raleigh
shortage
ranged
stanton
encouragement
firstly
conceded
overs
temporal
##uke
cbe
##bos
woo
certainty
pumps
##pton
stalked
##uli
lizzie
periodic
thieves
weaker
##night
gases
shoving
chooses
wc
##chemical
prompting
weights
##kill
robust
flanked
sticky
hu
tuberculosis
##eb
##eal
christchurch
resembled
wallet
reese
inappropriate
pictured
distract
fixing
fiddle
giggled
burger
heirs
hairy
mechanic
torque
apache
obsessed
chiefly
cheng
logging
##tag
extracted
meaningful
numb
##vsky
gloucestershire
reminding
##bay
unite
##lit
breeds
diminished
clown
glove
1860s
##ن
##ug
archibald
focal
freelance
sliced
depiction
##yk
organism
switches
sights
stray
crawling
##ril
lever
leningrad
interpretations
loops
anytime
reel
alicia
delighted
##ech
inhaled
xiv
suitcase
bernie
vega
licenses
northampton
exclusion
induction
monasteries
racecourse
homosexuality
##right
##sfield
##rky
dimitri
michele
alternatives
ions
commentators
genuinely
objected
pork
hospitality
fencing
stephan
warships
peripheral
wit
drunken
wrinkled
quentin
spends
departing
chung
numerical
spokesperson
##zone
johannesburg
caliber
killers
##udge
assumes
neatly
demographic
abigail
bloc
##vel
mounting
##lain
bentley
slightest
xu
recipients
##jk
merlin
##writer
seniors
prisons
blinking
hindwings
flickered
kappa
##hel
80s
strengthening
appealing
brewing
gypsy
mali
lashes
hulk
unpleasant
harassment
bio
treaties
predict
instrumentation
pulp
troupe
boiling
mantle
##ffe
ins
##vn
dividing
handles
verbs
##onal
coconut
senegal
340
thorough
gum
momentarily
##sto
cocaine
panicked
destined
##turing
teatro
denying
weary
captained
mans
##hawks
##code
wakefield
bollywood
thankfully
##16
cyril
##wu
amendments
##bahn
consultation
stud
reflections
kindness
1787
internally
##ovo
tex
mosaic
distribute
paddy
seeming
143
##hic
piers
##15
##mura
##verse
popularly
winger
kang
sentinel
mccoy
##anza
covenant
##bag
verge
fireworks
suppress
thrilled
dominate
##jar
swansea
##60
142
reconciliation
##ndi
stiffened
cue
dorian
##uf
damascus
amor
ida
foremost
##aga
porsche
unseen
dir
##had
##azi
stony
lexi
melodies
##nko
angular
integer
podcast
ants
inherent
jaws
justify
persona
##olved
josephine
##nr
##ressed
customary
flashes
gala
cyrus
glaring
backyard
ariel
physiology
greenland
html
stir
avon
atletico
finch
methodology
ked
##lent
mas
catholicism
townsend
branding
quincy
fits
containers
1777
ashore
aragon
##19
forearm
poisoning
##sd
adopting
conquer
grinding
amnesty
keller
finances
evaluate
forged
lankan
instincts
##uto
guam
bosnian
photographed
workplace
desirable
protector
##dog
allocation
intently
encourages
willy
##sten
bodyguard
electro
brighter
##ν
bihar
##chev
lasts
opener
amphibious
sal
verde
arte
##cope
captivity
vocabulary
yields
##tted
agreeing
desmond
pioneered
##chus
strap
campaigned
railroads
##ович
emblem
##dre
stormed
501
##ulous
marijuana
northumberland
##gn
##nath
bowen
landmarks
beaumont
##qua
danube
##bler
attorneys
th
ge
flyers
critique
villains
cass
mutation
acc
##0s
colombo
mckay
motif
sampling
concluding
syndicate
##rell
neon
stables
ds
warnings
clint
mourning
wilkinson
##tated
merrill
leopard
evenings
exhaled
emil
sonia
ezra
discrete
stove
farrell
fifteenth
prescribed
superhero
##rier
worms
helm
wren
##duction
##hc
expo
##rator
hq
unfamiliar
antony
prevents
acceleration
fiercely
mari
painfully
calculations
cheaper
ign
clifton
irvine
davenport
mozambique
##np
pierced
##evich
wonders
##wig
##cate
##iling
crusade
ware
##uel
enzymes
reasonably
mls
##coe
mater
ambition
bunny
eliot
kernel
##fin
asphalt
headmaster
torah
aden
lush
pins
waived
##care
##yas
joao
substrate
enforce
##grad
##ules
alvarez
selections
epidemic
tempted
##bit
bremen
translates
ensured
waterfront
29th
forrest
manny
malone
kramer
reigning
cookies
simpler
absorption
205
engraved
##ffy
evaluated
1778
haze
146
comforting
crossover
##abe
thorn
##rift
##imo
##pop
suppression
fatigue
cutter
##tr
201
wurttemberg
##orf
enforced
hovering
proprietary
gb
samurai
syllable
ascent
lacey
tick
lars
tractor
merchandise
rep
bouncing
defendants
##yre
huntington
##ground
##oko
standardized
##hor
##hima
assassinated
nu
predecessors
rainy
liar
assurance
lyrical
##uga
secondly
flattened
ios
parameter
undercover
##mity
bordeaux
punish
ridges
markers
exodus
inactive
hesitate
debbie
nyc
pledge
savoy
nagar
offset
organist
##tium
hesse
marin
converting
##iver
diagram
propulsion
pu
validity
reverted
supportive
##dc
ministries
clans
responds
proclamation
##inae
##ø
##rea
ein
pleading
patriot
sf
birch
islanders
strauss
hates
##dh
brandenburg
concession
rd
##ob
1900s
killings
textbook
antiquity
cinematography
wharf
embarrassing
setup
creed
farmland
inequality
centred
signatures
fallon
370
##ingham
##uts
ceylon
gazing
directive
laurie
##tern
globally
##uated
##dent
allah
excavation
threads
##cross
148
frantically
icc
utilize
determines
respiratory
thoughtful
receptions
##dicate
merging
chandra
seine
147
builders
builds
diagnostic
dev
visibility
goddamn
analyses
dhaka
cho
proves
chancel
concurrent
curiously
canadians
pumped
restoring
1850s
turtles
jaguar
sinister
spinal
traction
declan
vows
1784
glowed
capitalism
swirling
install
universidad
##lder
##oat
soloist
##genic
##oor
coincidence
beginnings
nissan
dip
resorts
caucasus
combustion
infectious
##eno
pigeon
serpent
##itating
conclude
masked
salad
jew
##gr
surreal
toni
##wc
harmonica
151
##gins
##etic
##coat
fishermen
intending
bravery
##wave
klaus
titan
wembley
taiwanese
ransom
40th
incorrect
hussein
eyelids
jp
cooke
dramas
utilities
##etta
##print
eisenhower
principally
granada
lana
##rak
openings
concord
##bl
bethany
connie
morality
sega
##mons
##nard
earnings
##kara
##cine
wii
communes
##rel
coma
composing
softened
severed
grapes
##17
nguyen
analyzed
warlord
hubbard
heavenly
behave
slovenian
##hit
##ony
hailed
filmmakers
trance
caldwell
skye
unrest
coward
likelihood
##aging
bern
sci
taliban
honolulu
propose
##wang
1700
browser
imagining
cobra
contributes
dukes
instinctively
conan
violinist
##ores
accessories
gradual
##amp
quotes
sioux
##dating
undertake
intercepted
sparkling
compressed
139
fungus
tombs
haley
imposing
rests
degradation
lincolnshire
retailers
wetlands
tulsa
distributor
dungeon
nun
greenhouse
convey
atlantis
aft
exits
oman
dresser
lyons
##sti
joking
eddy
judgement
omitted
digits
##cts
##game
juniors
##rae
cents
stricken
une
##ngo
wizards
weir
breton
nan
technician
fibers
liking
royalty
##cca
154
persia
terribly
magician
##rable
##unt
vance
cafeteria
booker
camille
warmer
##static
consume
cavern
gaps
compass
contemporaries
foyer
soothing
graveyard
maj
plunged
blush
##wear
cascade
demonstrates
ordinance
##nov
boyle
##lana
rockefeller
shaken
banjo
izzy
##ense
breathless
vines
##32
##eman
alterations
chromosome
dwellings
feudal
mole
153
catalonia
relics
tenant
mandated
##fm
fridge
hats
honesty
patented
raul
heap
cruisers
accusing
enlightenment
infants
wherein
chatham
contractors
zen
affinity
hc
osborne
piston
156
traps
maturity
##rana
lagos
##zal
peering
##nay
attendant
dealers
protocols
subset
prospects
biographical
##cre
artery
##zers
insignia
nuns
endured
##eration
recommend
schwartz
serbs
berger
cromwell
crossroads
##ctor
enduring
clasped
grounded
##bine
marseille
twitched
abel
choke
https
catalyst
moldova
italians
##tist
disastrous
wee
##oured
##nti
wwf
nope
##piration
##asa
expresses
thumbs
167
##nza
coca
1781
cheating
##ption
skipped
sensory
heidelberg
spies
satan
dangers
semifinal
202
bohemia
whitish
confusing
shipbuilding
relies
surgeons
landings
ravi
baku
moor
suffix
alejandro
##yana
litre
upheld
##unk
rajasthan
##rek
coaster
insists
posture
scenarios
etienne
favoured
appoint
transgender
elephants
poked
greenwood
defences
fulfilled
militant
somali
1758
chalk
potent
##ucci
migrants
wink
assistants
nos
restriction
activism
niger
##ario
colon
shaun
##sat
daphne
##erated
swam
congregations
reprise
considerations
magnet
playable
xvi
##р
overthrow
tobias
knob
chavez
coding
##mers
propped
katrina
orient
newcomer
##suke
temperate
##pool
farmhouse
interrogation
##vd
committing
##vert
forthcoming
strawberry
joaquin
macau
ponds
shocking
siberia
##cellular
chant
contributors
##nant
##ologists
sped
absorb
hail
1782
spared
##hore
barbados
karate
opus
originates
saul
##xie
evergreen
leaped
##rock
correlation
exaggerated
weekday
unification
bump
tracing
brig
afb
pathways
utilizing
##ners
mod
mb
disturbance
kneeling
##stad
##guchi
100th
pune
##thy
decreasing
168
manipulation
miriam
academia
ecosystem
occupational
rbi
##lem
rift
##14
rotary
stacked
incorporation
awakening
generators
guerrero
racist
##omy
cyber
derivatives
culminated
allie
annals
panzer
sainte
wikipedia
pops
zu
austro
##vate
algerian
politely
nicholson
mornings
educate
tastes
thrill
dartmouth
##gating
db
##jee
regan
differing
concentrating
choreography
divinity
##media
pledged
alexandre
routing
gregor
madeline
##idal
apocalypse
##hora
gunfire
culminating
elves
fined
liang
lam
programmed
tar
guessing
transparency
gabrielle
##gna
cancellation
flexibility
##lining
accession
shea
stronghold
nets
specializes
##rgan
abused
hasan
sgt
ling
exceeding
##₄
admiration
supermarket
##ark
photographers
specialised
tilt
resonance
hmm
perfume
380
sami
threatens
garland
botany
guarding
boiled
greet
puppy
russo
supplier
wilmington
vibrant
vijay
##bius
paralympic
grumbled
paige
faa
licking
margins
hurricanes
##gong
fest
grenade
ripping
##uz
counseling
weigh
##sian
needles
wiltshire
edison
costly
##not
fulton
tramway
redesigned
staffordshire
cache
gasping
watkins
sleepy
candidacy
##group
monkeys
timeline
throbbing
##bid
##sos
berth
uzbekistan
vanderbilt
bothering
overturned
ballots
gem
##iger
sunglasses
subscribers
hooker
compelling
ang
exceptionally
saloon
stab
##rdi
carla
terrifying
rom
##vision
coil
##oids
satisfying
vendors
31st
mackay
deities
overlooked
ambient
bahamas
felipe
olympia
whirled
botanist
advertised
tugging
##dden
disciples
morales
unionist
rites
foley
morse
motives
creepy
##₀
soo
##sz
bargain
highness
frightening
turnpike
tory
reorganization
##cer
depict
biographer
##walk
unopposed
manifesto
##gles
institut
emile
accidental
kapoor
##dam
kilkenny
cortex
lively
##13
romanesque
jain
shan
cannons
##ood
##ske
petrol
echoing
amalgamated
disappears
cautious
proposes
sanctions
trenton
##ر
flotilla
aus
contempt
tor
canary
cote
theirs
##hun
conceptual
deleted
fascinating
paso
blazing
elf
honourable
hutchinson
##eiro
##outh
##zin
surveyor
tee
amidst
wooded
reissue
intro
##ono
cobb
shelters
newsletter
hanson
brace
encoding
confiscated
dem
caravan
marino
scroll
melodic
cows
imam
##adi
##aneous
northward
searches
biodiversity
cora
310
roaring
##bers
connell
theologian
halo
compose
pathetic
unmarried
dynamo
##oot
az
calculation
toulouse
deserves
humour
nr
forgiveness
tam
undergone
martyr
pamela
myths
whore
counselor
hicks
290
heavens
battleship
electromagnetic
##bbs
stellar
establishments
presley
hopped
##chin
temptation
90s
wills
nas
##yuan
nhs
##nya
seminars
##yev
adaptations
gong
asher
lex
indicator
sikh
tobago
cites
goin
##yte
satirical
##gies
characterised
correspond
bubbles
lure
participates
##vid
eruption
skate
therapeutic
1785
canals
wholesale
defaulted
sac
460
petit
##zzled
virgil
leak
ravens
256
portraying
##yx
ghetto
creators
dams
portray
vicente
##rington
fae
namesake
bounty
##arium
joachim
##ota
##iser
aforementioned
axle
snout
depended
dismantled
reuben
480
##ibly
gallagher
##lau
##pd
earnest
##ieu
##iary
inflicted
objections
##llar
asa
gritted
##athy
jericho
##sea
##was
flick
underside
ceramics
undead
substituted
195
eastward
undoubtedly
wheeled
chimney
##iche
guinness
cb
##ager
siding
##bell
traitor
baptiste
disguised
inauguration
149
tipperary
choreographer
perched
warmed
stationary
eco
##ike
##ntes
bacterial
##aurus
flores
phosphate
##core
attacker
invaders
alvin
intersects
a1
indirectly
immigrated
businessmen
cornelius
valves
narrated
pill
sober
ul
nationale
monastic
applicants
scenery
##jack
161
motifs
constitutes
cpu
##osh
jurisdictions
sd
tuning
irritation
woven
##uddin
fertility
gao
##erie
antagonist
impatient
glacial
hides
boarded
denominations
interception
##jas
cookie
nicola
##tee
algebraic
marquess
bahn
parole
buyers
bait
turbines
paperwork
bestowed
natasha
renee
oceans
purchases
157
vaccine
215
##tock
fixtures
playhouse
integrate
jai
oswald
intellectuals
##cky
booked
nests
mortimer
##isi
obsession
sept
##gler
##sum
440
scrutiny
simultaneous
squinted
##shin
collects
oven
shankar
penned
remarkably
##я
slips
luggage
spectral
1786
collaborations
louie
consolidation
##ailed
##ivating
420
hoover
blackpool
harness
ignition
vest
tails
belmont
mongol
skinner
##nae
visually
mage
derry
##tism
##unce
stevie
transitional
##rdy
redskins
drying
prep
prospective
##21
annoyance
oversee
##loaded
fills
##books
##iki
announces
fda
scowled
respects
prasad
mystic
tucson
##vale
revue
springer
bankrupt
1772
aristotle
salvatore
habsburg
##geny
dal
natal
nut
pod
chewing
darts
moroccan
walkover
rosario
lenin
punjabi
##ße
grossed
scattering
wired
invasive
hui
polynomial
corridors
wakes
gina
portrays
##cratic
arid
retreating
erich
irwin
sniper
##dha
linen
lindsey
maneuver
butch
shutting
socio
bounce
commemorative
postseason
jeremiah
pines
275
mystical
beads
bp
abbas
furnace
bidding
consulted
assaulted
empirical
rubble
enclosure
sob
weakly
cancel
polly
yielded
##emann
curly
prediction
battered
70s
vhs
jacqueline
render
sails
barked
detailing
grayson
riga
sloane
raging
##yah
herbs
bravo
##athlon
alloy
giggle
imminent
suffers
assumptions
waltz
##itate
accomplishments
##ited
bathing
remixed
deception
prefix
##emia
deepest
##tier
##eis
balkan
frogs
##rong
slab
##pate
philosophers
peterborough
grains
imports
dickinson
rwanda
##atics
1774
dirk
lan
tablets
##rove
clone
##rice
caretaker
hostilities
mclean
##gre
regimental
treasures
norms
impose
tsar
tango
diplomacy
variously
complain
192
recognise
arrests
1779
celestial
pulitzer
##dus
bing
libretto
##moor
adele
splash
##rite
expectation
lds
confronts
##izer
spontaneous
harmful
wedge
entrepreneurs
buyer
##ope
bilingual
translate
rugged
conner
circulated
uae
eaton
##gra
##zzle
lingered
lockheed
vishnu
reelection
alonso
##oom
joints
yankee
headline
cooperate
heinz
laureate
invading
##sford
echoes
scandinavian
##dham
hugging
vitamin
salute
micah
hind
trader
##sper
radioactive
##ndra
militants
poisoned
ratified
remark
campeonato
deprived
wander
prop
##dong
outlook
##tani
##rix
##eye
chiang
darcy
##oping
mandolin
spice
statesman
babylon
182
walled
forgetting
afro
##cap
158
giorgio
buffer
##polis
planetary
##gis
overlap
terminals
kinda
centenary
##bir
arising
manipulate
elm
ke
1770
ak
##tad
chrysler
mapped
moose
pomeranian
quad
macarthur
assemblies
shoreline
recalls
stratford
##rted
noticeable
##evic
imp
##rita
##sque
accustomed
supplying
tents
disgusted
vogue
sipped
filters
khz
reno
selecting
luftwaffe
mcmahon
tyne
masterpiece
carriages
collided
dunes
exercised
flare
remembers
muzzle
##mobile
heck
##rson
burgess
lunged
middleton
boycott
bilateral
##sity
hazardous
lumpur
multiplayer
spotlight
jackets
goldman
liege
porcelain
rag
waterford
benz
attracts
hopeful
battling
ottomans
kensington
baked
hymns
cheyenne
lattice
levine
borrow
polymer
clashes
michaels
monitored
commitments
denounced
##25
##von
cavity
##oney
hobby
akin
##holders
futures
intricate
cornish
patty
##oned
illegally
dolphin
##lag
barlow
yellowish
maddie
apologized
luton
plagued
##puram
nana
##rds
sway
fanny
łodz
##rino
psi
suspicions
hanged
##eding
initiate
charlton
##por
nak
competent
235
analytical
annex
wardrobe
reservations
##rma
sect
162
fairfax
hedge
piled
buckingham
uneven
bauer
simplicity
snyder
interpret
accountability
donors
moderately
byrd
continents
##cite
##max
disciple
hr
jamaican
ping
nominees
##uss
mongolian
diver
attackers
eagerly
ideological
pillows
miracles
apartheid
revolver
sulfur
clinics
moran
163
##enko
ile
katy
rhetoric
##icated
chronology
recycling
##hrer
elongated
mughal
pascal
profiles
vibration
databases
domination
##fare
##rant
matthias
digest
rehearsal
polling
weiss
initiation
reeves
clinging
flourished
impress
ngo
##hoff
##ume
buckley
symposium
rhythms
weed
emphasize
transforming
##taking
##gence
##yman
accountant
analyze
flicker
foil
priesthood
voluntarily
decreases
##80
##hya
slater
sv
charting
mcgill
##lde
moreno
##iu
besieged
zur
robes
##phic
admitting
api
deported
turmoil
peyton
earthquakes
##ares
nationalists
beau
clair
brethren
interrupt
welch
curated
galerie
requesting
164
##ested
impending
steward
viper
##vina
complaining
beautifully
brandy
foam
nl
1660
##cake
alessandro
punches
laced
explanations
##lim
attribute
clit
reggie
discomfort
##cards
smoothed
whales
##cene
adler
countered
duffy
disciplinary
widening
recipe
reliance
conducts
goats
gradient
preaching
##shaw
matilda
quasi
striped
meridian
cannabis
cordoba
certificates
##agh
##tering
graffiti
hangs
pilgrims
repeats
##ych
revive
urine
etat
##hawk
fueled
belts
fuzzy
susceptible
##hang
mauritius
salle
sincere
beers
hooks
##cki
arbitration
entrusted
advise
sniffed
seminar
junk
donnell
processors
principality
strapped
celia
mendoza
everton
fortunes
prejudice
starving
reassigned
steamer
##lund
tuck
evenly
foreman
##ffen
dans
375
envisioned
slit
##xy
baseman
liberia
rosemary
##weed
electrified
periodically
potassium
stride
contexts
sperm
slade
mariners
influx
bianca
subcommittee
##rane
spilling
icao
estuary
##nock
delivers
iphone
##ulata
isa
mira
bohemian
dessert
##sbury
welcoming
proudly
slowing
##chs
musee
ascension
russ
##vian
waits
##psy
africans
exploit
##morphic
gov
eccentric
crab
peck
##ull
entrances
formidable
marketplace
groom
bolted
metabolism
patton
robbins
courier
payload
endure
##ifier
andes
refrigerator
##pr
ornate
##uca
ruthless
illegitimate
masonry
strasbourg
bikes
adobe
##³
apples
quintet
willingly
niche
bakery
corpses
energetic
##cliffe
##sser
##ards
177
centimeters
centro
fuscous
cretaceous
rancho
##yde
andrei
telecom
tottenham
oasis
ordination
vulnerability
presiding
corey
cp
penguins
sims
##pis
malawi
piss
##48
correction
##cked
##ffle
##ryn
countdown
detectives
psychiatrist
psychedelic
dinosaurs
blouse
##get
choi
vowed
##oz
randomly
##pol
49ers
scrub
blanche
bruins
dusseldorf
##using
unwanted
##ums
212
dominique
elevations
headlights
om
laguna
##oga
1750
famously
ignorance
shrewsbury
##aine
ajax
breuning
che
confederacy
greco
overhaul
##screen
paz
skirts
disagreement
cruelty
jagged
phoebe
shifter
hovered
viruses
##wes
mandy
##lined
##gc
landlord
squirrel
dashed
##ι
ornamental
gag
wally
grange
literal
spurs
undisclosed
proceeding
yin
##text
billie
orphan
spanned
humidity
indy
weighted
presentations
explosions
lucian
##tary
vaughn
hindus
##anga
##hell
psycho
171
daytona
protects
efficiently
rematch
sly
tandem
##oya
rebranded
impaired
hee
metropolis
peach
godfrey
diaspora
ethnicity
prosperous
gleaming
dar
grossing
playback
##rden
stripe
pistols
##tain
births
labelled
##cating
172
rudy
alba
##onne
aquarium
hostility
##gb
##tase
shudder
sumatra
hardest
lakers
consonant
creeping
demos
homicide
capsule
zeke
liberties
expulsion
pueblo
##comb
trait
transporting
##ddin
##neck
##yna
depart
gregg
mold
ledge
hangar
oldham
playboy
termination
analysts
gmbh
romero
##itic
insist
cradle
filthy
brightness
slash
shootout
deposed
bordering
##truct
isis
microwave
tumbled
sheltered
cathy
werewolves
messy
andersen
convex
clapped
clinched
satire
wasting
edo
vc
rufus
##jak
mont
##etti
poznan
##keeping
restructuring
transverse
##rland
azerbaijani
slovene
gestures
roommate
choking
shear
##quist
vanguard
oblivious
##hiro
disagreed
baptism
##lich
coliseum
##aceae
salvage
societe
cory
locke
relocation
relying
versailles
ahl
swelling
##elo
cheerful
##word
##edes
gin
sarajevo
obstacle
diverted
##nac
messed
thoroughbred
fluttered
utrecht
chewed
acquaintance
assassins
dispatch
mirza
##wart
nike
salzburg
swell
yen
##gee
idle
ligue
samson
##nds
##igh
playful
spawned
##cise
tease
##case
burgundy
##bot
stirring
skeptical
interceptions
marathi
##dies
bedrooms
aroused
pinch
##lik
preferences
tattoos
buster
digitally
projecting
rust
##ital
kitten
priorities
addison
pseudo
##guard
dusk
icons
sermon
##psis
##iba
bt
##lift
##xt
ju
truce
rink
##dah
##wy
defects
psychiatry
offences
calculate
glucose
##iful
##rized
##unda
francaise
##hari
richest
warwickshire
carly
1763
purity
redemption
lending
##cious
muse
bruises
cerebral
aero
carving
##name
preface
terminology
invade
monty
##int
anarchist
blurred
##iled
rossi
treats
guts
shu
foothills
ballads
undertaking
premise
cecilia
affiliates
blasted
conditional
wilder
minors
drone
rudolph
buffy
swallowing
horton
attested
##hop
rutherford
howell
primetime
livery
penal
##bis
minimize
hydro
wrecked
wrought
palazzo
##gling
cans
vernacular
friedman
nobleman
shale
walnut
danielle
##ection
##tley
sears
##kumar
chords
lend
flipping
streamed
por
dracula
gallons
sacrifices
gamble
orphanage
##iman
mckenzie
##gible
boxers
daly
##balls
##ان
208
##ific
##rative
##iq
exploited
slated
##uity
circling
hillary
pinched
goldberg
provost
campaigning
lim
piles
ironically
jong
mohan
successors
usaf
##tem
##ught
autobiographical
haute
preserves
##ending
acquitted
comparisons
203
hydroelectric
gangs
cypriot
torpedoes
rushes
chrome
derive
bumps
instability
fiat
pets
##mbe
silas
dye
reckless
settler
##itation
info
heats
##writing
176
canonical
maltese
fins
mushroom
stacy
aspen
avid
##kur
##loading
vickers
gaston
hillside
statutes
wilde
gail
kung
sabine
comfortably
motorcycles
##rgo
169
pneumonia
fetch
##sonic
axel
faintly
parallels
##oop
mclaren
spouse
compton
interdisciplinary
miner
##eni
181
clamped
##chal
##llah
separates
versa
##mler
scarborough
labrador
##lity
##osing
rutgers
hurdles
como
166
burt
divers
##100
wichita
cade
coincided
##erson
bruised
mla
##pper
vineyard
##ili
##brush
notch
mentioning
jase
hearted
kits
doe
##acle
pomerania
##ady
ronan
seizure
pavel
problematic
##zaki
domenico
##ulin
catering
penelope
dependence
parental
emilio
ministerial
atkinson
##bolic
clarkson
chargers
colby
grill
peeked
arises
summon
##aged
fools
##grapher
faculties
qaeda
##vial
garner
refurbished
##hwa
geelong
disasters
nudged
bs
shareholder
lori
algae
reinstated
rot
##ades
##nous
invites
stainless
183
inclusive
##itude
diocesan
til
##icz
denomination
##xa
benton
floral
registers
##ider
##erman
##kell
absurd
brunei
guangzhou
hitter
retaliation
##uled
##eve
blanc
nh
consistency
contamination
##eres
##rner
dire
palermo
broadcasters
diaries
inspire
vols
brewer
tightening
ky
mixtape
hormone
##tok
stokes
##color
##dly
##ssi
pg
##ometer
##lington
sanitation
##tility
intercontinental
apps
##adt
¹⁄₂
cylinders
economies
favourable
unison
croix
gertrude
odyssey
vanity
dangling
##logists
upgrades
dice
middleweight
practitioner
##ight
206
henrik
parlor
orion
angered
lac
python
blurted
##rri
sensual
intends
swings
angled
##phs
husky
attain
peerage
precinct
textiles
cheltenham
shuffled
dai
confess
tasting
bhutan
##riation
tyrone
segregation
abrupt
ruiz
##rish
smirked
blackwell
confidential
browning
amounted
##put
vase
scarce
fabulous
raided
staple
guyana
unemployed
glider
shay
##tow
carmine
troll
intervene
squash
superstar
##uce
cylindrical
len
roadway
researched
handy
##rium
##jana
meta
lao
declares
##rring
##tadt
##elin
##kova
willem
shrubs
napoleonic
realms
skater
qi
volkswagen
##ł
tad
hara
archaeologist
awkwardly
eerie
##kind
wiley
##heimer
##24
titus
organizers
cfl
crusaders
lama
usb
vent
enraged
thankful
occupants
maximilian
##gaard
possessing
textbooks
##oran
collaborator
quaker
##ulo
avalanche
mono
silky
straits
isaiah
mustang
surged
resolutions
potomac
descend
cl
kilograms
plato
strains
saturdays
##olin
bernstein
##ype
holstein
ponytail
##watch
belize
conversely
heroine
perpetual
##ylus
charcoal
piedmont
glee
negotiating
backdrop
prologue
##jah
##mmy
pasadena
climbs
ramos
sunni
##holm
##tner
##tri
anand
deficiency
hertfordshire
stout
##avi
aperture
orioles
##irs
doncaster
intrigued
bombed
coating
otis
##mat
cocktail
##jit
##eto
amir
arousal
sar
##proof
##act
##ories
dixie
pots
##bow
whereabouts
159
##fted
drains
bullying
cottages
scripture
coherent
fore
poe
appetite
##uration
sampled
##ators
##dp
derrick
rotor
jays
peacock
installment
##rro
advisors
##coming
rodeo
scotch
##mot
##db
##fen
##vant
ensued
rodrigo
dictatorship
martyrs
twenties
##н
towed
incidence
marta
rainforest
sai
scaled
##cles
oceanic
qualifiers
symphonic
mcbride
dislike
generalized
aubrey
colonization
##iation
##lion
##ssing
disliked
lublin
salesman
##ulates
spherical
whatsoever
sweating
avalon
contention
punt
severity
alderman
atari
##dina
##grant
##rop
scarf
seville
vertices
annexation
fairfield
fascination
inspiring
launches
palatinate
regretted
##rca
feral
##iom
elk
nap
olsen
reddy
yong
##leader
##iae
garment
transports
feng
gracie
outrage
viceroy
insides
##esis
breakup
grady
organizer
softer
grimaced
222
murals
galicia
arranging
vectors
##rsten
bas
##sb
##cens
sloan
##eka
bitten
ara
fender
nausea
bumped
kris
banquet
comrades
detector
persisted
##llan
adjustment
endowed
cinemas
##shot
sellers
##uman
peek
epa
kindly
neglect
simpsons
talon
mausoleum
runaway
hangul
lookout
##cic
rewards
coughed
acquainted
chloride
##ald
quicker
accordion
neolithic
##qa
artemis
coefficient
lenny
pandora
tx
##xed
ecstasy
litter
segunda
chairperson
gemma
hiss
rumor
vow
nasal
antioch
compensate
patiently
transformers
##eded
judo
morrow
penis
posthumous
philips
bandits
husbands
denote
flaming
##any
##phones
langley
yorker
1760
walters
##uo
##kle
gubernatorial
fatty
samsung
leroy
outlaw
##nine
unpublished
poole
jakob
##ᵢ
##ₙ
crete
distorted
superiority
##dhi
intercept
crust
mig
claus
crashes
positioning
188
stallion
301
frontal
armistice
##estinal
elton
aj
encompassing
camel
commemorated
malaria
woodward
calf
cigar
penetrate
##oso
willard
##rno
##uche
illustrate
amusing
convergence
noteworthy
##lma
##rva
journeys
realise
manfred
##sable
410
##vocation
hearings
fiance
##posed
educators
provoked
adjusting
##cturing
modular
stockton
paterson
vlad
rejects
electors
selena
maureen
##tres
uber
##rce
swirled
##num
proportions
nanny
pawn
naturalist
parma
apostles
awoke
ethel
wen
##bey
monsoon
overview
##inating
mccain
rendition
risky
adorned
##ih
equestrian
germain
nj
conspicuous
confirming
##yoshi
shivering
##imeter
milestone
rumours
flinched
bounds
smacked
token
##bei
lectured
automobiles
##shore
impacted
##iable
nouns
nero
##leaf
ismail
prostitute
trams
##lace
bridget
sud
stimulus
impressions
reins
revolves
##oud
##gned
giro
honeymoon
##swell
criterion
##sms
##uil
libyan
prefers
##osition
211
preview
sucks
accusation
bursts
metaphor
diffusion
tolerate
faye
betting
cinematographer
liturgical
specials
bitterly
humboldt
##ckle
flux
rattled
##itzer
archaeologists
odor
authorised
marshes
discretion
##ов
alarmed
archaic
inverse
##leton
explorers
##pine
drummond
tsunami
woodlands
##minate
##tland
booklet
insanity
owning
insert
crafted
calculus
##tore
receivers
##bt
stung
##eca
##nched
prevailing
travellers
eyeing
lila
graphs
##borne
178
julien
##won
morale
adaptive
therapist
erica
cw
libertarian
bowman
pitches
vita
##ional
crook
##ads
##entation
caledonia
mutiny
##sible
1840s
automation
##ß
flock
##pia
ironic
pathology
##imus
remarried
##22
joker
withstand
energies
##att
shropshire
hostages
madeleine
tentatively
conflicting
mateo
recipes
euros
ol
mercenaries
nico
##ndon
albuquerque
augmented
mythical
bel
freud
##child
cough
##lica
365
freddy
lillian
genetically
nuremberg
calder
209
bonn
outdoors
paste
suns
urgency
vin
restraint
tyson
##cera
##selle
barrage
bethlehem
kahn
##par
mounts
nippon
barony
happier
ryu
makeshift
sheldon
blushed
castillo
barking
listener
taped
bethel
fluent
headlines
pornography
rum
disclosure
sighing
mace
doubling
gunther
manly
##plex
rt
interventions
physiological
forwards
emerges
##tooth
##gny
compliment
rib
recession
visibly
barge
faults
connector
exquisite
prefect
##rlin
patio
##cured
elevators
brandt
italics
pena
173
wasp
satin
ea
botswana
graceful
respectable
##jima
##rter
##oic
franciscan
generates
##dl
alfredo
disgusting
##olate
##iously
sherwood
warns
cod
promo
cheryl
sino
##ة
##escu
twitch
##zhi
brownish
thom
ortiz
##dron
densely
##beat
carmel
reinforce
##bana
187
anastasia
downhill
vertex
contaminated
remembrance
harmonic
homework
##sol
fiancee
gears
olds
angelica
loft
ramsay
quiz
colliery
sevens
##cape
autism
##hil
walkway
##boats
ruben
abnormal
ounce
khmer
##bbe
zachary
bedside
morphology
punching
##olar
sparrow
convinces
##35
hewitt
queer
remastered
rods
mabel
solemn
notified
lyricist
symmetric
##xide
174
encore
passports
wildcats
##uni
baja
##pac
mildly
##ease
bleed
commodity
mounds
glossy
orchestras
##omo
damian
prelude
ambitions
##vet
awhile
remotely
##aud
asserts
imply
##iques
distinctly
modelling
remedy
##dded
windshield
dani
xiao
##endra
audible
powerplant
1300
invalid
elemental
acquisitions
##hala
immaculate
libby
plata
smuggling
ventilation
denoted
minh
##morphism
430
differed
dion
kelley
lore
mocking
sabbath
spikes
hygiene
drown
runoff
stylized
tally
liberated
aux
interpreter
righteous
aba
siren
reaper
pearce
millie
##cier
##yra
gaius
##iso
captures
##ttering
dorm
claudio
##sic
benches
knighted
blackness
##ored
discount
fumble
oxidation
routed
##ς
novak
perpendicular
spoiled
fracture
splits
##urt
pads
topology
##cats
axes
fortunate
offenders
protestants
esteem
221
broadband
convened
frankly
hound
prototypes
isil
facilitated
keel
##sher
sahara
awaited
bubba
orb
prosecutors
186
hem
520
##xing
relaxing
remnant
romney
sorted
slalom
stefano
ulrich
##active
exemption
folder
pauses
foliage
hitchcock
epithet
204
criticisms
##aca
ballistic
brody
hinduism
chaotic
youths
equals
##pala
pts
thicker
analogous
capitalist
improvised
overseeing
sinatra
ascended
beverage
##tl
straightforward
##kon
curran
##west
bois
325
induce
surveying
emperors
sax
unpopular
##kk
cartoonist
fused
##mble
unto
##yuki
localities
##cko
##ln
darlington
slain
academie
lobbying
sediment
puzzles
##grass
defiance
dickens
manifest
tongues
alumnus
arbor
coincide
184
appalachian
mustafa
examiner
cabaret
traumatic
yves
bracelet
draining
heroin
magnum
baths
odessa
consonants
mitsubishi
##gua
kellan
vaudeville
##fr
joked
null
straps
probation
##ław
ceded
interfaces
##pas
##zawa
blinding
viet
224
rothschild
museo
640
huddersfield
##vr
tactic
##storm
brackets
dazed
incorrectly
##vu
reg
glazed
fearful
manifold
benefited
irony
##sun
stumbling
##rte
willingness
balkans
mei
wraps
##aba
injected
##lea
gu
syed
harmless
##hammer
bray
takeoff
poppy
timor
cardboard
astronaut
purdue
weeping
southbound
cursing
stalls
diagonal
##neer
lamar
bryce
comte
weekdays
harrington
##uba
negatively
##see
lays
grouping
##cken
##henko
affirmed
halle
modernist
##lai
hodges
smelling
aristocratic
baptized
dismiss
justification
oilers
##now
coupling
qin
snack
healer
##qing
gardener
layla
battled
formulated
stephenson
gravitational
##gill
##jun
1768
granny
coordinating
suites
##cd
##ioned
monarchs
##cote
##hips
sep
blended
apr
barrister
deposition
fia
mina
policemen
paranoid
##pressed
churchyard
covert
crumpled
creep
abandoning
tr
transmit
conceal
barr
understands
readiness
spire
##cology
##enia
##erry
610
startling
unlock
vida
bowled
slots
##nat
##islav
spaced
trusting
admire
rig
##ink
slack
##70
mv
207
casualty
##wei
classmates
##odes
##rar
##rked
amherst
furnished
evolve
foundry
menace
mead
##lein
flu
wesleyan
##kled
monterey
webber
##vos
wil
##mith
##на
bartholomew
justices
restrained
##cke
amenities
191
mediated
sewage
trenches
ml
mainz
##thus
1800s
##cula
##inski
caine
bonding
213
converts
spheres
superseded
marianne
crypt
sweaty
ensign
historia
##br
spruce
##post
##ask
forks
thoughtfully
yukon
pamphlet
ames
##uter
karma
##yya
bryn
negotiation
sighs
incapable
##mbre
##ntial
actresses
taft
##mill
luce
prevailed
##amine
1773
motionless
envoy
testify
investing
sculpted
instructors
provence
kali
cullen
horseback
##while
goodwin
##jos
gaa
norte
##ldon
modify
wavelength
abd
214
skinned
sprinter
forecast
scheduling
marries
squared
tentative
##chman
boer
##isch
bolts
swap
fisherman
assyrian
impatiently
guthrie
martins
murdoch
194
tanya
nicely
dolly
lacy
med
##45
syn
decks
fashionable
millionaire
##ust
surfing
##ml
##ision
heaved
tammy
consulate
attendees
routinely
197
fuse
saxophonist
backseat
malaya
##lord
scowl
tau
##ishly
193
sighted
steaming
##rks
303
911
##holes
##hong
ching
##wife
bless
conserved
jurassic
stacey
unix
zion
chunk
rigorous
blaine
198
peabody
slayer
dismay
brewers
nz
##jer
det
##glia
glover
postwar
int
penetration
sylvester
imitation
vertically
airlift
heiress
knoxville
viva
##uin
390
macon
##rim
##fighter
##gonal
janice
##orescence
##wari
marius
belongings
leicestershire
196
blanco
inverted
preseason
sanity
sobbing
##due
##elt
##dled
collingwood
regeneration
flickering
shortest
##mount
##osi
feminism
##lat
sherlock
cabinets
fumbled
northbound
precedent
snaps
##mme
researching
##akes
guillaume
insights
manipulated
vapor
neighbour
sap
gangster
frey
f1
stalking
scarcely
callie
barnett
tendencies
audi
doomed
assessing
slung
panchayat
ambiguous
bartlett
##etto
distributing
violating
wolverhampton
##hetic
swami
histoire
##urus
liable
pounder
groin
hussain
larsen
popping
surprises
##atter
vie
curt
##station
mute
relocate
musicals
authorization
richter
##sef
immortality
tna
bombings
##press
deteriorated
yiddish
##acious
robbed
colchester
cs
pmid
ao
verified
balancing
apostle
swayed
recognizable
oxfordshire
retention
nottinghamshire
contender
judd
invitational
shrimp
uhf
##icient
cleaner
longitudinal
tanker
##mur
acronym
broker
koppen
sundance
suppliers
##gil
4000
clipped
fuels
petite
##anne
landslide
helene
diversion
populous
landowners
auspices
melville
quantitative
##xes
ferries
nicky
##llus
doo
haunting
roche
carver
downed
unavailable
##pathy
approximation
hiroshima
##hue
garfield
valle
comparatively
keyboardist
traveler
##eit
congestion
calculating
subsidiaries
##bate
serb
modernization
fairies
deepened
ville
averages
##lore
inflammatory
tonga
##itch
co₂
squads
##hea
gigantic
serum
enjoyment
retailer
verona
35th
cis
##phobic
magna
technicians
##vati
arithmetic
##sport
levin
##dation
amtrak
chow
sienna
##eyer
backstage
entrepreneurship
##otic
learnt
tao
##udy
worcestershire
formulation
baggage
hesitant
bali
sabotage
##kari
barren
enhancing
murmur
pl
freshly
putnam
syntax
aces
medicines
resentment
bandwidth
##sier
grins
chili
guido
##sei
framing
implying
gareth
lissa
genevieve
pertaining
admissions
geo
thorpe
proliferation
sato
bela
analyzing
parting
##gor
awakened
##isman
huddled
secrecy
##kling
hush
gentry
540
dungeons
##ego
coasts
##utz
sacrificed
##chule
landowner
mutually
prevalence
programmer
adolescent
disrupted
seaside
gee
trusts
vamp
georgie
##nesian
##iol
schedules
sindh
##market
etched
hm
sparse
bey
beaux
scratching
gliding
unidentified
216
collaborating
gems
jesuits
oro
accumulation
shaping
mbe
anal
##xin
231
enthusiasts
newscast
##egan
janata
dewey
parkinson
179
ankara
biennial
towering
dd
inconsistent
950
##chet
thriving
terminate
cabins
furiously
eats
advocating
donkey
marley
muster
phyllis
leiden
##user
grassland
glittering
iucn
loneliness
217
memorandum
armenians
##ddle
popularized
rhodesia
60s
lame
##illon
sans
bikini
header
orbits
##xx
##finger
##ulator
sharif
spines
biotechnology
strolled
naughty
yates
##wire
fremantle
milo
##mour
abducted
removes
##atin
humming
wonderland
##chrome
##ester
hume
pivotal
##rates
armand
grams
believers
elector
rte
apron
bis
scraped
##yria
endorsement
initials
##llation
eps
dotted
hints
buzzing
emigration
nearer
##tom
indicators
##ulu
coarse
neutron
protectorate
##uze
directional
exploits
pains
loire
1830s
proponents
guggenheim
rabbits
ritchie
305
hectare
inputs
hutton
##raz
verify
##ako
boilers
longitude
##lev
skeletal
yer
emilia
citrus
compromised
##gau
pokemon
prescription
paragraph
eduard
cadillac
attire
categorized
kenyan
weddings
charley
##bourg
entertain
monmouth
##lles
nutrients
davey
mesh
incentive
practised
ecosystems
kemp
subdued
overheard
##rya
bodily
maxim
##nius
apprenticeship
ursula
##fight
lodged
rug
silesian
unconstitutional
patel
inspected
coyote
unbeaten
##hak
34th
disruption
convict
parcel
##cl
##nham
collier
implicated
mallory
##iac
##lab
susannah
winkler
##rber
shia
phelps
sediments
graphical
robotic
##sner
adulthood
mart
smoked
##isto
kathryn
clarified
##aran
divides
convictions
oppression
pausing
burying
##mt
federico
mathias
eileen
##tana
kite
hunched
##acies
189
##atz
disadvantage
liza
kinetic
greedy
paradox
yokohama
dowager
trunks
ventured
##gement
gupta
vilnius
olaf
##thest
crimean
hopper
##ej
progressively
arturo
mouthed
arrondissement
##fusion
rubin
simulcast
oceania
##orum
##stra
##rred
busiest
intensely
navigator
cary
##vine
##hini
##bies
fife
rowe
rowland
posing
insurgents
shafts
lawsuits
activate
conor
inward
culturally
garlic
265
##eering
eclectic
##hui
##kee
##nl
furrowed
vargas
meteorological
rendezvous
##aus
culinary
commencement
##dition
quota
##notes
mommy
salaries
overlapping
mule
##iology
##mology
sums
wentworth
##isk
##zione
mainline
subgroup
##illy
hack
plaintiff
verdi
bulb
differentiation
engagements
multinational
supplemented
bertrand
caller
regis
##naire
##sler
##arts
##imated
blossom
propagation
kilometer
viaduct
vineyards
##uate
beckett
optimization
golfer
songwriters
seminal
semitic
thud
volatile
evolving
ridley
##wley
trivial
distributions
scandinavia
jiang
##ject
wrestled
insistence
##dio
emphasizes
napkin
##ods
adjunct
rhyme
##ricted
##eti
hopeless
surrounds
tremble
32nd
smoky
##ntly
oils
medicinal
padded
steer
wilkes
219
255
concessions
hue
uniquely
blinded
landon
yahoo
##lane
hendrix
commemorating
dex
specify
chicks
##ggio
intercity
1400
morley
##torm
highlighting
##oting
pang
oblique
stalled
##liner
flirting
newborn
1769
bishopric
shaved
232
currie
##ush
dharma
spartan
##ooped
favorites
smug
novella
sirens
abusive
creations
espana
##lage
paradigm
semiconductor
sheen
##rdo
##yen
##zak
nrl
renew
##pose
##tur
adjutant
marches
norma
##enity
ineffective
weimar
grunt
##gat
lordship
plotting
expenditure
infringement
lbs
refrain
av
mimi
mistakenly
postmaster
1771
##bara
ras
motorsports
tito
199
subjective
##zza
bully
stew
##kaya
prescott
1a
##raphic
##zam
bids
styling
paranormal
reeve
sneaking
exploding
katz
akbar
migrant
syllables
indefinitely
##ogical
destroys
replaces
applause
##phine
pest
##fide
218
articulated
bertie
##thing
##cars
##ptic
courtroom
crowley
aesthetics
cummings
tehsil
hormones
titanic
dangerously
##ibe
stadion
jaenelle
auguste
ciudad
##chu
mysore
partisans
##sio
lucan
philipp
##aly
debating
henley
interiors
##rano
##tious
homecoming
beyonce
usher
henrietta
prepares
weeds
##oman
ely
plucked
##pire
##dable
luxurious
##aq
artifact
password
pasture
juno
maddy
minsk
##dder
##ologies
##rone
assessments
martian
royalist
1765
examines
##mani
##rge
nino
223
parry
scooped
relativity
##eli
##uting
##cao
congregational
noisy
traverse
##agawa
strikeouts
nickelodeon
obituary
transylvania
binds
depictions
polk
trolley
##yed
##lard
breeders
##under
dryly
hokkaido
1762
strengths
stacks
bonaparte
connectivity
neared
prostitutes
stamped
anaheim
gutierrez
sinai
##zzling
bram
fresno
madhya
##86
proton
##lena
##llum
##phon
reelected
wanda
##anus
##lb
ample
distinguishing
##yler
grasping
sermons
tomato
bland
stimulation
avenues
##eux
spreads
scarlett
fern
pentagon
assert
baird
chesapeake
ir
calmed
distortion
fatalities
##olis
correctional
pricing
##astic
##gina
prom
dammit
ying
collaborate
##chia
welterweight
33rd
pointer
substitution
bonded
umpire
communicating
multitude
paddle
##obe
federally
intimacy
##insky
betray
ssr
##lett
##lean
##lves
##therapy
airbus
##tery
functioned
ud
bearer
biomedical
netflix
##hire
##nca
condom
brink
ik
##nical
macy
##bet
flap
gma
experimented
jelly
lavender
##icles
##ulia
munro
##mian
##tial
rye
##rle
60th
gigs
hottest
rotated
predictions
fuji
bu
##erence
##omi
barangay
##fulness
##sas
clocks
##rwood
##liness
cereal
roe
wight
decker
uttered
babu
onion
xml
forcibly
##df
petra
sarcasm
hartley
peeled
storytelling
##42
##xley
##ysis
##ffa
fibre
kiel
auditor
fig
harald
greenville
##berries
geographically
nell
quartz
##athic
cemeteries
##lr
crossings
nah
holloway
reptiles
chun
sichuan
snowy
660
corrections
##ivo
zheng
ambassadors
blacksmith
fielded
fluids
hardcover
turnover
medications
melvin
academies
##erton
ro
roach
absorbing
spaniards
colton
##founded
outsider
espionage
kelsey
245
edible
##ulf
dora
establishes
##sham
##tries
contracting
##tania
cinematic
costello
nesting
##uron
connolly
duff
##nology
mma
##mata
fergus
sexes
gi
optics
spectator
woodstock
banning
##hee
##fle
differentiate
outfielder
refinery
226
312
gerhard
horde
lair
drastically
##udi
landfall
##cheng
motorsport
odi
##achi
predominant
quay
skins
##ental
edna
harshly
complementary
murdering
##aves
wreckage
##90
ono
outstretched
lennox
munitions
galen
reconcile
470
scalp
bicycles
gillespie
questionable
rosenberg
guillermo
hostel
jarvis
kabul
volvo
opium
yd
##twined
abuses
decca
outpost
##cino
sensible
neutrality
##64
ponce
anchorage
atkins
turrets
inadvertently
disagree
libre
vodka
reassuring
weighs
##yal
glide
jumper
ceilings
repertory
outs
stain
##bial
envy
##ucible
smashing
heightened
policing
hyun
mixes
lai
prima
##ples
celeste
##bina
lucrative
intervened
kc
manually
##rned
stature
staffed
bun
bastards
nairobi
priced
##auer
thatcher
##kia
tripped
comune
##ogan
##pled
brasil
incentives
emanuel
hereford
musica
##kim
benedictine
biennale
##lani
eureka
gardiner
rb
knocks
sha
##ael
##elled
##onate
efficacy
ventura
masonic
sanford
maize
leverage
##feit
capacities
santana
##aur
novelty
vanilla
##cter
##tour
benin
##oir
##rain
neptune
drafting
tallinn
##cable
humiliation
##boarding
schleswig
fabian
bernardo
liturgy
spectacle
sweeney
pont
routledge
##tment
cosmos
ut
hilt
sleek
universally
##eville
##gawa
typed
##dry
favors
allegheny
glaciers
##rly
recalling
aziz
##log
parasite
requiem
auf
##berto
##llin
illumination
##breaker
##issa
festivities
bows
govern
vibe
vp
333
sprawled
larson
pilgrim
bwf
leaping
##rts
##ssel
alexei
greyhound
hoarse
##dler
##oration
seneca
##cule
gaping
##ulously
##pura
cinnamon
##gens
##rricular
craven
fantasies
houghton
engined
reigned
dictator
supervising
##oris
bogota
commentaries
unnatural
fingernails
spirituality
tighten
##tm
canadiens
protesting
intentional
cheers
sparta
##ytic
##iere
##zine
widen
belgarath
controllers
dodd
iaaf
navarre
##ication
defect
squire
steiner
whisky
##mins
560
inevitably
tome
##gold
chew
##uid
##lid
elastic
##aby
streaked
alliances
jailed
regal
##ined
##phy
czechoslovak
narration
absently
##uld
bluegrass
guangdong
quran
criticizing
hose
hari
##liest
##owa
skier
streaks
deploy
##lom
raft
bose
dialed
huff
##eira
haifa
simplest
bursting
endings
ib
sultanate
##titled
franks
whitman
ensures
sven
##ggs
collaborators
forster
organising
ui
banished
napier
injustice
teller
layered
thump
##otti
roc
battleships
evidenced
fugitive
sadie
robotics
##roud
equatorial
geologist
##iza
yielding
##bron
##sr
internationale
mecca
##diment
sbs
skyline
toad
uploaded
reflective
undrafted
lal
leafs
bayern
##dai
lakshmi
shortlisted
##stick
##wicz
camouflage
donate
af
christi
lau
##acio
disclosed
nemesis
1761
assemble
straining
northamptonshire
tal
##asi
bernardino
premature
heidi
42nd
coefficients
galactic
reproduce
buzzed
sensations
zionist
monsieur
myrtle
##eme
archery
strangled
musically
viewpoint
antiquities
bei
trailers
seahawks
cured
pee
preferring
tasmanian
lange
sul
##mail
##working
colder
overland
lucivar
massey
gatherings
haitian
##smith
disapproval
flaws
##cco
##enbach
1766
npr
##icular
boroughs
creole
forums
techno
1755
dent
abdominal
streetcar
##eson
##stream
procurement
gemini
predictable
##tya
acheron
christoph
feeder
fronts
vendor
bernhard
jammu
tumors
slang
##uber
goaltender
twists
curving
manson
vuelta
mer
peanut
confessions
pouch
unpredictable
allowance
theodor
vascular
##factory
bala
authenticity
metabolic
coughing
nanjing
##cea
pembroke
##bard
splendid
36th
ff
hourly
##ahu
elmer
handel
##ivate
awarding
thrusting
dl
experimentation
##hesion
##46
caressed
entertained
steak
##rangle
biologist
orphans
baroness
oyster
stepfather
##dridge
mirage
reefs
speeding
##31
barons
1764
227
inhabit
preached
repealed
##tral
honoring
boogie
captives
administer
johanna
##imate
gel
suspiciously
1767
sobs
##dington
backbone
hayward
garry
##folding
##nesia
maxi
##oof
##ppe
ellison
galileo
##stand
crimea
frenzy
amour
bumper
matrices
natalia
baking
garth
palestinians
##grove
smack
conveyed
ensembles
gardening
##manship
##rup
##stituting
1640
harvesting
topography
jing
shifters
dormitory
##carriage
##lston
ist
skulls
##stadt
dolores
jewellery
sarawak
##wai
##zier
fences
christy
confinement
tumbling
credibility
fir
stench
##bria
##plication
##nged
##sam
virtues
##belt
marjorie
pba
##eem
##made
celebrates
schooner
agitated
barley
fulfilling
anthropologist
##pro
restrict
novi
regulating
##nent
padres
##rani
##hesive
loyola
tabitha
milky
olson
proprietor
crambidae
guarantees
intercollegiate
ljubljana
hilda
##sko
ignorant
hooded
##lts
sardinia
##lidae
##vation
frontman
privileged
witchcraft
##gp
jammed
laude
poking
##than
bracket
amazement
yunnan
##erus
maharaja
linnaeus
264
commissioning
milano
peacefully
##logies
akira
rani
regulator
##36
grasses
##rance
luzon
crows
compiler
gretchen
seaman
edouard
tab
buccaneers
ellington
hamlets
whig
socialists
##anto
directorial
easton
mythological
##kr
##vary
rhineland
semantic
taut
dune
inventions
succeeds
##iter
replication
branched
##pired
jul
prosecuted
kangaroo
penetrated
##avian
middlesbrough
doses
bleak
madam
predatory
relentless
##vili
reluctance
##vir
hailey
crore
silvery
1759
monstrous
swimmers
transmissions
hawthorn
informing
##eral
toilets
caracas
crouch
kb
##sett
295
cartel
hadley
##aling
alexia
yvonne
##biology
cinderella
eton
superb
blizzard
stabbing
industrialist
maximus
##gm
##orus
groves
maud
clade
oversized
comedic
##bella
rosen
nomadic
fulham
montane
beverages
galaxies
redundant
swarm
##rot
##folia
##llis
buckinghamshire
fen
bearings
bahadur
##rom
gilles
phased
dynamite
faber
benoit
vip
##ount
##wd
booking
fractured
tailored
anya
spices
westwood
cairns
auditions
inflammation
steamed
##rocity
##acion
##urne
skyla
thereof
watford
torment
archdeacon
transforms
lulu
demeanor
fucked
serge
##sor
mckenna
minas
entertainer
##icide
caress
originate
residue
##sty
1740
##ilised
##org
beech
##wana
subsidies
##ghton
emptied
gladstone
ru
firefighters
voodoo
##rcle
het
nightingale
tamara
edmond
ingredient
weaknesses
silhouette
285
compatibility
withdrawing
hampson
##mona
anguish
giggling
##mber
bookstore
##jiang
southernmost
tilting
##vance
bai
economical
rf
briefcase
dreadful
hinted
projections
shattering
totaling
##rogate
analogue
indicted
periodical
fullback
##dman
haynes
##tenberg
##ffs
##ishment
1745
thirst
stumble
penang
vigorous
##ddling
##kor
##lium
octave
##ove
##enstein
##inen
##ones
siberian
##uti
cbn
repeal
swaying
##vington
khalid
tanaka
unicorn
otago
plastered
lobe
riddle
##rella
perch
##ishing
croydon
filtered
graeme
tripoli
##ossa
crocodile
##chers
sufi
mined
##tung
inferno
lsu
##phi
swelled
utilizes
£2
cale
periodicals
styx
hike
informally
coop
lund
##tidae
ala
hen
qui
transformations
disposed
sheath
chickens
##cade
fitzroy
sas
silesia
unacceptable
odisha
1650
sabrina
pe
spokane
ratios
athena
massage
shen
dilemma
##drum
##riz
##hul
corona
doubtful
niall
##pha
##bino
fines
cite
acknowledging
bangor
ballard
bathurst
##resh
huron
mustered
alzheimer
garments
kinase
tyre
warship
##cp
flashback
pulmonary
braun
cheat
kamal
cyclists
constructions
grenades
ndp
traveller
excuses
stomped
signalling
trimmed
futsal
mosques
relevance
##wine
wta
##23
##vah
##lter
hoc
##riding
optimistic
##´s
deco
sim
interacting
rejecting
moniker
waterways
##ieri
##oku
mayors
gdansk
outnumbered
pearls
##ended
##hampton
fairs
totals
dominating
262
notions
stairway
compiling
pursed
commodities
grease
yeast
##jong
carthage
griffiths
residual
amc
contraction
laird
sapphire
##marine
##ivated
amalgamation
dissolve
inclination
lyle
packaged
altitudes
suez
canons
graded
lurched
narrowing
boasts
guise
wed
enrico
##ovsky
rower
scarred
bree
cub
iberian
protagonists
bargaining
proposing
trainers
voyages
vans
fishes
##aea
##ivist
##verance
encryption
artworks
kazan
sabre
cleopatra
hepburn
rotting
supremacy
mecklenburg
##brate
burrows
hazards
outgoing
flair
organizes
##ctions
scorpion
##usions
boo
234
chevalier
dunedin
slapping
##34
ineligible
pensions
##38
##omic
manufactures
emails
bismarck
238
weakening
blackish
ding
mcgee
quo
##rling
northernmost
xx
manpower
greed
sampson
clicking
##ange
##horpe
##inations
##roving
torre
##eptive
##moral
symbolism
38th
asshole
meritorious
outfits
splashed
biographies
sprung
astros
##tale
302
737
filly
raoul
nw
tokugawa
linden
clubhouse
##apa
tracts
romano
##pio
putin
tags
##note
chained
dickson
gunshot
moe
gunn
rashid
##tails
zipper
##bas
##nea
contrasted
##ply
##udes
plum
pharaoh
##pile
aw
comedies
ingrid
sandwiches
subdivisions
1100
mariana
nokia
kamen
hz
delaney
veto
herring
##words
possessive
outlines
##roup
siemens
stairwell
rc
gallantry
messiah
palais
yells
233
zeppelin
##dm
bolivar
##cede
smackdown
mckinley
##mora
##yt
muted
geologic
finely
unitary
avatar
hamas
maynard
rees
bog
contrasting
##rut
liv
chico
disposition
pixel
##erate
becca
dmitry
yeshiva
narratives
##lva
##ulton
mercenary
sharpe
tempered
navigate
stealth
amassed
keynes
##lini
untouched
##rrie
havoc
lithium
##fighting
abyss
graf
southward
wolverine
balloons
implements
ngos
transitions
##icum
ambushed
concacaf
dormant
economists
##dim
costing
csi
rana
universite
boulders
verity
##llon
collin
mellon
misses
cypress
fluorescent
lifeless
spence
##ulla
crewe
shepard
pak
revelations
##م
jolly
gibbons
paw
##dro
##quel
freeing
##test
shack
fries
palatine
##51
##hiko
accompaniment
cruising
recycled
##aver
erwin
sorting
synthesizers
dyke
realities
sg
strides
enslaved
wetland
##ghan
competence
gunpowder
grassy
maroon
reactors
objection
##oms
carlson
gearbox
macintosh
radios
shelton
##sho
clergyman
prakash
254
mongols
trophies
oricon
228
stimuli
twenty20
cantonese
cortes
mirrored
##saurus
bhp
cristina
melancholy
##lating
enjoyable
nuevo
##wny
downfall
schumacher
##ind
banging
lausanne
rumbled
paramilitary
reflex
ax
amplitude
migratory
##gall
##ups
midi
barnard
lastly
sherry
##hp
##nall
keystone
##kra
carleton
slippery
##53
coloring
foe
socket
otter
##rgos
mats
##tose
consultants
bafta
bison
topping
##km
490
primal
abandonment
transplant
atoll
hideous
mort
pained
reproduced
tae
howling
##turn
unlawful
billionaire
hotter
poised
lansing
##chang
dinamo
retro
messing
nfc
domesday
##mina
blitz
timed
##athing
##kley
ascending
gesturing
##izations
signaled
tis
chinatown
mermaid
savanna
jameson
##aint
catalina
##pet
##hers
cochrane
cy
chatting
##kus
alerted
computation
mused
noelle
majestic
mohawk
campo
octagonal
##sant
##hend
241
aspiring
##mart
comprehend
iona
paralyzed
shimmering
swindon
rhone
##eley
reputed
configurations
pitchfork
agitation
francais
gillian
lipstick
##ilo
outsiders
pontifical
resisting
bitterness
sewer
rockies
##edd
##ucher
misleading
1756
exiting
galloway
##nging
risked
##heart
246
commemoration
schultz
##rka
integrating
##rsa
poses
shrieked
##weiler
guineas
gladys
jerking
owls
goldsmith
nightly
penetrating
##unced
lia
##33
ignited
betsy
##aring
##thorpe
follower
vigorously
##rave
coded
kiran
knit
zoology
tbilisi
##28
##bered
repository
govt
deciduous
dino
growling
##bba
enhancement
unleashed
chanting
pussy
biochemistry
##eric
kettle
repression
toxicity
nrhp
##arth
##kko
##bush
ernesto
commended
outspoken
242
mca
parchment
sms
kristen
##aton
bisexual
raked
glamour
navajo
a2
conditioned
showcased
##hma
spacious
youthful
##esa
usl
appliances
junta
brest
layne
conglomerate
enchanted
chao
loosened
picasso
circulating
inspect
montevideo
##centric
##kti
piazza
spurred
##aith
bari
freedoms
poultry
stamford
lieu
##ect
indigo
sarcastic
bahia
stump
attach
dvds
frankenstein
lille
approx
scriptures
pollen
##script
nmi
overseen
##ivism
tides
proponent
newmarket
inherit
milling
##erland
centralized
##rou
distributors
credentials
drawers
abbreviation
##lco
##xon
downing
uncomfortably
ripe
##oes
erase
franchises
##ever
populace
##bery
##khar
decomposition
pleas
##tet
daryl
sabah
##stle
##wide
fearless
genie
lesions
annette
##ogist
oboe
appendix
nair
dripped
petitioned
maclean
mosquito
parrot
rpg
hampered
1648
operatic
reservoirs
##tham
irrelevant
jolt
summarized
##fp
medallion
##taff
##−
clawed
harlow
narrower
goddard
marcia
bodied
fremont
suarez
altering
tempest
mussolini
porn
##isms
sweetly
oversees
walkers
solitude
grimly
shrines
hk
ich
supervisors
hostess
dietrich
legitimacy
brushes
expressive
##yp
dissipated
##rse
localized
systemic
##nikov
gettysburg
##js
##uaries
dialogues
muttering
251
housekeeper
sicilian
discouraged
##frey
beamed
kaladin
halftime
kidnap
##amo
##llet
1754
synonymous
depleted
instituto
insulin
reprised
##opsis
clashed
##ctric
interrupting
radcliffe
insisting
medici
1715
ejected
playfully
turbulent
##47
starvation
##rini
shipment
rebellious
petersen
verification
merits
##rified
cakes
##charged
1757
milford
shortages
spying
fidelity
##aker
emitted
storylines
harvested
seismic
##iform
cheung
kilda
theoretically
barbie
lynx
##rgy
##tius
goblin
mata
poisonous
##nburg
reactive
residues
obedience
##евич
conjecture
##rac
401
hating
sixties
kicker
moaning
motown
##bha
emancipation
neoclassical
##hering
consoles
ebert
professorship
##tures
sustaining
assaults
obeyed
affluent
incurred
tornadoes
##eber
##zow
emphasizing
highlanders
cheated
helmets
##ctus
internship
terence
bony
executions
legislators
berries
peninsular
tinged
##aco
1689
amplifier
corvette
ribbons
lavish
pennant
##lander
worthless
##chfield
##forms
mariano
pyrenees
expenditures
##icides
chesterfield
mandir
tailor
39th
sergey
nestled
willed
aristocracy
devotees
goodnight
raaf
rumored
weaponry
remy
appropriations
harcourt
burr
riaa
##lence
limitation
unnoticed
guo
soaking
swamps
##tica
collapsing
tatiana
descriptive
brigham
psalm
##chment
maddox
##lization
patti
caliph
##aja
akron
injuring
serra
##ganj
basins
##sari
astonished
launcher
##church
hilary
wilkins
sewing
##sf
stinging
##fia
##ncia
underwood
startup
##ition
compilations
vibrations
embankment
jurist
##nity
bard
juventus
groundwater
kern
palaces
helium
boca
cramped
marissa
soto
##worm
jae
princely
##ggy
faso
bazaar
warmly
##voking
229
pairing
##lite
##grate
##nets
wien
freaked
ulysses
rebirth
##alia
##rent
mummy
guzman
jimenez
stilled
##nitz
trajectory
tha
woken
archival
professions
##pts
##pta
hilly
shadowy
shrink
##bolt
norwood
glued
migrate
stereotypes
devoid
##pheus
625
evacuate
horrors
infancy
gotham
knowles
optic
downloaded
sachs
kingsley
parramatta
darryl
mor
##onale
shady
commence
confesses
kan
##meter
##placed
marlborough
roundabout
regents
frigates
io
##imating
gothenburg
revoked
carvings
clockwise
convertible
intruder
##sche
banged
##ogo
vicky
bourgeois
##mony
dupont
footing
##gum
pd
##real
buckle
yun
penthouse
sane
720
serviced
stakeholders
neumann
bb
##eers
comb
##gam
catchment
pinning
rallies
typing
##elles
forefront
freiburg
sweetie
giacomo
widowed
goodwill
worshipped
aspirations
midday
##vat
fishery
##trick
bournemouth
turk
243
hearth
ethanol
guadalajara
murmurs
sl
##uge
afforded
scripted
##hta
wah
##jn
coroner
translucent
252
memorials
puck
progresses
clumsy
##race
315
candace
recounted
##27
##slin
##uve
filtering
##mac
howl
strata
heron
leveled
##ays
dubious
##oja
##т
##wheel
citations
exhibiting
##laya
##mics
##pods
turkic
##lberg
injunction
##ennial
##mit
antibodies
##44
organise
##rigues
cardiovascular
cushion
inverness
##zquez
dia
cocoa
sibling
##tman
##roid
expanse
feasible
tunisian
algiers
##relli
rus
bloomberg
dso
westphalia
bro
tacoma
281
downloads
##ours
konrad
duran
##hdi
continuum
jett
compares
legislator
secession
##nable
##gues
##zuka
translating
reacher
##gley
##ła
aleppo
##agi
tc
orchards
trapping
linguist
versatile
drumming
postage
calhoun
superiors
##mx
barefoot
leary
##cis
ignacio
alfa
kaplan
##rogen
bratislava
mori
##vot
disturb
haas
313
cartridges
gilmore
radiated
salford
tunic
hades
##ulsive
archeological
delilah
magistrates
auditioned
brewster
charters
empowerment
blogs
cappella
dynasties
iroquois
whipping
##krishna
raceway
truths
myra
weaken
judah
mcgregor
##horse
mic
refueling
37th
burnley
bosses
markus
premio
query
##gga
dunbar
##economic
darkest
lyndon
sealing
commendation
reappeared
##mun
addicted
ezio
slaughtered
satisfactory
shuffle
##eves
##thic
##uj
fortification
warrington
##otto
resurrected
fargo
mane
##utable
##lei
##space
foreword
ox
##aris
##vern
abrams
hua
##mento
sakura
##alo
uv
sentimental
##skaya
midfield
##eses
sturdy
scrolls
macleod
##kyu
entropy
##lance
mitochondrial
cicero
excelled
thinner
convoys
perceive
##oslav
##urable
systematically
grind
burkina
287
##tagram
ops
##aman
guantanamo
##cloth
##tite
forcefully
wavy
##jou
pointless
##linger
##tze
layton
portico
superficial
clerical
outlaws
##hism
burials
muir
##inn
creditors
hauling
rattle
##leg
calais
monde
archers
reclaimed
dwell
wexford
hellenic
falsely
remorse
##tek
dough
furnishings
##uttered
gabon
neurological
novice
##igraphy
contemplated
pulpit
nightstand
saratoga
##istan
documenting
pulsing
taluk
##firmed
busted
marital
##rien
disagreements
wasps
##yes
hodge
mcdonnell
mimic
fran
pendant
dhabi
musa
##nington
congratulations
argent
darrell
concussion
losers
regrets
thessaloniki
reversal
donaldson
hardwood
thence
achilles
ritter
##eran
demonic
jurgen
prophets
goethe
eki
classmate
buff
##cking
yank
irrational
##inging
perished
seductive
qur
sourced
##crat
##typic
mustard
ravine
barre
horizontally
characterization
phylogenetic
boise
##dit
##runner
##tower
brutally
intercourse
seduce
##bbing
fay
ferris
ogden
amar
nik
unarmed
##inator
evaluating
kyrgyzstan
sweetness
##lford
##oki
mccormick
meiji
notoriety
stimulate
disrupt
figuring
instructional
mcgrath
##zoo
groundbreaking
##lto
flinch
khorasan
agrarian
bengals
mixer
radiating
##sov
ingram
pitchers
nad
tariff
##cript
tata
##codes
##emi
##ungen
appellate
lehigh
##bled
##giri
brawl
duct
texans
##ciation
##ropolis
skipper
speculative
vomit
doctrines
stresses
253
davy
graders
whitehead
jozef
timely
cumulative
haryana
paints
appropriately
boon
cactus
##ales
##pid
dow
legions
##pit
perceptions
1730
picturesque
##yse
periphery
rune
wr
##aha
celtics
sentencing
whoa
##erin
confirms
variance
425
moines
mathews
spade
rave
m1
fronted
fx
blending
alleging
reared
##gl
237
##paper
grassroots
eroded
##free
##physical
directs
ordeal
##sław
accelerate
hacker
rooftop
##inia
lev
buys
cebu
devote
##lce
specialising
##ulsion
choreographed
repetition
warehouses
##ryl
paisley
tuscany
analogy
sorcerer
hash
huts
shards
descends
exclude
nix
chaplin
gaga
ito
vane
##drich
causeway
misconduct
limo
orchestrated
glands
jana
##kot
u2
##mple
##sons
branching
contrasts
scoop
longed
##virus
chattanooga
##75
syrup
cornerstone
##tized
##mind
##iaceae
careless
precedence
frescoes
##uet
chilled
consult
modelled
snatch
peat
##thermal
caucasian
humane
relaxation
spins
temperance
##lbert
occupations
lambda
hybrids
moons
mp3
##oese
247
rolf
societal
yerevan
ness
##ssler
befriended
mechanized
nominate
trough
boasted
cues
seater
##hom
bends
##tangle
conductors
emptiness
##lmer
eurasian
adriatic
tian
##cie
anxiously
lark
propellers
chichester
jock
ev
2a
##holding
credible
recounts
tori
loyalist
abduction
##hoot
##redo
nepali
##mite
ventral
tempting
##ango
##crats
steered
##wice
javelin
dipping
laborers
prentice
looming
titanium
##ː
badges
emir
tensor
##ntation
egyptians
rash
denies
hawthorne
lombard
showers
wehrmacht
dietary
trojan
##reus
welles
executing
horseshoe
lifeboat
##lak
elsa
infirmary
nearing
roberta
boyer
mutter
trillion
joanne
##fine
##oked
sinks
vortex
uruguayan
clasp
sirius
##block
accelerator
prohibit
sunken
byu
chronological
diplomats
ochreous
510
symmetrical
1644
maia
##tology
salts
reigns
atrocities
##ия
hess
bared
issn
##vyn
cater
saturated
##cycle
##isse
sable
voyager
dyer
yusuf
##inge
fountains
wolff
##39
##nni
engraving
rollins
atheist
ominous
##ault
herr
chariot
martina
strung
##fell
##farlane
horrific
sahib
gazes
saetan
erased
ptolemy
##olic
flushing
lauderdale
analytic
##ices
530
navarro
beak
gorilla
herrera
broom
guadalupe
raiding
sykes
311
bsc
deliveries
1720
invasions
carmichael
tajikistan
thematic
ecumenical
sentiments
onstage
##rians
##brand
##sume
catastrophic
flanks
molten
##arns
waller
aimee
terminating
##icing
alternately
##oche
nehru
printers
outraged
##eving
empires
template
banners
repetitive
za
##oise
vegetarian
##tell
guiana
opt
cavendish
lucknow
synthesized
##hani
##mada
finalized
##ctable
fictitious
mayoral
unreliable
##enham
embracing
peppers
rbis
##chio
##neo
inhibition
slashed
togo
orderly
embroidered
safari
salty
236
barron
benito
totaled
##dak
pubs
simulated
caden
devin
tolkien
momma
welding
sesame
##ept
gottingen
hardness
630
shaman
temeraire
620
adequately
pediatric
##kit
ck
assertion
radicals
composure
cadence
seafood
beaufort
lazarus
mani
warily
cunning
kurdistan
249
cantata
##kir
ares
##41
##clusive
nape
townland
geared
insulted
flutter
boating
violate
draper
dumping
malmo
##hh
##romatic
firearm
alta
bono
obscured
##clave
exceeds
panorama
unbelievable
##train
preschool
##essed
disconnected
installing
rescuing
secretaries
accessibility
##castle
##drive
##ifice
##film
bouts
slug
waterway
mindanao
##buro
##ratic
halves
##ل
calming
liter
maternity
adorable
bragg
electrification
mcc
##dote
roxy
schizophrenia
##body
munoz
kaye
whaling
239
mil
tingling
tolerant
##ago
unconventional
volcanoes
##finder
deportivo
##llie
robson
kaufman
neuroscience
wai
deportation
masovian
scraping
converse
##bh
hacking
bulge
##oun
administratively
yao
580
amp
mammoth
booster
claremont
hooper
nomenclature
pursuits
mclaughlin
melinda
##sul
catfish
barclay
substrates
taxa
zee
originals
kimberly
packets
padma
##ality
borrowing
ostensibly
solvent
##bri
##genesis
##mist
lukas
shreveport
veracruz
##ь
##lou
##wives
cheney
tt
anatolia
hobbs
##zyn
cyclic
radiant
alistair
greenish
siena
dat
independents
##bation
conform
pieter
hyper
applicant
bradshaw
spores
telangana
vinci
inexpensive
nuclei
322
jang
nme
soho
spd
##ign
cradled
receptionist
pow
##43
##rika
fascism
##ifer
experimenting
##ading
##iec
##region
345
jocelyn
maris
stair
nocturnal
toro
constabulary
elgin
##kker
msc
##giving
##schen
##rase
doherty
doping
sarcastically
batter
maneuvers
##cano
##apple
##gai
##git
intrinsic
##nst
##stor
1753
showtime
cafes
gasps
lviv
ushered
##thed
fours
restart
astonishment
transmitting
flyer
shrugs
##sau
intriguing
cones
dictated
mushrooms
medial
##kovsky
##elman
escorting
gaped
##26
godfather
##door
##sell
djs
recaptured
timetable
vila
1710
3a
aerodrome
mortals
scientology
##orne
angelina
mag
convection
unpaid
insertion
intermittent
lego
##nated
endeavor
kota
pereira
##lz
304
bwv
glamorgan
insults
agatha
fey
##cend
fleetwood
mahogany
protruding
steamship
zeta
##arty
mcguire
suspense
##sphere
advising
urges
##wala
hurriedly
meteor
gilded
inline
arroyo
stalker
##oge
excitedly
revered
##cure
earle
introductory
##break
##ilde
mutants
puff
pulses
reinforcement
##haling
curses
lizards
stalk
correlated
##fixed
fallout
macquarie
##unas
bearded
denton
heaving
802
##ocation
winery
assign
dortmund
##lkirk
everest
invariant
charismatic
susie
##elling
bled
lesley
telegram
sumner
bk
##ogen
##к
wilcox
needy
colbert
duval
##iferous
##mbled
allotted
attends
imperative
##hita
replacements
hawker
##inda
insurgency
##zee
##eke
casts
##yla
680
ives
transitioned
##pack
##powering
authoritative
baylor
flex
cringed
plaintiffs
woodrow
##skie
drastic
ape
aroma
unfolded
commotion
nt
preoccupied
theta
routines
lasers
privatization
wand
domino
ek
clenching
nsa
strategically
showered
bile
handkerchief
pere
storing
christophe
insulting
316
nakamura
romani
asiatic
magdalena
palma
cruises
stripping
405
konstantin
soaring
##berman
colloquially
forerunner
havilland
incarcerated
parasites
sincerity
##utus
disks
plank
saigon
##ining
corbin
homo
ornaments
powerhouse
##tlement
chong
fastened
feasibility
idf
morphological
usable
##nish
##zuki
aqueduct
jaguars
keepers
##flies
aleksandr
faust
assigns
ewing
bacterium
hurled
tricky
hungarians
integers
wallis
321
yamaha
##isha
hushed
oblivion
aviator
evangelist
friars
##eller
monograph
ode
##nary
airplanes
labourers
charms
##nee
1661
hagen
tnt
rudder
fiesta
transcript
dorothea
ska
inhibitor
maccabi
retorted
raining
encompassed
clauses
menacing
1642
lineman
##gist
vamps
##ape
##dick
gloom
##rera
dealings
easing
seekers
##nut
##pment
helens
unmanned
##anu
##isson
basics
##amy
##ckman
adjustments
1688
brutality
horne
##zell
sui
##55
##mable
aggregator
##thal
rhino
##drick
##vira
counters
zoom
##01
##rting
mn
montenegrin
packard
##unciation
##♭
##kki
reclaim
scholastic
thugs
pulsed
##icia
syriac
quan
saddam
banda
kobe
blaming
buddies
dissent
##lusion
##usia
corbett
jaya
delle
erratic
lexie
##hesis
435
amiga
hermes
##pressing
##leen
chapels
gospels
jamal
##uating
compute
revolving
warp
##sso
##thes
armory
##eras
##gol
antrim
loki
##kow
##asian
##good
##zano
braid
handwriting
subdistrict
funky
pantheon
##iculate
concurrency
estimation
improper
juliana
##his
newcomers
johnstone
staten
communicated
##oco
##alle
sausage
stormy
##stered
##tters
superfamily
##grade
acidic
collateral
tabloid
##oped
##rza
bladder
austen
##ellant
mcgraw
##hay
hannibal
mein
aquino
lucifer
wo
badger
boar
cher
christensen
greenberg
interruption
##kken
jem
244
mocked
bottoms
cambridgeshire
##lide
sprawling
##bbly
eastwood
ghent
synth
##buck
advisers
##bah
nominally
hapoel
qu
daggers
estranged
fabricated
towels
vinnie
wcw
misunderstanding
anglia
nothin
unmistakable
##dust
##lova
chilly
marquette
truss
##edge
##erine
reece
##lty
##chemist
##connected
272
308
41st
bash
raion
waterfalls
##ump
##main
labyrinth
queue
theorist
##istle
bharatiya
flexed
soundtracks
rooney
leftist
patrolling
wharton
plainly
alleviate
eastman
schuster
topographic
engages
immensely
unbearable
fairchild
1620
dona
lurking
parisian
oliveira
ia
indictment
hahn
bangladeshi
##aster
vivo
##uming
##ential
antonia
expects
indoors
kildare
harlan
##logue
##ogenic
##sities
forgiven
##wat
childish
tavi
##mide
##orra
plausible
grimm
successively
scooted
##bola
##dget
##rith
spartans
emery
flatly
azure
epilogue
##wark
flourish
##iny
##tracted
##overs
##oshi
bestseller
distressed
receipt
spitting
hermit
topological
##cot
drilled
subunit
francs
##layer
eel
##fk
##itas
octopus
footprint
petitions
ufo
##say
##foil
interfering
leaking
palo
##metry
thistle
valiant
##pic
narayan
mcpherson
##fast
gonzales
##ym
##enne
dustin
novgorod
solos
##zman
doin
##raph
##patient
##meyer
soluble
ashland
cuffs
carole
pendleton
whistling
vassal
##river
deviation
revisited
constituents
rallied
rotate
loomed
##eil
##nting
amateurs
augsburg
auschwitz
crowns
skeletons
##cona
bonnet
257
dummy
globalization
simeon
sleeper
mandal
differentiated
##crow
##mare
milne
bundled
exasperated
talmud
owes
segregated
##feng
##uary
dentist
piracy
props
##rang
devlin
##torium
malicious
paws
##laid
dependency
##ergy
##fers
##enna
258
pistons
rourke
jed
grammatical
tres
maha
wig
512
ghostly
jayne
##achal
##creen
##ilis
##lins
##rence
designate
##with
arrogance
cambodian
clones
showdown
throttle
twain
##ception
lobes
metz
nagoya
335
braking
##furt
385
roaming
##minster
amin
crippled
##37
##llary
indifferent
hoffmann
idols
intimidating
1751
261
influenza
memo
onions
1748
bandage
consciously
##landa
##rage
clandestine
observes
swiped
tangle
##ener
##jected
##trum
##bill
##lta
hugs
congresses
josiah
spirited
##dek
humanist
managerial
filmmaking
inmate
rhymes
debuting
grimsby
ur
##laze
duplicate
vigor
##tf
republished
bolshevik
refurbishment
antibiotics
martini
methane
newscasts
royale
horizons
levant
iain
visas
##ischen
paler
##around
manifestation
snuck
alf
chop
futile
pedestal
rehab
##kat
bmg
kerman
res
fairbanks
jarrett
abstraction
saharan
##zek
1746
procedural
clearer
kincaid
sash
luciano
##ffey
crunch
helmut
##vara
revolutionaries
##tute
creamy
leach
##mmon
1747
permitting
nes
plight
wendell
##lese
contra
ts
clancy
ipa
mach
staples
autopsy
disturbances
nueva
karin
pontiac
##uding
proxy
venerable
haunt
leto
bergman
expands
##helm
wal
##pipe
canning
celine
cords
obesity
##enary
intrusion
planner
##phate
reasoned
sequencing
307
harrow
##chon
##dora
marred
mcintyre
repay
tarzan
darting
248
harrisburg
margarita
repulsed
##hur
##lding
belinda
hamburger
novo
compliant
runways
bingham
registrar
skyscraper
ic
cuthbert
improvisation
livelihood
##corp
##elial
admiring
##dened
sporadic
believer
casablanca
popcorn
##29
asha
shovel
##bek
##dice
coiled
tangible
##dez
casper
elsie
resin
tenderness
rectory
##ivision
avail
sonar
##mori
boutique
##dier
guerre
bathed
upbringing
vaulted
sandals
blessings
##naut
##utnant
1680
306
foxes
pia
corrosion
hesitantly
confederates
crystalline
footprints
shapiro
tirana
valentin
drones
45th
microscope
shipments
texted
inquisition
wry
guernsey
unauthorized
resigning
760
ripple
schubert
stu
reassure
felony
##ardo
brittle
koreans
##havan
##ives
dun
implicit
tyres
##aldi
##lth
magnolia
##ehan
##puri
##poulos
aggressively
fei
gr
familiarity
##poo
indicative
##trust
fundamentally
jimmie
overrun
395
anchors
moans
##opus
britannia
armagh
##ggle
purposely
seizing
##vao
bewildered
mundane
avoidance
cosmopolitan
geometridae
quartermaster
caf
415
chatter
engulfed
gleam
purge
##icate
juliette
jurisprudence
guerra
revisions
##bn
casimir
brew
##jm
1749
clapton
cloudy
conde
hermitage
278
simulations
torches
vincenzo
matteo
##rill
hidalgo
booming
westbound
accomplishment
tentacles
unaffected
##sius
annabelle
flopped
sloping
##litz
dreamer
interceptor
vu
##loh
consecration
copying
messaging
breaker
climates
hospitalized
1752
torino
afternoons
winfield
witnessing
##teacher
breakers
choirs
sawmill
coldly
##ege
sipping
haste
uninhabited
conical
bibliography
pamphlets
severn
edict
##oca
deux
illnesses
grips
##pl
rehearsals
sis
thinkers
tame
##keepers
1690
acacia
reformer
##osed
##rys
shuffling
##iring
##shima
eastbound
ionic
rhea
flees
littered
##oum
rocker
vomiting
groaning
champ
overwhelmingly
civilizations
paces
sloop
adoptive
##tish
skaters
##vres
aiding
mango
##joy
nikola
shriek
##ignon
pharmaceuticals
##mg
tuna
calvert
gustavo
stocked
yearbook
##urai
##mana
computed
subsp
riff
hanoi
kelvin
hamid
moors
pastures
summons
jihad
nectar
##ctors
bayou
untitled
pleasing
vastly
republics
intellect
##η
##ulio
##tou
crumbling
stylistic
sb
##ی
consolation
frequented
h₂o
walden
widows
##iens
404
##ignment
chunks
improves
288
grit
recited
##dev
snarl
sociological
##arte
##gul
inquired
##held
bruise
clube
consultancy
homogeneous
hornets
multiplication
pasta
prick
savior
##grin
##kou
##phile
yoon
##gara
grimes
vanishing
cheering
reacting
bn
distillery
##quisite
##vity
coe
dockyard
massif
##jord
escorts
voss
##valent
byte
chopped
hawke
illusions
workings
floats
##koto
##vac
kv
annapolis
madden
##onus
alvaro
noctuidae
##cum
##scopic
avenge
steamboat
forte
illustrates
erika
##trip
570
dew
nationalities
bran
manifested
thirsty
diversified
muscled
reborn
##standing
arson
##lessness
##dran
##logram
##boys
##kushima
##vious
willoughby
##phobia
286
alsace
dashboard
yuki
##chai
granville
myspace
publicized
tricked
##gang
adjective
##ater
relic
reorganisation
enthusiastically
indications
saxe
##lassified
consolidate
iec
padua
helplessly
ramps
renaming
regulars
pedestrians
accents
convicts
inaccurate
lowers
mana
##pati
barrie
bjp
outta
someplace
berwick
flanking
invoked
marrow
sparsely
excerpts
clothed
rei
##ginal
wept
##straße
##vish
alexa
excel
##ptive
membranes
aquitaine
creeks
cutler
sheppard
implementations
ns
##dur
fragrance
budge
concordia
magnesium
marcelo
##antes
gladly
vibrating
##rral
##ggles
montrose
##omba
lew
seamus
1630
cocky
##ament
##uen
bjorn
##rrick
fielder
fluttering
##lase
methyl
kimberley
mcdowell
reductions
barbed
##jic
##tonic
aeronautical
condensed
distracting
##promising
huffed
##cala
##sle
claudius
invincible
missy
pious
balthazar
ci
##lang
butte
combo
orson
##dication
myriad
1707
silenced
##fed
##rh
coco
netball
yourselves
##oza
clarify
heller
peg
durban
etudes
offender
roast
blackmail
curvature
##woods
vile
309
illicit
suriname
##linson
overture
1685
bubbling
gymnast
tucking
##mming
##ouin
maldives
##bala
gurney
##dda
##eased
##oides
backside
pinto
jars
racehorse
tending
##rdial
baronetcy
wiener
duly
##rke
barbarian
cupping
flawed
##thesis
bertha
pleistocene
puddle
swearing
##nob
##tically
fleeting
prostate
amulet
educating
##mined
##iti
##tler
75th
jens
respondents
analytics
cavaliers
papacy
raju
##iente
##ulum
##tip
funnel
271
disneyland
##lley
sociologist
##iam
2500
faulkner
louvre
menon
##dson
276
##ower
afterlife
mannheim
peptide
referees
comedians
meaningless
##anger
##laise
fabrics
hurley
renal
sleeps
##bour
##icle
breakout
kristin
roadside
animator
clover
disdain
unsafe
redesign
##urity
firth
barnsley
portage
reset
narrows
268
commandos
expansive
speechless
tubular
##lux
essendon
eyelashes
smashwords
##yad
##bang
##claim
craved
sprinted
chet
somme
astor
wrocław
orton
266
bane
##erving
##uing
mischief
##amps
##sund
scaling
terre
##xious
impairment
offenses
undermine
moi
soy
contiguous
arcadia
inuit
seam
##tops
macbeth
rebelled
##icative
##iot
590
elaborated
frs
uniformed
##dberg
259
powerless
priscilla
stimulated
980
qc
arboretum
frustrating
trieste
bullock
##nified
enriched
glistening
intern
##adia
locus
nouvelle
ollie
ike
lash
starboard
ee
tapestry
headlined
hove
rigged
##vite
pollock
##yme
thrive
clustered
cas
roi
gleamed
olympiad
##lino
pressured
regimes
##hosis
##lick
ripley
##ophone
kickoff
gallon
rockwell
##arable
crusader
glue
revolutions
scrambling
1714
grover
##jure
englishman
aztec
263
contemplating
coven
ipad
preach
triumphant
tufts
##esian
rotational
##phus
328
falkland
##brates
strewn
clarissa
rejoin
environmentally
glint
banded
drenched
moat
albanians
johor
rr
maestro
malley
nouveau
shaded
taxonomy
v6
adhere
bunk
airfields
##ritan
1741
encompass
remington
tran
##erative
amelie
mazda
friar
morals
passions
##zai
breadth
vis
##hae
argus
burnham
caressing
insider
rudd
##imov
##mini
##rso
italianate
murderous
textual
wainwright
armada
bam
weave
timer
##taken
##nh
fra
##crest
ardent
salazar
taps
tunis
##ntino
allegro
gland
philanthropic
##chester
implication
##optera
esq
judas
noticeably
wynn
##dara
inched
indexed
crises
villiers
bandit
royalties
patterned
cupboard
interspersed
accessory
isla
kendrick
entourage
stitches
##esthesia
headwaters
##ior
interlude
distraught
draught
1727
##basket
biased
sy
transient
triad
subgenus
adapting
kidd
shortstop
##umatic
dimly
spiked
mcleod
reprint
nellie
pretoria
windmill
##cek
singled
##mps
273
reunite
##orous
747
bankers
outlying
##omp
##ports
##tream
apologies
cosmetics
patsy
##deh
##ocks
##yson
bender
nantes
serene
##nad
lucha
mmm
323
##cius
##gli
cmll
coinage
nestor
juarez
##rook
smeared
sprayed
twitching
sterile
irina
embodied
juveniles
enveloped
miscellaneous
cancers
dq
gulped
luisa
crested
swat
donegal
ref
##anov
##acker
hearst
mercantile
##lika
doorbell
ua
vicki
##alla
##som
bilbao
psychologists
stryker
sw
horsemen
turkmenistan
wits
##national
anson
mathew
screenings
##umb
rihanna
##agne
##nessy
aisles
##iani
##osphere
hines
kenton
saskatoon
tasha
truncated
##champ
##itan
mildred
advises
fredrik
interpreting
inhibitors
##athi
spectroscopy
##hab
##kong
karim
panda
##oia
##nail
##vc
conqueror
kgb
leukemia
##dity
arrivals
cheered
pisa
phosphorus
shielded
##riated
mammal
unitarian
urgently
chopin
sanitary
##mission
spicy
drugged
hinges
##tort
tipping
trier
impoverished
westchester
##caster
267
epoch
nonstop
##gman
##khov
aromatic
centrally
cerro
##tively
##vio
billions
modulation
sedimentary
283
facilitating
outrageous
goldstein
##eak
##kt
ld
maitland
penultimate
pollard
##dance
fleets
spaceship
vertebrae
##nig
alcoholism
als
recital
##bham
##ference
##omics
m2
##bm
trois
##tropical
##в
commemorates
##meric
marge
##raction
1643
670
cosmetic
ravaged
##ige
catastrophe
eng
##shida
albrecht
arterial
bellamy
decor
harmon
##rde
bulbs
synchronized
vito
easiest
shetland
shielding
wnba
##glers
##ssar
##riam
brianna
cumbria
##aceous
##rard
cores
thayer
##nsk
brood
hilltop
luminous
carts
keynote
larkin
logos
##cta
##ا
##mund
##quay
lilith
tinted
277
wrestle
mobilization
##uses
sequential
siam
bloomfield
takahashi
274
##ieving
presenters
ringo
blazed
witty
##oven
##ignant
devastation
haydn
harmed
newt
therese
##peed
gershwin
molina
rabbis
sudanese
001
innate
restarted
##sack
##fus
slices
wb
##shah
enroll
hypothetical
hysterical
1743
fabio
indefinite
warped
##hg
exchanging
525
unsuitable
##sboro
gallo
1603
bret
cobalt
homemade
##hunter
mx
operatives
##dhar
terraces
durable
latch
pens
whorls
##ctuated
##eaux
billing
ligament
succumbed
##gly
regulators
spawn
##brick
##stead
filmfare
rochelle
##nzo
1725
circumstance
saber
supplements
##nsky
##tson
crowe
wellesley
carrot
##9th
##movable
primate
drury
sincerely
topical
##mad
##rao
callahan
kyiv
smarter
tits
undo
##yeh
announcements
anthologies
barrio
nebula
##islaus
##shaft
##tyn
bodyguards
2021
assassinate
barns
emmett
scully
##mah
##yd
##eland
##tino
##itarian
demoted
gorman
lashed
prized
adventist
writ
##gui
alla
invertebrates
##ausen
1641
amman
1742
align
healy
redistribution
##gf
##rize
insulation
##drop
adherents
hezbollah
vitro
ferns
yanking
269
php
registering
uppsala
cheerleading
confines
mischievous
tully
##ross
49th
docked
roam
stipulated
pumpkin
##bry
prompt
##ezer
blindly
shuddering
craftsmen
frail
scented
katharine
scramble
shaggy
sponge
helix
zaragoza
279
##52
43rd
backlash
fontaine
seizures
posse
cowan
nonfiction
telenovela
wwii
hammered
undone
##gpur
encircled
irs
##ivation
artefacts
oneself
searing
smallpox
##belle
##osaurus
shandong
breached
upland
blushing
rankin
infinitely
psyche
tolerated
docking
evicted
##col
unmarked
##lving
gnome
lettering
litres
musique
##oint
benevolent
##jal
blackened
##anna
mccall
racers
tingle
##ocene
##orestation
introductions
radically
292
##hiff
##باد
1610
1739
munchen
plead
##nka
condo
scissors
##sight
##tens
apprehension
##cey
##yin
hallmark
watering
formulas
sequels
##llas
aggravated
bae
commencing
##building
enfield
prohibits
marne
vedic
civilized
euclidean
jagger
beforehand
blasts
dumont
##arney
##nem
740
conversions
hierarchical
rios
simulator
##dya
##lellan
hedges
oleg
thrusts
shadowed
darby
maximize
1744
gregorian
##nded
##routed
sham
unspecified
##hog
emory
factual
##smo
##tp
fooled
##rger
ortega
wellness
marlon
##oton
##urance
casket
keating
ley
enclave
##ayan
char
influencing
jia
##chenko
412
ammonia
erebidae
incompatible
violins
cornered
##arat
grooves
astronauts
columbian
rampant
fabrication
kyushu
mahmud
vanish
##dern
mesopotamia
##lete
ict
##rgen
caspian
kenji
pitted
##vered
999
grimace
roanoke
tchaikovsky
twinned
##analysis
##awan
xinjiang
arias
clemson
kazakh
sizable
1662
##khand
##vard
plunge
tatum
vittorio
##nden
cholera
##dana
##oper
bracing
indifference
projectile
superliga
##chee
realises
upgrading
299
porte
retribution
##vies
nk
stil
##resses
ama
bureaucracy
blackberry
bosch
testosterone
collapses
greer
##pathic
ioc
fifties
malls
##erved
bao
baskets
adolescents
siegfried
##osity
##tosis
mantra
detecting
existent
fledgling
##cchi
dissatisfied
gan
telecommunication
mingled
sobbed
6000
controversies
outdated
taxis
##raus
fright
slams
##lham
##fect
##tten
detectors
fetal
tanned
##uw
fray
goth
olympian
skipping
mandates
scratches
sheng
unspoken
hyundai
tracey
hotspur
restrictive
##buch
americana
mundo
##bari
burroughs
diva
vulcan
##6th
distinctions
thumping
##ngen
mikey
sheds
fide
rescues
springsteen
vested
valuation
##ece
##ely
pinnacle
rake
sylvie
##edo
almond
quivering
##irus
alteration
faltered
##wad
51st
hydra
ticked
##kato
recommends
##dicated
antigua
arjun
stagecoach
wilfred
trickle
pronouns
##pon
aryan
nighttime
##anian
gall
pea
stitch
##hei
leung
milos
##dini
eritrea
nexus
starved
snowfall
kant
parasitic
cot
discus
hana
strikers
appleton
kitchens
##erina
##partisan
##itha
##vius
disclose
metis
##channel
1701
tesla
##vera
fitch
1735
blooded
##tila
decimal
##tang
##bai
cyclones
eun
bottled
peas
pensacola
basha
bolivian
crabs
boil
lanterns
partridge
roofed
1645
necks
##phila
opined
patting
##kla
##lland
chuckles
volta
whereupon
##nche
devout
euroleague
suicidal
##dee
inherently
involuntary
knitting
nasser
##hide
puppets
colourful
courageous
southend
stills
miraculous
hodgson
richer
rochdale
ethernet
greta
uniting
prism
umm
##haya
##itical
##utation
deterioration
pointe
prowess
##ropriation
lids
scranton
billings
subcontinent
##koff
##scope
brute
kellogg
psalms
degraded
##vez
stanisław
##ructured
ferreira
pun
astonishing
gunnar
##yat
arya
prc
gottfried
##tight
excursion
##ographer
dina
##quil
##nare
huffington
illustrious
wilbur
gundam
verandah
##zard
naacp
##odle
constructive
fjord
kade
##naud
generosity
thrilling
baseline
cayman
frankish
plastics
accommodations
zoological
##fting
cedric
qb
motorized
##dome
##otted
squealed
tackled
canucks
budgets
situ
asthma
dail
gabled
grasslands
whimpered
writhing
judgments
##65
minnie
pv
##carbon
bananas
grille
domes
monique
odin
maguire
markham
tierney
##estra
##chua
libel
poke
speedy
atrium
laval
notwithstanding
##edly
fai
kala
##sur
robb
##sma
listings
luz
supplementary
tianjin
##acing
enzo
jd
ric
scanner
croats
transcribed
##49
arden
cv
##hair
##raphy
##lver
##uy
357
seventies
staggering
alam
horticultural
hs
regression
timbers
blasting
##ounded
montagu
manipulating
##cit
catalytic
1550
troopers
##meo
condemnation
fitzpatrick
##oire
##roved
inexperienced
1670
castes
##lative
outing
314
dubois
flicking
quarrel
ste
learners
1625
iq
whistled
##class
282
classify
tariffs
temperament
355
folly
liszt
##yles
immersed
jordanian
ceasefire
apparel
extras
maru
fished
##bio
harta
stockport
assortment
craftsman
paralysis
transmitters
##cola
blindness
##wk
fatally
proficiency
solemnly
##orno
repairing
amore
groceries
ultraviolet
##chase
schoolhouse
##tua
resurgence
nailed
##otype
##×
ruse
saliva
diagrams
##tructing
albans
rann
thirties
1b
antennas
hilarious
cougars
paddington
stats
##eger
breakaway
ipod
reza
authorship
prohibiting
scoffed
##etz
##ttle
conscription
defected
trondheim
##fires
ivanov
keenan
##adan
##ciful
##fb
##slow
locating
##ials
##tford
cadiz
basalt
blankly
interned
rags
rattling
##tick
carpathian
reassured
sync
bum
guildford
iss
staunch
##onga
astronomers
sera
sofie
emergencies
susquehanna
##heard
duc
mastery
vh1
williamsburg
bayer
buckled
craving
##khan
##rdes
bloomington
##write
alton
barbecue
##bians
justine
##hri
##ndt
delightful
smartphone
newtown
photon
retrieval
peugeot
hissing
##monium
##orough
flavors
lighted
relaunched
tainted
##games
##lysis
anarchy
microscopic
hopping
adept
evade
evie
##beau
inhibit
sinn
adjustable
hurst
intuition
wilton
cisco
44th
lawful
lowlands
stockings
thierry
##dalen
##hila
##nai
fates
prank
tb
maison
lobbied
provocative
1724
4a
utopia
##qual
carbonate
gujarati
purcell
##rford
curtiss
##mei
overgrown
arenas
mediation
swallows
##rnik
respectful
turnbull
##hedron
##hope
alyssa
ozone
##ʻi
ami
gestapo
johansson
snooker
canteen
cuff
declines
empathy
stigma
##ags
##iner
##raine
taxpayers
gui
volga
##wright
##copic
lifespan
overcame
tattooed
enactment
giggles
##ador
##camp
barrington
bribe
obligatory
orbiting
peng
##enas
elusive
sucker
##vating
cong
hardship
empowered
anticipating
estrada
cryptic
greasy
detainees
planck
sudbury
plaid
dod
marriott
kayla
##ears
##vb
##zd
mortally
##hein
cognition
radha
319
liechtenstein
meade
richly
argyle
harpsichord
liberalism
trumpets
lauded
tyrant
salsa
tiled
lear
promoters
reused
slicing
trident
##chuk
##gami
##lka
cantor
checkpoint
##points
gaul
leger
mammalian
##tov
##aar
##schaft
doha
frenchman
nirvana
##vino
delgado
headlining
##eron
##iography
jug
tko
1649
naga
intersections
##jia
benfica
nawab
##suka
ashford
gulp
##deck
##vill
##rug
brentford
frazier
pleasures
dunne
potsdam
shenzhen
dentistry
##tec
flanagan
##dorff
##hear
chorale
dinah
prem
quezon
##rogated
relinquished
sutra
terri
##pani
flaps
##rissa
poly
##rnet
homme
aback
##eki
linger
womb
##kson
##lewood
doorstep
orthodoxy
threaded
westfield
##rval
dioceses
fridays
subsided
##gata
loyalists
##biotic
##ettes
letterman
lunatic
prelate
tenderly
invariably
souza
thug
winslow
##otide
furlongs
gogh
jeopardy
##runa
pegasus
##umble
humiliated
standalone
tagged
##roller
freshmen
klan
##bright
attaining
initiating
transatlantic
logged
viz
##uance
1723
combatants
intervening
stephane
chieftain
despised
grazed
317
cdc
galveston
godzilla
macro
simulate
##planes
parades
##esses
960
##ductive
##unes
equator
overdose
##cans
##hosh
##lifting
joshi
epstein
sonora
treacherous
aquatics
manchu
responsive
##sation
supervisory
##christ
##llins
##ibar
##balance
##uso
kimball
karlsruhe
mab
##emy
ignores
phonetic
reuters
spaghetti
820
almighty
danzig
rumbling
tombstone
designations
lured
outset
##felt
supermarkets
##wt
grupo
kei
kraft
susanna
##blood
comprehension
genealogy
##aghan
##verted
redding
##ythe
1722
bowing
##pore
##roi
lest
sharpened
fulbright
valkyrie
sikhs
##unds
swans
bouquet
merritt
##tage
##venting
commuted
redhead
clerks
leasing
cesare
dea
hazy
##vances
fledged
greenfield
servicemen
##gical
armando
blackout
dt
sagged
downloadable
intra
potion
pods
##4th
##mism
xp
attendants
gambia
stale
##ntine
plump
asteroids
rediscovered
buds
flea
hive
##neas
1737
classifications
debuts
##eles
olympus
scala
##eurs
##gno
##mute
hummed
sigismund
visuals
wiggled
await
pilasters
clench
sulfate
##ances
bellevue
enigma
trainee
snort
##sw
clouded
denim
##rank
##rder
churning
hartman
lodges
riches
sima
##missible
accountable
socrates
regulates
mueller
##cr
1702
avoids
solids
himalayas
nutrient
pup
##jevic
squat
fades
nec
##lates
##pina
##rona
##ου
privateer
tequila
##gative
##mpton
apt
hornet
immortals
##dou
asturias
cleansing
dario
##rries
##anta
etymology
servicing
zhejiang
##venor
##nx
horned
erasmus
rayon
relocating
£10
##bags
escalated
promenade
stubble
2010s
artisans
axial
liquids
mora
sho
yoo
##tsky
bundles
oldies
##nally
notification
bastion
##ths
sparkle
##lved
1728
leash
pathogen
highs
##hmi
immature
880
gonzaga
ignatius
mansions
monterrey
sweets
bryson
##loe
polled
regatta
brightest
pei
rosy
squid
hatfield
payroll
addict
meath
cornerback
heaviest
lodging
##mage
capcom
rippled
##sily
barnet
mayhem
ymca
snuggled
rousseau
##cute
blanchard
284
fragmented
leighton
chromosomes
risking
##md
##strel
##utter
corinne
coyotes
cynical
hiroshi
yeomanry
##ractive
ebook
grading
mandela
plume
agustin
magdalene
##rkin
bea
femme
trafford
##coll
##lun
##tance
52nd
fourier
upton
##mental
camilla
gust
iihf
islamabad
longevity
##kala
feldman
netting
##rization
endeavour
foraging
mfa
orr
##open
greyish
contradiction
graz
##ruff
handicapped
marlene
tweed
oaxaca
spp
campos
miocene
pri
configured
cooks
pluto
cozy
pornographic
##entes
70th
fairness
glided
jonny
lynne
rounding
sired
##emon
##nist
remade
uncover
##mack
complied
lei
newsweek
##jured
##parts
##enting
##pg
293
finer
guerrillas
athenian
deng
disused
stepmother
accuse
gingerly
seduction
521
confronting
##walker
##going
gora
nostalgia
sabres
virginity
wrenched
##minated
syndication
wielding
eyre
##56
##gnon
##igny
behaved
taxpayer
sweeps
##growth
childless
gallant
##ywood
amplified
geraldine
scrape
##ffi
babylonian
fresco
##rdan
##kney
##position
1718
restricting
tack
fukuoka
osborn
selector
partnering
##dlow
318
gnu
kia
tak
whitley
gables
##54
##mania
mri
softness
immersion
##bots
##evsky
1713
chilling
insignificant
pcs
##uis
elites
lina
purported
supplemental
teaming
##americana
##dding
##inton
proficient
rouen
##nage
##rret
niccolo
selects
##bread
fluffy
1621
gruff
knotted
mukherjee
polgara
thrash
nicholls
secluded
smoothing
thru
corsica
loaf
whitaker
inquiries
##rrier
##kam
indochina
289
marlins
myles
peking
##tea
extracts
pastry
superhuman
connacht
vogel
##ditional
##het
##udged
##lash
gloss
quarries
refit
teaser
##alic
##gaon
20s
materialized
sling
camped
pickering
tung
tracker
pursuant
##cide
cranes
soc
##cini
##typical
##viere
anhalt
overboard
workout
chores
fares
orphaned
stains
##logie
fenton
surpassing
joyah
triggers
##itte
grandmaster
##lass
##lists
clapping
fraudulent
ledger
nagasaki
##cor
##nosis
##tsa
eucalyptus
tun
##icio
##rney
##tara
dax
heroism
ina
wrexham
onboard
unsigned
##dates
moshe
galley
winnie
droplets
exiles
praises
watered
noodles
##aia
fein
adi
leland
multicultural
stink
bingo
comets
erskine
modernized
canned
constraint
domestically
chemotherapy
featherweight
stifled
##mum
darkly
irresistible
refreshing
hasty
isolate
##oys
kitchener
planners
##wehr
cages
yarn
implant
toulon
elects
childbirth
yue
##lind
##lone
cn
rightful
sportsman
junctions
remodeled
specifies
##rgh
291
##oons
complimented
##urgent
lister
ot
##logic
bequeathed
cheekbones
fontana
gabby
##dial
amadeus
corrugated
maverick
resented
triangles
##hered
##usly
nazareth
tyrol
1675
assent
poorer
sectional
aegean
##cous
296
nylon
ghanaian
##egorical
##weig
cushions
forbid
fusiliers
obstruction
somerville
##scia
dime
earrings
elliptical
leyte
oder
polymers
timmy
atm
midtown
piloted
settles
continual
externally
mayfield
##uh
enrichment
henson
keane
persians
1733
benji
braden
pep
324
##efe
contenders
pepsi
valet
##isches
298
##asse
##earing
goofy
stroll
##amen
authoritarian
occurrences
adversary
ahmedabad
tangent
toppled
dorchester
1672
modernism
marxism
islamist
charlemagne
exponential
racks
unicode
brunette
mbc
pic
skirmish
##bund
##lad
##powered
##yst
hoisted
messina
shatter
##ctum
jedi
vantage
##music
##neil
clemens
mahmoud
corrupted
authentication
lowry
nils
##washed
omnibus
wounding
jillian
##itors
##opped
serialized
narcotics
handheld
##arm
##plicity
intersecting
stimulating
##onis
crate
fellowships
hemingway
casinos
climatic
fordham
copeland
drip
beatty
leaflets
robber
brothel
madeira
##hedral
sphinx
ultrasound
##vana
valor
forbade
leonid
villas
##aldo
duane
marquez
##cytes
disadvantaged
forearms
kawasaki
reacts
consular
lax
uncles
uphold
##hopper
concepcion
dorsey
lass
##izan
arching
passageway
1708
researches
tia
internationals
##graphs
##opers
distinguishes
javanese
divert
##uven
plotted
##listic
##rwin
##erik
##tify
affirmative
signifies
validation
##bson
kari
felicity
georgina
zulu
##eros
##rained
##rath
overcoming
##dot
argyll
##rbin
1734
chiba
ratification
windy
earls
parapet
##marks
hunan
pristine
astrid
punta
##gart
brodie
##kota
##oder
malaga
minerva
rouse
##phonic
bellowed
pagoda
portals
reclamation
##gur
##odies
##⁄₄
parentheses
quoting
allergic
palette
showcases
benefactor
heartland
nonlinear
##tness
bladed
cheerfully
scans
##ety
##hone
1666
girlfriends
pedersen
hiram
sous
##liche
##nator
1683
##nery
##orio
##umen
bobo
primaries
smiley
##cb
unearthed
uniformly
fis
metadata
1635
ind
##oted
recoil
##titles
##tura
##ια
406
hilbert
jamestown
mcmillan
tulane
seychelles
##frid
antics
coli
fated
stucco
##grants
1654
bulky
accolades
arrays
caledonian
carnage
optimism
puebla
##tative
##cave
enforcing
rotherham
seo
dunlop
aeronautics
chimed
incline
zoning
archduke
hellenistic
##oses
##sions
candi
thong
##ople
magnate
rustic
##rsk
projective
slant
##offs
danes
hollis
vocalists
##ammed
congenital
contend
gesellschaft
##ocating
##pressive
douglass
quieter
##cm
##kshi
howled
salim
spontaneously
townsville
buena
southport
##bold
kato
1638
faerie
stiffly
##vus
##rled
297
flawless
realising
taboo
##7th
bytes
straightening
356
jena
##hid
##rmin
cartwright
berber
bertram
soloists
411
noses
417
coping
fission
hardin
inca
##cen
1717
mobilized
vhf
##raf
biscuits
curate
##85
##anial
331
gaunt
neighbourhoods
1540
##abas
blanca
bypassed
sockets
behold
coincidentally
##bane
nara
shave
splinter
terrific
##arion
##erian
commonplace
juris
redwood
waistband
boxed
caitlin
fingerprints
jennie
naturalized
##ired
balfour
craters
jody
bungalow
hugely
quilt
glitter
pigeons
undertaker
bulging
constrained
goo
##sil
##akh
assimilation
reworked
##person
persuasion
##pants
felicia
##cliff
##ulent
1732
explodes
##dun
##inium
##zic
lyman
vulture
hog
overlook
begs
northwards
ow
spoil
##urer
fatima
favorably
accumulate
sargent
sorority
corresponded
dispersal
kochi
toned
##imi
##lita
internacional
newfound
##agger
##lynn
##rigue
booths
peanuts
##eborg
medicare
muriel
nur
##uram
crates
millennia
pajamas
worsened
##breakers
jimi
vanuatu
yawned
##udeau
carousel
##hony
hurdle
##ccus
##mounted
##pod
rv
##eche
airship
ambiguity
compulsion
recapture
##claiming
arthritis
##osomal
1667
asserting
ngc
sniffing
dade
discontent
glendale
ported
##amina
defamation
rammed
##scent
fling
livingstone
##fleet
875
##ppy
apocalyptic
comrade
lcd
##lowe
cessna
eine
persecuted
subsistence
demi
hoop
reliefs
710
coptic
progressing
stemmed
perpetrators
1665
priestess
##nio
dobson
ebony
rooster
itf
tortricidae
##bbon
##jian
cleanup
##jean
##øy
1721
eighties
taxonomic
holiness
##hearted
##spar
antilles
showcasing
stabilized
##nb
gia
mascara
michelangelo
dawned
##uria
##vinsky
extinguished
fitz
grotesque
£100
##fera
##loid
##mous
barges
neue
throbbed
cipher
johnnie
##a1
##mpt
outburst
##swick
spearheaded
administrations
c1
heartbreak
pixels
pleasantly
##enay
lombardy
plush
##nsed
bobbie
##hly
reapers
tremor
xiang
minogue
substantive
hitch
barak
##wyl
kwan
##encia
910
obscene
elegance
indus
surfer
bribery
conserve
##hyllum
##masters
horatio
##fat
apes
rebound
psychotic
##pour
iteration
##mium
##vani
botanic
horribly
antiques
dispose
paxton
##hli
##wg
timeless
1704
disregard
engraver
hounds
##bau
##version
looted
uno
facilitates
groans
masjid
rutland
antibody
disqualification
decatur
footballers
quake
slacks
48th
rein
scribe
stabilize
commits
exemplary
tho
##hort
##chison
pantry
traversed
##hiti
disrepair
identifiable
vibrated
baccalaureate
##nnis
csa
interviewing
##iensis
##raße
greaves
wealthiest
343
classed
jogged
£5
##58
##atal
illuminating
knicks
respecting
##uno
scrubbed
##iji
##dles
kruger
moods
growls
raider
silvia
chefs
kam
vr
cree
percival
##terol
gunter
counterattack
defiant
henan
ze
##rasia
##riety
equivalence
submissions
##fra
##thor
bautista
mechanically
##heater
cornice
herbal
templar
##mering
outputs
ruining
ligand
renumbered
extravagant
mika
blockbuster
eta
insurrection
##ilia
darkening
ferocious
pianos
strife
kinship
##aer
melee
##anor
##iste
##may
##oue
decidedly
weep
##jad
##missive
##ppel
354
puget
unease
##gnant
1629
hammering
kassel
ob
wessex
##lga
bromwich
egan
paranoia
utilization
##atable
##idad
contradictory
provoke
##ols
##ouring
##tangled
knesset
##very
##lette
plumbing
##sden
##¹
greensboro
occult
sniff
338
zev
beaming
gamer
haggard
mahal
##olt
##pins
mendes
utmost
briefing
gunnery
##gut
##pher
##zh
##rok
1679
khalifa
sonya
##boot
principals
urbana
wiring
##liffe
##minating
##rrado
dahl
nyu
skepticism
np
townspeople
ithaca
lobster
somethin
##fur
##arina
##−1
freighter
zimmerman
biceps
contractual
##herton
amend
hurrying
subconscious
##anal
336
meng
clermont
spawning
##eia
##lub
dignitaries
impetus
snacks
spotting
twigs
##bilis
##cz
##ouk
libertadores
nic
skylar
##aina
##firm
gustave
asean
##anum
dieter
legislatures
flirt
bromley
trolls
umar
##bbies
##tyle
blah
parc
bridgeport
crank
negligence
##nction
46th
constantin
molded
bandages
seriousness
00pm
siegel
carpets
compartments
upbeat
statehood
##dner
##edging
marko
730
platt
##hane
paving
##iy
1738
abbess
impatience
limousine
nbl
##talk
441
lucille
mojo
nightfall
robbers
##nais
karel
brisk
calves
replicate
ascribed
telescopes
##olf
intimidated
##reen
ballast
specialization
##sit
aerodynamic
caliphate
rainer
visionary
##arded
epsilon
##aday
##onte
aggregation
auditory
boosted
reunification
kathmandu
loco
robyn
402
acknowledges
appointing
humanoid
newell
redeveloped
restraints
##tained
barbarians
chopper
1609
italiana
##lez
##lho
investigates
wrestlemania
##anies
##bib
690
##falls
creaked
dragoons
gravely
minions
stupidity
volley
##harat
##week
musik
##eries
##uously
fungal
massimo
semantics
malvern
##ahl
##pee
discourage
embryo
imperialism
1910s
profoundly
##ddled
jiangsu
sparkled
stat
##holz
sweatshirt
tobin
##iction
sneered
##cheon
##oit
brit
causal
smyth
##neuve
diffuse
perrin
silvio
##ipes
##recht
detonated
iqbal
selma
##nism
##zumi
roasted
##riders
tay
##ados
##mament
##mut
##rud
840
completes
nipples
cfa
flavour
hirsch
##laus
calderon
sneakers
moravian
##ksha
1622
rq
294
##imeters
bodo
##isance
##pre
##ronia
anatomical
excerpt
##lke
dh
kunst
##tablished
##scoe
biomass
panted
unharmed
gael
housemates
montpellier
##59
coa
rodents
tonic
hickory
singleton
##taro
451
1719
aldo
breaststroke
dempsey
och
rocco
##cuit
merton
dissemination
midsummer
serials
##idi
haji
polynomials
##rdon
gs
enoch
prematurely
shutter
taunton
£3
##grating
##inates
archangel
harassed
##asco
326
archway
dazzling
##ecin
1736
sumo
wat
##kovich
1086
honneur
##ently
##nostic
##ttal
##idon
1605
403
1716
blogger
rents
##gnan
hires
##ikh
##dant
howie
##rons
handler
retracted
shocks
1632
arun
duluth
kepler
trumpeter
##lary
peeking
seasoned
trooper
##mara
laszlo
##iciencies
##rti
heterosexual
##inatory
##ssion
indira
jogging
##inga
##lism
beit
dissatisfaction
malice
##ately
nedra
peeling
##rgeon
47th
stadiums
475
vertigo
##ains
iced
restroom
##plify
##tub
illustrating
pear
##chner
##sibility
inorganic
rappers
receipts
watery
##kura
lucinda
##oulos
reintroduced
##8th
##tched
gracefully
saxons
nutritional
wastewater
rained
favourites
bedrock
fisted
hallways
likeness
upscale
##lateral
1580
blinds
prequel
##pps
##tama
deter
humiliating
restraining
tn
vents
1659
laundering
recess
rosary
tractors
coulter
federer
##ifiers
##plin
persistence
##quitable
geschichte
pendulum
quakers
##beam
bassett
pictorial
buffet
koln
##sitor
drills
reciprocal
shooters
##57
##cton
##tees
converge
pip
dmitri
donnelly
yamamoto
aqua
azores
demographics
hypnotic
spitfire
suspend
wryly
roderick
##rran
sebastien
##asurable
mavericks
##fles
##200
himalayan
prodigy
##iance
transvaal
demonstrators
handcuffs
dodged
mcnamara
sublime
1726
crazed
##efined
##till
ivo
pondered
reconciled
shrill
sava
##duk
bal
cad
heresy
jaipur
goran
##nished
341
lux
shelly
whitehall
##hre
israelis
peacekeeping
##wled
1703
demetrius
ousted
##arians
##zos
beale
anwar
backstroke
raged
shrinking
cremated
##yck
benign
towing
wadi
darmstadt
landfill
parana
soothe
colleen
sidewalks
mayfair
tumble
hepatitis
ferrer
superstructure
##gingly
##urse
##wee
anthropological
translators
##mies
closeness
hooves
##pw
mondays
##roll
##vita
landscaping
##urized
purification
sock
thorns
thwarted
jalan
tiberius
##taka
saline
##rito
confidently
khyber
sculptors
##ij
brahms
hammersmith
inspectors
battista
fivb
fragmentation
hackney
##uls
arresting
exercising
antoinette
bedfordshire
##zily
dyed
##hema
1656
racetrack
variability
##tique
1655
austrians
deteriorating
madman
theorists
aix
lehman
weathered
1731
decreed
eruptions
1729
flaw
quinlan
sorbonne
flutes
nunez
1711
adored
downwards
fable
rasped
1712
moritz
mouthful
renegade
shivers
stunts
dysfunction
restrain
translit
327
pancakes
##avio
##cision
##tray
351
vial
##lden
bain
##maid
##oxide
chihuahua
malacca
vimes
##rba
##rnier
1664
donnie
plaques
##ually
337
bangs
floppy
huntsville
loretta
nikolay
##otte
eater
handgun
ubiquitous
##hett
eras
zodiac
1634
##omorphic
1820s
##zog
cochran
##bula
##lithic
warring
##rada
dalai
excused
blazers
mcconnell
reeling
bot
este
##abi
geese
hoax
taxon
##bla
guitarists
##icon
condemning
hunts
inversion
moffat
taekwondo
##lvis
1624
stammered
##rest
##rzy
sousa
fundraiser
marylebone
navigable
uptown
cabbage
daniela
salman
shitty
whimper
##kian
##utive
programmers
protections
rm
##rmi
##rued
forceful
##enes
fuss
##tao
##wash
brat
oppressive
reykjavik
spartak
ticking
##inkles
##kiewicz
adolph
horst
maui
protege
straighten
cpc
landau
concourse
clements
resultant
##ando
imaginative
joo
reactivated
##rem
##ffled
##uising
consultative
##guide
flop
kaitlyn
mergers
parenting
somber
##vron
supervise
vidhan
##imum
courtship
exemplified
harmonies
medallist
refining
##rrow
##ка
amara
##hum
780
goalscorer
sited
overshadowed
rohan
displeasure
secretive
multiplied
osman
##orth
engravings
padre
##kali
##veda
miniatures
mis
##yala
clap
pali
rook
##cana
1692
57th
antennae
astro
oskar
1628
bulldog
crotch
hackett
yucatan
##sure
amplifiers
brno
ferrara
migrating
##gree
thanking
turing
##eza
mccann
ting
andersson
onslaught
gaines
ganga
incense
standardization
##mation
sentai
scuba
stuffing
turquoise
waivers
alloys
##vitt
regaining
vaults
##clops
##gizing
digger
furry
memorabilia
probing
##iad
payton
rec
deutschland
filippo
opaque
seamen
zenith
afrikaans
##filtration
disciplined
inspirational
##merie
banco
confuse
grafton
tod
##dgets
championed
simi
anomaly
biplane
##ceptive
electrode
##para
1697
cleavage
crossbow
swirl
informant
##lars
##osta
afi
bonfire
spec
##oux
lakeside
slump
##culus
##lais
##qvist
##rrigan
1016
facades
borg
inwardly
cervical
xl
pointedly
050
stabilization
##odon
chests
1699
hacked
ctv
orthogonal
suzy
##lastic
gaulle
jacobite
rearview
##cam
##erted
ashby
##drik
##igate
##mise
##zbek
affectionately
canine
disperse
latham
##istles
##ivar
spielberg
##orin
##idium
ezekiel
cid
##sg
durga
middletown
##cina
customized
frontiers
harden
##etano
##zzy
1604
bolsheviks
##66
coloration
yoko
##bedo
briefs
slabs
debra
liquidation
plumage
##oin
blossoms
dementia
subsidy
1611
proctor
relational
jerseys
parochial
ter
##ici
esa
peshawar
cavalier
loren
cpi
idiots
shamrock
1646
dutton
malabar
mustache
##endez
##ocytes
referencing
terminates
marche
yarmouth
##sop
acton
mated
seton
subtly
baptised
beige
extremes
jolted
kristina
telecast
##actic
safeguard
waldo
##baldi
##bular
endeavors
sloppy
subterranean
##ensburg
##itung
delicately
pigment
tq
##scu
1626
##ound
collisions
coveted
herds
##personal
##meister
##nberger
chopra
##ricting
abnormalities
defective
galician
lucie
##dilly
alligator
likened
##genase
burundi
clears
complexion
derelict
deafening
diablo
fingered
champaign
dogg
enlist
isotope
labeling
mrna
##erre
brilliance
marvelous
##ayo
1652
crawley
ether
footed
dwellers
deserts
hamish
rubs
warlock
skimmed
##lizer
870
buick
embark
heraldic
irregularities
##ajan
kiara
##kulam
##ieg
antigen
kowalski
##lge
oakley
visitation
##mbit
vt
##suit
1570
murderers
##miento
##rites
chimneys
##sling
condemn
custer
exchequer
havre
##ghi
fluctuations
##rations
dfb
hendricks
vaccines
##tarian
nietzsche
biking
juicy
##duced
brooding
scrolling
selangor
##ragan
352
annum
boomed
seminole
sugarcane
##dna
departmental
dismissing
innsbruck
arteries
ashok
batavia
daze
kun
overtook
##rga
##tlan
beheaded
gaddafi
holm
electronically
faulty
galilee
fractures
kobayashi
##lized
gunmen
magma
aramaic
mala
eastenders
inference
messengers
bf
##qu
407
bathrooms
##vere
1658
flashbacks
ideally
misunderstood
##jali
##weather
mendez
##grounds
505
uncanny
##iii
1709
friendships
##nbc
sacrament
accommodated
reiterated
logistical
pebbles
thumped
##escence
administering
decrees
drafts
##flight
##cased
##tula
futuristic
picket
intimidation
winthrop
##fahan
interfered
339
afar
francoise
morally
uta
cochin
croft
dwarfs
##bruck
##dents
##nami
biker
##hner
##meral
nano
##isen
##ometric
##pres
##ан
brightened
meek
parcels
securely
gunners
##jhl
##zko
agile
hysteria
##lten
##rcus
bukit
champs
chevy
cuckoo
leith
sadler
theologians
welded
##section
1663
jj
plurality
xander
##rooms
##formed
shredded
temps
intimately
pau
tormented
##lok
##stellar
1618
charred
ems
essen
##mmel
alarms
spraying
ascot
blooms
twinkle
##abia
##apes
internment
obsidian
##chaft
snoop
##dav
##ooping
malibu
##tension
quiver
##itia
hays
mcintosh
travers
walsall
##ffie
1623
beverley
schwarz
plunging
structurally
m3
rosenthal
vikram
##tsk
770
ghz
##onda
##tiv
chalmers
groningen
pew
reckon
unicef
##rvis
55th
##gni
1651
sulawesi
avila
cai
metaphysical
screwing
turbulence
##mberg
augusto
samba
56th
baffled
momentary
toxin
##urian
##wani
aachen
condoms
dali
steppe
##3d
##app
##oed
##year
adolescence
dauphin
electrically
inaccessible
microscopy
nikita
##ega
atv
##cel
##enter
##oles
##oteric
##ы
accountants
punishments
wrongly
bribes
adventurous
clinch
flinders
southland
##hem
##kata
gough
##ciency
lads
soared
##ה
undergoes
deformation
outlawed
rubbish
##arus
##mussen
##nidae
##rzburg
arcs
##ingdon
##tituted
1695
wheelbase
wheeling
bombardier
campground
zebra
##lices
##oj
##bain
lullaby
##ecure
donetsk
wylie
grenada
##arding
##ης
squinting
eireann
opposes
##andra
maximal
runes
##broken
##cuting
##iface
##ror
##rosis
additive
britney
adultery
triggering
##drome
detrimental
aarhus
containment
jc
swapped
vichy
##ioms
madly
##oric
##rag
brant
##ckey
##trix
1560
1612
broughton
rustling
##stems
##uder
asbestos
mentoring
##nivorous
finley
leaps
##isan
apical
pry
slits
substitutes
##dict
intuitive
fantasia
insistent
unreasonable
##igen
##vna
domed
hannover
margot
ponder
##zziness
impromptu
jian
lc
rampage
stemming
##eft
andrey
gerais
whichever
amnesia
appropriated
anzac
clicks
modifying
ultimatum
cambrian
maids
verve
yellowstone
##mbs
conservatoire
##scribe
adherence
dinners
spectra
imperfect
mysteriously
sidekick
tatar
tuba
##aks
##ifolia
distrust
##athan
##zle
c2
ronin
zac
##pse
celaena
instrumentalist
scents
skopje
##mbling
comical
compensated
vidal
condor
intersect
jingle
wavelengths
##urrent
mcqueen
##izzly
carp
weasel
422
kanye
militias
postdoctoral
eugen
gunslinger
##ɛ
faux
hospice
##for
appalled
derivation
dwarves
##elis
dilapidated
##folk
astoria
philology
##lwyn
##otho
##saka
inducing
philanthropy
##bf
##itative
geek
markedly
sql
##yce
bessie
indices
rn
##flict
495
frowns
resolving
weightlifting
tugs
cleric
contentious
1653
mania
rms
##miya
##reate
##ruck
##tucket
bien
eels
marek
##ayton
##cence
discreet
unofficially
##ife
leaks
##bber
1705
332
dung
compressor
hillsborough
pandit
shillings
distal
##skin
381
##tat
##you
nosed
##nir
mangrove
undeveloped
##idia
textures
##inho
##500
##rise
ae
irritating
nay
amazingly
bancroft
apologetic
compassionate
kata
symphonies
##lovic
airspace
##lch
930
gifford
precautions
fulfillment
sevilla
vulgar
martinique
##urities
looting
piccolo
tidy
##dermott
quadrant
armchair
incomes
mathematicians
stampede
nilsson
##inking
##scan
foo
quarterfinal
##ostal
shang
shouldered
squirrels
##owe
344
vinegar
##bner
##rchy
##systems
delaying
##trics
ars
dwyer
rhapsody
sponsoring
##gration
bipolar
cinder
starters
##olio
##urst
421
signage
##nty
aground
figurative
mons
acquaintances
duets
erroneously
soyuz
elliptic
recreated
##cultural
##quette
##ssed
##tma
##zcz
moderator
scares
##itaire
##stones
##udence
juniper
sighting
##just
##nsen
britten
calabria
ry
bop
cramer
forsyth
stillness
##л
airmen
gathers
unfit
##umber
##upt
taunting
##rip
seeker
streamlined
##bution
holster
schumann
tread
vox
##gano
##onzo
strive
dil
reforming
covent
newbury
predicting
##orro
decorate
tre
##puted
andover
ie
asahi
dept
dunkirk
gills
##tori
buren
huskies
##stis
##stov
abstracts
bets
loosen
##opa
1682
yearning
##glio
##sir
berman
effortlessly
enamel
napoli
persist
##peration
##uez
attache
elisa
b1
invitations
##kic
accelerating
reindeer
boardwalk
clutches
nelly
polka
starbucks
##kei
adamant
huey
lough
unbroken
adventurer
embroidery
inspecting
stanza
##ducted
naia
taluka
##pone
##roids
chases
deprivation
florian
##jing
##ppet
earthly
##lib
##ssee
colossal
foreigner
vet
freaks
patrice
rosewood
triassic
upstate
##pkins
dominates
ata
chants
ks
vo
##400
##bley
##raya
##rmed
555
agra
infiltrate
##ailing
##ilation
##tzer
##uppe
##werk
binoculars
enthusiast
fujian
squeak
##avs
abolitionist
almeida
boredom
hampstead
marsden
rations
##ands
inflated
334
bonuses
rosalie
patna
##rco
329
detachments
penitentiary
54th
flourishing
woolf
##dion
##etched
papyrus
##lster
##nsor
##toy
bobbed
dismounted
endelle
inhuman
motorola
tbs
wince
wreath
##ticus
hideout
inspections
sanjay
disgrace
infused
pudding
stalks
##urbed
arsenic
leases
##hyl
##rrard
collarbone
##waite
##wil
dowry
##bant
##edance
genealogical
nitrate
salamanca
scandals
thyroid
necessitated
##!
##"
###
##$
##%
##&
##'
##(
##)
##*
##+
##,
##-
##.
##/
##:
##;
##<
##=
##>
##?
##@
##[
##\
##]
##^
##_
##`
##{
##|
##}
##~
##¡
##¢
##£
##¤
##¥
##¦
##§
##¨
##©
##ª
##«
##¬
##®
##±
##´
##µ
##¶
##·
##º
##»
##¼
##¾
##¿
##æ
##ð
##÷
##þ
##đ
##ħ
##ŋ
##œ
##ƒ
##ɐ
##ɑ
##ɒ
##ɔ
##ɕ
##ə
##ɡ
##ɣ
##ɨ
##ɪ
##ɫ
##ɬ
##ɯ
##ɲ
##ɴ
##ɹ
##ɾ
##ʀ
##ʁ
##ʂ
##ʃ
##ʉ
##ʊ
##ʋ
##ʌ
##ʎ
##ʐ
##ʑ
##ʒ
##ʔ
##ʰ
##ʲ
##ʳ
##ʷ
##ʸ
##ʻ
##ʼ
##ʾ
##ʿ
##ˈ
##ˡ
##ˢ
##ˣ
##ˤ
##β
##γ
##δ
##ε
##ζ
##θ
##κ
##λ
##μ
##ξ
##ο
##π
##ρ
##σ
##τ
##υ
##φ
##χ
##ψ
##ω
##б
##г
##д
##ж
##з
##м
##п
##с
##у
##ф
##х
##ц
##ч
##ш
##щ
##ъ
##э
##ю
##ђ
##є
##і
##ј
##љ
##њ
##ћ
##ӏ
##ա
##բ
##գ
##դ
##ե
##թ
##ի
##լ
##կ
##հ
##մ
##յ
##ն
##ո
##պ
##ս
##վ
##տ
##ր
##ւ
##ք
##־
##א
##ב
##ג
##ד
##ו
##ז
##ח
##ט
##י
##ך
##כ
##ל
##ם
##מ
##ן
##נ
##ס
##ע
##ף
##פ
##ץ
##צ
##ק
##ר
##ש
##ת
##،
##ء
##ب
##ت
##ث
##ج
##ح
##خ
##ذ
##ز
##س
##ش
##ص
##ض
##ط
##ظ
##ع
##غ
##ـ
##ف
##ق
##ك
##و
##ى
##ٹ
##پ
##چ
##ک
##گ
##ں
##ھ
##ہ
##ے
##अ
##आ
##उ
##ए
##क
##ख
##ग
##च
##ज
##ट
##ड
##ण
##त
##थ
##द
##ध
##न
##प
##ब
##भ
##म
##य
##र
##ल
##व
##श
##ष
##स
##ह
##ा
##ि
##ी
##ो
##।
##॥
##ং
##অ
##আ
##ই
##উ
##এ
##ও
##ক
##খ
##গ
##চ
##ছ
##জ
##ট
##ড
##ণ
##ত
##থ
##দ
##ধ
##ন
##প
##ব
##ভ
##ম
##য
##র
##ল
##শ
##ষ
##স
##হ
##া
##ি
##ী
##ে
##க
##ச
##ட
##த
##ந
##ன
##ப
##ம
##ய
##ர
##ல
##ள
##வ
##ா
##ி
##ு
##ே
##ை
##ನ
##ರ
##ಾ
##ක
##ය
##ර
##ල
##ව
##ා
##ก
##ง
##ต
##ท
##น
##พ
##ม
##ย
##ร
##ล
##ว
##ส
##อ
##า
##เ
##་
##།
##ག
##ང
##ད
##ན
##པ
##བ
##མ
##འ
##ར
##ལ
##ས
##မ
##ა
##ბ
##გ
##დ
##ე
##ვ
##თ
##ი
##კ
##ლ
##მ
##ნ
##ო
##რ
##ს
##ტ
##უ
##ᄀ
##ᄂ
##ᄃ
##ᄅ
##ᄆ
##ᄇ
##ᄉ
##ᄊ
##ᄋ
##ᄌ
##ᄎ
##ᄏ
##ᄐ
##ᄑ
##ᄒ
##ᅡ
##ᅢ
##ᅥ
##ᅦ
##ᅧ
##ᅩ
##ᅪ
##ᅭ
##ᅮ
##ᅯ
##ᅲ
##ᅳ
##ᅴ
##ᅵ
##ᆨ
##ᆫ
##ᆯ
##ᆷ
##ᆸ
##ᆼ
##ᴬ
##ᴮ
##ᴰ
##ᴵ
##ᴺ
##ᵀ
##ᵃ
##ᵇ
##ᵈ
##ᵉ
##ᵍ
##ᵏ
##ᵐ
##ᵒ
##ᵖ
##ᵗ
##ᵘ
##ᵣ
##ᵤ
##ᵥ
##ᶜ
##ᶠ
##‐
##‑
##‒
##–
##—
##―
##‖
##‘
##’
##‚
##“
##”
##„
##†
##‡
##•
##…
##‰
##′
##″
##›
##‿
##⁄
##⁰
##ⁱ
##⁴
##⁵
##⁶
##⁷
##⁸
##⁹
##⁻
##ⁿ
##₅
##₆
##₇
##₈
##₉
##₊
##₍
##₎
##ₐ
##ₑ
##ₒ
##ₓ
##ₕ
##ₖ
##ₗ
##ₘ
##ₚ
##ₛ
##ₜ
##₤
##₩
##€
##₱
##₹
##ℓ
##№
##ℝ
##™
##⅓
##⅔
##←
##↑
##→
##↓
##↔
##↦
##⇄
##⇌
##⇒
##∂
##∅
##∆
##∇
##∈
##∗
##∘
##√
##∞
##∧
##∨
##∩
##∪
##≈
##≡
##≤
##≥
##⊂
##⊆
##⊕
##⊗
##⋅
##─
##│
##■
##▪
##●
##★
##☆
##☉
##♠
##♣
##♥
##♦
##♯
##⟨
##⟩
##ⱼ
##⺩
##⺼
##⽥
##、
##。
##〈
##〉
##《
##》
##「
##」
##『
##』
##〜
##あ
##い
##う
##え
##お
##か
##き
##く
##け
##こ
##さ
##し
##す
##せ
##そ
##た
##ち
##っ
##つ
##て
##と
##な
##に
##ぬ
##ね
##の
##は
##ひ
##ふ
##へ
##ほ
##ま
##み
##む
##め
##も
##や
##ゆ
##よ
##ら
##り
##る
##れ
##ろ
##を
##ん
##ァ
##ア
##ィ
##イ
##ウ
##ェ
##エ
##オ
##カ
##キ
##ク
##ケ
##コ
##サ
##シ
##ス
##セ
##タ
##チ
##ッ
##ツ
##テ
##ト
##ナ
##ニ
##ノ
##ハ
##ヒ
##フ
##ヘ
##ホ
##マ
##ミ
##ム
##メ
##モ
##ャ
##ュ
##ョ
##ラ
##リ
##ル
##レ
##ロ
##ワ
##ン
##・
##ー
##一
##三
##上
##下
##不
##世
##中
##主
##久
##之
##也
##事
##二
##五
##井
##京
##人
##亻
##仁
##介
##代
##仮
##伊
##会
##佐
##侍
##保
##信
##健
##元
##光
##八
##公
##内
##出
##分
##前
##劉
##力
##加
##勝
##北
##区
##十
##千
##南
##博
##原
##口
##古
##史
##司
##合
##吉
##同
##名
##和
##囗
##四
##国
##國
##土
##地
##坂
##城
##堂
##場
##士
##夏
##外
##大
##天
##太
##夫
##奈
##女
##子
##学
##宀
##宇
##安
##宗
##定
##宣
##宮
##家
##宿
##寺
##將
##小
##尚
##山
##岡
##島
##崎
##川
##州
##巿
##帝
##平
##年
##幸
##广
##弘
##張
##彳
##後
##御
##德
##心
##忄
##志
##忠
##愛
##成
##我
##戦
##戸
##手
##扌
##政
##文
##新
##方
##日
##明
##星
##春
##昭
##智
##曲
##書
##月
##有
##朝
##木
##本
##李
##村
##東
##松
##林
##森
##楊
##樹
##橋
##歌
##止
##正
##武
##比
##氏
##民
##水
##氵
##氷
##永
##江
##沢
##河
##治
##法
##海
##清
##漢
##瀬
##火
##版
##犬
##王
##生
##田
##男
##疒
##発
##白
##的
##皇
##目
##相
##省
##真
##石
##示
##社
##神
##福
##禾
##秀
##秋
##空
##立
##章
##竹
##糹
##美
##義
##耳
##良
##艹
##花
##英
##華
##葉
##藤
##行
##街
##西
##見
##訁
##語
##谷
##貝
##貴
##車
##軍
##辶
##道
##郎
##郡
##部
##都
##里
##野
##金
##鈴
##镇
##長
##門
##間
##阝
##阿
##陳
##陽
##雄
##青
##面
##風
##食
##香
##馬
##高
##龍
##龸
##fi
##fl
##!
##(
##)
##,
##-
##.
##/
##:
##?
##~
|
Tools/DGLPyTorch/SyntheticGraphGeneration/syngen/cli | cli | __init__ | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import argparse
from syngen.cli.commands.synthesize import SynthesizeCommand
from syngen.cli.commands.preprocess import PreprocessingCommand
from syngen.cli.commands.mimic_dataset import MimicDatasetCommand
from syngen.cli.commands.pretrain import PretrainCommand
def get_parser():
parser = argparse.ArgumentParser(
description="Synthetic Graph Generation Tool",
formatter_class=argparse.ArgumentDefaultsHelpFormatter
)
command = parser.add_subparsers(title="command")
command.required = True
SynthesizeCommand().init_parser(command)
PreprocessingCommand().init_parser(command)
MimicDatasetCommand().init_parser(command)
PretrainCommand().init_parser(command)
return parser
|
CUDA-Optimized/FastSpeech/fastspeech/trt/plugins/repeat | repeat | test_repeat_plugin | # Copyright (c) 2020, NVIDIA CORPORATION. All rights reserved.
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright
# notice, this list of conditions and the following disclaimer in the
# documentation and/or other materials provided with the distribution.
# * Neither the name of the NVIDIA CORPORATION nor the
# names of its contributors may be used to endorse or promote products
# derived from this software without specific prior written permission.
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
# ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
# WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
# DISCLAIMED. IN NO EVENT SHALL NVIDIA CORPORATION BE LIABLE FOR ANY
# DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
# (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
# LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
# ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
# SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
from __future__ import print_function
import numpy as np
import tensorrt as trt
import pycuda.autoinit
import pycuda.driver as cuda
import ctypes
import os
import time
import sys
logger = trt.Logger(trt.Logger.INFO)
PLUGIN_PATH = '/home/dahn/git/fastspeech/fastspeech/trt/plugins/repeat/RepeatPlugin.so'
ctypes.cdll.LoadLibrary(PLUGIN_PATH)
def get_plugin_creator(plugin_name):
trt.init_libnvinfer_plugins(logger, '')
plugin_creator_list = trt.get_plugin_registry().plugin_creator_list
plugin_creator = None
for c in plugin_creator_list:
if c.name == plugin_name:
plugin_creator = c
return plugin_creator
def build_engine(shape, shape2):
plugin_creator = get_plugin_creator('RepeatPlugin')
if plugin_creator == None:
print('Plugin not found. Exiting')
exit()
builder = trt.Builder(logger)
builder.max_batch_size = 1024
builder.max_workspace_size = 1 << 20
builder.fp16_mode = use_fp16
network = builder.create_network()
tensor = network.add_input('input1', trt.DataType.FLOAT, shape)
tensor2 = network.add_input('input2', trt.DataType.FLOAT, shape2)
tensor = network.add_plugin_v2(
[tensor, tensor2],
plugin_creator.create_plugin('RepeatPlugin', trt.PluginFieldCollection([
trt.PluginField('maxOutputLength', np.array([MAX_OUTPUT_LENGTH], dtype=np.int32), trt.PluginFieldType.INT32)
]))
).get_output(0)
network.mark_output(tensor)
return builder.build_cuda_engine(network)
def run_trt(input1, input2):
batch_size = input1.shape[0]
engine = build_engine(input1.shape[1:], input2.shape[1:])
context = engine.create_execution_context()
d_input1 = cuda.mem_alloc(input1.nbytes)
d_input2 = cuda.mem_alloc(input2.nbytes)
output = np.zeros(shape=(batch_size, MAX_OUTPUT_LENGTH, input1.shape[2]), dtype=np.float32)
d_output = cuda.mem_alloc(output.nbytes)
cuda.memcpy_htod(d_input1, input1)
cuda.memcpy_htod(d_input2, input2)
bindings = [int(d_input1), int(d_input2), int(d_output)]
start = time.time()
context.execute(batch_size, bindings)
end = time.time()
time_elapsed = end - start
print("time elapsed: {:06f}".format(time_elapsed))
cuda.memcpy_dtoh(output, d_output)
return output
use_fp16 = len(sys.argv) > 1 and sys.argv[1].isdigit() and int(sys.argv[1]) == 1
print('Use FP16:', use_fp16)
##
# accuray test
##
MAX_OUTPUT_LENGTH=8
inputs = np.array([
[[1, 2], [4, 5], [7, 8]],
[[3, 4], [5, 6], [8, 9]]
], np.float32)
masks = np.ones((2,3,1), np.float32)
repeats = np.array([
[[0, 2, 10]],
[[1, 2, 1]]
], np.float32)
output = run_trt(inputs, repeats)
print(output)
print(output.shape)
print(type(output))
output_mask = run_trt(masks, repeats)
print(output_mask)
print(output_mask.shape)
print(type(output_mask))
##
# latency test
##
# MAX_OUTPUT_LENGTH=1024
# inputs = np.full((16, 256, 384), 2, np.float32)
# masks = np.ones((16, 256, 384), np.float32)
# repeats = np.full((16, 256), 4, np.float32)
# output = run_trt(inputs, repeats)
# output_mask = run_trt(masks, repeats) |
PyTorch/Classification/ConvNets/se-resnext101-32x4d/training/AMP | AMP | DGX1V_se-resnext101-32x4d_AMP_90E | python ./multiproc.py --nproc_per_node 8 ./launch.py --model se-resnext101-32x4d --precision AMP --mode convergence --platform DGX1V /imagenet --epochs 90 --mixup 0.0 --workspace ${1:-./} --raport-file raport.json
|
PyTorch/LanguageModeling/BERT/triton/deployment_toolkit/bermuda | bermuda | tensorrt | # Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import logging
import sys
from pathlib import Path
from typing import Dict, NamedTuple, Optional, Union
import numpy as np
# pytype: disable=import-error
try:
import pycuda.autoinit
import pycuda.driver as cuda
except Exception as e:
logging.getLogger(__name__).warning(f"Problems with importing pycuda package; {e}")
# pytype: enable=import-error
import tensorrt as trt # pytype: disable=import-error
from ..core import BaseLoader, BaseRunner, BaseRunnerSession, Format, Model, TensorSpec
from ..extensions import loaders, runners
LOGGER = logging.getLogger(__name__)
TRT_LOGGER = trt.Logger(trt.Logger.INFO)
# documentation:
# https://docs.nvidia.com/deeplearning/tensorrt/api/python_api/index.html
# https://docs.nvidia.com/deeplearning/tensorrt/developer-guide/index.html#python_samples_section
_NP_DTYPE2TRT_DTYPE = {
np.dtype("float32"): trt.DataType.FLOAT,
np.dtype("float16"): trt.DataType.HALF,
np.dtype("int8"): trt.DataType.INT8,
np.dtype("int32"): trt.DataType.INT32,
np.dtype("bool"): trt.DataType.BOOL,
}
class TensorRTLoader(BaseLoader):
def load(self, model_path: Union[str, Path], **_) -> Model:
model_path = Path(model_path)
LOGGER.debug(f"Loading TensorRT engine from {model_path}")
engine = self._load_engine(model_path)
if engine is None:
LOGGER.debug("Unable to load engine without plugins. Loading plugins.")
trt.init_libnvinfer_plugins(logger=TRT_LOGGER, namespace="")
LOGGER.debug(f"Loading TensorRT engine with plugins from {model_path}")
engine = self._load_engine(model_path)
if engine is None:
raise RuntimeError(f"Could not load ICudaEngine from {model_path}")
inputs = {}
outputs = {}
for binding_idx in range(engine.num_bindings):
name = engine.get_binding_name(binding_idx)
is_input = engine.binding_is_input(binding_idx)
dtype = np.dtype(trt.nptype(engine.get_binding_dtype(binding_idx))).name
shape = engine.get_binding_shape(binding_idx)
if is_input:
inputs[name] = TensorSpec(name, dtype, shape)
else:
outputs[name] = TensorSpec(name, dtype, shape)
return Model(engine, None, inputs, outputs)
def _load_engine(self, model_path: Path):
with model_path.open("rb") as fh, trt.Runtime(TRT_LOGGER) as runtime:
engine = runtime.deserialize_cuda_engine(fh.read())
return engine
class TRTBuffers(NamedTuple):
x_host: Optional[Dict[str, object]]
x_dev: Dict[str, object]
y_pred_host: Dict[str, object]
y_pred_dev: Dict[str, object]
class TensorRTRunner(BaseRunner):
def __init__(self):
pass
def init_inference(self, model: Model):
return TensorRTRunnerSession(model=model)
class TensorRTRunnerSession(BaseRunnerSession):
def __init__(self, model: Model):
super().__init__(model)
assert isinstance(model.handle, trt.ICudaEngine)
self._model = model
self._has_dynamic_shapes = None
self._context = None
self._engine: trt.ICudaEngine = self._model.handle
self._cuda_context = pycuda.autoinit.context
self._input_names = None
self._output_names = None
self._buffers = None
def __enter__(self):
self._context = self._engine.create_execution_context()
self._context.__enter__()
self._input_names = [
self._engine[idx] for idx in range(self._engine.num_bindings) if self._engine.binding_is_input(idx)
]
self._output_names = [
self._engine[idx] for idx in range(self._engine.num_bindings) if not self._engine.binding_is_input(idx)
]
# all_binding_shapes_specified is True for models without dynamic shapes
# so initially this variable is False for models with dynamic shapes
self._has_dynamic_shapes = not self._context.all_binding_shapes_specified
return self
def __exit__(self, exc_type, exc_value, traceback):
self._context.__exit__(exc_type, exc_value, traceback)
self._input_names = None
self._output_names = None
# TODO: are cuda buffers dealloc automatically?
self._buffers = None
def __call__(self, x):
buffers = self._prepare_buffers_if_needed(x)
bindings = self._update_bindings(buffers)
for name in self._input_names:
cuda.memcpy_htod(buffers.x_dev[name], buffers.x_host[name])
self._cuda_context.push()
self._context.execute_v2(bindings=bindings)
self._cuda_context.pop()
for name in self._output_names:
cuda.memcpy_dtoh(buffers.y_pred_host[name], buffers.y_pred_dev[name])
return buffers.y_pred_host
def _update_bindings(self, buffers: TRTBuffers):
bindings = [None] * self._engine.num_bindings
for name in buffers.y_pred_dev:
binding_idx: int = self._engine[name]
bindings[binding_idx] = buffers.y_pred_dev[name]
for name in buffers.x_dev:
binding_idx: int = self._engine[name]
bindings[binding_idx] = buffers.x_dev[name]
return bindings
def _set_dynamic_input_shapes(self, x_host):
def _is_shape_dynamic(input_shape):
return any([dim is None or dim == -1 for dim in input_shape])
for name in self._input_names:
bindings_idx = self._engine[name]
data_shape = x_host[name].shape # pytype: disable=attribute-error
if self._engine.is_shape_binding(bindings_idx):
input_shape = self._context.get_shape(bindings_idx)
if _is_shape_dynamic(input_shape):
self._context.set_shape_input(bindings_idx, data_shape)
else:
input_shape = self._engine.get_binding_shape(bindings_idx)
if _is_shape_dynamic(input_shape):
self._context.set_binding_shape(bindings_idx, data_shape)
assert self._context.all_binding_shapes_specified and self._context.all_shape_inputs_specified
def _prepare_buffers_if_needed(self, x_host: Dict[str, object]):
# pytype: disable=attribute-error
new_batch_size = list(x_host.values())[0].shape[0]
current_batch_size = list(self._buffers.y_pred_host.values())[0].shape[0] if self._buffers else 0
# pytype: enable=attribute-error
if self._has_dynamic_shapes or new_batch_size != current_batch_size:
# TODO: are CUDA buffers dealloc automatically?
self._set_dynamic_input_shapes(x_host)
y_pred_host = {}
for name in self._output_names:
shape = self._context.get_binding_shape(self._engine[name])
binding_idx: int = self._engine[name]
dtype_from_trt_binding = np.dtype(trt.nptype(self._engine.get_binding_dtype(binding_idx)))
dtype_from_model_spec = np.dtype(self._model.outputs[name].dtype)
assert dtype_from_model_spec == dtype_from_trt_binding
y_pred_host[name] = np.zeros(shape, dtype=dtype_from_model_spec)
y_pred_dev = {name: cuda.mem_alloc(data.nbytes) for name, data in y_pred_host.items()}
# cast host input into binding dtype
def _cast_input(name, data):
binding_idx: int = self._engine[name]
np_dtype = trt.nptype(self._engine.get_binding_dtype(binding_idx))
return data.astype(np_dtype)
x_host = {name: _cast_input(name, host_input) for name, host_input in x_host.items()}
x_dev = {
name: cuda.mem_alloc(host_input.nbytes)
for name, host_input in x_host.items()
if name in self._input_names # pytype: disable=attribute-error
}
self._buffers = TRTBuffers(None, x_dev, y_pred_host, y_pred_dev)
return self._buffers._replace(x_host=x_host)
if "pycuda.driver" in sys.modules:
loaders.register_extension(Format.TRT.value, TensorRTLoader)
runners.register_extension(Format.TRT.value, TensorRTRunner)
else:
LOGGER.warning("Do not register TensorRT extension due problems with importing pycuda.driver package.")
|
PyTorch/Forecasting/TFT | TFT | tft_torchhub | # Copyright (c) 2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import os
import sys
import urllib.request
from zipfile import ZipFile
import torch
from torch.utils.data import DataLoader
NGC_CHECKPOINT_URLS = {}
NGC_CHECKPOINT_URLS["electricity"] = "https://api.ngc.nvidia.com/v2/models/nvidia/dle/tft_base_pyt_ckpt_ds-electricity/versions/22.11.0_amp/zip"
NGC_CHECKPOINT_URLS["traffic"] = "https://api.ngc.nvidia.com/v2/models/nvidia/dle/tft_base_pyt_ckpt_ds-traffic/versions/22.11.0_amp/zip"
def _download_checkpoint(checkpoint, force_reload):
model_dir = os.path.join(torch.hub._get_torch_home(), 'checkpoints')
if not os.path.exists(model_dir):
os.makedirs(model_dir)
ckpt_file = os.path.join(model_dir, os.path.basename(checkpoint))
if not os.path.exists(ckpt_file) or force_reload:
sys.stderr.write('Downloading checkpoint from {}\n'.format(checkpoint))
urllib.request.urlretrieve(checkpoint, ckpt_file)
with ZipFile(ckpt_file, "r") as zf:
zf.extractall(path=model_dir)
return os.path.join(model_dir, "checkpoint.pt")
def nvidia_tft(pretrained=True, **kwargs):
from .modeling import TemporalFusionTransformer
"""Constructs a TFT model.
For detailed information on model input and output, training recipies, inference and performance
visit: github.com/NVIDIA/DeepLearningExamples and/or ngc.nvidia.com
Args (type[, default value]):
pretrained (bool, True): If True, returns a pretrained model.
dataset (str, 'electricity'): loads selected model type electricity or traffic. Defaults to electricity
"""
ds_type = kwargs.get("dataset", "electricity")
ckpt = _download_checkpoint(NGC_CHECKPOINT_URLS[ds_type], True)
state_dict = torch.load(ckpt)
config = state_dict['config']
model = TemporalFusionTransformer(config)
if pretrained:
model.load_state_dict(state_dict['model'])
model.eval()
return model
def nvidia_tft_data_utils(**kwargs):
from .data_utils import TFTDataset
from .configuration import ElectricityConfig
class Processing:
@staticmethod
def download_data(path):
if not os.path.exists(os.path.join(path, "raw")):
os.makedirs(os.path.join(path, "raw"), exist_ok=True)
dataset_url = "https://archive.ics.uci.edu/ml/machine-learning-databases/00321/LD2011_2014.txt.zip"
ckpt_file = os.path.join(path, "raw/electricity.zip")
if not os.path.exists(ckpt_file):
sys.stderr.write('Downloading checkpoint from {}\n'.format(dataset_url))
urllib.request.urlretrieve(dataset_url, ckpt_file)
with ZipFile(ckpt_file, "r") as zf:
zf.extractall(path=os.path.join(path, "raw/electricity/"))
@staticmethod
def preprocess(path):
config = ElectricityConfig()
if not os.path.exists(os.path.join(path, "processed")):
os.makedirs(os.path.join(path, "processed"), exist_ok=True)
from data_utils import standarize_electricity as standarize
from data_utils import preprocess
standarize(os.path.join(path, "raw/electricity"))
preprocess(os.path.join(path, "raw/electricity/standarized.csv"), os.path.join(path, "processed/electricity_bin/"), config)
@staticmethod
def get_batch(path):
config = ElectricityConfig()
test_split = TFTDataset(os.path.join(path, "processed/electricity_bin/", "test.csv"), config)
data_loader = DataLoader(test_split, batch_size=16, num_workers=0)
for i, batch in enumerate(data_loader):
if i == 40:
break
return batch
return Processing()
|
PyTorch/SpeechRecognition/wav2vec2/scripts/docker | docker | build | #!/usr/bin/env bash
# Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
docker build . --rm -t wav2vec2
|
TensorFlow2/Classification/ConvNets/efficientnet_v1 | efficientnet_v1 | README | # EfficientNet v1 For TensorFlow 2.6
This repository provides scripts and recipes to train EfficientNet v1-B0 and v1-B4 to achieve state-of-the-art accuracy.
The content of the repository is maintained by NVIDIA and is tested against each NGC monthly released container to ensure consistent accuracy and performance over time.
## Table Of Contents
- [Model overview](#model-overview)
* [Model architecture](#model-architecture)
* [Default configuration](#default-configuration)
* [Feature support matrix](#feature-support-matrix)
* [Features](#features)
* [Mixed precision training](#mixed-precision-training)
* [Enabling mixed precision](#enabling-mixed-precision)
* [Enabling TF32](#enabling-tf32)
- [Setup](#setup)
* [Requirements](#requirements)
- [Quick Start Guide](#quick-start-guide)
- [Advanced](#advanced)
* [Scripts and sample code](#scripts-and-sample-code)
* [Parameters](#parameters)
* [Command-line options](#command-line-options)
* [Getting the data](#getting-the-data)
* [Training process](#training-process)
* [Multi-node](#multi-node)
* [Inference process](#inference-process)
- [Performance](#performance)
* [Benchmarking](#benchmarking)
* [Training performance benchmark](#training-performance-benchmark)
* [Inference performance benchmark](#inference-performance-benchmark)
* [Results](#results)
* [Training accuracy results for EfficientNet v1-B0](#training-accuracy-results-for-efficientnet-v1-b0)
* [Training accuracy: NVIDIA DGX A100 (8x A100 80GB)](#training-accuracy-nvidia-dgx-a100-8x-a100-80gb)
* [Training accuracy: NVIDIA DGX-1 (8x V100 32GB)](#training-accuracy-nvidia-dgx-1-8x-v100-32gb)
* [Training accuracy results for EfficientNet v1-B4](#training-accuracy-results-for-efficientnet-v1-b4)
* [Training accuracy: NVIDIA DGX A100 (8x A100 80GB)](#training-accuracy-nvidia-dgx-a100-8x-a100-80gb)
* [Training accuracy: NVIDIA DGX-1 (8x V100 32GB)](#training-accuracy-nvidia-dgx-1-8x-v100-32gb)
* [Training performance results for EfficientNet v1-B0](#training-performance-results-for-efficientnet-v1-b0)
* [Training performance: NVIDIA DGX A100 (8x A100 80GB)](#training-performance-nvidia-dgx-a100-8x-a100-80gb)
* [Training performance: NVIDIA DGX-1 (8x V100 32GB)](#training-performance-nvidia-dgx-1-8x-v100-32gb)
* [Training performance results for EfficientNet v1-B4](#training-performance-results-for-efficientnet-v1-b4)
* [Training performance: NVIDIA DGX A100 (8x A100 80GB)](#training-performance-nvidia-dgx-a100-8x-a100-80gb)
* [Training performance: NVIDIA DGX-1 (8x V100 32GB)](#training-performance-nvidia-dgx-1-8x-v100-32gb)
* [Inference performance results for EfficientNet v1-B0](#inference-performance-results-for-efficientnet-v1-b0)
* [Inference performance: NVIDIA DGX A100 (1x A100 80GB)](#inference-performance-nvidia-dgx-a100-1x-a100-80gb)
* [Inference performance: NVIDIA DGX-1 (1x V100 32GB)](#inference-performance-nvidia-dgx-1-1x-v100-32gb)
* [Inference performance results for EfficientNet v1-B4](#inference-performance-results-for-efficientnet-v1-b4)
* [Inference performance: NVIDIA DGX A100 (1x A100 80GB)](#inference-performance-nvidia-dgx-a100-1x-a100-80gb)
* [Inference performance: NVIDIA DGX-1 (1x V100 32GB)](#inference-performance-nvidia-dgx-1-1x-v100-32gb)
- [Release notes](#release-notes)
* [Changelog](#changelog)
* [Known issues](#known-issues)
## Model overview
### Model architecture
EfficientNet v1 is developed based on AutoML and Compound Scaling. In particular,
a mobile-size baseline network called EfficientNet v1-B0 is developed from AutoML MNAS Mobile
framework, the building block is mobile inverted bottleneck MBConv with squeeze-and-excitation optimization.
Then, through a compound scaling method, this baseline is scaled up to obtain EfficientNet v1-B1
to B7.
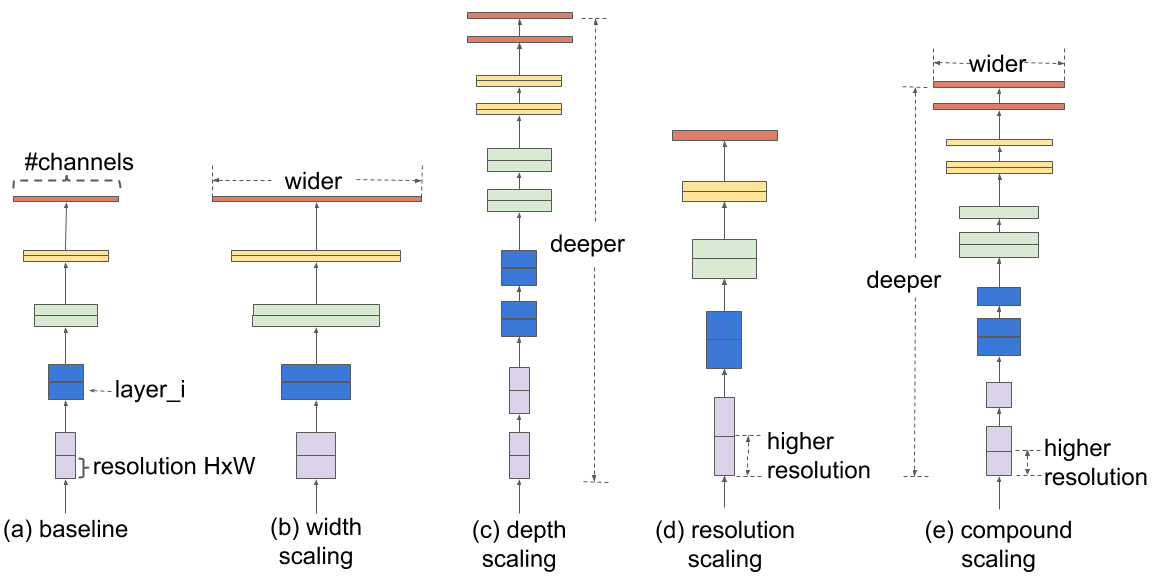
### Default configuration
Here is the Baseline EfficientNet v1-B0 structure.

The following features are supported by this implementation:
- General:
- XLA support
- Mixed precision support
- Multi-GPU support using Horovod
- Multi-node support using Horovod
- Cosine LR Decay
- Inference:
- Support for inference on a single image is included
- Support for inference on a batch of images is included
### Feature support matrix
| Feature | EfficientNet
|-----------------------|-------------------------- |
|Horovod Multi-GPU training (NCCL) | Yes |
|Multi-GPU training | Yes |
|Multi-node training | Yes |
|Automatic mixed precision (AMP) | Yes |
|XLA | Yes |
|Gradient Accumulation| Yes |
|Stage-wise Training| Yes |
#### Features
**Multi-GPU training with Horovod**
Our model uses Horovod to implement efficient multi-GPU training with NCCL. For details, refer to example sources in this repository or refer to the [TensorFlow tutorial](https://github.com/horovod/horovod/#usage).
**Multi-node training with Horovod**
Our model also uses Horovod to implement efficient multi-node training.
**Automatic Mixed Precision (AMP)**
Computation graphs can be modified by TensorFlow on runtime to support mixed precision training. A detailed explanation of mixed precision can be found in Appendix.
**Gradient Accumulation**
Gradient Accumulation is supported through a custom train_step function. This feature is enabled only when grad_accum_steps is greater than 1.
### Mixed precision training
Mixed precision is the combined use of different numerical precisions in a computational method. [Mixed precision](https://arxiv.org/abs/1710.03740) training offers significant computational speedup by performing operations in half-precision format while storing minimal information in single-precision to retain as much information as possible in critical parts of the network. Since the introduction of [Tensor Cores](https://developer.nvidia.com/tensor-cores) in NVIDIA Volta, and following with both the NVIDIA Turing and NVIDIA Ampere architectures, significant training speedups are experienced by switching to mixed precision -- up to 3x overall speedup on the most arithmetically intense model architectures. Using [mixed precision training](https://docs.nvidia.com/deeplearning/performance/mixed-precision-training/index.html) previously required two steps:
1. Porting the model to use the FP16 data type where appropriate.
2. Adding loss scaling to preserve small gradient values.
This can now be achieved using Automatic Mixed Precision (AMP) for TensorFlow to enable the full [mixed precision methodology](https://docs.nvidia.com/deeplearning/sdk/mixed-precision-training/index.html#tensorflow) in your existing TensorFlow model code. AMP enables mixed precision training on NVIDIA Volta, NVIDIA Turing, and NVIDIA Ampere GPU architectures automatically. The TensorFlow framework code makes all necessary model changes internally.
In TF-AMP, the computational graph is optimized to use as few casts as necessary and maximize the use of FP16, and the loss scaling is automatically applied inside of supported optimizers. AMP can be configured to work with the existing tf.contrib loss scaling manager by disabling the AMP scaling with a single environment variable to perform only the automatic mixed-precision optimization. It accomplishes this by automatically rewriting all computation graphs with the necessary operations to enable mixed precision training and automatic loss scaling.
For information about:
- How to train using mixed precision, refer to the [Mixed Precision Training](https://arxiv.org/abs/1710.03740) paper and [Training With Mixed Precision](https://docs.nvidia.com/deeplearning/performance/mixed-precision-training/index.html) documentation.
- Techniques used for mixed precision training, refer to the [Mixed-Precision Training of Deep Neural Networks](https://devblogs.nvidia.com/mixed-precision-training-deep-neural-networks/) blog.
- How to access and enable AMP for TensorFlow, refer to [Using TF-AMP](https://docs.nvidia.com/deeplearning/dgx/tensorflow-user-guide/index.html#tfamp) from the TensorFlow User Guide.
#### Enabling mixed precision
Mixed precision is enabled in TensorFlow by using the Automatic Mixed Precision (TF-AMP) extension, which casts variables to half-precision upon retrieval while storing variables in single-precision format. Furthermore, to preserve small gradient magnitudes in backpropagation, a [loss scaling](https://docs.nvidia.com/deeplearning/sdk/mixed-precision-training/index.html#lossscaling) step must be included when applying gradients. In TensorFlow, loss scaling can be applied statically by using simple multiplication of loss by a constant value or automatically, by TF-AMP. Automatic mixed precision makes all the adjustments internally in TensorFlow, providing two benefits over manual operations. First, programmers need not modify network model code, reducing development and maintenance effort. Second, using AMP maintains forward and backward compatibility with all the APIs for defining and running TensorFlow models.
To enable mixed precision, you can simply add the `--use_amp` to the command-line used to run the model. This will enable the following code:
```
if params.use_amp:
policy = tf.keras.mixed_precision.experimental.Policy('mixed_float16', loss_scale='dynamic')
tf.keras.mixed_precision.experimental.set_policy(policy)
```
#### Enabling TF32
TensorFloat-32 (TF32) is the new math mode in [NVIDIA A100](https://www.nvidia.com/en-us/data-center/a100/) GPUs for handling the matrix math, also called tensor operations. TF32 running on Tensor Cores in A100 GPUs can provide up to 10x speedups compared to single-precision floating-point math (FP32) on NVIDIA Volta GPUs.
TF32 Tensor Cores can speed up networks using FP32, typically with no loss of accuracy. It is more robust than FP16 for models which require a high dynamic range for weights or activations.
For more information, refer to the [TensorFloat-32 in the A100 GPU Accelerates AI Training, HPC up to 20x](https://blogs.nvidia.com/blog/2020/05/14/tensorfloat-32-precision-format/) blog post.
TF32 is supported in the NVIDIA Ampere GPU architecture and is enabled by default.
## Setup
The following section lists the requirements that you need to meet in order to start training the EfficientNet model.
# Requirements
This repository contains Dockerfile which extends the TensorFlow NGC container and encapsulates some dependencies. Aside from these dependencies, ensure you have the following components:
- [NVIDIA Docker](https://github.com/NVIDIA/nvidia-docker)
- [TensorFlow 21.09-py3] NGC container or later
- Supported GPUs:
- [NVIDIA Volta architecture](https://www.nvidia.com/en-us/data-center/volta-gpu-architecture/)
- [NVIDIA Turing architecture](https://www.nvidia.com/en-us/geforce/turing/)
- [NVIDIA Ampere architecture](https://www.nvidia.com/en-us/data-center/nvidia-ampere-gpu-architecture/)
For more information about how to get started with NGC containers, refer to the following sections from the NVIDIA GPU Cloud Documentation and the Deep Learning Documentation:
- [Getting Started Using NVIDIA GPU Cloud](https://docs.nvidia.com/ngc/ngc-getting-started-guide/index.html)
- [Accessing And Pulling From The NGC Container Registry](https://docs.nvidia.com/deeplearning/frameworks/user-guide/index.html#accessing_registry)
- [Running TensorFlow](https://docs.nvidia.com/deeplearning/frameworks/tensorflow-release-notes/running.html#running)
As an alternative to the use of the Tensorflow2 NGC container, to set up the required environment or create your own container, refer to the versioned [NVIDIA Container Support Matrix](https://docs.nvidia.com/deeplearning/frameworks/support-matrix/index.html).
For multi-node, the sample provided in this repository requires [Enroot](https://github.com/NVIDIA/enroot) and [Pyxis](https://github.com/NVIDIA/pyxis) set up on a [SLURM](https://slurm.schedmd.com) cluster.
## Quick Start Guide
To train your model using mixed or TF32 precision with Tensor Cores or using FP32, perform the following steps using the default parameters of the EfficientNet model on the ImageNet dataset. For the specifics concerning training and inference, refer to the [Advanced](#advanced) section.
1. Clone the repository.
```
git clone https://github.com/NVIDIA/DeepLearningExamples.git
cd DeepLearningExamples/TensorFlow2/Classification/ConvNets/efficientnet
```
2. Download and prepare the dataset.
`Runner.py` supports ImageNet with [TensorFlow Datasets (TFDS)](https://www.tensorflow.org/datasets/overview). Refer to the [TFDS ImageNet readme](https://github.com/tensorflow/datasets/blob/master/docs/catalog/imagenet2012.md) for manual download instructions.
3. Build EfficientNet on top of the NGC container.
`bash ./scripts/docker/build.sh YOUR_DESIRED_CONTAINER_NAME`
4. Start an interactive session in the NGC container to run training/inference. **Ensure that `launch.sh` has the correct path to ImageNet on your machine and that this path is mounted onto the `/data` directory because this is where training and evaluation scripts search for data.**
`bash ./scripts/docker/launch.sh YOUR_DESIRED_CONTAINER_NAME`
5. Start training.
To run training for a standard configuration, **under the container default entry point `/workspace`**, run one of the scripts in the `./efficinetnet_v1/{B0,B4}/training/{AMP,TF32,FP32}/convergence_8x{A100-80G, V100-32G}.sh`. For example:
`bash ./efficinetnet_v1/B0/training/AMP/convergence_8xA100-80G.sh`
6. Start validation/evaluation.
To run validation/evaluation for a standard configuration, **under the container default entry point `/workspace`**, run one of the scripts in the `./efficinetnet_v1/{B0,B4}/evaluation/evaluation_{AMP,FP32,TF32}_8x{A100-80G, V100-32G}.sh`. The evaluation script is configured to use the checkpoint specified in `checkpoint` for evaluation. The specified checkpoint will be read from the location passed by `--model_dir'.For example:
`bash ./efficinetnet_v1/B0/evaluation/evaluation_AMP_A100-80G.sh`
7. Start inference/predictions.
To run inference for a standard configuration, **under the container default entry point `/workspace`**, run one of the scripts in the `./efficinetnet_v1/{B0,B4}/inference/inference_{AMP,FP32,TF32}.sh`.
Ensure your JPEG images used to run inference on are mounted in the `/infer_data` directory with this folder structure :
```
infer_data
| ├── images
| | ├── image1.JPEG
| | ├── image2.JPEG
```
For example:
`bash ./efficinetnet_v1/B0/inference/inference_AMP.sh`
Now that you have your model trained and evaluated, you can choose to compare your training results with our [Training accuracy results](#training-accuracy-results). You can also choose to benchmark your performance to [Training performance benchmark](#training-performance-results) or [Inference performance benchmark](#inference-performance-results). Following the steps in these sections will ensure that you achieve the same accuracy and performance results as stated in the [Results](#results) section.
## Advanced
The following sections provide greater details of the dataset, running training and inference, and the training results.
### Scripts and sample code
The repository is structured as follows:
- `scripts/` - shell scripts to build and launch EfficientNet container on top of NGC container
- `efficientnet_{v1,v2}` scripts to launch training, evaluation and inference
- `model/` - building blocks and EfficientNet model definitions
- `runtime/` - detailed procedure for each running mode
- `utils/` - support util functions for learning rates, optimizers, etc.
- `dataloader/` provides data pipeline utils
- `config/` contains model definitions
### Parameters
The hyper parameters can be grouped into model-specific hyperparameters (e.g., #layers ) and general hyperparameters (e.g., #training epochs).
The model-specific hyperparameters are to be defined in a python module, which must be passed in the command line via --cfg ( `python main.py --cfg config/efficientnet_v1/b0_cfg.py`). To override model-specific hyperparameters, you can use a comma-separated list of k=v pairs (e.g., `python main.py --cfg config/efficientnet_v1/b0_cfg.py --mparams=bn_momentum=0.9,dropout=0.5`).
The general hyperparameters and their default values can be found in `utils/cmdline_helper.py`. The user can override these hyperparameters in the command line (e.g., `python main.py --cfg config/efficientnet_v1/b0_cfg.py --data_dir xx --train_batch_size 128`). Here is a list of important hyperparameters:
- `--mode` (`train_and_eval`,`train`,`eval`,`prediction`) - the default is `train_and_eval`.
- `--use_amp` Set to True to enable AMP
- `--use_xla` Set to True to enable XLA
- `--model_dir` The folder where model checkpoints are saved (the default is `/workspace/output`)
- `--data_dir` The folder where data resides (the default is `/data/`)
- `--log_steps` The interval of steps between logging of batch level stats.
- `--augmenter_name` Type of data augmentation
- `--raug_num_layers` Number of layers used in the random data augmentation scheme
- `--raug_magnitude` Strength of transformations applied in the random data augmentation scheme
- `--cutmix_alpha` Cutmix parameter used in the last stage of training.
- `--mixup_alpha` Mixup parameter used in the last stage of training.
- `--defer_img_mixing` Move image mixing ops from the data loader to the model/GPU (faster training)
- `--eval_img_size` Size of images used for evaluation
- `--eval_batch_size` The evaluation batch size per GPU
- `--n_stages` Number of stages used for stage-wise training
- `--train_batch_size` The training batch size per GPU
- `--train_img_size` Size of images used in the last stage of training
- `--base_img_size` Size of images used in the first stage of training
- `--max_epochs` The number of training epochs
- `--warmup_epochs` The number of epochs of warmup
- `--moving_average_decay` The decay weight used for EMA
- `--lr_init` The learning rate for a batch size of 128, effective learning rate will be automatically scaled according to the global training batch size: `lr=lr_init * global_BS/128 where global_BS=train_batch_size*n_GPUs`
- `--lr_decay` Learning rate decay policy
- `--weight_decay` Weight decay coefficient
- `--save_checkpoint_freq` Number of epochs to save checkpoints
**NOTE**: Avoid changing the default values of the general hyperparameters provided in `utils/cmdline_helper.py`. The reason is that some other models supported by this repository may rely on such default values. If you want to change the values, override them via the command line.
### Command-line options
To display the full list of available options and their descriptions, use the `-h` or `--help` command-line option, for example:
`python main.py --help`
### Getting the data
Refer to the [TFDS ImageNet readme](https://github.com/tensorflow/datasets/blob/master/docs/catalog/imagenet2012.md) for manual download instructions.
To train on the ImageNet dataset, pass `$path_to_ImageNet_tfrecords` to `$data_dir` in the command-line.
Name the TFRecords in the following scheme:
- Training images - `/data/train-*`
- Validation images - `/data/validation-*`
### Training process
The training process can start from scratch or resume from a checkpoint.
By default, bash script `scripts/{B0,B4}/training/{AMP,FP32,TF32}/convergence_8x{A100-80G,V100-32G}.sh` will start the training process with the following settings.
- Use 8 GPUs by Horovod
- Has XLA enabled
- Saves checkpoints after every 10 epochs to `/workspace/output/` folder
- AMP or FP32 or TF32 based on the folder `scripts/{B0,B4}/training/{AMP, FP32, TF32}`
The training starts from scratch if `--model_dir` has no checkpoints in it. To resume from a checkpoint, place the checkpoint into `--model_dir` and make sure the `checkpoint` file points to it.
#### Multi-node
Multi-node runs can be launched on a Pyxis/enroot Slurm cluster (refer to [Requirements](#requirements)) with the `run_{B0,B4}_multinode.sub` script with the following command for a 4-node NVIDIA DGX A100 example:
```
PARTITION=<partition_name> sbatch N 4 --ntasks-per-node=8 run_B0_multinode.sub
PARTITION=<partition_name> sbatch N 4 --ntasks-per-node=8 run_B4_multinode.sub
```
Checkpoints will be saved after `--save_checkpoint_freq` epochs at `checkpointdir`. The latest checkpoint will be automatically picked up to resume training in case it needs to be resumed. Cluster partition name has to be provided `<partition_name>`.
Note that the `run_{B0,B4}_multinode.sub` script is a starting point that has to be adapted depending on the environment. In particular, pay attention to the variables such as `--container-image`, which handles the container image to train, and `--datadir`, which handles the location of the ImageNet data.
Refer to the scripts to find the full list of variables to adjust for your system.
## Inference process
Validation can be done either during training (when `--mode train_and_eval` is used) or in a post-training setting (`--mode eval`) on a checkpointed model. The evaluation script expects data in the tfrecord format.
`bash ./scripts/{B0,B4}/evaluation/evaluation_{AMP,FP32,TF32}_{A100-80G,V100-32G}.sh`
Metrics gathered through this process are as follows:
```
- eval_loss
- eval_accuracy_top_1
- eval_accuracy_top_5
- avg_exp_per_second_eval
- avg_exp_per_second_eval_per_GPU
- avg_time_per_exp_eval : Average Latency
- latency_90pct : 90% Latency
- latency_95pct : 95% Latency
- latency_99pct : 99% Latency
```
The scripts used for inference expect the inference data in the following directory structure:
```
infer_data
| ├── images
| | ├── image1.JPEG
| | ├── image2.JPEG
```
Run:
`bash ./scripts/{B0,B4}/inference/inference_{AMP,FP32,TF32}.sh`
## Performance
The performance measurements in this document were conducted at the time of publication and may not reflect the performance achieved from NVIDIA’s latest software release. For the most up-to-date performance measurements, go to [NVIDIA Data Center Deep Learning Product Performance](https://developer.nvidia.com/deep-learning-performance-training-inference).
### Benchmarking
The following section shows how to run benchmarks measuring the model performance in training and inference modes.
#### Training performance benchmark
Training benchmark for EfficientNet v1-B0 was run on NVIDIA DGX A100 80GB and NVIDIA DGX-1 V100 32GB.
To benchmark training performance with other parameters, run:
`bash ./scripts/B0/training/{AMP, FP32, TF32}/train_benchmark_8x{A100-80G, V100-32G}.sh`
Training benchmark for EfficientNet v1-B4 was run on NVIDIA DGX A100 80GB and NVIDIA DGX-1 V100 32GB.
`bash ./scripts/B4/training/{AMP, FP32, TF32}/train_benchmark_8x{A100-80G, V100-32G}.sh`
#### Inference performance benchmark
Inference benchmark for EfficientNet v1-B0 was run on NVIDIA DGX A100 80GB and NVIDIA DGX-1 V100 32GB.
Inference benchmark for EfficientNet v1-B4 was run on NVIDIA DGX A100 80GB and NVIDIA DGX-1 V100 32GB.
### Results
The following sections provide details on how we achieved our performance and accuracy in training and inference.
#### Training accuracy results for EfficientNet v1-B0
##### Training accuracy: NVIDIA DGX A100 (8x A100 80GB)
Our results were obtained by running the training scripts in the tensorflow:21.09-tf2-py3 NGC container on NVIDIA DGX A100 (8x A100 80GB) GPUs. We evaluated the models using both the original and EMA weights and selected the higher accuracy to report.
| GPUs | Accuracy - TF32 | Accuracy - mixed precision | Time to train - TF32 | Time to train - mixed precision | Time to train speedup (TF32 to mixed precision) |
|----------|------------------|-----------------------------|-------------------------|----------------------------------|--------------------------------------------------------|
| 8 | 77.60% | 77.59% | 19.5hrs | 8.5hrs | 2.29 |
| 16 | 77.51% | 77.48% | 10hrs | 4.5hrs | 2.22 |
##### Training accuracy: NVIDIA DGX-1 (8x V100 32GB)
Our results were obtained by running the training scripts in the tensorflow:21.09-tf2-py3 NGC container on NVIDIA DGX V100 (8x V100 32GB) GPUs. We evaluated the models using both the original and EMA weights and selected the higher accuracy to report.
| GPUs | Accuracy - FP32 | Accuracy - mixed precision | Time to train - FP32 | Time to train - mixed precision | Time to train speedup (FP32 to mixed precision) |
|----------|------------------|-----------------------------|-------------------------|----------------------------------|--------------------------------------------------------|
| 8 | 77.67% | 77.69% | 49.0hrs | 38.0hrs | 1.29 |
| 32 | 77.55% | 77.53% | 11.5hrs | 10hrs | 1.15 |
#### Training accuracy results for EfficientNet v1-B4
##### Training accuracy: NVIDIA DGX A100 (8x A100 80GB)
Our results were obtained by running the training scripts in the tensorflow:21.09-tf2-py3 NGC container on multi-node NVIDIA DGX A100 (8x A100 80GB) GPUs. We evaluated the models using both the original and EMA weights and selected the higher accuracy to report.
| GPUs | Accuracy - TF32 | Accuracy - mixed precision | Time to train - TF32 | Time to train - mixed precision | Time to train speedup (TF32 to mixed precision) |
|----------|------------------|-----------------------------|-------------------------|----------------------------------|--------------------------------------------------------|
| 32 | 82.98% | 83.13% | 38hrs | 14hrs | 2.00 |
| 64 | 83.14% | 83.05% | 19hrs | 7hrs | 2.00 |
##### Training accuracy: NVIDIA DGX V100 (8x V100 32GB)
Our results were obtained by running the training scripts in the tensorflow:21.09-tf2-py3 NGC container on NVIDIA DGX V100 (8x A100 32GB) GPUs. We evaluated the models using both the original and EMA weights and selected the higher accuracy to report.
| GPUs | Accuracy - FP32 | Accuracy - mixed precision | Time to train - TF32 | Time to train - mixed precision | Time to train speedup (FP32 to mixed precision) |
|----------|------------------|-----------------------------|-------------------------|----------------------------------|--------------------------------------------------------|
| 32 | 82.64% | 82.88% | 97.0hrs | 41.0hrs | 2.37 |
| 64 | 82.74% | 83.16% | 50.0hrs | 20.5hrs | 2.43 |
#### Training performance results for EfficientNet v1-B0
##### Training performance: NVIDIA DGX A100 (8x A100 80GB)
| GPUs | Throughput - TF32 | Throughput - mixed precision | Throughput speedup (TF32 - mixed precision) | Weak scaling - TF32 | Weak scaling - mixed precision |
|-----|------------------------|---------------------------------|-----------------------------------------------|------------------------|----------------------------------|
| 1 | 1209 | 3454 | 2.85 | 1 | 1 |
| 8 | 9119 | 20647 | 2.26 | 7.54 | 5.98 |
| 16 | 17815 | 40644 | 2.28 | 14.74 | 11.77 |
##### Training performance: NVIDIA DGX-1 (8x V100 32GB)
| GPUs | Throughput - FP32 | Throughput - mixed precision | Throughput speedup (FP32 - mixed precision) | Weak scaling - FP32 | Weak scaling - mixed precision |
|-----|------------------------|---------------------------------|-----------------------------------------------|------------------------|----------------------------------|
| 1 | 752 | 868 | 1.15 | 1 | 1 |
| 8 | 4504 | 4880 | 1.08 | 5.99 | 5.62 |
| 32 | 15309 | 18424 | 1.20 | 20.36 | 21.23 |
#### Training performance results for EfficientNet v1-B4
##### Training performance: NVIDIA DGX A100 (8x A100 80GB)
| GPUs | Throughput - TF32 | Throughput - mixed precision | Throughput speedup (TF32 - mixed precision) | Weak scaling - TF32 | Weak scaling - mixed precision |
|-----|------------------------|---------------------------------|-----------------------------------------------|------------------------|----------------------------------|
| 1 | 165 | 470 | 2.85 | 1 | 1 |
| 8 | 1308 | 3550 | 2.71 | 7.93 | 7.55 |
| 32 | 4782 | 12908 | 2.70 | 28.98 | 27.46 |
| 64 | 9473 | 25455 | 2.69 | 57.41 | 54.16 |
##### Training performance: NVIDIA DGX-1 (8x V100 32GB)
| GPUs | Throughput - FP32 | Throughput - mixed precision | Throughput speedup (FP32 - mixed precision) | Weak scaling - TF32 | Weak scaling - mixed precision |
|-----|------------------------|---------------------------------|-----------------------------------------------|------------------------|----------------------------------|
| 1 | 79 | 211 | 2.67 | 1 | 1 |
| 8 | 570 | 1258 | 2.21 | 7.22 | 5.96 |
| 32 | 1855 | 4325 | 2.33 | 23.48 | 20.50 |
| 64 | 3568 | 8643 | 2.42 | 45.16 | 40.96 |
#### Inference performance results for EfficientNet v1-B0
##### Inference performance: NVIDIA DGX A100 (1x A100 80GB)
Our results were obtained by running the inferencing benchmarking script in the tensorflow:21.09-tf2-py3 NGC container on the NVIDIA DGX A100 (1x A100 80GB) GPU.
FP16 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) |Latency 95% (ms) |Latency 99% (ms) |
|-------------|------------------|--------|--------|--------|--------|--------|
| 1 | 224x224 | 110.97 | 9.09 | 9.02 | 9.04 | 9.09 | #| 95.71 | 10.45 | 10.34 | 10.38 | 10.42 |
| 8 | 224x224 | 874.91 | 9.12 | 9.04 | 9.08 | 9.12 | #| 616.02 | 12.99 | 12.81 | 12.88 | 12.98 |
| 32 | 224x224 | 2188.84| 14.62 | 14.35 | 14.43 | 14.52 |
| 1024 | 224x224 | 9729.85| 105.24 | 101.50 | 103.20 | 105.24 |
TF32 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) | Latency 95% (ms) | Latency 99% (ms) |
|------------|-----------------|---------|--------|--------|--------|--------|
| 1 | 224x224 | 127.95 | 7.88 | 7.83 | 7.84 | 7.87 | # 119.26 | 8.38 | 8.30 | 8.34 | 8.37 |
| 8 | 224x224 | 892.27 | 8.97 | 8.88 | 8.91 | 8.94 | # | 803.61 | 9.96 | 9.82 | 9.87 | 9.93
| 32 | 224x224 | 2185.02 | 14.65 | 14.33 | 14.43 | 14.54 |
| 512 | 224x224 | 5253.19 | 97.46 | 96.57 | 97.03 | 97.46 |
##### Inference performance: NVIDIA DGX-1 (1x V100 32GB)
FP16 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) |Latency 95% (ms) |Latency 99% (ms) |
|-------------|------------------|--------|--------|--------|--------|--------|
| 1 | 224x224 | 97.53 | 10.25 | 10.11 | 10.13 | 10.21 |
| 8 | 224x224 | 752.72 | 10.63 | 10.49 | 10.54 | 10.59 |
| 32 | 224x224 | 1768.05| 18.10 | 17.88 | 17.96 | 18.04 |
| 512 | 224x224 | 5399.88| 94.82 | 92.85 | 93.89 | 94.82 |
FP32 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) | Latency 95% (ms) | Latency 99% (ms) |
|------------|-----------------|---------|--------|--------|--------|--------|
| 1 | 224x224 | 97.01 | 10.31 | 10.17 | 10.22 | 10.28 |
| 8 | 224x224 | 649.79 | 12.31 | 12.16 | 12.22 | 12.28 |
| 32 | 224x224 | 1861.65 | 17.19 | 16.98 | 17.03 | 17.10 |
| 256 | 224x224 | 2829.34 | 90.48 | 89.80 | 90.13 | 90.43 |
#### Inference performance results for EfficientNet v1-B4
##### Inference performance: NVIDIA DGX A100 (1x A100 80GB)
Our results were obtained by running the inferencing benchmarking script in the tensorflow:21.09-tf2-py3 NGC container on the NVIDIA DGX A100 (1x A100 80GB) GPU.
FP16 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) |Latency 95% (ms) |Latency 99% (ms) |
|------------|------------------|--------|--------|--------|--------|--------|
| 1 | 380x380 | 61.36 | 16.30 | 16.20 | 16.24 | 16.28 | #61.36 16.30 16.20 16.24 16.28| 45.40 | 22.03 | 21.82 | 21.90 | 21.99 |
| 8 | 380x380 | 338.60 | 23.63 | 23.34 | 23.46 | 23.58 |
| 32 | 380x380 | 971.68 | 32.93 | 32.46 | 32.61 | 32.76 |
| 128 | 380x380 | 1497.21| 85.28 | 83.01 | 83.68 | 84.70 |
TF32 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) | Latency 95% (ms) | Latency 99% (ms) |
|------------|-----------------|---------|--------|--------|--------|--------|
| 1 | 380x380 | 60.54 | 16.52 | 16.34 | 16.41 | 16.49 |
| 8 | 380x380 | 366.82 | 21.81 | 21.48 | 21.61 | 21.75 |
| 32 | 380x380 | 642.78 | 49.78 | 49.41 | 49.53 | 49.65 |
| 64 | 380x380 | 714.55 | 89.54 | 89.00 | 89.17 | 89.34 |
##### Inference performance: NVIDIA DGX-1 (1x V100 32GB)
FP16 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) |Latency 95% (ms) |Latency 99% (ms) |
|------------|------------------|--------|--------|--------|--------|--------|
| 1 | 380x380 | 55.71 | 17.95 | 17.68 | 17.93 | 17.86 | #61.36 16.30 16.20 16.24 16.28| 45.40 | 22.03 | 21.82 | 21.90 | 21.99 |
| 8 | 380x380 | 256.72 | 31.16 | 30.92 | 31.02 | 31.12 |
| 16 | 380x380 | 350.14 | 45.75 | 45.44 | 45.57 | 45.68 |
| 64 | 380x380 | 805.21 | 79.46 | 78.74 | 78.86 | 79.01 |
TF32 Inference Latency
| Batch size | Resolution | Throughput Avg | Latency Avg (ms) | Latency 90% (ms) | Latency 95% (ms) | Latency 99% (ms) |
|------------|-----------------|---------|--------|--------|--------|--------|
| 1 | 380x380 | 49.03 | 20.40 | 20.03 | 20.18 | 20.34 |
| 8 | 380x380 | 258.21 | 30.98 | 30.83 | 30.89 | 30.95 |
| 16 | 380x380 | 310.84 | 51.47 | 51.26 | 51.34 | 51.42 |
| 32 | 380x380 | 372.23 | 85.97 | 85.70 | 85.79 | 85.89 |
## Release notes
### Changelog
February 2022
- Second release
- Code Refactoring
- Add support of graduate accumulation
- Add support of exponential moving average evaluation
- Update all accuracy and performance tables on V100 and A100 results
March 2021
- Initial release
### Known issues
- EfficientNet v1-B0 does not improve training speed by using AMP as compared to FP32, because of the CPU-bound Auto-augmentation.
|
TensorFlow/LanguageModeling/BERT/data | data | BooksDownloader | # Copyright (c) 2019 NVIDIA CORPORATION. All rights reserved.
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import subprocess
class BooksDownloader:
def __init__(self, save_path):
self.save_path = save_path
pass
def download(self):
bookscorpus_download_command = 'python3 /workspace/bookcorpus/download_files.py --list /workspace/bookcorpus/url_list.jsonl --out'
bookscorpus_download_command += ' ' + self.save_path + '/bookscorpus'
bookscorpus_download_command += ' --trash-bad-count'
bookscorpus_download_process = subprocess.run(bookscorpus_download_command, shell=True, check=True) |
TensorFlow/Detection/SSD/models/research/object_detection/samples/configs | configs | faster_rcnn_resnet101_coco | # Faster R-CNN with Resnet-101 (v1), configuration for MSCOCO Dataset.
# Users should configure the fine_tune_checkpoint field in the train config as
# well as the label_map_path and input_path fields in the train_input_reader and
# eval_input_reader. Search for "PATH_TO_BE_CONFIGURED" to find the fields that
# should be configured.
model {
faster_rcnn {
num_classes: 90
image_resizer {
keep_aspect_ratio_resizer {
min_dimension: 600
max_dimension: 1024
}
}
feature_extractor {
type: 'faster_rcnn_resnet101'
first_stage_features_stride: 16
}
first_stage_anchor_generator {
grid_anchor_generator {
scales: [0.25, 0.5, 1.0, 2.0]
aspect_ratios: [0.5, 1.0, 2.0]
height_stride: 16
width_stride: 16
}
}
first_stage_box_predictor_conv_hyperparams {
op: CONV
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
truncated_normal_initializer {
stddev: 0.01
}
}
}
first_stage_nms_score_threshold: 0.0
first_stage_nms_iou_threshold: 0.7
first_stage_max_proposals: 300
first_stage_localization_loss_weight: 2.0
first_stage_objectness_loss_weight: 1.0
initial_crop_size: 14
maxpool_kernel_size: 2
maxpool_stride: 2
second_stage_box_predictor {
mask_rcnn_box_predictor {
use_dropout: false
dropout_keep_probability: 1.0
fc_hyperparams {
op: FC
regularizer {
l2_regularizer {
weight: 0.0
}
}
initializer {
variance_scaling_initializer {
factor: 1.0
uniform: true
mode: FAN_AVG
}
}
}
}
}
second_stage_post_processing {
batch_non_max_suppression {
score_threshold: 0.0
iou_threshold: 0.6
max_detections_per_class: 100
max_total_detections: 300
}
score_converter: SOFTMAX
}
second_stage_localization_loss_weight: 2.0
second_stage_classification_loss_weight: 1.0
}
}
train_config: {
batch_size: 1
optimizer {
momentum_optimizer: {
learning_rate: {
manual_step_learning_rate {
initial_learning_rate: 0.0003
schedule {
step: 900000
learning_rate: .00003
}
schedule {
step: 1200000
learning_rate: .000003
}
}
}
momentum_optimizer_value: 0.9
}
use_moving_average: false
}
gradient_clipping_by_norm: 10.0
fine_tune_checkpoint: "PATH_TO_BE_CONFIGURED/model.ckpt"
from_detection_checkpoint: true
data_augmentation_options {
random_horizontal_flip {
}
}
}
train_input_reader: {
tf_record_input_reader {
input_path: "PATH_TO_BE_CONFIGURED/mscoco_train.record-?????-of-00100"
}
label_map_path: "PATH_TO_BE_CONFIGURED/mscoco_label_map.pbtxt"
}
eval_config: {
num_examples: 8000
# Note: The below line limits the evaluation process to 10 evaluations.
# Remove the below line to evaluate indefinitely.
max_evals: 10
}
eval_input_reader: {
tf_record_input_reader {
input_path: "PATH_TO_BE_CONFIGURED/mscoco_val.record-?????-of-00010"
}
label_map_path: "PATH_TO_BE_CONFIGURED/mscoco_label_map.pbtxt"
shuffle: false
num_readers: 1
}
|
PyTorch/Classification/GPUNet/triton/deployment_toolkit/library | library | utils | # Copyright (c) 2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
from collections import Counter
from typing import Callable, Dict, List, Optional
import networkx as nx
from ..core import ShapeSpec
def infer_precision(
nx_graph: nx.Graph,
input_names: List[str],
output_names: List[str],
get_node_dtype_fn: Callable,
):
node_dtypes = [nx_graph.nodes[node_name].get("dtype", None) for node_name in nx_graph.nodes]
node_dtypes = [dt for dt in node_dtypes if dt is None or dt.kind not in ["i", "b"]]
dtypes_counter = Counter(node_dtypes)
return dtypes_counter.most_common()[0][0]
def get_shapes_with_dynamic_axes(dataloader, batch_size_dim: Optional[int] = None):
def _set_dynamic_shapes(t, shapes):
for k, v in t.items():
shape = list(v.shape)
for dim, s in enumerate(shape):
if shapes[k][dim] != -1 and shapes[k][dim] != s:
shapes[k][dim] = -1
def _mark_batch_axis(shape, batch_axis: int):
shape = list(shape)
shape[batch_axis] = -1
return tuple(shape)
## get all shapes from input and output tensors
input_shapes = {}
output_shapes = {}
for batch in dataloader:
_, x, y = batch
for k, v in x.items():
input_shapes[k] = list(v.shape)
for k, v in y.items():
output_shapes[k] = list(v.shape)
break
# based on max <max_num_iters> iterations, check which
# dimensions differ to determine dynamic_axes
max_num_iters = 100
for idx, batch in enumerate(dataloader):
if idx >= max_num_iters:
break
_, x, y = batch
_set_dynamic_shapes(x, input_shapes)
_set_dynamic_shapes(y, output_shapes)
if batch_size_dim is not None:
input_shapes = {name: _mark_batch_axis(shape, batch_size_dim) for name, shape in input_shapes.items()}
output_shapes = {name: _mark_batch_axis(shape, batch_size_dim) for name, shape in output_shapes.items()}
return input_shapes, output_shapes
def get_dynamic_axes(dataloader, batch_size_dim: Optional[int] = None):
input_shapes, output_shapes = get_shapes_with_dynamic_axes(dataloader, batch_size_dim=batch_size_dim)
all_shapes = {**input_shapes, **output_shapes}
dynamic_axes = {}
for k, shape in all_shapes.items():
for idx, s in enumerate(shape):
if s == -1:
dynamic_axes[k] = {idx: k + "_" + str(idx)}
for k in all_shapes:
if k in dynamic_axes:
dynamic_axes[k].update({batch_size_dim: "batch_size_" + str(batch_size_dim)})
else:
dynamic_axes[k] = {batch_size_dim: "batch_size_" + str(batch_size_dim)}
return dynamic_axes
def get_input_shapes(dataloader, max_batch_size=1) -> Dict[str, ShapeSpec]:
def init_counters_and_shapes(x, counters, min_shapes, max_shapes):
for k, v in x.items():
counters[k] = Counter()
min_shapes[k] = [float("inf")] * v.ndim
max_shapes[k] = [float("-inf")] * v.ndim
counters = {}
min_shapes: Dict[str, tuple] = {}
max_shapes: Dict[str, tuple] = {}
for idx, batch in enumerate(dataloader):
ids, x, y = batch
if idx == 0:
init_counters_and_shapes(x, counters, min_shapes, max_shapes)
for k, v in x.items():
shape = v.shape
counters[k][shape] += 1
min_shapes[k] = tuple(min(a, b) for a, b in zip(min_shapes[k], shape))
max_shapes[k] = tuple(max(a, b) for a, b in zip(max_shapes[k], shape))
opt_shapes: Dict[str, tuple] = {}
for k, v in counters.items():
opt_shapes[k] = v.most_common(1)[0][0]
shapes = {}
for k in opt_shapes.keys(): # same keys in min_shapes and max_shapes
shapes[k] = ShapeSpec(
min=(1,) + min_shapes[k][1:],
max=(max_batch_size,) + max_shapes[k][1:],
opt=(max_batch_size,) + opt_shapes[k][1:],
)
return shapes
|
Tools/DGLPyTorch/SyntheticGraphGeneration/syngen/generator/graph | graph | base_graph_generator | # Copyright (c) 2023, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import abc
import json
import pickle
from abc import ABC
from typing import List, Optional, Set, Tuple
import numpy as np
from syngen.generator.graph.utils import BaseLogger
from syngen.generator.graph.seeder import BaseSeeder
class BaseGenerator(abc.ABC):
""" BaseGenerator class """
JSON_ASSERTION = "Expected file to be json"
@classmethod
def get_generators(cls, include_parents=True):
""" Recursively find subclasses
Args:
include_parents (bool): whether to include parents to other classes. (default: `True`)
Returns:
generators: dictionary with all the subclasses
"""
generators = dict()
for child in cls.__subclasses__():
children = child.get_generators(include_parents)
generators.update(children)
if include_parents or not children:
if abc.ABC not in child.__bases__ and BaseGenerator not in child.__bases__:
generators[child.__name__] = child
return generators
def save(self, path):
raise NotImplementedError()
@classmethod
def load(cls, path):
raise NotImplementedError()
class BaseGraphGenerator(BaseGenerator, ABC):
""" Base class for all graph generators
Args:
*args: optional positional args
**kwargs: optional key-word args
"""
def __init__(
self,
seed: Optional[int] = None,
logdir: str = "./logs",
gpu: bool = True,
verbose: bool = False,
*args,
**kwargs,
):
self._fit_results = None
self.seeder = BaseSeeder(seed)
self.seeder.reseed()
self.logger = BaseLogger(logdir)
self.logger.log(f"Using seed: {self.seeder.seed}")
self.gpu = gpu
self.verbose = verbose
def fit(
self, graph: List[Tuple[int, int]], is_directed: bool, *args, **kwargs
):
""" Fits generator on the graph
Args:
graph (List[Tuple[int, int]]): graph to be fitted on
is_directed (bool): flag indicating whether the graph is directed
*args: optional positional args
**kwargs: optional key-word args
"""
raise NotImplementedError()
def generate(
self,
num_nodes: int,
num_edges: int,
is_directed: bool,
*args,
return_node_ids: bool = False,
**kwargs,
) -> np.ndarray:
""" Generates graph with approximately `num_nodes` and exactly `num_edges` from generator
Args:
num_nodes (int): approximate number of nodes to be generated
num_edges (int): exact number of edges to be generated
is_directed (bool): flag indicating whether the generated graph has to be directed
return_node_ids (bool): flag indicating whether the generator has to return nodes_ids as the second output
*args: optional positional args
**kwargs: optional key-word args
"""
raise NotImplementedError()
def set_fit_results(self, fit_results):
self._fit_results = fit_results
def get_fit_results(self):
return self._fit_results
def save_fit_results(self, save_path: str = "./fit_results.json"):
""" Store fitted results into json file
Args:
save_path (str): path to the json file with the fitted result
"""
assert (
self._fit_results
), "There are no fit results to be saved, \
call fit method first or load the results from the file"
assert save_path.endswith(".json"), self.JSON_ASSERTION
with open(save_path, "w") as fjson:
json.dump(self._fit_results, fjson)
def load_fit_results(self, load_path: str = "./fit_results.json"):
"""load fitted results from json file
Args:
load_path (str): path to the json file with the fitted result
"""
assert load_path.endswith(".json"), self.JSON_ASSERTION
with open(load_path, "r") as fjson:
self._fit_results = json.load(fjson)
def save(self, path):
with open(path, 'wb') as file_handler:
pickle.dump(self, file_handler, protocol=pickle.HIGHEST_PROTOCOL)
@classmethod
def load(cls, path):
with open(path, 'rb') as file_handler:
model = pickle.load(file_handler)
return model
@staticmethod
def add_args(parser):
return parser
class BaseBipartiteGraphGenerator(BaseGenerator, ABC):
""" Base class for all bipartite graph generators
Args:
*args: optional positional args
**kwargs: optional key-word args
"""
def __init__(
self,
seed: Optional[int] = None,
logdir: str = "./logs",
gpu: bool = True,
verbose: bool = False,
*args,
**kwargs,
):
self._fit_src_dst_results = None
self._fit_dst_src_results = None
self.seeder = BaseSeeder(seed)
self.seeder.reseed()
self.logger = BaseLogger(logdir)
self.logger.log(f"Using seed: {self.seeder.seed}")
self.gpu = gpu
self.verbose = verbose
def fit(
self,
graph: List[Tuple[int, int]],
src_set: Set[int],
dst_set: Set[int],
is_directed: bool,
transform_graph: bool,
*args,
**kwargs,
):
""" Fits generator on the graph
Args:
graph (List[Tuple[int, int]]): graph to be fitted on
src_set (Set[int]): set of source nodes
dst_set (Set[int]): set of destination nodes
is_directed (bool): flag indicating whether the graph is directed
*args: optional positional args
**kwargs: optional key-word args
"""
raise NotImplementedError()
def generate(
self,
num_nodes_src_set: int,
num_nodes_dst_set: int,
num_edges_src_dst: int,
num_edges_dst_src: int,
is_directed: bool,
return_node_ids: bool = False,
transform_graph: bool = True,
*args,
**kwargs,
):
""" Generates graph with approximately `num_nodes_src_set`/`num_nodes_dst_set` nodes
and exactly `num_edges_src_dst`/`num_edges_dst_src` edges from generator
Args:
num_nodes_src_set (int): approximate number of source nodes to be generated
num_nodes_dst_set (int): approximate number of destination nodes to be generated
num_edges_src_dst (int): exact number of source->destination edges to be generated
num_edges_dst_src (int): exact number of destination->source to be generated
is_directed (bool) flag indicating whether the generated graph has to be directed
return_node_ids (bool): flag indicating whether the generator has to return nodes_ids as the second output
*args: optional positional args
**kwargs: optional key-word args
"""
raise NotImplementedError()
def set_fit_results(self, fit_results):
self._fit_src_dst_results, self._fit_dst_src_results = fit_results
def get_fit_results(self):
return self._fit_src_dst_results, self._fit_dst_src_results
def save_fit_results(self, save_path: str = "./fit_results.json"):
""" Stores fitted results into json file
Args:
save_path (str): path to the json file with the fitted result
"""
assert (
self._fit_src_dst_results or self._fit_dst_src_results
), "There are no fit results to be saved, \
call fit method first or load the results from the file"
assert save_path.endswith(".json"), self.JSON_ASSERTION
wrapped_results = {
"fit_src_dst_results": self._fit_src_dst_results,
"fit_dst_src_results": self._fit_dst_src_results,
}
with open(save_path, "w") as fjson:
json.dump(wrapped_results, fjson)
def load_fit_results(self, load_path: str = "./fit_results.json"):
""" Loads fitted results from json file
Args:
load_path (str): path to the json file with the fitted result
"""
assert load_path.endswith(".json"), self.JSON_ASSERTION
with open(load_path, "r") as fjson:
wrapped_results = json.load(fjson)
assert (
"fit_src_dst_results" in wrapped_results
and "fit_dst_src_results" in wrapped_results
), "Required keys fit_src_dst_results and fit_dst_src_results keys in the json not found"
self._fit_src_dst_results = wrapped_results["fit_src_dst_results"]
self._fit_dst_src_results = wrapped_results["fit_dst_src_results"]
def save(self, path):
with open(path, 'wb') as file_handler:
pickle.dump(self, file_handler, protocol=pickle.HIGHEST_PROTOCOL)
@classmethod
def load(cls, path):
with open(path, 'rb') as file_handler:
model = pickle.load(file_handler)
return model
@staticmethod
def add_args(parser):
return parser
|
CUDA-Optimized/FastSpeech/tacotron2 | tacotron2 | distributed | # BSD 3-Clause License
# Copyright (c) 2018-2020, NVIDIA Corporation
# All rights reserved.
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
# * Redistributions of source code must retain the above copyright notice, this
# list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright notice,
# this list of conditions and the following disclaimer in the documentation
# and/or other materials provided with the distribution.
# * Neither the name of the copyright holder nor the names of its
# contributors may be used to endorse or promote products derived from
# this software without specific prior written permission.
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
# DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
# FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
# SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
# CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
# OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
# OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
"""https://github.com/NVIDIA/tacotron2"""
import torch
import torch.distributed as dist
from torch.nn.modules import Module
from torch.autograd import Variable
def _flatten_dense_tensors(tensors):
"""Flatten dense tensors into a contiguous 1D buffer. Assume tensors are of
same dense type.
Since inputs are dense, the resulting tensor will be a concatenated 1D
buffer. Element-wise operation on this buffer will be equivalent to
operating individually.
Arguments:
tensors (Iterable[Tensor]): dense tensors to flatten.
Returns:
A contiguous 1D buffer containing input tensors.
"""
if len(tensors) == 1:
return tensors[0].contiguous().view(-1)
flat = torch.cat([t.contiguous().view(-1) for t in tensors], dim=0)
return flat
def _unflatten_dense_tensors(flat, tensors):
"""View a flat buffer using the sizes of tensors. Assume that tensors are of
same dense type, and that flat is given by _flatten_dense_tensors.
Arguments:
flat (Tensor): flattened dense tensors to unflatten.
tensors (Iterable[Tensor]): dense tensors whose sizes will be used to
unflatten flat.
Returns:
Unflattened dense tensors with sizes same as tensors and values from
flat.
"""
outputs = []
offset = 0
for tensor in tensors:
numel = tensor.numel()
outputs.append(flat.narrow(0, offset, numel).view_as(tensor))
offset += numel
return tuple(outputs)
'''
This version of DistributedDataParallel is designed to be used in conjunction with the multiproc.py
launcher included with this example. It assumes that your run is using multiprocess with 1
GPU/process, that the model is on the correct device, and that torch.set_device has been
used to set the device.
Parameters are broadcasted to the other processes on initialization of DistributedDataParallel,
and will be allreduced at the finish of the backward pass.
'''
class DistributedDataParallel(Module):
def __init__(self, module):
super(DistributedDataParallel, self).__init__()
#fallback for PyTorch 0.3
if not hasattr(dist, '_backend'):
self.warn_on_half = True
else:
self.warn_on_half = True if dist._backend == dist.dist_backend.GLOO else False
self.module = module
for p in self.module.state_dict().values():
if not torch.is_tensor(p):
continue
dist.broadcast(p, 0)
def allreduce_params():
if(self.needs_reduction):
self.needs_reduction = False
buckets = {}
for param in self.module.parameters():
if param.requires_grad and param.grad is not None:
tp = type(param.data)
if tp not in buckets:
buckets[tp] = []
buckets[tp].append(param)
if self.warn_on_half:
if torch.cuda.HalfTensor in buckets:
print("WARNING: gloo dist backend for half parameters may be extremely slow." +
" It is recommended to use the NCCL backend in this case. This currently requires" +
"PyTorch built from top of tree master.")
self.warn_on_half = False
for tp in buckets:
bucket = buckets[tp]
grads = [param.grad.data for param in bucket]
coalesced = _flatten_dense_tensors(grads)
dist.all_reduce(coalesced)
coalesced /= dist.get_world_size()
for buf, synced in zip(grads, _unflatten_dense_tensors(coalesced, grads)):
buf.copy_(synced)
for param in list(self.module.parameters()):
def allreduce_hook(*unused):
param._execution_engine.queue_callback(allreduce_params)
if param.requires_grad:
param.register_hook(allreduce_hook)
def forward(self, *inputs, **kwargs):
self.needs_reduction = True
return self.module(*inputs, **kwargs)
'''
def _sync_buffers(self):
buffers = list(self.module._all_buffers())
if len(buffers) > 0:
# cross-node buffer sync
flat_buffers = _flatten_dense_tensors(buffers)
dist.broadcast(flat_buffers, 0)
for buf, synced in zip(buffers, _unflatten_dense_tensors(flat_buffers, buffers)):
buf.copy_(synced)
def train(self, mode=True):
# Clear NCCL communicator and CUDA event cache of the default group ID,
# These cache will be recreated at the later call. This is currently a
# work-around for a potential NCCL deadlock.
if dist._backend == dist.dist_backend.NCCL:
dist._clear_group_cache()
super(DistributedDataParallel, self).train(mode)
self.module.train(mode)
'''
'''
Modifies existing model to do gradient allreduce, but doesn't change class
so you don't need "module"
'''
def apply_gradient_allreduce(module):
if not hasattr(dist, '_backend'):
module.warn_on_half = True
else:
module.warn_on_half = True if dist._backend == dist.dist_backend.GLOO else False
for p in module.state_dict().values():
if not torch.is_tensor(p):
continue
dist.broadcast(p, 0)
def allreduce_params():
if(module.needs_reduction):
module.needs_reduction = False
buckets = {}
for param in module.parameters():
if param.requires_grad and param.grad is not None:
tp = param.data.dtype
if tp not in buckets:
buckets[tp] = []
buckets[tp].append(param)
if module.warn_on_half:
if torch.cuda.HalfTensor in buckets:
print("WARNING: gloo dist backend for half parameters may be extremely slow." +
" It is recommended to use the NCCL backend in this case. This currently requires" +
"PyTorch built from top of tree master.")
module.warn_on_half = False
for tp in buckets:
bucket = buckets[tp]
grads = [param.grad.data for param in bucket]
coalesced = _flatten_dense_tensors(grads)
dist.all_reduce(coalesced)
coalesced /= dist.get_world_size()
for buf, synced in zip(grads, _unflatten_dense_tensors(coalesced, grads)):
buf.copy_(synced)
for param in list(module.parameters()):
def allreduce_hook(*unused):
Variable._execution_engine.queue_callback(allreduce_params)
if param.requires_grad:
param.register_hook(allreduce_hook)
def set_needs_reduction(self, input, output):
self.needs_reduction = True
module.register_forward_hook(set_needs_reduction)
return module
|
TensorFlow/Detection/SSD/models/research/slim/preprocessing | preprocessing | inception_preprocessing | # Copyright 2016 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Provides utilities to preprocess images for the Inception networks."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import tensorflow as tf
from tensorflow.python.ops import control_flow_ops
def apply_with_random_selector(x, func, num_cases):
"""Computes func(x, sel), with sel sampled from [0...num_cases-1].
Args:
x: input Tensor.
func: Python function to apply.
num_cases: Python int32, number of cases to sample sel from.
Returns:
The result of func(x, sel), where func receives the value of the
selector as a python integer, but sel is sampled dynamically.
"""
sel = tf.random_uniform([], maxval=num_cases, dtype=tf.int32)
# Pass the real x only to one of the func calls.
return control_flow_ops.merge([
func(control_flow_ops.switch(x, tf.equal(sel, case))[1], case)
for case in range(num_cases)])[0]
def distort_color(image, color_ordering=0, fast_mode=True, scope=None):
"""Distort the color of a Tensor image.
Each color distortion is non-commutative and thus ordering of the color ops
matters. Ideally we would randomly permute the ordering of the color ops.
Rather then adding that level of complication, we select a distinct ordering
of color ops for each preprocessing thread.
Args:
image: 3-D Tensor containing single image in [0, 1].
color_ordering: Python int, a type of distortion (valid values: 0-3).
fast_mode: Avoids slower ops (random_hue and random_contrast)
scope: Optional scope for name_scope.
Returns:
3-D Tensor color-distorted image on range [0, 1]
Raises:
ValueError: if color_ordering not in [0, 3]
"""
with tf.name_scope(scope, 'distort_color', [image]):
if fast_mode:
if color_ordering == 0:
image = tf.image.random_brightness(image, max_delta=32. / 255.)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
else:
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
image = tf.image.random_brightness(image, max_delta=32. / 255.)
else:
if color_ordering == 0:
image = tf.image.random_brightness(image, max_delta=32. / 255.)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
image = tf.image.random_hue(image, max_delta=0.2)
image = tf.image.random_contrast(image, lower=0.5, upper=1.5)
elif color_ordering == 1:
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
image = tf.image.random_brightness(image, max_delta=32. / 255.)
image = tf.image.random_contrast(image, lower=0.5, upper=1.5)
image = tf.image.random_hue(image, max_delta=0.2)
elif color_ordering == 2:
image = tf.image.random_contrast(image, lower=0.5, upper=1.5)
image = tf.image.random_hue(image, max_delta=0.2)
image = tf.image.random_brightness(image, max_delta=32. / 255.)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
elif color_ordering == 3:
image = tf.image.random_hue(image, max_delta=0.2)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
image = tf.image.random_contrast(image, lower=0.5, upper=1.5)
image = tf.image.random_brightness(image, max_delta=32. / 255.)
else:
raise ValueError('color_ordering must be in [0, 3]')
# The random_* ops do not necessarily clamp.
return tf.clip_by_value(image, 0.0, 1.0)
def distorted_bounding_box_crop(image,
bbox,
min_object_covered=0.1,
aspect_ratio_range=(0.75, 1.33),
area_range=(0.05, 1.0),
max_attempts=100,
scope=None):
"""Generates cropped_image using a one of the bboxes randomly distorted.
See `tf.image.sample_distorted_bounding_box` for more documentation.
Args:
image: 3-D Tensor of image (it will be converted to floats in [0, 1]).
bbox: 3-D float Tensor of bounding boxes arranged [1, num_boxes, coords]
where each coordinate is [0, 1) and the coordinates are arranged
as [ymin, xmin, ymax, xmax]. If num_boxes is 0 then it would use the whole
image.
min_object_covered: An optional `float`. Defaults to `0.1`. The cropped
area of the image must contain at least this fraction of any bounding box
supplied.
aspect_ratio_range: An optional list of `floats`. The cropped area of the
image must have an aspect ratio = width / height within this range.
area_range: An optional list of `floats`. The cropped area of the image
must contain a fraction of the supplied image within in this range.
max_attempts: An optional `int`. Number of attempts at generating a cropped
region of the image of the specified constraints. After `max_attempts`
failures, return the entire image.
scope: Optional scope for name_scope.
Returns:
A tuple, a 3-D Tensor cropped_image and the distorted bbox
"""
with tf.name_scope(scope, 'distorted_bounding_box_crop', [image, bbox]):
# Each bounding box has shape [1, num_boxes, box coords] and
# the coordinates are ordered [ymin, xmin, ymax, xmax].
# A large fraction of image datasets contain a human-annotated bounding
# box delineating the region of the image containing the object of interest.
# We choose to create a new bounding box for the object which is a randomly
# distorted version of the human-annotated bounding box that obeys an
# allowed range of aspect ratios, sizes and overlap with the human-annotated
# bounding box. If no box is supplied, then we assume the bounding box is
# the entire image.
sample_distorted_bounding_box = tf.image.sample_distorted_bounding_box(
tf.shape(image),
bounding_boxes=bbox,
min_object_covered=min_object_covered,
aspect_ratio_range=aspect_ratio_range,
area_range=area_range,
max_attempts=max_attempts,
use_image_if_no_bounding_boxes=True)
bbox_begin, bbox_size, distort_bbox = sample_distorted_bounding_box
# Crop the image to the specified bounding box.
cropped_image = tf.slice(image, bbox_begin, bbox_size)
return cropped_image, distort_bbox
def preprocess_for_train(image, height, width, bbox,
fast_mode=True,
scope=None,
add_image_summaries=True):
"""Distort one image for training a network.
Distorting images provides a useful technique for augmenting the data
set during training in order to make the network invariant to aspects
of the image that do not effect the label.
Additionally it would create image_summaries to display the different
transformations applied to the image.
Args:
image: 3-D Tensor of image. If dtype is tf.float32 then the range should be
[0, 1], otherwise it would converted to tf.float32 assuming that the range
is [0, MAX], where MAX is largest positive representable number for
int(8/16/32) data type (see `tf.image.convert_image_dtype` for details).
height: integer
width: integer
bbox: 3-D float Tensor of bounding boxes arranged [1, num_boxes, coords]
where each coordinate is [0, 1) and the coordinates are arranged
as [ymin, xmin, ymax, xmax].
fast_mode: Optional boolean, if True avoids slower transformations (i.e.
bi-cubic resizing, random_hue or random_contrast).
scope: Optional scope for name_scope.
add_image_summaries: Enable image summaries.
Returns:
3-D float Tensor of distorted image used for training with range [-1, 1].
"""
with tf.name_scope(scope, 'distort_image', [image, height, width, bbox]):
if bbox is None:
bbox = tf.constant([0.0, 0.0, 1.0, 1.0],
dtype=tf.float32,
shape=[1, 1, 4])
if image.dtype != tf.float32:
image = tf.image.convert_image_dtype(image, dtype=tf.float32)
# Each bounding box has shape [1, num_boxes, box coords] and
# the coordinates are ordered [ymin, xmin, ymax, xmax].
image_with_box = tf.image.draw_bounding_boxes(tf.expand_dims(image, 0),
bbox)
if add_image_summaries:
tf.summary.image('image_with_bounding_boxes', image_with_box)
distorted_image, distorted_bbox = distorted_bounding_box_crop(image, bbox)
# Restore the shape since the dynamic slice based upon the bbox_size loses
# the third dimension.
distorted_image.set_shape([None, None, 3])
image_with_distorted_box = tf.image.draw_bounding_boxes(
tf.expand_dims(image, 0), distorted_bbox)
if add_image_summaries:
tf.summary.image('images_with_distorted_bounding_box',
image_with_distorted_box)
# This resizing operation may distort the images because the aspect
# ratio is not respected. We select a resize method in a round robin
# fashion based on the thread number.
# Note that ResizeMethod contains 4 enumerated resizing methods.
# We select only 1 case for fast_mode bilinear.
num_resize_cases = 1 if fast_mode else 4
distorted_image = apply_with_random_selector(
distorted_image,
lambda x, method: tf.image.resize_images(x, [height, width], method),
num_cases=num_resize_cases)
if add_image_summaries:
tf.summary.image('cropped_resized_image',
tf.expand_dims(distorted_image, 0))
# Randomly flip the image horizontally.
distorted_image = tf.image.random_flip_left_right(distorted_image)
# Randomly distort the colors. There are 1 or 4 ways to do it.
num_distort_cases = 1 if fast_mode else 4
distorted_image = apply_with_random_selector(
distorted_image,
lambda x, ordering: distort_color(x, ordering, fast_mode),
num_cases=num_distort_cases)
if add_image_summaries:
tf.summary.image('final_distorted_image',
tf.expand_dims(distorted_image, 0))
distorted_image = tf.subtract(distorted_image, 0.5)
distorted_image = tf.multiply(distorted_image, 2.0)
return distorted_image
def preprocess_for_eval(image, height, width,
central_fraction=0.875, scope=None):
"""Prepare one image for evaluation.
If height and width are specified it would output an image with that size by
applying resize_bilinear.
If central_fraction is specified it would crop the central fraction of the
input image.
Args:
image: 3-D Tensor of image. If dtype is tf.float32 then the range should be
[0, 1], otherwise it would converted to tf.float32 assuming that the range
is [0, MAX], where MAX is largest positive representable number for
int(8/16/32) data type (see `tf.image.convert_image_dtype` for details).
height: integer
width: integer
central_fraction: Optional Float, fraction of the image to crop.
scope: Optional scope for name_scope.
Returns:
3-D float Tensor of prepared image.
"""
with tf.name_scope(scope, 'eval_image', [image, height, width]):
if image.dtype != tf.float32:
image = tf.image.convert_image_dtype(image, dtype=tf.float32)
# Crop the central region of the image with an area containing 87.5% of
# the original image.
if central_fraction:
image = tf.image.central_crop(image, central_fraction=central_fraction)
if height and width:
# Resize the image to the specified height and width.
image = tf.expand_dims(image, 0)
image = tf.image.resize_bilinear(image, [height, width],
align_corners=False)
image = tf.squeeze(image, [0])
image = tf.subtract(image, 0.5)
image = tf.multiply(image, 2.0)
return image
def preprocess_image(image, height, width,
is_training=False,
bbox=None,
fast_mode=True,
add_image_summaries=True):
"""Pre-process one image for training or evaluation.
Args:
image: 3-D Tensor [height, width, channels] with the image. If dtype is
tf.float32 then the range should be [0, 1], otherwise it would converted
to tf.float32 assuming that the range is [0, MAX], where MAX is largest
positive representable number for int(8/16/32) data type (see
`tf.image.convert_image_dtype` for details).
height: integer, image expected height.
width: integer, image expected width.
is_training: Boolean. If true it would transform an image for train,
otherwise it would transform it for evaluation.
bbox: 3-D float Tensor of bounding boxes arranged [1, num_boxes, coords]
where each coordinate is [0, 1) and the coordinates are arranged as
[ymin, xmin, ymax, xmax].
fast_mode: Optional boolean, if True avoids slower transformations.
add_image_summaries: Enable image summaries.
Returns:
3-D float Tensor containing an appropriately scaled image
Raises:
ValueError: if user does not provide bounding box
"""
if is_training:
return preprocess_for_train(image, height, width, bbox, fast_mode,
add_image_summaries=add_image_summaries)
else:
return preprocess_for_eval(image, height, width)
|
TensorFlow/Detection/SSD/models/research/object_detection/utils | utils | test_utils | # Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Contains functions which are convenient for unit testing."""
import numpy as np
import tensorflow as tf
from object_detection.core import anchor_generator
from object_detection.core import box_coder
from object_detection.core import box_list
from object_detection.core import box_predictor
from object_detection.core import matcher
from object_detection.utils import shape_utils
# Default size (both width and height) used for testing mask predictions.
DEFAULT_MASK_SIZE = 5
class MockBoxCoder(box_coder.BoxCoder):
"""Simple `difference` BoxCoder."""
@property
def code_size(self):
return 4
def _encode(self, boxes, anchors):
return boxes.get() - anchors.get()
def _decode(self, rel_codes, anchors):
return box_list.BoxList(rel_codes + anchors.get())
class MockMaskHead(object):
"""Simple maskhead that returns all zeros as mask predictions."""
def __init__(self, num_classes):
self._num_classes = num_classes
def predict(self, features):
batch_size = tf.shape(features)[0]
return tf.zeros((batch_size, 1, self._num_classes, DEFAULT_MASK_SIZE,
DEFAULT_MASK_SIZE),
dtype=tf.float32)
class MockBoxPredictor(box_predictor.BoxPredictor):
"""Simple box predictor that ignores inputs and outputs all zeros."""
def __init__(self, is_training, num_classes, add_background_class=True):
super(MockBoxPredictor, self).__init__(is_training, num_classes)
self._add_background_class = add_background_class
def _predict(self, image_features, num_predictions_per_location):
image_feature = image_features[0]
combined_feature_shape = shape_utils.combined_static_and_dynamic_shape(
image_feature)
batch_size = combined_feature_shape[0]
num_anchors = (combined_feature_shape[1] * combined_feature_shape[2])
code_size = 4
zero = tf.reduce_sum(0 * image_feature)
num_class_slots = self.num_classes
if self._add_background_class:
num_class_slots = num_class_slots + 1
box_encodings = zero + tf.zeros(
(batch_size, num_anchors, 1, code_size), dtype=tf.float32)
class_predictions_with_background = zero + tf.zeros(
(batch_size, num_anchors, num_class_slots), dtype=tf.float32)
predictions_dict = {
box_predictor.BOX_ENCODINGS:
box_encodings,
box_predictor.CLASS_PREDICTIONS_WITH_BACKGROUND:
class_predictions_with_background
}
return predictions_dict
class MockKerasBoxPredictor(box_predictor.KerasBoxPredictor):
"""Simple box predictor that ignores inputs and outputs all zeros."""
def __init__(self, is_training, num_classes, add_background_class=True):
super(MockKerasBoxPredictor, self).__init__(
is_training, num_classes, False, False)
self._add_background_class = add_background_class
def _predict(self, image_features, **kwargs):
image_feature = image_features[0]
combined_feature_shape = shape_utils.combined_static_and_dynamic_shape(
image_feature)
batch_size = combined_feature_shape[0]
num_anchors = (combined_feature_shape[1] * combined_feature_shape[2])
code_size = 4
zero = tf.reduce_sum(0 * image_feature)
num_class_slots = self.num_classes
if self._add_background_class:
num_class_slots = num_class_slots + 1
box_encodings = zero + tf.zeros(
(batch_size, num_anchors, 1, code_size), dtype=tf.float32)
class_predictions_with_background = zero + tf.zeros(
(batch_size, num_anchors, num_class_slots), dtype=tf.float32)
predictions_dict = {
box_predictor.BOX_ENCODINGS:
box_encodings,
box_predictor.CLASS_PREDICTIONS_WITH_BACKGROUND:
class_predictions_with_background
}
return predictions_dict
class MockAnchorGenerator(anchor_generator.AnchorGenerator):
"""Mock anchor generator."""
def name_scope(self):
return 'MockAnchorGenerator'
def num_anchors_per_location(self):
return [1]
def _generate(self, feature_map_shape_list):
num_anchors = sum([shape[0] * shape[1] for shape in feature_map_shape_list])
return box_list.BoxList(tf.zeros((num_anchors, 4), dtype=tf.float32))
class MockMatcher(matcher.Matcher):
"""Simple matcher that matches first anchor to first groundtruth box."""
def _match(self, similarity_matrix, valid_rows):
return tf.constant([0, -1, -1, -1], dtype=tf.int32)
def create_diagonal_gradient_image(height, width, depth):
"""Creates pyramid image. Useful for testing.
For example, pyramid_image(5, 6, 1) looks like:
# [[[ 5. 4. 3. 2. 1. 0.]
# [ 6. 5. 4. 3. 2. 1.]
# [ 7. 6. 5. 4. 3. 2.]
# [ 8. 7. 6. 5. 4. 3.]
# [ 9. 8. 7. 6. 5. 4.]]]
Args:
height: height of image
width: width of image
depth: depth of image
Returns:
pyramid image
"""
row = np.arange(height)
col = np.arange(width)[::-1]
image_layer = np.expand_dims(row, 1) + col
image_layer = np.expand_dims(image_layer, 2)
image = image_layer
for i in range(1, depth):
image = np.concatenate((image, image_layer * pow(10, i)), 2)
return image.astype(np.float32)
def create_random_boxes(num_boxes, max_height, max_width):
"""Creates random bounding boxes of specific maximum height and width.
Args:
num_boxes: number of boxes.
max_height: maximum height of boxes.
max_width: maximum width of boxes.
Returns:
boxes: numpy array of shape [num_boxes, 4]. Each row is in form
[y_min, x_min, y_max, x_max].
"""
y_1 = np.random.uniform(size=(1, num_boxes)) * max_height
y_2 = np.random.uniform(size=(1, num_boxes)) * max_height
x_1 = np.random.uniform(size=(1, num_boxes)) * max_width
x_2 = np.random.uniform(size=(1, num_boxes)) * max_width
boxes = np.zeros(shape=(num_boxes, 4))
boxes[:, 0] = np.minimum(y_1, y_2)
boxes[:, 1] = np.minimum(x_1, x_2)
boxes[:, 2] = np.maximum(y_1, y_2)
boxes[:, 3] = np.maximum(x_1, x_2)
return boxes.astype(np.float32)
def first_rows_close_as_set(a, b, k=None, rtol=1e-6, atol=1e-6):
"""Checks if first K entries of two lists are close, up to permutation.
Inputs to this assert are lists of items which can be compared via
numpy.allclose(...) and can be sorted.
Args:
a: list of items which can be compared via numpy.allclose(...) and are
sortable.
b: list of items which can be compared via numpy.allclose(...) and are
sortable.
k: a non-negative integer. If not provided, k is set to be len(a).
rtol: relative tolerance.
atol: absolute tolerance.
Returns:
boolean, True if input lists a and b have the same length and
the first k entries of the inputs satisfy numpy.allclose() after
sorting entries.
"""
if not isinstance(a, list) or not isinstance(b, list) or len(a) != len(b):
return False
if not k:
k = len(a)
k = min(k, len(a))
a_sorted = sorted(a[:k])
b_sorted = sorted(b[:k])
return all([
np.allclose(entry_a, entry_b, rtol, atol)
for (entry_a, entry_b) in zip(a_sorted, b_sorted)
])
|
TensorFlow/LanguageModeling/BERT/biobert/scripts | scripts | run_pretraining_pubmed_base_phase_2 | #! /bin/bash
echo "Container nvidia build = " $NVIDIA_BUILD_ID
init_checkpoint=${1}
train_batch_size=${2:-16}
learning_rate=${3:-"2.9e-4"}
cased=${4:-false}
precision=${5:-"fp16"}
use_xla=${6:-true}
num_gpu=${7:-16}
warmup_steps=${8:-"434"}
train_steps=${9:-4340}
num_accumulation_steps=${10:-128}
save_checkpoint_steps=${11:-5000}
eval_batch_size=${12:-26}
use_fp16=""
if [ "$precision" = "fp16" ] ; then
echo "fp16 activated!"
use_fp16="--amp"
else
echo "fp32/tf32 activated!"
use_fp16="--noamp"
fi
if [ "$use_xla" = "true" ] ; then
use_xla_tag="--use_xla"
echo "XLA activated"
else
use_xla_tag="--nouse_xla"
fi
if [ "$cased" = "true" ] ; then
DO_LOWER_CASE=0
CASING_DIR_PREFIX="cased"
else
DO_LOWER_CASE=1
CASING_DIR_PREFIX="uncased"
fi
BERT_CONFIG=/workspace/bert/data/download/google_pretrained_weights/${CASING_DIR_PREFIX}_L-12_H-768_A-12/bert_config.json
RESULTS_DIR=/results
CHECKPOINTS_DIR=${RESULTS_DIR}/biobert_phase_2
mkdir -p ${CHECKPOINTS_DIR}
INPUT_FILES_DIR="/workspace/bert/data/tfrecord/lower_case_${DO_LOWER_CASE}_seq_len_512_max_pred_80_masked_lm_prob_0.15_random_seed_12345_dupe_factor_5_shard_1472_test_split_10/pubmed_baseline/training"
EVAL_FILES_DIR="/workspace/bert/data/tfrecord/lower_case_${DO_LOWER_CASE}_seq_len_512_max_pred_80_masked_lm_prob_0.15_random_seed_12345_dupe_factor_5_shard_1472_test_split_10/pubmed_baseline/test"
if [ $num_gpu -gt 1 ] ; then
mpi_command="mpirun -np $num_gpu -H localhost:$num_gpu \
--allow-run-as-root -bind-to none -map-by slot \
-x NCCL_DEBUG=INFO \
-x LD_LIBRARY_PATH \
-x PATH -mca pml ob1 -mca btl ^openib"
use_hvd="--horovod"
else
mpi_command=""
use_hvd=""
fi
export GBS=$(expr $train_batch_size \* $num_gpus \* num_accumulation_steps)
printf -v TAG "tf_bert_bio_1n_phase2_cased_%s_%s_gbs%d" "$cased" "$precision" $GBS
DATESTAMP=`date +'%y%m%d%H%M%S'`
LOGFILE=$RESULTS_DIR/$TAG.$DATESTAMP.log
printf "Logs written to %s\n" "$LOGFILE"
$mpi python3 /workspace/bert/run_pretraining.py \
--input_files_dir=$INPUT_FILES_DIR \
--eval_files_dir=$EVAL_FILES_DIR \
--output_dir=$CHECKPOINTS_DIR \
--bert_config_file=$BERT_CONFIG \
--do_train=True \
--do_eval=True \
--train_batch_size=$train_batch_size \
--eval_batch_size=$eval_batch_size \
--max_seq_length=512 \
--max_predictions_per_seq=80 \
--num_train_steps=$train_steps \
--num_warmup_steps=$warmup_steps \
--save_checkpoints_steps=$save_checkpoint_steps \
--num_accumulation_steps=$num_accumulation_steps \
--learning_rate=$learning_rate \
--report_loss \
$use_hvd $use_xla_tag $use_fp16 \
--init_checkpoint=$INIT_CHECKPOINT |& tee $LOGFILE |
PyTorch/Recommendation/DLRM/preproc/gpu | gpu | get_gpu_resources | #! /bin/bash
ADDRS=`nvidia-smi --query-gpu=index --format=csv,noheader | sed -e ':a' -e 'N' -e'$!ba' -e 's/\n/","/g'`
echo {\"name\": \"gpu\", \"addresses\":[\"$ADDRS\"]}
|
TensorFlow2/Recommendation/WideAndDeep/tests/feature_specs | feature_specs | less_numerical | channel_spec:
label:
- clicked
map: []
multihot_categorical:
- topic_id_list
- entity_id_list
- category_id_list
numerical:
- document_id_promo_ctr
- publisher_id_promo_ctr
- source_id_promo_ctr
- document_id_promo_count
- publish_time_days_since_published
- ad_id_ctr
- advertiser_id_ctr
- campaign_id_ctr
- ad_id_count
- publish_time_promo_days_since_published
onehot_categorical:
- ad_id
- document_id
- platform
- document_id_promo
- campaign_id
- advertiser_id
- source_id
- geo_location
- geo_location_country
- geo_location_state
- publisher_id
- source_id_promo
- publisher_id_promo
feature_spec:
ad_id:
cardinality: 250000
ad_id_count: {}
ad_id_ctr: {}
advertiser_id:
cardinality: 2500
advertiser_id_ctr: {}
campaign_id:
cardinality: 5000
campaign_id_ctr: {}
category_id_list:
cardinality: 100
max_hotness: 3
clicked: {}
document_id:
cardinality: 300000
document_id_promo:
cardinality: 100000
document_id_promo_count: {}
document_id_promo_ctr: {}
entity_id_list:
cardinality: 10000
max_hotness: 3
geo_location:
cardinality: 2500
geo_location_country:
cardinality: 300
geo_location_state:
cardinality: 2000
platform:
cardinality: 4
publish_time_days_since_published: {}
publish_time_promo_days_since_published: {}
publisher_id:
cardinality: 1000
publisher_id_promo:
cardinality: 1000
publisher_id_promo_ctr: {}
source_id:
cardinality: 4000
source_id_promo:
cardinality: 4000
source_id_promo_ctr: {}
topic_id_list:
cardinality: 350
max_hotness: 3
metadata: {}
source_spec:
test:
- features:
- clicked
- ad_id
- document_id
- platform
- document_id_promo
- campaign_id
- advertiser_id
- source_id
- geo_location
- geo_location_country
- geo_location_state
- publisher_id
- source_id_promo
- publisher_id_promo
- topic_id_list
- entity_id_list
- category_id_list
- document_id_promo_ctr
- publisher_id_promo_ctr
- source_id_promo_ctr
- document_id_promo_count
- publish_time_days_since_published
- ad_id_ctr
- advertiser_id_ctr
- campaign_id_ctr
- ad_id_count
- publish_time_promo_days_since_published
files:
- valid.csv
type: csv
train:
- features:
- clicked
- ad_id
- document_id
- platform
- document_id_promo
- campaign_id
- advertiser_id
- source_id
- geo_location
- geo_location_country
- geo_location_state
- publisher_id
- source_id_promo
- publisher_id_promo
- topic_id_list
- entity_id_list
- category_id_list
- document_id_promo_ctr
- publisher_id_promo_ctr
- source_id_promo_ctr
- document_id_promo_count
- publish_time_days_since_published
- ad_id_ctr
- advertiser_id_ctr
- campaign_id_ctr
- ad_id_count
- publish_time_promo_days_since_published
files:
- train.csv
type: csv
|
Tools/PyTorch/TimeSeriesPredictionPlatform | TimeSeriesPredictionPlatform | launch_preproc | # Copyright (c) 2021-2022, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import warnings
import hydra
warnings.filterwarnings("ignore")
@hydra.main(config_path="conf/", config_name="preproc_config")
def main(cfg):
print(cfg)
preprocessor = hydra.utils.instantiate(cfg, _recursive_=False)
train, valid, test = preprocessor.preprocess()
preprocessor.fit_scalers(train)
train = preprocessor.apply_scalers(train)
valid = preprocessor.apply_scalers(valid)
test = preprocessor.apply_scalers(test)
train = preprocessor.impute(train)
valid = preprocessor.impute(valid)
test = preprocessor.impute(test)
preprocessor.save_state()
preprocessor.save_datasets(train, valid, test)
if __name__ == "__main__":
main()
|
PyTorch/LanguageModeling/BERT/triton/large/runner | runner | start_NVIDIA-DGX-1-(1x-V100-32GB) | # Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#!/bin/bash
# Install Docker
. /etc/os-release && \
curl -fsSL https://download.docker.com/linux/debian/gpg | apt-key add - && \
echo "deb [arch=amd64] https://download.docker.com/linux/debian buster stable" > /etc/apt/sources.list.d/docker.list && \
curl -s -L https://nvidia.github.io/nvidia-docker/gpgkey| apt-key add - && \
curl -s -L https://nvidia.github.io/nvidia-docker/$ID$VERSION_ID/nvidia-docker.list > /etc/apt/sources.list.d/nvidia-docker.list && \
apt-get update && \
apt-get install -y docker-ce docker-ce-cli containerd.io nvidia-docker2
# Install packages
pip install -r triton/runner/requirements.txt
# Evaluate Runner
python3 -m "triton.large.runner.__main__" \
--config-path "triton/large/runner/config_NVIDIA-DGX-1-(1x-V100-32GB).yaml" \
--device 0 |