filename
stringlengths 7
140
| content
stringlengths 0
76.7M
|
---|---|
code/numerical_analysis/integral/src/integral_rectangle.c | #include <stdio.h>
/*Part of Cosmos by OpenGenus Foundation*/
double
integral_rectangle(double a, double b, double step, double (*f)(double))
{
double fragment = (b - a) / step;
double res = step * (f(a) + f(b)) / 6.0;
int i;
for (i = 1; i <= fragment; ++i)
res += 4.0 / 6.0 * step * f(a + step * (i - 0.5));
for (i = 1; i <= fragment - 1; ++i)
res += 2.0 / 6.0 * step * f(a + step * i);
return (res);
}
int
main(void)
{
double a, b, step, res;
printf("Enter interval: \n");
printf("a: \n");
scanf("%lf", &a);
printf("b: \n");
scanf("%lf", &b);
printf("Enter integration step: ");
scanf("%lf", &step);
res = integral_rectangle(a, b, step,
({
double f(double x)
{
return x * x + x;
}
f;}));
printf("Result = %lf \n", res);
return (0);
}
|
code/numerical_analysis/integral/src/integral_rectangle.cpp | #include <iostream>
#include <functional>
//Part of Cosmos by OpenGenus Foundation
double integralRectangle(double a, double b, double step, std::function<double(double)> f)
{
double fragment, res;
fragment = (b - a) / step;
res = step * (f(a) + f(b)) / 6.0;
for (int i = 1; i <= fragment; ++i)
res = res + 4.0 / 6.0 * step * f(a + step * (i - 0.5));
for (int i = 1; i <= fragment - 1; ++i)
res = res + 2.0 / 6.0 * step * f(a + step * i);
return res;
}
int main()
{
double a, b, step, res;
std::cout << "Enter interval: " << std::endl;
std::cout << "a: " << std::endl;
std::cin >> a;
std::cout << "b: " << std::endl;
std::cin >> b;
std::cout << "Enter integration step: " << std::endl;
std::cin >> step;
res = integralRectangle(a, b, step, [](double x) -> double
{
return x;
});
std::cout << "Result: " << res << std::endl;
return 0;
}
|
code/numerical_analysis/integral/src/integral_rectangle.java | package cosmos;
//Part of Cosmos by OpenGenus Foundation
public class IntegralRectangle {
public static void main(String[] args) {
double a = 0.0;
double b = 1.0;
int step = 1000;
IntegralFunction integralFunction = (x) -> x * x * x; // a∫b x^3 dx
double sum = calculateIntegral(a, b, step, integralFunction);
System.out.println("Approximate integral value = " + sum); // exact answer = 0.25
}
public static double calculateIntegral(
double a,
double b,
int numOfRectangles,
IntegralFunction function) {
double widthOfRectangle = (b - a) / numOfRectangles; // Compute width
double sum = 0.0; // Clear running sum
for(int i = 1; i <= numOfRectangles; i++) {
double xOfRectangle = a + (i - 1) * widthOfRectangle; // Use xOfRectangle to simplify formula...
double heightOfRectangle = function.valueAt(xOfRectangle); // Height of rectangle is value of function at that point
sum += (widthOfRectangle * heightOfRectangle);
}
return sum;
}
public interface IntegralFunction {
public double valueAt(double value);
}
}
|
code/numerical_analysis/integral/src/integral_rectangle.py | # Part of Cosmos by OpenGenus Foundation
def integral_rectangle(a, b, step, func):
fragment = (b - a) / step
res = step * (func(a) + func(b)) / 6.0
i = 1
while i <= fragment:
res += 4.0 / 6.0 * step * func(a + step * (i - 0.5))
i += 1
i = 1
while i <= fragment - 1:
res += 2.0 / 6.0 * step * func(a + step * i)
i += 1
return res
print("Enter interval: ")
start = float(input("a: "))
finish = float(input("b: "))
move = float(input("Enter integration step: "))
result = integral_rectangle(start, finish, move, lambda x: x ** 2 + x)
print("Result: ", result)
|
code/numerical_analysis/integral/src/integral_trapezoid.c | #include <stdio.h>
/*Part of Cosmos by OpenGenus Foundation*/
double
integral_trapezoid(double a, double b, double step, double (*f)(double))
{
double fragment = (b - a) / step;
double res = step * (f(a) + f(b)) / 2.0;
int i;
for (i = 1; i <= fragment - 1; ++i)
res = res + step * f(a + step * i);
return (res);
}
int
main(void)
{
double a, b, step, res;
printf("Enter interval: \n");
printf("a: \n");
scanf("%lf", &a);
printf("b: \n");
scanf("%lf", &b);
printf("Enter integration step: ");
scanf("%lf", &step);
res = integral_trapezoid(a, b, step,
({
double f(double x)
{
return x * x + x;
}
f;}));
printf("Result = %lf \n", res);
return (0);
}
|
code/numerical_analysis/integral/src/integral_trapezoid.cpp | #include <iostream>
#include <functional>
//Part of Cosmos by OpenGenus Foundation
double integralTrapezoid(double a, double b, double step, std::function<double(double)> f)
{
double fragment, res;
fragment = (b - a) / step;
res = step * (f(a) + f(b)) / 2.0;
for (int i = 1; i <= fragment - 1; ++i)
res = res + step * f(a + step * i);
return res;
}
int main()
{
double a, b, step, res;
std::cout << "Enter interval: " << std::endl;
std::cout << "a: " << std::endl;
std::cin >> a;
std::cout << "b: " << std::endl;
std::cin >> b;
std::cout << "Enter integration step: " << std::endl;
std::cin >> step;
res = integralTrapezoid(a, b, step, [](double x) -> double
{
return x;
});
std::cout << "Result: " << res << std::endl;
return 0;
}
|
code/numerical_analysis/integral/src/integral_trapezoid.java | package cosmos;
public class IntegralTrapezoidal {
public static void main(String[] args) {
double a = 0.0;
double b = 1.0;
int step = 1000;
IntegralFunction integralFunction = (x) -> x * x * x; // a∫b x^3 dx
double sum = calculateIntegral(a, b, step, integralFunction);
System.out.println("Approximate integral value = " + sum); // exact answer = 0.25
}
public static double calculateIntegral(
double a,
double b,
int numofTrapezoids,
IntegralFunction function) {
double h = (b - a) / numofTrapezoids; // Compute width
double area = (function.valueAt(a) + function.valueAt(b)) / 2.0;
for(int i = 1; i < numofTrapezoids; i++) {
double y = a + i * h;
area += function.valueAt(y);
}
area *= h;
return area;
}
public interface IntegralFunction {
public double valueAt(double value);
}
}
|
code/numerical_analysis/integral/src/integral_trapezoid.py | # Part of Cosmos by OpenGenus Foundation
def integral_trapezoid(a, b, step, func):
fragment = (b - a) / step
res = step * (func(a) + func(b)) / 2.0
i = 1
while i <= fragment - 1:
res = res + step * func(a + step * i)
i += 1
return res
print("Enter interval: ")
start = float(input("a: "))
finish = float(input("b: "))
move = float(input("Enter integration step: "))
result = integral_trapezoid(start, finish, move, lambda x: x ** 2 + x)
print("Result: ", result)
|
code/numerical_analysis/iteration/src/Iteration Method.cpp | #include<bits/stdc++.h>
using namespace std;
/*
Program to Find Root of the equation x*x*x+x-5=0 by Iteration Method
By simplified this equation we got eq=pow(5-x,(1/3)) then differentiate eq we got (-1/3*pow(5-x,(2/3)))
then we checked if we put natural number in x, will it lower than 1 for all natural number? if no we can't
apply iteration method else we can apply iteration method
*/
int main()
{
float x=1;
float eq=pow(5-x,(1/3));
float diff=(-1/3*pow(5-x,(2/3)));
int flag=0;
cout<<" Here us The Equation : \n";
cout<<" x*x*x+x-5=0 \n\n\n";
while(x<100)
{
if((-1/3*pow(5-x,(2/3)))>1)
{
flag=1;
break;
}
x++;
}
if(flag==1)
{
cout<<"Iteration Method Not Applicable in This Equation\n";
}
else
{
x=1;
for(int i=1;i<=10;i++)
{
x=sqrt(1/x+1);
cout<<"Step Taken "<<i<<" X="<<x<<endl;
}
}
return 0;
}
|
code/numerical_analysis/monte_carlo/src/integral_monte_carlo.cpp | #include <iostream>
#include <functional>
#include <random>
//Part of Cosmos by OpenGenus Foundation
double integralMonteCarlo(double a, double b, double pointCount, std::function<double(double)> f)
{
double res, randX, randY;
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_real_distribution<> dis;
for (int i = 0; i < pointCount; ++i)
{
randX = ((b - a) * dis(gen)) + a;
randY += f(randX);
}
res = (b - a) * (randY / pointCount);
return res;
}
int main()
{
double a, b, pointCount, res;
std::cout << "Enter interval: " << std::endl;
std::cout << "a: " << std::endl;
std::cin >> a;
std::cout << "b: " << std::endl;
std::cin >> b;
std::cout << "Enter point count: " << std::endl;
std::cin >> pointCount;
res = integralMonteCarlo(a, b, pointCount, [](double x) -> double
{
return x * x + x;
});
std::cout << "Result: " << res << std::endl;
return 0;
}
|
code/numerical_analysis/monte_carlo/src/integral_montecarlo.c | #include <stdio.h>
#include <stdlib.h>
/*Part of Cosmos by OpenGenus Foundation*/
double
integral_monte_carlo(double a, double b, double point_count, double (*f)(double))
{
double x, y = 0;
int i;
for (i = 0; i < point_count; ++i){
x = ((b - a) * ((double)random() / RAND_MAX)) + a;
y += f(x);
}
return ((b - a) * (y / point_count));
}
int
main(void)
{
double a, b, point_count, res;
printf("Enter interval: \n");
printf("a: \n");
scanf("%lf", &a);
printf("b: \n");
scanf("%lf", &b);
printf("Enter point count: ");
scanf("%lf", &point_count);
res = integral_monte_carlo(a, b, point_count, ({
double f(double x)
{
return (x * x + x);
}
f;}));
printf("Result = %lf \n", res);
return (0);
}
|
code/numerical_analysis/monte_carlo/src/integral_montecarlo.py | import random
# Part of Cosmos by OpenGenus Foundation
def integral_monte_carlo(a, b, point_count, func):
rand_y = 0.0
i = 0
while i < point_count:
rand_x = (b - a) * random.random() + a
rand_y += func(rand_x)
i += 1
res = (b - a) * (rand_y / point_count)
return res
print("Enter interval: ")
start = float(input("a: "))
finish = float(input("b: "))
count = float(input("Enter point count: "))
result = integral_monte_carlo(start, finish, count, lambda x: x ** 2 + x)
print("Result: ", result)
|
code/numerical_analysis/monte_carlo/src/pi_monte_carlo.cpp | #include <iostream>
#include <random>
//Part of Cosmos by OpenGenus Foundation
double piMonteCarlo(double pointCount)
{
double circlePoints = 0;
double pi, x, y;
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_real_distribution<> dis;
for (int i = 0; i < pointCount; ++i)
{
x = dis(gen);
y = dis(gen);
if (x * x + y * y < 1)
circlePoints++;
}
pi = (circlePoints / pointCount) * 4;
return pi;
}
int main()
{
double pointCount, pi;
std::cout << "Enter point count: " << std::endl;
std::cin >> pointCount;
pi = piMonteCarlo(pointCount);
std::cout << "Pi = " << pi << std::endl;
return 0;
}
|
code/numerical_analysis/monte_carlo/src/pi_montecarlo.c | #include <stdio.h>
#include <stdlib.h>
/*Part of Cosmos by OpenGenus Foundation*/
double
pi_monte_carlo(double point_count)
{
double x, y, circle_points = 0;
int i;
for (i = 0; i < point_count; ++i){
x = (double)random() / RAND_MAX;
y = (double)random() / RAND_MAX;
if (x * x + y * y <= 1)
++circle_points;
}
return ((circle_points / point_count) * 4);
}
int
main(void)
{
double point_count = 0;
printf("Enter point count: ");
scanf("%lf", &point_count);
printf("Pi = %lf \n", pi_monte_carlo(point_count));
return (0);
}
|
code/numerical_analysis/monte_carlo/src/pi_montecarlo.py | import random
# Part of Cosmos by OpenGenus Foundation
def pi_monte_carlo(point_count):
circle_points = 0.0
i = 0
while i < point_count:
x = random.random()
y = random.random()
if x * x + y * y < 1:
circle_points += 1
i += 1
pi = (circle_points / point_count) * 4
return pi
count = float(input("Enter point count: "))
res = pi_monte_carlo(count)
print("Pi = ", res)
|
code/numerical_analysis/newton_rapson/src/Newton Rapson Method.cpp | #include<bits/stdc++.h>
using namespace std;
/*
Program to Find Root of the equation x*x*x-18=0 by Newton Rapson Method
First we take 2 values from function of the Equation (negative/positive) then place it in x and y
then we Differentiate This equation;
then apply formula
*/
double func(double x)
{
return x*x*x-18;
}
int main()
{
int i;
float a,b;
cout<<"Here is The Equation : \n\n x*x*x-18=0 \n\n";
cout<<"Enter Right Value for A and B : \n";
cin>>a>>b;
if(func(a)<0 && func(b)>0)
{
for(i=1; i<=10; i++)
{
float equation = ((a*a*a)-18);
float differentiate = (3*(a*a));
a-=equation/differentiate;
cout<<"Steps Taken "<<i<<" X="<<a<<endl;
}
}
else
cout<<"You have not Assigned right A and B\n";
return 0;
}
|
code/numerical_analysis/polynomial_interpolations/src/lagrange_interpolation.py | """
This program provides functionalities to carry out polynomial
interpolation using Lagrange's polynomials.
Reference: https://en.wikipedia.org/wiki/Lagrange_polynomial.
"""
class Lagrange:
"""
This class implements interpolation using Lagrange's polynomial,
For a given set of points (xj, yj) with no two xj values equal,
the Lagrange polynomial is the polynomial of lowest degree that
assumes at each value xj the corresponding value yj, so that the
functions coincide at each point.
>>> lagrange = Lagrange()
>>> lagrange.fit([1, 2, 3], [7, 8, 9])
>>> lagrange.interpolate(4)
10.0
>>> lagrange.interpolate(-2)
4.0
"""
def __init__(self):
self.build_str = ""
def fit(self, x_points: list, y_points: list) -> None:
"""
Set up a the appropriate polynomial string for x_points, y_points
values.
Arguments:
x_points, y_points: A list of x and corresponding y points
through which the polynomial passes.
"""
k = len(x_points)
if k != len(y_points):
raise Exception("x_points and y_points must be of the same length")
build_str = ""
for j in range(k):
build_l = ""
el = "((x - {xm}) / ({xj} - {xm}))"
for m in range(k):
if j != m:
build_l += el.format(xm=x_points[m], xj=x_points[j]) + " * "
build_l = build_l[:-2]
build_str += str(y_points[j]) + "*" + build_l + " + "
self.build_str = build_str[:-2]
def interpolate(self, x: float) -> float:
"""
Interpolate the value of the polynomial at x for the fitted values.
Returns the interpolated value.
"""
ev = self.build_str.replace("x", str(x))
return eval(ev)
if __name__ == "__main__":
import doctest
doctest.testmod()
|
code/numerical_analysis/polynomial_interpolations/src/nevilles_method.py | """
Python program to show how to interpolate and evaluate a polynomial
using Neville's method.
Neville’s method evaluates a polynomial that passes through a
given set of x and y points for a particular x value (x0) using the
Newton polynomial form.
Reference:
https://rpubs.com/aaronsc32/nevilles-method-polynomial-interpolation
"""
def neville_interpolate(x_points: list, y_points: list, x0: int) -> list:
"""
Interpolate and evaluate a polynomial using Neville's method.
Arguments:
x_points, y_points: Iterables of x and corresponding y points through
which the polynomial passes.
x0: The value of x to evaluate the polynomial for.
Return Value: A list of the approximated value and the Neville iterations
table respectively.
>>> import pprint
>>> neville_interpolate((1,2,3,4,6), (6,7,8,9,11), 5)[0]
10.0
>>> pprint.pprint(neville_interpolate((1,2,3,4,6), (6,7,8,9,11), 99)[1])
[[0, 6, 0, 0, 0],
[0, 7, 0, 0, 0],
[0, 8, 104.0, 0, 0],
[0, 9, 104.0, 104.0, 0],
[0, 11, 104.0, 104.0, 104.0]]
>>> neville_interpolate((1,2,3,4,6), (6,7,8,9,11), 99)[0]
104.0
>>> neville_interpolate((1,2,3,4,6), (6,7,8,9,11), '')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/mattwisdom/thealgo/nevilles_method.py", line 35, in neville_interpo\
late
for i in range(2, n):
TypeError: unsupported operand type(s) for -: 'str' and 'int'
"""
n = len(x_points)
q = [[0] * n for i in range(n)]
for i in range(n):
q[i][1] = y_points[i]
for i in range(2, n):
for j in range(i, n):
q[j][i] = (
(x0 - x_points[j - i + 1]) * q[j][i - 1]
- (x0 - x_points[j]) * q[j - 1][i - 1]
) / (x_points[j] - x_points[j - i + 1])
return [q[n - 1][n - 1], q]
if __name__ == "__main__":
import doctest
doctest.testmod()
|
code/numerical_analysis/runge_kutt/src/rk4.c | #include <stdio.h>
#include <stdlib.h>
#include <math.h>
/*Part of Cosmos by OpenGenus Foundation*/
struct xy{
double x;
double y;
};
struct xy *
runge_kutt(struct xy start_conditions,
double start_interval,
double finish_interval,
double step,
int *size_array,
double (*f)(double, double))
{
double k1, k2, k3, k4;
int number_steps = (finish_interval - start_interval) / step;
*size_array = number_steps + 1;
struct xy *res = malloc(sizeof(struct xy) * (*size_array));
res[0] = start_conditions;
int i;
for (i = 1; i < (*size_array); ++i){
k1 = step * f(start_conditions.x, start_conditions.y);
k2 = step * f(start_conditions.x + step / 2.0, start_conditions.y + k1 / 2.0);
k3 = step * f(start_conditions.x + step / 2.0, start_conditions.y + k2 / 2.0);
k4 = step * f(start_conditions.x + step, start_conditions.y + k3);
start_conditions.y += (k1 + 2.0 * k2 + 2.0 * k3 + k4) / 6.0;
start_conditions.x += step;
res[i] = start_conditions;
}
return (res);
}
int
main(void)
{
struct xy start_conditions;
double start_interval, finish_interval, step;
int size_array = 0;
printf("Enter start conditions: ");
printf("X = ");
scanf("%lf", &start_conditions.x);
printf("Y = ");
scanf("%lf", &start_conditions.y);
printf("Enter interval: ");
printf("Start = ");
scanf("%lf", &start_interval);
printf("Finish = ");
scanf("%lf", &finish_interval);
printf("Enter step: ");
scanf("%lf", &step);
struct xy *res = runge_kutt(start_conditions,
start_interval,
finish_interval,
step,
&size_array,
({
double f(double x, double y)
{
return (pow(x, 2) * (3 * y + exp(pow(x, 3))));
}
f;}));
int i;
for (i = 0; i < size_array; ++i){
printf("X = %lf Y = %lf \n", res[i].x, res[i].y);
}
return (0);
}
|
code/numerical_analysis/runge_kutt/src/rk4.cpp | #include <iostream>
#include <vector>
#include <functional>
#include <cmath>
using xy = std::pair<double, double>;
std::vector<xy> rungeKutt(xy startConditions,
double startInterval,
double finishInterval,
double step,
std::function<double(double, double)> f)
{
std::vector<xy> res;
unsigned int numberSteps = std::ceil((finishInterval - startInterval) / step);
double k1, k2, k3, k4;
res.reserve(numberSteps + 1);
res.push_back(startConditions);
for (unsigned int i = 0; i < numberSteps; ++i)
{
k1 = step * f(startConditions.first, startConditions.second);
k2 = step * f(startConditions.first + step / 2.0, startConditions.second + k1 / 2.0);
k3 = step * f(startConditions.first + step / 2.0, startConditions.second + k2 / 2.0);
k4 = step * f(startConditions.first + step, startConditions.second + k3 );
startConditions.second += (k1 + 2.0 * k2 + 2.0 * k3 + k4) / 6.0;
startConditions.first += step;
res.push_back(startConditions);
}
return res;
}
int main()
{
xy startConditions;
double startInterval, finishInterval, step;
std::vector<xy> res;
std::cout << "Enter start condition:" << "\n";
std::cout << "X = " << std::endl;
std::cin >> startConditions.first;
std::cout << "Y = " << std::endl;
std::cin >> startConditions.second;
std::cout << "Enter interval:" << "\n";
std::cout << "Start = " << std::endl;
std::cin >> startInterval;
std::cout << "Finish = " << std::endl;
std::cin >> finishInterval;
std::cout << "Enter step: " << std::endl;
std::cin >> step;
auto func = [](double x, double y) -> double
{
return std::pow(x, 2) * (3 * y + std::exp(std::pow(x, 3)));
};
res = rungeKutt(startConditions, startInterval, finishInterval, step, func);
for (auto it = res.begin(); it != res.end(); ++it)
std::cout << "X = " << it->first << " Y = " << it->second << std::endl;
return 0;
}
|
code/numerical_analysis/runge_kutt/src/rk4.py | import math
def runge_kutt(start_conditions, finish_interval, step, f):
res = []
res_x = start_conditions[0]
res_y = start_conditions[1]
while res_x < finish_interval:
res.append((res_x, res_y))
k1 = step * f(res_x, res_y)
k2 = step * f(res_x + step / 2.0, res_y + k1 / 2.0)
k3 = step * f(res_x + step / 2.0, res_y + k2 / 2.0)
k4 = step * f(res_x + step, res_y + k3)
res_y += (k1 + 2.0 * k2 + 2.0 * k3 + k4) / 6.0
res_x += step
return res
print("Enter start condition: ")
x0 = float(input("X= "))
y0 = float(input("Y= "))
st_cond = [x0, y0]
fin_int = float(input("Enter finish interval: "))
stp = float(input("Enter step: "))
res = runge_kutt(
st_cond,
fin_int,
stp,
lambda x, y: math.pow(x, 2) * (3 * y + math.exp(math.pow(x, 3))),
)
for result_x, result_y in res:
print("X= %8.6f, Y= %8.6f" % (result_x, result_y))
|
code/online_challenges/src/README.md | # Cosmos Programming Challenge Solutions
> Your personal library of every algorithm and data structure code that you will ever encounter
This folder contains solutions to various programming problems from a variety of websites, such as [Codechef](https://www.codechef.com/), [Project Euler](https://projecteuler.net/), [HackerRank](https://www.hackerrank.com/),[Codeforces](https://codeforces.com/) and [LeetCode](https://leetcode.com/). The problem solutions are implemented in a myriad of languages.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/online_challenges/src/codechef/AMSGAME1/AMSGAME1.c | #include <stdio.h>
long long int gcd(long long int a, long long int b)
{
if (b == 0)
{
return a;
}
return gcd(b, a % b);
}
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
long long int n;
scanf("%lld", & n);
long long int a;
scanf("%lld", & a);
if (n == 1)
{
printf("%lld\n", a);
continue;
}
while (--n)
{
long long int b;
scanf("%lld", & b);
a = a < b ? gcd(a, b) : gcd(b, a);
}
printf("%lld\n", a);
}
return 0;
}
|
code/online_challenges/src/codechef/AMSGAME1/README.md | # Problem Link:
[AMSGAME1](https://www.codechef.com/problems/AMSGAME1)
# Description
T test cases. The first line of each test case contains a single integer N, the length of the sequence. The second line contains N positive integers, each separated by a single space. |
code/online_challenges/src/codechef/BACREP/BACREP.cpp | #include <iostream>
using namespace std;
#define ll long long
#define dd double
#define endl "\n"
#define pb push_back
#define all(v) v.begin(),v.end()
#define mp make_pair
#define fi first
#define se second
#define fo(i,n) for(int i=0;i<n;i++)
#define fo1(i,n) for(int i=1;i<=n;i++)
ll mod=1000000007;
const ll N=500050;
bool vis[N];
vector <int> adj[N];
ll depth[N]={0};
vector <int> atd[N];
ll maxdepth=1;
ll par[N]={0};
ll po(ll k ,ll n,ll ans,ll temp,ll ans1)
{
if(n==0)return ans;
while(temp<=n)
{
ans*=ans1;ans%=mod;ans1=ans1*ans1;ans1%=mod;n=n-temp;temp*=2;
}
return po(k,n,ans,1,k)%mod;
//eg. po(2,78,1,1,2);
}
void bfs(int root)
{
vis[root]=1;
depth[root]=1;
atd[1].pb(root);
queue <int> q;
q.push(root);
while(!q.empty())
{
ll a=q.front();
// vis[a]=1;
q.pop();
for(int i:adj[a])
{
if(vis[i])
continue;
vis[i]=1;
depth[i]=depth[a]+1;
atd[depth[i]].pb(i);
par[i]=a;
maxdepth=depth[i];
q.push(i);
}
}
}
ll min(ll a,ll b)
{
if(a>b)return b;else return a;
}
ll max(ll a,ll b)
{
if(a>b)return a;else return b;
}
int main()
{
ios_base::sync_with_stdio(0);
cin.tie(0); cout.tie(0);
ll t;
//cin>>t;
t=1;
while(t--)
{
ll n,q;
cin>>n>>q;
fo(i,n-1)
{
ll a,b;
cin>>a>>b;
adj[a].pb(b);
adj[b].pb(a);
}
bfs(1);
ll store[n+1]={0};
for(int i=1;i<=n;i++)
{
ll c;
cin>>c;
store[i]=c;
}
while(q--)
{
for(int i=maxdepth;i>=2;i--)
{
for(int j:atd[i])
{
if(adj[j].size()!=1)
store[j]=0;
store[j]+=store[par[j]];
}
}
store[1]=0;
char ch;
cin>>ch;
if(ch=='?')
{
ll a;
cin>>a;
cout<<store[a]<<'\n';
}
else
{
ll a,b;
cin>>a>>b;
store[a]+=b;
}
}
}
return 0;
}
|
code/online_challenges/src/codechef/BACREP/README.md | # Problem Link
[BACREP](https://www.codechef.com/OCT19A/problems/BACREP)
# Description
Chef has a rooted tree with N vertices (numbered 1 through N); vertex 1 is the root of the tree. Initially, there are some bacteria in its vertices. Let's denote the number of sons of vertex v by sv; a leaf is a vertex without sons. During each second, the following events happen:
For each non-leaf vertex v, if there are x bacteria in this vertex at the start of this second, they divide into sv⋅x bacteria. At the end of this second, x of these bacteria instantly move to each of its sons (none of them stay in vertex v). The original x bacteria do not exist any more.
Zero or more bacteria appear in one vertex. These bacteria do not divide or move at this second.
Initially, we are at the start of second 0. You should process Q queries ― one query during each of the seconds 0 through Q−1. There are two types of queries:
+ v k: During this second, k bacteria appear in vertex v.
? v: Find the number of bacteria in vertex v at the end of this second ― after the divided bacteria have moved. |
code/online_challenges/src/codechef/BRKBKS/Brkbks.java | import java.io.*;
import java.util.*;
class Brkbks {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
int t = s.nextInt();
while (t-- > 0) {
int strength = s.nextInt();
int w1 = s.nextInt();
int w2 = s.nextInt();
int w3 = s.nextInt();
ArrayList<Integer> arrLL = new ArrayList<>();
arrLL.add(w1);
arrLL.add(w2);
arrLL.add(w3);
int hits = 0;
while (arrLL.size() != 0) {
int sum = 0;
// Calculating the sum of widths of bricks
while (sum < strength) {
sum += arrLL.get(0);
if (sum <= strength) {
arrLL.remove(0);
}
if (arrLL.size() == 0) {
break;
}
}
// Hits required by Ada to break the given stack of bricks
hits++;
}
System.out.println(hits);
}
}
}
/*
TEST CASE
INPUT
3
3 1 2 2
2 1 1 1
3 2 2 1
OUTPUT
2
2
2
*/
|
code/online_challenges/src/codechef/BRKBKS/README.md | # Problem Link:
[BRKBKS](https://www.codechef.com/JAN20B/problems/BRKBKS/)
# Description
Ada's strength is SS. Whenever she hits a stack of bricks, consider the largest k≥0k≥0 such that the sum of widths of the topmost kk bricks does not exceed SS; the topmost kk bricks break and are removed from the stack. Before each hit, Ada may also decide to reverse the current stack of bricks, with no cost.
Find the minimum number of hits Ada needs in order to break all bricks if she performs the reversals optimally. You are not required to minimize the number of reversals. |
code/online_challenges/src/codechef/CARVANS/CARVANS.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
int n;
scanf("%d", & n);
int arr[n], i;
for (i = 0; i < n; ++i)
{
scanf("%d", & arr[i]);
}
int top, c = 0;
top = arr[0];
for (i = 0; i < n; ++i)
{
if (top >= arr[i])
{
c++;
top = arr[i];
}
}
printf("%d\n", c);
}
return 0;
}
|
code/online_challenges/src/codechef/CARVANS/README.md | # Problem Link:
[CARVANS](https://www.codechef.com/problems/CARVANS)
# Description
Most problems on CodeChef highlight chef's love for food and cooking but little is known about his love for racing sports. He is an avid Formula 1 fan. He went to watch this year's Indian Grand Prix at New Delhi. He noticed that one segment of the circuit was a long straight road. It was impossible for a car to overtake other cars on this segment. Therefore, a car had to lower down its speed if there was a slower car in front of it. While watching the race, Chef started to wonder how many cars were moving at their maximum speed.
Formally, you're given the maximum speed of N cars in the order they entered the long straight segment of the circuit. Each car prefers to move at its maximum speed. If that's not possible because of the front car being slow, it might have to lower its speed. It still moves at the fastest possible speed while avoiding any collisions. For the purpose of this problem, you can assume that the straight segment is infinitely long.
Count the number of cars which were moving at their maximum speed on the straight segment. |
code/online_challenges/src/codechef/CASH/HardCash.java | import java.util.Scanner;
class Hardcash {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
int t = s.nextInt();
while (t-- > 0) {
int n = s.nextInt();
int k = s.nextInt();
int[] arr = new int[n];
//input
for (int i = 0; i < n; i++) {
arr[i] = s.nextInt();
}
int bag = 0;
for (int i = 0; i < n; i++) {
if (arr[i] % k == 0) {
continue;
} else {
int step = arr[i] % k;
if (k - step <= bag) {
bag -= (k - step);
} else {
bag += step;
}
}
}
System.out.println(bag);
}
}
}
/*
TEST CASE
2
5 7
1 14 4 41 1
3 9
1 10 19
OUTPUT
5
3
*/
/*
TEST CASE
INPUT
2
600000
250000
OUTPUT
577500
250000
*/
|
code/online_challenges/src/codechef/CASH/README.md | # Problem Link:
[CASH](https://www.codechef.com/FEB20B/problems/CASH/)
# Description
There are N bags with coins in a row (numbered 1 through N); for each valid i, the i - th bag contains A(i) coins. Chef should make the number of coins in each bag divisible by a given integer K in the following way:
- choose an integer c between 0 and N (inclusive)
- take some coins from the first c bags ― formally, for each i (1 ≤ i ≤ c), he may choose any number of coins between 0 and A(i) inclusive and take them out of the i-th bag
- move some of these coins to some of the last N−c bags ― formally, for each i ( c+1 ≤ i ≤ N), he may place a non-negative number of coins in the i-th bag
The number of coins placed in the last N−c bags must not exceed the number of coins taken out from the first c bags, but there may be some coins left over. Let's denote the number of these coins by RR. You should find the smallest possible value of RR. |
code/online_challenges/src/codechef/CASH/hard_cash.cpp | #include <iostream>
int main() {
int t;
std::cin >> t;
while (t--) {
int n, k, x, sum = 0;
std::cin >> n >> k;
for (int i = 0; i < n; ++i) {
std::cin >> x;
sum += x;
}
sum %= k;
std::cout << sum << "\n";
}
}
|
code/online_challenges/src/codechef/CHDIGER/CHDIGER.cpp | #include <iostream>
#include <algorithm>
#include <list>
#include <stack>
#include <string>
int main ()
{
int t;
std::cin >> t;
while (t--)
{
std::string n;
int d;
std::cin >> n >> d;
std::list<char>ans;
std::stack<char>st;
for (int i = 0; i < n.size(); ++i)
{
if (n[i] - '0' < d)
{
while (!st.empty() && st.top() > n[i])
st.pop();
st.push(n[i]);
}
}
while (!st.empty())
{
ans.push_front(st.top());
st.pop();
}
int sz = n.size() - ans.size();
for (int i = 0; i < sz; ++i)
{
ans.push_back(char(d + '0'));
}
for (auto i : ans)
{
std::cout << i;
}
std::cout << "\n";
}
return 0;
}
|
code/online_challenges/src/codechef/CHDIGER/README.md | # Problem Link:
[CHDIGER](https://www.codechef.com/MARCH19B/problems/CHDIGER)
# Description
Chef Leonardo has a decimal integer N and a non-zero decimal digit d. N does not contain the digit zero; specifically, N should always be treated as a decimal integer without leading zeroes.
Chef likes d and does not like any other digit, so he decided to change N. He may apply the following operation any number of times (including zero): append the digit d to the decimal representation of N (d becomes the least significant digit of N), then remove one occurrence of one digit from the decimal representation of N.
Chef has already changed a lot of numbers and he got bored. Now, he wants to know the smallest possible value of N
which can be obtained by performing the operation described above. Can you help him?
|
code/online_challenges/src/codechef/CHEALG/CHEALG.java | // Part of Cosmos by OpenGenus
import java.util.*;
import java.lang.*;
import java.io.*;
class chealg
{
public static void main (String[] args) throws java.lang.Exception
{Scanner sc=new Scanner(System.in);
int T=sc.nextInt();
while(T>0)
{ String s=sc.next();
String str="";
int l=s.length();
int count=1;
for(int i=0;i<l;i++)
{ char c=s.charAt(i);
if(l==1)
str+=c+"1";
else if(i!=l-1)
{
char a=s.charAt(i+1);
if(a==c)
count++;
else
{ str+=c+String.valueOf(count);
count=1;
}
}
else
{
char b=s.charAt(i-1);
if(b==c)
str+=c+String.valueOf(count);
else
{ str+=c+"1";
count=1;
}
}
}
if(str.length()>=l)
System.out.println("NO");
else
System.out.println("YES");
T--;
}
}
}
|
code/online_challenges/src/codechef/CHEALG/README.md | Problem Statement:
One day, Saeed was teaching a string compression algorithm.
This algorithm finds all maximal substrings which contains only one character repeated one or more times (a substring is maximal if it we cannot add one character to its left or right without breaking this property) and replaces each such substring by the string "cK", where K is the length of the substring and c is the only character it contains.
For example, "aabaaa" is compressed to "a2b1a3".
Saeed wanted to check if the students understood the algorithm, so he wrote a string S on the board and asked the students if the algorithm is effective on S, i.e. if the string created by compressing S is strictly shorter than S.
Help them answer this question.
|
code/online_challenges/src/codechef/CHEFING/CHEFING.cpp | #include<bits/stdc++.h>
using namespace std;
int main()
{
int t,n,cnt,i,j;
string s;
cin >> t;
while(t--)
{
cnt = 0;
cin >> n;
int hash[n][26] = {0};
for(i = 0 ; i < n ; i++)
{
cin >> s;
for(j = 0 ; j < s.length() ; j++)
{
hash[i][s[j]-97]++;
}
}
for(i = 0 ; i < 26 ; i++)
{
for(j = 0 ; j < n ; j++)
{
if(hash[j][i] == 0)
{
cnt++;
break;
}
}
}
cout << 26 - cnt << "\n";
}
return 0;
} |
code/online_challenges/src/codechef/CHEFING/README.md | # Problem Link:
[CHEFING](https://www.codechef.com/FEB19B/problems/CHEFING/)
# Description
Chef recently visited ShareChat Cafe and was highly impressed by the food. Being a food enthusiast, he decided to enquire about the ingredients of each dish.
There are N dishes represented by strings S1,S2,…,SN. Each ingredient used for making dishes in ShareChat Cafe is represented by a lowercase English letter. For each valid i, the ingredients used to make dish i correspond to characters in the string Si (note that ingredients may be used multiple times). A special ingredient is an ingredient which is present in each dish at least once.
Chef wants to know the number of special ingredients in ShareChat Cafe. Since Chef is too busy with work, can you help him?
|
code/online_challenges/src/codechef/CHFING/CHFING.cpp | #include<bits/stdc++.h>
using namespace std;
#define ll long long int
#define FLASH ios_base::sync_with_stdio(false);cin.tie(0);cout.tie(0)
#define mod 1000000007
int main()
{
int t;
long long k,n,ans,x,y,z,a;
cin >> t;
while(t--)
{
ans = x = y = z = a = 0;
cin >> n >> k;
k = k-1;
x = (k/(n-1));
y = (n%mod - 1%mod)%mod;
if(x%2 == 0)
{
a = x/2;
z = ((a%mod)*((x+1)%mod))%mod;
}
else
{
a = (x+1)/2;
z = ((a%mod)*(x%mod))%mod;
}
ans = ((y%mod)*(z%mod))%mod;
if(k % (n-1) != 0)
{
ans = ans + (k%(n-1))*(x+1);
}
cout << ans%mod << "\n";
}
return 0;
} |
code/online_challenges/src/codechef/CHFING/README.md | # Problem Link:
[CHFING](https://www.codechef.com/JUNE19A/problems/CHFING/)
# Description
Chef wants to make a feast. In order to do that, he needs a lot of different ingredients. Each ingredient has a certain tastiness; the tastiness of each ingredient may be any positive integer. Initially, for each tastiness between K and K+N−1 (inclusive), Chef has an infinite supply of ingredients with this tastiness.
The ingredients have a special property: any two of them can be mixed to create a new ingredient. If the original ingredients had tastiness x and y (possibly x=y), the new ingredient has tastiness x+y. The ingredients created this way may be used to mix other ingredients as well. Chef is free to mix ingredients in any way he chooses any number of times.
Let's call a tastiness v (v>0) unreachable if there is no way to obtain an ingredient with tastiness v; otherwise, tastiness v is reachable. Chef wants to make ingredients with all reachable values of tastiness and he would like to know the number of unreachable values. Help him solve this problem. Since the answer may be large, compute it modulo 1,000,000,007 (109+7).
Note that there is an infinite number of reachable values of tastiness, but it can be proven that the number of unreachable values is always finite for N≥2. |
code/online_challenges/src/codechef/CHNUM/CHNUM.cpp | #include <iostream>
#include <algorithm>
#include <list>
#include <stack>
#include <string>
int main ()
{
int t;
std::cin >> t;
while (t--)
{
int n;
std::cin >> n;
int a;
int cnt_ps = 0, cnt_nt = 0;
for (int i = 0; i < n; ++i)
{
std::cin >> a;
if (a < 0)
cnt_nt++;
else cnt_ps++;
}
if (cnt_nt == 0)
cnt_nt = cnt_ps;
else if (cnt_ps == 10)
cnt_ps = cnt_nt;
std::cout << std::max(cnt_ps, cnt_nt) << " " << std::min(cnt_ps, cnt_nt) << "\n";
}
return 0;
}
|
code/online_challenges/src/codechef/CHNUM/README.md | # Problem Link
[CHNUM](https://www.codechef.com/MARCH19B/problems/CHNUM)
# Description
Recently, Chef hosted a strange competition at the Byteland annual fair. There were N participants in the competition (numbered 1 through N); at the end of the competition, their scores were A1,A2,…,AN. Since it was a strange competition, negative scores were allowed too.
The total score of the competition is calculated as follows:
Divide the participants into one or more non-empty groups (subsets);
if there are K groups, let's denote them by G1,G2,…,GK. Each participant should be a member of exactly one group.
Sum up the scores of participants in each individual group. For each valid i, let's denote the sum of scores of participants in group i by Xi.
The total score of the competition is the sum of squares of these summed up scores, i.e. X1^2 + X2^2+…+XK^2.
Chef wants to choose the groups in such a way that the total score is maximum possible. Since there may be many ways to form the groups that lead to the maximum total score, Chef wants to know just the size of the largest group and the size of the smallest group which could be formed while still maximising the total score. These sizes are independent - formally, they are the maximum and minimum size sof a group such that there is a way to form groups which maximises the total score and contains a group with size s.
|
code/online_challenges/src/codechef/CLEANUP/CLEANUP.c | #include <stdio.h>
int main()
{
int t;
scanf("%d", & t);
while (t != 0)
{
int i;
int n, m;
scanf("%d%d", & n, & m);
int a[n];
for (i = 1; i <= n; ++i)
a[i] = 1;
for (i = 1; i <= m; ++i)
{
int o;
scanf("%d", & o);
a[o] = 0;
}
for (i = 1; i <= n; ++i)
{
if (a[i] != 0)
{
printf("%d ", i);
a[i] = 0;
while (a[i] == 0)
i++;
}
}
printf("\n");
for (i = 1; i <= n; ++i)
{
if (a[i] != 0)
{
printf("%d ", i);
}
}
t--;
printf("\n");
}
return 0;
}
|
code/online_challenges/src/codechef/CLEANUP/README.md | # Problem Link:
[CLEANUP](https://www.codechef.com/problems/CLEANUP/)
# Description
After a long and successful day of preparing food for the banquet, it is time to clean up. There is a list of n jobs to do before the kitchen can be closed for the night. These jobs are indexed from 1 to n.
Most of the cooks have already left and only the Chef and his assistant are left to clean up. Thankfully, some of the cooks took care of some of the jobs before they left so only a subset of the n jobs remain. The Chef and his assistant divide up the remaining jobs in the following manner. The Chef takes the unfinished job with least index, the assistant takes the unfinished job with the second least index, the Chef takes the unfinished job with the third least index, etc. That is, if the unfinished jobs were listed in increasing order of their index then the Chef would take every other one starting with the first job in the list and the assistant would take every other one starting with the second job on in the list.
The cooks logged which jobs they finished before they left. Unfortunately, these jobs were not recorded in any particular order. Given an unsorted list of finished jobs, you are to determine which jobs the Chef must complete and which jobs his assitant must complete before closing the kitchen for the evening. |
code/online_challenges/src/codechef/CNOTE/CNOTE.c | #include<stdio.h>
int main()
{
int t;
scanf("%d", & t);
while (t--)
{
int x, y, k, n;
scanf("%d%d%d%d", & x, & y, & k, & n);
int p[n], c[n], flag = 0, i;
for (i = 0; i < n; ++i)
{
scanf("%d%d", & p[i], & c[i]);
if (p[i] >= x - y && c[i] <= k)
{
++flag;
}
}
if (flag == 0)
{
printf("UnluckyChef\n");
} else
{
printf("LuckyChef\n");
}
}
}
|
code/online_challenges/src/codechef/CNOTE/README.md | # Problem Link:
[CNOTE](https://www.codechef.com/problems/CNOTE/)
# Description
Chef likes to write poetry. Today, he has decided to write a X pages long poetry, but unfortunately his notebook has only Y pages left in it. Thus he decided to buy a new CHEFMATE notebook and went to the stationary shop. Shopkeeper showed him some N notebooks, where the number of pages and price of the ith one are Pi pages and Ci rubles respectively. Chef has spent some money preparing for Ksen's birthday, and then he has only K rubles left for now.
Chef wants to buy a single notebook such that the price of the notebook should not exceed his budget and he is able to complete his poetry.
Help Chef accomplishing this task. You just need to tell him whether he can buy such a notebook or not. Note that Chef can use all of the Y pages in the current notebook, and Chef can buy only one notebook because Chef doesn't want to use many notebooks. |
code/online_challenges/src/codechef/COINS/COINS.py | a = {}
def dollar(n):
if n <= 11:
a[n] = n
return a[n]
if n in a:
return a[n]
a[n] = max(n, dollar(int(n / 2)) + dollar(int(n / 3)) + dollar(int(n / 4)))
return a[n]
while True:
try:
n = int(input())
except EOFError:
break
if n <= 11:
print(n)
else:
print(dollar(n))
|
code/online_challenges/src/codechef/COINS/README.md | # Problem Link:
[COINS] (https://www.codechef.com/problems/COINS)
# Description
In Byteland they have a very strange monetary system.
Each Bytelandian gold coin has an integer number written on it. A coin n can be exchanged in a bank into three coins: n/2, n/3 and n/4. But these numbers are all rounded down (the banks have to make a profit).
You can also sell Bytelandian coins for American dollars. The exchange rate is 1:1. But you can not buy Bytelandian coins.
You have one gold coin. What is the maximum amount of American dollars you can get for it?
Input
The input will contain several test cases (not more than 10). Each testcase is a single line with a number n, 0 <= n <= 1 000 000 000. It is the number written on your coin.
Output
For each test case output a single line, containing the maximum amount of American dollars you can make.
Example
Input:
12
2
Output:
13
2
You can change 12 into 6, 4 and 3, and then change these into 6+4+3=13. If you try changing the coin 2 into 3 smaller coins, you will get 1, 0 and 0, and later you can get no more than 1 out of them. It is better just to change the 2 coin directly into 2. |
code/online_challenges/src/codechef/COINS/coins.cpp | #include <iostream>
#include <unordered_map>
#define lli long long int
std::unordered_map<lli, lli> mpp;
lli check(lli n)
{
if (n == 0) {
mpp[0] = 0;
return 0;
}
if (mpp[n] != 0)
return mpp[n];
else {
mpp[n] = check(n / 2) + check(n / 3) + check(n / 4);
mpp[n] = std::max(n, mpp[n]);
return mpp[n];
}
}
int main()
{
for (lli n; std::cin >> n;) {
lli d = check(n);
std::cout<< std::max(n, d) << "\n";
}
return 0;
}
|
code/online_challenges/src/codechef/CONFLIP/CONFLIP.c | #include<stdio.h>
int main()
{
int t;
scanf("%d", & t);
while (t--)
{
int u;
scanf("%d", & u);
while (u--)
{
int i, n, q;
scanf("%d%d%d", & i, & n, & q);
if (i == q)
{
printf("%d\n", n / 2);
} else
{
printf("%d\n", n / 2 + n % 2);
}
}
}
}
|
code/online_challenges/src/codechef/CONFLIP/README.md | # Problem Link:
[CONFLIP](https://www.codechef.com/problems/CONFLIP/)
# Description
Little Elephant was fond of inventing new games. After a lot of research, Little Elephant came to know that most of the animals in the forest were showing less interest to play the multi-player games.Little Elephant had started to invent single player games, and succeeded in inventing the new single player game named COIN FLIP.
In this game the player will use N coins numbered from 1 to N, and all the coins will be facing in "Same direction" (Either Head or Tail),which will be decided by the player before starting of the game.
The player needs to play N rounds.In the k-th round the player will flip the face of the all coins whose number is less than or equal to k. That is, the face of coin i will be reversed, from Head to Tail, or, from Tail to Head, for i ≤ k.
Elephant needs to guess the total number of coins showing a particular face after playing N rounds. Elephant really becomes quite fond of this game COIN FLIP, so Elephant plays G times. Please help the Elephant to find out the answer. |
code/online_challenges/src/codechef/COVID19/COVID19.cpp | #include<bits/stdc++.h>
using namespace std;
int main()
{
int T;
cin>>T;
while(T--)
{
int n;
cin>>n;
std::vector<int> x(n);
for(int &i:x)
cin>>i;
int smallest=10,largest=0,infected=1;
for(int i=1;i<n;i++)
{
if(x[i]-x[i-1]<=2)
infected++;
else
{
largest=max(largest,infected);
smallest=min(smallest,infected);
infected=1;
}
}
if(infected!=0)
{
largest=max(largest,infected);
smallest=min(smallest,infected);
}
smallest=min(smallest,largest);
cout<<smallest<<" "<<largest<<"\n";
}
return 0;
}
|
code/online_challenges/src/codechef/COVID19/README.md | # Problem Link:
[COVID19](https://www.codechef.com/problems/COVID19)
# Description
There are N people on a street (numbered 1 through N). For simplicity, we'll view them as points on a line. For each valid i, the position of the i-th person is Xi.
It turns out that exactly one of these people is infected with the virus COVID-19, but we do not know which one. The virus will spread from an infected person to a non-infected person whenever the distance between them is at most 2. If we wait long enough, a specific set of people (depending on the person that was infected initially) will become infected; let's call the size of this set the final number of infected people.
Your task is to find the smallest and largest value of the final number of infected people, i.e. this number in the best and in the worst possible scenario.
|
code/online_challenges/src/codechef/DIVIDING/DIVIDING.c | #include <stdio.h>
int main(void)
{
long int n;
scanf("%ld", & n);
long int i;
long long int a[n];
long long int s = 0;
for (i = 0; i < n; ++i)
{
scanf("%lld", & a[i]);
s = s + a[i];
}
for (i = 1; i <= n; ++i)
s -= i;
if (s == 0)
printf("YES\n");
else
printf("NO\n");
return 0;
}
|
code/online_challenges/src/codechef/DIVIDING/README.md | # Problem Link:
[DIVIDING](https://www.codechef.com/problems/DIVIDING/)
# Description
Are you fond of collecting some kind of stuff? Mike is crazy about collecting stamps. He is an active member of Stamp Collecting Сommunity(SCC).
SCC consists of N members which are fond of philately. A few days ago Mike argued with the others from SCC. Mike told them that all stamps of the members could be divided in such a way that i'th member would get i postage stamps. Now Mike wants to know if he was right. The next SCC meeting is tomorrow. Mike still has no answer.
So, help Mike! There are N members in the SCC, i'th member has Ci stamps in his collection. Your task is to determine if it is possible to redistribute C1 + C2 + ... + Cn stamps among the members of SCC thus that i'th member would get i stamps.
|
code/online_challenges/src/codechef/EGGFREE/EGGFREE.cpp | #include <iostream>
using namespace std;
int tt,n,m,old,it,i,j,k,x[222],y[222],u[222];
bool g[222][222],q;
char r[222];
int main()
{
scanf("%d",&tt);
while (tt--)
{
scanf("%d%d",&n,&m);
memset(u,0,sizeof(u));
memset(g,0,sizeof(g));
memset(r,0,sizeof(r));
for (i=0; i<m; i++)
{
scanf("%d%d",&x[i],&y[i]);
g[x[i]][y[i]]=true;
g[y[i]][x[i]]=true;
}
for (it=1; it<=n; )
{
old=it;
for (i=1; i<=n; i++) if (u[i]==0)
{
q=true;
for (j=1; j<=n && q; j++) if (g[i][j]) for (k=1; k<j; k++) if (g[i][k])
{
if (!g[j][k]) { q=false; break;
}
}
if (q)
{
u[i]=it++;
for (j=1; j<=n; j++) if (g[i][j]) g[i][j]=g[j][i]=0;
break;
}
}
if (old==it) break;
}
if (it<=n) { puts("No solution"); continue;
}
for (i=0; i<m; i++) r[i]=(u[x[i]]<u[y[i]])?'^':'v';
puts(r);
}
return 0;
}
|
code/online_challenges/src/codechef/EGGFREE/README.md | # Problem Link
[EGGFREE](https://www.codechef.com/MARCH20A/problems/EGGFREE)
# Description
Let's call a directed graph egg-free if it is acyclic and for any three pairwise distinct vertices x, y and z, if the graph contains edges x→y and x→z, then it also contains an edge y→z and/or an edge z→y.
You are given an undirected graph with N vertices (numbered 1 through N) and M edges (numbered 1 through M). Find a way to assign a direction to each of its edges such that the resulting directed graph is egg-free, or determine that it is impossible. If there are multiple solutions, you may find any one. |
code/online_challenges/src/codechef/EID2/README.md | # Problem Link:
[EID2](https://www.codechef.com/problems/EID2/)
# Description
Eidi gifts are a tradition in which children receive money from elder relatives during the Eid celebration.
Chef has three children (numbered 1,2,3) and he wants to give them Eidi gifts. The oldest child, Chefu, thinks that a distribution of money is fair only if an older child always receives more money than a younger child; if two children have the same age, they should receive the same amounts of money.
For each valid i, the i-th child is Ai years old and it received Ci units of money. Determine whether this distribution of money is fair.
|
code/online_challenges/src/codechef/EID2/eid2.cpp | #include <algorithm>
#include <iostream>
#include <vector>
bool istrue(std::vector<int> a, std::vector<int> c) {
std::pair<int, int> pairt[3];
bool x = true;
int i;
for (i = 0; i < 3; ++i) {
pairt[i].first = a[i];
pairt[i].second = c[i];
}
std::sort(pairt, pairt + 3);
for (i = 0; i < 2; ++i) {
if (pairt[i].first == pairt[i + 1].first) {
if (pairt[i].second != pairt[i + 1].second) {
x = false;
break;
}
}
if (pairt[i].second > pairt[i + 1].second) {
x = false;
break;
}
if (pairt[i].second == pairt[i + 1].second) {
if (pairt[i].first != pairt[i + 1].first) {
x = false;
break;
}
}
}
return x;
}
int main() {
int t;
std::cin >> t;
while (t--) {
std::vector<int> a(3), c(3);
int i;
for (i = 0; i < 3; ++i) {
std::cin >> a[i];
}
for (i = 0; i < 3; ++i) {
std::cin >> c[i];
}
bool x = istrue(a, c);
if (x) {
std::cout << "FAIR" << std::endl;
} else {
std::cout << "NOT FAIR" << std::endl;
}
}
}
|
code/online_challenges/src/codechef/ERROR/ERROR.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
char feedback[100000];
scanf("%s", feedback);
int i, d = 0;
for (i = 2; feedback[i] != '\0'; ++i)
{
if ((feedback[i] == '0' && feedback[i - 1] == '1' && feedback[i - 2] == '0') || (feedback[i] == '1' && feedback[i - 1] == '0' && feedback[i - 2] == '1'))
{
d = 1;
break;
}
}
if (d == 0)
printf("Bad\n");
else
printf("Good\n");
}
return 0;
}
|
code/online_challenges/src/codechef/ERROR/README.md | # Problem Link:
[ERROR](https://www.codechef.com/problems/ERROR/)
# Description
Lots of geeky customers visit our chef's restaurant everyday. So, when asked to fill the feedback form, these customers represent the feedback using a binary string (i.e a string that contains only characters '0' and '1'.
Now since chef is not that great in deciphering binary strings, he has decided the following criteria to classify the feedback as Good or Bad :
If the string contains the substring "010" or "101", then the feedback is Good, else it is Bad. Note that, to be Good it is not necessary to have both of them as substring.
So given some binary strings, you need to output whether according to the chef, the strings are Good or Bad. |
code/online_challenges/src/codechef/FCTRL/Factorial.c | #include <stdio.h>
#include <stdlib.h>
int main()
{
long t;
scanf("%ld", & t);
while (t--)
{
long n;
scanf("%ld", & n);
long s = 1, sum = 0;
while (1)
{
s *= 5;
if (s > n)
break;
else
{
long k = n / s;
sum += k;
}
}
printf("%ld\n", sum);
}
}
|
code/online_challenges/src/codechef/FCTRL/README.md | # Problem Link
[FCTRL](https://www.codechef.com/problems/FCTRL/)
# Description
The most important part of a GSM network is so called Base Transceiver Station (BTS). These transceivers form the areas called cells (this term gave the name to the cellular phone) and every phone connects to the BTS with the strongest signal (in a little simplified view). Of course, BTSes need some attention and technicians need to check their function periodically.
The technicians faced a very interesting problem recently. Given a set of BTSes to visit, they needed to find the shortest path to visit all of the given points and return back to the central company building. Programmers have spent several months studying this problem but with no results. They were unable to find the solution fast enough. After a long time, one of the programmers found this problem in a conference article. Unfortunately, he found that the problem is so called "Traveling Salesman Problem" and it is very hard to solve. If we have N BTSes to be visited, we can visit them in any order, giving us N! possibilities to examine. The function expressing that number is called factorial and can be computed as a product
1.2.3.4....N. The number is very high even for a relatively small N.
The programmers understood they had no chance to solve the problem. But because they have already received the research grant from the government, they needed to continue with their studies and produce at least some results. So they started to study behavior of the factorial function.
For example, they defined the function Z. For any positive integer N, Z(N) is the number of zeros at the end of the decimal form of number N!. They noticed that this function never decreases. If we have two numbers N1 < N2, then Z(N1) <= Z(N2). It is because we can never "lose" any trailing zero by multiplying by any positive number. We can only get new and new zeros. The function Z is very interesting, so we need a computer program that can determine its value efficiently.
|
code/online_challenges/src/codechef/GCD2/GCD2.c | #include <stdio.h>
#include <string.h>
int gcd(int a, int b)
{
return b == 0 ? a : gcd(b, a % b);
}
int main()
{
int t;
scanf("%d", & t);
while (t--)
{
char arr[250];
int a;
scanf("%d", & a);
scanf("%s", arr);
if (a == 0)
printf("%s\n", arr);
else {
int i, m = 0;
for (i = 0; i < strlen(arr); ++i)
{
m = (m * 10 + arr[i] - '0') % a;
}
int n = gcd(a, m);
printf("%d\n", n);
}
}
return 0;
}
|
code/online_challenges/src/codechef/GCD2/README.md | # Problem Link:
[GCD2](https://www.codechef.com/problems/GCD2/)
# Description
Frank explained its friend Felman the algorithm of Euclides to calculate the GCD of two numbers. Then Felman implements it algorithm
int gcd(int a, int b)
{
if (b==0)
return a;
else
return gcd(b,a%b);
}
and it proposes to Frank that makes it but with a little integer and another integer that has up to 250 digits.
Your task is to help Frank programming an efficient code for the challenge of Felman. |
code/online_challenges/src/codechef/GDOG/GDOG.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
int n, k;
scanf("%d%d", & n, & k);
int i, max = 0, c;
for (i = 2; i <= k; ++i)
{
c = n % i;
if (c >= max)
{
max = c;
}
}
printf("%d\n", max);
}
return 0;
}
|
code/online_challenges/src/codechef/GDOG/README.md | # Problem Link:
[GDOG](https://www.codechef.com/problems/GDOG/)
# Description
Tuzik is a little dog. But despite the fact he is still a puppy he already knows about the pretty things that coins are. He knows that for every coin he can get very tasty bone from his master. He believes that some day he will find a treasure and have loads of bones.
And finally he found something interesting. A wooden chest containing N coins! But as you should remember, Tuzik is just a little dog, and so he can't open it by himself. Actually, the only thing he can really do is barking. He can use his barking to attract nearby people and seek their help. He can set the loudness of his barking very precisely, and therefore you can assume that he can choose to call any number of people, from a minimum of 1, to a maximum of K.
When people come and open the chest they divide all the coins between them in such a way that everyone will get the same amount of coins and this amount is maximal possible. If some coins are not used they will leave it on the ground and Tuzik will take them after they go away. Since Tuzik is clearly not a fool, he understands that his profit depends on the number of people he will call. While Tuzik works on his barking, you have to find the maximum possible number of coins he can get. |
code/online_challenges/src/codechef/GUESSNUM/Guessnum.java | import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
public class Guessnum {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
int t = s.nextInt();
while (t-- > 0) {
long a = s.nextLong();
long m = s.nextLong();
ArrayList<Long> arrLL = new ArrayList<>();
ArrayList<Long> newarrLL = new ArrayList<>();
// finding factors of m
for (long i = 1; i <= Math.sqrt(m); i++) {
if (m % i == 0) {
if (m / i == i) {
// Storing all factors of m in arrLL
arrLL.add(i);
} else {
arrLL.add(i);
arrLL.add((m / i));
}
}
}
int count = 0;
// f is number of factors
for (long f : arrLL) {
if ((f - 1) % a == 0) {
long q = (f - 1) / a;
long d = m / f;
long n = q * d;
if (n != 0) {
newarrLL.add(n);
count++;
}
}
}
System.out.println(count);
Collections.sort(newarrLL);
for (long i : newarrLL) {
System.out.print(i + " ");
}
System.out.println();
}
}
}
/*
TEST CASE
INPUT
3
3 35
5 50
4 65
OUTPUT
1
10
0
3
13 15 16
*/
|
code/online_challenges/src/codechef/GUESSNUM/README.md | # Problem Link:
[GUESSNUM](https://www.codechef.com/problems/GUESSNUM)
# Description
Chef is playing a game with his brother Chefu. He asked Chefu to choose a positive integer N, multiply it by a given integer A, then choose a divisor of N (possibly N itself) and add it to the product. Let's denote the resulting integer by M; more formally, M=A⋅N+d, where d is some divisor of N.
Chefu told Chef the value of M and now, Chef should guess N. Help him find all values of N which Chefu could have chosen.
|
code/online_challenges/src/codechef/Greedy Puppy/Greedy_Pupy.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
int n, k;
scanf("%d%d", & n, & k);
int i, max = 0, c;
for (i = 2; i <= k; ++i)
{
c = n % i;
if (c >= max)
{
max = c;
}
}
printf("%d\n", max);
}
return 0;
}
|
code/online_challenges/src/codechef/Greedy Puppy/README.md | # Problem Link:
[GDOG](https://www.codechef.com/problems/GDOG/)
# Description
Tuzik is a little dog. But despite the fact he is still a puppy he already knows about the pretty things that coins are. He knows that for every coin he can get very tasty bone from his master. He believes that some day he will find a treasure and have loads of bones.
And finally he found something interesting. A wooden chest containing N coins! But as you should remember, Tuzik is just a little dog, and so he can't open it by himself. Actually, the only thing he can really do is barking. He can use his barking to attract nearby people and seek their help. He can set the loudness of his barking very precisely, and therefore you can assume that he can choose to call any number of people, from a minimum of 1, to a maximum of K.
When people come and open the chest they divide all the coins between them in such a way that everyone will get the same amount of coins and this amount is maximal possible. If some coins are not used they will leave it on the ground and Tuzik will take them after they go away. Since Tuzik is clearly not a fool, he understands that his profit depends on the number of people he will call. While Tuzik works on his barking, you have to find the maximum possible number of coins he can get. |
code/online_challenges/src/codechef/HILLJUMP/HILLJUMP.cpp | #include<iostream>
using namespace std;
void jump(int[], int);
void inc(int[], int);
int main()
{
std::ios::sync_with_stdio(false);
//int j;for(j=0;j<100;j++)printf("1 ");
int n, q; cin>>n>>q;
int arr[n]; arr[0]=0;
int i; for(i=0;i<n;i++)
{
int temp; cin>>temp; arr[i]+=temp; arr[i+1]=-temp;
}
while(q--)
{
int a;
cin>>a;
if(a==1) jump(arr, n);
else inc(arr, n);
}
return 0;
}
void jump(int arr[], int n)
{
int start, num, curr;
cin>>start>>num;
curr = start-1;
int i; int sum=0;
int f=1;
while(num-- && f==1)
{
int i; f=0; int sum=0;
for(i = curr+1; i<(n<curr+101?n:curr+101); i++)
{
sum+=arr[i];
if(sum>0){curr = i;f=1;break;}
}
}
cout<<curr+1<<endl;
}
void inc(int arr[], int n)
{
int start, end, lol;
cin>>start>>end>>lol;
arr[start-1]+=lol; if(end<n)arr[end]-=lol;
}
|
code/online_challenges/src/codechef/HILLJUMP/README.md | # Problem Link
[HILLJUMP](https://www.codechef.com/AUG17/status/HILLJUMP)
# Description
Chef is going to organize a hill jumping competition and he is going to be one of the judges in it. In this competition there are N hills in a row, and the initial height of i-th hill is Ai. Participants are required to demonstrate their jumping skills by doing what the judges tell them.
Judges will give each participant a card which has two numbers, i and k, which means that the participant should start at the i-th hill and jump k times, where one jump should be from the current hill to the nearest hill to the right which is strictly higher (in height) than the current one. If there is no such hill or its distance (i.e. difference between their indices) is more than 100 then the participant should remain in his current hill.
Please help Chef by creating a program to use it during the competitions. It should read the initial heights of the hill and should support two kinds of operations:
Type 1: Given a two numbers: i and k, your program should output the index of the hill the participant is expected to finish if he starts from the i-th hill (as explained above).
Type 2: Given three numbers: L, R, X, the heights of all the hills between L and R, both end points inclusive, should be increased by X (if X is negative then their height is decreased). |
code/online_challenges/src/codechef/HORSES/HORSES.c | #include <stdio.h>
#include<stdlib.h>
int main(void)
{
int t, i, j;
scanf("%d", & t);
for (i = 1; i <= t; ++i)
{
int n;
scanf("%d", & n);
int s[n];
for (j = 0; j < n; ++j)
scanf("%d", & s[j]);
int min = abs(s[0] - s[1]);
for (j = 0; j < n; ++j)
{
int k;
for (k = j + 1; k < n; ++k)
{
if (abs(s[j] - s[k]) < min)
min = abs(s[j] - s[k]);
}
}
printf("%d\n", min);
}
return 0;
}
|
code/online_challenges/src/codechef/HORSES/README.md | # Problem Link:
[HORSES](https://www.codechef.com/problems/HORSES/)
# Description
Chef is very fond of horses. He enjoys watching them race. As expected, he has a stable full of horses. He, along with his friends, goes to his stable during the weekends to watch a few of these horses race. Chef wants his friends to enjoy the race and so he wants the race to be close. This can happen only if the horses are comparable on their skill i.e. the difference in their skills is less.
There are N horses in the stable. The skill of the horse i is represented by an integer S[i]. The Chef needs to pick 2 horses for the race such that the difference in their skills is minimum. This way, he would be able to host a very interesting race. Your task is to help him do this and report the minimum difference that is possible between 2 horses in the race. |
code/online_challenges/src/codechef/J7/J7.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
int p, s;
scanf("%d%d", & p, & s);
double x1 = (p + sqrt(p * p - (24 * s))) / 12;
double x2 = (p - sqrt(p * p - (24 * s))) / 12;
double y1 = (p - (8 * x1)) / 4;
double y2 = (p - (8 * x2)) / 4;
double v1 = x1 * x1 * y1;
double v2 = x2 * x2 * y2;
printf("%.2f\n", (v1 > v2) ? v1 : v2);
}
return 0;
}
|
code/online_challenges/src/codechef/J7/README.md | # Problem Link:
[J7](https://www.codechef.com/problems/J7)
# Description
Johnny needs to make a rectangular box for his physics class project. He has bought P cm of wire and S cm2 of special paper. He would like to use all the wire (for the 12 edges) and paper (for the 6 sides) to make the box.
What is the largest volume of the box that Johnny can make? |
code/online_challenges/src/codechef/JAIN/JAIN.cpp | #include <iostream>
#include <algorithm>
#include <list>
#include <stack>
#include <string>
#include <map>
#include <vector>
#include <set>
#include <utility>
std::map<char,int>g_mp;
void build ()
{
g_mp.insert(std::make_pair('a',16));
g_mp.insert(std::make_pair('e',8));
g_mp.insert(std::make_pair('i',4));
g_mp.insert(std::make_pair('o',2));
g_mp.insert(std::make_pair('u',1));
}
int main()
{
build();
int t;
std::cin >> t;
while (t--)
{
int n;
std::cin >> n;
std::string a[n];
for (int i = 0; i < n; ++i)
std::cin >> a[i];
std::vector<short>value;
std::set<short>s;
std::vector<long long>count(33,0);
for (int i = 0; i < n; i++)
{
int x = 0;
for(int j = 0; j < a[i].size(); ++j)
{
x = x | g_mp[a[i][j]];
}
if (s.find(x) == s.end())
{
value.push_back(x);
count[x] = 1;
s.insert(x);
}
else
{
count[x]++;
}
}
long long ans = 0;
for (int i = 0; i < value.size(); ++i)
{
for (int j = i + 1; j < value.size(); ++j)
if ((value[i] | value[j]) == 31)
ans = ans + (count[value[i]] * count[value[j]]);
}
ans = ans + ((count[31] * (count[31] - 1)) / 2);
std::cout << ans << "\n";
}
return 0;
}
|
code/online_challenges/src/codechef/JAIN/README.md | # Problem Link
[JAIN](https://www.codechef.com/MARCH19B/problems/JAIN)
# Description
Chef has N dishes, numbered 1 through N. For each valid i, dish i is described by a string Di containing only lowercase vowels, i.e. characters 'a', 'e', 'i', 'o', 'u'.
A meal consists of exactly two dishes. Preparing a meal from dishes i and j (i≠j) means concatenating the strings Di and Dj in an arbitrary order into a string M describing the meal. Chef likes this meal if the string M contains each lowercase vowel at least once.
Now, Chef is wondering - what is the total number of (unordered) pairs of dishes such that he likes the meal prepared from these dishes?
|
code/online_challenges/src/codechef/JOHNY/JOHNY.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
int i, j;
int n;
scanf("%d", & n);
long long int a[100];
long long int pj = 0;
for (i = 0; i < n; ++i)
{
scanf("%lld", & a[i]);
}
int x;
scanf("%d", & x);
int cr = a[x - 1];
for (i = 0; i < n - 1; ++i)
{
for (j = 0; j < n - 1; ++j)
{
if (a[j] > a[j + 1])
{
pj = a[j + 1];
a[j + 1] = a[j];
a[j] = pj;
}
}
}
for (i = 0; i < n; ++i)
{
if (a[i] == cr)
{
printf("%d\n", i + 1);
}
}
}
return 0;
}
|
code/online_challenges/src/codechef/JOHNY/JOHNY.cpp | #include <iostream>
int main() {
int t;
std::cin >> t;
while (t--) {
int n;
std::cin >> n;
int a[100];
for (int i = 0; i < n; ++i) {
std::cin >> a[i];
}
int k;
std::cin >> k;
int ans = 0;
for (int i = 0; i < n; ++i) {
ans += (a[k - 1] > a[i]);
}
std::cout << ans + 1 << '\n';
}
return 0;
}
|
code/online_challenges/src/codechef/JOHNY/JOHNY.py | t = int(input())
for x in range(t):
n = int(input())
p = list(map(int, input().split()))
k = int(input())
a = p[k - 1]
p.sort()
print(p.index(a) + 1)
|
code/online_challenges/src/codechef/JOHNY/README.md | # Problem Link:
[JOHNY](https://www.codechef.com/problems/JOHNY/)
# Description
Vlad enjoys listening to music. He lives in Sam's Town. A few days ago he had a birthday, so his parents gave him a gift: MP3-player! Vlad was the happiest man in the world! Now he can listen his favorite songs whenever he wants!
Vlad built up his own playlist. The playlist consists of N songs, each has a unique positive integer length. Vlad likes all the songs from his playlist, but there is a song, which he likes more than the others. It's named "Uncle Johny".
After creation of the playlist, Vlad decided to sort the songs in increasing order of their lengths. For example, if the lengths of the songs in playlist was {1, 3, 5, 2, 4} after sorting it becomes {1, 2, 3, 4, 5}. Before the sorting, "Uncle Johny" was on K-th position (1-indexing is assumed for the playlist) in the playlist.
Vlad needs your help! He gives you all the information of his playlist. Your task is to find the position of "Uncle Johny" in the sorted playlist. |
code/online_challenges/src/codechef/LAZERTST/LAZERTST.cpp | #include<iostream>
using namespace std;
typedef long long ll;
void solve()
{
ll n,m,k,q;
cin>>n>>m>>k>>q;
vector<pair<int,int>> qs(q);
vector<ll> ans(q,0);
for (int i = 0; i < q; i++)
{
cin>>qs[i].first>>qs[i].second;
}
if(k==3)
{
cout<<2<<' ';
for (int i = 0; i < q; i++)
{
cout<<m-1<<' ';
}
cout<<endl;
int h;
cin>>h;
if(h==-1)
{
exit(1);
}
}
else if(k==100)
{
ll gm = 0;
for (int i = 0; i < q; i++)
{
cout<<1<<' '<<qs[i].first<<' '<<qs[i].second<<' '<<(m*i)/10<<endl;
ll h;
cin>>h;
if(h==-1)
{
exit(1);
}
gm = max(gm,h);
}
cout<<2<<' ';
for (int i = 0; i < q; i++)
{
cout<<gm<<' ';
}
cout<<endl;
int h;
cin>>h;
if(h==-1)
{
exit(1);
}
}
}
int main()
{
ios_base::sync_with_stdio(0);
cin.tie(0);
int t;
cin>>t;
while(t--)
solve();
return 0;
}
|
code/online_challenges/src/codechef/LAZERTST/README.md | # Problem Link
[EGGFREE](https://www.codechef.com/MARCH20A/problems/LAZERTST)
# Description
### This is an interactive problem.
When Vivek was creating the problem LAZER, he realised that it can be used to create a different, interactive problem. Can you solve this problem too?
Let's frame this problem formally. You are given integers N, M, K and Q. Consider a random sequence A1,A2,…,AN, where each element is generated independently and uniformly randomly between 1 and M (inclusive). As described in the problem statement of LAZER, we create line segments between pairs of points (i,Ai) and (i+1,Ai+1) for each valid i.
The height of a line segment connecting points (x1,y1) and (x2,y2) is defined as |y1−y2|. You are given Q queries. In each query, you are given integers L and R; you should consider the line segments such that the x-coordinates of their endpoints lie between L and R inclusive, and you should find the maximum of heights of these segments.
Your task is to answer these queries. However, the sequence A is hidden and you may only ask at most K questions (of a different type from the queries you should answer). In each of your questions:
You should choose integers x1, x2 and y, where 1≤x1<x2≤N and 1≤y≤109.
Consider a laser beam fired horizontally to the right from the point (x1,y), which stops at the point (x2,y) and does not propagate further.
This beam collides with zero or more of the line segments described above. The beam is considered to collide with a line segment if it intersects or touches this segment, except when the left endpoint of this segment is (x2,y) or its right endpoint is (x1,y).
The answer to this question is the maximum of heights of the segments with which it collides or the information that it does not collide with any segments |
code/online_challenges/src/codechef/LEPERMUT/LEPERMUT.c | #include <stdio.h>
int main(void)
{
int t;
scanf("%d", & t);
while (t--)
{
int n;
scanf("%d", & n);
int a[100];
int i;
for (i = 0; i < n; ++i)
{
scanf("%d", & a[i]);
}
int c = 0;
for (i = 0; i < n; ++i)
{
int j;
for (j = i + 2; j < n; ++j)
{
if (a[i] > a[j])
{
c = 1;
break;
}
}
}
if (c == 0)
printf("YES\n");
else
printf("NO\n");
}
return 0;
}
|
code/online_challenges/src/codechef/LEPERMUT/README.md | # Problem Link:
[LEPERMUT](https://www.codechef.com/problems/LEPERMUT/)
# Description
The Little Elephant likes permutations. This time he has a permutation A[1], A[2], ..., A[N] of numbers 1, 2, ..., N.
He calls a permutation A good, if the number of its inversions is equal to the number of its local inversions. The number of inversions is equal to the number of pairs of integers (i; j) such that 1 ≤ i < j ≤ N and A[i] > A[j], and the number of local inversions is the number of integers i such that 1 ≤ i < N and A[i] > A[i+1].
The Little Elephant has several such permutations. Help him to find for each permutation whether it is good or not. Print YES for a corresponding test case if it is good and NO otherwise. |
code/online_challenges/src/codechef/MARBLES/MARBLES.c | #include <stdio.h>
int main(void) {
int t;
scanf("%d", &t);
while(t > 0)
{
t--;
int n, k;
long long int ans = 1;
scanf("%d", &n);
scanf("%d", &k);
if(n == k)
printf("%lld\n", ans);
else
{
n--;
k--;
ans = 1;
if(k > n/2)
k = n - k;
for(int i = 0; i < k; ++i)
{
ans *= n - i;
ans /= i + 1;
}
printf("%lld\n", ans);
}
}
return 0;
}
|
code/online_challenges/src/codechef/MARBLES/MARBLES.py | t = int(input())
while t > 0:
t -= 1
ans = 1
n, k = map(int, input().split())
if n == k:
print(ans)
else:
n -= 1
k -= 1
if k > n / 2:
k = n - k
ans = 1
for i in range(k):
ans *= n - i
ans /= i + 1
print(int(ans))
|
code/online_challenges/src/codechef/MARBLES/README.md | # Problem Link:
[MARBLES](https://www.codechef.com/problems/MARBLES)
# Description
Rohit dreams he is in a shop with an infinite amount of marbles. He is allowed to select n marbles. There are marbles of k different colors. From each color there are also infinitely many marbles. Rohit wants to have at least one marble of each color, but still there are a lot of possibilities for his selection. In his effort to make a decision he wakes up. Now he asks you how many possibilities for his selection he would have had. Assume that marbles of equal color can't be distinguished, and the order of the marbles is irrelevant.
Input
The first line of input contains a number T <= 100 that indicates the number of test cases to follow. Each test case consists of one line containing n and k, where n is the number of marbles Rohit selects and k is the number of different colors of the marbles. You can assume that 1<=k<=n<=1000000.
Output
For each test case print the number of possibilities that Rohit would have had. You can assume that this number fits into a signed 64 bit integer.
Example
Input:
2
10 10
30 7
Output:
1
475020
|
code/online_challenges/src/codechef/MARCHA1/MARCHA1.c | #include <stdio.h>
int main()
{
int t;
scanf("%d", & t);
while (t--)
{
int m, n, i, j;
scanf("%d %d", & n, & m);
int b[m + 1];
for (i = 1; i <= m; ++i)
b[i] = 0;
b[0] = 1;
for (i = 0; i < n; ++i)
{
int k;
scanf("%d", & k);
for (j = m - k; j >= 0; --j)
{
if (b[j] > 0)
b[j + k] = 1;
}
}
if (b[m] > 0)
printf("Yes\n");
else
printf("No\n");
}
return 0;
}
|
code/online_challenges/src/codechef/MARCHA1/README.md | # Problem Link:
[MARCHA1](https://www.codechef.com/problems/MARCHA1/)
# Description
In the mysterious country of Byteland, everything is quite different from what you'd normally expect. In most places, if you were approached by two mobsters in a dark alley, they would probably tell you to give them all the money that you have. If you refused, or didn't have any - they might even beat you up.
In Byteland the government decided that even the slightest chance of someone getting injured has to be ruled out. So, they introduced a strict policy. When a mobster approaches you in a dark alley, he asks you for a specific amount of money. You are obliged to show him all the money that you have, but you only need to pay up if he can find a subset of your banknotes whose total value matches his demand. Since banknotes in Byteland can have any positive integer value smaller than one thousand you are quite likely to get off without paying.
Both the citizens and the gangsters of Byteland have very positive feelings about the system. No one ever gets hurt, the gangsters don't lose their jobs, and there are quite a few rules that minimize that probability of getting mugged (the first one is: don't go into dark alleys - and this one is said to work in other places also).
|
code/online_challenges/src/codechef/MATCHES/Mathces.cpp | #include <iostream>
#include <vector>
int main() {
std::vector<int> m = {6, 2, 5, 5, 4, 5, 6, 3, 7, 6};
int t;
std::cin >> t;
while (t--) {
long long int a, b, sum = 0;
std::cin >> a >> b;
a += b;
while (a > 0) {
sum += m[a % 10];
a /= 10;
}
std::cout << sum << "\n";
}
}
|
code/online_challenges/src/codechef/MATCHES/README.md | # Problem Link:
[MATCHES](https://www.codechef.com/problems/MATCHES/)
# Description
Chef's son Chefu found some matches in the kitchen and he immediately starting playing with them.
The first thing Chefu wanted to do was to calculate the result of his homework — the sum of A and B, and write it using matches. Help Chefu and tell him the number of matches needed to write the result.
Digits are formed using matches in the following way:
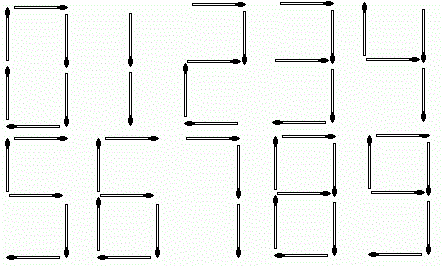
|
code/online_challenges/src/codechef/MATCHES/matches.c | #include <stdio.h>
int main() {
int m[] = {6, 2, 5, 5, 4, 5, 6, 3, 7, 6};
int t;
scanf("%d", &t);
while (t--) {
long long int a, b, sum = 0;
scanf("%lld%lld", &a, &b);
a += b;
while (a > 0) {
sum += m[a % 10];
a /= 10;
}
printf("%lld\n", sum);
}
}
|
code/online_challenges/src/codechef/MAXDIFF/MAXDIFF.c | #include <stdio.h>
int main()
{
int t;
scanf("%d", & t);
while (t--)
{
int i, j, n, k;
scanf("%d %d", & n, & k);
int a[n];
for (i = 0; i < n; ++i)
scanf("%d", & a[i]);
for (i = 0; i < n - 1; ++i)
{
for (j = 0; j < n - i - 1; ++j)
{
if (a[j] > a[j + 1])
{
int temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
}
}
}
int ch = 0, sn = 0;
if (n - k > k)
{
for (i = 0; i < k; ++i)
sn += a[i];
for (i = k; i < n; ++i)
ch += a[i];
} else
{
for (i = 0; i < n - k; ++i)
sn += a[i];
for (i = n - k; i < n; ++i)
ch += a[i];
}
printf("%d\n", ch - sn);
}
return 0;
}
|
code/online_challenges/src/codechef/MAXDIFF/README.md | # Problem Link:
[MAXDIFF](https://www.codechef.com/problems/MAXDIFF/)
# Description
Chef has gone shopping with his 5-year old son. They have bought N items so far. The items are numbered from 1 to N, and the item i weighs Wi grams.
Chef's son insists on helping his father in carrying the items. He wants his dad to give him a few items. Chef does not want to burden his son. But he won't stop bothering him unless he is given a few items to carry. So Chef decides to give him some items. Obviously, Chef wants to give the kid less weight to carry.
However, his son is a smart kid. To avoid being given the bare minimum weight to carry, he suggests that the items are split into two groups, and one group contains exactly K items. Then Chef will carry the heavier group, and his son will carry the other group.
Help the Chef in deciding which items should the son take. Your task will be simple. Tell the Chef the maximum possible difference between the weight carried by him and the weight carried by the kid. |
code/online_challenges/src/codechef/MEETUP/MEETUP.cpp | #include <iostream>
#include <fstream>
#include <set>
#include <map>
#include <string>
#include <vector>
#include <bitset>
#include <algorithm>
#include <cstring>
#include <cstdlib>
#include <cmath>
#include <cassert>
#include <queue>
#define mp make_pair
#define pb push_back
typedef long long ll;
typedef long double ld;
using namespace std;
#ifndef LOCAL
#define cerr _cer
struct _cert
{
template <typename T> _cert& operator << (T)
{
return *this;
}
};
_cert _cer;
#endif
template <typename T> void dprint(T begin, T end)
{
for (auto i = begin; i != end; i++)
{
cerr << (*i) << " ";
}
cerr << "\n";
}
char buf[100];
int ka, kb;
map<string, int > mm1;
map<string, int > mm2;
int cc1, cc2;
set<int> eds1[1200];
set<int> eds2[1200];
string s1[1200];
string s2[1200];
int rd1()
{
scanf(" %s", buf);
if (mm1.count(buf))
return mm1[buf];
else
{
s1[cc1] = buf;
return mm1[buf] = cc1++;
}
}
int rd2()
{
scanf(" %s", buf);
if (mm2.count(buf))
return mm2[buf];
else
{
s2[cc2] = buf;
return mm2[buf] = cc2++;
}
}
void del1(int v)
{
for (int i: eds1[v])
{
eds1[i].erase(v);
}
eds1[v].clear();
}
void del2(int v)
{
for (int i: eds2[v])
{
eds2[i].erase(v);
}
eds2[v].clear();
}
int ask1(int v)
{
cout << "A " << s1[v] << "\n";
cout.flush();
return rd2();
}
int ask2(int v)
{
cout << "B " << s2[v] << "\n";
cout.flush();
return rd1();
}
void solve(vector<int> av1, vector<int> av2)
{
set<int> ss;
for (int i: av1)
if (i < ka)
ss.insert(eds1[i].size());
int bst = -1;
for (int i: av2)
{
if (i < kb)
{
if (ss.count(eds2[i].size()))
{
bst = eds2[i].size();
break;
}
}
}
if (bst == -1)
{
cout << "C No\n";
cout.flush();
exit(0);
}
int n = av1.size();
int c1 = bst;
int c2 = n - 1 - bst;
if (c1 < c2)
{
int a = -1;
for (int i: av1)
{
if (eds1[i].size() == bst && i < ka)
{
a = i;
break;
}
}
int b = ask1(a);
if (b < kb) {
cout << "C Yes\n";
cout.flush();
exit(0);
}
vector<int> avi;
vector<int> avii;
for (int i: av1)
if (i != a && eds1[a].count(i) == 1)
{
avi.push_back(i);
}
else if (i != a)
{
del1(i);
}
del1(a);
for (int i: av2)
if (i != b && eds2[b].count(i) == 1)
{
avii.push_back(i);
}
else if (i != b)
{
del2(i);
}
del2(b);
solve(avi, avii);
}
else
{
int b = -1;
for (int i: av2)
{
if (eds2[i].size() == bst && i < kb)
{
b = i;
break;
}
}
int a = ask2(b);
if (a < ka) {
cout << "C Yes\n";
cout.flush();
exit(0);
}
vector<int> avi;
vector<int> avii;
for (int i: av1)
if (i != a && eds1[a].count(i) == 0)
{
avi.push_back(i);
}
else if (i != a)
{
del1(i);
}
del1(a);
for (int i: av2)
if (i != b && eds2[b].count(i) == 0)
{
avii.push_back(i);
}
else if (i != b)
{
del2(i);
}
del2(b);
solve(avi, avii);
}
}
int n, m;
int main()
{
scanf("%d%d%d%d", &n, &m, &ka, &kb);
for (int i = 0; i < n; ++i)
assert(rd1() == i);
for (int j = 0; j < m; ++j)
{
int a = rd1();
int b = rd1();
eds1[a].insert(b);
eds1[b].insert(a);
}
for (int i = 0; i < n; ++i)
assert(rd2() == i);
for (int j = 0; j < m; ++j)
{
int a = rd2();
int b = rd2();
eds2[a].insert(b);
eds2[b].insert(a);
}
vector<int> av1;
for (int i = 0; i < n; ++i)
av1.push_back(i);
vector<int> av2;
for (int i = 0; i < n; ++i)
av2.push_back(i);
solve(av1, av2);
return 0;
}
|
code/online_challenges/src/codechef/MEETUP/README.md | # Problem Link
[MEETUP](https://www.codechef.com/problems/MEETUP)
# Description
This is an interactive problem. It means that every time you output something, you must finish with a newline character and flush the output. For example, in C++ use the fflush(stdout) function, in Java — call System.out.flush(), in Python — sys.stdout.flush(), and in Pascal — flush(output). Only after flushing the output will you be able to read from the input again.
Alice and Bob are taking a trip to Byteland. The country can be modeled as an undirected graph with n cities and m bidirectional roads. There are at most 1,000 cities, and between 0 and n * (n-1) / 2 roads. The graph is not necessarily connected.
Alice likes visiting cities that are directly connected. She chose ka nodes that form a clique (i.e. every pair of cities has a direct road between them). Bob on the other hand likes to visit cities that are not directly connected. He chose kb nodes that form an independent set of the graph (i.e. no pair of cities has a direct road between them).
Alice and Bob would like to know if their itineraries have any common cities. This seemingly simple task is complicated by the fact that Alice and Bob have maps in different languages.
Alice’s map has the cities with names a1, a2, …, an. Bob’s map has cities with names b1, b2, …, bn. These names are all distinct.
Luckily for you, the judge knows how to translate strings between the languages. Formally, there is a hidden function f that the judge keeps such that for every i there exists a unique j such that f(ai) = bj, and ap, aq have a direct edge if and only if f(ap),f(aq) have a direct edge. We will denote f-1(x) to denote the inverse of the function f. Intuitively, f translates strings from language A to language B, and f-1 translates them from language B to language A.
Translating words takes a lot of effort. You would like to help Alice and Bob by translating as few as words as possible. You can ask the judge what the value of f(ai) is, or what the value of f-1(bj) is. You may only ask the judge at most 10 questions. After asking questions, you must be able to determine if Alice and Bob will visit any common cities. Formally, this means checking whether there exists a city ai that Alice visits and a city bj that Bob visits such that f(ai) = bj. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.