instance_id
stringlengths 12
53
| patch
stringlengths 278
33.9k
| repo
stringlengths 7
48
| base_commit
stringlengths 40
40
| hints_text
stringclasses 189
values | test_patch
stringlengths 212
264k
| problem_statement
stringlengths 23
37.7k
| version
stringclasses 1
value | FAIL_TO_PASS
sequencelengths 1
902
| PASS_TO_PASS
sequencelengths 0
7.6k
| created_at
stringlengths 25
25
| __index_level_0__
int64 1.89k
4.13k
|
---|---|---|---|---|---|---|---|---|---|---|---|
devopshq__artifactory-cleanup-100 | diff --git a/README.md b/README.md
index c0a63ec..ee5afde 100644
--- a/README.md
+++ b/README.md
@@ -135,6 +135,12 @@ artifactory-cleanup --days-in-future=10
# Not satisfied with built-in rules? Write your own rules in python and connect them!
artifactory-cleanup --load-rules=myrule.py
docker run -v "$(pwd)":/app devopshq/artifactory-cleanup artifactory-cleanup --load-rules=myrule.py
+
+# Save the table summary in a file
+artifactory-cleanup --output=myfile.txt
+
+# Save the summary in a Json file
+artifactory-cleanup --output=myfile.txt --output-format=json
```
# Rules
diff --git a/artifactory_cleanup/cli.py b/artifactory_cleanup/cli.py
index 312c999..b168ecf 100644
--- a/artifactory_cleanup/cli.py
+++ b/artifactory_cleanup/cli.py
@@ -1,3 +1,4 @@
+import json
import logging
import sys
from datetime import timedelta, date
@@ -5,6 +6,7 @@ from datetime import timedelta, date
import requests
from hurry.filesize import size
from plumbum import cli
+from plumbum.cli.switches import Set
from prettytable import PrettyTable
from requests.auth import HTTPBasicAuth
@@ -62,6 +64,19 @@ class ArtifactoryCleanupCLI(cli.Application):
mandatory=False,
)
+ _output_file = cli.SwitchAttr(
+ "--output", help="Choose the output file", mandatory=False
+ )
+
+ _output_format = cli.SwitchAttr(
+ "--output-format",
+ Set("table", "json", case_sensitive=False),
+ help="Choose the output format",
+ default="table",
+ requires=["--output"],
+ mandatory=False,
+ )
+
@property
def VERSION(self):
# To prevent circular imports
@@ -83,6 +98,36 @@ class ArtifactoryCleanupCLI(cli.Application):
print(f"Simulating cleanup actions that will occur on {today}")
return today
+ def _format_table(self, result) -> PrettyTable:
+ table = PrettyTable()
+ table.field_names = ["Cleanup Policy", "Files count", "Size"]
+ table.align["Cleanup Policy"] = "l"
+
+ for policy_result in result["policies"]:
+ row = [
+ policy_result["name"],
+ policy_result["file_count"],
+ size(policy_result["size"]),
+ ]
+ table.add_row(row)
+
+ table.add_row(["", "", ""])
+ table.add_row(["Total size: {}".format(size(result["total_size"])), "", ""])
+ return table
+
+ def _print_table(self, result: dict):
+ print(self._format_table(result))
+
+ def _create_output_file(self, result, filename, format):
+ text = None
+ if format == "table":
+ text = self._format_table(result).get_string()
+ else:
+ text = json.dumps(result)
+
+ with open(filename, "w") as file:
+ file.write(text)
+
def main(self):
today = self._get_today()
if self._load_rules:
@@ -112,9 +157,7 @@ class ArtifactoryCleanupCLI(cli.Application):
if self._policy:
cleanup.only(self._policy)
- table = PrettyTable()
- table.field_names = ["Cleanup Policy", "Files count", "Size"]
- table.align["Cleanup Policy"] = "l"
+ result = {"policies": [], "total_size": 0}
total_size = 0
block_ctx_mgr, test_ctx_mgr = get_context_managers()
@@ -124,16 +167,21 @@ class ArtifactoryCleanupCLI(cli.Application):
if summary is None:
continue
total_size += summary.artifacts_size
- row = [
- summary.policy_name,
- summary.artifacts_removed,
- size(summary.artifacts_size),
- ]
- table.add_row(row)
- table.add_row(["", "", ""])
- table.add_row(["Total size: {}".format(size(total_size)), "", ""])
- print(table)
+ result["policies"].append(
+ {
+ "name": summary.policy_name,
+ "file_count": summary.artifacts_removed,
+ "size": summary.artifacts_size,
+ }
+ )
+
+ result["total_size"] = total_size
+
+ self._print_table(result)
+
+ if self._output_file:
+ self._create_output_file(result, self._output_file, self._output_format)
if __name__ == "__main__":
| devopshq/artifactory-cleanup | c523f319dc7835c5b3bd583f5ba187184df24dbc | diff --git a/tests/data/expected_output.txt b/tests/data/expected_output.txt
new file mode 100644
index 0000000..2471489
--- /dev/null
+++ b/tests/data/expected_output.txt
@@ -0,0 +1,8 @@
++--------------------------------------------------------+-------------+------+
+| Cleanup Policy | Files count | Size |
++--------------------------------------------------------+-------------+------+
+| Remove all files from repo-name-here older then 7 days | 1 | 528B |
+| Use your own rules! | 1 | 528B |
+| | | |
+| Total size: 1K | | |
++--------------------------------------------------------+-------------+------+
\ No newline at end of file
diff --git a/tests/test_cli.py b/tests/test_cli.py
index f5c5afe..2869e23 100644
--- a/tests/test_cli.py
+++ b/tests/test_cli.py
@@ -1,4 +1,6 @@
+import json
import pytest
+from filecmp import cmp
from artifactory_cleanup import ArtifactoryCleanupCLI
@@ -69,3 +71,101 @@ def test_destroy(capsys, shared_datadir, requests_mock):
last_request.url
== "https://repo.example.com/artifactory/repo-name-here/path/to/file/filename1.json"
)
+
+
+@pytest.mark.usefixtures("requests_repo_name_here")
+def test_output_table(capsys, shared_datadir, requests_mock):
+ _, code = ArtifactoryCleanupCLI.run(
+ [
+ "ArtifactoryCleanupCLI",
+ "--config",
+ str(shared_datadir / "cleanup.yaml"),
+ "--load-rules",
+ str(shared_datadir / "myrule.py"),
+ ],
+ exit=False,
+ )
+ stdout, stderr = capsys.readouterr()
+ print(stdout)
+ assert code == 0, stdout
+ assert (
+ "| Cleanup Policy | Files count | Size |"
+ in stdout
+ )
+
+
+@pytest.mark.usefixtures("requests_repo_name_here")
+def test_output_table(capsys, shared_datadir, requests_mock, tmp_path):
+ output_file = tmp_path / "output.txt"
+ _, code = ArtifactoryCleanupCLI.run(
+ [
+ "ArtifactoryCleanupCLI",
+ "--config",
+ str(shared_datadir / "cleanup.yaml"),
+ "--load-rules",
+ str(shared_datadir / "myrule.py"),
+ "--output-format",
+ "table",
+ "--output",
+ str(output_file),
+ ],
+ exit=False,
+ )
+ stdout, stderr = capsys.readouterr()
+ print(stdout)
+ assert code == 0, stdout
+ assert cmp(output_file, shared_datadir / "expected_output.txt") is True
+
+
+@pytest.mark.usefixtures("requests_repo_name_here")
+def test_output_json(capsys, shared_datadir, requests_mock, tmp_path):
+ output_json = tmp_path / "output.json"
+ _, code = ArtifactoryCleanupCLI.run(
+ [
+ "ArtifactoryCleanupCLI",
+ "--config",
+ str(shared_datadir / "cleanup.yaml"),
+ "--load-rules",
+ str(shared_datadir / "myrule.py"),
+ "--output-format",
+ "json",
+ "--output",
+ str(output_json),
+ ],
+ exit=False,
+ )
+ stdout, stderr = capsys.readouterr()
+ assert code == 0, stdout
+ with open(output_json, "r") as file:
+ assert json.load(file) == {
+ "policies": [
+ {
+ "name": "Remove all files from repo-name-here older then 7 days",
+ "file_count": 1,
+ "size": 528,
+ },
+ {"name": "Use your own rules!", "file_count": 1, "size": 528},
+ ],
+ "total_size": 1056,
+ }
+
+
+@pytest.mark.usefixtures("requests_repo_name_here")
+def test_require_output_json(capsys, shared_datadir, requests_mock):
+ _, code = ArtifactoryCleanupCLI.run(
+ [
+ "ArtifactoryCleanupCLI",
+ "--config",
+ str(shared_datadir / "cleanup.yaml"),
+ "--load-rules",
+ str(shared_datadir / "myrule.py"),
+ "--output-format",
+ "json",
+ ],
+ exit=False,
+ )
+ assert code == 2, stdout
+ stdout, stderr = capsys.readouterr()
+ assert (
+ "Error: Given --output-format, the following are missing ['output']" in stdout
+ )
| Output format to Json
We would like a feature request to have the output table to Json format.
The PrettyTable libraries can do it : https://pypi.org/project/prettytable/
If I have time, I would propose a PR | 0.0 | [
"tests/test_cli.py::test_output_json",
"tests/test_cli.py::test_require_output_json",
"tests/test_cli.py::test_output_table"
] | [
"tests/test_cli.py::test_dry_mode",
"tests/test_cli.py::test_destroy",
"tests/test_cli.py::test_help"
] | 2023-03-17 06:28:51+00:00 | 1,893 |
|
devopsspiral__KubeLibrary-124 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index b7b2f42..e8800dd 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -5,8 +5,14 @@ The format is based on [Keep a Changelog](http://keepachangelog.com/)
and this project adheres to [Semantic Versioning](http://semver.org/).
## In progress
+
+## [0.8.1] - 2022-12-16
+### Added
- Add proxy configuration fetched from `HTTP_PROXY` or `http_proxy` environment variable
+### Fixed
+Fix disabling cert validation [#124](https://github.com/devopsspiral/KubeLibrary/pull/124)
+
## [0.8.0] - 2022-10-27
### Added
- Add function list_namespaced_stateful_set_by_pattern [#114](https://github.com/devopsspiral/KubeLibrary/pull/113) by [@siaomingjeng](https://github.com/siaomingjeng)
diff --git a/src/KubeLibrary/KubeLibrary.py b/src/KubeLibrary/KubeLibrary.py
index 3f73adc..ebff896 100755
--- a/src/KubeLibrary/KubeLibrary.py
+++ b/src/KubeLibrary/KubeLibrary.py
@@ -1,6 +1,5 @@
import json
import re
-import ssl
import urllib3
from os import environ
@@ -276,7 +275,7 @@ class KubeLibrary:
def _add_api(self, reference, class_name):
self.__dict__[reference] = class_name(self.api_client)
if not self.cert_validation:
- self.__dict__[reference].api_client.rest_client.pool_manager.connection_pool_kw['cert_reqs'] = ssl.CERT_NONE
+ self.__dict__[reference].api_client.configuration.verify_ssl = False
def k8s_api_ping(self):
"""Performs GET on /api/v1/ for simple check of API availability.
diff --git a/src/KubeLibrary/version.py b/src/KubeLibrary/version.py
index 8675559..73baf8f 100644
--- a/src/KubeLibrary/version.py
+++ b/src/KubeLibrary/version.py
@@ -1,1 +1,1 @@
-version = "0.8.0"
+version = "0.8.1"
| devopsspiral/KubeLibrary | 7f4037c283a38751f9a31160944a17e7b80ec97b | diff --git a/test/test_KubeLibrary.py b/test/test_KubeLibrary.py
index b99291b..7cae67e 100644
--- a/test/test_KubeLibrary.py
+++ b/test/test_KubeLibrary.py
@@ -1,7 +1,6 @@
import json
import mock
import re
-import ssl
import unittest
from KubeLibrary import KubeLibrary
from KubeLibrary.exceptions import BearerTokenWithPrefixException
@@ -306,7 +305,7 @@ class TestKubeLibrary(unittest.TestCase):
kl = KubeLibrary(kube_config='test/resources/k3d', cert_validation=False)
for api in TestKubeLibrary.apis:
target = getattr(kl, api)
- self.assertEqual(target.api_client.rest_client.pool_manager.connection_pool_kw['cert_reqs'], ssl.CERT_NONE)
+ self.assertEqual(target.api_client.configuration.verify_ssl, False)
@responses.activate
def test_KubeLibrary_inits_with_bearer_token(self):
| certificate verify failed issue when using Get Namespaced Pod Exec
When using the Get Namespaced Pod Exec keywork on a k8s cluster using a custom CA, the following error occurs :
```
ssl.SSLCertVerificationError: [SSL: CERTIFICATE_VERIFY_FAILED] certificate verify failed: unable to get issuer certificate (_ssl.c:1129)
```
Other keywords (Read Namespaced Pod Status, List Namespaced Pod By Pattern ...) are working as expected.
As a quick fix, I'm adding the following line in the _add_api method of the library :
```
def _add_api(self, reference, class_name):
self.__dict__[reference] = class_name(self.api_client)
if not self.cert_validation:
self.__dict__[reference].api_client.rest_client.pool_manager.connection_pool_kw['cert_reqs'] = ssl.CERT_NONE
self.__dict__[reference].api_client.configuration.verify_ssl = False
```
Am I missing something regarding the library configuration ?
Versions :
```
KubeLibrary: 0.8.0
Python: 3.9.13
Kubernetes: 1.24
```
KubeLibrary :
```
Library KubeLibrary kube_config=${KUBECONFIG_FILE} cert_validation=False
KubeLibrary.Get Namespaced Pod Exec
... name=my-pod
... namespace=${namespace}
... argv_cmd=${command}
``` | 0.0 | [
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_deployment_by_pattern"
] | [
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_dynamic_delete",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespace",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_inits_with_bearer_token",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_endpoints",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_daemon_set",
"test/test_KubeLibrary.py::TestKubeLibrary::test_inits_all_api_clients",
"test/test_KubeLibrary.py::TestKubeLibrary::test_assert_pod_has_annotations",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_secret_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_dynamic_create",
"test/test_KubeLibrary.py::TestKubeLibrary::test_get_namespaced_exec_without_container",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_cron_job",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_ingress",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_service_account_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_horizontal_pod_autoscaler",
"test/test_KubeLibrary.py::TestKubeLibrary::test_assert_container_has_env_vars",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_ingress",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_dynamic_replace",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_job_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_inits_with_context",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_daemon_set",
"test/test_KubeLibrary.py::TestKubeLibrary::test_get_matching_pods_in_namespace",
"test/test_KubeLibrary.py::TestKubeLibrary::test_get_namespaced_exec_not_argv_and_list",
"test/test_KubeLibrary.py::TestKubeLibrary::test_get_configmaps_in_namespace",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_cluster_role_binding",
"test/test_KubeLibrary.py::TestKubeLibrary::test_filter_containers_images",
"test/test_KubeLibrary.py::TestKubeLibrary::test_filter_pods_containers_statuses_by_name",
"test/test_KubeLibrary.py::TestKubeLibrary::test_evaluate_callable_from_k8s_client",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_persistent_volume_claim_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_dynamic_init",
"test/test_KubeLibrary.py::TestKubeLibrary::test_get_kubelet_version",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_role_binding",
"test/test_KubeLibrary.py::TestKubeLibrary::test_generate_alphanumeric_str",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_inits_from_kubeconfig",
"test/test_KubeLibrary.py::TestKubeLibrary::test_assert_pod_has_labels",
"test/test_KubeLibrary.py::TestKubeLibrary::test_filter_pods_containers_by_name",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_dynamic_patch",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_inits_with_bearer_token_with_ca_crt",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_role",
"test/test_KubeLibrary.py::TestKubeLibrary::test_filter_containers_resources",
"test/test_KubeLibrary.py::TestKubeLibrary::test_get_namespaced_exec_with_container",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_fails_for_wrong_context",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_stateful_set_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_persistent_volume_claim",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_horizontal_pod_autoscaler",
"test/test_KubeLibrary.py::TestKubeLibrary::test_KubeLibrary_dynamic_get",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_service",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_service",
"test/test_KubeLibrary.py::TestKubeLibrary::test_inits_with_bearer_token_raises_BearerTokenWithPrefixException",
"test/test_KubeLibrary.py::TestKubeLibrary::test_read_namespaced_pod_status",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_replica_set_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_cron_job",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_namespaced_pod_by_pattern",
"test/test_KubeLibrary.py::TestKubeLibrary::test_list_cluster_role"
] | 2022-12-16 14:17:09+00:00 | 1,894 |
|
devsnd__tinytag-156 | diff --git a/README.md b/README.md
index 39bfda9..cec3e9d 100644
--- a/README.md
+++ b/README.md
@@ -66,7 +66,7 @@ List of possible attributes you can get with TinyTag:
tag.title # title of the song
tag.track # track number as string
tag.track_total # total number of tracks as string
- tag.year # year or data as string
+ tag.year # year or date as string
For non-common fields and fields specific to single file formats use extra
diff --git a/tinytag/tinytag.py b/tinytag/tinytag.py
index 66fff62..a581e66 100644
--- a/tinytag/tinytag.py
+++ b/tinytag/tinytag.py
@@ -484,7 +484,7 @@ class ID3(TinyTag):
FRAME_ID_TO_FIELD = { # Mapping from Frame ID to a field of the TinyTag
'COMM': 'comment', 'COM': 'comment',
'TRCK': 'track', 'TRK': 'track',
- 'TYER': 'year', 'TYE': 'year',
+ 'TYER': 'year', 'TYE': 'year', 'TDRC': 'year',
'TALB': 'album', 'TAL': 'album',
'TPE1': 'artist', 'TP1': 'artist',
'TIT2': 'title', 'TT2': 'title',
| devsnd/tinytag | 5107f8611192a166dfea4f75619377ab2d5bda49 | diff --git a/tinytag/tests/test_all.py b/tinytag/tests/test_all.py
index abbb5dc..743e48b 100644
--- a/tinytag/tests/test_all.py
+++ b/tinytag/tests/test_all.py
@@ -73,7 +73,7 @@ testfiles = OrderedDict([
('samples/utf-8-id3v2.mp3',
{'extra': {}, 'genre': 'Acustico',
'track_total': '21', 'track': '01', 'filesize': 2119, 'title': 'Gran día',
- 'artist': 'Paso a paso', 'album': 'S/T', 'disc': '', 'disc_total': '0'}),
+ 'artist': 'Paso a paso', 'album': 'S/T', 'disc': '', 'disc_total': '0', 'year': '2003'}),
('samples/empty_file.mp3',
{'extra': {}, 'filesize': 0}),
('samples/silence-44khz-56k-mono-1s.mp3',
@@ -88,10 +88,11 @@ testfiles = OrderedDict([
'track_total': '12', 'genre': 'AlternRock',
'title': 'Out of the Woodwork', 'artist': 'Courtney Barnett',
'albumartist': 'Courtney Barnett', 'disc': '1',
- 'comment': 'Amazon.com Song ID: 240853806', 'composer': 'Courtney Barnett'}),
+ 'comment': 'Amazon.com Song ID: 240853806', 'composer': 'Courtney Barnett',
+ 'year': '2013'}),
('samples/utf16be.mp3',
{'extra': {}, 'title': '52-girls', 'filesize': 2048, 'track': '6', 'album': 'party mix',
- 'artist': 'The B52s', 'genre': 'Rock'}),
+ 'artist': 'The B52s', 'genre': 'Rock', 'year': '1981'}),
('samples/id3v22_image.mp3',
{'extra': {}, 'title': 'Kids (MGMT Cover) ', 'filesize': 35924,
'album': 'winniecooper.net ', 'artist': 'The Kooks', 'year': '2008',
@@ -189,7 +190,7 @@ testfiles = OrderedDict([
('samples/id3v24_genre_null_byte.mp3',
{'extra': {}, 'filesize': 256, 'album': '\u79d8\u5bc6', 'albumartist': 'aiko',
'artist': 'aiko', 'disc': '1', 'genre': 'Pop',
- 'title': '\u661f\u306e\u306a\u3044\u4e16\u754c', 'track': '10'}),
+ 'title': '\u661f\u306e\u306a\u3044\u4e16\u754c', 'track': '10', 'year': '2008'}),
('samples/vbr_xing_header_short.mp3',
{'filesize': 432, 'audio_offset': 133, 'bitrate': 24.0, 'channels': 1, 'duration': 0.144,
'extra': {}, 'samplerate': 8000}),
@@ -376,7 +377,7 @@ testfiles = OrderedDict([
{'extra': {}, 'channels': 2, 'duration': 0.2999546485260771, 'filesize': 6892,
'artist': 'Serhiy Storchaka', 'title': 'Pluck', 'album': 'Python Test Suite',
'bitrate': 176.4, 'samplerate': 11025, 'audio_offset': 116,
- 'comment': 'Audacity Pluck + Wahwah'}),
+ 'comment': 'Audacity Pluck + Wahwah', 'year': '2013'}),
('samples/M1F1-mulawC-AFsp.afc',
{'extra': {}, 'channels': 2, 'duration': 2.936625, 'filesize': 47148,
'bitrate': 256.0, 'samplerate': 8000, 'audio_offset': 154,
| [BUG] Parsing mp3 file w/ valid TDRC tag does not populate year
**Describe the bug**
When parsing a mp3 file w/ valid v2.4 metadata and a TDRC frame, TinyTag does not populate the year attribute in the output.
**To Reproduce**
Steps to reproduce the behavior:
1. Open a valid mp3 file w/ TinyTag
2. See `Found id3 Frame TDRC` in the program output
3. Resulting dict still has `"year": null`
**Expected behavior**
Valid timestamps (yyyy, yyyy-MM, yyyy-MM-dd, yyyy-MM-ddTHH, yyyy-MM-ddTHH:mm
and yyyy-MM-ddTHH:mm:ss) should result in year attribute populated.
**Sample File**
https://www.dropbox.com/s/lz6vlzhw18znqp8/cdwal5Kw3Fc.mp3?dl=0 | 0.0 | [
"tinytag/tests/test_all.py::test_file_reading[samples/utf-8-id3v2.mp3-expected8]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v24-long-title.mp3-expected12]",
"tinytag/tests/test_all.py::test_file_reading[samples/utf16be.mp3-expected13]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v24_genre_null_byte.mp3-expected39]",
"tinytag/tests/test_all.py::test_file_reading[samples/pluck-pcm8.aiff-expected77]"
] | [
"tinytag/tests/test_all.py::test_file_reading[samples/vbri.mp3-expected0]",
"tinytag/tests/test_all.py::test_file_reading[samples/cbr.mp3-expected1]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header.mp3-expected2]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header_2channel.mp3-expected3]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22-test.mp3-expected4]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-44-s-v1.mp3-expected5]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v1-latin1.mp3-expected6]",
"tinytag/tests/test_all.py::test_file_reading[samples/UTF16.mp3-expected7]",
"tinytag/tests/test_all.py::test_file_reading[samples/empty_file.mp3-expected9]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-44khz-56k-mono-1s.mp3-expected10]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-22khz-mono-1s.mp3-expected11]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22_image.mp3-expected14]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22.TCO.genre.mp3-expected15]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_comment_utf_16_with_bom.mp3-expected16]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_comment_utf_16_double_bom.mp3-expected17]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_genre_id_out_of_bounds.mp3-expected18]",
"tinytag/tests/test_all.py::test_file_reading[samples/image-text-encoding.mp3-expected19]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v1_does_not_overwrite_id3v2.mp3-expected20]",
"tinytag/tests/test_all.py::test_file_reading[samples/nicotinetestdata.mp3-expected21]",
"tinytag/tests/test_all.py::test_file_reading[samples/chinese_id3.mp3-expected22]",
"tinytag/tests/test_all.py::test_file_reading[samples/cut_off_titles.mp3-expected23]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_xxx_lang.mp3-expected24]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr8.mp3-expected25]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr8stereo.mp3-expected26]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr11.mp3-expected27]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr11stereo.mp3-expected28]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr16.mp3-expected29]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr16stereo.mp3-expected30]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr22.mp3-expected31]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr22stereo.mp3-expected32]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr32.mp3-expected33]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr32stereo.mp3-expected34]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr44.mp3-expected35]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr44stereo.mp3-expected36]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr48.mp3-expected37]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr48stereo.mp3-expected38]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header_short.mp3-expected40]",
"tinytag/tests/test_all.py::test_file_reading[samples/empty.ogg-expected41]",
"tinytag/tests/test_all.py::test_file_reading[samples/multipagecomment.ogg-expected42]",
"tinytag/tests/test_all.py::test_file_reading[samples/multipage-setup.ogg-expected43]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.ogg-expected44]",
"tinytag/tests/test_all.py::test_file_reading[samples/corrupt_metadata.ogg-expected45]",
"tinytag/tests/test_all.py::test_file_reading[samples/composer.ogg-expected46]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.opus-expected47]",
"tinytag/tests/test_all.py::test_file_reading[samples/8khz_5s.opus-expected48]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.wav-expected49]",
"tinytag/tests/test_all.py::test_file_reading[samples/test3sMono.wav-expected50]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-tagged.wav-expected51]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-riff-tags.wav-expected52]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-22khz-mono-1s.wav-expected53]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_header_with_a_zero_byte.wav-expected54]",
"tinytag/tests/test_all.py::test_file_reading[samples/adpcm.wav-expected55]",
"tinytag/tests/test_all.py::test_file_reading[samples/riff_extra_zero.wav-expected56]",
"tinytag/tests/test_all.py::test_file_reading[samples/riff_extra_zero_2.wav-expected57]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac1sMono.flac-expected58]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac453sStereo.flac-expected59]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac1.5sStereo.flac-expected60]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac_application.flac-expected61]",
"tinytag/tests/test_all.py::test_file_reading[samples/no-tags.flac-expected62]",
"tinytag/tests/test_all.py::test_file_reading[samples/variable-block.flac-expected63]",
"tinytag/tests/test_all.py::test_file_reading[samples/106-invalid-streaminfo.flac-expected64]",
"tinytag/tests/test_all.py::test_file_reading[samples/106-short-picture-block-size.flac-expected65]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_id3_header.flac-expected66]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_padded_id3_header.flac-expected67]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_padded_id3_header2.flac-expected68]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac_with_image.flac-expected69]",
"tinytag/tests/test_all.py::test_file_reading[samples/test2.wma-expected70]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.m4a-expected71]",
"tinytag/tests/test_all.py::test_file_reading[samples/test2.m4a-expected72]",
"tinytag/tests/test_all.py::test_file_reading[samples/iso8859_with_image.m4a-expected73]",
"tinytag/tests/test_all.py::test_file_reading[samples/alac_file.m4a-expected74]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-tagged.aiff-expected75]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.aiff-expected76]",
"tinytag/tests/test_all.py::test_file_reading[samples/M1F1-mulawC-AFsp.afc-expected78]",
"tinytag/tests/test_all.py::test_pathlib_compatibility",
"tinytag/tests/test_all.py::test_binary_path_compatibility",
"tinytag/tests/test_all.py::test_override_encoding",
"tinytag/tests/test_all.py::test_mp3_length_estimation",
"tinytag/tests/test_all.py::test_unpad",
"tinytag/tests/test_all.py::test_mp3_image_loading",
"tinytag/tests/test_all.py::test_mp3_id3v22_image_loading",
"tinytag/tests/test_all.py::test_mp3_image_loading_without_description",
"tinytag/tests/test_all.py::test_mp3_image_loading_with_utf8_description",
"tinytag/tests/test_all.py::test_mp3_image_loading2",
"tinytag/tests/test_all.py::test_mp3_utf_8_invalid_string_raises_exception",
"tinytag/tests/test_all.py::test_mp3_utf_8_invalid_string_can_be_ignored",
"tinytag/tests/test_all.py::test_mp4_image_loading",
"tinytag/tests/test_all.py::test_flac_image_loading",
"tinytag/tests/test_all.py::test_aiff_image_loading",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp3_id3.x-ID3]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp3_fffb.x-ID3]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_ogg.x-Ogg]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_wav.x-Wave]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_flac.x-Flac]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_wma.x-Wma]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp4_m4a.x-MP4]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_aiff.x-Aiff]",
"tinytag/tests/test_all.py::test_show_hint_for_wrong_usage",
"tinytag/tests/test_all.py::test_to_str"
] | 2022-09-02 01:45:25+00:00 | 1,895 |
|
devsnd__tinytag-171 | diff --git a/tinytag/tinytag.py b/tinytag/tinytag.py
index a7a8a21..8ba376d 100644
--- a/tinytag/tinytag.py
+++ b/tinytag/tinytag.py
@@ -924,8 +924,10 @@ class Ogg(TinyTag):
'artist': 'artist',
'date': 'year',
'tracknumber': 'track',
+ 'tracktotal': 'track_total',
'totaltracks': 'track_total',
'discnumber': 'disc',
+ 'disctotal': 'disc_total',
'totaldiscs': 'disc_total',
'genre': 'genre',
'description': 'comment',
| devsnd/tinytag | 85a16fa18bcc534d1d61f10ca539955823945d5d | diff --git a/tinytag/tests/test_all.py b/tinytag/tests/test_all.py
index 8f4421c..0b70517 100644
--- a/tinytag/tests/test_all.py
+++ b/tinytag/tests/test_all.py
@@ -230,7 +230,7 @@ testfiles = OrderedDict([
'track': '1', 'disc': '1', 'title': 'Bad Apple!!', 'duration': 2.0, 'year': '2008.05.25',
'filesize': 10000, 'artist': 'nomico',
'album': 'Exserens - A selection of Alstroemeria Records',
- 'comment': 'ARCD0018 - Lovelight'}),
+ 'comment': 'ARCD0018 - Lovelight', 'disc_total': '1', 'track_total': '13'}),
('samples/8khz_5s.opus',
{'extra': {}, 'filesize': 7251, 'channels': 1, 'samplerate': 48000, 'duration': 5.0}),
| Fail to read track_total, but it is ok in foobar
I got some tracks from qobuz.
I have some problems in reading the track_total. It showed 'null' in track_total. The others is OK, such as artist, album etc....
But its track_total seems OK, when checked in foobar.
Why?
The attachment are an example flac file
[19. I m Not The Man You Think I Am.zip](https://github.com/devsnd/tinytag/files/10875229/19.I.m.Not.The.Man.You.Think.I.Am.zip)
| 0.0 | [
"tinytag/tests/test_all.py::test_file_reading[samples/test.opus-expected47]"
] | [
"tinytag/tests/test_all.py::test_file_reading[samples/vbri.mp3-expected0]",
"tinytag/tests/test_all.py::test_file_reading[samples/cbr.mp3-expected1]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header.mp3-expected2]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header_2channel.mp3-expected3]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22-test.mp3-expected4]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-44-s-v1.mp3-expected5]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v1-latin1.mp3-expected6]",
"tinytag/tests/test_all.py::test_file_reading[samples/UTF16.mp3-expected7]",
"tinytag/tests/test_all.py::test_file_reading[samples/utf-8-id3v2.mp3-expected8]",
"tinytag/tests/test_all.py::test_file_reading[samples/empty_file.mp3-expected9]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-44khz-56k-mono-1s.mp3-expected10]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-22khz-mono-1s.mp3-expected11]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v24-long-title.mp3-expected12]",
"tinytag/tests/test_all.py::test_file_reading[samples/utf16be.mp3-expected13]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22_image.mp3-expected14]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22.TCO.genre.mp3-expected15]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_comment_utf_16_with_bom.mp3-expected16]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_comment_utf_16_double_bom.mp3-expected17]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_genre_id_out_of_bounds.mp3-expected18]",
"tinytag/tests/test_all.py::test_file_reading[samples/image-text-encoding.mp3-expected19]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v1_does_not_overwrite_id3v2.mp3-expected20]",
"tinytag/tests/test_all.py::test_file_reading[samples/nicotinetestdata.mp3-expected21]",
"tinytag/tests/test_all.py::test_file_reading[samples/chinese_id3.mp3-expected22]",
"tinytag/tests/test_all.py::test_file_reading[samples/cut_off_titles.mp3-expected23]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_xxx_lang.mp3-expected24]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr8.mp3-expected25]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr8stereo.mp3-expected26]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr11.mp3-expected27]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr11stereo.mp3-expected28]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr16.mp3-expected29]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr16stereo.mp3-expected30]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr22.mp3-expected31]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr22stereo.mp3-expected32]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr32.mp3-expected33]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr32stereo.mp3-expected34]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr44.mp3-expected35]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr44stereo.mp3-expected36]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr48.mp3-expected37]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr48stereo.mp3-expected38]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v24_genre_null_byte.mp3-expected39]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header_short.mp3-expected40]",
"tinytag/tests/test_all.py::test_file_reading[samples/empty.ogg-expected41]",
"tinytag/tests/test_all.py::test_file_reading[samples/multipagecomment.ogg-expected42]",
"tinytag/tests/test_all.py::test_file_reading[samples/multipage-setup.ogg-expected43]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.ogg-expected44]",
"tinytag/tests/test_all.py::test_file_reading[samples/corrupt_metadata.ogg-expected45]",
"tinytag/tests/test_all.py::test_file_reading[samples/composer.ogg-expected46]",
"tinytag/tests/test_all.py::test_file_reading[samples/8khz_5s.opus-expected48]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.wav-expected49]",
"tinytag/tests/test_all.py::test_file_reading[samples/test3sMono.wav-expected50]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-tagged.wav-expected51]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-riff-tags.wav-expected52]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-22khz-mono-1s.wav-expected53]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_header_with_a_zero_byte.wav-expected54]",
"tinytag/tests/test_all.py::test_file_reading[samples/adpcm.wav-expected55]",
"tinytag/tests/test_all.py::test_file_reading[samples/riff_extra_zero.wav-expected56]",
"tinytag/tests/test_all.py::test_file_reading[samples/riff_extra_zero_2.wav-expected57]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac1sMono.flac-expected58]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac453sStereo.flac-expected59]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac1.5sStereo.flac-expected60]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac_application.flac-expected61]",
"tinytag/tests/test_all.py::test_file_reading[samples/no-tags.flac-expected62]",
"tinytag/tests/test_all.py::test_file_reading[samples/variable-block.flac-expected63]",
"tinytag/tests/test_all.py::test_file_reading[samples/106-invalid-streaminfo.flac-expected64]",
"tinytag/tests/test_all.py::test_file_reading[samples/106-short-picture-block-size.flac-expected65]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_id3_header.flac-expected66]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_padded_id3_header.flac-expected67]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_padded_id3_header2.flac-expected68]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac_with_image.flac-expected69]",
"tinytag/tests/test_all.py::test_file_reading[samples/test2.wma-expected70]",
"tinytag/tests/test_all.py::test_file_reading[samples/lossless.wma-expected71]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.m4a-expected72]",
"tinytag/tests/test_all.py::test_file_reading[samples/test2.m4a-expected73]",
"tinytag/tests/test_all.py::test_file_reading[samples/iso8859_with_image.m4a-expected74]",
"tinytag/tests/test_all.py::test_file_reading[samples/alac_file.m4a-expected75]",
"tinytag/tests/test_all.py::test_file_reading[samples/mpeg4_desc_cmt.m4a-expected76]",
"tinytag/tests/test_all.py::test_file_reading[samples/mpeg4_xa9des.m4a-expected77]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-tagged.aiff-expected78]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.aiff-expected79]",
"tinytag/tests/test_all.py::test_file_reading[samples/pluck-pcm8.aiff-expected80]",
"tinytag/tests/test_all.py::test_file_reading[samples/M1F1-mulawC-AFsp.afc-expected81]",
"tinytag/tests/test_all.py::test_file_reading[samples/invalid_sample_rate.aiff-expected82]",
"tinytag/tests/test_all.py::test_pathlib_compatibility",
"tinytag/tests/test_all.py::test_binary_path_compatibility",
"tinytag/tests/test_all.py::test_override_encoding",
"tinytag/tests/test_all.py::test_mp3_length_estimation",
"tinytag/tests/test_all.py::test_unpad",
"tinytag/tests/test_all.py::test_mp3_image_loading",
"tinytag/tests/test_all.py::test_mp3_id3v22_image_loading",
"tinytag/tests/test_all.py::test_mp3_image_loading_without_description",
"tinytag/tests/test_all.py::test_mp3_image_loading_with_utf8_description",
"tinytag/tests/test_all.py::test_mp3_image_loading2",
"tinytag/tests/test_all.py::test_mp3_utf_8_invalid_string_raises_exception",
"tinytag/tests/test_all.py::test_mp3_utf_8_invalid_string_can_be_ignored",
"tinytag/tests/test_all.py::test_mp4_image_loading",
"tinytag/tests/test_all.py::test_flac_image_loading",
"tinytag/tests/test_all.py::test_ogg_image_loading",
"tinytag/tests/test_all.py::test_aiff_image_loading",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp3_id3.x-ID3]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp3_fffb.x-ID3]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_ogg.x-Ogg]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_wav.x-Wave]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_flac.x-Flac]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_wma.x-Wma]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp4_m4a.x-MP4]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_aiff.x-Aiff]",
"tinytag/tests/test_all.py::test_show_hint_for_wrong_usage",
"tinytag/tests/test_all.py::test_to_str"
] | 2023-03-20 22:28:45+00:00 | 1,896 |
|
devsnd__tinytag-178 | diff --git a/tinytag/tinytag.py b/tinytag/tinytag.py
index a3f1305..595a101 100644
--- a/tinytag/tinytag.py
+++ b/tinytag/tinytag.py
@@ -170,40 +170,48 @@ class TinyTag(object):
return parser
@classmethod
- def get_parser_class(cls, filename, filehandle):
+ def get_parser_class(cls, filename=None, filehandle=None):
if cls != TinyTag: # if `get` is invoked on TinyTag, find parser by ext
return cls # otherwise use the class on which `get` was invoked
- parser_class = cls._get_parser_for_filename(filename)
- if parser_class is not None:
- return parser_class
+ if filename:
+ parser_class = cls._get_parser_for_filename(filename)
+ if parser_class is not None:
+ return parser_class
# try determining the file type by magic byte header
- parser_class = cls._get_parser_for_file_handle(filehandle)
- if parser_class is not None:
- return parser_class
+ if filehandle:
+ parser_class = cls._get_parser_for_file_handle(filehandle)
+ if parser_class is not None:
+ return parser_class
raise TinyTagException('No tag reader found to support filetype! ')
@classmethod
- def get(cls, filename, tags=True, duration=True, image=False, ignore_errors=False,
- encoding=None):
- try: # cast pathlib.Path to str
- import pathlib
- if isinstance(filename, pathlib.Path):
- filename = str(filename.absolute())
- except ImportError:
- pass
+ def get(cls, filename=None, tags=True, duration=True, image=False,
+ ignore_errors=False, encoding=None, file_obj=None):
+ should_open_file = (file_obj is None)
+ if should_open_file:
+ try:
+ file_obj = io.open(filename, 'rb')
+ except TypeError:
+ file_obj = io.open(str(filename.absolute()), 'rb') # Python 3.4/3.5 pathlib support
+ filename = file_obj.name
else:
- filename = os.path.expanduser(filename)
- size = os.path.getsize(filename)
- if not size > 0:
- return TinyTag(None, 0)
- with io.open(filename, 'rb') as af:
- parser_class = cls.get_parser_class(filename, af)
- tag = parser_class(af, size, ignore_errors=ignore_errors)
+ file_obj = io.BufferedReader(file_obj) # buffered reader to support peeking
+ try:
+ file_obj.seek(0, os.SEEK_END)
+ filesize = file_obj.tell()
+ file_obj.seek(0)
+ if filesize <= 0:
+ return TinyTag(None, filesize)
+ parser_class = cls.get_parser_class(filename, file_obj)
+ tag = parser_class(file_obj, filesize, ignore_errors=ignore_errors)
tag._filename = filename
tag._default_encoding = encoding
tag.load(tags=tags, duration=duration, image=image)
tag.extra = dict(tag.extra) # turn default dict into dict so that it can throw KeyError
return tag
+ finally:
+ if should_open_file:
+ file_obj.close()
def __str__(self):
return json.dumps(OrderedDict(sorted(self.as_dict().items())))
| devsnd/tinytag | 337c044ce51f9e707b8cd31d02896c9041a98afa | diff --git a/tinytag/tests/test_all.py b/tinytag/tests/test_all.py
index 0b70517..aa3a38e 100644
--- a/tinytag/tests/test_all.py
+++ b/tinytag/tests/test_all.py
@@ -510,6 +510,13 @@ def test_pathlib_compatibility():
TinyTag.get(filename)
+def test_bytesio_compatibility():
+ testfile = next(iter(testfiles.keys()))
+ filename = os.path.join(testfolder, testfile)
+ with io.open(filename, 'rb') as file_handle:
+ TinyTag.get(file_obj=io.BytesIO(file_handle.read()))
+
+
@pytest.mark.skipif(sys.platform == "win32", reason='Windows does not support binary paths')
def test_binary_path_compatibility():
binary_file_path = os.path.join(os.path.dirname(__file__).encode('utf-8'), b'\x01.mp3')
| Support BytesIo objects for tagging
It would be awesome to support BytesIO or any other IO types with TinyTag. This way it can be used in some scenarios where files are packaged and do not have a normal path. | 0.0 | [
"tinytag/tests/test_all.py::test_bytesio_compatibility"
] | [
"tinytag/tests/test_all.py::test_file_reading[samples/vbri.mp3-expected0]",
"tinytag/tests/test_all.py::test_file_reading[samples/cbr.mp3-expected1]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header.mp3-expected2]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header_2channel.mp3-expected3]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22-test.mp3-expected4]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-44-s-v1.mp3-expected5]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v1-latin1.mp3-expected6]",
"tinytag/tests/test_all.py::test_file_reading[samples/UTF16.mp3-expected7]",
"tinytag/tests/test_all.py::test_file_reading[samples/utf-8-id3v2.mp3-expected8]",
"tinytag/tests/test_all.py::test_file_reading[samples/empty_file.mp3-expected9]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-44khz-56k-mono-1s.mp3-expected10]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-22khz-mono-1s.mp3-expected11]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v24-long-title.mp3-expected12]",
"tinytag/tests/test_all.py::test_file_reading[samples/utf16be.mp3-expected13]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22_image.mp3-expected14]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v22.TCO.genre.mp3-expected15]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_comment_utf_16_with_bom.mp3-expected16]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_comment_utf_16_double_bom.mp3-expected17]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_genre_id_out_of_bounds.mp3-expected18]",
"tinytag/tests/test_all.py::test_file_reading[samples/image-text-encoding.mp3-expected19]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v1_does_not_overwrite_id3v2.mp3-expected20]",
"tinytag/tests/test_all.py::test_file_reading[samples/nicotinetestdata.mp3-expected21]",
"tinytag/tests/test_all.py::test_file_reading[samples/chinese_id3.mp3-expected22]",
"tinytag/tests/test_all.py::test_file_reading[samples/cut_off_titles.mp3-expected23]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_xxx_lang.mp3-expected24]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr8.mp3-expected25]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr8stereo.mp3-expected26]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr11.mp3-expected27]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr11stereo.mp3-expected28]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr16.mp3-expected29]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr16stereo.mp3-expected30]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr22.mp3-expected31]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr22stereo.mp3-expected32]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr32.mp3-expected33]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr32stereo.mp3-expected34]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr44.mp3-expected35]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr44stereo.mp3-expected36]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr48.mp3-expected37]",
"tinytag/tests/test_all.py::test_file_reading[samples/mp3/vbr/vbr48stereo.mp3-expected38]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3v24_genre_null_byte.mp3-expected39]",
"tinytag/tests/test_all.py::test_file_reading[samples/vbr_xing_header_short.mp3-expected40]",
"tinytag/tests/test_all.py::test_file_reading[samples/empty.ogg-expected41]",
"tinytag/tests/test_all.py::test_file_reading[samples/multipagecomment.ogg-expected42]",
"tinytag/tests/test_all.py::test_file_reading[samples/multipage-setup.ogg-expected43]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.ogg-expected44]",
"tinytag/tests/test_all.py::test_file_reading[samples/corrupt_metadata.ogg-expected45]",
"tinytag/tests/test_all.py::test_file_reading[samples/composer.ogg-expected46]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.opus-expected47]",
"tinytag/tests/test_all.py::test_file_reading[samples/8khz_5s.opus-expected48]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.wav-expected49]",
"tinytag/tests/test_all.py::test_file_reading[samples/test3sMono.wav-expected50]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-tagged.wav-expected51]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-riff-tags.wav-expected52]",
"tinytag/tests/test_all.py::test_file_reading[samples/silence-22khz-mono-1s.wav-expected53]",
"tinytag/tests/test_all.py::test_file_reading[samples/id3_header_with_a_zero_byte.wav-expected54]",
"tinytag/tests/test_all.py::test_file_reading[samples/adpcm.wav-expected55]",
"tinytag/tests/test_all.py::test_file_reading[samples/riff_extra_zero.wav-expected56]",
"tinytag/tests/test_all.py::test_file_reading[samples/riff_extra_zero_2.wav-expected57]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac1sMono.flac-expected58]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac453sStereo.flac-expected59]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac1.5sStereo.flac-expected60]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac_application.flac-expected61]",
"tinytag/tests/test_all.py::test_file_reading[samples/no-tags.flac-expected62]",
"tinytag/tests/test_all.py::test_file_reading[samples/variable-block.flac-expected63]",
"tinytag/tests/test_all.py::test_file_reading[samples/106-invalid-streaminfo.flac-expected64]",
"tinytag/tests/test_all.py::test_file_reading[samples/106-short-picture-block-size.flac-expected65]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_id3_header.flac-expected66]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_padded_id3_header.flac-expected67]",
"tinytag/tests/test_all.py::test_file_reading[samples/with_padded_id3_header2.flac-expected68]",
"tinytag/tests/test_all.py::test_file_reading[samples/flac_with_image.flac-expected69]",
"tinytag/tests/test_all.py::test_file_reading[samples/test2.wma-expected70]",
"tinytag/tests/test_all.py::test_file_reading[samples/lossless.wma-expected71]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.m4a-expected72]",
"tinytag/tests/test_all.py::test_file_reading[samples/test2.m4a-expected73]",
"tinytag/tests/test_all.py::test_file_reading[samples/iso8859_with_image.m4a-expected74]",
"tinytag/tests/test_all.py::test_file_reading[samples/alac_file.m4a-expected75]",
"tinytag/tests/test_all.py::test_file_reading[samples/mpeg4_desc_cmt.m4a-expected76]",
"tinytag/tests/test_all.py::test_file_reading[samples/mpeg4_xa9des.m4a-expected77]",
"tinytag/tests/test_all.py::test_file_reading[samples/test-tagged.aiff-expected78]",
"tinytag/tests/test_all.py::test_file_reading[samples/test.aiff-expected79]",
"tinytag/tests/test_all.py::test_file_reading[samples/pluck-pcm8.aiff-expected80]",
"tinytag/tests/test_all.py::test_file_reading[samples/M1F1-mulawC-AFsp.afc-expected81]",
"tinytag/tests/test_all.py::test_file_reading[samples/invalid_sample_rate.aiff-expected82]",
"tinytag/tests/test_all.py::test_pathlib_compatibility",
"tinytag/tests/test_all.py::test_binary_path_compatibility",
"tinytag/tests/test_all.py::test_override_encoding",
"tinytag/tests/test_all.py::test_mp3_length_estimation",
"tinytag/tests/test_all.py::test_unpad",
"tinytag/tests/test_all.py::test_mp3_image_loading",
"tinytag/tests/test_all.py::test_mp3_id3v22_image_loading",
"tinytag/tests/test_all.py::test_mp3_image_loading_without_description",
"tinytag/tests/test_all.py::test_mp3_image_loading_with_utf8_description",
"tinytag/tests/test_all.py::test_mp3_image_loading2",
"tinytag/tests/test_all.py::test_mp3_utf_8_invalid_string_raises_exception",
"tinytag/tests/test_all.py::test_mp3_utf_8_invalid_string_can_be_ignored",
"tinytag/tests/test_all.py::test_mp4_image_loading",
"tinytag/tests/test_all.py::test_flac_image_loading",
"tinytag/tests/test_all.py::test_ogg_image_loading",
"tinytag/tests/test_all.py::test_aiff_image_loading",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp3_id3.x-ID3]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp3_fffb.x-ID3]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_ogg.x-Ogg]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_wav.x-Wave]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_flac.x-Flac]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_wma.x-Wma]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_mp4_m4a.x-MP4]",
"tinytag/tests/test_all.py::test_detect_magic_headers[samples/detect_aiff.x-Aiff]",
"tinytag/tests/test_all.py::test_show_hint_for_wrong_usage",
"tinytag/tests/test_all.py::test_to_str"
] | 2023-06-02 19:58:04+00:00 | 1,897 |
|
dfm__emcee-295 | diff --git a/.travis.yml b/.travis.yml
index fa02161..4aa919c 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -23,7 +23,7 @@ install:
- travis_retry python setup.py develop
script:
- - py.test -v emcee/tests --cov emcee
+ - pytest -v emcee/tests --cov emcee
after_success:
- coveralls
diff --git a/emcee/ensemble.py b/emcee/ensemble.py
index ae6a976..7d30afc 100644
--- a/emcee/ensemble.py
+++ b/emcee/ensemble.py
@@ -478,8 +478,8 @@ class EnsembleSampler(object):
@property
@deprecated("get_log_prob()")
def lnprobability(self): # pragma: no cover
- return self.get_log_prob()
-
+ log_prob = self.get_log_prob()
+ return np.swapaxes(log_prob, 0, 1)
@property
@deprecated("get_log_prob(flat=True)")
def flatlnprobability(self): # pragma: no cover
| dfm/emcee | 593a6b134170c47af6e31106244df78b6fb194db | diff --git a/emcee/tests/unit/test_sampler.py b/emcee/tests/unit/test_sampler.py
index 48a351a..ca38a56 100644
--- a/emcee/tests/unit/test_sampler.py
+++ b/emcee/tests/unit/test_sampler.py
@@ -47,11 +47,15 @@ def test_shapes(backend, moves, nwalkers=32, ndim=3, nsteps=10, seed=1234):
assert tau.shape == (ndim,)
# Check the shapes.
- assert sampler.chain.shape == (nwalkers, nsteps, ndim), \
- "incorrect coordinate dimensions"
+ with pytest.warns(DeprecationWarning):
+ assert sampler.chain.shape == (nwalkers, nsteps, ndim), \
+ "incorrect coordinate dimensions"
+ with pytest.warns(DeprecationWarning):
+ assert sampler.lnprobability.shape == (nwalkers, nsteps), \
+ "incorrect probability dimensions"
assert sampler.get_chain().shape == (nsteps, nwalkers, ndim), \
"incorrect coordinate dimensions"
- assert sampler.lnprobability.shape == (nsteps, nwalkers), \
+ assert sampler.get_log_prob().shape == (nsteps, nwalkers), \
"incorrect probability dimensions"
assert sampler.acceptance_fraction.shape == (nwalkers,), \
@@ -77,14 +81,14 @@ def test_errors(backend, nwalkers=32, ndim=3, nsteps=5, seed=1234):
# Test for not running.
with pytest.raises(AttributeError):
- sampler.chain
+ sampler.get_chain()
with pytest.raises(AttributeError):
- sampler.lnprobability
+ sampler.get_log_prob()
# What about not storing the chain.
sampler.run_mcmc(coords, nsteps, store=False)
with pytest.raises(AttributeError):
- sampler.chain
+ sampler.get_chain()
# Now what about if we try to continue using the sampler with an
# ensemble of a different shape.
@@ -121,12 +125,15 @@ def run_sampler(backend, nwalkers=32, ndim=3, nsteps=25, seed=1234,
def test_thin(backend):
with backend() as be:
with pytest.raises(ValueError):
- run_sampler(be, thin=-1)
+ with pytest.warns(DeprecationWarning):
+ run_sampler(be, thin=-1)
with pytest.raises(ValueError):
- run_sampler(be, thin=0.1)
+ with pytest.warns(DeprecationWarning):
+ run_sampler(be, thin=0.1)
thinby = 3
sampler1 = run_sampler(None)
- sampler2 = run_sampler(be, thin=thinby)
+ with pytest.warns(DeprecationWarning):
+ sampler2 = run_sampler(be, thin=thinby)
for k in ["get_chain", "get_log_prob"]:
a = getattr(sampler1, k)()[thinby-1::thinby]
b = getattr(sampler2, k)()
| sampler.lnprobability is transposed in emcee3?
tl;dr it looks like `sampler.lnprobability` is transposed in emcee 3 from what I would expect from emcee 2.
Sample code (copy pasta'd from the old docs):
```python
import numpy as np
import emcee
def lnprob(x, ivar):
return -0.5 * np.sum(ivar * x ** 2)
ndim, nwalkers = 10, 100
ivar = 1. / np.random.rand(ndim)
p0 = [np.random.rand(ndim) for i in range(nwalkers)]
sampler = emcee.EnsembleSampler(nwalkers, ndim, lnprob, args=[ivar])
_ = sampler.run_mcmc(p0, 1000)
print(emcee.__version__)
print(sampler.chain.shape, sampler.lnprobability.shape)
```
## Output from emcee 2.2.1
```
2.2.1
(100, 1000, 10) (100, 1000)
```
## Output from emcee 3.0rc2
```
3.0rc2
(100, 1000, 10) (1000, 100)
``` | 0.0 | [
"emcee/tests/unit/test_sampler.py::test_shapes[Backend-None]",
"emcee/tests/unit/test_sampler.py::test_shapes[Backend-moves1]",
"emcee/tests/unit/test_sampler.py::test_shapes[Backend-moves2]",
"emcee/tests/unit/test_sampler.py::test_shapes[Backend-moves3]"
] | [
"emcee/tests/unit/test_sampler.py::test_thin[Backend]",
"emcee/tests/unit/test_sampler.py::test_thin_by[Backend-True]",
"emcee/tests/unit/test_sampler.py::test_thin_by[Backend-False]",
"emcee/tests/unit/test_sampler.py::test_restart[Backend]",
"emcee/tests/unit/test_sampler.py::test_vectorize",
"emcee/tests/unit/test_sampler.py::test_pickle[Backend]"
] | 2019-04-11 00:18:44+00:00 | 1,898 |
|
dfm__emcee-354 | diff --git a/src/emcee/autocorr.py b/src/emcee/autocorr.py
index 5156372..4a804ff 100644
--- a/src/emcee/autocorr.py
+++ b/src/emcee/autocorr.py
@@ -49,7 +49,7 @@ def integrated_time(x, c=5, tol=50, quiet=False):
"""Estimate the integrated autocorrelation time of a time series.
This estimate uses the iterative procedure described on page 16 of
- `Sokal's notes <http://www.stat.unc.edu/faculty/cji/Sokal.pdf>`_ to
+ `Sokal's notes <https://www.semanticscholar.org/paper/Monte-Carlo-Methods-in-Statistical-Mechanics%3A-and-Sokal/0bfe9e3db30605fe2d4d26e1a288a5e2997e7225>`_ to
determine a reasonable window size.
Args:
diff --git a/src/emcee/backends/hdf.py b/src/emcee/backends/hdf.py
index d4e6d6c..9503512 100644
--- a/src/emcee/backends/hdf.py
+++ b/src/emcee/backends/hdf.py
@@ -2,7 +2,7 @@
from __future__ import division, print_function
-__all__ = ["HDFBackend", "TempHDFBackend"]
+__all__ = ["HDFBackend", "TempHDFBackend", "does_hdf5_support_longdouble"]
import os
from tempfile import NamedTemporaryFile
@@ -19,6 +19,25 @@ except ImportError:
h5py = None
+def does_hdf5_support_longdouble():
+ if h5py is None:
+ return False
+ with NamedTemporaryFile(prefix="emcee-temporary-hdf5",
+ suffix=".hdf5",
+ delete=False) as f:
+ f.close()
+
+ with h5py.File(f.name, "w") as hf:
+ g = hf.create_group("group")
+ g.create_dataset("data", data=np.ones(1, dtype=np.longdouble))
+ if g["data"].dtype != np.longdouble:
+ return False
+ with h5py.File(f.name, "r") as hf:
+ if hf["group"]["data"].dtype != np.longdouble:
+ return False
+ return True
+
+
class HDFBackend(Backend):
"""A backend that stores the chain in an HDF5 file using h5py
diff --git a/tox.ini b/tox.ini
index 6c09ae3..9f72a99 100644
--- a/tox.ini
+++ b/tox.ini
@@ -1,5 +1,5 @@
[tox]
-envlist = py{36,37}
+envlist = py{36,37,38}
[testenv]
description = Unit tests
| dfm/emcee | c14b212964cfc1543c41f0bd186cafb399e847f7 | diff --git a/src/emcee/tests/integration/test_longdouble.py b/src/emcee/tests/integration/test_longdouble.py
index a2142d5..b8d2c7a 100644
--- a/src/emcee/tests/integration/test_longdouble.py
+++ b/src/emcee/tests/integration/test_longdouble.py
@@ -2,6 +2,7 @@ import numpy as np
import pytest
import emcee
+from emcee.backends.hdf import does_hdf5_support_longdouble, TempHDFBackend
def test_longdouble_doesnt_crash_bug_312():
@@ -17,9 +18,11 @@ def test_longdouble_doesnt_crash_bug_312():
sampler.run_mcmc(p0, 100)
-@pytest.mark.parametrize("cls", [emcee.backends.Backend,
- emcee.backends.TempHDFBackend])
+@pytest.mark.parametrize("cls", emcee.backends.get_test_backends())
def test_longdouble_actually_needed(cls):
+ if (issubclass(cls, TempHDFBackend)
+ and not does_hdf5_support_longdouble()):
+ pytest.xfail("HDF5 does not support long double on this platform")
mjd = np.longdouble(58000.)
sigma = 100*np.finfo(np.longdouble).eps*mjd
diff --git a/src/emcee/tests/unit/test_backends.py b/src/emcee/tests/unit/test_backends.py
index e5466ec..370008e 100644
--- a/src/emcee/tests/unit/test_backends.py
+++ b/src/emcee/tests/unit/test_backends.py
@@ -2,12 +2,18 @@
import os
from itertools import product
+from tempfile import NamedTemporaryFile
-import h5py
import numpy as np
import pytest
from emcee import EnsembleSampler, backends, State
+from emcee.backends.hdf import does_hdf5_support_longdouble
+
+try:
+ import h5py
+except ImportError:
+ h5py = None
__all__ = ["test_backend", "test_reload"]
@@ -55,6 +61,7 @@ def _custom_allclose(a, b):
assert np.allclose(a[n], b[n])
+@pytest.mark.skipif(h5py is None, reason="HDF5 not available")
def test_uninit(tmpdir):
fn = str(tmpdir.join("EMCEE_TEST_FILE_DO_NOT_USE.h5"))
if os.path.exists(fn):
@@ -208,6 +215,7 @@ def test_restart(backend, dtype):
assert np.allclose(a, b), "inconsistent acceptance fraction"
+@pytest.mark.skipif(h5py is None, reason="HDF5 not available")
def test_multi_hdf5():
with backends.TempHDFBackend() as backend1:
run_sampler(backend1)
@@ -227,6 +235,9 @@ def test_multi_hdf5():
@pytest.mark.parametrize("backend", all_backends)
def test_longdouble_preserved(backend):
+ if (issubclass(backend, backends.TempHDFBackend)
+ and not does_hdf5_support_longdouble()):
+ pytest.xfail("HDF5 does not support long double on this platform")
nwalkers = 10
ndim = 2
nsteps = 5
@@ -252,14 +263,3 @@ def test_longdouble_preserved(backend):
assert s.log_prob.dtype == np.longdouble
assert np.all(s.log_prob == lp)
-
-
-def test_hdf5_dtypes():
- nwalkers = 10
- ndim = 2
- with backends.TempHDFBackend(dtype=np.longdouble) as b:
- assert b.dtype == np.longdouble
- b.reset(nwalkers, ndim)
- with h5py.File(b.filename, "r") as f:
- g = f["test"]
- assert g["chain"].dtype == np.longdouble
| long double tests fail on ppc64el
**General information:**
- emcee version: 3.0.2
- platform: ppc64el
- installation method (pip/conda/source/other?): Ubuntu/Debian package
**Problem description:**
Version 3.0.2 of emcee is not migrating to the release pocket of Ubuntu because tests are failing on ppc64el: https://autopkgtest.ubuntu.com/packages/e/emcee/groovy/ppc64el
### Expected behavior
tests pass
### Actual behavior:
test_longdouble_actually_needed[TempHDFBackend] and test_longdouble_preserved[TempHDFBackend] fail:
```
_______________ test_longdouble_actually_needed[TempHDFBackend] ________________
cls = <class 'emcee.backends.hdf.TempHDFBackend'>
@pytest.mark.parametrize("cls", [emcee.backends.Backend,
emcee.backends.TempHDFBackend])
def test_longdouble_actually_needed(cls):
mjd = np.longdouble(58000.)
sigma = 100*np.finfo(np.longdouble).eps*mjd
def log_prob(x):
assert x.dtype == np.longdouble
return -0.5 * np.sum(((x-mjd)/sigma) ** 2)
ndim, nwalkers = 1, 20
steps = 1000
p0 = sigma*np.random.randn(nwalkers, ndim).astype(np.longdouble) + mjd
assert not all(p0 == mjd)
with cls(dtype=np.longdouble) as backend:
sampler = emcee.EnsembleSampler(nwalkers,
ndim,
log_prob,
backend=backend)
sampler.run_mcmc(p0, steps)
samples = sampler.get_chain().reshape((-1,))
> assert samples.dtype == np.longdouble
E AssertionError: assert dtype('<f8') == <class 'numpy.float128'>
E + where dtype('<f8') = array([ 5.80000000e+04, -4.60105223e-27, 5.80000000e+04, ...,\n -6.24580733e-26, 5.80000000e+04, -7.38930048e-27]).dtype
E + and <class 'numpy.float128'> = np.longdouble
```
(the other failure is similar)
### What have you tried so far?
Googling and getting a headache :)
I know that "long double" on PowerPC / POWER is a strange pair-of-64-bit-doubles type so I'm not surprised that it's on POWER that I see the problem. I also see from searching that hd5/h5py/numpy have a history of problems in this area (e.g. https://github.com/h5py/h5py/issues/817) so I'm not sure what the resolution should be. Skipping these tests on ppc64el for now (maybe in an Ubuntu specific patch) might make sense -- it's not like the failure of these new tests indicates any kind of regression. | 0.0 | [
"src/emcee/tests/integration/test_longdouble.py::test_longdouble_actually_needed[Backend]",
"src/emcee/tests/unit/test_backends.py::test_longdouble_preserved[Backend]"
] | [] | 2020-08-18 14:48:36+00:00 | 1,899 |
|
dfm__emcee-386 | diff --git a/src/emcee/ensemble.py b/src/emcee/ensemble.py
index 45cc4ba..24faba3 100644
--- a/src/emcee/ensemble.py
+++ b/src/emcee/ensemble.py
@@ -1,9 +1,10 @@
# -*- coding: utf-8 -*-
import warnings
+from itertools import count
+from typing import Dict, List, Optional, Union
import numpy as np
-from itertools import count
from .backends import Backend
from .model import Model
@@ -61,6 +62,10 @@ class EnsembleSampler(object):
to accept a list of position vectors instead of just one. Note
that ``pool`` will be ignored if this is ``True``.
(default: ``False``)
+ parameter_names (Optional[Union[List[str], Dict[str, List[int]]]]):
+ names of individual parameters or groups of parameters. If
+ specified, the ``log_prob_fn`` will recieve a dictionary of
+ parameters, rather than a ``np.ndarray``.
"""
@@ -76,6 +81,7 @@ class EnsembleSampler(object):
backend=None,
vectorize=False,
blobs_dtype=None,
+ parameter_names: Optional[Union[Dict[str, int], List[str]]] = None,
# Deprecated...
a=None,
postargs=None,
@@ -157,6 +163,49 @@ class EnsembleSampler(object):
# ``args`` and ``kwargs`` pickleable.
self.log_prob_fn = _FunctionWrapper(log_prob_fn, args, kwargs)
+ # Save the parameter names
+ self.params_are_named: bool = parameter_names is not None
+ if self.params_are_named:
+ assert isinstance(parameter_names, (list, dict))
+
+ # Don't support vectorizing yet
+ msg = "named parameters with vectorization unsupported for now"
+ assert not self.vectorize, msg
+
+ # Check for duplicate names
+ dupes = set()
+ uniq = []
+ for name in parameter_names:
+ if name not in dupes:
+ uniq.append(name)
+ dupes.add(name)
+ msg = f"duplicate paramters: {dupes}"
+ assert len(uniq) == len(parameter_names), msg
+
+ if isinstance(parameter_names, list):
+ # Check for all named
+ msg = "name all parameters or set `parameter_names` to `None`"
+ assert len(parameter_names) == ndim, msg
+ # Convert a list to a dict
+ parameter_names: Dict[str, int] = {
+ name: i for i, name in enumerate(parameter_names)
+ }
+
+ # Check not too many names
+ msg = "too many names"
+ assert len(parameter_names) <= ndim, msg
+
+ # Check all indices appear
+ values = [
+ v if isinstance(v, list) else [v]
+ for v in parameter_names.values()
+ ]
+ values = [item for sublist in values for item in sublist]
+ values = set(values)
+ msg = f"not all values appear -- set should be 0 to {ndim-1}"
+ assert values == set(np.arange(ndim)), msg
+ self.parameter_names = parameter_names
+
@property
def random_state(self):
"""
@@ -251,8 +300,9 @@ class EnsembleSampler(object):
raise ValueError("'store' must be False when 'iterations' is None")
# Interpret the input as a walker state and check the dimensions.
state = State(initial_state, copy=True)
- if np.shape(state.coords) != (self.nwalkers, self.ndim):
- raise ValueError("incompatible input dimensions")
+ state_shape = np.shape(state.coords)
+ if state_shape != (self.nwalkers, self.ndim):
+ raise ValueError(f"incompatible input dimensions {state_shape}")
if (not skip_initial_state_check) and (
not walkers_independent(state.coords)
):
@@ -416,6 +466,10 @@ class EnsembleSampler(object):
if np.any(np.isnan(p)):
raise ValueError("At least one parameter value was NaN")
+ # If the parmaeters are named, then switch to dictionaries
+ if self.params_are_named:
+ p = ndarray_to_list_of_dicts(p, self.parameter_names)
+
# Run the log-probability calculations (optionally in parallel).
if self.vectorize:
results = self.log_prob_fn(p)
@@ -427,9 +481,7 @@ class EnsembleSampler(object):
map_func = self.pool.map
else:
map_func = map
- results = list(
- map_func(self.log_prob_fn, (p[i] for i in range(len(p))))
- )
+ results = list(map_func(self.log_prob_fn, p))
try:
log_prob = np.array([float(l[0]) for l in results])
@@ -444,8 +496,9 @@ class EnsembleSampler(object):
else:
try:
with warnings.catch_warnings(record=True):
- warnings.simplefilter("error",
- np.VisibleDeprecationWarning)
+ warnings.simplefilter(
+ "error", np.VisibleDeprecationWarning
+ )
try:
dt = np.atleast_1d(blob[0]).dtype
except Warning:
@@ -455,7 +508,8 @@ class EnsembleSampler(object):
"placed in an object array. Numpy has "
"deprecated this automatic detection, so "
"please specify "
- "blobs_dtype=np.dtype('object')")
+ "blobs_dtype=np.dtype('object')"
+ )
dt = np.dtype("object")
except ValueError:
dt = np.dtype("object")
@@ -557,8 +611,8 @@ class _FunctionWrapper(object):
def __init__(self, f, args, kwargs):
self.f = f
- self.args = [] if args is None else args
- self.kwargs = {} if kwargs is None else kwargs
+ self.args = args or []
+ self.kwargs = kwargs or {}
def __call__(self, x):
try:
@@ -605,3 +659,22 @@ def _scaled_cond(a):
return np.inf
c = b / bsum
return np.linalg.cond(c.astype(float))
+
+
+def ndarray_to_list_of_dicts(
+ x: np.ndarray,
+ key_map: Dict[str, Union[int, List[int]]],
+) -> List[Dict[str, Union[np.number, np.ndarray]]]:
+ """
+ A helper function to convert a ``np.ndarray`` into a list
+ of dictionaries of parameters. Used when parameters are named.
+
+ Args:
+ x (np.ndarray): parameter array of shape ``(N, n_dim)``, where
+ ``N`` is an integer
+ key_map (Dict[str, Union[int, List[int]]):
+
+ Returns:
+ list of dictionaries of parameters
+ """
+ return [{key: xi[val] for key, val in key_map.items()} for xi in x]
| dfm/emcee | f0489d74250e2034d76c3a812b9afae74c9ca191 | diff --git a/src/emcee/tests/unit/test_ensemble.py b/src/emcee/tests/unit/test_ensemble.py
new file mode 100644
index 0000000..f6f5ad2
--- /dev/null
+++ b/src/emcee/tests/unit/test_ensemble.py
@@ -0,0 +1,184 @@
+"""
+Unit tests of some functionality in ensemble.py when the parameters are named
+"""
+import string
+from unittest import TestCase
+
+import numpy as np
+import pytest
+
+from emcee.ensemble import EnsembleSampler, ndarray_to_list_of_dicts
+
+
+class TestNP2ListOfDicts(TestCase):
+ def test_ndarray_to_list_of_dicts(self):
+ # Try different numbers of keys
+ for n_keys in [1, 2, 10, 26]:
+ keys = list(string.ascii_lowercase[:n_keys])
+ key_set = set(keys)
+ key_dict = {key: i for i, key in enumerate(keys)}
+ # Try different number of walker/procs
+ for N in [1, 2, 3, 10, 100]:
+ x = np.random.rand(N, n_keys)
+
+ LOD = ndarray_to_list_of_dicts(x, key_dict)
+ assert len(LOD) == N, "need 1 dict per row"
+ for i, dct in enumerate(LOD):
+ assert dct.keys() == key_set, "keys are missing"
+ for j, key in enumerate(keys):
+ assert dct[key] == x[i, j], f"wrong value at {(i, j)}"
+
+
+class TestNamedParameters(TestCase):
+ """
+ Test that a keyword-based log-probability function instead of
+ a positional.
+ """
+
+ # Keyword based lnpdf
+ def lnpdf(self, pars) -> np.float64:
+ mean = pars["mean"]
+ var = pars["var"]
+ if var <= 0:
+ return -np.inf
+ return (
+ -0.5 * ((mean - self.x) ** 2 / var + np.log(2 * np.pi * var)).sum()
+ )
+
+ def lnpdf_mixture(self, pars) -> np.float64:
+ mean1 = pars["mean1"]
+ var1 = pars["var1"]
+ mean2 = pars["mean2"]
+ var2 = pars["var2"]
+ if var1 <= 0 or var2 <= 0:
+ return -np.inf
+ return (
+ -0.5
+ * (
+ (mean1 - self.x) ** 2 / var1
+ + np.log(2 * np.pi * var1)
+ + (mean2 - self.x - 3) ** 2 / var2
+ + np.log(2 * np.pi * var2)
+ ).sum()
+ )
+
+ def lnpdf_mixture_grouped(self, pars) -> np.float64:
+ mean1, mean2 = pars["means"]
+ var1, var2 = pars["vars"]
+ const = pars["constant"]
+ if var1 <= 0 or var2 <= 0:
+ return -np.inf
+ return (
+ -0.5
+ * (
+ (mean1 - self.x) ** 2 / var1
+ + np.log(2 * np.pi * var1)
+ + (mean2 - self.x - 3) ** 2 / var2
+ + np.log(2 * np.pi * var2)
+ ).sum()
+ + const
+ )
+
+ def setUp(self):
+ # Draw some data from a unit Gaussian
+ self.x = np.random.randn(100)
+ self.names = ["mean", "var"]
+
+ def test_named_parameters(self):
+ sampler = EnsembleSampler(
+ nwalkers=10,
+ ndim=len(self.names),
+ log_prob_fn=self.lnpdf,
+ parameter_names=self.names,
+ )
+ assert sampler.params_are_named
+ assert list(sampler.parameter_names.keys()) == self.names
+
+ def test_asserts(self):
+ # ndim name mismatch
+ with pytest.raises(AssertionError):
+ _ = EnsembleSampler(
+ nwalkers=10,
+ ndim=len(self.names) - 1,
+ log_prob_fn=self.lnpdf,
+ parameter_names=self.names,
+ )
+
+ # duplicate names
+ with pytest.raises(AssertionError):
+ _ = EnsembleSampler(
+ nwalkers=10,
+ ndim=3,
+ log_prob_fn=self.lnpdf,
+ parameter_names=["a", "b", "a"],
+ )
+
+ # vectorize turned on
+ with pytest.raises(AssertionError):
+ _ = EnsembleSampler(
+ nwalkers=10,
+ ndim=len(self.names),
+ log_prob_fn=self.lnpdf,
+ parameter_names=self.names,
+ vectorize=True,
+ )
+
+ def test_compute_log_prob(self):
+ # Try different numbers of walkers
+ for N in [4, 8, 10]:
+ sampler = EnsembleSampler(
+ nwalkers=N,
+ ndim=len(self.names),
+ log_prob_fn=self.lnpdf,
+ parameter_names=self.names,
+ )
+ coords = np.random.rand(N, len(self.names))
+ lnps, _ = sampler.compute_log_prob(coords)
+ assert len(lnps) == N
+ assert lnps.dtype == np.float64
+
+ def test_compute_log_prob_mixture(self):
+ names = ["mean1", "var1", "mean2", "var2"]
+ # Try different numbers of walkers
+ for N in [8, 10, 20]:
+ sampler = EnsembleSampler(
+ nwalkers=N,
+ ndim=len(names),
+ log_prob_fn=self.lnpdf_mixture,
+ parameter_names=names,
+ )
+ coords = np.random.rand(N, len(names))
+ lnps, _ = sampler.compute_log_prob(coords)
+ assert len(lnps) == N
+ assert lnps.dtype == np.float64
+
+ def test_compute_log_prob_mixture_grouped(self):
+ names = {"means": [0, 1], "vars": [2, 3], "constant": 4}
+ # Try different numbers of walkers
+ for N in [8, 10, 20]:
+ sampler = EnsembleSampler(
+ nwalkers=N,
+ ndim=5,
+ log_prob_fn=self.lnpdf_mixture_grouped,
+ parameter_names=names,
+ )
+ coords = np.random.rand(N, 5)
+ lnps, _ = sampler.compute_log_prob(coords)
+ assert len(lnps) == N
+ assert lnps.dtype == np.float64
+
+ def test_run_mcmc(self):
+ # Sort of an integration test
+ n_walkers = 4
+ sampler = EnsembleSampler(
+ nwalkers=n_walkers,
+ ndim=len(self.names),
+ log_prob_fn=self.lnpdf,
+ parameter_names=self.names,
+ )
+ guess = np.random.rand(n_walkers, len(self.names))
+ n_steps = 50
+ results = sampler.run_mcmc(guess, n_steps)
+ assert results.coords.shape == (n_walkers, len(self.names))
+ chain = sampler.chain
+ assert chain.shape == (n_walkers, n_steps, len(self.names))
| [ENH] support for dictionaries and/or namedtuples
## Proposal
The sampler API shares a lot of features with `scipy.optimize.minimize` -- given a model that can be typed as `Callable(List,...)` keep proposing samples to improve the model. This is fine for many problems, but one can imagine a scenario where it would be useful for model developers to handle samples with more than just a `List`. Handling parameters in a `List` means that writing a posterior *requires defining parameters positionally*. In contrast, in packages like pytorch one can refer to model parameters by name (eg. a `torch.nn.Linear` has a `weight` and `bias` named parameters).
This issue proposes enhancing the emcee API to allow for dictionary and/or namedtuple parameter constructs. Looking at the API for the `EnsembleSampler`, this would require an additional constructor argument, since the sampler does not inspect the state of the `log_prob_fn`.
Here is a minimum proposed implementation for supporting a dictionary:
```python
class EnsembleSampler(object):
def __init__(
self,
nwalkers: int,
ndim: int,
log_prob_fn: Callable(Union[List, Dict]),
parameter_names: Optional[List[str]] = None,
...
):
...
# Save the names
self.parameter_names = parameter_names
self.name_parameters: bool = parameter_names is not None
if self.name_parameters:
assert len(parameter_names) == ndim, f"names must match dimensions"
...
def sample(...):
...
with get_progress_bar(progress, total) as pbar:
i = 0
for _ in count() if iterations is None else range(iterations):
for _ in range(yield_step):
...
# If our parameters are named, return them in a named format
if self.name_parameters:
state = {key: value for key, value in zip(self.parameter_names, state)}
yield state
```
This allows for a lot more flexibility downstream for model developers. As a simple example, consider a model composed of two parts, that each can be updated in a way that doesn't affect the higher level model:
```python
class PartA:
__slots__ = ["param_a"]
param_a: float
def __call__(self):
...
class PartB:
__slots__ = ["param_b"]
param_b: float
def __call__(self):
...
class MyModel:
def __init__(self, a = PartA(), b = PartB()):
self.a = a
self.b = b
def log_likelihood_fn(self, params: Dict[str, float]):
# Update the composed parts of the model
for key in self.a.__slots__:
setattr(self.a, params[key])
for key in self.b.__slots__:
setattr(self.b, params[key])
# Final likelihood uses the results of A and B, but we don't
# care how they are implemented
return f(self.a(), self.b())
```
If this sounds like an attractive feature I would be happy to work on a PR. | 0.0 | [
"src/emcee/tests/unit/test_ensemble.py::TestNP2ListOfDicts::test_ndarray_to_list_of_dicts",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_asserts",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_compute_log_prob",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_compute_log_prob_mixture",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_compute_log_prob_mixture_grouped",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_named_parameters",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_run_mcmc"
] | [] | 2021-04-24 22:21:11+00:00 | 1,900 |
|
dfm__emcee-510 | diff --git a/src/emcee/ensemble.py b/src/emcee/ensemble.py
index 3212099..cfef4a5 100644
--- a/src/emcee/ensemble.py
+++ b/src/emcee/ensemble.py
@@ -489,10 +489,19 @@ class EnsembleSampler(object):
results = list(map_func(self.log_prob_fn, p))
try:
- log_prob = np.array([float(l[0]) for l in results])
- blob = [l[1:] for l in results]
+ # perhaps log_prob_fn returns blobs?
+
+ # deal with the blobs first
+ # if l does not have a len attribute (i.e. not a sequence, no blob)
+ # then a TypeError is raised. However, no error will be raised if
+ # l is a length-1 array, np.array([1.234]). In that case blob
+ # will become an empty list.
+ blob = [l[1:] for l in results if len(l) > 1]
+ if not len(blob):
+ raise IndexError
+ log_prob = np.array([_scalar(l[0]) for l in results])
except (IndexError, TypeError):
- log_prob = np.array([float(l) for l in results])
+ log_prob = np.array([_scalar(l) for l in results])
blob = None
else:
# Get the blobs dtype
@@ -502,7 +511,7 @@ class EnsembleSampler(object):
try:
with warnings.catch_warnings(record=True):
warnings.simplefilter(
- "error", np.VisibleDeprecationWarning
+ "error", np.exceptions.VisibleDeprecationWarning
)
try:
dt = np.atleast_1d(blob[0]).dtype
@@ -682,3 +691,16 @@ def ndarray_to_list_of_dicts(
list of dictionaries of parameters
"""
return [{key: xi[val] for key, val in key_map.items()} for xi in x]
+
+
+def _scalar(fx):
+ # Make sure a value is a true scalar
+ # 1.0, np.float64(1.0), np.array([1.0]), np.array(1.0)
+ if not np.isscalar(fx):
+ try:
+ fx = np.asarray(fx).item()
+ except (TypeError, ValueError) as e:
+ raise ValueError("log_prob_fn should return scalar") from e
+ return float(fx)
+ else:
+ return float(fx)
diff --git a/tox.ini b/tox.ini
index d759fa6..c566174 100644
--- a/tox.ini
+++ b/tox.ini
@@ -1,12 +1,12 @@
[tox]
-envlist = py{37,38,39,310}{,-extras},lint
+envlist = py{39,310,311,312}{,-extras},lint
[gh-actions]
python =
- 3.7: py37
- 3.8: py38
- 3.9: py39-extras
+ 3.9: py39
3.10: py310
+ 3.11: py311-extras
+ 3.12: py312
[testenv]
deps = coverage[toml]
| dfm/emcee | b68933df3df80634cf133cab7e92db0458fffe13 | diff --git a/.github/workflows/tests.yml b/.github/workflows/tests.yml
index 3a3dc22..063f0c0 100644
--- a/.github/workflows/tests.yml
+++ b/.github/workflows/tests.yml
@@ -16,7 +16,7 @@ jobs:
runs-on: ${{ matrix.os }}
strategy:
matrix:
- python-version: ["3.7", "3.8", "3.9", "3.10"]
+ python-version: ["3.9", "3.10", "3.11", "3.12"]
os: ["ubuntu-latest"]
include:
- python-version: "3.9"
@@ -49,6 +49,30 @@ jobs:
COVERALLS_PARALLEL: true
COVERALLS_FLAG_NAME: ${{ matrix.python-version }}-${{ matrix.os }}
+ leading_edge:
+ runs-on: ${{ matrix.os }}
+ strategy:
+ matrix:
+ python-version: ["3.12"]
+ os: ["ubuntu-latest"]
+
+ steps:
+ - name: Checkout
+ uses: actions/checkout@v4
+ with:
+ fetch-depth: 0
+ - name: Setup Python
+ uses: actions/setup-python@v5
+ with:
+ python-version: ${{ matrix.python-version }}
+ - name: Install dependencies
+ run: |
+ python -m pip install -U pip
+ python -m pip install pip install pytest "numpy>=2.0.0rc1"
+ python -m pip install -e.
+ - name: Run tests
+ run: pytest
+
coverage:
needs: tests
runs-on: ubuntu-latest
diff --git a/src/emcee/tests/unit/test_ensemble.py b/src/emcee/tests/unit/test_ensemble.py
index f0568b2..e7981c8 100644
--- a/src/emcee/tests/unit/test_ensemble.py
+++ b/src/emcee/tests/unit/test_ensemble.py
@@ -183,3 +183,37 @@ class TestNamedParameters(TestCase):
assert results.coords.shape == (n_walkers, len(self.names))
chain = sampler.chain
assert chain.shape == (n_walkers, n_steps, len(self.names))
+
+
+class TestLnProbFn(TestCase):
+ # checks that the log_prob_fn can deal with a variety of 'scalar-likes'
+ def lnpdf(self, x):
+ v = np.log(np.sqrt(np.pi) * np.exp(-((x / 2.0) ** 2)))
+ v = float(v[0])
+ assert np.isscalar(v)
+ return v
+
+ def lnpdf_arr1(self, x):
+ v = self.lnpdf(x)
+ return np.array([v])
+
+ def lnpdf_float64(self, x):
+ v = self.lnpdf(x)
+ return np.float64(v)
+
+ def lnpdf_arr0D(self, x):
+ v = self.lnpdf(x)
+ return np.array(v)
+
+ def test_deal_with_scalar_likes(self):
+ rng = np.random.default_rng()
+ fns = [
+ self.lnpdf,
+ self.lnpdf_arr1,
+ self.lnpdf_float64,
+ self.lnpdf_arr0D,
+ ]
+ for fn in fns:
+ init = rng.random((50, 1))
+ sampler = EnsembleSampler(50, 1, fn)
+ _ = sampler.run_mcmc(initial_state=init, nsteps=20)
| Incompatible with numpy 2.0.0rc1
Numpy 2 is around the corner with having its first release candidate: https://pypi.org/project/numpy/2.0.0rc1
`emcee` at least uses `VisibleDeprecationWarning` from numpy that will be removed with numpy 2: https://numpy.org/devdocs/release/2.0.0-notes.html
> Warnings and exceptions present in [numpy.exceptions](https://numpy.org/devdocs/reference/routines.exceptions.html#module-numpy.exceptions) (e.g, [ComplexWarning](https://numpy.org/devdocs/reference/generated/numpy.exceptions.ComplexWarning.html#numpy.exceptions.ComplexWarning), [VisibleDeprecationWarning](https://numpy.org/devdocs/reference/generated/numpy.exceptions.VisibleDeprecationWarning.html#numpy.exceptions.VisibleDeprecationWarning)) are no longer exposed in the main namespace.
So with the release of numpy 2, emcee will run into some troubles. | 0.0 | [
"src/emcee/tests/unit/test_ensemble.py::TestLnProbFn::test_deal_with_scalar_likes"
] | [
"src/emcee/tests/unit/test_ensemble.py::TestNP2ListOfDicts::test_ndarray_to_list_of_dicts",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_asserts",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_compute_log_prob",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_compute_log_prob_mixture",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_compute_log_prob_mixture_grouped",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_named_parameters",
"src/emcee/tests/unit/test_ensemble.py::TestNamedParameters::test_run_mcmc"
] | 2024-04-04 22:01:34+00:00 | 1,901 |
|
dfm__tess-atlas-108 | diff --git a/setup.py b/setup.py
index 0a7c68b..dabe2f7 100644
--- a/setup.py
+++ b/setup.py
@@ -31,15 +31,17 @@ INSTALL_REQUIRES = [
"lightkurve>=2.0.11",
"plotly>=4.9.0",
"arviz>=0.10.0",
- "corner",
+ "corner>=2.2.1",
"pandas",
"jupyter",
"ipykernel",
- "jupytext",
+ "jupytext<1.11,>=1.8", # pinned for jypyter-book
"kaleido",
"aesara-theano-fallback",
"theano-pymc>=1.1.2",
"jupyter-book",
+ "seaborn",
+ "jupyter_client==6.1.12", # pinned beacuse of nbconvert bug https://github.com/jupyter/nbconvert/pull/1549#issuecomment-818734169
]
EXTRA_REQUIRE = {"test": ["pytest>=3.6", "testbook>=0.2.3"]}
EXTRA_REQUIRE["dev"] = EXTRA_REQUIRE["test"] + [
@@ -101,6 +103,7 @@ if __name__ == "__main__":
"run_tois=tess_atlas.notebook_preprocessors.run_tois:main",
"runs_stats_plotter=tess_atlas.analysis.stats_plotter:main",
"make_webpages=tess_atlas.webbuilder.build_pages:main",
+ "make_slurm_job=tess_atlas.batch_job_generator.slurm_job_generator:main",
]
},
)
diff --git a/src/tess_atlas/batch_job_generator/__init__.py b/src/tess_atlas/batch_job_generator/__init__.py
new file mode 100644
index 0000000..e69de29
diff --git a/src/tess_atlas/batch_job_generator/slurm_job_generator.py b/src/tess_atlas/batch_job_generator/slurm_job_generator.py
new file mode 100644
index 0000000..a1ed5a4
--- /dev/null
+++ b/src/tess_atlas/batch_job_generator/slurm_job_generator.py
@@ -0,0 +1,67 @@
+import argparse
+import os
+import shutil
+from typing import List
+
+import pandas as pd
+
+TEMPLATE_FILE = os.path.join(os.path.dirname(__file__), "slurm_template.sh")
+
+
+def make_slurm_file(outdir: str, toi_numbers: List[int], module_loads: str):
+ with open(TEMPLATE_FILE, "r") as f:
+ file_contents = f.read()
+ outdir = os.path.abspath(outdir)
+ logfile_name = os.path.join(outdir, "toi_slurm_jobs.log")
+ jobfile_name = os.path.join(outdir, "slurm_job.sh")
+ path_to_python = shutil.which("python")
+ path_to_env_activate = path_to_python.replace("python", "activate")
+ file_contents = file_contents.replace(
+ "{{{TOTAL NUM}}}", str(len(toi_numbers) - 1)
+ )
+ file_contents = file_contents.replace("{{{MODULE LOADS}}}", module_loads)
+ file_contents = file_contents.replace("{{{OUTDIR}}}", outdir)
+ file_contents = file_contents.replace(
+ "{{{LOAD ENV}}}", f"source {path_to_env_activate}"
+ )
+ file_contents = file_contents.replace("{{{LOG FILE}}}", logfile_name)
+ toi_str = " ".join([str(toi) for toi in toi_numbers])
+ file_contents = file_contents.replace("{{{TOI NUMBERS}}}", toi_str)
+ with open(jobfile_name, "w") as f:
+ f.write(file_contents)
+ print(f"Jobfile created, to run job: \nsbatch {jobfile_name}")
+
+
+def get_toi_numbers(toi_csv: str):
+ df = pd.read_csv(toi_csv)
+ return list(df.toi_numbers.values)
+
+
+def get_cli_args():
+ parser = argparse.ArgumentParser(
+ description="Create slurm job for analysing TOIs"
+ )
+ parser.add_argument(
+ "--toi_csv",
+ help="CSV with the toi numbers to analyse (csv needs a column with `toi_numbers`)",
+ )
+ parser.add_argument(
+ "--outdir", help="outdir for jobs", default="notebooks"
+ )
+ parser.add_argument(
+ "--module_loads",
+ help="String containing all module loads in one line (each module separated by a space)",
+ )
+ args = parser.parse_args()
+ return args.toi_csv, args.outdir, args.module_loads
+
+
+def main():
+ toi_csv, outdir, module_loads = get_cli_args()
+ os.makedirs(outdir, exist_ok=True)
+ toi_numbers = get_toi_numbers(toi_csv)
+ make_slurm_file(outdir, toi_numbers, module_loads)
+
+
+if __name__ == "__main__":
+ main()
diff --git a/src/tess_atlas/batch_job_generator/slurm_template.sh b/src/tess_atlas/batch_job_generator/slurm_template.sh
new file mode 100644
index 0000000..250c930
--- /dev/null
+++ b/src/tess_atlas/batch_job_generator/slurm_template.sh
@@ -0,0 +1,18 @@
+#!/bin/bash
+#
+#SBATCH --job-name=run_tois
+#SBATCH --output={{{LOG FILE}}}
+#
+#SBATCH --ntasks=1
+#SBATCH --time=300:00
+#SBATCH --mem-per-cpu=500MB
+#
+#SBATCH --array=0-{{{TOTAL NUM}}}
+
+module load {{{MODULE LOADS}}}
+{{{LOAD ENV}}}
+
+
+TOI_NUMBERS=({{{TOI NUMBERS}}})
+
+srun run_toi ${TOI_NUMBERS[$SLURM_ARRAY_TASK_ID]} --outdir {{{OUTDIR}}}
diff --git a/src/tess_atlas/notebook_preprocessors/run_toi.py b/src/tess_atlas/notebook_preprocessors/run_toi.py
index 1f17ba7..a76c4f5 100644
--- a/src/tess_atlas/notebook_preprocessors/run_toi.py
+++ b/src/tess_atlas/notebook_preprocessors/run_toi.py
@@ -87,7 +87,7 @@ def execute_toi_notebook(notebook_filename):
def get_cli_args():
"""Get the TOI number from the CLI and return it"""
- parser = argparse.ArgumentParser(prog="run_toi_in_pool")
+ parser = argparse.ArgumentParser(prog="run_toi")
default_outdir = os.path.join(os.getcwd(), "notebooks")
parser.add_argument(
"toi_number", type=int, help="The TOI number to be analysed (e.g. 103)"
| dfm/tess-atlas | 6274de545082661de3677fb609fa7274f26afb47 | diff --git a/.github/workflows/tests.yml b/.github/workflows/tests.yml
index d45d0c3..ca420da 100644
--- a/.github/workflows/tests.yml
+++ b/.github/workflows/tests.yml
@@ -26,7 +26,7 @@ jobs:
- name: Install dependencies
run: |
python -m pip install --upgrade pip
- pip install --use-feature=2020-resolver -U -e ".[dev]"
+ pip install -U -e ".[dev]"
# Test the py:light -> ipynb -> py:light round trip conversion
- name: roundtrip conversion test
diff --git a/tests/test_slurm_job_generator.py b/tests/test_slurm_job_generator.py
new file mode 100644
index 0000000..59a582a
--- /dev/null
+++ b/tests/test_slurm_job_generator.py
@@ -0,0 +1,24 @@
+import os
+import unittest
+
+from tess_atlas.batch_job_generator.slurm_job_generator import make_slurm_file
+
+
+class JobgenTest(unittest.TestCase):
+ def setUp(self):
+ self.start_dir = os.getcwd()
+ self.outdir = f"test_jobgen"
+ os.makedirs(self.outdir, exist_ok=True)
+
+ def tearDown(self):
+ import shutil
+
+ if os.path.exists(self.outdir):
+ shutil.rmtree(self.outdir)
+
+ def test_slurmfile(self):
+ make_slurm_file(self.outdir, [100, 101, 102], "module load 1")
+
+
+if __name__ == "__main__":
+ unittest.main()
| Cluster jobs failing to pre-process notebooks: TypeError: 'coroutine' object is not subscriptable | 0.0 | [
"tests/test_slurm_job_generator.py::JobgenTest::test_slurmfile"
] | [] | 2021-10-06 02:54:35+00:00 | 1,902 |
|
dgasmith__opt_einsum-11 | diff --git a/README.md b/README.md
index a792df7..9e4ed20 100644
--- a/README.md
+++ b/README.md
@@ -131,6 +131,35 @@ True
By contracting terms in the correct order we can see that this expression can be computed with N^4 scaling. Even with the overhead of finding the best order or 'path' and small dimensions, opt_einsum is roughly 900 times faster than pure einsum for this expression.
+
+## Reusing paths using ``contract_expression``
+
+If you expect to repeatedly use a particular contraction it can make things simpler and more efficient to not compute the path each time. Instead, supplying ``contract_expression`` with the contraction string and the shapes of the tensors generates a ``ContractExpression`` which can then be repeatedly called with any matching set of arrays. For example:
+
+```python
+>>> my_expr = oe.contract_expression("abc,cd,dbe->ea", (2, 3, 4), (4, 5), (5, 3, 6))
+>>> print(my_expr)
+<ContractExpression> for 'abc,cd,dbe->ea':
+ 1. 'dbe,cd->bce' [GEMM]
+ 2. 'bce,abc->ea' [GEMM]
+```
+
+Now we can call this expression with 3 arrays that match the original shapes without having to compute the path again:
+
+```python
+>>> x, y, z = (np.random.rand(*s) for s in [(2, 3, 4), (4, 5), (5, 3, 6)])
+>>> my_expr(x, y, z)
+array([[ 3.08331541, 4.13708916],
+ [ 2.92793729, 4.57945185],
+ [ 3.55679457, 5.56304115],
+ [ 2.6208398 , 4.39024187],
+ [ 3.66736543, 5.41450334],
+ [ 3.67772272, 5.46727192]])
+```
+
+Note that few checks are performed when calling the expression, and while it will work for a set of arrays with the same ranks as the original shapes but differing sizes, it might no longer be optimal.
+
+
## More details on paths
Finding the optimal order of contraction is not an easy problem and formally scales factorially with respect to the number of terms in the expression. First, lets discuss what a path looks like in opt_einsum:
diff --git a/opt_einsum/__init__.py b/opt_einsum/__init__.py
index 2b3430f..16c844a 100644
--- a/opt_einsum/__init__.py
+++ b/opt_einsum/__init__.py
@@ -1,4 +1,4 @@
-from .contract import contract, contract_path
+from .contract import contract, contract_path, contract_expression
from . import paths
from . import blas
from . import helpers
diff --git a/opt_einsum/contract.py b/opt_einsum/contract.py
index 43b78e9..4efb1a9 100644
--- a/opt_einsum/contract.py
+++ b/opt_einsum/contract.py
@@ -259,7 +259,7 @@ def contract_path(*operands, **kwargs):
def contract(*operands, **kwargs):
"""
contract(subscripts, *operands, out=None, dtype=None, order='K',
- casting='safe', use_blas=False, optimize=True, memory_limit=None)
+ casting='safe', use_blas=True, optimize=True, memory_limit=None)
Evaluates the Einstein summation convention on the operands. A drop in
replacment for NumPy's einsum function that optimizes the order of contraction
@@ -323,14 +323,10 @@ def contract(*operands, **kwargs):
See opt_einsum.contract_path or numpy.einsum
"""
-
- # Grab non-einsum kwargs
optimize_arg = kwargs.pop('optimize', True)
if optimize_arg is True:
optimize_arg = 'greedy'
- use_blas = kwargs.pop('use_blas', True)
-
valid_einsum_kwargs = ['out', 'dtype', 'order', 'casting']
einsum_kwargs = {k: v for (k, v) in kwargs.items() if k in valid_einsum_kwargs}
@@ -338,25 +334,38 @@ def contract(*operands, **kwargs):
if optimize_arg is False:
return np.einsum(*operands, **einsum_kwargs)
- # Make sure all keywords are valid
- valid_contract_kwargs = ['memory_limit', 'use_blas'] + valid_einsum_kwargs
- unknown_kwargs = [k for (k, v) in kwargs.items() if k not in valid_contract_kwargs]
+ # Grab non-einsum kwargs
+ use_blas = kwargs.pop('use_blas', True)
+ memory_limit = kwargs.pop('memory_limit', None)
+ gen_expression = kwargs.pop('gen_expression', False)
+
+ # Make sure remaining keywords are valid for einsum
+ unknown_kwargs = [k for (k, v) in kwargs.items() if k not in valid_einsum_kwargs]
if len(unknown_kwargs):
raise TypeError("Did not understand the following kwargs: %s" % unknown_kwargs)
- # Special handeling if out is specified
- specified_out = False
- out_array = einsum_kwargs.pop('out', None)
- if out_array is not None:
- specified_out = True
+ if gen_expression:
+ full_str = operands[0]
# Build the contraction list and operand
- memory_limit = kwargs.pop('memory_limit', None)
-
operands, contraction_list = contract_path(
*operands, path=optimize_arg, memory_limit=memory_limit, einsum_call=True, use_blas=use_blas)
- handle_out = False
+ # check if performing contraction or just building expression
+ if gen_expression:
+ return ContractExpression(full_str, contraction_list, **einsum_kwargs)
+
+ return _core_contract(operands, contraction_list, **einsum_kwargs)
+
+
+def _core_contract(operands, contraction_list, **einsum_kwargs):
+ """Inner loop used to perform an actual contraction given the output
+ from a ``contract_path(..., einsum_call=True)`` call.
+ """
+
+ # Special handeling if out is specified
+ out_array = einsum_kwargs.pop('out', None)
+ specified_out = out_array is not None
# Start contraction loop
for num, contraction in enumerate(contraction_list):
@@ -366,8 +375,7 @@ def contract(*operands, **kwargs):
tmp_operands.append(operands.pop(x))
# Do we need to deal with the output?
- if specified_out and ((num + 1) == len(contraction_list)):
- handle_out = True
+ handle_out = specified_out and ((num + 1) == len(contraction_list))
# Call tensordot
if blas:
@@ -412,3 +420,104 @@ def contract(*operands, **kwargs):
return out_array
else:
return operands[0]
+
+
+class ContractExpression:
+ """Helper class for storing an explicit ``contraction_list`` which can
+ then be repeatedly called solely with the array arguments.
+ """
+
+ def __init__(self, contraction, contraction_list, **einsum_kwargs):
+ self.contraction = contraction
+ self.contraction_list = contraction_list
+ self.einsum_kwargs = einsum_kwargs
+ self.num_args = len(contraction.split('->')[0].split(','))
+
+ def __call__(self, *arrays, **kwargs):
+ if len(arrays) != self.num_args:
+ raise ValueError("This `ContractExpression` takes exactly %s array arguments "
+ "but received %s." % (self.num_args, len(arrays)))
+
+ out = kwargs.pop('out', None)
+ if kwargs:
+ raise ValueError("The only valid keyword argument to a `ContractExpression` "
+ "call is `out=`. Got: %s." % kwargs)
+
+ try:
+ return _core_contract(list(arrays), self.contraction_list, out=out, **self.einsum_kwargs)
+ except ValueError as err:
+ original_msg = "".join(err.args) if err.args else ""
+ msg = ("Internal error while evaluating `ContractExpression`. Note that few checks are performed"
+ " - the number and rank of the array arguments must match the original expression. "
+ "The internal error was: '%s'" % original_msg, )
+ err.args = msg
+ raise
+
+ def __repr__(self):
+ return "ContractExpression('%s')" % self.contraction
+
+ def __str__(self):
+ s = "<ContractExpression> for '%s':" % self.contraction
+ for i, c in enumerate(self.contraction_list):
+ s += "\n %i. " % (i + 1)
+ s += "'%s'" % c[2] + (" [%s]" % c[-1] if c[-1] else "")
+ if self.einsum_kwargs:
+ s += "\neinsum_kwargs=%s" % self.einsum_kwargs
+ return s
+
+
+class _ShapeOnly(np.ndarray):
+ """Dummy ``numpy.ndarray`` which has a shape only - for generating
+ contract expressions.
+ """
+
+ def __init__(self, shape):
+ self.shape = shape
+
+
+def contract_expression(subscripts, *shapes, **kwargs):
+ """Generate an reusable expression for a given contraction with
+ specific shapes, which can for example be cached.
+
+ Parameters
+ ----------
+ subscripts : str
+ Specifies the subscripts for summation.
+ shapes : sequence of integer tuples
+ Shapes of the arrays to optimize the contraction for.
+ kwargs :
+ Passed on to ``contract_path`` or ``einsum``. See ``contract``.
+
+ Returns
+ -------
+ expr : ContractExpression
+ Callable with signature ``expr(*arrays)`` where the array's shapes
+ should match ``shapes``.
+
+ Notes
+ -----
+ - The `out` keyword argument should be supplied to the generated expression
+ rather than this function.
+ - The generated expression will work with any arrays which have
+ the same rank (number of dimensions) as the original shapes, however, if
+ the actual sizes are different, the expression may no longer be optimal.
+
+ Examples
+ --------
+
+ >>> expr = contract_expression("ab,bc->ac", (3, 4), (4, 5))
+ >>> a, b = np.random.rand(3, 4), np.random.rand(4, 5)
+ >>> c = expr(a, b)
+ >>> np.allclose(c, a @ b)
+ True
+
+ """
+ if not kwargs.get('optimize', True):
+ raise ValueError("Can only generate expressions for optimized contractions.")
+
+ if kwargs.get('out', None) is not None:
+ raise ValueError("`out` should only be specified when calling a `ContractExpression`, not when building it.")
+
+ dummy_arrays = [_ShapeOnly(s) for s in shapes]
+
+ return contract(subscripts, *dummy_arrays, gen_expression=True, **kwargs)
| dgasmith/opt_einsum | fd612f0966a555f8a9783669ad3842f2dbfd1462 | diff --git a/tests/test_contract.py b/tests/test_contract.py
index 016d784..6885522 100644
--- a/tests/test_contract.py
+++ b/tests/test_contract.py
@@ -5,7 +5,7 @@ Tets a series of opt_einsum contraction paths to ensure the results are the same
from __future__ import division, absolute_import, print_function
import numpy as np
-from opt_einsum import contract, contract_path, helpers
+from opt_einsum import contract, contract_path, helpers, contract_expression
import pytest
tests = [
@@ -127,3 +127,73 @@ def test_printing():
ein = contract_path(string, *views)
assert len(ein[1]) == 729
+
+
+@pytest.mark.parametrize("string", tests)
+@pytest.mark.parametrize("optimize", ['greedy', 'optimal'])
+@pytest.mark.parametrize("use_blas", [False, True])
+@pytest.mark.parametrize("out_spec", [False, True])
+def test_contract_expressions(string, optimize, use_blas, out_spec):
+ views = helpers.build_views(string)
+ shapes = [view.shape for view in views]
+ expected = contract(string, *views, optimize=False, use_blas=False)
+
+ expr = contract_expression(
+ string, *shapes, optimize=optimize, use_blas=use_blas)
+
+ if out_spec and ("->" in string) and (string[-2:] != "->"):
+ out, = helpers.build_views(string.split('->')[1])
+ expr(*views, out=out)
+ else:
+ out = expr(*views)
+
+ assert np.allclose(out, expected)
+
+ # check representations
+ assert string in expr.__repr__()
+ assert string in expr.__str__()
+
+
+def test_contract_expression_checks():
+ # check optimize needed
+ with pytest.raises(ValueError):
+ contract_expression("ab,bc->ac", (2, 3), (3, 4), optimize=False)
+
+ # check sizes are still checked
+ with pytest.raises(ValueError):
+ contract_expression("ab,bc->ac", (2, 3), (3, 4), (42, 42))
+
+ # check if out given
+ out = np.empty((2, 4))
+ with pytest.raises(ValueError):
+ contract_expression("ab,bc->ac", (2, 3), (3, 4), out=out)
+
+ # check still get errors when wrong ranks supplied to expression
+ expr = contract_expression("ab,bc->ac", (2, 3), (3, 4))
+
+ # too few arguments
+ with pytest.raises(ValueError) as err:
+ expr(np.random.rand(2, 3))
+ assert "`ContractExpression` takes exactly 2" in str(err)
+
+ # too many arguments
+ with pytest.raises(ValueError) as err:
+ expr(np.random.rand(2, 3), np.random.rand(2, 3), np.random.rand(2, 3))
+ assert "`ContractExpression` takes exactly 2" in str(err)
+
+ # wrong shapes
+ with pytest.raises(ValueError) as err:
+ expr(np.random.rand(2, 3, 4), np.random.rand(3, 4))
+ assert "Internal error while evaluating `ContractExpression`" in str(err)
+ with pytest.raises(ValueError) as err:
+ expr(np.random.rand(2, 4), np.random.rand(3, 4, 5))
+ assert "Internal error while evaluating `ContractExpression`" in str(err)
+ with pytest.raises(ValueError) as err:
+ expr(np.random.rand(2, 3), np.random.rand(3, 4),
+ out=np.random.rand(2, 4, 6))
+ assert "Internal error while evaluating `ContractExpression`" in str(err)
+
+ # should only be able to specify out
+ with pytest.raises(ValueError) as err:
+ expr(np.random.rand(2, 3), np.random.rand(3, 4), order='F')
+ assert "only valid keyword argument to a `ContractExpression`" in str(err)
| reusable einsum expressions
Essentially, I'm using ``opt_einsum`` for some tensor network calculations, and a significant proportion of time is spent in ``contract_path``, even for relatively large sized tensors and pre-calculated paths.
Specifically, a huge number of contractions with the same indices and shapes are performed over and over again and I already cache these using the path from ``contract_path``. However, it seems even with the path supplied, a large amount of time is spent parsing the input, in ``can_blas``, ``parse_einsum_input`` etc.
My suggestion would be something like:
```python
shapes = [(2, 3), (3, 4), (4, 5)]
my_expr = einsum_expression("ab,bc,cd->ad", *shapes)
for _ in many_repeats:
...
x, y, z = (rand(s) for s in shapes)
out = my_expr(x, y, z)
...
```
I.e. it would only accept arrays of the same dimensions (and probably an ``out`` argument) and would otherwise skip all parsing, using a ``contraction_list`` stored within the function.
Anyway, I'm about to knock something up to test for myself, but thought it might be of more general interest. | 0.0 | [
"tests/test_contract.py::test_compare[a,ab,abc->abc]",
"tests/test_contract.py::test_compare[a,b,ab->ab]",
"tests/test_contract.py::test_compare[ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_compare[ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_compare[abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_compare[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_compare[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_compare[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_compare[abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_compare[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_compare[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_compare[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_compare[bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_compare[ab,ab,c->]",
"tests/test_contract.py::test_compare[ab,ab,c->c]",
"tests/test_contract.py::test_compare[ab,ab,cd,cd->]",
"tests/test_contract.py::test_compare[ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_compare[ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_compare[ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_compare[ab,cd,ef->abcdef]",
"tests/test_contract.py::test_compare[ab,cd,ef->acdf]",
"tests/test_contract.py::test_compare[ab,cd,de->abcde]",
"tests/test_contract.py::test_compare[ab,cd,de->be]",
"tests/test_contract.py::test_compare[ab,bcd,cd->abcd]",
"tests/test_contract.py::test_compare[ab,bcd,cd->abd]",
"tests/test_contract.py::test_compare[eb,cb,fb->cef]",
"tests/test_contract.py::test_compare[dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_compare[bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_compare[dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_compare[fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_compare[abcd,ad]",
"tests/test_contract.py::test_compare[ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_compare[baa,dcf,af,cde->be]",
"tests/test_contract.py::test_compare[bd,db,eac->ace]",
"tests/test_contract.py::test_compare[fff,fae,bef,def->abd]",
"tests/test_contract.py::test_compare[efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_compare[ab,ab]",
"tests/test_contract.py::test_compare[ab,ba]",
"tests/test_contract.py::test_compare[abc,abc]",
"tests/test_contract.py::test_compare[abc,bac]",
"tests/test_contract.py::test_compare[abc,cba]",
"tests/test_contract.py::test_compare[ab,bc]",
"tests/test_contract.py::test_compare[ab,cb]",
"tests/test_contract.py::test_compare[ba,bc]",
"tests/test_contract.py::test_compare[ba,cb]",
"tests/test_contract.py::test_compare[abcd,cd]",
"tests/test_contract.py::test_compare[abcd,ab]",
"tests/test_contract.py::test_compare[abcd,cdef]",
"tests/test_contract.py::test_compare[abcd,cdef->feba]",
"tests/test_contract.py::test_compare[abcd,efdc]",
"tests/test_contract.py::test_compare[aab,bc->ac]",
"tests/test_contract.py::test_compare[ab,bcc->ac]",
"tests/test_contract.py::test_compare[aab,bcc->ac]",
"tests/test_contract.py::test_compare[baa,bcc->ac]",
"tests/test_contract.py::test_compare[aab,ccb->ac]",
"tests/test_contract.py::test_compare[aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_compare[ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_compare[bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_compare[bb,ff,be->e]",
"tests/test_contract.py::test_compare[bcb,bb,fc,fff->]",
"tests/test_contract.py::test_compare[fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_compare[afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_compare[adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_compare[bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_compare[dba,ead,cad->bce]",
"tests/test_contract.py::test_compare[aef,fbc,dca->bde]",
"tests/test_contract.py::test_compare_blas[a,ab,abc->abc]",
"tests/test_contract.py::test_compare_blas[a,b,ab->ab]",
"tests/test_contract.py::test_compare_blas[ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_compare_blas[ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_compare_blas[abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_compare_blas[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_compare_blas[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_compare_blas[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_compare_blas[abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_compare_blas[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_compare_blas[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_compare_blas[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_compare_blas[bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_compare_blas[ab,ab,c->]",
"tests/test_contract.py::test_compare_blas[ab,ab,c->c]",
"tests/test_contract.py::test_compare_blas[ab,ab,cd,cd->]",
"tests/test_contract.py::test_compare_blas[ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_compare_blas[ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_compare_blas[ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_compare_blas[ab,cd,ef->abcdef]",
"tests/test_contract.py::test_compare_blas[ab,cd,ef->acdf]",
"tests/test_contract.py::test_compare_blas[ab,cd,de->abcde]",
"tests/test_contract.py::test_compare_blas[ab,cd,de->be]",
"tests/test_contract.py::test_compare_blas[ab,bcd,cd->abcd]",
"tests/test_contract.py::test_compare_blas[ab,bcd,cd->abd]",
"tests/test_contract.py::test_compare_blas[eb,cb,fb->cef]",
"tests/test_contract.py::test_compare_blas[dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_compare_blas[bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_compare_blas[dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_compare_blas[fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_compare_blas[abcd,ad]",
"tests/test_contract.py::test_compare_blas[ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_compare_blas[baa,dcf,af,cde->be]",
"tests/test_contract.py::test_compare_blas[bd,db,eac->ace]",
"tests/test_contract.py::test_compare_blas[fff,fae,bef,def->abd]",
"tests/test_contract.py::test_compare_blas[efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_compare_blas[ab,ab]",
"tests/test_contract.py::test_compare_blas[ab,ba]",
"tests/test_contract.py::test_compare_blas[abc,abc]",
"tests/test_contract.py::test_compare_blas[abc,bac]",
"tests/test_contract.py::test_compare_blas[abc,cba]",
"tests/test_contract.py::test_compare_blas[ab,bc]",
"tests/test_contract.py::test_compare_blas[ab,cb]",
"tests/test_contract.py::test_compare_blas[ba,bc]",
"tests/test_contract.py::test_compare_blas[ba,cb]",
"tests/test_contract.py::test_compare_blas[abcd,cd]",
"tests/test_contract.py::test_compare_blas[abcd,ab]",
"tests/test_contract.py::test_compare_blas[abcd,cdef]",
"tests/test_contract.py::test_compare_blas[abcd,cdef->feba]",
"tests/test_contract.py::test_compare_blas[abcd,efdc]",
"tests/test_contract.py::test_compare_blas[aab,bc->ac]",
"tests/test_contract.py::test_compare_blas[ab,bcc->ac]",
"tests/test_contract.py::test_compare_blas[aab,bcc->ac]",
"tests/test_contract.py::test_compare_blas[baa,bcc->ac]",
"tests/test_contract.py::test_compare_blas[aab,ccb->ac]",
"tests/test_contract.py::test_compare_blas[aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_compare_blas[ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_compare_blas[bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_compare_blas[bb,ff,be->e]",
"tests/test_contract.py::test_compare_blas[bcb,bb,fc,fff->]",
"tests/test_contract.py::test_compare_blas[fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_compare_blas[afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_compare_blas[adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_compare_blas[bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_compare_blas[dba,ead,cad->bce]",
"tests/test_contract.py::test_compare_blas[aef,fbc,dca->bde]",
"tests/test_contract.py::test_printing",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ba]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,abc]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,bac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,cba]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bc]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cb]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,bc]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,cb]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[False-False-greedy-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ba]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,abc]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,bac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,cba]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bc]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cb]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,bc]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,cb]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[False-False-optimal-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ba]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,abc]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,bac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,cba]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bc]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cb]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,bc]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,cb]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[False-True-greedy-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ba]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,abc]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,bac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,cba]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bc]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cb]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,bc]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,cb]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[False-True-optimal-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ba]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,abc]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,bac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,cba]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bc]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cb]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,bc]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,cb]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[True-False-greedy-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ba]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,abc]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,bac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,cba]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bc]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cb]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,bc]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,cb]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[True-False-optimal-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ba]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,abc]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,bac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,cba]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bc]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cb]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,bc]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,cb]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[True-True-greedy-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-a,ab,abc->abc]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-a,b,ab->ab]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abhe,hidj,jgba,hiab,gab]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bdhe,acad,hiab,agac,hibd]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->c]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->cd]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd,ef,ef->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->abcdef]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->acdf]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->abcde]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->be]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abcd]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abd]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-eb,cb,fb->cef]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-dd,fb,be,cdb->cef]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bca,cdb,dbf,afc->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-dcc,fce,ea,dbf->ab]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-fdf,cdd,ccd,afe->ae]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ad]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ed,fcd,ff,bcf->be]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,dcf,af,cde->be]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bd,db,eac->ace]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-fff,fae,bef,def->abd]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-efc,dbc,acf,fd->abe]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ba]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,abc]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,bac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,cba]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bc]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cb]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,bc]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,cb]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cd]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ab]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef->feba]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,efdc]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,bcc->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,ccb->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,fa,df,ecc->bde]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-ecb,fef,bad,ed->ac]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bcf,bbb,fbf,fc->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bb,ff,be->e]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bcb,bb,fc,fff->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-fbb,dfd,fc,fc->]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-afd,ba,cc,dc->bf]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-adb,bc,fa,cfc->d]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-bbd,bda,fc,db->acf]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-dba,ead,cad->bce]",
"tests/test_contract.py::test_contract_expressions[True-True-optimal-aef,fbc,dca->bde]",
"tests/test_contract.py::test_contract_expression_checks"
] | [] | 2017-12-04 01:27:21+00:00 | 1,903 |
|
dgasmith__opt_einsum-141 | diff --git a/opt_einsum/contract.py b/opt_einsum/contract.py
index b837b21..6e7550a 100644
--- a/opt_einsum/contract.py
+++ b/opt_einsum/contract.py
@@ -214,7 +214,7 @@ def contract_path(*operands, **kwargs):
indices = set(input_subscripts.replace(',', ''))
# Get length of each unique dimension and ensure all dimensions are correct
- dimension_dict = {}
+ size_dict = {}
for tnum, term in enumerate(input_list):
sh = input_shps[tnum]
@@ -224,18 +224,18 @@ def contract_path(*operands, **kwargs):
for cnum, char in enumerate(term):
dim = int(sh[cnum])
- if char in dimension_dict:
+ if char in size_dict:
# For broadcasting cases we always want the largest dim size
- if dimension_dict[char] == 1:
- dimension_dict[char] = dim
- elif dim not in (1, dimension_dict[char]):
+ if size_dict[char] == 1:
+ size_dict[char] = dim
+ elif dim not in (1, size_dict[char]):
raise ValueError("Size of label '{}' for operand {} ({}) does not match previous "
- "terms ({}).".format(char, tnum, dimension_dict[char], dim))
+ "terms ({}).".format(char, tnum, size_dict[char], dim))
else:
- dimension_dict[char] = dim
+ size_dict[char] = dim
# Compute size of each input array plus the output array
- size_list = [helpers.compute_size_by_dict(term, dimension_dict) for term in input_list + [output_subscript]]
+ size_list = [helpers.compute_size_by_dict(term, size_dict) for term in input_list + [output_subscript]]
memory_arg = _choose_memory_arg(memory_limit, size_list)
num_ops = len(input_list)
@@ -245,7 +245,7 @@ def contract_path(*operands, **kwargs):
# indices_in_input = input_subscripts.replace(',', '')
inner_product = (sum(len(x) for x in input_sets) - len(indices)) > 0
- naive_cost = helpers.flop_count(indices, inner_product, num_ops, dimension_dict)
+ naive_cost = helpers.flop_count(indices, inner_product, num_ops, size_dict)
# Compute the path
if not isinstance(path_type, (str, paths.PathOptimizer)):
@@ -256,10 +256,10 @@ def contract_path(*operands, **kwargs):
path = [tuple(range(num_ops))]
elif isinstance(path_type, paths.PathOptimizer):
# Custom path optimizer supplied
- path = path_type(input_sets, output_set, dimension_dict, memory_arg)
+ path = path_type(input_sets, output_set, size_dict, memory_arg)
else:
path_optimizer = paths.get_path_fn(path_type)
- path = path_optimizer(input_sets, output_set, dimension_dict, memory_arg)
+ path = path_optimizer(input_sets, output_set, size_dict, memory_arg)
cost_list = []
scale_list = []
@@ -275,10 +275,10 @@ def contract_path(*operands, **kwargs):
out_inds, input_sets, idx_removed, idx_contract = contract_tuple
# Compute cost, scale, and size
- cost = helpers.flop_count(idx_contract, idx_removed, len(contract_inds), dimension_dict)
+ cost = helpers.flop_count(idx_contract, idx_removed, len(contract_inds), size_dict)
cost_list.append(cost)
scale_list.append(len(idx_contract))
- size_list.append(helpers.compute_size_by_dict(out_inds, dimension_dict))
+ size_list.append(helpers.compute_size_by_dict(out_inds, size_dict))
tmp_inputs = [input_list.pop(x) for x in contract_inds]
tmp_shapes = [input_shps.pop(x) for x in contract_inds]
@@ -312,7 +312,7 @@ def contract_path(*operands, **kwargs):
return operands, contraction_list
path_print = PathInfo(contraction_list, input_subscripts, output_subscript, indices, path, scale_list, naive_cost,
- opt_cost, size_list, dimension_dict)
+ opt_cost, size_list, size_dict)
return path, path_print
@@ -393,15 +393,26 @@ def contract(*operands, **kwargs):
- ``'optimal'`` An algorithm that explores all possible ways of
contracting the listed tensors. Scales factorially with the number of
terms in the contraction.
+ - ``'dp'`` A faster (but essentially optimal) algorithm that uses
+ dynamic programming to exhaustively search all contraction paths
+ without outer-products.
+ - ``'greedy'`` An cheap algorithm that heuristically chooses the best
+ pairwise contraction at each step. Scales linearly in the number of
+ terms in the contraction.
+ - ``'random-greedy'`` Run a randomized version of the greedy algorithm
+ 32 times and pick the best path.
+ - ``'random-greedy-128'`` Run a randomized version of the greedy
+ algorithm 128 times and pick the best path.
- ``'branch-all'`` An algorithm like optimal but that restricts itself
to searching 'likely' paths. Still scales factorially.
- ``'branch-2'`` An even more restricted version of 'branch-all' that
only searches the best two options at each step. Scales exponentially
with the number of terms in the contraction.
- - ``'greedy'`` An algorithm that heuristically chooses the best pair
- contraction at each step.
- ``'auto'`` Choose the best of the above algorithms whilst aiming to
keep the path finding time below 1ms.
+ - ``'auto-hq'`` Aim for a high quality contraction, choosing the best
+ of the above algorithms whilst aiming to keep the path finding time
+ below 1sec.
memory_limit : {None, int, 'max_input'} (default: None)
Give the upper bound of the largest intermediate tensor contract will build.
@@ -412,7 +423,7 @@ def contract(*operands, **kwargs):
The default is None. Note that imposing a limit can make contractions
exponentially slower to perform.
- backend : str, optional (default: ``numpy``)
+ backend : str, optional (default: ``auto``)
Which library to use to perform the required ``tensordot``, ``transpose``
and ``einsum`` calls. Should match the types of arrays supplied, See
:func:`contract_expression` for generating expressions which convert
diff --git a/opt_einsum/path_random.py b/opt_einsum/path_random.py
index a49c06c..94b29a8 100644
--- a/opt_einsum/path_random.py
+++ b/opt_einsum/path_random.py
@@ -163,6 +163,8 @@ class RandomOptimizer(paths.PathOptimizer):
raise NotImplementedError
def __call__(self, inputs, output, size_dict, memory_limit):
+ self._check_args_against_first_call(inputs, output, size_dict)
+
# start a timer?
if self.max_time is not None:
t0 = time.time()
diff --git a/opt_einsum/paths.py b/opt_einsum/paths.py
index 4103224..b952bc2 100644
--- a/opt_einsum/paths.py
+++ b/opt_einsum/paths.py
@@ -43,6 +43,19 @@ class PathOptimizer(object):
where ``path`` is a list of int-tuples specifiying a contraction order.
"""
+
+ def _check_args_against_first_call(self, inputs, output, size_dict):
+ """Utility that stateful optimizers can use to ensure they are not
+ called with different contractions across separate runs.
+ """
+ args = (inputs, output, size_dict)
+ if not hasattr(self, '_first_call_args'):
+ # simply set the attribute as currently there is no global PathOptimizer init
+ self._first_call_args = args
+ elif args != self._first_call_args:
+ raise ValueError("The arguments specifiying the contraction that this path optimizer "
+ "instance was called with have changed - try creating a new instance.")
+
def __call__(self, inputs, output, size_dict, memory_limit=None):
raise NotImplementedError
@@ -336,6 +349,7 @@ class BranchBound(PathOptimizer):
>>> optimal(isets, oset, idx_sizes, 5000)
[(0, 2), (0, 1)]
"""
+ self._check_args_against_first_call(inputs, output, size_dict)
inputs = tuple(map(frozenset, inputs))
output = frozenset(output)
| dgasmith/opt_einsum | 95c1c80b081cce3f50b55422511d362e6fe1f0cd | diff --git a/opt_einsum/tests/test_paths.py b/opt_einsum/tests/test_paths.py
index 5105bbe..04769f6 100644
--- a/opt_einsum/tests/test_paths.py
+++ b/opt_einsum/tests/test_paths.py
@@ -299,6 +299,12 @@ def test_custom_random_greedy():
assert path_info.largest_intermediate == optimizer.best['size']
assert path_info.opt_cost == optimizer.best['flops']
+ # check error if we try and reuse the optimizer on a different expression
+ eq, shapes = oe.helpers.rand_equation(10, 4, seed=41)
+ views = list(map(np.ones, shapes))
+ with pytest.raises(ValueError):
+ path, path_info = oe.contract_path(eq, *views, optimize=optimizer)
+
def test_custom_branchbound():
eq, shapes = oe.helpers.rand_equation(8, 4, seed=42)
@@ -320,6 +326,12 @@ def test_custom_branchbound():
assert path_info.largest_intermediate == optimizer.best['size']
assert path_info.opt_cost == optimizer.best['flops']
+ # check error if we try and reuse the optimizer on a different expression
+ eq, shapes = oe.helpers.rand_equation(8, 4, seed=41)
+ views = list(map(np.ones, shapes))
+ with pytest.raises(ValueError):
+ path, path_info = oe.contract_path(eq, *views, optimize=optimizer)
+
@pytest.mark.skipif(sys.version_info < (3, 2), reason="requires python3.2 or higher")
def test_parallel_random_greedy():
| Possible bug in RandomGreedy path
I have found an interesting quirk of the RandomGreedy optimizer that may not have been intended. In my application, I need to repeat two contractions several times. I use the same optimizer to compute the paths in advance and cache the result. If I use RandomGreedy, then I get the wrong shape for the second contraction, which leads me to believe that there's some internal state of RandomGreedy that persists into computing the second path. Below is a MWE.
```python
import torch
import opt_einsum as oe
eqn1 = 'abc,bef,ahi,ehk,uvc,vwf,uxi,wxk->'
eqn2 = 'abd,beg,ahj,ehl,mndo,npgq,mrjs,prlt,uvo,vwq,uxs,wxt->'
shapes1 = [(1, 1, 2), (1, 1, 2), (1, 1, 2), (1, 1, 2),
(1, 1, 2), (1, 1, 2), (1, 1, 2), (1, 1, 2)]
shapes2 = [(1, 1, 2), (1, 1, 2), (1, 1, 2), (1, 1, 2), (1, 1, 2, 2), (1, 1, 2, 2),
(1, 1, 2, 2), (1, 1, 2, 2), (1, 1, 2), (1, 1, 2), (1, 1, 2), (1, 1, 2)]
tens1 = [torch.randn(*sh) for sh in shapes1]
tens2 = [torch.randn(*sh) for sh in shapes2]
for Opt in [oe.DynamicProgramming, oe.RandomGreedy]:
opt = Opt(minimize='flops')
expr1 = oe.contract_expression(eqn1, *[ten.shape for ten in tens1], optimize=opt)
expr2 = oe.contract_expression(eqn2, *[ten.shape for ten in tens2], optimize=opt)
print(expr1(*tens1))
print(expr2(*tens2))
```
The output of this program, using the latest PyPI release, is:
```
tensor(-0.0187)
tensor(0.4303)
tensor(-0.0187)
tensor([[[[[-0.4418]],
[[ 1.2737]]]]])
```
Lines 1 and 3 should match (and do), but so should 2 and 4 (and don't). | 0.0 | [
"opt_einsum/tests/test_paths.py::test_custom_random_greedy",
"opt_einsum/tests/test_paths.py::test_custom_branchbound"
] | [
"opt_einsum/tests/test_paths.py::test_size_by_dict",
"opt_einsum/tests/test_paths.py::test_flop_cost",
"opt_einsum/tests/test_paths.py::test_bad_path_option",
"opt_einsum/tests/test_paths.py::test_explicit_path",
"opt_einsum/tests/test_paths.py::test_path_optimal",
"opt_einsum/tests/test_paths.py::test_path_greedy",
"opt_einsum/tests/test_paths.py::test_memory_paths",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-eb,cb,fb->cef-order0]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-eb,cb,fb->cef-order1]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-eb,cb,fb->cef-order2]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-eb,cb,fb->cef-order3]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-eb,cb,fb->cef-order4]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dd,fb,be,cdb->cef-order5]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-dd,fb,be,cdb->cef-order6]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-dd,fb,be,cdb->cef-order7]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dd,fb,be,cdb->cef-order8]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dd,fb,be,cdb->cef-order9]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-dd,fb,be,cdb->cef-order10]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-bca,cdb,dbf,afc->-order11]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-bca,cdb,dbf,afc->-order12]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-bca,cdb,dbf,afc->-order13]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-bca,cdb,dbf,afc->-order14]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-bca,cdb,dbf,afc->-order15]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dcc,fce,ea,dbf->ab-order16]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-dcc,fce,ea,dbf->ab-order17]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-dcc,fce,ea,dbf->ab-order18]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dcc,fce,ea,dbf->ab-order19]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-dcc,fce,ea,dbf->ab-order20]",
"opt_einsum/tests/test_paths.py::test_optimal_edge_cases",
"opt_einsum/tests/test_paths.py::test_greedy_edge_cases",
"opt_einsum/tests/test_paths.py::test_dp_edge_cases_dimension_1",
"opt_einsum/tests/test_paths.py::test_dp_edge_cases_all_singlet_indices",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_optimize_for_outer_products",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_optimize_for_size",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_cost_cap",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[greedy]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[branch-2]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[branch-all]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[optimal]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[dp]",
"opt_einsum/tests/test_paths.py::test_large_path[2]",
"opt_einsum/tests/test_paths.py::test_large_path[3]",
"opt_einsum/tests/test_paths.py::test_large_path[26]",
"opt_einsum/tests/test_paths.py::test_large_path[52]",
"opt_einsum/tests/test_paths.py::test_large_path[116]",
"opt_einsum/tests/test_paths.py::test_large_path[300]",
"opt_einsum/tests/test_paths.py::test_parallel_random_greedy",
"opt_einsum/tests/test_paths.py::test_custom_path_optimizer",
"opt_einsum/tests/test_paths.py::test_custom_random_optimizer",
"opt_einsum/tests/test_paths.py::test_optimizer_registration"
] | 2020-05-12 21:47:13+00:00 | 1,904 |
|
dgasmith__opt_einsum-145 | diff --git a/docs/source/backends.rst b/docs/source/backends.rst
index d297b19..7e48678 100644
--- a/docs/source/backends.rst
+++ b/docs/source/backends.rst
@@ -325,3 +325,47 @@ so again just the ``backend`` keyword needs to be supplied:
[-462.52362 , -121.12659 , -67.84769 , 624.5455 ],
[ 5.2839584, 36.44155 , 81.62852 , 703.15784 ]],
dtype=float32)
+
+
+Contracting arbitrary objects
+=============================
+
+There is one more explicit backend that can handle arbitrary arrays of objects,
+so long the *objects themselves* just support multiplication and addition (
+``__mul__`` and ``__add__`` dunder methods respectively). Use it by supplying
+``backend='object'``.
+
+For example, imagine we want to perform a contraction of arrays made up of
+`sympy <www.sympy.org>`_ symbols:
+
+.. code-block:: python
+
+ >>> import opt_einsum as oe
+ >>> import numpy as np
+ >>> import sympy
+
+ >>> # define the symbols
+ >>> a, b, c, d, e, f, g, h, i, j, k, l = [sympy.symbols(oe.get_symbol(i)) for i in range(12)]
+ >>> a * b + c * d
+ 𝑎𝑏+𝑐𝑑
+
+ >>> # define the tensors (you might explicitly specify ``dtype=object``)
+ >>> X = np.array([[a, b], [c, d]])
+ >>> Y = np.array([[e, f], [g, h]])
+ >>> Z = np.array([[i, j], [k, l]])
+
+ >>> # contract the tensors!
+ >>> oe.contract('uv,vw,wu->u', X, Y, Z, backend='object')
+ array([i*(a*e + b*g) + k*(a*f + b*h), j*(c*e + d*g) + l*(c*f + d*h)],
+ dtype=object)
+
+There are a few things to note here:
+
+* The returned array is a ``numpy.ndarray`` but since it has ``dtype=object``
+ it can really hold *any* python objects
+* We had to explicitly use ``backend='object'``, since :func:`numpy.einsum`
+ would have otherwise been dispatched to, which can't handle ``dtype=object``
+ (though :func:`numpy.tensordot` in fact can)
+* Although an optimized pairwise contraction order is used, the looping in each
+ single contraction is **performed in python so performance will be
+ drastically lower than for numeric dtypes!**
diff --git a/opt_einsum/backends/dispatch.py b/opt_einsum/backends/dispatch.py
index 85a6ceb..f5777ae 100644
--- a/opt_einsum/backends/dispatch.py
+++ b/opt_einsum/backends/dispatch.py
@@ -8,6 +8,7 @@ import importlib
import numpy
+from . import object_arrays
from . import cupy as _cupy
from . import jax as _jax
from . import tensorflow as _tensorflow
@@ -49,6 +50,10 @@ _cached_funcs = {
('tensordot', 'numpy'): numpy.tensordot,
('transpose', 'numpy'): numpy.transpose,
('einsum', 'numpy'): numpy.einsum,
+ # also pre-populate with the arbitrary object backend
+ ('tensordot', 'object'): numpy.tensordot,
+ ('transpose', 'object'): numpy.transpose,
+ ('einsum', 'object'): object_arrays.object_einsum,
}
diff --git a/opt_einsum/backends/object_arrays.py b/opt_einsum/backends/object_arrays.py
new file mode 100644
index 0000000..3b394e1
--- /dev/null
+++ b/opt_einsum/backends/object_arrays.py
@@ -0,0 +1,60 @@
+"""
+Functions for performing contractions with array elements which are objects.
+"""
+
+import numpy as np
+import functools
+import operator
+
+
+def object_einsum(eq, *arrays):
+ """A ``einsum`` implementation for ``numpy`` arrays with object dtype.
+ The loop is performed in python, meaning the objects themselves need
+ only to implement ``__mul__`` and ``__add__`` for the contraction to be
+ computed. This may be useful when, for example, computing expressions of
+ tensors with symbolic elements, but note it will be very slow when compared
+ to ``numpy.einsum`` and numeric data types!
+
+ Parameters
+ ----------
+ eq : str
+ The contraction string, should specify output.
+ arrays : sequence of arrays
+ These can be any indexable arrays as long as addition and
+ multiplication is defined on the elements.
+
+ Returns
+ -------
+ out : numpy.ndarray
+ The output tensor, with ``dtype=object``.
+ """
+
+ # when called by ``opt_einsum`` we will always be given a full eq
+ lhs, output = eq.split('->')
+ inputs = lhs.split(',')
+
+ sizes = {}
+ for term, array in zip(inputs, arrays):
+ for k, d in zip(term, array.shape):
+ sizes[k] = d
+
+ out_size = tuple(sizes[k] for k in output)
+ out = np.empty(out_size, dtype=object)
+
+ inner = tuple(k for k in sizes if k not in output)
+ inner_size = tuple(sizes[k] for k in inner)
+
+ for coo_o in np.ndindex(*out_size):
+
+ coord = dict(zip(output, coo_o))
+
+ def gen_inner_sum():
+ for coo_i in np.ndindex(*inner_size):
+ coord.update(dict(zip(inner, coo_i)))
+ locs = (tuple(coord[k] for k in term) for term in inputs)
+ elements = (array[loc] for array, loc in zip(arrays, locs))
+ yield functools.reduce(operator.mul, elements)
+
+ out[coo_o] = functools.reduce(operator.add, gen_inner_sum())
+
+ return out
| dgasmith/opt_einsum | e88983faeb1221cc802ca8cefe2b425cfebe4880 | diff --git a/opt_einsum/tests/test_backends.py b/opt_einsum/tests/test_backends.py
index 905d2ba..2c05f35 100644
--- a/opt_einsum/tests/test_backends.py
+++ b/opt_einsum/tests/test_backends.py
@@ -431,3 +431,25 @@ def test_auto_backend_custom_array_no_tensordot():
# Shaped is an array-like object defined by opt_einsum - which has no TDOT
assert infer_backend(x) == 'opt_einsum'
assert parse_backend([x], 'auto') == 'numpy'
+
+
+@pytest.mark.parametrize("string", tests)
+def test_object_arrays_backend(string):
+ views = helpers.build_views(string)
+ ein = contract(string, *views, optimize=False, use_blas=False)
+ assert ein.dtype != object
+
+ shps = [v.shape for v in views]
+ expr = contract_expression(string, *shps, optimize=True)
+
+ obj_views = [view.astype(object) for view in views]
+
+ # try raw contract
+ obj_opt = contract(string, *obj_views, backend='object')
+ assert obj_opt.dtype == object
+ assert np.allclose(ein, obj_opt.astype(float))
+
+ # test expression
+ obj_opt = expr(*obj_views, backend='object')
+ assert obj_opt.dtype == object
+ assert np.allclose(ein, obj_opt.astype(float))
| Potential bug (outer tensor product)
This works...:
```python
>>> import mpmath
>>> import numpy
>>> m0 = numpy.eye(2)
>>> opt_einsum.contract('bc,ad->abcd', m0, m0)
array([[[[1., 0.], ...)
```
...but this breaks:
```python
>>> m1 = m0 + mpmath.mpc(0)
>>> opt_einsum.contract('bc,ad->abcd', m1, m1)
Traceback (most recent call last): (...)
TypeError: invalid data type for einsum
```
However, using opt_einsum with mpmath is otherwise OK in principle:
```python
>>> opt_einsum.contract('bc,cd->bd', m1, m1)
array([[mpc(real='1.0', imag='0.0'), mpc(real='0.0', imag='0.0')],
[mpc(real='0.0', imag='0.0'), mpc(real='1.0', imag='0.0')]],
dtype=object)
``` | 0.0 | [
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[ab,bc->ca]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[abc,bcd,dea]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[abc,def->fedcba]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[abc,bcd,df->fa]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[ijk,ikj]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[i,j->ij]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[ijk,k->ij]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[AB,BC->CA]"
] | [
"opt_einsum/tests/test_backends.py::test_auto_backend_custom_array_no_tensordot"
] | 2020-07-02 04:12:10+00:00 | 1,905 |
|
dgasmith__opt_einsum-149 | diff --git a/opt_einsum/backends/jax.py b/opt_einsum/backends/jax.py
index 6bc1a1f..aefd941 100644
--- a/opt_einsum/backends/jax.py
+++ b/opt_einsum/backends/jax.py
@@ -13,18 +13,18 @@ _JAX = None
def _get_jax_and_to_jax():
- global _JAX
- if _JAX is None:
- import jax
+ global _JAX
+ if _JAX is None:
+ import jax
- @to_backend_cache_wrap
- @jax.jit
- def to_jax(x):
- return x
+ @to_backend_cache_wrap
+ @jax.jit
+ def to_jax(x):
+ return x
- _JAX = jax, to_jax
+ _JAX = jax, to_jax
- return _JAX
+ return _JAX
def build_expression(_, expr): # pragma: no cover
diff --git a/opt_einsum/contract.py b/opt_einsum/contract.py
index 6e7550a..1403f98 100644
--- a/opt_einsum/contract.py
+++ b/opt_einsum/contract.py
@@ -51,16 +51,22 @@ class PathInfo(object):
" Complete contraction: {}\n".format(self.eq), " Naive scaling: {}\n".format(len(self.indices)),
" Optimized scaling: {}\n".format(max(self.scale_list)), " Naive FLOP count: {:.3e}\n".format(
self.naive_cost), " Optimized FLOP count: {:.3e}\n".format(self.opt_cost),
- " Theoretical speedup: {:3.3f}\n".format(self.speedup),
+ " Theoretical speedup: {:.3e}\n".format(self.speedup),
" Largest intermediate: {:.3e} elements\n".format(self.largest_intermediate), "-" * 80 + "\n",
"{:>6} {:>11} {:>22} {:>37}\n".format(*header), "-" * 80
]
for n, contraction in enumerate(self.contraction_list):
inds, idx_rm, einsum_str, remaining, do_blas = contraction
- remaining_str = ",".join(remaining) + "->" + self.output_subscript
- path_run = (self.scale_list[n], do_blas, einsum_str, remaining_str)
- path_print.append("\n{:>4} {:>14} {:>22} {:>37}".format(*path_run))
+
+ if remaining is not None:
+ remaining_str = ",".join(remaining) + "->" + self.output_subscript
+ else:
+ remaining_str = "..."
+ size_remaining = max(0, 56 - max(22, len(einsum_str)))
+
+ path_run = (self.scale_list[n], do_blas, einsum_str, remaining_str, size_remaining)
+ path_print.append("\n{:>4} {:>14} {:>22} {:>{}}".format(*path_run))
return "".join(path_print)
@@ -303,7 +309,14 @@ def contract_path(*operands, **kwargs):
einsum_str = ",".join(tmp_inputs) + "->" + idx_result
- contraction = (contract_inds, idx_removed, einsum_str, input_list[:], do_blas)
+ # for large expressions saving the remaining terms at each step can
+ # incur a large memory footprint - and also be messy to print
+ if len(input_list) <= 20:
+ remaining = tuple(input_list)
+ else:
+ remaining = None
+
+ contraction = (contract_inds, idx_removed, einsum_str, remaining, do_blas)
contraction_list.append(contraction)
opt_cost = sum(cost_list)
@@ -529,7 +542,7 @@ def _core_contract(operands, contraction_list, backend='auto', evaluate_constant
# Start contraction loop
for num, contraction in enumerate(contraction_list):
- inds, idx_rm, einsum_str, remaining, blas_flag = contraction
+ inds, idx_rm, einsum_str, _, blas_flag = contraction
# check if we are performing the pre-pass of an expression with constants,
# if so, break out upon finding first non-constant (None) operand
| dgasmith/opt_einsum | 2cbd28e612ff0af54656c34d7f6c239efe524b12 | diff --git a/opt_einsum/tests/test_contract.py b/opt_einsum/tests/test_contract.py
index e6dfb04..8268e88 100644
--- a/opt_einsum/tests/test_contract.py
+++ b/opt_einsum/tests/test_contract.py
@@ -171,7 +171,7 @@ def test_printing():
views = helpers.build_views(string)
ein = contract_path(string, *views)
- assert len(str(ein[1])) == 726
+ assert len(str(ein[1])) == 728
@pytest.mark.parametrize("string", tests)
diff --git a/opt_einsum/tests/test_input.py b/opt_einsum/tests/test_input.py
index e5c1677..f3b6c06 100644
--- a/opt_einsum/tests/test_input.py
+++ b/opt_einsum/tests/test_input.py
@@ -34,7 +34,8 @@ def test_type_errors():
contract("", 0, out='test')
# order parameter must be a valid order
- with pytest.raises(TypeError):
+ # changed in Numpy 1.19, see https://github.com/numpy/numpy/commit/35b0a051c19265f5643f6011ee11e31d30c8bc4c
+ with pytest.raises((TypeError, ValueError)):
contract("", 0, order='W')
# casting parameter must be a valid casting
| Remove 'remaining' from PathInfo? (incurs n^2 memory overhead)
When building the ``contraction_list``, ``opt_einsum`` handles a list of 'remaining' terms, and at each new step these are copied (``input_list`` below):
https://github.com/dgasmith/opt_einsum/blob/2cbd28e612ff0af54656c34d7f6c239efe524b12/opt_einsum/contract.py#L306
A single, consumed version of this list is needed for constructing the items in the contraction list. However all the copies at each stage are not needed in the actual contraction (``_core_contract``) function - they are just for printing in the ``PathInfo`` object.
The problem is that each copied list of remaining terms is of average length `n / 2` and there are generally `n - 1` of them, incurring a `n^2` memory overhead, which caused problems for us (10s of gigabytes of problems!) in some large contractions. Here's an extreme sample of such a gigantic ``PathInfo``:
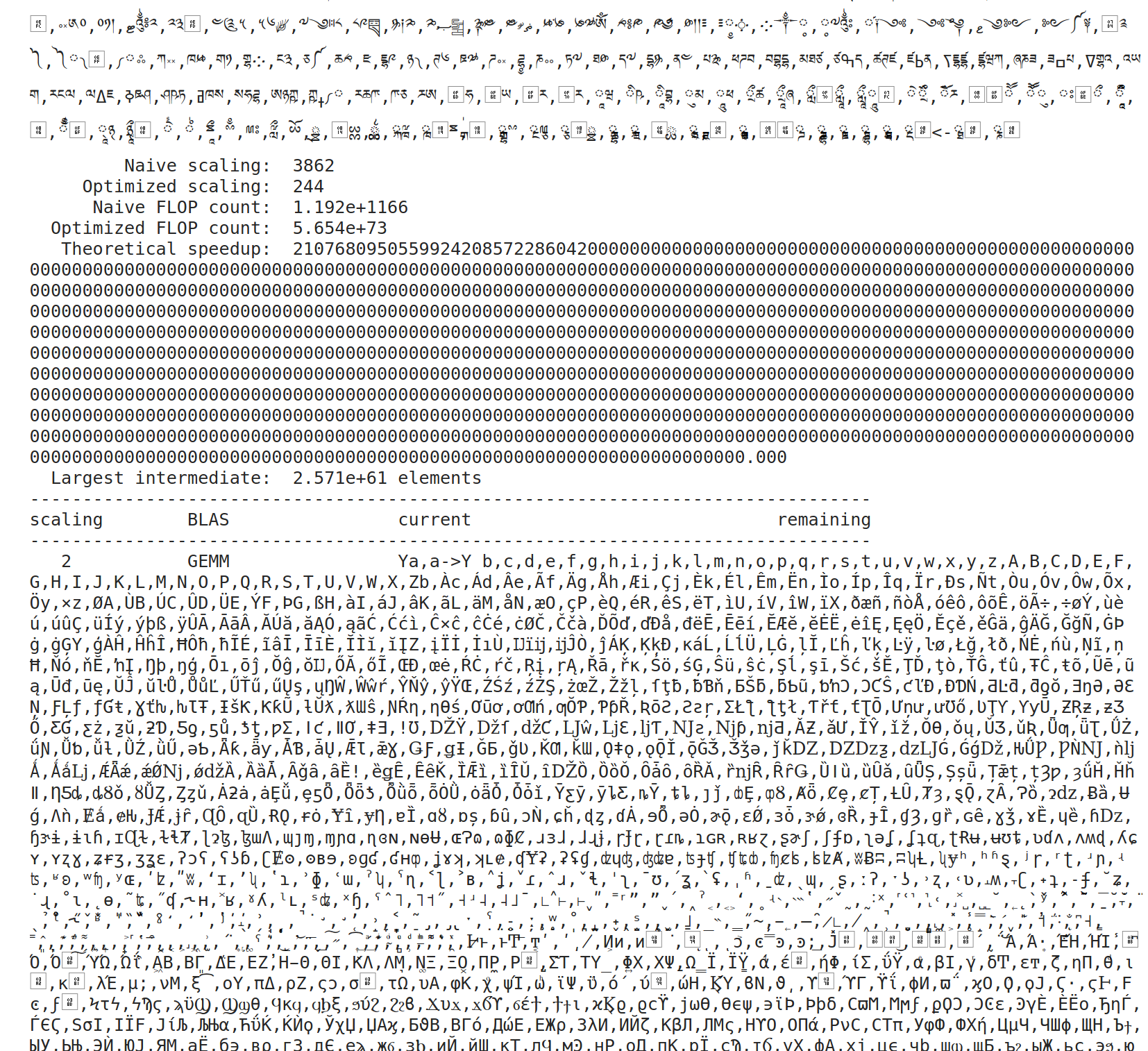
With 50 contractions each of several thousand tensors there was a total of nearly 100,000,000 'remaining' lists of strings.
I'd suggest either:
* a) *Easiest* - we just remove the 'remaining' terms from the ``PathInfo`` object, I'd be fine with this but possibly its useful information for others?
* b) *Medium* - the 'remaining' terms are only accumulated below a certain threshold size
* c) *Hardest* - they are generated lazily (and maybe optionally) by the ``PathInfo`` object upon request only (e.g. printing) | 0.0 | [
"opt_einsum/tests/test_contract.py::test_printing"
] | [
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,bac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,cba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_some_non_alphabet_maintains_order",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expression_interleaved_input",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants0]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[bdef,cdkj,ji,ikeh,hbc,lfo-constants1]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants2]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants3]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ijab,acd,bce,df,ef->ji-constants4]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,cd,ad,cb-constants5]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,bc,cd-constants6]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,abc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,bac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,cba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ba,bc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ba,cb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_path_supply_shapes",
"opt_einsum/tests/test_input.py::test_type_errors",
"opt_einsum/tests/test_input.py::test_value_errors",
"opt_einsum/tests/test_input.py::test_contract_inputs",
"opt_einsum/tests/test_input.py::test_compare[...a->...]",
"opt_einsum/tests/test_input.py::test_compare[a...->...]",
"opt_einsum/tests/test_input.py::test_compare[a...a->...a]",
"opt_einsum/tests/test_input.py::test_compare[...,...]",
"opt_einsum/tests/test_input.py::test_compare[a,b]",
"opt_einsum/tests/test_input.py::test_compare[...a,...b]",
"opt_einsum/tests/test_input.py::test_ellipse_input1",
"opt_einsum/tests/test_input.py::test_ellipse_input2",
"opt_einsum/tests/test_input.py::test_ellipse_input3",
"opt_einsum/tests/test_input.py::test_ellipse_input4",
"opt_einsum/tests/test_input.py::test_singleton_dimension_broadcast",
"opt_einsum/tests/test_input.py::test_large_int_input_format",
"opt_einsum/tests/test_input.py::test_hashable_object_input_format"
] | 2020-07-16 19:23:46+00:00 | 1,906 |
|
dgasmith__opt_einsum-196 | diff --git a/opt_einsum/contract.py b/opt_einsum/contract.py
index 0df94d0..0f70379 100644
--- a/opt_einsum/contract.py
+++ b/opt_einsum/contract.py
@@ -4,6 +4,7 @@ Contains the primary optimization and contraction routines.
from collections import namedtuple
from decimal import Decimal
+from functools import lru_cache
from typing import Any, Dict, Iterable, List, Optional, Sequence, Tuple
from . import backends, blas, helpers, parser, paths, sharing
@@ -542,15 +543,20 @@ def contract(*operands_: Any, **kwargs: Any) -> ArrayType:
return _core_contract(operands, contraction_list, backend=backend, **einsum_kwargs)
+@lru_cache(None)
+def _infer_backend_class_cached(cls: type) -> str:
+ return cls.__module__.split(".")[0]
+
+
def infer_backend(x: Any) -> str:
- return x.__class__.__module__.split(".")[0]
+ return _infer_backend_class_cached(x.__class__)
-def parse_backend(arrays: Sequence[ArrayType], backend: str) -> str:
+def parse_backend(arrays: Sequence[ArrayType], backend: Optional[str]) -> str:
"""Find out what backend we should use, dipatching based on the first
array if ``backend='auto'`` is specified.
"""
- if backend != "auto":
+ if (backend != "auto") and (backend is not None):
return backend
backend = infer_backend(arrays[0])
@@ -565,7 +571,7 @@ def parse_backend(arrays: Sequence[ArrayType], backend: str) -> str:
def _core_contract(
operands_: Sequence[ArrayType],
contraction_list: ContractionListType,
- backend: str = "auto",
+ backend: Optional[str] = "auto",
evaluate_constants: bool = False,
**einsum_kwargs: Any,
) -> ArrayType:
@@ -703,7 +709,7 @@ class ContractExpression:
self._evaluated_constants: Dict[str, Any] = {}
self._backend_expressions: Dict[str, Any] = {}
- def evaluate_constants(self, backend: str = "auto") -> None:
+ def evaluate_constants(self, backend: Optional[str] = "auto") -> None:
"""Convert any constant operands to the correct backend form, and
perform as many contractions as possible to create a new list of
operands, stored in ``self._evaluated_constants[backend]``. This also
@@ -746,7 +752,7 @@ class ContractExpression:
self,
arrays: Sequence[ArrayType],
out: Optional[ArrayType] = None,
- backend: str = "auto",
+ backend: Optional[str] = "auto",
evaluate_constants: bool = False,
) -> ArrayType:
"""The normal, core contraction."""
diff --git a/opt_einsum/paths.py b/opt_einsum/paths.py
index eac3630..ac8ccd5 100644
--- a/opt_einsum/paths.py
+++ b/opt_einsum/paths.py
@@ -1217,7 +1217,7 @@ class DynamicProgramming(PathOptimizer):
output = frozenset(symbol2int[c] for c in output_)
size_dict_canonical = {symbol2int[c]: v for c, v in size_dict_.items() if c in symbol2int}
size_dict = [size_dict_canonical[j] for j in range(len(size_dict_canonical))]
- naive_cost = naive_scale * len(inputs) * functools.reduce(operator.mul, size_dict)
+ naive_cost = naive_scale * len(inputs) * functools.reduce(operator.mul, size_dict, 1)
inputs, inputs_done, inputs_contractions = _dp_parse_out_single_term_ops(inputs, all_inds, ind_counts)
| dgasmith/opt_einsum | a274599c87c6224382e2ce328c7439b7b713e363 | diff --git a/opt_einsum/tests/test_backends.py b/opt_einsum/tests/test_backends.py
index 87d2ba2..7c81c67 100644
--- a/opt_einsum/tests/test_backends.py
+++ b/opt_einsum/tests/test_backends.py
@@ -443,6 +443,7 @@ def test_auto_backend_custom_array_no_tensordot():
# Shaped is an array-like object defined by opt_einsum - which has no TDOT
assert infer_backend(x) == "opt_einsum"
assert parse_backend([x], "auto") == "numpy"
+ assert parse_backend([x], None) == "numpy"
@pytest.mark.parametrize("string", tests)
diff --git a/opt_einsum/tests/test_contract.py b/opt_einsum/tests/test_contract.py
index 453a779..ef3ee5d 100644
--- a/opt_einsum/tests/test_contract.py
+++ b/opt_einsum/tests/test_contract.py
@@ -13,6 +13,7 @@ tests = [
"a,->a",
"ab,->ab",
",ab,->ab",
+ ",,->",
# Test hadamard-like products
"a,ab,abc->abc",
"a,b,ab->ab",
diff --git a/opt_einsum/tests/test_paths.py b/opt_einsum/tests/test_paths.py
index c6fef4f..eb4b18b 100644
--- a/opt_einsum/tests/test_paths.py
+++ b/opt_einsum/tests/test_paths.py
@@ -58,6 +58,7 @@ path_scalar_tests = [
["ab,->ab", 1],
[",a,->a", 2],
[",,a,->a", 3],
+ [",,->", 2],
]
| allow `backend=None` as shorthand for 'auto'
A couple of times I've be caught out (e.g. https://github.com/jcmgray/quimb/issues/130) that the special value for inferring the backend is not `backend=None`. Might be nice to allow both?
If so would just require a simple:
```python
if backend not in ("auto", None):
return backend
```
in `parse_backend` of `contract.py`. | 0.0 | [
"opt_einsum/tests/test_backends.py::test_auto_backend_custom_array_no_tensordot",
"opt_einsum/tests/test_contract.py::test_compare[dp-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-,,->]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dp-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dynamic-programming-,,->-2]"
] | [
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[ab,bc->ca]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[abc,bcd,dea]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[abc,def->fedcba]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[abc,bcd,df->fa]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[ijk,ikj]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[i,j->ij]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[ijk,k->ij]",
"opt_einsum/tests/test_backends.py::test_object_arrays_backend[AB,BC->CA]",
"opt_einsum/tests/test_contract.py::test_compare[auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,->a]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[,,->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,bac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,cba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_some_non_alphabet_maintains_order",
"opt_einsum/tests/test_contract.py::test_printing",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-auto-hq-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-eager-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-opportunistic-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dp-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-dynamic-programming-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-a,->a]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-,,->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-128-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expression_interleaved_input",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants0]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[bdef,cdkj,ji,ikeh,hbc,lfo-constants1]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants2]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants3]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ijab,acd,bce,df,ef->ji-constants4]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,cd,ad,cb-constants5]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,bc,cd-constants6]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,->a]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[,ab,->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[,,->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,abc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,bac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,cba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ba,bc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ba,cb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_path_supply_shapes",
"opt_einsum/tests/test_paths.py::test_size_by_dict",
"opt_einsum/tests/test_paths.py::test_flop_cost",
"opt_einsum/tests/test_paths.py::test_bad_path_option",
"opt_einsum/tests/test_paths.py::test_explicit_path",
"opt_einsum/tests/test_paths.py::test_path_optimal",
"opt_einsum/tests/test_paths.py::test_path_greedy",
"opt_einsum/tests/test_paths.py::test_memory_paths",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-eb,cb,fb->cef-order0]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-eb,cb,fb->cef-order1]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-eb,cb,fb->cef-order2]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-eb,cb,fb->cef-order3]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-eb,cb,fb->cef-order4]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dd,fb,be,cdb->cef-order5]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-dd,fb,be,cdb->cef-order6]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-dd,fb,be,cdb->cef-order7]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dd,fb,be,cdb->cef-order8]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dd,fb,be,cdb->cef-order9]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-dd,fb,be,cdb->cef-order10]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-bca,cdb,dbf,afc->-order11]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-bca,cdb,dbf,afc->-order12]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-bca,cdb,dbf,afc->-order13]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-bca,cdb,dbf,afc->-order14]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-bca,cdb,dbf,afc->-order15]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dcc,fce,ea,dbf->ab-order16]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-dcc,fce,ea,dbf->ab-order17]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-dcc,fce,ea,dbf->ab-order18]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dcc,fce,ea,dbf->ab-order19]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[dp-dcc,fce,ea,dbf->ab-order20]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-hq-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-hq-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-hq-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-hq-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[auto-hq-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[optimal-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[optimal-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[optimal-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[optimal-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[optimal-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-all-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-all-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-all-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-all-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-all-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-2-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-2-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-2-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-2-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-2-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-1-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-1-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-1-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-1-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[branch-1-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[greedy-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[greedy-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[greedy-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[greedy-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[greedy-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[eager-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[eager-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[eager-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[eager-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[eager-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[opportunistic-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[opportunistic-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[opportunistic-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[opportunistic-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[opportunistic-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dp-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dp-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dp-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dp-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dynamic-programming-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dynamic-programming-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dynamic-programming-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[dynamic-programming-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-,,->-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-128-a,->a-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-128-ab,->ab-1]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-128-,a,->a-2]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-128-,,a,->a-3]",
"opt_einsum/tests/test_paths.py::test_path_scalar_cases[random-greedy-128-,,->-2]",
"opt_einsum/tests/test_paths.py::test_optimal_edge_cases",
"opt_einsum/tests/test_paths.py::test_greedy_edge_cases",
"opt_einsum/tests/test_paths.py::test_dp_edge_cases_dimension_1",
"opt_einsum/tests/test_paths.py::test_dp_edge_cases_all_singlet_indices",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_optimize_for_outer_products",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_optimize_for_size",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_cost_cap",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[flops-663054-18900-path0]",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[size-1114440-2016-path1]",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[write-983790-2016-path2]",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[combo-973518-2016-path3]",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[limit-983832-2016-path4]",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[combo-256-983790-2016-path5]",
"opt_einsum/tests/test_paths.py::test_custom_dp_can_set_minimize[limit-256-983832-2016-path6]",
"opt_einsum/tests/test_paths.py::test_dp_errors_when_no_contractions_found",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[greedy]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[branch-2]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[branch-all]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[optimal]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[dp]",
"opt_einsum/tests/test_paths.py::test_large_path[2]",
"opt_einsum/tests/test_paths.py::test_large_path[3]",
"opt_einsum/tests/test_paths.py::test_large_path[26]",
"opt_einsum/tests/test_paths.py::test_large_path[52]",
"opt_einsum/tests/test_paths.py::test_large_path[116]",
"opt_einsum/tests/test_paths.py::test_large_path[300]",
"opt_einsum/tests/test_paths.py::test_custom_random_greedy",
"opt_einsum/tests/test_paths.py::test_custom_branchbound",
"opt_einsum/tests/test_paths.py::test_branchbound_validation",
"opt_einsum/tests/test_paths.py::test_parallel_random_greedy",
"opt_einsum/tests/test_paths.py::test_custom_path_optimizer",
"opt_einsum/tests/test_paths.py::test_custom_random_optimizer",
"opt_einsum/tests/test_paths.py::test_optimizer_registration"
] | 2022-07-06 09:27:11+00:00 | 1,907 |
|
dgasmith__opt_einsum-48 | diff --git a/opt_einsum/compat.py b/opt_einsum/compat.py
new file mode 100644
index 0000000..38cf55d
--- /dev/null
+++ b/opt_einsum/compat.py
@@ -0,0 +1,10 @@
+# Python 2/3 compatability shim
+
+try:
+ # Python 2
+ get_chr = unichr
+ strings = (str, type(get_chr(300)))
+except NameError:
+ # Python 3
+ get_chr = chr
+ strings = str
diff --git a/opt_einsum/contract.py b/opt_einsum/contract.py
index a7932f0..01998dd 100644
--- a/opt_einsum/contract.py
+++ b/opt_einsum/contract.py
@@ -6,6 +6,7 @@ import numpy as np
from . import backends
from . import blas
+from . import compat
from . import helpers
from . import parser
from . import paths
@@ -176,7 +177,7 @@ def contract_path(*operands, **kwargs):
naive_cost = helpers.flop_count(indices, inner_product, num_ops, dimension_dict)
# Compute the path
- if not isinstance(path_type, str):
+ if not isinstance(path_type, compat.strings):
path = path_type
elif num_ops == 1:
# Nothing to be optimized
@@ -274,7 +275,7 @@ def _einsum(*operands, **kwargs):
"""
fn = backends.get_func('einsum', kwargs.pop('backend', 'numpy'))
- if not isinstance(operands[0], str):
+ if not isinstance(operands[0], compat.strings):
return fn(*operands, **kwargs)
einsum_str, operands = operands[0], operands[1:]
diff --git a/opt_einsum/parser.py b/opt_einsum/parser.py
index c5370b1..adfc19f 100644
--- a/opt_einsum/parser.py
+++ b/opt_einsum/parser.py
@@ -8,6 +8,8 @@ from collections import OrderedDict
import numpy as np
+from . import compat
+
einsum_symbols_base = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
@@ -40,7 +42,7 @@ def get_symbol(i):
"""
if i < 52:
return einsum_symbols_base[i]
- return chr(i + 140)
+ return compat.get_chr(i + 140)
def gen_unused_symbols(used, n):
@@ -133,7 +135,7 @@ def parse_einsum_input(operands):
if len(operands) == 0:
raise ValueError("No input operands")
- if isinstance(operands[0], str):
+ if isinstance(operands[0], compat.strings):
subscripts = operands[0].replace(" ", "")
operands = [possibly_convert_to_numpy(x) for x in operands[1:]]
diff --git a/opt_einsum/sharing.py b/opt_einsum/sharing.py
index 504ba05..54c94bd 100644
--- a/opt_einsum/sharing.py
+++ b/opt_einsum/sharing.py
@@ -129,7 +129,7 @@ def einsum_cache_wrap(einsum):
canonical = sorted(zip(inputs, map(id, operands)), key=lambda x: x[1])
canonical_ids = tuple(id_ for _, id_ in canonical)
canonical_inputs = ','.join(input_ for input_, _ in canonical)
- canonical_equation = alpha_canonicalize('{}->{}'.format(canonical_inputs, output))
+ canonical_equation = alpha_canonicalize(canonical_inputs + "->" + output)
key = 'einsum', backend, canonical_equation, canonical_ids
return _memoize(key, einsum, equation, *operands, backend=backend)
| dgasmith/opt_einsum | 9e646be16b0a7be8ee88becefc1bba267956bc3b | diff --git a/opt_einsum/tests/test_contract.py b/opt_einsum/tests/test_contract.py
index 43b5061..fdfacc7 100644
--- a/opt_einsum/tests/test_contract.py
+++ b/opt_einsum/tests/test_contract.py
@@ -7,7 +7,7 @@ import sys
import numpy as np
import pytest
-from opt_einsum import contract, contract_path, helpers, contract_expression
+from opt_einsum import compat, contract, contract_path, helpers, contract_expression
tests = [
# Test hadamard-like products
@@ -117,7 +117,6 @@ def test_drop_in_replacement(string):
assert np.allclose(opt, np.einsum(string, *views))
-@pytest.mark.skipif(sys.version_info[0] < 3, reason='requires python3')
@pytest.mark.parametrize("string", tests)
def test_compare_greek(string):
views = helpers.build_views(string)
@@ -125,7 +124,7 @@ def test_compare_greek(string):
ein = contract(string, *views, optimize=False, use_blas=False)
# convert to greek
- string = ''.join(chr(ord(c) + 848) if c not in ',->.' else c for c in string)
+ string = ''.join(compat.get_chr(ord(c) + 848) if c not in ',->.' else c for c in string)
opt = contract(string, *views, optimize='greedy', use_blas=False)
assert np.allclose(ein, opt)
@@ -146,7 +145,6 @@ def test_compare_blas(string):
assert np.allclose(ein, opt)
-@pytest.mark.skipif(sys.version_info[0] < 3, reason='requires python3')
@pytest.mark.parametrize("string", tests)
def test_compare_blas_greek(string):
views = helpers.build_views(string)
@@ -154,7 +152,7 @@ def test_compare_blas_greek(string):
ein = contract(string, *views, optimize=False)
# convert to greek
- string = ''.join(chr(ord(c) + 848) if c not in ',->.' else c for c in string)
+ string = ''.join(compat.get_chr(ord(c) + 848) if c not in ',->.' else c for c in string)
opt = contract(string, *views, optimize='greedy')
assert np.allclose(ein, opt)
@@ -163,10 +161,9 @@ def test_compare_blas_greek(string):
assert np.allclose(ein, opt)
-@pytest.mark.skipif(sys.version_info[0] < 3, reason='requires python3')
def test_some_non_alphabet_maintains_order():
# 'c beta a' should automatically go to -> 'a c beta'
- string = 'c' + chr(ord('b') + 848) + 'a'
+ string = 'c' + compat.get_chr(ord('b') + 848) + 'a'
# but beta will be temporarily replaced with 'b' for which 'cba->abc'
# so check manual output kicks in:
x = np.random.rand(2, 3, 4)
diff --git a/opt_einsum/tests/test_input.py b/opt_einsum/tests/test_input.py
index 022a078..311356e 100644
--- a/opt_einsum/tests/test_input.py
+++ b/opt_einsum/tests/test_input.py
@@ -230,7 +230,6 @@ def test_singleton_dimension_broadcast():
assert np.allclose(res2, np.full((1, 5), 5))
-@pytest.mark.skipif(sys.version_info.major == 2, reason="Requires python 3.")
def test_large_int_input_format():
string = 'ab,bc,cd'
x, y, z = build_views(string)
diff --git a/opt_einsum/tests/test_paths.py b/opt_einsum/tests/test_paths.py
index 8087dd2..da39e23 100644
--- a/opt_einsum/tests/test_paths.py
+++ b/opt_einsum/tests/test_paths.py
@@ -3,6 +3,8 @@ Tests the accuracy of the opt_einsum paths in addition to unit tests for
the various path helper functions.
"""
+import itertools
+
import numpy as np
import pytest
@@ -174,3 +176,14 @@ def test_can_optimize_outer_products():
a, b, c = [np.random.randn(10, 10) for _ in range(3)]
d = np.random.randn(10, 2)
assert oe.contract_path("ab,cd,ef,fg", a, b, c, d, path='greedy')[0] == [(2, 3), (0, 2), (0, 1)]
+
+
+@pytest.mark.parametrize('num_symbols', [2, 3, 26, 26 + 26, 256 - 140, 300])
+def test_large_path(num_symbols):
+ symbols = ''.join(oe.get_symbol(i) for i in range(num_symbols))
+ dimension_dict = dict(zip(symbols, itertools.cycle([2, 3, 4])))
+ expression = ','.join(symbols[t:t+2] for t in range(num_symbols - 1))
+ tensors = oe.helpers.build_views(expression, dimension_dict=dimension_dict)
+
+ # Check that path construction does not crash
+ oe.contract_path(expression, *tensors, path='greedy')
| Support for get_symbol(116) in Python 2
`get_symbol(x)` currently fails in Python 2 for any `x >= 116`:
```
i = 116
def get_symbol(i):
"""Get the symbol corresponding to int ``i`` - runs through the usual 52
letters before resorting to unicode characters, starting at ``chr(192)``.
Examples
--------
>>> get_symbol(2)
'c'
>>> oe.get_symbol(200)
'Ŕ'
>>> oe.get_symbol(20000)
'京'
"""
if i < 52:
return einsum_symbols_base[i]
> return chr(i + 140)
E ValueError: chr() arg not in range(256)
```
Can we fix this by use `unichr()` and unicode strings in Python 2? | 0.0 | [
"opt_einsum/tests/test_contract.py::test_compare[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,bac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,cba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_some_non_alphabet_maintains_order",
"opt_einsum/tests/test_contract.py::test_printing",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants0]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[bdef,cdkj,ji,ikeh,hbc,lfo-constants1]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants2]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants3]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ijab,acd,bce,df,ef->ji-constants4]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,cd,ad,cb-constants5]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,bc,cd-constants6]",
"opt_einsum/tests/test_input.py::test_value_errors",
"opt_einsum/tests/test_input.py::test_compare[...a->...]",
"opt_einsum/tests/test_input.py::test_compare[a...->...]",
"opt_einsum/tests/test_input.py::test_compare[a...a->...a]",
"opt_einsum/tests/test_input.py::test_compare[...,...]",
"opt_einsum/tests/test_input.py::test_compare[a,b]",
"opt_einsum/tests/test_input.py::test_compare[...a,...b]",
"opt_einsum/tests/test_input.py::test_ellipse_input1",
"opt_einsum/tests/test_input.py::test_ellipse_input2",
"opt_einsum/tests/test_input.py::test_ellipse_input3",
"opt_einsum/tests/test_input.py::test_ellipse_input4",
"opt_einsum/tests/test_input.py::test_singleton_dimension_broadcast",
"opt_einsum/tests/test_input.py::test_large_int_input_format",
"opt_einsum/tests/test_paths.py::test_size_by_dict",
"opt_einsum/tests/test_paths.py::test_flop_cost",
"opt_einsum/tests/test_paths.py::test_path_optimal",
"opt_einsum/tests/test_paths.py::test_path_greedy",
"opt_einsum/tests/test_paths.py::test_memory_paths",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-eb,cb,fb->cef-order0]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-eb,cb,fb->cef-order1]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dd,fb,be,cdb->cef-order2]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dd,fb,be,cdb->cef-order3]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-bca,cdb,dbf,afc->-order4]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-bca,cdb,dbf,afc->-order5]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dcc,fce,ea,dbf->ab-order6]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dcc,fce,ea,dbf->ab-order7]",
"opt_einsum/tests/test_paths.py::test_optimal_edge_cases",
"opt_einsum/tests/test_paths.py::test_greedy_edge_cases",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products",
"opt_einsum/tests/test_paths.py::test_large_path[2]",
"opt_einsum/tests/test_paths.py::test_large_path[3]",
"opt_einsum/tests/test_paths.py::test_large_path[26]",
"opt_einsum/tests/test_paths.py::test_large_path[52]",
"opt_einsum/tests/test_paths.py::test_large_path[116]",
"opt_einsum/tests/test_paths.py::test_large_path[300]"
] | [] | 2018-08-23 19:50:31+00:00 | 1,908 |
|
dgasmith__opt_einsum-53 | diff --git a/opt_einsum/sharing.py b/opt_einsum/sharing.py
index 54c94bd..038309f 100644
--- a/opt_einsum/sharing.py
+++ b/opt_einsum/sharing.py
@@ -3,11 +3,43 @@
import contextlib
import functools
import numbers
-from collections import Counter
+from collections import Counter, defaultdict
+import threading
from .parser import alpha_canonicalize, parse_einsum_input
-_SHARING_STACK = []
+
+_SHARING_STACK = defaultdict(list)
+
+
+try:
+ get_thread_id = threading.get_ident
+except AttributeError:
+ def get_thread_id():
+ return threading.current_thread().ident
+
+
+def currently_sharing():
+ """Check if we are currently sharing a cache -- thread specific.
+ """
+ return get_thread_id() in _SHARING_STACK
+
+
+def get_sharing_cache():
+ """Return the most recent sharing cache -- thread specific.
+ """
+ return _SHARING_STACK[get_thread_id()][-1]
+
+
+def _add_sharing_cache(cache):
+ _SHARING_STACK[get_thread_id()].append(cache)
+
+
+def _remove_sharing_cache():
+ tid = get_thread_id()
+ _SHARING_STACK[tid].pop()
+ if not _SHARING_STACK[tid]:
+ del _SHARING_STACK[tid]
@contextlib.contextmanager
@@ -34,11 +66,11 @@ def shared_intermediates(cache=None):
"""
if cache is None:
cache = {}
+ _add_sharing_cache(cache)
try:
- _SHARING_STACK.append(cache)
yield cache
finally:
- _SHARING_STACK.pop()
+ _remove_sharing_cache()
def count_cached_ops(cache):
@@ -52,7 +84,7 @@ def _save_tensors(*tensors):
"""Save tensors in the cache to prevent their ids from being recycled.
This is needed to prevent false cache lookups.
"""
- cache = _SHARING_STACK[-1]
+ cache = get_sharing_cache()
for tensor in tensors:
cache['tensor', id(tensor)] = tensor
@@ -62,7 +94,7 @@ def _memoize(key, fn, *args, **kwargs):
Results will be stored in the innermost ``cache`` yielded by
:func:`shared_intermediates`.
"""
- cache = _SHARING_STACK[-1]
+ cache = get_sharing_cache()
if key in cache:
return cache[key]
result = fn(*args, **kwargs)
@@ -77,7 +109,7 @@ def transpose_cache_wrap(transpose):
@functools.wraps(transpose)
def cached_transpose(a, axes, backend='numpy'):
- if not _SHARING_STACK:
+ if not currently_sharing():
return transpose(a, axes, backend=backend)
# hash by axes
@@ -96,7 +128,7 @@ def tensordot_cache_wrap(tensordot):
@functools.wraps(tensordot)
def cached_tensordot(x, y, axes=2, backend='numpy'):
- if not _SHARING_STACK:
+ if not currently_sharing():
return tensordot(x, y, axes, backend=backend)
# hash based on the (axes_x,axes_y) form of axes
@@ -117,7 +149,7 @@ def einsum_cache_wrap(einsum):
@functools.wraps(einsum)
def cached_einsum(*args, **kwargs):
- if not _SHARING_STACK:
+ if not currently_sharing():
return einsum(*args, **kwargs)
# hash modulo commutativity by computing a canonical ordering and names
@@ -143,7 +175,7 @@ def to_backend_cache_wrap(to_backend):
@functools.wraps(to_backend)
def cached_to_backend(array):
- if not _SHARING_STACK:
+ if not currently_sharing():
return to_backend(array)
# hash by id
| dgasmith/opt_einsum | 284f233a4057e57ba8a3c1eef28e6bb6b8bfe2b1 | diff --git a/opt_einsum/tests/test_sharing.py b/opt_einsum/tests/test_sharing.py
index b0b860a..ea7f00e 100644
--- a/opt_einsum/tests/test_sharing.py
+++ b/opt_einsum/tests/test_sharing.py
@@ -6,11 +6,11 @@ import numpy as np
import pytest
from opt_einsum import (contract_expression, contract_path, get_symbol,
- helpers, shared_intermediates)
+ contract, helpers, shared_intermediates)
from opt_einsum.backends import to_cupy, to_torch
from opt_einsum.contract import _einsum
from opt_einsum.parser import parse_einsum_input
-from opt_einsum.sharing import count_cached_ops
+from opt_einsum.sharing import count_cached_ops, currently_sharing, get_sharing_cache
try:
import cupy
@@ -362,3 +362,23 @@ def test_chain_sharing(size, backend):
print('Without sharing: {} expressions'.format(num_exprs_nosharing))
print('With sharing: {} expressions'.format(num_exprs_sharing))
assert num_exprs_nosharing > num_exprs_sharing
+
+
+def test_multithreaded_sharing():
+ from multiprocessing.pool import ThreadPool
+
+ def fn():
+ X, Y, Z = helpers.build_views('ab,bc,cd')
+
+ with shared_intermediates():
+ contract('ab,bc,cd->a', X, Y, Z)
+ contract('ab,bc,cd->b', X, Y, Z)
+
+ return len(get_sharing_cache())
+
+ expected = fn()
+ pool = ThreadPool(8)
+ fs = [pool.apply_async(fn) for _ in range(16)]
+ assert not currently_sharing()
+ assert [f.get() for f in fs] == [expected] * 16
+ pool.close()
| Support multi-threading in shared_intermediates
Following up on https://github.com/dgasmith/opt_einsum/pull/43#issuecomment-414661988, the `shared_intermediates` cache is currently not locked.
One option would be to assign the thread id of a unique owner of the cache, such that at least thread conflicts could be detected and reported, rather than mere silent performance degradation. | 0.0 | [
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-ab,bc->ca]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-abc,bcd,dea]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-abc,def->fedcba]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-abc,bcd,df->fa]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-ijk,ikj]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-i,j->ij]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-ijk,k->ij]",
"opt_einsum/tests/test_sharing.py::test_sharing_value[numpy-AB,BC->CA]",
"opt_einsum/tests/test_sharing.py::test_complete_sharing[numpy]",
"opt_einsum/tests/test_sharing.py::test_sharing_reused_cache[numpy]",
"opt_einsum/tests/test_sharing.py::test_no_sharing_separate_cache[numpy]",
"opt_einsum/tests/test_sharing.py::test_sharing_nesting[numpy]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-ab,bc->ca]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-abc,bcd,dea]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-abc,def->fedcba]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-abc,bcd,df->fa]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-ijk,ikj]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-i,j->ij]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-ijk,k->ij]",
"opt_einsum/tests/test_sharing.py::test_sharing_modulo_commutativity[numpy-AB,BC->CA]",
"opt_einsum/tests/test_sharing.py::test_partial_sharing[numpy]",
"opt_einsum/tests/test_sharing.py::test_sharing_with_constants[numpy]",
"opt_einsum/tests/test_sharing.py::test_chain[numpy-3]",
"opt_einsum/tests/test_sharing.py::test_chain[numpy-4]",
"opt_einsum/tests/test_sharing.py::test_chain[numpy-5]",
"opt_einsum/tests/test_sharing.py::test_chain_2[numpy-3]",
"opt_einsum/tests/test_sharing.py::test_chain_2[numpy-4]",
"opt_einsum/tests/test_sharing.py::test_chain_2[numpy-5]",
"opt_einsum/tests/test_sharing.py::test_chain_2[numpy-10]",
"opt_einsum/tests/test_sharing.py::test_chain_2_growth[numpy]",
"opt_einsum/tests/test_sharing.py::test_chain_sharing[numpy-3]",
"opt_einsum/tests/test_sharing.py::test_chain_sharing[numpy-4]",
"opt_einsum/tests/test_sharing.py::test_chain_sharing[numpy-5]",
"opt_einsum/tests/test_sharing.py::test_multithreaded_sharing"
] | [] | 2018-08-24 18:55:58+00:00 | 1,909 |
|
dgasmith__opt_einsum-84 | diff --git a/opt_einsum/__init__.py b/opt_einsum/__init__.py
index 3d8b47b..0ec9eb6 100644
--- a/opt_einsum/__init__.py
+++ b/opt_einsum/__init__.py
@@ -6,15 +6,18 @@ from . import blas
from . import helpers
from . import paths
from . import path_random
-# Handle versioneer
-from ._version import get_versions
from .contract import contract, contract_path, contract_expression
from .parser import get_symbol
from .sharing import shared_intermediates
from .paths import BranchBound
from .path_random import RandomGreedy
+# Handle versioneer
+from ._version import get_versions
versions = get_versions()
__version__ = versions['version']
__git_revision__ = versions['full-revisionid']
del get_versions, versions
+
+
+paths.register_path_fn('random-greedy', path_random.random_greedy)
diff --git a/opt_einsum/contract.py b/opt_einsum/contract.py
index 2f2d262..f7d2d91 100644
--- a/opt_einsum/contract.py
+++ b/opt_einsum/contract.py
@@ -13,10 +13,9 @@ from . import compat
from . import helpers
from . import parser
from . import paths
-from . import path_random
from . import sharing
-__all__ = ["contract_path", "contract", "format_const_einsum_str", "ContractExpression", "shape_only", "shape_only"]
+__all__ = ["contract_path", "contract", "format_const_einsum_str", "ContractExpression", "shape_only"]
class PathInfo(object):
@@ -93,6 +92,9 @@ def _choose_memory_arg(memory_limit, size_list):
return int(memory_limit)
+_VALID_CONTRACT_KWARGS = {'optimize', 'path', 'memory_limit', 'einsum_call', 'use_blas', 'shapes'}
+
+
def contract_path(*operands, **kwargs):
"""
Find a contraction order 'path', without performing the contraction.
@@ -124,6 +126,9 @@ def contract_path(*operands, **kwargs):
Use BLAS functions or not
memory_limit : int, optional (default: None)
Maximum number of elements allowed in intermediate arrays.
+ shapes : bool, optional
+ Whether ``contract_path`` should assume arrays (the default) or array
+ shapes have been supplied.
Returns
-------
@@ -193,8 +198,7 @@ def contract_path(*operands, **kwargs):
"""
# Make sure all keywords are valid
- valid_contract_kwargs = ['optimize', 'path', 'memory_limit', 'einsum_call', 'use_blas']
- unknown_kwargs = [k for (k, v) in kwargs.items() if k not in valid_contract_kwargs]
+ unknown_kwargs = set(kwargs) - _VALID_CONTRACT_KWARGS
if len(unknown_kwargs):
raise TypeError("einsum_path: Did not understand the following kwargs: {}".format(unknown_kwargs))
@@ -206,6 +210,7 @@ def contract_path(*operands, **kwargs):
path_type = kwargs.pop('optimize', 'auto')
memory_limit = kwargs.pop('memory_limit', None)
+ shapes = kwargs.pop('shapes', False)
# Hidden option, only einsum should call this
einsum_call_arg = kwargs.pop("einsum_call", False)
@@ -217,7 +222,10 @@ def contract_path(*operands, **kwargs):
# Build a few useful list and sets
input_list = input_subscripts.split(',')
input_sets = [set(x) for x in input_list]
- input_shps = [x.shape for x in operands]
+ if shapes:
+ input_shps = operands
+ else:
+ input_shps = [x.shape for x in operands]
output_set = set(output_subscript)
indices = set(input_subscripts.replace(',', ''))
@@ -257,29 +265,17 @@ def contract_path(*operands, **kwargs):
# Compute the path
if not isinstance(path_type, (compat.strings, paths.PathOptimizer)):
+ # Custom path supplied
path = path_type
- elif num_ops == 1:
- # Nothing to be optimized
- path = [(0, )]
- elif num_ops == 2:
+ elif num_ops <= 2:
# Nothing to be optimized
- path = [(0, 1)]
+ path = [tuple(range(num_ops))]
elif isinstance(path_type, paths.PathOptimizer):
+ # Custom path optimizer supplied
path = path_type(input_sets, output_set, dimension_dict, memory_arg)
- elif path_type == "optimal" or (path_type == "auto" and num_ops <= 4):
- path = paths.optimal(input_sets, output_set, dimension_dict, memory_arg)
- elif path_type == 'branch-all' or (path_type == "auto" and num_ops <= 6):
- path = paths.branch(input_sets, output_set, dimension_dict, memory_arg, nbranch=None)
- elif path_type == 'branch-2' or (path_type == "auto" and num_ops <= 8):
- path = paths.branch(input_sets, output_set, dimension_dict, memory_arg, nbranch=2)
- elif path_type == 'branch-1' or (path_type == "auto" and num_ops <= 14):
- path = paths.branch(input_sets, output_set, dimension_dict, memory_arg, nbranch=1)
- elif path_type == 'random-greedy':
- path = path_random.random_greedy(input_sets, output_set, dimension_dict, memory_arg)
- elif path_type in ("auto", "greedy", "eager", "opportunistic"):
- path = paths.greedy(input_sets, output_set, dimension_dict, memory_arg)
else:
- raise KeyError("Path name '{}' not found".format(path_type))
+ path_optimizer = paths.get_path_fn(path_type)
+ path = path_optimizer(input_sets, output_set, dimension_dict, memory_arg)
cost_list = []
scale_list = []
diff --git a/opt_einsum/path_random.py b/opt_einsum/path_random.py
index 5f40cc8..49bf95e 100644
--- a/opt_einsum/path_random.py
+++ b/opt_einsum/path_random.py
@@ -11,13 +11,13 @@ import functools
from collections import deque
from . import helpers
-from .paths import PathOptimizer, ssa_greedy_optimize, get_better_fn, ssa_to_linear, calc_k12_flops
+from . import paths
__all__ = ["RandomGreedy", "random_greedy"]
-class RandomOptimizer(PathOptimizer):
+class RandomOptimizer(paths.PathOptimizer):
"""Base class for running any random path finder that benefits
from repeated calling, possibly in a parallel fashion. Custom random
optimizers should subclass this, and the ``setup`` method should be
@@ -81,7 +81,7 @@ class RandomOptimizer(PathOptimizer):
self.max_repeats = max_repeats
self.max_time = max_time
self.minimize = minimize
- self.better = get_better_fn(minimize)
+ self.better = paths.get_better_fn(minimize)
self.parallel = parallel
self.pre_dispatch = pre_dispatch
@@ -95,7 +95,7 @@ class RandomOptimizer(PathOptimizer):
def path(self):
"""The best path found so far.
"""
- return ssa_to_linear(self.best['ssa_path'])
+ return paths.ssa_to_linear(self.best['ssa_path'])
@property
def parallel(self):
@@ -256,7 +256,7 @@ def thermal_chooser(queue, remaining, nbranch=8, temperature=1, rel_temperature=
# compute relative probability for each potential contraction
if temperature == 0.0:
- energies = [1 if cost == cmin else 0 for cost in costs]
+ energies = [1 if c == cmin else 0 for c in costs]
else:
# shift by cmin for numerical reasons
energies = [math.exp(-(c - cmin) / temperature) for c in costs]
@@ -282,7 +282,7 @@ def ssa_path_compute_cost(ssa_path, inputs, output, size_dict):
max_size = 0
for i, j in ssa_path:
- k12, flops12 = calc_k12_flops(inputs, output, remaining, i, j, size_dict)
+ k12, flops12 = paths.calc_k12_flops(inputs, output, remaining, i, j, size_dict)
remaining.discard(i)
remaining.discard(j)
remaining.add(len(inputs))
@@ -302,7 +302,7 @@ def _trial_greedy_ssa_path_and_cost(r, inputs, output, size_dict, choose_fn, cos
else:
random.seed(r)
- ssa_path = ssa_greedy_optimize(inputs, output, size_dict, choose_fn, cost_fn)
+ ssa_path = paths.ssa_greedy_optimize(inputs, output, size_dict, choose_fn, cost_fn)
cost, size = ssa_path_compute_cost(ssa_path, inputs, output, size_dict)
return ssa_path, cost, size
diff --git a/opt_einsum/paths.py b/opt_einsum/paths.py
index df800bf..8ce6a67 100644
--- a/opt_einsum/paths.py
+++ b/opt_einsum/paths.py
@@ -5,6 +5,7 @@ from __future__ import absolute_import, division, print_function
import heapq
import random
+import functools
import itertools
from collections import defaultdict
@@ -12,7 +13,8 @@ import numpy as np
from . import helpers
-__all__ = ["optimal", "BranchBound", "branch", "greedy"]
+
+__all__ = ["optimal", "BranchBound", "branch", "greedy", "auto", "get_path_fn"]
_UNLIMITED_MEM = {-1, None, float('inf')}
@@ -444,6 +446,11 @@ def branch(inputs, output, size_dict, memory_limit=None, **optimizer_kwargs):
return optimizer(inputs, output, size_dict, memory_limit)
+branch_all = functools.partial(branch, nbranch=None)
+branch_2 = functools.partial(branch, nbranch=2)
+branch_1 = functools.partial(branch, nbranch=1)
+
+
def _get_candidate(output, sizes, remaining, footprints, dim_ref_counts, k1, k2, cost_fn):
either = k1 | k2
two = k1 & k2
@@ -645,3 +652,53 @@ def greedy(inputs, output, size_dict, memory_limit=None, choose_fn=None, cost_fn
ssa_path = ssa_greedy_optimize(inputs, output, size_dict, cost_fn=cost_fn, choose_fn=choose_fn)
return ssa_to_linear(ssa_path)
+
+
+_AUTO_CHOICES = {}
+for i in range(1, 5):
+ _AUTO_CHOICES[i] = optimal
+for i in range(5, 7):
+ _AUTO_CHOICES[i] = branch_all
+for i in range(7, 9):
+ _AUTO_CHOICES[i] = branch_2
+for i in range(9, 15):
+ _AUTO_CHOICES[i] = branch_1
+
+
+def auto(inputs, output, size_dict, memory_limit=None):
+ """Finds the contraction path by automatically choosing the method based on
+ how many input arguments there are.
+ """
+ N = len(inputs)
+ return _AUTO_CHOICES.get(N, greedy)(inputs, output, size_dict, memory_limit)
+
+
+_PATH_OPTIONS = {
+ 'auto': auto,
+ 'optimal': optimal,
+ 'branch-all': branch_all,
+ 'branch-2': branch_2,
+ 'branch-1': branch_1,
+ 'greedy': greedy,
+ 'eager': greedy,
+ 'opportunistic': greedy,
+}
+
+
+def register_path_fn(name, fn):
+ """Add path finding function ``fn`` as an option with ``name``.
+ """
+ if name in _PATH_OPTIONS:
+ raise KeyError("Path optimizer '{}' already exists.".format(name))
+
+ _PATH_OPTIONS[name.lower()] = fn
+
+
+def get_path_fn(path_type):
+ """Get the correct path finding function from str ``path_type``.
+ """
+ if path_type not in _PATH_OPTIONS:
+ raise KeyError("Path optimizer '{}' not found, valid options are {}."
+ .format(path_type, set(_PATH_OPTIONS.keys())))
+
+ return _PATH_OPTIONS[path_type]
| dgasmith/opt_einsum | ff86c2c9d2bb51d9816b2a92c08787379d512eb2 | diff --git a/opt_einsum/tests/test_backends.py b/opt_einsum/tests/test_backends.py
index ca046e4..b596cf1 100644
--- a/opt_einsum/tests/test_backends.py
+++ b/opt_einsum/tests/test_backends.py
@@ -2,6 +2,7 @@ import numpy as np
import pytest
from opt_einsum import contract, helpers, contract_expression, backends, sharing
+from opt_einsum.contract import infer_backend, parse_backend, Shaped
try:
import cupy
@@ -337,3 +338,10 @@ def test_torch_with_constants():
assert isinstance(res_got3, torch.Tensor)
res_got3 = res_got3.numpy() if res_got3.device.type == 'cpu' else res_got3.cpu().numpy()
assert np.allclose(res_exp, res_got3)
+
+
+def test_auto_backend_custom_array_no_tensordot():
+ x = Shaped((1, 2, 3))
+ # Shaped is an array-like object defined by opt_einsum - which has no TDOT
+ assert infer_backend(x) == 'opt_einsum'
+ assert parse_backend([x], 'auto') == 'numpy'
diff --git a/opt_einsum/tests/test_contract.py b/opt_einsum/tests/test_contract.py
index 7227e2d..775aac0 100644
--- a/opt_einsum/tests/test_contract.py
+++ b/opt_einsum/tests/test_contract.py
@@ -257,3 +257,9 @@ def test_linear_vs_ssa(equation):
ssa_path = linear_to_ssa(linear_path)
linear_path2 = ssa_to_linear(ssa_path)
assert linear_path2 == linear_path
+
+
+def test_contract_path_supply_shapes():
+ eq = 'ab,bc,cd'
+ shps = [(2, 3), (3, 4), (4, 5)]
+ contract_path(eq, *shps, shapes=True)
diff --git a/opt_einsum/tests/test_paths.py b/opt_einsum/tests/test_paths.py
index a860ee7..33b876b 100644
--- a/opt_einsum/tests/test_paths.py
+++ b/opt_einsum/tests/test_paths.py
@@ -108,6 +108,16 @@ def test_flop_cost():
assert 2000 == oe.helpers.flop_count("abc", True, 2, size_dict)
+def test_bad_path_option():
+ with pytest.raises(KeyError):
+ oe.contract("a,b,c", [1], [2], [3], optimize='optimall')
+
+
+def test_explicit_path():
+ x = oe.contract("a,b,c", [1], [2], [3], optimize=[(1, 2), (0, 1)])
+ assert x.item() == 6
+
+
def test_path_optimal():
test_func = oe.paths.optimal
@@ -353,3 +363,22 @@ def test_custom_random_optimizer():
assert optimizer.was_used
assert len(optimizer.costs) == 16
+
+
+def test_optimizer_registration():
+
+ def custom_optimizer(inputs, output, size_dict, memory_limit):
+ return [(0, 1)] * (len(inputs) - 1)
+
+ with pytest.raises(KeyError):
+ oe.paths.register_path_fn('optimal', custom_optimizer)
+
+ oe.paths.register_path_fn('custom', custom_optimizer)
+ assert 'custom' in oe.paths._PATH_OPTIONS
+
+ eq = 'ab,bc,cd'
+ shapes = [(2, 3), (3, 4), (4, 5)]
+ path, path_info = oe.contract_path(eq, *shapes, shapes=True,
+ optimize='custom')
+ assert path == [(0, 1), (0, 1)]
+ del oe.paths._PATH_OPTIONS['custom']
| FR: separate 'auto' strategy out of contract_path
The 'auto' strategy is really nice, but it requires actual arrays because it is based into `contract_path`. Sometimes I want to get a path based merely on shapes. It should be easy to factor out the logic from `contract_path` to a new `paths.auto` that conforms to the standard shape-based path interface. | 0.0 | [
"opt_einsum/tests/test_contract.py::test_contract_path_supply_shapes",
"opt_einsum/tests/test_paths.py::test_optimizer_registration"
] | [
"opt_einsum/tests/test_backends.py::test_auto_backend_custom_array_no_tensordot",
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,ba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,abc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,bac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abc,cba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,bc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ba,cb]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_drop_in_replacement[aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_greek[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_compare_blas_greek[random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_some_non_alphabet_maintains_order",
"opt_einsum/tests/test_contract.py::test_printing",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-False-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[False-True-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-False-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-optimal-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-all-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-2-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-branch-1-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ad]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,ba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,abc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,bac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abc,cba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ba,bc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ba,cb]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cd]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,ab]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_contract_expressions[True-True-random-greedy-aef,fbc,dca->bde]",
"opt_einsum/tests/test_contract.py::test_contract_expression_interleaved_input",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants0]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[bdef,cdkj,ji,ikeh,hbc,lfo-constants1]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants2]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[hbc,bdef,cdkj,ji,ikeh,lfo-constants3]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ijab,acd,bce,df,ef->ji-constants4]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,cd,ad,cb-constants5]",
"opt_einsum/tests/test_contract.py::test_contract_expression_with_constants[ab,bc,cd-constants6]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-0-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-2-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[False-4-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-0-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-2-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-2-5-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-4-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-4-optimal]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-5-greedy]",
"opt_einsum/tests/test_contract.py::test_rand_equation[True-4-3-5-optimal]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,ab,abc->abc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[a,b,ab->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ea,fb,gc,hd,abcd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ea,fb,abcd,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ea,fb,gc,hd->efgh]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[acdf,jbje,gihb,hfac,gfac,gifabc,hfac0]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[acdf,jbje,gihb,hfac,gfac,gifabc,hfac1]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[cd,bdhe,aidb,hgca,gc,hgibcd,hgac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abhe,hidj,jgba,hiab,gab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bde,cdh,agdb,hica,ibd,hgicd,hiac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[chd,bde,agbc,hiad,hgc,hgi,hiad]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[chd,bde,agbc,hiad,bdi,cgh,agdb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bdhe,acad,hiab,agac,hibd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,c->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,c->c]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd->cd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab,cd,cd,ef,ef->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,ef->abcdef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,ef->acdf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,de->abcde]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cd,de->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcd,cd->abcd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcd,cd->abd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[eb,cb,fb->cef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dd,fb,be,cdb->cef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bca,cdb,dbf,afc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dcc,fce,ea,dbf->ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fdf,cdd,ccd,afe->ae]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ad]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ed,fcd,ff,bcf->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[baa,dcf,af,cde->be]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bd,db,eac->ace]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fff,fae,bef,def->abd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[efc,dbc,acf,fd->abe]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,ba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,abc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,bac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abc,cba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,cb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ba,bc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ba,cb]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cd]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,ab]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cdef]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,cdef->feba]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[abcd,efdc]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,bc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[baa,bcc->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,ccb->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aab,fa,df,ecc->bde]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[ecb,fef,bad,ed->ac]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bcf,bbb,fbf,fc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bb,ff,be->e]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bcb,bb,fc,fff->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[fbb,dfd,fc,fc->]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[afd,ba,cc,dc->bf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[adb,bc,fa,cfc->d]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[bbd,bda,fc,db->acf]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[dba,ead,cad->bce]",
"opt_einsum/tests/test_contract.py::test_linear_vs_ssa[aef,fbc,dca->bde]",
"opt_einsum/tests/test_paths.py::test_size_by_dict",
"opt_einsum/tests/test_paths.py::test_flop_cost",
"opt_einsum/tests/test_paths.py::test_bad_path_option",
"opt_einsum/tests/test_paths.py::test_explicit_path",
"opt_einsum/tests/test_paths.py::test_path_optimal",
"opt_einsum/tests/test_paths.py::test_path_greedy",
"opt_einsum/tests/test_paths.py::test_memory_paths",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-eb,cb,fb->cef-order0]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-eb,cb,fb->cef-order1]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-eb,cb,fb->cef-order2]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-eb,cb,fb->cef-order3]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dd,fb,be,cdb->cef-order4]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-dd,fb,be,cdb->cef-order5]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-dd,fb,be,cdb->cef-order6]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dd,fb,be,cdb->cef-order7]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-bca,cdb,dbf,afc->-order8]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-bca,cdb,dbf,afc->-order9]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-bca,cdb,dbf,afc->-order10]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-bca,cdb,dbf,afc->-order11]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[greedy-dcc,fce,ea,dbf->ab-order12]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-all-dcc,fce,ea,dbf->ab-order13]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[branch-2-dcc,fce,ea,dbf->ab-order14]",
"opt_einsum/tests/test_paths.py::test_path_edge_cases[optimal-dcc,fce,ea,dbf->ab-order15]",
"opt_einsum/tests/test_paths.py::test_optimal_edge_cases",
"opt_einsum/tests/test_paths.py::test_greedy_edge_cases",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[greedy]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[branch-2]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[branch-all]",
"opt_einsum/tests/test_paths.py::test_can_optimize_outer_products[optimal]",
"opt_einsum/tests/test_paths.py::test_large_path[2]",
"opt_einsum/tests/test_paths.py::test_large_path[3]",
"opt_einsum/tests/test_paths.py::test_large_path[26]",
"opt_einsum/tests/test_paths.py::test_large_path[52]",
"opt_einsum/tests/test_paths.py::test_large_path[116]",
"opt_einsum/tests/test_paths.py::test_large_path[300]",
"opt_einsum/tests/test_paths.py::test_custom_random_greedy",
"opt_einsum/tests/test_paths.py::test_custom_branchbound",
"opt_einsum/tests/test_paths.py::test_parallel_random_greedy",
"opt_einsum/tests/test_paths.py::test_custom_path_optimizer",
"opt_einsum/tests/test_paths.py::test_custom_random_optimizer"
] | 2019-02-20 14:10:04+00:00 | 1,910 |
|
dgilland__pydash-164 | diff --git a/AUTHORS.rst b/AUTHORS.rst
index a1843ad..d167ec9 100644
--- a/AUTHORS.rst
+++ b/AUTHORS.rst
@@ -24,3 +24,4 @@ Contributors
- Gonzalo Naveira, `gonzalonaveira@github <https://github.com/gonzalonaveira>`_
- Wenbo Zhao, zhaowb@gmail.com, `zhaowb@github <https://github.com/zhaowb>`_
- Mervyn Lee, `mervynlee94@github <https://github.com/mervynlee94>`_
+- Weineel Lee, `weineel@github <https://github.com/weineel>`_
diff --git a/src/pydash/objects.py b/src/pydash/objects.py
index f3bed2b..6949e8e 100644
--- a/src/pydash/objects.py
+++ b/src/pydash/objects.py
@@ -934,7 +934,7 @@ def _merge_with(obj, *sources, **kwargs):
if _result is not None:
result = _result
elif all_sequences or all_mappings:
- result = _merge_with(obj_value, src_value, _setter=setter)
+ result = _merge_with(obj_value, src_value, iteratee=iteratee, _setter=setter)
else:
result = src_value
| dgilland/pydash | fa61732c01b39cec0de66f958cef27e7f31bcac2 | diff --git a/tests/test_objects.py b/tests/test_objects.py
index 2217438..b218043 100644
--- a/tests/test_objects.py
+++ b/tests/test_objects.py
@@ -573,11 +573,11 @@ def test_merge_no_link_list():
[
(
(
- {"fruits": ["apple"], "vegetables": ["beet"]},
- {"fruits": ["banana"], "vegetables": ["carrot"]},
- lambda a, b: a + b if isinstance(a, list) else b,
+ {"fruits": ["apple"], "others": {"vegetables": ["beet"]}},
+ {"fruits": ["banana"], "others": {"vegetables": ["carrot"]}},
+ lambda a, b: a + b if isinstance(a, list) else None,
),
- {"fruits": ["apple", "banana"], "vegetables": ["beet", "carrot"]},
+ {"fruits": ["apple", "banana"], "others": {"vegetables": ["beet", "carrot"]}},
),
],
)
| merge_with: iteratee is lost when _merge_with is recursing.
```python
def merge_array(obj_val, src_val):
if isinstance(obj_val, list) and isinstance(src_val, list):
return difference(obj_val + src_val)
merge_with({ 'd': { 'x': [2] } }, { 'd': { 'x': [5] } }, merge_array)
```
### expect
```
{ 'd': { 'x': [2, 5] } }
```
### actual
```
{ 'd': { 'x': [5] } }
```
| 0.0 | [
"tests/test_objects.py::test_merge_with[case0-expected0]"
] | [
"tests/test_objects.py::test_assign[case0-expected0]",
"tests/test_objects.py::test_assign[case1-expected1]",
"tests/test_objects.py::test_assign_with[case0-expected0]",
"tests/test_objects.py::test_callables[case0-expected0]",
"tests/test_objects.py::test_callables[case1-expected1]",
"tests/test_objects.py::test_clone[case0]",
"tests/test_objects.py::test_clone[case1]",
"tests/test_objects.py::test_clone_with[case0-<lambda>-expected0]",
"tests/test_objects.py::test_clone_with[case1-<lambda>-expected1]",
"tests/test_objects.py::test_clone_deep[case0]",
"tests/test_objects.py::test_clone_deep[case1]",
"tests/test_objects.py::test_clone_deep[case2]",
"tests/test_objects.py::test_clone_deep_with[case0-<lambda>-expected0]",
"tests/test_objects.py::test_clone_deep_with[case1-<lambda>-expected1]",
"tests/test_objects.py::test_clone_deep_with[case2-<lambda>-expected2]",
"tests/test_objects.py::test_clone_deep_with[a-<lambda>-a]",
"tests/test_objects.py::test_defaults[case0-expected0]",
"tests/test_objects.py::test_defaults_deep[case0-expected0]",
"tests/test_objects.py::test_defaults_deep[case1-expected1]",
"tests/test_objects.py::test_defaults_deep[case2-expected2]",
"tests/test_objects.py::test_defaults_deep[case3-expected3]",
"tests/test_objects.py::test_defaults_deep[case4-expected4]",
"tests/test_objects.py::test_to_dict[case0-expected0]",
"tests/test_objects.py::test_to_dict[case1-expected1]",
"tests/test_objects.py::test_invert[case0-expected0]",
"tests/test_objects.py::test_invert[case1-expected1]",
"tests/test_objects.py::test_invert_by[case0-expected0]",
"tests/test_objects.py::test_invert_by[case1-expected1]",
"tests/test_objects.py::test_invert_by[case2-expected2]",
"tests/test_objects.py::test_invoke[case0-1]",
"tests/test_objects.py::test_invoke[case1-2]",
"tests/test_objects.py::test_invoke[case2-None]",
"tests/test_objects.py::test_find_key[case0-expected0]",
"tests/test_objects.py::test_find_key[case1-expected1]",
"tests/test_objects.py::test_find_key[case2-expected2]",
"tests/test_objects.py::test_find_last_key[case0-expected0]",
"tests/test_objects.py::test_find_last_key[case1-expected1]",
"tests/test_objects.py::test_find_last_key[case2-expected2]",
"tests/test_objects.py::test_for_in[case0-expected0]",
"tests/test_objects.py::test_for_in[case1-expected1]",
"tests/test_objects.py::test_for_in[case2-expected2]",
"tests/test_objects.py::test_for_in_right[case0-expected0]",
"tests/test_objects.py::test_for_in_right[case1-expected1]",
"tests/test_objects.py::test_for_in_right[case2-expected2]",
"tests/test_objects.py::test_get[case0-expected0]",
"tests/test_objects.py::test_get[case1-4]",
"tests/test_objects.py::test_get[case2-expected2]",
"tests/test_objects.py::test_get[case3-4]",
"tests/test_objects.py::test_get[case4-None]",
"tests/test_objects.py::test_get[case5-expected5]",
"tests/test_objects.py::test_get[case6-expected6]",
"tests/test_objects.py::test_get[case7-expected7]",
"tests/test_objects.py::test_get[case8-None]",
"tests/test_objects.py::test_get[case9-None]",
"tests/test_objects.py::test_get[case10-2]",
"tests/test_objects.py::test_get[case11-2]",
"tests/test_objects.py::test_get[case12-expected12]",
"tests/test_objects.py::test_get[case13-expected13]",
"tests/test_objects.py::test_get[case14-haha]",
"tests/test_objects.py::test_get[case15-haha]",
"tests/test_objects.py::test_get[case16-None]",
"tests/test_objects.py::test_get[case17-5]",
"tests/test_objects.py::test_get[case18-5]",
"tests/test_objects.py::test_get[case19-5]",
"tests/test_objects.py::test_get[case20-4]",
"tests/test_objects.py::test_get[case21-5]",
"tests/test_objects.py::test_get[case22-42]",
"tests/test_objects.py::test_get[case23-42]",
"tests/test_objects.py::test_get[case24-42]",
"tests/test_objects.py::test_get[case25-42]",
"tests/test_objects.py::test_get[case26-value]",
"tests/test_objects.py::test_get[case27-expected27]",
"tests/test_objects.py::test_get[case28-None]",
"tests/test_objects.py::test_get[case29-1]",
"tests/test_objects.py::test_get[case30-1]",
"tests/test_objects.py::test_get[case31-1]",
"tests/test_objects.py::test_get[case32-None]",
"tests/test_objects.py::test_get[case33-None]",
"tests/test_objects.py::test_get[case34-expected34]",
"tests/test_objects.py::test_get[case35-3]",
"tests/test_objects.py::test_get[case36-1]",
"tests/test_objects.py::test_get[case37-1]",
"tests/test_objects.py::test_get[case38-John",
"tests/test_objects.py::test_get__should_not_populate_defaultdict",
"tests/test_objects.py::test_has[case0-True]",
"tests/test_objects.py::test_has[case1-True]",
"tests/test_objects.py::test_has[case2-True]",
"tests/test_objects.py::test_has[case3-False]",
"tests/test_objects.py::test_has[case4-True]",
"tests/test_objects.py::test_has[case5-True]",
"tests/test_objects.py::test_has[case6-True]",
"tests/test_objects.py::test_has[case7-False]",
"tests/test_objects.py::test_has[case8-True]",
"tests/test_objects.py::test_has[case9-True]",
"tests/test_objects.py::test_has[case10-True]",
"tests/test_objects.py::test_has[case11-True]",
"tests/test_objects.py::test_has[case12-False]",
"tests/test_objects.py::test_has[case13-False]",
"tests/test_objects.py::test_has[case14-False]",
"tests/test_objects.py::test_has[case15-False]",
"tests/test_objects.py::test_has[case16-True]",
"tests/test_objects.py::test_has[case17-True]",
"tests/test_objects.py::test_has[case18-True]",
"tests/test_objects.py::test_has[case19-True]",
"tests/test_objects.py::test_has[case20-True]",
"tests/test_objects.py::test_has__should_not_populate_defaultdict",
"tests/test_objects.py::test_keys[case0-expected0]",
"tests/test_objects.py::test_keys[case1-expected1]",
"tests/test_objects.py::test_map_values[case0-expected0]",
"tests/test_objects.py::test_map_values[case1-expected1]",
"tests/test_objects.py::test_map_values_deep[case0-expected0]",
"tests/test_objects.py::test_map_values_deep[case1-expected1]",
"tests/test_objects.py::test_merge[case0-expected0]",
"tests/test_objects.py::test_merge[case1-expected1]",
"tests/test_objects.py::test_merge[case2-expected2]",
"tests/test_objects.py::test_merge[case3-expected3]",
"tests/test_objects.py::test_merge[case4-expected4]",
"tests/test_objects.py::test_merge[case5-expected5]",
"tests/test_objects.py::test_merge[case6-expected6]",
"tests/test_objects.py::test_merge[case7-expected7]",
"tests/test_objects.py::test_merge[case8-expected8]",
"tests/test_objects.py::test_merge[case9-expected9]",
"tests/test_objects.py::test_merge[case10-None]",
"tests/test_objects.py::test_merge[case11-None]",
"tests/test_objects.py::test_merge[case12-None]",
"tests/test_objects.py::test_merge[case13-expected13]",
"tests/test_objects.py::test_merge[case14-expected14]",
"tests/test_objects.py::test_merge[case15-expected15]",
"tests/test_objects.py::test_merge_no_link_dict",
"tests/test_objects.py::test_merge_no_link_list",
"tests/test_objects.py::test_omit[case0-expected0]",
"tests/test_objects.py::test_omit[case1-expected1]",
"tests/test_objects.py::test_omit[case2-expected2]",
"tests/test_objects.py::test_omit[case3-expected3]",
"tests/test_objects.py::test_omit[case4-expected4]",
"tests/test_objects.py::test_omit[case5-expected5]",
"tests/test_objects.py::test_omit[case6-expected6]",
"tests/test_objects.py::test_omit[case7-expected7]",
"tests/test_objects.py::test_omit[case8-expected8]",
"tests/test_objects.py::test_omit_by[case0-expected0]",
"tests/test_objects.py::test_omit_by[case1-expected1]",
"tests/test_objects.py::test_omit_by[case2-expected2]",
"tests/test_objects.py::test_omit_by[case3-expected3]",
"tests/test_objects.py::test_parse_int[case0-1]",
"tests/test_objects.py::test_parse_int[case1-1]",
"tests/test_objects.py::test_parse_int[case2-1]",
"tests/test_objects.py::test_parse_int[case3-1]",
"tests/test_objects.py::test_parse_int[case4-11]",
"tests/test_objects.py::test_parse_int[case5-10]",
"tests/test_objects.py::test_parse_int[case6-8]",
"tests/test_objects.py::test_parse_int[case7-16]",
"tests/test_objects.py::test_parse_int[case8-10]",
"tests/test_objects.py::test_parse_int[case9-None]",
"tests/test_objects.py::test_pick[case0-expected0]",
"tests/test_objects.py::test_pick[case1-expected1]",
"tests/test_objects.py::test_pick[case2-expected2]",
"tests/test_objects.py::test_pick[case3-expected3]",
"tests/test_objects.py::test_pick[case4-expected4]",
"tests/test_objects.py::test_pick[case5-expected5]",
"tests/test_objects.py::test_pick[case6-expected6]",
"tests/test_objects.py::test_pick[case7-expected7]",
"tests/test_objects.py::test_pick[case8-expected8]",
"tests/test_objects.py::test_pick[case9-expected9]",
"tests/test_objects.py::test_pick[case10-expected10]",
"tests/test_objects.py::test_pick_by[case0-expected0]",
"tests/test_objects.py::test_pick_by[case1-expected1]",
"tests/test_objects.py::test_pick_by[case2-expected2]",
"tests/test_objects.py::test_pick_by[case3-expected3]",
"tests/test_objects.py::test_pick_by[case4-expected4]",
"tests/test_objects.py::test_pick_by[case5-expected5]",
"tests/test_objects.py::test_pick_by[case6-expected6]",
"tests/test_objects.py::test_rename_keys[case0-expected0]",
"tests/test_objects.py::test_rename_keys[case1-expected1]",
"tests/test_objects.py::test_rename_keys[case2-expected2]",
"tests/test_objects.py::test_set_[case0-expected0]",
"tests/test_objects.py::test_set_[case1-expected1]",
"tests/test_objects.py::test_set_[case2-expected2]",
"tests/test_objects.py::test_set_[case3-expected3]",
"tests/test_objects.py::test_set_[case4-expected4]",
"tests/test_objects.py::test_set_[case5-expected5]",
"tests/test_objects.py::test_set_[case6-expected6]",
"tests/test_objects.py::test_set_[case7-expected7]",
"tests/test_objects.py::test_set_[case8-expected8]",
"tests/test_objects.py::test_set_[case9-expected9]",
"tests/test_objects.py::test_set_[case10-expected10]",
"tests/test_objects.py::test_set_[case11-expected11]",
"tests/test_objects.py::test_set_[case12-expected12]",
"tests/test_objects.py::test_set_[case13-expected13]",
"tests/test_objects.py::test_set_[case14-expected14]",
"tests/test_objects.py::test_set_with[case0-expected0]",
"tests/test_objects.py::test_set_with[case1-expected1]",
"tests/test_objects.py::test_set_with[case2-expected2]",
"tests/test_objects.py::test_set_with[case3-expected3]",
"tests/test_objects.py::test_to_boolean[case0-True]",
"tests/test_objects.py::test_to_boolean[case1-False]",
"tests/test_objects.py::test_to_boolean[case2-True]",
"tests/test_objects.py::test_to_boolean[case3-True]",
"tests/test_objects.py::test_to_boolean[case4-False]",
"tests/test_objects.py::test_to_boolean[case5-False]",
"tests/test_objects.py::test_to_boolean[case6-None]",
"tests/test_objects.py::test_to_boolean[case7-None]",
"tests/test_objects.py::test_to_boolean[case8-False]",
"tests/test_objects.py::test_to_boolean[case9-True]",
"tests/test_objects.py::test_to_boolean[case10-False]",
"tests/test_objects.py::test_to_boolean[case11-True]",
"tests/test_objects.py::test_to_boolean[case12-False]",
"tests/test_objects.py::test_to_boolean[case13-False]",
"tests/test_objects.py::test_to_boolean[case14-True]",
"tests/test_objects.py::test_to_boolean[case15-False]",
"tests/test_objects.py::test_to_boolean[case16-True]",
"tests/test_objects.py::test_to_boolean[case17-None]",
"tests/test_objects.py::test_to_boolean[case18-False]",
"tests/test_objects.py::test_to_boolean[case19-None]",
"tests/test_objects.py::test_to_integer[1.4-1_0]",
"tests/test_objects.py::test_to_integer[1.9-1_0]",
"tests/test_objects.py::test_to_integer[1.4-1_1]",
"tests/test_objects.py::test_to_integer[1.9-1_1]",
"tests/test_objects.py::test_to_integer[foo-0]",
"tests/test_objects.py::test_to_integer[None-0]",
"tests/test_objects.py::test_to_integer[True-1]",
"tests/test_objects.py::test_to_integer[False-0]",
"tests/test_objects.py::test_to_integer[case8-0]",
"tests/test_objects.py::test_to_integer[case9-0]",
"tests/test_objects.py::test_to_integer[case10-0]",
"tests/test_objects.py::test_to_number[case0-3.0]",
"tests/test_objects.py::test_to_number[case1-2.6]",
"tests/test_objects.py::test_to_number[case2-990.0]",
"tests/test_objects.py::test_to_number[case3-None]",
"tests/test_objects.py::test_to_pairs[case0-expected0]",
"tests/test_objects.py::test_to_pairs[case1-expected1]",
"tests/test_objects.py::test_to_string[1-1]",
"tests/test_objects.py::test_to_string[1.25-1.25]",
"tests/test_objects.py::test_to_string[True-True]",
"tests/test_objects.py::test_to_string[case3-[1]]",
"tests/test_objects.py::test_to_string[d\\xc3\\xa9j\\xc3\\xa0",
"tests/test_objects.py::test_to_string[-]",
"tests/test_objects.py::test_to_string[None-]",
"tests/test_objects.py::test_to_string[case7-2024-08-02]",
"tests/test_objects.py::test_transform[case0-expected0]",
"tests/test_objects.py::test_transform[case1-expected1]",
"tests/test_objects.py::test_transform[case2-expected2]",
"tests/test_objects.py::test_update[case0-expected0]",
"tests/test_objects.py::test_update[case1-expected1]",
"tests/test_objects.py::test_update[case2-expected2]",
"tests/test_objects.py::test_update_with[case0-expected0]",
"tests/test_objects.py::test_update_with[case1-expected1]",
"tests/test_objects.py::test_update_with[case2-expected2]",
"tests/test_objects.py::test_unset[obj0-a.0.b.c-True-new_obj0]",
"tests/test_objects.py::test_unset[obj1-1-True-new_obj1]",
"tests/test_objects.py::test_unset[obj2-1-True-new_obj2]",
"tests/test_objects.py::test_unset[obj3-path3-True-new_obj3]",
"tests/test_objects.py::test_unset[obj4-[0][0]-False-new_obj4]",
"tests/test_objects.py::test_unset[obj5-[0][0][0]-False-new_obj5]",
"tests/test_objects.py::test_values[case0-expected0]",
"tests/test_objects.py::test_values[case1-expected1]"
] | 2021-06-26 09:07:43+00:00 | 1,911 |
|
dgilland__pydash-222 | diff --git a/src/pydash/arrays.py b/src/pydash/arrays.py
index d9540a5..03b1847 100644
--- a/src/pydash/arrays.py
+++ b/src/pydash/arrays.py
@@ -98,6 +98,9 @@ __all__ = (
T = t.TypeVar("T")
T2 = t.TypeVar("T2")
+T3 = t.TypeVar("T3")
+T4 = t.TypeVar("T4")
+T5 = t.TypeVar("T5")
SequenceT = t.TypeVar("SequenceT", bound=t.Sequence)
MutableSequenceT = t.TypeVar("MutableSequenceT", bound=t.MutableSequence)
@@ -2387,9 +2390,34 @@ def unshift(array: t.List[T], *items: T2) -> t.List[t.Union[T, T2]]:
return array # type: ignore
-def unzip(array: t.Iterable[t.Iterable[T]]) -> t.List[t.List[T]]:
+@t.overload
+def unzip(array: t.Iterable[t.Tuple[T, T2]]) -> t.List[t.Tuple[T, T2]]:
+ ...
+
+
+@t.overload
+def unzip(array: t.Iterable[t.Tuple[T, T2, T3]]) -> t.List[t.Tuple[T, T2, T3]]:
+ ...
+
+
+@t.overload
+def unzip(array: t.Iterable[t.Tuple[T, T2, T3, T4]]) -> t.List[t.Tuple[T, T2, T3, T4]]:
+ ...
+
+
+@t.overload
+def unzip(array: t.Iterable[t.Tuple[T, T2, T3, T4, T5]]) -> t.List[t.Tuple[T, T2, T3, T4, T5]]:
+ ...
+
+
+@t.overload
+def unzip(array: t.Iterable[t.Iterable[t.Any]]) -> t.List[t.Tuple[t.Any, ...]]:
+ ...
+
+
+def unzip(array):
"""
- The inverse of :func:`zip_`, this method splits groups of elements into lists composed of
+ The inverse of :func:`zip_`, this method splits groups of elements into tuples composed of
elements from each group at their corresponding indexes.
Args:
@@ -2400,24 +2428,38 @@ def unzip(array: t.Iterable[t.Iterable[T]]) -> t.List[t.List[T]]:
Example:
- >>> unzip([[1, 4, 7], [2, 5, 8], [3, 6, 9]])
- [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
+ >>> unzip([(1, 4, 7), (2, 5, 8), (3, 6, 9)])
+ [(1, 2, 3), (4, 5, 6), (7, 8, 9)]
.. versionadded:: 1.0.0
+
+ .. versionchanged:: 8.0.0
+ Support list of tuples instead.
"""
return zip_(*array)
@t.overload
def unzip_with(
- array: t.Iterable[t.Iterable[T]],
+ array: t.Iterable[t.Tuple[T, T2]],
iteratee: t.Union[
- t.Callable[[T, T, int, t.List[T]], T2],
- t.Callable[[T, T, int], T2],
- t.Callable[[T, T], T2],
- t.Callable[[T], T2],
+ t.Callable[[t.Union[T, T2, T3], t.Union[T, T2], int], T3],
+ t.Callable[[t.Union[T, T2, T3], t.Union[T, T2]], T3],
+ t.Callable[[t.Union[T, T2, T3]], T3],
],
-) -> t.List[T2]:
+) -> t.List[T3]:
+ ...
+
+
+@t.overload
+def unzip_with(
+ array: t.Iterable[t.Iterable[t.Any]],
+ iteratee: t.Union[
+ t.Callable[[t.Any, t.Any, int], T3],
+ t.Callable[[t.Any, t.Any], T3],
+ t.Callable[[t.Any], T3],
+ ],
+) -> t.List[T3]:
...
@@ -2425,15 +2467,15 @@ def unzip_with(
def unzip_with(
array: t.Iterable[t.Iterable[T]],
iteratee: None = None,
-) -> t.List[t.List[T]]:
+) -> t.List[t.Tuple[T]]:
...
def unzip_with(array, iteratee=None):
"""
This method is like :func:`unzip` except that it accepts an iteratee to specify how regrouped
- values should be combined. The iteratee is invoked with four arguments: ``(accumulator, value,
- index, group)``.
+ values should be combined. The iteratee is invoked with three arguments: ``(accumulator, value,
+ index)``.
Args:
array: List to process.
@@ -2445,7 +2487,7 @@ def unzip_with(array, iteratee=None):
Example:
>>> from pydash import add
- >>> unzip_with([[1, 10, 100], [2, 20, 200]], add)
+ >>> unzip_with([(1, 10, 100), (2, 20, 200)], add)
[3, 30, 300]
.. versionadded:: 3.3.0
@@ -2624,7 +2666,43 @@ def xor_with(array, *lists, **kwargs):
)
-def zip_(*arrays: t.Iterable[T]) -> t.List[t.List[T]]:
+@t.overload
+def zip_(array1: t.Iterable[T], array2: t.Iterable[T2], /) -> t.List[t.Tuple[T, T2]]:
+ ...
+
+
+@t.overload
+def zip_(
+ array1: t.Iterable[T], array2: t.Iterable[T2], array3: t.Iterable[T3], /
+) -> t.List[t.Tuple[T, T2, T3]]:
+ ...
+
+
+@t.overload
+def zip_(
+ array1: t.Iterable[T], array2: t.Iterable[T2], array3: t.Iterable[T3], array4: t.Iterable[T4], /
+) -> t.List[t.Tuple[T, T2, T3, T4]]:
+ ...
+
+
+@t.overload
+def zip_(
+ array1: t.Iterable[T],
+ array2: t.Iterable[T2],
+ array3: t.Iterable[T3],
+ array4: t.Iterable[T4],
+ array5: t.Iterable[T5],
+ /,
+) -> t.List[t.Tuple[T, T2, T3, T4, T5]]:
+ ...
+
+
+@t.overload
+def zip_(*arrays: t.Iterable[t.Any]) -> t.List[t.Tuple[t.Any, ...]]:
+ ...
+
+
+def zip_(*arrays):
"""
Groups the elements of each array at their corresponding indexes. Useful for separate data
sources that are coordinated through matching array indexes.
@@ -2638,12 +2716,14 @@ def zip_(*arrays: t.Iterable[T]) -> t.List[t.List[T]]:
Example:
>>> zip_([1, 2, 3], [4, 5, 6], [7, 8, 9])
- [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
+ [(1, 4, 7), (2, 5, 8), (3, 6, 9)]
.. versionadded:: 1.0.0
+
+ .. versionchanged:: 8.0.0
+ Return list of tuples instead of list of lists.
"""
- # zip returns as a list of tuples so convert to list of lists
- return [list(item) for item in zip(*arrays)]
+ return list(zip(*arrays))
@t.overload
@@ -2724,40 +2804,47 @@ def zip_object_deep(keys: t.Iterable[t.Any], values: t.Union[t.List[t.Any], None
@t.overload
def zip_with(
- *arrays: t.Iterable[T],
+ array1: t.Iterable[T],
+ array2: t.Iterable[T2],
+ *,
iteratee: t.Union[
- t.Callable[[T, T, int, t.List[T]], T2],
- t.Callable[[T, T, int], T2],
- t.Callable[[T, T], T2],
- t.Callable[[T], T2],
+ t.Callable[[T, T2, int], T3],
+ t.Callable[[T, T2], T3],
+ t.Callable[[T], T3],
],
-) -> t.List[T2]:
+) -> t.List[T3]:
...
@t.overload
-def zip_with(*arrays: t.Iterable[T]) -> t.List[t.List[T]]:
+def zip_with(
+ *arrays: t.Iterable[t.Any],
+ iteratee: t.Union[
+ t.Callable[[t.Any, t.Any, int], T2],
+ t.Callable[[t.Any, t.Any], T2],
+ t.Callable[[t.Any], T2],
+ ],
+) -> t.List[T2]:
...
@t.overload
def zip_with(
*arrays: t.Union[
- t.Iterable[T],
- t.Callable[[T, T, int, t.List[T]], T2],
- t.Callable[[T, T, int], T2],
- t.Callable[[T, T], T2],
- t.Callable[[T], T2],
+ t.Iterable[t.Any],
+ t.Callable[[t.Any, t.Any, int], T2],
+ t.Callable[[t.Any, t.Any], T2],
+ t.Callable[[t.Any], T2],
],
-) -> t.List[t.Union[t.List[T], T2]]:
+) -> t.List[T2]:
...
def zip_with(*arrays, **kwargs):
"""
This method is like :func:`zip` except that it accepts an iteratee to specify how grouped values
- should be combined. The iteratee is invoked with four arguments: ``(accumulator, value, index,
- group)``.
+ should be combined. The iteratee is invoked with three arguments:
+ ``(accumulator, value, index)``.
Args:
*arrays: Lists to process.
diff --git a/src/pydash/chaining/all_funcs.pyi b/src/pydash/chaining/all_funcs.pyi
index c1e3145..3d7f90a 100644
--- a/src/pydash/chaining/all_funcs.pyi
+++ b/src/pydash/chaining/all_funcs.pyi
@@ -562,23 +562,49 @@ class AllFuncs:
def unshift(self: "Chain[t.List[T]]", *items: T2) -> "Chain[t.List[t.Union[T, T2]]]":
return self._wrap(pyd.unshift)(*items)
- def unzip(self: "Chain[t.Iterable[t.Iterable[T]]]") -> "Chain[t.List[t.List[T]]]":
+ @t.overload
+ def unzip(self: "Chain[t.Iterable[t.Tuple[T, T2]]]") -> "Chain[t.List[t.Tuple[T, T2]]]": ...
+ @t.overload
+ def unzip(
+ self: "Chain[t.Iterable[t.Tuple[T, T2, T3]]]",
+ ) -> "Chain[t.List[t.Tuple[T, T2, T3]]]": ...
+ @t.overload
+ def unzip(
+ self: "Chain[t.Iterable[t.Tuple[T, T2, T3, T4]]]",
+ ) -> "Chain[t.List[t.Tuple[T, T2, T3, T4]]]": ...
+ @t.overload
+ def unzip(
+ self: "Chain[t.Iterable[t.Tuple[T, T2, T3, T4, T5]]]",
+ ) -> "Chain[t.List[t.Tuple[T, T2, T3, T4, T5]]]": ...
+ @t.overload
+ def unzip(
+ self: "Chain[t.Iterable[t.Iterable[t.Any]]]",
+ ) -> "Chain[t.List[t.Tuple[t.Any, ...]]]": ...
+ def unzip(self):
return self._wrap(pyd.unzip)()
@t.overload
def unzip_with(
- self: "Chain[t.Iterable[t.Iterable[T]]]",
+ self: "Chain[t.Iterable[t.Tuple[T, T2]]]",
iteratee: t.Union[
- t.Callable[[T, T, int, t.List[T]], T2],
- t.Callable[[T, T, int], T2],
- t.Callable[[T, T], T2],
- t.Callable[[T], T2],
+ t.Callable[[t.Union[T, T2, T3], t.Union[T, T2], int], T3],
+ t.Callable[[t.Union[T, T2, T3], t.Union[T, T2]], T3],
+ t.Callable[[t.Union[T, T2, T3]], T3],
],
- ) -> "Chain[t.List[T2]]": ...
+ ) -> "Chain[t.List[T3]]": ...
+ @t.overload
+ def unzip_with(
+ self: "Chain[t.Iterable[t.Iterable[t.Any]]]",
+ iteratee: t.Union[
+ t.Callable[[t.Any, t.Any, int], T3],
+ t.Callable[[t.Any, t.Any], T3],
+ t.Callable[[t.Any], T3],
+ ],
+ ) -> "Chain[t.List[T3]]": ...
@t.overload
def unzip_with(
self: "Chain[t.Iterable[t.Iterable[T]]]", iteratee: None = None
- ) -> "Chain[t.List[t.List[T]]]": ...
+ ) -> "Chain[t.List[t.Tuple[T]]]": ...
def unzip_with(self, iteratee=None):
return self._wrap(pyd.unzip_with)(iteratee)
@@ -612,7 +638,11 @@ class AllFuncs:
def xor_with(self, *lists, **kwargs):
return self._wrap(pyd.xor_with)(*lists, **kwargs)
- def zip_(self: "Chain[t.Iterable[T]]", *arrays: t.Iterable[T]) -> "Chain[t.List[t.List[T]]]":
+ @t.overload
+ def zip_(
+ self: "Chain[t.Iterable[t.Any]]", *arrays: t.Iterable[t.Any]
+ ) -> "Chain[t.List[t.Tuple[t.Any, ...]]]": ...
+ def zip_(self, *arrays):
return self._wrap(pyd.zip_)(*arrays)
zip = zip_
@@ -638,29 +668,32 @@ class AllFuncs:
@t.overload
def zip_with(
self: "Chain[t.Iterable[T]]",
- *arrays: t.Iterable[T],
+ array2: t.Iterable[T2],
+ *,
iteratee: t.Union[
- t.Callable[[T, T, int, t.List[T]], T2],
- t.Callable[[T, T, int], T2],
- t.Callable[[T, T], T2],
- t.Callable[[T], T2],
+ t.Callable[[T, T2, int], T3], t.Callable[[T, T2], T3], t.Callable[[T], T3]
],
- ) -> "Chain[t.List[T2]]": ...
+ ) -> "Chain[t.List[T3]]": ...
@t.overload
def zip_with(
- self: "Chain[t.Iterable[T]]", *arrays: t.Iterable[T]
- ) -> "Chain[t.List[t.List[T]]]": ...
+ self: "Chain[t.Iterable[t.Any]]",
+ *arrays: t.Iterable[t.Any],
+ iteratee: t.Union[
+ t.Callable[[t.Any, t.Any, int], T2],
+ t.Callable[[t.Any, t.Any], T2],
+ t.Callable[[t.Any], T2],
+ ],
+ ) -> "Chain[t.List[T2]]": ...
@t.overload
def zip_with(
- self: "Chain[t.Union[t.Iterable[T], t.Callable[[T, T, int, t.List[T]], T2], t.Callable[[T, T, int], T2], t.Callable[[T, T], T2], t.Callable[[T], T2]]]",
+ self: "Chain[t.Union[t.Iterable[t.Any], t.Callable[[t.Any, t.Any, int], T2], t.Callable[[t.Any, t.Any], T2], t.Callable[[t.Any], T2]]]",
*arrays: t.Union[
- t.Iterable[T],
- t.Callable[[T, T, int, t.List[T]], T2],
- t.Callable[[T, T, int], T2],
- t.Callable[[T, T], T2],
- t.Callable[[T], T2],
+ t.Iterable[t.Any],
+ t.Callable[[t.Any, t.Any, int], T2],
+ t.Callable[[t.Any, t.Any], T2],
+ t.Callable[[t.Any], T2],
],
- ) -> "Chain[t.List[t.Union[t.List[T], T2]]]": ...
+ ) -> "Chain[t.List[T2]]": ...
def zip_with(self, *arrays, **kwargs):
return self._wrap(pyd.zip_with)(*arrays, **kwargs)
@@ -2689,9 +2722,9 @@ class AllFuncs:
return self._wrap(pyd.to_number)(precision)
@t.overload
- def to_pairs(self: "Chain[t.Mapping[T, T2]]") -> "Chain[t.List[t.List[t.Union[T, T2]]]]": ...
+ def to_pairs(self: "Chain[t.Mapping[T, T2]]") -> "Chain[t.List[t.Tuple[T, T2]]]": ...
@t.overload
- def to_pairs(self: "Chain[t.Iterable[T]]") -> "Chain[t.List[t.List[t.Union[int, T]]]]": ...
+ def to_pairs(self: "Chain[t.Iterable[T]]") -> "Chain[t.List[t.Tuple[int, T]]]": ...
@t.overload
def to_pairs(self: "Chain[t.Any]") -> "Chain[t.List]": ...
def to_pairs(self):
diff --git a/src/pydash/objects.py b/src/pydash/objects.py
index d5a7c66..c325cb9 100644
--- a/src/pydash/objects.py
+++ b/src/pydash/objects.py
@@ -2114,12 +2114,12 @@ def to_number(obj: t.Any, precision: int = 0) -> t.Union[float, None]:
@t.overload
-def to_pairs(obj: t.Mapping[T, T2]) -> t.List[t.List[t.Union[T, T2]]]:
+def to_pairs(obj: t.Mapping[T, T2]) -> t.List[t.Tuple[T, T2]]:
...
@t.overload
-def to_pairs(obj: t.Iterable[T]) -> t.List[t.List[t.Union[int, T]]]:
+def to_pairs(obj: t.Iterable[T]) -> t.List[t.Tuple[int, T]]:
...
@@ -2130,28 +2130,31 @@ def to_pairs(obj: t.Any) -> t.List:
def to_pairs(obj):
"""
- Creates a two-dimensional list of an object's key-value pairs, i.e., ``[[key1, value1], [key2,
- value2]]``.
+ Creates a list of tuples of an object's key-value pairs, i.e.,
+ ``[(key1, value1), (key2, value2)]``.
Args:
obj: Object to process.
Returns:
- Two dimensional list of object's key-value pairs.
+ List of tuples of the object's key-value pairs.
Example:
>>> to_pairs([1, 2, 3, 4])
- [[0, 1], [1, 2], [2, 3], [3, 4]]
+ [(0, 1), (1, 2), (2, 3), (3, 4)]
>>> to_pairs({"a": 1})
- [['a', 1]]
+ [('a', 1)]
.. versionadded:: 1.0.0
.. versionchanged:: 4.0.0
Renamed from ``pairs`` to ``to_pairs``.
+
+ .. versionchanged:: 8.0.0
+ Returning list of tuples instead of list of lists.
"""
- return [[key, value] for key, value in iterator(obj)]
+ return [(key, value) for key, value in iterator(obj)]
def to_string(obj: t.Any) -> str:
| dgilland/pydash | ef48d55fb5eecf5d3da3a69cab337e6345b1fc29 | diff --git a/tests/pytest_mypy_testing/test_arrays.py b/tests/pytest_mypy_testing/test_arrays.py
index 2e30951..d7d6cb3 100644
--- a/tests/pytest_mypy_testing/test_arrays.py
+++ b/tests/pytest_mypy_testing/test_arrays.py
@@ -408,8 +408,7 @@ def test_mypy_unshift() -> None:
@pytest.mark.mypy_testing
def test_mypy_unzip() -> None:
- reveal_type(_.unzip([[1, 4, 7], [2, 5, 8], [3, 6, 9]])) # R: builtins.list[builtins.list[builtins.int]]
- reveal_type(_.unzip([(1, 4, 7), (2, 5, 8), (3, 6, 9)])) # R: builtins.list[builtins.list[builtins.int]]
+ reveal_type(_.unzip([(1, 4, 7), (2, 5, 8), (3, 6, 9)])) # R: builtins.list[Tuple[builtins.int, builtins.int, builtins.int]]
@pytest.mark.mypy_testing
@@ -447,7 +446,8 @@ def test_mypy_xor_with() -> None:
@pytest.mark.mypy_testing
def test_mypy_zip_() -> None:
- reveal_type(_.zip_([1, 2, 3], [4, 5, 6], [7, 8, 9])) # R: builtins.list[builtins.list[builtins.int]]
+ reveal_type(_.zip_([1, 2, 3], [4, 5, 6], [7, 8, 9])) # R: builtins.list[Tuple[builtins.int, builtins.int, builtins.int]]
+ reveal_type(_.zip_([1, 2, 3], ["one", "two", "three"])) # R: builtins.list[Tuple[builtins.int, builtins.str]]
@pytest.mark.mypy_testing
@@ -469,5 +469,10 @@ def test_mypy_zip_with() -> None:
def add(x: int, y: int) -> int:
return x + y
- reveal_type(_.zip_with([1, 2], [10, 20], [100, 200], add)) # R: builtins.list[Union[builtins.list[builtins.int], builtins.int]]
+ reveal_type(_.zip_with([1, 2], [10, 20], add)) # R: builtins.list[builtins.int]
reveal_type(_.zip_with([1, 2], [10, 20], [100, 200], iteratee=add)) # R: builtins.list[builtins.int]
+
+ def more_hello(s: str, n: int) -> str:
+ return s * n
+
+ reveal_type(_.zip_with(["hello", "hello", "hello"], [1, 2, 3], iteratee=more_hello)) # R: builtins.list[builtins.str]
diff --git a/tests/pytest_mypy_testing/test_objects.py b/tests/pytest_mypy_testing/test_objects.py
index b4df527..28645e0 100644
--- a/tests/pytest_mypy_testing/test_objects.py
+++ b/tests/pytest_mypy_testing/test_objects.py
@@ -298,8 +298,8 @@ def test_mypy_to_number() -> None:
@pytest.mark.mypy_testing
def test_mypy_to_pairs() -> None:
- reveal_type(_.to_pairs([1, 2, 3, 4])) # R: builtins.list[builtins.list[builtins.int]]
- reveal_type(_.to_pairs({'a': 1})) # R: builtins.list[builtins.list[Union[builtins.str, builtins.int]]]
+ reveal_type(_.to_pairs([1, 2, 3, 4])) # R: builtins.list[Tuple[builtins.int, builtins.int]]
+ reveal_type(_.to_pairs({'a': 1})) # R: builtins.list[Tuple[builtins.str, builtins.int]]
reveal_type(_.to_pairs(MyClass())) # R: builtins.list[Any]
diff --git a/tests/test_arrays.py b/tests/test_arrays.py
index cdf6217..a0070cf 100644
--- a/tests/test_arrays.py
+++ b/tests/test_arrays.py
@@ -853,8 +853,8 @@ def test_unshift(case, expected):
"case,expected",
[
(
- [["moe", 30, True], ["larry", 40, False], ["curly", 35, True]],
- [["moe", "larry", "curly"], [30, 40, 35], [True, False, True]],
+ [("moe", 30, True), ("larry", 40, False), ("curly", 35, True)],
+ [("moe", "larry", "curly"), (30, 40, 35), (True, False, True)],
)
],
)
@@ -866,7 +866,7 @@ def test_unzip(case, expected):
"case,expected",
[
(([],), []),
- (([[1, 10, 100], [2, 20, 200]],), [[1, 2], [10, 20], [100, 200]]),
+ (([[1, 10, 100], [2, 20, 200]],), [(1, 2), (10, 20), (100, 200)]),
(([[2, 4, 6], [2, 2, 2]], _.power), [4, 16, 36]),
],
)
@@ -902,7 +902,7 @@ def test_xor_with(case, expected):
[
(
(["moe", "larry", "curly"], [30, 40, 35], [True, False, True]),
- [["moe", 30, True], ["larry", 40, False], ["curly", 35, True]],
+ [("moe", 30, True), ("larry", 40, False), ("curly", 35, True)],
)
],
)
@@ -935,7 +935,7 @@ def test_zip_object_deep(case, expected):
@parametrize(
"case,expected",
[
- (([1, 2],), [[1], [2]]),
+ (([1, 2],), [(1,), (2,)]),
(([1, 2], [3, 4], _.add), [4, 6]),
],
)
diff --git a/tests/test_objects.py b/tests/test_objects.py
index d3328cf..d0f0ea4 100644
--- a/tests/test_objects.py
+++ b/tests/test_objects.py
@@ -847,8 +847,8 @@ def test_to_number(case, expected):
@parametrize(
"case,expected",
[
- ({"a": 1, "b": 2, "c": 3}, [["a", 1], ["b", 2], ["c", 3]]),
- ([1, 2, 3], [[0, 1], [1, 2], [2, 3]]),
+ ({"a": 1, "b": 2, "c": 3}, [("a", 1), ("b", 2), ("c", 3)]),
+ ([1, 2, 3], [(0, 1), (1, 2), (2, 3)]),
],
)
def test_to_pairs(case, expected):
| Embrace the tuple
Would you be open to PR that switches `zip_`, `zip_with` and `to_pairs` to return list of tuples instead?
Using a list in python is very similar to using a tuple but in static typing having lists is a bit annoying.
For example:
```py
import pydash
d: dict[str, int] = {"key1": 1, "key2": 2}
pairs = pydash.to_pairs(d) # this is list[list[str | int]]
first_key, first_value = pairs[0] # first_key is `str | int` and first_value is `str | int`
```
This doesn't make much sense, we know `first_key` should be `str` and `first_value` should be `int`. If `to_pairs` returned list of tuples instead we could type it better and not have this problem.
Same with `zip_`:
```py
import pydash
zipped = pydash.zip_([1, 2, 3], ["hello", "hello", "hello"])
```
`zipped` is a list of lists of `str | int`, we lost the order on the type level, int and str are blend in the lists.
And I think the hardest to work with might be `zip_with`
```py
import pydash
def more_hello(s: str, time: int) -> str:
return s.upper() * time
zipped = pydash.zip_with(
["hello", "hello", "hello"],
[1, 2, 3],
iteratee=more_hello # type error here: cannot assign `str | int` to `str` and `str | int` to `int`
)
```
while this is valid at runtime, the type checker doesn't know that the first argument is `str` and second is `int` because it is all blend into the `list[str | int]`
This makes them really hard to use in a type checked context. Chaining functions with the result of these functions is very impractical. I think it would make a lot more sense for these functions to return tuples. | 0.0 | [
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_unzip",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_zip_",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_zip_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_pairs",
"tests/test_arrays.py::test_unzip[case0-expected0]",
"tests/test_arrays.py::test_unzip_with[case1-expected1]",
"tests/test_arrays.py::test_zip_[case0-expected0]",
"tests/test_arrays.py::test_zip_with[case0-expected0]"
] | [
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_chunk",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_compact",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_concat",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_difference",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_difference_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_difference_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_drop",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_drop_right",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_drop_right_while",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_drop_while",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_duplicates",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_fill",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_find_index",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_find_last_index",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_flatten",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_flatten_deep",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_flatten_depth",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_from_pairs",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_head",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_index_of",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_initial",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_intercalate",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_interleave",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_intersection",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_intersection_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_intersection_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_intersperse",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_last",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_last_index_of",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_mapcat",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_nth",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_pop",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_pull",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_pull_all",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_pull_all_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_pull_all_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_pull_at",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_push",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_remove",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_reverse",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_shift",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_slice_",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sort",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_index",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_index_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_index_of",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_last_index",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_last_index_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_last_index_of",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_uniq",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_sorted_uniq_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_splice",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_split_at",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_tail",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_take",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_take_right",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_take_right_while",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_take_while",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_union",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_union_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_union_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_uniq",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_uniq_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_uniq_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_unshift",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_unzip_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_without",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_xor",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_xor_by",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_xor_with",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_zip_object",
"tests/pytest_mypy_testing/test_arrays.py::[mypy]test_mypy_zip_object_deep",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_chunk",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_compact",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_concat",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_difference",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_difference_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_difference_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_drop",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_drop_right",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_drop_right_while",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_drop_while",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_duplicates",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_fill",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_find_index",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_find_last_index",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_flatten",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_flatten_deep",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_flatten_depth",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_from_pairs",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_head",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_index_of",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_initial",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_intercalate",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_interleave",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_intersection",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_intersection_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_intersection_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_intersperse",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_last",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_last_index_of",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_mapcat",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_nth",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_pop",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_pull",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_pull_all",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_pull_all_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_pull_all_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_pull_at",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_push",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_remove",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_reverse",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_shift",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_slice_",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sort",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_index",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_index_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_index_of",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_last_index",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_last_index_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_last_index_of",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_uniq",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_sorted_uniq_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_splice",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_split_at",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_tail",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_take",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_take_right",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_take_right_while",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_take_while",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_union",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_union_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_union_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_uniq",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_uniq_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_uniq_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_unshift",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_unzip",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_unzip_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_without",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_xor",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_xor_by",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_xor_with",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_zip_",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_zip_object",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_zip_object_deep",
"tests/pytest_mypy_testing/test_arrays.py::test_mypy_zip_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_assign",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_assign_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_callables",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_clone",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_clone_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_clone_deep",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_clone_deep_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_defaults",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_defaults_deep",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_find_key",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_find_last_key",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_for_in",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_for_in_right",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_get",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_has",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_invert",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_invert_by",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_invoke",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_keys",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_map_keys",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_map_values",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_map_values_deep",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_merge",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_merge_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_omit",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_omit_by",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_parse_int",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_pick",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_pick_by",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_rename_keys",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_set_",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_set_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_boolean",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_dict",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_integer",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_list",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_number",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_to_string",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_transform",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_update",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_update_with",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_unset",
"tests/pytest_mypy_testing/test_objects.py::[mypy]test_mypy_values",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_assign",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_assign_with",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_callables",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_clone",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_clone_with",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_clone_deep",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_clone_deep_with",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_defaults",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_defaults_deep",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_find_key",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_find_last_key",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_for_in",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_for_in_right",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_get",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_has",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_invert",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_invert_by",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_invoke",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_keys",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_map_keys",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_map_values",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_map_values_deep",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_merge",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_merge_with",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_omit",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_omit_by",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_parse_int",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_pick",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_pick_by",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_rename_keys",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_set_",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_set_with",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_boolean",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_dict",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_integer",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_list",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_number",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_pairs",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_to_string",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_transform",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_update",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_update_with",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_unset",
"tests/pytest_mypy_testing/test_objects.py::test_mypy_values",
"tests/test_arrays.py::test_chunk[case0-expected0]",
"tests/test_arrays.py::test_chunk[case1-expected1]",
"tests/test_arrays.py::test_chunk[case2-expected2]",
"tests/test_arrays.py::test_chunk[case3-expected3]",
"tests/test_arrays.py::test_chunk[case4-expected4]",
"tests/test_arrays.py::test_chunk[case5-expected5]",
"tests/test_arrays.py::test_compact[case0-expected0]",
"tests/test_arrays.py::test_compact[case1-expected1]",
"tests/test_arrays.py::test_concat[case0-expected0]",
"tests/test_arrays.py::test_concat[case1-expected1]",
"tests/test_arrays.py::test_concat[case2-expected2]",
"tests/test_arrays.py::test_concat[case3-expected3]",
"tests/test_arrays.py::test_concat[case4-expected4]",
"tests/test_arrays.py::test_concat[case5-expected5]",
"tests/test_arrays.py::test_difference[case0-expected0]",
"tests/test_arrays.py::test_difference[case1-expected1]",
"tests/test_arrays.py::test_difference[case2-expected2]",
"tests/test_arrays.py::test_difference[case3-expected3]",
"tests/test_arrays.py::test_difference_by[case0-expected0]",
"tests/test_arrays.py::test_difference_by[case1-expected1]",
"tests/test_arrays.py::test_difference_by[case2-expected2]",
"tests/test_arrays.py::test_difference_with[case0-expected0]",
"tests/test_arrays.py::test_difference_with[case1-expected1]",
"tests/test_arrays.py::test_difference_with[case2-expected2]",
"tests/test_arrays.py::test_drop[case0-expected0]",
"tests/test_arrays.py::test_drop[case1-expected1]",
"tests/test_arrays.py::test_drop[case2-expected2]",
"tests/test_arrays.py::test_drop[case3-expected3]",
"tests/test_arrays.py::test_drop[case4-expected4]",
"tests/test_arrays.py::test_drop_while[case0-expected0]",
"tests/test_arrays.py::test_drop_right[case0-expected0]",
"tests/test_arrays.py::test_drop_right[case1-expected1]",
"tests/test_arrays.py::test_drop_right[case2-expected2]",
"tests/test_arrays.py::test_drop_right[case3-expected3]",
"tests/test_arrays.py::test_drop_right[case4-expected4]",
"tests/test_arrays.py::test_drop_right_while[case0-expected0]",
"tests/test_arrays.py::test_duplicates[case0-expected0]",
"tests/test_arrays.py::test_duplicates[case1-expected1]",
"tests/test_arrays.py::test_fill[case0-expected0]",
"tests/test_arrays.py::test_fill[case1-expected1]",
"tests/test_arrays.py::test_fill[case2-expected2]",
"tests/test_arrays.py::test_fill[case3-expected3]",
"tests/test_arrays.py::test_fill[case4-expected4]",
"tests/test_arrays.py::test_fill[case5-expected5]",
"tests/test_arrays.py::test_find_index[case0-<lambda>-1]",
"tests/test_arrays.py::test_find_index[case1-filter_by1-1]",
"tests/test_arrays.py::test_find_index[case2-<lambda>--1]",
"tests/test_arrays.py::test_find_last_index[case0-<lambda>-2]",
"tests/test_arrays.py::test_find_last_index[case1-filter_by1-1]",
"tests/test_arrays.py::test_find_last_index[case2-<lambda>--1]",
"tests/test_arrays.py::test_flatten[case0-expected0]",
"tests/test_arrays.py::test_flatten_deep[case0-expected0]",
"tests/test_arrays.py::test_flatten_depth[case0-expected0]",
"tests/test_arrays.py::test_flatten_depth[case1-expected1]",
"tests/test_arrays.py::test_flatten_depth[case2-expected2]",
"tests/test_arrays.py::test_flatten_depth[case3-expected3]",
"tests/test_arrays.py::test_from_pairs[case0-expected0]",
"tests/test_arrays.py::test_from_pairs[case1-expected1]",
"tests/test_arrays.py::test_head[case0-1]",
"tests/test_arrays.py::test_head[case1-None]",
"tests/test_arrays.py::test_index_of[case0-2-0-1]",
"tests/test_arrays.py::test_index_of[case1-2-3-4]",
"tests/test_arrays.py::test_index_of[case2-2-True-2]",
"tests/test_arrays.py::test_index_of[case3-4-0--1]",
"tests/test_arrays.py::test_index_of[case4-2-10--1]",
"tests/test_arrays.py::test_index_of[case5-0-0--1]",
"tests/test_arrays.py::test_initial[case0-expected0]",
"tests/test_arrays.py::test_initial[case1-expected1]",
"tests/test_arrays.py::test_intercalate[case0-expected0]",
"tests/test_arrays.py::test_intercalate[case1-expected1]",
"tests/test_arrays.py::test_interleave[case0-expected0]",
"tests/test_arrays.py::test_interleave[case1-expected1]",
"tests/test_arrays.py::test_interleave[case2-expected2]",
"tests/test_arrays.py::test_interleave[case3-expected3]",
"tests/test_arrays.py::test_intersection[case0-expected0]",
"tests/test_arrays.py::test_intersection[case1-expected1]",
"tests/test_arrays.py::test_intersection[case2-expected2]",
"tests/test_arrays.py::test_intersection[case3-expected3]",
"tests/test_arrays.py::test_intersection[case4-expected4]",
"tests/test_arrays.py::test_intersection[case5-expected5]",
"tests/test_arrays.py::test_intersection_by[case0-expected0]",
"tests/test_arrays.py::test_intersection_by[case1-expected1]",
"tests/test_arrays.py::test_intersection_by[case2-expected2]",
"tests/test_arrays.py::test_intersection_by[case3-expected3]",
"tests/test_arrays.py::test_intersection_by[case4-expected4]",
"tests/test_arrays.py::test_intersection_by[case5-expected5]",
"tests/test_arrays.py::test_intersection_by[case6-expected6]",
"tests/test_arrays.py::test_intersection_with[case0-expected0]",
"tests/test_arrays.py::test_intersection_with[case1-expected1]",
"tests/test_arrays.py::test_intersection_with[case2-expected2]",
"tests/test_arrays.py::test_intersection_with[case3-expected3]",
"tests/test_arrays.py::test_intersection_with[case4-expected4]",
"tests/test_arrays.py::test_intersection_with[case5-expected5]",
"tests/test_arrays.py::test_intersperse[case0-expected0]",
"tests/test_arrays.py::test_intersperse[case1-expected1]",
"tests/test_arrays.py::test_intersperse[case2-expected2]",
"tests/test_arrays.py::test_last[case0-3]",
"tests/test_arrays.py::test_last[case1-None]",
"tests/test_arrays.py::test_last_index_of[case0-2-0--1]",
"tests/test_arrays.py::test_last_index_of[case1-2-3-1]",
"tests/test_arrays.py::test_last_index_of[case2-0-0--1]",
"tests/test_arrays.py::test_last_index_of[case3-3-0--1]",
"tests/test_arrays.py::test_last_index_of[case4-3-1--1]",
"tests/test_arrays.py::test_last_index_of[case5-3-2--1]",
"tests/test_arrays.py::test_last_index_of[case6-3-3-3]",
"tests/test_arrays.py::test_last_index_of[case7-3-4-3]",
"tests/test_arrays.py::test_last_index_of[case8-3-5-3]",
"tests/test_arrays.py::test_last_index_of[case9-3-6-3]",
"tests/test_arrays.py::test_last_index_of[case10-3--1-3]",
"tests/test_arrays.py::test_last_index_of[case11-3--2-3]",
"tests/test_arrays.py::test_last_index_of[case12-3--3-3]",
"tests/test_arrays.py::test_last_index_of[case13-3--4--1]",
"tests/test_arrays.py::test_last_index_of[case14-3--5--1]",
"tests/test_arrays.py::test_last_index_of[case15-3--6--1]",
"tests/test_arrays.py::test_last_index_of[case16-3-None-3]",
"tests/test_arrays.py::test_mapcat[case0-expected0]",
"tests/test_arrays.py::test_nth[case0-2-33]",
"tests/test_arrays.py::test_nth[case1-0-11]",
"tests/test_arrays.py::test_nth[case2--1-33]",
"tests/test_arrays.py::test_nth[case3-4-None]",
"tests/test_arrays.py::test_pop[case0-3-after0]",
"tests/test_arrays.py::test_pop[case1-1-after1]",
"tests/test_arrays.py::test_pop[case2-2-after2]",
"tests/test_arrays.py::test_pull[case0-values0-expected0]",
"tests/test_arrays.py::test_pull_all[case0-values0-expected0]",
"tests/test_arrays.py::test_pull_all[case1-values1-expected1]",
"tests/test_arrays.py::test_pull_all[case2-values2-expected2]",
"tests/test_arrays.py::test_pull_all_by[case0-values0-None-expected0]",
"tests/test_arrays.py::test_pull_all_by[case1-values1-<lambda>-expected1]",
"tests/test_arrays.py::test_pull_all_with[case0-values0-None-expected0]",
"tests/test_arrays.py::test_pull_all_with[case1-values1-<lambda>-expected1]",
"tests/test_arrays.py::test_pull_all_with[case2-values2-<lambda>-expected2]",
"tests/test_arrays.py::test_pull_at[case0-expected0]",
"tests/test_arrays.py::test_pull_at[case1-expected1]",
"tests/test_arrays.py::test_pull_at[case2-expected2]",
"tests/test_arrays.py::test_push[case0-expected0]",
"tests/test_arrays.py::test_push[case1-expected1]",
"tests/test_arrays.py::test_push[case2-expected2]",
"tests/test_arrays.py::test_remove[case0-<lambda>-expected0]",
"tests/test_arrays.py::test_remove[case1-<lambda>-expected1]",
"tests/test_arrays.py::test_reverse[case0-expected0]",
"tests/test_arrays.py::test_reverse[abcdef-fedcba]",
"tests/test_arrays.py::test_shift[case0-1-after0]",
"tests/test_arrays.py::test_slice_[case0-expected0]",
"tests/test_arrays.py::test_slice_[case1-expected1]",
"tests/test_arrays.py::test_slice_[case2-expected2]",
"tests/test_arrays.py::test_slice_[case3-expected3]",
"tests/test_arrays.py::test_slice_[case4-expected4]",
"tests/test_arrays.py::test_slice_[case5-expected5]",
"tests/test_arrays.py::test_slice_[case6-expected6]",
"tests/test_arrays.py::test_slice_[case7-expected7]",
"tests/test_arrays.py::test_slice_[case8-expected8]",
"tests/test_arrays.py::test_slice_[case9-expected9]",
"tests/test_arrays.py::test_sort[case0-expected0]",
"tests/test_arrays.py::test_sort[case1-expected1]",
"tests/test_arrays.py::test_sort[case2-expected2]",
"tests/test_arrays.py::test_sort[case3-expected3]",
"tests/test_arrays.py::test_sort_comparator_key_exception",
"tests/test_arrays.py::test_sorted_index[case0-2]",
"tests/test_arrays.py::test_sorted_index[case1-2]",
"tests/test_arrays.py::test_sorted_index[case2-2]",
"tests/test_arrays.py::test_sorted_index[case3-0]",
"tests/test_arrays.py::test_sorted_index_by[case0-2]",
"tests/test_arrays.py::test_sorted_index_by[case1-2]",
"tests/test_arrays.py::test_sorted_index_of[array0-10-3]",
"tests/test_arrays.py::test_sorted_index_of[array1-11--1]",
"tests/test_arrays.py::test_sorted_last_index[case0-4]",
"tests/test_arrays.py::test_sorted_last_index[case1-4]",
"tests/test_arrays.py::test_sorted_last_index[case2-0]",
"tests/test_arrays.py::test_sorted_last_index_by[case0-2]",
"tests/test_arrays.py::test_sorted_last_index_by[case1-2]",
"tests/test_arrays.py::test_sorted_last_index_of[array0-10-4]",
"tests/test_arrays.py::test_sorted_last_index_of[array1-11--1]",
"tests/test_arrays.py::test_sorted_uniq[case0-expected0]",
"tests/test_arrays.py::test_sorted_uniq[case1-expected1]",
"tests/test_arrays.py::test_sorted_uniq_by[case0-<lambda>-expected0]",
"tests/test_arrays.py::test_sorted_uniq_by[case1-<lambda>-expected1]",
"tests/test_arrays.py::test_splice[case0-expected0-after0]",
"tests/test_arrays.py::test_splice[case1-expected1-after1]",
"tests/test_arrays.py::test_splice[case2-expected2-after2]",
"tests/test_arrays.py::test_splice[case3-expected3-after3]",
"tests/test_arrays.py::test_splice[case4-expected4-after4]",
"tests/test_arrays.py::test_splice_string[case0-1splice23]",
"tests/test_arrays.py::test_split_at[case0-expected0]",
"tests/test_arrays.py::test_split_at[case1-expected1]",
"tests/test_arrays.py::test_tail[case0-expected0]",
"tests/test_arrays.py::test_tail[case1-expected1]",
"tests/test_arrays.py::test_take[case0-expected0]",
"tests/test_arrays.py::test_take[case1-expected1]",
"tests/test_arrays.py::test_take[case2-expected2]",
"tests/test_arrays.py::test_take[case3-expected3]",
"tests/test_arrays.py::test_take[case4-expected4]",
"tests/test_arrays.py::test_take_while[case0-expected0]",
"tests/test_arrays.py::test_take_right[case0-expected0]",
"tests/test_arrays.py::test_take_right[case1-expected1]",
"tests/test_arrays.py::test_take_right[case2-expected2]",
"tests/test_arrays.py::test_take_right[case3-expected3]",
"tests/test_arrays.py::test_take_right[case4-expected4]",
"tests/test_arrays.py::test_take_right_while[case0-expected0]",
"tests/test_arrays.py::test_uniq[case0-expected0]",
"tests/test_arrays.py::test_uniq[case1-expected1]",
"tests/test_arrays.py::test_uniq_by[case0-<lambda>-expected0]",
"tests/test_arrays.py::test_uniq_by[case1-iteratee1-expected1]",
"tests/test_arrays.py::test_uniq_by[case2-x-expected2]",
"tests/test_arrays.py::test_uniq_by[case3-<lambda>-expected3]",
"tests/test_arrays.py::test_uniq_with[case0-<lambda>-expected0]",
"tests/test_arrays.py::test_uniq_with[case1-<lambda>-expected1]",
"tests/test_arrays.py::test_union[case0-expected0]",
"tests/test_arrays.py::test_union[case1-expected1]",
"tests/test_arrays.py::test_union_by[case0-<lambda>-expected0]",
"tests/test_arrays.py::test_union_by[case1-<lambda>-expected1]",
"tests/test_arrays.py::test_union_by[case2-None-expected2]",
"tests/test_arrays.py::test_union_by[case3-None-expected3]",
"tests/test_arrays.py::test_union_with[case0-expected0]",
"tests/test_arrays.py::test_union_with[case1-expected1]",
"tests/test_arrays.py::test_union_with[case2-expected2]",
"tests/test_arrays.py::test_unshift[case0-expected0]",
"tests/test_arrays.py::test_unshift[case1-expected1]",
"tests/test_arrays.py::test_unshift[case2-expected2]",
"tests/test_arrays.py::test_unzip_with[case0-expected0]",
"tests/test_arrays.py::test_unzip_with[case2-expected2]",
"tests/test_arrays.py::test_without[case0-expected0]",
"tests/test_arrays.py::test_xor[case0-expected0]",
"tests/test_arrays.py::test_xor[case1-expected1]",
"tests/test_arrays.py::test_xor_by[case0-expected0]",
"tests/test_arrays.py::test_xor_with[case0-expected0]",
"tests/test_arrays.py::test_zip_object[case0-expected0]",
"tests/test_arrays.py::test_zip_object[case1-expected1]",
"tests/test_arrays.py::test_zip_object_deep[case0-expected0]",
"tests/test_arrays.py::test_zip_object_deep[case1-expected1]",
"tests/test_arrays.py::test_zip_with[case1-expected1]",
"tests/test_objects.py::test_assign[case0-expected0]",
"tests/test_objects.py::test_assign[case1-expected1]",
"tests/test_objects.py::test_assign_with[case0-expected0]",
"tests/test_objects.py::test_callables[case0-expected0]",
"tests/test_objects.py::test_callables[case1-expected1]",
"tests/test_objects.py::test_clone[case0]",
"tests/test_objects.py::test_clone[case1]",
"tests/test_objects.py::test_clone_with[case0-<lambda>-expected0]",
"tests/test_objects.py::test_clone_with[case1-<lambda>-expected1]",
"tests/test_objects.py::test_clone_deep[case0]",
"tests/test_objects.py::test_clone_deep[case1]",
"tests/test_objects.py::test_clone_deep[case2]",
"tests/test_objects.py::test_clone_deep_with[case0-<lambda>-expected0]",
"tests/test_objects.py::test_clone_deep_with[case1-<lambda>-expected1]",
"tests/test_objects.py::test_clone_deep_with[case2-<lambda>-expected2]",
"tests/test_objects.py::test_clone_deep_with[a-<lambda>-a]",
"tests/test_objects.py::test_defaults[case0-expected0]",
"tests/test_objects.py::test_defaults_deep[case0-expected0]",
"tests/test_objects.py::test_defaults_deep[case1-expected1]",
"tests/test_objects.py::test_defaults_deep[case2-expected2]",
"tests/test_objects.py::test_defaults_deep[case3-expected3]",
"tests/test_objects.py::test_defaults_deep[case4-expected4]",
"tests/test_objects.py::test_to_dict[case0-expected0]",
"tests/test_objects.py::test_to_dict[case1-expected1]",
"tests/test_objects.py::test_invert[case0-expected0]",
"tests/test_objects.py::test_invert[case1-expected1]",
"tests/test_objects.py::test_invert_by[case0-expected0]",
"tests/test_objects.py::test_invert_by[case1-expected1]",
"tests/test_objects.py::test_invert_by[case2-expected2]",
"tests/test_objects.py::test_invoke[case0-1]",
"tests/test_objects.py::test_invoke[case1-2]",
"tests/test_objects.py::test_invoke[case2-None]",
"tests/test_objects.py::test_find_key[case0-expected0]",
"tests/test_objects.py::test_find_key[case1-expected1]",
"tests/test_objects.py::test_find_key[case2-expected2]",
"tests/test_objects.py::test_find_last_key[case0-expected0]",
"tests/test_objects.py::test_find_last_key[case1-expected1]",
"tests/test_objects.py::test_find_last_key[case2-expected2]",
"tests/test_objects.py::test_for_in[case0-expected0]",
"tests/test_objects.py::test_for_in[case1-expected1]",
"tests/test_objects.py::test_for_in[case2-expected2]",
"tests/test_objects.py::test_for_in_right[case0-expected0]",
"tests/test_objects.py::test_for_in_right[case1-expected1]",
"tests/test_objects.py::test_for_in_right[case2-expected2]",
"tests/test_objects.py::test_get[case0-expected0]",
"tests/test_objects.py::test_get[case1-4]",
"tests/test_objects.py::test_get[case2-expected2]",
"tests/test_objects.py::test_get[case3-4]",
"tests/test_objects.py::test_get[case4-None]",
"tests/test_objects.py::test_get[case5-expected5]",
"tests/test_objects.py::test_get[case6-expected6]",
"tests/test_objects.py::test_get[case7-expected7]",
"tests/test_objects.py::test_get[case8-None]",
"tests/test_objects.py::test_get[case9-None]",
"tests/test_objects.py::test_get[case10-2]",
"tests/test_objects.py::test_get[case11-2]",
"tests/test_objects.py::test_get[case12-expected12]",
"tests/test_objects.py::test_get[case13-expected13]",
"tests/test_objects.py::test_get[case14-haha]",
"tests/test_objects.py::test_get[case15-haha]",
"tests/test_objects.py::test_get[case16-None]",
"tests/test_objects.py::test_get[case17-5]",
"tests/test_objects.py::test_get[case18-5]",
"tests/test_objects.py::test_get[case19-5]",
"tests/test_objects.py::test_get[case20-4]",
"tests/test_objects.py::test_get[case21-5]",
"tests/test_objects.py::test_get[case22-5]",
"tests/test_objects.py::test_get[case23-42]",
"tests/test_objects.py::test_get[case24-49]",
"tests/test_objects.py::test_get[case25-42]",
"tests/test_objects.py::test_get[case26-42]",
"tests/test_objects.py::test_get[case27-42]",
"tests/test_objects.py::test_get[case28-value]",
"tests/test_objects.py::test_get[case29-expected29]",
"tests/test_objects.py::test_get[case30-None]",
"tests/test_objects.py::test_get[case31-1]",
"tests/test_objects.py::test_get[case32-1]",
"tests/test_objects.py::test_get[case33-1]",
"tests/test_objects.py::test_get[case34-None]",
"tests/test_objects.py::test_get[case35-None]",
"tests/test_objects.py::test_get[case36-expected36]",
"tests/test_objects.py::test_get[case37-3]",
"tests/test_objects.py::test_get[case38-1]",
"tests/test_objects.py::test_get[case39-1]",
"tests/test_objects.py::test_get[case40-John",
"tests/test_objects.py::test_get[case41-None]",
"tests/test_objects.py::test_get__should_not_populate_defaultdict",
"tests/test_objects.py::test_get__raises_for_objects_when_path_restricted[obj0-__init__.__globals__]",
"tests/test_objects.py::test_get__raises_for_objects_when_path_restricted[obj1-__globals__]",
"tests/test_objects.py::test_get__raises_for_objects_when_path_restricted[obj2-subobj.__builtins__]",
"tests/test_objects.py::test_get__raises_for_objects_when_path_restricted[obj3-__builtins__]",
"tests/test_objects.py::test_get__does_not_raise_for_dict_or_list_when_path_restricted[obj0-__globals__]",
"tests/test_objects.py::test_get__does_not_raise_for_dict_or_list_when_path_restricted[obj1-__builtins__]",
"tests/test_objects.py::test_get__does_not_raise_for_dict_or_list_when_path_restricted[obj2-__globals__]",
"tests/test_objects.py::test_get__does_not_raise_for_dict_or_list_when_path_restricted[obj3-__builtins__]",
"tests/test_objects.py::test_get__does_not_raise_for_objects_when_path_is_unrestricted[obj0-__name__]",
"tests/test_objects.py::test_get__does_not_raise_for_objects_when_path_is_unrestricted[obj1-foo.__dict__]",
"tests/test_objects.py::test_get__does_not_raise_for_objects_when_path_is_unrestricted[obj2-__len__]",
"tests/test_objects.py::test_has[case0-True]",
"tests/test_objects.py::test_has[case1-True]",
"tests/test_objects.py::test_has[case2-True]",
"tests/test_objects.py::test_has[case3-False]",
"tests/test_objects.py::test_has[case4-True]",
"tests/test_objects.py::test_has[case5-True]",
"tests/test_objects.py::test_has[case6-True]",
"tests/test_objects.py::test_has[case7-False]",
"tests/test_objects.py::test_has[case8-True]",
"tests/test_objects.py::test_has[case9-True]",
"tests/test_objects.py::test_has[case10-True]",
"tests/test_objects.py::test_has[case11-True]",
"tests/test_objects.py::test_has[case12-False]",
"tests/test_objects.py::test_has[case13-False]",
"tests/test_objects.py::test_has[case14-False]",
"tests/test_objects.py::test_has[case15-False]",
"tests/test_objects.py::test_has[case16-True]",
"tests/test_objects.py::test_has[case17-True]",
"tests/test_objects.py::test_has[case18-True]",
"tests/test_objects.py::test_has[case19-True]",
"tests/test_objects.py::test_has[case20-True]",
"tests/test_objects.py::test_has__should_not_populate_defaultdict",
"tests/test_objects.py::test_keys[case0-expected0]",
"tests/test_objects.py::test_keys[case1-expected1]",
"tests/test_objects.py::test_map_values[case0-expected0]",
"tests/test_objects.py::test_map_values[case1-expected1]",
"tests/test_objects.py::test_map_values_deep[case0-expected0]",
"tests/test_objects.py::test_map_values_deep[case1-expected1]",
"tests/test_objects.py::test_merge[case0-expected0]",
"tests/test_objects.py::test_merge[case1-expected1]",
"tests/test_objects.py::test_merge[case2-expected2]",
"tests/test_objects.py::test_merge[case3-expected3]",
"tests/test_objects.py::test_merge[case4-expected4]",
"tests/test_objects.py::test_merge[case5-expected5]",
"tests/test_objects.py::test_merge[case6-expected6]",
"tests/test_objects.py::test_merge[case7-expected7]",
"tests/test_objects.py::test_merge[case8-expected8]",
"tests/test_objects.py::test_merge[case9-expected9]",
"tests/test_objects.py::test_merge[case10-None]",
"tests/test_objects.py::test_merge[case11-None]",
"tests/test_objects.py::test_merge[case12-None]",
"tests/test_objects.py::test_merge[case13-expected13]",
"tests/test_objects.py::test_merge[case14-expected14]",
"tests/test_objects.py::test_merge[case15-expected15]",
"tests/test_objects.py::test_merge_no_link_dict",
"tests/test_objects.py::test_merge_no_link_list",
"tests/test_objects.py::test_merge_with[case0-expected0]",
"tests/test_objects.py::test_omit[case0-expected0]",
"tests/test_objects.py::test_omit[case1-expected1]",
"tests/test_objects.py::test_omit[case2-expected2]",
"tests/test_objects.py::test_omit[case3-expected3]",
"tests/test_objects.py::test_omit[case4-expected4]",
"tests/test_objects.py::test_omit[case5-expected5]",
"tests/test_objects.py::test_omit[case6-expected6]",
"tests/test_objects.py::test_omit[case7-expected7]",
"tests/test_objects.py::test_omit[case8-expected8]",
"tests/test_objects.py::test_omit_by[case0-expected0]",
"tests/test_objects.py::test_omit_by[case1-expected1]",
"tests/test_objects.py::test_omit_by[case2-expected2]",
"tests/test_objects.py::test_omit_by[case3-expected3]",
"tests/test_objects.py::test_parse_int[case0-1]",
"tests/test_objects.py::test_parse_int[case1-1]",
"tests/test_objects.py::test_parse_int[case2-1]",
"tests/test_objects.py::test_parse_int[case3-1]",
"tests/test_objects.py::test_parse_int[case4-11]",
"tests/test_objects.py::test_parse_int[case5-10]",
"tests/test_objects.py::test_parse_int[case6-8]",
"tests/test_objects.py::test_parse_int[case7-16]",
"tests/test_objects.py::test_parse_int[case8-10]",
"tests/test_objects.py::test_parse_int[case9-None]",
"tests/test_objects.py::test_pick[case0-expected0]",
"tests/test_objects.py::test_pick[case1-expected1]",
"tests/test_objects.py::test_pick[case2-expected2]",
"tests/test_objects.py::test_pick[case3-expected3]",
"tests/test_objects.py::test_pick[case4-expected4]",
"tests/test_objects.py::test_pick[case5-expected5]",
"tests/test_objects.py::test_pick[case6-expected6]",
"tests/test_objects.py::test_pick[case7-expected7]",
"tests/test_objects.py::test_pick[case8-expected8]",
"tests/test_objects.py::test_pick[case9-expected9]",
"tests/test_objects.py::test_pick[case10-expected10]",
"tests/test_objects.py::test_pick_by[case0-expected0]",
"tests/test_objects.py::test_pick_by[case1-expected1]",
"tests/test_objects.py::test_pick_by[case2-expected2]",
"tests/test_objects.py::test_pick_by[case3-expected3]",
"tests/test_objects.py::test_pick_by[case4-expected4]",
"tests/test_objects.py::test_pick_by[case5-expected5]",
"tests/test_objects.py::test_pick_by[case6-expected6]",
"tests/test_objects.py::test_rename_keys[case0-expected0]",
"tests/test_objects.py::test_rename_keys[case1-expected1]",
"tests/test_objects.py::test_rename_keys[case2-expected2]",
"tests/test_objects.py::test_set_[case0-expected0]",
"tests/test_objects.py::test_set_[case1-expected1]",
"tests/test_objects.py::test_set_[case2-expected2]",
"tests/test_objects.py::test_set_[case3-expected3]",
"tests/test_objects.py::test_set_[case4-expected4]",
"tests/test_objects.py::test_set_[case5-expected5]",
"tests/test_objects.py::test_set_[case6-expected6]",
"tests/test_objects.py::test_set_[case7-expected7]",
"tests/test_objects.py::test_set_[case8-expected8]",
"tests/test_objects.py::test_set_[case9-expected9]",
"tests/test_objects.py::test_set_[case10-expected10]",
"tests/test_objects.py::test_set_[case11-expected11]",
"tests/test_objects.py::test_set_[case12-expected12]",
"tests/test_objects.py::test_set_[case13-expected13]",
"tests/test_objects.py::test_set_[case14-expected14]",
"tests/test_objects.py::test_set_on_class_works_the_same_with_string_and_list",
"tests/test_objects.py::test_set_with[case0-expected0]",
"tests/test_objects.py::test_set_with[case1-expected1]",
"tests/test_objects.py::test_set_with[case2-expected2]",
"tests/test_objects.py::test_set_with[case3-expected3]",
"tests/test_objects.py::test_to_boolean[case0-True]",
"tests/test_objects.py::test_to_boolean[case1-False]",
"tests/test_objects.py::test_to_boolean[case2-True]",
"tests/test_objects.py::test_to_boolean[case3-True]",
"tests/test_objects.py::test_to_boolean[case4-False]",
"tests/test_objects.py::test_to_boolean[case5-False]",
"tests/test_objects.py::test_to_boolean[case6-None]",
"tests/test_objects.py::test_to_boolean[case7-None]",
"tests/test_objects.py::test_to_boolean[case8-False]",
"tests/test_objects.py::test_to_boolean[case9-True]",
"tests/test_objects.py::test_to_boolean[case10-False]",
"tests/test_objects.py::test_to_boolean[case11-True]",
"tests/test_objects.py::test_to_boolean[case12-False]",
"tests/test_objects.py::test_to_boolean[case13-False]",
"tests/test_objects.py::test_to_boolean[case14-True]",
"tests/test_objects.py::test_to_boolean[case15-False]",
"tests/test_objects.py::test_to_boolean[case16-True]",
"tests/test_objects.py::test_to_boolean[case17-None]",
"tests/test_objects.py::test_to_boolean[case18-False]",
"tests/test_objects.py::test_to_boolean[case19-None]",
"tests/test_objects.py::test_to_integer[1.4-1_0]",
"tests/test_objects.py::test_to_integer[1.9-1_0]",
"tests/test_objects.py::test_to_integer[1.4-1_1]",
"tests/test_objects.py::test_to_integer[1.9-1_1]",
"tests/test_objects.py::test_to_integer[foo-0]",
"tests/test_objects.py::test_to_integer[None-0]",
"tests/test_objects.py::test_to_integer[True-1]",
"tests/test_objects.py::test_to_integer[False-0]",
"tests/test_objects.py::test_to_integer[case8-0]",
"tests/test_objects.py::test_to_integer[case9-0]",
"tests/test_objects.py::test_to_integer[case10-0]",
"tests/test_objects.py::test_to_number[case0-3.0]",
"tests/test_objects.py::test_to_number[case1-2.6]",
"tests/test_objects.py::test_to_number[case2-990.0]",
"tests/test_objects.py::test_to_number[case3-None]",
"tests/test_objects.py::test_to_pairs[case0-expected0]",
"tests/test_objects.py::test_to_pairs[case1-expected1]",
"tests/test_objects.py::test_to_string[1-1]",
"tests/test_objects.py::test_to_string[1.25-1.25]",
"tests/test_objects.py::test_to_string[True-True]",
"tests/test_objects.py::test_to_string[case3-[1]]",
"tests/test_objects.py::test_to_string[d\\xc3\\xa9j\\xc3\\xa0",
"tests/test_objects.py::test_to_string[-]",
"tests/test_objects.py::test_to_string[None-]",
"tests/test_objects.py::test_to_string[case7-2024-08-08]",
"tests/test_objects.py::test_transform[case0-expected0]",
"tests/test_objects.py::test_transform[case1-expected1]",
"tests/test_objects.py::test_transform[case2-expected2]",
"tests/test_objects.py::test_update[case0-expected0]",
"tests/test_objects.py::test_update[case1-expected1]",
"tests/test_objects.py::test_update[case2-expected2]",
"tests/test_objects.py::test_update_with[case0-expected0]",
"tests/test_objects.py::test_update_with[case1-expected1]",
"tests/test_objects.py::test_update_with[case2-expected2]",
"tests/test_objects.py::test_unset[obj0-a.0.b.c-True-new_obj0]",
"tests/test_objects.py::test_unset[obj1-1-True-new_obj1]",
"tests/test_objects.py::test_unset[obj2-1-True-new_obj2]",
"tests/test_objects.py::test_unset[obj3-path3-True-new_obj3]",
"tests/test_objects.py::test_unset[obj4-[0][0]-False-new_obj4]",
"tests/test_objects.py::test_unset[obj5-[0][0][0]-False-new_obj5]",
"tests/test_objects.py::test_values[case0-expected0]",
"tests/test_objects.py::test_values[case1-expected1]"
] | 2024-02-20 03:09:03+00:00 | 1,912 |
|
dhatim__python-license-check-75 | diff --git a/liccheck/requirements.py b/liccheck/requirements.py
index 8bfc446..8c8e106 100644
--- a/liccheck/requirements.py
+++ b/liccheck/requirements.py
@@ -25,6 +25,10 @@ def parse_requirements(requirement_file):
for req in pip_parse_requirements(requirement_file, session=PipSession()):
install_req = install_req_from_parsed_requirement(req)
if install_req.markers and not pkg_resources.evaluate_marker(str(install_req.markers)):
+ # req should not installed due to env markers
+ continue
+ elif install_req.editable:
+ # skip editable req as they are failing in the resolve phase
continue
requirements.append(pkg_resources.Requirement.parse(str(install_req.req)))
return requirements
| dhatim/python-license-check | 6f804d1314997075883a57ce24baa5a8ee4afe98 | diff --git a/tests/test_get_packages_info.py b/tests/test_get_packages_info.py
index 1ec99b0..8daadbe 100644
--- a/tests/test_get_packages_info.py
+++ b/tests/test_get_packages_info.py
@@ -11,6 +11,7 @@ def test_license_strip(tmpfile):
tmpfh.close()
assert get_packages_info(tmppath)[0]["licenses"] == ["MIT"]
+
def test_requirements_markers(tmpfile):
tmpfh, tmppath = tmpfile
tmpfh.write(
@@ -24,6 +25,19 @@ def test_requirements_markers(tmpfile):
assert len(get_packages_info(tmppath)) == 1
+def test_editable_requirements_get_ignored(tmpfile):
+ tmpfh, tmppath = tmpfile
+ tmpfh.write(
+ "-e file:some_editable_req\n"
+ "pip\n"
+ )
+ tmpfh.close()
+
+ packages_info = get_packages_info(tmppath)
+ assert len(packages_info) == 1
+ assert packages_info[0]["name"] == "pip"
+
+
@pytest.mark.parametrize(
('no_deps', 'expected_packages'), (
pytest.param(
| Editable requirements (-e) raises "DistributionNotFound: The 'None' distribution was not found"
Editable requirements, i.e: rows like this one
`-e file:some_internal_package # via -r requirements.in`
Are currently making this library fail with `pkg_resources.DistributionNotFound: The 'None' distribution was not found and is required by the application`
This is kind of related to https://github.com/dhatim/python-license-check/issues/33 but although error message is the same, it is actually a different problem as that one relates to `git+https://` construct, and this one, to the `-e` construct | 0.0 | [
"tests/test_get_packages_info.py::test_editable_requirements_get_ignored"
] | [
"tests/test_get_packages_info.py::test_license_strip",
"tests/test_get_packages_info.py::test_deps[with",
"tests/test_get_packages_info.py::test_deps[without"
] | 2021-07-12 08:57:27+00:00 | 1,913 |
|
dicompyler__dicompyler-core-96 | diff --git a/.travis.yml b/.travis.yml
index 8de7b4f..d399453 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -1,7 +1,6 @@
language: python
python:
- 2.7
- - 3.4
- 3.5
- 3.6
install:
diff --git a/CONTRIBUTING.rst b/CONTRIBUTING.rst
index e37af2c..048bc4b 100644
--- a/CONTRIBUTING.rst
+++ b/CONTRIBUTING.rst
@@ -101,7 +101,7 @@ Before you submit a pull request, check that it meets these guidelines:
2. If the pull request adds functionality, the docs should be updated. Put
your new functionality into a function with a docstring, and add the
feature to the list in README.rst.
-3. The pull request should work for Python 2.7, 3.3, 3.4 and 3.5, and for PyPy. Check
+3. The pull request should work for Python 2.7, 3.5, and 3.6. Check
https://travis-ci.org/dicompyler/dicompyler-core/pull_requests
and make sure that the tests pass for all supported Python versions.
diff --git a/README.rst b/README.rst
index cf7c59f..c24a703 100644
--- a/README.rst
+++ b/README.rst
@@ -15,7 +15,7 @@ Other information
- Free software: `BSD license <https://github.com/dicompyler/dicompyler-core/blob/master/LICENSE>`__
- Documentation: `Read the docs <https://dicompyler-core.readthedocs.io>`__
-- Tested on Python 2.7, 3.4, 3.5, 3.6
+- Tested on Python 2.7, 3.5, 3.6
Dependencies
------------
diff --git a/dicompylercore/dicomparser.py b/dicompylercore/dicomparser.py
index 3be8daa..2aa8346 100755
--- a/dicompylercore/dicomparser.py
+++ b/dicompylercore/dicomparser.py
@@ -410,7 +410,7 @@ class DicomParser:
if (pixel_array.min() < wmin):
wmin = pixel_array.min()
# Default window is the range of the data array
- window = int(abs(wmax) + abs(wmin))
+ window = int(wmax - wmin)
# Default level is the range midpoint minus the window minimum
level = int(window / 2 - abs(wmin))
return window, level
diff --git a/dicompylercore/dvh.py b/dicompylercore/dvh.py
index 24155a4..14c2777 100644
--- a/dicompylercore/dvh.py
+++ b/dicompylercore/dvh.py
@@ -262,7 +262,7 @@ class DVH(object):
@property
def max(self):
"""Return the maximum dose."""
- if self.counts.size == 1:
+ if self.counts.size <= 1 or max(self.counts) == 0:
return 0
diff = self.differential
# Find the the maximum non-zero dose bin
@@ -271,7 +271,7 @@ class DVH(object):
@property
def min(self):
"""Return the minimum dose."""
- if self.counts.size == 1:
+ if self.counts.size <= 1 or max(self.counts) == 0:
return 0
diff = self.differential
# Find the the minimum non-zero dose bin
@@ -280,7 +280,7 @@ class DVH(object):
@property
def mean(self):
"""Return the mean dose."""
- if self.counts.size == 1:
+ if self.counts.size <= 1 or max(self.counts) == 0:
return 0
diff = self.differential
# Find the area under the differential histogram
@@ -462,7 +462,7 @@ class DVH(object):
else:
volume_counts = self.absolute_volume(self.volume).counts
- if volume > volume_counts.max():
+ if volume_counts.size == 0 or volume > volume_counts.max():
return DVHValue(0.0, self.dose_units)
# D100 case
diff --git a/requirements_dev.txt b/requirements_dev.txt
index 8193cbd..7af08f4 100644
--- a/requirements_dev.txt
+++ b/requirements_dev.txt
@@ -1,8 +1,8 @@
bumpversion==0.5.3
-wheel==0.32.3
-flake8==3.6.0
-tox==3.6.1
+wheel==0.33.1
+flake8==3.7.7
+tox==3.7.0
coverage==4.5.2
-Sphinx==1.8.3
-sphinx-rtd-theme==0.4.2
-cryptography==2.4.2
+Sphinx==1.8.4
+sphinx-rtd-theme==0.4.3
+cryptography==2.6.1
diff --git a/setup.py b/setup.py
index 59ad242..82124af 100644
--- a/setup.py
+++ b/setup.py
@@ -62,8 +62,6 @@ setup(
"Programming Language :: Python :: 2",
'Programming Language :: Python :: 2.7',
'Programming Language :: Python :: 3',
- 'Programming Language :: Python :: 3.3',
- 'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Topic :: Scientific/Engineering :: Medical Science Apps.',
| dicompyler/dicompyler-core | f5f9fa770d0573373510135863fa2933919cdba7 | diff --git a/tests/test_dvh.py b/tests/test_dvh.py
index 6c9d403..3e64782 100644
--- a/tests/test_dvh.py
+++ b/tests/test_dvh.py
@@ -204,6 +204,27 @@ class TestDVH(unittest.TestCase):
self.dvh.name = "test"
self.assertEqual(self.dvh.plot(), self.dvh)
+ def test_dvh_statistics_with_no_counts(self):
+ subject = dvh.DVH(array([]), array([0]))
+ self.assertEqual(subject.max, 0)
+ self.assertEqual(subject.min, 0)
+ self.assertEqual(subject.mean, 0)
+
+ def test_dose_constraint_with_no_counts(self):
+ subject = dvh.DVH(array([]), array([0]))
+ subject.dose_constraint(1)
+
+ def test_dvh_statistics_with_zero_volume(self):
+ subject = dvh.DVH(array([0, 0]), array([0, 1]))
+ self.assertEqual(subject.max, 0)
+ self.assertEqual(subject.min, 0)
+ self.assertEqual(subject.mean, 0)
+
+ def test_dose_constraint_with_zero_volume(self):
+ subject = dvh.DVH(array([0, 0]), array([0, 1]))
+ subject.dose_constraint(1)
+
+
if __name__ == '__main__':
import sys
sys.exit(unittest.main())
| dvh.max gives IndexError
Hello and thank you for the project.
I am using dicompyler-core 0.5.4 to extract statistics and I have been running into an exception with certain DCM files. When calculating `dvh.max`, the following error is thrown:
```
File "/home/nico/.local/share/virtualenvs/dcm-extractor-8Y6EYVZw/lib/python3.7/site-packages/dicompylercore/dvh.py", line 269, in max
return diff.bins[1:][diff.counts > 0][-1]
IndexError: boolean index did not match indexed array along dimension 0; dimension is 0 but corresponding boolean dimension is 1
```
This seems to happen whenever dvh.counts is either empty, or has all same values (such that dvh.diff.counts is all 0s).
Please let me know if I can assist in troubleshooting.
| 0.0 | [
"tests/test_dvh.py::TestDVH::test_dose_constraint_with_no_counts",
"tests/test_dvh.py::TestDVH::test_dose_constraint_with_zero_volume",
"tests/test_dvh.py::TestDVH::test_dvh_statistics_with_no_counts",
"tests/test_dvh.py::TestDVH::test_dvh_statistics_with_zero_volume"
] | [
"tests/test_dvh.py::TestDVH::test_absolute_relative_dose_dvh",
"tests/test_dvh.py::TestDVH::test_absolute_relative_full_conversion",
"tests/test_dvh.py::TestDVH::test_absolute_relative_volume_dvh",
"tests/test_dvh.py::TestDVH::test_cumulative_dvh",
"tests/test_dvh.py::TestDVH::test_differential_dvh",
"tests/test_dvh.py::TestDVH::test_dvh_compare",
"tests/test_dvh.py::TestDVH::test_dvh_describe",
"tests/test_dvh.py::TestDVH::test_dvh_properties",
"tests/test_dvh.py::TestDVH::test_dvh_statistics",
"tests/test_dvh.py::TestDVH::test_dvh_statistics_shorthand",
"tests/test_dvh.py::TestDVH::test_dvh_statistics_shorthand_fail",
"tests/test_dvh.py::TestDVH::test_dvh_value",
"tests/test_dvh.py::TestDVH::test_plotting",
"tests/test_dvh.py::TestDVH::test_raw_data_dvh_max_bins"
] | 2019-02-25 13:56:03+00:00 | 1,914 |
|
differint__differint-12 | diff --git a/differint/differint.py b/differint/differint.py
index b503322..f001e13 100644
--- a/differint/differint.py
+++ b/differint/differint.py
@@ -145,6 +145,49 @@ def Beta(x,y):
return Gamma(x)*Gamma(y)/Gamma(x+y)
+def MittagLeffler(a, b, x, num_terms=50, *, ignore_special_cases=False):
+ ''' Calculate the Mittag-Leffler function by checking for special cases, and trying to
+ reduce the parameters. If neither of those work, it just brute forces it.
+
+ Parameters
+ ==========
+ a : float
+ The first parameter of the Mittag-Leffler function.
+ b : float
+ The second parameter of the Mittag-Leffler function
+ x : float or 1D-array of floats
+ The value or values to be evaluated at.
+ num_terms : int
+ The number of terms to calculate in the sum. Ignored if
+ a special case can be used instead. Default value is 100.
+ ignore_special_cases : bool
+ Don't use the special cases, use the series definition.
+ Probably only useful for testing. Default value is False.
+ '''
+ # check for quick special cases
+ if not ignore_special_cases:
+ if a == 0:
+ if (np.abs(x) < 1).all():
+ return 1 / Gamma(b) * 1 / (1 - x)
+ return x * np.inf
+ elif a == 0.5 and b == 1:
+ # requires calculation of the complementary error function
+ pass
+ elif a == 1 and b == 1:
+ return np.exp(x)
+ elif a == 2 and b == 1:
+ return np.cosh(np.sqrt(x))
+ elif a == 1 and b == 2:
+ return (np.exp(x) - 1) / x
+ elif a == 2 and b == 2:
+ return np.sinh(np.sqrt(x)) / np.sqrt(x)
+ # manually calculate with series definition
+ exponents = np.arange(num_terms)
+ exp_vals = np.array([x]).T ** exponents
+ gamma_vals = np.array([Gamma(exponent * a + b) for exponent in exponents])
+ return np.sum(exp_vals / gamma_vals, axis=1)
+
+
def GLcoeffs(alpha,n):
""" Computes the GL coefficient array of size n.
@@ -489,3 +532,93 @@ class GLIinterpolat:
self.nxt = alpha*(2+alpha)/8
self.crr = (4-alpha*alpha)/4
self.prv = alpha*(alpha-2)/8
+
+def PCcoeffs(alpha, j, n):
+ if 1 < alpha:
+ if j == 0:
+ return (n+1)**alpha * (alpha - n) + n**alpha * (2 * n - alpha - 1) - (n - 1)**(alpha + 1)
+ elif j == n:
+ return 2 ** (alpha + 1) - alpha - 3
+ return (n - j + 2) ** (alpha + 1) + 3 * (n - j) ** (alpha + 1) - 3 * (n - j + 1) ** (alpha + 1) - (n - j - 1) ** (alpha + 1)
+
+def PCsolver(initial_values, alpha, f_name, domain_start=0, domain_end=1, num_points=100):
+ """ Solve an equation of the form D[y(x)]=f(x, y(x)) using the predictor-corrector
+ method, modified to be compatible with fractional derivatives.
+
+ see Deng, W. (2007) Short memory principle and a predictor–corrector approach for
+ fractional differential equations. Journal of Computational and Applied
+ Mathematics.
+
+ test examples from
+ Baskonus, H.M., Bulut, H. (2015) On the numerical solutions of some fractional
+ ordinary differential equations by fractional Adams-Bashforth-Moulton method.
+ De Gruyter.
+ Weilbeer, M. (2005) Efficient Numerical Methods for Fractional Differential
+ Equations and their Analytical Background.
+
+
+
+ Parameters
+ ==========
+ initial_values : float 1d-array
+ A list of initial values for the IVP. There should be as many IVs
+ as ceil(alpha).
+ alpha : float
+ The order of the differintegral in the equation to be computed.
+ f_name : function handle or lambda function
+ This is the function on the right side of the equation, and should
+ accept two variables; first the independant variable, and second
+ the equation to be solved.
+ domain_start : float
+ The left-endpoint of the function domain. Default value is 0.
+ domain_end : float
+ The right-endpoint of the function domain; the point at which the
+ differintegral is being evaluated. Default value is 1.
+ num_points : integer
+ The number of points in the domain. Default value is 100.
+
+ Output
+ ======
+ y_correction : float 1d-array
+ The calculated solution to the IVP at each of the points
+ between the left and right endpoint.
+
+ Examples:
+ >>> f_name = lambda x, y : y - x - 1
+ >>> initial_values = [1, 1]
+ >>> y_solved = PCsolver(initial_values, 1.5, f_name)
+ >>> theoretical = np.linspace(0, 1, 100) + 1
+ >>> np.allclose(y_solved, theoretical)
+ True
+ """
+ x_points = np.linspace(domain_start, domain_end, num_points)
+ step_size = x_points[1] - x_points[0]
+ y_correction = np.zeros(num_points, dtype='complex_')
+ y_prediction = np.zeros(num_points, dtype='complex_')
+
+ y_prediction[0] = initial_values[0]
+ y_correction[0] = initial_values[0]
+ for x_index in range(num_points - 1):
+ initial_value_contribution = 0
+ if 1 < alpha and alpha < 2:
+ initial_value_contribution = initial_values[1] * step_size
+ elif 2 < alpha:
+ for k in range(1, int(np.ceil(alpha))):
+ initial_value_contribution += initial_values[k] / np.math.factorial(k) * (x_points[x_index + 1] ** k - x_points[x_index] ** k)
+ elif alpha < 1:
+ raise ValueError('Not yet supported!')
+ y_prediction[x_index + 1] += initial_value_contribution
+ y_prediction[x_index + 1] += y_correction[x_index]
+ y_prediction[x_index + 1] += step_size ** alpha / Gamma(alpha + 1) * f_name(x_points[x_index], y_correction[x_index])
+ subsum = 0
+ for j in range(x_index + 1):
+ subsum += PCcoeffs(alpha, j, x_index) * f_name(x_points[j], y_correction[j])
+ y_prediction[x_index + 1] += step_size ** alpha / Gamma(alpha + 2) * subsum
+
+ y_correction[x_index + 1] += initial_value_contribution
+ y_correction[x_index + 1] += y_correction[x_index]
+ y_correction[x_index + 1] += step_size ** alpha / Gamma(alpha + 2) * alpha * f_name(x_points[x_index], y_correction[x_index])
+ y_correction[x_index + 1] += step_size ** alpha / Gamma(alpha + 2) * f_name(x_points[x_index + 1], y_prediction[x_index + 1])
+ y_correction[x_index + 1] += step_size ** alpha / Gamma(alpha + 2) * subsum
+
+ return y_correction
| differint/differint | 6b0a4813a37b6734fb9733dd769f340ecb74aa27 | diff --git a/tests/test.py b/tests/test.py
index d663249..af03cbd 100644
--- a/tests/test.py
+++ b/tests/test.py
@@ -12,6 +12,7 @@ size_coefficient_array = 20
test_N = 512
sqrtpi2 = 0.88622692545275794
truevaluepoly = 0.94031597258
+PC_x_power = np.linspace(0, 1, 100) ** 5.5
INTER = GLIinterpolat(1)
@@ -33,6 +34,10 @@ RL_r = RL(0.5, lambda x: np.sqrt(x), 0, 1, test_N)
RL_result = RL_r[-1]
RL_length = len(RL_r)
+# Get FODE function for solving.
+PC_func_power = lambda x, y : 1/24 * Gamma(5 + 1.5) * x**4 + x**(8 + 2 * 1.5) - y**2
+PC_func_ML = lambda x,y : y
+
class HelperTestCases(unittest.TestCase):
""" Tests for helper functions. """
@@ -89,6 +94,23 @@ class HelperTestCases(unittest.TestCase):
def testComplexValue(self):
self.assertEqual(np.round(Gamma(1j), 4), -0.1549-0.498j)
+
+ """ Unit tests for Mittag-Leffler function. """
+
+ def test_ML_cosh_root(self):
+ xs = np.arange(10, 0.1)
+ self.assertTrue((np.abs(MittagLeffler(2, 1, xs, ignore_special_cases=True)\
+ - np.cosh(np.sqrt(xs))) <= 1e-3).all())
+
+ def test_ML_exp(self):
+ xs = np.arange(10, 0.1)
+ self.assertTrue((np.abs(MittagLeffler(1, 1, xs, ignore_special_cases=True)\
+ - np.exp(xs)) <= 1e-3).all())
+
+ def test_ML_geometric(self):
+ xs = np.arange(1, 0.05)
+ self.assertTrue((np.abs(MittagLeffler(0, 1, xs, ignore_special_cases=True)\
+ - 1 / (1 - xs)) <= 1e-3).all())
class TestInterpolantCoefficients(unittest.TestCase):
""" Test the correctness of the interpolant coefficients. """
@@ -131,7 +153,21 @@ class TestAlgorithms(unittest.TestCase):
def test_RL_accuracy_sqrt(self):
self.assertTrue(abs(RL_result - sqrtpi2) <= 1e-4)
-
+
+class TestSolvers(unittest.TestCase):
+ """ Tests for the correct solution to the equations. """
+ def test_PC_solution_three_halves(self):
+ self.assertTrue((np.abs(PCsolver([0, 0], 1.5, PC_func_power, 0, 1, 100)-PC_x_power) <= 1e-2).all())
+
+ def test_PC_solution_ML(self):
+ xs = np.linspace(0, 1, 100)
+ ML_alpha = MittagLeffler(5.5, 1, xs ** 5.5)
+ self.assertTrue((np.abs(PCsolver([1, 0, 0, 0, 0, 0], 5.5, PC_func_ML)-ML_alpha) <= 1e-2).all())
+
+ def test_PC_solution_linear(self):
+ xs = np.linspace(0, 1, 100)
+ self.assertTrue((np.abs(PCsolver([1, 1], 1.5, lambda x,y : y-x-1)-(xs+1)) <= 1e-2).all())
+
if __name__ == '__main__':
unittest.main(argv=['first-arg-is-ignored'], exit=False)
| Caputo Fractional Derivative - reg
HI! Good Morning!
Is it applicable for caputo derivative? In specific, predictor-corrector algorithm. | 0.0 | [
"tests/test.py::HelperTestCases::test_ML_cosh_root",
"tests/test.py::HelperTestCases::test_ML_exp",
"tests/test.py::HelperTestCases::test_ML_geometric"
] | [
"tests/test.py::HelperTestCases::testComplexValue",
"tests/test.py::HelperTestCases::testFiveFactorial",
"tests/test.py::HelperTestCases::testNegativePoles",
"tests/test.py::HelperTestCases::testRealValue",
"tests/test.py::HelperTestCases::test_GL_binomial_coefficient_array_size",
"tests/test.py::HelperTestCases::test_checkValues",
"tests/test.py::HelperTestCases::test_functionCheck",
"tests/test.py::HelperTestCases::test_isInteger",
"tests/test.py::HelperTestCases::test_isPositiveInteger",
"tests/test.py::HelperTestCases::test_pochhammer",
"tests/test.py::TestAlgorithms::test_GLI_accuracy_sqrt",
"tests/test.py::TestAlgorithms::test_GLI_result_length",
"tests/test.py::TestAlgorithms::test_GL_accuracy_sqrt",
"tests/test.py::TestAlgorithms::test_GLpoint_accuracy_polynomial",
"tests/test.py::TestAlgorithms::test_GLpoint_sqrt_accuracy",
"tests/test.py::TestAlgorithms::test_RL_accuracy_sqrt",
"tests/test.py::TestAlgorithms::test_RL_matrix_shape",
"tests/test.py::TestAlgorithms::test_RL_result_length",
"tests/test.py::TestAlgorithms::test_RLpoint_accuracy_polynomial",
"tests/test.py::TestAlgorithms::test_RLpoint_sqrt_accuracy"
] | 2022-11-16 05:58:58+00:00 | 1,915 |
|
diogommartins__simple_json_logger-14 | diff --git a/setup.py b/setup.py
index a1dddaa..db14acc 100644
--- a/setup.py
+++ b/setup.py
@@ -1,7 +1,7 @@
from setuptools import setup, find_packages
-VERSION = '0.2.2'
+VERSION = '0.2.3'
setup(name='simple_json_logger',
diff --git a/simple_json_logger/formatter.py b/simple_json_logger/formatter.py
index b4eb58a..629eecb 100644
--- a/simple_json_logger/formatter.py
+++ b/simple_json_logger/formatter.py
@@ -1,7 +1,7 @@
import logging
try:
from logging import _levelToName
-except ImportError:
+except ImportError: # pragma: no cover
from logging import _levelNames as _levelToName
import traceback
@@ -32,7 +32,7 @@ class JsonFormatter(logging.Formatter):
return obj.strftime(DATETIME_FORMAT)
elif istraceback(obj):
tb = ''.join(traceback.format_tb(obj))
- return tb.strip()
+ return tb.strip().split('\n')
elif isinstance(obj, Exception):
return "Exception: %s" % str(obj)
elif callable(obj):
| diogommartins/simple_json_logger | 1bc3c8d12784d55417e8d46b4fe9b44bd8cd1f99 | diff --git a/tests/test_logger.py b/tests/test_logger.py
index a98e7f4..8094814 100644
--- a/tests/test_logger.py
+++ b/tests/test_logger.py
@@ -78,12 +78,11 @@ class LoggerTests(unittest.TestCase):
json_log = json.loads(logged_content)
exc_class, exc_message, exc_traceback = json_log['exc_info']
- self.assertIn(member=exception_message,
- container=exc_message)
+ self.assertEqual('Exception: {}'.format(exception_message), exc_message)
current_func_name = inspect.currentframe().f_code.co_name
- self.assertIn(member=current_func_name,
- container=exc_traceback)
+ self.assertIn(current_func_name, exc_traceback[0])
+ self.assertIn('raise Exception(exception_message)', exc_traceback[1])
def test_it_logs_datetime_objects(self):
message = {
| Fix multiline log for stacktraces
Multiline stack traces must be formatted as a list of strings | 0.0 | [
"tests/test_logger.py::LoggerTests::test_it_logs_exceptions_tracebacks"
] | [
"tests/test_logger.py::LoggerTests::test_flatten_method_parameter_does_nothing_is_message_isnt_a_dict",
"tests/test_logger.py::LoggerTests::test_extra_param_adds_content_to_document_root",
"tests/test_logger.py::LoggerTests::test_it_escapes_strings",
"tests/test_logger.py::LoggerTests::test_extra_parameter_on_log_method_function_call_updates_extra_parameter_on_init",
"tests/test_logger.py::LoggerTests::test_flatten_instance_attr_adds_messages_to_document_root",
"tests/test_logger.py::LoggerTests::test_flatten_param_adds_message_to_document_root",
"tests/test_logger.py::LoggerTests::test_it_forwards_serializer_kwargs_parameter_to_serializer",
"tests/test_logger.py::LoggerTests::test_it_logs_valid_json_string_if_message_is_json_serializeable",
"tests/test_logger.py::LoggerTests::test_flatten_instance_attr_overwrites_default_attributes",
"tests/test_logger.py::LoggerTests::test_callable_values_are_called_before_serialization",
"tests/test_logger.py::LoggerTests::test_it_logs_valid_json_string_if_message_isnt_json_serializeable",
"tests/test_logger.py::LoggerTests::test_extra_parameter_adds_content_to_root_of_all_messages",
"tests/test_logger.py::LoggerTests::test_it_forwards_serializer_kwargs_instance_attr_to_serializer",
"tests/test_logger.py::LoggerTests::test_it_logs_datetime_objects",
"tests/test_logger.py::LoggerTests::test_flatten_method_parameter_overwrites_default_attributes",
"tests/test_logger.py::LoggerTests::test_it_logs_current_log_time"
] | 2018-05-31 14:58:34+00:00 | 1,916 |
|
dirty-cat__dirty_cat-197 | diff --git a/dirty_cat/super_vectorizer.py b/dirty_cat/super_vectorizer.py
index 84b39c9..420d00b 100644
--- a/dirty_cat/super_vectorizer.py
+++ b/dirty_cat/super_vectorizer.py
@@ -106,10 +106,9 @@ class SuperVectorizer(ColumnTransformer):
None to apply `remainder`, 'drop' for dropping the columns,
or 'passthrough' to return the unencoded columns.
- auto_cast: bool, default=False
+ auto_cast: bool, default=True
If set to `True`, will try to convert each column to the best possible
data type (dtype).
- Experimental. It is advised to cast them beforehand, and leave this false.
handle_missing: str, default=''
One of the following values: 'error' or '' (empty).
@@ -144,7 +143,7 @@ class SuperVectorizer(ColumnTransformer):
high_card_cat_transformer: Optional[Union[BaseEstimator, str]] = GapEncoder(),
numerical_transformer: Optional[Union[BaseEstimator, str]] = None,
datetime_transformer: Optional[Union[BaseEstimator, str]] = None,
- auto_cast: bool = False,
+ auto_cast: bool = True,
# Following parameters are inherited from ColumnTransformer
handle_missing: str = '',
remainder='passthrough',
@@ -171,8 +170,6 @@ class SuperVectorizer(ColumnTransformer):
self.transformer_weights = transformer_weights
self.verbose = verbose
- self.columns_ = []
-
def _auto_cast_array(self, X):
"""
Takes an array and tries to convert its columns to the best possible
diff --git a/examples/03_automatic_preprocessing_with_the_supervectorizer.py b/examples/03_automatic_preprocessing_with_the_supervectorizer.py
index 69ed18a..b9f6d88 100644
--- a/examples/03_automatic_preprocessing_with_the_supervectorizer.py
+++ b/examples/03_automatic_preprocessing_with_the_supervectorizer.py
@@ -82,7 +82,7 @@ from sklearn.pipeline import Pipeline
from dirty_cat import SuperVectorizer
pipeline = Pipeline([
- ('vectorizer', SuperVectorizer(auto_cast=True)),
+ ('vectorizer', SuperVectorizer()),
('clf', HistGradientBoostingRegressor(random_state=42))
])
@@ -111,7 +111,6 @@ print(f'std={np.std(scores)}')
# Let us perform the same workflow, but without the `Pipeline`, so we can
# analyze its mechanisms along the way.
sup_vec = SuperVectorizer(
- auto_cast=True,
high_card_str_transformer=GapEncoder(n_components=50),
high_card_cat_transformer=GapEncoder(n_components=50)
)
| dirty-cat/dirty_cat | 766aa5c905a737d0a90e8432bed84aac6101754a | diff --git a/dirty_cat/test/test_super_vectorizer.py b/dirty_cat/test/test_super_vectorizer.py
index 2f34d8e..b70a26b 100644
--- a/dirty_cat/test/test_super_vectorizer.py
+++ b/dirty_cat/test/test_super_vectorizer.py
@@ -3,6 +3,8 @@ import sklearn
import pandas as pd
from sklearn.preprocessing import StandardScaler
+from sklearn.utils.validation import check_is_fitted
+from sklearn.exceptions import NotFittedError
from distutils.version import LooseVersion
@@ -79,7 +81,6 @@ def test_super_vectorizer():
# Test casting values
vectorizer_cast = SuperVectorizer(
cardinality_threshold=3,
- auto_cast=True,
# we must have n_samples = 5 >= n_components
high_card_str_transformer=GapEncoder(n_components=2),
high_card_cat_transformer=GapEncoder(n_components=2),
@@ -130,6 +131,18 @@ def test_get_feature_names():
assert vectorizer_w_drop.get_feature_names() == expected_feature_names_drop
+def test_fit():
+ # Simply checks sklearn's `check_is_fitted` function raises an error if
+ # the SuperVectorizer is instantiated but not fitted.
+ # See GH#193
+ sup_vec = SuperVectorizer()
+ with pytest.raises(NotFittedError):
+ if LooseVersion(sklearn.__version__) >= LooseVersion('0.22'):
+ assert check_is_fitted(sup_vec)
+ else:
+ assert check_is_fitted(sup_vec, attributes=dir(sup_vec))
+
+
if __name__ == '__main__':
print('start test_super_vectorizer')
test_super_vectorizer()
@@ -137,5 +150,8 @@ if __name__ == '__main__':
print('start test_get_feature_names')
test_get_feature_names()
print('test_get_feature_names passed')
+ print('start test_fit')
+ test_fit()
+ print('test_fit passed')
print('Done')
| Better error message when SuperVectorizer's transform method is called before fit
Scikit-learn has a mechanism to check that an estimator is fitted, and if not raise a good error message. We should use it. | 0.0 | [
"dirty_cat/test/test_super_vectorizer.py::test_fit"
] | [] | 2021-07-21 13:30:28+00:00 | 1,917 |
|
dirty-cat__dirty_cat-261 | diff --git a/CHANGES.rst b/CHANGES.rst
index 3b92fa9..027a15b 100644
--- a/CHANGES.rst
+++ b/CHANGES.rst
@@ -30,6 +30,7 @@ Release 0.2.1
Major changes
-------------
+
* Improvements to the :class:`SuperVectorizer`
- Type detection works better: handles dates, numerics columns encoded as strings,
@@ -51,6 +52,8 @@ Bug-fixes
* GapEncoder's `get_feature_names_out` now accepts all iterators, not just lists.
+* Fixed `DeprecationWarning` raised by the usage of `distutils.version.LooseVersion`
+
Notes
-----
diff --git a/build_tools/circle/list_versions.py b/build_tools/circle/list_versions.py
index 19fa8aa..0322cda 100755
--- a/build_tools/circle/list_versions.py
+++ b/build_tools/circle/list_versions.py
@@ -5,9 +5,10 @@ import json
import re
import sys
-from distutils.version import LooseVersion
+from dirty_cat.utils import Version
from urllib.request import urlopen
+
def json_urlread(url):
try:
return json.loads(urlopen(url).read().decode('utf8'))
@@ -80,7 +81,7 @@ for src, dst in symlinks.items():
seen = set()
for name in (NAMED_DIRS +
sorted((k for k in dirs if k[:1].isdigit()),
- key=LooseVersion, reverse=True)):
+ key=Version, reverse=True)):
version_num, pdf_size = dirs[name]
if version_num in seen:
# symlink came first
diff --git a/dirty_cat/datasets/fetching.py b/dirty_cat/datasets/fetching.py
index f42dd59..8b28c93 100644
--- a/dirty_cat/datasets/fetching.py
+++ b/dirty_cat/datasets/fetching.py
@@ -24,8 +24,8 @@ import pandas as pd
from pathlib import Path
from collections import namedtuple
from typing import Union, Dict, Any
-from distutils.version import LooseVersion
+from dirty_cat.utils import Version
from dirty_cat.datasets.utils import get_data_dir
@@ -165,7 +165,7 @@ def _download_and_write_openml_dataset(dataset_id: int,
from sklearn.datasets import fetch_openml
fetch_kwargs = {}
- if LooseVersion(sklearn.__version__) >= LooseVersion('0.22'):
+ if Version(sklearn.__version__) >= Version('0.22'):
fetch_kwargs.update({'as_frame': True})
# The ``fetch_openml()`` function returns a Scikit-Learn ``Bunch`` object,
diff --git a/dirty_cat/gap_encoder.py b/dirty_cat/gap_encoder.py
index dea1cdc..772c6d2 100644
--- a/dirty_cat/gap_encoder.py
+++ b/dirty_cat/gap_encoder.py
@@ -16,7 +16,6 @@ The principle is as follows:
"""
import warnings
import numpy as np
-from distutils.version import LooseVersion
from scipy import sparse
from sklearn import __version__ as sklearn_version
from sklearn.utils import check_random_state, gen_batches
@@ -28,15 +27,16 @@ from sklearn.neighbors import NearestNeighbors
from sklearn.utils.fixes import _object_dtype_isnan
import pandas as pd
from .utils import check_input
+from dirty_cat.utils import Version
-if LooseVersion(sklearn_version) <= LooseVersion('0.22'):
+if Version(sklearn_version) <= Version('0.22'):
from sklearn.cluster.k_means_ import _k_init
-elif LooseVersion(sklearn_version) < LooseVersion('0.24'):
+elif Version(sklearn_version) < Version('0.24'):
from sklearn.cluster._kmeans import _k_init
else:
from sklearn.cluster import kmeans_plusplus
-if LooseVersion(sklearn_version) <= LooseVersion('0.22'):
+if Version(sklearn_version) <= Version('0.22'):
from sklearn.decomposition.nmf import _beta_divergence
else:
from sklearn.decomposition._nmf import _beta_divergence
@@ -106,12 +106,12 @@ class GapEncoderColumn(BaseEstimator, TransformerMixin):
unq_V = sparse.hstack((unq_V, unq_V2), format='csr')
if not self.hashing: # Build n-grams/word vocabulary
- if LooseVersion(sklearn_version) < LooseVersion('1.0'):
+ if Version(sklearn_version) < Version('1.0'):
self.vocabulary = self.ngrams_count_.get_feature_names()
else:
self.vocabulary = self.ngrams_count_.get_feature_names_out()
if self.add_words:
- if LooseVersion(sklearn_version) < LooseVersion('1.0'):
+ if Version(sklearn_version) < Version('1.0'):
self.vocabulary = np.concatenate((
self.vocabulary,
self.word_count_.get_feature_names()
@@ -153,7 +153,7 @@ class GapEncoderColumn(BaseEstimator, TransformerMixin):
n-grams counts.
"""
if self.init == 'k-means++':
- if LooseVersion(sklearn_version) < LooseVersion('0.24'):
+ if Version(sklearn_version) < Version('0.24'):
W = _k_init(
V, self.n_components,
x_squared_norms=row_norms(V, squared=True),
@@ -179,7 +179,7 @@ class GapEncoderColumn(BaseEstimator, TransformerMixin):
W = np.hstack((W, W2))
# if k-means doesn't find the exact number of prototypes
if W.shape[0] < self.n_components:
- if LooseVersion(sklearn_version) < LooseVersion('0.24'):
+ if Version(sklearn_version) < Version('0.24'):
W2 = _k_init(
V, self.n_components - W.shape[0],
x_squared_norms=row_norms(V, squared=True),
@@ -284,7 +284,7 @@ class GapEncoderColumn(BaseEstimator, TransformerMixin):
"""
vectorizer = CountVectorizer()
vectorizer.fit(list(self.H_dict_.keys()))
- if LooseVersion(sklearn_version) < LooseVersion('1.0'):
+ if Version(sklearn_version) < Version('1.0'):
vocabulary = np.array(vectorizer.get_feature_names())
else:
vocabulary = np.array(vectorizer.get_feature_names_out())
diff --git a/dirty_cat/similarity_encoder.py b/dirty_cat/similarity_encoder.py
index c0cfe8b..18b9f4e 100644
--- a/dirty_cat/similarity_encoder.py
+++ b/dirty_cat/similarity_encoder.py
@@ -15,7 +15,6 @@ The principle is as follows:
import warnings
import numpy as np
-from distutils.version import LooseVersion
from joblib import Parallel, delayed
from scipy import sparse
import sklearn
@@ -26,6 +25,7 @@ from sklearn.preprocessing import OneHotEncoder
from sklearn.utils import check_random_state
from sklearn.utils.fixes import _object_dtype_isnan
+from dirty_cat.utils import Version
from . import string_distances
from .string_distances import get_ngram_count, preprocess
@@ -400,7 +400,7 @@ class SimilarityEncoder(OneHotEncoder):
self.vocabulary_ngram_counts_.append(vocabulary_ngram_count)
self.drop_idx_ = self._compute_drop_idx()
- if LooseVersion(sklearn.__version__) >= LooseVersion('1.1.0'):
+ if Version(sklearn.__version__) >= Version('1.1.0'):
self._infrequent_enabled = False
return self
diff --git a/dirty_cat/super_vectorizer.py b/dirty_cat/super_vectorizer.py
index e31e00a..219d117 100644
--- a/dirty_cat/super_vectorizer.py
+++ b/dirty_cat/super_vectorizer.py
@@ -15,15 +15,15 @@ import pandas as pd
from warnings import warn
from typing import Union, Optional, List
-from distutils.version import LooseVersion
from sklearn.base import BaseEstimator
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import OneHotEncoder
from dirty_cat import GapEncoder, DatetimeEncoder
+from dirty_cat.utils import Version
-_sklearn_loose_version = LooseVersion(sklearn.__version__)
+_sklearn_loose_version = Version(sklearn.__version__)
def _has_missing_values(df: Union[pd.DataFrame, pd.Series]) -> bool:
@@ -402,7 +402,7 @@ class SuperVectorizer(ColumnTransformer):
impute: bool = False
if isinstance(trans, OneHotEncoder) \
- and _sklearn_loose_version < LooseVersion('0.24'):
+ and _sklearn_loose_version < Version('0.24'):
impute = True
if impute:
@@ -446,9 +446,9 @@ class SuperVectorizer(ColumnTransformer):
e.g. "job_title_Police officer",
or "<column_name>" if not encoded.
"""
- if _sklearn_loose_version < LooseVersion('0.23'):
+ if _sklearn_loose_version < Version('0.23'):
try:
- if _sklearn_loose_version < LooseVersion('1.0'):
+ if _sklearn_loose_version < Version('1.0'):
ct_feature_names = super().get_feature_names()
else:
ct_feature_names = super().get_feature_names_out()
@@ -460,7 +460,7 @@ class SuperVectorizer(ColumnTransformer):
'transformers, or update your copy of scikit-learn.'
)
else:
- if _sklearn_loose_version < LooseVersion('1.0'):
+ if _sklearn_loose_version < Version('1.0'):
ct_feature_names = super().get_feature_names()
else:
ct_feature_names = super().get_feature_names_out()
@@ -478,7 +478,7 @@ class SuperVectorizer(ColumnTransformer):
if not hasattr(trans, 'get_feature_names'):
all_trans_feature_names.extend(cols)
else:
- if _sklearn_loose_version < LooseVersion('1.0'):
+ if _sklearn_loose_version < Version('1.0'):
trans_feature_names = trans.get_feature_names(cols)
else:
trans_feature_names = trans.get_feature_names_out(cols)
diff --git a/dirty_cat/utils.py b/dirty_cat/utils.py
index 4891f7a..920e0ad 100644
--- a/dirty_cat/utils.py
+++ b/dirty_cat/utils.py
@@ -2,6 +2,8 @@ import collections
import numpy as np
+from typing import Tuple, Union
+
class LRUDict:
""" dict with limited capacity
@@ -44,3 +46,81 @@ def check_input(X):
'array.reshape(1, -1) if it contains a single sample.'
)
return X
+
+
+class Version:
+ """
+ Replacement for `distutil.version.LooseVersion` and `packaging.version.Version`.
+ Implemented to avoid `DeprecationWarning`s raised by the former,
+ and avoid adding a dependency for the latter.
+
+ It is therefore very bare-bones, so its code shouldn't be too
+ hard to understand.
+ It currently only supports major and minor versions.
+
+ Inspired from https://stackoverflow.com/a/11887825/9084059
+ Should eventually dissapear.
+
+ Examples:
+ >>> # Standard usage
+ >>> Version(sklearn.__version__) > Version('0.22')
+ >>> Version(sklearn.__version__) > '0.22'
+ >>> # In general, pass the version as numbers separated by dots.
+ >>> Version('1.5') <= Version('1.6.5')
+ >>> Version('1.5') <= '1.6.5'
+ >>> # You can also pass the separator for specific cases
+ >>> Version('1-5', separator='-') == Version('1-6-5', separator='-')
+ >>> Version('1-5', separator='-') == '1-6-5'
+ >>> Version('1-5', separator='-') == '1.6.5' # Won't work !
+ """
+
+ def __init__(self, value: str, separator: str = '.'):
+ self.separator = separator
+ self.major, self.minor = self._parse_version(value)
+
+ def __repr__(self):
+ return f'Version({self.major}.{self.minor})'
+
+ def _parse_version(self, value: str) -> Tuple[int, int]:
+ raw_parts = value.split(self.separator)
+ if len(raw_parts) == 0:
+ raise ValueError(f'Could not extract version from {value!r} '
+ f'(separator: {self.separator!r})')
+ elif len(raw_parts) == 1:
+ major = int(raw_parts[0])
+ minor = 0
+ else:
+ major = int(raw_parts[0])
+ minor = int(raw_parts[1])
+ # Ditch the rest
+ return major, minor
+
+ def _cast_to_version(self, other: Union["Version", str]) -> "Version":
+ if isinstance(other, str):
+ # We pass our separator, as we expect they are the same
+ other = Version(other, self.separator)
+ return other
+
+ def __eq__(self, other: Union["Version", str]):
+ other = self._cast_to_version(other)
+ return (self.major, self.minor) == (other.major, other.minor)
+
+ def __ne__(self, other: Union["Version", str]):
+ other = self._cast_to_version(other)
+ return (self.major, self.minor) != (other.major, other.minor)
+
+ def __lt__(self, other: Union["Version", str]):
+ other = self._cast_to_version(other)
+ return (self.major, self.minor) < (other.major, other.minor)
+
+ def __le__(self, other: Union["Version", str]):
+ other = self._cast_to_version(other)
+ return (self.major, self.minor) <= (other.major, other.minor)
+
+ def __gt__(self, other: Union["Version", str]):
+ other = self._cast_to_version(other)
+ return (self.major, self.minor) > (other.major, other.minor)
+
+ def __ge__(self, other: Union["Version", str]):
+ other = self._cast_to_version(other)
+ return (self.major, self.minor) >= (other.major, other.minor)
| dirty-cat/dirty_cat | 4c5b7892f5be9bf070690bba302dbc9a60164349 | diff --git a/dirty_cat/datasets/tests/test_fetching.py b/dirty_cat/datasets/tests/test_fetching.py
index b0056c4..a85f425 100644
--- a/dirty_cat/datasets/tests/test_fetching.py
+++ b/dirty_cat/datasets/tests/test_fetching.py
@@ -15,7 +15,7 @@ import warnings
import pandas as pd
from pathlib import Path
-from distutils.version import LooseVersion
+from dirty_cat.utils import Version
from unittest import mock
from unittest.mock import mock_open
@@ -152,7 +152,7 @@ def test__download_and_write_openml_dataset(mock_fetch_openml):
test_data_dir = get_test_data_dir()
_download_and_write_openml_dataset(1, test_data_dir)
- if LooseVersion(sklearn.__version__) >= LooseVersion('0.22'):
+ if Version(sklearn.__version__) >= Version('0.22'):
mock_fetch_openml.assert_called_once_with(data_id=1,
data_home=str(test_data_dir),
as_frame=True)
diff --git a/dirty_cat/test/test_super_vectorizer.py b/dirty_cat/test/test_super_vectorizer.py
index d299f19..a836dcc 100644
--- a/dirty_cat/test/test_super_vectorizer.py
+++ b/dirty_cat/test/test_super_vectorizer.py
@@ -7,10 +7,10 @@ from sklearn.preprocessing import StandardScaler
from sklearn.utils.validation import check_is_fitted
from sklearn.exceptions import NotFittedError
-from distutils.version import LooseVersion
from dirty_cat import SuperVectorizer
from dirty_cat import GapEncoder
+from dirty_cat.utils import Version
def check_same_transformers(expected_transformers: dict, actual_transformers: list):
@@ -336,7 +336,7 @@ def test_get_feature_names_out():
vectorizer_w_pass = SuperVectorizer(remainder='passthrough')
vectorizer_w_pass.fit(X)
- if LooseVersion(sklearn.__version__) < LooseVersion('0.23'):
+ if Version(sklearn.__version__) < Version('0.23'):
with pytest.raises(NotImplementedError):
# Prior to sklearn 0.23, ColumnTransformer.get_feature_names
# with "passthrough" transformer(s) raises a NotImplementedError
@@ -370,7 +370,7 @@ def test_fit():
# See GH#193
sup_vec = SuperVectorizer()
with pytest.raises(NotFittedError):
- if LooseVersion(sklearn.__version__) >= LooseVersion('0.22'):
+ if Version(sklearn.__version__) >= Version('0.22'):
assert check_is_fitted(sup_vec)
else:
assert check_is_fitted(sup_vec, attributes=dir(sup_vec))
diff --git a/dirty_cat/test/test_utils.py b/dirty_cat/test/test_utils.py
index 70caf4a..a339c34 100644
--- a/dirty_cat/test/test_utils.py
+++ b/dirty_cat/test/test_utils.py
@@ -1,4 +1,6 @@
-from dirty_cat.utils import LRUDict
+import pytest
+
+from dirty_cat.utils import LRUDict, Version
def test_lrudict():
@@ -13,3 +15,28 @@ def test_lrudict():
for x in range(5):
assert (x not in dict_)
+
+
+def test_version():
+ # Test those specified in its docstring
+ assert Version('1.5') <= Version('1.6.5')
+ assert Version('1.5') <= '1.6.5'
+
+ assert (Version('1-5', separator='-') == Version('1-6-5', separator='-')) is False
+ assert (Version('1-5', separator='-') == '1-6-5') is False
+ with pytest.raises(ValueError):
+ assert not (Version('1-5', separator='-') == '1.6.5')
+
+ # Test all comparison methods
+ assert Version('1.0') == Version('1.0')
+ assert (Version('1.0') != Version('1.0')) is False
+
+ assert Version('1.0') < Version('1.1')
+ assert Version('1.1') <= Version('1.5')
+ assert Version('1.1') <= Version('1.1')
+ assert (Version('1.1') < Version('1.1')) is False
+
+ assert Version('1.1') > Version('0.5')
+ assert Version('1.1') >= Version('0.9')
+ assert Version('1.1') >= Version('1.1')
+ assert (Version('1.1') >= Version('1.9')) is False
| DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
<!--Provide a brief description of the bug.-->
Since december 2021, `distutils.version.Version` (and thus `LooseVersion`) [is deprecated](https://github.com/pypa/setuptools/commit/1701579e0827317d8888c2254a17b5786b6b5246).
We use it in [several components](https://github.com/dirty-cat/dirty_cat/search?q=LooseVersion), and therefore we have a number of warnings in the CI logs ([for example](https://github.com/dirty-cat/dirty_cat/runs/6667648188?check_suite_focus=true), under "Run tests")
Sample:
```
dirty_cat/test/test_similarity_encoder.py: 118 warnings
/Users/runner/work/dirty_cat/dirty_cat/dirty_cat/similarity_encoder.py:328: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
if LooseVersion(sklearn.__version__) > LooseVersion('0.21'):
```
as suggested by the warning, we could use `packaging.version.Version` instead. | 0.0 | [
"dirty_cat/datasets/tests/test_fetching.py::test_fetch_openml_dataset",
"dirty_cat/datasets/tests/test_fetching.py::test_fetch_openml_dataset_mocked",
"dirty_cat/datasets/tests/test_fetching.py::test__download_and_write_openml_dataset",
"dirty_cat/datasets/tests/test_fetching.py::test__read_json_from_gz",
"dirty_cat/datasets/tests/test_fetching.py::test__get_details",
"dirty_cat/datasets/tests/test_fetching.py::test__get_features",
"dirty_cat/datasets/tests/test_fetching.py::test__export_gz_data_to_csv",
"dirty_cat/datasets/tests/test_fetching.py::test__features_to_csv_format",
"dirty_cat/datasets/tests/test_fetching.py::test_import_all_datasets",
"dirty_cat/test/test_super_vectorizer.py::test_with_clean_data",
"dirty_cat/test/test_super_vectorizer.py::test_with_dirty_data",
"dirty_cat/test/test_super_vectorizer.py::test_with_arrays",
"dirty_cat/test/test_super_vectorizer.py::test_fit",
"dirty_cat/test/test_super_vectorizer.py::test_transform",
"dirty_cat/test/test_super_vectorizer.py::test_fit_transform_equiv",
"dirty_cat/test/test_utils.py::test_lrudict",
"dirty_cat/test/test_utils.py::test_version"
] | [] | 2022-06-07 13:06:50+00:00 | 1,918 |
|
dirty-cat__dirty_cat-300 | diff --git a/CHANGES.rst b/CHANGES.rst
index a1f7651..da2fc09 100644
--- a/CHANGES.rst
+++ b/CHANGES.rst
@@ -7,14 +7,21 @@ Major changes
* New encoder: :class:`DatetimeEncoder` can transform a datetime column into several numerical
columns (year, month, day, hour, minute, second, ...). It is now the default transformer used
in the :class:`SuperVectorizer` for datetime columns.
-* The :class:`SuperVectorizer` has seen some major improvements and bug fixes.
+* The :class:`SuperVectorizer` has seen some major improvements and bug fixes
+ - Fixes the automatic casting logic in ``transform``.
+ - **Behavior change** To avoid dimensionality explosion when a feature has two unique values,
+ the default encoder (:class:`OneHotEncoder`) now drops one of the two
+ vectors (see parameter `drop="if_binary"`).
+ - ``fit_transform`` and ``transform`` can now return unencoded features,
+ like the :class:`ColumnTransformer`'s behavior.
+ Previously, a ``RuntimeError`` was raised.
* **Backward-incompatible change in the SuperVectorizer**: to apply ``remainder``
to features (with the ``*_transformer`` parameters), the value ``'remainder'``
must be passed, instead of ``None`` in previous versions.
``None`` now indicates that we want to use the default transformer.
* Support for Python 3.6 and 3.7 has been dropped. Python >= 3.8 is now required.
* Bumped minimum dependencies:
- - sklearn>=0.22
+ - sklearn>=0.23
- scipy>=1.4.0
- numpy>=1.17.3
- pandas>=1.2.0
diff --git a/README.rst b/README.rst
index e0e232c..26a7a14 100644
--- a/README.rst
+++ b/README.rst
@@ -38,7 +38,7 @@ dirty_cat requires:
- Python (>= 3.8)
- NumPy (>= 1.17.3)
- SciPy (>= 1.4.0)
-- scikit-learn (>= 0.22.0)
+- scikit-learn (>= 0.23.0)
- pandas (>= 1.2.0)
User installation
diff --git a/dirty_cat/gap_encoder.py b/dirty_cat/gap_encoder.py
index a0471af..b06d622 100644
--- a/dirty_cat/gap_encoder.py
+++ b/dirty_cat/gap_encoder.py
@@ -35,21 +35,12 @@ from dirty_cat.utils import Version
from .utils import check_input
-if Version(sklearn_version) == Version("0.22"):
- with warnings.catch_warnings():
- warnings.simplefilter("ignore")
- from sklearn.cluster.k_means_ import _k_init
-elif Version(sklearn_version) < Version("0.24"):
+if Version(sklearn_version) < Version("0.24"):
from sklearn.cluster._kmeans import _k_init
else:
from sklearn.cluster import kmeans_plusplus
-if Version(sklearn_version) == Version("0.22"):
- with warnings.catch_warnings():
- warnings.simplefilter("ignore")
- from sklearn.decomposition.nmf import _beta_divergence
-else:
- from sklearn.decomposition._nmf import _beta_divergence
+from sklearn.decomposition._nmf import _beta_divergence
class GapEncoderColumn(BaseEstimator, TransformerMixin):
diff --git a/dirty_cat/super_vectorizer.py b/dirty_cat/super_vectorizer.py
index 6643dc7..6c4e8b4 100644
--- a/dirty_cat/super_vectorizer.py
+++ b/dirty_cat/super_vectorizer.py
@@ -96,16 +96,18 @@ class SuperVectorizer(ColumnTransformer):
cardinality_threshold : int, default=40
Two lists of features will be created depending on this value: strictly
- under this value, the low cardinality categorical values, and above or
- equal, the high cardinality categorical values.
+ under this value, the low cardinality categorical features, and above or
+ equal, the high cardinality categorical features.
Different transformers will be applied to these two groups,
defined by the parameters `low_card_cat_transformer` and
`high_card_cat_transformer` respectively.
+ Note: currently, missing values are counted as a single unique value
+ (so they count in the cardinality).
low_card_cat_transformer : typing.Optional[typing.Union[sklearn.base.TransformerMixin, typing.Literal["drop", "remainder", "passthrough"]]], default=None # noqa
Transformer used on categorical/string features with low cardinality
(threshold is defined by `cardinality_threshold`).
- Can either be a transformer object instance (e.g. `OneHotEncoder()`),
+ Can either be a transformer object instance (e.g. `OneHotEncoder(drop="if_binary")`),
a `Pipeline` containing the preprocessing steps,
'drop' for dropping the columns,
'remainder' for applying `remainder`,
@@ -226,6 +228,16 @@ class SuperVectorizer(ColumnTransformer):
imputed_columns_: typing.List[str]
The list of columns in which we imputed the missing values.
+ Notes
+ -----
+ The column order of the input data is not guaranteed to be the same
+ as the output data (returned by `transform`).
+ This is a due to the way the ColumnTransformer works.
+ However, the output column order will always be the same for different
+ calls to `transform` on a same fitted SuperVectorizer instance.
+ For example, if input data has columns ['name', 'job', 'year], then output
+ columns might be shuffled, e.g., ['job', 'year', 'name'], but every call
+ to `transform` will return this order.
"""
transformers_: List[Tuple[str, Union[str, TransformerMixin], List[str]]]
@@ -290,7 +302,7 @@ class SuperVectorizer(ColumnTransformer):
if isinstance(self.low_card_cat_transformer, sklearn.base.TransformerMixin):
self.low_card_cat_transformer_ = clone(self.low_card_cat_transformer)
elif self.low_card_cat_transformer is None:
- self.low_card_cat_transformer_ = OneHotEncoder()
+ self.low_card_cat_transformer_ = OneHotEncoder(drop="if_binary")
elif self.low_card_cat_transformer == "remainder":
self.low_card_cat_transformer_ = self.remainder
else:
@@ -406,6 +418,11 @@ class SuperVectorizer(ColumnTransformer):
def fit_transform(self, X, y=None):
"""
Fit all transformers, transform the data, and concatenate the results.
+ In practice, it (1) converts features to their best possible types
+ if `auto_cast=True`, (2) classify columns based on their data type,
+ (3) replaces "false missing" (see function `_replace_false_missing`),
+ and imputes categorical columns depending on `impute_missing`, and
+ finally, transforms X.
Parameters
----------
@@ -470,16 +487,21 @@ class SuperVectorizer(ColumnTransformer):
).columns.to_list()
# Classify categorical columns by cardinality
+ _nunique_values = { # Cache results
+ col: X[col].nunique() for col in categorical_columns
+ }
low_card_cat_columns = [
col
for col in categorical_columns
- if X[col].nunique() < self.cardinality_threshold
+ if _nunique_values[col] < self.cardinality_threshold
]
high_card_cat_columns = [
col
for col in categorical_columns
- if X[col].nunique() >= self.cardinality_threshold
+ if _nunique_values[col] >= self.cardinality_threshold
]
+ # Clear cache
+ del _nunique_values
# Next part: construct the transformers
# Create the list of all the transformers.
@@ -603,24 +625,10 @@ class SuperVectorizer(ColumnTransformer):
typing.List[str]
Feature names.
"""
- if Version(sklearn_version) < Version("0.23"):
- try:
- if Version(sklearn_version) < Version("1.0"):
- ct_feature_names = super().get_feature_names()
- else:
- ct_feature_names = super().get_feature_names_out()
- except NotImplementedError:
- raise NotImplementedError(
- "Prior to sklearn 0.23, get_feature_names with "
- '"passthrough" is unsupported. To use the method, '
- 'either make sure there is no "passthrough" in the '
- "transformers, or update your copy of scikit-learn. "
- )
+ if Version(sklearn_version) < Version("1.0"):
+ ct_feature_names = super().get_feature_names()
else:
- if Version(sklearn_version) < Version("1.0"):
- ct_feature_names = super().get_feature_names()
- else:
- ct_feature_names = super().get_feature_names_out()
+ ct_feature_names = super().get_feature_names_out()
all_trans_feature_names = []
for name, trans, cols, _ in self._iter(fitted=True):
diff --git a/dirty_cat/utils.py b/dirty_cat/utils.py
index c5b8442..d7f780f 100644
--- a/dirty_cat/utils.py
+++ b/dirty_cat/utils.py
@@ -77,8 +77,8 @@ class Version:
Examples:
>>> # Standard usage
- >>> Version(sklearn.__version__) > Version('0.22')
- >>> Version(sklearn.__version__) > '0.22'
+ >>> Version(sklearn.__version__) > Version('0.23')
+ >>> Version(sklearn.__version__) > '0.23'
>>> # In general, pass the version as numbers separated by dots.
>>> Version('1.5') <= Version('1.6.5')
>>> Version('1.5') <= '1.6.5'
diff --git a/requirements-min.txt b/requirements-min.txt
index eff1e8c..75702d6 100644
--- a/requirements-min.txt
+++ b/requirements-min.txt
@@ -1,6 +1,6 @@
numpy==1.17.3
scipy==1.4.0
-scikit-learn==0.22.0
+scikit-learn==0.23.0
pytest
pytest-cov
coverage
diff --git a/setup.py b/setup.py
index 73bf659..a015caa 100755
--- a/setup.py
+++ b/setup.py
@@ -39,7 +39,7 @@ if __name__ == "__main__":
packages=find_packages(),
package_data={"dirty_cat": ["VERSION.txt"]},
install_requires=[
- "scikit-learn>=0.21",
+ "scikit-learn>=0.23",
"numpy>=1.16",
"scipy>=1.2",
"pandas>=1.2.0",
| dirty-cat/dirty_cat | 9e59a1ae72abfb453768d6cd41daca9cbb4cc942 | diff --git a/dirty_cat/test/test_super_vectorizer.py b/dirty_cat/test/test_super_vectorizer.py
index 4b04c77..c97e826 100644
--- a/dirty_cat/test/test_super_vectorizer.py
+++ b/dirty_cat/test/test_super_vectorizer.py
@@ -1,4 +1,4 @@
-from typing import Any, Dict, List, Tuple
+from typing import Any, Tuple
import numpy as np
import pandas as pd
@@ -145,15 +145,7 @@ def _get_datetimes_dataframe() -> pd.DataFrame:
)
-def _test_possibilities(
- X,
- expected_transformers_df: Dict[str, List[str]],
- expected_transformers_2: Dict[str, List[str]],
- expected_transformers_np_no_cast: Dict[str, List[int]],
- expected_transformers_series: Dict[str, List[str]],
- expected_transformers_plain: Dict[str, List[str]],
- expected_transformers_np_cast: Dict[str, List[int]],
-):
+def _test_possibilities(X):
"""
Do a bunch of tests with the SuperVectorizer.
We take some expected transformers results as argument. They're usually
@@ -167,10 +159,18 @@ def _test_possibilities(
numerical_transformer=StandardScaler(),
)
# Warning: order-dependant
+ expected_transformers_df = {
+ "numeric": ["int", "float"],
+ "low_card_cat": ["str1", "cat1"],
+ "high_card_cat": ["str2", "cat2"],
+ }
vectorizer_base.fit_transform(X)
check_same_transformers(expected_transformers_df, vectorizer_base.transformers)
# Test with higher cardinality threshold and no numeric transformer
+ expected_transformers_2 = {
+ "low_card_cat": ["str1", "str2", "cat1", "cat2"],
+ }
vectorizer_default = SuperVectorizer() # Using default values
vectorizer_default.fit_transform(X)
check_same_transformers(expected_transformers_2, vectorizer_default.transformers)
@@ -178,12 +178,20 @@ def _test_possibilities(
# Test with a numpy array
arr = X.to_numpy()
# Instead of the columns names, we'll have the column indices.
+ expected_transformers_np_no_cast = {
+ "low_card_cat": [2, 4],
+ "high_card_cat": [3, 5],
+ "numeric": [0, 1],
+ }
vectorizer_base.fit_transform(arr)
check_same_transformers(
expected_transformers_np_no_cast, vectorizer_base.transformers
)
# Test with pandas series
+ expected_transformers_series = {
+ "low_card_cat": ["cat1"],
+ }
vectorizer_base.fit_transform(X["cat1"])
check_same_transformers(expected_transformers_series, vectorizer_base.transformers)
@@ -196,9 +204,19 @@ def _test_possibilities(
)
X_str = X.astype("object")
# With pandas
+ expected_transformers_plain = {
+ "high_card_cat": ["str2", "cat2"],
+ "low_card_cat": ["str1", "cat1"],
+ "numeric": ["int", "float"],
+ }
vectorizer_cast.fit_transform(X_str)
check_same_transformers(expected_transformers_plain, vectorizer_cast.transformers)
# With numpy
+ expected_transformers_np_cast = {
+ "numeric": [0, 1],
+ "low_card_cat": [2, 4],
+ "high_card_cat": [3, 5],
+ }
vectorizer_cast.fit_transform(X_str.to_numpy())
check_same_transformers(expected_transformers_np_cast, vectorizer_cast.transformers)
@@ -208,43 +226,7 @@ def test_with_clean_data():
Defines the expected returns of the vectorizer in different settings,
and runs the tests with a clean dataset.
"""
- X = _get_clean_dataframe()
- # Define the transformers we'll use throughout the test.
- expected_transformers_df = {
- "numeric": ["int", "float"],
- "low_card_cat": ["str1", "cat1"],
- "high_card_cat": ["str2", "cat2"],
- }
- expected_transformers_2 = {
- "low_card_cat": ["str1", "str2", "cat1", "cat2"],
- }
- expected_transformers_np_no_cast = {
- "low_card_cat": [2, 4],
- "high_card_cat": [3, 5],
- "numeric": [0, 1],
- }
- expected_transformers_series = {
- "low_card_cat": ["cat1"],
- }
- expected_transformers_plain = {
- "high_card_cat": ["str2", "cat2"],
- "low_card_cat": ["str1", "cat1"],
- "numeric": ["int", "float"],
- }
- expected_transformers_np_cast = {
- "numeric": [0, 1],
- "low_card_cat": [2, 4],
- "high_card_cat": [3, 5],
- }
- _test_possibilities(
- X,
- expected_transformers_df,
- expected_transformers_2,
- expected_transformers_np_no_cast,
- expected_transformers_series,
- expected_transformers_plain,
- expected_transformers_np_cast,
- )
+ _test_possibilities(_get_clean_dataframe())
def test_with_dirty_data() -> None:
@@ -252,44 +234,7 @@ def test_with_dirty_data() -> None:
Defines the expected returns of the vectorizer in different settings,
and runs the tests with a dataset containing missing values.
"""
- X = _get_dirty_dataframe()
- # Define the transformers we'll use throughout the test.
- expected_transformers_df = {
- "numeric": ["int", "float"],
- "low_card_cat": ["str1", "cat1"],
- "high_card_cat": ["str2", "cat2"],
- }
- expected_transformers_2 = {
- "low_card_cat": ["str1", "str2", "cat1", "cat2"],
- }
- expected_transformers_np_no_cast = {
- "low_card_cat": [2, 4],
- "high_card_cat": [3, 5],
- "numeric": [0, 1],
- }
- expected_transformers_series = {
- "low_card_cat": ["cat1"],
- }
- expected_transformers_plain = {
- "high_card_cat": ["str2", "cat2"],
- "low_card_cat": ["str1", "cat1"],
- "numeric": ["int", "float"],
- }
- expected_transformers_np_cast = {
- "numeric": [0, 1],
- "low_card_cat": [2, 4],
- "high_card_cat": [3, 5],
- }
-
- _test_possibilities(
- X,
- expected_transformers_df,
- expected_transformers_2,
- expected_transformers_np_no_cast,
- expected_transformers_series,
- expected_transformers_plain,
- expected_transformers_np_cast,
- )
+ _test_possibilities(_get_dirty_dataframe())
def test_auto_cast() -> None:
@@ -376,49 +321,39 @@ def test_get_feature_names_out() -> None:
vec_w_pass = SuperVectorizer(remainder="passthrough")
vec_w_pass.fit(X)
- if Version(sklearn.__version__) < Version("0.23"):
- with pytest.raises(NotImplementedError):
- # Prior to sklearn 0.23, ColumnTransformer.get_feature_names
- # with "passthrough" transformer(s) raises a NotImplementedError
- assert vec_w_pass.get_feature_names_out()
+ # In this test, order matters. If it doesn't, convert to set.
+ expected_feature_names_pass = [
+ "str1_public",
+ "str2_chef",
+ "str2_lawyer",
+ "str2_manager",
+ "str2_officer",
+ "str2_teacher",
+ "cat1_yes",
+ "cat2_20K+",
+ "cat2_30K+",
+ "cat2_40K+",
+ "cat2_50K+",
+ "cat2_60K+",
+ "int",
+ "float",
+ ]
+ if Version(sklearn.__version__) < Version("1.0"):
+ assert vec_w_pass.get_feature_names() == expected_feature_names_pass
else:
- # In this test, order matters. If it doesn't, convert to set.
- expected_feature_names_pass = [
- "str1_private",
- "str1_public",
- "str2_chef",
- "str2_lawyer",
- "str2_manager",
- "str2_officer",
- "str2_teacher",
- "cat1_no",
- "cat1_yes",
- "cat2_20K+",
- "cat2_30K+",
- "cat2_40K+",
- "cat2_50K+",
- "cat2_60K+",
- "int",
- "float",
- ]
- if Version(sklearn.__version__) < Version("1.0"):
- assert vec_w_pass.get_feature_names() == expected_feature_names_pass
- else:
- assert vec_w_pass.get_feature_names_out() == expected_feature_names_pass
+ assert vec_w_pass.get_feature_names_out() == expected_feature_names_pass
vec_w_drop = SuperVectorizer(remainder="drop")
vec_w_drop.fit(X)
# In this test, order matters. If it doesn't, convert to set.
expected_feature_names_drop = [
- "str1_private",
"str1_public",
"str2_chef",
"str2_lawyer",
"str2_manager",
"str2_officer",
"str2_teacher",
- "cat1_no",
"cat1_yes",
"cat2_20K+",
"cat2_30K+",
@@ -448,7 +383,11 @@ def test_transform() -> None:
s = [34, 5.5, "private", "manager", "yes", "60K+"]
x = np.array(s).reshape(1, -1)
x_trans = sup_vec.transform(x)
- assert (x_trans == [[1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 34, 5.5]]).all()
+ assert x_trans.tolist() == [
+ [0.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 0.0, 0.0, 1.0, 34.0, 5.5]
+ ]
+ # To understand the list above:
+ # print(dict(zip(sup_vec.get_feature_names_out(), x_trans.tolist()[0])))
def test_fit_transform_equiv() -> None:
@@ -492,13 +431,20 @@ def test_passthrough():
auto_cast=False,
)
- X_enc_dirty = sv.fit_transform(X_dirty)
- X_enc_clean = sv.fit_transform(X_clean)
+ X_enc_dirty = pd.DataFrame(
+ sv.fit_transform(X_dirty), columns=sv.get_feature_names_out()
+ )
+ X_enc_clean = pd.DataFrame(
+ sv.fit_transform(X_clean), columns=sv.get_feature_names_out()
+ )
+ # Reorder encoded arrays' columns (see SV's doc "Notes" section as to why)
+ X_enc_dirty = X_enc_dirty[X_dirty.columns]
+ X_enc_clean = X_enc_clean[X_clean.columns]
dirty_flat_df = X_dirty.to_numpy().ravel().tolist()
- dirty_flat_trans_df = X_enc_dirty.ravel().tolist()
+ dirty_flat_trans_df = X_enc_dirty.to_numpy().ravel().tolist()
assert all(map(_is_equal, zip(dirty_flat_df, dirty_flat_trans_df)))
- assert (X_clean.to_numpy() == X_enc_clean).all()
+ assert (X_clean.to_numpy() == X_enc_clean.to_numpy()).all()
if __name__ == "__main__":
| One hot encoding of Supervectorizer for columns with 2 values
Just something I observed using SuperVectorizer, on a dataset with columns having mostly 2 categorical values. As documented, it runs one hot encoding on them, and this blows up the feature space. This is probably an edge case, and the example I have here is maybe an extreme one. For just two values it seemed excessive to do one-hot encoding.
```
from dirty_cat import SuperVectorizer
from sklearn.datasets import fetch_openml
X, y = fetch_openml("kr-vs-kp", return_X_y=True)
X.shape
(3196, 36)
X.describe()
bkblk bknwy bkon8 bkona bkspr bkxbq bkxcr bkxwp blxwp bxqsq cntxt dsopp dwipd ... simpl skach skewr skrxp spcop stlmt thrsk wkcti wkna8 wknck wkovl wkpos wtoeg
count 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 ... 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196 3196
unique 2 2 2 2 2 2 2 2 2 2 2 2 2 ... 2 2 2 2 2 2 2 2 2 2 2 2 2
top f f f f f f f f f f f f l ... f f t f f f f f f f t t n
freq 2839 2971 3076 2874 2129 1722 2026 2500 1980 2225 1817 2860 2205 ... 1975 3185 2216 3021 3195 3149 3060 2631 3021 1984 2007 2345 2407
[4 rows x 36 columns]
sv = SuperVectorizer()
X_tx = sv.fit_transform(X)
X_tx.shape
(3196, 73)
``` | 0.0 | [
"dirty_cat/test/test_super_vectorizer.py::test_transform"
] | [
"dirty_cat/test/test_super_vectorizer.py::test_with_clean_data",
"dirty_cat/test/test_super_vectorizer.py::test_fit",
"dirty_cat/test/test_super_vectorizer.py::test_with_dirty_data"
] | 2022-08-08 12:14:01+00:00 | 1,919 |
|
dirty-cat__dirty_cat-378 | diff --git a/CHANGES.rst b/CHANGES.rst
index f774809..49769fe 100644
--- a/CHANGES.rst
+++ b/CHANGES.rst
@@ -3,12 +3,21 @@
Release 0.4.0
=============
-Minor changes
+Major changes
-------------
* Unnecessary API has been made private: everything (files, functions, classes)
starting with an underscore shouldn't be imported in your code.
+Minor changes
+-------------
+
+Bug fixes
+---------
+
+* :class:`MinHashEncoder` now considers `None` as missing values, rather
+ than raising an error.
+
Release 0.3.0
=============
@@ -55,7 +64,7 @@ Notes
Release 0.2.2
=============
-Bug-fixes
+Bug fixes
---------
* Fixed a bug in the :class:`SuperVectorizer` causing a `FutureWarning`
diff --git a/dirty_cat/_minhash_encoder.py b/dirty_cat/_minhash_encoder.py
index 96a9a6e..8c6c86c 100644
--- a/dirty_cat/_minhash_encoder.py
+++ b/dirty_cat/_minhash_encoder.py
@@ -26,6 +26,7 @@ from ._fast_hash import ngram_min_hash
from ._string_distances import get_unique_ngrams
from ._utils import LRUDict, check_input
+NoneType = type(None)
class MinHashEncoder(BaseEstimator, TransformerMixin):
"""
@@ -208,7 +209,8 @@ class MinHashEncoder(BaseEstimator, TransformerMixin):
for k in range(X.shape[1]):
X_in = X[:, k].reshape(-1)
for i, x in enumerate(X_in):
- if isinstance(x, float): # true if x is a missing value
+ if (isinstance(x, float) # true if x is a missing value
+ or x is None):
is_nan_idx = True
elif x not in self.hash_dict_:
X_out[i, k * self.n_components : counter] = self.hash_dict_[
| dirty-cat/dirty_cat | 9eb591250ab9dfd756ab08ef764278f256bf9d3c | diff --git a/dirty_cat/tests/test_minhash_encoder.py b/dirty_cat/tests/test_minhash_encoder.py
index b55f56d..c640fb0 100644
--- a/dirty_cat/tests/test_minhash_encoder.py
+++ b/dirty_cat/tests/test_minhash_encoder.py
@@ -133,6 +133,15 @@ def test_missing_values(input_type: str, missing: str, hashing: str) -> None:
return
+def test_missing_values_none():
+ # Test that "None" is also understood as a missing value
+ a = np.array([['a', 'b', None, 'c']], dtype=object).T
+
+ enc = MinHashEncoder()
+ d = enc.fit_transform(a)
+ np.testing.assert_array_equal(d[2], 0)
+
+
def test_cache_overflow() -> None:
# Regression test for cache overflow resulting in -1s in encoding
def get_random_string(length):
| MinHashEncoder does not understand "None" as missing value
The following raises an error because the None is not captured as a NaN:
```python
import numpy as np
a = np.array([['a', 'b', None, 'c']], dtype=object).T
from dirty_cat import MinHashEncoder
enc = MinHashEncoder()
enc.fit_transform(a)
```
| 0.0 | [
"dirty_cat/tests/test_minhash_encoder.py::test_missing_values_none"
] | [
"dirty_cat/tests/test_minhash_encoder.py::test_multiple_columns",
"dirty_cat/tests/test_minhash_encoder.py::test_input_type",
"dirty_cat/tests/test_minhash_encoder.py::test_missing_values[numpy-error-fast]",
"dirty_cat/tests/test_minhash_encoder.py::test_missing_values[numpy-zero_impute-fast]",
"dirty_cat/tests/test_minhash_encoder.py::test_cache_overflow",
"dirty_cat/tests/test_minhash_encoder.py::test_min_hash_encoder_hashing_fast_minmax_hash[0]",
"dirty_cat/tests/test_minhash_encoder.py::test_min_hash_encoder_hashing_fast_minmax_hash[1]",
"dirty_cat/tests/test_minhash_encoder.py::test_min_hash_encoder_hashing_fast_minmax_hash[2]",
"dirty_cat/tests/test_minhash_encoder.py::test_min_hash_encoder_hashing_fast[0]",
"dirty_cat/tests/test_minhash_encoder.py::test_min_hash_encoder_hashing_fast[1]",
"dirty_cat/tests/test_minhash_encoder.py::test_min_hash_encoder_hashing_fast[2]"
] | 2022-10-06 09:24:23+00:00 | 1,920 |
|
dirty-cat__dirty_cat-401 | diff --git a/dirty_cat/_super_vectorizer.py b/dirty_cat/_super_vectorizer.py
index db8f862..d5bac42 100644
--- a/dirty_cat/_super_vectorizer.py
+++ b/dirty_cat/_super_vectorizer.py
@@ -4,7 +4,6 @@ transformers/encoders to different types of data, without the need to
manually categorize them beforehand, or construct complex Pipelines.
"""
-
from typing import Dict, List, Literal, Optional, Tuple, Union
from warnings import warn
@@ -20,6 +19,9 @@ from sklearn.preprocessing import OneHotEncoder
from dirty_cat import DatetimeEncoder, GapEncoder
from dirty_cat._utils import Version
+# Required for ignoring lines too long in the docstrings
+# flake8: noqa: E501
+
def _has_missing_values(df: Union[pd.DataFrame, pd.Series]) -> bool:
"""
@@ -82,19 +84,21 @@ OptionalTransformer = Optional[
class SuperVectorizer(ColumnTransformer):
- """
- Easily transforms a heterogeneous data table (such as a dataframe) to
- a numerical array for machine learning. For this it transforms each
- column depending on its data type.
- It provides a simplified interface for the :class:`sklearn.compose.ColumnTransformer` ;
- more documentation of attributes and functions are available in its doc.
+ """Easily transform a heterogeneous array to a numerical one.
+
+ Easily transforms a heterogeneous data table
+ (such as a :class:`pandas.DataFrame`) to a numerical array for machine
+ learning. For this it transforms each column depending on its data type.
+ It provides a simplified interface for the
+ :class:`sklearn.compose.ColumnTransformer`; more documentation of
+ attributes and functions are available in its doc.
.. versionadded:: 0.2.0
Parameters
----------
- cardinality_threshold : int, default=40
+ cardinality_threshold : int, optional, default=40
Two lists of features will be created depending on this value: strictly
under this value, the low cardinality categorical features, and above or
equal, the high cardinality categorical features.
@@ -104,19 +108,19 @@ class SuperVectorizer(ColumnTransformer):
Note: currently, missing values are counted as a single unique value
(so they count in the cardinality).
- low_card_cat_transformer : typing.Optional[typing.Union[sklearn.base.TransformerMixin, typing.Literal["drop", "remainder", "passthrough"]]], default=None # noqa
+ low_card_cat_transformer : {"drop", "remainder", "passthrough"} or Transformer, optional
Transformer used on categorical/string features with low cardinality
(threshold is defined by `cardinality_threshold`).
- Can either be a transformer object instance (e.g. `OneHotEncoder(drop="if_binary")`),
+ Can either be a transformer object instance (e.g. `OneHotEncoder()`),
a `Pipeline` containing the preprocessing steps,
'drop' for dropping the columns,
'remainder' for applying `remainder`,
'passthrough' to return the unencoded columns,
- or None to use the default transformer (`OneHotEncoder()`).
+ or None to use the default transformer (`OneHotEncoder(drop="if_binary")`).
Features classified under this category are imputed based on the
strategy defined with `impute_missing`.
- high_card_cat_transformer : typing.Optional[typing.Union[sklearn.base.TransformerMixin, typing.Literal["drop", "remainder", "passthrough"]]], default=None # noqa
+ high_card_cat_transformer : {"drop", "remainder", "passthrough"} or Transformer, optional
Transformer used on categorical/string features with high cardinality
(threshold is defined by `cardinality_threshold`).
Can either be a transformer object instance (e.g. `GapEncoder()`),
@@ -128,7 +132,7 @@ class SuperVectorizer(ColumnTransformer):
Features classified under this category are imputed based on the
strategy defined with `impute_missing`.
- numerical_transformer : typing.Optional[typing.Union[sklearn.base.TransformerMixin, typing.Literal["drop", "remainder", "passthrough"]]], default=None # noqa
+ numerical_transformer : {"drop", "remainder", "passthrough"} or Transformer, optional
Transformer used on numerical features.
Can either be a transformer object instance (e.g. `StandardScaler()`),
a `Pipeline` containing the preprocessing steps,
@@ -139,7 +143,7 @@ class SuperVectorizer(ColumnTransformer):
Features classified under this category are not imputed at all
(regardless of `impute_missing`).
- datetime_transformer : typing.Optional[typing.Union[sklearn.base.TransformerMixin, typing.Literal["drop", "remainder", "passthrough"]]], default=None
+ datetime_transformer : {"drop", "remainder", "passthrough"} or Transformer, optional
Transformer used on datetime features.
Can either be a transformer object instance (e.g. `DatetimeEncoder()`),
a `Pipeline` containing the preprocessing steps,
@@ -150,11 +154,11 @@ class SuperVectorizer(ColumnTransformer):
Features classified under this category are not imputed at all
(regardless of `impute_missing`).
- auto_cast : bool, default=True
+ auto_cast : bool, optional, default=True
If set to `True`, will try to convert each column to the best possible
data type (dtype).
- impute_missing : str, default='auto'
+ impute_missing : {"auto", "force", "skip"}, optional, default='auto'
When to impute missing values in categorical (textual) columns.
'auto' will impute missing values if it is considered appropriate
(we are using an encoder that does not support missing values and/or
@@ -166,7 +170,7 @@ class SuperVectorizer(ColumnTransformer):
it is left to the user to manage.
See also attribute `imputed_columns_`.
- remainder : typing.Union[typing.Literal["drop", "passthrough"], sklearn.base.TransformerMixin], default='drop' # noqa
+ remainder : {"drop", "passthrough"} or Transformer, optional, default='drop'
By default, only the specified columns in `transformers` are
transformed and combined in the output, and the non-specified
columns are dropped. (default ``'drop'``).
@@ -180,31 +184,32 @@ class SuperVectorizer(ColumnTransformer):
Note that using this feature requires that the DataFrame columns
input at :term:`fit` and :term:`transform` have identical order.
- sparse_threshold: float, default=0.3
+ sparse_threshold : float, optional, default=0.3
If the output of the different transformers contains sparse matrices,
these will be stacked as a sparse matrix if the overall density is
lower than this value. Use sparse_threshold=0 to always return dense.
When the transformed output consists of all dense data, the stacked
result will be dense, and this keyword will be ignored.
- n_jobs : int, default=None
+ n_jobs : int, optional
Number of jobs to run in parallel.
- ``None`` means 1 unless in a :obj:`joblib.parallel_backend` context.
+ ``None`` (the default) means 1 unless in a
+ :obj:`joblib.parallel_backend` context.
``-1`` means using all processors.
- transformer_weights : dict, default=None
+ transformer_weights : dict, optional
Multiplicative weights for features per transformer. The output of the
transformer is multiplied by these weights. Keys are transformer names,
values the weights.
- verbose : bool, default=False
+ verbose : bool, optional, default=False
If True, the time elapsed while fitting each transformer will be
- printed as it is completed
+ printed as it is completed.
Attributes
----------
- transformers_: typing.List[typing.Tuple[str, typing.Union[str, sklearn.base.TransformerMixin], typing.List[str]]] # noqa
+ transformers_: list of 3-tuples (str, Transformer or str, list of str)
The collection of fitted transformers as tuples of
(name, fitted_transformer, column). `fitted_transformer` can be an
estimator, 'drop', or 'passthrough'. In case there were no columns
@@ -220,24 +225,27 @@ class SuperVectorizer(ColumnTransformer):
The fitted array's columns. They are applied to the data passed
to the `transform` method.
- types_: typing.Dict[str, type]
+ types_: dict mapping str to type
A mapping of inferred types per column.
Key is the column name, value is the inferred dtype.
Exists only if `auto_cast=True`.
- imputed_columns_: typing.List[str]
+ imputed_columns_: list of str
The list of columns in which we imputed the missing values.
Notes
-----
The column order of the input data is not guaranteed to be the same
- as the output data (returned by `transform`).
- This is a due to the way the ColumnTransformer works.
+ as the output data (returned by :func:`SuperVectorizer.transform`).
+ This is a due to the way the :class:`sklearn.compose.ColumnTransformer`
+ works.
However, the output column order will always be the same for different
- calls to `transform` on a same fitted SuperVectorizer instance.
- For example, if input data has columns ['name', 'job', 'year], then output
+ calls to :func:`SuperVectorizer.transform` on a same fitted
+ :class:`SuperVectorizer` instance.
+ For example, if input data has columns ['name', 'job', 'year'], then output
columns might be shuffled, e.g., ['job', 'year', 'name'], but every call
- to `transform` will return this order.
+ to :func:`SuperVectorizer.transform` on this instance will return this
+ order.
"""
transformers_: List[Tuple[str, Union[str, TransformerMixin], List[str]]]
@@ -257,7 +265,7 @@ class SuperVectorizer(ColumnTransformer):
numerical_transformer: OptionalTransformer = None,
datetime_transformer: OptionalTransformer = None,
auto_cast: bool = True,
- impute_missing: str = "auto",
+ impute_missing: Literal["auto", "force", "skip"] = "auto",
# The next parameters are inherited from ColumnTransformer
remainder: Union[
Literal["drop", "passthrough"], TransformerMixin
| dirty-cat/dirty_cat | 9bc0f1fa93a151fc599f6198960ba6d1fabdf5a3 | diff --git a/dirty_cat/tests/test_docstrings.py b/dirty_cat/tests/test_docstrings.py
index 7177f69..c8772bb 100644
--- a/dirty_cat/tests/test_docstrings.py
+++ b/dirty_cat/tests/test_docstrings.py
@@ -35,7 +35,6 @@ DOCSTRING_TEMP_IGNORE_SET = {
"dirty_cat._similarity_encoder.SimilarityEncoder.fit",
"dirty_cat._similarity_encoder.SimilarityEncoder.transform",
"dirty_cat._similarity_encoder.SimilarityEncoder.fit_transform",
- "dirty_cat._super_vectorizer.SuperVectorizer",
"dirty_cat._super_vectorizer.SuperVectorizer.fit_transform",
"dirty_cat._super_vectorizer.SuperVectorizer.transform",
"dirty_cat._super_vectorizer.SuperVectorizer._auto_cast",
@@ -148,17 +147,18 @@ def filter_errors(errors, method, Estimator=None):
# - GL02: If there's a blank line, it should be before the
# first line of the Returns section, not after (it allows to have
# short docstrings for properties).
+ # - SA01: See Also section not found
+ # - EX01: No examples section found; FIXME: remove when #373 resolved
- if code in ["RT02", "GL01", "GL02"]:
+ if code in ["RT02", "GL01", "GL02", "SA01", "EX01"]:
continue
# Following codes are only taken into account for the
# top level class docstrings:
# - ES01: No extended summary found
- # - SA01: See Also section not found
# - EX01: No examples section found
- if method is not None and code in ["EX01", "SA01", "ES01"]:
+ if method is not None and code in ["EX01", "ES01"]:
continue
yield code, message
diff --git a/dirty_cat/tests/test_gap_encoder.py b/dirty_cat/tests/test_gap_encoder.py
index 3c4ecc0..f891508 100644
--- a/dirty_cat/tests/test_gap_encoder.py
+++ b/dirty_cat/tests/test_gap_encoder.py
@@ -2,10 +2,10 @@ import numpy as np
import pandas as pd
import pytest
from sklearn import __version__ as sklearn_version
-from sklearn.datasets import fetch_20newsgroups
from dirty_cat import GapEncoder
from dirty_cat._utils import Version
+from dirty_cat.tests.utils import generate_data
def test_analyzer():
@@ -15,8 +15,7 @@ def test_analyzer():
"""
add_words = False
n_samples = 70
- X_txt = fetch_20newsgroups(subset="train")["data"][:n_samples]
- X = np.array([X_txt, X_txt]).T
+ X = generate_data(n_samples)
n_components = 10
# Test first analyzer output:
encoder = GapEncoder(
@@ -56,8 +55,7 @@ def test_analyzer():
def test_gap_encoder(
hashing: bool, init: str, analyzer: str, add_words: bool, n_samples: int = 70
) -> None:
- X_txt = fetch_20newsgroups(subset="train")["data"][:n_samples]
- X = np.array([X_txt, X_txt]).T
+ X = generate_data(n_samples)
n_components = 10
# Test output shape
encoder = GapEncoder(
@@ -117,8 +115,7 @@ def test_input_type() -> None:
def test_partial_fit(n_samples=70) -> None:
- X_txt = fetch_20newsgroups(subset="train")["data"][:n_samples]
- X = np.array([X_txt, X_txt]).T
+ X = generate_data(n_samples)
# Gap encoder with fit on one batch
enc = GapEncoder(random_state=42, batch_size=n_samples, max_iter=1)
X_enc = enc.fit_transform(X)
@@ -131,8 +128,7 @@ def test_partial_fit(n_samples=70) -> None:
def test_get_feature_names_out(n_samples=70) -> None:
- X_txt = fetch_20newsgroups(subset="train")["data"][:n_samples]
- X = np.array([X_txt, X_txt]).T
+ X = generate_data(n_samples)
enc = GapEncoder(random_state=42)
enc.fit(X)
# Expect a warning if sklearn >= 1.0
@@ -164,8 +160,7 @@ def test_overflow_error() -> None:
def test_score(n_samples: int = 70) -> None:
- X_txt = fetch_20newsgroups(subset="train")["data"][:n_samples]
- X1 = np.array(X_txt)[:, None]
+ X1 = generate_data(n_samples)
X2 = np.hstack([X1, X1])
enc = GapEncoder(random_state=42)
enc.fit(X1)
diff --git a/dirty_cat/tests/utils.py b/dirty_cat/tests/utils.py
index b710d5b..15da3e1 100644
--- a/dirty_cat/tests/utils.py
+++ b/dirty_cat/tests/utils.py
@@ -5,7 +5,6 @@ import numpy as np
def generate_data(n_samples, as_list=False):
MAX_LIMIT = 255 # extended ASCII Character set
- i = 0
str_list = []
for i in range(n_samples):
random_string = "category "
@@ -14,7 +13,6 @@ def generate_data(n_samples, as_list=False):
random_string += chr(random_integer)
if random_integer < 50:
random_string += " "
- i += 1
str_list += [random_string]
if as_list is True:
X = str_list
| Make our tests more robust to flawky downloads
Some tests use data downloaded from internet:
https://github.com/dirty-cat/dirty_cat/blob/master/dirty_cat/tests/test_minhash_encoder.py
As a result, the tests are fragile (see https://github.com/dirty-cat/dirty_cat/actions/runs/3175021096/jobs/5176906979#step:6:907 for instance).
It seems to me that some of these tests could be rewritten on generated data.
### Tests with flawky downloads
- [X] `test_fast_hash.py` #402
- [X] `test_gap_encoder.py` #401
- [X] `test_minhash_encoder.py` #379 | 0.0 | [
"dirty_cat/tests/test_docstrings.py::test_docstring[SuperVectorizer-None]"
] | [
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-fit]",
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-fit_transform]",
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-get_metadata_routing]",
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-get_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-set_output]",
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-set_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[DatetimeEncoder-transform]",
"dirty_cat/tests/test_docstrings.py::test_docstring[GapEncoder-fit_transform]",
"dirty_cat/tests/test_docstrings.py::test_docstring[GapEncoder-get_metadata_routing]",
"dirty_cat/tests/test_docstrings.py::test_docstring[GapEncoder-get_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[GapEncoder-set_output]",
"dirty_cat/tests/test_docstrings.py::test_docstring[GapEncoder-set_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[MinHashEncoder-fit_transform]",
"dirty_cat/tests/test_docstrings.py::test_docstring[MinHashEncoder-get_metadata_routing]",
"dirty_cat/tests/test_docstrings.py::test_docstring[MinHashEncoder-get_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[MinHashEncoder-set_output]",
"dirty_cat/tests/test_docstrings.py::test_docstring[MinHashEncoder-set_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-get_feature_names_out]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-get_metadata_routing]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-get_most_frequent]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-get_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-infrequent_categories_]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-inverse_transform]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-set_output]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-set_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SimilarityEncoder-set_transform_request]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SuperVectorizer-get_metadata_routing]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SuperVectorizer-get_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[SuperVectorizer-set_output]",
"dirty_cat/tests/test_docstrings.py::test_docstring[TargetEncoder-fit_transform]",
"dirty_cat/tests/test_docstrings.py::test_docstring[TargetEncoder-get_metadata_routing]",
"dirty_cat/tests/test_docstrings.py::test_docstring[TargetEncoder-get_params]",
"dirty_cat/tests/test_docstrings.py::test_docstring[TargetEncoder-set_output]",
"dirty_cat/tests/test_docstrings.py::test_docstring[TargetEncoder-set_params]",
"dirty_cat/tests/test_gap_encoder.py::test_analyzer",
"dirty_cat/tests/test_gap_encoder.py::test_gap_encoder[False-k-means++-word-True]",
"dirty_cat/tests/test_gap_encoder.py::test_gap_encoder[True-random-char-False]",
"dirty_cat/tests/test_gap_encoder.py::test_gap_encoder[True-k-means-char_wb-True]",
"dirty_cat/tests/test_gap_encoder.py::test_input_type",
"dirty_cat/tests/test_gap_encoder.py::test_partial_fit",
"dirty_cat/tests/test_gap_encoder.py::test_get_feature_names_out",
"dirty_cat/tests/test_gap_encoder.py::test_overflow_error",
"dirty_cat/tests/test_gap_encoder.py::test_score",
"dirty_cat/tests/test_gap_encoder.py::test_missing_values[zero_impute]",
"dirty_cat/tests/test_gap_encoder.py::test_missing_values[error]",
"dirty_cat/tests/test_gap_encoder.py::test_missing_values[aaa]"
] | 2022-10-28 09:16:09+00:00 | 1,921 |
|
disqus__python-phabricator-64 | diff --git a/CHANGES b/CHANGES
index 2fd4c34..64c95a0 100644
--- a/CHANGES
+++ b/CHANGES
@@ -1,3 +1,7 @@
+0.8.1
+
+* Fixed bug where built-in interface dict overwrote `interface` methods from Almanac (`interface.search`, `interface.edit`). The `Resource` attribute `interface` is renamed `_interface`.
+
0.8.0
* Switch to using requests
diff --git a/phabricator/__init__.py b/phabricator/__init__.py
index b01d167..6d53748 100644
--- a/phabricator/__init__.py
+++ b/phabricator/__init__.py
@@ -224,7 +224,7 @@ class Result(MutableMapping):
class Resource(object):
def __init__(self, api, interface=None, endpoint=None, method=None, nested=False):
self.api = api
- self.interface = interface or copy.deepcopy(parse_interfaces(INTERFACES))
+ self._interface = interface or copy.deepcopy(parse_interfaces(INTERFACES))
self.endpoint = endpoint
self.method = method
self.nested = nested
@@ -232,7 +232,7 @@ class Resource(object):
def __getattr__(self, attr):
if attr in getattr(self, '__dict__'):
return getattr(self, attr)
- interface = self.interface
+ interface = self._interface
if self.nested:
attr = "%s.%s" % (self.endpoint, attr)
submethod_exists = False
@@ -254,7 +254,7 @@ class Resource(object):
def _request(self, **kwargs):
# Check for missing variables
- resource = self.interface
+ resource = self._interface
def validate_kwarg(key, target):
# Always allow list
@@ -391,4 +391,4 @@ class Phabricator(Resource):
interfaces = query()
- self.interface = parse_interfaces(interfaces)
+ self._interface = parse_interfaces(interfaces)
diff --git a/setup.py b/setup.py
index c7586bc..ec3f0e5 100644
--- a/setup.py
+++ b/setup.py
@@ -14,7 +14,7 @@ if sys.version_info[:2] <= (3, 3):
setup(
name='phabricator',
- version='0.8.0',
+ version='0.8.1',
author='Disqus',
author_email='opensource@disqus.com',
url='http://github.com/disqus/python-phabricator',
| disqus/python-phabricator | a922ba404a4578f9f909e241539b1fb5a99585b2 | diff --git a/phabricator/tests/test_phabricator.py b/phabricator/tests/test_phabricator.py
index cad5a8e..e12b285 100644
--- a/phabricator/tests/test_phabricator.py
+++ b/phabricator/tests/test_phabricator.py
@@ -163,7 +163,7 @@ class PhabricatorTest(unittest.TestCase):
def test_endpoint_shadowing(self):
- shadowed_endpoints = [e for e in self.api.interface.keys() if e in self.api.__dict__]
+ shadowed_endpoints = [e for e in self.api._interface.keys() if e in self.api.__dict__]
self.assertEqual(
shadowed_endpoints,
[],
| Conflict between Resource class attribute and new almanac interface endpoints
With [rPe502df509d5f](https://secure.phabricator.com/rPe502df509d5f8dd3106be50d7d83ac244ff96cb4), upstream phab implements almanac conduit endpoints: `almanc.interface.search` and `almanc.interface.edit`.
This now conflicts with the existing Resource class attribute `self.interface`. If you reproduce this against a Phab instance with the new `almanac.interface.*` conduit endpoints:
```
from phabricator import Phabricator
phab = Phabricator()
phab.almanac.interface.search
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'dict' object has no attribute 'search'
```
it throws an error because it is using the Resource class attribute `self.interface` instead of treating it like a normal method. This does not occur when the method name does not conflict with the Resource attributes:
```
phab.almanac.device.search
<phabricator.Resource object at 0x7f760699cf60>
```
The Resource class should not rely on certain keywords *not* becoming conduit methods. | 0.0 | [
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_endpoint_shadowing"
] | [
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_bad_status",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_classic_resources",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_connect",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_generate_hash",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_maniphest_find",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_map_param_type",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_nested_resources",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_user_whoami",
"phabricator/tests/test_phabricator.py::PhabricatorTest::test_validation"
] | 2021-02-09 01:47:25+00:00 | 1,922 |
|
dixudx__rtcclient-184 | diff --git a/examples/how_to/workitem/get_workitem.py b/examples/how_to/workitem/get_workitem.py
index ef095f8..7064f40 100644
--- a/examples/how_to/workitem/get_workitem.py
+++ b/examples/how_to/workitem/get_workitem.py
@@ -9,7 +9,7 @@ if __name__ == "__main__":
url = "https://your_domain:9443/jazz"
username = "your_username"
password = "your_password"
- myclient = RTCClient(url, username, password)
+ myclient = RTCClient(url, username, password) # ends_with_jazz , old_rtc_authentication kwargs
# get all workitems
# If both projectarea_id and projectarea_name are None, all the workitems
diff --git a/poetry.lock b/poetry.lock
index 739b595..7f9ad3d 100644
--- a/poetry.lock
+++ b/poetry.lock
@@ -747,6 +747,18 @@ files = [
{file = "six-1.16.0.tar.gz", hash = "sha256:1e61c37477a1626458e36f7b1d82aa5c9b094fa4802892072e49de9c60c4c926"},
]
+[[package]]
+name = "toml"
+version = "0.10.2"
+description = "Python Library for Tom's Obvious, Minimal Language"
+category = "dev"
+optional = false
+python-versions = ">=2.6, !=3.0.*, !=3.1.*, !=3.2.*"
+files = [
+ {file = "toml-0.10.2-py2.py3-none-any.whl", hash = "sha256:806143ae5bfb6a3c6e736a764057db0e6a0e05e338b5630894a5f779cabb4f9b"},
+ {file = "toml-0.10.2.tar.gz", hash = "sha256:b3bda1d108d5dd99f4a20d24d9c348e91c4db7ab1b749200bded2f839ccbe68f"},
+]
+
[[package]]
name = "tomli"
version = "2.0.1"
@@ -932,4 +944,4 @@ testing = ["big-O", "flake8 (<5)", "jaraco.functools", "jaraco.itertools", "more
[metadata]
lock-version = "2.0"
python-versions = ">=3.7.0,<4.0"
-content-hash = "e34c2dbb238bb43211cc83b01a6fef54cfed2547b3f034dd4c7e47940cc84f95"
+content-hash = "d1b93aabe50ca296f8324af41db0ba3b97b09444730854b7ebc3497f6e70fbdb"
diff --git a/pyproject.toml b/pyproject.toml
index bd2a89d..7fe23fe 100644
--- a/pyproject.toml
+++ b/pyproject.toml
@@ -53,6 +53,7 @@
]
yapf = "*"
tox = "*"
+ toml = "*"
[build-system]
diff --git a/rtcclient/client.py b/rtcclient/client.py
index aba7afb..5d2be51 100644
--- a/rtcclient/client.py
+++ b/rtcclient/client.py
@@ -8,7 +8,7 @@ import six
import xmltodict
from rtcclient import exception
-from rtcclient import urlparse, urlquote, OrderedDict
+from rtcclient import urlencode, urlparse, urlquote, OrderedDict
from rtcclient.base import RTCBase
from rtcclient.models import FiledAgainst, FoundIn, Comment, Action, State # noqa: F401
from rtcclient.models import IncludedInBuild, ChangeSet, Attachment # noqa: F401
@@ -58,7 +58,8 @@ class RTCClient(RTCBase):
proxies=None,
searchpath=None,
ends_with_jazz=True,
- verify: Union[bool, str] = False):
+ verify: Union[bool, str] = False,
+ old_rtc_authentication=False):
"""Initialization
See params above
@@ -68,6 +69,7 @@ class RTCClient(RTCBase):
self.password = password
self.proxies = proxies
self.verify = verify
+ self.old_rtc_authentication = old_rtc_authentication
RTCBase.__init__(self, url)
if not isinstance(ends_with_jazz, bool):
@@ -99,6 +101,24 @@ class RTCClient(RTCBase):
proxies=self.proxies,
allow_redirects=_allow_redirects)
+ if self.old_rtc_authentication:
+ # works with server that needs 0.6.0 version
+ _headers["Content-Type"] = self.CONTENT_URL_ENCODED
+ if resp.headers.get("set-cookie") is not None:
+ _headers["Cookie"] = resp.headers.get("set-cookie")
+
+ credentials = urlencode({
+ "j_username": self.username,
+ "j_password": self.password
+ })
+
+ resp = self.post(self.url + "/authenticated/j_security_check",
+ data=credentials,
+ verify=False,
+ headers=_headers,
+ proxies=self.proxies,
+ allow_redirects=_allow_redirects)
+
# authfailed
authfailed = resp.headers.get("x-com-ibm-team-repository-web-auth-msg")
if authfailed == "authfailed":
| dixudx/rtcclient | da253d2d1eedb8e9e88c98cfba2828daa2a81f60 | diff --git a/tests/test_client.py b/tests/test_client.py
index ad6309f..49e7fd5 100644
--- a/tests/test_client.py
+++ b/tests/test_client.py
@@ -2,6 +2,8 @@ from rtcclient import RTCClient
import requests
import pytest
import utils_test
+from unittest.mock import call
+
from rtcclient.project_area import ProjectArea
from rtcclient.models import Severity, Priority, FoundIn, FiledAgainst
from rtcclient.models import TeamArea, Member, PlannedFor
@@ -42,6 +44,254 @@ def test_headers(mocker):
assert client.headers == expected_headers
+def test_client_rest_calls_new_auth(mocker):
+ mocked_get = mocker.patch("requests.get")
+ mocked_post = mocker.patch("requests.post")
+
+ mock_rsp = mocker.MagicMock(spec=requests.Response)
+ mock_rsp.status_code = 200
+
+ mocked_get.return_value = mock_rsp
+ mocked_post.return_value = mock_rsp
+
+ mock_rsp.headers = {"set-cookie": "cookie-id"}
+ _ = RTCClient(url="http://test.url:9443/jazz",
+ username="user",
+ password="password")
+
+ # assert GET calls
+ assert mocked_get.call_count == 2
+ mocked_get.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'text/xml',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True),
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'text/xml',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True)
+ ])
+
+ # assert POST calls
+ assert mocked_post.call_count == 0
+
+
+def test_client_rest_calls_old_auth(mocker):
+ mocked_get = mocker.patch("requests.get")
+ mocked_post = mocker.patch("requests.post")
+
+ mock_rsp = mocker.MagicMock(spec=requests.Response)
+ mock_rsp.status_code = 200
+
+ mocked_get.return_value = mock_rsp
+ mocked_post.return_value = mock_rsp
+
+ mock_rsp.headers = {"set-cookie": "cookie-id"}
+ _ = RTCClient(url="http://test.url:9443/jazz",
+ username="user",
+ password="password",
+ old_rtc_authentication=True)
+
+ # assert GET calls
+ assert mocked_get.call_count == 2
+ mocked_get.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'application/x-www-form-urlencoded',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True),
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'application/x-www-form-urlencoded',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True)
+ ])
+
+ # assert POST calls
+ assert mocked_post.call_count == 1
+ mocked_post.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/j_security_check',
+ data='j_username=user&j_password=password',
+ json=None,
+ verify=False,
+ headers={
+ 'Content-Type': 'application/x-www-form-urlencoded',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ allow_redirects=True)
+ ])
+
+
+def test_headers_auth_required_new_auth(mocker):
+ mocked_get = mocker.patch("requests.get")
+ mocked_post = mocker.patch("requests.post")
+
+ mock_rsp = mocker.MagicMock(spec=requests.Response)
+ mock_rsp.status_code = 200
+
+ # auth required
+ mock_rsp.headers = {
+ "set-cookie": "cookie-id",
+ "X-com-ibm-team-repository-web-auth-msg": "authrequired"
+ }
+
+ mocked_get.return_value = mock_rsp
+ mocked_post.return_value = mock_rsp
+
+ _ = RTCClient(url="http://test.url:9443/jazz",
+ username="user",
+ password="password")
+
+ # assert GET calls
+ assert mocked_get.call_count == 2
+ mocked_get.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'text/xml',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True),
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'text/xml',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True)
+ ])
+
+ # assert POST calls
+ assert mocked_post.call_count == 1
+ mocked_post.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/j_security_check',
+ data={
+ 'j_username': 'user',
+ 'j_password': 'password'
+ },
+ json=None,
+ verify=False,
+ headers={'Content-Type': 'application/x-www-form-urlencoded'},
+ proxies=None,
+ timeout=60,
+ allow_redirects=True)
+ ])
+
+
+def test_headers_auth_required_old_auth(mocker):
+ mocked_get = mocker.patch("requests.get")
+ mocked_post = mocker.patch("requests.post")
+
+ mock_rsp = mocker.MagicMock(spec=requests.Response)
+ mock_rsp.status_code = 200
+
+ # auth required
+ mock_rsp.headers = {
+ "set-cookie": "cookie-id",
+ "X-com-ibm-team-repository-web-auth-msg": "authrequired"
+ }
+
+ mocked_get.return_value = mock_rsp
+ mocked_post.return_value = mock_rsp
+
+ _ = RTCClient(url="http://test.url:9443/jazz",
+ username="user",
+ password="password",
+ old_rtc_authentication=True)
+
+ # assert GET calls
+ assert mocked_get.call_count == 2
+ mocked_get.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'application/x-www-form-urlencoded',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True),
+ call('http://test.url:9443/jazz/authenticated/identity',
+ verify=False,
+ headers={
+ 'Content-Type': 'application/x-www-form-urlencoded',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ auth=('user', 'password'),
+ allow_redirects=True)
+ ])
+
+ # assert POST calls
+ assert mocked_post.call_count == 2
+ mocked_post.assert_has_calls([
+ call('http://test.url:9443/jazz/authenticated/j_security_check',
+ data='j_username=user&j_password=password',
+ json=None,
+ verify=False,
+ headers={
+ 'Content-Type': 'application/x-www-form-urlencoded',
+ 'Cookie': 'cookie-id',
+ 'Accept': 'text/xml'
+ },
+ proxies=None,
+ timeout=60,
+ allow_redirects=True),
+ call('http://test.url:9443/jazz/authenticated/j_security_check',
+ data={
+ 'j_username': 'user',
+ 'j_password': 'password'
+ },
+ json=None,
+ verify=False,
+ headers={'Content-Type': 'application/x-www-form-urlencoded'},
+ proxies=None,
+ timeout=60,
+ allow_redirects=True)
+ ])
+
+
class TestRTCClient:
@pytest.fixture(autouse=True)
| url not found: jazz/authenticated
Hi,
I was trying to use your plugin to, at least, connect to a jazz server using your `get_workitem.py` script into `examples/how_to/workitem` folder.
I've changed the file with :
```python
url = "https://__the_url__:443/ccm/jazz"
username = "my_username"
password = "my_password"
myclient = RTCClient(url, username, password, ends_with_jazz=True)
```
Adding also `ends_with_jazz=True` that I saw from docs/other issues.
This is the trace I get:
```python
2023-03-22 14:49:42,753 DEBUG client.RTCClient: Get response from https://__the_url__:443/ccm/jazz/authenticated/identity
2023-03-22 14:49:42,764 DEBUG urllib3.connectionpool: Starting new HTTPS connection (1): __the_url__:443
2023-03-22 14:49:42,979 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/jazz/authenticated/identity HTTP/1.1" 302 0
2023-03-22 14:49:43,000 DEBUG urllib3.connectionpool: Resetting dropped connection: __the_url__
2023-03-22 14:49:43,153 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/secure/authenticated/identity?redirectPath=%2Fccm%2Fjazz%2Fauthenticated%2Fidentity HTTP/1.1" 302 0
2023-03-22 14:49:43,167 DEBUG urllib3.connectionpool: Resetting dropped connection: __the_url__
2023-03-22 14:49:43,325 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/auth/authrequired HTTP/1.1" 200 1072
2023-03-22 14:49:48,130 DEBUG client.RTCClient: Get response from https://__the_url__:443/ccm/jazz/authenticated/identity
2023-03-22 14:49:48,141 DEBUG urllib3.connectionpool: Starting new HTTPS connection (1): __the_url__:443
2023-03-22 14:49:48,368 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/jazz/authenticated/identity HTTP/1.1" 302 0
2023-03-22 14:49:48,381 DEBUG urllib3.connectionpool: Resetting dropped connection: __the_url__
2023-03-22 14:49:48,543 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/secure/authenticated/identity?redirectPath=%2Fccm%2Fjazz%2Fauthenticated%2Fidentity HTTP/1.1" 302 0
2023-03-22 14:49:48,583 DEBUG urllib3.connectionpool: Resetting dropped connection: __the_url__
2023-03-22 14:49:48,837 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/auth/authrequired HTTP/1.1" 200 1072
2023-03-22 14:49:56,708 DEBUG client.RTCClient: Post a request to https://__the_url__:443/ccm/jazz/authenticated/j_security_check with data: {'j_username': 'my_username', 'j_password': 'my_password'} and json: None
2023-03-22 14:49:56,717 DEBUG urllib3.connectionpool: Starting new HTTPS connection (1): __the_url__:443
2023-03-22 14:49:57,598 DEBUG urllib3.connectionpool: https://__the_url__:443 "POST /ccm/jazz/authenticated/j_security_check HTTP/1.1" 302 0
2023-03-22 14:49:57,610 DEBUG urllib3.connectionpool: Resetting dropped connection: __the_url__
2023-03-22 14:49:57,768 DEBUG urllib3.connectionpool: https://__the_url__:443 "GET /ccm/jazz/authenticated/ HTTP/1.1" 404 None
2023-03-22 14:49:57,773 ERROR client.RTCClient: Failed POST request at <https://__the_url__:443/ccm/jazz/authenticated/j_security_check> with response: b'Error 404: Not Found\n'
2023-03-22 14:49:57,773 INFO client.RTCClient: 404
Traceback (most recent call last):
File "...\rtcclient\examples\how_to\workitem\get_workitem.py", line 13, in <module>
myclient = RTCClient(url, username, password, ends_with_jazz=True)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "...\rtcclient\rtcclient\client.py", line 77, in __init__
self.headers = self._get_headers()
^^^^^^^^^^^^^^^^^^^
File "...\rtcclient\rtcclient\client.py", line 155, in _get_headers
resp = self.post(self.url + "/authenticated/j_security_check",
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "...\rtcclient\rtcclient\utils.py", line 27, in wrapper
return func(*args, **kwargs)
^^^^^^^^^^^^^^^^^^^^^
File "...\rtcclient\rtcclient\base.py", line 144, in post
response.raise_for_status()
File "...\rtcclient\venv\Lib\site-packages\requests\models.py", line 1021, in raise_for_status
raise HTTPError(http_error_msg, response=self)
requests.exceptions.HTTPError: 404 Client Error: Not Found for url: https://__the_url__/ccm/jazz/authenticated/
python-BaseException
```
So the point that raises exception is [this line](https://github.com/dixudx/rtcclient/blob/master/rtcclient/client.py#L136), the line number differs because of some tests I made related to cookies (from a fork), but those changes are commented.
Some information on my environment:
- Python3.11
- rtcclient checkout of main branch
- IBM Jazz 7.0.2
Thank you! | 0.0 | [
"tests/test_client.py::test_client_rest_calls_old_auth",
"tests/test_client.py::test_headers_auth_required_old_auth"
] | [
"tests/test_client.py::test_headers",
"tests/test_client.py::test_client_rest_calls_new_auth",
"tests/test_client.py::test_headers_auth_required_new_auth",
"tests/test_client.py::TestRTCClient::test_get_projectareas_unarchived",
"tests/test_client.py::TestRTCClient::test_get_projectareas_archived",
"tests/test_client.py::TestRTCClient::test_get_projectarea_unarchived",
"tests/test_client.py::TestRTCClient::test_get_projectarea_exception",
"tests/test_client.py::TestRTCClient::test_get_projectarea_archived",
"tests/test_client.py::TestRTCClient::test_get_projectarea_byid",
"tests/test_client.py::TestRTCClient::test_get_projectarea_id",
"tests/test_client.py::TestRTCClient::test_get_projectarea_id_exception",
"tests/test_client.py::TestRTCClient::test_get_projectarea_byid_exception",
"tests/test_client.py::TestRTCClient::test_get_projectarea_ids",
"tests/test_client.py::TestRTCClient::test_get_projectarea_ids_exception",
"tests/test_client.py::TestRTCClient::test_check_projectarea_id",
"tests/test_client.py::TestRTCClient::test_get_teamareas_unarchived",
"tests/test_client.py::TestRTCClient::test_get_teamareas_archived",
"tests/test_client.py::TestRTCClient::test_get_teamarea_unarchived",
"tests/test_client.py::TestRTCClient::test_get_teamarea_archived",
"tests/test_client.py::TestRTCClient::test_get_owned_by",
"tests/test_client.py::TestRTCClient::test_get_plannedfors_unarchived",
"tests/test_client.py::TestRTCClient::test_get_plannedfors_archived",
"tests/test_client.py::TestRTCClient::test_get_plannedfor_unarchived",
"tests/test_client.py::TestRTCClient::test_get_plannedfor_archived",
"tests/test_client.py::TestRTCClient::test_get_severities",
"tests/test_client.py::TestRTCClient::test_get_severity",
"tests/test_client.py::TestRTCClient::test_get_priorities",
"tests/test_client.py::TestRTCClient::test_get_priority",
"tests/test_client.py::TestRTCClient::test_get_foundins_unarchived",
"tests/test_client.py::TestRTCClient::test_get_foundins_archived",
"tests/test_client.py::TestRTCClient::test_get_foundin_unarchived",
"tests/test_client.py::TestRTCClient::test_get_foundin_archived",
"tests/test_client.py::TestRTCClient::test_get_filedagainsts_unarchived",
"tests/test_client.py::TestRTCClient::test_get_filedagainsts_archived",
"tests/test_client.py::TestRTCClient::test_get_filedagainst_unarchived",
"tests/test_client.py::TestRTCClient::test_get_filedagainst_archived",
"tests/test_client.py::TestRTCClient::test_get_workitem",
"tests/test_client.py::TestRTCClient::test_get_workitems_unarchived",
"tests/test_client.py::TestRTCClient::test_get_workitems_archived",
"tests/test_client.py::TestRTCClient::test_list_fields",
"tests/test_client.py::TestRTCClient::test_list_fields_from_workitem",
"tests/test_client.py::TestRTCClient::test_create_workitem",
"tests/test_client.py::TestRTCClient::test_copy_workitem",
"tests/test_client.py::TestRTCClient::test_update_workitem",
"tests/test_client.py::TestRTCClient::test_check_itemtype"
] | 2023-03-28 16:13:18+00:00 | 1,923 |
|
django-json-api__django-rest-framework-json-api-1074 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index 91faffd..91551dc 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -14,6 +14,7 @@ any parts of the framework not mentioned in the documentation should generally b
* Fixed invalid relationship pointer in error objects when field naming formatting is used.
* Properly resolved related resource type when nested source field is defined.
+* Prevented overwriting of pointer in custom error object
### Added
diff --git a/docs/usage.md b/docs/usage.md
index 939e4e6..952fe80 100644
--- a/docs/usage.md
+++ b/docs/usage.md
@@ -237,7 +237,7 @@ class MyViewset(ModelViewSet):
```
-### Exception handling
+### Error objects / Exception handling
For the `exception_handler` class, if the optional `JSON_API_UNIFORM_EXCEPTIONS` is set to True,
all exceptions will respond with the JSON:API [error format](https://jsonapi.org/format/#error-objects).
@@ -245,6 +245,21 @@ all exceptions will respond with the JSON:API [error format](https://jsonapi.org
When `JSON_API_UNIFORM_EXCEPTIONS` is False (the default), non-JSON:API views will respond
with the normal DRF error format.
+In case you need a custom error object you can simply raise an `rest_framework.serializers.ValidationError` like the following:
+
+```python
+raise serializers.ValidationError(
+ {
+ "id": "your-id",
+ "detail": "your detail message",
+ "source": {
+ "pointer": "/data/attributes/your-pointer",
+ }
+
+ }
+)
+```
+
### Performance Testing
If you are trying to see if your viewsets are configured properly to optimize performance,
diff --git a/rest_framework_json_api/utils.py b/rest_framework_json_api/utils.py
index 8317c10..2e86e76 100644
--- a/rest_framework_json_api/utils.py
+++ b/rest_framework_json_api/utils.py
@@ -417,21 +417,20 @@ def format_error_object(message, pointer, response):
if isinstance(message, dict):
# as there is no required field in error object we check that all fields are string
- # except links and source which might be a dict
+ # except links, source or meta which might be a dict
is_custom_error = all(
[
isinstance(value, str)
for key, value in message.items()
- if key not in ["links", "source"]
+ if key not in ["links", "source", "meta"]
]
)
if is_custom_error:
if "source" not in message:
message["source"] = {}
- message["source"] = {
- "pointer": pointer,
- }
+ if "pointer" not in message["source"]:
+ message["source"]["pointer"] = pointer
errors.append(message)
else:
for k, v in message.items():
| django-json-api/django-rest-framework-json-api | 46551a9aada19c503e363b20b47765009038d996 | diff --git a/tests/test_utils.py b/tests/test_utils.py
index 9b47e9f..038e8ce 100644
--- a/tests/test_utils.py
+++ b/tests/test_utils.py
@@ -7,6 +7,7 @@ from rest_framework.views import APIView
from rest_framework_json_api import serializers
from rest_framework_json_api.utils import (
+ format_error_object,
format_field_name,
format_field_names,
format_link_segment,
@@ -389,3 +390,45 @@ def test_get_resource_type_from_serializer_without_resource_name_raises_error():
"can not detect 'resource_name' on serializer "
"'SerializerWithoutResourceName' in module 'tests.test_utils'"
)
+
+
+@pytest.mark.parametrize(
+ "message,pointer,response,result",
+ [
+ # Test that pointer does not get overridden in custom error
+ (
+ {
+ "status": "400",
+ "source": {
+ "pointer": "/data/custom-pointer",
+ },
+ "meta": {"key": "value"},
+ },
+ "/data/default-pointer",
+ Response(status.HTTP_400_BAD_REQUEST),
+ [
+ {
+ "status": "400",
+ "source": {"pointer": "/data/custom-pointer"},
+ "meta": {"key": "value"},
+ }
+ ],
+ ),
+ # Test that pointer gets added to custom error
+ (
+ {
+ "detail": "custom message",
+ },
+ "/data/default-pointer",
+ Response(status.HTTP_400_BAD_REQUEST),
+ [
+ {
+ "detail": "custom message",
+ "source": {"pointer": "/data/default-pointer"},
+ }
+ ],
+ ),
+ ],
+)
+def test_format_error_object(message, pointer, response, result):
+ assert result == format_error_object(message, pointer, response)
| Avoid overwriting of pointer when using custom errors
The code that produces JSON:API error objects assumes that all fields being validated are under `/data/attributes`:
https://github.com/django-json-api/django-rest-framework-json-api/blob/b0257c065ac2439036ac0543139e7f5913755177/rest_framework_json_api/utils.py#L339
and there doesn't appear to be a way to override the `pointer` value when specifying the field on a serializer.
Use case: I would like to be able to specify a serializer for the top-level `"meta"` field and have any validation errors on that data generate responses properly populated with `"source": {"pointer": "/meta/foo"}`. | 0.0 | [
"tests/test_utils.py::test_format_error_object[message0-/data/default-pointer-response0-result0]"
] | [
"tests/test_utils.py::test_get_related_resource_type_from_nested_source[m2m_source.targets-ManyToManyTarget-related_field_kwargs0]",
"tests/test_utils.py::test_get_related_resource_type_from_nested_source[m2m_target.sources.-ManyToManySource-related_field_kwargs1]",
"tests/test_utils.py::test_get_related_resource_type_from_nested_source[fk_source.target-ForeignKeyTarget-related_field_kwargs2]",
"tests/test_utils.py::test_get_related_resource_type_from_nested_source[fk_target.source-ForeignKeySource-related_field_kwargs3]",
"tests/test_utils.py::test_get_resource_name_no_view",
"tests/test_utils.py::test_get_resource_name_from_view[False-False-APIView]",
"tests/test_utils.py::test_get_resource_name_from_view[False-True-APIViews]",
"tests/test_utils.py::test_get_resource_name_from_view[dasherize-False-api-view]",
"tests/test_utils.py::test_get_resource_name_from_view[dasherize-True-api-views]",
"tests/test_utils.py::test_get_resource_name_from_view_custom_resource_name[False-False]",
"tests/test_utils.py::test_get_resource_name_from_view_custom_resource_name[False-True]",
"tests/test_utils.py::test_get_resource_name_from_view_custom_resource_name[dasherize-False]",
"tests/test_utils.py::test_get_resource_name_from_view_custom_resource_name[dasherize-True]",
"tests/test_utils.py::test_get_resource_name_from_model[False-False-BasicModel]",
"tests/test_utils.py::test_get_resource_name_from_model[False-True-BasicModels]",
"tests/test_utils.py::test_get_resource_name_from_model[dasherize-False-basic-model]",
"tests/test_utils.py::test_get_resource_name_from_model[dasherize-True-basic-models]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class[False-False-BasicModel]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class[False-True-BasicModels]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class[dasherize-False-basic-model]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class[dasherize-True-basic-models]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class_custom_resource_name[False-False]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class_custom_resource_name[False-True]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class_custom_resource_name[dasherize-False]",
"tests/test_utils.py::test_get_resource_name_from_model_serializer_class_custom_resource_name[dasherize-True]",
"tests/test_utils.py::test_get_resource_name_from_plain_serializer_class[False-False]",
"tests/test_utils.py::test_get_resource_name_from_plain_serializer_class[False-True]",
"tests/test_utils.py::test_get_resource_name_from_plain_serializer_class[dasherize-False]",
"tests/test_utils.py::test_get_resource_name_from_plain_serializer_class[dasherize-True]",
"tests/test_utils.py::test_get_resource_name_with_errors[400]",
"tests/test_utils.py::test_get_resource_name_with_errors[403]",
"tests/test_utils.py::test_get_resource_name_with_errors[500]",
"tests/test_utils.py::test_format_field_names[False-output0]",
"tests/test_utils.py::test_format_field_names[camelize-output1]",
"tests/test_utils.py::test_format_field_names[capitalize-output2]",
"tests/test_utils.py::test_format_field_names[dasherize-output3]",
"tests/test_utils.py::test_format_field_names[underscore-output4]",
"tests/test_utils.py::test_undo_format_field_names[False-output0]",
"tests/test_utils.py::test_undo_format_field_names[camelize-output1]",
"tests/test_utils.py::test_format_field_name[False-full_name]",
"tests/test_utils.py::test_format_field_name[camelize-fullName]",
"tests/test_utils.py::test_format_field_name[capitalize-FullName]",
"tests/test_utils.py::test_format_field_name[dasherize-full-name]",
"tests/test_utils.py::test_format_field_name[underscore-full_name]",
"tests/test_utils.py::test_undo_format_field_name[False-fullName]",
"tests/test_utils.py::test_undo_format_field_name[camelize-full_name]",
"tests/test_utils.py::test_format_link_segment[False-first_Name]",
"tests/test_utils.py::test_format_link_segment[camelize-firstName]",
"tests/test_utils.py::test_format_link_segment[capitalize-FirstName]",
"tests/test_utils.py::test_format_link_segment[dasherize-first-name]",
"tests/test_utils.py::test_format_link_segment[underscore-first_name]",
"tests/test_utils.py::test_undo_format_link_segment[False-fullName]",
"tests/test_utils.py::test_undo_format_link_segment[camelize-full_name]",
"tests/test_utils.py::test_format_value[False-first_name]",
"tests/test_utils.py::test_format_value[camelize-firstName]",
"tests/test_utils.py::test_format_value[capitalize-FirstName]",
"tests/test_utils.py::test_format_value[dasherize-first-name]",
"tests/test_utils.py::test_format_value[underscore-first_name]",
"tests/test_utils.py::test_format_resource_type[None-None-ResourceType]",
"tests/test_utils.py::test_format_resource_type[camelize-False-resourceType]",
"tests/test_utils.py::test_format_resource_type[camelize-True-resourceTypes]",
"tests/test_utils.py::test_get_related_resource_type[ManyToManySource-targets-ManyToManyTarget]",
"tests/test_utils.py::test_get_related_resource_type[ManyToManyTarget-sources-ManyToManySource]",
"tests/test_utils.py::test_get_related_resource_type[ForeignKeySource-target-ForeignKeyTarget]",
"tests/test_utils.py::test_get_related_resource_type[ForeignKeyTarget-sources-ForeignKeySource]",
"tests/test_utils.py::test_get_related_resource_type_from_plain_serializer_class[related_field_kwargs0-BasicModel]",
"tests/test_utils.py::test_get_related_resource_type_from_plain_serializer_class[related_field_kwargs1-BasicModel]",
"tests/test_utils.py::test_get_related_resource_type_from_plain_serializer_class[related_field_kwargs2-BasicModel]",
"tests/test_utils.py::test_get_resource_type_from_serializer_without_resource_name_raises_error",
"tests/test_utils.py::test_format_error_object[message1-/data/default-pointer-response1-result1]"
] | 2022-07-20 11:13:20+00:00 | 1,924 |
|
django-json-api__django-rest-framework-json-api-1137 | diff --git a/AUTHORS b/AUTHORS
index e27ba5f..797ab52 100644
--- a/AUTHORS
+++ b/AUTHORS
@@ -3,6 +3,7 @@ Adam Ziolkowski <adam@adsized.com>
Alan Crosswell <alan@columbia.edu>
Alex Seidmann <alex@leanpnt.de>
Anton Shutik <shutikanton@gmail.com>
+Arttu Perälä <arttu@perala.me>
Ashley Loewen <github@ashleycodes.tech>
Asif Saif Uddin <auvipy@gmail.com>
Beni Keller <beni@matraxi.ch>
diff --git a/CHANGELOG.md b/CHANGELOG.md
index 7a1c344..3f9c175 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -23,6 +23,7 @@ any parts of the framework not mentioned in the documentation should generally b
### Fixed
* Refactored handling of the `sort` query parameter to fix duplicate declaration in the generated schema definition
+* Non-field serializer errors are given a source.pointer value of "/data".
## [6.0.0] - 2022-09-24
diff --git a/example/api/serializers/identity.py b/example/api/serializers/identity.py
index 069259d..5e2a42e 100644
--- a/example/api/serializers/identity.py
+++ b/example/api/serializers/identity.py
@@ -23,6 +23,13 @@ class IdentitySerializer(serializers.ModelSerializer):
)
return data
+ def validate(self, data):
+ if data["first_name"] == data["last_name"]:
+ raise serializers.ValidationError(
+ "First name cannot be the same as last name!"
+ )
+ return data
+
class Meta:
model = auth_models.User
fields = (
diff --git a/rest_framework_json_api/serializers.py b/rest_framework_json_api/serializers.py
index a288471..4b619ac 100644
--- a/rest_framework_json_api/serializers.py
+++ b/rest_framework_json_api/serializers.py
@@ -155,7 +155,12 @@ class IncludedResourcesValidationMixin:
class ReservedFieldNamesMixin:
"""Ensures that reserved field names are not used and an error raised instead."""
- _reserved_field_names = {"meta", "results", "type"}
+ _reserved_field_names = {
+ "meta",
+ "results",
+ "type",
+ api_settings.NON_FIELD_ERRORS_KEY,
+ }
def get_fields(self):
fields = super().get_fields()
diff --git a/rest_framework_json_api/utils.py b/rest_framework_json_api/utils.py
index 3d374ee..dab8a3b 100644
--- a/rest_framework_json_api/utils.py
+++ b/rest_framework_json_api/utils.py
@@ -13,6 +13,7 @@ from django.utils import encoding
from django.utils.translation import gettext_lazy as _
from rest_framework import exceptions, relations
from rest_framework.exceptions import APIException
+from rest_framework.settings import api_settings
from .settings import json_api_settings
@@ -381,10 +382,14 @@ def format_drf_errors(response, context, exc):
]
for field, error in response.data.items():
+ non_field_error = field == api_settings.NON_FIELD_ERRORS_KEY
field = format_field_name(field)
pointer = None
- # pointer can be determined only if there's a serializer.
- if has_serializer:
+ if non_field_error:
+ # Serializer error does not refer to a specific field.
+ pointer = "/data"
+ elif has_serializer:
+ # pointer can be determined only if there's a serializer.
rel = "relationships" if field in relationship_fields else "attributes"
pointer = f"/data/{rel}/{field}"
if isinstance(exc, Http404) and isinstance(error, str):
| django-json-api/django-rest-framework-json-api | ad5a793b54ca2922223352a58830515d25fa4807 | diff --git a/example/tests/test_generic_viewset.py b/example/tests/test_generic_viewset.py
index c8a28f2..40812d6 100644
--- a/example/tests/test_generic_viewset.py
+++ b/example/tests/test_generic_viewset.py
@@ -129,3 +129,35 @@ class GenericViewSet(TestBase):
)
assert expected == response.json()
+
+ def test_nonfield_validation_exceptions(self):
+ """
+ Non-field errors should be attributed to /data source.pointer.
+ """
+ expected = {
+ "errors": [
+ {
+ "status": "400",
+ "source": {
+ "pointer": "/data",
+ },
+ "detail": "First name cannot be the same as last name!",
+ "code": "invalid",
+ },
+ ]
+ }
+ response = self.client.post(
+ "/identities",
+ {
+ "data": {
+ "type": "users",
+ "attributes": {
+ "email": "miles@example.com",
+ "first_name": "Miles",
+ "last_name": "Miles",
+ },
+ }
+ },
+ )
+
+ assert expected == response.json()
| Object-level validation errors given an incorrect source.pointer
## Description of the Bug Report
When raising a non-field `ValidationError` in a serializer [in accordance with the DRF documentation](https://www.django-rest-framework.org/api-guide/serializers/#object-level-validation), the error is attributed to a non-existing `/data/attributes/non_field_errors` path instead of `/data`.
```python
from rest_framework_json_api import serializers
class ProductSerializer(serializers.ModelSerializer):
def validate(self, data):
if data.get("best_before_date") and data.get("expiry_date"):
raise serializers.ValidationError(
"Specify either a best before date or an expiry date."
)
return data
```
```json
{
"errors": [
{
"detail": "Specify either a best before date or an expiry date.",
"status": "400",
"source": {
"pointer": "/data/attributes/non_field_errors"
},
"code": "invalid"
}
]
}
```
Complete minimal example available [here](https://github.com/arttuperala/jsonapi_non_field_errors).
### Discussed in https://github.com/django-json-api/django-rest-framework-json-api/discussions/1135
## Checklist
- [x] Certain that this is a bug (if unsure or you have a question use [discussions](https://github.com/django-json-api/django-rest-framework-json-api/discussions) instead)
- [x] Code snippet or unit test added to reproduce bug
| 0.0 | [
"example/tests/test_generic_viewset.py::GenericViewSet::test_nonfield_validation_exceptions"
] | [
"example/tests/test_generic_viewset.py::GenericViewSet::test_ember_expected_renderer",
"example/tests/test_generic_viewset.py::GenericViewSet::test_default_validation_exceptions",
"example/tests/test_generic_viewset.py::GenericViewSet::test_default_rest_framework_behavior",
"example/tests/test_generic_viewset.py::GenericViewSet::test_custom_validation_exceptions"
] | 2023-03-07 08:33:04+00:00 | 1,925 |
|
django-json-api__django-rest-framework-json-api-1162 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index d494fc2..a9dc65d 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -40,6 +40,8 @@ any parts of the framework not mentioned in the documentation should generally b
return value.name
```
+* `SerializerMethodResourceRelatedField(many=True)` relationship data now includes a meta section.
+
### Fixed
* Refactored handling of the `sort` query parameter to fix duplicate declaration in the generated schema definition
diff --git a/rest_framework_json_api/renderers.py b/rest_framework_json_api/renderers.py
index f766020..9a0d9c0 100644
--- a/rest_framework_json_api/renderers.py
+++ b/rest_framework_json_api/renderers.py
@@ -19,6 +19,7 @@ import rest_framework_json_api
from rest_framework_json_api import utils
from rest_framework_json_api.relations import (
HyperlinkedMixin,
+ ManySerializerMethodResourceRelatedField,
ResourceRelatedField,
SkipDataMixin,
)
@@ -152,6 +153,11 @@ class JSONRenderer(renderers.JSONRenderer):
if not isinstance(field, SkipDataMixin):
relation_data.update({"data": resource.get(field_name)})
+ if isinstance(field, ManySerializerMethodResourceRelatedField):
+ relation_data.update(
+ {"meta": {"count": len(resource.get(field_name))}}
+ )
+
data.update({field_name: relation_data})
continue
| django-json-api/django-rest-framework-json-api | 71508efa3fee8ff75c9cfcd092844801aeb407e1 | diff --git a/example/tests/integration/test_non_paginated_responses.py b/example/tests/integration/test_non_paginated_responses.py
index 60376a8..92d26de 100644
--- a/example/tests/integration/test_non_paginated_responses.py
+++ b/example/tests/integration/test_non_paginated_responses.py
@@ -51,6 +51,7 @@ def test_multiple_entries_no_pagination(multiple_entries, client):
"related": "http://testserver/entries/1/suggested/",
"self": "http://testserver/entries/1/relationships/suggested",
},
+ "meta": {"count": 1},
},
"suggestedHyperlinked": {
"links": {
@@ -106,6 +107,7 @@ def test_multiple_entries_no_pagination(multiple_entries, client):
"related": "http://testserver/entries/2/suggested/",
"self": "http://testserver/entries/2/relationships/suggested",
},
+ "meta": {"count": 1},
},
"suggestedHyperlinked": {
"links": {
diff --git a/example/tests/integration/test_pagination.py b/example/tests/integration/test_pagination.py
index 4c60e96..1a4bd05 100644
--- a/example/tests/integration/test_pagination.py
+++ b/example/tests/integration/test_pagination.py
@@ -51,6 +51,7 @@ def test_pagination_with_single_entry(single_entry, client):
"related": "http://testserver/entries/1/suggested/",
"self": "http://testserver/entries/1/relationships/suggested",
},
+ "meta": {"count": 0},
},
"suggestedHyperlinked": {
"links": {
diff --git a/example/tests/test_filters.py b/example/tests/test_filters.py
index ab74dd0..87f9d05 100644
--- a/example/tests/test_filters.py
+++ b/example/tests/test_filters.py
@@ -512,6 +512,7 @@ class DJATestFilters(APITestCase):
{"type": "entries", "id": "11"},
{"type": "entries", "id": "12"},
],
+ "meta": {"count": 11},
},
"suggestedHyperlinked": {
"links": {
| SerializerMethodResourceRelatedField does not include meta section in relationship response.
`SerializerMethodResourceRelatedField` does not include a meta section in the relationship response like `ResourceRelatedField` does. This doesn't make sense, because `SerializerMethodResourceRelatedField` extends `ResourceRelatedField` and both seem to return every related record in the response (for the record I've only tried with a little over a thousand related records).
So for example if you had an ObjectA with a has many relationship with ObjectB:
```python
objectbs = SerializerMethodResourceRelatedField(
source='get_objectbs',
many=True,
model=ObjectB,
read_only=True
)
def get_objectbs(self, obj):
return ObjectB.objects.filter(objecta=obj)
```
then your response would look like:
```json
"relationships": {
"objectb": {
"data": [...]
}
}
```
instead of:
```json
"relationships": {
"objectb": {
"meta": {
"count": 2
},
"data": [...]
}
}
``` | 0.0 | [
"example/tests/integration/test_non_paginated_responses.py::test_multiple_entries_no_pagination",
"example/tests/integration/test_pagination.py::test_pagination_with_single_entry",
"example/tests/test_filters.py::DJATestFilters::test_search_keywords"
] | [
"example/tests/test_filters.py::DJATestFilters::test_filter_empty_association_name",
"example/tests/test_filters.py::DJATestFilters::test_filter_exact",
"example/tests/test_filters.py::DJATestFilters::test_filter_exact_fail",
"example/tests/test_filters.py::DJATestFilters::test_filter_fields_intersection",
"example/tests/test_filters.py::DJATestFilters::test_filter_fields_union_list",
"example/tests/test_filters.py::DJATestFilters::test_filter_in",
"example/tests/test_filters.py::DJATestFilters::test_filter_invalid_association_name",
"example/tests/test_filters.py::DJATestFilters::test_filter_isempty",
"example/tests/test_filters.py::DJATestFilters::test_filter_isnull",
"example/tests/test_filters.py::DJATestFilters::test_filter_malformed_left_bracket",
"example/tests/test_filters.py::DJATestFilters::test_filter_missing_right_bracket",
"example/tests/test_filters.py::DJATestFilters::test_filter_missing_rvalue",
"example/tests/test_filters.py::DJATestFilters::test_filter_missing_rvalue_equal",
"example/tests/test_filters.py::DJATestFilters::test_filter_no_brackets",
"example/tests/test_filters.py::DJATestFilters::test_filter_no_brackets_equal",
"example/tests/test_filters.py::DJATestFilters::test_filter_no_brackets_rvalue",
"example/tests/test_filters.py::DJATestFilters::test_filter_not_null",
"example/tests/test_filters.py::DJATestFilters::test_filter_related",
"example/tests/test_filters.py::DJATestFilters::test_filter_related_fieldset_class",
"example/tests/test_filters.py::DJATestFilters::test_filter_related_missing_fieldset_class",
"example/tests/test_filters.py::DJATestFilters::test_filter_repeated_relations",
"example/tests/test_filters.py::DJATestFilters::test_filter_single_relation",
"example/tests/test_filters.py::DJATestFilters::test_many_params",
"example/tests/test_filters.py::DJATestFilters::test_param_duplicate_page",
"example/tests/test_filters.py::DJATestFilters::test_param_duplicate_sort",
"example/tests/test_filters.py::DJATestFilters::test_param_invalid",
"example/tests/test_filters.py::DJATestFilters::test_search_multiple_keywords",
"example/tests/test_filters.py::DJATestFilters::test_sort",
"example/tests/test_filters.py::DJATestFilters::test_sort_camelcase",
"example/tests/test_filters.py::DJATestFilters::test_sort_double_negative",
"example/tests/test_filters.py::DJATestFilters::test_sort_invalid",
"example/tests/test_filters.py::DJATestFilters::test_sort_related",
"example/tests/test_filters.py::DJATestFilters::test_sort_reverse",
"example/tests/test_filters.py::DJATestFilters::test_sort_underscore"
] | 2023-06-28 06:04:41+00:00 | 1,926 |
|
django-json-api__django-rest-framework-json-api-1220 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index 964f6b1..0b05600 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -22,6 +22,7 @@ any parts of the framework not mentioned in the documentation should generally b
* `ModelSerializer` fields are now returned in the same order than DRF
* Avoided that an empty attributes dict is rendered in case serializer does not
provide any attribute fields.
+* Avoided shadowing of exception when rendering errors (regression since 4.3.0).
### Removed
diff --git a/rest_framework_json_api/utils.py b/rest_framework_json_api/utils.py
index 2e57fbb..e12080a 100644
--- a/rest_framework_json_api/utils.py
+++ b/rest_framework_json_api/utils.py
@@ -381,11 +381,7 @@ def format_drf_errors(response, context, exc):
errors.extend(format_error_object(message, "/data", response))
# handle all errors thrown from serializers
else:
- # Avoid circular deps
- from rest_framework import generics
-
- has_serializer = isinstance(context["view"], generics.GenericAPIView)
- if has_serializer:
+ try:
serializer = context["view"].get_serializer()
fields = get_serializer_fields(serializer) or dict()
relationship_fields = [
@@ -393,6 +389,11 @@ def format_drf_errors(response, context, exc):
for name, field in fields.items()
if is_relationship_field(field)
]
+ except Exception:
+ # ignore potential errors when retrieving serializer
+ # as it might shadow error which is currently being
+ # formatted
+ serializer = None
for field, error in response.data.items():
non_field_error = field == api_settings.NON_FIELD_ERRORS_KEY
@@ -401,7 +402,7 @@ def format_drf_errors(response, context, exc):
if non_field_error:
# Serializer error does not refer to a specific field.
pointer = "/data"
- elif has_serializer:
+ elif serializer:
# pointer can be determined only if there's a serializer.
rel = "relationships" if field in relationship_fields else "attributes"
pointer = f"/data/{rel}/{field}"
| django-json-api/django-rest-framework-json-api | 2568417f1940834c058ccf8319cf3e4bc0913bee | diff --git a/tests/test_views.py b/tests/test_views.py
index de5d1b7..acba7e6 100644
--- a/tests/test_views.py
+++ b/tests/test_views.py
@@ -154,6 +154,17 @@ class TestModelViewSet:
"included"
]
+ @pytest.mark.urls(__name__)
+ def test_list_with_invalid_include(self, client, foreign_key_source):
+ url = reverse("foreign-key-source-list")
+ response = client.get(url, data={"include": "invalid"})
+ assert response.status_code == status.HTTP_400_BAD_REQUEST
+ result = response.json()
+ assert (
+ result["errors"][0]["detail"]
+ == "This endpoint does not support the include parameter for path invalid"
+ )
+
@pytest.mark.urls(__name__)
def test_list_with_default_included_resources(self, client, foreign_key_source):
url = reverse("default-included-resources-list")
| Validation of include parameter causes unhandled exception
## Description of the Bug Report
When requesting an API endpoint with the `include` query param, specifying an unsupported value results in an uncaught exception and traceback (below). Note: this only seems to happen when querying using the browseable API. When I use [Insomnia](https://insomnia.rest/), I get a 400 response as I'd expect.
Versions:
- Python: 3.6.15
- Django: 3.1.8
- DRF: 3.12.4
- DRF-JA: 3.1.0
<details><summary>Stack trace (browseable API)</summary>
<pre><code>
Traceback (most recent call last):
File "/opt/python/django/core/handlers/exception.py", line 47, in inner
response = get_response(request)
File "/opt/python/django/core/handlers/base.py", line 204, in _get_response
response = response.render()
File "/opt/python/django/template/response.py", line 105, in render
self.content = self.rendered_content
File "/opt/python/rest_framework/response.py", line 70, in rendered_content
ret = renderer.render(self.data, accepted_media_type, context)
File "/opt/python/rest_framework/renderers.py", line 724, in render
context = self.get_context(data, accepted_media_type, renderer_context)
File "/opt/python/rest_framework/renderers.py", line 655, in get_context
raw_data_post_form = self.get_raw_data_form(data, view, 'POST', request)
File "/opt/python/rest_framework/renderers.py", line 554, in get_raw_data_form
serializer = view.get_serializer()
File "/opt/python/rest_framework/generics.py", line 110, in get_serializer
return serializer_class(*args, **kwargs)
File "/opt/python/rest_framework_json_api/serializers.py", line 113, in __init__
validate_path(this_serializer_class, included_field_path, included_field_name)
File "/opt/python/rest_framework_json_api/serializers.py", line 99, in validate_path
path
</code></pre>
</details>
<details><summary>400 response (Insomnia)</summary>
<pre><code>
{
"errors": [
{
"detail": "This endpoint does not support the include parameter for path foo",
"status": "400",
"source": {
"pointer": "/data"
},
"code": "parse_error"
}
],
"meta": {
"version": "unversioned"
}
}
</code></pre>
</details>
## Checklist
- [x] Certain that this is a bug (if unsure or you have a question use [discussions](https://github.com/django-json-api/django-rest-framework-json-api/discussions) instead)
- [ ] Code snippet or unit test added to reproduce bug
- Should be reproducible in any minimal app with no include serializers configured
| 0.0 | [
"tests/test_views.py::TestModelViewSet::test_list_with_invalid_include"
] | [
"tests/test_views.py::TestModelViewSet::test_list",
"tests/test_views.py::TestModelViewSet::test_list_with_include_foreign_key",
"tests/test_views.py::TestModelViewSet::test_list_with_include_many_to_many_field",
"tests/test_views.py::TestModelViewSet::test_list_with_include_nested_related_field",
"tests/test_views.py::TestModelViewSet::test_list_with_default_included_resources",
"tests/test_views.py::TestModelViewSet::test_retrieve",
"tests/test_views.py::TestModelViewSet::test_retrieve_with_include_foreign_key",
"tests/test_views.py::TestModelViewSet::test_patch",
"tests/test_views.py::TestModelViewSet::test_delete",
"tests/test_views.py::TestModelViewSet::test_get_related_field_name_handles_formatted_link_segments[False]",
"tests/test_views.py::TestModelViewSet::test_get_related_field_name_handles_formatted_link_segments[dasherize]",
"tests/test_views.py::TestModelViewSet::test_get_related_field_name_handles_formatted_link_segments[camelize]",
"tests/test_views.py::TestModelViewSet::test_get_related_field_name_handles_formatted_link_segments[capitalize]",
"tests/test_views.py::TestModelViewSet::test_get_related_field_name_handles_formatted_link_segments[underscore]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[list_action-action_kwargs0-get]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[list_action-action_kwargs0-post]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[list_action-action_kwargs0-patch]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[list_action-action_kwargs0-delete]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[detail_action-action_kwargs1-get]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[detail_action-action_kwargs1-post]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[detail_action-action_kwargs1-patch]",
"tests/test_views.py::TestReadonlyModelViewSet::test_custom_action_allows_all_methods[detail_action-action_kwargs1-delete]",
"tests/test_views.py::TestAPIView::test_patch",
"tests/test_views.py::TestAPIView::test_post_with_missing_id",
"tests/test_views.py::TestAPIView::test_patch_with_custom_id"
] | 2024-04-18 19:46:32+00:00 | 1,927 |
|
django-money__django-money-594 | diff --git a/djmoney/money.py b/djmoney/money.py
index 70bea0b..b744c66 100644
--- a/djmoney/money.py
+++ b/djmoney/money.py
@@ -106,7 +106,6 @@ class Money(DefaultMoney):
# we overwrite the 'targets' so the wrong synonyms are called
# Example: we overwrite __add__; __radd__ calls __add__ on DefaultMoney...
__radd__ = __add__
- __rsub__ = __sub__
__rmul__ = __mul__
diff --git a/docs/changes.rst b/docs/changes.rst
index 2643b4d..8c0b272 100644
--- a/docs/changes.rst
+++ b/docs/changes.rst
@@ -15,6 +15,7 @@ Changelog
**Fixed**
- Pin ``pymoneyed<1.0`` as it changed the ``repr`` output of the ``Money`` class.
+- Subtracting ``Money`` from ``moneyed.Money``. Regression, introduced in ``1.2``. `#593`_
`1.2.2`_ - 2020-12-29
---------------------
@@ -694,6 +695,7 @@ wrapping with ``money_manager``.
.. _0.3: https://github.com/django-money/django-money/compare/0.2...0.3
.. _0.2: https://github.com/django-money/django-money/compare/0.2...a6d90348085332a393abb40b86b5dd9505489b04
+.. _#593: https://github.com/django-money/django-money/issues/593
.. _#586: https://github.com/django-money/django-money/issues/586
.. _#585: https://github.com/django-money/django-money/pull/585
.. _#583: https://github.com/django-money/django-money/issues/583
| django-money/django-money | bb222ed619f72a0112aef8c0fd9a4823b1e6e388 | diff --git a/tests/test_money.py b/tests/test_money.py
index 65199c4..bb7ea79 100644
--- a/tests/test_money.py
+++ b/tests/test_money.py
@@ -2,7 +2,7 @@ from django.utils.translation import override
import pytest
-from djmoney.money import Money, get_current_locale
+from djmoney.money import DefaultMoney, Money, get_current_locale
def test_repr():
@@ -114,3 +114,12 @@ def test_decimal_places_display_overwrite():
assert str(number) == "$1.23457"
number.decimal_places_display = None
assert str(number) == "$1.23"
+
+
+def test_sub_negative():
+ # See GH-593
+ total = DefaultMoney(0, "EUR")
+ bills = (Money(8, "EUR"), Money(25, "EUR"))
+ for bill in bills:
+ total -= bill
+ assert total == Money(-33, "EUR")
| Money should not override `__rsub__` method
As [reported](https://github.com/py-moneyed/py-moneyed/issues/144) in pymoneyed the following test case fails:
```python
from moneyed import Money as PyMoney
from djmoney.money import Money
def test_sub_negative():
total = PyMoney(0, "EUR")
bills = (Money(8, "EUR"), Money(25, "EUR"))
for bill in bills:
total -= bill
assert total == Money(-33, "EUR")
# AssertionError: assert <Money: -17 EUR> == <Money: -33 EUR>
```
This is caused by `djmoney.money.Money` overriding `__rsub__`. | 0.0 | [
"tests/test_money.py::test_sub_negative"
] | [
"tests/test_money.py::test_repr",
"tests/test_money.py::test_html_safe",
"tests/test_money.py::test_html_unsafe",
"tests/test_money.py::test_default_mul",
"tests/test_money.py::test_default_truediv",
"tests/test_money.py::test_reverse_truediv_fails",
"tests/test_money.py::test_get_current_locale[pl-PL_PL]",
"tests/test_money.py::test_get_current_locale[pl_PL-pl_PL]",
"tests/test_money.py::test_round",
"tests/test_money.py::test_configurable_decimal_number",
"tests/test_money.py::test_localize_decimal_places_default",
"tests/test_money.py::test_localize_decimal_places_overwrite",
"tests/test_money.py::test_localize_decimal_places_both",
"tests/test_money.py::test_add_decimal_places",
"tests/test_money.py::test_add_decimal_places_zero",
"tests/test_money.py::test_mul_decimal_places",
"tests/test_money.py::test_fix_decimal_places",
"tests/test_money.py::test_fix_decimal_places_none",
"tests/test_money.py::test_fix_decimal_places_multiple",
"tests/test_money.py::test_decimal_places_display_overwrite"
] | 2021-01-10 12:54:57+00:00 | 1,928 |
|
django-money__django-money-596 | diff --git a/djmoney/money.py b/djmoney/money.py
index b744c66..f109fdc 100644
--- a/djmoney/money.py
+++ b/djmoney/money.py
@@ -39,17 +39,27 @@ class Money(DefaultMoney):
""" Set number of digits being displayed - `None` resets to `DECIMAL_PLACES_DISPLAY` setting """
self._decimal_places_display = value
- def _fix_decimal_places(self, *args):
- """ Make sure to user 'biggest' number of decimal places of all given money instances """
- candidates = (getattr(candidate, "decimal_places", 0) for candidate in args)
- return max([self.decimal_places, *candidates])
+ def _copy_attributes(self, source, target):
+ """Copy attributes to the new `Money` instance.
+
+ This class stores extra bits of information about string formatting that the parent class doesn't have.
+ The problem is that the parent class creates new instances of `Money` without in some of its methods and
+ it does so without knowing about `django-money`-level attributes.
+ For this reason, when this class uses some methods of the parent class that have this behavior, the resulting
+ instances lose those attribute values.
+
+ When it comes to what number of decimal places to choose, we take the maximum number.
+ """
+ for attribute_name in ("decimal_places", "decimal_places_display"):
+ value = max([getattr(candidate, attribute_name, 0) for candidate in (self, source)])
+ setattr(target, attribute_name, value)
def __add__(self, other):
if isinstance(other, F):
return other.__radd__(self)
other = maybe_convert(other, self.currency)
result = super().__add__(other)
- result.decimal_places = self._fix_decimal_places(other)
+ self._copy_attributes(other, result)
return result
def __sub__(self, other):
@@ -57,14 +67,14 @@ class Money(DefaultMoney):
return other.__rsub__(self)
other = maybe_convert(other, self.currency)
result = super().__sub__(other)
- result.decimal_places = self._fix_decimal_places(other)
+ self._copy_attributes(other, result)
return result
def __mul__(self, other):
if isinstance(other, F):
return other.__rmul__(self)
result = super().__mul__(other)
- result.decimal_places = self._fix_decimal_places(other)
+ self._copy_attributes(other, result)
return result
def __truediv__(self, other):
@@ -72,11 +82,11 @@ class Money(DefaultMoney):
return other.__rtruediv__(self)
result = super().__truediv__(other)
if isinstance(result, self.__class__):
- result.decimal_places = self._fix_decimal_places(other)
+ self._copy_attributes(other, result)
return result
def __rtruediv__(self, other):
- # Backported from py-moneyd, non released bug-fix
+ # Backported from py-moneyed, non released bug-fix
# https://github.com/py-moneyed/py-moneyed/blob/c518745dd9d7902781409daec1a05699799474dd/moneyed/classes.py#L217-L218
raise TypeError("Cannot divide non-Money by a Money instance.")
@@ -100,7 +110,34 @@ class Money(DefaultMoney):
def __round__(self, n=None):
amount = round(self.amount, n)
- return self.__class__(amount, self.currency)
+ new = self.__class__(amount, self.currency)
+ self._copy_attributes(self, new)
+ return new
+
+ def round(self, ndigits=0):
+ new = super().round(ndigits)
+ self._copy_attributes(self, new)
+ return new
+
+ def __pos__(self):
+ new = super().__pos__()
+ self._copy_attributes(self, new)
+ return new
+
+ def __neg__(self):
+ new = super().__neg__()
+ self._copy_attributes(self, new)
+ return new
+
+ def __abs__(self):
+ new = super().__abs__()
+ self._copy_attributes(self, new)
+ return new
+
+ def __rmod__(self, other):
+ new = super().__rmod__(other)
+ self._copy_attributes(self, new)
+ return new
# DefaultMoney sets those synonym functions
# we overwrite the 'targets' so the wrong synonyms are called
diff --git a/docs/changes.rst b/docs/changes.rst
index 8c0b272..f526c12 100644
--- a/docs/changes.rst
+++ b/docs/changes.rst
@@ -16,6 +16,7 @@ Changelog
- Pin ``pymoneyed<1.0`` as it changed the ``repr`` output of the ``Money`` class.
- Subtracting ``Money`` from ``moneyed.Money``. Regression, introduced in ``1.2``. `#593`_
+- Missing the right ``Money.decimal_places`` and ``Money.decimal_places_display`` values after some arithmetic operations. `#595`_
`1.2.2`_ - 2020-12-29
---------------------
@@ -695,6 +696,7 @@ wrapping with ``money_manager``.
.. _0.3: https://github.com/django-money/django-money/compare/0.2...0.3
.. _0.2: https://github.com/django-money/django-money/compare/0.2...a6d90348085332a393abb40b86b5dd9505489b04
+.. _#595: https://github.com/django-money/django-money/issues/595
.. _#593: https://github.com/django-money/django-money/issues/593
.. _#586: https://github.com/django-money/django-money/issues/586
.. _#585: https://github.com/django-money/django-money/pull/585
| django-money/django-money | 9ac8d8b1f7060437bfb1912c4182cb0b553c1519 | diff --git a/tests/test_money.py b/tests/test_money.py
index bb7ea79..e736854 100644
--- a/tests/test_money.py
+++ b/tests/test_money.py
@@ -80,31 +80,31 @@ def test_add_decimal_places_zero():
assert result.decimal_places == 3
-def test_mul_decimal_places():
- """ Test __mul__ and __rmul__ """
- two = Money("1.0000", "USD", decimal_places=4)
-
- result = 2 * two
- assert result.decimal_places == 4
-
- result = two * 2
- assert result.decimal_places == 4
-
-
-def test_fix_decimal_places():
- one = Money(1, "USD", decimal_places=7)
- assert one._fix_decimal_places(Money(2, "USD", decimal_places=3)) == 7
- assert one._fix_decimal_places(Money(2, "USD", decimal_places=30)) == 30
-
-
-def test_fix_decimal_places_none():
- one = Money(1, "USD", decimal_places=7)
- assert one._fix_decimal_places(None) == 7
-
-
-def test_fix_decimal_places_multiple():
- one = Money(1, "USD", decimal_places=7)
- assert one._fix_decimal_places(None, Money(3, "USD", decimal_places=8)) == 8
+@pytest.mark.parametrize("decimal_places", (1, 4))
+@pytest.mark.parametrize(
+ "operation",
+ (
+ lambda a, d: a * 2,
+ lambda a, d: 2 * a,
+ lambda a, d: a / 5,
+ lambda a, d: a - Money("2", "USD", decimal_places=d, decimal_places_display=d),
+ lambda a, d: Money("2", "USD", decimal_places=d, decimal_places_display=d) - a,
+ lambda a, d: a + Money("2", "USD", decimal_places=d, decimal_places_display=d),
+ lambda a, d: Money("2", "USD", decimal_places=d, decimal_places_display=d) + a,
+ lambda a, d: -a,
+ lambda a, d: +a,
+ lambda a, d: abs(a),
+ lambda a, d: 5 % a,
+ lambda a, d: round(a),
+ lambda a, d: a.round(),
+ ),
+)
+def test_keep_decimal_places(operation, decimal_places):
+ # Arithmetic operations should keep the `decimal_places` value
+ amount = Money("1.0000", "USD", decimal_places=decimal_places, decimal_places_display=decimal_places)
+ new = operation(amount, decimal_places)
+ assert new.decimal_places == decimal_places
+ assert new.decimal_places_display == decimal_places
def test_decimal_places_display_overwrite():
| `Money.decimal_places` are not always the same after arithmetic operations
`djmoney.money.Money` uses some methods from `moneyed.Money`. In these methods (e.g. `__neg__`) new instances are created with only `amount` and `currency`. For this reason, our `django-money` specific attributes are not copied.
Example:
```python
amount = -Money(1, "USD", decimal_places=7)
amount.decimal_places # 2
``` | 0.0 | [
"tests/test_money.py::test_keep_decimal_places[<lambda>0-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>0-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>1-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>1-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>2-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>2-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>3-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>3-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>4-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>4-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>5-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>5-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>6-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>6-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>7-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>7-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>8-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>8-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>9-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>9-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>10-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>10-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>11-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>11-4]",
"tests/test_money.py::test_keep_decimal_places[<lambda>12-1]",
"tests/test_money.py::test_keep_decimal_places[<lambda>12-4]"
] | [
"tests/test_money.py::test_repr",
"tests/test_money.py::test_html_safe",
"tests/test_money.py::test_html_unsafe",
"tests/test_money.py::test_default_mul",
"tests/test_money.py::test_default_truediv",
"tests/test_money.py::test_reverse_truediv_fails",
"tests/test_money.py::test_get_current_locale[pl-PL_PL]",
"tests/test_money.py::test_get_current_locale[pl_PL-pl_PL]",
"tests/test_money.py::test_round",
"tests/test_money.py::test_configurable_decimal_number",
"tests/test_money.py::test_localize_decimal_places_default",
"tests/test_money.py::test_localize_decimal_places_overwrite",
"tests/test_money.py::test_localize_decimal_places_both",
"tests/test_money.py::test_add_decimal_places",
"tests/test_money.py::test_add_decimal_places_zero",
"tests/test_money.py::test_decimal_places_display_overwrite",
"tests/test_money.py::test_sub_negative"
] | 2021-01-10 14:14:52+00:00 | 1,929 |
|
django-money__django-money-604 | diff --git a/djmoney/models/fields.py b/djmoney/models/fields.py
index 8b812be..e24af66 100644
--- a/djmoney/models/fields.py
+++ b/djmoney/models/fields.py
@@ -35,7 +35,7 @@ def get_value(obj, expr):
else:
expr = expr.value
if isinstance(expr, OldMoney):
- expr.__class__ = Money
+ expr = Money(expr.amount, expr.currency)
return expr
@@ -206,7 +206,7 @@ class MoneyField(models.DecimalField):
elif isinstance(default, (float, Decimal, int)):
default = Money(default, default_currency)
elif isinstance(default, OldMoney):
- default.__class__ = Money
+ default = Money(default.amount, default.currency)
if default is not None and default is not NOT_PROVIDED and not isinstance(default, Money):
raise ValueError("default value must be an instance of Money, is: %s" % default)
return default
diff --git a/docs/changes.rst b/docs/changes.rst
index 1622376..49d3aa1 100644
--- a/docs/changes.rst
+++ b/docs/changes.rst
@@ -4,6 +4,10 @@ Changelog
`Unreleased`_ - TBD
-------------------
+**Fixed**
+
+- Do not mutate the input ``moneyed.Money`` class to ``djmoney.money.Money`` in ``MoneyField.default`` and F-expressions. `#603`_ (`moser`_)
+
`1.3`_ - 2021-01-10
-------------------
@@ -700,6 +704,7 @@ wrapping with ``money_manager``.
.. _0.3: https://github.com/django-money/django-money/compare/0.2...0.3
.. _0.2: https://github.com/django-money/django-money/compare/0.2...a6d90348085332a393abb40b86b5dd9505489b04
+.. _#603: https://github.com/django-money/django-money/issues/603
.. _#595: https://github.com/django-money/django-money/issues/595
.. _#593: https://github.com/django-money/django-money/issues/593
.. _#586: https://github.com/django-money/django-money/issues/586
@@ -852,6 +857,7 @@ wrapping with ``money_manager``.
.. _lobziik: https://github.com/lobziik
.. _mattions: https://github.com/mattions
.. _mithrilstar: https://github.com/mithrilstar
+.. _moser: https://github.com/moser
.. _MrFus10n: https://github.com/MrFus10n
.. _msgre: https://github.com/msgre
.. _mstarostik: https://github.com/mstarostik
| django-money/django-money | 8014bed7968665eb55cc298108fd1922667aec24 | diff --git a/tests/test_models.py b/tests/test_models.py
index d13ec03..3641356 100644
--- a/tests/test_models.py
+++ b/tests/test_models.py
@@ -83,6 +83,31 @@ class TestVanillaMoneyField:
retrieved = model_class.objects.get(pk=instance.pk)
assert retrieved.money == expected
+ def test_old_money_not_mutated_default(self):
+ # See GH-603
+ money = OldMoney(1, "EUR")
+
+ # When `moneyed.Money` is passed as a default value to a model
+ class Model(models.Model):
+ price = MoneyField(default=money, max_digits=10, decimal_places=2)
+
+ class Meta:
+ abstract = True
+
+ # Its class should remain the same
+ assert type(money) is OldMoney
+
+ def test_old_money_not_mutated_f_object(self):
+ # See GH-603
+ money = OldMoney(1, "EUR")
+
+ instance = ModelWithVanillaMoneyField.objects.create(money=Money(5, "EUR"), integer=2)
+ # When `moneyed.Money` is a part of an F-expression
+ instance.money = F("money") + money
+
+ # Its class should remain the same
+ assert type(money) is OldMoney
+
def test_old_money_defaults(self):
instance = ModelWithDefaultAsOldMoney.objects.create()
assert instance.money == Money(".01", "RUB")
| djmoney alters existing objects
We have an application that uses `moneyed` and (sub-classes of) its `Money` type in a non-Django environment. We recently started integrating this into a Django app that uses djmoney and started getting weird errors in seemingly unrelated cases because instances of `djmoney.money.Money` started showing up there.
We tracked this down to a behavior of the djmoney package in `djmoney.models.fields`:
https://github.com/django-money/django-money/blob/bb222ed619f72a0112aef8c0fd9a4823b1e6e388/djmoney/models/fields.py#L38
https://github.com/django-money/django-money/blob/bb222ed619f72a0112aef8c0fd9a4823b1e6e388/djmoney/models/fields.py#L209
The two places alter **the type of an existing object**. Can we change this to copying the objects before altering them? | 0.0 | [
"tests/test_models.py::TestVanillaMoneyField::test_old_money_not_mutated_default",
"tests/test_models.py::TestVanillaMoneyField::test_old_money_not_mutated_f_object"
] | [
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithVanillaMoneyField-kwargs0-expected0]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithVanillaMoneyField-kwargs1-expected1]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs2-expected2]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs3-expected3]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs4-expected4]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs5-expected5]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs6-expected6]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs7-expected7]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsMoney-kwargs8-expected8]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsFloat-kwargs9-expected9]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsStringWithCurrency-kwargs10-expected10]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsString-kwargs11-expected11]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsInt-kwargs12-expected12]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsDecimal-kwargs13-expected13]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[CryptoModel-kwargs14-expected14]",
"tests/test_models.py::TestVanillaMoneyField::test_old_money_defaults",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithVanillaMoneyField-other_value0]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[BaseModel-other_value1]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithDefaultAsMoney-other_value2]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithDefaultAsFloat-other_value3]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithDefaultAsFloat-other_value4]",
"tests/test_models.py::TestVanillaMoneyField::test_invalid_values[value0]",
"tests/test_models.py::TestVanillaMoneyField::test_invalid_values[value1]",
"tests/test_models.py::TestVanillaMoneyField::test_invalid_values[value2]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[money-Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[money-Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[second_money-Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[second_money-Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_rounding",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters0-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters1-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters3-0]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters6-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters7-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters8-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters9-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters10-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters0-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters1-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters3-0]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters6-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters7-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters8-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters9-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters10-2]",
"tests/test_models.py::TestVanillaMoneyField::test_date_lookup",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-startswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-regex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-iregex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-istartswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-contains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-lt-5-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-endswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-iendswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-gte-4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-iexact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-exact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-isnull-True-0]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-range-rhs12-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-lte-2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-gt-3-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-icontains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-in-rhs16-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-startswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-regex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-iregex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-istartswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-contains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-lt-5-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-endswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-iendswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-gte-4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-iexact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-exact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-isnull-True-0]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-range-rhs12-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-lte-2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-gt-3-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-icontains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-in-rhs16-1]",
"tests/test_models.py::TestVanillaMoneyField::test_exact_match",
"tests/test_models.py::TestVanillaMoneyField::test_issue_300_regression",
"tests/test_models.py::TestVanillaMoneyField::test_range_search",
"tests/test_models.py::TestVanillaMoneyField::test_filter_chaining",
"tests/test_models.py::TestVanillaMoneyField::test_currency_querying[ModelWithVanillaMoneyField]",
"tests/test_models.py::TestVanillaMoneyField::test_currency_querying[ModelWithChoicesMoneyField]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup[Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup[Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup_f_expression[Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup_f_expression[Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_isnull_lookup",
"tests/test_models.py::TestVanillaMoneyField::test_null_default",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithVanillaMoneyField-money-kwargs0-PLN]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithVanillaMoneyField-money-kwargs1-EUR]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithVanillaMoneyField-money-kwargs2-EUR]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithSharedCurrency-first-kwargs3-CZK]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_defaults",
"tests/test_models.py::TestGetOrCreate::test_defaults",
"tests/test_models.py::TestGetOrCreate::test_currency_field_lookup",
"tests/test_models.py::TestGetOrCreate::test_no_default_model[NullMoneyFieldModel-create_kwargs0-get_kwargs0]",
"tests/test_models.py::TestGetOrCreate::test_no_default_model[ModelWithSharedCurrency-create_kwargs1-get_kwargs1]",
"tests/test_models.py::TestGetOrCreate::test_shared_currency",
"tests/test_models.py::TestNullableCurrency::test_create_nullable",
"tests/test_models.py::TestNullableCurrency::test_create_default",
"tests/test_models.py::TestNullableCurrency::test_fails_with_null_currency",
"tests/test_models.py::TestNullableCurrency::test_fails_with_nullable_but_no_default",
"tests/test_models.py::TestNullableCurrency::test_query_not_null",
"tests/test_models.py::TestNullableCurrency::test_query_null",
"tests/test_models.py::TestFExpressions::test_save[f_obj0-expected0]",
"tests/test_models.py::TestFExpressions::test_save[f_obj1-expected1]",
"tests/test_models.py::TestFExpressions::test_save[f_obj2-expected2]",
"tests/test_models.py::TestFExpressions::test_save[f_obj3-expected3]",
"tests/test_models.py::TestFExpressions::test_save[f_obj4-expected4]",
"tests/test_models.py::TestFExpressions::test_save[f_obj5-expected5]",
"tests/test_models.py::TestFExpressions::test_save[f_obj6-expected6]",
"tests/test_models.py::TestFExpressions::test_save[f_obj7-expected7]",
"tests/test_models.py::TestFExpressions::test_save[f_obj8-expected8]",
"tests/test_models.py::TestFExpressions::test_save[f_obj9-expected9]",
"tests/test_models.py::TestFExpressions::test_save[f_obj10-expected10]",
"tests/test_models.py::TestFExpressions::test_save[f_obj11-expected11]",
"tests/test_models.py::TestFExpressions::test_save[f_obj12-expected12]",
"tests/test_models.py::TestFExpressions::test_save[f_obj13-expected13]",
"tests/test_models.py::TestFExpressions::test_save[f_obj14-expected14]",
"tests/test_models.py::TestFExpressions::test_save[f_obj15-expected15]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj0-expected0]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj1-expected1]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj2-expected2]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj3-expected3]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj4-expected4]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj5-expected5]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj6-expected6]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj7-expected7]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj8-expected8]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj9-expected9]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj10-expected10]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj11-expected11]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj12-expected12]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj13-expected13]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj14-expected14]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj15-expected15]",
"tests/test_models.py::TestFExpressions::test_default_update",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs0-filter_value0-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs1-filter_value1-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs2-filter_value2-False]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs3-filter_value3-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs4-filter_value4-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs5-filter_value5-False]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs6-filter_value6-False]",
"tests/test_models.py::TestFExpressions::test_update_fields_save",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj0]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj1]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj2]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj3]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj4]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj5]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj6]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj7]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj8]",
"tests/test_models.py::TestExpressions::test_bulk_update",
"tests/test_models.py::TestExpressions::test_conditional_update",
"tests/test_models.py::TestExpressions::test_create_func",
"tests/test_models.py::TestExpressions::test_value_create[None-None]",
"tests/test_models.py::TestExpressions::test_value_create[10-expected1]",
"tests/test_models.py::TestExpressions::test_value_create[value2-expected2]",
"tests/test_models.py::TestExpressions::test_value_create_invalid",
"tests/test_models.py::TestExpressions::test_expressions_for_non_money_fields",
"tests/test_models.py::test_find_models_related_to_money_models",
"tests/test_models.py::test_allow_expression_nodes_without_money",
"tests/test_models.py::test_base_model",
"tests/test_models.py::TestInheritance::test_model[InheritedModel]",
"tests/test_models.py::TestInheritance::test_model[InheritorModel]",
"tests/test_models.py::TestInheritance::test_fields[InheritedModel]",
"tests/test_models.py::TestInheritance::test_fields[InheritorModel]",
"tests/test_models.py::TestManager::test_manager",
"tests/test_models.py::TestManager::test_objects_creation",
"tests/test_models.py::TestProxyModel::test_instances",
"tests/test_models.py::TestProxyModel::test_patching",
"tests/test_models.py::TestDifferentCurrencies::test_add_default",
"tests/test_models.py::TestDifferentCurrencies::test_sub_default",
"tests/test_models.py::TestDifferentCurrencies::test_eq",
"tests/test_models.py::TestDifferentCurrencies::test_ne",
"tests/test_models.py::TestDifferentCurrencies::test_ne_currency",
"tests/test_models.py::test_manager_instance_access[ModelWithNonMoneyField]",
"tests/test_models.py::test_manager_instance_access[InheritorModel]",
"tests/test_models.py::test_manager_instance_access[InheritedModel]",
"tests/test_models.py::test_manager_instance_access[ProxyModel]",
"tests/test_models.py::test_different_hashes",
"tests/test_models.py::test_migration_serialization",
"tests/test_models.py::test_clear_meta_cache[ModelWithVanillaMoneyField-objects]",
"tests/test_models.py::test_clear_meta_cache[ModelWithCustomDefaultManager-custom]",
"tests/test_models.py::TestFieldAttributes::test_missing_attributes",
"tests/test_models.py::TestFieldAttributes::test_default_currency",
"tests/test_models.py::TestCustomManager::test_method",
"tests/test_models.py::test_package_is_importable",
"tests/test_models.py::test_hash_uniqueness",
"tests/test_models.py::test_override_decorator",
"tests/test_models.py::TestSharedCurrency::test_attributes",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_match[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_match[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_no_match[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_no_match[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_f_query[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_f_query[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_in_lookup[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_in_lookup[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_create_with_money",
"tests/test_models.py::test_order_by",
"tests/test_models.py::test_distinct_through_wrapper",
"tests/test_models.py::test_mixer_blend"
] | 2021-02-02 14:03:40+00:00 | 1,930 |
|
django-money__django-money-647 | diff --git a/djmoney/models/fields.py b/djmoney/models/fields.py
index 9b7195e..9d42575 100644
--- a/djmoney/models/fields.py
+++ b/djmoney/models/fields.py
@@ -298,8 +298,11 @@ class MoneyField(models.DecimalField):
if self._has_default:
kwargs["default"] = self.default.amount
- if self.default_currency is not None and self.default_currency != DEFAULT_CURRENCY:
- kwargs["default_currency"] = str(self.default_currency)
+ if self.default_currency != DEFAULT_CURRENCY:
+ if self.default_currency is not None:
+ kwargs["default_currency"] = str(self.default_currency)
+ else:
+ kwargs["default_currency"] = None
if self.currency_choices != CURRENCY_CHOICES:
kwargs["currency_choices"] = self.currency_choices
if self.currency_field_name:
| django-money/django-money | 2040eff83fef2d4a1d882c016ab2b5e0853d2f84 | diff --git a/tests/test_models.py b/tests/test_models.py
index f573ed3..506fac4 100644
--- a/tests/test_models.py
+++ b/tests/test_models.py
@@ -787,3 +787,11 @@ def test_mixer_blend():
instance = mixer.blend(ModelWithTwoMoneyFields)
assert isinstance(instance.amount1, Money)
assert isinstance(instance.amount2, Money)
+
+
+def test_deconstruct_includes_default_currency_as_none():
+ instance = ModelWithNullableCurrency()._meta.get_field("money")
+ __, ___, args, kwargs = instance.deconstruct()
+ new = MoneyField(*args, **kwargs)
+ assert new.default_currency == instance.default_currency
+ assert new.default_currency is None
| default_currency=None keeps detecting migration changes
Based on the comment in the discussion found here: https://github.com/django-money/django-money/issues/530#issuecomment-650166087
If `MoneyField(..., default_currency=None)` is added to an already existing model, so that the field is triggering migration operations `AddField` or `AlterField`. Django keeps detecting changes on the field/model when running `python manage.py makemigrations`. | 0.0 | [
"tests/test_models.py::test_deconstruct_includes_default_currency_as_none"
] | [
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithVanillaMoneyField-kwargs0-expected0]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithVanillaMoneyField-kwargs1-expected1]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs2-expected2]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs3-expected3]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs4-expected4]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs5-expected5]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs6-expected6]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[BaseModel-kwargs7-expected7]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsMoney-kwargs8-expected8]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsFloat-kwargs9-expected9]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsStringWithCurrency-kwargs10-expected10]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsString-kwargs11-expected11]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsInt-kwargs12-expected12]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[ModelWithDefaultAsDecimal-kwargs13-expected13]",
"tests/test_models.py::TestVanillaMoneyField::test_create_defaults[CryptoModel-kwargs14-expected14]",
"tests/test_models.py::TestVanillaMoneyField::test_old_money_not_mutated_default",
"tests/test_models.py::TestVanillaMoneyField::test_old_money_not_mutated_f_object",
"tests/test_models.py::TestVanillaMoneyField::test_old_money_defaults",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithVanillaMoneyField-other_value0]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[BaseModel-other_value1]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithDefaultAsMoney-other_value2]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithDefaultAsFloat-other_value3]",
"tests/test_models.py::TestVanillaMoneyField::test_revert_to_default[ModelWithDefaultAsFloat-other_value4]",
"tests/test_models.py::TestVanillaMoneyField::test_invalid_values[value0]",
"tests/test_models.py::TestVanillaMoneyField::test_invalid_values[value1]",
"tests/test_models.py::TestVanillaMoneyField::test_invalid_values[value2]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[money-Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[money-Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[second_money-Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_save_new_value[second_money-Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_rounding",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters0-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters1-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters3-0]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters6-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters7-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters8-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters9-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money0-filters10-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters0-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters1-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters3-0]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters6-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters7-3]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters8-2]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters9-1]",
"tests/test_models.py::TestVanillaMoneyField::test_comparison_lookup[Money1-filters10-2]",
"tests/test_models.py::TestVanillaMoneyField::test_date_lookup",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-startswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-regex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-iregex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-istartswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-contains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-lt-5-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-endswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-iendswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-gte-4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-iexact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-exact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-isnull-True-0]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-range-rhs12-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-lte-2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-gt-3-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-icontains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money0-in-rhs16-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-startswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-regex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-iregex-^[134]-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-istartswith-2-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-contains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-lt-5-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-endswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-iendswith-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-gte-4-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-iexact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-exact-3-1]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-isnull-True-0]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-range-rhs12-4]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-lte-2-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-gt-3-3]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-icontains-5-2]",
"tests/test_models.py::TestVanillaMoneyField::test_all_lookups[Money1-in-rhs16-1]",
"tests/test_models.py::TestVanillaMoneyField::test_exact_match",
"tests/test_models.py::TestVanillaMoneyField::test_issue_300_regression",
"tests/test_models.py::TestVanillaMoneyField::test_range_search",
"tests/test_models.py::TestVanillaMoneyField::test_filter_chaining",
"tests/test_models.py::TestVanillaMoneyField::test_currency_querying[ModelWithVanillaMoneyField]",
"tests/test_models.py::TestVanillaMoneyField::test_currency_querying[ModelWithChoicesMoneyField]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup[Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup[Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup_f_expression[Money0]",
"tests/test_models.py::TestVanillaMoneyField::test_in_lookup_f_expression[Money1]",
"tests/test_models.py::TestVanillaMoneyField::test_isnull_lookup",
"tests/test_models.py::TestVanillaMoneyField::test_null_default",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithVanillaMoneyField-money-kwargs0-PLN]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithVanillaMoneyField-money-kwargs1-EUR]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithVanillaMoneyField-money-kwargs2-EUR]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_currency[ModelWithSharedCurrency-first-kwargs3-CZK]",
"tests/test_models.py::TestGetOrCreate::test_get_or_create_respects_defaults",
"tests/test_models.py::TestGetOrCreate::test_defaults",
"tests/test_models.py::TestGetOrCreate::test_currency_field_lookup",
"tests/test_models.py::TestGetOrCreate::test_no_default_model[NullMoneyFieldModel-create_kwargs0-get_kwargs0]",
"tests/test_models.py::TestGetOrCreate::test_no_default_model[ModelWithSharedCurrency-create_kwargs1-get_kwargs1]",
"tests/test_models.py::TestGetOrCreate::test_shared_currency",
"tests/test_models.py::TestNullableCurrency::test_create_nullable",
"tests/test_models.py::TestNullableCurrency::test_create_default",
"tests/test_models.py::TestNullableCurrency::test_fails_with_null_currency",
"tests/test_models.py::TestNullableCurrency::test_fails_with_nullable_but_no_default",
"tests/test_models.py::TestNullableCurrency::test_query_not_null",
"tests/test_models.py::TestNullableCurrency::test_query_null",
"tests/test_models.py::TestFExpressions::test_save[f_obj0-expected0]",
"tests/test_models.py::TestFExpressions::test_save[f_obj1-expected1]",
"tests/test_models.py::TestFExpressions::test_save[f_obj2-expected2]",
"tests/test_models.py::TestFExpressions::test_save[f_obj3-expected3]",
"tests/test_models.py::TestFExpressions::test_save[f_obj4-expected4]",
"tests/test_models.py::TestFExpressions::test_save[f_obj5-expected5]",
"tests/test_models.py::TestFExpressions::test_save[f_obj6-expected6]",
"tests/test_models.py::TestFExpressions::test_save[f_obj7-expected7]",
"tests/test_models.py::TestFExpressions::test_save[f_obj8-expected8]",
"tests/test_models.py::TestFExpressions::test_save[f_obj9-expected9]",
"tests/test_models.py::TestFExpressions::test_save[f_obj10-expected10]",
"tests/test_models.py::TestFExpressions::test_save[f_obj11-expected11]",
"tests/test_models.py::TestFExpressions::test_save[f_obj12-expected12]",
"tests/test_models.py::TestFExpressions::test_save[f_obj13-expected13]",
"tests/test_models.py::TestFExpressions::test_save[f_obj14-expected14]",
"tests/test_models.py::TestFExpressions::test_save[f_obj15-expected15]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj0-expected0]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj1-expected1]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj2-expected2]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj3-expected3]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj4-expected4]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj5-expected5]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj6-expected6]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj7-expected7]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj8-expected8]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj9-expected9]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj10-expected10]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj11-expected11]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj12-expected12]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj13-expected13]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj14-expected14]",
"tests/test_models.py::TestFExpressions::test_f_update[f_obj15-expected15]",
"tests/test_models.py::TestFExpressions::test_default_update",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs0-filter_value0-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs1-filter_value1-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs2-filter_value2-False]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs3-filter_value3-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs4-filter_value4-True]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs5-filter_value5-False]",
"tests/test_models.py::TestFExpressions::test_filtration[create_kwargs6-filter_value6-False]",
"tests/test_models.py::TestFExpressions::test_update_fields_save",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj0]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj1]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj2]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj3]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj4]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj5]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj6]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj7]",
"tests/test_models.py::TestFExpressions::test_invalid_expressions_access[f_obj8]",
"tests/test_models.py::TestExpressions::test_bulk_update",
"tests/test_models.py::TestExpressions::test_conditional_update",
"tests/test_models.py::TestExpressions::test_create_func",
"tests/test_models.py::TestExpressions::test_value_create[None-None]",
"tests/test_models.py::TestExpressions::test_value_create[10-expected1]",
"tests/test_models.py::TestExpressions::test_value_create[value2-expected2]",
"tests/test_models.py::TestExpressions::test_value_create_invalid",
"tests/test_models.py::TestExpressions::test_expressions_for_non_money_fields",
"tests/test_models.py::test_find_models_related_to_money_models",
"tests/test_models.py::test_allow_expression_nodes_without_money",
"tests/test_models.py::test_base_model",
"tests/test_models.py::TestInheritance::test_model[InheritedModel]",
"tests/test_models.py::TestInheritance::test_model[InheritorModel]",
"tests/test_models.py::TestInheritance::test_fields[InheritedModel]",
"tests/test_models.py::TestInheritance::test_fields[InheritorModel]",
"tests/test_models.py::TestManager::test_manager",
"tests/test_models.py::TestManager::test_objects_creation",
"tests/test_models.py::TestProxyModel::test_instances",
"tests/test_models.py::TestProxyModel::test_patching",
"tests/test_models.py::TestDifferentCurrencies::test_add_default",
"tests/test_models.py::TestDifferentCurrencies::test_sub_default",
"tests/test_models.py::TestDifferentCurrencies::test_eq",
"tests/test_models.py::TestDifferentCurrencies::test_ne",
"tests/test_models.py::TestDifferentCurrencies::test_ne_currency",
"tests/test_models.py::test_manager_instance_access[ModelWithNonMoneyField]",
"tests/test_models.py::test_manager_instance_access[InheritorModel]",
"tests/test_models.py::test_manager_instance_access[InheritedModel]",
"tests/test_models.py::test_manager_instance_access[ProxyModel]",
"tests/test_models.py::test_different_hashes",
"tests/test_models.py::test_migration_serialization",
"tests/test_models.py::test_clear_meta_cache[ModelWithVanillaMoneyField-objects]",
"tests/test_models.py::test_clear_meta_cache[ModelWithCustomDefaultManager-custom]",
"tests/test_models.py::TestFieldAttributes::test_missing_attributes",
"tests/test_models.py::TestFieldAttributes::test_default_currency",
"tests/test_models.py::TestCustomManager::test_method",
"tests/test_models.py::test_package_is_importable",
"tests/test_models.py::test_hash_uniqueness",
"tests/test_models.py::test_override_decorator",
"tests/test_models.py::TestSharedCurrency::test_attributes",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_match[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_match[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_no_match[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_filter_by_money_no_match[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_f_query[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_f_query[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_in_lookup[args0-kwargs0]",
"tests/test_models.py::TestSharedCurrency::test_in_lookup[args1-kwargs1]",
"tests/test_models.py::TestSharedCurrency::test_create_with_money",
"tests/test_models.py::test_order_by",
"tests/test_models.py::test_distinct_through_wrapper",
"tests/test_models.py::test_mixer_blend"
] | 2021-12-27 14:25:56+00:00 | 1,931 |
|
django-money__django-money-689 | diff --git a/djmoney/contrib/exchange/models.py b/djmoney/contrib/exchange/models.py
index 4d97846..7963967 100644
--- a/djmoney/contrib/exchange/models.py
+++ b/djmoney/contrib/exchange/models.py
@@ -40,6 +40,8 @@ def get_rate(source, target, backend=None):
Converts exchange rate on the DB side if there is no backends with given base currency.
Uses data from the default backend if the backend is not specified.
"""
+ if str(source) == str(target):
+ return 1
if backend is None:
backend = get_default_backend_name()
key = f"djmoney:get_rate:{source}:{target}:{backend}"
@@ -53,8 +55,6 @@ def get_rate(source, target, backend=None):
def _get_rate(source, target, backend):
source, target = str(source), str(target)
- if str(source) == target:
- return 1
rates = Rate.objects.filter(currency__in=(source, target), backend=backend).select_related("backend")
if not rates:
raise MissingRate(f"Rate {source} -> {target} does not exist")
| django-money/django-money | 94f279562b252a0ce631d3929534a68f83e93711 | diff --git a/tests/contrib/exchange/test_model.py b/tests/contrib/exchange/test_model.py
index 4bd6b75..4d37c78 100644
--- a/tests/contrib/exchange/test_model.py
+++ b/tests/contrib/exchange/test_model.py
@@ -64,8 +64,10 @@ def test_string_representation(backend):
def test_cache():
with patch("djmoney.contrib.exchange.models._get_rate", wraps=_get_rate) as original:
assert get_rate("USD", "USD") == 1
+ assert original.call_count == 0
+ assert get_rate("USD", "EUR") == 2
assert original.call_count == 1
- assert get_rate("USD", "USD") == 1
+ assert get_rate("USD", "EUR") == 2
assert original.call_count == 1
diff --git a/tests/contrib/test_django_rest_framework.py b/tests/contrib/test_django_rest_framework.py
index 4a7e4a2..5372a91 100644
--- a/tests/contrib/test_django_rest_framework.py
+++ b/tests/contrib/test_django_rest_framework.py
@@ -106,7 +106,7 @@ class TestMoneyField:
def test_serializer_with_fields(self):
serializer = self.get_serializer(ModelWithVanillaMoneyField, data={"money": "10.00"}, fields_=("money",))
- serializer.is_valid(True)
+ serializer.is_valid(raise_exception=True)
assert serializer.data == {"money": "10.00"}
@pytest.mark.parametrize(
| calling get_rate with identical source and target currency should be a noop
- it is always exactly 1
- that doesn't even have to hit the cache ;) | 0.0 | [
"tests/contrib/exchange/test_model.py::test_cache"
] | [
"tests/contrib/exchange/test_model.py::test_unknown_currency_with_partially_exiting_currencies[NOK-ZAR]",
"tests/contrib/exchange/test_model.py::test_unknown_currency_with_partially_exiting_currencies[USD-ZAR]",
"tests/contrib/exchange/test_model.py::test_get_rate[EUR-USD-expected2-1]",
"tests/contrib/exchange/test_model.py::test_bad_configuration",
"tests/contrib/exchange/test_model.py::test_rates_via_base[SEK-NOK-expected1]",
"tests/contrib/exchange/test_model.py::test_unknown_currency[SEK-ZWL]",
"tests/contrib/exchange/test_model.py::test_rates_via_base[NOK-SEK-expected0]",
"tests/contrib/exchange/test_model.py::test_without_installed_exchange",
"tests/contrib/exchange/test_model.py::test_get_rate[source3-USD-1-0]",
"tests/contrib/exchange/test_model.py::test_get_rate[USD-USD-1-0]",
"tests/contrib/exchange/test_model.py::test_unknown_currency_with_partially_exiting_currencies[ZAR-USD]",
"tests/contrib/exchange/test_model.py::test_get_rate[USD-target4-1-0]",
"tests/contrib/exchange/test_model.py::test_get_rate[USD-EUR-2-1]",
"tests/contrib/exchange/test_model.py::test_string_representation",
"tests/contrib/exchange/test_model.py::test_unknown_currency_with_partially_exiting_currencies[ZAR-NOK]",
"tests/contrib/exchange/test_model.py::test_unknown_currency[USD-EUR]"
] | 2022-09-27 10:03:46+00:00 | 1,932 |
|
django-treebeard__django-treebeard-186 | diff --git a/CHANGES b/CHANGES
index 4b8acb7..ef16948 100644
--- a/CHANGES
+++ b/CHANGES
@@ -1,3 +1,13 @@
+Release 4.4 (Oct 26, 2020)
+----------------------------
+
+* Implement a non-destructive path-fixing algorithm for ``MP_Node.fix_tree``.
+* Ensure ``post_save`` is triggered *after* the parent node is updated in ``MP_AddChildHandler``.
+* Fix static URL generation to use ``static`` template tag instead of constructing the URL manually.
+* Declare support for Django 2.2, 3.0 and 3.1.
+* Drop support for Django 2.1 and lower.
+* Drop support for Python 2.7 and Python 3.5.
+
Release 4.3.1 (Dec 25, 2019)
----------------------------
@@ -221,7 +231,7 @@ New features added
- script to build documentation
- updated numconv.py
-
+
Bugs fixed
~~~~~~~~~~
@@ -230,7 +240,7 @@ Bugs fixed
Solves bug in postgres when the table isn't created by syncdb.
* Removing unused method NS_Node._find_next_node
-
+
* Fixed MP_Node.get_tree to include the given parent when given a leaf node
diff --git a/README.rst b/README.rst
index 8e31be6..688ea51 100644
--- a/README.rst
+++ b/README.rst
@@ -16,6 +16,9 @@ Status
.. image:: https://travis-ci.org/django-treebeard/django-treebeard.svg?branch=master
:target: https://travis-ci.org/django-treebeard/django-treebeard
+.. image:: https://ci.appveyor.com/api/projects/status/mwbf062v68lhw05c?svg=true
+ :target: https://ci.appveyor.com/project/mvantellingen/django-treebeard
+
.. image:: https://img.shields.io/pypi/v/django-treebeard.svg
:target: https://pypi.org/project/django-treebeard/
diff --git a/appveyor.yml b/appveyor.yml
index 5ee9a8f..807ce95 100644
--- a/appveyor.yml
+++ b/appveyor.yml
@@ -1,7 +1,25 @@
-# Do a dummy build so that AppVeyor doesn't fail
-# while we're waiting for it to be disconnected altogether
+services:
+ - mssql2016
-branches:
- only: []
+environment:
+ matrix:
+ - TOXENV: py36-dj22-mssql
+ - TOXENV: py37-dj22-mssql
+ - TOXENV: py38-dj22-mssql
+ - TOXENV: py36-dj30-mssql
+ - TOXENV: py37-dj30-mssql
+ - TOXENV: py38-dj30-mssql
+ - TOXENV: py36-dj31-mssql
+ - TOXENV: py37-dj31-mssql
+ - TOXENV: py38-dj31-mssql
-build: false # i.e. do nut run msbuild
+matrix:
+ fast_finish: true
+
+install:
+ - C:\Python36\python -m pip install tox
+
+build: false # Not a C# project
+
+test_script:
+ - C:\Python36\scripts\tox
diff --git a/tox.ini b/tox.ini
index d909363..2105bd3 100644
--- a/tox.ini
+++ b/tox.ini
@@ -6,7 +6,7 @@
[tox]
envlist =
- py{36,37,38}-dj{22,30,31}-{sqlite,postgres,mysql}
+ py{36,37,38}-dj{22,30,31}-{sqlite,postgres,mysql,mssql}
[testenv:docs]
basepython = python
@@ -25,9 +25,11 @@ deps =
dj31: Django>=3.1,<3.2
postgres: psycopg2>=2.6
mysql: mysqlclient>=1.3.9
+ mssql: django-mssql-backend>=2.8.1
setenv =
sqlite: DATABASE_ENGINE=sqlite
postgres: DATABASE_ENGINE=psql
mysql: DATABASE_ENGINE=mysql
+ mssql: DATABASE_ENGINE=mssql
commands = pytest
diff --git a/treebeard/__init__.py b/treebeard/__init__.py
index 00d37f5..80f4727 100644
--- a/treebeard/__init__.py
+++ b/treebeard/__init__.py
@@ -10,10 +10,13 @@ Release logic:
5. git push
6. assure that all tests pass on https://travis-ci.org/django-treebeard/django-treebeard/builds/
7. git push --tags
- 8. python setup.py sdist upload
- 9. bump the version, append ".dev0" to __version__
-10. git add treebeard/__init__.py
-11. git commit -m 'Start with <version>'
-12. git push
+ 8. pip install --upgrade pip wheel twine
+ 9. python setup.py clean --all
+ 9. python setup.py sdist bdist_wheel
+10. twine upload dist/*
+11. bump the version, append ".dev0" to __version__
+12. git add treebeard/__init__.py
+13. git commit -m 'Start with <version>'
+14. git push
"""
-__version__ = '4.3.1'
+__version__ = '4.4.0'
diff --git a/treebeard/forms.py b/treebeard/forms.py
index 4f9ef11..547c6d9 100644
--- a/treebeard/forms.py
+++ b/treebeard/forms.py
@@ -178,11 +178,8 @@ class MoveNodeForm(forms.ModelForm):
def add_subtree(cls, for_node, node, options):
""" Recursively build options tree. """
if cls.is_loop_safe(for_node, node):
- options.append(
- (node.pk,
- mark_safe(cls.mk_indent(node.get_depth()) + escape(node))))
- for subnode in node.get_children():
- cls.add_subtree(for_node, subnode, options)
+ for item, _ in node.get_annotated_list(node):
+ options.append((item.pk, mark_safe(cls.mk_indent(item.get_depth()) + escape(item))))
@classmethod
def mk_dropdown_tree(cls, model, for_node=None):
| django-treebeard/django-treebeard | c3abc0c812da30a9ab5b2a8bcf976c860a44ee95 | diff --git a/treebeard/tests/settings.py b/treebeard/tests/settings.py
index c8a2745..47cc1c8 100644
--- a/treebeard/tests/settings.py
+++ b/treebeard/tests/settings.py
@@ -36,6 +36,19 @@ def get_db_conf():
'HOST': '127.0.0.1',
'PORT': '',
}
+ elif database_engine == "mssql":
+ return {
+ 'ENGINE': 'sql_server.pyodbc',
+ 'NAME': 'master',
+ 'USER': 'sa',
+ 'PASSWORD': 'Password12!',
+ 'HOST': '(local)\\SQL2016',
+ 'PORT': '',
+ 'OPTIONS': {
+ 'driver': 'SQL Server Native Client 11.0',
+ 'MARS_Connection': 'True',
+ },
+ }
DATABASES = {'default': get_db_conf()}
SECRET_KEY = '7r33b34rd'
diff --git a/treebeard/tests/test_treebeard.py b/treebeard/tests/test_treebeard.py
index 03e7619..c68963d 100644
--- a/treebeard/tests/test_treebeard.py
+++ b/treebeard/tests/test_treebeard.py
@@ -2164,8 +2164,8 @@ class TestMoveNodeForm(TestNonEmptyTree):
def test_form_leaf_node(self, model):
nodes = list(model.get_tree())
- node = nodes.pop()
safe_parent_nodes = self._get_node_ids_and_depths(nodes)
+ node = nodes.pop()
self._move_node_helper(node, safe_parent_nodes)
def test_form_admin(self, model):
@@ -2725,3 +2725,16 @@ class TestTreeAdmin(TestNonEmptyTree):
('4', 1, 1),
('41', 2, 0)]
assert self.got(model) == expected
+
+
+class TestMPFormPerformance(TestCase):
+ @classmethod
+ def setup_class(cls):
+ cls.model = models.MP_TestNode
+ cls.model.load_bulk(BASE_DATA)
+
+ def test_form_add_subtree_no_of_queries(self):
+ form_class = movenodeform_factory(self.model)
+ form = form_class()
+ with self.assertNumQueries(len(self.model.get_root_nodes()) + 1):
+ form.mk_dropdown_tree(self.model)
| Publish releases as wheels?
I see on PyPI that the package’s releases only contain source distribution, for example for the latest [4.3.1](https://pypi.org/project/django-treebeard/4.3.1/#files). Would it be possible to additionally publish wheels?
The project is already set up for universal wheels since #130, and will switch to Python3-only wheels once #153 is merged – so I think this should just be a matter of adding `bdist_wheel` to the release process as described here: https://github.com/django-treebeard/django-treebeard/blob/3a8062cf4584336cc63551f332e9926e1af207ed/treebeard/__init__.py#L14
More general info on wheels: https://pythonwheels.com/. For me I’m particularly interested in speeding up installations. | 0.0 | [
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_leaf_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_leaf_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_leaf_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_leaf_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_leaf_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_leaf_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMPFormPerformance::test_form_add_subtree_no_of_queries"
] | [
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_load_bulk_empty[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_load_bulk_empty[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_load_bulk_empty[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_load_bulk_empty[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_load_bulk_empty[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_load_bulk_empty[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_dump_bulk_empty[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_dump_bulk_empty[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_dump_bulk_empty[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_dump_bulk_empty[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_dump_bulk_empty[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_dump_bulk_empty[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_add_root_empty[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_add_root_empty[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_add_root_empty[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_add_root_empty[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_add_root_empty[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_add_root_empty[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_root_nodes_empty[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_root_nodes_empty[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_root_nodes_empty[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_root_nodes_empty[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_root_nodes_empty[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_root_nodes_empty[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_first_root_node_empty[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_first_root_node_empty[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_first_root_node_empty[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_first_root_node_empty[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_first_root_node_empty[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_first_root_node_empty[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_last_root_node_empty[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_last_root_node_empty[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_last_root_node_empty[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_last_root_node_empty[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_last_root_node_empty[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_last_root_node_empty[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_tree[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_tree[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_tree[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_tree[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_tree[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_tree[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_annotated_list[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_annotated_list[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_annotated_list[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_annotated_list[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_annotated_list[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestEmptyTree::test_get_annotated_list[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_bulk_existing[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_bulk_existing[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_bulk_existing[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_bulk_existing[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_bulk_existing[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_bulk_existing[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_all[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_all[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_all[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_all[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_all[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_all[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_all[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_all[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_all[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_all[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_all[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_all[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_leaf[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_leaf[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_leaf[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_leaf[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_leaf[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_tree_leaf[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_all[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_all[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_all[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_all[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_all[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_all[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_leaf[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_leaf[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_leaf[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_leaf[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_leaf[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_annotated_list_leaf[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_dump_bulk_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_keeping_ids[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_keeping_ids[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_keeping_ids[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_keeping_ids[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_keeping_ids[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_keeping_ids[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_with_fk[AL_TestNodeRelated]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_with_fk[MP_TestNodeRelated]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_load_and_dump_bulk_with_fk[NS_TestNodeRelated]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_root_nodes[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_root_nodes[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_root_nodes[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_root_nodes[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_root_nodes[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_root_nodes[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_first_root_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_first_root_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_first_root_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_first_root_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_first_root_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_first_root_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_last_root_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_last_root_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_last_root_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_last_root_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_last_root_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_get_last_root_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_passed_instance[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_passed_instance[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_passed_instance[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_passed_instance[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_passed_instance[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_passed_instance[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_already_saved_instance[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_already_saved_instance[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_already_saved_instance[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_already_saved_instance[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_already_saved_instance[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestClassMethods::test_add_root_with_already_saved_instance[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_leaf[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_leaf[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_leaf[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_leaf[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_leaf[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_leaf[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_parent[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_parent[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_parent[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_parent[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_parent[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_parent[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children_count[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children_count[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children_count[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children_count[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children_count[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_children_count[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_siblings[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_siblings[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_siblings[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_siblings[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_siblings[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_siblings[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_prev_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_prev_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_prev_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_prev_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_prev_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_prev_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_next_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_next_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_next_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_next_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_next_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_next_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_first_child[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_last_child[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_ancestors[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_ancestors[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_ancestors[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_ancestors[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_ancestors[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_ancestors[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendants[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendants[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendants[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendants[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendants[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendants[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendant_count[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendant_count[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendant_count[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendant_count[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendant_count[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_get_descendant_count[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_sibling_of[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_sibling_of[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_sibling_of[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_sibling_of[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_sibling_of[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_sibling_of[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_child_of[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_child_of[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_child_of[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_child_of[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_child_of[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_child_of[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_descendant_of[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_descendant_of[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_descendant_of[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_descendant_of[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_descendant_of[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSimpleNodeMethods::test_is_descendant_of[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_leaf[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_leaf[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_leaf[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_leaf[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_leaf[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_leaf[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_to_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_passed_instance[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_passed_instance[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_passed_instance[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_passed_instance[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_passed_instance[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_passed_instance[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_already_saved_instance[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_already_saved_instance[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_already_saved_instance[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_already_saved_instance[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_already_saved_instance[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_with_already_saved_instance[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_post_save[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_post_save[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_post_save[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_post_save[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_post_save[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddChild::test_add_child_post_save[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_invalid_pos[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_invalid_pos[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_invalid_pos[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_invalid_pos[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_invalid_pos[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_invalid_pos[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_missing_nodeorderby[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_missing_nodeorderby[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_missing_nodeorderby[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_missing_nodeorderby[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_missing_nodeorderby[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_missing_nodeorderby[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_last[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_first[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_left_noleft[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_right_noright[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_with_passed_instance[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_with_passed_instance[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_with_passed_instance[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_with_passed_instance[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_with_passed_instance[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_with_passed_instance[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_already_saved_instance[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_already_saved_instance[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_already_saved_instance[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_already_saved_instance[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_already_saved_instance[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAddSibling::test_add_sibling_already_saved_instance[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_leaf[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_leaf[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_leaf[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_leaf[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_leaf[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_leaf[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_root_nodes[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_root_nodes[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_root_nodes[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_root_nodes[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_root_nodes[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_root_nodes[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_children[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_children[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_children[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_children[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_children[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_filter_children[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_nonexistant_nodes[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_nonexistant_nodes[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_nonexistant_nodes[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_nonexistant_nodes[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_nonexistant_nodes[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_nonexistant_nodes[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_same_node_twice[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_same_node_twice[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_same_node_twice[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_same_node_twice[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_same_node_twice[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_same_node_twice[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_root_nodes[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_root_nodes[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_root_nodes[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_root_nodes[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_root_nodes[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_root_nodes[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_nodes[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_nodes[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_nodes[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_nodes[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_nodes[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestDelete::test_delete_all_nodes[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_invalid_pos[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_invalid_pos[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_invalid_pos[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_invalid_pos[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_invalid_pos[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_invalid_pos[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_to_descendant[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_to_descendant[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_to_descendant[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_to_descendant[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_to_descendant[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_to_descendant[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_missing_nodeorderby[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_missing_nodeorderby[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_missing_nodeorderby[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_missing_nodeorderby[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_missing_nodeorderby[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveErrors::test_move_missing_nodeorderby[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveSortedErrors::test_nonsorted_move_in_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestMoveSortedErrors::test_nonsorted_move_in_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestMoveSortedErrors::test_nonsorted_move_in_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_left_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_left_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_left_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_left_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_left_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_left_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_right_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_right_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_right_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_right_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_right_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_right_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_child_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_child_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_child_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_child_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_child_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_last_child_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_child_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_child_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_child_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_child_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_child_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeafRoot::test_move_leaf_first_child_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_right_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_right_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_right_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_right_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_right_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_right_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling_itself[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling_itself[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling_itself[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling_itself[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling_itself[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_left_sibling_itself[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_last_child[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveLeaf::test_move_leaf_first_child[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_noleft_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_noleft_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_noleft_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_noleft_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_noleft_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_left_noleft_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_noright_sibling_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_noright_sibling_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_noright_sibling_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_noright_sibling_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_noright_sibling_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_right_noright_sibling_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_child_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_child_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_child_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_child_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_child_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_first_child_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_child_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_child_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_child_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_child_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_child_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranchRoot::test_move_branch_last_child_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_noleft_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_noleft_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_noleft_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_noleft_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_noleft_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_noleft_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_noright_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_noright_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_noright_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_noright_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_noright_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_right_noright_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_itself_sibling[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_itself_sibling[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_itself_sibling[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_itself_sibling[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_itself_sibling[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_left_itself_sibling[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_first_child[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveBranch::test_move_branch_last_child[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_root_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_root_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_root_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_child_root_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_child_root_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_child_root_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_child_nonroot_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_child_nonroot_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_add_child_nonroot_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_move_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_move_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_move_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_move_sortedsibling[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_move_sortedsibling[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestTreeSorted::test_move_sortedsibling[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_tree_all[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_tree_all[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_tree_all[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_tree_node[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_tree_node[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_tree_node[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_root_nodes[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_root_nodes[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_root_nodes[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_root_node[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_root_node[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_root_node[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_root_node[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_root_node[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_root_node[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_is_root[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_is_root[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_is_root[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_is_leaf[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_is_leaf[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_is_leaf[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_root[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_root[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_root[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_parent[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_parent[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_parent[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_children[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_children[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_children[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_children_count[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_children_count[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_children_count[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_siblings[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_siblings[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_siblings[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_sibling[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_sibling[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_sibling[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_prev_sibling[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_prev_sibling[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_prev_sibling[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_next_sibling[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_next_sibling[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_next_sibling[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_sibling[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_sibling[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_sibling[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_child[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_child[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_first_child[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_child[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_child[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_last_child[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_ancestors[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_ancestors[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_ancestors[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_descendants[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_descendants[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_descendants[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_descendant_count[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_descendant_count[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_get_descendant_count[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_cascading_deletion[AL_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_cascading_deletion[MP_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestInheritedModels::test_cascading_deletion[NS_TestNodeInherited]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_root[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_root[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_root[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_root[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_root[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_root[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestHelpers::test_descendants_group_count_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMP_TreeSortedAutoNow::test_sorted_by_autonow_workaround[MP_TestNodeSortedAutoNow]",
"treebeard/tests/test_treebeard.py::TestMP_TreeSortedAutoNow::test_sorted_by_autonow_FAIL[MP_TestNodeSortedAutoNow]",
"treebeard/tests/test_treebeard.py::TestMP_TreeStepOverflow::test_add_root[MP_TestNodeSmallStep]",
"treebeard/tests/test_treebeard.py::TestMP_TreeStepOverflow::test_add_child[MP_TestNodeSmallStep]",
"treebeard/tests/test_treebeard.py::TestMP_TreeStepOverflow::test_add_sibling[MP_TestNodeSmallStep]",
"treebeard/tests/test_treebeard.py::TestMP_TreeStepOverflow::test_move[MP_TestNodeSmallStep]",
"treebeard/tests/test_treebeard.py::TestMP_TreeShortPath::test_short_path[MP_TestNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFindProblems::test_find_problems[MP_TestNodeAlphabet]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFix::test_fix_tree_non_destructive[MP_TestNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFix::test_fix_tree_non_destructive[MP_TestSortedNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFix::test_fix_tree_destructive[MP_TestNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFix::test_fix_tree_destructive[MP_TestSortedNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFix::test_fix_tree_with_fix_paths[MP_TestNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestMP_TreeFix::test_fix_tree_with_fix_paths[MP_TestSortedNodeShortPath]",
"treebeard/tests/test_treebeard.py::TestIssues::test_many_to_many_django_user_anonymous[MP_TestManyToManyWithUser]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_root_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_root_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_root_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_root_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_root_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_root_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_admin[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_admin[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_admin[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_admin[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_admin[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestMoveNodeForm::test_form_admin[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestModelAdmin::test_default_fields[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestModelAdmin::test_default_fields[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestModelAdmin::test_default_fields[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestModelAdmin::test_default_fields[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestModelAdmin::test_default_fields[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestModelAdmin::test_default_fields[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_root_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_root_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_root_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_child_root_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_child_root_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_child_root_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_child_nonroot_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_child_nonroot_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_add_child_nonroot_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_move_sorted[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_move_sorted[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_move_sorted[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_move_sortedsibling[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_move_sortedsibling[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_move_sortedsibling[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_sorted_form[AL_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_sorted_form[MP_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestSortedForm::test_sorted_form[NS_TestNodeSorted]",
"treebeard/tests/test_treebeard.py::TestForm::test_form[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_form[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_form[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_form[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_form[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_form[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_move_node_form[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_move_node_form[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_move_node_form[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_move_node_form[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_move_node_form[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_move_node_form[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_get_position_ref_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_get_position_ref_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_get_position_ref_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_get_position_ref_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_get_position_ref_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_get_position_ref_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_clean_cleaned_data[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_clean_cleaned_data[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_clean_cleaned_data[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_clean_cleaned_data[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_clean_cleaned_data[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_clean_cleaned_data[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_edit[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_edit[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_edit[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_edit[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_edit[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_edit[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_new[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_new[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_new[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_new[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_new[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestForm::test_save_new[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestAdminTreeTemplateTags::test_treebeard_css",
"treebeard/tests/test_treebeard.py::TestAdminTreeTemplateTags::test_treebeard_js",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_changelist_view",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_get_node[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_get_node[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_get_node[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_get_node[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_get_node[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_get_node[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_keyerror[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_keyerror[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_keyerror[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_keyerror[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_keyerror[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_keyerror[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_valueerror[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_valueerror[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_valueerror[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_valueerror[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_valueerror[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_node_validate_valueerror[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_missing_nodeorderby[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_missing_nodeorderby[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_missing_nodeorderby[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_missing_nodeorderby[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_missing_nodeorderby[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_missing_nodeorderby[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_invalid_pos[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_invalid_pos[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_invalid_pos[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_invalid_pos[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_invalid_pos[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_invalid_pos[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_to_descendant[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_to_descendant[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_to_descendant[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_to_descendant[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_to_descendant[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_validate_to_descendant[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_left[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_left[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_left[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_left[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_left[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_left[NS_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_last_child[AL_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_last_child[MP_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_last_child[NS_TestNode]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_last_child[AL_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_last_child[MP_TestNode_Proxy]",
"treebeard/tests/test_treebeard.py::TestTreeAdmin::test_move_last_child[NS_TestNode_Proxy]"
] | 2020-10-26 06:31:31+00:00 | 1,933 |
|
django__asgiref-183 | diff --git a/asgiref/wsgi.py b/asgiref/wsgi.py
index 7155ab2..8811118 100644
--- a/asgiref/wsgi.py
+++ b/asgiref/wsgi.py
@@ -55,8 +55,8 @@ class WsgiToAsgiInstance:
"""
environ = {
"REQUEST_METHOD": scope["method"],
- "SCRIPT_NAME": scope.get("root_path", ""),
- "PATH_INFO": scope["path"],
+ "SCRIPT_NAME": scope.get("root_path", "").encode("utf8").decode("latin1"),
+ "PATH_INFO": scope["path"].encode("utf8").decode("latin1"),
"QUERY_STRING": scope["query_string"].decode("ascii"),
"SERVER_PROTOCOL": "HTTP/%s" % scope["http_version"],
"wsgi.version": (1, 0),
| django/asgiref | 3020b4a309033ad0b7041dda7712b0ed729e338f | diff --git a/tests/test_wsgi.py b/tests/test_wsgi.py
index 647f465..3490573 100644
--- a/tests/test_wsgi.py
+++ b/tests/test_wsgi.py
@@ -50,6 +50,48 @@ async def test_basic_wsgi():
assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+@pytest.mark.asyncio
+async def test_wsgi_path_encoding():
+ """
+ Makes sure the WSGI wrapper has basic functionality.
+ """
+ # Define WSGI app
+ def wsgi_application(environ, start_response):
+ assert environ["SCRIPT_NAME"] == "/中国".encode("utf8").decode("latin-1")
+ assert environ["PATH_INFO"] == "/中文".encode("utf8").decode("latin-1")
+ start_response("200 OK", [])
+ yield b""
+
+ # Wrap it
+ application = WsgiToAsgi(wsgi_application)
+ # Launch it as a test application
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/中文",
+ "root_path": "/中国",
+ "query_string": b"bar=baz",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ # Check they send stuff
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 200,
+ "headers": [],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
@pytest.mark.asyncio
async def test_wsgi_empty_body():
"""
| WSGI Error in non-ascii path
https://github.com/encode/starlette/issues/997
I noticed that the ASGI document clearly states that utf8 encoding is required, but WSGI seems to require latin-1 encoding.
https://www.python.org/dev/peps/pep-3333/#a-note-on-string-types
https://asgi.readthedocs.io/en/latest/specs/www.html#connection-scope | 0.0 | [
"tests/test_wsgi.py::test_wsgi_path_encoding"
] | [
"tests/test_wsgi.py::test_basic_wsgi",
"tests/test_wsgi.py::test_wsgi_empty_body",
"tests/test_wsgi.py::test_wsgi_multi_body"
] | 2020-07-14 02:53:15+00:00 | 1,934 |
|
django__asgiref-191 | diff --git a/README.rst b/README.rst
index ab1abc7..d1a9369 100644
--- a/README.rst
+++ b/README.rst
@@ -43,7 +43,11 @@ ASGI server.
Note that exactly what threads things run in is very specific, and aimed to
keep maximum compatibility with old synchronous code. See
-"Synchronous code & Threads" below for a full explanation.
+"Synchronous code & Threads" below for a full explanation. By default,
+``sync_to_async`` will run all synchronous code in the program in the same
+thread for safety reasons; you can disable this for more performance with
+``@sync_to_async(thread_sensitive=False)``, but make sure that your code does
+not rely on anything bound to threads (like database connections) when you do.
Threadlocal replacement
diff --git a/asgiref/sync.py b/asgiref/sync.py
index a46f7d4..97b5d31 100644
--- a/asgiref/sync.py
+++ b/asgiref/sync.py
@@ -61,9 +61,17 @@ class AsyncToSync:
except RuntimeError:
# There's no event loop in this thread. Look for the threadlocal if
# we're inside SyncToAsync
- self.main_event_loop = getattr(
- SyncToAsync.threadlocal, "main_event_loop", None
+ main_event_loop_pid = getattr(
+ SyncToAsync.threadlocal, "main_event_loop_pid", None
)
+ # We make sure the parent loop is from the same process - if
+ # they've forked, this is not going to be valid any more (#194)
+ if main_event_loop_pid and main_event_loop_pid == os.getpid():
+ self.main_event_loop = getattr(
+ SyncToAsync.threadlocal, "main_event_loop", None
+ )
+ else:
+ self.main_event_loop = None
def __call__(self, *args, **kwargs):
# You can't call AsyncToSync from a thread with a running event loop
@@ -247,7 +255,7 @@ class SyncToAsync:
# Single-thread executor for thread-sensitive code
single_thread_executor = ThreadPoolExecutor(max_workers=1)
- def __init__(self, func, thread_sensitive=False):
+ def __init__(self, func, thread_sensitive=True):
self.func = func
functools.update_wrapper(self, func)
self._thread_sensitive = thread_sensitive
@@ -312,6 +320,7 @@ class SyncToAsync:
"""
# Set the threadlocal for AsyncToSync
self.threadlocal.main_event_loop = loop
+ self.threadlocal.main_event_loop_pid = os.getpid()
# Set the task mapping (used for the locals module)
current_thread = threading.current_thread()
if AsyncToSync.launch_map.get(source_task) == current_thread:
@@ -356,6 +365,11 @@ class SyncToAsync:
return None
-# Lowercase is more sensible for most things
-sync_to_async = SyncToAsync
+# Lowercase aliases (and decorator friendliness)
async_to_sync = AsyncToSync
+
+
+def sync_to_async(func=None, thread_sensitive=True):
+ if func is None:
+ return lambda f: SyncToAsync(f, thread_sensitive=thread_sensitive)
+ return SyncToAsync(func, thread_sensitive=thread_sensitive)
diff --git a/asgiref/wsgi.py b/asgiref/wsgi.py
index 8811118..40fba20 100644
--- a/asgiref/wsgi.py
+++ b/asgiref/wsgi.py
@@ -29,6 +29,7 @@ class WsgiToAsgiInstance:
def __init__(self, wsgi_application):
self.wsgi_application = wsgi_application
self.response_started = False
+ self.response_content_length = None
async def __call__(self, scope, receive, send):
if scope["type"] != "http":
@@ -114,6 +115,11 @@ class WsgiToAsgiInstance:
(name.lower().encode("ascii"), value.encode("ascii"))
for name, value in response_headers
]
+ # Extract content-length
+ self.response_content_length = None
+ for name, value in response_headers:
+ if name.lower() == "content-length":
+ self.response_content_length = int(value)
# Build and send response start message.
self.response_start = {
"type": "http.response.start",
@@ -130,14 +136,25 @@ class WsgiToAsgiInstance:
# Translate the scope and incoming request body into a WSGI environ
environ = self.build_environ(self.scope, body)
# Run the WSGI app
+ bytes_sent = 0
for output in self.wsgi_application(environ, self.start_response):
# If this is the first response, include the response headers
if not self.response_started:
self.response_started = True
self.sync_send(self.response_start)
+ # If the application supplies a Content-Length header
+ if self.response_content_length is not None:
+ # The server should not transmit more bytes to the client than the header allows
+ bytes_allowed = self.response_content_length - bytes_sent
+ if len(output) > bytes_allowed:
+ output = output[:bytes_allowed]
self.sync_send(
{"type": "http.response.body", "body": output, "more_body": True}
)
+ bytes_sent += len(output)
+ # The server should stop iterating over the response when enough data has been sent
+ if bytes_sent == self.response_content_length:
+ break
# Close connection
if not self.response_started:
self.response_started = True
diff --git a/docs/conf.py b/docs/conf.py
index 40499c5..6935f11 100644
--- a/docs/conf.py
+++ b/docs/conf.py
@@ -55,9 +55,9 @@ author = 'ASGI Team'
# built documents.
#
# The short X.Y version.
-version = '2.0'
+version = '3.0'
# The full version, including alpha/beta/rc tags.
-release = '2.0'
+release = '3.0'
# The language for content autogenerated by Sphinx. Refer to documentation
# for a list of supported languages.
diff --git a/docs/extensions.rst b/docs/extensions.rst
index 372ba20..aaf5764 100644
--- a/docs/extensions.rst
+++ b/docs/extensions.rst
@@ -77,3 +77,54 @@ and treat it as if the client had made a request.
The ASGI server should set the pseudo ``:authority`` header value to
be the same value as the request that triggered the push promise.
+
+Zero Copy Send
+--------------
+
+Zero Copy Send allows you to send the contents of a file descriptor to the
+HTTP client with zero copy (where the underlying OS directly handles the data
+transfer from a source file or socket without loading it into Python and
+writing it out again).
+
+ASGI servers that implement this extension will provide
+``http.response.zerocopysend`` in the extensions part of the scope::
+
+ "scope": {
+ ...
+ "extensions": {
+ "http.response.zerocopysend": {},
+ },
+ }
+
+The ASGI framework can initiate a zero-copy send by sending a message with
+the following keys. This message can be sent at any time after the
+*Response Start* message but before the final *Response Body* message,
+and can be mixed with ``http.response.body``. It can also be called
+multiple times in one response. Except for the characteristics of
+zero-copy, it should behave the same as ordinary ``http.response.body``.
+
+Keys:
+
+* ``type`` (*Unicode string*): ``"http.response.zerocopysend"``
+
+* ``file`` (*file descriptor object*): An opened file descriptor object
+ with an underlying OS file descriptor that can be used to call
+ ``os.sendfile``. (e.g. not BytesIO)
+
+* ``offset`` (*int*): Optional. If this value exists, it will specify
+ the offset at which sendfile starts to read data from ``file``.
+ Otherwise, it will be read from the current position of ``file``.
+
+* ``count`` (*int*): Optional. ``count`` is the number of bytes to
+ copy between the file descriptors. If omitted, the file will be read until
+ its end.
+
+* ``more_body`` (*bool*): Signifies if there is additional content
+ to come (as part of a Response Body message). If ``False``, response
+ will be taken as complete and closed, and any further messages on
+ the channel will be ignored. Optional; if missing defaults to
+ ``False``.
+
+After calling this extension to respond, the ASGI application itself should
+actively close the used file descriptor - ASGI servers are not responsible for
+closing descriptors.
diff --git a/docs/implementations.rst b/docs/implementations.rst
index 8b4da65..b4043af 100644
--- a/docs/implementations.rst
+++ b/docs/implementations.rst
@@ -75,3 +75,14 @@ Starlette
Starlette is a minimalist ASGI library for writing against basic but powerful
``Request`` and ``Response`` classes. Supports HTTP.
+
+
+rpc.py
+------
+
+*Beta* / https://github.com/abersheeran/rpc.py
+
+An easy-to-use and powerful RPC framework. RPC server base on WSGI & ASGI, client base
+on ``httpx``. Supports synchronous functions, asynchronous functions, synchronous
+generator functions, and asynchronous generator functions. Optional use of Type hint
+for type conversion. Optional OpenAPI document generation.
| django/asgiref | 1c9d06329dbd679a7c564b0652147855a721edb4 | diff --git a/tests/test_sync.py b/tests/test_sync.py
index 5a4b342..911e719 100644
--- a/tests/test_sync.py
+++ b/tests/test_sync.py
@@ -1,4 +1,5 @@
import asyncio
+import multiprocessing
import threading
import time
from concurrent.futures import ThreadPoolExecutor
@@ -279,11 +280,10 @@ def test_thread_sensitive_outside_sync():
await inner()
# Inner sync function
+ @sync_to_async
def inner():
result["thread"] = threading.current_thread()
- inner = sync_to_async(inner, thread_sensitive=True)
-
# Run it
middle()
assert result["thread"] == threading.current_thread()
@@ -300,22 +300,20 @@ async def test_thread_sensitive_outside_async():
result_2 = {}
# Outer sync function
+ @sync_to_async
def outer(result):
middle(result)
- outer = sync_to_async(outer, thread_sensitive=True)
-
# Middle async function
@async_to_sync
async def middle(result):
await inner(result)
# Inner sync function
+ @sync_to_async
def inner(result):
result["thread"] = threading.current_thread()
- inner = sync_to_async(inner, thread_sensitive=True)
-
# Run it (in supposed parallel!)
await asyncio.wait([outer(result_1), inner(result_2)])
@@ -338,22 +336,20 @@ def test_thread_sensitive_double_nested_sync():
await level2()
# Sync level 2
+ @sync_to_async
def level2():
level3()
- level2 = sync_to_async(level2, thread_sensitive=True)
-
# Async level 3
@async_to_sync
async def level3():
await level4()
# Sync level 2
+ @sync_to_async
def level4():
result["thread"] = threading.current_thread()
- level4 = sync_to_async(level4, thread_sensitive=True)
-
# Run it
level1()
assert result["thread"] == threading.current_thread()
@@ -369,22 +365,20 @@ async def test_thread_sensitive_double_nested_async():
result = {}
# Sync level 1
+ @sync_to_async
def level1():
level2()
- level1 = sync_to_async(level1, thread_sensitive=True)
-
# Async level 2
@async_to_sync
async def level2():
await level3()
# Sync level 3
+ @sync_to_async
def level3():
level4()
- level3 = sync_to_async(level3, thread_sensitive=True)
-
# Async level 4
@async_to_sync
async def level4():
@@ -395,6 +389,29 @@ async def test_thread_sensitive_double_nested_async():
assert result["thread"] == threading.current_thread()
+def test_thread_sensitive_disabled():
+ """
+ Tests that we can disable thread sensitivity and make things run in
+ separate threads.
+ """
+
+ result = {}
+
+ # Middle async function
+ @async_to_sync
+ async def middle():
+ await inner()
+
+ # Inner sync function
+ @sync_to_async(thread_sensitive=False)
+ def inner():
+ result["thread"] = threading.current_thread()
+
+ # Run it
+ middle()
+ assert result["thread"] != threading.current_thread()
+
+
class ASGITest(TestCase):
"""
Tests collection of async cases inside classes
@@ -415,3 +432,32 @@ def test_sync_to_async_detected_as_coroutinefunction():
assert not asyncio.iscoroutinefunction(sync_to_async)
assert asyncio.iscoroutinefunction(sync_to_async(sync_func))
+
+
+@pytest.mark.asyncio
+async def test_multiprocessing():
+ """
+ Tests that a forked process can use async_to_sync without it looking for
+ the event loop from the parent process.
+ """
+
+ test_queue = multiprocessing.Queue()
+
+ async def async_process():
+ test_queue.put(42)
+
+ def sync_process():
+ """Runs async_process synchronously"""
+ async_to_sync(async_process)()
+
+ def fork_first():
+ """Forks process before running sync_process"""
+ fork = multiprocessing.Process(target=sync_process)
+ fork.start()
+ fork.join(3)
+ # Force cleanup in failed test case
+ if fork.is_alive():
+ fork.terminate()
+ return test_queue.get(True, 1)
+
+ assert await sync_to_async(fork_first)() == 42
diff --git a/tests/test_wsgi.py b/tests/test_wsgi.py
index 3490573..6dcee1c 100644
--- a/tests/test_wsgi.py
+++ b/tests/test_wsgi.py
@@ -1,3 +1,5 @@
+import sys
+
import pytest
from asgiref.testing import ApplicationCommunicator
@@ -126,6 +128,130 @@ async def test_wsgi_empty_body():
assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+@pytest.mark.asyncio
+async def test_wsgi_clamped_body():
+ """
+ Makes sure WsgiToAsgi clamps a body response longer than Content-Length
+ """
+
+ def wsgi_application(environ, start_response):
+ start_response("200 OK", [("Content-Length", "8")])
+ return [b"0123", b"45", b"6789"]
+
+ application = WsgiToAsgi(wsgi_application)
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/",
+ "query_string": b"",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 200,
+ "headers": [(b"content-length", b"8")],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"0123",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"45",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"67",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
+@pytest.mark.asyncio
+async def test_wsgi_stops_iterating_after_content_length_bytes():
+ """
+ Makes sure WsgiToAsgi does not iterate after than Content-Length bytes
+ """
+
+ def wsgi_application(environ, start_response):
+ start_response("200 OK", [("Content-Length", "4")])
+ yield b"0123"
+ pytest.fail("WsgiToAsgi should not iterate after Content-Length bytes")
+ yield b"4567"
+
+ application = WsgiToAsgi(wsgi_application)
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/",
+ "query_string": b"",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 200,
+ "headers": [(b"content-length", b"4")],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"0123",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
+@pytest.mark.asyncio
+async def test_wsgi_multiple_start_response():
+ """
+ Makes sure WsgiToAsgi only keep Content-Length from the last call to start_response
+ """
+
+ def wsgi_application(environ, start_response):
+ start_response("200 OK", [("Content-Length", "5")])
+ try:
+ raise ValueError("Application Error")
+ except ValueError:
+ start_response("500 Server Error", [], sys.exc_info())
+ return [b"Some long error message"]
+
+ application = WsgiToAsgi(wsgi_application)
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/",
+ "query_string": b"",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 500,
+ "headers": [],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"Some long error message",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
@pytest.mark.asyncio
async def test_wsgi_multi_body():
"""
| Proposal: "http.response.sendfile" extension.
Add the sendfile extension to allow ASGI programs to return response through `sendfile`.
In `scope`:
```python
"scope": {
...
"extensions": {
"http.response.sendfile": {},
},
}
```
Response:
```python
await send({"type": "http.response.start", ...})
await send({"type": "http.response.sendfile", "file": file-obj})
```
Some related links:
https://docs.python.org/3.7/library/asyncio-eventloop.html#asyncio.loop.sendfile
https://github.com/encode/uvicorn/issues/35 | 0.0 | [
"tests/test_sync.py::test_thread_sensitive_outside_sync",
"tests/test_sync.py::test_thread_sensitive_outside_async",
"tests/test_sync.py::test_thread_sensitive_double_nested_sync",
"tests/test_sync.py::test_thread_sensitive_disabled",
"tests/test_wsgi.py::test_wsgi_clamped_body",
"tests/test_wsgi.py::test_wsgi_stops_iterating_after_content_length_bytes"
] | [
"tests/test_sync.py::test_sync_to_async",
"tests/test_sync.py::test_sync_to_async_decorator",
"tests/test_sync.py::test_nested_sync_to_async_retains_wrapped_function_attributes",
"tests/test_sync.py::test_sync_to_async_method_decorator",
"tests/test_sync.py::test_sync_to_async_method_self_attribute",
"tests/test_sync.py::test_async_to_sync_to_async",
"tests/test_sync.py::test_async_to_sync",
"tests/test_sync.py::test_async_to_sync_decorator",
"tests/test_sync.py::test_async_to_sync_method_decorator",
"tests/test_sync.py::test_async_to_sync_in_async",
"tests/test_sync.py::test_async_to_sync_in_thread",
"tests/test_sync.py::test_async_to_async_method_self_attribute",
"tests/test_sync.py::test_thread_sensitive_double_nested_async",
"tests/test_sync.py::ASGITest::test_wrapped_case_is_collected",
"tests/test_sync.py::test_sync_to_async_detected_as_coroutinefunction",
"tests/test_sync.py::test_multiprocessing",
"tests/test_wsgi.py::test_basic_wsgi",
"tests/test_wsgi.py::test_wsgi_path_encoding",
"tests/test_wsgi.py::test_wsgi_empty_body",
"tests/test_wsgi.py::test_wsgi_multiple_start_response",
"tests/test_wsgi.py::test_wsgi_multi_body"
] | 2020-09-01 16:18:43+00:00 | 1,935 |
|
django__asgiref-196 | diff --git a/asgiref/wsgi.py b/asgiref/wsgi.py
index 8811118..40fba20 100644
--- a/asgiref/wsgi.py
+++ b/asgiref/wsgi.py
@@ -29,6 +29,7 @@ class WsgiToAsgiInstance:
def __init__(self, wsgi_application):
self.wsgi_application = wsgi_application
self.response_started = False
+ self.response_content_length = None
async def __call__(self, scope, receive, send):
if scope["type"] != "http":
@@ -114,6 +115,11 @@ class WsgiToAsgiInstance:
(name.lower().encode("ascii"), value.encode("ascii"))
for name, value in response_headers
]
+ # Extract content-length
+ self.response_content_length = None
+ for name, value in response_headers:
+ if name.lower() == "content-length":
+ self.response_content_length = int(value)
# Build and send response start message.
self.response_start = {
"type": "http.response.start",
@@ -130,14 +136,25 @@ class WsgiToAsgiInstance:
# Translate the scope and incoming request body into a WSGI environ
environ = self.build_environ(self.scope, body)
# Run the WSGI app
+ bytes_sent = 0
for output in self.wsgi_application(environ, self.start_response):
# If this is the first response, include the response headers
if not self.response_started:
self.response_started = True
self.sync_send(self.response_start)
+ # If the application supplies a Content-Length header
+ if self.response_content_length is not None:
+ # The server should not transmit more bytes to the client than the header allows
+ bytes_allowed = self.response_content_length - bytes_sent
+ if len(output) > bytes_allowed:
+ output = output[:bytes_allowed]
self.sync_send(
{"type": "http.response.body", "body": output, "more_body": True}
)
+ bytes_sent += len(output)
+ # The server should stop iterating over the response when enough data has been sent
+ if bytes_sent == self.response_content_length:
+ break
# Close connection
if not self.response_started:
self.response_started = True
| django/asgiref | cfd82e48a2059ff93ce20b94e1755c455e3c3d29 | diff --git a/tests/test_wsgi.py b/tests/test_wsgi.py
index 3490573..6dcee1c 100644
--- a/tests/test_wsgi.py
+++ b/tests/test_wsgi.py
@@ -1,3 +1,5 @@
+import sys
+
import pytest
from asgiref.testing import ApplicationCommunicator
@@ -126,6 +128,130 @@ async def test_wsgi_empty_body():
assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+@pytest.mark.asyncio
+async def test_wsgi_clamped_body():
+ """
+ Makes sure WsgiToAsgi clamps a body response longer than Content-Length
+ """
+
+ def wsgi_application(environ, start_response):
+ start_response("200 OK", [("Content-Length", "8")])
+ return [b"0123", b"45", b"6789"]
+
+ application = WsgiToAsgi(wsgi_application)
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/",
+ "query_string": b"",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 200,
+ "headers": [(b"content-length", b"8")],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"0123",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"45",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"67",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
+@pytest.mark.asyncio
+async def test_wsgi_stops_iterating_after_content_length_bytes():
+ """
+ Makes sure WsgiToAsgi does not iterate after than Content-Length bytes
+ """
+
+ def wsgi_application(environ, start_response):
+ start_response("200 OK", [("Content-Length", "4")])
+ yield b"0123"
+ pytest.fail("WsgiToAsgi should not iterate after Content-Length bytes")
+ yield b"4567"
+
+ application = WsgiToAsgi(wsgi_application)
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/",
+ "query_string": b"",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 200,
+ "headers": [(b"content-length", b"4")],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"0123",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
+@pytest.mark.asyncio
+async def test_wsgi_multiple_start_response():
+ """
+ Makes sure WsgiToAsgi only keep Content-Length from the last call to start_response
+ """
+
+ def wsgi_application(environ, start_response):
+ start_response("200 OK", [("Content-Length", "5")])
+ try:
+ raise ValueError("Application Error")
+ except ValueError:
+ start_response("500 Server Error", [], sys.exc_info())
+ return [b"Some long error message"]
+
+ application = WsgiToAsgi(wsgi_application)
+ instance = ApplicationCommunicator(
+ application,
+ {
+ "type": "http",
+ "http_version": "1.0",
+ "method": "GET",
+ "path": "/",
+ "query_string": b"",
+ "headers": [],
+ },
+ )
+ await instance.send_input({"type": "http.request"})
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.start",
+ "status": 500,
+ "headers": [],
+ }
+ assert (await instance.receive_output(1)) == {
+ "type": "http.response.body",
+ "body": b"Some long error message",
+ "more_body": True,
+ }
+ assert (await instance.receive_output(1)) == {"type": "http.response.body"}
+
+
@pytest.mark.asyncio
async def test_wsgi_multi_body():
"""
| WsgiToAsgi should stop sending response body after content-length bytes
Hi,
I was having issues when serving partial requests (using Range headers) from [whitenoise](https://github.com/evansd/whitenoise) wrapped in WsgiToAsgi. Some HTTP and ASGI servers in front of that complained about extra content in the response body (I've had the issue with traefik in front of uvicorn and with [uvicorn itself when using the httptools protocol handler](https://github.com/encode/uvicorn/blob/master/uvicorn/protocols/http/httptools_impl.py#L517)).
One contributing factor is whitenoise which returns the file handle to the whole file in a [wsgiref.FileWrapper](https://github.com/python/cpython/blob/master/Lib/wsgiref/util.py#L11), seeked to the correct position for the range request. It then expects the server to only read as many bytes as defined in the Content-Length header.
The [WSGI specs allows for this behavior](https://www.python.org/dev/peps/pep-3333/#handling-the-content-length-header) and [gunicorn implements it](https://github.com/benoitc/gunicorn/blob/master/gunicorn/http/wsgi.py#L333) and stops reading after as many bytes as specified in Content-Length.
The [ASGI spec](https://asgi.readthedocs.io/en/latest/specs/www.html#http) does not say much about the consistency between Content-Length header and the "http.response.body" events. The relevant parts could be :
> Protocol servers must flush any data passed to them into the send buffer before returning from a send call.
> Yielding content from the WSGI application maps to sending http.response.body messages.
The first one suggests that any piece of body from an "http.response.body" event must be sent, the other one could mean that all behavior defined for WSGI should apply to ASGI, including not-sending extra body, maybe ?
[uvicorn](https://github.com/encode/uvicorn/blob/master/uvicorn/protocols/http/h11_impl.py#L475), [daphe](https://github.com/django/daphne/blob/master/daphne/http_protocol.py#L255) and [hypercorn](https://gitlab.com/pgjones/hypercorn/-/blob/master/src/hypercorn/protocol/http_stream.py#L147) lean more towards the first interpretation and pass through the whole readable content or simply raise an exception.
If you agree that the WsgiToAsgi adapter should clamp the body I can work on a patch.
Aside from this, the ASGI spec could probably be improved by defining this point. I don't really know if ASGI servers should prefer clamping or forwarding extra body responses but I have the vague feeling that allowing ASGI events to generate incorrectly framed HTTP messages could generate hard to diagnose issues in the future without providing any benefit.
If the sendfile extension from #184 is added, the same point will probably arise, it would be very inconvenient to only be able to use sendfile when serving whole files. WSGI also specifies the clamping behavior [when using sendfile](https://www.python.org/dev/peps/pep-3333/#optional-platform-specific-file-handling) and [gunicorn](https://github.com/benoitc/gunicorn/blob/master/gunicorn/http/wsgi.py#L365) implements it.
| 0.0 | [
"tests/test_wsgi.py::test_wsgi_clamped_body",
"tests/test_wsgi.py::test_wsgi_stops_iterating_after_content_length_bytes"
] | [
"tests/test_wsgi.py::test_basic_wsgi",
"tests/test_wsgi.py::test_wsgi_path_encoding",
"tests/test_wsgi.py::test_wsgi_empty_body",
"tests/test_wsgi.py::test_wsgi_multiple_start_response",
"tests/test_wsgi.py::test_wsgi_multi_body"
] | 2020-09-16 19:11:25+00:00 | 1,936 |
|
django__asgiref-211 | diff --git a/asgiref/server.py b/asgiref/server.py
index 9fd2e0c..a83b7bf 100644
--- a/asgiref/server.py
+++ b/asgiref/server.py
@@ -3,6 +3,8 @@ import logging
import time
import traceback
+from .compatibility import guarantee_single_callable
+
logger = logging.getLogger(__name__)
@@ -84,10 +86,11 @@ class StatelessServer:
self.delete_oldest_application_instance()
# Make an instance of the application
input_queue = asyncio.Queue()
- application_instance = self.application(scope=scope)
+ application_instance = guarantee_single_callable(self.application)
# Run it, and stash the future for later checking
future = asyncio.ensure_future(
application_instance(
+ scope=scope,
receive=input_queue.get,
send=lambda message: self.application_send(scope, message),
)
| django/asgiref | 5c249e818bd5e7acef957e236535098c3aff7b42 | diff --git a/tests/test_server.py b/tests/test_server.py
new file mode 100644
index 0000000..77bbd4d
--- /dev/null
+++ b/tests/test_server.py
@@ -0,0 +1,11 @@
+from asgiref.server import StatelessServer
+
+
+def test_stateless_server():
+ """StatlessServer can be instantiated with an ASGI 3 application."""
+
+ async def app(scope, receive, send):
+ pass
+
+ server = StatelessServer(app)
+ server.get_or_create_application_instance("scope_id", {})
| Has StatelessServer been updated to the ASGI 3.0 spec?
I'm currently trying to upgrade my app from Channels 2.4.0 to Channels 3.0.0 and I'm having issues with running my worker.
After some investigation, I'm under the impression that the `StatelessServer` provided in `asgiref` hasn't been updated to the new Application specifications in ASGI 3.0.
Part of StatelessServer that is trying to instantiate an application with a scope, then call it using the receive and send arguments:
https://github.com/django/asgiref/blob/5c249e818bd5e7acef957e236535098c3aff7b42/asgiref/server.py#L87-L94
Specification for the new Application format:
https://asgi.readthedocs.io/en/latest/specs/main.html#applications
That is: `coroutine application(scope, receive, send)`
Since the Worker in Channels is based on `StatelessServer`, this is breaking the worker functionality in Channels 3.0.0.
Is this something that is in the works? Anything I can do to help with this? | 0.0 | [
"tests/test_server.py::test_stateless_server"
] | [] | 2020-11-05 02:42:27+00:00 | 1,937 |
|
django__asgiref-239 | diff --git a/CHANGELOG.txt b/CHANGELOG.txt
index 3c617e8..e4ed96f 100644
--- a/CHANGELOG.txt
+++ b/CHANGELOG.txt
@@ -1,3 +1,9 @@
+Pending
+-------
+
+* async_to_sync and sync_to_async now check their arguments are functions of
+ the correct type.
+
3.3.1 (2020-11-09)
------------------
diff --git a/asgiref/sync.py b/asgiref/sync.py
index 869fb48..a8d91ea 100644
--- a/asgiref/sync.py
+++ b/asgiref/sync.py
@@ -101,6 +101,8 @@ class AsyncToSync:
executors = Local()
def __init__(self, awaitable, force_new_loop=False):
+ if not callable(awaitable) or not asyncio.iscoroutinefunction(awaitable):
+ raise TypeError("async_to_sync can only be applied to async functions.")
self.awaitable = awaitable
try:
self.__self__ = self.awaitable.__self__
@@ -325,6 +327,8 @@ class SyncToAsync:
)
def __init__(self, func, thread_sensitive=True):
+ if not callable(func) or asyncio.iscoroutinefunction(func):
+ raise TypeError("sync_to_async can only be applied to sync functions.")
self.func = func
functools.update_wrapper(self, func)
self._thread_sensitive = thread_sensitive
| django/asgiref | 6469d0f3486dae0b6229e12d32ae6695600ed3ec | diff --git a/tests/test_sync.py b/tests/test_sync.py
index 5e9c171..5f9d9ec 100644
--- a/tests/test_sync.py
+++ b/tests/test_sync.py
@@ -45,6 +45,34 @@ async def test_sync_to_async():
loop.set_default_executor(old_executor)
+def test_sync_to_async_fail_non_function():
+ """
+ async_to_sync raises a TypeError when called with a non-function.
+ """
+ with pytest.raises(TypeError) as excinfo:
+ sync_to_async(1)
+
+ assert excinfo.value.args == (
+ "sync_to_async can only be applied to sync functions.",
+ )
+
+
+@pytest.mark.asyncio
+async def test_sync_to_async_fail_async():
+ """
+ sync_to_async raises a TypeError when applied to a sync function.
+ """
+ with pytest.raises(TypeError) as excinfo:
+
+ @sync_to_async
+ async def test_function():
+ pass
+
+ assert excinfo.value.args == (
+ "sync_to_async can only be applied to sync functions.",
+ )
+
+
@pytest.mark.asyncio
async def test_sync_to_async_decorator():
"""
@@ -152,6 +180,33 @@ async def test_async_to_sync_to_async():
assert result["thread"] == threading.current_thread()
+def test_async_to_sync_fail_non_function():
+ """
+ async_to_sync raises a TypeError when applied to a non-function.
+ """
+ with pytest.raises(TypeError) as excinfo:
+ async_to_sync(1)
+
+ assert excinfo.value.args == (
+ "async_to_sync can only be applied to async functions.",
+ )
+
+
+def test_async_to_sync_fail_sync():
+ """
+ async_to_sync raises a TypeError when applied to a sync function.
+ """
+ with pytest.raises(TypeError) as excinfo:
+
+ @async_to_sync
+ def test_function(self):
+ pass
+
+ assert excinfo.value.args == (
+ "async_to_sync can only be applied to async functions.",
+ )
+
+
def test_async_to_sync():
"""
Tests we can call async_to_sync outside of an outer event loop.
| Prevent accidental mixup of sync_to_async / async_to_sync
Putting `sync_to_async` onto an `async def` silently breaks it. Instead, it would be good if that raised an error at decorator application time - and likewise for the opposite case. | 0.0 | [
"tests/test_sync.py::test_sync_to_async_fail_non_function",
"tests/test_sync.py::test_sync_to_async_fail_async",
"tests/test_sync.py::test_async_to_sync_fail_non_function",
"tests/test_sync.py::test_async_to_sync_fail_sync"
] | [
"tests/test_sync.py::test_sync_to_async",
"tests/test_sync.py::test_sync_to_async_decorator",
"tests/test_sync.py::test_nested_sync_to_async_retains_wrapped_function_attributes",
"tests/test_sync.py::test_sync_to_async_method_decorator",
"tests/test_sync.py::test_sync_to_async_method_self_attribute",
"tests/test_sync.py::test_async_to_sync_to_async",
"tests/test_sync.py::test_async_to_sync",
"tests/test_sync.py::test_async_to_sync_decorator",
"tests/test_sync.py::test_async_to_sync_method_decorator",
"tests/test_sync.py::test_async_to_sync_in_async",
"tests/test_sync.py::test_async_to_sync_in_thread",
"tests/test_sync.py::test_async_to_async_method_self_attribute",
"tests/test_sync.py::test_thread_sensitive_outside_sync",
"tests/test_sync.py::test_thread_sensitive_outside_async",
"tests/test_sync.py::test_thread_sensitive_with_context_matches",
"tests/test_sync.py::test_thread_sensitive_nested_context",
"tests/test_sync.py::test_thread_sensitive_context_without_sync_work",
"tests/test_sync.py::test_thread_sensitive_double_nested_sync",
"tests/test_sync.py::test_thread_sensitive_double_nested_async",
"tests/test_sync.py::test_thread_sensitive_disabled",
"tests/test_sync.py::ASGITest::test_wrapped_case_is_collected",
"tests/test_sync.py::test_sync_to_async_detected_as_coroutinefunction",
"tests/test_sync.py::test_multiprocessing"
] | 2021-02-09 09:21:06+00:00 | 1,938 |
|
django__asgiref-320 | diff --git a/.pre-commit-config.yaml b/.pre-commit-config.yaml
index 8a05077..0379a31 100644
--- a/.pre-commit-config.yaml
+++ b/.pre-commit-config.yaml
@@ -6,7 +6,7 @@ repos:
args: ["--py37-plus"]
- repo: https://github.com/psf/black
- rev: 20.8b1
+ rev: 22.3.0
hooks:
- id: black
args: ["--target-version=py37"]
diff --git a/asgiref/sync.py b/asgiref/sync.py
index b71b379..a70dac1 100644
--- a/asgiref/sync.py
+++ b/asgiref/sync.py
@@ -1,3 +1,4 @@
+import asyncio
import asyncio.coroutines
import contextvars
import functools
@@ -101,6 +102,10 @@ class AsyncToSync:
# Local, not a threadlocal, so that tasks can work out what their parent used.
executors = Local()
+ # When we can't find a CurrentThreadExecutor from the context, such as
+ # inside create_task, we'll look it up here from the running event loop.
+ loop_thread_executors: "Dict[asyncio.AbstractEventLoop, CurrentThreadExecutor]" = {}
+
def __init__(self, awaitable, force_new_loop=False):
if not callable(awaitable) or not _iscoroutinefunction_or_partial(awaitable):
# Python does not have very reliable detection of async functions
@@ -164,6 +169,7 @@ class AsyncToSync:
old_current_executor = None
current_executor = CurrentThreadExecutor()
self.executors.current = current_executor
+ loop = None
# Use call_soon_threadsafe to schedule a synchronous callback on the
# main event loop's thread if it's there, otherwise make a new loop
# in this thread.
@@ -175,6 +181,7 @@ class AsyncToSync:
if not (self.main_event_loop and self.main_event_loop.is_running()):
# Make our own event loop - in a new thread - and run inside that.
loop = asyncio.new_event_loop()
+ self.loop_thread_executors[loop] = current_executor
loop_executor = ThreadPoolExecutor(max_workers=1)
loop_future = loop_executor.submit(
self._run_event_loop, loop, awaitable
@@ -194,6 +201,8 @@ class AsyncToSync:
current_executor.run_until_future(call_result)
finally:
# Clean up any executor we were running
+ if loop is not None:
+ del self.loop_thread_executors[loop]
if hasattr(self.executors, "current"):
del self.executors.current
if old_current_executor:
@@ -378,6 +387,9 @@ class SyncToAsync:
# Create new thread executor in current context
executor = ThreadPoolExecutor(max_workers=1)
self.context_to_thread_executor[thread_sensitive_context] = executor
+ elif loop in AsyncToSync.loop_thread_executors:
+ # Re-use thread executor for running loop
+ executor = AsyncToSync.loop_thread_executors[loop]
elif self.deadlock_context and self.deadlock_context.get(False):
raise RuntimeError(
"Single thread executor already being used, would deadlock"
| django/asgiref | cde961b13c69b90216c4c1c81d1ad1ca1bc22b48 | diff --git a/tests/test_server.py b/tests/test_server.py
index 8f86096..616ccf2 100644
--- a/tests/test_server.py
+++ b/tests/test_server.py
@@ -21,7 +21,8 @@ class Server(StatelessServer):
application,
max_applications=max_applications,
)
- self._sock = sock.socket(sock.AF_INET, sock.SOCK_DGRAM | sock.SOCK_NONBLOCK)
+ self._sock = sock.socket(sock.AF_INET, sock.SOCK_DGRAM)
+ self._sock.setblocking(False)
self._sock.bind(("127.0.0.1", 0))
@property
@@ -54,7 +55,8 @@ class Server(StatelessServer):
class Client:
def __init__(self, name):
- self._sock = sock.socket(sock.AF_INET, sock.SOCK_DGRAM | sock.SOCK_NONBLOCK)
+ self._sock = sock.socket(sock.AF_INET, sock.SOCK_DGRAM)
+ self._sock.setblocking(False)
self.name = name
async def register(self, server_addr, name=None):
diff --git a/tests/test_sync.py b/tests/test_sync.py
index 8f563d9..2837423 100644
--- a/tests/test_sync.py
+++ b/tests/test_sync.py
@@ -397,15 +397,21 @@ def test_thread_sensitive_outside_sync():
@async_to_sync
async def middle():
await inner()
+ await asyncio.create_task(inner_task())
- # Inner sync function
+ # Inner sync functions
@sync_to_async
def inner():
result["thread"] = threading.current_thread()
+ @sync_to_async
+ def inner_task():
+ result["thread2"] = threading.current_thread()
+
# Run it
middle()
assert result["thread"] == threading.current_thread()
+ assert result["thread2"] == threading.current_thread()
@pytest.mark.asyncio
| sync_to_async does not find root thread inside a task
When you use `create_task` (or any way of making a coroutine not through asgiref), and the root thread is synchronous, then `sync_to_async` in thread-sensitive mode will use the root thread outside of tasks and a single, specific new thread inside of tasks, ruining the thread-sensitive guarantee.
Instead, we should make `sync_to_async` always first look for an executor on the root thread and, if it finds it, use that to run code. | 0.0 | [
"tests/test_sync.py::test_thread_sensitive_outside_sync"
] | [
"tests/test_server.py::test_stateless_server",
"tests/test_server.py::test_server_delete_instance",
"tests/test_sync.py::test_sync_to_async",
"tests/test_sync.py::test_sync_to_async_fail_non_function",
"tests/test_sync.py::test_sync_to_async_fail_async",
"tests/test_sync.py::test_async_to_sync_fail_partial",
"tests/test_sync.py::test_sync_to_async_decorator",
"tests/test_sync.py::test_nested_sync_to_async_retains_wrapped_function_attributes",
"tests/test_sync.py::test_sync_to_async_method_decorator",
"tests/test_sync.py::test_sync_to_async_method_self_attribute",
"tests/test_sync.py::test_async_to_sync_to_async",
"tests/test_sync.py::test_async_to_sync_fail_non_function",
"tests/test_sync.py::test_async_to_sync_fail_sync",
"tests/test_sync.py::test_async_to_sync",
"tests/test_sync.py::test_async_to_sync_decorator",
"tests/test_sync.py::test_async_to_sync_method_decorator",
"tests/test_sync.py::test_async_to_sync_in_async",
"tests/test_sync.py::test_async_to_sync_in_thread",
"tests/test_sync.py::test_async_to_sync_in_except",
"tests/test_sync.py::test_async_to_sync_partial",
"tests/test_sync.py::test_async_to_sync_method_self_attribute",
"tests/test_sync.py::test_thread_sensitive_outside_async",
"tests/test_sync.py::test_thread_sensitive_with_context_matches",
"tests/test_sync.py::test_thread_sensitive_nested_context",
"tests/test_sync.py::test_thread_sensitive_context_without_sync_work",
"tests/test_sync.py::test_thread_sensitive_double_nested_sync",
"tests/test_sync.py::test_thread_sensitive_double_nested_async",
"tests/test_sync.py::test_thread_sensitive_disabled",
"tests/test_sync.py::ASGITest::test_wrapped_case_is_collected",
"tests/test_sync.py::test_sync_to_async_detected_as_coroutinefunction",
"tests/test_sync.py::test_multiprocessing",
"tests/test_sync.py::test_sync_to_async_uses_executor",
"tests/test_sync.py::test_sync_to_async_deadlock_raises",
"tests/test_sync.py::test_sync_to_async_deadlock_ignored_with_exception",
"tests/test_sync.py::test_sync_to_async_with_blocker_non_thread_sensitive"
] | 2022-03-18 19:33:50+00:00 | 1,939 |
|
django__asgiref-325 | diff --git a/asgiref/sync.py b/asgiref/sync.py
index a70dac1..d02bd4a 100644
--- a/asgiref/sync.py
+++ b/asgiref/sync.py
@@ -107,7 +107,12 @@ class AsyncToSync:
loop_thread_executors: "Dict[asyncio.AbstractEventLoop, CurrentThreadExecutor]" = {}
def __init__(self, awaitable, force_new_loop=False):
- if not callable(awaitable) or not _iscoroutinefunction_or_partial(awaitable):
+ if not callable(awaitable) or (
+ not _iscoroutinefunction_or_partial(awaitable)
+ and not _iscoroutinefunction_or_partial(
+ getattr(awaitable, "__call__", awaitable)
+ )
+ ):
# Python does not have very reliable detection of async functions
# (lots of false negatives) so this is just a warning.
warnings.warn(
| django/asgiref | 12f6355d50c271aab821a37dbf2cde5863cc2643 | diff --git a/tests/test_sync.py b/tests/test_sync.py
index 2837423..c5677fc 100644
--- a/tests/test_sync.py
+++ b/tests/test_sync.py
@@ -3,6 +3,7 @@ import functools
import multiprocessing
import threading
import time
+import warnings
from concurrent.futures import ThreadPoolExecutor
from functools import wraps
from unittest import TestCase
@@ -365,6 +366,29 @@ def test_async_to_sync_partial():
assert result["worked"]
+def test_async_to_sync_on_callable_object():
+ """
+ Tests async_to_sync on a callable class instance
+ """
+
+ result = {}
+
+ class CallableClass:
+ async def __call__(self, value):
+ await asyncio.sleep(0)
+ result["worked"] = True
+ return value
+
+ # Run it (without warnings)
+ with warnings.catch_warnings():
+ warnings.simplefilter("error")
+ sync_function = async_to_sync(CallableClass())
+ out = sync_function(42)
+
+ assert out == 42
+ assert result["worked"] is True
+
+
def test_async_to_sync_method_self_attribute():
"""
Tests async_to_sync on a method copies __self__.
| `sync.async_to_sync` incorrectly warns "a non-async-marked callable" for async callable class instance
Repro
```python
from asgiref.sync import async_to_sync
class CallableClass:
async def __call__(self):
return None
async_to_sync(CallableClass())
```
Yields the `UserWarning`
```
UserWarning: async_to_sync was passed a non-async-marked callable
```
Though the code works at runtime. | 0.0 | [
"tests/test_sync.py::test_async_to_sync_on_callable_object"
] | [
"tests/test_sync.py::test_sync_to_async",
"tests/test_sync.py::test_sync_to_async_fail_non_function",
"tests/test_sync.py::test_sync_to_async_fail_async",
"tests/test_sync.py::test_async_to_sync_fail_partial",
"tests/test_sync.py::test_sync_to_async_decorator",
"tests/test_sync.py::test_nested_sync_to_async_retains_wrapped_function_attributes",
"tests/test_sync.py::test_sync_to_async_method_decorator",
"tests/test_sync.py::test_sync_to_async_method_self_attribute",
"tests/test_sync.py::test_async_to_sync_to_async",
"tests/test_sync.py::test_async_to_sync_fail_non_function",
"tests/test_sync.py::test_async_to_sync_fail_sync",
"tests/test_sync.py::test_async_to_sync",
"tests/test_sync.py::test_async_to_sync_decorator",
"tests/test_sync.py::test_async_to_sync_method_decorator",
"tests/test_sync.py::test_async_to_sync_in_async",
"tests/test_sync.py::test_async_to_sync_in_thread",
"tests/test_sync.py::test_async_to_sync_in_except",
"tests/test_sync.py::test_async_to_sync_partial",
"tests/test_sync.py::test_async_to_sync_method_self_attribute",
"tests/test_sync.py::test_thread_sensitive_outside_sync",
"tests/test_sync.py::test_thread_sensitive_outside_async",
"tests/test_sync.py::test_thread_sensitive_with_context_matches",
"tests/test_sync.py::test_thread_sensitive_nested_context",
"tests/test_sync.py::test_thread_sensitive_context_without_sync_work",
"tests/test_sync.py::test_thread_sensitive_double_nested_sync",
"tests/test_sync.py::test_thread_sensitive_double_nested_async",
"tests/test_sync.py::test_thread_sensitive_disabled",
"tests/test_sync.py::ASGITest::test_wrapped_case_is_collected",
"tests/test_sync.py::test_sync_to_async_detected_as_coroutinefunction",
"tests/test_sync.py::test_multiprocessing",
"tests/test_sync.py::test_sync_to_async_uses_executor",
"tests/test_sync.py::test_sync_to_async_deadlock_raises",
"tests/test_sync.py::test_sync_to_async_deadlock_ignored_with_exception",
"tests/test_sync.py::test_sync_to_async_with_blocker_non_thread_sensitive"
] | 2022-05-10 11:58:24+00:00 | 1,940 |
|
django__channels_redis-274 | diff --git a/channels_redis/core.py b/channels_redis/core.py
index af288bd..94531e9 100644
--- a/channels_redis/core.py
+++ b/channels_redis/core.py
@@ -1,6 +1,5 @@
import asyncio
import base64
-import binascii
import collections
import functools
import hashlib
@@ -18,6 +17,8 @@ import msgpack
from channels.exceptions import ChannelFull
from channels.layers import BaseChannelLayer
+from .utils import _consistent_hash
+
logger = logging.getLogger(__name__)
AIOREDIS_VERSION = tuple(map(int, aioredis.__version__.split(".")))
@@ -858,15 +859,7 @@ class RedisChannelLayer(BaseChannelLayer):
### Internal functions ###
def consistent_hash(self, value):
- """
- Maps the value to a node value between 0 and 4095
- using CRC, then down to one of the ring nodes.
- """
- if isinstance(value, str):
- value = value.encode("utf8")
- bigval = binascii.crc32(value) & 0xFFF
- ring_divisor = 4096 / float(self.ring_size)
- return int(bigval / ring_divisor)
+ return _consistent_hash(value, self.ring_size)
def make_fernet(self, key):
"""
diff --git a/channels_redis/pubsub.py b/channels_redis/pubsub.py
index 62907d5..b8d549e 100644
--- a/channels_redis/pubsub.py
+++ b/channels_redis/pubsub.py
@@ -8,6 +8,8 @@ import uuid
import aioredis
import msgpack
+from .utils import _consistent_hash
+
logger = logging.getLogger(__name__)
@@ -106,11 +108,7 @@ class RedisPubSubLoopLayer:
"""
Return the shard that is used exclusively for this channel or group.
"""
- if len(self._shards) == 1:
- # Avoid the overhead of hashing and modulo when it is unnecessary.
- return self._shards[0]
- shard_index = abs(hash(channel_or_group_name)) % len(self._shards)
- return self._shards[shard_index]
+ return self._shards[_consistent_hash(channel_or_group_name, len(self._shards))]
def _get_group_channel_name(self, group):
"""
diff --git a/channels_redis/utils.py b/channels_redis/utils.py
new file mode 100644
index 0000000..7b30fdc
--- /dev/null
+++ b/channels_redis/utils.py
@@ -0,0 +1,17 @@
+import binascii
+
+
+def _consistent_hash(value, ring_size):
+ """
+ Maps the value to a node value between 0 and 4095
+ using CRC, then down to one of the ring nodes.
+ """
+ if ring_size == 1:
+ # Avoid the overhead of hashing and modulo when it is unnecessary.
+ return 0
+
+ if isinstance(value, str):
+ value = value.encode("utf8")
+ bigval = binascii.crc32(value) & 0xFFF
+ ring_divisor = 4096 / float(ring_size)
+ return int(bigval / ring_divisor)
| django/channels_redis | 49e419dce642b7aef12daa3fa8495f6b9beb1fbe | diff --git a/tests/test_utils.py b/tests/test_utils.py
new file mode 100644
index 0000000..3a78330
--- /dev/null
+++ b/tests/test_utils.py
@@ -0,0 +1,20 @@
+import pytest
+
+from channels_redis.utils import _consistent_hash
+
+
+@pytest.mark.parametrize(
+ "value,ring_size,expected",
+ [
+ ("key_one", 1, 0),
+ ("key_two", 1, 0),
+ ("key_one", 2, 1),
+ ("key_two", 2, 0),
+ ("key_one", 10, 6),
+ ("key_two", 10, 4),
+ (b"key_one", 10, 6),
+ (b"key_two", 10, 4),
+ ],
+)
+def test_consistent_hash_result(value, ring_size, expected):
+ assert _consistent_hash(value, ring_size) == expected
| PubSub: Sharding broken with multiple workers or worker restarts
The "classic" implementation selects shards like this:
```
def consistent_hash(self, value):
"""
Maps the value to a node value between 0 and 4095
using CRC, then down to one of the ring nodes.
"""
if isinstance(value, str):
value = value.encode("utf8")
bigval = binascii.crc32(value) & 0xFFF
ring_divisor = 4096 / float(self.ring_size)
return int(bigval / ring_divisor)
```
The PUBSUB implementation does this:
```
def _get_shard(self, channel_or_group_name):
"""
Return the shard that is used exclusively for this channel or group.
"""
if len(self._shards) == 1:
# Avoid the overhead of hashing and modulo when it is unnecessary.
return self._shards[0]
shard_index = abs(hash(channel_or_group_name)) % len(self._shards)
return self._shards[shard_index]
```
I was surprised that there's a difference when I first looked at this, but now this started to really bite us in production after we introduced sharding: `hash()` is not guaranteed to return the same thing across multiple invocations of `python`, even on the same machine, unless `PYTHONHASHSEED` is set manually, which probably no-one ever does. See https://docs.python.org/3/using/cmdline.html#envvar-PYTHONHASHSEED
I think we should just use the same hash function across implementations. I'll send a PR :) | 0.0 | [
"tests/test_utils.py::test_consistent_hash_result[key_one-1-0]",
"tests/test_utils.py::test_consistent_hash_result[key_two-1-0]",
"tests/test_utils.py::test_consistent_hash_result[key_one-2-1]",
"tests/test_utils.py::test_consistent_hash_result[key_two-2-0]",
"tests/test_utils.py::test_consistent_hash_result[key_one-10-6_0]",
"tests/test_utils.py::test_consistent_hash_result[key_two-10-4_0]",
"tests/test_utils.py::test_consistent_hash_result[key_one-10-6_1]",
"tests/test_utils.py::test_consistent_hash_result[key_two-10-4_1]"
] | [] | 2021-09-01 20:09:19+00:00 | 1,941 |
|
django__daphne-396 | diff --git a/CHANGELOG.txt b/CHANGELOG.txt
index 630c0fa..31e4650 100644
--- a/CHANGELOG.txt
+++ b/CHANGELOG.txt
@@ -11,7 +11,14 @@ Unreleased
range of versions does not represent a good use of maintainer time. Going
forward the latest Twisted version will be required.
-* Added `log-fmt` CLI argument.
+* Set ``daphne`` as default ``Server`` header.
+
+ This can be configured with the ``--server-name`` CLI argument.
+
+ Added the new ``--no-server-name`` CLI argument to disable the ``Server``
+ header, which is equivalent to ``--server-name=` (an empty name).
+
+* Added ``--log-fmt`` CLI argument.
3.0.2 (2021-04-07)
------------------
diff --git a/daphne/cli.py b/daphne/cli.py
index 2e83a5c..accafe1 100755
--- a/daphne/cli.py
+++ b/daphne/cli.py
@@ -93,7 +93,7 @@ class CommandLineInterface:
self.parser.add_argument(
"--log-fmt",
help="Log format to use",
- default="%(asctime)-15s %(levelname)-8s %(message)s"
+ default="%(asctime)-15s %(levelname)-8s %(message)s",
)
self.parser.add_argument(
"--ping-interval",
@@ -162,7 +162,10 @@ class CommandLineInterface:
"--server-name",
dest="server_name",
help="specify which value should be passed to response header Server attribute",
- default="Daphne",
+ default="daphne",
+ )
+ self.parser.add_argument(
+ "--no-server-name", dest="server_name", action="store_const", const=""
)
self.server = None
diff --git a/daphne/http_protocol.py b/daphne/http_protocol.py
index a289e93..f0657fd 100755
--- a/daphne/http_protocol.py
+++ b/daphne/http_protocol.py
@@ -249,8 +249,8 @@ class WebRequest(http.Request):
# Write headers
for header, value in message.get("headers", {}):
self.responseHeaders.addRawHeader(header, value)
- if self.server.server_name and self.server.server_name.lower() != "daphne":
- self.setHeader(b"server", self.server.server_name.encode("utf-8"))
+ if self.server.server_name and not self.responseHeaders.hasHeader("server"):
+ self.setHeader(b"server", self.server.server_name.encode())
logger.debug(
"HTTP %s response started for %s", message["status"], self.client_addr
)
diff --git a/daphne/server.py b/daphne/server.py
index 0d463d0..4334217 100755
--- a/daphne/server.py
+++ b/daphne/server.py
@@ -56,7 +56,7 @@ class Server:
websocket_handshake_timeout=5,
application_close_timeout=10,
ready_callable=None,
- server_name="Daphne",
+ server_name="daphne",
# Deprecated and does not work, remove in version 2.2
ws_protocols=None,
):
| django/daphne | 87bc5a7975e3e77ec64a183058b6e875cf744cf4 | diff --git a/tests/test_cli.py b/tests/test_cli.py
index 17335ed..51eab2e 100644
--- a/tests/test_cli.py
+++ b/tests/test_cli.py
@@ -240,3 +240,18 @@ class TestCLIInterface(TestCase):
exc.exception.message,
"--proxy-headers has to be passed for this parameter.",
)
+
+ def test_custom_servername(self):
+ """
+ Passing `--server-name` will set the default server header
+ from 'daphne' to the passed one.
+ """
+ self.assertCLI([], {"server_name": "daphne"})
+ self.assertCLI(["--server-name", ""], {"server_name": ""})
+ self.assertCLI(["--server-name", "python"], {"server_name": "python"})
+
+ def test_no_servername(self):
+ """
+ Passing `--no-server-name` will set server name to '' (empty string)
+ """
+ self.assertCLI(["--no-server-name"], {"server_name": ""})
diff --git a/tests/test_http_response.py b/tests/test_http_response.py
index 1fc2439..22f6480 100644
--- a/tests/test_http_response.py
+++ b/tests/test_http_response.py
@@ -13,9 +13,12 @@ class TestHTTPResponse(DaphneTestCase):
Lowercases and sorts headers, and strips transfer-encoding ones.
"""
return sorted(
- (name.lower(), value.strip())
- for name, value in headers
- if name.lower() != b"transfer-encoding"
+ [(b"server", b"daphne")]
+ + [
+ (name.lower(), value.strip())
+ for name, value in headers
+ if name.lower() not in (b"server", b"transfer-encoding")
+ ]
)
def encode_headers(self, headers):
| `Daphne` sets `server='daphne'` header for `websocket` connections, but not for `http-requests`
# Hi there,
( Correct me if i am wrong here )
Daphne ( by default ) doesn't set a server header.
By default these servers sets a header to identify backend technologies:
- [Uvicorn](github.com/encode/uvicorn) : uvicorn
- [Hypercorn](https://gitlab.com/pgjones/hypercorn) : hypercorn-{implementation}
- [Gunicorn](https://github.com/benoitc/gunicorn) : gunicorn/{version}
# Why should we do it ?
To allow technology detection by extensions like [Wappalyzer](https://github.com/AliasIO/wappalyzer/) | 0.0 | [
"tests/test_cli.py::TestCLIInterface::test_custom_servername",
"tests/test_cli.py::TestCLIInterface::test_no_servername"
] | [
"tests/test_cli.py::TestEndpointDescriptions::testBasics",
"tests/test_cli.py::TestEndpointDescriptions::testFileDescriptorBinding",
"tests/test_cli.py::TestEndpointDescriptions::testMultipleEnpoints",
"tests/test_cli.py::TestEndpointDescriptions::testTcpPortBindings",
"tests/test_cli.py::TestEndpointDescriptions::testUnixSocketBinding",
"tests/test_cli.py::TestCLIInterface::testCLIBasics",
"tests/test_cli.py::TestCLIInterface::testCustomEndpoints",
"tests/test_cli.py::TestCLIInterface::testMixedCLIEndpointCreation",
"tests/test_cli.py::TestCLIInterface::testUnixSockets",
"tests/test_cli.py::TestCLIInterface::test_custom_proxyhost",
"tests/test_cli.py::TestCLIInterface::test_custom_proxyport",
"tests/test_cli.py::TestCLIInterface::test_default_proxyheaders",
"tests/test_http_response.py::TestHTTPResponse::test_body",
"tests/test_http_response.py::TestHTTPResponse::test_chunked_response",
"tests/test_http_response.py::TestHTTPResponse::test_chunked_response_empty",
"tests/test_http_response.py::TestHTTPResponse::test_custom_status_code",
"tests/test_http_response.py::TestHTTPResponse::test_headers",
"tests/test_http_response.py::TestHTTPResponse::test_headers_type",
"tests/test_http_response.py::TestHTTPResponse::test_headers_type_raw",
"tests/test_http_response.py::TestHTTPResponse::test_minimal_response",
"tests/test_http_response.py::TestHTTPResponse::test_status_code_required"
] | 2021-12-28 12:08:39+00:00 | 1,942 |
|
django__daphne-406 | diff --git a/daphne/http_protocol.py b/daphne/http_protocol.py
index 7df7bae..a289e93 100755
--- a/daphne/http_protocol.py
+++ b/daphne/http_protocol.py
@@ -50,6 +50,8 @@ class WebRequest(http.Request):
) # Shorten it a bit, bytes wise
def __init__(self, *args, **kwargs):
+ self.client_addr = None
+ self.server_addr = None
try:
http.Request.__init__(self, *args, **kwargs)
# Easy server link
@@ -77,9 +79,6 @@ class WebRequest(http.Request):
# requires unicode string.
self.client_addr = [str(self.client.host), self.client.port]
self.server_addr = [str(self.host.host), self.host.port]
- else:
- self.client_addr = None
- self.server_addr = None
self.client_scheme = "https" if self.isSecure() else "http"
| django/daphne | 6a5093982ca1eaffbc41f0114ac5bea7cb3902be | diff --git a/tests/test_http_protocol.py b/tests/test_http_protocol.py
new file mode 100644
index 0000000..024479d
--- /dev/null
+++ b/tests/test_http_protocol.py
@@ -0,0 +1,49 @@
+import unittest
+
+from daphne.http_protocol import WebRequest
+
+
+class MockServer:
+ """
+ Mock server object for testing.
+ """
+
+ def protocol_connected(self, *args, **kwargs):
+ pass
+
+
+class MockFactory:
+ """
+ Mock factory object for testing.
+ """
+
+ def __init__(self):
+ self.server = MockServer()
+
+
+class MockChannel:
+ """
+ Mock channel object for testing.
+ """
+
+ def __init__(self):
+ self.factory = MockFactory()
+ self.transport = None
+
+ def getPeer(self, *args, **kwargs):
+ return "peer"
+
+ def getHost(self, *args, **kwargs):
+ return "host"
+
+
+class TestHTTPProtocol(unittest.TestCase):
+ """
+ Tests the HTTP protocol classes.
+ """
+
+ def test_web_request_initialisation(self):
+ channel = MockChannel()
+ request = WebRequest(channel)
+ self.assertIsNone(request.client_addr)
+ self.assertIsNone(request.server_addr)
| 'WebRequest' object has no attribute 'client_addr'
Hi, I've recently migrated my Django project from WSGI + gunicorn to ASGI + daphne. It's working great apart from an occasional error in my Sentry/logs `builtins.AttributeError: 'WebRequest' object has no attribute 'client_addr'`.
It just seems to be the problem in the `log.debug` call on `connectionLost` in https://github.com/django/daphne/blob/main/daphne/http_protocol.py#L213
Looking at the class it seems that there is a chance that the `client_addr` is not set until later in the `process` method https://github.com/django/daphne/blob/main/daphne/http_protocol.py#L81 so there's a chance that the `self.client_addr` is simply not set.
I can't replicate this error by any means, it happens only occasionally (I guess that's why it pops up in the `connectionLost` method). Should the `client_addr` be set to None by default so that this log message doesn't cause fatals?
BTW I've seen https://github.com/django/daphne/issues/304 and https://github.com/django/daphne/issues/244. First one is giving 404 on SO, second one seems similar but they're mentioning websockets whereas I haven't even got to the point where I'm supporting websockets in my app.
- Your OS and runtime environment, and browser if applicable
Presumably platform browser agnostic. I don't have a reproduction method.
- A `pip freeze` output showing your package versions
channels==3.0.3
channels_redis==3.3.0
daphne==3.0.2
Django==3.2.6
- How you're running Channels (runserver? daphne/runworker? Nginx/Apache in front?)
daphne on Heroku with `daphne my_app.asgi:application --port $PORT --bind 0.0.0.0 --verbosity 2` in Procfile
- Console logs and full tracebacks of any errors
```
Jan 28 11:55:38 app/web.3 Unhandled Error
Jan 28 11:55:38 app/web.3 Traceback (most recent call last):
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/internet/asyncioreactor.py", line 257, in run
Jan 28 11:55:38 app/web.3 self._asyncioEventloop.run_forever()
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/asyncio/base_events.py", line 570, in run_forever
Jan 28 11:55:38 app/web.3 self._run_once()
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/asyncio/base_events.py", line 1859, in _run_once
Jan 28 11:55:38 app/web.3 handle._run()
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/asyncio/events.py", line 81, in _run
Jan 28 11:55:38 app/web.3 self._context.run(self._callback, *self._args)
Jan 28 11:55:38 app/web.3 --- <exception caught here> ---
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/python/log.py", line 101, in callWithLogger
Jan 28 11:55:38 app/web.3 return callWithContext({"system": lp}, func, *args, **kw)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/python/log.py", line 85, in callWithContext
Jan 28 11:55:38 app/web.3 return context.call({ILogContext: newCtx}, func, *args, **kw)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/python/context.py", line 118, in callWithContext
Jan 28 11:55:38 app/web.3 return self.currentContext().callWithContext(ctx, func, *args, **kw)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/python/context.py", line 83, in callWithContext
Jan 28 11:55:38 app/web.3 return func(*args, **kw)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/internet/asyncioreactor.py", line 145, in _readOrWrite
Jan 28 11:55:38 app/web.3 self._disconnectSelectable(selectable, why, read)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/internet/posixbase.py", line 300, in _disconnectSelectable
Jan 28 11:55:38 app/web.3 selectable.readConnectionLost(f)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/internet/tcp.py", line 308, in readConnectionLost
Jan 28 11:55:38 app/web.3 self.connectionLost(reason)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/internet/tcp.py", line 325, in connectionLost
Jan 28 11:55:38 app/web.3 protocol.connectionLost(reason)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/twisted/web/http.py", line 2508, in connectionLost
Jan 28 11:55:38 app/web.3 request.connectionLost(reason)
Jan 28 11:55:38 app/web.3 File "/app/.heroku/python/lib/python3.8/site-packages/daphne/http_protocol.py", line 213, in connectionLost
Jan 28 11:55:38 app/web.3 logger.debug("HTTP disconnect for %s", self.client_addr)
Jan 28 11:55:38 app/web.3 builtins.AttributeError: 'WebRequest' object has no attribute 'client_addr'
```
| 0.0 | [
"tests/test_http_protocol.py::TestHTTPProtocol::test_web_request_initialisation"
] | [] | 2022-02-13 22:18:11+00:00 | 1,943 |
|
django__daphne-500 | diff --git a/daphne/http_protocol.py b/daphne/http_protocol.py
index f0657fd..b7da1bf 100755
--- a/daphne/http_protocol.py
+++ b/daphne/http_protocol.py
@@ -9,7 +9,7 @@ from twisted.protocols.policies import ProtocolWrapper
from twisted.web import http
from zope.interface import implementer
-from .utils import parse_x_forwarded_for
+from .utils import HEADER_NAME_RE, parse_x_forwarded_for
logger = logging.getLogger(__name__)
@@ -69,6 +69,13 @@ class WebRequest(http.Request):
def process(self):
try:
self.request_start = time.time()
+
+ # Validate header names.
+ for name, _ in self.requestHeaders.getAllRawHeaders():
+ if not HEADER_NAME_RE.fullmatch(name):
+ self.basic_error(400, b"Bad Request", "Invalid header name")
+ return
+
# Get upgrade header
upgrade_header = None
if self.requestHeaders.hasHeader(b"Upgrade"):
diff --git a/daphne/utils.py b/daphne/utils.py
index 81f1f9d..0699314 100644
--- a/daphne/utils.py
+++ b/daphne/utils.py
@@ -1,7 +1,12 @@
import importlib
+import re
from twisted.web.http_headers import Headers
+# Header name regex as per h11.
+# https://github.com/python-hyper/h11/blob/a2c68948accadc3876dffcf979d98002e4a4ed27/h11/_abnf.py#L10-L21
+HEADER_NAME_RE = re.compile(rb"[-!#$%&'*+.^_`|~0-9a-zA-Z]+")
+
def import_by_path(path):
"""
| django/daphne | 9a282dd6277f4b921e494a9fc30a534a7f0d6ca6 | diff --git a/tests/test_http_request.py b/tests/test_http_request.py
index 52f6dd1..dca5bd7 100644
--- a/tests/test_http_request.py
+++ b/tests/test_http_request.py
@@ -310,3 +310,15 @@ class TestHTTPRequest(DaphneTestCase):
b"GET /?\xc3\xa4\xc3\xb6\xc3\xbc HTTP/1.0\r\n\r\n"
)
self.assertTrue(response.startswith(b"HTTP/1.0 400 Bad Request"))
+
+ def test_invalid_header_name(self):
+ """
+ Tests that requests with invalid header names fail.
+ """
+ # Test cases follow those used by h11
+ # https://github.com/python-hyper/h11/blob/a2c68948accadc3876dffcf979d98002e4a4ed27/h11/tests/test_headers.py#L24-L35
+ for header_name in [b"foo bar", b"foo\x00bar", b"foo\xffbar", b"foo\x01bar"]:
+ response = self.run_daphne_raw(
+ f"GET / HTTP/1.0\r\n{header_name}: baz\r\n\r\n".encode("ascii")
+ )
+ self.assertTrue(response.startswith(b"HTTP/1.0 400 Bad Request"))
| Daphne allows invalid characters within header names
# The bug
RFC 9110 defines the header names must consist of a single token, which is defined as follows:
```
token = 1*tchar
tchar = "!" / "#" / "$" / "%" / "&" / "'" / "*"
/ "+" / "-" / "." / "^" / "_" / "`" / "|" / "~"
/ DIGIT / ALPHA
; any VCHAR, except delimiters
```
When Daphne receives a request with a header name containing any or all of the following characters, it does not reject the message:
```
\x00\x01\x02\x03\x04\x05\x06\x07\x08\t\x0b\x0c\x0e\x0f\x10\x11\x12\x13\x14\x15\x16\x17\x18\x19\x1a\x1b\x1c\x1d\x1e\x1f "(),/;<=>?@[/]{}\xc0\xc1\xc2\xc3\xc4\xc5\xc6\xc7\xc8\xc9\xca\xcb\xcc\xcd\xce\xcf\xd0\xd1\xd2\xd3\xd4\xd5\xd6\xd7\xd8\xd9\xda\xdb\xdc\xdd\xde\xdf\xe0\xe1\xe2\xe3\xe4\xe5\xe6\xe7\xe8\xe9\xea\xeb\xec\xed\xee\xef
```
Thus, when you send the following request:
```
GET / HTTP/1.1\r\n
\x00\x01\x02\x03\x04\x05\x06\x07\x08\t\x0b\x0c\x0e\x0f\x10\x11\x12\x13\x14\x15\x16\x17\x18\x19\x1a\x1b\x1c\x1d\x1e\x1f "(),/;<=>?@[/]{}\xc0\xc1\xc2\xc3\xc4\xc5\xc6\xc7\xc8\xc9\xca\xcb\xcc\xcd\xce\xcf\xd0\xd1\xd2\xd3\xd4\xd5\xd6\xd7\xd8\xd9\xda\xdb\xdc\xdd\xde\xdf\xe0\xe1\xe2\xe3\xe4\xe5\xe6\xe7\xe8\xe9\xea\xeb\xec\xed\xee\xef: whatever\r\n
\r\n
```
Daphne's interpretation is as follows:
```
[
HTTPRequest(
method=b'GET', uri=b'/', version=b'1.1',
headers=[
(b'\x00\x01\x02\x03\x04\x05\x06\x07\x08\t\x0b\x0c\x0e\x0f\x10\x11\x12\x13\x14\x15\x16\x17\x18\x19\x1a\x1b\x1c\x1d\x1e\x1f "(),/;<=>?@[/]{}\xc0\xc1\xc2\xc3\xc4\xc5\xc6\xc7\xc8\xc9\xca\xcb\xcc\xcd\xce\xcf\xd0\xd1\xd2\xd3\xd4\xd5\xd6\xd7\xd8\xd9\xda\xdb\xdc\xdd\xde\xdf\xe0\xe1\xe2\xe3\xe4\xe5\xe6\xe7\xe8\xe9\xea\xeb\xec\xed\xee\xef', b'whatever'),
],
body=b'',
),
]
```
(i.e. the invalid header name made it into the request unchanged)
Nearly all other HTTP implementations reject the above request, including Uvicorn, Hypercorn, AIOHTTP, Apache httpd, Bun, CherryPy, Deno, FastHTTP, Go net/http, Gunicorn, H2O, Hyper, Jetty, libsoup, Lighttpd, Mongoose, Nginx, Node.js LiteSpeed, Passenger, Puma, Tomcat, OpenWrt uhttpd, Uvicorn, Waitress, WEBrick, and OpenBSD httpd.
## Your OS and runtime environment:
```
$ uname -a
Linux de8a63fbb2f4 6.7.2-arch1-2 #1 SMP PREEMPT_DYNAMIC Wed, 31 Jan 2024 09:22:15 +0000 x86_64 GNU/Linux
```
## `pip freeze`:
```
asgiref==3.7.2
attrs==23.2.0
autobahn==23.6.2
Automat==22.10.0
cffi==1.16.0
constantly==23.10.4
cryptography==42.0.2
Cython==0.29.32
daphne @ file:///app/daphne
h2==4.1.0
hpack==4.0.0
hyperframe==6.0.1
hyperlink==21.0.0
idna==3.6
incremental==22.10.0
priority==1.3.0
pyasn1==0.5.1
pyasn1-modules==0.3.0
pycparser==2.21
Pygments==2.14.0
pyOpenSSL==24.0.0
python-afl==0.7.4
PyYAML==6.0
service-identity==24.1.0
six==1.16.0
Twisted==23.10.0
txaio==23.1.1
typing_extensions==4.9.0
zope.interface==6.1
```
(Using Daphne built from main, with the latest commit being https://github.com/django/daphne/commit/993efe62ce9e9c1cd64c81b6ee7dfa7b819482c7 at the time of writing)
## How you're running Channels (runserver? daphne/runworker? Nginx/Apache in front?)
Directly invoking Daphne from the command line.
## Console logs and full tracebacks of any errors
N/A | 0.0 | [
"tests/test_http_request.py::TestHTTPRequest::test_invalid_header_name"
] | [
"tests/test_http_request.py::TestHTTPRequest::test_bad_requests",
"tests/test_http_request.py::TestHTTPRequest::test_duplicate_headers",
"tests/test_http_request.py::TestHTTPRequest::test_get_request",
"tests/test_http_request.py::TestHTTPRequest::test_headers",
"tests/test_http_request.py::TestHTTPRequest::test_headers_are_lowercased_and_stripped",
"tests/test_http_request.py::TestHTTPRequest::test_kitchen_sink",
"tests/test_http_request.py::TestHTTPRequest::test_minimal_request",
"tests/test_http_request.py::TestHTTPRequest::test_post_request",
"tests/test_http_request.py::TestHTTPRequest::test_raw_path",
"tests/test_http_request.py::TestHTTPRequest::test_request_body_chunking",
"tests/test_http_request.py::TestHTTPRequest::test_root_path_header",
"tests/test_http_request.py::TestHTTPRequest::test_x_forwarded_for_ignored",
"tests/test_http_request.py::TestHTTPRequest::test_x_forwarded_for_no_port",
"tests/test_http_request.py::TestHTTPRequest::test_x_forwarded_for_parsed"
] | 2024-02-10 14:23:01+00:00 | 1,944 |
|
django__daphne-84 | diff --git a/daphne/utils.py b/daphne/utils.py
index b066433..8fc339b 100644
--- a/daphne/utils.py
+++ b/daphne/utils.py
@@ -1,6 +1,13 @@
from twisted.web.http_headers import Headers
+def header_value(headers, header_name):
+ value = headers[header_name]
+ if isinstance(value, list):
+ value = value[0]
+ return value.decode("utf-8")
+
+
def parse_x_forwarded_for(headers,
address_header_name='X-Forwarded-For',
port_header_name='X-Forwarded-Port',
@@ -27,7 +34,7 @@ def parse_x_forwarded_for(headers,
address_header_name = address_header_name.lower().encode("utf-8")
result = original
if address_header_name in headers:
- address_value = headers[address_header_name][0].decode("utf-8")
+ address_value = header_value(headers, address_header_name)
if ',' in address_value:
address_value = address_value.split(",")[-1].strip()
@@ -47,7 +54,7 @@ def parse_x_forwarded_for(headers,
# header to avoid inconsistent results.
port_header_name = port_header_name.lower().encode("utf-8")
if port_header_name in headers:
- port_value = headers[port_header_name][0].decode("utf-8")
+ port_value = header_value(headers, port_header_name)
try:
result[1] = int(port_value)
except ValueError:
| django/daphne | 412d9a48dc30b0d43d8348f246f4b61efb6fca82 | diff --git a/daphne/tests/test_utils.py b/daphne/tests/test_utils.py
index 61fe1a3..10a10f3 100644
--- a/daphne/tests/test_utils.py
+++ b/daphne/tests/test_utils.py
@@ -7,9 +7,9 @@ from twisted.web.http_headers import Headers
from ..utils import parse_x_forwarded_for
-class TestXForwardedForParsing(TestCase):
+class TestXForwardedForHttpParsing(TestCase):
"""
- Tests that the parse_x_forwarded_for util correcly parses headers.
+ Tests that the parse_x_forwarded_for util correctly parses twisted Header.
"""
def test_basic(self):
@@ -59,3 +59,57 @@ class TestXForwardedForParsing(TestCase):
def test_no_original(self):
headers = Headers({})
self.assertIsNone(parse_x_forwarded_for(headers))
+
+
+class TestXForwardedForWsParsing(TestCase):
+ """
+ Tests that the parse_x_forwarded_for util correctly parses dict headers.
+ """
+
+ def test_basic(self):
+ headers = {
+ b'X-Forwarded-For': b'10.1.2.3',
+ b'X-Forwarded-Port': b'1234',
+ }
+ self.assertEqual(
+ parse_x_forwarded_for(headers),
+ ['10.1.2.3', 1234]
+ )
+
+ def test_address_only(self):
+ headers = {
+ b'X-Forwarded-For': b'10.1.2.3',
+ }
+ self.assertEqual(
+ parse_x_forwarded_for(headers),
+ ['10.1.2.3', 0]
+ )
+
+ def test_port_in_address(self):
+ headers = {
+ b'X-Forwarded-For': b'10.1.2.3:5123',
+ }
+ self.assertEqual(
+ parse_x_forwarded_for(headers),
+ ['10.1.2.3', 5123]
+ )
+
+ def test_multiple_proxys(self):
+ headers = {
+ b'X-Forwarded-For': b'10.1.2.3, 10.1.2.4',
+ }
+ self.assertEqual(
+ parse_x_forwarded_for(headers),
+ ['10.1.2.4', 0]
+ )
+
+ def test_original(self):
+ headers = {}
+ self.assertEqual(
+ parse_x_forwarded_for(headers, original=['127.0.0.1', 80]),
+ ['127.0.0.1', 80]
+ )
+
+ def test_no_original(self):
+ headers = {}
+ self.assertIsNone(parse_x_forwarded_for(headers))
| --proxy-headers error
Hi,
I just tested `daphne` with `--proxy-headers` behind a nginx server, and got the following exception when my browser initiates the websocket connection:
```
2017-02-07 15:13:43,115 ERROR Traceback (most recent call last):
File "/path/ve/local/lib/python2.7/site-packages/daphne/ws_protocol.py", line 62, in onConnect
self.requestHeaders,
AttributeError: 'WebSocketProtocol' object has no attribute 'requestHeaders'
```
I'm using the channels-examples/databinding app with the following virtualenv content:
```
appdirs==1.4.0
asgi-redis==1.0.0
asgiref==1.0.0
autobahn==0.17.1
channels==1.0.3
constantly==15.1.0
daphne==1.0.2
Django==1.10.5
incremental==16.10.1
msgpack-python==0.4.8
packaging==16.8
pyparsing==2.1.10
redis==2.10.5
six==1.10.0
Twisted==16.6.0
txaio==2.6.0
uWSGI==2.0.14
zope.interface==4.3.3
```
Is it a version compatibility issue, or a bug maybe ?
Thanks ! | 0.0 | [
"daphne/tests/test_utils.py::TestXForwardedForWsParsing::test_address_only",
"daphne/tests/test_utils.py::TestXForwardedForWsParsing::test_basic",
"daphne/tests/test_utils.py::TestXForwardedForWsParsing::test_multiple_proxys",
"daphne/tests/test_utils.py::TestXForwardedForWsParsing::test_port_in_address"
] | [
"daphne/tests/test_utils.py::TestXForwardedForHttpParsing::test_address_only",
"daphne/tests/test_utils.py::TestXForwardedForHttpParsing::test_basic",
"daphne/tests/test_utils.py::TestXForwardedForHttpParsing::test_multiple_proxys",
"daphne/tests/test_utils.py::TestXForwardedForHttpParsing::test_no_original",
"daphne/tests/test_utils.py::TestXForwardedForHttpParsing::test_original",
"daphne/tests/test_utils.py::TestXForwardedForHttpParsing::test_port_in_address",
"daphne/tests/test_utils.py::TestXForwardedForWsParsing::test_no_original",
"daphne/tests/test_utils.py::TestXForwardedForWsParsing::test_original"
] | 2017-02-13 08:54:21+00:00 | 1,945 |
|
dlce-eva__python-newick-45 | diff --git a/CHANGELOG.md b/CHANGELOG.md
new file mode 100644
index 0000000..ed832ef
--- /dev/null
+++ b/CHANGELOG.md
@@ -0,0 +1,9 @@
+# Changes
+
+The `newick` package adheres to [Semantic Versioning](http://semver.org/spec/v2.0.0.html).
+
+## [Unreleased]
+
+## [v1.3.0] - 2021-05-04
+
+Added support for reading and writing of node annotations (in comments).
diff --git a/README.md b/README.md
index f9cd4c8..4f6f695 100644
--- a/README.md
+++ b/README.md
@@ -39,6 +39,44 @@ a `list` of `newick.Node` objects.
>>> trees = read(pathlib.Path('fname'))
```
+### Supported Newick dialects
+
+The ["Newick specification"](http://biowiki.org/wiki/index.php/Newick_Format) states
+
+> Comments are enclosed in square brackets and may appear anywhere
+
+This has spawned a host of ad-hoc mechanisms to insert additional data into Newick trees.
+
+The `newick` package allows to deal with comments in two ways.
+
+- Ignoring comments:
+ ```python
+ >>> newick.loads('[a comment](a,b)c;', strip_comments=True)[0].newick
+ '(a,b)c'
+ ```
+- Reading comments as node annotations: Several software packages use Newick comments to
+ store node annotations, e.g. *BEAST, MrBayes or TreeAnnotator. Provided there are no
+ comments in places where they cannot be interpreted as node annotations, `newick` supports
+ reading and writing these annotations:
+ ```python
+ >>> newick.loads('(a[annotation],b)c;')[0].descendants[0].name
+ 'a'
+ >>> newick.loads('(a[annotation],b)c;')[0].descendants[0].comment
+ 'annotation'
+ >>> newick.loads('(a[annotation],b)c;')[0].newick
+ '(a[annotation],b)c'
+ ```
+
+Note that square brackets inside *quoted labels* will **not** be interpreted as comments
+or annotations:
+```python
+>>> newick.loads("('a[label]',b)c;")[0].descendants[0].name
+"'a[label]'"
+>>> newick.loads("('a[label]',b)c;")[0].newick
+"('a[label]',b)c"
+```
+
+
## Writing Newick
In parallel to the read operations there are three functions to serialize a single `Node` object or a `list` of `Node`
diff --git a/src/newick.py b/src/newick.py
index ac31951..b27c958 100644
--- a/src/newick.py
+++ b/src/newick.py
@@ -28,7 +28,7 @@ class Node(object):
descendants. It further has an ancestor, which is *None* if the node is the
root node of a tree.
"""
- def __init__(self, name=None, length=None, **kw):
+ def __init__(self, name=None, length=None, comment=None, **kw):
"""
:param name: Node label.
:param length: Branch length from the new node to its parent.
@@ -42,6 +42,7 @@ class Node(object):
raise ValueError(
'Node names or branch lengths must not contain "%s"' % char)
self.name = name
+ self.comment = comment
self._length = length
self.descendants = []
self.ancestor = None
@@ -63,7 +64,7 @@ class Node(object):
self._length = self._length_formatter(length_)
@classmethod
- def create(cls, name=None, length=None, descendants=None, **kw):
+ def create(cls, name=None, length=None, descendants=None, comment=None, **kw):
"""
Create a new `Node` object.
@@ -73,7 +74,7 @@ class Node(object):
:param kw: Additonal keyword arguments are passed through to `Node.__init__`.
:return: `Node` instance.
"""
- node = cls(name=name, length=length, **kw)
+ node = cls(name=name, length=length, comment=comment, **kw)
for descendant in descendants or []:
node.add_descendant(descendant)
return node
@@ -86,6 +87,8 @@ class Node(object):
def newick(self):
"""The representation of the Node in Newick format."""
label = self.name or ''
+ if self.comment:
+ label += '[{}]'.format(self.comment)
if self._length:
label += ':' + self._length
descendants = ','.join([n.newick for n in self.descendants])
@@ -423,10 +426,16 @@ def write(tree, fname, encoding='utf8'):
def _parse_name_and_length(s):
- length = None
+ length, comment = None, None
if ':' in s:
- s, length = s.split(':', 1)
- return s or None, length or None
+ parts = s.split(':')
+ if ']' not in parts[-1]: # A ] in length doesn't make sense - the : must be in a comment.
+ s = ':'.join(parts[:-1])
+ length = parts[-1]
+ if '[' in s and s.endswith(']'): # This looks like a node annotation in a comment.
+ s, comment = s.split('[', maxsplit=1)
+ comment = comment[:-1]
+ return s or None, length or None, comment
def _parse_siblings(s, **kw):
@@ -434,11 +443,12 @@ def _parse_siblings(s, **kw):
http://stackoverflow.com/a/26809037
"""
bracket_level = 0
+ square_bracket_level = 0
current = []
# trick to remove special-case of trailing chars
for c in (s + ","):
- if c == "," and bracket_level == 0:
+ if c == "," and bracket_level == 0 and square_bracket_level == 0:
yield parse_node("".join(current), **kw)
current = []
else:
@@ -446,6 +456,10 @@ def _parse_siblings(s, **kw):
bracket_level += 1
elif c == ")":
bracket_level -= 1
+ elif c == "[":
+ square_bracket_level += 1
+ elif c == "]":
+ square_bracket_level -= 1
current.append(c)
@@ -470,5 +484,5 @@ def parse_node(s, strip_comments=False, **kw):
raise ValueError('unmatched braces %s' % parts[0][:100])
descendants = list(_parse_siblings(')'.join(parts[:-1])[1:], **kw))
label = parts[-1]
- name, length = _parse_name_and_length(label)
- return Node.create(name=name, length=length, descendants=descendants, **kw)
+ name, length, comment = _parse_name_and_length(label)
+ return Node.create(name=name, length=length, comment=comment, descendants=descendants, **kw)
| dlce-eva/python-newick | da9132522ba05700fc0af50e5431eab36c294b3d | diff --git a/tests/test_newick.py b/tests/test_newick.py
index 93dcace..24b4078 100644
--- a/tests/test_newick.py
+++ b/tests/test_newick.py
@@ -95,6 +95,7 @@ class TestNodeDescendantsFunctionality(unittest.TestCase):
def test_read_write(tmp_path):
trees = read(pathlib.Path(__file__).parent / 'fixtures' / 'tree-glottolog-newick.txt')
+ assert '[' in trees[0].descendants[0].name
descs = [len(tree.descendants) for tree in trees]
# The bookkeeping family has 391 languages
assert descs[0] == 391
@@ -372,3 +373,22 @@ def test_prune_node():
t2 = loads(tree)[0]
t2.prune_by_names(["E"])
assert t1.newick == t2.newick
+
+
+def test_with_comments():
+ nwk = "(1[x&dmv={1},dmv1=0.260,dmv1_95%_hpd={0.003,0.625},dmv1_median=0.216,dmv1_range=" \
+ "{0.001,1.336},height=1.310e-15,height_95%_hpd={0.0,3.552e-15},height_median=0.0," \
+ "height_range={0.0,7.105e-15},length=2.188,length_95%_hpd={1.725,2.634}," \
+ "length_median=2.182,length_range={1.307,3.236}]:1.14538397925438," \
+ "2[&dmv={1},dmv1=0.260,dmv1_95%_hpd={0.003,0.625},dmv1_median=0.216,dmv1_range=" \
+ "{0.001,1.336},height=1.310e-15,height_95%_hpd={0.0,3.552e-15},height_median=0.0," \
+ "height_range={0.0,7.105e-15},length=2.188,length_95%_hpd={1.725,2.634}," \
+ "length_median=2.182,length_range={1.307,3.236}]:1.14538397925438)[y&dmv={1}," \
+ "dmv1=0.260,dmv1_95%_hpd={0.003,0.625},dmv1_median=0.216,dmv1_range={0.001,1.336}," \
+ "height=1.310e-15,height_95%_hpd={0.0,3.552e-15},height_median=0.0," \
+ "height_range={0.0,7.105e-15},length=2.188,length_95%_hpd={1.725,2.634}," \
+ "length_median=2.182,length_range={1.307,3.236}]"
+ tree = loads(nwk)[0]
+ assert tree.comment.startswith('y')
+ assert tree.descendants[0].name == '1' and tree.descendants[0].comment.startswith('x')
+ assert tree.newick == nwk
\ No newline at end of file
| Extended newick support
I'd like to process trees output by StarBEAST, but it uses nested annotations that aren't supported. An example 2 leaf tree:
```python
import newick
tree = "(1[&dmv={1},dmv1=0.260,dmv1_95%_hpd={0.003,0.625},dmv1_median=0.216,dmv1_range={0.001,1.336},height=1.310e-15,height_95%_hpd={0.0,3.552e-15},height_median=0.0,height_range={0.0,7.105e-15},length=2.188,length_95%_hpd={1.725,2.634},length_median=2.182,length_range={1.307,3.236}]:1.14538397925438,2[&dmv={1},dmv1=0.260,dmv1_95%_hpd={0.003,0.625},dmv1_median=0.216,dmv1_range={0.001,1.336},height=1.310e-15,height_95%_hpd={0.0,3.552e-15},height_median=0.0,height_range={0.0,7.105e-15},length=2.188,length_95%_hpd={1.725,2.634},length_median=2.182,length_range={1.307,3.236}]:1.14538397925438)[&dmv={1},dmv1=0.260,dmv1_95%_hpd={0.003,0.625},dmv1_median=0.216,dmv1_range={0.001,1.336},height=1.310e-15,height_95%_hpd={0.0,3.552e-15},height_median=0.0,height_range={0.0,7.105e-15},length=2.188,length_95%_hpd={1.725,2.634},length_median=2.182,length_range={1.307,3.236}]"
newick.loads(tree)
```
This fails with:
```
newick.loads(tree)
File "/home/jk/.local/lib/python3.8/site-packages/newick.py", line 369, in loads
return [parse_node(ss.strip(), **kw) for ss in s.split(';') if ss.strip()]
File "/home/jk/.local/lib/python3.8/site-packages/newick.py", line 369, in <listcomp>
return [parse_node(ss.strip(), **kw) for ss in s.split(';') if ss.strip()]
File "/home/jk/.local/lib/python3.8/site-packages/newick.py", line 471, in parse_node
return Node.create(name=name, length=length, descendants=descendants, **kw)
File "/home/jk/.local/lib/python3.8/site-packages/newick.py", line 76, in create
node = cls(name=name, length=length, **kw)
File "/home/jk/.local/lib/python3.8/site-packages/newick.py", line 42, in __init__
raise ValueError(
ValueError: Node names or branch lengths must not contain ","
```
It should be possible to make the parser a bit more sophisticated using regex so that stuff nested in the comment brackets isn't split.
Would it be possible to add this feature, or is it outside the remit of the package? Thanks. | 0.0 | [
"tests/test_newick.py::test_with_comments"
] | [
"tests/test_newick.py::test_empty_node",
"tests/test_newick.py::test_empty_node_newick_representation",
"tests/test_newick.py::test_empty_node_as_descendants_list",
"tests/test_newick.py::TestNodeBasicFunctionality::test_node_length_changeability",
"tests/test_newick.py::TestNodeBasicFunctionality::test_node_newick_representation_with_length",
"tests/test_newick.py::TestNodeBasicFunctionality::test_node_newick_representation_without_length",
"tests/test_newick.py::TestNodeBasicFunctionality::test_node_parameters_changeability",
"tests/test_newick.py::TestNodeBasicFunctionality::test_node_with_parameters_1",
"tests/test_newick.py::TestNodeBasicFunctionality::test_node_with_parameters_2",
"tests/test_newick.py::TestNodeDescendantsFunctionality::test_node_as_descendants_list",
"tests/test_newick.py::TestNodeDescendantsFunctionality::test_node_representation_with_deeper_descendants_1___D1_1____D1_2____D1_3__",
"tests/test_newick.py::TestNodeDescendantsFunctionality::test_node_representation_with_deeper_descendants_2___D__________",
"tests/test_newick.py::TestNodeDescendantsFunctionality::test_node_representation_with_deeper_descendants_3_____________",
"tests/test_newick.py::test_read_write",
"tests/test_newick.py::test_Node",
"tests/test_newick.py::test_repr",
"tests/test_newick.py::test_Node_custom_length",
"tests/test_newick.py::test_Node_ascii_art",
"tests/test_newick.py::test_Node_ascii_art_singleton",
"tests/test_newick.py::test_loads",
"tests/test_newick.py::test_dumps",
"tests/test_newick.py::test_clone",
"tests/test_newick.py::test_leaf_functions",
"tests/test_newick.py::test_prune[(A,((B,C),(D,E)))-A",
"tests/test_newick.py::test_prune[((A,B),((C,D),(E,F)))-A",
"tests/test_newick.py::test_prune[(b,(c,(d,(e,(f,g))h)i)a)-b",
"tests/test_newick.py::test_prune[(b,(c,(d,(e,(f,g))h)i)a)-c",
"tests/test_newick.py::test_prune_single_node_tree",
"tests/test_newick.py::test_redundant_node_removal[((B:0.2,(C:0.3,D:0.4)E:0.5)F:0.1)A;-kw0-(B:0.2,(C:0.3,D:0.4)E:0.5)A:0.1]",
"tests/test_newick.py::test_redundant_node_removal[((C)B)A-kw1-A]",
"tests/test_newick.py::test_redundant_node_removal[((C)B)A-kw2-C]",
"tests/test_newick.py::test_redundant_node_removal[((aiw),((aas,(kbt)),((abg),abf)))-kw3-(((aas,kbt),(abf,abg)),aiw)]",
"tests/test_newick.py::test_prune_and_node_removal",
"tests/test_newick.py::test_stacked_redundant_node_removal",
"tests/test_newick.py::test_polytomy_resolution",
"tests/test_newick.py::test_name_removal",
"tests/test_newick.py::test_internal_name_removal",
"tests/test_newick.py::test_leaf_name_removal",
"tests/test_newick.py::test_length_removal",
"tests/test_newick.py::test_all_removal",
"tests/test_newick.py::test_singletons",
"tests/test_newick.py::test_comments",
"tests/test_newick.py::test_get_node",
"tests/test_newick.py::test_prune_node"
] | 2021-05-04 18:11:44+00:00 | 1,946 |
|
dlce-eva__python-nexus-27 | diff --git a/src/nexus/reader.py b/src/nexus/reader.py
index e42d8a8..8df69cd 100644
--- a/src/nexus/reader.py
+++ b/src/nexus/reader.py
@@ -161,10 +161,18 @@ class NexusReader(object):
:return: String
"""
out = ["#NEXUS\n"]
- for block in self.blocks:
- out.append(self.blocks[block].write())
+ blocks = []
+ for block in self.blocks.values():
+ if block in blocks:
+ # We skip copies of blocks - which might have happened to provide shortcuts for
+ # semantically identical but differently named blocks, such as "data" and
+ # "characters".
+ continue
+ blocks.append(block)
+ for block in blocks:
+ out.append(block.write())
# empty line after block if needed
- if len(self.blocks) > 1:
+ if len(blocks) > 1:
out.append("\n")
return "\n".join(out)
| dlce-eva/python-nexus | 071aaca665cc9bfde15201a31c836c6587d04483 | diff --git a/tests/test_reader.py b/tests/test_reader.py
index 41e72e4..df9bd01 100644
--- a/tests/test_reader.py
+++ b/tests/test_reader.py
@@ -19,6 +19,11 @@ def test_reader_from_blocks():
assert hasattr(n, 'custom')
+def test_roundtrip(examples):
+ nex = NexusReader(examples.joinpath('example2.nex'))
+ _ = NexusReader.from_string(nex.write())
+
+
def test_read_file(nex, examples):
"""Test the Core functionality of NexusReader"""
assert 'data' in nex.blocks
| Round tripping a nexus with a characters block leads to two character blocks
...which means the resulting nexus can't be read. Example [here](https://github.com/phlorest/walker_and_ribeiro2011/blob/main/cldf/data.nex).
...which means:
```python
>>> from nexus import NexusReader
>>> NexusReader("walker_and_riberio2015/cldf/data.nex")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/Users/simon/.pyenv/versions/3.9.6/lib/python3.9/site-packages/nexus/reader.py", line 42, in __init__
self._set_blocks(NexusReader._blocks_from_file(filename))
File "/Users/simon/.pyenv/versions/3.9.6/lib/python3.9/site-packages/nexus/reader.py", line 77, in _set_blocks
raise NexusFormatException("Duplicate Block %s" % block)
nexus.exceptions.NexusFormatException: Duplicate Block characters
```
This is because of this:
https://github.com/dlce-eva/python-nexus/blob/071aaca665cc9bfde15201a31c836c6587d04483/src/nexus/reader.py#L80-L81
(which, I admit, I implemented as cheap way to always have a `data` block rather than looking for `data` or `characters`).
What's the best solution here @xrotwang ?
1. remove these two lines so this change doesn't happen (simplest solution, but means we need to keep a closer eye in e.g. phlorest, and add standardisation there (nex.blocks['data'] = nex.blocks['characters'], del(nex.blocks['characters'])).
2. delete blocks['characters'] once it's been copied to blocks['data']. This keeps the status quo but adds a magic invisible change so seems like a bad idea.
3. fix the handlers to create a data and a characters block. Round-tripping will duplicate data and characters but they'll be named differently at least.
4. revise `NexusWriter` to check for duplicate blocks, and perhaps allow writing of specific blocks only (nex.write(filename, blocks=['data'])
The fact that the moved block still thinks of itself as a characters block rather than a data block is a bug.
| 0.0 | [
"tests/test_reader.py::test_roundtrip"
] | [
"tests/test_reader.py::test_reader_from_blocks",
"tests/test_reader.py::test_read_file",
"tests/test_reader.py::test_error_on_missing_file",
"tests/test_reader.py::test_read_gzip_file",
"tests/test_reader.py::test_from_string",
"tests/test_reader.py::test_read_string_returns_self",
"tests/test_reader.py::test_write",
"tests/test_reader.py::test_write_to_file",
"tests/test_reader.py::test_error_on_duplicate_block"
] | 2021-12-02 06:58:22+00:00 | 1,947 |
|
dlr-eoc__ukis-pysat-156 | diff --git a/CHANGELOG.rst b/CHANGELOG.rst
index f0e3b22..d331e63 100644
--- a/CHANGELOG.rst
+++ b/CHANGELOG.rst
@@ -7,10 +7,11 @@ Changelog
Changed
^^^^^^^
-``requirements``: Pystac version updated to 1.0.0, STAC version 1.0.0 #147
+- ``data``: Removed pylandsat dependency and added methods for downloading landsat products from GCS in data module #106
Fixed
^^^^^
-- ``data``: Removed pylandsat dependency and added methods for downloading landsat products from GCS in data module #106
+- ``data``: Consistency for Landsat & Sentinel #151
Added
^^^^^
diff --git a/ukis_pysat/data.py b/ukis_pysat/data.py
index 507609b..63ff25b 100644
--- a/ukis_pysat/data.py
+++ b/ukis_pysat/data.py
@@ -306,6 +306,20 @@ class Source:
f"It is much easier to stream the asset yourself now using `requests.get(url, stream=True)`"
)
+ def _get_srcid_from_product_uuid_ee(self, product_uuid):
+ """
+ query EarthExplorer for srcid and meta (e.g. for url and bounds of product)
+ :param product_uuid: UUID of the satellite image product (String).
+
+ :return: product_uuid, meta_src of product
+ """
+ from landsatxplore.util import parse_scene_id, landsat_dataset
+
+ meta = parse_scene_id(product_uuid)
+ metadata = self.api.metadata(product_uuid, landsat_dataset(int(meta["satellite"])))
+
+ return metadata["display_id"], metadata
+
def download_image(self, product_uuid, target_dir):
"""Downloads satellite image data to a target directory for a specific product_id.
Incomplete downloads are continued and complete files are skipped.
@@ -322,19 +336,19 @@ class Source:
)
elif self.src == Datahub.EarthExplorer:
- if not Path(target_dir.joinpath(product_uuid + ".zip")).is_file():
-
+ product_srcid, _ = self._get_srcid_from_product_uuid_ee(product_uuid)
+ if not Path(target_dir.joinpath(product_srcid + ".zip")).is_file():
# download data from GCS if file does not already exist
- product = Product(product_uuid)
+ product = Product(product_srcid)
product.download(out_dir=target_dir, progressbar=False)
# compress download directory and remove original files
shutil.make_archive(
- target_dir.joinpath(product_uuid),
+ target_dir.joinpath(product_srcid),
"zip",
- root_dir=target_dir.joinpath(product_uuid),
+ root_dir=target_dir.joinpath(product_srcid),
)
- shutil.rmtree(target_dir.joinpath(product_uuid))
+ shutil.rmtree(target_dir.joinpath(product_srcid))
else:
self.api.download(product_uuid, target_dir, checksum=True)
@@ -356,17 +370,9 @@ class Source:
)
elif self.src == Datahub.EarthExplorer:
- # query EarthExplorer for url, srcid and bounds of product
- meta_src = self.api.request(
- "metadata",
- **{
- "datasetName": self.src.value,
- "entityIds": [product_uuid],
- },
- )
+ product_srcid, meta_src = self._get_srcid_from_product_uuid_ee(product_uuid)
url = meta_src[0]["browseUrl"]
bounds = geometry.shape(meta_src[0]["spatialFootprint"]).bounds
- product_srcid = meta_src[0]["displayId"]
else:
# query Scihub for url, srcid and bounds of product
| dlr-eoc/ukis-pysat | a079d5f05a8205d1b492e41c70841912b29bb684 | diff --git a/tests/test_data.py b/tests/test_data.py
index 0b3c903..7fea666 100644
--- a/tests/test_data.py
+++ b/tests/test_data.py
@@ -220,6 +220,38 @@ class DataTest(unittest.TestCase):
product._url("B1.tif"),
)
+ @requests_mock.Mocker(real_http=True)
+ def test_get_srcid_from_product_uuid_ee(self, m):
+ m.post(
+ "https://m2m.cr.usgs.gov/api/api/json/stable/login",
+ content=b'{"requestId": 241064318, "version": "stable", "data": "token", "errorCode": null, "errorMessage": null, "sessionId": 51372983}',
+ )
+ m.get(
+ "https://m2m.cr.usgs.gov/api/api/json/stable/scene-list-add",
+ content=b'{"requestId": 241159456, "version": "stable", "sessionId": 51393323, "data": {"browse":[{"id":"5e83d0b86f2c3061","browseRotationEnabled":false,"browseName":"LandsatLook Natural Color Preview Image","browsePath":"https:\\/\\/ims.cr.usgs.gov\\/browse\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1.jpg","overlayPath":"https:\\/\\/ims.cr.usgs.gov\\/wms\\/landsat_8_c1?sceneId=lc81930242020082lgn00","overlayType":"dmid_wms","thumbnailPath":"https:\\/\\/ims.cr.usgs.gov\\/thumbnail\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1.jpg"},{"id":"5e83d0b85da62c02","browseRotationEnabled":false,"browseName":"LandsatLook Thermal Preview Image","browsePath":"https:\\/\\/ims.cr.usgs.gov\\/browse\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_TIR.jpg","overlayPath":"https:\\/\\/ims.cr.usgs.gov\\/wms\\/landsat_8_c1?sceneId=lc81930242020082lgn00_tir","overlayType":"dmid_wms","thumbnailPath":"https:\\/\\/ims.cr.usgs.gov\\/thumbnail\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_TIR.jpg"},{"id":"5e83d0b8b814a0e6","browseRotationEnabled":false,"browseName":"LandsatLook Quality Preview Image","browsePath":"https:\\/\\/ims.cr.usgs.gov\\/browse\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_QB.jpg","overlayPath":"https:\\/\\/ims.cr.usgs.gov\\/wms\\/landsat_8_c1?sceneId=lc81930242020082lgn00_qb","overlayType":"dmid_wms","thumbnailPath":"https:\\/\\/ims.cr.usgs.gov\\/thumbnail\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_QB.jpg"}],"cloudCover":"12.47","entityId":"LC81930242020082LGN00","displayId":"LC08_L1TP_193024_20200322_20200326_01_T1","orderingId":"LC81930242020082LGN00","metadata":[{"id":"5e83d0b82af07b21","fieldName":"Landsat Product Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#landsat_product_id","value":"LC08_L1TP_193024_20200322_20200326_01_T1"},{"id":"5e83d0b88275745","fieldName":"Landsat Scene Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#landsat_scene_id","value":"LC81930242020082LGN00"},{"id":"5e83d0b92ff6b5e8","fieldName":"Acquisition Date","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":"2020\\/03\\/22"},{"id":"5e83d0b9332fd122","fieldName":"Collection Category","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":"T1"},{"id":"5e83d0b9948e2596","fieldName":"Collection Number","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":1},{"id":"5e83d0b922c5f981","fieldName":"WRS Path","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 193"},{"id":"5e83d0b94328e34e","fieldName":"WRS Row","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 024"},{"id":"5e83d0b9a34ebe01","fieldName":"Target WRS Path","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 193"},{"id":"5e83d0b8852f2e23","fieldName":"Target WRS Row","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 024"},{"id":"5e83d0b9173b715a","fieldName":"Nadir\\/Off Nadir","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":"NADIR"},{"id":"5e83d0b820eaa311","fieldName":"Roll Angle","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#roll_angle","value":"-0.001"},{"id":"5e83d0b8379c9dae","fieldName":"Date L-1 Generated","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#date_l1_generated","value":"2020\\/03\\/26"},{"id":"5e83d0b9a78d1dbe","fieldName":"Start Time","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#start_time","value":"2020:082:10:02:29.1296010"},{"id":"5e83d0b887e40f8e","fieldName":"Stop Time","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#stop_time","value":"2020:082:10:03:00.8996000"},{"id":"5e83d0b926d7d304","fieldName":"Station Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#distribution_site","value":"LGN"},{"id":"5e83d0b91ad332e3","fieldName":"Day\\/Night Indicator","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#day_or_night","value":"DAY"},{"id":"5e83d0b864f5722e","fieldName":"Land Cloud Cover","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#cloud_cover_land","value":"12.47"},{"id":"5e83d0b92e9d1b11","fieldName":"Scene Cloud Cover","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#cloud_cover","value":"12.47"},{"id":"5e83d0b991213d01","fieldName":"Ground Control Points Model","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#ground_control_points_model","value":253},{"id":"5e83d0b9e4a26b2a","fieldName":"Ground Control Points Version","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#ground_control_points_version","value":4},{"id":"5e83d0b9bb19d4cc","fieldName":"Geometric RMSE Model (meters)","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#geometric_rmse_model","value":"8.296"},{"id":"5e83d0b8f922f1d3","fieldName":"Geometric RMSE Model X","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#geometric_rmse_model_x","value":"5.809"},{"id":"5e83d0b93b83213d","fieldName":"Geometric RMSE Model Y","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#geometric_rmse_model_y","value":"5.924"},{"id":"5e83d0b9c9fa1556","fieldName":"Image Quality","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#image_quality_landsat_8","value":9},{"id":"5e83d0b8a926bb3e","fieldName":"Processing Software Version","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#processing_software_version","value":"LPGS_13.1.0"},{"id":"5e83d0b84092b361","fieldName":"Sun Elevation L1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#sun_elevation","value":"36.94009856"},{"id":"5e83d0b8bf763033","fieldName":"Sun Azimuth L1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#sun_azimuth","value":"156.99969272"},{"id":"5e83d0b97cb734c3","fieldName":"TIRS SSM Model","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#tirs_ssm_model","value":"FINAL"},{"id":"5e83d0b861614fa4","fieldName":"Data Type Level-1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#data_type_l1","value":"OLI_TIRS_L1TP"},{"id":"5e83d0b993a4fa4a","fieldName":"Sensor Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#sensor_id ","value":"OLI_TIRS"},{"id":"5e83d0b9de23d772","fieldName":"Panchromatic Lines","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#panchromatic_lines","value":16301},{"id":"5e83d0b8100f0577","fieldName":"Panchromatic Samples","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#panchromatic_samples","value":16101},{"id":"5e83d0b92bc96899","fieldName":"Reflective Lines","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#reflective_lines","value":8151},{"id":"5e83d0b8bbb70baf","fieldName":"Reflective Samples","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#reflective_samples","value":8051},{"id":"5e83d0b955642ca4","fieldName":"Thermal Lines","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#thermal_lines","value":8151},{"id":"5e83d0b96b1a0d35","fieldName":"Thermal Samples","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#thermal_samples","value":8051},{"id":"5e83d0b9104800de","fieldName":"Map Projection Level-1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#map_projection_l1","value":"UTM"},{"id":"5e83d0b9fb0dce2d","fieldName":"UTM Zone","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#utm_zone","value":33},{"id":"5e83d0b8fd64f557","fieldName":"Datum","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#datum","value":"WGS84"},{"id":"5e83d0b84cc2632e","fieldName":"Ellipsoid","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#ellipsoid","value":"WGS84"},{"id":"5e83d0b98f651440","fieldName":"Grid Cell Size Panchromatic","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#grid_cell_size_panchromatic","value":"15.00"},{"id":"5e83d0b9c6eaa87d","fieldName":"Grid Cell Size Reflective","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#grid_cell_size_reflective","value":"30.00"},{"id":"5e83d0b8c810ced","fieldName":"Grid Cell Size Thermal","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#grid_cell_size_thermal","value":"30.00"},{"id":"5e83d0b8a40bafa4","fieldName":"Bias Parameter File Name OLI","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#bpf_name_oli","value":"LO8BPF20200322095233_20200322113039.01"},{"id":"5e83d0b964a91d93","fieldName":"Bias Parameter File Name TIRS","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#bpf_name_tirs","value":"LT8BPF20200310060739_20200324104153.01"},{"id":"5e83d0b9b5d81214","fieldName":"Calibration Parameter File","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#cpf_name","value":"LC08CPF_20200101_20200331_01.04"},{"id":"5e83d0b9c67b22a3","fieldName":"RLUT File Name","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#rlut_file_name","value":"LC08RLUT_20150303_20431231_01_12.h5"},{"id":"5e83d0b9a610a996","fieldName":"Center Latitude","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"51°41\'35.34\\"N"},{"id":"5e83d0b94fad23df","fieldName":"Center Longitude","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"12°48\'15.73\\"E"},{"id":"5e83d0b9c1d54551","fieldName":"UL Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"52°45\'50.58\\"N"},{"id":"5e83d0b8d91068e","fieldName":"UL Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"11°49\'23.52\\"E"},{"id":"5e83d0b94ff6f17e","fieldName":"UR Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"52°17\'44.92\\"N"},{"id":"5e83d0b9a76119fe","fieldName":"UR Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"14°32\'32.78\\"E"},{"id":"5e83d0b9f29120e3","fieldName":"LL Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"51°03\'53.57\\"N"},{"id":"5e83d0b988d2162b","fieldName":"LL Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"11°06\'35.75\\"E"},{"id":"5e83d0b96162233","fieldName":"LR Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"50°36\'17.24\\"N"},{"id":"5e83d0b894a09772","fieldName":"LR Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"13°43\'57.40\\"E"},{"id":"5e83d0b87f203a10","fieldName":"Center Latitude dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"51.69315"},{"id":"5e83d0b9b2a9a299","fieldName":"Center Longitude dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"12.80437"},{"id":"5e83d0b8bc51bf5b","fieldName":"UL Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"52.76405"},{"id":"5e83d0b95071b3bf","fieldName":"UL Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"11.82320"},{"id":"5e83d0b9842b0429","fieldName":"UR Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"52.29581"},{"id":"5e83d0b931a16a9","fieldName":"UR Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"14.54244"},{"id":"5e83d0b8abad8ec9","fieldName":"LL Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"51.06488"},{"id":"5e83d0b92a9532e0","fieldName":"LL Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"11.10993"},{"id":"5e83d0b834fea374","fieldName":"LR Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"50.60479"},{"id":"5e83d0b9f619dbbe","fieldName":"LR Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"13.73261"}],"hasCustomizedMetadata":false,"options":{"bulk":true,"download":true,"order":true,"secondary":false},"selected":null,"spatialBounds":{"type":"Polygon","coordinates":[[[11.10993,50.60479],[11.10993,52.76405],[14.54244,52.76405],[14.54244,50.60479],[11.10993,50.60479]]]},"spatialCoverage":{"type":"Polygon","coordinates":[[[11.10993,51.06488],[13.73261,50.60479],[14.54244,52.29581],[11.8232,52.76405],[11.10993,51.06488]]]},"temporalCoverage":{"endDate":"2020-03-22 00:00:00","startDate":"2020-03-22 00:00:00"},"publishDate":"2020-03-22T09:56:49"}, "errorCode": null, "errorMessage": null}',
+ )
+ m.get(
+ "https://m2m.cr.usgs.gov/api/api/json/stable/scene-list-get",
+ content=b'{"requestId": 241160510, "version": "stable", "sessionId": 51393558, "data": [{"entityId":"LC81930242020082LGN00","datasetName":"landsat_8_c1"}], "errorCode": null, "errorMessage": null}',
+ )
+ m.get(
+ "https://m2m.cr.usgs.gov/api/api/json/stable/scene-list-remove",
+ content=b'{"requestId": 241161482, "version": "stable", "sessionId": 51393761, "data": null, "errorCode": null, "errorMessage": null}',
+ )
+ m.get(
+ "https://m2m.cr.usgs.gov/api/api/json/stable/scene-metadata",
+ content=b'{"requestId": 241162488, "version": "stable", "sessionId": 51393950, "data": {"browse":[{"id":"5e83d0b86f2c3061","browseRotationEnabled":false,"browseName":"LandsatLook Natural Color Preview Image","browsePath":"https:\\/\\/ims.cr.usgs.gov\\/browse\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1.jpg","overlayPath":"https:\\/\\/ims.cr.usgs.gov\\/wms\\/landsat_8_c1?sceneId=lc81930242020082lgn00","overlayType":"dmid_wms","thumbnailPath":"https:\\/\\/ims.cr.usgs.gov\\/thumbnail\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1.jpg"},{"id":"5e83d0b85da62c02","browseRotationEnabled":false,"browseName":"LandsatLook Thermal Preview Image","browsePath":"https:\\/\\/ims.cr.usgs.gov\\/browse\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_TIR.jpg","overlayPath":"https:\\/\\/ims.cr.usgs.gov\\/wms\\/landsat_8_c1?sceneId=lc81930242020082lgn00_tir","overlayType":"dmid_wms","thumbnailPath":"https:\\/\\/ims.cr.usgs.gov\\/thumbnail\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_TIR.jpg"},{"id":"5e83d0b8b814a0e6","browseRotationEnabled":false,"browseName":"LandsatLook Quality Preview Image","browsePath":"https:\\/\\/ims.cr.usgs.gov\\/browse\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_QB.jpg","overlayPath":"https:\\/\\/ims.cr.usgs.gov\\/wms\\/landsat_8_c1?sceneId=lc81930242020082lgn00_qb","overlayType":"dmid_wms","thumbnailPath":"https:\\/\\/ims.cr.usgs.gov\\/thumbnail\\/landsat_8_c1\\/2020\\/193\\/024\\/LC08_L1TP_193024_20200322_20200326_01_T1_QB.jpg"}],"cloudCover":"12.47","entityId":"LC81930242020082LGN00","displayId":"LC08_L1TP_193024_20200322_20200326_01_T1","orderingId":"LC81930242020082LGN00","metadata":[{"id":"5e83d0b82af07b21","fieldName":"Landsat Product Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#landsat_product_id","value":"LC08_L1TP_193024_20200322_20200326_01_T1"},{"id":"5e83d0b88275745","fieldName":"Landsat Scene Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#landsat_scene_id","value":"LC81930242020082LGN00"},{"id":"5e83d0b92ff6b5e8","fieldName":"Acquisition Date","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":"2020\\/03\\/22"},{"id":"5e83d0b9332fd122","fieldName":"Collection Category","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":"T1"},{"id":"5e83d0b9948e2596","fieldName":"Collection Number","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":1},{"id":"5e83d0b922c5f981","fieldName":"WRS Path","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 193"},{"id":"5e83d0b94328e34e","fieldName":"WRS Row","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 024"},{"id":"5e83d0b9a34ebe01","fieldName":"Target WRS Path","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 193"},{"id":"5e83d0b8852f2e23","fieldName":"Target WRS Row","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":" 024"},{"id":"5e83d0b9173b715a","fieldName":"Nadir\\/Off Nadir","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_c2_dictionary.html#acquisition_date","value":"NADIR"},{"id":"5e83d0b820eaa311","fieldName":"Roll Angle","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#roll_angle","value":"-0.001"},{"id":"5e83d0b8379c9dae","fieldName":"Date L-1 Generated","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#date_l1_generated","value":"2020\\/03\\/26"},{"id":"5e83d0b9a78d1dbe","fieldName":"Start Time","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#start_time","value":"2020:082:10:02:29.1296010"},{"id":"5e83d0b887e40f8e","fieldName":"Stop Time","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#stop_time","value":"2020:082:10:03:00.8996000"},{"id":"5e83d0b926d7d304","fieldName":"Station Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#distribution_site","value":"LGN"},{"id":"5e83d0b91ad332e3","fieldName":"Day\\/Night Indicator","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#day_or_night","value":"DAY"},{"id":"5e83d0b864f5722e","fieldName":"Land Cloud Cover","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#cloud_cover_land","value":"12.47"},{"id":"5e83d0b92e9d1b11","fieldName":"Scene Cloud Cover","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#cloud_cover","value":"12.47"},{"id":"5e83d0b991213d01","fieldName":"Ground Control Points Model","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#ground_control_points_model","value":253},{"id":"5e83d0b9e4a26b2a","fieldName":"Ground Control Points Version","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#ground_control_points_version","value":4},{"id":"5e83d0b9bb19d4cc","fieldName":"Geometric RMSE Model (meters)","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#geometric_rmse_model","value":"8.296"},{"id":"5e83d0b8f922f1d3","fieldName":"Geometric RMSE Model X","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#geometric_rmse_model_x","value":"5.809"},{"id":"5e83d0b93b83213d","fieldName":"Geometric RMSE Model Y","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#geometric_rmse_model_y","value":"5.924"},{"id":"5e83d0b9c9fa1556","fieldName":"Image Quality","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#image_quality_landsat_8","value":9},{"id":"5e83d0b8a926bb3e","fieldName":"Processing Software Version","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#processing_software_version","value":"LPGS_13.1.0"},{"id":"5e83d0b84092b361","fieldName":"Sun Elevation L1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#sun_elevation","value":"36.94009856"},{"id":"5e83d0b8bf763033","fieldName":"Sun Azimuth L1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#sun_azimuth","value":"156.99969272"},{"id":"5e83d0b97cb734c3","fieldName":"TIRS SSM Model","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#tirs_ssm_model","value":"FINAL"},{"id":"5e83d0b861614fa4","fieldName":"Data Type Level-1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#data_type_l1","value":"OLI_TIRS_L1TP"},{"id":"5e83d0b993a4fa4a","fieldName":"Sensor Identifier","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#sensor_id ","value":"OLI_TIRS"},{"id":"5e83d0b9de23d772","fieldName":"Panchromatic Lines","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#panchromatic_lines","value":16301},{"id":"5e83d0b8100f0577","fieldName":"Panchromatic Samples","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#panchromatic_samples","value":16101},{"id":"5e83d0b92bc96899","fieldName":"Reflective Lines","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#reflective_lines","value":8151},{"id":"5e83d0b8bbb70baf","fieldName":"Reflective Samples","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#reflective_samples","value":8051},{"id":"5e83d0b955642ca4","fieldName":"Thermal Lines","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#thermal_lines","value":8151},{"id":"5e83d0b96b1a0d35","fieldName":"Thermal Samples","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#thermal_samples","value":8051},{"id":"5e83d0b9104800de","fieldName":"Map Projection Level-1","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#map_projection_l1","value":"UTM"},{"id":"5e83d0b9fb0dce2d","fieldName":"UTM Zone","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#utm_zone","value":33},{"id":"5e83d0b8fd64f557","fieldName":"Datum","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#datum","value":"WGS84"},{"id":"5e83d0b84cc2632e","fieldName":"Ellipsoid","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#ellipsoid","value":"WGS84"},{"id":"5e83d0b98f651440","fieldName":"Grid Cell Size Panchromatic","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#grid_cell_size_panchromatic","value":"15.00"},{"id":"5e83d0b9c6eaa87d","fieldName":"Grid Cell Size Reflective","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#grid_cell_size_reflective","value":"30.00"},{"id":"5e83d0b8c810ced","fieldName":"Grid Cell Size Thermal","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#grid_cell_size_thermal","value":"30.00"},{"id":"5e83d0b8a40bafa4","fieldName":"Bias Parameter File Name OLI","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#bpf_name_oli","value":"LO8BPF20200322095233_20200322113039.01"},{"id":"5e83d0b964a91d93","fieldName":"Bias Parameter File Name TIRS","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#bpf_name_tirs","value":"LT8BPF20200310060739_20200324104153.01"},{"id":"5e83d0b9b5d81214","fieldName":"Calibration Parameter File","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#cpf_name","value":"LC08CPF_20200101_20200331_01.04"},{"id":"5e83d0b9c67b22a3","fieldName":"RLUT File Name","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#rlut_file_name","value":"LC08RLUT_20150303_20431231_01_12.h5"},{"id":"5e83d0b9a610a996","fieldName":"Center Latitude","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"51°41\'35.34\\"N"},{"id":"5e83d0b94fad23df","fieldName":"Center Longitude","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"12°48\'15.73\\"E"},{"id":"5e83d0b9c1d54551","fieldName":"UL Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"52°45\'50.58\\"N"},{"id":"5e83d0b8d91068e","fieldName":"UL Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"11°49\'23.52\\"E"},{"id":"5e83d0b94ff6f17e","fieldName":"UR Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"52°17\'44.92\\"N"},{"id":"5e83d0b9a76119fe","fieldName":"UR Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"14°32\'32.78\\"E"},{"id":"5e83d0b9f29120e3","fieldName":"LL Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"51°03\'53.57\\"N"},{"id":"5e83d0b988d2162b","fieldName":"LL Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"11°06\'35.75\\"E"},{"id":"5e83d0b96162233","fieldName":"LR Corner Lat","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"50°36\'17.24\\"N"},{"id":"5e83d0b894a09772","fieldName":"LR Corner Long","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_degrees","value":"13°43\'57.40\\"E"},{"id":"5e83d0b87f203a10","fieldName":"Center Latitude dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"51.69315"},{"id":"5e83d0b9b2a9a299","fieldName":"Center Longitude dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"12.80437"},{"id":"5e83d0b8bc51bf5b","fieldName":"UL Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"52.76405"},{"id":"5e83d0b95071b3bf","fieldName":"UL Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"11.82320"},{"id":"5e83d0b9842b0429","fieldName":"UR Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"52.29581"},{"id":"5e83d0b931a16a9","fieldName":"UR Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"14.54244"},{"id":"5e83d0b8abad8ec9","fieldName":"LL Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"51.06488"},{"id":"5e83d0b92a9532e0","fieldName":"LL Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"11.10993"},{"id":"5e83d0b834fea374","fieldName":"LR Corner Lat dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"50.60479"},{"id":"5e83d0b9f619dbbe","fieldName":"LR Corner Long dec","dictionaryLink":"https:\\/\\/lta.cr.usgs.gov\\/DD\\/landsat_dictionary.html#coordinates_decimal","value":"13.73261"}],"hasCustomizedMetadata":false,"options":{"bulk":true,"download":true,"order":true,"secondary":false},"selected":null,"spatialBounds":{"type":"Polygon","coordinates":[[[11.10993,50.60479],[11.10993,52.76405],[14.54244,52.76405],[14.54244,50.60479],[11.10993,50.60479]]]},"spatialCoverage":{"type":"Polygon","coordinates":[[[11.10993,51.06488],[13.73261,50.60479],[14.54244,52.29581],[11.8232,52.76405],[11.10993,51.06488]]]},"temporalCoverage":{"endDate":"2020-03-22 00:00:00","startDate":"2020-03-22 00:00:00"},"publishDate":"2020-03-22T09:56:49"}, "errorCode": null, "errorMessage": null}',
+ )
+ m.get(
+ "https://m2m.cr.usgs.gov/api/api/json/stable/logout",
+ content=b'{"errorCode":null,"error":"","data":true,"api_version":"1.4.1","access_level":"user","catalog_id":"EE","executionTime":0.4}',
+ )
+ with Source(datahub=Datahub.EarthExplorer) as src:
+ product_srcid, _ = src._get_srcid_from_product_uuid_ee(
+ product_uuid="LC81930242020082LGN00",
+ )
+ self.assertEqual(product_srcid, "LC08_L1TP_193024_20200322_20200326_01_T1")
+
if __name__ == "__main__":
unittest.main()
| Download Method Conflict in SCIHUB and EARTHEXPLORER
**Describe the bug**
when the item is created we set a value for the [ID's](https://github.com/dlr-eoc/ukis-pysat/blob/master/ukis_pysat/data.py#L394),
But when we query the hub we take another value of the item ìtem.id for Landsat and ```item. Properties['uuid'] for sentinel to download.
**To Reproduce**
To download we can give the ```item.id``` (product "ID") [here ](https://github.com/dlr-eoc/ukis-pysat/blob/master/ukis_pysat/data.py#L448) always and solve this internally.
**Expected behavior**
```UKIS_PYSAT``` download method for ```SCIHUB``` and ```EARTHEXPLORER``` both should have used either```product_uuid``` or ``product_id"``` . | 0.0 | [
"tests/test_data.py::DataTest::test_get_srcid_from_product_uuid_ee"
] | [
"tests/test_data.py::DataTest::test_aoi_geointerface",
"tests/test_data.py::DataTest::test_compute_md5",
"tests/test_data.py::DataTest::test_exception_false_aoi",
"tests/test_data.py::DataTest::test_init_exception_other_enum",
"tests/test_data.py::DataTest::test_init_exception_other_hub",
"tests/test_data.py::DataTest::test_init_stac_catalog",
"tests/test_data.py::DataTest::test_init_stac_url",
"tests/test_data.py::DataTest::test_meta_from_pid",
"tests/test_data.py::DataTest::test_product_available",
"tests/test_data.py::DataTest::test_url"
] | 2021-07-27 10:10:48+00:00 | 1,948 |
|
dls-controls__aioca-25 | diff --git a/aioca/_catools.py b/aioca/_catools.py
index 91b5012..bc41e02 100644
--- a/aioca/_catools.py
+++ b/aioca/_catools.py
@@ -412,7 +412,7 @@ class Subscription(object):
self.dropped_callbacks: int = 0
self.__event_loop = asyncio.get_event_loop()
self.__values: Deque[AugmentedValue] = collections.deque(
- maxlen=0 if all_updates else 1
+ maxlen=None if all_updates else 1
)
self.__lock = asyncio.Lock()
| dls-controls/aioca | 69ea466daf84a8bd371411c9902d1ba6fbee87f8 | diff --git a/tests/test_aioca.py b/tests/test_aioca.py
index ec7938f..2a5fbb0 100644
--- a/tests/test_aioca.py
+++ b/tests/test_aioca.py
@@ -46,6 +46,8 @@ BAD_EGUS = PV_PREFIX + "bad_egus"
WAVEFORM = PV_PREFIX + "waveform"
# A read only PV
RO = PV_PREFIX + "waveform.NELM"
+# Longer test timeouts as GHA has slow runners on Mac
+TIMEOUT = 10
def boom(value, *args) -> None:
@@ -80,7 +82,7 @@ def ioc():
pass
-def wait_for_ioc(ioc, timeout=5):
+def wait_for_ioc(ioc, timeout=TIMEOUT):
start = time.time()
while True:
assert time.time() - start < timeout
@@ -91,7 +93,7 @@ def wait_for_ioc(ioc, timeout=5):
@pytest.mark.asyncio
async def test_connect(ioc: subprocess.Popen) -> None:
- conn = await connect(LONGOUT)
+ conn = await connect(LONGOUT, timeout=TIMEOUT)
assert type(conn) is CANothing
conn2 = await connect([SI, NE], throw=False, timeout=1.0)
assert len(conn2) == 2
@@ -103,7 +105,7 @@ async def test_connect(ioc: subprocess.Popen) -> None:
@pytest.mark.asyncio
async def test_cainfo(ioc: subprocess.Popen) -> None:
- conn2 = await cainfo([WAVEFORM, SI])
+ conn2 = await cainfo([WAVEFORM, SI], timeout=TIMEOUT)
assert conn2[0].datatype == 1 # array
assert conn2[1].datatype == 0 # string
conn = await cainfo(LONGOUT)
@@ -157,20 +159,20 @@ async def test_get_non_existent_pvs_no_throw(ioc: subprocess.Popen) -> None:
async def test_get_two_pvs(
ioc: subprocess.Popen, pvs: Union[List[str], Tuple[str]]
) -> None:
- value = await caget(pvs)
+ value = await caget(pvs, timeout=TIMEOUT)
assert [42, "me"] == value
@pytest.mark.asyncio
async def test_get_pv_with_bad_egus(ioc: subprocess.Popen) -> None:
- value = await caget(BAD_EGUS, format=FORMAT_CTRL)
+ value = await caget(BAD_EGUS, format=FORMAT_CTRL, timeout=TIMEOUT)
assert 32 == value
assert value.units == "\ufffd" # unicode REPLACEMENT CHARACTER
@pytest.mark.asyncio
async def test_get_waveform_pv(ioc: subprocess.Popen) -> None:
- value = await caget(WAVEFORM)
+ value = await caget(WAVEFORM, timeout=TIMEOUT)
assert len(value) == 0
assert isinstance(value, dbr.ca_array)
await caput(WAVEFORM, [1, 2, 3, 4])
@@ -183,7 +185,7 @@ async def test_get_waveform_pv(ioc: subprocess.Popen) -> None:
@pytest.mark.asyncio
async def test_caput(ioc: subprocess.Popen) -> None:
# Need to test the timeout=None branch, but wrap with wait_for in case it fails
- v1 = await asyncio.wait_for(caput(LONGOUT, 43, wait=True, timeout=None), 5)
+ v1 = await asyncio.wait_for(caput(LONGOUT, 43, wait=True, timeout=None), TIMEOUT)
assert isinstance(v1, CANothing)
v2 = await caget(LONGOUT)
assert 43 == v2
@@ -192,7 +194,7 @@ async def test_caput(ioc: subprocess.Popen) -> None:
@pytest.mark.asyncio
async def test_caput_on_ro_pv_fails(ioc: subprocess.Popen) -> None:
with pytest.raises(cadef.CAException):
- await caput(RO, 43)
+ await caput(RO, 43, timeout=TIMEOUT)
result = await caput(RO, 43, throw=False)
assert str(result).endswith("Write access denied")
@@ -202,7 +204,7 @@ async def test_caput_on_ro_pv_fails(ioc: subprocess.Popen) -> None:
async def test_caput_two_pvs_same_value(
ioc: subprocess.Popen, pvs: Union[List[str], Tuple[str]]
) -> None:
- await caput([LONGOUT, SI], 43)
+ await caput([LONGOUT, SI], 43, timeout=TIMEOUT)
value = await caget([LONGOUT, SI])
assert [43, "43"] == value
await caput([LONGOUT, SI], "44")
@@ -212,7 +214,7 @@ async def test_caput_two_pvs_same_value(
@pytest.mark.asyncio
async def test_caput_two_pvs_different_value(ioc: subprocess.Popen) -> None:
- await caput([LONGOUT, SI], [44, "blah"])
+ await caput([LONGOUT, SI], [44, "blah"], timeout=TIMEOUT)
value = await caget([LONGOUT, SI])
assert [44, "blah"] == value
@@ -232,7 +234,7 @@ async def test_caget_non_existent() -> None:
@pytest.mark.asyncio
async def test_caget_non_existent_and_good(ioc: subprocess.Popen) -> None:
- await caput(WAVEFORM, [1, 2, 3, 4])
+ await caput(WAVEFORM, [1, 2, 3, 4], timeout=TIMEOUT)
try:
await caget([NE, WAVEFORM], timeout=1.0)
except CANothing:
@@ -246,7 +248,7 @@ async def test_caget_non_existent_and_good(ioc: subprocess.Popen) -> None:
assert len(x) == 0
-async def poll_length(array, gt=0, timeout=5):
+async def poll_length(array, gt=0, timeout=TIMEOUT):
start = time.time()
while not len(array) > gt:
await asyncio.sleep(0.01)
@@ -307,7 +309,7 @@ async def test_monitor_with_failing_dbr(ioc: subprocess.Popen, capsys) -> None:
@pytest.mark.asyncio
async def test_monitor_two_pvs(ioc: subprocess.Popen) -> None:
values: List[Tuple[AugmentedValue, int]] = []
- await caput(WAVEFORM, [1, 2], wait=True)
+ await caput(WAVEFORM, [1, 2], wait=True, timeout=TIMEOUT)
ms = camonitor([WAVEFORM, LONGOUT], lambda v, n: values.append((v, n)), count=-1)
# Wait for connection
@@ -369,6 +371,35 @@ async def test_long_monitor_callback(ioc: subprocess.Popen) -> None:
assert m.dropped_callbacks == 1
+@pytest.mark.asyncio
+async def test_long_monitor_all_updates(ioc: subprocess.Popen) -> None:
+ # Like above, but check all_updates gives us everything
+ values = []
+ wait_for_ioc(ioc)
+
+ async def cb(value):
+ values.append(value)
+ await asyncio.sleep(0.4)
+
+ m = camonitor(LONGOUT, cb, connect_timeout=(time.time() + 0.5,), all_updates=True)
+ # Wait for connection, calling first cb
+ await poll_length(values)
+ assert values == [42]
+ assert m.dropped_callbacks == 0
+ # These two caputs happen during the sleep of the first cb,
+ # they shouldn't be squashed as we ask for all_updates
+ await caput(LONGOUT, 43)
+ await caput(LONGOUT, 44)
+ # Wait until the second cb has finished
+ await asyncio.sleep(0.6)
+ assert [42, 43] == values
+ assert m.dropped_callbacks == 0
+ # Wait until the third cb (which is not dropped) has finished
+ await asyncio.sleep(0.6)
+ assert [42, 43, 44] == values
+ assert m.dropped_callbacks == 0
+
+
@pytest.mark.asyncio
async def test_exception_raising_monitor_callback(
ioc: subprocess.Popen, capsys
| camonitor all_updates=True not doing what it should?
After noticing I was missing some (very close) updates I decided that this was due to the default merging behavior of `camonitor`, so I tried monitoring with `all_updates=True`. But then I got **no** updates at all. Turns out that `all_updates` has effects on the size of the underlying deque. Relevant code:
```
self.__values: Deque[AugmentedValue] = collections.deque(
maxlen=0 if all_updates else 1
)
```
Now, `collections.deque(maxlen=0)` gives us a deque that effectively is **always full**.
Maybe the original intention was to make it `collections.deque(maxlen=None)` (the default)? | 0.0 | [
"tests/test_aioca.py::test_long_monitor_all_updates"
] | [
"tests/test_aioca.py::flake-8::FLAKE8",
"tests/test_aioca.py::mypy",
"tests/test_aioca.py::mypy-status",
"tests/test_aioca.py::BLACK",
"tests/test_aioca.py::test_connect",
"tests/test_aioca.py::test_cainfo",
"tests/test_aioca.py::test_get_non_existent_pvs_no_throw",
"tests/test_aioca.py::test_get_two_pvs[pvs0]",
"tests/test_aioca.py::test_get_two_pvs[pvs1]",
"tests/test_aioca.py::test_get_pv_with_bad_egus",
"tests/test_aioca.py::test_get_waveform_pv",
"tests/test_aioca.py::test_caput",
"tests/test_aioca.py::test_caput_on_ro_pv_fails",
"tests/test_aioca.py::test_caput_two_pvs_same_value[pvs0]",
"tests/test_aioca.py::test_caput_two_pvs_same_value[pvs1]",
"tests/test_aioca.py::test_caput_two_pvs_different_value",
"tests/test_aioca.py::test_caget_non_existent",
"tests/test_aioca.py::test_caget_non_existent_and_good",
"tests/test_aioca.py::test_monitor",
"tests/test_aioca.py::test_monitor_with_failing_dbr",
"tests/test_aioca.py::test_monitor_two_pvs",
"tests/test_aioca.py::test_long_monitor_callback",
"tests/test_aioca.py::test_exception_raising_monitor_callback",
"tests/test_aioca.py::test_camonitor_non_existent",
"tests/test_aioca.py::test_monitor_gc",
"tests/test_aioca.py::test_closing_event_loop",
"tests/test_aioca.py::test_value_event_raises",
"tests/test_aioca.py::test_ca_nothing_dunder_methods",
"tests/test_aioca.py::test_run_forever",
"tests/test_aioca.py::test_import_in_a_different_thread"
] | 2022-04-26 08:20:56+00:00 | 1,949 |
|
dls-controls__aioca-27 | diff --git a/CHANGELOG.rst b/CHANGELOG.rst
index 6c5a2eb..2dd7afa 100644
--- a/CHANGELOG.rst
+++ b/CHANGELOG.rst
@@ -12,6 +12,7 @@ Unreleased_
Fixed:
- `camonitor(all_updates=True) now works <../../pull/24>`_
+- `Fixed memory leak in camonitor <../../pull/26>`
1.3_ - 2021-10-15
-----------------
diff --git a/aioca/_catools.py b/aioca/_catools.py
index bc41e02..7892f9e 100644
--- a/aioca/_catools.py
+++ b/aioca/_catools.py
@@ -330,7 +330,8 @@ class Subscription(object):
def __create_signal_task(self, value):
task = asyncio.ensure_future(self.__signal(value))
- self.__tasks.append(task)
+ self.__tasks.add(task)
+ task.add_done_callback(self.__tasks.remove)
async def __signal(self, value):
"""Wrapper for performing callbacks safely: only performs the callback
@@ -428,13 +429,13 @@ class Subscription(object):
# background, as we may have to wait for the channel to become
# connected.
self.state = self.OPENING
- self.__tasks = [
+ self.__tasks = {
asyncio.ensure_future(
self.__create_subscription(
events, datatype, format, count, connect_timeout
)
)
- ]
+ }
async def __wait_for_channel(self, timeout):
try:
| dls-controls/aioca | 47155dce291e30195997b5c51b1487ce79b80771 | diff --git a/tests/test_aioca.py b/tests/test_aioca.py
index 2a5fbb0..9710564 100644
--- a/tests/test_aioca.py
+++ b/tests/test_aioca.py
@@ -270,6 +270,9 @@ async def test_monitor(ioc: subprocess.Popen) -> None:
ioc.communicate("exit")
await asyncio.sleep(0.1)
+ # Check that all callback tasks have terminated, and all that is left
+ # is the original __create_subscription task (which has now completed)
+ assert len(m._Subscription__tasks) == 1 # type: ignore
m.close()
assert [42, 43, 44] == values[:3]
| Possible memory leak
In __create_signal_task(), a task is appended to the list self.__tasks, but never removed from the list.
This list grows without bound.
The list of tasks is needed so that we can cancel them when closing.
But do we need that for events?
| 0.0 | [
"tests/test_aioca.py::test_monitor"
] | [
"tests/test_aioca.py::flake-8::FLAKE8",
"tests/test_aioca.py::mypy",
"tests/test_aioca.py::mypy-status",
"tests/test_aioca.py::BLACK",
"tests/test_aioca.py::test_connect",
"tests/test_aioca.py::test_cainfo",
"tests/test_aioca.py::test_get_non_existent_pvs_no_throw",
"tests/test_aioca.py::test_get_two_pvs[pvs0]",
"tests/test_aioca.py::test_get_two_pvs[pvs1]",
"tests/test_aioca.py::test_get_pv_with_bad_egus",
"tests/test_aioca.py::test_get_waveform_pv",
"tests/test_aioca.py::test_caput",
"tests/test_aioca.py::test_caput_on_ro_pv_fails",
"tests/test_aioca.py::test_caput_two_pvs_same_value[pvs0]",
"tests/test_aioca.py::test_caput_two_pvs_same_value[pvs1]",
"tests/test_aioca.py::test_caput_two_pvs_different_value",
"tests/test_aioca.py::test_caget_non_existent",
"tests/test_aioca.py::test_caget_non_existent_and_good",
"tests/test_aioca.py::test_monitor_with_failing_dbr",
"tests/test_aioca.py::test_monitor_two_pvs",
"tests/test_aioca.py::test_long_monitor_callback",
"tests/test_aioca.py::test_long_monitor_all_updates",
"tests/test_aioca.py::test_exception_raising_monitor_callback",
"tests/test_aioca.py::test_camonitor_non_existent",
"tests/test_aioca.py::test_monitor_gc",
"tests/test_aioca.py::test_closing_event_loop",
"tests/test_aioca.py::test_value_event_raises",
"tests/test_aioca.py::test_ca_nothing_dunder_methods",
"tests/test_aioca.py::test_run_forever",
"tests/test_aioca.py::test_import_in_a_different_thread"
] | 2022-06-07 16:01:46+00:00 | 1,950 |
|
dls-controls__aioca-38 | diff --git a/.github/workflows/code.yml b/.github/workflows/code.yml
index b26b076..95e0c7c 100644
--- a/.github/workflows/code.yml
+++ b/.github/workflows/code.yml
@@ -42,7 +42,7 @@ jobs:
- name: Install Python Dependencies
run: |
- pip install pipenv build
+ pip install pipenv==2022.4.8 build
pipenv install --dev --${{ matrix.pipenv }} --python $(python -c 'import sys; print(sys.executable)') && pipenv graph
- name: Create Sdist and Wheel
diff --git a/CHANGELOG.rst b/CHANGELOG.rst
index 9522c2b..8245674 100644
--- a/CHANGELOG.rst
+++ b/CHANGELOG.rst
@@ -9,7 +9,9 @@ and this project adheres to `Semantic Versioning <https://semver.org/spec/v2.0.0
Unreleased_
-----------
-Nothing yet
+Added:
+
+- `Add methods to allow inspecting Channels <../../pull/38>`_
1.6_ - 2023-04-06
-----------------
diff --git a/aioca/__init__.py b/aioca/__init__.py
index 11d056e..90ba0a4 100644
--- a/aioca/__init__.py
+++ b/aioca/__init__.py
@@ -24,12 +24,14 @@ from epicscorelibs.ca.dbr import (
from ._catools import (
CAInfo,
CANothing,
+ ChannelInfo,
Subscription,
caget,
cainfo,
camonitor,
caput,
connect,
+ get_channel_infos,
purge_channel_caches,
run,
)
@@ -45,6 +47,8 @@ __all__ = [
"cainfo", # Returns ca_info describing PV connection
"CAInfo", # Ca info object
"CANothing", # No value
+ "ChannelInfo", # Information about a particular channel
+ "get_channel_infos", # Return information about all channels
"purge_channel_caches", # Get rid of old channels
"run", # Run one aioca coroutine and clean up
# The version of aioca
diff --git a/aioca/_catools.py b/aioca/_catools.py
index 6b99166..dfb96a8 100644
--- a/aioca/_catools.py
+++ b/aioca/_catools.py
@@ -54,6 +54,9 @@ class ValueEvent(Generic[T]):
self._event.set()
self.value = value
+ def is_set(self) -> bool:
+ return self._event.is_set()
+
def clear(self):
self._event.clear()
self.value = RuntimeError("No value set")
@@ -252,6 +255,14 @@ class Channel(object):
"""Removes the given subscription from the list of receivers."""
self.__subscriptions.remove(subscription)
+ def count_subscriptions(self) -> int:
+ """Return the number of currently active subscriptions for this Channel"""
+ return len(self.__subscriptions)
+
+ def connected(self) -> bool:
+ """Return whether this Channel is currently connected to a PV"""
+ return self.__connect_event.is_set()
+
async def wait(self):
"""Waits for the channel to become connected if not already connected."""
await self.__connect_event.wait()
@@ -278,6 +289,9 @@ class ChannelCache(object):
self.__channels[name] = channel
return channel
+ def get_channels(self) -> List[Channel]:
+ return list(self.__channels.values())
+
def purge(self):
"""Purges all the channels in the cache: closes them right now. Will
cause other channel access to fail, so only to be done on shutdown."""
@@ -1081,6 +1095,32 @@ def get_channel(pv: str) -> Channel:
return channel
+class ChannelInfo:
+ """Information about a particular Channel
+
+ Attributes:
+ name: Process Variable name the Channel is targeting
+ connected: True if the Channel is currently connected
+ subscriber_count: Number of clients subscribed to this Channel"""
+
+ name: str
+ connected: bool
+ subscriber_count: int
+
+ def __init__(self, name, connected, subscriber_count):
+ self.name = name
+ self.connected = connected
+ self.subscriber_count = subscriber_count
+
+
+def get_channel_infos() -> List[ChannelInfo]:
+ """Return information about all Channels"""
+ return [
+ ChannelInfo(channel.name, channel.connected(), channel.count_subscriptions())
+ for channel in _Context.get_channel_cache().get_channels()
+ ]
+
+
# Another delicacy arising from relying on asynchronous CA event dispatching is
# that we need to manually flush IO events such as caget commands. To ensure
# that large blocks of channel access activity really are aggregated we used to
diff --git a/docs/api.rst b/docs/api.rst
index 2a58dc4..ec0888a 100644
--- a/docs/api.rst
+++ b/docs/api.rst
@@ -141,6 +141,11 @@ event loop. A convenience function is provided to do this:
.. autofunction:: run
+.. autoclass:: ChannelInfo()
+ :members:
+
+.. autofunction:: get_channel_infos
+
.. _Values:
Working with Values
| dls-controls/aioca | a5500d0ba11b3a91610fc449e5ed6b12530a4b4c | diff --git a/tests/test_aioca.py b/tests/test_aioca.py
index bd1c28b..56ef8aa 100644
--- a/tests/test_aioca.py
+++ b/tests/test_aioca.py
@@ -24,6 +24,7 @@ from aioca import (
camonitor,
caput,
connect,
+ get_channel_infos,
purge_channel_caches,
run,
)
@@ -610,3 +611,35 @@ def test_import_in_a_different_thread(ioc: subprocess.Popen) -> None:
]
)
assert output.strip() == b"42"
+
+
+@pytest.mark.asyncio
+async def test_channel_connected(ioc: subprocess.Popen) -> None:
+ values: List[AugmentedValue] = []
+ m = camonitor(LONGOUT, values.append, notify_disconnect=True)
+
+ # Wait for connection
+ await poll_length(values)
+
+ channels = get_channel_infos()
+ assert len(channels) == 1
+
+ channel = channels[0]
+ # Initially the PV is connected and has one monitor
+ assert channel.name == LONGOUT
+ assert channel.connected
+ assert channel.subscriber_count == 1
+
+ ioc.communicate("exit")
+ await asyncio.sleep(0.1)
+
+ # After IOC exits the channel is disconnected but still has one monitor
+ channel = get_channel_infos()[0]
+ assert not channel.connected
+ assert channel.subscriber_count == 1
+
+ m.close()
+
+ # Once the monitor is closed the subscription disappears
+ channel = get_channel_infos()[0]
+ assert channel.subscriber_count == 0
| Provide mechanism to access Channels
It would be useful to be able to interrogate the currently active Channels in `aioca`, to enable things like counting the number of open/closed connections.
This will need some new public getter functions, and probably a small amount of re-naming of various files and objects to make them public. | 0.0 | [
"tests/test_aioca.py::mypy",
"tests/test_aioca.py::mypy-status",
"tests/test_aioca.py::BLACK",
"tests/test_aioca.py::test_connect",
"tests/test_aioca.py::test_cainfo",
"tests/test_aioca.py::test_get_non_existent_pvs_no_throw",
"tests/test_aioca.py::test_get_two_pvs[pvs0]",
"tests/test_aioca.py::test_get_two_pvs[pvs1]",
"tests/test_aioca.py::test_get_pv_with_bad_egus",
"tests/test_aioca.py::test_get_waveform_pv",
"tests/test_aioca.py::test_caput",
"tests/test_aioca.py::test_caput_on_ro_pv_fails",
"tests/test_aioca.py::test_caput_two_pvs_same_value[pvs0]",
"tests/test_aioca.py::test_caput_two_pvs_same_value[pvs1]",
"tests/test_aioca.py::test_caput_two_pvs_different_value",
"tests/test_aioca.py::test_caget_non_existent",
"tests/test_aioca.py::test_caget_non_existent_and_good",
"tests/test_aioca.py::test_monitor",
"tests/test_aioca.py::test_monitor_with_failing_dbr",
"tests/test_aioca.py::test_monitor_two_pvs",
"tests/test_aioca.py::test_long_monitor_callback",
"tests/test_aioca.py::test_long_monitor_all_updates",
"tests/test_aioca.py::test_exception_raising_monitor_callback",
"tests/test_aioca.py::test_async_exception_raising_monitor_callback",
"tests/test_aioca.py::test_camonitor_non_existent",
"tests/test_aioca.py::test_camonitor_non_existent_async",
"tests/test_aioca.py::test_monitor_gc",
"tests/test_aioca.py::test_closing_event_loop",
"tests/test_aioca.py::test_value_event_raises",
"tests/test_aioca.py::test_ca_nothing_dunder_methods",
"tests/test_aioca.py::test_run_forever",
"tests/test_aioca.py::test_import_in_a_different_thread",
"tests/test_aioca.py::test_channel_connected"
] | [] | 2023-05-04 12:43:21+00:00 | 1,951 |
|
dmvass__sqlalchemy-easy-profile-17 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index 58e2c0a..d446327 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -6,6 +6,9 @@ and this project adheres to [Semantic Versioning](http://semver.org/spec/v2.0.0.
## [Unreleased]
+## [1.1.1] - 2020-07-26
+- Fixed deprecated time.clock [issue-16]
+
## [1.1.0] - 2020-06-29
- Removed support of python 2.7, 3.5
- Updated documentation
diff --git a/easy_profile/profiler.py b/easy_profile/profiler.py
index bfefff7..bc91152 100644
--- a/easy_profile/profiler.py
+++ b/easy_profile/profiler.py
@@ -3,20 +3,13 @@ import functools
import inspect
from queue import Queue
import re
-import sys
-import time
+from time import perf_counter
from sqlalchemy import event
from sqlalchemy.engine.base import Engine
from .reporters import StreamReporter
-# Optimize timer function for the platform
-if sys.platform == "win32": # pragma: no cover
- _timer = time.clock
-else:
- _timer = time.time
-
SQL_OPERATORS = ["select", "insert", "update", "delete"]
OPERATOR_REGEX = re.compile("(%s) *." % "|".join(SQL_OPERATORS), re.IGNORECASE)
@@ -177,10 +170,10 @@ class SessionProfiler:
def _before_cursor_execute(self, conn, cursor, statement, parameters,
context, executemany):
- context._query_start_time = _timer()
+ context._query_start_time = perf_counter()
def _after_cursor_execute(self, conn, cursor, statement, parameters,
context, executemany):
self.queries.put(DebugQuery(
- statement, parameters, context._query_start_time, _timer()
+ statement, parameters, context._query_start_time, perf_counter()
))
| dmvass/sqlalchemy-easy-profile | e98f169203de3478a4999376aac03323c5f54786 | diff --git a/tests/test_profiler.py b/tests/test_profiler.py
index 1a64f49..eee3a40 100644
--- a/tests/test_profiler.py
+++ b/tests/test_profiler.py
@@ -142,9 +142,9 @@ class TestSessionProfiler(unittest.TestCase):
self.assertListEqual(debug_queries, stats["call_stack"])
self.assertDictEqual(stats["duplicates"], duplicates)
- @mock.patch("easy_profile.profiler._timer")
+ @mock.patch("easy_profile.profiler.perf_counter")
def test__before_cursor_execute(self, mocked):
- expected_time = time.time()
+ expected_time = time.perf_counter()
mocked.return_value = expected_time
profiler = SessionProfiler()
context = mock.Mock()
@@ -158,7 +158,7 @@ class TestSessionProfiler(unittest.TestCase):
)
self.assertEqual(context._query_start_time, expected_time)
- @mock.patch("easy_profile.profiler._timer")
+ @mock.patch("easy_profile.profiler.perf_counter")
def test__after_cursor_execute(self, mocked):
expected_query = debug_queries[0]
mocked.return_value = expected_query.end_time
| Does not work on python 3.8
Exception raises when trying to use time.clock on profiler.py.
timer.clock was deprecated since Python 3.3 and removed in 3.8.
From Python DOCs:
Deprecated since version 3.3, will be removed in version 3.8: The behaviour of this function depends on the platform: use perf_counter() or process_time() instead, depending on your requirements, to have a well defined behaviour.
| 0.0 | [
"tests/test_profiler.py::TestSessionProfiler::test__after_cursor_execute",
"tests/test_profiler.py::TestSessionProfiler::test__before_cursor_execute"
] | [
"tests/test_profiler.py::TestSessionProfiler::test__get_stats",
"tests/test_profiler.py::TestSessionProfiler::test__reset_stats",
"tests/test_profiler.py::TestSessionProfiler::test_begin",
"tests/test_profiler.py::TestSessionProfiler::test_begin_alive",
"tests/test_profiler.py::TestSessionProfiler::test_commit",
"tests/test_profiler.py::TestSessionProfiler::test_commit_alive",
"tests/test_profiler.py::TestSessionProfiler::test_contextmanager_interface",
"tests/test_profiler.py::TestSessionProfiler::test_decorator",
"tests/test_profiler.py::TestSessionProfiler::test_decorator_path",
"tests/test_profiler.py::TestSessionProfiler::test_decorator_path_and_path_callback",
"tests/test_profiler.py::TestSessionProfiler::test_decorator_path_callback",
"tests/test_profiler.py::TestSessionProfiler::test_initialization_custom",
"tests/test_profiler.py::TestSessionProfiler::test_initialization_default",
"tests/test_profiler.py::TestSessionProfiler::test_stats"
] | 2020-07-26 10:50:58+00:00 | 1,952 |
|
dmvass__sqlalchemy-easy-profile-19 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index d446327..90450c4 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -6,6 +6,9 @@ and this project adheres to [Semantic Versioning](http://semver.org/spec/v2.0.0.
## [Unreleased]
+## [1.1.2] - 2020-10-21
+- Fixed queries for UNIX platforms [issue-18]
+
## [1.1.1] - 2020-07-26
- Fixed deprecated time.clock [issue-16]
diff --git a/easy_profile/profiler.py b/easy_profile/profiler.py
index bc91152..ce5793a 100644
--- a/easy_profile/profiler.py
+++ b/easy_profile/profiler.py
@@ -3,13 +3,20 @@ import functools
import inspect
from queue import Queue
import re
-from time import perf_counter
+import sys
+import time
from sqlalchemy import event
from sqlalchemy.engine.base import Engine
from .reporters import StreamReporter
+# Optimize timer function for the platform
+if sys.platform == "win32": # pragma: no cover
+ _timer = time.perf_counter
+else:
+ _timer = time.time
+
SQL_OPERATORS = ["select", "insert", "update", "delete"]
OPERATOR_REGEX = re.compile("(%s) *." % "|".join(SQL_OPERATORS), re.IGNORECASE)
@@ -170,10 +177,10 @@ class SessionProfiler:
def _before_cursor_execute(self, conn, cursor, statement, parameters,
context, executemany):
- context._query_start_time = perf_counter()
+ context._query_start_time = _timer()
def _after_cursor_execute(self, conn, cursor, statement, parameters,
context, executemany):
self.queries.put(DebugQuery(
- statement, parameters, context._query_start_time, perf_counter()
+ statement, parameters, context._query_start_time, _timer()
))
| dmvass/sqlalchemy-easy-profile | 889e2dbf43f78743e9d822cbe10c7b843ee234fe | diff --git a/tests/test_profiler.py b/tests/test_profiler.py
index eee3a40..1a64f49 100644
--- a/tests/test_profiler.py
+++ b/tests/test_profiler.py
@@ -142,9 +142,9 @@ class TestSessionProfiler(unittest.TestCase):
self.assertListEqual(debug_queries, stats["call_stack"])
self.assertDictEqual(stats["duplicates"], duplicates)
- @mock.patch("easy_profile.profiler.perf_counter")
+ @mock.patch("easy_profile.profiler._timer")
def test__before_cursor_execute(self, mocked):
- expected_time = time.perf_counter()
+ expected_time = time.time()
mocked.return_value = expected_time
profiler = SessionProfiler()
context = mock.Mock()
@@ -158,7 +158,7 @@ class TestSessionProfiler(unittest.TestCase):
)
self.assertEqual(context._query_start_time, expected_time)
- @mock.patch("easy_profile.profiler.perf_counter")
+ @mock.patch("easy_profile.profiler._timer")
def test__after_cursor_execute(self, mocked):
expected_query = debug_queries[0]
mocked.return_value = expected_query.end_time
| No report shown in console in 1.1.1
**Describe the bug**
A clear and concise description of what the bug is.
I have updated to 1.1.1 today.
The SQL report no longer shows in console output.
I am using a mac but not sure if it has anything to do with it.
The setup to enable app - wide console logging of SQL statements is the same.
This is the function that is run if env variable SQL_PROFILING = True. The app is simply flask app passed into the function. All of this works fine in 1.0.3 (and worked for last year) and is as per instructions manual. In the newest version nothing is logged to the console.
```python
def add_sql_profiling(app, config_: Config):
"""
Adds profiling of the SQL queries performed by sqlalchemy with number of duplicates and execution times.
"""
if config_.SQL_PROFILING:
try:
from easy_profile import EasyProfileMiddleware
app.wsgi_app = EasyProfileMiddleware(app.wsgi_app)
except ModuleNotFoundError:
logging.warning(
'Easy profile not installed, SQL profiling not available. Install using requirements-for-dev.txt'
)
```
**To Reproduce**
Steps to reproduce the behavior or test case.
pip install sqlalchemy-easy-profile==1.1.1
**Expected behavior**
A clear and concise description of what you expected to happen.
SQL statements report is logged in the console.
**Additional context**
Add any other context about the problem here.
| 0.0 | [
"tests/test_profiler.py::TestSessionProfiler::test__after_cursor_execute",
"tests/test_profiler.py::TestSessionProfiler::test__before_cursor_execute"
] | [
"tests/test_profiler.py::TestSessionProfiler::test__get_stats",
"tests/test_profiler.py::TestSessionProfiler::test__reset_stats",
"tests/test_profiler.py::TestSessionProfiler::test_begin",
"tests/test_profiler.py::TestSessionProfiler::test_begin_alive",
"tests/test_profiler.py::TestSessionProfiler::test_commit",
"tests/test_profiler.py::TestSessionProfiler::test_commit_alive",
"tests/test_profiler.py::TestSessionProfiler::test_contextmanager_interface",
"tests/test_profiler.py::TestSessionProfiler::test_decorator",
"tests/test_profiler.py::TestSessionProfiler::test_decorator_path",
"tests/test_profiler.py::TestSessionProfiler::test_decorator_path_and_path_callback",
"tests/test_profiler.py::TestSessionProfiler::test_decorator_path_callback",
"tests/test_profiler.py::TestSessionProfiler::test_initialization_custom",
"tests/test_profiler.py::TestSessionProfiler::test_initialization_default",
"tests/test_profiler.py::TestSessionProfiler::test_stats"
] | 2020-10-15 08:45:19+00:00 | 1,953 |
|
dmytrostriletskyi__pdbe-15 | diff --git a/.pylintrc b/.pylintrc
index 2a8fb44..5a5467d 100644
--- a/.pylintrc
+++ b/.pylintrc
@@ -304,7 +304,7 @@ function-name-hint=(([a-z][a-z0-9_]{2,30})|(_[a-z0-9_]*))$
function-rgx=(([a-z][a-z0-9_]{2,30})|(_[a-z0-9_]*))$
# Good variable names which should always be accepted, separated by a comma
-good-names=i,j,k,ex,Run,_,app_name,logger,urlpatterns,application,server_port
+good-names=i,j,k,ex,Run,_,app_name,logger,urlpatterns,application,server_port,multiple_function_declaration_begging_spaces,stored_import_pdb_line_begging_spaces
# Include a hint for the correct naming format with invalid-name
include-naming-hint=no
diff --git a/pdbe/commits.py b/pdbe/commits.py
index b5e31a5..425af66 100644
--- a/pdbe/commits.py
+++ b/pdbe/commits.py
@@ -1,8 +1,9 @@
"""
Pdbe commits logic.
"""
-from datetime import datetime
import binascii
+import keyword
+from datetime import datetime
from os import fdopen, getcwd, path, mkdir, walk, urandom, listdir
from os.path import join
from tempfile import mkstemp
@@ -24,48 +25,89 @@ def get_commit_functions(file_path: str) -> list:
with open(file_path, 'r') as file:
content = [line.strip() for line in file.readlines()]
+ suggested_function = ''
+
# pylint:disable=consider-using-enumerate
- for index in range(len(content)):
- line = content[index]
+ for line in content:
- if utils.does_line_contains_import_pdb(line):
- suggested_function = content[index - 1]
+ if utils.is_one_line_function_declaration_line(line) and not utils.is_commended_function(line):
+ function_open_bracket_index = line.index('(')
+ suggested_function = line[4:function_open_bracket_index]
- if utils.is_function_sign_in_line(suggested_function):
- function_open_bracket_index = suggested_function.index('(')
- function_name = suggested_function[4:function_open_bracket_index]
+ elif 'def' in line and '(' in line and not utils.is_commended_function(line):
+ function_open_bracket_index = line.index('(')
+ suggested_function = line[4:function_open_bracket_index]
- commit_functions.append(function_name)
+ if utils.does_line_contains_import_pdb(line):
+ commit_functions.append(suggested_function)
return commit_functions
+# too-many-locals, too-many-branches, refactor
+# pylint:disable=too-many-locals,too-many-branches
def restore_import_pdb_from_commit(commit_content: List[str], call_commit_path: str) -> None:
"""
Restore import pdb statements from specified commit.
"""
- file_to_restore = ''
+ commit_content.append('.py') # mock for algorithm below
+
+ files_to_restore = []
+ functions_to_restore = []
+ temp_restore = []
+
+ for content in commit_content:
+
+ if '.py' not in content:
+ temp_restore.append(content)
- for python_file in commit_content:
+ if '.py' in content:
+ if temp_restore:
+ functions_to_restore.append(temp_restore)
+ temp_restore = []
- if '.py' in python_file:
- file_to_restore = call_commit_path + python_file
+ file_to_restore = call_commit_path + content
+ files_to_restore.append(file_to_restore)
- else:
- fh, abs_path = mkstemp() # pylint:disable=invalid-name
+ for file_to_restore, functions in zip(files_to_restore, functions_to_restore):
- with fdopen(fh, 'w') as new_file:
- with open(file_to_restore) as old_file:
- for line in old_file:
- new_file.write(line)
+ fh, abs_path = mkstemp() # pylint:disable=invalid-name
- if 'def ' + python_file + '(' in line:
+ with fdopen(fh, 'w') as new_file:
+ with open(file_to_restore) as old_file:
+ stored_import_pdb_line_begging_spaces = ''
+ stored_function_name = ''
+ for line in old_file:
+ keywords_in_line = list(set(keyword.kwlist).intersection(line.split()))
+ new_file.write(line)
+
+ if 'def ' in line and '(' in line and '):' in line and not utils.is_commended_function(line):
+
+ strip_line = line.strip()
+ function_open_bracket_index = strip_line.index('(')
+ function_name = strip_line[4:function_open_bracket_index]
+
+ if function_name in functions:
# pylint:disable=invalid-name
import_pdb_line_begging_spaces = utils.get_import_pdb_line_begging_spaces(line)
new_file.write(import_pdb_line_begging_spaces + utils.IMPORT_PDB_LINE)
- utils.change_files_data(file_to_restore, abs_path)
+ elif 'def ' in line and '(' in line and '):' not in line:
+ function_name = line.split()[1][:-1]
+
+ if function_name in functions:
+ import_pdb_line_begging_spaces = utils.get_import_pdb_line_begging_spaces(line)
+ stored_import_pdb_line_begging_spaces = import_pdb_line_begging_spaces
+ stored_function_name = function_name
+ else:
+ stored_function_name = ''
+
+ elif 'def' not in line and '):' in line and not keywords_in_line:
+ if stored_function_name in functions:
+ new_file.write(stored_import_pdb_line_begging_spaces + utils.IMPORT_PDB_LINE)
+
+ utils.change_files_data(file_to_restore, abs_path)
def handle_commits_log() -> None:
diff --git a/pdbe/main.py b/pdbe/main.py
index a30afcd..d6d26b5 100644
--- a/pdbe/main.py
+++ b/pdbe/main.py
@@ -21,14 +21,36 @@ def put_import_pdb(file_path: str) -> None:
with fdopen(fh, 'w') as new_file:
with open(file_path) as old_file:
+
+ # for multiple function declaration
+ import_pdb_statement_to_write = ''
+ multiple_function_declaration_begging_spaces = ''
+
for line in old_file:
- if utils.is_function_sign_in_line(line) and not utils.is_commended_function(line):
+ if multiple_function_declaration_begging_spaces:
+ if 'def' not in line and '):' in line:
+ import_pdb_line_begging_spaces = multiple_function_declaration_begging_spaces
+ import_pdb_statement_to_write = import_pdb_line_begging_spaces + utils.IMPORT_PDB_LINE
+ multiple_function_declaration_begging_spaces = ''
+
+ if utils.is_one_line_function_declaration_line(line) and not utils.is_commended_function(line):
indents_space_count = utils.get_function_indent(line)
import_pdb_line_begging_spaces = utils.get_import_pdb_line_st_spaces(indents_space_count)
new_file.write(line + import_pdb_line_begging_spaces + utils.IMPORT_PDB_LINE)
+
else:
+ if 'def' in line and not utils.is_commended_function(line):
+ indents_space_count = utils.get_function_indent(line)
+ multiple_function_declaration_begging_spaces = utils.get_import_pdb_line_st_spaces(
+ indents_space_count
+ )
+
new_file.write(line)
+ if import_pdb_statement_to_write:
+ new_file.write(import_pdb_statement_to_write)
+ import_pdb_statement_to_write = ''
+
utils.change_files_data(file_path, abs_path)
diff --git a/pdbe/utils.py b/pdbe/utils.py
index 327aa9c..687ac76 100644
--- a/pdbe/utils.py
+++ b/pdbe/utils.py
@@ -8,7 +8,7 @@ IMPORT_PDB_LINE = 'import pdb; pdb.set_trace()\n'
LINE_FEED = '\n'
-def is_function_sign_in_line(line: str) -> bool:
+def is_one_line_function_declaration_line(line: str) -> bool: # pylint:disable=invalid-name
"""
Check if line contains function declaration.
"""
@@ -19,7 +19,7 @@ def does_line_contains_import_pdb(line: str) -> bool:
"""
Check if line contains import pdb statement.
"""
- return 'import pdb; pdb.set_trace()' in line
+ return ['import', 'pdb;', 'pdb.set_trace()'] == line.split()
def is_commended_function(line: str) -> bool:
| dmytrostriletskyi/pdbe | 8a611655496b306206ac9e7b4e9ba933ad66ab5b | diff --git a/tests/test_utils.py b/tests/test_utils.py
index 17480bc..bd96a5c 100644
--- a/tests/test_utils.py
+++ b/tests/test_utils.py
@@ -8,7 +8,7 @@ from ddt import data, ddt, unpack
from pdbe.utils import (
get_import_pdb_line_st_spaces,
get_function_indent,
- is_function_sign_in_line,
+ is_one_line_function_declaration_line,
)
@@ -29,7 +29,7 @@ class TestUtils(unittest.TestCase):
Case: needs to detect function declaration.
Expected: only line, that contains `def`, `(` and `):` substring confirmed.
"""
- result = is_function_sign_in_line(line)
+ result = is_one_line_function_declaration_line(line)
self.assertEqual(expected, result)
@data(
| Possible bug in multi-line function definition
https://github.com/dmytrostriletskyi/pdbe/blob/e103eb47de9961a1c4f7757cc37ca40eb98c0165/pdbe/utils.py#L11
This propably won't work with multiline function definitions. | 0.0 | [
"tests/test_utils.py::TestUtils::test_get_function_indent_1___def_function__args____kwargs_____0_",
"tests/test_utils.py::TestUtils::test_get_import_pdb_line_start_spaces_3__8_________________",
"tests/test_utils.py::TestUtils::test_get_function_indent_3___________def_function__args____kwargs_____8_",
"tests/test_utils.py::TestUtils::test_is_function_sign_in_line_3___def_function__args____kwargs_____True_",
"tests/test_utils.py::TestUtils::test_is_function_sign_in_line_2___def_fake_function____False_",
"tests/test_utils.py::TestUtils::test_get_import_pdb_line_start_spaces_1__0_________",
"tests/test_utils.py::TestUtils::test_get_function_indent_2_______def_function__args____kwargs_____4_",
"tests/test_utils.py::TestUtils::test_is_function_sign_in_line_1___import_sys___False_",
"tests/test_utils.py::TestUtils::test_get_import_pdb_line_start_spaces_2__4_____________"
] | [] | 2018-02-10 23:15:03+00:00 | 1,954 |
|
doccano__doccano-1557 | diff --git a/backend/api/views/download/data.py b/backend/api/views/download/data.py
index 68978184..79bfe7e8 100644
--- a/backend/api/views/download/data.py
+++ b/backend/api/views/download/data.py
@@ -1,3 +1,4 @@
+import json
from typing import Any, Dict, List
@@ -16,4 +17,10 @@ class Record:
self.metadata = metadata
def __str__(self):
- return f'{self.data}\t{self.label}'
+ return json.dumps({
+ 'id': self.id,
+ 'data': self.data,
+ 'label': self.label,
+ 'user': self.user,
+ 'metadata': self.metadata
+ })
diff --git a/backend/api/views/download/writer.py b/backend/api/views/download/writer.py
index dd0e88b4..5de1264e 100644
--- a/backend/api/views/download/writer.py
+++ b/backend/api/views/download/writer.py
@@ -90,7 +90,7 @@ class CsvWriter(BaseWriter):
def create_header(self, records: List[Record]) -> Iterable[str]:
header = ['id', 'data', 'label']
- header += list(itertools.chain(*[r.metadata.keys() for r in records]))
+ header += sorted(set(itertools.chain(*[r.metadata.keys() for r in records])))
return header
| doccano/doccano | 217cc85348972fcf38f3c58284dd2168db2bd3bb | diff --git a/backend/api/tests/download/__init__.py b/backend/api/tests/download/__init__.py
new file mode 100644
index 00000000..e69de29b
diff --git a/backend/api/tests/download/test_writer.py b/backend/api/tests/download/test_writer.py
new file mode 100644
index 00000000..2c48cb92
--- /dev/null
+++ b/backend/api/tests/download/test_writer.py
@@ -0,0 +1,53 @@
+import unittest
+from unittest.mock import call, patch
+
+from ...views.download.data import Record
+from ...views.download.writer import CsvWriter
+
+
+class TestCSVWriter(unittest.TestCase):
+
+ def setUp(self):
+ self.records = [
+ Record(id=0, data='exampleA', label=['labelA'], user='admin', metadata={'hidden': 'secretA'}),
+ Record(id=1, data='exampleB', label=['labelB'], user='admin', metadata={'hidden': 'secretB'}),
+ Record(id=2, data='exampleC', label=['labelC'], user='admin', metadata={'meta': 'secretC'})
+ ]
+
+ def test_create_header(self):
+ writer = CsvWriter('.')
+ header = writer.create_header(self.records)
+ expected = ['id', 'data', 'label', 'hidden', 'meta']
+ self.assertEqual(header, expected)
+
+ def test_create_line(self):
+ writer = CsvWriter('.')
+ record = self.records[0]
+ line = writer.create_line(record)
+ expected = {
+ 'id': record.id,
+ 'data': record.data,
+ 'label': record.label[0],
+ 'hidden': 'secretA'
+ }
+ self.assertEqual(line, expected)
+
+ @patch('os.remove')
+ @patch('zipfile.ZipFile')
+ @patch('csv.DictWriter.writerow')
+ @patch('builtins.open')
+ def test_dump(self, mock_open_file, csv_io, zip_io, mock_remove_file):
+ writer = CsvWriter('.')
+ writer.write(self.records)
+
+ self.assertEqual(mock_open_file.call_count, 1)
+ mock_open_file.assert_called_with('./admin.csv', mode='a', encoding='utf-8')
+
+ self.assertEqual(csv_io.call_count, len(self.records) + 1) # +1 is for a header
+ calls = [
+ call({'id': 'id', 'data': 'data', 'label': 'label', 'hidden': 'hidden', 'meta': 'meta'}),
+ call({'id': 0, 'data': 'exampleA', 'label': 'labelA', 'hidden': 'secretA'}),
+ call({'id': 1, 'data': 'exampleB', 'label': 'labelB', 'hidden': 'secretB'}),
+ call({'id': 2, 'data': 'exampleC', 'label': 'labelC', 'meta': 'secretC'})
+ ]
+ csv_io.assert_has_calls(calls)
| Metadata column repeated when exported as csv
Hi I have recently come across a bug when you export data as csv
<environment.-->
* Operating System:MacOS 10.14
* Python Version Used: 3.9.5
* Doccano installed through pip3 install doccano
I have created a DocumentClassification project and have imported some json data.
The json data is in the format of
```bash
{"text":"The ravioli was excellent" , "hidden":"The FOOD was excellent"}
```
When these sentences are imported, the "hidden" : "The FOOD was excellent" becomes part of the Metadata. I have quite a few of these sentences and have labelled them with my own labels
The issue is when I export the dataset as csv, the Metadata column repeats. For example if I have 10 labelled sentences, the Metadata column is repeated 10 times per row of data in excel. | 0.0 | [
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_create_header"
] | [
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_create_line",
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_dump"
] | 2021-11-11 01:37:39+00:00 | 1,955 |
|
doccano__doccano-1558 | diff --git a/backend/api/views/download/writer.py b/backend/api/views/download/writer.py
index 5de1264e..a4d5293a 100644
--- a/backend/api/views/download/writer.py
+++ b/backend/api/views/download/writer.py
@@ -84,7 +84,7 @@ class CsvWriter(BaseWriter):
return {
'id': record.id,
'data': record.data,
- 'label': '#'.join(record.label),
+ 'label': '#'.join(sorted(record.label)),
**record.metadata
}
@@ -144,6 +144,7 @@ class FastTextWriter(LineWriter):
def create_line(self, record):
line = [f'__label__{label}' for label in record.label]
+ line.sort()
line.append(record.data)
line = ' '.join(line)
return line
| doccano/doccano | 0d7bf054e619c144ec84fcf18f9457af8822a204 | diff --git a/backend/api/tests/download/test_writer.py b/backend/api/tests/download/test_writer.py
index 2c48cb92..720244dd 100644
--- a/backend/api/tests/download/test_writer.py
+++ b/backend/api/tests/download/test_writer.py
@@ -32,6 +32,16 @@ class TestCSVWriter(unittest.TestCase):
}
self.assertEqual(line, expected)
+ def test_label_order(self):
+ writer = CsvWriter('.')
+ record1 = Record(id=0, data='', label=['labelA', 'labelB'], user='', metadata={})
+ record2 = Record(id=0, data='', label=['labelB', 'labelA'], user='', metadata={})
+ line1 = writer.create_line(record1)
+ line2 = writer.create_line(record2)
+ expected = 'labelA#labelB'
+ self.assertEqual(line1['label'], expected)
+ self.assertEqual(line2['label'], expected)
+
@patch('os.remove')
@patch('zipfile.ZipFile')
@patch('csv.DictWriter.writerow')
| Mutli-label text classification export issues: same classes but in different orders
How to reproduce the behaviour
---------
<!-- Before submitting an issue, make sure to check the docs and closed issues and FAQ to see if any of the solutions work for you. https://github.com/doccano/doccano/wiki/Frequently-Asked-Questions -->
We are two annotators on a multi-label classification project. When I export the annotations, for some examples, me and my co-annotator have put the same labels, but on the exported CSV, they do not appear in the same order:
Annotator 1:
| text | labels |
| example 1 | label1#label2#label3 |
Annotator 2:
| text | labels |
| example 1 | label2#label3#label1 |
As I try to use these CSVs for comparing our annotations, this brings more difficulty.
<!-- Include a code example or the steps that led to the problem. Please try to be as specific as possible. -->
Your Environment
---------
<!-- Include details of your environment.-->
* Operating System: Debian
* Python Version Used: Don't know, I pulled the latest version from Docker Hub
* When you install doccano: 3 days ago
* How did you install doccano (Heroku button etc): Docker
| 0.0 | [
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_label_order"
] | [
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_create_header",
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_create_line",
"backend/api/tests/download/test_writer.py::TestCSVWriter::test_dump"
] | 2021-11-12 01:01:49+00:00 | 1,956 |
|
docker__docker-py-1022 | diff --git a/docker/auth/auth.py b/docker/auth/auth.py
index eedb7944..d23e6f3c 100644
--- a/docker/auth/auth.py
+++ b/docker/auth/auth.py
@@ -117,7 +117,7 @@ def parse_auth(entries, raise_on_error=False):
conf = {}
for registry, entry in six.iteritems(entries):
- if not (isinstance(entry, dict) and 'auth' in entry):
+ if not isinstance(entry, dict):
log.debug(
'Config entry for key {0} is not auth config'.format(registry)
)
@@ -130,6 +130,16 @@ def parse_auth(entries, raise_on_error=False):
'Invalid configuration for registry {0}'.format(registry)
)
return {}
+ if 'auth' not in entry:
+ # Starting with engine v1.11 (API 1.23), an empty dictionary is
+ # a valid value in the auths config.
+ # https://github.com/docker/compose/issues/3265
+ log.debug(
+ 'Auth data for {0} is absent. Client might be using a '
+ 'credentials store instead.'
+ )
+ return {}
+
username, password = decode_auth(entry['auth'])
log.debug(
'Found entry (registry={0}, username={1})'
@@ -189,6 +199,9 @@ def load_config(config_path=None):
if data.get('HttpHeaders'):
log.debug("Found 'HttpHeaders' section")
res.update({'HttpHeaders': data['HttpHeaders']})
+ if data.get('credsStore'):
+ log.debug("Found 'credsStore' section")
+ res.update({'credsStore': data['credsStore']})
if res:
return res
else:
| docker/docker-py | e743254b42080e6d199fc10f4812a42ecb8d536f | diff --git a/tests/unit/auth_test.py b/tests/unit/auth_test.py
index 921aae00..4ea40477 100644
--- a/tests/unit/auth_test.py
+++ b/tests/unit/auth_test.py
@@ -459,6 +459,5 @@ class LoadConfigTest(base.Cleanup, base.BaseTestCase):
with open(dockercfg_path, 'w') as f:
json.dump(config, f)
- self.assertRaises(
- errors.InvalidConfigFile, auth.load_config, dockercfg_path
- )
+ cfg = auth.load_config(dockercfg_path)
+ assert cfg == {}
| Empty auth dictionary should be valid
docker/compose#3265 | 0.0 | [
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_invalid_auth_dict"
] | [
"tests/unit/auth_test.py::RegressionTest::test_803_urlsafe_encode",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_explicit_hub_index_library_image",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_explicit_legacy_hub_index_library_image",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_invalid_index_name",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_dotted_hub_library_image",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_hub_image",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_hub_library_image",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_localhost",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_localhost_with_username",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_no_dots_but_port",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_no_dots_but_port_and_username",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_private_registry",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_private_registry_with_port",
"tests/unit/auth_test.py::ResolveRepositoryNameTest::test_resolve_repository_name_private_registry_with_username",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_default_explicit_none",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_default_registry",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_fully_explicit",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_hostname_only",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_legacy_config",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_no_match",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_no_path",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_no_path_trailing_slash",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_no_path_wrong_insecure_proto",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_no_path_wrong_secure_proto",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_no_protocol",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_authconfig_path_wrong_proto",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_registry_and_auth_explicit_hub",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_registry_and_auth_explicit_legacy_hub",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_registry_and_auth_hub_image",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_registry_and_auth_library_image",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_registry_and_auth_private_registry",
"tests/unit/auth_test.py::ResolveAuthTest::test_resolve_registry_and_auth_unauthenticated_registry",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_custom_config_env",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_custom_config_env_utf8",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_custom_config_env_with_auths",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_custom_config_env_with_headers",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_no_file",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_unknown_keys",
"tests/unit/auth_test.py::LoadConfigTest::test_load_config_with_random_name"
] | 2016-04-05 19:54:10+00:00 | 1,957 |
|
docker__docker-py-1130 | diff --git a/docker/api/container.py b/docker/api/container.py
index 9cc14dbd..b8507d85 100644
--- a/docker/api/container.py
+++ b/docker/api/container.py
@@ -15,12 +15,18 @@ class ContainerApiMixin(object):
'logs': logs and 1 or 0,
'stdout': stdout and 1 or 0,
'stderr': stderr and 1 or 0,
- 'stream': stream and 1 or 0,
+ 'stream': stream and 1 or 0
}
+
+ headers = {
+ 'Connection': 'Upgrade',
+ 'Upgrade': 'tcp'
+ }
+
u = self._url("/containers/{0}/attach", container)
- response = self._post(u, params=params, stream=stream)
+ response = self._post(u, headers=headers, params=params, stream=stream)
- return self._get_result(container, stream, response)
+ return self._read_from_socket(response, stream)
@utils.check_resource
def attach_socket(self, container, params=None, ws=False):
@@ -34,9 +40,18 @@ class ContainerApiMixin(object):
if ws:
return self._attach_websocket(container, params)
+ headers = {
+ 'Connection': 'Upgrade',
+ 'Upgrade': 'tcp'
+ }
+
u = self._url("/containers/{0}/attach", container)
- return self._get_raw_response_socket(self.post(
- u, None, params=self._attach_params(params), stream=True))
+ return self._get_raw_response_socket(
+ self.post(
+ u, None, params=self._attach_params(params), stream=True,
+ headers=headers
+ )
+ )
@utils.check_resource
def commit(self, container, repository=None, tag=None, message=None,
diff --git a/docker/api/exec_api.py b/docker/api/exec_api.py
index f0e4afa6..6e499960 100644
--- a/docker/api/exec_api.py
+++ b/docker/api/exec_api.py
@@ -56,8 +56,6 @@ class ExecApiMixin(object):
def exec_start(self, exec_id, detach=False, tty=False, stream=False,
socket=False):
# we want opened socket if socket == True
- if socket:
- stream = True
if isinstance(exec_id, dict):
exec_id = exec_id.get('Id')
@@ -66,10 +64,18 @@ class ExecApiMixin(object):
'Detach': detach
}
+ headers = {} if detach else {
+ 'Connection': 'Upgrade',
+ 'Upgrade': 'tcp'
+ }
+
res = self._post_json(
- self._url('/exec/{0}/start', exec_id), data=data, stream=stream
+ self._url('/exec/{0}/start', exec_id),
+ headers=headers,
+ data=data,
+ stream=True
)
if socket:
return self._get_raw_response_socket(res)
- return self._get_result_tty(stream, res, tty)
+ return self._read_from_socket(res, stream)
diff --git a/docker/client.py b/docker/client.py
index b96a78ce..6ca9e57a 100644
--- a/docker/client.py
+++ b/docker/client.py
@@ -29,6 +29,7 @@ from .ssladapter import ssladapter
from .tls import TLSConfig
from .transport import UnixAdapter
from .utils import utils, check_resource, update_headers, kwargs_from_env
+from .utils.socket import frames_iter
try:
from .transport import NpipeAdapter
except ImportError:
@@ -305,6 +306,14 @@ class Client(
for out in response.iter_content(chunk_size=1, decode_unicode=True):
yield out
+ def _read_from_socket(self, response, stream):
+ socket = self._get_raw_response_socket(response)
+
+ if stream:
+ return frames_iter(socket)
+ else:
+ return six.binary_type().join(frames_iter(socket))
+
def _disable_socket_timeout(self, socket):
""" Depending on the combination of python version and whether we're
connecting over http or https, we might need to access _sock, which
diff --git a/docker/utils/socket.py b/docker/utils/socket.py
new file mode 100644
index 00000000..ed343507
--- /dev/null
+++ b/docker/utils/socket.py
@@ -0,0 +1,68 @@
+import errno
+import os
+import select
+import struct
+
+import six
+
+
+class SocketError(Exception):
+ pass
+
+
+def read(socket, n=4096):
+ """
+ Reads at most n bytes from socket
+ """
+ recoverable_errors = (errno.EINTR, errno.EDEADLK, errno.EWOULDBLOCK)
+
+ # wait for data to become available
+ select.select([socket], [], [])
+
+ try:
+ if hasattr(socket, 'recv'):
+ return socket.recv(n)
+ return os.read(socket.fileno(), n)
+ except EnvironmentError as e:
+ if e.errno not in recoverable_errors:
+ raise
+
+
+def read_exactly(socket, n):
+ """
+ Reads exactly n bytes from socket
+ Raises SocketError if there isn't enough data
+ """
+ data = six.binary_type()
+ while len(data) < n:
+ next_data = read(socket, n - len(data))
+ if not next_data:
+ raise SocketError("Unexpected EOF")
+ data += next_data
+ return data
+
+
+def next_frame_size(socket):
+ """
+ Returns the size of the next frame of data waiting to be read from socket,
+ according to the protocol defined here:
+
+ https://docs.docker.com/engine/reference/api/docker_remote_api_v1.24/#/attach-to-a-container
+ """
+ try:
+ data = read_exactly(socket, 8)
+ except SocketError:
+ return 0
+
+ _, actual = struct.unpack('>BxxxL', data)
+ return actual
+
+
+def frames_iter(socket):
+ """
+ Returns a generator of frames read from socket
+ """
+ n = next_frame_size(socket)
+ while n > 0:
+ yield read(socket, n)
+ n = next_frame_size(socket)
| docker/docker-py | b511352bea79aff12d565b11662bebee36e362fc | diff --git a/tests/helpers.py b/tests/helpers.py
index 21036ace..94ea3887 100644
--- a/tests/helpers.py
+++ b/tests/helpers.py
@@ -1,9 +1,6 @@
-import errno
import os
import os.path
-import select
import shutil
-import struct
import tarfile
import tempfile
import unittest
@@ -54,7 +51,7 @@ def exec_driver_is_native():
c = docker_client()
EXEC_DRIVER = c.info()['ExecutionDriver']
c.close()
- return EXEC_DRIVER.startswith('native')
+ return EXEC_DRIVER.startswith('native') or EXEC_DRIVER == ''
def docker_client(**kwargs):
@@ -67,49 +64,6 @@ def docker_client_kwargs(**kwargs):
return client_kwargs
-def read_socket(socket, n=4096):
- """ Code stolen from dockerpty to read the socket """
- recoverable_errors = (errno.EINTR, errno.EDEADLK, errno.EWOULDBLOCK)
-
- # wait for data to become available
- select.select([socket], [], [])
-
- try:
- if hasattr(socket, 'recv'):
- return socket.recv(n)
- return os.read(socket.fileno(), n)
- except EnvironmentError as e:
- if e.errno not in recoverable_errors:
- raise
-
-
-def next_packet_size(socket):
- """ Code stolen from dockerpty to get the next packet size """
- data = six.binary_type()
- while len(data) < 8:
- next_data = read_socket(socket, 8 - len(data))
- if not next_data:
- return 0
- data = data + next_data
-
- if data is None:
- return 0
-
- if len(data) == 8:
- _, actual = struct.unpack('>BxxxL', data)
- return actual
-
-
-def read_data(socket, packet_size):
- data = six.binary_type()
- while len(data) < packet_size:
- next_data = read_socket(socket, packet_size - len(data))
- if not next_data:
- assert False, "Failed trying to read in the dataz"
- data += next_data
- return data
-
-
class BaseTestCase(unittest.TestCase):
tmp_imgs = []
tmp_containers = []
diff --git a/tests/integration/container_test.py b/tests/integration/container_test.py
index 56b648a3..61b33983 100644
--- a/tests/integration/container_test.py
+++ b/tests/integration/container_test.py
@@ -3,6 +3,8 @@ import signal
import tempfile
import docker
+from docker.utils.socket import next_frame_size
+from docker.utils.socket import read_exactly
import pytest
import six
@@ -1025,9 +1027,9 @@ class AttachContainerTest(helpers.BaseTestCase):
self.client.start(ident)
- next_size = helpers.next_packet_size(pty_stdout)
+ next_size = next_frame_size(pty_stdout)
self.assertEqual(next_size, len(line))
- data = helpers.read_data(pty_stdout, next_size)
+ data = read_exactly(pty_stdout, next_size)
self.assertEqual(data.decode('utf-8'), line)
diff --git a/tests/integration/exec_test.py b/tests/integration/exec_test.py
index 9f548080..8bf2762a 100644
--- a/tests/integration/exec_test.py
+++ b/tests/integration/exec_test.py
@@ -1,5 +1,8 @@
import pytest
+from docker.utils.socket import next_frame_size
+from docker.utils.socket import read_exactly
+
from .. import helpers
BUSYBOX = helpers.BUSYBOX
@@ -107,9 +110,9 @@ class ExecTest(helpers.BaseTestCase):
socket = self.client.exec_start(exec_id, socket=True)
self.addCleanup(socket.close)
- next_size = helpers.next_packet_size(socket)
+ next_size = next_frame_size(socket)
self.assertEqual(next_size, len(line))
- data = helpers.read_data(socket, next_size)
+ data = read_exactly(socket, next_size)
self.assertEqual(data.decode('utf-8'), line)
def test_exec_inspect(self):
diff --git a/tests/unit/api_test.py b/tests/unit/api_test.py
index 23fd1913..34bf14f6 100644
--- a/tests/unit/api_test.py
+++ b/tests/unit/api_test.py
@@ -93,6 +93,10 @@ def fake_put(self, url, *args, **kwargs):
def fake_delete(self, url, *args, **kwargs):
return fake_request('DELETE', url, *args, **kwargs)
+
+def fake_read_from_socket(self, response, stream):
+ return six.binary_type()
+
url_base = 'http+docker://localunixsocket/'
url_prefix = '{0}v{1}/'.format(
url_base,
@@ -103,7 +107,8 @@ class DockerClientTest(base.Cleanup, base.BaseTestCase):
def setUp(self):
self.patcher = mock.patch.multiple(
'docker.Client', get=fake_get, post=fake_post, put=fake_put,
- delete=fake_delete
+ delete=fake_delete,
+ _read_from_socket=fake_read_from_socket
)
self.patcher.start()
self.client = docker.Client()
diff --git a/tests/unit/exec_test.py b/tests/unit/exec_test.py
index 3007799c..6ba2a3dd 100644
--- a/tests/unit/exec_test.py
+++ b/tests/unit/exec_test.py
@@ -51,8 +51,36 @@ class ExecTest(DockerClientTest):
}
)
- self.assertEqual(args[1]['headers'],
- {'Content-Type': 'application/json'})
+ self.assertEqual(
+ args[1]['headers'], {
+ 'Content-Type': 'application/json',
+ 'Connection': 'Upgrade',
+ 'Upgrade': 'tcp'
+ }
+ )
+
+ def test_exec_start_detached(self):
+ self.client.exec_start(fake_api.FAKE_EXEC_ID, detach=True)
+
+ args = fake_request.call_args
+ self.assertEqual(
+ args[0][1], url_prefix + 'exec/{0}/start'.format(
+ fake_api.FAKE_EXEC_ID
+ )
+ )
+
+ self.assertEqual(
+ json.loads(args[1]['data']), {
+ 'Tty': False,
+ 'Detach': True
+ }
+ )
+
+ self.assertEqual(
+ args[1]['headers'], {
+ 'Content-Type': 'application/json'
+ }
+ )
def test_exec_inspect(self):
self.client.exec_inspect(fake_api.FAKE_EXEC_ID)
| The api client should send connection upgrade headers
To hint proxies about connection hijacking, docker clients should send connection upgrade headers like the docker cli does.
From https://docs.docker.com/engine/reference/api/docker_remote_api_v1.24/#/4-2-hijacking:
> In this version of the API, /attach, uses hijacking to transport stdin, stdout, and stderr on the same socket.
>
> To hint potential proxies about connection hijacking, Docker client sends connection upgrade headers similarly to websocket.
```
Upgrade: tcp
Connection: Upgrade
```
On Docker for Desktop, the proxy that sits between Docker Compose and the daemon will not be aware that the connection will be hijacked. This can lead to an issue where the proxy will install a CloseNotifier and just after that will hijack the connection, which is know to be incompatible. See https://github.com/docker/compose/issues/3685
See also https://github.com/docker/compose/issues/3700
| 0.0 | [
"tests/unit/api_test.py::DockerApiTest::test_auto_retrieve_server_version",
"tests/unit/api_test.py::DockerApiTest::test_create_host_config_secopt",
"tests/unit/api_test.py::DockerApiTest::test_ctor",
"tests/unit/api_test.py::DockerApiTest::test_events",
"tests/unit/api_test.py::DockerApiTest::test_events_with_filters",
"tests/unit/api_test.py::DockerApiTest::test_events_with_since_until",
"tests/unit/api_test.py::DockerApiTest::test_info",
"tests/unit/api_test.py::DockerApiTest::test_remove_link",
"tests/unit/api_test.py::DockerApiTest::test_retrieve_server_version",
"tests/unit/api_test.py::DockerApiTest::test_search",
"tests/unit/api_test.py::DockerApiTest::test_url_compatibility_http",
"tests/unit/api_test.py::DockerApiTest::test_url_compatibility_http_unix_triple_slash",
"tests/unit/api_test.py::DockerApiTest::test_url_compatibility_tcp",
"tests/unit/api_test.py::DockerApiTest::test_url_compatibility_unix",
"tests/unit/api_test.py::DockerApiTest::test_url_compatibility_unix_triple_slash",
"tests/unit/api_test.py::DockerApiTest::test_url_invalid_resource",
"tests/unit/api_test.py::DockerApiTest::test_url_no_resource",
"tests/unit/api_test.py::DockerApiTest::test_url_unversioned_api",
"tests/unit/api_test.py::DockerApiTest::test_url_valid_resource",
"tests/unit/api_test.py::DockerApiTest::test_version",
"tests/unit/api_test.py::DockerApiTest::test_version_no_api_version",
"tests/unit/exec_test.py::ExecTest::test_exec_create",
"tests/unit/exec_test.py::ExecTest::test_exec_inspect",
"tests/unit/exec_test.py::ExecTest::test_exec_resize",
"tests/unit/exec_test.py::ExecTest::test_exec_start",
"tests/unit/exec_test.py::ExecTest::test_exec_start_detached"
] | [] | 2016-07-13 21:07:00+00:00 | 1,958 |
|
docker__docker-py-1143 | diff --git a/docker/utils/utils.py b/docker/utils/utils.py
index 4d218692..1cfc8acc 100644
--- a/docker/utils/utils.py
+++ b/docker/utils/utils.py
@@ -22,8 +22,8 @@ import tarfile
import tempfile
import warnings
from distutils.version import StrictVersion
-from fnmatch import fnmatch
from datetime import datetime
+from fnmatch import fnmatch
import requests
import six
@@ -33,6 +33,10 @@ from .. import errors
from .. import tls
from .types import Ulimit, LogConfig
+if six.PY2:
+ from urllib import splitnport
+else:
+ from urllib.parse import splitnport
DEFAULT_HTTP_HOST = "127.0.0.1"
DEFAULT_UNIX_SOCKET = "http+unix://var/run/docker.sock"
@@ -387,7 +391,6 @@ def parse_repository_tag(repo_name):
# Protocol translation: tcp -> http, unix -> http+unix
def parse_host(addr, is_win32=False, tls=False):
proto = "http+unix"
- host = DEFAULT_HTTP_HOST
port = None
path = ''
@@ -427,32 +430,27 @@ def parse_host(addr, is_win32=False, tls=False):
)
proto = "https" if tls else "http"
- if proto != "http+unix" and ":" in addr:
- host_parts = addr.split(':')
- if len(host_parts) != 2:
- raise errors.DockerException(
- "Invalid bind address format: {0}".format(addr)
- )
- if host_parts[0]:
- host = host_parts[0]
+ if proto in ("http", "https"):
+ address_parts = addr.split('/', 1)
+ host = address_parts[0]
+ if len(address_parts) == 2:
+ path = '/' + address_parts[1]
+ host, port = splitnport(host)
- port = host_parts[1]
- if '/' in port:
- port, path = port.split('/', 1)
- path = '/{0}'.format(path)
- try:
- port = int(port)
- except Exception:
+ if port is None:
raise errors.DockerException(
"Invalid port: {0}".format(addr)
)
- elif proto in ("http", "https") and ':' not in addr:
- raise errors.DockerException(
- "Bind address needs a port: {0}".format(addr))
+ if not host:
+ host = DEFAULT_HTTP_HOST
else:
host = addr
+ if proto in ("http", "https") and port == -1:
+ raise errors.DockerException(
+ "Bind address needs a port: {0}".format(addr))
+
if proto == "http+unix" or proto == 'npipe':
return "{0}://{1}".format(proto, host)
return "{0}://{1}:{2}{3}".format(proto, host, port, path)
| docker/docker-py | 2d3bda84de39a75e560fc79512143d43e5d61226 | diff --git a/tests/unit/utils_test.py b/tests/unit/utils_test.py
index 68484fe5..0f7a58c9 100644
--- a/tests/unit/utils_test.py
+++ b/tests/unit/utils_test.py
@@ -404,10 +404,18 @@ class ParseHostTest(base.BaseTestCase):
'https://kokia.jp:2375': 'https://kokia.jp:2375',
'unix:///var/run/docker.sock': 'http+unix:///var/run/docker.sock',
'unix://': 'http+unix://var/run/docker.sock',
+ '12.234.45.127:2375/docker/engine': (
+ 'http://12.234.45.127:2375/docker/engine'
+ ),
'somehost.net:80/service/swarm': (
'http://somehost.net:80/service/swarm'
),
'npipe:////./pipe/docker_engine': 'npipe:////./pipe/docker_engine',
+ '[fd12::82d1]:2375': 'http://[fd12::82d1]:2375',
+ 'https://[fd12:5672::12aa]:1090': 'https://[fd12:5672::12aa]:1090',
+ '[fd12::82d1]:2375/docker/engine': (
+ 'http://[fd12::82d1]:2375/docker/engine'
+ ),
}
for host in invalid_hosts:
@@ -415,7 +423,7 @@ class ParseHostTest(base.BaseTestCase):
parse_host(host, None)
for host, expected in valid_hosts.items():
- self.assertEqual(parse_host(host, None), expected, msg=host)
+ assert parse_host(host, None) == expected
def test_parse_host_empty_value(self):
unix_socket = 'http+unix://var/run/docker.sock'
| Support IPv6 addresses in DOCKER_HOST
Raised in https://github.com/docker/compose/issues/2879.
See https://github.com/docker/docker/pull/16950 for the Engine implementation. | 0.0 | [
"tests/unit/utils_test.py::ParseHostTest::test_parse_host"
] | [
"tests/unit/utils_test.py::HostConfigTest::test_create_endpoint_config_with_aliases",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_invalid_cpu_cfs_types",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options_newer_api_version",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_blkio_constraints",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_period",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_quota",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_kill_disable",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_score_adj",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size_in_mb",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit_capitals",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_obj_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_ulimit_invalid_type",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_dict_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_obj_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_logconfig_invalid_config_type",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_alternate_env",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_empty",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_no_cert_path",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false_no_cert",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_compact",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_complete",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_empty",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_list",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_no_mode",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_bytes_input",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_unicode_input",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_commented_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_invalid_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_proper",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_with_equals_character",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_empty_value",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls_tcp_proto",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_tag",
"tests/unit/utils_test.py::ParseDeviceTest::test_dict",
"tests/unit/utils_test.py::ParseDeviceTest::test_full_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_hybrid_list",
"tests/unit/utils_test.py::ParseDeviceTest::test_partial_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_permissionless_string_definition",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_float",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_invalid",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_maxint",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_valid",
"tests/unit/utils_test.py::UtilsTest::test_convert_filters",
"tests/unit/utils_test.py::UtilsTest::test_create_ipam_config",
"tests/unit/utils_test.py::UtilsTest::test_decode_json_header",
"tests/unit/utils_test.py::SplitCommandTest::test_split_command_with_unicode",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_one_port",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_port_range",
"tests/unit/utils_test.py::PortsTest::test_host_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_non_matching_length_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_port_and_range_invalid",
"tests/unit/utils_test.py::PortsTest::test_port_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_split_port_invalid",
"tests/unit/utils_test.py::PortsTest::test_split_port_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_protocol",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_protocol",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_single_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_subdir_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_wildcard_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_custom_dockerfile",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_child",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_dockerignore",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_dupes",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_excludes",
"tests/unit/utils_test.py::ExcludePathsTest::test_question_mark",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_leading_dot_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_with_path_traversal",
"tests/unit/utils_test.py::ExcludePathsTest::test_subdirectory",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_exclude",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_end",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_start",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_wildcard_exception",
"tests/unit/utils_test.py::TarTest::test_tar_with_directory_symlinks",
"tests/unit/utils_test.py::TarTest::test_tar_with_empty_directory",
"tests/unit/utils_test.py::TarTest::test_tar_with_excludes",
"tests/unit/utils_test.py::TarTest::test_tar_with_file_symlinks"
] | 2016-07-28 01:43:06+00:00 | 1,959 |
|
docker__docker-py-1150 | diff --git a/docker/utils/decorators.py b/docker/utils/decorators.py
index 7c41a5f8..46c28a80 100644
--- a/docker/utils/decorators.py
+++ b/docker/utils/decorators.py
@@ -40,7 +40,7 @@ def minimum_version(version):
def update_headers(f):
def inner(self, *args, **kwargs):
if 'HttpHeaders' in self._auth_configs:
- if 'headers' not in kwargs:
+ if not kwargs.get('headers'):
kwargs['headers'] = self._auth_configs['HttpHeaders']
else:
kwargs['headers'].update(self._auth_configs['HttpHeaders'])
| docker/docker-py | 650cc70e934044fcb5dfd27fd27777f91c337b6c | diff --git a/tests/unit/utils_test.py b/tests/unit/utils_test.py
index 0f7a58c9..47ced433 100644
--- a/tests/unit/utils_test.py
+++ b/tests/unit/utils_test.py
@@ -20,9 +20,11 @@ from docker.utils import (
create_host_config, Ulimit, LogConfig, parse_bytes, parse_env_file,
exclude_paths, convert_volume_binds, decode_json_header, tar,
split_command, create_ipam_config, create_ipam_pool, parse_devices,
+ update_headers,
)
-from docker.utils.utils import create_endpoint_config
+
from docker.utils.ports import build_port_bindings, split_port
+from docker.utils.utils import create_endpoint_config
from .. import base
from ..helpers import make_tree
@@ -34,6 +36,37 @@ TEST_CERT_DIR = os.path.join(
)
+class DecoratorsTest(base.BaseTestCase):
+ def test_update_headers(self):
+ sample_headers = {
+ 'X-Docker-Locale': 'en-US',
+ }
+
+ def f(self, headers=None):
+ return headers
+
+ client = Client()
+ client._auth_configs = {}
+
+ g = update_headers(f)
+ assert g(client, headers=None) is None
+ assert g(client, headers={}) == {}
+ assert g(client, headers={'Content-type': 'application/json'}) == {
+ 'Content-type': 'application/json',
+ }
+
+ client._auth_configs = {
+ 'HttpHeaders': sample_headers
+ }
+
+ assert g(client, headers=None) == sample_headers
+ assert g(client, headers={}) == sample_headers
+ assert g(client, headers={'Content-type': 'application/json'}) == {
+ 'Content-type': 'application/json',
+ 'X-Docker-Locale': 'en-US',
+ }
+
+
class HostConfigTest(base.BaseTestCase):
def test_create_host_config_no_options(self):
config = create_host_config(version='1.19')
| Client.build crashes when trying to pull a new image if HttpHeaders are set in config file
```python
import docker
c = docker.Client()
c.build('https://github.com/docker/compose.git')
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
<ipython-input-3-d78c607c9627> in <module>()
----> 1 c.build('https://github.com/docker/compose.git')
/home/joffrey/.envs/pydocker/local/lib/python2.7/site-packages/docker/api/build.pyc in build(self, path, tag, quiet, fileobj, nocache, rm, stream, timeout, custom_context, encoding, pull, forcerm, dockerfile, container_limits, decode, buildargs, gzip)
102 headers=headers,
103 stream=stream,
--> 104 timeout=timeout,
105 )
106
/home/joffrey/.envs/pydocker/local/lib/python2.7/site-packages/docker/utils/decorators.pyc in inner(self, *args, **kwargs)
44 kwargs['headers'] = self._auth_configs['HttpHeaders']
45 else:
---> 46 kwargs['headers'].update(self._auth_configs['HttpHeaders'])
47 return f(self, *args, **kwargs)
48 return inner
AttributeError: 'NoneType' object has no attribute 'update'
``` | 0.0 | [
"tests/unit/utils_test.py::DecoratorsTest::test_update_headers"
] | [
"tests/unit/utils_test.py::HostConfigTest::test_create_endpoint_config_with_aliases",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_invalid_cpu_cfs_types",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options_newer_api_version",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_blkio_constraints",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_period",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_quota",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_kill_disable",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_score_adj",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size_in_mb",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit_capitals",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_obj_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_ulimit_invalid_type",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_dict_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_obj_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_logconfig_invalid_config_type",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_alternate_env",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_empty",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_no_cert_path",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false_no_cert",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_compact",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_complete",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_empty",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_list",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_no_mode",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_bytes_input",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_unicode_input",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_commented_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_invalid_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_proper",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_with_equals_character",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_empty_value",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls_tcp_proto",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_tag",
"tests/unit/utils_test.py::ParseDeviceTest::test_dict",
"tests/unit/utils_test.py::ParseDeviceTest::test_full_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_hybrid_list",
"tests/unit/utils_test.py::ParseDeviceTest::test_partial_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_permissionless_string_definition",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_float",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_invalid",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_maxint",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_valid",
"tests/unit/utils_test.py::UtilsTest::test_convert_filters",
"tests/unit/utils_test.py::UtilsTest::test_create_ipam_config",
"tests/unit/utils_test.py::UtilsTest::test_decode_json_header",
"tests/unit/utils_test.py::SplitCommandTest::test_split_command_with_unicode",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_one_port",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_port_range",
"tests/unit/utils_test.py::PortsTest::test_host_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_non_matching_length_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_port_and_range_invalid",
"tests/unit/utils_test.py::PortsTest::test_port_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_split_port_invalid",
"tests/unit/utils_test.py::PortsTest::test_split_port_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_protocol",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_protocol",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_single_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_subdir_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_wildcard_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_custom_dockerfile",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_child",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_dockerignore",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_dupes",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_excludes",
"tests/unit/utils_test.py::ExcludePathsTest::test_question_mark",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_leading_dot_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_with_path_traversal",
"tests/unit/utils_test.py::ExcludePathsTest::test_subdirectory",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_exclude",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_end",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_start",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_wildcard_exception",
"tests/unit/utils_test.py::TarTest::test_tar_with_directory_symlinks",
"tests/unit/utils_test.py::TarTest::test_tar_with_empty_directory",
"tests/unit/utils_test.py::TarTest::test_tar_with_excludes",
"tests/unit/utils_test.py::TarTest::test_tar_with_file_symlinks"
] | 2016-08-03 00:27:54+00:00 | 1,960 |
|
docker__docker-py-1167 | diff --git a/docker/client.py b/docker/client.py
index d1c6ee5f..ef718a72 100644
--- a/docker/client.py
+++ b/docker/client.py
@@ -114,7 +114,8 @@ class Client(
@classmethod
def from_env(cls, **kwargs):
- return cls(**kwargs_from_env(**kwargs))
+ version = kwargs.pop('version', None)
+ return cls(version=version, **kwargs_from_env(**kwargs))
def _retrieve_server_version(self):
try:
| docker/docker-py | 2ef02df2f06fafe7d71c96bac1e18d68217703ab | diff --git a/tests/unit/client_test.py b/tests/unit/client_test.py
index b21f1d6a..6ceb8cbb 100644
--- a/tests/unit/client_test.py
+++ b/tests/unit/client_test.py
@@ -25,6 +25,14 @@ class ClientTest(base.BaseTestCase):
client = Client.from_env()
self.assertEqual(client.base_url, "https://192.168.59.103:2376")
+ def test_from_env_with_version(self):
+ os.environ.update(DOCKER_HOST='tcp://192.168.59.103:2376',
+ DOCKER_CERT_PATH=TEST_CERT_DIR,
+ DOCKER_TLS_VERIFY='1')
+ client = Client.from_env(version='2.32')
+ self.assertEqual(client.base_url, "https://192.168.59.103:2376")
+ self.assertEqual(client._version, '2.32')
+
class DisableSocketTest(base.BaseTestCase):
class DummySocket(object):
| Feature Request: docker.from_env(version='auto')
Feature request to add auto api version support for ```docker.from_env()``` similar to ```docker.Client(version='auto')```?
I noticed that one of the suggestions from #402 for the ```version='auto'``` option was now available for ```docker.Client()``` but doesn't work for ```docker.from_env()```. | 0.0 | [
"tests/unit/client_test.py::ClientTest::test_from_env_with_version"
] | [
"tests/unit/client_test.py::ClientTest::test_from_env",
"tests/unit/client_test.py::DisableSocketTest::test_disable_socket_timeout",
"tests/unit/client_test.py::DisableSocketTest::test_disable_socket_timeout2",
"tests/unit/client_test.py::DisableSocketTest::test_disable_socket_timout_non_blocking"
] | 2016-08-23 23:53:03+00:00 | 1,961 |
|
docker__docker-py-1178 | diff --git a/docker/api/network.py b/docker/api/network.py
index 34cd8987..0ee0dab6 100644
--- a/docker/api/network.py
+++ b/docker/api/network.py
@@ -22,7 +22,8 @@ class NetworkApiMixin(object):
@minimum_version('1.21')
def create_network(self, name, driver=None, options=None, ipam=None,
- check_duplicate=None, internal=False):
+ check_duplicate=None, internal=False, labels=None,
+ enable_ipv6=False):
if options is not None and not isinstance(options, dict):
raise TypeError('options must be a dictionary')
@@ -34,6 +35,22 @@ class NetworkApiMixin(object):
'CheckDuplicate': check_duplicate
}
+ if labels is not None:
+ if version_lt(self._version, '1.23'):
+ raise InvalidVersion(
+ 'network labels were introduced in API 1.23'
+ )
+ if not isinstance(labels, dict):
+ raise TypeError('labels must be a dictionary')
+ data["Labels"] = labels
+
+ if enable_ipv6:
+ if version_lt(self._version, '1.23'):
+ raise InvalidVersion(
+ 'enable_ipv6 was introduced in API 1.23'
+ )
+ data['EnableIPv6'] = True
+
if internal:
if version_lt(self._version, '1.22'):
raise InvalidVersion('Internal networks are not '
@@ -76,8 +93,15 @@ class NetworkApiMixin(object):
@check_resource
@minimum_version('1.21')
- def disconnect_container_from_network(self, container, net_id):
- data = {"container": container}
+ def disconnect_container_from_network(self, container, net_id,
+ force=False):
+ data = {"Container": container}
+ if force:
+ if version_lt(self._version, '1.22'):
+ raise InvalidVersion(
+ 'Forced disconnect was introduced in API 1.22'
+ )
+ data['Force'] = force
url = self._url("/networks/{0}/disconnect", net_id)
res = self._post_json(url, data=data)
self._raise_for_status(res)
diff --git a/docs/api.md b/docs/api.md
index 895d7d45..1699344a 100644
--- a/docs/api.md
+++ b/docs/api.md
@@ -283,22 +283,25 @@ The utility can be used as follows:
```python
>>> import docker.utils
>>> my_envs = docker.utils.parse_env_file('/path/to/file')
->>> docker.utils.create_container_config('1.18', '_mongodb', 'foobar', environment=my_envs)
+>>> client.create_container('myimage', 'command', environment=my_envs)
```
-You can now use this with 'environment' for `create_container`.
-
-
## create_network
-Create a network, similar to the `docker network create` command.
+Create a network, similar to the `docker network create` command. See the
+[networks documentation](networks.md) for details.
**Params**:
* name (str): Name of the network
* driver (str): Name of the driver used to create the network
-
* options (dict): Driver options as a key-value dictionary
+* ipam (dict): Optional custom IP scheme for the network
+* check_duplicate (bool): Request daemon to check for networks with same name.
+ Default: `True`.
+* internal (bool): Restrict external access to the network. Default `False`.
+* labels (dict): Map of labels to set on the network. Default `None`.
+* enable_ipv6 (bool): Enable IPv6 on the network. Default `False`.
**Returns** (dict): The created network reference object
@@ -352,6 +355,8 @@ Inspect changes on a container's filesystem.
* container (str): container-id/name to be disconnected from a network
* net_id (str): network id
+* force (bool): Force the container to disconnect from a network.
+ Default: `False`
## events
| docker/docker-py | 24bfb99e05d57a7a098a81fb86ea7b93cff62661 | diff --git a/tests/integration/network_test.py b/tests/integration/network_test.py
index 27e1b14d..6726db4b 100644
--- a/tests/integration/network_test.py
+++ b/tests/integration/network_test.py
@@ -115,7 +115,8 @@ class TestNetworks(helpers.BaseTestCase):
network_data = self.client.inspect_network(net_id)
self.assertEqual(
list(network_data['Containers'].keys()),
- [container['Id']])
+ [container['Id']]
+ )
with pytest.raises(docker.errors.APIError):
self.client.connect_container_to_network(container, net_id)
@@ -127,6 +128,33 @@ class TestNetworks(helpers.BaseTestCase):
with pytest.raises(docker.errors.APIError):
self.client.disconnect_container_from_network(container, net_id)
+ @requires_api_version('1.22')
+ def test_connect_and_force_disconnect_container(self):
+ net_name, net_id = self.create_network()
+
+ container = self.client.create_container('busybox', 'top')
+ self.tmp_containers.append(container)
+ self.client.start(container)
+
+ network_data = self.client.inspect_network(net_id)
+ self.assertFalse(network_data.get('Containers'))
+
+ self.client.connect_container_to_network(container, net_id)
+ network_data = self.client.inspect_network(net_id)
+ self.assertEqual(
+ list(network_data['Containers'].keys()),
+ [container['Id']]
+ )
+
+ self.client.disconnect_container_from_network(container, net_id, True)
+ network_data = self.client.inspect_network(net_id)
+ self.assertFalse(network_data.get('Containers'))
+
+ with pytest.raises(docker.errors.APIError):
+ self.client.disconnect_container_from_network(
+ container, net_id, force=True
+ )
+
@requires_api_version('1.22')
def test_connect_with_aliases(self):
net_name, net_id = self.create_network()
@@ -300,7 +328,8 @@ class TestNetworks(helpers.BaseTestCase):
net_name, net_id = self.create_network()
with self.assertRaises(docker.errors.APIError):
self.client.create_network(net_name, check_duplicate=True)
- self.client.create_network(net_name, check_duplicate=False)
+ net_id = self.client.create_network(net_name, check_duplicate=False)
+ self.tmp_networks.append(net_id['Id'])
@requires_api_version('1.22')
def test_connect_with_links(self):
@@ -387,3 +416,27 @@ class TestNetworks(helpers.BaseTestCase):
_, net_id = self.create_network(internal=True)
net = self.client.inspect_network(net_id)
assert net['Internal'] is True
+
+ @requires_api_version('1.23')
+ def test_create_network_with_labels(self):
+ _, net_id = self.create_network(labels={
+ 'com.docker.py.test': 'label'
+ })
+
+ net = self.client.inspect_network(net_id)
+ assert 'Labels' in net
+ assert len(net['Labels']) == 1
+ assert net['Labels'] == {
+ 'com.docker.py.test': 'label'
+ }
+
+ @requires_api_version('1.23')
+ def test_create_network_with_labels_wrong_type(self):
+ with pytest.raises(TypeError):
+ self.create_network(labels=['com.docker.py.test=label', ])
+
+ @requires_api_version('1.23')
+ def test_create_network_ipv6_enabled(self):
+ _, net_id = self.create_network(enable_ipv6=True)
+ net = self.client.inspect_network(net_id)
+ assert net['EnableIPv6'] is True
diff --git a/tests/unit/network_test.py b/tests/unit/network_test.py
index 5bba9db2..2521688d 100644
--- a/tests/unit/network_test.py
+++ b/tests/unit/network_test.py
@@ -184,4 +184,4 @@ class NetworkTest(DockerClientTest):
self.assertEqual(
json.loads(post.call_args[1]['data']),
- {'container': container_id})
+ {'Container': container_id})
| Support create network EnableIPv6 and Labels options
Check the remote API:
https://docs.docker.com/engine/reference/api/docker_remote_api_v1.23/#create-a-network
There are two missing JSON parameters:
```
EnableIPv6 - Enable IPv6 on the network
Labels - Labels to set on the network, specified as a map: {"key":"value" [,"key2":"value2"]}
``` | 0.0 | [
"tests/unit/network_test.py::NetworkTest::test_disconnect_container_from_network"
] | [
"tests/unit/network_test.py::NetworkTest::test_connect_container_to_network",
"tests/unit/network_test.py::NetworkTest::test_create_network",
"tests/unit/network_test.py::NetworkTest::test_inspect_network",
"tests/unit/network_test.py::NetworkTest::test_list_networks",
"tests/unit/network_test.py::NetworkTest::test_remove_network"
] | 2016-09-01 01:42:42+00:00 | 1,962 |
|
docker__docker-py-1250 | diff --git a/Dockerfile b/Dockerfile
index 012a1259..993ac012 100644
--- a/Dockerfile
+++ b/Dockerfile
@@ -1,5 +1,4 @@
FROM python:2.7
-MAINTAINER Joffrey F <joffrey@docker.com>
RUN mkdir /home/docker-py
WORKDIR /home/docker-py
diff --git a/Dockerfile-py3 b/Dockerfile-py3
index 21e713bb..c7466517 100644
--- a/Dockerfile-py3
+++ b/Dockerfile-py3
@@ -1,5 +1,4 @@
FROM python:3.5
-MAINTAINER Joffrey F <joffrey@docker.com>
RUN mkdir /home/docker-py
WORKDIR /home/docker-py
diff --git a/docker/api/image.py b/docker/api/image.py
index 2c8cbb23..c1ebc69c 100644
--- a/docker/api/image.py
+++ b/docker/api/image.py
@@ -469,6 +469,11 @@ class ImageApiMixin(object):
Raises:
:py:class:`docker.errors.APIError`
If the server returns an error.
+
+ Example:
+
+ >>> client.tag('ubuntu', 'localhost:5000/ubuntu', 'latest',
+ force=True)
"""
params = {
'tag': tag,
diff --git a/docker/utils/socket.py b/docker/utils/socket.py
index 164b845a..4080f253 100644
--- a/docker/utils/socket.py
+++ b/docker/utils/socket.py
@@ -69,7 +69,11 @@ def frames_iter(socket):
"""
Returns a generator of frames read from socket
"""
- n = next_frame_size(socket)
- while n > 0:
- yield read(socket, n)
+ while True:
n = next_frame_size(socket)
+ if n == 0:
+ break
+ while n > 0:
+ result = read(socket, n)
+ n -= len(result)
+ yield result
diff --git a/docker/utils/utils.py b/docker/utils/utils.py
index b107f22e..823894c3 100644
--- a/docker/utils/utils.py
+++ b/docker/utils/utils.py
@@ -735,9 +735,9 @@ def create_host_config(binds=None, port_bindings=None, lxc_conf=None,
host_config['ShmSize'] = shm_size
- if pid_mode not in (None, 'host'):
- raise host_config_value_error('pid_mode', pid_mode)
- elif pid_mode:
+ if pid_mode:
+ if version_lt(version, '1.24') and pid_mode != 'host':
+ raise host_config_value_error('pid_mode', pid_mode)
host_config['PidMode'] = pid_mode
if ipc_mode:
@@ -1052,7 +1052,11 @@ def parse_env_file(env_file):
if line[0] == '#':
continue
- parse_line = line.strip().split('=', 1)
+ line = line.strip()
+ if not line:
+ continue
+
+ parse_line = line.split('=', 1)
if len(parse_line) == 2:
k, v = parse_line
environment[k] = v
diff --git a/setup.py b/setup.py
index edf4b0e5..3f2e3c4a 100644
--- a/setup.py
+++ b/setup.py
@@ -1,4 +1,5 @@
#!/usr/bin/env python
+import codecs
import os
import sys
@@ -35,7 +36,7 @@ with open('./test-requirements.txt') as test_reqs_txt:
long_description = ''
try:
- with open('./README.rst') as readme_rst:
+ with codecs.open('./README.rst', encoding='utf-8') as readme_rst:
long_description = readme_rst.read()
except IOError:
# README.rst is only generated on release. Its absence should not prevent
| docker/docker-py | f36c28926cf9d0e3bfe0f2275c084d63cd3c6169 | diff --git a/tests/integration/api_build_test.py b/tests/integration/api_build_test.py
index 9ae74f4d..3dac0e93 100644
--- a/tests/integration/api_build_test.py
+++ b/tests/integration/api_build_test.py
@@ -15,7 +15,6 @@ class BuildTest(BaseAPIIntegrationTest):
def test_build_streaming(self):
script = io.BytesIO('\n'.join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
@@ -32,7 +31,6 @@ class BuildTest(BaseAPIIntegrationTest):
return
script = io.StringIO(six.text_type('\n').join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
@@ -54,7 +52,6 @@ class BuildTest(BaseAPIIntegrationTest):
with open(os.path.join(base_dir, 'Dockerfile'), 'w') as f:
f.write("\n".join([
'FROM busybox',
- 'MAINTAINER docker-py',
'ADD . /test',
]))
@@ -182,7 +179,6 @@ class BuildTest(BaseAPIIntegrationTest):
with open(os.path.join(base_dir, 'Dockerfile'), 'w') as f:
f.write("\n".join([
'FROM busybox',
- 'MAINTAINER docker-py',
'ADD . /test',
]))
diff --git a/tests/integration/api_container_test.py b/tests/integration/api_container_test.py
index a5be6e76..f09e75ad 100644
--- a/tests/integration/api_container_test.py
+++ b/tests/integration/api_container_test.py
@@ -361,13 +361,6 @@ class CreateContainerTest(BaseAPIIntegrationTest):
host_config = inspect['HostConfig']
self.assertIn('MemorySwappiness', host_config)
- def test_create_host_config_exception_raising(self):
- self.assertRaises(TypeError,
- self.client.create_host_config, mem_swappiness='40')
-
- self.assertRaises(ValueError,
- self.client.create_host_config, pid_mode='40')
-
def test_create_with_environment_variable_no_value(self):
container = self.client.create_container(
BUSYBOX,
diff --git a/tests/unit/api_build_test.py b/tests/unit/api_build_test.py
index 8146fee7..927aa974 100644
--- a/tests/unit/api_build_test.py
+++ b/tests/unit/api_build_test.py
@@ -11,7 +11,6 @@ class BuildTest(BaseAPIClientTest):
def test_build_container(self):
script = io.BytesIO('\n'.join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
@@ -23,7 +22,6 @@ class BuildTest(BaseAPIClientTest):
def test_build_container_pull(self):
script = io.BytesIO('\n'.join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
@@ -35,7 +33,6 @@ class BuildTest(BaseAPIClientTest):
def test_build_container_stream(self):
script = io.BytesIO('\n'.join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
@@ -47,7 +44,6 @@ class BuildTest(BaseAPIClientTest):
def test_build_container_custom_context(self):
script = io.BytesIO('\n'.join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
@@ -60,7 +56,6 @@ class BuildTest(BaseAPIClientTest):
def test_build_container_custom_context_gzip(self):
script = io.BytesIO('\n'.join([
'FROM busybox',
- 'MAINTAINER docker-py',
'RUN mkdir -p /tmp/test',
'EXPOSE 8080',
'ADD https://dl.dropboxusercontent.com/u/20637798/silence.tar.gz'
diff --git a/tests/unit/utils_test.py b/tests/unit/utils_test.py
index 57aa226d..19d52c9f 100644
--- a/tests/unit/utils_test.py
+++ b/tests/unit/utils_test.py
@@ -205,6 +205,19 @@ class HostConfigTest(unittest.TestCase):
version='1.24', isolation={'isolation': 'hyperv'}
)
+ def test_create_host_config_pid_mode(self):
+ with pytest.raises(ValueError):
+ create_host_config(version='1.23', pid_mode='baccab125')
+
+ config = create_host_config(version='1.23', pid_mode='host')
+ assert config.get('PidMode') == 'host'
+ config = create_host_config(version='1.24', pid_mode='baccab125')
+ assert config.get('PidMode') == 'baccab125'
+
+ def test_create_host_config_invalid_mem_swappiness(self):
+ with pytest.raises(TypeError):
+ create_host_config(version='1.24', mem_swappiness='40')
+
class UlimitTest(unittest.TestCase):
def test_create_host_config_dict_ulimit(self):
@@ -465,10 +478,18 @@ class ParseEnvFileTest(unittest.TestCase):
def test_parse_env_file_commented_line(self):
env_file = self.generate_tempfile(
file_content='USER=jdoe\n#PASS=secret')
- get_parse_env_file = parse_env_file((env_file))
+ get_parse_env_file = parse_env_file(env_file)
self.assertEqual(get_parse_env_file, {'USER': 'jdoe'})
os.unlink(env_file)
+ def test_parse_env_file_newline(self):
+ env_file = self.generate_tempfile(
+ file_content='\nUSER=jdoe\n\n\nPASS=secret')
+ get_parse_env_file = parse_env_file(env_file)
+ self.assertEqual(get_parse_env_file,
+ {'USER': 'jdoe', 'PASS': 'secret'})
+ os.unlink(env_file)
+
def test_parse_env_file_invalid_line(self):
env_file = self.generate_tempfile(
file_content='USER jdoe')
| attach is causing an "Invalid Argument" exception from os.read
``` python
stream = client.attach(container, stream=True, stdout=True, stderr=True)
for chunk in stream:
pass
```
Results in:
```
File "/Users/michael/work/oss/marina/marina/build.py", line 695, in watcher
for chunk in stream:
File ".venv/lib/python3.5/site-packages/docker/utils/socket.py", line 67, in frames_iter
yield read(socket, n)
File ".venv/lib/python3.5/site-packages/docker/utils/socket.py", line 25, in read
return os.read(socket.fileno(), n)
OSError: [Errno 22] Invalid argument
```
Using docker-py 1.10.2 on OS X 10.11.6 with docker for mac 1.12.0-rc3. Reverting to 1.9.0 fixes the issue.
| 0.0 | [
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_pid_mode",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_newline"
] | [
"tests/unit/api_build_test.py::BuildTest::test_build_container",
"tests/unit/api_build_test.py::BuildTest::test_build_container_custom_context",
"tests/unit/api_build_test.py::BuildTest::test_build_container_custom_context_gzip",
"tests/unit/api_build_test.py::BuildTest::test_build_container_invalid_container_limits",
"tests/unit/api_build_test.py::BuildTest::test_build_container_pull",
"tests/unit/api_build_test.py::BuildTest::test_build_container_stream",
"tests/unit/api_build_test.py::BuildTest::test_build_container_with_container_limits",
"tests/unit/api_build_test.py::BuildTest::test_build_container_with_named_dockerfile",
"tests/unit/api_build_test.py::BuildTest::test_build_remote_with_registry_auth",
"tests/unit/api_build_test.py::BuildTest::test_set_auth_headers_with_dict_and_auth_configs",
"tests/unit/api_build_test.py::BuildTest::test_set_auth_headers_with_dict_and_no_auth_configs",
"tests/unit/api_build_test.py::BuildTest::test_set_auth_headers_with_empty_dict_and_auth_configs",
"tests/unit/utils_test.py::DecoratorsTest::test_update_headers",
"tests/unit/utils_test.py::HostConfigTest::test_create_endpoint_config_with_aliases",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_invalid_cpu_cfs_types",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_invalid_mem_swappiness",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options_newer_api_version",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_blkio_constraints",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_period",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_quota",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_dns_opt",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_isolation",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_kernel_memory",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_mem_reservation",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_kill_disable",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_score_adj",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_pids_limit",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size_in_mb",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_userns_mode",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit_capitals",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_obj_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_ulimit_invalid_type",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_dict_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_obj_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_logconfig_invalid_config_type",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_alternate_env",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_empty",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_no_cert_path",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false_no_cert",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_compact",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_complete",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_empty",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_list",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_no_mode",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_bytes_input",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_unicode_input",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_commented_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_invalid_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_proper",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_with_equals_character",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_empty_value",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls_tcp_proto",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_trailing_slash",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_tag",
"tests/unit/utils_test.py::ParseDeviceTest::test_dict",
"tests/unit/utils_test.py::ParseDeviceTest::test_full_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_hybrid_list",
"tests/unit/utils_test.py::ParseDeviceTest::test_partial_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_permissionless_string_definition",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_float",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_invalid",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_maxint",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_valid",
"tests/unit/utils_test.py::UtilsTest::test_convert_filters",
"tests/unit/utils_test.py::UtilsTest::test_create_ipam_config",
"tests/unit/utils_test.py::UtilsTest::test_decode_json_header",
"tests/unit/utils_test.py::SplitCommandTest::test_split_command_with_unicode",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_one_port",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_port_range",
"tests/unit/utils_test.py::PortsTest::test_host_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_non_matching_length_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_port_and_range_invalid",
"tests/unit/utils_test.py::PortsTest::test_port_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_split_port_invalid",
"tests/unit/utils_test.py::PortsTest::test_split_port_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_protocol",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_protocol",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_single_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_subdir_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_wildcard_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_custom_dockerfile",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_child",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_dockerignore",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_dupes",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_excludes",
"tests/unit/utils_test.py::ExcludePathsTest::test_question_mark",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_leading_dot_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_with_path_traversal",
"tests/unit/utils_test.py::ExcludePathsTest::test_subdirectory",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_exclude",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_end",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_start",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_wildcard_exception",
"tests/unit/utils_test.py::TarTest::test_tar_with_directory_symlinks",
"tests/unit/utils_test.py::TarTest::test_tar_with_empty_directory",
"tests/unit/utils_test.py::TarTest::test_tar_with_excludes",
"tests/unit/utils_test.py::TarTest::test_tar_with_file_symlinks",
"tests/unit/utils_test.py::FormatEnvironmentTest::test_format_env_binary_unicode_value",
"tests/unit/utils_test.py::FormatEnvironmentTest::test_format_env_no_value"
] | 2016-10-11 07:42:57+00:00 | 1,963 |
|
docker__docker-py-1255 | diff --git a/docker/api/image.py b/docker/api/image.py
index 7f25f9d9..262910cd 100644
--- a/docker/api/image.py
+++ b/docker/api/image.py
@@ -88,9 +88,6 @@ class ImageApiMixin(object):
u, data=data, params=params, headers=headers, timeout=None
)
)
- return self.import_image(
- src=data, repository=repository, tag=tag, changes=changes
- )
def import_image_from_file(self, filename, repository=None, tag=None,
changes=None):
diff --git a/docker/utils/utils.py b/docker/utils/utils.py
index b565732d..e1c7ad0c 100644
--- a/docker/utils/utils.py
+++ b/docker/utils/utils.py
@@ -453,8 +453,8 @@ def parse_host(addr, is_win32=False, tls=False):
"Bind address needs a port: {0}".format(addr))
if proto == "http+unix" or proto == 'npipe':
- return "{0}://{1}".format(proto, host)
- return "{0}://{1}:{2}{3}".format(proto, host, port, path)
+ return "{0}://{1}".format(proto, host).rstrip('/')
+ return "{0}://{1}:{2}{3}".format(proto, host, port, path).rstrip('/')
def parse_devices(devices):
| docker/docker-py | 008730c670afb2f88c7db308901586fb24f1a60c | diff --git a/tests/unit/utils_test.py b/tests/unit/utils_test.py
index 2a2759d0..059c82d3 100644
--- a/tests/unit/utils_test.py
+++ b/tests/unit/utils_test.py
@@ -522,6 +522,11 @@ class ParseHostTest(base.BaseTestCase):
expected_result = 'https://myhost.docker.net:3348'
assert parse_host(host_value, tls=True) == expected_result
+ def test_parse_host_trailing_slash(self):
+ host_value = 'tcp://myhost.docker.net:2376/'
+ expected_result = 'http://myhost.docker.net:2376'
+ assert parse_host(host_value) == expected_result
+
class ParseRepositoryTagTest(base.BaseTestCase):
sha = 'e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855'
| Client should tolerate trailing slashes in base_url
docker/compose#3869 | 0.0 | [
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_trailing_slash"
] | [
"tests/unit/utils_test.py::DecoratorsTest::test_update_headers",
"tests/unit/utils_test.py::HostConfigTest::test_create_endpoint_config_with_aliases",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_invalid_cpu_cfs_types",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_no_options_newer_api_version",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_blkio_constraints",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_period",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_cpu_quota",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_dns_opt",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_kernel_memory",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_mem_reservation",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_kill_disable",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_oom_score_adj",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_pids_limit",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_shm_size_in_mb",
"tests/unit/utils_test.py::HostConfigTest::test_create_host_config_with_userns_mode",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_dict_ulimit_capitals",
"tests/unit/utils_test.py::UlimitTest::test_create_host_config_obj_ulimit",
"tests/unit/utils_test.py::UlimitTest::test_ulimit_invalid_type",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_dict_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_create_host_config_obj_logconfig",
"tests/unit/utils_test.py::LogConfigTest::test_logconfig_invalid_config_type",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_alternate_env",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_empty",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_no_cert_path",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false_no_cert",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_compact",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_complete",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_empty",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_list",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_no_mode",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_bytes_input",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_unicode_input",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_commented_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_invalid_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_proper",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_with_equals_character",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_empty_value",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls_tcp_proto",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_tag",
"tests/unit/utils_test.py::ParseDeviceTest::test_dict",
"tests/unit/utils_test.py::ParseDeviceTest::test_full_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_hybrid_list",
"tests/unit/utils_test.py::ParseDeviceTest::test_partial_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_permissionless_string_definition",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_float",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_invalid",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_maxint",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_valid",
"tests/unit/utils_test.py::UtilsTest::test_convert_filters",
"tests/unit/utils_test.py::UtilsTest::test_create_ipam_config",
"tests/unit/utils_test.py::UtilsTest::test_decode_json_header",
"tests/unit/utils_test.py::SplitCommandTest::test_split_command_with_unicode",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_one_port",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_port_range",
"tests/unit/utils_test.py::PortsTest::test_host_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_non_matching_length_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_port_and_range_invalid",
"tests/unit/utils_test.py::PortsTest::test_port_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_split_port_invalid",
"tests/unit/utils_test.py::PortsTest::test_split_port_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_protocol",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_protocol",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_single_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_subdir_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_wildcard_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_custom_dockerfile",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_child",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_dockerignore",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_dupes",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_excludes",
"tests/unit/utils_test.py::ExcludePathsTest::test_question_mark",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_leading_dot_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_with_path_traversal",
"tests/unit/utils_test.py::ExcludePathsTest::test_subdirectory",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_exclude",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_end",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_start",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_wildcard_exception",
"tests/unit/utils_test.py::TarTest::test_tar_with_directory_symlinks",
"tests/unit/utils_test.py::TarTest::test_tar_with_empty_directory",
"tests/unit/utils_test.py::TarTest::test_tar_with_excludes",
"tests/unit/utils_test.py::TarTest::test_tar_with_file_symlinks"
] | 2016-10-12 23:07:38+00:00 | 1,964 |
|
docker__docker-py-1385 | diff --git a/docker/types/services.py b/docker/types/services.py
index b52afd27..93503dc0 100644
--- a/docker/types/services.py
+++ b/docker/types/services.py
@@ -1,6 +1,7 @@
import six
from .. import errors
+from ..constants import IS_WINDOWS_PLATFORM
from ..utils import format_environment, split_command
@@ -175,8 +176,17 @@ class Mount(dict):
else:
target = parts[1]
source = parts[0]
+ mount_type = 'volume'
+ if source.startswith('/') or (
+ IS_WINDOWS_PLATFORM and source[0].isalpha() and
+ source[1] == ':'
+ ):
+ # FIXME: That windows condition will fail earlier since we
+ # split on ':'. We should look into doing a smarter split
+ # if we detect we are on Windows.
+ mount_type = 'bind'
read_only = not (len(parts) == 2 or parts[2] == 'rw')
- return cls(target, source, read_only=read_only)
+ return cls(target, source, read_only=read_only, type=mount_type)
class Resources(dict):
| docker/docker-py | 07b20ce660f4a4b1e64ef3ede346eef9ec08635a | diff --git a/tests/unit/dockertypes_test.py b/tests/unit/dockertypes_test.py
index 5cf5f4e7..d11e4f03 100644
--- a/tests/unit/dockertypes_test.py
+++ b/tests/unit/dockertypes_test.py
@@ -10,6 +10,11 @@ from docker.types import (
EndpointConfig, HostConfig, IPAMConfig, IPAMPool, LogConfig, Mount, Ulimit,
)
+try:
+ from unittest import mock
+except:
+ import mock
+
def create_host_config(*args, **kwargs):
return HostConfig(*args, **kwargs)
@@ -258,28 +263,48 @@ class IPAMConfigTest(unittest.TestCase):
class TestMounts(unittest.TestCase):
def test_parse_mount_string_ro(self):
mount = Mount.parse_mount_string("/foo/bar:/baz:ro")
- self.assertEqual(mount['Source'], "/foo/bar")
- self.assertEqual(mount['Target'], "/baz")
- self.assertEqual(mount['ReadOnly'], True)
+ assert mount['Source'] == "/foo/bar"
+ assert mount['Target'] == "/baz"
+ assert mount['ReadOnly'] is True
def test_parse_mount_string_rw(self):
mount = Mount.parse_mount_string("/foo/bar:/baz:rw")
- self.assertEqual(mount['Source'], "/foo/bar")
- self.assertEqual(mount['Target'], "/baz")
- self.assertEqual(mount['ReadOnly'], False)
+ assert mount['Source'] == "/foo/bar"
+ assert mount['Target'] == "/baz"
+ assert not mount['ReadOnly']
def test_parse_mount_string_short_form(self):
mount = Mount.parse_mount_string("/foo/bar:/baz")
- self.assertEqual(mount['Source'], "/foo/bar")
- self.assertEqual(mount['Target'], "/baz")
- self.assertEqual(mount['ReadOnly'], False)
+ assert mount['Source'] == "/foo/bar"
+ assert mount['Target'] == "/baz"
+ assert not mount['ReadOnly']
def test_parse_mount_string_no_source(self):
mount = Mount.parse_mount_string("foo/bar")
- self.assertEqual(mount['Source'], None)
- self.assertEqual(mount['Target'], "foo/bar")
- self.assertEqual(mount['ReadOnly'], False)
+ assert mount['Source'] is None
+ assert mount['Target'] == "foo/bar"
+ assert not mount['ReadOnly']
def test_parse_mount_string_invalid(self):
with pytest.raises(InvalidArgument):
Mount.parse_mount_string("foo:bar:baz:rw")
+
+ def test_parse_mount_named_volume(self):
+ mount = Mount.parse_mount_string("foobar:/baz")
+ assert mount['Source'] == 'foobar'
+ assert mount['Target'] == '/baz'
+ assert mount['Type'] == 'volume'
+
+ def test_parse_mount_bind(self):
+ mount = Mount.parse_mount_string('/foo/bar:/baz')
+ assert mount['Source'] == "/foo/bar"
+ assert mount['Target'] == "/baz"
+ assert mount['Type'] == 'bind'
+
+ @pytest.mark.xfail
+ def test_parse_mount_bind_windows(self):
+ with mock.patch('docker.types.services.IS_WINDOWS_PLATFORM', True):
+ mount = Mount.parse_mount_string('C:/foo/bar:/baz')
+ assert mount['Source'] == "C:/foo/bar"
+ assert mount['Target'] == "/baz"
+ assert mount['Type'] == 'bind'
| swarm mode create service does not support volumn bind type?
hi all, i use docker py create service catch some error. my docker py version is
```
root@node-19:~# pip freeze | grep docker
docker==2.0.0
docker-compose==1.9.0
docker-pycreds==0.2.1
dockerpty==0.4.1
```
my docker version is
```
Client:
Version: 1.12.5
API version: 1.24
Go version: go1.6.4
Git commit: 7392c3b
Built: Fri Dec 16 02:30:42 2016
OS/Arch: linux/amd64
Server:
Version: 1.12.5
API version: 1.24
Go version: go1.6.4
Git commit: 7392c3b
Built: Fri Dec 16 02:30:42 2016
OS/Arch: linux/amd64
```
my use docker py create service code is
```python
import docker
client = docker.DockerClient(base_url='unix://var/run/docker.sock')
client.services.create(name="bab", command=["sleep", "30000"], image="busybox", mounts=["/data:/data:rw"])
```
look my create service code i just wand to create service and volumn local host dir to container, and dir path is absolute path. but i got some error like this
`
invalid volume mount source, must not be an absolute path: /data`
so i check docker py code find this
```python
def __init__(self, target, source, type='volume', read_only=False,
propagation=None, no_copy=False, labels=None,
driver_config=None):
self['Target'] = target
self['Source'] = source
if type not in ('bind', 'volume'):
raise errors.DockerError(
'Only acceptable mount types are `bind` and `volume`.'
)
self['Type'] = type
```
so i want to know what can i create service with volumn bind type . i need help. | 0.0 | [
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_bind"
] | [
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_invalid_cpu_cfs_types",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_invalid_mem_swappiness",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_no_options",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_no_options_newer_api_version",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_pid_mode",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_blkio_constraints",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_cpu_period",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_cpu_quota",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_dns_opt",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_isolation",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_kernel_memory",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_mem_reservation",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_oom_kill_disable",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_oom_score_adj",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_pids_limit",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_shm_size",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_shm_size_in_mb",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_userns_mode",
"tests/unit/dockertypes_test.py::UlimitTest::test_create_host_config_dict_ulimit",
"tests/unit/dockertypes_test.py::UlimitTest::test_create_host_config_dict_ulimit_capitals",
"tests/unit/dockertypes_test.py::UlimitTest::test_create_host_config_obj_ulimit",
"tests/unit/dockertypes_test.py::UlimitTest::test_ulimit_invalid_type",
"tests/unit/dockertypes_test.py::LogConfigTest::test_create_host_config_dict_logconfig",
"tests/unit/dockertypes_test.py::LogConfigTest::test_create_host_config_obj_logconfig",
"tests/unit/dockertypes_test.py::LogConfigTest::test_logconfig_invalid_config_type",
"tests/unit/dockertypes_test.py::EndpointConfigTest::test_create_endpoint_config_with_aliases",
"tests/unit/dockertypes_test.py::IPAMConfigTest::test_create_ipam_config",
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_named_volume",
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_string_invalid",
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_string_no_source",
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_string_ro",
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_string_rw",
"tests/unit/dockertypes_test.py::TestMounts::test_parse_mount_string_short_form"
] | 2017-01-09 23:14:24+00:00 | 1,965 |
|
docker__docker-py-1390 | diff --git a/docker/api/service.py b/docker/api/service.py
index 0d8421ec..d2621e68 100644
--- a/docker/api/service.py
+++ b/docker/api/service.py
@@ -1,5 +1,6 @@
import warnings
from .. import auth, errors, utils
+from ..types import ServiceMode
class ServiceApiMixin(object):
@@ -18,8 +19,8 @@ class ServiceApiMixin(object):
name (string): User-defined name for the service. Optional.
labels (dict): A map of labels to associate with the service.
Optional.
- mode (string): Scheduling mode for the service (``replicated`` or
- ``global``). Defaults to ``replicated``.
+ mode (ServiceMode): Scheduling mode for the service (replicated
+ or global). Defaults to replicated.
update_config (UpdateConfig): Specification for the update strategy
of the service. Default: ``None``
networks (:py:class:`list`): List of network names or IDs to attach
@@ -49,6 +50,9 @@ class ServiceApiMixin(object):
raise errors.DockerException(
'Missing mandatory Image key in ContainerSpec'
)
+ if mode and not isinstance(mode, dict):
+ mode = ServiceMode(mode)
+
registry, repo_name = auth.resolve_repository_name(image)
auth_header = auth.get_config_header(self, registry)
if auth_header:
@@ -191,8 +195,8 @@ class ServiceApiMixin(object):
name (string): New name for the service. Optional.
labels (dict): A map of labels to associate with the service.
Optional.
- mode (string): Scheduling mode for the service (``replicated`` or
- ``global``). Defaults to ``replicated``.
+ mode (ServiceMode): Scheduling mode for the service (replicated
+ or global). Defaults to replicated.
update_config (UpdateConfig): Specification for the update strategy
of the service. Default: ``None``.
networks (:py:class:`list`): List of network names or IDs to attach
@@ -222,6 +226,8 @@ class ServiceApiMixin(object):
if labels is not None:
data['Labels'] = labels
if mode is not None:
+ if not isinstance(mode, dict):
+ mode = ServiceMode(mode)
data['Mode'] = mode
if task_template is not None:
image = task_template.get('ContainerSpec', {}).get('Image', None)
diff --git a/docker/types/__init__.py b/docker/types/__init__.py
index 7230723e..8e2fc174 100644
--- a/docker/types/__init__.py
+++ b/docker/types/__init__.py
@@ -4,6 +4,6 @@ from .healthcheck import Healthcheck
from .networks import EndpointConfig, IPAMConfig, IPAMPool, NetworkingConfig
from .services import (
ContainerSpec, DriverConfig, EndpointSpec, Mount, Resources, RestartPolicy,
- TaskTemplate, UpdateConfig
+ ServiceMode, TaskTemplate, UpdateConfig
)
from .swarm import SwarmSpec, SwarmExternalCA
diff --git a/docker/types/services.py b/docker/types/services.py
index 6e1ad321..ec0fcb15 100644
--- a/docker/types/services.py
+++ b/docker/types/services.py
@@ -348,3 +348,38 @@ def convert_service_ports(ports):
result.append(port_spec)
return result
+
+
+class ServiceMode(dict):
+ """
+ Indicate whether a service should be deployed as a replicated or global
+ service, and associated parameters
+
+ Args:
+ mode (string): Can be either ``replicated`` or ``global``
+ replicas (int): Number of replicas. For replicated services only.
+ """
+ def __init__(self, mode, replicas=None):
+ if mode not in ('replicated', 'global'):
+ raise errors.InvalidArgument(
+ 'mode must be either "replicated" or "global"'
+ )
+ if mode != 'replicated' and replicas is not None:
+ raise errors.InvalidArgument(
+ 'replicas can only be used for replicated mode'
+ )
+ self[mode] = {}
+ if replicas:
+ self[mode]['Replicas'] = replicas
+
+ @property
+ def mode(self):
+ if 'global' in self:
+ return 'global'
+ return 'replicated'
+
+ @property
+ def replicas(self):
+ if self.mode != 'replicated':
+ return None
+ return self['replicated'].get('Replicas')
diff --git a/docs/api.rst b/docs/api.rst
index 110b0a7f..b5c1e929 100644
--- a/docs/api.rst
+++ b/docs/api.rst
@@ -110,5 +110,6 @@ Configuration types
.. autoclass:: Mount
.. autoclass:: Resources
.. autoclass:: RestartPolicy
+.. autoclass:: ServiceMode
.. autoclass:: TaskTemplate
.. autoclass:: UpdateConfig
| docker/docker-py | 5f0b469a09421b0d6140661de9466af74ac3e9ec | diff --git a/tests/integration/api_service_test.py b/tests/integration/api_service_test.py
index fc794002..77d7d28f 100644
--- a/tests/integration/api_service_test.py
+++ b/tests/integration/api_service_test.py
@@ -251,3 +251,31 @@ class ServiceTest(BaseAPIIntegrationTest):
con_spec = svc_info['Spec']['TaskTemplate']['ContainerSpec']
assert 'Env' in con_spec
assert con_spec['Env'] == ['DOCKER_PY_TEST=1']
+
+ def test_create_service_global_mode(self):
+ container_spec = docker.types.ContainerSpec(
+ 'busybox', ['echo', 'hello']
+ )
+ task_tmpl = docker.types.TaskTemplate(container_spec)
+ name = self.get_service_name()
+ svc_id = self.client.create_service(
+ task_tmpl, name=name, mode='global'
+ )
+ svc_info = self.client.inspect_service(svc_id)
+ assert 'Mode' in svc_info['Spec']
+ assert 'Global' in svc_info['Spec']['Mode']
+
+ def test_create_service_replicated_mode(self):
+ container_spec = docker.types.ContainerSpec(
+ 'busybox', ['echo', 'hello']
+ )
+ task_tmpl = docker.types.TaskTemplate(container_spec)
+ name = self.get_service_name()
+ svc_id = self.client.create_service(
+ task_tmpl, name=name,
+ mode=docker.types.ServiceMode('replicated', 5)
+ )
+ svc_info = self.client.inspect_service(svc_id)
+ assert 'Mode' in svc_info['Spec']
+ assert 'Replicated' in svc_info['Spec']['Mode']
+ assert svc_info['Spec']['Mode']['Replicated'] == {'Replicas': 5}
diff --git a/tests/unit/dockertypes_test.py b/tests/unit/dockertypes_test.py
index d11e4f03..5c470ffa 100644
--- a/tests/unit/dockertypes_test.py
+++ b/tests/unit/dockertypes_test.py
@@ -7,7 +7,8 @@ import pytest
from docker.constants import DEFAULT_DOCKER_API_VERSION
from docker.errors import InvalidArgument, InvalidVersion
from docker.types import (
- EndpointConfig, HostConfig, IPAMConfig, IPAMPool, LogConfig, Mount, Ulimit,
+ EndpointConfig, HostConfig, IPAMConfig, IPAMPool, LogConfig, Mount,
+ ServiceMode, Ulimit,
)
try:
@@ -260,7 +261,35 @@ class IPAMConfigTest(unittest.TestCase):
})
-class TestMounts(unittest.TestCase):
+class ServiceModeTest(unittest.TestCase):
+ def test_replicated_simple(self):
+ mode = ServiceMode('replicated')
+ assert mode == {'replicated': {}}
+ assert mode.mode == 'replicated'
+ assert mode.replicas is None
+
+ def test_global_simple(self):
+ mode = ServiceMode('global')
+ assert mode == {'global': {}}
+ assert mode.mode == 'global'
+ assert mode.replicas is None
+
+ def test_global_replicas_error(self):
+ with pytest.raises(InvalidArgument):
+ ServiceMode('global', 21)
+
+ def test_replicated_replicas(self):
+ mode = ServiceMode('replicated', 21)
+ assert mode == {'replicated': {'Replicas': 21}}
+ assert mode.mode == 'replicated'
+ assert mode.replicas == 21
+
+ def test_invalid_mode(self):
+ with pytest.raises(InvalidArgument):
+ ServiceMode('foobar')
+
+
+class MountTest(unittest.TestCase):
def test_parse_mount_string_ro(self):
mount = Mount.parse_mount_string("/foo/bar:/baz:ro")
assert mount['Source'] == "/foo/bar"
| Unable to create Service in `global` mode
Attempting to create a service with `mode="global"` returns an error from the Docker API:
```
cannot unmarshal string into Go value of type swarm.ServiceMode
``` | 0.0 | [
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_invalid_cpu_cfs_types",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_invalid_mem_swappiness",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_no_options",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_no_options_newer_api_version",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_pid_mode",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_blkio_constraints",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_cpu_period",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_cpu_quota",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_dns_opt",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_isolation",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_kernel_memory",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_mem_reservation",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_oom_kill_disable",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_oom_score_adj",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_pids_limit",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_shm_size",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_shm_size_in_mb",
"tests/unit/dockertypes_test.py::HostConfigTest::test_create_host_config_with_userns_mode",
"tests/unit/dockertypes_test.py::UlimitTest::test_create_host_config_dict_ulimit",
"tests/unit/dockertypes_test.py::UlimitTest::test_create_host_config_dict_ulimit_capitals",
"tests/unit/dockertypes_test.py::UlimitTest::test_create_host_config_obj_ulimit",
"tests/unit/dockertypes_test.py::UlimitTest::test_ulimit_invalid_type",
"tests/unit/dockertypes_test.py::LogConfigTest::test_create_host_config_dict_logconfig",
"tests/unit/dockertypes_test.py::LogConfigTest::test_create_host_config_obj_logconfig",
"tests/unit/dockertypes_test.py::LogConfigTest::test_logconfig_invalid_config_type",
"tests/unit/dockertypes_test.py::EndpointConfigTest::test_create_endpoint_config_with_aliases",
"tests/unit/dockertypes_test.py::IPAMConfigTest::test_create_ipam_config",
"tests/unit/dockertypes_test.py::ServiceModeTest::test_global_replicas_error",
"tests/unit/dockertypes_test.py::ServiceModeTest::test_global_simple",
"tests/unit/dockertypes_test.py::ServiceModeTest::test_invalid_mode",
"tests/unit/dockertypes_test.py::ServiceModeTest::test_replicated_replicas",
"tests/unit/dockertypes_test.py::ServiceModeTest::test_replicated_simple",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_bind",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_named_volume",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_string_invalid",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_string_no_source",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_string_ro",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_string_rw",
"tests/unit/dockertypes_test.py::MountTest::test_parse_mount_string_short_form"
] | [] | 2017-01-12 02:32:06+00:00 | 1,966 |
|
docker__docker-py-1393 | diff --git a/docker/api/client.py b/docker/api/client.py
index a9fe7d08..22c32b44 100644
--- a/docker/api/client.py
+++ b/docker/api/client.py
@@ -18,16 +18,20 @@ from .service import ServiceApiMixin
from .swarm import SwarmApiMixin
from .volume import VolumeApiMixin
from .. import auth
-from ..constants import (DEFAULT_TIMEOUT_SECONDS, DEFAULT_USER_AGENT,
- IS_WINDOWS_PLATFORM, DEFAULT_DOCKER_API_VERSION,
- STREAM_HEADER_SIZE_BYTES, DEFAULT_NUM_POOLS,
- MINIMUM_DOCKER_API_VERSION)
-from ..errors import (DockerException, TLSParameterError,
- create_api_error_from_http_exception)
+from ..constants import (
+ DEFAULT_TIMEOUT_SECONDS, DEFAULT_USER_AGENT, IS_WINDOWS_PLATFORM,
+ DEFAULT_DOCKER_API_VERSION, STREAM_HEADER_SIZE_BYTES, DEFAULT_NUM_POOLS,
+ MINIMUM_DOCKER_API_VERSION
+)
+from ..errors import (
+ DockerException, TLSParameterError,
+ create_api_error_from_http_exception
+)
from ..tls import TLSConfig
from ..transport import SSLAdapter, UnixAdapter
from ..utils import utils, check_resource, update_headers
from ..utils.socket import frames_iter
+from ..utils.json_stream import json_stream
try:
from ..transport import NpipeAdapter
except ImportError:
@@ -274,27 +278,20 @@ class APIClient(
def _stream_helper(self, response, decode=False):
"""Generator for data coming from a chunked-encoded HTTP response."""
+
if response.raw._fp.chunked:
- reader = response.raw
- while not reader.closed:
- # this read call will block until we get a chunk
- data = reader.read(1)
- if not data:
- break
- if reader._fp.chunk_left:
- data += reader.read(reader._fp.chunk_left)
- if decode:
- if six.PY3:
- data = data.decode('utf-8')
- # remove the trailing newline
- data = data.strip()
- # split the data at any newlines
- data_list = data.split("\r\n")
- # load and yield each line seperately
- for data in data_list:
- data = json.loads(data)
- yield data
- else:
+ if decode:
+ for chunk in json_stream(self._stream_helper(response, False)):
+ yield chunk
+ else:
+ reader = response.raw
+ while not reader.closed:
+ # this read call will block until we get a chunk
+ data = reader.read(1)
+ if not data:
+ break
+ if reader._fp.chunk_left:
+ data += reader.read(reader._fp.chunk_left)
yield data
else:
# Response isn't chunked, meaning we probably
diff --git a/docker/models/images.py b/docker/models/images.py
index 32068e69..6f8f4fe2 100644
--- a/docker/models/images.py
+++ b/docker/models/images.py
@@ -30,10 +30,10 @@ class Image(Model):
"""
The image's tags.
"""
- return [
- tag for tag in self.attrs.get('RepoTags', [])
- if tag != '<none>:<none>'
- ]
+ tags = self.attrs.get('RepoTags')
+ if tags is None:
+ tags = []
+ return [tag for tag in tags if tag != '<none>:<none>']
def history(self):
"""
diff --git a/docker/models/resource.py b/docker/models/resource.py
index 95712aef..ed3900af 100644
--- a/docker/models/resource.py
+++ b/docker/models/resource.py
@@ -23,6 +23,9 @@ class Model(object):
def __eq__(self, other):
return isinstance(other, self.__class__) and self.id == other.id
+ def __hash__(self):
+ return hash("%s:%s" % (self.__class__.__name__, self.id))
+
@property
def id(self):
"""
| docker/docker-py | aed1af6f6f8c97658ad8d11619ba0e7fce7af240 | diff --git a/tests/unit/models_images_test.py b/tests/unit/models_images_test.py
index 392c58d7..efb21166 100644
--- a/tests/unit/models_images_test.py
+++ b/tests/unit/models_images_test.py
@@ -83,6 +83,11 @@ class ImageTest(unittest.TestCase):
})
assert image.tags == []
+ image = Image(attrs={
+ 'RepoTags': None
+ })
+ assert image.tags == []
+
def test_history(self):
client = make_fake_client()
image = client.images.get(FAKE_IMAGE_ID)
diff --git a/tests/unit/models_resources_test.py b/tests/unit/models_resources_test.py
index 25c6a3ed..5af24ee6 100644
--- a/tests/unit/models_resources_test.py
+++ b/tests/unit/models_resources_test.py
@@ -12,3 +12,17 @@ class ModelTest(unittest.TestCase):
container.reload()
assert client.api.inspect_container.call_count == 2
assert container.attrs['Name'] == "foobar"
+
+ def test_hash(self):
+ client = make_fake_client()
+ container1 = client.containers.get(FAKE_CONTAINER_ID)
+ my_set = set([container1])
+ assert len(my_set) == 1
+
+ container2 = client.containers.get(FAKE_CONTAINER_ID)
+ my_set.add(container2)
+ assert len(my_set) == 1
+
+ image1 = client.images.get(FAKE_CONTAINER_ID)
+ my_set.add(image1)
+ assert len(my_set) == 2
| Exception retrieving untagged images on api >= 1.24
Docker API >= 1.24 will return a null object if image tags DNE instead of not including it in the response. This makes the dict.get fail to catch the null case and the list comprehension to iterate over a non-iterable.
```
File "<stdin>", line 1, in <module>
File "docker/models/images.py", line 16, in __repr__
return "<%s: '%s'>" % (self.__class__.__name__, "', '".join(self.tags))
File "docker/models/images.py", line 34, in tags
tag for tag in self.attrs.get('RepoTags', [])
TypeError: 'NoneType' object is not iterable
```
This is similar to an issue seen in [salt](https://github.com/saltstack/salt/pull/35447/commits/b833b5f9587534d3b843a026ef91abc4ec929d0f)
Was able to get things working with a pretty quick change:
```
diff --git a/docker/models/images.py b/docker/models/images.py
index 32068e6..39a640d 100644
--- a/docker/models/images.py
+++ b/docker/models/images.py
@@ -30,9 +30,11 @@ class Image(Model):
"""
The image's tags.
"""
+ tags = self.attrs.get('RepoTags', [])
+ if tags is None:
+ return []
return [
- tag for tag in self.attrs.get('RepoTags', [])
- if tag != '<none>:<none>'
+ tag for tag in tags if tag != '<none>:<none>'
]
def history(self):
``` | 0.0 | [
"tests/unit/models_images_test.py::ImageTest::test_tags",
"tests/unit/models_resources_test.py::ModelTest::test_hash"
] | [
"tests/unit/models_images_test.py::ImageCollectionTest::test_build",
"tests/unit/models_images_test.py::ImageCollectionTest::test_get",
"tests/unit/models_images_test.py::ImageCollectionTest::test_list",
"tests/unit/models_images_test.py::ImageCollectionTest::test_load",
"tests/unit/models_images_test.py::ImageCollectionTest::test_pull",
"tests/unit/models_images_test.py::ImageCollectionTest::test_push",
"tests/unit/models_images_test.py::ImageCollectionTest::test_remove",
"tests/unit/models_images_test.py::ImageCollectionTest::test_search",
"tests/unit/models_images_test.py::ImageTest::test_history",
"tests/unit/models_images_test.py::ImageTest::test_save",
"tests/unit/models_images_test.py::ImageTest::test_short_id",
"tests/unit/models_images_test.py::ImageTest::test_tag",
"tests/unit/models_resources_test.py::ModelTest::test_reload"
] | 2017-01-16 07:57:41+00:00 | 1,967 |
|
docker__docker-py-1399 | diff --git a/docker/models/images.py b/docker/models/images.py
index 32068e69..6f8f4fe2 100644
--- a/docker/models/images.py
+++ b/docker/models/images.py
@@ -30,10 +30,10 @@ class Image(Model):
"""
The image's tags.
"""
- return [
- tag for tag in self.attrs.get('RepoTags', [])
- if tag != '<none>:<none>'
- ]
+ tags = self.attrs.get('RepoTags')
+ if tags is None:
+ tags = []
+ return [tag for tag in tags if tag != '<none>:<none>']
def history(self):
"""
diff --git a/setup.py b/setup.py
index b82a74f7..9fc4ad66 100644
--- a/setup.py
+++ b/setup.py
@@ -1,10 +1,20 @@
#!/usr/bin/env python
+from __future__ import print_function
+
import codecs
import os
import sys
+import pip
+
from setuptools import setup, find_packages
+if 'docker-py' in [x.project_name for x in pip.get_installed_distributions()]:
+ print(
+ 'ERROR: "docker-py" needs to be uninstalled before installing this'
+ ' package:\npip uninstall docker-py', file=sys.stderr
+ )
+ sys.exit(1)
ROOT_DIR = os.path.dirname(__file__)
SOURCE_DIR = os.path.join(ROOT_DIR)
| docker/docker-py | 7db5f7ebcc615db903e3007c05495a82bb319810 | diff --git a/tests/unit/models_images_test.py b/tests/unit/models_images_test.py
index 392c58d7..efb21166 100644
--- a/tests/unit/models_images_test.py
+++ b/tests/unit/models_images_test.py
@@ -83,6 +83,11 @@ class ImageTest(unittest.TestCase):
})
assert image.tags == []
+ image = Image(attrs={
+ 'RepoTags': None
+ })
+ assert image.tags == []
+
def test_history(self):
client = make_fake_client()
image = client.images.get(FAKE_IMAGE_ID)
| docker-py installation breaks docker-compose
im not quite sure if this is correct, but trying to install `docker-py` through pip after i've installed `docker-compose` breaks `docker-compose` with
```
Traceback (most recent call last):
File "/usr/local/bin/docker-compose", line 7, in <module>
from compose.cli.main import main
File "/usr/local/lib/python2.7/site-packages/compose/cli/main.py", line 20, in <module>
from ..bundle import get_image_digests
File "/usr/local/lib/python2.7/site-packages/compose/bundle.py", line 13, in <module>
from .network import get_network_defs_for_service
File "/usr/local/lib/python2.7/site-packages/compose/network.py", line 7, in <module>
from docker.types import IPAMConfig
ImportError: cannot import name IPAMConfig
```
To fix that error, i just need to do the installations in this order:
```
pip install docker-py
pip install docker-compose
```
gist:
https://gist.github.com/serialdoom/3a443c420aa29f9422f8c5fc73f46602
python/pip versions tried:
```
docker run -it python:2.7.13 bash -c 'pip --version'
pip 9.0.1 from /usr/local/lib/python2.7/site-packages (python 2.7)
docker run -it python:2.7.12 bash -c 'pip --version'
pip 8.1.2 from /usr/local/lib/python2.7/site-packages (python 2.7)
``` | 0.0 | [
"tests/unit/models_images_test.py::ImageTest::test_tags"
] | [
"tests/unit/models_images_test.py::ImageCollectionTest::test_build",
"tests/unit/models_images_test.py::ImageCollectionTest::test_get",
"tests/unit/models_images_test.py::ImageCollectionTest::test_list",
"tests/unit/models_images_test.py::ImageCollectionTest::test_load",
"tests/unit/models_images_test.py::ImageCollectionTest::test_pull",
"tests/unit/models_images_test.py::ImageCollectionTest::test_push",
"tests/unit/models_images_test.py::ImageCollectionTest::test_remove",
"tests/unit/models_images_test.py::ImageCollectionTest::test_search",
"tests/unit/models_images_test.py::ImageTest::test_history",
"tests/unit/models_images_test.py::ImageTest::test_save",
"tests/unit/models_images_test.py::ImageTest::test_short_id",
"tests/unit/models_images_test.py::ImageTest::test_tag"
] | 2017-01-19 00:38:50+00:00 | 1,968 |
|
docker__docker-py-1710 | diff --git a/README.md b/README.md
index 747b98b2..3ff124d7 100644
--- a/README.md
+++ b/README.md
@@ -10,6 +10,10 @@ The latest stable version [is available on PyPI](https://pypi.python.org/pypi/do
pip install docker
+If you are intending to connect to a docker host via TLS, add `docker[tls]` to your requirements instead, or install with pip:
+
+ pip install docker[tls]
+
## Usage
Connect to Docker using the default socket or the configuration in your environment:
diff --git a/appveyor.yml b/appveyor.yml
index 1fc67cc0..41cde625 100644
--- a/appveyor.yml
+++ b/appveyor.yml
@@ -3,7 +3,7 @@ version: '{branch}-{build}'
install:
- "SET PATH=C:\\Python27-x64;C:\\Python27-x64\\Scripts;%PATH%"
- "python --version"
- - "pip install tox==2.1.1 virtualenv==13.1.2"
+ - "pip install tox==2.7.0 virtualenv==15.1.0"
# Build the binary after tests
build: false
diff --git a/docker/api/build.py b/docker/api/build.py
index cbef4a8b..5d4e7720 100644
--- a/docker/api/build.py
+++ b/docker/api/build.py
@@ -274,7 +274,10 @@ class BuildApiMixin(object):
self._auth_configs, registry
)
else:
- auth_data = self._auth_configs
+ auth_data = self._auth_configs.copy()
+ # See https://github.com/docker/docker-py/issues/1683
+ if auth.INDEX_NAME in auth_data:
+ auth_data[auth.INDEX_URL] = auth_data[auth.INDEX_NAME]
log.debug(
'Sending auth config ({0})'.format(
diff --git a/docker/auth.py b/docker/auth.py
index ec9c45b9..c3fb062e 100644
--- a/docker/auth.py
+++ b/docker/auth.py
@@ -10,7 +10,7 @@ from . import errors
from .constants import IS_WINDOWS_PLATFORM
INDEX_NAME = 'docker.io'
-INDEX_URL = 'https://{0}/v1/'.format(INDEX_NAME)
+INDEX_URL = 'https://index.{0}/v1/'.format(INDEX_NAME)
DOCKER_CONFIG_FILENAME = os.path.join('.docker', 'config.json')
LEGACY_DOCKER_CONFIG_FILENAME = '.dockercfg'
TOKEN_USERNAME = '<token>'
@@ -118,7 +118,7 @@ def _resolve_authconfig_credstore(authconfig, registry, credstore_name):
if not registry or registry == INDEX_NAME:
# The ecosystem is a little schizophrenic with index.docker.io VS
# docker.io - in that case, it seems the full URL is necessary.
- registry = 'https://index.docker.io/v1/'
+ registry = INDEX_URL
log.debug("Looking for auth entry for {0}".format(repr(registry)))
store = dockerpycreds.Store(credstore_name)
try:
diff --git a/docker/utils/build.py b/docker/utils/build.py
index 79b72495..d4223e74 100644
--- a/docker/utils/build.py
+++ b/docker/utils/build.py
@@ -26,6 +26,7 @@ def exclude_paths(root, patterns, dockerfile=None):
if dockerfile is None:
dockerfile = 'Dockerfile'
+ patterns = [p.lstrip('/') for p in patterns]
exceptions = [p for p in patterns if p.startswith('!')]
include_patterns = [p[1:] for p in exceptions]
diff --git a/requirements.txt b/requirements.txt
index 37541312..f3c61e79 100644
--- a/requirements.txt
+++ b/requirements.txt
@@ -1,6 +1,16 @@
-requests==2.11.1
-six>=1.4.0
-websocket-client==0.32.0
-backports.ssl_match_hostname>=3.5 ; python_version < '3.5'
-ipaddress==1.0.16 ; python_version < '3.3'
+appdirs==1.4.3
+asn1crypto==0.22.0
+backports.ssl-match-hostname==3.5.0.1
+cffi==1.10.0
+cryptography==1.9
docker-pycreds==0.2.1
+enum34==1.1.6
+idna==2.5
+ipaddress==1.0.18
+packaging==16.8
+pycparser==2.17
+pyOpenSSL==17.0.0
+pyparsing==2.2.0
+requests==2.14.2
+six==1.10.0
+websocket-client==0.40.0
diff --git a/setup.py b/setup.py
index 31180d23..4a33c8df 100644
--- a/setup.py
+++ b/setup.py
@@ -35,6 +35,16 @@ extras_require = {
# ssl_match_hostname to verify hosts match with certificates via
# ServerAltname: https://pypi.python.org/pypi/backports.ssl_match_hostname
':python_version < "3.3"': 'ipaddress >= 1.0.16',
+
+ # If using docker-py over TLS, highly recommend this option is
+ # pip-installed or pinned.
+
+ # TODO: if pip installing both "requests" and "requests[security]", the
+ # extra package from the "security" option are not installed (see
+ # https://github.com/pypa/pip/issues/4391). Once that's fixed, instead of
+ # installing the extra dependencies, install the following instead:
+ # 'requests[security] >= 2.5.2, != 2.11.0, != 2.12.2'
+ 'tls': ['pyOpenSSL>=0.14', 'cryptography>=1.3.4', 'idna>=2.0.0'],
}
version = None
| docker/docker-py | 5e4a69bbdafef6f1036b733ce356c6692c65e775 | diff --git a/tests/unit/utils_test.py b/tests/unit/utils_test.py
index 7045d23c..4a391fac 100644
--- a/tests/unit/utils_test.py
+++ b/tests/unit/utils_test.py
@@ -768,6 +768,11 @@ class ExcludePathsTest(unittest.TestCase):
self.all_paths - set(['foo/a.py'])
)
+ def test_single_subdir_single_filename_leading_slash(self):
+ assert self.exclude(['/foo/a.py']) == convert_paths(
+ self.all_paths - set(['foo/a.py'])
+ )
+
def test_single_subdir_with_path_traversal(self):
assert self.exclude(['foo/whoops/../a.py']) == convert_paths(
self.all_paths - set(['foo/a.py'])
| using private image in FROM during build is broken
Example:
```
import docker
from io import BytesIO
dockerfile="""
FROM <some-private-image-on-docker-hub>
CMD ["ls"]
"""
f = BytesIO(dockerfile.encode('utf-8'))
client = docker.APIClient(version='auto')
client.login(username='<user>', password='<pass>')
for l in client.build(fileobj=f, rm=True, tag='test', stream=True, pull=True):
print(l)
```
This example will error saying that it failed to find the image in FROM. If you add the full registry:
```
client = docker.APIClient(version='auto')
client.login(username='<user>', password='<pass>', registry='https://index.docker.io/v1/')
```
It will succeed. If you leave off the trailing slash on the registry url, it will fail.
python 3.5.2
docker-py 2.4.2 | 0.0 | [
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_single_filename_leading_slash"
] | [
"tests/unit/utils_test.py::DecoratorsTest::test_update_headers",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_alternate_env",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_empty",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_no_cert_path",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false",
"tests/unit/utils_test.py::KwargsFromEnvTest::test_kwargs_from_env_tls_verify_false_no_cert",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_compact",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_complete",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_empty",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_list",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_no_mode",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_bytes_input",
"tests/unit/utils_test.py::ConverVolumeBindsTest::test_convert_volume_binds_unicode_unicode_input",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_commented_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_invalid_line",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_newline",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_proper",
"tests/unit/utils_test.py::ParseEnvFileTest::test_parse_env_file_with_equals_character",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_empty_value",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_tls_tcp_proto",
"tests/unit/utils_test.py::ParseHostTest::test_parse_host_trailing_slash",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_index_user_image_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_no_tag",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_sha",
"tests/unit/utils_test.py::ParseRepositoryTagTest::test_private_reg_image_tag",
"tests/unit/utils_test.py::ParseDeviceTest::test_dict",
"tests/unit/utils_test.py::ParseDeviceTest::test_full_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_hybrid_list",
"tests/unit/utils_test.py::ParseDeviceTest::test_partial_string_definition",
"tests/unit/utils_test.py::ParseDeviceTest::test_permissionless_string_definition",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_float",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_invalid",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_maxint",
"tests/unit/utils_test.py::ParseBytesTest::test_parse_bytes_valid",
"tests/unit/utils_test.py::UtilsTest::test_convert_filters",
"tests/unit/utils_test.py::UtilsTest::test_decode_json_header",
"tests/unit/utils_test.py::SplitCommandTest::test_split_command_with_unicode",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_matching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_nonmatching_internal_ports",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_one_port",
"tests/unit/utils_test.py::PortsTest::test_build_port_bindings_with_port_range",
"tests/unit/utils_test.py::PortsTest::test_host_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_non_matching_length_port_ranges",
"tests/unit/utils_test.py::PortsTest::test_port_and_range_invalid",
"tests/unit/utils_test.py::PortsTest::test_port_only_with_colon",
"tests/unit/utils_test.py::PortsTest::test_split_port_empty_string",
"tests/unit/utils_test.py::PortsTest::test_split_port_invalid",
"tests/unit/utils_test.py::PortsTest::test_split_port_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_non_string",
"tests/unit/utils_test.py::PortsTest::test_split_port_random_port_range_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_no_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_range_with_protocol",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_ip_no_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_host_port",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_ipv6_address",
"tests/unit/utils_test.py::PortsTest::test_split_port_with_protocol",
"tests/unit/utils_test.py::PortsTest::test_with_no_container_port",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_single_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_subdir_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_directory_with_wildcard_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_double_wildcard",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_custom_dockerfile",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_child",
"tests/unit/utils_test.py::ExcludePathsTest::test_exclude_dockerfile_dockerignore",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_dupes",
"tests/unit/utils_test.py::ExcludePathsTest::test_no_excludes",
"tests/unit/utils_test.py::ExcludePathsTest::test_question_mark",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_and_double_wildcard",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_leading_dot_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_filename_trailing_slash",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_single_subdir_with_path_traversal",
"tests/unit/utils_test.py::ExcludePathsTest::test_subdirectory",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_exclude",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_end",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_filename_start",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_single_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_subdir_wildcard_filename",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_exception",
"tests/unit/utils_test.py::ExcludePathsTest::test_wildcard_with_wildcard_exception",
"tests/unit/utils_test.py::TarTest::test_tar_socket_file",
"tests/unit/utils_test.py::TarTest::test_tar_with_directory_symlinks",
"tests/unit/utils_test.py::TarTest::test_tar_with_empty_directory",
"tests/unit/utils_test.py::TarTest::test_tar_with_excludes",
"tests/unit/utils_test.py::TarTest::test_tar_with_file_symlinks",
"tests/unit/utils_test.py::ShouldCheckDirectoryTest::test_shoud_check_parent_directories_of_excluded",
"tests/unit/utils_test.py::ShouldCheckDirectoryTest::test_should_check_directory_not_excluded",
"tests/unit/utils_test.py::ShouldCheckDirectoryTest::test_should_check_excluded_directory_with_exceptions",
"tests/unit/utils_test.py::ShouldCheckDirectoryTest::test_should_check_subdirectories_of_exceptions",
"tests/unit/utils_test.py::ShouldCheckDirectoryTest::test_should_not_check_excluded_directories_with_no_exceptions",
"tests/unit/utils_test.py::ShouldCheckDirectoryTest::test_should_not_check_siblings_of_exceptions",
"tests/unit/utils_test.py::FormatEnvironmentTest::test_format_env_binary_unicode_value",
"tests/unit/utils_test.py::FormatEnvironmentTest::test_format_env_no_value"
] | 2017-08-15 22:39:49+00:00 | 1,969 |
|
docker__docker-py-2008 | diff --git a/docker/api/config.py b/docker/api/config.py
index b46b09c7..767bef26 100644
--- a/docker/api/config.py
+++ b/docker/api/config.py
@@ -6,7 +6,7 @@ from .. import utils
class ConfigApiMixin(object):
- @utils.minimum_version('1.25')
+ @utils.minimum_version('1.30')
def create_config(self, name, data, labels=None):
"""
Create a config
@@ -35,7 +35,7 @@ class ConfigApiMixin(object):
self._post_json(url, data=body), True
)
- @utils.minimum_version('1.25')
+ @utils.minimum_version('1.30')
@utils.check_resource('id')
def inspect_config(self, id):
"""
@@ -53,7 +53,7 @@ class ConfigApiMixin(object):
url = self._url('/configs/{0}', id)
return self._result(self._get(url), True)
- @utils.minimum_version('1.25')
+ @utils.minimum_version('1.30')
@utils.check_resource('id')
def remove_config(self, id):
"""
@@ -73,7 +73,7 @@ class ConfigApiMixin(object):
self._raise_for_status(res)
return True
- @utils.minimum_version('1.25')
+ @utils.minimum_version('1.30')
def configs(self, filters=None):
"""
List configs
diff --git a/docker/api/container.py b/docker/api/container.py
index cb97b794..05676f11 100644
--- a/docker/api/container.py
+++ b/docker/api/container.py
@@ -1018,7 +1018,10 @@ class ContainerApiMixin(object):
"""
params = {'t': timeout}
url = self._url("/containers/{0}/restart", container)
- res = self._post(url, params=params)
+ conn_timeout = self.timeout
+ if conn_timeout is not None:
+ conn_timeout += timeout
+ res = self._post(url, params=params, timeout=conn_timeout)
self._raise_for_status(res)
@utils.check_resource('container')
@@ -1107,9 +1110,10 @@ class ContainerApiMixin(object):
else:
params = {'t': timeout}
url = self._url("/containers/{0}/stop", container)
-
- res = self._post(url, params=params,
- timeout=(timeout + (self.timeout or 0)))
+ conn_timeout = self.timeout
+ if conn_timeout is not None:
+ conn_timeout += timeout
+ res = self._post(url, params=params, timeout=conn_timeout)
self._raise_for_status(res)
@utils.check_resource('container')
| docker/docker-py | accb9de52f6e383ad0335807f73c8c35bd6e7426 | diff --git a/tests/integration/api_container_test.py b/tests/integration/api_container_test.py
index e2125186..afd439f9 100644
--- a/tests/integration/api_container_test.py
+++ b/tests/integration/api_container_test.py
@@ -1165,6 +1165,15 @@ class RestartContainerTest(BaseAPIIntegrationTest):
assert info2['State']['Running'] is True
self.client.kill(id)
+ def test_restart_with_low_timeout(self):
+ container = self.client.create_container(BUSYBOX, ['sleep', '9999'])
+ self.client.start(container)
+ self.client.timeout = 1
+ self.client.restart(container, timeout=3)
+ self.client.timeout = None
+ self.client.restart(container, timeout=3)
+ self.client.kill(container)
+
def test_restart_with_dict_instead_of_id(self):
container = self.client.create_container(BUSYBOX, ['sleep', '9999'])
assert 'Id' in container
diff --git a/tests/unit/api_container_test.py b/tests/unit/api_container_test.py
index c33f129e..a7e183c8 100644
--- a/tests/unit/api_container_test.py
+++ b/tests/unit/api_container_test.py
@@ -1335,7 +1335,7 @@ class ContainerTest(BaseAPIClientTest):
'POST',
url_prefix + 'containers/3cc2351ab11b/restart',
params={'t': 2},
- timeout=DEFAULT_TIMEOUT_SECONDS
+ timeout=(DEFAULT_TIMEOUT_SECONDS + 2)
)
def test_restart_container_with_dict_instead_of_id(self):
@@ -1345,7 +1345,7 @@ class ContainerTest(BaseAPIClientTest):
'POST',
url_prefix + 'containers/3cc2351ab11b/restart',
params={'t': 2},
- timeout=DEFAULT_TIMEOUT_SECONDS
+ timeout=(DEFAULT_TIMEOUT_SECONDS + 2)
)
def test_remove_container(self):
| ContainerApiMixin::restart(timeout=...) uses default read timeout which results in ReadTimeout exception
ReadTimeout is thrown when you restart or stop a container with a high timeout (e.g. 120sec) in case when the container needs over 60 seconds to stop. This requires users to write code like this:
```
try:
my_container.client.api.timeout = 130
my_container.restart(timeout=110)
finally:
my_container.client.api.timeout = docker.constants.DEFAULT_TIMEOUT_SECONDS
```
or write their own `restart` method.
IMO better solution would be to use read timeout that is `max(docker.constants.DEFAULT_TIMEOUT_SECONDS, user_timeout + SOME_CONSTANT)`, because when someone stops a container with specified timeout (`user_timeout`) it's expected that there might be no data to read from the socket for at least `user_timeout` | 0.0 | [
"tests/unit/api_container_test.py::ContainerTest::test_restart_container",
"tests/unit/api_container_test.py::ContainerTest::test_restart_container_with_dict_instead_of_id"
] | [
"tests/unit/api_container_test.py::StartContainerTest::test_start_container",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_none",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_privileged",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_regression_573",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_binds_ro",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_binds_rw",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_links",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_links_as_list_of_tuples",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_lxc_conf",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_lxc_conf_compat",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_multiple_links",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_port_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_privileged",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_added_capabilities",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_aliases",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_mode",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_mode_and_ro_error",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_ro",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_rw",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_cgroup_parent",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_devices",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_dropped_capabilities",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_entrypoint",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpu_shares",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpus",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpuset",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpuset_mems",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_labels_dict",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_labels_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_links",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_links_as_list_of_tuples",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_lxc_conf",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_lxc_conf_compat",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mac_address",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_int",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_g_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_k_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_m_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_wrong_value",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_multiple_links",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_named_volume",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_port_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_ports",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_restart_policy",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_stdin_open",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_stop_signal",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_sysctl",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_tmpfs_dict",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_tmpfs_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_unicode_envvars",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_volume_string",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_working_dir",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_named_container",
"tests/unit/api_container_test.py::ContainerTest::test_container_stats",
"tests/unit/api_container_test.py::ContainerTest::test_container_top",
"tests/unit/api_container_test.py::ContainerTest::test_container_top_with_psargs",
"tests/unit/api_container_test.py::ContainerTest::test_container_update",
"tests/unit/api_container_test.py::ContainerTest::test_diff",
"tests/unit/api_container_test.py::ContainerTest::test_diff_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_export",
"tests/unit/api_container_test.py::ContainerTest::test_export_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_inspect_container",
"tests/unit/api_container_test.py::ContainerTest::test_inspect_container_undefined_id",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container_with_signal",
"tests/unit/api_container_test.py::ContainerTest::test_list_containers",
"tests/unit/api_container_test.py::ContainerTest::test_log_following",
"tests/unit/api_container_test.py::ContainerTest::test_log_following_backwards",
"tests/unit/api_container_test.py::ContainerTest::test_log_since",
"tests/unit/api_container_test.py::ContainerTest::test_log_since_with_datetime",
"tests/unit/api_container_test.py::ContainerTest::test_log_since_with_invalid_value_raises_error",
"tests/unit/api_container_test.py::ContainerTest::test_log_streaming",
"tests/unit/api_container_test.py::ContainerTest::test_log_streaming_and_following",
"tests/unit/api_container_test.py::ContainerTest::test_log_tail",
"tests/unit/api_container_test.py::ContainerTest::test_log_tty",
"tests/unit/api_container_test.py::ContainerTest::test_logs",
"tests/unit/api_container_test.py::ContainerTest::test_logs_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_pause_container",
"tests/unit/api_container_test.py::ContainerTest::test_port",
"tests/unit/api_container_test.py::ContainerTest::test_remove_container",
"tests/unit/api_container_test.py::ContainerTest::test_remove_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_rename_container",
"tests/unit/api_container_test.py::ContainerTest::test_resize_container",
"tests/unit/api_container_test.py::ContainerTest::test_stop_container",
"tests/unit/api_container_test.py::ContainerTest::test_stop_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_unpause_container",
"tests/unit/api_container_test.py::ContainerTest::test_wait",
"tests/unit/api_container_test.py::ContainerTest::test_wait_with_dict_instead_of_id"
] | 2018-04-25 22:20:48+00:00 | 1,970 |
|
docker__docker-py-2186 | diff --git a/docker/api/image.py b/docker/api/image.py
index a9f801e9..d3fed5c0 100644
--- a/docker/api/image.py
+++ b/docker/api/image.py
@@ -334,7 +334,8 @@ class ImageApiMixin(object):
Args:
repository (str): The repository to pull
tag (str): The tag to pull
- stream (bool): Stream the output as a generator
+ stream (bool): Stream the output as a generator. Make sure to
+ consume the generator, otherwise pull might get cancelled.
auth_config (dict): Override the credentials that
:py:meth:`~docker.api.daemon.DaemonApiMixin.login` has set for
this request. ``auth_config`` should contain the ``username``
diff --git a/docker/models/images.py b/docker/models/images.py
index 4578c0bd..30e86f10 100644
--- a/docker/models/images.py
+++ b/docker/models/images.py
@@ -1,5 +1,6 @@
import itertools
import re
+import warnings
import six
@@ -425,7 +426,21 @@ class ImageCollection(Collection):
if not tag:
repository, tag = parse_repository_tag(repository)
- self.client.api.pull(repository, tag=tag, **kwargs)
+ if 'stream' in kwargs:
+ warnings.warn(
+ '`stream` is not a valid parameter for this method'
+ ' and will be overridden'
+ )
+ del kwargs['stream']
+
+ pull_log = self.client.api.pull(
+ repository, tag=tag, stream=True, **kwargs
+ )
+ for _ in pull_log:
+ # We don't do anything with the logs, but we need
+ # to keep the connection alive and wait for the image
+ # to be pulled.
+ pass
if tag:
return self.get('{0}{2}{1}'.format(
repository, tag, '@' if tag.startswith('sha256:') else ':'
| docker/docker-py | e1e4048753aafc96571752cf54d96df7b24156d3 | diff --git a/tests/unit/models_containers_test.py b/tests/unit/models_containers_test.py
index 22dd2410..39e409e4 100644
--- a/tests/unit/models_containers_test.py
+++ b/tests/unit/models_containers_test.py
@@ -232,7 +232,9 @@ class ContainerCollectionTest(unittest.TestCase):
container = client.containers.run('alpine', 'sleep 300', detach=True)
assert container.id == FAKE_CONTAINER_ID
- client.api.pull.assert_called_with('alpine', platform=None, tag=None)
+ client.api.pull.assert_called_with(
+ 'alpine', platform=None, tag=None, stream=True
+ )
def test_run_with_error(self):
client = make_fake_client()
diff --git a/tests/unit/models_images_test.py b/tests/unit/models_images_test.py
index 67832795..fd894ab7 100644
--- a/tests/unit/models_images_test.py
+++ b/tests/unit/models_images_test.py
@@ -1,6 +1,8 @@
+import unittest
+import warnings
+
from docker.constants import DEFAULT_DATA_CHUNK_SIZE
from docker.models.images import Image
-import unittest
from .fake_api import FAKE_IMAGE_ID
from .fake_api_client import make_fake_client
@@ -43,7 +45,9 @@ class ImageCollectionTest(unittest.TestCase):
def test_pull(self):
client = make_fake_client()
image = client.images.pull('test_image:latest')
- client.api.pull.assert_called_with('test_image', tag='latest')
+ client.api.pull.assert_called_with(
+ 'test_image', tag='latest', stream=True
+ )
client.api.inspect_image.assert_called_with('test_image:latest')
assert isinstance(image, Image)
assert image.id == FAKE_IMAGE_ID
@@ -51,7 +55,9 @@ class ImageCollectionTest(unittest.TestCase):
def test_pull_multiple(self):
client = make_fake_client()
images = client.images.pull('test_image')
- client.api.pull.assert_called_with('test_image', tag=None)
+ client.api.pull.assert_called_with(
+ 'test_image', tag=None, stream=True
+ )
client.api.images.assert_called_with(
all=False, name='test_image', filters=None
)
@@ -61,6 +67,16 @@ class ImageCollectionTest(unittest.TestCase):
assert isinstance(image, Image)
assert image.id == FAKE_IMAGE_ID
+ def test_pull_with_stream_param(self):
+ client = make_fake_client()
+ with warnings.catch_warnings(record=True) as w:
+ client.images.pull('test_image', stream=True)
+
+ assert len(w) == 1
+ assert str(w[0].message).startswith(
+ '`stream` is not a valid parameter'
+ )
+
def test_push(self):
client = make_fake_client()
client.images.push('foobar', insecure_registry=True)
| Can't pull image with stream=True
Hi,
The scenario is as follows:
Mac 10.13.6
docker version v18.06.0-ce
Python 3.6
(python package) docker==3.5.0
private docker registry (docker hub, private repo)
docker login works ✔️
docker pull $image works ✔️
however, pulling via the docker python api fails when using the parameter `stream=True`:
In terminal window I run :
```
python
>>> client = docker.DockerClient(base_url='unix://var/run/docker.sock')
>>> client.login(username='XXXX', password='XXXX', registry='https://index.docker.io/v1/')
>>> client.images.pull('docker.io/vdmtl/portail-datamigration-worker-gcs-lib:latest', stream=True)
Traceback (most recent call last):
File "/Users/XXXX/apps/open-data-etl/venv/lib/python3.6/site-packages/docker/api/client.py", line 229, in _raise_for_status
response.raise_for_status()
File "/Users/XXX/apps/open-data-etl/venv/lib/python3.6/site-packages/requests/models.py", line 937, in raise_for_status
raise HTTPError(http_error_msg, response=self)
requests.exceptions.HTTPError: 404 Client Error: Not Found for url: http+docker://localhost/v1.35/images/docker.io/vdmtl/portail-datamigration-worker-gcs-lib:latest/json
>>> client.images.pull('docker.io/vdmtl/portail-datamigration-worker-gcs-lib:latest')
<Image: 'vdmtl/portail-datamigration-worker-gcs-lib:latest'>
```
As you can see removing `stream=True` is necessary in order to download my image.
| 0.0 | [
"tests/unit/models_images_test.py::ImageCollectionTest::test_pull",
"tests/unit/models_images_test.py::ImageCollectionTest::test_pull_multiple",
"tests/unit/models_images_test.py::ImageCollectionTest::test_pull_with_stream_param",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_run_pull"
] | [
"tests/unit/models_images_test.py::ImageCollectionTest::test_load",
"tests/unit/models_images_test.py::ImageCollectionTest::test_get",
"tests/unit/models_images_test.py::ImageCollectionTest::test_search",
"tests/unit/models_images_test.py::ImageCollectionTest::test_push",
"tests/unit/models_images_test.py::ImageCollectionTest::test_build",
"tests/unit/models_images_test.py::ImageCollectionTest::test_remove",
"tests/unit/models_images_test.py::ImageCollectionTest::test_list",
"tests/unit/models_images_test.py::ImageCollectionTest::test_labels",
"tests/unit/models_images_test.py::ImageTest::test_history",
"tests/unit/models_images_test.py::ImageTest::test_tag",
"tests/unit/models_images_test.py::ImageTest::test_short_id",
"tests/unit/models_images_test.py::ImageTest::test_save",
"tests/unit/models_images_test.py::ImageTest::test_tags",
"tests/unit/models_containers_test.py::ContainerTest::test_put_archive",
"tests/unit/models_containers_test.py::ContainerTest::test_restart",
"tests/unit/models_containers_test.py::ContainerTest::test_diff",
"tests/unit/models_containers_test.py::ContainerTest::test_update",
"tests/unit/models_containers_test.py::ContainerTest::test_exec_run",
"tests/unit/models_containers_test.py::ContainerTest::test_image",
"tests/unit/models_containers_test.py::ContainerTest::test_labels",
"tests/unit/models_containers_test.py::ContainerTest::test_attach",
"tests/unit/models_containers_test.py::ContainerTest::test_logs",
"tests/unit/models_containers_test.py::ContainerTest::test_start",
"tests/unit/models_containers_test.py::ContainerTest::test_pause",
"tests/unit/models_containers_test.py::ContainerTest::test_top",
"tests/unit/models_containers_test.py::ContainerTest::test_wait",
"tests/unit/models_containers_test.py::ContainerTest::test_status",
"tests/unit/models_containers_test.py::ContainerTest::test_resize",
"tests/unit/models_containers_test.py::ContainerTest::test_name",
"tests/unit/models_containers_test.py::ContainerTest::test_get_archive",
"tests/unit/models_containers_test.py::ContainerTest::test_rename",
"tests/unit/models_containers_test.py::ContainerTest::test_stop",
"tests/unit/models_containers_test.py::ContainerTest::test_unpause",
"tests/unit/models_containers_test.py::ContainerTest::test_remove",
"tests/unit/models_containers_test.py::ContainerTest::test_export",
"tests/unit/models_containers_test.py::ContainerTest::test_exec_run_failure",
"tests/unit/models_containers_test.py::ContainerTest::test_commit",
"tests/unit/models_containers_test.py::ContainerTest::test_kill",
"tests/unit/models_containers_test.py::ContainerTest::test_stats",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_run_remove",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_get",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_create",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_run_with_image_object",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_run",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_list",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_list_ignore_removed",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_run_detach",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_create_with_image_object",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_run_with_error",
"tests/unit/models_containers_test.py::ContainerCollectionTest::test_create_container_args"
] | 2018-11-28 19:26:48+00:00 | 1,971 |
|
docker__docker-py-2917 | diff --git a/docker/api/plugin.py b/docker/api/plugin.py
index 57110f11..10210c1a 100644
--- a/docker/api/plugin.py
+++ b/docker/api/plugin.py
@@ -51,19 +51,20 @@ class PluginApiMixin:
return True
@utils.minimum_version('1.25')
- def disable_plugin(self, name):
+ def disable_plugin(self, name, force=False):
"""
Disable an installed plugin.
Args:
name (string): The name of the plugin. The ``:latest`` tag is
optional, and is the default if omitted.
+ force (bool): To enable the force query parameter.
Returns:
``True`` if successful
"""
url = self._url('/plugins/{0}/disable', name)
- res = self._post(url)
+ res = self._post(url, params={'force': force})
self._raise_for_status(res)
return True
diff --git a/docker/models/plugins.py b/docker/models/plugins.py
index 69b94f35..16f5245e 100644
--- a/docker/models/plugins.py
+++ b/docker/models/plugins.py
@@ -44,16 +44,19 @@ class Plugin(Model):
self.client.api.configure_plugin(self.name, options)
self.reload()
- def disable(self):
+ def disable(self, force=False):
"""
Disable the plugin.
+ Args:
+ force (bool): Force disable. Default: False
+
Raises:
:py:class:`docker.errors.APIError`
If the server returns an error.
"""
- self.client.api.disable_plugin(self.name)
+ self.client.api.disable_plugin(self.name, force)
self.reload()
def enable(self, timeout=0):
diff --git a/docker/models/services.py b/docker/models/services.py
index 200dd333..92550681 100644
--- a/docker/models/services.py
+++ b/docker/models/services.py
@@ -320,6 +320,7 @@ CREATE_SERVICE_KWARGS = [
'labels',
'mode',
'update_config',
+ 'rollback_config',
'endpoint_spec',
]
| docker/docker-py | b2a18d7209f827d83cc33acb80aa31bf404ffd4b | diff --git a/tests/integration/api_plugin_test.py b/tests/integration/api_plugin_test.py
index 38f9d12d..3ecb0283 100644
--- a/tests/integration/api_plugin_test.py
+++ b/tests/integration/api_plugin_test.py
@@ -22,13 +22,13 @@ class PluginTest(BaseAPIIntegrationTest):
def teardown_method(self, method):
client = self.get_client_instance()
try:
- client.disable_plugin(SSHFS)
+ client.disable_plugin(SSHFS, True)
except docker.errors.APIError:
pass
for p in self.tmp_plugins:
try:
- client.remove_plugin(p, force=True)
+ client.remove_plugin(p)
except docker.errors.APIError:
pass
diff --git a/tests/integration/models_services_test.py b/tests/integration/models_services_test.py
index 982842b3..f1439a41 100644
--- a/tests/integration/models_services_test.py
+++ b/tests/integration/models_services_test.py
@@ -30,13 +30,18 @@ class ServiceTest(unittest.TestCase):
# ContainerSpec arguments
image="alpine",
command="sleep 300",
- container_labels={'container': 'label'}
+ container_labels={'container': 'label'},
+ rollback_config={'order': 'start-first'}
)
assert service.name == name
assert service.attrs['Spec']['Labels']['foo'] == 'bar'
container_spec = service.attrs['Spec']['TaskTemplate']['ContainerSpec']
assert "alpine" in container_spec['Image']
assert container_spec['Labels'] == {'container': 'label'}
+ spec_rollback = service.attrs['Spec'].get('RollbackConfig', None)
+ assert spec_rollback is not None
+ assert ('Order' in spec_rollback and
+ spec_rollback['Order'] == 'start-first')
def test_create_with_network(self):
client = docker.from_env(version=TEST_API_VERSION)
diff --git a/tests/unit/models_services_test.py b/tests/unit/models_services_test.py
index b9192e42..94a27f0e 100644
--- a/tests/unit/models_services_test.py
+++ b/tests/unit/models_services_test.py
@@ -11,6 +11,7 @@ class CreateServiceKwargsTest(unittest.TestCase):
'labels': {'key': 'value'},
'hostname': 'test_host',
'mode': 'global',
+ 'rollback_config': {'rollback': 'config'},
'update_config': {'update': 'config'},
'networks': ['somenet'],
'endpoint_spec': {'blah': 'blah'},
@@ -37,6 +38,7 @@ class CreateServiceKwargsTest(unittest.TestCase):
'name': 'somename',
'labels': {'key': 'value'},
'mode': 'global',
+ 'rollback_config': {'rollback': 'config'},
'update_config': {'update': 'config'},
'endpoint_spec': {'blah': 'blah'},
}
| Missed rollback_config in service's create/update methods.
Hi, in [documentation](https://docker-py.readthedocs.io/en/stable/services.html) for service written that it support `rollback_config` parameter, but in `models/services.py`'s `CREATE_SERVICE_KWARGS` list doesn't contain it.
So, I got this error:
`TypeError: create() got an unexpected keyword argument 'rollback_config'`
Can someone tell me, is this done intentionally, or is it a bug?
**Version:** `4.4.4, 5.0.0 and older`
**My diff:**
```
diff --git a/docker/models/services.py b/docker/models/services.py
index a29ff13..0f26626 100644
--- a/docker/models/services.py
+++ b/docker/models/services.py
@@ -314,6 +314,7 @@ CREATE_SERVICE_KWARGS = [
'labels',
'mode',
'update_config',
+ 'rollback_config',
'endpoint_spec',
]
```
PS. Full stacktrace:
```
In [54]: service_our = client.services.create(
...: name=service_name,
...: image=image_full_name,
...: restart_policy=restart_policy,
...: update_config=update_config,
...: rollback_config=rollback_config
...: )
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-54-8cc6a8a6519b> in <module>
----> 1 service_our = client.services.create(
2 name=service_name,
3 image=image_full_name,
4 restart_policy=restart_policy,
5 update_config=update_config,
/usr/local/lib/python3.9/site-packages/docker/models/services.py in create(self, image, command, **kwargs)
224 kwargs['image'] = image
225 kwargs['command'] = command
--> 226 create_kwargs = _get_create_service_kwargs('create', kwargs)
227 service_id = self.client.api.create_service(**create_kwargs)
228 return self.get(service_id)
/usr/local/lib/python3.9/site-packages/docker/models/services.py in _get_create_service_kwargs(func_name, kwargs)
369 # All kwargs should have been consumed by this point, so raise
370 # error if any are left
--> 371 if kwargs:
372 raise create_unexpected_kwargs_error(func_name, kwargs)
373
TypeError: create() got an unexpected keyword argument 'rollback_config'
``` | 0.0 | [
"tests/unit/models_services_test.py::CreateServiceKwargsTest::test_get_create_service_kwargs"
] | [] | 2021-11-23 07:33:12+00:00 | 1,972 |
|
docker__docker-py-3120 | diff --git a/docker/api/container.py b/docker/api/container.py
index fef76030..40607e79 100644
--- a/docker/api/container.py
+++ b/docker/api/container.py
@@ -1164,8 +1164,9 @@ class ContainerApiMixin:
'one_shot is only available in conjunction with '
'stream=False'
)
- return self._stream_helper(self._get(url, params=params),
- decode=decode)
+ return self._stream_helper(
+ self._get(url, stream=True, params=params), decode=decode
+ )
else:
if decode:
raise errors.InvalidArgument(
| docker/docker-py | 576e47aaacf690a3fdd6cf98c345d48ecf834b7d | diff --git a/tests/unit/api_container_test.py b/tests/unit/api_container_test.py
index c605da37..c4e2250b 100644
--- a/tests/unit/api_container_test.py
+++ b/tests/unit/api_container_test.py
@@ -1528,10 +1528,21 @@ class ContainerTest(BaseAPIClientTest):
fake_request.assert_called_with(
'GET',
url_prefix + 'containers/' + fake_api.FAKE_CONTAINER_ID + '/stats',
+ stream=True,
timeout=60,
params={'stream': True}
)
+ def test_container_stats_without_streaming(self):
+ self.client.stats(fake_api.FAKE_CONTAINER_ID, stream=False)
+
+ fake_request.assert_called_with(
+ 'GET',
+ url_prefix + 'containers/' + fake_api.FAKE_CONTAINER_ID + '/stats',
+ timeout=60,
+ params={'stream': False}
+ )
+
def test_container_stats_with_one_shot(self):
self.client.stats(
fake_api.FAKE_CONTAINER_ID, stream=False, one_shot=True)
| Containers stats is broken in Docker-py 6.1.0
Look like Docker-Py breaks the API to retrieve stats from containers.
With Docker 6.0.1 (on Ubuntu 22.04):
```
>>> import docker
>>> c = docker..from_env()
>>> for i in c.containers.list():
... i.stats(decode=True)
...
<generator object APIClient._stream_helper at 0x7f236f354eb0>
```
With Docker 6.1.0:
```
>>> import docker
>>> c = docker..from_env()
>>> for i in c.containers.list():
... i.stats(decode=True)
...
Never return anything...
```
Additional information:
```
$ cat /etc/os-release
PRETTY_NAME="Ubuntu 22.04.2 LTS"
NAME="Ubuntu"
VERSION_ID="22.04"
VERSION="22.04.2 LTS (Jammy Jellyfish)"
VERSION_CODENAME=jammy
ID=ubuntu
ID_LIKE=debian
HOME_URL="https://www.ubuntu.com/"
SUPPORT_URL="https://help.ubuntu.com/"
BUG_REPORT_URL="https://bugs.launchpad.net/ubuntu/"
PRIVACY_POLICY_URL="https://www.ubuntu.com/legal/terms-and-policies/privacy-policy"
UBUNTU_CODENAME=jammy
$ docker version
Client: Docker Engine - Community
Version: 23.0.1
API version: 1.42
Go version: go1.19.5
Git commit: a5ee5b1
Built: Thu Feb 9 19:46:56 2023
OS/Arch: linux/amd64
Context: default
Server: Docker Engine - Community
Engine:
Version: 23.0.1
API version: 1.42 (minimum version 1.12)
Go version: go1.19.5
Git commit: bc3805a
Built: Thu Feb 9 19:46:56 2023
OS/Arch: linux/amd64
Experimental: false
containerd:
Version: 1.6.19
GitCommit: 1e1ea6e986c6c86565bc33d52e34b81b3e2bc71f
runc:
Version: 1.1.4
GitCommit: v1.1.4-0-g5fd4c4d
docker-init:
Version: 0.19.0
```
Source: https://github.com/nicolargo/glances/issues/2366 | 0.0 | [
"tests/unit/api_container_test.py::ContainerTest::test_container_stats"
] | [
"tests/unit/api_container_test.py::StartContainerTest::test_start_container",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_none",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_privileged",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_regression_573",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_binds_ro",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_binds_rw",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_links",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_links_as_list_of_tuples",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_lxc_conf",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_lxc_conf_compat",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_multiple_links",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_port_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_privileged",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_added_capabilities",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_aliases",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_mode",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_mode_and_ro_error",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_ro",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_rw",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_cgroup_parent",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_cgroupns",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_device_requests",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_devices",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_dropped_capabilities",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_entrypoint",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpu_shares",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpus",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpuset",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpuset_mems",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_labels_dict",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_labels_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_links",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_links_as_list_of_tuples",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_lxc_conf",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_lxc_conf_compat",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mac_address",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_int",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_g_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_k_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_m_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_wrong_value",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_multiple_links",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_named_volume",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_platform",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_port_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_ports",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_restart_policy",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_stdin_open",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_stop_signal",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_sysctl",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_tmpfs_dict",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_tmpfs_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_unicode_envvars",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_volume_string",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_working_dir",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_named_container",
"tests/unit/api_container_test.py::ContainerTest::test_container_stats_with_one_shot",
"tests/unit/api_container_test.py::ContainerTest::test_container_stats_without_streaming",
"tests/unit/api_container_test.py::ContainerTest::test_container_top",
"tests/unit/api_container_test.py::ContainerTest::test_container_top_with_psargs",
"tests/unit/api_container_test.py::ContainerTest::test_container_update",
"tests/unit/api_container_test.py::ContainerTest::test_diff",
"tests/unit/api_container_test.py::ContainerTest::test_diff_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_export",
"tests/unit/api_container_test.py::ContainerTest::test_export_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_inspect_container",
"tests/unit/api_container_test.py::ContainerTest::test_inspect_container_undefined_id",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container_with_signal",
"tests/unit/api_container_test.py::ContainerTest::test_list_containers",
"tests/unit/api_container_test.py::ContainerTest::test_log_following",
"tests/unit/api_container_test.py::ContainerTest::test_log_following_backwards",
"tests/unit/api_container_test.py::ContainerTest::test_log_since",
"tests/unit/api_container_test.py::ContainerTest::test_log_since_with_datetime",
"tests/unit/api_container_test.py::ContainerTest::test_log_since_with_float",
"tests/unit/api_container_test.py::ContainerTest::test_log_since_with_invalid_value_raises_error",
"tests/unit/api_container_test.py::ContainerTest::test_log_streaming",
"tests/unit/api_container_test.py::ContainerTest::test_log_streaming_and_following",
"tests/unit/api_container_test.py::ContainerTest::test_log_tail",
"tests/unit/api_container_test.py::ContainerTest::test_log_tty",
"tests/unit/api_container_test.py::ContainerTest::test_logs",
"tests/unit/api_container_test.py::ContainerTest::test_logs_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_pause_container",
"tests/unit/api_container_test.py::ContainerTest::test_port",
"tests/unit/api_container_test.py::ContainerTest::test_remove_container",
"tests/unit/api_container_test.py::ContainerTest::test_remove_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_rename_container",
"tests/unit/api_container_test.py::ContainerTest::test_resize_container",
"tests/unit/api_container_test.py::ContainerTest::test_restart_container",
"tests/unit/api_container_test.py::ContainerTest::test_restart_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_stop_container",
"tests/unit/api_container_test.py::ContainerTest::test_stop_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_unpause_container",
"tests/unit/api_container_test.py::ContainerTest::test_wait",
"tests/unit/api_container_test.py::ContainerTest::test_wait_with_dict_instead_of_id"
] | 2023-05-06 19:23:04+00:00 | 1,973 |
|
docker__docker-py-3200 | diff --git a/docker/models/configs.py b/docker/models/configs.py
index 3588c8b5..5ef13778 100644
--- a/docker/models/configs.py
+++ b/docker/models/configs.py
@@ -30,6 +30,7 @@ class ConfigCollection(Collection):
def create(self, **kwargs):
obj = self.client.api.create_config(**kwargs)
+ obj.setdefault("Spec", {})["Name"] = kwargs.get("name")
return self.prepare_model(obj)
create.__doc__ = APIClient.create_config.__doc__
diff --git a/docker/utils/build.py b/docker/utils/build.py
index a5c4b0c2..86a4423f 100644
--- a/docker/utils/build.py
+++ b/docker/utils/build.py
@@ -10,8 +10,9 @@ from ..constants import IS_WINDOWS_PLATFORM
_SEP = re.compile('/|\\\\') if IS_WINDOWS_PLATFORM else re.compile('/')
_TAG = re.compile(
- r"^[a-z0-9]+((\.|_|__|-+)[a-z0-9]+)*(\/[a-z0-9]+((\.|_|__|-+)[a-z0-9]+)*)*" \
- + "(:[a-zA-Z0-9_][a-zA-Z0-9._-]{0,127})?$"
+ r"^[a-z0-9]+((\.|_|__|-+)[a-z0-9]+)*"
+ r"(?::[0-9]+)?(/[a-z0-9]+((\.|_|__|-+)[a-z0-9]+)*)*"
+ r"(:[a-zA-Z0-9_][a-zA-Z0-9._-]{0,127})?$"
)
diff --git a/docker/utils/utils.py b/docker/utils/utils.py
index 759ddd2f..dbd51303 100644
--- a/docker/utils/utils.py
+++ b/docker/utils/utils.py
@@ -5,7 +5,7 @@ import os
import os.path
import shlex
import string
-from datetime import datetime
+from datetime import datetime, timezone
from packaging.version import Version
from .. import errors
@@ -394,8 +394,8 @@ def convert_filters(filters):
def datetime_to_timestamp(dt):
- """Convert a UTC datetime to a Unix timestamp"""
- delta = dt - datetime.utcfromtimestamp(0)
+ """Convert a datetime to a Unix timestamp"""
+ delta = dt.astimezone(timezone.utc) - datetime(1970, 1, 1, tzinfo=timezone.utc)
return delta.seconds + delta.days * 24 * 3600
| docker/docker-py | 6ceb08273c157cbab7b5c77bd71e7389f1a6acc5 | diff --git a/tests/unit/fake_api.py b/tests/unit/fake_api.py
index 0524becd..03e53cc6 100644
--- a/tests/unit/fake_api.py
+++ b/tests/unit/fake_api.py
@@ -19,6 +19,8 @@ FAKE_VOLUME_NAME = 'perfectcherryblossom'
FAKE_NODE_ID = '24ifsmvkjbyhk'
FAKE_SECRET_ID = 'epdyrw4tsi03xy3deu8g8ly6o'
FAKE_SECRET_NAME = 'super_secret'
+FAKE_CONFIG_ID = 'sekvs771242jfdjnvfuds8232'
+FAKE_CONFIG_NAME = 'super_config'
# Each method is prefixed with HTTP method (get, post...)
# for clarity and readability
@@ -512,6 +514,11 @@ def post_fake_secret():
response = {'ID': FAKE_SECRET_ID}
return status_code, response
+def post_fake_config():
+ status_code = 200
+ response = {'ID': FAKE_CONFIG_ID}
+ return status_code, response
+
# Maps real api url to fake response callback
prefix = 'http+docker://localhost'
@@ -630,4 +637,6 @@ fake_responses = {
post_fake_network_disconnect,
f'{prefix}/{CURRENT_VERSION}/secrets/create':
post_fake_secret,
+ f'{prefix}/{CURRENT_VERSION}/configs/create':
+ post_fake_config,
}
diff --git a/tests/unit/fake_api_client.py b/tests/unit/fake_api_client.py
index 95cf63b4..79799421 100644
--- a/tests/unit/fake_api_client.py
+++ b/tests/unit/fake_api_client.py
@@ -37,6 +37,7 @@ def make_fake_api_client(overrides=None):
'create_host_config.side_effect': api_client.create_host_config,
'create_network.return_value': fake_api.post_fake_network()[1],
'create_secret.return_value': fake_api.post_fake_secret()[1],
+ 'create_config.return_value': fake_api.post_fake_config()[1],
'exec_create.return_value': fake_api.post_fake_exec_create()[1],
'exec_start.return_value': fake_api.post_fake_exec_start()[1],
'images.return_value': fake_api.get_fake_images()[1],
diff --git a/tests/unit/models_configs_test.py b/tests/unit/models_configs_test.py
new file mode 100644
index 00000000..6960397f
--- /dev/null
+++ b/tests/unit/models_configs_test.py
@@ -0,0 +1,10 @@
+import unittest
+
+from .fake_api_client import make_fake_client
+from .fake_api import FAKE_CONFIG_NAME
+
+class CreateConfigsTest(unittest.TestCase):
+ def test_create_config(self):
+ client = make_fake_client()
+ config = client.configs.create(name="super_config", data="config")
+ assert config.__repr__() == "<Config: '{}'>".format(FAKE_CONFIG_NAME)
diff --git a/tests/unit/utils_build_test.py b/tests/unit/utils_build_test.py
index fa7d833d..5f1bb1ec 100644
--- a/tests/unit/utils_build_test.py
+++ b/tests/unit/utils_build_test.py
@@ -6,11 +6,10 @@ import tarfile
import tempfile
import unittest
+import pytest
from docker.constants import IS_WINDOWS_PLATFORM
-from docker.utils import exclude_paths, tar
-
-import pytest
+from docker.utils import exclude_paths, tar, match_tag
from ..helpers import make_tree
@@ -489,3 +488,51 @@ class TarTest(unittest.TestCase):
assert member in names
assert 'a/c/b' in names
assert 'a/c/b/utils.py' not in names
+
+
+# selected test cases from https://github.com/distribution/reference/blob/8507c7fcf0da9f570540c958ea7b972c30eeaeca/reference_test.go#L13-L328
+@pytest.mark.parametrize("tag,expected", [
+ ("test_com", True),
+ ("test.com:tag", True),
+ # N.B. this implicitly means "docker.io/library/test.com:5000"
+ # i.e. the `5000` is a tag, not a port here!
+ ("test.com:5000", True),
+ ("test.com/repo:tag", True),
+ ("test:5000/repo", True),
+ ("test:5000/repo:tag", True),
+ ("test:5000/repo", True),
+ ("", False),
+ (":justtag", False),
+ ("Uppercase:tag", False),
+ ("test:5000/Uppercase/lowercase:tag", False),
+ ("lowercase:Uppercase", True),
+ # length limits not enforced
+ pytest.param("a/"*128 + "a:tag", False, marks=pytest.mark.xfail),
+ ("a/"*127 + "a:tag-puts-this-over-max", True),
+ ("aa/asdf$$^/aa", False),
+ ("sub-dom1.foo.com/bar/baz/quux", True),
+ ("sub-dom1.foo.com/bar/baz/quux:some-long-tag", True),
+ ("b.gcr.io/test.example.com/my-app:test.example.com", True),
+ ("xn--n3h.com/myimage:xn--n3h.com", True),
+ ("foo_bar.com:8080", True),
+ ("foo/foo_bar.com:8080", True),
+ ("192.168.1.1", True),
+ ("192.168.1.1:tag", True),
+ ("192.168.1.1:5000", True),
+ ("192.168.1.1/repo", True),
+ ("192.168.1.1:5000/repo", True),
+ ("192.168.1.1:5000/repo:5050", True),
+ # regex does not properly handle ipv6
+ pytest.param("[2001:db8::1]", False, marks=pytest.mark.xfail),
+ ("[2001:db8::1]:5000", False),
+ pytest.param("[2001:db8::1]/repo", True, marks=pytest.mark.xfail),
+ pytest.param("[2001:db8:1:2:3:4:5:6]/repo:tag", True, marks=pytest.mark.xfail),
+ pytest.param("[2001:db8::1]:5000/repo", True, marks=pytest.mark.xfail),
+ pytest.param("[2001:db8::1]:5000/repo:tag", True, marks=pytest.mark.xfail),
+ pytest.param("[2001:db8::]:5000/repo", True, marks=pytest.mark.xfail),
+ pytest.param("[::1]:5000/repo", True, marks=pytest.mark.xfail),
+ ("[fe80::1%eth0]:5000/repo", False),
+ ("[fe80::1%@invalidzone]:5000/repo", False),
+])
+def test_match_tag(tag: str, expected: bool):
+ assert match_tag(tag) == expected
| Can't create config object
Much like https://github.com/docker/docker-py/issues/2025 the config model is failing to create a new object due to 'name' KeyError
```
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "docker\models\configs.py", line 10, in __repr__
return f"<{self.__class__.__name__}: '{self.name}'>"
File "docker\models\configs.py", line 14, in name
return self.attrs['Spec']['Name']
```
This https://github.com/docker/docker-py/pull/2793 appears to be the fix that was implemented and should likely be implements for configs as well (if not other models that might have this issue) | 0.0 | [
"tests/unit/models_configs_test.py::CreateConfigsTest::test_create_config",
"tests/unit/utils_build_test.py::test_match_tag[test:5000/repo-True0]",
"tests/unit/utils_build_test.py::test_match_tag[test:5000/repo:tag-True]",
"tests/unit/utils_build_test.py::test_match_tag[test:5000/repo-True1]",
"tests/unit/utils_build_test.py::test_match_tag[192.168.1.1:5000/repo-True]",
"tests/unit/utils_build_test.py::test_match_tag[192.168.1.1:5000/repo:5050-True]"
] | [
"tests/unit/utils_build_test.py::ExcludePathsTest::test_directory",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_directory_with_single_exception",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_directory_with_subdir_exception",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_directory_with_trailing_slash",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_directory_with_wildcard_exception",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_double_wildcard",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_double_wildcard_with_exception",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_exclude_custom_dockerfile",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_exclude_dockerfile_child",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_exclude_dockerfile_dockerignore",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_exclude_include_absolute_path",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_include_wildcard",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_last_line_precedence",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_no_dupes",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_no_excludes",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_parent_directory",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_question_mark",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_and_double_wildcard",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_filename",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_filename_leading_dot_slash",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_filename_trailing_slash",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_subdir_single_filename",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_subdir_single_filename_leading_slash",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_subdir_wildcard_filename",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_single_subdir_with_path_traversal",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_subdirectory",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_trailing_double_wildcard",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_exclude",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_filename_end",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_filename_start",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_subdir_single_filename",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_subdir_wildcard_filename",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_with_exception",
"tests/unit/utils_build_test.py::ExcludePathsTest::test_wildcard_with_wildcard_exception",
"tests/unit/utils_build_test.py::TarTest::test_tar_directory_link",
"tests/unit/utils_build_test.py::TarTest::test_tar_socket_file",
"tests/unit/utils_build_test.py::TarTest::test_tar_with_broken_symlinks",
"tests/unit/utils_build_test.py::TarTest::test_tar_with_directory_symlinks",
"tests/unit/utils_build_test.py::TarTest::test_tar_with_empty_directory",
"tests/unit/utils_build_test.py::TarTest::test_tar_with_excludes",
"tests/unit/utils_build_test.py::TarTest::test_tar_with_file_symlinks",
"tests/unit/utils_build_test.py::test_match_tag[test_com-True]",
"tests/unit/utils_build_test.py::test_match_tag[test.com:tag-True]",
"tests/unit/utils_build_test.py::test_match_tag[test.com:5000-True]",
"tests/unit/utils_build_test.py::test_match_tag[test.com/repo:tag-True]",
"tests/unit/utils_build_test.py::test_match_tag[-False]",
"tests/unit/utils_build_test.py::test_match_tag[:justtag-False]",
"tests/unit/utils_build_test.py::test_match_tag[Uppercase:tag-False]",
"tests/unit/utils_build_test.py::test_match_tag[test:5000/Uppercase/lowercase:tag-False]",
"tests/unit/utils_build_test.py::test_match_tag[lowercase:Uppercase-True]",
"tests/unit/utils_build_test.py::test_match_tag[a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a/a:tag-puts-this-over-max-True]",
"tests/unit/utils_build_test.py::test_match_tag[aa/asdf$$^/aa-False]",
"tests/unit/utils_build_test.py::test_match_tag[sub-dom1.foo.com/bar/baz/quux-True]",
"tests/unit/utils_build_test.py::test_match_tag[sub-dom1.foo.com/bar/baz/quux:some-long-tag-True]",
"tests/unit/utils_build_test.py::test_match_tag[b.gcr.io/test.example.com/my-app:test.example.com-True]",
"tests/unit/utils_build_test.py::test_match_tag[xn--n3h.com/myimage:xn--n3h.com-True]",
"tests/unit/utils_build_test.py::test_match_tag[foo_bar.com:8080-True]",
"tests/unit/utils_build_test.py::test_match_tag[foo/foo_bar.com:8080-True]",
"tests/unit/utils_build_test.py::test_match_tag[192.168.1.1-True]",
"tests/unit/utils_build_test.py::test_match_tag[192.168.1.1:tag-True]",
"tests/unit/utils_build_test.py::test_match_tag[192.168.1.1:5000-True]",
"tests/unit/utils_build_test.py::test_match_tag[192.168.1.1/repo-True]",
"tests/unit/utils_build_test.py::test_match_tag[[2001:db8::1]:5000-False]",
"tests/unit/utils_build_test.py::test_match_tag[[fe80::1%eth0]:5000/repo-False]",
"tests/unit/utils_build_test.py::test_match_tag[[fe80::1%@invalidzone]:5000/repo-False]"
] | 2023-12-17 15:00:52+00:00 | 1,974 |
|
docker__docker-py-822 | diff --git a/docker/api/container.py b/docker/api/container.py
index 72c5852d..953a5f52 100644
--- a/docker/api/container.py
+++ b/docker/api/container.py
@@ -997,19 +997,16 @@ class ContainerApiMixin(object):
self._raise_for_status(res)
@utils.check_resource
- def start(self, container, binds=None, port_bindings=None, lxc_conf=None,
- publish_all_ports=None, links=None, privileged=None,
- dns=None, dns_search=None, volumes_from=None, network_mode=None,
- restart_policy=None, cap_add=None, cap_drop=None, devices=None,
- extra_hosts=None, read_only=None, pid_mode=None, ipc_mode=None,
- security_opt=None, ulimits=None):
+ def start(self, container, *args, **kwargs):
"""
Start a container. Similar to the ``docker start`` command, but
doesn't support attach options.
- **Deprecation warning:** For API version > 1.15, it is highly
- recommended to provide host config options in the ``host_config``
- parameter of :py:meth:`~ContainerApiMixin.create_container`.
+ **Deprecation warning:** Passing configuration options in ``start`` is
+ no longer supported. Users are expected to provide host config options
+ in the ``host_config`` parameter of
+ :py:meth:`~ContainerApiMixin.create_container`.
+
Args:
container (str): The container to start
@@ -1017,6 +1014,8 @@ class ContainerApiMixin(object):
Raises:
:py:class:`docker.errors.APIError`
If the server returns an error.
+ :py:class:`docker.errors.DeprecatedMethod`
+ If any argument besides ``container`` are provided.
Example:
@@ -1025,64 +1024,14 @@ class ContainerApiMixin(object):
... command='/bin/sleep 30')
>>> cli.start(container=container.get('Id'))
"""
- if utils.compare_version('1.10', self._version) < 0:
- if dns is not None:
- raise errors.InvalidVersion(
- 'dns is only supported for API version >= 1.10'
- )
- if volumes_from is not None:
- raise errors.InvalidVersion(
- 'volumes_from is only supported for API version >= 1.10'
- )
-
- if utils.compare_version('1.15', self._version) < 0:
- if security_opt is not None:
- raise errors.InvalidVersion(
- 'security_opt is only supported for API version >= 1.15'
- )
- if ipc_mode:
- raise errors.InvalidVersion(
- 'ipc_mode is only supported for API version >= 1.15'
- )
-
- if utils.compare_version('1.17', self._version) < 0:
- if read_only is not None:
- raise errors.InvalidVersion(
- 'read_only is only supported for API version >= 1.17'
- )
- if pid_mode is not None:
- raise errors.InvalidVersion(
- 'pid_mode is only supported for API version >= 1.17'
- )
-
- if utils.compare_version('1.18', self._version) < 0:
- if ulimits is not None:
- raise errors.InvalidVersion(
- 'ulimits is only supported for API version >= 1.18'
- )
-
- start_config_kwargs = dict(
- binds=binds, port_bindings=port_bindings, lxc_conf=lxc_conf,
- publish_all_ports=publish_all_ports, links=links, dns=dns,
- privileged=privileged, dns_search=dns_search, cap_add=cap_add,
- cap_drop=cap_drop, volumes_from=volumes_from, devices=devices,
- network_mode=network_mode, restart_policy=restart_policy,
- extra_hosts=extra_hosts, read_only=read_only, pid_mode=pid_mode,
- ipc_mode=ipc_mode, security_opt=security_opt, ulimits=ulimits,
- )
- start_config = None
-
- if any(v is not None for v in start_config_kwargs.values()):
- if utils.compare_version('1.15', self._version) > 0:
- warnings.warn(
- 'Passing host config parameters in start() is deprecated. '
- 'Please use host_config in create_container instead!',
- DeprecationWarning
- )
- start_config = self.create_host_config(**start_config_kwargs)
-
+ if args or kwargs:
+ raise errors.DeprecatedMethod(
+ 'Providing configuration in the start() method is no longer '
+ 'supported. Use the host_config param in create_container '
+ 'instead.'
+ )
url = self._url("/containers/{0}/start", container)
- res = self._post_json(url, data=start_config)
+ res = self._post(url)
self._raise_for_status(res)
@utils.minimum_version('1.17')
| docker/docker-py | 4c8c761bc15160be5eaa76d81edda17b067aa641 | diff --git a/tests/unit/api_container_test.py b/tests/unit/api_container_test.py
index 6c080641..abf36138 100644
--- a/tests/unit/api_container_test.py
+++ b/tests/unit/api_container_test.py
@@ -34,10 +34,7 @@ class StartContainerTest(BaseAPIClientTest):
args[0][1],
url_prefix + 'containers/3cc2351ab11b/start'
)
- self.assertEqual(json.loads(args[1]['data']), {})
- self.assertEqual(
- args[1]['headers'], {'Content-Type': 'application/json'}
- )
+ assert 'data' not in args[1]
self.assertEqual(
args[1]['timeout'], DEFAULT_TIMEOUT_SECONDS
)
@@ -63,25 +60,21 @@ class StartContainerTest(BaseAPIClientTest):
self.client.start(**{'container': fake_api.FAKE_CONTAINER_ID})
def test_start_container_with_lxc_conf(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(
fake_api.FAKE_CONTAINER_ID,
lxc_conf={'lxc.conf.k': 'lxc.conf.value'}
)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_lxc_conf_compat(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(
fake_api.FAKE_CONTAINER_ID,
lxc_conf=[{'Key': 'lxc.conf.k', 'Value': 'lxc.conf.value'}]
)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_binds_ro(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(
fake_api.FAKE_CONTAINER_ID, binds={
'/tmp': {
@@ -91,22 +84,18 @@ class StartContainerTest(BaseAPIClientTest):
}
)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_binds_rw(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(
fake_api.FAKE_CONTAINER_ID, binds={
'/tmp': {"bind": '/mnt', "ro": False}
}
)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_port_binds(self):
self.maxDiff = None
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(fake_api.FAKE_CONTAINER_ID, port_bindings={
1111: None,
2222: 2222,
@@ -116,18 +105,14 @@ class StartContainerTest(BaseAPIClientTest):
6666: [('127.0.0.1',), ('192.168.0.1',)]
})
- pytest.deprecated_call(call_start)
-
def test_start_container_with_links(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(
fake_api.FAKE_CONTAINER_ID, links={'path': 'alias'}
)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_multiple_links(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(
fake_api.FAKE_CONTAINER_ID,
links={
@@ -136,21 +121,15 @@ class StartContainerTest(BaseAPIClientTest):
}
)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_links_as_list_of_tuples(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(fake_api.FAKE_CONTAINER_ID,
links=[('path', 'alias')])
- pytest.deprecated_call(call_start)
-
def test_start_container_privileged(self):
- def call_start():
+ with pytest.raises(docker.errors.DeprecatedMethod):
self.client.start(fake_api.FAKE_CONTAINER_ID, privileged=True)
- pytest.deprecated_call(call_start)
-
def test_start_container_with_dict_instead_of_id(self):
self.client.start({'Id': fake_api.FAKE_CONTAINER_ID})
@@ -159,10 +138,7 @@ class StartContainerTest(BaseAPIClientTest):
args[0][1],
url_prefix + 'containers/3cc2351ab11b/start'
)
- self.assertEqual(json.loads(args[1]['data']), {})
- self.assertEqual(
- args[1]['headers'], {'Content-Type': 'application/json'}
- )
+ assert 'data' not in args[1]
self.assertEqual(
args[1]['timeout'], DEFAULT_TIMEOUT_SECONDS
)
| Passing host_config parameters in start() overrides the host_config that was passed in create()?
I had a `host_config` with `extra_hosts` defined. I used this `host_config` to create a container. When starting container, I passed extra volumes_from parameter. However, this lead to `extra_hosts` seemingly not doing their job.
After I moved `volumes_from` from `start()` to `create_host_config` everything started working OK.
I figured, I should report this, since I spent a couple of hours figuring this out. IMO, start() should override the necessary parts of host_config(and not the whole thing). Or raise an exception.
| 0.0 | [
"tests/unit/api_container_test.py::StartContainerTest::test_start_container",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_privileged",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_binds_ro",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_binds_rw",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_links",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_links_as_list_of_tuples",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_lxc_conf",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_lxc_conf_compat",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_multiple_links",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_with_port_binds"
] | [
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_none",
"tests/unit/api_container_test.py::StartContainerTest::test_start_container_regression_573",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_empty_volumes_from",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_privileged",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_added_capabilities",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_aliases",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_mode",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_mode_and_ro_error",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_ro",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_binds_rw",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_cgroup_parent",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_cpu_shares",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_cpuset",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_devices",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_dropped_capabilities",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_entrypoint",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpu_shares",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_host_config_cpuset",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_labels_dict",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_labels_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_links",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_links_as_list_of_tuples",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_lxc_conf",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_lxc_conf_compat",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mac_address",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_int",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_g_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_k_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_m_unit",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_mem_limit_as_string_with_wrong_value",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_multiple_links",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_named_volume",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_port_binds",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_ports",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_restart_policy",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_stdin_open",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_stop_signal",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_sysctl",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_tmpfs_dict",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_tmpfs_list",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_unicode_envvars",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_volume_string",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_volumes_from",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_container_with_working_dir",
"tests/unit/api_container_test.py::CreateContainerTest::test_create_named_container",
"tests/unit/api_container_test.py::ContainerTest::test_container_stats",
"tests/unit/api_container_test.py::ContainerTest::test_container_top",
"tests/unit/api_container_test.py::ContainerTest::test_container_top_with_psargs",
"tests/unit/api_container_test.py::ContainerTest::test_container_update",
"tests/unit/api_container_test.py::ContainerTest::test_diff",
"tests/unit/api_container_test.py::ContainerTest::test_diff_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_export",
"tests/unit/api_container_test.py::ContainerTest::test_export_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_inspect_container",
"tests/unit/api_container_test.py::ContainerTest::test_inspect_container_undefined_id",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_kill_container_with_signal",
"tests/unit/api_container_test.py::ContainerTest::test_list_containers",
"tests/unit/api_container_test.py::ContainerTest::test_log_following",
"tests/unit/api_container_test.py::ContainerTest::test_log_following_backwards",
"tests/unit/api_container_test.py::ContainerTest::test_log_since",
"tests/unit/api_container_test.py::ContainerTest::test_log_since_with_datetime",
"tests/unit/api_container_test.py::ContainerTest::test_log_streaming",
"tests/unit/api_container_test.py::ContainerTest::test_log_streaming_and_following",
"tests/unit/api_container_test.py::ContainerTest::test_log_tail",
"tests/unit/api_container_test.py::ContainerTest::test_log_tty",
"tests/unit/api_container_test.py::ContainerTest::test_logs",
"tests/unit/api_container_test.py::ContainerTest::test_logs_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_pause_container",
"tests/unit/api_container_test.py::ContainerTest::test_port",
"tests/unit/api_container_test.py::ContainerTest::test_remove_container",
"tests/unit/api_container_test.py::ContainerTest::test_remove_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_rename_container",
"tests/unit/api_container_test.py::ContainerTest::test_resize_container",
"tests/unit/api_container_test.py::ContainerTest::test_restart_container",
"tests/unit/api_container_test.py::ContainerTest::test_restart_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_stop_container",
"tests/unit/api_container_test.py::ContainerTest::test_stop_container_with_dict_instead_of_id",
"tests/unit/api_container_test.py::ContainerTest::test_unpause_container",
"tests/unit/api_container_test.py::ContainerTest::test_wait",
"tests/unit/api_container_test.py::ContainerTest::test_wait_with_dict_instead_of_id"
] | 2015-10-21 22:58:11+00:00 | 1,975 |
|
dogsheep__twitter-to-sqlite-24 | diff --git a/twitter_to_sqlite/cli.py b/twitter_to_sqlite/cli.py
index 56ba167..ad44655 100644
--- a/twitter_to_sqlite/cli.py
+++ b/twitter_to_sqlite/cli.py
@@ -5,7 +5,7 @@ import pathlib
import time
import click
-import sqlite_utils
+
from twitter_to_sqlite import archive
from twitter_to_sqlite import utils
@@ -102,7 +102,7 @@ def followers(db_path, auth, user_id, screen_name, silent):
"Save followers for specified user (defaults to authenticated user)"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
fetched = []
# Get the follower count, so we can have a progress bar
count = 0
@@ -152,7 +152,7 @@ def favorites(db_path, auth, user_id, screen_name, stop_after):
"Save tweets favorited by specified user"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
profile = utils.get_profile(db, session, user_id, screen_name)
with click.progressbar(
utils.fetch_favorites(session, user_id, screen_name, stop_after),
@@ -193,7 +193,7 @@ def user_timeline(db_path, auth, stop_after, user_id, screen_name, since, since_
raise click.ClickException("Use either --since or --since_id, not both")
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
profile = utils.get_profile(db, session, user_id, screen_name)
expected_length = profile["statuses_count"]
@@ -209,7 +209,9 @@ def user_timeline(db_path, auth, stop_after, user_id, screen_name, since, since_
pass
with click.progressbar(
- utils.fetch_user_timeline(session, user_id, screen_name, stop_after, since_id=since_id),
+ utils.fetch_user_timeline(
+ session, user_id, screen_name, stop_after, since_id=since_id
+ ),
length=expected_length,
label="Importing tweets",
show_pos=True,
@@ -253,7 +255,7 @@ def home_timeline(db_path, auth, since, since_id):
raise click.ClickException("Use either --since or --since_id, not both")
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
profile = utils.get_profile(db, session)
expected_length = 800
if since and db["timeline_tweets"].exists:
@@ -310,7 +312,7 @@ def users_lookup(db_path, identifiers, attach, sql, auth, ids):
"Fetch user accounts"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
identifiers = utils.resolve_identifiers(db, identifiers, attach, sql)
for batch in utils.fetch_user_batches(session, identifiers, ids):
utils.save_users(db, batch)
@@ -338,7 +340,7 @@ def statuses_lookup(db_path, identifiers, attach, sql, auth, skip_existing, sile
"Fetch tweets by their IDs"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
identifiers = utils.resolve_identifiers(db, identifiers, attach, sql)
if skip_existing:
existing_ids = set(
@@ -381,7 +383,7 @@ def list_members(db_path, identifiers, auth, ids):
"Fetch lists - accepts one or more screen_name/list_slug identifiers"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
for identifier in identifiers:
utils.fetch_and_save_list(db, session, identifier, ids)
@@ -477,7 +479,7 @@ def track(db_path, track, auth, verbose):
"Experimental: Save tweets matching these keywords in real-time"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
for tweet in utils.stream_filter(session, track=track):
if verbose:
print(json.dumps(tweet, indent=2))
@@ -505,7 +507,7 @@ def follow(db_path, identifiers, attach, sql, ids, auth, verbose):
"Experimental: Follow these Twitter users and save tweets in real-time"
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
identifiers = utils.resolve_identifiers(db, identifiers, attach, sql)
# Make sure we have saved these users to the database
for batch in utils.fetch_user_batches(session, identifiers, ids):
@@ -528,7 +530,7 @@ def _shared_friends_ids_followers_ids(
):
auth = json.load(open(auth))
session = utils.session_for_auth(auth)
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
identifiers = utils.resolve_identifiers(db, identifiers, attach, sql)
for identifier in identifiers:
# Make sure this user is saved
@@ -568,7 +570,7 @@ def import_(db_path, paths):
Import data from a Twitter exported archive. Input can be the path to a zip
file, a directory full of .js files or one or more direct .js files.
"""
- db = sqlite_utils.Database(db_path)
+ db = utils.open_database(db_path)
for filepath in paths:
path = pathlib.Path(filepath)
if path.suffix == ".zip":
diff --git a/twitter_to_sqlite/migrations.py b/twitter_to_sqlite/migrations.py
new file mode 100644
index 0000000..13d7c65
--- /dev/null
+++ b/twitter_to_sqlite/migrations.py
@@ -0,0 +1,22 @@
+from .utils import extract_and_save_source
+
+MIGRATIONS = []
+
+
+def migration(fn):
+ MIGRATIONS.append(fn)
+ return fn
+
+
+@migration
+def convert_source_column(db):
+ tables = set(db.table_names())
+ if "tweets" not in tables:
+ return
+ # Now we extract any '<a href=...' records from the source
+ for id, source in db.conn.execute(
+ "select id, source from tweets where source like '<%'"
+ ).fetchall():
+ db["tweets"].update(id, {"source": extract_and_save_source(db, source)})
+ db["tweets"].create_index(["source"])
+ db["tweets"].add_foreign_key("source")
diff --git a/twitter_to_sqlite/utils.py b/twitter_to_sqlite/utils.py
index 6f2a44e..1755c51 100644
--- a/twitter_to_sqlite/utils.py
+++ b/twitter_to_sqlite/utils.py
@@ -2,16 +2,46 @@ import datetime
import html
import json
import pathlib
+import re
import time
import urllib.parse
import zipfile
from dateutil import parser
from requests_oauthlib import OAuth1Session
+import sqlite_utils
# Twitter API error codes
RATE_LIMIT_ERROR_CODE = 88
+source_re = re.compile('<a href="(?P<url>.*?)".*?>(?P<name>.*?)</a>')
+
+
+def open_database(db_path):
+ db = sqlite_utils.Database(db_path)
+ # Only run migrations if this is an existing DB (has tables)
+ if db.tables:
+ migrate(db)
+ return db
+
+
+def migrate(db):
+ from twitter_to_sqlite.migrations import MIGRATIONS
+
+ if "migrations" not in db.table_names():
+ db["migrations"].create({"name": str, "applied": str}, pk="name")
+ applied_migrations = {
+ m[0] for m in db.conn.execute("select name from migrations").fetchall()
+ }
+ for migration in MIGRATIONS:
+ name = migration.__name__
+ if name in applied_migrations:
+ continue
+ migration(db)
+ db["migrations"].insert(
+ {"name": name, "applied": datetime.datetime.utcnow().isoformat()}
+ )
+
def session_for_auth(auth):
return OAuth1Session(
@@ -186,6 +216,8 @@ def ensure_tables(db):
table_names = set(db.table_names())
if "places" not in table_names:
db["places"].create({"id": str}, pk="id")
+ if "sources" not in table_names:
+ db["sources"].create({"id": str, "name": str, "url": str}, pk="id")
if "users" not in table_names:
db["users"].create(
{
@@ -210,9 +242,14 @@ def ensure_tables(db):
"retweeted_status": int,
"quoted_status": int,
"place": str,
+ "source": str,
},
pk="id",
- foreign_keys=(("user", "users", "id"), ("place", "places", "id")),
+ foreign_keys=(
+ ("user", "users", "id"),
+ ("place", "places", "id"),
+ ("source", "sources", "id"),
+ ),
)
db["tweets"].enable_fts(["full_text"], create_triggers=True)
db["tweets"].add_foreign_key("retweeted_status", "tweets")
@@ -235,6 +272,7 @@ def save_tweets(db, tweets, favorited_by=None):
user = tweet.pop("user")
transform_user(user)
tweet["user"] = user["id"]
+ tweet["source"] = extract_and_save_source(db, tweet["source"])
if tweet.get("place"):
db["places"].upsert(tweet["place"], pk="id", alter=True)
tweet["place"] = tweet["place"]["id"]
@@ -472,3 +510,9 @@ def read_archive_js(filepath):
for zi in zf.filelist:
if zi.filename.endswith(".js"):
yield zi.filename, zf.open(zi.filename).read()
+
+
+def extract_and_save_source(db, source):
+ m = source_re.match(source)
+ details = m.groupdict()
+ return db["sources"].upsert(details, hash_id="id").last_pk
| dogsheep/twitter-to-sqlite | 619f724a722b3f23f4364f67d3164b93e8ba2a70 | diff --git a/tests/test_migrations.py b/tests/test_migrations.py
new file mode 100644
index 0000000..5877d34
--- /dev/null
+++ b/tests/test_migrations.py
@@ -0,0 +1,49 @@
+import sqlite_utils
+from click.testing import CliRunner
+import sqlite_utils
+from twitter_to_sqlite import cli, migrations
+
+from .test_import import zip_contents_path
+
+
+def test_no_migrations_on_first_run(tmpdir, zip_contents_path):
+ output = str(tmpdir / "output.db")
+ args = ["import", output, str(zip_contents_path / "follower.js")]
+ result = CliRunner().invoke(cli.cli, args)
+ assert 0 == result.exit_code, result.stdout
+ db = sqlite_utils.Database(output)
+ assert ["archive_follower"] == db.table_names()
+ # Re-running the command again should also run the migrations
+ result = CliRunner().invoke(cli.cli, args)
+ db = sqlite_utils.Database(output)
+ assert {"archive_follower", "migrations"} == set(db.table_names())
+
+
+def test_convert_source_column():
+ db = sqlite_utils.Database(memory=True)
+ db["tweets"].insert_all(
+ [
+ {"id": 1, "source": '<a href="URL">NAME</a>'},
+ {"id": 2, "source": '<a href="URL2">NAME2</a>'},
+ {"id": 3, "source": "d3c1d39c57fecfc09202f20ea5e2db30262029fd"},
+ ],
+ pk="id",
+ )
+ migrations.convert_source_column(db)
+ assert [
+ {
+ "id": "d3c1d39c57fecfc09202f20ea5e2db30262029fd",
+ "url": "URL",
+ "name": "NAME",
+ },
+ {
+ "id": "000e4c4db71278018fb8c322f070d051e76885b1",
+ "url": "URL2",
+ "name": "NAME2",
+ },
+ ] == list(db["sources"].rows)
+ assert [
+ {"id": 1, "source": "d3c1d39c57fecfc09202f20ea5e2db30262029fd"},
+ {"id": 2, "source": "000e4c4db71278018fb8c322f070d051e76885b1"},
+ {"id": 3, "source": "d3c1d39c57fecfc09202f20ea5e2db30262029fd"},
+ ] == list(db["tweets"].rows)
diff --git a/tests/test_save_tweets.py b/tests/test_save_tweets.py
index 4bbe7ae..dc87a71 100644
--- a/tests/test_save_tweets.py
+++ b/tests/test_save_tweets.py
@@ -20,6 +20,7 @@ def db(tweets):
def test_tables(db):
assert {
+ "sources",
"users_fts_idx",
"users_fts_data",
"tweets_fts",
@@ -182,9 +183,9 @@ def test_tweets(db):
"retweeted_status": None,
"quoted_status": None,
"place": None,
+ "source": "e6528b505bcfd811fdd40ff2d46665dbccba2024",
"truncated": 0,
"display_text_range": "[0, 139]",
- "source": '<a href="http://itunes.apple.com/us/app/twitter/id409789998?mt=12" rel="nofollow">Twitter for Mac</a>',
"in_reply_to_status_id": None,
"in_reply_to_user_id": None,
"in_reply_to_screen_name": None,
@@ -207,9 +208,9 @@ def test_tweets(db):
"retweeted_status": None,
"quoted_status": 861696799362478100,
"place": None,
+ "source": "1f89d6a41b1505a3071169f8d0d028ba9ad6f952",
"truncated": 0,
"display_text_range": "[0, 239]",
- "source": '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>',
"in_reply_to_status_id": None,
"in_reply_to_user_id": None,
"in_reply_to_screen_name": None,
@@ -232,9 +233,9 @@ def test_tweets(db):
"retweeted_status": None,
"quoted_status": None,
"place": "01a9a39529b27f36",
+ "source": "95f3aaaddaa45937ac94765e0ddb68ba2be92d20",
"truncated": 0,
"display_text_range": "[45, 262]",
- "source": '<a href="http://twitter.com/download/iphone" rel="nofollow">Twitter for iPhone</a>',
"in_reply_to_status_id": "1169079390577320000",
"in_reply_to_user_id": "82016165",
"in_reply_to_screen_name": "scientiffic",
@@ -257,9 +258,9 @@ def test_tweets(db):
"retweeted_status": None,
"quoted_status": None,
"place": None,
+ "source": "942cfc2bf9f290ddbe3d78f1907dc084a00ed23f",
"truncated": 0,
"display_text_range": "[0, 235]",
- "source": '<a href="http://www.voxmedia.com" rel="nofollow">Vox Media</a>',
"in_reply_to_status_id": None,
"in_reply_to_user_id": None,
"in_reply_to_screen_name": None,
@@ -282,9 +283,9 @@ def test_tweets(db):
"retweeted_status": 1169242008432644000,
"quoted_status": None,
"place": None,
+ "source": "95f3aaaddaa45937ac94765e0ddb68ba2be92d20",
"truncated": 0,
"display_text_range": "[0, 143]",
- "source": '<a href="http://twitter.com/download/iphone" rel="nofollow">Twitter for iPhone</a>',
"in_reply_to_status_id": None,
"in_reply_to_user_id": None,
"in_reply_to_screen_name": None,
@@ -302,6 +303,32 @@ def test_tweets(db):
] == tweet_rows
+def test_sources(db):
+ source_rows = list(db["sources"].rows)
+ assert [
+ {
+ "id": "942cfc2bf9f290ddbe3d78f1907dc084a00ed23f",
+ "name": "Vox Media",
+ "url": "http://www.voxmedia.com",
+ },
+ {
+ "id": "95f3aaaddaa45937ac94765e0ddb68ba2be92d20",
+ "name": "Twitter for iPhone",
+ "url": "http://twitter.com/download/iphone",
+ },
+ {
+ "id": "1f89d6a41b1505a3071169f8d0d028ba9ad6f952",
+ "name": "Twitter Web App",
+ "url": "https://mobile.twitter.com",
+ },
+ {
+ "id": "e6528b505bcfd811fdd40ff2d46665dbccba2024",
+ "name": "Twitter for Mac",
+ "url": "http://itunes.apple.com/us/app/twitter/id409789998?mt=12",
+ },
+ ] == source_rows
+
+
def test_places(db):
place_rows = list(db["places"].rows)
assert [
| Extract "source" into a separate lookup table
It's pretty bulky and ugly at the moment:
<img width="334" alt="trump__tweets__1_820_rows" src="https://user-images.githubusercontent.com/9599/66264630-df23a080-e7bd-11e9-9154-403c2e69f841.png">
| 0.0 | [
"tests/test_migrations.py::test_no_migrations_on_first_run",
"tests/test_migrations.py::test_convert_source_column",
"tests/test_save_tweets.py::test_tables",
"tests/test_save_tweets.py::test_users",
"tests/test_save_tweets.py::test_tweets",
"tests/test_save_tweets.py::test_sources",
"tests/test_save_tweets.py::test_places",
"tests/test_save_tweets.py::test_media"
] | [] | 2019-10-17 15:24:56+00:00 | 1,976 |
|
dopefishh__pympi-39 | diff --git a/MANIFEST b/MANIFEST
index 5965331..0b43bb0 100644
--- a/MANIFEST
+++ b/MANIFEST
@@ -1,4 +1,5 @@
# file GENERATED by distutils, do NOT edit
+setup.cfg
setup.py
pympi/Elan.py
pympi/Praat.py
diff --git a/pympi/Elan.py b/pympi/Elan.py
index 4b4553e..0a3975c 100644
--- a/pympi/Elan.py
+++ b/pympi/Elan.py
@@ -1,10 +1,8 @@
-# -*- coding: utf-8 -*-
-
from xml.etree import cElementTree as etree
-import os
import re
import sys
import time
+import pathlib
import warnings
VERSION = '1.7'
@@ -663,7 +661,8 @@ class Eaf:
:returns: Tuple of the form: ``(min_time, max_time)``.
"""
return (0, 0) if not self.timeslots else\
- (min(self.timeslots.values()), max(self.timeslots.values()))
+ (min(v for v in self.timeslots.values() if v is not None),
+ max(v for v in self.timeslots.values() if v is not None))
def get_gaps_and_overlaps(self, tier1, tier2, maxlen=-1):
"""Give gaps and overlaps. The return types are shown in the table
@@ -1460,7 +1459,8 @@ def parse_eaf(file_path, eaf_obj, suppress_version_warning=False):
file_path = sys.stdin
# Annotation document
try:
- tree_root = etree.parse(file_path).getroot()
+ # py3.5 compat: etree.parse does not support pathlib.Path objects in py3.5.
+ tree_root = etree.parse(str(file_path)).getroot()
except etree.ParseError:
raise Exception('Unable to parse eaf, can you open it in ELAN?')
@@ -1623,15 +1623,7 @@ def to_eaf(file_path, eaf_obj, pretty=True):
:param bool pretty: Flag to set pretty printing.
"""
def rm_none(x):
- try: # Ugly hack to test if s is a string in py3 and py2
- basestring
-
- def isstr(s):
- return isinstance(s, basestring)
- except NameError:
- def isstr(s):
- return isinstance(s, str)
- return {k: v if isstr(v) else str(v) for k, v in x.items()
+ return {k: v if isinstance(v, str) else str(v) for k, v in x.items()
if v is not None}
# Annotation Document
ADOCUMENT = etree.Element('ANNOTATION_DOCUMENT', eaf_obj.adocument)
@@ -1717,7 +1709,8 @@ def to_eaf(file_path, eaf_obj, pretty=True):
except LookupError:
sys.stdout.write(etree.tostring(ADOCUMENT, encoding='UTF-8'))
else:
- if os.access(file_path, os.F_OK):
- os.rename(file_path, '{}.bak'.format(file_path))
+ file_path = pathlib.Path(file_path)
+ if file_path.exists():
+ file_path.rename(file_path.with_suffix('.bak'))
etree.ElementTree(ADOCUMENT).write(
- file_path, xml_declaration=True, encoding='UTF-8')
+ str(file_path), xml_declaration=True, encoding='UTF-8')
diff --git a/pympi/Praat.py b/pympi/Praat.py
index eba3c5d..9f1ecb6 100644
--- a/pympi/Praat.py
+++ b/pympi/Praat.py
@@ -1,6 +1,3 @@
-#!/usr/bin/env python
-# -*- coding: utf-8 -*-
-
import codecs
import re
import struct
@@ -63,11 +60,9 @@ class TextGrid:
elif textlen == -1:
textlen = struct.unpack('>h', ifile.read(2))[0]
data = ifile.read(textlen*2)
- # Hack to go from number to unicode in python3 and python2
- fun = unichr if 'unichr' in __builtins__ else chr
charlist = (data[i:i+2] for i in range(0, len(data), 2))
- return u''.join(
- fun(struct.unpack('>h', i)[0]) for i in charlist)
+ return ''.join(
+ chr(struct.unpack('>h', i)[0]) for i in charlist)
ifile.read(ord(ifile.read(1))) # skip oo type
self.xmin = struct.unpack('>d', ifile.read(8))[0]
@@ -98,9 +93,9 @@ class TextGrid:
line = next(ifile).decode(codec)
return pat.search(line).group(1)
- regfloat = re.compile('([\d.]+)\s*$', flags=re.UNICODE)
- regint = re.compile('([\d]+)\s*$', flags=re.UNICODE)
- regstr = re.compile('"(.*)"\s*$', flags=re.UNICODE)
+ regfloat = re.compile(r'([\d.]+)\s*$', flags=re.UNICODE)
+ regint = re.compile(r'([\d]+)\s*$', flags=re.UNICODE)
+ regstr = re.compile(r'"(.*)"\s*$', flags=re.UNICODE)
# Skip the Headers and empty line
next(ifile), next(ifile), next(ifile)
self.xmin = float(nn(ifile, regfloat))
@@ -159,7 +154,7 @@ class TextGrid:
elif number < 1 or number > len(self.tiers):
raise ValueError('Number not in [1..{}]'.format(len(self.tiers)))
elif tier_type not in Tier.P_TIERS:
- raise ValueError('tier_type has to be in {}'.format(self.P_TIERS))
+ raise ValueError('tier_type has to be in {}'.format(Tier.P_TIERS))
self.tiers.insert(number-1,
Tier(self.xmin, self.xmax, name, tier_type))
return self.tiers[number-1]
@@ -254,7 +249,8 @@ class TextGrid:
itier and f.write(struct.pack('>d', c[1]))
writebstr(c[2 if itier else 1])
elif mode in ['normal', 'n', 'short', 's']:
- with codecs.open(filepath, 'w', codec) as f:
+ # py3.5 compat: codecs.open does not support pathlib.Path objects in py3.5.
+ with codecs.open(str(filepath), 'w', codec) as f:
short = mode[0] == 's'
def wrt(indent, prefix, value, ff=''):
diff --git a/pympi/__init__.py b/pympi/__init__.py
index 53f76cc..791f39a 100644
--- a/pympi/__init__.py
+++ b/pympi/__init__.py
@@ -1,5 +1,5 @@
# Import the packages
from pympi.Praat import TextGrid
-from pympi.Elan import Eaf
+from pympi.Elan import Eaf, eaf_from_chat
__all__ = ['Praat', 'Elan', 'eaf_from_chat']
diff --git a/tox.ini b/tox.ini
index 142ca55..1ff9207 100644
--- a/tox.ini
+++ b/tox.ini
@@ -1,5 +1,5 @@
[tox]
-envlist = py{36,37,38}
+envlist = py{35,36,37,38}
skip_missing_interpreters = true
[testenv]
| dopefishh/pympi | fd924ffca6ea8ca2ef81384f97ce4396334ef850 | diff --git a/test/test_elan.py b/test/test_elan.py
index 0249154..34ee7da 100644
--- a/test/test_elan.py
+++ b/test/test_elan.py
@@ -1153,10 +1153,14 @@ class Elan(unittest.TestCase):
]
)
def test_to_file_to_eaf(eaf, schema, test_dir, tmp_path):
- filepath = tmp_path / 'test.eaf'
- eaf = Eaf(test_dir / eaf)
+ filepath = str(tmp_path / 'test.eaf')
+ eaf = Eaf(str(test_dir / eaf))
eaf.to_file(filepath)
schema = etree.XMLSchema(etree.XML(test_dir.joinpath(schema).read_text(encoding='utf8')))
xmlparser = etree.XMLParser(schema=schema)
etree.parse(str(filepath), xmlparser)
+
+
+def test_to_textgrid(test_dir):
+ _ = Eaf(str(test_dir / 'sample_2.7.eaf')).to_textgrid()
diff --git a/test/test_praat.py b/test/test_praat.py
index f71afc8..1c94c41 100644
--- a/test/test_praat.py
+++ b/test/test_praat.py
@@ -1,9 +1,7 @@
-#!/bin/env python
-# -*- coding: utf-8 -*-
-
+import pathlib
import unittest
-import tempfile
-import os
+import pytest
+
from pympi.Praat import TextGrid
@@ -126,38 +124,6 @@ class PraatTest(unittest.TestCase):
self.assertEqual([(1, 'tier1'), (2, 'tier3'), (3, 'tier2')],
list(self.tg.get_tier_name_num()))
- def test_to_file(self):
- for codec in ['utf-8', 'latin_1', 'mac_roman']:
- self.tg = TextGrid(xmax=20)
- tier1 = self.tg.add_tier('tier')
- tier1.add_interval(1, 2, 'i1')
- tier1.add_interval(2, 3, 'i2')
- tier1.add_interval(4, 5, 'i3')
-
- tier4 = self.tg.add_tier('tier')
- tier4.add_interval(1, 2, u'i1ü')
- tier4.add_interval(2.0, 3, 'i2')
- tier4.add_interval(4, 5.0, 'i3')
-
- tier2 = self.tg.add_tier('tier2', tier_type='TextTier')
- tier2.add_point(1, u'p1ü')
- tier2.add_point(2, 'p1')
- tier2.add_point(3, 'p1')
-
- tempf = tempfile.mkstemp()[1]
-
-# Normal mode
- self.tg.to_file(tempf, codec=codec)
- TextGrid(tempf, codec=codec)
-# Short mode
- self.tg.to_file(tempf, codec=codec, mode='s')
- TextGrid(tempf, codec=codec)
-# Binary mode
- self.tg.to_file(tempf, mode='b')
- TextGrid(tempf)
-
- os.remove(tempf)
-
def test_to_eaf(self):
tier1 = self.tg.add_tier('tier1')
tier2 = self.tg.add_tier('tier2', tier_type='TextTier')
@@ -267,5 +233,33 @@ class PraatTest(unittest.TestCase):
self.tier2.clear_intervals()
self.assertEqual([], self.tier2.intervals)
-if __name__ == '__main__':
- unittest.main()
+
+@pytest.mark.parametrize('codec', ['utf-8', 'latin_1', 'mac_roman'])
+def test_to_file(codec, tmp_path):
+ tg = TextGrid(xmax=20)
+ tier1 = tg.add_tier('tier')
+ tier1.add_interval(1, 2, 'i1')
+ tier1.add_interval(2, 3, 'i2')
+ tier1.add_interval(4, 5, 'i3')
+
+ tier4 = tg.add_tier('tier')
+ tier4.add_interval(1, 2, u'i1ü')
+ tier4.add_interval(2.0, 3, 'i2')
+ tier4.add_interval(4, 5.0, 'i3')
+
+ tier2 = tg.add_tier('tier2', tier_type='TextTier')
+ tier2.add_point(1, u'p1ü')
+ tier2.add_point(2, 'p1')
+ tier2.add_point(3, 'p1')
+
+ tempf = str(tmp_path / 'test')
+
+ # Normal mode
+ tg.to_file(pathlib.Path(tempf), codec=codec)
+ TextGrid(tempf, codec=codec)
+ # Short mode
+ tg.to_file(tempf, codec=codec, mode='s')
+ TextGrid(tempf, codec=codec)
+ # Binary mode
+ tg.to_file(tempf, mode='b')
+ TextGrid(tempf)
| Support pathlib.Path objects in addition to str file paths
I propose to additionally accept `pathlib.Path` objects wherever file paths as `str` are accepted now. This makes tests simpler (when using pytest's `tmp_path`) and is also becoming the standard behaviour of most python stdlib modules. | 0.0 | [
"test/test_elan.py::test_to_textgrid"
] | [
"test/test_elan.py::Elan::test_add_annotation",
"test/test_elan.py::Elan::test_add_controlled_vocabulary",
"test/test_elan.py::Elan::test_add_cv_description",
"test/test_elan.py::Elan::test_add_cv_entry",
"test/test_elan.py::Elan::test_add_external_ref",
"test/test_elan.py::Elan::test_add_language",
"test/test_elan.py::Elan::test_add_lexicon_ref",
"test/test_elan.py::Elan::test_add_license",
"test/test_elan.py::Elan::test_add_linguistic_type",
"test/test_elan.py::Elan::test_add_linked_file",
"test/test_elan.py::Elan::test_add_locale",
"test/test_elan.py::Elan::test_add_nested_reference_annotations",
"test/test_elan.py::Elan::test_add_property",
"test/test_elan.py::Elan::test_add_ref_annotation",
"test/test_elan.py::Elan::test_add_secondary_linked_file",
"test/test_elan.py::Elan::test_add_tier",
"test/test_elan.py::Elan::test_clean_time_slots",
"test/test_elan.py::Elan::test_copy_tier",
"test/test_elan.py::Elan::test_create_gaps_and_overlaps_tier",
"test/test_elan.py::Elan::test_eaf_from_chat",
"test/test_elan.py::Elan::test_filter_annotations",
"test/test_elan.py::Elan::test_get_annotation_data_after_time",
"test/test_elan.py::Elan::test_get_annotation_data_at_time",
"test/test_elan.py::Elan::test_get_annotation_data_before_time",
"test/test_elan.py::Elan::test_get_annotation_data_between_times",
"test/test_elan.py::Elan::test_get_annotation_data_for_tier",
"test/test_elan.py::Elan::test_get_child_tiers_for",
"test/test_elan.py::Elan::test_get_controlled_vocabulary_names",
"test/test_elan.py::Elan::test_get_cv_descriptions",
"test/test_elan.py::Elan::test_get_cv_entry",
"test/test_elan.py::Elan::test_get_external_ref",
"test/test_elan.py::Elan::test_get_external_ref_names",
"test/test_elan.py::Elan::test_get_full_time_interval",
"test/test_elan.py::Elan::test_get_gaps_and_overlaps2",
"test/test_elan.py::Elan::test_get_languages",
"test/test_elan.py::Elan::test_get_lexicon_ref",
"test/test_elan.py::Elan::test_get_lexicon_ref_names",
"test/test_elan.py::Elan::test_get_licenses",
"test/test_elan.py::Elan::test_get_linguistic_types_names",
"test/test_elan.py::Elan::test_get_linked_files",
"test/test_elan.py::Elan::test_get_locales",
"test/test_elan.py::Elan::test_get_parameters_for_linguistic_type",
"test/test_elan.py::Elan::test_get_parameters_for_tier",
"test/test_elan.py::Elan::test_get_properties",
"test/test_elan.py::Elan::test_get_ref_annotation_at_time",
"test/test_elan.py::Elan::test_get_ref_annotation_data_between_times",
"test/test_elan.py::Elan::test_get_ref_annotation_data_for_tier",
"test/test_elan.py::Elan::test_get_secondary_linked_files",
"test/test_elan.py::Elan::test_get_tier_ids_for_linguistic_type",
"test/test_elan.py::Elan::test_get_tier_names",
"test/test_elan.py::Elan::test_merge_tiers",
"test/test_elan.py::Elan::test_parse_eaf",
"test/test_elan.py::Elan::test_ref_get_annotation_data_after_time",
"test/test_elan.py::Elan::test_ref_get_annotation_data_before_time",
"test/test_elan.py::Elan::test_remove_all_annotations_from_tier",
"test/test_elan.py::Elan::test_remove_annotation",
"test/test_elan.py::Elan::test_remove_controlled_vocabulary",
"test/test_elan.py::Elan::test_remove_cv_description",
"test/test_elan.py::Elan::test_remove_cv_entry",
"test/test_elan.py::Elan::test_remove_external_ref",
"test/test_elan.py::Elan::test_remove_language",
"test/test_elan.py::Elan::test_remove_lexicon_ref",
"test/test_elan.py::Elan::test_remove_license",
"test/test_elan.py::Elan::test_remove_linguistic_type",
"test/test_elan.py::Elan::test_remove_linked_files",
"test/test_elan.py::Elan::test_remove_locale",
"test/test_elan.py::Elan::test_remove_property",
"test/test_elan.py::Elan::test_remove_ref_annotation",
"test/test_elan.py::Elan::test_remove_secondary_linked_files",
"test/test_elan.py::Elan::test_remove_tier",
"test/test_elan.py::Elan::test_remove_tiers",
"test/test_elan.py::Elan::test_rename_tier",
"test/test_elan.py::Elan::test_shift_annotations",
"test/test_elan.py::Elan::test_to_textgrid",
"test/test_elan.py::test_to_file_to_eaf[sample_2.8.eaf-EAFv2.8.xsd]",
"test/test_elan.py::test_to_file_to_eaf[sample_2.7.eaf-EAFv2.8.xsd]",
"test/test_elan.py::test_to_file_to_eaf[sample_3.0.eaf-EAFv3.0.xsd]",
"test/test_praat.py::PraatTest::test_add_interval",
"test/test_praat.py::PraatTest::test_add_point",
"test/test_praat.py::PraatTest::test_add_tier",
"test/test_praat.py::PraatTest::test_change_tier_name",
"test/test_praat.py::PraatTest::test_clear_intervals",
"test/test_praat.py::PraatTest::test_get_intervals",
"test/test_praat.py::PraatTest::test_get_tier",
"test/test_praat.py::PraatTest::test_get_tier_name_num",
"test/test_praat.py::PraatTest::test_get_tiers",
"test/test_praat.py::PraatTest::test_remove_interval",
"test/test_praat.py::PraatTest::test_remove_point",
"test/test_praat.py::PraatTest::test_remove_tier",
"test/test_praat.py::PraatTest::test_sort_tiers",
"test/test_praat.py::PraatTest::test_to_eaf",
"test/test_praat.py::test_to_file[utf-8]",
"test/test_praat.py::test_to_file[latin_1]",
"test/test_praat.py::test_to_file[mac_roman]"
] | 2021-02-04 11:28:45+00:00 | 1,977 |
|
dotmesh-io__dotscience-python-37 | diff --git a/dotscience/__init__.py b/dotscience/__init__.py
index e12da54..c2c3ac3 100644
--- a/dotscience/__init__.py
+++ b/dotscience/__init__.py
@@ -253,6 +253,9 @@ class Dotscience:
currentRun = None
def __init__(self):
+ self._reset()
+
+ def _reset(self):
self._mode = None
self._workload_file = None
self._root = os.getenv('DOTSCIENCE_PROJECT_DOT_ROOT', default=os.getcwd())
@@ -269,6 +272,7 @@ class Dotscience:
# TODO: make publish etc fail if we're not connected in remote mode.
if not project:
raise Exception("Please specify a project name as the third argument to ds.connect()")
+ self._reset()
self._dotmesh_client = DotmeshClient(
cluster_url=hostname + "/v2/dotmesh/rpc",
username=username,
| dotmesh-io/dotscience-python | 7e2ec1fceacd0bd8ec2517a2dd6cc9fd74a0f82a | diff --git a/dotscience/test_dotscience.py b/dotscience/test_dotscience.py
index 92790c7..89ab7e9 100644
--- a/dotscience/test_dotscience.py
+++ b/dotscience/test_dotscience.py
@@ -857,3 +857,39 @@ def test_multi_publish_2():
assert m1["__ID"] != m2["__ID"]
assert m1["start"] != m2["start"]
assert m1["end"] != m2["end"]
+
+
+def test_reconnect_resets_internal_state(monkeypatch):
+ class FakeDotmeshClient:
+ def __init__(self, cluster_url, username, api_key):
+ self.cluster_url = cluster_url
+ self.username = username
+ self.api_key = api_key
+
+ def ping(self):
+ pass
+
+ monkeypatch.setattr(dotscience, "DotmeshClient", FakeDotmeshClient)
+
+ ds = dotscience.Dotscience()
+ ds.connect("me", "pass", "myproj", "https://example.com")
+ assert ds._dotmesh_client.__dict__ == {
+ "cluster_url": "https://example.com/v2/dotmesh/rpc",
+ "username": "me",
+ "api_key": "pass",
+ }
+ assert ds._project_name == "myproj"
+
+ # Pretend we have a cached project:
+ ds._cached_project = "not empty"
+ assert ds._get_project_or_create("myproj") == "not empty"
+
+ # Now, reconnect:
+ ds.connect("me2", "pass2", "myproj2", "https://2.example.com")
+ assert ds._dotmesh_client.__dict__ == {
+ "cluster_url": "https://2.example.com/v2/dotmesh/rpc",
+ "username": "me2",
+ "api_key": "pass2",
+ }
+ assert ds._project_name == "myproj2"
+ assert ds._cached_project == None
| ds.connect() only connects to single project and runs overwritten [fusemachines]
Fuse machines run ds.connect() from a local machine but it only connects to a single project.
Also runs are getting overwritten when running on the same machine.
This text is just a placeholder: it was decided on the Fuse machines call Oct 21st 4-5pm BST that they may need to do a call with the support engineer to fully explain. And/or send a video/screenshots.
Link to description from Fuse Machines: https://docs.google.com/document/d/1ZLhKlW6AG3khlPVyrINXD3LaS1fSTilyEorrj66w9ow | 0.0 | [
"dotscience/test_dotscience.py::test_reconnect_resets_internal_state"
] | [
"dotscience/test_dotscience.py::test_input_1b",
"dotscience/test_dotscience.py::test_run_parameter_1",
"dotscience/test_dotscience.py::test_run_output_2",
"dotscience/test_dotscience.py::test_multi_publish_2",
"dotscience/test_dotscience.py::test_notice_jupyter_mode",
"dotscience/test_dotscience.py::test_explicit_script_name",
"dotscience/test_dotscience.py::test_notice_command_mode",
"dotscience/test_dotscience.py::test_label_1a",
"dotscience/test_dotscience.py::test_summary_1a",
"dotscience/test_dotscience.py::test_parameter_n",
"dotscience/test_dotscience.py::test_run_input_n",
"dotscience/test_dotscience.py::test_parameter_1a",
"dotscience/test_dotscience.py::test_run_summary_1",
"dotscience/test_dotscience.py::test_conflicting_mode_2",
"dotscience/test_dotscience.py::test_run_input_relative",
"dotscience/test_dotscience.py::test_run_input_2",
"dotscience/test_dotscience.py::test_run_summary_multi",
"dotscience/test_dotscience.py::test_run_basics",
"dotscience/test_dotscience.py::test_output_n",
"dotscience/test_dotscience.py::test_output_1a",
"dotscience/test_dotscience.py::test_run_start_1",
"dotscience/test_dotscience.py::test_start_end",
"dotscience/test_dotscience.py::test_run_start_2",
"dotscience/test_dotscience.py::test_summary_1b",
"dotscience/test_dotscience.py::test_description_a",
"dotscience/test_dotscience.py::test_non_conflicting_mode_2",
"dotscience/test_dotscience.py::test_description_c",
"dotscience/test_dotscience.py::test_conflicting_mode_1",
"dotscience/test_dotscience.py::test_parameter_1b",
"dotscience/test_dotscience.py::test_run_end_1",
"dotscience/test_dotscience.py::test_run_input_recursive",
"dotscience/test_dotscience.py::test_run_output_1",
"dotscience/test_dotscience.py::test_run_labels_1",
"dotscience/test_dotscience.py::test_run_labels_multi",
"dotscience/test_dotscience.py::test_run_input_1",
"dotscience/test_dotscience.py::test_run_output_n",
"dotscience/test_dotscience.py::test_error_b",
"dotscience/test_dotscience.py::test_label_1b",
"dotscience/test_dotscience.py::test_input_1a",
"dotscience/test_dotscience.py::test_run_null",
"dotscience/test_dotscience.py::test_multi_publish_1",
"dotscience/test_dotscience.py::test_output_1b",
"dotscience/test_dotscience.py::test_error_a",
"dotscience/test_dotscience.py::test_no_script_name_when_interactive",
"dotscience/test_dotscience.py::test_label_n",
"dotscience/test_dotscience.py::test_summary_n",
"dotscience/test_dotscience.py::test_run_output_relative",
"dotscience/test_dotscience.py::test_no_mode",
"dotscience/test_dotscience.py::test_input_n",
"dotscience/test_dotscience.py::test_run_tensorflow_model",
"dotscience/test_dotscience.py::test_null",
"dotscience/test_dotscience.py::test_run_end_2",
"dotscience/test_dotscience.py::test_non_conflicting_mode_1",
"dotscience/test_dotscience.py::test_run_output_recursive",
"dotscience/test_dotscience.py::test_run_tensorflow_model_with_classes",
"dotscience/test_dotscience.py::test_run_parameter_multi",
"dotscience/test_dotscience.py::test_description_b"
] | 2019-10-29 17:39:37+00:00 | 1,978 |
|
dotpot__InAppPy-54 | diff --git a/README.rst b/README.rst
index 9ea1223..771796a 100644
--- a/README.rst
+++ b/README.rst
@@ -17,10 +17,11 @@ Table of contents
2. Installation
3. Google Play (`receipt` + `signature`)
4. Google Play (verification)
-5. App Store (`receipt` + using optional `shared-secret`)
-6. App Store Response (`validation_result` / `raw_response`) example
-7. App Store, **asyncio** version (available in the inapppy.asyncio package)
-8. Development
+5. Google Play (verification with result)
+6. App Store (`receipt` + using optional `shared-secret`)
+7. App Store Response (`validation_result` / `raw_response`) example
+8. App Store, **asyncio** version (available in the inapppy.asyncio package)
+9. Development
1. Introduction
@@ -88,7 +89,42 @@ In-app purchase validation library for `Apple AppStore` and `GooglePlay` (`App S
return response
-5. App Store (validates `receipt` using optional `shared-secret` against iTunes service)
+5. Google Play verification (with result)
+=========================================
+Alternative to `.verify` method, instead of raising an error result class will be returned.
+
+.. code:: python
+
+ from inapppy import GooglePlayVerifier, errors
+
+
+ def google_validator(receipt):
+ """
+ Accepts receipt, validates in Google.
+ """
+ purchase_token = receipt['purchaseToken']
+ product_sku = receipt['productId']
+ verifier = GooglePlayVerifier(
+ GOOGLE_BUNDLE_ID,
+ GOOGLE_SERVICE_ACCOUNT_KEY_FILE,
+ )
+ response = {'valid': False, 'transactions': []}
+
+ result = verifier.verify_with_result(
+ purchase_token,
+ product_sku,
+ is_subscription=True
+ )
+
+ # result contains data
+ raw_response = result.raw_response
+ is_canceled = result.is_canceled
+ is_expired = result.is_expired
+
+ return result
+
+
+6. App Store (validates `receipt` using optional `shared-secret` against iTunes service)
========================================================================================
.. code:: python
@@ -110,7 +146,7 @@ In-app purchase validation library for `Apple AppStore` and `GooglePlay` (`App S
-6. App Store Response (`validation_result` / `raw_response`) example
+7. App Store Response (`validation_result` / `raw_response`) example
====================================================================
.. code:: json
@@ -190,7 +226,7 @@ In-app purchase validation library for `Apple AppStore` and `GooglePlay` (`App S
}
-7. App Store, asyncio version (available in the inapppy.asyncio package)
+8. App Store, asyncio version (available in the inapppy.asyncio package)
========================================================================
.. code:: python
@@ -213,7 +249,7 @@ In-app purchase validation library for `Apple AppStore` and `GooglePlay` (`App S
-8. Development
+9. Development
==============
.. code:: bash
diff --git a/inapppy/errors.py b/inapppy/errors.py
index 6436945..bad7e22 100644
--- a/inapppy/errors.py
+++ b/inapppy/errors.py
@@ -18,5 +18,4 @@ class InAppPyValidationError(Exception):
class GoogleError(InAppPyValidationError):
- def __init__(self, message: str = None, raw_response: dict = None, *args, **kwargs):
- super().__init__(message, raw_response, *args, **kwargs)
+ pass
diff --git a/inapppy/googleplay.py b/inapppy/googleplay.py
index 25a849c..eee64a8 100644
--- a/inapppy/googleplay.py
+++ b/inapppy/googleplay.py
@@ -72,6 +72,27 @@ class GooglePlayValidator:
return False
+class GoogleVerificationResult:
+ """Google verification result class."""
+
+ raw_response: dict = {}
+ is_expired: bool = False
+ is_canceled: bool = False
+
+ def __init__(self, raw_response: dict, is_expired: bool, is_canceled: bool):
+ self.raw_response = raw_response
+ self.is_expired = is_expired
+ self.is_canceled = is_canceled
+
+ def __repr__(self):
+ return (
+ f"GoogleVerificationResult("
+ f"raw_response={self.raw_response}, "
+ f"is_expired={self.is_expired}, "
+ f"is_canceled={self.is_canceled})"
+ )
+
+
class GooglePlayVerifier:
def __init__(self, bundle_id: str, private_key_path: str, http_timeout: int = 15) -> None:
"""
@@ -159,3 +180,32 @@ class GooglePlayVerifier:
raise GoogleError("Purchase cancelled", result)
return result
+
+ def verify_with_result(
+ self, purchase_token: str, product_sku: str, is_subscription: bool = False
+ ) -> GoogleVerificationResult:
+ """Verifies by returning verification result instead of raising an error,
+ basically it's and better alternative to verify method."""
+ service = build("androidpublisher", "v3", http=self.http)
+ verification_result = GoogleVerificationResult({}, False, False)
+
+ if is_subscription:
+ result = self.check_purchase_subscription(purchase_token, product_sku, service)
+ verification_result.raw_response = result
+
+ cancel_reason = int(result.get("cancelReason", 0))
+ if cancel_reason != 0:
+ verification_result.is_canceled = True
+
+ ms_timestamp = result.get("expiryTimeMillis", 0)
+ if self._ms_timestamp_expired(ms_timestamp):
+ verification_result.is_expired = True
+ else:
+ result = self.check_purchase_product(purchase_token, product_sku, service)
+ verification_result.raw_response = result
+
+ purchase_state = int(result.get("purchaseState", 1))
+ if purchase_state != 0:
+ verification_result.is_canceled = True
+
+ return verification_result
| dotpot/InAppPy | 662179362b679dbd3250cc759299bd454842b6dc | diff --git a/tests/test_google_verifier.py b/tests/test_google_verifier.py
index 0e213a7..ed517e2 100644
--- a/tests/test_google_verifier.py
+++ b/tests/test_google_verifier.py
@@ -32,6 +32,48 @@ def test_google_verify_subscription():
verifier.verify("test-token", "test-product", is_subscription=True)
+def test_google_verify_with_result_subscription():
+ with patch.object(googleplay, "build", return_value=None):
+ with patch.object(googleplay.GooglePlayVerifier, "_authorize", return_value=None):
+ verifier = googleplay.GooglePlayVerifier("test-bundle-id", "private_key_path", 30)
+
+ # expired
+ with patch.object(verifier, "check_purchase_subscription", return_value={"expiryTimeMillis": 666}):
+ result = verifier.verify_with_result("test-token", "test-product", is_subscription=True)
+ assert result.is_canceled is False
+ assert result.is_expired
+ assert result.raw_response == {"expiryTimeMillis": 666}
+ assert (
+ str(result) == "GoogleVerificationResult(raw_response="
+ "{'expiryTimeMillis': 666}, "
+ "is_expired=True, "
+ "is_canceled=False)"
+ )
+
+ # canceled
+ with patch.object(verifier, "check_purchase_subscription", return_value={"cancelReason": 666}):
+ result = verifier.verify_with_result("test-token", "test-product", is_subscription=True)
+ assert result.is_canceled
+ assert result.is_expired
+ assert result.raw_response == {"cancelReason": 666}
+ assert (
+ str(result) == "GoogleVerificationResult("
+ "raw_response={'cancelReason': 666}, "
+ "is_expired=True, "
+ "is_canceled=True)"
+ )
+
+ # norm
+ now = datetime.datetime.utcnow().timestamp()
+ exp_value = now * 1000 + 10 ** 10
+ with patch.object(verifier, "check_purchase_subscription", return_value={"expiryTimeMillis": exp_value}):
+ result = verifier.verify_with_result("test-token", "test-product", is_subscription=True)
+ assert result.is_canceled is False
+ assert result.is_expired is False
+ assert result.raw_response == {"expiryTimeMillis": exp_value}
+ assert str(result) is not None
+
+
def test_google_verify_product():
with patch.object(googleplay, "build", return_value=None):
with patch.object(googleplay.GooglePlayVerifier, "_authorize", return_value=None):
@@ -47,6 +89,33 @@ def test_google_verify_product():
verifier.verify("test-token", "test-product")
+def test_google_verify_with_result_product():
+ with patch.object(googleplay, "build", return_value=None):
+ with patch.object(googleplay.GooglePlayVerifier, "_authorize", return_value=None):
+ verifier = googleplay.GooglePlayVerifier("test-bundle-id", "private_key_path", 30)
+
+ # purchase
+ with patch.object(verifier, "check_purchase_product", return_value={"purchaseState": 0}):
+ result = verifier.verify_with_result("test-token", "test-product")
+ assert result.is_canceled is False
+ assert result.is_expired is False
+ assert result.raw_response == {"purchaseState": 0}
+ assert str(result) is not None
+
+ # cancelled
+ with patch.object(verifier, "check_purchase_product", return_value={"purchaseState": 1}):
+ result = verifier.verify_with_result("test-token", "test-product")
+ assert result.is_canceled
+ assert result.is_expired is False
+ assert result.raw_response == {"purchaseState": 1}
+ assert (
+ str(result) == "GoogleVerificationResult("
+ "raw_response={'purchaseState': 1}, "
+ "is_expired=False, "
+ "is_canceled=True)"
+ )
+
+
DATA_DIR = os.path.join(os.path.dirname(__file__), "data")
| cancelled android purchases do not get caught correctly
in lines 109-110 of googleplay.py:
```
cancel_reason = int(result.get('cancelReason', 0))
if cancel_reason != 0:
raise GoogleError('Subscription is canceled', result)
```
If we look at [the docs](https://developers.google.com/android-publisher/api-ref/purchases/subscriptions) we see that a value of `cancelReason = 0` also indicates cancellation by user. Therefore I do not understand what was the original intention of this snippet of code. If we look at the lines following this snippet:
```
ms_timestamp = result.get('expiryTimeMillis', 0)
if self._ms_timestamp_expired(ms_timestamp):
raise GoogleError('Subscription expired', result)
else:
result = self.check_purchase_product(purchase_token, product_sku, service)
purchase_state = int(result.get('purchaseState', 1))
if purchase_state != 0:
raise GoogleError('Purchase cancelled', result)
```
I think the general issue here is, that a subscription can be
a) not cancelled and not expired
b) cancelled and not expired
c) cancelled and expired
So even if we fixed the issue with the code above, we would have to rethink how we throw errors / catch google certificate expiry (see also #25).
I suggest we remove the current mechanism of throwing errors an instead return some sort of datastructure containing: `raw_response`, `is_expired`, `is_cancelled`.
That way we describe all 3 scenarios above. What do you think? | 0.0 | [
"tests/test_google_verifier.py::test_google_verify_with_result_subscription",
"tests/test_google_verifier.py::test_google_verify_with_result_product"
] | [
"tests/test_google_verifier.py::test_google_verify_subscription",
"tests/test_google_verifier.py::test_google_verify_product",
"tests/test_google_verifier.py::test_bad_request_subscription",
"tests/test_google_verifier.py::test_bad_request_product"
] | 2019-07-25 13:24:02+00:00 | 1,979 |
|
dougthor42__pynuget-41 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index 464e237..4caff42 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -6,6 +6,9 @@
+ Optionally run coverage when running in localhost development.
+ Fixed an issue where files with dependencies would break the "List" nuget
command (#39)
++ Fixed an issue where deleting the last remaining version of a package
+ would not delete the row in the Package table, thus causing subsequet pushes
+ of the same version to fail. (#38)
## 0.2.1 (2018-07-25)
diff --git a/src/pynuget/db.py b/src/pynuget/db.py
index cb7caf7..668c652 100644
--- a/src/pynuget/db.py
+++ b/src/pynuget/db.py
@@ -73,7 +73,7 @@ class Version(Base):
package = relationship("Package", backref="versions")
def __repr__(self):
- return "<Version({}, {})>".format(self.package.name, self.version)
+ return "<Version({}, {}, {})>".format(self.version_id, self.package.name, self.version)
@hybrid_property
def thing(self):
@@ -330,18 +330,19 @@ def delete_version(session, package_name, version):
.filter(Package.name == package_name)
.filter(Version.version == version)
)
- package = sql.one().package
- logger.debug(package)
+ version = sql.one()
+ pkg = version.package
- session.delete(sql.one())
- session.commit()
+ session.delete(version)
# update the Package.latest_version value, or delete the Package
versions = (session.query(Version)
+ .join(Package)
.filter(Package.name == package_name)
).all()
if len(versions) > 0:
- package.latest_version = max(v.version for v in versions)
+ pkg.latest_version = max(v.version for v in versions)
else:
- session.delete(package)
+ logger.info("No more versions exist. Deleting package %s" % pkg)
+ session.delete(pkg)
session.commit()
| dougthor42/pynuget | 4540549e0118c1e044799f2c8df5e48223216703 | diff --git a/tests/test_db.py b/tests/test_db.py
index 9f31f43..e2f4fb5 100644
--- a/tests/test_db.py
+++ b/tests/test_db.py
@@ -150,22 +150,46 @@ def test_insert_version(session):
def test_delete_version(session):
+ # Add additional dummy data.
+ pkg = db.Package(name="Foo", latest_version="0.9.6")
+ session.add(pkg)
+ session.commit()
+
+ session.add(db.Version(package_id=pkg.package_id, version="0.9.6"))
+ session.add(db.Version(package_id=pkg.package_id, version="0.9.7"))
+ session.commit()
+
+ # The package we're interested in.
+ pkg_id = 'dummy'
+
# get our initial counts
- version_count = session.query(sa.func.count(db.Version.version_id))
+ version_count = (session.query(sa.func.count(db.Version.version_id))
+ .join(db.Package)
+ .filter(db.Package.name == pkg_id))
package_count = session.query(sa.func.count(db.Package.package_id))
initial_version_count = version_count.scalar()
initial_package_count = package_count.scalar()
- # Delete a version
- pkg_id = 'dummy'
db.delete_version(session, pkg_id, '0.0.2')
+ # The number of versions for our package should have decreased by 1.
assert initial_version_count - 1 == version_count.scalar()
+
+ # We should still have 2 packages
+ assert initial_package_count == 2
assert initial_package_count == package_count.scalar()
+
+ # The deleted version should not show up at all. (Note this particular
+ # test only works because our dummy data only has 1 instance of "0.0.2")
assert '0.0.2' not in session.query(db.Version.version).all()
- # twice more, the 2nd of which should delete the package
+ # Deleting the highest version should change the `latest_version` value
+ qry = session.query(db.Package).filter(db.Package.name == 'dummy')
db.delete_version(session, pkg_id, '0.0.3')
+ assert qry.one().latest_version == '0.0.1'
+
+ # Deleting the last version of a package should remove the row from the
+ # Packages table.
db.delete_version(session, pkg_id, '0.0.1')
assert version_count.scalar() == 0
- assert package_count.scalar() == 0
+ assert package_count.scalar() == 1
| Deleting the last version of a package does not delete the entry in the "package" table.
Deleting the last version of a package does not delete the entry in the "package" table.
Steps to reproduce:
1. Upload `foo.0.0.1.nupkg`
2. Delete `foo.0.0.2.nupkg`
3. Attempt to upload `foo.0.0.1.nupkg` again
4. See that you get a 'package already exists' error.
5. View the sqlite file. table package contains 'foo' while table version does not. | 0.0 | [
"tests/test_db.py::test_delete_version"
] | [
"tests/test_db.py::test_count_packages",
"tests/test_db.py::test_search_packages",
"tests/test_db.py::test_package_updates",
"tests/test_db.py::test_find_by_pkg_name",
"tests/test_db.py::test_validate_id_and_version",
"tests/test_db.py::test_increment_download_count",
"tests/test_db.py::test_insert_or_update_package",
"tests/test_db.py::test_insert_version"
] | 2018-07-26 22:21:10+00:00 | 1,980 |
|
dpkp__kafka-python-1507 | diff --git a/kafka/cluster.py b/kafka/cluster.py
index 45f25ad..8078eb7 100644
--- a/kafka/cluster.py
+++ b/kafka/cluster.py
@@ -214,7 +214,8 @@ class ClusterMetadata(object):
return self.failed_update(error)
if not metadata.brokers:
- log.warning("No broker metadata found in MetadataResponse")
+ log.warning("No broker metadata found in MetadataResponse -- ignoring.")
+ return self.failed_update(Errors.MetadataEmptyBrokerList(metadata))
_new_brokers = {}
for broker in metadata.brokers:
diff --git a/kafka/conn.py b/kafka/conn.py
index f67edfb..a2d5ee6 100644
--- a/kafka/conn.py
+++ b/kafka/conn.py
@@ -292,11 +292,7 @@ class BrokerConnection(object):
# First attempt to perform dns lookup
# note that the underlying interface, socket.getaddrinfo,
# has no explicit timeout so we may exceed the user-specified timeout
- while time.time() < timeout:
- if self._dns_lookup():
- break
- else:
- return False
+ self._dns_lookup()
# Loop once over all returned dns entries
selector = None
@@ -903,6 +899,7 @@ class BrokerConnection(object):
Returns: version tuple, i.e. (0, 10), (0, 9), (0, 8, 2), ...
"""
+ timeout_at = time.time() + timeout
log.info('Probing node %s broker version', self.node_id)
# Monkeypatch some connection configurations to avoid timeouts
override_config = {
@@ -932,7 +929,7 @@ class BrokerConnection(object):
]
for version, request in test_cases:
- if not self.connect_blocking(timeout):
+ if not self.connect_blocking(timeout_at - time.time()):
raise Errors.NodeNotReadyError()
f = self.send(request)
# HACK: sleeping to wait for socket to send bytes
diff --git a/kafka/errors.py b/kafka/errors.py
index f4c8740..93a9f40 100644
--- a/kafka/errors.py
+++ b/kafka/errors.py
@@ -54,6 +54,10 @@ class StaleMetadata(KafkaError):
invalid_metadata = True
+class MetadataEmptyBrokerList(KafkaError):
+ retriable = True
+
+
class UnrecognizedBrokerVersion(KafkaError):
pass
| dpkp/kafka-python | c9d783a8211337205bc90c27d1f67beb65ac5d9e | diff --git a/test/test_cluster.py b/test/test_cluster.py
new file mode 100644
index 0000000..f010c4f
--- /dev/null
+++ b/test/test_cluster.py
@@ -0,0 +1,22 @@
+# pylint: skip-file
+from __future__ import absolute_import
+
+import pytest
+
+from kafka.cluster import ClusterMetadata
+from kafka.protocol.metadata import MetadataResponse
+
+
+def test_empty_broker_list():
+ cluster = ClusterMetadata()
+ assert len(cluster.brokers()) == 0
+
+ cluster.update_metadata(MetadataResponse[0](
+ [(0, 'foo', 12), (1, 'bar', 34)], []))
+ assert len(cluster.brokers()) == 2
+
+ # empty broker list response should be ignored
+ cluster.update_metadata(MetadataResponse[0](
+ [], # empty brokers
+ [(17, 'foo', []), (17, 'bar', [])])) # topics w/ error
+ assert len(cluster.brokers()) == 2
| Invalid bootstrap servers list blocks during startup
```python
>>> kafka.KafkaProducer(bootstrap_servers='can-not-resolve:9092')
# never returns
``` | 0.0 | [
"test/test_cluster.py::test_empty_broker_list"
] | [] | 2018-05-25 07:16:51+00:00 | 1,981 |
|
dr-prodigy__python-holidays-1087 | diff --git a/.github/workflows/ci-cd.yml b/.github/workflows/ci-cd.yml
index 5d982e6a..b1b98965 100644
--- a/.github/workflows/ci-cd.yml
+++ b/.github/workflows/ci-cd.yml
@@ -100,7 +100,7 @@ jobs:
name: dist
path: dist
- name: Publish Package
- uses: pypa/gh-action-pypi-publish@v1.8.5
+ uses: pypa/gh-action-pypi-publish@v1.8.6
with:
user: __token__
password: ${{ secrets.pypi_password }}
diff --git a/holidays/__init__.py b/holidays/__init__.py
index bc268a05..294c676e 100644
--- a/holidays/__init__.py
+++ b/holidays/__init__.py
@@ -10,9 +10,12 @@
# License: MIT (see LICENSE file)
from holidays.constants import *
-from holidays.countries import *
-from holidays.financial import *
from holidays.holiday_base import *
+from holidays.registry import EntityLoader
from holidays.utils import *
__version__ = "0.25"
+
+
+EntityLoader.load("countries", globals())
+EntityLoader.load("financial", globals())
diff --git a/holidays/registry.py b/holidays/registry.py
new file mode 100644
index 00000000..ca8ee397
--- /dev/null
+++ b/holidays/registry.py
@@ -0,0 +1,257 @@
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Authors: dr-prodigy <dr.prodigy.github@gmail.com> (c) 2017-2023
+# ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+import importlib
+from typing import Any, Dict, Iterable, Optional, Tuple, Union
+
+from holidays.holiday_base import HolidayBase
+
+RegistryDict = Dict[str, Tuple[str, ...]]
+
+COUNTRIES: RegistryDict = {
+ "albania": ("Albania", "AL", "ALB"),
+ "american_samoa": ("AmericanSamoa", "AS", "ASM", "HolidaysAS"),
+ "andorra": ("Andorra", "AD", "AND"),
+ "angola": ("Angola", "AO", "AGO"),
+ "argentina": ("Argentina", "AR", "ARG"),
+ "armenia": ("Armenia", "AM", "ARM"),
+ "aruba": ("Aruba", "AW", "ABW"),
+ "australia": ("Australia", "AU", "AUS"),
+ "austria": ("Austria", "AT", "AUT"),
+ "azerbaijan": ("Azerbaijan", "AZ", "AZE"),
+ "bahrain": ("Bahrain", "BH", "BAH"),
+ "bangladesh": ("Bangladesh", "BD", "BGD"),
+ "belarus": ("Belarus", "BY", "BLR"),
+ "belgium": ("Belgium", "BE", "BEL"),
+ "bolivia": ("Bolivia", "BO", "BOL"),
+ "bosnia_and_herzegovina": ("BosniaAndHerzegovina", "BA", "BIH"),
+ "botswana": ("Botswana", "BW", "BWA"),
+ "brazil": ("Brazil", "BR", "BRA"),
+ "bulgaria": ("Bulgaria", "BG", "BLG"),
+ "burundi": ("Burundi", "BI", "BDI"),
+ "canada": ("Canada", "CA", "CAN"),
+ "chile": ("Chile", "CL", "CHL"),
+ "china": ("China", "CN", "CHN"),
+ "colombia": ("Colombia", "CO", "COL"),
+ "costa_rica": ("CostaRica", "CR", "CRI"),
+ "croatia": ("Croatia", "HR", "HRV"),
+ "cuba": ("Cuba", "CU", "CUB"),
+ "curacao": ("Curacao", "CW", "CUW"),
+ "cyprus": ("Cyprus", "CY", "CYP"),
+ "czechia": ("Czechia", "CZ", "CZE"),
+ "denmark": ("Denmark", "DK", "DNK"),
+ "djibouti": ("Djibouti", "DJ", "DJI"),
+ "dominican_republic": ("DominicanRepublic", "DO", "DOM"),
+ "ecuador": ("Ecuador", "EC", "ECU"),
+ "egypt": ("Egypt", "EG", "EGY"),
+ "estonia": ("Estonia", "EE", "EST"),
+ "eswatini": ("Eswatini", "SZ", "SZW", "Swaziland"),
+ "ethiopia": ("Ethiopia", "ET", "ETH"),
+ "finland": ("Finland", "FI", "FIN"),
+ "france": ("France", "FR", "FRA"),
+ "georgia": ("Georgia", "GE", "GEO"),
+ "germany": ("Germany", "DE", "DEU"),
+ "greece": ("Greece", "GR", "GRC"),
+ "guam": ("Guam", "GU", "GUM", "HolidaysGU"),
+ "honduras": ("Honduras", "HN", "HND"),
+ "hongkong": ("HongKong", "HK", "HKG"),
+ "hungary": ("Hungary", "HU", "HUN"),
+ "iceland": ("Iceland", "IS", "ISL"),
+ "india": ("India", "IN", "IND"),
+ "indonesia": ("Indonesia", "ID", "IDN"),
+ "ireland": ("Ireland", "IE", "IRL"),
+ "isle_of_man": ("IsleOfMan", "IM", "IMN"),
+ "israel": ("Israel", "IL", "ISR"),
+ "italy": ("Italy", "IT", "ITA"),
+ "jamaica": ("Jamaica", "JM", "JAM"),
+ "japan": ("Japan", "JP", "JPN"),
+ "kazakhstan": ("Kazakhstan", "KZ", "KAZ"),
+ "kenya": ("Kenya", "KE", "KEN"),
+ "kyrgyzstan": ("Kyrgyzstan", "KG", "KGZ"),
+ "latvia": ("Latvia", "LV", "LVA"),
+ "lesotho": ("Lesotho", "LS", "LSO"),
+ "liechtenstein": ("Liechtenstein", "LI", "LIE"),
+ "lithuania": ("Lithuania", "LT", "LTU"),
+ "luxembourg": ("Luxembourg", "LU", "LUX"),
+ "madagascar": ("Madagascar", "MG", "MDG"),
+ "malawi": ("Malawi", "MW", "MWI"),
+ "malaysia": ("Malaysia", "MY", "MYS"),
+ "malta": ("Malta", "MT", "MLT"),
+ "marshall_islands": ("MarshallIslands", "MH", "MHL", "HolidaysMH"),
+ "mexico": ("Mexico", "MX", "MEX"),
+ "moldova": ("Moldova", "MD", "MDA"),
+ "monaco": ("Monaco", "MC", "MCO"),
+ "montenegro": ("Montenegro", "ME", "MNE"),
+ "morocco": ("Morocco", "MA", "MOR"),
+ "mozambique": ("Mozambique", "MZ", "MOZ"),
+ "namibia": ("Namibia", "NA", "NAM"),
+ "netherlands": ("Netherlands", "NL", "NLD"),
+ "new_zealand": ("NewZealand", "NZ", "NZL"),
+ "nicaragua": ("Nicaragua", "NI", "NIC"),
+ "nigeria": ("Nigeria", "NG", "NGA"),
+ "north_macedonia": ("NorthMacedonia", "MK", "MKD"),
+ "northern_mariana_islands": (
+ "NorthernMarianaIslands",
+ "MP",
+ "MNP",
+ "HolidaysMP",
+ ),
+ "norway": ("Norway", "NO", "NOR"),
+ "pakistan": ("Pakistan", "PK", "PAK"),
+ "panama": ("Panama", "PA", "PAN"),
+ "paraguay": ("Paraguay", "PY", "PRY"),
+ "peru": ("Peru", "PE", "PER"),
+ "philippines": ("Philippines", "PH", "PHL"),
+ "poland": ("Poland", "PL", "POL"),
+ "portugal": ("Portugal", "PT", "PRT"),
+ "puerto_rico": ("PuertoRico", "PR", "PRI", "HolidaysPR"),
+ "romania": ("Romania", "RO", "ROU"),
+ "russia": ("Russia", "RU", "RUS"),
+ "san_marino": ("SanMarino", "SM", "SMR"),
+ "saudi_arabia": ("SaudiArabia", "SA", "SAU"),
+ "serbia": ("Serbia", "RS", "SRB"),
+ "singapore": ("Singapore", "SG", "SGP"),
+ "slovakia": ("Slovakia", "SK", "SVK"),
+ "slovenia": ("Slovenia", "SI", "SVN"),
+ "south_africa": ("SouthAfrica", "ZA", "ZAF"),
+ "south_korea": ("SouthKorea", "KR", "KOR", "Korea"),
+ "spain": ("Spain", "ES", "ESP"),
+ "sweden": ("Sweden", "SE", "SWE"),
+ "switzerland": ("Switzerland", "CH", "CHE"),
+ "taiwan": ("Taiwan", "TW", "TWN"),
+ "thailand": ("Thailand", "TH", "THA"),
+ "tunisia": ("Tunisia", "TN", "TUN"),
+ "turkey": ("Turkey", "TR", "TUR"),
+ "ukraine": ("Ukraine", "UA", "UKR"),
+ "united_arab_emirates": ("UnitedArabEmirates", "AE", "ARE"),
+ "united_kingdom": ("UnitedKingdom", "GB", "GBR", "UK"),
+ "united_states_minor_outlying_islands": (
+ "UnitedStatesMinorOutlyingIslands",
+ "UM",
+ "UMI",
+ "HolidaysUM",
+ ),
+ "united_states_virgin_islands": (
+ "UnitedStatesVirginIslands",
+ "VI",
+ "VIR",
+ "HolidaysVI",
+ ),
+ "united_states": ("UnitedStates", "US", "USA"),
+ "uruguay": ("Uruguay", "UY", "URY"),
+ "uzbekistan": ("Uzbekistan", "UZ", "UZB"),
+ "vatican_city": ("VaticanCity", "VA", "VAT"),
+ "venezuela": ("Venezuela", "VE", "VEN"),
+ "vietnam": ("Vietnam", "VN", "VNM"),
+ "zambia": ("Zambia", "ZM", "ZMB"),
+ "zimbabwe": ("Zimbabwe", "ZW", "ZWE"),
+}
+
+FINANCIAL: RegistryDict = {
+ "european_central_bank": ("EuropeanCentralBank", "ECB", "TAR"),
+ "ny_stock_exchange": ("NewYorkStockExchange", "NYSE", "XNYS"),
+}
+
+
+class EntityLoader:
+ """Country and financial holidays entities lazy loader."""
+
+ __slots__ = ("entity", "entity_name", "module_name")
+
+ def __init__(self, path: str, *args, **kwargs) -> None:
+ """Set up a lazy loader."""
+ self.entity = None
+
+ entity_path = path.split(".")
+ self.entity_name = entity_path[-1]
+ self.module_name = ".".join(entity_path[0:-1])
+
+ super().__init__(*args, **kwargs)
+
+ def __call__(self, *args, **kwargs) -> HolidayBase:
+ """Create a new instance of a lazy-loaded entity."""
+ cls = self.get_entity()
+ return cls(*args, **kwargs) # type: ignore[misc, operator]
+
+ def __getattr__(self, name: str) -> Optional[Any]:
+ """Return attribute of a lazy-loaded entity."""
+ cls = self.get_entity()
+ return getattr(cls, name)
+
+ def __str__(self) -> str:
+ """Return lazy loader object string representation."""
+ return (
+ f"A lazy loader for {self.get_entity()}. For inheritance please "
+ f"use the '{self.module_name}.{self.entity_name}' class directly."
+ )
+
+ def get_entity(self) -> Optional[HolidayBase]:
+ """Return lazy-loaded entity."""
+ if self.entity is None:
+ self.entity = getattr(
+ importlib.import_module(self.module_name),
+ self.entity_name,
+ )
+
+ return self.entity
+
+ @staticmethod
+ def _get_entity_codes(
+ container: RegistryDict,
+ entity_length: Union[int, Iterable[int]],
+ include_aliases: bool = True,
+ ) -> Iterable[str]:
+ entity_length = (
+ {entity_length}
+ if isinstance(entity_length, int)
+ else set(entity_length)
+ )
+ for entities in container.values():
+ for entity in entities:
+ if len(entity) in entity_length:
+ yield entity
+ # Assuming that the alpha-2 code goes first.
+ if not include_aliases:
+ break
+
+ @staticmethod
+ def get_country_codes(include_aliases: bool = True) -> Iterable[str]:
+ """Get supported country codes.
+
+ :param include_aliases:
+ Whether to include entity aliases (e.g. UK for GB).
+ """
+ return EntityLoader._get_entity_codes(COUNTRIES, 2, include_aliases)
+
+ @staticmethod
+ def get_financial_codes(include_aliases: bool = True) -> Iterable[str]:
+ """Get supported financial codes.
+
+ :param include_aliases:
+ Whether to include entity aliases(e.g. TAR for ECB, XNYS for NYSE).
+ """
+ return EntityLoader._get_entity_codes(
+ FINANCIAL, (3, 4), include_aliases
+ )
+
+ @staticmethod
+ def load(prefix: str, scope: Dict) -> None:
+ """Load country or financial entities."""
+ entity_mapping = COUNTRIES if prefix == "countries" else FINANCIAL
+ for module, entities in entity_mapping.items():
+ scope.update(
+ {
+ entity: EntityLoader(
+ f"holidays.{prefix}.{module}.{entity}"
+ )
+ for entity in entities
+ }
+ )
diff --git a/holidays/utils.py b/holidays/utils.py
index 0c527f8e..8df9d96a 100755
--- a/holidays/utils.py
+++ b/holidays/utils.py
@@ -17,13 +17,12 @@ __all__ = (
"list_supported_financial",
)
-import inspect
import warnings
from functools import lru_cache
from typing import Dict, Iterable, List, Optional, Union
-from holidays import countries, financial
from holidays.holiday_base import HolidayBase
+from holidays.registry import EntityLoader
def country_holidays(
@@ -176,8 +175,10 @@ def country_holidays(
:class:`HolidayBase` class and define your own :meth:`_populate` method.
See documentation for examples.
"""
+ import holidays
+
try:
- return getattr(countries, country)(
+ return getattr(holidays, country)(
years=years,
subdiv=subdiv,
expand=expand,
@@ -238,8 +239,10 @@ def financial_holidays(
See :py:func:`country_holidays` documentation for further details and
examples.
"""
+ import holidays
+
try:
- return getattr(financial, market)(
+ return getattr(holidays, market)(
years=years,
subdiv=subdiv,
expand=expand,
@@ -286,10 +289,11 @@ def list_supported_countries(include_aliases=True) -> Dict[str, List[str]]:
A dictionary where the key is the ISO 3166-1 Alpha-2 country codes and
the value is a list of supported subdivision codes.
"""
+ import holidays
+
return {
- name if include_aliases else cls.country: list(cls.subdivisions)
- for name, cls in inspect.getmembers(countries, inspect.isclass)
- if len(name) == 2 and issubclass(cls, HolidayBase)
+ country_code: list(getattr(holidays, country_code).subdivisions)
+ for country_code in EntityLoader.get_country_codes(include_aliases)
}
@@ -305,8 +309,9 @@ def list_supported_financial(include_aliases=True) -> Dict[str, List[str]]:
A dictionary where the key is the market codes and
the value is a list of supported subdivision codes.
"""
+ import holidays
+
return {
- name if include_aliases else cls.market: list(cls.subdivisions)
- for name, cls in inspect.getmembers(financial, inspect.isclass)
- if len(name) in {3, 4} and issubclass(cls, HolidayBase)
+ financial_code: list(getattr(holidays, financial_code).subdivisions)
+ for financial_code in EntityLoader.get_financial_codes(include_aliases)
}
| dr-prodigy/python-holidays | 773afed052a3286cfc7a948ce7db2c626c061129 | diff --git a/tests/test_holiday_base.py b/tests/test_holiday_base.py
index 5d4af3e9..7bfa8ae4 100644
--- a/tests/test_holiday_base.py
+++ b/tests/test_holiday_base.py
@@ -20,7 +20,7 @@ from dateutil.relativedelta import MO
from dateutil.relativedelta import relativedelta as rd
import holidays
-from holidays.constants import JAN, FEB, MON, TUE, SAT, SUN
+from holidays.constants import JAN, FEB, OCT, MON, TUE, SAT, SUN
class TestBasics(unittest.TestCase):
@@ -449,10 +449,10 @@ class TestBasics(unittest.TestCase):
self.assertRaises(TypeError, lambda: 1 + holidays.US())
def test_inheritance(self):
- class NoColumbusHolidays(holidays.US):
+ class NoColumbusHolidays(holidays.countries.US):
def _populate(self, year):
- holidays.US._populate(self, year)
- self.pop(date(year, 10, 1) + rd(weekday=MO(+2)))
+ super()._populate(year)
+ self.pop(date(year, OCT, 1) + rd(weekday=MO(+2)))
hdays = NoColumbusHolidays()
self.assertIn(date(2014, 10, 13), self.holidays)
@@ -462,9 +462,9 @@ class TestBasics(unittest.TestCase):
self.assertNotIn(date(2020, 10, 12), hdays)
self.assertIn(date(2020, 1, 1), hdays)
- class NinjaTurtlesHolidays(holidays.US):
+ class NinjaTurtlesHolidays(holidays.countries.US):
def _populate(self, year):
- holidays.US._populate(self, year)
+ super()._populate(year)
self[date(year, 7, 13)] = "Ninja Turtle's Day"
hdays = NinjaTurtlesHolidays()
diff --git a/tests/test_registry.py b/tests/test_registry.py
new file mode 100644
index 00000000..66b93f6f
--- /dev/null
+++ b/tests/test_registry.py
@@ -0,0 +1,112 @@
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Authors: dr-prodigy <dr.prodigy.github@gmail.com> (c) 2017-2023
+# ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+import importlib
+import inspect
+import warnings
+from unittest import TestCase
+
+from holidays import countries, financial, registry
+
+
+class TestEntityLoader(TestCase):
+ # TODO(ark): consider running this just once for the latest Python version.
+ def test_countries_imports(self):
+ warnings.simplefilter("ignore")
+
+ import holidays
+
+ loader_entities = set()
+ for module, entities in registry.COUNTRIES.items():
+ module = importlib.import_module(f"holidays.countries.{module}")
+ for entity in entities:
+ countries_cls = getattr(countries, entity)
+ loader_cls = getattr(holidays, entity)
+ module_cls = getattr(module, entity)
+
+ self.assertIsNotNone(countries_cls, entity)
+ self.assertIsNotNone(loader_cls, entity)
+ self.assertIsNotNone(module_cls, entity)
+ self.assertEqual(countries_cls, module_cls)
+ self.assertTrue(isinstance(loader_cls, registry.EntityLoader))
+ self.assertTrue(isinstance(loader_cls(), countries_cls))
+ self.assertTrue(isinstance(loader_cls(), module_cls))
+
+ loader_entities.add(loader_cls.__name__)
+
+ countries_entities = set(
+ entity[0]
+ for entity in inspect.getmembers(countries, inspect.isclass)
+ )
+ self.assertEqual(
+ countries_entities,
+ loader_entities,
+ "Registry entities and countries entities don't match: %s"
+ % countries_entities.difference(loader_entities),
+ )
+
+ def test_country_str(self):
+ self.assertEqual(
+ str(
+ registry.EntityLoader(
+ "holidays.countries.united_states.US",
+ )
+ ),
+ "A lazy loader for <class 'holidays.countries.united_states.US'>. "
+ "For inheritance please use the "
+ "'holidays.countries.united_states.US' class directly.",
+ )
+
+ # TODO(ark): consider running this just once for the latest Python version.
+ def test_financial_imports(self):
+ import holidays
+
+ loader_entities = set()
+ for module, entities in registry.FINANCIAL.items():
+ module = importlib.import_module(f"holidays.financial.{module}")
+ for entity in entities:
+ financial_cls = getattr(financial, entity)
+ loader_cls = getattr(holidays, entity)
+ module_cls = getattr(module, entity)
+
+ self.assertIsNotNone(financial_cls, entity)
+ self.assertIsNotNone(loader_cls, entity)
+ self.assertIsNotNone(module_cls, entity)
+ self.assertEqual(financial_cls, module_cls)
+ self.assertTrue(isinstance(loader_cls, registry.EntityLoader))
+ self.assertTrue(isinstance(loader_cls(), financial_cls))
+ self.assertTrue(isinstance(loader_cls(), module_cls))
+
+ loader_entities.add(loader_cls.__name__)
+
+ financial_entities = set(
+ entity[0]
+ for entity in inspect.getmembers(financial, inspect.isclass)
+ )
+ self.assertEqual(
+ financial_entities,
+ loader_entities,
+ "Registry entities and financial entities don't match: %s"
+ % financial_entities.difference(loader_entities),
+ )
+
+ def test_financial_str(self):
+ self.assertEqual(
+ str(
+ registry.EntityLoader(
+ "holidays.financial.ny_stock_exchange.NYSE"
+ )
+ ),
+ "A lazy loader for "
+ "<class 'holidays.financial.ny_stock_exchange.NYSE'>. "
+ "For inheritance please use the "
+ "'holidays.financial.ny_stock_exchange.NYSE' class directly.",
+ )
| Maximize load performance
We advertise this library as "fast, efficient", and is performance or memory footprint affected by loading every single class for every single country (that's 360+ classes) even when the user needs holidays only for a single country, which I assume this to be 90%+ of use cases, or just a few countries? What about on small footprints (e.g. Raspberry Pi)? I would assume so, but am not very familiar with Python innards.
If so, are there architectures that we can implement to maximize load performance especially when only one (or a few) countries are needed?
_Originally posted by @mborsetti in https://github.com/dr-prodigy/python-holidays/issues/948#issuecomment-1432382359_
| 0.0 | [
"tests/test_holiday_base.py::TestBasics::test_add_countries",
"tests/test_holiday_base.py::TestBasics::test_add_financial",
"tests/test_holiday_base.py::TestBasics::test_add_holiday",
"tests/test_holiday_base.py::TestBasics::test_append",
"tests/test_holiday_base.py::TestBasics::test_contains",
"tests/test_holiday_base.py::TestBasics::test_copy",
"tests/test_holiday_base.py::TestBasics::test_eq_",
"tests/test_holiday_base.py::TestBasics::test_get",
"tests/test_holiday_base.py::TestBasics::test_get_list",
"tests/test_holiday_base.py::TestBasics::test_get_named_contains",
"tests/test_holiday_base.py::TestBasics::test_get_named_exact",
"tests/test_holiday_base.py::TestBasics::test_get_named_icontains",
"tests/test_holiday_base.py::TestBasics::test_get_named_iexact",
"tests/test_holiday_base.py::TestBasics::test_get_named_istartswith",
"tests/test_holiday_base.py::TestBasics::test_get_named_lookup_invalid",
"tests/test_holiday_base.py::TestBasics::test_get_named_startswith",
"tests/test_holiday_base.py::TestBasics::test_getitem",
"tests/test_holiday_base.py::TestBasics::test_inheritance",
"tests/test_holiday_base.py::TestBasics::test_is_leap_year",
"tests/test_holiday_base.py::TestBasics::test_is_weekend",
"tests/test_holiday_base.py::TestBasics::test_ne",
"tests/test_holiday_base.py::TestBasics::test_pop",
"tests/test_holiday_base.py::TestBasics::test_pop_named",
"tests/test_holiday_base.py::TestBasics::test_radd",
"tests/test_holiday_base.py::TestBasics::test_repr_country",
"tests/test_holiday_base.py::TestBasics::test_repr_market",
"tests/test_holiday_base.py::TestBasics::test_setitem",
"tests/test_holiday_base.py::TestBasics::test_str_country",
"tests/test_holiday_base.py::TestBasics::test_str_market",
"tests/test_holiday_base.py::TestBasics::test_update",
"tests/test_holiday_base.py::TestArgs::test_country",
"tests/test_holiday_base.py::TestArgs::test_deprecation_warnings",
"tests/test_holiday_base.py::TestArgs::test_expand",
"tests/test_holiday_base.py::TestArgs::test_observed",
"tests/test_holiday_base.py::TestArgs::test_serialization",
"tests/test_holiday_base.py::TestArgs::test_years",
"tests/test_holiday_base.py::TestKeyTransforms::test_dates",
"tests/test_holiday_base.py::TestKeyTransforms::test_datetimes",
"tests/test_holiday_base.py::TestKeyTransforms::test_exceptions",
"tests/test_holiday_base.py::TestKeyTransforms::test_strings",
"tests/test_holiday_base.py::TestKeyTransforms::test_timestamp",
"tests/test_holiday_base.py::TestCountryHolidayDeprecation::test_deprecation",
"tests/test_holiday_base.py::TestCountrySpecialHolidays::test_populate_special_holidays",
"tests/test_holiday_base.py::TestHolidaysTranslation::test_language_unavailable",
"tests/test_registry.py::TestEntityLoader::test_countries_imports",
"tests/test_registry.py::TestEntityLoader::test_country_str",
"tests/test_registry.py::TestEntityLoader::test_financial_imports",
"tests/test_registry.py::TestEntityLoader::test_financial_str"
] | [] | 2023-04-07 17:51:48+00:00 | 1,982 |
|
dr-prodigy__python-holidays-1257 | diff --git a/holidays/countries/greece.py b/holidays/countries/greece.py
index 28b24919..fae02e99 100644
--- a/holidays/countries/greece.py
+++ b/holidays/countries/greece.py
@@ -9,13 +9,12 @@
# Website: https://github.com/dr-prodigy/python-holidays
# License: MIT (see LICENSE file)
-from datetime import date
from datetime import timedelta as td
from gettext import gettext as tr
from holidays.calendars import _get_nth_weekday_from, JULIAN_CALENDAR
from holidays.calendars import GREGORIAN_CALENDAR
-from holidays.constants import MAR, MAY, OCT, MON
+from holidays.constants import MAR, OCT, MON
from holidays.holiday_base import HolidayBase
from holidays.holiday_groups import ChristianHolidays, InternationalHolidays
@@ -52,6 +51,9 @@ class Greece(HolidayBase, ChristianHolidays, InternationalHolidays):
# Independence Day.
self._add_holiday(tr("Εικοστή Πέμπτη Μαρτίου"), MAR, 25)
+ # Good Friday.
+ self._add_good_friday(tr("Μεγάλη Παρασκευή"))
+
# Easter Monday.
self._add_easter_monday(tr("Δευτέρα του Πάσχα"))
@@ -62,8 +64,7 @@ class Greece(HolidayBase, ChristianHolidays, InternationalHolidays):
name = self.tr("Εργατική Πρωτομαγιά")
name_observed = self.tr("%s (παρατηρήθηκε)")
- dt = date(year, MAY, 1)
- self._add_holiday(name, dt)
+ dt = self._add_labor_day(name)
if self.observed and self._is_weekend(dt):
# https://en.wikipedia.org/wiki/Public_holidays_in_Greece
labour_day_observed_date = _get_nth_weekday_from(1, MON, dt)
diff --git a/holidays/locale/el/LC_MESSAGES/GR.po b/holidays/locale/el/LC_MESSAGES/GR.po
index 78316365..11ba671e 100644
--- a/holidays/locale/el/LC_MESSAGES/GR.po
+++ b/holidays/locale/el/LC_MESSAGES/GR.po
@@ -3,10 +3,10 @@
#
msgid ""
msgstr ""
-"Project-Id-Version: Python Holidays 0.20\n"
-"POT-Creation-Date: 2023-02-15 14:53-0800\n"
-"PO-Revision-Date: 2023-02-16 08:50-0800\n"
-"Last-Translator: Arkadii Yakovets <ark@cho.red>\n"
+"Project-Id-Version: Python Holidays 0.26\n"
+"POT-Creation-Date: 2023-06-01 17:41+0300\n"
+"PO-Revision-Date: 2023-06-01 17:43+0300\n"
+"Last-Translator: ~Jhellico <jhellico@gmail.com>\n"
"Language-Team: Python Holidays localization team\n"
"Language: el\n"
"MIME-Version: 1.0\n"
@@ -16,60 +16,65 @@ msgstr ""
"X-Generator: Poedit 3.2.2\n"
#. New Year's Day.
-#: ./holidays/countries/greece.py:44
+#: ./holidays/countries/greece.py:43
msgid "Πρωτοχρονιά"
msgstr ""
#. Epiphany.
-#: ./holidays/countries/greece.py:47
+#: ./holidays/countries/greece.py:46
msgid "Θεοφάνεια"
msgstr ""
#. Clean Monday.
-#: ./holidays/countries/greece.py:50
+#: ./holidays/countries/greece.py:49
msgid "Καθαρά Δευτέρα"
msgstr ""
#. Independence Day.
-#: ./holidays/countries/greece.py:53
+#: ./holidays/countries/greece.py:52
msgid "Εικοστή Πέμπτη Μαρτίου"
msgstr ""
+#. Good Friday.
+#: ./holidays/countries/greece.py:55
+msgid "Μεγάλη Παρασκευή"
+msgstr ""
+
#. Easter Monday.
-#: ./holidays/countries/greece.py:56
+#: ./holidays/countries/greece.py:58
msgid "Δευτέρα του Πάσχα"
msgstr ""
#. Monday of the Holy Spirit.
-#: ./holidays/countries/greece.py:59
+#: ./holidays/countries/greece.py:61
msgid "Δευτέρα του Αγίου Πνεύματος"
msgstr ""
#. Labour Day.
-#: ./holidays/countries/greece.py:62
+#: ./holidays/countries/greece.py:64
msgid "Εργατική Πρωτομαγιά"
msgstr ""
-#: ./holidays/countries/greece.py:63
+#: ./holidays/countries/greece.py:65
#, c-format
msgid "%s (παρατηρήθηκε)"
msgstr ""
#. Assumption of Mary.
-#: ./holidays/countries/greece.py:77
+#: ./holidays/countries/greece.py:78
msgid "Κοίμηση της Θεοτόκου"
msgstr ""
#. Ochi Day.
-#: ./holidays/countries/greece.py:80
+#: ./holidays/countries/greece.py:81
msgid "Ημέρα του Όχι"
msgstr ""
#. Christmas Day.
-#: ./holidays/countries/greece.py:83
+#: ./holidays/countries/greece.py:84
msgid "Χριστούγεννα"
msgstr ""
-#: ./holidays/countries/greece.py:87
+#: ./holidays/countries/greece.py:88
msgid "Επόμενη ημέρα των Χριστουγέννων"
msgstr ""
diff --git a/holidays/locale/en_US/LC_MESSAGES/GR.po b/holidays/locale/en_US/LC_MESSAGES/GR.po
index 96288f05..d52e5c34 100644
--- a/holidays/locale/en_US/LC_MESSAGES/GR.po
+++ b/holidays/locale/en_US/LC_MESSAGES/GR.po
@@ -3,10 +3,10 @@
#
msgid ""
msgstr ""
-"Project-Id-Version: Python Holidays 0.20\n"
-"POT-Creation-Date: 2023-02-15 14:53-0800\n"
-"PO-Revision-Date: 2023-02-15 14:59-0800\n"
-"Last-Translator: Arkadii Yakovets <ark@cho.red>\n"
+"Project-Id-Version: Python Holidays 0.26\n"
+"POT-Creation-Date: 2023-06-01 17:41+0300\n"
+"PO-Revision-Date: 2023-06-01 17:44+0300\n"
+"Last-Translator: ~Jhellico <jhellico@gmail.com>\n"
"Language-Team: Python Holidays localization team\n"
"Language: en_US\n"
"MIME-Version: 1.0\n"
@@ -16,60 +16,65 @@ msgstr ""
"X-Generator: Poedit 3.2.2\n"
#. New Year's Day.
-#: ./holidays/countries/greece.py:44
+#: ./holidays/countries/greece.py:43
msgid "Πρωτοχρονιά"
msgstr "New Year’s Day"
#. Epiphany.
-#: ./holidays/countries/greece.py:47
+#: ./holidays/countries/greece.py:46
msgid "Θεοφάνεια"
msgstr "Epiphany"
#. Clean Monday.
-#: ./holidays/countries/greece.py:50
+#: ./holidays/countries/greece.py:49
msgid "Καθαρά Δευτέρα"
msgstr "Clean Monday"
#. Independence Day.
-#: ./holidays/countries/greece.py:53
+#: ./holidays/countries/greece.py:52
msgid "Εικοστή Πέμπτη Μαρτίου"
msgstr "Independence Day"
+#. Good Friday.
+#: ./holidays/countries/greece.py:55
+msgid "Μεγάλη Παρασκευή"
+msgstr "Good Friday"
+
#. Easter Monday.
-#: ./holidays/countries/greece.py:56
+#: ./holidays/countries/greece.py:58
msgid "Δευτέρα του Πάσχα"
msgstr "Easter Monday"
#. Monday of the Holy Spirit.
-#: ./holidays/countries/greece.py:59
+#: ./holidays/countries/greece.py:61
msgid "Δευτέρα του Αγίου Πνεύματος"
msgstr "Easter Monday"
#. Labour Day.
-#: ./holidays/countries/greece.py:62
+#: ./holidays/countries/greece.py:64
msgid "Εργατική Πρωτομαγιά"
msgstr "Labor Day"
-#: ./holidays/countries/greece.py:63
+#: ./holidays/countries/greece.py:65
#, c-format
msgid "%s (παρατηρήθηκε)"
msgstr "%s (Observed)"
#. Assumption of Mary.
-#: ./holidays/countries/greece.py:77
+#: ./holidays/countries/greece.py:78
msgid "Κοίμηση της Θεοτόκου"
msgstr "Assumption of Mary Day"
#. Ochi Day.
-#: ./holidays/countries/greece.py:80
+#: ./holidays/countries/greece.py:81
msgid "Ημέρα του Όχι"
msgstr "Ochi Day"
#. Christmas Day.
-#: ./holidays/countries/greece.py:83
+#: ./holidays/countries/greece.py:84
msgid "Χριστούγεννα"
msgstr "Christmas Day"
-#: ./holidays/countries/greece.py:87
+#: ./holidays/countries/greece.py:88
msgid "Επόμενη ημέρα των Χριστουγέννων"
msgstr "Day After Christmas"
| dr-prodigy/python-holidays | 4c691d998d1f31285d1a564e5ababcd3b00e973a | diff --git a/tests/countries/test_greece.py b/tests/countries/test_greece.py
index 93c1b87a..1d043600 100644
--- a/tests/countries/test_greece.py
+++ b/tests/countries/test_greece.py
@@ -9,8 +9,6 @@
# Website: https://github.com/dr-prodigy/python-holidays
# License: MIT (see LICENSE file)
-from datetime import date
-
from holidays.countries.greece import Greece, GR, GRC
from tests.common import TestCase
@@ -18,93 +16,122 @@ from tests.common import TestCase
class TestGreece(TestCase):
@classmethod
def setUpClass(cls):
- super().setUpClass(Greece)
+ super().setUpClass(Greece, years=range(2000, 2025))
def test_country_aliases(self):
self.assertCountryAliases(Greece, GR, GRC)
def test_fixed_holidays(self):
years = range(2000, 2025)
- for y in years:
- fdays = (
- (date(y, 1, 1), "Πρωτοχρονιά"),
- (date(y, 1, 6), "Θεοφάνεια"),
- (date(y, 3, 25), "Εικοστή Πέμπτη Μαρτίου"),
- (date(y, 5, 1), "Εργατική Πρωτομαγιά"),
- (date(y, 8, 15), "Κοίμηση της Θεοτόκου"),
- (date(y, 10, 28), "Ημέρα του Όχι"),
- (date(y, 12, 25), "Χριστούγεννα"),
- (date(y, 12, 26), "Επόμενη ημέρα των Χριστουγέννων"),
+ for m, d, name in (
+ (1, 1, "Πρωτοχρονιά"),
+ (1, 6, "Θεοφάνεια"),
+ (3, 25, "Εικοστή Πέμπτη Μαρτίου"),
+ (5, 1, "Εργατική Πρωτομαγιά"),
+ (8, 15, "Κοίμηση της Θεοτόκου"),
+ (10, 28, "Ημέρα του Όχι"),
+ (12, 25, "Χριστούγεννα"),
+ (12, 26, "Επόμενη ημέρα των Χριστουγέννων"),
+ ):
+ self.assertHolidaysName(
+ name, (f"{year}-{m}-{d}" for year in years)
)
- for d, dstr in fdays:
- self.assertIn(d, self.holidays)
- self.assertIn(dstr, self.holidays[d])
-
- def test_gr_clean_monday(self):
- checkdates = (
- date(2018, 2, 19),
- date(2019, 3, 11),
- date(2020, 3, 2),
- date(2021, 3, 15),
- date(2022, 3, 7),
- date(2023, 2, 27),
- date(2024, 3, 18),
+ def test_clean_monday(self):
+ self.assertHolidaysName(
+ "Καθαρά Δευτέρα",
+ "2018-02-19",
+ "2019-03-11",
+ "2020-03-02",
+ "2021-03-15",
+ "2022-03-07",
+ "2023-02-27",
+ "2024-03-18",
)
- for d in checkdates:
- self.assertIn(d, self.holidays)
- self.assertIn("Καθαρά Δευτέρα", self.holidays[d])
-
- def test_gr_easter_monday(self):
- checkdates = (
- date(2018, 4, 9),
- date(2019, 4, 29),
- date(2020, 4, 20),
- date(2021, 5, 3),
- date(2022, 4, 25),
- date(2023, 4, 17),
- date(2024, 5, 6),
+ def test_good_friday(self):
+ self.assertHolidaysName(
+ "Μεγάλη Παρασκευή",
+ "2018-04-06",
+ "2019-04-26",
+ "2020-04-17",
+ "2021-04-30",
+ "2022-04-22",
+ "2023-04-14",
+ "2024-05-03",
)
- for d in checkdates:
- self.assertIn(d, self.holidays)
- self.assertIn("Δευτέρα του Πάσχα", self.holidays[d])
-
- def test_gr_monday_of_the_holy_spirit(self):
- checkdates = (
- date(2018, 5, 28),
- date(2019, 6, 17),
- date(2020, 6, 8),
- date(2021, 6, 21),
- date(2022, 6, 13),
- date(2023, 6, 5),
- date(2024, 6, 24),
+ def test_easter_monday(self):
+ self.assertHolidaysName(
+ "Δευτέρα του Πάσχα",
+ "2018-04-09",
+ "2019-04-29",
+ "2020-04-20",
+ "2021-05-03",
+ "2022-04-25",
+ "2023-04-17",
+ "2024-05-06",
)
- for d in checkdates:
- self.assertIn(d, self.holidays)
- self.assertIn("Δευτέρα του Αγίου Πνεύματος", self.holidays[d])
-
- def test_gr_labour_day_observed(self):
- # Dates when labour day was observed on a different date
- checkdates = (
- date(2016, 5, 3),
- date(2021, 5, 4),
- date(2022, 5, 2),
- date(2033, 5, 2),
+ def test_monday_of_the_holy_spirit(self):
+ self.assertHolidaysName(
+ "Δευτέρα του Αγίου Πνεύματος",
+ "2018-05-28",
+ "2019-06-17",
+ "2020-06-08",
+ "2021-06-21",
+ "2022-06-13",
+ "2023-06-05",
+ "2024-06-24",
)
- # Years when labour date was observed on May 1st
- checkyears = (2017, 2018, 2019, 2020, 2023)
- for d in checkdates:
- self.assertIn(d, self.holidays)
- self.assertIn("Εργατική Πρωτομαγιά", self.holidays[d])
+ def test_labour_day_observed(self):
+ name_observed = "Εργατική Πρωτομαγιά (παρατηρήθηκε)"
+ dt = (
+ "2011-05-02",
+ "2016-05-03",
+ "2021-05-04",
+ "2022-05-02",
+ )
+ self.assertHolidaysName(name_observed, dt)
+ self.assertNoNonObservedHoliday(dt)
+ self.assertNoHolidayName(name_observed, 2017, 2018, 2019, 2020, 2023)
+
+ def test_l10n_default(self):
+ self.assertLocalizedHolidays(
+ (
+ ("2022-01-01", "Πρωτοχρονιά"),
+ ("2022-01-06", "Θεοφάνεια"),
+ ("2022-03-07", "Καθαρά Δευτέρα"),
+ ("2022-03-25", "Εικοστή Πέμπτη Μαρτίου"),
+ ("2022-04-22", "Μεγάλη Παρασκευή"),
+ ("2022-04-25", "Δευτέρα του Πάσχα"),
+ ("2022-05-01", "Εργατική Πρωτομαγιά"),
+ ("2022-05-02", "Εργατική Πρωτομαγιά (παρατηρήθηκε)"),
+ ("2022-06-13", "Δευτέρα του Αγίου Πνεύματος"),
+ ("2022-08-15", "Κοίμηση της Θεοτόκου"),
+ ("2022-10-28", "Ημέρα του Όχι"),
+ ("2022-12-25", "Χριστούγεννα"),
+ ("2022-12-26", "Επόμενη ημέρα των Χριστουγέννων"),
+ )
+ )
- # Check that there is no observed day created for years
- # when Labour Day was on May 1st
- for year in checkyears:
- for day in (2, 3, 4):
- d = date(year, 5, day)
- if d in self.holidays:
- self.assertNotIn("Εργατική Πρωτομαγιά", self.holidays[d])
+ def test_l10n_en_us(self):
+ self.assertLocalizedHolidays(
+ (
+ ("2022-01-01", "New Year’s Day"),
+ ("2022-01-06", "Epiphany"),
+ ("2022-03-07", "Clean Monday"),
+ ("2022-03-25", "Independence Day"),
+ ("2022-04-22", "Good Friday"),
+ ("2022-04-25", "Easter Monday"),
+ ("2022-05-01", "Labor Day"),
+ ("2022-05-02", "Labor Day (Observed)"),
+ ("2022-06-13", "Easter Monday"),
+ ("2022-08-15", "Assumption of Mary Day"),
+ ("2022-10-28", "Ochi Day"),
+ ("2022-12-25", "Christmas Day"),
+ ("2022-12-26", "Day After Christmas"),
+ ),
+ "en_US",
+ )
| Missing Orthodox Good Friday in Greece national holiday
Hello team,
I was looking at your package's data and noticed one missing day for Greece.
Source: https://www.officeholidays.com/countries/greece/2022
Missing day: 2023 Fri, Apr 14 National Holiday (Orthodox Good Friday)
Would it be possible to include it in your package, please?
Best regards,
David Boudart | 0.0 | [
"tests/countries/test_greece.py::TestGreece::test_good_friday",
"tests/countries/test_greece.py::TestGreece::test_l10n_default"
] | [
"tests/countries/test_greece.py::TestGreece::test_clean_monday",
"tests/countries/test_greece.py::TestGreece::test_country_aliases",
"tests/countries/test_greece.py::TestGreece::test_easter_monday",
"tests/countries/test_greece.py::TestGreece::test_fixed_holidays",
"tests/countries/test_greece.py::TestGreece::test_labour_day_observed",
"tests/countries/test_greece.py::TestGreece::test_monday_of_the_holy_spirit"
] | 2023-06-01 15:32:22+00:00 | 1,983 |
|
dr-prodigy__python-holidays-1278 | diff --git a/README.rst b/README.rst
index 88119e36..211fceee 100644
--- a/README.rst
+++ b/README.rst
@@ -108,7 +108,7 @@ Available Countries
.. _ISO 3166-2 code: https://en.wikipedia.org/wiki/ISO_3166-2
.. _ISO 639-1 code: https://en.wikipedia.org/wiki/List_of_ISO_639-1_codes
-We currently support 127 country codes. The standard way to refer to a country
+We currently support 128 country codes. The standard way to refer to a country
is by using its `ISO 3166-1 alpha-2 code`_, the same used for domain names, and
for a subdivision its `ISO 3166-2 code`_. Some of the countries support more
than one language for holiday names output.
@@ -219,6 +219,10 @@ The list of supported countries, their subdivisions and supported languages
- BG
-
- **bg**, en_US
+ * - Burkina Faso
+ - BF
+ -
+ -
* - Burundi
- BI
-
diff --git a/holidays/countries/__init__.py b/holidays/countries/__init__.py
index 65ad53c0..e75e4960 100644
--- a/holidays/countries/__init__.py
+++ b/holidays/countries/__init__.py
@@ -31,6 +31,7 @@ from .botswana import Botswana, BW, BWA
from .brazil import Brazil, BR, BRA
from .brunei import Brunei, BN, BRN
from .bulgaria import Bulgaria, BG, BLG
+from .burkina_faso import BurkinaFaso, BF, BFA
from .burundi import Burundi, BI, BDI
from .cameroon import Cameroon, CM, CMR
from .canada import Canada, CA, CAN
diff --git a/holidays/countries/burkina_faso.py b/holidays/countries/burkina_faso.py
new file mode 100644
index 00000000..34c1fbc4
--- /dev/null
+++ b/holidays/countries/burkina_faso.py
@@ -0,0 +1,144 @@
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Authors: dr-prodigy <dr.prodigy.github@gmail.com> (c) 2017-2023
+# ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+from datetime import date
+from datetime import timedelta as td
+
+from holidays.calendars import _CustomIslamicCalendar
+from holidays.constants import JAN, APR, MAY, JUN, JUL, AUG, SEP, OCT, NOV, DEC
+from holidays.holiday_base import HolidayBase
+from holidays.holiday_groups import ChristianHolidays, InternationalHolidays
+from holidays.holiday_groups import IslamicHolidays
+
+
+class BurkinaFaso(
+ HolidayBase, ChristianHolidays, InternationalHolidays, IslamicHolidays
+):
+ """
+ References:
+ - https://en.wikipedia.org/wiki/Public_holidays_in_Burkina_Faso
+ """
+
+ country = "BF"
+
+ def __init__(self, *args, **kwargs) -> None:
+ ChristianHolidays.__init__(self)
+ InternationalHolidays.__init__(self)
+ IslamicHolidays.__init__(self, calendar=BurkinaFasoIslamicCalendar())
+ super().__init__(*args, **kwargs)
+
+ def _add_observed(self, dt: date) -> None:
+ if self.observed and self._is_sunday(dt):
+ self._add_holiday("%s (Observed)" % self[dt], dt + td(days=+1))
+
+ def _populate(self, year):
+ # On 5 August 1960, Burkina Faso (Republic of Upper Volta at that time)
+ # gained independence from France.
+ if year <= 1960:
+ return None
+
+ super()._populate(year)
+
+ # New Year's Day.
+ self._add_observed(self._add_new_years_day("New Year's Day"))
+
+ if year >= 1967:
+ # Revolution Day.
+ self._add_observed(self._add_holiday("Revolution Day", JAN, 3))
+
+ # International Women's Day.
+ self._add_observed(self._add_womens_day("International Women's Day"))
+
+ # Easter Monday.
+ self._add_easter_monday("Easter Monday")
+
+ # Labour Day.
+ self._add_observed(self._add_labor_day("Labour Day"))
+
+ # Ascension Day.
+ self._add_ascension_thursday("Ascension Day")
+
+ # Independence Day.
+ self._add_observed(self._add_holiday("Independence Day", AUG, 5))
+
+ # Assumption Day.
+ self._add_observed(self._add_assumption_of_mary_day("Assumption Day"))
+
+ if year >= 2016:
+ # Martyrs' Day.
+ self._add_observed(self._add_holiday("Martyrs' Day", OCT, 31))
+
+ # All Saints' Day.
+ self._add_observed(self._add_all_saints_day("All Saints' Day"))
+
+ self._add_observed(
+ # Proclamation of Independence Day.
+ self._add_holiday("Proclamation of Independence Day", DEC, 11)
+ )
+
+ # Christmas Day.
+ self._add_observed(self._add_christmas_day("Christmas Day"))
+
+ # Eid al-Fitr.
+ self._add_eid_al_fitr_day("Eid al-Fitr")
+
+ # Eid al-Adha.
+ self._add_eid_al_adha_day("Eid al-Adha")
+
+ # Mawlid.
+ self._add_mawlid_day("Mawlid")
+
+
+class BF(BurkinaFaso):
+ pass
+
+
+class BFA(BurkinaFaso):
+ pass
+
+
+class BurkinaFasoIslamicCalendar(_CustomIslamicCalendar):
+ EID_AL_ADHA_DATES = {
+ 2014: ((OCT, 5),),
+ 2015: ((SEP, 24),),
+ 2016: ((SEP, 13),),
+ 2017: ((SEP, 2),),
+ 2018: ((AUG, 21),),
+ 2019: ((AUG, 11),),
+ 2020: ((JUL, 31),),
+ 2021: ((JUL, 20),),
+ 2022: ((JUL, 9),),
+ }
+
+ EID_AL_FITR_DATES = {
+ 2014: ((JUL, 29),),
+ 2015: ((JUL, 18),),
+ 2016: ((JUL, 7),),
+ 2017: ((JUN, 26),),
+ 2018: ((JUN, 15),),
+ 2019: ((JUN, 4),),
+ 2020: ((MAY, 24),),
+ 2021: ((MAY, 13),),
+ 2022: ((MAY, 2),),
+ 2023: ((APR, 21),),
+ }
+
+ MAWLID_DATES = {
+ 2014: ((JAN, 14),),
+ 2015: ((JAN, 3), (DEC, 24)),
+ 2016: ((DEC, 12),),
+ 2017: ((DEC, 1),),
+ 2018: ((NOV, 21),),
+ 2019: ((NOV, 10),),
+ 2020: ((OCT, 29),),
+ 2021: ((OCT, 19),),
+ 2022: ((OCT, 9),),
+ }
diff --git a/holidays/registry.py b/holidays/registry.py
index 241b8fa0..698be8e0 100644
--- a/holidays/registry.py
+++ b/holidays/registry.py
@@ -39,6 +39,7 @@ COUNTRIES: RegistryDict = {
"brazil": ("Brazil", "BR", "BRA"),
"brunei": ("Brunei", "BN", "BRN"),
"bulgaria": ("Bulgaria", "BG", "BLG"),
+ "burkina_faso": ("BurkinaFaso", "BF", "BFA"),
"burundi": ("Burundi", "BI", "BDI"),
"cameroon": ("Cameroon", "CM", "CMR"),
"canada": ("Canada", "CA", "CAN"),
| dr-prodigy/python-holidays | d44a0535262939d0146d2e107643d77b7f2043ba | diff --git a/tests/countries/test_burkina_faso.py b/tests/countries/test_burkina_faso.py
new file mode 100644
index 00000000..4758e192
--- /dev/null
+++ b/tests/countries/test_burkina_faso.py
@@ -0,0 +1,101 @@
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Authors: dr-prodigy <dr.prodigy.github@gmail.com> (c) 2017-2023
+# ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+from holidays.countries.burkina_faso import BurkinaFaso, BF, BFA
+from tests.common import TestCase
+
+
+class TestBurkinaFaso(TestCase):
+ @classmethod
+ def setUpClass(cls):
+ super().setUpClass(BurkinaFaso)
+
+ def test_country_aliases(self):
+ self.assertCountryAliases(BurkinaFaso, BF, BFA)
+
+ def test_no_holidays(self):
+ self.assertNoHolidays(BurkinaFaso(years=1960))
+
+ def test_revolution_day(self):
+ name = "Revolution Day"
+ self.assertHolidaysName(
+ name, (f"{year}-01-03" for year in range(1967, 2050))
+ )
+ self.assertNoHolidayName(name, BurkinaFaso(years=range(1961, 1967)))
+ self.assertNoHoliday(f"{year}-01-03" for year in range(1961, 1967))
+
+ def test_martyrs_day(self):
+ name = "Martyrs' Day"
+ self.assertHolidaysName(
+ name, (f"{year}-10-31" for year in range(2016, 2050))
+ )
+ self.assertNoHolidayName(name, BurkinaFaso(years=range(1961, 2016)))
+ self.assertNoHoliday(
+ f"{year}-10-31"
+ for year in set(range(1961, 2016)).difference({1979})
+ )
+
+ def test_observed(self):
+ dt = (
+ # New Year's Day
+ "2012-01-02",
+ "2017-01-02",
+ "2023-01-02",
+ # Revolution Day
+ "2010-01-04",
+ "2016-01-04",
+ "2021-01-04",
+ # International Women's Day
+ "2015-03-09",
+ "2020-03-09",
+ # Labour Day
+ "2011-05-02",
+ "2016-05-02",
+ # Independence Day
+ "2012-08-06",
+ "2018-08-06",
+ # Assumption Day
+ "2010-08-16",
+ "2021-08-16",
+ # All Saints' Day
+ "2015-11-02",
+ "2020-11-02",
+ # Proclamation of Independence Day
+ "2011-12-12",
+ "2022-12-12",
+ # Christmas Day
+ "2011-12-26",
+ "2016-12-26",
+ "2022-12-26",
+ )
+ self.assertHoliday(dt)
+ self.assertNoNonObservedHoliday(dt)
+
+ def test_2022(self):
+ self.assertHolidays(
+ ("2022-01-01", "New Year's Day"),
+ ("2022-01-03", "Revolution Day"),
+ ("2022-03-08", "International Women's Day"),
+ ("2022-04-18", "Easter Monday"),
+ ("2022-05-01", "Labour Day"),
+ ("2022-05-02", "Eid al-Fitr; Labour Day (Observed)"),
+ ("2022-05-26", "Ascension Day"),
+ ("2022-07-09", "Eid al-Adha"),
+ ("2022-08-05", "Independence Day"),
+ ("2022-08-15", "Assumption Day"),
+ ("2022-10-09", "Mawlid"),
+ ("2022-10-31", "Martyrs' Day"),
+ ("2022-11-01", "All Saints' Day"),
+ ("2022-12-11", "Proclamation of Independence Day"),
+ ("2022-12-12", "Proclamation of Independence Day (Observed)"),
+ ("2022-12-25", "Christmas Day"),
+ ("2022-12-26", "Christmas Day (Observed)"),
+ )
| Add Burkina Faso holidays
Useful links:
- https://en.wikipedia.org/wiki/Public_holidays_in_Burkina_Faso
- https://www.timeanddate.com/holidays/burkina-faso/ | 0.0 | [
"tests/countries/test_burkina_faso.py::TestBurkinaFaso::test_2022",
"tests/countries/test_burkina_faso.py::TestBurkinaFaso::test_country_aliases",
"tests/countries/test_burkina_faso.py::TestBurkinaFaso::test_martyrs_day",
"tests/countries/test_burkina_faso.py::TestBurkinaFaso::test_no_holidays",
"tests/countries/test_burkina_faso.py::TestBurkinaFaso::test_observed",
"tests/countries/test_burkina_faso.py::TestBurkinaFaso::test_revolution_day"
] | [] | 2023-06-03 16:07:51+00:00 | 1,984 |
|
dr-prodigy__python-holidays-1279 | diff --git a/README.rst b/README.rst
index 211fceee..c7cae973 100644
--- a/README.rst
+++ b/README.rst
@@ -108,7 +108,7 @@ Available Countries
.. _ISO 3166-2 code: https://en.wikipedia.org/wiki/ISO_3166-2
.. _ISO 639-1 code: https://en.wikipedia.org/wiki/List_of_ISO_639-1_codes
-We currently support 128 country codes. The standard way to refer to a country
+We currently support 129 country codes. The standard way to refer to a country
is by using its `ISO 3166-1 alpha-2 code`_, the same used for domain names, and
for a subdivision its `ISO 3166-2 code`_. Some of the countries support more
than one language for holiday names output.
@@ -235,6 +235,10 @@ The list of supported countries, their subdivisions and supported languages
- CA
- Provinces and territories: AB, BC, MB, NB, NL, NS, NT, NU, **ON**, PE, QC, SK, YT
- ar, **en**, en_US, fr, th
+ * - Chad
+ - TD
+ -
+ -
* - Chile
- CL
- Regions: AI, AN, AP, AR, AT, BI, CO, LI, LL, LR, MA, ML, NB, RM, TA, VS
diff --git a/holidays/countries/__init__.py b/holidays/countries/__init__.py
index e75e4960..aa860a45 100644
--- a/holidays/countries/__init__.py
+++ b/holidays/countries/__init__.py
@@ -35,6 +35,7 @@ from .burkina_faso import BurkinaFaso, BF, BFA
from .burundi import Burundi, BI, BDI
from .cameroon import Cameroon, CM, CMR
from .canada import Canada, CA, CAN
+from .chad import Chad, TD, TCD
from .chile import Chile, CL, CHL
from .china import China, CN, CHN
from .colombia import Colombia, CO, COL
diff --git a/holidays/countries/chad.py b/holidays/countries/chad.py
new file mode 100644
index 00000000..ffb0576d
--- /dev/null
+++ b/holidays/countries/chad.py
@@ -0,0 +1,136 @@
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Authors: dr-prodigy <dr.prodigy.github@gmail.com> (c) 2017-2023
+# ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+from datetime import date
+from datetime import timedelta as td
+
+from holidays.calendars import _CustomIslamicCalendar
+from holidays.constants import JAN, APR, MAY, JUN, JUL, AUG, SEP, OCT, NOV, DEC
+from holidays.holiday_base import HolidayBase
+from holidays.holiday_groups import ChristianHolidays, InternationalHolidays
+from holidays.holiday_groups import IslamicHolidays
+
+
+class Chad(
+ HolidayBase, ChristianHolidays, InternationalHolidays, IslamicHolidays
+):
+ """
+ References:
+ - https://en.wikipedia.org/wiki/Public_holidays_in_Chad
+ - https://www.ilo.org/dyn/natlex/docs/ELECTRONIC/97323/115433/F-316075167/TCD-97323.pdf # noqa: E501
+ """
+
+ country = "TD"
+ special_holidays = {
+ 2021: ((APR, 23, "Funeral of Idriss Déby Itno"),),
+ }
+
+ def __init__(self, *args, **kwargs) -> None:
+ ChristianHolidays.__init__(self)
+ InternationalHolidays.__init__(self)
+ IslamicHolidays.__init__(self, calendar=ChadIslamicCalendar())
+ super().__init__(*args, **kwargs)
+
+ def _add_observed(self, dt: date) -> None:
+ if self.observed and self._is_sunday(dt):
+ self._add_holiday("%s (Observed)" % self[dt], dt + td(days=+1))
+
+ def _populate(self, year):
+ # On 11 August 1960, Chad gained independence from France.
+ if year <= 1960:
+ return None
+
+ super()._populate(year)
+
+ # New Year's Day.
+ self._add_observed(self._add_new_years_day("New Year's Day"))
+
+ # International Women's Day.
+ self._add_observed(self._add_womens_day("International Women's Day"))
+
+ # Easter Monday.
+ self._add_easter_monday("Easter Monday")
+
+ # Labour Day.
+ self._add_observed(self._add_labor_day("Labour Day"))
+
+ # Independence Day.
+ self._add_observed(self._add_holiday("Independence Day", AUG, 11))
+
+ # All Saints' Day.
+ self._add_all_saints_day("All Saints' Day")
+
+ self._add_observed(
+ # Republic Day.
+ self._add_holiday("Republic Day", NOV, 28)
+ )
+
+ if year >= 1991:
+ self._add_observed(
+ # Freedom and Democracy Day.
+ self._add_holiday("Freedom and Democracy Day", DEC, 1)
+ )
+
+ # Christmas Day.
+ self._add_christmas_day("Christmas Day")
+
+ # Eid al-Fitr.
+ self._add_eid_al_fitr_day("Eid al-Fitr")
+
+ # Eid al-Adha.
+ self._add_eid_al_adha_day("Eid al-Adha")
+
+ # Mawlid.
+ self._add_mawlid_day("Mawlid")
+
+
+class TD(Chad):
+ pass
+
+
+class TCD(Chad):
+ pass
+
+
+class ChadIslamicCalendar(_CustomIslamicCalendar):
+ EID_AL_ADHA_DATES = {
+ 2015: ((SEP, 24),),
+ 2016: ((SEP, 13),),
+ 2017: ((SEP, 2),),
+ 2018: ((AUG, 22),),
+ 2019: ((AUG, 11),),
+ 2020: ((JUL, 31),),
+ 2021: ((JUL, 20),),
+ 2022: ((JUL, 9),),
+ }
+
+ EID_AL_FITR_DATES = {
+ 2015: ((JUL, 18),),
+ 2016: ((JUL, 7),),
+ 2017: ((JUN, 26),),
+ 2018: ((JUN, 15),),
+ 2019: ((JUN, 4),),
+ 2020: ((MAY, 24),),
+ 2021: ((MAY, 13),),
+ 2022: ((MAY, 2),),
+ 2023: ((APR, 21),),
+ }
+
+ MAWLID_DATES = {
+ 2015: ((JAN, 3), (DEC, 24)),
+ 2016: ((DEC, 12),),
+ 2017: ((DEC, 1),),
+ 2018: ((NOV, 21),),
+ 2019: ((NOV, 9),),
+ 2020: ((OCT, 29),),
+ 2021: ((OCT, 18),),
+ 2022: ((OCT, 8),),
+ }
diff --git a/holidays/registry.py b/holidays/registry.py
index 698be8e0..3b6324f1 100644
--- a/holidays/registry.py
+++ b/holidays/registry.py
@@ -43,6 +43,7 @@ COUNTRIES: RegistryDict = {
"burundi": ("Burundi", "BI", "BDI"),
"cameroon": ("Cameroon", "CM", "CMR"),
"canada": ("Canada", "CA", "CAN"),
+ "chad": ("Chad", "TD", "TCD"),
"chile": ("Chile", "CL", "CHL"),
"china": ("China", "CN", "CHN"),
"colombia": ("Colombia", "CO", "COL"),
| dr-prodigy/python-holidays | e8c53e3c61cd5c1277d17d1dee6fde378ad37680 | diff --git a/tests/countries/test_chad.py b/tests/countries/test_chad.py
new file mode 100644
index 00000000..3d61d8c2
--- /dev/null
+++ b/tests/countries/test_chad.py
@@ -0,0 +1,80 @@
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Authors: dr-prodigy <dr.prodigy.github@gmail.com> (c) 2017-2023
+# ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+from holidays.countries.chad import Chad, TD, TCD
+from tests.common import TestCase
+
+
+class TestChad(TestCase):
+ @classmethod
+ def setUpClass(cls):
+ super().setUpClass(Chad)
+
+ def test_country_aliases(self):
+ self.assertCountryAliases(Chad, TD, TCD)
+
+ def test_no_holidays(self):
+ self.assertNoHolidays(Chad(years=1960))
+
+ def test_special_holidays(self):
+ self.assertHoliday("2021-04-23")
+
+ def test_freedom_and_democracy_day(self):
+ name = "Freedom and Democracy Day"
+ self.assertHolidaysName(
+ name, (f"{year}-12-01" for year in range(1991, 2050))
+ )
+ self.assertNoHolidayName(name, Chad(years=range(1961, 1991)))
+ self.assertNoHoliday(
+ f"{year}-12-01"
+ for year in set(range(1961, 1991)).difference({1976})
+ )
+
+ def test_observed(self):
+ dt = (
+ # New Year's Day
+ "2012-01-02",
+ "2017-01-02",
+ "2023-01-02",
+ # International Women's Day
+ "2015-03-09",
+ "2020-03-09",
+ # Labour Day
+ "2011-05-02",
+ "2016-05-02",
+ # Independence Day
+ "2013-08-12",
+ "2019-08-12",
+ # Republic Day
+ "2010-11-29",
+ "2021-11-29",
+ # Freedom and Democracy Day
+ "2013-12-02",
+ "2019-12-02",
+ )
+ self.assertHoliday(dt)
+ self.assertNoNonObservedHoliday(dt)
+
+ def test_2022(self):
+ self.assertHolidays(
+ ("2022-01-01", "New Year's Day"),
+ ("2022-03-08", "International Women's Day"),
+ ("2022-04-18", "Easter Monday"),
+ ("2022-05-01", "Labour Day"),
+ ("2022-05-02", "Eid al-Fitr; Labour Day (Observed)"),
+ ("2022-07-09", "Eid al-Adha"),
+ ("2022-08-11", "Independence Day"),
+ ("2022-10-08", "Mawlid"),
+ ("2022-11-01", "All Saints' Day"),
+ ("2022-11-28", "Republic Day"),
+ ("2022-12-01", "Freedom and Democracy Day"),
+ ("2022-12-25", "Christmas Day"),
+ )
| Add Chad holidays
Useful links:
- https://en.wikipedia.org/wiki/Public_holidays_in_Chad
- https://www.timeanddate.com/holidays/chad/ | 0.0 | [
"tests/countries/test_chad.py::TestChad::test_2022",
"tests/countries/test_chad.py::TestChad::test_country_aliases",
"tests/countries/test_chad.py::TestChad::test_freedom_and_democracy_day",
"tests/countries/test_chad.py::TestChad::test_no_holidays",
"tests/countries/test_chad.py::TestChad::test_observed",
"tests/countries/test_chad.py::TestChad::test_special_holidays"
] | [] | 2023-06-03 18:35:59+00:00 | 1,985 |
|
dr-prodigy__python-holidays-1306 | diff --git a/holidays/holiday_base.py b/holidays/holiday_base.py
index 0690d59c..ccffa40c 100644
--- a/holidays/holiday_base.py
+++ b/holidays/holiday_base.py
@@ -724,7 +724,12 @@ class HolidayBase(Dict[date, str]):
if name
]
- def get_named(self, holiday_name: str, lookup="icontains") -> List[date]:
+ def get_named(
+ self,
+ holiday_name: str,
+ lookup="icontains",
+ split_multiple_names=True,
+ ) -> List[date]:
"""Return a list of all holiday dates matching the provided holiday
name. The match will be made case insensitively and partial matches
will be included by default.
@@ -739,12 +744,17 @@ class HolidayBase(Dict[date, str]):
icontains - case insensitive contains match;
iexact - case insensitive exact match;
istartswith - case insensitive starts with match;
+ :param split_multiple_names:
+ Either use the exact name for each date or split it by holiday
+ name delimiter.
:return:
A list of all holiday dates matching the provided holiday name.
"""
holiday_date_names_mapping: Dict[date, List[str]] = {
key: value.split(HOLIDAY_NAME_DELIMITER)
+ if split_multiple_names
+ else [value]
for key, value in self.items()
}
@@ -849,14 +859,24 @@ class HolidayBase(Dict[date, str]):
:raise:
KeyError if date is not a holiday and default is not given.
"""
- dates = self.get_named(name)
- if not dates:
+ use_exact_name = HOLIDAY_NAME_DELIMITER in name
+ dts = self.get_named(name, split_multiple_names=not use_exact_name)
+ if len(dts) == 0:
raise KeyError(name)
- for dt in dates:
+ popped = []
+ for dt in dts:
+ holiday_names = self[dt].split(HOLIDAY_NAME_DELIMITER)
self.pop(dt)
+ popped.append(dt)
- return dates
+ # Keep the rest of holidays falling on the same date.
+ if not use_exact_name:
+ holiday_names.remove(name)
+ if len(holiday_names) > 0:
+ self[dt] = HOLIDAY_NAME_DELIMITER.join(holiday_names)
+
+ return popped
def update( # type: ignore[override]
self, *args: Union[Dict[DateLike, str], List[DateLike], DateLike]
| dr-prodigy/python-holidays | 522a9951f4457c68977016ecc8d8d9e08011f8d2 | diff --git a/tests/countries/test_isle_of_man.py b/tests/countries/test_isle_of_man.py
index 157f6956..28a72bec 100644
--- a/tests/countries/test_isle_of_man.py
+++ b/tests/countries/test_isle_of_man.py
@@ -21,6 +21,17 @@ class TestIM(TestCase):
def test_country_aliases(self):
self.assertCountryAliases(IsleOfMan, IM, IMN)
+ def test_1970(self):
+ self.assertHolidays(
+ ("1970-03-27", "Good Friday"),
+ ("1970-03-30", "Easter Monday"),
+ ("1970-06-05", "TT Bank Holiday"),
+ ("1970-07-05", "Tynwald Day"),
+ ("1970-12-25", "Christmas Day"),
+ ("1970-12-26", "Boxing Day"),
+ ("1970-12-28", "Boxing Day (Observed)"),
+ )
+
def test_2022(self):
self.assertHolidays(
("2022-01-01", "New Year's Day"),
diff --git a/tests/test_holiday_base.py b/tests/test_holiday_base.py
index f6d36473..ffd27a9a 100644
--- a/tests/test_holiday_base.py
+++ b/tests/test_holiday_base.py
@@ -21,6 +21,7 @@ from dateutil.relativedelta import relativedelta as rd
import holidays
from holidays.constants import JAN, FEB, OCT, MON, TUE, SAT, SUN
+from holidays.constants import HOLIDAY_NAME_DELIMITER
class TestBasics(unittest.TestCase):
@@ -119,15 +120,60 @@ class TestBasics(unittest.TestCase):
self.assertNotIn(date(2014, 1, 1), self.holidays)
self.assertIn(date(2014, 7, 4), self.holidays)
- def test_pop_named(self):
+ def test_pop_named_single(self):
self.assertIn(date(2014, 1, 1), self.holidays)
- self.holidays.pop_named("New Year's Day")
- self.assertNotIn(date(2014, 1, 1), self.holidays)
+ dts = self.holidays.pop_named("New Year's Day")
+ for dt in dts:
+ self.assertNotIn(dt, self.holidays)
+
+ def test_pop_named_multiple(self):
+ dt = date(2022, 2, 22)
+ holiday_name_1 = "Holiday Name 1"
+ holiday_name_2 = "Holiday Name 2"
+ holiday_name_3 = "Holiday Name 3"
+ combined_name = HOLIDAY_NAME_DELIMITER.join(
+ (holiday_name_1, holiday_name_2, holiday_name_3)
+ )
+ self.holidays[dt] = holiday_name_1
+ self.holidays[dt] = holiday_name_2
+ self.holidays[dt] = holiday_name_3
+ self.assertEqual(self.holidays[dt], combined_name)
+
+ # Pop the entire date by multiple holidays exact name.
+ self.holidays.pop_named(combined_name)
+ self.assertNotIn(dt, self.holidays)
+
+ # Pop only one holiday by a single name.
+ self.holidays[dt] = holiday_name_1
+ self.holidays[dt] = holiday_name_2
+ self.holidays[dt] = holiday_name_3
+ self.assertEqual(self.holidays[dt], combined_name)
+
+ self.holidays.pop_named(holiday_name_1)
+ self.assertEqual(
+ self.holidays[dt],
+ HOLIDAY_NAME_DELIMITER.join((holiday_name_2, holiday_name_3)),
+ )
+
+ self.holidays.pop_named(holiday_name_3)
+ self.assertEqual(self.holidays[dt], holiday_name_2)
+
+ self.holidays.pop_named(holiday_name_2)
+ self.assertNotIn(dt, self.holidays)
+
+ def test_pop_named_exception(self):
self.assertRaises(
KeyError,
lambda: self.holidays.pop_named("New Year's Dayz"),
)
+ self.assertIn(date(2022, 1, 1), self.holidays)
+ self.holidays.pop_named("New Year's Day")
+ self.assertRaises(
+ KeyError,
+ lambda: self.holidays.pop_named("New Year's Day"),
+ )
+
def test_setitem(self):
self.holidays = holidays.US(years=[2014])
self.assertEqual(len(self.holidays), 10)
| Cannot "Pop" Combined Texas Emancipation/Juneteenth Holiday
Creating a holiday using TX as the state, there is the combined holiday 'Emancipation Day In Texas; Juneteenth National Independence Day'
Previous versions of python-holidays seemed to treat this concatenation with a comma a such: 'Emancipation Day In Texas, Juneteenth National Independence Day'
I am able to 'pop_named' the holiday in the older version, not in the newer with the semicolon seperator:
python3.6/holidays 0.11.3.1:

python 3.10/holidays .25:

| 0.0 | [
"tests/test_holiday_base.py::TestBasics::test_pop_named_multiple"
] | [
"tests/countries/test_isle_of_man.py::TestIM::test_1970",
"tests/countries/test_isle_of_man.py::TestIM::test_2022",
"tests/countries/test_isle_of_man.py::TestIM::test_country_aliases",
"tests/test_holiday_base.py::TestBasics::test_add_countries",
"tests/test_holiday_base.py::TestBasics::test_add_financial",
"tests/test_holiday_base.py::TestBasics::test_add_holiday",
"tests/test_holiday_base.py::TestBasics::test_append",
"tests/test_holiday_base.py::TestBasics::test_contains",
"tests/test_holiday_base.py::TestBasics::test_copy",
"tests/test_holiday_base.py::TestBasics::test_eq_",
"tests/test_holiday_base.py::TestBasics::test_get",
"tests/test_holiday_base.py::TestBasics::test_get_list",
"tests/test_holiday_base.py::TestBasics::test_get_named_contains",
"tests/test_holiday_base.py::TestBasics::test_get_named_exact",
"tests/test_holiday_base.py::TestBasics::test_get_named_icontains",
"tests/test_holiday_base.py::TestBasics::test_get_named_iexact",
"tests/test_holiday_base.py::TestBasics::test_get_named_istartswith",
"tests/test_holiday_base.py::TestBasics::test_get_named_lookup_invalid",
"tests/test_holiday_base.py::TestBasics::test_get_named_startswith",
"tests/test_holiday_base.py::TestBasics::test_getitem",
"tests/test_holiday_base.py::TestBasics::test_inheritance",
"tests/test_holiday_base.py::TestBasics::test_is_leap_year",
"tests/test_holiday_base.py::TestBasics::test_is_weekend",
"tests/test_holiday_base.py::TestBasics::test_ne",
"tests/test_holiday_base.py::TestBasics::test_pop",
"tests/test_holiday_base.py::TestBasics::test_pop_named_exception",
"tests/test_holiday_base.py::TestBasics::test_pop_named_single",
"tests/test_holiday_base.py::TestBasics::test_radd",
"tests/test_holiday_base.py::TestBasics::test_repr_country",
"tests/test_holiday_base.py::TestBasics::test_repr_market",
"tests/test_holiday_base.py::TestBasics::test_setitem",
"tests/test_holiday_base.py::TestBasics::test_str_country",
"tests/test_holiday_base.py::TestBasics::test_str_market",
"tests/test_holiday_base.py::TestBasics::test_update",
"tests/test_holiday_base.py::TestArgs::test_country",
"tests/test_holiday_base.py::TestArgs::test_deprecation_warnings",
"tests/test_holiday_base.py::TestArgs::test_expand",
"tests/test_holiday_base.py::TestArgs::test_observed",
"tests/test_holiday_base.py::TestArgs::test_serialization",
"tests/test_holiday_base.py::TestArgs::test_years",
"tests/test_holiday_base.py::TestKeyTransforms::test_date",
"tests/test_holiday_base.py::TestKeyTransforms::test_date_derived",
"tests/test_holiday_base.py::TestKeyTransforms::test_datetime",
"tests/test_holiday_base.py::TestKeyTransforms::test_exception",
"tests/test_holiday_base.py::TestKeyTransforms::test_string",
"tests/test_holiday_base.py::TestKeyTransforms::test_timestamp",
"tests/test_holiday_base.py::TestCountryHolidayDeprecation::test_deprecation",
"tests/test_holiday_base.py::TestCountrySpecialHolidays::test_populate_special_holidays",
"tests/test_holiday_base.py::TestHolidaysTranslation::test_language_unavailable"
] | 2023-06-10 01:43:28+00:00 | 1,986 |
|
dr-prodigy__python-holidays-1325 | diff --git a/CHANGES b/CHANGES
index 38bd8937..04d3b680 100644
--- a/CHANGES
+++ b/CHANGES
@@ -1,3 +1,10 @@
+Version 0.27.1
+==============
+
+Released June 21, 2023
+
+- Fix HolidayBase::pop_named partial holiday names removal (#1325 by @arkid15r)
+
Version 0.27
============
diff --git a/holidays/__init__.py b/holidays/__init__.py
index 12bda76a..02182bc9 100644
--- a/holidays/__init__.py
+++ b/holidays/__init__.py
@@ -14,7 +14,7 @@ from holidays.holiday_base import *
from holidays.registry import EntityLoader
from holidays.utils import *
-__version__ = "0.27"
+__version__ = "0.27.1"
EntityLoader.load("countries", globals())
diff --git a/holidays/holiday_base.py b/holidays/holiday_base.py
index 5c568e22..c25cf69c 100644
--- a/holidays/holiday_base.py
+++ b/holidays/holiday_base.py
@@ -822,7 +822,13 @@ class HolidayBase(Dict[date, str]):
# Keep the rest of holidays falling on the same date.
if not use_exact_name:
- holiday_names.remove(name)
+ name_lower = name.lower()
+ holiday_names = [
+ holiday_name
+ for holiday_name in holiday_names
+ if name_lower not in holiday_name.lower()
+ ]
+
if len(holiday_names) > 0:
self[dt] = HOLIDAY_NAME_DELIMITER.join(holiday_names)
diff --git a/setup.cfg b/setup.cfg
index 4c1e185c..f0505dea 100644
--- a/setup.cfg
+++ b/setup.cfg
@@ -37,7 +37,7 @@ python_requires = >=3.7
include_package_data = True
[bumpversion]
-current_version = 0.27
+current_version = 0.27.1
[flake8]
extend-ignore = F821
| dr-prodigy/python-holidays | 2b0463684d23adacb331c03fda7b794534c16c6d | diff --git a/tests/test_holiday_base.py b/tests/test_holiday_base.py
index c57af796..bfe86e01 100644
--- a/tests/test_holiday_base.py
+++ b/tests/test_holiday_base.py
@@ -156,6 +156,16 @@ class TestBasics(unittest.TestCase):
self.holidays.pop_named(holiday_name_2)
self.assertNotIn(dt, self.holidays)
+ def test_pop_named_partial(self):
+ self.assertIn(date(2014, 1, 1), self.holidays)
+ dts = self.holidays.pop_named("N")
+ for dt in dts:
+ self.assertNotIn(dt, self.holidays)
+ self.assertRaises(
+ KeyError,
+ lambda: self.holidays.pop_named("New Year"),
+ )
+
def test_pop_named_exception(self):
self.assertRaises(
KeyError,
| HolidayBase::pop_named won't remove holiday by a partial name
```
File ".../home-assistant-core/venv/lib/python3.10/site-packages/holidays/holiday_base.py", line 825, in pop_named
holiday_names.remove(name)
ValueError: list.remove(x): x not in list
``` | 0.0 | [
"tests/test_holiday_base.py::TestBasics::test_pop_named_partial"
] | [
"tests/test_holiday_base.py::TestBasics::test_add_countries",
"tests/test_holiday_base.py::TestBasics::test_add_financial",
"tests/test_holiday_base.py::TestBasics::test_add_holiday",
"tests/test_holiday_base.py::TestBasics::test_append",
"tests/test_holiday_base.py::TestBasics::test_contains",
"tests/test_holiday_base.py::TestBasics::test_copy",
"tests/test_holiday_base.py::TestBasics::test_eq_",
"tests/test_holiday_base.py::TestBasics::test_get",
"tests/test_holiday_base.py::TestBasics::test_get_list",
"tests/test_holiday_base.py::TestBasics::test_get_named_contains",
"tests/test_holiday_base.py::TestBasics::test_get_named_exact",
"tests/test_holiday_base.py::TestBasics::test_get_named_icontains",
"tests/test_holiday_base.py::TestBasics::test_get_named_iexact",
"tests/test_holiday_base.py::TestBasics::test_get_named_istartswith",
"tests/test_holiday_base.py::TestBasics::test_get_named_lookup_invalid",
"tests/test_holiday_base.py::TestBasics::test_get_named_startswith",
"tests/test_holiday_base.py::TestBasics::test_getitem",
"tests/test_holiday_base.py::TestBasics::test_inheritance",
"tests/test_holiday_base.py::TestBasics::test_is_leap_year",
"tests/test_holiday_base.py::TestBasics::test_is_weekend",
"tests/test_holiday_base.py::TestBasics::test_ne",
"tests/test_holiday_base.py::TestBasics::test_pop",
"tests/test_holiday_base.py::TestBasics::test_pop_named_exception",
"tests/test_holiday_base.py::TestBasics::test_pop_named_multiple",
"tests/test_holiday_base.py::TestBasics::test_pop_named_single",
"tests/test_holiday_base.py::TestBasics::test_radd",
"tests/test_holiday_base.py::TestBasics::test_repr_country",
"tests/test_holiday_base.py::TestBasics::test_repr_market",
"tests/test_holiday_base.py::TestBasics::test_setitem",
"tests/test_holiday_base.py::TestBasics::test_str_country",
"tests/test_holiday_base.py::TestBasics::test_str_market",
"tests/test_holiday_base.py::TestBasics::test_update",
"tests/test_holiday_base.py::TestArgs::test_country",
"tests/test_holiday_base.py::TestArgs::test_deprecation_warnings",
"tests/test_holiday_base.py::TestArgs::test_expand",
"tests/test_holiday_base.py::TestArgs::test_observed",
"tests/test_holiday_base.py::TestArgs::test_serialization",
"tests/test_holiday_base.py::TestArgs::test_years",
"tests/test_holiday_base.py::TestKeyTransforms::test_date",
"tests/test_holiday_base.py::TestKeyTransforms::test_date_derived",
"tests/test_holiday_base.py::TestKeyTransforms::test_datetime",
"tests/test_holiday_base.py::TestKeyTransforms::test_exception",
"tests/test_holiday_base.py::TestKeyTransforms::test_string",
"tests/test_holiday_base.py::TestKeyTransforms::test_timestamp",
"tests/test_holiday_base.py::TestCountryHolidayDeprecation::test_deprecation",
"tests/test_holiday_base.py::TestCountrySpecialHolidays::test_populate_special_holidays",
"tests/test_holiday_base.py::TestHolidaysTranslation::test_language_unavailable"
] | 2023-06-21 15:53:34+00:00 | 1,987 |
|
dr-prodigy__python-holidays-371 | diff --git a/holidays/countries/croatia.py b/holidays/countries/croatia.py
index 519fe18b..0136f1e3 100644
--- a/holidays/countries/croatia.py
+++ b/holidays/countries/croatia.py
@@ -11,18 +11,18 @@
# Website: https://github.com/dr-prodigy/python-holidays
# License: MIT (see LICENSE file)
-from datetime import date
+from datetime import date, timedelta
from dateutil.easter import easter
-from dateutil.relativedelta import relativedelta as rd
-from holidays.constants import JAN, MAY, JUN, AUG, OCT, \
- NOV, DEC
+from holidays.constants import JAN, MAY, JUN, AUG, OCT, NOV, DEC
from holidays.holiday_base import HolidayBase
class Croatia(HolidayBase):
+ # Updated with act 022-03 / 19-01 / 219 of 14 November 2019
+ # https://narodne-novine.nn.hr/clanci/sluzbeni/2019_11_110_2212.html
# https://en.wikipedia.org/wiki/Public_holidays_in_Croatia
def __init__(self, **kwargs):
@@ -32,6 +32,7 @@ class Croatia(HolidayBase):
def _populate(self, year):
# New years
self[date(year, JAN, 1)] = "Nova Godina"
+
# Epiphany
self[date(year, JAN, 6)] = "Sveta tri kralja"
easter_date = easter(year)
@@ -39,23 +40,23 @@ class Croatia(HolidayBase):
# Easter
self[easter_date] = "Uskrs"
# Easter Monday
- self[easter_date + rd(days=1)] = "Uskršnji ponedjeljak"
+ self[easter_date + timedelta(days=1)] = "Uskrsni ponedjeljak"
# Corpus Christi
- self[easter_date + rd(days=60)] = "Tijelovo"
+ self[easter_date + timedelta(days=60)] = "Tijelovo"
# International Workers' Day
self[date(year, MAY, 1)] = "Međunarodni praznik rada"
+ # Statehood day (new)
if year >= 2020:
- # Statehood day
self[date(year, MAY, 30)] = "Dan državnosti"
# Anti-fascist struggle day
self[date(year, JUN, 22)] = "Dan antifašističke borbe"
+ # Statehood day (old)
if year < 2020:
- # Statehood day
self[date(year, JUN, 25)] = "Dan državnosti"
# Victory and Homeland Thanksgiving Day
@@ -64,17 +65,16 @@ class Croatia(HolidayBase):
# Assumption of Mary
self[date(year, AUG, 15)] = "Velika Gospa"
+ # Independence Day (old)
if year < 2020:
- # Independence Day
self[date(year, OCT, 8)] = "Dan neovisnosti"
# All Saints' Day
- self[date(year, NOV, 1)] = "Dan svih svetih"
+ self[date(year, NOV, 1)] = "Svi sveti"
if year >= 2020:
# Memorial day
- self[date(year, NOV, 18)] =\
- "Dan sjećanja na žrtve Domovinskog rata"
+ self[date(year, NOV, 18)] = "Dan sjećanja"
# Christmas day
self[date(year, DEC, 25)] = "Božić"
diff --git a/holidays/countries/russia.py b/holidays/countries/russia.py
index 8e935295..41d36c92 100644
--- a/holidays/countries/russia.py
+++ b/holidays/countries/russia.py
@@ -53,8 +53,12 @@ class Russia(HolidayBase):
self[date(year, MAY, 9)] = "День Победы"
# Russia's Day
self[date(year, JUN, 12)] = "День России"
- # Unity Day
- self[date(year, NOV, 4)] = "День народного единства"
+ if year >= 2005:
+ # Unity Day
+ self[date(year, NOV, 4)] = "День народного единства"
+ else:
+ # October Revolution Day
+ self[date(year, NOV, 7)] = "День Октябрьской революции"
class RU(Russia):
diff --git a/holidays/countries/united_kingdom.py b/holidays/countries/united_kingdom.py
index 4054b559..dfa92fae 100644
--- a/holidays/countries/united_kingdom.py
+++ b/holidays/countries/united_kingdom.py
@@ -166,6 +166,8 @@ class UnitedKingdom(HolidayBase):
# Boxing Day
name = "Boxing Day"
+ if self.country == "Ireland":
+ name = "St. Stephen's Day"
self[date(year, DEC, 26)] = name
if self.observed and date(year, DEC, 26).weekday() == SAT:
self[date(year, DEC, 28)] = name + " (Observed)"
diff --git a/holidays/countries/united_states.py b/holidays/countries/united_states.py
index 595005e5..428eac78 100644
--- a/holidays/countries/united_states.py
+++ b/holidays/countries/united_states.py
@@ -452,12 +452,12 @@ class UnitedStates(HolidayBase):
# American Indian Heritage Day
# Family Day
# New Mexico Presidents' Day
- if (self.state in ('DE', 'FL', 'NH', 'NC', 'OK', 'TX', 'WV') and
+ if (self.state in ('CA', 'DE', 'FL', 'NH', 'NC', 'OK', 'TX', 'WV') and
year >= 1975) \
or (self.state == 'IN' and year >= 2010) \
or (self.state == 'MD' and year >= 2008) \
or self.state in ('NV', 'NM'):
- if self.state in ('DE', 'NH', 'NC', 'OK', 'WV'):
+ if self.state in ('CA', 'DE', 'NH', 'NC', 'OK', 'WV'):
name = "Day After Thanksgiving"
elif self.state in ('FL', 'TX'):
name = "Friday After Thanksgiving"
| dr-prodigy/python-holidays | 5ead4697d65317cf22d17571f2843ea323733a75 | diff --git a/tests.py b/tests.py
index 6832fdd4..43adb74c 100644
--- a/tests.py
+++ b/tests.py
@@ -1937,6 +1937,7 @@ class TestUS(unittest.TestCase):
self.assertIn(date(2017, 11, 20), pr_holidays)
def test_thanksgiving_day(self):
+ ca_holidays = holidays.US(state='CA')
de_holidays = holidays.US(state='DE')
fl_holidays = holidays.US(state='FL')
in_holidays = holidays.US(state='IN')
@@ -1955,6 +1956,8 @@ class TestUS(unittest.TestCase):
self.assertNotIn(dt + relativedelta(days=-1), self.holidays)
self.assertNotIn(dt + relativedelta(days=+1), self.holidays)
self.assertIn(dt + relativedelta(days=+1), de_holidays)
+ self.assertEqual(ca_holidays.get(dt + relativedelta(days=+1)),
+ "Day After Thanksgiving")
self.assertEqual(de_holidays.get(dt + relativedelta(days=+1)),
"Day After Thanksgiving")
self.assertEqual(nh_holidays.get(dt + relativedelta(days=+1)),
@@ -4949,6 +4952,9 @@ class TestCroatia(unittest.TestCase):
self.assertIn(date(2018, 11, 1), self.holidays)
self.assertIn(date(2018, 12, 25), self.holidays)
self.assertIn(date(2018, 12, 26), self.holidays)
+
+ def test_2020_new(self):
+ self.assertIn(date(2020, 5, 30), self.holidays)
self.assertIn(date(2020, 11, 18), self.holidays)
@@ -5282,6 +5288,10 @@ class TestRussia(unittest.TestCase):
def setUp(self):
self.holidays = holidays.RU()
+
+ def test_before_2005(self):
+ self.assertIn(date(2004, 11, 7), self.holidays)
+ self.assertNotIn(date(2004, 11, 4), self.holidays)
def test_2018(self):
# https://en.wikipedia.org/wiki/Public_holidays_in_Russia
@@ -5299,6 +5309,7 @@ class TestRussia(unittest.TestCase):
self.assertIn(date(2018, 5, 9), self.holidays)
self.assertIn(date(2018, 6, 12), self.holidays)
self.assertIn(date(2018, 11, 4), self.holidays)
+ self.assertNotIn(date(2018, 11, 7), self.holidays)
class TestLatvia(unittest.TestCase):
| Wrong workday info for country HR
Today (Oct. 8, 2020) my alarmclock automation did not go off, because my workday sensor gave the wrong info (no workday). This day used to be a holiday in Croatia, but is not anymore.
binary_sensor:
- platform: workday
country: HR
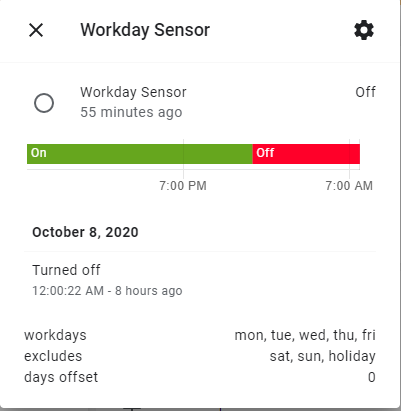
| 0.0 | [
"tests.py::TestUS::test_thanksgiving_day",
"tests.py::TestRussia::test_before_2005"
] | [
"tests.py::TestBasics::test_add",
"tests.py::TestBasics::test_append",
"tests.py::TestBasics::test_contains",
"tests.py::TestBasics::test_eq_ne",
"tests.py::TestBasics::test_get",
"tests.py::TestBasics::test_get_list",
"tests.py::TestBasics::test_get_named",
"tests.py::TestBasics::test_getitem",
"tests.py::TestBasics::test_inheritance",
"tests.py::TestBasics::test_list_supported_countries",
"tests.py::TestBasics::test_pop",
"tests.py::TestBasics::test_pop_named",
"tests.py::TestBasics::test_radd",
"tests.py::TestBasics::test_setitem",
"tests.py::TestBasics::test_update",
"tests.py::TestArgs::test_country",
"tests.py::TestArgs::test_expand",
"tests.py::TestArgs::test_observed",
"tests.py::TestArgs::test_years",
"tests.py::TestKeyTransforms::test_dates",
"tests.py::TestKeyTransforms::test_datetimes",
"tests.py::TestKeyTransforms::test_exceptions",
"tests.py::TestKeyTransforms::test_strings",
"tests.py::TestKeyTransforms::test_timestamp",
"tests.py::TestCountryHoliday::test_country",
"tests.py::TestCountryHoliday::test_country_province",
"tests.py::TestCountryHoliday::test_country_state",
"tests.py::TestCountryHoliday::test_country_years",
"tests.py::TestCountryHoliday::test_exceptions",
"tests.py::TestAruba::test_2017",
"tests.py::TestAruba::test_anthem_and_flag_day",
"tests.py::TestAruba::test_ascension_day",
"tests.py::TestAruba::test_betico_day",
"tests.py::TestAruba::test_carnaval_monday",
"tests.py::TestAruba::test_christmas",
"tests.py::TestAruba::test_easter_monday",
"tests.py::TestAruba::test_good_friday",
"tests.py::TestAruba::test_kings_day_after_2014",
"tests.py::TestAruba::test_kings_day_after_2014_substituted_earlier",
"tests.py::TestAruba::test_labour_day",
"tests.py::TestAruba::test_new_years",
"tests.py::TestAruba::test_queens_day_between_1891_and_1948",
"tests.py::TestAruba::test_queens_day_between_1891_and_1948_substituted_later",
"tests.py::TestAruba::test_queens_day_between_1949_and_1980_substituted_later",
"tests.py::TestAruba::test_queens_day_between_1949_and_2013",
"tests.py::TestAruba::test_queens_day_between_1980_and_2013_substituted_earlier",
"tests.py::TestAruba::test_second_christmas",
"tests.py::TestBulgaria::test_before_1990",
"tests.py::TestBulgaria::test_christmas",
"tests.py::TestBulgaria::test_easter",
"tests.py::TestBulgaria::test_independence_day",
"tests.py::TestBulgaria::test_labour_day",
"tests.py::TestBulgaria::test_liberation_day",
"tests.py::TestBulgaria::test_national_awakening_day",
"tests.py::TestBulgaria::test_new_years_day",
"tests.py::TestBulgaria::test_saint_georges_day",
"tests.py::TestBulgaria::test_twenty_fourth_of_may",
"tests.py::TestBulgaria::test_unification_day",
"tests.py::TestCA::test_boxing_day",
"tests.py::TestCA::test_canada_day",
"tests.py::TestCA::test_christmas_day",
"tests.py::TestCA::test_civic_holiday",
"tests.py::TestCA::test_discovery_day",
"tests.py::TestCA::test_easter_monday",
"tests.py::TestCA::test_family_day",
"tests.py::TestCA::test_good_friday",
"tests.py::TestCA::test_islander_day",
"tests.py::TestCA::test_labour_day",
"tests.py::TestCA::test_national_aboriginal_day",
"tests.py::TestCA::test_new_years",
"tests.py::TestCA::test_nunavut_day",
"tests.py::TestCA::test_remembrance_day",
"tests.py::TestCA::test_st_georges_day",
"tests.py::TestCA::test_st_jean_baptiste_day",
"tests.py::TestCA::test_st_patricks_day",
"tests.py::TestCA::test_thanksgiving",
"tests.py::TestCA::test_victoria_day",
"tests.py::TestCO::test_2016",
"tests.py::TestCO::test_others",
"tests.py::TestMX::test_benito_juarez",
"tests.py::TestMX::test_change_of_government",
"tests.py::TestMX::test_christmas",
"tests.py::TestMX::test_constitution_day",
"tests.py::TestMX::test_independence_day",
"tests.py::TestMX::test_labor_day",
"tests.py::TestMX::test_new_years",
"tests.py::TestMX::test_revolution_day",
"tests.py::TestNetherlands::test_2017",
"tests.py::TestNetherlands::test_ascension_day",
"tests.py::TestNetherlands::test_easter",
"tests.py::TestNetherlands::test_easter_monday",
"tests.py::TestNetherlands::test_first_christmas",
"tests.py::TestNetherlands::test_kings_day_after_2014",
"tests.py::TestNetherlands::test_kings_day_after_2014_substituted_earlier",
"tests.py::TestNetherlands::test_liberation_day",
"tests.py::TestNetherlands::test_liberation_day_after_1990_in_lustrum_year",
"tests.py::TestNetherlands::test_liberation_day_after_1990_non_lustrum_year",
"tests.py::TestNetherlands::test_new_years",
"tests.py::TestNetherlands::test_queens_day_between_1891_and_1948",
"tests.py::TestNetherlands::test_queens_day_between_1891_and_1948_substituted_later",
"tests.py::TestNetherlands::test_queens_day_between_1949_and_1980_substituted_later",
"tests.py::TestNetherlands::test_queens_day_between_1949_and_2013",
"tests.py::TestNetherlands::test_queens_day_between_1980_and_2013_substituted_earlier",
"tests.py::TestNetherlands::test_second_christmas",
"tests.py::TestNetherlands::test_whit_monday",
"tests.py::TestNetherlands::test_whit_sunday",
"tests.py::TestUS::test_alaska_day",
"tests.py::TestUS::test_all_souls_day",
"tests.py::TestUS::test_arbor_day",
"tests.py::TestUS::test_bennington_battle_day",
"tests.py::TestUS::test_casimir_pulaski_day",
"tests.py::TestUS::test_cesar_chavez_day",
"tests.py::TestUS::test_christmas_day",
"tests.py::TestUS::test_christmas_eve",
"tests.py::TestUS::test_columbus_day",
"tests.py::TestUS::test_confederate_memorial_day",
"tests.py::TestUS::test_constitution_day",
"tests.py::TestUS::test_day_after_christmas",
"tests.py::TestUS::test_discovery_day",
"tests.py::TestUS::test_easter_monday",
"tests.py::TestUS::test_election_day",
"tests.py::TestUS::test_emancipation_day",
"tests.py::TestUS::test_emancipation_day_in_puerto_rico",
"tests.py::TestUS::test_emancipation_day_in_texas",
"tests.py::TestUS::test_emancipation_day_in_virgin_islands",
"tests.py::TestUS::test_epiphany",
"tests.py::TestUS::test_evacuation_day",
"tests.py::TestUS::test_good_friday",
"tests.py::TestUS::test_guam_discovery_day",
"tests.py::TestUS::test_holy_thursday",
"tests.py::TestUS::test_inauguration_day",
"tests.py::TestUS::test_independence_day",
"tests.py::TestUS::test_jefferson_davis_birthday",
"tests.py::TestUS::test_kamehameha_day",
"tests.py::TestUS::test_labor_day",
"tests.py::TestUS::test_lady_of_camarin_day",
"tests.py::TestUS::test_lee_jackson_day",
"tests.py::TestUS::test_liberation_day_guam",
"tests.py::TestUS::test_liberty_day",
"tests.py::TestUS::test_lincolns_birthday",
"tests.py::TestUS::test_lyndon_baines_johnson_day",
"tests.py::TestUS::test_mardi_gras",
"tests.py::TestUS::test_martin_luther",
"tests.py::TestUS::test_memorial_day",
"tests.py::TestUS::test_nevada_day",
"tests.py::TestUS::test_new_years",
"tests.py::TestUS::test_new_years_eve",
"tests.py::TestUS::test_patriots_day",
"tests.py::TestUS::test_pioneer_day",
"tests.py::TestUS::test_primary_election_day",
"tests.py::TestUS::test_prince_jonah_kuhio_kalanianaole_day",
"tests.py::TestUS::test_robert_lee_birthday",
"tests.py::TestUS::test_san_jacinto_day",
"tests.py::TestUS::test_statehood_day",
"tests.py::TestUS::test_stewards_day",
"tests.py::TestUS::test_susan_b_anthony_day",
"tests.py::TestUS::test_texas_independence_day",
"tests.py::TestUS::test_three_kings_day",
"tests.py::TestUS::test_town_meeting_day",
"tests.py::TestUS::test_transfer_day",
"tests.py::TestUS::test_truman_day",
"tests.py::TestUS::test_veterans_day",
"tests.py::TestUS::test_victory_day",
"tests.py::TestUS::test_washingtons_birthday",
"tests.py::TestUS::test_west_virginia_day",
"tests.py::TestNZ::test_all_holidays_present",
"tests.py::TestNZ::test_anzac_day",
"tests.py::TestNZ::test_auckland_anniversary_day",
"tests.py::TestNZ::test_boxing_day",
"tests.py::TestNZ::test_canterbury_anniversary_day",
"tests.py::TestNZ::test_chatham_islands_anniversary_day",
"tests.py::TestNZ::test_christmas_day",
"tests.py::TestNZ::test_day_after_new_years",
"tests.py::TestNZ::test_easter_monday",
"tests.py::TestNZ::test_good_friday",
"tests.py::TestNZ::test_hawkes_bay_anniversary_day",
"tests.py::TestNZ::test_labour_day",
"tests.py::TestNZ::test_marlborough_anniversary_day",
"tests.py::TestNZ::test_nelson_anniversary_day",
"tests.py::TestNZ::test_new_years",
"tests.py::TestNZ::test_otago_anniversary_day",
"tests.py::TestNZ::test_south_canterbury_anniversary_day",
"tests.py::TestNZ::test_southland_anniversary_day",
"tests.py::TestNZ::test_sovereigns_birthday",
"tests.py::TestNZ::test_taranaki_anniversary_day",
"tests.py::TestNZ::test_waitangi_day",
"tests.py::TestNZ::test_wellington_anniversary_day",
"tests.py::TestNZ::test_westland_anniversary_day",
"tests.py::TestAU::test_adelaide_cup",
"tests.py::TestAU::test_all_holidays",
"tests.py::TestAU::test_anzac_day",
"tests.py::TestAU::test_australia_day",
"tests.py::TestAU::test_bank_holiday",
"tests.py::TestAU::test_boxing_day",
"tests.py::TestAU::test_christmas_day",
"tests.py::TestAU::test_easter_monday",
"tests.py::TestAU::test_easter_saturday",
"tests.py::TestAU::test_easter_sunday",
"tests.py::TestAU::test_family_and_community_day",
"tests.py::TestAU::test_good_friday",
"tests.py::TestAU::test_grand_final_day",
"tests.py::TestAU::test_labour_day",
"tests.py::TestAU::test_melbourne_cup",
"tests.py::TestAU::test_new_years",
"tests.py::TestAU::test_picnic_day",
"tests.py::TestAU::test_queens_birthday",
"tests.py::TestAU::test_reconciliation_day",
"tests.py::TestAU::test_royal_queensland_show",
"tests.py::TestAU::test_western_australia_day",
"tests.py::TestDE::test_75_jahrestag_beendigung_zweiter_weltkrieg",
"tests.py::TestDE::test_all_holidays_present",
"tests.py::TestDE::test_allerheiligen",
"tests.py::TestDE::test_buss_und_bettag",
"tests.py::TestDE::test_christi_himmelfahrt",
"tests.py::TestDE::test_fixed_holidays",
"tests.py::TestDE::test_frauentag",
"tests.py::TestDE::test_fronleichnam",
"tests.py::TestDE::test_heilige_drei_koenige",
"tests.py::TestDE::test_internationaler_frauentag",
"tests.py::TestDE::test_karfreitag",
"tests.py::TestDE::test_mariae_himmelfahrt",
"tests.py::TestDE::test_no_data_before_1990",
"tests.py::TestDE::test_ostermontag",
"tests.py::TestDE::test_ostersonntag",
"tests.py::TestDE::test_pfingstmontag",
"tests.py::TestDE::test_pfingstsonntag",
"tests.py::TestDE::test_reformationstag",
"tests.py::TestDE::test_tag_der_deutschen_einheit_in_1990",
"tests.py::TestDE::test_weltkindertag",
"tests.py::TestAT::test_all_holidays_present",
"tests.py::TestAT::test_christmas",
"tests.py::TestAT::test_easter_monday",
"tests.py::TestAT::test_national_day",
"tests.py::TestAT::test_new_years",
"tests.py::TestDK::test_2016",
"tests.py::TestUK::test_all_holidays_present",
"tests.py::TestUK::test_boxing_day",
"tests.py::TestUK::test_christmas_day",
"tests.py::TestUK::test_easter_monday",
"tests.py::TestUK::test_good_friday",
"tests.py::TestUK::test_may_day",
"tests.py::TestUK::test_new_years",
"tests.py::TestUK::test_royal_wedding",
"tests.py::TestUK::test_spring_bank_holiday",
"tests.py::TestScotland::test_2017",
"tests.py::TestIsleOfMan::test_2018",
"tests.py::TestIreland::test_2020",
"tests.py::TestES::test_fixed_holidays",
"tests.py::TestES::test_province_specific_days",
"tests.py::TestES::test_variable_days_in_2016",
"tests.py::TestTAR::test_26_december_day",
"tests.py::TestTAR::test_all_holidays_present",
"tests.py::TestTAR::test_christmas_day",
"tests.py::TestTAR::test_easter_monday",
"tests.py::TestTAR::test_good_friday",
"tests.py::TestTAR::test_labour_day",
"tests.py::TestTAR::test_new_years",
"tests.py::TestECB::test_new_years",
"tests.py::TestCZ::test_2017",
"tests.py::TestCZ::test_czech_deprecated",
"tests.py::TestCZ::test_others",
"tests.py::TestSK::test_2018",
"tests.py::TestSK::test_slovak_deprecated",
"tests.py::TestPL::test_2017",
"tests.py::TestPL::test_polish_deprecated",
"tests.py::TestPT::test_2017",
"tests.py::TestPortugalExt::test_2017",
"tests.py::TestNorway::test_christmas",
"tests.py::TestNorway::test_constitution_day",
"tests.py::TestNorway::test_easter",
"tests.py::TestNorway::test_new_years",
"tests.py::TestNorway::test_not_holiday",
"tests.py::TestNorway::test_pentecost",
"tests.py::TestNorway::test_sundays",
"tests.py::TestNorway::test_workers_day",
"tests.py::TestItaly::test_2017",
"tests.py::TestItaly::test_christmas",
"tests.py::TestItaly::test_easter",
"tests.py::TestItaly::test_easter_monday",
"tests.py::TestItaly::test_liberation_day_after_1946",
"tests.py::TestItaly::test_liberation_day_before_1946",
"tests.py::TestItaly::test_new_years",
"tests.py::TestItaly::test_province_specific_days",
"tests.py::TestItaly::test_republic_day_after_1948",
"tests.py::TestItaly::test_republic_day_before_1948",
"tests.py::TestItaly::test_saint_stephan",
"tests.py::TestSweden::test_christmas",
"tests.py::TestSweden::test_constitution_day",
"tests.py::TestSweden::test_easter",
"tests.py::TestSweden::test_new_years",
"tests.py::TestSweden::test_not_holiday",
"tests.py::TestSweden::test_pentecost",
"tests.py::TestSweden::test_sundays",
"tests.py::TestSweden::test_workers_day",
"tests.py::TestJapan::test_autumnal_equinox_day",
"tests.py::TestJapan::test_childrens_day",
"tests.py::TestJapan::test_coming_of_age",
"tests.py::TestJapan::test_constitution_memorial_day",
"tests.py::TestJapan::test_culture_day",
"tests.py::TestJapan::test_emperors_birthday",
"tests.py::TestJapan::test_foundation_day",
"tests.py::TestJapan::test_greenery_day",
"tests.py::TestJapan::test_health_and_sports_day",
"tests.py::TestJapan::test_invalid_years",
"tests.py::TestJapan::test_labour_thanks_giving_day",
"tests.py::TestJapan::test_marine_day",
"tests.py::TestJapan::test_mountain_day",
"tests.py::TestJapan::test_new_years_day",
"tests.py::TestJapan::test_reiwa_emperor_holidays",
"tests.py::TestJapan::test_respect_for_the_aged_day",
"tests.py::TestJapan::test_showa_day",
"tests.py::TestJapan::test_vernal_equinox_day",
"tests.py::TestFrance::test_2017",
"tests.py::TestFrance::test_alsace_moselle",
"tests.py::TestFrance::test_guadeloupe",
"tests.py::TestFrance::test_guyane",
"tests.py::TestFrance::test_la_reunion",
"tests.py::TestFrance::test_martinique",
"tests.py::TestFrance::test_mayotte",
"tests.py::TestFrance::test_nouvelle_caledonie",
"tests.py::TestFrance::test_others",
"tests.py::TestFrance::test_polynesie_francaise",
"tests.py::TestFrance::test_saint_barthelemy",
"tests.py::TestFrance::test_wallis_et_futuna",
"tests.py::TestBelgium::test_2017",
"tests.py::TestSouthAfrica::test_easter",
"tests.py::TestSouthAfrica::test_elections",
"tests.py::TestSouthAfrica::test_historic",
"tests.py::TestSouthAfrica::test_new_years",
"tests.py::TestSouthAfrica::test_not_holiday",
"tests.py::TestSouthAfrica::test_onceoff",
"tests.py::TestSouthAfrica::test_static",
"tests.py::TestSI::test_holidays",
"tests.py::TestSI::test_missing_years",
"tests.py::TestSI::test_non_holidays",
"tests.py::TestIE::test_august_bank_holiday",
"tests.py::TestIE::test_christmas_period",
"tests.py::TestIE::test_easter_monday",
"tests.py::TestIE::test_june_bank_holiday",
"tests.py::TestIE::test_may_bank_holiday",
"tests.py::TestIE::test_new_year_day",
"tests.py::TestIE::test_october_bank_holiday",
"tests.py::TestIE::test_st_patricks_day",
"tests.py::TestFinland::test_Juhannus",
"tests.py::TestFinland::test_fixed_holidays",
"tests.py::TestFinland::test_relative_holidays",
"tests.py::TestHungary::test_2018",
"tests.py::TestHungary::test_additional_day_off",
"tests.py::TestHungary::test_all_saints_day_since_1999",
"tests.py::TestHungary::test_christian_holidays_2nd_day_was_not_held_in_1955",
"tests.py::TestHungary::test_foundation_day_renamed_during_communism",
"tests.py::TestHungary::test_good_friday_since_2017",
"tests.py::TestHungary::test_holidays_during_communism",
"tests.py::TestHungary::test_labour_day_since_1946",
"tests.py::TestHungary::test_labour_day_was_doubled_in_early_50s",
"tests.py::TestHungary::test_monday_new_years_eve_day_off",
"tests.py::TestHungary::test_national_day_was_not_celebrated_during_communism",
"tests.py::TestHungary::test_october_national_day_since_1991",
"tests.py::TestHungary::test_whit_monday_since_1992",
"tests.py::TestSwitzerland::test_all_holidays_present",
"tests.py::TestSwitzerland::test_allerheiligen",
"tests.py::TestSwitzerland::test_auffahrt",
"tests.py::TestSwitzerland::test_berchtoldstag",
"tests.py::TestSwitzerland::test_bruder_chlaus",
"tests.py::TestSwitzerland::test_fest_der_unabhaengikeit",
"tests.py::TestSwitzerland::test_fixed_holidays",
"tests.py::TestSwitzerland::test_fronleichnam",
"tests.py::TestSwitzerland::test_heilige_drei_koenige",
"tests.py::TestSwitzerland::test_jahrestag_der_ausrufung_der_republik",
"tests.py::TestSwitzerland::test_jeune_genevois",
"tests.py::TestSwitzerland::test_josefstag",
"tests.py::TestSwitzerland::test_karfreitag",
"tests.py::TestSwitzerland::test_lundi_du_jeune",
"tests.py::TestSwitzerland::test_mariae_himmelfahrt",
"tests.py::TestSwitzerland::test_naefelser_fahrt",
"tests.py::TestSwitzerland::test_ostermontag",
"tests.py::TestSwitzerland::test_ostern",
"tests.py::TestSwitzerland::test_peter_und_paul",
"tests.py::TestSwitzerland::test_pfingsten",
"tests.py::TestSwitzerland::test_pfingstmontag",
"tests.py::TestSwitzerland::test_stephanstag",
"tests.py::TestSwitzerland::test_wiedererstellung_der_republik",
"tests.py::TestAR::test_belgrano_day",
"tests.py::TestAR::test_carnival_day",
"tests.py::TestAR::test_christmas",
"tests.py::TestAR::test_cultural_day",
"tests.py::TestAR::test_guemes_day",
"tests.py::TestAR::test_holy_week_day",
"tests.py::TestAR::test_independence_day",
"tests.py::TestAR::test_inmaculate_conception_day",
"tests.py::TestAR::test_labor_day",
"tests.py::TestAR::test_malvinas_war_day",
"tests.py::TestAR::test_may_revolution_day",
"tests.py::TestAR::test_memory_national_day",
"tests.py::TestAR::test_national_sovereignty_day",
"tests.py::TestAR::test_new_years",
"tests.py::TestAR::test_san_martin_day",
"tests.py::TestIND::test_2018",
"tests.py::TestBelarus::test_2018",
"tests.py::TestBelarus::test_before_1998",
"tests.py::TestBelarus::test_new_year",
"tests.py::TestBelarus::test_radunitsa",
"tests.py::TestHonduras::test_2014",
"tests.py::TestHonduras::test_2018",
"tests.py::TestCroatia::test_2018",
"tests.py::TestCroatia::test_2020_new",
"tests.py::TestUkraine::test_2018",
"tests.py::TestUkraine::test_before_1918",
"tests.py::TestUkraine::test_old_holidays",
"tests.py::TestBrazil::test_AC_holidays",
"tests.py::TestBrazil::test_AL_holidays",
"tests.py::TestBrazil::test_AM_holidays",
"tests.py::TestBrazil::test_AP_holidays",
"tests.py::TestBrazil::test_BA_holidays",
"tests.py::TestBrazil::test_BR_holidays",
"tests.py::TestBrazil::test_CE_holidays",
"tests.py::TestBrazil::test_DF_holidays",
"tests.py::TestBrazil::test_ES_holidays",
"tests.py::TestBrazil::test_GO_holidays",
"tests.py::TestBrazil::test_MA_holidays",
"tests.py::TestBrazil::test_MG_holidays",
"tests.py::TestBrazil::test_MS_holidays",
"tests.py::TestBrazil::test_MT_holidays",
"tests.py::TestBrazil::test_PA_holidays",
"tests.py::TestBrazil::test_PB_holidays",
"tests.py::TestBrazil::test_PE_holidays",
"tests.py::TestBrazil::test_PI_holidays",
"tests.py::TestBrazil::test_PR_holidays",
"tests.py::TestBrazil::test_RJ_holidays",
"tests.py::TestBrazil::test_RN_holidays",
"tests.py::TestBrazil::test_RO_holidays",
"tests.py::TestBrazil::test_RR_holidays",
"tests.py::TestBrazil::test_RS_holidays",
"tests.py::TestBrazil::test_SC_holidays",
"tests.py::TestBrazil::test_SE_holidays",
"tests.py::TestBrazil::test_SP_holidays",
"tests.py::TestBrazil::test_TO_holidays",
"tests.py::TestLU::test_2019",
"tests.py::TestRomania::test_2020",
"tests.py::TestRomania::test_children_s_day",
"tests.py::TestRussia::test_2018",
"tests.py::TestLatvia::test_2020",
"tests.py::TestLithuania::test_2018",
"tests.py::TestLithuania::test_day_of_dew",
"tests.py::TestLithuania::test_easter",
"tests.py::TestLithuania::test_fathers_day",
"tests.py::TestLithuania::test_mothers_day",
"tests.py::TestEstonia::test_boxing_day",
"tests.py::TestEstonia::test_christmas_day",
"tests.py::TestEstonia::test_christmas_eve",
"tests.py::TestEstonia::test_easter_sunday",
"tests.py::TestEstonia::test_good_friday",
"tests.py::TestEstonia::test_independence_day",
"tests.py::TestEstonia::test_midsummers_day",
"tests.py::TestEstonia::test_new_years",
"tests.py::TestEstonia::test_pentecost",
"tests.py::TestEstonia::test_restoration_of_independence_day",
"tests.py::TestEstonia::test_spring_day",
"tests.py::TestEstonia::test_victory_day",
"tests.py::TestIceland::test_commerce_day",
"tests.py::TestIceland::test_first_day_of_summer",
"tests.py::TestIceland::test_holy_friday",
"tests.py::TestIceland::test_maundy_thursday",
"tests.py::TestIceland::test_new_year",
"tests.py::TestKenya::test_2019",
"tests.py::TestHongKong::test_ching_ming_festival",
"tests.py::TestHongKong::test_christmas_day",
"tests.py::TestHongKong::test_chung_yeung_festival",
"tests.py::TestHongKong::test_common",
"tests.py::TestHongKong::test_easter",
"tests.py::TestHongKong::test_first_day_of_january",
"tests.py::TestHongKong::test_hksar_day",
"tests.py::TestHongKong::test_labour_day",
"tests.py::TestHongKong::test_lunar_new_year",
"tests.py::TestHongKong::test_mid_autumn_festival",
"tests.py::TestHongKong::test_national_day",
"tests.py::TestHongKong::test_tuen_ng_festival",
"tests.py::TestPeru::test_2019",
"tests.py::TestNigeria::test_fixed_holidays",
"tests.py::TestChile::test_2019",
"tests.py::TestDominicanRepublic::test_do_holidays_2020",
"tests.py::TestNicaragua::test_ni_holidays_2020",
"tests.py::TestSingapore::test_Singapore",
"tests.py::TestSerbia::test_armistice_day",
"tests.py::TestSerbia::test_labour_day",
"tests.py::TestSerbia::test_new_year",
"tests.py::TestSerbia::test_religious_holidays",
"tests.py::TestSerbia::test_statehood_day",
"tests.py::TestEgypt::test_2019",
"tests.py::TestEgypt::test_25_jan",
"tests.py::TestEgypt::test_25_jan_from_2009",
"tests.py::TestEgypt::test_coptic_christmas",
"tests.py::TestEgypt::test_hijri_based",
"tests.py::TestEgypt::test_labour_day",
"tests.py::TestGreece::test_fixed_holidays",
"tests.py::TestGreece::test_gr_clean_monday",
"tests.py::TestGreece::test_gr_easter_monday",
"tests.py::TestGreece::test_gr_monday_of_the_holy_spirit",
"tests.py::TestParaguay::test_easter",
"tests.py::TestParaguay::test_fixed_holidays",
"tests.py::TestParaguay::test_no_observed",
"tests.py::TestTurkey::test_2019",
"tests.py::TestTurkey::test_hijri_based",
"tests.py::TestKorea::test_birthday_of_buddha",
"tests.py::TestKorea::test_childrens_day",
"tests.py::TestKorea::test_christmas_day",
"tests.py::TestKorea::test_chuseok",
"tests.py::TestKorea::test_common",
"tests.py::TestKorea::test_constitution_day",
"tests.py::TestKorea::test_first_day_of_january",
"tests.py::TestKorea::test_hangeul_day",
"tests.py::TestKorea::test_independence_movement_day",
"tests.py::TestKorea::test_labour_day",
"tests.py::TestKorea::test_liberation_day",
"tests.py::TestKorea::test_lunar_new_year",
"tests.py::TestKorea::test_memorial_day",
"tests.py::TestKorea::test_national_foundation_day",
"tests.py::TestKorea::test_tree_planting_day",
"tests.py::TestKorea::test_years_range",
"tests.py::TestVietnam::test_common",
"tests.py::TestVietnam::test_first_day_of_january",
"tests.py::TestVietnam::test_independence_day",
"tests.py::TestVietnam::test_international_labor_day",
"tests.py::TestVietnam::test_king_hung_day",
"tests.py::TestVietnam::test_liberation_day",
"tests.py::TestVietnam::test_lunar_new_year",
"tests.py::TestVietnam::test_years_range",
"tests.py::TestMorocco::test_1961",
"tests.py::TestMorocco::test_1999",
"tests.py::TestMorocco::test_2019",
"tests.py::TestMorocco::test_hijri_based",
"tests.py::TestBurundi::test_all_saints_Day",
"tests.py::TestBurundi::test_ascension_day",
"tests.py::TestBurundi::test_assumption_Day",
"tests.py::TestBurundi::test_christmas_Day",
"tests.py::TestBurundi::test_eid_al_adha",
"tests.py::TestBurundi::test_independence_day",
"tests.py::TestBurundi::test_labour_day",
"tests.py::TestBurundi::test_ndadaye_day",
"tests.py::TestBurundi::test_new_year_day",
"tests.py::TestBurundi::test_ntaryamira_day",
"tests.py::TestBurundi::test_rwagasore_day",
"tests.py::TestBurundi::test_unity_day",
"tests.py::UnitedArabEmirates::test_2020",
"tests.py::UnitedArabEmirates::test_commemoration_day_since_2015",
"tests.py::UnitedArabEmirates::test_hijri_based",
"tests.py::TestDjibouti::test_2019",
"tests.py::TestDjibouti::test_hijri_based",
"tests.py::TestDjibouti::test_labour_day"
] | 2020-10-08 07:14:22+00:00 | 1,988 |
|
dr-prodigy__python-holidays-469 | diff --git a/CHANGES b/CHANGES
index 4d38f383..3f990929 100644
--- a/CHANGES
+++ b/CHANGES
@@ -3,6 +3,7 @@ Version 0.11.2
Released ????, ????
+- Support for Venezuela #470 (antusystem, dr-p)
- Poland fix #464 (m-ganko)
- Singapore updates for 2022 #456 (mborsetti)
- .gitignore fix #462 (TheLastProject)
diff --git a/README.rst b/README.rst
index 4e8df697..cb44ba7c 100644
--- a/README.rst
+++ b/README.rst
@@ -191,6 +191,7 @@ UnitedStates US/USA state = AL, AK, AS, AZ, AR, CA, CO, CT, DE, DC, FL
FM, MN, MS, MO, MT, NE, NV, NH, NJ, NM, NY, NC, ND, MP,
OH, OK, OR, PW, PA, PR, RI, SC, SD, TN, TX, UT, VT, VA,
VI, WA, WV, WI, WY
+Venezuela YV/VEN
Vietnam VN/VNM
Wales None
=================== ========= =============================================================
diff --git a/holidays/countries/__init__.py b/holidays/countries/__init__.py
index 7bb1c290..3584e388 100644
--- a/holidays/countries/__init__.py
+++ b/holidays/countries/__init__.py
@@ -92,4 +92,5 @@ from .united_kingdom import (
GBR,
)
from .united_states import UnitedStates, US, USA
+from .venezuela import Venezuela, YV, VEN
from .vietnam import Vietnam, VN, VNM
diff --git a/holidays/countries/spain.py b/holidays/countries/spain.py
index 794032b1..6b2c8dd0 100644
--- a/holidays/countries/spain.py
+++ b/holidays/countries/spain.py
@@ -117,7 +117,7 @@ class Spain(HolidayBase):
self._is_observed(date(year, MAR, 19), "San José")
if self.prov and self.prov not in ["CT", "VC"]:
self[easter(year) + rd(weeks=-1, weekday=TH)] = "Jueves Santo"
- self[easter(year) + rd(weeks=-1, weekday=FR)] = "Viernes Santo"
+ self[easter(year) + rd(weeks=-1, weekday=FR)] = "Viernes Santo"
if self.prov and self.prov in ["CT", "PV", "NC", "VC", "IB", "CM"]:
self[easter(year) + rd(weekday=MO)] = "Lunes de Pascua"
self._is_observed(date(year, MAY, 1), "Día del Trabajador")
diff --git a/holidays/countries/venezuela.py b/holidays/countries/venezuela.py
new file mode 100644
index 00000000..020a0122
--- /dev/null
+++ b/holidays/countries/venezuela.py
@@ -0,0 +1,97 @@
+# -*- coding: utf-8 -*-
+
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Author: ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# dr-prodigy <maurizio.montel@gmail.com> (c) 2017-2021
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+from datetime import date
+
+from dateutil.easter import easter
+from dateutil.relativedelta import relativedelta as rd, TH, FR
+
+from holidays.constants import (
+ JAN,
+ MAR,
+ APR,
+ MAY,
+ JUN,
+ JUL,
+ AUG,
+ SEP,
+ OCT,
+ NOV,
+ DEC,
+)
+from holidays.holiday_base import HolidayBase
+
+
+class Venezuela(HolidayBase):
+ """
+ https://dias-festivos.eu/dias-festivos/venezuela/#
+ """
+
+ def __init__(self, **kwargs):
+ self.country = "YV"
+ HolidayBase.__init__(self, **kwargs)
+
+ def _populate(self, year):
+ # New Year's Day
+ self[date(year, JAN, 1)] = "Año Nuevo [New Year's Day]"
+
+ self[date(year, MAY, 1)] = "Dia Mundial del Trabajador"
+
+ self[date(year, JUN, 24)] = "Batalla de Carabobo"
+
+ self[date(year, JUL, 5)] = "Dia de la Independencia"
+
+ self[date(year, JUL, 24)] = "Natalicio de Simón Bolívar"
+
+ self[date(year, OCT, 12)] = "Día de la Resistencia Indígena"
+
+ # Christmas Day
+ self[date(year, DEC, 24)] = "Nochebuena"
+
+ self[date(year, DEC, 25)] = "Día de Navidad"
+
+ self[date(year, DEC, 31)] = "Fiesta de Fin de Año"
+
+ # Semana Santa y Carnaval
+
+ if date(year, APR, 19) == (easter(year) - rd(days=2)):
+ self[
+ easter(year) - rd(days=2)
+ ] = "Viernes Santo y Declaración de la Independencia"
+ else:
+ # self[easter(year) - rd(weekday=FR(-1))] = "Viernes Santo"
+ self[date(year, APR, 19)] = "Declaración de la Independencia"
+ self[easter(year) - rd(days=2)] = "Viernes Santo"
+
+ # self[easter(year) - rd(weekday=TH(-1))] = "Jueves Santo"
+
+ if date(year, APR, 19) == (easter(year) - rd(days=3)):
+ self[easter(year) - rd(days=3)] = (
+ "Jueves Santo y Declaración " "de la Independencia"
+ )
+ else:
+ # self[easter(year) - rd(weekday=FR(-1))] = "Viernes Santo"
+ self[date(year, APR, 19)] = "Declaración de la Independencia"
+ self[easter(year) - rd(days=3)] = "Jueves Santo"
+
+ self[easter(year) - rd(days=47)] = "Martes de Carnaval"
+
+ self[easter(year) - rd(days=48)] = "Lunes de Carnaval"
+
+
+class YV(Venezuela):
+ pass
+
+
+class VEN(Venezuela):
+ pass
| dr-prodigy/python-holidays | a33745d6c356770a0c48949fdad262f71da403f2 | diff --git a/test/countries/__init__.py b/test/countries/__init__.py
index d0d28686..321e65f1 100644
--- a/test/countries/__init__.py
+++ b/test/countries/__init__.py
@@ -82,4 +82,5 @@ from .test_ukraine import *
from .test_united_arab_emirates import *
from .test_united_kingdom import *
from .test_united_states import *
+from .test_venezuela import *
from .test_vietnam import *
diff --git a/test/countries/test_spain.py b/test/countries/test_spain.py
index 7e8baaa5..355e1359 100644
--- a/test/countries/test_spain.py
+++ b/test/countries/test_spain.py
@@ -63,9 +63,7 @@ class TestES(unittest.TestCase):
self.assertEqual(
date(2016, 3, 24) in prov_holidays, prov not in ["CT", "VC"]
)
- self.assertEqual(
- date(2016, 3, 25) in prov_holidays, prov not in ["CT", "VC"]
- )
+ assert date(2016, 3, 25) in prov_holidays
self.assertEqual(
date(2016, 3, 28) in prov_holidays,
prov in ["CT", "PV", "NC", "VC", "IB", "CM"],
diff --git a/test/countries/test_venezuela.py b/test/countries/test_venezuela.py
new file mode 100644
index 00000000..c7ab4631
--- /dev/null
+++ b/test/countries/test_venezuela.py
@@ -0,0 +1,68 @@
+# -*- coding: utf-8 -*-
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Author: ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# dr-prodigy <maurizio.montel@gmail.com> (c) 2017-2021
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+import unittest
+
+from datetime import date
+
+import holidays
+
+
+class TestVenezuela(unittest.TestCase):
+ def test_YV_holidays(self):
+ self.holidays = holidays.YV(years=2019)
+ self.assertIn("2019-01-01", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 1, 1)], "Año Nuevo [New Year's Day]"
+ )
+ self.assertIn("2019-03-04", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 3, 4)],
+ "Lunes de Carnaval",
+ )
+ self.assertIn("2019-03-05", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 3, 5)], "Martes de Carnaval")
+ self.assertIn("2019-04-18", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 4, 18)], "Jueves Santo")
+ self.assertIn("2019-04-19", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 4, 19)],
+ "Viernes Santo y Declaración de la Independencia",
+ )
+ self.assertIn("2019-05-01", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 5, 1)], "Dia Mundial del Trabajador"
+ )
+ self.assertIn("2019-06-24", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 6, 24)], "Batalla de Carabobo"
+ )
+ self.assertIn("2019-05-01", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 7, 5)], "Dia de la Independencia"
+ )
+ self.assertIn("2019-07-24", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 7, 24)], "Natalicio de Simón Bolívar"
+ )
+ self.assertIn("2019-10-12", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 10, 12)], "Día de la Resistencia Indígena"
+ )
+ self.assertIn("2019-12-24", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 12, 24)], "Nochebuena")
+ self.assertIn("2019-12-25", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 12, 25)], "Día de Navidad")
+ self.assertIn("2019-12-31", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 12, 31)], "Fiesta de Fin de Año"
+ )
| 2 april 2021 in spain
hi,
dt.date(2021, 4, 2) in holidays.ES() don't work | 0.0 | [
"test/countries/__init__.py::TestES::test_variable_days_in_2016",
"test/countries/__init__.py::TestVenezuela::test_YV_holidays",
"test/countries/test_spain.py::TestES::test_variable_days_in_2016",
"test/countries/test_venezuela.py::TestVenezuela::test_YV_holidays"
] | [
"test/countries/__init__.py::TestAngola::test_easter",
"test/countries/__init__.py::TestAngola::test_long_weekend",
"test/countries/__init__.py::TestAngola::test_new_years",
"test/countries/__init__.py::TestAngola::test_not_holiday",
"test/countries/__init__.py::TestAngola::test_static",
"test/countries/__init__.py::TestAR::test_belgrano_day",
"test/countries/__init__.py::TestAR::test_carnival_day",
"test/countries/__init__.py::TestAR::test_christmas",
"test/countries/__init__.py::TestAR::test_cultural_day",
"test/countries/__init__.py::TestAR::test_guemes_day",
"test/countries/__init__.py::TestAR::test_holy_week_day",
"test/countries/__init__.py::TestAR::test_independence_day",
"test/countries/__init__.py::TestAR::test_inmaculate_conception_day",
"test/countries/__init__.py::TestAR::test_labor_day",
"test/countries/__init__.py::TestAR::test_malvinas_war_day",
"test/countries/__init__.py::TestAR::test_may_revolution_day",
"test/countries/__init__.py::TestAR::test_memory_national_day",
"test/countries/__init__.py::TestAR::test_national_sovereignty_day",
"test/countries/__init__.py::TestAR::test_new_years",
"test/countries/__init__.py::TestAR::test_san_martin_day",
"test/countries/__init__.py::TestAruba::test_2017",
"test/countries/__init__.py::TestAruba::test_anthem_and_flag_day",
"test/countries/__init__.py::TestAruba::test_ascension_day",
"test/countries/__init__.py::TestAruba::test_betico_day",
"test/countries/__init__.py::TestAruba::test_carnaval_monday",
"test/countries/__init__.py::TestAruba::test_christmas",
"test/countries/__init__.py::TestAruba::test_easter_monday",
"test/countries/__init__.py::TestAruba::test_good_friday",
"test/countries/__init__.py::TestAruba::test_kings_day_after_2014",
"test/countries/__init__.py::TestAruba::test_kings_day_after_2014_substituted_earlier",
"test/countries/__init__.py::TestAruba::test_labour_day",
"test/countries/__init__.py::TestAruba::test_new_years",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1891_and_1948",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1891_and_1948_substituted_later",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1949_and_1980_substituted_later",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1949_and_2013",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1980_and_2013_substituted_earlier",
"test/countries/__init__.py::TestAruba::test_second_christmas",
"test/countries/__init__.py::TestAU::test_adelaide_cup",
"test/countries/__init__.py::TestAU::test_all_holidays",
"test/countries/__init__.py::TestAU::test_anzac_day",
"test/countries/__init__.py::TestAU::test_australia_day",
"test/countries/__init__.py::TestAU::test_bank_holiday",
"test/countries/__init__.py::TestAU::test_boxing_day",
"test/countries/__init__.py::TestAU::test_christmas_day",
"test/countries/__init__.py::TestAU::test_easter_monday",
"test/countries/__init__.py::TestAU::test_easter_saturday",
"test/countries/__init__.py::TestAU::test_easter_sunday",
"test/countries/__init__.py::TestAU::test_family_and_community_day",
"test/countries/__init__.py::TestAU::test_good_friday",
"test/countries/__init__.py::TestAU::test_grand_final_day",
"test/countries/__init__.py::TestAU::test_labour_day",
"test/countries/__init__.py::TestAU::test_melbourne_cup",
"test/countries/__init__.py::TestAU::test_new_years",
"test/countries/__init__.py::TestAU::test_picnic_day",
"test/countries/__init__.py::TestAU::test_queens_birthday",
"test/countries/__init__.py::TestAU::test_reconciliation_day",
"test/countries/__init__.py::TestAU::test_royal_queensland_show",
"test/countries/__init__.py::TestAU::test_western_australia_day",
"test/countries/__init__.py::TestAT::test_all_holidays_present",
"test/countries/__init__.py::TestAT::test_christmas",
"test/countries/__init__.py::TestAT::test_easter_monday",
"test/countries/__init__.py::TestAT::test_national_day",
"test/countries/__init__.py::TestAT::test_new_years",
"test/countries/__init__.py::TestBangladesh::test_2020",
"test/countries/__init__.py::TestBelarus::test_2018",
"test/countries/__init__.py::TestBelarus::test_before_1998",
"test/countries/__init__.py::TestBelarus::test_new_year",
"test/countries/__init__.py::TestBelarus::test_radunitsa",
"test/countries/__init__.py::TestBelgium::test_2017",
"test/countries/__init__.py::TestBrazil::test_AC_holidays",
"test/countries/__init__.py::TestBrazil::test_AL_holidays",
"test/countries/__init__.py::TestBrazil::test_AM_holidays",
"test/countries/__init__.py::TestBrazil::test_AP_holidays",
"test/countries/__init__.py::TestBrazil::test_BA_holidays",
"test/countries/__init__.py::TestBrazil::test_BR_holidays",
"test/countries/__init__.py::TestBrazil::test_CE_holidays",
"test/countries/__init__.py::TestBrazil::test_DF_holidays",
"test/countries/__init__.py::TestBrazil::test_ES_holidays",
"test/countries/__init__.py::TestBrazil::test_GO_holidays",
"test/countries/__init__.py::TestBrazil::test_MA_holidays",
"test/countries/__init__.py::TestBrazil::test_MG_holidays",
"test/countries/__init__.py::TestBrazil::test_MS_holidays",
"test/countries/__init__.py::TestBrazil::test_MT_holidays",
"test/countries/__init__.py::TestBrazil::test_PA_holidays",
"test/countries/__init__.py::TestBrazil::test_PB_holidays",
"test/countries/__init__.py::TestBrazil::test_PE_holidays",
"test/countries/__init__.py::TestBrazil::test_PI_holidays",
"test/countries/__init__.py::TestBrazil::test_PR_holidays",
"test/countries/__init__.py::TestBrazil::test_RJ_holidays",
"test/countries/__init__.py::TestBrazil::test_RN_holidays",
"test/countries/__init__.py::TestBrazil::test_RO_holidays",
"test/countries/__init__.py::TestBrazil::test_RR_holidays",
"test/countries/__init__.py::TestBrazil::test_RS_holidays",
"test/countries/__init__.py::TestBrazil::test_SC_holidays",
"test/countries/__init__.py::TestBrazil::test_SE_holidays",
"test/countries/__init__.py::TestBrazil::test_SP_holidays",
"test/countries/__init__.py::TestBrazil::test_TO_holidays",
"test/countries/__init__.py::TestBulgaria::test_before_1990",
"test/countries/__init__.py::TestBulgaria::test_christmas",
"test/countries/__init__.py::TestBulgaria::test_easter",
"test/countries/__init__.py::TestBulgaria::test_independence_day",
"test/countries/__init__.py::TestBulgaria::test_labour_day",
"test/countries/__init__.py::TestBulgaria::test_liberation_day",
"test/countries/__init__.py::TestBulgaria::test_national_awakening_day",
"test/countries/__init__.py::TestBulgaria::test_new_years_day",
"test/countries/__init__.py::TestBulgaria::test_saint_georges_day",
"test/countries/__init__.py::TestBulgaria::test_twenty_fourth_of_may",
"test/countries/__init__.py::TestBulgaria::test_unification_day",
"test/countries/__init__.py::TestBurundi::test_all_saints_Day",
"test/countries/__init__.py::TestBurundi::test_ascension_day",
"test/countries/__init__.py::TestBurundi::test_assumption_Day",
"test/countries/__init__.py::TestBurundi::test_christmas_Day",
"test/countries/__init__.py::TestBurundi::test_eid_al_adha",
"test/countries/__init__.py::TestBurundi::test_independence_day",
"test/countries/__init__.py::TestBurundi::test_labour_day",
"test/countries/__init__.py::TestBurundi::test_ndadaye_day",
"test/countries/__init__.py::TestBurundi::test_new_year_day",
"test/countries/__init__.py::TestBurundi::test_ntaryamira_day",
"test/countries/__init__.py::TestBurundi::test_rwagasore_day",
"test/countries/__init__.py::TestBurundi::test_unity_day",
"test/countries/__init__.py::TestCA::test_boxing_day",
"test/countries/__init__.py::TestCA::test_canada_day",
"test/countries/__init__.py::TestCA::test_christmas_day",
"test/countries/__init__.py::TestCA::test_civic_holiday",
"test/countries/__init__.py::TestCA::test_discovery_day",
"test/countries/__init__.py::TestCA::test_easter_monday",
"test/countries/__init__.py::TestCA::test_family_day",
"test/countries/__init__.py::TestCA::test_good_friday",
"test/countries/__init__.py::TestCA::test_islander_day",
"test/countries/__init__.py::TestCA::test_labour_day",
"test/countries/__init__.py::TestCA::test_national_aboriginal_day",
"test/countries/__init__.py::TestCA::test_new_years",
"test/countries/__init__.py::TestCA::test_nunavut_day",
"test/countries/__init__.py::TestCA::test_remembrance_day",
"test/countries/__init__.py::TestCA::test_st_georges_day",
"test/countries/__init__.py::TestCA::test_st_jean_baptiste_day",
"test/countries/__init__.py::TestCA::test_st_patricks_day",
"test/countries/__init__.py::TestCA::test_thanksgiving",
"test/countries/__init__.py::TestCA::test_victoria_day",
"test/countries/__init__.py::TestChile::test_2009",
"test/countries/__init__.py::TestChile::test_2017",
"test/countries/__init__.py::TestChile::test_2018",
"test/countries/__init__.py::TestChile::test_2019",
"test/countries/__init__.py::TestChile::test_2020",
"test/countries/__init__.py::TestChile::test_2021",
"test/countries/__init__.py::TestChile::test_2024",
"test/countries/__init__.py::TestChile::test_2029",
"test/countries/__init__.py::TestCO::test_2016",
"test/countries/__init__.py::TestCO::test_others",
"test/countries/__init__.py::TestCroatia::test_2018",
"test/countries/__init__.py::TestCroatia::test_2020_new",
"test/countries/__init__.py::TestCuracao::test_2016",
"test/countries/__init__.py::TestCuracao::test_ascension_day",
"test/countries/__init__.py::TestCuracao::test_carnaval_monday",
"test/countries/__init__.py::TestCuracao::test_curacao_day",
"test/countries/__init__.py::TestCuracao::test_easter_monday",
"test/countries/__init__.py::TestCuracao::test_first_christmas",
"test/countries/__init__.py::TestCuracao::test_kings_day_after_2014",
"test/countries/__init__.py::TestCuracao::test_kings_day_after_2014_substituted_earlier",
"test/countries/__init__.py::TestCuracao::test_labour_day",
"test/countries/__init__.py::TestCuracao::test_national_anthem_flagday",
"test/countries/__init__.py::TestCuracao::test_new_years",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1891_and_1948",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1891_and_1948_substituted_later",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1949_and_1980_substituted_later",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1949_and_2013",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1980_and_2013_substituted_earlier",
"test/countries/__init__.py::TestCuracao::test_second_christmas",
"test/countries/__init__.py::TestCZ::test_2017",
"test/countries/__init__.py::TestCZ::test_czech_deprecated",
"test/countries/__init__.py::TestCZ::test_others",
"test/countries/__init__.py::TestDK::test_2016",
"test/countries/__init__.py::TestDjibouti::test_2019",
"test/countries/__init__.py::TestDjibouti::test_hijri_based",
"test/countries/__init__.py::TestDjibouti::test_labour_day",
"test/countries/__init__.py::TestDominicanRepublic::test_do_holidays_2020",
"test/countries/__init__.py::TestEgypt::test_2019",
"test/countries/__init__.py::TestEgypt::test_25_jan",
"test/countries/__init__.py::TestEgypt::test_25_jan_from_2009",
"test/countries/__init__.py::TestEgypt::test_coptic_christmas",
"test/countries/__init__.py::TestEgypt::test_hijri_based",
"test/countries/__init__.py::TestEgypt::test_labour_day",
"test/countries/__init__.py::TestEstonia::test_boxing_day",
"test/countries/__init__.py::TestEstonia::test_christmas_day",
"test/countries/__init__.py::TestEstonia::test_christmas_eve",
"test/countries/__init__.py::TestEstonia::test_easter_sunday",
"test/countries/__init__.py::TestEstonia::test_good_friday",
"test/countries/__init__.py::TestEstonia::test_independence_day",
"test/countries/__init__.py::TestEstonia::test_midsummers_day",
"test/countries/__init__.py::TestEstonia::test_new_years",
"test/countries/__init__.py::TestEstonia::test_pentecost",
"test/countries/__init__.py::TestEstonia::test_restoration_of_independence_day",
"test/countries/__init__.py::TestEstonia::test_spring_day",
"test/countries/__init__.py::TestEstonia::test_victory_day",
"test/countries/__init__.py::TestTAR::test_26_december_day",
"test/countries/__init__.py::TestTAR::test_all_holidays_present",
"test/countries/__init__.py::TestTAR::test_christmas_day",
"test/countries/__init__.py::TestTAR::test_easter_monday",
"test/countries/__init__.py::TestTAR::test_good_friday",
"test/countries/__init__.py::TestTAR::test_labour_day",
"test/countries/__init__.py::TestTAR::test_new_years",
"test/countries/__init__.py::TestECB::test_new_years",
"test/countries/__init__.py::TestFinland::test_Juhannus",
"test/countries/__init__.py::TestFinland::test_fixed_holidays",
"test/countries/__init__.py::TestFinland::test_relative_holidays",
"test/countries/__init__.py::TestFrance::test_2017",
"test/countries/__init__.py::TestFrance::test_alsace_moselle",
"test/countries/__init__.py::TestFrance::test_guadeloupe",
"test/countries/__init__.py::TestFrance::test_guyane",
"test/countries/__init__.py::TestFrance::test_la_reunion",
"test/countries/__init__.py::TestFrance::test_martinique",
"test/countries/__init__.py::TestFrance::test_mayotte",
"test/countries/__init__.py::TestFrance::test_nouvelle_caledonie",
"test/countries/__init__.py::TestFrance::test_others",
"test/countries/__init__.py::TestFrance::test_polynesie_francaise",
"test/countries/__init__.py::TestFrance::test_saint_barthelemy",
"test/countries/__init__.py::TestFrance::test_wallis_et_futuna",
"test/countries/__init__.py::TestGeorgia::test_2020",
"test/countries/__init__.py::TestGeorgia::test_easter",
"test/countries/__init__.py::TestGeorgia::test_not_holiday",
"test/countries/__init__.py::TestDE::test_75_jahrestag_beendigung_zweiter_weltkrieg",
"test/countries/__init__.py::TestDE::test_all_holidays_present",
"test/countries/__init__.py::TestDE::test_allerheiligen",
"test/countries/__init__.py::TestDE::test_buss_und_bettag",
"test/countries/__init__.py::TestDE::test_christi_himmelfahrt",
"test/countries/__init__.py::TestDE::test_fixed_holidays",
"test/countries/__init__.py::TestDE::test_frauentag",
"test/countries/__init__.py::TestDE::test_fronleichnam",
"test/countries/__init__.py::TestDE::test_heilige_drei_koenige",
"test/countries/__init__.py::TestDE::test_internationaler_frauentag",
"test/countries/__init__.py::TestDE::test_karfreitag",
"test/countries/__init__.py::TestDE::test_mariae_himmelfahrt",
"test/countries/__init__.py::TestDE::test_no_data_before_1990",
"test/countries/__init__.py::TestDE::test_ostermontag",
"test/countries/__init__.py::TestDE::test_ostersonntag",
"test/countries/__init__.py::TestDE::test_pfingstmontag",
"test/countries/__init__.py::TestDE::test_pfingstsonntag",
"test/countries/__init__.py::TestDE::test_reformationstag",
"test/countries/__init__.py::TestDE::test_tag_der_deutschen_einheit_in_1990",
"test/countries/__init__.py::TestDE::test_weltkindertag",
"test/countries/__init__.py::TestGreece::test_fixed_holidays",
"test/countries/__init__.py::TestGreece::test_gr_clean_monday",
"test/countries/__init__.py::TestGreece::test_gr_easter_monday",
"test/countries/__init__.py::TestGreece::test_gr_monday_of_the_holy_spirit",
"test/countries/__init__.py::TestHonduras::test_2014",
"test/countries/__init__.py::TestHonduras::test_2018",
"test/countries/__init__.py::TestHongKong::test_ching_ming_festival",
"test/countries/__init__.py::TestHongKong::test_christmas_day",
"test/countries/__init__.py::TestHongKong::test_chung_yeung_festival",
"test/countries/__init__.py::TestHongKong::test_common",
"test/countries/__init__.py::TestHongKong::test_easter",
"test/countries/__init__.py::TestHongKong::test_first_day_of_january",
"test/countries/__init__.py::TestHongKong::test_hksar_day",
"test/countries/__init__.py::TestHongKong::test_labour_day",
"test/countries/__init__.py::TestHongKong::test_lunar_new_year",
"test/countries/__init__.py::TestHongKong::test_mid_autumn_festival",
"test/countries/__init__.py::TestHongKong::test_national_day",
"test/countries/__init__.py::TestHongKong::test_tuen_ng_festival",
"test/countries/__init__.py::TestHungary::test_2018",
"test/countries/__init__.py::TestHungary::test_additional_day_off",
"test/countries/__init__.py::TestHungary::test_all_saints_day_since_1999",
"test/countries/__init__.py::TestHungary::test_christian_holidays_2nd_day_was_not_held_in_1955",
"test/countries/__init__.py::TestHungary::test_foundation_day_renamed_during_communism",
"test/countries/__init__.py::TestHungary::test_good_friday_since_2017",
"test/countries/__init__.py::TestHungary::test_holidays_during_communism",
"test/countries/__init__.py::TestHungary::test_labour_day_since_1946",
"test/countries/__init__.py::TestHungary::test_labour_day_was_doubled_in_early_50s",
"test/countries/__init__.py::TestHungary::test_monday_new_years_eve_day_off",
"test/countries/__init__.py::TestHungary::test_national_day_was_not_celebrated_during_communism",
"test/countries/__init__.py::TestHungary::test_october_national_day_since_1991",
"test/countries/__init__.py::TestHungary::test_whit_monday_since_1992",
"test/countries/__init__.py::TestIceland::test_commerce_day",
"test/countries/__init__.py::TestIceland::test_first_day_of_summer",
"test/countries/__init__.py::TestIceland::test_holy_friday",
"test/countries/__init__.py::TestIceland::test_maundy_thursday",
"test/countries/__init__.py::TestIceland::test_new_year",
"test/countries/__init__.py::TestIND::test_2018",
"test/countries/__init__.py::TestIreland::test_2020",
"test/countries/__init__.py::TestIreland::test_may_day",
"test/countries/__init__.py::TestIreland::test_st_stephens_day",
"test/countries/__init__.py::TestIsrael::test_independence_day",
"test/countries/__init__.py::TestIsrael::test_memorial_day",
"test/countries/__init__.py::TestIsrael::test_purim_day",
"test/countries/__init__.py::TestItaly::test_2017",
"test/countries/__init__.py::TestItaly::test_christmas",
"test/countries/__init__.py::TestItaly::test_easter",
"test/countries/__init__.py::TestItaly::test_easter_monday",
"test/countries/__init__.py::TestItaly::test_liberation_day_after_1946",
"test/countries/__init__.py::TestItaly::test_liberation_day_before_1946",
"test/countries/__init__.py::TestItaly::test_new_years",
"test/countries/__init__.py::TestItaly::test_province_specific_days",
"test/countries/__init__.py::TestItaly::test_republic_day_after_1948",
"test/countries/__init__.py::TestItaly::test_republic_day_before_1948",
"test/countries/__init__.py::TestItaly::test_saint_stephan",
"test/countries/__init__.py::TestJamaica::test_ash_wednesday",
"test/countries/__init__.py::TestJamaica::test_boxing_day",
"test/countries/__init__.py::TestJamaica::test_christmas_day",
"test/countries/__init__.py::TestJamaica::test_easter",
"test/countries/__init__.py::TestJamaica::test_easter_monday",
"test/countries/__init__.py::TestJamaica::test_emancipation_day",
"test/countries/__init__.py::TestJamaica::test_fathers_day",
"test/countries/__init__.py::TestJamaica::test_good_friday",
"test/countries/__init__.py::TestJamaica::test_independence_day",
"test/countries/__init__.py::TestJamaica::test_labour_day",
"test/countries/__init__.py::TestJamaica::test_mothers_day",
"test/countries/__init__.py::TestJamaica::test_national_heroes_day",
"test/countries/__init__.py::TestJamaica::test_new_years_day",
"test/countries/__init__.py::TestJamaica::test_valentines_day",
"test/countries/__init__.py::TestJapan::test_autumnal_equinox_day",
"test/countries/__init__.py::TestJapan::test_childrens_day",
"test/countries/__init__.py::TestJapan::test_coming_of_age",
"test/countries/__init__.py::TestJapan::test_constitution_memorial_day",
"test/countries/__init__.py::TestJapan::test_culture_day",
"test/countries/__init__.py::TestJapan::test_emperors_birthday",
"test/countries/__init__.py::TestJapan::test_foundation_day",
"test/countries/__init__.py::TestJapan::test_greenery_day",
"test/countries/__init__.py::TestJapan::test_health_and_sports_day",
"test/countries/__init__.py::TestJapan::test_heisei_emperor_holidays",
"test/countries/__init__.py::TestJapan::test_invalid_years",
"test/countries/__init__.py::TestJapan::test_labour_thanks_giving_day",
"test/countries/__init__.py::TestJapan::test_marine_day",
"test/countries/__init__.py::TestJapan::test_mountain_day",
"test/countries/__init__.py::TestJapan::test_new_years_day",
"test/countries/__init__.py::TestJapan::test_reiwa_emperor_holidays",
"test/countries/__init__.py::TestJapan::test_respect_for_the_aged_day",
"test/countries/__init__.py::TestJapan::test_showa_day",
"test/countries/__init__.py::TestJapan::test_showa_emperor_holidays",
"test/countries/__init__.py::TestJapan::test_vernal_equinox_day",
"test/countries/__init__.py::TestKenya::test_2019",
"test/countries/__init__.py::TestKorea::test_birthday_of_buddha",
"test/countries/__init__.py::TestKorea::test_childrens_day",
"test/countries/__init__.py::TestKorea::test_christmas_day",
"test/countries/__init__.py::TestKorea::test_chuseok",
"test/countries/__init__.py::TestKorea::test_common",
"test/countries/__init__.py::TestKorea::test_constitution_day",
"test/countries/__init__.py::TestKorea::test_first_day_of_january",
"test/countries/__init__.py::TestKorea::test_hangeul_day",
"test/countries/__init__.py::TestKorea::test_independence_movement_day",
"test/countries/__init__.py::TestKorea::test_labour_day",
"test/countries/__init__.py::TestKorea::test_liberation_day",
"test/countries/__init__.py::TestKorea::test_lunar_new_year",
"test/countries/__init__.py::TestKorea::test_memorial_day",
"test/countries/__init__.py::TestKorea::test_national_foundation_day",
"test/countries/__init__.py::TestKorea::test_tree_planting_day",
"test/countries/__init__.py::TestKorea::test_years_range",
"test/countries/__init__.py::TestLatvia::test_2020",
"test/countries/__init__.py::TestLithuania::test_2018",
"test/countries/__init__.py::TestLithuania::test_day_of_dew",
"test/countries/__init__.py::TestLithuania::test_easter",
"test/countries/__init__.py::TestLithuania::test_fathers_day",
"test/countries/__init__.py::TestLithuania::test_mothers_day",
"test/countries/__init__.py::TestLU::test_2019",
"test/countries/__init__.py::TestMalawi::test_easter",
"test/countries/__init__.py::TestMalawi::test_good_friday",
"test/countries/__init__.py::TestMalawi::test_new_years",
"test/countries/__init__.py::TestMalawi::test_not_holiday",
"test/countries/__init__.py::TestMalawi::test_static",
"test/countries/__init__.py::TestMX::test_benito_juarez",
"test/countries/__init__.py::TestMX::test_change_of_government",
"test/countries/__init__.py::TestMX::test_christmas",
"test/countries/__init__.py::TestMX::test_constitution_day",
"test/countries/__init__.py::TestMX::test_independence_day",
"test/countries/__init__.py::TestMX::test_labor_day",
"test/countries/__init__.py::TestMX::test_new_years",
"test/countries/__init__.py::TestMX::test_revolution_day",
"test/countries/__init__.py::TestMorocco::test_1961",
"test/countries/__init__.py::TestMorocco::test_1999",
"test/countries/__init__.py::TestMorocco::test_2019",
"test/countries/__init__.py::TestMorocco::test_hijri_based",
"test/countries/__init__.py::TestMozambique::test_easter",
"test/countries/__init__.py::TestMozambique::test_new_years",
"test/countries/__init__.py::TestMozambique::test_not_holiday",
"test/countries/__init__.py::TestNetherlands::test_2017",
"test/countries/__init__.py::TestNetherlands::test_ascension_day",
"test/countries/__init__.py::TestNetherlands::test_easter",
"test/countries/__init__.py::TestNetherlands::test_easter_monday",
"test/countries/__init__.py::TestNetherlands::test_first_christmas",
"test/countries/__init__.py::TestNetherlands::test_kings_day_after_2014",
"test/countries/__init__.py::TestNetherlands::test_kings_day_after_2014_substituted_earlier",
"test/countries/__init__.py::TestNetherlands::test_liberation_day",
"test/countries/__init__.py::TestNetherlands::test_liberation_day_after_1990_in_lustrum_year",
"test/countries/__init__.py::TestNetherlands::test_liberation_day_after_1990_non_lustrum_year",
"test/countries/__init__.py::TestNetherlands::test_new_years",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1891_and_1948",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1891_and_1948_substituted_later",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1949_and_1980_substituted_later",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1949_and_2013",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1980_and_2013_substituted_earlier",
"test/countries/__init__.py::TestNetherlands::test_second_christmas",
"test/countries/__init__.py::TestNetherlands::test_whit_monday",
"test/countries/__init__.py::TestNetherlands::test_whit_sunday",
"test/countries/__init__.py::TestNZ::test_all_holidays_present",
"test/countries/__init__.py::TestNZ::test_anzac_day",
"test/countries/__init__.py::TestNZ::test_auckland_anniversary_day",
"test/countries/__init__.py::TestNZ::test_boxing_day",
"test/countries/__init__.py::TestNZ::test_canterbury_anniversary_day",
"test/countries/__init__.py::TestNZ::test_chatham_islands_anniversary_day",
"test/countries/__init__.py::TestNZ::test_christmas_day",
"test/countries/__init__.py::TestNZ::test_day_after_new_years",
"test/countries/__init__.py::TestNZ::test_easter_monday",
"test/countries/__init__.py::TestNZ::test_good_friday",
"test/countries/__init__.py::TestNZ::test_hawkes_bay_anniversary_day",
"test/countries/__init__.py::TestNZ::test_labour_day",
"test/countries/__init__.py::TestNZ::test_marlborough_anniversary_day",
"test/countries/__init__.py::TestNZ::test_nelson_anniversary_day",
"test/countries/__init__.py::TestNZ::test_new_years",
"test/countries/__init__.py::TestNZ::test_otago_anniversary_day",
"test/countries/__init__.py::TestNZ::test_south_canterbury_anniversary_day",
"test/countries/__init__.py::TestNZ::test_southland_anniversary_day",
"test/countries/__init__.py::TestNZ::test_sovereigns_birthday",
"test/countries/__init__.py::TestNZ::test_taranaki_anniversary_day",
"test/countries/__init__.py::TestNZ::test_waitangi_day",
"test/countries/__init__.py::TestNZ::test_wellington_anniversary_day",
"test/countries/__init__.py::TestNZ::test_westland_anniversary_day",
"test/countries/__init__.py::TestNicaragua::test_ni_holidays_2020",
"test/countries/__init__.py::TestNigeria::test_fixed_holidays",
"test/countries/__init__.py::TestNorway::test_christmas",
"test/countries/__init__.py::TestNorway::test_constitution_day",
"test/countries/__init__.py::TestNorway::test_easter",
"test/countries/__init__.py::TestNorway::test_new_years",
"test/countries/__init__.py::TestNorway::test_not_holiday",
"test/countries/__init__.py::TestNorway::test_pentecost",
"test/countries/__init__.py::TestNorway::test_sundays",
"test/countries/__init__.py::TestNorway::test_workers_day",
"test/countries/__init__.py::TestParaguay::test_easter",
"test/countries/__init__.py::TestParaguay::test_fixed_holidays",
"test/countries/__init__.py::TestParaguay::test_no_observed",
"test/countries/__init__.py::TestPeru::test_2019",
"test/countries/__init__.py::TestPL::test_2017",
"test/countries/__init__.py::TestPL::test_2018",
"test/countries/__init__.py::TestPL::test_polish_deprecated",
"test/countries/__init__.py::TestPT::test_2017",
"test/countries/__init__.py::TestPortugalExt::test_2017",
"test/countries/__init__.py::TestRomania::test_2020",
"test/countries/__init__.py::TestRomania::test_children_s_day",
"test/countries/__init__.py::TestRussia::test_2018",
"test/countries/__init__.py::TestRussia::test_before_2005",
"test/countries/__init__.py::TestSaudiArabia::test_2020",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based_not_observed",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based_observed",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based_with_two_holidays_in_one_year",
"test/countries/__init__.py::TestSaudiArabia::test_national_day",
"test/countries/__init__.py::TestSaudiArabia::test_national_day_not_observed",
"test/countries/__init__.py::TestSaudiArabia::test_national_day_observed",
"test/countries/__init__.py::TestSerbia::test_armistice_day",
"test/countries/__init__.py::TestSerbia::test_labour_day",
"test/countries/__init__.py::TestSerbia::test_new_year",
"test/countries/__init__.py::TestSerbia::test_religious_holidays",
"test/countries/__init__.py::TestSerbia::test_statehood_day",
"test/countries/__init__.py::TestSingapore::test_Singapore",
"test/countries/__init__.py::TestSK::test_2018",
"test/countries/__init__.py::TestSK::test_slovak_deprecated",
"test/countries/__init__.py::TestSI::test_holidays",
"test/countries/__init__.py::TestSI::test_missing_years",
"test/countries/__init__.py::TestSI::test_non_holidays",
"test/countries/__init__.py::TestSouthAfrica::test_easter",
"test/countries/__init__.py::TestSouthAfrica::test_elections",
"test/countries/__init__.py::TestSouthAfrica::test_historic",
"test/countries/__init__.py::TestSouthAfrica::test_new_years",
"test/countries/__init__.py::TestSouthAfrica::test_not_holiday",
"test/countries/__init__.py::TestSouthAfrica::test_onceoff",
"test/countries/__init__.py::TestSouthAfrica::test_static",
"test/countries/__init__.py::TestES::test_fixed_holidays",
"test/countries/__init__.py::TestES::test_fixed_holidays_observed",
"test/countries/__init__.py::TestES::test_province_specific_days",
"test/countries/__init__.py::TestSweden::test_christmas",
"test/countries/__init__.py::TestSweden::test_constitution_day",
"test/countries/__init__.py::TestSweden::test_easter",
"test/countries/__init__.py::TestSweden::test_new_years",
"test/countries/__init__.py::TestSweden::test_not_holiday",
"test/countries/__init__.py::TestSweden::test_pentecost",
"test/countries/__init__.py::TestSweden::test_sundays",
"test/countries/__init__.py::TestSweden::test_workers_day",
"test/countries/__init__.py::TestSwitzerland::test_all_holidays_present",
"test/countries/__init__.py::TestSwitzerland::test_allerheiligen",
"test/countries/__init__.py::TestSwitzerland::test_auffahrt",
"test/countries/__init__.py::TestSwitzerland::test_berchtoldstag",
"test/countries/__init__.py::TestSwitzerland::test_bruder_chlaus",
"test/countries/__init__.py::TestSwitzerland::test_fest_der_unabhaengikeit",
"test/countries/__init__.py::TestSwitzerland::test_fixed_holidays",
"test/countries/__init__.py::TestSwitzerland::test_fronleichnam",
"test/countries/__init__.py::TestSwitzerland::test_heilige_drei_koenige",
"test/countries/__init__.py::TestSwitzerland::test_jahrestag_der_ausrufung_der_republik",
"test/countries/__init__.py::TestSwitzerland::test_jeune_genevois",
"test/countries/__init__.py::TestSwitzerland::test_josefstag",
"test/countries/__init__.py::TestSwitzerland::test_karfreitag",
"test/countries/__init__.py::TestSwitzerland::test_lundi_du_jeune",
"test/countries/__init__.py::TestSwitzerland::test_mariae_himmelfahrt",
"test/countries/__init__.py::TestSwitzerland::test_naefelser_fahrt",
"test/countries/__init__.py::TestSwitzerland::test_ostermontag",
"test/countries/__init__.py::TestSwitzerland::test_ostern",
"test/countries/__init__.py::TestSwitzerland::test_peter_und_paul",
"test/countries/__init__.py::TestSwitzerland::test_pfingsten",
"test/countries/__init__.py::TestSwitzerland::test_pfingstmontag",
"test/countries/__init__.py::TestSwitzerland::test_stephanstag",
"test/countries/__init__.py::TestSwitzerland::test_wiedererstellung_der_republik",
"test/countries/__init__.py::TestTurkey::test_2019",
"test/countries/__init__.py::TestTurkey::test_hijri_based",
"test/countries/__init__.py::TestUkraine::test_2018",
"test/countries/__init__.py::TestUkraine::test_before_1918",
"test/countries/__init__.py::TestUkraine::test_old_holidays",
"test/countries/__init__.py::UnitedArabEmirates::test_2020",
"test/countries/__init__.py::UnitedArabEmirates::test_commemoration_day_since_2015",
"test/countries/__init__.py::UnitedArabEmirates::test_hijri_based",
"test/countries/__init__.py::TestUK::test_all_holidays_present",
"test/countries/__init__.py::TestUK::test_boxing_day",
"test/countries/__init__.py::TestUK::test_christmas_day",
"test/countries/__init__.py::TestUK::test_easter_monday",
"test/countries/__init__.py::TestUK::test_good_friday",
"test/countries/__init__.py::TestUK::test_may_day",
"test/countries/__init__.py::TestUK::test_new_years",
"test/countries/__init__.py::TestUK::test_queens_jubilees",
"test/countries/__init__.py::TestUK::test_royal_weddings",
"test/countries/__init__.py::TestUK::test_spring_bank_holiday",
"test/countries/__init__.py::TestScotland::test_2017",
"test/countries/__init__.py::TestIsleOfMan::test_2018",
"test/countries/__init__.py::TestNorthernIreland::test_2018",
"test/countries/__init__.py::TestUS::test_alaska_day",
"test/countries/__init__.py::TestUS::test_all_souls_day",
"test/countries/__init__.py::TestUS::test_arbor_day",
"test/countries/__init__.py::TestUS::test_bennington_battle_day",
"test/countries/__init__.py::TestUS::test_casimir_pulaski_day",
"test/countries/__init__.py::TestUS::test_cesar_chavez_day",
"test/countries/__init__.py::TestUS::test_christmas_day",
"test/countries/__init__.py::TestUS::test_christmas_eve",
"test/countries/__init__.py::TestUS::test_columbus_day",
"test/countries/__init__.py::TestUS::test_confederate_memorial_day",
"test/countries/__init__.py::TestUS::test_constitution_day",
"test/countries/__init__.py::TestUS::test_day_after_christmas",
"test/countries/__init__.py::TestUS::test_discovery_day",
"test/countries/__init__.py::TestUS::test_easter_monday",
"test/countries/__init__.py::TestUS::test_election_day",
"test/countries/__init__.py::TestUS::test_emancipation_day",
"test/countries/__init__.py::TestUS::test_emancipation_day_in_puerto_rico",
"test/countries/__init__.py::TestUS::test_emancipation_day_in_texas",
"test/countries/__init__.py::TestUS::test_emancipation_day_in_virgin_islands",
"test/countries/__init__.py::TestUS::test_epiphany",
"test/countries/__init__.py::TestUS::test_evacuation_day",
"test/countries/__init__.py::TestUS::test_good_friday",
"test/countries/__init__.py::TestUS::test_guam_discovery_day",
"test/countries/__init__.py::TestUS::test_holy_thursday",
"test/countries/__init__.py::TestUS::test_inauguration_day",
"test/countries/__init__.py::TestUS::test_independence_day",
"test/countries/__init__.py::TestUS::test_jefferson_davis_birthday",
"test/countries/__init__.py::TestUS::test_kamehameha_day",
"test/countries/__init__.py::TestUS::test_labor_day",
"test/countries/__init__.py::TestUS::test_lady_of_camarin_day",
"test/countries/__init__.py::TestUS::test_lee_jackson_day",
"test/countries/__init__.py::TestUS::test_liberation_day_guam",
"test/countries/__init__.py::TestUS::test_liberty_day",
"test/countries/__init__.py::TestUS::test_lincolns_birthday",
"test/countries/__init__.py::TestUS::test_lyndon_baines_johnson_day",
"test/countries/__init__.py::TestUS::test_mardi_gras",
"test/countries/__init__.py::TestUS::test_martin_luther",
"test/countries/__init__.py::TestUS::test_memorial_day",
"test/countries/__init__.py::TestUS::test_nevada_day",
"test/countries/__init__.py::TestUS::test_new_years",
"test/countries/__init__.py::TestUS::test_new_years_eve",
"test/countries/__init__.py::TestUS::test_patriots_day",
"test/countries/__init__.py::TestUS::test_pioneer_day",
"test/countries/__init__.py::TestUS::test_primary_election_day",
"test/countries/__init__.py::TestUS::test_prince_jonah_kuhio_kalanianaole_day",
"test/countries/__init__.py::TestUS::test_robert_lee_birthday",
"test/countries/__init__.py::TestUS::test_san_jacinto_day",
"test/countries/__init__.py::TestUS::test_statehood_day",
"test/countries/__init__.py::TestUS::test_stewards_day",
"test/countries/__init__.py::TestUS::test_susan_b_anthony_day",
"test/countries/__init__.py::TestUS::test_texas_independence_day",
"test/countries/__init__.py::TestUS::test_thanksgiving_day",
"test/countries/__init__.py::TestUS::test_three_kings_day",
"test/countries/__init__.py::TestUS::test_town_meeting_day",
"test/countries/__init__.py::TestUS::test_transfer_day",
"test/countries/__init__.py::TestUS::test_truman_day",
"test/countries/__init__.py::TestUS::test_veterans_day",
"test/countries/__init__.py::TestUS::test_victory_day",
"test/countries/__init__.py::TestUS::test_washingtons_birthday",
"test/countries/__init__.py::TestUS::test_west_virginia_day",
"test/countries/__init__.py::TestVietnam::test_common",
"test/countries/__init__.py::TestVietnam::test_first_day_of_january",
"test/countries/__init__.py::TestVietnam::test_independence_day",
"test/countries/__init__.py::TestVietnam::test_international_labor_day",
"test/countries/__init__.py::TestVietnam::test_king_hung_day",
"test/countries/__init__.py::TestVietnam::test_liberation_day",
"test/countries/__init__.py::TestVietnam::test_lunar_new_year",
"test/countries/__init__.py::TestVietnam::test_years_range",
"test/countries/test_spain.py::TestES::test_fixed_holidays",
"test/countries/test_spain.py::TestES::test_fixed_holidays_observed",
"test/countries/test_spain.py::TestES::test_province_specific_days"
] | 2021-06-07 17:00:48+00:00 | 1,989 |
|
dr-prodigy__python-holidays-474 | diff --git a/CHANGES b/CHANGES
index 4d38f383..3f990929 100644
--- a/CHANGES
+++ b/CHANGES
@@ -3,6 +3,7 @@ Version 0.11.2
Released ????, ????
+- Support for Venezuela #470 (antusystem, dr-p)
- Poland fix #464 (m-ganko)
- Singapore updates for 2022 #456 (mborsetti)
- .gitignore fix #462 (TheLastProject)
diff --git a/README.rst b/README.rst
index 4e8df697..cb44ba7c 100644
--- a/README.rst
+++ b/README.rst
@@ -191,6 +191,7 @@ UnitedStates US/USA state = AL, AK, AS, AZ, AR, CA, CO, CT, DE, DC, FL
FM, MN, MS, MO, MT, NE, NV, NH, NJ, NM, NY, NC, ND, MP,
OH, OK, OR, PW, PA, PR, RI, SC, SD, TN, TX, UT, VT, VA,
VI, WA, WV, WI, WY
+Venezuela YV/VEN
Vietnam VN/VNM
Wales None
=================== ========= =============================================================
diff --git a/holidays/countries/__init__.py b/holidays/countries/__init__.py
index 7bb1c290..3584e388 100644
--- a/holidays/countries/__init__.py
+++ b/holidays/countries/__init__.py
@@ -92,4 +92,5 @@ from .united_kingdom import (
GBR,
)
from .united_states import UnitedStates, US, USA
+from .venezuela import Venezuela, YV, VEN
from .vietnam import Vietnam, VN, VNM
diff --git a/holidays/countries/spain.py b/holidays/countries/spain.py
index 794032b1..6b2c8dd0 100644
--- a/holidays/countries/spain.py
+++ b/holidays/countries/spain.py
@@ -117,7 +117,7 @@ class Spain(HolidayBase):
self._is_observed(date(year, MAR, 19), "San José")
if self.prov and self.prov not in ["CT", "VC"]:
self[easter(year) + rd(weeks=-1, weekday=TH)] = "Jueves Santo"
- self[easter(year) + rd(weeks=-1, weekday=FR)] = "Viernes Santo"
+ self[easter(year) + rd(weeks=-1, weekday=FR)] = "Viernes Santo"
if self.prov and self.prov in ["CT", "PV", "NC", "VC", "IB", "CM"]:
self[easter(year) + rd(weekday=MO)] = "Lunes de Pascua"
self._is_observed(date(year, MAY, 1), "Día del Trabajador")
diff --git a/holidays/countries/united_states.py b/holidays/countries/united_states.py
index fa3a23da..4be7f73c 100644
--- a/holidays/countries/united_states.py
+++ b/holidays/countries/united_states.py
@@ -375,6 +375,10 @@ class UnitedStates(HolidayBase):
elif date(year, JUN, 11).weekday() == SUN:
self[date(year, JUN, 12)] = "Kamehameha Day (Observed)"
+ # Juneteenth National Independence Day
+ if year >= 2021:
+ self[date(year, JUN, 19)] = "Juneteenth National Independence Day"
+
# Emancipation Day In Texas
if self.state == "TX" and year >= 1980:
self[date(year, JUN, 19)] = "Emancipation Day In Texas"
diff --git a/holidays/countries/venezuela.py b/holidays/countries/venezuela.py
new file mode 100644
index 00000000..020a0122
--- /dev/null
+++ b/holidays/countries/venezuela.py
@@ -0,0 +1,97 @@
+# -*- coding: utf-8 -*-
+
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Author: ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# dr-prodigy <maurizio.montel@gmail.com> (c) 2017-2021
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+from datetime import date
+
+from dateutil.easter import easter
+from dateutil.relativedelta import relativedelta as rd, TH, FR
+
+from holidays.constants import (
+ JAN,
+ MAR,
+ APR,
+ MAY,
+ JUN,
+ JUL,
+ AUG,
+ SEP,
+ OCT,
+ NOV,
+ DEC,
+)
+from holidays.holiday_base import HolidayBase
+
+
+class Venezuela(HolidayBase):
+ """
+ https://dias-festivos.eu/dias-festivos/venezuela/#
+ """
+
+ def __init__(self, **kwargs):
+ self.country = "YV"
+ HolidayBase.__init__(self, **kwargs)
+
+ def _populate(self, year):
+ # New Year's Day
+ self[date(year, JAN, 1)] = "Año Nuevo [New Year's Day]"
+
+ self[date(year, MAY, 1)] = "Dia Mundial del Trabajador"
+
+ self[date(year, JUN, 24)] = "Batalla de Carabobo"
+
+ self[date(year, JUL, 5)] = "Dia de la Independencia"
+
+ self[date(year, JUL, 24)] = "Natalicio de Simón Bolívar"
+
+ self[date(year, OCT, 12)] = "Día de la Resistencia Indígena"
+
+ # Christmas Day
+ self[date(year, DEC, 24)] = "Nochebuena"
+
+ self[date(year, DEC, 25)] = "Día de Navidad"
+
+ self[date(year, DEC, 31)] = "Fiesta de Fin de Año"
+
+ # Semana Santa y Carnaval
+
+ if date(year, APR, 19) == (easter(year) - rd(days=2)):
+ self[
+ easter(year) - rd(days=2)
+ ] = "Viernes Santo y Declaración de la Independencia"
+ else:
+ # self[easter(year) - rd(weekday=FR(-1))] = "Viernes Santo"
+ self[date(year, APR, 19)] = "Declaración de la Independencia"
+ self[easter(year) - rd(days=2)] = "Viernes Santo"
+
+ # self[easter(year) - rd(weekday=TH(-1))] = "Jueves Santo"
+
+ if date(year, APR, 19) == (easter(year) - rd(days=3)):
+ self[easter(year) - rd(days=3)] = (
+ "Jueves Santo y Declaración " "de la Independencia"
+ )
+ else:
+ # self[easter(year) - rd(weekday=FR(-1))] = "Viernes Santo"
+ self[date(year, APR, 19)] = "Declaración de la Independencia"
+ self[easter(year) - rd(days=3)] = "Jueves Santo"
+
+ self[easter(year) - rd(days=47)] = "Martes de Carnaval"
+
+ self[easter(year) - rd(days=48)] = "Lunes de Carnaval"
+
+
+class YV(Venezuela):
+ pass
+
+
+class VEN(Venezuela):
+ pass
| dr-prodigy/python-holidays | a33745d6c356770a0c48949fdad262f71da403f2 | diff --git a/test/countries/__init__.py b/test/countries/__init__.py
index d0d28686..321e65f1 100644
--- a/test/countries/__init__.py
+++ b/test/countries/__init__.py
@@ -82,4 +82,5 @@ from .test_ukraine import *
from .test_united_arab_emirates import *
from .test_united_kingdom import *
from .test_united_states import *
+from .test_venezuela import *
from .test_vietnam import *
diff --git a/test/countries/test_spain.py b/test/countries/test_spain.py
index 7e8baaa5..6108ad57 100644
--- a/test/countries/test_spain.py
+++ b/test/countries/test_spain.py
@@ -19,7 +19,7 @@ from datetime import date
import holidays
-class TestES(unittest.TestCase):
+class TestSpain(unittest.TestCase):
def setUp(self):
self.holidays = holidays.ES(observed=False)
self.holidays_observed = holidays.ES()
@@ -63,9 +63,7 @@ class TestES(unittest.TestCase):
self.assertEqual(
date(2016, 3, 24) in prov_holidays, prov not in ["CT", "VC"]
)
- self.assertEqual(
- date(2016, 3, 25) in prov_holidays, prov not in ["CT", "VC"]
- )
+ assert date(2016, 3, 25) in prov_holidays
self.assertEqual(
date(2016, 3, 28) in prov_holidays,
prov in ["CT", "PV", "NC", "VC", "IB", "CM"],
diff --git a/test/countries/test_united_states.py b/test/countries/test_united_states.py
index 4fb4fbf2..1e1a344f 100644
--- a/test/countries/test_united_states.py
+++ b/test/countries/test_united_states.py
@@ -667,9 +667,15 @@ class TestUS(unittest.TestCase):
def test_emancipation_day_in_texas(self):
tx_holidays = holidays.US(state="TX")
self.assertNotIn(date(1979, 6, 19), tx_holidays)
- for year in (1980, 2050):
+ for year in (1980, 2020):
self.assertNotIn(date(year, 6, 19), self.holidays)
self.assertIn(date(year, 6, 19), tx_holidays)
+ for year in (2021, 2050):
+ self.assertIn(date(year, 6, 19), tx_holidays)
+
+ def test_juneteenth(self):
+ self.assertNotIn(date(2020, 6, 19), self.holidays)
+ self.assertIn(date(2021, 6, 19), self.holidays)
def test_west_virginia_day(self):
wv_holidays = holidays.US(state="WV")
diff --git a/test/countries/test_venezuela.py b/test/countries/test_venezuela.py
new file mode 100644
index 00000000..c7ab4631
--- /dev/null
+++ b/test/countries/test_venezuela.py
@@ -0,0 +1,68 @@
+# -*- coding: utf-8 -*-
+# python-holidays
+# ---------------
+# A fast, efficient Python library for generating country, province and state
+# specific sets of holidays on the fly. It aims to make determining whether a
+# specific date is a holiday as fast and flexible as possible.
+#
+# Author: ryanss <ryanssdev@icloud.com> (c) 2014-2017
+# dr-prodigy <maurizio.montel@gmail.com> (c) 2017-2021
+# Website: https://github.com/dr-prodigy/python-holidays
+# License: MIT (see LICENSE file)
+
+import unittest
+
+from datetime import date
+
+import holidays
+
+
+class TestVenezuela(unittest.TestCase):
+ def test_YV_holidays(self):
+ self.holidays = holidays.YV(years=2019)
+ self.assertIn("2019-01-01", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 1, 1)], "Año Nuevo [New Year's Day]"
+ )
+ self.assertIn("2019-03-04", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 3, 4)],
+ "Lunes de Carnaval",
+ )
+ self.assertIn("2019-03-05", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 3, 5)], "Martes de Carnaval")
+ self.assertIn("2019-04-18", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 4, 18)], "Jueves Santo")
+ self.assertIn("2019-04-19", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 4, 19)],
+ "Viernes Santo y Declaración de la Independencia",
+ )
+ self.assertIn("2019-05-01", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 5, 1)], "Dia Mundial del Trabajador"
+ )
+ self.assertIn("2019-06-24", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 6, 24)], "Batalla de Carabobo"
+ )
+ self.assertIn("2019-05-01", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 7, 5)], "Dia de la Independencia"
+ )
+ self.assertIn("2019-07-24", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 7, 24)], "Natalicio de Simón Bolívar"
+ )
+ self.assertIn("2019-10-12", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 10, 12)], "Día de la Resistencia Indígena"
+ )
+ self.assertIn("2019-12-24", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 12, 24)], "Nochebuena")
+ self.assertIn("2019-12-25", self.holidays)
+ self.assertEqual(self.holidays[date(2019, 12, 25)], "Día de Navidad")
+ self.assertIn("2019-12-31", self.holidays)
+ self.assertEqual(
+ self.holidays[date(2019, 12, 31)], "Fiesta de Fin de Año"
+ )
| Add Juneteenth
https://www.nytimes.com/2021/06/17/us/politics/juneteenth-holiday-biden.html | 0.0 | [
"test/countries/__init__.py::TestSpain::test_variable_days_in_2016",
"test/countries/__init__.py::TestUS::test_juneteenth",
"test/countries/__init__.py::TestVenezuela::test_YV_holidays",
"test/countries/test_spain.py::TestSpain::test_variable_days_in_2016",
"test/countries/test_united_states.py::TestUS::test_juneteenth",
"test/countries/test_venezuela.py::TestVenezuela::test_YV_holidays"
] | [
"test/countries/__init__.py::TestAngola::test_easter",
"test/countries/__init__.py::TestAngola::test_long_weekend",
"test/countries/__init__.py::TestAngola::test_new_years",
"test/countries/__init__.py::TestAngola::test_not_holiday",
"test/countries/__init__.py::TestAngola::test_static",
"test/countries/__init__.py::TestAR::test_belgrano_day",
"test/countries/__init__.py::TestAR::test_carnival_day",
"test/countries/__init__.py::TestAR::test_christmas",
"test/countries/__init__.py::TestAR::test_cultural_day",
"test/countries/__init__.py::TestAR::test_guemes_day",
"test/countries/__init__.py::TestAR::test_holy_week_day",
"test/countries/__init__.py::TestAR::test_independence_day",
"test/countries/__init__.py::TestAR::test_inmaculate_conception_day",
"test/countries/__init__.py::TestAR::test_labor_day",
"test/countries/__init__.py::TestAR::test_malvinas_war_day",
"test/countries/__init__.py::TestAR::test_may_revolution_day",
"test/countries/__init__.py::TestAR::test_memory_national_day",
"test/countries/__init__.py::TestAR::test_national_sovereignty_day",
"test/countries/__init__.py::TestAR::test_new_years",
"test/countries/__init__.py::TestAR::test_san_martin_day",
"test/countries/__init__.py::TestAruba::test_2017",
"test/countries/__init__.py::TestAruba::test_anthem_and_flag_day",
"test/countries/__init__.py::TestAruba::test_ascension_day",
"test/countries/__init__.py::TestAruba::test_betico_day",
"test/countries/__init__.py::TestAruba::test_carnaval_monday",
"test/countries/__init__.py::TestAruba::test_christmas",
"test/countries/__init__.py::TestAruba::test_easter_monday",
"test/countries/__init__.py::TestAruba::test_good_friday",
"test/countries/__init__.py::TestAruba::test_kings_day_after_2014",
"test/countries/__init__.py::TestAruba::test_kings_day_after_2014_substituted_earlier",
"test/countries/__init__.py::TestAruba::test_labour_day",
"test/countries/__init__.py::TestAruba::test_new_years",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1891_and_1948",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1891_and_1948_substituted_later",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1949_and_1980_substituted_later",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1949_and_2013",
"test/countries/__init__.py::TestAruba::test_queens_day_between_1980_and_2013_substituted_earlier",
"test/countries/__init__.py::TestAruba::test_second_christmas",
"test/countries/__init__.py::TestAU::test_adelaide_cup",
"test/countries/__init__.py::TestAU::test_all_holidays",
"test/countries/__init__.py::TestAU::test_anzac_day",
"test/countries/__init__.py::TestAU::test_australia_day",
"test/countries/__init__.py::TestAU::test_bank_holiday",
"test/countries/__init__.py::TestAU::test_boxing_day",
"test/countries/__init__.py::TestAU::test_christmas_day",
"test/countries/__init__.py::TestAU::test_easter_monday",
"test/countries/__init__.py::TestAU::test_easter_saturday",
"test/countries/__init__.py::TestAU::test_easter_sunday",
"test/countries/__init__.py::TestAU::test_family_and_community_day",
"test/countries/__init__.py::TestAU::test_good_friday",
"test/countries/__init__.py::TestAU::test_grand_final_day",
"test/countries/__init__.py::TestAU::test_labour_day",
"test/countries/__init__.py::TestAU::test_melbourne_cup",
"test/countries/__init__.py::TestAU::test_new_years",
"test/countries/__init__.py::TestAU::test_picnic_day",
"test/countries/__init__.py::TestAU::test_queens_birthday",
"test/countries/__init__.py::TestAU::test_reconciliation_day",
"test/countries/__init__.py::TestAU::test_royal_queensland_show",
"test/countries/__init__.py::TestAU::test_western_australia_day",
"test/countries/__init__.py::TestAT::test_all_holidays_present",
"test/countries/__init__.py::TestAT::test_christmas",
"test/countries/__init__.py::TestAT::test_easter_monday",
"test/countries/__init__.py::TestAT::test_national_day",
"test/countries/__init__.py::TestAT::test_new_years",
"test/countries/__init__.py::TestBangladesh::test_2020",
"test/countries/__init__.py::TestBelarus::test_2018",
"test/countries/__init__.py::TestBelarus::test_before_1998",
"test/countries/__init__.py::TestBelarus::test_new_year",
"test/countries/__init__.py::TestBelarus::test_radunitsa",
"test/countries/__init__.py::TestBelgium::test_2017",
"test/countries/__init__.py::TestBrazil::test_AC_holidays",
"test/countries/__init__.py::TestBrazil::test_AL_holidays",
"test/countries/__init__.py::TestBrazil::test_AM_holidays",
"test/countries/__init__.py::TestBrazil::test_AP_holidays",
"test/countries/__init__.py::TestBrazil::test_BA_holidays",
"test/countries/__init__.py::TestBrazil::test_BR_holidays",
"test/countries/__init__.py::TestBrazil::test_CE_holidays",
"test/countries/__init__.py::TestBrazil::test_DF_holidays",
"test/countries/__init__.py::TestBrazil::test_ES_holidays",
"test/countries/__init__.py::TestBrazil::test_GO_holidays",
"test/countries/__init__.py::TestBrazil::test_MA_holidays",
"test/countries/__init__.py::TestBrazil::test_MG_holidays",
"test/countries/__init__.py::TestBrazil::test_MS_holidays",
"test/countries/__init__.py::TestBrazil::test_MT_holidays",
"test/countries/__init__.py::TestBrazil::test_PA_holidays",
"test/countries/__init__.py::TestBrazil::test_PB_holidays",
"test/countries/__init__.py::TestBrazil::test_PE_holidays",
"test/countries/__init__.py::TestBrazil::test_PI_holidays",
"test/countries/__init__.py::TestBrazil::test_PR_holidays",
"test/countries/__init__.py::TestBrazil::test_RJ_holidays",
"test/countries/__init__.py::TestBrazil::test_RN_holidays",
"test/countries/__init__.py::TestBrazil::test_RO_holidays",
"test/countries/__init__.py::TestBrazil::test_RR_holidays",
"test/countries/__init__.py::TestBrazil::test_RS_holidays",
"test/countries/__init__.py::TestBrazil::test_SC_holidays",
"test/countries/__init__.py::TestBrazil::test_SE_holidays",
"test/countries/__init__.py::TestBrazil::test_SP_holidays",
"test/countries/__init__.py::TestBrazil::test_TO_holidays",
"test/countries/__init__.py::TestBulgaria::test_before_1990",
"test/countries/__init__.py::TestBulgaria::test_christmas",
"test/countries/__init__.py::TestBulgaria::test_easter",
"test/countries/__init__.py::TestBulgaria::test_independence_day",
"test/countries/__init__.py::TestBulgaria::test_labour_day",
"test/countries/__init__.py::TestBulgaria::test_liberation_day",
"test/countries/__init__.py::TestBulgaria::test_national_awakening_day",
"test/countries/__init__.py::TestBulgaria::test_new_years_day",
"test/countries/__init__.py::TestBulgaria::test_saint_georges_day",
"test/countries/__init__.py::TestBulgaria::test_twenty_fourth_of_may",
"test/countries/__init__.py::TestBulgaria::test_unification_day",
"test/countries/__init__.py::TestBurundi::test_all_saints_Day",
"test/countries/__init__.py::TestBurundi::test_ascension_day",
"test/countries/__init__.py::TestBurundi::test_assumption_Day",
"test/countries/__init__.py::TestBurundi::test_christmas_Day",
"test/countries/__init__.py::TestBurundi::test_eid_al_adha",
"test/countries/__init__.py::TestBurundi::test_independence_day",
"test/countries/__init__.py::TestBurundi::test_labour_day",
"test/countries/__init__.py::TestBurundi::test_ndadaye_day",
"test/countries/__init__.py::TestBurundi::test_new_year_day",
"test/countries/__init__.py::TestBurundi::test_ntaryamira_day",
"test/countries/__init__.py::TestBurundi::test_rwagasore_day",
"test/countries/__init__.py::TestBurundi::test_unity_day",
"test/countries/__init__.py::TestCA::test_boxing_day",
"test/countries/__init__.py::TestCA::test_canada_day",
"test/countries/__init__.py::TestCA::test_christmas_day",
"test/countries/__init__.py::TestCA::test_civic_holiday",
"test/countries/__init__.py::TestCA::test_discovery_day",
"test/countries/__init__.py::TestCA::test_easter_monday",
"test/countries/__init__.py::TestCA::test_family_day",
"test/countries/__init__.py::TestCA::test_good_friday",
"test/countries/__init__.py::TestCA::test_islander_day",
"test/countries/__init__.py::TestCA::test_labour_day",
"test/countries/__init__.py::TestCA::test_national_aboriginal_day",
"test/countries/__init__.py::TestCA::test_new_years",
"test/countries/__init__.py::TestCA::test_nunavut_day",
"test/countries/__init__.py::TestCA::test_remembrance_day",
"test/countries/__init__.py::TestCA::test_st_georges_day",
"test/countries/__init__.py::TestCA::test_st_jean_baptiste_day",
"test/countries/__init__.py::TestCA::test_st_patricks_day",
"test/countries/__init__.py::TestCA::test_thanksgiving",
"test/countries/__init__.py::TestCA::test_victoria_day",
"test/countries/__init__.py::TestChile::test_2009",
"test/countries/__init__.py::TestChile::test_2017",
"test/countries/__init__.py::TestChile::test_2018",
"test/countries/__init__.py::TestChile::test_2019",
"test/countries/__init__.py::TestChile::test_2020",
"test/countries/__init__.py::TestChile::test_2021",
"test/countries/__init__.py::TestChile::test_2024",
"test/countries/__init__.py::TestChile::test_2029",
"test/countries/__init__.py::TestCO::test_2016",
"test/countries/__init__.py::TestCO::test_others",
"test/countries/__init__.py::TestCroatia::test_2018",
"test/countries/__init__.py::TestCroatia::test_2020_new",
"test/countries/__init__.py::TestCuracao::test_2016",
"test/countries/__init__.py::TestCuracao::test_ascension_day",
"test/countries/__init__.py::TestCuracao::test_carnaval_monday",
"test/countries/__init__.py::TestCuracao::test_curacao_day",
"test/countries/__init__.py::TestCuracao::test_easter_monday",
"test/countries/__init__.py::TestCuracao::test_first_christmas",
"test/countries/__init__.py::TestCuracao::test_kings_day_after_2014",
"test/countries/__init__.py::TestCuracao::test_kings_day_after_2014_substituted_earlier",
"test/countries/__init__.py::TestCuracao::test_labour_day",
"test/countries/__init__.py::TestCuracao::test_national_anthem_flagday",
"test/countries/__init__.py::TestCuracao::test_new_years",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1891_and_1948",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1891_and_1948_substituted_later",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1949_and_1980_substituted_later",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1949_and_2013",
"test/countries/__init__.py::TestCuracao::test_queens_day_between_1980_and_2013_substituted_earlier",
"test/countries/__init__.py::TestCuracao::test_second_christmas",
"test/countries/__init__.py::TestCZ::test_2017",
"test/countries/__init__.py::TestCZ::test_czech_deprecated",
"test/countries/__init__.py::TestCZ::test_others",
"test/countries/__init__.py::TestDK::test_2016",
"test/countries/__init__.py::TestDjibouti::test_2019",
"test/countries/__init__.py::TestDjibouti::test_hijri_based",
"test/countries/__init__.py::TestDjibouti::test_labour_day",
"test/countries/__init__.py::TestDominicanRepublic::test_do_holidays_2020",
"test/countries/__init__.py::TestEgypt::test_2019",
"test/countries/__init__.py::TestEgypt::test_25_jan",
"test/countries/__init__.py::TestEgypt::test_25_jan_from_2009",
"test/countries/__init__.py::TestEgypt::test_coptic_christmas",
"test/countries/__init__.py::TestEgypt::test_hijri_based",
"test/countries/__init__.py::TestEgypt::test_labour_day",
"test/countries/__init__.py::TestEstonia::test_boxing_day",
"test/countries/__init__.py::TestEstonia::test_christmas_day",
"test/countries/__init__.py::TestEstonia::test_christmas_eve",
"test/countries/__init__.py::TestEstonia::test_easter_sunday",
"test/countries/__init__.py::TestEstonia::test_good_friday",
"test/countries/__init__.py::TestEstonia::test_independence_day",
"test/countries/__init__.py::TestEstonia::test_midsummers_day",
"test/countries/__init__.py::TestEstonia::test_new_years",
"test/countries/__init__.py::TestEstonia::test_pentecost",
"test/countries/__init__.py::TestEstonia::test_restoration_of_independence_day",
"test/countries/__init__.py::TestEstonia::test_spring_day",
"test/countries/__init__.py::TestEstonia::test_victory_day",
"test/countries/__init__.py::TestTAR::test_26_december_day",
"test/countries/__init__.py::TestTAR::test_all_holidays_present",
"test/countries/__init__.py::TestTAR::test_christmas_day",
"test/countries/__init__.py::TestTAR::test_easter_monday",
"test/countries/__init__.py::TestTAR::test_good_friday",
"test/countries/__init__.py::TestTAR::test_labour_day",
"test/countries/__init__.py::TestTAR::test_new_years",
"test/countries/__init__.py::TestECB::test_new_years",
"test/countries/__init__.py::TestFinland::test_Juhannus",
"test/countries/__init__.py::TestFinland::test_fixed_holidays",
"test/countries/__init__.py::TestFinland::test_relative_holidays",
"test/countries/__init__.py::TestFrance::test_2017",
"test/countries/__init__.py::TestFrance::test_alsace_moselle",
"test/countries/__init__.py::TestFrance::test_guadeloupe",
"test/countries/__init__.py::TestFrance::test_guyane",
"test/countries/__init__.py::TestFrance::test_la_reunion",
"test/countries/__init__.py::TestFrance::test_martinique",
"test/countries/__init__.py::TestFrance::test_mayotte",
"test/countries/__init__.py::TestFrance::test_nouvelle_caledonie",
"test/countries/__init__.py::TestFrance::test_others",
"test/countries/__init__.py::TestFrance::test_polynesie_francaise",
"test/countries/__init__.py::TestFrance::test_saint_barthelemy",
"test/countries/__init__.py::TestFrance::test_wallis_et_futuna",
"test/countries/__init__.py::TestGeorgia::test_2020",
"test/countries/__init__.py::TestGeorgia::test_easter",
"test/countries/__init__.py::TestGeorgia::test_not_holiday",
"test/countries/__init__.py::TestDE::test_75_jahrestag_beendigung_zweiter_weltkrieg",
"test/countries/__init__.py::TestDE::test_all_holidays_present",
"test/countries/__init__.py::TestDE::test_allerheiligen",
"test/countries/__init__.py::TestDE::test_buss_und_bettag",
"test/countries/__init__.py::TestDE::test_christi_himmelfahrt",
"test/countries/__init__.py::TestDE::test_fixed_holidays",
"test/countries/__init__.py::TestDE::test_frauentag",
"test/countries/__init__.py::TestDE::test_fronleichnam",
"test/countries/__init__.py::TestDE::test_heilige_drei_koenige",
"test/countries/__init__.py::TestDE::test_internationaler_frauentag",
"test/countries/__init__.py::TestDE::test_karfreitag",
"test/countries/__init__.py::TestDE::test_mariae_himmelfahrt",
"test/countries/__init__.py::TestDE::test_no_data_before_1990",
"test/countries/__init__.py::TestDE::test_ostermontag",
"test/countries/__init__.py::TestDE::test_ostersonntag",
"test/countries/__init__.py::TestDE::test_pfingstmontag",
"test/countries/__init__.py::TestDE::test_pfingstsonntag",
"test/countries/__init__.py::TestDE::test_reformationstag",
"test/countries/__init__.py::TestDE::test_tag_der_deutschen_einheit_in_1990",
"test/countries/__init__.py::TestDE::test_weltkindertag",
"test/countries/__init__.py::TestGreece::test_fixed_holidays",
"test/countries/__init__.py::TestGreece::test_gr_clean_monday",
"test/countries/__init__.py::TestGreece::test_gr_easter_monday",
"test/countries/__init__.py::TestGreece::test_gr_monday_of_the_holy_spirit",
"test/countries/__init__.py::TestHonduras::test_2014",
"test/countries/__init__.py::TestHonduras::test_2018",
"test/countries/__init__.py::TestHongKong::test_ching_ming_festival",
"test/countries/__init__.py::TestHongKong::test_christmas_day",
"test/countries/__init__.py::TestHongKong::test_chung_yeung_festival",
"test/countries/__init__.py::TestHongKong::test_common",
"test/countries/__init__.py::TestHongKong::test_easter",
"test/countries/__init__.py::TestHongKong::test_first_day_of_january",
"test/countries/__init__.py::TestHongKong::test_hksar_day",
"test/countries/__init__.py::TestHongKong::test_labour_day",
"test/countries/__init__.py::TestHongKong::test_lunar_new_year",
"test/countries/__init__.py::TestHongKong::test_mid_autumn_festival",
"test/countries/__init__.py::TestHongKong::test_national_day",
"test/countries/__init__.py::TestHongKong::test_tuen_ng_festival",
"test/countries/__init__.py::TestHungary::test_2018",
"test/countries/__init__.py::TestHungary::test_additional_day_off",
"test/countries/__init__.py::TestHungary::test_all_saints_day_since_1999",
"test/countries/__init__.py::TestHungary::test_christian_holidays_2nd_day_was_not_held_in_1955",
"test/countries/__init__.py::TestHungary::test_foundation_day_renamed_during_communism",
"test/countries/__init__.py::TestHungary::test_good_friday_since_2017",
"test/countries/__init__.py::TestHungary::test_holidays_during_communism",
"test/countries/__init__.py::TestHungary::test_labour_day_since_1946",
"test/countries/__init__.py::TestHungary::test_labour_day_was_doubled_in_early_50s",
"test/countries/__init__.py::TestHungary::test_monday_new_years_eve_day_off",
"test/countries/__init__.py::TestHungary::test_national_day_was_not_celebrated_during_communism",
"test/countries/__init__.py::TestHungary::test_october_national_day_since_1991",
"test/countries/__init__.py::TestHungary::test_whit_monday_since_1992",
"test/countries/__init__.py::TestIceland::test_commerce_day",
"test/countries/__init__.py::TestIceland::test_first_day_of_summer",
"test/countries/__init__.py::TestIceland::test_holy_friday",
"test/countries/__init__.py::TestIceland::test_maundy_thursday",
"test/countries/__init__.py::TestIceland::test_new_year",
"test/countries/__init__.py::TestIND::test_2018",
"test/countries/__init__.py::TestIreland::test_2020",
"test/countries/__init__.py::TestIreland::test_may_day",
"test/countries/__init__.py::TestIreland::test_st_stephens_day",
"test/countries/__init__.py::TestIsrael::test_independence_day",
"test/countries/__init__.py::TestIsrael::test_memorial_day",
"test/countries/__init__.py::TestIsrael::test_purim_day",
"test/countries/__init__.py::TestItaly::test_2017",
"test/countries/__init__.py::TestItaly::test_christmas",
"test/countries/__init__.py::TestItaly::test_easter",
"test/countries/__init__.py::TestItaly::test_easter_monday",
"test/countries/__init__.py::TestItaly::test_liberation_day_after_1946",
"test/countries/__init__.py::TestItaly::test_liberation_day_before_1946",
"test/countries/__init__.py::TestItaly::test_new_years",
"test/countries/__init__.py::TestItaly::test_province_specific_days",
"test/countries/__init__.py::TestItaly::test_republic_day_after_1948",
"test/countries/__init__.py::TestItaly::test_republic_day_before_1948",
"test/countries/__init__.py::TestItaly::test_saint_stephan",
"test/countries/__init__.py::TestJamaica::test_ash_wednesday",
"test/countries/__init__.py::TestJamaica::test_boxing_day",
"test/countries/__init__.py::TestJamaica::test_christmas_day",
"test/countries/__init__.py::TestJamaica::test_easter",
"test/countries/__init__.py::TestJamaica::test_easter_monday",
"test/countries/__init__.py::TestJamaica::test_emancipation_day",
"test/countries/__init__.py::TestJamaica::test_fathers_day",
"test/countries/__init__.py::TestJamaica::test_good_friday",
"test/countries/__init__.py::TestJamaica::test_independence_day",
"test/countries/__init__.py::TestJamaica::test_labour_day",
"test/countries/__init__.py::TestJamaica::test_mothers_day",
"test/countries/__init__.py::TestJamaica::test_national_heroes_day",
"test/countries/__init__.py::TestJamaica::test_new_years_day",
"test/countries/__init__.py::TestJamaica::test_valentines_day",
"test/countries/__init__.py::TestJapan::test_autumnal_equinox_day",
"test/countries/__init__.py::TestJapan::test_childrens_day",
"test/countries/__init__.py::TestJapan::test_coming_of_age",
"test/countries/__init__.py::TestJapan::test_constitution_memorial_day",
"test/countries/__init__.py::TestJapan::test_culture_day",
"test/countries/__init__.py::TestJapan::test_emperors_birthday",
"test/countries/__init__.py::TestJapan::test_foundation_day",
"test/countries/__init__.py::TestJapan::test_greenery_day",
"test/countries/__init__.py::TestJapan::test_health_and_sports_day",
"test/countries/__init__.py::TestJapan::test_heisei_emperor_holidays",
"test/countries/__init__.py::TestJapan::test_invalid_years",
"test/countries/__init__.py::TestJapan::test_labour_thanks_giving_day",
"test/countries/__init__.py::TestJapan::test_marine_day",
"test/countries/__init__.py::TestJapan::test_mountain_day",
"test/countries/__init__.py::TestJapan::test_new_years_day",
"test/countries/__init__.py::TestJapan::test_reiwa_emperor_holidays",
"test/countries/__init__.py::TestJapan::test_respect_for_the_aged_day",
"test/countries/__init__.py::TestJapan::test_showa_day",
"test/countries/__init__.py::TestJapan::test_showa_emperor_holidays",
"test/countries/__init__.py::TestJapan::test_vernal_equinox_day",
"test/countries/__init__.py::TestKenya::test_2019",
"test/countries/__init__.py::TestKorea::test_birthday_of_buddha",
"test/countries/__init__.py::TestKorea::test_childrens_day",
"test/countries/__init__.py::TestKorea::test_christmas_day",
"test/countries/__init__.py::TestKorea::test_chuseok",
"test/countries/__init__.py::TestKorea::test_common",
"test/countries/__init__.py::TestKorea::test_constitution_day",
"test/countries/__init__.py::TestKorea::test_first_day_of_january",
"test/countries/__init__.py::TestKorea::test_hangeul_day",
"test/countries/__init__.py::TestKorea::test_independence_movement_day",
"test/countries/__init__.py::TestKorea::test_labour_day",
"test/countries/__init__.py::TestKorea::test_liberation_day",
"test/countries/__init__.py::TestKorea::test_lunar_new_year",
"test/countries/__init__.py::TestKorea::test_memorial_day",
"test/countries/__init__.py::TestKorea::test_national_foundation_day",
"test/countries/__init__.py::TestKorea::test_tree_planting_day",
"test/countries/__init__.py::TestKorea::test_years_range",
"test/countries/__init__.py::TestLatvia::test_2020",
"test/countries/__init__.py::TestLithuania::test_2018",
"test/countries/__init__.py::TestLithuania::test_day_of_dew",
"test/countries/__init__.py::TestLithuania::test_easter",
"test/countries/__init__.py::TestLithuania::test_fathers_day",
"test/countries/__init__.py::TestLithuania::test_mothers_day",
"test/countries/__init__.py::TestLU::test_2019",
"test/countries/__init__.py::TestMalawi::test_easter",
"test/countries/__init__.py::TestMalawi::test_good_friday",
"test/countries/__init__.py::TestMalawi::test_new_years",
"test/countries/__init__.py::TestMalawi::test_not_holiday",
"test/countries/__init__.py::TestMalawi::test_static",
"test/countries/__init__.py::TestMX::test_benito_juarez",
"test/countries/__init__.py::TestMX::test_change_of_government",
"test/countries/__init__.py::TestMX::test_christmas",
"test/countries/__init__.py::TestMX::test_constitution_day",
"test/countries/__init__.py::TestMX::test_independence_day",
"test/countries/__init__.py::TestMX::test_labor_day",
"test/countries/__init__.py::TestMX::test_new_years",
"test/countries/__init__.py::TestMX::test_revolution_day",
"test/countries/__init__.py::TestMorocco::test_1961",
"test/countries/__init__.py::TestMorocco::test_1999",
"test/countries/__init__.py::TestMorocco::test_2019",
"test/countries/__init__.py::TestMorocco::test_hijri_based",
"test/countries/__init__.py::TestMozambique::test_easter",
"test/countries/__init__.py::TestMozambique::test_new_years",
"test/countries/__init__.py::TestMozambique::test_not_holiday",
"test/countries/__init__.py::TestNetherlands::test_2017",
"test/countries/__init__.py::TestNetherlands::test_ascension_day",
"test/countries/__init__.py::TestNetherlands::test_easter",
"test/countries/__init__.py::TestNetherlands::test_easter_monday",
"test/countries/__init__.py::TestNetherlands::test_first_christmas",
"test/countries/__init__.py::TestNetherlands::test_kings_day_after_2014",
"test/countries/__init__.py::TestNetherlands::test_kings_day_after_2014_substituted_earlier",
"test/countries/__init__.py::TestNetherlands::test_liberation_day",
"test/countries/__init__.py::TestNetherlands::test_liberation_day_after_1990_in_lustrum_year",
"test/countries/__init__.py::TestNetherlands::test_liberation_day_after_1990_non_lustrum_year",
"test/countries/__init__.py::TestNetherlands::test_new_years",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1891_and_1948",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1891_and_1948_substituted_later",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1949_and_1980_substituted_later",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1949_and_2013",
"test/countries/__init__.py::TestNetherlands::test_queens_day_between_1980_and_2013_substituted_earlier",
"test/countries/__init__.py::TestNetherlands::test_second_christmas",
"test/countries/__init__.py::TestNetherlands::test_whit_monday",
"test/countries/__init__.py::TestNetherlands::test_whit_sunday",
"test/countries/__init__.py::TestNZ::test_all_holidays_present",
"test/countries/__init__.py::TestNZ::test_anzac_day",
"test/countries/__init__.py::TestNZ::test_auckland_anniversary_day",
"test/countries/__init__.py::TestNZ::test_boxing_day",
"test/countries/__init__.py::TestNZ::test_canterbury_anniversary_day",
"test/countries/__init__.py::TestNZ::test_chatham_islands_anniversary_day",
"test/countries/__init__.py::TestNZ::test_christmas_day",
"test/countries/__init__.py::TestNZ::test_day_after_new_years",
"test/countries/__init__.py::TestNZ::test_easter_monday",
"test/countries/__init__.py::TestNZ::test_good_friday",
"test/countries/__init__.py::TestNZ::test_hawkes_bay_anniversary_day",
"test/countries/__init__.py::TestNZ::test_labour_day",
"test/countries/__init__.py::TestNZ::test_marlborough_anniversary_day",
"test/countries/__init__.py::TestNZ::test_nelson_anniversary_day",
"test/countries/__init__.py::TestNZ::test_new_years",
"test/countries/__init__.py::TestNZ::test_otago_anniversary_day",
"test/countries/__init__.py::TestNZ::test_south_canterbury_anniversary_day",
"test/countries/__init__.py::TestNZ::test_southland_anniversary_day",
"test/countries/__init__.py::TestNZ::test_sovereigns_birthday",
"test/countries/__init__.py::TestNZ::test_taranaki_anniversary_day",
"test/countries/__init__.py::TestNZ::test_waitangi_day",
"test/countries/__init__.py::TestNZ::test_wellington_anniversary_day",
"test/countries/__init__.py::TestNZ::test_westland_anniversary_day",
"test/countries/__init__.py::TestNicaragua::test_ni_holidays_2020",
"test/countries/__init__.py::TestNigeria::test_fixed_holidays",
"test/countries/__init__.py::TestNorway::test_christmas",
"test/countries/__init__.py::TestNorway::test_constitution_day",
"test/countries/__init__.py::TestNorway::test_easter",
"test/countries/__init__.py::TestNorway::test_new_years",
"test/countries/__init__.py::TestNorway::test_not_holiday",
"test/countries/__init__.py::TestNorway::test_pentecost",
"test/countries/__init__.py::TestNorway::test_sundays",
"test/countries/__init__.py::TestNorway::test_workers_day",
"test/countries/__init__.py::TestParaguay::test_easter",
"test/countries/__init__.py::TestParaguay::test_fixed_holidays",
"test/countries/__init__.py::TestParaguay::test_no_observed",
"test/countries/__init__.py::TestPeru::test_2019",
"test/countries/__init__.py::TestPL::test_2017",
"test/countries/__init__.py::TestPL::test_2018",
"test/countries/__init__.py::TestPL::test_polish_deprecated",
"test/countries/__init__.py::TestPT::test_2017",
"test/countries/__init__.py::TestPortugalExt::test_2017",
"test/countries/__init__.py::TestRomania::test_2020",
"test/countries/__init__.py::TestRomania::test_children_s_day",
"test/countries/__init__.py::TestRussia::test_2018",
"test/countries/__init__.py::TestRussia::test_before_2005",
"test/countries/__init__.py::TestSaudiArabia::test_2020",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based_not_observed",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based_observed",
"test/countries/__init__.py::TestSaudiArabia::test_hijri_based_with_two_holidays_in_one_year",
"test/countries/__init__.py::TestSaudiArabia::test_national_day",
"test/countries/__init__.py::TestSaudiArabia::test_national_day_not_observed",
"test/countries/__init__.py::TestSaudiArabia::test_national_day_observed",
"test/countries/__init__.py::TestSerbia::test_armistice_day",
"test/countries/__init__.py::TestSerbia::test_labour_day",
"test/countries/__init__.py::TestSerbia::test_new_year",
"test/countries/__init__.py::TestSerbia::test_religious_holidays",
"test/countries/__init__.py::TestSerbia::test_statehood_day",
"test/countries/__init__.py::TestSingapore::test_Singapore",
"test/countries/__init__.py::TestSK::test_2018",
"test/countries/__init__.py::TestSK::test_slovak_deprecated",
"test/countries/__init__.py::TestSI::test_holidays",
"test/countries/__init__.py::TestSI::test_missing_years",
"test/countries/__init__.py::TestSI::test_non_holidays",
"test/countries/__init__.py::TestSouthAfrica::test_easter",
"test/countries/__init__.py::TestSouthAfrica::test_elections",
"test/countries/__init__.py::TestSouthAfrica::test_historic",
"test/countries/__init__.py::TestSouthAfrica::test_new_years",
"test/countries/__init__.py::TestSouthAfrica::test_not_holiday",
"test/countries/__init__.py::TestSouthAfrica::test_onceoff",
"test/countries/__init__.py::TestSouthAfrica::test_static",
"test/countries/__init__.py::TestSpain::test_fixed_holidays",
"test/countries/__init__.py::TestSpain::test_fixed_holidays_observed",
"test/countries/__init__.py::TestSpain::test_province_specific_days",
"test/countries/__init__.py::TestSweden::test_christmas",
"test/countries/__init__.py::TestSweden::test_constitution_day",
"test/countries/__init__.py::TestSweden::test_easter",
"test/countries/__init__.py::TestSweden::test_new_years",
"test/countries/__init__.py::TestSweden::test_not_holiday",
"test/countries/__init__.py::TestSweden::test_pentecost",
"test/countries/__init__.py::TestSweden::test_sundays",
"test/countries/__init__.py::TestSweden::test_workers_day",
"test/countries/__init__.py::TestSwitzerland::test_all_holidays_present",
"test/countries/__init__.py::TestSwitzerland::test_allerheiligen",
"test/countries/__init__.py::TestSwitzerland::test_auffahrt",
"test/countries/__init__.py::TestSwitzerland::test_berchtoldstag",
"test/countries/__init__.py::TestSwitzerland::test_bruder_chlaus",
"test/countries/__init__.py::TestSwitzerland::test_fest_der_unabhaengikeit",
"test/countries/__init__.py::TestSwitzerland::test_fixed_holidays",
"test/countries/__init__.py::TestSwitzerland::test_fronleichnam",
"test/countries/__init__.py::TestSwitzerland::test_heilige_drei_koenige",
"test/countries/__init__.py::TestSwitzerland::test_jahrestag_der_ausrufung_der_republik",
"test/countries/__init__.py::TestSwitzerland::test_jeune_genevois",
"test/countries/__init__.py::TestSwitzerland::test_josefstag",
"test/countries/__init__.py::TestSwitzerland::test_karfreitag",
"test/countries/__init__.py::TestSwitzerland::test_lundi_du_jeune",
"test/countries/__init__.py::TestSwitzerland::test_mariae_himmelfahrt",
"test/countries/__init__.py::TestSwitzerland::test_naefelser_fahrt",
"test/countries/__init__.py::TestSwitzerland::test_ostermontag",
"test/countries/__init__.py::TestSwitzerland::test_ostern",
"test/countries/__init__.py::TestSwitzerland::test_peter_und_paul",
"test/countries/__init__.py::TestSwitzerland::test_pfingsten",
"test/countries/__init__.py::TestSwitzerland::test_pfingstmontag",
"test/countries/__init__.py::TestSwitzerland::test_stephanstag",
"test/countries/__init__.py::TestSwitzerland::test_wiedererstellung_der_republik",
"test/countries/__init__.py::TestTurkey::test_2019",
"test/countries/__init__.py::TestTurkey::test_hijri_based",
"test/countries/__init__.py::TestUkraine::test_2018",
"test/countries/__init__.py::TestUkraine::test_before_1918",
"test/countries/__init__.py::TestUkraine::test_old_holidays",
"test/countries/__init__.py::UnitedArabEmirates::test_2020",
"test/countries/__init__.py::UnitedArabEmirates::test_commemoration_day_since_2015",
"test/countries/__init__.py::UnitedArabEmirates::test_hijri_based",
"test/countries/__init__.py::TestUK::test_all_holidays_present",
"test/countries/__init__.py::TestUK::test_boxing_day",
"test/countries/__init__.py::TestUK::test_christmas_day",
"test/countries/__init__.py::TestUK::test_easter_monday",
"test/countries/__init__.py::TestUK::test_good_friday",
"test/countries/__init__.py::TestUK::test_may_day",
"test/countries/__init__.py::TestUK::test_new_years",
"test/countries/__init__.py::TestUK::test_queens_jubilees",
"test/countries/__init__.py::TestUK::test_royal_weddings",
"test/countries/__init__.py::TestUK::test_spring_bank_holiday",
"test/countries/__init__.py::TestScotland::test_2017",
"test/countries/__init__.py::TestIsleOfMan::test_2018",
"test/countries/__init__.py::TestNorthernIreland::test_2018",
"test/countries/__init__.py::TestUS::test_alaska_day",
"test/countries/__init__.py::TestUS::test_all_souls_day",
"test/countries/__init__.py::TestUS::test_arbor_day",
"test/countries/__init__.py::TestUS::test_bennington_battle_day",
"test/countries/__init__.py::TestUS::test_casimir_pulaski_day",
"test/countries/__init__.py::TestUS::test_cesar_chavez_day",
"test/countries/__init__.py::TestUS::test_christmas_day",
"test/countries/__init__.py::TestUS::test_christmas_eve",
"test/countries/__init__.py::TestUS::test_columbus_day",
"test/countries/__init__.py::TestUS::test_confederate_memorial_day",
"test/countries/__init__.py::TestUS::test_constitution_day",
"test/countries/__init__.py::TestUS::test_day_after_christmas",
"test/countries/__init__.py::TestUS::test_discovery_day",
"test/countries/__init__.py::TestUS::test_easter_monday",
"test/countries/__init__.py::TestUS::test_election_day",
"test/countries/__init__.py::TestUS::test_emancipation_day",
"test/countries/__init__.py::TestUS::test_emancipation_day_in_puerto_rico",
"test/countries/__init__.py::TestUS::test_emancipation_day_in_texas",
"test/countries/__init__.py::TestUS::test_emancipation_day_in_virgin_islands",
"test/countries/__init__.py::TestUS::test_epiphany",
"test/countries/__init__.py::TestUS::test_evacuation_day",
"test/countries/__init__.py::TestUS::test_good_friday",
"test/countries/__init__.py::TestUS::test_guam_discovery_day",
"test/countries/__init__.py::TestUS::test_holy_thursday",
"test/countries/__init__.py::TestUS::test_inauguration_day",
"test/countries/__init__.py::TestUS::test_independence_day",
"test/countries/__init__.py::TestUS::test_jefferson_davis_birthday",
"test/countries/__init__.py::TestUS::test_kamehameha_day",
"test/countries/__init__.py::TestUS::test_labor_day",
"test/countries/__init__.py::TestUS::test_lady_of_camarin_day",
"test/countries/__init__.py::TestUS::test_lee_jackson_day",
"test/countries/__init__.py::TestUS::test_liberation_day_guam",
"test/countries/__init__.py::TestUS::test_liberty_day",
"test/countries/__init__.py::TestUS::test_lincolns_birthday",
"test/countries/__init__.py::TestUS::test_lyndon_baines_johnson_day",
"test/countries/__init__.py::TestUS::test_mardi_gras",
"test/countries/__init__.py::TestUS::test_martin_luther",
"test/countries/__init__.py::TestUS::test_memorial_day",
"test/countries/__init__.py::TestUS::test_nevada_day",
"test/countries/__init__.py::TestUS::test_new_years",
"test/countries/__init__.py::TestUS::test_new_years_eve",
"test/countries/__init__.py::TestUS::test_patriots_day",
"test/countries/__init__.py::TestUS::test_pioneer_day",
"test/countries/__init__.py::TestUS::test_primary_election_day",
"test/countries/__init__.py::TestUS::test_prince_jonah_kuhio_kalanianaole_day",
"test/countries/__init__.py::TestUS::test_robert_lee_birthday",
"test/countries/__init__.py::TestUS::test_san_jacinto_day",
"test/countries/__init__.py::TestUS::test_statehood_day",
"test/countries/__init__.py::TestUS::test_stewards_day",
"test/countries/__init__.py::TestUS::test_susan_b_anthony_day",
"test/countries/__init__.py::TestUS::test_texas_independence_day",
"test/countries/__init__.py::TestUS::test_thanksgiving_day",
"test/countries/__init__.py::TestUS::test_three_kings_day",
"test/countries/__init__.py::TestUS::test_town_meeting_day",
"test/countries/__init__.py::TestUS::test_transfer_day",
"test/countries/__init__.py::TestUS::test_truman_day",
"test/countries/__init__.py::TestUS::test_veterans_day",
"test/countries/__init__.py::TestUS::test_victory_day",
"test/countries/__init__.py::TestUS::test_washingtons_birthday",
"test/countries/__init__.py::TestUS::test_west_virginia_day",
"test/countries/__init__.py::TestVietnam::test_common",
"test/countries/__init__.py::TestVietnam::test_first_day_of_january",
"test/countries/__init__.py::TestVietnam::test_independence_day",
"test/countries/__init__.py::TestVietnam::test_international_labor_day",
"test/countries/__init__.py::TestVietnam::test_king_hung_day",
"test/countries/__init__.py::TestVietnam::test_liberation_day",
"test/countries/__init__.py::TestVietnam::test_lunar_new_year",
"test/countries/__init__.py::TestVietnam::test_years_range",
"test/countries/test_spain.py::TestSpain::test_fixed_holidays",
"test/countries/test_spain.py::TestSpain::test_fixed_holidays_observed",
"test/countries/test_spain.py::TestSpain::test_province_specific_days",
"test/countries/test_united_states.py::TestUS::test_alaska_day",
"test/countries/test_united_states.py::TestUS::test_all_souls_day",
"test/countries/test_united_states.py::TestUS::test_arbor_day",
"test/countries/test_united_states.py::TestUS::test_bennington_battle_day",
"test/countries/test_united_states.py::TestUS::test_casimir_pulaski_day",
"test/countries/test_united_states.py::TestUS::test_cesar_chavez_day",
"test/countries/test_united_states.py::TestUS::test_christmas_day",
"test/countries/test_united_states.py::TestUS::test_christmas_eve",
"test/countries/test_united_states.py::TestUS::test_columbus_day",
"test/countries/test_united_states.py::TestUS::test_confederate_memorial_day",
"test/countries/test_united_states.py::TestUS::test_constitution_day",
"test/countries/test_united_states.py::TestUS::test_day_after_christmas",
"test/countries/test_united_states.py::TestUS::test_discovery_day",
"test/countries/test_united_states.py::TestUS::test_easter_monday",
"test/countries/test_united_states.py::TestUS::test_election_day",
"test/countries/test_united_states.py::TestUS::test_emancipation_day",
"test/countries/test_united_states.py::TestUS::test_emancipation_day_in_puerto_rico",
"test/countries/test_united_states.py::TestUS::test_emancipation_day_in_texas",
"test/countries/test_united_states.py::TestUS::test_emancipation_day_in_virgin_islands",
"test/countries/test_united_states.py::TestUS::test_epiphany",
"test/countries/test_united_states.py::TestUS::test_evacuation_day",
"test/countries/test_united_states.py::TestUS::test_good_friday",
"test/countries/test_united_states.py::TestUS::test_guam_discovery_day",
"test/countries/test_united_states.py::TestUS::test_holy_thursday",
"test/countries/test_united_states.py::TestUS::test_inauguration_day",
"test/countries/test_united_states.py::TestUS::test_independence_day",
"test/countries/test_united_states.py::TestUS::test_jefferson_davis_birthday",
"test/countries/test_united_states.py::TestUS::test_kamehameha_day",
"test/countries/test_united_states.py::TestUS::test_labor_day",
"test/countries/test_united_states.py::TestUS::test_lady_of_camarin_day",
"test/countries/test_united_states.py::TestUS::test_lee_jackson_day",
"test/countries/test_united_states.py::TestUS::test_liberation_day_guam",
"test/countries/test_united_states.py::TestUS::test_liberty_day",
"test/countries/test_united_states.py::TestUS::test_lincolns_birthday",
"test/countries/test_united_states.py::TestUS::test_lyndon_baines_johnson_day",
"test/countries/test_united_states.py::TestUS::test_mardi_gras",
"test/countries/test_united_states.py::TestUS::test_martin_luther",
"test/countries/test_united_states.py::TestUS::test_memorial_day",
"test/countries/test_united_states.py::TestUS::test_nevada_day",
"test/countries/test_united_states.py::TestUS::test_new_years",
"test/countries/test_united_states.py::TestUS::test_new_years_eve",
"test/countries/test_united_states.py::TestUS::test_patriots_day",
"test/countries/test_united_states.py::TestUS::test_pioneer_day",
"test/countries/test_united_states.py::TestUS::test_primary_election_day",
"test/countries/test_united_states.py::TestUS::test_prince_jonah_kuhio_kalanianaole_day",
"test/countries/test_united_states.py::TestUS::test_robert_lee_birthday",
"test/countries/test_united_states.py::TestUS::test_san_jacinto_day",
"test/countries/test_united_states.py::TestUS::test_statehood_day",
"test/countries/test_united_states.py::TestUS::test_stewards_day",
"test/countries/test_united_states.py::TestUS::test_susan_b_anthony_day",
"test/countries/test_united_states.py::TestUS::test_texas_independence_day",
"test/countries/test_united_states.py::TestUS::test_thanksgiving_day",
"test/countries/test_united_states.py::TestUS::test_three_kings_day",
"test/countries/test_united_states.py::TestUS::test_town_meeting_day",
"test/countries/test_united_states.py::TestUS::test_transfer_day",
"test/countries/test_united_states.py::TestUS::test_truman_day",
"test/countries/test_united_states.py::TestUS::test_veterans_day",
"test/countries/test_united_states.py::TestUS::test_victory_day",
"test/countries/test_united_states.py::TestUS::test_washingtons_birthday",
"test/countries/test_united_states.py::TestUS::test_west_virginia_day"
] | 2021-06-22 21:22:30+00:00 | 1,990 |
|
dr-prodigy__python-holidays-555 | diff --git a/holidays/countries/spain.py b/holidays/countries/spain.py
index 6b2c8dd0..3fd94870 100644
--- a/holidays/countries/spain.py
+++ b/holidays/countries/spain.py
@@ -185,7 +185,10 @@ class Spain(HolidayBase):
elif self.prov == "NC":
self._is_observed(date(year, SEP, 27), "Día de Navarra")
elif self.prov == "PV":
- self._is_observed(date(year, OCT, 25), "Día del Páis Vasco")
+ if 2011 <= year <= 2013:
+ self._is_observed(
+ date(year, OCT, 25), "Día del Páis Vasco"
+ )
elif self.prov == "RI":
self._is_observed(date(year, JUN, 9), "Día de La Rioja")
| dr-prodigy/python-holidays | 5d89951a3390a8cf3ff73580e93aaace0bca0071 | diff --git a/test/countries/test_spain.py b/test/countries/test_spain.py
index 6108ad57..822ced95 100644
--- a/test/countries/test_spain.py
+++ b/test/countries/test_spain.py
@@ -84,7 +84,6 @@ class TestSpain(unittest.TestCase):
(9, 11): ["CT"],
(9, 27): ["NC"],
(10, 9): ["VC"],
- (10, 25): ["PV"],
}
for prov, prov_holidays in self.prov_holidays.items():
for year in range(2010, 2025):
@@ -142,3 +141,11 @@ class TestSpain(unittest.TestCase):
date(year, *fest_day) in prov_holidays,
prov in fest_prov,
)
+
+ def test_change_of_province_specific_days(self):
+ prov_holidays = self.prov_holidays["PV"]
+ self.assertNotIn(date(2010, 10, 25), prov_holidays)
+ self.assertIn(date(2011, 10, 25), prov_holidays)
+ self.assertIn(date(2012, 10, 25), prov_holidays)
+ self.assertIn(date(2013, 10, 25), prov_holidays)
+ self.assertNotIn(date(2014, 10, 25), prov_holidays)
| October 25 is no longer holiday in Spain Prov=PV
I'm using the WORKDAY integration of Home Assistant to obtain a binary sensor for workdays.
AFAIK python-holidays is being used "under the hood" in this integration
Yesterday October 25th was wrongly marked as holiday.
It's not holiday since 2014.
More info (sorry, in spanish): [https://es.wikipedia.org/wiki/D%C3%ADa_del_Pa%C3%ADs_Vasco#Creaci%C3%B3n_y_desaparici%C3%B3n_de_la_festividad] | 0.0 | [
"test/countries/test_spain.py::TestSpain::test_change_of_province_specific_days"
] | [
"test/countries/test_spain.py::TestSpain::test_fixed_holidays",
"test/countries/test_spain.py::TestSpain::test_fixed_holidays_observed",
"test/countries/test_spain.py::TestSpain::test_province_specific_days",
"test/countries/test_spain.py::TestSpain::test_variable_days_in_2016"
] | 2021-11-17 13:26:13+00:00 | 1,991 |
|
dr-prodigy__python-holidays-794 | diff --git a/holidays/countries/eswatini.py b/holidays/countries/eswatini.py
index 00ca75bf..798bd495 100644
--- a/holidays/countries/eswatini.py
+++ b/holidays/countries/eswatini.py
@@ -80,11 +80,13 @@ class Eswatini(HolidayBase):
class Swaziland(Eswatini):
- warnings.warn(
- "Swaziland is deprecated, use Eswatini instead.",
- DeprecationWarning,
- )
- pass
+ def __init__(self, *args, **kwargs) -> None:
+ warnings.warn(
+ "Swaziland is deprecated, use Eswatini instead.",
+ DeprecationWarning,
+ )
+
+ super().__init__(*args, **kwargs)
class SZ(Eswatini):
| dr-prodigy/python-holidays | 6f3242d6e357c00dd5878e1f21d24cbe1aaa25ed | diff --git a/test/countries/test_eswatini.py b/test/countries/test_eswatini.py
index 29d3d34e..038c2b92 100644
--- a/test/countries/test_eswatini.py
+++ b/test/countries/test_eswatini.py
@@ -10,6 +10,7 @@
# License: MIT (see LICENSE file)
import unittest
+import warnings
from datetime import date
import holidays
@@ -59,3 +60,12 @@ class TestEswatini(unittest.TestCase):
self.assertIn(date(2021, 4, 26), self.holidays)
self.assertIn(date(2021, 12, 27), self.holidays)
self.assertIn(date(2023, 1, 2), self.holidays)
+
+ def test_swaziland_deprecation_warning(self):
+ warnings.simplefilter("default")
+ with self.assertWarns(Warning):
+ holidays.Swaziland()
+
+ warnings.simplefilter("error")
+ with self.assertRaises(Warning):
+ holidays.Swaziland()
| DeprecationWarning upon "import holidays" in version 0.17
The implementation of deprecating the Swaziland calendar contains a bug. Just importing the holidays package is enough to fire the `DeprecationWarning`.
**Steps to reproduce (in bash):**
```bash
# Setup
python -m venv demo
source demo/bin/activate
pip install --upgrade pip
# Bad version
pip install holidays==0.17
# Expose bug
python -W error::DeprecationWarning -c 'import holidays'
# Workoround
pip uninstall -y holidays
pip install holidays!=0.17
python -W error::DeprecationWarning -c 'import holidays'
# Cleanup
deactivate
rm -rf demo
```
**Expected behavior:**
The `DeprecationWarning` should only fire when the user constructs an instance of the `Swaziland` or a subclass.
| 0.0 | [
"test/countries/test_eswatini.py::TestEswatini::test_swaziland_deprecation_warning"
] | [
"test/countries/test_eswatini.py::TestEswatini::test_easter",
"test/countries/test_eswatini.py::TestEswatini::test_holidays",
"test/countries/test_eswatini.py::TestEswatini::test_kings_birthday",
"test/countries/test_eswatini.py::TestEswatini::test_national_flag_day",
"test/countries/test_eswatini.py::TestEswatini::test_new_years",
"test/countries/test_eswatini.py::TestEswatini::test_observed",
"test/countries/test_eswatini.py::TestEswatini::test_once_off",
"test/countries/test_eswatini.py::TestEswatini::test_out_of_range"
] | 2022-11-15 00:30:03+00:00 | 1,992 |
Subsets and Splits